branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>abalarin/Swift-Calculator<file_sep>/Calculator/ViewController.swift
//
// ViewController.swift
// Calculator
//
// Created by <NAME> on 2/17/17.
// Copyright © 2017 Stanford Projects. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet private weak var display: UILabel!
private var displayValue: Double {
get {
return Double(display.text!)!
}
set {
display.text = String(newValue)
}
}
private var userIsInTheMiddleOfTyping = false
// Function for controlling number presses
@IBAction private func touchDigit(_ sender: UIButton) {
let digit = sender.currentTitle!
let currentText = display!.text!
if userIsInTheMiddleOfTyping {
display.text = currentText + digit
}
else{
display.text = digit
}
userIsInTheMiddleOfTyping = true
}
// Init the model
private var model = CalculatorModel()
var savedProgram: CalculatorModel.PropertyList?
@IBAction private func saveProgram() {
savedProgram = model.program
}
@IBAction func restoreProgram() {
if savedProgram != nil{
model.program = savedProgram!
displayValue = model.result
}
}
// Function for controlling math operations
@IBAction private func operation(_ sender: UIButton) {
if userIsInTheMiddleOfTyping {
model.setOperand(operand: displayValue)
userIsInTheMiddleOfTyping = false
}
if let mathmaticalSymbol = sender.currentTitle {
model.performOperation(symbol: mathmaticalSymbol)
}
displayValue = model.result
}
}
| 098db34a8652708a0d26e8a4c9525804990fdea2 | [
"Swift"
] | 1 | Swift | abalarin/Swift-Calculator | 19cc6ba8529612ccb956428636dc9e90977738d4 | 6eae9e41b1ef43fc8972d2be14a9e9a6fe5d6fe9 | |
refs/heads/master | <repo_name>fossabot/moocisen<file_sep>/app/includes/wizard_ajax.php
<?php
session_start();
$meschoix=$_POST["dataForm"]; //Mes choix
$solutions=$_POST["dataForm2"]; //Les solutions
$idm=$_POST["dataidm"]; //id mooc
$idc=$_POST["dataidc"]; //id chap
$ide=$_POST["dataide"]; //id exo
$tabide=$_POST["datatabide"]; //id tab des partie exo
$malus=$_POST["datamalus"]; //id tab des partie exo
//Insert le score sur l'exercice (score basé sur l'algorithme de levenshtein)
//Remarque : une fois le score rentré impossible de réécrire dessus avec cette fonction
function insertFaitToBDD($score,$id_user,$id_exercice){
include 'connect.inc.php'; //connexion bdd
try {
$requete_prepare= $bdd->prepare("INSERT INTO faire(score,id_user,id_exercice) VALUES('$score', '$id_user', '$id_exercice')"); // on prépare notre requête
$requete_prepare->execute();
//var_dump($requete_prepare);
// echo "->Sauvegarde du score<br>";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
//Insert le score sur l'exercice (score basé sur l'algorithme de levenshtein)
//Remarque : update le score si il est déjà présent
function insertFaitToBDDwithUpdate($score,$id_user,$id_exercice){
include 'connect.inc.php'; //connexion bdd
try {
$requete_prepare= $bdd->prepare("INSERT INTO faire(score,id_user,id_exercice) VALUES('$score', '$id_user', '$id_exercice') ON DUPLICATE KEY UPDATE score='$score',id_user='$id_user',id_exercice='$id_exercice'"); // on prépare notre requête
$requete_prepare->execute();
//var_dump($requete_prepare);
// echo "->Sauvegarde du score<br>";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
function insertFaitToBDDwithUpdateTabExo($score,$id_user,$tabide){
include 'connect.inc.php'; //connexion bdd
$tabide=json_decode($tabide);
// var_dump(count($tabide));
for($i=0;$i<count($tabide);$i++){
$id_exercice = $tabide[$i];
try {
$requete_prepare= $bdd->prepare("INSERT INTO faire(score,id_user,id_exercice) VALUES('$score', '$id_user', '$id_exercice') ON DUPLICATE KEY UPDATE score='$score',id_user='$id_user',id_exercice='$id_exercice'"); // on prépare notre requête
$requete_prepare->execute();
//var_dump($requete_prepare);
//echo ".";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
//echo "----".$tabide[$i];
}
// echo "<br>->Sauvegarde du score<br>";
}
function unlockTrophyAdvancement($id_user,$id_mooc){
include 'connect.inc.php'; //connexion bdd
//Permet de récuperer l'avancement d'un mooc pour l'utilisateur courant
$avancementMooc = $bdd->query('SELECT avancement AS avc FROM user INNER JOIN suivre ON user.id_user= suivre.id_user INNER JOIN mooc ON suivre.id_mooc=mooc.id_mooc WHERE user.id_user = "'.$id_user.'" AND mooc.id_mooc = "'.$id_mooc.'"');
$donnees6 = $avancementMooc->fetch();
$avancementMooc->closeCursor();
// Permet de récuperer le nombre de chapitre du MOOC
$nbChapitreMooc = $bdd->query('SELECT nb_chap FROM mooc WHERE id_mooc ="'.$id_mooc.'"');
$donnees7 = $nbChapitreMooc->fetch();
$nbChapitreMooc->closeCursor();
// Calcul du % d'avancement
$tab = Array();
$tab = preg_split('[-]', $donnees6["avc"]);
$avancement = sizeof($tab)-1;
$pourcentage = ceil($avancement/ $donnees7["nb_chap"]*100);
if($pourcentage>=30){
$nbSucces = 4; // nombre de succes par MOOC
$id_succes = $id_mooc*$nbSucces-($nbSucces-2);
try {
$requete_prepare= $bdd->prepare("INSERT INTO `mooc`.`debloquer` (`date_obtention`, `id_succes`, `id_user`) VALUES (current_date, '$id_succes', '$id_user')");
$requete_prepare->execute();
} catch (Exception $e) {
//echo $e->errorMessage();
}
}
if($pourcentage>=60){
$nbSucces = 4; // nombre de succes par MOOC
$id_succes = $id_mooc*$nbSucces-($nbSucces-3);
try {
$requete_prepare= $bdd->prepare("INSERT INTO `mooc`.`debloquer` (`date_obtention`, `id_succes`, `id_user`) VALUES (current_date, '$id_succes', '$id_user')");
$requete_prepare->execute();
} catch (Exception $e) {
//echo $e->errorMessage();
}
}
if($pourcentage==100){
$nbSucces = 4; // nombre de succes par MOOC
$id_succes = $id_mooc*$nbSucces-($nbSucces-4);
try {
$requete_prepare= $bdd->prepare("INSERT INTO `mooc`.`debloquer` (`date_obtention`, `id_succes`, `id_user`) VALUES (current_date, '$id_succes', '$id_user')");
$requete_prepare->execute();
} catch (Exception $e) {
//echo $e->errorMessage();
}
}
}
function updateSuivreChap($id_user,$id_chap,$id_mooc){
include 'connect.inc.php'; //connexion bdd
try {
$select = $bdd->prepare("SELECT * FROM suivre WHERE id_user = '$id_user' AND id_mooc = '$id_mooc'");
$select->execute();
$lignes = $select->fetchAll();
//var_dump($lignes);
$stringAvancement = $lignes[0]["avancement"];
// echo "avancement -> ".$stringAvancement;
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
if (strpos($stringAvancement, $id_chap) !== false) {
//valeur deja présente
$stringAvancement = $stringAvancement; //ajout du nouveau chapitre
}else{
$stringAvancement = $stringAvancement."-".$id_chap; //ajout du nouveau chapitre
}
// echo "avancement -> ".$stringAvancement;
try {
//$requete_prepare= $bdd->prepare("INSERT INTO suivre(avancement) VALUES '$stringAvancement' "); // on prépare notre requête
$requete_prepare= $bdd->prepare("UPDATE suivre SET avancement='$stringAvancement' WHERE id_user = '$id_user' AND id_mooc = '$id_mooc'"); // on prépare notre requête
$requete_prepare->execute();
unlockTrophyAdvancement($id_user,$id_mooc);
//var_dump($requete_prepare);
// echo "->updateSuivreChap<br>";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
//Fonction permettant de calculer la similitude entre 2 chaines (algorithme de levenshtein)
//Attention il y une limite pour la longueur de la chaine
function similaire($str1, $str2) {
$strlen1=strlen($str1);
$strlen2=strlen($str2);
$max=max($strlen1, $strlen2);
$splitSize=250;
if($max>$splitSize)
{
$lev=0;
for($cont=0;$cont<$max;$cont+=$splitSize)
{
if($strlen1<=$cont || $strlen2<=$cont)
{
$lev=$lev/($max/min($strlen1,$strlen2));
break;
}
$lev+=levenshtein(substr($str1,$cont,$splitSize), substr($str2,$cont,$splitSize));
}
}
else
$lev=levenshtein($str1, $str2);
$porcentage= -100*$lev/$max+100;
/*if($porcentage>75)
similar_text($str1,$str2,$porcentage);*/
return $porcentage;
}
//compare 2 chaine au format json
function compareTab($strChoix, $strSoluce){
$strSoluce=str_replace(',','","',$strSoluce);//car la structure du json n'est pas pareil
$strSoluce=str_replace('"",""','","',$strSoluce);//car la structure du json n'est pas pareil
//var_dump($strChoix);
//var_dump($strSoluce);
$strJsonChoix = json_decode($strChoix);
$strJsonSoluce= json_decode($strSoluce);
$compteur = 0; //nombre de bonne réponse
$sizeArraySoluce = count($strJsonSoluce); //nb de solution
$sizeArrayChoix = count($strJsonChoix); //nb de choix
$lePourcentage = 0;
//echo '<br>taille'.$sizeArraySoluce;
foreach($strJsonChoix as $keyChoix){
//echo '+'.$keyChoix.'+';
foreach($strJsonSoluce as $keySoluce){
if($keyChoix==$keySoluce){
$compteur = $compteur + 1;
}
}
}
if($sizeArrayChoix==0){
echo 'aucun choix';
}else if($sizeArraySoluce==$compteur && $sizeArraySoluce==$sizeArrayChoix){
echo '100% juste';
$lePourcentage = 100;
}else if($sizeArraySoluce==$compteur && $sizeArraySoluce<$sizeArrayChoix){
//100% juste mais
echo 'juste mais trop de réponse';
$lePourcentage = ($sizeArraySoluce/$sizeArrayChoix)*100;
}else if($sizeArraySoluce==$compteur && $sizeArraySoluce>$sizeArrayChoix){
//100% juste mais
echo 'juste mais trop de réponse';
$lePourcentage = ($sizeArrayChoix/$sizeArraySoluce)*100;
}else if($sizeArraySoluce>$compteur){
echo $compteur.' réponse(s) juste sur '.$sizeArraySoluce;
/*if($sizeArrayChoix==0)
$sizeArrayChoix=0.1;
$lePourcentage = (($compteur/$sizeArraySoluce)*100)/(($sizeArrayChoix/$sizeArraySoluce)); //sort 100 si aucun choix different
echo 'ici';
if($lePourcentage==100){
$lePourcentage = (($sizeArrayChoix/$sizeArraySoluce)*100);
}*/
// $lePourcentage=(($compteur*100)/$sizeArraySoluce);
if($compteur!=$sizeArrayChoix){
//malus 1 réponse fausse = (100*le nb de faux) / nb de soulution
$lePourcentage=(($compteur*100)/$sizeArraySoluce) - (100*($sizeArrayChoix-$compteur)/$sizeArraySoluce);
}else{
//pas de reponse fausse
$lePourcentage=(($compteur*100)/$sizeArraySoluce);
}
}else{
echo 'trop de réponse';
$lePourcentage = ($sizeArraySoluce/$compteur)*100;
}
if($lePourcentage<0){
$lePourcentage=0;
}
echo '<br>score inseré : '.$lePourcentage;
return $lePourcentage;
}
$lepourcentage=compareTab($meschoix,$solutions);
//echo '<br><br><br>Debug<br> les réponses sont :'.$solutions."<br>info débug : ".$idm." ".$idc." ".$tabide." -solution ".$solutions." -choix".$meschoix;
//Calcul du %
$lepourcentage=intval($lepourcentage); //Cast en entier
//Utilisation du malus
if(($lepourcentage-$malus)<0){
$lepourcentage = 0;
}else{
$lepourcentage = $lepourcentage-$malus;
}
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user']))){
//ok session
$id_user=$_SESSION['id_user'];
$score = $lepourcentage;
insertFaitToBDDwithUpdate($score,$id_user,$ide); //Insertion en BDD
insertFaitToBDDwithUpdateTabExo($score,$id_user,$tabide);
updateSuivreChap($_SESSION['id_user'],$idc,$idm);
//echo "passage en bdd";
}else{
echo '<br>test hors-ligne';
}
?><file_sep>/app/mooc/modele_chapitreX.php
<?php
$idMooc;
$idChap;
if(isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 ">
<!-- CONTENU COURS -->
<div class="animated slideInUp">
<div class="x_panel">
<!-- TITRE COURS -->
<div class="x_title">
<!-- ///////////////////////////////////////
/////////METTRE Nom chapitre ICI////////////
////////////////////////////////////////////-->
<h2> </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<!-- COURS -->
<div class="x_content">
<div class="my_step_box">
<div class='row'>
<div class="col-md-3"></div>
<div class="col-md-6">
<div class="btn-group" role="group" aria-label="...">
<button type="button" class="btn btn-default myBtnBack"><span class="glyphicon glyphicon-chevron-left"></span> Retour</button>
<button type="button" class="btn btn-default myBtnAll"><span class="glyphicon glyphicon-eye-open"></span> </button>
<button type="button" class="btn btn-default myBtnNext">Suivant<span class="glyphicon glyphicon-chevron-right"></span></button>
</div>
</div>
<div class="col-md-3"></div>
</div>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////// DEBUT CONTENU COURS ////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<!-- Debut box numéro 1 -->
<div class="box1" id="1">
<ol start="1">
<li dir="ltr">
<h2 dir="ltr">
</h2>
</li>
</ol>
<p dir="ltr">
</p>
<br/>
</div>
<!-- Fin box numéro 1 -->
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/////////////////////////////////// FIN CONTENU COURS //////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<br/>
</div>
</div>
</div>
</div>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////// DEBUT VIDEO /////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<div class="col-md-12 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Vidéo </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="videocontainer lg6">
<!-- METTRE ICI LE LIEN DE LA VIDEO -->
<iframe src=" " frameborder="0" ></iframe>
</div>
</div>
</div>
</div>
</div>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////// FIN VIDEO ///////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
-->
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//////////////////////////////////////// DEBUT EXERCICES ////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////-->
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInRight">
<div class="x_panel">
<div class="x_title">
<?php
nomChapitre($idMooc,$bdd,$numChap);
?>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Smart Wizard -->
<h3>Exercices</h3>
<div id="wizard" class="form_wizard wizard_horizontal">
<?php
creationWizardStep($idMooc,$numChap,$idChap,$bdd);
?>
</div>
<!-- IMPORTANT AFFICHE LES INDICE -->
<div id="indicebox"></div>
<!-- IMPORTANT AFFICHE LES SOLUTIONS -->
<div id="solucebox"></div>
</div>
</div>
</div>
<!--
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////// FIN EXERCICES ////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////-->
</div><file_sep>/app/includes/indice_ajax.php
<?php
//indice_ajax
session_start();
$dataSoluce=$_POST["dataSoluce"]; //la solution
$dataId=$_POST["dataId"]; //id soluce
//$ide=$_POST["dataide"]; //id exo
//echo $dataSoluce.'+++'.$dataId;
//Insert le score sur l'exercice (score basé sur l'algorithme de levenshtein)
//Remarque : update le score si il est déjà présent
function insertFaitToBDDwithUpdate($score,$id_user,$id_exercice){
include 'connect.inc.php'; //connexion bdd
try {
$requete_prepare= $bdd->prepare("INSERT INTO faire(score,id_user,id_exercice) VALUES('$score', '$id_user', '$id_exercice') ON DUPLICATE KEY UPDATE score='$score',id_user='$id_user',id_exercice='$id_exercice'"); // on prépare notre requête
$requete_prepare->execute();
//var_dump($requete_prepare);
echo "->Sauvegarde du score<br>";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user']))){
//ok session
$id_user=$_SESSION['id_user'];
$score=10;
insertFaitToBDDwithUpdate($score,$id_user,$dataId); //Insertion en BDD
//echo "passage en bdd";
}else{
echo '<br>test hors-ligne';
}
?>
<file_sep>/app/includes/profil_update_password.php
<?php
session_start();
include "../includes/connect.inc.php"; /// Connection bdd
/*
Modification du mot de passe depuis la page profif.php
*/
//ok=1 ko=0
function sessionValid(){
$verif = 1;
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user'])))
{
//session ok
}
else
{
$verif = 0;
}
return $verif;
}
//ok=1 ko=0
function formValid(){
$verif = 1;
if (isset($_POST['oldPassword'])) {
echo '<br>'.$_POST['oldPassword'];
}else{
$verif=0;
}
if (isset($_POST['newPassword'])) {
echo '<br>'.$_POST['newPassword'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
//Verifie si l id existe et mot de passe
//ok=1 ko=0 mdp pas le meme = 2
function verifId(){
include "../includes/connect.inc.php";
$verif = 1;
$id = $_SESSION['id_user'];
$oldPassword = $_POST['oldPassword'];
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user WHERE id_user='$id'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
$oldPasswordInBdd=$result[0]['password'];
var_dump($result);
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
if($result==NULL){
$verif = 0; //L'id de reset password
}
$oldPassword = md5($oldPassword);
var_dump($oldPasswordInBdd);
var_dump($oldPassword);
if($oldPasswordInBdd===$oldPassword){
$verif = 2;
}
return $verif;
}
//Met a jour l id pour le reset du mdp
function updateIdResetPwd(){
include "../includes/connect.inc.php";
$valPwd = $_POST['newPassword'];
$valPwd = md5($valPwd);
$id = $_SESSION['id_user'];
try {
$requete_prepare= $bdd->prepare("UPDATE user SET password='$<PASSWORD>' WHERE id_user='$id'"); // on prépare notre requête
$requete_prepare->execute();
echo "->OK pwd update";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
//Valide que le formulaire
$verif = formValid();
$verifSession = sessionValid();
if($verifSession==1){
if($verif==1){
$verifId = verifId();
if($verifId==0){
echo "->id n'existe pas";
header ("location: ../modules/connected/profil.php?erreur=Erreur mdp");
}else if($verifId==1){
echo "->mdp ne corresponde pas";
header ("location: ../modules/connected/profil.php?erreur=mdp non identique");
}else if($verif==1){
updateIdResetPwd();
header ("location: ../modules/connected/profil.php?ok=success");
}
}
else{
echo '<br>wrong form';
header ("location: ../modules/connected/profil.php?erreur=Erreur formulaire");
}
}else{
echo '<br>aucune session';
header ("location: ../index.php?erreur=Erreur session");
}
?><file_sep>/app/mooc/mooc1/chapitres/chapitre7.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 7. Expérience extra professionnelle</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false"><i class="fa fa-wrench"></i></a>
<ul class="dropdown-menu" role="menu">
<li><a href="#">Settings 1</a>
</li>
<li><a href="#">Settings 2</a>
</li>
</ul>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
N’hésitez surtout pas à lister vos compétences particulières et vos occupations associatives et bénévoles.
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/includes/request_reset.php
<?php
include 'connect.inc.php'; /// Connection bdd
//ok=1 ko=0
function formValid(){
$verif = 1;
if (isset($_POST['email'])) {
echo $_POST['email'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
//Evite d'avoir 2 mail identique en bdd ok=1 ko=0
function emailExist(){
include 'connect.inc.php';
$verif = 1; //existant par defaut
$email = $_POST['email'];
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user where email='$email'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
if (empty($result)){
$verif = 0;
}
return $verif;
}
$code =" ";
//Fonction qui genere une chaine aléatoire
function generateRandomString($length = 5) {
$characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
$charactersLength = strlen($characters);
$randomString = '';
for ($i = 0; $i < $length; $i++) {
$randomString .= $characters[rand(0, $charactersLength - 1)];
}
return $randomString;
}
//Met a jour l id pour le reset du mdp
function updateIdResetPwd(){
include 'connect.inc.php';
$valEmail = $_POST['email'];
$valEmailMd5 = (generateRandomString(3).$valEmail);
$valEmailMd5 = md5($valEmailMd5);
var_dump($valEmailMd5);
try {
$requete_prepare= $bdd->prepare("UPDATE user SET reset_password='$<PASSWORD>' WHERE email='$valEmail'"); // on prépare notre requête
$requete_prepare->execute();
echo "->OK";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
$code=$valEmailMd5;
return $valEmailMd5;
}
function sendEmail($urlLink){
// Check for empty fields
if(empty($_POST['email']))
{
echo "Erreur pas de mail";
return false;
}
$name = "MOOC Mot de passe";
$url = 'http://'.$_SERVER['SERVER_NAME'];
$email_address = $_POST['email']; //email de destination
$urlLink = $urlLink;
$message = "A Bientot";
//$email_address = '"$email_address"';
// Create the email and send the message
$to = "$email_address"; // Add your email address inbetween the '' replacing <EMAIL> - This is where the form will send a message to.
$email_subject = "Website Contact : $name";
//$email_body = "Voici votre le lien pour le nouveau mot de passe\n\n"."\n\nEmail: <a href='mooc/reset_password?id=$email_address'>$email_address</a>\n\nUrl: $urlLink\n\n$message";
$email_body = '
<html>
<head>
<title>Voici votre lien pour le nouveau mot de passe</title>
</head>
<body>
<p>Voici votre lien pour le nouveau mot de passe</p>
Email : '.$email_address.'<br>
Lien: <a href='.$url.'/app/modules/not-connected/changerMdp.php?id='.$urlLink.'>'.$url.'/app/modules/not-connected/changerMdp.php?id='.$urlLink.'</a> <br>
URL: '.$url.'/app/modules/not-connected/changerMdp.php?id='.$urlLink.'</a> <br>
'.$message.' <br>
Sinon vous pouvez vous rendre sur la page "mot de passe oublié" et rentrer le code : '.$code.'<br>
</body>
</html>
';
$headers = "From: <EMAIL>.fr\n"; // This is the email address the generated message will be from. We recommend using something like <EMAIL>.
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'Content-type: text/html; charset=utf-8' . "\r\n";
$headers .= "Reply-To: $email_address";
//$email_body->isHTML(true);
try{
mail($to,$email_subject,$email_body,$headers);
}
catch(Exception $Excep){
echo $e->errorMessage();
echo "->erreur mail";
}
return true;
}
$url = 'http://'.$_SERVER['SERVER_NAME'];
$verifMail = emailExist();
$verif = formValid();
if($verifMail==0){
//echo '<br>Mail inconnu';
header ("location: ../modules/not-connected/mdpOublie.php?erreur=Mail inconnu");
}else if($verif==1){
$urlResetPwd=updateIdResetPwd();
echo '<br>Url a envoyer = '.$url.'/moocisen/app/modules/not-connected/changerMdp.php?id='.$urlResetPwd;
sendEmail($urlResetPwd); // envoie de l'email
header ("location: ../index.php?ok=Mot de passe envoyé");
}
else{
//echo '<br>wrong form';
header ("location: ../modules/not-connected/mdpOublie.php?erreur=Formulaire erreur");
}
?><file_sep>/app/scripts/not-connected/connexion.js
/*!
*
* Google
* Copyright 2015 Google Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*
*/
// --------------- REGEX --------------------
$.validator.addMethod("usernameRegex", function(value, element) {
return this.optional(element) || /^[a-z\u00E0-\u00FC_.+-]+$/i.test(value);
});
$.validator.addMethod("mailRegex", function(value, element) {
return this.optional(element) || /^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$/i.test(value);
});
// --------------- Formulaire JqueryValidator ---------
var form = $("#myform");
form.validate({
//prendre le name
errorElement: 'span',
errorClass: 'help-block',
highlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').addClass("has-error");
},
unhighlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').removeClass("has-error");
},
rules: {
name: {
required: true,
usernameRegex: true,
minlength: 2,
},
surname: {
required: true,
usernameRegex: true,
minlength: 2,
},
pseudo: {
required: true,
minlength: 2,
},
password : {
required: true,
minlength: 4,
},
city:{
required: true,
},
name: {
required: true,
usernameRegex: true,
minlength: 3,
},
email: {
required: true,
mailRegex: true,
minlength: 3,
},
},
messages: {
username: {
required: "Username required",
},
name: {
required: "name required",
},
password : {
required: "<PASSWORD>",
},
name: {
required: "Name required",
},
email: {
required: "Email required",
},
}
});
// --------------- Login JqueryValidator ---------
var login = $("#myLogin");
login.validate({
//prendre le name
errorElement: 'span',
errorClass: 'help-block',
highlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').addClass("has-error");
},
unhighlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').removeClass("has-error");
},
rules: {
name: {
required: true,
usernameRegex: true,
minlength: 2,
},
password : {
required: true,
minlength: 4,
},
email: {
required: true,
mailRegex: true,
minlength: 3,
},
},
messages: {
password : {
required: "<PASSWORD>",
},
email: {
required: "Email required",
},
}
});
<file_sep>/app/includes/fonctionChap.php
<?php
function readchap($filename) {
if(!file_exists($filename))
throw new Exception ("not found");
$tab=file($filename);
$content="";
$l=0;
$total=count($tab);
while($l<$total) {
if(strpos($tab[$l],"===========")!==false)
break;
$l++;
}
$l++;
while($l<$total) {
if(strpos($tab[$l],"===========")!==false)
break;
$content.=$tab[$l];
$l++;
}
return $content;
}
function readchaps($max) {
$tab=array();
for($i=1;$i<=$max;$i++)
try{
$tab[]=readchap("mooc/chapitres/chapitre$i.php");
}catch(Exception $e){}
return $tab;
}
<file_sep>/app/scripts/not-connected/aPropos.js
/*!
*
* Google
* Copyright 2015 Google Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*
*/
/**
* gear-toy.js
* http://brm.io/gears-d3-js/
* License: MIT
*/
var _svg,
_allGears = [],
_randomiseInterval,
_canvasWidth = 1280,
_canvasHeight = 768,
_xOffset = _canvasWidth * 0.5,
_yOffset = _canvasHeight * 0.4,
_gearFactors = [64, 64, 32, 48, 48, 96, 112, 256],
_gearStyles = ['style-0', 'style-1', 'style-2', 'style-3', 'style-4'],
_autoShuffle = true;
var _options = {
radius: 16,
holeRadius: 0.4,
transition: true,
speed: 0.01,
autoShuffle: true,
number: 20,
addendum: 8,
dedendum: 3,
thickness: 0.7,
profileSlope: 0.5
};
var main = function() {
// set up our d3 svg element
_svg = d3.select('.gears-d3-canvas')
.append('svg')
.attr('viewBox', '0 0 ' + _canvasWidth + ' ' + _canvasHeight)
.attr('preserveAspectRatio', 'xMinYMin slice');
// generate and randomise scene
_generateScene(_options);
_randomiseScene(false);
// start a timer to randomise every few secs
_randomiseInterval = setInterval(function() {
if (_autoShuffle)
_randomiseScene(true);
}, 4000);
setTimeout(function() {
_randomiseScene(true);
}, 100);
// start the d3 animation timer
d3.timer(function () {
_svg.selectAll('.gear-path')
.attr('transform', function (d) {
d.angle += d.speed;
return 'rotate(' + d.angle * (180 / Math.PI) + ')';
});
});
};
var _generateScene = function(options) {
var holeRadius,
teeth,
radius,
factor,
newGear,
innerRadius;
_gearStyles = Gear.Utility.arrayShuffle(_gearStyles);
for (var i = 0; i < options.number; i++) {
factor = _gearFactors[i % _gearFactors.length];
radius = factor / 2;
teeth = radius / 4;
innerRadius = radius - options.addendum - options.dedendum;
holeRadius = factor > 96 ? innerRadius * 0.5 + innerRadius * 0.5 * options.holeRadius : innerRadius * options.holeRadius;
_allGears.push(newGear = Gear.create(_svg, {
radius: radius,
teeth: teeth,
x: 0,
y: 0,
holeRadius: holeRadius,
addendum: options.addendum,
dedendum: options.dedendum,
thickness: options.thickness,
profileSlope: options.profileSlope
}));
newGear.classed(_gearStyles[i % _gearStyles.length], true);
}
};
var _randomiseScene = function(transition) {
_allGears = Gear.Utility.arrayShuffle(_allGears);
Gear.randomArrange(_allGears, _xOffset, _yOffset);
Gear.setPower(_allGears[0], 0.01);
Gear.updateGears(_allGears);
_svg.selectAll('.gear')
.each(function(d, i) {
if (transition) {
d3.select(this)
.transition()
.ease('elastic')
.delay(i * 80 + Math.random() * 80)
.duration(1500)
.attr('transform', function(d) {
return 'translate(' + [ d.x, d.y ] + ')';
});
} else {
d3.select(this)
.attr('transform', function(d) {
return 'translate(' + [ d.x, d.y ] + ')';
});
}
});
};
main();<file_sep>/app/includes/inscription.php
<?php
include "connect.inc.php"; /// Connection bdd
//echo "inscription.php";
//ok=1 ko=0
function formValid(){
$verif = 1;
if (isset($_POST['surname'])) {
echo "}".$_POST['surname'];
}else{
$verif=0;
}
if (isset($_POST['name'])) {
echo "}".$_POST['name'];
}else{
$verif=0;
}
if (isset($_POST['pseudo'])) {
echo "}".$_POST['pseudo'];
}else{
$verif=0;
}
if (isset($_POST['email'])) {
echo "}".$_POST['email'];
}else{
$verif=0;
}
if (isset($_POST['password'])) {
echo "}".$_POST['password'];
echo "-->".md5($_POST['password']);
}else{
$verif=0;
}
if (isset($_POST['selectPays'])) {
echo "}".$_POST['selectPays'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
//Evite d'avoir 2 mail identique en bdd ok=1 ko=0
function emailExist(){
include "connect.inc.php";
$verif = 1; //existant par defaut
$email = $_POST['email'];
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user where email='$email'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
if (empty($result)){
$verif = 0;
}
return $verif;
}
function insertUsertoBDD(){
include "connect.inc.php";
$valSurname = $_POST['surname'];
$valName = $_POST['name'];
$valPseudo = $_POST['pseudo'];
$valEmail = $_POST['email'];
$valPassword = md5($_POST['password']);
$valPays = $_POST['selectPays'];
$valJob = $_POST['selectJob'];
$linkAvatar = '../../assets/images/profil/0user.png';
try {
//$requete_prepare= $bdd->prepare("INSERT INTO user(nom,prenom,pseudo,email,password,pays,grade) VALUES('$valSurname', '$valName', '$valPseudo', '$valEmail', '$valPassword', '$valPays', 1)"); // on prépare notre requête
$requete_prepare= $bdd->prepare("INSERT INTO user(nom,prenom,pseudo,email,password,pays,grade,avatar) VALUES('$valSurname', '$valName', '$valPseudo', '$valEmail', '$valPassword', '$valPays', 1, '$linkAvatar')"); // on prépare notre requête
$requete_prepare->execute();
var_dump($requete_prepare);
echo "->OK insertUsertoBDD";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
function startSession(){
include "connect.inc.php";
$email = $_POST['email'];
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user WHERE email='$email'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
session_start();
$_SESSION['login'] = $result[0]['email'];
$_SESSION['pseudo'] = $result[0]['pseudo'];
$_SESSION['id_user'] = $result[0]['id_user'];
$_SESSION['email'] = $result[0]['email'];
$_SESSION['pays'] = $result[0]['pays'];
$_SESSION['grade'] = $result[0]['grade'];
$_SESSION['nom'] = $result[0]['nom'];
$_SESSION['prenom'] = $result[0]['prenom'];
$_SESSION['professeur'] = $result[0]['professeur'];
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
$verifMail = emailExist();
$verif = formValid();
if($verifMail==1){
echo '<br>Mail déjà present';
header("Location: ../modules/not-connected/inscription.php?erreur=mail deja present");
}else if($verif==1){
insertUsertoBDD();
//Mettre startSession();
startSession();
header("Location: ../index.php?succes");
}
else{
echo '<br>wrong form';
header("Location: ../modules/not-connected/inscription.php?erreur= erreur formulaire");
}
?><file_sep>/app/includes/connect.inc.php
<?php
$connect_str= "mysql:host=127.0.0.1;dbname=mooc";
$connect_user= 'root';
$connect_pass= '';
try
{
$bdd= new PDO($connect_str, $connect_user, $connect_pass,array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION));
$utf8 = $bdd->prepare("SET NAMES UTF8");
$utf8->execute();
}
catch(PDOException $e)
{
echo "Erreur au niveau de la BDD (verifier si la BDD existe) voir includes/connect.inc.php pour configuration";
exit();
}
?><file_sep>/app/includes/login.php
<?php
include "connect.inc.php";
function formValid(){
$verif = 1;
if (isset($_POST['email'])) {
echo $_POST['email'];
}else{
$verif=0;
}
if (isset($_POST['password'])) {
//echo $_POST['password'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
function insertUsertoBDD(){
include "connect.inc.php";
$email = $_POST['email'];
$valPassword = $_POST['password'];
$valPassword = md5($valPassword);
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user WHERE email='$email'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
/*echo '--->'.$result[0]['email'];
echo '--->'.$valPassword;
var_dump($result);*/
if (empty($result)) {
header ("location: ../modules/not-connected/connexion.php?erreur=Aucun compte");
}else if($result[0]['email']==$email && $result[0]['password']==$valPassword){
session_start();
$_SESSION['login'] = $result[0]['email'];
$_SESSION['pseudo'] = $result[0]['pseudo'];
$_SESSION['id_user'] = $result[0]['id_user'];
$_SESSION['email'] = $result[0]['email'];
$_SESSION['pays'] = $result[0]['pays'];
$_SESSION['grade'] = $result[0]['grade'];
$_SESSION['professeur'] = $result[0]['professeur'];
$_SESSION['nom'] = $result[0]['nom'];
$_SESSION['prenom'] = $result[0]['prenom'];
$_SESSION['avatar'] = $result[0]['avatar'];
//------HISTORIQUE DES CONNEXIONS --------
include "insert_log.php";
//--------------------------------------
header('location: ../modules/connected/catalogue.php');
}else if($result[0]['email']==$email && $result[0]['password']!=$valPassword){
header ("location: ../modules/not-connected/connexion.php?erreur=Mot de passe faux");
}else{
header ("location: ../modules/not-connected/connexion.php?erreur=Erreur de saisie");
}
}
$verif = formValid();
if($verif==1){
insertUsertoBDD();
}
else{
echo '<br>wrong form';
}
?><file_sep>/app/mooc/mooc1/chapitres/chapitre10.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 10 : Exam Final avec rendu du travail</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false"><i class="fa fa-wrench"></i></a>
<ul class="dropdown-menu" role="menu">
<li><a href="#">Settings 1</a>
</li>
<li><a href="#">Settings 2</a>
</li>
</ul>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Send email -->
<h4>Envoi du travail
</h4>
<form class="form-horizontal form-label-left" enctype="multipart/form-data" action="_model/send_attachement.php" method="post" id="myform3" novalidate>
<div class="item form-group">
<label for="password" class="control-label col-md-3">Nom</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="oldPassword" type="text" name="name" required="required">
</div>
</div>
<div class="item form-group">
<label for="password" class="control-label col-md-3">Email</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="newPassword" type="email" name="email" data-validate-length-range="4" class="form-control col-md-7 col-xs-12" required="required">
</div>
</div>
<div class="item form-group">
<label for="password" class="control-label col-md-3">Pièces jointes</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input type="file" name="my_file" >
</div>
</div>
<div class="ln_solid"></div>
<div class="form-group">
<div class="col-md-6 col-md-offset-3">
<button id="send" type="submit" class="btn btn-success">Confirmer</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/includes/insert_log.php
<?php
include "connect.inc.php"; /// Connection bdd
//ok=1 ko=0
function sessionValid(){
$verif = 1;
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user'])))
{
//session ok
}
else
{
$verif = 0;
}
return $verif;
}
function insertLogtoBDD(){
include "connect.inc.php";
/* Récupération date est heure */
$date = date("d-m-Y");
$heure = date("H:i");
$connect_time = $date." ".$heure;
/* Récupération session */
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user']))){
$id_user = $_SESSION['id_user'];//ok
}else{
$id_user = 0;
}
if ((isset($_SESSION['email'])) && (!empty($_SESSION['email']))){
$email = $_SESSION['email'];//ok
}else{
$email = 'unknow';
}
/* Récupération ip */
$ip = $_SERVER["REMOTE_ADDR"];
/* Récupération url */
$lien = 'http://'.$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI'];
$bool = sessionValid();
/* Récupération info navigateur */
$browser = $_SERVER['HTTP_USER_AGENT'];
//echo "---->".$browser;
/* Récuperation avant derniere url */
$dernierlien = $_SERVER['REQUEST_URI'];
if($bool == 0)
{
try {
$requete_prepare= $bdd->prepare("INSERT INTO log(connect_time,ip,lien,dernierlien,browser) VALUES('$connect_time', '$ip', '$lien', '$dernierlien', '$browser')"); // on prépare notre requête
$requete_prepare->execute();
echo "->OK sans session";
} catch (Exception $e) {
//echo $e->errorMessage();
echo "->erreur";
}
}else{
try {
$requete_prepare= $bdd->prepare("INSERT INTO log(id_user,connect_time,email,ip,lien,dernierlien,browser) VALUES('$id_user', '$connect_time', '$email', '$ip', '$lien', '$dernierlien', '$browser')"); // on prépare notre requête
$requete_prepare->execute();
echo "->OK avec session";
} catch (Exception $e) {
//echo $e->errorMessage();
echo "->erreur";
}
}
}
insertLogtoBDD();
?><file_sep>/app/modules/not-connected/connexion.php
<?php
session_start();
if((isset($_SESSION['id_user']))) {
header ("location: ../connected/catalogue.php");
}
?>
<!DOCTYPE html>
<!--
Material Design Lite
Copyright 2015 Google Inc. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License
-->
<html lang="fr">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="og:title" content="Mooc Isen">
<meta property="og:image" content="">
<meta property="og:url" content="http://colombies.com/app">
<meta property="og:description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="robots" content="">
<!-- Title -->
<title>Connexion | Mooc Isen</title>
<!-- favicon.ico -->
<link rel="shortcut icon" href="../../favicon.ico">
<!-- Disable tap highlight on IE -->
<meta name="msapplication-tap-highlight" content="no">
<!-- Web Application Manifest -->
<link rel="manifest" href="../../manifest.json">
<!-- Add to homescreen for Chrome on Android -->
<meta name="mobile-web-app-capable" content="yes">
<meta name="application-name" content="Mooc Isen">
<link rel="icon" sizes="192x192" href="../../images/touch/chrome-touch-icon-192x192.png">
<!-- Add to homescreen for Safari on iOS -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Mooc Isen">
<link rel="apple-touch-icon" href="../../images/touch/apple-touch-icon.png">
<!-- Tile icon for Win8 (144x144 + tile color) -->
<meta name="msapplication-TileImage" content="../../images/touch/ms-touch-icon-144x144-precomposed.png">
<meta name="msapplication-TileColor" content="#457871">
<!-- Color the status bar on mobile devices -->
<meta name="theme-color" content="#457871">
<!-- Material Design Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:100,300,400,500,700">
<!-- Material Design Icons -->
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<!-- Material Design Lite -->
<link rel="stylesheet" href="../../styles/material.min.css">
<!-- Your styles -->
<link rel="stylesheet" href="../../styles/not-connected/connexion.css">
</head>
<body>
<!-- header -->
<header class="site-header">
<div class="aux cf">
<h2 class="site-header__title">
<a class="site-header__root-link" href="../../index.php">
<img class="site-header__wallet-logo" alt="Mooc Isen" src="../../images/header/mooc-logo.png">
</a>
</h2>
<nav class="site-header__main-nav" id="HeaderNav">
<ul>
<li>
<a class="nav-link" href="connexion.php">
Connexion
</a>
</li>
<li>
<a class="nav-link" href="inscription.php">
Inscription
</a>
</li>
<li>
<div class="site-header__cta site-header__cta--desktop">
<a href="catalogue.php">
<button id="header-cta" class="mdl-button mdl-button--raised mdl-button--accent mdl-color--teal mdl-js-button mdl-js-ripple-effect">
Catalogue
</button>
</a>
</li>
</ul>
</nav>
<div class="site-header__cta site-header__cta--mobile">
<button id="get-app--large" class="mdl-button mdl-button--raised mdl-button--colored mdl-color--teal js-get-app-button mdl-js-button mdl-js-ripple-effect" onclick="location.replace('catalogue.php');">
Catalogue
</button>
</div>
</header>
<!-- section -->
<div class="form-box">
<div class="head">Connexion</div>
<form action="../../includes/login.php" method="post" id="myLogin">
<main class="mdl-layout__content">
<div class="mdl-textfield mdl-js-textfield mdl-textfield--floating-label">
<input type="email" class="mdl-textfield__input" id="exampleInputEmail1" name="email"/>
<label class="mdl-textfield__label" for="exampleInputEmail1">Email</label>
<span class="mdl-textfield__error">Email incorrecte</span>
</div>
</main>
<main class="mdl-layout__content">
<div class="mdl-textfield mdl-js-textfield mdl-textfield--floating-label">
<input type="<PASSWORD>" class="mdl-textfield__input" id="exampleInputPassword1" name="password"/>
<label class="mdl-textfield__label" for="exampleInputPassword1">Mot de passe</label>
<span class="mdl-textfield__error">Mot de passe requiert</span>
</div>
</main>
<input type="submit" class="btn" value="Connexion"/>
</form>
<p class="text-p"><a href="mdpOublie.php">Mot de passe oublié ?</a></p>
</div>
<div aria-live="assertive" aria-atomic="true" aria-relevant="text" class="mdl-snackbar mdl-js-snackbar">
<div class="mdl-snackbar__text"></div>
<button type="button" class="mdl-snackbar__action"></button>
</div>
</br></br></br></br>
<!-- footer -->
<footer class="mdl-mega-footer">
<div class="mdl-mega-footer__middle-section">
<div class="mdl-mega-footer__drop-down-section" style="top:30px">
<img class="site-header__wallet-logo" alt="Mooc Isen" src="../../images/footer/logo-footer.png">
<ul class="mdl-mega-footer__link-list">
<li>
<h4>Apprendre avec l'ISEN</h4>
</li>
<li>
<a href="https://www.youtube.com/user/isentoulon" target="_blank">
<img class="site-header__wallet-logo" alt="YouTube" src="../../images/social/youtube.png">
</a>
<a href="https://twitter.com/isentoulon?lang=fr" target="_blank">
<img class="site-header__wallet-logo" alt="Twitter" src="../../images/social/twitter-white.png">
</a>
<a href="https://www.facebook.com/ISEN.Toulon" target="_blank">
<img class="site-header__wallet-logo" alt="Facebook" src="../../images/social/facebook-white.png">
</a>
<a href="https://www.instagram.com/isen.fr/" target="_blank">
<img class="site-header__wallet-logo" alt="Instagram" src="../../images/social/instagram-white.png">
</a>
<a href="https://plus.google.com/104791520528769416386/about" target="_blank">
<img class="site-header__wallet-logo" alt="Google+" src="../../images/social/google-white.png">
</a>
</li>
</br>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading"> Ressources</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="faq.php">Aide & FAQ</a></li>
<li><a href="catalogue.php">Catalogue</a></li>
<li><a href="proposerCours.php">Proposer un MOOC</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">MOOC Isen</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="aPropos.php">A propos</a></li>
<li><a href="actualitees.php">Actualités</a></li>
<li><a href="temoignages.php">Témoignages</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">Informations</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="contact.php">Contact</a></li>
<li><a href="legales.php">Légales</a></li>
</ul>
</div>
</div>
<div class="mdl-mega-footer__bottom-section">
<ul class="mdl-mega-footer__link-list">
<li>
<a href="legales.php">Politique de confidentialité</a>
|
<a href="legales.php">Modalités</a>
</li>
</ul>
</div>
<!-- Material Design lite -->
<script src="../../scripts/material.min.js"></script>
<!-- jquery -->
<script src="../../scripts/jquery/jquery.js"></script>
<!-- Validation -->
<script src="../../scripts/jqueryvalidate/jquery.validate.min.js"></script>
<script src="../../scripts/jqueryvalidate/additional-methods.min.js"></script>
<!-- Custom Theme JavaScript -->
<script src="../../scripts/not-connected/connexion.js"></script>
<!-- Custom Theme JavaScript -->
<script src="../../scripts/catch_error.js"></script>
<!-- Google Analytics: change UA-XXXXX-X to be your site's ID -->
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-77911480-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html><file_sep>/app/index.php
<?php
session_start();
if((isset($_SESSION['id_user']))) {
header ("location: modules/connected/catalogue.php");
}
?>
<!DOCTYPE html>
<html lang="fr">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="og:title" content="Mooc Isen">
<meta property="og:image" content="">
<meta property="og:url" content="http://colombies.com/app">
<meta property="og:description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="robots" content="">
<!-- Title -->
<title>Accueil | Mooc Isen</title>
<!-- favicon.ico -->
<link rel="shortcut icon" href="favicon.ico">
<!-- Disable tap highlight on IE -->
<meta name="msapplication-tap-highlight" content="no">
<!-- Web Application Manifest -->
<link rel="manifest" href="manifest.json">
<!-- Add to homescreen for Chrome on Android -->
<meta name="mobile-web-app-capable" content="yes">
<meta name="application-name" content="Mooc Isen">
<link rel="icon" sizes="192x192" href="images/touch/chrome-touch-icon-192x192.png">
<!-- Add to homescreen for Safari on iOS -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Mooc Isen">
<link rel="apple-touch-icon" href="images/touch/apple-touch-icon.png">
<!-- Tile icon for Win8 (144x144 + tile color) -->
<meta name="msapplication-TileImage" content="images/touch/ms-touch-icon-144x144-precomposed.png">
<meta name="msapplication-TileColor" content="#457871">
<!-- Color the status bar on mobile devices -->
<meta name="theme-color" content="#457871">
<!-- Material Design Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:100,300,400,500,700">
<!-- Material Design Icons -->
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<!-- Material Design Lite -->
<link rel="stylesheet" href="styles/material.min.css">
<!-- Your styles -->
<link rel="stylesheet" href="styles/not-connected/index.css">
</head>
<body>
<!-- header -->
<header class="site-header">
<div class="aux cf">
<h2 class="site-header__title">
<a class="site-header__root-link" href="index.php">
<img class="site-header__wallet-logo" alt="Mooc Isen" src="images/header/mooc-logo.png">
</a>
</h2>
<nav class="site-header__main-nav" id="HeaderNav">
<ul>
<li>
<a class="nav-link" href="modules/not-connected/connexion.php">
Connexion
</a>
</li>
<li>
<a class="nav-link" href="modules/not-connected/inscription.php">
Inscription
</a>
</li>
<li>
<div class="site-header__cta site-header__cta--desktop">
<a href="modules/not-connected/catalogue.php">
<button id="header-cta" class="mdl-button mdl-button--raised mdl-button--accent mdl-color--teal mdl-js-button mdl-js-ripple-effect">
Catalogue
</button>
</a>
</li>
</ul>
</nav>
<div class="site-header__cta site-header__cta--mobile">
<button id="get-app--large" class="mdl-button mdl-button--raised mdl-button--colored mdl-color--teal js-get-app-button mdl-js-button mdl-js-ripple-effect" onclick="location.replace('modules/not-connected/catalogue.php');">
Catalogue
</button>
</div>
</header>
<!-- banniere -->
<div class="hero hero--home">
<div class="hero__bg-container">
<div class="hero__bg-container-overlay">
<!-- <img src="images/allisdigital.png"> -->
<h1>ALL IS DIGITAL</h1>
<div class="hero__get-app">
<a href="modules/not-connected/catalogue.php">
<button id="get-app--hero__button" class="get-app hero__btn mdl-button mdl-js-button mdl-color--teal mdl-button--raised mdl-button--colored mdl-js-ripple-effect">
Catalogue
</button>
</a>
</div>
<a href="modules/not-connected/inscription.php" class="hero__video-btn mdl-button mdl-js-button">
Inscription
</a>
</div>
<li class="page-scroll">
<a href="#welcome">
<button class="hero__fab mdl-button mdl-js-button mdl-js-ripple-effect mdl-button--fab mdl-shadow--4dp">
<i class="material-icons"></i>
</button>
</a>
</li>
</div>
</div>
<div aria-live="assertive" aria-atomic="true" aria-relevant="text" class="mdl-snackbar mdl-js-snackbar">
<div class="mdl-snackbar__text"></div>
<button type="button" class="mdl-snackbar__action"></button>
</div>
<!-- section 1 -->
<section id="welcome" class="section section--welcome section--text-left section--rev section--align-image-bottom">
<div class="aux">
<div class="grid grid--bottom">
<div class="section__content grid__item palm--one-whole lap--one-half desk--one-half">
<header class="recesection__header" >
<h2 class="section__title">Bienvenue sur la plateforme MoocISEN</h2>
<p> La plateforme héberge des MOOCs destinés aux étudiants</p>
<p>Notre site sera accessible à un grand nombre de personnes que ce soit des élèves ingénieurs, des étudiants en faculté supérieure, des lycéens ou même des entreprises qui souhaitent former leurs employés à de nouvelles technologies.</p>
</header>
</div><!--
--><div class="section__content grid__item palm--one-whole lap--one-half desk--one-half">
<!-- iframe-responsive.css full css -->
<div class="videocontainer" >
<iframe src="https://www.youtube.com/embed/lX7kYDRIZO4?modestbranding=0&autohide=0&showinfo=1&controls=1" frameborder="0" allowfullscreen></iframe>
</div>
</div>
</div>
</div>
</section>
<!-- section 2 -->
<section class="section section--notifications section--grey section--align-image-bottom">
<div class="aux">
<div class="grid grid--middle">
<div class="section__content grid__item palm--one-whole lap--one-half desk--one-half">
<header class="section__header">
<h2 class="section__title">Accessible sur mobile<br></h2>
<p> </p>
</header>
</div><!--
--><div class="section__image grid__item palm--one-whole lap--one-half desk--one-half">
<picture>
<!--[if IE 9]><video style="display: none;"><![endif]-->
<source srcset="images/index/notifications-desktop.png" media="(min-width: 769px)">
<source srcset="images/index/notifications-mobile.png" media="(max-width: 768px)">
<!--[if IE 9]></video><![endif]-->
<img srcset="images/index/notifications-desktop.png" alt="">
</picture>
</div>
</div>
</div>
</section>
<!-- section3 -->
<section class="section section--safety section--rev">
<div class="aux">
<div class="grid grid--middle">
<div class="section__content grid__item palm--one-whole lap--one-half desk--one-half">
<header class="section__header">
<h2 class="section__title">MOOC accessible sans compte</h2>
<p>Inscrition permettra de suivre votre avancement et votre score</p>
<p>Page admin pour suivre l'avancement de tous les comptes</p>
</header>
</div><!--
--><div class="section__image grid__item palm--one-whole lap--one-half desk--one-half">
<picture>
<!--[if IE 9]><video style="display: none;"><![endif]-->
<source srcset="images/index/safety-desktop.png" media="(min-width: 481px)">
<source srcset="images/index/safety-mobile.png" media="(max-width: 480px)">
<!--[if IE 9]></video><![endif]-->
<img srcset="images/index/safety-desktop.png" alt="">
</picture>
</div>
</div>
</div>
</section>
<!-- section4 -->
<section class="section section--support section--grey">
<div class="aux">
<div class="grid">
<div class="help-item grid__item one-whole lap--one-half desk--one-half">
<h3>Questions?</h3>
<p>Visiter le <a href="modules/not-connected/faq.php">FAQs</a>.</p>
</div><!--
--><div class="help-item grid__item one-whole lap--one-half desk--one-half">
<h3>Besoin d'aide?</h3>
<p>Aller à <a href="modules/not-connected/faq.php">Aide</a>.</p>
</div>
</div>
</div>
</section>
<!-- footer -->
<footer class="mdl-mega-footer">
<div class="mdl-mega-footer__middle-section">
<div class="mdl-mega-footer__drop-down-section" style="top:30px">
<img class="site-header__wallet-logo" alt="<NAME>" src="images/footer/logo-footer.png">
<ul class="mdl-mega-footer__link-list">
<li>
<h4>Apprendre avec l'ISEN</h4>
</li>
<li>
<a href="https://www.youtube.com/user/isentoulon" target="_blank">
<img class="site-header__wallet-logo" alt="YouTube" src="images/social/youtube.png">
</a>
<a href="https://twitter.com/isentoulon?lang=fr" target="_blank">
<img class="site-header__wallet-logo" alt="Twitter" src="images/social/twitter-white.png">
</a>
<a href="https://www.facebook.com/ISEN.Toulon" target="_blank">
<img class="site-header__wallet-logo" alt="Facebook" src="images/social/facebook-white.png">
</a>
<a href="https://www.instagram.com/isen.fr/" target="_blank">
<img class="site-header__wallet-logo" alt="Instagram" src="images/social/instagram-white.png">
</a>
<a href="https://plus.google.com/104791520528769416386/about" target="_blank">
<img class="site-header__wallet-logo" alt="Google+" src="images/social/google-white.png">
</a>
</li>
</br>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading"> Ressources</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="modules/not-connected/faq.php">Aide & FAQ</a></li>
<li><a href="modules/not-connected/catalogue.php">Catalogue</a></li>
<li><a href="modules/not-connected/proposerCours.php">Proposer un MOOC</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">MOOC Isen</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="modules/not-connected/aPropos.php">A propos</a></li>
<li><a href="modules/not-connected/actualitees.php">Actualités</a></li>
<li><a href="modules/not-connected/temoignages.php">Témoignages</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">Informations</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="modules/not-connected/contact.php">Contact</a></li>
<li><a href="modules/not-connected/legales.php">Légales</a></li>
</ul>
</div>
</div>
<div class="mdl-mega-footer__bottom-section">
<ul class="mdl-mega-footer__link-list">
<li>
<a href="modules/not-connected/legales.php">Politique de confidentialité</a>
|
<a href="modules/not-connected/legales.php">Modalités</a>
</li>
</ul>
</div>
<!-- Material Design lite -->
<script src="scripts/material.min.js"></script>
<!-- jQuery -->
<script src="scripts/jquery/jquery.js"></script>
<!-- Plugin JavaScript -->
<script src="scripts/jqueryeasing/jquery.easing.min.js"></script>
<!-- Custom Theme JavaScript -->
<script src="scripts/not-connected/index.js"></script>
<script src="scripts/not-connected/scroll-down-button.js"></script>
<!-- Custom Theme JavaScript -->
<script src="scripts/catch_error.js"></script>
<!-- Google Analytics: change UA-XXXXX-X to be your site's ID -->
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-77911480-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html><file_sep>/app/mooc/mooc1/chapitres/chapitre1.php
<?php
$idMooc;
$idChap;
if(isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
//afficheChapitreExos($idMooc,$idChap,$bdd,0);
//echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
?>
<!-- 1ER BOITE -->
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 ">
<div class="animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 1 : Identité </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="my_step_box">
<!-- Cours -->
<div class='row'>
<div class="col-md-3"></div>
<div class="col-md-6">
<div class="btn-group" role="group" aria-label="...">
<button type="button" class="btn btn-default myBtnBack"><span class="glyphicon glyphicon-chevron-left"></span> Retour</button>
<button type="button" class="btn btn-default myBtnAll"><span class="glyphicon glyphicon-eye-open"></span> </button>
<button type="button" class="btn btn-default myBtnNext">Suivant<span class="glyphicon glyphicon-chevron-right"></span></button>
</div>
</div>
<div class="col-md-3"></div>
</div>
<!-- METTRE ICI LE CONTENU -->
<div class="box1" id="1">
<ol start="1">
<li dir="ltr">
<h2 dir="ltr">
Nom prénom
</h2>
</li>
</ol>
<p dir="ltr">
Mettre le nom et le prénom est indispensable, sauf si un CV anonyme est demandé. Si c’est le cas le recruteur aura soit la lettre de motivation soit votre mail pour savoir qui vous êtes (de toute façon il vous verra en entretien).
</p>
<p dir="ltr">
En général, le prénom se met avant le nom. Ce dernier doit être mis en capitale surtout pour ceux qui ont un nom qui peut être un prénom ou lorsque vos nom et prénom sont à consonance étrangère méconnue.
</p>
<p dir="ltr">
Le nom et le prénom doit être placé en début de CV, plutôt en haut et à gauche ou en haut centré.
</p>
<br/>
<p dir="ltr">
Exemple:
</p>
<p dir="ltr">
C’est bien de mettre : <NAME>, <NAME> (même si le nom écrit systématiquement en capital est mieux afin d’aider à la mémorisation).
</p>
<p dir="ltr">
Il faut éviter: Monsieur <NAME>, JACQUES Patrick, M. <NAME>
</p>
<br/>
</div>
<!-- FIN METTRE ICI LE CONTENU -->
</div>
<div class="box1" id="2">
<ol start="2">
<li dir="ltr">
<h2 dir="ltr">
Adresse et mobilité
</h2>
</li>
</ol>
<p dir="ltr">
Cette information est indispensable mais moins prioritaire (tout se fait souvent par internet, cela est moins vrai pour les administrations), en dehors de savoir où vous habitez par rapport au lieu de travail. Il y a un risque que votre
adresse peut être utilisée par un recruteur pour éliminer votre candidature si votre lieu de domicile est jugé trop éloigné de l'entreprise.
Pour diminuer ce risque, si vous êtes mobile géographiquement, spécifier votre niveau de mobilité: régionale (à préciser),
nationale, internationale.
</p>
<p dir="ltr">
En ce qui concerne la mise en forme, il vaut mieux éviter de mettre la ville tout en capitale, peu d'intérêt d'attirer le regard.
</p>
<br/>
</div>
<div class="box1" id="3">
<ol start="3">
<li dir="ltr">
<h2 dir="ltr">
Téléphone(s)
</h2>
</li>
</ol>
<p dir="ltr">
En ce qui concerne le numéro du téléphone, il faut mettre le numéro de téléphone où l’on peut vous joindre facilement, par exemple, le numéro de téléphone
portable. Faites attention à votre message en cas d’absence. Pensez à mettre (+33) si vous postulez à l’étranger.
</p>
<br/>
<p dir="ltr">
Si vous mettez deux numéros de téléphone ou plus sur votre CV, il est conseillé de placer le principal en premier. Par contre il est fort probable qu’en
cas de non réponse, le recruteur risque de passer directement au second numéro au lieu de laisser un message sur votre répondeur.
</p>
<br/>
<p dir="ltr">
Il est important de mettre au moins un numéro de téléphone, car il y a beaucoup de chances que le récruteur veuille vous contacter par téléphone.
</p>
<br/>
</div>
<div class="box1" id="4">
<ol start="4">
<li dir="ltr">
<h2 dir="ltr">
Mail(s)
</h2>
</li>
</ol>
<p dir="ltr">
Il faut avoir une adresse mail professionnelle, c’est à dire une adresse de type: <a href="mailto:<EMAIL>"><EMAIL></a>. Si vous
êtes étudiant, pensez à utiliser l’adresse mail de votre école.
</p>
<p dir="ltr">
Il faut éviter les pseudos et les adresses mails de type: «<EMAIL> » ou « <EMAIL> » qui feront au mieux sourire, au pire
fuir le recruteur. Votre professionnalisme risque de prendre un coup d’entrée de jeu. Faites attention aux providers qui sont souvent spammés (Hotmail ou
Yahoo par exemple).
</p>
<br/>
</div>
<div class="box1" id="5">
<ol start="5">
<li dir="ltr">
<h2 dir="ltr">
Date de naissance et âge
</h2>
</li>
</ol>
<p dir="ltr">
La date de naissance est conseillé à mettre sous la forme jj/mm/aaaa, vous pouvez mettre votre âge entre parentèses, attention à rectifier chaque année.
</p>
<br/>
<p dir="ltr">
Il est inutile de mentir sur votre âge, car il peut être facilement évalué, grâce aux dates d’obtention de vos diplômes.
</p>
<br/>
</div>
<div class="box1" id="6">
<ol start="6">
<li dir="ltr">
<h2 dir="ltr">
Lieu de naissance(optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez mettre votre lieu de naissance. Si votre nom à une consonance étrangère et que vous êtes français. Il y a peu d'intérêt à le mettre si votre
nom sonne bien Français.
</p>
<p dir="ltr">
C’est peut-être une indication intéressante pour le recruteur, notamment si elle témoigne d’une mobilité géographique internationale et – surtout – de la
parfaite connaissance d’une langue
</p>
<ol start="7">
<li dir="ltr">
<h2 dir="ltr">
Nationalité (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez spécifier votre nationalité si vous avez double nationalité. Ainsi que si vous êtes étranger, ou si votre nom prête à confusion (si vous avez un nom aux résonances étrangères, tout en étant de nationalité française, par exemple). Il y a peu d'intérêt si votre nom sonne bien français.
</p>
<ol start="8">
<li dir="ltr">
<h2 dir="ltr">
Réseau social (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez mettre un lien vers votre réseau social mais que si les informations accessibles sont présentées de façon professionnelle. Pensez à mettre régulièrement à jour vos informations. Les réseaux utilisés par les recruteurs sont: LinkedIn, Viadeo, twitter, Facebook (professionnel).
</p>
<p dir="ltr">
Généralement Viadeo est utilisé dans les métiers de la création, d’Internet et de la communication. LinkedIn est plutôt utilisé dans les métiers de l’industrie.
En ce qui concerne votre compte Twitter et votre page Facebook, faites très attention à ce que ce soient des comptes professionnels où il n’y a pas de contenu de votre vie privé.
</p>
<p dir="ltr">
Vous pouvez mettre un lien vers votre site internet si c’est en cohérence avec ce que vous souhaitez mettre en avant. Vérifiez qu'il fonctionne bien car recruteur fera sûrement un clic dessus.
</p>
<p dir="ltr">
Faites attention à votre e-réputation! Chaque lien que vous mentionnez doit apporter une réelle valeur ajoutée au CV et non le pénaliser. Un lien non valide, un blog d’humeur politique, un profil avec des commentaires acerbes ne feront que porter préjudice à la candidature.
</p>
<br/>
</div>
<div class="box1" id="7">
<ol start="10">
<li dir="ltr">
<h2 dir="ltr">
Bilingue (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez mettre que vous êtes bilingue, trilingue (ou plus). Il faut bien spécifier les langues. Forcément le recruteur pourra très bien entamer le dialogue dans la langue que vous maitrisez en dehors du Français.
</p>
<ol start="11">
<li dir="ltr">
<h2 dir="ltr">
Phrase d’accroche, titre du CV et objectifs (optionnel) Partie 1
</h2>
</li>
</ol>
<p dir="ltr">
La phrase d’accroche doit traduire un objectif, une ambition, elle doit être le plus factuelle possible. Elle induit la façon dont sera lu votre CV par la suite. Le recruteur va être influencé. Soit il arrête de lire, soit il va contrôler sa lecture en fonction de la phrase d'accroche.
</p>
<p dir="ltr">
Le titre fut longtemps présenté comme facultatif. Aujourd’hui la majorité des recruteurs considèrent qu’il est indispensable. Il doit cependant être manié avec intelligence : un titre trop flou donne l’impression d’un projet professionnel pas très clair tandis qu’un titre trop précis peut fermer des portes.
</p>
<p dir="ltr">
Le titre indique généralement un poste mais il peut aussi prendre l’intitulé d’un département. Quand on manque d’expérience, cela a l’avantage de désigner les métiers vers lesquels on s’oriente tout en montrant qu’on est prêt à étudier différents degrés hiérarchiques. Il est également possible d’ajouter le secteur d’activité si celui-ci est déterminant. En fait, c’est à chacun de placer le curseur en fonction de ses ambitions et de son parcours. Toutefois, il faut bannir les intitulés trop généraux (par exemple : communication).
</p>
<p dir="ltr">
Exemple de titre de CV:
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
Ingénieur électronique spécialisation téléphonie - séjour en Chine de 2 ans
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Ingénieur en informatique - bilingue anglais - mobilité internationale
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Ingénieur en électronique - mobilité internationale - 15 ans d’expérience
</p>
</li>
</ul>
</div>
<div class="box1" id="8">
<ol start="11">
<li dir="ltr">
<h2 dir="ltr">
Phrase d’accroche, titre du CV et objectifs (optionnel) Partie 2
</h2>
</li>
</ol>
<p dir="ltr">
La lecture du CV peut se faire avant ou après la lecture de la lettre de motivation, en règle générale, c’est cette dernière qui est lue en premier. C’est elle qui va donner du sens pour la lecture du CV, en précisant l’objet, les objectifs et les enjeux de la demande.
</p>
<p dir="ltr">
Attention un CV n’est pas une lettre de motivation. Si cette dernière traduit le présent et le futur, le CV traduit le passé et le présent, ils se complètent donc. Donc pas de longue phrase explicative en début de CV, on doit rester sur une accroche qui donne envie de lire et rend curieux de la suite ou qui synthétise les éléments clés et spécifiques du CV.
</p>
<p dir="ltr">
Les qualificatifs sur son CV ne doivent pas être choisis à la légère. LinkedIn a sorti les 10 mots les plus utilisés par ses membres. Les recruteurs se
lassent de ne voir que des candidats créatifs, stratégiques et motivés.
<img
src="https://lh4.googleusercontent.com/4wTBry7_9wSQ-yryybV4vaFaf1EF52MVkh_lttjnkEeyjHQWUD6rbdAY3RJg79nYBTFHSMryb0LGSRpQFP4chieqczRlkcD5pOdSnQjdxPruVTaqjvoLb31c59xcE4t5ZL7I66pk"
width="472"
height="301"
/>
</p>
<br/>
<p dir="ltr">
Ces mots ne donc pas des plus-values. Rappelez-vous d’un principe simple de communication, la méthode SOFA. Nous échangeons sur 4 niveaux :
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
Sentiment
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Opinion
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Faits
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Actions
</p>
</li>
</ul>
</div>
<div class="box1" id="9">
<ol start="11">
<li dir="ltr">
<h2 dir="ltr">
Phrase d’accroche, titre du CV et objectifs (optionnel) Partie 3
</h2>
</li>
</ol>
<p dir="ltr">
Les deux premiers sont des interprétations issues de faits observés personnellement mais qui sont généralisés, réduits ou transformés et ne constituent pas de preuve de ce que l’on dit.
</p>
<p dir="ltr">
Ex : je suis motivé, dynamique et expert : qu’est-ce qui le prouve ?
</p>
<p dir="ltr">
Les deux derniers sont des informations factuelles qui vont justement induire chez l’auditeur des sentiments et opinions.
</p>
<p dir="ltr">
Ex : 20X1 : responsable de projet développement site commercial chez X (6 mois) pour le client Z avec une équipe de 5 ingénieurs. Projet réalisé avec 1 mois d’avance et un budget optimisé de 23%.
</p>
<p dir="ltr">
Le lecteur pourra déduire, que vous êtes motivé, dynamique et expert.
</p>
<p dir="ltr">
Il faut privilégier les verbes d’action pour décrire ses missions passées : vous avez décidé, fixé, géré, dirigé, coordonné, organisé, formé, contrôler. Les recruteurs préfèrent des mots d’action pour définir l’expérience, les compétences et les réalisations. D'après l'étude de CareerBuilder, les recruteurs apprécieraient particulièrement les expressions :
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
Obtenu/réalisé
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Amélioré
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Formé, parrainé
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Managé
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Créé
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Résolu
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Portés volontaires
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Augmenter / réduire
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Idées
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Négocié
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Lancer, mettre en place
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Chiffre d’affaires / profits
</p>
</li>
<li dir="ltr">
<p dir="ltr">
En deçà du budget
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Remporté / gagné
</p>
</li>
</ul>
<br/>
</div>
<div class="box1" id="10">
<ol start="12">
<li dir="ltr">
<h2 dir="ltr">
Photo (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
La photo est un élément fortement sujet à émotion donc à interprétation. Votre photo doit donc donner envie de vous rencontrer, donc sourire, netteté, contraste, vêtement professionnel, attitude ouverte et couleurs sont de mises.
</p>
<p dir="ltr">
Concrètement, se mettre en avant sur son CV en ligne commence par présenter une bonne photo. Les profils avec photo ont 14 fois plus de chances d’être consultés. Si vous avez rencontré des recruteurs par exemple sur un forum ou dans un salon professionnel, elle peut être un moyen pour eux de vous reconnaître.
</p>
<p dir="ltr">
La photo n’est pas obligatoire dans un CV, surtout si elle ne vous met pas en valeur ou manque de professionnalisme. Mieux vaut ne pas afficher de photo du tout que d’insérer un portrait livide, décalé, voir raté sur votre CV. Evitez de mettre les scans de photo d'identité, les vieilles photos, les photos de soirées, de vacances ou de famille, qui manquent de pertinence et de maturité.
</p>
<p dir="ltr">
Préférez des photos dans des contextes professionnels. Une photo en décolleté est également à proscrire, miser sur l’atout physique dans une candidature est une faute de goût et révèle un manque flagrant d’arguments professionnels.
</p>
<p dir="ltr">
Enfin, vous postuler pour un poste d’ingénieur. Si c’est dans le développement web, la photo a moins d’importance qui si vous postulez sur un poste d’ingénieur d’affaires. La fréquence du contact avec le client souligne la différence sur la prise en compte de la photo.
</p>
<br/>
</div>
<div class="box1" id="11">
<ol start="13">
<li dir="ltr">
<h2 dir="ltr">
A eviter
</h2>
</li>
</ol>
<p dir="ltr">
Il y a certaines choses qu’il vaut mieux éviter de mettre dans un CV: sexe, situation familiale, religion, et bien sur les fausses informations.
</p>
<br/>
<p dir="ltr">
Si vous mentionnez votre sexe, attention au risque de discrimination à l'embauche si spécifiée. Puis dans la plupart des cas votre prénom indique le sexe en général sinon ce sera la surprise pour le recruteur, donc ce n’est pas la peine de le préciser en plus.
</p>
<p dir="ltr">
En ce qui concerne votre situation familiale, ce n'est plus comme avant, les situations sont nombreuses (célibataire, marié, pacsé, compagnon, divorcé …) et aucune ne traduit votre mobilité réelle.
</p>
<p dir="ltr">
Parfois il peut être tentant, pour les candidats, d'« embellir » leur CV, à coup de faux diplômes, de compétences surévaluées ou de hobbies trafiqués. Pourtant, ces petits arrangements avec la vérité peuvent vous coûter cher en vous faisant manquer le poste de vos rêves si le recruteur vient à découvrir la supercherie.
</p>
<br/>
</div>
<div class="box1" id="12">
<ol start="14">
<li dir="ltr">
<h2 dir="ltr">
Récapitulatif du chapitre
</h2>
</li>
</ol>
<p dir="ltr">
Nous pouvons résumer le chapitre en 12 points essentiels suivants:
</p>
<ol>
<li>
Le <b>nom</b> est mis en capitale lorsque le nom peut être un <b>prénom</b> ou lorsqu’ils sont à consonance étrangère.
</li>
<li>
Dans tous les cas pensez à mettre votre <b>mobilité</b>: régionale, nationale, internationale.
</li>
<li>
Mettre le <b>numéro de téléphone</b>portable est très conseillé. Si vous mettez plusieurs numéros de téléphone (on considère que 2 numéros c’est largement suffisant), placer le principal en premier.
</li>
<li>
<b>L’adresse mail</b> doit être professionnelle du type : <EMAIL>. Evitez les boites mails Yahoo et Hotmail.
</li>
<li>
<b>La date de naissance</b> est sous la forme jj/mm/aaaa. Il est très conseillé de mettre l’âge entre les parenthèses et ne pas oublier à rectifier chaque année.
</li>
<li>
<b>Le lieu de naissance</b> et la <b>nationalité</b> sont à mettre si votre nom est à consonance étrangère. Cela peut aussi indiquer une mobilité géographique intéressante.
</li>
<li>
Vous pouvez mettre le lien vers les <b>réseaux</b> sociaux suivants : Twitter, Facebook, Linkedin, Viadéo à conditions que ce sont les comptes professionnels qui ne contiennent pas les éléments de votre vie privée.
</li>
<li>
Il est vivement conseillé à mettre que vous êtes <b>bilingue</b> (voir même trilingue).
</li>
<li>
<b>La phrase d’accroche</b> traduit un objectif, une ambition. <b>Le titre de votre CV</b> doit indiquer un poste ou l’intitulé d’un département. Vous pouvez mettre un élément qui peut vous démarquer (mobilité, bilingue, etc.). N’oubliez pas à mettre les <b>objectifs</b> en utilisant les bons qualificatifs.
</li>
<li>
La <b>photo</b> n’est pas obligatoire. Si vous la mettez elle doit être prise dans le contexte professionnel.
</li>
<li>
<b>Evitez</b> de mentionner votre sexe, situation familiale et les mensonges.
</li>
</ol>
<br/>
</div>
</div>
</div>
</div>
<div class="row">
<!-- 1ER BOITE -->
<div class="col-md-12 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Vidéo </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="videocontainer lg6">
<!--<iframe src="https://www.youtube.com/embed/lX7kYDRIZO4" frameborder="0" ></iframe>-->
<iframe width="560" height="315" src="https://www.youtube.com/embed/_r7bht-LgvU" frameborder="0" allowfullscreen></iframe>
</div>
</div>
</div>
</div>
</div>
<div class="row">
<!-- 1ER BOITE -->
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Exemple </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Cours -->
<br>
<div >
<address>
<strong><NAME> ,<NAME></strong>
<br>La Citadelle, Présidium
<br>Nos Astra, Illium
<br>Phone: 1 (804) 123-9876
<br>Email: <EMAIL>
</address>
</div>
</div>
</div>
</div>
<!-- 2EME BOITE -->
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Icônes gratuit </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Cours -->
<strong>Voici un pack d'icônes en 512 pixels en png pour votre profil</strong> <br><br>
<!--<div class="row">
<div class="col-md-6 col-xs-6"><img class="" src="../mooc/mooc1/chapitres/images/chapitre1.jpg" width="150px" alt=""></div>
<div class="col-md-6 col-xs-6"><a href="../mooc/mooc1/chapitres/images/chapitre1.7z" target="_blank"><button id="send" type="submit" class="btn btn-success"><span class="glyphicon glyphicon-save-file"></span> Télécharger</button></a></div>
</div>-->
<div class="row">
<div class="left"><img class="" src="../mooc/mooc1/chapitres/images/chapitre1.jpg" width="150px" alt=""></div>
<div class="right"><br><div class="text-center"><a href="../mooc/mooc1/chapitres/images/chapitre1.7z" target="_blank"><button id="send" type="submit" class="btn btn-success"><span class="glyphicon glyphicon-save-file"></span> Télécharger</button></a></div></div>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInRight">
<div class="x_panel">
<div class="x_title">
<?php
nomChapitre($idMooc,$bdd,$numChap);
?>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Smart Wizard -->
<h3>Exercices</h3>
<div id="wizard" class="form_wizard wizard_horizontal">
<?php
creationWizardStep($idMooc,$numChap,$idChap,$bdd);
?>
</div>
<!-- IMPORTANT AFFICHE LES INDICE -->
<div id="indicebox"></div>
<!-- IMPORTANT AFFICHE LES SOLUTIONS -->
<div id="solucebox"></div>
<!-- IMPORTANT POUR LES REPONSE AU QCM -->
<?php
/*if(isset($_GET['idM']) && isset($_GET['idC'])) {
//$idMooc = $_GET['idM'];
echo '<div id="'.$_GET['idM'].'"></div>';
$idChap = $_GET['idC'];
}*/
?>
</div>
</div>
</div>
<file_sep>/app/includes/include_connected.php
<?php
function afficheMyProfilHeader($bdd){
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user']))){
$myid = $_SESSION['id_user'];
try {
//$select3 = $bdd->prepare("SELECT * FROM log");
$select3 = $bdd->prepare("SELECT * from user WHERE id_user=$myid");
$select3->execute();
$lignes3 = $select3->fetchAll();
echo '
<header class="demo-drawer-header">
<img src="'.$lignes3[0]["avatar"].'" class="demo-avatar">
<div class="demo-avatar-dropdown">
<span>'.$lignes3[0]["email"].'</span>
<div class="mdl-layout-spacer"></div>
<button id="accbtn" class="mdl-button mdl-js-button mdl-js-ripple-effect mdl-button--icon">
<i class="material-icons" role="presentation">arrow_drop_down</i>
<span class="visuallyhidden">Accounts</span>
</button>
<ul class="mdl-menu mdl-menu--bottom-right mdl-js-menu mdl-js-ripple-effect" for="accbtn">
<a class="mdl-navigation__link" href="../../includes/logout.php"><li class="mdl-menu__item">Se deconnecter<i class="material-icons">exit_to_app</i></li></a>
</ul>
</div>
</header>
';
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}else{
echo '
<header class="demo-drawer-header">
<img src="../../images/user.jpg" class="demo-avatar">
<div class="demo-avatar-dropdown">
<span>Hors ligne</span>
<div class="mdl-layout-spacer"></div>
<button id="accbtn" class="mdl-button mdl-js-button mdl-js-ripple-effect mdl-button--icon">
<i class="material-icons" role="presentation">arrow_drop_down</i>
<span class="visuallyhidden">Accounts</span>
</button>
</div>
</header>
';
}
}
function afficheMyNavLink(){
if(($_SESSION['professeur'])==1) {
echo '
<nav class="demo-navigation mdl-navigation mdl-color--blue-grey-800">
<a class="mdl-navigation__link" href="profil.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">person</i>Profil</a>
<a class="mdl-navigation__link" href="admin.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">dashboard</i>Admin</a>
<a class="mdl-navigation__link" href="catalogue.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">book</i>Catalogue</a>
<a class="mdl-navigation__link" href="actualitees.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">flag</i>Actualitées</a>
<a class="mdl-navigation__link" href="temoignages.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">local_offer</i>Témoignages</a>
<a class="mdl-navigation__link" href="proposerCours.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">shopping_cart</i>Proposer un MOOC</a>
<div class="mdl-layout-spacer"></div>
<a class="mdl-navigation__link" href="faq.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">help_outline</i>Aide & FAQ</a>
</nav>';
}else{
echo '
<nav class="demo-navigation mdl-navigation mdl-color--blue-grey-800">
<a class="mdl-navigation__link" href="profil.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">person</i>Profil</a>
<a class="mdl-navigation__link" href="catalogue.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">book</i>Catalogue</a>
<a class="mdl-navigation__link" href="actualitees.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">flag</i>Actualitées</a>
<a class="mdl-navigation__link" href="temoignages.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">local_offer</i>Témoignages</a>
<a class="mdl-navigation__link" href="proposerCours.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">shopping_cart</i>Proposer un MOOC</a>
<div class="mdl-layout-spacer"></div>
<a class="mdl-navigation__link" href="faq.php"><i class="mdl-color-text--blue-grey-400 material-icons" role="presentation">help_outline</i>Aide & FAQ</a>
</nav>';
}
}
?><file_sep>/app/mooc/mooc3/chapitres/chapitre0.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
//echo'erreur';
}
?>
<div class="row">
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Avant propos</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false"><i class="fa fa-wrench"></i></a>
<ul class="dropdown-menu" role="menu">
<li><a href="#">Settings 1</a>
</li>
<li><a href="#">Settings 2</a>
</li>
</ul>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Send email -->
<blockquote>
<p>
</p>
</blockquote>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/scripts/connected/profil.js
// --------------- REGEX --------------------
$.validator.addMethod("usernameRegex", function(value, element) {
return this.optional(element) || /^[a-z\u00E0-\u00FC_.+-]+$/i.test(value);
});
$.validator.addMethod("mailRegex", function(value, element) {
return this.optional(element) || /^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$/i.test(value);
});
// --------------- Login JqueryValidator ---------
var login = $("#myform1");
login.validate({
//prendre le name
errorElement: 'span',
errorClass: 'help-block',
highlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').addClass("has-error");
},
unhighlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').removeClass("has-error");
},
rules: {
name: {
required: true,
usernameRegex: true,
minlength: 2,
},
surname: {
required: true,
usernameRegex: true,
minlength: 2,
},
pseudo : {
required: true,
minlength: 2,
},
email: {
required: true,
mailRegex: true,
minlength: 3,
},
city:{
required: true,
},
},
messages: {
email: {
required: "email required",
},
surname : {
required: "surname required",
},
name: {
required: "name required",
},
name: {
required: "name required",
},
}
});
// --------------- Login JqueryValidator ---------
var myform2 = $("#myform2");
myform2.validate({
//prendre le name
errorElement: 'span',
errorClass: 'help-block',
highlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').addClass("has-error");
},
unhighlight: function(element, errorClass, validClass) {
$(element).closest('.form-group').removeClass("has-error");
},
rules: {
oldPassword : {
required: true,
minlength: 4,
},
newPassword: {
required: true,
minlength: 4,
},
},
messages: {
oldPassword: {
required: "<PASSWORD> de passe required",
},
newPassword : {
required: "<PASSWORD> mot de passe required",
},
}
});
function goMooc(idmooc){
location.href = "../mooc.php?idM="+idmooc+"&idC=0&numC=0";
}
function callGraph(idmooc){
//alert(idmooc);
var ctx = document.getElementById("myChart");
$.ajax({
type: "POST",
url: "../../includes/requestgraph.php",
dataType: 'json',
data: { data:idmooc },
success: function(data) {
//alert("success");
//console.log(data); // REGARDER DEBUG
console.log(data[0][0]["titre"]); // EXEMPLE
console.log(data[1][0]); // EXEMPLE
var titles = new Array();
data[0].forEach(function(elem,index){
titles[index] = elem.titre;
})
var pourcentage = new Array();
data[1].forEach(function(elem,index){
pourcentage[index] = elem;
})
//Get context with jQuery - using jQuery's .get() method.
$(".removechart").html('<canvas id="myChart" height="200" width="400" ></canvas>');
// reinit canvas
var ctx = $("#myChart");
//This will get the first returned node in the jQuery collection.
var myChart = new Chart(ctx, {
type: 'bar',
data: {
labels: titles,
datasets: [{
label: "% de réussite",
backgroundColor: "rgba(26,187,156,0.4)",
data: pourcentage
}],
},
options: {
scales: {
yAxes: [{
ticks: {
beginAtZero:true,
max:100
}
}]
}
}
});
}
});
}<file_sep>/app/mooc/mooc1/chapitres/chapitre5.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 5 : Langue</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
</div>
</div>
</div>
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Exemple</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
</div>
</div>
</div>
<file_sep>/app/modules/not-connected/faq.php
<?php
session_start();
if((isset($_SESSION['id_user']))) {
header ("location: ../connected/catalogue.php");
}
?>
<!DOCTYPE html>
<!--
Material Design Lite
Copyright 2015 Google Inc. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License
-->
<html lang="fr">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="og:title" content="Mooc Isen">
<meta property="og:image" content="">
<meta property="og:url" content="http://colombies.com/app">
<meta property="og:description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="robots" content="">
<!-- Title -->
<title>FAQ & Aide | Mooc Isen</title>
<!-- favicon.ic o -->
<link rel="shortcut icon" href="../../favicon.ico">
<!-- Disable tap highlight on IE -->
<meta name="msapplication-tap-highlight" content="no">
<!-- Web Application Manifest -->
<link rel="manifest" href="../../manifest.json">
<!-- Add to homescreen for Chrome on Android -->
<meta name="mobile-web-app-capable" content="yes">
<meta name="application-name" content="Mooc Isen">
<link rel="icon" sizes="192x192" href="../../images/touch/chrome-touch-icon-192x192.png">
<!-- Add to homescreen for Safari on iOS -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Mooc Isen">
<link rel="apple-touch-icon" href="../../images/touch/apple-touch-icon.png">
<!-- Tile icon for Win8 (144x144 + tile color) -->
<meta name="msapplication-TileImage" content="../../images/touch/ms-touch-icon-144x144-precomposed.png">
<meta name="msapplication-TileColor" content="#457871">
<!-- Color the status bar on mobile devices -->
<meta name="theme-color" content="#457871">
<!-- Material Design Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:100,300,400,500,700">
<!-- Material Design Icons -->
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons">
<!-- Material Design Lite -->
<link rel="stylesheet" href="../../styles/material.min.css">
<!-- Your styles -->
<link rel="stylesheet" href="../../styles/not-connected/faq.css">
</head>
<body>
<!-- header -->
<header class="site-header">
<div class="aux cf">
<h2 class="site-header__title">
<a class="site-header__root-link" href="../../index.php">
<img class="site-header__wallet-logo" alt="Mooc Isen" src="../../images/header/mooc-logo.png">
</a>
</h2>
<nav class="site-header__main-nav" id="HeaderNav">
<ul>
<li class="">
<a class="nav-link " href="connexion.php">
Connexion
</a>
</li>
<li>
<a class="nav-link" href="inscription.php">
Inscription
</a>
</li>
<li>
<div class="site-header__cta site-header__cta--desktop">
<a href="catalogue.php">
<button id="header-cta" class="mdl-button mdl-button--raised mdl-button--accent mdl-color--teal mdl-js-button mdl-js-ripple-effect">
Catalogue
</button>
</a>
</li>
</ul>
</nav>
<div class="site-header__cta site-header__cta--mobile">
<button id="get-app--large" class="mdl-button mdl-button--raised mdl-button--colored mdl-color--teal js-get-app-button mdl-js-button mdl-js-ripple-effect" onclick="location.replace('catalogue.php');">
Catalogue
</button>
</div>
</header>
<!-- FAQ -->
<div class="container">
<div class="aux">
<div class="grid page">
<aside class="grid__item desk--one-third">
<nav class="sidebar-nav">
<ul>
<li class="js-scroll-spy-link" data-scroll-spy-group="sidebar" data-scroll-spy-target="categorie1">
<a href="#categorie1" data-scroll-to="categorie1" data-g-event="TOC" data-g-label="categorie1">Découverte de la plateforme MOOC ISEN</a>
</li>
<li class="js-scroll-spy-link" data-scroll-spy-group="sidebar" data-scroll-spy-target="categorie2">
<a href="#categorie2" data-scroll-to="categorie2" data-g-event="TOC" data-g-label="categorie2">Les cours / MOOC sur MOOC ISEN</a>
</li>
<li class="js-scroll-spy-link" data-scroll-spy-group="sidebar" data-scroll-spy-target="categorie3">
<a href="#categorie3" data-scroll-to="categorie3" data-g-event="TOC" data-g-label="categorie3">Évaluation, attestation, certificat, diplôme</a>
</li>
</ul>
</nav>
</aside><!--
--><div class="grid__item desk--two-thirds">
<h1 class="page-title">Questions fréquement posées</h1>
<section class="question__section js-scroll-spy-target categorie1" data-scroll-spy-group="sidebar" data-scroll-spy-section="categorie1">
<a id="categorie1" class="question__anchor"></a>
<h4 class="faq-section__title">Découverte de la plateforme MOOC ISEN</h4>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="0:1">
<h5>Pourquoi suivre des cours sur MOOC’ISEN ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="0:1">
<p>
MOOC’ISEN propose des MOOC de qualité puisqu’ils sont fourni par l’ISEN (L’institut Supérieur de L’Electronique et du Numérique) une école d’ingénieur reconnue en France
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="0:2">
<h5>Comment faire pour m’inscrire sur MOOC’ISEN ? </h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="0:2">
<p>
Lorsque vous arrivez sur la page d’accueil, vous pourrez voir le bouton « Inscription » qui se trouve dans le menu en haut de la page, cliquez dessus et vous serez amené à remplir un formulaire, une fois ce formulaire rempli correctement vous serez inscrit au site et directement connecté à votre compte.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="0:3">
<h5>Comment puis-je changer mes informations sur MOOC’ISEN ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="0:3">
<p>
Pour cela il faudra vous rendre sur votre page de profil par le biais du menu latéral gauche, une fois arrivé, allez à la section « Modifier mon profil ». Vos informations seront déjà complétées, il ne vous reste plus qu’à changer ce que vous voulez, taper votre email dans le champ associé et confirmer.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="0:4">
<h5>Comment suivre un cours sur MOOC’ISEN ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="0:4">
<p>
Pour suivre un cours il vous suffit d’aller dans le « catalogue » des MOOC, une fois dedans vous verrez la liste des MOOC disponibles, si l’un d’eux vous intéresse, cliquez sur « Description », vous verrez une description plus détaillée de ce dernier. Enfin s’il vous intéresse vraiment cliquez sur le bouton « Inscription au cours ».
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="0:5">
<h5>Ais-je besoin d’être inscrit pour suivre un cours ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="0:5">
<p>
L’inscription n’est pas obligatoire pour suivre des cours sur MOOC’ISEN, néanmoins si vous ne possédez pas de compte, vous ne pourrez pas sauvegarder votre progression, vos scores et accéder à vos statistiques via la page profil.
</p>
</div>
</div>
</section>
<section class="question__section js-scroll-spy-target categorie2" data-scroll-spy-group="sidebar" data-scroll-spy-section="categorie2">
<a id="categorie2" class="question__anchor"></a>
<h4 class="faq-section__title">Les cours / MOOC sur MOOC ISEN</h4>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="1:1">
<h5>Vos cours sont-ils exclusivement en français ? </h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="1:1">
<p>
A ce jour, le site ne contient que des MOOC en français, peut-être d’autres langues viendront s’ajouter par la suite, mais si c’est le cas nous vous en tiendrons informé.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="1:2">
<h5>Suivre un cours, combien ça coute ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="1:2">
<p>
Les cours disponibles sur MOOC’ISEN sont tous et totalement gratuit pour tous.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="1:3">
<h5>Puis-je accéder aux cours à tout moment ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="1:3">
<p>
Les cours sont disponibles à tout moment et sans limite de durée.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="1:4">
<h5>Y a-t-il une certification à la clef ? </h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="1:4">
<p>
Pour le moment aucune certification n’est disponible, mais après tout on ne travaille pas toujours pour avoir une récompense.
</p>
</div>
</div>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="1:5">
<h5>Comment puis-je réinitialiser mon mot de passe sur MOOC’ISEN ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="1:5">
<p>
Si vous avez oublié votre mot de passe pour vous connecter, il vous suffit de cliquer sur « Mot de passe oublié », vous recevrez alors un email avec un lien qui vous permettra de réinitialiser votre mot de passe.
</p>
</div>
</div>
</section>
<section class="question__section js-scroll-spy-target categorie3" data-scroll-spy-group="sidebar" data-scroll-spy-section="categorie3">
<a id="categorie3" class="question__anchor"></a>
<h4 class="faq-section__title">Évaluation, attestation, certificat, diplôme</h4>
<div class="question">
<div class="question__question control js-toggle" data-toggle-target="2:1">
<h5>Comment puis-je consulter mon score, mon avancement et mes statistiques sur un MOOC ?</h5>
<span class="question__arrow">
<i class="material-icons question__icon"></i>
</span>
</div>
<div class="question__answer" aria-expanded="false" data-toggle-id="2:1">
<p>
Pour cela il faudra vous rendre sur votre page de profil par le biais du menu latéral gauche, une fois arrivé, allez à la section « Mes cours ». Vous pourrez ainsi voir les cours où vous êtes inscrit, ainsi que les scores réalisés et votre pourcentage d’avancement.
</p>
<p>
Pour voir vos statistiques sur ce MOOC il vous suffit de cliquer sur le bouton pour le cours de votre choix, le graphe s’afficherait dans la section « Statistiques » juste en dessous. Vous pourrez y trouver votre pourcentage de réussite pour chaque chapitre
</p>
</div>
</div>
</section>
</div>
</div>
</div>
</div>
<!-- footer -->
<footer class="mdl-mega-footer">
<div class="mdl-mega-footer__middle-section">
<div class="mdl-mega-footer__drop-down-section" style="top:30px">
<img class="site-header__wallet-logo" alt="Mooc Isen" src="../../images/footer/logo-footer.png">
<ul class="mdl-mega-footer__link-list">
<li>
<h4 style="color: #AFAFAF;">Apprendre avec l'ISEN</h4>
</li>
<li>
<a href="https://www.youtube.com/user/isentoulon" target="_blank">
<img class="site-header__wallet-logo" alt="YouTube" src="../../images/social/youtube.png">
</a>
<a href="https://twitter.com/isentoulon?lang=fr" target="_blank">
<img class="site-header__wallet-logo" alt="Twitter" src="../../images/social/twitter-white.png">
</a>
<a href="https://www.facebook.com/ISEN.Toulon" target="_blank">
<img class="site-header__wallet-logo" alt="Facebook" src="../../images/social/facebook-white.png">
</a>
<a href="https://www.instagram.com/isen.fr/" target="_blank">
<img class="site-header__wallet-logo" alt="Instagram" src="../../images/social/instagram-white.png">
</a>
<a href="https://plus.google.com/104791520528769416386/about" target="_blank">
<img class="site-header__wallet-logo" alt="Google+" src="../../images/social/google-white.png">
</a>
</li>
</br>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading"> Ressources</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="faq.php">Aide & FAQ</a></li>
<li><a href="catalogue.php">Catalogue</a></li>
<li><a href="proposerCours.php">Proposer un MOOC</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">MOOC Isen</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="aPropos.php">A propos</a></li>
<li><a href="actualitees.php">Actualités</a></li>
<li><a href="temoignages.php">Témoignages</a></li>
</ul>
</div>
<div class="mdl-mega-footer__drop-down-section">
<input class="mdl-mega-footer__heading-checkbox" type="checkbox" checked>
<h1 class="mdl-mega-footer__heading">Informations</h1>
<ul class="mdl-mega-footer__link-list">
<li><a href="contact.php">Contact</a></li>
<li><a href="legales.php">Légales</a></li>
</ul>
</div>
</div>
<div class="mdl-mega-footer__bottom-section">
<ul class="mdl-mega-footer__link-list">
<li>
<a href="legales.php">Politique de confidentialité</a>
|
<a href="legales.php">Modalités</a>
</li>
</ul>
</div>
<!-- Material Design lite -->
<script src="../../scripts/material.min.js"></script>
<!-- Custom Theme JavaScript -->
<script src="../../scripts/not-connected/faq.js"></script>
<script>
wallet.init('faq');
</script>
<!-- Google Analytics: change UA-XXXXX-X to be your site's ID -->
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-77911480-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html><file_sep>/app/modules/connected/profil.php
<?php
session_start();
include '../../includes/connect.inc.php';
include '../../includes/verif_session.php';
try {
$select3 = $bdd->prepare("SELECT nom,prenom,email,pseudo,pays FROM user WHERE id_user = ".$_SESSION["id_user"]."");
$select3->execute();
$lignes3 = $select3->fetchAll();
$nom = $lignes3[0]["nom"];
$prenom = $lignes3[0]["prenom"];
$email = $lignes3[0]["email"];
$pseudo = $lignes3[0]["pseudo"];
$pays = $lignes3[0]["pays"];
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
?>
<!DOCTYPE html>
<!--
Material Design Lite
Copyright 2015 Google Inc. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License
-->
<html lang="fr">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="og:title" content="Mooc Isen">
<meta property="og:image" content="">
<meta property="og:url" content="http://colombies.com/app">
<meta property="og:description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="robots" content="">
<!-- Title -->
<title>Profil | Mooc Isen</title>
<!-- favicon.ico -->
<link rel="shortcut icon" href="../../favicon.ico">
<!-- Disable tap highlight on IE -->
<meta name="msapplication-tap-highlight" content="no">
<!-- Web Application Manifest -->
<link rel="manifest" href="../../manifest.json">
<!-- Add to homescreen for Chrome on Android -->
<meta name="mobile-web-app-capable" content="yes">
<meta name="application-name" content="Mooc Isen">
<link rel="icon" sizes="192x192" href="../../images/touch/chrome-touch-icon-192x192.png">
<!-- Add to homescreen for Safari on iOS -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Mooc Isen">
<link rel="apple-touch-icon" href="../../images/touch/apple-touch-icon.png">
<!-- Tile icon for Win8 (144x144 + tile color) -->
<meta name="msapplication-TileImage" content="../../images/touch/ms-touch-icon-144x144-precomposed.png">
<meta name="msapplication-TileColor" content="#457871">
<!-- Color the status bar on mobile devices -->
<meta name="theme-color" content="#457871">
<!-- Bootstrap core CSS -->
<link href="../../assets/css/bootstrap.min.css" rel="stylesheet">
<!-- font awesome -->
<link href="../../assets/fonts/css/font-awesome.min.css" rel="stylesheet">
<!-- animate -->
<link href="../../assets/css/animate.min.css" rel="stylesheet">
<!-- Custom styling plus plugins -->
<link href="../../assets/css/custom.css" rel="stylesheet">
<link href="../../assets/css/maps/jquery-jvectormap-2.0.1.css" rel="stylesheet">
<link href="../../assets/css/icheck/flat/green.css" rel="stylesheet">
<link href="../../assets/css/floatexamples.css" rel="stylesheet">
<link href="../../assets/css/style.css" rel="stylesheet">
<link href="../../assets/css/progressbar/bootstrap-progressbar-3.3.0.css" rel="stylesheet">
<!-- slide horizontal img et avatar-->
<link href="../../assets/css/horizontal-slide.css" rel="stylesheet">
</head>
<body class="nav-md">
<div class="container body">
<div class="main_container">
<div class="col-md-3 left_col">
<div class="left_col scroll-view">
<div class="navbar nav_title" style="border: 0;">
<a href="../../index.php" class="site_title"><i class="glyphicon glyphicon-education"></i> <span>MOOCs</span></a>
</div>
<div class="clearfix"></div>
<!-- sidebar menu drawer-->
<div id="sidebar-menu" class="main_menu_side hidden-print main_menu">
</br>
<div class="menu_section">
<ul class="nav side-menu">
<?php
try{
//Affiche ou l'utilisateur est inscrit
$selectMySub = $bdd->prepare("SELECT * FROM mooc NATURAL JOIN suivre WHERE suivre.id_user = ".$_SESSION["id_user"]."");
$selectMySub->execute();
$lignesMySub = $selectMySub->fetchAll();
/* for($i=0;$i<count($lignesMySub);$i++){
echo "
<li><a><i class='glyphicon glyphicon-file'></i> ".$lignesMySub[$i]['nom_mooc']." <span class='fa fa-chevron-down'></span></a>
<ul class='nav child_menu' style='display: none'>
<li><a href='../mooc.php?idM=".$lignesMySub[$i]['id_mooc']."'><span class='glyphicon glyphicon-calendar' aria-hidden='true'></span> Inscrit = ".$lignesMySub[$i]['date_suivi']."</a>
</li>
<li><a href='../mooc.php?idM=".$lignesMySub[$i]['id_mooc']."&idC=0&numC=0'><span class='glyphicon glyphicon-sort-by-attributes' aria-hidden='true'></span> Avancement = ".$lignesMySub[$i]['avancement']."</a>
</li>
</ul>
</li>";
}*/
}catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur mes cours";
}
?>
</ul>
</div>
<div class="menu_section">
<h3>Menu</h3>
<ul class="nav side-menu">
<li><a><i class="fa fa-wrench"></i> Paramètres <span class="fa fa-chevron-down"></span></a>
<ul class="nav child_menu" style="display: none">
<li><a href="profil.php"><span class="glyphicon glyphicon-user" aria-hidden="true"></span> Profil</a>
</li>
<li><a href="catalogue.php"><span class="glyphicon glyphicon-dashboard" aria-hidden="true"></span> Dashboard</a>
</li>
</ul>
</li>
</ul>
</div>
</div>
</div>
</div>
<!-- top navigation -->
<div class="top_nav">
<div class="nav_menu">
<nav class="" role="navigation">
<div class="nav toggle">
<a id="menu_toggle"><i class="fa fa-bars"></i></a>
</div>
<ul class="nav navbar-nav navbar-right">
<!-- AFFICHAGE MENU -->
<li class="">
<?php
if ((isset($_SESSION['email'])) && (!empty($_SESSION['email']))){
//Si Connecter
?>
<a href="javascript:;" class="user-profile dropdown-toggle" data-toggle="dropdown" aria-expanded="false">
<?php
if ((isset($_SESSION['avatar'])) && (!empty($_SESSION['avatar']))){
$avatar=$_SESSION['avatar'];
echo '<img src="'.$avatar.'" alt="">';
}else{
echo '<img src="../../assets/images/user.png" alt="">';
}
?>
<?php
$short_string= $_SESSION['email']; //On va afficher juste les 5 premiers pour regler le pb d'affichage sur mobile
echo substr($short_string, 0, 10)."..";
?>
<span class=" fa fa-angle-down"></span>
<ul class="dropdown-menu dropdown-usermenu animated fadeIn pull-right">
<li><a href="profil.php"><i class="fa fa-user pull-right"></i>Profil</a>
</li>
<li><a href="../../includes/logout.php"><i class="fa fa-sign-out pull-right"></i>Déconnexion</a>
</li>
</ul>
</a>
<?php
//Si pas connecter
}else{
echo "<a href='../not-connected/inscription.php' class='user-profile dropdown-toggle'>";
echo "<img src='../../assets/images/loadpulseX60.gif' alt=''/>";
echo "<span class=' fa fa-angle-down'></span>";
echo "</a>";
}
?>
</li>
</ul>
</nav>
</div>
</div>
<!-- /top navigation -->
<!-- page content -->
<div class="right_col" role="main">
<div class="">
<div class="page-title">
<div class="title_left">
<h3>Mon profil</h3>
</div>
</div>
<div class="clearfix"></div>
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Modifier mon profil <small></small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<form class="form-horizontal form-label-left" action="../../includes/profil_update_user.php" method="post" id="myform1">
<!--<span class="section">Informations personnelles</span> -->
<!-- Photo d'avatar -->
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="Avatar"> Avatar<span class="required"></span>
</label>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="Avatar"> <span class="required"></span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12 ">
<ul class="horizontal-slide">
<!-- Genration des images -->
<?php
$dirname = "../../assets/images/profil/";
$images = glob($dirname."*.png");
foreach($images as $image) {
//echo '<img src="'.$image.'" /><br />';
echo '<label class="avatars">';
echo '<input type="radio" name="avatar" value="'.$image.'"/>';
//echo '<li class="col-md-2"><img class="" width="" src="'.$image.'"/></li>';
echo '<img class="img-circle" width="80px" src="'.$image.'"/>';
echo '</label>';
}
?>
</ul>
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="name">Nom <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="name" class="form-control col-md-7 col-xs-12" data-validate-length-range="6" data-validate-words="1" name="name" value=<?php echo $nom ?> required="required" type="text">
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="surname">Prénom <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="surname" class="form-control col-md-7 col-xs-12" data-validate-length-range="6" data-validate-words="1" name="surname" value=<?php echo $prenom ?> required="required" type="text">
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="pseudo">Pseudo <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="pseudo" class="form-control col-md-7 col-xs-12" data-validate-length-range="6" data-validate-words="1" name="pseudo" value=<?php echo $pseudo ?> required="required" type="text">
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="email">Email <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input type="email" id="email" name="email" placeholder=<?php echo $email ?> required="required" class="form-control col-md-7 col-xs-12">
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="occupation">Pays <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<select class="form-control" id="exampleSelect1" name="selectPays">
<option value="AF">Afghanistan</option>
<option value="AX">Åland Islands</option>
<option value="AL">Albania</option>
<option value="DZ">Algeria</option>
<option value="AS">American Samoa</option>
<option value="AD">Andorra</option>
<option value="AO">Angola</option>
<option value="AI">Anguilla</option>
<option value="AQ">Antarctica</option>
<option value="AG">Antigua and Barbuda</option>
<option value="AR">Argentina</option>
<option value="AM">Armenia</option>
<option value="AW">Aruba</option>
<option value="AU">Australia</option>
<option value="AT">Austria</option>
<option value="AZ">Azerbaijan</option>
<option value="BS">Bahamas</option>
<option value="BH">Bahrain</option>
<option value="BD">Bangladesh</option>
<option value="BB">Barbados</option>
<option value="BY">Belarus</option>
<option value="BE">Belgium</option>
<option value="BZ">Belize</option>
<option value="BJ">Benin</option>
<option value="BM">Bermuda</option>
<option value="BT">Bhutan</option>
<option value="BO">Bolivia, Plurinational State of</option>
<option value="BQ">Bonaire, Sint Eustatius and Saba</option>
<option value="BA">Bosnia and Herzegovina</option>
<option value="BW">Botswana</option>
<option value="BV">Bouvet Island</option>
<option value="BR">Brazil</option>
<option value="IO">British Indian Ocean Territory</option>
<option value="BN">Brunei Darussalam</option>
<option value="BG">Bulgaria</option>
<option value="BF">Burkina Faso</option>
<option value="BI">Burundi</option>
<option value="KH">Cambodia</option>
<option value="CM">Cameroon</option>
<option value="CA">Canada</option>
<option value="CV">Cape Verde</option>
<option value="KY">Cayman Islands</option>
<option value="CF">Central African Republic</option>
<option value="TD">Chad</option>
<option value="CL">Chile</option>
<option value="CN">China</option>
<option value="CX">Christmas Island</option>
<option value="CC">Cocos (Keeling) Islands</option>
<option value="CO">Colombia</option>
<option value="KM">Comoros</option>
<option value="CG">Congo</option>
<option value="CD">Congo, the Democratic Republic of the</option>
<option value="CK">Cook Islands</option>
<option value="CR">Costa Rica</option>
<option value="CI">Côte d'Ivoire</option>
<option value="HR">Croatia</option>
<option value="CU">Cuba</option>
<option value="CW">Curaçao</option>
<option value="CY">Cyprus</option>
<option value="CZ">Czech Republic</option>
<option value="DK">Denmark</option>
<option value="DJ">Djibouti</option>
<option value="DM">Dominica</option>
<option value="DO">Dominican Republic</option>
<option value="EC">Ecuador</option>
<option value="EG">Egypt</option>
<option value="SV">El Salvador</option>
<option value="GQ">Equatorial Guinea</option>
<option value="ER">Eritrea</option>
<option value="EE">Estonia</option>
<option value="ET">Ethiopia</option>
<option value="FK">Falkland Islands (Malvinas)</option>
<option value="FO">Faroe Islands</option>
<option value="FJ">Fiji</option>
<option value="FI">Finland</option>
<option value="FR" selected>France</option>
<option value="GF">French Guiana</option>
<option value="PF">French Polynesia</option>
<option value="TF">French Southern Territories</option>
<option value="GA">Gabon</option>
<option value="GM">Gambia</option>
<option value="GE">Georgia</option>
<option value="DE">Germany</option>
<option value="GH">Ghana</option>
<option value="GI">Gibraltar</option>
<option value="GR">Greece</option>
<option value="GL">Greenland</option>
<option value="GD">Grenada</option>
<option value="GP">Guadeloupe</option>
<option value="GU">Guam</option>
<option value="GT">Guatemala</option>
<option value="GG">Guernsey</option>
<option value="GN">Guinea</option>
<option value="GW">Guinea-Bissau</option>
<option value="GY">Guyana</option>
<option value="HT">Haiti</option>
<option value="HM">Heard Island and McDonald Islands</option>
<option value="VA">Holy See (Vatican City State)</option>
<option value="HN">Honduras</option>
<option value="HK">Hong Kong</option>
<option value="HU">Hungary</option>
<option value="IS">Iceland</option>
<option value="IN">India</option>
<option value="ID">Indonesia</option>
<option value="IR">Iran, Islamic Republic of</option>
<option value="IQ">Iraq</option>
<option value="IE">Ireland</option>
<option value="IM">Isle of Man</option>
<option value="IL">Israel</option>
<option value="IT">Italy</option>
<option value="JM">Jamaica</option>
<option value="JP">Japan</option>
<option value="JE">Jersey</option>
<option value="JO">Jordan</option>
<option value="KZ">Kazakhstan</option>
<option value="KE">Kenya</option>
<option value="KI">Kiribati</option>
<option value="KP">Korea, Democratic People's Republic of</option>
<option value="KR">Korea, Republic of</option>
<option value="KW">Kuwait</option>
<option value="KG">Kyrgyzstan</option>
<option value="LA">Lao People's Democratic Republic</option>
<option value="LV">Latvia</option>
<option value="LB">Lebanon</option>
<option value="LS">Lesotho</option>
<option value="LR">Liberia</option>
<option value="LY">Libya</option>
<option value="LI">Liechtenstein</option>
<option value="LT">Lithuania</option>
<option value="LU">Luxembourg</option>
<option value="MO">Macao</option>
<option value="MK">Macedonia, the former Yugoslav Republic of</option>
<option value="MG">Madagascar</option>
<option value="MW">Malawi</option>
<option value="MY">Malaysia</option>
<option value="MV">Maldives</option>
<option value="ML">Mali</option>
<option value="MT">Malta</option>
<option value="MH">Marshall Islands</option>
<option value="MQ">Martinique</option>
<option value="MR">Mauritania</option>
<option value="MU">Mauritius</option>
<option value="YT">Mayotte</option>
<option value="MX">Mexico</option>
<option value="FM">Micronesia, Federated States of</option>
<option value="MD">Moldova, Republic of</option>
<option value="MC">Monaco</option>
<option value="MN">Mongolia</option>
<option value="ME">Montenegro</option>
<option value="MS">Montserrat</option>
<option value="MA">Morocco</option>
<option value="MZ">Mozambique</option>
<option value="MM">Myanmar</option>
<option value="NA">Namibia</option>
<option value="NR">Nauru</option>
<option value="NP">Nepal</option>
<option value="NL">Netherlands</option>
<option value="NC">New Caledonia</option>
<option value="NZ">New Zealand</option>
<option value="NI">Nicaragua</option>
<option value="NE">Niger</option>
<option value="NG">Nigeria</option>
<option value="NU">Niue</option>
<option value="NF">Norfolk Island</option>
<option value="MP">Northern Mariana Islands</option>
<option value="NO">Norway</option>
<option value="OM">Oman</option>
<option value="PK">Pakistan</option>
<option value="PW">Palau</option>
<option value="PS">Palestinian Territory, Occupied</option>
<option value="PA">Panama</option>
<option value="PG">Papua New Guinea</option>
<option value="PY">Paraguay</option>
<option value="PE">Peru</option>
<option value="PH">Philippines</option>
<option value="PN">Pitcairn</option>
<option value="PL">Poland</option>
<option value="PT">Portugal</option>
<option value="PR">Puerto Rico</option>
<option value="QA">Qatar</option>
<option value="RE">Réunion</option>
<option value="RO">Romania</option>
<option value="RU">Russian Federation</option>
<option value="RW">Rwanda</option>
<option value="BL">Saint Barthélemy</option>
<option value="SH">Saint Helena, Ascension and Tristan da Cunha</option>
<option value="KN">Saint Kitts and Nevis</option>
<option value="LC">Saint Lucia</option>
<option value="MF">Saint Martin (French part)</option>
<option value="PM">Saint Pierre and Miquelon</option>
<option value="VC">Saint Vincent and the Grenadines</option>
<option value="WS">Samoa</option>
<option value="SM">San Marino</option>
<option value="ST">Sao Tome and Principe</option>
<option value="SA">Saudi Arabia</option>
<option value="SN">Senegal</option>
<option value="RS">Serbia</option>
<option value="SC">Seychelles</option>
<option value="SL">Sierra Leone</option>
<option value="SG">Singapore</option>
<option value="SX">Sint Maarten (Dutch part)</option>
<option value="SK">Slovakia</option>
<option value="SI">Slovenia</option>
<option value="SB">Solomon Islands</option>
<option value="SO">Somalia</option>
<option value="ZA">South Africa</option>
<option value="GS">South Georgia and the South Sandwich Islands</option>
<option value="SS">South Sudan</option>
<option value="ES">Spain</option>
<option value="LK">Sri Lanka</option>
<option value="SD">Sudan</option>
<option value="SR">Suriname</option>
<option value="SJ">Svalbard and Jan Mayen</option>
<option value="SZ">Swaziland</option>
<option value="SE">Sweden</option>
<option value="CH">Switzerland</option>
<option value="SY">Syrian Arab Republic</option>
<option value="TW">Taiwan, Province of China</option>
<option value="TJ">Tajikistan</option>
<option value="TZ">Tanzania, United Republic of</option>
<option value="TH">Thailand</option>
<option value="TL">Timor-Leste</option>
<option value="TG">Togo</option>
<option value="TK">Tokelau</option>
<option value="TO">Tonga</option>
<option value="TT">Trinidad and Tobago</option>
<option value="TN">Tunisia</option>
<option value="TR">Turkey</option>
<option value="TM">Turkmenistan</option>
<option value="TC">Turks and Caicos Islands</option>
<option value="TV">Tuvalu</option>
<option value="UG">Uganda</option>
<option value="UA">Ukraine</option>
<option value="AE">United Arab Emirates</option>
<option value="GB">United Kingdom</option>
<option value="US">United States</option>
<option value="UM">United States Minor Outlying Islands</option>
<option value="UY">Uruguay</option>
<option value="UZ">Uzbekistan</option>
<option value="VU">Vanuatu</option>
<option value="VE">Venezuela, Bolivarian Republic of</option>
<option value="VN">Viet Nam</option>
<option value="VG">Virgin Islands, British</option>
<option value="VI">Virgin Islands, U.S.</option>
<option value="WF">Wallis and Futuna</option>
<option value="EH">Western Sahara</option>
<option value="YE">Yemen</option>
<option value="ZM">Zambia</option>
<option value="ZW">Zimbabwe</option>
</select>
</div>
</div>
<div class="item form-group">
<label class="control-label col-md-3 col-sm-3 col-xs-12" for="occupation">Occupation <span class="required">*</span>
</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<select class="form-control" id="exampleSelect2" name="selectJob">
<option value="Etudiant">Etudiant</option>
<option value="En recherche d'emploi">En recherche d'emploi</option>
<option value="Actif">Actif</option>
</select>
</div>
</div>
<div class="ln_solid"></div>
<div class="form-group">
<div class="col-md-6 col-md-offset-3">
<button id="send" type="submit" class="btn btn-success">Confirmer</button>
<?php
//erreur venant du traitement
if(isset($_GET['erreur'])) {
echo $_GET['erreur'];
}
if(isset($_GET['ok'])) {
echo $_GET['ok'];
}
?>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Modifier mot de passe <small></small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<form class="form-horizontal form-label-left" action="../../includes/profil_update_password.php" method="post" id="myform2" novalidate>
<div class="item form-group">
<label for="password" class="control-label col-md-3">Ancien mot de passe</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="oldPassword" type="password" name="oldPassword" data-validate-length-range="4" class="form-control col-md-7 col-xs-12" required="required">
</div>
</div>
<div class="item form-group">
<label for="password" class="control-label col-md-3">Nouveau mot de passe</label>
<div class="col-md-6 col-sm-6 col-xs-12">
<input id="<PASSWORD>" type="<PASSWORD>" name="newPassword" data-validate-length-range="4" class="form-control col-md-7 col-xs-12" required="required">
</div>
</div>
<div class="ln_solid"></div>
<div class="form-group">
<div class="col-md-6 col-md-offset-3">
<button id="send" type="submit" class="btn btn-success">Confirmer</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
<!-- /fin row -->
<?php // count nombre trophées débloqués et nombre total de trophées
$countUnlockTrophy = $bdd->query('SELECT COUNT(id_succes) AS cpt FROM debloquer WHERE id_user= "'.$_SESSION['id_user'].'"');
$donnees = $countUnlockTrophy->fetch();
$countUnlockTrophy->closeCursor();
$countAllTrophy = $bdd->query('SELECT COUNT(id_succes) AS total FROM succes');
$donnees1 = $countAllTrophy->fetch();
$countAllTrophy->closeCursor();
?>
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Mes trophées <small><?php echo $donnees['cpt'].'/'.$donnees1['total'].' succès débloqué(s)';?> </small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<?php // trophées débloqués par l'utilisateur
$trophy = $bdd->prepare('SELECT debloquer.date_obtention ,succes.nom_succes, succes.description_succes, succes.type_succes FROM succes INNER JOIN debloquer ON succes.id_succes = debloquer.id_succes INNER JOIN user ON user.id_user = debloquer.id_user WHERE debloquer.id_user = "'.$_SESSION['id_user'].'"');
$trophy->execute();
$resuTrophy = $trophy->fetchAll();
for($i = 0; $i<sizeof($resuTrophy); $i++){
echo'<div class="col-md-3">';
if($resuTrophy[$i]["type_succes"]=="P"){
echo'<img src="../../assets/images/trophyP2.png" width="128" height="109" class="trophyUnlock" style="margin:auto;display:block">';
}
else if($resuTrophy[$i]["type_succes"]=="G"){
echo'<img src="../../assets/images/trophyG2.png" width="128" height="109" class="trophyUnlock" style="margin:auto;display:block">';
}
else if($resuTrophy[$i]["type_succes"]=="S"){
echo'<img src="../../assets/images/trophyS2.png" width="128" height="109" class="trophyUnlock" style="margin:auto;display:block">';
}
else{
echo'<img src="../../assets/images/trophyB2.png" width="128" height="109" class="trophyUnlock" style="margin:auto;display:block">';
}
echo'
<p class="text-center"><b>'.$resuTrophy[$i]["nom_succes"].'</b></p>
<p class="text-center">'.$resuTrophy[$i]["description_succes"].'</p>';
$date = date_create($resuTrophy[$i]["date_obtention"]);
echo'<p class="text-center">Débloqué le : '.date_format($date, 'd F Y').'</p>
</div>';
}
?>
</div>
</div>
</div>
</div>
<!-- /fin row -->
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Trophées restants <small><?php echo ($donnees1['total']-$donnees['cpt']).' succès non débloqué(s)';?></small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<?php // trophées non débloqués par l'utilisateur
$trophy1 = $bdd->prepare('SELECT * FROM succes WHERE id_succes NOT IN( SELECT id_succes FROM debloquer WHERE id_user= "'.$_SESSION['id_user'].'")');
$trophy1->execute();
$resuTrophy1 = $trophy1->fetchAll();
for($i = 0; $i<sizeof($resuTrophy1); $i++){
echo'<div class="col-md-3">';
if($resuTrophy1[$i]["type_succes"] =="P"){
echo'<img src="../../assets/images/trophyP2.png" width="128" height="109" class="trophyLock" style="margin:auto;display:block">';
}
else{
echo'<img src="../../assets/images/trophyG.png" width="128" height="109" class="trophyLock" style="margin:auto;display:block">';
}
echo'
<p class="text-center"><b>'.$resuTrophy1[$i]["nom_succes"].'</b></p>
<p class="text-center">'.$resuTrophy1[$i]["description_succes"].'</p>
</div>';
// <p class="text-center">Type : '.$resuTrophy1[$i]["type_succes"].'</p>
}
?>
</div>
</div>
</div>
</div>
<!-- /fin row -->
<?php // count nombre de cours suivis
$countCorseFollow = $bdd->query('SELECT COUNT(id_user) AS cpt2 FROM suivre WHERE id_user= "'.$_SESSION['id_user'].'"');
$donnees2 = $countCorseFollow->fetch();
$countCorseFollow->closeCursor();
?>
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Mes cours <small><?php echo ($donnees2["cpt2"]).' cours suivis';?></small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<table class="table table-striped projects">
<thead>
<tr>
<th style="width: 1%">#</th>
<th style="width: 20%">Nom du cours</th>
<th style="width: 30%">Avancement</th>
<th style="width: 10%">Score</th>
<th style="width: 20%">Actions</th>
</tr>
</thead>
<tbody>
<?php
// Affichage du tableau récapitulatif pour chaque projet
// Permet d'avoir les renseignements sur les cours suivis
try{
$corseFollow = $bdd->query('SELECT avancement, date_suivi, id_mooc,nom_mooc FROM user NATURAL JOIN suivre NATURAL JOIN mooc WHERE id_user= "'.$_SESSION['id_user'].'"');
$corseFollow->execute();
$donnees3 = $corseFollow->fetchAll();
}catch(Exception $Excep){
echo "->erreur donnees ";
}
for($i = 0; $i<sizeof($donnees3); $i++){
echo'<tr>
<td>#</td>
<td>
<a href="description.php?idM='.$donnees3[$i]['id_mooc'].'">'.$donnees3[$i]['nom_mooc'].'</a>
<br />
<small>Inscrit le '.$donnees3[$i]["date_suivi"].'</small>
</td>';
//Permet de récuperer l'avancement d'un mooc pour l'utilisateur courant
$avancementMooc = $bdd->query('SELECT avancement AS avc FROM user INNER JOIN suivre ON user.id_user= suivre.id_user INNER JOIN mooc ON suivre.id_mooc=mooc.id_mooc WHERE user.id_user = "'.$_SESSION["id_user"].'" AND mooc.id_mooc = "'.$donnees3[$i]["id_mooc"].'"');
$donnees6 = $avancementMooc->fetch();
$avancementMooc->closeCursor();
// Permet de récuperer le nombre de chapitre du MOOC
$nbChapitreMooc = $bdd->query('SELECT nb_chap FROM mooc WHERE id_mooc ="'.$donnees3[$i]["id_mooc"].'"');
$donnees7 = $nbChapitreMooc->fetch();
$nbChapitreMooc->closeCursor();
// Calcul du % d'avancement
$tab = Array();
$tab = preg_split('[-]', $donnees6["avc"]);
$avancement = sizeof($tab)-1;
$pourcentage = ceil($avancement/ $donnees7["nb_chap"]*100);
echo'
<td class="project_progress">
<div class="progress progress_sm">
<div class="progress-bar bg-green" role="progressbar" data-transitiongoal="'.$pourcentage.'"></div>
</div>
<small>'.$pourcentage.'% Terminé</small>
</td>';
//Permet de calculer le score de l'utilisateur courant sur un mooc
$scoreMooc = $bdd->query('SELECT sum(score) AS score FROM faire WHERE id_user= "'.$_SESSION["id_user"].'" AND id_exercice IN (SELECT id_exercice FROM mooc INNER JOIN chapitre ON mooc.id_mooc = chapitre.id_mooc INNER JOIN exercice ON chapitre.id_chapitre=exercice.id_chapitre WHERE mooc.id_mooc = "'.$donnees3[$i]["id_mooc"].'")');
$donnees4 = $scoreMooc->fetch();
$scoreMooc->closeCursor();
// Permet de calculer le score maximal possible en fonction des exercices réalisés par l'utilisateur pour ce MOOC
$scoreTotalMooc = $bdd->query('SELECT sum(valeur_exo) AS total FROM mooc INNER JOIN chapitre ON mooc.id_mooc = chapitre.id_mooc INNER JOIN exercice ON chapitre.id_chapitre=exercice.id_chapitre WHERE mooc.id_mooc = "'.$donnees3[$i]["id_mooc"].'" AND id_exercice IN(SELECT id_exercice FROM faire WHERE id_user="'.$_SESSION["id_user"].'")');
$donnees5 = $scoreTotalMooc->fetch();
$scoreTotalMooc->closeCursor();
echo'<td>
<p><span class="glyphicon glyphicon-star" aria-hidden="true"></span>';
if($donnees4["score"]==NULL){
echo'0';
}
else{
echo $donnees4["score"];
}
echo'/';
if($donnees5["total"]==NULL){
echo'0';
}
else{
echo $donnees5["total"];
}
echo'</p>
</td>
<td>
<button onclick="goMooc(this.name);" type="button" name="'.$donnees3[$i]['id_mooc'].'" class="btn btn-primary btn-xs"><i class="fa fa-mail-forward"></i> Aller </button>
<button onclick="callGraph(this.name);" type="button" name="'.$donnees3[$i]['id_mooc'].'" class="btn btn-success btn-xs"><i class="fa fa-bar-chart"></i> Statistiques </button>
</td>
</tr>';
}
?>
</tbody>
</table>
<!-- end project list -->
</div>
</div>
</div>
</div>
<!-- /fin row -->
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Statistiques<small></small></h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="removechart">
<canvas id="myChart" height="200" width="400" ></canvas>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- /page content -->
</div>
</div>
<div id="custom_notifications" class="custom-notifications dsp_none">
<ul class="list-unstyled notifications clearfix" data-tabbed_notifications="notif-group">
</ul>
<div class="clearfix"></div>
<div id="notif-group" class="tabbed_notifications"></div>
</div>
<!-- jQuery -->
<script src="../../assets/js/jquery.js"></script>
<script src="../../assets/js/jquery.min.js"></script>
<!-- Bootstrap -->
<script src="../../assets/js/bootstrap.min.js"></script>
<!-- chart js -->
<script src="../../assets/js/chartjs/Chart.js"></script>
<!-- bootstrap progress js -->
<script src="../../assets/js/progressbar/bootstrap-progressbar.min.js"></script>
<script src="../../assets/js/nicescroll/jquery.nicescroll.min.js"></script>
<!-- icheck -->
<script src="../../assets/js/icheck/icheck.min.js"></script>
<!-- daterangepicker -->
<script src="../../assets/js/moment.min.js"></script>
<script src="../../assets/js/datepicker/daterangepicker.js"></script>
<script src="../../assets/js/custom.js"></script>
<!-- form validation -->
<script src="../../assets/js/validator/validator.js"></script>
<!-- Validation -->
<script src="../../assets/js/jqueryvalidate/jquery.validate.min.js"></script>
<script src="../../assets/js/jqueryvalidate/additional-methods.min.js"></script>
<!-- flot js -->
<!--[if lte IE 8]><script type="text/javascript" src="js/excanvas.min.js"></script><![endif]-->
<script src="../../assets/js/flot/jquery.flot.js"></script>
<script src="../../assets/js/flot/jquery.flot.pie.js"></script>
<script src="../../assets/js/flot/jquery.flot.orderBars.js"></script>
<script src="../../assets/js/flot/jquery.flot.time.min.js"></script>
<script src="../../assets/js/flot/date.js"></script>
<script src="../../assets/js/flot/jquery.flot.spline.js"></script>
<script src="../../assets/js/flot/jquery.flot.stack.js"></script>
<script src="../../assets/js/flot/curvedLines.js"></script>
<script src="../../assets/js/flot/jquery.flot.resize.js"></script>
<!-- Custom JS-->
<script src="../../scripts/connected/profil.js"></script>
<!-- Catch error -->
<script src="../../scripts/catch_error.js"></script>
<!-- Google Analytics: change UA-XXXXX-X to be your site's ID -->
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-77911480-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html><file_sep>/app/mooc/mooc1/chapitres/chapitre2.php
<?php
$idMooc;
$idChap;
if(isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
//afficheChapitreExos($idMooc,$idChap,$bdd,0);
//echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 ">
<!-- CONTENU COURS -->
<div class="animated slideInUp">
<div class="x_panel">
<!-- TITRE COURS -->
<div class="x_title">
<h2> Chapitre 2 : Formation </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<!-- COURS -->
<div class="x_content">
<div class="my_step_box">
<div class='row'>
<div class="col-md-3"></div>
<div class="col-md-6">
<div class="btn-group" role="group" aria-label="...">
<button type="button" class="btn btn-default myBtnBack"><span class="glyphicon glyphicon-chevron-left"></span> Retour</button>
<button type="button" class="btn btn-default myBtnAll"><span class="glyphicon glyphicon-eye-open"></span> </button>
<button type="button" class="btn btn-default myBtnNext">Suivant<span class="glyphicon glyphicon-chevron-right"></span></button>
</div>
</div>
<div class="col-md-3"></div>
</div>
<div class="box1" id="1">
<ol start="1">
<li dir="ltr">
<h2 dir="ltr">
Introduction
</h2>
</li>
</ol>
<p dir="ltr">
Il est utile de rédiger la partie formation aux jeunes diplômés et jeunes expérimentés jusqu’à 5 ans d’expérience. Globalement, plus la partie expérience professionnelle se développe, plus la partie formation diminue.
</p>
<p dir="ltr">
Pour chaque formation il faut indiquer: l’intitulé exact du diplôme, le nom et le lieu de l’école, les années de formation. Pour structurer les informations, n’hésitez pas à utiliser des puces pour présenter chacune des formations suivies.
</p>
<br/>
</div>
<div class="box1" id="2">
<ol start="2">
<li dir="ltr">
<h2 dir="ltr">
Titre
</h2>
</li>
</ol>
<p dir="ltr">
En tant que titre il faut mettre l’intitulé exact de votre diplôme. Faites attention, car certains diplômes n’ont peut-être pas leur place. C’est le cas du Brevet des collèges si vous êtes titulaire d’un Baccalauréat, ou d’une première année de Master si vous possédez déjà le diplôme de Master 2.
</p>
<p dir="ltr">
En revanche, n’hésitez pas à détailler les projets pertinents développés au cours de cette formation. Vous pouvez indiquer les spécialités relatives aux diplômes que vous aurez pu acquérir. Lorsque vous faites les études en alternance, vous pouvez le spécifier.
</p>
<br/>
</div>
<div class="box1" id="3">
<ol start="3">
<li dir="ltr">
<h2 dir="ltr">
Dates
</h2>
</li>
</ol>
<p dir="ltr">
Vous remontez donc le temps, en commençant par votre formation la plus récente. La logique de présentation est donc anti chronologique.
</p>
<ol start="4">
<li dir="ltr">
<h2 dir="ltr">
Sigles
</h2>
</li>
</ol>
<p dir="ltr">
Lorsqu’il s’agit de sigles communément employés (BTS, DUT, IEP, etc.), il n’est pas utile de les expliciter, au contraire, cela vous fera gagner de la place. En revanche, pour tous les autres, il est important de les écrire en toutes lettres, qu’il s’agisse du nom de votre école, de votre diplôme, etc.
</p>
<br/>
</div>
<div class="box1" id="4">
<ol start="5">
<li dir="ltr">
<h2 dir="ltr">
Lieu
</h2>
</li>
</ol>
<p dir="ltr">
Précisez bien les noms et villes des établissements fréquentés.
</p>
<p dir="ltr">
Suivant l’établissement, vous serez classé différemment par les recruteurs et les grilles de salaire à l’embauche pour le même poste ne sont pas les mêmes.
</p>
<p dir="ltr">
Si vous avez fait des études à l’étranger, pensez à préciser le nom du pays.
</p>
<ol start="6">
<li dir="ltr">
<h2 dir="ltr">
Spécialités et gratifications
</h2>
</li>
</ol>
<p dir="ltr">
C’est le moment de préciser les mentions obtenus ou les félicitations du jury décroché à votre soutenance de mémoire ! Vos gratifications scolaires marquent votre capacité à vous couler dans un système, à aller au bout d’un projet. Le cas échéant, elles sont le garant de vos qualités d’abstraction, de mémoire, d’analyse et de synthèse.
</p>
<p dir="ltr">
Si lors de votre parcours vous avez fait des spécialisations dans certains domaines, n’hésitez pas à le mentionner.
</p>
<br/>
</div>
<div class="box1" id="5">
<ol start="7">
<li dir="ltr">
<h2 dir="ltr">
Exemple
</h2>
</li>
</ol>
<p dir="ltr">
Exemple de la partie “Formation” d’un CV:
Dans cet exemple il faut faire attention et ne pas oublier de mettre au saut de ligne qui coupe l’intitulé d’un sigle,
sinon cela rend la lecture difficile.
</p>
<p dir="ltr">
<img class="" src="../mooc/mooc1/chapitres/images/formation.png" width="500px" alt="">
</p>
<br/>
</div>
<div class="box1" id="6">
<ol start="8">
<li dir="ltr">
<h2 dir="ltr">
Récapitulatif du chapitre
</h2>
</li>
</ol>
<p dir="ltr">
<ol>
<li>
La partie formation est à mettre pour les jeunes diplômes et les personnes qui ont jusqu’à 15 ans d’expérience.
</li>
<li>
Pour chaque ligne de formation, il faut : date (anti chronologiques), titre, nom, lieu.
</li>
<li>
Evitez de mettre les diplômes qui n’ont pas leur place (BAC si vous avez Master, Master 1 si vous avez le diplôme de Master 2)
</li>
<li>
N’hésitez pas à mettre les spécialités relatives à votre formation.
</li>
<li>
Employez que des sigles communément employés.
</li>
<li>
Mettez les mentions que vous avez obtenues pour vos diplômes..
</li>
</ol>
</p>
<br/>
</div>
</div>
</div>
</div>
</div>
<!-- VIDEO -->
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Vidéo </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="videocontainer lg6">
<!--<iframe src="https://www.youtube.com/embed/lX7kYDRIZO4" frameborder="0" ></iframe>-->
<iframe width="560" height="315" src="https://www.youtube.com/embed/_r7bht-LgvU" frameborder="0" allowfullscreen></iframe>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- EXERCICES -->
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInRight">
<div class="x_panel">
<div class="x_title">
<?php
nomChapitre($idMooc,$bdd,$numChap);
?>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Smart Wizard -->
<h3>Exercices</h3>
<div id="wizard" class="form_wizard wizard_horizontal">
<?php
creationWizardStep($idMooc,$numChap,$idChap,$bdd);
?>
</div>
<!-- IMPORTANT AFFICHE LES INDICE -->
<div id="indicebox"></div>
<!-- IMPORTANT AFFICHE LES SOLUTIONS -->
<div id="solucebox"></div>
<!-- IMPORTANT POUR LES REPONSE AU QCM -->
<?php
/*if(isset($_GET['idM']) && isset($_GET['idC'])) {
//$idMooc = $_GET['idM'];
echo '<div id="'.$_GET['idM'].'"></div>';
$idChap = $_GET['idC'];
}*/
?>
</div>
</div>
</div>
</div><file_sep>/app/mooc/mooc1/chapitres/chapitre6.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 6 : Expérience professionnelle</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<p dir="ltr">
En clair, préciser la fonction principale (votre métier ou le poste que vous souhaitez occuper) de manière bien visible. Cette information remplacera
avantageusement une éventuelle mention »Curriculum Vitae ». Par ailleurs, vous dire qu’en lisant les diplômes et l’ expérience professionnelle le recruteur
pourra y comprendre ce qu’il souhaite est une grosse erreur. Votre CV doit être très précis et ne laisser aucun doute sur la nature de votre profil car le
recruteur cherche à être rassuré.
</p>
<p dir="ltr">
<strong id="docs-internal-guid-6f124f1b-c8ff-90eb-e200-f32594a02d16">
<br/>
</strong>
</p>
<p dir="ltr">
Les expériences sont le cœur du CV. Il faut donc les mettre en valeur.
</p>
<p dir="ltr">
Les informations de bases à faire apparaître sont les suivantes :
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
dates de l’expérience (bien sûr, les expériences sont présentées dans un ordre anté-chronologique, c’est-à-dire de la plus récente à la plus
ancienne) ;
</p>
</li>
<li dir="ltr">
<p dir="ltr">
intitulé du poste (à éventuellement reformuler si l’intitulé interne n’est pas clair. Dans ce cas, il faut bien sûr choisir un nom de poste
strictement équivalent) ;
</p>
</li>
<li dir="ltr">
<p dir="ltr">
nom de l’entreprise ;
</p>
</li>
<li dir="ltr">
<p dir="ltr">
ville, en précisant si nécessaire le pays ;
</p>
</li>
<li dir="ltr">
<p dir="ltr">
type de contrat (facultatif).
</p>
</li>
</ul>
<p dir="ltr">
Mais c’est la description du poste qui peut faire la différence. Détaillez entre deux et quatre missions par poste. Toutefois, la quantité d’informations
est subordonnée à la durée de l’expérience et à l’importance que vous souhaitez lui donner.
</p>
<div>
<br/>
</div>
</div>
</div>
</div>
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Exemple</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
</div>
</div>
</div>
<file_sep>/app/mooc/mooc1/chapitres/chapitre0.php
<?php
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
}
?>
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Avant propos</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li class="dropdown">
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<img class="img left" src="../mooc/mooc1/chapitres/images/mooc.png" style="margin: 20px;" width="300px" alt="">
 <br>
<p dir="ltr">
Un CV - Curriculum vitae est votre publicité, c’est votre reflet et il
est capital. Il n'existe pas de CV miracle mais en revanche un CV mal conçu et mal utilisé fait toujours de gros dégâts, même si le candidat possède un
excellent profil. Les recruteurs, submergés de candidatures et disposant de très peu de temps, sont obligés d'aller à l'essentiel. Résultat : de simples
détails dans la présentation du CV peuvent provoquer l'élimination d'un candidat. C’est pourquoi nous avons crée un MOOC “Comment réaliser un CV
d’ingénieur” qui vous permettra de créer votre propre CV et d’accéder au poste dont vous revez!
</p>
<p dir="ltr">
Il n'y a pas de vérité sur le CV, il y a des informations utiles ou non. Le CV est un document qui peut et est critiqué par toutes personnes à qui vous le
montrez (vous pouvez le refaire 10 fois après l'avoir montré à 10 personnes). Pourtant, personne ne se pose la question de qui est compétent pour l'évaluer
(recruter est aussi un métier, ce n'est pas que de l'intuition, ou de la logique (chacun a la sienne)). Donc montrez votre CV, écoutez les conseils, faites
préciser le pourquoi de leur remarques. Si ils n'ont pas d'explications autres que "cela ne se fait pas", où "c'est comme ça que l'on fait", remerciez
poliment et passez à autres chose.
</p>
<br/>
<p dir="ltr">
Avant d’attaquer la rédaction de votre CV, reflechissez à ce que vous allez mettre dedans, pour cela il faut:
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
Bien se connaître: savoir qui vous êtes, ce que vous voulez, par quoi vous êtes motivé, ce que vous cherchez, ce que vous savez faire et dans quoi
vous serez bien.
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Se faire connaître: envoyer un " message " qui vous soit fidèle et qui ne trompe pas son destinataire, c'est le rôle de votre CV : vous allez en
travailler le contenu et la forme jusqu'à en être content et fier et donc bien savoir le défendre en entretien.
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Connaître son " marché ": Identifier les entreprises qui recrutent dans votre métier. Répondre aux offres qui vous correspondent vraiment et qui
correspondent à ce que vous voulez faire. Pour le recruteur: Le recruteur a aussi ses lubies sur le CV. Si c'est possible, recherchez des
informations sur ces attendus. La lecture des sites internet permet de voir ce qui est important pour l'entreprise, la technique, les RH, les
valeurs, etc. Et cela vous donnera aussi un aperçu de leur sémantique (vocabulaire utilisé) à intégrer intelligemment dans votre CV .
</p>
</li>
</ul>
<p dir="ltr">
Le but n'est pas de tout dire mais noter ce qui peut intéresser. Informations réelles (pas de mensonge) en fonction de vos projets personnels et
professionnels (stage, emploi, job d'été, publicité...) et de l'objectif visé (réponse à une offre d'emploi, envoi d'une candidature spontané, outil de
veille sur les sites d'emploi …). Il faut faciliter la vie au recruteur pour qu'il trouve la perle rare qu'il recherche. Rappelez-vous qu'il a aussi peur
que vous, il ne faut pas qu'il se trompe vis-à-vis du demandeur.
</p>
<p dir="ltr">
<b>Pour accéder au cours, appuyer sur un des chapitres dans la barre de gauche</b>
</p>
<div>
<br/>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/includes/verif_session.php
<?php
//ok=1 ko=0
function sessionValid(){
$verif = 1;
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user'])))
{
//session ok
//echo $_SESSION['id_user'];
}
else
{
$verif = 0;
}
return $verif;
}
$verifSession = sessionValid();
if($verifSession == 1){
//echo ("ok session valide");
}else{
header ("location: ../includes/logout.php?erreur=Probleme de session");
}
?><file_sep>/app/includes/profil_update_user.php
<?php
session_start();
include "connect.inc.php"; /// Connection bdd
/*
Modification du mot de passe depuis la page profif.php
*/
//ok=1 ko=0
function sessionValid(){
$verif = 1;
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user'])))
{
//session ok
}
else
{
$verif = 0;
}
return $verif;
}
//ok=1 ko=0
function formValid(){
$verif = 1;
if (isset($_POST['surname'])) {
echo $_POST['surname'];
}else{
$verif=0;
}
if (isset($_POST['name'])) {
echo $_POST['name'];
}else{
$verif=0;
}
if (isset($_POST['pseudo'])) {
echo $_POST['pseudo'];
}else{
$verif=0;
}
if (isset($_POST['email'])) {
echo $_POST['email'];
}else{
$verif=0;
}
if (isset($_POST['selectPays'])) {
echo $_POST['selectPays'];
}else{
$verif=0;
}
if (isset($_POST['selectJob'])) {
echo $_POST['selectJob'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
//Met a jour l id pour le reset du mdp
function updateUser(){
include "connect.inc.php";
$id = $_SESSION['id_user'];
$name = $_POST['name'];
$surname = $_POST['surname'];
$pseudo = $_POST['pseudo'];
$pays = $_POST['selectPays'];
$email = $_POST['email'];
$selectJob = $_POST['selectJob'];
$avatar = $_POST['avatar'];
$_SESSION['avatar'] = $_POST['avatar'];
try {
$requete_prepare= $bdd->prepare("UPDATE user SET nom='$name', prenom='$surname', pseudo='$pseudo', email='$email', pays='$pays', statut='$selectJob', avatar='$avatar' WHERE id_user='$id'"); // on prépare notre requête
$requete_prepare->execute();
echo "->OK user update";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
//Valide que le formulaire
$verif = formValid();
$verifSession = sessionValid();
if($verifSession ==1){
if($verif==1){
updateUser();
header ("location: ../modules/connected/profil.php?ok=success");
}
else{
echo '<br>wrong form';
header ("location: ../modules/connected/profil.php?erreur=Erreur formulaire");
}
}else{
echo '<br>aucune session';
header ("location: ../modules/connected/profil.php?erreur=Erreur session");
}
?><file_sep>/app/includes/fonctionsDescription.php
<?php
//session start utilisé dans description.php
$valid = 1;
if (isset($_GET['idM'])) {
$idMooc = $_GET['idM'];
//echo $idMooc;
}else{
$valid = 0;
echo'erreur methode GET';
}
function afficheClassementMooc($idMooc,$bdd) {
try{
$findUser = $bdd->prepare('SELECT suivre.id_user,pseudo FROM suivre INNER JOIN user ON user.id_user=suivre.id_user WHERE id_mooc = "'.$idMooc.'"');
$findUser->execute();
$resufindUser = $findUser->fetchAll();
$classement = array();
for($j=0;$j<sizeof($resufindUser);$j++){
$scoreMooc = $bdd->query('SELECT sum(score) AS score FROM faire WHERE id_user= "'.$resufindUser[$j]["id_user"].'" AND id_exercice IN (SELECT id_exercice FROM mooc INNER JOIN chapitre ON mooc.id_mooc = chapitre.id_mooc INNER JOIN exercice ON chapitre.id_chapitre=exercice.id_chapitre WHERE mooc.id_mooc = "'.$idMooc.'")');
$donnees4 = $scoreMooc->fetch();
$scoreMooc->closeCursor();
$classement[$j][0] = $donnees4["score"];
$classement[$j][1] = $resufindUser[$j]["pseudo"];
}
rsort($classement);
echo 'Classement <br> <br>';
if(sizeof($resufindUser) <=5){
for($i=0;$i<sizeof($classement);$i++){
echo $classement[$i][1];
echo ' ';
if($classement[$i][0]==NULL){
echo'0';
}
else {
echo $classement[$i][0];
}
echo '<br><br>';
}
}
else{
for($i=0;$i<5;$i++){
echo $classement[$i][1];
echo ' ';
if($classement[$i][0]==NULL){
echo'0';
}
else {
echo $classement[$i][0];
}
echo '<br><br>';
}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur afficheClassementMooc()";
}
}
function getInfos()
{
global $idMooc;
include 'connect.inc.php';
try {
$select = $bdd->prepare("SELECT * FROM mooc WHERE id_mooc = $idMooc ");
$select->execute();
$lignes = $select->fetchAll();
echo'<div class="content col-md-6">
<div class="main">
<h3 class="name"> Nom : '.$lignes[0]["nom_mooc"].' </h3>
<h3 class="name"> Matière : '.$lignes[0]["matiere"].' </h3>
<h3 class="name text-justify"> Description : '.$lignes[0]["description"].' </h3>
<h3 class="name"> Prérequis : '.$lignes[0]["prerequis"].' </h3>
<h3 class="name"> Durée estimée: '.$lignes[0]["duree"].' heures </h3>
<h3 class="name"> Note : '.$lignes[0]["note"].' / 5 </h3>
</div>
</div>
<div class="content col-md-6">
<div class="main">
<div class="videocontainer">
<iframe src="https://www.youtube.com/embed/lX7kYDRIZO4" frameborder="0" allowfullscreen></iframe>
</div>
</div>
</div>
<br>
';
if ((isset($_SESSION['login'])) && (!empty($_SESSION['login']))){
echo'
<div class="col-sm-4 col-sm-offset-4 animated zoomIn">
<br>
<div class="">
<a href="mooc.php?idM='.$idMooc.'&insert='.$idMooc.'"><button name="id" class="btn btn-block btn-md btn-warning">Accéder au cours</button> </a>
</div>
</div>';
}else{
echo '
<div class="col-sm-4 col-sm-offset-4 animated zoomIn">
<br>
<div class="">
<a href="mooc.php?idM='.$idMooc.'"><button name="id" class="btn btn-block btn-md btn-info">Accéder au cours</button> </a>
</div>
</div>
';
}
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur getInfos()";
}
}
function getInfos2()
{
global $idMooc;
include 'connect.inc.php';
try {
$select = $bdd->prepare("SELECT * FROM mooc WHERE id_mooc = $idMooc ");
$select->execute();
$lignes = $select->fetchAll();
$scope_nom = $lignes[0]["nom_mooc"];
$scope_description = $lignes[0]["description"];
$scope_prerequis = $lignes[0]["prerequis"];
$scope_duree = $lignes[0]["duree"];
$scope_note = $lignes[0]["note"];
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur getInfos2()";
}
}
function getInfo3MDL(){
global $idMooc;
include 'connect.inc.php';
try {
$select = $bdd->prepare("SELECT * FROM mooc WHERE id_mooc = $idMooc ");
$select->execute();
$lignes = $select->fetchAll();
$scope_nom = $lignes[0]["nom_mooc"];
$scope_description = $lignes[0]["description"];
$scope_prerequis = $lignes[0]["prerequis"];
$scope_duree = $lignes[0]["duree"];
$scope_note = $lignes[0]["note"];
$scope_linkyoutube= $lignes[0]["linkyoutube"];
echo '
<div class="mdl-card__title">
<h2 class="mdl-card__title-text">Description</h2>
</div>
<div class="mdl-card__media mdl-color--white">
<img class="article-image" src="../../images/promo2.JPG" border="0" alt="">
</div>
<div class="mdl-card__supporting-text">
</div>
<div class="mdl-grid portfolio-copy">
<h3 class="mdl-cell mdl-cell--12-col mdl-typography--headline"> '.$scope_nom.'</h3>
<div class="mdl-cell mdl-cell--6-col mdl-card__supporting-text no-padding">
<h3 class="mdl-cell mdl-cell--12-col mdl-typography--headline"> <i class="material-icons">info_outline</i> Description : </h3>
<p>'.$scope_description.'</p>
<h3 class="mdl-cell mdl-cell--12-col mdl-typography--headline"> <i class="material-icons">extension</i> Prérequis : </h3>
<p>'.$scope_prerequis.'</p>
<h3 class="mdl-cell mdl-cell--12-col mdl-typography--headline"> <i class="material-icons">alarm</i> Durée : </h3>
<p>'.$scope_duree.' heures</p>
</div>
<div class="mdl-cell mdl-cell--6-col">';
if($scope_linkyoutube==null){
echo '<div class="">
<img class="article-image" src="../../images/social.jpg" width="80%" border="0" alt="">
</div>';
}else{
echo '<div class="videocontainer" >
<iframe src="'.$scope_linkyoutube.'" frameborder="0" allowfullscreen></iframe>
</div>';
}
echo '</div>
</div>';
/*<div class="mdl-cell mdl-cell--6-col">
<img class="article-image" src="../../images/social.jpg" width="400px" border="0" alt="">
</div>*/
if ((isset($_SESSION['login'])) && (!empty($_SESSION['login']))){
echo'
<a href="../mooc.php?idM='.$idMooc.'&insert='.$idMooc.'" style="margin: 50px;">
<button class="mdl-button mdl-js-button mdl-button--raised mdl-js-ripple-effect mdl-color--teal" style="height: 80px;">
<p style="color: white;padding-top: 10px;">Inscription au cours</p>
</button>
</a>
';
}else{
echo '
<a href="../mooc.php?idM='.$idMooc.'" style="margin: 50px;">
<button class="mdl-button mdl-js-button mdl-button--raised mdl-js-ripple-effect mdl-color--teal" style="height: 80px;">
<p style="color: white;padding-top: 10px;">Acceder au cours</p>
</button>
</a>
';
}
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur getInfos2()";
}
}
function callGetInfo3MDL(){
$valid2 = 1;
if (isset($_GET['idM'])) {
$idMooc = $_GET['idM'];
//echo $idMooc;
}else{
$valid2 = 0;
echo'erreur methode GET';
}
if($valid2==1){
getInfo3MDL(); //<------------ voir en haut
//echo "okok";
}
}
?><file_sep>/app/includes/cv_interactif.php
<?php
session_start();
$passe = 0;
if(isset($_SESSION['id_user'])) {
$id_user = $_SESSION['id_user'];
$passe=1;
}else{
$passe=0;
}
$scoreGlobal = 0;
if(isset($_POST["scoreGlobal"])) {
$scoreGlobal=$_POST["scoreGlobal"]; //id mooc
}
$id_exercice = 32;
if($passe == 1){
include 'connect.inc.php'; //connexion bdd
try {
$requete_prepare= $bdd->prepare("INSERT INTO faire(score,id_user,id_exercice) VALUES('$scoreGlobal', '$id_user', '$id_exercice') ON DUPLICATE KEY UPDATE score='$scoreGlobal',id_user='$id_user',id_exercice='$id_exercice'"); // on prépare notre requête
$requete_prepare->execute();
//echo "Votre score est de ".$scoreGlobal;
//var_dump($requete_prepare);
// echo "->Sauvegarde du score<br>";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
?><file_sep>/app/mooc/mooc4/chapitres/chapitre4.php
<?php
$idMooc;
$idChap;
if(isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
//afficheChapitreExos($idMooc,$idChap,$bdd,0);
//echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Cours </h2>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="my_step_box">
<!-- Cours -->
<div class='row'>
<div class="col-md-3"></div>
<div class="col-md-3"></div>
</div>
<div class="box1" id="1">
<p dir="ltr">
Structure de découpage du projet (SDP) / Work Breakdown Structure (WBS). Décomposition
hiérarchique du contenu total du projet, qui définit le travail que l'équipe de projet doit réaliser pour
atteindre les objectifs du projet et produire les livrables requis.
</p>
<p dir="ltr">
Référence de base du contenu [donnée de sortie] / Scope baseline [output]. Version approuvée
d'un énoncé du contenu, de la structure de découpage du projet et de son dictionnaire de la SDP
associé, qui ne peut être modifiée que par des procédures formelles de maîtrise des modifications
et qui est utilisée comme base de comparaison.
</p>
</div>
</div>
<div class="box1" id="2">
En ce qui concerne la mise en forme, il vaut mieux éviter de mettre la ville tout en capitale, peu d'intérêt d'attirer le regard.
</p>
<br/>
</div>
<div class="box1" id="3">
<ol start="3">
<li dir="ltr">
<h2 dir="ltr">
Téléphone(s)
</h2>
</li>
</ol>
<p dir="ltr">
En ce qui concerne le numéro du téléphone, il faut mettre le numéro de téléphone où l’on peut vous joindre facilement, par exemple, le numéro de téléphone
portable. Faites attention à votre message en cas d’absence. Pensez à mettre (+33) si vous postulez à l’étranger.
</p>
<br/>
<p dir="ltr">
Si vous mettez deux numéros de téléphone ou plus sur votre CV, il est conseillé de placer le principal en premier. Par contre il est fort probable qu’en
cas de non réponse, le recruteur risque de passer directement au second numéro au lieu de laisser un message sur votre répondeur.
</p>
<br/>
<p dir="ltr">
Il est important de mettre au moins un numéro de téléphone, car il y a beaucoup de chances que le récruteur veuille vous contacter par téléphone.
</p>
<br/>
</div>
<div class="box1" id="4">
<ol start="4">
<li dir="ltr">
<h2 dir="ltr">
Mail(s)
</h2>
</li>
</ol>
<p dir="ltr">
Il faut avoir une adresse mail professionnelle, c’est à dire une adresse de type: <a href="mailto:<EMAIL>"><EMAIL></a>. Si vous
êtes étudiant, pensez à utiliser l’adresse mail de votre école.
</p>
<p dir="ltr">
Il vaut mieux éviter les pseudos et les adresses mails de type: «<EMAIL> » ou « <EMAIL> » qui feront au mieux sourire, au pire
fuir le recruteur. Votre professionnalisme risque de prendre un coup d’entrée de jeu. Faites attention aux providers qui sont souvent spammés (Hotmail ou
Yahoo par exemple).
</p>
<br/>
</div>
<div class="box1" id="5">
<ol start="5">
<li dir="ltr">
<h2 dir="ltr">
Date de naissance et âge
</h2>
</li>
</ol>
<p dir="ltr">
La date de naissance est conseillé à mettre sous la forme jj/mm/aaaa, vous pouvez mettre votre âge entre parentèses, attention à rectifier chaque année.
</p>
<br/>
<p dir="ltr">
Il est inutile de mentir sur votre âge, car il peut être facilement évalué, grâce aux dates d’obtention de vos diplômes.
</p>
<br/>
</div>
<div class="box1" id="6">
<ol start="6">
<li dir="ltr">
<h2 dir="ltr">
Lieu de naissance(optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez mettre votre lieu de naissance. Si votre nom à une consonance étrangère et que vous êtes français. Il y a peu d'intérêt à le mettre si votre
nom sonne bien Français.
</p>
<p dir="ltr">
C’est peut-être une indication intéressante pour le recruteur, notamment si elle témoigne d’une mobilité géographique internationale et – surtout – de la
parfaite connaissance d’une langue
</p>
<ol start="7">
<li dir="ltr">
<h2 dir="ltr">
Nationalité (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Vous pouvez specifier votre nationalité si vous avez double nationalité. Il y a peu d'intérêt si votre nom sonne bien français.
</p>
<p dir="ltr">
Oui, si vous êtes étranger, ou si votre nom prête à confusion (si vous avez un nom aux résonances étrangères, tout en étant de nationalité française, par
exemple).
</p>
<ol start="8">
<li dir="ltr">
<h2 dir="ltr">
Réseau social (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
si les informations accessibles sont présentées de façons professionnelles. Pensez à mettre régulièrement à jour vos informations. Certains recruteurs
(30%) surfent sur les réseaux sociaux en quête d'informations, voir prennent contact avec vous via ces réseaux.
</p>
<p dir="ltr">
réseaux utilisés par les recruteurs : linkedin, viadéo, tweeter, à la rigueur facebook (professionnel), mettre les statistiques.
</p>
<p dir="ltr">
Site internet: Si en cohérence avec ce que vous souhaitez mettre en avant. Vérifiez qu'il fonctionne bien. Le recruteur fera sûrement un clic dessus.
</p>
<p dir="ltr">
Un lien vers son profil sur un réseau social ?
</p>
<p dir="ltr">
Il est judicieux de mentionner un lien vers sa page sur un réseau social si son contenu est à caractère strictement professionnel. Selon <NAME> : « les
réseaux sociaux professionnels nous apportent un éclairage sur les relations et les contacts du candidat ». Ils ont d’ailleurs la cote parmi les
recruteurs. « Viadeo est beaucoup utilisé dans les métiers de la création, d’Internet et de la communication, alors que les professionnels de l’industrie
ont davantage recours à LinkedIn », précise <NAME>. Et les liens vers son compte Twitter ou sa page Facebook ? Là encore, ils peuvent apparaître
sur un CV à condition que leur contenu ne versent pas dans le perso.
</p>
<p dir="ltr">
Une référence immédiatement vérifiable
</p>
<p dir="ltr">
Le book d’un créatif, la galerie photo d’un photographe, les sites créés par un chef de projet web… Les expériences et réalisations professionnelles
peuvent être mises en relief grâce aux liens hypertextes. « Il s’agit là d’une démonstration par l’exemple, d’une référence immédiatement vérifiable qui
apporte une réelle valeur ajoutée à la candidature », explique <NAME>, fondatrice du cabinet Elaee.
</p>
<p dir="ltr">
Cet avis, bon nombre de recruteurs le partagent. En revanche, ils sont moins unanimes en ce qui concerne les liens renvoyant aux sites des anciens
employeurs. Selon <NAME>, « le CV n’a pas vocation à faire la promo des entreprises pour lesquelles on a travaillé. » Pour <NAME>, ces liens
peuvent cependant apporter un complément d’information intéressant dans un secteur où il est difficile de connaître tous les acteurs.
</p>
<p dir="ltr">
Et qu’en est-il pour les liens renvoyant aux formations suivies ? Jugés inutiles par <NAME>, <NAME> estime qu’« ils peuvent être intéressants
pour un étudiant en recherche de stage ou de contrat en alternance ».
</p>
<p dir="ltr">
Attention à votre e-réputation !
</p>
<p dir="ltr">
Quels que soient les liens mentionnés, tous doivent faire l’objet d’une scrupuleuse attention. Chacun d’entre eux se doit d’apporter une réelle valeur
ajoutée au CV et non le pénaliser. Un lien non valide, un blog d’humeur politique, un profil avec des commentaires acerbes ne feront que porter préjudice à
la candidature. « Le candidat doit s’assurer qu’aucun lien mentionné ne porte atteinte à sa e-réputation », conseille ainsi <NAME>.
</p>
<p dir="ltr">
Méfiance donc avec les blogs et les sites personnels, certains peuvent agrémenter la rubrique « divers » du CV mais à condition d’apporter une plus-value
et de ne pas être trop personnel. « Le renvoi vers un blog tenu durant un tour du monde me fournira des éléments intéressants concernant la patience, la
méticulosité, la maîtrise d’Internet du candidat », illustre <NAME>.
</p>
<p dir="ltr">
Outil intéressant pour apporter la démonstration concrète de ses réalisations, le lien hypertexte doit être manié avec précaution et parcimonie. Car « trop
de liens tuent le lien », tranche <NAME>.
</p>
<br/>
</div>
<div class="box1" id="7">
<ol start="9">
<li dir="ltr">
<h2 dir="ltr">
Mobilité (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Nationale ou internationale si vous en êtes sûr. Information pouvant être utile pour le recruteur
</p>
<ol start="10">
<li dir="ltr">
<h2 dir="ltr">
Bilingue (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Il faut mettre que si c’est vrai. Spécifier les langues. Plus value évidente. Attention, le recruteur peut entamer le dialogue directement dans la langue
que vous maitrisez en dehors du Français
</p>
<ol start="11">
<li dir="ltr">
<h2 dir="ltr">
Phrase d’accroche (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Elle doit traduire un objectif, une ambition, elle doit être le plus factuelle possible. Elle induit la façon dont sera lu votre cv par la suite. Le
recruteur va être influencé. Soit il arrête de lire, soit il va contrôler sa lecture en fonction de la phrase d'accroche
</p>
<p dir="ltr">
Comme votre lettre de motivation ne sera lue qu’après de sévères sélections sur les CV. Ne vous privez pas de préciser sur votre CV vos objectifs. C’est en
quelque sorte un préambule de votre lettre d’accompagnement et ce préambule peut pousser le recruteur à analyser d’avantage votre candidature. De plus en
plus de cabinets indexent les CV dans divers outils, certains étant de simples index de recherche full-texte et d’autres sont équipés d’analyse sémantique,
il vous faut donc utiliser dans votre CV le vocabulaire courant propre à votre secteur d’activité en évitant les acronymes et, si votre parcours
professionnel un peu pauvre ne le permet pas, le paragraphe souhait et objectifs, vous donne toutes latitudes pour le faire.
</p>
<p dir="ltr">
Les qualificatifs sur son CV ne doivent pas être choisis à la légère. LinkedIn a sorti les 10 mots les plus utilisés par ses membres. Les recruteurs se
lassent de ne voir que des candidats créatifs, stratégiques et motivés.
<img
src="https://lh4.googleusercontent.com/4wTBry7_9wSQ-yryybV4vaFaf1EF52MVkh_lttjnkEeyjHQWUD6rbdAY3RJg79nYBTFHSMryb0LGSRpQFP4chieqczRlkcD5pOdSnQjdxPruVTaqjvoLb31c59xcE4t5ZL7I66pk"
width="472"
height="301"
/>
</p>
<br/>
<p dir="ltr">
Plutôt que de répéter les mêmes buzzwords, nous invitons nos membres à rendre compte de ce qu’ils ont accompli au travers de photos, de présentations ou
d’autres exemples afin d’apporter la preuve qu’ils sont effectivement "passionnés", "experts" et "créatifs". »
</p>
<br/>
</div>
<div class="box1" id="8">
<ol start="12">
<li dir="ltr">
<h2 dir="ltr">
Photo (optionnel)
</h2>
</li>
</ol>
<p dir="ltr">
Votre photo doit donner envie de vous rencontrer, sourire, netteté, contraste, vêtement professionnel, attitude ouverte, couleurs. (évitez les scans de
photo d'identité, les vieilles photos …). Elément fortement sujet à émotion donc à interprétation. Mieux vaut ne pas afficher de photo du tout que
d’insérer un portrait livide, décalé, voir raté sur votre CV.
</p>
<p dir="ltr">
Concrètement, se mettre en avant sur son CV en ligne commence par présenter une bonne photo. Les profils avec photo ont 14 fois plus de chances d’être
consultés. Bien sûr, sourire professionnel et tenue adéquate sont de mise.
</p>
<p dir="ltr">
Cependant, si vous avez rencontré des recruteurs par exemple sur un forum ou dans un salon professionnel, elle peut être un moyen pour eux de vous
reconnaître.
</p>
<p dir="ltr">
La photo n’est pas obligatoire dans un CV, surtout si elle ne vous met pas en valeur ou manque de professionnalisme. Evitez les photos de soirées, de
vacances ou de famille, qui manquent de pertinence et de maturité.
</p>
<p dir="ltr">
Préférez des photos dans des contextes professionnels. Une photo en décolleté est également à proscrire, miser sur l’atout physique dans une candidature
est une faute de goût et révèle un manque flagrant d’arguments professionnels.
</p>
<br/>
</div>
<div class="box1" id="9">
<ol start="13">
<li dir="ltr">
<h2 dir="ltr">
A eviter
</h2>
</li>
</ol>
<p dir="ltr">
Sexe: attention risque de discrimination à l'embauche si spécifiée (le prénom indique le sexe en général sinon ce sera la surprise pour le recruteur). Lui
faire la surprise lors de l'entretien
</p>
<br/>
<p dir="ltr">
Situation familiale: Ce n'est plus comme avant, les situations sont nombreuses (célibataire, marié, pacsé, compagnon, divorcé …) et aucune ne traduit votre
mobilité réelle.
</p>
<p dir="ltr">
Les mensonges: Il peut être tentant, pour les candidats, d'« embellir » leur CV, à coup de faux diplômes, de compétences surévaluées ou de hobbies
trafiqués. Selon une étude de Verifdiploma,
<a href="http://www.terrafemina.com/emploi-a-carrieres/actu/articles/26253-quels-sont-les-mensonges-les-plus-courants-sur-un-cv-.html">
26% des candidats n'hésiteraient d'ailleurs pas à mentir sur leur CV
</a>
. Pourtant, ces petits arrangements avec la vérité peuvent leur coûter cher en leur faisant manquer le poste de leurs rêves si le recruteur vient à
découvrir la supercherie.
</p>
<br/>
</div>
<div class="box1" id="10">
<ol start="14">
<li dir="ltr">
<h2 dir="ltr">
Titre du CV
</h2>
</li>
</ol>
<p dir="ltr">
Le titre fut longtemps présenté comme facultatif. Aujourd’hui la majorité des recruteurs considèrent qu’il est indispensable. Il doit cependant être manié
avec intelligence : un titre trop flou donne l’impression d’un projet professionnel pas très clair tandis qu’un titre trop précis peut fermer des portes.
</p>
<p>
Le titre indique généralement un poste (directeur administratif et financier) mais il peut aussi prendre l’intitulé d’un département (Direction
administrative et financière). Quand on manque d’expérience, cela a l’avantage de désigner les métiers vers lesquels on s’oriente tout en montrant qu’on
est prêt à étudier différents degrés hiérarchiques. Il est également possible d’ajouter le secteur d’activité si celui-ci est déterminant. En fait, c’est à
chacun de placer le curseur en fonction de ses ambitions et de son parcours. Toutefois, il faut bannir les intitulés trop généraux (par exemple :
communication).
</p>
<br/>
</div>
</div>
</div>
</div>
<div class="col-md-3 col-sm-12 col-xs-12 animated slideInRight">
</div>
<div class="col-md-3 col-sm-12 col-xs-12 animated slideInRight">
</div>
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInRight">
<div class="x_panel">
<div class="x_title">
<?php
nomChapitre($idMooc,$bdd,$numChap);
?>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Smart Wizard -->
<h3>Exercices</h3>
<div id="wizard" class="form_wizard wizard_horizontal">
<?php
creationWizardStep($idMooc,$numChap,$idChap,$bdd);
?>
</div>
<!-- IMPORTANT AFFICHE LES INDICE -->
<div id="indicebox"></div>
<!-- IMPORTANT AFFICHE LES SOLUTIONS -->
<div id="solucebox"></div>
<!-- IMPORTANT POUR LES REPONSE AU QCM -->
<?php
/*if(isset($_GET['idM']) && isset($_GET['idC'])) {
//$idMooc = $_GET['idM'];
echo '<div id="'.$_GET['idM'].'"></div>';
$idChap = $_GET['idC'];
}*/
?>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/includes/qcm.php
<?php
function exoQcm($idMooc,$idChap,$idExo,$bdd,$numeroExo)
{
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
$selectExo = $bdd->prepare("SELECT * FROM exercice WHERE id_chapitre = $idChap");
$selectExo->execute();
$lignesExo = $selectExo->fetchAll();
$i = 0;
$selectqcm = $bdd->prepare("SELECT * FROM qcm WHERE id_exercice = $idExo");
$selectqcm->execute();
$lignesQcm = $selectqcm->fetchAll();
//Reponse du qcm
$tabHint = array();
$tabSolution = array();
$numCaseTab=0;
$tabIndiceQcm = array();
for($i = 0; $i < 60; $i++)
{
$tabRandArray[$i] = rand(1, 8000);
//echo ','.$tabRandArray[$i];
}
//echo "size".$lignesQcm;
//var_dump($lignesQcm);
for($i = 0; $i < sizeof($lignesQcm) ; $i++)
{
$solution = $lignesQcm[$i]["solution"];
$solution=htmlentities($solution, ENT_QUOTES, "UTF-8");
$tabHint = preg_split('[-]', $solution);
echo '<input type="hidden" id="indice" class="'.$i.$idExo.'" name="indice" value="' . htmlspecialchars(stripslashes($lignesQcm[$i]["indice_qcm"])). '" />'; //indice_qcm
echo '<input type="hidden" id="explication" class="'.$i.$idExo.$idExo.'" name="explication" value="' . htmlspecialchars(stripslashes($lignesQcm[$i]["explication_qcm"])). '" />'; //explication_qcm
for($itab = 0; $itab < sizeof($tabHint) ; $itab++)
{
//var_dump($tabHint);
//echo sizeof($tabHint);
//echo "**".$tabHint[$itab];
//print_r("**".$tabHint[$itab]);
//obligatoire si plusieurs reponse de meme nom
//echo "<br>".$tabHint[$itab]."<br>";
$valint = intval($tabHint[$itab]);
//var_dump($valint );
$ez = $tabRandArray[$valint];
$lowercase = strtolower($tabHint[$itab]);
if($lowercase == 'oui'){
$tabSolution[$numCaseTab]=$ez.$i.$idExo; //rajoute $i si doublon
//print_r("+*".$tabSolution[$numCaseTab]);
}else if($lowercase == 'non'){
$tabSolution[$numCaseTab]=$ez.$i.$idExo; //rajoute $i si doublon
//print_r("-*".$tabSolution[$numCaseTab]);
}
else{
$tabSolution[$numCaseTab]=$ez.$i.$idExo;
//print_r("-*".$tabSolution[$numCaseTab]);
}
$numCaseTab++;
}
}
//var_dump($tabSolution);
//Type hidden qui correpond au reponse pour le qcm
//$temp = $tabSolution;
$temp = preg_replace("/\s+/","", $tabSolution);
$temp = str_replace( ',', '', $temp );
//$temp = preg_replace("/é/","xxx", $temp );
//print_r($temp);
//$tabSolution=implode(",",$tabSolution);
//$tabSolution=json_encode($tabSolution);
//echo 'tab réponse='.$tabSolution;
//Réponse présent
//echo "<input type='hidden' class='soluce' name='zyx' value='".implode(",", $tabSolution)."'/>";
echo '<input type="hidden" class="soluce" name="zyx" value="'.implode(",", $temp).'"/>';
//echo "<input type='hidden' id='idexo' name='nameexo' value='".$tabSolution."'/>";
//echo '<div id="solucebox"></div>'; //affichage sur show
/*if(isset($idMooc) && isset($_GET['idC'])){
echo "<input type='hidden' id='idm' name='idm' value='".$tabSolution."'/>";
}*/
echo "<input type='hidden' id='idm' name='idm' value='".$idMooc."'/>";
echo "<input type='hidden' id='idc' name='idc' value='".$idChap."'/>";
echo "<input type='hidden' id='ide' name='ide' value='".$idExo."'/>";
//echo "<input type='hidden' id='indice' class='".$idExo."' name='indice' value='".htmlspecialchars(stripslashes($lignesQcm[0]["indice_qcm"]))."'/>";//indice
echo '<input type="hidden" id="indice" class="'.$idExo.'" name="indice" value="' . htmlspecialchars(stripslashes($lignesQcm[0]["indice_qcm"])) . '" />';
//affichage du QCM
$ouinon = 0;
for($i = 0; $i < sizeof($lignesQcm) ; $i++)
{
$reponse = $lignesQcm[$i]["reponse_qcm"];
$tab = array();
$tab = preg_split('[-]', $reponse);
//var_dump($lignesExo);
echo'<div class="form-group">
<h2 class="StepTitle center">'.$lignesQcm[$i]["question"].'</h2><br>';
for($itab = 0; $itab < sizeof($tab) ; $itab++)
{
if( strtolower($tab[$itab]) == "oui" || strtolower($tab[$itab]) == "non")
{
if( strtolower($tab[$itab]) == "oui")
{
echo'<div class="ck-button">
<label>
<input type="checkbox" name="'.$tabRandArray[$itab].$i.$idExo.'" value="" style="display:none;"><span>'.$tab[$itab].'</span>
</label>
</div>';
}
else if( strtolower($tab[$itab]) == "non")
{
echo'<div class="ck-button">
<label>
<input type="checkbox"name="'.$tabRandArray[$itab].$i.$idExo.'" value="" style="display:none;"><span>'.$tab[$itab].'</span>
</label>
</div><br>';
}
}
else{
$affichageetvalue=htmlspecialchars(stripslashes($tab[$itab]));
$affichageetvalue=htmlentities($affichageetvalue, ENT_QUOTES, "UTF-8");
$affichageetvalue = preg_replace("/\s+/","",$affichageetvalue);
$affichageetvalue = str_replace( ',', '',$affichageetvalue);
echo '<div class="checkbox center">
<label class="hover">
<div class="icheckbox_flat-green checked hover" style="position: relative;"><input type="checkbox" name="'.$tabRandArray[$itab].$i.$idExo.'" class="flat" style="position: absolute; opacity: 0;"><ins class="iCheck-helper" style="position: absolute; top: 0%; left: 0%; display: block; width: 100%; height: 100%; margin: 0px; padding: 0px; border: 0px; opacity: 0; background: rgb(255, 255, 255);"></ins></div>
'.$tab[$itab].'<br>
</label>
</div>';
//echo 'sok';
}
}
echo' </div><br>';
echo '<button type="button" class="myindice btn btn-round btn-success btn-xs" value="'.$i.$idExo.'">Indice</button>'; // indice
echo '<button type="button" class="myexplication btn btn-round btn-success btn-xs" value="'.$i.$idExo.$idExo.'">Explication</button>'; // indice
//}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur afficheChapitreExos()";
}
}
?><file_sep>/app/mooc/mooc4/chapitres/chapitre0.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
//echo'erreur';
}
?>
<div class="row">
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Avant propos</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown" role="button" aria-expanded="false"><i class="fa fa-wrench"></i></a>
<ul class="dropdown-menu" role="menu">
<li><a href="#">Settings 1</a>
</li>
<li><a href="#">Settings 2</a>
</li>
</ul>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<img class="img left" src="../mooc/mooc1/chapitres/images/mooc.png" style="margin: 20px;" width="300px" alt="">
 <br>
<p dir="ltr">
L’acronyme MOOC signifie « Massive Open Online Course » que l’on peut traduire par « cours en ligne ouvert et massif ». Il s’agit donc :
- De cours et non pas de conférences ou de reportages. Ces cours sont d’un niveau universitaire.
- De cours diffusés sur internet.
- De cours gratuits et libre d’accès. Aucun prérequis n’est exigé de la part des participants. De plus, l’inscription sur les différentes plateformes de MOOCs est entièrement gratuite. Seule la délivrance de certificats (facultatifs) est payante. Cela ne signifie pas pour autant que le contenu délivré sur ces plateformes soit libre de droit. D’une manière générale, il ne peut être ni réutilisé, ni rediffusé sans le consentement de leurs auteurs.
- De cours massifs. Le nombre d'inscrits par cours peut varier de quelques milliers à plus de 100'000 participants. Néanmoins, tous les étudiants n’ont pas forcément l’intention de suivre l'enseignement du début jusqu'à la fin, et seule un petite proportion d’étudiants est véritablement active.
</p>
<div>
<br/>
</div>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/includes/requeteMooc.php
<?php
function titreMooc($idMooc,$bdd)
{
$selectChap = $bdd->prepare("SELECT * FROM mooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
echo'<h2><a style="text-decoration:none; color:white;" href="../../app/modules/mooc.php?idM='.$idMooc.'">'.$lignesChap[$idMooc-1]["nom_mooc"].'</a></h2>';
}
function creationWizardStep($idMooc,$numChap,$idChap,$bdd) {
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
if(sizeof($lignesChap) == 0){
echo 'Aucun chapitre présent';
}
else{
$partie = $lignesChap[$numChap-1]["partie"];
$tabPartie = array();
$tabPartie = preg_split('[-]', $partie);
//var_dump($lignesExo);
echo'<ul class="wizard_steps" style="padding-left: 0px;">';
for($ipart = 0; $ipart < sizeof($tabPartie) ; $ipart++)
{
echo '<li>
<a style="text-decoration:none; cursor:default;" href="#step-'.($ipart+1).' ">
<span class="step_no">'.($ipart+1).'</span>
<span class="step_descr">
</span>
</a>
</li>';
}
echo'</ul></li>';
$i = 0;
if ($idMooc !=0 && $numChap !=0)
{
for($i = 0; $i < sizeof($tabPartie) ; $i++)
{
echo '<div id="step-'.($i+1).'">
<div class="panel-body" style="display: block;">';
//echo nombreExoParChapitre($idMooc,$idChap,$bdd);
$erreur = afficheChapitreExos($idMooc,$idChap,$bdd,$i);
if($erreur == -1)
{
echo '<h2 class="StepTitle">Exercice en construction</h2>';
}
echo '</div>
</div>';
}
}
else{
$valid = 0;
echo'erreur';
}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur creationWizardStep()";
}
}
function chapitresplusSousPartie($idMooc,$bdd)
{
//var_dump($idMooc);
$stringAvancement=''; //contient les chapitre fais par un user
if ((isset($_SESSION['login'])) && (!empty($_SESSION['login']))){
$user=$_SESSION['id_user'];
//var_dump($user);
try {
$select = $bdd->prepare("SELECT * FROM suivre WHERE id_user = '$user' AND id_mooc = '$idMooc'");
$select->execute();
$lignes = $select->fetchAll();
//var_dump($lignes);
$stringAvancement = $lignes[0]["avancement"];
// echo "avancement -> ".$stringAvancement;
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur<br>";
}
}
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
if(sizeof($lignesChap) == 0){
echo 'Aucun chapitre présent';
}
else
{
for($i = 0; $i<sizeof($lignesChap); $i++)
{
$id_chap_tiret = '-'.($i+1); //verifie la présence
//var_dump($stringAvancement);
//var_dump($id_chap_tiret);
if (strpos($stringAvancement, $id_chap_tiret) !== false) {
echo '<li><a style="" ><i class="fa fa-check-circle"></i>'.$lignesChap[$i]["titre"].'<br><span class="fa fa-chevron-down"></span><br></a>';
$partie = $lignesChap[$i]["partie"];
$tabPartie = array();
$tabPartie = preg_split('[-]', $partie);
//var_dump($lignesExo);
echo' <ul class="nav child_menu" style="display: none">';
for($ipart = 0; $ipart < sizeof($tabPartie) ; $ipart++)
{
echo '<li><a href="../../app/modules/mooc.php?idM='.$idMooc.'&idC='.$lignesChap[$i]["id_chapitre"].
'&numC='.$lignesChap[$i]["numero"].'"">'.$tabPartie[$ipart].'</a></li>';
}
echo'</ul></li>';
}else{
echo '<li><a><i class="fa fa-book"></i>'.$lignesChap[$i]["titre"].'<br><span class="fa fa-chevron-down"></span><br></a>';
$partie = $lignesChap[$i]["partie"];
$tabPartie = array();
$tabPartie = preg_split('[-]', $partie);
//var_dump($lignesExo);
echo' <ul class="nav child_menu" style="display: none">';
for($ipart = 0; $ipart < sizeof($tabPartie) ; $ipart++)
{
echo '<li><a href="../../app/modules/mooc.php?idM='.$idMooc.'&idC='.$lignesChap[$i]["id_chapitre"].
'&numC='.$lignesChap[$i]["numero"].'"">'.$tabPartie[$ipart].'</a></li>';
}
echo'</ul></li>';
}
}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur chapitresplusSousPartie()";
}
}
function nomChapitre($idMooc,$bdd,$numChap)
{
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
echo '<h2> '.$lignesChap[$numChap-1]["titre"].' </h2>';
}
catch (Exception $e){
echo $e->errorMessage();
echo "->erreur idParNumeroChapitre()";
}
}
function idParNumeroExo($idMooc,$idChap,$bdd,$numeroExo)
{
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
$selectExo = $bdd->prepare("SELECT * FROM exercice WHERE id_chapitre = $idChap");
$selectExo->execute();
$lignesExo = $selectExo->fetchAll();
if(sizeof($lignesExo) != 0 && $numeroExo<sizeof($lignesExo))
{
//echo'<div><h3 class="name"> Exercice n°'.$lignesExo[$numeroExo]["numero"].' </h3></div>';
$idExo = $lignesExo[$numeroExo]["id_exercice"];
if(sizeof($lignesExo) != 0)
{
return $idExo;
}
}
else
{
echo 'Aucun exercice présent';
return -1;
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur idParNumeroExo()";
}
}
function nombreExoParChapitre($idMooc,$idChap,$bdd)
{
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
$selectExo = $bdd->prepare("SELECT * FROM exercice WHERE id_chapitre = $idChap");
$selectExo->execute();
$lignesExo = $selectExo->fetchAll();
if(sizeof($lignesExo) != 0)
{
return sizeof($lignesExo);
}
else
{
echo 'Aucun exercice présent';
return -1;
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur nombreExoParChapitre()";
}
}
function afficheChapitreExos($idMooc,$idChapitre,$bdd,$numeroExercice)
{
try{
if($idChapitre != -1) // -1 signifie que le chapitre n'existe pas
{
$idExercice = idParNumeroExo($idMooc,$idChapitre,$bdd,$numeroExercice); // -1 signifie que l'exercice n'existe pas
if($idExercice != -1)
{
exoQcm($idMooc,$idChapitre,$idExercice,$bdd,$numeroExercice);
}
else{
return -1;
}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur afficheChapitreExos()";
}
}
?><file_sep>/app/mooc/mooc1/chapitres/chapitre9.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 9 : Autres infos</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<p dir="ltr">
Le recruteur reçoit des centaines de « CV.doc » par jour. Simplifiez-lui la vie avec un « CV-NOM-prenom.doc ».
</p>
<ol>
<li dir="ltr">
<p dir="ltr">
Format
</p>
</li>
</ol>
<p dir="ltr">
Format CV: Format A4, il est possible de faire d’autres formats. Format portrait (standard), plutôt que paysage. Grammage 90g. Texture standard. Souvent le
recruteur est habitué à un mode de lecture et de rangement, tous formats originaux risquent de le géner dans son organisation.
</p>
<p dir="ltr">
Une idée reçue consiste à dire que le CV doit tenir sur une seule page. Faux. Votre CV la plupart du temps immatériel peut tenir sur plusieurs pages et
donc avoir une présentation aérée. N’hésitez pas non plus à développer ce qui vous paraît fondamental dans votre parcours, d’utiliser des couleurs, des
polices de caractères différents pour gagner en lisibilité. C’est votre CV. Il est le reflet de votre image. Appropriez-vous le. S’il dégage du caractère
le recruteur le sentira. Si vous souhaitez que votre CV soit conforme et très sobre alors c’est que vous êtes conformiste et le recruteur le retiendra
seulement si c’est ce qu’il recherche ! En d’autres termes, plus votre CV vous correspond, plus le recruteur sensible à votre CV sera celui qui vous
conviendra.
</p>
<div>
<br/>
</div>
</div>
</div>
</div>
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Exemple</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
</div>
</div>
</div>
<file_sep>/app/mooc/mooc1/chapitres/chapitre3.php
<?php
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
//afficheChapitreExos($idMooc,$idChap,$bdd,0);
//echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
?>
<script>
var nbClickMax=5;
var nbClick=0;
var scoreGlobal;
function compter()
{
nbClick++;
var nbTentativesrestantes = parseInt(document.getElementById("tentative").innerHTML);
nbTentativesrestantes=nbTentativesrestantes-1;
document.getElementById("tentative").innerHTML =nbTentativesrestantes;
if(nbClick>=nbClickMax)
{
// alert('Nombre de clic maximum atteint');
//
$.ajax({
url: '../includes/cv_interactif.php',
type: 'POST',
data: {
scoreGlobal: scoreGlobal, //la solution
},
success: function(data) {
var ndata=data; //jsondata c'est le callback de wizard.jss
//alert (ndata);
},
error: function(json) {
//alert('false');
//$('.alert-danger').show();
}
});
//scoreGlobal;
// On désactive les clics sur les areas
$("area").each(function() {
$(this).attr("onclick","inutile()");
});
// On désactive les clics sur l'image
$("#fauxcv").attr("onclick","inutile()");
}
}
function afficheTitle(title){
alert(title);
}
function augmenteScore(title){
var score = parseInt(document.getElementById("score").innerHTML);
score = score+20;
scoreGlobal=score;
document.getElementById("score").innerHTML =score;
$("#"+title).attr("onclick","dejaTrouve()");
}
function dejaTrouve(){
alert("Cette erreur à déjà été trouvée");
}
function inutile(){
}
function diminueScore(){
var score = parseInt(document.getElementById("score").innerHTML);
if(score>=20){
score=score-20;
scoreGlobal=score;
}
document.getElementById("score").innerHTML =score;
}
</script>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 ">
<!-- CONTENU COURS -->
<div class="animated slideInUp">
<div class="x_panel">
<!-- TITRE COURS -->
<div class="x_title">
<h2> Chapitre 3 : Projets </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<!-- COURS -->
<div class="x_content">
<div class="my_step_box">
<div class='row'>
<div class="col-md-3"></div>
<div class="col-md-6">
<div class="btn-group" role="group" aria-label="...">
<button type="button" class="btn btn-default myBtnBack"><span class="glyphicon glyphicon-chevron-left"></span> Retour</button>
<button type="button" class="btn btn-default myBtnAll"><span class="glyphicon glyphicon-eye-open"></span> </button>
<button type="button" class="btn btn-default myBtnNext">Suivant<span class="glyphicon glyphicon-chevron-right"></span></button>
</div>
</div>
<div class="col-md-3"></div>
</div>
<div class="box1" id="1">
<ol start="1">
<li dir="ltr">
<h2 dir="ltr">
Introduction
</h2>
</li>
</ol>
<p dir="ltr">
Dans cette section de votre CV, vous pouvez mettre les projets réalisés dans le cadre de vos études ou des projets personnels qui ont un rapport avec votre formation. Si vous n’avez pas beaucoup d’experience professionnelle, il est conseillé de mettre cette partie entre la formation et l’experience professionnelle. En revanche si vous avez plus de 5 ans d’experience en entreprise, cette partie n’est pas très utile. Dans ce cas la il est préferable de developper les projets effectués au sein d’une entreprise dans la description de vos experiences professionnelles.
</p>
<p dir="ltr">
Dans le cas ou vous avez effectué beaucoup de projets durant votre formation, selectionnez les projets que vous voulez mettre en avant par rapport au poste à lequel vous postulez.
</p>
<p dir="ltr">
Pour chaque projet il faut indiquer le titre, la date, courte description et preciser si c’est un projet d’équipe ou individuel. Pour structurer les informations, il est important d’utiliser les puces pour presénter chaque projet/groupe de projet suivi.
</p>
<br/>
</div>
<div class="box1" id="2">
<ol start="2">
<li dir="ltr">
<h2 dir="ltr">
Titre et Date
</h2>
</li>
</ol>
<p dir="ltr">
Tout d’abord, il faut mettre le titre de votre projet et la date. En ce qui concerne l’ordre, vous pouvez les classer soit par date anti-chronologique ou par criteres des projets. Par exemple, si vous avez effectué plusieurs projets informatiques en plusieurs langages, vous pouvez classer vos projets par langages informatiques.
</p>
<p dir="ltr">
Exemple de la partie “Projets” d’un CV:
Dans cet exemple il faut faire attention et ne pas oublier de mettre au saut de ligne qui coupe l’intitulé d’un sigle,
sinon cela rend la lecture difficile.
</p>
<p dir="ltr">
<img class="" src="../mooc/mooc1/chapitres/images/projets.png" width="500px" alt="">
</p>
<br/>
</div>
<div class="box1" id="3">
<ol start="3">
<li dir="ltr">
<h2 dir="ltr">
Description (Résultats-Objectifs)
</h2>
</li>
</ol>
<p dir="ltr">
Il est important de mettre la description et les objectifs de votre projet et les mots-clés importants. Cela permettra au recruteur de comprendre les compétences que vous avez pu developper durant la réalisation de ce projet et de quoi il s’agit exactement. Par exemple, si vous avez crée un site web et qu’il est disponible en ligne vous pouvez mettre un lien vers ce site.
</p>
<p dir="ltr">
Si votre projet faisait partie d’un concours, il est important de le mettre en avant et de mentionner les résultats, comme votre position finale parmi d’autres projets.
</p>
<br/>
</div>
<div class="box1" id="4">
<ol start="4">
<li dir="ltr">
<h2 dir="ltr">
Projet d’équipe/projet individuel (positions dans l’équipe)
</h2>
</li>
</ol>
<p dir="ltr">
</p>
Pour chaque projet precisez si c’était un projet individuel ou un projet d’équipe. Dans le cas du projet individuel, cela peut montrer que vous savez gérer un projet de A à Z tout seul. Dans le cas d’un projet d’équipe, vous pouvez mettre en valeur le fait que vous savez travailler en groupe, que vous savez diviser le travail du projet en plusieurs tâches entre les membres de l’équipe.
<br/>
</div>
<div class="box1" id="5">
<ol start="5">
<li dir="ltr">
<h2 dir="ltr">
Récapitulatif du chapitre
</h2>
</li>
</ol>
<p dir="ltr">
<ol>
<li>
Mettre la partie “Projets” si votre expérience professionnelle est inférieure à 5 ans d’expérience.
</li>
<li>
Si vous avez effectué beaucoup de projets, sélectionner ceux qui peuvent vous mettre en valeur en fonction du poste recherché.
</li>
<li>
Pour chaque projet, indiquer: Date/Titre/Description/Type Projet (individuel ou projet d’équipe)/Résultat
</li>
<li>
Classement par ordre anti chronologique ou par critères.
</li>
<li>
Dans la description mettez les points clés du projet.
</li>
</ol>
</p>
<br/>
</div>
</div>
</div>
</div>
</div>
<!-- VIDEO -->
<div class="row">
<div class="col-md-12 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Vidéo </h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<div class="videocontainer lg6">
<!--<iframe src="https://www.youtube.com/embed/lX7kYDRIZO4" frameborder="0" ></iframe>-->
<iframe width="560" height="315" src="https://www.youtube.com/embed/_r7bht-LgvU" frameborder="0" allowfullscreen></iframe>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- EXERCICES -->
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInRight">
<div class="x_panel">
<div class="x_title">
<?php
nomChapitre($idMooc,$bdd,$numChap);
?>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<!-- Smart Wizard -->
<h3>Exercices</h3>
<div id="wizard" class="form_wizard wizard_horizontal">
<?php
creationWizardStep($idMooc,$numChap,$idChap,$bdd);
?>
</div>
<!-- IMPORTANT AFFICHE LES INDICE -->
<div id="indicebox"></div>
<!-- IMPORTANT AFFICHE LES SOLUTIONS -->
<div id="solucebox"></div>
<!-- IMPORTANT POUR LES REPONSE AU QCM -->
<?php
/*if(isset($_GET['idM']) && isset($_GET['idC'])) {
//$idMooc = $_GET['idM'];
echo '<div id="'.$_GET['idM'].'"></div>';
$idChap = $_GET['idC'];
}*/
?>
</div>
</div>
</div>
<div class="col-md-12 col-sm-12 col-xs-12 animated slideInUp" >
<div class="x_panel">
<div class="x_title">
<h4> CV Intéractif </h4>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content" style="overflow:auto;" >
<p style="font-size:14px;"><b> Cliquez sur les erreurs de ce CV pour marquer des points, attention à ne pas cliquer de partout sinon vous en perdrez. Il y a 5 erreurs et vous disposez de 5 clics. A vous de jouer !</b><p>
<br>
<img style="margin:0 auto; display:block;" id="fauxcv" src="../mooc/mooc1/chapitres/images/fauxcv2.png" usemap="#map1" onclick="diminueScore();compter();">
<map name="map1">
<area style="text-decoration:none; cursor:default;" shape="rect" id="area1" coords="28,8,154,146" onclick="augmenteScore(this.id);compter();" />
<area style="text-decoration:none; cursor:default;" shape="rect" id="area2" coords="172,35,375,54" onclick="augmenteScore(this.id);compter();" />
<area style="text-decoration:none; cursor:default;" shape="rect" id="area3" coords="203,183,531,201" onclick="augmenteScore(this.id);compter();" />
<area style="text-decoration:none; cursor:default;" shape="rect" id="area4" coords="170,132,334,148" onclick="augmenteScore(this.id);compter();" />
<area style="text-decoration:none; cursor:default;" shape="rect" id="area5" coords="13,552,141,572" onclick="augmenteScore(this.id);compter();" />
</map>
<div>
<br>
<p>Score : <span id="score">0</span></p>
<p>Tentatives restantes : <span id="tentative">5</span></p>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<file_sep>/app/modules/mooc.php
<?php
session_start();
include '../includes/connect.inc.php';
include '../includes/requeteMooc.php';
include '../includes/qcm.php';
include '../includes/dragdrop.php';
//inscrition au mooc (voir description.php car method get ->fonctionsDescription.php)
if(isset($_SESSION['login']) && isset($_GET['insert']) && isset($_GET['idM'])){
$unlockTrophy = $bdd->query('SELECT COUNT(id_user) AS cpt FROM suivre WHERE id_user= "'.$_SESSION['id_user'].'" AND id_mooc="'.$_GET['idM'].'"');
$donnees = $unlockTrophy->fetch();
$unlockTrophy->closeCursor();
// echo $donnees['cpt'];
if($donnees['cpt']==0){
$iduser = $_SESSION['id_user'];
$idM = $_GET['idM'];
try {
$requete_prepare= $bdd->prepare("INSERT INTO suivre(date_suivi,avancement,id_user,id_mooc) VALUES(current_date, 0,'$iduser', '$idM')"); // on prépare notre requête
$requete_prepare->execute();
//echo "->OK inscrition au mooc";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}else{
// echo 'deja inscrit';
}
}
/** Function qui insere le succes lors de l'inscription au mooc*/
function unlockSuccessInscription($bdd){
if ((isset($_SESSION['id_user'])) && (!empty($_SESSION['id_user'])) && isset($_GET['idM']))
{
//session ok
$myid=$_SESSION['id_user'];
$idMooc = $_GET['idM'];
$nbSucces = 4; // nombre de succes par MOOC
$id_succes = $idMooc*$nbSucces-($nbSucces-1);
try {
$requete_prepare= $bdd->prepare("INSERT INTO `debloquer` (`date_obtention`, `id_succes`, `id_user`) VALUES (current_date, '$id_succes', '$myid')"); // on prépare notre requête
$requete_prepare->execute();
} catch (Exception $e) {
//echo $e->errorMessage();
}
}
}
unlockSuccessInscription($bdd);
$idMooc;
if(isset($_GET['idM'])) {
$idMooc = $_GET['idM'];
}else{
$valid = 0;
echo'erreur';
}
?>
<!--
Material Design Lite
Copyright 2015 Google Inc. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License
-->
<!DOCTYPE html>
<html lang="fr">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="og:title" content="Mooc Isen">
<meta property="og:image" content="">
<meta property="og:url" content="http://colombies.com/app">
<meta property="og:description" content="Une plateforme pour apprendre gratuitement des cours d'ingénieur à l'ISEN.">
<meta property="robots" content="">
<!-- Title -->
<title>Mooc Isen</title>
<!-- favicon.ico -->
<link rel="shortcut icon" href="../favicon.ico">
<!-- Disable tap highlight on IE -->
<meta name="msapplication-tap-highlight" content="no">
<!-- Web Application Manifest -->
<link rel="manifest" href="../manifest.json">
<!-- Add to homescreen for Chrome on Android -->
<meta name="mobile-web-app-capable" content="yes">
<meta name="application-name" content="<NAME>">
<link rel="icon" sizes="192x192" href="../images/touch/chrome-touch-icon-192x192.png">
<!-- Add to homescreen for Safari on iOS -->
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta name="apple-mobile-web-app-title" content="Mooc Isen">
<link rel="apple-touch-icon" href="../images/touch/apple-touch-icon.png">
<!-- Tile icon for Win8 (144x144 + tile color) -->
<meta name="msapplication-TileImage" content="../images/touch/ms-touch-icon-144x144-precomposed.png">
<meta name="msapplication-TileColor" content="#457871">
<!-- Color the status bar on mobile devices -->
<meta name="theme-color" content="#457871">
<!-- Bootstrap core CSS -->
<link href="../assets/css/bootstrap.min.css" rel="stylesheet">
<!-- font awesome -->
<link href="../assets/fonts/css/font-awesome.min.css" rel="stylesheet">
<!-- animate -->
<link href="../assets/css/animate.min.css" rel="stylesheet">
<!-- Custom styling plus plugins -->
<link href="../assets/css/custom.css" rel="stylesheet">
<link href="../assets/css/style.css" rel="stylesheet">
<link href="../assets/css/icheck/flat/green.css" rel="stylesheet">
<link href="../assets/css/floatexamples.css" rel="stylesheet">
<!-- iframe -->
<link href="../assets/css/iframe-responsive.css" rel="stylesheet">
</head>
<body class="nav-md">
<div class="container body">
<div class="main_container ">
<div class="col-md-3 left_col ">
<div class="left_col mygrid-wrapper-div">
<div class="navbar nav_title" style="border: 0;">
<a href="../index.php" class="site_title"><i class="glyphicon glyphicon-education"></i> <span>MOOCs</span></a>
</div>
<div class="clearfix"></div>
<!-- menu prile quick info -->
<div class="profile">
<div class="profile_pic">
<img src="../assets/images/user.png" style="margin: 10px;" alt="..." class="img-circle profile_img">
</div>
<div class="profile_info">
<span>MOOC,</span>
<?php
titreMooc($idMooc,$bdd);
?>
</div>
</div>
<!-- /menu prile quick info -->
</br>
<!-- sidebar menu -->
<div id="sidebar-menu" class="main_menu_side hidden-print main_menu ">
<div class="menu_section ">
<h3>Chapitres</h3>
<ul class="nav side-menu ">
<?php
$valid = 1;
$idMooc;
if (isset($_GET['idM'])) {
$idMooc = $_GET['idM'];
//requeteMooc.php
chapitresplusSousPartie($idMooc,$bdd);
//echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
?>
</div>
<div class="menu_section">
<h3>Menu</h3>
<ul class="nav side-menu">
<li><a><i class="fa fa-wrench"></i> Paramètres<span class="fa fa-chevron-down"></span></a>
<ul class="nav child_menu" style="display: none">
<li><a href="connected/profil.php"><span class="glyphicon glyphicon-user" aria-hidden="true"></span> Profil</a>
</li>
<li><a href="connected/admin.php"><span class="glyphicon glyphicon-dashboard" aria-hidden="true"></span> Dashboard</a>
</li>
</ul>
</li>
</ul>
</div>
</div>
<!-- /sidebar menu -->
</div>
</div>
<!-- top navigation -->
<div class="top_nav">
<div class="nav_menu">
<nav class="" role="navigation">
<div class="nav toggle">
<a id="menu_toggle"><i class="fa fa-bars"></i></a>
</div>
<ul class="nav navbar-nav navbar-right">
<!-- AFFICHAGE MENU -->
<li class="">
<?php
if ((isset($_SESSION['email'])) && (!empty($_SESSION['email']))){
//Si Connecter
?>
<a href="javascript:;" class="user-profile dropdown-toggle" data-toggle="dropdown" aria-expanded="false">
<?php
if ((isset($_SESSION['avatar'])) && (!empty($_SESSION['avatar']))){
$avatar=$_SESSION['avatar'];
$str = str_replace('../', '', $avatar);
echo '<img src="../'.$str.'" alt="">';
}else{
echo '<img src="../assets/images/user.png" alt="">';
}
?>
<?php
$short_string= $_SESSION['email']; //On va afficher juste les 5 premiers pour regler le pb d'affichage sur mobile
echo substr($short_string, 0, 10)."..";
?>
<span class=" fa fa-angle-down"></span>
<ul class="dropdown-menu dropdown-usermenu animated fadeIn pull-right">
<li><a href=" connected/profil.php"><i class="fa fa-user pull-right"></i>Profil</a>
</li>
<li><a href="../includes/logout.php"><i class="fa fa-sign-out pull-right"></i>Déconnexion</a>
</li>
</ul>
</a>
<?php
//Si pas connecter
}else{
echo "<a href='not-connected/inscription.php' class='user-profile dropdown-toggle'>";
echo "<img src='../assets/images/loadpulseX60.gif' alt=''/>";
echo "<span class=' fa fa-angle-down'></span>";
echo "</a>";
}
?>
</li>
</ul>
</nav>
</div>
</div>
<!-- /top navigation -->
<!-- page content -->
<div class="right_col" role="main">
<?php
if(isset($_GET['idC']) && isset($_GET['idM'])) {
$idChap = $_GET['idC'];
$idMooc = $_GET['idM'];
$numChap = $_GET['numC'];
include '../mooc/mooc'.$idMooc.'/chapitres/chapitre'.$numChap.'.php';
}
else if(isset($_GET['idM'])){
$idMooc = $_GET['idM'];
echo'<h3>Introduction</h3>';
include '../mooc/mooc'.$idMooc.'/chapitres/chapitre0.php';
}
?>
<div class="row">
</div>
</div>
<!-- /page content -->
</div>
</div>
<div id="custom_notifications" class="custom-notifications dsp_none">
<ul class="list-unstyled notifications clearfix" data-tabbed_notifications="notif-group">
</ul>
<div class="clearfix"></div>
<div id="notif-group" class="tabbed_notifications"></div>
</div>
<!-- jQuery -->
<script src="../assets/js/jquery.js"></script>
<script src="../assets/js/jquery.min.js"></script>
<script src="../assets/js/jquery-sortable.js"></script>
<!-- jQuery UI DRAG AND DROP-->
<script src="../assets/js/jquery-ui-1.11.4.custom/jquery-ui.min.js"></script>
<script src="../assets/js/bootstrap.min.js"></script>
<!-- gauge js -->
<script src="../assets/js/gauge/gauge.min.js"></script>
<!-- chart js -->
<script src="../assets/js/chartjs/chart.min.js"></script>
<!-- bootstrap progress js -->
<script src="../assets/js/progressbar/bootstrap-progressbar.min.js"></script>
<script src="../assets/js/nicescroll/jquery.nicescroll.min.js"></script>
<!-- icheck -->
<script src="../assets/js/icheck/icheck.min.js"></script>
<!-- daterangepicker -->
<script src="../assets/js/moment.min.js"></script>
<script src="../assets/js/datepicker/daterangepicker.js"></script>
<script src="../assets/js/custom.js"></script>
<!-- form validation -->
<script src="../assets/js/validator/validator.js"></script>
<!-- Validation -->
<script src="../assets/js/jqueryvalidate/jquery.validate.min.js"></script>
<script src="../assets/js/jqueryvalidate/additional-methods.min.js"></script>
<!-- flot js -->
<!--[if lte IE 8]><script type="text/javascript" src="js/excanvas.min.js"></script><![endif]-->
<script src="../assets/js/flot/jquery.flot.js"></script>
<script src="../assets/js/flot/jquery.flot.pie.js"></script>
<script src="../assets/js/flot/jquery.flot.orderBars.js"></script>
<script src="../assets/js/flot/jquery.flot.time.min.js"></script>
<script src="../assets/js/flot/date.js"></script>
<script src="../assets/js/flot/jquery.flot.spline.js"></script>
<script src="../assets/js/flot/jquery.flot.stack.js"></script>
<script src="../assets/js/flot/curvedLines.js"></script>
<script src="../assets/js/flot/jquery.flot.resize.js"></script>
<!-- skycons -->
<script src="../assets/js/skycons/skycons.js"></script>
<!-- form wizard -->
<script src="../assets/js/wizard/jquery.smartWizard.js"></script>
<!-- Custom JS -->
<script src="../scripts/mooc.js"></script>
<!-- Google Analytics: change UA-XXXXX-X to be your site's ID -->
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','//www.google-analytics.com/analytics.js','ga');
ga('create', 'UA-77911480-1', 'auto');
ga('send', 'pageview');
</script>
</body>
</html><file_sep>/app/mooc/mooc1/chapitres/chapitre4.php
<?php
/*
Chapitre où l'eleve envoie son CV par mail au prof
*/
$idMooc;
$idChap;
if (isset($_GET['idM']) && isset($_GET['idC'])) {
$idMooc = $_GET['idM'];
$idChap = $_GET['idC'];
}else{
$valid = 0;
echo'erreur';
}
?>
<div class="row">
<div class="col-md-6 col-sm-12 col-xs-12 animated slideInUp">
<div class="x_panel">
<div class="x_title">
<h2> Chapitre 4 : Compétences</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
<li><a class="close-link"><i class="fa fa-close"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
<div class="x_content">
<p dir="ltr">
Trop souvent négligées, les compétences sont pourtant fondamentales. Et elles ne se limitent surtout pas aux langues et autres logiciels informatiques.
</p>
<p dir="ltr">
Les compétences permettent de présenter une synthèse de ce que vous savez faire. Elles donnent un point de vue transversal sur votre carrière : vous
insistez sur ce que vous avez fait et appris à faire au cours de vos différents postes et formations. C’est grâce à elles qu’un recruteur peut rapidement
déterminer si votre profil correspond à une fiche de poste.
</p>
<ul>
<li dir="ltr">
<p dir="ltr">
Les compétences doivent être classées par domaine. Cela facilite leur lecture, prouve votre professionnalisme et votre esprit de synthèse.
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Elles doivent bien sûr correspondre aux expériences.
</p>
</li>
<li dir="ltr">
<p dir="ltr">
Il faut se méfier des redites entre les détails des expériences et les compétences : ceux-ci doivent se faire écho, pas se répéter.
</p>
</li>
</ul>
<p>
Une fois tous ces éléments bien en main, vous devez vous les réapproprier. En effet, le CV met en scène votre parcours et vos projets pour convaincre les
recruteurs de vos qualités. Comme dans toute mise en scène, le choix de l’organisation est fondamental. Indiquer discrètement ou au contraire souligner
votre formation, mettre en valeur une expérience plutôt qu’une autre sont autant de choix qui en diront long sur la perception que vous avez de votre
propre parcours.
</p>
</div>
</div>
</div>
<div class="col-md-6 col-sm-12 col-xs-12">
<div class="x_panel">
<div class="x_title">
<h2>Exemple</h2>
<ul class="nav navbar-right panel_toolbox">
<li><a class="collapse-link"><i class="fa fa-chevron-up"></i></a>
</li>
</ul>
<div class="clearfix"></div>
</div>
</div>
</div>
</div>
<file_sep>/db/log.sql
-- phpMyAdmin SQL Dump
-- version 4.1.14
-- http://www.phpmyadmin.net
--
-- Client : 127.0.0.1
-- Généré le : Mar 01 Mars 2016 à 21:52
-- Version du serveur : 5.6.17
-- Version de PHP : 5.5.12
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8 */;
--
-- Base de données : `mooc`
--
-- --------------------------------------------------------
--
-- Structure de la table `log`
--
CREATE TABLE IF NOT EXISTS `log` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`id_user` int(11) DEFAULT NULL,
`connect_time` varchar(50) DEFAULT NULL,
`email` varchar(60) DEFAULT NULL,
`ip` varchar(50) DEFAULT NULL,
`lien` varchar(100) DEFAULT NULL,
`dernierlien` varchar(300) DEFAULT NULL,
`pays` varchar(100) DEFAULT NULL,
`browser` varchar(300) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=24 ;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/app/includes/requestgraph.php
<?php
session_start();
include 'connect.inc.php'; // Connection bdd
if((isset($_POST["data"]))) {
$idMooc = $_POST["data"];
$titreMooc = $bdd->prepare('SELECT numero, titre,id_chapitre FROM chapitre WHERE id_mooc="'.$idMooc.'" ORDER BY numero');
$titreMooc->execute();
$resuTitreMooc = $titreMooc->fetchAll();
$scoreUser = array();
$scoreMax = array();
$pourcentage = array();
//Permet de calculer le score de l'utilisateur courant sur un chapitre sur un mooc
for($i=0;$i<sizeof($resuTitreMooc);$i++){
$scoreMooc = $bdd->query('SELECT sum(score) AS score FROM faire WHERE id_user= "'.$_SESSION["id_user"].'" AND id_exercice IN (SELECT id_exercice FROM mooc INNER JOIN chapitre ON mooc.id_mooc = chapitre.id_mooc INNER JOIN exercice ON "'.$resuTitreMooc[$i]["id_chapitre"].'"=exercice.id_chapitre WHERE mooc.id_mooc = "'.$idMooc.'")');
$donnees1 = $scoreMooc->fetch();
$scoreMooc->closeCursor();
if($donnees1["score"] == NULL){
$scoreUser[$i] = 0;
}
else{
$scoreUser[$i]= (int)$donnees1["score"];
}
}
// Permet de calculer le score maximal possible en fonction des exercices réalisés par l'utilisateur pour ce MOOC
for($i=0;$i<sizeof($resuTitreMooc);$i++){
$scoreMaxMooc = $bdd->query('SELECT sum(valeur_exo) AS total FROM mooc INNER JOIN chapitre ON mooc.id_mooc = chapitre.id_mooc INNER JOIN exercice ON chapitre.id_chapitre=exercice.id_chapitre WHERE mooc.id_mooc = "'.$idMooc.'" AND chapitre.id_chapitre="'.$resuTitreMooc[$i]["id_chapitre"].'" AND id_exercice IN(SELECT id_exercice FROM faire WHERE id_user= "'.$_SESSION["id_user"].'")');
$donnees2 = $scoreMaxMooc->fetch();
$scoreMaxMooc->closeCursor();
if($donnees2["total"] == NULL){
$scoreMax[$i] = 0;
}
else{
$scoreMax[$i]= (int)$donnees2["total"];
}
}
for($i=0;$i<sizeof($resuTitreMooc);$i++){
if($scoreMax[$i]==0){
$pourcentage[$i]=0;
}
else{
$pourcentage[$i]=(int)($scoreUser[$i]/$scoreMax[$i]*100);
}
}
// remplir pourcentage
$all = array();
$all[0] = $resuTitreMooc;
$all[1] = $pourcentage;
echo json_encode($all);
}else{
}
?><file_sep>/app/scripts/connected/catalogue.js
/*!
*
* Google
* Copyright 2015 Google Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License
*
*/
$(document).keydown(function(e) {
//console.log("---------"+e.which);
var retour =e.which;
if(retour==8){
// $(".mdl-cell").css('width', '30%');
$(".mdl-cell").show();
}
});
function filter(){
}
function filter2(){
var chaine = $(".search").val().toLowerCase();
//console.log(chaine);
if(chaine.length == 0){
$(".namesearch").each(function(){
$(this).parents(".mdl-cell").removeClass("hide").addClass("show");
});
}
if (chaine.length > 1){
if(true == true){
//$(".mdl-cell").show();
$(".namesearch").each(function(){
var n = $(this).text().toLowerCase().search(chaine);
if(n != -1){
$(this).parent().parent().show();
}
else{
//$(this).parent().parent().css('width', '10px');
$(this).parent().parent(".mdl-cell").hide();
}
});
}
/* else{
$(".matiere").each(function(){
var n = $(this).text().toLowerCase().search(chaine);
if(window.greeting == 8){
$(this).parents(".mdl-cell").removeClass("hide").addClass("show");
}
else{
$(this).parents(".mdl-cell").removeClass("show").addClass("hide");
console.log($(this).text().toLowerCase());
}
});
}*/
}
}<file_sep>/app/assets/js/cvinteractif.js
var nbClickMax=3;
var nbClick=0;
function compter()
{
nbClick++;
var nbTentativesrestantes = parseInt(document.getElementById("tentative").innerHTML);
nbTentativesrestantes=nbTentativesrestantes-1;
document.getElementById("tentative").innerHTML =nbTentativesrestantes;
if(nbClick>=nbClickMax)
{
alert('Nombre de clic maximum atteint');
// On désactive les clics sur les areas
$( "area" ).each(function() {
$(this).attr("onclick","inutile()");
});
// On désactive les clics sur l'image
$("#fauxcv").attr("onclick","inutile()");
}
}
function afficheTitle(title){
alert(title);
}
function augmenteScore(title){
var score = parseInt(document.getElementById("score").innerHTML);
score = score+20;
document.getElementById("score").innerHTML =score;
$("#"+title).attr("onclick","dejaTrouve()");
}
function dejaTrouve(){
alert("Cette erreur à déjà été trouvée");
}
function inutile(){
}
function diminueScore(){
var score = parseInt(document.getElementById("score").innerHTML);
if(score>=20){
score=score-20;
}
document.getElementById("score").innerHTML =score;
}<file_sep>/app/includes/dragdrop.php
<?php
function exoDragDrop($idMooc,$idChap,$idExo,$bdd,$numeroExo)
{
try{
$selectChap = $bdd->prepare("SELECT * FROM chapitre WHERE id_mooc = $idMooc");
$selectChap->execute();
$lignesChap = $selectChap->fetchAll();
$selectExo = $bdd->prepare("SELECT * FROM exercice WHERE id_chapitre = $idChap");
$selectExo->execute();
$lignesExo = $selectExo->fetchAll();
$i = 0;
$selectqcm = $bdd->prepare("SELECT * FROM dragdrop WHERE id_exercice = $idExo");
$selectqcm->execute();
$lignesQcm = $selectqcm->fetchAll();
for($i = 0; $i < sizeof($lignesQcm) ; $i++)
{
$reponse = $lignesQcm[$i]["reponse_dd"];
echo'<div class="form-group">
<h2 class="StepTitle center">'.$lignesQcm[$i]["texte"].'</h2><br>';
echo' </div><br>';
//}
}
}
catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur afficheChapitreExos()";
}
}
?><file_sep>/app/includes/affichages/menuChap.php
<div class="main_container">
<div class="col-md-3 left_col">
<div class="left_col scroll-view">
<div class="navbar nav_title" style="border: 0;">
<a href="../../index.php" class="site_title"><i class="glyphicon glyphicon-education"></i> <span>MOOCs</span></a>
</div>
<div class="clearfix"></div>
<!-- menu prile quick info -->
<div class="profile">
<div class="profile_pic">
<img src="../../assets/images/user.png" alt="..." class="img-circle profile_img">
</div>
<div class="profile_info">
<span>MOOC,</span>
<h2>Comment faire un CV d'ingénieur</h2>
</div>
</div>
<!-- /menu prile quick info -->
<br />
<!-- sidebar menu -->
<div id="sidebar-menu" class="main_menu_side hidden-print main_menu">
<div class="menu_section">
<h3>Chapitres</h3>
<ul class="nav side-menu">
<?php
$valid = 1;
$idMooc;
if (isset($_GET['idM'])) {
$idMooc = $_GET['idM'];
echo $idMooc;
}else{
$valid = 0;
echo'erreur';
}
chapitresplusSousPartie($idMooc,$bdd);
?>
</div>
<div class="menu_section">
<h3>Menu</h3>
<ul class="nav side-menu">
<li><a><i class="fa fa-question-circle"></i> Autre <span class="fa fa-chevron-down"></span></a>
<ul class="nav child_menu" style="display: none">
<!-- <li><a href="e_commerce.html">E-commerce</a>
</li>
<li><a href="projects.html">Projects</a>
</li>
<li><a href="project_detail.html">Project Detail</a>
</li>
<li><a href="contacts.html">Contacts</a>
</li>
<li><a href="profile.html">Profile</a>
</li>
-->
</ul>
</li>
<li><a><i class="fa fa-wrench"></i> Paramètres <span class="fa fa-chevron-down"></span></a>
<ul class="nav child_menu" style="display: none">
<!-- <li><a href="page_404.html">404 Error</a>
</li>
<li><a href="page_500.html">500 Error</a>
</li>
<li><a href="plain_page.html">Plain Page</a>
</li>
<li><a href="login.html">Login Page</a>
</li>
<li><a href="pricing_tables.html">Pricing Tables</a>
</li>
-->
</ul>
</li>
<li><a><i class="fa fa-envelope"></i> Tchat <span class="label label-success pull-right">Coming Soon</span></a>
</li>
</ul>
</div>
</div>
<!-- /sidebar menu -->
<!-- /menu footer buttons -->
<div class="sidebar-footer hidden-small">
<a data-toggle="tooltip" data-placement="top" title="Settings">
<span class="glyphicon glyphicon-cog" aria-hidden="true"></span>
</a>
<a data-toggle="tooltip" data-placement="top" title="FullScreen">
<span class="glyphicon glyphicon-fullscreen" aria-hidden="true"></span>
</a>
<a data-toggle="tooltip" data-placement="top" title="Lock">
<span class="glyphicon glyphicon-eye-close" aria-hidden="true"></span>
</a>
<a data-toggle="tooltip" data-placement="top" title="Logout">
<span class="glyphicon glyphicon-off" aria-hidden="true"></span>
</a>
</div>
<!-- /menu footer buttons -->
</div>
</div> <file_sep>/app/includes/update_pwd.php
<?php
include 'connect.inc.php'; /// Connection bdd
//ok=1 ko=0
function formValid(){
$verif = 1;
if (isset($_POST['password'])) {
echo '<br>'.$_POST['password'];
}else{
$verif=0;
}
if (isset($_POST['id'])) {
echo '<br>'.$_POST['id'];
}else{
$verif=0;
}
if($verif==0){
return 0;
}else{
return 1;
}
}
//Verifie si l id de reset_password existe
//ok=1 ko=0
function verifId(){
include 'connect.inc.php';
$verif = 1;
$id = $_POST['id'];
try {
$requete_prepare = $bdd->prepare("SELECT * FROM user WHERE reset_password='$id'"); // on prépare notre req
$requete_prepare->execute();
$result = $requete_prepare->fetchAll( PDO::FETCH_ASSOC );
var_dump($result);
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
if($result==NULL){
$verif = 0; //L'id de reset password
}
return $verif;
}
//Met a jour l id pour le reset du mdp
function updateIdResetPwd(){
include 'connect.inc.php';
$valPwd = $_POST['<PASSWORD>'];
$valPwd = md5($valPwd);
$id = $_POST['id'];
try {
$requete_prepare= $bdd->prepare("UPDATE user SET password='$<PASSWORD>' WHERE reset_password='$id'"); // on prépare notre requête
$requete_prepare->execute();
var_dump($requete_prepare);
echo "->OK pwd update";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
function resetId(){
include 'connect.inc.php';
$id = $_POST['id'];
try {
$requete_prepare= $bdd->prepare("UPDATE user SET reset_password='0' WHERE reset_password='$id'"); // on prépare notre requête
$requete_prepare->execute();
var_dump($requete_prepare);
echo "->OK reset_password update";
} catch (Exception $e) {
echo $e->errorMessage();
echo "->erreur";
}
}
$verif = formValid();
if(verifId()==0){
//header ("location: ../index.php?erreur=id n'existe pas (Lien déja utilisé)");
header ("location: ../modules/not-connected/changerMdp.php?erreur=id n'existe pas (Lien déja utilisé)");
//echo "->id n'existe pas (Lien déja utilisé)";
}else if($verif==1){
updateIdResetPwd();
resetId();
header ("location: ../index.php?ok=mdp reinit success");
}
else{
header ("location: ../modules/not-connected/changerMdp.php?erreur=Formulaire erreur");
//header ("location: ../index.php?erreur=formulaire erreur");
}
?> | ddd1297d876af5142ac4f106909f3e2fb7f75e92 | [
"JavaScript",
"SQL",
"PHP"
] | 45 | PHP | fossabot/moocisen | dc60efe38759add021fe61017adb277bb3a74caa | 6f39aeddc4bbeccd357069a757d324150f3f591e | |
refs/heads/master | <file_sep>function WalletService($resource) {
/**
* @name WalletService
*
* @description
* A service providing wallet data.
*/
var that = this;
/**
* A resource for retrieving game data.
*/
that.WalletResource = $resource(_urlPrefixes.API + "viewWallet");
/**
* A convenience method for retrieving a wallet object.
* Retrieval is done via a GET request to the /api/viewWallet endpoint.
* @param {object} params - the query string object used for a GET request to ../games/ endpoint
* @returns {object} WalletResource - wallet-related data
*/
that.viewWallet = function() {
return that.WalletResource;
};
}
angular.module("Wallet")
.service("WalletService", ["$resource", WalletService]);<file_sep>function WalletViewController(WalletService) {
var that = this;
/* Stored wallet objects. */
that.wallet = null;
/**
* Initialize the wallet list controller.
*/
that.init = function() {
that.wallet = WalletService.viewWallet().get();
};
}
angular.module("Wallet")
.controller("WalletViewController", [
"WalletService",
WalletViewController
]);<file_sep>var gulp = require('gulp'),
webserver = require('gulp-webserver'),
del = require('del'),
sass = require('gulp-sass'),
jshint = require('gulp-jshint'),
sourcemaps = require('gulp-sourcemaps'),
browserify = require('browserify'),
source = require('vinyl-source-stream'),
buffer = require('vinyl-buffer'),
uglify = require('gulp-uglify'),
gutil = require('gulp-util'),
ngAnnotate = require('browserify-ngannotate');
var CacheBuster = require('gulp-cachebust');
var cachebust = new CacheBuster();
gulp.task('clean', function (cb) {
del([
'dist'
], cb);
});
gulp.task('bower', function() {
var install = require("gulp-install");
return gulp.src(['./app/bower.json'])
.pipe(install());
});
gulp.task('sass', function(){
return gulp.src('app/styles/app.scss')
.pipe(sass()) // Converts Sass to CSS with gulp-sass
.pipe(gulp.dest('dist/css/'))
});
//gulp.task('build-css', function() {
// return gulp.src('./app/css/app.css')
// .pipe(sourcemaps.init())
//// .pipe(sass())
// .pipe(cachebust.resources())
//// .on('error', gutil.log)
// .pipe(sourcemaps.write('./maps'))
// .pipe(gulp.dest('./dist/css/'));
//});
gulp.task('jshint', function() {
gulp.src(['./app/*.js', './app/components/**/*.js'])
.pipe(jshint())
.pipe(jshint.reporter('default'));
});
gulp.task('build-js', function() {
var b = browserify({
entries: './app/app.js',
debug: true,
paths: ['./app','./app/components/**'],
transform: [ngAnnotate]
});
return b.bundle()
.pipe(source('bundle.js'))
.pipe(buffer())
.pipe(cachebust.resources())
.pipe(sourcemaps.init({loadMaps: true}))
.pipe(uglify())
.on('error', gutil.log)
.pipe(sourcemaps.write('./'))
.pipe(gulp.dest('./dist/js/'));
});
gulp.task('build', [ 'clean', 'sass', 'build-js'], function() {
return gulp.src('./app/index.html')
.pipe(cachebust.references())
.pipe(gulp.dest('./dist'));
});
gulp.task('watch', function() {
return gulp.watch(['./app/index.html','./app/components/**/*.html', './app/styles/*.*css', './app/**/*.js'], ['build']);
});
gulp.task('webserver', ['watch','build'], function() {
gulp.src('.')
.pipe(webserver({
livereload: false,
directoryListing: true,
open: "http://localhost:8001/dist/index.html"
}));
});
gulp.task('default', ['build']);<file_sep>django
djangorestservice
django-angular
google-cloud-datastore
<file_sep>module.exports = {
entry: "./app/app.js",
output: {
path: "/home/tyler/workspace/CryptoWalletService/frontend/dist/js/",
filename: "bundle.js",
sourceMapFilename: "bundle.js.map",
},
watch: true,
module: {
rules: [
{
test: /\.js$/,
enforce: "pre",
exclude: /node_modules/,
loader: "eslint-loader",
options: {
configFile: "./config/eslint.json"
}
},
{ test: /\.css$/, use: [ "style-loader", "css-loader" ]
}],
loaders: [
{ test: /\.html$/, loader: "mustache-loader" },
{ test: /\.json$/, loader: "json-loader" }]
},
resolve: {
extensions: [".js"]
}
};<file_sep>from django.http import HttpResponse
from django.shortcuts import render
from google.cloud import datastore
import json
# Create your views here.\
def view_wallet(request):
# Make a call to Google Cloud Datastore
datastore_client = datastore.Client(project='cryptowalletmanager')
kind = 'WalletHistory'
q = datastore_client.query(kind=kind, order=['-Timestamp'])
r = q.fetch(limit=1)
for data in r:
wallet_entity = data
print(data)
wallet_summary = {}
if wallet_entity is not None:
wallet_summary['USD'] = wallet_entity['TotalUSD']
wallet_summary['BTC'] = wallet_entity['TotalBTC']
# coin_summaries = {}
# exchange_summaries = {}
# exchange_details = wallet_summary['ExchangeDetails']
# for exhange_name in exchange_details.keys():
# e_data = exchange_details[exhange_name]
# e_summary = {'USD': e_data['TotalUSD'], 'BTC': e_data['TotalBTC']}
# exchange_summaries[exhange_name] = e_summary
#
# coin_details = exchange_details
return HttpResponse(json.dumps(wallet_summary))
<file_sep>/* Libs */
require("angular/angular");
require("angular-route/angular-route");
require("angular-resource/angular-resource");
/* Globals */
_ = require("lodash");
_urlPrefixes = {
API: "api/",
TEMPLATES: "static/app/"
};
/* Components */
require("./components/home/home");
require("./components/wallet/wallet");
/* App Dependencies */
angular.module("cws", [
"Home", // this is our component
"Wallet", // also ours
"ngResource",
"ngRoute"
]);
/* Config Vars */
var routesConfig = require("./routes");
/* App Config */
angular.module("cws").config(routesConfig); | 2a511082405c63c82369506170ed236219afeecb | [
"JavaScript",
"Python",
"Text"
] | 7 | JavaScript | techtyler/CryptoWalletService | 600ca1d8c41c517793076c433e1bbce7e922c4ce | 69ab23d4f7333037569b4f37b6c28cbe4e51b4eb | |
refs/heads/master | <file_sep>#!/usr/bin/env python
#<NAME>
#CSE 597C
import sys, os
def read_graph(file_el):
print 'reading graph'
fp = open(file_el, 'r')
n, m = fp.readline().split()
n = int(n)
m = int(m)
print 'Number of vertices: %d' % n
print 'Number of edges: %d' % m
print 'first open for degrees...'
with open('./DOT/'+file_el[:-4]+'.dot', 'w+') as output:
print 'opened new dot:'+'./DOT/'+file_el[:-4]+'.dot'
output.write('strict graph {\n')
for line in fp:
u, v = line.split()
u = int(u)
v = int(v)
if v > u:
output.write("\t%d -- %d\n" % (u, v))
output.write('}')
fp.close()
if __name__ == "__main__":
input_file_el = sys.argv[-1]
print 'making dot file'
read_graph(input_file_el)
<file_sep>#ifndef GRAPH_H
#define GRAPH_H
#include <igraph/igraph.h>
typedef struct {
int n; /* number of vertices */
int m; /* number of edges */
int *adj; /* adj[num_edges[i]..num_edges[i+1]-1] gives
vertices adjacent to vertex i */
int *num_edges; /* num_edges[i+1]-num_edges[i] gives degree of
vertex i */
} graph_t;
int read_graph_file(char *filename, graph_t *g);
int read_to_igraph(char *filename, igraph_t *ig);
int read_vertex_names(char* vertex_ids_filename, char** v_names, int** name_offsets, int n);
#endif
<file_sep>import os, sys, json
import pickle
import numpy as np
import datetime
import Quandl
atoke = open("../../api_key.ig", 'r').read().strip()
def run():
# Read in the symbols
syms = []
with open("../../WIKI_tickers.csv", 'r') as fp:
fp.readline()
for line in fp:
syms.append(line.split(',')[0].strip())
# Request the data for each symbol and store them in a dictionary
for sym in syms:
with open(os.path.join('../../data', sym.split('/')[1]), 'w+') as output:
sym_data = Quandl.get(sym + ".1",returns="numpy",authtoken=atoke)
pickle.dump(sym_data, output)
if __name__ == "__main__":
run()
<file_sep>#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <assert.h>
#include <math.h>
#include "graph.h"
#include "community_metrics.h"
#define SWAP_INTP(a,b) {int * t3mp; t3mp=(a); (a)=(b); (b)=t3mp;}
#define MAX_ITERS 100
// Structure and requisite comparison function //
typedef struct score_t{
int edge;
double score;
} score_t;
int score_cmp(const void* a, const void* b){
score_t* A = (score_t*)a;
score_t* B = (score_t*)b;
return (int)(B->score - A->score);
}
///////////////////////////////////////////////////////////////////////////////
//////////////////////////// BEGIN ADAMIC /////////////////////////////////////
///////////////////////////////////////////////////////////////////////////////
score_t* calc_adamic(graph_t* g){
score_t* edges = calloc(g->n*g->n, sizeof(score_t));
double* t_scores = calloc(g->n, sizeof(double));
int* visited = calloc(g->n, sizeof(int));
int i,j,k,x,y,z,numVisited,n=g->n;
int numE=0;
double t_score=0;
int* has_edge = calloc(n, sizeof(int));
for(x = 0; x < n; x++){
numVisited=0;
for(k = g->num_edges[x]; k < g->num_edges[x+1]; k++){
z=g->adj[k];
t_score = 1.0/log(g->num_edges[z+1]-g->num_edges[z]);
for(j = g->num_edges[z]; j < g->num_edges[z+1]; j++){
y = g->adj[j];
if(y!=x && t_score!=0 && t_scores[y]==0) {
visited[numVisited++]=y;
}
t_scores[y] += t_score;
}
}
for(k = g->num_edges[x]; k < g->num_edges[x+1]; k++){
has_edge[g->adj[k]] = 1;
}
//For each edge visited we now have calculated it's complete score so store
//in the scores array
for(i=0; i<numVisited; i++){
y=visited[i];
if(t_scores[y]==0 || has_edge[y]) continue;
edges[numE++].edge = x*n + y;
edges[numE].score = t_scores[y];
//Clear data
visited[i] = 0;
t_scores[y] = 0;
}
for(k = g->num_edges[x]; k < g->num_edges[x+1]; k++){
has_edge[g->adj[k]] = 0;
}
}
qsort(edges, numE, sizeof(score_t), score_cmp);
free(t_scores);
free(visited);
return edges;
}
///////////////////////////////////////////////////////////////////////////////
///////////////////////////// BEGIN KATZ //////////////////////////////////////
///////////////////////////////////////////////////////////////////////////////
int *list_to_matrix(graph_t *g) {
int n = g->n;
int *Adj = calloc(n*n, sizeof(int));
int i, j;
for (i=0; i < n; i++) {
for (j=g->num_edges[i]; j < g->num_edges[i+1]; j++) {
int u = g->adj[j];
Adj[i*n + u] = 1;
}
}
return Adj;
}
int *matrix_mult(int *A, int *B, int n) {
int i, j, k;
int *Adj = calloc(n*n, sizeof(int));
for (i=0; i < n; i++) {
for (j=0; j < n; j++) {
int sum = 0;
for (k=0; k < n; k++) {
sum = sum + (A[i*n+k]*B[k*n+j]);
}
Adj[i*n+j] = sum;
}
}
return Adj;
}
score_t *katz_it(graph_t *g, double beta) {
int n = g->n;
int i, j, k;
double exp = beta;
int* Adj = list_to_matrix(g);
int* cpy = malloc(n*n*sizeof(int));
int* temp = cpy;
memcpy(cpy, Adj, n*n*sizeof(int));
//initialize scores
score_t *scores = calloc(n*n, sizeof(score_t));
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
scores[i*n + j].edge = i*n + j;
}
}
// count paths of length 1 through 10
for(i = 0; i < 10; i++) {
fprintf(stderr, "Iter: %d\n", i);
for (j = 0; j < n; j++) {
for (k = 0; k < n; k++) {
scores[j*n + k].score += temp[j*n + k] * exp;
}
}
exp = exp * beta;
temp = matrix_mult(Adj, cpy, n);
free(cpy);
cpy = temp;
}
//Filter out edges in existence
for(i = 0; i < n; i++){
scores[i*(n+1)].score = 0;
for(j = g->num_edges[i]; j < g->num_edges[i+1]; j++){
scores[i*n + g->adj[j]].score = 0;
}
}
// sort the scores
qsort(scores, n*n, sizeof(score_t), score_cmp);
free(Adj);
Adj = NULL;
free(temp);
temp = NULL;
cpy = NULL;
return scores;
}
//matrix_mult(graph_t* a, )
int main(int argc, char **argv) {
char *input_graph_filename;
char* vertex_ids_filename;
char* v_names = 0x0;
int* name_offsets = 0x0;
graph_t g;
char* files[4] = {"results/adamic_10.dat", "results/adamic_100.dat", "results/katz_10.dat", "results/katz_100.dat"};
/* parse input args */
if (argc != 4) {
fprintf(stderr, "%s <edge list file> <vertex ids file> <beta>\n",
argv[0]);
exit(1);
}
double beta = atof(argv[3]);
input_graph_filename = argv[1];
vertex_ids_filename = argv[2];
read_graph_file(input_graph_filename, &g);
read_vertex_names(vertex_ids_filename, &v_names, &name_offsets, g.n);
score_t* links = calc_adamic(&g);
////////// Begin print code (ugly don't look) /////////////////
FILE* f;
f = fopen(files[0], "w");
for(int i=0; i<10; i++){
fprintf(f,"Edge: (%s,%s); Score: %f\n",
v_names+name_offsets[links[i].edge/g.n],
v_names+name_offsets[links[i].edge%g.n], links[i].score);
}
fclose(f);
f = fopen(files[1], "w");
for(int i=0; i<100; i++){
fprintf(f,"Edge: (%s,%s); Score: %f\n",
v_names+name_offsets[links[i].edge/g.n],
v_names+name_offsets[links[i].edge%g.n], links[i].score);
}
// Give the probability for edges to not be connected to neighbors
score_t *t;
t = katz_it(&g, beta);
fprintf(stderr, "The top predicted links and their scores:\n");
for (int i = 0; i < 10; i++) {
fprintf(stderr, "Edge: (%d,%d); Score: %f\n",
t[i].edge/g.n, t[i].edge%g.n, t[i].score);
}
f = fopen(files[2], "w");
for(int i=0; i<10; i++){
fprintf(f,"Edge: (%s,%s); Score: %f\n",
v_names+name_offsets[t[i].edge/g.n],
v_names+name_offsets[t[i].edge%g.n], t[i].score);
}
fclose(f);
f = fopen(files[3], "w");
for(int i=0; i<100; i++){
fprintf(f,"Edge: (%s,%s); Score: %f\n",
v_names+name_offsets[t[i].edge/g.n],
v_names+name_offsets[t[i].edge%g.n], t[i].score);
}
fclose(f);
free(v_names);
free(name_offsets);
return 0;
}
<file_sep>#ifndef COMMUNITY_METRICS_H
#define COMMUNITY_METRICS_H
typedef struct {
int size;
int intra;
int inter;
} c_density;
double modularity(const graph_t* g, const int* coms);
double min_intra_density(const graph_t* g, const c_density* densities);
double avg_conductance (const graph_t* g, const c_density* densities);
double coverage(const graph_t* g, const c_density* densities);
//Helper that calculates the number of intra and inter
//cluster edges per community
c_density* calc_edge_densities(const graph_t* g, const int* coms);
#endif
<file_sep>#<NAME>
#CSE 597C
import numpy as np
import matplotlib.pyplot as plt
def plot_deg_dist(x_list):
x = np.array(x_list)
fig = plt.figure()
plt.hist(x)
fig.suptitle('Degree Distribution')
plt.xlabel('Degree (d)')
plt.ylabel('Frequency')
plt.yscale('log', nonposy='clip')
plt.show()
def plot_assort(x_list, avg_list):
x = np.array(x_list)
y = np.array(avg_list)
fig = plt.figure()
plt.plot(x, y, 'o')
fig.suptitle('Assortativity')
plt.xlabel('Degree')
plt.ylabel('Average neighbor degree')
#plt.ylim([0,max(y)])
plt.yscale('log', nonposy='clip')
plt.xscale('log', nonposy='clip')
plt.show()
def plot_cc(cc_list):
prob = []
cc = []
for i in np.arange(0, 1, 0.01):
cc.append(i)
prob.append(float(sum(j < i for j in cc_list))/len(cc_list))
fig = plt.figure()
plt.plot(prob, cc, linewidth=2)
fig.suptitle('Cluster Coefficient')
plt.xlabel('Cluster Coefficient (c)')
plt.ylabel('P(x <= c)')
plt.show()
<file_sep>CC = gcc
EXEC = link_pred
GDB_EXEC = $(EXEC:%=g_%)
PROF_EXEC = $(EXEC:%=p_%)
HDIRS = -Ih -I/opt/local/include
CDIR = c/
CFLAGS = -Wall -std=gnu99 $(HDIRS)
LLIBS = -L/opt/local/lib -ligraph
LIBS = $(CDIR)graph.c $(CDIR)community_metrics.c
RM = -rm -f
all: $(EXEC)
$(EXEC): % : $(CDIR)%.c $(LIBS)
$(CC) -o $@ $(CFLAGS) -O3 $< $(LIBS) $(LLIBS)
$(GDB_EXEC): g_% : $(CDIR)%.c $(LIBS)
$(CC) -o $@ $(CFLAGS) -ggdb3 $< $(LIBS) $(LLIBS)
$(PROF_EXEC): p_% : $(CDIR)%.c $(LIBS)
$(CC) -o $@ $(CFLAGS) -O3 -pg $< $(LIBS) $(LLIBS)
clean:
$(RM) $(EXEC) $(GDB_EXEC) $(PROF_EXEC)
#Print variable (for debugging Makefile)
#PHONY: all
#all: ; $(info $$GDB_EXEC is [${GDB_EXEC}])
<file_sep>Project Team: <NAME> and <NAME>
We implemented Adamic/Adar and Katz algorithm. Performance wise, Adamic/Adar
was far superior to Katz. For this reason, our implementation of Katz was
truncated to 10 iterations, i.e. up to paths of length 10. We got some
interesting results between the two of them. The top 10/100 list for
Adamic/Adar are included as adamic_10.dat and adamic_100.dat. The top 10/100
list of Katz is inclded as katz_10.dat and katz_100.dat. We ran the Katz link
prediction algorithm with a beta value of 0.05. In our test, beta = 0.05,
preformed the most sensibly.
<file_sep>import os, sys
import numpy as np
import pickle
import datetime
import math
# N is the number of days
# start is the first day
# load the symbol data into memory
def load_sym_data(N, start):
data_path = '../data'
syms = {}
files = os.listdir(data_path)
for f in files:
with open(os.path.join(data_path, f), 'r') as fp:
the_file = pickle.load(fp)
found_start = False
failed = False
line = ''
index = 0
while not found_start and not failed:
try:
line = the_file[index]
if line[0] >= start:
found_start = True
index += 1
except IndexError:
failed = True
print the_file[index-1], index-1
if not failed:
syms[f] = []
for i in range(index-1,index+N):
try:
syms[f].append(the_file[i][1])
except IndexError:
syms.pop(f, None)
break
# syms should now hold the correct dates and the associated prices for all the
# stock symbols
for sym in syms:
print sym
print syms[sym]
return syms
# t is an index in the list of prices
# t = 0 represents the starting date
# t = 1 should be the starting point because of t-1
def R(sym, t):
return math.log(syms[sym][t]/syms[sym][t-1])
# magic?
def Cij(sym_i, sym_j, N):
RiRj = sum([R(sym_i, t)*R(sym_j,t) for t in range(1, N)])/N
Ri_Rj = (sum([R(sym_i,t) for t in range(1, N)])/N)*(sum([R(sym_j,t) for t in range(1, N)])/N)
top = RiRj - Ri_Rj
vsRi = math.pow(sum([R(sym_i,t) for t in range(1, N)])/N, 2)
vsRj = math.pow(sum([R(sym_j,t) for t in range(1, N)])/N, 2)
bl = sum([math.pow(R(sym_i,t), 2) - vsRi for t in range(1, N)])/N
br = sum([math.pow(R(sym_j,t), 2) - vsRj for t in range(1, N)])/N
bottom = math.sqrt(bl*br)
return top/bottom
if __name__ == "__main__":
N = 250
print 'N =', N, 'loading to memory'
syms = load_sym_data(N, datetime.datetime(2012, 1, 2, 0, 0))
theta = -0.1
vertID = {}
id = 0
graph_path = '../graphs/'
sample = 'dissim_first_250'
with open(os.path.join(graph_path, sample+'_names.txt'), 'w+') as fp:
for sym in syms:
fp.write('%s\n' % sym)
vertID[sym] = id
id += 1
m = 0
with open(os.path.join(graph_path, sample+'_el.tmp'), 'w+') as fp:
for sym_i in syms:
for sym_j in syms:
if sym_i != sym_j:
#print 'Cij =', Cij(sym_i, sym_j, N), 'for i =', sym_i, 'and j =', sym_j
temp = Cij(sym_i, sym_j, N)
if temp < theta:
print temp
# add an edge
m += 1
fp.write('%d %d\n' % (vertID[sym_i], vertID[sym_j]))
# the graph is now there, but we need the n and m values
n = id
with open(os.path.join(graph_path, sample+'_el.txt'), 'w+') as fp:
fp.write('%s %s\n' % (n, m))
fp2 = open(os.path.join(graph_path, sample+'_el.tmp'), 'r')
for edge in fp2:
fp.write(edge)
os.system('rm '+os.path.join(graph_path, sample+'_el.tmp'))
<file_sep>#!/usr/bin/env python
#<NAME>
#CSE 597C
import sys, os
import my_plot
# design of g
# n; /* number of vertices */
# m; /* number of edges */
# adj; /* adj[num_edges[i]..num_edges[i+1]-1] gives
# vertices adjacent to vertex i */
# num_edges; /* num_edges[i+1]-num_edges[i] gives degree of
# vertex i */
def analyze(g):
n = g['n']
m = g['m']
print 'finding degree distribution and assortitivity'
# find degree distribution
deg = []
avg_deg = []
for i in range(n):
degree_i = g['num_edges'][i+1] - g['num_edges'][i]
deg.append(degree_i)
temp_degs = []
for j in range(g['num_edges'][i], g['num_edges'][i+1]-1):
u = g['adj'][j]
degree_u = g['num_edges'][u+1] - g['num_edges'][u]
temp_degs.append(degree_u)
if len(temp_degs) > 0:
avg_deg.append(sum(temp_degs)/len(temp_degs))
else:
avg_deg.append(0)
my_plot.plot_deg_dist(deg)
my_plot.plot_assort(deg, avg_deg)
print 'finding clustering coefficient distribution'
cluster_co = []
for i in range(n):
k_i = g['num_edges'][i+1] - g['num_edges'][i]
t_g = (k_i*(k_i - 1))/2
num_tris = 0.0
temp_adj = {}
for j in range(g['num_edges'][i], g['num_edges'][i+1]-1):
temp_adj[g['adj'][j]] = True # use dict for const time check in N(i)
for vert in temp_adj:
for k in range(g['num_edges'][vert], g['num_edges'][vert+1]-1):
u = g['adj'][k]
if u in temp_adj:
if temp_adj[u]:
num_tris += 1
temp_adj[vert] = False
if t_g == 0:
cluster_co.append(0)
else:
cc = num_tris/t_g
cluster_co.append(cc)
#print cluster_co
print 'plotting cluster coefficient'
my_plot.plot_cc(cluster_co)
return 0
#essentially the python version of the read_graph function from centralities.c
def read_graph(file_el, file_names):
print 'reading graph'
fp = open(file_el, 'r')
n, m = fp.readline().split()
n = int(n)
m = int(m)
print 'Number of vertices: %d' % n
print 'Number of edges: %d' % m
print 'first open for degrees...'
degrees = []
for i in range(n):
degrees.append(0)
for line in fp:
u, v = line.split()
u = int(u)
v = int(v)
degrees[u] += 1
degrees[v] += 1
fp.close()
num_edges = []
adj = []
num_edges.append(0)
for i in range(1, n+1):
num_edges.append(num_edges[i-1] + degrees[i-1])
print 'second open for adjacency list'
fp = open(file_el, 'r')
fp.readline()
for i in range(n):
degrees[i] = 0
for i in range(2*m):
adj.append(0)
for line in fp:
u = int(line.split()[0])
v = int(line.split()[1])
uv_loc = num_edges[u] + degrees[u]
vu_loc = num_edges[v] + degrees[v]
adj[uv_loc] = v
adj[vu_loc] = u
degrees[u] += 1
degrees[v] += 1
g = {}
g['n'] = n
g['m'] = m
g['num_edges'] = num_edges
g['adj'] = adj
print 'finished reading in graph'
return g
if __name__ == "__main__":
input_file_el = sys.argv[-2]
input_file_names = sys.argv[-1]
g = read_graph(input_file_el, input_file_names)
analyze(g)
<file_sep>#include <stdlib.h>
#include <assert.h>
#include <omp.h>
#include "graph.h"
#include "assortivity_metrics.h"
#define NUM_THREADS 4
/*
int count_triangle_e(graph_t* g){
//For each edge <u,v>
// adj_intersect = Adj(u) \cap Adj(v)
// For each w \in adj_intersect
// output {u,v,w}; count++
// Note each triangle will be outputted/counted 3 times (remove u adj list as loop through maybe? aka <a,b> is valid edge iff a<b (no self loops))
// O(m*d_{max}*n)
return 0;
}
*/
static int edge_intersect_cnt(int* adj1, int len1, int* adj2, int len2){
int v_i1=0, v_i2=0, count=0;
int v_1=adj1[v_i1], v_2 = adj2[v_i2];
while(v_i1<len1 && v_i2<len2){
v_1 = adj1[v_i1];
v_2 = adj2[v_i2];
if(v_1<v_2){ v_i1++;}
else if(v_1==v_2) { count++; v_i1++; v_i2++;}
else { v_i2++; }
}
return count;
}
double* local_cluster_coeffs(graph_t* g){
//For each node u
// For each neighbor v
// neigh_edges+=|Adj(u)\cap Adj(v)|
//O(n*deg_{max}^2)?
double* cluster_coeffs = malloc(g->n*sizeof(double));
#pragma omp parallel num_threads(NUM_THREADS)
{
int deg_v, neigh_edges, u, deg_u, count;
int tId = omp_get_thread_num();
int loopFrom=g->n/NUM_THREADS*tId;
int loopTo=(tId==NUM_THREADS-1) ? g->n : g->n/NUM_THREADS*(tId+1);
for(int v = loopFrom; v<loopTo; v++){
deg_v = DEG(g,v);
neigh_edges=0;
for(int u_i=g->num_edges[v]; u_i<g->num_edges[v+1]; u_i++){
u=g->adj[u_i];
deg_u = DEG(g,u);
count = edge_intersect_cnt(&g->adj[g->num_edges[u]], deg_u,
&g->adj[g->num_edges[v]], deg_v);
neigh_edges+=count;
}
if(deg_v!=0 && deg_v-1!=0)
cluster_coeffs[v]=((double)neigh_edges)/(deg_v*(deg_v-1));
}
}
return cluster_coeffs;
}
#define CNT_IND(v) ((v)*2+1)
#define E_IND(v) ((v)*2)
double calc_assort(graph_t* g, double* avg_neigh_deg){
//Find max degree so we can alloc. an array of that size*2 for e_{ii}'s' and a count of verts with each deg
int max_deg=0;
for(int v=0; v<g->n; v++){
int cur_deg = DEG(g,v);
max_deg = (max_deg<cur_deg) ? cur_deg : max_deg;
}
int* assocs = calloc((max_deg+1)*2, sizeof(int));
//Scan vert's incrementing the appropriate count by it's degree
for(int v=0; v<g->n; v++){
int cur_deg = DEG(g,v);
assocs[CNT_IND(cur_deg)]++;
//Scan the vert's neighbors, incrementing the appropriate e_{ii} if they are of the same degree
for(int n_i=g->num_edges[v]; n_i<g->num_edges[v+1]; n_i++){
int n = g->adj[n_i];
int n_deg = DEG(g,n);
if(cur_deg!=0) {avg_neigh_deg[v]+=((double)n_deg)/cur_deg;}
//Count edges only once
if(v<n){
if(n_deg==cur_deg){
assocs[E_IND(cur_deg)]++;
}
}
}
}
//Calculate degree-based assortativity coefficient
//See Newman, "Mixing patterns in networks" Section II
double e_sum=0;
double a_sum=0;
for(int i=0; i<max_deg; i++){
double a_i=0;
e_sum += assocs[E_IND(i)];
a_i = ((double)assocs[CNT_IND(i)])*i/g->m;
a_sum += a_i*a_i;
}
e_sum/=g->m;
free(assocs);
return (e_sum - a_sum) /(1-a_sum);
}
#undef E_IND
#undef CNT_IND
<file_sep>#include <stdlib.h>
#include <assert.h>
#include "graph.h"
#include "community_metrics.h"
double modularity(const graph_t* g, const int* coms)
{
double q=0;
int* ttl_degs = calloc(g->n, sizeof(int));
// k_i = degree of vertex i, A_{ij} = # of edges between i and j; m is number of edges
// SUM(A_{ij} - k_i * k_j/2m) = SUM(A_{ij}) - SUM(k_i * SUM(k_j/2m)), where i,j are in the same community
// SUM(k_j) = [total degree of community of vertex i] - k_i
// So we store the total degrees of all the communities here => O(n) time
for(int v=0; v<g->n; v++){
ttl_degs[coms[v]] += g->num_edges[v+1]-g->num_edges[v];
}
//Here we calculate k_i * SUM(k_j/2m) for all vertices i and subtracting each to their respective communities
//In effect calculating the -SUM(k_i * SUM(k_j/2m))
//O(n) time
for(int v=0; v<g->n; v++){
int deg = g->num_edges[v+1] - g->num_edges[v];
q -= deg*((ttl_degs[coms[v]]-deg)/(2.0*g->num_edges[g->n]));
}
//Add in 1 for each edge between two vertices in the same community
//O(m)
for(int s=0; s<g->n; s++){
for(int t_i=g->num_edges[s]; t_i<g->num_edges[s+1]; t_i++){
int t=g->adj[t_i];
q += (coms[s]==coms[t]) ? 1 : 0;
}
}
q/=g->num_edges[g->n];
free(ttl_degs);
return q;
}
//Helper that calculates the number of intra and inter
//cluster edges per community
c_density* calc_edge_densities(const graph_t* g, const int* coms){
int n = g->n;
c_density* densities = calloc(n, sizeof(c_density));
for(int i=0; i<n; i++){
densities[coms[i]].size++; //
for(int j=g->num_edges[i]; j<g->num_edges[i+1]; j++){
int vert=g->adj[j];
(coms[i]==coms[vert]) ? densities[coms[i]].intra++ : densities[coms[i]].inter++;
}
}
for(int i=0; i<n; i++){
assert(densities[i].intra%2==0);
densities[i].intra/=2; //Counted each intra cluster edge twice
}
return densities;
}
double min_intra_density(const graph_t* g, const c_density* densities)
{
double min = 1.0/0.0; //INFINITY
double d;
for(int i=0; i<g->n; i++){
int sz = densities[i].size;
if(sz!=0){
d = (sz==1) ? 1 : (((double)densities[i].intra)/sz)/(sz-1);
min = (min<d) ? min : d;
}
}
return min;
}
double avg_conductance (const graph_t* g, const c_density* densities)
{
int num_com=0;
int g_deg = g->n;
double total=0.0;
//TODO: Parallel sum this
for(int i = 0; i<g->n; i++){
int sz=densities[i].size;
if(sz!=0){
int comm_deg = 2*densities[i].intra+densities[i].inter;//community degree
int comp_deg = g_deg - comm_deg; //complement degree
int min_deg = (comm_deg<comp_deg) ? comm_deg : comp_deg;
if(min_deg!=0){
total+=((double)densities[i].inter)/min_deg;
num_com++;
}
}
}
return total/num_com;
}
double coverage(const graph_t* g, const c_density* densities)
{
int num_intra = 0;
int num_edges = g->num_edges[g->n];
//TODO: Parallel sum this
for(int i=0; i<g->n; i++){
num_intra+=densities[i].intra;
}
return ((double)num_intra)/num_edges;
}
| c30e0869d42dd9cb93f61c3c80735c9a073224c9 | [
"Text",
"C",
"Python",
"Makefile"
] | 12 | Python | nlageman/CSE597C_Term_Project | 9684221422b39da3084225586b7975bcaa922c1f | cfba8edab21eaafb3ed538f6b4fbe607c3b1f34f | |
refs/heads/master | <repo_name>komtex/cs50-18<file_sep>/pset3/recover/recover.c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <cs50.h>
int main(int argc, char *argv[])
{
char *infile = argv[1];
if (argc != 2)
{
fprintf(stderr, "usage: ./recover image\n");
return 1;
}
//open memory card
FILE *inptr = fopen(infile, "r");
if (inptr == NULL)
{
fprintf(stderr, "Could not open %s.\n", infile);
return 2;
}
unsigned char buffer[512];
bool openFile = false;
int jpegStart = 0;
char filename [10];
FILE *img_file;
while (fread(&buffer, 512, 1, inptr))
{
if (buffer[0] == 0xff && buffer[1] == 0xd8 && buffer[2] == 0xff && (buffer[3] & 0xf0) == 0xe0)
//yes started jpeg
{
if (openFile)
{
//close prev. opened file
fclose(img_file);
//set to false
openFile = false;
sprintf(filename, "%03d.jpg", jpegStart);
img_file = fopen(filename, "a");
openFile = true;
jpegStart ++;
}
//do not have an open file
if (!openFile)
{
//name a new one
sprintf(filename, "%03d.jpg", jpegStart);
img_file = fopen(filename, "w");
openFile = true;
jpegStart ++;
}
fwrite(&buffer, 512, 1, img_file);
}
else
{
if (openFile)
{
fwrite(&buffer, 512, 1, img_file);
}
if (!openFile)
{
//does nothing
}
}
}
fclose(inptr);
fclose(img_file);
return 0;
}
<file_sep>/pset6/vigenere/vigenere3.py
import cs50
import sys
# Implements a Caesar cipher.
def main():
# Gets key as int; validates
cipher = sys.argv[1]
if not cipher.isalpha or not len(sys.argv) == 2:
exit(1)
# Gets plaintext
print("Plaintext: ", end="")
ptext = cs50.get_string()
print("Ciphertext: ", end="")
# Counter for cipher
n = 0
# Enciphers while preserving case, prints
for c in ptext:
key = get_key(cipher, n)
# Character is uppercase
if c.isupper():
upper = (((ord(c) - ord('A')) + key) % 26) + ord('A')
print(chr(upper), end="")
n += 1
# Character is lowercase
elif c.islower():
lower = (((ord(c) - ord('a')) + key) % 26) + ord('a')
print(chr(lower), end="")
n += 1
elif not c.isalpha():
print("{}".format(c), end="")
# Prints newline
print()
import sys
from cs50 import get_string
def is_valid(k):
for ch in k:
if not ch.isalpha():
return False
return True
def main():
if len(sys.argv) != 2 or not is_valid(sys.argv[1]):
print("Usage: ./vigenere k")
sys.exit(1)
k = sys.argv[1]
plaintext = get_string("plaintext: ")
j = 0
print("ciphertext: ", end="")
for ch in plaintext:
if not ch.isalpha():
print(ch, end="")
continue
ascii_offset = 65 if ch.isupper() else 97
pi = ord(ch) - ascii_offset
kj = ord(k[j % len(k)].upper()) - 65
ci = (pi + kj) % 26
j += 1
print(chr(ci + ascii_offset), end="")
print()
return 0
if __name__ == "__main__":
main()<file_sep>/pset6/caesar/caesar.py
from cs50 import get_string
from sys import argv
import sys
import cs50
def main():
# validate right command line
if len(sys.argv) != 2:
print("Usage: python caesar.py k")
sys.exit(1)
elif sys.argv[1].isdigit == 0:
print(f"Usage: python caesar.py k")
# get key as int, gets plaintext
else:
k = int(sys.argv[1])
s = get_string("plaintext: ")
print("ciphertext: ", end="")
# enciphers plaintext
for i in range(len(s)):
# Character is uppercase
if str.isupper(s[i]):
upper = (((ord(s[i]) - 65) + k) % 26) + 65
print(chr(upper), end="")
# Characters is lowercase
elif str.islower(s[i]):
lower = (((ord(s[i]) - 97) + k) % 26) + 97
print(chr(lower), end="")
else:
print("{}".format(s[i]), end="")
# print new line
print()
# print ciphertext
if __name__ == "__main__":
main()
<file_sep>/pset4/speller/dictionary3.c
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <strings.h>
#include <ctype.h>
#include "dictionary.h"
/**
* Returns true if word is in dictionary else false.
*/
int hashvalue(const char* a);
int word_count=0;
char word[LENGTH+1];
typedef struct node
{
char* word;
struct node* next;
}
node;
//each element in htable will contain an address to a node
node* htable[HT_SIZE];
bool check(const char* word)
{
int hv = hashvalue(word);
node* ptr = malloc(sizeof(node));
ptr = htable[hv]->next;
while(ptr!=NULL)
{
//if ( (int)toupper(ptr->word[1])<(int)toupper(word[1]))
// return false;
if (strcasecmp(ptr->word,word)==0)
{
return true;
}
else
{
ptr = ptr->next;
}
}
return false;
}
/**
* Loads dictionary into memory. Returns true if successful else false.
*/
bool load(const char* dictionary)
{
// Open the given file location
FILE* inptr = fopen(dictionary,"r");
// create a keeper for the word which is a character array of length Large
for (int i = 0; i < HT_SIZE; i++)
{
htable[i] = malloc(sizeof(node));
}
if (inptr == NULL)
{
printf("Could not open %s.\n", dictionary);
return false;
}
while (fscanf(inptr,"%s\n",word) != EOF)
{
node* newword = malloc(sizeof(node));
newword->word = malloc(strlen(word)+1);
strcpy(newword->word,word);
newword->next = NULL;
int hv = hashvalue(word);
word_count++;
if (htable[hv]==NULL)
{
htable[hv]->next = newword;
}
else
{
node* nodenext = malloc(sizeof(node));
nodenext = htable[hv]->next;
htable[hv]->next = newword;
newword->next = nodenext;
}
}
return true;
}
/**
Returns number of words in dictionary if loaded else 0 if not yet loaded.
*/
unsigned int size(void)
{
return word_count;
return 0;
}
/**
* Unloads dictionary from memory. Returns true if successful else false.
*/
bool unload(void)
{
node* pnode;
node* ptr;
for(int i=0;i<HT_SIZE;i++)
{
ptr = htable[i];
while(ptr!=NULL)
{
pnode=ptr;
ptr = ptr->next;
free(pnode);
}
}
free(ptr);
return true;
}
int hashvalue(const char* a)
{
int x = a[0];
int y = a[1];
int hash = 0 ;
if (x > 64 && x < 91 )
{
x = x + 32;
}
if (y > 64 && y < 91 )
{
y =y+32;
}
hash = (x*y)%(HT_SIZE);
return hash;
}
#include <stdbool.h>
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include "dictionary.h"
// size of hashtable
#define SIZE 1000000
// create nodes for linked list
typedef struct node
{
char word[LENGTH+1];
struct node* next;
}
node;
// create hashtable
node* hashtable[SIZE] = {NULL};
// create hash function
int hash (const char* word)
{
int hash = 0;
int n;
for (int i = 0; word[i] != '\0'; i++)
{
// alphabet case
if(isalpha(word[i]))
n = word [i] - 'a' + 1;
// comma case
else
n = 27;
hash = ((hash << 3) + n) % SIZE;
}
return hash;
}
// create global variable to count size
int dictionarySize = 0;
/**
* Loads dictionary into memory. Returns true if successful else false.
*/
bool load(const char* dictionary)
{
// TODO
// opens dictionary
FILE* file = fopen(dictionary, "r");
if (file == NULL)
return false;
// create an array for word to be stored in
char word[LENGTH+1];
// scan through the file, loading each word into the hash table
while (fscanf(file, "%s\n", word)!= EOF)
{
// increment dictionary size
dictionarySize++;
// allocate memory for new word
node* newWord = malloc(sizeof(node));
// put word in the new node
strcpy(newWord->word, word);
// find what index of the array the word should go in
int index = hash(word);
// if hashtable is empty at index, insert
if (hashtable[index] == NULL)
{
hashtable[index] = newWord;
newWord->next = NULL;
}
// if hashtable is not empty at index, append
else
{
newWord->next = hashtable[index];
hashtable[index] = newWord;
}
}
// close file
fclose(file);
// return true if successful
return true;
}
/**
* Returns true if word is in dictionary else false.
*/
bool check(const char* word)
{
// TODO
// creates a temp variable that stores a lower-cased version of the word
char temp[LENGTH + 1];
int len = strlen(word);
for(int i = 0; i < len; i++)
temp[i] = tolower(word[i]);
temp[len] = '\0';
// find what index of the array the word should be in
int index = hash(temp);
// if hashtable is empty at index, return false
if (hashtable[index] == NULL)
{
return false;
}
// create cursor to compare to word
node* cursor = hashtable[index];
// if hashtable is not empty at index, iterate through words and compare
while (cursor != NULL)
{
if (strcmp(temp, cursor->word) == 0)
{
return true;
}
cursor = cursor->next;
}
// if you don't find the word, return false
return false;
}
/**
* Returns number of words in dictionary if loaded else 0 if not yet loaded.
*/
unsigned int size(void)
{
// TODO
// if dictionary is loaded, return number of words
if (dictionarySize > 0)
{
return dictionarySize;
}
// if dictionary hasn't been loaded, return 0
else
return 0;
}
/**
* Unloads dictionary from memory. Returns true if successful else false.
*/
bool unload(void)
{
// TODO
// create a variable to go through index
int index = 0;
// iterate through entire hashtable array
while (index < SIZE)
{
// if hashtable is empty at index, go to next index
if (hashtable[index] == NULL)
{
index++;
}
// if hashtable is not empty, iterate through nodes and start freeing
else
{
while(hashtable[index] != NULL)
{
node* cursor = hashtable[index];
hashtable[index] = cursor->next;
free(cursor);
}
// once hashtable is empty at index, go to next index
index++;
}
}
// return true if successful
return true;
}
//hash function
int hash = 0;
int n;
for (int i = 0; word[i] != '\0'; i++)
{
// alphabet case
if(isalpha(word[i]))
n = word [i] - 'a' + 1;
// comma case
else
n = 27;
hash = ((hash << 3) + n) % N;
}
return hash;<file_sep>/README.md
CS50 is an outstanding resource which makes the fundementals of computer science available to everyone, for free.
Hats off to Harvard, EDX and everyone involved in putting it all together.
This repository is where I'm storing my personal progress on the course,
which I'm taking in 2019. In the spirit of academic honesty,
I would encourage you to not look if you're also taking the course or looking to enroll.
<file_sep>/pset6/caesar/caesar2.py
import cs50
import sys
# Implements a Caesar cipher.
def main():
# Gets key as int; validates
while True:
key = int(sys.argv[1])
if key > 0:
break
# Gets plaintext
print("Plaintext: ", end="")
ptext = cs50.get_string()
print("Ciphertext: ", end="")
# Enciphers while preserving case
for i in range(len(ptext)):
# Character is uppercase
if str.isupper(ptext[i]):
upper = (((ord(ptext[i]) - 65) + key) % 26) + 65
print(chr(upper), end="")
# Character is lowercase
elif str.islower(ptext[i]):
lower = (((ord(ptext[i]) - 97 ) + key ) % 26 ) + 97
print(chr(lower), end="")
else:
print("{}".format(ptext[i]), end="")
# Prints newline
print()
# print ciphertext
if __name__ == "__main__":
main()
<file_sep>/pset6/mario/less/mario.py
from cs50 import get_int
while True:
n = get_int("height:")
if n > 0 and n <= 8:
break
for i in range(n):
for j in range(i, n-1):
print(" ", end="")
for k in range(n, i+n+1):
print("#", end="")
print()
<file_sep>/pset6/vigenere/vigenere.py
from cs50 import get_string
from sys import argv
import sys
import cs50
def main():
# validate right command line
if len(sys.argv) != 2 or sys.argv[1].isalpha() == 0:
print("Usage: python vigenere.py k")
sys.exit(1)
# get key as int, gets plaintext
else:
key = sys.argv[1]
s = get_string("plaintext: ")
# counter for cipher
j = 0
print("ciphertext: ", end="")
# Enciphers casa, print
for i in s:
# character is uppercase
if i.isupper():
upper = (((ord(i) - 65) + (ord(key[j % len(key)].upper()) - 65)) % 26) + 65
j += 1
print(chr(upper), end="")
# character is lowercase
elif i.islower():
lower = (((ord(i) - 97) + (ord(key[j % len(key)].upper()) - 65)) % 26) + 97
j += 1
print(chr(lower), end="")
elif not i.isalpha():
print("{}".format(i), end="")
# print new line
print()
if __name__ == "__main__":
main()
<file_sep>/pset6/bleep/bleep.py
from cs50 import get_string
from sys import argv
import sys
def main():
#check command-line argument
if len(sys.argv) != 2:
print("Usage: python bleep.py dictionary")
sys.exit(1)
else:
#load txt file
load(argv[1])
#prompt user input
user_in = get_string("What message would you like to censor?")
def load(banned):
file = open("banned.txt","r")
words = file.read()
print(words)
file.close()
if __name__ == "__main__":
main()
<file_sep>/pset6/cash/cash.py
from cs50 import get_float
x = 0
while True:
n = get_float("Change owed:")
if n > 0:
break
coins = (round(n * 100))
while coins >= 25:
coins = coins - 25
x += 1
while coins >= 10:
coins = coins - 10
x += 1
while coins >= 5:
coins = coins - 5
x += 1
while coins >= 1:
coins = coins - 1
x += 1
print(x)<file_sep>/pset6/credit/credit.py
from cs50 import get_int
from math import floor
from cs50 import get_string
def main():
digit1 = 0
digit2 = 0
num_digits = 0
double_odds = 0
evens = 0
numbers = get_int("Number: ")
while numbers > 0:
# first and second digits at the beginning are equals
digit2 = digit1
digit1 = numbers % 10
# multiply each second digit and sum odd and even
if num_digits % 2 == 0:
evens += digit1
else:
m = 2 * digit1
double_odds += (m // 10) + (m % 10)
# loop till the end of digits
numbers //= 10
num_digits += 1
# test for validity by dividing to 10
is_valid = (evens + double_odds) % 10 == 0
first_two_digits = (digit1 * 10) + digit2
if digit1 == 4 and num_digits >= 13 and num_digits <= 16 and is_valid:
print("VISA\n")
elif first_two_digits >= 51 and first_two_digits <= 55 and num_digits == 16 and is_valid:
print("MASTERCARD\n")
elif (first_two_digits == 34 or first_two_digits == 37) and num_digits == 15 and is_valid:
print("AMEX\n")
else:
print("INVALID\n")
if __name__ == "__main__":
main()<file_sep>/pset7/survey/application.py
import cs50
import csv
import os
from flask import Flask, jsonify, redirect, render_template, request
# Configure application
app = Flask(__name__)
# Reload templates when they are changed
app.config["TEMPLATES_AUTO_RELOAD"] = True
@app.after_request
def after_request(response):
"""Disable caching"""
response.headers["Cache-Control"] = "no-cache, no-store, must-revalidate"
response.headers["Expires"] = 0
response.headers["Pragma"] = "no-cache"
return response
@app.route("/", methods=["GET"])
def get_index():
return redirect("/form")
@app.route("/form", methods=["GET"])
def get_form():
return render_template("form.html")
@app.route("/form", methods=["POST"])
def post_form():
if not request.form.get("name") or not request.form.get("group") or not request.form.get("phone"):
return render_template("error.html", message = "You must fill input field")
with open("survey.csv", "a") as file:
writer = csv.DictWriter(file, fieldnames = ["name", "phone", "group", "k1", "k2"])
writer.writerow({"name": request.form.get("name"), "phone": request.form.get("phone"), "group": request.form.get("group"), "k1": request.form.get("k1"), "k2": request.form.get("k2")})
return redirect("/sheet")
@app.route("/sheet", methods=["GET"])
def get_sheet():
with open('survey.csv', 'r') as file:
reader = csv.reader(file)
table_rows = list(reader)
return render_template("sheet.html", table_rows=table_rows)
<file_sep>/pset6/bleep/bleep1.py
from cs50 import get_string
from sys import argv
import sys
def main():
words = 0
#check command-line argument
if len(sys.argv) != 2:
print("Usage: python bleep.py dictionary")
sys.exit(1)
else:
#load txt file
load(argv[1])
#prompt user input
user_in = get_string("What message would you like to censor?")
#tokenize input
tokens = user_in.split()
#check with txt
for i in tokens:
if check(i):
for j in i:
print("*", end="")
else:
print(i, end="")
print(" ", end="")
return True
def load(banned.txt):
#load word in txt file
file = open(banned.txt, "r")
for line in file:
words.add(line.rstrip("\n"))
file.close()
return True
def check(word):
#Return true if word is in dictionary else false
return word.lower() in words
if __name__ == "__main__":
main()
| bbb7e83d171c2de403d9c01f8a96df4a1f804e18 | [
"Markdown",
"C",
"Python"
] | 13 | C | komtex/cs50-18 | 27c1e20e157e4ec32925878a5cafa1717ba06c20 | 5e535b9147e4c247c306a3c56c93e6c7793f2fac | |
refs/heads/master | <file_sep><?php
include "inclui.inc";
$name = $_POST['name'];
$email = $_POST['email'];
$message = $_POST['message'];
$human = intval($_POST['human'])
$sql = "INSERT INTO form VALUES";
$sql = "("$name","$email","$message","$human")";
$resultado = mysql_query ($sql);
echo "Mensagem enviada com sucesso";
mysql_close($conexao);
?>
<file_sep><?php
$conexao = mysql connect ("name","email","message","human");
mysql_select_db(bancodedados);
?>
| 66e325c2dfd88ebd6548d05e404619ee04d7f33f | [
"PHP"
] | 2 | PHP | MN4R4/testeonline2016 | ab21994798e2d9d38e1e5caccc4afd70fd7ac93f | a47a81a0ca5345273a8287ec134e1021cd74efbc | |
refs/heads/master | <repo_name>daq-db/eRPC<file_sep>/third_party/masstree-beta/README.md
From https://github.com/anujkaliaiitd/masstree-beta.
Do not use bootstrap.sh. Use cmake.
<file_sep>/src/rpc_impl/rpc_reset_handlers.cc
/**
* @file rpc_reset_handlers.cc
* @brief Handlers for session resets
*/
#include "rpc.h"
namespace erpc {
template <class TTr>
bool Rpc<TTr>::handle_reset_client_st(Session *session) {
assert(in_dispatch());
char issue_msg[kMaxIssueMsgLen];
sprintf(issue_msg,
"Rpc %u, lsn %u: Trying to reset client session. State = %s. Issue",
rpc_id, session->local_session_num,
session_state_str(session->state).c_str());
// Erase session slots from credit stall queue
for (const SSlot &sslot : session->sslot_arr) {
stallq.erase(std::remove(stallq.begin(), stallq.end(), &sslot),
stallq.end());
}
// Invoke continuation-with-failure for all active requests
for (SSlot &sslot : session->sslot_arr) {
if (sslot.tx_msgbuf == nullptr) {
sslot.tx_msgbuf = nullptr;
delete_from_active_rpc_list(sslot);
session->client_info.sslot_free_vec.push_back(sslot.index);
MsgBuffer *resp_msgbuf = sslot.client_info.resp_msgbuf;
resize_msg_buffer(resp_msgbuf, 0); // 0 response size marks the error
sslot.client_info.cont_func(context, sslot.client_info.tag);
}
}
assert(session->client_info.sslot_free_vec.size() == kSessionReqWindow);
// Change state before failure continuations
session->state = SessionState::kDisconnectInProgress;
// Act similar to handling a disconnect response
LOG_INFO("%s: None. Session resetted.\n", issue_msg);
free_ring_entries(); // Free before callback to allow creating new session
sm_handler(session->local_session_num, SmEventType::kDisconnected,
SmErrType::kSrvDisconnected, context);
bury_session_st(session);
return true;
}
template <class TTr>
bool Rpc<TTr>::handle_reset_server_st(Session *session) {
assert(in_dispatch());
assert(session->is_server());
session->state = SessionState::kResetInProgress;
char issue_msg[kMaxIssueMsgLen];
sprintf(issue_msg,
"Rpc %u, lsn %u: Trying to reset server session. State = %s. Issue",
rpc_id, session->local_session_num,
session_state_str(session->state).c_str());
size_t pending_enqueue_resps = 0;
for (const SSlot &sslot : session->sslot_arr) {
if (sslot.server_info.req_type != kInvalidReqType) pending_enqueue_resps++;
}
if (pending_enqueue_resps == 0) {
// Act similar to handling a disconnect request, but don't send SM response
LOG_INFO("%s: None. Session resetted.\n", issue_msg);
free_ring_entries();
bury_session_st(session);
return true;
} else {
LOG_WARN(
"Rpc %u, lsn %u: Cannot reset server session. "
"%zu enqueue_response pending.\n",
rpc_id, session->local_session_num, pending_enqueue_resps);
return false;
}
}
FORCE_COMPILE_TRANSPORTS
} // namespace erpc
<file_sep>/tests/client_tests/multi_process_test.cc
#include "client_tests.h"
std::atomic<size_t> num_processes_ready;
void req_handler(erpc::ReqHandle *req_handle, void *_c) {
auto *c = reinterpret_cast<BasicAppContext *>(_c);
auto &resp = req_handle->pre_resp_msgbuf;
c->rpc->resize_msg_buffer(&resp, sizeof(size_t));
c->rpc->enqueue_response(req_handle, &req_handle->pre_resp_msgbuf);
}
void cont_func(void *_c, void *) {
auto *c = static_cast<BasicAppContext *>(_c);
c->num_rpc_resps++;
}
// This threads acts as a process with a Nexus
void process_proxy_thread_func(size_t process_id, size_t num_processes) {
auto uri = "localhost:" + std::to_string(kBaseSmUdpPort + process_id);
Nexus nexus(uri, 0, 0);
nexus.register_req_func(kTestReqType, req_handler);
BasicAppContext c;
Rpc<CTransport> rpc(&nexus, &c, 0, basic_sm_handler);
c.rpc = &rpc;
// Barrier
num_processes_ready++;
while (num_processes_ready != num_processes) {
// Wait for Rpc objects to be registered with their Nexus
}
c.session_num_arr = new int[num_processes];
c.req_msgbufs.resize(num_processes);
c.resp_msgbufs.resize(num_processes);
for (size_t i = 0; i < num_processes; i++) {
if (i == process_id) continue;
auto remote_uri = "localhost:" + std::to_string(kBaseSmUdpPort + i);
c.session_num_arr[i] = c.rpc->create_session(remote_uri, 0);
c.req_msgbufs[i] = c.rpc->alloc_msg_buffer_or_die(sizeof(size_t));
c.resp_msgbufs[i] = c.rpc->alloc_msg_buffer_or_die(sizeof(size_t));
}
wait_for_sm_resps_or_timeout(c, num_processes - 1);
assert(c.num_sm_resps == num_processes - 1);
printf("Process %zu: All sessions connected\n", process_id);
for (size_t i = 0; i < num_processes; i++) {
if (i == process_id) continue;
c.rpc->enqueue_request(c.session_num_arr[i], kTestReqType,
&c.req_msgbufs[i], &c.resp_msgbufs[i], cont_func,
nullptr);
}
wait_for_rpc_resps_or_timeout(c, num_processes - 1);
assert(c.num_rpc_resps == num_processes - 1);
}
TEST(MultiProcessTest, TwoProcesses) {
num_processes_ready = 0;
std::thread process_proxy_thread_thread_1(process_proxy_thread_func, 0, 2);
std::thread process_proxy_thread_thread_2(process_proxy_thread_func, 1, 2);
process_proxy_thread_thread_1.join();
process_proxy_thread_thread_2.join();
}
TEST(MultiProcessTest, MaxProcesses) {
num_processes_ready = 0;
const size_t num_processes = kMaxNumERpcProcesses;
std::vector<std::thread> process_proxy_thread_vec(num_processes);
for (size_t i = 0; i < num_processes; i++) {
process_proxy_thread_vec[i] =
std::thread(process_proxy_thread_func, i, num_processes);
}
for (size_t i = 0; i < num_processes; i++) {
process_proxy_thread_vec[i].join();
}
}
int main(int argc, char **argv) {
testing::InitGoogleTest(&argc, argv);
return RUN_ALL_TESTS();
}
<file_sep>/src/heartbeat_mgr.h
#pragma once
#include <queue>
#include <sstream>
#include <unordered_map>
#include "common.h"
#include "rpc_constants.h"
#include "sm_types.h"
#include "util/autorun_helpers.h"
#include "util/timer.h"
#include "util/udp_client.h"
namespace erpc {
/**
* @brief A thread-safe heartbeat manager
*
* This class has two main tasks: First, it sends heartbeat messages to remote
* processes at fixed intervals. Second, it checks for timeouts, also at fixed
* intervals. These tasks are scheduled using a time-based priority queue.
*
* For efficiency, if a process has multiple sessions to a remote process,
* only one instance of the remote URI is tracked.
*
* This heartbeat manager is designed to keep the CPU use of eRPC's management
* thread close to zero in the steady state. An earlier version of eRPC's
* timeout detection used a reliable UDP library called ENet, which had
* non-negligible CPU use.
*/
class HeartbeatMgr {
private:
static constexpr bool kVerbose = true;
enum class EventType : bool {
kSend, // Send a heartbeat to the remote URI
kCheck // Check that we have received a heartbeat from the remote URI
};
/// Heartbeat info in the priority queue
class Event {
public:
EventType type;
std::string rem_uri; // The remote process for receiving/sending heartbeats
uint64_t tsc; // The time at which this event is triggered
Event(EventType type, const std::string &rem_uri, uint64_t tsc)
: type(type), rem_uri(rem_uri), tsc(tsc) {}
};
struct EventComparator {
bool operator()(const Event &p1, const Event &p2) {
return p1.tsc > p2.tsc;
}
};
public:
HeartbeatMgr(std::string hostname, uint16_t sm_udp_port, double freq_ghz,
size_t machine_failure_timeout_ms)
: hostname(hostname),
sm_udp_port(sm_udp_port),
freq_ghz(freq_ghz),
creation_tsc(rdtsc()),
failure_timeout_tsc(ms_to_cycles(machine_failure_timeout_ms, freq_ghz)),
hb_send_delta_tsc(failure_timeout_tsc / 10),
hb_check_delta_tsc(failure_timeout_tsc / 2) {}
/// Add a remote URI to the tracking set
void unlocked_add_remote(const std::string &remote_uri) {
std::lock_guard<std::mutex> lock(heartbeat_mutex);
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Starting tracking URI %s\n",
us_since_creation(rdtsc()), remote_uri.c_str());
}
size_t cur_tsc = rdtsc();
map_last_hb_rx.emplace(remote_uri, cur_tsc);
schedule_hb_send(remote_uri);
schedule_hb_check(remote_uri);
}
/// Receive a heartbeat
void unlocked_receive_hb(const SmPkt &sm_pkt) {
std::lock_guard<std::mutex> lock(heartbeat_mutex);
if (map_last_hb_rx.count(sm_pkt.client.uri()) == 0) {
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Ignoring heartbeat from URI %s\n",
us_since_creation(rdtsc()), sm_pkt.client.uri().c_str());
}
return;
}
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Receiving heartbeat from URI %s\n",
us_since_creation(rdtsc()), sm_pkt.client.uri().c_str());
}
map_last_hb_rx.emplace(sm_pkt.client.uri(), rdtsc());
}
/**
* @brief The main heartbeat work: Send heartbeats, and check expired timers
*
* @param failed_uris The list of failed remote URIs to fill-in
*/
void do_one(std::vector<std::string> &failed_uris) {
while (true) {
if (hb_event_pqueue.empty()) break;
const auto next_ev = hb_event_pqueue.top(); // Copy-out
// Stop processing the event queue when the top event is in the future
if (in_future(next_ev.tsc)) {
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Event %s is in the future\n",
us_since_creation(rdtsc()), ev_to_string(next_ev).c_str());
}
break;
}
if (map_last_hb_rx.count(next_ev.rem_uri) == 0) {
if (kVerbose) {
printf(
"heartbeat_mgr (%.0f us): Remote URI for event %s is not "
"in tracking set. Ignoring.\n",
us_since_creation(rdtsc()), ev_to_string(next_ev).c_str());
}
break;
}
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Handling event %s\n",
us_since_creation(rdtsc()), ev_to_string(next_ev).c_str());
}
hb_event_pqueue.pop(); // Consume the event
switch (next_ev.type) {
case EventType::kSend: {
SmPkt heartbeat =
make_heartbeat(hostname, sm_udp_port, next_ev.rem_uri);
hb_udp_client.send(heartbeat.server.hostname,
heartbeat.server.sm_udp_port, heartbeat);
schedule_hb_send(next_ev.rem_uri);
break;
}
case EventType::kCheck: {
size_t last_ping_rx = map_last_hb_rx[next_ev.rem_uri];
if (rdtsc() - last_ping_rx > failure_timeout_tsc) {
failed_uris.push_back(next_ev.rem_uri);
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Remote URI %s failed\n",
us_since_creation(rdtsc()), next_ev.rem_uri.c_str());
}
map_last_hb_rx.erase(map_last_hb_rx.find(next_ev.rem_uri));
} else {
schedule_hb_check(next_ev.rem_uri.c_str());
}
break;
}
}
}
}
private:
// Create a heartbeat packet send by the local URI to the remote URI.
//
// A heartbeat packet is a session management packet where most fields are
// invalid. The local sender acts as the SmPkt's client.
static SmPkt make_heartbeat(const std::string &local_hostname,
uint16_t local_sm_udp_port,
const std::string &remote_uri) {
SmPkt sm_hb;
sm_hb.pkt_type = SmPktType::kPingReq;
sm_hb.err_type = SmErrType::kNoError;
sm_hb.uniq_token = 0;
std::string remote_hostname;
uint16_t remote_sm_udp_port;
split_uri(remote_uri, remote_hostname, remote_sm_udp_port);
// In sm_hb's session endpoints, the transport type, Rpc ID, and session
// number are already invalid.
strcpy(sm_hb.client.hostname, local_hostname.c_str());
sm_hb.client.sm_udp_port = local_sm_udp_port;
strcpy(sm_hb.server.hostname, remote_hostname.c_str());
sm_hb.server.sm_udp_port = remote_sm_udp_port;
return sm_hb;
}
/// Return the microseconds between tsc and this manager's creation time
double us_since_creation(size_t tsc) const {
return to_usec(tsc - creation_tsc, freq_ghz);
}
/// Return a string representation of a ping event. This is outside the
/// Event class because we need creation_tsc.
std::string ev_to_string(const Event &e) const {
std::ostringstream ret;
ret << "[Type: " << (e.type == EventType::kSend ? "send" : "check")
<< ", URI " << e.rem_uri << ", time "
<< static_cast<size_t>(us_since_creation(e.tsc)) << " us]";
return ret.str();
}
/// Return true iff a timestamp is in the future
static bool in_future(size_t tsc) { return tsc > rdtsc(); }
void schedule_hb_send(const std::string &rem_uri) {
Event e(EventType::kSend, rem_uri, rdtsc() + hb_send_delta_tsc);
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Scheduling event %s\n",
us_since_creation(rdtsc()), ev_to_string(e).c_str());
}
hb_event_pqueue.push(e);
}
void schedule_hb_check(const std::string &rem_uri) {
Event e(EventType::kCheck, rem_uri, rdtsc() + hb_check_delta_tsc);
if (kVerbose) {
printf("heartbeat_mgr (%.0f us): Scheduling event %s\n",
us_since_creation(rdtsc()), ev_to_string(e).c_str());
}
hb_event_pqueue.push(e);
}
const std::string hostname; /// This process's local hostname
const uint16_t sm_udp_port; /// This process's management UDP port
const double freq_ghz; /// TSC frequency
const size_t creation_tsc; /// Time at which this manager was created
const size_t failure_timeout_tsc; /// Machine failure timeout in TSC cycles
/// We send heartbeats every every hb_send_delta_tsc cycles. This duration is
/// around a tenth of the failure timeout.
const size_t hb_send_delta_tsc;
/// We check heartbeats every every hb_check_delta_tsc cycles. This duration
/// is around half of the failure timeout #nyquist.
const size_t hb_check_delta_tsc;
std::priority_queue<Event, std::vector<Event>, EventComparator>
hb_event_pqueue;
// This map servers two purposes:
//
// 1. Its value for a remote URI is the timestamp when we last received a
// heartbeat from the remote URI.
// 2. The set of remote URIs in the map is our remote tracking set. Since we
// cannot delete efficiently from the event priority queue, events for
// remote URIs not in this map are ignored when they are dequeued.
std::unordered_map<std::string, uint64_t> map_last_hb_rx;
UDPClient<SmPkt> hb_udp_client;
std::mutex heartbeat_mutex; // Protects this heartbeat manager
};
}
| 82b042577a6db26b94a6c588e0c9358ac6bae02e | [
"Markdown",
"C++"
] | 4 | Markdown | daq-db/eRPC | 35270933836410d6779f9f2d4e1d8aaa1291a5d8 | 682631e7dc4d74a8b940d17c7c19b628dc74da54 | |
refs/heads/master | <repo_name>FerBayley/placoss<file_sep>/header.php
<!DOCTYPE html>
<!--[if lt IE 7 ]> <html class="ie ie6 no-js" lang="en"> <![endif]-->
<!--[if IE 7 ]> <html class="ie ie7 no-js" lang="en"> <![endif]-->
<!--[if IE 8 ]> <html class="ie ie8 no-js" lang="en"> <![endif]-->
<!--[if IE 9 ]> <html class="ie ie9 no-js" lang="en"> <![endif]-->
<!--[if gt IE 9]><!--><html class="no-js" lang="es"><!--<![endif]-->
<html lang="es">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title><?php bloginfo('name');?><?php wp_title();?></title>
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="description" content="Placoss muebles a medida, calida y diseño" />
<meta name="keywords" content="muebles, muebles a medida, muebles modernos" />
<!-- CSS -->
<link href="<?php bloginfo('template_directory')?>/style.css" rel="stylesheet" type="text/css" media="screen" />
<!-- CSS -->
<!--[if lt IE 9]>
<script src="dist/html5shiv.js"></script>
<![endif]-->
<!-- FAVICON -->
<link rel="shortcut icon" href="../favicon.ico">
<!-- FAVICON -->
<!-- JS -->
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.1/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.easing.min.js"></script>
<script type="text/javascript" src="js/supersized.3.2.7.min.js"></script>
<script type="text/javascript" src="js/modernizr.custom.18389.js"></script>
<!-- JS -->
<?php wp_head(); ?>
<?php wp_footer(); ?>
</head>
<body>
<section id="wrapper">
<!-- HEADER -->
<header>
<!-- CAJA -->
<div class="caja">
<nav>
<ul>
<li>
<a href="">INICIO</a>
</li>
<li>
<a href="">CONTACTENOS</a>
</li>
<a href="">
<div class="face"></div> <!-- End of face -->
</a>
</ul>
</nav>
</div> <!-- End caja -->
<!-- CAJA -->
<div class="clearfix"></div>
</header>
<!-- HEADER --><file_sep>/footer.php
<!-- FOOTER -->
<footer>
<ul>
<?php wp_list_pages('title_li=');?>
</ul>
<a href="">
<img src="<?php bloginfo('template_directory')?>/img/logo.jpg" alt="Placoss Muebles a medida" />
</a>
</footer>
<!-- FOOTER -->
<div class="clearfix"></div>
</section> <!-- End of wrapper -->
</body>
</html><file_sep>/index.php
<?php get_header(); ?>
<!-- CONTENIDO -->
<section class="contenido">
<a href="">
<div class="cuadro">
<?php if (have_posts()) : ?>
<?php while (have_posts()) : the_post(); ?>
<div class="item entry" id="post-<?php the_ID(); ?>">
<div class="itemhead">
<h3><a href="<?php the_permalink() ?>" rel="bookmark"><?php the_title(); ?></a></h3>
</div>
<p><?php the_content('Read more »'); ?></p>
</div>
<?php endwhile; ?>
<?php else : ?>
<?php endif; ?>
</div> <!-- End of cuador -->
</a>
</section>
<div class="clearfix"></div>
<!-- CONTENIDO -->
<?php get_footer(); ?> | 6808f248886759561b3a937f7aafd4dd09408e0e | [
"PHP"
] | 3 | PHP | FerBayley/placoss | 5a2ffe77c47d05784a22ae092702e3f3b9b2ebfc | 986d26b3ab73c7c560899c233a3eeb95feda3dcf | |
refs/heads/master | <file_sep><?php
/**
* @copyright JDuo responsive template © 2014 adhocgraFX / <NAME> - All Rights Reserved.
* @license GNU/GPL
**/
/**
* joomskeleton, joomfluid, joomflex, jduo, pasodoble, carcass module chrome
**/
// No direct access.
defined('_JEXEC') or die;
$module = $displayData['module'];
$params = $displayData['params'];
$attribs = $displayData['attribs'];
$modulePos = $module->position;
$moduleTag = $params->get('module_tag', 'div');
$headerTag = htmlspecialchars($params->get('header_tag', 'h4'));
$headerClass = htmlspecialchars($params->get('header_class', 'module-title'));
?>
<?php if ($module->content) : ?>
<<?php echo $moduleTag; ?> class="<?php echo $modulePos; ?> module <?php echo htmlspecialchars($params->get('moduleclass_sfx')); ?>">
<?php if ($module->showtitle && $headerClass !== 'module-title') : ?>
<div class="<?php echo $headerClass; ?>">
<<?php echo $headerTag; ?> class="title"><?php echo $module->title; ?></<?php echo $headerTag; ?>>
</div>
<?php endif; ?>
<?php if ($module->showtitle && $headerClass === 'module-title') : ?>
<div class="<?php echo $headerClass; ?>">
<<?php echo $headerTag; ?> class="title"><?php echo $module->title; ?></<?php echo $headerTag; ?>>
</div>
<?php endif; ?>
<div class="module-body">
<?php echo $module->content; ?>
</div>
</<?php echo $moduleTag; ?>>
<?php endif; ?>
<file_sep><?php
// get template params
// analytics
$analytics = $this->params->get('analytics');
// cookies
$c_accept = $this->params->get('acceptcookie');
$c_infotext = $this->params->get('cookieinfotext');
$c_buttontext = $this->params->get('cookiebuttontext');
$c_linktext = $this->params->get('cookielinktext');
$c_link = $this->params->get('cookielink');
// slideshow slider
$slider = $this->params->get('slideshow');
// code display
$prism = $this->params->get('js-prism');
?>
<!-- cookie info -->
<?php if ($c_accept == 1) : ?>
<?php if(isset($_POST['set_cookie'])):
if($_POST['set_cookie']==1)
setcookie("acceptcookie", "yes", time()+3600*24*365, "/");
?>
<?php endif; ?>
<div id="accept-cookie-container" class="box clouds" style="display:none;position:fixed;bottom:-1em;font-size:.75em;width:100%;text-align:center;z-index:99999">
<p><?php echo htmlspecialchars($c_infotext); ?>
<?php if (($c_linktext != "Cookie link caption") and ($c_link != "http://my_site.de")): ?>
<a href="<?php echo htmlspecialchars($c_link); ?>">
<?php echo htmlspecialchars($c_linktext); ?></a>
<?php endif; ?></p>
<button id="accept" class="btn btn-primary"><?php echo htmlspecialchars($c_buttontext); ?></button>
</div>
<script type="text/javascript">
jQuery(document).ready(function () {
function setCookie(c_name,value,exdays)
{
var exdate=new Date();
exdate.setDate(exdate.getDate() + exdays);
var c_value=escape(value) + ((exdays==null) ? "" : "; expires="+exdate.toUTCString()) + "; path=/";
document.cookie=c_name + "=" + c_value;
}
function readCookie(name) {
var nameEQ = name + "=";
var ca = document.cookie.split(';');
for(var i=0;i < ca.length;i++) {
var c = ca[i];
while (c.charAt(0)==' ') c = c.substring(1,c.length);
if (c.indexOf(nameEQ) == 0) return c.substring(nameEQ.length,c.length);
}
return null;
}
var $ca_container = jQuery('#accept-cookie-container');
var $ca_accept = jQuery('#accept');
var acceptcookie = readCookie('acceptcookie');
if(!(acceptcookie == "yes")){
$ca_container.delay(1000).slideDown('slow');
$ca_accept.click(function(){
setCookie("acceptcookie","yes",365);
jQuery.post('<?php echo JURI::current(); ?>', 'set_cookie=1', function(){});
$ca_container.slideUp('slow');
});
}
});
</script>
<?php endif; ?>
<!-- script für nav -->
<?php if ($this->countModules('nav')): ?>
<script type="text/javascript" src="<?php echo $tpath . '/scripts/nav.js'; ?>"></script>
<?php endif; ?>
<!-- script für aside -->
<?php if ($this->countModules('sidebar')): ?>
<script type="text/javascript" src="<?php echo $tpath . '/scripts/sidebar.js'; ?>"></script>
<?php endif; ?>
<!-- go to top plus sticky nav plus double tap to go-->
<script type="text/javascript" src="<?php echo $tpath . '/scripts/plugins.js'; ?>"></script>
<!-- google analytics code asynchron + anonym -->
<?php if ($analytics != "UA-XXXXX-X"): ?>
<script type="text/javascript">
var _gaq = _gaq || [];
_gaq.push(['_setAccount', '<?php echo htmlspecialchars($analytics); ?>']);
_gaq.push(['_gat._anonymizeIp']);
_gaq.push(['_trackPageview']);
(function() {
var ga = document.createElement('script'); ga.type = 'text/javascript'; ga.async = true;
ga.src = ('https:' == document.location.protocol ? 'https://ssl' : 'http://www') + '.google-analytics.com/ga.js';
var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(ga, s);
})();
</script>
<?php endif; ?>
<!-- script für flickity -->
<?php if ($slider): ?>
<script type="text/javascript" src="<?php echo $tpath . '/scripts/flickity.js'; ?>"></script>
<?php endif; ?>
<!-- script für prism -->
<?php if ($prism): ?>
<script type="text/javascript" src="<?php echo $tpath . '/scripts/prism.js'; ?>"></script>
<?php endif; ?><file_sep><?php
// no direct access
defined('_JEXEC') or die('Restricted access');
/** @var JDocumentError $this */
if (!isset($this->error))
{
$this->error = JError::raiseWarning(404, JText::_('JERROR_ALERTNOAUTHOR'));
$this->debug = false;
}
$app = JFactory::getApplication();
$tpath = $this->baseurl . '/templates/' . $this->template;
// get template params
$sitename = htmlspecialchars($app->get('sitename'), ENT_QUOTES, 'UTF-8');
// meta data
$this->setMetaData('viewport', 'width=device-width, initial-scale=1');
?>
<!DOCTYPE html>
<html lang="<?php echo $this->language; ?>" dir="<?php echo $this->direction; ?>">
<head>
<meta charset="utf-8"/>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="">
<title>Carcass RWD Joomla! template</title>
<!-- Custom styles for this template -->
<link href="<?php echo $tpath; ?>/css/template.css" rel="stylesheet">
<title><?php echo $this->error->getCode(); ?>
- <?php echo htmlspecialchars($this->error->getMessage(), ENT_QUOTES, 'UTF-8'); ?></title>
<?php if ($app->get('debug_lang', '0') == '1' || $app->get('debug', '0') == '1') : ?>
<link href="<?php echo JUri::root(true); ?>/media/cms/css/debug.css" rel="stylesheet"/>
<?php endif; ?>
<!--[if lt IE 9]>
<script src="<?php echo JUri::root(true); ?>/media/jui/js/html5.js"></script><![endif]-->
</head>
<body>
<header class="app-bar promote-layer" role="banner">
<section class="app-bar-container">
<h1 class="logo-text">
<a href="<?php echo $this->baseurl ?>"><?php echo htmlspecialchars($sitename); ?></a>
</h1>
</section>
</header>
<section class="layout block-group">
<section class="main-container">
<main role="main">
<section class="content">
<article>
<h1>
<?php echo $this->error->getCode(); ?> <?php echo htmlspecialchars($this->error->getMessage(), ENT_QUOTES, 'UTF-8'); ?>
</h1>
<h2>
<?php if ($this->error->getCode() == '404'): ?>
Leider haben wir diese Seite nicht gefunden.
<?php endif; ?>
Am Besten gehen wir zurück zur:
<a href="<?php echo JUri::root(true); ?>/index.php"
title="<?php echo JText::_('JERROR_LAYOUT_GO_TO_THE_HOME_PAGE'); ?>"><?php echo JText::_('JERROR_LAYOUT_HOME_PAGE'); ?>
</a>
</h2>
<br>
<p><?php echo JText::_('JERROR_LAYOUT_PLEASE_CONTACT_THE_SYSTEM_ADMINISTRATOR'); ?></p>
</article>
</section>
</main>
</section>
</section>
</body>
</html>
<file_sep><?php
/**
* @package Joomla.Site
* @subpackage Templates.carcass
*
* @copyright Copyright (C) 2005 - 2019 Open Source Matters, Inc. All rights reserved.
* @license GNU General Public License version 2 or later; see LICENSE.txt
*/
defined('_JEXEC') or die;
use Joomla\CMS\Factory;
use Joomla\CMS\HTML\HTMLHelper;
use Joomla\CMS\Language\Text;
use Joomla\CMS\Uri\Uri;
/** @var JDocumentHtml $this */
$app = Factory::getApplication();
$lang = $app->getLanguage();
$wa = $this->getWebAssetManager();
// Detecting Active Variables
$option = $app->input->getCmd('option', '');
$view = $app->input->getCmd('view', '');
$layout = $app->input->getCmd('layout', '');
$task = $app->input->getCmd('task', '');
$itemid = $app->input->getCmd('Itemid', '');
$sitename = htmlspecialchars($app->get('sitename'), ENT_QUOTES, 'UTF-8');
$menu = $app->getMenu()->getActive();
$pageclass = $menu->params->get('pageclass_sfx');
$tpath = $this->baseurl . '/templates/' . $this->template;
// get template params
// logo
$logo = $this->params->get('logo');
// Enable assets
$wa->enableAsset('template.carcass.base');
// meta data
$this->setMetaData('viewport', 'width=device-width, initial-scale=1');
?>
<!DOCTYPE html>
<html lang="<?php echo $this->language; ?>" dir="<?php echo $this->direction; ?>">
<head>
<jdoc:include type="metas"/>
<jdoc:include type="styles"/>
<jdoc:include type="scripts"/>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- icons -->
<link rel="apple-touch-icon" sizes="180x180" href="images/icons/apple-touch-icon.png">
<link rel="shortcut icon" href="favicon.ico" type="image/x-icon">
<link rel="icon" href="favicon.ico" type="image/x-icon">
</head>
<body class=" <?php echo $pageclass?>
<?php echo $option . ' view-' . $view . ($layout ? ' layout-' . $layout : ' no-layout') . ($task ? ' task-' . $task : ' no-task'); ?>
<?php echo ($itemid ? ' itemid-' . $itemid : '' ); ?>" role="document">
<header class="app-bar promote-layer" role="banner">
<section class="app-bar-container">
<?php if ($this->countModules('nav')): ?>
<button class="menu" aria-label="Navigation"></button>
<?php endif; ?>
<h1 class="logo-text">
<a href="<?php echo $this->baseurl ?>"><?php echo htmlspecialchars($sitename); ?></a>
</h1>
<?php if ($this->countModules('sidebar')): ?>
<button class="sidebar-menu" aria-label="Sidebar"></button>
<?php endif; ?>
</section>
</header>
<nav class="navdrawer-container promote-layer" id="navdrawer" role="navigation">
<?php if ($logo): ?>
<div class="logo-image">
<a href="<?php echo $this->baseurl ?>">
<img src="<?php echo $this->baseurl ?>/<?php echo htmlspecialchars($logo); ?>"
alt="<?php echo htmlspecialchars($sitename); ?>"/>
</a>
</div>
<?php else: ?>
<h4>Navigation</h4>
<?php endif; ?>
<?php if ($this->countModules('nav')): ?>
<jdoc:include type="modules" name="nav"/>
<?php endif; ?>
<?php if ($this->countModules('search')): ?>
<div class="search-container">
<jdoc:include type="modules" name="search" style=" none"/>
</div>
<?php endif; ?>
</nav>
<section class="layout block-group">
<?php if ($this->countModules('slideshow')): ?>
<section class="slider-container" role="complementary">
<jdoc:include type="modules" name="slideshow" style="flickity"/>
</section>
<?php endif; ?>
<?php if ($this->countModules('top_row')): ?>
<section class="top" role="complementary">
<jdoc:include type="modules" name="top_row" style="jduo"/>
</section>
<?php endif; ?>
<section class="main-container">
<main role="main">
<section class="content">
<jdoc:include type="message"/>
<jdoc:include type="component"/>
</section>
</main>
<?php if ($this->countModules('sidebar')): ?>
<aside class="sidebar-container" role="complementary">
<h4 class="sidebar-text">Sidebar</h4>
<jdoc:include type="modules" name="sidebar" style="jduo"/>
</aside>
<?php endif; ?>
</section>
<?php if ($this->countModules('bottom_row')): ?>
<section class="bottom" role="complementary">
<jdoc:include type="modules" name="bottom_row" style="jduo"/>
</section>
<?php endif; ?>
</section>
<section class="footer-wrapper block-group">
<?php if ($this->countModules('breadcrumbs')): ?>
<section class="breadcrumbs-container block-group" role="navigation">
<jdoc:include type="modules" name="breadcrumbs"style="none" />
</section>
<?php endif; ?>
<?php if ($this->countModules('footer')): ?>
<footer class="block-group" role="contentinfo">
<jdoc:include type="modules" name="footer" style="footer"/>
</footer>
<?php endif; ?>
</section>
<!-- to top -->
<a href="#" class="go-top"><span class="icon-chevron-up"></span><p hidden>Top</p></a>
<jdoc:include type="modules" name="debug" style="none"/>
<!-- load scripts -->
<?php include_once('scripts/scripts.php'); ?>
<!-- double Tap to go by <NAME> -->
<script type="text/javascript">
jQuery('nav li:has(ul)').doubleTapToGo();
</script>
</body>
</html>
<file_sep># Carcass
## responsive Joomla! 4 alpha 12 template
### work in progress
###Features:
* adhocgrafx template styleguide:
* pasodoble > scss
* bootstrap > scss
* skeleton made with Pasodoble RWD Joomla! 3.x template:
* lightweight off-canvas nav
* aside
* flexbox layout
* Options:
* responsive touchable slides: flickity
* code display: prism
* analytics
* accept cookies info
* instagram-like css img filter-classes
* category blog with cards styling; page-class-suffix | 0f5b21ccb36166a0c7853a7e8c9cb410b1fcae06 | [
"Markdown",
"PHP"
] | 5 | PHP | adhocgraFX/carcass | 3d7461f66eb37a289ccddc0913b366b1cd09c82e | 14b1d2cbba5b527ba563d92194749c1b9adced30 | |
refs/heads/main | <repo_name>melflyhope1/quizzzzz<file_sep>/ქუიზი.py
import requests
from bs4 import BeautifulSoup
from time import sleep
for each in range(1,6):
url_link = 'https://alta.ge/notebooks-page-{}.html'.format(each)
request = requests.get(url_link)
print(request)
text = request.text
soup = BeautifulSoup(text, 'html.parser')
x = soup.find('div', {'class': 'grid-list'})
f = open('content.csv', 'a')
for i in x.find_all('div'):
try:
notebook = i.find('a', {'class': 'product-title'})
print(notebook.text)
f.write(notebook.text + '\n')
except:
continue
sleep(20)<file_sep>/README.md
# quizzzzz
მოცემულ კოდს მოაქვს ლეპტოპები საიტიდან "alta.ge", თავისი დასახელებითა და ფერით, 20 წამის დაგვიანებით სხვადასხვა გვერდებიდან ინფორმაცია.
| de927896f547a0c0d361bac5797f58e716bfd0af | [
"Markdown",
"Python"
] | 2 | Python | melflyhope1/quizzzzz | 991fa74db3030bb279364bac9336008e6674ed33 | 24dec99389d58374915bb9132d27e475cc60e297 | |
refs/heads/master | <repo_name>yangaoyong2020/aoao-cms<file_sep>/src/main/java/com/aoao/cms/service/impl/ChannelServiceImpl.java
package com.aoao.cms.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.aoao.cms.dao.ChannelDao;
import com.aoao.cms.domain.Category;
import com.aoao.cms.domain.Channel;
import com.aoao.cms.service.ChannelService;
@Service
public class ChannelServiceImpl implements ChannelService {
@Autowired
private ChannelDao channeldao;
@Override
public List<Channel> selects() {
return channeldao.selects();
}
@Override
public List<Category> selectsByChannelId(Integer channelId) {
// TODO Auto-generated method stub
return channeldao.selectsByChannelId(channelId);
}
}
<file_sep>/src/main/java/com/aoao/cms/service/impl/CommentServiceImpl.java
package com.aoao.cms.service.impl;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.aoao.cms.dao.CommentDao;
import com.aoao.cms.domain.Article;
import com.aoao.cms.domain.Comment;
import com.aoao.cms.service.CommentService;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
@Service
public class CommentServiceImpl implements CommentService {
@Autowired
private CommentDao commentdao;
@Override
public int insert(Comment comment) {
try {
//增加评论
commentdao.insert(comment);
//改变文章的评论数据+1
int i = commentdao.updateArticle(comment.getArticleId());
System.out.println(i+"11111111111111111111111111");
return 1;
} catch (Exception e) {
e.printStackTrace();
}
return 0;
}
@Override
public PageInfo<Comment> selects(Article article, Integer page, Integer pagesize) {
PageHelper.startPage(page, pagesize);
List<Comment> list = commentdao.selects(article);
return new PageInfo<Comment>(list);
}
@Override
public PageInfo<Article> selectsByCommentNum(Integer page, Integer pagesize) {
PageHelper.startPage(page, pagesize);
List<Article> list = commentdao.selectsByCommentNum();
return new PageInfo<Article>(list) ;
}
}
<file_sep>/src/main/java/com/aoao/cms/service/UserService.java
package com.aoao.cms.service;
import java.util.List;
import com.aoao.cms.domain.User;
import com.github.pagehelper.PageInfo;
public interface UserService {
//用户列表
PageInfo<User> selects(User user,Integer page,Integer pagesize);
//更新用户
int update(User user);
//增加
int insert(User user);
//根据用户查询 用户是否存在
User selectByUsername(String username);
//登录
User login(User user);
}
<file_sep>/src/main/java/com/aoao/cms/controller/ChannelController.java
package com.aoao.cms.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.aoao.cms.domain.Category;
import com.aoao.cms.domain.Channel;
import com.aoao.cms.service.ChannelService;
/**
*
* @ClassName: ChannelController
* @Description:栏目控制器
* @author: Administrator
* @date: 2020年3月5日 上午10:23:20
*/
@Controller
@RequestMapping("channel")
public class ChannelController {
//你好啊
@Autowired
private ChannelService channelservice;
//查询所有的栏目
@ResponseBody
@RequestMapping("channels")
public List<Channel> channels(){
return channelservice.selects();
}
@ResponseBody
@RequestMapping("selectsByChannelId")
public List<Category> selectsByChannelId(Integer channelId){
return channelservice.selectsByChannelId(channelId);
}
}
<file_sep>/src/main/java/com/aoao/cms/service/CommentService.java
package com.aoao.cms.service;
import java.util.List;
import com.aoao.cms.domain.Article;
import com.aoao.cms.domain.Comment;
import com.github.pagehelper.PageInfo;
public interface CommentService {
//增加评论
int insert(Comment comment);
//查询评论 根据文章
PageInfo<Comment> selects(Article article,Integer page,Integer pagesize);
//查询评论 按照评论数量排序
PageInfo<Article> selectsByCommentNum(Integer page,Integer pagesize);
}
<file_sep>/src/main/java/com/aoao/cms/controller/IndexController.java
package com.aoao.cms.controller;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.aoao.cms.domain.Article;
import com.aoao.cms.domain.Category;
import com.aoao.cms.domain.Channel;
import com.aoao.cms.domain.Collect;
import com.aoao.cms.domain.Comment;
import com.aoao.cms.domain.Slide;
import com.aoao.cms.domain.User;
import com.aoao.cms.service.ArticleService;
import com.aoao.cms.service.ChannelService;
import com.aoao.cms.service.CollectService;
import com.aoao.cms.service.CommentService;
import com.aoao.cms.service.SlideService;
import com.github.pagehelper.PageInfo;
/**
*
* @ClassName: IndexController
* @Description: 系统首页入口
* @author: Administrator
* @date: 2020年3月9日 上午11:19:53
*/
@Controller
public class IndexController {
@Autowired
private ChannelService channelservice;
@Autowired
private ArticleService articleService;
@Autowired
private SlideService slideService;
@Autowired
private CommentService commentService;
@Autowired
private CollectService collectService;
@RequestMapping(value={"","/","index"})
public String index(Model m,Article article,@RequestParam(defaultValue="1")Integer page,@RequestParam(defaultValue="5")Integer pagesize){
article.setStatus(1);//只显示审核过的文章
article.setDeleted(0);//只显示未删除的
//封装article查询条件
m.addAttribute("article",article);
//查询左侧栏目
List<Channel> channels = channelservice.selects();
m.addAttribute("channels",channels);
//如果栏目ID 不为空 则查询其下所有的分类
if(article.getChannelId()!=null){
List<Category> categorys = channelservice.selectsByChannelId(article.getChannelId());
System.out.println(categorys);
m.addAttribute("categorys",categorys);
}
//如果栏目ID 为空 则查询其下默认的热点文章
if(article.getChannelId()==null){
article.setHot(1);
//查询轮播图
List<Slide> slides = slideService.selects();
m.addAttribute("slides",slides);
}
//查询所有的文章
PageInfo<Article> info = articleService.selects(article, page, pagesize);
m.addAttribute("info",info);
//在右侧显示10篇文章
Article article2 = new Article();
article2.setStatus(1);
article2.setDeleted(0);
PageInfo<Article> lastArticles = articleService.selects(article2, 1, 10);
m.addAttribute("lastArticles",lastArticles);
return "index/index";
}
/**
*
* @Title: articleDetail
* @Description: 文章详情
* @param id
* @return
* @return: String
*/
@RequestMapping("articleDetail")
public String articleDetail(HttpSession session,Model m,Integer id,@RequestParam(defaultValue="1")Integer page,@RequestParam(defaultValue="5")Integer pagesize){
Article article = articleService.select(id);
m.addAttribute("article",article);
//查询处当前文章的评论信息
PageInfo<Comment> info = commentService.selects(article, page, pagesize);
//查询所有文章的评论
PageInfo<Article> info2 = commentService.selectsByCommentNum( 1, 10);
m.addAttribute("info",info);
m.addAttribute("info2",info2);
//查询该文章是否被收藏过
User user = (User) session.getAttribute("user");
Collect collect =null;
if(null!=user){//如果用户已经登录 则查询是否被收藏过
collect = collectService.selectByTitleAndUserId(article.getTitle(), user.getId());
}
m.addAttribute("collect",collect);
return "index/articleDetail";
}
//增加评论'
@ResponseBody
@RequestMapping("addComment")
public boolean addConmment(Comment comment,Integer articleId,HttpSession session){
User user = (User) session.getAttribute("user");
if(null==user)
return false;//没有登录的用户不能评论
comment.setUserId(user.getId());
comment.setArticleId(articleId);
comment.setCreated(new Date());
return commentService.insert(comment)>0;
}
//增加收藏'
@ResponseBody
@RequestMapping("collect")
public boolean collect(Collect collect,HttpSession session){
User user = (User) session.getAttribute("user");
if(null==user)
return false;//没有登录的用户不能收藏
collect.setUser(user);//收藏人
collect.setCreated(new Date());//收藏时间
return collectService.insert(collect)>0;
}
//取消收藏'
@ResponseBody
@RequestMapping("deletecollect")
public boolean deletecollect(Integer id){
return collectService.delete(id)>0;
}
}
| 76c23859ebc1d68baa01dbe48a5786a71c8d1c7b | [
"Java"
] | 6 | Java | yangaoyong2020/aoao-cms | 9ec7225c85194502c0d9aaa9ce2cc36c5ef526a3 | c954b17ce711edf3e2ce3bce5bb7205a648068f7 | |
refs/heads/master | <file_sep>"""
Created on Sep 19, 2014
@author: memery
"""
import re
import csv
import time
import collections
import os
import codecs
class Newsletter(object):
"""The Newsletter class simply requires a plaintext to function.
[ADD MORE HERE]"""
content = []
flag_library = {}
output = []
def __init__(self, plaintext):
self.plaintext = plaintext
assert type(self.plaintext) == file, 'plaintext is not a file!'
def _content_from_plaintext(self):
"""This method reads the Newsletter's plaintext and populates the flag
library"""
with self.plaintext as source:
self.content = [line.decode('utf-8').strip('\n') for line in source]
self.plaintext.close()
def _find_plaintext_directory(self):
"""Returns directory where self.plaintext is located"""
return os.path.dirname(self.plaintext)
def _index_flags(self):
"""Creates a default dictionary that returns a tuple containing a
sentinal string and all indices where that string appears"""
flag_dict = {
'>>-->>Banner 1 Name>>': 'banners',
'>>-->>Banner 2 Name>>': 'banners',
'>>-->>Banner 3 Name>>': 'banners',
'>>-->>MP_Banner Name>>': 'banners',
'>>-->>Text Banner Name>>': 'textbanner',
'>>-->>Publication>>': 'pubs',
'>>-->>Review>>': 'revs',
'>>-->>Industry Title>>': 'inds',
'>>-->>Science Title>>': 'scis',
'>>-->>Policy Title>>': 'pols',
'>>-->>NIH Title>>': 'regs',
'>>-->>CBER Title>>': 'regs',
'>>-->>Regulatory Title>>': 'regs',
'>>-->>Event Title>>': 'events',
'>>-->>Job Title>>': 'jobs',
'>>-->>Newsletter Name>>': 'nl',
'>>-->>Newsletter Issue Number>>': 'issue',
'>>-->>Connexon Banner Name>>': 'cxnbanner',
'>>-->>Newsletter Issue Date>>': 'date',
'>>-->>Subject Line>>': 'subjectline',
'>>-->>Homepage Banner Name>>': 'hpbanner',
'>>-->>Old Jobs>>': 'oldjobs',
">>-->>Insert last week's jobs' HTML here>>": 'prevjobs',
'>>-->>end of plaintext>>-->>': 'end',
}
# Could still be better (Nested loop is ugly)
line_count = 0
self.flag_library = collections.defaultdict(list)
for line in self.content:
for flag in flag_dict:
if flag in line:
self.flag_library[flag_dict[flag]].append(line_count)
line_count += 1
def _index_content(self, index, offset=0):
"""returns the line in self.content equal to the value of index plus
the value of offset. Default offset is 0."""
return self.content[index + offset]
def _object_builder(self, index, theclass, offset_sequence):
"""Magic. Constructs any type of object (Banner/Article etc.) given
a starting index, the class and the sequence the offsets"""
attributes = [self._index_content(index, offset) for offset in offset_sequence]
return theclass(*attributes)
def _object_builder_dict(self):
"""A dictionary of which contains the flag_library keys and a tuple
containing the key's class object and a tuple of the offset sequence"""
builder_dict = {
'banners': (Banner, (1, 5, 3, 7)),
'pubs': (Publication, ()),
# 'revs':rev_gen(source, sentinals['revs']),
# 'inds':ind_gen(source, sentinals['inds']),
# 'scis':sci_gen(source, sentinals['scis']),
# 'pols':pol_gen(source, sentinals['pols']),
'events': (Event, (1, 3, 5, 7)),
'jobs': (Job, (1, 3, 5, 7, 9, 11, 13))
}
# for flag_type in self.flag_library:
# for flag_index in flag_type:
# flag_dict[flag_type]
def write_output(self):
"""Writes the properly formatted output to output.htm"""
outfile = codecs.open(self._find_plaintext_directory + r'\output.htm',
'w',
'utf-8-sig',
'replace')
out = [line + u'\n' for line in self.output]
outfile.writelines(out)
outfile.close()
class Article(object):
"""A subclass of Entry that provides a more specialized architecture for
Publications, Industry News etc."""
def __init__(self, date, title, summary, source, anchor_list,
link_list, name):
"""Initiate an Article instance"""
assert len(anchor_list) == len(link_list), \
'Anchor and Link lists unequal: ' + title
for link in link_list:
assert re.match(r'https?', link) is not None, \
'URL Malformed: ' + title
self.date = date
self.title = title
self.summary = summary
self.source = source
self.anchor_list = anchor_list
self.link_list = link_list
self.name = name
# Assign Newsletter Type
# call all newsletters of type, in last x days
def lineout(self, newsletter, strip=False):
"""Defines a recursive function that builds a list that will become a
Publication in the full Eloqua HTML"""
temp_links = self.link_list
temp_anchors = self.anchor_list
# Should give a better explanation of this
def recursive_string(links, anchors, newsletter):
"""A recursive function for adding all links and anchors in proper
order"""
core = [
'<!-- ' + self.name + ' SECTION Start -->',
'<a style="text-decoration: none" href="' +
links[0] +
'"><strong><font color="' +
newsletter['primary_color'] +
'" size="2" face="Arial">',
'<!-- ' + self.name + ' TITLE-->' +
self.title +
'<!-- End --></font></strong></a><br><font color="' +
newsletter['text_color'] +
'" size="2" face="Arial">',
'<!-- ' + self.name + ' CONTENT-->' +
self.summary +
' [' +
self.source +
'] <!-- End --></font>' +
'<a style="text-decoration: none" href="' +
links[0] +
'"><font color="' +
newsletter['secondary_color'] +
'" size="2" face="Arial"><strong>' +
anchors[0] +
'</strong></font></a>']
if len(links) == 1:
return core
else:
addon = [' <font color="' +
newsletter['secondary_color'] +
'" size="2" face="Arial"><strong>|</strong></font>',
' <a style="text-decoration: none" href="' +
temp_links.pop(-1) +
'"><font color="' +
newsletter['secondary_color'] +
'" size="2" face="Arial"><strong>' +
temp_anchors.pop(-1) +
'</strong></font></a>']
return recursive_string(links, anchors, newsletter) + addon
br_add = recursive_string(temp_links, temp_anchors, newsletter)
if not strip:
br_add[-1] += '<br><br>'
return br_add + ['<!-- ' + self.name + ' SECTION End -->']
class Publication(Article):
"""Subclass of Article. All Articles in the Publications Section go here"""
def __init__(self, date, title, summary, source, anchor_list,
link_list, impact, division, topstory):
"""Initiating"""
Article.__init__(self, date, title, summary, source, anchor_list,
link_list, '#PUBLICATIONS')
self.impact = impact
self.division = division
self.topstory = topstory
if 'Press release from' not in source:
self.source = '<em>' + source + '</em>'
# Perhaps this could be sorted by date
def __lt__(self, other):
"""How Publications are sorted"""
return self.impact < other.impact
def __repr__(self):
"""Representation of publication"""
return self.title
def __str__(self):
return self.title
def lineout_top(self, newsletter):
"""A version of nl.lineout that strips <br><br> and replaces it
with the Top Story border markup"""
top_list = self.lineout(newsletter, strip=True)
top_list[0] = '<!-- #TOPSTORY Start -->'
del top_list[-1]
top_list[-1] = (top_list[-1] +
('</td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom:1px solid; border-bottom-color: '
'#808080;">'))
return top_list
# set an if statement if IF already set
def lookup_impact(self):
"""opens the impact factor CSV and checks a impact factorless article
against the list of known impact factors. If the impact factor can't
be called, it calls new_impact() to input a new entry"""
with open(r'S:\CXN - Connexon\Connexogen\impactfactors.csv') as fin:
csvin = csv.reader(fin)
lookup = {row[0]: row for row in csvin}
to_find = re.sub('</?em>', '', self.source)
try:
return float(lookup[to_find][2])
except (KeyError, IndexError):
print '[' + self.source + '] ' + self.title
if self.anchor_list[0] == 'Press Release':
in_name = raw_input('Input Publication Abbreviation: ')
else:
in_name = to_find
in_issn = raw_input('Input ISSN: ')
in_impact = raw_input('Input Impact Factor: ')
new_impact(in_name, in_issn, in_impact)
return float(in_impact)
finally:
fin.close()
def set_impact(self, impact):
"""Sets the impact factor. Used when looking up impact factors from
impact factor csv."""
self.impact = impact
def new_impact(name, issn, impact):
"""Adds new impact factor information to the csv"""
with open(r'S:\CXN - Connexon\Connexogen\impactfactors.csv', 'ab') as fin:
if_sheet = csv.writer(fin)
if_sheet.writerow((name, issn, impact))
fin.close()
class Review(Publication):
"""A Review Publication. Contains author information"""
def __init__(self, date, title, summary, source, anchor_list, link_list,
impact, authors):
Article.__init__(self, date, title, summary, source, anchor_list,
link_list, '#REVIEWS')
self.authors = authors
self.impact = impact
self.source = '<em>' + source + '</em>'
def __lt__(self, other):
"""Sort by impact factor"""
return self.impact < other.impact
def __repr__(self):
"""Reviews are represented by titles"""
return self.title
def __str__(self):
return self.title
def archive(self, newsletter, volume, nl_date):
"""Provides HTML in Wordpress format"""
volume_dash = re.sub(r'\.', '-', volume)
date = time.strftime('%b %d', nl_date).replace(' 0', ' ')
date_dash = date.replace(' ', '-')
url = (newsletter['url'] +
u'issue/volume-' +
volume_dash +
u'-' +
date_dash +
u'/">')
out = [
'<tr>',
'<td class="reviewtitle"><a target="_blank" href="' +
self.link_list[0] + '">' + self.title + '</a><br>' +
self.authors + '</td>',
'<td><em>' + self.source + '</em></a></td>',
'<td>' + self.date + '</td>',
'<td><a target="_blank" href="' + url + volume + ' | ' +
date + '</a>' + '</td>',
'</tr>',
'']
return out
class ScienceNews(Article):
def __init__(self, date, title, summary, source, anchor_list, link_list):
Article.__init__(self, date, title, summary, source, anchor_list,
link_list, '#SCIENCE')
def __repr__(self):
return self.title
def __str__(self):
return self.title
class IndustryNews(Article):
def __init__(self, date, title, summary, source, anchor_list, link_list):
Article.__init__(
self,
date,
title,
summary,
source,
anchor_list,
link_list,
'#INDUSTRY')
def __repr__(self):
return self.title
def __str__(self):
return self.title
class PolicyNews(Article):
"""Creates a Policy News article. This is similar to an Industry News
article except for the <em> tags on the source and the use of 'Editorial'
as opposed to 'Press Release'"""
def __init__(self, date, title, summary, source, anchor_list, link_list):
Article.__init__(
self,
date,
title,
summary,
source,
anchor_list,
link_list,
'#POLICY')
def __repr__(self):
return self.title
def __str__(self):
return self.title
class Banner(object):
"""A Banner object contains a Name, URL, Alt-Text and Image URL
A Name is the internal reference used on the Connexon spreadsheet"""
def __init__(self, name, url, text, img):
self.name = name
self.text = text
self.url = url
self.img = img
def __repr__(self):
return self.name
def __str__(self):
return (self.url + '<img border="0" alt="' + self.text + '" src="'
+ self.img + '" width="645" height="110"></a>')
def banner_out(self, newsletter, issue, banner_number):
"""Formats a banner for use in a newsletter, appends all appropriate
HTML to banner"""
if banner_number == 1:
append = 'i'
elif banner_number == 2:
append = 'ii'
elif banner_number == 3:
append = 'iii'
else:
append = '_mp'
# need to revert back to 3 for the URL
banner_number = 3
return (
('<a style="text-decoration: none" href="'
'http://secure.eloqua.com/e/f2.aspx?elqFormName=') +
newsletter['name_short'] +
str(banner_number) +
'&elqSiteID=1832&e=<SPAN class=eloquaemail>EmailAddress</SPAN>&u=' +
self.url +
'&t=(A)' +
newsletter['name_short'].upper() +
issue +
append +
'"><img border="0" alt="' +
self.text +
'" src="' +
self.img +
'" width="645" height="110"></a>')
def homebanner_out(self):
"""Formats banner in HTML to be placed in the Wordpress website"""
return (
('<!--Banner Start--><div align="center">'
'<a style="text-decoration: none" href="') +
self.url + '"><img src="' + self.img + '" alt="' + self.text +
'"></a></div><!--Banner End-->')
class TextBanner(object):
"""TextBanner Object"""
def __init__(self, text, url):
"""Initiate Text Banner"""
self.text = text
self.url = url
def text_massage(self, newsletter, issue):
"""Provides the Newsletter with a fully formatted Text Banner"""
self.utm_replace(newsletter)
stringer = self.text.split('strong>')
stringer[0] = ('<font size="2" face="Arial" color="' +
newsletter['primary_color'] +
'">' + stringer[0])
stringer[2] = ('<font size="2" face="Arial" color="' +
newsletter['text_color'] + '">' +
stringer[2])
stringer[3] = ('<font size="2" face="Arial" color="' +
newsletter['primary_color'] + '">' +
stringer[3])
textad = 'strong>'.join(stringer)
textad += '</a>'
return (
'<a style="text-decoration: none" href="http://secure.eloqua.com/'
'e/f2.aspx?elqFormName=' + newsletter['name_short'] +
'3&elqSiteID=1832&e=<SPAN class=eloquaemail>EmailAddress</SPAN>' +
'&u=' + self.url + '&t=(A)' + newsletter['name_short'].upper() +
issue + 'iiitext">' + textad)
def __repr__(self):
"""Returns text banner representation"""
return self.text
def __str__(self):
"""returns generalized TextBanner string"""
return (self.url + '<font size="2" face="Arial" color="$primary">'
+ self.text + '</font></a>')
def _seturl(self, url):
"""Sets the URL to a new string"""
self.url = url
def utm_replace(self, newsletter):
"""Replaces the generic 'cxn' with the current newsletter name"""
self._seturl(re.sub(r'(\?utm_source=)\w+',
r'?utm_source=' + newsletter['name_short'],
self.url))
return self.url
class Event(object):
"""An Event Entry, can be formatted for a newsletter or archive entry"""
def __init__(self, title, link, date, location, name):
self.title = title
self.link = link
self.date = date
self.location = location
self.name = '#EVENT'
def __lt__(self, other):
return self.date < other.date
# Need single date as well
def date_conversion(self):
"""Converts time strings of format "%Y-%m-%d" to "%B %d, %Y".
Used to convert the archival format to newsletter format"""
input_date_strs = self.date.split(' to ')
if len(input_date_strs) == 1:
date = time.strptime(input_date_strs[0], "%Y-%m-%d")
return time.strftime("%B %d, %Y", date).replace(' 0', ' ')
else:
# use a list comprehension and tuple expansion
start, end = [
time.strptime(s, "%Y-%m-%d") for s in input_date_strs]
# if both in the same year, omit it from start date
start_fmt = "%B %d" if start.tm_year == end.tm_year else "%B %d, %Y"
# if both in the same month and year, omit month from end date
end_fmt = "%d, %Y" if (start.tm_mon, start.tm_year) == (end.tm_mon, end.tm_year) else "%B %d, %Y"
return (time.strftime(start_fmt, start) +
'-' +
time.strftime(end_fmt, end)).replace(' 0', ' ').replace('-0', '-')
def nl_location(self):
"""Generates a string from the event's city and country"""
loc_list = self.location.split(', ')
if len(loc_list) == 1 or len(loc_list) == 2:
return self.location
if len(loc_list) == 3:
return ', '.join((loc_list[0], loc_list[2]))
def arch_location(self):
"""Generates a string from the event's city and province/state"""
loc_list = self.location.split(', ')
if len(loc_list) == 1 or len(loc_list) == 2:
return self.location
if len(loc_list) == 3:
return ', '.join((loc_list[0], loc_list[1]))
def __repr__(self):
return self.title
def __str__(self):
return self.title
def nlout(self, newsletter):
"""Generates a list of lines to be included in a Newsletter"""
if newsletter['name_short'] != 'hn':
event = [
'<!-- ' + self.name + ' SECTION Start -->',
'<a style="text-decoration: none" href="' +
self.link +
'"><strong><font color="' +
newsletter['primary_color'] +
'" size="2" face="Arial">',
'<!-- #EVENT TITLE--><font color="' +
newsletter['secondary_color'] +
'">NEW</font> ' +
self.title +
'<!-- End --></font></strong></a><br><font color="' +
newsletter['text_color'] +
'" size="2" face="Arial">',
'<!-- #EVENT CONTENT-->' +
self.date_conversion() +
'<br>' +
self.nl_location() +
'<!-- End --><br><br>',
'<!-- ' + self.name + ' SECTION End -->']
else:
event = [
'<!-- ' + self.name + ' SECTION Start -->',
'<a style="text-decoration: none" href="' +
self.link +
'"><strong><font color="' +
newsletter['primary_color'] +
'" size="2" face="Arial">',
'<!-- #EVENT TITLE--><font color="#e36f1e">NEW</font> ' +
self.title +
'<!-- End --></font></strong></a><br><font color="' +
newsletter['text_color'] +
'" size="2" face="Arial">',
'<!-- #EVENT CONTENT-->' +
self.date_conversion() +
'<br>' +
self.nl_location() +
'<!-- End --><br><br>',
'<!-- ' + self.name + ' SECTION End -->']
return event
def archive(self):
"""Generates a list of lines to be printed in archiving"""
return [
u'<tr>',
u'<td><a href="' +
self.link +
u'" target="_blank">' +
self.title +
u'</a></td>',
u'<td>' +
self.date +
u'</td>',
u'<td>' +
self.arch_location() +
u'</td>',
u'</tr>',
u'']
class Job(object):
"""A Job Entry"""
name = '#JOB'
def __init__(self, title, org, location, date, job_type, requirement,
link):
self.title = title
self.org = org
self.location = location
self.date = date
self.job_type = job_type
self.requirement = requirement
self.link = link
def __repr__(self):
return self.title
def __str__(self):
return self.title
def nlout(self, newsletter):
"""Creates newsletter ready text for a given job"""
if newsletter['name_short'] != 'hn':
job = [
'<!-- ' + self.name + ' SECTION Start -->',
'<a style="text-decoration: none" href="' +
self.link +
'"><strong><font color="' +
newsletter['primary_color'] +
'" size="2" face="Arial">',
'<!-- #JOB TITLE--><font color="' +
newsletter['secondary_color'] +
'">NEW</font> ' +
self.title +
' (' +
self.org +
')',
'<!-- End --></font></strong></a><br><br>',
'<!-- ' + self.name + ' SECTION End -->']
else:
job = [
'<!-- ' + self.name + ' SECTION Start -->',
'<a style="text-decoration: none" href="' +
self.link +
'"><strong><font color="' +
newsletter['primary_color'] +
'" size="2" face="Arial">',
'<!-- #JOB TITLE--><font color="#e36f1e">NEW</font> ' +
self.title +
' (' +
self.org +
')',
'<!-- End --></font></strong></a><br><br>',
'<!-- ' + self.name + ' SECTION End -->']
return job
def archive(self, newsletter):
"""Create HTML for Job archive"""
out = [
'<tr>',
'<td><a href="' + self.link +
('" target="_blank"><span style="color: #2bb675;"><em>NEW </em>'
'</span>') + self.title + '</a></td>',
'<td>' + self.org + '</td>',
'<td>' + self.job_type + '</td>',
'<td>' + self.location + '</td>',
'<td>' + self.requirement + '</td>',
('<td><a target="_blank" href="' + newsletter['url'] + '">' +
newsletter['name_long'] + '</a></td>'),
'<td>' + self.date + '</td>',
'</tr>',
'']
return out
<file_sep>"""
Created on Aug 21, 2014
@author: memery
"""
import collections
import os
import time
import Connexogen
class Regulatory(object):
def __init__(self, name, doc_number, link, agency):
self.agency = agency
self.name = name
self.doc_number = doc_number
self.link = link
def _set_agency(self, agency):
self.agency = agency
def __repr__(self):
if self.doc_number != '':
return '[' + self.agency + '] ' + self.name + ' ' + '(FR Doc. No: ' + self.doc_number + ')'
else:
return '[' + self.agency + '] ' + self.name
def __str__(self):
if self.doc_number != '':
return '[' + self.agency + '] ' + self.name + ' ' + '(FR Doc. No: ' + self.doc_number + ')'
else:
return '[' + self.agency + '] ' + self.name
def lineout(self):
if self.doc_number != '':
return [
'<!-- #' + self.agency + ' SECTION Start -->',
('<a style="text-decoration: none" href="' + self.link +
'"><strong><font color="#e36f1e" size="2" face="Arial">'),
('<!-- #' + self.agency + ' TITLE-->' + self.name +
' (FR Doc. No: ' + self.doc_number +
')<!-- End --></font></strong></a><br><br>'),
'<!-- #' + self.agency + ' SECTION End -->']
else:
return [
'<!-- #' + self.agency + ' SECTION Start -->',
('<a style="text-decoration: none" href="' + self.link +
'"><strong><font color="#e36f1e" size="2" face="Arial">'),
('<!-- #' + self.agency + ' TITLE-->' + self.name +
' <!-- End --></font></strong></a><br><br>'),
'<!-- #' + self.agency + ' SECTION End -->']
def linebrstrip(self):
if self.doc_number != '':
return [
'<!-- #' + self.agency + ' SECTION Start -->',
('<a style="text-decoration: none" href="' + self.link +
'"><strong><font color="#e36f1e" size="2" face="Arial">'),
('<!-- #' + self.agency + ' TITLE-->' + self.name +
' (FR Doc. No: ' + self.doc_number +
')<!-- End --></font></strong></a>'),
'<!-- #' + self.agency + ' SECTION End -->']
else:
return [
'<!-- #' + self.agency + ' SECTION Start -->',
('<a style="text-decoration: none" href="' + self.link +
'"><strong><font color="#e36f1e" size="2" face="Arial">'),
('<!-- #' + self.agency + ' TITLE-->' + self.name +
' <!-- End --></font></strong></a>'),
'<!-- #' + self.agency + ' SECTION End -->']
def ctn_main():
source = Connexogen.sourcereader()
# Make sure to change this later
entries = ctn_entry_mapper(source[0], ctn_full_gen(source[0]))
flags = ctn_flag_mapper(source[0], ctn_nl_flags(source[0]))
out = []
out.extend(header(flags))
out.extend(menu(entries))
out.extend(topstory(flags, entries))
out.extend(publications(flags, entries))
out.extend(reviews(flags, entries))
out.extend(sciencenews(flags, entries))
out.extend(policynews(flags, entries))
out.extend(industrynews(flags, entries))
out.extend(nih(entries))
out.extend(cber(entries))
out.extend(regulatory(entries))
out.extend(eventsnl(flags, entries))
out.extend(jobsnl(flags, entries))
out.extend(footer())
Connexogen.writetofile(out, source[1])
Connexogen.wordpress(flags, entries, source[1])
return out
def ctn_full_gen(source):
"""Creates a default dictionary that returns a tuple containing a
sentinal string and all indices where that string appears"""
query = {'>>-->>Banner 1 Name>>': 'banners',
'>>-->>Banner 2 Name>>': 'banners',
'>>-->>Banner 3 Name>>': 'banners',
'>>-->>MP_Banner Name>>': 'banners',
'>>-->>Text Banner Name>>': 'textbanner',
'>>-->>Publication>>': 'pubs',
'>>-->>Review>>': 'revs',
'>>-->>Industry Title>>': 'inds',
'>>-->>Science Title>>': 'scis',
'>>-->>Policy Title>>': 'pols',
'>>-->>NIH Title>>': 'regs',
'>>-->>CBER Title>>': 'regs',
'>>-->>Regulatory Title>>': 'regs',
'>>-->>Event Title>>': 'events',
'>>-->>Job Title>>': 'jobs'}
line_count = 0
sentinals = collections.defaultdict(list)
for line in source:
for cue in query:
if cue in line:
sentinals[query[cue]].append(line_count)
line_count += 1
return sentinals
def ctn_entry_mapper(source, sentinals):
"""A very important function. This function takes makes all the entries
to their appropriate function and returns a dictionary of all entries in the
newsletter"""
func_map = {
'banners': Connexogen.banner_gen(source, sentinals['banners']),
'textbanner': Connexogen.textbanner_gen(source, sentinals['textbanner']),
'pubs': Connexogen.pub_gen(source, sentinals['pubs']),
'revs': Connexogen.rev_gen(source, sentinals['revs']),
'inds': Connexogen.ind_gen(source, sentinals['inds']),
'scis': Connexogen.sci_gen(source, sentinals['scis']),
'pols': Connexogen.pol_gen(source, sentinals['pols']),
'events': Connexogen.event_gen(source, sentinals['events']),
'jobs': Connexogen.job_gen(source, sentinals['jobs']),
'regs': reg_gen(source, sentinals['regs'])
}
return func_map
def reg_gen(source, indices):
"Generates Regulatory entries from source"""
regs = collections.defaultdict(list)
for index in indices:
if source[index + 1] != '':
if 'NIH' in source[index]:
reg = Regulatory(name=source[index + 1],
doc_number=source[index + 3],
link=source[index + 5],
agency=u'NIH')
elif 'CBER' in source[index]:
reg = Regulatory(name=source[index + 1],
doc_number='',
link=source[index + 3],
agency=u'CBER')
else:
reg = Regulatory(name=source[index + 1],
doc_number=source[index + 3],
link=source[index + 5],
agency=source[index + 7])
regs[reg.agency].append(reg)
return regs
def ctn_nl_flags(source):
"""Creates a default dictionary that returns a tuple containing a
sentinal string and all indices where that string appears"""
query = {'>>-->>Newsletter Issue Number>>': 'issue',
'>>-->>Newsletter Issue Date>>': 'date',
# '>>-->>Subject Line>>':'subjectline',
'>>-->>Homepage Banner Name>>': 'hpbanner',
'>>-->>Connexon Banner Name>>': 'cxnbanner',
'>>-->>Old Jobs>>': 'oldjobs',
">>-->>Insert last week's jobs' HTML here>>": 'prevjobs',
'>>-->>end of plaintext>>-->>': 'end'}
line_count = 0
# should probably convert to a regular dictionary
sentinals = collections.defaultdict(list)
for line in source:
for cue in query:
if cue in line:
sentinals[query[cue]].append(line_count)
line_count += 1
return sentinals
def ctn_flag_mapper(source, sentinals):
"""Maps the ctn_nl_flags sentinals to the appropriate function and stores that
information in a dictionary. When creating a new function dependent on a
flag, call it from here"""
func_map = {
'nl': ctn_info(),
'issue': Connexogen.issue_number(source, sentinals['issue']),
'date': Connexogen.issue_date(source, sentinals['date']),
# 'subjectline':subjectline(source, sentinals['subjectline']),
'hpbanner': Connexogen.homepagebanner(source, sentinals['hpbanner']),
'cxnbanner': Connexogen.homepagebanner(source, sentinals['cxnbanner']),
'oldjobs': Connexogen.job_grab(source, (sentinals['oldjobs'],
sentinals['prevjobs'])),
'prevjobs': Connexogen.job_grab(source, (sentinals['prevjobs'],
sentinals['end'])),
'end': Connexogen.end_init()}
return func_map
def ctn_info():
return {
'name_long': 'Cell Therapy News',
'name_short': 'ctn',
'primary_color': '#e36f1e',
'secondary_color': '#003366',
'text_color': '#666666',
'header_image':
('http://img.en25.com/eloquaimages/clients/StemcellTech'
'nologiesInc/%7B214e9670-0241-429d-8cfb-d1978ddcf8ae%'
'7D_Header-BottomCorner.jpg'),
'twitter_url': 'http://twitter.com/celltherapynews',
'background_color': '',
'footer_border_color': '',
'footer_background_color': '',
'division_list': (),
'url': 'http://www.celltherapynews.com/'}
def sourcereader_test():
"""A simple version of sourcereader that is used in unit testing"""
with open(r'C:\Users\memery\Documents\macro\macro\connexogen\ctn_plaintext_tester.txt', 'r') as source:
outdir = os.path.dirname(source.name)
source_list = []
for line in source:
line = line.decode('utf-8')
line = line.strip('\n')
source_list.append(line)
source.close()
return source_list, outdir
def header(flag_map):
nl_issue = str(flag_map['issue'])
nl_date = flag_map['date']
out = [
'<html>',
'<head>',
'<title>Newsletter Issue</title>',
('<style type="text/css">a:link {text-decoration: none; color:'
'#e36f1e;}a:visited {text-decoration: none; color:#e36f1e;}a:active'
' {text-decoration: none; color:#e36f1e;}a:hover {text-decoration: '
'underline; color:#e36f1e;}td {font-family: arial; font-size: '
'12px; color: #666666;}</style>'),
'</head>',
'<body>',
('<table align="center" width="715" border="0" cellpadding="0" '
'cellspacing="0"><tr><td width="100%" valign="top"><div align='
'"right"><font color="#003366" size="1" face="arial">'),
'<!-- #ISSUE Start -->',
('Issue ' + nl_issue + ' ' +
time.strftime("%B %d, %Y", nl_date).replace(' 0', ' ')),
'<!-- #ISSUE End-->',
'',
'</font></div>',
('<table width="715" cellspacing="0" cellpadding="0" border="0">'
'<tbody><tr><td width="25" valign="top" bgcolor="#f89021" align='
'"left" style="line-height:0;"><img width="16" height="14" border="0"'
' src="http://img.en25.com/eloquaimages/clients/Stemcell'
'TechnologiesInc/%7B0117c1a8-0f84-4cfe-b19c-c18be7f47d4e%7D_Header-'
'TopCorner.jpg"></td>'),
'',
'',
'<td bgcolor="#f89021" valign="top" colspan="2" align="right">',
'',
'',
('<table border="0" cellspacing="0" cellpadding="0" width="100%" '
'height="85"><tr><td height="85" valign="top" rowspan="2" width="205"'
' align="left"><a href="http://www.celltherapynews.com/" target="'
'_blank"><img border="0" alt="Cell Therapy News - Your Industry in '
'an Instant" src="http://img.en25.com/eloquaimages/clients/Stemcell'
'TechnologiesInc/%7B8ad845f3-c815-42df-9bbb-eae2d1d8868a%7D_Header-'
'Logo.gif" width="196" height="85"></a></td><td height="52" '
'colspan="3" align="right">'),
'',
('<img border="0" src="http://img.en25.com/eloquaimages/clients/'
'stemcelltechnologiesinc/%7b188a1c2d-dd54-4c7b-8e9c-c8bec8dadd39%7d_'
'header-circles.gif" width="215" height="52"></td></tr><tr><td '
'height="33" valign="bottom" align="left">'),
'<a href="http://www.celltherapynews.com/" target="_blank">',
('<img border="0" alt="Your Industry in an Instant" src="http://img.'
'en25.com/eloquaimages/clients/stemcelltechnologiesinc/%7b35d54b1e-'
'caf2-4402-8746-09ff65abbb71%7d_header-tagline-smaller.jpg" width='
'"276" height="21"></a></td><td height="33" valign="bottom" align='
'"right"><a href="http://twitter.com/celltherapynews" target="'
'_blank">'),
'',
('<img border="0" alt="Twitter" src="http://img.en25.com/eloquaimages/'
'clients/stemcelltechnologiesinc/%7b8759c6c2-521e-48c1-9f19-'
'a614a33e6faa%7d_header-twitter.gif" width="22" height="23"></a>'
' <a href="http://www.facebook.com/CellTherapyNews" '
'target="_blank">'),
('<img border="0" alt="Facebook" src="http://img.en25.com/eloquaimages'
'/clients/stemcelltechnologiesinc/%7b57429e4a-4426-492b-a48a-67d20bd7'
'87e1%7d_header-facebook.gif" width="22" height="24"></a></td>'),
'<td width=20> </td></tr></table>',
('</td></tr><tr><td bgcolor="#f89021" height="10" colspan="3"></td>'
'</tr><tr><td bgcolor="#ffe2c5" height="40" width="25"></td><td '
'bgcolor="#ffe2c5" valign="middle" width="670" align="left"><table '
'border="0" cellspacing="0" cellpadding="0" width="100%"><tr><td>'),
'',
('<font style="font-size: 13px; font-weight: bold;" face="Arial" '
'color="#e36f1e">'),
]
return out
def menu(entry_map):
"""Creates the menu, will not produce science news or review links if
you do not have any of those type of articles."""
out = ['', '<!-- #MENU Start -->']
html = ('<a style="text-decoration: none" href="#currentpublications">'
'<font color="#e36f1e">Publications</font></a> | ')
if len(entry_map['revs']) != 0:
html = (html +
'<a style="text-decoration: none" href="#reviews">'
'<font color="#e36f1e">Reviews</font></a> | ')
if len(entry_map['scis']) != 0:
html = (html +
'<a style="text-decoration: none" href="#sciencenews">'
'<font color="#e36f1e">Science</font></a> | ')
html = (html +
'<a style="text-decoration: none" href="#policy">'
'<font color="#e36f1e">Policy</font></a> | '
'<a style="text-decoration: none" href="#business">'
'<font color="#e36f1e">Business</font></a> | ')
if 'NIH' in entry_map['regs']:
html = (html +
'<a style="text-decoration: none" href="#NIH">'
'<font color="#e36f1e">NIH</font></a> | ')
if 'CBER' in entry_map['regs']:
html = (html +
'<a style="text-decoration: none" href="#CBER">'
'<font color="#e36f1e">CBER</font></a> | ')
temp_keys = entry_map['regs'].keys()
if 'NIH' in temp_keys:
temp_keys.remove('NIH')
if 'CBER' in temp_keys:
temp_keys.remove('CBER')
if len(temp_keys) == 1:
html = (html +
'<a style="text-decoration: none" href="#regulatory">'
'<font color="#e36f1e">Regulatory</font></a> | ')
html = (html +
'<a style="text-decoration: none" href="#events">'
'<font color="#e36f1e">Events</font></a> | '
'<a style="text-decoration: none" href="#jobs">'
'<font color="#e36f1e">Jobs</font></a>')
out.extend((html,
'<!-- #MENU End -->',
'',
('</font></td></tr></table></td><td bgcolor="#ffe2c5" valign="'
'bottom" width="20" align="right" style="line-height:0;">'
'<img border="0" src="http://img.en25.com/eloquaimages/clients/'
'StemcellTechnologiesInc/%7B214e9670-0241-429d-8cfb-d1978ddcf8'
'ae%7D_Header-BottomCorner.jpg" width="15" height="15" style="'
'float:right;"></td></tr></tbody></table></td></tr><tr>'
'<td align="middle">'),
''))
return out
def topstory(flag_map, entry_map):
"""Returns formatted Top Story as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
pubs = entry_map['pubs']
banners = entry_map['banners']
out = ['<!-- #TOPSTORY Start -->',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>TOP STORY</strong></font>'
'</td></tr><tr><td style="padding-bottom: 15px;" width="100%">')]
out.extend(pubs[0][0].lineout_top(newsletter))
out.extend(
('',
'<!-- #TOPSTORY BANNER Start-->',
banners[0].banner_out(newsletter, nl_issue, 1),
'<!-- #TOPSTORY BANNER End-->'))
out.extend(('</td></tr></table>', '<!-- #TOPSTORY End -->', ''))
return out
def publications(flag_map, entry_map):
"""Returns formatted Publications as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
pubs = entry_map['pubs']
banners = entry_map['banners']
out = [
'<!-- #PUBLICATIONS Start --><a name="currentpublications"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color='
'"#003366" face="Arial" size="2"><strong>PUBLICATIONS (<a style="'
'text-decoration: none" href="http://thomsonreuters.com/products_'
'services/science/free/essays/impact_factor/"><font color="'
'#003366">Ranked by impact factor of the journal</font></a>)'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: silver;">')]
for pub in pubs[1]:
out.extend(pub.lineout(newsletter))
out.append('')
# Adds the second banner
out.extend(
('<!-- #PUBLICATIONS BANNER Start-->',
banners[1].banner_out(newsletter, nl_issue, 2),
'<!-- #PUBLICATIONS BANNER End-->'))
# Adds footer
out.extend(
('</td></tr></table>',
'<!-- #PUBLICATIONS End -->',
'',
''))
return out
def reviews(flag_map, entry_map):
"""Returns formatted Review Articles as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
revs = entry_map['revs']
banners = entry_map['banners']
out = [
'<!-- #REVIEWS Start --><a name="reviews"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr>'),
('<td style="padding-bottom: 15px;" width="100%" height="20">'
'<font color="#003366" face="Arial" size="2"><strong>REVIEWS'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: silver;">'),
'']
for rev in revs:
out.extend(rev.lineout(newsletter))
out.append('')
out.extend(
('<font color="#666666" face="Arial" size="2">Visit our <a style="text-'
'decoration: none" href="' + newsletter['url'] + 'reviews/?utm_source='
'newsletter&utm_medium=link&utm_campaign=' + newsletter[
'name_short'] +
'newsletterreviews"><font color="' + newsletter['primary_color'] +
'"><strong>reviews page</strong></font></a> to see a complete list of'
' reviews in the cell, gene '
'and immunotherapy research field.<br><br></font>', ''))
out.extend(
('<!-- #REVIEWS BANNER Start-->',
banners[2].banner_out(newsletter, nl_issue, 3),
'<!-- #REVIEWS BANNER End-->'))
out.extend(('</td></tr></table>', '<!-- #REVIEWS End -->', '', ''))
return out
def sciencenews(flag_map, entry_map):
"""Returns formatted Science News articles as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
scis = entry_map['scis']
banners = entry_map['banners']
textbanner = entry_map['textbanner']
out = [
'<!-- #SCIENCE Start --><a name="sciencenews"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20">'
'<font color="#003366" face="Arial" size="2"><strong>SCIENCE NEWS'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: silver;">'),
'']
for sci in scis:
out.extend(sci.lineout(newsletter))
out.append('')
if len(banners) == 4:
out.extend(
('<!-- #SCIENCE BANNER Start-->',
banners[3].banner_out(newsletter, nl_issue, 4),
'<!-- #SCIENCE BANNER End-->'))
else:
out.extend(('<!-- #SCIENCE BANNER Start-->',
textbanner.text_massage(newsletter, nl_issue),
'<!-- #SCIENCE BANNER End-->'))
out.extend(('</td></tr></table>', '<!-- #SCIENCE End -->', '', ''))
return out
def policynews(flag_map, entry_map):
"""Returns formatted Policy News articles as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
pols = entry_map['pols']
banners = entry_map['banners']
textbanner = entry_map['textbanner']
out = [
'<!-- #POLICY Start --><a name="policy"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>POLICY</strong></font></td>'
'</tr><tr><td style="padding-bottom: 15px; border-bottom: 1px '
'solid; border-bottom-color: silver;">'),
'']
for pol in pols[:-1]:
out.extend(pol.lineout(newsletter))
out.append('')
if len(banners) == 4:
out.extend(pols[-1].lineout(newsletter))
out.extend(('<!-- #POLICY BANNER Start-->',
textbanner.text_massage(newsletter, nl_issue),
'<!-- #POLICY BANNER End-->'))
else:
out.extend(pols[-1].lineout(newsletter, strip=True))
out.append('')
out.extend(('</td></tr></table>', '<!-- #POLICY End -->', ''))
return out
def industrynews(flag_map, entry_map):
"""Returns formatted Industry News articles a list"""
newsletter = flag_map['nl']
inds = entry_map['inds']
out = [
'<!-- #BUSINESS Start --><a name="business"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>BUSINESS</strong></font>'
'</td></tr><tr><td style="padding-bottom: 15px; border-bottom: '
'1px solid; border-bottom-color: silver;">'),
'']
for ind in inds[:-1]:
out.extend(ind.lineout(newsletter))
out.append('')
out.extend(inds[-1].lineout(newsletter, strip=True))
out.append('')
out.extend(('</td></tr></table>', '<!-- #BUSINESS End -->', '', ''))
return out
def nih(entry_map):
regs = entry_map['regs']['NIH']
out = ['<!-- #NIH Start --><a name="NIH"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>NIH</strong></font></td>'
'</tr><tr><td style="padding-bottom: 15px; border-bottom: 1px '
'solid; border-bottom-color: silver;">'),
'']
for reg in regs[:-1]:
out.extend(reg.lineout())
out.append('')
out.extend(regs[-1].linebrstrip())
out.append('')
out.extend(('</td></tr></table>',
'<!-- #NIH End -->',
''))
return out
def cber(entry_map):
regs = entry_map['regs']['CBER']
out = ['<!-- #CBER Start --><a name="CBER"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>CBER</strong></font></td>'
'</tr><tr><td style="padding-bottom: 15px; border-bottom: 1px '
'solid; border-bottom-color: silver;">'),
'']
for reg in regs[:-1]:
out.extend(reg.lineout())
out.append('')
out.extend(regs[-1].linebrstrip())
out.append('')
out.extend(('</td></tr></table>',
'<!-- #CBER End -->',
''))
return out
def regulatory(entry_map):
regs = entry_map['regs']
reg_dir = {
'FDA': 'Food and Drug Administration (United States)',
'EMA': 'European Medicines Agency (European Union)',
'MHRA': 'Medicines and Healthcare Products Regulatory Agency',
'TGA': 'Therapeutic Goods Administration'}
out = ['<!-- #REGULATORY Start --><a name="regulatory"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>REGULATORY</strong></font>'
'</td></tr><tr><td style="padding-bottom: 15px; border-bottom: 1px '
'solid; border-bottom-color: silver;">')]
ag_list = []
for agency in regs.keys():
if agency in reg_dir:
ag_list.append(agency)
for agency in ag_list[:-1]:
out.extend(['<font color="#003366" face="Arial" size="2"><strong>' +
reg_dir[agency] + '</strong></font><br><br>',
''])
for reg in regs[agency]:
out.extend(reg.lineout())
out.append('')
out.extend(['<font color="#003366" face="Arial" size="2"><strong>' +
reg_dir[ag_list[-1]] + '</strong></font><br><br>',
''])
for reg in regs[ag_list[-1]][:-1]:
out.extend(reg.lineout())
out.append('')
out.extend(regs[ag_list[-1]][-1].linebrstrip())
out.append('')
out.extend(('</td></tr></table>',
'<!-- #REGULATORY End -->',
''))
return out
def eventsnl(flag_map, entry_map):
"""Returns formatted events as a list"""
newsletter = flag_map['nl']
events = entry_map['events']
out = ['<!-- #EVENT Start --><a name="events"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>EVENTS</strong></font>'
'</td></tr><tr><td style="padding-bottom: 15px; border-bottom: '
'1px solid; border-bottom-color: silver;">'),
'']
for event in events:
out.extend(event.nlout(newsletter))
out.append('')
out.extend(
(('<font face="Arial" size="2" color="#666666">'
'Visit our <a style="text-decoration: '
'none" href="http://www.celltherapynews.com/events/?utm_source='
'newsletter&utm_medium=link&utm_campaign=ctnnewsletterevents">'
'<font color="#003366"><strong>events page</strong></font></a> to '
'see a complete list of events in the cell, gene and immunotherapy '
'community.</font>'),
'</td></tr></table>',
'<!-- #EVENT End -->',
''))
return out
def jobsnl(flag_map, entry_map):
"""Returns formatted jobs as a list"""
newsletter = flag_map['nl']
prevjobs = flag_map['prevjobs']
jobs = entry_map['jobs']
out = ['<!-- #JOB Start --><a name="jobs"></a>',
('<table width="93%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="'
'#003366" face="Arial" size="2"><strong>JOB OPPORTUNITIES</strong>'
'</font></td></tr><tr><td style="padding-bottom: 15px;">'),
'']
for job in jobs:
out.extend(job.nlout(newsletter))
out.append('')
out.extend(prevjobs)
out.extend(
('',
('<br><font size="2" face="Arial" color="#666666"><a style="text-'
'decoration: none" href="http://connexoncreative.com/job/?utm_source='
'newsletter&utm_medium=link&utm_campaign=' + newsletter['name_short'] +
'newslettersubmitjobs"><font color="' + newsletter['primary_color'] +
'"><strong>Recruit Top Talent</strong></font></a>: Reach more than '
'50,000 potential candidates by <a href="http://connexoncreative.com/'
'job/?utm_source=newsletter&utm_medium=link&utm_campaign=' +
newsletter['name_short'] + 'newslettersubmitjobs"><strong><font color="' +
newsletter['primary_color'] + '"><u>posting</u></font></strong></a>'),
(' your organization\'s career opportunities on the Connexon Creative '
'<a href="http://connexoncreative.com/jobs/?utm_source=newsletter&utm'
'_medium=link&utm_campaign=' + newsletter['name_short'] + 'newsletterjobs"><strong>'
'<font color="' + newsletter[
'primary_color'] + '"><u>Job Board</u></font></strong></a>'
' at no cost.'),
'</font></td></tr></table>',
'<!-- #JOB End -->',
''))
return out
def footer():
out = [
'<!-- #FOOTER Start -->',
('<br></td></tr><tr><td width="100%" valign="top"><div align="center">'
'<table width="715" cellspacing="0" cellpadding="0" border="0" '
'style="float:left;"><tbody><tr><td width="25" valign="top" '
'bgcolor="#ffe2c5" align="left" style="height: 40px; line-height:0;">'
'<img width="13" height="13" border="0" src="http://img.en25.com/'
'eloquaimages/clients/StemcellTechnologiesInc/%7Bd24e7bcc-00c8-'
'45b3-bc7e-714c8f097275%7D_Footer-TopCorner.jpg"></td>'),
('<td bgcolor="#ffe2c5" align="left"><font size="2" face="arial" '
'color="#005581"><strong>SUBSCRIBER TOOLS:</strong></font></td>'
'<td valign="middle" bgcolor="#ffe2c5" align="right" style="text-'
'align:right;"><a target="_blank" href="http://www.connexoncreative'
'.com/subscription/?email=<span class=eloquaemail>EmailAddress'
'</span>"><img width="104" height="20" border="0" src="http://img.'
'en25.com/eloquaimages/clients/StemcellTechnologiesInc/%7B37a164a1-'
'e0c8-49ed-b729-430daa18f0e5%7D_Footer-Subscribe.jpg" alt='
'"Subscribe"></a>'),
'<a target="_blank" href="http://www.celltherapynews.com/archives/">',
('<img width="103" height="20" border="0" src="http://img.en25.com/'
'eloquaimages/clients/StemcellTechnologiesInc/%7B07d62e04-14e3-4673-'
'970d-a07eb9ecdb1e%7D_Footer-Archives.jpg" alt="Archives"></a> '
'<a target="_blank" href="http://twitter.com/celltherapynews">'),
('<img width="27" height="27" border="0" src="http://img.en25.com/'
'eloquaimages/clients/StemcellTechnologiesInc/%7B9f944301-ed95-42ab-'
'95ab-d810a3630148%7D_Header-Twitter.jpg" alt="Twitter"></a> '
'<a target="_blank" href="http://www.facebook.com/CellTherapyNews">'
'<img width="26" height="27" border="0" src="http://img.en25.com/'
'eloquaimages/clients/StemcellTechnologiesInc/%7Bf961867c-1b7a-4426-'
'8fd0-0c61f1898fe6%7D_Header-Facebook.jpg" alt="Facebook"></a></td>'
'<td bgcolor="#ffe2c5"> </td></tr><tr><td bgcolor="#f89021" '
'align="left" colspan="2" style="line-height:0;">'),
('<img width="123" height="43" border="0" src="http://img.en25.com/'
'eloquaimages/clients/StemcellTechnologiesInc/%7Bdc45feb5-fb9e-4fde-'
'bf04-a8e4c400093e%7D_Footer-Circles.gif"></td>'),
('<td bgcolor="#f89021" align="right" style="text-align:right;"><font '
'color="#ffffff"><a target="_blank" href="http://www.connexoncreative'
'.com/subscription/?email=<span class=eloquaemail>EmailAddress'
'</span>" style="text-decoration: none"><font size="2" face="arial" '
'color="#ffffff" style="font-weight: bold">Profile Center</font></a>'),
(' | <a target="_blank" href="http://www.connexoncreative.com" '
'style="text-decoration: none"><font size="2" face="arial" '
'color="#ffffff" style="font-weight: bold">Connexon Publications'
'</font></a>'),
(' | <a target="_blank" href="http://www.connexoncreative.com/'
'publication/" style="text-decoration: none"><font size="2" '
'face="arial" color="#ffffff" style="font-weight: bold">Article '
'Submission</font></a> | <a target="_blank" '
'href="http://connexoncreative.com/contact-us/" '
'style="text-decoration: none">'),
('<font size="2" face="arial" color="#ffffff" style="font-weight: '
'bold">Contact Us</font></a></font></td><td width="20" valign='
'"bottom" bgcolor="#f89021" align="right" style="line-height:0; '
'text-align:right;"><img width="13" height="15" border="0" src="'
'http://img.en25.com/eloquaimages/clients/StemcellTechnologiesInc/'
'%7B190374c8-37fe-445f-abc3-96e9a1cd0c6a%7D_Footer-BottomCorner.jpg">'
'</td></tr></tbody></table></div>'),
'<!-- #FOOTER End -->',
'',
'</td></tr></table>',
'</body></html>']
return out
if __name__ == "__main__":
tester = ctn_main()
Connexogen.writetofile(tester, r'C:\Users\memery\Documents\macro\macro\connexogen\\')
# BUG: CXN Banner does not appear
# TODO: Integrate archival function
# TODO: Reviews don't sort
# BUG: Can't not have CBER
<file_sep>'''
This module holds functions that contain a large amount of data that will not
be modified (e.g. the Newsletter or Textad info).
'''
from ConnexogenClasses import TextBanner
def newsletter_info(newsletter):
"""Retrieves the newsletter information in dictionary format based on
the newsletter's short name (e.g. 'esc')"""
info = {
'cscn': {
'name_long': 'Cancer Stem Cell News',
'name_short': 'cscn',
'primary_color': '#0096d6',
'secondary_color': '#8dc63f',
'text_color': '#000000',
'header_image': ('http://img.en25.com/EloquaImages/clients/'
'StemcellTechnologiesInc/{79506c2c-f80b-41be-b7bf-734393b1f1b6}'
'_NewsletterEmHeader_CSCN.png'),
'twitter_url': 'http://twitter.com/cancerscnews',
'background_color': '#cccccc',
'footer_border_color': '#0096d6',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.cancerstemcellnews.com/'},
'cbn': {
'name_long': 'Cord B<NAME>',
'name_short': 'cbn',
'primary_color': '#bc8d0a',
'secondary_color': '#c41230',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{d6fd8fee-0a6f-46b4-8f78-e61e09b8947e}'
'_NewsletterEmHeader_CBN.png'),
'twitter_url': 'http://twitter.com/Cord_Blood_News',
'background_color': '#fbe6ad',
'footer_border_color': '#666666',
'footer_background_color': '#fdf6e2',
'division_list': (),
'url': 'http://www.cordbloodresearchnews.com/'},
'ecm': {
'name_long': 'Extracellular Matrix News',
'name_short': 'ecm',
'primary_color': '#91278f',
'secondary_color': '#6cb33f',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{ca3ccc30-2caa-48bb-bddd-ba92c80b981c}'
'_NewsletterEmHeader_ECM.png'),
'twitter_url': 'http://twitter.com/ExtracellMatrix',
'background_color': '#cccccc',
'footer_border_color': '#91278f',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.extracellularmatrixnews.com/'},
'hin': {
'name_long': 'Human Immunology News',
'name_short': 'hin',
'primary_color': '#5d245a',
'secondary_color': '#765dc6',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{1431f70f-ebb9-455c-84f1-2fbcac72b7d7}'
'_NewsletterEmHeader_HIN.png'),
'twitter_url': 'http://twitter.com/humanimmunenews',
'background_color': '#cccccc',
'footer_border_color': '#5d245a',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.humanimmunologynews.com/'},
'irn': {
'name_long': 'Immune Regulation News',
'name_short': 'irn',
'primary_color': '#4f2683',
'secondary_color': '#009fc2',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{f9995aac-ea5c-41f2-ba83-5ebb839427cd}'
'_NewsletterEmHeader_IRN.png'),
'twitter_url': 'http://twitter.com/Immune_News',
'background_color': '#cccccc',
'footer_border_color': '#4f2683',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.immuneregulationnews.com/'},
'iidn': {
'name_long': 'Immunology of Infectious Disease News',
'name_short': 'iidn',
'primary_color': '#512698',
'secondary_color': '#765dc6',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{5aa8e6c6-5073-453b-8afa-40cd6ca59ffa}'
'_NewsletterEmHeader_IIDN.png'),
'twitter_url': 'http://twitter.com/infdisease_news',
'background_color': '#cccccc',
'footer_border_color': '#512698',
'footer_background_color': '#dddddd',
'division_list': ('',
'HIV'),
'url': 'http://www.immunologyofinfectiousdiseasenews.com/'},
'mcn': {
'name_long': 'Mammary Cell News',
'name_short': 'mcn',
'primary_color': '#006699',
'secondary_color': '#f8981d',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{adc50aa8-9287-454e-a2a3-ac4ef53d77ca}'
'_NewsletterEmHeader_MCN.png'),
'twitter_url': 'http://twitter.com/MammaryCell',
'background_color': '#b8b8b8',
'footer_border_color': '#006699',
'footer_background_color': '#cccccc',
'division_list': ('LABORATORY RESEARCH',
'CLINICAL RESEARCH'),
'url': 'http://www.mammarycellnews.com/'},
'mescn': {
'name_long': 'Mesenchymal Cell News',
'name_short': 'mescn',
'primary_color': '#004813',
'secondary_color': '#e36f1e',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{b9f69ff7-263d-4283-aee6-b15488206cc4}'
'_NewsletterEmHeader_MESCN.png'),
'twitter_url': 'http://twitter.com/MesenchymalCell',
'background_color': '#b8b8b8',
'footer_border_color': '#004813',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.mesenchymalcellnews.com/'},
'esc': {
'name_long': 'ESC & iPSC News',
'name_short': 'esc',
'primary_color': '#3366ff',
'secondary_color': '#ff9900',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{c3e1525d-7e28-472b-90fe-2254d6bbd7ba}'
'_NewsletterEmHeader_ESC.png'),
'twitter_url': 'http://twitter.com/ESC_iPSC_News',
'background_color': '#b8b8b8',
'footer_border_color': '#666666',
'footer_background_color': '#cccccc',
'division_list': (),
'url': 'http://www.escellnews.com/'},
'ncn': {
'name_long': 'Neural Cell News',
'name_short': 'ncn',
'primary_color': '#00853f',
'secondary_color': '#ff6600',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{e3376acd-afea-4df8-8f89-e098e1ccaa67}'
'_NewsletterEmHeader_NCN.png'),
'twitter_url': 'http://twitter.com/NeuralCell',
'background_color': '#b8b8b8',
'footer_border_color': '#666666',
'footer_background_color': '#dddddd',
'division_list': (),
'url': 'http://www.neuralcellnews.com/'},
'pacn': {
'name_long': 'Pancreatic Cell News',
'name_short': 'pacn',
'primary_color': '#e36f1e',
'secondary_color': '#a25018',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{82e32703-bd89-4603-ba3d-3fcf5f32564b}'
'_NewsletterEmHeader_PACN.png'),
'twitter_url': 'http://twitter.com/PancreaticCell',
'background_color': '#cccccc',
'footer_border_color': '#e36f1e',
'footer_background_color': '#dddddd',
'division_list': ('DIABETES & PANCREATITIS',
'PANCREATIC CANCER'),
'url': 'http://www.pancreaticcellnews.com/'},
'pcn': {
'name_long': 'Prostate Cell News',
'name_short': 'pcn',
'primary_color': '#0055a4',
'secondary_color': '#e36f1e',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{4900c798-bfa4-4776-9ce7-fafd716040e0}'
'_NewsletterEmHeader_PCN.png'),
'twitter_url': 'http://twitter.com/ProstateCell',
'background_color': '#e8e8e8',
'footer_border_color': '#0055a4',
'footer_background_color': '#dddddd',
'division_list': ('LABORATORY RESEARCH',
'CLINICAL RESEARCH'),
'url': 'http://www.prostatecellnews.com/'},
'pulcn': {
'name_long': 'Pulmonary Cell News',
'name_short': 'pulcn',
'primary_color': '#5d245a',
'secondary_color': '#98012e',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{32370387-17b3-46a9-a784-880a31a7b19f}'
'_NewsletterEmHeader_PULCN.png'),
'twitter_url': 'http://twitter.com/pulmonary_news',
'background_color': '#cccccc',
'footer_border_color': '#5d245a',
'footer_background_color': '#dddddd',
'division_list': ('',
'LUNG CANCER'),
'url': 'http://www.pulmonarycellnews.com/'},
'hn': {
'name_long': '<NAME>',
'name_short': 'hn',
'primary_color': '#990033',
'secondary_color': '#69321f',
'text_color': '#000000',
'header_image':
('http://img.en25.com/EloquaImages/clients/StemcellTechnologiesInc/'
'{c3621d49-7491-408f-93a3-1f2692fc9915}'
'_NewsletterEmHeader_HN.png'),
'twitter_url': 'http://twitter.com/Hema_News',
'background_color': '#cccccc',
'footer_border_color': '#990033',
'footer_background_color': '#dddddd',
'division_list': ('',
'CLINICAL RESEARCH'),
'url': 'http://www.hematopoiesisnews.com/'}}
return info[newsletter]
def get_textbanner(text_banner_name):
"""Return a textbanner based solely on it's name as it appears in
advertising schedule. Default utm_source is cxn"""
text_banners ={
'HIP_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong><br> View immunology lectures, '
'protocols and other resources on the </font><strong><em>Human '
'Immunology Portal</em>.</strong>'),
('http://www.humanimmunologyportal.com/?utm_source=cxn%26utm_medium='
'link%26utm_campaign=hipgeneral')),
'HIV_wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Free <em>Nature Reviews Immunology'
'</em> poster - Immune Response to HIV.</font><strong> Request a copy'
'.</strong>'),
('http://www.stemcell.com/Home/Forms/Wallcharts/Wallchart%20Immune%20'
'Response%20to%20HIV.aspx?utm_source=cxn%26utm_medium=link%26utm_'
'campaign=cshivposter')),
'HIV_profiles_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Learn how <em>ex vivo</em> models '
'drive progress in HIV research. </font><strong>Read the research '
'profiles.</strong>'),
('http://www.stemcell.com/en/Pages/HIV-Case-Studies.aspx?utm_source='
'cxn%26utm_medium=link%26utm_campaign=hivresearchprofiles')),
'Mouse_hema_wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Free mouse hematopoietic '
'progenitors wallchart. </font><strong>Request your copy.</strong>'),
('http://www.stemcell.com/Home/Forms/Wallcharts/Wallchart%20Mouse%20'
'Hematopoietic%20Progenitors.aspx?id={16CC3A92-DE41-46B0-A84C-'
'7AD83E7F8CB3}%26utm_source=cxn%26utm_medium=link%26utm_campaign='
'MethoCultWallchart')),
'Human_hema_wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Free human hematopoietic '
'progenitors wallchart.</font><strong> Request your copy.</strong>'),
('http://www.stemcell.com/Home/Forms/Wallcharts/Wallchart%20Human%20'
'Hematopoietic%20Progenitors.aspx?id={6B493CC5-5E99-45AF-A607-'
'7476868E88C0}%26utm_source=cxn%26utm_medium=link%26utm_campaign='
'ON225-HemaHumanWallchart_645x110')),
'Mesenchymal_wallchart_CXN_textad':TextBanner(
('<strong>New wallchart from our sponsor:</strong><br> Mesenchymal Stem'
' Cells (a <em>Nature Reviews Molecular Cell Biology</em> '
'collaboration).</font><strong>Request your free copy.</strong>'),
('http://www.stemcell.com/forms/wallcharts/wallchart-mesenchymal-stem-'
'cells?utm_source=cxn%26utm_medium=link%26utm_campaign='
'naturemscwallchart_645x110 ')),
'Neural_wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Free wallchart from <em>Nature '
'Neuroscience</em> - Neural Stem Cells. </font><strong>Request your '
'copy.</strong>'),
('http://www.stemcell.com/Home/Forms/Wallcharts/Wallchart Neural Stem '
'Cells.aspx?utm_source=cxn%26utm_medium=link%26utm_campaign='
'NeuralWallchart_645x110-RequestWallchart')),
'Regen_Med_Wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Pluripotent cell isolation for '
'regenerative medicine.</font> <strong>Request a free wallchart from '
'<em>Nature</em>.</strong>'),
('http://www.stemcell.com/Home/Forms/Wallcharts/Wallchart%20Pluripotent'
'%20Stem%20Cell%20Biology.aspx?utm_source=cxn%26utm_medium=link%26'
'utm_campaign=PluriWall_645x110')),
'Cord_blood_techbull_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong> Learn about assays for cord blood.'
' </font><strong>Download the free technical bulletin.</strong>'),
('http://www.stemcell.com/en/Technical-Resources/228ae/29331-The-CFC-'
'Assay-For-Cord-Blood.aspx?utm_source=cxn%26utm_medium=link%26utm_'
'campaign=ON218-CordBloodTechBull_645x110')),
'hESC_iPSC_webinar_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong><br> Learn About Groundbreaking '
'Applications for Feeder-Free hESC & hiPSC Culture. </font><strong>'
'Watch Webinar Now</strong>'),
('http://www.stemcell.com/en/Technical-Resources/0009e/Groundbreaking-'
'Applications-of-Feeder-Free-hESC-hiPSC-Culture.aspx?utm_source=cxn'
'%26utm_medium=link%26utm_campaign=recordedwebinar_mtesr1applications'
'_645x110')),
'ALDEFLUOR_vid_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong><br> Looking for ALDH<sup>+</sup> '
'cancer stem cells? </font><strong>Watch a video to see how '
'ALDEFLUOR™ can help.</strong>'),
('http://www.stemcell.com/en/Technical-Resources/c9294/ALDEFLUOR-Detect'
'-normal-and-cancer-precursor-cells.aspx?utm_source=cxn%26utm_medium='
'link%26utm_campaign=aldefluorquickvid')),
'Mamary_wallchart_CXN_textad':TextBanner(
('<strong>From our sponsor:</strong><br> Interested in assays for human'
' mammary stem and progenitor cells? </font><strong>Request your free'
' wallchart.</strong>'),
('http://www.stemcell.com/en/Forms/Wallcharts/Wallchart-Assays-for-'
'Human-Mammary-Stem-and-Progenitor-Cells.aspx?utm_source=cxn%26utm_'
'medium=link%26utm_campaign=%09ON107-MammaryWallchart-645x110_CTA_'
'RequestYH'))
}
return text_banners[text_banner_name]
<file_sep># -*- coding: utf-8 -*-
"""
The Connexogen Module generates a fully formed HTML Connexon Newsletter from
an appropriate a properly formatted text file"""
import Tkinter
import tkFileDialog
import os
import codecs
import collections
import shutil
import re
import time
import csv
from ConnexogenRetrieval import newsletter_info, get_textbanner
from ConnexogenClasses import Job, Event, \
Banner, PolicyNews, Publication, ScienceNews, Review, IndustryNews
def foi(newsletter):
"""Field of interest. changes the '_____ community' sentence at the
bottom of publications and events"""
# Regex expression. Note that string.strip could strip the 's' off of
# 'Hematopoiesis'
field = re.sub(' News', '', newsletter['name_long'])
# ESC & iPSC are always capitalized
if newsletter['name_short'] != 'esc':
field = field.lower()
return field
def full_gen(source):
"""Creates a default dictionary that returns a tuple containing a
sentinal string and all indices where that string appears"""
query = {'>>-->>Banner 1 Name>>': 'banners',
'>>-->>Banner 2 Name>>': 'banners',
'>>-->>MP_Banner Name>>': 'banners',
'>>-->>Text Banner Name>>': 'textbanner',
'>>-->>Publication>>': 'pubs',
'>>-->>Review>>': 'revs',
'>>-->>Industry Title>>': 'inds',
'>>-->>Science Title>>': 'scis',
'>>-->>Policy Title>>': 'pols',
'>>-->>Event Title>>': 'events',
'>>-->>Job Title>>': 'jobs',
}
line_count = 0
sentinals = collections.defaultdict(list)
for line in source:
for cue in query:
if cue in line:
sentinals[query[cue]].append(line_count)
line_count += 1
return sentinals
def entry_mapper(source, sentinals):
"""A very important function. This function takes makes all the entries
to their appropriate function and returns a dictionary of all entries in the
newsletter"""
func_map = {
'banners': banner_gen(source, sentinals['banners']),
'textbanner': textbanner_gen(source,
sentinals['textbanner']),
'pubs': pub_gen(source, sentinals['pubs']),
'revs': rev_gen(source, sentinals['revs']),
'inds': ind_gen(source, sentinals['inds']),
'scis': sci_gen(source, sentinals['scis']),
'pols': pol_gen(source, sentinals['pols']),
'events': event_gen(source, sentinals['events']),
'jobs': job_gen(source, sentinals['jobs'])}
return func_map
def pub_gen(source, indices):
"""Generates list of Publications from source"""
top = []
div1 = []
div2 = []
pubs = []
for index in indices:
if source[index + 8] != '':
# splits the anchor and link list strings into lists
anchor_list = source[index + 14].split(', ')
link_list = source[index + 16].split(', ')
topstory = False
if source[index + 4] == 'Y':
topstory = True
pub = Publication(
source[index + 2],
source[index + 8],
source[index + 10],
source[index + 12],
anchor_list,
link_list,
source[index + 6], # Impact Factor
source[index + 18],
topstory)
if pub.topstory or anchor_list[0] == 'Press Release':
pub.set_impact(100)
elif pub.impact == '':
pub.set_impact(pub.lookup_impact())
else:
pub.set_impact(float(pub.impact))
if topstory:
top.extend([pub])
elif pub.division == '1' or pub.division == '':
div1.extend([pub])
elif pub.division == '2':
div2.extend([pub])
div1.sort(reverse=True)
div2.sort(reverse=True)
# if Top Story section is empty then pop the highest impact publication
# from div1
if not top:
story = div1.pop(0)
top.append(story)
assert (len(top) == 1), "More than one top story!"
pubs.extend([top])
pubs.extend([div1])
pubs.extend([div2])
return pubs
def banner_gen(source, indices):
"""Generates banners from Source"""
banners = []
for index in indices:
if source[index + 3] != '':
banner = Banner(
source[index + 1],
source[index + 5],
source[index + 3],
source[index + 7])
banners.append(banner)
return banners
def textbanner_gen(source, index):
return get_textbanner(source[index[0] + 1])
def contestbanner_gen():
"""Provide Contest Banner.I haven't seen one in a while so it hasn't been
implemented"""
print 'Not implemented yet!'
# All generators after pub_gen are less complicated versions of that generator
# It may be worth it to actually have all objects feed through a single generator
def rev_gen(source, indices):
"""Generates Review articles from source"""
revs = []
for index in indices:
if source[index + 6] != '':
anchor_list = source[index + 14].split(', ')
link_list = source[index + 16].split(', ')
rev = Review(
source[index + 12],
source[index + 4],
source[index + 6],
source[index + 8],
anchor_list,
link_list,
source[index + 2],
source[index + 10])
if rev.impact == '':
rev.lookup_impact()
else:
rev.set_impact(float(rev.impact))
revs.extend([rev])
revs.sort(reverse=True)
return revs
def sci_gen(source, indices):
"""Generates Science News articles from source"""
scis = []
for index in indices:
if source[index + 1] != '':
sci = ScienceNews(None,
source[index + 1],
source[index + 3],
source[index + 5],
['Press Release'],
[source[index + 7]])
scis.extend([sci])
return scis
def ind_gen(source, indices):
"""Generates Industry News articles from source"""
inds = []
for index in indices:
if source[index + 1] != '':
if source[index + 9] == '':
anchors = ['Press Release']
else:
anchors = source[index + 9].split(', ')
ind = IndustryNews(None, source[index + 1], source[index + 3],
source[index + 5], anchors, [source[index + 7]])
inds.extend([ind])
return inds
def pol_gen(source, indices):
"""Generates Policy News articles from source"""
pols = []
for index in indices:
if source[index + 1] != '':
pol = PolicyNews(None,
source[index + 1],
source[index + 3],
'<em>' + source[index + 5] + '</em>',
['Editorial'],
[source[index + 7]])
pols.extend([pol])
return pols
def event_gen(source, indices):
"""Generates events from source"""
events = []
for index in indices:
if source[index + 1] != '':
event = Event(title=source[index + 1],
link=source[index + 3],
date=source[index + 5],
location=source[index + 7],
name='#EVENT')
events.extend([event])
events.sort()
return events
def job_gen(source, indices):
"Generates jobs from source"""
jobs = []
for index in indices:
if source[index + 1] != '':
job = Job(title=source[index + 1],
org=source[index + 3],
location=source[index + 5],
date=source[index + 7],
job_type=source[index + 9],
requirement=source[index + 11],
link=source[index + 13])
jobs.extend([job])
return jobs
def sourcereader():
"""Creates a file dialog so that you may select the appropriate
newsletter"""
# Calls an Tkinter UI object then immediately hides it
# Need to do this for tkFileDialog
root = Tkinter.Tk()
root.withdraw()
source = tkFileDialog.askopenfile(
parent=root,
initialdir=r"S:\CXN - Connexon\CXN Publications",
title="Select Plaintext")
outdir = os.path.dirname(source.name)
source_list = []
for line in source:
line = line.decode('utf-8')
line = line.strip('\n')
source_list.append(line)
source.close()
assert '>>-->>Newsletter Name>>' in source_list[0], \
'You have not selected a Connexon template textfile.'
return source_list, outdir
def sourcereader_test():
"""A simple version of sourcereader that is used in unit testing"""
with open(
r'S:\CXN - Connexon\CXN Publications\Extracellular Matrix News\Text Versions\''
r'Extracellular Matrix News 5.XX (2014)\ECM 5.30.htm',
'r') as source:
outdir = os.path.dirname(source.name)
source_list = []
for line in source:
line = line.decode('utf-8')
line = line.strip('\n')
source_list.append(line)
source.close()
return source_list, outdir
def true_main(mode):
"""The Main function, executes all operations except archival()"""
if mode == 'test':
source = sourcereader_test()
else:
source = sourcereader()
twitter_csv = os.path.expanduser(r"~\Desktop\twitter.csv")
twitter = open(twitter_csv, 'wb')
twitter.close()
entries = entry_mapper(source[0], full_gen(source[0]))
flags = flag_mapper(source[0], nl_flags(source[0]))
out = []
out.extend(header(flags))
out.extend(menu(flags, entries))
out.extend(topstory(flags, entries))
out.extend(publications(flags, entries))
if len(entries['revs']) != 0:
out.extend(reviews(flags, entries))
if len(entries['scis']) != 0:
out.extend(sciencenews(flags, entries))
out.extend(industrynews(flags, entries))
out.extend(policynews(flags, entries))
out.extend(eventsnl(flags, entries))
out.extend(jobsnl(flags, entries))
out.extend(footer(flags))
writetofile(out, source[1])
wordpress(flags, entries, source[1])
return out
def nl_flags(source):
"""Creates a default dictionary that returns a tuple containing a
sentinal string and all indices where that string appears"""
query = {'>>-->>Newsletter Name>>': 'nl',
'>>-->>Newsletter Issue Number>>': 'issue',
'>>-->>Newsletter Issue Date>>': 'date',
# '>>-->>Subject Line>>':'subjectline',
'>>-->>Homepage Banner Name>>': 'hpbanner',
'>>-->>Old Jobs>>': 'oldjobs',
">>-->>Insert last week's jobs' HTML here>>": 'prevjobs',
'>>-->>end of plaintext>>-->>': 'end'}
line_count = 0
# should probably convert to a regular dictionary
sentinals = collections.defaultdict(list)
for line in source:
for cue in query:
if cue in line:
sentinals[query[cue]].append(line_count)
line_count += 1
return sentinals
def flag_mapper(source, sentinals):
"""Maps the nl_flags sentinals to the appropriate function and stores that
information in a dictionary. When creating a new function dependent on a
flag, call it from here"""
func_map = {
'nl': nl(source, sentinals['nl']),
'issue': issue_number(source, sentinals['issue']),
'date': issue_date(source, sentinals['date']),
# 'subjectline':subjectline(source, sentinals['subjectline']),
'hpbanner': homepagebanner(source, sentinals['hpbanner']),
'oldjobs': job_grab(source, (sentinals['oldjobs'],
sentinals['prevjobs'])),
'prevjobs': job_grab(source, (sentinals['prevjobs'],
sentinals['end'])),
'end': end_init()}
return func_map
def nl(source, index):
"""returns all the newsletter information"""
newsletter = source[index[0] + 1].lower()
return newsletter_info(newsletter)
def issue_number(source, index):
"""returns the Issue Number"""
nl_issue = float(source[index[0] + 1])
# need format for issues ending in 0
return format(nl_issue, '.2f')
def issue_date(source, index):
"""Returns the issue date"""
nl_date = str(source[index[0] + 1])
return time.strptime(nl_date, '%B %d, %Y')
def subjectline(source, index):
"""Returns the subject line. This will be integrated into a csv writing
function some time in the future"""
return source[index[0] + 1]
def homepagebanner(source, index):
"""Returns the homepage banner in the proper format"""
hpbanner = banner_gen(source, index)
return hpbanner[0].homebanner_out()
def job_grab(source, indices):
"""This function is used to grab all the lines between the Old Jobs
tag and the Previous Jobs tag, or all the lines between the Previous Jobs
tag"""
return [line for line in source[indices[0][0] + 1:indices[1][0] - 1]]
def end_init():
"""I put this here so that the end tag would have a function mapped to it
This doesn't do anything for now."""
print "All flags loaded"
def header(flag_map):
"""Creates the header"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
nl_date = flag_map['date']
out = [
'<html>',
'<head>',
'<title>Newsletter Issue</title>',
('<style type="text/css">a:link {text-decoration: none; color:' +
newsletter['primary_color'] + ';}a:visited {text-decoration: none; color:' +
newsletter['primary_color'] + ';}a:active {text-decoration: none; color:' +
newsletter['primary_color'] +
';}a:hover {text-decoration: underline; color:' + newsletter['primary_color'] +
';}td {font-family: arial; font-size: 12px;}</style>'),
'</head>',
'<body>',
('<table class="webfix" style="border: 10px solid ' + newsletter['background_color'] +
'; horizontal-align: center;" bgcolor="' + newsletter['background_color'] +
'" align="center" width="755" border="0" cellpadding="0" '
'cellspacing="0"><tr><td width="100%"><div align="center">'
'<font color="#666666" size="1" face="Verdana">'),
'',
'<!-- #ISSUE Start -->',
(newsletter['name_long'] + ' ' + nl_issue + ' ' +
time.strftime("%B %d, %Y", nl_date).replace(' 0', ' ')),
'<!-- #ISSUE End-->',
'',
'</font></div><img border="0" alt="' + newsletter['name_long'] +
'" src="' + newsletter['header_image'] +
('" width="749" height="115"></td></tr><tr><td style="border-top: 1px '
'solid ') + newsletter['primary_color'] + '; border-bottom: 1px solid ' +
newsletter['primary_color'] + '; background-color: ' +
newsletter['footer_background_color'] + '; vertical-align: middle;" bgcolor="' +
newsletter['footer_background_color'] +
('" valign="middle" width="100%" height="30"><table border="0"'
' cellspacing="0" cellpadding="0" width="100%" height="30"><tr>'
'<td valign="middle"><font size="2" face="arial" color="#666666">'),
]
return out
def menu(flag_map, entry_map):
"""Creates the menu, will not produce science news or review links if
you do not have any of those type of articles."""
newsletter = flag_map['nl']
out = ['', '<!-- #MENU Start -->']
menu = ('<strong> In this issue:</strong> '
'<a href="#currentpublications" style="text-decoration: none">'
'<font color="' + newsletter['primary_color'] + '"><strong>Publications</strong>'
'</font></a> |')
if len(entry_map['revs']) != 0:
menu = (
menu + ' <a href="#reviews" style="text-decoration: none">'
'<font color="' + newsletter['primary_color'] + '"><strong>Reviews</strong>'
'</font></a> |')
if len(entry_map['scis']) != 0:
menu = (
menu + ' <a href="#sciencenews" style="text-decoration: '
'none"><font color="' + newsletter['primary_color'] + '"><strong>Science '
'News</strong></font></a> |')
menu = (
menu + ' <a href="#industrynews" style="text-decoration: none">'
'<font color="' + newsletter['primary_color'] + '"><strong>Industry News</strong>'
'</font></a> | <a href="#policynews" style="text-decoration:none"><font color="' +
newsletter[
'primary_color'] + '"><strong>Policy News'
'</strong></font></a> | <a href="#events" style='
'"text-decoration: none"><font color="' + newsletter['primary_color'] +
'"><strong>Events</strong></font></a> | <a href='
'"#jobopportunities" style="text-decoration: none"><font color'
'="' + newsletter['primary_color'] + '"><strong>Jobs</strong></font></a>')
out.extend(
(menu,
'<!-- #MENU End -->',
'',
(
'</font></td><td><div align="center"><a href="http://www.facebook'
'.com/CellTherapyNews"><img border=0 alt="Cell Therapy News on '
'Facebook" src="http://img.en25.com/eloquaimages/clients/'
'stemcelltechnologiesinc/%7B5d3d9c70-66c9-4e82-b056-abd80aef045e'
'%7D_social_default_facebook_icon.jpg" width="16" height="16">'
'</a> <a href="' + newsletter['twitter_url'] + '"><img border="0"'
' alt="' + str(newsletter['name_short']).upper()
+ ' on Twitter" src="http://img.en25.com/eloquaimages/clients/stemcelltechnologiesinc/'
'%7B361a5c5c-663f-40fa-82b7-01c545266032%7D_social_default_'
'twitter_icon.jpg" width="16" height="16"></a></div></td></tr>'
'</table></td></tr><tr>'),
('<td style="padding-left: 50px; padding-right: 50px; background-'
'color: #ffffff;" bgcolor="#ffffff">'),
'',
'<!-- #TOPSTORY Start -->',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td '
'style="padding-bottom: 15px;" width="100%" height="20"><font '
'color="' + newsletter['primary_color'] + '" face="Arial" size="3"><strong>'
'TOP STORY</strong></font></td></tr><tr><td style="padding-'
'bottom: 15px;" width="100%">')))
return out
def topstory(flag_map, entry_map):
"""Returns formatted Top Story as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
pubs = entry_map['pubs']
banners = entry_map['banners']
out = []
out.extend(pubs[0][0].lineout_top(newsletter))
out.extend(
('',
'<!-- #TOPSTORY BANNER Start-->',
banners[0].banner_out(newsletter, nl_issue, 1),
'<!-- #TOPSTORY BANNER End-->'))
out.extend(('</td></tr></table>', '<!-- #TOPSTORY End -->', ''))
return out
def publications(flag_map, entry_map):
"""Returns formatted Publications as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
pubs = entry_map['pubs']
banners = entry_map['banners']
out = []
out.extend(
('<!-- #PUBLICATIONS Start --><a name="currentpublications"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style='
'"padding-bottom: 15px;" width="100%" height="20"><strong>'
'<font color="' + newsletter['primary_color'] +
'" face="Arial" size="3">PUBLICATIONS</font></strong> <strong><font size="2" color="' +
newsletter['primary_color'] + '">(<a style="text-decoration: none" href="'
'http://thomsonreuters.com/products_services/science/free/essays/'
'impact_factor/"><font color="' + newsletter['primary_color'] + '">Ranked by '
'impact factor of the journal</font></a>)</font></strong></td>'
'</tr><tr><td style="padding-bottom: 15px; border-bottom: 1px '
'solid; border-bottom-color: #808080;">'),
''))
if len(newsletter['division_list']) == 2 and newsletter['division_list'][0] != '': # for named primaries
out.extend(
('<font color="' +
newsletter['primary_color'] +
'" face="Arial" size="2"><strong>' +
newsletter['division_list'][0] +
'</strong></font><br><br>',
''))
for pub in pubs[1]:
out.extend(pub.lineout(newsletter))
out.append('')
# if a second division exists, fill second division
if len(pubs[2]) > 0:
out.extend(
('<font color="' +
newsletter['primary_color'] +
'" face="Arial" size="2"><strong>' +
newsletter['division_list'][1] +
'</strong></font><br><br>',
''))
for pub in pubs[2]:
out.extend(pub.lineout(newsletter))
out.append('')
# Subscribe text for Immunology newsletters
if newsletter['name_short'] == 'hin' or newsletter['name_short'] == 'iidn' or newsletter['name_short'] == 'irn':
if newsletter['name_short'] == 'hin':
out.extend(
[
('<font size="2" color="#000000"><a target="_blank" style="'
'text-decoration: none" href="http://connexoncreative.com/'
'subscribe/?utm_source=newsletter&utm_medium=link&utm_camp'
'aign=hinnewslettersubscribe"><strong><font color="' +
newsletter['primary_color'] + '">Subscribe</font></strong></a> to our sister'
' publications: <a target="_blank" style="text-decoration:'
' none" href="http://www.immunologyofinfectiousdiseasenews'
'.com/"><strong><em><font color="' + newsletter['primary_color'] +
'">Immunology of Infectious Disease News</font></em>'
'</strong></a> and <a target="_blank" style="text-'
'decoration: none" href="http://www.immuneregulationnews.'
'com/"><em><strong><font color="' + newsletter['primary_color'] +
'">Immune Regulation News</font></strong></em></a></font>!'
'<br><br>'), ''])
elif newsletter['name_short'] == 'iidn':
out.extend(
[
('<font size="2" color="#000000">Don\'t forget to <a target'
'="_blank" style="text-decoration: none" href="http://connexon'
'creative.com/subscribe/?utm_source=newsletter&utm_medium='
'link&utm_campaign=iidnnewslettersubscribe"><strong><font '
'color="' + newsletter['primary_color'] + '">subscribe</font></strong></a> to '
'our sister publications: <a target="_blank" style="text-decoration: none" href="'
'http://www.humanimmunologynews.com/"><strong><em><font color="' +
newsletter[
'primary_color'] + '">Human Immunology'
' News</font></em></strong></a> and <a target="_blank" style="'
'text-decoration: none" href="http://www.immuneregulationnews.'
'com/"><em><strong><font color="' + newsletter[
'primary_color'] + '">Immune '
'Regulation News</font></strong></em></a></font>!<br><br>'),
''])
else:
out.extend(
[
('<font size="2" color="#000000"><a target="_blank" style="text'
'-decoration: none" href="http://connexoncreative.com/subscrib'
'e/?utm_source=newsletter&utm_medium=link&utm_campaign=irnnews'
'lettersubscribe"><strong><font color="' + newsletter['primary_color'] +
'">Subscribe</font></strong></a> to our sister publications:'
'<br> <a target="_blank" style="text-decoration: none" href="'
'http://www.humanimmunologynews.com/"><strong><em><font color="' +
newsletter['primary_color'] + '">Human Immunology News</font></em></strong></a>'
' and <a target="_blank" style="text-decoration: none" href="'
'http://www.immunologyofinfectiousdiseasenews.com/"><em>'
'<strong><font color="' + newsletter[
'primary_color'] + '">Immunology of Infect'
'ious Disease News</font></strong></em></a></font>!<br><br>'),
''])
# Adds the second banner
out.extend(
('<!-- #PUBLICATIONS BANNER Start-->',
banners[1].banner_out(newsletter, nl_issue, 2),
'<!-- #PUBLICATIONS BANNER End-->'))
# Adds footer
out.extend(
('</td></tr></table>',
'<!-- #PUBLICATIONS End -->',
'',
''))
for pub_set in pubs:
for pub in pub_set:
make_twitter_csv(pub, "pub")
return out
def reviews(flag_map, entry_map):
"""Returns formatted Review Articles as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
revs = entry_map['revs']
banners = entry_map['banners']
out = [
'<!-- #REVIEWS Start --><a name="reviews"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>REVIEWS</strong>'
'</font></td></tr><tr><td style="padding-bottom: 15px; border-'
'bottom: 1px solid; border-bottom-color: #808080;">'),
'']
for rev in revs:
out.extend(rev.lineout(newsletter))
out.append('')
out.extend(
('<font color="#000000" face="Arial" size="2">Visit our <a style="text-'
'decoration: none" href="' + newsletter['url'] + 'reviews/?utm_source='
'newsletter&utm_medium=link&utm_campaign=' + newsletter[
'name_short'] +
'newsletterreviews"><font color="' + newsletter['primary_color'] +
'"><strong>reviews page</strong></font></a> to see a complete list of '
'reviews in the ' + foi(newsletter) + ' research field.<br><br></font>', ''))
if len(banners) > 2:
# Note that I use '4' as the banner number to avoid appending iii
# Instead of _mp
out.extend(
('<!-- #REVIEWS BANNER Start-->',
banners[2].banner_out(newsletter, nl_issue, 4),
'<!-- #REVIEWS BANNER End-->'))
else:
out[-2] = re.sub('<br><br>', '', out[-2])
out.extend(('</td></tr></table>', '<!-- #REVIEWS End -->', '', ''))
space_in_csv(["Reviews"])
for rev in revs:
make_twitter_csv(rev, "rev")
return out
def sciencenews(flag_map, entry_map):
"""Returns formatted Science News articles as a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
scis = entry_map['scis']
textbanner = entry_map['textbanner']
out = [
'<!-- #SCIENCE Start --><a name="sciencenews"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>SCIENCE NEWS'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: #808080;">'),
'']
for sci in scis:
out.extend(sci.lineout(newsletter))
out.append('')
out.extend(('<!-- #SCIENCE BANNER Start-->',
textbanner.text_massage(newsletter, nl_issue),
'<!-- #SCIENCE BANNER End-->'))
out.extend(('</td></tr></table>', '<!-- #SCIENCE End -->', '', ''))
space_in_csv(["Science News"])
for sci in scis:
make_twitter_csv(sci, "sci")
return out
def industrynews(flag_map, entry_map):
"""Returns formatted Industry News articles a list"""
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
scis = entry_map['scis']
inds = entry_map['inds']
textbanner = entry_map['textbanner']
out = [
'<!-- #INDUSTRY Start --><a name="industrynews"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>INDUSTRY NEWS'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: #808080;">'),
'']
for ind in inds[:-1]:
out.extend(ind.lineout(newsletter))
out.append('')
if len(scis) > 0:
out.extend(inds[-1].lineout(newsletter, strip=True))
out.append('')
else:
out.extend(inds[-1].lineout(newsletter))
out.extend(('<!-- #INDUSTRY BANNER Start-->',
textbanner.text_massage(newsletter, nl_issue),
'<!-- #INDUSTRY BANNER End-->',
''))
out.extend(('</td></tr></table>', '<!-- #INDUSTRY End -->', '', ''))
space_in_csv(["Industry News"])
for ind in inds:
make_twitter_csv(ind, "ind")
return out
def policynews(flag_map, entry_map):
"""Returns formatted Policy News articles as a list"""
newsletter = flag_map['nl']
pols = entry_map['pols']
out = [
'<!-- #POLICY Start --><a name="policynews"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>POLICY NEWS</strong>'
'</font></td></tr><tr><td style="padding-bottom: 15px; border-'
'bottom: 1px solid; border-bottom-color: #808080;">'),
'']
if len(pols) > 0 and newsletter['name_short'] != 'esc':
for pol in pols:
out.extend(pol.lineout(newsletter))
out.append('')
if newsletter['name_short'] != 'esc':
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="http://grants.nih.gov'
'/grants/guide/index.html"><strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->National Institutes of Health '
'(United States)<!-- End --></font></strong></a><br><br>'),
'<!-- #POLICY SECTION End -->',
''))
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="'
'http://www.fda.gov/Drugs/GuidanceComplianceRegulatory'
'Information/Guidances/ucm121568.htm"><strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->Food and Drug Administration '
'(United States)<!-- End --></font></strong></a><br><br>'),
'<!-- #POLICY SECTION End -->',
''))
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="http://www.fda.gov/'
'BiologicsBloodVaccines/NewsEvents/default.htm">'
'<strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->Center for Biologics Evaluation and '
'Research (United States)<!-- End --></font></strong></a>'
'<br><br>'),
'<!-- #POLICY SECTION End -->',
''))
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="http://bit.ly/'
'1j4Ivvu"><strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->European Medicines Agency '
'(European Union)<!-- End --></font></strong></a><br><br>'),
'<!-- #POLICY SECTION End -->',
''))
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="http://www.mhra.gov.uk'
'/NewsCentre/index.htm"><strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->Medicines and Healthcare Products '
'Regulatory Agency (United Kingdom)<!-- End --></font>'
'</strong></a><br><br>'),
'<!-- #POLICY SECTION End -->',
''))
out.extend(
('<!-- #POLICY SECTION Start -->',
('<a style="text-decoration: none" href="http://www.tga.gov.'
'au/newsroom/news-latest.htm"><strong><font color="') +
newsletter['primary_color'] +
'" size="2" face="Arial">',
('<!-- #POLICY TITLE-->Therapeutic Goods Administration '
'(Australia)<!-- End --></font></strong></a>'),
'<!-- #POLICY SECTION End -->'))
if newsletter['name_short'] == 'esc':
for pol in pols[:-1]:
out.extend(pol.lineout(newsletter))
out.append('')
out.extend(pols[-1].lineout(newsletter, strip=True))
out.append('')
out.extend(('</td></tr></table>', '<!-- #POLICY End -->', ''))
if len(pols) != 0:
space_in_csv(["Policy News"])
for pol in pols:
make_twitter_csv(pol, "pol")
return out
def eventsnl(flag_map, entry_map):
"""Returns formatted events as a list"""
newsletter = flag_map['nl']
events = entry_map['events']
out = [
'<!-- #EVENT Start --><a name="events"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>EVENTS</strong>'
'</font></td></tr><tr><td style="padding-bottom: 15px; border-'
'bottom: 1px solid; border-bottom-color: #808080;">'),
'']
for event in events:
out.extend(event.nlout(newsletter))
out.append('')
out.extend(
(('<font face="Arial" size="2">Visit our '
'<a style="text-decoration: none" href="') +
newsletter['url'] +
'events/?utm_source=newsletter&utm_medium=link&utm_campaign=' +
newsletter['name_short'] +
'newsletterevents"><font color="' +
newsletter['primary_color'] +
('"><strong>events page</strong></font></a> to see a complete '
'list of events in the ') +
foi(newsletter) +
' community.</font>',
'</font></font></font></td></tr></table>',
'<!-- #EVENT End -->',
'',
''))
return out
# Should has persistance
def jobsnl(flag_map, entry_map):
"""Returns formatted jobs as a list"""
newsletter = flag_map['nl']
prevjobs = flag_map['prevjobs']
jobs = entry_map['jobs']
out = ['<!-- #JOB Start --><a name="jobopportunities"></a>',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;" width="100%" height="20"><font color="' +
newsletter['primary_color'] + '" face="Arial" size="3"><strong>JOB OPPORTUNITIES'
'</strong></font></td></tr><tr><td style="padding-bottom: 15px; '
'border-bottom: 1px solid; border-bottom-color: #808080;">'),
'']
for job in jobs:
out.extend(job.nlout(newsletter))
out.append('')
out.extend(prevjobs)
out.extend(
('',
('<br><font size="2" face="Arial" color="#000000"><a style="text-'
'decoration: none" href="http://connexoncreative.com/job/?utm_source='
'newsletter&utm_medium=link&utm_campaign=' + newsletter['name_short'] +
'newslettersubmitjobs"><font color="' + newsletter['primary_color'] +
'"><strong>Recruit Top Talent</strong></font></a>: Reach more than '
'50,000 potential candidates by <a href="http://connexoncreative.com/'
'job/?utm_source=newsletter&utm_medium=link&utm_campaign=' +
newsletter['name_short'] + 'newslettersubmitjobs"><strong><font color="' +
newsletter['primary_color'] + '"><u>posting</u></font></strong></a>'),
(' your organization\'s career opportunities on the Connexon Creative '
'<a href="http://connexoncreative.com/jobs/?utm_source=newsletter&utm'
'_medium=link&utm_campaign=' + newsletter['name_short'] + 'newsletterjobs"><strong>'
'<font color="' + newsletter[
'primary_color'] + '"><u>Job Board</u></font></strong></a>'
' at no cost.'),
'</font></td></tr></table>',
'<!-- #JOB End -->',
''))
return out
def footer(flag_map):
newsletter = flag_map['nl']
out = [
'<!-- #FOOTER Start -->',
('<table width="100%" border="0" cellspacing="0" cellpadding="0">'
'<tr><td width="100%" height="20"> </td></tr><tr><td style="'
'padding-bottom: 15px;"><br><div align="center"><font size="2" '
'face="Arial" color="' + newsletter['primary_color'] + '"><strong>Have we missed an'
' important article or publication in ' + newsletter[
'name_long'] + '? <a style="'
'text-decoration: none" href="http://connexoncreative.com/publication'
'/"><font color="' + newsletter['primary_color'] + '">Click here to submit!</font></a>'
'<br><br><a style="text-decoration: none" href="http://www.'
'connexoncreative.com/give-feedback/"><font color="' +
newsletter['primary_color'] +
'">Comments or suggestions? Submit your feedback here</font></a>'
'</strong></font>'),
('</div></td></tr></table></td></tr><tr><td style="border-top: 1px '
'solid; border-top-color: ' + newsletter['footer_border_color'] + '; border-bottom: 1px '
'solid; border-bottom-color: ' + newsletter[
'footer_border_color'] + '; background-color: ' +
newsletter['footer_background_color'] + '; vertical-align: middle;" bgcolor="' +
newsletter['footer_background_color'] + '" valign="middle" width="100%" height="30"><table '
'width="100%" height="30"><tr><td valign="middle"><font color='
'"#666666" size="2" face="Arial"><strong>Learn more about ' +
newsletter['name_long'] + ': </strong><a style="text-decoration: none" href="' +
newsletter['url'] + 'issue/"><font color="' + newsletter['primary_color'] +
'">Archives</font></a> | <a style="text-decoration: none" href="' +
newsletter['url'] + 'events/">'),
'<font color="' + newsletter['primary_color'] + '">Events</font></a>',
(' | <a style="text-decoration: none" href=" http://connexoncreative.'
'com/contact-us/">'),
'<font color="' + newsletter['primary_color'] +
'">Contact Us</font></a></font></td></tr></table></td></tr></table>',
'<!-- #FOOTER End -->',
'',
'</body></html>']
return out
def writetofile(out, outdir):
outfile = codecs.open(outdir + r'\output.htm', 'w', 'utf-8-sig', 'replace')
out = [line + u'\n' for line in out]
outfile.writelines(out)
outfile.close()
def wordpress(flag_map, entry_map, outdir):
hpbanner = flag_map['hpbanner']
newsletter = flag_map['nl']
nl_issue = str(flag_map['issue'])
nl_date = flag_map['date']
revs = entry_map['revs']
events = entry_map['events']
jobs = entry_map['jobs']
altfile = codecs.open(outdir + r'\wordpress.htm', 'w', 'utf-8-sig', 'replace')
out = []
out.extend((hpbanner, ''))
[out.extend(rev.archive(newsletter, nl_issue, nl_date)) for rev in revs]
[out.extend(event.archive()) for event in events]
[out.extend(job.archive(newsletter)) for job in jobs]
out = [line + u'\n' for line in out]
altfile.writelines(out)
print "Wordpress write successful"
altfile.close()
def archival():
"""Archival Process. Prompts for the issue , but really uses the outfile"""
root = Tkinter.Tk()
root.withdraw()
source = tkFileDialog.askopenfile(
parent=root,
initialdir=r"S:\CXN - Connexon\CXN Publications",
title="Select Plaintext")
outdir = os.path.dirname(source.name)
html = codecs.open(outdir + r'\output.htm', 'r', 'utf-8-sig', 'replace')
archive = codecs.open(
outdir +
'\\archive.htm',
'w',
'utf-8-sig',
'replace')
source.close()
html = html.read()
html = re.sub(
(ur'[\?&]elq=~~eloqua\.\.type--emailfield\.\.syntax--recipientid~~&elq'
ur'CampaignId=~~eloqua\.\.type--campaign\.\.campaignid--0\.\.'
ur'fieldname--id~~'),
u'',
html)
html = re.sub(
ur'[\?&]elq=<span class=eloquaemail>recipientid</span>',
u'',
html)
html = re.sub(ur'elqFormName=[a-z]+\d', u'elqFormName=cxn', html)
html = re.sub(
u'=<SPAN class=eloquaemail>EmailAddress</SPAN>',
u'=<EMAIL>',
html)
html = re.sub(r"\(A\)", '(Archive)', html)
html = re.sub(u'<a href="', u'<a target="_blank" href="', html)
html = re.sub(
u'<a style="text-decoration: none;" href="',
u'<a target="_blank" style="text-decoration: none" href="',
html,
flags=re.IGNORECASE)
html = re.sub(u'<a target="_blank" href="#', u'<a href="#', html)
html = re.sub(
u'<a target="_blank" style="text-decoration: none" href="#',
u'<a style="text-decoration: none" href="#',
html)
html = re.sub(u'(?:<html>((?s).*)<body>)', u'<body>', html)
html = re.sub(
u'(?:<!-- #FOOTER End -->((?s).*)</html>)',
u'<!-- #FOOTER End -->',
html)
archive.write(html)
source.close()
archive.close()
def make_twitter_csv(pub, section):
"""Builds a CSV out of the titles and Abstracts of each Publication and Review"""
open_access = ''
if "Full Article" in pub.anchor_list:
open_access = "open"
out = os.path.expanduser(r"~\Desktop\twitter.csv")
with open(out, 'ab') as twitter_out:
csv_out = csv.writer(twitter_out)
csv_out.writerow([pub.title.encode('utf-8'), pub.link_list[0].encode('utf-8'), section, pub.source.encode('utf-8'), open_access])
def space_in_csv(title):
out = os.path.expanduser(r"~\Desktop\twitter.csv")
with open(out, 'ab') as twitter_out:
csv_out = csv.writer(twitter_out)
csv_out.writerow(title)
# In the future, this function could fill in basic info in the template
def give_template():
"""give_template() generates a new plaintext file in the directory the
user selects."""
root = Tkinter.Tk()
root.withdraw()
source = tkFileDialog.askdirectory(
parent=root,
initialdir=r"S:\CXN - Connexon\CXN Publications",
title="Select Directory")
shutil.copy('plaintext_template.txt', source)
if __name__ == "__main__":
true_main('')
PROMPT = raw_input('Would you like archive now? (Say "Y") ')
if PROMPT.upper() == 'Y':
archival()
give_template()
# Extra Line in New Jobs
# TODO: Note when old job readded
# TODO: Combine Top Story and Division Number into a "flag" category
# TODO: URLLib to assess dead job links?
# TODO: Red tags when first put in Eloqua. Why?
# TODO: re.sub %26/& in banners, also banner source
# TODO: Dir persistence between nl and arch
# TODO: Auto openaccess tag for tweets
# TODO: Concurrent Access to the CSV (Perhaps JSON instead)
# TODO: Event banner generator?
# TODO: Sort equal IF Publications by number of anchor links
# TODO: Prompt error if event occurs before newsletter date
# BUG: Banners did not appear in HN 5.42 Archival Version (Did not change
# span class to <EMAIL>)
# BUG: Washingon DC still has United States in archival version
# TODO: Run true_main again prompt
# BUG: cxn not replaced in textad of CSCN 3.43
# TODO: Trailing Space Removal
# BUG: Double <em> tag on Review article in CBN 6.43
# TODO: Sort Science News by Conference Date?<file_sep>'''
Created on Aug 11, 2014
@author: memery
'''
from Bio import Entrez
Entrez.email = "<EMAIL>"
handle = Entrez.esearch("pubmed",term='"embryonic stem" or "induced pluripotent stem" and "last 5 days"[EPDAT]',sort='relevance')
record = Entrez.read(handle)
print record<file_sep>'''
Created on Aug 12, 2014
@author: memery
'''
import xlutils
print help(xlutils) | 20aa56f993ae3ca26e8d20bca72066a65b80117f | [
"Python"
] | 6 | Python | DeadHand49/Connexogen | 1529640fdbb2a02b35264c31c253857821adb9be | 331d22460a8a35bfe6f134011e6fa4379e499fe5 | |
refs/heads/master | <repo_name>opendatacity/YouTube<file_sep>/html/assets/js/helper.js
$(document).ready(function(){
/* embed overlay code */
$('body').append($($embed_overlay));
$('.embed-button').click(function(evt){
if ($('#embed-overlay:visible').length === 0) {
$('#embed-overlay').fadeIn('fast');
}
});
var $clickIn = false;
$('#embed-overlay').click(function(evt){
if (!$clickIn) {
$('#embed-overlay').fadeOut('fast');
}
$clickIn = false;
});
$('#embed-form').click(function(evt){
$clickIn = true;
});
/* embed code*/
if ($('#embed-form').length) {
var $f = $('#embed-form');
var $url = 'https://opendatacity.github.io/youtube';
var embedCode = function(){
var $size = $('input:radio[name=size]:checked',$f).val();
var $lang = $('input:radio[name=lang]:checked',$f).val();
$lang = ($lang === "") ? "de" : $lang;
$size = ($size === "") ? "large" : $size;
if ($lang == "de") {
var text = 'Die geblockten Top-1000-YouTube-Videos';
var suffix = 'Unterstützt durch <a href="http://www.myvideo.de">MyVideo</a>. Realisiert von <a href="http://www.opendatacity.de/">OpenDataCity</a>. Anwendung steht unter <a rel="license" href="http://creativecommons.org/licenses/by/3.0/de/">CC-BY 3.0</a>.';
//var file = 'frame.de.html';
} else {
var text = 'Top 1000 YouTube Videos';
var suffix = 'Supported by <a href="http://www.myvideo.de">MyVideo</a>. Made by <a href="http://www.opendatacity.de/">OpenDataCity</a>. This App is under <a rel="license" href="http://creativecommons.org/licenses/by/3.0/us/">CC-BY 3.0</a>.';
//var file = 'frame.en.html';
}
$('#embed-size',$f).show();
switch ($size) {
case 'large': var $wh = 'width="860" height="610"'; break;
case 'medium': var $wh = 'width="640" height="490"'; break;
case 'small': var $wh = 'width="520" height="370"'; break;
case 'verysmall': var $wh = 'width="420" height="740"'; break;
}
var $code = '<iframe src="'+$url+'" '+$wh+' scrolling="no" frameborder="0" style="margin:0"><a href="'+$url+'">'+text+'</a></iframe><br><small>'+suffix+'</small>';
$('#embed-code', $f).text($code);
};
embedCode();
$(":input", $f).change(function(){
embedCode();
});
}
});
| a4d017d333019deb7c125bad0e4be68053723137 | [
"JavaScript"
] | 1 | JavaScript | opendatacity/YouTube | 393f4b0f6adb849a525dc3961870877a5474bccb | ee350e81d61192d71aa5924a64a271eaa75a9287 | |
refs/heads/master | <repo_name>huxiutao/J2EE<file_sep>/FirstProject/src/com/delta/my/learn/Order.java
package com.delta.my.learn;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
/**
* 线程的调度顺序
*
* A --> B --> C
* A打印二次
* B打印四次
* B打印六次
* 循环十次
*
* @author Xuekao.Hu
*
*/
public class Order {
/**
*
* @param args
*/
public static void main(String[] args) {
ShareResource sr = new ShareResource();
new Thread(() -> {
for (int i = 1; i <= 10; i++) {
sr.printA(i);
}
}, "AA").start();
new Thread(() -> {
for (int i = 1; i <= 10; i++) {
sr.printB(i);
}
}, "BB").start();
new Thread(() -> {
for (int i = 1; i <= 10; i++) {
sr.printC(i);
}
}, "CC").start();
}
}
/**
* 资源类
* @author Xuekao.Hu
*/
class ShareResource{
private String flag = "A";
private Lock lock = new ReentrantLock();
private Condition conditionA = lock.newCondition();
private Condition conditionB = lock.newCondition();
private Condition conditionC = lock.newCondition();
public void printA(int totalNumber) {
lock.lock();
try {
// 1.判断
while ( !flag.equals("A")) {
conditionA.await();
}
// 2.操作
for (int i = 1; i <= 2; i++) {
System.out.println(Thread.currentThread().getName() + "\t " + flag + "_" + String.valueOf(i) + "\t" + totalNumber);
}
flag = "B";
// 3.通知
conditionB.signal();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printB(int totalNumber) {
lock.lock();
try {
// 1.判断
while (!flag.equals("B")) {
conditionB.await();
}
// 2.操作
for (int i = 1; i <= 4; i++) {
System.out.println(Thread.currentThread().getName() + "\t " + flag + "_" + String.valueOf(i) + "\t" + totalNumber);
}
flag = "C";
// 3.通知
conditionC.signal();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
lock.unlock();
}
}
public void printC(int totalNumber) {
lock.lock();
try {
// 1.判断
while (!flag.equals("C")) {
conditionC.await();
}
// 2.操作
for (int i = 1; i <= 6; i++) {
System.out.println(Thread.currentThread().getName() + "\t " + flag + "_" + String.valueOf(i) + "\t" + totalNumber);
}
flag = "A";
// 3.通知
conditionA.signal();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
<file_sep>/FirstProject/src/com/delta/my/learn/TestCode.java
package com.delta.my.learn;
/**
* Test Class
*
* @author xuekao.hu
*
*/
public class TestCode {
static {
System.out.println("1111");
}
public TestCode() {
System.out.println(333);
}
public static void main(String[] args) {
System.out.println(2222);
new TestCode();
}
}
| bc077a994d5bf016a2edbeb2cb23e3fd7f676683 | [
"Java"
] | 2 | Java | huxiutao/J2EE | 42fbb2ab0e16d575f7f586f409aac69f1325e5a5 | ed9f97636b9dcb6f21c614ad581b5a39b59e8420 | |
refs/heads/master | <repo_name>vuqueth/rob-angular-mentions<file_sep>/README.md
# Angular Mentions - Rob fork
Forked from [dmacfarlane/angular-mentions](https://github.com/dmacfarlane/angular-mentions)
Forked from [dessalines/angular-mentions](https://github.com/dessalines/angular-mentions)
Forked from [francisvgi/fvi-angular-mentions](https://github.com/francisvgi/fvi-angular-mentions)
Angular mentions inspired by [Ment.io](https://github.com/jeff-collins/ment.io).
This fork fixes several bugs that were present in older forks. The fixed bug list includes:
* Fixed issue with android keyboard causing mentions to not funciton at all on android.
* Fixed issue that prevented the mentions list from opening if the user deletes part of the entry.
Provides auto-complete suggestions for @mentions in text input fields, text areas,
and content editable fields. Not fully browser tested and comes without warranty!
git clone https://github.com/rob-moore/rob-angular-mentions.git
cd angular-mentions
ng serve
### Usage
Add the package as a dependency to your project using:
npm install --save rob-angular-mentions
Add the CSS to your index.html:
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">
Add the module to your app.module imports:
import { MentionModule } from 'rob-angular-mentions/mention';
...
@NgModule({
imports: [ MentionModule ],
...
})
Add the `[mentions]` directive to your input element:
<input type="text" [mentions]="mentionItems">
Where `mentionItems` is an an array of `MentionItem`:
```
mentionItems: Array<MentionItem> = [
{
items: {"jerry", "ben", "tom"},
triggerChar: '@',
mentionSelect: formatMention
},
{
items: {"happy", "sad", "trending"},
triggerChar: '#',
},
{
items: [
{
id: 1,
name: "community_A"
},
{
id: 2,
name: "community_B"
}
],
labelKey: "name",
triggerChar: "~"
}
];
```
#### Configuration Options
```
export interface MentionItem {
// The list of items to be searched on.
items: Array<{}>;
// the character that will trigger the menu behavior
triggerChar: string;
// option to specify the field in the objects to be used as the item label
labelKey?: string;
// option to limit the number of items shown in the pop-up menu
maxItems?: number;
// option to diable internal filtering. can be used to show the full list returned
// from an async operation (or allows a custom filter function to be used - in future)
disableSearch?: boolean;
// template to use for rendering list items
mentionListTemplate?: TemplateRef<any>;
// internal use
searchList? : MentionListComponent;
// optional function to format the selected item before inserting the text
selectMention? : any;
}
```
#### Output Events
- `(searchTerm)=""` event emitted whenever the search term changes. Can be used to trigger async search.
- `(selectedTerm)=""` event emitted whenever a term is selected.
THANKS TO DESSALINES (https://github.com/dessalines/angular-mentions) AND DMACFARLANE(https://github.com/dmacfarlane/angular-mentions).
<file_sep>/src/app/demo-simple/demo-simple.component.ts
import { Component } from '@angular/core';
import { MentionItem } from '../../mention/mention-item';
import { COMMON_NAMES } from '../common-names';
import { COMMON_TAGS } from '../common-tags';
/**
* Demo app showing usage of the mentions directive.
*/
@Component({
selector: 'demo-simple',
templateUrl: './demo-simple.component.html'
})
export class DemoSimpleComponent {
mentionItems: Array<MentionItem> = [];
ngOnInit(): void {
const self: this = this;
this.mentionItems = [
{
items: COMMON_NAMES,
triggerChar: '@',
},
{
items: COMMON_TAGS,
triggerChar: '#',
},
{
items: COMMON_NAMES,
triggerChar: '',
},
{
items: COMMON_NAMES,
triggerChar: ' ',
},
{
items: [
{
id: 1,
name: 'community_A'
},
{
id: 2,
name: 'community_B'
}
],
labelKey: 'name',
triggerChar: '~',
}
];
}
selectedTerm(item: any) {
console.log(item);
}
}
| 03870a5708a2cd81e137ecda241aea8e6d72c1b9 | [
"Markdown",
"TypeScript"
] | 2 | Markdown | vuqueth/rob-angular-mentions | 39b78ddbda968563bb88ae6a0c4ce36c194bf2d7 | 22c7636a668362cae487db80b3951542a1cfecd0 | |
refs/heads/master | <file_sep>import React, { Component, Fragment } from 'react';
import Header from './components/Header'
import PizzaForm from './components/PizzaForm'
import PizzaList from './containers/PizzaList'
const pizzaUrl = 'http://localhost:3000/pizzas'
class App extends Component {
constructor() {
super()
this.state = {
pizzas: [],
thisPizza: {
topping: '',
vegetarian: true,
size: ''
}
// thisPizza: {}
}
}
componentDidMount() {
fetch(pizzaUrl)
.then(resp => resp.json())
.then(pizzaData => this.setState({pizzas: pizzaData}))
.then(console.log(this.state.pizzas))
}
editPizza = pizza => {
this.setState({
thisPizza: pizza
})
}
chooseTopping = (e) => {
this.setState({
thisPizza: {...this.state.thisPizza,
topping: e.target.value
}
// this.setState({thisPizza: {topping: e.target.value})
})
console.log(this.state)
}
chooseSize = (e) => {
this.setState({
thisPizza: {...this.state.thisPizza,
size: e.target.value
}
})
console.log(e.target.value)
}
handleCheck = (boolean) => {
this.setState({
thisPizza: {...this.state.thisPizza,
vegetarian: boolean
}
})
console.log(boolean)
}
handleSubmit = (newPizza) => {
fetch(`http://localhost:3000/pizzas/${newPizza.id}`, {
method: 'PATCH',
headers: {
'Content-Type': 'application/json',
'Accept': 'application/json'
},
body: JSON.stringify(newPizza)
})
.then(resp => resp.json())
.then(pizzaData => {
let changedPizzas = this.state.pizzas.map(pizza => {
if (pizza.id === pizzaData.id){
return pizzaData
} else {
return pizza
}
})
this.setState({pizzas: changedPizzas})
})
}
render() {
return (
<Fragment>
<Header/>
<PizzaForm
pizza={this.state.thisPizza}
chooseTopping={this.chooseTopping}
chooseSize={this.chooseSize}
handleCheck={this.handleCheck}
handleSubmit={this.handleSubmit} />
<PizzaList
pizzas={this.state.pizzas}
editPizza={this.editPizza} />
</Fragment>
);
}
}
export default App;
| ab7306918bd7b17553045aa1212ca8746b0144f4 | [
"JavaScript"
] | 1 | JavaScript | jwkuchta/React-Pizza-atlanta-web-82619 | e8daf43c7817192cfbddfe8749f191f0634c175a | d064c8a50917331c534284c00162895f795c95cd | |
refs/heads/master | <repo_name>skipjacktuna/file-slack-to-google-drive<file_sep>/README.md
# file-slack-to-google-drive
<file_sep>/entrypoint.gs
// executed when receive a post.
function doPost(e) {
var jsonString = e.postData.getDataAsString();
var data = JSON.parse(jsonString);
return eventProcessing(data);
}
// for Test or save token.
function myFunction() {
doPost({
postData: {
getDataAsString: function () {
return '{"type":"event_callback", "event":{"type":"file_created","file":{"id":"XXXXXXXXX"},"file_id":"XXXXXXXXX","user_id":"XXXXXXXXX","event_ts":"1526713669.000085"}}';
}
}
});
}
// Function Map
var slackEventMap = {
file_created: processFileCreated
};
// set your google drive folder id
// If the folder URL is https://drive.google.com/drive/folders/xxxxxxxxxxxxxxxxxxxxxxxx
// id is xxxxxxxxxxxxxxxxxxxxxxxx.
var applicationFileFolderId = 'xxxxxxxxxxxxxxxxxxxxxxxx';
// executed when receive file created event.
function processFileCreated(event) {
// Get file info from slack.
var res = getFileInfo(event.file.id);
// Download Image
var blob = getPrivateImage(res.file.url_private_download);
// save my googel driver
var folder = DriveApp.getFolderById(applicationFileFolderId);
folder.createFile(blob);
}
<file_sep>/slack.gs
// slack
var slackSettings = {};
// save token
// Before using the script, specify your SLACK TOKEN as a parameter and execute once.
function saveSlackToken(token) {
var userProperties = PropertiesService.getUserProperties();
userProperties.setProperty('SLACK_ACCESS_TOKEN', token);
}
// load token
function loadSlackToken() {
if (slackSettings.SLACK_ACCESS_TOKEN === undefined) {
var userProperties = PropertiesService.getUserProperties();
slackSettings.SLACK_ACCESS_TOKEN = userProperties.getProperty('SLACK_ACCESS_TOKEN');
}
}
// slack event processing
function eventProcessing(data) {
loadSlackToken();
var resData = {};
if (data.type === 'event_callback') {
if (slackEventMap[data.event.type] !== undefined) {
console.info('receive event = %s.', data.event.type);
slackEventMap[data.event.type](data.event);
}
} else if (data.type === 'url_verification') {
resData.challenge = data.challenge;
}
return ContentService.createTextOutput(JSON.stringify(resData)).setMimeType(ContentService.MimeType.JSON);
}
function getPrivateImage(url) {
// set token
var headers = {
'Authorization': 'Bearer '+ slackSettings.SLACK_ACCESS_TOKEN
};
var options = {
'method' : 'get',
'headers' : headers
};
var response = UrlFetchApp.fetch(url, options);
return response.getBlob();
}
function getFileInfo(id) {
console.info('getFileInfo call. id = %s', id);
var response = UrlFetchApp.fetch('https://slack.com/api/files.info?token=' + slackSettings.SLACK_ACCESS_TOKEN + '&file=' + id);
if (response.getResponseCode() === 200) {
console.log(response.getContentText());
return JSON.parse(response.getContentText());
} else {
return null;
}
}
| 73904a352e1af61835ff6c8678211927464a9eb0 | [
"Markdown",
"JavaScript"
] | 3 | Markdown | skipjacktuna/file-slack-to-google-drive | 2f7c476e1848c2d01088432f814ad4ddf35ec8f7 | 9dbd7b218b1a465684c666e06788220ddf40e3ee | |
refs/heads/main | <repo_name>GabeGerdes/FirstResponsiveWebAppGerdes<file_sep>/Models/AgeFinder.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.ComponentModel.DataAnnotations;
namespace FirstResponsiveWebAppGerdes.Models
{
public class AgeFinder
{
public string UserName { get; set; }
[Required(ErrorMessage = "Please use a number.")]
[Range(1900,2021, ErrorMessage ="Please use a number between 2021 and 1900.")]
public int? BirthYear { get; set; }
public int? AgeThisYear()
{
int? age = 0;
const int thisYear = 2021;
age = thisYear - BirthYear;
return age;
}
}
}
<file_sep>/Controllers/HomeController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using FirstResponsiveWebAppGerdes.Models;
namespace FirstResponsiveWebAppGerdes.Controllers
{
public class HomeController : Controller
{
[HttpGet]
public IActionResult Index()
{
ViewBag.YOB = 0;
return View();
}
[HttpPost]
public IActionResult Index(AgeFinder model)
{
ViewBag.YOB = model.AgeThisYear();
return View(model);
}
}
}
| 8d0e6e117594de3ed6fe6a55c9425d5320e42751 | [
"C#"
] | 2 | C# | GabeGerdes/FirstResponsiveWebAppGerdes | ce2684c20c6f58ad3b688365673352571c29a4a9 | 8e016592413436d18b52655878951841717fc32a | |
refs/heads/main | <file_sep>import java.util.Scanner;
public class RockPaperSc {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("Lets play Rock, Paper or Scissors!");
System.out.println("Do you want to play? YES/NO");
String ready= scan.nextLine();
if(ready.equalsIgnoreCase("Yes")){
System.out.println("OKAY! Pick Rock, Paper or Scissors");
System.out.println("Rock=1 Paper=2 Scissors=3");
String hand= scan.nextLine();
if(hand.equalsIgnoreCase("Rock")|| hand.equalsIgnoreCase("Paper")|| hand.equalsIgnoreCase("Scissors")){
String computerHand= pickGenerate();
System.out.println("You has picked: "+hand);
System.out.println("Machine has picked: "+ computerHand);
if(hand.equalsIgnoreCase(computerHand)){
System.out.println("DRAW!");
}else if(hand.equalsIgnoreCase("Rock")&& computerHand.equalsIgnoreCase("Paper")||
hand.equalsIgnoreCase("Scissors")&& computerHand.equalsIgnoreCase("Rock")||
hand.equalsIgnoreCase("Paper")&& computerHand.equalsIgnoreCase("Scissors")){
System.out.println("Ohh You lost!");
}else if(hand.equalsIgnoreCase("Paper")&& computerHand.equalsIgnoreCase("Rock")||
hand.equalsIgnoreCase("Rock")&& computerHand.equalsIgnoreCase("Scissors")||
hand.equalsIgnoreCase("Scissors")&& computerHand.equalsIgnoreCase("Paper")){
System.out.println("Yeyy You won!!");
}
}else{
System.out.println("ERROR, Only accepted Rock Paper or Scissors");
}
}else{
System.out.println("Okay maybe next time. See you soon");
System.exit(0);
}
scan.close();
}
public static String pickGenerate(){
double randomHand= Math.random()*3;
int integer =(int)randomHand;
switch (integer) {
case 0: return "Rock";
case 1: return "Paper";
case 2: return "Scissors";
default: return "--";
}
}
}<file_sep># RockPaperScissors
Basic Rock Paper Scissors Game player 1 against machine- Java
| 3248bd3ea0a985921f31f761e1b48009b102b294 | [
"Markdown",
"Java"
] | 2 | Java | brevers/RockPaperScissors | b6c322b982eade298bf396175441b4c92a9284ec | 8c1f9d6fd7a9f3f79bada1532e895ca57b6d1ef8 | |
refs/heads/master | <repo_name>Xanewok/WWWWybory<file_sep>/wybory/__init__.py
# http://prezydent2000.pkw.gov.pl/gminy/index.html
# Należy przygotować generator stron HTML, który weźmie wyniki
# (pliki w excelu lub skonwertowane csv) wyborów i przygotuje zestaw stron:
# Wyniki wyborów w całym kraju plus mapka z województwami, na których można klikać
# Wyniki wyborów w każdym z województw plus odnośniki do okręgów
# Wyniki wyborów w każdym okręgu plus odnośniki do gmin
# Wyniki wyborów w każdej gminie w podziale na obwody
# Wymagania dodatkowe
# Powinien być generowany poprawny HTML
# Powinien być generowany porawny CSS
# Strona powinna być responsywna
from jinja2 import Environment, PackageLoader, select_autoescape
from wybory import data # Read and parse resources/*.xls data
env = Environment(
loader=PackageLoader('wybory', 'templates'),
autoescape=select_autoescape(['html', 'xml']),
trim_blocks=True,
lstrip_blocks=True,
)<file_sep>/wybory/data.py
import glob
from functools import reduce
import xlrd
from xlrd.timemachine import xrange
class Obwod:
objects = []
def __init__(self):
self.wojewodztwo = ""
self.wojewodztwo_criteria_id = 0
self.nr_okregu = 0
self.kod_gminy = 0
self.gmina = ""
self.powiat = ""
self.nr_obwodu = 0
self.typ_obwodu = ""
self.adres = ""
self.wyniki = [] # size of 12, starting from index 12 (0-indexed)
def __str__(self):
return "Wojewodztwo: " + str(self.wojewodztwo_criteria_id) + ", gmina: " + self.gmina
# https://developers.google.com/adwords/api/docs/appendix/geotargeting
# Target Type: Region
# Country Code: PL
# Parent ID: 2616
# Criteria ID, Name
# 20847, Lower Silesian Voivodeship
# 20848, Kuyavian-Pomeranian
# 20849, Lubusz Voivodeship
# 20850, Lodz Voivodeship
# 20851, Lublin Voivodeship
# 20852, Lesser Poland Voivodeship
# 20853, Masovian Voivodeship
# 20854, Opole Voivodeship
# 20855, Podlaskie Voivodeship
# 20856, Podkarpackie Voivodeship
# 20857, Pomeranian Voivodeship
# 20858, Swietokrzyskie
# 20859, Silesian Voivodeship
# 20860, Warmian-Masurian Voivodeship
# 20861, Greater Poland Voivodeship
# 20862, West Pomeranian Voivodeship
def okreg_to_geotarget_criteria_id(num):
if 1 <= num <= 4: # województwo DOLNOŚLĄSKIE: 1 Wrocław, 2 <NAME>, 3 Legnica, 4 Wałbrzych
return 20847
if 5 <= num <= 7: # województwo KUJAWSKO-POMORSKIE: 5 Bydgoszcz, 6 Toruń, 7 Włocławek
return 20848
if 13 <= num <= 14: # województwo LUBUSKIE: 13 <NAME>, 14 <NAME>
return 20849
if 15 <= num <= 19: # województwo ŁÓDZKIE: 15 Łodź, 16 Łodź, 17 <NAME>, 18 Sieradz, 19 Skierniewice
return 20850
if 8 <= num <= 12: # województwo LUBELSKIE: 8 Lublin, 9 B<NAME>, 10 Chełm, 11 Puławy, 12 Zamość
return 20851
if 20 <= num <= 27: # województwo MAŁOPOLSKIE: 20 Kraków, 21 Kraków, 22 Kraków, 23 Chrzanów, 24 Myślenice: 25 Nowy Sącz, 26 Nowy Targ, 27 Tarnów
return 20852
if 28 <= num <= 36: # województwo MAZOWIECKIE: 28 Warszawa, 29 Warszawa, 30 Ciechanów, 31 Legionów, 32 Ostrołęka, 33 Piaseczno, 34 Płock, 35 Radom, 36 Siedlce
return 20853
if 37 <= num <= 38: # województwo OPOLSKIE: 37 Opole: 38 Opole
return 20854
if 43 <= num <= 45: # województwo PODLASKIE: 43 Białystok, 44 Łomża, 45 Suwałki
return 20855
if 39 <= num <= 42: # województwo PODKARPACKIE: 39 Rzeszów, 40 Krosno, 41 Przemyśl, 42 Tarnobrzeg
return 20856
if 46 <= num <= 48: # województwo POMORSKIE: 46 Gdańsk, 47 Gdańsk, 48 Słupsk
return 20857
if 55 <= num <= 56: # województwo ŚWIĘTOKRZYSKIE: 55 Kielce: 56 Kielce
return 20858
if 49 <= num <= 54: # województwo ŚLĄSKIE: 49 Katowice: 50 Bielsko-Biała, 51 Bytom, 52 Częstochowa, 53 Gliwice: 54 Sosnowiec
return 20859
if 57 <= num <= 59: # województwo WARMIŃSKO-MAZURSKIE: 57 Olsztyn, 58 Elbląg, 59 Ełk
return 20860
if 60 <= num <= 64: # województwo WIELKOPOLSKIE: 60 Poznań, 61 Kalisz, 62 Konin, 63 Leszno, 64 Piła
return 20861
if 65 <= num <= 68: # województwo ZACHODNIOPOMORSKIE: 65 Szczecin, 66 Koszalin, 67 <NAME>, 68 Szczecinek
return 20862
def okreg_to_province_name(num):
if 1 <= num <= 4: return "Dolnośląskie"
if 5 <= num <= 7: return "Kujawsko-pomorskie"
if 13 <= num <= 14: return "Lubuskie"
if 15 <= num <= 19: return "Łódzkie"
if 8 <= num <= 12: return "Lubelskie"
if 20 <= num <= 27: return "Małopolskie"
if 28 <= num <= 36: return "Mazowieckie"
if 37 <= num <= 38: return "Opolskie"
if 43 <= num <= 45: return "Podlaskie"
if 39 <= num <= 42: return "Podkarpackie"
if 46 <= num <= 48: return "Pomorskie"
if 55 <= num <= 56: return "Świętokrzyskie"
if 49 <= num <= 54: return "Śląskie"
if 57 <= num <= 59: return "Warmińsko-mazurskie"
if 60 <= num <= 64: return "Wielkopolskie"
if 65 <= num <= 68: return "Zachodniopomorskie"
return "Nieznane"
def polish_province_ids():
return list(range(20847, 20863))
def candidate_count():
return 12
def candidate_name(id):
if id == 0:
return "<NAME>"
if id == 1:
return "<NAME>"
if id == 2:
return "<NAME>"
if id == 3:
return "<NAME>"
if id == 4:
return "<NAME>"
if id == 5:
return "<NAME>"
if id == 6:
return "<NAME>"
if id == 7:
return "<NAME>"
if id == 8:
return "<NAME>"
if id == 9:
return "<NAME>"
if id == 10:
return "<NAME>"
if id == 11:
return "<NAME>"
return "Candidate name out of bounds"
def empty_result_set():
results = [];
for i in range(0, candidate_count()):
results.append(0)
return results
def sum_results(results, sum):
for i in range(0, candidate_count()):
results[i] += sum[i]
return results
def calculate_result_set(obwod_obj_list):
results = reduce(sum_results, map(lambda x: x.wyniki, obwod_obj_list), empty_result_set())
total_count = int(sum(results))
if total_count == 0: total_count = 1 # Used only for percentage, if sum is 0, then vote/sum still will be 0
return map(lambda x: (candidate_name(x), int(results[x]), int(results[x])/total_count), range(0, candidate_count()))
def read_data():
obw_files = glob.glob('resources/obw*.xls')
for obw_file in obw_files:
workbook = xlrd.open_workbook(obw_file)
worksheet = workbook.sheet_by_index(0) # Assume only single sheet
for rownum in xrange(worksheet.nrows):
if rownum == 0: # Header
continue
obwod = Obwod()
values = worksheet.row_values(rownum)
obwod.wojewodztwo = okreg_to_province_name(values[0])
obwod.wojewodztwo_criteria_id = okreg_to_geotarget_criteria_id(values[0]) # num
obwod.nr_okregu = int(values[0]) # num
obwod.kod_gminy = int(values[1]) # num
obwod.gmina = values[2]
obwod.powiat = values[3]
obwod.nr_obwodu = values[4] # num
obwod.typ_obwodu = values[5]
obwod.adres = values[6]
for i in range(0, candidate_count()): # 12 candidates
obwod.wyniki.append(values[12 + i]) # num, values are values[12...23]
Obwod.objects.append(obwod)
read_data()
<file_sep>/wybory/build.py
# generate the page at some point
import os
import errno
import shutil
from collections import OrderedDict
from wybory import env, data
from more_itertools import unique_everseen
# Wyniki wyborów w całym kraju plus mapka z województwami, na których można klikać
# Wyniki wyborów w każdym z województw plus odnośniki do okręgów
# Wyniki wyborów w każdym okręgu plus odnośniki do gmin
# Wyniki wyborów w każdej gminie w podziale na obwody
def create_navigation(obwod, path_len):
path = [("Kraj", "kraj.html")]
if path_len == 1: return path
path.append( (obwod.wojewodztwo, "woj{0}.html".format(obwod.wojewodztwo_criteria_id)) )
if path_len == 2: return path
path.append( ("Okręg {0}".format(obwod.nr_okregu), "okr{0}.html".format(obwod.nr_okregu)) )
if path_len == 3: return path
path.append((obwod.gmina, "gm{0}.html".format(obwod.kod_gminy)))
return path
def build_kraj():
html_name = "kraj.html"
for woj_id in data.polish_province_ids():
build_wojewodztwo(woj_id)
result_set = list(data.calculate_result_set(data.Obwod.objects))
template = env.get_template('kraj.html')
out = template.render(result_set=result_set)
with open("build/" + html_name, "w+") as f:
f.write(out)
f.close()
def build_wojewodztwo(wojewodztwo_id):
html_name = "woj{0}.html".format(wojewodztwo_id)
if os.path.isfile("build/" + html_name): # Don't rerender if not needed
return html_name
wojewodztwo_list = filter(lambda x: x.wojewodztwo_criteria_id == wojewodztwo_id, data.Obwod.objects)
children = sorted(list(unique_everseen(wojewodztwo_list, lambda x: x.nr_okregu)), key=lambda x: x.nr_okregu)
children_links = OrderedDict(map(lambda x: ("Okręg {0}".format(x.nr_okregu), build_okreg(x.nr_okregu)), children))
wojewodztwo_list = filter(lambda x: x.wojewodztwo_criteria_id == wojewodztwo_id, data.Obwod.objects)
result_set = list(data.calculate_result_set(wojewodztwo_list))
next_obwod = next(obj for obj in data.Obwod.objects if obj.wojewodztwo_criteria_id == wojewodztwo_id)
nav_list = create_navigation(next_obwod, 2)
template = env.get_template('wojewodztwo.html')
out = template.render(my_dict=children_links, result_set=result_set, nav=nav_list)
with open("build/" + html_name, "w+") as f:
f.write(out)
f.close()
return html_name
def build_okreg(nr_okregu):
html_name = "okr{0}.html".format(nr_okregu)
if os.path.isfile("build/" + html_name): # Don't rerender if not needed
return html_name
okreg_list = filter(lambda x: x.nr_okregu == nr_okregu, data.Obwod.objects)
children = sorted(list(unique_everseen(okreg_list, lambda x: x.kod_gminy)), key=lambda x: x.gmina)
children_links = OrderedDict(map(lambda x: (x.gmina, build_gmina(x.kod_gminy)), children))
okreg_list = filter(lambda x: x.nr_okregu == nr_okregu, data.Obwod.objects)
result_set = list(data.calculate_result_set(okreg_list))
next_obwod = next(obj for obj in data.Obwod.objects if obj.nr_okregu == nr_okregu)
nav_list = create_navigation(next_obwod, 3)
template = env.get_template('okreg.html')
out = template.render(my_dict=children_links, result_set=result_set, nav=nav_list)
with open("build/" + html_name, "w+") as f:
f.write(out)
f.close()
return html_name
def build_gmina(kod_gminy):
html_name = "gm{0}.html".format(kod_gminy)
if os.path.isfile("build/" + html_name): # Don't rerender if not needed
return html_name
gmina_list = filter(lambda x: x.kod_gminy == kod_gminy, data.Obwod.objects)
results = OrderedDict(map(lambda x: (x, list(data.calculate_result_set([x]))), gmina_list))
next_obwod = next(obj for obj in data.Obwod.objects if obj.kod_gminy == kod_gminy)
nav_list = create_navigation(next_obwod, 4)
template = env.get_template('gmina.html')
out = template.render(my_list=gmina_list, result_set=results, nav=nav_list)
with open("build/" + html_name, "w+") as f:
f.write(out)
f.close()
return html_name
def make_sure_path_exists(path):
try:
os.makedirs(path)
except OSError as exception:
if exception.errno != errno.EEXIST:
raise
if __name__ == "__main__":
shutil.rmtree("build/", ignore_errors=True)
make_sure_path_exists("build/")
make_sure_path_exists("build/static/")
shutil.copyfile("static/style.css", "build/static/style.css")
shutil.copyfile("static/logo.png", "build/static/logo.png")
build_kraj()
<file_sep>/wybory/resources/download_data.sh
#!/bin/bash
for i in {1..68}; do wget `echo "http://prezydent2000.pkw.gov.pl/gminy/obwody/obw"$i".xls"`; done
| 29b45f50351ea00553bfd4430166ce8365d30603 | [
"Python",
"Shell"
] | 4 | Python | Xanewok/WWWWybory | 8b1119086d4e5149f789b8c4b2c379c8b4de0d0d | 277a7c4e0f559b10b55f69d468ba07e1c4007bf5 | |
refs/heads/master | <file_sep>READ ME ----------------------------------------------------
main()
No changes just remember to add os.chdir() if your files are not in the same directory as this script
global totdf will need to reflect the columns in which you plan on merging
mergeData()
Will use logic to merge csv and txt files using a similar format. You can change the individual code logic for if 'txt' or if 'csv' to match what your new files have
mtotdf & df will need to reflect the colums in which you plan on merging
sentimentAnalysis(sentimentcolumn, keycolumn)
**inputs**
sentimentcolumn = whatever dataframe column you would like to run sentiment on
keycolumn = key that is associated with that record
example for this HW sentimentAnalysis('Purpose:','Name:')
can change these variables to output more than one record if needed using last 2 iloc commands
**output**
best sentiment , best key, worst sentiment, worst key(of highest & lowest both)
mostUsedTokens(num, column)
**inputs**
num = how many tokens you want to retrieve
column = which column you would like to get the tokens from
example for this HW mostUsedTokens(10, 'Purpose:')
**output**
an array of value_counts() which shows the token and amount it shows up
<file_sep>#!/usr/bin/env python
# coding: utf-8
# In[192]:
import os
import pandas as pd
import numpy as np
import re
from textblob import TextBlob
import nltk
#corpus
nltk.download('punkt')
#merged DataFrame to be used in each function
totdf = pd.DataFrame(columns = ['Name:','Purpose:'])
files = []
def main():
return 0
def mergeData():
#local dataframe
mtotdf = pd.DataFrame(columns = ['Name:','Purpose:'])
#array of files within this directory
files = os.listdir()
for f in files:
#logic for all text files
if 'txt' in f:
file = open(f,'r')
#splitting text file into individual lines that go into an array
dataframe = file.read().splitlines()
#specific logic for individual file
if f == 'hw2a2.txt':
#use --- as delimeter
dataframe = [re.split('---',x) for x in dataframe]
#popout array of array into one array
dataframe = [item for elem in dataframe for item in elem]
#delete empty entries
dataframe = [x for x in dataframe if x]
#create a dataframe that has 2 columns (reshape) while deleting all special characters and deleting Name and Purpose while creating columns for Name and Purpose
df = pd.DataFrame(np.reshape(np.array([re.sub(r"[^a-zA-Z0-9]+", ' ', x.replace('Name','').replace('Purpose','')) for x in dataframe]),(-1,2)), columns = ['Name:','Purpose:'])
#csv logic
elif 'csv' in f:
df = pd.read_csv(f)
#logic for csv with index as first column
if len(df.columns) == 3:
df = df.drop(columns = 'Unnamed: 0')
columns = df.columns.values
#standardize column names
df = df.rename(columns = {columns[0]:'Name:',columns[1]:'Purpose:'})
else:
df = pd.DataFrame(columns = ['Name:','Purpose:'])
mtotdf = mtotdf.append(df,ignore_index = True)
#make global df equal to local df
global totdf
totdf = mtotdf
return 'Data has been merged'
def sentimentAnalysis(sentimentcolumn, keycolumn):
global totdf
#polarity ranges from -1 (negative) to 1 (positive)
polarity = []
#subjectivity ranges from 0 (factual in messaging) to 1 (mostly opinion phrases)
subjectivity = []
index = 0
#iterating through global dataframe for specified column
for x in totdf[sentimentcolumn]:
phrase = TextBlob(x)
#Textblob values for polarity and sentiment are put in seperate arrays
polarity = polarity + [phrase.sentiment.polarity]
subjectivity = subjectivity + [phrase.sentiment.subjectivity]
#after iteration these arrays are added to the dataframe
totdf['subjectivity'] = subjectivity
totdf['polarity'] = polarity
#bestsentiment is based on the highest polarity with the lowest subjectivity (most positive and most factual)
bestsentiment = totdf[sentimentcolumn].iloc[totdf.sort_values(by = ['polarity','subjectivity'], ascending = [False,True]).index[0]]
worstsentiment = totdf[sentimentcolumn].iloc[totdf.sort_values(by = ['polarity','subjectivity'], ascending = [False,True]).index[-1]]
#key is returned as well to say which company in this case has that purpose
bestkey = totdf[keycolumn].iloc[totdf.sort_values(by = ['polarity','subjectivity'], ascending = [False,True]).index[0]]
worstkey = totdf[keycolumn].iloc[totdf.sort_values(by = ['polarity','subjectivity'], ascending = [False,True]).index[-1]]
return bestsentiment, bestkey, worstsentiment, worstkey
def mostUsedTokens(num, column):
global totdf
tottokens = []
#iterate through dataframe for a given column
for x in totdf[column]:
#iterate through each purpose in order to retrieve individual words
for token in TextBlob(x).words:
tottokens = tottokens + [token]
#put tokens into dataframe
tokendf = pd.DataFrame(tottokens,columns = ['Tokens'])
#use value_counts to find the top num amount of tokens within that column
topnum = tokendf['Tokens'].value_counts()[:num]
#return the array of tokens with their values
return topnum
if __name__ == "__main__":
main()
| 8f3a028a69d8a3aafd66990af31cf58bbd0fe52c | [
"Python",
"Text"
] | 2 | Text | AGalli98/hw3 | 45e0df2e506555bd9b5d946fad7b93ee63b262af | 6ccdd148fbf3acc2d42c7847b26f87a8c12bf2b8 | |
refs/heads/master | <file_sep>#!/usr/bin/env python
"""
@author: jakub
"""
from src import Creator
wood = [
"T6_PLANKS",
"T7_PLANKS",
"T6_PLANKS_LEVEL1@1",
"T7_PLANKS_LEVEL1@1",
"T6_PLANKS_LEVEL2@2",
"T7_PLANKS_LEVEL2@2",
"T6_WOOD",
"T7_WOOD",
"T6_WOOD_LEVEL1@1",
"T7_WOOD_LEVEL1@1",
"T6_WOOD_LEVEL2@2",
"T7_WOOD_LEVEL2@2",
]
hides = [
"T6_HIDE",
"T7_HIDE",
"T6_HIDE_LEVEL1@1",
"T7_HIDE_LEVEL1@1",
"T6_HIDE_LEVEL2@2",
"T7_HIDE_LEVEL2@2",
"T6_LEATHER",
"T5_LEATHER",
"T5_LEATHER_LEVEL1@1",
"T6_LEATHER_LEVEL1@1",
"T5_LEATHER_LEVEL2@2",
"T6_LEATHER_LEVEL2@2",
]
animals = [
"T6_FARM_HORSE_BABY",
"T4_FARM_HORSE_BABY",
"T5_FARM_HORSE_BABY",
"T3_FARM_HORSE_BABY",
"T6_FARM_HORSE_GROWN",
"T4_FARM_HORSE_GROWN",
"T5_FARM_HORSE_GROWN",
"T3_FARM_HORSE_GROWN",
"T6_FARM_DIREWOLF_BABY",
"T6_FARM_DIREWOLF_GROWN",
]
stone = [
"T6_ROCK",
"T7_ROCK",
"T5_ROCK",
"T6_STONEBLOCK",
"T7_STONEBLOCK",
"T5_STONEBLOCK",
]
consumables = [
"T5_MEAL_OMELETTE",
"T7_MEAL_OMELETTE",
"T6_MEAL_STEW",
"T4_MEAL_STEW",
"T7_MEAL_PIE",
"T5_MEAL_PIE",
"T6_MEAL_SANDWICH",
"T4_MEAL_SANDWICH",
]
crops = [
"T1_CARROT",
"T1_FARM_CARROT_SEED",
"T7_FARM_CORN_SEED",
"T6_FARM_POTATO_SEED",
"T6_POTATO",
"T7_CORN",
"T8_PUMPKIN",
"T8_FARM_PUMPKIN_SEED",
]
Creator(
subset_name="wood", item_of_interest=wood
).update_frame()
Creator(
subset_name="hides", item_of_interest=hides
).update_frame()
Creator(
subset_name="animals", item_of_interest=animals
).update_frame()
Creator(
subset_name="stone", item_of_interest=stone
).update_frame()
Creator(
subset_name="consumables", item_of_interest=consumables
).update_frame()
Creator(
subset_name="crops", item_of_interest=crops
).update_frame()
<file_sep># Albion
Auction house project
<file_sep>#!/usr/bin/env python
"""
@author: jakub
"""
import requests
import json
import pandas as pd
from time import sleep
class Creator:
def __init__(
self, subset_name=None, item_of_interest=None
):
self.subset_name = subset_name
self.item_of_interest = item_of_interest
def create_frame(self):
dataframe = pd.DataFrame(
columns=[
"buy_price_max",
"buy_price_max_date",
"buy_price_min",
"buy_price_min_date",
"city",
"item_id",
"quality",
"sell_price_max",
"sell_price_max_date",
"sell_price_min",
"sell_price_min_date",
]
)
for j in self.item_of_interest:
response = requests.get(
f"https://www.albion-online-data.com/api/v2/stats/prices/{j}"
).json()
pre_df = pd.DataFrame.from_dict(response)
dataframe = pd.concat(
[dataframe, pre_df], sort=False
)
dataframe.drop(
[
"buy_price_max_date",
"buy_price_min_date",
],
axis=1,
inplace=True,
)
dataframe.to_csv(
f"{self.subset_name}.csv", index=False
)
def update_frame(self):
dataframe = pd.read_csv(f"{self.subset_name}.csv")
for j in self.item_of_interest:
response = requests.get(
f"https://www.albion-online-data.com/api/v2/stats/prices/{j}"
).json()
pre_df = pd.DataFrame.from_dict(response)
pre_df.drop(
[
"buy_price_max_date",
"buy_price_min_date",
],
axis=1,
inplace=True,
)
dataframe = pd.concat(
[dataframe, pre_df], sort=False
)
dataframe.drop_duplicates(
[
"city",
"item_id",
"sell_price_min",
"sell_price_min_date",
],
inplace=True,
)
dataframe.to_csv(
f"{self.subset_name}.csv", index=True
)
| 8e246822aa3b86ce9d23d11eeba94ee4c934f50c | [
"Markdown",
"Python"
] | 3 | Python | Plesoun/Albion | 354e434721a7d1117d0975d87784dc7fe71ffdfa | 1e82c024e059c5ef978e6cfa63bc999b243f4fa9 | |
refs/heads/master | <file_sep>#!/bin/sh
pacman -Syyu --noconfirm
pacman -S linux linux-firmware --noconfirm
systemctl disable netctl || /bin/true
pacman -Rns --noconfirm netctl || /bin/true
pacman -S --noconfirm networkmanager
systemctl enable NetworkManager
echo 'HOOKS=(base systemd autodetect sd-vconsole modconf block keyboard keymap sd-encrypt sd-lvm2 fsck filesystems' >> /etc/mkinitcpio.conf
rot=$(cryptsetup status luks|grep device|awk '{print $2}')
cryptrot="$(blkid $rot|awk '{print $2}'|sed 's/^.\{6\}//'|sed 's/.\{1\}$//')"
cat <<EOF > /boot/loader/entries/arch.conf
title Arch Linux
linux /vmlinuz-linux
initrd /intel-ucode.img
initrd /initramfs-linux.img
options rd.luks.uuid=${cryptrot} rd.luks.options=discard root=/dev/mapper/vg0-root quiet loglevel=3 rd.udev.log_priority=3 rd.systemd.show_status=auto vga=current fan_control=1
EOF
mkinitcpio -p linux
bootctl --path=/boot/ install
echo 'MAKEFLAGS="-j$(nproc)"' >> /etc/makepkg.conf
ln -sf /usr/share/zoneinfo/Europe/London /etc/localtime
hwclock --systohc
echo 'store /dev/md127 none luks,timeout=180' >> /etc/crypttab
echo '/dev/mapper/vg1-store /store ext4 rw,relatime,noauto,x-systemd.automount 0 2' >> /etc/fstab
<file_sep># Installtion notes
Previously, I had planned to make a modular metadistribution for Arch. Trying to do this taught me a few things:
- Automating an install is not always desirable.
- It is rare that I will not want to change something by hand that is best done early in the setup process. (Ie. I would be writing a script for other, hypothetical people; something I have no current interest in doing.)
- I do not often do installs at the moment. I have no need for a quick setup for remote machines.
- NixOS offers a good solution for automating server installs; it also lacks the rolling release issues associated with Arch. (Though, the install is a lot less minimal than a barebones Arch install.)
- I actually enjoy installing Arch, as it offers some moments for reflection on my system.
<file_sep>#!/bin/sh
# License: MIT
set -uo pipefail
trap 's=$?; echo "$0: Error on line "$LINENO": $BASH_COMMAND"; exit $s' ERR
MIRRORLIST_URL="https://www.archlinux.org/mirrorlist/?country=GB&protocol=https&use_mirror_status=on"
pacman -Sy --noconfirm pacman-contrib dialog archlinux-keyring
pacman-key --refresh-keys
curl -s "$MIRRORLIST_URL" | \
sed -e 's/^#Server/Server/' -e '/^#/d' | \
rankmirrors -n 5 - > /etc/pacman.d/mirrorlist
hostname=$(dialog --stdout --inputbox "Enter hostname" 0 0) || exit 1
clear
: ${hostname:?"hostname cannot be empty"}
user=$(dialog --stdout --inputbox "Enter admin username" 0 0) || exit 1
clear
: ${user:?"user cannot be empty"}
password=$(dialog --stdout --passwordbox "Enter admin password" 0 0) || exit 1
clear
: ${password:?"password <PASSWORD>"}
password2=$(dialog --stdout --passwordbox "Enter again" 0 0) || exit 1
clear
[[ "$password" == "$<PASSWORD>" ]] || ( echo "Passwords did not match"; exit 1; )
clear
devicelist=$(lsblk -dplnx size -o name,size | grep -Ev "boot|rpmb|loop" | tac)
device=$(dialog --stdout --menu "Select installation disk" 0 0 0 ${devicelist}) || exit 1
clear
timedatectl set-ntp true
umount --force -R /mnt || /bin/true
wipefs -af ${device}
wipefs -f -a ${device}
dd if=/dev/zero of=${device} bs=1M count=1024
parted --script "${device}" mklabel msdos \
mkpart primary ext4 1Mib 98% \
mkpart primary ext4 98% 100% \
set 2 boot on \
part_boot="$(ls ${device}* | grep -E "^${device}p?2$")"
part_crypt="$(ls ${device}* | grep -E "^${device}p?1$")"
wipefs "${part_boot}"
wipefs "${part_crypt}"
mkfs.ext4 "${part_boot}"
cryptsetup -c aes-xts-plain64 -y --use-random luksFormat "${part_crypt}"
cryptsetup luksOpen "${part_crypt}" luks
pvcreate /dev/mapper/luks
vgcreate vg0 /dev/mapper/luks
lvcreate --size 6G vg0 --name swap
lvcreate -l +100%FREE vg0 --name root
mkswap /dev/mapper/vg0-swap
mkfs.ext4 /dev/mapper/vg0-root
swapon /dev/mapper/vg0-swap
mount /dev/mapper/vg0-root /mnt
mkdir /mnt/boot
mount "${part_boot}" /mnt/boot
#mkdir -p /mnt/boot/loader/entries
pacman -Syy
pacstrap /mnt base base-devel mkinitcpio lvm2 sudo intel-ucode
genfstab -pU /mnt >> /mnt/etc/fstab
echo "${hostname}" > /mnt/etc/hostname
cat <<EOF > /mnt/etc/hosts
127.0.0.1 localhost
::1 localhost
127.0.1.1 $(echo $hostname).local $(echo $hostname)
EOF
mkdir /mnt/scripts &>/dev/null
cp *.sh /mnt/scripts &>/dev/null
cp /etc/pacman.d/mirrorlist /mnt/etc/pacman.d/
echo "LANG=en_GB.UTF-8" > /mnt/etc/locale.conf
echo "en_GB.UTF-8 UTF-8" > /mnt/etc/locale.gen
echo "en_GB ISO-8859-1" > /mnt/etc/locale.gen
arch-chroot /mnt useradd -mU -s /usr/bin/bash -G wheel "$user"
echo "$user:$password" | chpasswd --root /mnt
echo "root:$password" | chpasswd --root /mnt
echo "$user ALL=(ALL:ALL) ALL' >> /etc/sudoers"
arch-chroot /mnt /scripts/chrooted.sh
<file_sep>devicelist=$(lsblk -dplnx size -o name,size | grep -Ev "boot|rpmb|loop" | tac)
device=$(dialog --stdout --menu "Select installation disk" 0 0 0 ${devicelist}) || exit 1
clear
exec 1> >(tee "stdout.log")
exec 2> >(tee "stderr.log")
timedatectl set-ntp true
parted --script "${device}" --mklabel gpt \
mkpart ESP fat32 1Mib 129Mib \
set 1 boot on \
mkpart primary ext4 129MIB 100%
part_boot="$(ls ${device}* | grep -E "^${device}p?1$")"
part_crypt="$(ls ${device}* | grep -E "^${device}p?2$")"
wipefs "${part_boot}"
wipefs "${part_crypt}"
mkfs.vfat -F32 "${part_boot}"
cryptsetup -c aes-xts-plain64 -y --use-random luksFormat "${part_crypt}"
cryptsetup luksOpen "${part_crypt}" luks
pvcreate /dev/mapper/luks
vgcreate vg0 /dev/mapper/luks
lvcreate --size 6G vg0 --name swap
lvcreate -l +100%FREE vg0 --name root
mkswap /dev/mapper/vg0-swap
mkfs.ext4 /dev/mapper/vg0-root
swapon /dev/mapper/vg0-swap
mount /dev/mapper/vg0-root /mnt
mkdir /mnt/boot
mount "${part_boot}" /mnt/boot
pacstrap /mnt base base-devel git nvim linux-headers
genfstab -t PARTUUID /mnt >> /mnt/etc/fstab
echo "${hostname}" > /mnt/etc/hostname
arch-chroot /mnt bootctl install
cat <<EOF > /mnt/boot/loader/loader.conf
default arch
EOF
cat <<EOF > /mnt/boot/loader/entries/arch.conf
title Arch Linux
linux /vmlinuz-linux
initrd /initramfs-linux.img
options root=PARTUUID=$(blkid -s PARTUUID -o value "$part_root") rw
EOF
echo "LANG=en_GB.UTF-8" > /mnt/etc/locale.conf
arch-chroot /mnt useradd -mU -s /usr/bin/bash -G wheel "$user"
echo "$user:$password" | chpasswd --root /mnt
echo "$root:$password" | chpasswd --root /mnt
# below assumes admin user is setup
dialog --infobox "Refreshing Arch keyring..." 4 40
pacman --noconfirm -Sy archlinux-keyring >/dev/null 2>&1
dialog --infobox "Installing /`basedevel\` and \`git\`." 5 70
pacman --noconfirm --needed -S base-devel git >/dev/null 2>&1
[ -f /etc/sudoers.pacnew ] && cp /etc/sudoers.pacnew /etc/sudoers
newperms "%wheel ALL=(ALL) NOPASSWD: ALL"
grep "^Color" /etc/pacman.conf >/dev/null || sed -i "s/^#Color/Color/" /etc/pacman.conf
grep "ILoveCandy" /etc/pacman.conf >/dev/null || sed -i "/#VerbosePkgLists/a ILoveCandy" /etc/pacman.conf
sed -i "s/-j2/-j$(nproc)/;s/^#MAKEFLAGS/MAKEFLAGS/" /etc/makepkg.conf
[ -z "$aurhelper" ] && aurhelper="yay"
[ -f "/usr/bin/$aurhelper" ] || (
dialog --infobox "Installing \"$aurhelper\"..." 4 50
cd /tmp || exit
rm -rf /tmp/"$aurhelper"*
curl -s0 https://aur.archlinux.org/cgit/aur.git/snapshot/"$aurhelper".tar.gz &&
sudo -u "$name" tar -xvf "$aurhelper".tar.gz >/dev/null 2>&1 &&
cd "$aurhelper" &&
sudo -u "$name" makepkg --noconfirm -si >/dev/null 2>&1
cd /tmp || return
<file_sep>mount /dev/mapper/vg0-root /mnt/gentoo
ntpd -q -g
cd /mnt/gentoo
echo "If wget fails, go to lynx https://www.gentoo.org/downloads/mirrors/"
wget https://mirror.bytemark.co.uk/gentoo/releases/amd64/autobuilds/current-stage3-amd64/stage3-amd64-20191013T214502Z.tar.xz # this WILL change
tar xpvf stage3-*.tar.xz --xattrs-include='*.*' --numeric-owner
nano -w /mnt/gentoo/etc/portage/make.conf
| b9174b908827fa1d3446ee432d0138b074e933fa | [
"Markdown",
"Shell"
] | 5 | Shell | ashprice/arsch | 7eda0bcb9eea4bee4c5012b76fdfea35d45af5ea | 64f09a430eed3cb92e20fc18217afeafd88fdaa2 | |
refs/heads/master | <repo_name>xavieerbbc/Barrera-Bryam-ExamenFinal<file_sep>/Barrera-Bryam-ExamenFinal/src/ec/edu/ups/controlador/PacienteBean.java
package ec.edu.ups.controlador;
import java.io.Serializable;
import java.util.List;
import javax.annotation.PostConstruct;
import javax.ejb.EJB;
import javax.enterprise.context.SessionScoped;
import javax.faces.annotation.FacesConfig;
import javax.inject.Named;
import ec.edu.ups.ejb.PacienteFacade;
import ec.edu.ups.entidad.Paciente;
@FacesConfig(version = FacesConfig.Version.JSF_2_3)
@Named
@SessionScoped
public class PacienteBean implements Serializable{
private static final long serialVersionUID = 1L;
@EJB
private PacienteFacade ejbPacienteFacade;
private List<Paciente> list;
private String cedula;
private String nombres;
private String apellidos;
private String telefono;
private String direccion;
private String correoelectronico;
public PacienteFacade getEjbPacienteFacade() {
return ejbPacienteFacade;
}
public void setEjbPacienteFacade(PacienteFacade ejbPacienteFacade) {
this.ejbPacienteFacade = ejbPacienteFacade;
}
public List<Paciente> getList() {
return list;
}
public void setList(List<Paciente> list) {
this.list = list;
}
public String getCedula() {
return cedula;
}
public void setCedula(String cedula) {
this.cedula = cedula;
}
public String getNombres() {
return nombres;
}
public void setNombres(String nombres) {
this.nombres = nombres;
}
public String getApellidos() {
return apellidos;
}
public void setApellidos(String apellidos) {
this.apellidos = apellidos;
}
public String getTelefono() {
return telefono;
}
public void setTelefono(String telefono) {
this.telefono = telefono;
}
public String getDireccion() {
return direccion;
}
public void setDireccion(String direccion) {
this.direccion = direccion;
}
public String getCorreoelectronico() {
return correoelectronico;
}
public void setCorreoelectronico(String correoelectronico) {
this.correoelectronico = correoelectronico;
}
@PostConstruct
private void init() {
list= ejbPacienteFacade.findAll();
}
public String add() {
ejbPacienteFacade.create(new Paciente(this.cedula, this.nombres, this.apellidos, this.telefono, this.direccion, this.correoelectronico));
list = ejbPacienteFacade.findAll();
return null;
}
public String save(Paciente d) {
ejbPacienteFacade.edit(d);
d.setEditable(false);
return null;
}
}
<file_sep>/Barrera-Bryam-ExamenFinal/src/ec/edu/ups/controlador/CitaBean.java
package ec.edu.ups.controlador;
public class CitaBean {
}
<file_sep>/Barrera-Bryam-ExamenFinal/src/ec/edu/ups/ejb/SignosVFacade.java
package ec.edu.ups.ejb;
import java.util.List;
import javax.ejb.Stateless;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Query;
import ec.edu.ups.entidad.Paciente;
import ec.edu.ups.entidad.SignosVitales;
@Stateless
public class SignosVFacade extends AbstractFacade<SignosVitales>{
@PersistenceContext(unitName = "Barrera-Bryam-ExamenFinal")
private EntityManager em;
private SignosVFacade() {
super(SignosVitales.class);
}
@Override
protected EntityManager getEntityManager() {
// TODO Auto-generated method stub
return em;
}
public SignosVitales readSignos(String presion) {
Query query = em.createNamedQuery("getBySignos");
query.setParameter("presion", presion);
List result = query.getResultList();
SignosVitales respuesta = null;
if (!result.isEmpty()) {
respuesta = (SignosVitales)result.get(0);
}
return respuesta;
}
}
<file_sep>/Barrera-Bryam-ExamenFinal/src/ec/edu/ups/entidad/CitaMedica.java
package ec.edu.ups.entidad;
import java.io.Serializable;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
import javax.persistence.*;
/**
* Entity implementation class for Entity: CitaMedica
*
*/
@Entity
public class CitaMedica implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue()
private int codigo;
private Date fecha;
private String sintomas;
private String alergias;
private String enfermedadesPrevias;
//@OneToMany
//private Set<SignosVitales> listaSignos = new HashSet<SignosVitales>();
@ManyToOne
@JoinColumn
private Paciente paciente;
public CitaMedica() {
super();
}
public CitaMedica(Date fecha, String sintomas, String alergias, String enfermedadesPrevias, Paciente paciente) {
super();
this.fecha = fecha;
this.sintomas = sintomas;
this.alergias = alergias;
this.enfermedadesPrevias = enfermedadesPrevias;
this.paciente = paciente;
}
public int getCodigo() {
return codigo;
}
public void setCodigo(int codigo) {
this.codigo = codigo;
}
public Date getFecha() {
return fecha;
}
public void setFecha(Date fecha) {
this.fecha = fecha;
}
public String getSintomas() {
return sintomas;
}
public void setSintomas(String sintomas) {
this.sintomas = sintomas;
}
public String getAlergias() {
return alergias;
}
public void setAlergias(String alergias) {
this.alergias = alergias;
}
public String getEnfermedadesPrevias() {
return enfermedadesPrevias;
}
public void setEnfermedadesPrevias(String enfermedadesPrevias) {
this.enfermedadesPrevias = enfermedadesPrevias;
}
public Paciente getPaciente() {
return paciente;
}
public void setPaciente(Paciente paciente) {
this.paciente = paciente;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + codigo;
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
CitaMedica other = (CitaMedica) obj;
if (codigo != other.codigo)
return false;
return true;
}
}
| 53f33e29cf9cea2d9a2c69b14a80be4a0584d6c1 | [
"Java"
] | 4 | Java | xavieerbbc/Barrera-Bryam-ExamenFinal | 042b4d1032d0112de66195d07162467442031786 | d8a0493506c5919d9482c6d9ab21939734807601 | |
refs/heads/master | <repo_name>zkatemor/sentiment-analysis<file_sep>/models/dictionary.py
from sqlalchemy import Integer, String, ForeignKey, ARRAY
from db import session
class Dictionary(session.Model):
__tablename__ = 'dictionary'
id = session.Column(Integer, primary_key=True, autoincrement=True)
name = session.Column(String(50), nullable=False)
def __init__(self, name):
self.name = name
def __repr__(self):
return "<Dictionary(id={}, name={}".format(
self.id, self.name
)
def __str__(self):
return self.name
def serialize(self):
return {
'id': self.id,
'name': self.name
}
class Word(session.Model):
__tablename__ = 'word'
id = session.Column(Integer, primary_key=True, autoincrement=True)
word = session.Column(String(120), nullable=False)
part_of_speech = session.Column(String(50), nullable=False)
tone = session.Column(String(50), nullable=False, default='None')
dictionaryId = session.Column(Integer, ForeignKey('dictionary.id'), nullable=False)
dictionary = session.relationship('Dictionary', backref=session.backref('words', lazy=True))
def __init__(self, dictionaryId, word, tone, part_of_speech):
self.dictionaryId = dictionaryId
self.word = word
self.tone = tone
self.part_of_speech = part_of_speech
def __repr__(self):
return "<Word(id={}, word={}, tone={}, part_of_speech={}".format(
self.id, self.word, self.tone, self.part_of_speech
)
def __str__(self):
return "{}_{}".format(self.word, self.part_of_speech)
def serialize(self):
return {
'id': self.id,
'word': self.word,
'tone': self.tone,
'part_of_speech': self.part_of_speech,
'dictionary_id': self.dictionaryId
}
<file_sep>/scripts/get_test_review.py
import requests
import json
import os
from dotenv import load_dotenv
load_dotenv()
url = "https://market-scanner.ru/api/reviews"
key = os.environ.get('key')
reviews = []
def getReviewById(id):
data = {'key': key,
'id': id,
}
response = requests.post(url=url, data=data)
js = response.json().get('reviews')
print(js)
reviews.append(js)
# 1) леново 201 2) леново 438 3) сяоми 629 4) хонор 421 5) мейзу 330 = 2019
ids = [9323494, 10414225, 13527763, 12423732, 12748971]
for i in ids:
getReviewById(i)
with open('D:\\GitHub\\sentiment-analyzer\\app\\static\\reviews.json', 'w', encoding='utf-8') as f:
json.dump(reviews, f, ensure_ascii=False, indent=4)
<file_sep>/README.md
<h1>Классификация текстов по тональности
Lexicon-based approach</h1>
<h2>How to</h2>
Запуск проекта:
`virtualenv venv`
`source venv/bin/activate`
`pip install -r requirements.txt`
`python manage.py runserver`
<h2>Наборы данных</h2>
Источник: https://market-scanner.ru/doc/reviews
* **app/static/review_db.json** – набор данных для построения словаря оценочной лексики
* **app/static/reviews.json** – набор данных для тестирования метода классикации
* **app/dictionaries** - используемые словари оценочной лексики и слова-модификаторы
<h2>Ссылка на проект</h2>
https://sentiment-dictionary-based.herokuapp.com/sentiment
<h2>About</h2>
Забабурина Е.А. РАЗРАБОТКА ПРОГРАММЫ КЛАССИФИКАЦИИ ТЕКСТОВ ПО ТОНАЛЬНОСТИ: Выпускная квалификационная работа/ ВятГУ, каф. ПМиИ; рук. Разова Е.В. – Киров, 2020. – ПЗ 79 с., 13 рис., 40 источников, 5 приложений.
<file_sep>/migrations/versions/78f4d0125f95_.py
"""empty message
Revision ID: 78f4d0125f95
Revises: <KEY>
Create Date: 2020-04-13 19:37:18.613766
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = '<KEY>'
down_revision = '<KEY>'
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.drop_column('word', 'part_of_speech')
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.add_column('word', sa.Column('part_of_speech', sa.VARCHAR(length=50), autoincrement=False, nullable=False))
# ### end Alembic commands ###
<file_sep>/app/__init__.py
def create_app():
import os
from flask import Flask
from app.admin_panel import admin
from db import session
app = Flask(__name__)
app.config.from_object(os.environ['APP_SETTINGS'])
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
admin.init_app(app)
session.init_app(app)
from models.dictionary import Dictionary, Word
return app
<file_sep>/app/services/reporter.py
import datetime as dt
import json
class Reporter:
def __init__(self, text, result):
self.text = text
self.result = result
def get_dict(self):
report = {
'text': self.text,
'positive': list(self.result['words']['positive']),
'negative': list(self.result['words']['negative']),
'modifier': list(self.result['words']['modifier']),
'score': self.result['score'],
'sentiment': ""
}
if self.result['score'] > 0:
report['sentiment'] = 'Положительная тональность. Чистая положительная оценка: ' + str(
self.result['positive'])
else:
report['sentiment'] = 'Отрицательная тональность. Чистая отрицательная оценка: ' + str(
self.result['negative'])
return report
def get_report(self):
report = self.get_dict()
date = str(dt.datetime.now().date()) + str(dt.datetime.now().time()).replace(".", ":").replace(":", "-")
filename = "app/static/reports/Отчёт-" + date + ".json"
with open(filename, 'w', encoding='utf-8') as f:
json.dump(report, f, ensure_ascii=False, indent=4)
return filename.replace('app/static/', '')
@staticmethod
def get_reports(report):
date = str(dt.datetime.now().date()) + str(dt.datetime.now().time()).replace(".", ":").replace(":", "-")
filename = "app/static/reports/Отчёт-" + date + ".json"
with open(filename, 'w', encoding='utf-8') as f:
json.dump(report, f, ensure_ascii=False, indent=4)
return filename.replace('app/static/', '')
<file_sep>/scripts/score_rusentilex.py
"""формированиен весов слов для словаря rusentilex"""
import csv
from itertools import zip_longest
import pandas as pd
def get_weights(tones):
result = []
for i in tones:
if i == 'positive':
result.append(1)
else:
result.append(-1)
return result
df = pd.read_csv('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\rusentilex.csv',
encoding='utf-8')
term = df['term'].tolist()
weight = get_weights(df['tone'].tolist())
d = [term, weight]
export_data = zip_longest(*d, fillvalue='')
with open('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\rusentilex.csv', 'w',
newline='', encoding='utf-8') as f:
wr = csv.writer(f)
wr.writerow(("term", "weight"))
wr.writerows(export_data)
f.close()
<file_sep>/requirements.txt
alembic==1.4.2
aniso8601==8.0.0
atomicwrites==1.4.0
attrs==19.3.0
certifi==2020.4.5.2
chardet==3.0.4
Click==7.0
colorama==0.4.3
cycler==0.10.0
DAWG-Python==0.7.2
docopt==0.6.2
enum34==1.1.10
et-xmlfile==1.0.1
Flask==1.1.1
Flask-Admin==1.5.6
Flask-Ext==0.1
Flask-Migrate==2.5.3
Flask-RESTful==0.3.8
Flask-Script==2.0.6
Flask-SQLAlchemy==2.4.1
gunicorn==20.0.4
idna==2.9
importlib-metadata==1.6.1
itsdangerous==1.1.0
jdcal==1.4.1
Jinja2==2.11.1
joblib==0.15.1
kiwisolver==1.2.0
Mako==1.1.2
MarkupSafe==1.1.1
matplotlib==3.2.1
more-itertools==8.3.0
nltk==3.5
numpy==1.18.2
openpyxl==3.0.3
packaging==20.4
pandas==0.23.4
pandas-ml==0.6.1
pluggy==0.13.1
psycopg2-binary==2.8.5
py==1.8.1
pymorphy2==0.8
pymorphy2-dicts==2.4.393442.3710985
pyparsing==2.4.7
pytest==5.4.3
python-dateutil==2.8.1
python-dotenv==0.12.0
python-editor==1.0.4
pytz==2019.3
regex==2020.6.8
requests==2.23.0
scikit-learn==0.23.1
scipy==1.4.1
seaborn==0.10.1
six==1.14.0
sklearn==0.0
SQLAlchemy==1.3.16
threadpoolctl==2.1.0
tqdm==4.46.1
urllib3==1.25.9
wcwidth==0.2.4
Werkzeug==1.0.0
WTForms==2.2.1
xlrd==1.2.0
zipp==3.1.0
<file_sep>/db/__init__.py
from flask_sqlalchemy import SQLAlchemy
session = SQLAlchemy()
<file_sep>/scripts/test.py
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.metrics import classification_report
import seaborn as sn
from app.services.sentimental import Sentimental
def get_report_test(dictionary, is_unigram):
# результат классификации
prediction = []
# реальный результат
actual = []
sent = Sentimental(dictionary=dictionary,
negation='D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\negations.csv',
modifier='D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\modifier.csv',
is_unigram=is_unigram)
for i in range(0, len(texts)):
result = sent.analyze(texts[i])
if ratings[i] in range(4, 6):
actual.append('positive')
if result['score'] > 0:
prediction.append('positive')
elif result['score'] <= 0:
prediction.append('negative')
else:
actual.append('negative')
if result['score'] > 0:
prediction.append('positive')
elif result['score'] <= 0:
prediction.append('negative')
print(i)
print(classification_report(actual, prediction))
return prediction, actual
# загрузка тестового набора данных
df = pd.read_excel('D:\\GitHub\\sentiment-analyzer\\app\static\\reviews.xlsx', sheet_name="Sheet1")
texts = df['Text'].tolist()
ratings = df['Rating'].tolist()
dictionary_rusentilex = ['D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\rusentilex.csv']
dictionary_chi = ['D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\chi_minus.csv',
'D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\chi_plus.csv']
dictionary_cnn = ['D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\cnn_dict.csv']
dictionary_chi_collocations = ['D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\chi_collocations_minus.csv',
'D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\chi_collocations_plus.csv']
prediction, actual = get_report_test(dictionary_chi, True)
data = {'prediction': prediction,
'actual': actual
}
df = pd.DataFrame(data, columns=['actual', 'prediction'])
matrix = pd.crosstab(df['actual'], df['prediction'], rownames=['Actual'], colnames=['Predicted'])
print(matrix)
plt.figure(figsize=(10, 7))
sn.heatmap(matrix, annot=True, cmap="Greens")
plt.title("CHI UNIGRAM")
plt.show()
<file_sep>/app/api/views.py
from flask_restful import Resource, reqparse
from models.dictionary import Dictionary, Word
from db import session
class DictionaryView(Resource):
def get(self):
parser = reqparse.RequestParser()
parser.add_argument('id', required=False)
args = parser.parse_args()
if args['id']:
if Dictionary.query.filter(Dictionary.id == args['id']).count():
dictionaries = Dictionary.query.filter(Dictionary.id == args['id'])
js = Dictionary.serialize(dictionaries[0])
else:
return {'message': 'Dictionary not found', 'result': {}}, 404
else:
dictionaries = Dictionary.query.all()
js = [Dictionary.serialize(d) for d in dictionaries]
return {'message': 'Success', 'result': js}, 200
def post(self):
parser = reqparse.RequestParser()
parser.add_argument('name', required=True)
args = parser.parse_args()
dictionary = Dictionary(args['name'])
session.session.add(dictionary)
session.session.commit()
return {'message': 'Success', 'result': {}}, 201, {'Location': '/dictionary/:' + str(dictionary.id)}
class WordView(Resource):
def get(self):
parser = reqparse.RequestParser()
parser.add_argument('id', required=False)
args = parser.parse_args()
if args['id']:
if Word.query.filter(Word.id == args['id']).count():
words = Word.query.filter(Word.id == args['id'])
js = Word.serialize(words[0])
else:
return {'message': 'Word not found', 'result': {}}, 404
else:
words = Word.query.all()
js = [Word.serialize(d) for d in words]
return {'message': 'Success', 'result': js}, 200
def post(self):
parser = reqparse.RequestParser()
parser.add_argument('word', required=True)
parser.add_argument('part_of_speech', required=True)
parser.add_argument('tone', required=True)
parser.add_argument('dictionary_id', required=True)
args = parser.parse_args()
word = Word(args['dictionary_id'],
args['word'],
args['tone'],
args['part_of_speech']
)
session.session.add(word)
session.session.commit()
return {'message': 'Success', 'result': {}}, 201, {'Location': '/word/:' + str(word.id)}
<file_sep>/scripts/script.py
import json
import pandas as pd
with open('D:\\GitHub\\sentiment-analyzer\\app\static\\reviews.json', 'r', encoding='utf-8') as f:
js = json.load(f)
texts = []
ratings = []
for model in js:
for review in model:
texts.append(str(review['pluses']) + '\n' + str(review['minuses']) + '\n' + str(review['comment']))
ratings.append(review['rating'])
df = pd.DataFrame()
df['Text'] = texts
df['Rating'] = ratings
df.to_excel('D:\\GitHub\\sentiment-analyzer\\app\\static\\reviews.xlsx', index=False)
<file_sep>/scripts/get_weight_cnn.py
# загружаем неразмеченный словарь из json файла
import csv
import json
from itertools import zip_longest
import pandas as pd
import numpy as np
"""
Get new csv file with term, frequency, tone for next operations
with open('unallocated_dictionary.json', 'r', encoding='utf-8') as f:
js = json.load(f)
unallocated_words = [word[0] for word in js]
unallocated_frequency = [word[1] for word in js]
df = pd.read_csv('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\original\\dict_cnn.csv', encoding='utf-8')
tone = df['tone'].tolist()
d = [unallocated_words, unallocated_frequency, tone]
export_data = zip_longest(*d, fillvalue='')
with open('D:\\GitHub\\sentiment-analyzer\\scripts\\cnn_dict_tmp.csv', 'w', encoding='utf-8',
newline='') as f:
wr = csv.writer(f)
wr.writerow(("term", "frequency", "tone"))
wr.writerows(export_data)
f.close()
"""
def scale_positive(chi_square):
return 1 * (chi_square - np.min(chi_square)) / (np.max(chi_square) - np.min(chi_square))
def scale_negative(chi_square):
return -1 * (chi_square - np.min(chi_square)) / (np.max(chi_square) - np.min(chi_square))
df = pd.read_csv('D:\\GitHub\\sentiment-analyzer\\scripts\\cnn_dict_tmp.csv', encoding='utf-8')
tone = df['tone'].tolist()
term = df['term'].tolist()
frequency = df['frequency'].tolist()
positive_tone = []
positive_term = []
positive_f = []
negative_term = []
negative_f = []
for i in range(0, len(tone)):
if tone[i] == 'positive':
positive_term.append(term[i])
positive_f.append(frequency[i])
else:
negative_term.append(term[i])
negative_f.append(frequency[i])
negative = np.array(negative_f)
negative_scaled = scale_negative(negative)
positive = np.array(positive_f)
positive_scaled = scale_positive(positive)
scale = list(positive_scaled) + list(negative_scaled)
d = [positive_term + negative_term, scale]
export_data = zip_longest(*d, fillvalue='')
with open('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\cnn_dict.csv', 'w', encoding='utf-8',
newline='') as f:
wr = csv.writer(f)
wr.writerow(("term", "weight"))
wr.writerows(export_data)
f.close()
<file_sep>/app/services/sentimental.py
""" словарный метод классификации """
import os
import csv
import pymorphy2
import nltk
from collections import defaultdict
class Sentimental(object):
def __init__(self, dictionary=None, negation=None, modifier=None, is_unigram=True):
self.morph = pymorphy2.MorphAnalyzer()
if dictionary is None and negation is None and modifier is None:
base_dir = os.path.dirname(__file__)
dictionary = [os.path.join(base_dir, p) for p in ['dictionaries/rusentilex.csv']]
negation = os.path.join(base_dir, 'dictionaries/negations.csv')
modifier = os.path.join(base_dir, 'dictionaries/modifier.csv')
# словарь оценочной лексики
self.dictionary = {}
# слова-отрицатели
self.negations = set()
# слова-усилители
self.modifiers = {}
# является ли словарь - словарем униграм
self.is_unigram = is_unigram
# загрузка словаря и модификаторов из назначенных файлов
for wl_filename in self.__to_arg_list(dictionary):
self.load_dictionary(wl_filename)
for negations_filename in self.__to_arg_list(negation):
self.load_negations(negations_filename)
for modifier_filename in self.__to_arg_list(modifier):
self.load_modifiers(modifier_filename)
self.__negation_skip = {'очень', 'самый', 'достаточно', 'должен'}
@staticmethod
def __to_arg_list(obj):
if obj is not None:
if not isinstance(obj, list):
obj = [obj]
else:
obj = []
return obj
# распознавание слов-отрицателей
def __is_prefixed_by_negation(self, token_idx, tokens):
prev_idx = token_idx - 1
if tokens[prev_idx] in self.__negation_skip:
prev_idx -= 1
is_prefixed = False
if token_idx > 0 and prev_idx >= 0 and tokens[prev_idx] in self.negations:
is_prefixed = True
return is_prefixed, tokens[prev_idx]
# распознавание слов-усилителей
def __is_prefixed_by_modifier(self, token_idx, tokens):
prev_idx = token_idx - 1
percent = 0
is_prefixed = False
if token_idx > 0 and prev_idx >= 0 and tokens[prev_idx] in self.modifiers:
is_prefixed = True
percent = self.modifiers[tokens[prev_idx]]
return is_prefixed, tokens[prev_idx], percent
def load_negations(self, filename):
with open(filename, 'r', encoding='utf-8') as f:
reader = csv.DictReader(f)
negations = set([row['token'] for row in reader])
self.negations |= negations
def load_modifiers(self, filename):
with open(filename, 'r', encoding='utf-8') as f:
reader = csv.DictReader(f)
modifiers = {row['token']: row['percent'] for row in reader}
self.modifiers.update(modifiers)
def load_dictionary(self, filename):
with open(filename, 'r', encoding='utf-8') as f:
reader = csv.DictReader(f)
if 'app/dictionaries/rusentilex.csv' in filename \
or 'D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\rusentilex.csv' in filename:
dictionary = {row['term']: row['weight'] for row in reader}
else:
if self.is_unigram:
dictionary = {self.__delete_part_of_speech(row['term']): row['weight'] for row in reader}
else:
dictionary = {self.__delete_part_of_speech(row['term']): row['weight'] for row in reader}
self.dictionary.update(dictionary)
# разбивание текста на токены
def __get_tokens(self, sentence):
# удаляем все символы, кроме кириллицы
regex_tokenizer = nltk.tokenize.RegexpTokenizer('[а-яА-ЯЁё]+')
# приводим к нижнему регистру
words = regex_tokenizer.tokenize(sentence.lower())
# приводим каждое слово в нормальную форму и добавляем тег - часть речи
result = [self.morph.parse(w)[0].normal_form for w in words]
return result
# разбивание текста на биграмы
def __get_bigrams(self, sentence):
regex_tokenizer = nltk.tokenize.RegexpTokenizer('[а-яА-ЯЁё]+')
words = regex_tokenizer.tokenize(sentence.lower())
result = [self.morph.parse(words[i])[0].normal_form + ' ' + self.morph.parse(words[i + 1])[0].normal_form
for i in range(0, len(words) - 1)]
return result
# удаление части речи в слова или словосочетании
def __delete_part_of_speech(self, term):
if self.is_unigram:
new_term = term[:-5]
else:
terms = term.split()
new_term = terms[0][:-5] + ' ' + terms[1][:-5]
return new_term
# словарный метод
def analyze(self, sentence):
if self.is_unigram:
terms = self.__get_tokens(sentence)
else:
terms = self.__get_bigrams(sentence)
scores = defaultdict(float)
words = defaultdict(set)
comparative = 0
for i, term in enumerate(terms):
is_prefixed_by_negation, negation = self.__is_prefixed_by_negation(i, terms)
is_prefixed_by_modifier, modifier, mod_percent = self.__is_prefixed_by_modifier(i, terms)
if term in self.dictionary:
tone = 'positive' if float(self.dictionary[term]) > 0 else 'negative'
score = float(self.dictionary[term])
words[tone].add(term)
if is_prefixed_by_negation:
scores[tone] += score * -1
words['modifier'].add(negation)
else:
scores[tone] += score
if is_prefixed_by_modifier:
scores[tone] += score * float(mod_percent)
words['modifier'].add(modifier)
if len(terms) > 0:
comparative = (scores['positive'] + scores['negative']) / len(terms)
# результат - словарь с отчетом
result = {
'score': scores['positive'] + scores['negative'],
'positive': scores['positive'],
'negative': scores['negative'],
'comparative': comparative,
'words': words
}
return result
<file_sep>/manage.py
"""исходный код программы классификации текста по тональности"""
import csv
import os
import shutil
import pandas as pd
from werkzeug.utils import secure_filename
from dotenv import load_dotenv
from flask import render_template, request
from flask_restful import Api
from flask_script import Manager
from flask_migrate import Migrate, MigrateCommand
from app import create_app
from app.api.views import DictionaryView, WordView
from app.services.random import Random
from app.services.reporter import Reporter
from db import session
from app.services.sentimental import Sentimental
load_dotenv()
UPLOAD_FOLDER = 'app/static/uploads'
ALLOWED_EXTENSIONS = {'xlsx'}
app = create_app()
migrate = Migrate(app, session)
manager = Manager(app)
manager.add_command('db', MigrateCommand)
api = Api(app)
api.add_resource(DictionaryView, '/dictionary')
api.add_resource(WordView, '/word')
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
app.config['UPLOAD_FILENAME'] = ''
app.config['DICTIONARY'] = ['app/dictionaries/rusentilex.csv']
app.config['IS_UNIGRAM'] = True
# удаление старых файлов отчетов для экономии места на сервере
def delete_old_files(folder):
for filename in os.listdir(folder):
file_path = os.path.join(folder, filename)
try:
if os.path.isfile(file_path) or os.path.islink(file_path):
os.unlink(file_path)
elif os.path.isdir(file_path):
shutil.rmtree(file_path)
except Exception as e:
print('Failed to delete %s. Reason: %s' % (file_path, e))
@app.route('/sentiment', methods=['GET', 'POST'])
def index():
try:
if request.method == 'GET':
"""home page"""
result = {
'score': 0,
'positive': 0,
'negative': 0,
'comparative': 0,
'words': {}
}
random = Random('app/static/reviews.json')
text = random.get_review()
img = 'icons/hand.png'
positive = ''
negative = ''
modifier = ''
report = ''
else:
delete_old_files('app/static/reports')
text = request.form['text']
select = request.form.get('select')
dictionary = ['app/dictionaries/rusentilex.csv']
is_unigram = True
if str(select) == 'CHI unigram':
dictionary = ['app/dictionaries/chi_minus.csv', 'app/dictionaries/chi_plus.csv']
is_unigram = True
elif str(select) == 'CNN unigram':
dictionary = ['app/dictionaries/cnn_dict.csv']
is_unigram = True
elif str(select) == 'CHI bigram':
dictionary = ['app/dictionaries/chi_collocations_minus.csv',
'app/dictionaries/chi_collocations_plus.csv']
is_unigram = False
sent = Sentimental(dictionary=dictionary,
negation='app/dictionaries/negations.csv',
modifier='app/dictionaries/modifier.csv',
is_unigram=is_unigram)
result = sent.analyze(text)
if result['score'] > 0:
img = 'icons/positive.png'
elif result['score'] == 0:
img = 'icons/hand.png'
else:
img = 'icons/negative.png'
positive_str = [str(s) for s in result['words']['positive']]
positive = ", ".join(positive_str)
negative_str = [str(s) for s in result['words']['negative']]
negative = ", ".join(negative_str)
mod_str = [str(s) for s in result['words']['modifier']]
modifier = ", ".join(mod_str)
report = Reporter(text, result).get_report()
return render_template("index.html",
text=text,
result=result,
img=img,
positive=positive,
negative=negative,
modifier=modifier,
download_file=report)
except Exception as e:
print(e)
@app.route('/dictionaries/<name>', methods=['GET'])
def get_dictionaries(name):
about_dictionary = ""
name_dictionary = ""
dictionary = {}
html_file = ""
if name == 'chi_unigram':
name_dictionary = "<NAME>, построенный с помощью критерия согласия Пирсона (Хи-квадрат)"
about_dictionary = "Распределение тонально-окрашенных слов с помощью критерия анализа – оценки корреляции " \
"двух событий: «слово содержится в корпусе» и «слово содержится в подкорпусе " \
"достоинств или недостатков». Благодаря методу, основанному на распределении оценочных слов " \
"с помощью критерия согласия Пирсона, удалось получить 1260 положительно окрашенных слов и " \
"2267 с отрицательной окраской."
with open('app/dictionaries/chi_plus.csv', encoding='utf-8') as f:
dictionary = dict(filter(None, csv.reader(f)))
dictionary.pop('term')
with open('app/dictionaries/chi_minus.csv', encoding='utf-8') as f:
dictionary_minus = dict(filter(None, csv.reader(f)))
dictionary_minus.pop('term')
dictionary.update(dictionary_minus)
elif name == 'cnn_unigram':
name_dictionary = "<NAME>, построенный с помощью свёрточной нейронной сети"
about_dictionary = "Обученная с помощью сверточной нейросети модель и предобученной модели векторных " \
"представлений слов Word2Vec показала следующие результаты: удалось выявить 373 " \
"положительно-окрашенных слов, 5216 отрицательно-окрашенных слов и 114 " \
"нейтрально-окрашенных слов. Данное распределение связано с тем, что и размеченные данных, " \
"взятые из словаря RuSentiLex не были сбалансированными (отрицательных слов 7148, " \
"положительных – 2774 и нейтральных – 746)."
with open('app/dictionaries/cnn_dict.csv', encoding='utf-8') as f:
dictionary = dict(filter(None, csv.reader(f)))
dictionary.pop('term')
elif name == 'chi_bigram':
name_dictionary = "<NAME>, построенный с помощью критерия согласия Пирсона (Хи-квадрат)"
about_dictionary = "Распределение тонально-окрашенных словосочетаний с помощью критерия анализа – оценки " \
"корреляции " \
"двух событий: «слововочетание содержится в корпусе» и «словосочетание содержится в " \
"подкорпусе " \
"достоинств или недостатков». Благодаря методу, основанному на распределении оценочных слов " \
"с помощью критерия согласия Пирсона, удалось получить 20520 положительно окрашенных " \
"словосочетаний и " \
"19550 с отрицательной окраской."
with open('app/dictionaries/chi_collocations_plus.csv', encoding='utf-8') as f:
dictionary = dict(filter(None, csv.reader(f)))
dictionary.pop('term')
with open('app/dictionaries/chi_collocations_minus.csv', encoding='utf-8') as f:
dictionary_minus = dict(filter(None, csv.reader(f)))
dictionary_minus.pop('term')
dictionary.update(dictionary_minus)
elif name == 'all':
name_dictionary = "Словари оценочной лексики для классификации текстов по тональности"
html_file = "all_dictionary.html"
dictionary = dict(
хороший=1,
неплохой=0.5,
нехороший=-0.5,
плохой=-1
)
return render_template("dictionaries.html",
about_dictionary=about_dictionary,
dictionary=dictionary,
name_dictionary=name_dictionary,
html_file=html_file)
@app.route('/about', methods=['GET'])
def about_project():
return render_template("about.html")
def allowed_file(filename):
return '.' in filename and \
filename.rsplit('.', 1)[1] in ALLOWED_EXTENSIONS
@app.route('/analyze/files', methods=['GET', 'POST'])
def files_classifier():
delete_old_files('app/static/reports')
delete_old_files('app/static/uploads')
if request.method == 'POST':
file = request.files['file']
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
file.save(os.path.join(app.config['UPLOAD_FOLDER'], filename))
app.config['UPLOAD_FILENAME'] = filename
select = request.form.get('select')
if str(select) == 'CHI unigram':
dictionary = ['app/dictionaries/chi_minus.csv', 'app/dictionaries/chi_plus.csv']
is_unigram = True
elif str(select) == 'CNN unigram':
dictionary = ['app/dictionaries/cnn_dict.csv']
is_unigram = True
elif str(select) == 'RuSentiLex':
dictionary = ['app/dictionaries/rusentilex.csv']
is_unigram = True
elif str(select) == 'CHI bigram':
dictionary = ['app/dictionaries/chi_collocations_minus.csv',
'app/dictionaries/chi_collocations_plus.csv']
is_unigram = False
app.config['DICTIONARY'] = dictionary
app.config['IS_UNIGRAM'] = is_unigram
return render_template("files_analyze.html")
@app.route('/analyze/files/result', methods=['GET', 'POST'])
def ajax_files():
if os.listdir(app.config['UPLOAD_FOLDER']):
filename = 'app/static/uploads/' + app.config['UPLOAD_FILENAME']
df = pd.read_excel(filename, sheet_name="Лист1")
texts = df['text'].tolist()
dictionary = app.config['DICTIONARY']
is_unigram = app.config['IS_UNIGRAM']
sent = Sentimental(dictionary=dictionary,
negation='app/dictionaries/negations.csv',
modifier='app/dictionaries/modifier.csv', is_unigram=is_unigram)
report = []
for text in texts:
result = sent.analyze(text)
report.append(Reporter(text, result).get_dict())
download_file = Reporter.get_reports(report)
return render_template('include_files.html', download_file=download_file)
else:
return '<h3>Файл ещё не загружен</h3>'
if __name__ == '__main__':
manager.run()
<file_sep>/app/services/random.py
import json
import random
class Random:
def __init__(self, filename):
self.reviews = None
self.load_review(filename)
def load_review(self, filename):
with open(filename, 'r', encoding='utf-8') as f:
reviews = json.load(f)
self.reviews = reviews
def get_review(self):
texts = [str(review['pluses']) + '\n' + str(review['minuses']) + '\n' + str(review['comment'])
for model in self.reviews for review in model]
texts = list(map(lambda x: x.replace('None', ''), texts))
return random.choice(texts)
<file_sep>/app/admin_panel/__init__.py
from flask_admin import Admin
from flask_admin.contrib.sqla import ModelView
from db import session
from models.dictionary import Dictionary, Word
admin = Admin(name='Sentiment dashboard', template_mode='bootstrap3')
admin.add_view(ModelView(Dictionary, session.session))
admin.add_view(ModelView(Word, session.session))
<file_sep>/scripts/get_weight_minus.py
"""формирование весов слов для отлицательного словаря хи-квадрат"""
import csv
from itertools import zip_longest
import numpy as np
import pandas as pd
def scale(chi_square):
return -1 * (chi_square - np.min(chi_square)) / (np.max(chi_square) - np.min(chi_square))
df = pd.read_csv('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\original\\dict_collocations_minus.csv', encoding='utf-8')
chi = np.array(df['chi_square'])
chi_scaled = scale(chi)
words = df['term'].tolist()
d = [words, chi_scaled]
export_data = zip_longest(*d, fillvalue='')
with open('D:\\GitHub\\sentiment-analyzer\\app\\dictionaries\\chi_collocations_minus.csv', 'w', encoding='utf-8',
newline='') as f:
wr = csv.writer(f)
wr.writerow(("term", "weight"))
wr.writerows(export_data)
f.close()
| cfb1d8bce2c7dad1b7abf0fdaa9bc1f4518fdcae | [
"Markdown",
"Python",
"Text"
] | 18 | Python | zkatemor/sentiment-analysis | 1d87948b92eb3a44db4f6d384c26cfaaf50502d8 | 4c59aab84760ed2d80f302b23f473f6e7da37b4b | |
refs/heads/master | <repo_name>summerbuild/zabbix-conf<file_sep>/script/tcp_status
#!/bin/bash
#this script is used to get tcp and udp connetion status
#tcp status
case $1 in
closed)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/CLOSED/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
listen)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/LISTEN/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
synrecv)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/SYN_RECV/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
synsent)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/SYN_SENT/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
established)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/ESTAB/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
timewait)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/TIME_WAIT/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
closing)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/CLOSING/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
closewait)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/CLOSE_WAIT/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
lastack)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/LAST_ACK/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
finwait1)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/FIN_WAIT1/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
finwait2)
output=`ss -ant|awk '{++S[$1]}END{for(a in S) print a,S[a]}'|awk '/FIN_WAIT2/{print $2}'`
if [ "$output" == "" ];then
echo 0
else
echo $output
fi
;;
*)
echo -e "\e[033mUsage: sh $0 [closed|closing|closewait|synrecv|synsent|finwait1|finwait2|listen|established|lastack|timewait]\e[0m"
esac
<file_sep>/script/check_mem
#!/bin/bash
# check memory script
# sunny 2008.2.15
if [ -f /etc/redhat-release ];then
OS=`cat /etc/redhat-release |awk '{print $4}'|awk -F. '{print $1}'`
if [ $OS == 7 ];then
total_value=`free|grep Mem|awk '{print $2}'`
Free=`free|grep Mem|awk '{print $NF}'`
#cached_value=`free|grep Mem|awk '{print $6}'`
share_value=`free|grep Mem|awk '{print $5}'`
used_value=$[$total_value - $Free]
else
# Total memory
total_value=`cat /proc/meminfo |grep "^MemTotal"|awk '{print $2}'`
# Free memory
free_value=`cat /proc/meminfo |grep "^MemFree"|awk '{print $2}'`
buffers_value=`cat /proc/meminfo |grep "^Buffers"|awk '{print $2}'`
cached_value=`cat /proc/meminfo |grep "^Cached"|awk '{print $2}'`
Free=$[$free_value + $buffers_value + $cached_value]
# Used memory
used_value=$[$total_value - $Free]
fi
else
total_value=`free|grep Mem|awk '{print $2}'`
Free=`free|grep Mem|awk '{print $NF}'`
#cached_value=`free|grep Mem|awk '{print $6}'`
share_value=`free|grep Mem|awk '{print $5}'`
used_value=`expr $total_value - $Free`
fi
# to calculate used percent
# use the expression used * 100 / total
usedtmp=`expr $used_value \* 100`
usedent=`expr $usedtmp / $total_value`
#############################################################################################
total () {
#如果指定单位为M,则换算为M.默认是G为单位
if [ "$1" ];then
total=$[$total_value/1024]
if [ "$total" -le 1 ];then
total=`awk 'BEGIN{printf "%.2f\n",'$total_value/1024'}'|awk '{printf("%0.1f\n",$0)}'`
fi
else
#前一个awk保留两位小数,后一个awk4舍5入
total=`awk 'BEGIN{printf "%.2f\n",'$total_value/1024/1024'}'|awk '{printf("%0.1f\n",$0)}'`
fi
echo $total
}
#############################################################################################
free(){
if [ "$1" ];then
free=$[$Free/1024]
if [ "$free" -le 1 ];then
free=`awk 'BEGIN{printf "%.2f\n",'$Free/1024'}'`
fi
else
free=`awk 'BEGIN{printf "%.2f\n",'$Free/1024/1024'}'`
fi
echo $free
}
#############################################################################################
used (){
if [ "$1" ];then
used=$[$used_value/1024]
if [ "$used" -le 1 ];then
used=`awk 'BEGIN{printf "%.2f\n",'$used_value/1024'}'`
fi
else
used=`awk 'BEGIN{printf "%.2f\n",'$used_value/1024/1024'}'`
fi
echo $used
}
#############################################################################################
case $1 in
total)
total $2
;;
used)
used $2
;;
free)
free $2
;;
usedent)
echo $usedent
;;
--help|help)
echo " $1 [total|used|usedent];$2 [m|default is G]"
exit 0
esac
<file_sep>/script/zabbixDiscovery
#!/bin/bash
serviceDiscovery() {
project_dir="/home/deploy/projects/"
serviceList=($(ls $project_dir|grep "service"))
printf '{\n'
printf '\t"data":[\n'
for key in ${!serviceList[@]}
do
if [[ "$key" -lt "((${#serviceList[@]}-1))" ]];then
printf "\t\t{\n"
printf "\t\t\t\"{#SERVICENAME}\":\"${serviceList[$key]}\"},\n"
else
printf "\t\t{\n"
printf "\t\t\t\"{#SERVICENAME}\":\"${serviceList[$key]}\"}\n"
fi
done
printf '\t ]\n'
printf '}\n'
}
webDiscovery(){
webarray=(`ls /data/web-server/|grep -E "^tomcat.*"`)
length=${#webarray[@]}
printf "{\n"
printf '\t'"\"data\":["
for ((i=0;i<$length;i++))
do
printf '\n\t\t{'
printf "\"{#WEBNAME}\":\"${webarray[$i]}\"}"
if [ $i -lt $[$length-1] ];then
printf ','
fi
done
printf "\n\t]\n"
printf "}\n"
}
diskDiscovery(){
diskarray=(`cat /proc/diskstats |grep -E "\bsd[abcdefg]\b|\bxvd[abcdefg]\b|vd[abcdef]\b"|grep -i "\b$1\b"|awk '{print $3}'|sort|uniq 2>/dev/null`)
length=${#diskarray[@]}
printf "{\n"
printf '\t'"\"data\":["
for ((i=0;i<$length;i++))
do
printf '\n\t\t{'
printf "\"{#DISKNAME}\":\"${diskarray[$i]}\"}"
if [ $i -lt $[$length-1] ];then
printf ','
fi
done
printf "\n\t]\n"
printf "}\n"
}
zkDiscovery(){
process=$(ps -ef|grep "zookeeper"|grep -c "zoo.cfg")
if [ $process != 0 ];then
list=($(ps -ef|grep "zookeeper"|grep "zoo.cfg"|awk '{print $NF}'|sed 's#/bin/..##g'))
ipaddress=$(ifconfig|grep "inet addr"|grep -v "127.0.0.1"|awk -F: '{print $2}'|awk '{print $1}'|grep -E "^192|^10")
printf "{\n"
printf '\t'"\"data\":["
for key in ${!list[@]}
do
port=$(awk -F= '/clientPort/{print $2}' ${list[$key]})
printf '\n\t\t{'
printf "\"{#ZKNODES}\":\"$ipaddress:$port\"}"
if [[ "$key" -lt "((${#list[@]}-1))" ]];then
printf ","
fi
done
printf "\n\t]\n"
printf "}\n"
fi
}
redisDiscovery(){
redisarray=($(ps -ef|grep redis-server|grep -v grep|awk '{print $9}'))
length=${#redisarray[@]}
printf "{\n"
printf '\t'"\"data\":["
for ((i=0;i<$length;i++))
do
printf '\n\t\t{'
printf "\"{#REDISNODE}\":\"${redisarray[$i]}\"}"
if [ $i -lt $[$length-1] ];then
printf ','
fi
done
printf "\n\t]\n"
printf "}\n"
}
processDiscovery(){
if [ -f "/etc/zabbix/tmp/process" ];then
processarray=($(cat /etc/zabbix/tmp/process))
length=${#processarray[@]}
printf "{\n"
printf '\t'"\"data\":["
for ((i=0;i<$length;i++))
do
printf '\n\t\t{'
printf "\"{#PROCESS}\":\"${processarray[$i]}\"}"
if [ $i -lt $[$length-1] ];then
printf ','
fi
done
printf "\n\t]\n"
printf "}\n"
fi
}
case $1 in
service)
serviceDiscovery
;;
web)
webDiscovery
;;
disk)
diskDiscovery
;;
redis)
redisDiscovery
;;
process)
processDiscovery
;;
esac
<file_sep>/script/disk_io
#!/bin/bash
#
#
file="/tmp/disk_io"
if [ $1 ] && [ $2 ];then
`iostat -x -t 1 -d 2 > /tmp/disk_io`
fi
case $2 in
read)
data=`cat $file|grep $1|tail -1|awk '{print $6}'`
echo $data
;;
write)
data=`cat $file|grep $1|tail -1|awk '{print $7}'`
echo $data
;;
avgqu)
data=`cat $file|grep $1|tail -1|awk '{print $9}'`
echo $data
;;
wait)
data=`cat $file|grep $1|tail -1|awk '{print $10}'`
echo $data
;;
svctm)
data=`cat $file|grep $1|tail -1|awk '{print $11}'`
echo $data
;;
util)
if [ -f /etc/redhat-release ];then
data=`cat $file|grep $1|tail -1|awk '{print $12}'`
else
version=`cat /etc/issue|awk '{print $1}'|head -1`
if [ "$version" = "Ubuntu" ];then
data=`cat $file|grep $1|tail -1|awk '{print $14}'`
fi
fi
echo $data
;;
esac
| e9d4786f9dd89c27061a3aa2150e70348519de5e | [
"Shell"
] | 4 | Shell | summerbuild/zabbix-conf | f08ce13502f2859ae402e96cdb489c2e02ef178d | fbb7419f8ac0ac6c45de8bf6c611a313cfe01a73 | |
refs/heads/master | <repo_name>lailazaki/yodaish<file_sep>/app/models/page.rb
class Page < ActiveRecord::Base
def self.yoda(quote)
quote ||= "Lets Speak Some Yoda"
response = Unirest.get "https://yoda.p.mashape.com/yoda?sentence=#{quote}",
headers:{
"X-Mashape-Key" => "<KEY>",
"Accept" => "text/plain"
}
end
end
<file_sep>/spec/requests/authentication_pages_spec.rb
require 'rails_helper'
describe "Authentication" do
subject { page }
describe "signin page" do
before { visit signin_path }
it { should have_title('Sign In') }
it { should have_content('Sign In') }
describe 'signin process' do
let(:submit) { 'Sign In' }
let(:user) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
context 'valid information' do
before { sign_in user }
it { should have_title(user.name) }
end
context 'invalid information' do
end
end
end
end<file_sep>/spec/requests/user_pages_spec.rb
require 'rails_helper'
describe 'user pages' do
subject { page }
describe 'index' do
User.destroy_all
let(:user1) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
let(:user2) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
before { visit users_path }
it { should have_title('All Users') }
it { should have_selector('h1', text: 'All Users') }
it "lists each user" do
User.all.each do |user|
expect(page).to have_selector('li', text: user.id)
end
end
describe 'delete links' do
let!(:user1) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
let!(:user2) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
before { visit users_path }
it { should have_link('delete', href: user_path(User.first)) }
describe 'after clicking delete' do
before { click_link('delete', match: :first) }
it { should_not have_content('Laila Zaki') }
end
end
end
describe 'show' do
let(:user) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
before { visit user_path(user.id) }
it { should have_title(user.name) }
it { should have_selector('h1', text: user.name) }
end
describe 'new user page' do
before { visit signup_path }
it { should have_title('Sign Up') }
it { should have_selector('h1', 'Sign Up') }
describe 'create user' do
let(:submit) { 'Save' }
context 'valid information' do
before do
# fill in all necessary info in the fields
fill_in 'Name', with: '<NAME>'
fill_in 'Email', with: '<EMAIL>'
fill_in 'Password', with: '<PASSWORD>'
fill_in 'Confirm password', with: '<PASSWORD>'
end
it 'creates user' do
expect { click_button submit }.to change(User, :count).by(1)
end
describe 'after saving' do
before { click_button submit }
it { should have_title('Laila Zaki') }
end
end
context 'invalid information' do
it 'does not create user' do
expect { click_button submit }.not_to change(User, :count)
end
describe 'after submission' do
before { click_button submit }
it { should have_title('Sign Up') }
it { should have_content('error') }
end
end
end
end
describe 'edit user page' do
let(:user_for_edit) { User.create(name: "<NAME>", email: "<EMAIL>",
password: "<PASSWORD>", password_confirmation: "<PASSWORD>") }
before { visit edit_user_path(user_for_edit.id) }
it { should have_title('Edit Profile') }
it { should have_selector('h1', 'Edit Profile') }
describe 'update user' do
let(:submit) { 'Save' }
context 'valid information' do
before do
fill_in "Name", with: "<NAME>"
click_button submit
end
describe 'after saving changes' do
it { should have_title('<NAME>') }
specify { expect(user_for_edit.reload.name).to eq('<NAME>') }
end
end
context 'invalid information' do
before do
fill_in "Name", with: " "
click_button submit
end
describe 'after submission' do
it { should have_title('Edit Profile') }
it { should have_content('error') }
end
end
end
end
end<file_sep>/spec/controllers/pages_controller_spec.rb
require 'rails_helper'
describe PagesController, type: :controller do
describe '#index' do
it 'renders index' do
get :index
expect(response).to render_template(:index)
end
end
describe '#random' do
it 'renders random' do
get :random
expect(response).to render_template(:random)
end
end
describe '#about' do
it 'renders about' do
get :about
expect(response).to render_template(:about)
end
end
end<file_sep>/config/routes.rb
Rails.application.routes.draw do
root 'pages#index'
resources :pages
resources :users, except:[:new]
resources :sessions, only:[:create]
get '/', to: 'pages#index'
get '/random' , to: 'pages#random'
get '/about', to: 'pages#about'
get '/signup', to: 'users#new'
get '/signin', to: 'sessions#new'
delete '/signout', to: 'sessions#destroy'
end
<file_sep>/app/controllers/pages_controller.rb
class PagesController < ApplicationController
def index
@quote = Page.yoda(params[:quote])
end
def random
@active = 'random'
render :layout => false
end
def about
@active = 'about'
render :layout => false
end
end
| 6cd3dfa13d6849fa72d2111ff136e1c38b7443bf | [
"Ruby"
] | 6 | Ruby | lailazaki/yodaish | 9024da5ea4c93f26609eeb003289cd03c94451d2 | 17b7c689ae1676797e5c4cc1e917ba8d1f545d99 | |
refs/heads/master | <file_sep>-- 1. Write a query to display for each store its store ID, city, and country.
select s.store_id, c.city, co.country
from sakila.store s
inner join sakila.address a
using (address_id)
inner join sakila.city c
using (city_id)
inner join sakila.country co
using (country_id);
-- 2. Write a query to display how much business, in dollars, each store brought in.
select s.store_id, sum(p.amount)
from sakila.store s
inner join sakila.staff staff
using (store_id)
inner join sakila.payment p
using (staff_id)
group by s.store_id;
-- 3. What is the average running time of films by category?
select name, avg(length) as runTime
from sakila.category
inner join sakila.film_category fc
using (category_id)
join sakila.film f
using (film_id)
group by name
order by runTime desc;
-- 4. Which film categories are longest?
-- how do you define the longest? im gunna say top 3.
select name, avg(length) as runTime
from sakila.category
inner join sakila.film_category fc
using (category_id)
join sakila.film f
using (film_id)
group by name
order by runTime desc
limit 3;
-- 5. Display the most frequently rented movies in descending order.
select title, count(rental_id) as timesRented
from sakila.film
join sakila.inventory
using (film_id)
join sakila.rental
using (inventory_id)
group by title
order by timesRented desc;
-- 6. List the top five genres in gross revenue in descending order.
select name, sum(amount) as grossRevenue
from sakila.category
inner join sakila.film_category
using (category_id)
join sakila.inventory
using (film_id)
join sakila.rental
using (inventory_id)
join sakila.payment
using (rental_id)
group by name
order by grossRevenue desc
limit 5;
-- 7. Is "Academy Dinosaur" available for rent from Store 1?
select * from(
select i.inventory_id, f.film_id, f.title, i.store_id, r.rental_date, r.return_date
, rank() over(partition by i.inventory_id order by r.rental_date desc) as myRank
from sakila.film f
join sakila.inventory i
using (film_id)
join sakila.rental r
using (inventory_id)
where f.title = "Academy Dinosaur" and i.store_id = 1)sub
where myrank =1 and return_date is not null;
| 36dc03542f7a2d8250ad4c0cbf84d940d90d98ac | [
"SQL"
] | 1 | SQL | KaylaBolden/lab-sql-join-multiple-tables | cc6cabdf0d309336acb1e85c799f0055c7768c74 | 9b958267bb0c001db700a1df45dd3082628a81f7 | |
refs/heads/master | <file_sep>package main
import (
"context"
"flag"
"fmt"
"net/http"
"os/exec"
"runtime"
"golang.org/x/oauth2"
)
var (
configFilePath = flag.String("config", "client_secret.live.json", "Path to client configuration file")
)
func main() {
config, err := createConfig(configFilePath)
if err != nil {
fmt.Println(err)
}
var codeChannel chan string
codeChannel, err = startWebServer(config.RedirectURL)
if err != nil {
fmt.Println(err)
}
accessType := oauth2.SetAuthURLParam("access_type", "offline")
url := config.AuthCodeURL("dsadsadasdasd", accessType)
openURL(url)
code := <-codeChannel
context := context.Background()
token, err := config.Exchange(context, code)
if err != nil {
fmt.Println(err)
}
client := config.Client(context, token)
resp, err := client.Get("https://www.googleapis.com/youtube/v3/channels")
if err != nil {
fmt.Println(err)
}
fmt.Println(resp.Body)
}
func startWebServer(listenURI string) (codeCh chan string, err error) {
codeCh = make(chan string)
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
code := r.FormValue("code")
codeCh <- code // send code to OAuth flow
w.Header().Set("Content-Type", "text/plain")
fmt.Fprintf(w, "Received code: %v\r\nYou can now safely close this browser window.", code)
})
go http.ListenAndServe(":9090", nil)
return codeCh, nil
}
// openURL opens a browser window to the specified location.
// This code originally appeared at:
// http://stackoverflow.com/questions/10377243/how-can-i-launch-a-process-that-is-not-a-file-in-go
func openURL(url string) error {
var err error
switch runtime.GOOS {
case "linux":
err = exec.Command("xdg-open", url).Start()
case "windows":
err = exec.Command("rundll32", "url.dll,FileProtocolHandler", url).Start()
case "darwin":
err = exec.Command("open", url).Start()
default:
err = fmt.Errorf("Cannot open URL %s on this platform", url)
}
return err
}
<file_sep>package main
import (
"encoding/json"
"fmt"
"io/ioutil"
"os"
"path/filepath"
"golang.org/x/oauth2"
)
func createConfig(filename *string) (*oauth2.Config, error) {
data, err := ioutil.ReadFile(*filename)
if err != nil {
pwd, _ := os.Getwd()
fullPath := filepath.Join(pwd, *filename)
return nil, fmt.Errorf("Cannot read config file from %v", fullPath)
}
config := new(Config)
err = json.Unmarshal(data, &config)
if err != nil {
return nil, fmt.Errorf("Cannot read config file from %v", filename)
}
return &oauth2.Config{
Scopes: config.Web.Scopes,
ClientID: config.Web.ClientID,
ClientSecret: config.Web.ClientSecret,
RedirectURL: config.Web.RedirectURL,
Endpoint: oauth2.Endpoint{
AuthURL: config.Web.AuthURI,
TokenURL: config.Web.TokenURI,
},
}, nil
}
// Config struct
type Config struct {
Web ConfigSection `json:"web"`
Stored ConfigSection `json:"stored"`
}
// ConfigSection struct
type ConfigSection struct {
ClientID string `json:"client_id"`
ProjectID string `json:"project_id"`
AuthURI string `json:"auth_uri"`
TokenURI string `json:"token_uri"`
AuthProviderX509CertURL string `json:"auth_provider_x509_cert_url"`
ClientSecret string `json:"client_secret"`
RedirectURL string `json:"redirect_url"`
Scopes []string `json:"scopes"`
}
| 7af1b16e65bb7386acf4efda2bad61be5743780c | [
"Go"
] | 2 | Go | nsq6/f | d2889bd17e787ea7fc7fcf09fb2011a6c955cecd | 58beadb7f9ed473ecc0de7e1fd4ec6ef3caf4a85 | |
refs/heads/master | <repo_name>ezebran/aeromarket<file_sep>/src/components/Foot-bar.js
import React, { Component } from 'react';
const FootBar = (props) => {
let objSize = props.paginacion.cantidadProdus;
let artPosicion = props.paginacion.productosPorPag * props.paginacion.pagActual;
var pagActual = props.paginacion.pagActual;
const paginacionBarMas = (e) => {
e.preventDefault();
if(objSize != artPosicion){
var pagEnBar = pagActual + 1;
props.obtenerProductos(pagEnBar);
}
}
const paginacionBarMenos = (e) => {
e.preventDefault();
if(pagActual != 1){
var pagEnBar = pagActual - 1;
props.obtenerProductos(pagEnBar);
}
}
return(
<div>
<div className="m-bar">
<div className="m-bar-content">
<h2 className="n-products">
{artPosicion} of {objSize} products
<a href="#" className="arrow-cont arrow-resp">
<span className="icon-keyboard_arrow_right arrow-icon"></span>
</a>
</h2>
</div>
<div class="arrows-cont">
<a href="#" className="arrow-cont" onClick={paginacionBarMenos}>
<span className="icon-keyboard_arrow_left arrow-icon"></span>
</a>
<a href="#" className="arrow-cont" onClick={paginacionBarMas}>
<span className="icon-keyboard_arrow_right arrow-icon"></span>
</a>
</div>
</div>
<hr className="gray-line gl-foot" />
</div>
)
}
export default FootBar;<file_sep>/src/components/Header.js
import React from 'react';
import Cart from './Cart';
import Redeem from './ModalsContent/Redeem';
const Header = (props) => {
return(
<header>
<nav>
<img src="assets/images/aerolab-logo.svg" />
<div className="user-nav">
<Cart
toggleCart = {props.toggleCart}
/>
<h2 id="name" class="user-name">{props.usuarios.name}</h2>
<div className="btn btn-light">
<h2>{props.usuarios.points}</h2>
<img src="assets/images/icons/coin.svg" />
</div>
</div>
</nav>
<div className="h-banner">
<h1>Electronics</h1>
</div>
</header>
)
}
export default Header;<file_sep>/src/App.js
import React, { Component } from 'react';
import Header from './components/Header';
import MainBar from './components/Main-bar';
import Articles from './components/Articles';
import FootBar from './components/Foot-bar';
import Redeem from './components/ModalsContent/Redeem';
import Purchases from './components/ModalsContent/Purchases';
class App extends Component {
token = '<KEY>';
state = {
usuarios: {},
productos: [],
paginacion: {
pagActual: 1,
productosPorPag: 16,
cantidadProdus: 0,
ordenadoPor: 1
},
redeemContent: {},
redeemImgs: {}
}
//1 = reciente, 2 = porMenor, 3 = porMayor
componentDidMount() {
this.obtenerUsuario();
this.obtenerProductos();
this.ordenadoPor();
}
obtenerProductos = async (pagBar) => {
let url = `https://aerolab-challenge.now.sh/products/?token=${this.token}`;
const artPorPag = this.state.paginacion.productosPorPag;
await fetch(url)
.then(respuesta => {
return respuesta.json();
})
.then(productos => {
var pagEnMbar;
if(pagBar == undefined){
pagEnMbar = this.state.paginacion.pagActual;
}else{
pagEnMbar = pagBar;
}
var paginacionCopy = this.state.paginacion
paginacionCopy.cantidadProdus = productos.length
paginacionCopy.pagActual = pagEnMbar
this.setState({
paginacion: paginacionCopy,
productos: productos
})
})
let hasta = artPorPag * this.state.paginacion.pagActual;
let desde = hasta - artPorPag;
var produs = [];
let produsSinOrdenar = this.state.productos;
let botonMenor = document.getElementById('porMenor');
let botonMayor = document.getElementById('porMayor');
let botonReciente = document.getElementById('reciente');
if(this.state.paginacion.ordenadoPor == 2){
var produsXmenor = produsSinOrdenar.sort(function (prev, next){
return prev.cost - next.cost;
})
this.setState({
productos: produsXmenor
})
botonMenor.classList.remove("btn-light");
botonMenor.classList.add("btn-active");
botonMayor.classList.remove("btn-active");
botonMayor.classList.add("btn-light");
botonReciente.classList.remove("btn-active");
botonReciente.classList.add("btn-light");
} else if(this.state.paginacion.ordenadoPor == 3){
var produsXmayor = produsSinOrdenar.sort(function (prev, next){
return next.cost - prev.cost;
})
this.setState({
productos: produsXmayor
})
botonMenor.classList.remove("btn-active");
botonMenor.classList.add("btn-light");
botonMayor.classList.remove("btn-light");
botonMayor.classList.add("btn-active");
botonReciente.classList.remove("btn-active");
botonReciente.classList.add("btn-light");
}else{
var produsRecientes = produsSinOrdenar.sort(function (prev, next){
return prev._id - next._id;
})
this.setState({
productos: produsRecientes
})
botonMenor.classList.remove("btn-active");
botonMenor.classList.add("btn-light");
botonMayor.classList.remove("btn-active");
botonMayor.classList.add("btn-light");
botonReciente.classList.remove("btn-light");
botonReciente.classList.add("btn-active");
}
for(let i = 0;i < artPorPag;i++){
produs.push(this.state.productos[desde]);
desde++;
}
this.setState({
productos: produs
})
}
ordenadoPor = (num) => {
if(num == undefined){
num = this.state.paginacion.ordenadoPor;
}
var pagCopy = this.state.paginacion;
pagCopy.ordenadoPor = num;
this.setState({
paginacion: pagCopy
})
}
obtenerUsuario = async () => {
let url = `https://aerolab-challenge.now.sh/user/me/?token=${this.token}`;
await fetch(url)
.then(respuesta => {
return respuesta.json();
})
.then(usuarios => {
this.setState({
usuarios: usuarios
})
})
}
renderRedeem = (produ) => {
this.setState({
redeemContent: produ,
redeemImgs: produ.img
})
}
// toggleCart = e => this.setState({ showCart: !this.state.showCart });
render() {
return (
<div>
<Redeem
redeemContent = {this.state.redeemContent}
redeemImgs = {this.state.redeemImgs}
userPoints = {this.state.usuarios.points}
/>
<Purchases />
<Header
usuarios = {this.state.usuarios}
toggleCart = {this.toggleCart}
/>
<MainBar
paginacion = {this.state.paginacion}
obtenerProductos = {this.obtenerProductos}
ordenadoPor = {this.ordenadoPor}
/>
<Articles
productos = {this.state.productos}
usuarios = {this.state.usuarios}
renderRedeem = {this.renderRedeem}
/>
<FootBar
paginacion = {this.state.paginacion}
obtenerProductos = {this.obtenerProductos}
/>
</div>
);
}
}
export default App;
<file_sep>/src/components/ModalsContent/Purchases.js
import React, { Component } from 'react';
const Purchases = (props) => {
const toggleCartf = (e) => {
e.preventDefault();
let carrito = document.getElementById('purchaseModal');
carrito.classList.toggle("show-modal");
let modalContent = document.getElementById('modal-content-p');
modalContent.classList.toggle("form-e");
}
return(
<div class="modal" id="purchaseModal">
<form id="modal-content-p" class>
<a href="#" className="m-close" onClick={toggleCartf}><span className="icon-cross"></span></a>
<h4 className="m-title">list of products redeemed</h4>
<div className="m-product bor-bot">
<picture>
<source srcSet="assets/images/product-pics/AcerAspire-x2.png" media="(min-width: 600px)" />
<img src="assets/images/product-pics/AcerAspire-x1.png" />
</picture>
<div className="m-info-product">
<h5>Phones</h5>
<h4 className="m-p-name">iPhone 8</h4>
<div className="pb-price">
<h5>12.000</h5>
<img src="assets/images/icons/coin.svg" />
</div>
</div>
</div>
<div className="m-product bor-bot">
<picture>
<source srcSet="assets/images/product-pics/AcerAspire-x2.png" media="(min-width: 600px)" />
<img src="assets/images/product-pics/AcerAspire-x1.png" />
</picture>
<div className="m-info-product">
<h5>Phones</h5>
<h4 className="m-p-name">iPhone 8</h4>
<div className="pb-price">
<h5>12.000</h5>
<img src="assets/images/icons/coin.svg" />
</div>
</div>
</div>
<div className="m-product bor-bot">
<picture>
<source srcSet="assets/images/product-pics/AcerAspire-x2.png" media="(min-width: 600px)" />
<img src="assets/images/product-pics/AcerAspire-x1.png" />
</picture>
<div className="m-info-product">
<h5>Phones</h5>
<h4 className="m-p-name">iPhone 8</h4>
<div className="pb-price">
<h5>12.000</h5>
<img src="assets/images/icons/coin.svg" />
</div>
</div>
</div>
<div className="m-product bor-bot">
<picture>
<source srcSet="assets/images/product-pics/AcerAspire-x2.png" media="(min-width: 600px)" />
<img src="assets/images/product-pics/AcerAspire-x1.png" />
</picture>
<div className="m-info-product">
<h5>Phones</h5>
<h4 className="m-p-name">iPhone 8</h4>
<div className="pb-price">
<h5>12.000</h5>
<img src="assets/images/icons/coin.svg" />
</div>
</div>
</div>
</form>
</div>
)
}
export default Purchases;<file_sep>/src/components/Main-bar.js
import React from 'react';
const MainBar = (props) => {
let objSize = props.paginacion.cantidadProdus;
let artPosicion = props.paginacion.productosPorPag * props.paginacion.pagActual;
var pagActual = props.paginacion.pagActual;
const paginacionBarMas = (e) => {
e.preventDefault();
if(objSize != artPosicion){
var pagEnBar = pagActual + 1;
props.obtenerProductos(pagEnBar);
}
}
const paginacionBarMenos = (e) => {
e.preventDefault();
if(pagActual != 1){
var pagEnBar = pagActual - 1;
props.obtenerProductos(pagEnBar);
}
}
const ordenarPorReciente = (e) => {
e.preventDefault();
props.ordenadoPor(1);
props.obtenerProductos();
}
const ordenarPorMenorPrecio = (e) => {
e.preventDefault();
props.ordenadoPor(2);
props.obtenerProductos();
}
const ordenarPorMayorPrecio = (e) => {
e.preventDefault();
props.ordenadoPor(3);
props.obtenerProductos();
}
const ordenarProductos = (e) => {
e.preventDefault();
let orden = e.target.value;
if(orden == "reciente"){
props.ordenadoPor(1);
}else if(orden == "porMenor"){
props.ordenadoPor(2);
}else{
props.ordenadoPor(3);
}
props.obtenerProductos();
}
return(
<div>
<div className="m-bar">
<div className="m-bar-content">
<h2 className="n-products">
{artPosicion} of {objSize} products
<div class="arrows-cont">
<a href="#" className="arrow-cont arrow-resp" onClick={paginacionBarMenos}>
<span className="icon-keyboard_arrow_left arrow-icon"></span>
</a>
<a href="#" className="arrow-cont arrow-resp" onClick={paginacionBarMas}>
<span className="icon-keyboard_arrow_right arrow-icon"></span>
</a>
</div>
</h2>
<hr />
<ul>
<li className="p-sort">Sort by:</li>
<select id="sort" onChange={ordenarProductos}>
<option value="reciente" id="reciente">Most recent</option>
<option value="porMenor" id="porMenor">Lowest price</option>
<option value="porMayor" id="porMayor">Highest price</option>
</select>
</ul>
</div>
<div class="arrows-cont">
<a href="#" className="arrow-cont" onClick={paginacionBarMenos}>
<span className="icon-keyboard_arrow_left arrow-icon"></span>
</a>
<a href="#" className="arrow-cont" onClick={paginacionBarMas}>
<span className="icon-keyboard_arrow_right arrow-icon"></span>
</a>
</div>
</div>
<hr className="gray-line" />
</div>
)
}
export default MainBar;<file_sep>/src/components/Cart.js
import React, { Component } from 'react';
const Cart = (props) => {
const toggleCartf = (e) => {
e.preventDefault();
let carrito = document.getElementById('purchaseModal');
carrito.classList.toggle("show-modal");
let modalContent = document.getElementById('modal-content-p');
modalContent.classList.toggle("form-e");
}
return(
<a href="#" className="carrito-btn" onClick={toggleCartf}>
<img src="assets/images/icons/buy-gray.svg" />
</a>
)
}
export default Cart;<file_sep>/src/components/Article.js
import React, { Component } from 'react';
const Article = (props) => {
const toggleCartf = (e) => {
e.preventDefault();
let carrito = document.getElementById('redeemModal');
carrito.classList.toggle("show-modal");
let modalContent = document.getElementById('modal-content-r');
modalContent.classList.toggle("form-e");
const product = props.infoProductos;
props.renderRedeem(product)
}
if(props.infoProductos.cost > props.infoUsuarios.points){
return(
<a href="#">
<article>
<div class="btn miss-coin">
You need { props.infoProductos.cost - props.infoUsuarios.points }+ <img src="assets/images/icons/coin.svg" />
</div>
<picture>
<source srcSet={props.infoProductos.img.hdUrl} media="(min-width: 600px)" />
<img src={props.infoProductos.img.url} />
</picture>
<hr class="gray-line" />
<h5>{props.infoProductos.category}</h5>
<h4>{props.infoProductos.name}</h4>
</article>
</a>
)
}else{
return(
<a href="#">
<article>
<div className="buy-icon">
<img src="assets/images/icons/buy-blue.svg" />
</div>
<picture>
<source srcSet={props.infoProductos.img.hdUrl} media="(min-width: 600px)" />
<img src={props.infoProductos.img.url} />
</picture>
<hr className="gray-line" />
<h5>{props.infoProductos.category}</h5>
<h4>{props.infoProductos.name}</h4>
<div className="info-hover">
<div className="buy-icon-hover">
<img src="assets/images/icons/buy-white.svg" />
</div>
<div className="price">
<h3>{props.infoProductos.cost}</h3>
<img src="assets/images/icons/coin.svg" />
</div>
<a href="#" className="redeem" onClick={toggleCartf}>Redeem now</a>
</div>
</article>
</a>
)
}
}
export default Article;<file_sep>/src/components/article/ArticlePay.js
import React, { Component } from 'react';
const ArticlePay = (props) => {
return(
<a href="#">
<article>
<div className="buy-icon">
<img src="assets/images/icons/buy-blue.svg" />
</div>
<picture>
<source srcSet={props.infoProductos.img.hdUrl} media="(min-width: 600px)" />
<img src={props.infoProductos.img.url} />
</picture>
<hr className="gray-line" />
<h5>{props.infoProductos.category}</h5>
<h4>{props.infoProductos.name}</h4>
<div className="info-hover">
<div className="buy-icon-hover">
<img src="assets/images/icons/buy-white.svg" />
</div>
<div className="price">
<h3>{props.infoProductos.cost}</h3>
<img src="assets/images/icons/coin.svg" />
</div>
<a href="#" className="redeem">Redeem now</a>
</div>
</article>
</a>
)
}
export default ArticlePay;<file_sep>/src/components/article/ArticleNeed.js
import React, { Component } from 'react';
const ArticleNeed = (props) => {
return(
<a href="#">
<article>
<div class="btn miss-coin">
You need 1000 <img src="assets/images/icons/coin.svg" />
</div>
<picture>
<source srcSet={props.infoProductos.img.hdUrl} media="(min-width: 600px)" />
<img src={props.infoProductos.img.url} />
</picture>
<hr class="gray-line" />
<h5>{props.infoProductos.category}</h5>
<h4>{props.infoProductos.name}</h4>
</article>
</a>
)
}
export default ArticleNeed; | 9cdb288e43c28cd6978e9f73be185621feca9a92 | [
"JavaScript"
] | 9 | JavaScript | ezebran/aeromarket | 4d8053b1dffb93ad5ecd77d009f6317cbbbefc8a | 870d07e22ab102540dcd28a95cc6a10202621b12 | |
refs/heads/main | <repo_name>venanciomitidieri/Conta_Banco_OO<file_sep>/README.md
Criação de uma Conta em um banco qualquer e suas funções como ectrato, deposita, saca, transfere.
Usando como metodologia de estudo e prática o paradigma voltado a orientação a objetos.
Iniciamos o projeto com a class conta e fizemos seu construtor.
Em seguida fomos atribuindo todos os metodos necessário para que conta ficasse funcional.
<file_sep>/conta.py
""" No primeiro onde fica o construtor __initi__ é tudo que é a CONTA TEM, nos proximos def (metodos) será o que a
CONTA FAZ """
class Conta:
def __init__(self, numero, titular, saldo, limite): # Atributos
print(f'Construindo objeto... {self}')
self.__numero = numero # Colocar dois "Underscore" __ na frente da classe, significa que ela é privada.
self.__titular = titular
# Para acessar os atributos da função eu devo fazer ._Conta__saldo. Pra facilitar esse caminhos podemos criar
# os getter e setter mais conhecido no Python como @property e @setter
self.__saldo = saldo
self.__limite = limite
def extrato(self): # Metodos, Funções
print(f'Saldo {self.__saldo} do titular {self.__titular}')
def deposita(self, valor):
self.__saldo += valor
def __pode_sacar(self, valor_a_sacar):
valor_disponivel_a_sacar = self.__saldo + self.__limite
return valor_a_sacar <= valor_disponivel_a_sacar
def saca(self, valor):
if self.__pode_sacar(valor):
self.__saldo -= valor
else:
print(f'O valor {valor} passou o limite')
def transfere(self, valor, destino):
self.saca(valor)
destino.deposita(valor)
# O get serve para quando eu quero retornar um valor do objeto.
# O set serve para alterar um valor, como só o limite será alerado foi somente pra ele.
# No Python usamos muito o @property para facilitar ainda mais a chamada e edição das variaveis.
@property
def saldo(self):
return self.__saldo
@property
def titular(self):
return self.__titular
@property
def limite(self):
return self.__limite
@limite.setter
def limite(self, limite):
self.__limite = limite
@staticmethod
def codigo_banco():
return '001'
@staticmethod
def codigos_bancos():
return {'BB': '001', 'Caixa': '104', 'Bradesco': '237'}
'''
Além desse princípio de responsabilidade única existem outras que foram definidos através do <NAME> no
início dos anos 2000 e são conhecidos pelo acrônimo SOLID:
S - Single responsibility principle
O - Open/closed principle
L - Liskov substitution principle
I - Interface segregation principle
D - Dependency inversion principle
'''
'''
OBSERVAÇÕES:
1 - @staticmethod são metodos estaticos que usamos para quando queremos acessar um metodo sem ter criado um objeto
2 - @property utilizado para chamar um atributo atravez do metodo sem necessidade de colocar o get.
3 - Métodos estáticos devem ser usados com cautela caso contrário pode-se fugir do paradigma da orientação a objetos.
Métodos estáticos tem um cheiro de linguagem procedural já que independe de um objeto para ser chamado e não manipula
informações/atributos de objetos. Deve ser usado com bastante cautela!
4 -
''' | 2c200cef95399307a00bccfafee8b3e180cd3412 | [
"Markdown",
"Python"
] | 2 | Markdown | venanciomitidieri/Conta_Banco_OO | 95b4652bd24ac3072926e37d35bd25d3751923fb | 5aa739dce91a472b6036807c768cf6138c754c85 | |
refs/heads/main | <file_sep>const router = require('express').Router();
const Workout = require('../../models/workout.js');
router.post("/workouts", async ({ body }, res) => {
try {
let newWorkout = await Workout.create(body)
if (!newWorkout) {
res.status(400).json(newWorkout);
}
res.status(200).json(newWorkout);
} catch (error) {
res.status(400).json(error);
}
});
router.get('/workouts', async ({ body }, res) => {
try {
let allWorkouts = await Workout.aggregate([
{
$addFields: { totalDuration: { $sum: '$exercises.duration' } }
}
]);
if (!allWorkouts) {
res.status(500).json({ message: 'No workouts are in the database' })
}
res.status(200).json(allWorkouts);
} catch (error) {
res.status(400).json(error)
}
})
router.put('/workouts/:id', async ({ body, params }, res) => {
try {
let workoutData = await Workout.findOneAndUpdate({ _id: params.id }, {
$push: { exercises: body }
},
{ new: true });
if (!workoutData) {
res.status(404).json({ message: 'Could not find the workout.' })
}
res.status(200).json(workoutData);
} catch (error) {
res.status(500).json(error)
}
})
router.get('/workouts/range', async (req, res) => {
try {
const lastSevenWorkouts = await Workout.aggregate([
{
$addFields: { totalDuration: {$sum: '$exercises.duration'}}
}
]).sort({ date: -1}).limit(7)
if (!lastSevenWorkouts) {
res.status(400).json({ message: 'Your database does not have any workouts' });
}
res.status(200).json(lastSevenWorkouts);
} catch (error) {
res.status(500).json(error)
}
})
module.exports = router;<file_sep># Workout Tracker
## Description
Workout Tracker is an app to track user's daily resistance and cardio workouts. The user can also view their last 7 workouts in their dashboard through graphs to help visualize progress.
## Table of Contents
- [Workout Tracker](#workout-tracker)
- [Description](#description)
- [Table of Contents](#table-of-contents)
- [Installation](#installation)
- [Usage](#usage)
- [License](#license)
- [Tests](#tests)
- [Contributing](#contributing)
- [Questions](#questions)
- [Deployed Application](#deployed-application)
## Installation
***
Simply used the deployed version or run npm i to create a locally hosted version!
## Usage
***
Due to the nature of the app, the deployed version will not seperate your data from another user's data. For a personalized experience, please download and host a local version.
## License
***
Unlicensed
## Tests
***
None at this time
## Contributing
***
If you would like to contribute please email me
## Questions
If you have further questions, suggestions, or business inquiries please contact me via email.
## Deployed Application
[Link To Deployed App](https://evening-mountain-82600.herokuapp.com/)
<file_sep>const mongoose = require('mongoose');
const {Schema} = mongoose;
const workoutSchema = new Schema({
exercises: [{
name: {
type: String,
trim: true,
required: "Enter the name of the new exercise.",
},
type: {
type: String,
required: 'Enter what type of workout this is.'
},
weight: {
type: Number,
default: null,
},
sets: {
type: Number,
default: null,
},
reps: {
type: Number,
default: null,
},
duration: {
type: Number,
default: null,
required: 'Enter a duration for the exercise.'
},
distance: {
type: Number,
default: null,
}
}],
day: {
type: Date,
default: new Date(Date.now()).toISOString()
}
})
const Workout = mongoose.model('Workout', workoutSchema);
module.exports = Workout; | 8482be252e3b3ce1668b5c55f68cb6e6c8b597da | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | Juawito/workout-tracker | 4bae55899abcff6fcaf3971df00eb8d875b1948c | 0c6418ec20e0aed851c68d1bd14e709f36cea810 | |
refs/heads/main | <file_sep>select empno,ename,esal from Emp
<file_sep># internal-demo-2 | ba9ae4874986ca3a2d43c31832712712a2437c7a | [
"Markdown",
"SQL"
] | 2 | SQL | venugopalkpi/internal-demo-2 | d8cee0e15627ebe49cb2055cff1c1f6a479096b9 | 739b6d6131c14ad8b91a29419c636d74df3ef823 | |
refs/heads/master | <file_sep>package fr.lacombe;
public class ContractId {
public ContractId(String anyContractId) {
}
}
<file_sep>package fr.lacombe.Model.Request;
import fr.lacombe.Model.AdvisorId;
import fr.lacombe.Model.MovementDate;
import fr.lacombe.Model.MovementType;
import fr.lacombe.Model.SubscriberId;
public class HistoryRequest {
private MovementDate movementDate;
private AdvisorId advisorId;
private SubscriberId subscriberId;
private MovementType movementType;
public HistoryRequest(MovementDate movementDate, AdvisorId advisorId, SubscriberId subscriberId, MovementType movementType) {
this.movementDate = movementDate;
this.advisorId = advisorId;
this.subscriberId = subscriberId;
this.movementType = movementType;
}
public MovementDate getMovementDate() {
return movementDate;
}
public void setMovementDate(MovementDate movementDate) {
this.movementDate = movementDate;
}
public AdvisorId getAdvisorId() {
return advisorId;
}
public void setAdvisorId(AdvisorId advisorId) {
this.advisorId = advisorId;
}
public SubscriberId getSubscriberId() {
return subscriberId;
}
public void setSubscriberId(SubscriberId subscriberId) {
this.subscriberId = subscriberId;
}
public MovementType getMovementType() {
return movementType;
}
public void setMovementType(MovementType movementType) {
this.movementType = movementType;
}
}
<file_sep><?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>fr.lacombe.subscriber-address-kata</groupId>
<artifactId>subscriber-address-kata</artifactId>
<version>1.0-SNAPSHOT</version>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>8</source>
<target>8</target>
</configuration>
</plugin>
</plugins>
</build>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.1.7.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-contract-wiremock</artifactId>
<version>2.1.2.RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.assertj</groupId>
<artifactId>assertj-core</artifactId>
<version>3.13.2</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>info.cukes</groupId>
<artifactId>cucumber-java</artifactId>
<version>1.2.4</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>info.cukes</groupId>
<artifactId>cucumber-junit</artifactId>
<version>1.2.4</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>info.cukes</groupId>
<artifactId>cucumber-spring</artifactId>
<version>1.2.4</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-all</artifactId>
<version>1.9.5</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.github.tomakehurst</groupId>
<artifactId>wiremock-jre8</artifactId>
<version>2.24.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.1.5.RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot</artifactId>
<version>2.1.7.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-test</artifactId>
<version>2.1.3.RELEASE</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.7</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.github.tomakehurst</groupId>
<artifactId>wiremock-jre8</artifactId>
<version>2.24.1</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.9.9</version>
</dependency>
</dependencies>
</project><file_sep>package fr.lacombe.Utils;
import com.fasterxml.jackson.databind.ObjectMapper;
import fr.lacombe.Model.ContractList;
import fr.lacombe.Model.Country;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.util.ArrayList;
@Component
public class JsonMapper extends ObjectMapper {
public ContractList mapJsonToContractList(ResponseEntity<String> contractRepositoryResponse) throws IOException {
return new ContractList(new ArrayList<>());
}
public Country mapJsonToCountry(ResponseEntity<String> addressRepositoryResponse) throws IOException {
return this.readValue(addressRepositoryResponse.getBody(), Country.class);
}
}
<file_sep>package fr.lacombe.Model;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import static fr.lacombe.Model.CountryEnum.FRANCE;
public class Country {
private CountryEnum countryEnum;
@JsonCreator
public Country(@JsonProperty("country") CountryEnum countryEnum) {
this.countryEnum = countryEnum;
}
public boolean isFrance() {
return countryEnum.equals(FRANCE);
}
}
<file_sep>package fr.lacombe;
import cucumber.api.CucumberOptions;
import cucumber.api.junit.Cucumber;
import org.junit.runner.RunWith;
@RunWith(Cucumber.class)
@CucumberOptions(plugin = "pretty", features = "src/test/resources/cucumber")
public class RunCucumberTest extends SpringIntegrationTest{
}
<file_sep>package fr.lacombe.Model.Request;
import fr.lacombe.Model.ContractList;
public class ContractListRequest extends Request{
private ContractList contractList;
public ContractListRequest(ContractList contractList) {
this.contractList = contractList;
}
public ContractList getContractList() {
return contractList;
}
public void setContractList(ContractList contractList) {
this.contractList = contractList;
}
}
<file_sep>package fr.lacombe.Model;
import java.time.LocalDateTime;
public class MovementDate {
private LocalDateTime dateTime;
public MovementDate(LocalDateTime dateTime) {
this.dateTime = dateTime;
}
public LocalDateTime getDateTime() {
return dateTime;
}
public void setDateTime(LocalDateTime dateTime) {
this.dateTime = dateTime;
}
}
<file_sep>package fr.lacombe;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ContextConfiguration;
@ContextConfiguration
@SpringBootTest(classes = SpringDemoApplication.class, webEnvironment = SpringBootTest.WebEnvironment.DEFINED_PORT)
public class SpringIntegrationTest {
}
<file_sep>package fr.lacombe.Model;
import java.io.Serializable;
import java.util.Objects;
public class SubscriberAddress implements Serializable {
private CountryEnum countryEnum;
private int postalCode;
private String streetName;
private boolean isAddressActive;
private String city;
public SubscriberAddress(CountryEnum countryEnum, String city, int postalCode, String streetName, boolean isAddressActive) {
this.city = city;
this.countryEnum = countryEnum;
this.postalCode = postalCode;
this.streetName = streetName;
this.isAddressActive = isAddressActive;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SubscriberAddress that = (SubscriberAddress) o;
return postalCode == that.postalCode &&
isAddressActive == that.isAddressActive &&
countryEnum == that.countryEnum &&
streetName.equals(that.streetName) &&
city.equals(that.city);
}
@Override
public int hashCode() {
return Objects.hash(countryEnum, postalCode, streetName, isAddressActive, city);
}
public CountryEnum getCountryEnum() {
return countryEnum;
}
public void setCountryEnum(CountryEnum countryEnum) {
this.countryEnum = countryEnum;
}
public int getPostalCode() {
return postalCode;
}
public void setPostalCode(int postalCode) {
this.postalCode = postalCode;
}
public String getStreetName() {
return streetName;
}
public void setStreetName(String streetName) {
this.streetName = streetName;
}
public boolean isAddressActive() {
return isAddressActive;
}
public void setAddressActive(boolean addressActive) {
isAddressActive = addressActive;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
}
<file_sep>package fr.lacombe.Model.Request;
public abstract class Request {
}
<file_sep># suscriber-address-kata<file_sep>package fr.lacombe.Utils;
import fr.lacombe.Model.MovementDate;
import org.springframework.stereotype.Service;
@Service
public class TimeProvider implements TimeProviderInterface{
@Override
public MovementDate now() {
return null;
}
}
<file_sep>package fr.lacombe.Model.Request;
import fr.lacombe.Model.AdvisorId;
import fr.lacombe.Model.EffectiveDate;
import fr.lacombe.Model.SubscriberAddress;
import fr.lacombe.Model.SubscriberId;
import java.io.Serializable;
public class SubscriberModificationRequest extends Request implements Serializable {
private SubscriberAddress subscriberAddress;
private SubscriberId subscriberId;
private EffectiveDate effectiveDate;
private AdvisorId advisorId;
public SubscriberModificationRequest(SubscriberAddress subscriberAddress, SubscriberId subscriberId, EffectiveDate effectiveDate, AdvisorId advisorId) {
this.subscriberAddress = subscriberAddress;
this.subscriberId = subscriberId;
this.effectiveDate = effectiveDate;
this.advisorId = advisorId;
}
public SubscriberAddress getSubscriberAddress() {
return subscriberAddress;
}
public void setSubscriberAddress(SubscriberAddress subscriberAddress) {
this.subscriberAddress = subscriberAddress;
}
public EffectiveDate getEffectiveDate() {
return effectiveDate;
}
public void setEffectiveDate(EffectiveDate effectiveDate) {
this.effectiveDate = effectiveDate;
}
public AdvisorId getAdvisorId() {
return advisorId;
}
public void setAdvisorId(AdvisorId advisorId) {
this.advisorId = advisorId;
}
public SubscriberId getSubscriberId() {
return subscriberId;
}
public void setSubscriberId(SubscriberId subscriberId) {
this.subscriberId = subscriberId;
}
}
<file_sep>package fr.lacombe.Model;
public enum MovementType {
SUSBCRIBER_INFO
}
<file_sep>package fr.lacombe.Model;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class ContractList {
public List<SubscriberContract> contracts;
public ContractList(List<SubscriberContract> contracts) {
this.contracts = contracts;
}
public void modifySubscriberAddressOnAllContracts(SubscriberAddress subscriberAddress) {
for(SubscriberContract subscriberContract : contracts){
subscriberContract.modifySubscriberAddress(subscriberAddress);
}
}
}
| 9da543fb1128b710cf4733b66f54c197d469212b | [
"Markdown",
"Java",
"Maven POM"
] | 16 | Java | ClementBouchet/suscriber-address-kata | eff9d026c41f3c156e9c76453e6a31c1fed685d4 | 8bf7463cdf7c296b2662e0a1431e52d3aced6cc2 | |
refs/heads/master | <repo_name>atimos/advent2019<file_sep>/src/day2/error.rs
use std::{
error::Error as StdError,
fmt::{Display, Formatter, Result as FmtResult},
num::ParseIntError,
ops::{Add, Mul},
result::Result as StdResult,
string::ToString,
};
#[derive(Debug, PartialEq)]
pub enum Error {
Parse(ParseIntError),
Op { op: usize, pos: usize },
ArgCount(usize),
Eof,
}
pub type Result<T> = StdResult<T, Error>;
impl StdError for Error {
fn source(&self) -> Option<&(dyn std::error::Error + 'static)> {
match self {
Self::Parse(err) => Some(err),
_ => None,
}
}
}
impl Display for Error {
fn fmt(&self, f: &mut Formatter<'_>) -> FmtResult {
match self {
Self::Parse(err) => err.fmt(f),
Self::Op { op, pos } => write!(f, "Unknown op {}, in position {}", op, pos),
Self::ArgCount(pos) => write!(f, "Invalid argument count for op in position {}", pos),
Self::Eof => write!(f, "Unexpected end of file"),
}
}
}
impl From<ParseIntError> for Error {
fn from(err: ParseIntError) -> Self {
Self::Parse(err)
}
}
pub fn run(program: &str) -> Result<String> {
let mut pos = 0;
let mut program = program.split(',').map(str::parse).collect::<StdResult<Vec<usize>, _>>()?;
loop {
pos += match program.get(pos) {
Some(1) => apply(Add::add, pos, &mut program)?,
Some(2) => apply(Mul::mul, pos, &mut program)?,
Some(99) => break,
Some(op) => return Err(Error::Op { op: *op, pos }),
None => return Err(Error::Eof),
}
}
Ok(program.iter().map(ToString::to_string).collect::<Vec<String>>().join(","))
}
fn apply(op: impl Fn(usize, usize) -> usize, pos: usize, program: &mut [usize]) -> Result<usize> {
if let Some([first, second, output]) = &program.get(pos + 1..=pos + 3) {
program[*output] = op(program[*first], program[*second]);
Ok(4)
} else {
Err(Error::ArgCount(pos))
}
}
<file_sep>/src/day2/panic.rs
pub fn step1<R: std::io::Read>(input: R) {
println!("day 2 step 1 {}", run(parse(input))[0]);
}
pub fn step2<R: std::io::Read>(input: R) {
let program = parse(input);
let (verb, noun) = (0..100)
.flat_map(|verb| (0..100).map(move |noun| (verb, noun)))
.find(|(verb, noun)| {
let mut program = program.clone();
program[1] = *verb;
program[2] = *noun;
run(program)[0] == 19690720
})
.expect("Did not find result");
println!("day 2 step 2 {}", 100 * verb + noun);
}
pub fn parse<R: std::io::Read>(mut input: R) -> Vec<usize> {
let mut program = String::new();
input.read_to_string(&mut program).expect("Could not load program");
program.split(',').map(str::parse).collect::<Result<Vec<usize>, _>>().expect("Invalid program")
}
pub fn run(mut program: Vec<usize>) -> Vec<usize> {
let mut pos = 0;
loop {
if let Some(99) | None = program.get(pos) {
break;
}
match program.get(pos..=pos + 3) {
Some(&[1, in1, in2, out]) => program[out] = program[in1] + program[in2],
Some(&[2, in1, in2, out]) => program[out] = program[in1] * program[in2],
Some(&[op, _, _, _]) => panic!("Invalid operation {}", op),
_ => panic!("Unexpected end of file"),
};
pos += 4;
}
program
}
<file_sep>/src/day2/enum_.rs
use std::{
num::ParseIntError,
ops::{Add, Mul},
result::Result as StdResult,
string::ToString,
};
#[derive(Debug, PartialEq)]
pub enum Error {
Parse(ParseIntError),
Op { op: usize, pos: usize },
ArgCount(usize),
Eof,
}
pub type Result<T> = StdResult<T, Error>;
pub fn run(program: &str) -> Result<String> {
let mut pos = 0;
let mut program = program
.split(',')
.map(str::parse)
.collect::<StdResult<Vec<usize>, _>>()
.map_err(Error::Parse)?;
loop {
pos += match program.get(pos) {
Some(1) => apply(Add::add, pos, &mut program)?,
Some(2) => apply(Mul::mul, pos, &mut program)?,
Some(99) => break,
Some(op) => return Err(Error::Op { op: *op, pos }),
None => return Err(Error::Eof),
}
}
Ok(program.iter().map(ToString::to_string).collect::<Vec<String>>().join(","))
}
fn apply(op: impl Fn(usize, usize) -> usize, pos: usize, program: &mut [usize]) -> Result<usize> {
if let Some([first, second, output]) = &program.get(pos + 1..=pos + 3) {
program[*output] = op(program[*first], program[*second]);
Ok(4)
} else {
Err(Error::ArgCount(pos))
}
}
<file_sep>/src/day2/thiserror.rs
use joinery::Joinable;
#[derive(Debug, PartialEq, thiserror::Error, displaydoc::Display)]
pub enum Error {
/// {0}
Parse(#[source] std::num::ParseIntError),
/// Unknown op {op} in position {pos}
Op { op: usize, pos: usize },
/// Unexpected end of file
Eof,
/// Address {0} out of bounds
OutOfBounds(usize),
/// Could not finish program, max operations {0}
ToManyOperations(usize),
}
pub type Result<T> = std::result::Result<T, Error>;
const MAX_OPERATIONS: usize = 1000;
pub fn run(program: &str) -> Result<String> {
program
.split(',')
.map(str::parse)
.collect::<std::result::Result<_, _>>()
.map_err(Error::Parse)
.and_then(|mut program: Vec<usize>| {
let mut pos = 0;
for _ in 0..=MAX_OPERATIONS {
match program.get(pos) {
Some(99) | None => return Ok(program),
Some(op) if *op != 1 && *op != 2 => return Err(Error::Op { op: *op, pos }),
_ => {}
}
match program.get(pos..=pos + 3) {
Some(&[1, in1, in2, out]) => {
apply(std::ops::Add::add, in1, in2, out, &mut program)?
}
Some(&[2, in1, in2, out]) => {
apply(std::ops::Mul::mul, in1, in2, out, &mut program)?
}
None => return Err(Error::Eof),
Some(_) => unreachable!(),
};
dbg!(pos);
pos += 4;
}
Err(Error::ToManyOperations(MAX_OPERATIONS))
})
.map(|program| program.join_with(",").to_string())
}
fn apply(
op: impl Fn(usize, usize) -> usize,
in1: usize,
in2: usize,
out: usize,
program: &mut [usize],
) -> Result<()> {
let in1 = *program.get(in1).ok_or(Error::OutOfBounds(in1))?;
let in2 = *program.get(in2).ok_or(Error::OutOfBounds(in2))?;
let out = program.get_mut(out).ok_or(Error::OutOfBounds(out))?;
*out = op(in1, in2);
Ok(())
}
<file_sep>/Cargo.toml
[package]
name = "advent"
version = "0.1.0"
authors = ["atimos"]
edition = "2018"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
thiserror = "1.0.6"
displaydoc = "0.1"
joinery = "2.0.0"
<file_sep>/src/main.rs
#[allow(clippy::all)]
mod day2;
mod day3;
mod day4;
fn main() {
day4::step1();
day4::step2();
}
<file_sep>/src/day4.rs
pub fn step1() {
let result = (245318..=765747)
.map(|number| number.to_string().chars().collect::<Vec<char>>())
.filter(|d| d.windows(2).all(is_not_decr))
.filter(|d| d.iter().group_count().any(|c| c > 1))
.count();
dbg!(result);
}
pub fn step2() {
let result = (245318..=765747)
.map(|number| number.to_string().chars().collect::<Vec<char>>())
.filter(|d| d.windows(2).all(is_not_decr))
.filter(|d| d.iter().group_count().any(|c| c == 2))
.count();
dbg!(result);
}
fn is_not_decr(numbers: &[char]) -> bool {
match numbers {
&[n1, n2] => n1 <= n2,
_ => true,
}
}
struct GroupCount<I: Iterator>(std::iter::Peekable<I>);
impl<Iter: Iterator> Iterator for GroupCount<Iter>
where
Iter::Item: std::cmp::PartialEq,
{
type Item = usize;
fn next(&mut self) -> Option<Self::Item> {
let mut count = 1;
while let Some(item) = self.0.next() {
match self.0.peek() {
Some(next) if next == &item => count += 1,
_ => return Some(count),
}
}
None
}
}
trait GroupCountIter: Iterator {
fn group_count(self) -> GroupCount<Self>
where
Self: Sized,
{
GroupCount(self.peekable())
}
}
impl<T> GroupCountIter for T where T: Iterator {}
| 439446ecaa4ebebda90a69dc76bc82735872fe78 | [
"TOML",
"Rust"
] | 7 | Rust | atimos/advent2019 | 20def034d9b50dd9ecf6214cedeca853db47e5ba | e75fa1bbeb8befbe906311c20a67ce36f7d07b21 | |
refs/heads/master | <repo_name>Kapranov/mosaic<file_sep>/airlines/README.md
### Постановка задачи
У нас настаивают на том что бы отображать точку аэропорта
вылета или прилета. Задача выглядит следующим образом.
Есть image карты width x height, которые могут быть любыми.
Есть десятичные координаты аэропортов. Необходимо на плоской
карте (с любым разрешением) отобразить точку где находится аэропорт.
#### Список аэропортов
- London 51.5085300 longitude: -0.12574000
- New York 40.7142700 Longitude: -74.0059700
- Moskow 55.7522200 Longitude: 37.6155600
- Sydnay -33.8678500 Longitude: 151.2073200
- Buenos Aires -34.6131500 Longitude: -58.3772300
#### Что сделано
- Сделал маршрут от точки вылета к точке прибытия.
- Сделал список городов для отображения из списка.
- Добавлю позже больше функциональных возможностей.
### 27 april 2015 <NAME>
<file_sep>/estore-books/README.md
Synopsys
========
create single page application on Ember.js
feb 2015 <NAME>
<file_sep>/README.md
# README
7 May 2015 <NAME>
<file_sep>/airlines/js/app.js
App = Ember.Application.create();
App.Router.map(function() {
this.route("index", { path: "/" });
this.route("locations", { path: "/locations" });
this.route("about", { path: "/about" });
});
App.LocationsRoute = Ember.Route.extend({
model: function() {
return {
locations: [
Ember.Object.create({ name: 'London', latitude: 51.5085300, longitude: -0.1257400 }),
Ember.Object.create({ name: 'New York', latitude: 40.7142700 , longitude: -74.0059700 }),
Ember.Object.create({ name: 'Moskow', latitude: 55.7522200, longitude: 37.6155600 }),
Ember.Object.create({ name: 'Sydnay', latitude: -33.8678500, longitude: 151.2073200 }),
Ember.Object.create({ name: 'Buenos Aires', latitude: -34.6131500, longitude: -58.3772300 })
],
markers: [
Ember.Object.create({ latitude: 50.08703, longitude: 14.42024 }),
Ember.Object.create({ latitude: 50.08609, longitude: 14.42091 }),
Ember.Object.create({ latitude: 40.71356, longitude: -74.00632 }),
Ember.Object.create({ latitude: -33.86781, longitude: 151.20754 })
]
};
}
});
App.Marker = Ember.Object.extend({
});
App.InlineTextField = Ember.TextField.extend({
focusOut: function() {
this.set('blurredValue', this.get('value'));
}
});
App.MapView = Ember.View.extend({
id: 'map_canvas',
tagName: 'div',
attributeBindings: ['style'],
style:"width:100%; height:200px",
didInsertElement: function() {
var mapOptions = {
center: new google.maps.LatLng(37.871667, -122.272778),
zoom: 13,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
var controller = this.get("controller");
var map = new google.maps.Map(this.$().get(0),mapOptions);
var triggerButton = $('#' + this.get("triggerButton"));
console.log("found triggerButton" + triggerButton);
var that = this;
triggerButton.click(function () {
that.loadDirections();
return false;
});
directionsDisplay = new google.maps.DirectionsRenderer();
directionsDisplay.setMap(map);
this.set("map",map);
var that = this;
},
loadDirections: function() {
console.log("Observed content change in the index controller.... should update.....")
from = this.get("from");
to = this.get("to");
if (from && to) {
console.log("Loading up direction from " + from + " to " + to);
var directionsService = new google.maps.DirectionsService();
var request = {
origin:from,
destination:to,
travelMode: google.maps.DirectionsTravelMode.DRIVING
};
directionsService.route(request, function(response, status) {
if (status == google.maps.DirectionsStatus.OK) {
directionsDisplay.setDirections(response);
}
});
} else {
console.log("Need both a from and a to");
}
}.observes("from","to")
});
App.IndexController = Ember.ArrayController.extend({
fromToChanged: function() {
console.log("fromToChanged")
}.observes("from"),
directionsText: function() {
return "Going from " + this.get("from") + " to " + this.get("to");
}.property("from")
});
App.GoogleMapsComponent = Ember.Component.extend({
insertMap: function() {
var container = this.$('.map-canvas');
var options = {
center: new google.maps.LatLng(this.get('latitude'),
this.get('longitude')),
zoom: 17,
mapTypeId: google.maps.MapTypeId.ROADMAP
};
this.set('map', new google.maps.Map(container[0], options));
this.set('markerCache', []);
this.setMarkers();
}.on('didInsertElement'),
coordinatesChanged: function() {
var map = this.get('map');
if (map) map.setCenter(new google.maps.LatLng(this.get('latitude'), this.get('longitude')));
}.observes('latitude', 'longitude'),
setMarkers: function() {
var map = this.get('map'),
markers = this.get('markers'),
markerCache = this.get('markerCache');
markerCache.forEach(function(marker) { marker.setMap(null); });
markers.forEach(function(marker){
var gMapsMarker = new google.maps.Marker({
position: new google.maps.LatLng(marker.get('latitude'), marker.get('longitude')),
map: map
});
markerCache.pushObject(gMapsMarker);
}, this);
}.observes('[email protected]', '[email protected]')
});
| cfd926b9f465f4bbbd5265ad3124fc40b0f71063 | [
"Markdown",
"JavaScript"
] | 4 | Markdown | Kapranov/mosaic | 41d054aa75cebef449a6d30fc831da41f9e33a87 | fe3563d998c2296907a3fd5d8945b49fb045d69c | |
refs/heads/master | <file_sep>#!/usr/local/bin/python
import socket
from _thread import start_new_thread
def threaded(c):
while True:
data = c.recv(1024)
if not data or data.decode() == 'close':
break
c.send(data)
c.close()
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
s.bind(('0.0.0.0', 2222))
s.listen(10)
while True:
sock, addr = s.accept()
start_new_thread(threaded, (sock,))
# второй вариант
import socket
import threading
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('0.0.0.0', 2222))
s.listen(10)
def server(conn, addr):
while True:
data = conn.recv(1024)
if data == 'close' or not data: break
conn.send(data)
conn.close()
while True:
conn, addr = s.accept()
t = threading.Thread(target=server, args=(conn, addr))
t.start()
<file_sep>from sys import stdin
class MatrixError(BaseException):
def __init__(self, m1, m2):
self.matrix1 = m1
self.matrix2 = m2
class Matrix:
def __init__(self, d):
self.dim = [line[:] for line in d]
def __str__(self):
return '\n'.join(['\t'.join(map(str, line)) for line in self.dim])
def size(self):
return len(self.dim), len(self.dim[0])
def __add__(self, other):
if len(self.dim) == len(other.dim):
mas = []
for i in range(len(self.dim)):
if len(self.dim[i]) == len(other.dim[i]):
mas.append([self.dim[i][j] + other.dim[i][j]
for j in range(len(self.dim[i]))])
else:
raise MatrixError(self, other)
return Matrix(mas)
else:
raise MatrixError(self, other)
def mulmatrix(self, other):
mas = [[0 for i in range(len(other.dim))]
for j in range(len(self.dim))]
for i in range(len(self.dim)):
for j in range(len(other.dim)):
if len(self.dim[i]) == len(other.dim[j]):
mas[i][j] = Matrix.mulvec(self.dim[i], other.dim[j])
else:
tmp = Matrix.transposed(other)
raise MatrixError(self, tmp)
return Matrix(mas)
def __mul__(self, other):
if isinstance(other, (int, float)):
mas = [list(map(lambda x: x * other, y)) for y in self.dim]
return Matrix(mas)
elif isinstance(other, Matrix):
tmp = Matrix.transposed(other)
tmp = Matrix.mulmatrix(self, tmp)
return tmp
else:
raise MatrixError(self, other)
__rmul__ = __mul__
def transpose(self):
self.dim = list(zip(*self.dim))
return Matrix(self.dim)
def solve(self, vector):
if self.size()[0] != self.size()[1]:
raise Exception("Matrix not square!")
elif self.size()[1] != len(vector):
raise Exception("Matrix and vector different length!")
masd = []
for i in range(len(vector)):
mas = Matrix(self.dim)
for j in range(len(vector)):
mas.dim[j][i] = vector[j]
masd.append(Matrix.determinator(mas))
return list(map(lambda x: x / Matrix.determinator(self), masd))
@staticmethod
def mulvec(vec1, vec2):
return sum([i * j for i, j in zip(vec1, vec2)])
@staticmethod
def transposed(d):
return Matrix(list(zip(*d.dim)))
@staticmethod
def determinator(m):
if m.size()[0] == 2:
return m.dim[0][0] * m.dim[1][1] - m.dim[0][1] * m.dim[1][0]
elif m.size()[0] == 3:
return \
m.dim[0][0] * m.dim[1][1] * m.dim[2][2] \
+ m.dim[2][0] * m.dim[0][1] * m.dim[1][2] \
+ m.dim[1][0] * m.dim[2][1] * m.dim[0][2] \
- m.dim[2][0] * m.dim[1][1] * m.dim[0][2] \
- m.dim[0][0] * m.dim[2][1] * m.dim[1][2] \
- m.dim[1][0] * m.dim[0][1] * m.dim[2][2]
else:
raise Exception("Matrix range more than 3")
class SquareMatrix(Matrix):
def q__pow__(self, power, modulo=None): # def exp(x, a):
if power < 2:
return self
n = bin(power)[2:]
x_n = 1
for i in n:
if int(i) == 0:
x_n = x_n * x_n
else:
x_n = self * (x_n * x_n)
return x_n
def __pow__(self, p, modulo=None):
if p in [0, 1]:
return self
elif p == 2:
return self * self
if p % 2 != 0:
return self * (self ** (p - 1))
else:
return (self * self) ** (p // 2)
exec(stdin.read())
<file_sep>#!/usr/local/bin/python
import socket
import os
import sys
import select
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind(('0.0.0.0', 2222))
s.listen(10)
readsocks, writesocks = [], []
active_socks = []
active_socks.append(s)
while True:
readsocks = active_socks.copy()
res = select.select(readsocks, [], []) # readables, writeables, exceptions = select.select(readsocks, writesocks, [])
print(type(res))
print(type(res[0]))
readables, writeables = res[0:2]
for sockobj in readables:
if sockobj == s:
new_host = s.accept()
active_socks.append(new_host[0])
else:
data = sockobj.recv(1024)
if not data:
sockobj.close()
readsocks.remove(sockobj)
else:
sockobj.send(data)
sockobj.close()
active_socks.remove(sockobj)
<file_sep>import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('127.0.0.1', 8888))
msg = input("Message: ")
msg = msg.encode(encoding="utf-8")
# msg = bytearray(msg, 'UTF-8')
sock.send(msg)
sock.close()
<file_sep>import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
msg = input("Message: ")
msg = msg.encode(encoding="utf-8")
# msg = bytearray(msg, 'UTF-8')
sock.sendto(msg, ('127.0.0.1', 8888))
<file_sep>import requests
resp1 = requests.get('http://example.com')
print('Headers:', resp1.headers)
print(resp1.text)
resp2 = requests.post('http://example.com')
print(resp2)
print(resp2.headers)
<file_sep># Python
```
Files for my GitHub.
<file_sep>import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('127.0.0.1', 8888))
sock.send(b'Test massage')
result = sock.recv(1024)
print('Response: {}'.format(result))
sock.close()
| ad9f3148f0a7d0205df7703ed92fb0650b5a7784 | [
"Markdown",
"Python"
] | 8 | Python | RomAzhnak/Python | ad6cc5232920687d795c49047b0fd0a6143443b6 | 43e6dd4e721a1a33ff8c4d6ea02c1a620c25f08b | |
refs/heads/main | <file_sep>class Instrument:
"""
Class to create musical instruments
"""
def __init__(self, price, brand):
self.price = price
self.brand = brand
def __str__(self):
return f"{self.brand} - {self.price}$"
def play(self):
print("playing")
def keep_in_case(self):
print("keep in case")
def tune(self):
print("tuned")
class Guitar(Instrument):
"""
Class to create guitar
"""
def __init__(self, brand):
super().__init__(200, brand)
self.state = "new"
print(f"This is a {self.state} guitar - {self.price}$")
class Piano(Instrument):
"""
Class to create piano
"""
def __init__(self, brand):
super().__init__(1500, brand)
self.state = "used"
print(f"This is a {self.state} {brand} piano - {self.price}$")
def repair_action(self):
print("Piano refurbished")
<file_sep>from musical_instruments import Guitar, Piano
class FactoryInstrument:
@staticmethod
def get_instrument(type, brand):
if type == "guitar":
return Guitar(brand)
elif type == "piano":
return Piano(brand)
else:
return "Invalid type"
<file_sep># factory
Implementation of the factory design pattern
<file_sep>from factory_instruments import FactoryInstrument
def main():
guitar = FactoryInstrument.get_instrument("guitar", "Gibson L-5 CES")
piano = FactoryInstrument.get_instrument("piano", "Yamaha DX-7")
guitar.play()
piano.repair_action()
main()
| 0bcd063cdbaca8b8bfc4a7f1eeec05922954bf2a | [
"Markdown",
"Python"
] | 4 | Python | elciclon/factory | 6b8818b49bf7c220d909a921bad419446fd15e68 | ffbd1c8fb6c3d5703836c4170276ddf1cef1b86f | |
refs/heads/master | <file_sep>from flask import Flask, request
from newsapi import NewsApiClient
from gql import gql, Client
from gql.transport.aiohttp import AIOHTTPTransport
import logging, xmlrpc.client, requests, json, pandas as pd,pika
from flask import request
app = Flask(__name__)
#additional setup
def setup_logger(name, log_file, level=logging.INFO):
"""To setup as many loggers as you want"""
handler = logging.FileHandler(log_file)
handler.setFormatter(formatter)
logger = logging.getLogger(name)
logger.setLevel(level)
logger.addHandler(handler)
return logger
#log file setup
userLog = 'user.log'
cmdLog = 'calls.log'
formatter = logging.Formatter('%(asctime)s %(message)s', datefmt='%d/%m/%Y %H:%M:%S')
logging.basicConfig(filename=cmdLog,level=logging.DEBUG,format='%(asctime)s %(message)s', datefmt='%d/%m/%Y %H:%M:%S')
users = setup_logger('user', userLog)
@app.route('/')
def first():
return 'Hello, World!'
@app.route('/insertStudent')
def insertstudent():
studentid = request.args.get('studentid')
studentname = request.args.get('studentname')
studentdob = request.args.get('studentdob')
users.info('Insert student' + studentid + '-' + studentname + '-' + studentdob)
return 'Insert student' + studentid + '-' + studentname + '-' + studentdob
@app.route('/justweather')
def weather():
api_key = "<KEY>"
# base_url variable to store url
base_url = "http://api.openweathermap.org/data/2.5/forecast?"
# Give city name
city_name = request.args.get('city')
# complete_url variable to store
# complete url address
complete_url = base_url + "appid=" + api_key + "&q=" + city_name +"&units=metric"
# get method of requests module
# return response object
response = requests.get(complete_url)
# json method of response object
# convert json format data into
# python format data
x = response.json()
# declare rabbitmq queue
# Now x contains list of nested dictionaries
# Check the value of "cod" key is equal to
# "404", means city is found otherwise,
# city is not found
if x["cod"] != "404":
i=0
ret = "{"
while(i<= 39):
y=x["list"]
z=y[i]
main = z["main"]
curtemp = main["temp"]
weather = z["weather"]
q=weather[0]
desc = q["description"]
req = requests.post("http://localhost/rabbitmq/send.php/?date="+z["dt_txt"] +"&description="+desc+"&temperature="+ str(curtemp))
jsondata = '{"date" : "'+z["dt_txt"]+'", "current temp" : "'+str(curtemp)+'", "description" :"'+ desc+'"},'
ret = ret + jsondata
i = i+8
ret = json.dumps(ret[:-1]+"}")
ret = json.loads(ret)
return (ret)
else:
return(" City Not Found ")
@app.route('/updates')
def updates():
f = open('update.txt', 'r')
x = f.readlines()
output = '{'
for item in x:
# "line1": "item1",
output = output + '"line": "'+item + '",'
f.close()
output = output[:-1]
output = output + '}'
return output
@app.route('/ping')
def ping():
return 'Pong'
@app.route('/callClient')
def call_rpc():
temp = int(request.args.get('temp'))
with xmlrpc.client.ServerProxy("http://localhost:8001/") as proxy:
return proxy.temperature(temp)
@app.route('/students')
def call_graphql():
# Select your transport with a defined url endpoint
transport = AIOHTTPTransport(url="http://localhost:4000/graphql")
# Create a GraphQL client using the defined transport
client = Client(transport=transport, fetch_schema_from_transport=True)
studentid = request.args.get('studentid')
studentname = request.args.get('studentname')
studentdob = request.args.get('studentdob')
if(studentdob is not None):
#query by dob
query = gql(
"""
query QueryByDob($dob:String!)
{
studentQueryByDob(studentdob:$dob)
{
studentid
studentdob
studentname
}
}
""" )
params = {
"dob" : studentdob
}
result = client.execute(query, variable_values= params)
return(result)
elif(studentid is not None):
#query by id
query = gql(
"""
query QueryById($id:String!)
{
studentQueryById(studentid:$id)
{
studentid
studentdob
studentname
}
}
""" )
params = {
"id" : studentid
}
result = client.execute(query, variable_values= params)
return(result)
elif(studentname is not None):
query = gql(
"""
query QueryByName($name:String!)
{
studentQueryByName(studentname:$name)
{
studentid
studentdob
studentname
}
}
""" )
params = {
"name" : studentname
}
result = client.execute(query, variable_values= params)
return(result)
else:
return "please enter a single attribute" + studentid + '-' + studentname + '-' + studentdob<file_sep><?php
require_once __DIR__ . '/vendor/autoload.php';
use PhpAmqpLib\Connection\AMQPStreamConnection;
use PhpAmqpLib\Message\AMQPMessage;
$connection = new AMQPStreamConnection('localhost', 5672, 'guest', 'guest');
$channel = $connection->channel();
$channel->queue_declare('hello', false, false, false, false);
if(!isset($_REQUEST['date'],
$_REQUEST['description'],
$_REQUEST['temperature']))
return 0;
//sanitize data
$date = filter_var($_REQUEST['date'], FILTER_SANITIZE_SPECIAL_CHARS);
$description = filter_var($_REQUEST['description'], FILTER_SANITIZE_SPECIAL_CHARS);
$temp = filter_var($_REQUEST['temperature'], FILTER_SANITIZE_SPECIAL_CHARS);
$msg = new AMQPMessage("from php call Weather for " . $date . " is " . $description . "with temperature of " . (string)$temp);
$channel->basic_publish($msg, '', 'hello');<file_sep>from xmlrpc.server import SimpleXMLRPCServer
def temperature(temp):
if (0 <= temp <= 10):
return "cold"
elif(11 <= temp <=20):
return "warm"
else:
return "out of scope of specifications"
server = SimpleXMLRPCServer(("localhost", 8001))
print("Listening on port 8001...")
server.register_function(temperature, "temperature")
server.serve_forever()<file_sep>var express = require('express');
var { graphqlHTTP } = require('express-graphql');
var { buildSchema } = require('graphql');
var schema = buildSchema(`
type User {
id: String
name: String
}
type Student {
studentid: String
studentname: String
studentdob: String
}
type Query {
usercall(id: String): User
studentQueryById(studentid: String): Student
studentQueryByName(studentname: String): [Student]
studentQueryByDob(studentdob: String): [Student]
othercall: String
}
`);
// Maps id to User object
var fakeDatabase = {
'a': {
id: 'a',
name: 'alice',
},
'b': {
id: 'b',
name: 'bob',
},
'c': {
id: 'c',
name: 'carl',
},
};
var studentDatabase = {
'a': {
studentid: 'b00001',
studentname: 'alice',
studentdob: '99'
},
'b': {
studentid: 'b00002',
studentname: 'bob',
studentdob: '88'
},
'c': {
studentid: 'b00003',
studentname: 'tom',
studentdob: '91'
},
'd': {
studentid: 'b00004',
studentname: 'jim',
studentdob: '94'
},
'e': {
studentid: 'b00005',
studentname: 'bill',
studentdob: '99'
},
};
var root = {
usercall: ({id}) => {
return fakeDatabase[id]; // mapping to the data
},
studentQueryById: ({studentid}) => {
// send back the data
for (let [key, value] of Object.entries(studentDatabase)) {
if(value.studentid == studentid)
{
return studentDatabase[key]
}
}
},
studentQueryByName: ({studentname}) => {
// send back the data
var output = [];
for (let [key, value] of Object.entries(studentDatabase)) {
if(value.studentname == studentname)
{
output.push(studentDatabase[key])
}
}
return output
},
studentQueryByDob: ({studentdob}) => {
// send back the data
var output = [];
for (let [key, value] of Object.entries(studentDatabase)) {
if(value.studentdob == studentdob)
{
output.push(studentDatabase[key])
}
}
return output
},
othercall: ({}) => {
return 'This is the other call';
}
};
var app = express();
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
app.listen(4000);
console.log('Running a GraphQL API server at localhost:4000/graphql'); | 916461ca41f94be37536ee710f454c6a60b3511b | [
"JavaScript",
"Python",
"PHP"
] | 4 | Python | MechroLich/RESTfulAPI | f98fc95eccf5343a26f0ad0f77d04ad61899b5be | 5a93cfab6dc867653ff7bcdc99e4f6ee0a765761 | |
refs/heads/master | <repo_name>aurelchivu/NetflixClone<file_sep>/src/components/Nav.js
import React, { useState, useEffect } from 'react';
import '../style/Nav.css';
const Nav = () => {
const [showNav, handleShowNav] = useState(false);
const transitionNavbar = () => {
if (window.scrollY > 80) {
handleShowNav(true);
} else {
handleShowNav(false);
}
};
useEffect(() => {
window.addEventListener('scroll', transitionNavbar);
return () => window.removeEventListener('scroll', transitionNavbar);
}, []);
return (
<div className={`nav ${showNav && 'nav__black'}`}>
<div className='nav__contents'>
<img
className='nav__logo'
src='/netflix-transparent.png'
alt='netflix logo'
/>
<img
className='nav__avatar'
src='https://occ-0-3467-3466.1.nflxso.net/dnm/api/v6/K6hjPJd6cR6FpVELC5Pd6ovHRSk/AAAABXrmj4H9RUyDiO28L-KJxDGc9oDijd4jnl5RyCkiWMPB6xFxiVdRiKdOcNjG8kn4A3idJRTGAzuBuQ__c6yl1m0.png?r=fcc'
alt='netflix avatar'
/>
</div>
</div>
);
};
export default Nav;
<file_sep>/src/App.js
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import HomeScreen from './screens/HomeScreen';
import './style/App.css';
import LoginScreen from './screens/LoginScreen';
const App = () => {
return (
<div className='app'>
<Router>
<Route path='/' component={HomeScreen} exact />
<Route path='/login' component={LoginScreen}/>
</Router>
</div>
);
};
export default App;
| 7cc50c11cf480e953d4cc55204cc3c22f3103f41 | [
"JavaScript"
] | 2 | JavaScript | aurelchivu/NetflixClone | d4e5a68fda023a77f8fa7f11044919b64df85ef0 | 03f0d4926e5e1cff151503d6d343f2a89b789779 | |
refs/heads/master | <repo_name>TautvydasGec/TodoApp<file_sep>/src/index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter as Router, Route} from "react-router-dom";
import Welcome from './Welcome';
import App from './App';
import './index.css'
import './Welcome.css'
ReactDOM.render(
<React.StrictMode>
<Router>
<Route path="/" exact component={ Welcome }></Route>
<Route path="/app" exact component={ App }></Route>
</Router>
</React.StrictMode>,
document.getElementById('root')
);<file_sep>/src/Welcome.js
import React from 'react'
import { Link } from "react-router-dom";
import './Welcome.css'
export default function Welcome() {
return (
<div className = "Welcome">
<h1>Hello and Welcome to the Todo app</h1>
<Link to="/app"><button className = "app-button">App</button></Link>
</div>
)
}
<file_sep>/src/Task.js
import React from 'react'
export default function Task({ task, toggleComplete, handleDelete }) {
function handleCheckClick() {
toggleComplete(task.id)
}
function handleCheckDelete(){
handleDelete(task.id)
}
return (
<div>
<label className= {task.complete?"task-container done":"task-container"} >
<input
type="checkbox"
checked={task.complete}
onChange={handleCheckClick}
/>
<div className="task-name">{task.name}</div>
<div>{task.date}</div>
{task.late? <span role="img" aria-label="red dot">🔴</span> : <div></div>} {/*if a task is late a red indicator is shown
for styling purposes in default an empty span tag is generated*/}
<button className="btn delete-btn" onClick={handleCheckDelete}>Delete</button>
</label>
</div>
)
}
<file_sep>/src/App.js
import React, { useState, useRef, useEffect } from 'react';
import TodoList from './TodoList';
import uuid from 'uuid/dist/v4';
import './App.css';
const LOCAL_STORAGE_KEY = 'todoApp.task';
function App() {
const [tasks, setTasks] = useState([])
const taskNameRef = useRef()
const dueDateRef = useRef()
/*Loading tasks*/
useEffect(() => {
const storedTasks = JSON.parse(
localStorage.getItem(LOCAL_STORAGE_KEY)
);
storedTasks && setTasks(storedTasks);
}, []);
/*Saving tasks*/
useEffect(() => {
localStorage.setItem(LOCAL_STORAGE_KEY, JSON.stringify(tasks));
}, [tasks]);
/*List functions*/
function handleAdd(e) {
const name = taskNameRef.current.value
const date = dueDateRef.current.value
if (name ==="") return //does not allow empty task
setTasks(prevTasks => {
return [{ id: uuid(),
name: name,
complete: false,
date: date===""? "Date not specified" : date,
late: Date.parse(date)-Date.parse(new Date()) < 0 ? true : false, //date check should also happen every day, or every time the webpage is loaded
timeAdded: Date.parse(new Date()) //used for "Latest added" sort
},...prevTasks]
})
dueDateRef.current.value = null
taskNameRef.current.value = null
}
function toggleComplete(id) {
const newTasks = [...tasks];
const task = newTasks.find((task) => task.id === id);
task.complete = !task.complete;
setTasks(newTasks);
}
function handleDelete(id){
const newTasks = tasks.filter((task) => task.id !== id);
setTasks(newTasks);
}
/*sort functions*/
function sortByName(){
const newTasks = [...tasks];
newTasks.sort((a, b) => a.name.localeCompare(b.name));
setTasks(newTasks);
}
function sortByDate(){
const newTasks = [...tasks];
newTasks.sort((a, b) => a.date.localeCompare(b.date));
setTasks(newTasks);
}
function sortByTimeAdded(){
const newTasks = [...tasks];
newTasks.sort((a, b) => b.timeAdded-a.timeAdded);
setTasks(newTasks);
}
return (
<div className = "App">
<div className = "input-container">
<input ref={taskNameRef} type="text" />
<input type="date" ref ={dueDateRef} />
<button className="btn add-btn" onClick={handleAdd}>Add</button>
</div>
<div className = "sort-container">
<button className="btn" onClick={sortByName}>Sort By Name</button>
<button className="btn" onClick={sortByDate}>Sort By Date</button>
<button className="btn" onClick={sortByTimeAdded}>Sort By Latest Added</button>
</div>
<TodoList tasks={tasks} toggleComplete = {toggleComplete} handleDelete={handleDelete}/>
</div>
);
}
export default App;<file_sep>/README.md
# TodoApp
Todo App made with ReactJs
For preview [click here](https://relaxed-stonebraker-4132c2.netlify.app/)
<file_sep>/src/TodoList.js
import React from 'react';
import Task from './Task';
export default function TodoList({ tasks, toggleComplete, handleDelete }) {
return (
tasks.map(task =>{
return (<Task key={task.id} toggleComplete={toggleComplete} handleDelete={handleDelete} task={task} />)
})
)
} | 1cf842bf35550dd0f1045af1512d46329cb5cde8 | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | TautvydasGec/TodoApp | 561b17974b026cd4b8046b49b722f6998d00ee09 | 28cb3d2e8b1e66ac6384b1ab70caf64d3df75b8f | |
refs/heads/master | <file_sep>(function( window, $ ) {
var ns = window.componentNamespace = window.componentNamespace || "FG";
window[ns] = window[ns] || {};
// IE 8
Date.now = Date.now || function now() {
return new Date().getTime();
};
var Path = window[ns].Tau.Path = function() {
this.reset();
};
Path.prototype.isSufficient = function() {
return !!this.prevPoint && this.prevPrevPoint;
};
Path.prototype.distance = function() {
return this.prevPoint.x - this.prevPrevPoint.x;
};
Path.prototype.duration = function() {
return this.prevTime - this.prevPrevTime;
};
// TODO sort out variable names
Path.prototype.record = function( point ) {
this.prevPrevTime = this.prevTime;
this.prevPrevPoint = this.prevPoint;
// record the most recent drag point for decel on release
this.prevTime = Date.now();
this.prevPoint = point;
};
Path.prototype.velocity = function( timeStep ) {
var distance, time;
distance = this.distance();
time = this.duration();
return distance / ( time / timeStep );
};
Path.prototype.reset = function() {
this.prevPoint = undefined;
this.prevTime = undefined;
this.prevPrevTime = undefined;
this.prevPrevPoint = undefined;
};
Path.prototype.last = function() {
return this.prevPoint;
};
})(this, jQuery);
<file_sep>(function( $, window ) {
var Tau, $doc, $instance, instance, commonSetup, commonTeardown, config;
$doc = $( document );
Tau = window.FG.Tau;
commonSetup = function() {
// force default behavior
window.requestAnimationFrame = true;
$instance = $( "[data-tau='template-frames']" );
instance = new Tau( $instance[0], { canvas: false } );
// force the goto to pass the loaded image check
instance.$images.each(function() {
this.tauImageLoaded = true;
});
};
// TODO as needed
commonTeardown = function() {};
module( "constructor", {
setup: commonSetup,
teardown: commonTeardown
});
asyncTest( "starts auto-rotate", function() {
var oldIndex = instance.index;
setTimeout(function() {
ok(oldIndex <= instance.index);
start();
}, instance.autoRotateDelay() + instance.autoRotateStartDelay + 200);
});
test( "satisfies frame count", function() {
var frames = parseInt( $instance.find("[data-frames]").attr("data-frames"), 10 );
equal( $instance.children().filter( "img" ).length, frames );
});
test( "sets current", function() {
instance.goto(0);
ok( instance.$current[0]);
});
test( "focuses the first image", function() {
ok( instance.$images.eq( 0 ).attr( "class" ).indexOf( "focus" ) > -1 );
});
module( "change", config = {
setup: function() {
commonSetup();
instance.stopAutoRotate();
},
teardown: commonTeardown
});
test( "advances the index by the delta", function() {
instance.stopAutoRotate();
var index = instance.index;
instance.change( 2 );
instance.change( -1 );
equal( index + 2 - 1, instance.index );
});
module( "goto", config );
asyncTest( "changes focused class", function() {
var test = function(){
var oldFocused = instance.$element.find( ".focused" );
instance.goto( instance.index + 1 );
ok( oldFocused.attr("class").indexOf("focused") === -1 );
ok( instance.$current.attr("class").indexOf("focused") > -1 );
start();
};
if( instance.initialized ){
test();
} else {
$instance.bind("tau.init", function(){
test();
});
}
});
test( "wraps negative indices", function() {
instance.goto( 0 );
instance.change( -1 );
equal( instance.index, instance.$images.length - 1 );
});
test( "wraps positive indices ", function() {
instance.goto( instance.$images.length - 1 );
instance.change( 1 );
equal( instance.index, 0 );
});
module( "slow", {
setup: function() {
commonSetup();
instance.path.record({
x: 0
});
instance.path.record({
x: 100
});
}
});
test( "returns early when the path isn't sufficient", function() {
expect( 1 );
instance.path.reset();
ok( !instance.path.isSufficient() );
instance.rotate = function() {
ok( false );
};
instance.slow();
});
test( "calls rotate with updated coord", function() {
expect( 1 );
instance.velocity = 10;
// called with the right value
instance.rotate = function( point ) {
equal( point.x, 110 );
};
instance.slow();
});
test( "reduces velocity", function() {
var oldVelocity = instance.velocity = 10;
instance.slow();
// the velocity should be reduced
equal( instance.velocity, oldVelocity - instance.decelVal() );
});
test( "clears the slow interval when velocity <= 0", function() {
instance.velocity = instance.decelVal();
instance.slow();
ok( !instance.slowInterval );
equal( instance.velocity, 0 );
instance.velocity = instance.decelVal() - 1;
instance.slow();
ok( !instance.slowInterval );
equal( instance.velocity, 0 );
});
test( "clears the slow interval when velocity >= 0", function() {
instance.velocity = -1 * instance.decelVal();
instance.slow();
ok( !instance.slowInterval );
equal( instance.velocity, 0 );
instance.velocity = -1 * instance.decelVal() + 1;
instance.slow();
ok( !instance.slowInterval );
equal( instance.velocity, 0 );
});
module( "decel", {
setup: function() {
commonSetup();
instance.slow = function() {};
}
});
test( "returns early for insufficient paths", function() {
expect( 0 );
instance.path.isSufficient = function() { return false; };
instance.path.velocity = function() {
ok( false, "path velocity should not be called" );
};
instance.decel();
});
test( "keeps a lid on positive velocity", function() {
instance.path.isSufficient = function() { return true; };
instance.path.velocity = function() {
return 100;
};
instance.decel();
equal(instance.velocity, Tau.maxVelocity);
});
test( "keeps a lid on negative velocity", function() {
instance.path.isSufficient = function() { return true; };
instance.path.velocity = function() {
return -100;
};
instance.decel();
equal(instance.velocity, -1 * Tau.maxVelocity);
});
var path;
module( "path", {
setup: function() {
path = new Tau.Path();
}
});
test( "is only sufficient for calculations with two points", function() {
ok( !path.isSufficient(), "initially not sufficient" );
path.record( {} );
ok( !path.isSufficient(), "not sufficient after one record" );
path.record( {} );
ok( path.isSufficient(), "sufficient after two records" );
});
test( "distance is x, from second point to the first", function() {
path.record({ x: 5 });
path.record({ x: 0 });
equal( path.distance(), -5 );
});
asyncTest( "duration is from the second time to the first time", function() {
path.record({});
setTimeout(function() {
path.record({});
ok( path.duration() >= 100 );
start();
}, 100);
});
asyncTest( "velocity takes a time step", function() {
path.record({ x: 0 });
setTimeout(function() {
path.record({ x: 20 });
equal( Math.ceil(path.velocity( 20 )), 4 );
start();
}, 100);
});
test( "resets to inssufficient", function() {
path.record( {} );
path.record( {} );
ok( path.isSufficient(), "sufficient after two records" );
path.reset();
ok( !path.isSufficient(), "not sufficient after one record" );
});
var $noTmplInst, noTmplInst;
module("createImages", {
setup: function(){
commonSetup();
$noTmplInst = $( "[data-tau='no-template-frames']" );
noTmplInst = new Tau( $noTmplInst[0] );
$noImgs = $( "[data-tau='no-frames']" );
}
});
test( "doesn't add any images when the DOM has more than one already", function(){
equal(noTmplInst.$images.length, 2);
ok(instance.$images.length > 3);
});
test( "throws exception when there are no images", function(){
throws(function(){
new Tau( $noImgs[0] );
});
});
})( jQuery, this );
<file_sep>:warning: This project is archived and the repository is no longer maintained.
# Tau
[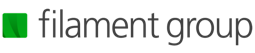 ](http://www.filamentgroup.com/)
Tau is a small and simple 360 gallery library. The primary goal is to start with a very light weight core and extend it with optional features as part of the build process. The core supports touch, mouse events, and automatic rotation. You can see a demo [here](https://filamentgroup.github.io/tau/demo).

## Setup
After including library in your document you can create an instance of Tau with:
```javascript
var tau = new window.componentNamespace.Tau( domElement );
```
Where `domElement` is an element conforming to the following markup pattern:
```html
<div class="tau" data-tau>
<div class="loading">loading...</div>
<img src="http://example.com/1.png"
data-src-template="http://example.com/$FRAME.png"
data-frames="72"
data-reduced-step-size="4"
data-auto-rotate-delay="200"></img>
</div>
```
The default `img` provides a fallback for browsers that fail to execute the instantiation. The `data-src-template` will be used to create the rest of the images images inside the parent element annotated with `data-tau`. Finally, an element tagged with the `loading` class will be displayed when the rotation hits an image that hasn't yet fired its loading event. It can be customized with additional markup and CSS as needs be.
## Quirks
Internet Explorer (up to and including 11) decodes the images slowly enough that they blink as they do the initial automatic rotation. To get IE to decode the images sooner we create a `div` inside the Tau element and load each of the images as 1px width and height, and then remove them once they are all loaded.
## Configuration
The following attributes are required on the initial `img` unless otherwise specified.
* `data-src-template` - the template for the additional `img` tags inserted by Tau
* `data-frames` - the number of images to be inserted
* `data-reduced-step-size` - factor of reduction for less capable browsers (ie, browsers with no raf)
* `data-auto-rotate-delay` - ms to wait after initialization to start auto-rotate
## Styles
The core styles can be found in `src/core.css`. The demo page also includes some styles for clarity that are not required by the library:
```css
.tau {
border: 3px solid #ccc;
margin-bottom: 2em;
position: relative;
}
.tau img {
width: 100%;
}
.tau .loading {
position: absolute;
z-index: 2;
left: 0;
top: 0;
}
```
<file_sep>(function( window, $ ) {
var $window, $doc;
$window = $(window);
$doc = $( document.documentElement );
var ns = window.componentNamespace = window.componentNamespace || "FG";
window[ns] = window[ns] || {};
Function.prototype.bind = Function.prototype.bind || function( context ) {
var self = this;
return function() {
self.apply( context, arguments );
};
};
var Tau = window[ns].Tau = function( element, options ) {
var startIndex, reducedStepSize;
this.element = element;
this.options = options || {};
this.$element = $( element );
this.$initial = this.$element.find( "img" );
this.$loading = this.$element.find( ".loading" );
this.index = 0;
// frame count by order of precendence
// 1. initial frames when they are specified explicitly
// 2. the data attribute on the initial image
// 3. the configured number of frames
this.frames =
this.$initial.length > 1 ? this.$initial.length :
parseInt( this.$initial.attr("data-frames"), 10 ) ||
this.options.frames;
// grab the user specified step size for when the browser is less-abled
reducedStepSize = parseInt( this.$initial.attr("data-reduced-step-size"), 10 ) || 4;
// TODO sort out a better qualification for the full set of images?
this.stepSize = window.requestAnimationFrame ? 1 : reducedStepSize;
// grab the user specified auto start delay
this.autoRotateStartDelay =
(this.options.autoplay || {}).delay ||
parseInt( this.$initial.attr("data-auto-rotate-delay"), 10 ) ||
Tau.autoRotateStartDelay;
this.mouseMoveBinding = this.rotateEvent.bind(this);
this.touchMoveBinding = this.rotateEvent.bind(this);
this.path = new Tau.Path();
// make sure the initial image stays visible after enhance
this.$initial.first().addClass( "focused" );
// hide all other images
this.$element.addClass( "tau-enhanced" );
// create a rendering spot to force decoding in IE and prevent blinking
this.$render = $( "<div data-render class=\"render\"></div>" )
.css( "position", "absolute" )
.css( "left", "0" )
.css( "top", "0" )
.prependTo( this.element );
if( this.options.canvas !== false ){
this.canvas = $( "<canvas/>").prependTo( this.element )[0];
if(this.canvas.getContext ){
this.canvasCtx = this.canvas.getContext("2d");
this.$element.addClass( "tau-canvas" );
$(window).bind("resize", function(){
clearTimeout(this.canvasResizeTimeout);
this.canvasResizeTimeout = setTimeout(this.renderCanvas.bind(this), 100);
}.bind(this));
}
}
if( this.options.controls ){
this.options.controls.text = this.options.controls.text || {
play: "Spin Object",
left: "Rotate Left",
right: "Rotate Right"
};
this.createControls();
}
// create the rest of the images
this.createImages();
// set the initial index and image
if( this.options.autoplay && this.options.autoplay.enabled ){
// start the automatic rotation
this.autostartTimeout = setTimeout(this.autoRotate.bind(this), this.autoRotateStartDelay);
}
// setup the event bindings for touch drag and mouse drag rotation
this.bind();
};
Tau.autoRotateTraversalTime = 4500;
Tau.autoRotateStartDelay = 100;
Tau.verticalScrollRatio = 4;
// Tau.decelTimeStep = Tau.autoRotateDelay / 2;
// Tau.decel = Tau.decelTimeStep / 8;
Tau.maxVelocity = 60;
Tau.prototype.createControls = function(){
this.$controls = $("<div class='tau-controls'></div>");
if(this.options.controls.play){
this.$controls.append(this.controlAnchorMarkup("play"));
}
if(this.options.controls.arrows){
this.$controls
.prepend(this.controlAnchorMarkup("left"))
.append(this.controlAnchorMarkup("right"));
}
this.$controls.bind("mousedown touchstart", this.onControlDown.bind(this));
this.$controls.bind("mouseup", this.onControlUp.bind(this));
// prevent link clicks from bubbling
this.$controls.bind("click", function(event){
if( $(event.target).is("a") ){
event.preventDefault();
}
});
this.$element.append(this.$controls);
};
Tau.prototype.controlAnchorMarkup = function(name){
var text = this.options.controls.text[name];
return "<a href='#' data-tau-controls='" + name +
"' title='" + text +
"'>" + text +
"</a>";
};
Tau.prototype.onControlDown = function(event){
var $link = $(event.target).closest("a");
switch($link.attr("data-tau-controls")){
case "left":
this.$element.addClass("control-left-down");
this.stopAutoRotate();
this.autoRotate();
break;
case "right":
this.$element.addClass("control-right-down");
this.stopAutoRotate();
this.autoRotate(true);
break;
}
};
Tau.prototype.onControlUp = function(event){
var $link = $(event.target).closest("a");
switch($link.attr("data-tau-controls")){
case "left":
case "right":
this.$element.removeClass("control-left-down");
this.$element.removeClass("control-right-down");
this.stopAutoRotate();
break;
case "play":
if( this.autoInterval ){
this.stopAutoRotate();
} else {
this.autoRotate();
}
break;
}
};
Tau.prototype.change = function( delta ) {
this.goto( this.options.reverse ? this.index - delta : this.index + delta );
};
Tau.prototype.goto = function( index ) {
var $next, normalizedIndex, imageCount = this.$images.length;
index = index % imageCount;
// stay within the bounds of the array
normalizedIndex = (imageCount + index) % imageCount;
// set the next image that's going to be shown/focused
$next = this.$images.eq( normalizedIndex );
// skip this action if the desired image isn't loaded yet
// TODO do something fancier here instead of just throwing up hands
if( !$next[0].tauImageLoaded ) {
this.showLoading();
return false;
}
// hide any image that happens to be visible (initial image when canvas)
if( this.$current ) {
this.$current.removeClass( "focused" );
} else {
this.$images.removeClass( "focused" );
}
// record the current focused image and make it visible
this.$current = $next;
// record the updated index only after advancing is possible
this.index = normalizedIndex;
if( this.canvasCtx ) {
return this.renderCanvas();
} else {
// show the new focused image
this.$current.addClass( "focused" );
return true;
}
};
Tau.prototype.renderCanvas = function() {
var $img = this.$current;
var img = $img[0];
var width = img.width;
var height = img.height;
var parentWidth = this.element.clientWidth;
var calcHeight = (parentWidth/width) * height;
if(!width || !height || !img.complete){
return false;
}
if( this.canvas.width !== parentWidth ||
this.canvas.height !== calcHeight || (parentWidth && calcHeight) ) {
this.canvas.width = parentWidth;
this.canvas.height = calcHeight;
}
this.canvasCtx.drawImage(img, 0, 0, parentWidth, calcHeight);
return true;
};
// TODO transplant the attributes from the initial image
Tau.prototype.createImages = function() {
var src, frames, html, $new, boundImageLoaded;
// if there are no image elements, raise an exception
if( this.$initial.length < 1 ){
throw new Error( "At least one image required" );
}
this.loadedCount = 0;
// if there is only one image element, assume it's a template
if( this.$initial.length == 1 ) {
this.markImageLoaded( this.$initial[0] );
src =
this.options.template ||
this.$initial.attr( "data-src-template" );
var imgs = [];
for( var i = this.stepSize + 1; i <= this.frames; i+= this.stepSize ) {
html = "<img src=" + src.replace("$FRAME", i) + "></img>";
$new = $( html );
imgs.push($new);
}
$.each(imgs, function(i, e){
var $img = $(e);
$img.bind("load error", function(e){ this.imageLoaded(i, e.target, e); }.bind(this));
this.$element.append( $img );
this.$render.append( $img.html() );
}.bind(this));
// take all the child images and use them as frames of the rotation
this.$images = this.$element.children().filter( "img" );
this.$current = this.$images;
this.goto(0);
} else {
// take all the child images and use them as frames of the rotation
this.$images = this.$element.children().filter( "img" );
this.$images.each(function(i, e){
// if the image height is greater than zero we assume the image is loaded
// otherwise we bind to onload and pray that we win the race
if( $(e).height() > 0 ){
this.imageLoaded( i, e );
} else {
$(e).bind("load error", function(event){
this.imageLoaded( i, event.target, event );
}.bind(this));
}
}.bind(this));
}
};
Tau.prototype.imageLoaded = function( index, element, event ) {
var initTriggered = false;
this.markImageLoaded( element );
// if the isn't going to play automatically and the first image is
// loaded make sure to render it
if( this.$element.find("img")[0] == element &&
(!event || event.type !== "error") &&
(!this.options.autoplay || !this.options.autoplay.enabled) ){
this.goto(0);
this.$element.trigger("tau.init");
initTriggered = true;
}
this.loadedCount++;
if( this.loadedCount >= this.frames - 1) {
this.hideLoading();
if(!initTriggered) {
this.$element.trigger("tau.init");
this.initialized = true;
}
}
};
Tau.prototype.markImageLoaded = function( element ) {
element.tauImageLoaded = true;
};
Tau.prototype.bind = function() {
this.$element.bind( "mousedown touchstart", this.track.bind(this) );
};
Tau.prototype.autoRotate = function( right ) {
// already rotating
if( this.autoInterval ) {
return;
}
this.$element.addClass("spinning");
// move once initially
this.change( right ? -1 : 1 );
// move after the interval
this.autoInterval = setInterval(function() {
this.change( right ? -1 : 1 );
}.bind(this), this.autoRotateDelay() * this.stepSize);
this.$element.trigger( "tau.auto-rotate-start" );
};
Tau.prototype.autoRotateDelay = function(){
return (this.options.interval || Tau.autoRotateTraversalTime) / this.frames;
};
Tau.prototype.stopAutoRotate = function() {
clearInterval( this.autoInterval );
clearInterval( this.autostartTimeout );
this.$element.removeClass("spinning");
this.autoInterval = undefined;
this.$element.trigger( "tau.auto-rotate-stop" );
};
Tau.prototype.track = function( event ) {
var point;
// ignore tracking on control clicks
if( $(event.target).closest(".tau-controls").length ){
return;
}
// prevent dragging behavior for mousedown
if( event.type === "mousedown" ){
event.preventDefault();
}
if( event.type === "touchstart" ) {
this.$element.trigger("tau.touch-tracking-start");
} else {
this.$element.trigger("tau.mouse-tracking-start");
}
if( this.tracking ) {
return;
}
$doc.one( "mouseup", this.release.bind(this) );
$doc.one( "touchend", this.release.bind(this) );
this.tracking = true;
// clean out the path since we'll need a new one for decel
this.path.reset();
// show the cursor as grabbing
this.cursorGrab();
// By default the number of pixels required to move the carousel by one
// frame is the ratio of the tau element width to the number of frames. That
// is, by default the user should be able to see the full rotation by moving
// their input device from one side of the tau element to the other.
var defaultThreshold = this.$element[0].clientWidth / this.frames ;
// divide the default by the sensitivity. If the senstivity is greater than
// 1 it will require less effort (smaller distance) to advance the rotation
// by a single slide. If the sensitivity is less than 1 it will require more
// effort
this.rotateThreshold = defaultThreshold / (this.options.sensitivity || 1);
// record the x for threshold calculations
point = this.getPoint( event );
this.downX = point.x;
this.downY = point.y;
this.downIndex = this.index;
$doc.bind( "mousemove", this.mouseMoveBinding );
$doc.bind( "touchmove", this.touchMoveBinding );
};
Tau.prototype.slow = function() {
// if the path gets broken during the decel just stop
if( !this.path.isSufficient() ) {
this.clearSlowInterval();
return;
}
this.rotate({
x: this.path.last().x + this.velocity,
y: this.path.last().y
});
if( this.velocity > 0 ){
this.velocity = this.velocity - this.decelVal();
if( this.velocity <= 0 ){
this.clearSlowInterval();
}
} else {
this.velocity = this.velocity + this.decelVal();
if( this.velocity >= 0 ){
this.clearSlowInterval();
}
}
};
Tau.prototype.decelVal = function(){
return this.decelTimeStep() / 8;
};
Tau.prototype.clearSlowInterval = function() {
clearInterval(this.slowInterval);
this.velocity = 0;
this.slowInterval = undefined;
};
Tau.prototype.decel = function() {
var velocity, sign;
// if we don't have two points of mouse or touch tracking this won't work
if( !this.path.isSufficient() ) {
return;
}
// determine the starting velocity based on the traced path
velocity = this.path.velocity( this.decelTimeStep() );
// borrowed from http://stackoverflow.com/questions/7624920/number-sign-in-javascript
sign = velocity > 0 ? 1 : velocity < 0 ? -1 : 0;
// keep a lid on how fast the rotation spins out
if( Math.abs(velocity) > Tau.maxVelocity ){
velocity = sign * Tau.maxVelocity;
}
this.velocity = velocity;
this.slowInterval = setInterval(this.slow.bind(this), this.decelTimeStep());
};
Tau.prototype.decelTimeStep = function(){
return this.autoRotateDelay() / 2;
};
Tau.prototype.release = function( event ) {
if( $(event.target).closest(".tau-controls").length ){
return;
}
if( !this.tracking ){
return;
}
if( event.type === "touchend" ) {
this.$element.trigger("tau.touch-tracking-stop");
} else {
this.$element.trigger("tau.mouse-tracking-stop");
}
this.decel();
this.cursorRelease();
// TODO sort out why shoestring borks when unbinding with a string split list
$doc.unbind( "mousemove", this.mouseMoveBinding );
$doc.unbind( "touchmove", this.touchMoveBinding );
this.tracking = false;
};
Tau.prototype.cursorGrab = function() {
$doc.addClass( "grabbing" );
};
Tau.prototype.cursorRelease = function() {
$doc.removeClass( "grabbing" );
};
Tau.prototype.showLoading = function() {
this.$loading.attr( "style" , "display: block" );
};
Tau.prototype.hideLoading = function() {
this.$loading.attr( "style" , "display: none" );
};
Tau.prototype.getPoint = function( event ) {
var touch = event.touches || (event.originalEvent && event.originalEvent.touches);
if( touch ){
return {
x: touch[0].pageX,
y: touch[0].pageY
};
}
return {
x: event.pageX || event.clientX,
y: event.pageY || event.clientY
};
};
Tau.prototype.rotateEvent = function( event ) {
// NOTE it might be better to prevent when the rotation returns anything BUT false
// so that slow drags still get the scroll prevented
if( this.rotate(this.getPoint(event)) ){
event.preventDefault();
}
};
Tau.prototype.rotate = function( point ) {
var deltaX, deltaY;
deltaX = point.x - this.downX;
deltaY = point.y - this.downY;
// if the movement on the Y dominates X then skip and allow scroll
if( Math.abs(deltaY) / Math.abs(deltaX) >= Tau.verticalScrollRatio ) {
return false;
}
// since we're rotating record the point for decel
this.path.record( point );
// NOTE to reverse the spin direction add the delta/thresh to the downIndex
if( Math.abs(deltaX) >= this.rotateThreshold ) {
// NOTE works better on mousedown, here allows autorotate to continue
this.stopAutoRotate();
var index;
if( this.options.reverse ) {
index = this.downIndex + Math.round(deltaX / this.rotateThreshold);
} else {
index = this.downIndex - Math.round(deltaX / this.rotateThreshold);
}
this.goto( index );
return true;
}
};
})(this, jQuery);
| 810c22a8bdd5551052ba44ec9e0580f7f24a9ee9 | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | filamentgroup/tau | 6310ed4e1afef765333ff24f94e1599a577e434b | f9050efe05a9da21b4ccaceca1ad6ed2e4409011 | |
refs/heads/master | <file_sep># Copyright (C) 2018 Fondazione Istituto Italiano di Tecnologia (IIT)
# All Rights Reserved.
# Authors: <NAME> <<EMAIL>>
cmake_minimum_required(VERSION 3.5)
project(find-superquadric)
set(CMAKE_CXX_EXTENSIONS OFF)
set(CMAKE_CXX_STANDARD 11)
set(CMAKE_CXX_STANDARD_REQUIRED 11)
if(NOT CMAKE_CONFIGURATION_TYPES)
if(NOT CMAKE_BUILD_TYPE)
message(STATUS "Setting build type to 'Release' as none was specified.")
set_property(CACHE CMAKE_BUILD_TYPE PROPERTY VALUE "Release")
endif()
endif()
if(NOT MSVC)
set(CMAKE_INSTALL_RPATH_USE_LINK_PATH TRUE)
endif()
find_package(YARP 3.0.0 REQUIRED)
find_package(ICUB REQUIRED)
list(APPEND CMAKE_MODULE_PATH ${ICUB_MODULE_PATH})
find_package(IPOPT REQUIRED)
find_package(VTK REQUIRED)
include(${VTK_USE_FILE})
add_definitions(${IPOPT_DEFINITIONS} -D_USE_MATH_DEFINES)
include_directories(${PROJECT_SOURCE_DIR}/src ${IPOPT_INCLUDE_DIRS})
add_executable(${PROJECT_NAME} src/nlp.h src/nlp.cpp src/main.cpp)
set_property(TARGET ${PROJECT_NAME} APPEND_STRING PROPERTY LINK_FLAGS " ${IPOPT_LINK_FLAGS}")
target_link_libraries(${PROJECT_NAME} ${YARP_LIBRARIES} ${IPOPT_LIBRARIES} ${VTK_LIBRARIES} ctrlLib)
install(TARGETS ${PROJECT_NAME} DESTINATION bin)
<file_sep>/******************************************************************************
* *
* Copyright (C) 2018 Fondazione Istituto Italiano di Tecnologia (IIT) *
* All Rights Reserved. *
* *
******************************************************************************/
/**
* @file main.cpp
* @authors: <NAME> <<EMAIL>>
*/
#include <cstdlib>
#include <memory>
#include <mutex>
#include <cmath>
#include <vector>
#include <set>
#include <map>
#include <algorithm>
#include <string>
#include <sstream>
#include <fstream>
#include <yarp/os/all.h>
#include <yarp/sig/all.h>
#include <yarp/math/Math.h>
#include <yarp/math/Rand.h>
#include <iCub/ctrl/clustering.h>
#include <vtkSmartPointer.h>
#include <vtkCommand.h>
#include <vtkProperty.h>
#include <vtkPolyDataMapper.h>
#include <vtkPointData.h>
#include <vtkSuperquadric.h>
#include <vtkUnsignedCharArray.h>
#include <vtkTransform.h>
#include <vtkSampleFunction.h>
#include <vtkContourFilter.h>
#include <vtkActor.h>
#include <vtkOrientationMarkerWidget.h>
#include <vtkAxesActor.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkRenderer.h>
#include <vtkVertexGlyphFilter.h>
#include <vtkCamera.h>
#include <vtkInteractorStyleSwitch.h>
#include "nlp.h"
using namespace std;
using namespace yarp::os;
using namespace yarp::sig;
using namespace yarp::math;
using namespace iCub::ctrl;
mutex mtx;
/****************************************************************/
class UpdateCommand : public vtkCommand
{
const bool *closing;
public:
/****************************************************************/
vtkTypeMacro(UpdateCommand, vtkCommand);
/****************************************************************/
static UpdateCommand *New()
{
return new UpdateCommand;
}
/****************************************************************/
UpdateCommand() : closing(nullptr) { }
/****************************************************************/
void set_closing(const bool &closing)
{
this->closing=&closing;
}
/****************************************************************/
void Execute(vtkObject *caller, unsigned long vtkNotUsed(eventId),
void *vtkNotUsed(callData))
{
lock_guard<mutex> lck(mtx);
vtkRenderWindowInteractor* iren=static_cast<vtkRenderWindowInteractor*>(caller);
if (closing!=nullptr)
{
if (*closing)
{
iren->GetRenderWindow()->Finalize();
iren->TerminateApp();
return;
}
}
iren->GetRenderWindow()->SetWindowName("Find Superquadric");
iren->Render();
}
};
/****************************************************************/
class Object
{
protected:
vtkSmartPointer<vtkPolyDataMapper> vtk_mapper;
vtkSmartPointer<vtkActor> vtk_actor;
public:
/****************************************************************/
vtkSmartPointer<vtkActor> &get_actor()
{
return vtk_actor;
}
};
/****************************************************************/
class Points : public Object
{
protected:
vtkSmartPointer<vtkPoints> vtk_points;
vtkSmartPointer<vtkUnsignedCharArray> vtk_colors;
vtkSmartPointer<vtkPolyData> vtk_polydata;
vtkSmartPointer<vtkVertexGlyphFilter> vtk_glyphFilter;
public:
/****************************************************************/
Points(const vector<Vector> &points, const int point_size)
{
vtk_points=vtkSmartPointer<vtkPoints>::New();
for (size_t i=0; i<points.size(); i++)
vtk_points->InsertNextPoint(points[i][0],points[i][1],points[i][2]);
vtk_polydata=vtkSmartPointer<vtkPolyData>::New();
vtk_polydata->SetPoints(vtk_points);
vtk_glyphFilter=vtkSmartPointer<vtkVertexGlyphFilter>::New();
vtk_glyphFilter->SetInputData(vtk_polydata);
vtk_glyphFilter->Update();
vtk_mapper=vtkSmartPointer<vtkPolyDataMapper>::New();
vtk_mapper->SetInputConnection(vtk_glyphFilter->GetOutputPort());
vtk_actor=vtkSmartPointer<vtkActor>::New();
vtk_actor->SetMapper(vtk_mapper);
vtk_actor->GetProperty()->SetPointSize(point_size);
}
/****************************************************************/
void set_points(const vector<Vector> &points)
{
vtk_points=vtkSmartPointer<vtkPoints>::New();
for (size_t i=0; i<points.size(); i++)
vtk_points->InsertNextPoint(points[i][0],points[i][1],points[i][2]);
vtk_polydata->SetPoints(vtk_points);
}
/****************************************************************/
bool set_colors(const vector<vector<unsigned char>> &colors)
{
if (colors.size()==vtk_points->GetNumberOfPoints())
{
vtk_colors=vtkSmartPointer<vtkUnsignedCharArray>::New();
vtk_colors->SetNumberOfComponents(3);
for (size_t i=0; i<colors.size(); i++)
vtk_colors->InsertNextTypedTuple(colors[i].data());
vtk_polydata->GetPointData()->SetScalars(vtk_colors);
return true;
}
else
return false;
}
/****************************************************************/
vtkSmartPointer<vtkPolyData> &get_polydata()
{
return vtk_polydata;
}
};
/****************************************************************/
class Superquadric : public Object
{
protected:
vtkSmartPointer<vtkSuperquadric> vtk_superquadric;
vtkSmartPointer<vtkSampleFunction> vtk_sample;
vtkSmartPointer<vtkContourFilter> vtk_contours;
vtkSmartPointer<vtkTransform> vtk_transform;
public:
/****************************************************************/
Superquadric(const Vector &r, const vector<double> &color,
const double opacity)
{
double bx=r[4];
double by=r[6];
double bz=r[5];
vtk_superquadric=vtkSmartPointer<vtkSuperquadric>::New();
vtk_superquadric->ToroidalOff();
vtk_superquadric->SetSize(1.0);
vtk_superquadric->SetCenter(zeros(3).data());
vtk_superquadric->SetScale(bx,by,bz);
vtk_superquadric->SetPhiRoundness(r[7]);
vtk_superquadric->SetThetaRoundness(r[8]);
vtk_sample=vtkSmartPointer<vtkSampleFunction>::New();
vtk_sample->SetSampleDimensions(50,50,50);
vtk_sample->SetImplicitFunction(vtk_superquadric);
vtk_sample->SetModelBounds(-bx,bx,-by,by,-bz,bz);
// The isosurface is defined at 0.0 as specified in
// https://github.com/Kitware/VTK/blob/master/Common/DataModel/vtkSuperquadric.cxx
vtk_contours=vtkSmartPointer<vtkContourFilter>::New();
vtk_contours->SetInputConnection(vtk_sample->GetOutputPort());
vtk_contours->GenerateValues(1,0.0,0.0);
vtk_mapper=vtkSmartPointer<vtkPolyDataMapper>::New();
vtk_mapper->SetInputConnection(vtk_contours->GetOutputPort());
vtk_mapper->ScalarVisibilityOff();
vtk_actor=vtkSmartPointer<vtkActor>::New();
vtk_actor->SetMapper(vtk_mapper);
vtk_actor->GetProperty()->SetColor(color[0],color[1],color[2]);
vtk_actor->GetProperty()->SetOpacity(opacity);
vtk_transform=vtkSmartPointer<vtkTransform>::New();
vtk_transform->Translate(r.subVector(0,2).data());
vtk_transform->RotateZ(r[3]);
vtk_transform->RotateX(-90.0);
vtk_actor->SetUserTransform(vtk_transform);
}
/****************************************************************/
void set_parameters(const Vector &r)
{
// Note: roundness parameter for axes x and y is shared in SQ model,
// but VTK shares axes x and z (ThetaRoundness).
// To get a good display, directions of axes y and z need to be swapped
// => parameters for y and z are inverted and a rotation of -90 degrees around x is added
double bx=r[4];
double by=r[6];
double bz=r[5];
vtk_superquadric->SetScale(bx,by,bz);
vtk_superquadric->SetPhiRoundness(r[7]); // roundness along model z axis (vtk y axis)
vtk_superquadric->SetThetaRoundness(r[8]); // common roundness along model x and y axes (vtk x and z axes)
vtk_sample->SetModelBounds(-bx,bx,-by,by,-bz,bz);
vtk_transform->Identity();
vtk_transform->Translate(r.subVector(0,2).data());
vtk_transform->RotateZ(r[3]); // rotate around vertical
vtk_transform->RotateX(-90.0); // rotate to invert y and z
}
};
/****************************************************************/
class Finder : public RFModule
{
Bottle outliersRemovalOptions;
unsigned int uniform_sample;
double random_sample;
bool from_file;
bool test_derivative;
bool viewer_enabled;
double inside_penalty;
bool closing;
class PointsProcessor : public PortReader {
Finder *finder;
bool read(ConnectionReader &connection) override {
PointCloud<DataXYZRGBA> points;
if (!points.read(connection))
return false;
Bottle reply;
finder->process(points,reply);
if (ConnectionWriter *writer=connection.getWriter())
reply.write(*writer);
return true;
}
public:
PointsProcessor(Finder *finder_) : finder(finder_) { }
} pointsProcessor;
RpcServer rpcPoints,rpcService;
vector<Vector> all_points,in_points,out_points,dwn_points;
vector<vector<unsigned char>> all_colors;
unique_ptr<Points> vtk_all_points,vtk_out_points,vtk_dwn_points;
unique_ptr<Superquadric> vtk_superquadric;
vtkSmartPointer<vtkRenderer> vtk_renderer;
vtkSmartPointer<vtkRenderWindow> vtk_renderWindow;
vtkSmartPointer<vtkRenderWindowInteractor> vtk_renderWindowInteractor;
vtkSmartPointer<vtkAxesActor> vtk_axes;
vtkSmartPointer<vtkOrientationMarkerWidget> vtk_widget;
vtkSmartPointer<vtkCamera> vtk_camera;
vtkSmartPointer<vtkInteractorStyleSwitch> vtk_style;
vtkSmartPointer<UpdateCommand> vtk_updateCallback;
/****************************************************************/
void removeOutliers()
{
double t0=Time::now();
if (outliersRemovalOptions.size()>=2)
{
double radius=outliersRemovalOptions.get(0).asDouble();
int minpts=outliersRemovalOptions.get(1).asInt();
Property options;
options.put("epsilon",radius);
options.put("minpts",minpts);
DBSCAN dbscan;
map<size_t,set<size_t>> clusters=dbscan.cluster(all_points,options);
size_t largest_class; size_t largest_size=0;
for (auto it=begin(clusters); it!=end(clusters); it++)
{
if (it->second.size()>largest_size)
{
largest_size=it->second.size();
largest_class=it->first;
}
}
auto &c=clusters[largest_class];
for (size_t i=0; i<all_points.size(); i++)
{
if (c.find(i)==end(c))
out_points.push_back(all_points[i]);
else
in_points.push_back(all_points[i]);
}
}
else
in_points=all_points;
double t1=Time::now();
yInfo()<<out_points.size()<<"outliers removed out of"
<<all_points.size()<<"points in"<<t1-t0<<"[s]";
}
/****************************************************************/
void sampleInliers()
{
double t0=Time::now();
if (random_sample>=1.0)
{
unsigned int cnt=0;
for (auto &p:in_points)
{
if ((cnt++%uniform_sample)==0)
dwn_points.push_back(p);
}
}
else
{
set<unsigned int> idx;
while (idx.size()<(size_t)(random_sample*in_points.size()))
{
unsigned int i=(unsigned int)(Rand::scalar(0.0,1.0)*in_points.size());
if (idx.find(i)==idx.end())
{
dwn_points.push_back(in_points[i]);
idx.insert(i);
}
}
}
double t1=Time::now();
yInfo()<<dwn_points.size()<<"samples out of"
<<in_points.size()<<"inliers in"<<t1-t0<<"[s]";
}
/****************************************************************/
Vector findSuperquadric() const
{
Ipopt::SmartPtr<Ipopt::IpoptApplication> app=new Ipopt::IpoptApplication;
app->Options()->SetNumericValue("tol",1e-6);
app->Options()->SetNumericValue("constr_viol_tol",1e-3);
app->Options()->SetIntegerValue("acceptable_iter",0);
app->Options()->SetStringValue("mu_strategy","adaptive");
app->Options()->SetIntegerValue("max_iter",1000);
app->Options()->SetStringValue("hessian_approximation","limited-memory");
app->Options()->SetStringValue("derivative_test",test_derivative?"first-order":"none");
app->Options()->SetIntegerValue("print_level",test_derivative?5:0);
app->Initialize();
double t0=Time::now();
Ipopt::SmartPtr<SuperQuadricNLP> nlp=new SuperQuadricNLP(dwn_points,inside_penalty);
Ipopt::ApplicationReturnStatus status=app->OptimizeTNLP(GetRawPtr(nlp));
double t1=Time::now();
Vector r=nlp->get_result();
yInfo()<<"center = ("<<r.subVector(0,2).toString(3,3)<<")";
yInfo()<<"angle ="<<r[3]<<"[deg]";
yInfo()<<"size = ("<<r.subVector(4,6).toString(3,3)<<")";
yInfo()<<"shape = ("<<r.subVector(7,8).toString(3,3)<<")";
yInfo()<<"found in ="<<t1-t0<<"[s]";
return r;
}
/****************************************************************/
bool configure(ResourceFinder &rf) override
{
Rand::init();
from_file=rf.check("file");
if (from_file)
{
string file=rf.find("file").asString();
ifstream fin(file.c_str());
if (!fin.is_open())
{
yError()<<"Unable to open file \""<<file<<"\"";
return false;
}
Vector p(3);
vector<unsigned int> c_(3);
vector<unsigned char> c(3);
string line;
while (getline(fin,line))
{
istringstream iss(line);
if (!(iss>>p[0]>>p[1]>>p[2]))
break;
all_points.push_back(p);
fill(c_.begin(),c_.end(),120);
iss>>c_[0]>>c_[1]>>c_[2];
c[0]=(unsigned char)c_[0];
c[1]=(unsigned char)c_[1];
c[2]=(unsigned char)c_[2];
all_colors.push_back(c);
}
}
else
{
rpcPoints.open("/find-superquadric/points:rpc");
rpcPoints.setReader(pointsProcessor);
rpcService.open("/find-superquadric/service:rpc");
attach(rpcService);
}
if (rf.check("remove-outliers"))
if (const Bottle *ptr=rf.find("remove-outliers").asList())
outliersRemovalOptions=*ptr;
uniform_sample=(unsigned int)rf.check("uniform-sample",Value(1)).asInt();
random_sample=rf.check("random-sample",Value(1.0)).asDouble();
inside_penalty=rf.check("inside-penalty",Value(1.0)).asDouble();
test_derivative=rf.check("test-derivative");
viewer_enabled=!rf.check("disable-viewer");
vector<double> color={0.0,0.3,0.6};
if (rf.check("color"))
{
if (const Bottle *ptr=rf.find("color").asList())
{
size_t len=std::min(color.size(),ptr->size());
for (size_t i=0; i<len; i++)
color[i]=ptr->get(i).asDouble();
}
}
double opacity=rf.check("opacity",Value(0.25)).asDouble();
vector<double> backgroundColor={0.7,0.7,0.7};
if (rf.check("background-color"))
{
if (const Bottle *ptr=rf.find("background-color").asList())
{
size_t len=std::min(backgroundColor.size(),ptr->size());
for (size_t i=0; i<len; i++)
backgroundColor[i]=ptr->get(i).asDouble();
}
}
removeOutliers();
sampleInliers();
vtk_all_points=unique_ptr<Points>(new Points(all_points,2));
vtk_out_points=unique_ptr<Points>(new Points(out_points,4));
vtk_dwn_points=unique_ptr<Points>(new Points(dwn_points,1));
vtk_all_points->set_colors(all_colors);
vtk_out_points->get_actor()->GetProperty()->SetColor(1.0,0.0,0.0);
vtk_dwn_points->get_actor()->GetProperty()->SetColor(1.0,1.0,0.0);
Vector r(9,0.0);
if (dwn_points.size()>0)
r=findSuperquadric();
vtk_superquadric=unique_ptr<Superquadric>(new Superquadric(r,color,opacity));
vtk_renderer=vtkSmartPointer<vtkRenderer>::New();
vtk_renderWindow=vtkSmartPointer<vtkRenderWindow>::New();
vtk_renderWindow->SetSize(600,600);
vtk_renderWindow->AddRenderer(vtk_renderer);
vtk_renderWindowInteractor=vtkSmartPointer<vtkRenderWindowInteractor>::New();
vtk_renderWindowInteractor->SetRenderWindow(vtk_renderWindow);
vtk_renderer->AddActor(vtk_all_points->get_actor());
vtk_renderer->AddActor(vtk_out_points->get_actor());
if (dwn_points.size()!=in_points.size())
vtk_renderer->AddActor(vtk_dwn_points->get_actor());
vtk_renderer->AddActor(vtk_superquadric->get_actor());
vtk_renderer->SetBackground(backgroundColor.data());
vtk_axes=vtkSmartPointer<vtkAxesActor>::New();
vtk_widget=vtkSmartPointer<vtkOrientationMarkerWidget>::New();
vtk_widget->SetOutlineColor(0.9300,0.5700,0.1300);
vtk_widget->SetOrientationMarker(vtk_axes);
vtk_widget->SetInteractor(vtk_renderWindowInteractor);
vtk_widget->SetViewport(0.0,0.0,0.2,0.2);
vtk_widget->SetEnabled(1);
vtk_widget->InteractiveOn();
vector<double> bounds(6),centroid(3);
vtk_all_points->get_polydata()->GetBounds(bounds.data());
for (size_t i=0; i<centroid.size(); i++)
centroid[i]=0.5*(bounds[i<<1]+bounds[(i<<1)+1]);
vtk_camera=vtkSmartPointer<vtkCamera>::New();
vtk_camera->SetPosition(centroid[0]+1.0,centroid[1],centroid[2]+0.5);
vtk_camera->SetFocalPoint(centroid.data());
vtk_camera->SetViewUp(0.0,0.0,1.0);
vtk_renderer->SetActiveCamera(vtk_camera);
vtk_style=vtkSmartPointer<vtkInteractorStyleSwitch>::New();
vtk_style->SetCurrentStyleToTrackballCamera();
vtk_renderWindowInteractor->SetInteractorStyle(vtk_style);
if (viewer_enabled)
{
vtk_renderWindowInteractor->Initialize();
vtk_renderWindowInteractor->CreateRepeatingTimer(10);
vtk_updateCallback=vtkSmartPointer<UpdateCommand>::New();
vtk_updateCallback->set_closing(closing);
vtk_renderWindowInteractor->AddObserver(vtkCommand::TimerEvent,vtk_updateCallback);
vtk_renderWindowInteractor->Start();
}
return true;
}
/****************************************************************/
double getPeriod() override
{
return 1.0;
}
/****************************************************************/
bool updateModule() override
{
return (!from_file && !viewer_enabled);
}
/****************************************************************/
void process(const PointCloud<DataXYZRGBA> &points, Bottle &reply)
{
reply.clear();
if (points.size()>0)
{
lock_guard<mutex> lck(mtx);
all_points.clear();
all_colors.clear();
in_points.clear();
out_points.clear();
dwn_points.clear();
Vector p(3);
vector<unsigned char> c(3);
for (int i=0; i<points.size(); i++)
{
p[0]=points(i).x;
p[1]=points(i).y;
p[2]=points(i).z;
c[0]=points(i).r;
c[1]=points(i).g;
c[2]=points(i).b;
all_points.push_back(p);
all_colors.push_back(c);
}
removeOutliers();
sampleInliers();
Vector r=findSuperquadric();
vtk_all_points->set_points(all_points);
vtk_all_points->set_colors(all_colors);
vtk_out_points->set_points(out_points);
vtk_dwn_points->set_points(dwn_points);
vtk_superquadric->set_parameters(r);
reply.read(r);
}
}
/****************************************************************/
bool respond(const Bottle &command, Bottle &reply) override
{
lock_guard<mutex> lck(mtx);
bool ok=false;
if (command.check("remove-outliers"))
{
if (const Bottle *ptr=command.find("remove-outliers").asList())
outliersRemovalOptions=*ptr;
ok=true;
}
if (command.check("uniform-sample"))
{
uniform_sample=(unsigned int)command.find("uniform-sample").asInt();
ok=true;
}
if (command.check("random-sample"))
{
random_sample=command.find("random-sample").asDouble();
ok=true;
}
if (command.check("inside-penalty"))
{
inside_penalty=command.find("inside-penalty").asDouble();
ok=true;
}
reply.addVocab(Vocab::encode(ok?"ack":"nack"));
return true;
}
/****************************************************************/
bool interruptModule() override
{
closing=true;
return true;
}
/****************************************************************/
bool close() override
{
if (rpcPoints.asPort().isOpen())
rpcPoints.close();
if (rpcService.asPort().isOpen())
rpcService.close();
return true;
}
public:
/****************************************************************/
Finder() : closing(false), pointsProcessor(this) { }
};
/****************************************************************/
int main(int argc, char *argv[])
{
Network yarp;
ResourceFinder rf;
rf.configure(argc,argv);
if (!rf.check("file"))
{
if (!yarp.checkNetwork())
{
yError()<<"Unable to find Yarp server!";
return EXIT_FAILURE;
}
}
Finder finder;
return finder.runModule(rf);
}
<file_sep>Fit a partial point cloud with a superquadric
=============================================
[](https://gitpod.io/#https://github.com/robotology/find-superquadric)
Solve an optimization problem to find out the best superquadric that fits a given partial point cloud.
### Note
The superquadric is parametrized in terms of its center, the shape, the principal axes and a rotation angle around the z-axis, in order to account for objects that are supposed to be lying on a table parallel to the x-y plane (to keep things simple :wink:).
:warning: If the input point cloud does not guarantee that the above assumptions hold, it will need to be first transformed through a convenient rototraslation.
The equation of the superquadric is the following:

### Dependencies
- [YARP](https://github.com/robotology/yarp)
- [iCub](https://github.com/robotology/icub-main)
- [Ipopt](https://github.com/coin-or/Ipopt)
- [VTK](https://github.com/Kitware/VTK)
### Command-line options
- `--file file-name`: specify the file containing the point cloud given in the following plain format:
```
x0 y0 z0 [r0 g0 b0]
x1 y1 z1 [r1 g1 b1]
...
```
RGB colors are optional.
- `--remove-outliers "(<radius> <minpts>)"`: outliers removal based on spatial density clustering. The aggregation of points in clusters is regulated through the distance _radius_, whereas _minpts_ represents the minimum number of points of a valid cluster. Only points belonging to the largest cluster will survive as inliers.
- `--uniform-sample <int>`: specify the integer step for performing uniform down-sampling as follows:
- `1` means no down-sampling
- `> 1` enables down-sampling
- `--random-sample <double>`: specify the percentage in [0,1] for performing random down-sampling.
- `--inside-penalty <double>`: specify how much to penalize points that will lie inside the superquadric's isosurface wrt points lying outside (default = 1.0).
- `--disable-viewer`: specify not to launch the viewer.
- `--color "(<r> <g> <b>)"`: change the color of the superquadric by specifying RGB components as double in the range [0,1].
- `--opacity <double>`: specify the opacity of the superquadric as double in the range [0,1].
- `--background-color "(<r> <g> <b>)"`: change background color by specifying RGB components as double in the range [0,1].
### Real-time mode
If no `--file` option is passed through the command line, the module will open up a port called `/find-superquadric/points:rpc` to which the point cloud can be sent as a `yarp::sig::PointCloud<yarp::sig::DataXYZRGBA>` object.
Then, the module will reply with the superquadric parameters:
```
center-x center-y center-z angle size-x size-y size-z epsilon-1 epsilon-2
```
The `angle` around the z-axis is returned in degrees, whereas `center-*` and `size-*` are expressed in the same length units of the input point cloud.
### Example
```sh
$ find-superquadric --remove-outliers "(0.01 10)" --random-sample 0.2 --file ./data/cylinder
```
### Output

| 9d5844cf773a70a186caf2648837518202fae5f2 | [
"Markdown",
"CMake",
"C++"
] | 3 | CMake | zzz622848/find-superquadric | 230ccf44207021ea0512ffe94083e4bc21d910e6 | fb6554d8c4754da008ea6134ec46e316c5e8907f | |
refs/heads/master | <repo_name>Hoofoo-WHU/memo-server<file_sep>/src/server.ts
import AV = require('leanengine')
import app from '@/app'
AV.init({
appId: process.env.LEANCLOUD_APP_ID!,
appKey: process.env.LEANCLOUD_APP_KEY!,
masterKey: process.env.LEANCLOUD_APP_MASTER_KEY!
});
(AV.Cloud as any).useMasterKey()
const PORT = parseInt(process.env.LEANCLOUD_APP_PORT || process.env.PORT || '3000')
app.listen(PORT, () => {
console.log('Memo app is running on port:', PORT)
// 注册全局未捕获异常处理器
process.on('uncaughtException', (err) => {
console.error('Caught exception:', err.stack)
})
process.on('unhandledRejection', (reason, p) => {
console.error('Unhandled Rejection at: Promise ', p, ' reason: ', reason.stack)
})
})
<file_sep>/docs/README.md
# 开发文档
## 项目目录
```
config
├── nodemon.config.json
├── webpack.config.js
src
├── app.ts
├── routes
│ ├── login.ts
│ └── memo.ts
├── server.ts
├── tslint.json
tsconfig.json
```
## 技术要点
### 使用nodemon自动重载
nodemon可以像node一样使用还可以监听项目内文件更新自动重载,但是使用typescript的就不那么方便了,需要将ts-node注册并监听*.ts文件
```shell
nodemon --inspect -r ts-node/register -r tsconfig-paths/register --config config/nodemon.config.json src/server.ts
```
由于tsc的编译bug会导致paths配置的相对路径不会被编译,从而使node找不到模块,注册tsconfig-path即可解决
### 打包
使用tsc构建会使node找不到模块,而且tsconfig-path在node无效,也没有提供命令行工具,又不想在服务端使用ts-node,所以产品打包改用webpack,其resolve路径的功能可以解决模块丢失问题。
### lint
使用ts-lint来检查代码风格,配合vscode的ts-lint插件可以维持代码的可维护性
### 自动化流程
使用precommit-hook在commit前进行lint和build,使用GitHub-Hook使master更新后自动部署到leanengine<file_sep>/src/routes/login.ts
import Axios from 'axios'
import Router = require('koa-router')
import jwt = require('jsonwebtoken')
const router = new Router({ prefix: '/login' })
const GITHUB_CLIENT_ID = process.env.GITHUB_CLIENT_ID
const GITHUB_CLIENT_SECRET = process.env.GITHUB_CLIENT_SECRET
router.post('/github/:code', async (ctx) => {
if (ctx.state.user) {
ctx.body = {
avatar: ctx.state.user.avatar,
name: ctx.state.user.name,
token: jwt.sign(
{
avatar: ctx.state.user.avatar,
name: ctx.state.user.name,
id: ctx.state.user.id
},
process.env.LEANCLOUD_APP_KEY!
)
}
return
}
if (ctx.params.code === 'undefined') {
ctx.status = 401
return
}
const res = await Axios({
data: {
client_id: GITHUB_CLIENT_ID,
client_secret: GITHUB_CLIENT_SECRET,
code: ctx.params.code
},
headers: {
Accept: 'application/json'
},
method: 'post',
url: 'https://github.com/login/oauth/access_token'
})
if (res.data.error) {
ctx.status = 401
ctx.body = {
error: res.data.error,
error_description: res.data.error_description
}
return
} else {
const res2 = await Axios.get('https://api.github.com/user', {
headers: {
Authorization: `token ${res.data.access_token}`
}
})
ctx.body = {
avatar: res2.data.avatar_url,
name: res2.data.name,
token: jwt.sign(
{
avatar: res2.data.avatar_url,
name: res2.data.name,
id: res2.data.id
},
process.env.LEANCLOUD_APP_KEY!,
{ expiresIn: '2h' }
)
}
}
})
export default router
<file_sep>/src/routes/memo.ts
import Router = require('koa-router')
import AV = require('leanengine')
const router = new Router()
router.prefix('/memo')
const Memo = AV.Object.extend('Memo')
router.get('/', async (ctx) => {
const query = new AV.Query('Memo')
query.equalTo('id', ctx.state.user.id)
const data = await query.find()
ctx.body = data
})
router.get('/:id', async (ctx) => {
try {
const query = new AV.Query('Memo')
const data = await query.get(ctx.params.id)
if (data.get('id') === ctx.state.user.id) {
ctx.body = data
} else {
ctx.status = 403
}
} catch (e) {
ctx.status = 404
}
})
router.post('/', async (ctx) => {
const memo = new Memo()
memo.set('id', ctx.state.user.id)
const data = await memo.save()
ctx.body = data
})
router.patch('/:id', async (ctx) => {
try {
const query = new AV.Query('Memo')
const memo = await query.get(ctx.params.id)
if (memo.get('id') !== ctx.state.user.id) {
ctx.status = 403
return
}
Reflect.ownKeys((ctx.request as any).body).forEach((val) => {
memo.set(val as string, (ctx.request as any).body[val])
})
const data = await memo.save()
ctx.body = data
} catch (e) {
ctx.status = 400
}
})
router.delete('/:id', async (ctx) => {
try {
const query = new AV.Query('Memo')
const memo = await query.get(ctx.params.id)
if (memo.get('id') !== ctx.state.user.id) {
ctx.status = 403
return
}
const data = await memo.destroy()
ctx.body = data
} catch (e) {
ctx.status = 400
}
})
export default router
<file_sep>/README.md
# memo-server
一个基于node.js的在线便签服务端
## 客户端项目
[Memo](https://github.com/Hoofoo-WHU/memo)
## 本地运行
首先确认本机已经安装 Node.js 运行环境
安装依赖
```shell
yarn install
```
启动项目
```shell
yarn dev
```
构建项目
```shell
yarn build
```
代码检查
```shell
yarn lint
```
使用leancloud启动项目,确保环境变量设置正确
```shell
brew install lean-cli -g
lean login
lean switch
lean up
```
Debug
```shell
chrome://inspect
```
## 相关文档
[开发文档](docs)
[API文档](docs/api.md)<file_sep>/src/app.ts
import login from '@/routes/login'
import memo from '@/routes/memo'
import Koa = require('koa')
import bodyParser = require('koa-bodyparser')
import convert = require('koa-convert')
import logger = require('koa-logger')
import Router = require('koa-router')
import AV = require('leanengine')
import jwt = require('koa-jwt')
const app = new Koa()
app.keys = ['memo']
app.use(convert(AV.koa() as any))
app.use(logger())
app.use(bodyParser())
app.use(async (ctx, next) => {
ctx.set('Access-Control-Allow-Origin', ctx.header.origin as any)
ctx.set('Access-Control-Allow-Methods', 'PATCH,OPTIONS,POST,GET,DELETE')
ctx.set('Access-Control-Allow-Headers', 'Content-Type,Authorization')
ctx.set('Access-Control-Allow-Credentials', 'true')
await next()
})
app.use((ctx, next) => {
if (ctx.method && /options/i.test(ctx.method)) {
ctx.body = ''
return
}
return next()
})
app.use(jwt({ secret: process.env.LEANCLOUD_APP_KEY! }).unless({ path: [/\/api\/login/] }))
const api = new Router({ prefix: '/api' })
api.use(memo.routes())
api.use(login.routes())
app.use(api.routes())
export default app
<file_sep>/docs/api.md
# API文档
## 响应格式
所有的响应格式均为application/json
## 登录
### 请求
```
POST /api/login/github/:code
```
其中code为GitHub OAuth提供的code
### 响应
403:登录失败
```json
{
"error": "错误",
"error_description": "错误描述"
}
```
200:登陆成功
```json
{
"avatar": "GitHub头像Url",
"name": "GitHub用户名"
}
```
## 获取所有便签
### 请求
```
GET /api/memo/
```
### 响应
403: 未登录
200: OK
```json
[
{
"position": {
"x": 429,
"y": 51,
"z": -2147483597
},
"color": "pink",
"text": "这是第一个标签",
"objectId": "5c52be4a17b54d0057295f66",
"createdAt": "2019-01-31T09:22:18.633Z",
"updatedAt": "2019-01-31T15:13:10.819Z"
}
]
```
## 根据id获取便签
### 请求
```
GET /api/memo/:id
```
其中id为objectId
### 响应
403: 没有权限访问或未登录
200: OK
```json
{
"position": {
"x": 429,
"y": 51,
"z": -2147483597
},
"color": "pink",
"text": "这是第一个标签",
"objectId": "5c52be4a17b54d0057295f66",
"createdAt": "2019-01-31T09:22:18.633Z",
"updatedAt": "2019-01-31T15:13:10.819Z"
}
```
## 新建标签
### 请求
```
POST /api/memo
```
### 响应
403: 未登录,没有权限
200: OK
```json
{
"objectId": "5c52be4a17b54d0057295f66",
"createdAt": "2019-01-31T09:22:18.633Z",
"updatedAt": "2019-01-31T15:13:10.819Z"
}
```
## 更新标签
### 请求
```
PATCH /api/memo/:id
```
```json
{
"position": {
"x": 429,
"y": 51,
"z": -2147483597
},
"color": "pink",
"text": "这是第一个标签修改后"
}
```
id为ObjectId,请求体参数可以任意组合
### 响应
403:没有权限
400: 请求错误
200:更新成功
## 删除标签
### 请求
```
DELETE /api/memo/:id
```
id为ObjectId
### 响应
400: 请求错误
403: 未登录或没有权限
200: 删除成功<file_sep>/config/webpack.config.js
const path = require('path')
const webpackModeExternals = require('webpack-node-externals')
const CleanWebpackPlugin = require('clean-webpack-plugin')
module.exports = {
entry: path.resolve('src/server.ts'),
mode: 'production',
target: 'node',
output: {
path: path.resolve('dist'),
filename: 'server.js'
},
resolve: {
alias: {
'@': path.resolve('src')
},
extensions: ['.ts']
},
externals: [
webpackModeExternals()
],
module: {
rules: [
{
test: /\.ts$/,
use: [
'ts-loader'
]
}
]
},
plugins: [
new CleanWebpackPlugin(path.resolve('dist'), { root: path.resolve('') })
]
} | 4385f40f0ae84b9fd4a5a0c35df035bcc67618ea | [
"Markdown",
"TypeScript",
"JavaScript"
] | 8 | TypeScript | Hoofoo-WHU/memo-server | 2cd4541914993216f621754c04cdfcba7d11eb51 | 6180d30b74fbdc41339b34afaeaf1a40c77d3bf8 | |
refs/heads/master | <file_sep>package edu.ucsf.rbvi.stringApp.internal.ui;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Window;
import java.awt.event.ActionEvent;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.text.DecimalFormat;
import java.text.NumberFormat;
import java.text.ParsePosition;
import java.util.ArrayList;
import java.util.Dictionary;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import javax.swing.AbstractAction;
import javax.swing.BorderFactory;
import javax.swing.Box;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JComboBox;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSlider;
import javax.swing.JTable;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.ListSelectionModel;
import javax.swing.ScrollPaneConstants;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.border.EtchedBorder;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
import javax.swing.table.TableRowSorter;
import org.apache.log4j.Logger;
import org.cytoscape.application.CyUserLog;
import org.cytoscape.model.CyNetwork;
import org.cytoscape.work.FinishStatus;
import org.cytoscape.work.ObservableTask;
import org.cytoscape.work.TaskFactory;
import org.cytoscape.work.TaskIterator;
import org.cytoscape.work.TaskObserver;
import edu.ucsf.rbvi.stringApp.internal.model.Databases;
import edu.ucsf.rbvi.stringApp.internal.model.Species;
import edu.ucsf.rbvi.stringApp.internal.model.StringManager;
import edu.ucsf.rbvi.stringApp.internal.model.StringNetwork;
import edu.ucsf.rbvi.stringApp.internal.tasks.GetAnnotationsTask;
import edu.ucsf.rbvi.stringApp.internal.tasks.ImportNetworkTaskFactory;
import edu.ucsf.rbvi.stringApp.internal.utils.ModelUtils;
// TODO: [Optional] Improve non-gui mode
public class GetTermsPanel extends JPanel {
StringNetwork stringNetwork = null;
StringNetwork initialStringNetwork = null;
final StringManager manager;
private final Logger logger = Logger.getLogger(CyUserLog.NAME);
// Map<String, List<String>> resolvedIdMap = null;
// Map<String, List<Annotation>> annotations = null;
JTextArea searchTerms;
JPanel mainSearchPanel;
JComboBox<Species> speciesCombo;
JComboBox<String> speciesPartnerCombo;
JSlider confidenceSlider;
JTextField confidenceValue;
JSlider additionalNodesSlider;
JTextField additionalNodesValue;
JCheckBox wholeOrgBox;
JButton importButton;
JButton backButton;
NumberFormat formatter = new DecimalFormat("#0.00");
NumberFormat intFormatter = new DecimalFormat("#0");
private boolean ignore = false;
private String useDATABASE = Databases.STRING.getAPIName();
private String netSpecies = "Homo sapiens";
private boolean queryAddNodes = false;
private int confidence = 40;
private int additionalNodes = 0;
public GetTermsPanel(final StringManager manager, final String useDATABASE, boolean queryAddNodes) {
super(new GridBagLayout());
this.manager = manager;
this.useDATABASE = useDATABASE;
this.queryAddNodes = queryAddNodes;
init();
}
public GetTermsPanel(final StringManager manager, StringNetwork stringNetwork,
String useDATABASE, String aNetSpecies, boolean queryAddNodes) {
this(manager, stringNetwork, useDATABASE, aNetSpecies, queryAddNodes, 40, 0);
}
public GetTermsPanel(final StringManager manager, StringNetwork stringNetwork,
String useDATABASE, String aNetSpecies, boolean queryAddNodes,
int confidence, int additionalNodes) {
super(new GridBagLayout());
this.manager = manager;
this.stringNetwork = stringNetwork;
this.initialStringNetwork = stringNetwork;
this.confidence = confidence;
this.additionalNodes = additionalNodes;
this.useDATABASE = useDATABASE;
if (aNetSpecies != null) {
this.netSpecies = aNetSpecies;
}
this.queryAddNodes = queryAddNodes;
init();
}
private void init() {
// Create the surrounding panel
setPreferredSize(new Dimension(800,650));
EasyGBC c = new EasyGBC();
// Create the species panel
List<Species> speciesList = Species.getSpecies();
if (speciesList == null) {
try {
speciesList = Species.readSpecies(manager);
} catch (Exception e) {
manager.error("Unable to get species: "+e.getMessage());
e.printStackTrace();
return;
}
}
JPanel organismBox = createOrgBox();
if (!queryAddNodes) {
JPanel speciesBox = createSpeciesComboBox(speciesList);
add(speciesBox, c.expandHoriz().insets(0,5,0,5));
// Create whole organism checkbox
if (!useDATABASE.equals(Databases.STITCH.getAPIName())) {
add(organismBox, c.down().expandBoth().insets(0, 5, 0, 5));
}
} else {
JPanel speciesBox = createSpeciesPartnerComboBox(ModelUtils.getAvailableInteractionPartners(manager.getCurrentNetwork()));
add(speciesBox, c.expandHoriz().insets(0,5,0,5));
}
// Create the search list panel
mainSearchPanel = createSearchPanel();
add(mainSearchPanel, c.down().expandBoth().insets(5,5,0,5));
// Create the slider for the confidence cutoff
JPanel confidenceSlider = createConfidenceSlider();
add(confidenceSlider, c.down().expandBoth().insets(5,5,0,5));
// Create the slider for the confidence cutoff
JPanel additionalNodesSlider = createAdditionalNodesSlider();
add(additionalNodesSlider, c.down().expandBoth().insets(5,5,0,5));
// Create the evidence types buttons
// createEvidenceButtons(manager.getEvidenceTypes());
// Add Query/Cancel buttons
JPanel buttonPanel = createControlButtons();
add(buttonPanel, c.down().expandHoriz().insets(0,5,5,5));
}
JPanel createSearchPanel() {
JPanel searchPanel = new JPanel(new GridBagLayout());
searchPanel.setPreferredSize(new Dimension(600,400));
EasyGBC c = new EasyGBC();
String label = "Enter protein names or identifiers:";
if (useDATABASE.equals(Databases.STITCH.getAPIName()))
label = "Enter protein or compound names or identifiers:";
JLabel searchLabel = new JLabel(label);
c.noExpand().anchor("northwest").insets(0,5,0,5);
searchPanel.add(searchLabel, c);
searchTerms = new JTextArea();
JScrollPane jsp = new JScrollPane(searchTerms);
c.down().expandBoth().insets(5,10,5,10);
searchPanel.add(jsp, c);
return searchPanel;
}
void replaceSearchPanel() {
mainSearchPanel.removeAll();
mainSearchPanel.revalidate();
mainSearchPanel.repaint();
mainSearchPanel.setLayout(new GridBagLayout());
EasyGBC c = new EasyGBC();
String label = "Enter protein names or identifiers:";
if (useDATABASE.equals(Databases.STITCH.getAPIName()))
label = "Enter protein or compound names or identifiers:";
JLabel searchLabel = new JLabel(label);
c.noExpand().anchor("northwest").insets(0,5,0,5);
mainSearchPanel.add(searchLabel, c);
searchTerms = new JTextArea();
JScrollPane jsp = new JScrollPane(searchTerms);
c.down().expandBoth().insets(5,10,5,10);
mainSearchPanel.add(jsp, c);
mainSearchPanel.revalidate();
mainSearchPanel.repaint();
}
JPanel createSpeciesComboBox(List<Species> speciesList) {
JPanel speciesPanel = new JPanel(new GridBagLayout());
EasyGBC c = new EasyGBC();
JLabel speciesLabel = new JLabel("Species:");
c.noExpand().insets(0,5,0,5);
speciesPanel.add(speciesLabel, c);
speciesCombo = new JComboBox<Species>(speciesList.toArray(new Species[1]));
// Set Human as the default
for (Species s: speciesList) {
if (s.toString().equals(netSpecies)) {
speciesCombo.setSelectedItem(s);
break;
}
}
JComboBoxDecorator.decorate(speciesCombo, true, true);
c.right().expandHoriz().insets(0,5,0,5);
speciesPanel.add(speciesCombo, c);
return speciesPanel;
}
JPanel createSpeciesPartnerComboBox(List<String> speciesList) {
JPanel speciesPanel = new JPanel(new GridBagLayout());
EasyGBC c = new EasyGBC();
JLabel speciesLabel = new JLabel("Species:");
c.noExpand().insets(0,5,0,5);
speciesPanel.add(speciesLabel, c);
speciesPartnerCombo = new JComboBox<String>(speciesList.toArray(new String[1]));
// Set Human as the default
for (String s: speciesList) {
if (s.equals(netSpecies)) {
speciesPartnerCombo.setSelectedItem(s);
break;
}
}
c.right().expandHoriz().insets(0,5,0,5);
speciesPanel.add(speciesPartnerCombo, c);
return speciesPanel;
}
JPanel createOrgBox() {
JPanel boxPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
// orgBox = new JCheckBox("All proteins of this species");
wholeOrgBox = new JCheckBox(new AbstractAction("All proteins of this species") {
@Override
public void actionPerformed(ActionEvent e) {
if (wholeOrgBox.isSelected()) {
searchTerms.setText("");
searchTerms.setEditable(false);
additionalNodesSlider.setValue(0);
additionalNodesSlider.setEnabled(false);
additionalNodesValue.setText("0");
additionalNodesValue.setEnabled(false);
} else {
searchTerms.setEditable(true);
additionalNodesSlider.setEnabled(true);
additionalNodesValue.setEnabled(true);
}
}
});
wholeOrgBox.setSelected(false);
boxPanel.add(wholeOrgBox);
return boxPanel;
}
JPanel createControlButtons() {
JPanel buttonPanel = new JPanel();
BoxLayout layout = new BoxLayout(buttonPanel, BoxLayout.LINE_AXIS);
buttonPanel.setLayout(layout);
JButton cancelButton = new JButton(new AbstractAction("Cancel") {
@Override
public void actionPerformed(ActionEvent e) {
cancel();
}
});
backButton = new JButton(new AbstractAction("Back") {
@Override
public void actionPerformed(ActionEvent e) {
stringNetwork.reset();
replaceSearchPanel();
importButton.setEnabled(true);
backButton.setEnabled(false);
importButton.setAction(new InitialAction());
speciesCombo.setEnabled(true);
wholeOrgBox.setEnabled(true);
getParent().revalidate();
}
});
backButton.setEnabled(false);
importButton = new JButton(new InitialAction());
buttonPanel.add(Box.createRigidArea(new Dimension(10,0)));
buttonPanel.add(cancelButton);
buttonPanel.add(Box.createHorizontalGlue());
// buttonPanel.add(Box.createRigidArea(new Dimension(10,0)));
buttonPanel.add(backButton);
buttonPanel.add(Box.createRigidArea(new Dimension(10,0)));
buttonPanel.add(importButton);
return buttonPanel;
}
JPanel createConfidenceSlider() {
JPanel confidencePanel = new JPanel(new GridBagLayout());
confidencePanel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.LOWERED));
EasyGBC c = new EasyGBC();
Font labelFont;
{
c.anchor("west").noExpand().insets(0,5,0,5);
JLabel confidenceLabel = new JLabel("Confidence (score) cutoff:");
labelFont = confidenceLabel.getFont();
confidenceLabel.setFont(new Font(labelFont.getFontName(), Font.BOLD, labelFont.getSize()));
confidencePanel.add(confidenceLabel, c);
}
{
confidenceSlider = new JSlider();
Dictionary<Integer, JLabel> labels = new Hashtable<Integer, JLabel>();
Font valueFont = new Font(labelFont.getFontName(), Font.BOLD, labelFont.getSize()-4);
for (int value = 0; value <= 100; value += 10) {
double labelValue = (double)value/100.0;
JLabel label = new JLabel(formatter.format(labelValue));
label.setFont(valueFont);
labels.put(value, label);
}
confidenceSlider.setLabelTable(labels);
confidenceSlider.setPaintLabels(true);
confidenceSlider.setValue(confidence);
confidenceSlider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
if (ignore) return;
ignore = true;
int value = confidenceSlider.getValue();
confidenceValue.setText(formatter.format(((double)value)/100.0));
ignore = false;
}
});
// c.anchor("southwest").expandHoriz().insets(0,5,0,5);
c.right().expandHoriz().insets(0,5,0,5);
confidencePanel.add(confidenceSlider, c);
}
{
confidenceValue = new JTextField(4);
confidenceValue.setHorizontalAlignment(JTextField.RIGHT);
confidenceValue.setText(formatter.format(((double)confidence)/100.0));
c.right().noExpand().insets(0,5,0,5);
confidencePanel.add(confidenceValue, c);
confidenceValue.addActionListener(new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
textFieldValueChanged();
}
});
confidenceValue.addFocusListener(new FocusAdapter() {
@Override
public void focusLost(FocusEvent e) {
textFieldValueChanged();
}
});
}
return confidencePanel;
}
JPanel createAdditionalNodesSlider() {
JPanel additionalNodesPanel = new JPanel(new GridBagLayout());
additionalNodesPanel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.LOWERED));
EasyGBC c = new EasyGBC();
Font labelFont;
{
c.anchor("west").noExpand().insets(0,5,0,5);
JLabel additionalNodesLabel = new JLabel("Maximum additional interactors:");
labelFont = additionalNodesLabel.getFont();
additionalNodesLabel.setFont(new Font(labelFont.getFontName(), Font.BOLD, labelFont.getSize()));
additionalNodesPanel.add(additionalNodesLabel, c);
}
{
additionalNodesSlider = new JSlider();
Dictionary<Integer, JLabel> labels = new Hashtable<Integer, JLabel>();
Font valueFont = new Font(labelFont.getFontName(), Font.BOLD, labelFont.getSize()-4);
for (int value = 0; value <= 100; value += 10) {
JLabel label = new JLabel(Integer.toString(value));
label.setFont(valueFont);
labels.put(value, label);
}
additionalNodesSlider.setLabelTable(labels);
additionalNodesSlider.setPaintLabels(true);
additionalNodesSlider.setValue(additionalNodes);
additionalNodesSlider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
if (ignore) return;
ignore = true;
int value = additionalNodesSlider.getValue();
additionalNodesValue.setText(Integer.toString(value));
ignore = false;
}
});
// c.anchor("southwest").expandHoriz().insets(0,5,0,5);
c.right().expandHoriz().insets(0,5,0,5);
additionalNodesPanel.add(additionalNodesSlider, c);
}
{
additionalNodesValue = new JTextField(4);
additionalNodesValue.setHorizontalAlignment(JTextField.RIGHT);
additionalNodesValue.setText(""+additionalNodes);
c.right().noExpand().insets(0,5,0,5);
additionalNodesPanel.add(additionalNodesValue, c);
additionalNodesValue.addActionListener(new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
addNodesFieldValueChanged();
}
});
additionalNodesValue.addFocusListener(new FocusAdapter() {
@Override
public void focusLost(FocusEvent e) {
addNodesFieldValueChanged();
}
});
}
return additionalNodesPanel;
}
private void addNodesFieldValueChanged() {
if (ignore) return;
ignore = true;
String text = additionalNodesValue.getText();
Number n = intFormatter.parse(text, new ParsePosition(0));
int val = 0;
if (n == null) {
try {
val = Integer.valueOf(additionalNodesValue.getText());
} catch (NumberFormatException nfe) {
val = addNodesInputError();
}
} else if (n.intValue() > 100 || n.intValue() < 0) {
val = addNodesInputError();
} else {
val = n.intValue();
}
val = val;
additionalNodesSlider.setValue(val);
ignore = false;
}
private int addNodesInputError() {
additionalNodesValue.setBackground(Color.RED);
JOptionPane.showMessageDialog(null,
"Please enter a number of additional nodes between 0 and 100",
"Alert", JOptionPane.ERROR_MESSAGE);
additionalNodesValue.setBackground(UIManager.getColor("TextField.background"));
// Reset the value to correspond to the current slider setting
int val = additionalNodesSlider.getValue();
additionalNodesValue.setText(Integer.toString(val));
return val;
}
private void textFieldValueChanged() {
if (ignore) return;
ignore = true;
String text = confidenceValue.getText();
Number n = formatter.parse(text, new ParsePosition(0));
double val = 0.0;
if (n == null) {
try {
val = Double.valueOf(confidenceValue.getText());
} catch (NumberFormatException nfe) {
val = inputError();
}
} else if (n.doubleValue() > 1.0 || n.doubleValue() < 0.0) {
val = inputError();
} else {
val = n.doubleValue();
}
val = val*100.0;
confidenceSlider.setValue((int)val);
ignore = false;
}
private double inputError() {
confidenceValue.setBackground(Color.RED);
JOptionPane.showMessageDialog(null,
"Please enter a confence cutoff between 0.0 and 1.0",
"Alert", JOptionPane.ERROR_MESSAGE);
confidenceValue.setBackground(UIManager.getColor("TextField.background"));
// Reset the value to correspond to the current slider setting
double val = ((double)confidenceSlider.getValue())/100.0;
confidenceValue.setText(formatter.format(val));
return val;
}
void importNetwork(int taxon, int confidence, int additionalNodes, boolean wholeOrg) {
Map<String, String> queryTermMap = new HashMap<>();
List<String> stringIds = null;
if (wholeOrg) {
// stringIds = ModelUtils.readOrganimsIDs(queryTermMap);
stringIds = null;
// stringIds = stringNetwork.combineIds(queryTermMap);
} else {
stringIds = stringNetwork.combineIds(queryTermMap);
}
// System.out.println("Importing "+stringIds);
TaskFactory factory = null;
if (!queryAddNodes) {
factory = new ImportNetworkTaskFactory(stringNetwork, speciesCombo.getSelectedItem().toString(),
taxon, confidence, additionalNodes, stringIds,
queryTermMap, useDATABASE);
} else {
factory = new ImportNetworkTaskFactory(stringNetwork, (String)speciesPartnerCombo.getSelectedItem(),
taxon, confidence, additionalNodes, stringIds,
queryTermMap, useDATABASE);
}
cancel();
manager.execute(factory.createTaskIterator());
}
public void createResolutionPanel() {
speciesCombo.setEnabled(false);
wholeOrgBox.setEnabled(false);
mainSearchPanel.removeAll();
mainSearchPanel.revalidate();
mainSearchPanel.repaint();
final Map<String, ResolveTableModel> tableModelMap = new HashMap<>();
for (String term: stringNetwork.getAnnotations().keySet()) {
tableModelMap.put(term, new ResolveTableModel(this, term, stringNetwork.getAnnotations().get(term)));
}
mainSearchPanel.setLayout(new GridBagLayout());
EasyGBC c = new EasyGBC();
{
String label = "<html><b>Multiple possible matches for some terms:</b> ";
label += "Select the term in the left column to see the possibilities, then select the correct term from the table";
label += "</html>";
JLabel lbl = new JLabel(label);
c.anchor("northeast").expandHoriz();
mainSearchPanel.add(lbl, c);
}
{
JPanel annPanel = new JPanel(new GridBagLayout());
EasyGBC ac = new EasyGBC();
final JTable table = new JTable();
table.setRowSelectionAllowed(false);
final JPanel selectPanel = new JPanel(new FlowLayout());
final JButton selectAllButton = new JButton(new SelectEverythingAction(tableModelMap));
final JButton clearAllButton = new JButton(new ClearEverythingAction(tableModelMap));
final JButton selectAllTermButton = new JButton("Select All in Term");
final JButton clearAllTermButton = new JButton("Clear All in Term");
selectAllTermButton.setEnabled(false);
clearAllTermButton.setEnabled(false);
selectPanel.add(selectAllButton);
selectPanel.add(clearAllButton);
selectPanel.add(selectAllTermButton);
selectPanel.add(clearAllTermButton);
Object[] terms = stringNetwork.getAnnotations().keySet().toArray();
final JList termList = new JList(terms);
termList.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
termList.addListSelectionListener(new ListSelectionListener() {
public void valueChanged(ListSelectionEvent e) {
String term = (String)termList.getSelectedValue();
showTableRow(table, term, tableModelMap);
selectAllTermButton.setAction(new SelectAllTermAction(term, tableModelMap));
selectAllTermButton.setEnabled(true);
clearAllTermButton.setAction(new ClearAllTermAction(term, tableModelMap));
clearAllTermButton.setEnabled(true);
}
});
termList.setFixedCellWidth(95);
termList.setMinimumSize(new Dimension(100,100));
JScrollPane termScroller = new JScrollPane(termList);
termScroller.setHorizontalScrollBarPolicy(ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
termScroller.setPreferredSize(new Dimension(100, 350));
termScroller.setMinimumSize(new Dimension(100, 350));
ac.anchor("east").expandVert();
annPanel.add(termScroller, ac);
JScrollPane tableScroller = new JScrollPane(table);
ac.right().expandBoth().insets(0,5,0,5);
annPanel.add(tableScroller, ac);
c.down().expandBoth().insets(5,0,5,0);
mainSearchPanel.add(annPanel, c);
// Now, select the first term
termList.setSelectedIndex(0);
c.down().spanHoriz(2).expandHoriz().insets(0,5,0,5);
mainSearchPanel.add(selectPanel, c);
}
importButton.setAction(new ResolvedAction());
backButton.setEnabled(true);
revalidate();
if (stringNetwork.haveResolvedNames()) {
importButton.setEnabled(true);
} else
importButton.setEnabled(false);
}
public void addResolvedStringID(String term, String id) {
stringNetwork.addResolvedStringID(term, id);
if (stringNetwork.haveResolvedNames()) {
importButton.setEnabled(true);
} else
importButton.setEnabled(false);
}
public void removeResolvedStringID(String term, String id) {
stringNetwork.removeResolvedStringID(term, id);
if (stringNetwork.haveResolvedNames()) {
importButton.setEnabled(true);
} else
importButton.setEnabled(false);
}
private void showTableRow(JTable table, String term, Map<String, ResolveTableModel> tableModelMap) {
TableRowSorter sorter = new TableRowSorter(tableModelMap.get(term));
sorter.setSortable(0, false);
sorter.setSortable(1, true);
sorter.setSortable(2, false);
table.setModel(tableModelMap.get(term));
table.setRowSorter(sorter);
table.setAutoResizeMode( JTable.AUTO_RESIZE_OFF );
table.getColumnModel().getColumn(2).setCellRenderer(new TextAreaRenderer());
table.getColumnModel().getColumn(0).setPreferredWidth(50);
table.getColumnModel().getColumn(1).setPreferredWidth(75);
table.getColumnModel().getColumn(2).setPreferredWidth(525);
}
public void cancel() {
stringNetwork = initialStringNetwork;
if (stringNetwork != null) stringNetwork.reset();
replaceSearchPanel();
importButton.setEnabled(true);
backButton.setEnabled(false);
importButton.setAction(new InitialAction());
((Window)getRootPane().getParent()).dispose();
}
class InitialAction extends AbstractAction implements TaskObserver {
public InitialAction() {
super("Import");
}
@Override
public void actionPerformed(ActionEvent e) {
// Start our task cascade
String speciesName = "";
if (!queryAddNodes) {
speciesName = speciesCombo.getSelectedItem().toString();
} else {
speciesName = (String)speciesPartnerCombo.getSelectedItem();
}
int taxon = Species.getSpeciesTaxId(speciesName);
if (taxon == -1) {
// Oops -- unknown species
JOptionPane.showMessageDialog(null, "Unknown species: '"+speciesName+"'",
"Unknown species", JOptionPane.ERROR_MESSAGE);
return;
}
if (stringNetwork == null)
stringNetwork = new StringNetwork(manager);
String terms = searchTerms.getText();
if (wholeOrgBox != null && wholeOrgBox.isSelected()) {
importNetwork(taxon, confidenceSlider.getValue(), 0, wholeOrgBox.isSelected());
return;
}
if (terms == null || terms.length() == 0) {
JOptionPane.showMessageDialog(null, "No terms were entered -- nothing to search for",
"Nothing entered", JOptionPane.ERROR_MESSAGE);
return;
}
// Strip off any blank lines as well as trailing spaces
terms = terms.replaceAll("(?m)^\\s*", "");
terms = terms.replaceAll("(?m)\\s*$", "");
manager.info("Getting annotations for "+speciesName+"terms: "+terms);
// Launch a task to get the annotations.
manager.execute(new TaskIterator(new GetAnnotationsTask(stringNetwork, taxon, terms, useDATABASE)),this);
}
@Override
public void allFinished(FinishStatus finishStatus) {
}
@Override
public void taskFinished(ObservableTask task) {
if (!(task instanceof GetAnnotationsTask)) {
return;
}
GetAnnotationsTask annTask = (GetAnnotationsTask)task;
final int taxon = annTask.getTaxon();
if (stringNetwork.getAnnotations() == null || stringNetwork.getAnnotations().size() == 0) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JOptionPane.showMessageDialog(null, "Your query returned no results",
"No results", JOptionPane.ERROR_MESSAGE);
}
});
return;
}
boolean noAmbiguity = stringNetwork.resolveAnnotations();
if (noAmbiguity) {
int additionalNodes = additionalNodesSlider.getValue();
// This mimics the String web site behavior
if (stringNetwork.getResolvedTerms() == 1 && additionalNodes == 0) {
/*
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JOptionPane.showMessageDialog(null,
"This will return only one node (Hint: increase maximum interactors slider?)",
"Hint", JOptionPane.WARNING_MESSAGE);
}
});
*/
additionalNodes = 10;
logger.warn("STRING: Only one protein or compound was selected -- additional interactions set to 10");
}
// additionalNodes = 10;
final int addNodes = additionalNodes;
SwingUtilities.invokeLater(new Runnable() {
public void run() {
importNetwork(taxon, confidenceSlider.getValue(), addNodes, wholeOrgBox.isSelected());
}
});
} else {
createResolutionPanel();
}
}
}
class ResolvedAction extends AbstractAction {
public ResolvedAction() {
super("Import");
}
@Override
public void actionPerformed(ActionEvent e) {
Species species = (Species)speciesCombo.getSelectedItem();
int additionalNodes = additionalNodesSlider.getValue();
if (stringNetwork.getResolvedTerms() == 1 && additionalNodes == 0) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JOptionPane.showMessageDialog(null,
"This will return only one node (Hint: increase maximum interactors slider?)",
"Hint", JOptionPane.WARNING_MESSAGE);
}
});
}
// if (stringNetwork.getResolvedTerms() == 1)
// additionalNodes = 10;
if (wholeOrgBox.isSelected()) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
JOptionPane.showMessageDialog(null,
"This will return a network for the whole organims and might take a while!",
"Hint", JOptionPane.WARNING_MESSAGE);
}
});
}
int taxon = species.getTaxId();
importNetwork(taxon, confidenceSlider.getValue(), additionalNodes, wholeOrgBox.isSelected());
}
}
}
<file_sep>package edu.ucsf.rbvi.stringApp.internal.ui;
import java.util.List;
import javax.swing.table.AbstractTableModel;
import org.cytoscape.model.CyRow;
import org.cytoscape.model.CyTable;
import edu.ucsf.rbvi.stringApp.internal.model.EnrichmentTerm;
public class EnrichmentTableModel extends AbstractTableModel {
private String[] columnNames;
private CyTable cyTable;
private Long[] rowNames;
public EnrichmentTableModel(CyTable cyTable, String[] columnNames) {
this.columnNames = columnNames;
this.cyTable = cyTable;
initData();
}
public int getColumnCount() {
return columnNames.length;
}
public int getRowCount() {
return cyTable.getRowCount();
}
public String getColumnName(int col) {
return columnNames[col];
}
public Object getValueAt(int row, int col) {
final String colName = columnNames[col];
final Long rowName = rowNames[row];
// swingColumns = new String[] { colShownChart, colName, colDescription, colFDR,
// colGenesCount, colGenes, colGenesSUID };
if (colName.equals(EnrichmentTerm.colShowChart)) {
return cyTable.getRow(rowName).get(colName, Boolean.class);
} else if (colName.equals(EnrichmentTerm.colFDR)) {
return cyTable.getRow(rowName).get(colName, Double.class);
} else if (colName.equals(EnrichmentTerm.colGenesCount)) {
return cyTable.getRow(rowName).get(colName, Integer.class);
} else if (colName.equals(EnrichmentTerm.colGenes)) {
return cyTable.getRow(rowName).getList(colName, String.class);
} else if (colName.equals(EnrichmentTerm.colGenesSUID)) {
return cyTable.getRow(rowName).getList(colName, Long.class);
} else {
return cyTable.getRow(rowName).get(colName, String.class);
}
}
public Object getValueAt(int row, String colName) {
// final String colName = columnNames[col];
final Long rowName = rowNames[row];
// swingColumns = new String[] { colShownChart, colName, colDescription, colFDR,
// colGenesCount, colGenes, colGenesSUID };
if (colName.equals(EnrichmentTerm.colShowChart)) {
return cyTable.getRow(rowName).get(colName, Boolean.class);
} else if (colName.equals(EnrichmentTerm.colFDR)) {
return cyTable.getRow(rowName).get(colName, Double.class);
} else if (colName.equals(EnrichmentTerm.colGenesCount)) {
return cyTable.getRow(rowName).get(colName, Integer.class);
} else if (colName.equals(EnrichmentTerm.colGenes)) {
return cyTable.getRow(rowName).getList(colName, String.class);
} else if (colName.equals(EnrichmentTerm.colGenesSUID)) {
return cyTable.getRow(rowName).getList(colName, Long.class);
} else {
return cyTable.getRow(rowName).get(colName, String.class);
}
}
public Class<?> getColumnClass(int c) {
final String colName = columnNames[c];
// return cyTable.getColumn(colName).getClass();
if (colName.equals(EnrichmentTerm.colShowChart)) {
return Boolean.class;
} else if (colName.equals(EnrichmentTerm.colFDR)) {
return Double.class;
} else if (colName.equals(EnrichmentTerm.colGenesCount)) {
return Integer.class;
} else if (colName.equals(EnrichmentTerm.colGenes)) {
return List.class;
} else if (colName.equals(EnrichmentTerm.colGenesSUID)) {
return List.class;
} else {
return String.class;
}
}
public boolean isCellEditable(int row, int col) {
if (columnNames[col].equals(EnrichmentTerm.colShowChart)) {
return true;
} else {
return false;
}
}
public void setValueAt(Object value, int row, int col) {
final String colName = columnNames[col];
final Long rowName = rowNames[row];
if (cyTable.getColumn(colName) == null) {
cyTable.createColumn(EnrichmentTerm.colShowChart, Boolean.class, false);
}
cyTable.getRow(rowName).set(colName, value);
fireTableCellUpdated(row, col);
}
private void initData() {
List<CyRow> rows = cyTable.getAllRows();
// Object[][] data = new Object[rows.size()][EnrichmentTerm.swingColumns.length];
rowNames = new Long[rows.size()];
int i = 0;
for (CyRow row : rows) {
rowNames[i] = row.get(EnrichmentTerm.colID, Long.class);
i++;
}
}
} | 4a4a9d70bdb77dfcf57b94846bda6c81b5888e70 | [
"Java"
] | 2 | Java | mkutmon/stringApp | 2b07ca75bd69b4cbc746dce862bcd07b109331b6 | 714fcee42e50fa3e1bc721048802f9815fbafd23 | |
refs/heads/master | <repo_name>tomstejskal/AbraDAO<file_sep>/src/components/mainWindow.js
// @flow
import React from 'react';
import Model from '../core/model';
import Message from '../core/message';
import QueryEditor from './queryEditor';
import AppBar from 'material-ui/AppBar';
import MuiThemeProvider from 'material-ui/styles/MuiThemeProvider';
import type { MessageType, MapType } from '../core/types';
const menuClick = Message.new();
const queryMsg = Message.new();
type TModel = MapType;
const MainWindow = {
init(): TModel {
return Model.newMap({
query: QueryEditor.init()
});
},
update(model: TModel, msg: MessageType): TModel {
switch (msg.type()) {
case menuClick.type(): {
alert('button clicked!');
return model;
}
case queryMsg.type(): {
return model.set('query',
QueryEditor.update(model.get('query'), msg.data()));
}
default:
return model;
}
},
view(props: Object): Object {
const { model, dispatch } = props;
const QueryEditorView = QueryEditor.view;
return (
<MuiThemeProvider>
<div>
<AppBar title="Abra DAO" />
<div style={{ padding: "0.5em 2em" }}>
<div className="row">
<div className="col-xs-12">
<QueryEditorView
model={model.get('query')}
dispatch={msg => dispatch(queryMsg(msg))}
/>
</div>
</div>
</div>
</div>
</MuiThemeProvider>
);
}
};
export default MainWindow;
<file_sep>/src/index.js
// @flow
import App from './core/app';
import MainWindow from './components/mainWindow';
import './index.css';
import 'roboto-fontface/css/roboto/roboto-fontface.css';
import 'flexboxgrid/css/flexboxgrid.min.css';
import injectTapEventPlugin from 'react-tap-event-plugin';
// Needed for onTouchTap
// http://stackoverflow.com/a/34015469/988941
injectTapEventPlugin();
App.start(MainWindow);
<file_sep>/src/core/action.js
// @flow
import Message from './message';
import type { ComputationType, MessageConsType, ActionType, DispatchType } from './types';
const none_: ActionType = (dispatch: DispatchType) => dispatch(Message.none());
const Action = {
none(): ActionType {
return none_;
},
new(computation: ComputationType, successMsg: MessageConsType, failureMsg: MessageConsType): ActionType {
return (dispatch: DispatchType) => {
computation(
successData => {
dispatch(successMsg(successData))
},
failureData => {
dispatch(failureMsg(failureData))
}
);
}
}
};
export default Action;
<file_sep>/src/core/app.js
// @flow
import React from 'react';
import ReactDOM from 'react-dom';
import Action from './action';
import type { AppType } from './types';
const App = {
start(app: AppType): void {
let model = app.init();
const rootElem = document.getElementById('root');
function render(dispatch) {
const Main = app.view;
ReactDOM.render(
<Main model={model} dispatch={dispatch} />,
rootElem
);
}
function dispatch(msg) {
const result = app.update(model, msg);
let action = Action.none();
if (Array.isArray(result)) {
[model, action] = result;
} else {
model = result;
}
render(dispatch);
if (action !== Action.none()) {
action(dispatch);
}
}
render(dispatch);
}
};
export default App;
<file_sep>/src/core/types.js
// @flow
import type { Map, List } from 'immutable';
export type MapType = Map<any, any>;
export type ListType = List<any>;
export type ModelType = any;
export type MessageType = {
type: () => Symbol,
data: () => any
};
export type MessageConsType = (data: any) => MessageType;
export type DispatchType = (msg: MessageType) => void;
export type ActionType = (dispatch: DispatchType) => void;
export type ComputationType = (successData: any, failureData: any) => void;
export type AppType = {
init: () => ModelType,
update: (model: ModelType, msg: MessageType) => ModelType | [ModelType, ActionType],
view: (props: Object) => Object
};
| e92465f3a43db9a506917935a6f90b2d428f9429 | [
"JavaScript"
] | 5 | JavaScript | tomstejskal/AbraDAO | 98a7e55bdce470031b062d145b8ad9bbd5f4f58f | 69b27994fd3a95f961bce579034d028ea7e564e2 | |
refs/heads/master | <file_sep>import React, { useState, useEffect } from "react";
const SearchInput = (props) => {
const [searchParams, setSearchParams] = useState("");
const [suggestions, setSuggestions] = useState([]);
const [display, setDisplay] = useState(false)
const displayBtn = () => {
if (searchParams.length > 2)
setDisplay(!display)
}
useEffect(() => {
const fetchAutoComplete = async () => {
let fetchedSuggestions = [];
console.log("asd");
if (searchParams.length > 2) {
try {
const response = await fetch(
`https://dataservice.accuweather.com/locations/v1/cities/autocomplete?apikey=<KEY>&q=${searchParams}`
);
console.log(response)
const responseData = await response.json();
console.log(responseData)
fetchedSuggestions = responseData.map((el) => el.LocalizedName);
fetchedSuggestions = [...new Set(fetchedSuggestions)];
console.log(fetchedSuggestions);
setSuggestions(fetchedSuggestions);
displayBtn();
} catch (err) {
return (
<div>
<h2>Some Error Happend!</h2>
</div>
);
}
}
};
if (searchParams.length > 2) {
fetchAutoComplete();
} else {
return;
}
}, [searchParams]);
return (
<div>
<div className="homepage">
<div className="homepage-search-bar">
<div className="homepage-search-bar--input-div"></div>
<input placeholder="City Location" className="homepage-search-input" value={searchParams} onChange={(e) => setSearchParams(e.target.value)} />
<div className="homepage-search--icon">
<i onClick={() => props.fetchData(searchParams)} className="gg-search" ></i>
</div>
</div>
{ display && <ul className="AutoComlete" >
{suggestions.map((city) => (
<li onClick={() => displayBtn() + props.fetchData(searchParams) + setSearchParams(city)} key={city} style={{cursor:'pointer'}}> {city} </li>
))}
</ul>}
</div>
</div>
);
};
export default SearchInput;<file_sep>import React from "react";
import { NavLink } from "react-router-dom";
const MainHeader = () => {
const openMenu = () => {
document.querySelector(".sidebar").classList.add("open");
};
const closeMenu = () => {
document.querySelector(".sidebar").classList.remove("open");
};
return (
<div className="navigation-header">
<aside className="sidebar">
<h3 className='menu-header'>Menu</h3>
<button className="sidebar-close-button" onClick={closeMenu}>
x
</button>
<ul>
<li>
<NavLink className='list-side-menu' onClick={closeMenu} to="/" exact>
Home
</NavLink>
</li>
<li>
<NavLink className='list-side-menu' onClick={closeMenu} to="/favorites">
Favorites
</NavLink>
</li>
</ul>
</aside>
<button className="hamburger-btn" onClick={openMenu}>
☰
</button>
<h2 className='main-header'>Weather App</h2>
<div className="navigation-header-nav-links">
<ul className="nav-links">
<li>
<NavLink to="/" exact>
Home
</NavLink>
</li>
<li>
<NavLink to="/favorites">Favorites</NavLink>
</li>
</ul>
</div>
</div>
);
};
export default MainHeader;
<file_sep>import React from "react";
import DailyBox from "../components/DailyBox";
import { useHistory } from "react-router-dom";
const Favorites = (props) => {
const history = useHistory();
const getDayFromDate = (date) => {
const localeDateString = date.slice(0, 10).replace(/-/g, "/");
return localeDateString;
};
const redirectToFavoriteLocation = (weatherObj, cityObj) => {
props.setHomeScreen(weatherObj, cityObj);
history.push("/");
};
return (
<div>
<h2>Favorites</h2>
<div>
<ul className="favorites-list">
{props.favorites.map((el, i) => (
<DailyBox
onClick={() => redirectToFavoriteLocation(el.daysData, el.city)}
key={el.Date + i}
city={el.city.LocalizedName}
date={getDayFromDate(el.Date)}
temp={el.Temperature.Maximum.Value}
weather={el.Day.IconPhrase}
/>
))}
</ul>
</div>
</div>
);
};
export default Favorites;<file_sep>import React from "react";
import LocationBoxData from "../components/LocationBoxData";
import SearchInput from "../components/SearchInput";
const HomePage = (props) => {
return (
<React.Fragment>
<SearchInput fetchData={props.fetchData} />
<LocationBoxData city={props.city} daysData={props.daysData} addToFavorites={props.addToFavorites} removeFromFavorites={props.removeFromFavorites}
favorites={props.favorites} />
</React.Fragment>
);
};
export default HomePage;
<file_sep>import React from "react";
const DailyBox = (props) => {
return (
<li onClick={props.onClick} >
{props.city ? <p>{props.city}</p> : null}
{props.weather === "Sunny"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/01-s.png" /> : null}
{props.weather === "Mostly sunny"? <img alt="suny" src="https://developer.accuweather.com/sites/default/files/02-s.png" /> : null}
{props.weather === "Partly sunny"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/03-s.png" /> : null}
{props.weather === "Intermittent clouds"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/04-s.png" /> : null}
{props.weather === "Hazy Sunshine"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/05-s.png" /> : null}
{props.weather === "Mostly cloudy"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/06-s.png" /> : null}
{props.weather === "Cloudy"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/07-s.png" /> : null}
{props.weather === "Dreary (Overcast)"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/08-s.png" /> : null}
{props.weather === "Fog"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/11-s.png" /> : null}
{props.weather === "Showers"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/12-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Showers"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/13-s.png" /> : null}
{props.weather === "Partly Sunny w/ Showers"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/14-s.png" /> : null}
{props.weather === "T-Storms"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/15-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ T-Storms"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/16-s.png" /> : null}
{props.weather === "Partly Sunny w/ T-Storms"? <img alt="sun" src="hhttps://developer.accuweather.com/sites/default/files/17-s.png" /> : null}
{props.weather === "Flurries"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/19-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Flurries"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/20-s.png" /> : null}
{props.weather === "Partly Sunny w/ Flurries"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/21-s.png" /> : null}
{props.weather === "Snow"? <img alt="suny" src="https://developer.accuweather.com/sites/default/files/22-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Snow"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/23-s.png" /> : null}
{props.weather === "Ice"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/24-s.png" /> : null}
{props.weather === "Sleet"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/25-s.png" /> : null}
{props.weather === "Freezing Rain"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/26-s.png" /> : null}
{props.weather === "Rain and Snow"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/29-s.png" /> : null}
{props.weather === "Hot"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/30-s.png" /> : null}
{props.weather === "Cold"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/31-s.png" /> : null}
{props.weather === "Windy"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/32-s.png" /> : null}
{props.weather === "Clear"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/33-s.png" /> : null}
{props.weather === "Mostly Clear"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/34-s.png" /> : null}
{props.weather === "Partly Cloudy"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/35-s.png" /> : null}
{props.weather === "Intermittent Clouds"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/36-s.png" /> : null}
{props.weather === "Hazy Moonlight"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/37-s.png" /> : null}
{props.weather === "Partly Cloudy w/ Showers"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/39-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Showers"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/40-s.png" /> : null}
{props.weather === "Partly Cloudy w/ T-Storms"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/41-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ T-Storms"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/42-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Flurries"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/43-s.png" /> : null}
{props.weather === "Mostly Cloudy w/ Snow"? <img alt="sun" src="https://developer.accuweather.com/sites/default/files/44-s.png" /> : null}
<p>{props.date}</p>
<b>
<p>{props.temp}* F</p>
</b>
<p > {props.weather} </p>
</li>
);
};
export default DailyBox;
<file_sep>import React, { useState, useEffect } from "react";
import "./App.css";
import MainHeader from "./components/MainHeader";
import { BrowserRouter, Switch, Route, Redirect } from "react-router-dom";
import HomePage from "./pages/HomePage";
import Favorites from "./pages/Favorites";
function App() {
const [city, setCity] = useState({});
const [daysData, setDaysData] = useState({});
const [favorites, setFavorites] = useState([]);
const [isLoading, setIsLoading] = useState(true);
const [error, setError] = useState(false);
const setHomeScreen = (favoriteWeatherObj, favoriteCityObj) => {
setCity(favoriteCityObj);
setDaysData(favoriteWeatherObj);
};
const fetchData = async (searchParams = "tel aviv") => {
setIsLoading(true);
try {
const response = await fetch(
`http://dataservice.accuweather.com/locations/v1/cities/autocomplete?apikey=<KEY>&q=${searchParams}`
);
const responseData = await response.json();
setCity(responseData[0]);
const weatherResponse = await fetch(
`http://dataservice.accuweather.com/forecasts/v1/daily/5day/${responseData[0].Key}?apikey=<KEY>`
);
const weatherResponseData = await weatherResponse.json();
setDaysData(weatherResponseData);
} catch (err) {
setError(err.message);
}
setIsLoading(false);
};
const addToFavorites = (dailyWeather, city) => {
setFavorites([...favorites, { ...dailyWeather, city, daysData }]);
};
const removeFromFavorites = (key) => {
const newFavorites = favorites.filter((el) => el.city.Key !== key);
setFavorites(newFavorites);
};
useEffect(() => {
fetchData();
}, []);
if (isLoading) return <div>Loading...</div>;
if (error) return <div>{error}</div>;
return (
<BrowserRouter>
<MainHeader />
<main>
<Switch>
<Route path="/" exact component={() => ( <HomePage city={city} daysData={daysData} addToFavorites={addToFavorites} removeFromFavorites={removeFromFavorites}
favorites={favorites} fetchData={fetchData} /> )} />
<Route path="/favorites" exact component={() => ( <Favorites setHomeScreen={setHomeScreen} favorites={favorites} /> )} />
<Redirect to="/" />
</Switch>
</main>
</BrowserRouter>
);
}
export default App;<file_sep>self.__precacheManifest = (self.__precacheManifest || []).concat([
{
"revision": "9ee83975ba7d3a152b0ba98f4de00e62",
"url": "/weather/index.html"
},
{
"revision": "9ac<KEY> <PASSWORD>",
"url": "/weather/static/css/main.4d16ea8a.chunk.css"
},
{
"revision": "ce99c7804a10e5cd6f9e",
"url": "/weather/static/js/2.f5727f7a.chunk.js"
},
{
"revision": "c64c486544348f10a6d6c716950bc223",
"url": "/weather/static/js/2.f5727f7a.chunk.js.LICENSE.txt"
},
{
"revision": "9<KEY>",
"url": "/weather/static/js/main.28ee2fc1.chunk.js"
},
{
"revision": "9eb98cf7fbd675a7fd34",
"url": "/weather/static/js/runtime-main.c0a41632.js"
}
]); | 3bb0ead8eb4529b9a8866d24f36baf1791cd3d96 | [
"JavaScript"
] | 7 | JavaScript | ohadleib/weather | 4315bed03624089b4fcd4125789f9a14c32fe1cd | f0bd230e7572dcad11de28ddfef18468d6f9731b | |
refs/heads/master | <file_sep># ReadExcelSheetDataCompare
Reading Excel sheet data from particular col and comparing with another excel sheet >> according to first col checking the value is present in another file
# Package required
1. xlrd
>> Fulture work - Data updation into particular col,row
<file_sep>import os
import xlrd
# read from resources folder
package_dir = os.path.dirname(os.path.abspath(__file__))
firstFile = os.path.join(package_dir+'/resources', 'sample.xlsx')
secondFile = os.path.join(package_dir+'/resources', 'sample1.xls')
# first file
workbook = xlrd.open_workbook(firstFile, "rb")
sheet = workbook.sheet_by_index(0)
# second file
workbook1 = xlrd.open_workbook(secondFile, "rb")
sheet1 = workbook1.sheet_by_index(0)
# print("Total no of columns: ", sheet.nrows)
# print("Total no of rows: ", sheet.ncols)
# find row index
def find_row_index(searchValue):
for i in range(1, sheet1.nrows):
if sheet1.cell_value(i, 0) == searchValue:
row_index = i
break
return row_index
for i in range(1, sheet.nrows):
name = sheet.cell_value(i, 0)
age = sheet.cell_value(i, 4)
row_index_from_second = find_row_index(name)
age1 = sheet1.cell_value(row_index_from_second, 4)
if age == age1:
print("True")
else:
print("False")
| 7559252dcc06c24d0f9cb1367cc9698e3d5ee57c | [
"Markdown",
"Python"
] | 2 | Markdown | prabhatv96/ReadExcelSheetDataCompare | 84416eeb998d4ff535952ae0711eb879c7f032fa | 6ffba3b512d202fe05a9a0a026d2f8466e2dcac6 | |
refs/heads/master | <file_sep>import { gql } from "apollo-server-express";
import { makeExecutableSchema } from "graphql-tools";
import Hello from "graphql/Hello";
import { merge } from "lodash";
const Query = gql`
type Query {
_empty: String
}
`;
const Mutation = gql`
type Mutation {
_empty: String
}
`;
const getTypes = () =>
Object.values({
Query,
Mutation,
...Hello.getTypes(),
});
const getResolvers = () => merge(Hello.getResolvers());
const schema = makeExecutableSchema({
typeDefs: getTypes(),
resolvers: getResolvers(),
});
export default schema;
<file_sep># GraphQL Easy Starter
## By EasyCoders
[EasyCoders.io](https://easycoders.io)<file_sep>import getResolvers from "./resolvers";
import getTypes from "./type";
export default { getTypes, getResolvers };
<file_sep>import { gql } from "apollo-server-express";
const Hello = gql`
extend type Query {
hello: Hello
}
type Hello {
message: String
}
`;
const getTypes = () => ({ Hello });
export default getTypes;
<file_sep>import { IResolvers } from "apollo-server-express";
const resolvers = {
Query: {
hello: () => ({
message: () => "Hello World!",
}),
},
};
const getResolvers = () =>
({
...resolvers,
} as IResolvers);
export default getResolvers;
| be516e69414b5dcecc4f18b70210e7dfee4660fe | [
"Markdown",
"TypeScript"
] | 5 | TypeScript | easycodersio/GraphQLStarter | 92bb6d602842a9a2e034ba2b76c8af840a7e3a4f | 68a3bfd5ecae12ab1d30732d3985a9162980ca13 | |
refs/heads/master | <file_sep>using System;
namespace Moving
{
class Program
{
static void Main(string[] args)
{
int freeWidth = int.Parse(Console.ReadLine());
int freeLength = int.Parse(Console.ReadLine());
int freeHeight = int.Parse(Console.ReadLine());
int size = freeHeight * freeLength * freeWidth;
int sum = 0;
bool overSize = false;
string command = Console.ReadLine();
while(command != "Done")
{
int neededSpace = int.Parse(command);
sum += neededSpace;
if(sum > size)
{
overSize = true;
break;
}
command = Console.ReadLine();
}
if (overSize)
{
Console.WriteLine($"No more free space! You need {sum - size} Cubic meters more.");
}
else
{
Console.WriteLine($"{size - sum } Cubic meters left.");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _02._Common_Elements
{
class Program
{
static void Main(string[] args)
{
// string input = Console.ReadLine();
////1
//Console.WriteLine(string.Join(" ", arr1));
/*foreach (var item in arr1)
{
Console.Write(item);
}
//Console.WriteLine(arr1[2]);
*/
string[] arr1 = Console.ReadLine().Split();
string[] arr2 = Console.ReadLine().Split();
for (int i = 0; i < arr2.Length; i++)
{
for (int j = 0; j < arr1.Length; j++)
{
if(arr2[i] == arr1[j])
{
Console.Write($"{arr2[i]} ");
}
}
}
}
}
}
<file_sep>using System;
namespace _01._SoftUni_Reception
{
class Program
{
static void Main(string[] args)
{
int studentsPerHour1 = int.Parse(Console.ReadLine());
int studentsPerHour2 = int.Parse(Console.ReadLine());
int studentsPerHour3 = int.Parse(Console.ReadLine());
int studentsCount = int.Parse(Console.ReadLine());
int allStudentsPerHour = studentsPerHour1 + studentsPerHour2 + studentsPerHour3;
int timeNeeded = 0;
while(studentsCount > 0)
{
timeNeeded += 1;
if (timeNeeded % 4 == 0)
{
timeNeeded += 1;
}
if (allStudentsPerHour >= studentsCount)
{
break;
}
else
{
studentsCount -= allStudentsPerHour;
}
}
Console.WriteLine($"Time needed: {timeNeeded}h.");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P01.BasicStackOperations
{
class Program
{
static void Main(string[] args)
{
int[] cmdArgs = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
int N = cmdArgs[0];
int S = cmdArgs[1];
int X = cmdArgs[2];
int[] numbers = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
Stack<int> stack = new Stack<int>(numbers);
//for(int i = 0; i < N; i++)
//{
// stack.Push(numbers[i]);
//}
for (int i = 0; i < S; i++)
{
stack.Pop();
}
if (!stack.Any())
{
Console.WriteLine(0);
}
else if(stack.Any(n => n == X))
{
Console.WriteLine("true");
}
else
{
Console.WriteLine(stack.Min());
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Inbox_Manager
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<string>> users = new Dictionary<string, List<string>>();
while (true)
{
string input = Console.ReadLine();
if(input == "Statistics")
{
break;
}
string[] command = input
.Split("->");
string user = command[1];
switch (command[0])
{
case "Add":
if (!users.ContainsKey(user))
{
users[user] = new List<string>();
}
else
{
Console.WriteLine($"{user} is already registered");
}
break;
case "Send":
string email = command[2];
if (users.ContainsKey(user))
{
users[user].Add(email);
}
break;
case "Delete":
if (users.ContainsKey(user))
{
users.Remove(user);
}
else
{
Console.WriteLine($"{user} not found!");
}
break;
}
}
users = users
.OrderByDescending(kvp => kvp.Value.Count)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
Console.WriteLine($"Users count: {users.Count}");
foreach (var kvp in users)
{
Console.WriteLine(kvp.Key);
foreach (string email in kvp.Value)
{
Console.WriteLine($"- {email}");
}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _07._Predicate_For_Names
{
class Program
{
static void Main(string[] args)
{
int length = int.Parse(Console.ReadLine());
string[] names = Console.ReadLine()
.Split();
Predicate<string> filterForNames = new Predicate<string>((name)
=> name.Length <= length);
Action<string[]> FiltersNames = new Action<string[]>((names) =>
{
foreach (string name in names)
{
if (filterForNames(name))
{
Console.WriteLine(name);
}
}
//return names;
});
FiltersNames(names);
}
}
}
<file_sep>using System;
using System.Linq;
using System.Text.RegularExpressions;
namespace _03._SoftUni_Bar_Income
{
class Program
{
static void Main(string[] args)
{
double income = 0;
//string pattern = @"%(?<name>[A-z][a-z]+)%[^|$%.]*<(?<productName>[\w]+)>[^|$%.]*\|(?<quantity>\d+)\|[^|$%.]*(?<price>\d+(\.\d+)?)\$";
// string pattern = @"^%(?<name>[A-Z][a-z]+)%[^|$%.]*<(?<productName>\w+)>[^|$%.]*\|(?<quantity>\d+)\|[^|$%.]*?(?<price>[-+]?[0-9]*\.?[0-9]+([eE][-+]?[0-9]+)?)\$";
string pattern = @"%(?<name>[A-z][a-z]+)%[^|$%.]*<(?<productName>\w+)>[^|$%.]*\|(?<quantity>\d+)\|[^|$%.]*?(?<price>\d+\.?\d*)\$";
//string pattern = @"^%(?<customer>[A-Z][a-z]+)%[^|$%.]*<(?<product>\w+)>[^|$%.]*\|(?<count>\d+)\|[^|$%.]*?(?<price>[-+]?[0-9]*\.?[0-9]+([eE][-+]?[0-9]+)?)\$";
while (true)
{
string input = Console.ReadLine();
if(input == "end of shift")
{
break;
}
if (Regex.IsMatch(input, pattern))
{
var match = Regex.Match(input, pattern);
string name = match.Groups["name"].Value;
string product = match.Groups["productName"].Value;
double totalPrice = int.Parse(match.Groups["quantity"].Value)
* double.Parse(match.Groups["price"].Value);
Console.WriteLine($"{name}: {product} - {totalPrice:F2}");
income += totalPrice;
}
}
Console.WriteLine($"Total income: {income:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace Polymorphism
{
class Program
{
static void Main(string[] args)
{
//Vehicle vehicle = new Airplane();
Vehicle airplane = new Airplane();
Vehicle truck = new Truck();
//PrintVehicleModel(airplane);
//PrintVehicleModel(truck);
var vehicles = new List<IVehicle> { airplane, truck, new Ship()};
PrintVrumVrum(airplane);
PrintVrumVrum(truck);
PrintVrumVrum(new AirFighter());
PrintVrumVrum(new CatOnJet());
var anotherAirplane = new Airplane();
Console.WriteLine(anotherAirplane.VrumVrum(10));
}
public static void PrintVrumVrum(Vehicle vehicle)
{
//if(vehicle is Airplane airplane)////Bad way of doing
//{
// Console.WriteLine(airplane.VrumVrum());
//}
//else if(vehicle is CatOnJet catOnJet)
//{
// Console.WriteLine(catOnJet.VrumVrum());
//}
Console.WriteLine(vehicle.VrumVrum());
}
public static void PrintVehicleModel(Vehicle vehicle)
{
Console.WriteLine(vehicle.Model);
}
}
}
<file_sep>using System;
namespace LeftAndRightSum
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int leftsum = 0;
int rightsum = 0;
for(int i=0;i<n*2;i++)
{
int num = int.Parse(Console.ReadLine());
if(i<n)
{
leftsum += num;
}
else if(i<n*2)
{
rightsum += num;
}
}
if(rightsum == leftsum)
{
Console.WriteLine($"Yes, sum = {rightsum}");
}
else
{
Console.WriteLine($"No, diff = {Math.Abs(rightsum-leftsum)}");
}
}
}
}
<file_sep>using P04.PizzaCalories.Ingredients;
using System;
namespace P04.PizzaCalories
{
public class StartUp
{
static void Main(string[] args)
{
try
{
string[] inputName = Console.ReadLine().Split();
string pizzaName = inputName[1];
string[] inputDough = Console.ReadLine().Split();
string flourType = inputDough[1];
string bakingTech = inputDough[2];
double weight = double.Parse(inputDough[3]);
Dough dough = new Dough(flourType, bakingTech, weight);
////Creating pizza
Pizza pizza = new Pizza(pizzaName, dough);
string command;
while((command=Console.ReadLine()) != "END")
{
string[] commandArgs = command
.Split();
string toppingType = commandArgs[1];
double weight1 = double.Parse(commandArgs[2]);
Topping topping = new Topping(toppingType, weight1);
pizza.AddTopping(topping);
// Console.WriteLine($"{topping.ToppingCalories():F2}");
}
Console.WriteLine($"{pizza.Name} - {pizza.TotalCalories:F2} Calories.");
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
}
}
}
<file_sep>using System;
namespace Vacation
{
class Program
{
static void Main(string[] args)
{
int peopleCount = int.Parse(Console.ReadLine());
string typeGroup = Console.ReadLine();
string day = Console.ReadLine();
double price = 0;
if(typeGroup == "Students")
{
switch (day)
{
case "Friday":
price = peopleCount * 8.45;
break;
case "Saturday":
price = peopleCount * 9.80;
break;
case "Sunday":
price = peopleCount * 10.46;
break;
}
if(peopleCount >= 30)
{
price -= price * 0.15;
}
}
else if(typeGroup == "Business")
{
if(peopleCount >= 100)
{
peopleCount -= 10;
}
switch (day)
{
case "Friday":
price = peopleCount * 10.90;
break;
case "Saturday":
price = peopleCount * 15.60;
break;
case "Sunday":
price = peopleCount * 16;
break;
}
}
else
{
switch (day)
{
case "Friday":
price = peopleCount * 15;
break;
case "Saturday":
price = peopleCount * 20;
break;
case "Sunday":
price = peopleCount * 22.50;
break;
}
if(peopleCount >= 10 && peopleCount <= 20)
{
price -= price * 0.05;
}
}
Console.WriteLine($"Total price: {price:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P06.SpeedRacing
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
HashSet<Car> cars = new HashSet<Car>();
for (int i = 0; i < n; i++)
{
string[] carArgs = Console.ReadLine()
.Split();
string model = carArgs[0];
double fuelAmount = double.Parse(carArgs[1]);
double fuelConsPerKm = double.Parse(carArgs[2]);
Car car = new Car(model, fuelAmount, fuelConsPerKm);
/////TO CHECK IF THERE IS A CAR WITH THE SAME MODEL
cars.Add(car);
}
string command;
while((command = Console.ReadLine()) != "End")
{
string[] driveArgs = command
.Split();
string carModel = driveArgs[1];
int amountOfKm = int.Parse(driveArgs[2]);
var searchedCar = cars.First(c => c.Model == carModel);
searchedCar.Drive(amountOfKm);
}
foreach (var car in cars)
{
Console.WriteLine($"{car.Model} {car.FuelAmount:F2} {car.TravelledDistance}");
}
}
}
}
<file_sep>using System;
namespace Methods
{
class Program
{
/// overloading func - 2 or more functions with the same name doing the same thing with different types of parameters
///recoursing - to call the func in the same func
static void Main(string[] args)
{
//int a = 4; int b = 7;
int sum = SumNumbers(5,6);
Console.WriteLine(sum);
}
static int SumNumbers(int num1 , int num2)
{
//Console.WriteLine("Hello!");
return num1 + num2;
}
static double SumNumbers(double num1,double num2)
{
return num1 + num2;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _09.Pokemon_Don_t_Go
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
List<int> removedList = new List<int>();
while (list.Count > 0)
{
int index = int.Parse(Console.ReadLine());
if (index < 0)
{
removedList.Add(list[0]);
int removed = list[0];
// index = 0;
list.RemoveAt(0);
list.Insert(0, list[list.Count - 1]);
for (int i = 0; i < list.Count; i++)
{
//if (i == index)
//{
// continue;
//}
if (list[i] <= removed)
{
list[i] += removed;
}
else if (list[i] > removed)
{
list[i] -= removed;
}
}
continue;
//
}
else if(index > list.Count - 1)
{
removedList.Add(list[list.Count - 1]);
int removed = list[list.Count - 1];
// index =
list.RemoveAt(list.Count - 1);
list.Add(list[0]);
for (int i = 0; i < list.Count; i++)
{
//if (i == index)
//{
// continue;
//}
if (list[i] <= removed)
{
list[i] += removed;
}
else if (list[i] > removed)
{
list[i] -= removed;
}
}
continue;
//
}
else{
for (int i = 0; i < list.Count; i++)
{
if (i == index)
{
continue;
}
if (list[i] <= list[index])
{
list[i] += list[index];
}
else if (list[i] > list[index])
{
list[i] -= list[index];
}
}
removedList.Add(list[index]);
list.RemoveAt(index);
}
}
Console.WriteLine(removedList.Sum());
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P05.BirthdayCelebrations
{
interface IIdentifiable
{
string GetId();
}
}
<file_sep>using System;
using System.Linq;
namespace MultiArraysTry
{
class Program
{
static void Main(string[] args)
{
int[,] matrix = { {1,2,3,4},{5,6,7,8} };
/////Accesing elements of the array
// Console.WriteLine(matrix[1, 2]);
///array.GetLenght(dimension)
/////Printing the multidimensional arrays
for (int row = 0; row < matrix.GetLength(0); row++)
{
for (int col = 0; col < matrix.GetLength(1); col++)
{
Console.Write($"{matrix[row, col]} ");
}
}
Console.WriteLine("");
Console.WriteLine("SECOND METHOD FOR PRINTING THIS TYPE OF ARRAY");
foreach(int el in matrix)
{
Console.Write($"{el} ");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _06._Reverse_And_Exclude
{
class Program
{
static void Main(string[] args)
{
List<int> numbers = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToList();
int n = int.Parse(Console.ReadLine());
Predicate<int> isDivisable = new Predicate<int>((num) => num % n == 0);
var ReverseAndRemovesDivisableNums = new Func<List<int>, List<int>>((list) =>
{
list.Reverse();
for (int i = 0; i < list.Count; i++)
{
if (isDivisable(list[i]))
{
list.Remove(list[i]);
i--;
}
}
return list;
});
numbers = ReverseAndRemovesDivisableNums(numbers);
Console.WriteLine(string.Join(" ",numbers));
}
}
}
<file_sep>using System;
namespace Yardgreening
{
class Program
{
static void Main(string[] args)
{
double kvMetri = double.Parse(Console.ReadLine());
double priceWithoutdiscount = kvMetri * 7.61;
double discount = priceWithoutdiscount * 0.18;
double res = priceWithoutdiscount - discount;
Console.WriteLine($"The final price is: {res} lv.");
Console.WriteLine($"The discount is: {discount} lv.");
}
}
}
<file_sep>using System;
using System.Linq;
namespace JaggedArray
{
class Program
{
static void Main(string[] args)
{
//int[][] array = new int[4][];
//array[0] = new int[3];
//array[1] = new int[2];
//array[2] = new int[4];
//array[3] = new int[1];
/////FILLING JAGGED ARRAY
///
/*
1 2 3 4
5 6
7 8 9 10 11
*/
int rows = int.Parse(Console.ReadLine());
int[][] array = new int[rows][];
for (int row = 0; row < rows; row++)
{
int[] currentRow = Console.ReadLine().Split(' ').Select(int.Parse).ToArray();
array[row] = currentRow;
}
for (int row = 0; row < array.Length; row++)
{
int[] currentRow = array[row];
for (int col = 0; col < currentRow.Length; col++)
{
Console.Write(array[row][col] + " ");
}
Console.WriteLine();
}
}
}
}
<file_sep>using System;
namespace Class_Abstraction
{
///abstract///keyword for methods and classes //abstract class - restricted class(to use it must be in inherited from
//another class) // abstract method - can be used only in abstract class and dont have a body ;the body is provided
// by the derived class
abstract class Animal
{
public abstract void animalSound();
public void sleep()
{
Console.WriteLine("zzzz");
}
}
class Pig : Animal
{
public override void animalSound()
{
Console.WriteLine("The pig says wee wee");
}
}
class Program
{
static void Main(string[] args)
{
Pig myPig = new Pig();
myPig.animalSound();
myPig.sleep();
}
}
}
<file_sep>namespace _3._Raiding.Contracts
{
public interface ICastAbilitable
{
public string CastAbility();
}
}
<file_sep>namespace Aquariums.Tests
{
using NUnit.Framework;
using System;
[TestFixture]
public class AquariumsTests
{
[Test]
public void ConstructorShouldInicialiseCorrectlyAllProperties()
{
Aquarium aquarium = new Aquarium("Za ribi", 4);
string expectedName = "Za ribi";
int expectedCapacity = 4;
Assert.AreEqual(expectedName, aquarium.Name);
Assert.AreEqual(expectedCapacity, aquarium.Capacity);
}
[TestCase("",4)]
[TestCase(null, 4)]
public void ConstructorShouldThrowExceptionIfNameIsNullOrEmpty(string name,int capacity)
{
Assert.Throws<ArgumentNullException>(() =>
{
Aquarium aquarium = new Aquarium(name, capacity);
});
}
[TestCase("Za ribi",-4)]
public void ConstructorShouldThrowExceptionIfTheGivenValueIsLowerThanZero(string name, int capacity)
{
Assert.Throws<ArgumentException>(() =>
{
Aquarium aquarium = new Aquarium(name, capacity);
});
}
[Test]
public void AddShouldAddFishToTheCollection()
{
Aquarium aquarium = new Aquarium("Za ribi", 4);
int expectedCount = 1;
aquarium.Add(new Fish("Joro"));
Assert.AreEqual(expectedCount, aquarium.Count);
}
[Test]
public void AddShouldThrowExceptionIfWetryToAddOverTheCapacity()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
aquarium.Add(new Fish("Joro"));
Assert.Throws<InvalidOperationException>(() =>
{
aquarium.Add(new Fish("Pesho"));
});
}
[Test]
public void RemoveFishShouldRemoveFishFromTheCollection()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
aquarium.Add(new Fish("Joro"));
aquarium.RemoveFish("Joro");
Assert.AreEqual(0, aquarium.Count);
}
[Test]
public void RemoveFishShouldThrowExcpetionForInvalidFish()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
Assert.Throws<InvalidOperationException>(() =>
{
aquarium.RemoveFish("Joro");
});
}
[Test]
public void SellFishShouldReturnRequestedFish()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
aquarium.Add(new Fish("Joro"));
Fish returnedFish = aquarium.SellFish("Joro");
Assert.AreEqual(returnedFish.Name, "Joro");
}
[Test]
public void SellFishShouldThrowExcpetionIfFishWithThisNameDoesntExist()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
aquarium.Add(new Fish("Joro"));
Assert.Throws<InvalidOperationException>(() =>
{
Fish returnedFish = aquarium.SellFish("Gosho");
});
}
[Test]
public void ReportShouldWorkCorrectly()
{
Aquarium aquarium = new Aquarium("Za ribi", 1);
aquarium.Add(new Fish("Joro"));
string report = aquarium.Report();
Assert.AreEqual("Fish available at Za ribi: Joro", report);
}
}
}
<file_sep># C# Related
Some of excercises I made, while learning C#
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04._BorderControl.Contracts
{
public interface IRobot
{
string Model { get; }
string Id { get; }
}
}
<file_sep>using System;
using System.Linq;
using System.Reflection;
namespace _02._Shoot_for_the_Win
{
class Program
{
static void Main(string[] args)
{
int[] targets = Console.ReadLine()
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
int countShotTargets = 0;
string command;
while((command = Console.ReadLine()) != "End")
{
int indexToShoot = int.Parse(command);
if(indexToShoot < 0 || indexToShoot >= targets.Length || targets[indexToShoot] == -1)
{
continue;
}
int shotTargetValue = targets[indexToShoot];
targets[indexToShoot] = -1;
countShotTargets++;
for(int i = 0; i < targets.Length; i++)
{
if(targets[i] > shotTargetValue)
{
targets[i] -= shotTargetValue;
}
else if(targets[i] <= shotTargetValue && targets[i] != -1)
{
targets[i] += shotTargetValue;
}
}
}
Console.WriteLine($"Shot targets: {countShotTargets} -> {string.Join(' ',targets)}");
}
}
}
<file_sep>using P07.MilitaryElite.Enumerations;
using System;
using System.Collections.Generic;
using System.Text;
namespace P07.MilitaryElite.Contracts
{
public interface ISpecialisedSoldier : IPrivate
{
Corps Corps { get; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Interfaces_and_Abstraction
{
public interface IMovable
{
void Move(int x, int y);
}
}
<file_sep>using System;
namespace Poke_Mon
{
class Program
{
static void Main(string[] args)
{
int N = int.Parse(Console.ReadLine());
int M = int.Parse(Console.ReadLine());
int Y = int.Parse(Console.ReadLine());
int nOrigin = N;
int countPoked = 0;
while (N >= M)
{
if (N == 0.5 * nOrigin)
{
if (Y > 0)
{
N /= Y;
if(N < M)
{
break;
}
}
}
N -= M;
countPoked++;
}
Console.WriteLine(N);
Console.WriteLine(countPoked);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P08.CollectionHierarchy.Contracts
{
public interface IRemovable : IAddable
{
void Remove();
}
}
<file_sep>using System;
using System.Linq;
namespace _03._Heart_Delivery
{
class Program
{
static void Main(string[] args)
{
int[] arr = Console.ReadLine().Split('@', StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
int indexOfCupid = 0;
string input;
while((input = Console.ReadLine()) != "Love!")
{
string[] command = input.Split();
int jumpLength = int.Parse(command[1]);
if(indexOfCupid + jumpLength < arr.Length && arr[indexOfCupid + jumpLength] != 0)
{
indexOfCupid += jumpLength;
arr[indexOfCupid] -= 2;
if(arr[indexOfCupid] == 0)
{
Console.WriteLine($"Place {indexOfCupid} has Valentine's day.");
}
}
else if(indexOfCupid + jumpLength < arr.Length && arr[indexOfCupid + jumpLength] == 0)
{
indexOfCupid += jumpLength;
Console.WriteLine($"Place {indexOfCupid} already had Valentine's day.");
}
else
{
indexOfCupid = 0;
if(arr[indexOfCupid] == 0)
{
Console.WriteLine($"Place {indexOfCupid} already had Valentine's day.");
}
else
{
arr[indexOfCupid] -= 2;
if (arr[indexOfCupid] == 0)
{
Console.WriteLine($"Place {indexOfCupid} has Valentine's day.");
}
}
}
}
Console.WriteLine($"Cupid's last position was {indexOfCupid}.");
if(arr.All(h => h == 0))
{
Console.WriteLine($"Mission was successful.");
}
else
{
int countFailedHouses = arr.Count(h => h != 0);
Console.WriteLine($"Cupid has failed {countFailedHouses} places.");
}
}
}
}
<file_sep>using System;
namespace PetShop
{
class Program
{
static void Main(string[] args)
{
int countDogs = int.Parse(Console.ReadLine());
int countOther = int.Parse(Console.ReadLine());
double neededMoney = countDogs * 2.50 + countOther * 4;
Console.WriteLine($"{neededMoney} lv.");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _07._The_V_Logger
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, HashSet<string>> vloggerWithHisFollowers = new
Dictionary<string, HashSet<string>>();
Dictionary<string, HashSet<string>> vloggerWithHisFowolling = new
Dictionary<string, HashSet<string>>();
string command;
while((command = Console.ReadLine()) != "Statistics")
{
string[] cmdArgs = command
.Split(" ", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
string operation = cmdArgs[1];
if (operation == "joined")
{
string vloggerToJoin = cmdArgs[0];
if (!vloggerWithHisFollowers.ContainsKey(vloggerToJoin))
{
vloggerWithHisFollowers[vloggerToJoin] = new HashSet<string>();
vloggerWithHisFowolling[vloggerToJoin] = new HashSet<string>();
}
}
else if(operation == "followed")
{
string vloggerWhichFollowed = cmdArgs[0];
string vloggerFollowed = cmdArgs[2];
if(vloggerWithHisFollowers.ContainsKey(vloggerWhichFollowed)
&& vloggerWithHisFowolling.ContainsKey(vloggerFollowed)
&& vloggerFollowed != vloggerWhichFollowed
&& !vloggerWithHisFowolling[vloggerWhichFollowed].Contains(vloggerFollowed))
{
///For the vlogger which followed the other one
vloggerWithHisFowolling[vloggerWhichFollowed].Add(vloggerFollowed);
///For the followed vlogger
vloggerWithHisFollowers[vloggerFollowed].Add(vloggerWhichFollowed);
}
}
}
//Ordering dict to be ordered by count of followers
vloggerWithHisFollowers = vloggerWithHisFollowers
.OrderByDescending(kvp => kvp.Value.Count)
.ThenBy(kvp => vloggerWithHisFowolling[kvp.Key].Count)
// .ThenBy(kvp => vloggerWithHisFowolling[kvp.Key].Count)
.ToDictionary(x => x.Key, y => y.Value);
//vloggerWithHisFowolling = vloggerWithHisFowolling
// .OrderBy(kvp => kvp.Value.Count)
// .ThenBy(kvp => kvp.Value)
// .ToDictionary(x => x.Key, y => y.Value);
/////
//Best vlogger
Console.WriteLine($"The V-Logger has a total of {vloggerWithHisFollowers.Count} vloggers in its logs.");
Console.WriteLine($"1. {vloggerWithHisFollowers.First().Key}" +
$" : {vloggerWithHisFollowers.First().Value.Count} followers," +
$" {vloggerWithHisFowolling[vloggerWithHisFollowers.First().Key].Count} following");
foreach (var follower in vloggerWithHisFollowers.First().Value.OrderBy(s => s))
{
Console.WriteLine($"* {follower}");
}
/////The other vloggers
int counter = 0;
foreach (var kvp in vloggerWithHisFollowers)
{
if(counter == 0)
{
counter++;
continue;
}
Console.WriteLine($"{counter + 1}. {kvp.Key} : {kvp.Value.Count} followers," +
$" {vloggerWithHisFowolling[kvp.Key].Count} following");
counter++;
}
}
}
}
<file_sep>using System;
namespace FishingBoat
{
class Program
{
static void Main(string[] args)
{
int budget = int.Parse(Console.ReadLine());
string season = Console.ReadLine();
int countFisherman = int.Parse(Console.ReadLine());
double moneyNeeded = 0.0;
switch(season)
{
case "Spring":
moneyNeeded = 3000;
break;
case "Summer":
// break;
case "Autumn":
moneyNeeded = 4200;
break;
case "Winter":
moneyNeeded = 2600;
break;
}
if (countFisherman <= 6)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.1;
}
else if (countFisherman >= 7 && countFisherman <= 11)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.15;
}
else if (countFisherman >= 12)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.25;
}
if (countFisherman % 2 == 0 && season!="Autumn")
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.05;
}
// Console.WriteLine(moneyNeeded);
if (budget >= moneyNeeded)
{
Console.WriteLine($"Yes! You have {budget - moneyNeeded:F2} leva left.");
}
else if(budget < moneyNeeded)
{
Console.WriteLine($"Not enough money! You need {moneyNeeded - budget:F2} leva.");
}
}
}
}
<file_sep>using System;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
// Console.WriteLine("Hello SoftUni");
/* string name = "Vladi ";
string family_name = "Kolev";
string fullname = name + family_name;
Console.WriteLine(fullname);
Console.WriteLine(name + family_name);*/
/* Console.WriteLine("ENter user:");
string user = Console.ReadLine();
Console.WriteLine("Username is " + user);*/
/* Console.WriteLine("Enter AGE:");
int age = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("Age e " + age);
*/
/* int x = 4;
// int y = 6;int z = 4;
Console.WriteLine(Math.Sqrt(x));
Console.WriteLine(Math.Abs(-4.7));
Console.WriteLine(Math.Round(9.99));
*/
string txt = "<NAME>";
Console.WriteLine(txt.Length);
txt = txt.ToUpper();
//Console.WriteLine(txt.ToUpper());
Console.WriteLine(txt);
Console.WriteLine(txt.IndexOf("K"));
int surnamPos = txt.IndexOf("K");
string lastname = txt.Substring(surnamPos);
Console.WriteLine(lastname);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04._BorderControl.Contracts
{
interface IIdentifiable
{
string GetId();
}
}
<file_sep>using System;
namespace Charity_campaign
{
class Program
{
static void Main(string[] args)
{
int dni = int.Parse(Console.ReadLine());
int brSladkari = int.Parse(Console.ReadLine());
int brTorti = int.Parse(Console.ReadLine());
int brGofreti = int.Parse(Console.ReadLine());
int brPancakes = int.Parse(Console.ReadLine());
double sumaZaDen = (brTorti * 45 + brGofreti * 5.80 + brPancakes * 3.20)*brSladkari;
double sumaOtCqlataKampaniq = sumaZaDen * dni;
double sumaSledPokrivaneNaRazhodi = sumaOtCqlataKampaniq - sumaOtCqlataKampaniq / 8;
Console.WriteLine(sumaSledPokrivaneNaRazhodi);
}
}
}
<file_sep>using System;
using System.Linq;
namespace _6._Jagged_Array_Manipulator
{
class Program
{
static void Main(string[] args)
{
int rows = int.Parse(Console.ReadLine());
double[][] arr = new double[rows][];
for (int row = 0; row < rows; row++)
{
int[] currRow = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
arr[row] = new double[currRow.Length];
for (int col = 0; col < arr[row].Length; col++)
{
arr[row][col] = currRow[col];
}
}
for (int row = 0; row < rows - 1; row++)
{
if (arr[row + 1].Length == arr[row].Length)
{
arr[row + 1] = arr[row + 1].Select(x => x * 2).ToArray();
arr[row] = arr[row].Select(x => x * 2).ToArray();
}
else
{
arr[row + 1] = arr[row + 1].Select(x => x / 2).ToArray();
arr[row] = arr[row].Select(x => x / 2).ToArray();
}
}
while (true)
{
string command = Console.ReadLine();
if(command == "End")
{
break;
}
string[] cmdArgs = command.Split();
if(cmdArgs[0] == "Add")
{
int row = int.Parse(cmdArgs[1]);
int col = int.Parse(cmdArgs[2]);
int value = int.Parse(cmdArgs[3]);
if(row >= 0 && row < rows && col >= 0 && col < arr[row].Length)
{
arr[row][col] += value;
}
}
else if(cmdArgs[0] == "Subtract")
{
int row = int.Parse(cmdArgs[1]);
int col = int.Parse(cmdArgs[2]);
int value = int.Parse(cmdArgs[3]);
if (row >= 0 && row < rows && col >= 0 && col < arr[row].Length)
{
arr[row][col] -= value;
}
}
}
PrintArr(arr);
}
private static void PrintArr(double[][] arr)
{
foreach (var row in arr)
{
Console.WriteLine(string.Join(" ", row));
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _12._TriFunction
{
class Program
{
static void Main(string[] args)
{
int targetSum = int.Parse(Console.ReadLine());
string[] names = Console.ReadLine()
.Split();
Func<string, int, bool> isValidWord = ((name, sum) => name.ToCharArray()
.Select(ch => (int)ch).Sum() >= sum);
Func<string[], int, Func<string, int, bool>, string> firstValidName =
(arr, sum, func) => arr.FirstOrDefault(str => func(str,sum));
var result = firstValidName(names, targetSum, isValidWord);
Console.WriteLine(result);
}
}
}
<file_sep>using System;
namespace HoneyWinterReserves
{
class Program
{
static void Main(string[] args)
{
double honeyNeeded = double.Parse(Console.ReadLine());
double sumHoney = 0;
string command = Console.ReadLine();
while(command != "Winter has come")
{
string nameBee = command;
for (int i = 0; i < 6; i++)
{
double honeyThisMonth = double.Parse(Console.ReadLine());
sumHoney += honeyThisMonth;
}
if(sumHoney < 0)
{
Console.WriteLine($"{nameBee} was banished for gluttony");
}
//else if(sumHoney>=honeyNeeded)
//{
// Console.WriteLine($"Well done! Honey surplus {sumHoney - honeyNeeded:F2}.");
//}
//else
//{
// Console.WriteLine($"Hard Winter! Honey needed {honeyNeeded - sumHoney:F2}.");
//}
if (sumHoney >= honeyNeeded)
{
Console.WriteLine($"Well done! Honey surplus {sumHoney - honeyNeeded:F2}.");
break;
}
command = Console.ReadLine();
}
if (sumHoney < honeyNeeded)
{
Console.WriteLine($"Hard Winter! Honey needed {honeyNeeded - sumHoney:F2}.");
}
}
}
}
<file_sep>using P08.CollectionHierarchy.Contracts;
using System;
using System.Collections.Generic;
using System.Reflection.Metadata.Ecma335;
using System.Text;
namespace P08.CollectionHierarchy.Models
{
public class AddRemoveCollection : IRemovable
{
private List<string> data;
private List<int> indexes;
private List<string> removedElements;
public AddRemoveCollection()
{
this.data = new List<string>();
this.indexes = new List<int>();
this.removedElements = new List<string>();
}
public void Add(string element)
{
this.indexes.Add(0);
this.data.Insert(0, element);
}
public void Remove()
{
string lastItem = this.data[this.data.Count - 1];
this.removedElements.Add(lastItem);
this.data.RemoveAt(this.data.Count - 1);
}
public void GetRemoved()
{
Console.WriteLine(string.Join(" ",this.removedElements));
}
public override string ToString()
{
return $"{string.Join(" ", this.indexes)}";
}
}
}
<file_sep>using System;
namespace PasswordGenerator
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int l = int.Parse(Console.ReadLine());
for(int i=1;i<=n;i++)
{
for(int j=1;j<=n;j++)
{
for(int k=97;k<97+l;k++)
{
for(int m=97;m<97+l;m++)
{
for(int o=1;o<=n;o++)
{
if(o > i && o > j)
{
Console.Write($"{i}{j}{Convert.ToChar(k)}{Convert.ToChar(m)}{o} ");
}
}
}
}
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Cars
{
public abstract class Car : ICar
{
protected Car(string model,string color)
{
this.Model = model;
this.Color = color;
}
public string Model { get; private set; }
public string Color { get; private set; }
public abstract string Start();
public string Stop()
{
return "Stopped";
}
}
}
<file_sep>using System;
namespace Deposit_calculator
{
class Program
{
static void Main(string[] args)
{
double deposiranasuma = double.Parse(Console.ReadLine());
int srok =int.Parse(Console.ReadLine());
double godLihvenProcent = double.Parse(Console.ReadLine());
// Console.WriteLine(godLihvenProcent*deposiranasuma/100);
double res = deposiranasuma + srok * ((deposiranasuma * godLihvenProcent/100) / 12);
Console.WriteLine(res);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Inheritance
{
public class Doctor:Employee
{
public List<string> Patients { get; set; }
}
}
<file_sep>using System;
using System.Text;
using System.Text.RegularExpressions;
namespace _02._Problem
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
string pattern = @"(.+)>(?<first>\d{3})\|(?<second>[a-z]{3})\|(?<third>[A-Z]{3})\|(?<fourth>[^\n]{3})<(\1)";
for (int i = 0; i < n; i++)
{
string currPass = Console.ReadLine();
if(Regex.IsMatch(currPass, pattern))
{
Match match = Regex.Match(currPass, pattern);
StringBuilder sb = new StringBuilder();
sb.Append(match.Groups["first"].Value);
sb.Append(match.Groups["second"].Value);
sb.Append(match.Groups["third"].Value);
sb.Append(match.Groups["fourth"].Value);
Console.WriteLine($"Password: {sb.ToString()}");
}
else
{
Console.WriteLine("Try another password!");
}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
///string - referent type
///string - immutable;///They are read only
string text = "Exam";
string newText = "Retake Exam";
string third= String.Concat(text, newText);
string txt = new string(new char[] { '1', '2', '3', '4' });
Console.WriteLine(txt.ToCharArray());
string texte = "Hello, from, Softuni, my name, is ,Pesho";
int indexOfComma = texte.LastIndexOf(',');
Console.WriteLine(indexOfComma);
///Substring
string substring = texte.Substring(texte.Length-6);
//text.IndexOf(', ', )
string substring2 = new string(texte.Reverse().ToArray());
Console.WriteLine(substring);
////Split()
///
char[] separators = { '!', ',' };
string[] words = text.Split(separators);
}
}
}
<file_sep>using System;
using System.Linq;
namespace Order_By
{
class Program
{
static void Main(string[] args)
{
var input = Console.ReadLine().Split().ToList();
input = input
.OrderBy(x => x[0])
.ThenByDescending(x => x.Length)
.ToList();
Console.WriteLine(string.Join(" ", input));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public class AirFighter : Airplane
{
public int Damage { get; set; }
public override string VrumVrum()
{
return "Fighter is fighting";
}
}
}
<file_sep>using System;
using System.Linq;
namespace Jagged___Array_Modification
{
class Program
{
static void Main(string[] args)
{
int rows = int.Parse(Console.ReadLine());
int[][] array = new int[rows][];
for (int row = 0; row < rows; row++)
{
int[] currentRow = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
array[row] = currentRow;
}
while (true)
{
string command = Console.ReadLine().ToLower();
if(command == "end")
{
break;
}
string[] cmdArgs = command.Split();
if(rows<)
if (cmdArgs[0] == "add")
{
}
else if(cmdArgs[0] == "subtract")
{
}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _03.Extract_File
{
class Program
{
static void Main(string[] args)
{
string path = Console.ReadLine();
string[] pathArgs = path
.Split('\\', StringSplitOptions.RemoveEmptyEntries)
.ToArray();
string[] fileInfo = pathArgs.Last()
.Split('.');
string[] filenameArgs =fileInfo.Take(fileInfo.Length - 1)
.ToArray();
string fileName = string.Join(".", filenameArgs);
string fileExtension = fileInfo.Last();
Console.WriteLine($"File name: {fileName}");
Console.WriteLine($"File extension: {fileExtension}");
}
}
}
<file_sep>using System;
using System.Numerics;
namespace _3.Big_Factorial
{
class Program
{
static void Main(string[] args)
{
int number = int.Parse(Console.ReadLine());
BigInteger bigInteger = 1;
//50
//50* 49 * 48
for (int i = number ; i > 0; i--)
{
bigInteger *= i;
}
Console.WriteLine(bigInteger);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _06._Courses
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<string>> courses = new Dictionary<string, List<string>>();
while (true)
{
string inp = Console.ReadLine();
if(inp == "end")
{
break;
}
string[] command = inp.Split(" : ");
string courseName = command[0];
string studentName = command[1];
if (!courses.ContainsKey(courseName))
{
courses[courseName] = new List<string>();
// courses[courseName].Add(studentName);
}
courses[courseName].Add(studentName);
}
Dictionary<string, List<string>> sortedCourses = courses
.OrderByDescending(kvp => kvp.Value.Count)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var kvp in sortedCourses)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value.Count}");
foreach (var student in kvp.Value.OrderBy(n => n))
{
Console.WriteLine($"-- {student}");
}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace SquareWithMaximumSum
{
class Program
{
static void Main(string[] args)
{
int[] sizes = Console.ReadLine().Split(", ").Select(int.Parse).ToArray();
int[,] matrix = new int[sizes[0],sizes[1]];
for(int row =0;row<matrix.GetLength(0);row++)
{
int[] colElem = Console.ReadLine().Split(", ").Select(int.Parse).ToArray();
for(int col = 0; col < matrix.GetLength(1);col++)
{
matrix[row, col] = colElem[col];
}
}
int maxSum = int.MinValue;
int maxRow = 0;
int maxCol = 0;
for(int row =0;row< matrix.GetLength(0)-1;row++)
{
for (int col = 0; col < matrix.GetLength(1)-1; col++)
{
int currentSum = matrix[row, col]
+ matrix[row, col + 1]
+ matrix[row + 1, col]
+ matrix[row + 1, col + 1];
if(currentSum> maxSum)
{
maxSum = currentSum;
maxRow = row;
maxCol = col;
}
}
} Console.WriteLine($"{matrix[maxRow, maxCol]} {matrix[maxRow, maxCol + 1]}");
Console.WriteLine($"{matrix[maxRow+1, maxCol]} {matrix[maxRow+1, maxCol + 1]}");
Console.WriteLine(maxSum);
}
}
}
<file_sep>using System;
using System.Linq;
namespace _02._Warships
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
string[] commmands = Console.ReadLine().Split(",", StringSplitOptions.RemoveEmptyEntries).ToArray();
for (int i = 0; i < n; i++)
{
char[] row = Console.ReadLine().Split(" ", StringSplitOptions.RemoveEmptyEntries).Select(char.Parse).ToArray();
for (int j = 0; j < n; j++)
{
field[i, j] = row[j];
}
}
// int countDestrByP1 = 0;
// int countDestrByP2 = 0;'
int countDestr = 0;
int countP1Ships = 0;
int countP2Ships = 0;
countP1Ships = GetCountP1Ships(n, field, countP1Ships);
countP2Ships = GetCountP2Ships(n, field, countP2Ships);
for (int i = 0; i < commmands.Length; i++)
{
int x, y;
/*if (commmands[i].Length == 1)
{
x = int.Parse(commmands[i]);
y = int.Parse(commmands[i]);
}
*/
// else
//{
int[] coordinates = commmands[i].Split(" ", StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray();
x = coordinates[0];
y = coordinates[1];
//}
if (x < 0 || x >= n || y < 0 || y >= n)
{
continue;
}
///player 1
if (i % 2 == 0)
{
commandsForP1(n, field, ref countDestr, ref countP1Ships, ref countP2Ships, x, y, true);
}
//p2
else
{
commandsForP1(n, field, ref countDestr, ref countP1Ships, ref countP2Ships, x, y, false);
}
if (countP1Ships == 0)
{
Console.WriteLine($"Player Two has won the game! {countDestr} ships have been sunk in the battle.");
break;
}
else if (countP2Ships == 0)
{
Console.WriteLine($"Player One has won the game! {countDestr} ships have been sunk in the battle.");
break;
}
/* Console.WriteLine();
PrintField(n, field);
*/
}
Console.WriteLine();
PrintField(n, field);
if (countP1Ships != 0 && countP2Ships != 0)
{
Console.WriteLine($"It's a draw! Player One has {countP1Ships} ships left. Player Two has {countP2Ships} ships left.");
}
}
private static void commandsForP1(int n, char[,] field, ref int countDestr, ref int countP1Ships, ref int countP2Ships, int x, int y, bool isP1)
{
if(isP1 == true)
{
if (field[x, y] == '>')
{
countDestr++;
field[x, y] = 'X';
countP2Ships--;
}
}
else
{
if (field[x, y] == '<')
{
countDestr++;
field[x, y] = 'X';
countP1Ships--;
}
}
if (field[x, y] == '#')
{
//up
if (x - 1 >= 0)
{
if (field[x - 1, y] == '>')
{
countDestr++;
countP2Ships--;
field[x - 1, y] = 'X';
}
else if (field[x - 1, y] == '<')
{
countDestr++;
countP1Ships--;
field[x - 1, y] = 'X';
}
//up right
if (y + 1 < n)
{
if (field[x - 1, y + 1] == '>')
{
countDestr++;
countP2Ships--;
field[x - 1, y + 1] = 'X';
}
else if (field[x - 1, y + 1] == '<')
{
countDestr++;
countP1Ships--;
field[x - 1, y + 1] = 'X';
}
}
//up left
if (y - 1 >= 0)
{
if (field[x - 1, y - 1] == '>')
{
countDestr++;
countP2Ships--;
field[x - 1, y - 1] = 'X';
}
else if (field[x - 1, y - 1] == '<')
{
countDestr++;
countP1Ships--;
field[x - 1, y - 1] = 'X';
}
}
}
//down
if (x + 1 < n)
{
if (field[x + 1, y] == '>')
{
countDestr++;
countP2Ships--;
field[x + 1, y] = 'X';
}
else if (field[x + 1, y] == '<')
{
countDestr++;
countP1Ships--;
field[x + 1, y] = 'X';
}
//down right
if (y + 1 < n)
{
if (field[x + 1, y + 1] == '>')
{
countDestr++;
countP2Ships--;
field[x + 1, y + 1] = 'X';
}
else if (field[x + 1, y + 1] == '<')
{
countDestr++;
countP1Ships--;
field[x + 1, y + 1] = 'X';
}
}
//down left
if (y - 1 >= 0)
{
if (field[x + 1, y - 1] == '>')
{
countDestr++;
countP2Ships--;
field[x + 1, y - 1] = 'X';
}
else if (field[x + 1, y - 1] == '<')
{
countDestr++;
countP1Ships--;
field[x + 1, y - 1] = 'X';
}
}
}
//left
if (y - 1 >= 0)
{
if (field[x, y - 1] == '>')
{
countDestr++;
countP2Ships--;
field[x, y - 1] = 'X';
}
else if (field[x, y - 1] == '<')
{
countDestr++;
countP1Ships--;
field[x, y - 1] = 'X';
}
}
//right
if (y + 1 < n)
{
if (field[x, y + 1] == '>')
{
countDestr++;
countP2Ships--;
field[x, y + 1] = 'X';
}
else if (field[x, y + 1] == '<')
{
countDestr++;
countP1Ships--;
field[x, y + 1] = 'X';
}
}
field[x, y] = 'X';
}
}
private static void PrintField(int n, char[,] field)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
Console.Write(field[i, j]+" ");
}
Console.WriteLine();
}
}
private static int GetCountP2Ships(int n, char[,] field, int countP2Ships)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == '>')
{
countP2Ships++;
}
}
}
return countP2Ships;
}
private static int GetCountP1Ships(int n, char[,] field, int countP1Ships)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == '<')
{
countP1Ships++;
}
}
}
return countP1Ships;
}
}
}
<file_sep>using System;
namespace _01._Disneyland_Journey
{
class Program
{
static void Main(string[] args)
{
double moneyNeeded = double.Parse(Console.ReadLine());
int months = int.Parse(Console.ReadLine());
double collectedMoney = 0;
for (int i = 0; i < months; i++)
{
if((i+1) != 1 && (i + 1) % 2 != 0)
{
collectedMoney -= collectedMoney * 0.16;
}
if((i+1) % 4 == 0)
{
collectedMoney += collectedMoney * 0.25;
}
collectedMoney += 0.25 * moneyNeeded;
}
if(collectedMoney >= moneyNeeded)
{
Console.WriteLine($"Bravo! You can go to Disneyland and you will have {collectedMoney-moneyNeeded:F2}lv. for souvenirs.");
}
else
{
Console.WriteLine($"Sorry. You need {moneyNeeded - collectedMoney:F2}lv. more.");
}
}
}
}
<file_sep>using System;
namespace ClassProperties
{
class Person
{
private string name = "Private";
public string Name
{
get { return name; }
set { name = value; }
}
}
class Program
{
static void Main(string[] args)
{
Person first = new Person();
// first.Name = "Public";
Console.WriteLine(first.Name);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _02._Odd_Occurrences
{
class Program
{
static void Main(string[] args)
{
string[] words = Console.ReadLine().Split();
Dictionary<string, int> counts = new Dictionary<string, int>();
for (int i = 0; i < words.Length; i++)
{
string word = words[i].ToLower();
if (!counts.ContainsKey(word))
{
counts[word] = 1;
}
else
{
counts[word]++;
}
}
foreach (var item in counts)
{
if(item.Value % 2 != 0)
{
Console.Write($"{item.Key} ");
}
}
}
}
}
<file_sep>using System;
using System.Linq;
using System.Collections.Generic;
namespace SumMatrixColumns
{
class Program
{
static void Main(string[] args)
{
int[] sizes = Console.ReadLine().Split(", ").Select(int.Parse).ToArray();
int[,] matrix = new int[sizes[0], sizes[1]];
for(int row=0;row<matrix.GetLength(0);row++)
{
var col = Console.ReadLine().Split().Select(int.Parse).ToArray();
for(int c = 0;c<matrix.GetLength(1);c++)
{
matrix[row, c] = col[c];
}
}
for(int c=0;c<matrix.GetLength(1);c++)
{
int sum = 0;
for(int r = 0;r<matrix.GetLength(0);r++)
{
sum += matrix[r, c];
}
Console.WriteLine(sum);
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _9._Miner
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
string[] commands = Console.ReadLine()
.Split(' ', StringSplitOptions.RemoveEmptyEntries)
.ToArray();
InicialisingField(n, field);
int minerRow = -1;
int minerCol = -1;
FindingWhereisTheMiner(n, field, ref minerRow, ref minerCol);
int coalsInField = 0;
coalsInField = FindCountOfCoalsInField(n, field, coalsInField);
int foundCoals = 0;
for (int i = 0; i < commands.Length; i++)
{
string currCommand = commands[i];
if (currCommand == "left")
{
if (minerCol - 1 >= 0)
{
CheckWhatCharItIs(field, minerRow, minerCol - 1,
ref minerRow, ref minerCol,ref foundCoals,n);
}
}
else if (currCommand == "right")
{
if (minerCol + 1 < n)
{
CheckWhatCharItIs(field, minerRow, minerCol + 1,
ref minerRow, ref minerCol,ref foundCoals,n);
}
}
else if (currCommand == "up")
{
if (minerRow - 1 >= 0)
{
CheckWhatCharItIs(field, minerRow - 1, minerCol,
ref minerRow, ref minerCol,ref foundCoals,n);
}
}
else if (currCommand == "down")
{
if (minerRow + 1 < n)
{
CheckWhatCharItIs(field, minerRow + 1, minerCol,
ref minerRow, ref minerCol,ref foundCoals,n);
}
}
if (foundCoals == coalsInField)
{
Console.WriteLine($"You collected all coals! ({minerRow}, {minerCol})");
return;
}
//Console.WriteLine();
//PrintField(n, field);
//Console.WriteLine();
}
Console.WriteLine($"{coalsInField - foundCoals} coals left. ({minerRow}, {minerCol})");
}
private static void PrintField(int n, char[,] field)
{
for (int row = 0; row < n; row++)
{
for (int col = 0; col < n; col++)
{
Console.Write(field[row, col] + " ");
}
Console.WriteLine();
}
}
private static void CheckWhatCharItIs(char[,] field,int charRow ,
int charCol ,ref int minerRow,ref int minerCol,ref int foundCoals,int n)
{
if(field[charRow,charCol] == '*')
{
field[minerRow, minerCol] = '*';
field[charRow, charCol] = 's';
minerRow = charRow;
minerCol = charCol;
// FindingWhereisTheMiner(n,field,ref minerRow, ref minerCol);
}
else if(field[charRow, charCol] == 'c')
{
foundCoals++;
field[minerRow, minerCol] = '*';
field[charRow, charCol] = 's';
minerRow = charRow;
minerCol = charCol;
//FindingWhereisTheMiner(n, field, ref minerRow, ref minerCol);
}
else if(field[charRow, charCol] == 'e')
{
minerRow = charRow;
minerCol = charCol;
field[charRow, charCol] = 's';
Console.WriteLine($"Game over! ({minerRow}, {minerCol})");
Environment.Exit(0);
}
}
private static void FindingWhereisTheMiner(int n, char[,] field, ref int minerRow, ref int minerCol)
{
for (int row = 0; row < n; row++)
{
for (int col = 0; col < n; col++)
{
if (field[row, col] == 's')
{
minerRow = row;
minerCol = col;
}
}
}
}
private static int FindCountOfCoalsInField(int n, char[,] field, int coalsInField)
{
for (int row = 0; row < n; row++)
{
for (int col = 0; col < n; col++)
{
if (field[row, col] == 'c')
{
coalsInField++;
}
}
}
return coalsInField;
}
private static void InicialisingField(int n, char[,] field)
{
for (int row = 0; row < n; row++)
{
char[] currRow = Console.ReadLine()
.Split(' ', StringSplitOptions.RemoveEmptyEntries)
.Select(char.Parse)
.ToArray();
for (int col = 0; col < n; col++)
{
field[row, col] = currRow[col];
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Cars
{
public class Battery
{
}
}
<file_sep>using System;
using System.Linq;
namespace _10.LadyBugs
{
class Program
{
static void Main(string[] args)
{
int size = int.Parse(Console.ReadLine());
int[] field = new int[size];
// field = Console.ReadLine().Split().Select(int.Parse).ToArray();
int[] IndexesWithBees = Console.ReadLine().Split().Select(int.Parse).ToArray();
for (int i = 0; i < IndexesWithBees.Length; i++)
{
if (IndexesWithBees[i] < field.Length&& IndexesWithBees[i] >= 0)
{
field[IndexesWithBees[i]] = 1;
}
}
while (true)
{
string input = Console.ReadLine();
if (input == "end")
{
break;
}
string[] command = input.Split();
int ladybugIndex = int.Parse(command[0]);
string direction = command[1];
int flyLength = int.Parse(command[2]);
// Console.WriteLine(ladybugIndex + direction + flyLength);
if (field[ladybugIndex] == 1 && ladybugIndex < field.Length && ladybugIndex >= 0
&& flyLength != 0)
{
field[ladybugIndex] = 0;
if (direction == "right")
{
if ((ladybugIndex + flyLength) < size
&& ladybugIndex + flyLength >= 0
&& field[ladybugIndex + flyLength] == 0)
{
//if (field[ladybugIndex + flyLength] != 1)
//{
field[ladybugIndex + flyLength] = 1;
//}
}
/////////Problemno
else if (ladybugIndex + flyLength < field.Length
&& ladybugIndex + flyLength >= 0 &&
field[ladybugIndex + flyLength] == 1)
{
//if ((ladybugIndex + flyLength + ladybugIndex+ flyLength) < size)
//{
/// field[ladybugIndex + flyLength + ladybugIndex + flyLength] = 1;
//}
while (ladybugIndex + flyLength < field.Length
&& ladybugIndex + flyLength >= 0
&& field[ladybugIndex + flyLength] == 1) //продължава напред със същия брой движения ДОКАТО не открие празно място
{
flyLength += flyLength; //затова увеличаваме хода със същия брой движения
if (ladybugIndex + flyLength < field.Length
&& ladybugIndex + flyLength >= 0 // а тази проверка е в случай, че числото е ОТРИЦАТЕЛНО, за да не излезе от полето НАЛЯВО
&& field[ladybugIndex + flyLength] == 0)
{
field[ladybugIndex + flyLength] = 1; //ако открие празна позиция, я заема
break; //и излизаме от while-цикъла
}
}
}
}
}
else if (direction == "left")
{
if ((ladybugIndex - flyLength) >= 0 && ladybugIndex - flyLength < field.Length
&& field[ladybugIndex - flyLength] == 0)
{
//if (field[ladybugIndex - flyLength] != 1)
//{
field[ladybugIndex - flyLength] = 1;
//}
}
/////////Problemno
else if (field[ladybugIndex - flyLength] == 1 && ladybugIndex - flyLength >= 0
&& ladybugIndex - flyLength < field.Length)
{
// if ((ladybugIndex - flyLength - ladybugIndex - flyLength) >= 0)
// {
// field[ladybugIndex - flyLength - ladybugIndex - flyLength] = 1;
// }
while (ladybugIndex - flyLength >= 0 // ДОКАТО (while) не открие празна позиция
&& ladybugIndex - flyLength < field.Length
&& field[ladybugIndex - flyLength] == 1)
{
flyLength += flyLength; // тук си е плюс, а не минус, въпреки че е наляво, но... за да не ходи напред-назад
if (ladybugIndex - flyLength >= 0
&& ladybugIndex - flyLength < field.Length
&& field[ladybugIndex - flyLength] == 0)
{
field[ladybugIndex - flyLength] = 1;
break;
}
}
}
}
}
Console.WriteLine(string.Join(" ", field));
}
}
}
<file_sep>using P04._BorderControl.Contracts;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04._BorderControl.Models
{
public class Citizen : IIdentifiable
{
private string name;
private int age;
private string id;
public Citizen(string name, int age, string id)
{
this.name = name;
this.age = age;
this.id = id;
}
// public string Name { get; }
//public int Age { get; }
//public string Id { get; }
public string GetId()
{
return this.id;
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace _10._Crossroads
{
class Program
{
static void Main(string[] args)
{
int durationOfGreenLight = int.Parse(Console.ReadLine());
int durationOfFreeWindow = int.Parse(Console.ReadLine());
Queue<string> cars = new Queue<string>();
bool crashed = false;
string crashedCar = string.Empty;
int hitIndex = -1;
int totalCarsPassed = 0;
string command;
while((command = Console.ReadLine()) != "END")
{
if (command == "green")
{
int currentGreenLight = durationOfGreenLight;
while (cars.Any() && currentGreenLight > 0)
{
string currCar = cars.Peek();
int carLength = currCar.Length;
if (carLength <= currentGreenLight )
{
totalCarsPassed++;
currentGreenLight -= currCar.Length;
cars.Dequeue();
}
else
{
carLength -= currentGreenLight;
if (carLength <= durationOfFreeWindow)
{
totalCarsPassed++;
cars.Dequeue();
}
else
{
crashed = true;
crashedCar = currCar;
hitIndex = currentGreenLight
+ durationOfFreeWindow;
}
break;
}
}
}
else
{
cars.Enqueue(command);
}
if (crashed)
{
break;
}
}
if (crashed)
{
Console.WriteLine("A crash happened!");
Console.WriteLine($"{crashedCar} was hit at {crashedCar[hitIndex]}.");
}
else
{
Console.WriteLine("Everyone is safe.");
Console.WriteLine($"{totalCarsPassed} total cars passed the crossroads.");
}
}
}
}
<file_sep>using P03.Raiding.Models;
using P03.Raiding.Models.Factory;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P03.Raiding.Core
{
public class Engine
{
private ICollection<BaseHero> heroes;
private HeroFactory heroFactory;
public Engine()
{
heroes = new List<BaseHero>();
heroFactory = new HeroFactory();
}
public void Run()
{
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string heroName = Console.ReadLine();
string heroType = Console.ReadLine();
//try
//{
// Validate(heroType);
//}
//catch (ArgumentException ae)
//{
// Console.WriteLine(ae.Message);
// i--;
// continue;
//}
BaseHero hero;
try
{
hero = heroFactory.MakeHero(heroName, heroType);
heroes.Add(hero);
}
catch (Exception e)
{
Console.WriteLine(e.Message);
i--;
}
}
int bossPower = int.Parse(Console.ReadLine());
int sumHeroPower = 0;
foreach (var hero in heroes)
{
Console.WriteLine(hero.CastAbility());
sumHeroPower += hero.Power;
}
// Console.WriteLine(sumHeroPower);
ResultOfFight(bossPower, sumHeroPower);
}
private static void ResultOfFight(int bossPower, int sumHeroPower)
{
if (sumHeroPower >= bossPower)
{
Console.WriteLine("Victory!");
}
else
{
Console.WriteLine("Defeat...");
}
}
//private static void Validate(string heroType)
//{
// if (heroType != "Druid" && heroType != "Paladin" && heroType != "Rogue"
// && heroType != "Warrior")
// {
// throw new ArgumentException("Invalid hero!");
// }
//}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace _02._Race
{
class Program
{
static void Main(string[] args)
{
List<string> names = Console.ReadLine()
.Split(", ")
.ToList();
Dictionary<string, int> racers = new Dictionary<string, int>();
foreach (string name in names)
{
if (!racers.ContainsKey(name))
{
racers[name] = 0;
}
}
while (true)
{
string input = Console.ReadLine();
int distance = 0;
if (input == "end of race")
{
break;
}
string wordPattern = @"[A-Za-z]";
string name = "";
var matches = Regex.Matches(input, wordPattern);
foreach (Match match in matches)
{
name += match.Value;
}
if (racers.ContainsKey(name))
{
var matchesDistance = Regex.Matches(input, @"[0-9]");
foreach (Match match in matchesDistance)
{
distance += int.Parse(match.Value);
}
racers[name] += distance;
}
}
racers = racers
.OrderByDescending(kvp => kvp.Value)
.Take(3)
.ToDictionary(a => a.Key, b => b.Value);
int counter = 1;
foreach (var kvp in racers)
{
if (counter == 1)
{
Console.WriteLine($"1st place: {kvp.Key}");
}
else if (counter == 2)
{
Console.WriteLine($"2nd place: {kvp.Key}");
}
else if (counter == 3)
{
Console.WriteLine($"3rd place: {kvp.Key}");
}
counter++;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _04.Orders
{
class Program
{
static void Main(string[] args)
{
// Dictionary<string,Dictionary<int,int>> d
Dictionary<string, double> productPrices = new Dictionary<string, double>();
Dictionary<string, int> productQuant = new Dictionary<string, int>();
while (true)
{
string inp = Console.ReadLine();
if(inp == "buy")
{
break;
}
string[] productArgs = inp.Split().ToArray();
string name = productArgs[0];
double price = double.Parse(productArgs[1]);
int quant = int.Parse(productArgs[2]);
if (!productQuant.ContainsKey(name))
{
productQuant[name] = 0;
productQuant[name] = 0;
}
productQuant[name] += quant;
productPrices[name] = price;
}
foreach (var kvp in productPrices)
{
string name = kvp.Key;
double price = kvp.Value;
int quantity = productQuant[name];
Console.WriteLine($"{name} -> {price*quantity:F2}");
}
}
}
}
<file_sep>using System;
namespace Vacation_books_list
{
class Program
{
static void Main(string[] args)
{
int broiStranici = int.Parse(Console.ReadLine());
double procheteniZaChas = double.Parse(Console.ReadLine());
int dniZaKoitoDaGiProchete = int.Parse(Console.ReadLine());
double ResbroichasovedaOtdeli = (broiStranici / procheteniZaChas)/dniZaKoitoDaGiProchete;
Console.WriteLine(ResbroichasovedaOtdeli);
}
}
}
<file_sep> using System;
using System.Collections.Generic;
namespace SortingList
{
class Program
{
static void Main(string[] args)
{
List<int> list = new List<int>()
{ 1,-1,3,645,34,233};
Console.WriteLine(string.Join(" ", list));
list.Sort();
Console.WriteLine(string.Join(" ", list));
list.Reverse();
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Encapsulation
{
public class CommonValidator
{
public static void ValidateNull(object value, string name = null)
{
if (value == null)
{
string message = name == null ? $"Value cannot be null" : "Name cannot be null";
throw new NullReferenceException();
}
}
public static void ValidateMin(int value, int minimum , string typeName , string properyName)
{
if(value < minimum)
{
throw new ArgumentOutOfRangeException($"{typeName}.{properyName} cannot be less than minimum");
}
}
public static void ValidateMin(decimal value, int minimum, string typeName, string properyName)
{
if (value < minimum)
{
throw new ArgumentOutOfRangeException($"{typeName}.{properyName} cannot be less than minimum");
}
}
}
}
<file_sep>using System;
using System.Text;
namespace StringBuilder_try
{
class Program
{
static void Main(string[] args)
{
StringBuilder sb = new StringBuilder(1000);
for (int i = 0; i < 10 ; i++)
{
sb.Append("Softuni");
}
sb.Clear();
Console.WriteLine(sb);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Stack_of_Strings
{
public static class CollectionExtensions
{
public static bool IsEmpty<T>(this ICollection<T> collection)
{
return collection.Count == 0;
}
public static void AddRange<T>(this ICollection<T> collection, IEnumerable<T> values)
{
foreach (var value in values)
{
collection.Add(value);
}
}
}
}
<file_sep>using System;
namespace ChristmasTournament
{
class Program
{
static void Main(string[] args)
{
int days = int.Parse(Console.ReadLine());
double moneyRaisedThisDay = 0;
double allMoney = 0;
int countWinDay = 0;
int countLostDay = 0;
for (int i = 0; i < days; i++)
{
int countWins = 0;
int countLosses = 0;
moneyRaisedThisDay = 0;
string command = Console.ReadLine();
while (command != "Finish")
{
string result = Console.ReadLine();
if (result == "win")
{
moneyRaisedThisDay += 20;
countWins++;
}
else
{
countLosses++;
}
command = Console.ReadLine();
}
if (countWins > countLosses)
{
moneyRaisedThisDay += moneyRaisedThisDay * 0.1;
allMoney += moneyRaisedThisDay;
countWinDay++;
}
else
{
allMoney += moneyRaisedThisDay;
countLostDay++;
}
}
if(countWinDay > countLostDay)
{
allMoney += allMoney * 0.2;
Console.WriteLine($"You won the tournament! Total raised money: {allMoney:F2}");
}
else
{
Console.WriteLine($"You lost the tournament! Total raised money: {allMoney:F2}");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.NeedForSpeed.Motorcycles
{
public class RaceMotorcycle : Motorcycle
{
private const double defaultFuelConsumption = 8;
public RaceMotorcycle(int horsePower, double fuel) : base(horsePower, fuel)
{
}
public override double FuelConsumption => defaultFuelConsumption;
//{
// get
// {
// return this.FuelConsumption;
// }
// set
// {
// this.FuelConsumption = defaultFuelConsumption;
// }
//}
public override void Drive(double kilometers)
{
double fuelAfterDrive = this.Fuel - kilometers * this.FuelConsumption;
if (fuelAfterDrive >= 0)
{
this.Fuel -= kilometers * this.FuelConsumption;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace CounterStrike.Models.Guns
{
public class Pistol : Gun
{
private const int pistolFireBulletsCount = 1;
public Pistol(string name, int bulletsCount)
: base(name, bulletsCount)
{
}
public override int Fire()
{
return pistolFireBulletsCount;
}
}
}
<file_sep>using System;
namespace Special_Number
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
bool isSpecial = true;
for(int i=1111;i<9999;i++)
{
isSpecial = true;
string command = i.ToString();
for(int j=0;j<command.Length;j++)
{
int num = int.Parse(command[j].ToString());
if (num == 0)
{
isSpecial = false;
continue;
}
if (n%num!=0)
{
isSpecial = false;
break;
}
}
if(isSpecial)
{
Console.Write($"{i} ");
}
}
}
}
}
<file_sep>using System;
namespace Hotel_Reservation
{
class Program
{
static void Main(string[] args)
{
var calculator = new PriceCalculator();
calculator.Calculate(10.5, Season.Spring);
}
}
}
<file_sep>using System;
namespace _04.Food_for_Pets
{
class Program
{
static void Main(string[] args)
{
int countDays = int.Parse(Console.ReadLine());
double allFood = double.Parse(Console.ReadLine());
// double eatenFoodADay = 0;
double eatenByDog = 0;
double eatenByCat = 0;
double sumDog = 0;
double sumCat = 0;
double sumEaten = 0;
double biscuits = 0;
for(int i=1;i<=countDays;i++)
{
eatenByDog = double.Parse(Console.ReadLine());
sumDog += eatenByDog;
eatenByCat = double.Parse(Console.ReadLine());
sumCat += eatenByCat;
//eatenFoodADay = eatenByDog + eatenByCat;
sumEaten += eatenByCat + eatenByDog;
if(i%3==0)
{
biscuits += (eatenByDog + eatenByCat) * 0.1;
}
}
Console.WriteLine($"Total eaten biscuits: {Math.Round(biscuits)}gr.");
Console.WriteLine($"{sumEaten*100 / allFood:F2}% of the food has been eaten.");
Console.WriteLine($"{sumDog*100/sumEaten:F2}% eaten from the dog.");
Console.WriteLine($"{sumCat*100/sumEaten:F2}% eaten from the cat.");
}
}
}
<file_sep>using System;
using System.Linq;
namespace _01._Activation_Keys
{
class Program
{
static void Main(string[] args)
{
string activationKey = Console.ReadLine();
string input;
while((input = Console.ReadLine()) != "Generate")
{
string[] command = input.Split(">>>").ToArray();
if(command[0] == "Contains")
{
string substr = command[1];
if (activationKey.Contains(substr))
{
Console.WriteLine($"{activationKey} contains {substr}");
}
else
{
Console.WriteLine("Substring not found!");
}
}
else if(command[0] == "Flip")
{
if(command[1] == "Upper")
{
int startIndex = int.Parse(command[2]);
int endIndex = int.Parse(command[3]);
string oldString = activationKey.Substring(startIndex, endIndex - startIndex);
string newString = oldString.ToUpper();
activationKey = activationKey.Replace(oldString, newString);
}
else
{
int startIndex = int.Parse(command[2]);
int endIndex = int.Parse(command[3]);
string oldString = activationKey.Substring(startIndex, endIndex - startIndex);
string newString = oldString.ToLower();
activationKey = activationKey.Replace(oldString, newString);
}
Console.WriteLine(activationKey);
}
else
{
int startIndex = int.Parse(command[1]);
int endIndex = int.Parse(command[2]);
string oldString = activationKey.Substring(startIndex, endIndex - startIndex);
activationKey = activationKey.Replace(oldString, "");
Console.WriteLine(activationKey);
}
}
Console.WriteLine($"Your activation key is: {activationKey}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text.RegularExpressions;
namespace _02._Mirror_Words
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
//string hiddenPattern = @"((#|@)(?<word1>[A-Za-z]{3,})(\2))((\2)(?<word2>[A-Za-z]{3,})(\4))";
string hiddenPattern = @"(@|#)(?<first>[A-Za-z]{3,})\1\1(?<second>[A-Za-z]{3,})\1";
List<string> mirrorWords = new List<string>();
var matches = Regex.Matches(text, hiddenPattern);
if(matches.Count == 0)
{
Console.WriteLine("No word pairs found!");
}
else
{
Console.WriteLine($"{matches.Count} word pairs found!");
//Dictionary<string, string> mirrorWords = new Dictionary<string, string>();
foreach (Match match in matches)
{
string str1 = match.Groups["first"].Value;
string str2 = match.Groups["second"].Value;
char[] str2Arr = str2.ToCharArray();
Array.Reverse(str2Arr);
string str2InStringReversed = new string(str2Arr);
if(str1 == str2InStringReversed)
{
string mirrorWord = str1 + " <=> " + str2;
mirrorWords.Add(mirrorWord);
}
}
}
if (mirrorWords.Count != 0)
{
Console.WriteLine("The mirror words are:");
Console.WriteLine(String.Join(", ", mirrorWords));
}
else
{
Console.WriteLine("No mirror words!");
}
}
}
}
<file_sep>using System;
namespace Class_Enum
{
class Program
{
static void Main(string[] args)
{
int[] arr = { 1, 2, 3, 4 ,6};
Console.WriteLine(arr.Length);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _08.Vehicle_Catalogue
{
class Truck
{
public string Brand { get; set; }
public string Model { get; set; }
public int Weight { get; set; }
}
class Car
{
public string Brand { get; set; }
public string Model { get; set; }
public int HorsePower { get; set; }
}
class CatalogVehicle
{
public CatalogVehicle()
{
Cars = new List<Car>();
Trucks = new List<Truck>();
}
public List<Car> Cars { get; set; }
public List<Truck> Trucks { get; set; }
}
class Program
{
static void Main(string[] args)
{
CatalogVehicle catalog = new CatalogVehicle();
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] info = input.Split("/");
string brand = info[1];
string model = info[2];
int HorsePowerOrWeight = int.Parse(info[3]);
if(info[0] == "Car")
{
Car car = new Car();
car.Brand = brand;
car.Model = model;
car.HorsePower = HorsePowerOrWeight;
catalog.Cars.Add(car);
}
else
{
Truck truck = new Truck();
truck.Brand = brand;
truck.Model = model;
truck.Weight = HorsePowerOrWeight;
catalog.Trucks.Add(truck);
}
}
if (catalog.Cars.Count > 0)
{
Console.WriteLine("Cars:");
foreach (Car car in catalog.Cars.OrderBy(x => x.Brand))
{
Console.WriteLine($"{car.Brand}: {car.Model} - {car.HorsePower}hp");
}
}
if (catalog.Trucks.Count > 0)
{
Console.WriteLine("Trucks:");
foreach (Truck truck in catalog.Trucks.OrderBy(x => x.Brand))
{
Console.WriteLine($"{truck.Brand}: {truck.Model} - {truck.Weight}kg");
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P07.MilitaryElite.Exceptions
{
public class InvalidCorpsException : Exception
{
private const string DEF_MESS = "Ivalid corps";
public InvalidCorpsException()
:base(DEF_MESS)
{
}
public InvalidCorpsException(string message)
: base(message)
{
}
// public static string
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P06.FoodShortage
{
public interface IBirthable
{
string GetBirth();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Collections.Specialized;
using System.Linq;
namespace _10._Predicate_Party_
{
class Program
{
static void Main(string[] args)
{
List<string> names = Console.ReadLine()
.Split()
.ToList();
string command;
while((command = Console.ReadLine()) != "Party!")
{
string[] cmdArgs = command.Split();
string cmdType = cmdArgs[0];
string[] predicateArgs = cmdArgs
.Skip(1)
.ToArray();
Predicate<string> predicate = GetPredicate(predicateArgs);
if(cmdType == "Remove")
{
names.RemoveAll(predicate);
}
else if(cmdType == "Double")
{
DoubleNames(names, predicate);
}
}
if(names.Count == 0)
{
Console.WriteLine("Nobody is going to the party!");
}
else
{
Console.WriteLine($"{string.Join(", ",names)} are going to the party!");
}
}
private static void DoubleNames(List<string> names, Predicate<string> predicate)
{
for (int i = 0; i < names.Count; i++)
{
string currName = names[i];
if (predicate(currName))
{
names.Insert(i + 1, currName);
i++;
}
}
}
static Predicate<string> GetPredicate(string[] predicateArgs)
{
string prType = predicateArgs[0];
string prArg = predicateArgs[1];
Predicate<string> predicate = null;
if (prType == "StartsWith")
{
predicate = new Predicate<string>((name) =>
{
return name.StartsWith(prArg);
});
}
else if(prType == "EndsWith")
{
predicate = new Predicate<string>((name) =>
{
return name.EndsWith(prArg);
});
}
else if(prType == "Length")
{
predicate = new Predicate<string>((name) =>
{
return name.Length == int.Parse(prArg);
});
}
return predicate;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _01._Flower_Wreaths
{
class Program
{
static void Main(string[] args)
{
Stack<int> lilies = new Stack<int>(Console.ReadLine().Split(", ").Select(int.Parse).ToArray());
Queue<int> roses = new Queue<int>(Console.ReadLine().Split(", ").Select(int.Parse).ToArray());
int count_wraiths = 0;
int stored_flowers = 0;
while(lilies.Any() && roses.Any())
{
int sum = lilies.Peek() + roses.Peek();
if(sum == 15)
{
count_wraiths++;
lilies.Pop();
roses.Dequeue();
}
else if(sum > 15)
{
int to_push = lilies.Pop() - 2;
lilies.Push(to_push);
}
else if(sum < 15)
{
lilies.Pop();
roses.Dequeue();
stored_flowers += sum;
}
}
while(stored_flowers > 0)
{
if(stored_flowers - 15 >= 0)
{
count_wraiths++;
stored_flowers -= 15;
}
else
{
break;
}
}
if(count_wraiths >= 5)
{
Console.WriteLine($"You made it, you are going to the competition with {count_wraiths} wreaths!");
}
else
{
Console.WriteLine($"You didn't make it, you need {5-count_wraiths} wreaths more!");
}
}
}
}
<file_sep>using P01.Vehicles.Common;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Text;
namespace P01.Vehicles.Models
{
public class Truck : Vehicle
{
private const double FUEL_CONS_INCREMENT = 1.6;
private const double REFUEL_EFIICIENCY_PERCENTSGE = 0.95;
public Truck(double fuelquantity, double fuelconsumption, double tankCapacity)
: base(fuelquantity, fuelconsumption, tankCapacity)
{
}
public override double FuelConsumption
{
get
{
return base.FuelConsumption;
}
protected set
{
base.FuelConsumption = value + FUEL_CONS_INCREMENT;
}
}
public override void Refuel(double liters)
{
double litersIn = liters * REFUEL_EFIICIENCY_PERCENTSGE;
if(liters > 0)
{
if (this.FuelQuantity + litersIn > this.TankCapacity)
{
string exc = string.Format(ExceptionMessage
.InvalidFuelTankExceptionMessage, liters);
throw new InvalidOperationException(exc);
}
this.FuelQuantity += litersIn;
}
else
{
throw new InvalidOperationException(ExceptionMessage.FuelIsNegativeOrZero);
}
}
}
}
<file_sep>using System;
namespace SupppliesForSchool
{
class Program
{
static void Main(string[] args)
{
int countPen = int.Parse(Console.ReadLine());
int countMarkers = int.Parse(Console.ReadLine());
double litre = double.Parse(Console.ReadLine());
int countSale = int.Parse(Console.ReadLine());
double neededMoney = countPen * 5.80 + countMarkers * 7.20 + litre * 1.20;
neededMoney = neededMoney - ((neededMoney * countSale) / 100);
Console.WriteLine($"{neededMoney:F3}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Hotel_Reservation
{
class PriceCalculator
{
public decimal Calculate(decimal pricePerDay, int days ,Season season,Discount discount = Discount.None)
{
var price = days * pricePerDay * (int)season;
price -= (int)discount * price/100;
return price;
}
}
}
<file_sep>using System;
using System.Linq;
using System.Text;
namespace P02.BookWorm
{
class Program
{
static void Main(string[] args)
{
string receivedString = Console.ReadLine();
StringBuilder initialString = new StringBuilder();
initialString.Append(receivedString);
int N = int.Parse(Console.ReadLine());
char[,] field = new char[N, N];
InitField(N, field);
int playerRow = -1;
int playerCol = -1;
//Finding player's row and col
FindPlayerRowCol(N, field, ref playerRow, ref playerCol);
string command;
while ((command = Console.ReadLine()) != "end")
{
if (command == "up")
{
if (playerRow - 1 < 0)
{
initialString.Remove(initialString.Length - 1, 1);
}
else
{
field[playerRow, playerCol] = '-';
char currChar = field[playerRow - 1, playerCol];
if (char.IsLetter(currChar))
{
initialString.Append(currChar);
}
field[playerRow - 1, playerCol] = 'P';
playerRow -= 1;
}
}
else if (command == "down")
{
if (playerRow + 1 == N)
{
initialString.Remove(initialString.Length - 1, 1);
}
else
{
field[playerRow, playerCol] = '-';
char currChar = field[playerRow + 1, playerCol];
if (char.IsLetter(currChar))
{
initialString.Append(currChar);
}
field[playerRow + 1, playerCol] = 'P';
playerRow += 1;
}
}
else if (command == "left")
{
if (playerCol - 1 < 0)
{
initialString.Remove(initialString.Length - 1, 1);
}
else
{
field[playerRow, playerCol] = '-';
char currChar = field[playerRow, playerCol - 1];
if (char.IsLetter(currChar))
{
initialString.Append(currChar);
}
field[playerRow, playerCol - 1] = 'P';
playerCol -= 1;
}
}
else if (command == "right")
{
if (playerCol + 1 == N)
{
initialString.Remove(initialString.Length - 1, 1);
}
else
{
field[playerRow, playerCol] = '-';
char currChar = field[playerRow, playerCol + 1];
if (char.IsLetter(currChar))
{
initialString.Append(currChar);
}
field[playerRow, playerCol + 1] = 'P';
playerCol += 1;
}
}
}
Console.WriteLine(initialString);
PrintMatrix(N, field);
}
private static void PrintMatrix(int N, char[,] field)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
Console.Write($"{field[row, col]}");
}
Console.WriteLine();
}
}
private static void FindPlayerRowCol(int N, char[,] field, ref int playerRow, ref int playerCol)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
if (field[row, col] == 'P')
{
playerRow = row;
playerCol = col;
}
}
}
}
private static void InitField(int N, char[,] field)
{
for (int row = 0; row < N; row++)
{
string currRow = Console.ReadLine();
for (int col = 0; col < N; col++)
{
field[row, col] = currRow[col];
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
namespace P01.GenericBoxofString
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
List<double> elements = new List<double>();
for (int i = 0; i < n; i++)
{
double element = double.Parse(Console.ReadLine());
elements.Add(element);
//Box<int> box = new Box<int>(value);
//Console.WriteLine(box);
}
Box<double> box = new Box<double>(elements);
double elementToCompare = double.Parse(Console.ReadLine());
Console.WriteLine(box.CountGreaterElements(elements,elementToCompare));
//int[] indexesToSwap = Console.ReadLine()
// .Split()
// .Select(int.Parse)
// .ToArray();
//box.SwapElementsByIndex(elements, indexesToSwap[0], indexesToSwap[1]);
//Console.WriteLine(box);
}
}
}
<file_sep>using System;
namespace _09._Palindrome_Integers
{
class Program
{
static void Main(string[] args)
{
while (true)
{
string command = Console.ReadLine();
if(command == "END")
{
break;
}
int num = int.Parse(command);
IsPalindrome(num);
}
}
static void IsPalindrome(int n)
{
string str = n.ToString();
string res = "";
int len = str.Length - 1;
while(len >= 0)
{
res += str[len];
len--;
}
if(str == res)
{
Console.WriteLine("true");
}
else
{
Console.WriteLine("false");
}
}
}
}
<file_sep>using System;
namespace _05.LoadSuitcases
{
class Program
{
static void Main(string[] args)
{
double capacity = double.Parse(Console.ReadLine());
string command = Console.ReadLine();
int countluggage = 0;
bool noSpace = false;
while(command != "End")
{
double capSuitcase = double.Parse(command);
if((countluggage+1)%3==0)
{
capSuitcase = capSuitcase + capSuitcase * 0.1;
}
if(capacity<capSuitcase)
{
noSpace = true;
break;
}
capacity = capacity - capSuitcase;
countluggage++;
command = Console.ReadLine();
}
if(command=="End")
{
Console.WriteLine("Congratulations! All suitcases are loaded!");
}
if(noSpace == true)
{
Console.WriteLine("No more space!");
}
Console.WriteLine($"Statistic: {countluggage} suitcases loaded.");
// Console.WriteLine(capacity);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Diagnostics.CodeAnalysis;
using System.Text;
namespace P05.ComparingObjects
{
public class Person : IComparable<Person>
{
public Person(string name,int age , string town)
{
this.Name = name;
this.Age = age;
this.Town = town;
}
public string Name { get; set; }
public int Age { get; set; }
public string Town { get; set; }
public int CompareTo([AllowNull] Person person)
{
int result = 1;
if(person != null)
{
result = this.Name.CompareTo(person.Name);
if(result == 0)
{
result = this.Age.CompareTo(person.Age);
if(result == 0)
{
result = this.Town.CompareTo(this.Town);
}
}
}
return result;
}
}
}
<file_sep>using System;
namespace Concatanate
{
class Program
{
static void Main(string[] args)
{
string firstname = Console.ReadLine();
string lastname = Console.ReadLine();
int age = int.Parse(Console.ReadLine());
string grad = Console.ReadLine();
// Console.WriteLine("You are"+ firstname +lastname, a -years old person from <town>.");
Console.WriteLine($"You are {firstname} {lastname}, a {age}-years old person from {grad}.");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public class CatOnJet : Vehicle
{
public string Name { get; set; }
public override string VrumVrum()
{
return "Cat is meowing";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _08._Balanced_Parenthesis
{
class Program
{
static void Main(string[] args)
{
string input = Console.ReadLine();
Stack<char> parenthessStack = new Stack<char>();
foreach (var symbol in input)
{
if (parenthessStack.Any())
{
char check = parenthessStack.Peek();
if(check == '{' && symbol == '}')
{
parenthessStack.Pop();
continue;
}
else if(check == '[' && symbol == ']')
{
parenthessStack.Pop();
continue;
}
else if(check == '(' && symbol == ')')
{
parenthessStack.Pop();
continue;
}
}
parenthessStack.Push(symbol);
}
Console.WriteLine(parenthessStack.Any() ? "NO" : "YES");
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
namespace P03.Stack
{
public class Stack<T> : IEnumerable<T>
{
private readonly List<T> data;
public Stack()
{
this.data = new List<T>();
}
public void Push(IEnumerable<T> elements)
{
foreach (var element in elements)
{
this.Push(element);
}
}
public void Push(T element)
{
this.data.Add(element);
}
public T Pop()
{
if(this.data.Count == 0)
{
throw new InvalidOperationException("No elements");
}
T removedElement = this.data[this.data.Count - 1];
this.data.RemoveAt(this.data.Count - 1);
return removedElement;
}
public IEnumerator<T> GetEnumerator()
{
for(int i = this.data.Count - 1;i >= 0; i--)
{
yield return this.data[i];
}
}
IEnumerator IEnumerable.GetEnumerator()
{
return this.GetEnumerator();
}
}
}
<file_sep>using System;
namespace _01._Bonus_Scoring_System
{
class Program
{
static void Main(string[] args)
{
int countStudents = int.Parse(Console.ReadLine());
int countLectures = int.Parse(Console.ReadLine());
int initialBonus = int.Parse(Console.ReadLine());
double maxBonus = 0;
int maxAttend = 0;
for (int i = 0; i < countStudents; i++)
{
int currAttendance = int.Parse(Console.ReadLine());
double currBonus = ((1.0*currAttendance / countLectures)) * (5 + initialBonus);
if(currBonus > maxBonus)
{
maxBonus = currBonus;
maxAttend = currAttendance;
}
}
Console.WriteLine($"Max Bonus: {Math.Ceiling(maxBonus)}.");
Console.WriteLine($"The student has attended {maxAttend} lectures.");
}
}
}
<file_sep>using System;
namespace TrekkingMania
{
class Program
{
static void Main(string[] args)
{
double countMus = 0;
double countMon = 0;
double countKili = 0;
double countK2 = 0;
double countEver = 0;
double peopleCount = 0;
int groups = int.Parse(Console.ReadLine());
for(int i=0;i<groups;i++)
{
int people = int.Parse(Console.ReadLine());
peopleCount += people;
if (people <= 5)
{
countMus += people;
}
else if (people >= 6 && people <= 12)
{
countMon += people;
}
else if (people >= 13 && people <= 25)
{
countKili += people;
}
else if (people >= 26 && people <= 40)
{
countK2 += people;
}
else if(people>=41)
{
countEver += people;
}
}
Console.WriteLine($"{countMus/peopleCount*100:F2}%");
Console.WriteLine($"{countMon / peopleCount * 100:F2}%");
Console.WriteLine($"{countKili / peopleCount * 100:F2}%");
Console.WriteLine($"{countK2 / peopleCount * 100:F2}%");
Console.WriteLine($"{countEver / peopleCount * 100:F2}%");
}
}
}
<file_sep>using System;
namespace MultiplicationTable
{
class Program
{
static void Main(string[] args)
{
for(int i=1;i<=10;i++)
{
for(int j=1;j<=10;j++)
{
Console.WriteLine($"{i} * {j} = {i*j}");
}
}
}
}
}
<file_sep>using System;
namespace _01._Computer_Store
{
class Program
{
static void Main(string[] args)
{
double totalPrice = 0;
double totalTaxes = 0;
string input;
while((input = Console.ReadLine()) != "special" && input != "regular")
{
double currPrice = double.Parse(input);
if (currPrice < 0)
{
Console.WriteLine("Invalid price!");
}
else
{
totalTaxes += currPrice * 0.2;
//currPrice += currPrice * 0.2;
totalPrice += currPrice;
}
}
double totalPriceWithDisc = totalPrice + totalTaxes;
if(input == "special")
{
totalPriceWithDisc -= (totalPrice + totalTaxes) * 0.1;
}
if(totalPrice == 0)
{
Console.WriteLine("Invalid order!");
}
else
{
Console.WriteLine($"Congratulations you've just bought a new computer!\n" +
$"Price without taxes: {totalPrice:F2}$\n" +
$"Taxes: {totalTaxes:F2}$\n" +
$"-----------\n" +
$"Total price: {totalPriceWithDisc:F2}$");
}
}
}
}
<file_sep>using System;
namespace _10._Radioactive_Mutant_Vampire_Bunnies
{
class Program
{
static void Main(string[] args)
{
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _06._Vehicle_Catalogue
{
class Program
{
static void Main(string[] args)
{
List<Vehicle> catalogue = new List<Vehicle>();
while (true)
{
string input = Console.ReadLine();
if(input == "End")
{
break;
}
string[] command = input.Split();
string type = command[0];
string model = command[1];
string color = command[2];
int horsePower = int.Parse(command[3]);
Vehicle vehicle = new Vehicle(type, model, color, horsePower);
catalogue.Add(vehicle);
}
while (true)
{
string input = Console.ReadLine();
if(input == "Close the Catalogue")
{
break;
}
Vehicle vehicleWithThatModel = catalogue.Find(v => v.Model == input);
Console.WriteLine(vehicleWithThatModel.ToString());
}
List<Vehicle> onlyCars = catalogue.Where(x => x.Type == "car").ToList();
List<Vehicle> onlyTrucks = catalogue.Where(x => x.Type == "truck").ToList();
double sumCarsHorse = 0;
double sumTrucksHorse = 0;
foreach (Vehicle car in onlyCars)
{
sumCarsHorse += car.HorsePower;
}
foreach (Vehicle truck in onlyTrucks)
{
sumTrucksHorse += truck.HorsePower;
}
double averageCars = sumCarsHorse / onlyCars.Count;
double averageTrucks = sumTrucksHorse / onlyTrucks.Count;
// Console.WriteLine($"Cars have average horsepower of: {Math.Round(averageCars,2):F2}.");
// Console.WriteLine($"Trucks have average horsepower of: {Math.Round(averageTrucks, 2):F2}.");
if (onlyCars.Count > 0)
{
Console.WriteLine($"Cars have average horsepower of: {Math.Round(averageCars, 2):F2}.");
}
else
{
Console.WriteLine($"Cars have average horsepower of: {0:f2}.");
}
if (onlyTrucks.Count > 0)
{
Console.WriteLine($"Trucks have average horsepower of: {Math.Round(averageTrucks, 2):F2}.");
}
else
{
Console.WriteLine($"Trucks have average horsepower of: {0:f2}.");
}
}
}
class Vehicle
{
public Vehicle(string type,string model,string color,int horsePower)
{
this.Type = type;
this.Model = model;
this.Color = color;
this.HorsePower = horsePower;
}
public string Type { get; set; }
public string Model { get; set; }
public string Color { get; set; }
public int HorsePower { get; set; }
public override string ToString()
{
if (this.Type == "car")
{
return $"Type: Car\n" +
$"Model: {this.Model}\n" +
$"Color: {this.Color}\n" +
$"Horsepower: {this.HorsePower}\n".Trim();
}
else
{
return $"Type: Truck\n" +
$"Model: {this.Model}\n" +
$"Color: {this.Color}\n" +
$"Horsepower: {this.HorsePower}\n".Trim();
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P05.DateModifier
{
public class DateModifier
{
public int CalculateDifference(string data1,string data2)
{
var date1Arr = data1.Split()
.Select(int.Parse)
.ToArray();
DateTime dateTime1 = new DateTime(date1Arr[0], date1Arr[1], date1Arr[2]);
var date2Arr = data2.Split()
.Select(int.Parse)
.ToArray();
DateTime dateTime2 = new DateTime(date2Arr[0], date2Arr[1], date2Arr[2]);
return Math.Abs((dateTime1 - dateTime2).Days);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _04.Songs
{
class Song
{
///Type list // Name // Duration
public string TypeList { get; set; }
public string Name { get; set; }
public string Time { get; set; }
}
class Program
{
static void Main(string[] args)
{
///
//3
//favourite_DownTown_3: 14
//favourite_Kiss_4: 16
//favourite_Smooth Criminal_4:01
//favourite
int n = int.Parse(Console.ReadLine());
List<Song> songs = new List<Song>();
for (int i = 0; i < n; i++)
{
string[] input = Console.ReadLine()
.Split('_');
string typeList = input[0];
string name = input[1];
string time = input[2];
Song song = new Song();
song.TypeList = typeList;
song.Name = name;
song.Time = time;
songs.Add(song);
}
string targetListType = Console.ReadLine();
if (targetListType == "all")
{
for (int i = 0; i < songs.Count; i++)
{
Console.WriteLine(songs[i].Name);
}
}
else
{
for (int i = 0; i < songs.Count; i++)
{
if (songs[i].TypeList == targetListType)
{
Console.WriteLine(songs[i].Name);
}
}
///2nd example
//songs = songs.FindAll(x => x.TypeList == targetListType);
//foreach (var currSong in songs)
//{
// Console.WriteLine(currSong.Name);
//}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _8._Bombs
{
class Program
{
static void Main(string[] args)
{
int N = int.Parse(Console.ReadLine());
int[,] matrix = new int[N, N];
InicialisingMatrix(N, matrix);
string[] bombInfo = Console.ReadLine().Split().ToArray();
for (int i = 0; i < bombInfo.Length; i++)
{
int[] bombArgs = bombInfo[i]
.Split(',', StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
int row = bombArgs[0];
int col = bombArgs[1];
if (matrix[row, col] <= 0)
{
continue;
}
int damage = matrix[row, col];
matrix[row, col] = 0;
if (row - 1 >= 0)
{
if (IsAlive(matrix, row - 1, col))
{
matrix[row - 1, col] -= damage;
}
}
if (row + 1 < N)
{
if (IsAlive(matrix, row + 1, col))
{
matrix[row + 1, col] -= damage;
}
}
if (col - 1 >= 0)
{
if (IsAlive(matrix, row, col - 1))
{
matrix[row, col - 1] -= damage;
}
}
if (col + 1 < N)
{
if (IsAlive(matrix, row, col + 1))
{
matrix[row, col + 1] -= damage;
}
}
if (col - 1 >= 0 && row - 1 >= 0)
{
if (IsAlive(matrix, row - 1, col - 1))
{
matrix[row - 1, col - 1] -= damage;
}
}
if (col + 1 < N && row - 1 >= 0)
{
if (IsAlive(matrix, row - 1, col + 1))
{
matrix[row - 1, col + 1] -= damage;
}
}
if (row + 1 < N && col - 1 >= 0)
{
if (IsAlive(matrix, row + 1, col - 1))
{
matrix[row + 1, col - 1] -= damage;
}
}
if (row + 1 < N && col + 1 < N)
{
if (IsAlive(matrix, row + 1, col + 1))
{
matrix[row + 1, col + 1] -= damage;
}
}
}
int aliveCells = 0;
int sumOfAliveCells = 0;
aliveCells = FindingAliveCells(N, matrix, aliveCells);
sumOfAliveCells = FindingSumOfAliveCells(N, matrix, sumOfAliveCells);
Console.WriteLine($"Alive cells: {aliveCells}");
Console.WriteLine($"Sum: {sumOfAliveCells}");
PrintMatrix(N, matrix);
}
private static int FindingSumOfAliveCells(int N, int[,] matrix, int sumOfAliveCells)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
if (IsAlive(matrix, row, col))
{
sumOfAliveCells += matrix[row, col];
}
}
}
return sumOfAliveCells;
}
private static int FindingAliveCells(int N, int[,] matrix, int aliveCells)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
if (IsAlive(matrix, row, col))
{
aliveCells++;
}
}
}
return aliveCells;
}
private static bool IsAlive(int [,] matrix , int row , int col)
{
if(matrix[row,col] > 0)
{
return true;
}
return false;
}
private static void PrintMatrix(int N, int[,] matrix)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
Console.Write(matrix[row, col] + " ");
}
Console.WriteLine();
}
}
private static void InicialisingMatrix(int N, int[,] matrix)
{
for (int row = 0; row < N; row++)
{
int[] currRow = Console.ReadLine()
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
for (int col = 0; col < N; col++)
{
matrix[row, col] = currRow[col];
}
}
}
}
}
<file_sep>using FightingArena;
using NUnit.Framework;
using System;
namespace Tests
{
public class ArenaTests
{
private Arena arena;
private Warrior w1;
private Warrior attacker;
private Warrior defender;
[SetUp]
public void Setup()
{
arena = new Arena();
w1 = new Warrior("Vlado",5,50);
attacker = new Warrior("Pesho", 10, 80);
defender = new Warrior("Gosho", 5, 60);
}
[Test]
public void TestIfConstructorWorksCorrectly()
{
Assert.IsNotNull(this.arena.Warriors);
}
[Test]
public void EnrollShouldAddTheWarriorToTheArenaAndIncreaseCount()
{
arena.Enroll(w1);
Assert.That(this.arena.Warriors, Has.Member(w1));
Assert.AreEqual(1, arena.Warriors.Count);
}
[Test]
public void EnrollingSameWarriorShouldThrowException()
{
arena.Enroll(w1);
Assert.Throws<InvalidOperationException>(() =>
{
arena.Enroll(w1);
});
}
[Test]
public void EnrollingSameWarriorShouldThrowException2()
{
arena.Enroll(w1);
Warrior w1Copy = new Warrior(w1.Name, w1.Damage, w1.HP);
Assert.Throws<InvalidOperationException>(() =>
{
arena.Enroll(w1Copy);
});
}
[Test]
public void TestFightingWithMissingAttacker()
{
arena.Enroll(defender);
Assert.Throws<InvalidOperationException>(() =>
{
arena.Fight(attacker.Name, defender.Name);
});
}
[Test]
public void TestFightingWithMissingDefender()
{
arena.Enroll(attacker);
Assert.Throws<InvalidOperationException>(() =>
{
arena.Fight(attacker.Name, defender.Name);
});
}
[Test]
public void TestFightingBetweenTwoWarrioirs()
{
int expectedAHP = attacker.HP - defender.Damage;
int expectedDHP = defender.HP - attacker.Damage;
arena.Enroll(attacker);
arena.Enroll(defender);
arena.Fight(attacker.Name, defender.Name);
Assert.AreEqual(expectedAHP, attacker.HP);
Assert.AreEqual(defender.HP, defender.HP);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Stack_of_Strings
{
class StackOfStrings:Stack<string>
{
public bool IsEmpty()
{
return this.Count == 0;
}
public void AddRange(IEnumerable<string> values)
{
foreach (var value in values)
{
this.Push(value);
}
}
}
}
<file_sep>using System;
using System.IO.Compression;
namespace _06.ZipAndExtract
{
class Program
{
static void Main(string[] args)
{
try
{
ZipFile.CreateFromDirectory(".","myArchive.zip");
// ZipFile.ExtractToDirectory()
}
catch (Exception)
{
throw;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _05._Fashion_Boutique
{
class Program
{
static void Main(string[] args)
{
int[] clothes = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
Stack<int> stack = new Stack<int>(clothes);
int rackCapacity = int.Parse(Console.ReadLine());
int racksCount = 1;
int sumOfClothes = 0;
while (stack.Any())
{
sumOfClothes += stack.Peek();
if(sumOfClothes < rackCapacity)
{
stack.Pop();
}
else if(sumOfClothes == rackCapacity)
{
//racksCount++;
stack.Pop();
//sumOfClothes = stack.Pop();
}
else
{
racksCount++;
sumOfClothes = stack.Pop();
}
}
Console.WriteLine(racksCount);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _01._The_Fight_for_Gondor
{
class Program
{
static void Main(string[] args)
{
int countWaves = int.Parse(Console.ReadLine());
Queue<int> plates = new Queue<int>(Console.ReadLine().Split(" ", StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray());
bool isDestr = false;
for (int i = 1; i <= countWaves; i++)
{
Stack<int> orcs = new Stack<int>(Console.ReadLine().Split(" ", StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray());
if (i % 3 == 0)
{
plates.Enqueue(int.Parse(Console.ReadLine()));
}
while (orcs.Any() && plates.Any())
{
if (orcs.Peek() > plates.Peek())
{
orcs.Push(orcs.Pop() - plates.Peek());
plates.Dequeue();
if (orcs.Peek() <= 0)
{
orcs.Pop();
}
}
else if (orcs.Peek() < plates.Peek())
{
plates.Enqueue(plates.Dequeue() - orcs.Peek());
for (int j = 0; j < plates.Count-1; j++)
{
plates.Enqueue(plates.Dequeue());
}
orcs.Pop();
}
else if (orcs.Peek() == plates.Peek())
{
orcs.Pop();
plates.Dequeue();
}
}
if (!plates.Any())
{
Console.WriteLine("The orcs successfully destroyed the Gondor's defense.");
isDestr = true;
if (orcs.Any())
{
Console.WriteLine($"Orcs left: {string.Join(", ", orcs)}");
}
break;
}
}
if (!isDestr)
{
Console.WriteLine("The people successfully repulsed the orc's attack.");
}
if (plates.Any())
{
Console.WriteLine($"Plates left: {string.Join(", ", plates)}");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace ReverseStringUsingStack
{
class Program
{
static void Main(string[] args)
{
var input = Console.ReadLine();
Stack<char> stack = new Stack<char>(input);
//foreach (var symbol in input)
//{
// stack.Push(symbol);
//}
while(stack.Any())
{
Console.Write(stack.Pop());
}
}
}
}
<file_sep>using System;
namespace SumOfTwoNumbers
{
class Program
{
static void Main(string[] args)
{
int first = int.Parse(Console.ReadLine());
int last = int.Parse(Console.ReadLine());
int magicNum = int.Parse(Console.ReadLine());
bool foundComb = false;
int combCounter = 0;
for(int i = first;i<=last;i++)
{
for(int j=first;j<=last;j++)
{
combCounter++;
if((i+j)==magicNum)
{
Console.WriteLine($"Combination N:{combCounter} ({i} + {j} = {magicNum})");
foundComb = true;
break;
}
}
if(foundComb)
{
break;
}
}
if(!foundComb)
{
Console.WriteLine($"{ combCounter} combinations - neither equals { magicNum}");
}
}
}
}
<file_sep>using P06.FoodShortage.Contracts;
namespace P06.FoodShortage
{
public class Citizen : IIdentifiable,IBirthable,IBuyer
{
//private string name;
private int age;
private string id;
private string birthdate;
private int food = 0;
public Citizen(string name, int age, string id,string birthdate)
{
this.Name = name;
this.age = age;
this.id = id;
this.birthdate = birthdate;
}
public string Name { get; }
public int Food
{
get
{
return this.food;
}
private set
{
this.food = value;
}
}
public void BuyFood()
{
this.Food += 10;
}
public string GetBirth()
{
return this.birthdate;
}
//public string Name { get; }
//public int Age { get; }
//public string Id { get; }
public string GetId()
{
return this.id;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _11._Key_Revolver
{
class Program
{
static void Main(string[] args)
{
int bulletPrice = int.Parse(Console.ReadLine());
int sizeGunBarrel = int.Parse(Console.ReadLine());
int[] bulletsInfo = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
int[] locksInfo = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
int intelligenceValue = int.Parse(Console.ReadLine());
Stack<int> bullets = new Stack<int>(bulletsInfo);
Queue<int> locks = new Queue<int>(locksInfo);
int countShooting = 0;
int bulletsCost = 0;
while(bullets.Any() && locks.Any())
{
int currBullet = bullets.Pop();
countShooting++;
if(currBullet <= locks.Peek())
{
Console.WriteLine("Bang!");
locks.Dequeue();
}
else
{
Console.WriteLine("Ping!");
}
if (countShooting == sizeGunBarrel && bullets.Any())
{
Console.WriteLine("Reloading!");
countShooting = 0;
}
bulletsCost += bulletPrice;
}
if (!locks.Any())
{
Console.WriteLine($"{bullets.Count} bullets left. Earned " +
$"${intelligenceValue - bulletsCost}");
}
else
{
Console.WriteLine($"Couldn't get through. Locks left: {locks.Count}");
}
}
}
}
<file_sep>using System;
using System.Text;
namespace _01._Email_Validator
{
class Program
{
static void Main(string[] args)
{
string email = Console.ReadLine();
while (true)
{
string input = Console.ReadLine();
if (input == "Complete")
{
break;
}
string[] command = input.Split();
switch (command[0])
{
case "Make":
if (command[1] == "Upper")
{
email = email.ToUpper();
Console.WriteLine(email);
}
else
{
email = email.ToLower();
Console.WriteLine(email);
}
break;
case "GetDomain":
int count = int.Parse(command[1]);
string sub = email.Substring(email.Length-count);
Console.WriteLine(sub);
break;
case "GetUsername":
if (email.Contains('@'))
{
int ind = email.IndexOf('@');
StringBuilder sb = new StringBuilder();
foreach (char c in email)
{
if (c == '@')
{
break;
}
sb.Append(c);
}
Console.WriteLine(sb);
}
else
{
Console.WriteLine($"The email {email} doesn't contain the @ symbol.");
}
break;
case "Replace":
char toReplace = char.Parse(command[1]);
email = email.Replace(toReplace, '-');
Console.WriteLine(email);
break;
case "Encrypt":
for (int i = 0; i < email.Length; i++)
{
char currCh = email[i];
Console.Write($"{(int)currCh} ");
}
break;
}
}
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace _12._Cups_and_Bottles
{
class Program
{
static void Main(string[] args)
{
int[] cupsInfo = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
int[] bottlesInfo = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
Queue<int> cups = new Queue<int>(cupsInfo);
Stack<int> bottles = new Stack<int>(bottlesInfo);
int wastedWater = 0;
while(cups.Any() && bottles.Any())
{
int currCup = cups.Peek();
int currBottle = bottles.Pop();
if (currBottle >= currCup)
{
cups.Dequeue();
wastedWater += currBottle - currCup;
}
else
{
currCup -= currBottle;
cups.Dequeue();
// cups.Enqueue(currCup);
while (true)
{
int nowBottle = bottles.Pop();
if (nowBottle < currCup)
{
currCup -= nowBottle;
}
else
{
wastedWater += nowBottle - currCup;
break;
}
}
}
}
if (!cups.Any())
{
Console.WriteLine($"Bottles: {string.Join(" ",bottles)}");
}
else
{
Console.WriteLine($"Cups: {string.Join(" ", cups)}");
}
Console.WriteLine($"Wasted litters of water: {wastedWater}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace CountUpperCaseWords
{
class Program
{
static void Main(string[] args)
{
Console
.ReadLine()
.Split()
.Where(word => char.IsUpper(word[0]))
.ToList()
.ForEach(Console.WriteLine);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P05.BirthdayCelebrations.Contracts
{
public interface IBirthable
{
string GetBirth();
}
}
<file_sep>using System;
using System.Linq;
namespace _02._MuOnline
{
class Program
{
static void Main(string[] args)
{
int initialHealth = 100;
int initialBitcoins = 0;
string[] dungeons = Console.ReadLine().Split('|', StringSplitOptions.RemoveEmptyEntries).ToArray();
int roomCount = 0;
for(int i = 0; i < dungeons.Length; i++)
{
roomCount++;
string[] currDungeon = dungeons[i].Split().ToArray();
string command = currDungeon[0];
if(command == "potion")
{
int amount = int.Parse(currDungeon[1]);
if(initialHealth < 100)
{
if(initialHealth + amount > 100)
{
Console.WriteLine($"You healed for {100 - initialHealth} hp.");
initialHealth = 100;
}
else
{
initialHealth += amount;
Console.WriteLine($"You healed for {amount} hp.");
}
}
Console.WriteLine($"Current health: {initialHealth} hp.");
}
else if(command == "chest")
{
int amount = int.Parse(currDungeon[1]);
initialBitcoins += amount;
Console.WriteLine($"You found {amount} bitcoins.");
}
else
{
int monsterAttack = int.Parse(currDungeon[1]);
initialHealth -= monsterAttack;
if(initialHealth > 0)
{
Console.WriteLine($"You slayed {command}.");
}
else
{
Console.WriteLine($"You died! Killed by {command}.");
Console.WriteLine($"Best room: {roomCount}");
return;
}
}
}
Console.WriteLine($"You've made it!");
Console.WriteLine($"Bitcoins: {initialBitcoins}");
Console.WriteLine($"Health: {initialHealth}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03.Numbers_2_
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine()
.Split(' ', StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToList();
var modifiedList = list
.Where(x => x > list.Average())
.OrderByDescending(x => x)
.Take(5)
.ToList();
if (modifiedList.Any())
{
Console.WriteLine(string.Join(' ', modifiedList));
}
else
{
Console.WriteLine("No");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Data.Common;
using System.Linq;
using System.Text;
namespace Rabbits
{
public class Cage
{
private List<Rabbit> data;
public Cage(string name , int capacity)
{
this.Name = name;
this.Capacity = capacity;
data = new List<Rabbit>();
}
public int Count
=> data.Count;
public string Name { get; private set; }
public int Capacity { get; private set; }
public void Add(Rabbit rabbit)
{
if(this.Count < this.Capacity)
{
data.Add(rabbit);
}
}
public bool RemoveRabbit(string name)
{
return data.Remove(data.FirstOrDefault(r => r.Name == name));
}
public void RemoveSpecies(string species)
{
data.RemoveAll(r => r.Species == species);
}
public Rabbit SellRabbit(string name)
{
Rabbit rabbitToSell = data.FirstOrDefault(r => r.Name == name);
if(rabbitToSell != null)
{
rabbitToSell.Available = false;
}
return rabbitToSell;
}
public Rabbit[] SellRabbitsBySpecies(string species)
{
var rabbitsToSell = data.Where(r => r.Species == species).ToArray();
foreach (var rabbit in rabbitsToSell)
{
rabbit.Available = false;
}
return rabbitsToSell;
}
public string Report()
{
StringBuilder sb = new StringBuilder();
sb
.AppendLine($"Rabbits available at {this.Name}:");
foreach (Rabbit rabbit in data.Where(r => r.Available == true))
{
sb.AppendLine(rabbit.ToString());
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace ReadingList_from_one_line
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
PrintList(list);
}
static void PrintList(List<int> list)
{
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P01.Vehicles.Models.Contracts
{
public interface IDriveable
{
string Drive(double kilometers);
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Legendary_Farming
{
class Program
{
static void Main(string[] args)
{
string[] keyMaterialNames = new string[]
{
"shards",
"fragments",
"motes"
};
Dictionary<string, int> keyMaterials = new Dictionary<string, int>();
Dictionary<string, int> junk = new Dictionary<string, int>();
string itemObtained = string.Empty;
bool obtained = false;
keyMaterials["shards"] = 0;
keyMaterials["fragments"] = 0;
keyMaterials["motes"] = 0;
while (!obtained)
{
string[] inputArgs = Console.ReadLine()
.Split()
.ToArray();
for (int i = 0; i < inputArgs.Length; i += 2)
{
int qty = int.Parse(inputArgs[i]);
string material = inputArgs[i + 1].ToLower();
if (keyMaterialNames.Contains(material))
{
keyMaterials[material] += qty;
if(keyMaterials.Any(m => m.Value >= 250))
{
if(material == "shards")
{
itemObtained = "Shadowmourne";
}
else if(material == "fragments")
{
itemObtained = "Valanyr";
}
else
{
itemObtained = "Dragonwrath";
}
keyMaterials[material] -= 250;
obtained = true;
break;
}
}
else
{
AddJunk(junk, qty, material);
}
}
}
Console.WriteLine($"{itemObtained} obtained!");
keyMaterials = keyMaterials.OrderByDescending(kvp => kvp.Value)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var kvp in keyMaterials)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
junk = junk
.OrderBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var kvp in junk)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
private static void AddJunk(Dictionary<string, int> junk, int qty, string material)
{
if (!junk.ContainsKey(material))
{
junk[material] = 0;
}
junk[material] += qty;
}
}
}
<file_sep>using P04.WildFarm.Models.Foods;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Models.Animals.Mammals.Felines
{
public class Tiger : Feline
{
private const double WEIGHT_MULT = 1.00;
public Tiger(string name, double weight, string livingRegion, string breed)
: base(name, weight, livingRegion, breed)
{
}
public override double WeightMultiplier => WEIGHT_MULT;
public override ICollection<Type> PrefferedFoods
=> new List<Type>() {typeof(Meat) };
public override void ProduceSound()
{
Console.WriteLine("ROAR!!!");
}
}
}
<file_sep>using System;
namespace _02._Snake
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
for (int i = 0; i < n; i++)
{
string currRow = Console.ReadLine();
for (int j = 0; j < n; j++)
{
field[i, j] = currRow[j];
}
}
int snakeRow = -1;
int snakeCol = -1;
getSnakeCoordinates(n, field, ref snakeRow, ref snakeCol);
int countFood = 0;
bool isOut = false;
while (countFood < 10 && isOut == false)
{
string command = Console.ReadLine();
field[snakeRow, snakeCol] = '.';
if(command == "up")
{
if(snakeRow - 1 < 0)
{
isOut = true;
continue;
}
checkPos(field, snakeRow - 1, snakeCol, ref countFood, n, ref snakeRow, ref snakeCol);
field[snakeRow, snakeCol] = 'S';
}
else if(command == "down")
{
if(snakeRow + 1 == n)
{
isOut = true;
continue;
}
checkPos(field, snakeRow + 1, snakeCol, ref countFood, n, ref snakeRow, ref snakeCol);
field[snakeRow, snakeCol] = 'S';
}
else if (command == "left")
{
if (snakeCol - 1 < 0)
{
isOut = true;
continue;
}
checkPos(field, snakeRow, snakeCol - 1, ref countFood, n, ref snakeRow, ref snakeCol);
field[snakeRow, snakeCol] = 'S';
}
else
{
if (snakeCol + 1 == n)
{
isOut = true;
continue;
}
checkPos(field, snakeRow, snakeCol + 1, ref countFood, n, ref snakeRow, ref snakeCol);
field[snakeRow, snakeCol] = 'S';
}
}
if(isOut == true)
{
Console.WriteLine("Game over!");
}
if(countFood >= 10)
{
Console.WriteLine("You won! You fed the snake.");
}
Console.WriteLine($"Food eaten: {countFood}");
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
Console.Write(field[i, j]);
}
Console.WriteLine();
}
}
public static void checkPos(char[,] field, int changedRow, int changedCol, ref int foodQuantity,
int n, ref int snakeRow, ref int snakeCol)
{
if(field[changedRow, changedCol] == '*')
{
foodQuantity++;
snakeRow = changedRow;
snakeCol = changedCol;
}
else if(field[changedRow, changedCol] == 'B')
{
field[changedRow, changedCol] = '.';
int burrowRow = -1;
int burrowCol = -1;
for(int row = 0; row < n; row++)
{
for(int col = 0; col < n; col++)
{
if(field[row, col] == 'B')
{
burrowRow = row;
burrowCol = col;
}
}
}
snakeRow = burrowRow;
snakeCol = burrowCol;
}
else
{
snakeRow = changedRow;
snakeCol = changedCol;
}
}
public static void getSnakeCoordinates(int n, char[,] field, ref int snakeRow, ref int snakeCol)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if(field[i, j] == 'S')
{
snakeRow = i;
snakeCol = j;
}
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _04._Largest_3_Numbers
{
class Program
{
static void Main(string[] args)
{
//List<int> nums = Console.ReadLine()
// .Split()
// .Select(int.Parse)
// .ToList();
//List<int> sortedNums = nums.OrderByDescending(x => x)
// .ToList();
////OTHER WAY
List<int> nums = Console.ReadLine()
.Split()
.Select(int.Parse)
.OrderByDescending(x=>x)
.Take(3)
.ToList();
//for (int i = 0; i < 3 && i<sortedNums.Count; i++)
//{
// Console.Write($"{sortedNums[i]} ");
//}
Console.WriteLine(string.Join(" ", nums));
}
}
}
<file_sep>using System;
namespace _01.Person
{
class Program
{
static void Main(string[] args)
{
}
}
}
<file_sep>using System;
using System.Linq;
namespace _02._Array_Modifier
{
class Program
{
static void Main(string[] args)
{
int[] arr = Console.ReadLine()
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
string input;
while((input = Console.ReadLine()) != "end")
{
string[] command = input
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.ToArray();
if(command[0] == "swap")
{
int index1 = int.Parse(command[1]);
int index2 = int.Parse(command[2]);
int tmp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = tmp;
}
else if(command[0] == "multiply")
{
int index1 = int.Parse(command[1]);
int index2 = int.Parse(command[2]);
arr[index1] = arr[index1] * arr[index2];
}
else
{
for(int i = 0; i < arr.Length; i++)
{
arr[i]--;
}
}
}
Console.WriteLine(string.Join(", ", arr));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace Try
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(LengthOfLongestSubstring("aab"));
}
public static int LengthOfLongestSubstring(string s)
{
Dictionary<char, int> countChar = new Dictionary<char, int>();
int counter = 0;
int maxCounter = 0;
foreach (char c in s)
{
counter++;
if (countChar.ContainsKey(c))
{
countChar[c]++;
countChar = null;
countChar = new Dictionary<char, int>();
counter = 0;
//continue;
}
else
{
countChar[c] = 0;
}
if (maxCounter < counter)
{
maxCounter = counter;
}
}
return maxCounter;
}
}
}
<file_sep>using System;
namespace _02._Vowels_Count
{
class Program
{
static void Main(string[] args)
{
string str = Console.ReadLine();
Console.WriteLine(VowelCount(str));
}
static int VowelCount(string str)
{
string newStr = str.ToLower();
int counter = 0;
foreach (char letter in newStr)
{
if(letter == 'a' || letter == 'e' || letter == 'i' || letter == 'o' || letter == 'u')
{
counter++;
}
}
return counter;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public abstract class Vehicle : IVehicle
{
public string Model { get; set; }
public virtual string VrumVrum()
{
return "Vehicle is moving";
}
}
}
<file_sep>using CounterStrike.Models.Maps.Contracts;
using CounterStrike.Models.Players.Contracts;
using CounterStrike.Utilities.Messages;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace CounterStrike.Models.Maps
{
public class Map : IMap
{
public string Start(ICollection<IPlayer> players)
{
var terrorists = players.Where(p => p.GetType().Name == "Terrorist");
var counterTerrorists = players.Where(p => p.GetType().Name == "CounterTerrorist");
////////
while(terrorists.Any(p => p.IsAlive) && counterTerrorists.Any(c => c.IsAlive))
{
foreach (IPlayer terrorist in terrorists.Where(t => t.IsAlive))
{
int damage = terrorist.Gun.Fire();
foreach (IPlayer counterTerrorist in counterTerrorists.Where(c => c.IsAlive))
{
counterTerrorist.TakeDamage(damage);
}
}
foreach (IPlayer counterTerrorist in counterTerrorists.Where(t => t.IsAlive))
{
int damage = counterTerrorist.Gun.Fire();
foreach (IPlayer terrorist in terrorists.Where(c => c.IsAlive))
{
terrorist.TakeDamage(damage);
}
}
}
if(terrorists.Any(t => t.IsAlive))
{
return "Terrorist wins!";
}
else
{
return "Counter Terrorist wins!";
}
}
}
}
<file_sep>using P01.Vehicles.Common;
using System;
using System.Collections.Generic;
using System.Text;
namespace P01.Vehicles.Models
{
public class Bus : Vehicle
{
public Bus(double fuelquantity, double fuelconsumption, double tankCapacity)
: base(fuelquantity, fuelconsumption, tankCapacity)
{
}
public override string Drive(double kilometers)
{
double fuelNeeded = kilometers * (this.FuelConsumption + 1.4);
if (this.FuelQuantity < fuelNeeded)
{
throw new InvalidOperationException(string
.Format(ExceptionMessage.NotEnoughFuelExceptionMessage
, this.GetType().Name));
}
this.FuelQuantity -= fuelNeeded;
return $"{this.GetType().Name} travelled {kilometers} km";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _09.ForceBook
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<string>> book = new Dictionary<string, List<string>>();
while (true)
{
string inp = Console.ReadLine();
if (inp == "Lumpawaroo")
{
break;
}
string[] inputArgs = inp
.Split(new string[] { " | ", " -> " }, StringSplitOptions.RemoveEmptyEntries)
.ToArray();
if (inp.Contains("|"))
{
string side = inputArgs[0];
string user = inputArgs[1];
AddUser(book, side, user);
}
else if (inp.Contains("->"))
{
string user = inputArgs[0];
string side = inputArgs[1];
MoveUserToSide(book, user, side);
}
}
PrintOutput(book);
}
private static void PrintOutput(Dictionary<string, List<string>> book)
{
Dictionary<string, List<string>> orderedBook = book
.Where(kvp => kvp.Value.Count > 0)
.OrderByDescending(kvp => kvp.Value.Count)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var kvp in orderedBook)
{
string currentSide = kvp.Key;
List<string> currentSideUsers = kvp.Value
.OrderBy(x => x)
.ToList();
Console.WriteLine($"Side: {currentSide}, Members: {currentSideUsers.Count}");
foreach (var name in currentSideUsers)
{
Console.WriteLine($"! {name}");
}
}
}
static void AddUser(Dictionary<string, List<string>> book, string side, string user)
{
if (!book.ContainsKey(side))
{
book[side] = new List<string>();
}
if (!book.Values.Any(l => l.Contains(user))) /////To try without Any just Contains
{
book[side].Add(user);
}
}
static void MoveUserToSide(Dictionary<string, List<string>> book, string user, string side)
{
foreach (var kvp in book)
{
if (kvp.Value.Contains(user))
{
kvp.Value.Remove(user);
}
}
if (!book.ContainsKey(side))
{
book[side] = new List<string>();
}
book[side].Add(user);
Console.WriteLine($"{user} joins the {side} side!");
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace _01._Cooking
{
class Program
{
static void Main(string[] args)
{
Queue<int> liquids = new Queue<int>(Console.ReadLine().Split(' ').Select(int.Parse).ToArray());
Stack<int> ingredients = new Stack<int>(Console.ReadLine().Split(' ').Select(int.Parse).ToArray());
Dictionary<int, string> itemsTable = new Dictionary<int, string>()
{
{25, "Bread" },
{50, "Cake" },
{75, "Pastry" },
{100, "Fruit Pie"}
};
Dictionary<string, int> cookedItems = new Dictionary<string, int>()
{
{"Bread", 0 },
{"Cake", 0 },
{"Pastry", 0 },
{"Fruit Pie", 0 }
};
while (liquids.Count > 0 && ingredients.Count > 0)
{
int sum = liquids.Peek() + ingredients.Peek();
if(itemsTable.ContainsKey(sum))
{
string itemName = itemsTable[sum];
cookedItems[itemName]++;
liquids.Dequeue();
ingredients.Pop();
}
else
{
liquids.Dequeue();
int popped = ingredients.Pop();
ingredients.Push(popped + 3);
}
}
if(cookedItems.All(kvp => kvp.Value >= 1))
{
Console.WriteLine($"Wohoo! You succeeded in cooking all the food!");
}
else
{
Console.WriteLine("Ugh, what a pity! You didn't have enough materials to cook everything.");
}
if(liquids.Any())
{
Console.WriteLine($"Liquids left: {string.Join(", ", liquids)}");
}
else
{
Console.WriteLine("Liquids left: none");
}
if (ingredients.Any())
{
Console.WriteLine($"Ingredients left: {string.Join(", ", ingredients)}");
}
else
{
Console.WriteLine("Ingredients left: none");
}
cookedItems = cookedItems.OrderBy(kvp => kvp.Key).ToDictionary(x => x.Key, y => y.Value);
foreach (var kvp in cookedItems)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
}
}
<file_sep>using System;
namespace _01._National_Court
{
class Program
{
static void Main(string[] args)
{
int countPeoplePerHour1 = int.Parse(Console.ReadLine());
int countPeoplePerHour2 = int.Parse(Console.ReadLine());
int countPeoplePerHour3 = int.Parse(Console.ReadLine());
int allPeoplePerHour = countPeoplePerHour1 + countPeoplePerHour2 + countPeoplePerHour3;
int peopleCount = int.Parse(Console.ReadLine());
int timeNeeded = 0;
while(peopleCount > 0)
{
timeNeeded++;
if(timeNeeded % 4 == 0)
{
timeNeeded++;
}
if (allPeoplePerHour >= peopleCount)
{
break;
}
else
{
peopleCount -= allPeoplePerHour;
}
}
Console.WriteLine($"Time needed: {timeNeeded}h.");
}
}
}
<file_sep>using System;
namespace OldBooks
{
class Program
{
static void Main(string[] args)
{
string searchedBook = Console.ReadLine();
string bookName = Console.ReadLine();
int countbooks = 0;
bool isfound = false;
while (bookName != "No More Books")
{
if (bookName == searchedBook)
{
isfound = true;
break;
}
else
{
countbooks++;
bookName = Console.ReadLine();
}
}
if(isfound == true)
{
Console.WriteLine($"You checked {countbooks} books and found it.");
}
else
{
Console.WriteLine("The book you search is not here!");
Console.WriteLine($"You checked {countbooks} books.");
}
}
}
}
<file_sep>using System;
namespace _10.Rage_Expenses
{
class Program
{
static void Main(string[] args)
{
int lostGames = int.Parse(Console.ReadLine());
float headsetPrice = float.Parse(Console.ReadLine());
float mousePrice = float.Parse(Console.ReadLine());
float keyboardPrice = float.Parse(Console.ReadLine());
float displayPrice = float.Parse(Console.ReadLine());
float expenses = 0;
int countTrashedKeyboard = 0;
for (int i = 1; i <= lostGames; i++)
{
if(i % 2 == 0)
{
expenses += headsetPrice;
}
if (i % 3 == 0)
{
expenses += mousePrice;
}
if(i % 2 == 0 && i % 3 == 0)
{
expenses += keyboardPrice;
countTrashedKeyboard++;
}
if(countTrashedKeyboard == 2)
{
expenses += displayPrice;
countTrashedKeyboard = 0;
}
}
Console.WriteLine($"Rage expenses: {expenses:F2} lv.");
}
}
}
<file_sep>using SantaWorkshop.Core.Contracts;
using SantaWorkshop.Models.Dwarfs;
using SantaWorkshop.Models.Dwarfs.Contracts;
using SantaWorkshop.Models.Instruments;
using SantaWorkshop.Models.Instruments.Contracts;
using SantaWorkshop.Models.Presents;
using SantaWorkshop.Models.Presents.Contracts;
using SantaWorkshop.Models.Workshops;
using SantaWorkshop.Repositories;
using SantaWorkshop.Repositories.Contracts;
using SantaWorkshop.Utilities.Messages;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace SantaWorkshop.Core
{
public class Controller : IController
{
private DwarfRepository dwarfs;
private PresentRepository presents;
public Controller()
{
this.dwarfs = new DwarfRepository();
this.presents = new PresentRepository();
}
public string AddDwarf(string dwarfType, string dwarfName)
{
//"HappyDwarf" and "SleepyDwarf".
IDwarf dwarf = null;
if(dwarfType == "HappyDwarf")
{
dwarf = new HappyDwarf(dwarfName);
}
else if(dwarfType == "SleepyDwarf")
{
dwarf = new SleepyDwarf(dwarfName);
}
else
{
throw new InvalidOperationException(ExceptionMessages.InvalidDwarfType);
}
dwarfs.Add(dwarf);
return string.Format(OutputMessages.DwarfAdded, dwarfType, dwarfName);
}
public string AddInstrumentToDwarf(string dwarfName, int power)
{
if(this.dwarfs.FindByName(dwarfName) == null)
{
throw new InvalidOperationException(ExceptionMessages.InexistentDwarf);
}
IDwarf dwarf = dwarfs.FindByName(dwarfName);
IInstrument instrument = new Instrument(power);
dwarf.AddInstrument(instrument);
return string.Format(OutputMessages.InstrumentAdded,power,dwarfName);
}
public string AddPresent(string presentName, int energyRequired)
{
IPresent present = new Present(presentName, energyRequired);
presents.Add(present);
return string.Format(OutputMessages.PresentAdded,presentName);
}
public string CraftPresent(string presentName)
{
IPresent present = presents.FindByName(presentName);
ICollection<IDwarf> dwarves = dwarfs
.Models
.Where(d => d.Energy >= 50)
.OrderByDescending(d => d.Energy)
.ToList();
if(dwarves.Count == 0)
{
throw new InvalidOperationException(ExceptionMessages.DwarfsNotReady);
}
Workshop workshop = new Workshop();
while(dwarves.Any())
{
IDwarf currDwarf = dwarves.First();
workshop.Craft(present, currDwarf);
if (!currDwarf.Instruments.Any())
{
dwarves.Remove(currDwarf);
}
if(currDwarf.Energy == 0)
{
dwarves.Remove(currDwarf);
this.dwarfs.Remove(currDwarf);
}
if (present.IsDone())
{
return string.Format(OutputMessages.PresentIsDone,presentName);
}
}
///////PresentISDone
return string.Format(OutputMessages.PresentIsNotDone, presentName);
}
public string Report()
{
StringBuilder sb = new StringBuilder();
int countCraftedPresents = this.presents
.Models
.Count(p => p.IsDone());
sb
.AppendLine($"{countCraftedPresents} presents are done!")
.AppendLine($"Dwarfs info:");
foreach (IDwarf dwarf in this.dwarfs.Models)
{
int countNotBrokenInstruments = dwarf
.Instruments
.Count(i => !i.IsBroken());
sb
.AppendLine($"Name: {dwarf.Name}")
.AppendLine($"Energy: {dwarf.Energy}")
.AppendLine($"Instruments: {countNotBrokenInstruments} not broken left");
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace _01._Bombs
{
class Program
{
static void Main(string[] args)
{
Queue<int> bombEffects = new Queue<int>(
Console.ReadLine()
.Split(", ")
.Select(int.Parse).ToList());
Stack<int> bombCasing = new Stack<int>(
Console.ReadLine()
.Split(", ")
.Select(int.Parse).ToList());
Dictionary<int, string> typeBombs = new Dictionary<int, string>()
{
{ 40, "Datura Bombs" },
{ 60, "Cherry Bombs" },
{ 120, "Smoke Decoy Bombs"}
};
Dictionary<string, int> bombPouch = new Dictionary<string, int>()
{
{ "Datura Bombs", 0 },
{ "Cherry Bombs", 0 },
{ "Smoke Decoy Bombs", 0}
};
while(bombEffects.Any() && bombCasing.Any() && bombPouch.Any(kvp => kvp.Value < 3))
{
int sum = bombEffects.Peek() + bombCasing.Peek();
if(typeBombs.Any(kvp => kvp.Key == sum))
{
string newBombName = typeBombs[sum];
bombPouch[newBombName]++;
bombEffects.Dequeue();
bombCasing.Pop();
}
else
{
int prevBombCasing = bombCasing.Pop();
bombCasing.Push(prevBombCasing - 5);
}
}
if(bombPouch.All(kvp => kvp.Value >= 3))
{
Console.WriteLine("Bene! You have successfully filled the bomb pouch!");
}
else
{
Console.WriteLine("You don't have enough materials to fill the bomb pouch.");
}
if(bombEffects.Any())
{
Console.WriteLine($"Bomb Effects: {string.Join(", ", bombEffects)}");
}
else
{
Console.WriteLine("Bomb Effects: empty");
}
if (bombCasing.Any())
{
Console.WriteLine($"Bomb Casings: {string.Join(", ", bombCasing)}");
}
else
{
Console.WriteLine("Bomb Casings: empty");
}
foreach(var kvp in bombPouch.OrderBy(kvp => kvp.Key))
{
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
}
}
<file_sep>using System;
using System.Text;
namespace _04.Caesar_Cipher
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < text.Length; i++)
{
char letter = text[i];
letter = (char)(letter + 3);
sb.Append(letter);
}
Console.WriteLine(sb);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _05.Softuni_Parking
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
Dictionary<string, string> parking = new Dictionary<string, string>();
for (int i = 0; i < n; i++)
{
string[] command = Console.ReadLine().Split();
if(command[0] == "register")
{
string user = command[1];
string plate = command[2];
if (!parking.ContainsKey(user))
{
parking[user] = plate;
Console.WriteLine($"{user} registered {plate} successfully");
}
else
{
Console.WriteLine($"ERROR: already registered with plate number {parking[user]}");
}
}
else if(command[0] == "unregister")
{
string user = command[1];
if (!parking.Any(c => c.Key == user))
{
Console.WriteLine($"ERROR: user {user} not found");
}
else
{
parking.Remove(user);
Console.WriteLine($"{user} unregistered successfully");
//foreach (var kvp in parking)
//{
// if(kvp.Key == user)
// {
// parking.Remove(kvp.Key);
// }
//}
}
}
}
foreach (var kvp in parking)
{
Console.WriteLine($"{kvp.Key} => {kvp.Value}");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _02._The_Lift
{
class Program
{
static void Main(string[] args)
{
int peopleWaiting = int.Parse(Console.ReadLine());
int[] lift = Console.ReadLine().Split(' ').Select(int.Parse).ToArray();
for (int i = 0; i < lift.Length && peopleWaiting != 0; i++)
{
if(lift[i] < 4)
{
int peopleCanEnter = 4 - lift[i];
if(peopleWaiting >= peopleCanEnter)
{
peopleWaiting -= peopleCanEnter;
lift[i] += peopleCanEnter;
}
else if(peopleWaiting < peopleCanEnter)
{
while(peopleWaiting > 0)
{
peopleWaiting--;
lift[i]++;
}
}
}
else
{
continue;
}
}
if(peopleWaiting == 0 && lift.Any(w => w < 4))
{
Console.WriteLine("The lift has empty spots!");
Console.WriteLine(string.Join(' ',lift));
}
else if(peopleWaiting > 0 && lift.All(w => w == 4))
{
Console.WriteLine($"There isn't enough space! {peopleWaiting} people in a queue!");
Console.WriteLine(string.Join(' ', lift));
}
else if(lift.All(w => w == 4) && peopleWaiting == 0)
{
Console.WriteLine(string.Join(' ', lift));
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace TestApp
{
public class Calculator
{
public int CalculateSum(params int[] numbers)
{
return numbers.Sum();
}
public int Product(params int[] numbers)
{
int result = 1;
for (int i = 0; i < numbers.Length; i++)
{
result *= numbers[i];
}
return result;
}
}
}
<file_sep>using P08.CollectionHierarchy.Contracts;
using System;
using System.Collections.Generic;
using System.Text;
namespace P08.CollectionHierarchy.Models
{
public class MyList : IMyList
{
private List<string> data;
private List<int> indexes;
private List<string> removedElements;
public MyList()
{
this.data = new List<string>();
this.indexes = new List<int>();
this.removedElements = new List<string>();
}
public int Used
{
get
{
return this.data.Count;
}
}
public void Add(string element)
{
this.indexes.Add(0);
this.data.Insert(0, element);
}
public void Remove()
{
string itemToRemove = this.data[0];
this.removedElements.Add(itemToRemove);
this.data.RemoveAt(0);
}
public void GetRemoved()
{
Console.WriteLine(string.Join(" ", this.removedElements));
}
public override string ToString()
{
return $"{string.Join(" ", this.indexes)}";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Inheritance
{
public class Programmer:Employee
{
public string Language { get; set; }
}
}
<file_sep>using System;
using System.Runtime.CompilerServices;
namespace P07.Tuple
{
class Program
{
static void Main(string[] args)
{
string[] personInfo = Console.ReadLine()
.Split();
string fullName = $"{personInfo[0]} {personInfo[1]}";
string address = personInfo[2];
string[] nameAndBeer = Console.ReadLine().Split();
string name = nameAndBeer[0];
int beerAmount = int.Parse(nameAndBeer[1]);
string[] thirdInput = Console.ReadLine().Split();
int firstArg = int.Parse(thirdInput[0]);
double secondArg = double.Parse(thirdInput[1]);
Tuple<string, string> firstTuple = new Tuple<string, string>(fullName, address);
Tuple<string, int> secondTuple = new Tuple<string, int>(name, beerAmount);
Tuple<int, double> thirdTuple = new Tuple<int, double>(firstArg, secondArg);
Console.WriteLine(firstTuple);
Console.WriteLine(secondTuple);
Console.WriteLine(thirdTuple);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace ReadingList
{
class Program
{
static void Main(string[] args)
{
List<int> list = new List<int>();
int n = int.Parse(Console.ReadLine());
ReadList(list, n);
PrintList(list);
}
static void PrintList(List <int> list)
{
//for (int i = 0; i < list.Count; i++)
//{
// Console.WriteLine(list[i]);
//}
Console.WriteLine(string.Join(" ", list));
}
static void ReadList(List<int> list, int n)
{
for (int i = 0; i < n; i++)
{
list.Add(int.Parse(Console.ReadLine()));
}
//return array;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Objects
{
class Cars
{
public string color = "red";
public int maxSpeed = 10;
public void Suobsht()
{
Console.WriteLine("BRUM BRUM");
}
}
}
<file_sep>using System;
using System.Linq;
namespace _09._List_Of_Predicates
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int[] dividers = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
Predicate<int> isDivisable = new Predicate<int>((n)
=> dividers.All(d => n % d == 0));
Action<int, int[]> FindDivisableNums = new Action<int, int[]>((n, dividers) =>
{
for (int i = 1; i <= n; i++)
{
if (isDivisable(i))
{
Console.Write(i + " ");
}
}
});
FindDivisableNums(n, dividers);
}
}
}
<file_sep>using System;
namespace Clock
{
class Program
{
static void Main(string[] args)
{
for(int i = 0;i<24;i++)
{
for(int j= 0;j<60;j++)
{
Console.WriteLine($"{i}:{j}");
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Globalization;
using System.Linq;
namespace Sorting
{
class Program
{
static void Main(string[] args)
{
///////Selection sort
List<int> list = new List<int>() { 4, 3, 2, 1 };
for (int i = 0; i < list.Count; i++)
{
int min = list[i];
int minIndex = i;
for (int j = i; j < list.Count; j++)
{
if(list[j] < min)
{
min = list[j];
minIndex = j;
}
}
int temp = list[i];
list[i] = list[minIndex];
list[minIndex] = temp;
}
Console.WriteLine(string.Join(" ", list));
/////////Bubble sort
List<int> list2 = new List<int>() { 4, 3, 2, 1 };
for (int i = 0; i < list2.Count; i++)
{
for (int j = i+1; j < list2.Count; j++)
{
if(list2[i] > list2[j])
{
int temp = list2[i];
list2[i] = list2[j];
list2[j] = temp;
}
}
}
Console.WriteLine(string.Join(" ", list2));
}
}
}
<file_sep>using P06.FoodShortage.Contracts;
using P06.FoodShortage.Models;
using System;
using System.Collections.Generic;
using System.Linq;
namespace P06.FoodShortage
{
public class Engine
{
public void Run()
{
// List<string> allIds = new List<string>();
// List<IIdentifiable> citizens = new List<IIdentifiable>();
// List<IIdentifiable> robots = new List<IIdentifiable>();
// List<IIdentifiable> robotsAndCitizens = new List<IIdentifiable>();
//List<IBirthable> birthables = new List<IBirthable>();
List<IBuyer> buyers = new List<IBuyer>();
List<Citizen> citizens = new List<Citizen>();
List<Rebel> rebels = new List<Rebel>();
//string command;
int N = int.Parse(Console.ReadLine());
for (int i = 0; i < N; i++)
{
string[] commandArgs = Console.ReadLine()
.Split()
.ToArray();
if(commandArgs.Length == 4)
{
string name = commandArgs[0];
int age = int.Parse(commandArgs[1]);
string id = commandArgs[2];
string birthdate = commandArgs[3];
if(!citizens.Any(c=>c.Name == name) && !rebels.Any(r=>r.Name == name))
{
Citizen citizen = new Citizen(name, age, id,birthdate);
citizens.Add(citizen);
buyers.Add(citizen);
}
// citizens.Add(citizen);
// robotsAndCitizens.Add(citizen);
// birthables.Add(citizen);
}
else if(commandArgs.Length == 3)
{
string name = commandArgs[0];
int age = int.Parse(commandArgs[1]);
string group = commandArgs[2];
if (!citizens.Any(c => c.Name == name) && !rebels.Any(r => r.Name == name))
{
Rebel rebel = new Rebel(name, age, group);
rebels.Add(rebel);
buyers.Add(rebel);
}
}
}
string command;
while((command = Console.ReadLine()) != "End")
{
string name = command;
if(citizens.Any(c => c.Name == name))
{
IBuyer buyer = citizens.Find(c => c.Name == name);
buyer.BuyFood();
}
else if(rebels.Any(r => r.Name == name))
{
IBuyer buyer = rebels.Find(r => r.Name == name);
buyer.BuyFood();
}
}
int sumFood = 0;
foreach (IBuyer buyer in buyers)
{
sumFood += buyer.Food;
}
Console.WriteLine(sumFood);
}
}
}
<file_sep>using System;
using System.Security.Cryptography;
namespace RandomType
{
class Program
{
static void Main(string[] args)
{
Random random = new Random();
int result = random.Next(0, 2);
Console.WriteLine(result);
}
}
}
<file_sep>using System;
namespace BirthdayParty
{
class Program
{
static void Main(string[] args)
{
double naem = double.Parse(Console.ReadLine());
double neobhodimBudget = naem + naem * 0.2 + (naem * 0.2 - naem * 0.2 * 0.45) + ( naem/3) ;
/* Console.WriteLine(naem * 0.2);
Console.WriteLine(naem * 0.2 - naem * 0.2 * 0.45);
Console.WriteLine(naem/3);
*/
Console.WriteLine(neobhodimBudget);
}
}
}
<file_sep>using System;
namespace OnTImeForExam
{
class Program
{
static void Main(string[] args)
{
int examhour = int.Parse(Console.ReadLine());
int examminutes = int.Parse(Console.ReadLine());
int hourThere = int.Parse(Console.ReadLine());
int minutesThere = int.Parse(Console.ReadLine());
int examInMinutes = examhour * 60 + examminutes;
int ThereInMinutes = hourThere * 60 + minutesThere;
// Console.WriteLine(examInMinutes);
// Console.WriteLine(ThereInMinutes);
if(ThereInMinutes > examInMinutes)
{
Console.WriteLine("Late");
if(ThereInMinutes - examInMinutes < 60)
{
Console.WriteLine($"{ThereInMinutes - examInMinutes} minutes after the start");
}
else
{
if ((ThereInMinutes - examInMinutes) % 60 >= 10)
{
Console.WriteLine($"{(ThereInMinutes - examInMinutes) / 60}:{(ThereInMinutes - examInMinutes) % 60} hours after the start");
}
else
{
Console.WriteLine($"{(ThereInMinutes - examInMinutes) / 60}:0{(ThereInMinutes - examInMinutes) % 60} hours after the start");
}
}
}
else if((ThereInMinutes == examInMinutes) || (ThereInMinutes >= examInMinutes - 30) )
{
Console.WriteLine("On time");
if(ThereInMinutes >= examInMinutes - 30)
{
Console.WriteLine($"{examInMinutes - ThereInMinutes} minutes before the start");
}
}
else if( ThereInMinutes < examInMinutes - 30)
{
Console.WriteLine("Early");
if(examInMinutes - ThereInMinutes >=60)
{ if ((examInMinutes - ThereInMinutes) % 60 >= 10)
Console.WriteLine($"{(examInMinutes - ThereInMinutes) / 60}:{(examInMinutes - ThereInMinutes) % 60} hours before the start");
else
{
Console.WriteLine($"{(examInMinutes - ThereInMinutes) / 60}:0{(examInMinutes - ThereInMinutes) % 60} hours before the start");
}
}
else
{
Console.WriteLine($"{examInMinutes - ThereInMinutes} minutes before the start");
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
namespace SoftUniParking
{
public class Parking
{
private readonly ICollection<Car> cars;
private int capacity;
public Parking(int capacity)
{
this.capacity = capacity;
this.cars = new List<Car>();
}
public IReadOnlyCollection<Car> Cars
=> (IReadOnlyCollection<Car>)this.cars;
public int Count
=> this.Cars.Count;
public string AddCar(Car car)
{
if(this.Cars.Any(c => c.RegistrationNumber == car.RegistrationNumber))
{
return "Car with that registration number, already exists!";
//return false;
}
else if(this.capacity == this.Count)
{
return "Parking is full!";
}
else
{
this.cars.Add(car);
return $"Successfully added new car {car.Make} {car.RegistrationNumber}";
}
}
public string RemoveCar(string registrNum)
{
if(!this.Cars.Any(c => c.RegistrationNumber == registrNum))
{
return "Car with that registration number, doesn't exist!";
}
else
{
var carToRemove = this.Cars.First(c => c.RegistrationNumber == registrNum);
this.cars.Remove(carToRemove);
return $"Successfully removed {registrNum}";
}
}
public Car GetCar(string registrNum)
{
Car carNeeded = null;
if(this.Cars.Any(c => c.RegistrationNumber == registrNum))
{
carNeeded = this.Cars.FirstOrDefault(c => c.RegistrationNumber == registrNum);
}
return carNeeded;
}
public void RemoveSetOfRegistrationNumber(List<string> RegistrationNumbers)
{
foreach (var rNum in RegistrationNumbers)
{
this.RemoveCar(rNum);
}
}
}
}
<file_sep>using System;
namespace SquareArea
{
class Program
{
static void Main(string[] args)
{
int side = int.Parse(Console.ReadLine());
int lice = side * side;
Console.WriteLine(lice);
}
}
}
<file_sep>using System;
namespace _02.Repeat_Strings
{
class Program
{
static void Main(string[] args)
{
string[] words = Console.ReadLine().Split();
string result = "";
for (int i = 0; i < words.Length; i++)
{
string word = words[i];
for (int j = 0; j < word.Length; j++)
{
result += word;
}
//Console.Write(new string(word.ToCharArray(),0, word.Length));
}
Console.WriteLine(result);
}
}
}
<file_sep>using System;
using System.Linq;
namespace _04._Password_Validator
{
class Program
{
static void Main(string[] args)
{
string pass = Console.ReadLine();
if(pass.Length >= 6 && pass.Length <= 10 && pass.All(char.IsLetterOrDigit) && pass.Count(char.IsDigit) >= 2)
{
Console.WriteLine("Password is valid");
}
else
{
IsPassBetweenRequirements(pass);
DoesConsistsOfLettersAndDigits(pass);
DoesHaveAtLeast2Digits(pass);
}
}
static void IsPassBetweenRequirements(string pass)
{
if(pass.Length < 6 || pass.Length > 10)
{
Console.WriteLine("Password must be between 6 and 10 characters");
}
}
static void DoesConsistsOfLettersAndDigits(string pass)
{
if(!pass.All(char.IsLetterOrDigit))
{
Console.WriteLine("Password must consist only of letters and digits");
}
}
static void DoesHaveAtLeast2Digits(string pass)
{
//int count = 0;
//for (int i = 0; i < pass.Length; i++)
//{
// if (char.IsDigit(pass[i]))
// {
// count++;
// }
//}
//if(count < 2)
//{
// Console.WriteLine("Password must have at least 2 digits");
//}
if (pass.Count(char.IsDigit) < 2)
{
Console.WriteLine("Password must have at least 2 digits");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _08._Ranking
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, string> contests = new Dictionary<string, string>();
string command1;
while((command1 = Console.ReadLine()) != "end of contests")
{
string[] cmdArgs = command1
.Split(":", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
string contestName = cmdArgs[0];
string pass = cmdArgs[1];
contests[contestName] = pass;
}
Dictionary<string, Dictionary<string, int>> users = new
Dictionary<string, Dictionary<string, int>>();
string command2;
while ((command2 = Console.ReadLine()) != "end of submissions")
{
string[] cmdArgs = command2
.Split("=>", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
string contestName = cmdArgs[0];
string pass = cmdArgs[1];
string username = cmdArgs[2];
int points = int.Parse(cmdArgs[3]);
if(contests.ContainsKey(contestName) && contests[contestName] == pass)
{
if (!users.ContainsKey(username))
{
users[username] = new Dictionary<string, int>();
}
if(users[username].ContainsKey(contestName)
&& users[username][contestName] < points)
{
users[username][contestName] = points;
}
else if(!users[username].ContainsKey(contestName))
{
users[username][contestName] = points;
}
}
}
///Finding best user
var bestUsers = users
.OrderByDescending(kvp => kvp.Value.Values.Sum())
.ToDictionary(x => x.Key, y => y.Value);
Console.WriteLine($"Best candidate is {bestUsers.First().Key} with total " +
$"{bestUsers.First().Value.Values.Sum()} points.");
//////Ordering the users
users = users
.OrderBy(kvp => kvp.Key)
.ToDictionary(x => x.Key, y => y.Value);
Console.WriteLine("Ranking:");
foreach (var user in users)
{
Console.WriteLine($"{user.Key}");
foreach (var contest in user.Value.OrderByDescending(kvp => kvp.Value))
{
Console.WriteLine($"# {contest.Key} -> {contest.Value}");
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace MergingLists
{
class Program
{
//static void Main(string[] args)
//{
// List<int> first = Console.ReadLine().Split().Select(int.Parse).ToList();
// List<int> second = Console.ReadLine().Split().Select(int.Parse).ToList();
// List<int> result = new List<int>();
// result = MergeLists(first, second);
// Console.WriteLine(string.Join(" ", result));
//}
//static List<int> MergeLists(List<int> first, List<int> second)
//{
// int count = Math.Max(first.Count, second.Count);
// List<int> newList = new List<int>();
// for (int i = 0; i < count; i++)
// {
// if( i < first.Count)
// {
// newList.Add(first[i]);
// }
// if (i < second.Count)
// {
// newList.Add(second[i]);
// }
// }
// return newList;
//}
static void Main(string[] args)
{
List<int> first = Console.ReadLine().Split().Select(int.Parse).ToList();
List<int> second = Console.ReadLine().Split().Select(int.Parse).ToList();
List<int> result = MergeLists(first, second);
Console.WriteLine(string.Join(" ", result));
}
static List<int> MergeLists(List<int> first , List<int> second)
{
List<int> newList = new List<int>();
int countNew = Math.Max(first.Count, second.Count);
for (int i = 0; i < countNew; i++)
{
if(i < first.Count)
{
newList.Add(first[i]);
}
if(i < second.Count)
{
newList.Add(second[i]);
}
}
return newList;
}
}
}
<file_sep>using P05.BirthdayCelebrations.Contracts;
using System;
using System.Collections.Generic;
using System.Text;
namespace _05._Birthday_Celebrations.Models
{
public class Pet : IBirthable
{
private string name;
private string birthDate;
public Pet(string name , string birthDate)
{
this.name = name;
this.birthDate = birthDate;
}
public string GetBirth()
{
return this.birthDate;
}
}
}
<file_sep>using System;
namespace _01._Smallest_of_Three_Numbers
{
class Program
{
static void Main(string[] args)
{
int n1 = int.Parse(Console.ReadLine());
int n2 = int.Parse(Console.ReadLine());
int n3 = int.Parse(Console.ReadLine());
Console.WriteLine(Min(n1, n2, n3));
}
static int Min(int n1 , int n2 , int n3)
{
// int min = int.MaxValue;
if(n1 < n2 && n1 < n3)
{
return n1;
}
else if(n2 < n1 && n2 < n3)
{
return n2;
}
else
{
return n3;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Contracts
{
public interface ISoundable
{
void ProduceSound();
}
}
<file_sep>using System;
namespace _07._Water_Overflow
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int capacity = 255;
int sumQuantity = 0;
for (int i = 0; i < n; i++)
{
int quantity = int.Parse(Console.ReadLine());
if (quantity > capacity)
{
Console.WriteLine("Insufficient capacity!");
}
else
{
capacity -= quantity;
sumQuantity += quantity;
}
}
Console.WriteLine(sumQuantity);
}
}
}
<file_sep>using _05._Birthday_Celebrations.Models;
using P05.BirthdayCelebrations.Contracts;
using System;
using System.Collections.Generic;
using System.Linq;
namespace P05.BirthdayCelebrations
{
public class Engine
{
public void Run()
{
// List<string> allIds = new List<string>();
// List<IIdentifiable> citizens = new List<IIdentifiable>();
// List<IIdentifiable> robots = new List<IIdentifiable>();
List<IIdentifiable> robotsAndCitizens = new List<IIdentifiable>();
List<IBirthable> birthables = new List<IBirthable>();
string command;
while((command = Console.ReadLine()) != "End")
{
string[] commandArgs = command
.Split()
.ToArray();
if(commandArgs.Length == 5)
{
string name = commandArgs[1];
int age = int.Parse(commandArgs[2]);
string id = commandArgs[3];
string birthdate = commandArgs[4];
Citizen citizen = new Citizen(name, age, id,birthdate);
// citizens.Add(citizen);
robotsAndCitizens.Add(citizen);
birthables.Add(citizen);
}
else if(commandArgs[0] == "Robot")
{
string model = commandArgs[1];
string id = commandArgs[2];
Robot robot = new Robot(model, id);
//robots.Add(robot);
robotsAndCitizens.Add(robot);
}
else
{
string name = commandArgs[1];
string birthdate = commandArgs[2];
Pet pet = new Pet(name, birthdate);
birthables.Add(pet);
}
}
// string lastDigitFakeId = Console.ReadLine();
// PrintFakeId(robotsAndCitizens, lastDigitFakeId);
string lastDigitYear = Console.ReadLine();
PrintListLastDigitThisYear(birthables, lastDigitYear);
}
private void PrintListLastDigitThisYear(List<IBirthable> birthables, string lastDigitYear)
{
foreach (IBirthable birthable in birthables)
{
string currBirthDate = birthable.GetBirth();
if (currBirthDate.EndsWith(lastDigitYear))
{
Console.WriteLine(currBirthDate);
}
}
}
private void PrintFakeId(List<IIdentifiable> robotsAndCitizens , string lastDigitFakeId)
{
foreach (IIdentifiable citizenOrRobot in robotsAndCitizens)
{
string currId = citizenOrRobot.GetId();
if (currId.EndsWith(lastDigitFakeId))
{
Console.WriteLine(currId);
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _01.CountSameValuesInArray
{
class Program
{
static void Main(string[] args)
{
//-2.5 4 3 -2.5 -5.5 4 3 3 -2.5 3
Dictionary<double, int> numbers = new Dictionary<double, int>();
double[] arr = Console.ReadLine()
.Split()
.Select(double.Parse)
.ToArray();
for (int i = 0; i < arr.Length; i++)
{
double num = arr[i];
if (!numbers.ContainsKey(num))
{
numbers[num] = 0;
}
numbers[num]++;
}
foreach (var kvp in numbers)
{
Console.WriteLine($"{kvp.Key} - {kvp.Value} times");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace FilterByAge
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
var people = new Dictionary<string,int>();
for (int i = 0; i < n; i++)
{
string[] cmdArgs = Console.ReadLine()
.Split(", ");
string name = cmdArgs[0];
int age = int.Parse(cmdArgs[1]);
people.Add(name, age);
}
string filterText = Console.ReadLine();
int ageToFilter = int.Parse(Console.ReadLine());
if (filterText == "older")
{
people = people
.Where(kvp => kvp.Value > ageToFilter)
.ToDictionary(x => x.Key, y => y.Value);
}
else if(filterText == "younger")
{
people = people
.Where(kvp => kvp.Value < ageToFilter)
.ToDictionary(x => x.Key, y => y.Value);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.CompilerServices;
namespace _03._Periodic_Table
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
var periodicTable = new SortedSet<string>();
for (int i = 0; i < n; i++)
{
string[] cmdArgs = Console.ReadLine()
.Split(" ", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
for (int j = 0; j < cmdArgs.Length; j++)
{
string currElem = cmdArgs[j];
periodicTable.Add(currElem);
}
}
//periodicTable = periodicTable
// .OrderBy(x => x)
// .ToHashSet();
Console.WriteLine(string.Join(" ", periodicTable));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace Hot_Potato
{
class Program
{
static void Main(string[] args)
{
//var numbers = new Queue<int>();
//numbers.Enqueue(1);
//numbers.Enqueue(2);
//numbers.Enqueue(3);
var names = Console.ReadLine().Split();
int toss = int.Parse(Console.ReadLine());
var queue = new Queue<string>(names);
int currentIndex = 1;
while(queue.Count>1)
{
var currentName = queue.Dequeue();
if(currentIndex == toss)
{
Console.WriteLine($"Remove {currentName}");
currentIndex = 0;
}
else
{
queue.Enqueue(currentName);
}
currentIndex++;
}
Console.WriteLine($"Last is {queue.Dequeue()}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _02.Change_List
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] command = input.Split();
switch (command[0])
{
case "Delete":
int num = int.Parse(command[1]);
for (int i = 0; i < list.Count; i++)
{
if(list[i] == num)
{
list.Remove(list[i]);
i--;
}
}
break;
case "Insert":
int number = int.Parse(command[1]);
int index = int.Parse(command[2]);
list.Insert(index, number);
break;
}
}
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
namespace CatWalking
{
class Program
{
static void Main(string[] args)
{
int minutesADay = int.Parse(Console.ReadLine());
int countRazhodki = int.Parse(Console.ReadLine());
int CaloriesIntake = int.Parse(Console.ReadLine());
int burnedCalories = countRazhodki * minutesADay * 5;
if (burnedCalories >= (0.5 * CaloriesIntake))
{
Console.WriteLine($"Yes, the walk for your cat is enough. Burned calories per day: {burnedCalories}.");
}
else
{
Console.WriteLine($"No, the walk for your cat is not enough. Burned calories per day: {burnedCalories}.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace SetsAndDictionaries
{
class Program
{
static void Main(string[] args)
{
//Dictionary<int, int> dictionary = new Dictionary<int, int>();
//dictionary.Add(1, 10);
//dictionary.Add(2, 10);
//foreach (var (key,value) in dictionary)
//{
// Console.WriteLine(key + " "+ value);
//}
/////Other type of dcitionary
///
var townWithNeighbourHoods = new Dictionary<string, List<string>>();
townWithNeighbourHoods.Add("Sofia", new List<string>());
townWithNeighbourHoods["Sofia"].Add("Vitosha");
townWithNeighbourHoods["Sofia"].Add("Gerena");
foreach (var town in townWithNeighbourHoods)
{
Console.WriteLine(town.Key);
foreach (var kvartal in town.Value)
{
Console.WriteLine(kvartal);
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _02._Shopping_List
{
class Program
{
static void Main(string[] args)
{
List<string> list = Console.ReadLine().Split('!').ToList();
string input;
while((input = Console.ReadLine()) != "Go Shopping!")
{
string[] command = input.Split().ToArray();
if(command[0] == "Urgent")
{
string itemToAdd = command[1];
if(!list.Any(item => item == itemToAdd))
{
list.Insert(0, itemToAdd);
}
}
else if(command[0] == "Unnecessary")
{
string itemToRemove = command[1];
if(list.Any(item => item == itemToRemove))
{
list.Remove(itemToRemove);
}
}
else if(command[0] == "Correct")
{
string oldItem = command[1];
string newItem = command[2];
if(list.Any(item => item == oldItem))
{
////////////
int indexofOldItem = list.FindIndex(item => item == oldItem);
list[indexofOldItem] = newItem;
}
}
else if(command[0] == "Rearrange")
{
string item = command[1];
if(list.Any(it => it == item))
{
list.Remove(item);
list.Add(item);
}
}
}
Console.WriteLine(string.Join(", ", list));
}
}
}
<file_sep>using P09.ExplicitInterfaces.Contracts;
using P09.ExplicitInterfaces.Models;
using System;
using System.Linq;
namespace P09.ExplicitInterfaces
{
class StartUp
{
static void Main(string[] args)
{
string command;
while((command = Console.ReadLine()) != "End")
{
string[] cmdArgs = command
.Split()
.ToArray();
string name = cmdArgs[0];
string country = cmdArgs[1];
int age = int.Parse(cmdArgs[2]);
Citizen citizen = new Citizen(name, country, age);
IPerson person = citizen;
IResident resident = citizen;
Console.WriteLine(person.GetName());
Console.WriteLine(resident.GetName());
}
}
}
}
<file_sep>using System;
namespace CinemaTIckets
{
class Program
{
static void Main(string[] args)
{
float ticketsCount = 0;
int standardCount = 0;
int studentCount = 0;
int kidCount = 0;
int sumStandard = 0;
int sumStudent = 0;
int sumKid = 0;
float sumTickets = 0;
string command = Console.ReadLine();
while(command != "Finish")
{
float freespace = float.Parse(Console.ReadLine());
string typeTicket = Console.ReadLine();
while(typeTicket != "End"&&typeTicket != "Finish")
{
ticketsCount++;
if(typeTicket == "standard")
{
standardCount++;
}
else if(typeTicket == "kid")
{
kidCount++;
}
else if(typeTicket == "student")
{
studentCount++;
}
typeTicket = Console.ReadLine();
// typeTicket = Console.ReadLine();
}
if (typeTicket == "Finish")
{
Console.WriteLine($"{command} - {(ticketsCount / freespace) * 100:F2}% full.");
sumTickets += ticketsCount;
sumStandard += standardCount;
sumKid += kidCount;
sumStudent += studentCount;
Console.WriteLine($"Total tickets: {sumTickets}");
Console.WriteLine($"{(sumStudent/sumTickets)*100:F2}% student tickets.");
Console.WriteLine($"{ (sumStandard/sumTickets)*100:F2}% standard tickets.");
Console.WriteLine($"{ (sumKid/sumTickets)*100:F2}% kids tickets.");
break;
}
else
{
Console.WriteLine($"{command} - {(ticketsCount / freespace) * 100:F2}% full.");
sumTickets += ticketsCount;
sumStandard += standardCount;
sumKid += kidCount;
sumStudent += studentCount;
standardCount = 0;
kidCount = 0;
studentCount = 0;
ticketsCount = 0;
command = Console.ReadLine();
}
}
}
}
}
<file_sep>using ExtendedDatabase;
using Microsoft.VisualStudio.TestPlatform.ObjectModel;
using NUnit.Framework;
using NUnit.Framework.Constraints;
using System;
using System.Threading;
namespace Tests
{
public class ExtendedDatabaseTests
{
private Person gosho;
private Person pesho;
private ExtendedDatabase.ExtendedDatabase database;
[SetUp]
public void Setup()
{
gosho = new Person(447788556699, "Gosho");
pesho = new Person(114560, "Pesho");
database = new ExtendedDatabase.ExtendedDatabase(gosho, pesho);
}
[Test]
public void ConstructorShouldInicialise()
{
var expected = new Person[] { gosho, pesho };
var actual = expected;
var database = new ExtendedDatabase.ExtendedDatabase(expected);
Assert.That(actual, Is.EqualTo(expected));
}
[Test]
public void AddShouldIncreaseDatabaseCount()
{
int expectedCount = 3;
database.Add(new Person(21323, "Stamo"));
int actualCount = this.database.Count;
Assert.AreEqual(expectedCount, actualCount);
}
[Test]
public void AddShouldThrowExceptionIfWeTryToAddMoreThan16People()
{
database = new ExtendedDatabase.ExtendedDatabase(gosho, pesho,
new Person(1323, "tamo"), new Person(13233, "amo"), new Person(132123, "Ramo")
, new Person(323, "tdmo"), new Person(21, "trmo"), new Person(23, "taqmo")
, new Person(4, "Gom"), new Person(133, "Tom"), new Person(123, "Seamo")
, new Person(512, "Feamo"),
new Person(234, "tadfhjjgmo"), new Person(1315623, "taasdfasmo"), new Person(3455, "tamsdo")
, new Person(2656, "ryttu"));
Assert.Throws<InvalidOperationException>(() =>
{
database.Add(new Person(21323, "Stamo"));
});
}
[Test]
public void AddShouldThrowExceptionIfWeTryToAddAlreadyExistingUsername()
{
Assert.Throws<InvalidOperationException>(() =>
{
database.Add(new Person(23, "Gosho"));
});
}
[Test]
public void AddShouldThrowExceptionIfWeTryToAddAlreadyExistingId()
{
Assert.Throws<InvalidOperationException>(() =>
{
database.Add(new Person(447788556699, "Gone"));
});
}
[Test]
public void RemoveShouldDecreaseDatabaseCount()
{
int expectedCount = 1;
database.Remove();
int actualCount = database.Count;
Assert.AreEqual(expectedCount, actualCount);
}
[Test]
public void RemoveShouldThrowExceptionIfCountIsZero()
{
database = new ExtendedDatabase.ExtendedDatabase();
Assert.Throws<InvalidOperationException>(() =>
{
database.Remove();
});
}
[Test]
public void FindByUsernameShouldThrowExceptionIfGivenStringIsNull()
{
Assert.Throws<ArgumentNullException>(() =>
{
database.FindByUsername("");
});
}
[Test]
public void FindByUsernameShouldThrowExceptionIfNoUserIsPresentByThisName()
{
Assert.Throws<InvalidOperationException>(() =>
{
database.FindByUsername("Stamo");
});
}
[Test]
public void FindByUSerNameShouldReturnValidUser()
{
var expected = pesho;
var actual = database.FindByUsername("Pesho");
Assert.AreEqual(expected, actual);
}
[Test]
public void FindByUserNameShouldBeCaseSensitive()
{
Assert.Throws<InvalidOperationException>(() =>
{
database.FindByUsername("gosho");
});
}
[Test]
public void FindByIdShouldThrowExceptionIfNegativeIdIsGiven()
{
Assert.Throws<ArgumentOutOfRangeException>(() =>
{
database.FindById(-10);
});
}
[Test]
public void FindByIdShouldThrowExceptionIfThereIsNotSuchId()
{
Assert.Throws<InvalidOperationException>(() =>
{
database.FindById(23324);
});
}
[Test]
public void FindByIdShouldReturnValidUser()
{
var expected = pesho;
var actual = database.FindById(114560);
Assert.AreEqual(expected, actual);
}
[Test]
public void AddRangeThrowExceptionIfYouTryToMakeDatabaseWithMoreThan16People()
{
Assert.Throws<ArgumentException>(() =>
{
database = new ExtendedDatabase.ExtendedDatabase(gosho, pesho,
new Person(1323, "tamo"), new Person(13233, "amo"), new Person(132123, "Ramo")
, new Person(323, "tdmo"), new Person(21, "trmo"), new Person(23, "taqmo")
, new Person(4, "Gom"), new Person(133, "Tom"), new Person(123, "Seamo")
, new Person(512, "Feamo"),
new Person(234, "tadfhjjgmo"), new Person(1315623, "taasdfasmo"), new Person(3455, "tamsdo")
, new Person(2656, "ryttu"), new Person(256, "Joro"));
});
}
}
}<file_sep>using System;
using System.Linq;
using System.Runtime.CompilerServices;
namespace _02._Archery_Tournament
{
class Program
{
static void Main(string[] args)
{
int[] targets = Console.ReadLine().Split("|").Select(int.Parse).ToArray();
int points = 0;
while (true)
{
string inp = Console.ReadLine();
if(inp == "Game over")
{
break;
}
string[] command = inp.Split("@");
switch (command[0])
{
case "Shoot Left":
int startIndex = int.Parse(command[1]);
int length = int.Parse(command[2]);
//int position = ;
if(startIndex >= 0 && startIndex < targets.Length)
{
for (int i = 0; i < length; i++)
{
startIndex--;
if(startIndex == -1)
{
startIndex = targets.Length - 1;
}
}
if(targets[startIndex] >= 5)
{
targets[startIndex] -= 5;
points += 5;
}
else if (targets[startIndex] > 0)
{
points += targets[startIndex];
targets[startIndex] = 0;
}
}
break;
case "Shoot Right":
int startInd = int.Parse(command[1]);
int len = int.Parse(command[2]);
//int position = ;
if (startInd >= 0 && startInd < targets.Length)
{
for (int i = 0; i < len; i++)
{
startInd++;
if (startInd == targets.Length)
{
startInd = 0;
}
}
if (targets[startInd] >= 5)
{
targets[startInd] -= 5;
points += 5;
}
else if(targets[startInd] > 0)
{
points += targets[startInd];
targets[startInd] = 0;
}
}
break;
case "Reverse":
targets = targets.Reverse().ToArray();
break;
}
//if (inp == "Reverse")
//{
// targets = targets.Reverse().ToArray();
//}
}
Console.WriteLine(string.Join(" - ", targets));
Console.WriteLine($"Iskren finished the archery tournament with {points} points!");
}
}
}
<file_sep>using System;
namespace Objects
{
class Program {
static void Main(string[] args)
{
Cars firstcar = new Cars();
Cars secondcar = new Cars();
firstcar.color = "blue";
secondcar.color = "red";
Console.WriteLine(firstcar.color);
Console.WriteLine(secondcar.color);
Console.WriteLine(firstcar.maxSpeed);
firstcar.Suobsht();
//Console.WriteLine("Hello World!");
}
}
}
<file_sep>namespace Presents.Tests
{
using NUnit.Framework;
using System;
using System.Diagnostics.Contracts;
public class PresentsTests
{
private Bag bag;
private Present present;
[SetUp]
public void SetUp()
{
bag = new Bag();
}
[Test]
public void TestIfCTorPresentWorksCorrectly()
{
present = new Present("Joro", 2);
Assert.AreEqual("Joro", present.Name);
Assert.AreEqual(2, present.Magic);
}
[Test]
public void CreateShouldAddPresentToTheBag()
{
int expectedCount = 1;
present = new Present("Joro", 2);
bag.Create(present);
Assert.AreEqual(expectedCount, bag.GetPresents().Count);
}
[Test]
public void CreateShouldThrowExceptionIfPresontIsNull()
{
present = null;
Assert.Throws<ArgumentNullException>(() =>
{
bag.Create(present);
});
}
[Test]
public void CreateShouldThrowExcpetionIfThisPresentAlreadyExists()
{
present = new Present("Joro", 2);
bag.Create(present);
Assert.Throws<InvalidOperationException>(() =>
{
bag.Create(present);
});
}
[Test]
public void RemoveShouldDecreaseCount()
{
present = new Present("Joro", 2);
bag.Create(present);
bag.Remove(present);
Assert.AreEqual(0, bag.GetPresents().Count);
}
[Test]
public void GetPresentWithLeastMagicReturnsCorrectly()
{
present = new Present("Joro", 6);
Present present1 = new Present("Vlado", 3);
Present present2 = new Present("Gosho", 5);
bag.Create(present);
bag.Create(present1);
bag.Create(present2);
Present returnedPresent = bag.GetPresentWithLeastMagic();
Assert.AreEqual(present1, returnedPresent);
}
[Test]
public void GetPresentShouldReturnPresentWithGivenName()
{
present = new Present("Joro", 6);
Present present1 = new Present("Vlado", 3);
Present present2 = new Present("Gosho", 5);
bag.Create(present);
bag.Create(present1);
bag.Create(present2);
Present returnedPresent = bag.GetPresent("Vlado");
Assert.AreEqual(present1,returnedPresent);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Wizard_Poker
{
class Program
{
static void Main(string[] args)
{
List<string> list = Console.ReadLine().Split(":").ToList();
List<string> deck = new List<string>();
while (true)
{
string input = Console.ReadLine();
if(input == "Ready")
{
break;
}
string[] command = input.Split();
switch (command[0])
{
case "Add":
if (list.Contains(command[1]))
{
deck.Add(command[1]);
}
else
{
Console.WriteLine("Card not found.");
}
break;
case "Insert":
string cardName = command[1];
int index = int.Parse(command[2]);
if (list.Contains(command[1]) && index >= 0 && index <= deck.Count)
{
deck.Insert(index, cardName);
}
else
{
Console.WriteLine("Error!");
}
break;
case "Remove":
if (deck.Contains(command[1]))
{
deck.Remove(command[1]);
}
else
{
Console.WriteLine("Card not found.");
}
break;
case "Swap":
string card1 = command[1];
string card2 = command[2];
int ind1 = deck.IndexOf(card1);
int ind2 = deck.IndexOf(card2);
string temp = deck[ind1];
deck[ind1] = deck[ind2];
deck[ind2] = temp;
break;
case "Shuffle":
deck.Reverse();
break;
}
}
Console.WriteLine(string.Join(" ", deck));
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
var input1 = Console.ReadLine().Split().Select(int.Parse).ToArray();
var input2 = Console.ReadLine().Split().Select(int.Parse).ToArray();
Stack<int> materialsForCrafting = new Stack<int>(input1);
Queue<int> magicLevelValues = new Queue<int>(input2);
Dictionary<int, string> presentsMagicLevel = new Dictionary<int, string>
{
{ 150,"Doll" },
{ 250,"Wooden train"},
{ 300,"Teddy bear" },
{ 400,"Bicycle"}
};
SortedDictionary<string, int> presentsMade = new SortedDictionary<string, int>();
//DOll ->1
//Bicycle ->2
while(materialsForCrafting.Count > 0 && magicLevelValues.Count>0)
{
int magicLevel = materialsForCrafting.Peek() * magicLevelValues.Peek();
if(presentsMagicLevel.ContainsKey(magicLevel))
{
if (!presentsMade.ContainsKey(presentsMagicLevel[magicLevel]))
{
presentsMade.Add(presentsMagicLevel[magicLevel], 0);
}
presentsMade[presentsMagicLevel[magicLevel]]++;
materialsForCrafting.Pop();
magicLevelValues.Dequeue();
}
else
{
if(magicLevel<0)
{
int sum = materialsForCrafting.Peek() + magicLevelValues.Peek();
materialsForCrafting.Pop();
magicLevelValues.Dequeue();
materialsForCrafting.Push(sum);
}
else if(magicLevel>0)
{
int material = materialsForCrafting.Peek() + 15;
materialsForCrafting.Pop();
magicLevelValues.Dequeue();
materialsForCrafting.Push(material);
}
else if(magicLevel==0)
{
if (materialsForCrafting.Peek() == 0)
{
materialsForCrafting.Pop();
}
if(magicLevelValues.Peek()==0)
{
magicLevelValues.Dequeue();
}
}
}
}
if((presentsMade.ContainsKey("Doll") && presentsMade.ContainsKey("Wooden train"))
|| (presentsMade.ContainsKey("Teddy bear")&& presentsMade.ContainsKey("Bicycle")))
{
Console.WriteLine("The presents are crafted! Merry Christmas!");
}
else
{
Console.WriteLine("No presents this Christmas!");
}
if(materialsForCrafting.Count>0)
{
Console.WriteLine("Materials left:"+ string.Join(", ",materialsForCrafting));
}
if(magicLevelValues.Count>0)
{
Console.WriteLine("Magic left:" + string.Join(", ", magicLevelValues));
}
foreach (var present in presentsMade)
{
Console.WriteLine($"{present.Key}: {present.Value}");
}
// 10 - 5 20 15 -30 10
// 40 60 10 4 10 0
// 10 - 5 20 15 - 30 10
// 40 60 10 4 10 0
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Models
{
public class Owner
{
private List<Cat> cats;
public Owner(string name)
{
this.Name = name;
this.cats = new List<Cat>();
}
public string Name { get; }
public void AddCat(string name , int age)
{
var cat = new Cat(name, age, this);/////We are giving as an argument the Owner Class with this keyword
this.cats.Add(cat);
}
}
}
<file_sep>using System;
using System.Linq;
namespace _03._Rounding_Numbers
{
class Program
{
static void Main(string[] args)
{
double[] arr = Console.ReadLine().Split().Select(double.Parse).ToArray();
int[] realarr = new int[arr.Length];
for (int i = 0; i < realarr.Length; i++)
{
realarr[i] = (int)Math.Round(arr[i], MidpointRounding.AwayFromZero);
}
for (int i = 0; i < realarr.Length; i++)
{
Console.WriteLine($"{arr[i]} => {realarr[i]}");
//Console.WriteLine(string.Join("=> ", realarr));
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _2.Loot
{
class Program
{
static void Main(string[] args)
{
List<string> loot = Console.ReadLine().Split('|', StringSplitOptions.RemoveEmptyEntries).ToList();
string input;
while ((input = Console.ReadLine()) != "Yohoho!")
{
string[] command = input.Split(' ', StringSplitOptions.RemoveEmptyEntries).ToArray();
if(command[0] == "Loot")
{
for (int i = 1; i < command.Length; i++)
{
string itemToAdd = command[i];
if (!loot.Contains(itemToAdd))
{
loot.Insert(0, itemToAdd);
}
}
}
else if(command[0] == "Drop")
{
int index = int.Parse(command[1]);
if(index >= 0 && index < loot.Count)
{
string itemToRemove = loot[index];
loot.RemoveAt(index);
loot.Add(itemToRemove);
}
}
else if(command[0] == "Steal")
{
int count = int.Parse(command[1]);
List<string> removedItems = new List<string>();
if(count >= loot.Count)
{
//loot.RemoveRange(0, loot.Count);
int length = loot.Count;
for (int i = 0; i < length; i++)
{
string item = loot[0];
removedItems.Add(item);
loot.RemoveAt(0);
}
}
else
{
for (int i = 0; i < count; i++)
{
string item = loot[loot.Count - count + i];
removedItems.Add(item);
loot.RemoveAt(loot.Count - count + i);
}
//loot.RemoveRange(loot.Count - count, count);
}
Console.WriteLine(string.Join(", ", removedItems));
}
}
if(loot.Count > 0)
{
double sumItemsLength = loot.Sum(i => i.Length);
Console.WriteLine($"Average treasure gain: {(sumItemsLength/loot.Count):F2} pirate credits.");
}
else
{
Console.WriteLine("Failed treasure hunt.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace BakeryOpenning
{
public class Bakery
{
private readonly ICollection<Employee> data;
public string Name { get; set; }
public int Capacity { get; set; }
public Bakery(string name, int capacity)
{
this.data = new List<Employee>();
this.Name = name;
this.Capacity = capacity;
}
public void Add(Employee employee)
{
if(this.data.Count < this.Capacity)
{
this.data.Add(employee);
}
}
public bool Remove(string name)
{
return this.data.Remove(this.data.FirstOrDefault(e => e.Name == name));
}
public Employee GetOldestEmployee()
{
return this.data.OrderByDescending(e => e.Age).First();
}
public Employee GetEmployee(string name)
{
return this.data.FirstOrDefault(e => e.Name == name);
}
public int Count { get => this.data.Count; }
public string Report()
{
StringBuilder sb = new StringBuilder();
sb.AppendLine($"Employees working at Bakery {this.Name}:");
foreach(var em in this.data)
{
sb.AppendLine(em.ToString());
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.PizzaCalories.Ingredients
{
public class Topping
{
private string toppingType;
private double weight;
private const double baseCaloriesPerGram = 2;
public Topping(string toppingType , double weight)
{
this.ToppingType = toppingType;
this.Weight = weight;
}
public string ToppingType
{
get
{
return this.toppingType;
}
private set
{
if(value.ToLower() != "meat" && value.ToLower() != "veggies" && value.ToLower() != "cheese" && value.ToLower() != "sauce")
{
throw new ArgumentException($"Cannot place {value} on top of your pizza.");
}
this.toppingType = value;
}
}
public double Weight
{
get
{
return this.weight;
}
private set
{
if(value < 1 || value > 50)
{
throw new ArgumentException($"{this.ToppingType} weight should be in the range [1..50].");
}
this.weight = value;
}
}
public double ToppingCalories()
{
double toppingModifier = FindModifier();
return baseCaloriesPerGram * this.Weight * toppingModifier;
}
private double FindModifier()
{
double toppingTypeModifier = 0;
switch (this.ToppingType.ToLower())
{
case "meat":
toppingTypeModifier = 1.2;
break;
case "veggies":
toppingTypeModifier = 0.8;
break;
case "cheese":
toppingTypeModifier = 1.1;
break;
case "sauce":
toppingTypeModifier = 0.9;
break;
}
return toppingTypeModifier;
}
}
}
<file_sep>using System;
using System.Linq;
namespace _01
{
class Program
{
static void Main(string[] args)
{
string username = Console.ReadLine();
string input;
while((input = Console.ReadLine()) != "Sign up")
{
string[] command = input.Split(" ", StringSplitOptions.RemoveEmptyEntries).ToArray();
if(command[0] == "Case")
{
if(command[1] == "lower")
{
username = username.ToLower();
}
else
{
username = username.ToUpper();
}
Console.WriteLine(username);
}
else if(command[0] == "Reverse")
{
int startIndex = int.Parse(command[1]);
int endIndex = int.Parse(command[2]);
if(startIndex >= 0 && endIndex < username.Length)
{
string substr = username.Substring(startIndex, endIndex - startIndex + 1);
char[] subsArray = substr.ToCharArray();
Array.Reverse(subsArray);
string res = new string(subsArray);
Console.WriteLine(res);
}
}
else if(command[0] == "Cut")
{
string subs = command[1];
if(username.Contains(subs))
{
int index = username.IndexOf(subs);
username = username.Remove(index, subs.Length);
Console.WriteLine(username);
////////not sure
}
else
{
Console.WriteLine($"The word {username} doesn't contain {subs}.");
}
}
else if(command[0] == "Replace")
{
char cToReplace = char.Parse(command[1]);
username = username.Replace(cToReplace, '*');
Console.WriteLine(username);
}
else
{
char cToCheck = char.Parse(command[1]);
if(username.Contains(cToCheck))
{
Console.WriteLine("Valid");
}
else
{
Console.WriteLine($"Your username must contain {cToCheck}.");
}
}
}
}
}
}
<file_sep>using System;
namespace _04._Sum_of_Chars
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int charSum = 0;
while (n > 0)
{
char letter = char.Parse(Console.ReadLine());
charSum += letter;
n--;
}
Console.WriteLine($"The sum equals: {charSum}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _10._SoftUni_Exam_Results
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> results = new Dictionary<string, int>();
Dictionary<string, int> countLanguages = new Dictionary<string, int>();
while (true)
{
string inp = Console.ReadLine();
if (inp == "exam finished")
{
break;
}
string[] command = inp.Split("-");
if(command[1] == "banned")
{
string userName = command[0];
results.Remove(userName);
}
else
{
string userName = command[0];
string language = command[1];
int points = int.Parse(command[2]);
if (!countLanguages.ContainsKey(language))
{
countLanguages[language] = 0;
}
if (!results.ContainsKey(userName))
{
results[userName] = 0;
}
if(points > results[userName])
{
results[userName] = points;
}
countLanguages[language]++;
}
}
var sortedResults = results
.OrderByDescending(kvp => kvp.Value)
.ThenBy(kvp => kvp.Key)
.ToDictionary( a => a.Key, b => b.Value);
countLanguages = countLanguages
.OrderByDescending(kvp => kvp.Value)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
Console.WriteLine("Results:");
foreach (var kvp in sortedResults)
{
Console.WriteLine($"{kvp.Key} | {kvp.Value}");
}
Console.WriteLine("Submissions:");
foreach (var lang in countLanguages)
{
Console.WriteLine($"{lang.Key} - {lang.Value}");
}
}
}
}
<file_sep>using System;
namespace _05._Add_and_Subtract
{
class Program
{
static void Main(string[] args)
{
int num1 = int.Parse(Console.ReadLine());
int num2 = int.Parse(Console.ReadLine());
int num3 = int.Parse(Console.ReadLine());
int res = Sum(num1, num2);
Console.WriteLine(Subtract(res, num3));
}
static int Sum(int n1,int n2)
{
return n1 + n2;
}
static int Subtract(int n1 , int n2)
{
return n1 - n2;
}
}
}
<file_sep>using System;
namespace _08._Factorial_Division
{
class Program
{
static void Main(string[] args)
{
int a = int.Parse(Console.ReadLine());
int b = int.Parse(Console.ReadLine());
double factoredA = Factor(a);
double factoredB = Factor(b);
double res = factoredA / factoredB;
Console.WriteLine($"{res:F2}");
}
static double Factor(int a)
{
double res = 1;
while (a > 0)
{
res *= a;
a--;
}
//Console.WriteLine(res);
return res;
}
}
}
<file_sep>using System;
namespace Volleyball
{
class Program
{
static void Main(string[] args)
{
string typeYear = Console.ReadLine();
double p = double.Parse(Console.ReadLine());
double h = double.Parse(Console.ReadLine());
double countPlayedGames = 0;
// Console.WriteLine(p * 2 / 3);
countPlayedGames = ((48 - h)* 0.75 + h + (p * 2/3) );
// Console.WriteLine(countPlayedGames);
if(typeYear == "leap")
{
countPlayedGames = countPlayedGames + countPlayedGames * 0.15;
}
Console.WriteLine(Math.Floor(countPlayedGames));
}
}
}
<file_sep>using P08.CollectionHierarchy.Models;
using System;
namespace P08.CollectionHierarchy
{
class StartUp
{
static void Main(string[] args)
{
AddCollection addCollection = new AddCollection();
AddRemoveCollection addRemoveCollection = new AddRemoveCollection();
MyList myList = new MyList();
string[] firstLine = Console.ReadLine()
.Split();
for (int i = 0; i < firstLine.Length; i++)
{
string element = firstLine[i];
addCollection.Add(element);
addRemoveCollection.Add(element);
myList.Add(element);
}
int countRemoveOperations = int.Parse(Console.ReadLine());
for (int i = 0; i < countRemoveOperations; i++)
{
addRemoveCollection.Remove();
myList.Remove();
}
Console.WriteLine(addCollection);
Console.WriteLine(addRemoveCollection);
Console.WriteLine(myList);
addRemoveCollection.GetRemoved();
myList.GetRemoved();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P07.MilitaryElite.Exceptions
{
public class InvalidMissionCompletionException : Exception
{
private const string DEF_MSG = "Mission already completed";
public InvalidMissionCompletionException()
:base(DEF_MSG)
{
}
public InvalidMissionCompletionException(string message) : base(message)
{
}
}
}
<file_sep>using System;
namespace Scholarship
{
class Program
{
static void Main(string[] args)
{
double income = double.Parse(Console.ReadLine());
double averageSuccess = double.Parse(Console.ReadLine());
double minimalSalary = double.Parse(Console.ReadLine());
double scholarshipForResults = averageSuccess * 25 ;
double socialScholarship = minimalSalary * 0.35;
if(averageSuccess>=5.5 && income < minimalSalary )
{
if(scholarshipForResults > socialScholarship)
{
Console.WriteLine($"You get a scholarship for excellent results {Math.Floor(scholarshipForResults)} BGN");
}
else if(scholarshipForResults < socialScholarship)
{
Console.WriteLine($"You get a Social scholarship {Math.Floor(socialScholarship)} BGN");
}
else
{
Console.WriteLine($"You get a scholarship for excellent results {Math.Floor(scholarshipForResults)} BGN");
}
}
else if(averageSuccess >= 5.5)
{
Console.WriteLine($"You get a scholarship for excellent results {Math.Floor(scholarshipForResults)} BGN");
}
else if(income<minimalSalary && averageSuccess>4.5 )
{
Console.WriteLine($"You get a Social scholarship {Math.Floor(socialScholarship)} BGN");
}
else
{
Console.WriteLine("You cannot get a scholarship!");
}
}
}
}
<file_sep>using System;
using System.Runtime.CompilerServices;
namespace Encapsulation
{
class Program
{
static void Main(string[] args)
{
// var cat = new Cat("Luchko",3,"Ivan");
// Console.WriteLine(cat.Details());
///////////////
//var person = new PersonBuilder()
// .WithFirstName("Ivan")
// .WithAge(15)
// .Build();
//var person = new Person();
//person
// .RegisterForExam()
// .ParticipateInExam()
// .CalculateScore();
//var name = SetValue("Ivan")
//}
//private static void SetValue(string name)
//{
// // name ??= throw new InvalidOperationException();
//}
var team = new Team("Top Team");
team.AddPlayer(new Person("Ivan", "Ivanov", 50));
team.AddPlayer(new Person("Ivan", "Peshov", 30));
Console.WriteLine(team.FirstTeam.Count);
Console.WriteLine(team.ReserveTeam.Count);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace Messaging
{
class Program
{
static void Main(string[] args)
{
List<string> log = new List<string>();
string input;
while((input = Console.ReadLine()) != "end")
{
string[] command = input.Split(' ',StringSplitOptions.RemoveEmptyEntries).ToArray();
if(command[0] == "Chat")
{
string message = command[1];
log.Add(message);
}
else if(command[0] == "Delete")
{
string messToDelete = command[1];
if(log.Any(m => m == messToDelete))
{
log.Remove(messToDelete);
}
}
else if(command[0] == "Edit")
{
string messToEdit = command[1];
string editedVers = command[2];
int index = log.FindIndex(i => i == messToEdit);
log[index] = editedVers;
}
else if(command[0] == "Pin")
{
string message = command[1];
log.Remove(message);
log.Add(message);
}
else if(command[0] == "Spam")
{
for (int i = 1; i < command.Length; i++)
{
log.Add(command[i]);
}
}
}
Console.WriteLine(string.Join('\n', log));
}
}
}
<file_sep>using System;
namespace _03._Elevator
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int capacity = int.Parse(Console.ReadLine());
int courses = 0;
while (n > 0)
{
n -= capacity;
courses++;
}
Console.WriteLine(courses);
}
}
}
<file_sep>using System;
using System.Linq;
namespace _08.Letters_Change_Numbers
{
class Program
{
static void Main(string[] args)
{
string[] words = Console.ReadLine()
.Split(" ", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
double sum = 0;
for (int i = 0; i < words.Length; i++)
{
sum = ProcessWord(words, sum, i);
}
Console.WriteLine($"{sum:F2}");
}
private static double ProcessWord(string[] words, double sum, int i)
{
double tempSum = 0;
string currWord = words[i];
char firstLetter = currWord[0];
char lastLetter = currWord[currWord.Length - 1];
ParseeNumberFromInput(currWord);
double number = ParseeNumberFromInput(currWord);
tempSum += number;
int firstLetterPos = GetAlphabeticalPosByLetter(firstLetter);
int lastLetterPos = GetAlphabeticalPosByLetter(lastLetter);
if (char.IsUpper(firstLetter) && firstLetterPos > 0)
{
tempSum /= firstLetterPos;
}
else if (char.IsLower(firstLetter) && firstLetterPos > 0)
{
tempSum = number * firstLetterPos;
}
if (char.IsUpper(lastLetter) && lastLetterPos > 0)
{
tempSum -= lastLetterPos;
}
else if (char.IsLower(lastLetter) && lastLetterPos > 0)
{
tempSum += lastLetterPos;
}
sum += tempSum;
return sum;
}
static int GetAlphabeticalPosByLetter(char letter)
{
int position = -1;
if (char.IsLetter(letter))
{
if (char.IsUpper(letter))
{
position = letter - 64;
}
else
{
position = letter - 96;
}
}
return position;
}
private static double ParseeNumberFromInput(string currWord)
{
char[] numberAsChar = currWord.Skip(1)
.Take(currWord.Length - 2)
.ToArray();
string numberAsString = String.Join("", numberAsChar);
double number = double.Parse(numberAsString);
return number;
}
// static int
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P06.SpeedRacing
{
public class Car
{
public Car(string model,double fuelAmount,double fuelCons)
{
this.Model = model;
this.FuelAmount = fuelAmount;
this.FuelConsumptionPerKilometer = fuelCons;
}
public string Model { get; private set; }
public double FuelAmount { get; private set; }
public double FuelConsumptionPerKilometer { get; private set; }
public double TravelledDistance { get; private set; }
public void Drive(int kilometers)
{
double fuelNeeded = kilometers * this.FuelConsumptionPerKilometer;
if(this.FuelAmount >= fuelNeeded)
{
this.FuelAmount -= fuelNeeded;
this.TravelledDistance += kilometers;
}
else
{
Console.WriteLine("Insufficient fuel for the drive");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
namespace _07._List_Manipulation_Advanced
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
while (true)
{
string input = Console.ReadLine();
if (input == "end")
{
break;
}
string[] command = input.Split();
switch (command[0])
{
case "Contains":
DoesContain(list, int.Parse(command[1]));
break;
case "PrintEven":
PrintEven(list);
break;
case "PrintOdd":
PrintOdd(list);
break;
case "GetSum":
int sum = GetSum(list);
Console.WriteLine(sum);
break;
case "Filter":
string condition = command[1];
int num = int.Parse(command[2]);
Filter(list, condition, num);
break;
}
}
}
static void DoesContain(List<int> list, int number)
{
if (list.Contains(number))
{
Console.WriteLine("Yes");
}
else
{
Console.WriteLine("No such number");
}
}
static void PrintEven(List<int> list)
{
List<int> evenList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
if (list[i] % 2 == 0)
{
evenList.Add(list[i]);
}
}
Console.WriteLine(string.Join(" ", evenList));
}
static void PrintOdd(List<int> list)
{
List<int> oddList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
if (list[i] % 2 != 0)
{
oddList.Add(list[i]);
}
}
Console.WriteLine(string.Join(" ", oddList));
}
static int GetSum(List<int> list)
{
return list.Sum();
}
static void Filter(List<int> list, string condtion, int num)
{
//List<int> filteredList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
switch (condtion)
{
case "<":
if (list[i] >= num)
{
list.Remove(list[i]);
i--;
}
break;
case ">":
if (list[i] <= num)
{
list.Remove(list[i]);
i--;
}
break;
case ">=":
if (list[i] < num)
{
list.Remove(list[i]);
i--;
}
break;
case "<=":
if (list[i] > num)
{
list.Remove(list[i]);
i--;
}
break;
}
}
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04._BorderControl.Contracts
{
public interface ICitizen
{
string Name { get; }
int Age { get; }
string Id { get; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._P_rates
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> citiesGold = new Dictionary<string, int>();
Dictionary<string, int> citiesPopulation = new Dictionary<string, int>();
string input;
while((input = Console.ReadLine()) != "Sail")
{
string[] currCity = input.Split("||").ToArray();
string cityName = currCity[0];
int population = int.Parse(currCity[1]);
int gold = int.Parse(currCity[2]);
if(!citiesGold.ContainsKey(cityName))
{
citiesGold[cityName] = 0;
citiesPopulation[cityName] = 0;
}
citiesGold[cityName] += gold;
citiesPopulation[cityName] += population;
}
string input2;
while((input2 = Console.ReadLine()) != "End")
{
string[] task = input2.Split("=>").ToArray();
if(task[0] == "Plunder")
{
string townName = task[1];
int peopleToKill = int.Parse(task[2]);
int goldToSteal = int.Parse(task[3]);
citiesGold[townName] -= goldToSteal;
citiesPopulation[townName] -= peopleToKill;
Console.WriteLine($"{townName} plundered! {goldToSteal} gold stolen, {peopleToKill} citizens killed.");
if(citiesPopulation[townName] <= 0 || citiesGold[townName] <= 0)
{
citiesGold.Remove(townName);
citiesPopulation.Remove(townName);
Console.WriteLine($"{townName} has been wiped off the map!");
}
}
else if(task[0] == "Prosper")
{
string townName = task[1];
int goldIncrease = int.Parse(task[2]);
if(goldIncrease < 0)
{
Console.WriteLine("Gold added cannot be a negative number!");
continue;
}
citiesGold[townName] += goldIncrease;
Console.WriteLine($"{goldIncrease} gold added to the city treasury. {townName} now has {citiesGold[townName]} gold.");
}
}
citiesGold = citiesGold
.OrderByDescending(kvp => kvp.Value)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
if(citiesGold.Any())
{
Console.WriteLine($"Ahoy, Captain! There are {citiesGold.Count} wealthy settlements to go to:");
foreach (string cityName in citiesGold.Keys)
{
Console.WriteLine($"{cityName} -> Population: {citiesPopulation[cityName]} citizens, Gold: {citiesGold[cityName]} kg");
}
}
else
{
Console.WriteLine("Ahoy, Captain! All targets have been plundered and destroyed!");
}
}
}
}
<file_sep>using System;
namespace HotelProject
{
class Program
{
static void Main(string[] args)
{
string month = Console.ReadLine();
int countDays = int.Parse(Console.ReadLine());
double moneyStudio = 0;
double moneyApartment = 0;
switch(month)
{
case "May":
case "October":
moneyStudio = countDays * 50;
moneyApartment = countDays * 65;
if(countDays > 14)
{
moneyStudio = moneyStudio - moneyStudio * 0.3;
moneyApartment = moneyApartment - moneyApartment * 0.1;
}
else if(countDays>7)
{
moneyStudio = moneyStudio - moneyStudio * 0.05;
}
break;
case "June":
case "September":
moneyStudio = countDays * 75.20;
moneyApartment = countDays * 68.70;
if(countDays > 14)
{
moneyStudio = moneyStudio - moneyStudio * 0.2;
moneyApartment = moneyApartment - moneyApartment * 0.1;
}
break;
case "July":
case "August":
moneyStudio = countDays * 76;
moneyApartment = countDays * 77;
if(countDays > 14)
{
moneyApartment = moneyApartment - moneyApartment * 0.1;
}
break;
}
Console.WriteLine($"Apartment: { moneyApartment:F2} lv.");
Console.WriteLine($"Studio: {moneyStudio:F2} lv.");
}
}
}
<file_sep>using System;
using System.Text;
namespace ex1
{
class Program
{
static void Main(string[] args)
{
int N = int.Parse(Console.ReadLine());
//StringBuilder sb = new StringBuilder();
double totalPrice = 0;
for (int i = 0; i < N; i++)
{
double pricePerCapsule = double.Parse(Console.ReadLine());
int days = int.Parse(Console.ReadLine());
int capsulesCount = int.Parse(Console.ReadLine());
double currPrice = Math.Round(((days * capsulesCount) * pricePerCapsule),2);
totalPrice += currPrice;
Console.WriteLine($"The price for the coffee is: ${currPrice:F2}");
//sb.AppendLine($"The price for the coffee is: ${Math.Round(currPrice,2):F2}");
}
//Console.WriteLine(sb.ToString().TrimEnd());
Console.WriteLine($"Total: ${Math.Round(totalPrice,2):F2}");
}
}
}
<file_sep>using System;
using System.Text;
namespace _01._Activation_Keys
{
class Program
{
static void Main(string[] args)
{
string key = Console.ReadLine();
while (true)
{
string input = Console.ReadLine();
if(input == "Generate")
{
break;
}
string[] command = input.Split(">>>");
if(command[0] == "Contains")
{
string sub = command[1];
if (key.Contains(sub))
{
Console.WriteLine($"{key} contains {sub}");
}
else
{
Console.WriteLine("Substring not found!");
}
}
else if(command[0] == "Flip")
{
int startIndex = int.Parse(command[2]);
int endIndex = int.Parse(command[3]);
if (command[1] == "Upper")
{
//string sub = key.Substring(startIndex, endIndex)
// //key = sub.ToUpper();
StringBuilder sb = new StringBuilder();
for (int i = 0; i < key.Length; i++)
{
//key[i] = key[i].ToString().ToUpper();
if (i >= startIndex && i < endIndex)
{
sb.Append(key[i].ToString().ToUpper());
}
else
{
sb.Append(key[i]);
}
}
key = sb.ToString();
}
else
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < key.Length; i++)
{
//key[i] = key[i].ToString().ToUpper();
if (i >= startIndex && i < endIndex)
{
sb.Append(key[i].ToString().ToLower());
}
else
{
sb.Append(key[i]);
}
}
key = sb.ToString();
}
Console.WriteLine(key);
}
else
{
int startIndex = int.Parse(command[1]);
int endIndex = int.Parse(command[2]);
int count = endIndex - startIndex;
key = key.Remove(startIndex, count);
Console.WriteLine(key);
}
}
Console.WriteLine($"Your activation key is: {key}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03.Numbers
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine()
.Split(' ')
.Select(int.Parse)
.ToList();
double average = list.Average();
var modifiedList = new List<int>();
foreach (var item in list)
{
if(item > average)
{
modifiedList.Add(item);
}
}
if (modifiedList.Any())
{
modifiedList = modifiedList
.OrderByDescending(x => x)
.Take(5)
.ToList();
Console.WriteLine(string.Join(' ', modifiedList));
}
else
{
Console.WriteLine("No");
}
}
}
}
<file_sep>using System;
using System.Collections.Specialized;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
namespace _01.Even_Lines
{
class Program
{
static void Main(string[] args)
{
char[] charactersToReplace = { '-', ',', '.', '!', '?' };
StreamReader streamReader = new StreamReader("text.txt");
int counter = 0;
while (!streamReader.EndOfStream)
{
string line = streamReader.ReadLine();
if(line == null)
{
break;
}
if(counter % 2 == 0)
{
line = ReplaceAll(charactersToReplace, '@', line);
line = ReverseWordsInText(line);
Console.WriteLine(line);
}
counter++;
}
}
static string ReplaceAll(char[] replace , char replacement, string str)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < str.Length; i++)
{
char currSymbol = str[i];
if (replace.Contains(currSymbol))
{
sb.Append(replacement);
}
else
{
sb.Append(currSymbol);
}
}
return sb.ToString().TrimEnd();
}
static string ReverseWordsInText(string str)
{
StringBuilder sb = new StringBuilder();
string[] words = str
.Split()
.ToArray();
for (int i = 0; i < words.Length; i++)
{
sb.Append(words[words.Length - i - 1]);
sb.Append(' ');
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>
namespace TestDemos.Test
{
using System;
using System.ComponentModel.DataAnnotations;
using System.Data;
using TestApp;
using Xunit;
public class CalculatorTest
{
[Fact]
public void SumShouldReturnCorrectResultWithTwoNumbers()
{
var calculator = new Calculator();
var result = calculator.CalculateSum(1, 2);
Assert.Equal(3, result);
//if(result != 3)
//{
// throw new InvalidOperationException
// ("SumShouldReturnCorrectResultWithTwoNumbers failed");
//}
}
[Fact]
public void SumShouldReturnCorrectResultWithManyNumbers()
{
var calculator = new Calculator();
var result = calculator.CalculateSum(1, 2,3,4,5,6);
//if (result != 21)
//{
// throw new InvalidOperationException
// ("SumShouldReturnCorrectResultWithManyNumbers failed");
//}
Assert.Equal(21, result);
}
[Fact]
public void SumShouldReturnCorrectResultWithNoNumbers()
{
var calculator = new Calculator();
var result = calculator.CalculateSum();
//if (result != 0)
//{
// throw new InvalidOperationException
// ("SumShouldReturnCorrectResultWithNoNumbers failed");
//}
Assert.Equal(0, result);
}
[Fact]
public void ProductShouldReturnCorrectResultWithTwoNumbers()
{
var calculator = new Calculator();
var result = calculator.Product(2, 3);
Assert.Equal(6, result);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Product_Shop
{
class Program
{
static void Main(string[] args)
{
var shops = new
Dictionary<string, Dictionary<string, double>>();
string command;
while ((command = Console.ReadLine()) != "Revision")
{
string[] cmdArgs = command
.Split(", ",StringSplitOptions.RemoveEmptyEntries);
string shopName = cmdArgs[0];
string productName = cmdArgs[1];
double price = double.Parse(cmdArgs[2]);
if (!shops.ContainsKey(shopName))
{
shops[shopName] = new Dictionary<string, double>();
}
if (!shops[shopName].ContainsKey(productName))
{
shops[shopName][productName] = 0;
}
shops[shopName][productName] = price;
// shops[shopName].Add(productName, price);
}
shops = shops
.OrderBy(kvp => kvp.Key)
.ToDictionary(x => x.Key, y => y.Value);
foreach (var kvp in shops)
{
Console.WriteLine($"{kvp.Key}->");
foreach (var product in kvp.Value)
{
Console.WriteLine($"Product: {product.Key}, Price: {product.Value}");
}
}
}
}
}
<file_sep>using System;
namespace HoneyHarvest
{
class Program
{
static void Main(string[] args)
{
string typeFlower = Console.ReadLine();
int countFlowers = int.Parse(Console.ReadLine());
string season = Console.ReadLine();
double result=0;
if(season == "Spring")
{
switch (typeFlower)
{
case "Sunflower":
result = countFlowers * 10;
break;
case "Daisy":
result = countFlowers * (12+12*0.1);
break;
case "Lavender":
result = countFlowers * 12;
break;
case "Mint":
result = countFlowers * (10+10*0.1);
break;
}
// result += result * 0.1;
}
if(season == "Summer")
{
switch (typeFlower)
{
case "Sunflower":
case "Daisy":
case "Lavender":
result = countFlowers * 8;
break;
case "Mint":
result = countFlowers * 12;
break;
}
result += result * 0.1;
}
if(season == "Autumn")
{
// Console.WriteLine("AAAAA");
switch (typeFlower)
{ //Console.WriteLine("AAAAA");
case "Sunflower":
result = countFlowers * 12;
//Console.WriteLine(result);
break;
case "Daisy":
result = countFlowers * 6;
break;
case "Lavender":
result = countFlowers * 6;
break;
case "Mint":
result = countFlowers * 6;
break;
}
result = result *0.95;
}
Console.WriteLine($"Total honey harvested: {result:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace DefiningClasses
{
public class Family
{
public List<Person> members;
public Family()
{
this.members = new List<Person>();
}
// public IReadOnlyCollection<Person> People
// => (IReadOnlyCollection<Person>) this.people;
public void AddMember(Person member)
{
this.members.Add(member);
}
public Person GetOldestMember()
{
return this.members.OrderByDescending(p => p.Age).First();
}
public void FilterMembersWithAgeLowerThanOrEqualThan30()
{
this.members = this.members
.Where(m => m.Age > 30)
.OrderBy(m => m.Name)
.ToList();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P01.DatingApp
{
class Program
{
static void Main(string[] args)
{
int[] malesInput = Console.ReadLine()
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
int[] femalesInput = Console.ReadLine()
.Split(' ',StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
Stack<int> males = new Stack<int>(malesInput);
Queue<int> females = new Queue<int>(femalesInput);
int succesfullMatches = 0;
while(males.Any() && females.Any())
{
int currMale = males.Peek();
int currFemale = females.Peek();
if(currMale <= 0)
{
males.Pop();
continue;
}
if(currFemale <= 0)
{
females.Dequeue();
continue;
}
if(currMale % 25 == 0)
{
males.Pop();
males.Pop();
continue;
}
if(currFemale % 25 == 0)
{
females.Dequeue();
females.Dequeue();
continue;
}
if(currMale == currFemale)
{
males.Pop();
females.Dequeue();
succesfullMatches++;
}
else
{
females.Dequeue();
int decreasedMale = males.Pop() - 2;
males.Push(decreasedMale);
}
}
Console.WriteLine($"Matches: {succesfullMatches}");
if (males.Any())
{
Console.WriteLine($"Males left: {string.Join(", ",males)}");
}
else
{
Console.WriteLine("Males left: none");
}
if (females.Any())
{
Console.WriteLine($"Females left: {string.Join(", ", females)}");
}
else
{
Console.WriteLine("Females left: none");
}
}
}
}
<file_sep>using P04._BorderControl.Contracts;
using P04._BorderControl.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P04._BorderControl.Core
{
public class Engine
{
public void Run()
{
// List<string> allIds = new List<string>();
// List<IIdentifiable> citizens = new List<IIdentifiable>();
// List<IIdentifiable> robots = new List<IIdentifiable>();
List<IIdentifiable> robotsAndCitizens = new List<IIdentifiable>();
string command;
while((command = Console.ReadLine()) != "End")
{
string[] commandArgs = command
.Split()
.ToArray();
if(commandArgs.Length == 3)
{
string name = commandArgs[0];
int age = int.Parse(commandArgs[1]);
string id = commandArgs[2];
Citizen citizen = new Citizen(name, age, id);
// citizens.Add(citizen);
robotsAndCitizens.Add(citizen);
}
else if(commandArgs.Length == 2)
{
string model = commandArgs[0];
string id = commandArgs[1];
Robot robot = new Robot(model, id);
//robots.Add(robot);
robotsAndCitizens.Add(robot);
}
}
string lastDigitFakeId = Console.ReadLine();
PrintFakeId(robotsAndCitizens, lastDigitFakeId);
}
private void PrintFakeId(List<IIdentifiable> robotsAndCitizens , string lastDigitFakeId)
{
foreach (IIdentifiable citizenOrRobot in robotsAndCitizens)
{
string currId = citizenOrRobot.GetId();
if (currId.EndsWith(lastDigitFakeId))
{
Console.WriteLine(currId);
}
}
}
}
}
<file_sep>using NUnit.Framework;
[TestFixture]
public class DummyTests
{
[Test]
public void DummyShouldLoseHealth()
{
//Arrange
var dummy = new Dummy(100, 200);
//Act
dummy.TakeAttack(50);
//Assert
Assert.That(dummy.Health, Is.EqualTo(50));
}
[Test]
public void DeadDummyShouldThrowExceptionIfAttacked()
{
//Arrange
var dummy = new Dummy(0, 200);
//Assert
Assert.That(() => dummy.TakeAttack(50),//Act
Throws.InvalidOperationException.With.Message.EqualTo("Dummy is dead.")
);
}
[Test]
public void DeadDummyShouldGiveExperience()
{
//Arrange
var dummy = new Dummy(0, 200);
//Act
var experience = dummy.GiveExperience();
//Assert
Assert.That(experience, Is.EqualTo(200));
}
[Test]
public void DummyShouldNotGiveExperienceIfDead()
{
//Arrange
var dummy = new Dummy(100, 200);
//Assert
Assert.That(() => dummy.GiveExperience(),//Act
Throws.InvalidOperationException.With.Message.EqualTo("Target is not dead."));
}
}
<file_sep>using System;
namespace ClassConsrtuctors
{
class Person
{
public string name;
public Person()
{
name = "Gosho";
}
}
class Program
{
static void Main(string[] args)
{
Person first = new Person();
// first.name = "Ivan";
Console.WriteLine(first.name);
}
}
}
<file_sep>using System;
namespace ConsoleApp1
{
class Program
{
static void Main(string[] args)
{
int biscuitsADay = int.Parse(Console.ReadLine());
int countWorkers = int.Parse(Console.ReadLine());
int otherFactoryBiscuitsfor30Days = int.Parse(Console.ReadLine());
int sumBiscuits = 0;
for (int i = 1; i <= 30; i++)
{
if(i % 3 == 0)
{
// sumBiscuits += countWorkers * (int)Math.Floor(biscuitsADay * 0.75);
sumBiscuits += (int)Math.Floor(countWorkers *biscuitsADay * 0.75);
//sumBiscuits += Math.Floor(countWorkers*biscuitsADay * 0.75);
}
else
{
sumBiscuits += countWorkers * biscuitsADay;
}
}
Console.WriteLine($"You have produced {sumBiscuits} biscuits for the past month.");
if(sumBiscuits > otherFactoryBiscuitsfor30Days)
{
int diff = sumBiscuits - otherFactoryBiscuitsfor30Days;
Console.WriteLine($"You produce {(double)diff / otherFactoryBiscuitsfor30Days * 100:F2} percent more biscuits.");
}
else
{
int diff = otherFactoryBiscuitsfor30Days - sumBiscuits;
Console.WriteLine($"You produce {(double)diff / otherFactoryBiscuitsfor30Days * 100:F2} percent less biscuits.");
}
}
}
}
<file_sep>using P03.Telephony.Contracts;
using P03.Telephony.Exceptions;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P03.Telephony.Models
{
public class StationaryPhone : ICallable
{
public StationaryPhone()
{
}
public string Call(string number)
{
if(!number.All(ch => char.IsDigit(ch)))
{
throw new InvalidNumberException();
}
return $"Dialing... {number}";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Team
{
public class Team
{
public string Name { get; }
public List<>
}
}
<file_sep>using System;
namespace CleverLily
{
class Program
{
static void Main(string[] args)
{
int age = int.Parse(Console.ReadLine());
double priceLaundry = double.Parse(Console.ReadLine());
double priceDolls = double.Parse(Console.ReadLine());
int countDolls = 0;
double moneyEarned = 0;
int countStealedMoney = 0;
int savedMoney = 0;
for(int i=1;i<=age;i++)
{
if(i % 2 == 0)
{
savedMoney += 10;
moneyEarned += savedMoney;
countStealedMoney++;
}
else
{
countDolls++;
}
}
moneyEarned = moneyEarned + countDolls * priceDolls -countStealedMoney*1;
if(moneyEarned >= priceLaundry)
{
Console.WriteLine($"Yes! {moneyEarned - priceLaundry:F2}");
}
else
{
Console.WriteLine($"No! {priceLaundry - moneyEarned:F2}");
}
}
}
}
<file_sep>using System;
using System.IO;
namespace Streams__Files_and_Directories___Demo
{
class Program
{
static void Main(string[] args)
{
// FileStream stream = new FileStream("text.txt", FileMode.OpenOrCreate);
//FileStream file = new FileStream(@"..\..\text.txt", FileMode.Open);
// FileStream file = new FileStream("text.txt", FileMode.Open);
//StreamWriter writer = new StreamWriter("result.txt");
/////using gurantees that the stream will be closed after stopping the process
//using (writer)
//{
// // writer.WriteLine("Test");
// // writer.Write("Another");
//}
//StreamReader reader = new StreamReader("text.txt");
//string result = reader.ReadLine();
//Console.WriteLine(result);
///// or other way
//// using StreamWriter writer = new StreamWriter("result.txt");
///// writer.Write("Test");c
//// writer.Write("Another");
/////
//FileStream stream2 = new FileStream("result.dat", FileMode.OpenOrCreate);
////using var writer2 = new BinaryWriter(stream2);
////writer2.Write(true);
////writer2.Write(false);
////writer2.Write(true);
//using var reader2 = new BinaryReader(stream2);
//var first = reader2.ReadBoolean();
//var second = reader2.ReadBoolean();
//var third = reader2.ReadBoolean();
//Console.WriteLine(first);
using var reader = new StreamReader("text.txt");
using var writer = new StreamWriter("output.txt");
int count = 1;
while (true)
{
var line = reader.ReadLine();
if (line == null)
{
break;
}
var convertedLine = $"{count}.{line}";
writer.WriteLine(convertedLine);
count++;
}
}
}
}
<file_sep>using System;
namespace Train_the_Trainers
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
double sumAllGrades = 0;
int countPresentations = 0;
string command = Console.ReadLine();
while(command != "Finish")
{
double sum = 0;
double avr = 0;
for(int i=0;i<n;i++)
{
double grade = double.Parse(Console.ReadLine());
sum += grade;
sumAllGrades += grade;
countPresentations++;
}
avr = sum / n;
Console.WriteLine($"{command} - {avr:F2}.");
command = Console.ReadLine();
}
Console.WriteLine($"Student's final assessment is {sumAllGrades/countPresentations:F2}.");
}
}
}
<file_sep>using System;
namespace NumsFromOneTO10
{
class Program
{
static void Main(string[] args)
{
/* Console.WriteLine(1);
Console.WriteLine(2);
Console.WriteLine(3);
Console.WriteLine(4);
Console.WriteLine(5);
Console.WriteLine(6);
Console.WriteLine(7);
Console.WriteLine(8);
Console.WriteLine(9);
Console.WriteLine(10);
*/
int input = int.Parse(Console.ReadLine());
Console.WriteLine(input);
}
}
}
<file_sep>using System;
namespace _06._Middle_Characters
{
class Program
{
static void Main(string[] args)
{
string str = Console.ReadLine();
PrintMiddleCharacters(str);
}
static void PrintMiddleCharacters(string str)
{
if(str.Length % 2 == 0)
{
Console.WriteLine($"{str[(str.Length-1)/2]}{str[(str.Length - 1) / 2 + 1]}");
}
else
{
Console.WriteLine(str[(str.Length - 1) / 2]);
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _04._Reverse_Array_of_Strings
{
class Program
{
static void Main(string[] args)
{
var items = Console.ReadLine().Split().ToArray();
int length = items.Length;
for (int i = 0; i <= length/2; i++,length--)
{
var old_el = items[i];
items[i] = items[length - 1];
items[length - 1] = old_el;
}
Console.WriteLine(string.Join(" ", items));
}
}
}
<file_sep>using System;
namespace _02.Bee
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
for (int i = 0; i < n; i++)
{
string row = Console.ReadLine();
for (int j = 0; j < n; j++)
{
field[i, j] = row[j];
}
}
int beeRow = -1;
int beeCol = -1;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == 'B')
{
beeRow = i;
beeCol = j;
}
}
}
int count_pollinated = 0;
bool isOut = false;
string command;
while (isOut != true)
{
if ((command = Console.ReadLine()) == "End")
{
break;
}
if (command == "up")
{
if (move_bee(n, "up", ref field, beeRow - 1, beeCol,
ref beeRow, ref beeCol, ref count_pollinated, ref isOut) == false)
{
continue;
}
}
else if (command == "down")
{
if (move_bee(n, "down", ref field, beeRow + 1, beeCol,
ref beeRow, ref beeCol, ref count_pollinated, ref isOut) == false)
{
continue;
}
}
else if (command == "left")
{
if (move_bee(n, "left", ref field, beeRow, beeCol - 1,
ref beeRow, ref beeCol, ref count_pollinated, ref isOut) == false)
{
continue;
}
}
else if (command == "right")
{
if (move_bee(n, "right", ref field, beeRow, beeCol + 1,
ref beeRow, ref beeCol, ref count_pollinated, ref isOut) == false)
{
continue;
}
}
PrintField(n, field);
Console.WriteLine();
}
if (isOut)
{
Console.WriteLine("The bee got lost!");
}
if (count_pollinated >= 5)
{
Console.WriteLine($"Great job, the bee managed to pollinate {count_pollinated} flowers!");
}
else
{
Console.WriteLine($"The bee couldn't pollinate the flowers, she needed {5 - count_pollinated} flowers more");
}
PrintField(n, field);
}
private static void PrintField(int n, char[,] field)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
Console.Write(field[i, j]);
}
Console.WriteLine();
}
}
public static bool move_bee(int n, string command, ref char[,] field, int changedRow, int changedCol,
ref int beeRow, ref int beeCow, ref int count_pollinated, ref bool isOut)
{
field[beeRow, beeCow] = '.';///no
if (changedRow < 0 || changedRow == n || changedCol < 0 || changedCol == n)
{
isOut = true;
return false;
}
if(field[changedRow, changedCol] == 'f')
{
count_pollinated++;
}
else if(field[changedRow, changedCol] == 'O')
{
field[changedRow, changedCol] = '.';
if (command == "up")/// check if it is out
{
move_bee(n, "up", ref field, changedRow - 1, changedCol, ref beeRow, ref beeCow, ref count_pollinated, ref isOut);
}
else if(command == "down")
{
move_bee(n, "down", ref field, changedRow + 1, changedCol, ref beeRow, ref beeCow, ref count_pollinated, ref isOut);
}
else if(command == "left")
{
move_bee(n, "left", ref field, changedRow, changedCol - 1, ref beeRow, ref beeCow, ref count_pollinated, ref isOut);
}
else
{
move_bee(n, "right", ref field, changedRow, changedCol + 1, ref beeRow, ref beeCow, ref count_pollinated, ref isOut);
}
}
else
{
//just move
}
field[changedRow, changedCol] = 'B';
beeRow = changedRow;
beeCow = changedCol;
return true;
}
}
}
<file_sep>using System;
using System.Linq;
namespace _01.Reverse_Strings
{
class Program
{
static void Main(string[] args)
{
string str = Console.ReadLine();
while(str != "end")
{
string reversed = "";
for (int i = str.Length - 1; i >= 0; i--)
{
reversed += str[i];
}
Console.WriteLine($"{str} = {reversed}");
str = Console.ReadLine();
}
}
}
}
<file_sep>using FightingArena;
using NUnit.Framework;
using System;
namespace Tests
{
public class WarriorTests
{
private Warrior warrior;
[SetUp]
public void Setup()
{
}
[Test]
public void ConstructorShouldInicialise()
{
string name = "Vlado";
int damage = 50;
int health = 100;
warrior = new Warrior(name, damage, health);
Assert.AreEqual(name, warrior.Name);
Assert.AreEqual(damage, warrior.Damage);
Assert.AreEqual(health, warrior.HP);
}
//[TestCase(string str = Strin, 50, 100)]
[TestCase("",50,100)]
[TestCase("Vlado",0,100)]
[TestCase("Vlado",-50,100)]
[TestCase("Vlado",50,-100)]
public void ConstructorShouldThrowExceptionIfGivenWrongArguments(string name,int damage,
int hp)
{
Assert.Throws<ArgumentException>(() =>
{
warrior = new Warrior(name, damage, hp);
});
}
[TestCase("Vlado", 50, 30)]
[TestCase("Vlado", 50, 29)]
public void AttackShouldThrowExceptionIfAttackersHpIsBelow30(string name, int damage,
int hp)
{
warrior = new Warrior(name, damage, hp);
Assert.Throws<InvalidOperationException>(() =>
{
warrior.Attack(new Warrior("Ivan", 10, 100));
});
}
[TestCase("Toni", 50, 30)]
[TestCase("Toni", 50, 29)]
public void AttackShouldThrowExceptionIfTheattackedWarriorsHpIsBElow30(string name, int damage,
int hp)
{
warrior = new Warrior("Vlado", 50, 100);
Warrior attacked = new Warrior(name, damage, hp);
Assert.Throws<InvalidOperationException>(() =>
{
warrior.Attack(attacked);
});
}
[Test]
public void AttackShouldThrowExceptionIfTheAttackedHaveMoreDamageThanTheAttacker()
{
warrior = new Warrior("Vlado", 50, 99);
Assert.Throws<InvalidOperationException>(() =>
{
warrior.Attack(new Warrior("Toni", 100, 100));
});
}
[Test]
public void AttackShouldLowerTheirHealth()
{
warrior = new Warrior("Vlado", 50, 100);
Warrior attacked = new Warrior("Toni", 50, 100);
warrior.Attack(attacked);
Assert.AreEqual(attacked.HP, 50);
Assert.AreEqual(warrior.HP, 50);
}
[Test]
public void AttackShouldLowerTheirHealth2()
{
warrior = new Warrior("Vlado", 50, 100);
Warrior attacked = new Warrior("Toni", 50, 40);
warrior.Attack(attacked);
Assert.AreEqual(attacked.HP, 0);
Assert.AreEqual(warrior.HP, 50);
}
}
}<file_sep>using System;
using System.Linq;
using System.Windows.Markup;
namespace JaggedArrayModification
{
class Program
{
static void Main(string[] args)
{
int rows = int.Parse(Console.ReadLine());
int[][] array = new int[rows][];
for (int row = 0; row < rows; row++)
{
int[] currentRow = Console.ReadLine().Split(' ').Select(int.Parse).ToArray();
array[row] = currentRow;
}
while(true)
{
string command = Console.ReadLine();
if(command == "END")
{
foreach(var currentRow in array)
{
Console.WriteLine(string.Join(" ", currentRow));
}
break;
}
string[] commandParts = command.Split();
int row = int.Parse(commandParts[1]);
int col = int.Parse(commandParts[2]);
int value = int.Parse(commandParts[3]);
if(row < 0 || row>= rows || col < 0 || col>= array[row].Length)
{
Console.WriteLine("Invalid cordinates");
}
if(commandParts[0] == "add")
{
array[row][col] += value;
}
else if(commandParts[0] == "substract")
{
array[row][col] -= value;
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
using System.Xml;
namespace P04.PizzaCalories.Ingredients
{
public class Dough
{
private string flourType;
private string bakingTechnique;
private double weight;
private const double caloriesPerGram = 2;
public Dough(string flourType, string bakingTechnique, double weight)
{
this.FlourType = flourType;
this.BakingTechnique = bakingTechnique;
this.Weight = weight;
}
public string FlourType
{
get
{
return this.flourType;
}
private set
{
if(value.ToLower() != "white" && value.ToLower() != "wholegrain")
{
throw new ArgumentException("Invalid type of dough.");
}
this.flourType = value;
}
}
public string BakingTechnique
{
get
{
return this.bakingTechnique;
}
private set
{
if (value.ToLower() != "crispy" && value.ToLower() != "chewy" && value.ToLower() != "homemade")
{
throw new ArgumentException("Invalid type of dough.");
}
this.bakingTechnique = value;
}
}
public double Weight
{
get
{
return this.weight;
}
private set
{
if(value < 1 || value > 200)
{
throw new ArgumentException("Dough weight should be in the range [1..200].");
}
this.weight = value;
}
}
public double DoughCalories()
{
double flourModifier = 0;
double bakingModifier = 0;
flourModifier = FindFlourModifier();
bakingModifier = FindBakingModifier();
return caloriesPerGram * this.Weight * flourModifier * bakingModifier;
}
private double FindFlourModifier()
{
double flourModifier = 0;
if (this.FlourType.ToLower() == "white")
{
flourModifier = 1.5;
}
else if(this.FlourType.ToLower() == "wholegrain")
{
flourModifier = 1;
}
return flourModifier;
}
private double FindBakingModifier()
{
double bakingModifier;
if (this.BakingTechnique.ToLower() == "crispy")
{
bakingModifier = 0.9;
}
else if (this.BakingTechnique.ToLower() == "chewy")
{
bakingModifier = 1.1;
}
else
{
bakingModifier = 1.0;
}
return bakingModifier;
}
}
}
<file_sep>using System;
using System.Globalization;
namespace DayOfWeek
{
class Program
{
static void Main(string[] args)
{
//18 - 04 - 2016
string date = Console.ReadLine();
DateTime dateTime = DateTime.ParseExact(date,"dd-MM-yyyy",CultureInfo.InvariantCulture);
Console.WriteLine(dateTime.DayOfWeek);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._P_rates
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> citiesPopulation = new Dictionary<string, int>();
Dictionary<string, int> citiesGold = new Dictionary<string, int>();
while (true)
{
string input = Console.ReadLine();
if(input == "Sail")
{
break;
}
string[] command = input.Split("||");
string city = command[0];
int population = int.Parse(command[1]);
int gold = int.Parse(command[2]);
if (!citiesPopulation.ContainsKey(city))
{
citiesPopulation[city] = 0;
citiesGold[city] = 0;
}
citiesPopulation[city] += population;
citiesGold[city] += gold;
}
while (true)
{
string input = Console.ReadLine();
if(input == "End")
{
break;
}
string[] command = input.Split("=>");
if(command[0] == "Plunder")
{
string city = command[1];
int peopleKilled = int.Parse(command[2]);
int goldStolen = int.Parse(command[3]);
Console.WriteLine($"{city} plundered! {goldStolen} gold stolen, {peopleKilled} citizens killed.");
if(citiesGold[city]-goldStolen > 0 && citiesPopulation[city] - peopleKilled > 0)
{
citiesGold[city] -= goldStolen;
citiesPopulation[city] -= peopleKilled;
}
else
{
citiesPopulation.Remove(city);
citiesGold.Remove(city);
Console.WriteLine($"{city} has been wiped off the map!");
}
}
else if(command[0] == "Prosper")
{
string city = command[1];
int goldIncome = int.Parse(command[2]);
if(goldIncome < 0)
{
Console.WriteLine("Gold added cannot be a negative number!");
}
else
{
citiesGold[city] += goldIncome;
Console.WriteLine($"{goldIncome} gold added to the city treasury. {city} now has {citiesGold[city]} gold.");
}
}
}
citiesGold = citiesGold
.OrderByDescending(kvp => kvp.Value)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
if(citiesGold.Count > 0)
{
Console.WriteLine($"Ahoy, Captain! There are {citiesGold.Count} wealthy settlements to go to:");
foreach (string city in citiesGold.Keys)
{
Console.WriteLine($"{city} -> Population: {citiesPopulation[city]} citizens, Gold: {citiesGold[city]} kg");
}
}
else
{
Console.WriteLine("Ahoy, Captain! All targets have been plundered and destroyed!");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace P04.OpinionPoll
{
public class Program
{
static void Main(string[] args)
{
Family family = new Family();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string[] personArgs = Console.ReadLine().Split();
string name = personArgs[0];
int age = int.Parse(personArgs[1]);
Person person = new Person(name, age);
family.AddMember(person);
}
//Person oldestPerson = family.GetOldestMember();
////Console.WriteLine(oldestPerson.ToString());
//Console.WriteLine($"{oldestPerson.Name} {oldestPerson.Age}");
var membersSorted = family.Members
.Where(m => m.Age > 30)
.OrderBy(m => m.Name)
.ToList();
foreach (var member in membersSorted)
{
Console.WriteLine($"{member.Name} - {member.Age}");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace List_of_Products
{
class Program
{
static void Main(string[] args)
{
List<string> list = new List<string>();
int n = int.Parse(Console.ReadLine());
list = AddProductsAndSort(list, n);
for (int i = 0; i < n ; i++)
{
Console.WriteLine($"{i + 1}.{list[i]}");
}
}
static List<string> AddProductsAndSort(List<string> list, int n)
{
for (int i = 0; i < n; i++)
{
string product = Console.ReadLine();
list.Add(product);
}
list.Sort();
return list;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Models
{
internal class Cat // internal - only for this project
{
private int age;
public Cat(string name,int age)
{
this.Name = name;
this.age = age;
}
public Cat(string name , int age , Owner owner)
:this(name,age)
{
this.Owner = owner;
}
public string Name { get; }
public Owner Owner { get;}
public string Details()
{
return $"{this.Name} - {this.age} - {this.Owner.Name}";
}
}
}
<file_sep>using System;
namespace _08.Beer_Kegs
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
double maxVolume = double.MinValue;
string maxModel = "";
for (int i = 0; i < n; i++)
{
string model = Console.ReadLine();
float radius = float.Parse(Console.ReadLine());
int height = int.Parse(Console.ReadLine());
double currVolume = Math.PI * Math.Pow(radius,2) * height;
if (currVolume > maxVolume)
{
maxVolume = currVolume;
maxModel = model;
}
}
Console.WriteLine(maxModel);
}
}
}
<file_sep>using P01.Vehicles.Models;
using P01.Vehicles.Models.Factorys;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices.ComTypes;
using System.Text;
namespace P01.Vehicles.Core
{
public class Engine
{
private VehicleFactory vehicleFactory ;
public Engine()
{
vehicleFactory = new VehicleFactory();
}
public void Run()
{
Vehicle car = ProduceVehicle();
Vehicle truck = ProduceVehicle();
Vehicle bus = ProduceVehicle();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string[] cmdArgs = Console.ReadLine()
.Split()
.ToArray();
try
{
ProcessCommand(car, truck,bus, cmdArgs);
}
catch (InvalidOperationException ioe)
{
Console.WriteLine(ioe.Message);
}
}
//Console.WriteLine($"{car.FuelQuantity:F2}");
//Console.WriteLine($"{truck.FuelQuantity:F2}");
Console.WriteLine(car.ToString());
Console.WriteLine(truck.ToString());
Console.WriteLine(bus);
}
private static void ProcessCommand(Vehicle car, Vehicle truck,Vehicle bus, string[] cmdArgs)
{
string action = cmdArgs[0];
string type = cmdArgs[1];
if (action == "Drive")
{
double kilometers = double.Parse(cmdArgs[2]);
if (type == "Car")
{
Console.WriteLine(car.Drive(kilometers));
}
else if (type == "Truck")
{
Console.WriteLine(truck.Drive(kilometers));
}
else if(type == "Bus")
{
Console.WriteLine(bus.Drive(kilometers));
}
}
else if (action == "Refuel")
{
double liters = double.Parse(cmdArgs[2]);
if (type == "Car")
{
car.Refuel(liters);
}
else if (type == "Truck")
{
truck.Refuel(liters);
}
else if (type == "Bus")
{
bus.Refuel(liters);
}
}
else if(action == "DriveEmpty")
{
double kilometers = double.Parse(cmdArgs[2]);
Console.WriteLine(bus.DriveEmpty(kilometers));
}
}
private Vehicle ProduceVehicle()
{
string[] vehicleArgs = Console.ReadLine()
.Split()
.ToArray();
string type = vehicleArgs[0];
double fuelQuantity = double.Parse(vehicleArgs[1]);
double fuelCons = double.Parse(vehicleArgs[2]);
double tankCapacity = double.Parse(vehicleArgs[3]);
Vehicle vehicle = vehicleFactory
.ProduceVehicle(type, fuelQuantity, fuelCons,tankCapacity);
return vehicle;
}
}
}
<file_sep>using System;
using System.Linq;
namespace P01.ListyIterator
{
class Program
{
static void Main(string[] args)
{
string[] creationData = Console.ReadLine().Split();
ListyIterator<string> collection;
if(creationData.Length > 1)
{
collection = new ListyIterator<string>(creationData.Skip(1));
}
else
{
collection = new ListyIterator<string>();
}
string command;
while((command = Console.ReadLine()) != "END")
{
if(command == "Move")
{
Console.WriteLine(collection.Move());
}
else if(command == "HasNext")
{
Console.WriteLine(collection.HasNext());
}
else if(command == "Print")
{
try
{
collection.Print();
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
}
else if(command == "PrintAll")
{
foreach (var item in collection)
{
Console.Write($"{item} ");
}
Console.WriteLine();
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace ProductShop
{
class Program
{
static void Main(string[] args)
{
var productShop = new Dictionary<string, Dictionary<string, double>>();
string input = Console.ReadLine();
while(input != "Revision")
{
string[] inputInfo = input
.Split(", ", StringSplitOptions.RemoveEmptyEntries);
string shopName = inputInfo[0];
string productName = inputInfo[1];
double productPrice = double.Parse(inputInfo[2]);
if(!productShop.ContainsKey(shopName))
{
productShop.Add(shopName, new Dictionary<string, double>());
}
if(!productShop[shopName].ContainsKey(productName))
{
productShop[shopName].Add(productName, 0);
}
productShop[shopName][productName] = productPrice;
input = Console.ReadLine();
}
foreach (var (shopName,products) in productShop.OrderBy(x => x.Key))
{
Console.WriteLine($"{shopName}->");
foreach (var currentProduct in products)
{
Console.WriteLine($"Product:{currentProduct.Key},price:{currentProduct.Value:F1}");
}
}
}
}
}
<file_sep>using System;
namespace fruitMarket
{
class Program
{
static void Main(string[] args)
{
double cenaQgodi = double.Parse(Console.ReadLine());
double brBananas = double.Parse(Console.ReadLine());
double brOranges = double.Parse(Console.ReadLine());
double brMalini = double.Parse(Console.ReadLine());
double brQgodi = double.Parse(Console.ReadLine());
double cenaMalini = cenaQgodi - cenaQgodi * 0.5;
double cenaOranges = cenaMalini - cenaMalini * 0.4;
double cenaBananas = cenaMalini - cenaMalini * 0.8;
double KolkoPariTrqbvat = cenaQgodi * brQgodi + cenaMalini * brMalini + cenaOranges * brOranges + cenaBananas * brBananas;
Console.WriteLine(KolkoPariTrqbvat);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Encapsulation
{
public class PersonBuilder
{
private Person person;
public PersonBuilder()
{
this.person = new Person();
}
public PersonBuilder WithFirstName(string firstName)
{
this.person.FirstName = firstName;
return this;
}
public PersonBuilder WithLastName(string lastName)
{
this.person.LastName = lastName;
return this;
}
public PersonBuilder WithAge(int age)
{
this.person.Age = age;
return this;
}
public Person Build()
{
return this.person;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _02.RandomiseWords
{
class Program
{
static void Main(string[] args)
{
Random random = new Random();
string[] input = Console.ReadLine().Split();
for (int i = 0; i < input.Length; i++)
{
int index = random.Next(0, input.Length);
string temp = input[i];
input[i] = input[index];
input[index] = temp;
// Console.WriteLine(input[index]);
}
foreach (var item in input)
{
Console.WriteLine(item);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _02._Sets_of_Elements
{
class Program
{
static void Main(string[] args)
{
int[] cmdArgs = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
int n = cmdArgs[0];
int m = cmdArgs[1];
HashSet<int> set1 = new HashSet<int>();
HashSet<int> set2 = new HashSet<int>();
for (int i = 0; i < n; i++)
{
int num = int.Parse(Console.ReadLine());
set1.Add(num);
}
for (int i = 0; i < m; i++)
{
int num = int.Parse(Console.ReadLine());
set2.Add(num);
}
foreach (var num in set1)
{
if(set2.Any(n => n == num))
{
Console.Write(num + " ");
}
}
}
}
}
<file_sep>using System;
namespace _10._4TopNumber
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
isTopNumber(n);
}
static void isTopNumber(int n)
{
for (int i = 1; i <= n; i++)
{
int sum = 0;
int num = i;
bool hasOddDigit = false;
while (num > 0)
{
sum += num % 10;
int digit = num % 10;
if(digit % 2 != 0)
{
hasOddDigit = true;
}
num /= 10;
}
if(sum % 8 == 0 && hasOddDigit)
{
Console.WriteLine(i);
}
}
}
}
}
<file_sep>using System;
namespace AreaOfFiqueres
{
class Program
{
static void Main(string[] args)
{
string fiquare = Console.ReadLine();
double result = 0;
switch (fiquare)
{
case "square":
double side = double.Parse(Console.ReadLine());
result = side * side;
break;
case "rectangle":
double length = double.Parse(Console.ReadLine());
double width = double.Parse(Console.ReadLine());
result = length * width;
break;
case "circle":
double radius = double.Parse(Console.ReadLine());
result = radius * radius * Math.PI;
break;
case "triangle":
double side1 = double.Parse(Console.ReadLine());
double side2 = double.Parse(Console.ReadLine());
result = (side1 * side2) / 2;
break;
}
Console.WriteLine($"{result:F3}");
}
}
}
<file_sep>using System;
namespace _04.BeeHive_Population
{
class Program
{
static void Main(string[] args)
{
double population = double.Parse(Console.ReadLine());
int years = int.Parse(Console.ReadLine());
for (int i = 1; i <= years; i++)
{
//Console.WriteLine($"Godini{i}");
population = population + Math.Floor(population / 10) * 2 ;
// Console.WriteLine($"Izlupeni:{population}");
if(i % 5 == 0)
{
population -= Math.Floor(population / 50) * 5;
// Console.WriteLine($"Imigraciq:{population}");
}
population = population - 2 * Math.Floor(population / 20);
// Console.WriteLine($"Umrqli:{population}");
}
Console.WriteLine($"Beehive population: {population}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace TestDemos.UnitTest1
{
using NUnit.Framework;
using TestApp1;
[TestFixture]
public class BankAccountTests
{
[Test]
public void CreatingBankAccountShouldSetInititalAccount()
{
var bankAccount = new BankAccount(2000);
Assert.AreEqual(2000, bankAccount.Amount);
}
}
}
<file_sep>using System;
namespace ArrWithMethods
{
class Program
{
static void Main(string[] args)
{
int[] arr = { 10, 20, 30 };
string[] tokens = Console.ReadLine().Split();
string command = tokens[0];
if(command == "add")
{
int num = int.Parse(tokens[1]);
int[] result = AddElement(arr,num);
Console.WriteLine(string.Join(" ", result));
}
//Add 10
//Remove
//Rotate Left
//Switch 1 2
}
static int[] AddElement(int[] arr, int num)
{
int[] arrNew = new int[arr.Length + 1];
Array.Copy(arr, arrNew,arr.Length);
arrNew[arrNew.Length - 1] = num;
return arrNew;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public class Ship : Vehicle
{
public int MaxDepth { get; set; }
public override string VrumVrum()
{
return "Ship is floating";
}
}
}
<file_sep>using System;
namespace MetricConverter
{
class Program
{
static void Main(string[] args)
{
double number = double.Parse(Console.ReadLine());
string unit = Console.ReadLine();
string toConvertUnit = Console.ReadLine();
if(unit == "mm" )
{
if(toConvertUnit == "cm" )
{
number = number / 10;
}
else
{
number = number / 1000;
}
}
else if(unit =="cm")
{
if(toConvertUnit == "mm")
{
number = number * 10;
}
else
{
number = number / 100;
}
}
else
{
if(toConvertUnit == "mm")
{
number = number * 1000;
}
else
{
number = number * 100;
}
}
Console.WriteLine($"{number:F3}");
}
}
}
<file_sep>using P04.WildFarm.Contracts;
using P04.WildFarm.Exceptions;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Models
{
public abstract class Animal : IAnimal,ISoundable
{
private string UneatableFoodMessage = "{0} does not eat {1}!";
protected Animal(string name,double weight)
{
this.Name = name;
this.Weight = weight;
}
public string Name { get; private set; }
public double Weight { get; private set; }
public int FoodEaten { get; private set; }
public abstract double WeightMultiplier { get; }
public abstract ICollection<Type> PrefferedFoods { get; }
public void Feed(IFood food)
{
if (!this.PrefferedFoods.Contains(food.GetType()))
{
throw new UneatableFoodException(string
.Format(UneatableFoodMessage,this.GetType().Name,food.GetType().Name));
}
this.Weight += this.WeightMultiplier * food.Quantity;
this.FoodEaten += food.Quantity;
}
public abstract void ProduceSound();
public override string ToString()
{
return $"{this.GetType().Name} [{this.Name},";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P03.ShoppingSpree.Common
{
public static class GlobalConstants
{
public static string InvalidNameExceptionMessage = "Name cannot be empty";
public static string InvalidMoneyException = "Money cannot be negative";
}
}
<file_sep>using System;
namespace Equal_Sums_Even_Odd_Position
{
class Program
{
static void Main(string[] args)
{
int first = int.Parse(Console.ReadLine());
int second = int.Parse(Console.ReadLine());
for(int i=first;i<=second;i++)
{
string currentNum = i.ToString();
int oddSum = 0;
int evenSum = 0;
for(int j=0;j<currentNum.Length;j++)
{
int currDigit = int.Parse(currentNum[j].ToString());
if(j%2==0)
{
evenSum += currDigit;
}
else
{
oddSum += currDigit;
}
}
if(oddSum == evenSum)
{
Console.Write(currentNum +" ");
}
}
}
}
}
<file_sep>using System;
namespace NewHouse
{
class Program
{
static void Main(string[] args)
{
string typeFlower = Console.ReadLine();
int countFlowers = int.Parse(Console.ReadLine());
int budget = int.Parse(Console.ReadLine());
double moneyNeeded = 0.00;
if (typeFlower == "Roses")
{
moneyNeeded = countFlowers * 5;
if (countFlowers > 80)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.1;
}
}
else if (typeFlower == "Dahlias")
{
moneyNeeded = countFlowers * 3.80;
if (countFlowers > 90)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.15;
}
}
else if(typeFlower == "Tulips")
{
moneyNeeded = countFlowers * 2.80;
if (countFlowers > 80)
{
moneyNeeded = moneyNeeded - moneyNeeded * 0.15;
}
//Console.WriteLine(moneyNeeded);
}
else if(typeFlower == "Narcissus")
{
moneyNeeded = countFlowers * 3;
if (countFlowers < 120)
{
moneyNeeded = moneyNeeded + moneyNeeded * 0.15;
}
}
else if(typeFlower == "Gladiolus")
{
moneyNeeded = countFlowers * 2.5;
if (countFlowers < 80)
{
moneyNeeded = moneyNeeded + moneyNeeded * 0.2;
}
}
if(budget>=moneyNeeded)
{
Console.WriteLine($"Hey, you have a great garden with {countFlowers} {typeFlower} and {budget - moneyNeeded:F2} leva left.");
}
else
{
Console.WriteLine($"Not enough money, you need {moneyNeeded-budget:F2} leva more.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
namespace _3.WordCount
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, int> wordAndFrequency = new Dictionary<string, int>();
string[] words = File.ReadAllText("../../../words.txt").Split();
using (StreamReader reader = new StreamReader("../../../text.txt"))
{
string currentLine = reader.ReadLine();
while (currentLine != null)
{
string[] wordsInCurrentLine = currentLine.ToLower()
.Split(new[] { ' ', '.', ',', '-', '?', '!', ':', ';' }, StringSplitOptions.RemoveEmptyEntries);
foreach (var word in words)
{
foreach (var item in wordsInCurrentLine)
{
if (word == item)
{
if (wordAndFrequency.ContainsKey(item) == false)
{
wordAndFrequency.Add(item, 0);
}
wordAndFrequency[item]++;
}
}
}
currentLine = reader.ReadLine();
}
}
using (StreamWriter writer = new StreamWriter("../../../output.txt"))
{
foreach (var item in wordAndFrequency.OrderByDescending(x => x.Value))
{
writer.WriteLine($"{item.Key} - {item.Value}");
}
}
}
}
}<file_sep>using System;
namespace Cake
{
class Program
{
static void Main(string[] args)
{
int width = int.Parse(Console.ReadLine());
int lenght = int.Parse(Console.ReadLine());
int size = width * lenght;
int sum = 0;
//bool iseaten = false;
while(sum < size)
{
string command = Console.ReadLine();
if(command == "STOP")
{
break;
}
int szcake = int.Parse(command);
sum = sum + szcake;
}
if(sum>=size)
{
Console.WriteLine($"No more cake left! You need {sum - size} pieces more.");
}
else
{
Console.WriteLine($"{size-sum} pieces are left.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Cars
{
public class Seat : Car
{
public Seat(string model,string color)
:base(model,color)
{
}
public override string Start()
{
return "Started";
}
}
}
<file_sep>using System;
namespace _09._Padawan_Equipment
{
class Program
{
static void Main(string[] args)
{
//double a= 23.34343543;
//Console.WriteLine(Math.Round(a, 2));
double ivanchoMoney = double.Parse(Console.ReadLine());
int studentsCount = int.Parse(Console.ReadLine());
double priceSabre = double.Parse(Console.ReadLine());
double priceRobe = double.Parse(Console.ReadLine());
double priceBelt = double.Parse(Console.ReadLine());
double countSabres = studentsCount + studentsCount * 0.1;
countSabres = Math.Ceiling(countSabres);
// Console.WriteLine($"Count sabres:{countSabres}");
int freeBelts = studentsCount / 6;
double countBelts = studentsCount - freeBelts;
// Console.WriteLine($"Count belts:{countBelts}");
// Console.WriteLine($"Count :{freeBelts}");
double res = (countSabres * priceSabre) + (countBelts * priceBelt) + (studentsCount * priceRobe);
// Console.WriteLine($"Resultat:{res}");
if (res <= ivanchoMoney)
{
Console.WriteLine($"The money is enough - it would cost {res:F2}lv.");
}
else
{
Console.WriteLine($"Ivan Cho will need {res - ivanchoMoney:F2}lv more.");
}
// Console.WriteLine(freeBelts);
}
}
}
<file_sep>using PlayersAndMonsters.Wizards;
using System;
namespace PlayersAndMonsters
{
public class StartUp
{
public static void Main(string[] args)
{
//SoulMaster sm = new SoulMaster("as", 300);
//DarkWizard da = new DarkWizard("Gosho", 20);
//Console.WriteLine(da.ToString());
//Console.WriteLine(sm.ToString());
}
}
}<file_sep>using P01.Vehicles.Common;
using System;
using System.Collections.Generic;
using System.Text;
namespace P01.Vehicles.Models.Factorys
{
public class VehicleFactory
{
public Vehicle ProduceVehicle(string type , double fuelQty , double fuelCons , double tankCapacity)
{
Vehicle vehicle = null;
if(type == "Car")
{
vehicle = new Car(fuelQty,fuelCons,tankCapacity);
}
else if(type == "Truck")
{
vehicle = new Truck(fuelQty, fuelCons,tankCapacity);
}
else if(type == "Bus")
{
vehicle = new Bus(fuelQty, fuelCons, tankCapacity);
}
if(vehicle == null)
{
throw new ArgumentException(ExceptionMessage
.InvalidTypeVehicleExceptionMessage);
}
return vehicle;
}
}
}
<file_sep>using System;
using System.Linq;
class Program
{
static void Main()
{
//Console.ReadLine();
//string[] bestDna = null;
//int bestLen = -1;
//int startIndex = -1;
//int bestDnaSum = 0;
//int bestSampleIndex = 0;
//int currentSampleIndex = 0;
//while (true)
//{
// string input = Console.ReadLine();
// if (input == "Clone them!")
// {
// break;
// }
// string[] currentDna = input.Split('!', StringSplitOptions.RemoveEmptyEntries);
// int currentLen = 0;
// int currentBestLen = 0;
// int currentEndIndex = 0;
// for (int a = 0; a < currentDna.Length; a++)
// {
// if (currentDna[a] == "1")
// {
// currentLen++;
// if (currentLen > currentBestLen)
// {
// currentEndIndex = a;
// currentBestLen = currentLen;
// }
// }
// else
// {
// currentLen = 0;
// }
// }
// int currentStartIndex = currentEndIndex - currentBestLen + 1;
// bool isCurrentDnaBetter = false;
// int currentDnaSum = currentDna.Select(int.Parse).Sum();
// if (currentBestLen > bestLen)
// {
// isCurrentDnaBetter = true;
// }
// else if (currentBestLen == bestLen)
// {
// if (currentStartIndex < startIndex)
// {
// isCurrentDnaBetter = true;
// }
// else if (currentStartIndex == startIndex)
// {
// if (currentDnaSum > bestDnaSum)
// {
// isCurrentDnaBetter = true;
// }
// }
// }
// currentSampleIndex++;
// if (isCurrentDnaBetter)
// {
// bestDna = currentDna;
// bestLen = currentBestLen;
// startIndex = currentStartIndex;
// bestDnaSum = currentDnaSum;
// bestSampleIndex = currentSampleIndex;
// }
//}
//Console.WriteLine($"Best DNA sample {bestSampleIndex} with sum: {bestDnaSum}.");
//Console.WriteLine(string.Join(' ', bestDna));
Console.ReadLine();
string[] bestDna = null;
int bestLen = -1;
int startIndex = -1;
int bestDnaSum = 0;
int bestSampleIndex = 0;
int currentSampleIndex = 0;
while (true)
{
string input = Console.ReadLine();
if(input == "Clone them!")
{
break;
}
string[] currentDna = input.Split("!").ToArray();
int currLen = 0;
int currBestLen = 0;
int currEndIndex = 0;
for (int i = 0; i < currentDna.Length; i++)
{
if(currentDna[i] == "1")
{
currLen++;
if(currLen > currBestLen)
{
currEndIndex = i;
currBestLen = currLen;
}
}
else
{
currLen = 0;
}
}
int currStartIndex = currEndIndex - currBestLen + 1;
bool isCurrentBetter = false;
int currSum = currentDna.Select(int.Parse).Sum();
if(currBestLen > bestLen)
{
isCurrentBetter = true;
}
else if(currBestLen == bestLen)
{
if(currStartIndex < startIndex)
{
isCurrentBetter = true;
}
else if(currSum > bestDnaSum)
{
isCurrentBetter = true;
}
}
currentSampleIndex++;
if(isCurrentBetter)
{
bestDna = currentDna;
bestDnaSum = currSum;
startIndex = currStartIndex;
bestLen = currBestLen;
bestSampleIndex = currentSampleIndex;
}
}
Console.WriteLine($"Best DNA sample {bestSampleIndex} with sum: {bestDnaSum}.");
Console.WriteLine(string.Join(" ", bestDna));
}
}<file_sep>using System;
namespace Vacation
{
class Program
{
static void Main(string[] args)
{
double moneyNeeded = double.Parse(Console.ReadLine());
double totalMoney = double.Parse(Console.ReadLine());
int days = 0;
int spendingCounter = 0;
while(totalMoney < moneyNeeded && spendingCounter < 5)
{
string operation = Console.ReadLine();
double num = double.Parse(Console.ReadLine());
days++;
if(operation == "spend")
{
totalMoney = totalMoney - num;
spendingCounter++;
if(totalMoney < 0)
{
totalMoney = 0;
}
}
else if(operation == "save")
{
totalMoney = totalMoney + num;
spendingCounter = 0;
}
}
if(spendingCounter == 5)
{
Console.WriteLine("You can't save the money.");
Console.WriteLine($"{days}");
}
else
{
Console.WriteLine($"You saved the money for {days} days.");
}
}
}
}
<file_sep>using System;
namespace _05.Login
{
class Program
{
static void Main(string[] args)
{
string username = Console.ReadLine();
string password = "";
int lenght = username.Length - 1;
while (lenght >= 0)
{
password = <PASSWORD> + username[lenght];
lenght--;
}
//Console.WriteLine(password);
string command = Console.ReadLine();
int counter = 0;
bool isBanned = false;
while(command != password)
{
if(counter+1 == 4)
{
isBanned = true;
break;
}
Console.WriteLine("Incorrect password. Try again.");
// Console.WriteLine("Counter: {counter}");
command = Console.ReadLine();
counter++;
}
if (isBanned)
{
Console.WriteLine($"User {username} blocked!");
}
else
{
Console.WriteLine($"User {username} logged in.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _07._Append_Arrays
{
class Program
{
static void Main(string[] args)
{
//1 2 3 |4 5 6 | 7 8
// string[] input = Console.ReadLine().Split("|").ToArray();
// Console.WriteLine(string.Join(" ",input));
//Console.WriteLine(input[0]);
//Console.WriteLine(input[1]);
//Console.WriteLine(input[2]);
//List<int> list = Console.ReadLine().Split("|").Select(int.Parse).ToList();
List<string> input = Console.ReadLine().Split("|").Reverse().ToList();
List<int> numbers = new List<int>();
for (int i = 0; i < input.Count; i++)
{
numbers.AddRange(input[i].Split(" ", StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToList());
}
// numbers.Sort();
Console.WriteLine(string.Join(" ", numbers));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._School_Library
{
class Program
{
static void Main(string[] args)
{
List<string> shelf = Console.ReadLine().Split("&").ToList();
while (true)
{
string input = Console.ReadLine();
if (input == "Done")
{
break;
}
string[] command = input.Split(" | ");
switch (command[0])
{
case "Add Book":
if (!shelf.Contains(command[1]))
{
shelf.Insert(0, command[1]);
}
break;
case "Take Book":
if (shelf.Contains(command[1]))
{
shelf.Remove(command[1]);
}
break;
case "Swap Books":
if (shelf.Contains(command[1]) && shelf.Contains(command[2]))
{
int ind1 = shelf.IndexOf(command[1]);
int ind2 = shelf.IndexOf(command[2]);
string temp = shelf[ind1];
shelf[ind1] = shelf[ind2];
shelf[ind2] = temp;
}
break;
case "Insert Book":
shelf.Add(command[1]);
break;
case "Check Book":
int index = int.Parse(command[1]);
if (index >= 0 && index < shelf.Count)
{
Console.WriteLine(shelf[index]);
}
break;
}
}
Console.WriteLine(string.Join(", ", shelf));
}
}
}
<file_sep>using System;
namespace _02.Bee_2_
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
for (int i = 0; i < n; i++)
{
string row = Console.ReadLine();
for (int j = 0; j < n; j++)
{
field[i, j] = row[j];
}
}
int beeRow = -1;
int beeCol = -1;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == 'B')
{
beeRow = i;
beeCol = j;
}
}
}
int countflowers = 0;
string command;
while((command = Console.ReadLine()) != "End")
{
field[beeRow, beeCol] = '.';
beeRow = MoveX(beeRow, command);
beeCol = MoveY(beeCol, command);
if (beeRow < 0 || beeRow >= n || beeCol < 0 || beeCol >= n)
{
Console.WriteLine("The bee got lost!");
break;
}
if(field[beeRow, beeCol] == 'f')
{
countflowers++;
}
else if(field[beeRow, beeCol] == 'O')
{
field[beeRow, beeCol] = '.';
beeRow = MoveX(beeRow, command);
beeCol = MoveY(beeCol, command);
if (field[beeRow, beeCol] == 'f')
{
countflowers++;
}
if (beeRow < 0 || beeRow >= n || beeCol < 0 || beeCol >= n)
{
Console.WriteLine("The bee got lost!");
break;
}
}
field[beeRow, beeCol] = 'B';
}
if(countflowers < 5)
{
Console.WriteLine($"The bee couldn't pollinate the flowers, she needed {5 - countflowers} flowers more");
}
else
{
Console.WriteLine($"Great job, the bee managed to pollinate {countflowers} flowers!");
}
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
Console.Write(field[i, j]);
}
Console.WriteLine();
}
}
private static int MoveX(int beeRow, string command)
{
if(command == "up")
{
beeRow -= 1;
}
else if(command == "down")
{
beeRow += 1;
}
return beeRow;
}
private static int MoveY(int beeCol, string command)
{
if (command == "left")
{
beeCol -= 1;
}
else if (command == "right")
{
beeCol += 1;
}
return beeCol;
}
}
}
<file_sep>using System;
namespace _02._Selling
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
char[,] field = new char[n, n];
for (int i = 0; i < n; i++)
{
string row = Console.ReadLine();
for (int j = 0; j < n; j++)
{
field[i, j] = row[j];
}
}
int yourRow = -1;
int yourCol = -1;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == 'S')
{
yourRow = i;
yourCol = j;
}
}
}
int collectedMoney = 0;
while(collectedMoney < 50)
{
string command = Console.ReadLine();
field[yourRow, yourCol] = '-';
yourRow = MoveX(yourRow, command);
yourCol = MoveY(yourCol, command);
if (yourRow < 0 || yourRow >= n || yourCol < 0 || yourCol >= n)
{
Console.WriteLine("Bad news, you are out of the bakery.");
break;
}
if(field[yourRow, yourCol] == 'O')
{
field[yourRow, yourCol] = '-';
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
if (field[i, j] == 'O')
{
yourRow = i;
yourCol = j;
}
}
}
}
else if(field[yourRow, yourCol] == '-')
{
}
else
{
collectedMoney += int.Parse(field[yourRow, yourCol].ToString());
}
field[yourRow, yourCol] = 'S';
}
if(collectedMoney >= 50)
{
Console.WriteLine("Good news! You succeeded in collecting enough money!");
}
Console.WriteLine($"Money: {collectedMoney}");
for (int i = 0; i < n; i++)
{
for (int j = 0; j < n; j++)
{
Console.Write(field[i, j]);
}
Console.WriteLine();
}
}
private static int MoveX(int beeRow, string command)
{
if (command == "up")
{
beeRow -= 1;
}
else if (command == "down")
{
beeRow += 1;
}
return beeRow;
}
private static int MoveY(int beeCol, string command)
{
if (command == "left")
{
beeCol -= 1;
}
else if (command == "right")
{
beeCol += 1;
}
return beeCol;
}
}
}
<file_sep>using System;
namespace P08.Threeuple
{
class Program
{
static void Main(string[] args)
{
string[] personInfo = Console.ReadLine()
.Split();
string fullName = $"{personInfo[0]} {personInfo[1]}";
string address = personInfo[2];
string town = personInfo[3];
string[] nameAndBeer = Console.ReadLine().Split();
string name = nameAndBeer[0];
int beerAmount = int.Parse(nameAndBeer[1]);
bool isDrunk = nameAndBeer[2] == "drunk" ? true : false;
string[] bankArgs = Console.ReadLine().Split();
string realName = bankArgs[0];
double accountBalance = double.Parse(bankArgs[1]);
string bankName = bankArgs[2];
Threeuple<string, string, string> firstThreeuple = new
Threeuple<string, string, string>(fullName, address, town);
Threeuple<string, int, bool> secondThreeuple = new
Threeuple<string, int, bool>(name, beerAmount, isDrunk);
Threeuple<string, double, string> thirdThreeuple = new
Threeuple<string, double, string>(realName, accountBalance, bankName);
Console.WriteLine(firstThreeuple);
Console.WriteLine(secondThreeuple);
Console.WriteLine(thirdThreeuple);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public class Motorcycle : Vehicle
{
public int MaxSpeed { get; set; }
}
}
<file_sep>using System;
namespace Inheritance
{
class Program
{
static void Main(string[] args)
{
var student = new Student();
var doctor = new Doctor();
}
}
}
<file_sep>using System;
using System.Numerics;
namespace _09._Spice_Must_Flow
{
class Program
{
static void Main(string[] args)
{
BigInteger startingYield = BigInteger.Parse(Console.ReadLine());
BigInteger days = 0;
BigInteger sum = 0;
if (startingYield < 100)
{
Console.WriteLine(days);
Console.WriteLine(sum);
}
else
{
while (startingYield >= 100)
{
// days++;
sum = sum + startingYield - 26;
startingYield -= 10;
days++;
}
sum -= 26;
Console.WriteLine(days);
Console.WriteLine(sum);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime;
namespace _04._List_Operations
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
while (true)
{
string input = Console.ReadLine();
if (input == "End")
{
break;
}
string[] command = input.Split();
switch (command[0])
{
case "Add":
list.Add(int.Parse(command[1]));
break;
case "Insert":
int num = int.Parse(command[1]);
int index = int.Parse(command[2]);
if (index >= 0 && index < list.Count)
{
list.Insert(index, num);
}
else
{
Console.WriteLine("Invalid index");
}
break;
case "Remove":
if (int.Parse(command[1]) < 0 || int.Parse(command[1]) >= list.Count)
{
Console.WriteLine("Invalid index");
}
else
{
list.RemoveAt(int.Parse(command[1]));
}
break;
case "Shift":
int times = int.Parse(command[2]);
if (command[1] == "left")
{
for (int i = 0; i < times; i++)
{
list.Add(list[0]);
list.RemoveAt(0);
}
}
else if(command[1] == "right")
{
for (int i = 0; i < times; i++)
{
list.Insert(0, list[list.Count-1]);
list.RemoveAt(list.Count - 1);
}
}
break;
}
}
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Contracts
{
public interface IAnimal
{
string Name { get; }
double Weight { get; }
int FoodEaten { get; }
void Feed(IFood food);
void ProduceSound();
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography;
namespace P09.PokemonTrainer
{
class Program
{
static void Main(string[] args)
{
List<Trainer> trainers = new List<Trainer>();
string command;
while((command = Console.ReadLine()) != "Tournament")
{
string[] cmdArgs = command
.Split(' ',StringSplitOptions.RemoveEmptyEntries);
string trainerName = cmdArgs[0];
string pokemonName = cmdArgs[1];
string pokemonElement = cmdArgs[2];
int pokemonHealth = int.Parse(cmdArgs[3]);
Pokemon pokemon = new Pokemon(pokemonName, pokemonElement, pokemonHealth);
if(!trainers.Any(t => t.Name == trainerName))
{
Trainer trainer = new Trainer(trainerName);
trainer.Pokemons.Add(pokemon);
trainers.Add(trainer);
}
else
{
Trainer trainer = trainers.Find(t => t.Name == trainerName);
trainer.Pokemons.Add(pokemon);
}
}
string command2;
while((command2 = Console.ReadLine()) != "End")
{
string wantedElement = command2;
foreach (var trainer in trainers)
{
if(trainer.Pokemons.Any(p => p.Element == wantedElement))
{
trainer.NumberOfBadges++;
}
else
{
DecreasePokemonsHealthBy10(trainer);
//trainer.Pokemons.Select(p => p.Health - 10);
trainer.Pokemons.RemoveAll(p => p.Health <= 0);
}
}
}
trainers = trainers
.OrderByDescending(t => t.NumberOfBadges)
.ToList();
foreach (var trainer in trainers)
{
Console.WriteLine($"{trainer.Name} {trainer.NumberOfBadges}" +
$" {trainer.Pokemons.Count}");
}
}
private static void DecreasePokemonsHealthBy10(Trainer trainer)
{
foreach (var pokemon in trainer.Pokemons)
{
pokemon.Health -= 10;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace MatchingBrackets
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
var stack = new Stack<int>();
for(int i=0;i< text.Length;i++)
{
var symbol = text[i];
if (symbol == '(')
{
stack.Push(i);
}
else if(symbol == ')')
{
int indexOfOpeningBracket = stack.Pop();
string result = text.Substring(indexOfOpeningBracket, i - indexOfOpeningBracket + 1);
Console.WriteLine(result);
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text.RegularExpressions;
namespace _02._Emoji_Detector
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
var matchesDigit = Regex.Matches(text, @"\d");
long coolTreshold = 1;
foreach (Match match in matchesDigit)
{
coolTreshold *= long.Parse(match.Value);
}
string emojiPattern = @"(:{2}|\*{2})(?<emoji>[A-z][a-z]{2,})\1";
var emojiMatches = Regex.Matches(text, emojiPattern);
int countValidEmojis = 0;
List<string> coolEmojis = new List<string>();
foreach (Match emoji in emojiMatches)
{
int coolness = 0;
foreach (char c in emoji.Groups["emoji"].Value)
{
coolness += (int)c;
}
if(coolness >= coolTreshold)
{
coolEmojis.Add(emoji.ToString());
}
countValidEmojis++;
}
Console.WriteLine($"Cool threshold: { coolTreshold}");
Console.WriteLine($"{countValidEmojis} emojis found in the text. The cool ones are:");
if(coolEmojis.Count > 0)
{
foreach (string emoji in coolEmojis)
{
Console.WriteLine(emoji);
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace _05._Nether_Realms
{
class Program
{
static void Main(string[] args)
{
List<string> names = Console.ReadLine()
.Split(new char[] { ' ', ',' }, StringSplitOptions.RemoveEmptyEntries).OrderBy(d => d)
.ToList();
Dictionary<string, int> health = new Dictionary<string, int>();
Dictionary<string, decimal> damage = new Dictionary<string, decimal>();
foreach (string name in names)
{
if (!health.ContainsKey(name) && !damage.ContainsKey(name))
{
health[name] = GetHealth(name);
damage[name] = GetDamage(name);
}
}
foreach (string name in names.OrderBy(n => n))
{
Console.WriteLine($"{name} - {health[name]} health, {damage[name]:F2} damage");
}
}
static decimal GetDamage(string name)
{
string damagePattern = @"([-+])?\d+(\.\d+)?";
//Problem with mine pattern;
// string damagePattern = @"[\-\+]?[\d]+(?:[\.]*[\d]+|[\d]*)";
var matches = Regex.Matches(name, damagePattern);
decimal damage = 0;
foreach (Match match in matches)
{
damage += decimal.Parse(match.Value);
}
foreach (char c in name)
{
if(c == '*')
{
damage *= 2;
}
else if(c == '/')
{
damage /= 2;
}
}
return damage;
}
static int GetHealth(string name)
{
string healthPatttern = @"[^0-9\+\-*/\.]";
// string healthPatttern = @"[^0-9\+\-\*\/\.]+";
var matches = Regex.Matches(name, healthPatttern);
int health = 0;
foreach (Match match in matches)
{
char currCh = char.Parse(match.Value);
health += (int)(currCh);
}
return health;
}
}
}
<file_sep>using System;
namespace TIme_15minutes
{
class Program
{
static void Main(string[] args)
{
int hour = int.Parse(Console.ReadLine());
int minutes = int.Parse(Console.ReadLine());
// Console.WriteLine(minutes);
//if minutes are like 01 they are used as 1
if(hour>=0 && hour<=23 && minutes>=0 && minutes<=59)
{
if(minutes+15<=59)
{
Console.WriteLine($"{hour}:{minutes+15}");
}
else
{
int sum = minutes + 15;
minutes = sum - 60;
if (hour + 1 == 24)
{
hour = 0;
}
else
{
hour++;
}
if(minutes<10)
{
Console.WriteLine($"{hour}:0{minutes}");
}
else
Console.WriteLine($"{hour}:{minutes}");
}
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace P03.Stack
{
class Program
{
static void Main(string[] args)
{
var stack = new Stack<int>();
string command;
while ((command = Console.ReadLine()) != "END")
{
var cmdArgs = command
.Split(new char[] { ' ', ',' }, StringSplitOptions.RemoveEmptyEntries);
//string[] commandArgs = command.Split(" ");
if (cmdArgs.Length > 1)
{
//commandArgs = commandArgs.Skip(1).Split(",");
//command = commandArgs.Skip(1).ToString();
//var cmdArgs = command.Split(", ").Select(int.Parse);
var elements = cmdArgs.Skip(1).Select(int.Parse);
stack.Push(elements);
}
else if (cmdArgs[0] == "Push")
{
int elemToPush = int.Parse(cmdArgs[1]);
stack.Push(elemToPush);
}
else if (command == "Pop")
{
try
{
stack.Pop();
}
catch (Exception e)
{
Console.WriteLine(e.Message);
}
}
}
PrintStack(stack);
PrintStack(stack);
}
private static void PrintStack(Stack<int> stack)
{
foreach (var item in stack)
{
Console.WriteLine(item);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace List
{
class Program
{
static void Main(string[] args)
{
List<int> list = new List<int>();
list.Add(5);
list.Add(6);
list.Add(-51);
list.Add(33);
//list.RemoveAt(1);
RemoveSoftuni(list,6);
Console.WriteLine($"list[0]={list[0]}");
Console.WriteLine($"Count:{list.Count}");
}
static void RemoveSoftuni(List<int> list, int element)
{
list.Remove(element);
}
}
}
<file_sep>using P04.PizzaCalories.Ingredients;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04.PizzaCalories
{
public class Pizza
{
private string name;
// private Dough dough;
private int numberOfToppings;
public Pizza(string name,Dough dough)
{
this.Name = name;
this.Dough = dough;
this.TotalCalories += this.Dough.DoughCalories();
}
public string Name
{
get
{
return this.name;
}
private set
{
if(string.IsNullOrEmpty(value) || value.Length > 15)
{
throw new ArgumentException("Pizza name should be between 1 and 15 symbols.");
}
this.name = value;
}
}
public int NumberOfToppings
{
get
{
return this.numberOfToppings;
}
private set
{
if(numberOfToppings < 0 || numberOfToppings > 10)
{
throw new ArgumentException("Number of toppings should be in range [0..10].");
}
this.numberOfToppings = value;
}
}
public Dough Dough { get; set; }
public double TotalCalories { get; private set; }
public void AddTopping(Topping topping)
{
this.TotalCalories += topping.ToppingCalories();
this.NumberOfToppings++;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Cars
{
public interface IElectricCar : ICar
{
Battery Battery { get; }
}
}
<file_sep>using System;
using System.Data;
using System.Linq;
namespace _09.KaminoFactory_Working_
{
class Program
{
static void Main(string[] args)
{
int length = int.Parse(Console.ReadLine());
int LeftMostStartingIndex = -1;
int MaxSeq = 0;
int maxSeqIndex = -1;
int[] MaxArray = new int[length];
int seqCounter = 0;
string command;
while((command = Console.ReadLine()) != "Clone them!")
{
int[] currSequence = command
.Split('!')
.Select(int.Parse)
.ToArray();
int startingIndex = 0;
int sequnceLength = 0;
for (int i = 0; i < currSequence.Length; i++)
{
if(currSequence[i] == 1)
{
startingIndex = i;
sequnceLength++;
}
else
{
if(MaxSeq < sequnceLength)
{
MaxSeq = sequnceLength;
LeftMostStartingIndex = startingIndex;
maxSeqIndex = seqCounter;
currSequence.CopyTo(MaxArray,0);
}
else if(MaxSeq == sequnceLength)
{
if(LeftMostStartingIndex > startingIndex)
{
MaxSeq = sequnceLength;
LeftMostStartingIndex = startingIndex;
maxSeqIndex = seqCounter;
currSequence.CopyTo(MaxArray, 0);
}
}
startingIndex = 0;
sequnceLength = 0;
}
}
seqCounter++;
}
Console.WriteLine($"Best DNA sample {maxSeqIndex + 1} with sum: {MaxArray.Sum()}.");
Console.WriteLine(string.Join(" ", MaxArray));
}
}
}
<file_sep>using P05.Restaurant_Again_.Foods.Starter;
using System;
using System.Collections.Generic;
using System.Text;
namespace P05.Restaurant_Again_.Foods.Starter
{
public class Soup : Starter
{
public Soup(string name, decimal price, double grams)
: base(name, price, grams)
{
}
}
}
<file_sep>using System;
namespace Graduation_pt2
{
class Program
{
static void Main(string[] args)
{
string name = Console.ReadLine();
int grade = 0;
double sumMarks = 0;
int timesBadGrades = 0;
bool isExcluded = false;
while(grade < 12)
{
double mark = double.Parse(Console.ReadLine());
if(mark >= 4)
{
sumMarks += mark;
grade++;
}
else
{
timesBadGrades++;
if(timesBadGrades > 1)
{
isExcluded = true;
break;
}
else
{
grade++;
}
}
}
if(isExcluded == true)
{
Console.WriteLine($"{name} has been excluded at {grade} grade");
}
else
{
Console.WriteLine($"{name} graduated. Average grade: {sumMarks / grade:F2}");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Memory_Game
{
class Program
{
static void Main(string[] args)
{
List<string> list = Console.ReadLine().Split(' ',StringSplitOptions.RemoveEmptyEntries).ToList();
int movesMade = 0;
string input;
bool haveWon = false;
while((input = Console.ReadLine()) != "end")
{
int[] indexes = input.Split(' ',StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToArray();
int index1 = indexes[0];
int index2 = indexes[1];
movesMade++;
if(index1 < 0 || index1 >= list.Count || index2 < 0 || index2 >= list.Count
|| index1 == index2)
{
int indexToAddTo = list.Count / 2;
string elemToAdd2Times = $"-{movesMade}a";
list.Insert(indexToAddTo, elemToAdd2Times);
list.Insert(indexToAddTo, elemToAdd2Times);
Console.WriteLine("Invalid input! Adding additional elements to the board");
}
else
{
if(list[index1] == list[index2])
{
string elToDelete = list[index1];
Console.WriteLine($"Congrats! You have found matching elements - {list[index1]}!");
list.RemoveAll(i => i == elToDelete);
}
else
{
Console.WriteLine("Try again!");
}
if (!list.Any())
{
haveWon = true;
break;
}
}
}
if (haveWon)
{
Console.WriteLine($"You have won in {movesMade} turns!");
}
else
{
Console.WriteLine($"Sorry you lose :(\n" +$"{string.Join(' ',list)}");
}
}
}
}
<file_sep>#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct player_t {
char name[10];
int poreden_num;
};
struct game_t {
int curr_round;
struct player_t* arr;
int board[16];
int size;
};
int roll_die()
{
int arr[] = { 1,2,3,4,5,6 };
int num = arr[rand() % 6];
return num;
}
void move_player(struct game_t* game, struct player_t* player)
{
//game->arr = malloc(4*sizeof(struct player_t));
int por_num = player->poreden_num;
int is_occupied = 0;
int moved_pos = roll_die();
printf("DICE:NOM:%d:%dpos\n", player->poreden_num, moved_pos);
for (int i = 0; i < 16; i++)
{
if (player->poreden_num == game->board[i])
{
game->board[i] = 0;
for (int j = 0; j < game->size; j++)
{
if ((game->board[i + moved_pos] == game->arr[j].poreden_num) || (game->board[((i + moved_pos) - 16)] == game->arr[j].poreden_num))
{
for (int k = 0; k < 16; k++)
{
if (game->board[k] == game->arr[j].poreden_num)
{
game->board[k] = 0;
}
}
//free(game->arr+j);
for (int z = j; z < game->size; z++)
{
game->arr[z] = game->arr[z + 1];
}
game->size--;
//free(game->arr[3]);
game->arr = realloc(game->arr, game->size * sizeof(struct player_t));
//memmove(game->arr+j,game->arr+j+1,(3-j)*sizeof(int));
//is_occupied = 1;
//struct player_t *tmp = game->arr[j+1];
//arr[j]=tmp;
break;
}
}
//if(is_occupied==0)
//{
if ((i + moved_pos) >= 16)
{
int index = (i + moved_pos) - 16;
game->board[index] = por_num;
break;
}
else
{
game->board[i + moved_pos] = por_num;
break;
}
// }
}
}
}
int play_round(struct game_t* game)
{
game->curr_round++;
//
for (int i = 0; i < 16; i++)
{
printf("%d\n", game->board[i]);
}
printf("\n");
for (int k = 0; k < game->size; k++)
{
printf("%d %s %d\n", k, game->arr[k].name, game->arr[k].poreden_num);
}
//
printf("Starting round %d\n", game->curr_round);
for (int i = 0; i < game->size; i++)
{
move_player(game, game->arr + i);
if (game->size == 1)
{
return 1;
}
}
if (game->size >= 2)
{
return 0;
}
}
void play_game(struct game_t* game)
{
int result = 0;
while (result != 1)
{
result = play_round(game);
}
}
int main()
{
//struct player_t fir={"Gosho",0};
//fir.poreden_num = roll_die();
//printf("%d",fir.poreden_num);
//struct game_t game;
// game.curr_round = 1;
// game.arr=malloc(4*sizeof(struct player_t));
struct player_t player1 = { "Vladi", 1 };
struct player_t player2 = { "Gosho", 2 };
struct player_t player3 = { "Mitko", 3 };
struct player_t player4 = { "Ivan", 4 };
struct player_t* players = malloc(4 * sizeof(struct player_t));
players[0] = player1;
players[1] = player2;
players[2] = player3;
players[3] = player4;
struct game_t game = { 0, players };
game.size = 4;
game.arr = players;
// struct player_t players[4] = {{"First",1},{"Second",2},{"Third",3},{"Fourth",4}};
// game.arr = players;
game.board[0] = 1;
game.board[1] = 2;
game.board[2] = 3;
game.board[3] = 4;
game.board[4] = 0;
game.board[5] = 0;
game.board[6] = 0;
game.board[7] = 0;
game.board[8] = 0;
game.board[9] = 0;
game.board[10] = 0;
game.board[11] = 0;
game.board[12] = 0;
game.board[13] = 0;
game.board[14] = 0;
game.board[15] = 0;
///MOVING PLAYERS
//move_player(&game, &game.arr[3]);
// move_player(&game, &game.arr[1]);
// move_player(&game, &game.arr[1]);
// move_player(&game, &game.arr[1]);
// for(int j=0;j<
/////ROUND
//printf("Resultat ot rounda:%d\n",play_round(&game));
/////GAME
printf("Game:\n");
play_game(&game);
for (int i = 0; i < 16; i++)
{
printf("%d\n", game.board[i]);
}
printf("\n");
for (int k = 0; k < game.size; k++)
{
printf("%d %s %d\n", k, game.arr[k].name, game.arr[k].poreden_num);
}
return 0;
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P01.GenericBoxofString
{
public class Box<T> where T: IComparable
{
public Box(List<T> value)
{
this.Values = value;
}
public List<T> Values { get; set; }
public void SwapElementsByIndex(List<T> items, int index1, int index2)
{
T temp = items[index1];
items[index1] = items[index2];
items[index2] = temp;
}
public int CountGreaterElements(List<T> list,T elementToCompare)
{
int counter = 0;
foreach (var element in list)
{
if(elementToCompare.CompareTo(element) < 0)
{
counter++;
}
}
return counter;
}
public override string ToString()
{
StringBuilder sb = new StringBuilder();
foreach (var item in this.Values)
{
sb.AppendLine($"{item.GetType()}: {item}");
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>using System;
namespace _02.Mountain_Rain
{
class Program
{
static void Main(string[] args)
{
double recordInSec = double.Parse(Console.ReadLine());
double recordInMet = double.Parse(Console.ReadLine());
double timeFor1m = double.Parse(Console.ReadLine());
double secGeorge = recordInMet * timeFor1m;
double timesSlow = Math.Floor(recordInMet / 50);
secGeorge = secGeorge + timesSlow * 30;
if(secGeorge < recordInSec)
{
Console.WriteLine($"Yes! The new record is {secGeorge:F2} seconds.");
}
else
{
Console.WriteLine($"No! He was {secGeorge-recordInSec:F2} seconds slower.");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _07._Max_Sequence_of_Equal_Elements
{
class Program
{
static void Main(string[] args)
{
// int[] arr = Console.ReadLine().Split().Select(int.Parse).ToArray();
// int startIndex = 0;
// int endIndex = 0;
// int countSeq = 0;
// for (int i = 0; i < 1; i++)
// {
// for (int j = 0; j < arr.Length; j++)
// {
// if(arr[i] == arr[j])
// {
// startIndex = j;
// countSeq++;
// }
// else
// {
// countSeq = 0;
// endIndex = j-1;
// }
// }
// }
// Console.WriteLine(startIndex);
// Console.WriteLine(endIndex);
//}
int[] numbers = Console.ReadLine().Split().Select(int.Parse).ToArray();
int counter = 0;
int winningCounter = 0;
int index = 0;
string number = string.Empty;
for (int i = 0; i<numbers.Length - 1; i++)
{
if (numbers[i] == numbers[i + 1])
{
counter++;
if (counter > winningCounter)
{
winningCounter = counter;
index = i;
number = numbers[i].ToString();
}
}
else
{
counter = 0;
}
}
for (int i = 0; i <= winningCounter; i++)
{
Console.Write(number + " ");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _04._Students
{
class Student
{
public Student(string firstName,string lastName ,float grade)
{
this.FirstName = firstName;
this.LastName = lastName;
this.Grade = grade;
}
public string FirstName { get; set; }
public string LastName { get; set; }
public float Grade { get; set; }
public override string ToString()
{
// return base.ToString();
return $"{this.FirstName} { this.LastName}: { this.Grade:F2}";
}
}
class Program
{
static void Main(string[] args)
{
int count = int.Parse(Console.ReadLine());
List<Student> students = new List<Student>();
for (int i = 0; i < count; i++)
{
string[] info = Console.ReadLine().Split();
string firstName = info[0];
string lastName = info[1];
float grade = float.Parse(info[2]);
Student student = new Student(firstName, lastName, grade);
students.Add(student);
}
//students.OrderByDescending(x => x.Grade);
//for (int i = 0; i < students.Count; i++)
//{
// Console.WriteLine(students[i].ToString());
//}
foreach (Student student in students.OrderByDescending(x => x.Grade))
{
Console.WriteLine(student.ToString());
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _07._Student_Academy
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<double>> students = new Dictionary<string, List<double>>();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string name = Console.ReadLine();
double grade = double.Parse(Console.ReadLine());
if (!students.ContainsKey(name))
{
students[name] = new List<double>();
}
students[name].Add(grade);
}
Dictionary<string, double> studentsAverage = new Dictionary<string, double>();
foreach (var kvp in students)
{
studentsAverage[kvp.Key] = kvp.Value.Average();
}
studentsAverage = studentsAverage
.Where(kvp => kvp.Value >= 4.50)
.OrderByDescending(kvp => kvp.Value)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var kvp in studentsAverage)
{
Console.WriteLine($"{kvp.Key} -> {kvp.Value:F2}");
}
}
}
}
<file_sep>using System;
namespace Combinations
{
class Program
{
static void Main(string[] args)
{
int counter = 0;
int n = int.Parse(Console.ReadLine());
for(int x1=0;x1<=n;x1++)
{
for(int x2 = 0; x2<=n;x2++)
{
for(int x3 = 0;x3<=n;x3++)
{
if ((x1 + x2 + x3) == n)
{
counter++;
}
}
}
}
Console.Write(counter);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _01._Count_Chars_in_a_String
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
Dictionary<char, int> dict = new Dictionary<char, int>();
for (int i = 0; i < text.Length; i++)
{
char currCh = text[i];
if(currCh == ' ')
{
continue;
}
if (!dict.ContainsKey(currCh))
{
dict[currCh] = 1;
}
else
{
dict[currCh]++;
}
}
foreach (var item in dict)
{
Console.WriteLine($"{item.Key} -> {item.Value}");
}
//for (int i = 0; i < word.Length; i++)
//{
// char symbol = char.Parse(word[i]);
// Console.WriteLine(symbol);
//}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P01.Vehicles.Common
{
public static class ExceptionMessage
{
public static string NotEnoughFuelExceptionMessage = "{0} needs refueling";
public static string InvalidTypeVehicleExceptionMessage = "Invalid vehicle type";
public static string InvalidFuelTankExceptionMessage = "Cannot fit {0} fuel in the tank";
public static string FuelIsNegativeOrZero = "Fuel must be a positive number";
}
}
<file_sep>using System;
using System.Linq;
namespace _02._Character_Multiplier
{
class Program
{
static void Main(string[] args)
{
string[] inpArgs = Console.ReadLine()
.Split()
.ToArray();
Console.WriteLine(CharMultiplier(inpArgs[0], inpArgs[1]));
}
public static int CharMultiplier(string f, string s)
{
int sum = 0;
int shorterLen = 0;
if(f.Length != s.Length)
{
shorterLen = f.Length > s.Length ? s.Length : f.Length;
}
else
{
shorterLen = f.Length;
}
for (int i = 0; i < shorterLen; i++)
{
sum += (int)(s[i] * f[i]);
}
if(shorterLen == f.Length && shorterLen < s.Length)
{
for (int i = f.Length; i < s.Length; i++)
{
sum += (int)(s[i]);
}
}
else if(shorterLen == s.Length && shorterLen < f.Length)
{
for (int i = s.Length; i < f.Length; i++)
{
sum += (int)(f[i]);
}
}
return sum;
}
}
}
<file_sep>using NUnit.Framework;
using System;
[TestFixture]
public class AxeTests
{
[Test]
public void AxeShouldLooseDurabilityAfterAttack()
{
//Arrange
var axe = new Axe(10, 5);
var target = new Dummy(100, 500);
//Act
axe.Attack(target);
//Assert
Assert.That(axe.DurabilityPoints, Is.EqualTo(4));
}
[Test]
public void AxeShouldThrowExceptionIfAttackIsMadeWithBrokenWeapon()
{
//Arrange
var axe = new Axe(10, 0);
var target = new Dummy(100, 500);
//Assert
Assert.That(() => axe.Attack(target),//Act
Throws.InvalidOperationException
.With.Message.EqualTo("Axe is broken."));
}
}<file_sep>using System;
namespace SkiTrip
{
class Program
{
static void Main(string[] args)
{
int countDays = int.Parse(Console.ReadLine());
string typeRoom = Console.ReadLine();
string grade =Console.ReadLine();
countDays = countDays - 1;
double price = 0;
switch(typeRoom)
{
case "room for one person":
price = countDays * 18;
break;
case "apartment":
price = countDays * 25;
if (countDays < 10)
{
price = price - price * 0.3;
}
else if (countDays >= 10 && countDays <= 15)
{
price = price - price * 0.35;
}
else
{
price = price - price * 0.5;
}
break;
case "president apartment":
price = countDays * 35;
if (countDays < 10)
{
price = price - price * 0.1;
}
else if (countDays >= 10 && countDays <= 15)
{
price = price - price * 0.15;
}
else
{
price = price - price * 0.2;
}
break;
}
if(grade == "positive")
{
price = price + price * 0.25;
}
else
{
price = price - price * 0.1;
}
Console.WriteLine($"{price:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _10.Softuni_Course_Planning
{
class Program
{
static void Main(string[] args)
{
List<string> list = Console.ReadLine().Split(", ").ToList();
while (true)
{
string input = Console.ReadLine();
if(input == "course start")
{
break;
}
string[] command = input.Split(":");
string lessonTitle = command[1];
switch (command[0])
{
case "Add":
//string lessonTitle = command[1];
if (!list.Contains(lessonTitle))
{
list.Add(lessonTitle);
}
break;
case "Insert":
// string lessonTitle2 = command[1];
int index = int.Parse(command[2]);
if (!list.Contains(lessonTitle))
{
list.Insert(index, lessonTitle);
}
break;
case "Remove":
if (list.Contains(lessonTitle))
{
list.Remove(lessonTitle);
if (list.Contains($"{lessonTitle}-Exercise"))
{
list.Remove($"{lessonTitle}-Exercise");
}
}
break;
case "Swap":
string lessonTitle2 = command[2];
if(list.Contains(lessonTitle) && list.Contains(lessonTitle2))
{
string temp = command[1];
command[1] = command[2];
command[2] = temp;
int i1 = list.IndexOf(command[1]);
int i2 = list.IndexOf(command[2]);
list[i1] = command[2];
list[i2] = command[1];
if (list.Contains($"{command[1]}-Exercise"))
{
int ind = list.IndexOf(command[1]);
list.Remove($"{command[1]}-Exercise");
list.Insert(ind+1, $"{command[1]}-Exercise");
}
if (list.Contains($"{command[2]}-Exercise"))
{
int ind2 = list.IndexOf(command[2]);
list.Remove($"{command[2]}-Exercise");
list.Insert(ind2 + 1, $"{command[2]}-Exercise");
}
}
break;
case "Exercise":
if(list.Contains(lessonTitle) && !list.Contains($"{lessonTitle}-Exercise"))
{
int i = list.IndexOf(lessonTitle);
list.Insert(i+1, $"{lessonTitle}-Exercise");
}
if (!list.Contains(lessonTitle))
{
list.Add(lessonTitle);
list.Add($"{lessonTitle}-Exercise");
}
break;
}
}
for (int i = 0; i < list.Count; i++)
{
Console.WriteLine($"{i + 1}.{list[i]}");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _02._Knights_of_Honor
{
class Program
{
static void Main(string[] args)
{
//Action<string> printString = Console.WriteLine;
//string[] names = Console.ReadLine()
// .Split();
//names
// .Select(n => "Sir " + n)
// .ToList()
// .ForEach(printString);
Action<string> printString = (name) =>
{
Console.WriteLine("Sir " + name);
};
Console.ReadLine()
.Split()
.ToList()
.ForEach(printString);
}
}
}
<file_sep>using P04.WildFarm.Models.Foods;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Models.Animals.Mammals.Felines
{
public class Cat : Feline
{
private const double WEIGHT_MULT = 0.30;
public Cat(string name, double weight, string livingRegion, string breed)
: base(name, weight, livingRegion, breed)
{
}
public override double WeightMultiplier
=> WEIGHT_MULT;
public override ICollection<Type> PrefferedFoods
=> new List<Type>() { typeof(Vegetable), typeof(Meat)};
public override void ProduceSound()
{
Console.WriteLine("Meow");
}
}
}
<file_sep>using System;
namespace VowelsSum
{
class Program
{
static void Main(string[] args)
{
string text = Console.ReadLine();
int sumVolews = 0;
for(int i=0; i < text.Length; i++)
{
char letter = text[i];
switch(letter)
{
case 'a':
sumVolews = sumVolews + 1;
break;
case 'e':
sumVolews = sumVolews + 2;
break;
case 'i':
sumVolews = sumVolews + 3;
break;
case 'o':
sumVolews = sumVolews + 4;
break;
case 'u':
sumVolews = sumVolews + 5;
break;
}
}
Console.WriteLine(sumVolews);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P07.RawData
{
class Program
{
static void Main(string[] args)
{
List<Car> cars = new List<Car>();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string[] carArgs = Console.ReadLine()
.Split();
string model = carArgs[0];
Engine engine = new Engine(int.Parse(carArgs[1]), int.Parse(carArgs[2]));
Cargo cargo = new Cargo(int.Parse(carArgs[3]), carArgs[4]);
Tire tire1 = new Tire(double.Parse(carArgs[5]), int.Parse(carArgs[6]));
Tire tire2 = new Tire(double.Parse(carArgs[7]), int.Parse(carArgs[8]));
Tire tire3 = new Tire(double.Parse(carArgs[9]), int.Parse(carArgs[10]));
Tire tire4 = new Tire(double.Parse(carArgs[11]), int.Parse(carArgs[12]));
List<Tire> tires = new List<Tire>() { tire1, tire2, tire3, tire4 };
Car car = new Car(model, engine, cargo, tires);
cars.Add(car);
}
string typeCars = Console.ReadLine();
if(typeCars == "fragile")
{
var sortedCars = cars
.Where(c => c.Cargo.Type == "fragile")
.Where(c => c.Tires.Any(t => t.Pressure < 1))
.ToList();
Console.WriteLine(string.Join(Environment.NewLine, sortedCars.Select(c => c.Model)));
}
else
{
var sortedCars = cars
.Where(c => c.Cargo.Type == "flamable")
.Where(c => c.Engine.Power > 250)
.ToList();
Console.WriteLine(string.Join(Environment.NewLine, sortedCars.Select(c => c.Model)));
}
}
}
}
<file_sep>using System;
namespace AccountBallance
{
class Program
{
static void Main(string[] args)
{
string command = Console.ReadLine();
double sum = 0;
while(command != "NoMoreMoney")
{
double num = double.Parse(command);
if(num < 0)
{
Console.WriteLine("Invalid operation!");
break;
}
else
{
sum += num;
Console.WriteLine($"Increase: {num:F2}");
}
command = Console.ReadLine();
}
Console.WriteLine($"Total: {sum:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace ReverseStrings
{
class Program
{
static void Main(string[] args)
{
var input = Console.ReadLine();
//Stack stack = new Stack();
var stack = new Stack<char>();
// Stack<int> stack = new;
// Stack<int>();
foreach(var ch in input)
{
stack.Push(ch);
}
////without foreach to fill the stack use this
//var stack = new Stack<char>(input);
////Even faster way is
//var stack = new Stack<char>(Console.ReadLine());
while (stack.Count != 0)
{
Console.Write(stack.Pop());
}
//Console.WriteLine();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Encapsulation
{
public class Car
{
public Car(string make,string model)//////immutable
{
this.Make = make;
this.Model = model;
}
public string Model { get; }
public string Make { get; }
}
}
<file_sep>using System;
namespace Beehive_Defence
{
class Program
{
static void Main(string[] args)
{
int beeCount = int.Parse(Console.ReadLine());
int healthBear = int.Parse(Console.ReadLine());
int attackBear = int.Parse(Console.ReadLine());
while(true)
{
beeCount = beeCount - attackBear;
if(beeCount<100)
{ if(beeCount<0)
{
beeCount = 0;
}
Console.WriteLine($"The bear stole the honey! Bees left {beeCount}.");
break;
}
healthBear -= beeCount * 5;
if(healthBear<=0)
{
Console.WriteLine($"Beehive won! Bees left {beeCount}.");
break;
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime;
namespace _02._Articles
{
class Article
{
public Article(string title, string content, string author)
{
this.Title = title;
this.Content = content;
this.Author = author;
}
public string Title { get; set; }
public string Content { get; set; }
public string Author { get; set; }
public void Edit(string newContent)
{
this.Content = newContent;
}
public void ChangeAuthor(string newAuthor)
{
this.Author = newAuthor;
}
public void Rename(string newTitle)
{
this.Title = newTitle;
}
public override string ToString()
{
//return base.ToString();
return $"{this.Title} - {this.Content}: {this.Author}";
}
}
class Catalog
{
public Catalog()
{
this.Articles = new List<Article>();
}
public List<Article> Articles { get; set; }
}
class Program
{
static void Main(string[] args)
{
Catalog catalog = new Catalog();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string[] input = Console.ReadLine().Split(", ");
string title = input[0];
string content = input[1];
string author = input[2];
Article article = new Article(title, content, author);
catalog.Articles.Add(article);
}
string criteria = Console.ReadLine();
if(criteria == "title")
{
foreach (Article article in catalog.Articles.OrderBy(x => x.Title))
{
Console.WriteLine(article.ToString());
}
}
else if(criteria == "content")
{
foreach (Article article in catalog.Articles.OrderBy(x => x.Content))
{
Console.WriteLine(article.ToString());
}
}
else
{
foreach (Article article in catalog.Articles.OrderBy(x => x.Author))
{
Console.WriteLine(article.ToString());
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace AverageStudentGrades
{
class Program
{
static void Main(string[] args)
{
var grades = new Dictionary<string, List<decimal>>();
int countOfStudents = int.Parse(Console.ReadLine());
for (int i = 0; i < countOfStudents; i++)
{
string[] inputInfo = Console.ReadLine().Split();
string name = inputInfo[0];
decimal grade = decimal.Parse(inputInfo[1]);
if(!grades.ContainsKey(name))
{
grades.Add(name, new List<decimal>());
}
grades[name].Add(grade);
}
foreach (var (nameKey,gradeValues) in grades)
{
//Console.WriteLine($"{nameKey}->{string.Join(" ",gradeValues)} (avg: {gradeValues.Average():F2})");
Console.Write($"{nameKey}->");
foreach (var gradeValue in gradeValues)
{
Console.Write($"{gradeValue:F2} ");
}
Console.Write($"(avg:{gradeValues.Average():F2})");
Console.WriteLine();
}
}
}
}
<file_sep>using System;
namespace ExamPreparation
{
class Program
{
static void Main(string[] args)
{
int countBadGradesNeeded = int.Parse(Console.ReadLine());
string nameOfExcercise = Console.ReadLine();
int grade = 0;
int countWorseGrades = 0;
int countAllGrades = 0;
double sumgrades = 0;
string lastExcercise = "";
while (nameOfExcercise != "Enough" && countBadGradesNeeded != countWorseGrades)
{
grade = int.Parse(Console.ReadLine());
lastExcercise = nameOfExcercise;
if (grade <= 4)
{
countWorseGrades++;
}
else
{
countAllGrades++;
}
sumgrades = sumgrades + grade;
nameOfExcercise = Console.ReadLine();
}
if(nameOfExcercise == "Enough")
{
Console.WriteLine($"Average score: {sumgrades /(countAllGrades + countWorseGrades):F2}");
Console.WriteLine($"Number of problems: {countWorseGrades+countAllGrades}");
Console.WriteLine($"Last problem: {lastExcercise}");
}
else
{
Console.WriteLine($"You need a break, { countWorseGrades} poor grades.");
}
}
}
}
<file_sep>using Moq;
using NUnit.Framework;
using Skeleton;
[TestFixture]
public class HeroTests
{
[Test]
public void HeroShouldGainXpIfTargetDies()
{
const int experience = 200;
//Arrange
var fakeWeapon = Mock.Of<IWeapon>();
var fakeTarget = new Mock<ITarget>();
fakeTarget
.Setup(t => t.IsDead())
.Returns(true);
fakeTarget
.Setup(t => t.GiveExperience())
.Returns(experience);
var hero = new Hero("TestHero", fakeWeapon);
//Act
hero.Attack(fakeTarget.Object);
//Assert
Assert.That(hero.Experience, Is.EqualTo(experience));
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P06.FoodShortage
{
interface IIdentifiable
{
string GetId();
}
}
<file_sep>using System;
namespace StrongNumber
{
class Program
{
static void Main(string[] args)
{
int num = int.Parse(Console.ReadLine());
int num1 = num;
int sum = 0;
while(num>0)
{
int subnum = num % 10;
num = num / 10;
// Console.WriteLine($"NUM:{num}");
int sumInside = 1;
for(int i = 1; i <= subnum; i++)
{
sumInside *= i;
}
sum += sumInside;
// Console.WriteLine($"SumInside:{sumInside}");
// Console.WriteLine($"Sum:{sum}");
}
if (sum == num1)
{
Console.WriteLine("yes");
}
else
{
Console.WriteLine("no");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text.RegularExpressions;
namespace _01.Furniture
{
class Program
{
static void Main(string[] args)
{
string pattern = @">>(?<name>\w+)<<(?<price>\d+(.\d+)?)!(?<quantity>\d+)";
List<string> purchasedFurniture = new List<string>();
decimal moneySpend = 0;
while (true)
{
string input = Console.ReadLine();
if(input == "Purchase")
{
break;
}
if (Regex.IsMatch(input, pattern))
{
Match match = Regex.Match(input, pattern);
string name = match.Groups["name"].Value;
decimal price = decimal.Parse(match.Groups["price"].Value);
int quantity = int.Parse(match.Groups["quantity"].Value);
purchasedFurniture.Add(name);
moneySpend += price * quantity;
}
}
Console.WriteLine("Bought furniture:");
if (purchasedFurniture.Count > 0)
{
Console.WriteLine(string.Join(Environment.NewLine, purchasedFurniture));
}
Console.WriteLine($"Total money spend: {moneySpend:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace TestDemos.UnitTest1
{
using NUnit.Framework;
[TestFixture]
public class CalculatorTests
{
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _08._Anonymous_Threat
{
class Program
{
static void Main(string[] args)
{
List<string> list = Console.ReadLine().Split().ToList();
while (true)
{
string input = Console.ReadLine();
if(input == "3:1")
{
break;
}
string[] command = input.Split();
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.InteropServices;
namespace _07._List_Manipulation_Advanced
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
bool isChanged = false;
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] command = input.Split();
if (command[0] == "Add")
{
list.Add(int.Parse(command[1]));
isChanged = true;
}
else if (command[0] == "Remove")
{
list.Remove(int.Parse(command[1]));
isChanged = true;
}
else if (command[0] == "RemoveAt")
{
list.RemoveAt(int.Parse(command[1]));
isChanged = true;
}
else if(command[0] == "Insert")
{
list.Insert(int.Parse(command[2]), int.Parse(command[1]));
isChanged = true;
}
switch (command[0])
{
case "Contains":
DoesContain(list, int.Parse(command[1]));
break;
case "PrintEven":
PrintEven(list);
break;
case "PrintOdd":
PrintOdd(list);
break;
case "GetSum":
int sum = GetSum(list);
Console.WriteLine(sum);
break;
case "Filter":
string condition = command[1];
int num = int.Parse(command[2]);
Filter(list, condition, num);
break;
}
}
if (isChanged)
{
Console.WriteLine(string.Join(" ", list));
}
}
static void DoesContain(List<int> list,int number)
{
if (list.Contains(number))
{
Console.WriteLine("Yes");
}
else
{
Console.WriteLine("No such number");
}
}
static void PrintEven(List<int> list)
{
List<int> evenList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
if (list[i] % 2 == 0)
{
evenList.Add(list[i]);
}
}
Console.WriteLine(string.Join(" ", evenList));
}
static void PrintOdd(List<int> list)
{
List<int> oddList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
if (list[i] % 2 != 0)
{
oddList.Add(list[i]);
}
}
Console.WriteLine(string.Join(" ", oddList));
}
static int GetSum(List<int> list)
{
return list.Sum();
}
static void Filter(List<int> list , string condtion , int num)
{
List<int> filteredList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
switch (condtion)
{
case "<":
if(list[i] < num)
{
filteredList.Add(list[i]);
}
break;
case ">":
if (list[i] > num)
{
filteredList.Add(list[i]);
}
break;
case ">=":
if (list[i] >= num)
{
filteredList.Add(list[i]);
}
break;
case "<=":
if (list[i] <= num)
{
filteredList.Add(list[i]);
}
break;
}
}
Console.WriteLine(string.Join(" ", filteredList));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P08.CollectionHierarchy.Contracts
{
public interface IMyList : IRemovable
{
int Used { get; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _01.Train
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
int maxCapacity = int.Parse(Console.ReadLine());
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] command = input.Split();
if(command[0] == "Add")
{
list.Add(int.Parse(command[1]));
}
else
{
int passeng = int.Parse(command[0]);
for (int i = 0; i < list.Count; i++)
{
if(list[i]+passeng <= maxCapacity)
{
list[i] += passeng;
break;
}
}
}
}
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
namespace GodzillavsKong
{
class Program
{
static void Main(string[] args)
{
double budget = double.Parse(Console.ReadLine());
int countStatist = int.Parse(Console.ReadLine());
double priceClothes = double.Parse(Console.ReadLine());
double deckorPrice = budget * 0.1;
if(countStatist > 150 )
{
priceClothes = priceClothes - priceClothes * 0.1;
}
double moneyNeeded = countStatist * priceClothes + deckorPrice ;
if(moneyNeeded > budget)
{
Console.WriteLine("Not enough money!");
Console.WriteLine($"Wingard needs {moneyNeeded - budget:F2} leva more.");
}
else
{
Console.WriteLine("Action!");
Console.WriteLine($"Wingard starts filming with {budget - moneyNeeded:F2} leva left.");
}
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Text;
namespace LibraryIterator
{
public class Library :IEnumerable<Book>
{
private List<Book> books;
public Library()
{
}
public void Add(Book book)
{
this.books.Add(book);
}
public IEnumerator<Book> GetEnumerator()
{
return new BookIterator(this.books);
}
IEnumerator IEnumerable.GetEnumerator()
{
return this.books.GetEnumerator();
}
private class BookIterator : IEnumerator<Book>
{
private List<Book> books;
private int index;
public BookIterator(List<Book> books)
{
this.books = books;
}
public Book Current
{
get
{
return this.books[this.index];
}
}
object IEnumerator.Current => this.Current;
public void Dispose()
{
throw new NotImplementedException();
}
public bool MoveNext()
{
return ++this.index < this.books.Count;
}
public void Reset()
{
this.index = -1;
}
}
}
}
<file_sep>using System;
namespace CareOfPuppy
{
class Program
{
static void Main(string[] args)
{
int purchasedFoodInKg = int.Parse(Console.ReadLine());
string command = Console.ReadLine();
int sumFoodInGrams = 0;
while(command!="Adopted")
{
int foodInGram = int.Parse(command);
sumFoodInGrams += foodInGram;
command = Console.ReadLine();
}
if(purchasedFoodInKg*1000>=sumFoodInGrams)
{
Console.WriteLine($"Food is enough! Leftovers: {purchasedFoodInKg*1000-sumFoodInGrams} grams.");
}
else
{
Console.WriteLine($"Food is not enough. You need {sumFoodInGrams - purchasedFoodInKg*1000} grams more.");
}
}
}
}
<file_sep>namespace P06.FoodShortage
{
public class Pet : IBirthable
{
private string name;
private string birthDate;
public Pet(string name , string birthDate)
{
this.name = name;
this.birthDate = birthDate;
}
public string GetBirth()
{
return this.birthDate;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _02._Average_Student_Grades
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
Dictionary<string, List<decimal>> students = new Dictionary<string, List<decimal>>();
for (int i = 0; i < n; i++)
{
string[] studentArgs = Console.ReadLine()
.Split();
string studentName = studentArgs[0];
decimal studentGrade = decimal.Parse(studentArgs[1]);
if (!students.ContainsKey(studentName))
{
students[studentName] = new List<decimal>();
}
students[studentName].Add(studentGrade);
}
foreach (var kvp in students)
{
Console.Write($"{kvp.Key} -> ");
foreach (var grade in kvp.Value)
{
Console.Write($"{grade:F2} ");
}
Console.WriteLine($"(avg: {kvp.Value.Average():F2})");
}
}
}
}
<file_sep>using System;
using System.ComponentModel;
using System.Runtime;
namespace _03.Calculations
{
class Program
{
static void Main(string[] args)
{
string command = Console.ReadLine();
int num1 = int.Parse(Console.ReadLine());
int num2 = int.Parse(Console.ReadLine());
int result = 0;
switch (command)
{
case "add":
result = Add(num1, num2);
break;
case "divide":
result = Divide(num1, num2);
break;
case "substract":
result = Substract(num1, num2);
break;
case "multiply":
result = Multiply(num1, num2);
break;
}
Console.WriteLine(result);
}
static int Add(int num1, int num2)
{
return (num1 + num2);
}
static int Substract(int num1, int num2)
{
return (num1 - num2);
}
static int Multiply(int num1, int num2)
{
return (num1 * num2);
}
static int Divide(int num1, int num2)
{
return (num1 / num2);
}
}
}
<file_sep>using System;
namespace Cinema
{
class Program
{
static void Main(string[] args)
{
string typeScene = Console.ReadLine();
int rows = int.Parse(Console.ReadLine());
int collons = int.Parse(Console.ReadLine());
double income = 0;
switch (typeScene)
{
case "Premiere":
income = rows * collons * 12.00;
break;
case "Normal":
income = rows * collons * 7.50;
break;
case "Discount":
income = rows * collons * 5.00;
break;
}
Console.WriteLine($"{income:F2} leva");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _06._Wardrobe
{
class Program
{
static void Main(string[] args)
{
var wardrobe = new Dictionary<string, Dictionary<string, int>>();
int n = int.Parse(Console.ReadLine());
for (int i = 0; i < n; i++)
{
string[] cmdArgs = Console.ReadLine()
.Split(" -> ", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
string color = cmdArgs[0];
string[] clothesWithThisColor = cmdArgs[1]
.Split(",", StringSplitOptions.RemoveEmptyEntries)
.ToArray();
if (!wardrobe.ContainsKey(color))
{
wardrobe[color] = new Dictionary<string, int>();
}
foreach (var cloth in clothesWithThisColor)
{
if (!wardrobe[color].ContainsKey(cloth))
{
wardrobe[color][cloth] = 0;
}
wardrobe[color][cloth]++;
}
}
string[] itemSearchedArgs = Console.ReadLine()
.Split(" ",StringSplitOptions.RemoveEmptyEntries)
.ToArray();
foreach (var kvp in wardrobe)
{
Console.WriteLine($"{kvp.Key} clothes:");
foreach (var cloth in kvp.Value)
{
if(kvp.Key == itemSearchedArgs[0] && cloth.Key == itemSearchedArgs[1])
{
Console.WriteLine($"* {cloth.Key} - {cloth.Value} (found!)");
}
else
{
Console.WriteLine($"* {cloth.Key} - {cloth.Value}");
}
}
}
}
}
}
<file_sep>using System;
using System.Data;
using System.Linq;
namespace SumMatrixElements
{
class Program
{
static void Main(string[] args)
{
int[] sizes = Console.ReadLine().Split(", ").Select(int.Parse).ToArray();
int[,] matrix = new int[sizes[0], sizes[1]];
for(int row = 0;row<matrix.GetLength(0);row++)
{
int[] colElements = Console.ReadLine().Split(", ").Select(int.Parse).ToArray();
for(int col = 0;col<matrix.GetLength(1);col++)
{
matrix[row, col] = colElements[col];
}
}
int sum = 0;
for(int row = 0; row < matrix.GetLength(0);row++)
{
for(int col = 0;col < matrix.GetLength(1);col++)
{
sum += matrix[row,col];
}
}
Console.WriteLine(matrix.GetLength(0));
Console.WriteLine(matrix.GetLength(1));
Console.WriteLine(sum);
}
}
}
<file_sep>using System;
using System.Linq;
namespace JaggedArraysDemo
{
class Program
{
static void Main(string[] args)
{
int rows = int.Parse(Console.ReadLine());
int[][] array = new int[rows][];
for (int row = 0; row < rows; row++)
{
int[] currentRow = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
array[row] = currentRow;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _03._Problem
{
class Program
{
static void Main(string[] args)
{
//Dictionary<string, List<string>> emails = new Dictionary<string, List<string>>();
//string input;
//while((input = Console.ReadLine()) != "Statistics")
//{
// string[] command = input.Split("->", StringSplitOptions.RemoveEmptyEntries);
// if(command[0] == "Add")
// {
// string username = command[1];
// if(emails.ContainsKey(username))
// {
// Console.WriteLine($"{username} is already registered");
// }
// else
// {
// emails[username] = new List<string>();
// }
// }
// else if(command[0] == "Send")
// {
// string username = command[1];
// string emailToSend = command[2];
// emails[username].Add(emailToSend);
// }
// else
// {
// string username = command[1];
// if(emails.ContainsKey(username))
// {
// emails.Remove(username);
// }
// else
// {
// Console.WriteLine($"{username} not found!");
// }
// }
//}
//emails = emails
// .OrderByDescending(kvp => kvp.Value.Count)
// .ThenBy(kvp => kvp.Key)
// .ToDictionary(a => a.Key, b => b.Value);
//Console.WriteLine($"Users count: {emails.Count}");
//foreach (string user in emails.Keys)
//{
// Console.WriteLine(user);
// foreach (string email in emails[user])
// {
// Console.WriteLine($"- {email}");
// }
//}
for (int i = 10; i > 3; i -= 2)
{
Console.Write($"{i }");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace _01.Person
{
class Child:Person
{
}
}
<file_sep>using System;
namespace NumbersEndingIn7
{
class Program
{
static void Main(string[] args)
{
for(int i=0;i<1000; i++)
{
if(i%10 == 7)
{
Console.WriteLine(i);
}
}
}
}
}
<file_sep>using System;
namespace Walking
{
class Program
{
static void Main(string[] args)
{
int sumSteps = 0;
bool goalReached = false;
while(sumSteps < 10000)
{
string command = Console.ReadLine();
if (command == "Going home")
{
int stepsback = int.Parse(Console.ReadLine());
sumSteps = sumSteps + stepsback;
if(sumSteps >= 10000)
{
goalReached = true;
break;
}
else
{
goalReached = false;
break;
}
}
else
{ int steps = int.Parse(command);
sumSteps = sumSteps + steps;
}
// Console.WriteLine(steps);
}
if(sumSteps >= 10000)
{
goalReached = true;
}
if(goalReached)
{
Console.WriteLine("Goal reached! Good job!");
Console.WriteLine($"{sumSteps - 10000} steps over the goal!");
}
else
{
Console.WriteLine($"{10000 - sumSteps} more steps to reach goal.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.NeedForSpeed.Car
{
public class SportCar : Car
{
private const double defaultFuelConsumption = 10;
public SportCar(int horsePower, double fuel) : base(horsePower, fuel)
{
}
public override double FuelConsumption => defaultFuelConsumption;
//{
// get
// {
// return this.FuelConsumption;
// }
// set
// {
// this.FuelConsumption = defaultFuelConsumption;
// }
//}
public override void Drive(double kilometers)
{
double fuelAfterDrive = this.Fuel - kilometers * this.FuelConsumption;
if (fuelAfterDrive >= 0)
{
this.Fuel -= kilometers * this.FuelConsumption;
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace CountSameValuesInArray
{
class Program
{
static void Main(string[] args)
{
double[] input = Console.ReadLine().Split().Select(double.Parse).ToArray();
Dictionary<double, int> dictionary = new Dictionary<double, int>();
foreach (var currentNumber in input)
{
if (!dictionary.ContainsKey(currentNumber))
{
dictionary.Add(currentNumber, 0);
}
dictionary[currentNumber]++;
}
foreach (var (key, value) in dictionary)
{
Console.WriteLine($"{key}-{value}times");
}
//double[] input = Console.ReadLine().Split().Select(double.Parse).ToArray();
//Dictionary<double, int> dictionary = new Dictionary<double, int>();
//foreach (var el in input)
//{
// if(!dictionary.ContainsKey(el))
// {
// dictionary.Add(el, 0);
// }
// dictionary[el]++;
//}
//foreach (var (key,value) in dictionary)
//{
// Console.WriteLine($"{key}-{value}");
//}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public class Airplane : Vehicle
{
public int MaxPeople { get; set; }
public override string VrumVrum()
{
return "Airplane is flying";
}
public string VrumVrum(int people)
{
return $"Airplane is flying with {people}";
}
}
}
<file_sep>using System;
namespace _05.Orders
{
class Program
{
static void Main(string[] args)
{
string product = Console.ReadLine();
}
}
}
<file_sep>using System;
namespace _01._Secret_Chat
{
class Program
{
static void Main(string[] args)
{
string message = Console.ReadLine();
string input;
while((input = Console.ReadLine()) != "Reveal")
{
string[] command = input.Split(":|:");
if(command[0] == "InsertSpace")
{
int index = int.Parse(command[1]);
message = message.Insert(index, " ");
Console.WriteLine(message);
}
else if(command[0] == "Reverse")
{
string subs = command[1];
if (message.Contains(subs))
{
int startIndex = message.IndexOf(subs);
message = message.Remove(startIndex, subs.Length);
char[] subsArr = subs.ToCharArray();
Array.Reverse(subsArr);
string reversedSubstr = new string(subsArr);
message += reversedSubstr;
Console.WriteLine(message);
}
else
{
Console.WriteLine("error");
}
}
else
{
string subs = command[1];
string replacement = command[2];
message = message.Replace(subs, replacement);
Console.WriteLine(message);
}
}
Console.WriteLine($"You have a new text message: {message}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P01.Bombs
{
class Program
{
static void Main(string[] args)
{
int[] bombEffectsInfo = Console.ReadLine()
.Split(", ")
.Select(int.Parse)
.ToArray();
int[] bombCasingInfo = Console.ReadLine()
.Split(", ")
.Select(int.Parse)
.ToArray();
Queue<int> bombEffects = new Queue<int>(bombEffectsInfo);
Stack<int> bombCasings = new Stack<int>(bombCasingInfo);
var bombs = new Dictionary<int, string>()
{
{ 40,"Datura Bombs" }
,{60 ,"Cherry Bombs"}
,{120,"Smoke Decoy Bombs"}
};
Dictionary<string, int> madeBombsCount = new Dictionary<string, int>()
{
{ "Datura Bombs",0 }
,{"Cherry Bombs",0 }
,{"Smoke Decoy Bombs",0}
};
while(bombEffects.Any() && bombCasings.Any() && madeBombsCount.Any(kvp => kvp.Value < 3))
{
int currBombEffect = bombEffects.Peek();
int currBombCasing = bombCasings.Peek();
if(bombs.Any(kvp => kvp.Key == currBombEffect + currBombCasing))
{
string bombMadeName = bombs[currBombCasing + currBombEffect];
madeBombsCount[bombMadeName]++;
bombEffects.Dequeue();
bombCasings.Pop();
}
else
{
bombCasings.Push(bombCasings.Pop() - 5);
}
}
if(madeBombsCount.All(kvp => kvp.Value >= 3))
{
Console.WriteLine("Bene! You have successfully filled the bomb pouch!");
}
else
{
Console.WriteLine("You don't have enough materials to fill the bomb pouch.");
}
if (bombEffects.Any())
{
Console.WriteLine($"Bomb Effects: {string.Join(", ",bombEffects)}");
}
else
{
Console.WriteLine("Bomb Effects: empty");
}
if (bombCasings.Any())
{
Console.WriteLine($"Bomb Casings: {string.Join(", ", bombCasings)}");
}
else
{
Console.WriteLine("Bomb Casings: empty");
}
madeBombsCount = madeBombsCount
.OrderBy(kvp => kvp.Key)
.ToDictionary(x => x.Key, y => y.Value);
foreach (var kvp in madeBombsCount)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value}");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _03._House_Party
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
List<string> listNames = new List<string>();
for (int i = 0; i < n; i++)
{
string[] input = Console.ReadLine().Split();
string name = input[0];
if(input[2] == "going!")
{
if (!listNames.Contains(name))
{
listNames.Add(name);
}
else
{
Console.WriteLine($"{name} is already in the list!");
}
}
else
{
if (listNames.Contains(name))
{
listNames.Remove(name);
}
else
{
Console.WriteLine($"{name} is not in the list!");
}
}
}
for (int i = 0; i < listNames.Count; i++)
{
Console.WriteLine(listNames[i]);
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _08.Magic_Sum
{
class Program
{
static void Main(string[] args)
{
int[] arr = Console.ReadLine().Split().Select(int.Parse).ToArray();
int magicNum = int.Parse(Console.ReadLine());
for (int i = 0; i < arr.Length; i++)
{
for (int j = i+1; j < arr.Length; j++)
{
if(arr[i] + arr[j] == magicNum)
{
Console.WriteLine($"{arr[i]} {arr[j]}");
}
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace Gauss_trick
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
GausTrick(list);
}
static void GausTrick(List<int> list)
{
for (int i = 0,j = list.Count-1; i < list.Count/2; i++, j--)
{
Console.Write($"{list[i] + list[j]} ");
}
if(list.Count % 2 != 0)
{
Console.Write(list[list.Count / 2]);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Inheritance
{
public class Person
{
private int age;
public string Name { get; set; }
public string Address { get; set; }
public override string ToString()
{
return $"{this.Name} - {this.age}";
}
}
}
<file_sep>using System;
namespace _1st
{
class Program
{
static void Main(string[] args)
{
int countBees = int.Parse(Console.ReadLine());
int countFlowers = int.Parse(Console.ReadLine());
double allHoney = countBees * countFlowers * 0.21;
double countPiti = allHoney / 100;
double unusedHoney = allHoney % 100;
Console.WriteLine($"{Math.Floor(countPiti)} honeycombs filled.");
Console.WriteLine($"{unusedHoney:F2} grams of honey left.");
}
}
}
<file_sep>using System;
using System.Linq;
namespace _4._Matrix_Shuffling
{
class Program
{
static void Main(string[] args)
{
int[] dimensions = Console.ReadLine()
.Split(' ', StringSplitOptions.RemoveEmptyEntries)
.Select(int.Parse)
.ToArray();
int rows = dimensions[0];
int cols = dimensions[1];
string[,] matrix = new string[rows, cols];
for (int row = 0; row < rows; row++)
{
string[] currRow = Console.ReadLine()
.Split(' ', StringSplitOptions.RemoveEmptyEntries)
// .Select(int.Parse)
.ToArray();
for (int col = 0; col < cols; col++)
{
matrix[row, col] = currRow[col];
}
}
while (true)
{
string command = Console.ReadLine();
if(command == "END")
{
break;
}
string[] cmdArgs = command.Split(' ', StringSplitOptions.RemoveEmptyEntries);
if(cmdArgs[0] == "swap" && cmdArgs.Length == 5)
{
int x1 = int.Parse(cmdArgs[1]);
int x2 = int.Parse(cmdArgs[2]);
int y1 = int.Parse(cmdArgs[3]);
int y2 = int.Parse(cmdArgs[4]);
if (!AreCoordinatesValid(x1, x2, y1, y2, rows, cols))
{
Console.WriteLine("Invalid input!");
continue;
}
string temp = matrix[x1, x2];
matrix[x1, x2] = matrix[y1, y2];
matrix[y1, y2] = temp;
for (int row = 0; row < rows; row++)
{
for (int col = 0; col < cols; col++)
{
Console.Write(matrix[row,col] + " ");
}
Console.WriteLine();
}
}
else
{
Console.WriteLine("Invalid input!");
}
}
}
private static bool AreCoordinatesValid(int x1,int x2,int y1, int y2,int rowsLength,int colsLength)
{
if(x1 < 0 || x1 > rowsLength -1 || x2 < 0 || x2 > colsLength - 1
|| y1 < 0 || y1 > rowsLength - 1 || y2 < 0 || y2 > colsLength - 1)
{
return false;
}
return true;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _05._Count_Symbols
{
class Program
{
static void Main(string[] args)
{
var dict = new Dictionary<char, int>();
char[] text = Console.ReadLine().ToCharArray();
//string text = Console.ReadLine();
foreach (var symbol in text)
{
if (!dict.ContainsKey(symbol))
{
dict[symbol] = 0;
}
dict[symbol]++;
}
dict = dict
.OrderBy(kvp => kvp.Key)
.ToDictionary(x => x.Key, y => y.Value);
foreach (var kvp in dict)
{
Console.WriteLine($"{kvp.Key}: {kvp.Value} time/s");
}
}
}
}
<file_sep>using System;
namespace Vending_Machine
{
class Program
{
static void Main(string[] args)
{
string command = Console.ReadLine();
double totalMoney = 0;
while(command != "Start")
{
double ins = double.Parse(command);
if(ins == 0.1 || ins == 0.2 || ins == 0.5 || ins == 1 || ins == 2)
{
totalMoney += ins;
}
else
{
Console.WriteLine($"Cannot accept {ins}");
}
command = Console.ReadLine();
}
string command2 = Console.ReadLine();
while(command2 != "End")
{
string product = command2;
switch (product)
{
case "Nuts":
if(totalMoney >= 2)
{
totalMoney -= 2;
Console.WriteLine($"Purchased {product.ToLower()}");
}
else
{
Console.WriteLine("Sorry, not enough money");
}
break;
case "Water":
if (totalMoney >= 0.7)
{
totalMoney -= 0.7;
Console.WriteLine($"Purchased {product.ToLower()}");
}
else
{
Console.WriteLine("Sorry, not enough money");
}
break;
case "Crisps":
if (totalMoney >= 1.5)
{
totalMoney -= 1.5;
Console.WriteLine($"Purchased {product.ToLower()}");
}
else
{
Console.WriteLine("Sorry, not enough money");
}
break;
case "Soda":
if (totalMoney >= 0.8)
{
totalMoney -= 0.8;
Console.WriteLine($"Purchased {product.ToLower()}");
}
else
{
Console.WriteLine("Sorry, not enough money");
}
break;
case "Coke":
if (totalMoney >= 1)
{
totalMoney -= 1;
Console.WriteLine($"Purchased {product.ToLower()}");
}
else
{
Console.WriteLine("Sorry, not enough money");
}
break;
default:
Console.WriteLine("Invalid product");
break;
} command2 = Console.ReadLine();
}
Console.WriteLine($"Change: {totalMoney:F2}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _05.Students_2
{
class Student
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public string HomeTown { get; set; }
}
class Program
{
static void Main(string[] args)
{
List<Student> students = new List<Student>();
while (true)
{
string input = Console.ReadLine();
if (input == "end")
{
break;
}
string[] info = input.Split();
if (IsStudentExisting(students, info[0], info[1]))
{
Student student = GetStudent(students, info[0], info[1]);
student.FirstName = info[0];
student.LastName = info[1];
student.Age = int.Parse(info[2]);
student.HomeTown = info[3];
}
else
{
Student student = new Student();
student.FirstName = info[0];
student.LastName = info[1];
student.Age = int.Parse(info[2]);
student.HomeTown = info[3];
students.Add(student);
}
}
string city = Console.ReadLine();
for (int i = 0; i < students.Count; i++)
{
if (students[i].HomeTown == city)
{
Console.WriteLine($"{students[i].FirstName} {students[i].LastName} is {students[i].Age} years old.");
}
}
}
static bool IsStudentExisting(List<Student> students,string firstName, string lastName)
{
foreach (Student student in students)
{
if(student.FirstName == firstName && student.LastName == lastName)
{
return true;
}
}
return false;
}
static Student GetStudent(List<Student> students , string firstName , string lastName)
{
Student existingStudent = null;
foreach (Student student in students)
{
if (student.FirstName == firstName && student.LastName == lastName)
{
existingStudent = student;
}
}
return existingStudent;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
namespace Heroes
{
public class HeroRepository
{
private readonly List<Hero> data;
public HeroRepository()
{
this.data = new List<Hero>();
}
public int Count => this.data.Count;
public void Add(Hero hero)
{
this.data.Add(hero);
}
public void Remove(string name)
{
this.data.Remove(this.data.FirstOrDefault(h => h.Name == name));
}
public Hero GetHeroWithHighestStrength()
{
// int maxItemStrength = this.data.Max(h => h.Item.Strength);
return this.data.FirstOrDefault(h => h.Item.Strength == this.data.Max(he => he.Item.Strength));
}
public Hero GetHeroWithHighestAbility()
{
return this.data.FirstOrDefault(h => h.Item.Ability == this.data.Max(he => he.Item.Ability));
}
public Hero GetHeroWithHighestIntelligence()
{
return this.data.FirstOrDefault(h => h.Item.Intelligence == this.data.Max(he => he.Item.Intelligence));
}
public override string ToString()
{
StringBuilder sb = new StringBuilder();
for(int i = 0; i < this.Count; i++)
{
sb.AppendLine(this.data[i].ToString());
}
return sb.ToString().TrimEnd();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P07.MilitaryElite.Exceptions
{
public class InvalidMissionStateException : Exception
{
private const string DEF_MSG = "Invalid mission state";
public InvalidMissionStateException()
:base(DEF_MSG)
{
}
public InvalidMissionStateException(string message)
: base(message)
{
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _08._Company_Users
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<string>> companies = new Dictionary<string, List<string>>();
while (true)
{
string inp = Console.ReadLine();
if(inp == "End")
{
break;
}
string[] command = inp.Split(" -> ").ToArray();
string companyName = command[0];
string id = command[1];
if (!companies.ContainsKey(companyName))
{
companies[companyName] = new List<string>();
}
if (!companies[companyName].Contains(id))
{
companies[companyName].Add(id);
}
}
foreach (var kvp in companies.OrderBy(c => c.Key))
{
Console.WriteLine(kvp.Key);
foreach (var id in kvp.Value)
{
Console.WriteLine($"-- {id}");
}
}
}
}
}
<file_sep>using System;
using System.ComponentModel.DataAnnotations;
namespace Salary
{
class Program
{
static void Main(string[] args)
{
int nBrowsers = int.Parse(Console.ReadLine());
int salary = int.Parse(Console.ReadLine());
for(int i=0; i < nBrowsers; i++)
{
if (salary <= 0)
{
break;
}
string nameBrowser = Console.ReadLine();
if(nameBrowser == "Facebook")
{
salary = salary - 150;
}
else if(nameBrowser == "Instagram")
{
salary = salary - 100;
}
else if(nameBrowser == "Reddit")
{
salary = salary - 50;
}
}
if (salary <= 0)
{
Console.WriteLine("You have lost your salary.");
}
else
{
Console.WriteLine(salary);
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace Lambda_Expressions
{
class Program
{
static void Main(string[] args)
{
int[] arr = new int[] { 1, 2, 3, 4, 5 };
///with Select
int[] arrayByFive = arr
.Select(x => x*5)
.ToArray();
///with Where
///
Console.WriteLine(string.Join(" ", arrayByFive));
}
}
}
<file_sep>using System;
using System.Diagnostics.Tracing;
using System.IO;
using System.Linq;
using System.Reflection.Metadata.Ecma335;
using System.Runtime.InteropServices;
namespace _02.LineNumbers
{
class Program
{
static void Main(string[] args)
{
string[] lines = File.ReadAllLines("text.txt");
for(int i = 0; i < lines.Length; i++)
{
string currLine = lines[i];
int lettersCnt = CountOfLetters(currLine);
int marksCnt = CountOfPunctuonalMarks(currLine);
lines[i] = $"Line {i + 1}: {currLine} ({lettersCnt})({marksCnt})";
}
File.WriteAllLines("../../../output.txt", lines);
}
static int CountOfLetters(string line)
{
int cnt = 0;
for (int i = 0; i < line.Length; i++)
{
char currChar = line[i];
if (char.IsLetter(currChar))
{
cnt++;
}
}
return cnt;
}
static int CountOfPunctuonalMarks(string line)
{
char[] marks = { '-', ',', '.', '!', '?' };
int cnt = 0;
for (int i = 0; i < line.Length; i++)
{
char curChar = line[i];
if (marks.Contains(curChar))
{
cnt++;
}
}
return cnt;
}
}
}
<file_sep>using System;
namespace Change_Bureau
{
class Program
{
static void Main(string[] args)
{
double bit = double.Parse(Console.ReadLine());
double chin = double.Parse(Console.ReadLine());
double komis = double.Parse(Console.ReadLine());
double resInLev = bit * 1168 + chin * 0.15 * 1.76;
resInLev = resInLev-(resInLev * (komis / 100));
double resInEvro = resInLev / 1.95;
Console.WriteLine($"{resInEvro:F2}");
}
}
}
<file_sep>using P01.Vehicles.Common;
using P01.Vehicles.Models.Contracts;
using System;
using System.Collections.Generic;
using System.Text;
namespace P01.Vehicles.Models
{
public abstract class Vehicle : IDriveable, IRefueable
{
private double fuelquantity;
private double tankCapacity;
public Vehicle(double fuelquantity,double fuelconsumption,double tankCapacity)
{
this.FuelQuantity = fuelquantity > tankCapacity ? 0 : fuelquantity;
this.FuelConsumption = fuelconsumption;
this.TankCapacity = tankCapacity;
}
public double FuelQuantity
{
get
{
return this.fuelquantity;
}
protected set
{
//if(value < 0)
//{
// throw new InvalidOperationException(ExceptionMessage.FuelIsNegativeOrZero);
//}
this.fuelquantity = value;
}
}
public virtual double FuelConsumption { get; protected set; }
public double TankCapacity {
get
{
return this.tankCapacity;
}
private set
{
this.tankCapacity = value;
}
}
public virtual string Drive(double kilometers)
{
double fuelNeeded = kilometers * this.FuelConsumption;
if(this.FuelQuantity < fuelNeeded)
{
throw new InvalidOperationException(string
.Format(ExceptionMessage.NotEnoughFuelExceptionMessage
,this.GetType().Name));
}
this.FuelQuantity -= fuelNeeded;
return $"{this.GetType().Name} travelled {kilometers} km";
}
public virtual void Refuel(double liters)
{
//if()
if(liters > 0)
{
if(this.FuelQuantity + liters > this.TankCapacity)
{
string exc = string.Format(ExceptionMessage
.InvalidFuelTankExceptionMessage, liters);
throw new InvalidOperationException(exc);
}
this.FuelQuantity += liters;
}
else
{
throw new InvalidOperationException(ExceptionMessage.FuelIsNegativeOrZero);
}
}
public string DriveEmpty(double kilometers)
{
return this.Drive(kilometers);
}
public override string ToString()
{
return $"{this.GetType().Name}: {this.FuelQuantity:F2}";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace Stacks_and_Queues_Demo
{
class Program
{
static void Main(string[] args)
{
var myStack = new Stack<string>();
myStack.Push("First");
myStack.Push("Second");
myStack.Push("Third");
Console.WriteLine(myStack.Peek());
Console.WriteLine(myStack.Peek());
var stack = new Stack<int>();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _09._ForceBook__My_solution_
{
class Program
{
static void Main(string[] args)
{
Dictionary<string, List<string>> book = new Dictionary<string, List<string>>();
while (true)
{
string inp = Console.ReadLine();
if(inp == "Lumpawaroo")
{
break;
}
string[] inputArgs = inp
.Split(new string[] { " | ", " -> " }, StringSplitOptions.RemoveEmptyEntries);
if(inp.Contains("|"))
{
string side = inputArgs[0];
string user = inputArgs[1];
AddUser(book, side, user);
}
else
{
string side = inputArgs[1];
string user = inputArgs[0];
MoveUser(book, side, user);
}
}
book = book
.Where(kvp => kvp.Value.Count>0)
.OrderByDescending(kvp => kvp.Value.Count)
.ThenBy(kvp => kvp.Key)
.ToDictionary(a => a.Key, b => b.Value);
foreach (var side in book)
{
Console.WriteLine($"Side: {side.Key}, Members: {side.Value.Count}");
foreach (var user in side.Value.OrderBy(u =>u))
{
Console.WriteLine($"! {user}");
}
}
}
private static void MoveUser(Dictionary<string, List<string>> book, string side, string user)
{
if (book.Any(b => b.Value.Contains(user)))
{
foreach (var kvp in book)
{
if (kvp.Value.Contains(user))
{
kvp.Value.Remove(user);
}
}
}
if (!book.ContainsKey(side))
{
book[side] = new List<string>();
}
book[side].Add(user);
Console.WriteLine($"{user} joins the {side} side!");
}
private static void AddUser(Dictionary<string, List<string>> book, string side, string user)
{
if (!book.ContainsKey(side))
{
book[side] = new List<string>();
}
if (!book.Any(b => b.Value.Contains(user)))
{
book[side].Add(user);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace _05.Students_2
{
class Student
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
public string HomeTown { get; set; }
}
class Program
{
static void Main(string[] args)
{
List<Student> list = new List<Student>();
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] info = input.Split();
Student student = new Student();
student.FirstName = info[0];
student.LastName = info[1];
student.Age = int.Parse(info[2]);
student.HomeTown = info[3];
list.Add(student);
}
string city = Console.ReadLine();
for (int i = 0; i < list.Count; i++)
{
if(list[i].HomeTown == city)
{
Console.WriteLine($"{list[i].FirstName} {list[i].LastName} is {list[i].Age} years old.");
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.CompilerServices;
namespace _03._Inventory
{
class Program
{
static void Main(string[] args)
{
List<string> inventory = Console.ReadLine().Split(", ", StringSplitOptions.RemoveEmptyEntries).ToList();
string input;
while((input = Console.ReadLine()) != "Craft!")
{
string[] command = input.Split(" - ", StringSplitOptions.RemoveEmptyEntries).ToArray();
if(command[0] == "Collect")
{
string itemToAdd = command[1];
if(!inventory.Any(item => item == itemToAdd))
{
inventory.Add(itemToAdd);
}
}
else if(command[0] == "Drop")
{
string itemToRemove = command[1];
if (inventory.Any(item => item == itemToRemove))
{
inventory.Remove(itemToRemove);
}
}
else if(command[0] == "Combine Items")
{
string[] items = command[1].Split(":", StringSplitOptions.RemoveEmptyEntries).ToArray();
string oldItem = items[0];
string newItem = items[1];
int indexOfOldItem;
if((indexOfOldItem = inventory.FindIndex(item => item == oldItem)) != -1)
{
inventory.Insert(indexOfOldItem + 1, newItem);
}
}
else
{
string itemToRenew = command[1];
if (inventory.Any(item => item == itemToRenew))
{
inventory.Remove(itemToRenew);
inventory.Add(itemToRenew);
}
}
}
Console.WriteLine(string.Join(", ", inventory));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using System.Text;
namespace Polymorphism
{
public class Truck : Vehicle
{
public int MaxLoad { get; set; }
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace P01.ClubParty
{
class Program
{
static void Main(string[] args)
{
int hallsMaxCapacity = int.Parse(Console.ReadLine());
string[] input = Console.ReadLine().Split();
Stack<string> inputLines = new Stack<string>(input);
Dictionary<char, List<int>> hallsAndPeople = new Dictionary<char, List<int>>();
Queue<char> halls = new Queue<char>();
while (inputLines.Any())
{
char hallName;
char.TryParse(inputLines.Peek(), out hallName);
if (char.IsLetter(hallName))
{
hallName = char.Parse(inputLines.Pop());
halls.Enqueue(hallName);
hallsAndPeople[hallName] = new List<int>();
}
else
{
if (halls.Any())
{
char currHall = halls.Peek();
int peopleToJoin = int.Parse(inputLines.Peek());
if (hallsAndPeople[currHall].Sum() + peopleToJoin == hallsMaxCapacity)
{
hallsAndPeople[currHall].Add(peopleToJoin);
halls.Dequeue();
inputLines.Pop();
PrintHall(hallsAndPeople, currHall);
}
else if (hallsAndPeople[currHall].Sum() + peopleToJoin > hallsMaxCapacity)
{
halls.Dequeue();
PrintHall(hallsAndPeople, currHall);
}
else
{
hallsAndPeople[currHall].Add(peopleToJoin);
//halls.Dequeue();
inputLines.Pop();
}
}
else
{
inputLines.Pop();
}
}
}
}
private static void PrintHall(Dictionary<char, List<int>> hallsAndPeople, char currHall)
{
Console.WriteLine($"{currHall} -> " +
$"{string.Join(", ", hallsAndPeople[currHall])}");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Globalization;
namespace Class
{
class Program
{
static void Main(string[] args)
{
//string[] myArray = new string[10];
//myArray.Length;
// var birthday = new { day = 22, month = 6 , year = 1990};////Making class on one line
//Cat cat = new Cat();
//cat.Name = "Maca";
//Console.WriteLine(cat.Name);
string input = "07-13-2020";
// DateTime dateTime = DateTime.Parse(input);
DateTime dateTime = DateTime.ParseExact(input, "MM-dd-yyyy", CultureInfo.InvariantCulture);
dateTime = dateTime.AddDays(2);
Console.WriteLine(dateTime.DayOfWeek);
////////////////////////////
///
Cat cat = new Cat();
cat.Eat();
Console.WriteLine(cat.Fat);
///////////
///CONSTRUCTORS
// var list = new List<string>(20);
Cat cat2 = new Cat();
cat2.Kittens.Add("Maci");
//cat2.Kittens = new List<string>(); WITHOUT CONSTUCTOR
foreach (var name in cat2.Kittens)
{
Console.WriteLine(name);
}
////////////////////
///
//// List<Cat>
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace _05._Bomb_Numbers
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
int[] input = Console.ReadLine().Split().Select(int.Parse).ToArray();
int bombNum = input[0];
int bombPower = input[1];
list = Detonate(list, bombNum, bombPower);
Console.WriteLine(list.Sum());
}
static List<int> Detonate(List<int> list,int bombNum,int bombPower)
{
int index = 0;
while (list.Contains(bombNum))
{
index = list.IndexOf(bombNum);
int leftRange = bombPower;
int rightRange = bombPower;
if(index - leftRange < 0)
{
leftRange = index;
}
if(index + rightRange >= list.Count)
{
rightRange = list.Count - index - 1;
}
list.RemoveRange(index - leftRange, leftRange + rightRange + 1);
}
return list;
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Class
{
class Cat
{
//public string Name{ get; set;}
//public string breed { get; set; }
//public int age { get; set; }
public Cat()//string name
{
//Name = name;///WHEN YOU TYPE SOME PARAMETER OF THE CONSTRUCTOR YOU SHOULD SET THE VALUE
//OR YOU DONT DO ANYTHING WITH IT (LIKE HERE)
///INICIALISING THE LIST IN THE CONSTRUCTOR SO WE DONT DO IT IN PROGRAM.CS
///BECAUSE OF THIS THE CONSTRUCTOR IS HELPFUL
Kittens = new List<string>();
}
public string Name { get; set; }
public List<string> Kittens { get; set; }
public int Age { get; set; }
public double Fat { get; set; }
public void Eat()
{
Fat += 200;
}
}
}
<file_sep>using System;
namespace FruitShop
{
class Program
{
static void Main(string[] args)
{
string fruit = Console.ReadLine();
string day = Console.ReadLine();
double count = double.Parse(Console.ReadLine());
double result = 0;
switch (day)
{
case "Monday":
case "Tuesday":
case "Wednesday":
case "Thursday":
case "Friday":
switch(fruit)
{
case "banana":
result = 2.50 * count;
break;
case "apple":
result = 1.20 * count;
break;
case "orange":
result = 0.85 * count;
break;
case "grapefruit":
result = 1.45 * count;
break;
case "kiwi":
result = 2.70 * count;
break;
case "pineapple":
result = 5.50 * count;
break;
case "grapes":
result = 3.85 * count;
break;
default:
Console.WriteLine("error");
break;
}
break;
case "Saturday":
case "Sunday":
switch (fruit)
{
case "banana":
result = 2.70 * count;
break;
case "apple":
result = 1.25 * count;
break;
case "orange":
result = 0.90 * count;
break;
case "grapefruit":
result = 1.60 * count;
break;
case "kiwi":
result = 3.00 * count;
break;
case "pineapple":
result = 5.60 * count;
break;
case "grapes":
result = 4.20 * count;
break;
default:
Console.WriteLine("error");
break;
}
break;
default:
Console.WriteLine("error");
break;
}
if (result != 0)
{
Console.WriteLine($"{result:f2}");
}
}
}
}
<file_sep>using System;
using System.Linq;
namespace _1._Diagonal_Difference
{
class Program
{
static void Main(string[] args)
{
int N = int.Parse(Console.ReadLine());
int[,] matrix = new int[N, N];
for (int row = 0; row < matrix.GetLength(0); row++)
{
int[] currRow = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
for (int col = 0; col < matrix.GetLength(1); col++)
{
matrix[row, col] = currRow[col];
}
}
int diag1 = 0;
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
if(row == col)
{
diag1 += matrix[row,col];
}
}
}
int diag2 = 0;
for (int i = N - 1; i >= 0; i--)
{
int row = N - i - 1;
int col = i;
diag2 += matrix[row,col];
}
Console.WriteLine(Math.Abs(diag1 - diag2));
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace StackSum
{
class Program
{
static void Main(string[] args)
{
int[] numbers = Console.ReadLine()
.Split()
.Select(int.Parse)
.ToArray();
var stack = new Stack<int>(numbers);
while(true)
{
string[] command = Console.ReadLine()
.ToLower()
.Split();
if (command[0] == "add")
{
stack.Push(int.Parse(command[1]));
stack.Push(int.Parse(command[2]));
}
else if (command[0] == "remove")
{
int totalElementsToRemove = int.Parse(command[1]);
if (stack.Count >= totalElementsToRemove)
{
for (int i = 0; i < totalElementsToRemove; i++)
{
if (stack.Any())
{
stack.Pop();
}
}
}
}
else
{
break;
}
}
Console.WriteLine($"Sum:{stack.Sum()} ");
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
namespace RemoveNegativesAndReverse
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
list = RemoveNegativesAndReverse(list);
if(list.Count == 0)
{
Console.WriteLine("empty");
}
else
{
Console.WriteLine(string.Join(" ", list));
}
}
static List<int> RemoveNegativesAndReverse(List<int> list)
{
List<int> resList = new List<int>();
for (int i = 0; i < list.Count; i++)
{
if (list[i] > 0)
{
resList.Add(list[i]);
}
}
resList.Reverse();
return resList;
}
}
}
<file_sep>using CarManager;
using NUnit.Framework;
using NUnit.Framework.Constraints;
using System;
namespace Tests
{
public class CarTests
{
private Car car;
[SetUp]
public void Setup()
{
}
[Test]
public void ConstructorInitEverything()
{
string make = "Mercedes";
string model = "Amg";
double fuelCons = 3;
double capacity = 100;
car = new Car(make, model, fuelCons, capacity);
Assert.AreEqual(make, car.Make);
Assert.AreEqual(model, car.Model);
Assert.AreEqual(fuelCons, car.FuelConsumption);
Assert.AreEqual(capacity, car.FuelCapacity);
}
[TestCase("","Amg",3,100)]
[TestCase("Mercedes", "", 3, 100)]
[TestCase("Mercedes", "Amg", -3, 100)]
[TestCase("Mercedes", "Amg", 0, 100)]
[TestCase("Mercedes", "Amg", 3, 0)]
[TestCase("Mercedes", "Amg", 3, -100)]
public void ConstructorInicializingExcpetions(string make, string model,
double fuelConsumption, double fuelCapacity)
{
Assert.Throws<ArgumentException>(() =>
{
car = new Car(make, model, fuelConsumption, fuelCapacity);
});
}
//[Test]
//public void FuelAmountShouldThrowExceptionIfItsLowerThanZero()
//{
//}
[TestCase(-3)]
[TestCase(0)]
public void RefuelShouldThrowExceptionIfItIsGivenZeroOrSmallerNumber(double fuelToRefuel)
{
car = new Car("Mercedes", "Amg", 3, 100);
Assert.Throws<ArgumentException>(() =>
{
car.Refuel(fuelToRefuel);
});
}
[Test]
public void RefuelShouldIncreaseFuelAmountByGiven()
{
car = new Car("Mercedes", "Amg", 3, 100);
double expectedFuelAmount = 10;
car.Refuel(10);
Assert.AreEqual(expectedFuelAmount, car.FuelAmount);
}
[Test]
public void RefuelShouldNotIncreaseOverTheCapacity()
{
car = new Car("Mercedes", "Amg", 3, 100);
double expectedFuel = 100;
car.Refuel(110);
Assert.AreEqual(expectedFuel, car.FuelAmount);
}
[Test]
public void DriveShouldThrowExceptionIfItDoesntHaveEnoughFuel()
{
car = new Car("Mercedes", "Amg", 3, 100);
Assert.Throws<InvalidOperationException>(() =>
{
car.Drive(23);
});
}
[Test]
public void DriveShouldDecreaseFuelAmountCorrectly()
{
car = new Car("Mercedes", "Amg", 3, 100);
double expectedFuelLeft = 97;
car.Refuel(100);
car.Drive(100);
Assert.That(expectedFuelLeft, Is.EqualTo(car.FuelAmount));
}
}
}<file_sep>using System;
namespace _1.Train
{
class Program
{
static void Main(string[] args)
{
int n = int.Parse(Console.ReadLine());
int[] train = new int[n];
int sumPeople = 0;
for (int i = 0; i < train.Length; i++)
{
train[i] = int.Parse(Console.ReadLine());
sumPeople += train[i];
}
foreach (var item in train)
{
Console.Write($"{item} ");
}
Console.WriteLine();
Console.WriteLine(sumPeople);
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Cars
{
public class Tesla : Car,IElectricCar
{
public Tesla(string model , string color)
:base(model,color)
{
}
public Battery Battery { get; set; }
public override string Start()
{
return "Tesla does not make any noise.";
}
}
}
<file_sep>using System;
using System.Text.RegularExpressions;
namespace Match_All_Words
{
class Program
{
static void Main(string[] args)
{
Regex regex = new Regex(@"\d{2}(\W)\w{3}\1[0-9]{4}");
MatchCollection matches= regex.Matches(@"30.Dec.1994 30/Dec/1994 33-Jul-1995.");
bool matches2 = regex.IsMatch(@"30.Dec.1994 30/Dec/1994 33-Jul-1995.");
Console.WriteLine(matches2);
Console.WriteLine(matches.Count);
foreach (Match match in matches)
{
Console.WriteLine(match.Value);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace P04.PizzaCalories.Core
{
public class Engine
{
public static void Run()
{
}
}
}
<file_sep>using System;
namespace Sum_Prime_Non_Prime
{
class Program
{
static void Main(string[] args)
{
string command = Console.ReadLine();
int primeSum = 0;
int nonprimeSum = 0;
bool is_non_prime = false;
while(command!="stop")
{
int number = int.Parse(command);
if(number<0)
{
Console.WriteLine("Number is negative.");
command = Console.ReadLine();
continue;
}
for(int i=2;i<number;i++)
{
if(number%i==0)
{
is_non_prime = true;
break;
}
}
if(is_non_prime && number!= 1)
{
nonprimeSum += number;
is_non_prime = false;
}
else
{
primeSum += number;
}
command = Console.ReadLine();
}
Console.WriteLine($"Sum of all prime numbers is: {primeSum}");
Console.WriteLine($"Sum of all non prime numbers is: {nonprimeSum}");
}
}
}
<file_sep>using System;
using System.Runtime.InteropServices;
namespace _02._Snake
{
class Program
{
static void Main(string[] args)
{
int N = int.Parse(Console.ReadLine());
char[,] field = new char[N, N];
InitField(N, field);
int snakeRow = -1;
int snakeCol = -1;
FindingSnakeCoordinates(N, field, ref snakeRow, ref snakeCol);
int foodQuantity = 0;
bool isOut = false;
while (foodQuantity < 10 && isOut == false)
{
string command = Console.ReadLine();
field[snakeRow, snakeCol] = '.';
if (command == "up")
{
if (snakeRow - 1 < 0)
{
isOut = true;
continue;
}
CheckingPositionChar(field, snakeRow - 1, snakeCol, ref foodQuantity, N,ref snakeRow,ref snakeCol);
//snakeRow -= 1;
}
else if (command == "down")
{
if (snakeRow + 1 == N)
{
isOut = true;
continue;
}
CheckingPositionChar(field, snakeRow + 1, snakeCol, ref foodQuantity, N, ref snakeRow, ref snakeCol);
//snakeRow += 1;
}
else if (command == "left")
{
if (snakeCol - 1 < 0)
{
isOut = true;
continue;
}
CheckingPositionChar(field, snakeRow, snakeCol - 1, ref foodQuantity, N, ref snakeRow, ref snakeCol);
//snakeCol -= 1;
}
else if (command == "right")
{
if (snakeCol + 1 == N)
{
isOut = true;
continue;
}
CheckingPositionChar(field, snakeRow, snakeCol + 1, ref foodQuantity, N, ref snakeRow, ref snakeCol);
//snakeCol += 1;
}
// PrintMatrix(N, field);
}
if (isOut)
{
Console.WriteLine("Game over!");
}
else
{
Console.WriteLine("You won! You fed the snake.");
}
Console.WriteLine($"Food eaten: {foodQuantity}");
PrintMatrix(N, field);
}
private static void PrintMatrix(int N, char[,] field)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
Console.Write(field[row, col]);
}
Console.WriteLine();
}
}
private static void CheckingPositionChar(char[,] field,int row,int col,
ref int foodQuantity,int N,ref int snakeRow,ref int snakeCol)
{
if (field[row, col] == '*')
{
foodQuantity++;
field[row, col] = 'S';
snakeRow = row;
snakeCol = col;
}
else if(field[row,col] == 'B')
{
field[row, col] = '.';
int burrowRow = -1;
int burrowCol = -1;
for (int row2 = 0; row2 < N; row2++)
{
for (int col2 = 0; col2 < N; col2++)
{
if(field[row2,col2] == 'B')
{
burrowRow = row2;
burrowCol = col2;
break;
}
}
}
field[burrowRow, burrowCol] = 'S';
//////
snakeRow = burrowRow;
snakeCol = burrowCol;
}
else
{
field[row, col] = 'S';
snakeRow = row;
snakeCol = col;
}
}
private static void FindingSnakeCoordinates(int N, char[,] field, ref int snakeRow, ref int snakeCol)
{
for (int row = 0; row < N; row++)
{
for (int col = 0; col < N; col++)
{
if (field[row, col] == 'S')
{
snakeRow = row;
snakeCol = col;
}
}
}
}
private static void InitField(int N, char[,] field)
{
for (int row = 0; row < N; row++)
{
string currRow = Console.ReadLine();
for (int col = 0; col < N; col++)
{
field[row, col] = currRow[col];
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Polymorphism
{
public interface IVehicle
{
string Model { get; }
string VrumVrum();
}
}
<file_sep>using System;
using System.Collections.Generic;
namespace Stack_of_Strings
{
class Program
{
static void Main(string[] args)
{
var hashSet = new HashSet<int>();
hashSet.AddRange(new[] { 2, 3, 4 });
var list = new List<string>();
var isEmpty = list.IsEmpty();
var dict = new Dictionary<int, string>();
dict.IsEmpty();
//var myCoolStack = new StackOfStrings();
//myCoolStack.AddRange(new List<string> { "Ivan", "Pesho" });
//myCoolStack.AddRange(new string[] { "<NAME>", "<NAME>" });
// myCoolStack.AddRange(new Stack<string>())
}
}
}
<file_sep>using System;
namespace Class_Inheritance
{
///sealed class Vehicle /// doesn't allow this class to be inherited - sealed
class Vehicle
{
public string brand = "Ford";
public void honk()
{
Console.WriteLine("BEEP BEEP");
}
}
class Car : Vehicle
{
public string modelName = "Mustang";
}
class Program
{
static void Main(string[] args)
{
Car myCar = new Car();
myCar.honk();
Console.WriteLine($"{myCar.brand} {myCar.modelName}");
}
}
}
<file_sep>using P04.WildFarm.Models.Foods;
using System;
using System.Collections.Generic;
using System.Text;
namespace P04.WildFarm.Models.Animals.Mammals
{
public class Mouse : Mammal
{
private const double WEIGHT_MULT = 0.10;
public Mouse(string name, double weight, string livingRegion)
: base(name, weight, livingRegion)
{
}
public override double WeightMultiplier => WEIGHT_MULT;
public override ICollection<Type> PrefferedFoods =>
new List<Type>() { typeof(Vegetable), typeof(Fruit) };
public override void ProduceSound()
{
Console.WriteLine("Squeak");
}
public override string ToString()
{
return base.ToString() + $" {this.Weight}, { this.LivingRegion}, { this.FoodEaten}]";
}
}
}
<file_sep>using P03.ShoppingSpree.Common;
using System;
using System.Collections.Generic;
using System.Text;
namespace P03.ShoppingSpree.Models
{
public class Person
{
private string name;
private decimal money;
private List<Product> bag;
private Person()
{
this.bag = new List<Product>();
}
public Person(string name , decimal money)
: this()
{
this.Name = name;
this.Money = money;
}
public string Name
{
get
{
return this.name;
}
private set
{
if (string.IsNullOrWhiteSpace(value))
{
throw new ArgumentException(GlobalConstants.InvalidNameExceptionMessage);
}
this.name = value;
}
}
public decimal Money
{
get
{
return this.money;
}
private set
{
if (value < 0)
{
throw new ArgumentException(GlobalConstants.InvalidMoneyException);
}
this.money = value;
}
}
public IReadOnlyCollection<Product> Bag
{
get
{
return this.bag;
///asReadOnly
}
}
public void BuyProduct(Product product)
{
if(this.Money < product.Cost)
{
throw new InvalidCastException($"{this.Name} can't afford {product.Name}");
}
else
{
// Console.WriteLine($"{this.Name} bought {this.Name}");
this.Money -= product.Cost;
this.bag.Add(product);
}
}
public override string ToString()
{
string productsOutput = this.Bag.Count > 0 ?
String.Join(", ", this.Bag) : "Nothing bought";
return $"{this.Name} - {productsOutput}";
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace _05.Multiply_Big_Number
{
class Program
{
static void Main(string[] args)
{
char[] firstNum = Console.ReadLine()
.ToCharArray();
int multiplier = int.Parse(Console.ReadLine());
if(multiplier == 0)
{
Console.WriteLine(0);
return;
}
StringBuilder sb = new StringBuilder();
int remainder = 0;
for (int i = firstNum.Length - 1; i >= 0; i--)
{
char currentCh = firstNum[i];
int currNum = int.Parse(currentCh.ToString());
int sum = (currNum * multiplier) + remainder;
sb.Append(sum % 10);
remainder = sum / 10;
}
if(remainder != 0)
{
sb.Append(remainder);
}
List<char> result = sb.ToString().Reverse()
.ToList();
RemoveTrailingZeros(result);
Console.WriteLine(string.Join("", result));
}
private static void RemoveTrailingZeros(List<char> resultArr)
{
if(resultArr[0] == '0')
{
int endIndex = 0;
for (int j = 1; j < resultArr.Count; j++)
{
if(resultArr[j] != '0')
{
endIndex = j;
}
}
for (int i = 0; i < endIndex; i++)
{
resultArr.RemoveAt(0);
}
}
}
}
}
<file_sep>using System;
namespace ToyShop
{
class Program
{
static void Main(string[] args)
{
double excursionPrize = double.Parse(Console.ReadLine());
int countPuzzels = int.Parse(Console.ReadLine());
int countDolls = int.Parse(Console.ReadLine());
int countBear = int.Parse(Console.ReadLine());
int countMinions = int.Parse(Console.ReadLine());
int countTrucks = int.Parse(Console.ReadLine());
double result = countPuzzels * 2.60 + countDolls * 3 + countBear * 4.10 + countMinions * 8.20 + countTrucks * 2;
// Console.WriteLine(result);
int countEverything = countPuzzels + countDolls + countBear + countMinions + countTrucks;
// Console.WriteLine(countEverything);
if (countEverything>= 50)
{
result = result - result * 0.25;
}
result = result - result * 0.1;
//Console.WriteLine(result);
if (result >= excursionPrize)
{
Console.WriteLine($"Yes! {result - excursionPrize:F2} lv left.");
}
else
{
Console.WriteLine($"Not enough money! {excursionPrize - result:F2} lv needed.");
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Text;
namespace Class
{
public class Shop
{
public List<string> Items { get; set; }
public decimal Price { get; set; }
public decimal TotalPrice
{
get
{
return this.Items.Count * Price;
}
// return this.Item
}
}
}
<file_sep>using System;
namespace MaxNumber
{
class Program
{
static void Main(string[] args)
{
string command = Console.ReadLine();
int max = int.MinValue;
while(command != "Stop")
{
int num = int.Parse(command);
if(num > max)
{
max = num;
}
command = Console.ReadLine();
}
Console.WriteLine(max);
}
}
}
<file_sep>using System;
namespace _03._Characters_in_Range
{
class Program
{
static void Main(string[] args)
{
char start = char.Parse(Console.ReadLine());
char end = char.Parse(Console.ReadLine());
PrintCharactersInRange(start, end);
}
static void PrintCharactersInRange(char start , char end)
{
int IntStart = (int)start;
int IntEnd = (int)end;
//Console.WriteLine(IntStart);
//Console.WriteLine(IntEnd);
if (IntStart < IntEnd)
{
for (int i = IntStart + 1; i < IntEnd; i++)
{
Console.Write($"{(char)i} ");
}
}
else
{
for (int i = IntEnd + 1; i < IntStart; i++)
{
Console.Write($"{(char)i} ");
}
}
}
}
}
<file_sep>using P03.Telephony.Contracts;
using P03.Telephony.Exceptions;
using P03.Telephony.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P03.Telephony.Core
{
class Engine : IEngine
{
public void Run()
{
string[] phoneNumbers = Console.ReadLine()
.Split()
.ToArray();
string[] sites = Console.ReadLine()
.Split()
.ToArray();
StationaryPhone stationaryPhone = new StationaryPhone();
Smartphone smartphone = new Smartphone();
CallNumbers(phoneNumbers, stationaryPhone, smartphone);
BrowseSites(sites, smartphone);
}
private static void BrowseSites(string[] sites, Smartphone smartphone)
{
foreach (var url in sites)
{
try
{
Console.WriteLine(smartphone.Browse(url));
}
catch (InvalidUrlException iue)
{
Console.WriteLine(iue.Message);
}
}
}
private static void CallNumbers(string[] phoneNumbers, StationaryPhone stationaryPhone, Smartphone smartphone)
{
foreach (var number in phoneNumbers)
{
try
{
if (number.Length == 7)
{
Console.WriteLine(stationaryPhone.Call(number));
}
else if (number.Length == 10)
{
Console.WriteLine(smartphone.Call(number));
}
else
{
throw new InvalidNumberException();
}
}
catch (InvalidNumberException ine)
{
Console.WriteLine(ine.Message);
}
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Net.Mail;
using System.Text;
namespace Encapsulation
{
public class Person
{
private string firstName;
private string lastName;
private int age;
private decimal salary;
public Person()
{
}
public Person(string firstname, string lastName, int age )
{
this.FirstName = firstname;
this.LastName = LastName;
this.Age = age;
}
public string FirstName
{
get
{
return this.firstName;
}
internal set
{
CommonValidator.ValidateNull(value, "Person first name");
this.firstName = value;
}
}
public string LastName
{
get
{
return this.lastName;
}
internal set
{
if (value == null)
{
throw new InvalidOperationException("Person Last name cannot be null");
}
//CommonValidator.ValidateNull(value, nameof(Person), nameof(this.LastName));
this.lastName = value;
}
}
public int Age
{
get
{
return this.age;
}
internal set
{
//if(value < 30)
//{
// throw new InvalidOperationException("Invalid age.");
//}
CommonValidator.ValidateMin(value, 0, nameof(Person), nameof(Person.Age));
this.age = value;
}
}
public string Gender { get; internal set; }
public DateTime BirthDay { get; internal set; }
public decimal Salary
{
get
{
return this.salary;
}
internal set
{
CommonValidator.ValidateMin(value, 460, nameof(Person), nameof(this.Salary));
this.salary = value;
}
}
public Person RegisterForExam()
{
//Logic registration
return this;
}
public Person ParticipateInExam()
{
//Logic participation
return this;
}
public Person CalculateScore()
{
//Logic Score
return this;
}
public override string ToString()
{
return $"{this.FirstName} {this.LastName} is {this.Age} years old.";
}
public void IncreaseSalary(decimal percentage)
{
var delimiter = 100;
if(this.Age < 30)
{
delimiter = 200;
}
this.Salary += this.Salary * percentage / delimiter;
}
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace P01.ListyIterator
{
public class ListyIterator<T> : IEnumerable<T>
{
private readonly List<T> data;
private int currInternalIndex;
public ListyIterator()
{
this.data = new List<T>();
this.currInternalIndex = 0;
}
public ListyIterator(IEnumerable<T> collectionData)
{
this.data = new List<T>(collectionData);
}
public bool Move()
{
if(this.currInternalIndex < this.data.Count - 1)
{
this.currInternalIndex++;
}
else
{
return false;
}
return true;
}
public bool HasNext()
{
return this.currInternalIndex < this.data.Count - 1;
}
public void Print()
{
if (!this.data.Any())
{
throw new InvalidOperationException("Invalid operation!");
}
Console.WriteLine(this.data[this.currInternalIndex]);
}
public IEnumerator<T> GetEnumerator()
{
for (int i = 0; i < this.data.Count; i++)
{
yield return this.data[i];
}
}
IEnumerator IEnumerable.GetEnumerator()
{
return this.GetEnumerator();
}
}
}
<file_sep>using System;
namespace Array
{
class Program
{
static void Main(string[] args)
{
int[] ages = new int[3];
ages[0] = 5;
ages[1] = 6;
ages[2] = 7;
/*foreach(int age in ages)
{
Console.WriteLine(age);
}*/
for (int i = 0; i < ages.Length; i++)
{
Console.WriteLine(ages[i]);
}
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
namespace _06._List_Manipulation_Basics
{
class Program
{
static void Main(string[] args)
{
List<int> list = Console.ReadLine().Split().Select(int.Parse).ToList();
while (true)
{
string input = Console.ReadLine();
if(input == "end")
{
break;
}
string[] command = input.Split();
if(command[0] == "Add")
{
list.Add(int.Parse(command[1]));
}
else if(command[0] == "Remove")
{
list.Remove(int.Parse(command[1]));
}
else if(command[0] == "RemoveAt")
{
list.RemoveAt(int.Parse(command[1]));
}
else
{
list.Insert(int.Parse(command[2]), int.Parse(command[1]));
}
}
Console.WriteLine(string.Join(" ", list));
}
}
}
<file_sep>using System;
using System.Diagnostics.CodeAnalysis;
namespace Travelling
{
class Program
{
static void Main(string[] args)
{
string destination = Console.ReadLine();
while(destination != "End")
{
double sum = 0;
double neededSum = double.Parse(Console.ReadLine());
while(sum<neededSum)
{
double savedMoney = double.Parse(Console.ReadLine());
sum += savedMoney;
}
Console.WriteLine($"Going to {destination}!");
destination = Console.ReadLine();
}
}
}
}
| 4d063b90786a75cb625705433ded0f6a2711afbc | [
"Markdown",
"C#",
"C"
] | 396 | C# | Vladikolev0321/CSharpRelated | ce667df9b47e34a5e092ecc2d33c740a8cf537e2 | 46aa825f8b36790703bdcb0a0a6148ba9d74f334 | |
refs/heads/master | <file_sep>import React, { Component } from 'react';
import './SearchBox.css';
class SearchBox extends Component {
render() {
return (
<div className="search-box">
<form className="search-box__form" onSubmit={(e) => this.props.movieRequest(e)}>
<label className="search-box__form-label">
Искать фильм по названию:
<input
onChange = {(e) => this.props.changeMovies(e)}
name = 'nameMuvei'
value={this.props.searchMovies}
type="text"
className="search-box__form-input"
placeholder="Например, <NAME>"
/>
</label>
<button
type="submit"
className="search-box__form-submit"
disabled={!this.props.searchMovies}
>
Искать
</button>
</form>
</div>
);
}
}
export default SearchBox;<file_sep>import React, { Component } from 'react';
import './Favorites.css';
class Favorites extends Component {
state = {
title: 'Новый список',
movies: [
{ imdbID: 'tt0068646', title: 'The Godfather', year: 1972 }
]
}
render() {
return (
<div className="favorites">
<input value= {this.props.titleNewList} className="favorites__name" onChange = {(e) => this.props.getTitle(e)}/>
<ul className="favorites__list">
{this.props.selectedListMovies.map((item) => {
return <li className="listItem" key={item.imdbID}>{item.Title} ({item.Year})<button className="but" onClick = {() => this.props.deleteFilms(item.imdbID)}>❌</button></li>;
})}
</ul>
<button type="button" className="favorites__save" onClick = {this.props.requestGeneration}>Сохранить список</button>
</div>
);
}
}
export default Favorites;<file_sep>export function GetListMovies(nameMovies) {
return fetch(`http://www.omdbapi.com/?apikey=ce6c89d6&s=${nameMovies}`)
.then(response => response.json())
}
export function creatingMovieList(arr, title) {
let info = {
title: title,
movies: arr
}
return fetch('https://acb-api.algoritmika.org/api/movies/list', {
method: 'POST',
headers: { 'Content-type': 'application/json' },
body: JSON.stringify(info)
}).then(response => response.json());
}
export function getSelectMovieList(id){
return fetch(`https://acb-api.algoritmika.org/api/movies/list/${id}`)
.then(response => response.json())
}
export function GetListIDMovies() {
return fetch(`http://www.omdbapi.com/?apikey=ce6c89d6&i=tt3896198`)
.then(response => response.json()).then(data => console.log(data))
}
export function renderingList(arr){
let arrObj = arr.map((item) => fetch(`http://www.omdbapi.com/?apikey=ce6c89d6&i=${item}`).then(response => response.json()))
return Promise.all(arrObj)
}
| 323647c479978ae46aeb39219d7fcb8a36d1e3f3 | [
"JavaScript"
] | 3 | JavaScript | rashadd777/bootcamp-m4-starter | 2206695d17e1164ba418d1fff80de5abbb0039ae | df206779be7abeb37e1ab3d4bf55b05cce43a426 | |
refs/heads/master | <file_sep>export default {
setModalOpen({ commit }, data) {
commit('SET_MODAL_OPEN', data)
},
setfullPictureItem({ commit }, data) {
commit('SET_FULL_PICTURE_ITEM', data)
}
}<file_sep>export default {
SET_MODAL_OPEN(state, data) {
state.isModalOpen = data
},
SET_FULL_PICTURE_ITEM(state, data) {
state.fullPictureItem = data
}
}<file_sep># agile-galery
## Build Setup
```bash
# install dependencies
$ npm install
# serve with hot reload at localhost:3000
$ npm run dev
# build for production and launch server
$ npm run build
$ npm run start
# generate static project
$ npm run generate
```
All the packages are registred in the package.json, so npm install will be enougth to install dependencies, after that npm run buid or npm run dev (edit mode) are the available commands.<file_sep>export default () => ({
isModalOpen: false,
fullPictureItem: undefined
})
| ae248b98f6388e2ece76cb38791b5673063637e0 | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | atooomik/agile-galery | ca5e73c114d340cf15eab6735292141b890d7c68 | 8b3031a54d0936a524c9bb287aa3a5a7c1905f3e | |
refs/heads/master | <file_sep>package com.example.userssp.interfaces
import com.example.userssp.User
interface OnClickListener {
fun onClick(user: User, position: Int)
}<file_sep>package com.example.userssp
import android.content.Context
import android.content.DialogInterface
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
import com.example.userssp.adapter.UserAdapter
import com.example.userssp.databinding.ActivityMainBinding
import com.example.userssp.interfaces.OnClickListener
import com.google.android.material.dialog.MaterialAlertDialogBuilder
import com.google.android.material.textfield.TextInputEditText
class MainActivity : AppCompatActivity(), OnClickListener {
private lateinit var userAdapter: UserAdapter
private lateinit var linearLayoutManager: RecyclerView.LayoutManager
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
handlePreferences()
initRecyclerView()
}
private fun handlePreferences() {
val preferences = getPreferences(Context.MODE_PRIVATE)
val isFirstTime = preferences.getBoolean(getString(R.string.sp_first_time), true)
if (isFirstTime) {
var dialogView = layoutInflater.inflate(R.layout.dialog_layout, null)
MaterialAlertDialogBuilder(this)
.setTitle(R.string.dialog_title)
.setView(dialogView)
.setCancelable(false)
.setPositiveButton(R.string.dialog_confirm) { _, _ ->
val username = dialogView.findViewById<TextInputEditText>(R.id.etUsername)
.text.toString()
with(preferences.edit()) {
putBoolean(getString(R.string.sp_first_time), false)
putString(getString(R.string.sp_username), username)
.apply() // este se ejecuta en seundo plano
}
}
.setNegativeButton("Cerrar", null)
.show()
} else {
val usernane = preferences.getString(getString(R.string.sp_username), "Si data en Sp")
Toast.makeText(this, "Dato guardado $usernane", Toast.LENGTH_SHORT).show()
}
}
private fun handleOnPressPositiveButton() {
}
private fun initRecyclerView() {
userAdapter = UserAdapter(getUsers(), this)
linearLayoutManager = LinearLayoutManager(this)
binding.recyclerView.apply {
layoutManager = linearLayoutManager
adapter = userAdapter
}
}
private fun getUsers(): MutableList<User> {
val user = mutableListOf<User>()
val giuseppe = User(
1,
"Giuseppe",
"Varas",
"https://www.bovinoapp.com/wp-content/uploads/2019/02/WhatsApp-Image-2019-02-26-at-11.14.30.jpeg"
)
val pepe = User(
2,
"Pepe",
"Varas",
"https://www.bovinoapp.com/wp-content/uploads/2019/02/WhatsApp-Image-2019-02-26-at-11.14.30.jpeg"
)
val jose = User(
3,
"Jose",
"Varas",
"https://www.bovinoapp.com/wp-content/uploads/2019/02/WhatsApp-Image-2019-02-26-at-11.14.30.jpeg"
)
user.add(giuseppe)
user.add(pepe)
user.add(jose)
user.add(giuseppe)
user.add(pepe)
user.add(jose)
user.add(giuseppe)
user.add(pepe)
user.add(jose)
user.add(giuseppe)
user.add(pepe)
user.add(jose)
user.add(giuseppe)
user.add(pepe)
user.add(jose)
return user
}
override fun onClick(user: User, position: Int) {
Toast.makeText(this, "$position: ${user.getFullName()}", Toast.LENGTH_SHORT).show()
}
} | 409a865536cea2893c6746c2517e8703654228ea | [
"Kotlin"
] | 2 | Kotlin | giuseppeabn/POC_SharedPreferences | 5b82b5031b63bb0680ef51d1973aa74d55994e98 | aff4180e1ae4526dc1a4db6db9be14d3723b7b0d | |
refs/heads/master | <file_sep>
public class Sentence {
private String text;
public Sentence(String aText)
{
this.text=aText;
}
public void reverse()
{
int length = text.length();
String result = " ";
for (int i = length-1; i >= 0; i--) {
result = result + text.charAt(i);
}
System.out.println(result);
}
public static String recReverse(String str) {
int localI = i++;
if ((null == str) || (str.length() <= 1)) {
return str;
}
System.out.println("Step " + localI + ": " + str.substring(1) + " / " + str.charAt(0));
String reversed = reverse(str.substring(1)) + str.charAt(0);
System.out.println("Step " + localI + " returns: " + reversed);
return reversed;
}
}
<file_sep>//import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
//import javax.swing.border.EmptyBorder;
import java.awt.GridBagLayout;
import javax.swing.JComboBox;
import java.awt.GridBagConstraints;
import javax.swing.JLabel;
import java.awt.Insets;
import javax.swing.JTextField;
import java.awt.Font;
import javax.swing.JButton;
//import javax.swing.border.LineBorder;
//import java.awt.Color;
import javax.swing.border.TitledBorder;
import javax.swing.ButtonGroup;
public class SortGUI extends JFrame {
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private JTextField textField_2;
private JLabel lblBubbleSortIn;
private JLabel lblSelectionSortIn;
private JLabel lblInsertionSortIn;
private JTextField textField_3;
private JLabel label;
private JTextField textField_4;
private JLabel label_1;
private JTextField textField_5;
private JLabel label_2;
private JButton button;
private JButton button_1;
private JButton button_2;
private JPanel panel;
private JPanel panel_1;
private JComboBox [] comboBox;
private final ButtonGroup buttonGroup = new ButtonGroup();
public static final long serialVersionUID =1L;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
SortGUI frame = new SortGUI();
//frame.pack();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public SortGUI() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 466, 325);
contentPane = new JPanel();
contentPane.setBorder(null);
setContentPane(contentPane);
GridBagLayout gbl_contentPane = new GridBagLayout();
gbl_contentPane.columnWidths = new int[]{0, 0, 0, 0};
gbl_contentPane.rowHeights = new int[]{0, 0, 0, 0, 0, 0, 0, 0, 0, 0};
gbl_contentPane.columnWeights = new double[]{1.0, 1.0, 1.0, Double.MIN_VALUE};
gbl_contentPane.rowWeights = new double[]{0.0, 0.0, 0.0, 0.0, 1.0, 0.0, 0.0, 0.0, 0.0, Double.MIN_VALUE};
contentPane.setLayout(gbl_contentPane);
JLabel lblPleaseSelectThe = new JLabel("Please select the");
GridBagConstraints gbc_lblPleaseSelectThe = new GridBagConstraints();
gbc_lblPleaseSelectThe.anchor = GridBagConstraints.SOUTH;
gbc_lblPleaseSelectThe.insets = new Insets(0, 0, 5, 5);
gbc_lblPleaseSelectThe.gridx = 0;
gbc_lblPleaseSelectThe.gridy = 0;
contentPane.add(lblPleaseSelectThe, gbc_lblPleaseSelectThe);
JLabel lblNumberOfElements = new JLabel("number of elements");
GridBagConstraints gbc_lblNumberOfElements = new GridBagConstraints();
gbc_lblNumberOfElements.insets = new Insets(0, 0, 5, 5);
gbc_lblNumberOfElements.gridx = 0;
gbc_lblNumberOfElements.gridy = 1;
contentPane.add(lblNumberOfElements, gbc_lblNumberOfElements);
//JComboBox comboBox = new JComboBox();
JComboBox comboBox = new JComboBox (new String[]{"100","1000","10000","100000"});
GridBagConstraints gbc_comboBox = new GridBagConstraints();
gbc_comboBox.fill = GridBagConstraints.HORIZONTAL;
gbc_comboBox.insets = new Insets(0, 0, 5, 5);
gbc_comboBox.gridx = 1;
gbc_comboBox.gridy = 1;
contentPane.add(comboBox, gbc_comboBox);
JLabel lblForYourArray = new JLabel("for your Array");
GridBagConstraints gbc_lblForYourArray = new GridBagConstraints();
gbc_lblForYourArray.insets = new Insets(0, 0, 5, 5);
gbc_lblForYourArray.gridx = 0;
gbc_lblForYourArray.gridy = 2;
contentPane.add(lblForYourArray, gbc_lblForYourArray);
panel_1 = new JPanel();
panel_1.setBorder(new TitledBorder(null, "Sorted Array Analysis", TitledBorder.LEADING, TitledBorder.TOP, null, null));
GridBagConstraints gbc_panel_1 = new GridBagConstraints();
gbc_panel_1.fill = GridBagConstraints.HORIZONTAL;
gbc_panel_1.gridwidth = 3;
gbc_panel_1.insets = new Insets(0, 0, 5, 0);
gbc_panel_1.gridx = 0;
gbc_panel_1.gridy = 4;
contentPane.add(panel_1, gbc_panel_1);
GridBagLayout gbl_panel_1 = new GridBagLayout();
gbl_panel_1.columnWidths = new int[]{0, 0, 0, 0};
gbl_panel_1.rowHeights = new int[]{0, 0, 0};
gbl_panel_1.columnWeights = new double[]{1.0, 1.0, 1.0, Double.MIN_VALUE};
gbl_panel_1.rowWeights = new double[]{0.0, 0.0, Double.MIN_VALUE};
panel_1.setLayout(gbl_panel_1);
lblBubbleSortIn = new JLabel("Bubble sort in millisecs");
GridBagConstraints gbc_lblBubbleSortIn = new GridBagConstraints();
gbc_lblBubbleSortIn.insets = new Insets(0, 0, 5, 5);
gbc_lblBubbleSortIn.gridx = 0;
gbc_lblBubbleSortIn.gridy = 0;
panel_1.add(lblBubbleSortIn, gbc_lblBubbleSortIn);
lblBubbleSortIn.setFont(new Font("Tahoma", Font.PLAIN, 8));
lblSelectionSortIn = new JLabel("Selection sort in millisecs");
GridBagConstraints gbc_lblSelectionSortIn = new GridBagConstraints();
gbc_lblSelectionSortIn.insets = new Insets(0, 0, 5, 5);
gbc_lblSelectionSortIn.gridx = 1;
gbc_lblSelectionSortIn.gridy = 0;
panel_1.add(lblSelectionSortIn, gbc_lblSelectionSortIn);
lblSelectionSortIn.setFont(new Font("Tahoma", Font.PLAIN, 8));
lblInsertionSortIn = new JLabel("Insertion sort in millisecs");
GridBagConstraints gbc_lblInsertionSortIn = new GridBagConstraints();
gbc_lblInsertionSortIn.insets = new Insets(0, 0, 5, 0);
gbc_lblInsertionSortIn.gridx = 2;
gbc_lblInsertionSortIn.gridy = 0;
panel_1.add(lblInsertionSortIn, gbc_lblInsertionSortIn);
lblInsertionSortIn.setFont(new Font("Tahoma", Font.PLAIN, 8));
textField = new JTextField();
GridBagConstraints gbc_textField = new GridBagConstraints();
gbc_textField.insets = new Insets(0, 0, 0, 5);
gbc_textField.gridx = 0;
gbc_textField.gridy = 1;
panel_1.add(textField, gbc_textField);
textField.setColumns(10);
textField_2 = new JTextField();
GridBagConstraints gbc_textField_2 = new GridBagConstraints();
gbc_textField_2.insets = new Insets(0, 0, 0, 5);
gbc_textField_2.gridx = 1;
gbc_textField_2.gridy = 1;
panel_1.add(textField_2, gbc_textField_2);
textField_2.setColumns(10);
textField_1 = new JTextField();
GridBagConstraints gbc_textField_1 = new GridBagConstraints();
gbc_textField_1.gridx = 2;
gbc_textField_1.gridy = 1;
panel_1.add(textField_1, gbc_textField_1);
textField_1.setColumns(10);
panel = new JPanel();
panel.setBorder(new TitledBorder(null, "Unsorted Array Analysis", TitledBorder.LEADING, TitledBorder.TOP, null, null));
GridBagConstraints gbc_panel = new GridBagConstraints();
gbc_panel.fill = GridBagConstraints.HORIZONTAL;
gbc_panel.gridwidth = 3;
gbc_panel.insets = new Insets(0, 0, 5, 0);
gbc_panel.gridx = 0;
gbc_panel.gridy = 5;
contentPane.add(panel, gbc_panel);
GridBagLayout gbl_panel = new GridBagLayout();
gbl_panel.columnWidths = new int[]{0, 0, 0, 0, 0, 0};
gbl_panel.rowHeights = new int[]{0, 0, 0};
gbl_panel.columnWeights = new double[]{1.0, 1.0, 1.0, 0.0, 1.0, Double.MIN_VALUE};
gbl_panel.rowWeights = new double[]{0.0, 0.0, Double.MIN_VALUE};
panel.setLayout(gbl_panel);
label = new JLabel("Bubble sort in millisecs");
GridBagConstraints gbc_label = new GridBagConstraints();
gbc_label.insets = new Insets(0, 0, 5, 5);
gbc_label.gridx = 0;
gbc_label.gridy = 1;
panel.add(label, gbc_label);
label.setFont(new Font("Tahoma", Font.PLAIN, 8));
label_1 = new JLabel("Selection sort in millisecs");
GridBagConstraints gbc_label_1 = new GridBagConstraints();
gbc_label_1.insets = new Insets(0, 0, 5, 5);
gbc_label_1.gridx = 2;
gbc_label_1.gridy = 1;
panel.add(label_1, gbc_label_1);
label_1.setFont(new Font("Tahoma", Font.PLAIN, 8));
label_2 = new JLabel("Insertion sort in millisecs");
GridBagConstraints gbc_label_2 = new GridBagConstraints();
gbc_label_2.insets = new Insets(0, 0, 5, 0);
gbc_label_2.gridx = 4;
gbc_label_2.gridy = 1;
panel.add(label_2, gbc_label_2);
label_2.setFont(new Font("Tahoma", Font.PLAIN, 8));
textField_3 = new JTextField();
GridBagConstraints gbc_textField_3 = new GridBagConstraints();
gbc_textField_3.insets = new Insets(0, 0, 0, 5);
gbc_textField_3.gridx = 0;
gbc_textField_3.gridy = 2;
panel.add(textField_3, gbc_textField_3);
textField_3.setColumns(10);
textField_4 = new JTextField();
GridBagConstraints gbc_textField_4 = new GridBagConstraints();
gbc_textField_4.insets = new Insets(0, 0, 0, 5);
gbc_textField_4.gridx = 2;
gbc_textField_4.gridy = 2;
panel.add(textField_4, gbc_textField_4);
textField_4.setColumns(10);
textField_5 = new JTextField();
GridBagConstraints gbc_textField_5 = new GridBagConstraints();
gbc_textField_5.gridx = 4;
gbc_textField_5.gridy = 2;
panel.add(textField_5, gbc_textField_5);
textField_5.setColumns(10);
button = new JButton("Bubble");
buttonGroup.add(button);
GridBagConstraints gbc_button = new GridBagConstraints();
gbc_button.fill = GridBagConstraints.HORIZONTAL;
gbc_button.anchor = GridBagConstraints.NORTH;
gbc_button.insets = new Insets(0, 0, 0, 5);
gbc_button.gridx = 0;
gbc_button.gridy = 8;
contentPane.add(button, gbc_button);
button_1 = new JButton("Selection");
buttonGroup.add(button_1);
GridBagConstraints gbc_button_1 = new GridBagConstraints();
gbc_button_1.fill = GridBagConstraints.HORIZONTAL;
gbc_button_1.anchor = GridBagConstraints.NORTH;
gbc_button_1.insets = new Insets(0, 0, 0, 5);
gbc_button_1.gridx = 1;
gbc_button_1.gridy = 8;
contentPane.add(button_1, gbc_button_1);
button_2 = new JButton("Insertion");
buttonGroup.add(button_2);
GridBagConstraints gbc_button_2 = new GridBagConstraints();
gbc_button_2.fill = GridBagConstraints.HORIZONTAL;
gbc_button_2.anchor = GridBagConstraints.NORTH;
gbc_button_2.gridx = 2;
gbc_button_2.gridy = 8;
contentPane.add(button_2, gbc_button_2);
}
}
<file_sep># QuatraticSorting
Algorithims
| 3a8ecd03ece3227b18edcdbcd85ee9e3d1fd0736 | [
"Markdown",
"Java"
] | 3 | Java | L00113302/QuatraticSorting | e811e078adf17e9a931b3dde47ca0613d12cd1b4 | f91c15c1d2e5228f55a973f219ed60556e8658eb | |
refs/heads/master | <file_sep>console.log("Hello XYZ!");
<file_sep>//Data Types
let name='Sumit';
console.log(name); | 640586e57abaacedb734ac7dd96dc4a5897302de | [
"JavaScript"
] | 2 | JavaScript | sumitsingh2503/TestRepo | c79d6fd0e13f8d703cecf5fb29ead1722c24c796 | 6ee74ce89c2b6ee3a06715f287291c5d71067bc7 | |
refs/heads/LinkedIn | <repo_name>arizona2014/social-media-api<file_sep>/README.md
This will be the readme page<file_sep>/linkedin_ok.php
<h1>PALO IT's Linkedin Informations</h1>
<?php
session_start();
require_once('linkedin_app.php');
//$strAccessToken = "<KEY>";
/*
$strAccessToken = $_SESSION['_oauth_token'];
$ObjLinkedIn = new LinkedInOAuth2($strAccessToken);
$context = stream_context_create(array(
'http' => array(
'header' => "Authorization: Bearer " . $strAccessToken
)
));
$homepage = file_get_contents('https://www.linkedin.com/company/palo-it/followers?page_num=2', false, $context);
var_dump( $homepage );
*/
//$strAccessToken = "<KEY>";
$strAccessToken = $_SESSION['_oauth_token'];
$ObjLinkedIn = new LinkedInOAuth2($strAccessToken);
$intCompanyId = 2452379; // This is PALO-IT's Linkedin Account ID
// GET Company's Info
$arrCompanyInfo = $ObjLinkedIn->getCompany($intCompanyId);
var_dump($arrCompanyInfo);
// GET Company's Number of Followers
$arrCompanyFollowers = $ObjLinkedIn->getCompanyFollowers($intCompanyId);
var_dump($arrCompanyFollowers);
?>
| 1fd5e0714e93b0a965efea40c58b1542886bfdbb | [
"Markdown",
"PHP"
] | 2 | Markdown | arizona2014/social-media-api | 209d2b20575ebfc602249110d39c635157ac4302 | 0951a86fba07e41126f9dcdafc7827121ff4216d | |
refs/heads/master | <file_sep>package novelcrawler
// ErrorCode code of error type
type ErrorCode int
const (
// Success success
Success ErrorCode = iota
// UnknownError error type
UnknownError
// RemoteNotExist remote url not exists
RemoteNotExist
// DBError the errors about database operation
DBError
// NovelName failed to get novel name
NovelName
// InternalError internal error
InternalError
)
// ErrorString get the description of error code
func (code *ErrorCode) ErrorString() string {
errors := [...]string{
"Success",
"Unknown error",
"Remote novel not exists",
"Database operation error",
"Failed to get novel name",
"Internal error",
}
if *code < Success || *code > InternalError {
return "Wrong error code"
}
return errors[*code]
}
<file_sep>// +build !windows
package config
import (
"os"
)
func getabspath() string {
return getdbpath() + getdbname()
}
func getdbname() string {
return "novel.db"
}
func getdbpath() string {
return os.Getenv("HOME") + "/.novelCrawler/"
}
func getTempDir() string {
return "/tmp/colly-cache"
}<file_sep>package novelcrawler
import (
"fmt"
"log"
"strings"
)
// Chstonum returns the int number of chinese number,
// if unexpected number presents, or larger than 99999999,
// an error will be returned
func Chstonum(strnum string) (result int, err error) {
var method func(int, *int)
var containsBits bool
if strings.Contains(strnum, "百") ||
strings.Contains(strnum, "千") ||
strings.Contains(strnum, "万") ||
strings.Contains(strnum, "十") {
method = withbits()
containsBits = true
} else {
method = withoutbits()
containsBits = false
}
pos := 0
for _, ch := range strnum {
pos, err = positionOf(ch)
if err != nil {
return pos, err
}
method(pos, &result)
if result == -1 {
log.Fatalf("Error: unexpcted number: %v(%v)", strnum, ch)
return -1, fmt.Errorf("Error: unexpcted number: %v(%v)", strnum, ch)
}
}
if containsBits && pos != 0 {
result += pos
}
return
}
func withbits() func(int, *int) {
tmp := -1
return func(pos int, result *int) {
if pos == 0 {
tmp = -1
} else if pos > 0 && pos < 10 {
tmp = pos
} else if pos >= 10 && pos < 10000 {
if tmp < 0 && *result <= 0 {
tmp = 1
}
*result += (tmp * pos)
tmp = -1
} else {
if *result == 0 && tmp <= 0 {
*result = -1
} else if tmp > 0 {
*result += tmp
}
*result *= pos
tmp = -1
}
}
}
func withoutbits() func(int, *int) {
return func(pos int, result *int) {
if pos == 0 {
*result *= 10
} else if pos > 0 && pos < 10 {
*result *= 10
*result += pos
} else {
*result = -1
}
}
}
// positionOf return the position of a rune,
// if not in the slice, return an error
func positionOf(ch rune) (int, error) {
if ch == '两' {
ch = '二'
}
chsnums := []rune{'零', '一', '二', '三', '四', '五', '六', '七', '八', '九'}
for pos, value := range chsnums {
if ch == value {
return pos, nil
}
}
return bitToNumber(ch)
}
func bitToNumber(ch rune) (result int, err error) {
switch ch {
case '十':
result = 10
case '百':
result = 100
case '千':
result = 1000
case '万':
result = 10000
default:
return -1, fmt.Errorf("Unexpcted chinese number: %v", ch)
}
return
}
// NumberToChs converts int number to chinese number
func NumberToChs(number int) string {
chs := []string{"", "十", "百", "千", "万"}
chsnums := []string{"零", "一", "二", "三", "四", "五", "六", "七", "八", "九"}
var result string
current := -1
last := -1
for index := 0; number > 0; index = (index + 1) % len(chs) {
if current != -1 {
last = current
if index == 0 {
index = 1
}
}
current = number % 10
number /= 10
if current == 0 && (last == -1 || last == 0) {
if index == 4 {
result += chs[index]
}
continue
} else if current == 0 && index != 4 {
result = chsnums[current] + result
} else if current == 0 && index == 4 {
result = chs[index] + chsnums[current] + result
} else {
result = chsnums[current] + chs[index] + result
}
}
return result
}
<file_sep>// +build windows
package config
import (
"os"
)
func getdbname() string {
return "novel.db"
}
func getdbpath() string {
return os.Getenv("APPDATA") + "\\novelCrawler\\"
}
func getabspath() string {
return Getdbpath() + getdbname()
}
func getTempDir() string {
return os.Getenv("TEMP") + "\\colly-cache"
}<file_sep>package crwaler
import (
"log"
"regexp"
"strconv"
"strings"
"time"
"github.com/imwyl/novelCrawler/config"
"github.com/imwyl/novelCrawler/pkg/novelCrawler"
"github.com/gocolly/colly"
"github.com/gocolly/colly/proxy"
"github.com/imwyl/novelCrawler/dao"
)
var chapterRegex, _ = regexp.Compile("(第[一二三四五六七八九零十百千万]+[章|节]\\s+.*)")
var novelNameRegex, _ = regexp.Compile("^(.*)最新章节$")
var novelURIRegex, _ = regexp.Compile(`\d+/\d+`)
var chapterURLRegex, _ = regexp.Compile("(\\d+)\\.html")
var firstChapterRegex, _ = regexp.Compile(`<a href="(\d+).html">第一[章|节]`)
var tempDir = config.GetTempDir()
func getChapterNumber(chapterURL string) int {
if chapterURL == "" {
return -1
}
chapterNumberArr := chapterURLRegex.FindStringSubmatch(chapterURL)
if len(chapterNumberArr) <= 1 {
log.Fatalln(chapterURL)
return -1
}
result, err := strconv.Atoi(chapterNumberArr[1])
if err != nil {
return -1
}
return result
}
func getChapters(URL string) {
var c = colly.NewCollector(
colly.AllowedDomains("piaotian.net", "www.piaotian.net", "www.piaotian.com", "piaotian.com" ),
colly.CacheDir(tempDir))
c.Limit(&colly.LimitRule{
Parallelism: 5,
})
enableProxy(c)
d := c.Clone()
enableProxy(d)
d.Limit(&colly.LimitRule{
Parallelism: 5,
})
db, err := dao.GetDB()
if err != nil {
log.Fatalln(err)
}
var chapter dao.Chapter
novel := dao.Novel{ID: getNovelURI(URL)}
db.First(&novel)
db.Where("novel_id = ?", novel.ID).Order("id desc").First(&chapter)
chapterMap := make(map[int]*dao.Chapter)
// get chapters of novel
c.OnHTML("ul", func(e *colly.HTMLElement) {
e.ForEach("li", func(_ int, el *colly.HTMLElement) {
if chapterRegex.MatchString(el.ChildText("a")) {
chapterURL := el.ChildAttr("a", "href")
if thisChapter := getChapterNumber(chapterURL); thisChapter-novel.First+1 > int(chapter.ID) {
if chapterMap[thisChapter] == nil {
chapterMap[thisChapter] = &dao.Chapter{
ID: uint(thisChapter),
NovelID: novel.ID,
Name: chapterRegex.FindStringSubmatch(el.ChildText("a"))[1],
}
log.Printf("Add %d %v to map", thisChapter, chapterMap[thisChapter])
}
d.Visit(e.Request.AbsoluteURL(el.ChildAttr("a", "href")))
}
}
})
})
// get content of novel
d.OnHTML("div#main", func(e *colly.HTMLElement) {
requestURL := chapterURLRegex.FindStringSubmatch(e.Request.URL.String())[1]
chapterURL := getChapterNumber(requestURL)
thisChapter := chapterMap[chapterURL]
log.Println("div#main", thisChapter)
thisChapter.Content = strings.Replace(e.Text, "\u00a0\u00a0\u00a0\u00a0", "<p>", -1)
thisChapter.Save()
//log.Println()
})
// div#main is add by javascript, so add it here
d.OnResponse(func(res *colly.Response) {
res.Body = []byte(strings.Replace(string(res.Body), "<br>", "<div id=\"main\">", 1))
log.Println("Response code: ", string(res.Body))
})
c.Visit(URL)
c.Wait()
}
// novelExits return the novel exits in database or not
func novelExists(URL string) bool {
novelURI := getNovelURI(URL)
log.Println(novelURI)
return dao.NovelExists(novelURI)
}
// createNovel create novel record in database
func createNovel(URL string, boolChan chan novelcrawler.ErrorCode) {
log.Println("URL", URL)
db, getDbErr := dao.GetDB()
if getDbErr != nil {
log.Fatalln("Can not open databse:\n", getDbErr)
panic(getDbErr)
}
defer db.Close()
var c = colly.NewCollector(
colly.AllowedDomains("www.piaotian.com", "piaotian.com", "piaotian.net", "www.piaotian.net"),
colly.CacheDir(tempDir))
enableProxy(c)
var remoteNovelExist bool
var firstChapter int
c.OnResponse(func(res *colly.Response) {
remoteNovelExist = res.StatusCode == 200
if !remoteNovelExist {
boolChan <- novelcrawler.RemoteNotExist
} else {
firstChapterSlice := firstChapterRegex.FindStringSubmatch(string(res.Body))
if len(firstChapterSlice) <= 1 {
boolChan <- novelcrawler.InternalError
} else {
firstChapter, _ = strconv.Atoi(firstChapterSlice[1])
}
}
})
c.OnHTML("h1", func(e *colly.HTMLElement) {
h1title := e.Text
// get novel's name and save to database
if novelName := novelNameRegex.FindStringSubmatch(h1title); len(novelName) > 1 && remoteNovelExist {
log.Println(novelName)
novel := dao.Novel{
ID: getNovelURI(URL),
Name: novelName[1],
First: firstChapter,
UpdateAt: time.Now(),
}
log.Println("create novel ", novel.Name)
db.Create(&novel)
boolChan <- novelcrawler.Success
} else {
boolChan <- novelcrawler.NovelName
log.Println("novelExists ", remoteNovelExist)
}
})
c.Visit(URL)
c.Wait()
}
// Start the crawler
func Start(URL string) novelcrawler.ErrorCode {
modifyURL(&URL)
if !novelExists(URL) {
errorChan := make(chan novelcrawler.ErrorCode)
go createNovel(URL, errorChan)
if createResult := <-errorChan; createResult != novelcrawler.Success {
return createResult
}
}
getChapters(URL)
return novelcrawler.Success
}
func modifyURL(URL *string) {
if !strings.Contains(*URL, "https://www.") {
*URL = "https://www." + *URL
}
if strings.HasSuffix(*URL, "/") {
*URL = string((*URL)[:len(*URL)-1])
} else {
*URL = strings.Replace(*URL, "/index.html", "", 1)
}
}
func enableProxy(c *colly.Collector) {
p, proxyErr := proxy.RoundRobinProxySwitcher("socks5://127.0.0.1:9050")
if proxyErr != nil {
panic(proxyErr)
}
c.SetProxyFunc(p);
}
func getNovelURI(URL string) string {
return novelURIRegex.FindString(URL)
}
<file_sep>package config
import (
"log"
"os"
)
// Getdbname returns the name of db file
func Getdbname() string {
return getdbname()
}
// Getdbpath returns the path of db file
func Getdbpath() string {
path := getdbpath()
_, err := os.Stat(path)
if err != nil {
result := os.MkdirAll(path, 777)
if result != nil {
log.Fatalf("Create dir %s failed", path)
return ""
}
log.Println("Create dir:", path)
}
return path
}
// Getabspath return absolute path of db file
func Getabspath() string {
return getabspath()
}
// GetTempDir returns temp directory on diffrent OS
func GetTempDir() string {
return getTempDir()
}<file_sep>package novelcrawler
import (
nc "github.com/imwyl/novelCrawler/config"
"testing"
)
func TestGetdbname(t *testing.T) {
tests := []struct {
name string
want string
}{
{"test1", ".novel.db"},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := nc.Getdbname(); got != tt.want {
t.Errorf("Getdbname() = %v, want %v", got, tt.want)
}
})
}
}
func TestGetdbpath(t *testing.T) {
tests := []struct {
name string
want string
}{
{"test2", "C:\\Users\\IMwyl\\AppData\\Roaming\\novelCrawler\\"},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := nc.Getdbpath(); got != tt.want {
t.Errorf("Getdbpath() = %v, want %v", got, tt.want)
}
})
}
}
func TestGetabspath(t *testing.T) {
tests := []struct {
name string
want string
}{
{"test3", "/home/wyl/.novel.db"},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := nc.Getabspath(); got != tt.want {
t.Errorf("Getabspath() = %v, want %v", got, tt.want)
}
})
}
}
func TestGetTempDir(t *testing.T) {
tests := []struct {
name string
want string
}{
{"test3", "C:\\Users\\IMwyl\\AppData\\Local\\Temp\\colly-cache"},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := nc.GetTempDir(); got != tt.want {
t.Errorf("Getabspath() = %v, want %v", got, tt.want)
}
})
}
}<file_sep>package novelcrawler
import (
"testing"
"github.com/imwyl/novelCrawler/pkg/novelCrawler"
)
func TestChstonum(t *testing.T) {
type args struct {
strnum string
}
tests := []struct {
name string
args args
wantResult int
wantErr bool
}{
{"0", args{"零"},
0, false},
{"1", args{"一"},
1, false},
{"2", args{"十二"},
12, false},
{"3", args{"一百零三"},
103, false},
{"3", args{"一零三"},
103, false},
{"4", args{"一百四十五"},
145, false},
{"5-1", args{"六千零七十八"},
6078, false},
{"5-3", args{"六千零一"},
6001, false},
{"5-2", args{"六零七八"},
6078, false},
{"6", args{"九千一百零二"},
9102, false},
{"7", args{"三千四百五十六"},
3456, false},
{"8", args{"七万零八"},
70008, false},
{"9", args{"九万零一百零二"},
90102, false},
{"10", args{"三万零四百五十六"},
30456, false},
{"11", args{"七万八千零九"},
78009, false},
{"12", args{"十万两千三百零四"},
102304, false},
{"13", args{"五十万六千七百八十九"},
506789, false},
{"13-1", args{"五零六七八九"},
506789, false},
{"14", args{"一百二十三万四千五百六十七"},
1234567, false},
{"err", args{"第一章"},
-1, true},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
gotResult, err := novelcrawler.Chstonum(tt.args.strnum)
if (err != nil) != tt.wantErr {
t.Errorf("Chstonum() error = %v, wantErr %v", err, tt.wantErr)
return
}
if gotResult != tt.wantResult {
t.Errorf("Chstonum() = %v, want %v", gotResult, tt.wantResult)
}
})
}
}
func TestNumberToChs(t *testing.T) {
type args struct {
number int
}
tests := []struct {
name string
args args
want string
}{
{"1", args{1200}, "一千二百"},
{"2", args{10}, "一十"},
{"3", args{14}, "一十四"},
{"4", args{20}, "二十"},
{"5", args{100}, "一百"},
{"6", args{104}, "一百零四"},
{"7", args{112}, "一百一十二"},
{"8", args{130}, "一百三十"},
{"9", args{1000}, "一千"},
{"10", args{1002}, "一千零二"},
{"11", args{1030}, "一千零三十"},
{"12", args{4056}, "四千零五十六"},
{"13", args{7890}, "七千八百九十"},
{"14", args{1200}, "一千二百"},
{"15", args{102345}, "一十万零二千三百四十五"},
{"16", args{40000}, "四万"},
{"17", args{500000}, "五十万"},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := novelcrawler.NumberToChs(tt.args.number); got != tt.want {
t.Errorf("NumberToChs() = %v, want %v", got, tt.want)
}
})
}
}
<file_sep>package dao
import (
"time"
"github.com/imwyl/novelCrawler/config"
"github.com/jinzhu/gorm"
)
// Novel the novels
type Novel struct {
ID string `grom:"primary_key; auto_increment:false"`
Name string `gorm:"type:varchar(100);not null"`
Chapters []Chapter `gorm:"foreignkey:NovelID"`
First int `gorm:"not null"`
UpdateAt time.Time `gorm:"not null"`
}
// Chapter the chapters of volumn
type Chapter struct {
ID uint `gorm:"primary_key;auto_increment:false"`
Name string
NovelID string `gorm:"not null"`
Content string `gorm:"type:Text(100000)"`
}
// GetDB returns a database connection
func GetDB() (db *gorm.DB, err error) {
db, err = gorm.Open("sqlite3", config.Getabspath())
return
}
// Save a chapter entity
func (chapter *Chapter) Save() (bool, error) {
db, err := GetDB()
if err != nil {
return false, err
}
var count int
if db.Model(chapter).Where("id = ?", chapter.ID).Count(&count); count == 0 {
db.Create(chapter)
} else {
db.Save(chapter)
}
var novel Novel
db.Model(chapter).Related(&novel)
db.Model(&novel).Update("update_at", time.Now())
return true, nil
}
// NovelExists check a record exists or not
func NovelExists(id string) bool {
db, err := GetDB()
if err != nil {
panic(err)
}
defer db.Close()
var count int
db.Model(&Novel{}).Where("id = ?", id).Count(&count)
return count > 0
}
<file_sep>package main
import (
"strings"
"flag"
"log"
"github.com/imwyl/novelCrawler/dao"
"github.com/imwyl/novelCrawler/crwaler"
_ "github.com/jinzhu/gorm/dialects/sqlite"
)
func main() {
var URL string
flag.StringVar(&URL, "URL", "", "飘天小说地址")
flag.Parse()
if URL == "" {
log.Fatalln("No URL")
return
} else if !strings.Contains(URL, "piaotian") {
log.Fatalln("Incorrent URL")
}
db, err := dao.GetDB()
if err != nil {
log.Fatalln(err)
return
}
defer db.Close()
db.AutoMigrate(&dao.Chapter{}, &dao.Novel{})
crwaler.Start(URL)
}
<file_sep>package crwaler
import "testing"
func Test_chapterOrder(t *testing.T) {
type args struct {
chapterURL string
}
tests := []struct {
name string
args args
want int
}{
{"1", args{"1234221.html"}, 1234221},
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := getChapterNumber(tt.args.chapterURL); got != tt.want {
t.Errorf("chapterOrder() = %v, want %v", got, tt.want)
}
})
}
}
| fb93d560e3bdea2759d3fa6d8ce770a7bac7b031 | [
"Go"
] | 11 | Go | imwyl/novelCrawler | 83f007aece7e672e210beefe2281f7a8f8fa4ef8 | 285027a9babf56a8437c214a19648eb66d560c86 | |
refs/heads/master | <file_sep>var express = require('express');
var router = express.Router();
var Obras= require('../controllers/obras')
router.get('/obras', function(req, res) {
let compositor= req.query.compositor
let instrumento = req.query.instrumento
//var chaves = req.params.id.split('&')
if(compositor){
Obras.listarFromCompositor(compositor)
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
}
else if(instrumento){
Obras.listarObrasInstrumento(instrumento)
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
}
else{
Obras.listar()
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
}
});
router.get('/obras/:id', function(req, res) {
//var chaves = req.params.id.split('&')
Obras.consultar(req.params.id)
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
});
router.get('/tipos', function(req, res) {
//var chaves = req.params.id.split('&')
Obras.listarTipos()
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
});
router.get('/obrasQuant', function(req, res) {
//var chaves = req.params.id.split('&')
Obras.listarObrasPartiturasQuantidade()
.then(dados => res.jsonp(dados))
.catch(error => res.status(500).jsonp(error))
});
router.get('/*', function(req, res) {
res.status(500).jsonp();
});
router.post('/*', function(req, res) {
res.status(500).jsonp();
});
router.put('/*', function(req, res) {
res.status(500).jsonp();
});
router.delete('/*', function(req, res) {
res.status(500).json();
});
module.exports = router;
<file_sep>function apagarMusica(id){
console.log('Vou tentar apagar o ' + id + '....')
axios.delete('/musica/' + id)
.then(response => window.location.assign('/'))
.catch(error => console.log(error))
}<file_sep>var Obras = require('../models/obras')
var mongoose = require('mongoose');
const ObjectId = mongoose.Types.ObjectId
module.exports.listar = () =>{
return Obras
.find()
.exec()
}
module.exports.listarByAno = (ano) => {
return Obras
.find({anoCriacao: ano})
.exec()
}
module.exports.listarByCompositorDuracao = (comp, dur) => {
return Obras
.find({$and : {compositor: comp, duracao: dur}})
.exec()
}
module.exports.listarByPeriodo = (ano) => {
return Obras
.find({perido: {$gt: data}})
.exec()
}
module.exports.consultar = (id) => {
return Obras
.findOne({_id: id})
.exec()
}
module.exports.listarCompositores = () => {
return Obras
.distinct("compositor")
.exec()
}
module.exports.listarPeriodos = () => {
return Obras
.distinct("periodo")
.exec()
}<file_sep>var Premios = require('../models/premios')
var mongoose = require('mongoose');
const ObjectId = mongoose.Types.ObjectId
//Devolve a lista de alunos
module.exports.listar = () =>{
return Premios
.find()
.select({year:1, category:1})
.exec()
}
module.exports.listarC = category =>{
return Premios
.find({category: category})
.exec()
}
module.exports.listarCD = (categoria, data) =>{
return Premios
.find({year: {$gt: data}, category: categoria})
.exec()
}
module.exports.listarLaureados = () =>{
return Premios
.aggregate([{$unwind: "$laureates"},
{$group:
{_id: "$laureates.id",
firstname:{$first: "$laureates.firstname"},
surname:{$first: "$laureates.surname"},
prize:
{$push:
{year:"$year", category:"$category"}
}
}
},
{$project:
{firstname:1, surname:1, prize:1, _id:0}
}
])
.sort({firstname:1, surname:1})
.exec()
}
module.exports.consultar = id =>{
return Premios
.findOne({_id: ObjectId(id)})
.exec()
}
module.exports.listarCategorias = () =>{
return Premios.distinct('category').exec()
}
<file_sep>var Filme = require('../models/filme')
const mongoose = require('mongoose')
const ObjectId = mongoose.Types.ObjectId
const Filmes = module.exports
Filmes.listar = () =>{
return Filme
.find()
.sort({title:1})
.exec()
}
Filmes.apagar = id => {
return Filme
.findOneAndDelete({_id: ObjectId(id)})
.exec()
}
Filmes.consultar = id =>{
return Filme
.findOne({_id: ObjectId(id)})
.exec()
}
Filmes.adicionar = dados => {
var filme = new Filme(dados)
return filme.save({useFindAndModify : false})
}
Filmes.modificar = (id, dados) => {
return Filme.findOneAndUpdate({_id: ObjectId(id)}, dados)
.exec()
//ver se dá
}
Filmes.contar = () =>{
return Film.countDocuments().exec()
}
Filmes.listarPagina = (page) => {
return Filme
.find({}, {})
.skip((page)*30)
.limit(30)
.sort({title:1})
.exec()
}
<file_sep>var http = require('http')
var url = require('url')
var fs = require('fs')
http.createServer(function (req, res) {
var partes = req.url.split('/')
var qstr = partes[partes.length - 1]
var str = qstr.split('.')
var contentType = str[partes.length - 1]
if(contentType == undefined)
sender(res, 'dataset/arq' + qstr + '.xml', "text/xml");
else
if(contentType == "ico" | contentType == "gif")
sender(res,'dataset/' + qstr, contentType)
else
sender(res, qstr, contentType )
}).listen(12345)
console.log('Servidor à escuta na porta 12345.....')
function sender(res, path, type){
fs.readFile(path, function(err, data){
if(err) {
console.log(path);
erro(res);
}
else{
res.writeHead(200, {'Content-Type': type})
res.write(data)
res.end()
}
})
}
function erro(res){
res.writeHead(404, {'Content-Type':'text/text'})
res.write("Não foi possível encontrar o ficheiro requirido")
res.end()
}<file_sep>var Obras = require('../models/obra')
//Devolve a lista de alunos
module.exports.listar = () =>{
return Obras
.find()
.select({id:1, title:1, compositor:1, tipo:1, _id:0})
.exec()
}
module.exports.consultar = id =>{
return Obras
.findOne({id: id})
.exec()
}
module.exports.listarTipos = () => {
return Obras
.distinct('tipo')
.exec()
}
module.exports.listarFromCompositor = compositor =>{
return Obras
.find({compositor: compositor})
.exec()
}
module.exports.listarObrasInstrumento = instrumento =>{
return Obras
.find({"instrumentos.designacao": instrumento})
.exec()
}
module.exports.listarObrasPartiturasQuantidade = () =>{
return Obras
.aggregate([{$unwind : "$instrumentos"},
{$group:
{_id : "$id",
partituras: {$sum: 1},
titulo: {$first: "$titulo"}}},
])
}
<file_sep><?xml version="1.0" encoding="UTF-8"?><html>
<head>
<title>Página de um arqueosítio</title>
<meta charset="UTF8"></meta>
<link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css"></link>
</head>
<body>
<div class="w3-container">
<h1>Monte da Saia; Cidade da citânia; Monte de Fralães </h1>
<h3>Imagem</h3>
<img src="" alt=" em falta!"></img>
<h3>Descrição</h3>
Povoado
fortificado
<h3>Informações de localização</h3>
<table class="w3-table">
<tr>
<th width="10%">Cronologia:</th>
<td> Proto-História / Romanização</td>
</tr>
<tr>
<th>Lugar:</th>
<td> Monte da Saia </td>
</tr>
<tr>
<th>Freguesia:</th>
<td></td>
</tr>
<tr>
<th>Concelho:</th>
<td> Barcelos </td>
</tr>
<tr>
<th>Latitude:</th>
<td> 498,8 </td>
</tr>
<tr>
<th>Longitude:</th>
<td> 162,1 </td>
</tr>
<tr>
<th>Altitude</th>
<td> 303m </td>
</tr>
</table>
<hr></hr>
<h3>Acesso</h3>
<p>O acesso pode fazer-se a partir de Chorente, ou Chavão, por caminho
carreteiro.
</p>
<hr></hr>
<h3>Quadro</h3>
<p>O Monte da Saia situa-se no limite da bacia do Cávado, com a bacia do rio
Este. Na sua vertente NE nasce o rio Covo, que corre para o Cávado; a Este,
Sul e Oeste correm vários subafluentes do rio Este. O monte corresponde a
uma elevação de altitude média, que ocupa uma posição de destaque no relevo
da região.
</p>
<hr></hr>
<h3>Autor</h3>
<p> <NAME> </p>
<hr></hr>
<h3>Bibliografia</h3>
<p><NAME>. (1876). As ruínas existentes no Monte da Saia, Comércio do Lima,
no 52, 22/11/1876.
</p>
<p><NAME>. (1877) As ruínas existentes no Monte da Saia, Comércio do Lima,
no 59, 10/1/1877.
</p>
<p><NAME>. (1903). Cidade velha de Santa Luzia, Arqueólogo
Português, 8, pp. 376, 379.
</p>
<p><NAME>. (1913). Religiões da Lusitânia, III, Lisboa. pp.510-512;</p>
<p><NAME>. (1948). O concelho de Barcelos Aquém e Além Cávado, Barcelos, 2
vol. pp. 121.
</p>
<p><NAME> (1968). Câmaras funerárias de la Cultura Castrena, Arquivo
Español de Arqueologia, Madrid, 44 (117-118), pp.33-34;
</p>
<p><NAME>. (1980b). Zur Atlantichen Bronzezeit in Portugal, Germania, 58,
Frankfurt, no. 17.
</p>
<p><NAME>. (1990). O Povoamento Proto-Histórico e a Romanização da Bacia do
Médio Cávado, Cadernos de Arqueologia - Monografias, 5, Universidade do
Minho, Braga, pp. 74.
</p>
<hr></hr>
<h3>Deposito</h3>
<p> Museu Nacional de Arqueologia e Etnologia.</p>
<hr></hr>
<h3>Desenho Arquitetural</h3>
<p>O povoado nunca foi objecto de escavações arqueológicas sistemáticas, sendo
conhecido, sobretudo, a partir de achados ocorridos, ou no seu interior, ou
nas suas imediações. Possui pelo menos três linhas de muralhas e na sua
superfície recolheram-se abundantes fragmentos de cerâmica micácea, de fabrico indígena e de cerâmica romana.
Nele foi ainda identificado um monumento
com forno, do mesmo género dos descobertos em Briteiros e em Santa
Maria dos Galegos, este último, também no concelho de Barcelos. J. Leite de
Vasconcelos publicou duas estelas aparecidas no
sítio do Forno dos Mouros, numa das encostas do monte. Possuem um nicho, com
figuras togadas; as peças deveriam estar relacionadas com o monumento e com
o forno.
</p>
<hr></hr>
<h3>Interesse</h3>
<hr></hr>
<h3>Interpretação</h3>
<p>
Povoado fortificado
romananizado.
</p>
<hr></hr>
<h3>Trabalhos no Arquiosítio</h3>
<hr></hr>
<h5>Data</h5>04-Fev-1998
<hr></hr>
<address>
<a href="../website.html#d1e795">Voltar à página principal</a>
</address>
<hr></hr>
</div>
</body>
</html><file_sep>var mongoose = require('mongoose');
var jsonfile = require('jsonfile');
mongoImport = function (dataBaseName, collectionName, fileName){
mongoose.connect('mongodb://127.0.0.1:27017/' + dataBaseName, {useNewUrlParser: true, useUnifiedTopology: true})
.then(() => {
jsonfile.readFile(fileName, (error, data) =>{
if(error){
console.log("Read file error")
return
}
else{
let newSchema = mongoose.Schema({}, {strict:false})
let newModel = mongoose.model(collectionName, newSchema)
let newObj = new newModel(data)
newObj.save()
.then(data => {
console.log("Success")
mongoose.connection.close()})
.catch(error => console.log(error.message))
}
})
})
.catch(error => console.log("Unable to connect: " + error.message))
}
main = function (){
if(process.argv.length != 5){
console.log("Number of arguments invalid")
return
}
let dataBaseName = process.argv[2]
let collectionName = process.argv[3]
let fileName = process.argv[4]
mongoImport(dataBaseName, collectionName, fileName)
}
main()
<file_sep>var http = require('http')
var url = require('url')
var pug = require('pug')
var fs = require('fs')
var jsonfile = require('jsonfile')
var {parse} = require('querystring') //só exporta o parsing
var myBD = "tarefas.json"
var myServer = http.createServer((req,res) => {
var purl = url.parse(req.url, true)
var query = purl.query
console.log(req.method + ' ' + purl.pathname)
if(req.method == 'GET'){
if((purl.pathname == '/') || (purl.pathname == '/gestaoTarefas')){
jsonfile.readFile(myBD, (erro, tarefas) =>{
res.writeHead(200, {'Content-Type': 'text/html; charset=utf-8'})
if(erro){
res.write(pug.renderFile('erro.pug', {e: "Erro na leitura da BD...."}))
res.end();
console.log("erro no get")
return
}
setExpireDate(tarefas)
res.write(pug.renderFile('front-page.pug', {lista: tarefas}))
res.end();
console.log("get com sucesso")
})
}
else if(purl.pathname == '/w3.css'){
res.writeHead(200, {'Content-Type': 'text/css'})
fs.readFile('stylesheets/w3.css', (erro, dados) =>{
if(erro){
res.write(pug.renderFile('erro.pug', {e: "Stylesheet não encontrada...."}))
res.end()
console.log("erro de styleshet")
return
}
console.log("getting stylesheets")
res.write(dados)
res.end()
})
}
else if(purl.pathname == '/apagaVelhas.js'){
res.writeHead(200, {'Content-Type': 'text/javascript'})
fs.readFile('apagaVelhas.js', (erro, dados) =>{
if(erro){
res.write(pug.renderFile('erro.pug', {e: "Script não encontrado...."}))
res.end()
console.log("erro de styleshet")
return
}
console.log("getting script")
res.write(dados)
res.end()
})
}
else {
console.log("erro de path 2")
res.writeHead(200, {
'Content-Type' : 'text/html; charset=utf-8'
})
res.write(pug.renderFile('erro.pug', {e: "ERRO: O método " + req.method + " não suporta o caminho " +
purl.pathname}))
res.end();
}
}
else if(req.method == 'POST'){
if(purl.pathname == '/tarefa'){
recuperaInfo(req, resultado => {
jsonfile.readFile(myBD, (erro, tarefas) =>{
if(!erro){
tarefas.push(resultado)
jsonfile.writeFile(myBD, tarefas, erro2 => {
if(erro2)
console.log(erro) //TODO mandar html e no de baixo tbm (DELETE)
else{
console.log("refresh")
res.writeHead(303, {'Location': '/'})
res.end()
}
})
}
})
})
}
else{
res.writeHead(200, {
'Content-Type' : 'text/html; charset=utf-8'
})
res.write(pug.renderFile('erro.pug', {e: "ERRO: O método " + req.method + " não suporta o caminho " +
purl.pathname}))
res.end();
}
}
else if(req.method == "DELETE"){
if(purl.pathname == '/tarefasvelhas'){
jsonfile.readFile(myBD, (erro, tarefas) =>{
if(!erro){
tarefasfaziveis = filter(tarefas)
jsonfile.writeFile(myBD, tarefasfaziveis, erro2 =>{
if(erro2)
console.log(erro)
else{
res.writeHead(303, {'Location': '/'})
res.end()
}
})
}
})
}
else{
res.writeHead(200, {
'Content-Type' : 'text/html; charset=utf-8'
})
res.write(pug.renderFile('erro.pug', {e: "ERRO: O método " + req.method + " não suporta o caminho " +
purl.pathname}))
res.end();
}
}
else{
console.log("ERRO: " + req.method + " não suportado....")
res.writeHead(200, {
'Content-Type' : 'text/html; charset=utf-8'
})
res.write(pug.renderFile('erro.pug', {e: "ERRO: " + req.method + " não suportado...."}))
res.end();
}
})
myServer.listen(12345, ()=>{
console.log("Servidor à escuta na porta 12345")
})
function setExpireDate(tarefas){
for(tarefa of tarefas){
var t = new Date(tarefa.data_limite) - Date.now()
var d = Math.abs(Math.ceil(t/(1000 * 60 * 60 * 24)))
if(d == 0){
d += " dias. Está quase a acabar o tempo!"
}
else{
d += " dias. Está tranquilo"
if(t < 0 ){
d += " atrás. Já foste..."
}
}
tarefa.tempo_restante = d
}
}
function recuperaInfo(request, callback){
if(request.headers['content-type'] == 'application/x-www-form-urlencoded'){
let body = ''
//enquanto conseguirmos ler dados do request
request.on('data', bloco => {
body += bloco.toString()
})
request.on('end', ()=> {
callback(parse(body))
})
}
}
function filter(tarefas){
var res = new Array();
var length = tarefas.length;
var localDate = new Date();
console.dir(localDate);
for(var j = 0; j < length; j++)
{
var objDate = Date.parse(tarefas[j].data_limite)
console.dir(objDate)
if(localDate <= objDate)
{
console.log("if")
res.push(tarefas[j])
}
}
console.log(res);
return res
}<file_sep>var express = require('express');
var router = express.Router();
var axios = require('axios')
router.get('/', function(req, res) {
let path = 'http://localhost:3000/api/laureados';
axios.get(path)
.then(dados =>{
res.render('lista-laureados', {lista : dados.data})
})
.catch(erro =>{
res.render('error', {error: erro})
})
})
router.get('/*', function(req, res) {
res.render('error', {error: "O método GET não suporta esse caminho"})
});
module.exports = router;<file_sep>var express = require('express');
var router = express.Router();
var axios = require('axios')
let apikey = <KEY>";
/* GET home page. */
router.get('/', function(req, res, next) {
axios.get('http://clav-api.dglab.gov.pt/api/entidades' + apikey)
.then(dados => {
res.render('lista-entidades', {entidades : dados.data})
})
.catch(erro => {
res.render('error', {error: erro})
})
});
router.get('/tipologia/:id', function(req, res, next) {
var id = req.params.id;
axios.get('http://clav-api.dglab.gov.pt/api/tipologias/'+ id + apikey)
.then(tipologia => {
axios.get('http://clav-api.dglab.gov.pt/api/tipologias/'+ id + '/elementos' + apikey)
.then(elementos => {
axios.get('http://clav-api.dglab.gov.pt/api/tipologias/'+ id + '/intervencao/dono' + apikey)
.then(dono => {
axios.get('http://clav-api.dglab.gov.pt/api/tipologias/'+ id + '/intervencao/participante' + apikey)
.then(participante => {
res.render('tipologia', {tipologia: tipologia.data, elementos: elementos.data, dono: dono.data, participante: participante.data})
})
.catch(erro => {res.render('error', {error: erro})})
}).catch(erro => {res.render('error', {error: erro})})
.catch(erro => {res.render('error', {error: erro})})
}).catch(erro => {res.render('error', {error: erro})
})
}).catch(erro => {res.render('error', {error: erro})
})
});
router.get('/entidade/:id', function(req, res, next) {
axios.get('http://clav-api.dglab.gov.pt/api/entidades/' + req.params.id + apikey)
.then(entidade =>{
axios.get('http://clav-api.dglab.gov.pt/api/entidades/' + req.params.id + '/intervencao/dono' + apikey)
.then(dono =>{
axios.get('http://clav-api.dglab.gov.pt/api/entidades/' + req.params.id + '/intervencao/participante' + apikey)
.then(participante =>{
axios.get('http://clav-api.dglab.gov.pt/api/entidades/' + req.params.id + '/tipologias' + apikey)
.then(tipologias =>{
res.render('entidade', {entidade: entidade.data, dono: dono.data, participante: participante.data, tipologias: tipologias.data})
}).catch(erro =>{res.render('error', {error: erro})
})
}).catch(erro =>{res.render('error', {error: erro})
})
}).catch(erro =>{res.render('error', {error: erro})
})
}).catch(erro =>{ res.render('error', {error: erro})
})
});
router.get('/*', function(req, res) {
res.render('error', {error: "O método GET não suporta esse caminho"})
});
router.post('/*', function(req, res) {
res.render('error', {error: "O método POST não suporta esse caminho"})
});
router.put('/*', function(req, res) {
res.render('error', {error: "O método PUT não suporta esse caminho"})
});
router.delete('/*', function(req, res) {
res.render('error', {error: "O método DELETE não suporta esse caminho"})
});
module.exports = router;
<file_sep><html>
<head>
<META http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>
Project-Record
</title>
<meta charset="UTF8">
<link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css">
</head>
<body>
<div class="w3-container">
<h1 class="w3-center">Project Record</h1>
</div>
<div class="w3-container">
<hr>
<table class="w3-table">
<tr>
<td><b>KEY NAME: </b>TP9</td>
</tr>
<tr>
<td><b>TITLE: </b>Implementação de uma ferramenta semelhante ao mongoimport, mas utilizando nodejs e mongoose</td>
</tr>
<tr>
<td><b>BEGIN DATE: </b>novembro 2019</td>
</tr>
<tr>
<td><b>End Date: </b>novembro 2019</td>
</tr>
<tr>
<td><b>SUPERVISOR: </b><a href="https://www.di.uminho.pt/~jcr"><NAME></a></td>
</tr>
</table>
<hr>
</div>
<div class="w3-container">
<h2>Workteam</h2>
<table class="w3-table w3-striped">
<tr>
<th>Identifier</th>
<th>Name</th>
<th>Email</th>
<th>Git</th>
</tr>
<tr>
<td>81736</td>
<td><NAME></td>
<td><EMAIL></td>
<td>https://github.com/Dantas198</td>
</tr>
</table>
<hr>
</div>
<div class="w3-container">
<h2>Abstract</h2>
<p>
Criação de um executável que irá importar uma base de dados a partir de um ficheiro JSON. Isto é feito através da criação de
um esquema vazio e com uma estrutura não rígida e a partir dele é criado um modelo com o nome da coleção, para depois ser guardado
com os dados obtidos a partir de um leitura do ficheiro JSON.
</p>
<hr>
</div>
<div class="w3-container">
<h2>Deliverables</h2>
<ol>
<li><a href="../mongoImport.js">Ficheiro com o código fonte para correr o comando</a></li>
</ol>
<hr>
</div>
</body>
</html>
<file_sep>var jsonfile = require('jsonfile')
var nanoid = require('nanoid')
var myDB = __dirname + '/../../arq.json'
jsonfile.readFile(myDB, (erro, musicas) => {
if(!erro){
putIds(musicas)
jsonfile.writeFile(myDB, musicas, erro =>{
if(erro){
console.log("Não foi possivel escrever")
console.dir(erro);
}
else
console.log("Sucesso")
})
}
else
console.log("Erro a abriro ficheiro")})
function putIds(lista){
for(musica of lista){
musica.id = nanoid();
}
}<file_sep>var mongoose = require('mongoose');
var partituraSchema = new mongoose.Schema({
path: String,
voz: String
})
var instrumentoSchema = new mongoose.Schema({
designacao: String,
partitura: partituraSchema
});
var obraSchema = new mongoose.Schema({
id: String,
titulo: String,
tipo:String,
compositor:String,
instrumentos: [instrumentoSchema]
});
module.exports = mongoose.model('obras', obraSchema);<file_sep><?xml version="1.0" encoding="UTF-8"?><html>
<head>
<title>Página de um arqueosítio</title>
<meta charset="UTF8"></meta>
<link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css"></link>
</head>
<body>
<div class="w3-container">
<h1> Paredes </h1>
<h3>Imagem</h3>
<img src="" alt=" em falta!"></img>
<h3>Descrição</h3> Ponte; Calçada
<h3>Informações de localização</h3>
<table class="w3-table">
<tr>
<th width="10%">Cronologia:</th>
<td> Idade Média</td>
</tr>
<tr>
<th>Lugar:</th>
<td> Ponte Velha </td>
</tr>
<tr>
<th>Freguesia:</th>
<td></td>
</tr>
<tr>
<th>Concelho:</th>
<td> Montalegre </td>
</tr>
<tr>
<th>Latitude:</th>
<td> 519,8 </td>
</tr>
<tr>
<th>Longitude:</th>
<td> 211,8 </td>
</tr>
<tr>
<th>Altitude</th>
<td> 830m </td>
</tr>
</table>
<hr></hr>
<h3>Acesso</h3>
<p>Sobre a Corga de Caniçó, a menos de 500 metros para Sul da aldeia de
Paredes. O acesso faz-se a pé (cerca de 10 minutos), por caminho carreteiro,
a partir da aldeia de Paredes. A esta acede-se por estradão de terra batida,
a partir da estrada municipal que liga Salto a Ruivães por Zebral. O
monumento não está sinalizado.
</p>
<hr></hr>
<h3>Quadro</h3>
<hr></hr>
<h3>Autor</h3>
<p> <NAME> </p>
<hr></hr>
<h3>Bibliografia</h3>
<p>COSTA, J.G.(1968). Montalegre e Terras de Barroso. Montalegre, 1968.</p>
<p> FONTES, L.(1992). Sítios e Achados Arqueológicos do Concelho de
Montalegre. Braga, 1992 (edição policopiada da CCRN - Porto).
</p>
<hr></hr>
<h3>Deposito</h3>
<hr></hr>
<h3>Desenho Arquitetural</h3>
<p>Entre Caniçó e Torrinheiras conserva-se o traçado da via medieval que dava
ligação a cabeceiras de Basto. Até à Senhora
dos Aflitos e sobretudo nas proximidades da aldeia de Paredes, conservam-se
amplos troços de via lajeada, com 2,5m de largura média. Passa sobre a Corga
de Caniçó, a cerca de 300 metros para Sul de Paredes, através de uma ponte
de pedra de tradição arquitectónica medieval, com tabuleiro em cavalete
sobre um arcode volta perfeita, bem aparelhado com
silhares. Exceptuando a ponte, que necessita
ser limpa e restaurada, a calçada e a envolvência paisagística, com relevo
para o tradicional ordenamento agrário da veiga, apresentam-se muito bem
conservadas.
</p>
<hr></hr>
<h3>Interesse</h3>
<p>Embora não possua um significativo valor patrimonial, este monumento tem
muito interesse para a compreensão da rede de comunicações medieval,
oferecendo simultaneamente um elevado potencial para a implementação de
circuitos pedonais.
</p>
<hr></hr>
<h3>Interpretação</h3>
<p>Ponte e calçada medievais.</p>
<hr></hr>
<h3>Trabalhos no Arquiosítio</h3>
<hr></hr>
<h5>Data</h5>04-FEV-1998
<hr></hr>
<address>
<a href="../website.html#d1e8298">Voltar à página principal</a>
</address>
<hr></hr>
</div>
</body>
</html><file_sep>function editaMusica(id){
console.log('Vou tentar apagar o ' + id + '....')
file = {
'@t': document.getElementById('6').value,
'#text': document.getElementById('7').value
}
params = {
id: id,
tit: document.getElementById('1').value,
duracao: document.getElementById('2').value,
prov: document.getElementById('3').value,
local: document.getElementById('4').value,
musico: document.getElementById('5').value,
file
}
axios.put('/musica/' + id, params)
.then(response => window.location.assign('/'))
.catch(error => console.log(error))
}
| 402dd99524917176d1bd27892c74d5a2ac24273f | [
"JavaScript",
"HTML"
] | 17 | JavaScript | Dantas198/DWeb2019 | a63c0a9643939c84fa5d0ec670ee18ba4a08548f | 78d3338ccce81fa4e1a551814ee9484d8a43bc15 | |
refs/heads/master | <repo_name>mobangjack/mqtt_led_iot<file_sep>/start_listen_led_cur_state.sh
mosquitto_sub -h mqtt -t 'led/curstate' -v
<file_sep>/io.cpp
#include <iostream>
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h> //define O_WRONLY and O_RDONLY
void help()
{
printf("Usage: io <file> <r/w? <value>\n");
}
int main(int argc, char** argv)
{
if (argc < 3)
{
help();
return -1;
}
char* file = argv[1];
char input = 0;
char* value = 0;
if (strcmp(argv[2], "w") == 0)
{
if (argc < 4)
{
help();
return -1;
}
else
{
input = 1;
value = argv[3];
}
}
else if (strcmp(argv[2], "r") == 0)
{
input = 0;
}
else
{
help();
return -1;
}
int fd = -1;
if (input)
{
fd = open(file, O_WRONLY);
}
else
{
fd = open(file, O_RDONLY);
}
if (fd == -1)
{
printf("[ERROR] Cannot open file\n");
return -1;
}
if (input)
{
write(fd, value, 1);
printf("Write val: %c\n", value[0]);
}
else
{
char buf[2];
memset(buf, 0, 2 * sizeof(char));
read(fd, buf, 1);
printf("Read val: %c\n", buf[0]);
}
close(fd);
fd = -1;
return 0;
}
<file_sep>/Makefile
CFLAGS=-Wall -ggdb -I../../lib -I../../lib/cpp
LDFLAGS=-L../../lib ../../lib/cpp/libmosquittopp.so.1 ../../lib/libmosquitto.so.1
.PHONY: all clean
all : mqtt_led_new_state_sub
mqtt_led_new_state_sub : main.o mqtt_led_new_state_sub.o
${CXX} $^ -o $@ ${LDFLAGS}
main.o : main.cpp
${CXX} -c $^ -o $@ ${CFLAGS}
mqtt_led_new_state_sub.o : mqtt_led_new_state_sub.cpp
${CXX} -c $^ -o $@ ${CFLAGS}
clean :
-rm -f *.o mqtt_led_new_state_sub
<file_sep>/test.cpp
#include "gpio.h"
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, char** argv)
{
if (argc < 2)
{
printf("Usage: gpio_read <port>\n");
return -1;
}
gpio line(argv[1]);
line.wire(O_RDONLY);
if (!line.isWired())
{
printf("[ERROR] Cannot wire gpio\n");
return -1;
}
int hz = 10;
int us = (1.0 / (double)hz) * 1e6;
while (1)
{
int result = line.stat();
printf("[INFO] gpio stat: %c\n", result);
//usleep(us);
int i = 1e8;
while(--i);
}
line.cutoff();
return 0;
}
<file_sep>/pub_led_new_state.sh
mosquitto_pub -h mqtt -t 'led/newstate' -m $1
<file_sep>/gpio_write.cpp
#include "gpio.h"
#include <stdio.h>
#include <fcntl.h>
int main(int argc, char** argv)
{
if (argc < 3)
{
printf("Usage: gpio_write <port> <value>\n");
return -1;
}
gpio line(argv[1]);
line.wire(O_WRONLY);
if (!line.isWired())
{
printf("[ERROR] Unable to wire gpio\n");
return -1;
}
line.assign(argv[2][0]);
line.cutoff();
return 0;
}
<file_sep>/mqtt_led_new_state_sub.h
#ifndef MQTT_LED_NEW_STATE_SUB_H
#define MQTT_LED_NEW_STATE_SUB_H
#include <mosquittopp.h>
#include <string>
#include "gpio.h"
class mqtt_led_new_state_sub : public mosqpp::mosquittopp
{
public:
mqtt_led_new_state_sub(const char *id, const char *topic, const char *device);
~mqtt_led_new_state_sub();
void on_connect(int rc);
void on_message(const struct mosquitto_message *message);
void on_subscribe(int mid, int qos_count, const int *granted_qos);
protected:
std::string m_topic;
gpio m_led;
};
#endif
<file_sep>/gpio.h
#ifndef GPIO_H
#define GPIO_H
#include <string>
class gpio
{
public:
gpio(std::string port);
~gpio();
bool wire(int flag);
bool isWired();
int stat();
bool assign(char val);
void cutoff();
protected:
int fd;
std::string port;
bool is_wired;
};
#endif
<file_sep>/mqtt_led_cur_state_pub.h
#ifndef MQTT_LED_CUR_STATE_PUB_H
#define MQTT_LED_CUR_STATE_PUB_H
#include <mosquittopp.h>
#include "gpio.h"
class mqtt_led_cur_state_pub : public mosqpp::mosquittopp
{
public:
mqtt_led_cur_state_pub(const char *id, const char *topic, const char *device);
~mqtt_led_cur_state_pub();
void on_connect(int rc);
void on_message(const struct mosquitto_message *message);
void on_subscribe(int mid, int qos_count, const int *granted_qos);
void advertise();
void spin(int hz);
protected:
std::string m_topic;
gpio m_led;
};
#endif
<file_sep>/mqtt_led_cur_state_pub.cpp
#include <cstdio>
#include <cstring>
#include <fcntl.h>
#include <unistd.h>
#include "mqtt_led_cur_state_pub.h"
#include <mosquittopp.h>
#include <string.h>
mqtt_led_cur_state_pub::mqtt_led_cur_state_pub(const char *id, const char *topic, const char *device) : mosquittopp(id), m_topic(topic), m_led(device)
{
m_led.wire(O_RDONLY);
if (!m_led.isWired())
{
printf("[ERROR] Cannot wire led\n");
}
};
mqtt_led_cur_state_pub::~mqtt_led_cur_state_pub()
{
if (m_led.isWired())
{
m_led.cutoff();
}
}
void mqtt_led_cur_state_pub::on_connect(int rc)
{
printf("Connected with code %d.\n", rc);
// if(rc == 0){
/* Only attempt to subscribe on a successful connect. */
// subscribe(NULL, m_topic.c_str());
// }
}
void mqtt_led_cur_state_pub::on_message(const struct mosquitto_message *message)
{
// char buf[2];
// if(!strcmp(message->topic, "led/brightness")){
// memset(buf, 0, 2*sizeof(char));
/* Copy N-1 bytes to ensure always 0 terminated. */
// memcpy(buf, message->payload, 1*sizeof(char));
// }
}
void mqtt_led_cur_state_pub::on_subscribe(int mid, int qos_count, const int *granted_qos)
{
printf("Subscription succeeded.\n");
}
void mqtt_led_cur_state_pub::advertise()
{
if (m_led.isWired())
{
int state = m_led.stat();
if (state == -1)
{
printf("[WARN] Led stat exception (would not be published)\n");
return;
}
char buf[2];
memset(buf, 0, 2 * sizeof(char));
sprintf( buf,"%c", state);
publish(NULL, m_topic.c_str(), 2, buf);
}
}
void mqtt_led_cur_state_pub::spin(int hz)
{
int us = (1.0 / (double)hz) * 1e6;
while (1)
{
advertise();
usleep(us);
}
}
<file_sep>/mqtt_led_cur_state_pub_main.cpp
#include "mqtt_led_cur_state_pub.h"
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
void help()
{
printf("Usage: mqtt_led_cur_state_pub_main <id> <topic> <device> <host> <port> <keepalive> <hz>\n");
}
int main(int argc, char *argv[])
{
if (argc < 8)
{
help();
return -1;
}
const char* id = argv[1];
const char* topic = argv[2];
const char* device = argv[3];
const char* host = argv[4];
const int port = atoi(argv[5]);
const int keepalive = atoi(argv[6]);
const int hz = atoi(argv[7]);
mqtt_led_cur_state_pub led_cur_state_pub(id, topic, device);
led_cur_state_pub.connect(host, port, keepalive);
// int hz = 1;
// int us = (1.0 / (double)hz) * 1e6;
// int rc;
mosqpp::lib_init();
led_cur_state_pub.spin(hz);
// while(1){
// rc = led_cur_state_pub.loop();
// if(rc){
// led_cur_state_pub.reconnect();
// }
// led_cur_state_pub.advertise();
// usleep(us);
// }
mosqpp::lib_cleanup();
return 0;
}
<file_sep>/gpio_read.cpp
#include "gpio.h"
#include <stdio.h>
#include <fcntl.h>
int main(int argc, char** argv)
{
if (argc < 2)
{
printf("Usage: gpio_read <port>\n");
return -1;
}
gpio line(argv[1]);
line.wire(O_RDONLY);
if (!line.isWired())
{
printf("[ERROR] Cannot wire gpio\n");
return -1;
}
int result = line.stat();
printf("[INFO] gpio stat: %c\n", result);
line.cutoff();
return 0;
}
<file_sep>/gpio.cpp
#include "gpio.h"
#include <iostream>
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h> //define O_WRONLY and O_RDONLY
gpio::gpio(std::string port)
{
this->fd = -1;
this->port = port;
this->is_wired = false;
}
bool gpio::wire(int flag)
{
fd = open(port.c_str(), flag);
if (fd != -1)
{
is_wired = true;
}
else
{
is_wired = false;
}
return is_wired;
}
bool gpio::isWired()
{
return this->is_wired;
}
int gpio::stat()
{
if (!is_wired)
{
return -1;
}
char buf[2];
memset(buf, 0, 2 * sizeof(char));
int len = read(fd, buf, 1);
if (len != 1)
{
return -1;
}
lseek(fd, 0, SEEK_SET); // important!!!
return buf[0];
}
bool gpio::assign(char val)
{
if (!is_wired)
{
return false;
}
int len = write(fd, &val, 1);
if (len != 1)
{
return false;
}
return true;
}
void gpio::cutoff()
{
if (fd != -1)
{
close(fd);
fd = -1;
}
is_wired = false;
}
gpio::~gpio()
{
cutoff();
}
<file_sep>/mqtt_led_new_state_sub.cpp
#include <cstdio>
#include <cstring>
#include <fcntl.h>
#include "mqtt_led_new_state_sub.h"
#include <mosquittopp.h>
mqtt_led_new_state_sub::mqtt_led_new_state_sub(const char *id, const char *topic, const char *device) : mosquittopp(id), m_topic(topic), m_led(device)
{
m_led.wire(O_WRONLY);
if (!m_led.isWired())
{
printf("[ERROR] Cannot wire led\n");
}
};
mqtt_led_new_state_sub::~mqtt_led_new_state_sub()
{
if (m_led.isWired())
{
m_led.cutoff();
}
}
void mqtt_led_new_state_sub::on_connect(int rc)
{
printf("Connected with code %d.\n", rc);
if(rc == 0){
/* Only attempt to subscribe on a successful connect. */
subscribe(NULL, m_topic.c_str());
}
}
void mqtt_led_new_state_sub::on_message(const struct mosquitto_message *message)
{
char buf[2];
if(!strcmp(message->topic, m_topic.c_str())){
memset(buf, 0, 2*sizeof(char));
/* Copy N-1 bytes to ensure always 0 terminated. */
memcpy(buf, message->payload, 1*sizeof(char));
bool success = false;
if (m_led.isWired())
{
success = m_led.assign(buf[0]);
printf("[INFO] Assign led to %c\n: %d", buf[0], success);
}
else
{
printf("[WARN] Led not wired, io operation was not available\n");
}
}
}
void mqtt_led_new_state_sub::on_subscribe(int mid, int qos_count, const int *granted_qos)
{
printf("Subscription succeeded.\n");
}
| 34eb68a92634ca9e5fafd607f4a39ad59a208f2f | [
"Makefile",
"C++",
"Shell"
] | 14 | Shell | mobangjack/mqtt_led_iot | 618550f9b41511d65842a2629f713c70f57de4b1 | 041bc26e9d76413c08c2ad8ab221187f4127e8bc | |
refs/heads/main | <file_sep># H1. Правильная скобочная последовательность
Рассмотрим последовательность, состоящую из круглых, квадратных и фигурных скобок. Программа дожна определить, является ли данная скобочная последовательность правильной.
## Формат ввода
В единственной строке записана скобочная последовательность, содержащая не более 100000 скобок.
## Формат вывода
Если данная последовательность правильная, то программа должна вывести строку **yes**, иначе строку *no*.
### Пример 1
| Ввод | Вывод |
|--------|--------|
| ()[] | yes |
### Пример 2
|Ввод | Вывод |
|-------|---------|
| ([)] | no |
<file_sep>#include <iostream>
#include <stack>
using namespace std;
void solve(string &s){
stack<char> cur;
for(int i = 0; i < s.size(); i++){
if(!cur.empty() && ((cur.top() == '(' && s[i] == ')') || (cur.top() == '{' && s[i] == '}') || (cur.top() == '[' && s[i] == ']')))
cur.pop();
else cur.push(s[i]);
}
if(cur.empty()) cout << "YES";
else cout << "NO";
}
signed main() {
string str;
cin >> str;
solve(str);
}
| fb4f7672a9440e3300447fa10d70b0203d621115 | [
"Markdown",
"C++"
] | 2 | Markdown | initmxself/Parenthesis-sequences | 9203b1956424ca708943a4599f020d4578161890 | b312792e4c965feceea6096d5073018d2fbf4eb3 | |
refs/heads/master | <repo_name>reetambhowmick/py-test-auto-framework<file_sep>/AutomationPractice/registration_and_login/pom/page_authentication.py
from selenium.webdriver.support.select import Select
from AutomationPractice.registration_and_login.pom.base import BasePage
from AutomationPractice.registration_and_login.pom.locators import Locators
class AuthenticationPage(BasePage):
""" Authentication Page Class
It contains multiple sub section: Login, Create Account and Personal Info section as class composition
"""
def __init__(self, driver):
BasePage.__init__(self, driver)
self.login_section = LoginSection(self)
self.create_account_section = CreateAccountSection(self)
self.personal_info_section = PersonalInfoSection(self)
class LoginSection:
""" Login section """
driver = None
def __init__(self, page):
self.page = page
# Below is creating class attribute for each locator in Locator class as one Element object and one locator_* string
for k, v in Locators.Authentication_login.items():
setattr(self, k, self.page.com.element(v))
setattr(self, "elem_ " + k, v)
def input_email_address(self, email):
self.textbox_email().send_keys(email)
def input_password(self, password):
self.textbox_password().send_keys(<PASSWORD>)
def click_sign_in_button(self):
self.button_sign_in().click()
def perform_login(self, email, password, wait_for_next_page_load=True):
self.input_email_address(email)
self.input_password(<PASSWORD>)
self.click_sign_in_button()
if wait_for_next_page_load:
self.page.wait_for_page_load("myaccountpage")
def get_error_list(self):
errors = [x.text for x in self.list_error_items(find_all=True)]
return errors
def is_login_error_occured(self):
return (len(self.get_error_list()) > 0)
class CreateAccountSection:
""" Create Account Section """
def __init__(self, page):
self.page = page
# Below is creating class attribute for each locator in Locator class as one Element object and one locator_* string
for k, v in Locators.Authentication_create_account.items():
setattr(self, k, self.page.com.element(v))
setattr(self, "elem_ " + k, v)
def input_email_address(self, email):
self.textbox_email().send_keys(email)
def click_button_create_account(self, wait_for_next_page_load=True):
self.button_create_account().click()
if wait_for_next_page_load:
self.page.wait_for_page_load("personalinfopage")
class PersonalInfoSection:
""" Personal Info fields section"""
def __init__(self, page):
self.page = page
# Below is creating class attribute for each locator in Locator class as one Element object and one locator_* string
for k, v in Locators.Authentication_personal_info.items():
setattr(self, k, self.page.com.element(v))
setattr(self, "elem_ " + k, v)
def get_section_heading(self):
return self.subheadings().text
def input_personal_info(self, fields_values):
if "title" in fields_values:
if fields_values["title"] == "mrs":
self.field_option_title_Mrs().click()
else:
self.field_option_title_Mr().click()
if "first_name" in fields_values:
self.field_textbox_first_name().send_keys(fields_values["first_name"])
# self.field_textbox_address_firstname().send_keys(fields_values["first_name"])
if "last_name" in fields_values:
self.field_textbox_last_name().send_keys(fields_values["last_name"])
# self.field_textbox_address_lastname().send_keys(fields_values["last_name"])
if "email" not in fields_values:
if self.field_textbox_email().get_attribute('value'):
self.field_textbox_email().clear()
if "password" in fields_values:
self.field_textbox_password().send_keys(fields_values["password"])
if "dob_date" in fields_values:
Select(self.field_select_dob_day()).select_by_value(str(fields_values["dob_date"]))
if "dob_month" in fields_values:
Select(self.field_select_dob_month()).select_by_value(str(fields_values["dob_month"]))
if "dob_year" in fields_values:
Select(self.field_select_dob_year()).select_by_value(str(fields_values["dob_year"]))
if "company" in fields_values:
self.field_textbox_address_company().send_keys(fields_values["company"])
if "address1" in fields_values:
self.field_textbox_address_address1().send_keys(fields_values["address1"])
if "address2" in fields_values:
self.field_textbox_address_address2().send_keys(fields_values["address2"])
if "city" in fields_values:
self.field_textbox_address_city().send_keys(fields_values["city"])
if "state_index" in fields_values:
Select(self.field_select_address_state()).select_by_value(str(fields_values["state_index"]))
if "zipcode" in fields_values:
self.field_textbox_address_postcode().send_keys(fields_values["zipcode"])
if "additional_info" in fields_values:
self.field_textbox_address_additionalinfo().send_keys(fields_values["additional_info"])
if "home_phone" in fields_values:
self.field_textbox_address_phone().send_keys(fields_values["home_phone"])
if "mobile_phone" in fields_values:
self.field_textbox_address_mobile().send_keys(fields_values["mobile_phone"])
if "address_alias" in fields_values:
el_ad_alias = self.field_textbox_address_alias()
el_ad_alias.clear()
el_ad_alias.send_keys(fields_values["address_alias"])
else:
el_ad_alias = self.field_textbox_address_alias()
el_ad_alias.clear()
def click_button_register(self, wait_for_next_page_load=True):
self.button_register().click()
if wait_for_next_page_load:
self.page.wait_for_page_load("myaccountpage")
def get_error_list(self):
errors = [x.text for x in self.list_error_items(find_all=True)]
return errors
def is_login_error_occured(self):
return (len(self.get_error_list()) > 0)
<file_sep>/AutomationPractice/registration_and_login/pom/locators.py
class Locators:
Home = {
'link_sign_in': 'css|a.login',
'link_sign_out': 'css|a.logout'
}
Authentication_create_account = {
'heading': 'tagname|h1',
'subheading': 'css|form#create-account_form h3',
'textbox_email': 'id|email_create',
'button_create_account': 'id|SubmitCreate',
'list_error_items': 'css|#create_account_error>ol>li'
}
Authentication_login = {
'heading': 'tagname|h1',
'subheadings': 'css|form#login_form h3',
'textbox_email': 'id|email',
'textbox_password': '<PASSWORD>',
'button_sign_in': 'id|SubmitLogin',
'list_error_items': 'css|div.center_column> :nth-child(2)>ol>li'
}
Authentication_personal_info = {
'heading': 'tagname|h1',
'subheadings': 'tagname|h3',
'div_account_creation': 'css|div.account_creation',
'field_option_title_Mr': 'id|uniform-id_gender1',
'field_option_title_Mrs': 'id|uniform-id_gender2',
'field_textbox_first_name': 'id|customer_firstname',
'field_textbox_last_name': 'id|customer_lastname',
'field_textbox_email': 'id|email',
'field_textbox_password': '<PASSWORD>',
'field_select_dob_day': 'id|days',
'field_select_dob_month': 'id|months',
'field_select_dob_year': 'id|years',
'field_checkbox_newsletter': 'id|uniform-newsletter',
'field_checkbox_offers': 'id|uniform-optin',
'field_textbox_address_firstname': 'id|firstname',
'field_textbox_address_lastname': 'id|lastname',
'field_textbox_address_company': 'id|company',
'field_textbox_address_address1': 'id|address1',
'field_textbox_address_address2': 'id|address2',
'field_textbox_address_city': 'id|city',
'field_select_address_state': 'id|id_state',
'field_textbox_address_postcode': 'id|postcode',
'field_select_address_country': 'id|id_country',
'field_textbox_address_additionalinfo': 'id|other',
'field_textbox_address_phone': 'id|phone',
'field_textbox_address_mobile': 'id|phone_mobile',
'field_textbox_address_alias': 'id|alias',
'field_textbox_tax_idno': 'id|dni',
'list_error_items': 'css|.alert-danger>ol>li',
'button_register': 'id|submitAccount'
}
Myaccount = {
'heading': 'tagname|h1',
'link_account': 'css|a.account'
}
<file_sep>/AutomationPractice/registration_and_login/pom/base.py
"""
This is base page to handle common elements,methods across the pages.
"""
from selenium.common.exceptions import ElementNotVisibleException
from core.com import Com
from AutomationPractice.registration_and_login.pom.locators import Locators
class BasePage(object):
""" This is Base Page class. For all common properties and methods. All page classes inherit this class."""
driver = None
Locators = Locators
def __init__(self, driver):
BasePage.driver = driver
self.com = Com(driver)
def open_url(self, url):
self.driver.get(url)
def close(self):
BasePage.driver.close()
def quit(self):
BasePage.driver.quit()
def get_title(self):
return self.driver.title
def get_page_h1_heading_text(self):
return self.com.findElement(self.Locators.Authentication_personal_info['heading']).text
def wait_for_page_load(self, page_name):
try:
if page_name == "homepage":
self.com.wait.wait_for_element_visible(self.Locators.Home["link_sign_in"])
elif page_name == "loginpage" or page_name == "createaccount":
self.com.wait.wait_for_element_visible(self.Locators.Authentication_create_account["subheading"])
elif page_name == "personalinfopage":
self.com.wait.wait_for_element_with_text_present(
self.Locators.Authentication_personal_info['subheadings'], "YOUR PERSONAL INFORMATION")
elif page_name == "myaccountpage":
self.com.wait.wait_for_element_visible(self.Locators.Myaccount["link_account"])
else:
raise Exception("Incorrect Page Name passed")
except ElementNotVisibleException as e:
print(e)
raise Exception("Page {} is unable to load".format(page_name))
<file_sep>/AutomationPractice/registration_and_login/tests/test_registration.py
import time
import pytest
from AutomationPractice.registration_and_login.testdata.test_data_provider import TestDataProvider
class TestRegistration:
@pytest.mark.positive
@pytest.mark.parametrize("new_user_data",
TestDataProvider.generate_user_reg_data(save_user=True))
def test_registration_success_scenario(self, app, new_user_data):
# TestDataProvider.save_created_account(new_user_data["email"], new_user_data["password"])
app.home_page.open_homepage()
app.home_page.click_sign_in()
app.authentication_page.create_account_section.input_email_address(new_user_data["email"])
app.authentication_page.create_account_section.click_button_create_account()
actual = app.authentication_page.get_page_h1_heading_text()
pytest.assume("CREATE AN ACCOUNT" == actual, "Actual:{} ,Expected: {}".format(actual, "CREATE AN ACCOUNT"))
actual = app.authentication_page.personal_info_section.get_section_heading()
pytest.assume("YOUR PERSONAL INFORMATION" == actual,
"Actual:{} ,Expected: {}".format(actual, "YOUR PERSONAL INFORMATION"))
app.authentication_page.personal_info_section.input_personal_info(new_user_data)
app.authentication_page.personal_info_section.click_button_register()
title = app.authentication_page.get_title()
expected_title = "My account - My Store"
pytest.assume(title == expected_title, "Actual:{} ,Expected: {}".format(title, expected_title))
full_name = app.myaccount_page.get_logged_in_full_name()
expected_full_name = new_user_data["first_name"] + " " + new_user_data["last_name"]
pytest.assume(full_name == expected_full_name.lower(),
"Actual:{} ,Expected: {}".format(full_name, expected_full_name))
@pytest.mark.positive
@pytest.mark.parametrize("new_user_data",
TestDataProvider.generate_user_reg_data(only_required_fields=True,save_user=True))
def test_registration_success_scenario_only_required_fields(self, app, new_user_data):
# TestDataProvider.save_created_account(new_user_data["email"], new_user_data["password"])
app.home_page.open_homepage()
app.home_page.click_sign_in()
app.authentication_page.create_account_section.input_email_address(new_user_data["email"])
app.authentication_page.create_account_section.click_button_create_account()
actual = app.authentication_page.get_page_h1_heading_text()
pytest.assume("CREATE AN ACCOUNT" == actual, "Actual:{} ,Expected: {}".format(actual, "CREATE AN ACCOUNT"))
actual = app.authentication_page.personal_info_section.get_section_heading()
pytest.assume("YOUR PERSONAL INFORMATION" == actual,
"Actual:{} ,Expected: {}".format(actual, "YOUR PERSONAL INFORMATION"))
app.authentication_page.personal_info_section.input_personal_info(new_user_data)
app.authentication_page.personal_info_section.click_button_register()
title = app.authentication_page.get_title()
expected_title = "My account - My Store"
pytest.assume(title == expected_title, "Actual:{} ,Expected: {}".format(title, expected_title))
full_name = app.myaccount_page.get_logged_in_full_name()
expected_full_name = new_user_data["first_name"] + " " + new_user_data["last_name"]
pytest.assume(full_name == expected_full_name.lower(),
"Actual:{} ,Expected: {}".format(full_name, expected_full_name))
@pytest.mark.negative
@pytest.mark.parametrize("new_user_data,errors",
TestDataProvider.get_user_reg_data_list_with_errors())
def test_registration_personal_info_page_all_negative_scenarios_without_required_field(self, app, new_user_data,
errors):
app.home_page.open_homepage()
app.home_page.click_sign_in()
app.authentication_page.create_account_section.input_email_address(
new_user_data["email_for_create_account_page"])
app.authentication_page.create_account_section.click_button_create_account()
app.authentication_page.personal_info_section.input_personal_info(new_user_data)
app.authentication_page.personal_info_section.click_button_register(wait_for_next_page_load=False)
actual_errors = app.authentication_page.personal_info_section.get_error_list()
pytest.assume(len(actual_errors) == len(errors),
"Actual:{} ,Expected: {}".format(len(actual_errors), len(errors)))
for i in range(len(errors)):
pytest.assume(actual_errors[i] == errors[i], "Actual:{} ,Expected: {}".format(actual_errors[i], errors[i]))
<file_sep>/README.md
# py-test-auto-framework
Sample Test Automation project to show automation by using Python, Pytest and Selenium in Page Object Model structure.
## Prerequisite
* Python 3.7 +
* pipenv should be installed (follow instruction here https://github.com/pypa/pipenv)
## Installation
```bash
> git clone https://github.com/abkabhishek/py-test-auto-framework.git
> cd py-test-auto-framework
> pipenv shell
> pipenv install
> export PYTHONPATH=$(pwd):$PYTHONPATH
```
## To Run Test
```python
#to run all test
pytest -v
#to run test function containing keyword (registration
pytest -v -k registration
#to run test function marked with mentioned marker (positive)
pytest -v -m positive
#to run all test and generate html report
pytest --html=report.html -v
```
* Root directory contains docker-compose file to run selenium hub and node. Running it and changing value of "environment" to "remote" in tests> config_test.json.
* Complete site's Pom and Tests should be placed inside site name folder - AutomationPractice > Module name - registration_and_login > then pom, tests, testdata.
* testdata folder contains the Test Data Provider class and maintaining created account details as well.
<file_sep>/core/com.py
"""
This is module to contain commonly used functionality like find elements
"""
import time
from sys import stdout as console
from selenium.webdriver.common.by import By
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
WAIT_TIME_OUT = 180
class Com:
driver = None
wait = None
windowTabs = {}
def __init__(self, driver):
Com.driver = driver
Com.wait = Wait(driver)
@classmethod
def is_exist(cls, locatr):
return cls.driver.find_elements(*Locatr.get_locatr_by(locatr))
@classmethod
def get_element_text_else_false(cls, elem):
if elem:
return elem.text
else:
return False
@classmethod
def findElement(cls, locatr, elem=None):
"""
locatr param should be of "css|string" style
elem param is optional selenium element, if provided then find_element will be done on that passed element
"""
try:
if elem:
return elem.find_element(*Locatr.get_locatr_by(locatr))
else:
return cls.driver.find_element(*Locatr.get_locatr_by(locatr))
except NoSuchElementException as e:
print(e)
print("Unable to find the element by: {}, locator: {}".format(*Locatr.get_locatr_by(locatr)))
raise Exception("Element not found")
@classmethod
def findElements(cls, locatr, elem=None):
"""
locatr param should be of "css|string" style
elem param is optional selenium element, if provided then find_element will be done on that passed element
"""
if elem:
return elem.find_elements(*Locatr.get_locatr_by(locatr))
else:
return cls.driver.find_elements(*Locatr.get_locatr_by(locatr))
@classmethod
def element(cls, locatr):
"""
This is the custom element function, in every pom class, every locator is created of this type function.
User can perform action direction on the element, no need to find element first.
Example:
Home.Search_box().send_keys("<PASSWORD>")
It can get multiple optional parameters at the time of actions:
Home.Results(True,1).click()
=> first param = find_all=True - To find list of elements
=> second param = element index to return, else it will return all list.
"""
def find_element(find_all=False, return_item=False):
try:
if find_all:
elems = cls.findElements(locatr)
else:
elem = cls.findElement(locatr)
if find_all and return_item == False and elems:
return elems
elif find_all and return_item != False and elems:
return elems[return_item - 1]
else:
return elem
except Exception as ex:
# console.write(str(ex))
console.write("---- Element not found, Exception occurred while finding the element ----")
print(ex)
raise Exception("Element Not found")
# return False
return find_element
@classmethod
def elements(cls, locatr):
"""
This is similar like element function above but its for list of elements specifically
"""
def find_elements(return_item=False):
try:
elems = cls.findElements(locatr)
if return_item == False and elems:
return elems
elif return_item != False and elems:
return elems[return_item - 1]
else:
return elems
except Exception as ex:
# console.write(str(ex))
console.write("---- Element not found, Exception occurred while finding the elements ----")
console.write(ex)
raise Exception("Elements Not found")
return find_elements
class Locatr:
"""
Helper class to convert "css|string" type locator to (CSS_SELECTOR,"string")
"""
@staticmethod
def get_locatr_by(locatr):
if "|" in locatr:
locatrBy, locatrStr = locatr.split("|")
if locatrBy.lower() == "xpath":
return By.XPATH, locatrStr
elif locatrBy.lower() == "id":
return By.ID, locatrStr
elif locatrBy.lower() == "css":
return By.CSS_SELECTOR, locatrStr
elif locatrBy.lower() == "name":
return By.NAME, locatrStr
elif locatrBy.lower() == "class":
return By.CLASS_NAME, locatrStr
elif locatrBy.lower() == "tagname":
return By.TAG_NAME, locatrStr
else:
return By.ID, locatrStr
else:
raise Exception("Invalid locatr string : {}".format(locatr))
class Wait:
def __init__(self, driver):
self.driver = driver
def wait_for_element_visible(self, locatr, timeout=WAIT_TIME_OUT):
try:
element = WebDriverWait(self.driver, timeout).until(
EC.visibility_of_element_located(Locatr.get_locatr_by(locatr))
)
return element
except Exception as e:
print(e)
raise Exception("Element ( by: {}, locator: {}) is not visible after waiting until timeout {}".format(*Locatr.get_locatr_by(locatr),timeout))
def wait_for_element_with_text_present(self,locatr,text,timeout=WAIT_TIME_OUT):
try:
element = WebDriverWait(self.driver, timeout).until(
EC.text_to_be_present_in_element(Locatr.get_locatr_by(locatr),text)
)
return element
except Exception as e:
print(e)
raise Exception("Element ( by: {}, locator: {}) is not present with text {} after waiting until timeout {}".format(*Locatr.get_locatr_by(locatr),text,timeout))
def is_element_present(self, locatr):
try:
self.driver.find_element(*Locatr.get_locatr_by(locatr))
return True
except Exception:
return False
def is_element_text_visible(self,locatr,text):
try:
element = self.driver.find_element(*Locatr.get_locatr_by(locatr))
return element.is_displayed() and text in element.text
except Exception:
return False<file_sep>/AutomationPractice/registration_and_login/pom/page_myaccount.py
from AutomationPractice.registration_and_login.pom.base import BasePage
from AutomationPractice.registration_and_login.pom.locators import Locators
class MyaccountPage(BasePage):
""" My Account Page Class """
def __init__(self, driver):
BasePage.__init__(self, driver)
# Below is creating class attribute for each locator in Locator class as one Element object and one locator_* string
for k, v in Locators.Myaccount.items():
setattr(self, k, self.com.element(v))
setattr(self, "elem_ " + k, v)
def get_logged_in_full_name(self):
return self.link_account().text.lower()
<file_sep>/AutomationPractice/registration_and_login/tests/test_login.py
import pytest
from AutomationPractice.registration_and_login.testdata.test_data_provider import TestDataProvider
class TestLogin:
@pytest.mark.positive
def test_open_homepage(self,app):
app.home_page.open_homepage()
assert("My Store" == app.home_page.get_title())
@pytest.mark.positive
@pytest.mark.parametrize("user_creds,page_title",
[({"username": "<EMAIL>", "password": "<PASSWORD>"},
"My account - My Store")])
def test_login_success(self, app, user_creds, page_title):
app.home_page.open_homepage()
app.home_page.click_sign_in()
app.authentication_page.login_section.perform_login(user_creds["username"], user_creds["password"])
assert (page_title == app.authentication_page.get_title())
@pytest.mark.negative
@pytest.mark.parametrize("user_creds,page_title,error_count,expected_errors",
TestDataProvider.get_creds_list_neg_combo1())
def test_login_failure_invalid_creds(self, app, user_creds, page_title, error_count, expected_errors):
app.home_page.open_homepage()
app.home_page.click_sign_in()
app.authentication_page.login_section.perform_login(user_creds["username"], user_creds["password"],
wait_for_next_page_load=False)
errors = app.authentication_page.login_section.get_error_list()
pytest.assume(error_count == len(errors))
for i in range(error_count):
pytest.assume(expected_errors[i] == errors[i])
pytest.assume(page_title == app.authentication_page.get_title())
<file_sep>/AutomationPractice/registration_and_login/pom/page_home.py
from AutomationPractice.registration_and_login.pom.base import BasePage
from AutomationPractice.registration_and_login.pom.locators import Locators
class HomePage(BasePage):
""" Home Page Class """
def __init__(self, driver, base_url=None):
BasePage.__init__(self, driver)
self.base_url = base_url
# Below is creating class attribute for each locator in Locator class as one Element object and one locator_* string
for k, v in Locators.Home.items():
setattr(self, k, self.com.element(v))
setattr(self, "elem_ " + k, v)
def open_homepage(self):
self.driver.get(self.base_url)
self.wait_for_page_load("homepage")
def click_sign_in(self):
self.link_sign_in().click()
self.wait_for_page_load("loginpage")
def click_sign_out(self):
self.link_sign_out().click()
<file_sep>/AutomationPractice/registration_and_login/testdata/test_data_provider.py
from faker import Faker
import os
from pprint import pprint
from sys import stdout as console
dir_path = os.path.dirname(os.path.realpath(__file__))
class TestDataProvider:
"""This is Test Data Provider class to supply test data after generating it as per requirement to test functions and saving created account in a csv file for later use."""
email_template = "<EMAIL>"
currentCount = None
@classmethod
def save_created_account(cls, email, password):
"""
To Save created account details for later use and not to generate same email account for new registration.
New created account should be saved through this
"""
with open(os.path.join(dir_path, "created_accounts.csv"), "a+") as fl:
fl.write("{}, {}\n".format(email, password))
console.write("====== Created User is Saved =======")
@classmethod
def get_created_account(cls, get_all=False):
""" To get account credentials for existing created account """
try:
with open(os.path.join(dir_path, "created_accounts.csv"), "r") as fl:
if get_all:
account = [[account.rstrip("\n").split(", ")] for account in fl.readlines()]
else:
account = fl.readline().rstrip("\n").split(", ")
except FileNotFoundError as e:
return 0, "No account is available"
return account
@classmethod
def generate_user_reg_data(cls, count=1, without_fields=None, only_required_fields=False,save_user=False):
"""
To Generate Personal Information Fields data for new account registration
"""
faker = Faker()
user_reg_data_list = []
for i in range(count):
user_reg_data = {}
user_reg_data["first_name"], user_reg_data["last_name"] = faker.first_name(), faker.last_name()
user_reg_data["email"] = cls.generate_email()
user_reg_data["password"] = "<PASSWORD>"
if save_user:
cls.save_created_account(user_reg_data["email"],user_reg_data["password"])
address = faker.address()
first_address, second_address = address.split("\n")
# _,state,zipcode = second_address.split()
user_reg_data["address1"] = first_address
user_reg_data["city"] = faker.city()
user_reg_data["state_index"] = faker.random_int(1, 53)
user_reg_data["zipcode"] = faker.zipcode()
user_reg_data["mobile_phone"] = 1111111112
user_reg_data["address_alias"] = str(user_reg_data["state_index"]) + str(user_reg_data["zipcode"])
if only_required_fields == False:
title = faker.boolean()
if title:
user_reg_data["title"] = "mrs"
else:
user_reg_data["title"] = "mr"
dob = str(faker.date_of_birth())
dob_year, dob_month, dob_date = dob.split("-")
user_reg_data["dob_year"], user_reg_data["dob_month"], user_reg_data["dob_date"] = int(dob_year), int(
dob_month), int(dob_date)
user_reg_data["company"] = faker.company()
user_reg_data["address2"] = second_address
user_reg_data["home_phone"] = 1111111111
user_reg_data["additional_info"] = faker.text()
if without_fields:
for item in without_fields:
del user_reg_data[item]
user_reg_data_list.append(user_reg_data)
return user_reg_data_list
@classmethod
def generate_email(cls):
"""To Generated new Email for new account creation as per saved account details """
return cls.email_template.format(cls.get_number_of_accounts() + 1)
@classmethod
def get_number_of_accounts(cls):
accounts = cls.get_created_account(get_all=True)
total_accounts = len(accounts)
TestDataProvider.currentCount = total_accounts
return total_accounts
@classmethod
def get_creds_list_neg_combo1(cls):
"""
For Login Tests,
It provide negative test data with expected error.
"""
user_creds_list = [({"username": "<EMAIL>", "password": "<PASSWORD>"},
"Login - My Store",
1, ["Authentication failed."]),
({"username": "<EMAIL>", "password": "<PASSWORD>"},
"Login - My Store",
1, ["Authentication failed."]),
({"username": "<EMAIL>", "password": "<PASSWORD>"},
"Login - My Store",
1, ["Authentication failed."]),
({"username": "", "password": ""},
"Login - My Store",
1, ["An email address required."]),
({"username": "<EMAIL>", "password": ""},
"Login - My Store",
1, ["Password is required."]),
({"username": "", "password": "<PASSWORD>"},
"Login - My Store",
1, ["An email address required."])
]
return user_creds_list
@classmethod
def get_user_reg_data_list_with_errors(cls):
"""
For Registration Tests.
This test function provides negative test data as required field.
One row of data is missing with one required field at a time and their expected error.
"""
required_fields_errors = {
"first_name": "firstname is required.",
"last_name": "lastname is required.",
"email": "email is required.",
"password": "<PASSWORD>.",
"address1": "address1 is required.",
"city": "city is required.",
"state_index": "This country requires you to choose a State.",
"zipcode": "The Zip/Postal code you've entered is invalid. It must follow this format: 00000",
"mobile_phone": "You must register at least one phone number.",
"address_alias": "alias is required."
}
generated_data = []
for k, v in required_fields_errors.items():
data1 = (cls.generate_user_reg_data(without_fields=[k], only_required_fields=True)[0],
[v])
data1[0]["email_for_create_account_page"] = cls.generate_email()
generated_data.append(data1)
return generated_data
<file_sep>/AutomationPractice/registration_and_login/tests/conftest.py
"""
Shared Fixtures
"""
import json
import os
import pytest
import selenium.webdriver
from AutomationPractice.registration_and_login.pom.page_home import HomePage
from AutomationPractice.registration_and_login.pom.page_authentication import AuthenticationPage
from AutomationPractice.registration_and_login.pom.page_myaccount import MyaccountPage
from core.browser import Browser
dir_path = os.path.dirname(os.path.realpath(__file__))
@pytest.fixture
def config(scope='session'):
# Read the file
with open(os.path.join(dir_path, 'config_test.json')) as config_file:
config = json.load(config_file)
# Assert values are acceptable
assert config['browser'] in ['firefox', 'chrome', 'Headless Chrome']
assert isinstance(config['implicit_wait'], int)
assert config['implicit_wait'] > 0
# Return config so it can be used
return config
@pytest.fixture
def app(config):
# get Web Driver
b = Browser.get_driver({"browser": config['browser'], "environment": config['environment'],"headless":config["headless"]})
# Make its calls wait for elements to appear
if config['environment']=="remote":
b.implicitly_wait(config['implicit_wait']+30)
else:
b.implicitly_wait(config['implicit_wait'])
app = App(b, config['base_url'])
yield app
b.quit()
del app
class App:
def __init__(self, driver, base_url):
self.home_page = HomePage(driver, base_url)
self.authentication_page = AuthenticationPage(driver)
self.myaccount_page = MyaccountPage(driver)
| deddc5bf97356c07439d9e7db678ea788472354d | [
"Markdown",
"Python"
] | 11 | Python | reetambhowmick/py-test-auto-framework | a9a2ce55d23dea9c53d05799f2b1f3380aa1d854 | 21c17526e272dbc83e7cef3e63ac8d6e8452623b | |
refs/heads/master | <repo_name>laurasimonp/oeb-documentation-frontend<file_sep>/src/app/app-routing.module.ts
import { NgModule } from "@angular/core";
import { RouterModule, Routes } from "@angular/router";
import { OebComponent } from "./oeb/oeb.component";
import { ScientificComponent } from "./scientific/scientific.component";
import { TechnicalComponent } from "./technical/technical.component";
import { TopicComponent } from "./topic/topic.component";
import { RepositoryComponent } from "./repository/repository.component";
import { RepositoryDataComponent } from "./repository-data/repository-data.component";
import { APIsComponent } from './apis/apis.component';
import { PageNotFoundComponent } from "./page-not-found/page-not-found.component";
const appRoutes: Routes = [
{ path: "oeb", component: OebComponent },
{ path: "scientific", component: ScientificComponent },
{ path: "technical", component: TechnicalComponent },
{ path: "topics", component: TopicComponent },
{ path: "repositories", component: RepositoryComponent },
{ path: "repositories/:name", component: RepositoryDataComponent },
{ path: "apis", component: APIsComponent },
{ path: "", redirectTo: "/oeb", pathMatch: "full" },
{ path: "**", component: PageNotFoundComponent },
];
@NgModule({
imports: [RouterModule.forRoot(appRoutes, { enableTracing: false })],
exports: [RouterModule],
})
export class AppRoutingModule { }
<file_sep>/src/app/model/Topic.ts
export class Topic {
private _name: String;
private _url: String;
private _description: String;
/**
*Creates an instance of Topic.
* @param {String} [name]
* @param {String} [url]
* @param {String} [description]
* @memberof Topic
*/
constructor(name?: String, url?: String, description?: String) {
this._name = name;
this._url = url;
this._description = description;
}
/**
* Getter name
* @return {String}
*/
public get name(): String {
return this._name;
}
/**
* Getter url
* @return {String}
*/
public get url(): String {
return this._url;
}
/**
* Getter description
* @return {String}
*/
public get description(): String {
return this._description;
}
/**
* Setter name
* @param {String} value
*/
public set name(value: String) {
this._name = value;
}
/**
* Setter url
* @param {String} value
*/
public set url(value: String) {
this._url = value;
}
/**
* Setter description
* @param {String} value
*/
public set description(value: String) {
this._description = value;
}
}<file_sep>/src/app/topic/topic.component.ts
import { Component, OnInit } from '@angular/core';
import { Topic } from '../model/Topic';
import { TopicService } from "../services/topic.service";
@Component({
selector: 'app-topic',
templateUrl: './topic.component.html',
styleUrls: ['./topic.component.css']
})
export class TopicComponent implements OnInit {
//attributes
topics: Topic[];
constructor(private topicService: TopicService) { }
ngOnInit(): void {
this.topicService.getTopics().subscribe(data => {
this.topics = data;
});
}
}
<file_sep>/src/app/app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { HttpClientModule } from "@angular/common/http";
import { NgxPaginationModule } from "ngx-pagination";
import { AppComponent } from "./app.component";
import { AppRoutingModule } from "./app-routing.module";
import { OebComponent } from "./oeb/oeb.component";
import { ScientificComponent } from "./scientific/scientific.component";
import { TechnicalComponent } from "./technical/technical.component";
import { TopicComponent } from "./topic/topic.component";
import { RepositoryComponent } from "./repository/repository.component";
import { RepositoryDataComponent } from "./repository-data/repository-data.component";
import { PageNotFoundComponent } from "./page-not-found/page-not-found.component";
import { MarkedPipe } from "./marked.pipe";
import { TopMenuComponent } from "./top-menu/top-menu.component";
import { NgSelectModule } from "@ng-select/ng-select";
import { APIsComponent } from './apis/apis.component';
@NgModule({
imports: [
BrowserModule,
FormsModule,
HttpClientModule,
NgxPaginationModule,
AppRoutingModule,
NgSelectModule,
],
declarations: [
AppComponent,
OebComponent,
ScientificComponent,
TechnicalComponent,
TopicComponent,
RepositoryComponent,
RepositoryDataComponent,
PageNotFoundComponent,
MarkedPipe,
TopMenuComponent,
APIsComponent,
],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule { }
<file_sep>/src/app/repository-data/repository-data.component.ts
import { Component, OnInit } from "@angular/core";
import { Repository } from "../model/Repository";
import { RepositoryService } from "../services/repository.service";
import { ActivatedRoute } from "@angular/router";
@Component({
selector: "app-repository-data",
templateUrl: "./repository-data.component.html",
styleUrls: ["./repository-data.component.css"],
})
export class RepositoryDataComponent implements OnInit {
//attributes
repo: Repository;
constructor(
private repoService: RepositoryService,
private route: ActivatedRoute
) { }
ngOnInit(): void {
this.viewRepoData(this.getParam("name"));
}
/**
* Get param from url
*/
public getParam(param: string): string {
return this.route.snapshot.paramMap.get(param);
}
viewRepoData(name: string) {
this.repoService.getRepoDataByName(name).subscribe((data) => {
this.repo = data;
});
}
}
<file_sep>/src/app/services/topic.service.ts
import { Injectable } from '@angular/core';
import { Topic } from "../model/Topic";
import { HttpClient } from "@angular/common/http";
import { Observable } from "rxjs";
import { environment } from "../../environments/environment";
@Injectable({
providedIn: 'root'
})
export class TopicService {
private topicsUrl = environment.TOPICS;
public topics: Observable<Topic[]>;
constructor(private http: HttpClient) { }
getTopics(): Observable<Topic[]> {
this.topics = this.http.get<Topic[]>(this.topicsUrl);
return this.topics;
}
}
<file_sep>/src/app/model/Contributor.ts
export class Contributor {
private _login: string;
private _url: string;
/**
*Creates an instance of Contributor.
* @param {string} login
* @param {string} url
* @memberof Contributor
*/
constructor(login: string, url: string) {
this._login = login;
this._url = url;
}
/**
* Getter login
* @return {string}
*/
public get login(): string {
return this._login;
}
/**
* Getter url
* @return {string}
*/
public get url(): string {
return this._url;
}
/**
* Setter login
* @param {string} value
*/
public set login(value: string) {
this._login = value;
}
/**
* Setter url
* @param {string} value
*/
public set url(value: string) {
this._url = value;
}
}<file_sep>/src/app/top-menu/top-menu.component.ts
import { Component, OnInit } from "@angular/core";
import { Location } from "@angular/common";
/**
* This component is where we specify the top menu paths
*/
@Component({
selector: "app-top-menu",
templateUrl: "./top-menu.component.html",
styleUrls: ["./top-menu.component.css"]
})
export class TopMenuComponent implements OnInit {
constructor(private location: Location) { }
/**
* Navigation links and labels for the menu on the left (LOGO)
*/
public dashboardLink = {
label: "OpenEBench",
path: "oeb"
};
/**
* Navigation links and labels for the menu on the right
*/
public navLinks: any[];
ngOnInit() {
this.navLinks = [
{
label: "OpenEBench",
path: "/oeb"
},
{
label: "Scientific Benchmarking",
path: "/scientific"
},
{
label: "Technical Monitoring",
path: "/technical"
},
{
label: "Topics",
path: "/topics"
},
{
label: "Repositories",
path: "/repositories"
},
{
label: "APIs",
path: "/apis"
}
];
}
/**
* Get URL path
*/
getPath() {
return this.location.isCurrentPathEqualTo(this.dashboardLink.path);
}
}
<file_sep>/src/app/services/repository.service.ts
import { Injectable } from "@angular/core";
import { Repository } from "../model/Repository";
import { HttpClient } from "@angular/common/http";
import { HttpParams } from "@angular/common/http";
import { Observable } from "rxjs";
import { environment } from "../../environments/environment";
@Injectable({
providedIn: "root",
})
export class RepositoryService {
private topicsUrl = environment.TOPICS;
private reposUrl = environment.REPOSITORIES;
private repoUrl = environment.REPOSITORY;
public topics: Observable<string[]>;
public repos: Observable<Repository[]>;
public filteredRepos: Observable<Repository[]>;
public repo: Observable<Repository>;
constructor(private http: HttpClient) { }
getTopics(): Observable<string[]> {
this.topics = this.http.get<string[]>(this.topicsUrl);
return this.topics;
}
getRepos(): Observable<Repository[]> {
this.repos = this.http.get<Repository[]>(this.reposUrl);
return this.repos;
}
getFilteredRepos(topicsArray: string[]): Observable<Repository[]> {
const options = topicsArray
? {
params: new HttpParams({
fromObject: { t: topicsArray },
}),
}
: {};
this.filteredRepos = this.http.get<Repository[]>(this.reposUrl, options);
return this.filteredRepos;
}
getRepoDataByName(name: string): Observable<Repository> {
this.repo = this.http.get<Repository>(this.repoUrl + name);
return this.repo;
}
}
<file_sep>/src/app/model/Repository.ts
export class Repository {
private _id: string;
private _name: string;
private _topics: string[];
private _description: string;
private _url: string;
private _contributors: string[];
private _languages: string[];
private _license: string;
private _readme: string;
/**
*Creates an instance of Repository.
* @param {string} id
* @param {string} name
* @param {string[]} topics
* @param {string} description
* @param {string} url
* @param {string[]} contributors
* @param {string[]} languages
* @param {string} license
* @param {string} readme
* @memberof Repository
*/
constructor(id?: string, name?: string, topics?: string[], description?: string, url?: string, contributors?: string[], languages?: string[], license?: string, readme?: string) {
this._id = id;
this._name = name;
this._topics = topics;
this._description = description;
this._url = url;
this._contributors = contributors;
this._languages = languages;
this._license = license;
this._readme = readme;
}
/**
* Getter id
* @return {string}
*/
public get id(): string {
return this._id;
}
/**
* Getter name
* @return {string}
*/
public get name(): string {
return this._name;
}
/**
* Getter topics
* @return {string[]}
*/
public get topics(): string[] {
return this._topics;
}
/**
* Getter description
* @return {string}
*/
public get description(): string {
return this._description;
}
/**
* Getter url
* @return {string}
*/
public get url(): string {
return this._url;
}
/**
* Getter contributors
* @return {string[]}
*/
public get contributors(): string[] {
return this._contributors;
}
/**
* Getter languages
* @return {string[]}
*/
public get languages(): string[] {
return this._languages;
}
/**
* Getter license
* @return {string}
*/
public get license(): string {
return this._license;
}
/**
* Getter readme
* @return {string}
*/
public get readme(): string {
return this._readme;
}
/**
* Setter id
* @param {string} value
*/
public set id(value: string) {
this._id = value;
}
/**
* Setter name
* @param {string} value
*/
public set name(value: string) {
this._name = value;
}
/**
* Setter topics
* @param {string[]} value
*/
public set topics(value: string[]) {
this._topics = value;
}
/**
* Setter description
* @param {string} value
*/
public set description(value: string) {
this._description = value;
}
/**
* Setter url
* @param {string} value
*/
public set url(value: string) {
this._url = value;
}
/**
* Setter contributors
* @param {string[]} value
*/
public set contributors(value: string[]) {
this._contributors = value;
}
/**
* Setter languages
* @param {string[]} value
*/
public set languages(value: string[]) {
this._languages = value;
}
/**
* Setter license
* @param {string} value
*/
public set license(value: string) {
this._license = value;
}
/**
* Setter readme
* @param {string} value
*/
public set readme(value: string) {
this._readme = value;
}
}
<file_sep>/src/app/repository/repository.component.ts
import { Component, OnInit } from "@angular/core";
import { Repository } from "../model/Repository";
import { RepositoryService } from "../services/repository.service";
import { Topic } from "../model/Topic";
@Component({
selector: "app-repository",
templateUrl: "./repository.component.html",
styleUrls: ["./repository.component.css"],
})
export class RepositoryComponent implements OnInit {
// attributes
topics: string[] = [];
repos: Repository[] = [];
// filter properties
nameFilter = "";
selectedTopics: Topic[] = [];
filteredRepos: Repository[] = [];
// pagination properties
currentPage: number;
itemsPerPage: number;
constructor(private repoService: RepositoryService) { }
ngOnInit(): void {
this.repoService.getTopics().subscribe((data) => {
this.topics = data;
});
this.repoService.getRepos().subscribe((data) => {
this.repos = data;
this.filteredRepos = this.repos;
});
this.itemsPerPage = 10;
this.currentPage = 1;
}
getSelectedTopics() {
const filterTopics: string[] = [];
this.selectedTopics
? this.selectedTopics.forEach((element) => {
filterTopics.push(element.url.toString());
})
: "";
this.repoService.getFilteredRepos(filterTopics).subscribe((data) => {
this.filteredRepos = data;
});
}
filter() {
this.filteredRepos = this.repos.filter((repository) => {
let nameValid = false;
for (let topic of repository.topics) {
if (repository.name.toLowerCase().indexOf(
this.nameFilter.toLowerCase()) != -1) {
nameValid = true;
} else if (repository.description.toLowerCase().indexOf(
this.nameFilter.toLowerCase()) != -1) {
nameValid = true;
} else if (topic.toLowerCase().indexOf(
this.nameFilter.toLowerCase()) != -1) {
nameValid = true;
}
}
return nameValid;
});
}
}
| 87d9db2c4ea4e3f7d7952fcca3dfe6662079a0fa | [
"TypeScript"
] | 11 | TypeScript | laurasimonp/oeb-documentation-frontend | fcbd7a2f9b5b927358a79cb60bbc6a3b12095213 | aea5347e91f4b3bd33324c09678cf0ce27023466 | |
refs/heads/master | <file_sep>var app = new Vue({
el: '.app',
data: {
title: 'Mastrangelo Sindaco',
showLegal: false,
showList: false,
elezionitrasparenti: true,
key: '',
min: 1,
max: 16,
coalizione: [
{
key: 'forzaitalia',
logo: 'images/forzaitalia.png',
name: '<NAME>'
},
{
key: 'fratelliditalia',
logo: 'images/fratelliditalia.png',
name: 'Fratelli d\'Italia'
},
{
key: 'lega',
logo: 'images/lega.png',
name: '<NAME>'
},
{
key: 'rinnova',
logo: 'images/rinnova.png',
name: '(r)INNova'
},
{
key: 'mastrangelo',
logo: 'images/mastrangelo.png',
name: '<NAME>'
},
{
key: 'primagioia',
logo: 'images/primagioia.png',
name: '<NAME>'
}
]
},
methods: {
goToLegal: function () {
this.showLegal = !this.showLegal;
this.showList = false;
console.log(this.showLegal);
},
changeLista: function (lista) {
this.key = lista;
this.showList = true;
console.log('lista changed to -> ' + this.key);
},
getListImage: function (index) {
var r = 'coalizione/' + this.key.key + '/' + index + '.jpg';
console.log(r);
return r;
},
getListCV: function (index) {
return 'coalizione/' + this.key.key + '/' + index + '-cv.pdf';
},
getListLegal: function (index) {
return 'coalizione/' + this.key.key + '/' + index + '-legal.pdf';
}
}
}) | 9cb9bf86115e90b52a1ba195897d2f7bcc7f7512 | [
"JavaScript"
] | 1 | JavaScript | followhiterabbit-www/mastrangelosindaco.it | abda743dd1779197157cc9058ccae1993347d5f0 | 456fc7f9f9cd6caf656df7be317fc273974e2483 | |
refs/heads/master | <file_sep>#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Tue Apr 9 11:38:55 2019
@author: <NAME>
"""
import numpy as np
import rdkit
from rdkit import Chem
from rdkit.Chem import Descriptors
import pandas as pd
def generate(smiles, verbose=False):
moldata= []
for elem in smiles:
mol=Chem.MolFromSmiles(elem)
moldata.append(mol)
baseData= np.arange(1,1)
i=0
for mol in moldata:
desc_MolWt = Descriptors.MolWt(mol)
desc_MolLogP = Descriptors.MolLogP(mol)
desc_MolMR = Descriptors.MolMR(mol)
desc_HeavyAtomCount = Descriptors.HeavyAtomCount(mol)
desc_NumHAcceptors = Descriptors.NumHAcceptors(mol)
desc_NumHDonors = Descriptors.NumHDonors(mol)
desc_NumHeteroatoms = Descriptors.NumHeteroatoms(mol)
desc_NumRotatableBonds = Descriptors.NumRotatableBonds(mol)
desc_NumValenceElectrons = Descriptors.NumValenceElectrons(mol)
desc_NumAromaticRings = Descriptors.NumAromaticRings(mol)
desc_NumSaturatedRings = Descriptors.NumSaturatedRings(mol)
desc_NumAliphaticRings = Descriptors.NumAliphaticRings(mol)
desc_RingCount = Descriptors.RingCount(mol)
desc_TPSA = Descriptors.TPSA(mol)
desc_LabuteASA = Descriptors.LabuteASA(mol)
desc_BalabanJ = Descriptors.BalabanJ(mol)
desc_BertzCT = Descriptors.BertzCT(mol)
row = np.array([desc_MolWt,desc_MolLogP,desc_MolMR,desc_HeavyAtomCount,desc_NumHAcceptors,desc_NumHDonors,desc_NumHeteroatoms,
desc_NumRotatableBonds,desc_NumValenceElectrons,desc_NumAromaticRings,desc_NumSaturatedRings,
desc_NumAliphaticRings,desc_RingCount,desc_TPSA,desc_LabuteASA,desc_BalabanJ,desc_BertzCT])
if(i==0):
baseData=row
else:
baseData=np.vstack([baseData, row])
i=i+1
columnNames=["MolWt","MolLogP","MolMR","HeavyAtomCount","NumHAcceptors","NumHDonors","NumHeteroatoms",
"NumRotatableBonds","NumValenceElectrons","NumAromaticRings","NumSaturatedRings",
"NumAliphaticRings","RingCount","TPSA","LabuteASA","BalabanJ","BertzCT"]
descriptors = pd.DataFrame(data=baseData,columns=columnNames)
print("Total descriptors generated: 17 x "+str(len(smiles)))
return descriptors<file_sep># Aqueous Solubility Data Curation
AqSolDB is created by the Autonomous Energy Materials Discovery [AMD] research group, consists of aqueous solubility values of 9,982 unique compounds curated from 9 different publicly available aqueous solubility datasets. This openly accessible dataset, which is the largest of its kind, and will not only serve as a useful reference source of measured solubility data, but also as a much improved and generalizable training data source for building data-driven models.
## Citation
If you use AqSolDB in your study, please cite the following paper.
Paper: [Nature Scientific Data](https://doi.org/10.1038/s41597-019-0151-1) - https://doi.org/10.1038/s41597-019-0151-1
## Other Platforms
> Online reproducible code: [Code Ocean](https://doi.org/10.24433/CO.1992938.v1)
> Data science kernels: [Kaggle](https://www.kaggle.com/sorkun/aqsoldb-a-curated-aqueous-solubility-dataset)
> Easy search from AqSolDB: [AMD Website](https://www.amdlab.nl/database/AqSolDB/)
> Data Repository: [Harvard Dataverse](https://doi.org/10.7910/DVN/OVHAW8)
## Overview
This repository has been developed in order to curate various aqueous solubility datasets into a broad and extensive dataset called AqSolDB.
The curation process in this work can be accomplished by executing two python scripts in the given sequence:
1. data-preprocess.py - for pre-processing the raw data set to a standardized format
2. data-curation.py - for merging the standardized datasets, assigning reliability lables and adding 2D descriptors
These two python scripts call upon functions from other python modules that are defined in:
- preprocess.py
- merge.py
- descriptors.py
Further information about curation process can be found in the associated manuscript.
# Examples
## data-preprocess.py
This file converts 2 example sub-datasets (25 instances from raw forms of dataset-A[1] and dataset-H[6]) which are then converted into a standardized format. (This is an example how to preprocess datasets. The preporcessed data files already in the data folder.)
**inputs:**
1. raw-dataset-A.csv (various solubility metrics (g/L, mg/L..) with Name and CAS Number)
2. raw-dataset-H.csv (has solubility values(LogS) with SLN representations)
**outputs:**
1. dataset-A.csv
2. dataset-H.csv
### Note
To apply this method to your own dataset, perform the following steps:
1. Check the available properties, representations, and solubility units of your dataset
2. Select the suitable preprocessing methods from the "preprocess.py" module.
## data-curation.py
This file curates, i.e., merges datasets, selects most reliable values among multiple occurences, and adds 2D descriptors from 9 different standardized datasets that are obtained after the pre-processing step.
**inputs:**
1. dataset-A.csv [1]
2. dataset-B.csv [2]
3. dataset-C.csv [3]
4. dataset-D.csv [4]
5. dataset-E.csv [5]
6. dataset-F.csv [6]
7. dataset-G.csv [7]
8. dataset-H.csv [6]
9. dataset-I.csv [8]
**outputs:**
1. dataset_curated.csv
### Note
To apply this method, your input dataset should be in the standardized format (output of preprocessing) having following columns:
- ID
- Name
- InChI
- InChIKey
- SMILES
- Solubility
- Prediction
# License and Copyright
MIT License
Copyright (c) 2019 <NAME>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
# References
[1] eChemPortal - The Global Portal to Information on Chemical Substances. https://www.echemportal.org/.
[2] <NAME>. Preliminary Report: Water Solubility Estimation by Base Compound Modification.Environmental Science Center, Syracuse, NY (1995).
[3] <NAME>., <NAME>., <NAME>., <NAME>.& <NAME>. Calculation of aqueous solubility of crystalline un-ionized organic chemicals and drugs based on structural similarity and physicochemical descriptors.Journal of Chemical Information and Computer Sciences 54, 683–691 (2014).
[4] <NAME>., <NAME>. Upgrade of PCGEMS Water Solubility Estimation Method. Environmental Science Center, Syracuse, NY(1994)
[5] <NAME>. Estimation of aqueous solubility for a diverse set of organic compounds based on molecular topology.Journal of Chemical Informationand Computer Sciences 40, 773–777 (2000).
[6] <NAME>., <NAME>. & <NAME>. Aqueous solubility prediction based on weighted atom type counts and solvent accessible surface areas. Journal of Chemical Information and Modeling 49, 571–581 (2009).
[7] <NAME>. S. ESOL: estimating aqueous solubility directly from molecular structure. Journal of Chemical Information and Computer Sciences 44,1000–1005 (2004).
[8] <NAME>., <NAME>. & <NAME>. Solubility challenge: can you predict solubilities of 32 molecules using a database of 100 reliable measurements?.Journal of Chemical Information and Modeling 48, 1289–1303 (2008).
<file_sep>#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon Apr 8 11:31:39 2019
@author: <NAME>
"""
import pandas as pd
import merge
import descriptors
data_a = pd.read_csv('data/dataset-A.csv', header=0)
data_b = pd.read_csv('data/dataset-B.csv', header=0)
data_c = pd.read_csv('data/dataset-C.csv', header=0)
data_d = pd.read_csv('data/dataset-D.csv', header=0)
data_e = pd.read_csv('data/dataset-E.csv', header=0)
data_f = pd.read_csv('data/dataset-F.csv', header=0)
data_g = pd.read_csv('data/dataset-G.csv', header=0)
data_h = pd.read_csv('data/dataset-H.csv', header=0)
data_i = pd.read_csv('data/dataset-I.csv', header=0)
# first merge data directly
data_merged= pd.concat([data_a,data_b,data_c,data_d,data_e,data_f,data_g,data_h,data_i])
data_merged=data_merged.reset_index(drop=True)
# curate the data
data_curated = merge.curate(data_merged)
# generate the descriptors
descriptors = descriptors.generate(data_curated['SMILES'].values)
# combine descriptors with
data_curated=pd.concat([data_curated, descriptors], axis=1)
# write into CSV file
data_curated.to_csv("results/data_curated.csv", sep=',', encoding='utf-8', index=False)
<file_sep>#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Tue Apr 9 11:30:41 2019
@author: <NAME>
"""
import numpy as np
import pandas as pd
import preprocess
def main():
data_path_a="data/raw-dataset-A.csv"
data_path_h="data/raw-dataset-H.csv"
#raw dataset various solubility metrics (g/L, mg/L..) with Name and CAS Number
preprocess_dataset_a(data_path_a)
#raw dataset has solubility values(LogS) with SLN representations
preprocess_dataset_h(data_path_h)
def preprocess_dataset_a(data_path):
print("\n\n**\nPreprocess of raw-dataset-A is started\n**")
data = pd.read_csv(data_path, header=0)
smiles_list=preprocess.collect_smiles_from_web(data['Name'].values,data['CAS'].values,verbose=0)
inchi_list,inchikey_list=preprocess.smiles_to_inchi_inchikey(smiles_list,verbose=0)
id_list=preprocess.generate_id_list("A",len(smiles_list))
name_list=preprocess.collect_names_from_web(inchikey_list,smiles_list,verbose=0)
logs_list=preprocess.convert_to_logs(smiles_list,data['Metric'].values,data['OriginalSol'].values,verbose=0)
prediction_list=preprocess.collect_predictions_from_web(smiles_list,verbose=0)
dataset_a_df = pd.DataFrame(np.column_stack([ id_list, name_list, inchi_list, inchikey_list, smiles_list, logs_list, prediction_list]),
columns=[ 'ID', 'Name', 'InChI', 'InChIKey', 'SMILES', 'Solubility', 'Prediction'])
#filter dataset by removing missing information. (for strings: "XXX" and for numeric: "999")
dataset_a_df_clean = preprocess.clean(dataset_a_df)
#update ID after filtering
id_list=preprocess.generate_id_list("A",len(dataset_a_df_clean.index))
id_clean_df = pd.DataFrame({'ID': id_list})
dataset_a_df_clean.update(id_clean_df)
#write dataset into CSV file
dataset_a_df_clean.to_csv('../results/dataset-A.csv', index=False)
print("**\nPreprocessed dataset-A is written into dataset-A.csv\n**")
return
def preprocess_dataset_h(data_path):
print("\n\n**\nPreprocess of raw-dataset-H is started\n**")
data = pd.read_csv(data_path, header=0)
smiles_list=preprocess.sln_to_smiles(data['SLN'].values,verbose=0)
inchi_list,inchikey_list=preprocess.smiles_to_inchi_inchikey(smiles_list,verbose=0)
id_list=preprocess.generate_id_list("H",len(smiles_list))
name_list=preprocess.collect_names_from_web(inchikey_list,smiles_list,verbose=0)
logs_list=data['Solubility'].values
prediction_list=preprocess.collect_predictions_from_web(smiles_list,verbose=0)
dataset_h_df = pd.DataFrame(np.column_stack([ id_list, name_list, inchi_list, inchikey_list, smiles_list, logs_list, prediction_list]),
columns=[ 'ID', 'Name', 'InChI', 'InChIKey', 'SMILES', 'Solubility', 'Prediction'])
#filter dataset by removing missing information. (for strings: "XXX" and for numeric: "999")
dataset_h_df_clean = preprocess.clean(dataset_h_df)
#update ID after filtering
id_list=preprocess.generate_id_list("H",len(dataset_h_df_clean.index))
id_clean_df = pd.DataFrame({'ID': id_list})
dataset_h_df_clean.update(id_clean_df)
#write dataset into CSV file
dataset_h_df_clean.to_csv('../results/dataset-H.csv', index=False)
print("**\nPreprocessed dataset-H is written into dataset-H.csv\n**")
return
if __name__== "__main__":
main()<file_sep>#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon Apr 8 13:52:29 2019
@author: <NAME>
"""
import numpy as np
import pandas as pd
import math
# Selects most reliable values among the multiple occurences
def curate(data_merged):
smiles_list= data_merged['SMILES'].tolist()
solubility_list= data_merged['Solubility'].tolist()
id_list= data_merged['ID'].tolist()
inchi_list= data_merged['InChI'].tolist()
name_list= data_merged['Name'].tolist()
prediction_list= data_merged['Prediction'].tolist()
# define variables and assign default values
dif_val_list = []
dif_sol_list = []
same_value_counter=0 #same molecules with same values
different_value_counter_2times=0 #same molecules with different values (2 occurences)
different_value_counter_mutiple=0 #same molecules with different values (more than 2 occurences)
ocurrence_count=[-999]*len(id_list)
SD=[-999]*len(id_list)
reliability_group=["-"]*len(id_list)
selected_list=[0]*len(id_list)
# First step: Remove same molecules with same solubility values (change their SMILES into "XXX")
for i in range(0,len(id_list)):
same_value_List=[]
if(smiles_list[i] != "XXX" ):
same_value_List.append(i)
# collect same molecules with in range of 0.01 solubility value
for j in range(i+1,len(id_list)):
if(inchi_list[i]==inchi_list[j]):
if(math.fabs(solubility_list[i]-solubility_list[j])<=0.01):
same_value_List.append(j)
# select the best source according to: 1:name existance 2: size of the dataset (already in ordered according to size)
if(len(same_value_List)>1):
bestId=same_value_List[0]
for sameId in same_value_List:
if((pd.isnull(name_list[bestId]) or name_list[bestId]=="-") and ( not pd.isnull(name_list[sameId]) and name_list[sameId]!="-")):
bestId=sameId
same_value_List.remove(bestId)
for sameId in same_value_List:
smiles_list[sameId]="XXX"
same_value_counter=same_value_counter+1
print ("Total removed same molecule with same value: "+str(same_value_counter))
# Second step: Select the most reliable solubility value among the same molecules (change unselected SMILES into XXX)
for i in range(0,len(id_list)):
same_molecule_List=[]
# collect same molecules with different solubility value
if(smiles_list[i] != "XXX" and selected_list[i]==0):
same_molecule_List.append(i)
for j in range(i+1,len(id_list)):
if(smiles_list[j] != "XXX" and inchi_list[i]==inchi_list[j]):
same_molecule_List.append(j)
# if occurrence count=1 (Group:G1)
if(len(same_molecule_List)==1):
selected_list[i]=1
reliability_group[i]="G1"
SD[i]=0
ocurrence_count[i]=1
# if occurrence count = 2 (closest to reference (prediction) method )
elif(len(same_molecule_List)==2):
# calculate difference betweeen values and prediction (tie breaker)
diff1=math.fabs(solubility_list[same_molecule_List[0]]-prediction_list[same_molecule_List[0]])
diff2=math.fabs(solubility_list[same_molecule_List[1]]-prediction_list[same_molecule_List[1]])
bestId=same_molecule_List[0]
if(diff1<=diff2):
smiles_list[same_molecule_List[1]]="XXX"
different_value_counter_2times=different_value_counter_2times+1
bestId=same_molecule_List[0]
selected_list[bestId]=1
else:
smiles_list[same_molecule_List[0]]="XXX"
different_value_counter_2times=different_value_counter_2times+1
bestId=same_molecule_List[1]
selected_list[bestId]=1
# decide reliability group (if SD>0.5 Group:G2, else Group:G3)
diff=math.fabs(solubility_list[same_molecule_List[0]]-solubility_list[same_molecule_List[1]])
if(diff>1):
reliability_group[bestId]="G2"
else:
reliability_group[bestId]="G3"
# store differences and SD and occurrence count
SD[bestId]=diff/2
ocurrence_count[bestId]=2
# if occurrence count > 2 (closest to mean method )
elif(len(same_molecule_List)>2):
total=0
different_solubility_values_list=[]
for sameId in same_molecule_List:
total=total+solubility_list[sameId]
different_solubility_values_list.append(solubility_list[sameId])
mean=total / len(same_molecule_List)
bestId=same_molecule_List[0]
bestDiff=999
for sameId in same_molecule_List:
diff=math.fabs(solubility_list[sameId]-mean)
if(diff<bestDiff):
bestId=sameId
bestDiff=diff
selected_list[bestId]=1
std=np.std(different_solubility_values_list, axis=0)
SD[bestId]=std
ocurrence_count[bestId]=len(same_molecule_List)
# decide reliability group (if SD>0.5 Group:G4, else Group:G5)
if(std>0.5):
reliability_group[bestId]="G4"
else:
reliability_group[bestId]="G5"
same_molecule_List.remove(bestId)
for sameId in same_molecule_List:
smiles_list[sameId]="XXX"
different_value_counter_mutiple=different_value_counter_mutiple+1
# add reliability information to curated dataset and filter duplicates
data_merged['SD']=pd.Series(SD)
data_merged['Occurrences']=pd.Series(ocurrence_count)
data_merged['Group']=pd.Series(reliability_group)
data_merged=data_merged.drop(columns=['Prediction'])
data_filtered=data_merged[data_merged['Group'] !="-"]
data_filtered=data_filtered.reset_index(drop=True)
return data_filtered
<file_sep>#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Wed Apr 3 17:23:41 2019
@authors: <NAME>, <NAME>
"""
import numpy as np
from rdkit import Chem
from rdkit.Chem import rdSLNParse
from rdkit.Chem import rdinchi
from rdkit.Chem import Descriptors
import urllib.request as urllib2
from time import sleep
#Convert SLN to SMILES
def sln_to_smiles(sln_list,verbose=0):
error_counter=0
warning_counter=0
smiles_list= []
inchis_from_sln=[]
inchis_from_smiles=[]
for elem in sln_list:
mol=rdSLNParse.MolFromQuerySLN(elem)
if(mol!=None):
smiles=Chem.MolToSmiles(mol)
smiles_list.append(smiles)
inchi, retcode, message, logs, aux =rdinchi.MolToInchi(mol)
inchis_from_sln.append(inchi)
mol=Chem.MolFromSmiles(smiles)
if(mol!=None):
inchi, retcode, message, logs, aux =rdinchi.MolToInchi(mol)
inchis_from_smiles.append(inchi)
else:
inchis_from_smiles.append("XXX")
if(verbose!=0):
print(elem+ ": SMILES cannot converted to mol object, added XXX instead!")
else:
smiles_list.append("XXX")
print(elem+ ": SLN can not converted to mol object, added XXX instead!")
error_counter=error_counter+1
for i1,i2 in zip(inchis_from_sln,inchis_from_smiles):
if(i1!=i2):
if(verbose!=0):
print("Warning:"+i1+" - "+i2)
warning_counter=warning_counter+1
print("\nConversion from SLN to SMILES is completed.")
print("Total errors:"+str(error_counter))
print("Total warnings:"+str(warning_counter)+"\n")
return smiles_list
#Returns InChI InChIKey from SMILES
def smiles_to_inchi_inchikey(smiles,verbose=0):
error_counter=0
warning_counter=0
inchis=[]
inchis2=[]
inchiKeys=[]
for elem in smiles:
mol=Chem.MolFromSmiles(elem)
if(mol!=None):
inchi, retcode, message, logs, aux =rdinchi.MolToInchi(mol)
inchiKey=rdinchi.InchiToInchiKey(inchi)
inchis.append(inchi)
inchiKeys.append(inchiKey)
try:
mol, retcode, message, logs=rdinchi.InchiToMol(inchi)
if(mol!=None):
inchi2, retcode, message, logs, aux =rdinchi.MolToInchi(mol)
inchis2.append(inchi2)
else:
inchis2.append("XXX")
if(verbose!=0):
print(elem+ ": InChI cannot converted to mol object, added XXX instead!")
except:
if(verbose!=0):
print(retcode)
print(message)
print("Smiles:"+elem)
inchis2.append("XXX")
else:
inchis.append("XXX")
inchiKeys.append("XXX")
inchis2.append("XXX")
if(verbose!=0):
print(elem+ ": can not converted added XXX instead! ")
error_counter=error_counter+1
for i1,i2 in zip(inchis,inchis2):
if(i1!=i2):
if(verbose!=0):
print("Warning:"+i1+" - "+i2)
warning_counter=warning_counter+1
print("\nGeneration of InChI and InChIKey from SMILES is completed.")
print("Total errors:"+str(error_counter))
print("Total warnings:"+str(warning_counter)+"\n")
return inchis,inchiKeys
#Returns ID list by dataset name and size
def generate_id_list(name,size):
id_list= []
for i in range(1, size+1):
id_list.append(name+"-"+str(i))
return id_list
#Converts different solubility units into the LogS
def convert_to_logs(smiles_list,unit_list,solubility_list,verbose=0):
error_counter=0
logs_list=[]
for i in range(len(smiles_list)):
try:
mw=Descriptors.MolWt(Chem.MolFromSmiles(smiles_list[i]))
try:
if(unit_list[i]=="g/L"):
logs_list.append(np.log10(float(solubility_list[i])/mw))
elif(unit_list[i]=="mg/L"):
logs_list.append(np.log10(float(solubility_list[i])/(mw*1000)))
elif(unit_list[i]=="µg/L"):
logs_list.append(np.log10(float(solubility_list[i])/(mw*1000000)))
elif(unit_list[i]=="M"):
logs_list.append(np.log10(float(solubility_list[i])))
elif(unit_list[i]=="µM"):
logs_list.append(np.log10(float(solubility_list[i])/1000000))
elif(unit_list[i]=="LogS"):
logs_list.append(solubility_list[i])
else:
if(verbose!=0):
print("Unknown metric:"+unit_list[i])
error_counter=error_counter+1
logs_list.append(999)
except:
if(verbose!=0):
print("Error during the calculation! Solubility:" +str(solubility_list[i]) +" unit:"+unit_list[i])
error_counter=error_counter+1
logs_list.append(999)
except:
if(verbose!=0):
print("Error during the creation of mol object! Smiles:" +smiles_list[i])
error_counter=error_counter+1
logs_list.append(999)
print("\nConversion of units into LogS is completed.")
print("Total instances:"+str(len(smiles_list)))
print("Total errors:"+str(error_counter)+"\n")
return logs_list
#Collects SMILES from the Chemical Identifier Resolver web service of the National Cancer Institute using Name and CAS number
def collect_smiles_from_web(name_list,cas_list,verbose=0):
error_counter=0
smiles_list = []
for i in range(len(name_list)):
if(verbose!=0):
print(name_list[i])
try:
sleep(0.04)
target_url="https://cactus.nci.nih.gov/chemical/structure/"+cas_list[i].lstrip("0").rstrip(" ")+"/smiles"
context = urllib2.urlopen(target_url)
smiles=context.read().decode('utf-8')
smiles_list.append(smiles)
except:
if(verbose!=0):
print("Cannot collected SMILES using CAS:"+cas_list[i])
print("Trying to collect using Name:"+name_list[i])
try:
sleep(0.04)
target_url="https://cactus.nci.nih.gov/chemical/structure/"+name_list[i].rstrip(" ").replace(" ", "%20")+"/smiles"
context = urllib2.urlopen(target_url)
smiles=context.read().decode('utf-8')
smiles_list.append(smiles)
except:
if(verbose!=0):
print("Cannot collected SMILES using Name:"+name_list[i])
smiles_list.append("XXX")
error_counter=error_counter+1
print("\nCollecting SMILES from webservice is completed.")
print("Total instances:"+str(len(name_list)))
print("Total errors:"+str(error_counter)+"\n")
return smiles_list
#Collects names from the Chemical Identifier Resolver web service of the National Cancer Institute using InChIKey and SMILES
def collect_names_from_web(incikey_list,smiles_list,verbose=0):
error_counter=0
name_list = []
for i in range(len(incikey_list)):
try:
sleep(0.04)
target_url="https://cactus.nci.nih.gov/chemical/structure/"+incikey_list[i]+"/iupac_name"
context = urllib2.urlopen(target_url)
name=context.read().decode('utf-8')
name_list.append(name)
except:
if(verbose!=0):
print("Cannot collected name using InChIKey:"+incikey_list[i])
print("Trying to collect using SMILES:"+smiles_list[i])
try:
sleep(0.04)
target_url="https://cactus.nci.nih.gov/chemical/structure/"+smiles_list[i].replace("#","%23")+"/iupac_name"
context = urllib2.urlopen(target_url)
name=context.read().decode('utf-8')
name_list.append(name)
except:
if(verbose!=0):
print("Cannot collected name using SMILES:"+smiles_list[i])
name_list.append("XXX")
error_counter=error_counter+1
print("\nCollecting names from webservice is completed.")
print("Total instances:"+str(len(incikey_list)))
print("Total errors:"+str(error_counter)+"\n")
return name_list
#Collects solubility predictions from the ALOGPS 2.1 using InChIKey and SMILES
def collect_predictions_from_web(smiles_list,verbose=0):
error_counter=0
prediction_list = []
for i in range(len(smiles_list)):
try:
sleep(0.04)
target_url="http://192.168.127.12/web/alogps/calc?SMILES="+smiles_list[i]
context = urllib2.urlopen(target_url)
text=context.read().decode('utf-8')
if("could not" not in text):
prediction=text.split(" ")[5]
prediction_list.append(prediction)
else:
raise Exception("Cannot collected prediction for:"+smiles_list[i])
except:
if(verbose!=0):
print("Cannot collected prediction for:"+smiles_list[i])
prediction_list.append(999)
error_counter=error_counter+1
print("\nCollecting predictions from webservice is completed.")
print("Total instances:"+str(len(smiles_list)))
print("Total errors:"+str(error_counter)+"\n")
return prediction_list
#filter dataset by removing missing information. (for strings: "XXX" and for numeric: "999")
def clean(dataset,verbose=1):
dataset_clean = dataset[(dataset.InChIKey != "XXX") & (dataset.Solubility != "999") ].reset_index(drop=True)
print("Total removed during the cleaning:"+str(len(dataset)-len(dataset_clean))+"\n")
return dataset_clean
| fadc902375231c4d070204110275788a87ad14fb | [
"Markdown",
"Python"
] | 6 | Python | mcsorkun/AqSolDB | 8e02b548fd9a78778ff89a5aa9a460d1a289cc3a | c241c73d49d81e4726f019af3130383a036d6680 | |
refs/heads/master | <file_sep># typeditor
对 typecho 自带编辑器的重新实现,寻找了很多,只有 typecho 的 markdown 标准深得我心
原版是 php + jq 实现,typeditor 使用原生 ts 来写,适配所有前端场景
### Use
npm:
```
yarn add typeditor -S
```
dom:
```html
<div id="typeditor"></div>
```
js:
```javascript
let te = new Typeditor({
el: '#typeditor', //目标容器
preview:`https://www.clicli.us/${gv}` //预览地址,可以根据 id 跳转到转义过的前端页面
})
```
#### get/set value
```JavaScript
te.value()
te.value('念念不忘,必有回响')
```
### Parse
关于 markdown 的解析库,typeditor 推荐同样小而美的 [snarkdown](https://github.com/developit/snarkdown)
### License
MIT © 132yse inspired by [typecho](https://github.com/typecho/typecho)
<file_sep>class Typeditor {
content: string
el: Element
template: string
constructor(options: Options) {
this.el = document.querySelector(options.el)
this.content = ''
this.init()
}
init() {
this.el.innerHTML = `
<style>
@import "https://at.alicdn.com/t/font_1151392_5hwi3w2ygqu.css";
li {
list-style: none;
}
* {
padding: 0;
margin: 0
}
#typeditor {
height: 100%;
width: 100%;
position: relative;
font: 14px 微软雅黑;
color: #334265;
}
#typeditor .menu li {
display: inline-block;
padding: 6px;
cursor: pointer;
}
#typeditor .menu{
margin: 6px 0
}
#typeditor .menu li:hover,
#typeditor .menu li.active {
background: #e0e4ef;
border-radius: 4px;
}
#typeditor .editor {
height: 100%;
box-sizing: border-box;
outline: 0;
padding: 6px;
}
</style>
<div class="menu">
<ul>
<li><i class="te te-bold"></i></li>
<li><i class="te te-italic"></i></li>
<li><i class="te te-header"></i></li>
<li><i class="te te-quote"></i></li>
<li><i class="te te-code"></i></li>
<li><i class="te te-link"></i></li>
<li><i class="te te-image"></i></li>
<li><i class="te te-preview"></i></li>
</ul>
</div>
<div class="editor" contenteditable="true"></div>
`
}
static tags = {
bold: { start: '**', end: '**', placeholder: '请输入加粗内容' },
italic: { start: '*', end: '*', placeholder: '请输入加斜内容' },
link: { start: '[', end: '][N]', placeholder: '请输入链接标题' },
image: { start: '![', end: '][N]', placeholder: '请输入图片描述' },
quote: { start: '>', end: '', placeholder: '请输入引用内容' },
code: { start: '`', end: '`', placeholder: '请输入代码内容' },
}
value(content: string) {
return content ? (this.el.innerHTML = content) : this.content
}
}
interface Options {
el: string
}
| 8997210c524854909754aac4d0a370afdcefead1 | [
"Markdown",
"TypeScript"
] | 2 | Markdown | yisar/typeditor | b46c88f38050149c2b0846300fb6870dd82504f5 | c33aa2f6cb675660ce56bf9777f2014c03729f45 | |
refs/heads/master | <file_sep>## OOAD Project
- 14302010038 王翀
- 14302010049 蒲实
1. 架构
- PJ实现了数据层和业务层,主要使用到Spring和Hibernate
- 数据层使用Hibernate封装,实现在`src\common`和`src\model`中
- 其中`model`实现了数据库的ORM映射
- `common`封装了基本的数据库操作,对外提供操作的接口
- 业务层实现在`src\service`中,对于每个用例提供了操作的接口,最后的测试也是在这里提供的接口上进行测试
- 业务层对数据库的操作完全通过数据层提供的接口实现
---
2. 数据层 `src\common`
- ORM `src\model`
- `Company`:企业
- `RiskCheckTemplateItem`:检查条目,为某一具体的检查条目
- `RiskCheckTemplate`:检查模版,包括了一个检查项的集合
- `RiskCheckPlan`:检查计划,包括了对应了检查模版
- `RiskCheck`:检查任务,一次检查包括了对应的企业、计划,并包含了具体的检查条目
- `RiskCheckItem`:企业可见的某一具体的检查条目,企业可以对其录入进度
- 其中`eunums`有`Company`和`RiskCheck`的`state`的枚举,以及相应的数据库转换器类
- 数据库操作`src\common`
- `src\common\IPersistenceManager`提供对数据库所有操作的接口
- `src\common\PMHibernateImpl`为上述接口的实现
- 数据库 Mysql
- host : 192.168.127.12:3306
- username: kadoufall
- password: <PASSWORD>
- table : ooad1
---
3. 业务逻辑层 `src\service`
- `ICompanyService` : 封装了企业的相关操作
- `IRiskCheckTemplateItemService` : 封装了具体的检查条目的添加和搜索
- `IRiskCheckTemplateService` : 封装了检查模版的添加、编辑、搜索和添加具体的检查条目
- `IRiskCheckPlanService` : 封装了检查计划的添加、编辑、搜索和删除
- `IRiskCheckService` : 封装了分配一次任务的操作
---
4. JUnit单元测试 `src-test`
- 测试的内容标注在注释中
---
5. 项目配置 `src-config`
- 配置数据源、`Hibernate`以及数据层和业务层的位置
---
PS : PJ的数据库持久化参考了张天戈老师的demo
<file_sep>package service;
import model.RiskCheckTemplateItem;
import java.util.List;
public interface IRiskCheckTemplateItemService {
RiskCheckTemplateItem add(String name, String content);
List<RiskCheckTemplateItem> search(String input);
}
<file_sep>package model;
import common.BaseModelObject;
import common.IPersistenceManager;
import javax.persistence.*;
import java.util.Set;
@Entity
@Table(name = "riskchecktemplate")
public class RiskCheckTemplate extends BaseModelObject {
private String name;
private String summary;
private Set<RiskCheckTemplateItem> riskCheckTemplateItems;
public static RiskCheckTemplate create(IPersistenceManager pm, String name, String summary, Set<RiskCheckTemplateItem> riskCheckTemplateItems) {
RiskCheckTemplate re = new RiskCheckTemplate();
re.setName(name);
re.setSummary(summary);
re.setRiskCheckTemplateItems(riskCheckTemplateItems);
pm.save(re);
return re;
}
@Column(name = "name", nullable = false, length = 45)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Column(name = "summary", nullable = false, length = 100)
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
@Access(AccessType.PROPERTY)
@ManyToMany(cascade = {
CascadeType.DETACH,
CascadeType.MERGE,
CascadeType.REFRESH,
CascadeType.PERSIST
}, fetch = FetchType.LAZY)
public Set<RiskCheckTemplateItem> getRiskCheckTemplateItems() {
return riskCheckTemplateItems;
}
public void setRiskCheckTemplateItems(Set<RiskCheckTemplateItem> riskCheckTemplateItems) {
this.riskCheckTemplateItems = riskCheckTemplateItems;
}
}
<file_sep>package service;
import model.Company;
import model.RiskCheckItem;
import model.enums.CompanyState;
import java.util.Set;
public interface ICompanyService {
void finishOneCheckRiskItem(RiskCheckItem riskCheckItem, String result);
Company add(String code, String name, CompanyState state, String organizationalCode,
String industryGenera, String industry, String businessCategory, String contact, String contactNumber);
void delete(Company company);
Company edit(Company company, String code, String name, CompanyState state, String organizationalCode,
String industryGenera, String industry, String businessCategory, String contact, String contactNumber);
Set<RiskCheckItem> getAllRiskCheckItem(Company company);
}
<file_sep>package model;
import common.BaseModelObject;
import common.IPersistenceManager;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Table;
@Entity
@Table(name = "riskchecktemplateitem")
public class RiskCheckTemplateItem extends BaseModelObject {
private String name;
private String content;
public static RiskCheckTemplateItem create(IPersistenceManager pm, String name, String content) {
RiskCheckTemplateItem re = new RiskCheckTemplateItem();
re.setName(name);
re.setContent(content);
pm.save(re);
return re;
}
@Column(name = "name", nullable = false, length = 100)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Column(name = "content", nullable = false, length = 100)
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
<file_sep>import common.BasePersistenceTest;
import common.IPersistenceManager;
import model.*;
import model.enums.CompanyState;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import service.IRiskCheckService;
import java.util.HashSet;
import static org.junit.Assert.*;
/**
* Created by asus on 2017/6/20.
*/
public class RiskCheckServiceTest extends BasePersistenceTest {
@Autowired
IRiskCheckService service;
@Autowired
IPersistenceManager pm;
@Before
public void setUp() throws Exception {
}
@After
public void tearDown() throws Exception {
pm.deleteAll(Company.class);
pm.deleteAll(RiskCheck.class);
pm.deleteAll(RiskCheckPlan.class);
pm.deleteAll(RiskCheckTemplate.class);
pm.deleteAll(RiskCheckTemplateItem.class);
}
// 测试将一个plan发放给一个company,与其他测试中的add类似
@Test
public void add() throws Exception {
Company company = Company.create(pm, "", "", CompanyState.NORMAL, "", "", "", "", "", "" );
RiskCheckTemplate template = RiskCheckTemplate.create(pm, "template","", new HashSet<RiskCheckTemplateItem>());
RiskCheckPlan plan = RiskCheckPlan.create(pm, "plan", template);
RiskCheck task = service.add(company, plan, "2017-06-30");
RiskCheck result = pm.get(RiskCheck.class, task.getId());
assertNotNull(result);
}
}<file_sep>/*
* Copyright 2006 Centenum Software Solutions, Inc. All rights reserved.
* CENTENUM PROPRIETARY/CONFIDENTIAL.
*/
package common;
import org.hibernate.Criteria;
import org.hibernate.Query;
import org.hibernate.Session;
import org.springframework.beans.BeansException;
import org.springframework.beans.factory.BeanFactory;
import org.springframework.beans.factory.BeanFactoryAware;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.springframework.orm.hibernate4.support.HibernateDaoSupport;
import org.springframework.transaction.annotation.Transactional;
import java.io.Serializable;
import java.lang.reflect.Field;
import java.util.List;
/**
* A hibernate/Spring framework implementation for IObjectFactory
*
* @author <NAME>
*
*/
@Transactional
public class PMHibernateImpl extends HibernateDaoSupport implements IPersistenceManager, BeanFactoryAware {
public static IPersistenceManager getInstance() {
ClassPathXmlApplicationContext appContext = new ClassPathXmlApplicationContext(
new String[] { "HibernateApplicationContext.xml" });
return (IPersistenceManager) ((BeanFactory) appContext)
.getBean("objectFactory");
}
BeanFactory beanFactory;
private Class getImplClass(Class clazz) {
if (clazz.isInterface()) {
return beanFactory.getBean(getBeanID(clazz)).getClass();
} else {
return clazz;
}
}
private String getBeanID(Class clazz) {
int pos = clazz.getName().lastIndexOf('.') + 1;
return clazz.getName().substring(pos);
}
@Override
public IModelObject create(Class clazz) {
IModelObject bean = (IModelObject) beanFactory
.getBean(getBeanID(clazz));
return bean;
}
@Override
public IModelObject get(Class clazz, Serializable id) {
IModelObject bean = (IModelObject) getHibernateTemplate().get(
getImplClass(clazz), id);
return bean;
}
@Override
public IModelObject load(IModelObject e) {
getHibernateTemplate().load(e, e.getId());
return e;
}
@Override
public void delete(IModelObject obj) {
getHibernateTemplate().delete(obj);
}
@Override
public IModelObject save(IModelObject obj) {
getHibernateTemplate().saveOrUpdate(obj);
return obj;
}
@Override
public IModelObject create(IModelObject obj) {
getHibernateTemplate().saveOrUpdate(obj);
return obj;
}
@Override
public <T extends IModelObject> List<T> findByProperty(Class<T> clazz, String propertyName, String value) {
System.out.println("from " + clazz.getName() +" clazz where clazz."+propertyName+" like '% :"+propertyName+"%'");
return (List<T>)getHibernateTemplate().find("from " + clazz.getName() +" clazz where clazz."+propertyName+" like '%"+value+"%'");
}
@Override
public <T extends IModelObject> List<T> findByFussyValue(Class<T> clazz, String value) {
Field[] fields = clazz.getDeclaredFields();
StringBuffer hql = new StringBuffer();
hql.append("from " + clazz.getName() +" clazz where ");
//String sql = "from " + clazz.getName() +" clazz where ";clazz."+propertyName+" like '%"+value+"%'"
if(fields[0].getType().getSimpleName().equals("String"))
hql.append("clazz."+fields[0].getName()+" like '%"+value+"%'");
for (int i = 1; i<fields.length; i++) {
if(fields[i].getType().getSimpleName().equals("String"))
hql.append(" or clazz."+fields[i].getName()+" like '%"+value+"%'");
}
return (List<T>)getHibernateTemplate().find(hql.toString());
}
@Override
public <T extends IModelObject> List<T> all(Class<T> clazz) {
return (List<T>)getHibernateTemplate().find("from " + clazz.getName());
}
@Override
public boolean exist(IModelObject value) {
throw new RuntimeException("not supported");
}
@Override
public void setBeanFactory(BeanFactory beanFactory) throws BeansException {
this.beanFactory = beanFactory;
}
/*
* (non-Javadoc)
*
* @see
* edu.fudan.framework.persistence.IObjectFactory#deleteAll(java.lang.Class)
*/
@Override
public void deleteAll(Class clazz) {
getHibernateTemplate().deleteAll(all(clazz));
}
@Override
public Criteria createCriteria(Class clazz) {
return getHibernateSession().createCriteria(clazz);
}
@Override
public void save(IModelObject model, Serializable id) {
// getSession().persist(model, id);
}
@Override
public Session getHibernateSession() {
return currentSession();
}
@Override
public void logicDelete(IModelObject modelObject) {
save(modelObject);
}
@Override
public Query createQuery(String hql) {
return getHibernateSession().createQuery(hql);
}
}
<file_sep>package service;
import model.RiskCheckTemplate;
import model.RiskCheckTemplateItem;
import java.util.List;
import java.util.Set;
public interface IRiskCheckTemplateService {
RiskCheckTemplate add(String name, String summary, Set<RiskCheckTemplateItem> riskCheckTemplateItems);
RiskCheckTemplate edit(RiskCheckTemplate riskCheckTemplate, String name, String summary, Set<RiskCheckTemplateItem> riskCheckTemplateItems);
RiskCheckTemplate addRiskCheckTemplateItem(RiskCheckTemplate riskCheckTemplate, RiskCheckTemplateItem riskCheckTemplateItem);
List<RiskCheckTemplate> search(String input);
}
| cafd8da5f599a3b932549a40c35915ad5e8d8fc6 | [
"Markdown",
"Java"
] | 8 | Markdown | FDU324/OOAD_1 | a3bec347383ef17b82672cddfbeb25ed41eab121 | 06bc7b1638a34b184955c81ba50b91ad7f052e5b | |
refs/heads/master | <file_sep>package ac.za.cput.streetstock.repository.payments;
public interface PaypalRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.transaction;
public class Buy
{
}
<file_sep>package ac.za.cput.streetstock.repository.user;
public interface CustomerRepository
{
}
<file_sep>package ac.za.cput.streetstock.factory.device;
public class LaptopFactory
{
}
<file_sep>package ac.za.cput.streetstock;
import org.springframework.boot.SpringApplication;
public class StreetStock
{
public static void main(String[] args)
{
SpringApplication.run(StreetStock.class, args);
}
}
<file_sep>package ac.za.cput.streetstock.domain.device;
public class Linux extends Laptop
{
private String xfce;
private String Gnome;
private String kde;
}
<file_sep>package ac.za.cput.streetstock.repository.transaction;
public interface BuyRepository
{
}
<file_sep>package ac.za.cput.streetstock.repository.login;
public interface LoginDetailsRepository
{
}
<file_sep>package ac.za.cput.streetstock.repository.user;
public interface AdministratorRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.product;
public class Update
{
}
<file_sep>package ac.za.cput.streetstock.repository.product;
public interface StockRepository
{
}
<file_sep>package ac.za.cput.streetstock.repository.System;
public interface WindowsRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.user;
public class Customer
{
private String userName;
private String phoneNumber;
}
<file_sep>package ac.za.cput.streetstock.repository.System;
public interface LinuxRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.payments;
public class PaymentStatus
{
private String paid;
private String pending;
}
<file_sep>package ac.za.cput.streetstock.domain.device;
public class PC
{
}
<file_sep>package ac.za.cput.streetstock.domain.user;
public class Distributor
{
private String userName;
private String phoneNumber;
}
<file_sep>package ac.za.cput.streetstock.repository.payments;
public interface PaymentStatusRepository
{
}
<file_sep>package ac.za.cput.streetstock.repository.device;
public interface PhonesRepository
{
}
<file_sep>package ac.za.cput.streetstock.factory.device;
import ac.za.cput.streetstock.domain.device.PC;
import ac.za.cput.streetstock.util.GenerateUserName;
public class PCFactory
{
public static PC buildPC(String pcName)
{
return new PC.Builder().pcID(GenerateUserName.generateUserName())
.pcName(pcName)
.build();
}
}
<file_sep>package ac.za.cput.streetstock.Service;
public interface PCService
{
}
<file_sep>package ac.za.cput.streetstock.Service;
public interface ConsolesService
{
}
<file_sep>package ac.za.cput.streetstock.repository.device;
public interface ConsolesInterface
{
}
<file_sep>package ac.za.cput.streetstock.repository.impl;
import ac.za.cput.streetstock.domain.device.Laptop;
import ac.za.cput.streetstock.domain.device.PC;
import ac.za.cput.streetstock.repository.device.PCRepository;
import java.util.HashSet;
import java.util.Set;
public class PCRepositoryImpl implements PCRepository
{
private static PCRepositoryImpl repository = null;
private Set<PC> pcs;
private PCRepositoryImpl()
{
this.pcs = new HashSet<>();
}
private PC findPC(String pcID)
{
return this.pcs.stream()
.filter(laptop -> laptop.getPcID().trim().equals(pcID))
.findAny()
.orElse(null);
}
public static PCRepositoryImpl getRepository()
{
if (repository == null) repository = new PCRepositoryImpl();
return repository;
}
public Laptop create(Laptop pc)
{
this.pcs.add(pc);
return pc;
}
public PC read(final String pcID)
{
PC pc = findPC(pcID);
return pc;
}
public void delete(String pcID)
{
PC pc = findPC(pcID);
if (pc != null) this.pcs.remove(pc);
}
public PC update(PC pc)
{
PC toDelete = findPC(pc.getPcID());
if(toDelete != null)
{
this.pcs.remove(toDelete);
return create(pc);
}
return null;
}
public Set<PC> getAll()
{
return this.pcs;
}
}<file_sep>package ac.za.cput.streetstock.repository.product;
public interface RemoveRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.System;
import ac.za.cput.streetstock.domain.device.Laptop;
public class Linux extends Laptop
{
private String xfce;
private String Gnome;
private String kde;
}
<file_sep>package ac.za.cput.streetstock.repository.transaction;
public interface SellRepository
{
}
<file_sep>package ac.za.cput.streetstock.domain.System;
import ac.za.cput.streetstock.domain.device.Laptop;
public class Windows extends Laptop
{
private String OSVersion;
private Windows(){}
private Windows(Builder builder)
{
this.OSVersion = builder.OSVersion;
}
public String getOSVersion()
{
return OSVersion;
}
public static class Builder
{
private String OSVersion;
public Builder OSVersion(String OSVersion)
{
this.OSVersion = OSVersion;
return this;
}
}
}<file_sep>package ac.za.cput.streetstock.factory.product;
import ac.za.cput.streetstock.domain.product.Products;
import ac.za.cput.streetstock.util.UserName;
public class ProductsFactory
{
public static Products getProduct(String productName)
{
return new Products.Builder().model(UserName.generateUserName())
.productName(productName)
.build();
}
}<file_sep>package ac.za.cput.streetstock.factory.transaction;
public class SaleStatusFactory
{
}
<file_sep>package ac.za.cput.streetstock.factory.payments;
public class PaymentStatusFactory
{
}
<file_sep>package ac.za.cput.streetstock.factory.user;
public class DistributorFactory
{
private String userName;
private String phoneNumber;
}
<file_sep>package ac.za.cput.streetstock.domain;
public class PC
{
}
<file_sep>package ac.za.cput.streetstock.factory.product;
public class StockFactory
{
}
<file_sep>package ac.za.cput.streetstock.repository.product;
public interface UpdateRepository
{
}
<file_sep>package ac.za.cput.streetstock.factory.System;
public class IOSFactory
{
private String S;
private String Plus;
private String normal;
private IOSFactory()
{
}
}
<file_sep>package ac.za.cput.streetstock.factory.device;
public class ConsolesFactory
{
}
<file_sep>package ac.za.cput.streetstock.domain.device;
public class Android extends Phones
{
private String AndroidVer;
}<file_sep>package ac.za.cput.streetstock.domain.device;
public class Laptop
{
}
<file_sep>package ac.za.cput.streetstock.factory.user;
public class AdministratorFactory
{
}
<file_sep>package ac.za.cput.streetstock.factory.payments;
public class PaypalFactory
{
}
<file_sep>package ac.za.cput.streetstock.factory.transaction;
public class SellFactory
{
}
<file_sep>package ac.za.cput.streetstock.domain.product;
public class Remove
{
}
<file_sep>package ac.za.cput.streetstock.Service;
public interface LaptopService
{
}
| 3bcd9f269fc61e946d697ec9c408be692663efe3 | [
"Java"
] | 44 | Java | ShamahSean/streetstock | 9ee78cd667cfa44b041800d0de5af50bba3a2fad | c31a52e87916fc4af9be7a2aa54effb9690697a6 | |
refs/heads/master | <file_sep>import React from "react";
import Counter from "./counter";
const Counters = ({ counters, onAdd, onDelete, onIncrement, onReset }) => {
return (
<div>
<button onClick={() => onAdd()} className="btn btn-info btn-sm m-2">
Add
</button>
{counters.map(counter => (
<Counter
key={counter.id}
counter={counter}
onDelete={() => onDelete(counter)}
onIncrement={() => onIncrement(counter)}
onReset={() => onReset(counter)}
/>
))}
</div>
);
};
export default Counters;
| 59777a5ffe57048d8497010054af106a63fc0960 | [
"JavaScript"
] | 1 | JavaScript | ufuk-techclass/counters-react-exercise | 479e60ad146e48dfda34460d249fac7491d8d2de | 7970387253b7103f488f4878efd17f97e15aa186 | |
refs/heads/master | <repo_name>gabriele-bonadiman/MS-01-multitenancy-microservice<file_sep>/src/main/java/com/gabrielebonadiman/multitenancy/service/impl/EventServiceImpl.java
package com.gabrielebonadiman.multitenancy.service.impl;
import com.gabrielebonadiman.multitenancy.client.UaaClient;
import com.gabrielebonadiman.multitenancy.domain.Event;
import com.gabrielebonadiman.multitenancy.repository.EventRepository;
import com.gabrielebonadiman.multitenancy.service.EventService;
import com.gabrielebonadiman.multitenancy.service.dto.EventDTO;
import com.gabrielebonadiman.multitenancy.service.mapper.EventMapper;
import com.gabrielebonadiman.multitenancy.web.rest.errors.BadRequestAlertException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.UUID;
/**
* Service Implementation for managing Event.
*/
@Service
@Transactional
public class EventServiceImpl implements EventService {
private final Logger log = LoggerFactory.getLogger(EventServiceImpl.class);
private final EventRepository eventRepository;
private final EventMapper eventMapper;
private final UaaClient uaaClient;
private final static String EXCEPTION_MESSAGE = "An error occurred with Event";
private final static String EXCEPTION_ENTITY = "Event";
private final static String EXCEPTION_HTTP_LABEL = "isBadRequest";
/*
@GetMapping("/aa")
public String asd(){
return uaaClient.getUserCompanyId().toString();
}
*/
public EventServiceImpl(EventRepository eventRepository, EventMapper eventMapper, UaaClient uaaClient) {
this.eventRepository = eventRepository;
this.eventMapper = eventMapper;
this.uaaClient = uaaClient;
}
/**
* Save a event.
*
* @param eventDTO the entity to save
* @return the persisted entity
*/
@Override
public EventDTO save(EventDTO eventDTO) {
UUID companyId =
uaaClient.getUserCompanyId();
if (companyId != null) {
log.debug("Request to save Event : {}", eventDTO);
eventDTO.setCompanyId(companyId);
Event event = eventMapper.toEntity(eventDTO);
event = eventRepository.save(event);
return eventMapper.toDto(event);
}
else{
log.debug("Unable to get the company ID");
throw new BadRequestAlertException(EXCEPTION_MESSAGE,EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
/**
* Get all the events.
*
* @param pageable the pagination information
* @return the list of entities
*/
@Override
@Transactional(readOnly = true)
public Page<EventDTO> findAll(Pageable pageable) {
log.debug("Request to get all Events");
UUID companyId =
uaaClient.getUserCompanyId();
if (companyId != null) {
return eventRepository.findAllByCompanyId(pageable, companyId)
.map(eventMapper::toDto);
} else {
log.debug("Unable to get the company ID");
throw new BadRequestAlertException(EXCEPTION_MESSAGE, EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
/**
* Get one event by id.
*
* @param id the id of the entity
* @return the entity
*/
@Override
@Transactional(readOnly = true)
public EventDTO findOne(UUID id) {
log.debug("Request to get Event : {}", id);
UUID companyId =
uaaClient.getUserCompanyId();
if (companyId != null) {
Event event = eventRepository.findOne(id);
if (event != null) {
if (event.getCompanyId() != companyId) {
log.error("The system has been compromised. Request to fetch from database an event" +
" that does not belong to the user: {} using companyID", id, companyId);
throw new SecurityException();
}else{
log.debug("Request to delete Event : {}", id);
return eventMapper.toDto(event);
}
}
else{
log.debug("Unable to find an event with UUID: {}", id);
throw new BadRequestAlertException(EXCEPTION_MESSAGE, EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
else {
log.debug("Unable to get the company ID");
throw new BadRequestAlertException(EXCEPTION_MESSAGE, EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
/**
* Delete the event by id.
*
* @param id the id of the entity
*/
@Override
public void delete(UUID id) {
UUID companyId =
uaaClient.getUserCompanyId();
if (companyId != null) {
Event event = eventRepository.findOne(id);
if (event != null) {
if (event.getCompanyId() != companyId){
log.error("The system has been compromised. Request to fetch from database an event" +
" that does not belong to the user: {} using companyID", id, companyId);
throw new SecurityException();
}
else{
log.debug("Request to delete Event : {}", id);
eventRepository.delete(id);
}
}
else{
log.debug("Unable to find an event with UUID: {}", id);
throw new BadRequestAlertException(EXCEPTION_MESSAGE, EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
else{
log.debug("Unable to get the company ID");
throw new BadRequestAlertException(EXCEPTION_MESSAGE, EXCEPTION_ENTITY, EXCEPTION_HTTP_LABEL);
}
}
}
<file_sep>/src/main/java/com/gabrielebonadiman/multitenancy/domain/Event.java
package com.gabrielebonadiman.multitenancy.domain;
import org.hibernate.annotations.*;
import org.hibernate.annotations.Cache;
import javax.persistence.*;
import javax.persistence.Entity;
import javax.persistence.Table;
import javax.validation.constraints.*;
import java.io.Serializable;
import java.util.Date;
import java.util.Objects;
import java.util.UUID;
/**
* A Event.
*/
@Entity
@Table(name = "event")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class Event implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(generator = "uuid2")
@GenericGenerator(name = "uuid2", strategy = "uuid2")
@Column(columnDefinition = "BINARY(16)")
private UUID id;
@NotNull
@Column(name = "name", nullable = false)
private String name;
@NotNull
@Column(name = "jhi_date", nullable = false)
private Date date;
@Column(name = "host")
private String host;
@Column(name = "company_id")
private UUID companyId;
@CreationTimestamp
@Temporal(TemporalType.TIMESTAMP)
@Column(name = "created_at")
private Date createdAt;
@UpdateTimestamp
@Temporal(TemporalType.TIMESTAMP)
@Column(name = "last_update")
private Date lastUpdate;
// jhipster-needle-entity-add-field - JHipster will add fields here, do not remove
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public String getName() {
return name;
}
public Event name(String name) {
this.name = name;
return this;
}
public void setName(String name) {
this.name = name;
}
public Date getDate() {
return date;
}
public Event date(Date date) {
this.date = date;
return this;
}
public void setDate(Date date) {
this.date = date;
}
public String getHost() {
return host;
}
public Event host(String host) {
this.host = host;
return this;
}
public void setHost(String host) {
this.host = host;
}
public Date getLastUpdate() {
return lastUpdate;
}
public Event lastUpdate(Date lastUpdate) {
this.lastUpdate = lastUpdate;
return this;
}
public void setLastUpdate(Date lastUpdate) {
this.lastUpdate = lastUpdate;
}
public Date getCreatedAt() {
return createdAt;
}
public Event createdAt(Date createdAt) {
this.createdAt = createdAt;
return this;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public UUID getCompanyId() {
return companyId;
}
public Event companyId(UUID companyId) {
this.companyId = companyId;
return this;
}
public void setCompanyId(UUID companyId) {
this.companyId = companyId;
}
// jhipster-needle-entity-add-getters-setters - JHipster will add getters and setters here, do not remove
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Event event = (Event) o;
if (event.getId() == null || getId() == null) {
return false;
}
return Objects.equals(getId(), event.getId());
}
@Override
public int hashCode() {
return Objects.hashCode(getId());
}
@Override
public String toString() {
return "Event{" +
"id=" + id +
", name='" + name + '\'' +
", date=" + date +
", host='" + host + '\'' +
", companyId=" + companyId +
", createdAt=" + createdAt +
", lastUpdate=" + lastUpdate +
'}';
}
}
<file_sep>/src/test/java/com/gabrielebonadiman/multitenancy/web/rest/EventResourceIntTest.java
package com.gabrielebonadiman.multitenancy.web.rest;
import com.gabrielebonadiman.multitenancy.MultitenancyApp;
import com.gabrielebonadiman.multitenancy.client.UaaClient;
import com.gabrielebonadiman.multitenancy.config.SecurityBeanOverrideConfiguration;
import com.gabrielebonadiman.multitenancy.domain.Event;
import com.gabrielebonadiman.multitenancy.repository.EventRepository;
import com.gabrielebonadiman.multitenancy.service.EventService;
import com.gabrielebonadiman.multitenancy.service.dto.EventDTO;
import com.gabrielebonadiman.multitenancy.service.mapper.EventMapper;
import com.gabrielebonadiman.multitenancy.web.rest.errors.BadRequestAlertException;
import com.gabrielebonadiman.multitenancy.web.rest.errors.ExceptionTranslator;
import org.apache.commons.lang.time.DateUtils;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.MockitoAnnotations;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.mock.mockito.MockBean;
import org.springframework.data.web.PageableHandlerMethodArgumentResolver;
import org.springframework.http.MediaType;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.transaction.annotation.Transactional;
import javax.persistence.EntityManager;
import java.util.Date;
import java.util.List;
import java.util.UUID;
import static com.gabrielebonadiman.multitenancy.web.rest.TestUtil.createFormattingConversionService;
import static org.assertj.core.api.Assertions.assertThat;
import static org.hamcrest.Matchers.hasItem;
import static org.mockito.BDDMockito.given;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
/**
* Test class for the EventResource REST controller.
*
* @see EventResource
*/
@RunWith(SpringRunner.class)
@SpringBootTest(classes = {MultitenancyApp.class, SecurityBeanOverrideConfiguration.class})
public class EventResourceIntTest {
private static final String DEFAULT_NAME = "AAAAAAAAAA";
private static final String UPDATED_NAME = "BBBBBBBBBB";
private static final Date DEFAULT_DATE = new Date();
private static final Date UPDATED_DATE = new Date();
private static final String DEFAULT_HOST = "AAAAAAAAAA";
private static final String UPDATED_HOST = "BBBBBBBBBB";
private static final UUID DEFAULT_EVENT_ID = UUID.randomUUID();
private static final UUID UPDATED_EVENT_ID= UUID.randomUUID();
private static final UUID DEFAULT_COMPANY_ID = UUID.randomUUID();
private static final UUID UPDATED_COMPANY_ID = UUID.randomUUID();
@Autowired
private EventRepository eventRepository;
@Autowired
private EventMapper eventMapper;
@Autowired
private EventService eventService;
@Autowired
private MappingJackson2HttpMessageConverter jacksonMessageConverter;
@Autowired
private PageableHandlerMethodArgumentResolver pageableArgumentResolver;
@Autowired
private ExceptionTranslator exceptionTranslator;
@Autowired
private EntityManager em;
@MockBean
private UaaClient uaaClient;
private MockMvc restEventMockMvc;
private Event event;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
final EventResource eventResource = new EventResource(eventService);
given(uaaClient.getUserCompanyId()).willReturn(DEFAULT_COMPANY_ID);
this.restEventMockMvc = MockMvcBuilders.standaloneSetup(eventResource)
.setCustomArgumentResolvers(pageableArgumentResolver)
.setControllerAdvice(exceptionTranslator)
.setConversionService(createFormattingConversionService())
.setMessageConverters(jacksonMessageConverter).build();
}
/**
* Create an entity for this test.
*
* This is a static method, as tests for other entities might also need it,
* if they test an entity which requires the current entity.
*/
public static Event createEntity(EntityManager em) {
Event event = new Event()
.name(DEFAULT_NAME)
.date(DEFAULT_DATE)
.host(DEFAULT_HOST);
return event;
}
@Before
public void initTest() {
event = createEntity(em);
}
@Test
@Transactional
public void createEvent() throws Exception {
int databaseSizeBeforeCreate = eventRepository.findAll().size();
// Create the Event
EventDTO eventDTO = eventMapper.toDto(event);
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isCreated());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeCreate + 1);
Event testEvent = eventList.get(eventList.size() - 1);
assertThat(testEvent.getName()).isEqualTo(DEFAULT_NAME);
assertThat(testEvent.getDate()).isEqualTo(DEFAULT_DATE);
assertThat(testEvent.getHost()).isEqualTo(DEFAULT_HOST);
assertThat(testEvent.getCompanyId()).isEqualTo(DEFAULT_COMPANY_ID);
}
@Test
@Transactional
public void createEventWithUaaReturnNullCompanyId() throws Exception {
int databaseSizeBeforeCreate = eventRepository.findAll().size();
given(uaaClient.getUserCompanyId()).willReturn(null);
// Create the Event
EventDTO eventDTO = eventMapper.toDto(event);
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().is4xxClientError());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void createEventWithCompanyIdNotNull() throws Exception {
int databaseSizeBeforeCreate = eventRepository.findAll().size();
event.setCompanyId(DEFAULT_COMPANY_ID);
// Create the Event
EventDTO eventDTO = eventMapper.toDto(event);
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().is4xxClientError());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void checkNameIsRequired() throws Exception {
int databaseSizeBeforeTest = eventRepository.findAll().size();
// set the field null
event.setName(null);
// Create the Event, which fails.
EventDTO eventDTO = eventMapper.toDto(event);
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isBadRequest());
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void checkDateIsRequired() throws Exception {
int databaseSizeBeforeTest = eventRepository.findAll().size();
// set the field null
event.setDate(null);
// Create the Event, which fails.
EventDTO eventDTO = eventMapper.toDto(event);
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isBadRequest());
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void updateNonExistingEvent() throws Exception {
int databaseSizeBeforeUpdate = eventRepository.findAll().size();
// Create the Event
EventDTO eventDTO = eventMapper.toDto(event);
// If the entity doesn't have an ID, it will be created instead of just being updated
restEventMockMvc.perform(put("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isCreated());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeUpdate + 1);
}
@Test
@Transactional
public void equalsVerifier() throws Exception {
TestUtil.equalsVerifier(Event.class);
Event event1 = new Event();
event1.setId(DEFAULT_EVENT_ID);
Event event2 = new Event();
event2.setId(event1.getId());
assertThat(event1).isEqualTo(event2);
event2.setId(UPDATED_EVENT_ID);
assertThat(event1).isNotEqualTo(event2);
event1.setId(null);
assertThat(event1).isNotEqualTo(event2);
}
@Test
@Transactional
public void dtoEqualsVerifier() throws Exception {
TestUtil.equalsVerifier(EventDTO.class);
EventDTO eventDTO1 = new EventDTO();
eventDTO1.setId(DEFAULT_EVENT_ID);
EventDTO eventDTO2 = new EventDTO();
assertThat(eventDTO1).isNotEqualTo(eventDTO2);
eventDTO2.setId(eventDTO1.getId());
assertThat(eventDTO1).isEqualTo(eventDTO2);
eventDTO2.setId(UPDATED_EVENT_ID);
assertThat(eventDTO1).isNotEqualTo(eventDTO2);
eventDTO1.setId(null);
assertThat(eventDTO1).isNotEqualTo(eventDTO2);
}
@Test
@Transactional
public void testEntityFromId() {
assertThat(eventMapper.fromId(DEFAULT_EVENT_ID).getId()).isEqualTo(DEFAULT_EVENT_ID);
assertThat(eventMapper.fromId(null)).isNull();
}
@Test
@Transactional
public void getEvent() throws Exception {
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
// Get the event
restEventMockMvc.perform(get("/api/events/{id}", event.getId()))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.id").value(event.getId().toString()))
.andExpect(jsonPath("$.name").value(DEFAULT_NAME))
.andExpect(jsonPath("$.host").value(DEFAULT_HOST))
.andExpect(jsonPath("$.companyId").value(DEFAULT_COMPANY_ID.toString()));
}
@Test
@Transactional
public void getEventUaaReturnNullCompanyId() throws Exception {
// Initialize the database
given(uaaClient.getUserCompanyId()).willReturn(null);
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
// Get the event
restEventMockMvc.perform(get("/api/events/{id}", event.getId()))
.andExpect(status().is4xxClientError());
}
@Test
@Transactional
public void getEventThatDoesNotExists() throws Exception {
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
// Get the event
restEventMockMvc.perform(get("/api/events/{id}", UPDATED_EVENT_ID))
.andExpect(status().is4xxClientError());
}
@Test
@Transactional
public void getEventWithDifferentCompanyId() throws Exception {
given(uaaClient.getUserCompanyId()).willReturn(UPDATED_COMPANY_ID);
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
// Get the event
restEventMockMvc.perform(get("/api/events/{id}", event.getId()))
.andExpect(status().is5xxServerError());
}
@Test
@Transactional
public void deleteEvent() throws Exception {
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
int databaseSizeBeforeDelete = eventRepository.findAll().size();
// Get the event
restEventMockMvc.perform(delete("/api/events/{id}", event.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().isOk());
// Validate the database is empty
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeDelete - 1);
}
@Test
@Transactional
public void deleteEventWithUaaReturnNullCompanyId() throws Exception {
given(uaaClient.getUserCompanyId()).willReturn(null);
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
int databaseSizeBeforeDelete = eventRepository.findAll().size();
// Get the event
restEventMockMvc.perform(delete("/api/events/{id}", event.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().is4xxClientError());
}
@Test
@Transactional
public void deleteEventWithDifferentCompanyId() throws Exception {
given(uaaClient.getUserCompanyId()).willReturn(UPDATED_COMPANY_ID);
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
int databaseSizeBeforeDelete = eventRepository.findAll().size();
// Get the event
restEventMockMvc.perform(delete("/api/events/{id}", event.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().is5xxServerError());
}
@Test
@Transactional
public void createEventWithExistingId() throws Exception {
int databaseSizeBeforeCreate = eventRepository.findAll().size();
// Create the Event with an existing ID
event.setId(DEFAULT_EVENT_ID);
EventDTO eventDTO = eventMapper.toDto(event);
// An entity with an existing ID cannot be created, so this API call must fail
restEventMockMvc.perform(post("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isBadRequest());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void getAllEvents() throws Exception {
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
// Get all the eventList
restEventMockMvc.perform(get("/api/events?sort=id,desc"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.[*].name").value(hasItem(DEFAULT_NAME)))
.andExpect(jsonPath("$.[*].host").value(hasItem(DEFAULT_HOST)))
.andExpect(jsonPath("$.[*].companyId").value(hasItem(DEFAULT_COMPANY_ID.toString())));
}
@Test
@Transactional
public void getNonExistingEvent() throws Exception {
// Get the event
restEventMockMvc.perform(get("/api/events/{id}", DEFAULT_EVENT_ID))
.andExpect(status().is4xxClientError());
}
@Test
@Transactional
public void updateEvent() throws Exception {
// Initialize the database
event.setCompanyId(DEFAULT_COMPANY_ID);
eventRepository.saveAndFlush(event);
int databaseSizeBeforeUpdate = eventRepository.findAll().size();
// Update the event
Event updatedEvent = eventRepository.findOne(event.getId());
// Disconnect from session so that the updates on updatedEvent are not directly saved in db
em.detach(updatedEvent);
updatedEvent
.name(UPDATED_NAME)
.date(UPDATED_DATE)
.host(UPDATED_HOST);
EventDTO eventDTO = eventMapper.toDto(updatedEvent);
restEventMockMvc.perform(put("/api/events")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(eventDTO)))
.andExpect(status().isOk());
// Validate the Event in the database
List<Event> eventList = eventRepository.findAll();
assertThat(eventList).hasSize(databaseSizeBeforeUpdate);
Event testEvent = eventList.get(eventList.size() - 1);
assertThat(testEvent.getName()).isEqualTo(UPDATED_NAME);
assertThat(testEvent.getDate()).isEqualTo(UPDATED_DATE);
assertThat(testEvent.getHost()).isEqualTo(UPDATED_HOST);
}
}
<file_sep>/src/main/java/com/gabrielebonadiman/multitenancy/service/dto/EventDTO.java
package com.gabrielebonadiman.multitenancy.service.dto;
import java.time.LocalDate;
import javax.validation.constraints.*;
import java.io.Serializable;
import java.util.Date;
import java.util.Objects;
import java.util.UUID;
/**
* A DTO for the Event entity.
*/
public class EventDTO implements Serializable {
private UUID id;
@NotNull
private String name;
@NotNull
private Date date;
private String host;
private UUID companyId;
private Date createdAt;
private Date lastUpdate;
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public UUID getCompanyId() {
return companyId;
}
public void setCompanyId(UUID companyId) {
this.companyId = companyId;
}
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
public Date getLastUpdate() {
return lastUpdate;
}
public void setLastUpdate(Date lastUpdate) {
this.lastUpdate = lastUpdate;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
EventDTO eventDTO = (EventDTO) o;
if(eventDTO.getId() == null || getId() == null) {
return false;
}
return Objects.equals(getId(), eventDTO.getId());
}
@Override
public int hashCode() {
return Objects.hashCode(getId());
}
@Override
public String toString() {
return "EventDTO{" +
"id=" + id +
", name='" + name + '\'' +
", date=" + date +
", host='" + host + '\'' +
", companyId=" + companyId +
", createdAt=" + createdAt +
", lastUpdate=" + lastUpdate +
'}';
}
}
<file_sep>/src/main/java/com/gabrielebonadiman/multitenancy/config/package-info.java
/**
* Spring Framework configuration files.
*/
package com.gabrielebonadiman.multitenancy.config;
| d1fbada60ea48192f3e6f8e4b6052fc9321509cb | [
"Java"
] | 5 | Java | gabriele-bonadiman/MS-01-multitenancy-microservice | 514081a25dcfb2d96cdd380cbc0f66e2d48c66cd | 96c513fdd06800ce210a7dd3476822423bb63b7c | |
refs/heads/master | <repo_name>lisunshiny/rails-lite<file_sep>/test.rb
require 'byebug'
require 'uri'
@params = {}
def parse_www_encoded_form(www_encoded_form)
return if www_encoded_form.nil?
resp_hash = {}
arr = URI.decode_www_form(www_encoded_form)
arr.map!{|el| el[0] = parse_key(el[0]); el}
# byebug
arr.each do |keyval|
keys = keyval[0]
hash = @params
if keys.length > 1
keys[0..-2].each do |key|
hash[key] = {} unless hash[key]
hash = hash[key]
end
end
hash[keys[-1]] = keyval[1]
end
end
def parse_key(key)
key.split(/\]\[|\[|\]/)
end
test = "{ 'user' => { 'address' => { 'street' => 'main', 'zip' => '89436' } } }"
<file_sep>/bin/p01_basic_server.rb
require 'webrick'
require 'byebug'
server = WEBrick::HTTPServer.new(Port: 3000)
server.mount_proc("/") do |request, response|
response.content_type = "text/text"
response.body = request.path
byebug
end
trap('INT') do
server.shutdown
end
server.start
| cf50e461dda7f362dc1c42ee4b0fc4d1140f900c | [
"Ruby"
] | 2 | Ruby | lisunshiny/rails-lite | b38167e7ffbc3c8cf1e3bf189843ea4101d96158 | 1b786d062de35db8fa526881f59af17d126dd5b3 | |
refs/heads/master | <repo_name>lishaodan/ESP8266<file_sep>/wifi_net/script2.lua
test git
commit
append text 2 1242342
asfdafsd
test the git
<file_sep>/wifi_net/init.lua
pin1 = 4
gpio.mode(pin1, gpio.OUTPUT)
pin2 = 3
gpio.mode(pin2, gpio.OUTPUT)
wifi.setmode(wifi.STATIONAP)
cfg={}
cfg.ssid="DANBAO"
cfg.pwd="<PASSWORD>"
wifi.ap.config(cfg)
sv = net.createServer(net.TCP, 30)
if sv then
sv:listen(9000, function(conn)
print(conn:getaddr())
conn:on("receive", function(sck, data)
print(data)
--conn:send(data)
--print(a)
if (data == "open\n") then
print("pin is open")
conn:send("ECHO: pin is open\n")
gpio.write(pin1, gpio.HIGH)
gpio.write(pin2, gpio.HIGH)
elseif (data == "close\n") then
print("pin is close")
conn:send("ECHO: pin is close\n")
gpio.write(pin1, gpio.LOW)
gpio.write(pin2, gpio.LOW)
end
end)
conn:send("hello world")
end)
end
<file_sep>/dht11/init.lua
wifi.setmode(wifi.STATIONAP)
cfg={}
cfg.ssid="DANBAO"
cfg.pwd="<PASSWORD>"
wifi.ap.config(cfg)
pin = 4
sv = net.createServer(net.TCP, 30)
if sv then
sv:listen(9000, function(conn)
print(conn:getaddr())
conn:on("receive", function(sck, data)
print(data)
conn:send(data)
if (data == "send\n") then
-------------------------------------
status, temp, humi, temp_dec, humi_dec = dht.read(pin)
if status == dht.OK then
-- Integer firmware using this example
text_data=string.format("DHT Temperature:%d.%03d;Humidity:%d.%03d\r\n",
math.floor(temp),
temp_dec,
math.floor(humi),
humi_dec
)
print(text_data)
elseif status == dht.ERROR_CHECKSUM then
print( "DHT Checksum error." )
elseif status == dht.ERROR_TIMEOUT then
print( "DHT timed out." )
end
------------------------------------------
conn:send(text_data)
end
end)
conn:send("hello world")
end)
end
| c10cc8f3a7ed8bde339023c736788eb27abd363f | [
"Lua"
] | 3 | Lua | lishaodan/ESP8266 | 447f75726d51c5a18e3366667abf2639b6310c08 | 1123c8c3716c39af31947b36a7852a4cacbffbbd | |
refs/heads/master | <file_sep>function produceDrivingRange(blockRange) {
return function(num1, num2) {
let number1;
let number2;
if (num1.length === 4) {
number1 = parseInt(num1.slice(0, 2), 10);
} else {
number1 = parseInt(num1.slice(0, 1), 10);
}
if (num2.length === 4) {
number2 = parseInt(num2.slice(0, 2));
} else {
number2 = parseInt(num2.slice(0, 1));
}
let total = number1 - number2;
let numberDiff = Math.abs(total);
console.log(numberDiff)
if (blockRange >= numberDiff) {
let diff = blockRange - numberDiff;
return `within range by ${diff}`;
} else {
let diff = numberDiff - blockRange;
return `${diff} blocks out of range`;
}
}
}
function produceTipCalculator(decimal) {
return function(base) {
return decimal * base;
}
}
| 74cb0c3d2a9140e475424fe540cf7b546d140d28 | [
"JavaScript"
] | 1 | JavaScript | Timothy-Eubank/js-advanced-scope-closures-lab-web-091817 | 183a578c0235273f44063ff53033ea9e0de3d0f3 | 20441495b20ca7fd871da01f58652de7710bd684 | |
refs/heads/master | <file_sep>-- inserindo registro na tabela mesas
insert into mesas (mesa_codigo, mesa_situacao, data_criacao, data_atualizacao) values ('00001', 'A', '01/01/2016', '01/01/2016');
insert into mesas (mesa_codigo, mesa_situacao, data_criacao, data_atualizacao) values ('00002', 'A', '01/01/2016', '01/01/2016');
select * from mesas;
-- inserindo registros na tabela funcionarios
insert into funcionarios (
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'00001',
'<NAME>',
'A',
5,
'GERENTE',
'01/01/2016'
);
insert into funcionarios (
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'00002',
'Souza',
'A',
2,
'GARÇOM',
'01/01/2016'
);
select * from funcionarios;
-- Inserindo registros na tabela produtos
insert into produtos (
produto_codigo,
produto_nome,
produto_valor,
produto_situacao,
data_criacao,
data_atualizacao
) values (
'001',
'REFRIGERANTE',
10,
'A',
'01/01/2016',
'01/01/2016'
);
insert into produtos (
produto_codigo,
produto_nome,
produto_valor,
produto_situacao,
data_criacao,
data_atualizacao
) values (
'002',
'AGUA',
3,
'A',
'01/01/2016',
'01/01/2016'
);
insert into produtos (
produto_codigo,
produto_nome,
produto_valor,
produto_situacao,
data_criacao,
data_atualizacao
) values (
'003',
'PASTEL',
7,
'A',
'01/01/2016',
'01/01/2016'
);
select * from produtos;
-- inserir registros na tabela de vendas
insert into vendas (
funcionario_id,
mesa_id,
venda_codigo,
venda_valor,
venda_total,
venda_desconto,
venda_situacao,
data_criacao,
data_atualizacao
) values (
2,
1,
'0001',
'20',
'20',
'0',
'A',
'01/01/2016',
'01/01/2016'
);
insert into vendas (
funcionario_id,
mesa_id,
venda_codigo,
venda_valor,
venda_total,
venda_desconto,
venda_situacao,
data_criacao,
data_atualizacao
) values (
2,
2,
'0002',
'21',
'21',
'0',
'A',
'01/01/2016',
'01/01/2016'
);
select * from vendas;
-- inserir registro na tabela itens_vendas
insert into itens_vendas (
produto_id,
vendas_id,
item_valor,
item_quantidade,
item_total,
data_criacao,
data_atualizacao
) values (
1,
1,
10,
2,
20,
'01/01/2016',
'01/01/2016'
);
insert into itens_vendas (
produto_id,
vendas_id,
item_valor,
item_quantidade,
item_total,
data_criacao,
data_atualizacao
) values (
1,
2,
7,
3,
21,
'01/01/2016',
'01/01/2016'
);
select * from itens_vendas;<file_sep>-- || para concatenar strings
select funcionario_codigo || ' ' || funcionario_nome
from funcionarios
where id = 1;
select (funcionario_codigo || 8 || funcionario_nome)
from funcionarios
where id = 1;
-- Contando os caracteres de uma string
select funcionario_nome nome from funcionarios where id = 2;
select char_length(funcionario_nome) from funcionarios where id = 2;
-- Transformando letras minúsculas em maúsculas com upper(string)
select upper(funcionario_nome) from funcionarios;
select upper('livro postgresql');
-- Transforma apenas as primeiras letras de cada palavra
select initcap('livro postgresql');
-- Transforma maiúsculas em minúsculas com lower(string)
select funcionario_nome from funcionarios;
select lower(funcionario_nome) from funcionarios;
-- Substituindo string com overlay() e extraindo com substring()
select overlay(funcionario_nome placing '******' from 3 for 5) from funcionarios where id = 1;
-- Extração de um techo da string
select substring(funcionario_nome from 3 for 5) from funcionarios where id = 1;
-- Localizando uma string position
select funcionario_nome from funcionarios where id = 1;
select position('cinius' in funcionario_nome) from funcionarios where id = 1;<file_sep>insert into funcionarios (
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0100',
'<NAME>',
'A',
'2',
'GARÇOM',
'01/03/2016'
);
insert into funcionarios (
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0101',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
insert into funcionarios(
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0102',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
insert into funcionarios(
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0103',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
insert into funcionarios(
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0104',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
insert into funcionarios(
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0105',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
insert into funcionarios(
funcionario_codigo,
funcionario_nome,
funcionario_situacao,
funcionario_comissao,
funcionario_cargo,
data_criacao
) values (
'0106',
'<NAME>',
'A',
2,
'GARÇOM',
'01/03/2016'
);
select * from funcionarios;
select * from funcionarios where funcionario_nome ilike 'VINICIUS%';
select * from funcionarios where funcionario_nome ilike '%souza%';<file_sep>-- consultar os registros mesas
select mesa_codigo, mesa_situacao from mesas;
select * from mesas where mesa_codigo = '00002';
-- ATUALIZANDO REGISTROS
select * from produtos;
update produtos set produto_valor = 4 where id = 2;
select * from vendas;
update vendas set mesa_id = 1 where id = 2;
-- DELETANDO REGISTROS
select * from mesas;
delete from mesas where id = 2;<file_sep>-- criando uma consulta relacionado duas tabelas com join
select distinct funcionario_nome
from funcionarios, vendas
where funcionarios.id = vendas.funcionario_id
order by funcionario_nome;
select distinct funcionario_nome from funcionarios, vendas order by funcionario_nome;
select distinct funcionario_nome
from funcionarios
inner join vendas
on (funcionarios.id = vendas.funcionario_id)
order by funcionario_nome;
select distinct funcionario_nome
from funcionarios f
join vendas v
on (f.id = v.funcionario_id)
order by funcionario_nome;
select funcionario_nome, v.id
from funcionarios f
left join vendas v
on f.id = v.funcionario_id
order by funcionario_nome desc;
select v.id, v.venda_total, funcionario_nome from vendas v
right join funcionarios f
on v.funcionario_id = f.id
order by v.venda_total;
create or replace view vendas_do_dia as
select distinct produto_nome,
sum(v.venda_total)
from produtos p, itens_vendas iv, vendas v
where p.id = iv.produto_id and v.id = iv.vendas_id and v.data_criacao = '01/01/2016'
group by produto_nome;
select * from vendas_do_dia;
select * from vendas_do_dia where produto_nome = 'PASTEL';
create or replace view produtos_vendas as
select p.id PRODUTO_ID,
p.produto_nome PRODUTO_NOME,
v.id VENDA_ID,
iv.id ITEM_ID,
iv.item_valor ITEM_VALOR,
v.data_criacao DATA_CRIACAO
from produtos p, vendas v, itens_vendas iv
where v.id = iv.vendas_id
and p.id = iv.produto_id
order by data_criacao desc;
select * from produtos_vendas;
select produto_nome from produtos_vendas where data_criacao = '01/01/2016';<file_sep>
-- relacionando duas tabelas por meio de uma subconsulta, na qual buscaremos os funcionários que possuem vínculo com uma ou mais vendas
select funcionario_nome from funcionarios where id in (select funcionario_id from vendas);
-- realizando uma consulta apenas buscando as vendas realizadas em 2016
-- passando o ano da data atual por parâmetro
select funcionario_nome from funcionarios where id in(select funcionario_id from vendas where date_part('year', data_criacao) = '2016');
select v.id, f.funcionario_nome from vendas v inner join funcionarios f on v.funcionario_id = f.id;
-- realizando uma consulta trazendo apenas os nomes distintos dos funcionarios e ordenando em ordem alfabética
select distinct funcionario_nome
from funcionarios
where id in(select funcionario_id
from vendas v
where date_part('year', data_criacao) = '2016')
order by funcionario_nome desc;
select distinct produto_nome
from produtos
where id in (select id from itens_vendas v);
select * from itens_vendas;<file_sep>-- criando toas sequencias (ids com autoincremento)
create sequence mesa_id_seq;
create sequence vendas_id_seq;
create sequence itens_vendas_id_seq;
create sequence produtos_id_seq;
create sequence funcionario_id_seq;
create sequence comissoes_id_seq;
-- vincular cada sequence com suas respectivas tabelas
alter table mesas alter column id set default nextval('mesa_id_seq');
alter table vendas alter column id set default nextval('vendas_id_seq');
alter table itens_vendas alter column id set default nextval('itens_vendas_id_seq');
alter table produtos alter column id set default nextval('produtos_id_seq');
alter table funcionarios alter column id set default nextval('funcionario_id_seq');
alter table comissoes alter column id set default nextval('comissoes_id_seq');
-- deletando uma sequence vinculado a uma tabela
drop sequence funcionario_id_seq cascade;
-- deletando uma sequence não vinculada a uma tabela
drop sequence funcionario_id_seq;
-- ALTERANDO TABELA
-- inserir um novo campo
alter table comissoes add column data_pagamento int;
-- alterando o tipo da coluna
-- por meio da exclusao da coluna e criando uma nova
alter table comissoes drop column data_pagamento;
alter table comissoes add column data_pagamento timestamp;
-- por meio do alter
alter table comissoes alter column data_pagamento type timestamp using data_pagamento_timestamp;
-- alterando o nome de uma coluna
ALTER TABLE vendas RENAME COLUMN vanda_valor TO venda_valor;
<file_sep>-- excluir uma table
drop table comissoes;
-- tabela para gravar registro do caculo das comissoes sem uma chave primaria e sem chave estrangeira
create table comissoe(
id int not null,
funcionario_id int,
comissao_valor real,
comissao_situacao varchar(1) default 'A',
data_criacao timestamp,
data_atualizacao timestamp
);
-- renomear uma table
ALTER TABLE public.comissoe RENAME TO comissoes;
-- criando uma constraints PK (chave primaria)
alter table comissoes add constraint comissoes_pkey primary key(id);
-- criando uma constraints FK (chave estrangeiras)
alter table comissoes add foreign key (funcionario_id) references funcionarios(id);
-- deletando uma constraint
-- removendo FK da tabela de comissoes (funcionario_id) que se relacionova com a tabela de funcionarios(id)
alter table comissoes drop constraint comissoes_funcionario_id_fkey;
-- criar uma constraint que dira para o banco testa o campo venda_total é maior que zero (positivo) a cada novo registro
alter table vendas add check ( venda_total > 0 );
-- criar uma cosntraint para não permitir inserir nulo no campo
alter table funcionarios add check ( funcionario_nome <> null );<file_sep>-- tabela para gravar registro das mesas
create table mesas (
id int not null primary key,
mesa_codigo varchar(20),
mesa_situacao varchar(1) default 'A',
data_criacao timestamp,
data_atualizacao timestamp
);
-- tabela para gravar registro dos funcionarios
create table funcionarios(
id int not null primary key,
funcionario_codigo varchar(20),
funcionario_nome varchar(100),
funcionario_situacao varchar(1) default 'A',
funcionario_comissao real,
funcionario_cargo varchar(30),
data_criacao timestamp,
data_atualizacao timestamp
);
-- tabela para gravar registro das vendas
create table vendas(
id int not null primary key,
funcionario_id int references funcionarios (id),
mesa_id int references mesas(id),
venda_codigo varchar(20),
vanda_valor real,
venda_total real,
venda_desconto real,
venda_situacao varchar(1) default 'A',
data_criacao timestamp,
data_atualizacao timestamp
);
-- tabela para gravar registro dos produtos
create table produtos(
id int not null primary key,
produto_codigo varchar(20),
produto_nome varchar(60),
produto_valor real,
produto_situacao varchar(1) default 'A',
data_criacao timestamp,
data_atualizacao timestamp
);
-- tabela para gravar registro dos itens das vendas
create table itens_vendas(
id int not null primary key,
produto_id int not null references produtos(id),
vendas_id int not null references vendas(id),
item_valor real,
item_quantidade int,
item_total real,
data_criacao timestamp,
data_atualizacao timestamp
);
-- tabela para gravar registro do c?culo das comissoes
create table comissoes(
id int not null primary key,
funcionario_id int references funcionarios(id),
comissao_valor real,
comissao_situacao varchar(1) default 'A',
data_criacao timestamp,
data_atualizacao timestamp
); | a515c112e1bfc195c640c3558fb77dc3021e5957 | [
"SQL"
] | 9 | SQL | icarolopes/exemplos_postgres_CDC | 51b3bb0017e48aa1ad1154f12cd33b3788b0e7a8 | 5f8e0f3c481f0a714887f9142464e7392f9f0cbc | |
refs/heads/master | <repo_name>thesultanster/SPG<file_sep>/app/src/main/java/spg/com/spg/SPGWifiActivity.java
package spg.com.spg;
import android.graphics.Color;
import android.graphics.PorterDuff;
import android.graphics.drawable.Drawable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v7.widget.Toolbar;
public class SPGWifiActivity extends AppCompatActivity {
Toolbar toolbar;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_spgwifi);
// Setting toolbar
toolbar = (Toolbar) findViewById(R.id.toolbar);
if (toolbar != null)
setSupportActionBar(toolbar);
toolbar.setTitleTextColor(Color.parseColor("#FFFFFF"));
toolbar.setTitle("SPG WIFI");
final Drawable upArrow = getResources().getDrawable(R.drawable.abc_ic_ab_back_mtrl_am_alpha);
upArrow.setColorFilter(Color.parseColor("#FFFFFF"), PorterDuff.Mode.SRC_ATOP);
getSupportActionBar().setHomeAsUpIndicator(upArrow);
getSupportActionBar().setDisplayHomeAsUpEnabled(true);
getSupportActionBar().setDisplayShowHomeEnabled(true);
}
}
<file_sep>/app/src/main/java/spg/com/spg/Home.java
package spg.com.spg;
import android.content.Intent;
import android.graphics.LinearGradient;
import android.support.design.widget.TabLayout;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.widget.LinearLayout;
import android.widget.TextView;
public class Home extends NavigationDrawerFramework {
TabLayout tabLayout;
TextView fadeTitle;
TextView fadeBody;
LinearLayout ciscoCard;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_home);
getToolbar().setTitle("HOME");
tabLayout = (TabLayout) findViewById(R.id.tabs);
tabLayout.addTab(tabLayout.newTab().setText("MY SPG"));
tabLayout.addTab(tabLayout.newTab().setText("MY STAYS"));
fadeTitle = (TextView) findViewById(R.id.fadeTitle);
fadeBody = (TextView) findViewById(R.id.fadeBody);
ciscoCard = (LinearLayout) findViewById(R.id.ciscoCard);
ciscoCard.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(getApplicationContext(), SPGWifiActivity.class);
startActivity(intent);
}
});
AlphaAnimation fadeIn = new AlphaAnimation(0.0f , 1.0f ) ;
fadeIn.setDuration(1200);
fadeIn.setFillAfter(true);
fadeIn.setStartOffset(800);
fadeTitle.setAnimation(fadeIn);
fadeBody.setAnimation(fadeIn);
}
}
| ae8fe668f1b70a0f087e3c1c12128d54fe3d0d34 | [
"Java"
] | 2 | Java | thesultanster/SPG | b44e7b9ebb112520cb56173b22dcc9acd6c39fdd | 9e822a39c9fa3ab2464d7462d65475a1327902ce | |
refs/heads/master | <repo_name>DChuchin/biref<file_sep>/README.md
# parcel builerplate
## Install dependencies
```
$ yarn
```
## Available tasks
```sh
# Run dev mode and watch the files
$ yarn watch
# Build and minify the code
$ yarn build
# Format the code (used prettier)
$ yarn format
# Remove dist folder
$ yarn clean
```
## Features
- scss, sass for styling
- autoprefixer
- prettier for code formatting (by pre-commit hook or manually use format command see above)
- babel for js transpilation
- image minification
<file_sep>/src/js/main.js
import render from './modules/render';
import model from './modules/model';
import resetRequired from './modules/resetRequired';
const form = document.querySelector('form');
const nextBtn = document.querySelector('#nextBtn');
const prevBtn = document.querySelector('#prevBtn');
const goNext = e => {
e.preventDefault();
if (form.reportValidity()) {
model.direction = 'forward';
model.nextStep();
}
render();
};
const goBack = e => {
e.preventDefault();
model.direction = 'backward';
model.prevStep();
render();
};
// listeners
form.addEventListener('input', () => render());
prevBtn.addEventListener('click', goBack);
nextBtn.addEventListener('click', goNext);
window.model = model; // TODO remove from prod
// resetRequired(form);
render();
<file_sep>/src/js/modules/checkboxValidity.js
const checkboxValidity = checkboxGroup => {
const requiredElements = Array.from(
checkboxGroup.querySelectorAll('input[type="checkbox"]')
);
return requiredElements.some(element => element.checked);
};
export default checkboxValidity;
<file_sep>/src/js/modules/updateRequired.js
const updateRequired = fieldset => {
Array.from(fieldset.elements).forEach(formElement => {
if (formElement.dataset.required) {
formElement.required = formElement.dataset.required;
}
});
};
export default updateRequired;
<file_sep>/src/js/modules/isValid.js
const isValid = fieldset => {};
<file_sep>/src/js/modules/model.js
class Model {
constructor(step = 0, maxStep = 0) {
Object.assign(this, { step, maxStep });
}
nextStep() {
if (this.step < this.maxStep) {
this.step += 1;
}
}
prevStep() {
if (this.step > 0) {
this.step -= 1;
}
}
}
const form = document.querySelector('form');
const stepSections = form && Array.from(form.querySelectorAll('[data-step]'));
const maxStep = Math.max(
...stepSections.map(step => Math.floor(step.dataset.step))
);
const model = new Model(0, maxStep);
export default model;
| ed060faa9c1a5eff8fe8266be933257c222d3e45 | [
"Markdown",
"JavaScript"
] | 6 | Markdown | DChuchin/biref | 3e59145340ffd0e40309c134d14db9960ac13bf3 | f4d7cec03f9d5c5f3e7d7ed0a007a8380b4c4985 | |
refs/heads/master | <file_sep>console.log('create feature login')
var a = 3 - 2
console.log(a) | 284ec421e9610796f71117518bde0c3317a56481 | [
"JavaScript"
] | 1 | JavaScript | nafis-laila/latihan_git_jwcm17 | 5102beb00cae6da196876e265b294d2d66e7ce32 | 5314ea763225ecc47ca4cfe758598e9573abf104 | |
refs/heads/master | <file_sep>import React, { Component } from 'react';
class Home extends Component {
constructor(){
super();
this.parallaxArray = [5];
for(let i = 0; i < 5; i++){
this.parallaxArray[i] = React.createRef();
}
//this.state = {parallaxArray: this.parallaxArray};
this.handleScroll = this.handleScroll.bind(this);
}
componentDidMount(){
window.addEventListener('scroll', this.handleScroll);
}
componentWillUnmount(){
window.removeEventListener('scroll', this.handleScroll);
}
handleScroll(e){
/*for(let i = 0; i < 5; i++){
this.parallaxArray[i].style = {transform: `translate3d(0px, ${i * 5}px, 0px)`};
}*/
console.log("hello world");
}
render() {
return (
<div id="homewrapper">
<div id="home">
<div className="parallax" ref={this.parallaxArray[0]}></div>
<div className="parallax" ref={this.parallaxArray[1]}></div>
<div className="parallax" ref={this.parallaxArray[2]}></div>
<div className="parallax" ref={this.parallaxArray[3]}></div>
<div className="parallax" ref={this.parallaxArray[4]}></div>
</div>
</div>
);
}
}
export default Home;
<file_sep>import React, { Component } from 'react';
class Tools extends Component {
render() {
return (
<div id="toolswrapper">
<div id="tools"></div>
</div>
);
}
}
export default Tools;
<file_sep>import React, { Component } from 'react';
import { NavLink } from 'react-router-dom';
class Header extends Component {
constructor(props){
super(props);
this.state = {breadcrumbs: []};
}
componentDidMount(){
let patharray = this.props.location.pathname.split('/');
this.setState({breadcrumbs: patharray});
}
componentDidUpdate(prevProps){
if(this.props.location.pathname !== prevProps.location.pathname){
let patharray = this.props.location.pathname.split('/');
this.setState({breadcrumbs: patharray});
}
}
render() {
for (let i = 0; i < this.state.breadcrumbs.length; i++){
if(this.state.breadcrumbs[i] !== ""){
/*let test = "";
for (let i2 = 0; i2 < i; i--){
//test += this.state.breadcrumbs[i];
console.log("aaa");
}*/
console.log(this.state.breadcrumbs[i]);
}
}
return (
<div id="header">
<div id="shimapanempty"></div>
<div id="shimapanblue">
</div>
<div id="shimapanlightblue"></div>
<div id="shimapanwhite"></div>
</div>
);
}
}
export default Header;
<file_sep>import React, { Component } from 'react';
class Editbar extends Component {
render() {
return (
<div id="editbarwrapper">
<div id="editbar"></div>
</div>
);
}
}
export default Editbar;<file_sep>import React, { Component } from 'react';
import {
UncontrolledDropdown,
DropdownToggle,
DropdownMenu,
DropdownItem
} from 'reactstrap';
import{
NavLink
} from 'react-router-dom';
class Navbar extends Component {
render() {
return (
<div id="navbar">
<NavLink to="/" id="logogroup">
<div id="logoimage"></div>
<div id="logotext">Vastestness</div>
</NavLink>
<div id="exploregroup">
<UncontrolledDropdown id="uncontrolleddropdown" nav inNavbar>
<DropdownToggle id="explorebutton" nav caret>
EXPLORE
</DropdownToggle>
<DropdownMenu left="true">
<NavLink to="/post/art" className="exploreoptions">
Art
</NavLink>
<NavLink to="/post/music" className="exploreoptions">
Music
</NavLink>
<NavLink to="/post/code" className="exploreoptions">
Code
</NavLink>
<DropdownItem className="exploreoptions" divider />
<NavLink to="/tools" className="exploreoptions">
Tools
</NavLink>
</DropdownMenu>
</UncontrolledDropdown>
</div>
<div id="searchgroup">
<form id="searchform">
<input type="text" placeholder="Search"></input>
<button id="searchbutton"></button>
</form>
</div>
</div>
);
}
}
export default Navbar;
| 827e7e706fca54f50bd0b261bee5d8c738453f22 | [
"JavaScript"
] | 5 | JavaScript | celepye/webclient | 2f6576349f6800b606dfa645774f5166cdbf89bc | 1c2f39bb3bc193c75818ee92e29c85a86b39ae8a | |
refs/heads/master | <file_sep>package com.example.bazile.morpion.game
val x = "X"
val o = "O"<file_sep>package com.example.bazile.morpion.Utils
import android.content.Context
import android.util.AttributeSet
import android.widget.TextView
import android.graphics.Typeface
class FippsTextView : TextView{
constructor(context: Context?) : super(context){
val face = Typeface.createFromAsset(context!!.assets, "fonts/fipps_regular.otf")
this.typeface = face
}
constructor(context: Context?, attrs: AttributeSet?) : super(context, attrs){
val face = Typeface.createFromAsset(context!!.assets, "fonts/fipps_regular.otf")
this.typeface = face
}
constructor(context: Context?, attrs: AttributeSet?, defStyleAttr: Int) : super(context, attrs, defStyleAttr){
val face = Typeface.createFromAsset(context!!.assets, "fonts/fipps_regular.otf")
this.typeface = face
}
}<file_sep>package com.example.bazile.morpion.game
val combinaison: Array<IntArray> = arrayOf(
intArrayOf(1, 2, 3),
intArrayOf(4, 5, 6),
intArrayOf(7, 8, 9),
intArrayOf(2, 5, 8),
intArrayOf(1, 4, 7),
intArrayOf(3, 6, 9),
intArrayOf(1, 5, 9),
intArrayOf(3, 5, 7)
)
<file_sep>package com.example.bazile.morpion.game
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.view.View
import android.widget.*
import com.example.bazile.morpion.R
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity() : AppCompatActivity(), View.OnClickListener {
private var board = mutableMapOf<Int, String>()
private var Player = true
private var winner: String = ""
private var totalBoard = 9
private val btn = arrayOfNulls<Button>(totalBoard)
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
initButon()
replay_button.setOnClickListener {
Toast.makeText(this, "Replay", Toast.LENGTH_LONG).show()
newGame()
}
}
private fun initButon() {
for (i in 1..totalBoard) {
val button = findViewById<Button>(resources.getIdentifier("button$i", "id", packageName))
button.setOnClickListener(this)
btn[i - 1] = button
}
}
override fun onClick(view: View) {
val buSelected: Button = view as Button
var selected = 0
when (buSelected.id) {
R.id.button1 -> selected = 1
R.id.button2 -> selected = 2
R.id.button3 -> selected = 3
R.id.button4 -> selected = 4
R.id.button5 -> selected = 5
R.id.button6 -> selected = 6
R.id.button7 -> selected = 7
R.id.button8 -> selected = 8
R.id.button9 -> selected = 9
}
jouer(selected, buSelected)
checkWinner()
}
private fun checkWinner(): Int {
if (board.isNotEmpty()) {
for (combine in combinaison) {
val (a, b, c) = combine
if (board[a] != null && board[a] == board[b] && board[a] == board[c] && board[a] == x) {
Toast.makeText(this, "Winner 1 ", Toast.LENGTH_LONG).show()
this.winner = board.toString()
return 10
}
if (board[a] != null && board[a] == board[b] && board[a] == board[c] && board[a] == o) {
Toast.makeText(this, "Winner 2 ", Toast.LENGTH_LONG).show()
this.winner = board.toString()
return 10
}
}
}
return 0
}
private fun jouer(selected: Int, buSelected: Button) {
var cpt = 0
if (!winner.isEmpty()) {
Toast.makeText(this, "The game is over", Toast.LENGTH_LONG).show()
return
}
for (i in 1..totalBoard) {
if (board[i] != null) {
cpt++
}
}
if (cpt == 8)
Toast.makeText(this, "Draw ", Toast.LENGTH_LONG).show()
if (board[selected] != null) {
Toast.makeText(this, "Don't play ", Toast.LENGTH_LONG).show()
return
}
when {
Player -> board[selected] = x
else -> board[selected] = o
}
buSelected.text = board[selected]
Player = !Player
}
private fun dejouer(selected: Int, buSelected: Button) {
if (board[selected] == x || board[selected] == o) {
board[selected] = ""
buSelected.text = board[selected]
}
}
private fun resetButton() {
for (i in 1..totalBoard) {
var button = btn[i - 1]
button?.text = ""
button?.isEnabled = true
}
}
private fun newGame() {
board = mutableMapOf()
Player = true
winner = ""
resetButton()
}
}
<file_sep>package com.example.bazile.morpion.game
import android.annotation.SuppressLint
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import android.widget.Toast
import com.example.bazile.morpion.R
import kotlinx.android.synthetic.main.activity_inscription.*
import java.util.regex.Pattern
class Inscription : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_inscription)
valider()
}
private fun checkMail():Boolean{
return !edit_text_email.text.isEmpty() && isEmailValid(edit_text_email.text.toString())
}
private fun isEmailValid(email: String): Boolean {
return Pattern.compile(
"^(([\\w-]+\\.)+[\\w-]+|([a-zA-Z]|[\\w-]{2,}))@"
+ "((([0-1]?[0-9]{1,2}|25[0-5]|2[0-4][0-9])\\.([0-1]?"
+ "[0-9]{1,2}|25[0-5]|2[0-4][0-9])\\."
+ "([0-1]?[0-9]{1,2}|25[0-5]|2[0-4][0-9])\\.([0-1]?"
+ "[0-9]{1,2}|25[0-5]|2[0-4][0-9]))|"
+ "([a-zA-Z]+[\\w-]+\\.)+[a-zA-Z]{2,4})$"
).matcher(email).matches()
}
private fun checkpassword():Boolean{
return !edit_text_password.text.isEmpty() && edit_text_password.text.length >=6
}
@SuppressLint("ShowToast")
private fun valider(){
valider.setOnClickListener {
if (checkMail() && checkpassword())
Toast.makeText(this, "Le mail correct and password", Toast.LENGTH_LONG).show()
else
Toast.makeText(this, "Veilliez mettre un mail correct et un password de 6 mots", Toast.LENGTH_LONG).show()
}
}
}<file_sep>package com.example.bazile.morpion.game
import android.annotation.SuppressLint
import android.content.Intent
import android.os.Bundle
import android.support.v7.app.AppCompatActivity
import com.example.bazile.morpion.R
import kotlinx.android.synthetic.main.activity_title.*
@SuppressLint("Registered")
class TitleActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_title)
onclick()
}
private fun onclick() {
single_player.setOnClickListener {
val intent = Intent(this, MainActivity::class.java)
startActivity(intent)
}
multi_player.setOnClickListener {
val intent = Intent(this, Inscription::class.java)
startActivity(intent)
}
}
} | d550a8cab8c61a4e016bae791d8ba2b2a0aab816 | [
"Kotlin"
] | 6 | Kotlin | OliverBazile/Morpion | 576d79ba758039902fc8c0026735e97cbc6d5406 | 0a7bc3998bc822f0219f74ccb1307511da5bec76 | |
refs/heads/master | <file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Service\WorkflowService;
/**
* WorkflowServiceAware trait.
*
* @author <NAME> <<EMAIL>>
*/
trait WorkflowServiceAwareTrait
{
/**
* @param string $key
* @param mixed $service
*
* @return $this
*/
protected abstract function setService($key, $service);
/**
* @param string $key
*
* @return mixed
*/
protected abstract function getService($key);
/**
* @return WorkflowService
*/
public function getWorkflowService()
{
return $this->getService('workflow');
}
/**
* @param WorkflowService $service
*
* @return $this
*/
public function setWorkflowService(WorkflowService $service)
{
return $this->setService('workflow', $service);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Action\Base;
use Velocity\Core\Bag;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
/**
* @author <NAME> <<EMAIL>>
*/
abstract class AbstractLetterAction extends AbstractTextNotificationAction
{
/**
* @param string $type
* @param Bag $params
* @param Bag $context
*
* @throws \Exception
*/
protected function sendLetterByType($type, Bag $params, Bag $context)
{
// @todo
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Validator\Constraints;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\Validator\Constraints\MongoId;
/**
* @author <NAME> <<EMAIL>>
*
* @group constraints
* @group validator
*/
class MongoIdTest extends PHPUnit_Framework_TestCase
{
/**
* @group unit
*/
public function testConstruct()
{
$v = new MongoId();
$this->assertNotNull($v);
$this->assertEquals('velocity_mongo_id', $v->validatedBy());
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Validator\Constraints;
use PHPUnit_Framework_TestCase;
use Symfony\Component\Validator\Context\ExecutionContextInterface;
use Velocity\Bundle\ApiBundle\Validator\Constraints\MongoId;
use Velocity\Bundle\ApiBundle\Validator\Constraints\MongoIdValidator;
/**
* @author <NAME> <<EMAIL>>
*
* @group constraints
* @group validator
*/
class MongoIdValidatorTest extends PHPUnit_Framework_TestCase
{
/**
* @var ExecutionContextInterface|\PHPUnit_Framework_MockObject_MockObject $context
*/
protected $context;
/**
* @var MongoIdValidator
*/
protected $v;
/**
*
*/
public function setUp()
{
$this->context = $this->getMock('Symfony\\Component\\Validator\\Context\\ExecutionContextInterface', [], [], '', false);
$this->v = new MongoIdValidator();
$this->v->initialize($this->context);
}
/**
* @group unit
*/
public function testConstruct()
{
$this->assertNotNull(new MongoIdValidator());
}
/**
* @group unit
*/
public function testValidateForNull()
{
$this->context->expects($this->once())->method('addViolation')->with('Not a valid Mongo ID');
$this->v->validate(null, new MongoId());
}
/**
* @group unit
*/
public function testValidateForMalformed()
{
$this->context->expects($this->once())->method('addViolation')->with('Not a valid Mongo ID');
$this->v->validate('abc12', new MongoId());
}
/**
* @group unit
*/
public function testValidate()
{
$this->context->expects($this->never())->method('addViolation');
$this->v->validate('aaaaaaaaaaaaaaaaaaaa0000', new MongoId());
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use Zend\Code\Generator\MethodGenerator;
use Velocity\Bundle\ApiBundle\Service\CallableService;
use Velocity\Bundle\ApiBundle\Service\CodeGeneratorService;
/**
* @author <NAME> <<EMAIL>>
*
* @group codeGenerator
*/
class CodeGeneratorServiceTest extends PHPUnit_Framework_TestCase
{
/**
* @var CodeGeneratorService
*/
protected $s;
/**
* @var CallableService|\PHPUnit_Framework_MockObject_MockObject
*/
protected $callableService;
/**
*
*/
public function setUp()
{
$this->callableService = $this->getMock("Velocity\\Bundle\\ApiBundle\\Service\\CallableService", [], [], '', false);
$this->s = new CodeGeneratorService($this->callableService);
}
/**
* @group unit
*/
public function testConstruct()
{
$this->assertNotNull($this->s);
}
/**
* @group unit
*/
public function testCreateClassForEmptyClassReturnZendClassWithNoMethods()
{
$zClass = $this->s->createClass('MyTestClass');
$this->assertEquals('MyTestClass', $zClass->getName());
$this->assertEquals(0, count($zClass->getMethods()));
}
/**
* @group unit
*/
public function testCreateClassForOneMethodWithoutTypeReturnZendClassWithThisBasicMethod()
{
$zClass = $this->s->createClass(
'MyTestClass',
[
'methods' => [
['name' => 'method1'],
],
]
);
$this->assertEquals(1, count($zClass->getMethods()));
}
/**
* @group integ
*/
public function testCreateClassForMethodWithExistingTypeReturnZendClassWithBuiltMethod()
{
$this->s->setCallableService(new CallableService());
$this->s->registerMethodType(
'basic2',
function (MethodGenerator $zMethod) {
$zMethod->setBody("return 'this is generated';");
}
);
$zClass = $this->s->createClass(
'MyTestClass',
[
'methods' => [
'method1' => [],
'method2' => ['type' => 'basic2'],
],
]
);
$this->assertEquals(2, count($zClass->getMethods()));
$zMethod = $zClass->getMethod('method2');
$this->assertEquals("return 'this is generated';", $zMethod->getBody());
}
/**
* @group integ
*/
public function testCreateClassForMethodWithUnknownTypeThrowException()
{
$this->s->setCallableService(new CallableService());
$this->setExpectedException('RuntimeException', "No 'iAmUnknown' in methodTypes list", 412);
$this->s->createClass(
'MyTestClass',
[
'methods' => [
'method1' => ['type' => 'iAmUnknown'],
],
]
);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Generator;
use Velocity\Core\Traits\ServiceTrait;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
/**
* String Generator Action.
*
* @author <NAME> <<EMAIL>>
*/
class StringGenerator
{
use ServiceTrait;
/**
* @return string
*
* @Velocity\Generator("random_string")
*/
public function generateString()
{
return rand(0, 1000).microtime(true).rand(rand(0, 100), 10000);
}
/**
* @return string
*
* @Velocity\Generator("random_sha1")
*/
public function generateRandomSha1String()
{
return $this->generateSha1String($this->generateRandomMd5String());
}
/**
* @param string $string
*
* @return string
*
* @Velocity\Generator("sha1")
*/
public function generateSha1String($string)
{
return sha1($string);
}
/**
* @return string
*
* @Velocity\Generator("random_md5")
*/
public function generateRandomMd5String()
{
return $this->generateMd5String($this->generateString());
}
/**
* @param string $string
*
* @return string
*
* @Velocity\Generator("md5")
*/
public function generateMd5String($string)
{
return md5($string);
}
/**
* @param mixed $data
*
* @return string
*
* @Velocity\Generator("serialized")
*/
public function generateSerializedString($data)
{
return serialize($data);
}
/**
* @param mixed $data
*
* @return string
*
* @Velocity\Generator("fingerprint")
*/
public function generateFingerPrintString($data)
{
if (is_string($data)) {
return $this->generateMd5String($data);
}
return $this->generateMd5String($this->generateSerializedString($data));
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\CodeGenerator;
use Zend\Code\Generator\ValueGenerator;
use Zend\Code\Generator\MethodGenerator;
use Zend\Code\Generator\DocBlockGenerator;
use Zend\Code\Generator\ParameterGenerator;
use Zend\Code\Generator\DocBlock\Tag\ParamTag;
use Zend\Code\Generator\DocBlock\Tag\ReturnTag;
use Zend\Code\Generator\DocBlock\Tag\ThrowsTag;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
/**
* @author <NAME> <<EMAIL>>
*/
class SdkMethodsCodeGenerator
{
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("sdk.construct")
*/
public function generateSdkConstructMethod(MethodGenerator $zMethod, array $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Construct a %s service', $definition['serviceName']),
null,
[
new ParamTag('sdk', ['SdkInterface'], 'Underlying SDK'),
]
));
$zMethod->setParameters([
new ParameterGenerator('sdk', 'SdkInterface'),
]);
$zMethod->setBody('$this->setSdk($sdk);');
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("sdk.service.test.testConstruct")
*/
public function generateSdkServiceTestTestConstructMethod(MethodGenerator $zMethod, array $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Test constructor for service %s', $definition['serviceName']),
null,
[]
));
$zMethod->setParameters([]);
$zMethod->setBody(
'$this->sdk = $this->getMock(\'Phppro\\\\Sdk\\\\Sdk\', [], [], \'\', false);'."\n".sprintf('$this->assertNotNull(new %sService($this->sdk));', ucfirst($definition['serviceName']))
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.get")
*/
public function generateCrudGetMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace('{id}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Return the specified %s', $definition['type']),
null,
[
new ParamTag('id', ['mixed'], sprintf('ID of the %s', $definition['type'])),
new ParamTag('fields', ['array'], 'List of fields to retrieve'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('id', 'string'),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->get(sprintf(\'%s\', $id), $fields, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.getBy")
*/
public function generateCrudGetByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition += ['field' => 'id'];
$definition['route'] = str_replace('{'.$definition['field'].'}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Return the specified %s by %s', $definition['type'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf(ucfirst($definition['field']).' of the %s', $definition['type'])),
new ParamTag('fields', ['array'], 'List of fields to retrieve'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'string'),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->get(sprintf(\'%s\', $%s), $fields, $options);', $definition['route'], $definition['field'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.getPropertyBy")
*/
public function generateCrudGetPropertyByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition += ['field' => 'id', 'property' => 'id'];
$definition['route'] = str_replace(['{'.$definition['field'].'}', '{'.$definition['property'].'}'], '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Return the specified %s %s by %s', $definition['type'], $definition['property'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf(ucfirst($definition['field']).' of the %s', $definition['type'])),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['mixed']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'string'),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->get(sprintf(\'%s\', $%s), [], $options);', $definition['route'], $definition['field'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.getPropertyPathBy")
*/
public function generateCrudGetPropertyPathByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition += ['field' => 'id', 'property' => 'id'];
$definition['route'] = str_replace(['{'.$definition['field'].'}', '{'.$definition['property'].'}'], '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Return the local path of the file containing the specified %s %s by %s', $definition['type'], $definition['property'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf(ucfirst($definition['field']).' of the %s', $definition['type'])),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['mixed']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'string'),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->getPath(sprintf(\'%s\', $%s), [], [\'format\' => \'%s\'] + $options);', $definition['route'], $definition['field'], $definition['format'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.create")
*/
public function generateCrudCreateMethod(MethodGenerator $zMethod, $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Create a new %s', $definition['type']),
null,
[
new ParamTag('data', ['array'], 'Data to store'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('data', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->create(\'%s\', $data, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.sub.createBy")
*/
public function generateCrudSubCreateByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition += ['field' => 'id', 'subType' => 'subType'];
$definition['route'] = str_replace('{'.$definition['field'].'}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Create a new %s %s by %s', $definition['type'], $definition['subType'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf('%s of the %s', ucfirst($definition['field']), $definition['type'])),
new ParamTag('data', ['array'], 'Data to store'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'mixed'),
new ParameterGenerator('data', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->create(sprintf(\'%s\', $%s), $data, $options);', $definition['route'], $definition['field'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.sub.getBy")
*/
public function generateCrudSubGetByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition += ['field' => 'id', 'subType' => 'subType'];
$definition['route'] = str_replace(['{'.$definition['field'].'}', '{id}'], '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Return the specified %s %s by %s %s', $definition['type'], $definition['subType'], $definition['type'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf('%s of the %s', ucfirst($definition['field']), $definition['type'])),
new ParamTag('id', ['string'], 'The id'),
new ParamTag('fields', ['array'], 'Data to store'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'mixed'),
new ParameterGenerator('id', 'string'),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->get(sprintf(\'%s\', $%s, $id), $fields, $options);', $definition['route'], $definition['field'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.delete")
*/
public function generateCrudDeleteMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace('{id}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Delete the specified %s', $definition['type']),
null,
[
new ParamTag('id', ['mixed'], sprintf('ID of the %s', $definition['type'])),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('id', 'string'),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->delete(sprintf(\'%s\', $id), $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.purge")
*/
public function generateCrudPurgeMethod(MethodGenerator $zMethod, $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Purge all %ss', $definition['type']),
null,
[
new ParamTag('criteria', ['array'], 'Optional criteria to filter deleteds'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('criteria', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->purge(\'%s\', $criteria, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.update")
*/
public function generateCrudUpdateMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace('{id}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Update the specified %s', $definition['type']),
null,
[
new ParamTag('id', ['mixed'], sprintf('ID of the %s', $definition['type'])),
new ParamTag('data', ['array'], 'Data to update'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('id', 'string'),
new ParameterGenerator('data', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->update(sprintf(\'%s\', $id), $data, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.sub.setPropertyBy")
*/
public function generateCrudSubSetPropertyByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace(['{id}', '{'.$definition['key'].'}'], ['%s', '%s'], $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Set the %s of the %s %s to %s', $definition['property'], $definition['type'], $definition['subType'], $definition['value']),
null,
[
new ParamTag($definition['key'], ['string'], sprintf($definition['key'].' of the %s', $definition['type'])),
new ParamTag('id', ['mixed'], sprintf('ID of the %s %s', $definition['type'], $definition['subType'])),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['key'], 'string'),
new ParameterGenerator('id', 'string'),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->update(sprintf(\'%s\', $%s, $id), [\'%s\' => %s], $options);', $definition['route'], $definition['key'], $definition['property'], var_export($definition['value'], true))
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.updateProperty")
*/
public function generateCrudUpdatePropertyMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace('{id}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Update %s of the specified %s', $definition['property'], $definition['type']),
null,
[
new ParamTag('id', ['mixed'], sprintf('ID of the %s', $definition['type'])),
new ParamTag('data', ['mixed'], sprintf('Value for the %s', $definition['property'])),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('id', 'string'),
new ParameterGenerator('data', 'mixed'),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->update(sprintf(\'%s\', $id), $data, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.find")
*/
public function generateCrudFindMethod(MethodGenerator $zMethod, $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Find %ss', $definition['type']),
null,
[
new ParamTag('criteria', ['array'], sprintf('Optional criteria to filter %ss', $definition['type'])),
new ParamTag('fields', ['array'], 'Optional fields to retrieve'),
new ParamTag('limit', ['int'], 'Optional limit'),
new ParamTag('offset', ['int'], 'Optional offset'),
new ParamTag('sorts', ['array'], 'Optional sorts'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('criteria', 'array', []),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('limit', 'int', new ValueGenerator(null)),
new ParameterGenerator('offset', 'int', 0),
new ParameterGenerator('sorts', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->find(\'%s\', $criteria, $fields, $limit, $offset, $sorts, $options);', $definition['route'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.sub.findBy")
*/
public function generateCrudSubFindByMethod(MethodGenerator $zMethod, $definition = [])
{
$definition['route'] = str_replace('{'.$definition['field'].'}', '%s', $definition['route']);
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Find %s %ss by %s', $definition['type'], $definition['subType'], $definition['field']),
null,
[
new ParamTag($definition['field'], ['mixed'], sprintf(ucfirst($definition['field']).' of the %s', $definition['type'])),
new ParamTag('criteria', ['array'], sprintf('Optional criteria to filter %ss', $definition['type'])),
new ParamTag('fields', ['array'], 'Optional fields to retrieve'),
new ParamTag('limit', ['int'], 'Optional limit'),
new ParamTag('offset', ['int'], 'Optional offset'),
new ParamTag('sorts', ['array'], 'Optional sorts'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator($definition['field'], 'mixed'),
new ParameterGenerator('criteria', 'array', []),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('limit', 'int', new ValueGenerator(null)),
new ParameterGenerator('offset', 'int', 0),
new ParameterGenerator('sorts', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->find(sprintf(\'%s\', $%s), $criteria, $fields, $limit, $offset, $sorts, $options);', $definition['route'], $definition['field'])
);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("crud.findPage")
*/
public function generateCrudFindPageMethod(MethodGenerator $zMethod, $definition = [])
{
$zMethod->setDocBlock(new DocBlockGenerator(
sprintf('Find a page of %ss', $definition['type']),
null,
[
new ParamTag('criteria', ['array'], sprintf('Optional criteria to filter %ss', $definition['type'])),
new ParamTag('fields', ['array'], 'Optional fields to retrieve'),
new ParamTag('page', ['int'], 'Rank of the page to retrieve'),
new ParamTag('size', ['int'], 'Size of pages'),
new ParamTag('sorts', ['array'], 'Optional sorts'),
new ParamTag('options', ['array'], 'Options'),
new ReturnTag(['array']),
new ThrowsTag(['\\Exception'], 'if an error occured'),
]
));
$zMethod->setParameters([
new ParameterGenerator('criteria', 'array', []),
new ParameterGenerator('fields', 'array', []),
new ParameterGenerator('page', 'int', 0),
new ParameterGenerator('size', 'int', 10),
new ParameterGenerator('sorts', 'array', []),
new ParameterGenerator('options', 'array', []),
]);
$zMethod->setBody(
sprintf('return $this->getSdk()->findPage(\'%s\', $criteria, $fields, $page, $size, $sorts, $options);', $definition['route'])
);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Service\StripeService;
/**
* StripeServiceAware trait.
*
* @author <NAME> <<EMAIL>>
*/
trait StripeServiceAwareTrait
{
/**
* @param string $key
* @param mixed $service
*
* @return $this
*/
protected abstract function setService($key, $service);
/**
* @param string $key
*
* @return mixed
*/
protected abstract function getService($key);
/**
* @return StripeService
*/
public function getStripeService()
{
return $this->getService('stripe');
}
/**
* @param StripeService $service
*
* @return $this
*/
public function setStripeService(StripeService $service)
{
return $this->setService('stripe', $service);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Service;
use Symfony\Component\HttpFoundation\Request;
use Velocity\Core\Traits\ServiceTrait;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\PaymentProviderInterface;
/**
* PaymentProvider Service.
*
* @author <NAME> <<EMAIL>>
*/
class PaymentProviderService
{
use ServiceTrait;
use ServiceAware\ExpressionServiceAwareTrait;
/**
* List of payment providers.
*
* @var PaymentProviderInterface[]
*/
protected $providers;
/**
* @var array
*/
protected $rules;
/**
* Construct a new service.
*
* @param ExpressionService $expressionService
* @param PaymentProviderInterface[] $providers
* @param array $rules
*/
public function __construct(ExpressionService $expressionService, $providers = [], $rules = [])
{
$this->setExpressionService($expressionService);
foreach ($providers as $name => $provider) {
$this->addProvider($provider, $name);
}
foreach ($rules as $condition => $providerName) {
$this->addRule($condition, $providerName);
}
}
/**
* Return the list of registered providers.
*
* @return PaymentProviderInterface[]
*/
public function getProviders()
{
return $this->providers;
}
/**
* Add a new provider for the specified name (replace if exist).
*
* @param PaymentProviderInterface $provider
* @param string $name
*
* @return $this
*/
public function addProvider(PaymentProviderInterface $provider, $name)
{
$this->providers[$name] = $provider;
return $this;
}
/**
* Add a new rule
*
* @param string $condition
* @param string $providerName
*
* @return $this
*
* @throws \Exception
*/
public function addRule($condition, $providerName)
{
$this->rules[] = ['condition' => $condition, 'provider' => $providerName];
return $this;
}
/**
* Return the provider registered for the specified name.
*
* @param string $name
*
* @return PaymentProviderInterface
*
* @throws \Exception if no provider registered for this name
*/
public function getProviderByName($name)
{
if (!isset($this->providers[$name])) {
throw $this->createRequiredException(
"No payment provider registered for name '%s'",
$name
);
}
return $this->providers[$name];
}
/**
* @param array $data
*
* @return string
*
* @throws \Exception
*/
public function selectProvider($data = [])
{
foreach ($this->rules as $rule) {
if ($this->isValidCondition($rule['condition'], $data)) {
return $rule['provider'];
}
}
throw $this->createRequiredException("No payment provider available");
}
/**
* @param string $condition
* @param array $data
*
* @return bool
*/
public function isValidCondition($condition, $data = [])
{
return (bool) $this->getExpressionService()->evaluate($condition, $data);
}
/**
* @param string $provider
* @param string $callback
* @param Request $request
*
* @return array
*
* @throws \Exception
*/
public function parseCallbackRequest($provider, $callback, Request $request)
{
return $this->getProviderByName($provider)->parseCallbackRequest($callback, $request);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Service\PaymentProviderService;
/**
* PaymentProviderServiceAware trait.
*
* @author <NAME> <<EMAIL>>
*/
trait PaymentProviderServiceAwareTrait
{
/**
* @param string $key
* @param mixed $service
*
* @return $this
*/
protected abstract function setService($key, $service);
/**
* @param string $key
*
* @return mixed
*/
protected abstract function getService($key);
/**
* @return PaymentProviderService
*/
public function getPaymentProviderService()
{
return $this->getService('paymentProvider');
}
/**
* @param PaymentProviderService $service
*
* @return $this
*/
public function setPaymentProviderService(PaymentProviderService $service)
{
return $this->setService('paymentProvider', $service);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Twig;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\Twig\ApiExtension;
/**
* @author <NAME> <<EMAIL>>
*
* @group twig
*/
class ApiExtensionTest extends PHPUnit_Framework_TestCase
{
/**
* @var ApiExtension
*/
protected $e;
/**
*
*/
public function setUp()
{
$this->e = new ApiExtension();
}
/**
* @group unit
*/
public function testConstruct()
{
$e = new ApiExtension(['a' => 'b', 'c' => 'd']);
$this->assertNotNull($e);
$this->assertEquals(['velocity' => ['a' => 'b', 'c' => 'd']], $e->getGlobals());
}
/**
* @group unit
*/
public function testGetFilters()
{
$this->assertNotEquals(0, count($this->e->getFilters()));
}
/**
* @group unit
*/
public function testGetBase64EncodedString()
{
$this->assertEquals(base64_encode('test'), $this->e->getBase64EncodedString('test'));
}
/**
* @group unit
*/
public function testGetName()
{
$this->assertEquals('velocity_api', $this->e->getName());
}
}
<file_sep>#!/bin/bash
while [ 1 ]; do
clear
bin/phpunit -c phpunit-basic.xml.dist $*
sleep 3
done;
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Action;
use Velocity\Core\Bag;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
use Velocity\Bundle\ApiBundle\Action\Base\AbstractMailAction;
/**
* @author <NAME> <<EMAIL>>
*/
class MailAction extends AbstractMailAction
{
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("mail", description="send a mail")
*/
public function sendMail(Bag $params, Bag $context)
{
$this->sendMailByType(null, $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("mail_user", description="send a user mail")
*/
public function sendUserMail(Bag $params, Bag $context)
{
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('mail_user', $params->get('template')));
$params->setDefault('_locale', $this->getCurrentLocale());
$params->setDefault('_tenant', $this->getTenant());
$this->sendMailByType('user', $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("mail_admin", description="send an admin mail")
*/
public function sendAdminMail(Bag $params, Bag $context)
{
$params->setDefault('recipients', $this->getDefaultRecipientsByTypeAndNature('mail_admins', $params->get('template')));
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('mail_admin', $params->get('template')));
$params->setDefault('_tenant', $this->getTenant());
$this->sendMailByType('admin', $params, $context);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use PHPUnit_Framework_MockObject_MockObject;
use Symfony\Component\ExpressionLanguage\ExpressionLanguage;
use Symfony\Component\Templating\EngineInterface;
use Velocity\Bundle\ApiBundle\Service\ExpressionService;
/**
* @author <NAME> <<EMAIL>>
*
* @group expression
*/
class ExpressionServiceTest extends PHPUnit_Framework_TestCase
{
/**
* @var ExpressionService
*/
protected $s;
/**
* @var EngineInterface|PHPUnit_Framework_MockObject_MockObject
*/
protected $templatingService;
/**
* @var ExpressionLanguage|PHPUnit_Framework_MockObject_MockObject
*/
protected $expressionLanguage;
/**
*
*/
public function setUp()
{
$this->templatingService = $this->getMock("Symfony\\Component\\Templating\\EngineInterface", [], [], '', false);
$this->expressionLanguage = $this->getMock("Symfony\\Component\\ExpressionLanguage\\ExpressionLanguage", [], [], '', false);
$this->s = new ExpressionService($this->templatingService, $this->expressionLanguage);
}
/**
* @group unit
*/
public function testConstruct()
{
$this->assertNotNull($this->s);
}
/**
* @group integ
*/
public function testEvaluateExpressionLanguage()
{
$this->s->setExpressionLanguage(new ExpressionLanguage());
$vars = ['a' => [1, 2], 'b' => 5, 'c' => 2];
$this->assertEquals([1, 2], $this->s->evaluate('$a', $vars));
$this->assertEquals(5, $this->s->evaluate('$b', $vars));
$this->assertEquals(2, $this->s->evaluate('$c', $vars));
$this->assertEquals(2.5, $this->s->evaluate('$ (b + c - 2) / 2', $vars));
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\CodeGenerator;
use Zend\Code\Generator\MethodGenerator;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
/**
* @author <NAME> <<EMAIL>>
*/
class BasicMethodsCodeGenerator
{
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("basic")
*/
public function generateBasicMethod(MethodGenerator $zMethod, array $definition = [])
{
unset($zMethod, $definition);
}
/**
* @param MethodGenerator $zMethod
* @param array $definition
*
* @Velocity\CodeGeneratorMethodType("get")
*/
public function generateGetMethod(MethodGenerator $zMethod, $definition = [])
{
unset($definition);
$zMethod->setParameters([]);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Action;
use Velocity\Core\Bag;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
use Velocity\Bundle\ApiBundle\Action\Base\AbstractLetterAction;
/**
* @author <NAME> <<EMAIL>>
*/
class LetterAction extends AbstractLetterAction
{
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("letter", description="send a letter")
*/
public function sendLetter(Bag $params, Bag $context)
{
$this->sendLetterByType(null, $params, $context);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\PaymentProvider;
use Symfony\Component\HttpFoundation\Request;
use Velocity\Core\Traits\ServiceTrait;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Service\PayPalService;
use Velocity\Bundle\ApiBundle\PaymentProviderInterface;
/**
* PayPal Payment Provider.
*
* @author <NAME> <<EMAIL>>
*/
class PayPalPaymentProvider implements PaymentProviderInterface
{
use ServiceTrait;
use ServiceAware\PayPalServiceAwareTrait;
/**
* PayPalPaymentProvider constructor.
*
* @param PayPalService $payPalService
*/
public function __construct(PayPalService $payPalService)
{
$this->setPayPalService($payPalService);
}
/**
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function create($data = [], $options = [])
{
$order = [
'successUrl' => $data['succeedUrl'],
'cancelUrl' => $data['canceledUrl'],
'currency' => $data['currency'],
'name' => $data['name'],
'amount' => $data['amount'],
'description' => $data['description'],
'items' => [
[
'name' => $data['name'],
'description' => $data['description'],
'amount' => $data['amount'],
'currency' => $data['currency'],
],
],
];
$payPalOrder = $this->getPayPalService()->createOrder($order, $options);
return [
'url' => $payPalOrder['paymentUrl'],
'transaction' => $payPalOrder['token'],
'correlation' => $payPalOrder['correlationId'],
];
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function confirm($id, $data = [], $options = [])
{
if (!isset($data['payerId'])) {
throw $this->createRequiredException('Payer id is missing');
}
return $this->getPayPalService()->confirmOrder($id, ['payerId' => $data['payerId']], $options);
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function fail($id, $data = [], $options = [])
{
if (!isset($data['payerId'])) {
throw $this->createRequiredException('Payer id is missing');
}
return $this->getPayPalService()->failOrder($id, ['payerId' => $data['payerId']], $options);
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function cancel($id, $data = [], $options = [])
{
if (!isset($data['payerId'])) {
throw $this->createRequiredException('Payer id is missing');
}
return $this->getPayPalService()->cancelOrder($id, ['payerId' => $data['payerId']], $options);
}
/**
* @param string|array $id
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function get($id, $options = [])
{
return $this->getPayPalService()->getOrder($id, $options);
}
/**
* @param string $callback
* @param Request $request
*
* @return array
*
* @throws \Exception
*/
public function parseCallbackRequest($callback, Request $request)
{
switch ($callback) {
case 'paid':
if (!$request->query->has('token')) {
throw $this->createMalformedException('Malformed callback parameters (#1)');
}
if (!$request->query->has('PayerID')) {
throw $this->createMalformedException('Malformed callback parameters (#2)');
}
return ['transaction' => $request->query->get('token'), 'payer' => $request->query->get('PayerID')];
default:
return [];
}
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Listener;
use Velocity\Core\Traits\ServiceTrait;
use Symfony\Component\HttpFoundation\JsonResponse;
use Symfony\Component\HttpKernel\Event\GetResponseEvent;
/**
* Request Listener.
*
* @author <NAME> <<EMAIL>>
*/
class RequestListener
{
use ServiceTrait;
/**
* @param array $allowedLocales
* @param string $defaultLocale
*/
public function __construct(array $allowedLocales, $defaultLocale)
{
$this->allowLocales($allowedLocales);
$this->setDefaultLocale($defaultLocale);
}
/**
* @param string $defaultLocale
*
* @return $this
*/
public function setDefaultLocale($defaultLocale)
{
return $this->setParameter('defaultLocale', $defaultLocale);
}
/**
* @return string
*
* @throws \Exception
*/
public function getDefaultLocale()
{
return $this->getParameter('defaultLocale');
}
/**
* @param array $locales
*
* @return $this
*/
public function allowLocales(array $locales)
{
foreach ($locales as $locale) {
$this->pushArrayParameterKeyItem('allowedLocales', strtolower($locale), true);
}
return $this;
}
/**
* @param string $locale
*
* @return bool
*/
public function isAllowedLocale($locale)
{
return $this->hasArrayParameterKey('allowedLocales', strtolower($locale));
}
/**
* Kernel request event callback.
*
* @param GetResponseEvent $event
*
* @return void
*/
public function onKernelRequest(GetResponseEvent $event)
{
$request = $event->getRequest();
if ($event->isMasterRequest()) {
$request->setLocale($this->getDefaultLocale());
foreach ($request->getLanguages() as $locale) {
if ($this->isAllowedLocale($locale)) {
$request->setLocale($locale);
break;
}
}
}
if ('json' !== $request->getContentType()) {
return;
}
$data = json_decode($request->getContent(), true);
if (json_last_error() !== JSON_ERROR_NONE) {
$event->setResponse(new JsonResponse(
[
'code' => 412,
'status' => 'exception',
'message' => 'Malformed data (json syntax)',
],
412
));
} elseif (is_array($data)) {
$request->request->replace($data);
}
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Action;
use Velocity\Core\Bag;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
use Velocity\Bundle\ApiBundle\Action\Base\AbstractSmsAction;
/**
* @author <NAME> <<EMAIL>>
*/
class SmsAction extends AbstractSmsAction
{
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("sms", description="send a sms")
*/
public function sendSms(Bag $params, Bag $context)
{
$this->sendSmsByType(null, $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("sms_user", description="send a user sms")
*/
public function sendUserSms(Bag $params, Bag $context)
{
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('sms_user', $params->get('template')));
$params->setDefault('_locale', $this->getCurrentLocale());
$params->setDefault('_tenant', $this->getTenant());
$this->sendSmsByType('user', $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("sms_admin", description="send an admin sms")
*/
public function sendAdminSms(Bag $params, Bag $context)
{
$params->setDefault('recipients', $this->getDefaultRecipientsByTypeAndNature('sms_admins', $params->get('template')));
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('sms_admin', $params->get('template')));
$params->setDefault('_tenant', $this->getTenant());
$this->sendSmsByType('admin', $params, $context);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle;
use Symfony\Component\HttpKernel\Bundle\Bundle;
use Doctrine\Common\Annotations\AnnotationReader;
use Symfony\Component\HttpKernel\KernelInterface;
use Velocity\Bundle\ApiBundle\Service\VelocityService;
use Symfony\Component\DependencyInjection\ContainerBuilder;
use Symfony\Bundle\SecurityBundle\DependencyInjection\SecurityExtension;
use Velocity\Bundle\ApiBundle\DependencyInjection\Security\Factory\ApiFactory;
use Velocity\Bundle\ApiBundle\DependencyInjection\Compiler\VelocityCompilerPass;
/**
* Velocity Api Bundle.
*
* @author <NAME> <<EMAIL>>
*/
class VelocityApiBundle extends Bundle
{
/**
* @var KernelInterface
*/
protected $kernel;
/**
* @var ApiFactory
*/
protected $apiFactory;
/**
* @var VelocityService
*/
protected $velocityService;
/**
* @param KernelInterface $kernel
* @param ApiFactory $apiFactory
* @param VelocityService $velocityService
*/
public function __construct(KernelInterface $kernel, $apiFactory = null, $velocityService = null)
{
if (null === $apiFactory) {
$apiFactory = new ApiFactory();
}
if (null === $velocityService) {
$velocityService = new VelocityService(new AnnotationReader());
}
$this->kernel = $kernel;
$this->apiFactory = $apiFactory;
$this->velocityService = $velocityService;
}
/**
* @return ApiFactory
*/
public function getApiFactory()
{
return $this->apiFactory;
}
/**
* @return KernelInterface
*/
public function getKernel()
{
return $this->kernel;
}
/**
* @return VelocityService
*/
public function getVelocityService()
{
return $this->velocityService;
}
/**
* @param ContainerBuilder $container
*/
public function build(ContainerBuilder $container)
{
parent::build($container);
if ($container->hasExtension('security')) {
$extension = $container->getExtension('security');
/** @var SecurityExtension $extension */
$extension->addSecurityListenerFactory($this->getApiFactory());
}
$container->addCompilerPass(new VelocityCompilerPass($this->getKernel(), $this->getVelocityService()));
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Action;
use Velocity\Core\Bag;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
use Velocity\Bundle\ApiBundle\Action\Base\AbstractFaxAction;
/**
* @author <NAME> <<EMAIL>>
*/
class FaxAction extends AbstractFaxAction
{
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("fax", description="send a fax")
*/
public function sendFax(Bag $params, Bag $context)
{
$this->sendFaxByType(null, $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("fax_user", description="send a user fax")
*/
public function sendUserFax(Bag $params, Bag $context)
{
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('fax_user', $params->get('template')));
$params->setDefault('_locale', $this->getCurrentLocale());
$params->setDefault('_tenant', $this->getTenant());
$this->sendFaxByType('user', $params, $context);
}
/**
* @param Bag $params
* @param Bag $context
*
* @Velocity\Action("fax_admin", description="send an admin fax")
*/
public function sendAdminFax(Bag $params, Bag $context)
{
$params->setDefault('recipients', $this->getDefaultRecipientsByTypeAndNature('fax_admins', $params->get('template')));
$params->setDefault('sender', $this->getDefaultSenderByTypeAndNature('fax_admin', $params->get('template')));
$params->setDefault('_tenant', $this->getTenant());
$this->sendFaxByType('admin', $params, $context);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Mock;
use Symfony\Component\DependencyInjection\Definition;
use Symfony\Component\DependencyInjection\ContainerBuilder;
/**
* Mock for easy velocity definition creation.
*
* @author <NAME> <<EMAIL>>
* @author <NAME> <<EMAIL>>
*/
class ContainerBuilderMock extends ContainerBuilder
{
/**
* @param string $id
*
* @return Definition
*
* @see \Symfony\Component\DependencyInjection\ContainerBuilder::getDefinition()
*/
public function getDefinition($id)
{
$id = strtolower($id);
if (!$this->hasDefinition($id) && 'velocity' === strstr($id, '.', true)) {
$this->setDefinition($id, new Definition());
}
parent::getDefinition($id);
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\Service\BusinessRuleService;
use Velocity\Bundle\ApiBundle\WorkflowInterface;
use Velocity\Bundle\ApiBundle\Service\WorkflowService;
/**
* @author <NAME> <<EMAIL>>
*
* @group workflow
*/
class WorkflowServiceTest extends PHPUnit_Framework_TestCase
{
/**
* @var WorkflowService
*/
protected $s;
/**
* @var BusinessRuleService|\PHPUnit_Framework_MockObject_MockObject
*/
protected $businessRule;
/**
*
*/
public function setUp()
{
$this->businessRule = $this->getMock('Velocity\\Bundle\\ApiBundle\\Service\\BusinessRuleService', [], [], '', false);
$this->s = new WorkflowService($this->businessRule);
}
/**
* @group unit
*/
public function testConstruct()
{
$this->assertNotNull($this->s);
}
/**
* @group unit
*/
public function testRegisterFromDefinition()
{
$this->assertFalse($this->s->has('workflow1'));
$this->s->registerFromDefinition('workflow1', []);
$this->assertTrue($this->s->has('workflow1'));
$workflow = $this->s->get('workflow1');
$this->assertTrue($workflow instanceof WorkflowInterface);
}
/**
* @group unit
*/
public function testHasTransition()
{
$this->s->registerFromDefinition('w', ['steps' => ['s1', 's2', 's3'], 'transitions' => ['s1' => ['s2'], 's2' => ['s3', 's1']]]);
$this->assertTrue($this->s->hasTransition('w', 's1', 's2'));
$this->assertFalse($this->s->hasTransition('w', 's1', 's3'));
$this->assertFalse($this->s->hasTransition('w', 's1', 'sX'));
$this->assertTrue($this->s->hasTransition('w', 's2', 's3'));
$this->assertTrue($this->s->hasTransition('w', 's2', 's1'));
$this->assertFalse($this->s->hasTransition('w', 's3', 's1'));
}
/**
* @group unit
*/
public function testTransition()
{
$this->businessRule->expects($this->exactly(2))->method('executeBusinessRulesForModelOperation');
$this->s->registerFromDefinition('w', ['steps' => ['s1', 's2', 's3'], 'transitions' => ['s1' => ['s2'], 's2' => ['s3', 's1']]]);
$docBefore = new \stdClass();
$docBefore->status = 's1';
$doc = new \stdClass();
$doc->status = 's2';
$this->s->transitionModelProperty('m', $doc, 'status', $docBefore, 'w');
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\GenericDocument;
/**
* @author <NAME> <<EMAIL>>
*
* @group document
*/
class GenericDocumentTest extends PHPUnit_Framework_TestCase
{
/**
* @group unit
*/
public function testConstruct()
{
$d = new GenericDocument('<a></a>', 'xml');
$this->assertNotNull($d);
$this->assertEquals('<a></a>', $d->getContent());
$this->assertEquals('application/xml', $d->getContentType());
}
/**
* @group unit
*/
public function testConstructForUnknownFormatLoadDefaultFormat()
{
$d = new GenericDocument('xyz', 'xyz');
$this->assertEquals('application/octet-stream', $d->getContentType());
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests;
use PHPUnit_Framework_TestCase;
use Symfony\Bundle\SecurityBundle\DependencyInjection\SecurityExtension;
use Symfony\Component\DependencyInjection\ContainerBuilder;
use Velocity\Bundle\ApiBundle\VelocityApiBundle;
use Symfony\Component\HttpKernel\KernelInterface;
/**
* @author <NAME> <<EMAIL>>
*
* @group velocityapibundle
*/
class VelocityApiBundleTest extends PHPUnit_Framework_TestCase
{
/**
* @var VelocityApiBundle
*/
protected $b;
/**
* @var KernelInterface|\PHPUnit_Framework_MockObject_MockObject
*/
protected $kernel;
/**
*
*/
public function setUp()
{
$this->kernel = $this->getMock('Symfony\\Component\\HttpKernel\\KernelInterface', [], [], '', false);
$this->b = new VelocityApiBundle($this->kernel);
}
/**
* @group unit
*/
public function testConstruct()
{
$b = new VelocityApiBundle($this->kernel);
$this->assertNotNull($b);
}
/**
* @group integ
*/
public function testBuild()
{
$containerBuilder = new ContainerBuilder();
/** @var SecurityExtension|\PHPUnit_Framework_MockObject_MockObject $extension */
$extension = $this->getMock('Symfony\\Bundle\\SecurityBundle\\DependencyInjection\\SecurityExtension', ['addSecurityListenerFactory', 'getAlias'], [], '', false);
$extension->expects($this->once())->method('getAlias')->will($this->returnValue('security'));
$extension->expects($this->once())->method('addSecurityListenerFactory')->with($this->b->getApiFactory());
$containerBuilder->registerExtension($extension);
$previousPassCount = count($containerBuilder->getCompilerPassConfig()->getPasses());
$this->b->build($containerBuilder);
$this->assertEquals($previousPassCount + 1, count($containerBuilder->getCompilerPassConfig()->getPasses()));
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Generator;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\Generator\StringGenerator;
/**
* @author <NAME> <<EMAIL>>
*
* @group generator
*/
class StringGeneratorTest extends PHPUnit_Framework_TestCase
{
/**
* @var StringGenerator
*/
protected $g;
/**
*
*/
public function setUp()
{
$this->g = new StringGenerator();
}
/**
* @group unit
*/
public function testGenerateMd5()
{
$this->assertEquals(32, strlen($this->g->generateRandomMd5String()));
$this->assertTrue(0 < preg_match('/^[a-f0-9]+$/', $g = $this->g->generateRandomMd5String()), sprintf("string '%s' is not valid md5 string", $g));
}
/**
* @group unit
*/
public function testGenerateSha1()
{
$this->assertEquals(40, strlen($this->g->generateRandomSha1String()));
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use Velocity\Bundle\ApiBundle\Document;
/**
* @author <NAME> <<EMAIL>>
*
* @group document
*/
class DocumentTest extends PHPUnit_Framework_TestCase
{
/**
* @group unit
*/
public function testConstruct()
{
$d = new Document('<a></a>', 'application/xml', 'x.xml');
$this->assertNotNull($d);
$this->assertEquals('<a></a>', $d->getContent());
$this->assertEquals('application/xml', $d->getContentType());
$this->assertEquals('x.xml', $d->getFileName());
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Service;
use MongoId;
use Exception;
use MongoClient;
use MongoCollection;
use Velocity\Core\Traits\ServiceTrait;
use Velocity\Bundle\ApiBundle\Event\DatabaseQueryEvent;
use Symfony\Component\EventDispatcher\EventDispatcherInterface;
/**
* Database Service.
*
* @author <NAME> <<EMAIL>>
*/
class DatabaseService
{
use ServiceTrait;
/**
* @var \DateTime[]
*/
protected $timers;
/**
* Constructs a new service
*
* @param MongoClient $mongoClient
* @param EventDispatcherInterface $eventDispatcher
* @param string $databaseName
* @param bool $randomDatabaseName
*
* @throws \Exception
*/
public function __construct(MongoClient $mongoClient, EventDispatcherInterface $eventDispatcher, $databaseName, $randomDatabaseName = false)
{
$this->timers = [];
$this->setEventDispatcher($eventDispatcher);
$this->setMongoClient($mongoClient);
if (true === $randomDatabaseName) {
$databaseName .= '_'.((int) microtime(true)).'_'.substr(md5(rand(0, 10000)), -8);
}
if (64 <= strlen($databaseName)) {
throw $this->createMalformedException(
"Database name is too long, maximum is 64 characters (found: %d)",
strlen($databaseName)
);
}
$this->setDatabaseName($databaseName);
}
/**
* Return the underlying database name.
*
* @return string
*/
public function getDatabaseName()
{
return $this->getParameter('databaseName');
}
/**
* Set the underlying database name.
*
* @param string $databaseName
*
* @return $this
*/
public function setDatabaseName($databaseName)
{
return $this->setParameter('databaseName', $databaseName);
}
/**
* Return the underlying Mongo Client (connection).
*
* @return MongoClient
*/
public function getMongoClient()
{
return $this->getService('mongoClient');
}
/**
* Set the underlying Mongo Client (connection).
*
* @param MongoClient $service
*
* @return $this
*/
public function setMongoClient(MongoClient $service)
{
return $this->setService('mongoClient', $service);
}
/**
* Drop the current (or the specified) database.
*
* @param string $name
*
* @return $this
*/
public function drop($name = null)
{
$this->getMongoClient()
->selectDB(null === $name ? $this->getDatabaseName() : $name)
->drop()
;
return $this;
}
/**
* Returns the specified Mongo Collection.
*
* @param string $name
* @param array $options
*
* @return MongoCollection
*/
public function getCollection($name, $options = [])
{
return $this->getMongoClient()->selectCollection(
isset($options['db']) ? $options['db'] : $this->getDatabaseName(),
$name
);
}
/**
* Update (the first matching criteria) a document of the specified collection.
*
* @param string $collection
* @param array $criteria
* @param array $data
* @param array $options
*
* @return bool
*
* @throws \Exception
*/
public function update($collection, $criteria = [], $data = [], $options = [])
{
$this->start();
try {
$builtCriteria = $this->buildCriteria($criteria);
$builtData = $this->buildData($data);
$result = $this->getCollection($collection, $options)->update(
$builtCriteria,
$builtData,
$options
);
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('update', 'db.'.$collection.'.update('.json_encode($builtCriteria).', '.json_encode($builtData).')', ['collection' => $collection, 'criteria' => $builtCriteria, 'data' => $builtData, 'options' => $options], $startDate, $endDate, $result));
return $result;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('update', 'db.'.$collection.'.update('.json_encode($criteria).', '.json_encode($data).')', ['rawCriteria' => $criteria, 'rawData' => $data, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Remove (the first matching criteria) a document of the specified collection.
*
* @param string $collection
* @param array $criteria
* @param array $options
* @return array|bool
*/
public function remove($collection, $criteria = [], $options = [])
{
$this->start();
try {
$builtCriteria = $this->buildCriteria($criteria);
$result = $this->getCollection($collection, $options)->remove(
$builtCriteria,
$options
);
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('remove', 'db.'.$collection.'.remove('.json_encode($builtCriteria).')', ['collection' => $collection, 'criteria' => $builtCriteria, 'options' => $options], $startDate, $endDate, $result));
return $result;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('remove', 'db.'.$collection.'.remove('.json_encode($criteria).')', ['rawCriteria' => $criteria, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Insert a single document into the specified collection.
*
* @param string $collection
* @param array $data
* @param array $options
*
* @return array|bool
*
* @throws \Exception
*/
public function insert($collection, $data = [], $options = [])
{
$this->start();
try {
$builtData = $this->buildData($data);
$result = $this->getCollection($collection, $options)->insert(
$builtData,
$options
);
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('insert', 'db.'.$collection.'.insert('.json_encode($builtData).')', ['data' => $builtData, 'options' => $options], $startDate, $endDate, $result));
return $result;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('insert', 'db.'.$collection.'.insert('.json_encode($data).')', ['rawData' => $data, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Insert a list of documents into the specified collection.
*
* @param string $collection
* @param array $bulkData
* @param array $options
*
* @return mixed
*/
public function bulkInsert($collection, $bulkData = [], $options = [])
{
$this->start();
try {
$builtData = $this->buildBulkData($bulkData);
$result = $this->getCollection($collection, $options)->batchInsert(
$builtData,
$options
);
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('bulkInsert', 'db.'.$collection.'.bulkInsert('.json_encode($builtData).')', ['data' => $builtData, 'options' => $options], $startDate, $endDate, $result));
return $result;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('bulkInsert', 'db.'.$collection.'.bulkIinsert('.json_encode($bulkData).')', ['rawData' => $bulkData, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Retrieve (if match criteria) a list of documents from the specified collection.
*
* @param string $collection
* @param array $criteria
* @param array $fields
* @param null|int $limit
* @param int $offset
* @param array $sorts
* @param array $options
*
* @return \MongoCursor
*
* @throws \Exception
*/
public function find($collection, $criteria = [], $fields = [], $limit = null, $offset = 0, $sorts = [], $options = [])
{
$this->start();
try {
$builtCriteria = $this->buildCriteria($criteria);
$builtFields = $this->buildFields($fields);
$builtSorts = $this->buildSorts($sorts);
$cursor = $this->getCollection($collection, $options)->find($builtCriteria);
if (count($builtFields)) {
$cursor->fields($builtFields);
}
if (count($builtSorts)) {
$cursor->sort($builtSorts);
}
if (is_numeric($offset) && $offset > 0) {
$cursor->skip($offset);
}
if (is_numeric($limit) && $limit > 0) {
$cursor->limit($limit);
}
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('find', 'db.'.$collection.'.find('.json_encode($builtCriteria).', '.json_encode($builtFields).')', ['criteria' => $builtCriteria, 'fields' => $builtFields, 'limit' => $limit, 'sort' => $builtSorts, 'skip' => $offset, 'options' => $options], $startDate, $endDate, $cursor));
return $cursor;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('find', 'db.'.$collection.'.find('.json_encode($criteria).', '.json_encode($fields).')', ['rawCriteria' => $criteria, 'rawFields' => $fields, 'limit' => $limit, 'rawSort' => $sorts, 'skip' => $offset, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Retrieve (if match criteria) one document from the specified collection.
*
* @param string $collection
* @param array $criteria
* @param array $fields
* @param array $options
*
* @return array|null
*
* @throws \Exception
*/
public function findOne($collection, $criteria = [], $fields = [], $options = [])
{
$this->start();
try {
$builtCriteria = $this->buildCriteria($criteria);
$builtFields = $this->buildFields($fields);
$result = $this->getCollection($collection, $options)->findOne(
$builtCriteria,
$builtFields
);
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('findOne', 'db.'.$collection.'.findOne('.json_encode($builtCriteria).', '.json_encode($builtFields).')', ['criteria' => $builtCriteria, 'fields' => $builtFields, 'options' => $options], $startDate, $endDate, $result));
return $result;
} catch (\Exception $e) {
list ($startDate, $endDate) = $this->stop();
$this->dispatch('database.query.executed', new DatabaseQueryEvent('findOne', 'db.'.$collection.'.findOne('.json_encode($criteria).', '.json_encode($fields).')', ['rawCriteria' => $criteria, 'rawFields' => $fields, 'options' => $options], $startDate, $endDate, null, $e));
throw $e;
}
}
/**
* Count the documents matching the criteria.
*
* @param string $collection
* @param array $criteria
* @param array $options
*
* @return int
*/
public function count($collection, $criteria = [], $options = [])
{
return $this->find($collection, $criteria, ['_id'], null, 0, [], $options)
->count(true);
}
/**
* Ensures the specified index is present on the specified fields of the collection.
*
* @param string $collection
* @param string|array $fields
* @param mixed $index
* @param array $options
*
* @return bool
*/
public function ensureIndex($collection, $fields, $index, $options = [])
{
return $this->getCollection($collection, $options)->createIndex(
is_array($fields) ? $fields : [$fields => true],
$index
);
}
/**
* @param string|null $name
*
* @return array
*/
public function getStatistics($name = null)
{
return $this->getMongoClient()
->selectDB(null === $name ? $this->getDatabaseName() : $name)
->command(['dbStats' => true])
;
}
/**
* Ensure specified id is a MongoId (convert to MongoId if is string).
*
* @param string $id
*
* @return \MongoId
*
* @throws Exception if malformed
*/
protected function ensureMongoId($id)
{
if (is_object($id) && $id instanceof MongoId) {
return $id;
}
if (!preg_match('/^[a-f0-9]{24}$/', $id)) {
throw $this->createMalformedException('Malformed id');
}
return new MongoId($id);
}
/**
* Ensure criteria are well formed (array).
*
* @param array $criteria
*
* @return array
*/
protected function buildCriteria($criteria)
{
if (!is_array($criteria)) {
return [];
}
if (isset($criteria['_id'])) {
$criteria['_id'] = $this->ensureMongoId($criteria['_id']);
}
if (isset($criteria['$or']) && is_array($criteria['$or'])) {
foreach ($criteria['$or'] as $a => $b) {
if (isset($b['_id'])) {
$criteria['$or'][$a]['_id'] = $this->ensureMongoId($b['_id']);
}
}
}
foreach ($criteria as $k => $v) {
if ('*notempty*' === $v) {
$criteria[$k] = ['$exists' => true];
}
if ('*empty*' === $v) {
$criteria[$k] = ['$exists' => false];
}
}
return $criteria;
}
/**
* Ensure fields are well formed (array).
*
* @param array|mixed $fields
*
* @return array
*/
protected function buildFields($fields)
{
$cleanedFields = [];
if (!is_array($fields)) {
return $cleanedFields;
}
foreach ($fields as $k => $v) {
if (is_numeric($k)) {
if (!is_string($v)) {
$v = (string) $v;
}
$cleanedFields[$v] = true;
} else {
if (!is_bool($v)) {
$v = (bool) $v;
}
$cleanedFields[$k] = $v;
}
}
return $cleanedFields;
}
/**
* Ensure sorts are well formed (array).
*
* @param array|mixed $sorts
*
* @return array
*/
protected function buildSorts($sorts)
{
$cleanedSorts = [];
if (!is_array($sorts)) {
return $cleanedSorts;
}
foreach ($sorts as $k => $v) {
if (is_numeric($k)) {
if (!is_string($v)) {
$v = (string) $v;
}
$cleanedSorts[$v] = 1;
} else {
$v = ((int) $v) === -1 ? -1 : 1;
$cleanedSorts[$k] = $v;
}
}
return $cleanedSorts;
}
/**
* Ensure document data are well formed (array).
*
* @param array $data
*
* @return array
*/
protected function buildData($data)
{
if (!is_array($data) || !count($data)) {
return [];
}
if (isset($data['_id'])) {
$data['_id'] = $this->ensureMongoId($data['_id']);
}
return $data;
}
/**
* Ensure documents data are well formed (array).
*
* @param array $bulkData
*
* @return array
*/
protected function buildBulkData($bulkData)
{
if (!is_array($bulkData) || !count($bulkData)) {
return [];
}
foreach ($bulkData as $a => $b) {
$bulkData[$a] = $this->buildData($b);
}
return $bulkData;
}
/**
* @return float
*/
protected function start()
{
$now = microtime(true);
$this->timers[] = $now;
return $now;
}
/**
* @return float[]
*
* @throws \Exception
*/
protected function stop()
{
if (!count($this->timers)) {
$this->start();
//throw $this->createRequiredException('No timer started'.(new \Exception("toto"))->getTraceAsString());
}
$endDate = microtime(true);
return [array_pop($this->timers), $endDate];
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\PaymentProvider;
use Symfony\Component\HttpFoundation\Request;
use Velocity\Bundle\ApiBundle\Service\MangoPayService;
use Velocity\Core\Traits\ServiceTrait;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
use Velocity\Bundle\ApiBundle\PaymentProviderInterface;
/**
* MangoPay Payment Provider.
*
* @author <NAME> <<EMAIL>>
*/
class MangoPayPaymentProvider implements PaymentProviderInterface
{
use ServiceTrait;
use ServiceAware\MangoPayServiceAwareTrait;
/**
* MangoPayPaymentProvider constructor.
*
* @param MangoPayService $mangoPayService
*/
public function __construct(MangoPayService $mangoPayService)
{
$this->setMangoPayService($mangoPayService);
}
/**
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function create($data = [], $options = [])
{
throw $this->createNotYetImplementedException('MangoPay payment provider not yet implemented');
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function confirm($id, $data = [], $options = [])
{
throw $this->createNotYetImplementedException('MangoPay payment provider not yet implemented');
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function fail($id, $data = [], $options = [])
{
throw $this->createNotYetImplementedException('MangoPay payment provider not yet implemented');
}
/**
* @param string|array $id
* @param array $data
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function cancel($id, $data = [], $options = [])
{
throw $this->createNotYetImplementedException('MangoPay payment provider not yet implemented');
}
/**
* @param string|array $id
* @param array $options
*
* @return array
*
* @throws \Exception
*/
public function get($id, $options = [])
{
throw $this->createNotYetImplementedException('MangoPay payment provider not yet implemented');
}
/**
* @param string $callback
* @param Request $request
*
* @return array
*/
public function parseCallbackRequest($callback, Request $request)
{
switch ($callback) {
default:
return [];
}
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Model;
use Velocity\Bundle\ApiBundle\Annotation as Velocity;
use /** @noinspection PhpUnusedAliasInspection */ Sensio\Bundle\FrameworkExtraBundle\Configuration\Route;
/**
* @author <NAME> <<EMAIL>>
* @Velocity\Model("modelWithAnnotatedMethods")
*/
class ModelWithAnnotatedMethods
{
/**
* @Route("/test-route")
*
* @Velocity\Sdk
*/
public function sdkMethod()
{
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Service;
use Velocity\Bundle\ApiBundle\RepositoryInterface;
use Velocity\Core\Traits\ServiceTrait;
use Symfony\Component\Form\Guess\Guess;
use Symfony\Component\Form\Guess\TypeGuess;
use Velocity\Bundle\ApiBundle\Traits\ServiceAware;
/**
* MetaData Service.
*
* @author <NAME> <<EMAIL>>
*/
class MetaDataService
{
use ServiceTrait;
use ServiceAware\StorageServiceAwareTrait;
use ServiceAware\WorkflowServiceAwareTrait;
use ServiceAware\GeneratorServiceAwareTrait;
/**
* @var array
*/
protected $callbacks = [];
/**
* @var array
*/
protected $models = [];
/**
* @var array
*/
protected $modelIds = [];
/**
* @var array
*/
protected $sdk = ['services' => []];
/**
* @param string $name
* @param DocumentServiceInterface|SubDocumentServiceInterface|SubSubDocumentServiceInterface|mixed $service
*
* @return $this
*/
public function addCrudService($name, $service)
{
return $this->setArrayParameterKey('crudServices', $name, $service);
}
/**
* @param string $name
*
* @return DocumentServiceInterface|SubDocumentServiceInterface|SubSubDocumentServiceInterface|mixed
*
* @throws \Exception
*/
public function getCrudService($name)
{
return $this->getArrayParameterKey('crudServices', $name);
}
/**
* @return DocumentServiceInterface[]|SubDocumentServiceInterface[]|SubSubDocumentServiceInterface[]|array
*/
public function getCrudServices()
{
return $this->getArrayParameter('crudServices');
}
/**
* @param StorageService $storageService
* @param GeneratorService $generatorService
* @param WorkflowService $workflowService
*/
public function __construct(StorageService $storageService, GeneratorService $generatorService, WorkflowService $workflowService)
{
$this->setStorageService($storageService);
$this->setGeneratorService($generatorService);
$this->setWorkflowService($workflowService);
}
/**
* @param string $class
*
* @return $this
*
* @throws \Exception
*/
public function checkModel($class)
{
if (!$this->isModel($class)) {
throw $this->createUnexpectedException("Class '%s' is not registered as a model", $class);
}
return $this;
}
/**
* @param string $class
* @param array $definition
*
* @return $this
*/
public function addModel($class, $definition)
{
if (!isset($this->models[$class])) {
$this->models[$class] = [
'embeddedReferences' => [],
'embeddedReferenceLists' => [],
'referenceLists' => [],
'refreshes' => [],
'generateds' => [],
'storages' => [],
'ids' => [],
'types' => [],
'fingerPrints' => [],
'workflows' => [],
'triggers' => [],
'cachedLists' => [],
];
}
$this->models[$class] += $definition;
$this->modelIds[strtolower($definition['id'])] = $class;
return $this;
}
/**
* @param $modelClass
*
* @return mixed|DocumentServiceInterface|SubDocumentServiceInterface|SubSubDocumentServiceInterface
*/
public function getCrudServiceByModelClass($modelClass)
{
return $this->getCrudService($this->getModel($modelClass)['id']);
}
/**
* @param string $type
* @param mixed $callback
*
* @return $this
*/
public function addCallback($type, $callback)
{
if (!isset($this->callbacks[$type])) {
$this->callbacks[$type] = [];
}
$this->callbacks[$type][] = $callback;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyEmbeddedReference($class, $property, $definition)
{
$this->checkModel($class);
$this->models[$class]['embeddedReferences'][$property] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyEmbeddedReferenceList($class, $property, $definition)
{
$this->checkModel($class);
$this->models[$class]['embeddedReferenceLists'][$property] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyCachedList($class, $property, $definition)
{
$this->checkModel($class);
$this->models[$class]['cachedLists'][$property] = $definition;
$modelId = $this->models[$class]['id'];
foreach ($definition['triggers'] as $triggerName => $trigger) {
$sourceClass = $this->getModelClassForId($trigger['model']);
unset($trigger['model']);
$this->checkModel($sourceClass);
if (!isset($this->models[$sourceClass]['triggers'])) {
$this->models[$sourceClass]['triggers'] = [];
}
$this->models[$sourceClass]['triggers'][$modelId.'.'.$triggerName] = ['targetDocType' => $modelId, 'targetDocProperty' => $property] + $trigger;
}
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyReferenceList($class, $property, $definition)
{
$this->checkModel($class);
$this->models[$class]['referenceLists'][$property] = $definition;
$values = $definition['value'];
if (!is_array($values)) {
$values = [];
}
if (!isset($this->models[$class]['types'][$property])) {
$this->models[$class]['types'][$property] = [];
}
$this->models[$class]['types'][$property]['type'] = 'referenceList';
$this->models[$class]['types'][$property]['values'] = $values;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyRefresh($class, $property, $definition)
{
$this->checkModel($class);
$operations = $definition['value'];
if (!is_array($operations)) {
$operations = [$operations];
}
foreach ($operations as $operation) {
if (!isset($this->models[$class]['refreshes'][$operation])) {
$this->models[$class]['refreshes'][$operation] = [];
}
$this->models[$class]['refreshes'][$operation][$property] = true;
}
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyEnum($class, $property, $definition)
{
$this->checkModel($class);
$values = $definition['value'];
if (!is_array($values)) {
$values = [];
}
if (!isset($this->models[$class]['types'][$property])) {
$this->models[$class]['types'][$property] = [];
}
$this->models[$class]['types'][$property]['type'] = 'enum';
$this->models[$class]['types'][$property]['values'] = $values;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyDefaultValue($class, $property, $definition)
{
$this->checkModel($class);
$value = isset($definition['value']) ? $definition['value'] : null;
if (!isset($this->models[$class]['types'][$property])) {
$this->models[$class]['types'][$property] = [];
}
$this->models[$class]['types'][$property]['default'] = $value;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyGenerated($class, $property, $definition)
{
$this->checkModel($class);
$definition['type'] = $definition['value'];
unset($definition['value']);
$this->models[$class]['generateds'][$property] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyFingerPrint($class, $property, $definition)
{
$this->checkModel($class);
unset($definition['value']);
if (!isset($definition['of'])) {
$definition['of'] = [];
}
if (!is_array($definition['of'])) {
$definition['of'] = [$definition['of']];
}
$this->models[$class]['fingerPrints'][$property] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyStorage($class, $property, $definition)
{
$this->checkModel($class);
$definition['key'] = $definition['value'];
unset($definition['value']);
$this->models[$class]['storages'][$property] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyWorkflow($class, $property, $definition)
{
$this->checkModel($class);
$this->models[$class]['workflows'][$property] = $definition;
if (!isset($this->models[$class]['types'][$property])) {
$this->models[$class]['types'][$property] = [];
}
$this->models[$class]['types'][$property]['type'] = 'workflow';
$this->models[$class]['types'][$property]['values'] = isset($definition['steps']) ? $definition['steps'] : [];
$this->getWorkflowService()->registerFromDefinition($definition['id'], $definition);
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function addModelPropertyId($class, $property, $definition)
{
$this->checkModel($class);
$definition['name'] = isset($definition['name']) ? $definition['name'] : '_id';
$definition['property'] = $property;
unset($definition['value']);
$this->models[$class]['ids'] = $definition;
return $this;
}
/**
* @param string $class
* @param string $property
* @param array $definition
*
* @return $this
*/
public function setModelPropertyType($class, $property, $definition)
{
$this->checkModel($class);
if (!isset($this->models[$class]['types'][$property])) {
$this->models[$class]['types'][$property] = [];
}
$this->models[$class]['types'][$property]['type'] = $definition['name'];
return $this;
}
/**
* @param string $sourceClass
* @param string $sourceMethod
* @param string $route
* @param string $service
* @param string $method
* @param string $type
* @param array $params
* @param array $options
*
* @return $this
*
* @throws \Exception
*/
public function addSdkMethod($sourceClass, $sourceMethod, $route, $service, $method, $type, $params = [], $options = [])
{
if (!isset($this->sdk['services'][$service])) {
$this->sdk['services'][$service] = ['methods' => []];
}
if (isset($this->sdk['services'][$service]['methods'][$method])) {
throw $this->createDuplicatedException("SDK Method '%s' already registered for service '%s'", $method, $service);
}
$this->sdk['services'][$service]['methods'][$method] = [
'sourceClass' => $sourceClass,
'sourceMethod' => $sourceMethod,
'type' => $type,
'route' => $route,
'params' => $params,
'options' => $options,
];
return $this;
}
/**
* @return array
*/
public function getSdkServices()
{
return $this->sdk['services'];
}
/**
* @param string $id
*
* @return string
*
* @throws \Exception
*/
public function getModelClassForId($id)
{
if (!isset($this->modelIds[strtolower($id)])) {
throw $this->createRequiredException("Unknown model '%s'", $id);
}
return $this->modelIds[strtolower($id)];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelEmbeddedReferences($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['embeddedReferences'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelReferenceLists($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['referenceLists'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelTriggers($class, array $options = [])
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
unset($options);
return $this->models[$class]['triggers'];
}
/**
* @param string|Object $class
* @param string $property
*
* @return array
*
* @throws \Exception
*/
public function getModelReferenceListByProperty($class, $property)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
if (!isset($this->models[$class]['referenceLists'][$property])) {
throw $this->createRequiredException("Property '%s' is a not a reference list", $property);
}
return $this->models[$class]['referenceLists'][$property];
}
/**
* @return array
*/
public function getModels()
{
return $this->models;
}
/**
* @param string|object $class
*
* @return bool
*/
public function isModel($class)
{
if (is_object($class)) {
$class = get_class($class);
}
return true === isset($this->models[$class]);
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelTypes($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['types'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelDefaults($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
$defaults = [];
foreach ($this->models[$class]['types'] as $property => $type) {
if (!isset($type['default'])) {
continue;
}
$defaults[$property] = $type['default'];
}
return $defaults;
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelGenerateds($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['generateds'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelFingerPrints($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['fingerPrints'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelStorages($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['storages'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelWorkflows($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['workflows'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelEmbeddedReferenceLists($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['embeddedReferenceLists'];
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModelCachedLists($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['cachedLists'];
}
/**
* @param string|Object $class
*
* @return array|null
*/
public function getModelIdProperty($class)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return $this->models[$class]['ids'];
}
/**
* @param string|Object $class
* @param string $operation
*
* @return array
*/
public function getModelRefreshablePropertiesByOperation($class, $operation)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return isset($this->models[$class]['refreshes'][$operation])
? array_keys($this->models[$class]['refreshes'][$operation])
: []
;
}
/**
* @param string|Object $class
* @param string $property
*
* @return null|string
*/
public function getModelPropertyType($class, $property)
{
if (is_object($class)) {
$class = get_class($class);
}
$this->checkModel($class);
return isset($this->models[$class]['types'][$property])
? $this->models[$class]['types'][$property]
: null;
}
/**
* @param string $class
* @param string $property
*
* @return TypeGuess
*/
public function getModelPropertyTypeGuess($class, $property)
{
$propertyType = $this->getModelPropertyType($class, $property);
if (null === $propertyType) {
return new TypeGuess(null, [], Guess::LOW_CONFIDENCE);
}
switch ($propertyType['type']) {
case 'enum':
return new TypeGuess('choice', ['choices' => $propertyType['values']], Guess::HIGH_CONFIDENCE);
case 'referenceList':
$referenceList = $this->getModelReferenceListByProperty($class, $property);
$choices = [];
foreach ($this->getCrudService($referenceList['type'])->find([], []) as $choice) {
$choice = (array) $choice;
$choices[$choice[$referenceList['key']]] = $choice[$referenceList['labelKey']];
}
return new TypeGuess('choice', ['multiple' => true, 'choices' => $choices], Guess::HIGH_CONFIDENCE);
case 'workflow':
return new TypeGuess('choice', ['choices' => $propertyType['steps']], Guess::HIGH_CONFIDENCE);
default:
return new TypeGuess(null, [], Guess::LOW_CONFIDENCE);
}
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
public function refresh($doc, $options = [])
{
$doc = $this->convertScalarProperties($doc, $options);
$doc = $this->fetchEmbeddedReferences($doc, $options);
$doc = $this->fetchEmbeddedReferenceLists($doc, $options);
$doc = $this->triggerRefreshes($doc, $options);
$doc = $this->buildGenerateds($doc, $options);
$doc = $this->computeFingerPrints($doc, $options);
$doc = $this->saveStorages($doc, $options);
$doc = $this->loadDefaultValues($doc, $options);
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
public function clean($doc, $options = [])
{
$doc = $this->populateStorages($doc, $options);
$this->refreshCached($doc, $options);
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*/
protected function refreshCached($doc, array $options = [])
{
$triggers = $this->getModelTriggers($doc, $options);
$options += ['operation' => null];
foreach ($triggers as $triggerName => $trigger) {
$targetId = $this->replaceProperty($doc, $trigger['targetId']);
$updateData = $this->replaceProperty($doc, $trigger['targetData']);
$requiredFields = [];
foreach ($trigger['targetData'] as $kkk => $vvv) {
if ('@' === $vvv{0}) {
$requiredFields[substr($vvv, 1)] = true;
}
}
$requiredFields = array_values($requiredFields);
$createData = $updateData;
$updateCriteria = [];
$createCriteria = [];
$deleteCriteria = [];
$skip = true;
$list = isset($trigger['joinFieldType']) && 'list' === $trigger['joinFieldType'];
$processNew = false;
$processUpdated = false;
$processDeleted = false;
switch ($options['operation']) {
case 'create':
if ($list) {
if (count($doc->{$trigger['joinField']})) {
$createCriteria['$or'] = [];
foreach ($doc->{$trigger['joinField']} as $kk => $vv) {
$createCriteria['$or'][] = ['_id' => $vv->id];
}
$processNew = true;
$skip = false;
}
} else {
$createCriteria['_id'] = $doc->{$trigger['joinField']}->id;
$processNew = true;
$skip = false;
}
break;
case 'update':
$updateData = array_filter($updateData, function ($v) { return null !== $v;});
if ($list) {
$expectedIds = [];
$existingIds = [];
if (count($doc->{$trigger['joinField']})) {
foreach ($doc->{$trigger['joinField']} as $kk => $vv) {
$expectedIds[$vv->id] = true;
}
}
$criteria = [
$trigger['targetDocProperty'] . '.' . $targetId => '*notempty*',
];
$docs = $this->getCrudService($trigger['targetDocType'])->find($criteria, ['id']);
if (count($docs)) {
foreach ($docs as $_doc) {
$existingIds[$_doc->id] = true;
}
}
$newIds = array_diff_key($expectedIds, $existingIds);
$deletedIds = array_diff_key($existingIds, $expectedIds);
$updatedIds = array_intersect_key($existingIds, $expectedIds);
if (count($newIds)) {
$createCriteria['$or'] = [];
foreach(array_keys($newIds) as $newId) {
$createCriteria['$or'][] = ['_id' => $newId];
}
$realDoc = $this->getCrudService($this->getModel($doc)['id'])->get($doc->id, $requiredFields);
foreach($requiredFields as $requiredField) {
if (isset($realDoc->$requiredField) && !isset($doc->$requiredField)) {
$doc->$requiredField = $realDoc->$requiredField;
}
}
$skip = false;
$processNew = true;
}
if (count($deletedIds)) {
$deleteCriteria['$or'] = [];
foreach(array_keys($deletedIds) as $deletedId) {
$deleteCriteria['$or'][] = ['_id' => $deletedId];
}
$skip = false;
$processDeleted = true;
}
if (count($updatedIds)) {
$updateCriteria['$or'] = [];
foreach(array_keys($updatedIds) as $updatedId) {
$updateCriteria['$or'][] = ['_id' => $updatedId];
}
$skip = false;
$processUpdated = true;
}
} else {
$criteria = [
$trigger['targetDocProperty'] . '.' . $targetId => '*notempty*',
];
$docs = $this->getCrudService($trigger['targetDocType'])->find($criteria, ['id']);
if (count($docs)) {
$updateCriteria['$or'] = [];
foreach ($docs as $_doc) {
$updateCriteria['$or'][] = ['_id' => $_doc->id];
}
$processUpdated = true;
$skip = false;
}
}
break;
case 'delete':
$criteria = [
$trigger['targetDocProperty'].'.'.$targetId => '*notempty*',
];
$docs = $this->getCrudService($trigger['targetDocType'])->find($criteria, ['id']);
if (count($docs)) {
$deleteCriteria['$or'] = [];
foreach ($docs as $_doc) {
$deleteCriteria['$or'][] = ['_id' => $_doc->id];
}
$skip = false;
$processDeleted = true;
}
break;
}
if (!$skip) {
/** @var RepositoryInterface $repo */
$repo = $this->getCrudService($trigger['targetDocType'])->getRepository();
if ($processDeleted) {
$repo->unsetProperty($deleteCriteria, $trigger['targetDocProperty'] . '.' . $targetId);
}
if ($processNew) {
$repo->alter($createCriteria, ['$set' => [$trigger['targetDocProperty'].'.'.$targetId => $createData]], ['multiple' => true]);
}
if ($processUpdated) {
$updates = [];
foreach ($updateData as $k => $v) {
$updates[$trigger['targetDocProperty'].'.'.$targetId.'.'.$k] = $v;
}
if (count($updates)) {
$repo->alter($updateCriteria, ['$set' => $updates], ['multiple' => true]);
}
}
}
}
}
/**
* @param mixed $doc
* @param mixed $value
*
* @return mixed
*/
protected function replaceProperty($doc, $value)
{
if (is_array($value)) {
foreach ($value as $k => $v) {
$value[$k] = $this->replaceProperty($doc, $v);
}
return $value;
}
if ('@' !== $value{0}) {
return $value;
}
$key = <EMAIL>($value, 1);
if (!property_exists($doc, $key)) {
return null;
}
return $doc->$key;
}
/**
* Process the registered callbacks.
*
* @param string $type
* @param mixed $subject
* @param array $options
*
* @return mixed
*/
public function callback($type, $subject = null, $options = [])
{
if (!isset($this->callbacks[$type]) || !count($this->callbacks[$type])) {
return $subject;
}
foreach ($this->callbacks[$type] as $callback) {
$r = call_user_func_array($callback, [$subject, $options]);
if (null !== $r) {
$subject = $r;
}
}
return $subject;
}
/**
* @param string|Object $class
*
* @return array
*/
public function getModel($class)
{
if (is_object($class)) {
$class = get_class($class);
}
if ('stdClass' === $class) {
// bypass check if stdClass (used for array to object cast)
return [];
}
$this->checkModel($class);
return $this->models[$class];
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*
* @throws \Exception
*/
public function convertObjectToArray($doc, $options = [])
{
$options += ['removeNulls' => true];
if (!is_object($doc)) {
throw $this->createMalformedException('Not a valid object');
}
$removeNulls = true === $options['removeNulls'];
$meta = $this->getModel($doc);
$data = get_object_vars($doc);
$globalObjectCast = false;
if (get_class($doc) === 'stdClass') {
$globalObjectCast = true;
}
foreach ($data as $k => $v) {
if ($removeNulls && null === $v) {
unset($data[$k]);
continue;
}
if (isset($meta['types'][$k]['type'])) {
switch (true) {
case 'DateTime' === substr($meta['types'][$k]['type'], 0, 8):
$data = $this->convertDataDateTimeFieldToMongoDateWithTimeZone($data, $k);
continue 2;
}
}
if (is_object($v)) {
$objectCast = false;
if ('stdClass' === get_class($v)) {
$objectCast = true;
}
$v = $this->convertObjectToArray($v, $options);
if (true === $objectCast) {
$v = (object) $v;
}
}
$data[$k] = $v;
}
if (true === $globalObjectCast) {
$data = (object) $data;
}
return $data;
}
/**
* @param mixed $doc
* @param array $data
* @param array $options
*
* @return mixed
*/
public function populateObject($doc, $data = [], $options = [])
{
if (isset($data['_id']) && !isset($data['id'])) {
$data['id'] = (string) $data['_id'];
unset($data['_id']);
} else {
if (isset($data['id'])) {
$data['id'] = (string) $data['id'];
}
}
$doc = $this->mutateArrayToObject($data, $doc, $options);
return $doc;
}
/**
* @param mixed $doc
* @param mixed $data
* @param string $propertyName
* @param array $options
*
* @return mixed
*
* @throws \Exception
*/
public function populateObjectProperty($doc, $data, $propertyName, $options = [])
{
if (!property_exists($doc, $propertyName)) {
throw $this->createRequiredException("Property '%s' does not exist on %s", $propertyName, get_class($doc));
}
$doc->$propertyName = $data;
$this->populateStorages($doc, $options);
return $doc->$propertyName;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function fetchEmbeddedReferences($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
foreach ($this->getModelEmbeddedReferences($doc) as $property => $embeddedReference) {
if (!$this->isPopulableModelProperty($doc, $property, $options)) {
continue;
}
$type = $this->getModelPropertyType($doc, $property);
$doc->$property = $this->convertIdToObject($doc->$property, isset($embeddedReference['class']) ? $embeddedReference['class'] : ($type ? $type['type'] : null), $embeddedReference['type']);
}
return $doc;
}
/**
* @param mixed $doc
* @param string $property
* @param array $options
*
* @return bool
*/
protected function isPopulableModelProperty($doc, $property, array $options = [])
{
return property_exists($doc, $property) && (!isset($options['populateNulls']) || (false === $options['populateNulls'] && null !== $doc->$property));
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*
* @throws \Exception
*/
protected function fetchEmbeddedReferenceLists($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
foreach ($this->getModelEmbeddedReferenceLists($doc) as $property => $embeddedReferenceList) {
if (!$this->isPopulableModelProperty($doc, $property, $options)) {
continue;
}
$type = $this->getModelPropertyType($doc, $property);
if (!is_array($doc->$property)) {
$doc->$property = [];
}
$subDocs = [];
foreach ($doc->$property as $kk => $subDocKey) {
$tt = isset($embeddedReferenceList['class']) ? $embeddedReferenceList['class'] : ($type ? $type['type'] : null);
if (null !== $tt) {
$tt = preg_replace('/^array<([^>]+)>$/', '\\1', $tt);
}
$subDoc = $this->convertIdToObject($subDocKey, $tt, $embeddedReferenceList['type']);
unset($doc->$property[$kk]);
if (!isset($subDoc->id)) {
throw $this->createRequiredException('Property id is empty for embedded reference', 500);
}
$subDocs[$subDoc->id] = $subDoc;
}
$doc->$property = (object) $subDocs;
}
return $doc;
}
/**
* @param string $id
* @param string $class
* @param string $type
*
* @return Object
*/
protected function convertIdToObject($id, $class, $type)
{
$model = $this->createModelInstance(['model' => $class]);
$fields = array_keys(get_object_vars($model));
return $this->getCrudService($type)->get($id, $fields, ['model' => $model]);
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function buildGenerateds($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
$generateds = $this->getModelGenerateds($doc);
foreach ($generateds as $k => $v) {
$generate = false;
if (isset($v['trigger'])) {
if (isset($doc->{$v['trigger']})) {
$generate = true;
}
} else {
if ($this->isPopulableModelProperty($doc, $k, $options)) {
$generate = true;
}
}
if (true === $generate) {
$doc->$k = $this->generateValue($v, $doc);
}
}
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function loadDefaultValues($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
if (!isset($options['operation']) || 'create' !== $options['operation']) {
return $doc;
}
$defaults = $this->getModelDefaults($doc);
foreach ($defaults as $k => $v) {
if (!$this->isPopulableModelProperty($doc, $k, $options)) {
continue;
}
$doc->$k = $v;
}
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function computeFingerPrints($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
$fingerPrints = $this->getModelFingerPrints($doc);
foreach ($fingerPrints as $k => $v) {
$values = [];
$found = false;
foreach ($v['of'] as $p) {
if (!isset($doc->$p)) {
$values[$p] = null;
continue;
} else {
$values[$p] = $doc->$p;
$found = true;
}
}
unset($v['of']);
if (true === $found) {
$doc->$k = $this->generateValue(['type' => 'fingerprint'], count($values) > 1 ? $values : array_shift($values));
}
}
unset($options);
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function saveStorages($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
$vars = ((array) $doc);
foreach ($options as $k => $v) {
if (!isset($vars[$k])) {
$vars[$k] = $v;
}
}
$storages = $this->getModelStorages($doc);
foreach ($storages as $k => $definition) {
if (!$this->isPopulableModelProperty($doc, $k, $options)) {
continue;
}
$doc->$k = $this->saveStorageValue($doc->$k, $definition, $vars);
}
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function populateStorages($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
unset($options);
$storages = $this->getModelStorages($doc);
foreach ($storages as $k => $definition) {
if (isset($doc->$k)) {
$doc->$k = $this->readStorageValue($doc->$k);
}
unset($definition);
}
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*
* @throws \Exception
*/
protected function triggerRefreshes($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
if (!isset($options['operation'])) {
return $doc;
}
foreach ($this->getModelRefreshablePropertiesByOperation($doc, $options['operation']) as $property) {
$type = $this->getModelPropertyType($doc, $property);
switch ($type['type']) {
case "DateTime<'c'>":
$doc->$property = new \DateTime();
break;
default:
throw $this->createUnexpectedException("Unable to refresh model property '%s': unsupported type '%s'", $property, $type);
}
}
return $doc;
}
/**
* @param mixed $doc
* @param array $options
*
* @return mixed
*/
protected function convertScalarProperties($doc, $options = [])
{
if (!is_object($doc)) {
return $doc;
}
$types = $this->getModelTypes($doc);
foreach ($types as $property => $type) {
if (!$this->isPopulableModelProperty($doc, $property, ['populateNulls' => false] + $options)) {
continue;
}
switch ($type['type']) {
case "DateTime<'c'>":
if ('' === $doc->$property) {
$doc->$property = null;
}
break;
}
}
return $doc;
}
/**
* @param array $definition
* @param mixed $data
*
* @return string
*
* @throws \Exception
*/
protected function generateValue($definition, $data)
{
return $this->getGeneratorService()->generate($definition['type'], is_object($data) ? (array) $data : $data);
}
/**
* @param mixed $value
* @param array $definition
* @param mixed $vars
*
* @return string
*
* @throws \Exception
*/
protected function saveStorageValue($value, $definition, $vars)
{
$key = $definition['key'];
if (0 < preg_match_all('/\{([^\}]+)\}/', $key, $matches)) {
foreach ($matches[1] as $i => $match) {
$key = str_replace($matches[0][$i], isset($vars[$match]) ? $vars[$match] : null, $key);
}
}
$this->getStorageService()->save($key, $value);
return $key;
}
/**
* @param mixed $key
*
* @return mixed
*
* @throws \Exception
*/
protected function readStorageValue($key)
{
return $this->getStorageService()->read($key);
}
/**
* @param array $options
*
* @return object
*/
protected function createModelInstance(array $options)
{
$class = $options['model'];
return new $class();
}
/**
* @param array $data
* @param mixed $doc
* @param array $options
*
* @return Object
*/
protected function mutateArrayToObject($data, $doc, array $options = [])
{
$embeddedReferences = $this->getModelEmbeddedReferences($doc);
$embeddedReferenceLists = $this->getModelEmbeddedReferenceLists($doc);
$cachedLists = $this->getModelCachedLists($doc);
$types = $this->getModelTypes($doc);
foreach ($data as $k => $v) {
if (!property_exists($doc, $k)) {
continue;
}
if (isset($embeddedReferences[$k])) {
$v = $this->mutateArrayToObject($v, $this->createModelInstance(['model' => $embeddedReferences[$k]['class']]), $options);
}
if (isset($embeddedReferenceLists[$k])) {
$tt = isset($embeddedReferenceLists[$k]['class']) ? $embeddedReferenceLists[$k]['class'] : (isset($types[$k]) ? $types[$k]['type'] : null);
if (null !== $tt) {
$tt = preg_replace('/^array<([^>]+)>$/', '\\1', $tt);
}
if (!is_array($v)) {
$v = [];
}
$subDocs = [];
foreach ($v as $kk => $vv) {
$subDocs[$kk] = $this->mutateArrayToObject($vv, $this->createModelInstance(['model' => $tt]), $options);
}
$v = $subDocs;
}
if (isset($cachedLists[$k])) {
$tt = isset($cachedLists[$k]['class']) ? $cachedLists[$k]['class'] : (isset($types[$k]) ? $types[$k]['type'] : null);
if (null !== $tt) {
$tt = preg_replace('/^array<([^>]+)>$/', '\\1', $tt);
}
if (!is_array($v)) {
$v = [];
}
$subDocs = [];
foreach ($v as $kk => $vv) {
$subDocs[$kk] = $this->mutateArrayToObject($vv, $this->createModelInstance(['model' => $tt]), $options);
}
$v = $subDocs;
}
if (isset($types[$k])) {
switch (true) {
case 'DateTime' === substr($types[$k]['type'], 0, 8):
$data = $this->revertDocumentMongoDateWithTimeZoneFieldToDateTime($data, $k);
$v = $data[$k];
}
$doc->$k = $v;
} else {
$doc->$k = $v;
}
}
$doc = $this->populateStorages($doc);
return $doc;
}
/**
* @param array $data
* @param string $fieldName
*
* @return array
*
* @throws \Exception
*/
protected function convertDataDateTimeFieldToMongoDateWithTimeZone($data, $fieldName)
{
if (!isset($data[$fieldName])) {
throw $this->createRequiredException("Missing date time field '%s'", $fieldName);
}
if (null !== $data[$fieldName] && !$data[$fieldName] instanceof \DateTime) {
throw $this->createRequiredException("Field '%s' must be a valid DateTime", $fieldName);
}
/** @var \DateTime $date */
$date = $data[$fieldName];
$data[$fieldName] = new \MongoDate($date->getTimestamp());
$data[sprintf('%s_tz', $fieldName)] = $date->getTimezone()->getName();
return $data;
}
/**
* @param array $doc
* @param string $fieldName
*
* @return array
*
* @throws \Exception
*/
protected function revertDocumentMongoDateWithTimeZoneFieldToDateTime($doc, $fieldName)
{
if (!isset($doc[$fieldName])) {
throw $this->createRequiredException("Missing mongo date field '%s'", $fieldName);
}
if (!isset($doc[sprintf('%s_tz', $fieldName)])) {
$doc[sprintf('%s_tz', $fieldName)] = date_default_timezone_get();
}
if (!$doc[$fieldName] instanceof \MongoDate) {
throw $this->createMalformedException("Field '%s' must be a valid MongoDate", $fieldName);
}
/** @var \MongoDate $mongoDate */
$mongoDate = $doc[$fieldName];
$date = new \DateTime(sprintf('@%d', $mongoDate->sec), new \DateTimeZone('UTC'));
$doc[$fieldName] = date_create_from_format(
\DateTime::ISO8601,
preg_replace('/(Z$|\+0000$)/', $doc[sprintf('%s_tz', $fieldName)], $date->format(\DateTime::ISO8601))
);
unset($doc[sprintf('%s_tz', $fieldName)]);
return $doc;
}
}
<file_sep><?php
/*
* This file is part of the VELOCITY package.
*
* (c) PHPPRO <<EMAIL>>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Velocity\Bundle\ApiBundle\Tests\Service;
use PHPUnit_Framework_TestCase;
use Doctrine\Common\Annotations\AnnotationReader;
use Symfony\Component\DependencyInjection\Definition;
use Velocity\Bundle\ApiBundle\Service\VelocityService;
use Symfony\Component\DependencyInjection\ContainerBuilder;
use Velocity\Bundle\ApiBundle\Tests\Mock\ContainerBuilderMock;
/**
* @author <NAME> <<EMAIL>>
*/
class VelocityServiceTest extends PHPUnit_Framework_TestCase
{
/**
* @var VelocityService
*/
protected $s;
/**
* @var ContainerBuilder
*/
protected $containerMock;
/**
*
*/
public function setUp()
{
$this->s = new VelocityService(new AnnotationReader());
}
/**
* @group unit
* @group velocity
*/
public function testSimpleModelClasses()
{
$d = new Definition();
$this->s->loadClassesMetaData([
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model1',
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model2',
], $d);
$this->assertEquals(
[
['addModel', ['Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model1', ['id' => 'm1']]],
['addModel', ['Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model2', ['id' => 'm2']]],
],
$d->getMethodCalls()
);
}
/**
* @group unit
* @group velocity
*/
public function testModelClassesWithAnnotatedMethods()
{
$d = new Definition();
$this->s->loadClassesMetaData([
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedMethods',
], $d);
$addedMethods = $d->getMethodCalls();
$this->assertCount(2, $addedMethods);
$this->assertEquals(
['addModel', ['Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedMethods', ['id' => 'modelWithAnnotatedMethods']]],
$addedMethods[0]
);
list($sourceClass, $sourceMethod) = $addedMethods[1];
$this->assertEquals('addSdkMethod', $sourceClass);
$this->assertContains('sdkMethod', $sourceMethod);
$this->assertContains('Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedMethods', $sourceMethod);
}
/**
* @group unit
* @group velocity
*/
public function testModelClassesWithAnnotatedProperties()
{
$d = new Definition();
$this->s->loadClassesMetaData([
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedProperties',
], $d);
$addedMethods = $d->getMethodCalls();
$this->assertCount(3, $addedMethods);
$this->assertEquals(
['addModel', ['Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedProperties', ['id' => 'modelWithAnnotatedProperties']]],
$addedMethods[0]
);
list($sourceClass, $sourceProperty) = $addedMethods[1];
$this->assertEquals('addModelPropertyEmbeddedReference', $sourceClass);
$this->assertContains('embeddedReference', $sourceProperty);
list($sourceClass, $sourceProperty) = $addedMethods[2];
$this->assertEquals('addModelPropertyId', $sourceClass);
$this->assertContains('id', $sourceProperty);
}
/**
* @group unit
*/
public function testIsVelocityAnnotatedClass()
{
$this->assertTrue($this->s->isVelocityAnnotatedClass('Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model1'));
$this->assertTrue($this->s->isVelocityAnnotatedClass('Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model2'));
$this->assertFalse($this->s->isVelocityAnnotatedClass(__CLASS__));
}
/**
* @group unit
*/
public function testFindVelocityAnnotatedClassesInDirectory()
{
$classes = $this->s->findVelocityAnnotatedClassesInDirectory(__DIR__.'/../Model');
$expected = [
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model1',
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\Model2',
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedMethods',
'Velocity\\Bundle\\ApiBundle\\Tests\\Model\\ModelWithAnnotatedProperties',
];
$this->assertEquals($expected, $classes);
$this->assertEquals(count($expected), count($classes));
}
/**
* @group unit
*/
public function testAnalyzeTags()
{
$container = new ContainerBuilderMock();
$this->s->analyzeTags($container);
$this->assertGreaterThan(10, count($container->getDefinitions()));
}
}
| 88a69d1f77f78e2c6e19cf89b170428f7af362c3 | [
"PHP",
"Shell"
] | 32 | PHP | michel-maier/velocity-api-bundle | eb183797892da973464d085b34492923b91d4a30 | 21706a6d3b22c71ec8a1c3fa656403e3b549af96 | |
refs/heads/master | <file_sep>const fs = require('fs');
const normalize = require('path').normalize;
module.exports = function requireDirectory(appDir, directoryPath) {
const dirToRead = (appDir) ? appDir +'/'+ directoryPath : directoryPath;
try {
if (!fs.existsSync(dirToRead)) return [];
return fs.readdirSync(dirToRead).map(function(file) {
if (file.endsWith('.map')) return null;
if (file.toLowerCase().indexOf('test')>=0) return null;
const fileParts = file.split('.');
if (fileParts.length > 1) fileParts.pop();
const moduleName = fileParts.join('.'); // don't worry about what type of extension
return {
moduleName,
requirePath: normalize(dirToRead + '/' + moduleName),
importPath: normalize(directoryPath + '/' + moduleName),
};
}).filter(Boolean);
} catch (e) {
return [];
}
};
| 6e7177b0e722c7a2bb8583008f33506aa5f8586e | [
"JavaScript"
] | 1 | JavaScript | ryanstevens/mods2require | a2097f6334283e7d13aff3b998c02e91a08607bd | 5528e0913e74cafac9aaf406e1ce02298a013ba5 | |
refs/heads/master | <file_sep>Market.git
<file_sep><!doctype html>
<html lang="en">
<head>
<title>Title</title>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="<KEY>" crossorigin="anonymous">
</head>
<body>
<div class="container" style="margin-top: 20px;">
<div class="row">
<div class="col-12">
<form method="POST">
<div class="form-group">
<input type="text" placeholder="<NAME>" name="name" value="<?php if (isset($_POST['name'])) {
echo $_POST['name'];
} ?>" class="form-control" id="exampleInputtext" aria-describedby="textHelp">
</div>
<div class="form-group">
<select name="city" class="form-control">
<option value="cairo" <?php if (isset($_POST['city']) && $_POST['city'] == 'cairo') { ?> selected='selected' <?php } ?>>Cairo</option>
<option value="giza" <?php if (isset($_POST['city']) && $_POST['city'] == 'giza') { ?> selected='selected' <?php } ?>>Giza</option>
<option value="alex" <?php if (isset($_POST['city']) && $_POST['city'] == 'alex') { ?> selected='selected' <?php } ?>>Alex</option>
<option value="others" <?php if (isset($_POST['city']) && $_POST['city'] == 'others') { ?> selected='selected' <?php } ?>>Others</option>
</select>
</div>
<div class="form-group">
<input type="number" name="products_num" value="<?php if (isset($_POST['products_num'])) {
echo $_POST['products_num'];
} ?>" placeholder="Number of Products" class="form-control" id="exampleInputtext">
</div>
<button type="submit" name="enter" class="btn btn-danger form-control"> Enter Products </button>
<?php if (isset($_POST['enter'])) {
$i;
?>
<div class="container">
<table class="table text-center">
<thead>
<tr>
<th scope="col">Product Name</th>
<th scope="col">Price</th>
<th scope="col">Quantity</th>
</tr>
</thead>
<tbody>
<?php
for ($i = 0; $i < $_POST['products_num']; $i++) { ?>
<tr>
<td><input type="text" name="products<?php echo $i ?>" class="form-control"></td>
<td><input type="text" name="price<?php echo $i ?>" class="form-control"></td>
<td><input type="number" name="quantity<?php echo $i ?>" class="form-control"></td>
</tr>
<?php } ?>
</tbody>
</table>
<button type="submit" name="calc" class="btn btn-danger form-control"> Calculate </button>
</div>
<?php } ?>
<div class="container">
<table class="table text-center" style="font-size: 18px;">
<?php
if (isset($_POST['calc'])) { ?>
<thead>
<tr>
<th scope="col">Product Name</th>
<th scope="col">Price</th>
<th scope="col">Quantity</th>
<th scope="col">Sub Total</th>
</tr>
</thead>
<tbody>
<?php
$sum = 0;
for ($i = 0; $i < $_POST['products_num']; $i++) { ?>
<tr>
<td> <?php echo $_POST['products' . $i] ?> </td>
<td> <?php echo $_POST['price' . $i] ?> </td>
<td> <?php echo $_POST['quantity' . $i] ?> </td>
<td> <?php echo ($sub_total = $_POST['price' . $i] * $_POST['quantity' . $i]) ?> </td>
</tr>
<?php
$sum += $sub_total;
$total_price = $sum;
} ?>
<?php
if ($total_price < 1000) {
$discountfees = $total_price * 1;
}
if ($total_price >= 1000 && $total_price < 3000) {
$discountfees = $total_price * 0.1;
}
if ($total_price >= 3000 && $total_price < 4500) {
$discountfees = $total_price * 0.15;
}
if ($total_price > 4500) {
$discountfees = $total_price * 0.2;
}
$priceafterdiscount = $total_price - $discountfees;
?>
<?php if ($_POST['city'] == 'cairo') {
$fees = 0 . ' EGP';
}
if ($_POST['city'] == 'giza') {
$fees = 30 . ' EGP';
}
if ($_POST['city'] == 'alex') {
$fees = 50 . ' EGP';
}
if ($_POST['city'] == 'others') {
$fees = 100 . ' EGP';
} ?>
<?php $nettotal = $priceafterdiscount + $fees; ?>
<?php
$bill = [
'Client name' => $_POST['name'],
'City' => $_POST['city'],
'Total' => $sum,
'Discount' => $discountfees,
'Delivery' => $fees,
'Total after Discount' => $priceafterdiscount
]; ?>
<?php
foreach ($bill as $key => $value) { ?>
<tr>
<th><?php echo $key ?></th>
<td> <?php echo $value ?> </td>
<td></td>
<td></td>
</tr>
<?php } ?>
<tr>
<th style="color: red;">Net Total</th>
<td></td>
<td></td>
<td style="color: red;font-weight:bold;"><?php echo $nettotal ?></td>
</tr>
<?php } ?>
</tbody>
</table>
</div>
</form>
</div>
</div>
</div>
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
</body>
</html> | 0286a497bc0eac1fdf6d02401991ff7b1f9a7b73 | [
"Markdown",
"PHP"
] | 2 | Markdown | TyseerElsayed/Market | 635954cb0e32be582f6993f35e6a087b758a36ee | cfc588cb7e8fa2f3c8bd8711a48f277a4c359886 | |
refs/heads/master | <repo_name>Alesh/Squall-CXX<file_sep>/demo/hello_cb.cxx
#include <iostream>
#include <squall/EventLoop.hxx>
using squall::Event;
using squall::EventLoop;
int main(int argc, char const* argv[]) {
int cnt = 0;
EventLoop event_loop;
event_loop.setup_timer([](int revents) {
if (revents == Event::Timeout)
std::cout << "Hello, Alesh! (" << revents << ")" << std::endl;
return true;
}, 1.0);
event_loop.setup_timer([&cnt](int revents) {
if (revents == Event::Timeout) {
std::cout << "Hello, World! (" << revents << ")" << std::endl;
cnt++;
}
return (cnt < 3);
}, 2.5);
event_loop.setup_signal([&event_loop](int revents) {
if (revents == Event::Signal) {
std::cout << "\nGot SIGINT. Bye! (" << revents << ")" << std::endl;
event_loop.stop();
}
return false;
}, SIGINT);
event_loop.start();
return 0;
}<file_sep>/include/squall/base/EventLoop.hxx
#ifndef SQUALL__BASE__EVENT_LOOP__HXX
#define SQUALL__BASE__EVENT_LOOP__HXX
#include <ev.h>
#include <memory>
#include <functional>
#include <unordered_map>
namespace squall {
/**
* Event codes
*/
enum Event : int {
Read = EV_READ,
Write = EV_WRITE,
Timeout = EV_TIMER,
Signal = EV_SIGNAL,
Error = EV_ERROR,
Cleanup = EV_CLEANUP,
};
namespace base {
/**
* Base template class that implement an event loop.
*/
template <typename Context>
class EventLoop {
using OnEvent = std::function<void(Context context, int revents) noexcept>;
OnEvent _on_event;
bool _running = false;
struct ev_loop* _p_loop;
std::unordered_map<Context, std::unique_ptr<ev_io>> _io_watchers;
std::unordered_map<Context, std::unique_ptr<ev_timer>> _timer_watchers;
std::unordered_map<Context, std::unique_ptr<ev_signal>> _signal_watchers;
template <typename Watcher>
static void callback(struct ev_loop* p_loop, Watcher* p_watcher, int revents) {
auto p_event_loop = static_cast<EventLoop<Context>*>(ev_userdata(p_loop));
auto p_context = static_cast<Context*>(p_watcher->data);
p_event_loop->_on_event(*p_context, revents);
}
public:
/* Constructor
* `on_event` is the callback that will be called to transmit occurred events. */
EventLoop(const OnEvent& on_event) : _on_event(on_event) {
_p_loop = ev_loop_new(0);
ev_set_userdata(_p_loop, static_cast<void*>(this));
};
/* Destructor */
virtual ~EventLoop() {
ev_loop_destroy(_p_loop);
}
/* Return true if an event dispatching is active. */
bool is_running() {
return _running;
}
/* Starts event dispatching.*/
void start() {
_running = true;
while (_running)
ev_run(_p_loop, EVRUN_ONCE);
while (_io_watchers.size())
cancel_io(_io_watchers.begin()->first);
while (_timer_watchers.size())
cancel_timer(_timer_watchers.begin()->first);
while (_signal_watchers.size())
cancel_signal(_signal_watchers.begin()->first);
}
/* Stops event dispatching.*/
void stop() {
if (_running) {
_running = false;
ev_break(_p_loop, EVBREAK_ONE);
}
}
/* Setup to call `on_event` for a given `context`
* when the I/O device with a given `fd` would be to read and/or write `mode`. */
bool setup_io(Context context, int fd, int mode) {
auto p_watcher = new ev_io();
ev_io_init(p_watcher, EventLoop::template callback<ev_io>, fd, mode);
ev_io_start(_p_loop, p_watcher);
if (ev_is_active(p_watcher)) {
auto result = _io_watchers.insert(std::make_pair(context, std::unique_ptr<ev_io>(p_watcher)));
if (!result.second) {
cancel_io(result.first->first);
result = _io_watchers.insert(std::make_pair(context, std::unique_ptr<ev_io>(p_watcher)));
}
if (result.second) {
result.first->second.get()->data = (void*)(&(result.first->first));
return true;
}
}
return false;
}
/* Updates I/O mode for event watchig extablished with method `setup_io` for a given `context`. */
bool update_io(Context context, int mode) {
auto found = _io_watchers.find(context);
if (found != _io_watchers.end()) {
auto p_watcher = found->second.get();
if (_running && ev_is_active(p_watcher))
ev_io_stop(_p_loop, p_watcher);
ev_io_set(p_watcher, p_watcher->fd, mode);
ev_io_start(_p_loop, p_watcher);
if (ev_is_active(p_watcher))
return true;
}
return false;
}
/* Cancels an event watchig extablished with method `setup_io` for a given `context`. */
bool cancel_io(Context context) {
auto found = _io_watchers.find(context);
if (found != _io_watchers.end()) {
auto p_watcher = found->second.get();
if (ev_is_active(p_watcher))
ev_io_stop(_p_loop, p_watcher);
_io_watchers.erase(found);
return true;
}
return false;
}
/* Setup to call `on_event` for a given `context` every `seconds`. */
bool setup_timer(Context context, double seconds) {
auto p_watcher = new ev_timer();
ev_timer_init(p_watcher, EventLoop::template callback<ev_timer>, seconds, seconds);
ev_timer_start(_p_loop, p_watcher);
if (ev_is_active(p_watcher)) {
auto result = _timer_watchers.insert(std::make_pair(context, std::unique_ptr<ev_timer>(p_watcher)));
if (!result.second) {
cancel_timer(result.first->first);
result = _timer_watchers.insert(std::make_pair(context, std::unique_ptr<ev_timer>(p_watcher)));
}
if (result.second) {
result.first->second.get()->data = (void*)(&(result.first->first));
return true;
}
}
return false;
}
/* Cancels an event watchig extablished with method `setup_timer` for a given `context`. */
bool cancel_timer(Context context) {
auto found = _timer_watchers.find(context);
if (found != _timer_watchers.end()) {
auto p_watcher = found->second.get();
if (ev_is_active(p_watcher))
ev_timer_stop(_p_loop, p_watcher);
_timer_watchers.erase(found);
return true;
}
return false;
}
/* Setup to call `on_event` for a given `context`
* when the system signal with a given `signum` recieved. */
bool setup_signal(Context context, int signum) {
auto p_watcher = new ev_signal();
ev_signal_init(p_watcher, EventLoop::template callback<ev_signal>, signum);
ev_signal_start(_p_loop, p_watcher);
if (ev_is_active(p_watcher)) {
auto result = _signal_watchers.insert(std::make_pair(context, std::unique_ptr<ev_signal>(p_watcher)));
if (!result.second) {
cancel_signal(result.first->first);
result = _signal_watchers.insert(std::make_pair(context, std::unique_ptr<ev_signal>(p_watcher)));
}
if (result.second) {
result.first->second.get()->data = (void*)(&(result.first->first));
return true;
}
}
return false;
}
/* Cancels an event watchig extablished with method `setup_signal` for a given `context`. */
bool cancel_signal(Context context) {
auto found = _signal_watchers.find(context);
if (found != _signal_watchers.end()) {
auto p_watcher = found->second.get();
if (ev_is_active(p_watcher))
ev_signal_stop(_p_loop, p_watcher);
_signal_watchers.erase(found);
return true;
}
return false;
}
};
} // end of base
} // end of squall
#endif
<file_sep>/Default.cmake
set(CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -g -std=c++11")
include(FindPackageHandleStandardArgs)
find_library(LIBEV_LIBRARY NAMES ev)
find_path(LIBEV_INCLUDE_DIR ev.h PATH_SUFFIXES include/ev)
find_package_handle_standard_args(libev DEFAULT_MSG LIBEV_LIBRARY LIBEV_INCLUDE_DIR)
include_directories(${CMAKE_HOME_DIRECTORY}/include)<file_sep>/setup.py
import sys
import os.path
from setuptools import setup, Extension
try:
from Cython.Build import cythonize
except ImportError:
cythonize = None
if sys.version_info[:2] < (3, 5):
raise NotImplementedError("Required python version 3.5 or greater")
include_dirs = [os.path.join(os.path.dirname(__file__), "include")]
settings = {
'name': 'Squall-Core.cython',
'version': '0.1.dev27',
'author': '<NAME>',
'author_email': '<EMAIL>',
'description': "This is Squall-Core optimized module which implements"
"the cooperative multitasking based on event-driven"
"switching async/await coroutines.",
'namespace_packages': ['squall'],
'package_dir': {'squall': 'python/squall'},
'ext_modules': cythonize([Extension('squall.core_cython',
['python/squall/core_cython.pyx'],
include_dirs=include_dirs,
language="c++", libraries=['ev'],
extra_compile_args=["-std=c++11"])]),
'zip_safe': False
}
setup(**settings)<file_sep>/tests/test_EventLoop.cpp
#include "../include/squall/base/EventLoop.hxx"
#include "../include/squall/EventLoop.hxx"
#include "catch.hpp"
TEST_CASE("squall::base::EventLoop<const char*>", "[EventLoop]") {
using squall::Event;
using EventLoop = squall::base::EventLoop<const char*>;
int cnt = 0;
std::string result;
const char* A = "A";
const char* B = "B";
const char* W = "W";
EventLoop event_loop([&cnt, &result, &B, &W, &event_loop](const char* Ch, int revents) {
result += Ch;
if (Ch[0] == 'A') {
cnt++;
if (cnt==1)
event_loop.setup_io(W, 0, Event::Write);
else if (cnt==6)
event_loop.cancel_timer(B);
else if (cnt==10)
event_loop.stop();
}
else if (Ch[0] == 'W')
event_loop.cancel_io(W);
});
event_loop.setup_timer(A, 0.1);
event_loop.setup_timer(B, 0.26);
event_loop.start();
REQUIRE(result == "AWABAAABAAAAA");
}
TEST_CASE("squall::EventLoop", "[EventLoop]") {
using squall::Event;
using squall::EventLoop;
int A = 0;
int B = 0;
std::string result;
EventLoop event_loop;
event_loop.setup_timer([&](int revents){
A++;
result += 'A';
if (A == 1) {
event_loop.setup_io([&](int revents){
result += 'W';
return false;
}, 0, Event::Write);
}
else if (A == 10)
event_loop.stop();
return true;
}, 0.1);
event_loop.setup_timer([&](int revents){
B = B + 1;
result += "B";
return (B < 2);
}, 0.26);
event_loop.start();
REQUIRE(result == "AWABAAABAAAAA");
}<file_sep>/include/Squall.hxx
/**
* The Squall is template library which provides
* the event-driven and async network primitives.
*/
#ifndef SQUALL__HXX
#define SQUALL__HXX
#endif<file_sep>/demo/hello_cp.cxx
#include <iostream>
#include <squall/base/EventLoop.hxx>
using squall::Event;
using EventLoop = squall::base::EventLoop<const char*>;
int main(int argc, char const* argv[]) {
EventLoop event_loop([&event_loop](const char* name, int revents) {
if (revents == Event::Timeout) {
std::cout << "Hello, " << name << "! (" << revents << ")" << std::endl;
} else
if (revents == Event::Signal) {
std::cout << "\nGot " << name << ". Bye! (" << revents << ")" << std::endl;
event_loop.stop();
}
});
event_loop.setup_timer("Alesh", 1.0);
event_loop.setup_timer("World", 2.5);
event_loop.setup_signal("SIGINT", SIGINT);
event_loop.start();
return 0;
}<file_sep>/include/squall/EventLoop.hxx
#ifndef SQUALL__EVENT_LOOP__HXX
#define SQUALL__EVENT_LOOP__HXX
#include "base/EventLoop.hxx"
namespace squall {
using namespace std::placeholders;
using Callback = std::function<bool(int revents) noexcept>;
/**
* Event loop implementation for callback.
*/
class EventLoop : base::EventLoop<std::shared_ptr<Callback>> {
using Base = base::EventLoop<std::shared_ptr<Callback>>;
void _base_on_event(std::shared_ptr<Callback> handle, int revents) noexcept {
auto callback = *handle.get();
if(!callback(revents) && is_running()) {
cancel_io(handle);
cancel_timer(handle);
cancel_signal(handle);
}
}
public:
EventLoop()
: Base(std::bind(&EventLoop::_base_on_event, this, _1, _2)) {}
/* Return true if an event dispatching is active. */
bool is_running() {
return Base::is_running();
}
/* Starts event dispatching.*/
void start() {
Base::start();
}
/* Stops event dispatching.*/
void stop() {
Base::stop();
}
/* Setup to call `callback` when the I/O device
* with a given `fd` would be to read and/or write `mode`. */
std::shared_ptr<Callback> setup_io(const Callback& callback, int fd, int mode) {
auto handle = std::shared_ptr<Callback>(new Callback(callback));
if (Base::setup_io(handle, fd, mode))
return handle;
else
return std::shared_ptr<Callback>(nullptr);
}
/* Setup to call `callback` every `seconds`. */
std::shared_ptr<Callback> setup_timer(const Callback& callback, double seconds) {
auto handle = std::shared_ptr<Callback>(new Callback(callback));
if (Base::setup_timer(handle, seconds))
return handle;
else
return std::shared_ptr<Callback>(nullptr);
}
/* Setup to call `callback` when the system signal
* with a given `signum` recieved. */
std::shared_ptr<Callback> setup_signal(const Callback& callback, int signum) {
auto handle = std::shared_ptr<Callback>(new Callback(callback));
if (Base::setup_signal(handle, signum))
return handle;
else
return std::shared_ptr<Callback>(nullptr);
}
/* Updates I/O mode for event watchig extablished with method `setup_io`. */
bool update_io(std::shared_ptr<Callback> handle, int mode) {
return Base::update_io(handle, mode);
}
/* Cancels event watchig extablished with method `setup_io`. */
bool cancel_io(std::shared_ptr<Callback> handle) {
return Base::cancel_io(handle);
}
/* Cancels event watchig extablished with method `setup_timer`. */
bool cancel_timer(std::shared_ptr<Callback> handle) {
return Base::cancel_timer(handle);
}
/* Cancels event watchig extablished with method `setup_signal`. */
bool cancel_signal(std::shared_ptr<Callback> handle) {
return Base::cancel_signal(handle);
}
};
} // end of squall
#endif
<file_sep>/CMakeLists.txt
cmake_minimum_required(VERSION 3.2)
project(Squall_CXX)
add_subdirectory(demo)
add_subdirectory(tests)<file_sep>/python/squall/common/EventLoop.hxx
#ifndef SQUALL__PYTHON__EVENT_LOOP__HXX
#define SQUALL__PYTHON__EVENT_LOOP__HXX
#include <Python.h>
#include <squall/base/EventLoop.hxx>
using squall::Event;
using namespace squall;
using namespace std::placeholders;
/* Event loop implementation for python extension. */
class EventLoop : base::EventLoop<PyObject*> {
using Base = base::EventLoop<PyObject*>;
PyThreadState* _thread_save;
void _base_on_event(PyObject* callback, int revents) noexcept {
PyEval_RestoreThread(_thread_save);
PyObject* py_arglist = Py_BuildValue("(i)", revents);
PyObject* py_result = PyObject_CallObject(callback, py_arglist);
Py_XDECREF(py_arglist);
Py_XDECREF(py_result);
_thread_save = PyEval_SaveThread();
}
public:
EventLoop()
: Base(std::bind(&EventLoop::_base_on_event, this, _1, _2)) {}
/* Return true if an event dispatching is active. */
bool is_running() {
return Base::is_running();
}
/* Starts event dispatching.*/
void start() {
_thread_save = PyEval_SaveThread();
Base::start();
PyEval_RestoreThread(_thread_save);
}
/* Stops event dispatching.*/
void stop() {
Base::stop();
}
/* Setup to call `callback` when the I/O device
* with a given `fd` would be to read and/or write `mode`. */
bool setup_io(PyObject* callback, int fd, int mode) {
if (Base::setup_io(callback, fd, mode)) {
Py_XINCREF(callback);
return true;
}
return false;
}
/* Setup to call `callback` every `seconds`. */
bool setup_timer(PyObject* callback, double seconds) {
if (Base::setup_timer(callback, seconds)) {
Py_XINCREF(callback);
return true;
}
return false;
}
/* Setup to call `callback` when the system signal
* with a given `signum` recieved. */
bool setup_signal(PyObject* callback, int signum) {
if (Base::setup_signal(callback, signum)) {
Py_XINCREF(callback);
return true;
}
return false;
}
/* Updates I/O mode for event watchig extablished with method `setup_io`. */
bool update_io(PyObject* callback, int mode) {
return Base::update_io(callback, mode);
}
/* Cancels event watchig extablished with method `setup_io`. */
bool cancel_io(PyObject* callback) {
if (Base::cancel_io(callback)) {
Py_XDECREF(callback);
return true;
}
return false;
}
/* Cancels event watchig extablished with method `setup_timer`. */
bool cancel_timer(PyObject* callback) {
if (Base::cancel_timer(callback)) {
Py_XDECREF(callback);
return true;
}
return false;
}
/* Cancels event watchig extablished with method `setup_signal`. */
bool cancel_signal(PyObject* callback) {
if (Base::cancel_signal(callback)) {
Py_XDECREF(callback);
return true;
}
return false;
}
};
#endif<file_sep>/README.md
# Squall #
The Squall is template library which provides
the event-driven and async network primitives. | 23adc044f03a123d247469b086bcedb4fec82c58 | [
"Markdown",
"Python",
"CMake",
"C++"
] | 11 | C++ | Alesh/Squall-CXX | 88b61e23c51d36fcd27211a1230de9a178646d63 | c7b2b2a7484698504184ccdee3b5e2a3969b482b | |
refs/heads/master | <file_sep>/**
* 获取数据类型
* @author shizhihuaxu 2019-04-10
* @param {[type]} elem 任意类型的元素
* @return {String} 描述元素类型的字符串,小写
*/
export const getType = (elem) => {
return Object.prototype.toString.call(elem).slice(8, -1).toLowerCase()
}
/**
* 判断一个变量是否为空 null、undefined、''、[]、{}
* @author shizhihuaxu 2019-08-26
* @param any elem 变量
* @return Boolean true/false
*/
export const isEmpty = (elem) => {
// 是否为空的标志位
let flag = false
// 变量的数据类型
let type = Object.prototype.toString.call(elem).slice(8, -1).toLowerCase()
switch (type) {
case 'undefined' || 'null':
flag = true
break;
case 'string':
flag = elem.length === 0
break
case 'array':
flag = (elem.length === 0)
break
case 'object':
flag = Object.keys(elem).length === 0
break
}
return flag
}
/**
* 浅拷贝 引用类型 一层复制
* @author shizhihuaxu 2019-08-09
* @param {Object} obj 源对象
* @return {Object} 新对象
*/
export const shallowClone = (obj) => {
let res = {}
for (let key in obj) {
if (Object.prototype.hasOwnProperty.call(obj, key)) { // 只复制自身属性,不包含原型链上的属性
res[key] = obj[key]
}
}
return res
}
/**
* 深拷贝 引用类型 深层次复制
* @author shizhihuaxu 2019-04-08
* @param {Object} obj 原对象
* @return {Object} 新对象
*/
/** 局限性:
1、层次过深导致堆栈溢出
优点:
1、JSON.stringify内部做了循环引用的检测
*/
export const deepClone1 = (obj) => {
let temp = JSON.stringfy(obj)
return JSON.parse(temp)
}
export const deepClone = (obj, res) => {
if(!(typeof obj === 'object' && obj !== null)) return obj // 排除掉 null 和 非对象的情况
var res = res || {}
for (let key in obj) {
if (Object.prototype.hasOwnProperty.call(obj, key)) { // 只复制自身属性,不包含原型链上的属性
if (typeof obj[key] === 'object') {
res[key] = (obj[key].constructor === "Array") ? [] : {}
deepClone(obj[key], res[key]) // 尾递归优化 防止层级过深爆栈的问题
} else {
res[key] = obj[key]
}
}
}
return res
}
/**
* 去除字符串前后空格
* @author shizhihuaxu 2019-04-08
* @param {String} str 原字符串
* @return {string} 去除前后空格的字符串
*/
export const trim = (str) => {
return (str || '').replace(/^(\s|00A0)+|(\s|00A0)+$/g, '')
}
/**
* 判断字符串是否是回文
* @author shizhihuaxu 2019-04-10
* @param {String} str [description]
* @return {Boolean} [description]
*/
export const isPalindrome = (str) => {
let left = 0
let right = str.length - 1
while (left < right) {
if (str[left] !== str[right]) {
return false
}
left++
right--
}
return true
}
// method 2
export const isPalindrome1 = (str) => {
let arr = str.split('')
if (arr.reverse().join('') === str) return true
return false
}
/**
* 对象深层取值优雅的解决办法,避免属性不存在报错
* @author shizhihuaxu 2019-06-24
* @param {Array} path 目标对象的属性路径形成的数组
* @param {Object} obj 目标对象
* @return {[type]} 如果属性存在则返回属性值,否则返回null
*
* @example
* const props = {
user: {
posts: [
{ title: 'Foo', comments: [ 'Good one!', 'Interesting...' ] },
{ title: 'Bar', comments: [ 'Ok' ] },
{ title: 'Baz', comments: []}
],
}
console.log(get(['user', 'posts', 0, 'comments'], props)) // [ 'Good one!', 'Interesting...' ]
}
*/
export const getDeepValue = (path, obj) => path.reduce((curObj, curVal) => (curObj && curObj[curVal]) ? curObj[curVal] : null, obj)
/**
* 不四舍五入的保留 n 位小数
* @author shizhihuaxu 2019-07-26
* @param {Number} num 操作的数值
* @param {Number} len 保留小数的长度
* @return {Number} 保留小数后的数值
*/
export const toNonroundingFixed = (num, len = 1) => {
if (typeof num !== 'number' || typeof len !== 'number')
throw new Error('Invalid arguments')
if (len >= 10)
throw new Error('The decimal length is too long')
return num.toFixed(len + 1).slice(0, -1) * 1
}
/**
* 基础数据类型的数组去重
* @author shizhihuaxu 2020-09-27
* @param Array 需要去重的数组
* @return Array 已去重的数组
*/
export const uniqueArr = (arr) => {
if (!arr.length) return []
let newArr = []
arr.forEach((cur, index, curArr) => {
// 处理 NaN 的情况
if (cur !== cur) {
for (let i = 0, len = index; i < len; i++) {
if (Number.isNaN(newArr[i])) { // 如果新数组中已经存在 NaN,不再寻找
break
} else {
newArr.push(cur)
}
}
} else {
// indexOf 会返回第一个存在的索引,当 arr.indexOf(self) 与 index 不相同的时候,说明之前存在过,即为重复
// indexOf 使用严格相等的模式判断
arr.indexOf(cur) === index ? newArr.push(cur) : null
}
})
return newArr
}
let arr1 = [NaN, NaN, undefined, undefined, null, null, 1, '1', +0, -0, 2, 2]
let arr2 = uniqueArr(arr1)
arr2 // [NaN, undefined, null, 1, '1', 0, 2]
/**
* 基础数据类型数组去重,未处理 NaN 的情况
*/
export const uniqueArr1 = (arr) => {
let newArr = arr.reduce((acc, cur) => {
if (acc.indexOf(cur) === -1) { // indexOf 使用严格相等的模式判断
acc.push(cur)
}
return acc
}, [])
return newArr
}
<file_sep>// dom 相关工具
/**
* 获取当前元素距离浏览器的绝对位置
* @author shizhihuaxu 2019-04-08
* @param {HTMLElement} obj Dom 对象
* @return {Object} 一个包含上侧与左侧距离的对象
*/
export const getObjPosition = (obj) => {
let left = 0
let top = 0
while (obj.offsetParent) {
left += obj.offsetLeft
top += obj.offsetTop
obj = obj.offsetParent
}
return { left: left, top: top }
}
/**
* 判断是否为 IE 浏览器
* @author shizhihuaxu 2019-04-08
* @return {Boolean} true or false
*/
export const isIE = () => {
return !!document.all
}
/**
* 兼容添加事件监听 惰性载入
* @author shizhihuaxu 2019-04-08
* @return {Function} 用于添加监听事件的兼容方法
*/
export const addEvent = (function() {
if (window.addEventListener) {
return function(el, type, fn, capture) {
el.addEventListener(type, function(e) {
fn.call(el, e)
}, (capture))
}
} else {
return function(el, type, fn, capture) {
el.attachEvent('on' + type, function(e) {
fn.call(el, e)
})
}
}
})()
/**
* 防抖 去弹跳
* @author shizhihuaxu 2019-04-23
* @param {Function} fn 回调函数
* @param {Number} dely 等待时间 ms
* @return {Function}
*/
export const debounce = (fn, dely) => {
let timeout
return function() {
clearTimeout(timeout)
let context = this
let args = arguments
timeout = setTimeout(function() {
fn.apply(context, args)
}, dely)
}
}
/**
* 节流
* @author shizhihuaxu 2019-04-23
* @param {Function} fn 回调函数
* @param {Number} gap 间隔时间 ms
* @return {Function} void
*/
export const threshold = (fn, gap) => {
let timeout
let start = new Date()
return function() {
let context = this
let args = arguments
let cur = new Date() - 0
clearTimeout(timeout)
if (cur - start >= gap) {
fn.apply(context, args)
start = cur
} else {
timeout = setTimeout(function() {
fn.apply(context, args)
}, gap)
}
}
} | 1654fa6063d6cb21f6d1566636ea8be2dc6e0814 | [
"JavaScript"
] | 2 | JavaScript | shizhihuaxu/js-utils | cc238bb534444be224eb76d1a9bf15c01c4833d8 | 47e2d8e0e0c680bd5e0c746ebad984756dfb192c | |
refs/heads/master | <repo_name>Wynand/comp3490<file_sep>/project/app.js
var app = require('express')();
var http = require('http').Server(app);
var io = require('socket.io')(http);
var THREE = require('three');
var bodyParser = require('body-parser');
app.use(bodyParser.json()); // for parsing application/json
var updatable = new Map();
var initialized = false;
var updates = new Array();
app.get('/',
function(req, res){
if(!initialized){
makeClawMachine();
}
res.sendFile(__dirname + '/a1/clawmachine.html');
}
);
app.get('/clawStart.js',
function(req, res){
res.sendFile(__dirname + '/a1/clawStart.js' );
}
);
app.get('/js/KeyboardState.js',
function(req, res){
res.sendFile(__dirname + '/a1/js/KeyboardState.js' );
}
);
app.post('/update',
function(req, res){
if(updatable.has(req.body.name) && updatable.get(req.body.name).has(req.body.direction)){
console.log(req.body.name + " " + req.body.direction);
updatable.get(req.body.name).get(req.body.direction)();
}
res.send('');
}
)
io.on('connection',
function(socket){
console.log("A user is connected");
socket.on('disconnect',
function(){
console.log("user disconnected");
}
);
}
);
http.listen(process.env.PORT || 3000,
function(){
console.log("Listening to on port %d", this.address().port);
}
);
function makeClawMachine(){
var arcadeMachine = new THREE.Group();
var bodyMaterial = new THREE.MeshLambertMaterial();
bodyMaterial.color.setRGB( 0.5, 0.5, 0.5 );
var crane = makeCrane( bodyMaterial ); // will move up/down
crane.position.y = 700;
crane.name = 'crane';
updatable.set('crane', new Map());
updatable.get('crane').set('incr',
function(){
if(crane.position.y < 700){
crane.position.y += 1;
io.sockets.emit('crane', {data: crane.position.y});
}
}
);
updatable.get('crane').set('decr',
function(){
if(crane.position.y > 500){
crane.position.y += -1;
io.sockets.emit('crane', {data: crane.position.y});
}
}
);
arcadeMachine.add( crane );
return arcadeMachine;
}
function makeCrane( bodyMaterial ){
var crane = new THREE.Group();
railShape = new THREE.BoxGeometry( 20, 20, 300 );
railL = new THREE.Mesh(
railShape , bodyMaterial );
railL.position.x = 125;
railL.position.y = 0;
railL.position.z = 0;
crane.add( railL );
railR = new THREE.Mesh(
railShape , bodyMaterial );
railR.position.x = -railL.position.x;
railR.position.y = railL.position.y;
railR.position.z = railL.position.z;
crane.add( railR );
railArm = makeRailArm( bodyMaterial ); // this will move forward and backward
railArm.name = 'railArm';
updatable.set('railArm',new Map())
var railRef = railArm;
var max = 100;
updatable.get('railArm').set('incr',
function(){
if(railRef.position.z < max){
railRef.position.z += 1;
io.sockets.emit('railArm', {data:railRef.position.z});
}
}
);
updatable.get('railArm').set('decr',
function(){
if(railRef.position.z > -max){
railRef.position.z += -1;
io.sockets.emit('railArm', {data: railRef.position.z});
}
}
);
crane.add( railArm );
return crane;
}
function makeRailArm( bodyMaterial ){
railArm = new THREE.Group();
railB = new THREE.Mesh(
new THREE.BoxGeometry( 300 , 20, 20 ), bodyMaterial );
railArm.add( railB );
hangingArm = makeHangingArm( bodyMaterial ); // this will move right/left
hangingArm.position.y = -20;
hangingArm.name = 'hangingArm';
updatable.set('hangingArm', new Map())
var haRef = hangingArm;
var max = 125;
updatable.get('hangingArm').set('incr',
function(){
if(haRef.position.x < max){
haRef.position.x += 1;
io.sockets.emit('hangingArm', {data: haRef.position.x});
}
}
);
updatable.get('hangingArm').set('decr',
function(){
if(haRef.position.x > -max){
haRef.position.x += -1;
io.sockets.emit('hangingArm', {data: haRef.position.x});
}
}
);
railArm.add( hangingArm );
return railArm;
}
function makeHangingArm( bodyMaterial ){
var hangingArm = new THREE.Group();
return hangingArm;
}
<file_sep>/project/README.txt
To run the server first you need to ensure that you have node, npm and yarn installed.
Once these are installed and you are in the directory with all the files, execute "yarn install"
After this succeeds, execute ifconfig or ipconfig and note your local IP address.
After getting your IP address, execute 'yarn start'. The server should now be running.
On a machine on the same network, enter whatever you ip address is into the url bar, and append port number ':3000'
If you run this on multiple machines, you will notice all the mobile devices are in vr mode and the desktop browsers are in the classic mode for the assignment.
From here you can move things around in one window and see the effect on a different one.
Another alternative is to go to "https://hidden-beyond-21959.herokuapp.com/" and see the app hosted there. There is only a single instance of this, so anyone on the site at the time can manipulate the arcade machine.
The joystick and camera only move in the instance where you enter commands. Controls to move the camera are asdw qe while the crane is moved with the arrow keys, shift and space.
In VR mode, the arcade machine may start behind you, so look around.<file_sep>/a1/README.txt
CS3490 Assignment 1: Claw Machine (Barebones Edition)
/**********************************************************************
* What is your name and student number?
***********************************************************************/
<NAME>
7754210
/**********************************************************************
* What browser and OS did you use to develop / test?
***********************************************************************/
Windows 10, insider preview w/ Chrome
Ubuntu 16.04LTS w/ Chrome
MacOSX w/ Chromed
/**********************************************************************
* Which components of the assignment do you feel you have completed.
**********************************************************************/
Keep one of the 3 options below, and delete the other two. The intention of this
is that nobody receives a grade that's way out of line with expectations
due to technical difficulties.
i. Box with supports and a top: (Fully Completed)
ii. Console, joystick, guards and prize bin: (Fully Completed)
iii. Arrow keys cause joystick to bend: (Fully Completed)
iv: Claw mechanism, created in a hierarchical manner: (Fully Completed)
v: Keyboard allows claw to move (in xz plane): (Fully Completed)
vi: Spacebar drops claw (in y plane): (Fully Completed)
vii. Pressing "v" changes viewpoint: (Fully Completed)
/**********************************************************************
* List any additional comments that you feel may be relevant.
**********************************************************************/
Other controls are :
Q = down
E = up
W = forward
S = backward
A = left
D = right
The guards are glass.
A hierarchy of the claw assembly was achieved with the THREE.js 'Groups' and also functions to create components.
The view is lock when in 'egocentric' mode, however you can still navigate with the mouse in 'generic' mode.
To dynamically update the components, the functions to update them were added to an array of functions called every iteration.<file_sep>/a2/clawStart.js
////////////////////////////////////////////////////////////////////////////////
/* COMP 3490 A1 Skeleton for Claw Machine (Barebones Edition)
* Note that you may make use of the skeleton provided, or start from scratch.
* The choice is up to you.
* Read the assignment directions carefully
* Your claw mechanism should be created such that it is represented hierarchically
* You might consider looking at THREE.Group and THREE.Object3D for reference
* If you want to play around with the canvas position, or other HTML/JS level constructs
* you are welcome to do so.
/*global variables, coordinates, clock etc. */
var keyboard;
var camera, scene, renderer;
var cameraControls;
var updates = new Array();
var clock = new THREE.Clock();
var phase = 0;
var jRef;
var reflection;
var view = 1;
xrot = 0;
zrot = 0;
function fillScene() {
scene = new Physijs.Scene();
scene.fog = new THREE.Fog( 0x808080, 2000, 4000 );
scene.setGravity(new THREE.Vector3( 0, -100, 0 ));
// Some basic default lighting - in A2 complexity will be added
//scene.add( new THREE.AmbientLight( 0x222222 ) );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( 200, 500, 500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( -200, 500, 500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( 200, 500, -500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( -200, 500, -500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( 200, -500, 500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( -200, -500, 500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( 200, -500, -500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
var light = new THREE.PointLight( 0xffffff, 0.2 );
light.position.set( -200, -500, -500 );
light.castShadow = true;
light.receiveShadow = true;
scene.add( light );
/*
light = new THREE.PointLight( 0xffffff, 0.9 );
light.position.set( -200, -100, -400 );
light.castShadow = true;
light.recieveShadow = true;
scene.add( light );
*/
//A simple grid floor, the variables hint at the plane that this lies within
// Later on we might install new flooring.
var linoleum = Physijs.createMaterial(new THREE.MeshPhysicalMaterial( {
transparent: true,
opacity: .97,
specular: 0x050505,
shininess: 1,
metalness: 1
} ))
linoleum.color.setRGB( 0.7, 0.5, 0.3);
var floor = new Physijs.BoxMesh( new THREE.BoxGeometry( 10000, 1, 10000), linoleum , 0 );
floor.receiveShadow = true;
//floor.castShadow = true;
scene.add(floor);
//Visualize the Axes - Useful for debugging, can turn this off if desired
var axes = new THREE.AxisHelper(150);
axes.position.y = 1;
scene.add(axes);
drawClawMachine();
}
function drawClawMachine() {
reflection = true;
var clawMachineReflection = makeClawMachine();
reflection = false;
var clawMachine = makeClawMachine();
scene.add( clawMachine );
clawMachineReflection.rotateX(Math.PI);
clawMachineReflection.rotateY(Math.PI);
scene.add( clawMachineReflection );
//clawMachineReflection.rotateX(3.14569);
//clawMachineReflection.rotateY(3.14569);
//scene.add( clawMachineReflection );
} // drawClawMachine
function makeClawMachine(){
var machineGeo = new THREE.BoxGeometry( 0, 0, 0 );
var bodyMaterial = Physijs.createMaterial(new THREE.MeshPhysicalMaterial());
bodyMaterial.color.setRGB( 0.5, 0.5, 0.5 );
var arcadeMachine = new Physijs.BoxMesh( machineGeo, bodyMaterial, 0 );
var frame = makeFrame( bodyMaterial );
var crane = makeCrane( bodyMaterial ); // will move up/down
crane.position.y = 700;
var maxHeight = 700;
var minHeight = 535;
if(!reflection){
updates.push(
function(){
if(keyboard.pressed("space")){
phase = 1
}
if(phase == 1){
crane.position.y += -1;/*
if(movingDown){
crane.position.y += -1;
} else{
crane.position.y += 1;
}*/
}
if(crane.position.y <= minHeight && phase == 1){
phase = 2;
} /*else if(!movingDown && moving && crane.position.y > maxHeight){
moving = false;
}*/
if(phase == 3){
crane.position.y += 1;
}
if(crane.position.y >= maxHeight && phase == 3){
phase = 4;
}
crane.__dirtyPosition = true;
}
);
}
var cPanel = makeControlPanel( bodyMaterial, 0 );
cPanel.position.y = 300;
cPanel.position.z = -200;
var prizeBucket = makeChute( bodyMaterial, 0 );
prizeBucket.position.y = 420;
prizeBucket.position.x = 0;
prizeBucket.position.z = -75;
var prizeChute = makeChute( bodyMaterial, 0 );
prizeChute.rotateX(1);
prizeChute.position.y = 200;
prizeChute.position.x = 0;
prizeChute.position.z = -150;
arcadeMachine.add( frame );
arcadeMachine.add( crane );
arcadeMachine.add( cPanel );
arcadeMachine.add( prizeBucket );
arcadeMachine.add( prizeChute );
arcadeMachine.castShadow = true;
arcadeMachine.recieveShadow = true;
var hOffset = 475;
var height = (maxHeight - 125) - hOffset;
var width = 200;
var depth = 200;
var ball;
var ballWidth = 30;
var ballGeometry = new THREE.SphereGeometry( ballWidth/2, 32, 32 );
for(k = 1; k < height/ballWidth; k++ ){
ball = new Physijs.SphereMesh( ballGeometry, bodyMaterial );
ball.position.y = ( k*ballWidth + hOffset);
scene.add(ball);
}
return arcadeMachine;
}
function makeControlPanel( bodyMaterial ){
var cPanel = new THREE.Group();
var baseMaterial = Physijs.createMaterial(new THREE.MeshPhysicalMaterial({ map: THREE.ImageUtils.loadTexture('src/wood2.jpg') }));
panel = new Physijs.BoxMesh( new THREE.BoxGeometry( 250, 20, 100), baseMaterial, 0 );
panel.position.x = 0;
panel.position.y = 0;
panel.position.z = 0;
panel.castShadow = true;
panel.recieveShadow = true;
cPanel.add( panel );
var slot = makeCoinSlot( bodyMaterial );
slot.position.y = 40;
slot.position.z = 50;
cPanel.add(slot);
var led = Physijs.createMaterial(new THREE.MeshPhysicalMaterial( {
transparent: true,
opacity: .6,
specular: 0x050505,
shininess: 1,
metalness: 0
} ))
led.color.setRGB( 0, 0, 1);
bbutton = new Physijs.BoxMesh( new THREE.BoxGeometry( 30, 10, 30), led, 0 );
bbutton.position.y = 15;
bbutton.position.x = 80;
bbutton.castShadow = true;
bbutton.recieveShadow = true;
bbLight = new THREE.PointLight( 0x0000ff, 1 , 40);
bbLight.position.y = 25;
bbutton.add(bbLight)
cPanel.add( bbutton );
if(!reflection){
updates.push(
function(){
if(phase != 0){
bbutton.position.y = 10;
} else {
bbutton.position.y = 15;
}
}
);
}
var jContainer = new THREE.Group(); // should rotate
var led2 = Physijs.createMaterial(new THREE.MeshPhysicalMaterial( {
transparent: true,
opacity: .60,
specular: 0x050505,
shininess: 1,
metalness: 0
} ))
led2.color.setRGB( 1, 0, 0);
button = new Physijs.BoxMesh( new THREE.BoxGeometry( 6, 2, 6), led2, 0 );
button.position.y = 81;
button.castShadow = true;
button.recieveShadow = true;
bLight = new THREE.PointLight( 0xcd0000, 1 , 10);
bLight.position.y = 2;
button.add(bLight)
jContainer.add( button );
if(!reflection){
updates.push(
function(){
if(phase != 0){
button.position.y = 81;
} else {
button.position.y = 82;
}
}
);
}
joyStick = new Physijs.BoxMesh( new THREE.BoxGeometry( 10, 80, 10), bodyMaterial, 0 );
joyStick.position.y = 40;
joyStick.castShadow = true;
joyStick.recieveShadow = true;
jContainer.add( joyStick );
jContainer.position.x = -75;
jContainer.position.y = 10;
jContainer.position.z = 0;
if(!reflection){
updates.push(
function(){
jRef = jContainer;
var rot = 0.3;
jRef.rotateX(-xrot);
xrot = 0;
jRef.rotateZ(-zrot);
zrot = 0;
if(keyboard.pressed("d") || keyboard.pressed("right")){
zrot += rot;
}
if(keyboard.pressed("a") || keyboard.pressed("left")){
zrot += -rot;
}
jRef.rotateZ(zrot);
if(keyboard.pressed("w") || keyboard.pressed("up")){
xrot += rot;
}
if(keyboard.pressed("s") || keyboard.pressed("down")){
xrot += -rot;
}
jRef.rotateX(xrot);
}
);
}
cPanel.add( jContainer );
return cPanel;
}
function makeCoinSlot( bodyMaterial ) {
var led = Physijs.createMaterial(new THREE.MeshPhysicalMaterial( {
transparent: true,
opacity: .6,
specular: 0x050505,
shininess: 1,
metalness: 0
} ))
led.color.setRGB( 0, 1, 0);
var back = new Physijs.BoxMesh( new THREE.BoxGeometry( 11.5, 20, 5), led, 0 );
var bottom = new Physijs.BoxMesh( new THREE.BoxGeometry( 11.5, 5, 5), led, 0 );
bottom.position.z = -5;
bottom.position.y = -7.5;
back.add(bottom);
var top = new Physijs.BoxMesh( new THREE.BoxGeometry( 11.5, 5, 5), led, 0 );
top.position.z = -5;
top.position.y = -bottom.position.y;
back.add(top);
var left = new Physijs.BoxMesh( new THREE.BoxGeometry( 5, 20, 5), led, 0 );
left.position.z = -5;
left.position.x = -3.5;
back.add(left);
var right = new Physijs.BoxMesh( new THREE.BoxGeometry( 5, 20, 5), led, 0 );
right.position.z = -5;
right.position.x = -left.position.x;
back.add(right);
var bbLight = new THREE.PointLight( 0x00cd00, 1 , 40);
bbLight.position.z = -2.5
back.add(bbLight)
return back;
}
function makeFrame( bodyMaterial ){
var frameGeo = new THREE.BoxGeometry( 0, 0, 0 );
var frame = new Physijs.BoxMesh( frameGeo, bodyMaterial, 0 );
var baseMaterial = Physijs.createMaterial(new THREE.MeshPhysicalMaterial({ map: THREE.ImageUtils.loadTexture('src/wood.jpg') }));
var base = new Physijs.BoxMesh( new THREE.BoxGeometry( 300, 400, 300 ), baseMaterial, 0 );
base.position.x = 0;
base.position.y = 200;
base.position.z = 0;
base.castShadow = true;
base.recieveShadow = true;
frame.add( base );
stands = makeStands( bodyMaterial, 0 );
stands.position.y = 600;
frame.add( stands );
glass = makeGlass();
glass.position.y = 600;
frame.add( glass );
boxTop = new Physijs.BoxMesh(
new THREE.BoxGeometry( 300, 50, 300 ), baseMaterial, 0 );
boxTop.position.x = 0;
boxTop.position.y = 800;
boxTop.position.z = 0;
var spot1 = makeChute(bodyMaterial);
spot1.castShadow = true;
var light1 = new THREE.PointLight( 0xffffff, 10 , 800);
light1.castShadow = true;
light1.receiveShadow = true;
light1.position.y = 5;
spot1.add(light1);
var spot2 = makeChute(bodyMaterial);
spot2.castShadow = true;
var light2 = new THREE.PointLight( 0xffffff, 10 , 800);
light2.castShadow = true;
light2.receiveShadow = true;
light1.position.y = 5;
spot2.add(light2);
spot1.position.z = -100;
spot1.position.y = -55;
spot2.position.z = -spot1.position.z;
spot2.position.y = spot1.position.y;
boxTop.add(spot1);
boxTop.add(spot2);
var marMaterial = Physijs.createMaterial(new THREE.MeshPhysicalMaterial({ map: THREE.ImageUtils.loadTexture('src/fun.jpg') }));
var marquis = new Physijs.BoxMesh(
new THREE.BoxGeometry( 250, 100, 30 ), marMaterial, 0 );
marquis.position.z = -80;
marquis.position.y = 60;
var mlight = new THREE.PointLight( 0xffffff, 5 , 200);
mlight.castShadow = true;
mlight.receiveShadow = true;
mlight.position.y = 5;
mlight.position.z = -20;
marquis.add(mlight);
boxTop.add(marquis);
frame.add( boxTop );
return frame;
}
function makeMarquis(){
}
function makeGlass(){
glassMaterial = new THREE.MeshPhysicalMaterial({transparent: true});
glassMaterial.opacity = 0.3;
glassMaterial.color.setRGB(70,130,180);
var glassGeo = new THREE.BoxGeometry( 0, 0, 0 );
var glass = new Physijs.BoxMesh( glassGeo, glassMaterial, 0 );
dist = 148;
shapeFB = new THREE.BoxGeometry( 2 , 400 , 300 );
shapeLR = new THREE.BoxGeometry( 300 , 400 , 2 );
panef = new Physijs.BoxMesh( shapeLR, glassMaterial , 0 );
panef.position.z = -dist;
panef.castShadow = true;
panef.recieveShadow = true;
paneb = new Physijs.BoxMesh( shapeLR, glassMaterial , 0 );
paneb.position.z = dist;
paneb.castShadow = true;
paneb.recieveShadow = true;
panel = new Physijs.BoxMesh( shapeFB, glassMaterial , 0 );
panel.position.x = -dist;
panel.castShadow = true;
panel.recieveShadow = true;
paner = new Physijs.BoxMesh( shapeFB, glassMaterial , 0 );
paner.position.x = dist;
paner.castShadow = true;
paner.recieveShadow = true;
glass.add( panef );
glass.add( paneb );
glass.add( panel );
glass.add( paner );
return glass;
}
function makeChute( bodyMaterial ){
var baseMaterial = Physijs.createMaterial(new THREE.MeshPhysicalMaterial({ map: THREE.ImageUtils.loadTexture('src/rust.jpg') }));
var chuteGeo = new THREE.BoxGeometry( 0, 0, 0 );
var chute = new Physijs.BoxMesh( chuteGeo, baseMaterial, 0 );
var sideLR = new THREE.BoxGeometry( 4, 80, 80);
var sideFB = new THREE.BoxGeometry( 80, 80, 4);
var disp = 38;
var front = new Physijs.BoxMesh( sideFB, baseMaterial, 0 );
front.position.z = -disp;
chute.add( front );
var back = new Physijs.BoxMesh( sideFB, baseMaterial, 0 );
back.position.z = disp;
chute.add( back );
var left = new Physijs.BoxMesh( sideLR, baseMaterial, 0 );
left.position.x = -disp;
chute.add( left );
var right = new Physijs.BoxMesh( sideLR, baseMaterial, 0 );
right.position.x = disp;
chute.add( right );
return chute;
}
function makeStands( bodyMaterial ){
var barGeo = new THREE.BoxGeometry( 0, 0, 0 );
var bars = new Physijs.BoxMesh( barGeo, bodyMaterial, 0 );
var barShape = new THREE.BoxGeometry( 25, 400, 25);
disp = 125;
left = -disp;
right = disp;
front = -disp;
back = disp;
bar1 = new Physijs.BoxMesh( barShape , bodyMaterial, 0 );
bar1.position.x = left;
bar1.position.z = front;
bar1.castShadow = true;
bar1.recieveShadow = true;
bar2 = new Physijs.BoxMesh( barShape , bodyMaterial, 0 );
bar2.position.x = right;
bar2.position.z = front;
bar2.castShadow = true;
bar2.recieveShadow = true;
bar3 = new Physijs.BoxMesh( barShape , bodyMaterial, 0 );
bar3.position.x = left;
bar3.position.z = back;
bar3.castShadow = true;
bar3.recieveShadow = true;
bar4 = new Physijs.BoxMesh( barShape , bodyMaterial, 0 );
bar4.position.x = right;
bar4.position.z = back;
bar4.castShadow = true;
bar4.recieveShadow = true;
bars.add( bar1 );
bars.add( bar2 );
bars.add( bar3 );
bars.add( bar4 );
return bars;
}
function makeCrane( bodyMaterial ){
var craneGeo = new THREE.BoxGeometry( 0, 0, 0 );
var crane = new Physijs.BoxMesh( craneGeo, bodyMaterial, 0 );
railShape = new THREE.BoxGeometry( 20, 20, 300 );
railL = new Physijs.BoxMesh(
railShape , bodyMaterial, 0 );
railL.position.x = 125;
railL.position.y = 0;
railL.position.z = 0;
railL.castShadow = true;
railL.recieveShadow = true;
crane.add( railL );
railR = new Physijs.BoxMesh(
railShape , bodyMaterial, 0 );
railR.position.x = -railL.position.x;
railR.position.y = railL.position.y;
railR.position.z = railL.position.z;
railR.castShadow = true;
railR.recieveShadow = true;
crane.add( railR );
railArm = makeRailArm( bodyMaterial, 0 ); // this will move forward and backward
if(!reflection){
updates.push(
function(){
var railRef = railArm;
var max = 100;
if((keyboard.pressed("w") || keyboard.pressed("up")) && railRef.position.z < max && phase == 0){
railRef.position.z += 1;
} else if((keyboard.pressed("s") || keyboard.pressed("down")) && railRef.position.z > -max && phase == 0){
railRef.position.z += -1;
}
if(phase == 5 && railRef.position.z != -75){
if(railRef.position.z > -75){
railRef.position.z += -1;
} else {
railRef.position.z += 1;
}
} else if(phase == 5){
phase = 6;
}
railArm.__dirtyPosition = true;
}
);
}
crane.add( railArm );
return crane;
}
function makeRailArm( bodyMaterial ){
var armGeo = new THREE.BoxGeometry( 0, 0, 0 );
var railArm = new Physijs.BoxMesh( armGeo, bodyMaterial, 0 );
railB = new Physijs.BoxMesh(
new THREE.BoxGeometry( 300 , 20, 20 ), bodyMaterial, 0 );
railB.castShadow = true;
railB.recieveShadow = true;
railArm.add( railB );
hangingArm = makeHangingArm( bodyMaterial, 0 ); // this will move right/left
hangingArm.position.y = -20;
if(!reflection){
updates.push(
function(){
var haRef = hangingArm;
var max = 125;
if((keyboard.pressed("a") || keyboard.pressed("left")) && haRef.position.x < max && phase == 0){
haRef.position.x += 1;
} else if((keyboard.pressed("d") || keyboard.pressed("right")) && haRef.position.x > -max && phase == 0){
haRef.position.x += -1;
}
if(phase == 4 && haRef.position.x != 0){
if(haRef.position.x > 0){
haRef.position.x += -1;
} else {
haRef.position.x += 1;
}
} else if(phase == 4){
phase = 5;
}
hangingArm.__dirtyPosition = true;
}
);
}
railArm.add( hangingArm );
return railArm;
}
function makeHangingArm( bodyMaterial ){
var armGeo = new THREE.BoxGeometry( 0, 0, 0 );
var hangingArm = new Physijs.BoxMesh( armGeo, bodyMaterial, 0 );
var arm = new Physijs.BoxMesh( new THREE.BoxGeometry( 15, 50, 15), bodyMaterial, 0 );
arm.position.y = -12;
arm.castShadow = true;
arm.recieveShadow = true;
hangingArm.add( arm );
var claw = makeClaw( bodyMaterial, 0 );
claw.position.y = -50;
hangingArm.add( claw );
return hangingArm;
}
function makeClaw( bodyMaterial ){
var claw = new THREE.Group();
var palm = new Physijs.BoxMesh( new THREE.BoxGeometry( 30, 30, 30 ), bodyMaterial, 0 );
palm.castShadow = true;
palm.recieveShadow = true;
var pHolder1 = makePhalange( bodyMaterial, 0 );
var pHolder2 = makePhalange( bodyMaterial, 0 );
pHolder2.rotateY(6.29/3);
var pHolder3 = makePhalange( bodyMaterial, 0 );
pHolder3.rotateY(2*6.29/3);
updates.push(
function(){
claw.__dirtyPosition = true;
palm.__dirtyPosition = true;
pHolder1.__dirtyPosition = true;
pHolder2.__dirtyPosition = true;
pHolder3.__dirtyPosition = true;
pHolder1.__dirtyRotation = true;
pHolder2.__dirtyRotation = true;
pHolder3.__dirtyRotation = true;
}
);
claw.add(palm);
claw.add(pHolder1);
claw.add(pHolder2);
claw.add(pHolder3);
return claw;
}
function makePhalange( bodyMaterial ){
var phalGeo = new THREE.BoxGeometry( 0, 0, 0 );
var phalange = new Physijs.BoxMesh( phalGeo, bodyMaterial, 0 );
var upper = new Physijs.BoxMesh( new THREE.BoxGeometry( 5, 30, 5 ), bodyMaterial, 1 );
upper.castShadow = true;
upper.receiveShadow = true;
upper.position.y = -15;
upper.position.z = 15
upper.rotateX(-1.3);
phalange.add(upper);
var lower = new Physijs.BoxMesh( new THREE.BoxGeometry( 5, 30, 5 ), bodyMaterial, 1 );
lower.castShadow = true;
lower.receiveShadow = true;
lower.position.y = -33;
lower.position.z = 30;
if(!reflection){
updates.push(
function(){
lower.__dirtyPosition = true;
lower.__dirtyRotation = true;
}
);
}
phalange.add(lower);
var closing = false;
var releasing = false;
var incrMax = 30;
var incr = 0;
if(!reflection){
updates.push(
function(){
if(phase == 2){
phalange.rotateX((1/incrMax)/2)
incr++;
if(incr >= incrMax){
phase = 3;
}
}
if(phase == 6){
phalange.rotateX((-1/incrMax)/2)
incr--;
if(incr <= 0){
phase = 0;
}
}
lower.__dirtyPosition = true;
}
);
}
return phalange;
}
// Initialization. Define the size of the canvas and store the aspect ratio
// You can change these as well
function init() {
var canvasWidth = window.innerWidth ||
document.documentElement.clientWidth ||
document.body.clientWidth;
var canvasHeight = window.innerHeight ||
document.documentElement.clientHeight ||
document.body.clientHeight; // found in a stack overflow answer by confile
var canvasRatio = canvasWidth / canvasHeight;
// Set up a renderer. This will allow WebGL to make your scene appear
renderer = new THREE.WebGLRenderer( { antialias: true } );
renderer.gammaInput = true;
renderer.gammaOutput = true;
renderer.shadowMap.enabled = true;
renderer.setSize(canvasWidth, canvasHeight);
renderer.setClearColor( 0xAAAAAA, 1.0 );
// You also want a camera. The camera has a default position, but you most likely want to change this.
// You'll also want to allow a viewpoint that is reminiscent of using the machine as described in the pdf
// This might include a different position and/or a different field of view etc.
camera = new THREE.PerspectiveCamera( 45, canvasRatio, 1, 4000 );
// Moving the camera with the mouse is simple enough - so this is provided. However, note that by default,
// the keyboard moves the viewpoint as well
cameraControls = new THREE.OrbitControls(camera, renderer.domElement);
camera.position.set( 800, 600, -1200);
cameraControls.target.set(0,400,0);
keyboard = new KeyboardState();
updates.push(keyboard.update);
updates.push(setCameraAngle);
}
// We want our document object model (a javascript / HTML construct) to include our canvas
// These allow for easy integration of webGL and HTML
function addToDOM() {
var canvas = document.getElementById('canvas');
canvas.appendChild(renderer.domElement);
}
// This is a browser callback for repainting
// Since you might change view, or move things
// We cant to update what appears
function animate() {
update();
window.requestAnimationFrame(animate);
render();
}
viewSet = false;
function update(){
for (i in updates){
updates[i]();
}
}
function setCameraAngle(){
if(keyboard.down("v")){
view = -view;
}
if(view == -1){
camera.position.set( 0, 800, -1200);
cameraControls.target.set(0,400,0);
viewSet = true;
}else if(view == 1 && viewSet){
camera.position.set( 800, 600, -1200);
cameraControls.target.set(0,400,0);
viewSet = false;
}
}
// getDelta comes from THREE.js - this tells how much time passed since this was last called
// This might be useful if time is needed to make things appear smooth, in any animation, or calculation
// The following function stores this, and also renders the scene based on the defined scene and camera
function render() {
var delta = clock.getDelta();
cameraControls.update(delta);
renderer.render(scene, camera);
scene.simulate();
}
// Since we're such talented programmers, we include some exception handeling in case we break something
// a try and catch accomplished this as it often does
// The sequence below includes initialization, filling up the scene, adding this to the DOM, and animating (updating what appears)
try {
init();
fillScene();
addToDOM();
animate();
} catch(error) {
console.log("You did something bordering on utter madness. Error was:");
console.log(error);
}
| 74b5306307c43326d0093c2a71bc6eee740b1315 | [
"JavaScript",
"Text"
] | 4 | JavaScript | Wynand/comp3490 | 12b78d8d047c2f72e827cc964bfa64629c7d098f | 5de3df2f6bb9cc6bfb526d574a0918a34b7d8003 | |
refs/heads/main | <file_sep>using System;
namespace Массивы_3
{
class Program
{
static void Main(string[] args)
{
int[] wc = new int[] { 1, 6, 3, 5, 7, 8, 9 };
Console.WriteLine("Стасик 1");
SnowOne(wc);
int t = SnowOne(wc);
Console.WriteLine(t);
Console.WriteLine("Стасик 2");
SnowTwo(wc);
int h = SnowTwo(wc);
Console.WriteLine(h);
Console.WriteLine("Стасик 3");
SnowFour(wc);
int r = SnowFour(wc);
Console.WriteLine(r);
}
public static int SnowOne(int[] wc)
{
int t = wc[0];
for (int a = 0; a < wc.Length; a++)
{
if (wc[a] > t)
{
t = wc[a];
}
}
return t;
}
public static int SnowTwo(int[] wc)
{
int h = 0;
for (int b = 0; b < wc.Length; b++)
{
if (wc[b] == h)
{
h = wc[b];
}
}
return h;
}
public static int SnowFour(int[] wc)
{
int r = 0;
for (int v = 0; v < wc.Length; v++)
{
r += wc[v];
}
return r;
}
}
}
| 45d23e462f87b99ea1d2e8acfa460d4360199701 | [
"C#"
] | 1 | C# | HornyFerret/practic-3 | fe317d40bdf53b607690eba205a73d8968d60104 | 9627f11510a25d1c04b5e584bea1d9f543345c37 | |
refs/heads/master | <repo_name>csantala/learn<file_sep>/application/views/components/ajax/synopsis_editor.php
<?php date_default_timezone_set($timezone);?>
<script type="text/javascript" src="/js/script.js"></script>
<span class="synopsis">
<div class="rows" data-assignment_id="<?php echo $assignment_id?>" data-step_id="<?php echo $step_id?>" data-session="0">
<table class="ExcelTable2013">
<tr style="color:#bbb">
<!--th></th>
<th>task
<span class="details pull-right">elapsed time: <span id="elapsed_time"></span> </span></th-->
</tr>
<?php
foreach ($rows as $row) { ?>
<tr class="rowx">
<td class="start">
<span data-time="<?php echo $row->time ?>"><?php echo date('g:i:s', $row->time);?></span>
</td>
<td>
<input data-time="<?php echo $row->time ?>" data-step_id="<?php echo $step_id?>" maxlength="300" class="task span10" type="text" data-i="<?php echo $row->position; ?>" <?php if ($row->task != '') { ?> value="<?php echo quotes_to_entities($row->task); ?>"<?php } ?>/>
</td>
</tr>
<?php } ?>
</table>
</div>
</span>
<br /><file_sep>/application/views/dashboard_view.php
<?php
$dashboard_tip = "Bookmark this page for future reference.";
$assignment_tip = "Email or Text this URL to your students.";
$progress_tip = "Student progress appears in this panel. Click a Report link to view a report of the student's synopsis. Report links only appear when a student has submitted their completed work.";
$student_tip = "Click name for student worksheet.";
?>
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>Dashboard</title>
<!-- Meta -->
<meta charset="UTF-8" />
<meta http-equiv="refresh" content="120">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<!--[if IE]><meta http-equiv='X-UA-Compatible' content='IE=edge,chrome=1'><![endif]-->
<!-- Bootstrap -->
<link href="/common/bootstrap/css/bootstrap.css" rel="stylesheet" />
<link href="/common/bootstrap/css/responsive.css" rel="stylesheet" />
<script type="application/javascript" src="/js/wysihtml5-0.3.0_rc2.js" ></script>
<script type="application/javascript" src="/js/jquery-1.7.1.min.js"></script>
<script type="application/javascript" src="/js/bootstrap.min.js"></script>
<script type="application/javascript" src="/js/bootstrap-wysihtml5.js"></script>
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script src="/common/theme/scripts/plugins/system/less.min.js"></script>
<script src="/js/bookmark.js"></script>
<script src="/js/learn.js"></script>
</head>
<body data-dashboard_id="<?php echo $dashboard_id;?>">
<h4 id="assignment_header"></h4>
<h5><?php echo $course . " " . $instructor;?></h5>
<br>
<h5>ASSIGNMENT URL • <a href="#" data-toggle="tooltip" title="" data-placement="bottom" data-original-title="<?php echo $assignment_tip;?>">?</a></h5>
<p>
<input onclick="select()" class="span9" style="color:#008000" type="text" value="<?php echo $assignment_url;?>">
</p>
<h5>OBJECTIVE</h5>
<p>
<textarea style="vertical-align: middle; color:#000;" tabindex="1" class="span9 objective" name="objective"><?php echo $objective;?></textarea>
<a id="upd_obj" class="label add_synopsis" data-dashboard_id="<?php echo $dashboard_id;?>">update</a>
</p>
<?php
if (count($steps) > 0) { ?>
<h5>STEPS</h5>
<?php } ?>
<?php
$i = 1;
foreach ($steps as $step) { ?>
<p>
<?php echo $i; $i++;?>. <input style="vertical-align: middle" class="span9 step<?php echo $step->id;?>" type="text" name="step<?php echo $step->id;?>" data-step_id="step<?php echo $step->id;?>" value="<?php echo quotes_to_entities($step->step);?>" >
<a class="upd_step label add_synopsis <?php echo $step->id;?>" data-step="<?php echo $step->id;?>">update</a>
</p>
<?php }
?>
<br>
<!--h4 id="progress">Progress • <a href="#" data-toggle="tooltip" title="" data-original-title="<?php echo $progress_tip;?>">?</a></h4-->
<table id="scanner_data" class="dynamicTable table table-striped table-bordered table-condensed dataTable">
<tr>
<th>Worksheet • <a href="#" data-toggle="tooltip" title="" data-placement="top" data-original-title="<?php echo $student_tip;?>">?</a></th>
<th>Elapsed Time</th>
<th>Status</th>
<th>Grade</th>
</tr>
<?php
if (! empty($scanner_data)) {
foreach($scanner_data as $data) { //ds($data,1);?>
<tr>
<td><a target="_blank" href="/home/<?php echo $data->assignment_id;?>/<?php echo $data->synopsis_id;?>"><?php echo $data->student_name;?></a></td>
<td><?php echo $data->elapsed_time;?></td>
<td><?php echo $data->status;?></td>
<td><?php echo $data->mark;?></td>
<td><a target="_blank" href="/home/<?php echo $data->assignment_id;?>/<?php echo $data->synopsis_id;?>/<?php echo $dashboard_id;?>">mark assignment</a></td>
<!--td><?php if ($data->report_url != '') { ?><a href="<?php echo $data->report_url;?>">Report</a><?php } else { echo "-----"; }?></td-->
</tr>
<?php } ?>
<?php } else { ?>
<?php for ($i = 0; $i < 4; $i++) { ?>
<tr>
<td class="scanner_cell" colspan="4"> </td>
</tr>
<?php } ?>
<?php } ?>
</table>
<hr>
<div id="getlost">
<?php $this->load->view('/components/footer') ?>
</div>
<script src="/common/bootstrap/js/bootstrap.min.js"></script>
<script src="/common/theme/scripts/demo/common.js?1384198042"></script>
<!--script src="//ajax.googleapis.com/ajax/libs/jqueryui/1.10.3/jquery-ui.min.js"></script -->
</body>
</html><file_sep>/readme.md
LEARN 1.0 Copyright 2013-2014 by <NAME>
This is an experiment to aid the human learning process through the breakdown of an object into multiple steps with student/teacher interaction available at each step. The system allows for an assignment to be created and then completed by students with their progress available at the assignment dashboard. As this is a hashed based system, user accounts are not required.
<file_sep>/application/views/submitted_view.php
<?php // date_default_timezone_set($timezone); ?>
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>Assignment</title>
<meta name="description" content="Assignment Objectives and URL">
<!-- Meta -->
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<!--[if IE]><meta http-equiv='X-UA-Compatible' content='IE=edge,chrome=1'><![endif]-->
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script src="/common/theme/scripts/plugins/system/less.min.js"></script>
</head>
<body>
<!-- Content -->
<div id="contentx">
<div class="innerLR innerT">
<h4>Your worksheet has been submitted to your instructor.</h4>
<h5>Worksheet permalink: <a href="<?php echo $worksheet_url;?>"><?php echo $worksheet_url;?></a></h5>
</div>
</div>
</body>
</html><file_sep>/application/controllers/home.php
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Home extends CI_Controller {
public function __construct() {
parent::__construct();
}
public function index() {
$this->load->library('typography');
$objective = '';
$assignment_id = $this->uri->segment(2);
$synopsis_id = $this->uri->segment(3);
$dashboard_id = $this->uri->segment(4);
if ($assignment_id) {
$assignment = $this->Objectives_model->get_assignment($assignment_id);
// verify dashboard id
if ($dashboard_id == $assignment->dashboard_id) { $is_instructor = true; } else { $is_instructor = false; }
if ($assignment == '') { show_404(); }
$student_meta = $this->Synopsis_model->get_student($synopsis_id);
$steps = $this->Step_model->get_steps_with_notes($assignment_id, $synopsis_id);
// get synopsis for steps
$rows = array();
foreach ($steps as $step) {
$rows[] = $this->Task_model->tasks($assignment_id,$step->id, $synopsis_id);
}
// get marked meta if any
$data = compact('assignment_id', 'synopsis_id');
$marked_meta = $this->Synopsis_model->get_mark($data);
$view_data = array(
'rows' => $rows,
'marked_meta' => $marked_meta,
'is_instructor' => $is_instructor,
'dashboard_id' => $dashboard_id,
'course' => $assignment->course,
'instructor' => $assignment->instructor,
'objective' => $assignment->objective,
'assignment_id' => $assignment_id,
'assignment_hash' => $assignment_id,
'steps' => $steps,
'date' => time(), //$rows[0]->time,
'synopsis_id' => $synopsis_id,
'assignment_hash' => $assignment_id,
'session' => null,
'project_id' => $assignment_id,
'timezone' => $student_meta->timezone,
'student_name' => $student_meta->student_name
);
//$this->load->view('editor_view', $view_data);
$this->load->view('worksheet_view', $view_data);
} else {
show_404();
// TODO: generate proper id
$assignment_id = time();
$view_data = array(
'assignment_id' => $assignment_id,
'objective' => $objective
);
$this->load->view('home_view', $view_data);
}
}
public function write_note() {
$this->Note_model->write_note($_POST);
}
public function edit_objective() {
$this->load->view('/components/ajax/edit_objective', $_POST);
}
public function update_objective() {
// update this objective in db
$data = array(
'project_id' => $_POST['project_id'],
'objective' => $_POST['objective']
);
$this->Objectives_model->update_objective($data);
$this->load->view('/components/ajax/updated_objective', $_POST);
}
// synopsis editor ajax
public function load_editor() {
$rows = $this->Task_model->tasks($_POST['assignment_id'],$_POST['step_id'],$_POST['synopsis_id']);
// create new synopses if !$rows
if (empty($rows)) {
$rows[] = (object)array(
'step_id' => $_POST['step_id'],
'assignment_id' => $_POST['assignment_id'],
'synopsis_id' => $_POST['synopsis_id'],
'position' => 1,
'session' => time(),
'time' => time(),
'task' => ''
);
}
$view_vars = array(
'assignment_id' => $_POST['assignment_id'],
'synopsis_id' => $_POST['synopsis_id'],
'step_id' => $_POST['step_id'],
'rows' => $rows,
'timezone' => $_POST['timezone']
);
$this->load->view('/components/ajax/synopsis_editor', $view_vars);
}
}<file_sep>/application/models/note_model.php
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Note_model extends CI_Model {
public function get_notes($assignment_id, $synopsis_id) {
$this->db->where('assignment_id', $assignment_id);
$this->db->where('synopsis_id', $synopsis_id);
$query = $this->db->get('note');
$data = $query->result();
if (! empty($data[0])) { return $data[0]; }
return array();
}
public function write_note($data) {
$query = $this->db->get_where('note', array(
'step_id' => $data['step_id'],
'assignment_id' => $data['assignment_id'],
'synopsis_id' => $data['synopsis_id']
));
// update if present, otherwise create a new row if unique ($objective_id)
if ($query->num_rows() > 0) {
$this->db->where('step_id', $data['step_id']);
$this->db->where('assignment_id', $data['assignment_id']);
$this->db->where('synopsis_id', $data['synopsis_id']);
$this->db->update('note', $data);
} else {
$this->db->insert('note', $data);
}
}
public function update_notes($post) {
foreach ($post as $name => $note) {
if (is_int($name)) {
$data = array(
'assignment_id' => $post['aid'],
'synopsis_id' => $post['pid'],
'step_id' => $name,
'note' => $note
);
$query = $this->db->get_where('note', array(
'step_id' => $name,
'assignment_id' => $post['aid'],
'synopsis_id' => $post['pid']
));
// update if present, otherwise create a new row if unique ($objective_id)
if ($query->num_rows() > 0) {
error_log('update!' . $name);
$this->db->where('step_id', $name);
$this->db->where('assignment_id', $data['assignment_id']);
$this->db->where('synopsis_id', $data['synopsis_id']);
$this->db->update('note', $data);
} else {error_log('write!');
$this->db->insert('note', $data);
}
}
}
}
}<file_sep>/misc/sql.php
-- phpMyAdmin SQL Dump
-- version 4.0.9
-- http://www.phpmyadmin.net
--
-- Host: localhost
-- Generation Time: Jan 30, 2014 at 07:45 AM
-- Server version: 5.5.15
-- PHP Version: 5.4.17
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET time_zone = "+00:00";
--
-- Database: `learn`
--
-- --------------------------------------------------------
--
-- Table structure for table `comments`
--
CREATE TABLE IF NOT EXISTS `comments` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user` varchar(25) NOT NULL,
`comments_container_id` varchar(20) NOT NULL,
`branch` int(11) NOT NULL DEFAULT '0',
`comment` blob NOT NULL,
`date` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
-- --------------------------------------------------------
--
-- Table structure for table `content`
--
CREATE TABLE IF NOT EXISTS `content` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`date` int(11) NOT NULL,
`content_id` varchar(32) NOT NULL,
`content` blob NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=4 ;
-- --------------------------------------------------------
--
-- Table structure for table `note`
--
CREATE TABLE IF NOT EXISTS `note` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`note` blob NOT NULL,
`assignment_id` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`date` int(11) NOT NULL,
`synopsis_id` varchar(20) COLLATE utf32_unicode_ci NOT NULL,
`step_id` int(11) NOT NULL,
`status` enum('open','closed') COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=13 ;
-- --------------------------------------------------------
--
-- Table structure for table `objectives`
--
CREATE TABLE IF NOT EXISTS `objectives` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`time` int(11) NOT NULL,
`objective` varchar(300) COLLATE utf32_unicode_ci NOT NULL,
`dashboard_id` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`user_id` int(11) NOT NULL,
`status` enum('open','closed') COLLATE utf32_unicode_ci NOT NULL DEFAULT 'open',
`steps` blob NOT NULL,
`teacher_email` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`assignment_id` varchar(200) COLLATE utf32_unicode_ci NOT NULL,
`instructor` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`course` varchar(200) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=36 ;
-- --------------------------------------------------------
--
-- Table structure for table `project`
--
CREATE TABLE IF NOT EXISTS `project` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL,
`project_id` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`date` int(10) NOT NULL,
`title` text COLLATE utf32_unicode_ci NOT NULL,
`description` blob NOT NULL,
`github_link` varchar(1024) COLLATE utf32_unicode_ci NOT NULL,
`local_link` varchar(300) COLLATE utf32_unicode_ci NOT NULL,
`staging_link` varchar(300) COLLATE utf32_unicode_ci NOT NULL,
`production_link` varchar(300) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=1 ;
-- --------------------------------------------------------
--
-- Table structure for table `report`
--
CREATE TABLE IF NOT EXISTS `report` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL,
`hash` varchar(20) COLLATE utf32_unicode_ci NOT NULL,
`project_id` varchar(200) COLLATE utf32_unicode_ci NOT NULL,
`project_title` varchar(200) COLLATE utf32_unicode_ci NOT NULL,
`elapsed_time` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`timezone` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`assignment_hash` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`student_name` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=28 ;
-- --------------------------------------------------------
--
-- Table structure for table `statistics`
--
CREATE TABLE IF NOT EXISTS `statistics` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`assignment_hash` varchar(40) COLLATE utf8_unicode_ci NOT NULL,
`synopsis_hash` varchar(40) COLLATE utf8_unicode_ci NOT NULL,
`tasks` int(11) NOT NULL,
`elapsed_time` int(11) NOT NULL,
`link` varchar(100) COLLATE utf8_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci AUTO_INCREMENT=1 ;
-- --------------------------------------------------------
--
-- Table structure for table `steps`
--
CREATE TABLE IF NOT EXISTS `steps` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`step` varchar(500) COLLATE utf32_unicode_ci NOT NULL,
`date` int(11) NOT NULL,
`dashboard_id` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`assignment_id` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=37 ;
-- --------------------------------------------------------
--
-- Table structure for table `synopsis`
--
CREATE TABLE IF NOT EXISTS `synopsis` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`assignment_id` varchar(11) COLLATE utf32_unicode_ci NOT NULL,
`synopsis_id` varchar(11) COLLATE utf32_unicode_ci NOT NULL,
`elapsed_time` varchar(100) CHARACTER SET utf8 COLLATE utf8_unicode_ci NOT NULL,
`status` enum('in_progress','submitted','returned','completed') CHARACTER SET utf8 COLLATE utf8_unicode_ci NOT NULL DEFAULT 'in_progress',
`student_name` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`report_url` varchar(200) COLLATE utf32_unicode_ci NOT NULL,
`timezone` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
`mark` varchar(2) COLLATE utf32_unicode_ci NOT NULL,
`comments` blob NOT NULL,
`dashboard_id` varchar(20) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=41 ;
-- --------------------------------------------------------
--
-- Table structure for table `task`
--
CREATE TABLE IF NOT EXISTS `task` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`assignment_id` varchar(50) COLLATE utf32_unicode_ci NOT NULL,
`synopsis_id` varchar(40) COLLATE utf32_unicode_ci NOT NULL,
`step_id` varchar(20) COLLATE utf32_unicode_ci NOT NULL,
`session` int(10) NOT NULL,
`time` int(10) NOT NULL,
`position` int(11) NOT NULL,
`task` varchar(100) COLLATE utf32_unicode_ci NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf32 COLLATE=utf32_unicode_ci AUTO_INCREMENT=21 ;
-- --------------------------------------------------------
--
-- Table structure for table `tracker`
--
CREATE TABLE IF NOT EXISTS `tracker` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`dt` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`referer` varchar(255) NOT NULL DEFAULT '',
`referer_is_local` tinyint(4) NOT NULL DEFAULT '0',
`url` varchar(255) NOT NULL DEFAULT '',
`page_title` varchar(255) NOT NULL DEFAULT '',
`search_terms` varchar(255) NOT NULL DEFAULT '',
`img_search` tinyint(4) NOT NULL DEFAULT '0',
`browser_family` varchar(255) NOT NULL DEFAULT '',
`browser_version` varchar(15) NOT NULL DEFAULT '',
`os` varchar(255) NOT NULL DEFAULT '',
`os_version` varchar(255) NOT NULL DEFAULT '',
`ip` varchar(15) NOT NULL DEFAULT '',
`user_agent` varchar(255) NOT NULL DEFAULT '',
`exec_time` float NOT NULL DEFAULT '0',
`num_queries` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=2590 ;
-- --------------------------------------------------------
--
-- Table structure for table `users`
--
CREATE TABLE IF NOT EXISTS `users` (
`user_id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`user_email` varchar(255) NOT NULL DEFAULT '',
`user_pass` varchar(60) NOT NULL DEFAULT '',
`user_date` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`user_modified` datetime NOT NULL DEFAULT '0000-00-00 00:00:00',
`user_last_login` datetime DEFAULT NULL,
`user_hash` varchar(255) DEFAULT NULL,
`user_level` enum('user','administrator') NOT NULL DEFAULT 'user',
`user_timezone` varchar(50) NOT NULL,
`user_name` varchar(50) NOT NULL,
`remember` varchar(20) NOT NULL,
`product` enum('half','full') NOT NULL DEFAULT 'half',
PRIMARY KEY (`user_id`),
UNIQUE KEY `user_email` (`user_email`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=1 ;
<file_sep>/application/views/whatis_view.php
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>LEARN</title>
<!-- Meta -->
<meta charset="UTF-8" />
<meta name="description" content="Instructor driven student assignment system.">
<meta name="keywords" content="learning aid, assignment generator">
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<meta http-equiv="X-UA-Compatible" content="IE=9; IE=8; IE=7; IE=EDGE" />
<!-- Excel-like css -->
<link href="/css/excel-2007.css" rel="stylesheet" type="text/css" />
<!-- Bootstrap -->
<link href="/common/bootstrap/css/bootstrap.css" rel="stylesheet" />
<link href="/common/bootstrap/css/responsive.css" rel="stylesheet" />
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- Excel-like css -->
<link href="/css/excel-2007.css" rel="stylesheet" type="text/css" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script src="/common/theme/scripts/plugins/system/less.min.js"></script>
</head>
<body class="">
<!-- Content -->
<div id="contentx">
<div class="innerLR innerT">
<div class="widget">
<div class="widget-head">
<h4 class="heading edit_objective">LEARN</h4>
<span class="details pull-right">
<a href="<?php echo site_url();?>create">Create An Assignment</a>
</span>
</div>
<div class="widget-body">
<div class="row-fluid">
<h5>What is this?</h5>
<p>This is a system which manages the creation, completion, and marking of an assignment conducted by an instructor with any number of students.<p>
<h5>Usage</h5>
<p><a href="<?php echo site_url();?>create">Create an assignment</a>, send its Assignment URL to your students, and then monitor their work from your dashboard.</p>
<p>The Assignment URL will provide each student with a unique worksheet of which you may monitor and mark.</p>
<h5>Demo</h5>
<p>Example A: <a href="http://learn.ablitica.com/dashboard/yd7dJ9l/Y8v8rQj">Instructor Dashboard</a></p>
<p>Example B: <a href="http://learn.ablitica.com/assignment/Y8v8rQj" target="_blank">Assigment URL for Students</a></p>
<p>Example C: <a href="http://learn.ablitica.com/home/Y8v8rQj/27w73YD" target="_blank">Student Worksheet</a></p>
</div>
</div>
</div>
</div>
<!-- // Content END -->
</div>
<?php $this->load->view('/components/footer') ?>
<?php $this->load->view('/components/themer') ?>
<?php $this->load->view('/components/js_includes') ?>
</body>
</html><file_sep>/application/views/create_view.php
<?php
$objective_tip = "Enter the main objective of this assignment here (required field).";
$step_tip = "Enter the steps for the student to complete. Students will checkmark each step upon completion.";
$email_tip = "Receive email notifications when when a student has submitted a report (not requried).";
?>
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>Create Assignment</title>
<!-- Meta -->
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<!--[if IE]><meta http-equiv='X-UA-Compatible' content='IE=edge,chrome=1'><![endif]-->
<!-- Bootstrap -->
<link href="/common/bootstrap/css/bootstrap.css" rel="stylesheet" />
<link href="/common/bootstrap/css/responsive.css" rel="stylesheet" />
<!-- Bootstrap Extended -->
<script src="/js/wysihtml5-0.3.0_rc2.js"></script>
<script src="/js/jquery-1.7.1.min.js"></script>
<script src="/js/bootstrap.min.js"></script>
<script src="/js/bootstrap-wysihtml5.js"></script>
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script src="/common/theme/scripts/plugins/system/less.min.js"></script>
<!-- Form validation -->
<script type="text/javascript" src="/js/jquery-1.10.2.min.js"></script>
<script type="text/javascript" src="/js/jquery-ui.js"></script>
<script type="text/javascript" src="/js/jquery.form.min.js"></script>
<script type="text/javascript" src="/js/jquery-validate.js"></script>
<script type="text/javascript" src="/js/learn.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('.objective').focus();
$('#submit').click(function() {
$('#assignment_form').submit();
});
$('#assignment_form').validate({
rules: {
objective: {
required: true
},
step1: {
// required: true,
required: true
},
},
submitHandler: function (form) {
form.submit()
}
});
});
</script>
</head>
<body>
<h4 id="assignment_header">LEARN 1.0</h4>
<br>
<form id="assignment_form" action="/create" method="post">
<p><input tabindex="1" type="text" class="span8 course" placeholder="course" name="course"></p>
<p><input tabindex="2" type="text" class="span8 instructor" placeholder="instructor" name="instructor"></p>
<h5>OBJECTIVE • <a href="#" data-toggle="tooltip" title="" data-original-title="<?php echo $objective_tip;?>">?</a></h5>
<textarea tabindex="3" class="span8 objective" style="color:#000" name="objective"></textarea>
<div id="objectives">
<h5>STEPS • <a href="#" data-toggle="tooltip" title="" data-original-title="<?php echo $step_tip;?>">?</a></h5>
<?php for ($i = 1; $i<=5; $i++) {?>
<p><?php echo $i;?>. <input tabindex="<?php echo $i + 3; ?>" data-i="<?php echo $i;?>" name="step<?php echo $i;?>" class="span8 rowx" type="text" value=""></p>
<?php } ?>
<button class="btn add_button">Add Step</button>
</div>
<br>
<p>
<a tabindex="100" id="submit" class="btn btn-icon btn-primary glyphicons parents"><i></i>Create Assignment</a>
</p>
</form>
<br>
<hr>
<div id="getlost">
<?php $this->load->view('/components/footer') ?>
</div>
<!-- Bootstrap -->
<script src="/common/bootstrap/js/bootstrap.min.js"></script>
</body>
</html><file_sep>/application/controllers/create.php
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Create extends CI_Controller {
public function __construct() {
parent::__construct();
}
public function index() {
$stats = $this->tracker_lib->track('create assignment');
$this->db->insert('tracker', $stats);
$dashboard_id = hashids_encrypt(time());
$assignment_id = hashids_encrypt(time() + rand(0,10000));
if (! empty($_POST)) {
$instructor = $_POST['instructor'];
$course = $_POST['course'];
$teacher_email = '<EMAIL>'; // $_POST['teacher_email'];
$objective = $_POST['objective'];
// bundle steps
$steps = array();
foreach($_POST as $name => $step) {
if ($name == 'objective' ||
$name == 'course' ||
$name == 'instructor') {
//$steps[] = $step;
} else { $steps[] = $step; }
}
// write steps to db
foreach($steps as $step) {
$data = compact('dashboard_id', 'assignment_id', 'step');
$this->Step_model->write_step($data);
}
$data = array(
'time' => time(),
'dashboard_id' => $dashboard_id,
'objective' => $objective,
'steps' => '',
'teacher_email' => $teacher_email,
'assignment_id' => $assignment_id,
'course' => $course,
'instructor' => $instructor
);
// write objective and hash to db
$this->Objectives_model->update_objective($data);
$data += array(
'assignment_url' => 'a url',
'synopsis_url' => 's url'
);
redirect(site_url() . 'dashboard/' . $dashboard_id . '/' . $assignment_id);
} else {
$this->load->view('create_view');
}
}
public function update_objective() {
if (! empty($_POST)) {
$this->Objectives_model->update_objective($_POST);
}
}
public function update_step() {
if (! empty($_POST)) {
$this->Step_model->update_step($_POST);
}
}
public function begin() {
$data = array(
'student_name' => $_POST['student_name'],
'assignment_id' => $_POST['assignment_id'],
'synopsis_id' => $_POST['synopsis_id'],
'timezone' => $_POST['timezone']
);
$this->Synopsis_model->label_synopsis($data);
redirect($_POST['synopsis_url']);
}
}<file_sep>/application/models/step_model.php
<?php if ( ! defined('BASEPATH')) exit('No direct script access allowed');
class Step_model extends CI_Model {
public function write_step($step) {
//ds($step,1);
if ($step['step'] != '') {
$this->db->insert('steps', $step);
}
}
public function get_steps($assignment_hash) {
$this->db->where('assignment_id', $assignment_hash);
$query = $this->db->get('steps');
$data = $query->result();
if (empty($data)) { return null; }
else { return $data; }
}
public function get_steps_with_notes($assignment_id, $synopsis_id) {
$this->db->select('*');
$this->db->where('steps.assignment_id', $assignment_id);
$this->db->from('note');
$this->db->join('steps', "note.step_id = steps.id AND note.synopsis_id = '{$synopsis_id}'", 'right');
$query = $this->db->get();
$result = $query->result();
// ds($result);
return $result;
}
public function update_step($post) {
$data = array(
'dashboard_id' => $post['dashboard_id'],
'step' => $post['step']
);
error_log($post['step']);
$this->db->where('dashboard_id', $post['dashboard_id']);
$this->db->where('id', $post['step_id']);
$this->db->update('steps', $data);
}
}<file_sep>/application/views/worksheet_view.php
<?php
date_default_timezone_set($timezone);
$synopsis_tip = "Log each task you take when working on steps. Checkmark the step when finished.";
$steps_tip = "Click 'log tasks' to log your work. Click the green checkbox when complete.";
?>
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>Worksheet</title>
<!-- Meta -->
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<!--[if IE]><meta http-equiv='X-UA-Compatible' content='IE=edge,chrome=1'><![endif]-->
<!-- Bootstrap -->
<link href="/common/bootstrap/css/bootstrap.css" rel="stylesheet" />
<link href="/common/bootstrap/css/responsive.css" rel="stylesheet" />
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script src="/common/theme/scripts/plugins/system/less.min.js"></script>
</head>
<body data-timezone="<?php echo $timezone;?>" data-synopsis_id="<?php echo $synopsis_id;?>">
<h4 id="assignment_header"></h4>
<h5><?php echo $course . " " . $instructor;?></h5>
<table>
<tr>
<td>
<div id="student_name_container">
<h5>STUDENT • <a href="#" data-toggle="tooltip" title="" data-placement="bottom" data-original-title="<?php echo $synopsis_tip;?>">?</a></h5>
<p>
<input id="student_name" value=" <?php echo $student_name; ?>" name="student_name" class="span3 student_name" type="text" readonly style="color:#000000" />
</p>
</div>
</td>
<td>
<div id="marked_meta">
<?php
if(! empty($marked_meta)) { ?>
<h3><?php echo $marked_meta->mark; ?></h3>
<h4><?php echo $marked_meta->comments;?></h4>
<?php } ?>
</div>
</td>
</tr>
</table>
<br>
<form id="begin" action="/generate/generate_report" method="post" data-sid="<?php echo $synopsis_id;?>" data-aid="<?php echo $assignment_id;?>">
<h5>OBJECTIVE</h5>
<p><textarea tabindex="1" class="span12 objective" name="objective" style="color:#000" readonly><?php echo $objective;?></textarea></p>
<h5>STEPS • <a href="#" data-toggle="tooltip" title="" data-placement="bottom" data-original-title="<?php echo $steps_tip;?>">?</a></h5>
<table>
<?php $s=1; foreach ($steps as $step) { ?>
<?php
// so this renders the steps with the notes from the join with css and readonly params set depending on step status
// step panels CSS and text area properties are controlled through js
$status = 'check'; $visual = "step_panel_sel"; $readonly = null;
if ($step->status == 'open' || $step->status == '') { $status = 'unchecked'; $visual="step_panel_unsel"; } else { $status = 'check'; $readonly = 'readonly';}
?>
<tr class="step_panel<?php echo $step->id;?> <?php echo $visual;?>">
<td class="step_number<?php echo $step->id;?> row<?php echo $step->id;?>">
<?php echo $s;?>.
</td>
<td>
<input class="span11 steps" type="text" readonly value="<?php echo quotes_to_entities($step->step);?>" style="color:#000;" />
</td>
<td class="checkbox c<?php echo $step->id;?>">
<a class="glyphicons <?php echo $status;?> begin begin<?php echo $step->id;?>" data-s="<?php echo $s;?>" data-step_id="<?php echo $step->id;?>" data-status="<?php echo $step->status;?>"><i></i></a>
</td>
</tr>
<tr class="step_panel<?php echo $step->id;?> <?php echo $visual;?>">
<td>
</td>
<td colspan="2">
<div class="synopsis" data-assignment_id="<?php echo $assignment_id;?>" data-step_id="<?php echo $step->id;?>">
<table>
<?php
if (count($rows[$s-1]) > 0) {
foreach ($rows[$s-1] as $row) { ?>
<?php if ($row->task != '') { ?>
<tr class="rowx">
<td class="start">
<span data-time="<?php echo $row->time ?>"><?php echo date('g:i:s', $row->time);?></span>
</td>
<td>
<?php echo quotes_to_entities($row->task); ?>
</td>
</tr>
<?php } ?>
<?php } ?>
<?php } else { ?>
<tr class="rowx synopsis">
<td class="start">
<span></span>
</td>
<td>
<i class="cta">click to log tasks</i>
</td>
</tr>
<?php } ?>
</table>
<br>
</div>
</td>
</tr>
<tr><td colspan="2"> </td>
<?php $s++; }?>
</table>
<div class="span12">
<div id="done">
<input type="hidden" name="pid" value="<?php echo $synopsis_id;?>">
<input type="hidden" name="aid" value="<?php echo $assignment_id;?>">
<?php if ($is_instructor) {?>
<!--input type="submit" class="btn btn-block btn-success confirm" value=" UPDATE ASSIGNMENT "-->
<?php } else { ?>
<input type="submit" class="btn btn-block btn-success" value=" SUBMIT ASSIGNMENT ">
<?php } ?>
</div>
</div>
</form>
<?php if ($is_instructor) {?>
<div class="clearfix"></div>
<div class="span12">
<form action="/generate/mark" method="post">
<p><input type="text" placeholder="grade" name="mark" class="span1" size="2" value="<?php echo $marked_meta->mark; ?>"></p>
<p><textarea placeholder="comments" name="comments" class="span12"><?php echo $marked_meta->comments;?></textarea></p>
<input type="hidden" name="dashboard_id" value="<?php echo $dashboard_id;?>">
<input type="hidden" name="assignment_id" value="<?php echo $assignment_id;?>">
<input type="hidden" name="synopsis_id" value="<?php echo $synopsis_id;?>">
<p><input type="submit" class="btn btn-block btn-success span12" value=" MARK ASSIGNMENT "></p>
</form>
</div>
<?php } ?>
<br /><br />
<?php $this->load->view('/components/js_includes') ?>
<script src="/common/bootstrap/js/bootstrap.min.js"></script>
</body>
</html><file_sep>/application/views/assignment_view.php
<!DOCTYPE html>
<!--[if lt IE 7]> <html class="ie lt-ie9 lt-ie8 lt-ie7"> <![endif]-->
<!--[if IE 7]> <html class="ie lt-ie9 lt-ie8"> <![endif]-->
<!--[if IE 8]> <html class="ie lt-ie9"> <![endif]-->
<!--[if gt IE 8]> <html class="ie gt-ie8"> <![endif]-->
<!--[if !IE]><!--><html><!-- <![endif]-->
<head>
<title>Assignment: <?php echo $objective;?></title>
<!-- Meta -->
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, user-scalable=0, minimum-scale=1.0, maximum-scale=1.0">
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="black">
<!--[if IE]><meta http-equiv='X-UA-Compatible' content='IE=edge,chrome=1'><![endif]-->
<!-- Bootstrap -->
<link href="/common/bootstrap/css/bootstrap.css" rel="stylesheet" />
<link href="/common/bootstrap/css/responsive.css" rel="stylesheet" />
<script src="/js/wysihtml5-0.3.0_rc2.js"></script>
<script src="/js/jquery-1.7.1.min.js"></script>
<script src="/js/bootstrap.min.js"></script>
<script src="/js/bootstrap-wysihtml5.js"></script>
<!-- Glyphicons Font Icons -->
<link href="/common/theme/css/glyphicons.css" rel="stylesheet" />
<!-- Uniform Pretty Checkboxes -->
<link href="/common/theme/scripts/plugins/forms/pixelmatrix-uniform/css/uniform.default.css" rel="stylesheet" />
<!-- Main Theme Stylesheet :: CSS -->
<link href="/common/theme/css/style-light.css?1369753444" rel="stylesheet" />
<!-- General css -->
<link href="/css/style.css" rel="stylesheet" type="text/css" />
<!-- LESS.js Library -->
<script type="text/javascript" src="/common/theme/scripts/plugins/system/less.min.js"></script>
<script src="/js/jstz.min.js"></script>
<script type="text/javascript" src="/js/jquery-1.10.2.min.js"></script>
<script>
$(document).ready(function() {
$("#student_name").focus();
var timezone = jstz.determine();
$("#timezone").val(timezone.name());
//$.cookie('timezone', timezone.name());
});
</script>
</head>
<body>
<h4 id="assignment_header"></h4>
<h5><?php echo $course . " " . $instructor;?></h5>
<br>
<form id="begin" action="/create/begin" method="post">
<h5>STUDENT NAME</h5>
<p>
<input tabindex="1" id="student_name" name="student_name" class="span3" type="text" style="color:#000000" />
</p>
<h5>OBJECTIVE</h5>
<p><textarea tabindex="1" class="span9 objective" name="objective" style="color:#000" readonly><?php echo $objective;?></textarea></p>
<h5>STEPS</h5>
<?php foreach ($steps as $step) { ?>
<p><input class="span9 step" tabindex="3" type="text" readonly value="<?php echo quotes_to_entities($step->step);?>" /></p>
<?php } ?>
<input type="hidden" value="<?php echo $synopsis_url?>" name="synopsis_url">
<input type="hidden" value="<?php echo $synopsis_id;?>" name="synopsis_id">
<input type="hidden" value="<?php echo $assignment_id;?>" name="assignment_id">
<input type="hidden" id="timezone" name="timezone">
<br>
<p><button tabindex="2" class="btn btn-icon btn-primary glyphicons lightbulb" type="submit" value="Assign"><i></i>Begin</button></p>
</form>
<hr>
<div id="x">
<?php //$this->load->view('/components/footer') ?>
</div>
<?php $this->load->view('/components/js_includes') ?>
<script src="/common/bootstrap/js/bootstrap.min.js"></script>
<script src="/common/theme/scripts/demo/common.js?1384198042"></script>
<script type="text/javascript" src="/js/jquery.cookie.js"></script>
</body>
</html> | eafe9f72a79a1576d01119b132f923eefd236199 | [
"Markdown",
"PHP"
] | 13 | PHP | csantala/learn | 470c04981d458c6223e8d86fbd32177f54836ac9 | 9493338e7a38d2250f2681ad84082a439632263d | |
refs/heads/master | <file_sep>package net.jorhlok.shootyshoot
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
/**
* Created by joshm on 6/15/2017.
*/
class Door(MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
companion object {val TileNum = 33; val TypeName = "Door"}
init {
Type = Door.TypeName
CollEntities = true
CollEntWhite = emptySet()
AABB.set(0f,0f,1f,1f)
}
override fun draw(deltatime: Float) {
MGR.drawRgb("_door",0f,Position.x+0.5f,Position.y+0.5f)
}
}<file_sep>package net.jorhlok.shootyshoot
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.Entity
/**
* Created by joshm on 6/15/2017.
*/
abstract class SSEntity(
var MGR: MultiGfxRegister,
var MAR: MultiAudioRegister) : Entity() {
companion object {
open fun getId() = 0
}
}<file_sep>package net.jorhlok.shootyshoot
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
/**
* Created by joshm on 6/15/2017.
*/
class Pedestal(MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
companion object {val TileNum = 34}
override fun draw(deltatime: Float) {
MGR.drawRgb("_pedestal",0f,Position.x+0.5f,Position.y+0.5f)
}
}<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.Gdx
import com.badlogic.gdx.Input
import com.badlogic.gdx.graphics.OrthographicCamera
import com.badlogic.gdx.maps.tiled.TiledMapTileLayer
import com.badlogic.gdx.math.Rectangle
import net.jorhlok.multiav.MTrack
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.DungeonMaster
import net.jorhlok.oops.LabelledObject
class TestDM(mapname: String,
var MGR: MultiGfxRegister,
var MAR: MultiAudioRegister) : DungeonMaster(mapname) {
var muz: MTrack? = null
var cam = OrthographicCamera()
var cambounds = Rectangle()
var statetime = 0f
var Player: TestPlatformer? = null
var enter = true
override fun begin() {
val LyrTileObj = Level!!.layers["TileObjects"] as TiledMapTileLayer?
if (LyrTileObj != null) for (y in 0..LyrTileObj.height-1)
for (x in 0..LyrTileObj.width-1) {
val obj = LyrTileObj.getCell(x,y)
if (obj != null && obj.tile != null) when (obj.tile.id) {
//71 black box
//72 moving
Door.TileNum -> {
val o = Door(MGR,MAR)
o.Position.set(x.toFloat(),y.toFloat())
Living.add(o)
}
Pedestal.TileNum -> {
val o = Pedestal(MGR,MAR)
o.Position.set(x.toFloat(),y.toFloat())
Living.add(o)
}
Alchemy.TileNum -> {
val o = Alchemy(MGR,MAR)
o.Position.set(x.toFloat(),y.toFloat())
Living.add(o)
}
ThruPlat.TileNum -> {
val o = ThruPlat(MGR,MAR)
o.Position.set(x.toFloat(),y.toFloat())
Living.add(o)
}
49 -> Living.add(Collectible("_mineral",x.toFloat(),y.toFloat(),MGR,MAR))
50 -> Living.add(Collectible("_herb",x.toFloat(),y.toFloat(),MGR,MAR))
51 -> Living.add(Collectible("_eyeball",x.toFloat(),y.toFloat(),MGR,MAR))
52 -> Living.add(Collectible("_worm",x.toFloat(),y.toFloat(),MGR,MAR))
53 -> Living.add(Collectible("_dollar",x.toFloat(),y.toFloat(),MGR,MAR))
54 -> Living.add(Collectible("_potion",x.toFloat(),y.toFloat(),MGR,MAR))
}
}
MGR.camera.setToOrtho(false,640f,360f)
MGR.updateCam()
MGR.setBufScalar("main",1/16f)
cam = MGR.getBufCam("main")!!
cam.setToOrtho(false,640/16f,360/16f)
cambounds.set(320/16f,180/16f,LyrTerrain!!.width-640/16f,LyrTerrain!!.height-360/16f)
MAR.setMusVolume(0.125f)
MAR.setSFXVolume(0.25f)
muz = MAR.getMus("frcasio")
muz?.Generate()
muz?.play(true)
Player = TestPlatformer(this,MGR,MAR)
Player!!.Parent = this
Player!!.Name = "Player"
Living.add(Player!!)
}
override fun end() {
muz?.stop()
muz?.dispose()
Living.clear()
Player = null
}
override fun prestep(deltatime: Float) {
if (Gdx.input.isKeyPressed(Input.Keys.ENTER)) {
if (enter == false) ScriptLaunch = "pause"
enter = true
} else enter = false
if (Gdx.input.isKeyPressed(Input.Keys.SPACE) || Gdx.input.isKeyPressed(Input.Keys.UP)
|| Gdx.input.isKeyPressed(Input.Keys.W))
Player!!.Mailbox.add(LabelledObject("CtrlJump",true))
else
Player!!.Mailbox.add(LabelledObject("CtrlJump",false))
if (Gdx.input.isKeyPressed(Input.Keys.RIGHT) || Gdx.input.isKeyPressed(Input.Keys.D))
Player!!.Mailbox.add(LabelledObject("CtrlRt",true))
else
Player!!.Mailbox.add(LabelledObject("CtrlRt",false))
if (Gdx.input.isKeyPressed(Input.Keys.LEFT) || Gdx.input.isKeyPressed(Input.Keys.A))
Player!!.Mailbox.add(LabelledObject("CtrlLf",true))
else
Player!!.Mailbox.add(LabelledObject("CtrlLf",false))
if (Gdx.input.isKeyPressed(Input.Keys.DOWN) || Gdx.input.isKeyPressed(Input.Keys.S))
Player!!.Mailbox.add(LabelledObject("CtrlDn",true))
else
Player!!.Mailbox.add(LabelledObject("CtrlDn",false))
}
override fun draw(deltatime: Float) {
statetime += deltatime
cam.position.set(keepPointInBox(Player!!.Position.cpy().add(0.5f,0.5f),cambounds),0f)
cam.update()
MGR.startBuffer("main")
// MGR.clear(0.1f,0.1f,0.1f,1f)
MGR.clear(0f,1f,1f,1f)
MGR.drawTileLayer(LyrTerrain!!,"sprites",1f,statetime)
for (e in Living) {
e.draw(deltatime)
}
MGR.stopBuffer()
// MGR.drawBuffer("main")
// MGR.flush()
}
override fun flip() {
MGR.drawBuffer("main")
MGR.flush()
}
override fun pause() {
muz?.stop()
muz?.dispose()
}
override fun unpause() {
val quitting = Parent!!.GlobalData["quitting"]
if (quitting != null && quitting.label != "") ScriptStop = true
muz?.Generate()
muz?.play(true)
MGR.camera.setToOrtho(false,640f,360f)
MGR.updateCam()
MGR.setBufScalar("main",1/16f)
cam = MGR.getBufCam("main")!!
cam.setToOrtho(false,640/16f,360/16f)
}
override fun clone() = TestDM(MapName,MGR,MAR)
}
<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.Gdx
import com.badlogic.gdx.Input
import com.badlogic.gdx.math.Vector2
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.DungeonMaster
import net.jorhlok.oops.LabelledObject
/**
* Created by joshm on 7/20/2017.
*/
class PauseDM(var mapname: String,
var MGR: MultiGfxRegister,
var MAR: MultiAudioRegister) : DungeonMaster(mapname) {
var enter = true
var x = 0f
var y = 0f
override fun begin() {
val cam = MGR.getBufCam("main")
if (cam != null) {
x = cam.position.x
y = cam.position.y
}
}
override fun prestep(deltatime: Float) {
if (Gdx.input.isKeyPressed(Input.Keys.ESCAPE)) {
Parent!!.GlobalData.put("quitting", LabelledObject("y"))
ScriptStop = true
}
if (Gdx.input.isKeyPressed(Input.Keys.ENTER)) {
if (!enter) ScriptStop = true
}
else enter = false
}
override fun draw(deltatime: Float) {
Parent!!.drawPrevious(deltatime)
MGR.startBuffer("main")
MGR.drawString("libmono","PAUSE",x,y)
MGR.stopBuffer()
}
override fun flip() {
MGR.drawBuffer("main")
MGR.flush()
}
override fun clone() = PauseDM(mapname, MGR,MAR)
}<file_sep># ShootyShoot
Small game, possibly.
jorhlok.net
<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.math.Vector2
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.Entity
class TestPlatformer(val DM: TestDM, MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
var Gravity = Vector2(0f, -24f)
var Grounded = false
var JumpVelo = 12f
var WalkVelo = 16f
var WalkAccl = 16f
var jump = false
var left = false
var right = false
var drawdir = false
var forcefall = false
var wobbletime = 0f
val wobbledepth = 20f
val wobbleperiod = 0.25f
val tolup = 0.5f/10f
val toldn = 0.5f/10f
init {
AABB.set(3/16f, 0f, 10/16f, 14/16f)
Tolerance.set(2/10f, 10/14f)
Position.set(5f, 8f)
Friction.set(16f,0f)
Physics = true
CollTiles = true
CollEntities = true
val ceg = HashSet<String>()
val ceat = HashSet<String>()
ceat.add(ThruPlat.TypeName)
CollEntAsTile = ceat
ceg.add(ThruPlat.TypeName)
ceg.add(Door.TypeName)
CollEntGray = ceg
Type = "TestPlatformer"
}
override fun prestep(deltatime: Float) {
Velocity.add(Gravity.cpy().scl(deltatime))
for (p in Mailbox) {
if (p.label.startsWith("Ctrl")) {
val b = p.obj as Boolean
when (p.label) {
"CtrlJump" -> {
val oldjump = jump
jump = b
if (/*Grounded &&*/ jump && !oldjump) {
//jump!
Velocity.add(0f, JumpVelo)
if (Velocity.y > JumpVelo) Velocity.y = JumpVelo
Grounded = false
MAR.playSFX("jump")
}
}
"CtrlLf" -> left = b
"CtrlRt" -> right = b
"CtrlDn" -> forcefall = b
}
if (left && !right) {
Velocity.x -= WalkAccl * deltatime
if (Velocity.x < -1*WalkVelo) Velocity.x = -1*WalkVelo
drawdir = false
} else if (right && !left) {
Velocity.x += WalkAccl * deltatime
if (Velocity.x > WalkVelo) Velocity.x = WalkVelo
drawdir = true
}
}
}
//easier to snap moving upward than downward
if (Velocity.y > 0) Tolerance.x = tolup
else Tolerance.x = toldn
Mailbox.clear()
}
override fun poststep(deltatime: Float) {
if (Velocity.y < 0)
Grounded = false
else if (Velocity.y == 0f) Grounded = true
for (m in Mailbox) {
when {
m.label.startsWith("CollEnt/${Door.TypeName}") -> DM.ScriptSwap = "title"
}
// System.out.println(m.label)
}
}
override fun checkCollEntity(deltatime: Float, e: Entity): Boolean {
when(e.Type) {
ThruPlat.TypeName -> return !forcefall && Velocity.y <= 0 && Position.y >= e.Position.y && mkVRect().overlaps(e.mkRect())
Door.TypeName -> return forcefall
}
return false
}
override fun draw(deltatime: Float) {
var rot = 0f
if (Velocity.x != 0f) {
wobbletime += deltatime*Velocity.x/WalkVelo
if (wobbletime > wobbleperiod) wobbletime -= wobbleperiod
rot = Math.sin(wobbletime/wobbleperiod*2*Math.PI).toFloat()*wobbledepth*-1
}
else wobbletime = 0f
if (drawdir) {
MGR.drawRgb("_guyrt", 0f, Position.x + 0.5f, Position.y,1f,1f,rot,Vector2(0.5f,0f))
MGR.drawRgb("_gunrt", 0f, Position.x + 10/16f, Position.y,1f,1f,0f,Vector2())
} else {
MGR.drawRgb("_guylf", 0f, Position.x + 0.5f, Position.y,1f,1f,rot,Vector2(0.5f,0f))
MGR.drawRgb("_gunlf", 0f, Position.x - 10/16f, Position.y,1f,1f,0f,Vector2())
}
}
}
<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.ApplicationAdapter
import com.badlogic.gdx.Gdx
import com.badlogic.gdx.assets.loaders.resolvers.InternalFileHandleResolver
import com.badlogic.gdx.graphics.Color
import com.badlogic.gdx.graphics.Texture
import com.badlogic.gdx.graphics.g2d.Animation
import com.badlogic.gdx.graphics.g2d.freetype.FreeTypeFontGenerator
import com.badlogic.gdx.maps.tiled.TmxMapLoader
import com.badlogic.gdx.utils.Array
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.ObjectOrientedPlaySet
class ShootyShoot : ApplicationAdapter() {
private var mgr: MultiGfxRegister? = null
private var audio: MultiAudioRegister? = null
private var oops: ObjectOrientedPlaySet? = null
var statetime = 0f
override fun create() {
var bigpal = Array<Color>()
bigpal.add(Color(0f,0f,0f,0f))
bigpal.add(Color(0f,0f,0f,1f))
bigpal.add(Color(4/15f,12/15f,2/15f,1f))
bigpal.add(Color(13/15f,13/15f,13/15f,1f))
bigpal.add(Color(7/15f,8/15f,1f,1f))
bigpal.add(Color(0f,0f,0f,1f))
bigpal.add(Color(4/15f,12/15f,2/15f,1f))
bigpal.add(Color(13/15f,13/15f,13/15f,1f))
mgr = MultiGfxRegister()
mgr!!.palette = bigpal
audio = MultiAudioRegister()
mkav()
mgr!!.Generate()
audio!!.Generate()
oops = ObjectOrientedPlaySet()
oops!!.addTileMap("test0", TmxMapLoader(InternalFileHandleResolver()).load("map/test0.tmx"))
oops!!.addTileMap("test1", TmxMapLoader(InternalFileHandleResolver()).load("map/test1.tmx"))
oops!!.addTileMap("test2", TmxMapLoader(InternalFileHandleResolver()).load("map/test2.tmx"))
val dm = TestDM("test2",mgr!!,audio!!)
oops!!.addMasterScript("title", Title("",mgr!!,audio!!))
oops!!.addMasterScript("pause", PauseDM("",mgr!!,audio!!))
oops!!.addMasterScript("testdm", dm)
oops!!.launchScript("title")
}
override fun render() {
val deltatime = Gdx.graphics.deltaTime
statetime += deltatime
if (statetime >= 4) {
System.out.println("${Gdx.graphics.framesPerSecond} FPS")
statetime -= 4f
}
// val c = mgr!!.getBufCam("main")
// c!!.translate(Math.sin(statetime*Math.PI/2).toFloat(),Math.cos(statetime*Math.PI/2).toFloat())
// c!!.rotate(Math.sin(statetime*Math.PI/2).toFloat())
// c!!.zoom = 0.5f
// c!!.update()
// mgr!!.fillShapes()
// mgr!!.drawCircle(320f,180f,Math.sin(statetime*Math.PI/2).toFloat()*4+12,Color(0.2f,0.3f,1f,1f))
//game logic
oops!!.step(deltatime)
try {
oops!!.draw(deltatime)
} catch (e: Exception) {
System.err.println("Error drawing!")
e.printStackTrace()
}
if (oops!!.Quit) Gdx.app.exit()
// mgr!!.drawPal("_girl",4,0f,64f,64f,2f,2f,statetime*90, Vector2())
// mgr!!.drawPal("_girl",0,0f,16f,16f,2f,2f,statetime*90)
// mgr!!.drawPal("_girl",0,0f,48f,48f,2f,2f,statetime*90)
// mgr!!.drawString("libmono","wubba lubba dub dub",Math.round(Math.sin(statetime*Math.PI/2)*64f+320f).toFloat(),Math.round(Math.cos(statetime*Math.PI/2)*64f+180f).toFloat(),1f,1f,0f,Vector2(0.5f,0.5f), mgr!!.palette[2])
// mgr!!.drawString("libmono","wubba lubba dub dub\n\n grass tastes bad",Math.sin(statetime*Math.PI/2).toFloat()*64f+320f,Math.cos(statetime*Math.PI/2).toFloat()*64f+180f,2f,1f,statetime*-90,Vector2(0.5f,0.5f), mgr!!.palette[2])
// mgr!!.drawRgb("pacrt",statetime*3,320f,180f,1f,1f,statetime*-90f+90f)
}
override fun resize(width: Int, height: Int) {
}
override fun dispose() {
oops?.dispose()
mgr?.dispose()
audio?.dispose()
}
fun mkav() {
mgr?.newBuffer("main",640,360,640f,360f)
mgr?.newImagePal("imgmap","gfx/imgmap.png",8,8)
mgr?.newSpritePal("girl","imgmap",0,0,2,2)
mgr?.newSpritePal("column","imgmap",30,4,2,2)
mgr?.newMapTileRgb("sprites",241,"_greenblock")
mgr?.newMapTileRgb("sprites",242,"_skyblueblock")
mgr?.newMapTileRgb("sprites",243,"_whiteblock")
mgr?.newMapTileRgb("sprites",244,"_greyblock")
audio?.newMusic("frcasio", "bgm/FriendlyCasiotone.ogg")
// audio?.newMusic("mkds", "bgm/mkdsintro.ogg", "bgm/mkds.ogg")
audio?.newSFX("pew", "sfx/pew.wav")
audio?.newSFX("jump", "sfx/jump.wav")
audio?.newSFX("dash", "sfx/dash.wav")
audio?.newSFX("growl", "sfx/growl.wav")
val generator = FreeTypeFontGenerator(Gdx.files.internal("gfx/libmono.ttf"))
val parameter = FreeTypeFontGenerator.FreeTypeFontParameter()
parameter.size = 14 * 1
parameter.magFilter = Texture.TextureFilter.Linear
parameter.minFilter = Texture.TextureFilter.Linear
parameter.hinting = FreeTypeFontGenerator.Hinting.Full
mgr?.newFont("libmono",generator.generateFont(parameter),1f)
generator.dispose()
mgr?.newImageRgb("sprites", "gfx/sprites.png", 16, 16)
mgr?.newSpriteRgb("guyrt", "sprites", 0, 0, 1, 1, false, false)
mgr?.newSpriteRgb("guylf", "sprites", 0, 0, 1, 1, true, false)
mgr?.newSpriteRgb("gunrt", "sprites", 1, 0, 1, 1, false, false)
mgr?.newSpriteRgb("gunlf", "sprites", 1, 0, 1, 1, true, false)
mgr?.newSpriteRgb("pac0lf", "sprites", 14, 0, 1, 1, false, false)
mgr?.newSpriteRgb("pac0rt", "sprites", 14, 0, 1, 1, true, false)
mgr?.newSpriteRgb("pac1lf", "sprites", 15, 0, 1, 1, false, false)
mgr?.newSpriteRgb("pac1rt", "sprites", 15, 0, 1, 1, true, false)
mgr?.newSpriteRgb("shot0", "sprites", 0, 1, 1, 1, false, false)
mgr?.newSpriteRgb("shot1", "sprites", 1, 1, 1, 1, false, false)
mgr?.newSpriteRgb("shot2", "sprites", 2, 1, 1, 1, false, false)
mgr?.newSpriteRgb("shot3", "sprites", 3, 1, 1, 1, false, false)
mgr?.newSpriteRgb("door", "sprites", 0, 2, 1, 1, false, false)
mgr?.newSpriteRgb("pedestal", "sprites", 1, 2, 1, 1, false, false)
mgr?.newSpriteRgb("alchemy", "sprites", 2, 2, 2, 1, false, false)
mgr?.newSpriteRgb("mineral", "sprites", 0, 3, 1, 1, false, false)
mgr?.newSpriteRgb("herb", "sprites", 1, 3, 1, 1, false, false)
mgr?.newSpriteRgb("eyeball", "sprites", 2, 3, 1, 1, false, false)
mgr?.newSpriteRgb("worm", "sprites", 3, 3, 1, 1, false, false)
mgr?.newSpriteRgb("dollar", "sprites", 4, 3, 1, 1, false, false)
mgr?.newSpriteRgb("potion", "sprites", 5, 3, 1, 1, false, false)
mgr?.newSpriteRgb("mana", "sprites", 6, 3, 1, 1, false, false)
mgr?.newSpriteRgb("coffee", "sprites", 7, 3, 1, 1, false, false)
mgr?.newSpriteRgb("redbar", "sprites", 0, 4, 1, 1, false, false)
mgr?.newSpriteRgb("darkredbar", "sprites", 1, 4, 1, 1, false, false)
mgr?.newSpriteRgb("bluebar", "sprites", 2, 4, 1, 1, false, false)
mgr?.newSpriteRgb("darkbluebar", "sprites", 3, 4, 1, 1, false, false)
mgr?.newSpriteRgb("yellowbar", "sprites", 4, 4, 1, 1, false, false)
mgr?.newSpriteRgb("darkyellowbar", "sprites", 5, 4, 1, 1, false, false)
mgr?.newSpriteRgb("uiback", "sprites", 6, 4, 1, 1, false, false)
mgr?.newSpriteRgb("uislot", "sprites", 7, 4, 1, 1, false, false)
mgr?.newSpriteRgb("cursor", "sprites", 8, 4, 1, 1, false, false)
mgr?.newSpriteRgb("greenblock", "sprites", 0, 15, 1, 1, false, false)
mgr?.newSpriteRgb("skyblueblock", "sprites", 1, 15, 1, 1, false, false)
mgr?.newSpriteRgb("whiteblock", "sprites", 2, 15, 1, 1, false, false)
mgr?.newSpriteRgb("greyblock", "sprites", 3, 15, 1, 1, false, false)
mgr?.newAnimRgb("newshot", Array<String>(arrayOf("shot0", "shot1", "shot2")), 0.125f, Animation.PlayMode.NORMAL)
mgr?.newAnimRgb("shot", Array<String>(arrayOf("shot3", "shot2")), 0.01f, Animation.PlayMode.LOOP)
mgr?.newAnimRgb("paclf", Array<String>(arrayOf("pac0lf", "pac1lf")), 0.5f, Animation.PlayMode.LOOP)
mgr?.newAnimRgb("pacrt", Array<String>(arrayOf("pac0rt", "pac1rt")), 0.5f, Animation.PlayMode.LOOP)
}
}
<file_sep>package net.jorhlok.shootyshoot
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
/**
* Created by joshm on 6/15/2017.
*/
class ThruPlat(MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
companion object {val TileNum = 67; val TypeName = "ThruPlat"}
init {
Type = ThruPlat.TypeName
CollEntities = true //allow collisions
CollEntWhite = emptySet() //but I don't pay attention
AABB.set(0f,6/16f,1f,4/16f)
}
override fun draw(deltatime: Float) {
MGR.drawRgb("_bluebar",0f,Position.x+0.5f,Position.y+0.5f)
}
}<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.math.Vector2
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
/**
* Created by joshm on 6/15/2017.
*/
class Alchemy(MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
companion object {val TileNum = 35}
override fun draw(deltatime: Float) {
MGR.drawRgb("_alchemy",0f,Position.x,Position.y,1f,1f,0f, Vector2())
}
}<file_sep>package net.jorhlok.shootyshoot
import com.badlogic.gdx.Gdx
import com.badlogic.gdx.Input
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
import net.jorhlok.oops.DungeonMaster
/**
* Created by joshm on 6/17/2017.
*/
class Title(mapname: String,
var MGR: MultiGfxRegister,
var MAR: MultiAudioRegister) : DungeonMaster(mapname) {
override fun begin() {
MGR.camera.setToOrtho(false,640f,360f)
MGR.updateCam()
MGR.getBufCam("main")?.setToOrtho(false,640f,360f)
MGR.setBufScalar("main")
}
override fun prestep(deltatime: Float) {
if (Gdx.input.isKeyPressed(Input.Keys.SPACE) || Gdx.input.isKeyPressed(Input.Keys.ENTER))
ScriptSwap = "testdm"
}
override fun draw(deltatime: Float) {
MGR.startBuffer("main")
MGR.clear(0.1f,0.1f,0.1f,1f)
MGR.drawString("libmono","Press Start",320f,180.5f)
MGR.stopBuffer()
// MGR.drawBuffer("main")
// MGR.flush()
}
override fun flip() {
MGR.drawBuffer("main")
MGR.flush()
}
override fun clone() = Title(MapName,MGR,MAR)
}<file_sep>package net.jorhlok.shootyshoot
import net.jorhlok.multiav.MultiAudioRegister
import net.jorhlok.multiav.MultiGfxRegister
/**
* Created by joshm on 6/16/2017.
*/
class Collectible(val anim: String, x: Float, y:Float, MGR: MultiGfxRegister, MAR: MultiAudioRegister) : SSEntity(MGR, MAR) {
val animlen = 2f
var statetime = Math.random().toFloat()*animlen
val hoverdepth = 4/16f
val hidelen = 5f
var hidetime = hidelen
init {
Position.set(x,y)
AABB.set(0f,0f,1f,1f)
val s = HashSet<String>()
s.add("TestPlatformer")
CollEntWhite = s
CollEntities = true
}
override fun poststep(deltatime: Float) {
for (m in Mailbox) {
if (hidetime >= hidelen && m.label.startsWith("CollEnt/")) {
hidetime = deltatime*-1
statetime = hidetime-hidelen
MAR.playSFX("pew")
}
}
if (hidetime < hidelen) hidetime += deltatime
}
override fun draw(deltatime: Float) {
statetime += deltatime
if (statetime > animlen) statetime -= animlen
if (hidetime >= hidelen) MGR.drawRgb(anim,0f,Position.x+0.5f,Position.y+0.5f+Math.sin(statetime/animlen*Math.PI*2).toFloat()*hoverdepth)
}
} | a62497da3e52397740001f8e587c87cb62552f47 | [
"Markdown",
"Kotlin"
] | 12 | Kotlin | Jorhlok/ShootyShoot | 05a6d3b9445f2d10bc0d1f4dba28a77fda47e47a | 4f65acce31befd2bbab0002eaa35e20a5eb1e652 | |
refs/heads/master | <file_sep>package com.woolfer.varteq.tasks.horseTrack.objectTypes;
import sun.invoke.empty.Empty;
import java.util.Currency;
import java.util.Iterator;
import java.util.Map;
/**
* Created by pavlenko on 3/14/2015.
*/
public class MoneyOT {
protected Map<Integer, Integer> cash;
protected Currency currency;
public MoneyOT(Currency currency) {
this.currency = currency;
}
public Map<Integer, Integer> getCash() {
return cash;
}
public void setCash(Map<Integer, Integer> cash) {
this.cash = cash;
}
public Currency getCurrency() {
return currency;
}
public void setCurrency(Currency currency) {
this.currency = currency;
}
@Override
public String toString() {
StringBuilder str = new StringBuilder();
Iterator iter = cash.entrySet().iterator();
String symbol = currency.getSymbol();
while (iter.hasNext()) {
Map.Entry entry = (Map.Entry) iter.next();
str.append(symbol);
str.append(entry.getKey() + ", ");
str.append(entry.getValue() + "\n");
}
return str.toString();
}
}
<file_sep>package com.woolfer.varteq.tasks.horseTrack.impl;
import com.woolfer.varteq.tasks.horseTrack.events.Payouts;
import com.woolfer.varteq.tasks.horseTrack.objectTypes.HorseOT;
import com.woolfer.varteq.tasks.horseTrack.commons.Horses;
import com.woolfer.varteq.tasks.horseTrack.objectTypes.MoneyOT;
import com.woolfer.varteq.tasks.horseTrack.services.MachineService;
import java.util.*;
/**
* Created by pavlenko on 3/14/2015.
*/
public class MachineServiceImpl implements MachineService {
private final Map<Integer, HorseOT> horsesList;
private DollarTellerMachine tellerMachine;
private int winHorseId;
private int amount;
private int winningAmount;
private MoneyOT gainings;
public MachineServiceImpl(DollarTellerMachine tellerMachine, Map<Integer, HorseOT> horsesList) {
this.tellerMachine = tellerMachine;
this.horsesList = horsesList;
}
@Override
public void setBet(int horseId, int amount) {
this.winHorseId = horseId;
this.amount = amount;
}
@Override
public String checkResult() {
HorseOT horse = horsesList.get(winHorseId);
if (horse.getLastResult() == Horses.WON) {
winningAmount = getWinningAmount(amount, horse.getOdds());
if (dispensing(winningAmount)) {
Payouts.payoutWin(horse.getName() + "," + tellerMachine.getCurrency().getSymbol() + winningAmount);
Payouts.dispensing(gainings.toString());
}
} else {
Payouts.noPayout(horse.getName());
}
return null;
}
private boolean dispensing(int winningAmount) {
Map<Integer, Integer> cash = new TreeMap<>(new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2.compareTo(o1);
}
});
Map<Integer, Integer> cashTellerMachine = tellerMachine.getMoney().getCash();
cash.putAll(cashTellerMachine);
Map<Integer, Integer> dispensingList = new TreeMap();
for (Map.Entry entry : cashTellerMachine.entrySet()) {
dispensingList.put((Integer) entry.getKey(), 0);
}
int payoutResult = 0;
int remainderPayment = winningAmount;
for (Map.Entry entry : cash.entrySet()) {
Integer denominationInt = (Integer) entry.getKey();
int denomination = denominationInt.intValue();
Integer inventoryInt = (Integer) entry.getValue();
int inventory = inventoryInt.intValue();
int count = remainderPayment / denomination;
if (inventory > 0) {
if (count > inventory) count = inventory;
payoutResult += count * denomination;
inventory -= count;
entry.setValue(Integer.valueOf(inventory));
dispensingList.put(denominationInt, count);
remainderPayment = remainderPayment - count * denomination;
}
if (payoutResult == winningAmount) {
gainings = new MoneyOT(tellerMachine.getCurrency());
gainings.setCash(dispensingList);
tellerMachine.getMoney().setCash(new TreeMap<>(cash));
return true;
}
}
if (payoutResult != winningAmount) {
Payouts.insufficientFunds(String.valueOf(winningAmount));
return false;
}
return false;
}
private int getWinningAmount(int amount, int odds) {
return amount * odds;
}
}
<file_sep>package com.woolfer.varteq.tasks.horseTrack.services;
/**
* Created by pavlenko on 3/14/2015.
*/
public interface MachineService {
public void setBet(int horseId, int amount);
public String checkResult();
}
<file_sep>package com.woolfer.varteq.tasks.horseTrack.services;
import java.util.Collections;
/**
* Created by pavlenko on 3/14/2015.
*/
public interface HorseService {
public void declareHorses();
public void setWinner(int horseId);
public String showHorsesList();
}
<file_sep>package com.woolfer.varteq.tasks.horseTrack.impl;
import com.woolfer.varteq.tasks.horseTrack.commons.Denominations;
import com.woolfer.varteq.tasks.horseTrack.objectTypes.MoneyOT;
import com.woolfer.varteq.tasks.horseTrack.objectTypes.TellerMachine;
import java.util.*;
/**
* Created by pavlenko on 3/15/2015.
*/
public class DollarTellerMachine implements TellerMachine {
private MoneyOT money;
private String currencyCode = "USD";
private Currency currency = Currency.getInstance(currencyCode);
public DollarTellerMachine() {
money = new MoneyOT(currency);
money.setCash(new TreeMap<Integer, Integer>());
generateCashInventory(money.getCash());
}
@Override
public MoneyOT getMoney() {
return money;
}
@Override
public void setMoney(MoneyOT money) {
this.money = money;
}
@Override
public void generateCashInventory(Map<Integer, Integer> cashInventory){
cashInventory.put(Denominations.ONE.getDenomination(), 10);
cashInventory.put(Denominations.FIVE.getDenomination(), 10);
cashInventory.put(Denominations.TEN.getDenomination(), 10);
cashInventory.put(Denominations.TWENTY.getDenomination(), 10);
cashInventory.put(Denominations.ONE_HUNDRED.getDenomination(), 10);
}
@Override
public void restockCashInventory(){
Map cashInventory = money.getCash();
if(cashInventory != null) {
cashInventory.clear();
generateCashInventory(cashInventory);
}
}
public String getCurrencyCode() {
return currencyCode;
}
public Currency getCurrency() {
return currency;
}
}
<file_sep>package com.woolfer.varteq.tasks.horseTrack.commons;
/**
* Created by pavlenko on 3/14/2015.
*/
public enum Commands {
RESTOCK("R"), QUIT("Q"), WIN("W");
private String command;
Commands(String command) {
this.command = command;
}
public String getCommand(){
return command;
}
}
| 059b9b533b75c9bc592de019dd5c92282b449ff2 | [
"Java"
] | 6 | Java | AndriiPavlenko/varteq | 13054b30cb979d28bab926803934c9231d6688e1 | 7324a4540b4f1c85c4fa13e5088a7d77a4a26989 | |
refs/heads/master | <file_sep>class Inverter{
initialBatteryCapacity = null;
finalBatteryCapacity = null;
initialBatteryPercentage = 0.7;
finalBatteryPercentage = 0.9;
getInitialBatteryCapacity(batteryAmp){
this.initialBatteryCapacity = batteryAmp * this.initialBatteryPercentage;
return this.initalBatteryCapacity;
}
getFinalBatteryCapacity(){
this.finalBatteryCapacity = this.initialBatteryCapacity * this.finalBatteryPercentage;
return this.finalBatteryCapacity;
}
}
n = new Inverter;
n.getInitialBatteryCapacity(200);
n.getFinalBatteryCapacity();
console.log(n);<file_sep><?php
namespace CsrfToken;
class CsrfToken
{
public static function generateCsrfToken()
{
$_SESSION['token'] = base64_encode(openssl_random_pseudo_bytes(64));
}
public static function checkCsrfToken($csrfToken)
{
if (isset($_SESSION['token']) && $csrfToken === $_SESSION['token']) {
unset($_SESSION['token']);
return true;
}
return false;
}
}
<file_sep><?php
$url = 'http://sourcesms.com/api/api-function.php';
$fields_string = null;
$fields = [
'from' => 'MOSCO',
'to' => '09036924798',
'message' => 'hello world',
'username' => '',
'pword' => '',
'hash' => '',
'formcountry' => '234',
'sourceinfo' => '1'
];
print_r($fields);
<file_sep><?php
require 'Interface.php';
class Model implements Test
{
public function call()
{
echo "hello world\n";
}
public function foo()
{
}
}
<file_sep>if ($('.cookie-banner').length) {
$('.cookie-banner').slideDown(5000);
}<file_sep><?php
if (isset($_GET['accept-cookies'])) {
setcookie('accept-cookies', 'true', time() + 31556925);
header('location: ./');
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>cookie banner</title>
<link rel="stylesheet" href="cookie.css">
</head>
<body>
<?php
if (!isset($_COOKIE['accept-cookies'])) {
?>
<div class="cookie-banner">
<div class="container">
<p>we use cookies on this website. By using this website, we will assume you consent to
<a href="/cookies">the cookies we set</a> .</p>
<a href="?accept-cookies" class="button">Ok, continue</a>
</div>
</div>
<?php
}
?>
<p>this is a cookie banner</p>
<script src="jquery.js"></script>
<script src="cookie.js"></script>
</body>
</html><file_sep>#include <iostream>
using namespace std;
double drainage_time;
int Qty,Battery_power,volt_out = 220;
double get_VA(int appliance_power){
double VA=appliance_power/0.8;
return VA;
}
double KVA(double VA) {
double KVA = VA/1000;
return KVA;
}
double get_DRAIN_CURR(double KVA){
double drain_current = (1000 * KVA) / volt_out;
return drain_current;
}
double get_battery_capacity(int Battery_power){
double battery_capacity = Battery_power*0.7;
return battery_capacity;
}
double get_real_battery_capacity( double battery_capacity){
double real_battery_capacity = battery_capacity -(0.2*battery_capacity);
return real_battery_capacity ;
}
int main(){
double appliance_power = 445;
Battery_power = 200;
double n = get_VA(appliance_power);
n = KVA(n);
n = get_DRAIN_CURR(n);
cout << n << endl ;
return 0;
}<file_sep><?php
require 'Model.php';
class Tester extends Model
{
public function __construct()
{
$this->call();
}
}
new Tester();
<file_sep><?php
namespace DB;
use PDO;
use PDOException;
class DB
{
private static $instance = null;
private $pdo;
private $query;
private $error = false;
private $results;
private $count = 0;
private $lastInsertID = '';
private function __construct()
{
try {
$this->pdo = new PDO('mysql:host=127.0.0.1;dbname=test', 'root', '');
} catch (PDOException $e) {
die($e->getMessage());
}
}
public static function getInstance()
{
if (!isset(self::$instance)) {
self::$instance = new DB();
}
return self::$instance;
}
public function query($sql, $params = [])
{
$this->error = false;
if ($this->query = $this->pdo->prepare($sql)) {
$x = 1;
if (count($params)) {
foreach ($params as $param) {
$this->query->bindValue($x, $param);
$x++;
}
}
}
return $this;
}
}
<file_sep><?php
interface Test
{
function call();
}
<file_sep><?php
namespace Test;
class Test
{
private $file = 'Connection.php';
private $host = '$host';
private $user = '$user';
private $pass = <PASSWORD>';
private $db = '$db';
private $link = '$link';
private $newhost = '$this->host';
private $newuser = '$this->user';
private $newpass = <PASSWORD>';
private $newdb = '$this->db';
private $newlink = '$this->link';
private $connection = [];
private $error = [];
public function __construct()
{
}
private function checkIfDatabaseConnectionFileExists()
{
if (file_exists($this->file)) {
echo "it exists";
} else {
$this->createDatabaseConnectionFile();
}
}
public function createDatabaseConnectionFile()
{
$fcontent = "<?php
namespace App\Core;
use mysqli;
class Connection
{
private $this->host;
private $this->user;
private $this->pass;
private $this->db;
protected $this->link;
public function __construct()
{
$this->newhost = 'localhost';
$this->newuser = 'root';
$this->newpass = '<PASSWORD>';
$this->newdb = 'db';
}
protected function connect()
{
$this->newlink = new mysqli($this->newhost, $this->newuser, $this->newpass, $this->newdb);
if (!$this->newlink) {
echo 'connection to database is down';
}
}
}
";
$file = fopen($this->file, 'w');
fwrite($file, $fcontent);
fclose($file);
}
}
$f = new Test();
$f->createDatabaseConnectionFile();
<file_sep><?php
require 'vendor/autoload.php';
// This will output the barcode as HTML output to display in the browser
$generator = new Picqer\Barcode\BarcodeGeneratorHTML();
echo "<div style='margin:0 auto; display:grid; grid-template-columns:20% 20% 20% 20% 20%;'>";
for ($i=0; $i < 50; $i++) {
echo $generator->getBarcode(rand('0123456789', '9999999999'), $generator::TYPE_CODE_128).'<br>';
}
echo "</div>";
<file_sep><?php
require_once 'DB.php';
$db = DB\DB::getInstance();
| d0d9d6ea2e871ed89d736b2bbe4ec4e5bdda9c7f | [
"JavaScript",
"C++",
"PHP"
] | 13 | JavaScript | geraldon1997/test | c6c93c7579d6006f93c259f9146558ee91d7ac33 | 1092cad5ad08ecca024addea7c3009ac53b33ca8 | |
refs/heads/master | <file_sep># grunt-jasmine-chromeapp
> Run jasmine specs in a Chrome Packaged App
[](https://app.shippable.com/projects/54d3c81a5ab6cc13528afac2/builds/latest)
Getting Started
---------------
This plugin requires Grunt ```~0.4.0```
If you haven't used Grunt before, be sure to check out the Getting Started guide, which explains how to create your grunt file.
Once you're familiar with the process, this plugin can be installed as:
```shell
npm install grunt-jasmine-chromeapp --save-dev
```
Once the plugin has been installed, it may be enabled with this line of JavaScript:
```javascript
grunt.loadNpmTasks('grunt-jasmine-chromeapp');
```
Jasmine Task
------------
Run this task with the ```grunt jasmine_chromeapp``` command.
Automatically builds and maintains the spec runner and reports results back to the grunt console.
Starts chrome with a dynamically created packaged application which runs jasmine specs. The package
reports results back to a web server run by the plugin, which then reports back to the console.
This structure is chosen becasue selenium is unable to debug or instrument packaged applications
directly.
Customize your SpecRunner
-------------------------
Use your own files in the app to customize your tests.
### Options
#### src
Type: `String|Array`
Your source files and specs. These are the files you are testing.
#### options.paths
Type: `String|Array`
Listing of which files should be included as script tags in the test runner. If not set, defaults
to all files listed as source files, but can define a subset of those files to support copying
non-js files, or other support files which should not be directly included.
#### options.keepRunner
Type: `Boolean`
Default: `false`
Prevents the auto-generated app from being automatically deleted, and leave the browser open.
#### options.binary
Type: `String`
Default: `undefined`
Specify the locations of `google-chrome` to run for testing. Defaults to the [per-platform
default locations](https://code.google.com/p/selenium/wiki/ChromeDriver) specified by
chromedriver if not specified.
#### options.flags
Type: `Array`
Default: `[]`
Additional command-line flags to pass to chrome. These are appended to the default flags
used for instantiation: `--no-first-run`, `--force-app-mode`, `--apps-keep-chrome-alive-in-tests`,
`--load-and-launch-app`, and `--user-data-dir`.
`--no-startup-window` is also used for the Mac platform.
#### options.timeout
Type: `Number`
Default: `30000`
How many milliseconds to wait for the browser to start up before failing.
#### options.outfile
Type: `String`
Default: `.build`
The directory to stage the chrome app into.
<file_sep>/*globals describe, it, expect, console*/
describe('jasmine-chromeapp', function () {
'use strict';
it('Runs & Reports', function () {
console.warn('A log Message');
expect(true).toBe(true);
});
it('Has helper files copied appropriately', function (done) {
var el = document.createElement('script');
window.callback = function (included) {
expect(included).toBe(true);
delete window.callback;
if (el.parentNode) {
el.parentNode.removeChild(el);
}
done();
};
el.addEventListener('error', window.callback, true);
el.src = '/scripts/test/helper.js';
document.body.appendChild(el);
});
}); | ebe0a1ae966fd758f1103f42fac89dad495247db | [
"Markdown",
"JavaScript"
] | 2 | Markdown | willscott/grunt-jasmine-chromeapp | 8582a56e0fd3c559948656fae157082910c45055 | 235ad8897f095f12bbffff5da970b0956cd3df7a | |
refs/heads/master | <repo_name>Tsukoon/monblog-V4<file_sep>/Vue/gabarit.php
<!doctype html>
<html lang="fr">
<head>
<meta charset="UTF-8" />
<link rel="stylesheet" href="./Css/style.css" />
<title>Mon Blog</title>
</head>
<body>
<div id="global">
<header>
<h1 id="titreBlog"><a href="index.php">Mon Blog</a></h1>
<p>Je vous souhaite la bienvenue sur ce modeste blog.</p>
</header>
<nav>
<section>
<h1>Billets</h1>
<ul>
<li><a href="todo">Billets récents</a></li>
<li><a href="todo">Tous les billets</a></li>
</ul>
</section>
<section>
<h1>Administration</h1>
<ul>
<li><a href="todo">Ecrire un billet</a></li>
</ul>
</section>
</nav>
<div id="contenu">
<?= $contenu ?>
</div> <!-- #contenu -->
<footer id="piedBlog">
Blog réalisé avec PHP, HTML5 et CSS.
</footer>
</div> <!-- #global -->
</body>
</html>
<file_sep>/index.php
<?php
require './Controleur/actions.php';
try {
if ((isset($_GET['action'])) && ($_GET['action']=='afficherBillet')) {
if (isset($_GET['id'])) {
// intval() renvoie la valeur numérique du paramètre ou 0 en cas d'échec
$id = intval($_GET['id']);
if ($id != 0) {
afficherBillets($id);
}
else
throw new Exception("Identifiant de billet incorrect");
}
else
throw new Exception("Aucun identifiant de billet");
}
else {
listerBillets();
}
}
catch (Exception $e) {
afficherErreur($e->getMessage());
}
<file_sep>/README.md
# monblog-V4
Logiciel de blogging
<file_sep>/Modele/modele.php
<?php
class Modele
{
private $monPdo;
private static $bdd=null;
private function __construct()
{
$this->monPdo = new PDO('mysql:host=localhost;dbname=monblog;charset=utf8', 'root', '', array(PDO::ATTR_ERRMODE=>PDO::ERRMODE_EXCEPTION));
}
public static function getBdd()
{
if(self::$bdd==null)
{
self::$bdd = new Modele();
}
return self::$bdd;
}
public function getBillets()
{
$requeteBillets = "select * from T_BILLET order by BIL_ID desc";
$stmtBillets = $this->monPdo->query($requeteBillets);
$billets = $stmtBillets->fetchAll();
return $billets;
}
public function getBillet($idBillet)
{
$requeteBillet = "select * from T_BILLET where BIL_ID= $idBillet";
$stmtBillet = $this->monPdo->query($requeteBillet);
$billet = $stmtBillet->fetch(); // Accès au premier élément résultat
return $billet;
}
}
<file_sep>/Vue/listeBillets.php
<?php ob_start()?>
<?php
foreach ($billets as $billet):
?>
<article>
<header>
<h1 class="titreBillet">
<a href="<?= $lienBillet.$billet['BIL_ID']?>">
<?= $billet['BIL_TITRE'] ?> </a></h1>
<time><?= $billet['BIL_DATE'] ?></time>
</header>
<p><?= $billet['BIL_CONTENU'] ?></p>
</article>
<hr />
<?php endforeach; ?>
<?php $contenu = ob_get_clean() ?>
<?php require 'gabarit.php'; ?>
| f0f1e1c33ef4c35e2b8f4d2df7bf33adc23c6729 | [
"Markdown",
"PHP"
] | 5 | PHP | Tsukoon/monblog-V4 | 9ebc428264884dfbdecd7a68814e438cca1b634c | b1567ab6c8b390a95e739b26c6a530d7f29f90dd | |
refs/heads/main | <repo_name>ChengYuHsieh/explanation_robustness<file_sep>/bbmp.py
import torch
from torch.autograd import Variable
from torchvision import models
import cv2
import sys
import numpy as np
def tv_norm(input, tv_beta):
img = input[0, 0, :, :]
row_grad = torch.mean(torch.abs((img[:-1 , :] - img[1 :, :])).pow(tv_beta))
col_grad = torch.mean(torch.abs((img[: , :-1] - img[: , 1 :])).pow(tv_beta))
return row_grad + col_grad
def bbmp(X, y, model, device):
print('Computing explanation by BBMP...')
tv_beta = 3
learning_rate = 0.1
max_iterations = 1000
l1_coeff = 10
tv_coeff = 0.01
img = X.cpu().numpy().reshape(28, 28)
blurred_img = cv2.GaussianBlur(img, (11, 11), 5)
mask_init = np.ones((28, 28), dtype = np.float32)
mask = torch.Tensor(mask_init).to(device).view(1, 1, 28, 28)
mask.requires_grad = True
optimizer = torch.optim.Adam([mask], lr=learning_rate)
img = torch.Tensor(img).to(device).view(1, 1, 28, 28)
blurred_img = torch.Tensor(blurred_img).to(device).view(1, 1, 28, 28)
category = y.item()
for i in range(max_iterations):
perturbated_input = img.mul(mask) + \
blurred_img.mul(1-mask)
noise = torch.randn(1, 1, 28, 28).to(device)
perturbated_input = perturbated_input + noise
outputs = model(perturbated_input)
loss = l1_coeff*torch.mean(mask) + \
tv_coeff*tv_norm(mask, tv_beta) + outputs[0, category]
optimizer.zero_grad()
loss.backward()
optimizer.step()
# Optional: clamping seems to give better results
mask.data.clamp_(0, 1)
return mask.data.cpu().numpy()<file_sep>/requirements.txt
absl-py==0.7.1
astor==0.8.0
astroid==2.3.1
attrs==19.1.0
backcall==0.1.0
bleach==3.1.0
certifi==2019.6.16
cffi==1.5.2
chardet==2.3.0
cleverhans==3.0.1
cloudpickle==1.2.2
command-not-found==0.3
cycler==0.10.0
dask==0.20.0
decorator==4.3.0
-e git+https://github.com/marcoancona/DeepExplain.git@<PASSWORD>#egg=deepexplain
defusedxml==0.6.0
entrypoints==0.3
fail2ban==0.9.3
future==0.17.1
gast==0.2.2
google-pasta==0.1.7
grpcio==1.21.1
gym==0.14.0
h5py==2.9.0
ipykernel==5.1.1
ipython==7.5.0
ipython-genutils==0.2.0
ipywidgets==7.4.2
isort==4.3.21
jedi==0.14.0
Jinja2==2.10.1
joblib==0.9.4
jsonschema==3.0.1
jupyter==1.0.0
jupyter-client==5.2.4
jupyter-console==6.0.0
jupyter-core==4.5.0
kaggle==1.5.5
Keras==2.2.4
Keras-Applications==1.0.8
Keras-Preprocessing==1.1.0
kiwisolver==1.0.1
language-selector==0.1
lazy-object-proxy==1.4.1
llvmlite==0.29.0
Markdown==3.1.1
MarkupSafe==1.1.1
matplotlib==3.0.1
mccabe==0.6.1
mistune==0.8.4
mnist==0.2.2
nbconvert==5.5.0
nbformat==4.4.0
networkx==2.2
nose==1.3.7
notebook==5.7.8
numba==0.44.1
numpy==1.15.3
olympic==0.1.5
opencv-python==4.1.1.26
pandas==0.24.2
pandocfilters==1.4.2
parso==0.5.0
pexpect==4.7.0
pickleshare==0.7.5
Pillow==5.3.0
ply==3.7
prometheus-client==0.7.1
prompt-toolkit==2.0.9
protobuf==3.8.0
ptyprocess==0.6.0
pycodestyle==2.5.0
pycparser==2.14
pycurl==7.43.0
pyglet==1.3.2
Pygments==2.4.2
pygobject==3.20.0
pyinotify==0.9.6
pylint==2.4.2
pyparsing==2.2.2
pyrsistent==0.15.2
python-apt==1.1.0b1+ubuntu0.16.4.8
python-dateutil==2.7.5
python-debian==0.1.27
python-slugify==3.0.3
python-systemd==231
pytz==2019.1
PyWavelets==1.0.1
PyYAML==5.1.1
pyzmq==18.0.1
qtconsole==4.5.1
requests==2.9.1
scikit-image==0.14.1
scikit-learn==0.17
scipy==1.1.0
screen-resolution-extra==0.0.0
Send2Trash==1.5.0
simplejson==3.8.1
six==1.12.0
ssh-import-id==5.5
tensorboard==1.14.0
tensorboardX==1.8
tensorflow-estimator==1.14.0
tensorflow-gpu==1.14.0
tensorflow-probability==0.7.0
termcolor==1.1.0
terminado==0.8.2
testpath==0.4.2
text-unidecode==1.2
toolz==0.9.0
torch==1.2.0
torchfile==0.1.0
torchvision==0.4.0
tornado==6.0.3
tqdm==4.34.0
traitlets==4.3.2
typed-ast==1.4.0
ufw==0.35
unattended-upgrades==0.1
urllib3==1.24.3
virtualenv==16.6.1
visdom==0.1.8.8
wcwidth==0.1.7
webencodings==0.5.1
websocket-client==0.56.0
Werkzeug==0.15.4
widgetsnbextension==3.4.2
wrapt==1.11.2
xkit==0.0.0
<file_sep>/pgd.py
import torch
from torch.autograd import Variable
import torch.nn as nn
import torch.optim as optim
import torchvision.transforms as transforms
import torchvision.datasets as datasets
import torchvision.transforms.functional as F
import numpy as np
def sample_eps_L2(num_dim, epsilon, N, anchor_masks, device):
if num_dim == 0:
return torch.zeros_like(anchor_masks).float()
normal_deviates = np.random.normal(size=(N, num_dim))
total_dim = normal_deviates.shape[1]
radius = np.linalg.norm(normal_deviates, axis=1, keepdims=True)
normal_deviates = normal_deviates * (np.random.rand(N, total_dim) ** (1.0 / total_dim))
points = normal_deviates * (epsilon) / radius
samples = torch.zeros_like(anchor_masks).float()
samples[~anchor_masks] = torch.FloatTensor(points).to(device).view(-1)
return samples
def pgd(Xs, ys, model, epsilons, norm, niters, step_size, anchor_masks, targeted, targets=None,
box_min=0., box_max=1., verbose=False, multi_start=False, device=None):
if multi_start: # currently only support only example at a time
assert Xs.shape == (1, 1, 28, 28)
assert ys.shape == (1,)
assert epsilons.shape == (1,)
num_start = 1000
anchor_masks = anchor_masks.repeat(num_start, 1, 1, 1)
epsilons = epsilons.repeat(num_start)
num_free_dim = 28*28 - anchor_masks[0].sum()
if norm == 2:
samples = sample_eps_L2(num_free_dim, epsilons[0].item(), num_start, anchor_masks, device)
Xs_pgd = Variable(Xs.data + samples, requires_grad=True)
Xs_pgd.data = torch.clamp(Xs_pgd.data, 0.0, 1.0)
else:
raise ValueError('norm not supported')
if targeted:
targets = targets.repeat(num_start).view(-1, 1)
else:
ys = ys.repeat(num_start).view(-1, 1)
else:
Xs_pgd = Variable(Xs.data, requires_grad=True)
if targeted:
targets = targets.view(-1, 1)
else:
ys = ys.view(-1, 1)
for i in range(niters):
outputs = model(Xs_pgd)
if targeted:
loss = (torch.gather(outputs, 1, targets)).sum()
else:
loss = -((torch.gather(outputs, 1, ys)).sum())
loss.backward()
cur_grad = Xs_pgd.grad.data
eta = cur_grad
eta[anchor_masks] = 0.
zero_mask = eta.view(eta.shape[0], -1).norm(norm, dim=-1) == 0.
eta = (eta * step_size /
eta.view(eta.shape[0], -1).norm(norm, dim=-1).view(-1, 1, 1, 1))
eta[zero_mask] = 0.
Xs_pgd = Variable(Xs_pgd.data + eta, requires_grad=True)
Xs_pgd.data = torch.clamp(Xs_pgd.data, 0.0, 1.0)
eta = Xs_pgd.data - Xs.data
mask = eta.view(eta.shape[0], -1).norm(norm, dim=1) <= epsilons
scaling_factor = eta.view(eta.shape[0], -1).norm(norm, dim=1)
scaling_factor[mask] = epsilons[mask]
eta *= (epsilons / scaling_factor).view(-1, 1, 1, 1)
Xs_pgd.data = Xs.data + eta
ys_pred_pgd = model(Xs_pgd)
if targeted:
successes = ys_pred_pgd.data.max(1)[1] == targets.data.view(-1)
else:
successes = ys_pred_pgd.data.max(1)[1] != ys.data.view(-1)
# print('attack success on {} of {}'.format(successes.sum().item(), len(Xs_pgd)))
if multi_start:
success = successes.any(dim=0, keepdim=True)
success_Xs_pgd = Xs_pgd.data[successes][[0]] if success.item() else Xs_pgd.data[[0]]
return success, success_Xs_pgd
else:
return successes, Xs_pgd.data
<file_sep>/README.txt
The main file is "mnist_example.ipynb". Please use Jupyter notebook to open the file.
We also provide an HTML file "mnist_example.html" containing the executed results of the notebook. The file could be open in standard web browsers.
The code is written in Python 3.
- Install python dependencies:
1. `pip install -r requirements.txt`
Reference:
1. https://github.com/PAIR-code/saliency
2. https://github.com/marcoancona/DeepExplain
3. https://github.com/ruthcfong/pytorch-explain-black-box
4. https://github.com/chihkuanyeh/saliency_evaluation<file_sep>/expl_utils.py
import os
import sys
import math
import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
from pgd import pgd
from sklearn import linear_model
def binary_search(cond, Xs, ys, epsilons, anchor_masks, targeted, targets,
upper=np.inf, lower=0.0, tol=0.00001, max_steps=100, upscale_mult=10.0,
downscale_mult=10.0, lower_limit=1e-8, upper_limit=1e+3):
"""batch binary search for attack approximation of robustness
"""
batch_size = len(Xs)
upper_not_found = np.full(batch_size, True)
lower_not_found = np.full(batch_size, True)
upper = np.full(batch_size, upper)
lower = np.full(batch_size, lower)
Xs_pgd = torch.zeros_like(Xs) # placeholder for perturbed images
step = 0
run_idx = np.arange(batch_size)
while len(run_idx) != 0:
if targeted:
cur_successes, cur_Xs_pgd = cond(Xs[run_idx], ys[run_idx], epsilons[run_idx], anchor_masks[run_idx],
targeted, targets[run_idx])
else:
cur_successes, cur_Xs_pgd = cond(Xs[run_idx], ys[run_idx], epsilons[run_idx], anchor_masks[run_idx],
targeted, None)
for cur_i, run_i in enumerate(run_idx):
if cur_successes[cur_i]: # success at current value (attack success)
Xs_pgd[run_i] = cur_Xs_pgd[cur_i]
if lower_not_found[run_i]: # always success, we have not found the true lower bound
upper[run_i] = epsilons[run_i]
epsilons[run_i] /= downscale_mult # downscale search range, try to find an pseudo lower bound
else:
upper[run_i] = epsilons[run_i]
epsilons[run_i] = 0.5 * (lower[run_i] + upper[run_i])
upper_not_found[run_i] = False # when initial is a success we always have a valid upper bound
else:
if upper_not_found[run_i]:
lower[run_i] = epsilons[run_i]
epsilons[run_i] *= upscale_mult
else:
lower[run_i] = epsilons[run_i]
epsilons[run_i] = 0.5 * (lower[run_i] + upper[run_i]) # when initial is a failure we have a pseudo lower bound
lower_not_found[run_i] = False
step += 1
not_meet_tolerance = (upper - lower) > tol # not meet tolerance
not_exceed_limit = np.logical_and((epsilons < upper_limit), (epsilons > lower_limit)) # not exceed limit
run_cond = np.logical_and(not_meet_tolerance, not_exceed_limit)
run_idx = np.arange(batch_size)[run_cond]
if step >= max_steps:
break
return upper, Xs_pgd
def uniform_sampling(X, num_samples, cur_anchor=None):
"""direct uniform sampling
"""
if cur_anchor is not None:
# sample from the rest of un-anchored features
samples = np.repeat(cur_anchor.reshape(1, -1), num_samples, axis=0)
samples_flatten = np.random.choice([0, 1], size=int(num_samples * (28*28 - cur_anchor.sum())), replace=True, p=[0.8, 0.2])
samples[samples!=1] = samples_flatten
else:
samples = np.random.choice([0,1], size=(num_samples, X.shape[1], X.shape[2], X.shape[3]), replace=True, p=[0.8, 0.2])
return samples, samples
def iterative_sampling(X, num_samples, cur_anchor, num_new_anchor):
samples = np.repeat(cur_anchor.reshape(1, -1), num_samples, axis=0)
samples_flatten = np.zeros((num_samples, int(28*28-cur_anchor.sum())))
samples_flatten[:, :num_new_anchor] = 1
samples_flatten = np.apply_along_axis(np.random.permutation, 1, samples_flatten)
samples[samples == 0] = samples_flatten.reshape(-1)
Zs = samples_flatten
return samples, Zs
def evaluate_robustness(Xs, ys, model, targeted, targets, norm, anchors, eps_start, step_size, niters, device,
reverse_anchor=False, batch_size=10000):
"""evaluating robustness for different anchors for a given X"""
assert Xs.shape == (1, 1, 28, 28)
assert ys.shape == (1,)
if targeted:
assert targets.shape == (1,)
total = range(len(anchors))
num_batch = len(total) // batch_size
if len(total) % batch_size != 0:
num_batch += 1
batches = [total[i*batch_size:(i+1)*batch_size] for i in range(num_batch)]
robust_ub = np.full(len(total), np.inf)
for batch in batches:
Xs_repeat = Xs.repeat(len(batch), 1, 1, 1)
ys_repeat = ys.repeat(len(batch))
eps_repeat = np.full(len(batch), eps_start)
if targeted:
targets_repeat = targets.repeat(len(batch))
cur_anchors = torch.BoolTensor(anchors[batch]).to(device)
if reverse_anchor:
cur_anchors = ~cur_anchors
def binary_search_cond(Xs, ys, epsilons, anchor_masks, targeted, targets):
epsilons = torch.FloatTensor(epsilons).to(device)
successes, Xs_pgd = pgd(Xs, ys, model, epsilons, norm, niters, step_size, anchor_masks, targeted, targets=targets,
box_min=0., box_max=1., verbose=False, multi_start=False, device=device)
return successes, Xs_pgd
if targeted:
robust_ub[batch], _ = binary_search(binary_search_cond, Xs_repeat, ys_repeat, eps_repeat, cur_anchors, targeted, targets_repeat, tol=0.001, max_steps=25)
else:
robust_ub[batch], _ = binary_search(binary_search_cond, Xs_repeat, ys_repeat, eps_repeat, cur_anchors, targeted, None, tol=0.001, max_steps=25)
return robust_ub
def greedy(X, y, model, targeted, target, norm, eps_start, step_size, niters, device, reverse_anchor, percentage=0.3, writer=None):
anchor_map = np.zeros(28 * 28)
X_np = X.cpu().numpy()
cur_anchor = np.zeros(28 * 28)
cur_val = 1000 # for greedy ranking
while cur_anchor.sum() < 784 * percentage:
n_sample = int(784 - cur_anchor.sum())
Zs = np.eye(n_sample)
anchor_samples = np.repeat(cur_anchor.reshape(1, -1), n_sample, axis=0)
anchor_samples[anchor_samples != 1] = Zs.reshape(-1)
anchor_samples = anchor_samples.reshape(n_sample, 1, 28, 28)
robust_ub = evaluate_robustness(X, y, model, targeted, target, norm, anchor_samples, eps_start,
step_size, niters, device, reverse_anchor=reverse_anchor)
robust_ub[robust_ub == np.inf] = np.linalg.norm(X_np - np.zeros_like(X_np))
ks = np.ones(n_sample)
feature_robust = kernel_regression(Zs, ks, robust_ub)
if reverse_anchor:
max_idx = feature_robust.argsort()[:int(784*0.05)]
else:
max_idx = feature_robust.argsort()[-int(784*0.05):] # select four features at a single greedy step (speed up)
max_idx = np.where(cur_anchor != 1)[0][max_idx]
cur_anchor[max_idx] = 1
anchor_map[max_idx] = cur_val
cur_val -= 2
anchor_map = anchor_map.reshape(1, 1, 28, 28)
return anchor_map
def one_step_banzhaf(X, y, model, targeted, target, norm, eps_start, step_size, niters, device, reverse_anchor, writer=None):
n_sample = 10000
# anchor_samples = uniform_sampling(X, num_samples)
X_np = X.cpu().numpy()
Xs = np.repeat(X_np.reshape(1, -1), n_sample, axis=0)
Zs = np.apply_along_axis(shap_sampling, 1, Xs, p=0.5)
anchor_samples = Zs.reshape(n_sample, 1, 28, 28)
robust_ub = evaluate_robustness(X, y, model, targeted, target, norm, anchor_samples, eps_start,
step_size, niters, device, reverse_anchor=reverse_anchor)
robust_ub[robust_ub == np.inf] = np.linalg.norm(X_np - np.zeros_like(X_np))
ks = np.ones(n_sample)
feature_robust = kernel_regression(Zs, ks, robust_ub)
if reverse_anchor:
feature_robust = -feature_robust
if writer is None:
return feature_robust
else:
raise NotImplementedError()
fig, name = vis(X, feature_robust, np.zeros_like(X), 'ls_regression', None, tensorboard=True, rescale=False)
writer.add_figure(name, fig)
def forward_batch(model, input, batchsize):
inputsize = input.shape[0]
for count in range((inputsize - 1) // batchsize + 1):
end = min(inputsize, (count + 1) * batchsize)
if count == 0:
tempinput = input[count * batchsize:end]
out = model(tempinput.cuda())
out = out.data.cpu().numpy()
else:
tempinput = input[count * batchsize:end]
temp = model(tempinput.cuda()).data
out = np.concatenate([out, temp.cpu().numpy()], axis=0)
return out
def shap(X, label, pdt, model, n_sample):
X = X.cpu().numpy()
Xs = np.repeat(X.reshape(1, -1), n_sample, axis=0)
Xs_img = Xs.reshape(n_sample, 1, 28, 28)
Zs = np.apply_along_axis(sample_shap_Z, 1, Xs)
Zs_real = np.copy(Zs)
Zs_real[Zs == 1] = Xs[Zs == 1]
Zs_real_img = Zs_real.reshape(n_sample, 1, 28, 28)
Zs_img = Variable(torch.tensor(Xs_img - Zs_real_img), requires_grad=False).float()
out = forward_batch(model, Zs_img, 5000)
ys = out[:, label]
ys = pdt.data.cpu().numpy() - ys
ks = np.apply_along_axis(shap_kernel, 1, Zs, X=X.reshape(-1))
expl = kernel_regression(Zs, ks, ys)
return expl
def kernel_regression(Is, ks, ys):
"""
*Inputs:
I: sample of perturbation of interest, shape = (n_sample, n_feature)
K: kernel weight
*Return:
expl: explanation minimizing the weighted least square
"""
n_sample, n_feature = Is.shape
IIk = np.matmul(np.matmul(Is.transpose(), np.diag(ks)), Is)
Iyk = np.matmul(np.matmul(Is.transpose(), np.diag(ks)), ys)
expl = np.matmul(np.linalg.pinv(IIk), Iyk)
return expl
def nCr(n, r):
f = math.factorial
return f(n) // f(r) // f(n-r)
def shap_sampling(X, p=0.5):
nz_ind = np.nonzero(X)[0]
nz_ind = np.arange(X.shape[0])
num_nz = len(nz_ind)
bb = 0
while bb == 0 or bb == num_nz:
aa = np.random.rand(num_nz)
bb = np.sum(aa > p)
sample_ind = np.where(aa > p)
Z = np.zeros(len(X))
Z[nz_ind[sample_ind]] = 1
return Z
def sample_shap_Z(X):
nz_ind = np.nonzero(X)[0]
nz_ind = np.arange(X.shape[0])
num_nz = len(nz_ind)
bb = 0
while bb == 0 or bb == num_nz:
aa = np.random.rand(num_nz)
bb = np.sum(aa > 0.5)
sample_ind = np.where(aa > 0.5)
Z = np.zeros(len(X))
Z[nz_ind[sample_ind]] = 1
return Z
def shap_kernel(Z, X):
M = X.shape[0]
z_ = np.count_nonzero(Z)
return (M-1) * 1.0 / (z_ * (M - 1 - z_) * nCr(M - 1, z_))
def greedy_as(X, y, model, targeted, target, norm, eps_start, step_size, niters, device, reverse_anchor, percentage=0.5):
print('Computing explanation by Greedy-AS...')
anchor_map = np.zeros(28 * 28)
X_np = X.cpu().numpy()
cur_anchor = np.zeros(28 * 28)
cur_val = 1000. # for greedy ranking
while cur_anchor.sum() < 784 * percentage:
print('number of relevant features selected:', cur_anchor.sum())
n_sample = 5000
anchor_samples, Zs = uniform_sampling(X_np, n_sample, cur_anchor)
anchor_samples = anchor_samples.reshape(n_sample, 1, 28, 28)
robust_ub = evaluate_robustness(X, y, model, targeted, target, norm, anchor_samples, eps_start,
step_size, niters, device, reverse_anchor=reverse_anchor)
robust_ub[robust_ub == np.inf] = np.linalg.norm(X_np - np.zeros_like(X_np))
ks = np.ones(n_sample)
feature_robust = kernel_regression(Zs, ks, robust_ub)
if reverse_anchor:
max_idx = feature_robust[cur_anchor != 1].argsort()[:int(784*0.05)]
else:
max_idx = feature_robust[cur_anchor != 1].argsort()[-int(784*0.05):]
max_idx = np.where(cur_anchor != 1)[0][max_idx]
cur_anchor[max_idx] = 1
anchor_map[max_idx] = cur_val
cur_val -= 2
anchor_map = anchor_map.reshape(1, 1, 28, 28)
return anchor_map
def plot_eval_curve(X, y, model, target, norm, eps_start, step_size, niters, writer, anchor, method, reverse_anchor):
plot_step = 0
for i in range(5, 50, 5):
threshold = np.percentile(anchor, 100-i)
cur_anchor = np.copy(anchor)
cur_anchor[anchor <= threshold] = 0
cur_anchor[anchor > threshold] = 1
if reverse_anchor:
cur_anchor = np.ones_like(cur_anchor) - cur_anchor
def binary_search_cond(current_epsilons, cur_anchor_masks):
success, X_pgd, margin = pgd(None, X.repeat(len(current_epsilons), 1, 1, 1), y.repeat(len(current_epsilons)),
[model], current_epsilons, norm, niters=niters, step_size=step_size, alpha=[1],
anchor_idx=cur_anchor_masks, target=target.repeat(len(current_epsilons)), multi_start=True, multi_success=False)
return success, X_pgd, margin
robust_ub, X_pgd, _ = binary_search(binary_search_cond, np.full((1, 1, 28, 28), eps_start), cur_anchor, tol=0.001, max_steps=25)
robust_ub = robust_ub[0]
print('robustness:', robust_ub)
fig, name = vis(X, cur_anchor, X_pgd, method, robust_ub, tensorboard=True, plot_curve=True)
writer.add_scalar(name+'_robust', robust_ub, plot_step)
writer.add_figure(name+'_vis', fig, plot_step)
plot_step += 1
def empty_expl(Xs):
anchor_maps = np.zeros_like(Xs)
return anchor_maps
def random_expl(Xs):
anchor_maps = np.random.rand(*Xs.shape)
return anchor_maps
def saliency_expl(expl_method, Xs, ys, model):
"""currently only suppor one example at a time"""
print('Computing explanation by {}...'.format(expl_method))
assert Xs.shape[0] == 1
assert ys.shape[0] == 1
if expl_method == 'SG':
sg_r = 0.2
sg_N = 500
given_expl = 'Grad'
else:
sg_r = None
sg_N = None
given_expl = None
anchor_maps, _ = get_explanation_pdt(Xs, model, ys, expl_method, sg_r=sg_r, sg_N=sg_N, given_expl=given_expl, binary_I=False)
anchor_maps = np.abs(anchor_maps)
return anchor_maps
def get_explanation_pdt(image, model, label, exp, sg_r=None, sg_N=None, given_expl=None, binary_I=False):
label = label[0]
image_v = Variable(image, requires_grad=True)
model.zero_grad()
out = model(image_v)
pdtr = out[:, label]
pdt = torch.sum(out[:, label])
if exp == 'Grad':
pdt.backward()
grad = image_v.grad
expl = grad.data.cpu().numpy()
if binary_I:
expl = expl * image.cpu().numpy()
elif exp == 'LOO':
mask = torch.eye(784).view(784, 1, 28, 28).bool()
image_loo = image.repeat(784, 1, 1, 1)
image_loo[mask] = 0
image_loo = Variable(image_loo, requires_grad=True)
pred = model(image_loo)[:, label]
expl = pred - pdtr.item()
expl = expl.data.cpu().numpy()
elif exp == 'SHAP':
expl = shap(image.cpu(), label, pdt, model, 20000)
elif exp == 'IG':
for i in range(10):
image_v = Variable(image * i/10, requires_grad=True)
model.zero_grad()
out = model(image_v)
pdt = torch.sum(out[:, label])
pdt.backward()
grad = image_v.grad
if i == 0:
expl = grad.data.cpu().numpy() / 10
else:
expl += grad.data.cpu().numpy() / 10
if binary_I:
expl = expl * image.cpu().numpy()
else:
raise NotImplementedError('Explanation method not supported.')
return expl, pdtr
| ab478434ffad1ad222ac4c0af4f8cad358c56fcc | [
"Python",
"Text"
] | 5 | Python | ChengYuHsieh/explanation_robustness | a8e5ef269ced75e34d554b71ad1dacc2c04088fc | aa58af55ba926a58310972ce219f10314ef6b8e7 | |
refs/heads/master | <repo_name>itsh01/BatcherService<file_sep>/spec/batcherSpec.js
describe("batcher test suit:", function () {
var batcher, dataStructure;
function setUpTest() {
dataStructure = buildDataStructure();
batcher = buildBatcher(dataStructure);
}
function generateDataSequence(length) {
return _.range(length).map(function (i) {
var obj = {};
obj[i] = i;
return obj;
});
}
function assumeAmountOfRegisteredCallbacks(num) {
var callbacks = _.range(num).map(function (i) {
return jasmine.createSpy('callback_' + i);
});
callbacks.forEach(function (f) {
batcher.uponCompletion(f);
});
return callbacks;
}
function setSequence(seq) {
seq.forEach(function (dataToSet) {
batcher.setData(dataToSet);
});
}
function assumeNumOfRegisteredOperation(numOfOperations) {
var dataObjectsArr = generateDataSequence(numOfOperations);
setSequence(dataObjectsArr);
}
function expectCallbacksNotHaveBeenCalled(callbacks) {
callbacks.forEach(function (f) {
expect(f).not.toHaveBeenCalled();
});
}
function expectToBeCalledOnce(callbacks) {
expectNumOfCalls(callbacks, 1);
}
function expectNumOfCalls(callbacks, numOfCalls) {
callbacks.forEach(function (f) {
expect(f.calls.count()).toBe(numOfCalls);
});
}
describe('flushing:', function () {
beforeEach(setUpTest);
it("should combine several operations to one setData invocation", function () {
assumeNumOfRegisteredOperation(3);
expect(dataStructure.getCounter()).toBe(0);
batcher.flush();
expect(dataStructure.getCounter()).toBe(1);
});
it("should return the number of operation flushed", function () {
var operationCount = 5;
assumeNumOfRegisteredOperation(operationCount);
expect(batcher.flush()).toBe(operationCount);
});
it("should merge the dataToSet into one object (on multiple keys remember only the last", function () {
setSequence([{'multipleKey': 'firstValue'}, {'multipleKey': 'lastValue'}]);
batcher.flush();
expect(dataStructure._data).toEqual({'multipleKey': 'lastValue'});
});
});
describe('UponCompletion:', function () {
beforeEach(setUpTest);
it("should call the callback after flushing", function () {
var callbacks = assumeAmountOfRegisteredCallbacks(1);
assumeNumOfRegisteredOperation(3);
expectCallbacksNotHaveBeenCalled(callbacks);
batcher.flush();
expectToBeCalledOnce(callbacks);
});
it("should allowed multiple callbacks", function () {
var callbacks = assumeAmountOfRegisteredCallbacks(2);
assumeNumOfRegisteredOperation(3);
expectCallbacksNotHaveBeenCalled(callbacks);
batcher.flush();
expectToBeCalledOnce(callbacks);
});
it("should not called the callbacks when nothing were flushed (even though flush() was called)", function () {
var callbacks = assumeAmountOfRegisteredCallbacks(2);
batcher.flush();
expectCallbacksNotHaveBeenCalled(callbacks);
});
});
describe('Async execution:', function () {
beforeEach(setUpTest);
it("without flushing, the setData operation is asynchronous", function () {
assumeNumOfRegisteredOperation(3);
expect(dataStructure._data).toEqual({});
expect(dataStructure.getCounter()).toBe(0);
});
it("setData should flush itself on the next tick", function (done) {
assumeNumOfRegisteredOperation(3);
expect(dataStructure.getCounter()).toBe(0);
setTimeout(function () {
expect(dataStructure.getCounter()).toBe(1);
done();
}, 200);
});
it("setData on different ticks should NOT be batched to one invocation", function (done) {
assumeNumOfRegisteredOperation(3);
setTimeout(function () {
assumeNumOfRegisteredOperation(3);
}, 0);
setTimeout(function () {
expect(dataStructure.getCounter()).toBe(2);
done();
}, 200);
});
it("calling flush() after self-flusing should have no effect (because there shouldn't be anything to flush)", function (done) {
assumeNumOfRegisteredOperation(3);
setTimeout(function () {
var numOfFlushedOperation = batcher.flush();
expect(dataStructure.getCounter()).toBe(1);
expect(numOfFlushedOperation).toBe(0);
done();
}, 200);
});
});
});
<file_sep>/README.md
# Batcher-Service
Write your implementation inside `project/src/batcher.js` file.
In order to run the tests, open `project/SpecRunner.html` in you favorite browser. To rerun the tests - refresh the page.
You can find the spec for this service [here](https://docs.google.com/a/wix.com/document/d/1UruXwGcvYDrfLKVre92<KEY>/pub)
| 5e8fcc83b3ca3597b536390f0de187259ff00331 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | itsh01/BatcherService | 4539e7b0f73ae3c61bf2ba43b007dbcf57cd7855 | 22f8c930c4eb93e8114b39e7fc0a26fcb0d3c34b | |
refs/heads/master | <repo_name>vj2014/ProgrammingAssignment2<file_sep>/cachematrix.R
## Calculating inverse of matrix(particularly big matrix) is a costly operation.The aim of this
## R script is to calculate the inverse of a matrix and cache it so that it wont be calculated
## everytime.
## This function get a matrix as input and returns a special vector containing function which is used
## to set and get matrix , set and get the inverse of the matrix. inverse of the matrix is cached
## in a different enviroment from the enviroment where the function is defined.
makeCacheMatrix <- function(x = matrix()) {
i <- NULL
set <- function(y) {
x <<- y
i <<- NULL
}
get <- function() x
setinverse <- function(inverse) i <<- inverse
getinverse <- function() i
list(set = set, get = get,
setinverse = setinverse,
getinverse = getinverse)
}
## This function gets the special vector created by makecacheMatrix function as input,returns the
## inverse of the matrix either by taking it from the special vector if already cached or by
## calculating it if not already cached.
cacheSolve <- function(x, ...) {
i <- x$getinverse()
if(!is.null(i)) {
message("getting cached data")
return(i)
}
data <- x$get()
i <- solve(data, ...)
x$setinverse(i)
i
}
| 64e2344b922c278101f02ef46a04ad956bfb94d1 | [
"R"
] | 1 | R | vj2014/ProgrammingAssignment2 | bda6b94230c3187686fbc93dc5d1ccfdcc3bbead | db7b497d93f4a7ec93f2327d6f556ca319b9e065 | |
refs/heads/master | <repo_name>edwinluisi/first_one<file_sep>/README.md
# first_oneBe sure to have your SSH key set up and that your public key is added to yout GitHub account.
<file_sep>/apache_dplymnt_bluink.sh
#!/bin/bash
############################################
# Script to deploy Apache at Bluink env
# Script name: apache_dplymnt_bluink.sh
# Author: <NAME>
# Date: Mar 19, 2022
#
############################################
# Redirect stdout/stderr to a file
echo -e "The output information regarding this execution can be found in the apache_dplymnt_bluink-`date +"%Y-%m-%d_%H%M%S"`.log file.\n"
exec > apache_dplymnt_bluink-`date +"%Y-%m-%d_%H%M%S"`.log 2>&1
LIGHT_MAGENTA='\033[0;95m'
MAGENTA='\033[1;35m'
GREEN='\033[1;32m'
RED='\033[1;31m'
NC='\033[0m' # No Color
APACHE_DROOT_FILE='/etc/apache2/sites-available/000-default.conf'
APACHE_SVC_STATUS='systemctl status apache2.service'
if [ "$EUID" -eq 0 ]
then
echo -e "Performing prerequisite verification...\n"
if grep -q 'DocumentRoot /var/www/html/public' ${APACHE_DROOT_FILE} ;
then
echo -e "${GREEN}SUCCESS${NC} - The 'DocumentRoot /var/www/html/public' string was found in the ${APACHE_DROOT_FILE} configuration file.\n"
else
echo -e "${RED}FAIL${NC} - The 'DocumentRoot /var/www/html/public' string was not found in the configuration file.\n"
exit
fi
if [[ $(apache2 -v) ]]
then
echo -e "${GREEN}SUCCESS${NC} - The following Apache version is installed:\n`apache2 -v`\n"
else
echo -e "${RED}FAIL${NC} - Apache installation not found!\n"
exit
fi
if [[ `${APACHE_SVC_STATUS}` == *"active (running)"* ]]
then
echo -e "${GREEN}SUCCESS${NC} - Apache is up and running!\n"
else
echo -e "${RED}FAIL${NC} - Apache is not running!\n"
exit
fi
else
echo "This script can only be executed by root!"
exit
fi
echo -e "\nDeploying..."
### BEGIN - Check and create the symlink ###
#
echo -e "${MAGENTA}Cheking symlink: /var/www/html/public -> /var/www/html/new-folder${NC}"
chk_symlink? () {
test "$(readlink "${1}")";
}
SYMLINK_FILE=/var/www/html/public
if chk_symlink? "${SYMLINK_FILE}"; then
echo -e "The $SYMLINK_FILE exists already and it is a valid symlink: \n`ls -ld ${SYMLINK_FILE}`"
else
echo -e "There is no $SYMLINK_FILE symlink pointing to /var/www/html/new-folder."
echo -e "${LIGHT_MAGENTA}Creating symlink: /var/www/html/public -> /var/www/html/new-folder${NC}"
ln -s /var/www/html/new-folder /var/www/html/public
echo -e "The $SYMLINK_FILE symlink is now created: \n`ls -ld ${SYMLINK_FILE}`"
fi
#
### END - Check and create the symlink ###
### BEGIN - Untar the new-folder ###
#
NFOLDER_FILE='/var/www/html/new-folder/index.html'
echo -e "${MAGENTA}\n\nChecking if the ${NFOLDER_FILE} file exists already...${NC}"
if [ -f "$NFOLDER_FILE" ]; then
echo "The $NFOLDER_FILE exists already."
else
echo -e "The $NFOLDER_FILE does not exist and will be created..."
echo -e "${LIGHT_MAGENTA}Untarring new-folder.tar.gz file with webserver files in it to /var/www/html...${NC}"
tar -xzvf new-folder.tar.gz -C /var/www/html/
ls -ltr /var/www/html/new-folder/
fi
#
### END - Untar the new-folder ###
### BEGIN - new-folder owner ###
#
echo -e "${MAGENTA}\n\nMaking sure root is the /var/www/html/new-folder owner...${NC}"
chown root:root /var/www/html/new-folder
ls -ld /var/www/html/new-folder
#
### END - new-folder owner ###
### BEGIN - Basic request to the deployed page ###
#
echo -e "${MAGENTA}\n\nCalling for the new webserver content...${NC}"
curl localhost
echo -e "\n\n"
#
### END - Basic request to the deployed page ### | 7d8f36724a9bd12db42b3940b50e0012fd74508b | [
"Markdown",
"Shell"
] | 2 | Markdown | edwinluisi/first_one | 67794ed1e9c0fa4b41ee881257f7fa36ce936b83 | c231c3ee199ed365f6bedfef7526f8211a9fb9da | |
refs/heads/master | <file_sep>"use strict";
var path = require('path');
var fs = require('fs-extra');
var cwd = process.cwd();
var toArr = function toArr(val) {
return Array.isArray(val) ? val : val ? [val] : [];
};
module.exports = function (options) {
var rendererPath = options.renderer ? options.renderer : path.resolve(cwd, './doc-scripts.renderer.js');
var hasRenderer = false;
try {
fs.accessSync(rendererPath);
hasRenderer = true;
} catch (e) {}
var code = "\n var React = require('react')\n var ReactDOM = require('react-dom')\n var ReactDocRenderer = require('" + (hasRenderer ? rendererPath : 'react-doc-renderer') + "')\n " + toArr(options.requires).map(function (path) {
return "require(\"" + path + "\")";
}) + "\n ReactDocRenderer = ReactDocRenderer.__esModule ? ReactDocRenderer.default : ReactDocRenderer\n\n var docs = [\n " + options.docs.map(function (path) {
return "(function(){\n var component = require(\"" + path + "\");\n return {\n path:\"" + path + "\",\n component:component,\n meta:component.meta\n }\n })()";
}).join(',') + "\n ];\n \n ReactDOM.render(\n React.createElement(ReactDocRenderer,{docs:docs}),\n document.getElementById('root')\n );\n ";
return {
code: code
};
};<file_sep>const path = require('path')
const fs = require('fs-extra')
const cwd = process.cwd()
const toArr = val => (Array.isArray(val) ? val : val ? [val] : [])
module.exports = function(options) {
const rendererPath = options.renderer
? options.renderer
: path.resolve(cwd, './doc-scripts.renderer.js')
let hasRenderer = false
try {
fs.accessSync(rendererPath)
hasRenderer = true
} catch (e) {}
const code = `
var React = require('react')
var ReactDOM = require('react-dom')
var ReactDocRenderer = require('${
hasRenderer ? rendererPath : 'react-doc-renderer'
}')
${toArr(options.requires).map(path => {
return `require("${path}")`
})}
ReactDocRenderer = ReactDocRenderer.__esModule ? ReactDocRenderer.default : ReactDocRenderer
var docs = [
${options.docs
.map(path => {
return `(function(){
var component = require("${path}");
return {
path:"${path}",
component:component,
meta:component.meta
}
})()`
})
.join(',')}
];
ReactDOM.render(
React.createElement(ReactDocRenderer,{docs:docs}),
document.getElementById('root')
);
`
return {
code
}
}
| 769f8e5bfb2041f847e34e7b5e45f6fb135fe1a5 | [
"JavaScript"
] | 2 | JavaScript | uncledb/doc-scripts | 00e4af2c28a0cd115025af2b32ef0edf8a9205a7 | 8520328c04ca0b5a85ed234b0b43be3ed999e50e | |
refs/heads/master | <file_sep>package com.minutes111.launchmode.ui;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.support.v7.app.AlertDialog;
import android.support.v7.app.AppCompatActivity;
import android.text.method.ScrollingMovementMethod;
import android.util.Log;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.MenuItem;
import android.view.View;
import android.widget.TextView;
import com.minutes111.launchmode.BaseApplication;
import com.minutes111.launchmode.R;
public abstract class BaseActivity extends AppCompatActivity {
private String[] intentFlagsText = {"CLEAR_TOP", "CLEAR_WHEN_TASK_RESET", "EXCLUDE_FROM_RECENTS",
"FORWARD_RESULT", "MULTIPLE_TASK", "NEW_TASK", "NO_HISTORY", "NO_USER_ACTION", "PREVIOUS_IS_TOP",
"REORDER_TO_FRONT", "RESET_TASK_IF_NEEDED", "SINGLE_TOP"};
private int[] intentFlags = {Intent.FLAG_ACTIVITY_CLEAR_TOP, Intent.FLAG_ACTIVITY_CLEAR_WHEN_TASK_RESET,
Intent.FLAG_ACTIVITY_EXCLUDE_FROM_RECENTS, Intent.FLAG_ACTIVITY_FORWARD_RESULT,
Intent.FLAG_ACTIVITY_MULTIPLE_TASK, Intent.FLAG_ACTIVITY_NEW_TASK, Intent.FLAG_ACTIVITY_NO_HISTORY,
Intent.FLAG_ACTIVITY_NO_USER_ACTION, Intent.FLAG_ACTIVITY_PREVIOUS_IS_TOP,
Intent.FLAG_ACTIVITY_REORDER_TO_FRONT, Intent.FLAG_ACTIVITY_RESET_TASK_IF_NEEDED,
Intent.FLAG_ACTIVITY_SINGLE_TOP};
private static final String LOG_TAG = "myLogs";
private static final String LOG_TAG_M = "mLog";
private TextView mLifeCycle;
private BaseApplication mApp;
private StringBuilder lifecycleActivity = new StringBuilder();
private Handler mHandler = new Handler();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.base_activity);
View activityLayout = findViewById(R.id.lay_main);
activityLayout.setBackgroundResource(getBackgroundColor());
mApp = (BaseApplication) getApplication();
TextView tvHeader = (TextView) findViewById(R.id.txt_header);
mLifeCycle = (TextView) findViewById(R.id.txt_lifecycle);
mLifeCycle.setMovementMethod(new ScrollingMovementMethod());
showMethodStack();
tvHeader.setText(getLaunchMode());
mApp = (BaseApplication) getApplication();
mApp.pushToStack(this);
}
@Override
protected void onResume() {
showMethodStack();
Runnable stackActivityDrawer = new StackActivityDrawer(this);
mHandler.postDelayed(stackActivityDrawer, 500);
super.onResume();
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
showMethodStack();
MenuInflater inflater = getMenuInflater();
inflater.inflate(R.menu.menu_base, menu);
return true;
}
@Override
public boolean onPrepareOptionsMenu(Menu menu) {
showMethodStack();
MenuItem item = menu.findItem(R.id.menu_item_filter_mode);
String title = "Turn IntentFilter mode " + (mApp.isIntentFilterMode() ? "OFF" : "ON");
item.setTitle(title);
return super.onPrepareOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
showMethodStack();
switch (item.getItemId()) {
case R.id.menu_item_filter_mode:
mApp.changeIntentFilterMode();
break;
}
return super.onOptionsItemSelected(item);
}
@Override
public void onContentChanged() {
showMethodStack();
super.onContentChanged();
}
@Override
protected void onPause() {
showMethodStack();
super.onPause();
}
@Override
protected void onRestart() {
showMethodStack();
super.onRestart();
}
@Override
protected void onStart() {
showMethodStack();
super.onStart();
}
@Override
protected void onStop() {
showMethodStack();
super.onStop();
}
@Override
protected void onDestroy() {
showMethodStack();
mApp.removeFromStack(this);
super.onDestroy();
}
private String getLaunchMode() {
return "[" + hashCode() + "] " + getClass().getSimpleName();
}
public void onClickButton(View view) {
if (mApp.isIntentFilterMode()) {
showButtonDialog(view);
} else {
startActivity(getNewIntent(view));
}
}
private Intent getNewIntent(View view) {
Intent intent;
switch (view.getId()) {
case R.id.btn_singleTop:
intent = new Intent(this, SingleTop.class);
break;
case R.id.btn_singleTask:
intent = new Intent(this, SingleTask.class);
break;
case R.id.btn_singleInstance:
intent = new Intent(this, SingleInstance.class);
break;
default:
intent = new Intent(this, Standard.class);
break;
}
return intent;
}
private void showButtonDialog(View view) {
AlertDialog.Builder adb = new AlertDialog.Builder(this);
adb.setTitle("Selection mode");
adb.setCancelable(true);
DialogInterface.OnMultiChoiceClickListener listener = new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
Log.d(LOG_TAG, String.valueOf(which));
}
};
adb.setMultiChoiceItems(intentFlagsText, null, listener);
adb.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.d(LOG_TAG, String.valueOf(which));
}
});
adb.show();
}
private void showMethodStack() {
String methodName = Thread.currentThread().getStackTrace()[3].getMethodName();
Log.d(LOG_TAG_M, methodName);
lifecycleActivity.append(methodName).append("\n");
if (mLifeCycle != null) {
mLifeCycle.setText(lifecycleActivity);
}
}
public abstract int getBackgroundColor();
}
<file_sep>package com.minutes111.launchmode.ui;
import com.minutes111.launchmode.R;
public class Standard extends BaseActivity{
@Override
public int getBackgroundColor() {
return R.color.standard;
}
}
| a8878778b7842296aba7961ba00c2270fadad7e5 | [
"Java"
] | 2 | Java | dan1603/LaunchMode | 729debc6b8ae4785ac17ac30628bb505dc11e2a3 | 0bc3907f0d17f36509285d1ed053821d6cbaacaa | |
refs/heads/master | <file_sep>import * as Msal from "msal";
import { msalConfig } from "./conf";
const loginRequest = {
scopes: ["openid", "profile", "User.Read"]
};
const graphConfig = {
graphMeEndpoint: "https://graph.microsoft.com/v1.0/me"
};
const myMSALObj = new Msal.UserAgentApplication(msalConfig);
const callMSGraph = (endpoint, token, callback) => {
const headers = new Headers();
const bearer = `Bearer ${token}`;
headers.append("Authorization", bearer);
const options = {
method: "GET",
headers: headers
};
alert("request made to Graph API at: " + new Date().toString());
fetch(endpoint, options)
.then(response => response.json())
.then(response => callback(response, endpoint))
.catch(error => alert(JSON.stringify(error)));
};
const updateUI = (data, endpoint) => {
alert(JSON.stringify(data));
alert(endpoint);
};
export const signIn = () => {
alert("signing in");
myMSALObj
.loginPopup(loginRequest)
.then(loginResponse => {
alert("success");
alert("id_token acquired at: " + new Date().toString());
alert(JSON.stringify(loginResponse));
if (myMSALObj.getAccount()) {
alert(JSON.stringify(myMSALObj.getAccount()));
} else {
alert("did get account");
}
})
.catch(error => {
alert("error");
alert(JSON.stringify(error));
});
};
export const signOut = () => {
myMSALObj.logout();
};
function getTokenPopup(request) {
return myMSALObj.acquireTokenSilent(request).catch(error => {
alert(JSON.stringify(error));
alert("silent token acquisition fails. acquiring token using popup");
// fallback to interaction when silent call fails
return myMSALObj
.acquireTokenPopup(request)
.then(tokenResponse => {
return tokenResponse;
})
.catch(error => {
alert(JSON.stringify(error));
});
});
}
export const seeProfile = () => {
if (myMSALObj.getAccount()) {
getTokenPopup(loginRequest)
.then(response => {
callMSGraph(
graphConfig.graphMeEndpoint,
response.accessToken,
updateUI
);
})
.catch(error => {
alert(JSON.stringify(error));
});
} else {
alert("not loggedin, please login first");
}
};
<file_sep>import React, { useState, Component } from "react";
import { Button, StyleSheet, View } from "react-native";
import { signIn, seeProfile, signOut } from "./helpers";
const App = () => {
return (
<View style={styles.app}>
<View style={styles.header} />
<Button onPress={() => signIn()} title="Login" />
<br />
<Button onPress={() => seeProfile()} title="See Profile" />
<br />
<Button onPress={() => signOut()} title="Logout" />
</View>
);
};
const styles = StyleSheet.create({
app: {
marginHorizontal: "auto",
maxWidth: 500
},
logo: {
height: 80
},
header: {
padding: 20
},
title: {
fontWeight: "bold",
fontSize: "1.5rem",
marginVertical: "1em",
textAlign: "center"
},
text: {
lineHeight: "1.5em",
fontSize: "1.125rem",
marginVertical: "1em",
textAlign: "center"
},
link: {
color: "#1B95E0"
},
code: {
fontFamily: "monospace, monospace"
}
});
export default App;
| c192b7ab8085623764f715e8f05a47c02367dc77 | [
"JavaScript"
] | 2 | JavaScript | naveenslog/react-native | 060f3f32a24a02f1cf47ef161b0d8f780159b1d3 | 2937e467693351829ead8d72b8c496310f11ec73 | |
refs/heads/master | <repo_name>varun21290/leetcode_solutions<file_sep>/Perfect Squares/Readme.md
# Problem Statement
Given a positive integer n, find the least number of perfect square numbers (for example, 1, 4, 9, 16, ...) which sum to n.
Example 1:
Input: n = 12
Output: 3
Explanation: 12 = 4 + 4 + 4.
<file_sep>/Possible Bipartition/Readme.md
# Problem Statement
Given a set of N people (numbered 1, 2, ..., N), we would like to split everyone into two groups of any size.
Each person may dislike some other people, and they should not go into the same group.
Formally, if dislikes[i] = [a, b], it means it is not allowed to put the people numbered a and b into the same group.
Return true if and only if it is possible to split everyone into two groups in this way.
Input: N = 4, dislikes = [[1,2],[1,3],[2,4]]
Output: true
Explanation: group1 [1,4], group2 [2,3]
Input: N = 5, dislikes = [[1,2],[2,3],[3,4],[4,5],[1,5]]
Output: false
<file_sep>/PerfectSquare/PerfectSquare.py
def isPerfectSquare(self, num: int) -> bool:
if(num<2): return True
x=2
y=num//2
while(y>=x):
z=(x+y)//2
srt=z*z
if(srt==num): return True
elif(srt>num): y=z-1
else: x=z+1
return False
nums = [4,9,144,399]
for x in nums:
print (x,isPerfectSquare(x))
<file_sep>/Two City Scheduling/solution.py
class Solution:
def twoCitySchedCost(self, costs: [[int]]) -> int:
l=sorted(costs,key=lambda x: x[0]-x[1])
n=len(costs)
return (sum(x[0] for x in l[0:n//2]) + sum(x[1] for x in l[n//2:]))
<file_sep>/Insert Delete GetRandom O(1)/solution.py
class RandomizedSet:
ds={}
size=0
import random
def __init__(self):
"""
Initialize your data structure here.
"""
self.ds={}
self.size=0
def insert(self, val: int) -> bool:
"""
Inserts a value to the set. Returns true if the set did not already contain the specified element.
"""
if val in self.ds:
return False
else:
self.ds[val]=val
self.size+=1
return True
def remove(self, val: int) -> bool:
"""
Removes a value from the set. Returns true if the set contained the specified element.
"""
if val in self.ds:
self.ds.pop(val)
self.size-=1
return True
else:
return False
def getRandom(self) -> int:
"""
Get a random element from the set.
"""
sel=random.randint(0,self.size-1)
return self.ds[list(self.ds.keys())[sel]]
# Your RandomizedSet object will be instantiated and called as such:
# obj = RandomizedSet()
# param_1 = obj.insert(val)
# param_2 = obj.remove(val)
# param_3 = obj.getRandom()
<file_sep>/Permutation In String/permutationInString.py
class Solution:
def checkInclusion(self, s1: str, s2: str) -> bool:
if(len(s2)<len(s1)): return False
count_s1=Counter(s1)
len_s1=len(s1)
len_s2=len(s2)
i=len_s1-1
while(i<len_s2):
if(s1.find(s2[i])!=-1):
if (count_s1==Counter(s2[i-len_s1+1:i+1])):
return True
i+=1
else:
i+=len_s1
return False
def checkInclusionHash(self, s1: str, s2: str) -> bool:
if(len(s2)<len(s1)): return False
len_s1=len(s1)
len_s2=len(s2)
shashs1=0
shashs2=0
for i in range(len_s1):
shashs1=shashs1+hash(s1[i])
shashs2=shashs2+hash(s2[i])
if(shashs1==shashs2): return True
for i in range(len_s1,len_s2):
shashs2=shashs2+hash(s2[i])-hash(s2[i-len_s1])
if(shashs1==shashs2): return True
return False
<file_sep>/Cousins in Binary Tree/Readme.md
# Problem Statement
In a binary tree, the root node is at depth 0, and children of each depth k node are at depth k+1.
Two nodes of a binary tree are cousins if they have the same depth, but have different parents.
We are given the root of a binary tree with unique values, and the values x and y of two different nodes in the tree.
Return true if and only if the nodes corresponding to the values x and y are cousins.
Input: root = [1,2,3,4], x = 4, y = 3
Output: false
Input: root = [1,2,3,null,4,null,5], x = 5, y = 4
Output: true
<file_sep>/Find All Anagrams/findAllAnagrams.py
class Solution:
def findAnagrams(self, s: str, p: str) -> [int]:
if(len(s)==0 or len(p)>len(s)): return []
find=[]
anagrams={}
lp=sorted(p)
len_p=len(p)
len_s=len(s)
i=len_p-1
while(i<len_s):
if(p.find(s[i])!=-1):
if (lp==sorted(s[i-len_p+1:i+1])):
find.append(i-len_p+1)
i+=1
else: i+=len_p
return find
def findAnagrams2(self, s: str, p: str) -> [int]:
if(len(s)==0 or len(p)>len(s)): return []
find=[]
count_p=Counter(p)
len_p=len(p)
len_s=len(s)
i=len_p-1
while(i<len_s):
if(p.find(s[i])!=-1):
if (count_p==Counter(s[i-len_p+1:i+1])):
find.append(i-len_p+1)
i+=1
else:
i+=len_p
return find
<file_sep>/Find the Town Judge/TownJudge.py
def townJudge(A,N):
a0 = {x[0] for x in A}
candidate=-1
trustedby=0
for i in range(N):
if (i+1 not in a0): can=i+1
for a in A:
if (a[1]==candidate): trustedby+=1
if(trustedby==N-1): return candidate
else : return -1
<file_sep>/Permutation In String/Readme.md
# Problem Statement
Given two strings s1 and s2, write a function to return true if s2 contains the permutation of s1.
In other words, one of the first string's permutations is the substring of the second string.
Input: s1 = "ab" s2 = "eidbaooo"
Output: True
Explanation: s2 contains one permutation of s1 ("ba").
<file_sep>/Reconstruct Itinerary/Solution.py
import bisect
class Solution:
def findItinerary(self, tickets: [[str]]) -> [str]:
graph={}
itinerary=[]
for ticket in tickets:
if ticket[0] not in graph:
graph[ticket[0]]=[ticket[1]]
else:
bisect.insort(graph[ticket[0]],ticket[1])
def dfs(graph,itinerary,src):
if src in graph:
while(len(graph[src])>0):
new_src=graph[src][0]
graph[src]=graph[src][1:]
dfs(graph,itinerary,new_src)
itinerary.append(src)
dfs(graph,itinerary,"JFK")
itinerary.reverse()
return itinerary
<file_sep>/Sort Characters By Frequency/frequencySort.py
class Solution:
def frequencySort(self, s: str) -> str:
freq={}
arr=[]
for i in range(len(string)):
if(string[i] in freq.keys()): freq[string[i]]=freq[string[i]]+1
else: freq[string[i]]=1
for key,item in freq.items():
arr.append([key,item])
from operator import itemgetter
res = sorted(arr, key = itemgetter(1))
s=''
for i in range(len(res),0,-1):
l=[res[i-1][0]]*res[i-1][1]
for x in l:
s=s+x
return s
<file_sep>/Word Search II/Solution.py
from collections import defaultdict
class Solution:
def __init__(self):
self.result=set()
def findWords(self, board: [[str]], words: [str]) -> [str]:
class Trie(object):
def __init__(self):
self.child = {'#':0}
def insert(self, word):
current = self.child
for l in word:
if l not in current:
current[l] = {'#':0}
current = current[l]
current['#']=1
def search(self,word):
current=self.child
for l in word:
if l in current:
current=current[l]
elif l not in current:
return -1
if(current['#']==1): return 1
else: return 0
trie=Trie()
for word in words:
trie.insert(word)
def dfs(i,j,graph,c):
ss=graph[i][j]
if (ss=='-1'): return
graph[i][j]='-1'
c=c+ss
t=trie.search(c)
# print(c,t)
if (t!=-1):
if t==1:
self.result.add(c)
dfs(i,j+1,graph,c)
dfs(i,j-1,graph,c)
dfs(i+1,j,graph,c)
dfs(i-1,j,graph,c)
graph[i][j]=ss
graph=[['-1']*(len(board[0])+2) for _ in range(len(board)+2)]
for i in range(len(board)):
for j in range(len(board[0])):
graph[i+1][j+1]=board[i][j]
for i in range(1,len(graph)-1):
for j in range(1,len(graph[0])-1):
dfs(i,j,graph,"")
return list(self.result)
<file_sep>/Delete Node in a List/Readme.md
# Problem Statement
Write a function to delete a node (except the tail) in a singly linked list, given only access to that node.
Input: head = [4,5,1,9], node = 5
Output: [4,1,9]
Explanation: You are given the second node with value 5, the linked list should become 4 -> 1 -> 9 after calling your function.
<file_sep>/MajorityElement/MajorityElement.py
def majority(nums,n,e,m,i):
if(nums.count(nums[n])>e):
return nums[n]
else:
i=i+1
if (n==0 or n==m-1):
return 'na'
if(n<=e):
o = majority(nums,0+int(m/(2**i)),e,m,i)
if o!='na' :
return o
if(n>=e):
o = majority(nums,n+int(m/(2**i)),e,m,i)
if o!='na' :
return o
return 'na'
nums=[1,0,1,1]
n=len(nums)
print (majority(nums,int(n/(2**1)),int(n/2),n,1))
<file_sep>/Odd Even Linked List/oddEvenLinkedList.py
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def oddEvenList(self, head: ListNode) -> ListNode:
if (head is None): return head
node=head
temp1=node.next
temp2=node.next
node.next=temp2.next
if(node.next is not None):
node=node.next
temp1.next=node.next
while(node.next is not None):
temp2=node.next
node.next=temp2.next
if(node.next is None): break
node=node.next
temp2.next=node.next
node.next=temp1
temp2.next=None
return head
def oddEvenListBetter(self, head: ListNode) -> ListNode:
if (head is None): return head
odd=head
evenHead=head.next
even=evenHead
while( even is not None and even.next is not None):
odd.next=even.next
odd=odd.next
even.next=odd.next
even=even.next
odd.next=evenHead
return head
<file_sep>/Cheapest flights within K stops/solution.py
class Solution:
def findCheapestPrice(self, n: int, flights: [[int]], src: int, dst: int, K: int) -> int:
# import collections
graph=collections.defaultdict(list)
for u,v,c in flights:
graph[u].append((v,c))
queue=collections.deque([(src,0,0)])
inf=float('inf')
minCost=inf
while queue:
node, steps, costSoFar=queue.popleft()
if(node==dst):
minCost=min(minCost,costSoFar)
continue
if(steps>K or costSoFar>minCost):
continue
for v,c in graph[node]:
queue.append((v,steps+1,costSoFar+c))
return minCost if minCost!=inf else -1
<file_sep>/FirstUniqueCharacter/FirstUniqueCharacter.java
import java.io.IOException;
public class FirstUniqueCharacter {
public static int firstUniqChar(String s) {
int map[] = new int[26];
int j = s.length();
for (char c : s.toCharArray()) {
map[c-97]+= 1;
}
for (int i = 0;i<26;i++) {
if(map[i]==1 && s.indexOf(i+97) < j) j = s.indexOf(i+97);
}
return j==s.length()?-1:j;
}
public static void main(String[] args) throws IOException {
String s = "leetccodel";
System.out.println(firstUniqChar(s));
}
}
<file_sep>/Coin Change 2/Readme.md
# Problem Statement
You are given coins of different denominations and a total amount of money.
Write a function to compute the number of combinations that make up that amount.
You may assume that you have infinite number of each kind of coin.
Input: amount = 5, coins = [1, 2, 5]
Output: 4
Explanation: there are four ways to make up the amount:
5=5
5=2+2+1
5=2+1+1+1
5=1+1+1+1+1
<file_sep>/Construct BST/bst.py
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
class Solution:
def bstFromPreorder(self, preorder: [int]) -> TreeNode:
root=TreeNode(preorder[0])
for i in range(1,len(preorder)):
node=root
while(node):
if(node.val>preorder[i]):
if(node.left): node = node.left
else:
node.left = TreeNode(preorder[i])
break
if(node.val<preorder[i]):
if(node.right): node=node.right
else:
node.right = TreeNode(preorder[i])
break
return root
<file_sep>/Invert Binary Tree/solution.py
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def invertTree(self, root: TreeNode) -> TreeNode:
def reverse(root1,root2):
if(root1.left):
root2.right=TreeNode(root1.left.val)
reverse(root1.left,root2.right)
if(root1.right):
root2.left=TreeNode(root1.right.val)
reverse(root1.right,root2.left)
if(root):
new_root = TreeNode(root.val)
reverse(root,new_root)
return new_root
<file_sep>/Uncrossed Lines/solution.py
class Solution:
def maxUncrossedLines(self, A: [int], B: [int]) -> int:
# dp=[0]*(len(A)+1)
# for i in range((len(A)+1)):
# dp[i]=[0]*(len(B)+1)
elem = set(A)&set(B)
A,B = [a for a in A if a in elem],[b for b in B if b in elem]
dp=[[0]*(len(B)+1) for _ in range(len(A)+1)]
i=len(A)-1
while(i>=0):
j=len(B)-1
while(j>=0):
if(A[i]==B[j]):
dp[i][j]=1+dp[i+1][j+1]
if(A[i]!=B[j]):
dp[i][j]=max(dp[i][j+1],dp[i+1][j])
j-=1
i-=1
return dp[0][0]
<file_sep>/Longest Duplicate Substring/Solution.py
class Solution:
def longestDupSubstring(self, S: str) -> str:
def rabinKarp(pattern, text, p_len, t_len):
hash_map={}
res=0
p=0
t=0
h=1
d=26
q=10000001
for i in range(p_len-1):
h = (h*d)%q
for i in range(p_len):
p = (d*p + ord(pattern[i]))%q
t = (d*t + ord(text[i+1]))%q
hash_map[p]=0
if(t==p and text[1:p_len+1] == pattern):
return pattern,0
hash_map[t]=1
for i in range(1,t_len-p_len+1):
t=(d*(t-ord(text[i])*h) + ord(text[i+p_len]))%q
if t<0: t+=q
if(t in hash_map and text[i+1:i+1+p_len] == text[hash_map[t]:hash_map[t]+p_len]):
return text[i+1:i+1+p_len],hash_map[t]
else:
hash_map[t]=i+1
return '',0
final_res=""
l=0
i=0
r=len(S)-1
while(l<=r):
S_len=len(S)
sub_res=''
m=(l+r)//2
pattern=S[i:i+m]
sub_res,new_i=rabinKarp(pattern,S[i:],m,len(S[i+1:]))
if sub_res!='':
final_res=sub_res
l=m+1
S=S[i:]
i=new_i
else:
r=m-1
return final_res
<file_sep>/Queue Reconstruction By Height/solution.py
class Solution:
def reconstructQueue(self, people: [[int]]) -> [[int]]:
people=sorted(people,key=lambda x: x[0])
sorted_people=[[-1,-1] for _ in range(len(people))]
for x in people:
i=x[1]
j=0
while i>0 or sorted_people[j][0]!=-1:
if sorted_people[j][0]==-1 or sorted_people[j][0]==x[0]:
i-=1
j+=1
sorted_people[j]=x
return sorted_people
def reconstructQueueBetter(self, people: [[int]]) -> [[int]]:
people.sort(key = lambda person: (person[0], -person[1]))
people.reverse()
queue = []
for person in people:
queue.insert(person[1],person)
return queue
<file_sep>/Trie/Trie.py
class Trie:
def __init__(self):
"""
Initialize your data structure here.
"""
self.key = ""
self.children = []
self.endOfWord = False
def insert(self, word: str) -> None:
"""
Inserts a word into the trie.
"""
trie = self
for s in word:
flag=0
for i in range(len(trie.children)):
if (trie.children[i].key==s):
trie = trie.children[i]
flag=1
break
if(flag==0):
new_node = Trie()
new_node.key=s
trie.children.append(new_node)
trie = new_node
trie.endOfWord = True
def search(self, word: str) -> bool:
"""
Returns if the word is in the trie.
"""
trie = self
for s in word:
flag=0
for i in range(len(trie.children)):
if (trie.children[i].key==s):
trie = trie.children[i]
flag=1
break
if (flag==0): return False
return True if trie.endOfWord else False
def startsWith(self, prefix: str) -> bool:
"""
Returns if there is any word in the trie that starts with the given prefix.
"""
trie = self
for s in prefix:
flag=0
for i in range(len(trie.children)):
if (trie.children[i].key==s):
trie = trie.children[i]
flag=1
break
if (flag==0): return False
return True
# Your Trie object will be instantiated and called as such:
# obj = Trie()
# obj.insert(word)
# param_2 = obj.search(word)
# param_3 = obj.startsWith(prefix)
<file_sep>/README.md
# leetcode solutions
contains solutions to leetcode problems
<file_sep>/Counting Bits/solution.py
class Solution:
def countBits(self, num: int) -> [int]:
import math
l=[0]
for i in range(1,num+1):
l.append(1+l[-2**(math.floor(math.log(i,2)))])
return l
<file_sep>/Perfect Squares/Solution.py
import math
class Solution:
def numSquares(self, n: int) -> int:
dp=[0,1,2,3]
for i in range(4,n+1):
dp.append(i)
for x in range(1,int(math.ceil(math.sqrt(i)))+1):
temp=x*x
if(temp<=i):
dp[i]=min(dp[i],1+dp[i-temp])
else:
break
return dp[n]
##########################################
def numSquaresBetter(self, n: int) -> int:
while( n % 4 == 0 ): # Reduction by factor of 4
n /= 4
if n % 8 == 7: # If n = 8k + 7, returns 4
return 4
for a in range( int(sqrt(n))+1 ): # Check if n = a^2 + b^2, return 0 / 1
b = int(sqrt(n - a**2))
if a**2 + b**2 == n:
return (a>0) + (b>0)
return 3
<file_sep>/Edit Distance/Readme.md
# Problem Statement
Given two words word1 and word2, find the minimum number of operations required to convert word1 to word2.
You have the following 3 operations permitted on a word:
Insert a character
Delete a character
Replace a character
<file_sep>/Sum Root to Leaf Numbers Solution/Readme.md
# Problem Statement
Given a binary tree containing digits from 0-9 only, each root-to-leaf path could represent a number.
An example is the root-to-leaf path 1->2->3 which represents the number 123.
Find the total sum of all root-to-leaf numbers.
Note: A leaf is a node with no children.
Input: [4,9,0,5,1]
Output: 1026
Explanation:
The root-to-leaf path 4->9->5 represents the number 495.
The root-to-leaf path 4->9->1 represents the number 491.
The root-to-leaf path 4->0 represents the number 40.
Therefore, sum = 495 + 491 + 40 = 1026.
<file_sep>/Odd Even Linked List/Readme.md
# Problem Statement
Given a singly linked list, group all odd nodes together followed by the even nodes.
Please note here we are talking about the node number and not the value in the nodes.
You should try to do it in place. The program should run in O(1) space complexity and O(nodes) time complexity.
Input: 2->1->3->5->6->4->7->NULL
Output: 2->3->6->7->1->5->4->NULL
<file_sep>/Edit Distance/Pythonic_Solution.py
from collections import deque
class Solution:
def minDistance(self, word1: str, word2: str) -> int:
visited = set()
q = deque([(word1, word2, 0)])
while q:
w1, w2, dist = q.popleft()
if (w1, w2) not in visited:
visited.add((w1, w2))
if w1 == w2:
return dist
while w1 and w2 and w1[0] == w2[0]:
w1 = w1[1:]
w2 = w2[1:]
dist += 1
q.extend([(
w1[1:], w2[1:], dist),
(w1, w2[1:], dist),
(w1[1:], w2, dist)])
<file_sep>/Count Square Submatrices with All Ones/Readme.md
# Problem Statement
Given a m * n matrix of ones and zeros, return how many square submatrices have all ones.
Input: matrix =
[
[0,1,1,1],
[1,1,1,1],
[0,1,1,1]
]
Output: 15
Explanation:
There are 10 squares of side 1.
There are 4 squares of side 2.
There is 1 square of side 3.
Total number of squares = 10 + 4 + 1 = 15.
<file_sep>/contiguous array/solution.py
class Solution:
def findMaxLength(self, nums: [int]) -> int:
max_length=0
length_map={}
zero=0
one=0
for i in range(len(nums)):
if(nums[i]==0): zero+=1
else: one+=1
diff=zero-one
if(diff==0):
max_length=i+1
if(diff in length_map):
if(max_length < i-length_map[diff]):
max_length = i-length_map[diff]
else:
length_map[diff] = i
return max_length
<file_sep>/Stock Span/stockSpan.py
class StockSpanner:
price_history=[]
price_map=[]
stack=[]
def __init__(self):
self.price_history=[]
self.price_map=[]
self.span=[]
def next(self, price: int) -> int:
j=len(self.price_history)-1
while(j!=-1 and self.price_history[j]<=price):
j=self.price_map[j]
self.price_history.append(price)
self.price_map.append(j)
return len(self.price_history)-j-1
def nextBetter(self, price: int) -> int:
span = 1
while self.stack and self.stack[-1][0] <= price:
span += self.stack.pop()[1]
self.stack.append((price, span))
return span
<file_sep>/MajorityElement/MajorityElement.java
import java.io.IOException;
public class MajorityElement {
public static int majorityElement(int[] nums) {
int e = 0;
int n = nums.length;
return (majority(nums,(int) Math.floor(n/Math.pow(2,1)),(int) Math.floor(n/2),n,1));
}
private static int frequency(int[] nums,int n) {
int count = 0;
for(int i =0;i<nums.length;i++) {
if(nums[i]==n) count+=1;
}
return count;
}
private static int majority(int[] nums,int n,int e,int m,int i) {
if(frequency(nums,nums[n])>e) return nums[n];
else {
i = i+1;
if(n==0 || n==m-1) return -1;
if(n<=e) {
int o = majority(nums,0+(int) Math.floor(m/Math.pow(2,i)),e,m,i);
if (o!=-1) return o;
}
if(n>=e) {
int o = majority(nums,n+(int) Math.floor(m/Math.pow(2,i)),e,m,i);
if (o!=-1) return o;
}
return -1;
}
}
public static int majorityElementBetter(int[] nums) {
if (nums == null || nums.length == 0) {
return 0;
}
return majorityElement(nums, 0);
}
private static int majorityElement(int[] nums, int start){
int count = 1;
int num = nums[start];
for(int i = start+1;i<nums.length;i++){
if(num == nums[i]) count++;
else count--;
if(count == 0) return majorityElement(nums,i+1);
}
return num;
}
public static void main(String[] args) throws IOException {
int s[] = {2,2,1,3,1,1,4,1,1,5,1,1,6};
System.out.println(majorityElement(s));
System.out.println(majorityElementBetter(s));
}
}
<file_sep>/Remove K Digits/RemoveKDigits.py
def removeKdigits(self, num: str, k: int)
new_num=''
i=len(num)-k
j=0
while(i>0):
minm=j
while(j<=len(num)-i):
if(num[j]<num[minm]):minm=j
j+=1
new_num = new_num+num[minm]
i=i-1
j=minm+1
return new_num.lstrip('0') or '0'
def removeKdigitsBetter(self, num: str, k: int) -> str:
new_num=[]
for n in num:
while k and new_num and new_num[-1]>n:
new_num.pop()
k-=1
new_num.append(n)
new_num = new_num[:-k] if k else new_num
return "".join(new_num).lstrip('0') or "0"
<file_sep>/Insert Delete GetRandom O(1)/Readme.md
# Problem Statement
Design a data structure that supports all following operations in average O(1) time.
insert(val): Inserts an item val to the set if not already present.
remove(val): Removes an item val from the set if present.
getRandom: Returns a random element from current set of elements.
Each element must have the same probability of being returned.
<file_sep>/Possible Bipartition/solution.py
class Solution:
def possibleBipartition(self, N: int, dislikes: [[int]]) -> bool:
matrix=[[0]*N for _ in range(N)]
for dislike in dislikes:
matrix[dislike[0]-1][dislike[1]-1]=1
matrix[dislike[1]-1][dislike[0]-1]=1
queue=[0]
color={}
color[0]=1
n=0
while len(queue)>0:
for i in range(len(matrix[0])):
if matrix[queue[0]][i]==1:
if i not in color:
color[i]= 1 if color[queue[0]]==0 else 0
queue.append(i)
elif color[i]==color[queue[0]]:
return False
queue=queue[1:]
n+=1
if(len(queue)==0 and n<N):
queue.append(n)
if (n not in color):
color[n]=1
return True
<file_sep>/Find All Anagrams/Readme.md
# Problem Statement
Given a string s and a non-empty string p, find all the start indices of p's anagrams in s.
Strings consists of lowercase English letters only and the length of both strings s and p will not be larger than 20,100.
The order of output does not matter.
Input:
s: "cbaebabacd" p: "abc"
Output:
[0, 6]
Explanation:
The substring with start index = 0 is "cba", which is an anagram of "abc".
The substring with start index = 6 is "bac", which is an anagram of "abc".
<file_sep>/Maximum Sum Circular Subarray/maxSumCircularSubarray.py
class Solution:
def maxSubarraySumCircular(self, arr: [int]) -> int:
new_arr=arr.copy()
new_arr.sort()
max_sum=0
if(new_arr[0]==new_arr[-1]):
if(new_arr[0]>0): return new_arr[0]*len(new_arr)
else: return new_arr[0]
if(new_arr[0]>0):
for x in new_arr:
max_sum+=x
return max_sum
def maxSum(A) :
n=len(A)//2
# included=[]
included_so_far=[]
max_sum=-30000*30
max_so_far=0
i=0
j=0
# print (A)
for i in range(n):
max_so_far+=A[i]
if(max_so_far>=max_sum):
max_sum=max_so_far
start=j
end=i
if(max_so_far<0):
max_so_far=0
j=i+1
i=start
j=start
max_so_far=0
while(i<2*n):
if(i-j==n):
max_so_far=0
i=i%n+1
j=i
continue
max_so_far+=A[i]
if(max_so_far>max_sum):
max_sum=max_so_far
if(max_so_far<0):
max_so_far=0
j=i+1
i+=1
return max_sum
new_arr = arr.copy()
new_arr.extend(arr)
return maxSum(new_arr)
<file_sep>/Course Schedule/Solution.py
class Solution:
def canFinish(self, numCourses: int, prerequisites: [[int]]) -> bool:
graph={}
vertices=[0]*numCourses
for edge in prerequisites:
if edge[0] in graph:
graph[edge[0]].append(edge[1])
else:
vertices[edge[0]]=1
graph[edge[0]]=[edge[1]]
visited=[0]*numCourses
reVisited=[0]*numCourses
def dfs(key):
visited[key]=1
reVisited[key]=1
if vertices[key]==1:
for req in graph[key]:
if reVisited[req]==1: return False
if dfs(req)==False: return False
reVisited[key]=0
return True
for par in graph.keys():
if visited[par]==0: flag=dfs(par)
if flag==False: return False
return True
def canFinishBetter(self, numCourses: int, prerequisites: [[int]]) -> bool:
graph = [[] for _ in range(numCourses)]
visit = [0 for _ in range(numCourses)]
for x, y in prerequisites:
graph[x].append(y)
for i in range(numCourses):
if not self.dfs(i, visit, graph):
return False
return True
def dfs(self, i, visit, graph):
if visit[i] == -1:
return False
if visit[i] == 1:
return True
visit[i] = -1
for j in graph[i]:
if not self.dfs(j, visit, graph):
return False
visit[i] = 1
return True
<file_sep>/Coin Change 2/solution.py
class Solution:
def change(self, amount: int, coins: [int]) -> int:
if amount==0: return 1
dp=[]
for _ in range(len(coins)+1):
l=[1]
l.extend([0]*amount)
dp.append(l)
dp[0][0]=0
for i in range(1,len(coins)+1):
for j in range(1,amount+1):
dp[i][j]=dp[i-1][j]+ (0 if j<coins[i-1] else dp[i][j-coins[i-1]])
return dp[-1][-1]
def changeBetter(self, amount: int, coins: [int]) -> int:
changes = [0] * (amount + 1)
changes[0] = 1
for coin in coins:
for i in range(0, amount - coin + 1):
if changes[i]:
changes[i + coin] += changes[i]
return changes[amount]
<file_sep>/FloodFill/floodFill.py
def floodFill(self, image: [[int]], sr: int, sc: int, newColor: int) -> [[int]]:
def fill(image,x,y,color,newColor,checked):
checked.append([sr,sc])
if(image[x][y]!=color or image[x][y]==newColor):
return image
else:
image[x][y]=newColor
if(y+1<len(image[0]) and [x,y+1] not in checked): #x,y+1
image = fill(image,x,y+1,color,newColor)
if(y-1>=0 and [x,y-1] not in checked): #x,y-1
image = fill(image,x,y-1,color,newColor)
if(x+1<len(image) and [x+1,y] not in checked): #x+1,y
image = fill(image,x+1,y,color,newColor)
if(x-1>=0 and [x-1,y] not in checked): #x-1,y
image = fill(image,x-1,y,color,newColor)
return image
checked=[]
return fill(image,sr,sc,image[sr][sc],newColor,checked)
<file_sep>/FirstUniqueCharacter/FirstUniqueCharacter.py
def firstUniqChar(s):
for i in range(len(s)):
if s[i]=='1': continue
if(i==s.rfind(s[i])): return i
else: s=s.replace(s[i],'1')
return -1
<file_sep>/Single Element in a Sorted Array/singleNonDuplicate.py
def singleNonDuplicate(self, nums: [int]) -> int:
if(len(nums)==1): return nums[0]
def check(nums,x,y):
m=(x+y)//2
if(m==0): return nums[m]
if(m==len(nums)-1): return nums[m]
if(nums[m-1]!=nums[m] and nums[m+1]!=nums[m]):
return nums[m]
elif (nums[m-1]==nums[m] and m%2==0):
return check(nums,x,m)
elif (nums[m+1]==nums[m] and m%2==0):
return check(nums,m,y)
elif (nums[m-1]==nums[m] and m%2!=0):
return check(nums,m+1,y)
elif (nums[m+1]==nums[m] and m%2!=0):
return check(nums,x,m-1)
return check(nums,0,len(nums)-1)
<file_sep>/K closest points/readme.md
# Problem Statement
We have a list of points on the plane. Find the K closest points to the origin (0, 0).
(Here, the distance between two points on a plane is the Euclidean distance.)
You may return the answer in any order. The answer is guaranteed to be unique (except for the order that it is in.)
Input: points = [[3,3],[5,-1],[-2,4]], K = 2
Output: [[3,3],[-2,4]]
(The answer [[-2,4],[3,3]] would also be accepted.)
<file_sep>/Sort Colors/solution.py
class Solution:
def sortColors(self, nums: [int]) -> None:
"""
Do not return anything, modify nums in-place instead.
"""
x,y=0,len(nums)-1
i=0
while(i<=y):
if(nums[i]==2):
nums[i]=nums[y]
nums[y]=2
y=y-1
elif(nums[i]==0):
nums[i]=nums[x]
nums[x]=0
x=x+1
i=i+1
else: i=i+1
<file_sep>/Permutation Sequence/Solution.py
class Solution:
def getPermutation(self, n: int, k: int) -> str:
if n==1: return str(n)
def factorial(x):
factorial=1
for i in range(1,x+1):
factorial*=i
return factorial
n_perm=[factorial(i) for i in range(n-1,0,-1)]
n_list=[_ for _ in range(1,n+1,1)]
perm=''
i=0
while (i<n):
if (k==0):
for x in range(len(n_list)-1,-1,-1):
perm=perm+str(n_list[x])
break
else:
index=k//n_perm[i]+ (1 if k%n_perm[i]>0 else 0)
perm=perm+str(n_list[index-1])
n_list.remove(n_list[index-1])
k=k-(k//n_perm[i])*n_perm[i]
i+=1
return perm
<file_sep>/K closest points/solution.py
class Solution:
def kClosest(self, points: [[int]], K: int) -> [[int]]:
dist_map={}
dist_list=[]
selected=[]
for point in points:
dist=point[0]*point[0]+point[1]*point[1]
dist_list.append(dist)
if dist in dist_map.keys():
dist_map[dist].append(point)
else: dist_map[dist]=[point]
dist_list.sort()
i=0
while(len(selected)<K):
selected.extend(dist_map[dist_list[i]])
i+=1
return selected
# more pythonic way of doing it....
def kClosest(self, points: List[List[int]], K: int) -> List[List[int]]:
def _euclidean(p):
return math.sqrt(p[0]*p[0] + p[1] * p[1])
return sorted(points, key=_euclidean)[0:K]
<file_sep>/Construct BST/Readme.md
Return the root node of a binary search tree that matches the given preorder traversal.
It's guaranteed that for the given test cases there is always possible to find a binary search tree with the given requirements.
Input: [8,5,1,7,10,12]
Output: [8,5,10,1,7,null,12]
Constraints:
1 <= preorder.length <= 100
1 <= preorder[i] <= 10^8
The values of preorder are distinct.
<file_sep>/PerfectSquare/Readme.md
# Problem Statement
Given a positive integer num, write a function which returns True if num is a perfect square else False.
Note: Do not use any built-in library function such as sqrt.
Input: 16
Output: true
Input: 14
Output: false
<file_sep>/Dungeon/Solution.py
class Solution:
def calculateMinimumHP(self, dungeon: [[int]]) -> int:
dp=[[float(inf)]*(len(dungeon[0])+1) for _ in range(len(dungeon)+1)]
for i in range(len(dungeon)-1,-1,-1):
for j in range(len(dungeon[0])-1,-1,-1):
if(i==len(dungeon)-1 and j==len(dungeon[0])-1):
dp[i][j]=abs(dungeon[-1][-1]) if dungeon[-1][-1]<0 else 0
else:
update=min(dp[i+1][j],dp[i][j+1])
if(dungeon[i][j]-update>=0): dp[i][j]=0
else: dp[i][j] = abs(dungeon[i][j]-update)
return dp[0][0]+1
<file_sep>/FirstUniqueCharacter/Readme.md
# Problem Statement -
Given a string, find the first non-repeating character in it and return it's index. If it doesn't exist, return -1.
s = "leetcode"
return 0.
s = "loveleetcode"
return 2.
<file_sep>/Trie/Readme.md
# Trie (Prefix Tree)
https://www.cs.bu.edu/teaching/c/tree/trie/
https://en.wikipedia.org/wiki/Trie
<file_sep>/Sum Root to Leaf Numbers Solution/Solution.py
class Solution:
def sumNumbers(self, root: TreeNode) -> int:
if(not root):return 0
stack_val=''
s=0
def dfs(node,stack_val,s):
if(not node):
return stack_val,s
stack_val=stack_val+str(node.val)
if(not node.left and not node.right):
s+=int(stack_val)
stack_val,s=dfs(node.left,stack_val,s)
stack_val,s=dfs(node.right,stack_val,s)
stack_val=stack_val[0:len(stack_val)-1]
return stack_val,s
_,s=dfs(root,stack_val,s)
return s
<file_sep>/Edit Distance/Solution.py
class Solution:
def minDistance(self, word1: str, word2: str) -> int:
dp=[[0]*(len(word2)+1) for _ in range(len(word1)+1)]
for i in range(len(word1)+1):
for j in range(len(word2)+1):
if i==0: dp[i][j]=j
elif j==0: dp[i][j]=i
elif word1[i-1]==word2[j-1]: dp[i][j] = dp[i-1][j-1]
else: dp[i][j]=1+min(dp[i-1][j-1],
dp[i-1][j],
dp[i][j-1])
return dp[-1][-1]
<file_sep>/Invert Binary Tree/Readme.md
# Problem Statement
Invert a binary tree.
Input: 4,2,7,1,3,6,9
Output: 4,7,2,9,6,3,1
<file_sep>/Counting Bits/Readme.md
# Problem Statement
Given a non negative integer number num.
For every numbers i in the range 0 ≤ i ≤ num calculate the number of 1's in their binary representation and return them as an array.
Input: 5
Output: [0,1,1,2,1,2]
<file_sep>/Single Element in a Sorted Array/Readme.md
# Problem Statement
You are given a sorted array consisting of only integers where every element appears exactly twice, except for one element which appears exactly once.
Find this single element that appears only once.
Input: [1,1,2,3,3,4,4,8,8]
Output: 2
<file_sep>/Cousins in Binary Tree/Cousins.py
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
leaf5 = TreeNode(val=5)
leaf4 = TreeNode(val=4)
node2 = TreeNode(val=2,left=None,right=leaf4)
node3 = TreeNode(val=3,left=None,right=leaf5)
root = TreeNode(val=1,left=node2,right=node3)
def cousins(root,x,i,parent=None):
j=-1
if(root.val==x):
return i,parent
if(root.left):
j,parent=cousins(root.left,x,i+1,root.val)
if(j!=-1):
return j,parent
if(root.right):
j,parent=cousins(root.right,x,i+1,root.val)
if(j!=-1):
return j,parent
return -1,-1
x_i,x_parent=cousins(root,4,0)
y_i,y_parent=cousins(root,5,0)
print (x_i,x_parent)
print (y_i,y_parent)
if(x_i==y_i and x_parent!=y_parent):
print ('cousins')
<file_sep>/Surrounded Regions/solution.py
class Solution:
def solve(self, board: [[str]]) -> None:
"""
Do not return anything, modify board in-place instead.
"""
row=len(board)
if row==0: return
col=len(board[0])
for i in range(row):
for j in range(col):
if board[i][j]=='O': board[i][j]='-'
def floodFill(a,b):
board[a][b]='O'
l,r,u,d=b-1,b+1,a-1,a+1
if l>=0 and board[a][l]=='-': floodFill(a,l)
if r<col and board[a][r]=='-': floodFill(a,r)
if u>=0 and board[u][b]=='-': floodFill(u,b)
if d<row and board[d][b]=='-': floodFill(d,b)
for i in [0,row-1]:
for j in range(col):
if board[i][j]=='-': floodFill(i,j)
for i in range(1,row-1):
for j in [0,col-1]:
if board[i][j]=='-': floodFill(i,j)
for i in range(row):
for j in range(col):
if board[i][j]=='-': board[i][j]='X'
<file_sep>/Kth Smallest Element in a BST/kthSmallestElement.py
# Definition for a binary tree node.
class Solution:
def kthSmallest(self, root: TreeNode, k: int) -> int:
self.k=k
def inorder(root):
inorder_list=[]
if(root.left and self.k>0):
inorder_list.extend(inorder(root.left))
if(self.k>0):
inorder_list.append(root.val)
self.k-=1
if(root.right and self.k>0):
inorder_list.extend(inorder(root.right))
return inorder_list
return inorder(root)[-1]
def kthSmallest1(self, root: TreeNode, k: int) -> int:
def inorder(root):
inorder_list=[]
if(root.left):
inorder_list.extend(inorder(root.left))
inorder_list.append(root.val)
if(root.right):
inorder_list.extend(inorder(root.right))
return inorder_list
return inorder(root)[k-1]
<file_sep>/Cheapest flights within K stops/Readme.md
# Problem Statement
There are n cities connected by m flights. Each flight starts from city u and arrives at v with a price w.
Now given all the cities and flights, together with starting city src and the destination dst,
your task is to find the cheapest price from src to dst with up to k stops. If there is no such route, output -1.
Example 1:
Input:
n = 3, edges = [[0,1,100],[1,2,100],[0,2,500]]
src = 0, dst = 2, k = 1
Output: 200
<file_sep>/Check Straight Line/checkStraightLine.py
def checkStraightLine(coordinates) -> bool:
def check(coordinates,m=None,b=None,c=None):
if(c==None):
for x in coordinates:
if(x[1]-x[0]*m!=b):
return False
elif (c=='x'):
for x in coordinates:
if(x[0]!=m):
return False
elif(c=='y'):
for x in coordinates:
if(x[1]!=m):
return False
return True
if (coordinates[1][1]-coordinates[0][1]==0):
return check(coordinates,m=coordinates[1][1],c='y')
elif (coordinates[1][0]-coordinates[0][0]==0):
return check(coordinates,m=coordinates[1][0],c='x')
else:
m = (coordinates[1][1]-coordinates[0][1])/(coordinates[1][0]-coordinates[0][0])
b = coordinates[0][1]-coordinates[0][0]*m
return check(coordinates,m=m,b=b)
c = [[-7,-3],[-7,-1],[-2,-2],[0,-8],[2,-2],[5,-6],[5,-5],[1,7]]
checkStraightLine(c)
<file_sep>/contiguous array/Readme.md
# Problem Statement
Given a binary array, find the maximum length of a contiguous subarray with equal number of 0 and 1.
Input: [0,1,0]
Output: 2
Explanation: [0, 1] (or [1, 0]) is a longest contiguous subarray with equal number of 0 and 1.
<file_sep>/Interval List Intersections/intervalIntersection.py
class Solution:
def intervalIntersection(self, A: [[int]], B: [[int]]) -> [[int]]:
out=[]
i=0
for a in A:
while(i<len(B)):
if(B[i][0]>a[1]): break
if(a[0]>B[i][1]):
i+=1
continue
out.append([max(a[0],B[i][0]),min(a[1],B[i][1])])
if(B[i][1]>a[1]):
break
i+=1
return out
<file_sep>/Queue Reconstruction By Height/Readme.md
# Problem Statement
Suppose you have a random list of people standing in a queue.
Each person is described by a pair of integers (h, k), where h is the height of the person and k is the
number of people in front of this person who have a height greater than or equal to h.
Write an algorithm to reconstruct the queue.
Input:
[[7,0], [4,4], [7,1], [5,0], [6,1], [5,2]]
Output:
[[5,0], [7,0], [5,2], [6,1], [4,4], [7,1]]
<file_sep>/Surrounded Regions/Readme.md
# Problem Statement
Given a 2D board containing 'X' and 'O' (the letter O), capture all regions surrounded by 'X'.
A region is captured by flipping all 'O's into 'X's in that surrounded region.
Input:
X X X X
X O O X
X X O X
X O X X
Output:
X X X X
X X X X
X X X X
X O X X
Surrounded regions shouldn’t be on the border, which means that any 'O' on the border of the board are not flipped to 'X'.
Any 'O' that is not on the border and it is not connected to an 'O' on the border will be flipped to 'X'.
Two cells are connected if they are adjacent cells connected horizontally or vertically.
| 88ae7af3e7d4a46acd35614556819a7c08609ad6 | [
"Markdown",
"Java",
"Python"
] | 69 | Markdown | varun21290/leetcode_solutions | 1a341180755dc678f1d33247a0d980e13c3d1d03 | e73303702ff98d81f1b6a2da32aeea6795d068ee | |
refs/heads/master | <repo_name>metrosierra/quVario<file_sep>/helium_marki/helium_marki.py
#!/usr/bin/env python3
# Made 2020, <NAME>
# mingsongwu [at] outlook [dot] sg
# github.com/starryblack/quVario
### READINGS IN readings FOLDER VERY USEFUL FOR HELIUM ATOM APPROXIMATIONS
import math
import sys
import os
import time
from datetime import datetime
### using sympy methods for symbolic integration. Potentially more precise and convenient (let's not numerically estimate yet)
import numpy as np
import scipy as sp
import scipy.constants as sc
import sympy as sy
from sympy import conjugate, simplify, lambdify, sqrt
from sympy import *
from IPython.display import display
from scipy import optimize, integrate
#### For future monte carlo integration work hopefully
import mcint
import random
### Honestly OOP isnt really shining in advantage now, other than me not caring about the order of functions and using global variables liberally.
class eiGen():
### initialisation protocol for eiGen object
def __init__(self):
self.hbar = sc.hbar
self.mass_e = sc.electron_mass
self.q = sc.elementary_charge
self.pi = sc.pi
self.perm = sc.epsilon_0
self.k = (self.q**2)/(4*self.pi*self.perm)
sy.init_printing()
self.macro1()
### important bit: generating sets of 3d spherical coordinate sympy symbols for each electron. In this case 2, but can be easily scaled arbitrarily. All generated symbols stored in master self.bases list. Somehow I can't assign each variable a self attribute directly????
def spawn_electrons(self, number, coord_sys = 's'):
self.bases = []
if coord_sys == 'spherical' or 's':
for i in range(number):
temp = []
for q in ['r', 'theta', 'phi']:
temp.append(sy.symbols(q + str(i)))
self.bases.append(temp)
elif coord_sys == 'cartesian' or 'cart':
for i in range(number):
temp = []
for q in ['x', 'y', 'z']:
temp.append(sy.symbols(q + str(i)))
self.bases.append(temp)
elif coord_sys == 'cylindrical' or 'cylin':
for i in range(number):
temp = []
for q in ['rho', 'phi', 'z']:
temp.append(sy.symbols(q + str(i)))
self.bases.append(temp)
### this is a kind of brute force laplace, because I cannot use the sympy.laplace function AND specify which electron it should operate wrt
def laplace(self, expr, index, coord_sys = 'spherical'):
if coord_sys == 'spherical' or 's':
r = self.bases[index][0]
t = self.bases[index][1]
p = self.bases[index][2]
grad = [sy.diff(expr, r), 1/r * sy.diff(expr, t), 1/(r*sin(t)) * sy.diff(expr, p)]
lap = (1/r**2)*sy.diff(r**2 * grad[0], r) + (1/(r*sin(t)))*(sy.diff(grad[1] * sin(t), t) + sy.diff(grad[2], p))
return lap
### Just makes the psi function variable. #TODO! augment this to read off a txt file (easier to see or find or collect the various trial forms)
def spawn_psi(self):
self.psi = -sy.exp(-self.alpha0*(self.r0 + self.r1))
### Just generating parameters. Only need 1 now.
def spawn_alphas(self, number):
for i in range(number):
setattr(self, 'alpha{}'.format(i), sy.symbols('alpha{}'.format(i), real = True))
### Automatically slaps the SPHERICAL VOLUME jacobian for self.symintegrate. Tightly paired with said function.
def jacob_gen(self, index, dimensions = 3, type = 's'):
if dimensions == 3 and type == 's':
return self.bases[index][0]**2*sin(self.bases[index][1])
## Symbolic integration (volume only for now) with spherical jacobian added for each set of coordinates
def symintegrate(self, expr, number, dimensions = 3, type = "spherical"):
if type == 'spherical' or type == 's':
if dimensions == 3:
for i in range(number):
temp = sy.integrate(expr*self.jacob_gen(index = i), (self.bases[i][0], 0, oo), (self.bases[i][1], 0, pi),(self.bases[i][2], 0, 2*pi), conds = "none")
expr = temp
return temp
### Final workhorse that implements scipy fmin function to minimise our expression. The processing is general but the output is specific to our current helium example (1 parameter)
def iterate(self, func, guess, args):
print('\nIterator initialised!! Please be patient!!\n')
starttime = time.time()
temp = optimize.fmin(func, guess, args = (args), full_output = 1)
endtime = time.time()
elapsedtime = endtime - starttime
now = datetime.now()
date_time = now.strftime('%d/%m/%Y %H:%M:%S')
# just returns datetime of attempt, elapsed time, optimised parameter, optimised value, number of iterations
return [date_time, elapsedtime, guess, temp[0], temp[1], temp[3]]
def lambdah(self, expr, var, type = 'scipy'):
return lambdify((var), expr, type)
################################################################
#These are situation specific functions, for our particular helium integration problem.
###specifically atomic units
def hamiltonian_he(self, trial):
self.ke = -0.5 * (self.laplace(trial, 0) + self.laplace(trial, 1))
self.nucleus = -(2/self.r0 + 2/self.r1)
self.repel = (1/ sqrt(self.r0**2 + self.r1**2 - 2*self.r0*self.r1*cos(self.theta0)))
print('\nKinetic Energy terms (self.ke), Nucleus Attraction terms (self.nucleus), Electron Repulsion terms (self.repel) generated!!!\n')
return self.ke, self.nucleus, self.repel
### This our custom situtaion, where the hydrogenic component is analytic (ie a nice expression with parameter as sole variable) and the cross repulsion term is a numerically evaluated integral. So programme must substitute trial parameter and THEN integrate to check if end value is minimum
def final_expr1(self, a, cross, hydrogenic):
integrand = integrate.nquad(cross, [[0, sp.inf], # r0
[0, sp.pi], # theta0
[0, sp.inf], # phi0
], args = (a,)) #phi1
print(hydrogenic(a) + integrand[0], 'heart trees')
return hydrogenic(a) + integrand[0]
### writes self.iterate results to a paired txr file. If file no exist it is spawned.
def custom_log(self, data = [], comments = None):
log = open('marki_log.txt', 'a+')
separator = '\n{}\n'.format(''.join('#' for i in range(10)))
info = separator + 'Datetime = {}\n'.format(data[0]) + 'Time taken (s) = {}\n'.format(data[1]) + 'Initial parameter guess (atomic untis) = {}\n'.format(data[2]) + 'Optimised parameter (atomic units) = {}\n'.format(data[3]) + 'Optimised function value (atomic units) = {}\n'.format(data[4]) + 'Number of iterations = {}\n'.format(data[5]) + 'Comments = ({})\n'.format(comments)
print(info)
log.write(info)
log.close()
### actual sequence of processing events. Technically this can be transfered to a separate macro script. Hence the name macro.
def macro1(self):
self.spawn_alphas(2)
self.spawn_electrons(2)
self.r0 = self.bases[0][0]
self.theta0 = self.bases[0][1]
self.phi0 = self.bases[0][2]
self.r1 = self.bases[1][0]
self.theta1 = self.bases[1][1]
self.phi1 = self.bases[1][2]
self.spawn_psi()
ke, attract, repel = self.hamiltonian_he(self.psi)
normal = self.symintegrate(conjugate(self.psi)*self.psi, 2)
result1 = self.symintegrate(conjugate(self.psi)*ke, 2)
result2 = self.symintegrate(conjugate(self.psi)*attract*self.psi, 2)
self.hydrogenic = self.lambdah(result1/normal + result2/normal, (self.alpha0))
jacob_special = 2*4*sp.pi**2*self.r0**2*self.r1**2*sin(self.theta0)
cross_vars = (self.r0, self.theta0, self.r1, self.alpha0)
self.cross_term = self.lambdah(simplify(jacob_special*conjugate(self.psi)*repel*self.psi/normal), (cross_vars))
result = self.iterate(self.final_expr1, guess = 1.6, args = (self.cross_term, self.hydrogenic))
self.custom_log(result, 'test run 2 with cross term, psi = -self.alpha0*(self.r0 + self.r1)')
def __enter__(self):
pass
def __exit__(self, e_type, e_val, traceback):
pass
### start script
e = eiGen()
<file_sep>/archives/optipack_deprecated.py
#!/usr/bin/env python3
# Made 2020, <NAME>, <NAME>
# mingsongwu [at] outlook [dot] sg
# github.com/starryblack/quVario
### This script provides the montypython object AND minimiss object that handles any calls to monte carlo integration functions and minimisation functions of our design. It interfaces with the python packages installed for basic functionalities (ie mcint)
### optipack should serve helium_markii.py which is the higher level script for direct user interface
### as of 27 may 2020 montypython uses mcint, and minimiss uses scipy fmin functions as main work horses.
### montypython removed, replaced with simple functions because this is the only sane way to be able to numba jit them
import math
import sys
import os
import time
from datetime import datetime
### using sympy methods for symbolic integration. Potentially more precise and convenient (let's not numerically estimate yet)
import numpy as np
import scipy as sp
import scipy.constants as sc
import sympy as sy
from sympy import conjugate, simplify, lambdify, sqrt
from sympy import *
from IPython.display import display
from scipy import optimize, integrate
import matplotlib.pyplot as plt
#### For future monte carlo integration work hopefully
import mcint
import random
from numba import jit, njit
def integrator_uniform(self, integrand, bounds, sample_iter):
''' using mcint package to determine the integral via uniform distribution
sampling over an interval
'''
# measure is the 'volume' over the region you are integrating over
result,error = mcint.integrate(integrand, self.sampler(bounds),
self.get_measure(bounds), sample_iter)
return result, error
def get_measure(self, bounds):
''' obtains n dimensional 'measure' for the integral, which effectively
is the volume in n dimensional space of the integral bounds. used for
multiplying the expected value.
inputs:
bounds: list of size n, indicating the n bounds of the definite
integral
outputs:
measure: float
'''
measure = 1
if bounds.ndim == 1:
dimlength = bounds[1] - bounds[0]
measure *= dimlength
else:
for i in bounds:
dimlength = i[1] - i[0]
measure *= dimlength
return measure
def sampler(self, bounds):
''' generates a tuple of n input values from a random uniform distribution
e.g. for three dimensions, outputs tuple = (x,y,z) where x,y,z are
floats from a uniorm distribution
inputs:
bounds
outputs:
sample (tuple)
'''
while True:
sample = ()
if bounds.ndim == 1:
dimsample = random.uniform(bounds[0],bounds[1])
x = list(sample)
x.append(dimsample)
sample = tuple(x)
else:
for i in bounds:
dimsample = random.uniform(i[0],i[1])
x = list(sample)
x.append(dimsample)
sample = tuple(x)
yield sample
def integrand(self, x):
''' this is the integrand function
inputs: x (array), where x[0] denotes the first variable
'''
return x[0]**2 * sp.exp(-(x[0]) ** 2)
def combine_pq(self, pfunc, qfunc):
''' multiplies p and q for the uniform integrator!
'''
return pfunc * qfunc
######## These helper functions concern the Metropolis algorithm implementation of the integral
@njit
def metropolis_hastings(pfunc, iter, alpha, dims):
# we make steps based on EACH electron, the number of which we calculate from dims/3
# 'scale' of the problem. this is arbitrary
s = 3.
# we discard some initial steps to allow the walkers to reach the distribution
equi_threshold = 0
initial_matrix = np.zeros((int(dims/3), 3))
reject_ratio = 0.
therm = 1
test = []
samples = []
for i in range(int(dims/3)):
initial_matrix[i] = 2.*s*np.random.rand(3) - s
# now sample iter number of points
for i in range(iter):
# choose which electron to take for a walk:
e_index = np.random.randint(0, dims/3)
trial_matrix = initial_matrix.copy()
trial_matrix[e_index] += (2.*s*np.random.rand(3) - s)/(dims/3)
# trial_matrix[e_index] = hi
proposed_pt = np.reshape(trial_matrix, (1, dims))[0]
initial_pt = np.reshape(initial_matrix, (1, dims))[0]
# print(initial_pt)
p = pfunc(proposed_pt, alpha) / pfunc(initial_pt, alpha)
if p > np.random.rand():
initial_matrix = trial_matrix.copy()
# print(initial_matrix == trial_matrix)
else:
reject_ratio += 1./iter
if i > equi_threshold:
if (i-therm)%therm == 0:
test.append(np.reshape(initial_matrix, (1, dims))[0][3])
samples.append(np.reshape(initial_matrix, (1, dims))[0])
return samples, reject_ratio, test
@njit
def integrator_mcmc(pfunc, qfunc, sample_iter, walkers, alpha, dims):
therm = 0
vals = np.zeros(walkers)
val_errors = 0.
test = []
for i in range(walkers):
mc_samples, rejects, p = metropolis_hastings(pfunc, sample_iter, alpha, dims)
sums = 0.
# obtain arithmetic average of sampled Q values
for array in mc_samples[therm:]:
sums += qfunc(array, alpha)
test.append(qfunc(array, alpha))
vals[i] = (sums/(sample_iter - therm))
# also calculate the variance
vals_squared = np.sum(vals**2)
vals_avg = np.sum(vals) /walkers
variance = vals_squared/walkers - (vals_avg) ** 2
std_error = np.sqrt(variance/walkers)
print('Iteration cycle complete, result = ', vals_avg, 'error = ', std_error, 'rejects = ', rejects)
return vals_avg, std_error, rejects, test, p
@njit
def mcmc_q(x, alpha):
''' this is the Q part of the integrand function for mcmc
inputs: x(array), denoting iter number of sample points, given by
'''
### helium local energy
r1 = x[0:2]
r2 = x[3:]
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r1_hat = r1 / r1_len
r2_hat = r2 / r2_len
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
return ((-4 + np.dot(r1_hat - r2_hat, r1 - r2 ) / (r12 * (1+alpha*r12)**2)
- 1/ (r12*(1+alpha*r12)**3)
- 1/ (4*(1+alpha*r12)**4)
+ 1/ r12 ))[0]
@njit
def mcmc_p(x, alpha):
'''
this is the integrand function for mcmc
'''
### helium wavefunction squared
r1 = x[0:3]
r2 = x[3:]
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
return ((np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
* np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2)[0]
#########################
class MiniMiss():
def __init__(self):
print('MiniMiss optimisation machine initialised and ready!\n')
def minimise(self, func, guess, ftol):
starttime = time.time()
temp = optimize.fmin(func, guess, full_output = 1, ftol = ftol)
endtime = time.time()
elapsedtime = endtime - starttime
now = datetime.now()
date_time = now.strftime('%d/%m/%Y %H:%M:%S')
# just returns datetime of attempt, elapsed time, optimised parameter, optimised value, number of iterations
return [date_time, elapsedtime, guess, temp[0], temp[1], temp[3]]
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('\n\nMiniMiss object self-destructing\n\n')
<file_sep>/helium_marki/hydr_var.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Mon May 18 10:42:53 2020
Variational Method for finding ground state energy of Hydrogen.
Exercise 1.19 in Quantum Chemistry.
The Hamiltonian is fixed at the moment.
@author: kenton
"""
#%% Import packages and initialisation of spaces
from sympy import (exp, conjugate, symbols, simplify, lambdify)
from sympy.vector import CoordSys3D, Del
from sympy.parsing.sympy_parser import parse_expr
from scipy.optimize import fmin
from scipy.integrate import quad
#import scipy as sp
#import matplotlib.pyplot as plt
# initialisation of coordinate systems for vector calculus
# we work in Spherical coordinates because of the nature of the problem
# this simplifies the need for the Laplacian as SymPy has sphericals built in
R = CoordSys3D('R', transformation = 'spherical')
delop = Del() #by convention
#Sympy help
#
#Note that R.r, R.theta, R.phi are the variables called base scalars
# Base scalars can be treated as symbols
#and R.i, R.j, R.k are the r, theta, phi basis vectors
#
#https://docs.sympy.org/latest/tutorial/basic_operations.html
#%% Define symbolic parameters and numerical infinity
alpha = symbols('alpha', real = 0)
inf = 1000
#%% Define functions
#Hamiltonian operator H operating on psi
def H(psi):
''' Function: the Hamiltonian operator operating on some trial function psi.
This is in atomic units.
Input:
some trial function psi(r)
Output:
another scalar field Sympy expression
'''
H = - 1 / 2 * del2(psi) - 1 / R.r * psi
return H
#Del-squared, the Laplacian for the Schrodinger equation
def del2(f):
'''Laplacian operator in spherical polar coordinates
Input:
some Sympy expression f to which the Laplacian is applied
Output:
A Sympy expression
'''
del2 = delop.dot(delop(f)).doit()
return del2
# We need to supply the Jacobian manually because we are no longer integrating
# with SymPy.
# The Jacobian of the spherical integral is r**2 sin(theta)
# ignore sin(theta) because of symmetry
jacobian = R.r ** 2
def readtrialfuncs(txtdoc = 'hydr_var_trialfuncs.txt'):
''' Reads a text document of trial functions and creates a sympy list
inputs:
txtdoc: text document filename
outputs:
trialfuns: a list of trial functions
'''
f = open(txtdoc, "r")
trialfuncs = []
for line in f:
x = parse_expr(line)
x = x.subs(symbols('r'), R.r)
trialfuncs.append(x)
f.close()
return trialfuncs
# Trial function psi defined using SymPy
# A decaying Gaussian
#psi = exp(-alpha * R.r**2)
#%% This part defines function related to the variational method
# definining a function such that SciPy Optimisation can be used
def expectation_intgrl(expectation_lamb, a, stpt, endpt):
''' Formualtes an integral of the lambdified expectation function
inputs:
a (float): parameter
stpt (float): starting point of the integral
endpt (float): endpoint of the integral
output:
expectation_integral[0]: numerical result of the integral
'''
expectation_integral = quad(expectation_lamb, 0, endpt,args=(a))
return expectation_integral[0]
def norm_intgrl(norm_lamb, a, stpt, endpt):
''' Finds the square of the Normalisation constant of the trial function.
Formualtes an integral of the lambdified normalisation function and uses
the fact that the trial functions must be normalised to 1.
inputs:
a (float):
stpt (float): starting point of the integral
endpt (float): endpoint of the integral
output:
N_squared: normalisation
'''
norm_intgrl = quad(norm_lamb, stpt, endpt, args = (a))
N_squared = 1 / norm_intgrl[0]
return N_squared
def var_intgrl(a, expectation_lamb, norm_lamb, stpt = 0, endpt = 1000):
''' Multiplies the normalisation with the expectation integral
inputs:
expectation_intgrl (function of a):
norm_intgrl (function of a): N_squared, normalisation for trial function
stpt (float): starting point of the integral (0 by default)
endpt (float): endpoint of the integral (1000 effectively infinity)
outputs:
var_intgrl (function of a): numerical result of the
variation integral
'''
var_intgrl = (norm_intgrl(norm_lamb, a, stpt, endpt) *
expectation_intgrl(expectation_lamb, a, stpt, endpt))
return var_intgrl
def energy(psi):
''' Obtains the optimised parameter and upper bound of the energy of the system
inputs:
trial function psi (sympy expression) that satisfies the BCs
outputs:
alpha_op and energy_max (tuple)
'''
#The expectation value <psi|H|psi>
expectation = simplify(conjugate(psi) * H(psi) )
expectation_lamb = lambdify((R.r, alpha), expectation * jacobian, 'scipy')
#the normalisation value <psi|psi>
norm = conjugate(psi) * psi
norm_lamb = lambdify((R.r, alpha), norm * jacobian, 'scipy')
#supply the initial guess for the variation intergral
initial_guess = 0.1
#minimise the variation integral with respect to the parameter
alpha_op, energy_max = fmin(var_intgrl,
initial_guess, (expectation_lamb, norm_lamb),
disp= 0, full_output = 1)[0:2]
return (alpha_op, energy_max)
def main():
''' Main function, obtains the lowest energy of all trial functions and prints
the intermediate energies
outputs: overall_energy_min
'''
energies = []
print('In atomic units,')
for psi in readtrialfuncs():
alpha_op, energy_min = energy(psi)
energies.append(energy_min)
print('\nFor psi = %s, \nThe optimised parameter is %f. \nThe energy upper bound is %f.' % (psi, float(alpha_op),energy_min))
overall_energy_min = min(energies)
return overall_energy_min
#%% Execution of the minimisation of the variation integral
print('\nThe overall maximum energy is %f.' %main())
<file_sep>/archives/metronumba.py
#!/usr/bin/env python3
#%%
import math
import sys
import os
import time
from datetime import datetime
import numpy as np
import scipy as sp
import scipy.constants as sc
#### For future monte carlo integration work hopefully
import mcint
import random
import numba as nb
from numba import jit, njit, prange
'''
https://jellis18.github.io/post/2018-01-02-mcmc-part1/
^ this is a really informative website about calibrating MCMC algorithms based on acceptance reject ratio
'''
#%%
@njit
def metropolis_hastings(pfunc, iter, alpha, dims):
# we make steps based on EACH electron, the number of which we calculate from dims/3
# 'scale' of the problem. this is arbitrary
s = 3.
# we discard some initial steps to allow the walkers to reach the distribution
equi_threshold = 0
initial_matrix = np.zeros((int(dims/3), 3))
reject_ratio = 0.
therm = 1
test = []
samples = []
for i in range(int(dims/3)):
initial_matrix[i] = 2.*s*np.random.rand(3) - s
# now sample iter number of points
for i in range(iter):
# choose which electron to take for a walk:
e_index = np.random.randint(0, dims/3)
trial_matrix = initial_matrix.copy()
trial_matrix[e_index] += (2.*s*np.random.rand(3) - s)/(dims/3)
# trial_matrix[e_index] = hi
proposed_pt = np.reshape(trial_matrix, (1, dims))[0]
initial_pt = np.reshape(initial_matrix, (1, dims))[0]
# print(initial_pt)
p = pfunc(proposed_pt, alpha) / pfunc(initial_pt, alpha)
if p > np.random.rand():
initial_matrix = trial_matrix.copy()
# print(initial_matrix == trial_matrix)
else:
reject_ratio += 1./iter
if i > equi_threshold:
if (i-therm)%therm == 0:
test.append(np.reshape(initial_matrix, (1, dims))[0][3])
samples.append(np.reshape(initial_matrix, (1, dims))[0])
return samples, reject_ratio, test
@njit
def integrator_mcmc(pfunc, qfunc, sample_iter, walkers, alpha, dims):
therm = 0
vals = np.zeros(walkers)
val_errors = 0.
test = []
for i in range(walkers):
mc_samples, rejects, p = metropolis_hastings(pfunc, sample_iter, alpha, dims)
sums = 0.
# obtain arithmetic average of sampled Q values
for array in mc_samples[therm:]:
sums += qfunc(array, alpha)
test.append(qfunc(array, alpha))
vals[i] = (sums/(sample_iter - therm))
# also calculate the variance
vals_squared = np.sum(vals**2)
vals_avg = np.sum(vals) /walkers
variance = vals_squared/walkers - (vals_avg) ** 2
std_error = np.sqrt(variance/walkers)
print('Iteration cycle complete, result = ', vals_avg, 'error = ', std_error, 'rejects = ', rejects)
return vals_avg, std_error, rejects, test, p
@njit
def mcmc_q(x, alpha):
''' this is the Q part of the integrand function for mcmc
inputs: x(array), denoting iter number of sample points, given by
'''
### helium local energy
r1 = x[0:2]
r2 = x[3:]
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r1_hat = r1 / r1_len
r2_hat = r2 / r2_len
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
return ((-4 + np.dot(r1_hat - r2_hat, r1 - r2 ) / (r12 * (1+alpha*r12)**2)
- 1/ (r12*(1+alpha*r12)**3)
- 1/ (4*(1+alpha*r12)**4)
+ 1/ r12 ))[0]
@njit
def mcmc_p(x, alpha):
'''
this is the integrand function for mcmc
'''
### helium wavefunction squared
r1 = x[0:3]
r2 = x[3:]
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
return ((np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
* np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2)[0]
<file_sep>/helium_markii/uniform.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Wed Jun 17 16:24:56 2020
Basic Uniform Monte Carlo Integrator
Applied to Variational Calculations
"""
import time
import numpy as np
from scipy.optimize import fmin
import matplotlib.pyplot as plt
from numba import njit, prange
@njit(parallel = True)
def Uint(integrand, sampler, bounds, measure, n, alpha):
''' Obtains integral result
Inputs:
integrand: function
sampler: uniform sampler, function
bounds: integral bounds, array
n: number of samples per dimension
'''
# this takes n samples from the integrand and stores it in values
values = np.zeros(n)
for i in prange(n):
sample = sampler(bounds)
val = integrand(sample, alpha)
values[i] = val
# this is the value of the sampled integrand values
result = measure * np.sum(values) / n
return result
@njit
def Uint_iter(integrand, sampler, bounds, measure, n, iters, alpha):
''' returns array of integral results
'''
# obtain iters integral results
results = np.zeros(iters)
for i in range(iters):
results[i] = Uint(integrand, sampler, bounds, measure, n, alpha)
return results
@njit
def get_measure(bounds):
''' obtains the volume of the dims dimensional hypercube.
Used for multiplying the expected value.
inputs:
bounds: array of size dims, indicating the dims bounds of the definite
integral
outputs:
measure: float
'''
measure = 1.
dims = len(bounds)
for i in range(dims):
b = bounds[i]
dimlength = float(b[1] - b[0])
measure *= dimlength
return measure
@njit
def sampler(bounds):
''' returns a uniformly distributed random array of size dims
inputs:
bounds is a 2D array, specifying the integration range of each dim
'''
dims = len(bounds)
samples = np.zeros(dims)
for i in range(dims):
b = bounds[i]
samples[i] = np.random.uniform(b[0], b[1])
return samples
@njit
def psiHpsi(x, alpha):
''' Local energy term
'''
r1 = x[0:3]
r2 = x[3:]
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r1_hat = r1 / r1_len
r2_hat = r2 / r2_len
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
EL = ((-4 + np.dot(r1_hat - r2_hat, r1 - r2 ) / (r12 * (1+alpha[0]*r12)**2)
- 1/ (r12*(1+alpha[0]*r12)**3)
- 1/ (4*(1+alpha[0]*r12)**4)
+ 1/ r12 ))
psisq = ((np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
* np.exp(r12 / (2 * (1+ alpha[0]* r12)))) ** 2)
return psisq* EL
@njit
def psisq(x, alpha):
''' Squared trial wavefunction
'''
r1_len = np.sqrt(x[0]**2 + x[1]**2 + x[2]**2)
r2_len = np.sqrt(x[3]**2 + x[4]**2 + x[5]**2)
r12 = np.sqrt((x[0]-x[3])**2 + (x[1]-x[4])**2 + (x[2]-x[5])**2)
psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
* np.exp(r12 / (2 * (1+ alpha[0]* r12)))) ** 2
return psisq
# I just can't jit this part for some odd reason? All functions that it calls are
# jitted though
@njit
def evalenergy(alpha):
#initialise settings
domain = 2.
dims = 6
bounds = []
for i in range(dims):
bounds.append([-domain, domain])
bounds = np.array(bounds)
n = 100000
iters = 30
measure = get_measure(bounds)
expresults = Uint_iter(psiHpsi, sampler, bounds, measure, n, iters, alpha)
normresults = Uint_iter(psisq, sampler, bounds, measure, n, iters, alpha)
#obtain average and variance
vals = expresults/normresults
avg = np.sum(vals) / iters
vals_squared = np.sum(vals**2)
var = (vals_squared/ iters - avg **2)
std = np.sqrt(var)
E = avg
print(E, std)
# print('When alpha is {}, the energy is {} with std {}' .format(alpha, E, std))
return E
### Minimisation algorithm
# start_time = time.time()
# fmin(evalenergy, 0.1, full_output = 1, ftol = 1)
# duration = (time.time() - start_time)
# print('Time taken:', duration)
#%%%%%%%%%%%%%%%%%%%%%%%%
### Graph Plotting stuff
def plot_iter_graphs(itegrand, bounds, iter_range, lit_val, plotval=1, plotnormval=1, plottime=1):
''' This function is used for plotting graphs
'''
results = []
variances = []
times = []
def get_lit(x):
return float(lit_val) + 0*x
for iters in iter_range:
iters = int(iters)
start_time = time.time()
x = Uint(integrand, sampler, bounds, iters)
duration = (time.time() - start_time)
results.append(x[0])
variances.append(x[1])
times.append(duration)
if plotval:
plt.plot(np.log10(iter_range), results)
plt.xscale = 'log'
plt.ylabel('Value')
plt.xlabel('Iterations log scale')
plt.grid()
plt.plot()
plt.show()
if plotnormval:
xrange = np.log10(iter_range)
plt.plot(xrange, results/get_lit(np.log10(iter_range)))
plt.xscale = 'log'
plt.ylabel('Normalised Value')
plt.xlabel('Iterations log scale')
plt.grid()
plt.plot()
plt.show()
if plottime:
plt.plot(np.log10(iter_range), times)
plt.xscale = 'log'
plt.ylabel('Time taken (s)')
plt.xlabel('Iterations log scale')
plt.grid()
plt.show()
pass
<file_sep>/helium_markii/optipack.py
#!/usr/bin/env python3
# Made 2020, <NAME>, <NAME>
# mingsongwu [at] outlook [dot] sg
# github.com/starryblack/quVario
### This script provides the montypython object AND minimiss object that handles any calls to monte carlo integration functions and minimisation functions of our design. It interfaces with the python packages installed for basic functionalities (ie mcint)
### optipack should serve helium_markii.py which is the higher level script for direct user interface
### as of 27 may 2020 montypython uses mcint, and minimiss uses scipy fmin functions as main work horses.
### montypython removed, replaced with simple functions because this is the only sane way to be able to numba jit them
import math
import sys
import os
import time
from datetime import datetime
import statistics
### using sympy methods for symbolic integration. Potentially more precise and convenient (let's not numerically estimate yet)
import numpy as np
import scipy as sp
import scipy.constants as sc
from scipy import optimize, integrate
import sympy as sy
from sympy import conjugate, simplify, lambdify, sqrt
from sympy import *
from IPython.display import display
import vegas
import matplotlib.pyplot as plt
import random
from numba import jit, njit, prange
@njit
def metropolis_hastings(pfunc, iter, alpha, dims):
# we make steps based on EACH electron, the number of which we calculate from dims/3
# 'scale' of the problem. this is arbitrary
s = 3.
# we discard some initial steps to allow the walkers to reach the distribution
equi_threshold = 0
initial_matrix = np.zeros((int(dims/3), 3))
reject_ratio = 0.
therm = 1
test = []
samples = []
for i in range(int(dims/3)):
initial_matrix[i] = 2.*s*np.random.rand(3) - s
# now sample iter number of points
for i in range(iter):
# choose which electron to take for a walk:
e_index = np.random.randint(0, dims/3)
trial_matrix = initial_matrix.copy()
trial_matrix[e_index] += (2.*s*np.random.rand(3) - s)/(dims/3)
# trial_matrix[e_index] = hi
proposed_pt = np.reshape(trial_matrix, (1, dims))[0]
initial_pt = np.reshape(initial_matrix, (1, dims))[0]
# print(initial_pt)
p = pfunc(proposed_pt, alpha) / pfunc(initial_pt, alpha)
if p > np.random.rand():
initial_matrix = trial_matrix.copy()
# print(initial_matrix == trial_matrix)
else:
reject_ratio += 1./iter
if i > equi_threshold:
if (i-therm)%therm == 0:
test.append(np.reshape(initial_matrix, (1, dims))[0][3])
samples.append(np.reshape(initial_matrix, (1, dims))[0])
return samples, reject_ratio, test
@njit
def integrator_mcmc(pfunc, qfunc, sample_iter, walkers, alpha, dims, verbose = True):
therm = 0
vals = np.zeros(walkers)
val_errors = 0.
test = []
for i in range(walkers):
mc_samples, rejects, p = metropolis_hastings(pfunc, sample_iter, alpha, dims)
sums = 0.
# obtain arithmetic average of sampled Q values
for array in mc_samples[therm:]:
sums += qfunc(array, alpha)
test.append(qfunc(array, alpha))
vals[i] = (sums/(sample_iter - therm))
# also calculate the variance
vals_squared = np.sum(vals**2)
vals_avg = np.sum(vals) /walkers
variance = vals_squared/walkers - (vals_avg) ** 2
std_error = np.sqrt(variance/walkers)
if verbose:
print('Iteration cycle complete, result = ', vals_avg, 'error = ', std_error, 'rejects = ', rejects, 'alpha current = ', alpha)
return vals_avg, std_error, rejects, test, p
#%%%%%%%%%%%%%%%%%%%%%%%
@njit(parallel = True)
def Uint(integrand, bounds, n, alpha):
# this takes n samples from the integrand and stores it in values
values = 0
for x in prange(n):
sample = np.zeros(len(bounds))
for i in range(len(bounds)):
sample[i] = np.random.uniform(bounds[i][0], bounds[i][1])
val = integrand(sample, alpha)
values += val
# this is the value of the sampled integrand values
result = values / n
return result
@njit
def Ueval(normalisation, expectation, n, iters, alpha, dimensions):
#initialise settings
domain = 3.
dims = dimensions
#origin centered symmetrical volume
bounds = []
for i in range(dims):
bounds.append([-domain, domain])
bounds = np.array(bounds)
measure = 1.
for i in range(dims):
dimlength = float(bounds[i][1] - bounds[i][0])
measure *= dimlength
results = np.zeros(iters)
normresults = np.zeros(iters)
for i in range(iters):
results[i] = Uint(expectation, bounds, n, alpha) * measure
normresults[i] = Uint(normalisation, bounds, n, alpha) * measure
#obtain average and variance
vals = results / normresults
avg = np.sum(vals) / iters
vals_squared = np.sum(vals**2)
var = (vals_squared/ iters - avg **2)
std = np.sqrt(var)
E = avg
print(E, std )
# print('When alpha is {}, the energy is {} with std {}' .format(alpha, E, std))
return E, std
#%%%%%%%%%%%%%%%%%%%%%%%
class LasVegas():
def __init__(self):
print('LasVegas up for business!')
def vegas_int(self, expec, norm, evals, iter, dimensions, volumespan, verbose = True):
self.final_results = {}
start_time = time.time()
# assign integration volume to integrator
bound = volumespan
dims = dimensions
# creates symmetric bounds specified by [-bound, bound] in dims dimensions
symm_bounds = dims * [[-bound,bound]]
# simultaneously initialises expectation and normalisation integrals
expinteg = vegas.Integrator(symm_bounds)
norminteg = vegas.Integrator(symm_bounds)
# adapt to the integrands; discard results
expinteg(expec, nitn = 5, neval = 1000)
norminteg(norm, nitn = 5, neval = 1000)
# do the final integrals
expresult = expinteg(expec, nitn = iter, neval = evals)
normresult = norminteg(norm, nitn = iter, neval = evals)
E = expresult.mean/normresult.mean
if verbose:
print('Energy is {} when alpha is'.format(E), ' with sdev = ', [expresult.sdev, normresult.sdev])
print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
self.final_results['energy'] = E
self.final_results['dev'] = [expresult.sdev, normresult.sdev]
self.final_results['pvalue'] = [expresult.Q, normresult.Q]
return self.final_results
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('\n\nLasVegas object self-destructing\n\n')
#%%%%%%%%%%%%%%%%%%%%%%%
class MiniMiss():
def __init__(self):
print('MiniMiss optimisation machine initialised and ready!\n')
def neldermead(self, func, guess, ftol, bounds = [(0., 3.),(0., 3.),(0., 0.3) ]):
starttime = time.time()
temp = optimize.fmin(func, guess, full_output = 1, ftol = ftol)
# temp = optimize.differential_evolution(func, bounds)
# temp = optimize.minimize(func, guess, method = 'BFGS', tol = 0.01)
endtime = time.time()
elapsedtime = endtime - starttime
now = datetime.now()
date_time = now.strftime('%d/%m/%Y %H:%M:%S')
# just returns datetime of attempt, elapsed time, optimised parameter, optimised value, number of iterations
return [date_time, elapsedtime, guess, temp]
def gradient(self, func, guess, tolerance, convergence = 'fuzzy', args = None):
print('\nGradient descent initiated, type of convergence selected as ' + convergence +'\n')
cycle_count = 0
position0 = guess
ep = 0.000001
step = 0.1
step_ratio = 0.5
epsilons = np.identity(len(guess))*ep
delta1 = 10.
point_collection = []
satisfied = False
while satisfied == False:
cycle_count+=1
print('Cycle', cycle_count)
value1 = func(position0)
vector = np.zeros(len(guess))
for i in range(len(guess)):
vector[i] = (func(position0 + epsilons[i]) - func(position0))/ep
vectornorm = vector/(np.linalg.norm(vector))
print(vectornorm)
position0 += -vectornorm * step
value2 = func(position0)
delta1 = value2 - value1
positionprime = position0 + vectornorm*(step*step_ratio)
value3 = func(positionprime)
delta2 = value3 - value1
if delta2 < delta1:
print('shrink!')
position0 = positionprime
step = step*step_ratio
delta1 = delta2
value2 = value3
point_collection.append(value2)
if convergence == 'strict':
satisfied = abs(delta1) < tolerance
finalvalue = value2
elif convergence == 'fuzzy':
if len(point_collection) >= 10:
data_set = point_collection[-10:]
print('std', statistics.pstdev(data_set))
print('mean', statistics.mean(data_set))
satisfied = abs(statistics.pstdev(data_set)/statistics.mean(data_set)) < tolerance
finalvalue = statistics.mean(data_set)
if cycle > 150:
print('Max iterations reached, did not converge according to tolerance!!')
break
print('Convergence sucess!')
return finalvalue, position0
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('\n\nMiniMiss object self-destructing\n\n')
<file_sep>/helium_markii/lasvegas.py
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
vegas example from the Basic Integrals section
of the Tutorial and Overview section of the
vegas documentation.
"""
from __future__ import print_function # makes this work for python2 and 3
import vegas
import numpy as np
import time
from numba import jit, njit
from scipy.optimize import fmin
import matplotlib.pyplot as plt
#from optipack import MiniMiss, integrator_mcmc, metropolis_hastings, mcmc_q, mcmc_p
import psiham
psilet_args = {'electrons': 2, 'alphas': 3, 'coordsys': 'cartesian'}
ham = psiham.HamLet(trial_expr = 'threepara1', **psilet_args)
variables, expr = ham.he_expect()
temp1 = ham.vegafy(expr, coordinates = variables, name = 'expec')
variables, expr = ham.he_norm()
temp2 = ham.vegafy(expr, coordinates = variables, name = 'norm')
exec(temp1, globals())
exec(temp2, globals())
final_results = {}
#SHOW_GRID = False
#OPTIM = True
def main(alpha):
global alpha0
# global alpha1
# global alpha2
alpha0 = alpha[0]
# alpha1 = alpha[1]
# alpha2 = alpha[2]
start_time = time.time()
# assign integration volume to integrator
bound = 8
dims = 6
# creates symmetric bounds specified by [-bound, bound] in dims dimensions
symm_bounds = dims * [[-bound,bound]]
# simultaneously initialises expectation and normalisation integrals
expinteg = vegas.Integrator(symm_bounds)
norminteg = vegas.Integrator(symm_bounds)
# adapt to the integrands; discard results
expinteg(expec, nitn=5, neval=1000)
norminteg(norm,nitn=5, neval=1000)
# do the final integrals
expresult = expinteg(expec, nitn=10, neval=100000)
normresult = norminteg(norm, nitn=10, neval=100000)
if not OPTIM:
E = expresult.mean/normresult.mean
else:
E = expresult[0].mean/ normresult[0].mean
# print('Energy is {} when alpha is {}'.format(E, alpha), ' with sdev = ', [expresult.sdev, normresult.sdev])
# print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
final_results['energy'] = E
final_results['dev'] = [expresult.sdev, normresult.sdev]
final_results['pvalue'] = [expresult.Q, normresult.Q]
return E
#
#### Optimisation ALgorithm
#start_time = time.time()
#OPTIM = False
#
##plt.figure(figsize=(16,10))
#
##print('Plotting function initialised!')
##energies = []
##alpha = np.linspace(0.1, 0.2, 20)
## for i in alpha:
## alpha0 = i
##
## energy_samples = []
## for j in range(1):
## energy_samples.append(evalenergy(i))
## avg = np.average(energy_samples)
## print('Averaged energy for %f is %f' %(i, avg))
## energies.append(avg)
## plt.plot(alpha, energies, color='dodgerblue')
## print("--- Total time: %s seconds ---" % (time.time() - start_time))
#
## plt.savefig('Vegas')
#
#OPTIM = False
#start_time = time.time()
#result = fmin(main, [0.15], ftol = 0.01, xtol = 0.001, full_output=True)
##e = evalenergy(0.5)
##print(e)
#print("--- Total time: %s seconds ---" % (time.time() - start_time))
#%%%%%%%%%%%%%%%%%%%%
#########################################################################
#def evalenergy(alpha):
# @vegas.batchintegrand
# def expec(x):
# ''' Expectation value function, used as numerator for variational integral
# '''
#
# r1 = np.array(x[:,0:3])
# r2 = np.array(x[:,3:])
#
# r1_len = np.sqrt(x[:,0]**2 + x[:,1]**2 + x[:,2]**2)
# r2_len = np.sqrt(x[:,3]**2 + x[:,4]**2 + x[:,5]**2)
#
# r1_hat = r1 / np.reshape(r1_len, (-1,1))
# r2_hat = r2 / np.reshape(r2_len, (-1,1))
#
# r12 = np.sqrt((x[:,0]-x[:,3])**2 + (x[:,1]-x[:,4])**2 + (x[:,2]-x[:,5])**2)
#
# EL = (-4 + np.sum((r1_hat-r2_hat)*(r1-r2), 1) / (r12 * (1 + alpha*r12)**2)
# - 1/ (r12*(1 + alpha*r12)**3)
# - 1/ (4*(1 + alpha*r12)**4)
# + 1/ r12 )
#
# psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
# * np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2
# return psisq * EL
#
# @vegas.batchintegrand
# def norm(x):
# ''' Squared trial wavefunction, used as denominator for variational integral
# '''
## x = np.reshape(x, (1,-1))
#
# r1_len = np.sqrt(x[:,0]**2 + x[:,1]**2 + x[:,2]**2)
# r2_len = np.sqrt(x[:,3]**2 + x[:,4]**2 + x[:,5]**2)
#
# r12 = np.sqrt((x[:,0]-x[:,3])**2 + (x[:,1]-x[:,4])**2 + (x[:,2]-x[:,5])**2)
#
# psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
# * np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2
# return psisq
#
# def main():
# start_time = time.time()
#
# # assign integration volume to integrator
# bound = 8
# dims = 6
# # creates symmetric bounds specified by [-bound, bound] in dims dimensions
# symm_bounds = dims * [[-bound,bound]]
#
# # simultaneously initialises expectation and normalisation integrals
# expinteg = vegas.Integrator(symm_bounds)
# norminteg = vegas.Integrator(symm_bounds)
#
# # adapt to the integrands; discard results
# expinteg(expec, nitn=5, neval=1000)
# norminteg(norm,nitn=5, neval=1000)
# # do the final integrals
# expresult = expinteg(expec, nitn=10, neval=10000)
# normresult = norminteg(norm, nitn=10, neval=10000)
#
#### Code for printing the grid
#### and diagnostics
## print(expresult.summary())
## print('expresult = %s Q = %.2f' % (expresult, expresult.Q))
## if SHOW_GRID:
## expinteg.map.show_grid(20)
##
## print(normresult.summary())
## print('normresult = %s Q = %.2f' % (normresult, normresult.Q))
## if SHOW_GRID:
## norminteg.map.show_grid(20)
#
# ### obtain numerical result
# ### Different expressions for plotting/ minimisation algorithm
# if not OPTIM:
# E = expresult.mean/normresult.mean
# else:
# E = expresult[0].mean/ normresult[0].mean
## print('Energy is %f when alpha is %f' %(E, alpha))
## print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
# final_results['energy'] = E
# final_results['dev'] = [expresult.sdev, normresult.sdev]
# final_results['pvalue'] = [expresult.Q, normresult.Q]
# return E
# E = main()
# return E
# #%% This is for one-parameter plotting
# #### Run plotting function, plot alpha against energies
# start_time = time.time()
# OPTIM = False
#
# plt.figure(figsize=(16,10))
# #high resolution bit closer to the minimum
# #alpha0 = np.linspace(0.001, 0.1, 5)
# #energies0 = []
# #for i in alpha0:
# # energies0.append(evalenergy(i))
#
# print('Plotting function initialised!')
# energies = []
# alpha = np.linspace(0.1, 0.2, 20)
# for i in alpha:
# energy_samples = []
# for j in range(1):
# energy_samples.append(evalenergy(i))
# avg = np.average(energy_samples)
# print('Averaged energy for %f is %f' %(i, avg))
# energies.append(avg)
# plt.plot(alpha, energies, color='dodgerblue')
# print("--- Total time: %s seconds ---" % (time.time() - start_time))
#
# plt.savefig('Vegas')
#
# #%%
# ##low resolution bit
# #alpha = np.linspace(0.25, 2, 10)
# #energies2 = []
# #for i in alpha:
# # energies2.append(evalenergy(i))
# #plt.plot(alpha, energies2, color = 'dodgerblue')
# #
# #### Use optimisation to obtain the minimum
# #### and plot a point
# ##OPTIM = False
# ##result = fmin(evalenergy, 0.2, ftol = 0.01, xtol = 0.001, full_output=True)
# ##plt.plot(result[0], result[1], 'ro', ms=5)
# #
# #plt.xlabel('alpha')
# #plt.ylabel('energy')
# #plt.grid()
# #plt.show()
# #print("--- Total time: %s seconds ---" % (time.time() - start_time))
#
##############################################################################
### THE FOLLOWING CODE IS FOR EXTRACTING DATA AND PLOTTING GRAPHS
#%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
#### To plot a histogram of evaluations
#### VEGAS PLOTS FOR ENERGIES AND HISTOGRAMS
#N = 50
#
#energies = []
#optparas = []
#start_time = time.time()
#print('Loop initialised!')
#
#for i in range(N):
# print(i)
# loop_time = time.time()
# result = fmin(evalenergy, [0.15], ftol = 0.01, xtol = 0.001, full_output=1, disp=0)
# energies.append(result[1])
# optparas.append(float(result[0]))
# print("--- Loop time: %s seconds ---" % (time.time() - loop_time))
#
#print("--- Total time: %s seconds ---" % (time.time() - start_time))
#
###########
#### Save data into a csv
#n = '1e5'
#name = 'vegas_1param_iters=%s_N=%i' %(n,N)
#np.savetxt('%s.csv' %name, np.transpose([energies,optparas]),
# fmt = '%s', delimiter=',', header = (name + '_t=2176'))
#
#
##########
#### Plot histograms
#avg = np.mean(energies)
#std = np.std(energies)
#plt.figure(figsize=(16,10))
#plt.rcParams.update({'font.size': 22})
#
#plt.hist(energies,10)
#plt.xlabel('Energy (Atomic Units)')
#plt.ylabel('N')
#plt.figtext(0.2,0.75,'oneparam, N=%i, \n'
# 'n=%s, bound=8 \n'
# 'avg E= %.5f \n'
# 'std = %.5f'%(N, n, avg,std))
#plt.title('Histogram of distributed optimised energy values for VEGAS')
##plt.savefig('vegas_energyhist_1param_%s' %n)
#plt.show()
#
#alphaavg = np.mean(optparas)
#alphastd = np.std(optparas)
#plt.figure(figsize=(16,10))
#plt.xlabel('Variational parameter alpha')
#plt.ylabel('N')
#plt.figtext(0.6,0.7,'oneparam, N=%i, \n n=%s, bound=8 \n'
# 'avg alpha = %.5f \n'
# 'std = %.5f'%(N, n, alphaavg,alphastd))
#plt.title('Histogram of distributed optimised parameters for VEGAS')
#plt.hist(optparas,10)
##plt.savefig('vegas_alphahist_1_param_%s' %n)
#plt.show()
#%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
### VEGAS analysis Code to analyse the Q values
#
#Qexp = np.zeros((50,5))
#Qnorm = np.zeros((50,5))
#
#N = 50
#n = 5e5
#alpha = [0.15606, 0.15387, 0.15156, 0.15109, 0.15336]
#
#index = 0
#
#qexp = []
#qnorm = []
#
#for i in range(N):
# print(i)
# loop_time = time.time()
# evalenergy(alpha[index])
# qexp.append(final_results['pvalue'][0])
# qnorm.append(final_results['pvalue'][1])
# print("--- Loop time: %s seconds ---" % (time.time() - loop_time))
#
#print('The average Qexp value is: ' ,np.average(qexp))
#print('The average Qnorm value is: ' ,np.average(qnorm))
#
##Qexp[:,index] = qexp
##Qnorm[:,index] = qnorm
#
#
###########
#### Save data into a csv
##Qe = np.zeros((50,5))
#
##name = 'vegas_1param_Qnorm'
##np.savetxt('%s.csv' %name, Qnorm,
## fmt = '%s', delimiter=',', header = (name + str(iters)))
#
#### Obtain the average
#iters = [1e4, 5e4, 1e5, 5e5, 1e6]
#x = []
#y = []
#for i in range(5):
# x.append(np.average(Qexp[:,i]))
# y.append(np.average(Qnorm[:,i]))
#
#plt.scatter(iters, x)
#plt.scatter(iters, y)
#plt.show()
#
#
#### Obtain the standard deviation
#xstd = []
#ystd = []
#for i in range(5):
# xstd.append(np.std(Qexp[:,i]))
# ystd.append(np.std(Qnorm[:,i]))
#
#plt.scatter(iters, xstd)
#plt.scatter(iters, ystd)
#plt.show()
#%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
#### Analysis of the impact of bound sizes
#
#bounds = [1.5, 1.6, 1.7,1.8, 1.9]
#B_energy_2 = np.zeros((50, 5))
#B_alpha_2 = np.zeros((50, 5))
#
#N = 50
#OPTIM = False
#
#for j in range(len(bounds)):
# bound = bounds[j]
# benergy = []
# bopt = []
# def evalenergy(alpha):
# @vegas.batchintegrand
# def expec(x):
# ''' Expectation value function, used as numerator for variational integral
# '''
# r1 = np.array(x[:,0:3])
# r2 = np.array(x[:,3:])
#
# r1_len = np.sqrt(x[:,0]**2 + x[:,1]**2 + x[:,2]**2)
# r2_len = np.sqrt(x[:,3]**2 + x[:,4]**2 + x[:,5]**2)
#
# r1_hat = r1 / np.reshape(r1_len, (-1,1))
# r2_hat = r2 / np.reshape(r2_len, (-1,1))
#
# r12 = np.sqrt((x[:,0]-x[:,3])**2 + (x[:,1]-x[:,4])**2 + (x[:,2]-x[:,5])**2)
#
# EL = (-4 + np.sum((r1_hat-r2_hat)*(r1-r2), 1) / (r12 * (1 + alpha*r12)**2)
# - 1/ (r12*(1 + alpha*r12)**3)
# - 1/ (4*(1 + alpha*r12)**4)
# + 1/ r12 )
#
# psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
# * np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2
# return psisq * EL
#
# @vegas.batchintegrand
# def norm(x):
# ''' Squared trial wavefunction, used as denominator for variational integral
# '''
#
# r1_len = np.sqrt(x[:,0]**2 + x[:,1]**2 + x[:,2]**2)
# r2_len = np.sqrt(x[:,3]**2 + x[:,4]**2 + x[:,5]**2)
#
# r12 = np.sqrt((x[:,0]-x[:,3])**2 + (x[:,1]-x[:,4])**2 + (x[:,2]-x[:,5])**2)
#
# psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
# * np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2
# return psisq
#
# def main():
# # assign integration volume to integrator
# dims = 6
# # creates symmetric bounds specified by [-bound, bound] in dims dimensions
# symm_bounds = dims * [[-bound,bound]]
#
# # simultaneously initialises expectation and normalisation integrals
# expinteg = vegas.Integrator(symm_bounds)
# norminteg = vegas.Integrator(symm_bounds)
#
# # adapt to the integrands; discard results
# expinteg(expec, nitn=5, neval=1000)
# norminteg(norm,nitn=5, neval=1000)
# # do the final integrals
# expresult = expinteg(expec, nitn=10, neval=100000)
# normresult = norminteg(norm, nitn=10, neval=100000)
#
# ### obtain numerical result
# ### Different expressions for plotting/ minimisation algorithm
# if not OPTIM:
# E = expresult.mean/normresult.mean
# else:
# E = expresult[0].mean/ normresult[0].mean
#
# final_results['energy'] = E
# final_results['dev'] = [expresult.sdev, normresult.sdev]
# final_results['pvalue'] = [expresult.Q, normresult.Q]
# print(E)
# return E
# E = main()
# return E
#
# for i in range(N):
# print(j, i)
# start_time = time.time()
# result = fmin(evalenergy, [0.15], ftol = 0.01, xtol = 0.001, full_output=1, disp=0)
# benergy.append(result[1])
# bopt.append(float(result[0]))
# print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
# B_energy_2[:,j] = benergy
# B_alpha_2[:,j] = bopt
# print(np.mean(benergy))
#
#name = 'vegas_bound_energy_comp_2'
#np.savetxt('%s.csv' %name, B_energy_2,
# fmt = '%s', delimiter=',', header = (name + str(bounds)))
#
#name = 'vegas_bound_ealpha_comp_2'
#np.savetxt('%s.csv' %name, B_alpha_2,
# fmt = '%s', delimiter=',', header = (name + str(bounds)))
#
#
#bounds = [1.5, 1.6, 1.7,1.8, 1.9]
#B_energy_mean = np.mean(B_energy_2, 0)
#B_alpha_mean = np.mean(B_alpha_2, 0)
#B_energy_std = np.std(B_energy_2, 0)
#B_alpha_std = np.std(B_alpha_2, 0)
#
#B_processed_2 = np.transpose([bounds, B_energy_mean, B_alpha_mean, B_energy_std, B_alpha_std])
#name = 'vegas_bound_processed_2'
#np.savetxt('%s.csv' %name, B_processed_2,
# fmt = '%s', delimiter=',', header = (name +'bound_energy_alpha_estd_astd'))
#%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%
# This is for testing with regards to the twopara and
# threepara case. Since the loop is slow,
#twopara_energy_new = np.zeros((10,1))
#twopara_energy_backup_2 = twopara_energy.copy()
threepara_energy= np.zeros((N, len(iters)))
#%%
energy = []
#def main(alpha):
## global alpha0
## alpha0 = alpha[0]
## global alpha1
## alpha1 = alpha[1]
# if len(alpha) >= 1:
# global alpha0
# alpha0 = alpha[0]
# if len(alpha) >= 2:
# global alpha1
# alpha1 = alpha[1]
# if len(alpha) == 3:
# global alpha2
# alpha2 = alpha[2]
#
# start_time = time.time()
# # assign integration volume to integrator
# bound = 8
# dims = 6
# # creates symmetric bounds specified by [-bound, bound] in dims dimensions
# symm_bounds = dims * [[-bound,bound]]
#
# # simultaneously initialises expectation and normalisation integrals
# expinteg = vegas.Integrator(symm_bounds)
# norminteg = vegas.Integrator(symm_bounds)
#
# # adapt to the integrands; discard results
# expinteg(expec, nitn=5, neval=1000)
# norminteg(norm,nitn=5, neval=1000)
# # do the final integrals
# expresult = expinteg(expec, nitn=10, neval=iters[indx])
# normresult = norminteg(norm, nitn=10, neval=iters[indx])
#
#
# E = expresult.mean/normresult.mean
#
# print(alpha, E)
# print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
# return E
def iterplot(data_arr, N, iters, indx):
s_time = time.time()
opt =[]
def main(alpha):
if len(alpha) >= 1:
global alpha0
alpha0 = alpha[0]
if len(alpha) >= 2:
global alpha1
alpha1 = alpha[1]
if len(alpha) == 3:
global alpha2
alpha2 = alpha[2]
start_time = time.time()
# assign integration volume to integrator
bound = 8
dims = 6
# creates symmetric bounds specified by [-bound, bound] in dims dimensions
symm_bounds = dims * [[-bound,bound]]
# simultaneously initialises expectation and normalisation integrals
expinteg = vegas.Integrator(symm_bounds)
norminteg = vegas.Integrator(symm_bounds)
# adapt to the integrands; discard results
expinteg(expec, nitn=5, neval=1000)
norminteg(norm,nitn=5, neval=1000)
# do the final integrals
expresult = expinteg(expec, nitn=10, neval=iters[indx])
normresult = norminteg(norm, nitn=10, neval=iters[indx])
E = expresult.mean/normresult.mean
print(alpha, E)
print("--- Iteration time: %s seconds ---" % (time.time() - start_time))
return E
for i in range(N):
print(indx, i)
repeat_time = time.time()
# start_time = time.time()
result = fmin(main, [2, 1.6, 0.15], ftol = 0.1, xtol = 0.01, full_output=1, disp=1)
energy.append(result[1])
print("--- Iteration time: %s seconds ---" % (time.time() - repeat_time))
dur = time.time() - s_time
print("--- Total time: %s seconds ---" % (dur))
data_arr[:,indx] = energy
return data_arr
def save_energy(data_arr, name):
''' Save data and processed data into a csv
'''
np.savetxt('%s.csv' %name, (data_arr),
fmt = '%s', delimiter=',', header = (name+ str(iters)))
### Process data and save into csv
eavg = np.mean(data_arr, 0)
estd = np.std(data_arr, 0)
np.savetxt('%s.csv' %name+'_processed', (np.transpose([iters, eavg, estd])),
fmt = '%s', delimiter=',', header = (name+ 'iters, eavg, estd'))
### Main code
iters = [1e4, 5e4, 1e5, 5e5, 1e6]
N = 10
indx = 2
threepara_energy = iterplot(threepara_energy, N, iters, indx)
#threepara_energy[:,0] = np.loadtxt(name+'.csv', usecols= 0)
#%%
#name = 'vegas_3param_iters'
#save_energy(threepara_energy, name)
#%%
def getpdf(bound, alpha, absv = 0.4135889547408346):
@vegas.batchintegrand
def norm(x):
''' Squared trial wavefunction, used as denominator for variational integral
'''
r1_len = np.sqrt(x[:,0]**2 + x[:,1]**2 + x[:,2]**2)
r2_len = np.sqrt(x[:,3]**2 + x[:,4]**2 + x[:,5]**2)
r12 = np.sqrt((x[:,0]-x[:,3])**2 + (x[:,1]-x[:,4])**2 + (x[:,2]-x[:,5])**2)
psisq = (np.exp(-2 * r1_len)* np.exp(-2 * r2_len)
* np.exp(r12 / (2 * (1+ alpha*r12)))) ** 2
return psisq / absv
dims = 6
# creates symmetric bounds specified by [-bound, bound] in dims dimensions
symm_bounds = dims * [[-bound,bound]]
# simultaneously initialises expectation and normalisation integrals
norminteg = vegas.Integrator(symm_bounds)
# adapt to the integrands; discard results
norminteg(norm,nitn=5, neval=1000)
# do the final integrals
normresult = norminteg(norm, nitn=10, neval=1000000)
norm = normresult.mean
return norm
#%%
#bound = np.linspace(1,10, 30)
#prob = []
#
#for i in bound:
# prob.append(getpdf(i, 0.155))
#
#%%
#plt.ylabel('probability located in 6 dimensional bound')
#plt.xlabel('bound')
#plt.grid()
#plt.plot(bound, prob, label = 'one para with alpha=0.155' )
#plt.legend()
#%%
#np.concatenate((bound, prob))
##x = np.reshape()
#np.savetxt('pdist.csv', np.array([bound, prob]).transpose(), delimiter= ',')
<file_sep>/README.md
## Welcome to quVario
This repository contains our attempt at coding a quantum variational method machine with Python3. The work is part of our Imperial College Physics Year 1 Summer Project. Due to the coronavirus, we were forced to choose a more computational project topic.
quVario is essentially a python ecosystem that can mathematically represent multi-electron (valance) atom system and find the system's ground state energy via the variational method. The integration techniques utilised range from deterministic quadrature methods to monte carlo methods. We can describe our iterative progress through two main prongs: Mark I (deterministic) and Mark II (monte carlo).
As of August 2020, the systems tested are the hydrogen and helium atoms.
### Helium_marki
```markdown
- First attempt at quantum method of variation problem
- Uses Sympy for symbolic differentiation
- Used out of the box SciPy integration numerical methods (numerical differentiation too, for hydrogen test case)
```
### Helium_markii
```markdown
- Monte Carlo progression of Mark I, with ecosystem further developed:
- psiham.py contains class objects that do the generation of the integrals (symbolic differentiation, algebra, etc.) in the form of a numba jit decorated function.
- optipack.py contains the actual integration functions: uniform monte carlo, metropolis algorithm, VEGAS algorithm (to be cited)
- psiham and optipack feeds helium_markii.py which does the actual execution.
```
<file_sep>/helium_markii/psiham.py
#!/usr/bin/env python3
# Made 2020, <NAME>, <NAME>
# mingsongwu [at] outlook [dot] sg
# github.com/starryblack/quVario
### This script provides the hamLet object which handles calls for functions pertaining to hamiltonian generation (supposed to be general, but this case our focus is helium 5 term hamiltonian)
### hamiltonian should serve helium_markii.py which is the higher level script for direct user interface
### This script also provides the psiLet object which handles calls for functions related to creating a trial wavefunction object. It MUST be compatible with hamiltonian such that the objects can interact to give expectation term (quantum chem)
### psifunction should serve helium_markii.py which is the higher level script for direct user interface
#%%%%%%%%%%%%%%%%%%
import math
import sys
import os
import time
from datetime import datetime
import numpy as np
import scipy as sp
import scipy.constants as sc
import sympy as sy
from sympy import conjugate, simplify, lambdify, sqrt
from sympy import *
from IPython.display import display
from scipy import optimize, integrate
from sympy.parsing.sympy_parser import parse_expr
from numba import jit, njit
from sympy.utilities.lambdify import lambdastr
'''
MS's note: I will recycle my previous script for the two sets of electron coordinates and use sympy to symbolically generate the laplacian for each electron. I think we can safe assume our trial functions are analytical indefinitely so finding the laplacian is easy?
everything will be kept to atomic units
'''
class HamLet():
def __init__(self, trial_expr, **kwargs):
starttime = time.time()
print('Hamiltonian Operator object initialised')
self.coordsys = 'cartesian'
with PsiLet(**kwargs) as psi:
self.bases = psi.bases
self.alphas = psi.alphas
func_selected = psi.getfunc(trial_expr)
self.trial = simplify(parse_expr(func_selected()))
#for convenience
self.r0 = parse_expr(psi.r0)
self.r1 = parse_expr(psi.r1)
self.r01 = parse_expr(psi.r01)
self.variables = [a for b in self.bases for a in b]
#custom 'numbafy' protocol. adapted from <NAME> (jankoslavic)
def numbafy(self, expression, coordinates = None, parameters = None, name='trial_func'):
if coordinates:
code_coord = []
for i, x in enumerate(coordinates):
code_coord.append(f'{x} = coordinates[{i}]')
code_coord = '\n '.join(code_coord)
if parameters:
code_param = []
for i, x in enumerate(parameters):
code_param.append(f'{x} = parameters[{i}]')
code_param = '\n '.join(code_param)
temp = lambdastr((), expression)
temp = temp[len('lambda : '):]
temp = temp.replace('math.exp', 'np.exp')
code_expression = f'{temp}'
template = f"""
@njit
def {name}(coordinates, parameters):
{code_coord}
{code_param}
return {code_expression}"""
print('Numba function template generated! Its name is ', name)
return template
def vegafy(self, expression, coordinates = None, name = 'vega_func'):
if coordinates:
code_coord = []
for i, x in enumerate(coordinates):
code_coord.append(f'{x} = coordinates[:,{i}]')
code_coord = '\n '.join(code_coord)
temp = lambdastr((), expression)
temp = temp[len('lambda : '):]
temp = temp.replace('math.exp', 'np.exp')
code_expression = f'{temp}'
template = f"""
@vegas.batchintegrand
def {name}(coordinates):
{code_coord}
return {code_expression}"""
print('Vega function template generated! Its name is ', name)
return template
def he_getfuncs(self):
return self.he_elocal(), self.he_norm(), self.he_trial()
def he_elocal(self):
operated = self.he_operate(self.trial)
return (self.variables, operated/self.trial)
def he_norm(self):
return (self.variables, self.trial*self.trial)
def he_expect(self):
operated = self.he_operate(self.trial)
return (self.variables, operated*self.trial)
def he_trial(self):
return (self.variables, self.trial)
def he_operate(self, expr):
lap1 = -0.5*self.laplace(expr, 0)
lap2 = -0.5*self.laplace(expr, 1)
attract = -2*(1/self.r0 + 1/self.r1)*expr
repel = (1/self.r01)*expr
return lap1 + lap2 + attract + repel
def laplace(self, expr, index, coordsys = 'c'):
if coordsys == 'cartesian' or coordsys == 'c':
x = self.bases[index][0]
y = self.bases[index][1]
z = self.bases[index][2]
grad = [sy.diff(expr, x), sy.diff(expr, y), sy.diff(expr, z)]
lap = sy.diff(grad[0], x) + sy.diff(grad[1], y) + sy.diff(grad[2], z)
elif coord_sys == 'spherical' or coordsys == 's':
r = self.bases[index][0]
t = self.bases[index][1]
p = self.bases[index][2]
grad = [sy.diff(expr, r), 1/r * sy.diff(expr, t), 1/(r*sin(t)) * sy.diff(expr, p)]
lap = (1/r**2)*sy.diff(r**2 * grad[0], r) + (1/(r*sin(t)))*(sy.diff(grad[1] * sin(t), t) + sy.diff(grad[2], p))
return lap
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('HamLet object self-destructing...')
class PsiLet():
def __init__(self, electrons = 2, alphas = 1, coordsys = 'cartesian'):
print('psiLet object initialised.')
self.spawn_electrons(electrons, coord_sys = coordsys)
print('{} {} electron coordinate set(s) spawned'.format(electrons, coordsys))
self.spawn_alphas(alphas)
print('{} alpha parameter(s) spawned'.format(alphas))
self.r0 = '(x0**2 + y0**2 + z0**2)**0.5'
self.r1 = '(x1**2 + y1**2 + z1**2)**0.5'
self.r01 = '((x0-x1)**2 + (y0-y1)**2 + (z0-z1)**2)**0.5'
self.dot01 = 'x0*x1 + y0*y1 + z0*z1'
def spawn_electrons(self, number, coord_sys = 'cartesian'):
self.bases = []
self.basis_names = {
'cartesian': ['x', 'y', 'z'],
'spherical': ['r', 'theta', 'phi'],
'cylindrical': ['rho', 'phi', 'z']
}
for i in range(number):
temp = []
for q in self.basis_names[coord_sys]:
temp.append(sy.symbols(q + str(i)))
self.bases.append(temp)
def spawn_alphas(self, number):
self.alphas = []
for i in range(number):
self.alphas.append(sy.symbols('alpha' + str(i)))
def getfunc(self, function):
self.func_dict = {
'onepara1': self.onepara1,
'twopara1': self.twopara1,
'threepara1': self.threepara1,
'threepara2': self.threepara2
}
return self.func_dict[function]
def onepara1(self):
expr = f'''exp(-2*{self.r0}-2*{self.r1}+{self.r01}/(2*(1+alpha0*{self.r01})))'''
return expr
def twopara1(self):
expr = f'''exp(-alpha0*({self.r0}+{self.r1})+{self.r01}/(2*(1+alpha1*{self.r01})))'''
return expr
def threepara1(self):
expr = f'''(exp(-alpha0*{self.r0}-alpha1*{self.r1}) + exp(-alpha1*{self.r0}-alpha0*{self.r1}))*(1 + alpha2*{self.r01})'''
return expr
def threepara2(self):
expr = f'''(exp(-alpha0*{self.r0}-alpha1*{self.r1}) + exp(-alpha1*{self.r0}-alpha0*{self.r1}))*exp({self.r01}/(2*(1 + alpha2*{self.r01})))'''
return expr
### this function is flawed, gives numerically incorrect result. Use with caution
def manual2(self):
expr = f'''-4 + ({self.r0} + {self.r1})*(1 - {self.dot01}/({self.r0}*{self.r1}))/({self.r01}*(1+alpha0*{self.r01})**2) - 1/({self.r01}*(1+alpha0*{self.r01})**3) - 1/(4*(1+alpha0*{self.r01})**4) + 1/{self.r01}'''
return expr
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('psiLet object self-destructing')
<file_sep>/helium_markii/helium_markii.py
#!/usr/bin/env python3
# Made 2020, <NAME>, <NAME>
# mingsongwu [at] outlook [dot] sg
# github.com/starryblack/quVario
import math
import time
import numpy as np
from numba import njit, prange
import vegas
import matplotlib.pyplot as plt
from optipack import MiniMiss, LasVegas
from optipack import integrator_mcmc, metropolis_hastings
from optipack import Uint, Ueval
import psiham
import matplotlib.pyplot as plt
class Noble():
def __init__(self, evals = 10000, iters = 40, verbose = True):
print('Noble initialising')
self.dims = 6
self.mini = MiniMiss()
self.vegas = LasVegas()
psilet_args = {'electrons': 2, 'alphas': 3, 'coordsys': 'cartesian'}
self.ham = psiham.HamLet(trial_expr = 'threepara2', **psilet_args)
self.verbose = verbose
self.numba_he_elocal()
self.numba_pdf()
self.numba_expec()
self.vega_expec()
self.vega_pdf()
self.evals = evals
self.iters = iters
# data = self.minimise(function = self.monte_uniform, guess = np.array([2.001, 2.0, 0.2]))
# data = self.minimise(function = self.mcmc_metro, guess = np.array([2.001, 2.0, 0.2]))
# mcmc_args = [pfunc, qfunc , self.evals, self.iters, 6]
# data = jit_grad_descent(integrator_mcmc, guess = np.array([1.9, 2.0, 0.2]), tolerance = 0.0005)
# print(data)
# data = self.grad_descent(self.monte_vegas, np.array([1.9, 2.0, 0.2]))
# print(data)
# for i in range(100):
# self.temp.append(self.monte_vegas(np.array([1.9, 2.0, 0.2])))
# for i in range(100):
# self.temp.append(self.mcmc_metro(np.array([1.9, 2.0, 0.2])))
# print(data[3])
# self.custom_log(data, comments = 'Uniform integrator, 2 para')
def numba_he_elocal(self):
variables, expr = self.ham.he_elocal()
temp = self.ham.numbafy(expr, coordinates = variables, parameters = self.ham.alphas, name = 'qfunc')
exec(temp, globals())
def numba_pdf(self):
variables, expr = self.ham.he_norm()
temp = self.ham.numbafy(expr, coordinates = variables, parameters = self.ham.alphas, name = 'pfunc')
exec(temp, globals())
def numba_expec(self):
variables, expr = self.ham.he_expect()
temp = self.ham.numbafy(expr, coordinates = self.ham.variables, parameters = self.ham.alphas, name = 'expec')
exec(temp, globals())
def vega_expec(self):
variables, expr = self.ham.he_expect()
temp = self.ham.vegafy(expr, coordinates = self.ham.variables, name = 'vega_expec')
exec(temp, globals())
def vega_pdf(self):
variables, expr = self.ham.he_norm()
temp = self.ham.vegafy(expr, coordinates = self.ham.variables, name = 'vega_pfunc')
exec(temp, globals())
def monte_metro(self, args):
temp = integrator_mcmc(pfunc, qfunc , self.evals, self.iters, alpha = args, dims = self.dims, verbose = self.verbose)
self.std = temp[1]
self.reject_ratio = temp[2]
return temp[0]
def monte_uniform(self, args):
temp = Ueval(pfunc, expec, self.evals, self.iters, alpha = args, dimensions = self.dims)
self.std = temp[1]
self.reject_ratio = 0.0
return temp[0]
def monte_vegas(self, alpha):
global alpha0
global alpha1
global alpha2
alpha0 = alpha[0]
alpha1 = alpha[1]
alpha2 = alpha[2]
temp = self.vegas.vegas_int(vega_expec, vega_pfunc, self.evals, self.iters, dimensions = self.dims, volumespan = 8., verbose = self.verbose)
return temp['energy']
def nead_min(self, function, guess):
temp = self.mini.neldermead(func = function, guess = guess, ftol = 0.01)
# temp = grad_des(func = function, guess = guess, tolerance = 0.0001)
return temp
def grad_descent(self, function, guess):
temp = self.mini.gradient(func = function, guess = guess, tolerance = 0.05)
return temp
def stability_protocol(self, fixedpoint, iterations):
temp_master = [[],[],[]]
functions = [self.monte_metro, self.monte_uniform, self.monte_vegas]
for index, func in enumerate(functions):
start = time.time()
for i in range(iterations):
temp_master[index].append(func(guess))
print('Function #{} done!'.format(index))
return temp_master
def custom_log(self, data = [], comments = None):
log = open('markii_log.txt', 'a+')
separator = '\n{}\n'.format(''.join('#' for i in range(10)))
info = separator + 'Datetime = {}\n'.format(data[0]) + 'Time taken (s) = {:.4f}\n'.format(data[1]) + 'Initial parameter guess (atomic untis) = {}\n'.format(data[2]) + 'Optimised parameter (atomic units) = {}\n'.format(data[3]) + 'Optimised function value (atomic units) = {:.4f} with std = {:.4f}\n'.format(data[4], self.std) + 'Iterations = {}, Evaluations/iter = {} with rejection ratio = {:.4f}\n'.format(self.iters, self.evals, self.reject_ratio) + 'Comments = ({})\n'.format(comments)
print(info)
log.write(info)
log.close()
def __enter__(self):
return self
def __exit__(self, e_type, e_val, traceback):
print('Noble object self-destructing')
@njit
def jit_grad_des(func, guess, tolerance):
cycle_count = 0
position0 = guess
ep = 0.00000001
step = 0.2
step_ratio = 0.5
epsilons = np.identity(len(guess))*ep
delta1 = 10.
satisfied = False
point_collection = []
while satisfied == False:
cycle_count+=1
print('Cycle', cycle_count)
value1 = func(pfunc, qfunc, sample_iter = 10000, walkers = 40, alpha = position0, dims = 6)[0]
vector = np.zeros(len(guess))
for i in range(len(guess)):
vector[i] = (func(pfunc, qfunc, sample_iter = 10000, walkers = 40, alpha = position0 + epsilons[i], dims = 6)[0] - func(pfunc, qfunc, sample_iter = 10000, walkers = 40, alpha = position0, dims = 6)[0])/ep
vectornorm = vector/(np.linalg.norm(vector))
print(vectornorm)
position0 += -vectornorm * step
value2 = func(pfunc, qfunc, sample_iter = 10000, walkers = 40, alpha = position0, dims = 6)[0]
delta1 = value2 - value1
positionprime = position0 + vectornorm*(step*step_ratio)
value3 = func(pfunc, qfunc, sample_iter = 10000, walkers = 40, alpha = positionprime, dims = 6)[0]
delta2 = value3 - value1
if delta2 < delta1:
print('shrink!')
position0 = positionprime
step = step*step_ratio
delta1 = delta2
value2 = value3
point_collection.append(value2)
if convergence == 'strict':
satisfied = abs(delta1) < tolerance
finalvalue = value2
elif convergence == 'fuzzy':
if len(point_collection) >= 5:
data_set = point_collection[-5:]
print('std', statistics.pstdev(data_set))
print('mean', statistics.mean(data_set))
satisfied = abs(statistics.pstdev(data_set)/statistics.mean(data_set)) < tolerance
finalvalue = statistics.mean(data_set)
print('Convergence sucess!')
return finalvalue, position0
n = Noble(evals = 10000, iters = 10, verbose = False)
evals = [10000, 50000, 100000, 500000, 1000000]
paras = [2,1]
guesses = [np.array([2.0,0.2]), np.array([0.2])]
trials = ['twopara1', 'onepara1']
for x, para in enumerate(paras):
data = []
for i in evals:
n.evals = i
psilet_args = {'electrons': 2, 'alphas': para, 'coordsys': 'cartesian'}
n.ham = psiham.HamLet(trial_expr = trials[x], **psilet_args)
n.numba_pdf()
n.numba_expec()
e_min = n.nead_min(n.monte_uniform, guesses[x])[3][1]
data.append(np.array([e_min, n.std]))
print(data)
print('{} and {}'.format(para,i), 'done')
np.savetxt('__results__/processed/uniform_test/{}para_{}iterations_10avg.txt'.format(para, i), np.c_[np.array(data)])
# # n.stability_protocol(np.array([1.9, 2.0, 0.2]), 100)
# temp =[]
# for i in range(100):
# start = time.time()
# result = n.grad_descent(n.monte_metro, np.array([1.6, 2.0, 0.2]))
# temp.append(result[0])
# print(time.time() - start)
#%%%%%%%%%%%%%
# print(np.mean(temp))
# plt.hist(temp, bins = 15)
# iterations = 100
# np.savetxt('__results__/stability_study/mcmc_{}iterations.txt'.format(iterations), np.c_[np.array(temp0)])
# np.savetxt('__results__/stability_study/uniform_{}iterations.txt'.format(iterations), np.c_[np.array(temp1)])
# np.savetxt('__results__/stability_study/vegas.txt_{}iterations.txt'.format(iterations), np.c_[np.array(temp2)])
#
#
# plt.hist(temp0, bins = 10, histtype=u'step')
# plt.hist(temp1, bins = 10, histtype=u'step')
# plt.hist(temp2, bins = 10, histtype=u'step')
# plt.legend(['Metro', 'Uniform', 'Vegas'])
#
#
# plt.show()
#%%%%%%%%%%%
| bb475a0925e23a0e074dff798bc41e2917951205 | [
"Markdown",
"Python"
] | 10 | Python | metrosierra/quVario | d32b18b0cb4ebc916ec13b38d65aaaede9a2841f | 91f546223a3368f5adadea82cf385a6ee9e2554e |