branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <file_sep>
fn main() {
let x = 5 / 10;
}<file_sep>#[deny(state_machine)]
struct Phone;
impl Phone {
fn begin_incoming_call(&mut self) {
unimplemented!()
}
fn accept_call(&mut self) {
unimplemented!()
}
fn end_call(&mut self) {
unimplemented!()
}
fn begin_outgoing_call(&mut self) {
unimplemented!()
}
fn finished_dialing(&mut self) {
unimplemented!()
}
fn call_accepted(&mut self) {
unimplemented!()
}
}
fn main() {
let mut x = Phone;
x.begin_incoming_call();
x.accept_call();
x.end_call();
}
<file_sep># Sample project for the static analysis workshop at rustfest Paris (2018)
## Prerequisites
```bash
rustup override set nightly-2018-12-21
```
## Installing the checker
```
cargo install
```
## Running the checker
We're changing the target-dir in order to prevent rebuilding when building normally without the checker
```bash
RUSTC_WRAPPER=rustfest2018_workshop cargo check --target-dir /tmp/checker/`pwd`
```
<file_sep>fn main() {
let footransmutebar = 42; //~ ERROR NOOOOO
}<file_sep>fn main() {
let transmute = 42;
println!("Hello, world! {}", transmute);
}
<file_sep>// This is just a sanity test that should always be successful
fn main() {}<file_sep>#![feature(box_syntax)]
#![feature(rustc_private)]
extern crate rustc;
extern crate rustc_data_structures;
extern crate rustc_driver;
extern crate syntax;
use rustc::{declare_lint, lint_array};
use rustc::hir::*;
use rustc::hir::intravisit::FnKind;
use rustc::lint::*;
use rustc::mir;
use rustc::ty;
use rustc_data_structures::indexed_vec::IndexVec;
use rustc_driver::driver;
use syntax::ast::{Ident, NodeId};
use syntax::source_map::Span;
use std::collections::{HashMap, HashSet};
use if_chain::if_chain;
declare_lint! {
pub NO_INT_DIV,
Forbid,
"integer division can panic, use checked_div"
}
pub struct Pass;
impl LintPass for Pass {
fn get_lints(&self) -> LintArray {
lint_array!(NO_INT_DIV)
}
}
declare_lint! {
pub TRANSMUTE,
Forbid,
"the interns keep taking shortcuts that bite us later"
}
impl<'a, 'tcx> LateLintPass<'a, 'tcx> for Pass {
fn check_expr(&mut self, cx: &LateContext<'a, 'tcx>, expr: &'tcx Expr) {
if_chain! {
if let ExprKind::Binary(ref op, _, _) = expr.node;
if BinOpKind::Div == op.node;
then {
let ty = cx.tables.expr_ty(expr);
match ty.sty {
ty::Int(_) | ty::Uint(_) => {
cx.span_lint(
NO_INT_DIV,
expr.span,
"This might panic",
);
},
_ => {},
}
}
}
}
}
pub struct NoTransmute;
impl LintPass for NoTransmute {
fn get_lints(&self) -> LintArray {
lint_array!(TRANSMUTE)
}
}
impl EarlyLintPass for NoTransmute {
fn check_ident(&mut self, cx: &EarlyContext, ident: Ident) {
if ident.to_string().contains("transmute") {
cx.span_lint(
TRANSMUTE,
ident.span,
"no. No. NO. NOOOOOO!!!! Like seriously, doesn't anyone read our coding guidelines?",
);
}
}
}
declare_lint! {
pub STATE_MACHINE,
Forbid,
"ensures that the code upholds the state machine models"
}
pub struct StateMachine {
start: &'static str,
transitions: HashMap<&'static str, (&'static str, &'static str)>,
}
impl LintPass for StateMachine {
fn get_lints(&self) -> LintArray {
lint_array!(STATE_MACHINE)
}
}
impl<'a, 'tcx> LateLintPass<'a, 'tcx> for StateMachine {
fn check_fn(
&mut self,
cx: &LateContext<'a, 'tcx>,
_: FnKind<'tcx>,
_: &'tcx FnDecl,
body: &'tcx Body,
_: Span,
_: NodeId,
) {
let def_id = cx.tcx.hir().body_owner_def_id(body.id());
let mir = cx.tcx.optimized_mir(def_id);
let mut states: IndexVec<mir::BasicBlock, HashSet<&'static str>> =
IndexVec::from_elem(HashSet::new(), mir.basic_blocks());
states[mir::START_BLOCK].insert(self.start);
for (bb, bbdata) in mir.basic_blocks().iter_enumerated() {
if let mir::TerminatorKind::Call {
func,
destination: Some((_, succ)),
..
} = &bbdata.terminator().kind
{
let transition = self.transitions.iter().find(|(&transition, _)| {
match func.ty(&mir.local_decls, cx.tcx).sty {
ty::FnDef(did, _) => cx.tcx.item_name(did) == transition,
_ => false,
}
});
if let Some((transition, (start, end))) = transition {
if states[bb].contains(start) {
states[*succ].insert(end);
} else {
cx.span_lint(
STATE_MACHINE,
bbdata.terminator().source_info.span,
&format!(
"state transition `{}` not applicable for states {:?}",
transition, states[bb]
),
);
}
}
} else {
// the next block contains all states that the previous one did
let new = states[bb].clone();
for &succ in bbdata.terminator().successors() {
states[succ].extend(&new)
}
}
}
}
}
pub fn main() {
let args: Vec<_> = std::env::args().collect();
rustc_driver::run(move || {
let mut compiler = driver::CompileController::basic();
compiler.after_parse.callback = Box::new(move |state| {
let mut ls = state.session.lint_store.borrow_mut();
ls.register_early_pass(None, false, box NoTransmute);
ls.register_late_pass(None, false, box Pass);
let transitions = vec![
("begin_incoming_call", ("HangedUp", "Ringing")),
("accept_call", ("Ringing", "Calling")),
("end_call", ("Calling", "HangedUp")),
("begin_outgoing_call", ("HangedUp", "Dialing")),
("finished_dialing", ("Dialing", "Waiting")),
("call_accepted", ("Waiting", "Calling")),
];
let transitions = transitions.into_iter().collect();
ls.register_late_pass(
None,
false,
box StateMachine {
start: "HangedUp",
transitions,
},
);
});
rustc_driver::run_compiler(&args, Box::new(compiler), None, None)
});
}
<file_sep>[package]
name = "rustfest2018_workshop"
version = "0.1.0"
authors = ["<NAME> <<EMAIL>>"]
edition = "2018"
[dependencies]
if_chain = "0.1.2"
[dev-dependencies]
compiletest_rs = "0.3.17"
| 36f923dfc6973f34c136e3a48da2d19f35e91109 | [
"Markdown",
"Rust",
"TOML"
] | 8 | Rust | oli-obk/rustfest2018_workshop | 2f672e708ca7ac688e311d43fa9c67461dc77665 | 8ca2db0cbfb307c9ff3c02b581f62baa7987fbca | |
refs/heads/master | <repo_name>jdalleau/acMark<file_sep>/README.md
# acMark
### acMark: General Generator for Attributed Graph with Community Structure
Users can control the characteristics of generated graphs by acMark.
## Requirements
- numpy >= 1.14.5
- scipy >= 1.1.0
## Example
> $ python acmark.py
'test.mat' is generated by the example.
## Usage
In a python code,
> import acmark
A, X, C = acmark.acmark(n=1000, m=4000, d=100)
Other parameters are described below:
## Parameter (default)
outpath : path to output file (.mat)
n (=1000) : number of nodes
m (=4000) : number of edges
d (=100) : number of attributes
k (=5) : number of clusters
k2 (=10) : number of clusters for attributes
alpha (=0.2) : parameters for balancing inter-edges and intra-edges
beta (=10) : parameters of separability for attribute cluster proportions
gamma (=1) : parameters of separability for cluster transfer proportions
node_d (=0) : choice for node degree (0:power law, 1:uniform, 2:normal)
com_s (=0) : choice for community size (0:power law, 1:uniform, 2:normal)
phi_d (=3) : parameters of exponent for power law distribution for node degree
phi_c (=2) : parameters of exponent for power law distribution for community size
delta_d (=3) : parameters for uniform distribution for node degree
delta_c (=2) : parameters for uniform distribution for community size
sigma_d (=0.1) : parameters for normal distribution for node degree
sigma_c (=0.1) : parameters for normal distribution for community size
r (=10) : number of iterations for edge construction
att_ber (=0.0) : ratio of attributes which takes discrete value
att_pow (=0.0) : ratio of attributes which follow power law distributions
att_uni (=0.0) : ratio of attributes which follow uniform distributions
att_nor (=0.5) : ratio of attributes which follow normal distributions
dev_power_max (=3) : upper bound of deviations for power law distribution for random attributes
dev_power_min (=2) : lower bound of deviations for power law distribution for random attributes
dev_normal_max (=0.3) : upper bound of deviations for normal distribution for random attributes
dev_normal_min (=0.1) : lower bound of deviations for normal distribution for random attributes
uni_att (=0.2) : range of paramters for uniform distribution for random attributes
## Citation
<file_sep>/acmark.py
# coding: utf-8
# # Core Program
import numpy as np
from scipy import sparse
import random
import scipy.io as sio
from scipy.stats import bernoulli
# ## You can see the details of the inputs on README
# ## Outputs
# ##### 1: adjacency matrix
# ##### 2: attribute matrix
# ##### 3: cluster assignment vector
def derived_from_dirichlet(n, m, d, k, k2, alpha, beta, gamma, node_d, com_s, phi_d, phi_c, sigma_d, sigma_c, delta_d, delta_c, att_power, att_uniform, att_normal):
def selectivity(pri=0, node_d=0, com_s=0):
# priority
priority_list = ["edge", "degree"]
priority = priority_list[pri]
# node degree
node_degree_list = ["power_law", "uniform", "normal", "zipfian"]
node_degree = node_degree_list[node_d]
# community size
com_size_list = ["power_law", "uniform", "normal", "zipfian"]
com_size = com_size_list[com_s]
return priority, node_degree, com_size
# (("edge","degree"),("power_law","uniform","normal","zipfian"),("power_law","uniform","normal","zipfian"))
priority, node_degree, com_size = selectivity(node_d=node_d, com_s=com_s)
# ## distribution generator
def distribution_generator(flag, para_pow, para_normal, para_zip, t):
if flag == "power_law":
dist = 1 - np.random.power(para_pow, t) # R^{k}
elif flag == "uniform":
dist = np.random.uniform(0, 1, t)
elif flag == "normal":
dist = np.random.normal(0.5, para_normal, t)
elif flag == "zipfian":
dist = np.random.zipf(para_zip, t)
return dist
# ## generate a community size list
def community_generation(n, k, com_size, phi_c, sigma_c, delta_c):
chi = distribution_generator(com_size, phi_c, sigma_c, delta_c, k)
# chi = chi*(chi_max-chi_min)+chi_min
chi = chi * alpha / sum(chi)
# Cluster assignment
# print(chi)
U = np.random.dirichlet(chi, n) # topology cluster matrix R^{n*k}
return U
# ## Cluster assignment
U = community_generation(n, k, com_size, phi_c, sigma_c, delta_c)
# ## Edge construction
# ## node degree generation
def node_degree_generation(n, m, priority, node_degree, phi_d, sigma_d, delta_d):
theta = distribution_generator(node_degree, phi_d, sigma_d, delta_d, n)
if priority == "edge":
theta = np.array(list(map(int, theta * m * 2 / sum(theta) + 1)))
# else:
# theta = np.array(list(map(int,theta*(theta_max-theta_min)+theta_min)))
return theta
theta = node_degree_generation(
n, m, priority, node_degree, phi_d, sigma_d, delta_d)
# Attribute generation
num_power = int(d*att_power)
num_uniform = int(d*att_uniform)
num_normal = int(d*att_normal)
num_random = d-num_power-num_uniform-num_normal
# Input4 for attribute
beta_dist = "normal" # 0:power-law, 1:normal, 2:uniform
gamma_dist = "normal" # 0:power-law, 1:normal, 2:uniform
phi_V = 2
sigma_V = 0.1
delta_V = 0.2
phi_H = 2
sigma_H = 0.1
delta_H = 0.2
# generating V
chi = distribution_generator(beta_dist, phi_V, sigma_V, delta_V, k2)
chi = np.array(chi)/sum(chi)*beta
# attribute cluster matrix R^{d*k2}
V = np.random.dirichlet(chi, num_power+num_uniform+num_normal)
# generating H
chi = distribution_generator(gamma_dist, phi_H, sigma_H, delta_H, k2)
chi = np.array(chi)/sum(chi)*gamma
H = np.random.dirichlet(chi, k) # cluster transfer matrix R^{k*k2}
return U, H, V, theta
def acmark(outpath="", n=1000, m=4000, d=100, k=5, k2=10, r=10, alpha=0.2, beta=10, gamma=1., node_d=0, com_s=0, phi_d=3, phi_c=2, sigma_d=0.1, sigma_c=0.1, delta_d=3, delta_c=2, att_power=0.0, att_uniform=0.0, att_normal=0.5, att_ber=0.0, dev_normal_max=0.3, dev_normal_min=0.1, dev_power_max=3, dev_power_min=2, uni_att=0.2):
U, H, V, theta = derived_from_dirichlet(n, m, d, k, k2, alpha, beta, gamma, node_d, com_s,
phi_d, phi_c, sigma_d, sigma_c, delta_d, delta_c, att_power, att_uniform, att_normal)
C = [] # cluster list (finally, R^{n})
for i in range(n):
C.append(np.argmax(U[i]))
r *= k
def edge_construction(n, U, theta, around=1.0, r=10*k):
# list of edge construction candidates
S = sparse.dok_matrix((n, n))
degree_list = np.zeros(n)
# print_count=0
for i in range(n):
# if 100*i/n+1 >= print_count:
# print(str(print_count)+"%",end="\r",flush=True)
# print_count+=1
count = 0
while count < r and degree_list[i] < theta[i]:
# step1 create candidate list
candidate = []
n_candidate = theta[i]
candidate = np.random.randint(
0, n-1, size=n_candidate) # for undirected graph
candidate = list(set(candidate))
# step2 create edges
for j in candidate:
if i < j:
i_ = i
j_ = j
else:
i_ = j
j_ = i
if i_ != j_ and S[i_, j_] == 0 and degree_list[i_] < around * theta[i_] and degree_list[j_] < around * theta[j_]:
S[i_, j_] = np.random.poisson(U[i_, :].transpose().dot(
U[j_, :]), 1) # ingoring node degree
if S[i_, j_] > 0:
degree_list[i_] += 1
degree_list[j_] += 1
count += 1
return S
S = edge_construction(n, U, theta)
### Attribute Generation ###
def sigmoid(x):
return 1.0 / (1.0 + np.exp(-x))
### Construct attribute matrix X from latent factors ###
X = U.dot(H.dot(V.T))
# X = sigmoid(U.dot(H)).dot(W.transpose())
# ### Variation of attribute
num_bernoulli = int(d*att_ber)
num_power = int(d*att_power)
num_uniform = int(d*att_uniform)
num_normal = int(d*att_normal)
num_random = d-num_power-num_uniform-num_normal
def variation_attribute(n, k, X, C, num_bernoulli, num_power, num_uniform, num_normal, dev_normal_min, dev_normal_max, dev_power_min, dev_power_max, uni_att):
for i in range(num_bernoulli):
for p in range(n):
X[p, i] = bernoulli.rvs(p=X[p, i], size=1)
dim = num_bernoulli
for i in range(num_power): # each demension
clus_dev = np.random.uniform(dev_normal_min, dev_normal_max-0.1, k)
exp = np.random.uniform(dev_power_min, dev_power_max, 1)
for p in range(n): # each node
# clus_dev[C[p]]
X[p, i] = (X[p, i] * np.random.normal(1.0,
clus_dev[C[p]], 1)) ** exp
dim += num_power
for i in range(dim, dim+num_uniform): # each demension
clus_dev = np.random.uniform(1.0-uni_att, 1.0+uni_att, n)
for p in range(n):
X[p, i] *= clus_dev[C[p]]
dim += num_uniform
for i in range(dim, dim+num_normal): # each demension
clus_dev = np.random.uniform(dev_normal_min, dev_normal_max, k)
for p in range(n): # each node
X[p, i] *= np.random.normal(1.0, clus_dev[C[p]], 1)
return X
### Apply probabilistic distributions to X ###
X = variation_attribute(n, k, X, C, num_bernoulli, num_power, num_uniform, num_normal,
dev_normal_min, dev_normal_max, dev_power_min, dev_power_max, uni_att)
# random attribute
def concat_random_attribute(n, X, num_random):
rand_att = []
for i in range(num_random):
random_flag = random.randint(0, 2)
if random_flag == 0:
rand_att.append(np.random.normal(0.5, 0.2, n))
elif random_flag == 1:
rand_att.append(np.random.uniform(0, 1, n))
else:
rand_att.append(1.0-np.random.power(2, n))
return np.concatenate((X, np.array(rand_att).T), axis=1)
if num_random != 0:
X = concat_random_attribute(n, X, num_random)
# ## Regularization for attributes
for i in range(d):
X[:, i] -= min(X[:, i])
X[:, i] /= max(X[:, i])
if outpath != "":
sio.savemat(outpath, {'S': S, 'X': X, 'C': C})
else:
return S, X, C
# acmark(outpath="test.mat")
| 7c488e3f3dfcb88ba4dbed97d60514e916575f60 | [
"Markdown",
"Python"
] | 2 | Markdown | jdalleau/acMark | c4987db14fee20e28b785534516c3a972b741fa1 | 3fb2d57510027079497054570571dbb0f04ed1a6 | |
refs/heads/master | <repo_name>Toushiat96/travel-lover<file_sep>/src/Components/Login/Login.js
import React, { useContext, useState } from 'react';
import * as firebase from "firebase/app";
import "firebase/auth";
import firebaseConfig from './firebase.config';
import { UserContext } from '../../App';
import { useHistory, useLocation } from 'react-router-dom';
import Header from '../Header/Header';
import { Form } from 'react-bootstrap';
import { FormGroup } from '@material-ui/core';
const Login = () => {
const [loggedInUser, setLoggedInUser] = useContext(UserContext);
const [newUser, setNewUser] = useState(false);
const history = useHistory();
const location = useLocation();
const { from } = location.state || { from: { pathname: "/" } };
if (firebase.apps.length === 0) {
firebase.initializeApp(firebaseConfig);
}
const handleBlur = (event) => {
let isFieldValid = true;
if (event.target.name === 'email') {
isFieldValid = /\S+@\S+\.\S+/.test(event.target.value); ////regular expression /\S+@\S+\.\S+/ diye email validation
}
if (event.target.name === 'password') {
const isPasswordValid = event.target.value.length > 6;
const passwordHasNumber = /\d{1}/.test(event.target.value); //regular expression /\d{1}/ diye pass validation
//console.log(isPasswordValid && passwordHasNumber);
isFieldValid = (isPasswordValid && passwordHasNumber);
}
if (isFieldValid) {
const newUserInfo = { ...loggedInUser };
newUserInfo[event.target.name] = event.target.value;
setLoggedInUser(newUserInfo);
}
}
const handleSubmit = (event) => {
if (newUser && loggedInUser.email && loggedInUser.password){
//console.log("submitting");
firebase.auth().createUserWithEmailAndPassword(loggedInUser.email, loggedInUser.password)
.then(res =>{
console.log(res);
const {displayName, email} = res.user;
const emailUser = {name: displayName, email: email};
//const newUserInfo = {...loggedInUser};
// newUserInfo.error = '';
// newUserInfo.success = true;
setLoggedInUser(emailUser);
updateUserName(loggedInUser.name);
// const signedInUser = {
// // isSignedIn: 'true',
// name: displayName,
// email: email
// };
history.replace(from);
})
.catch(error => {
// Handle Errors here.
const newUserInfo = {...loggedInUser};
newUserInfo.error = error.message;
newUserInfo.success = false;
setLoggedInUser(newUserInfo);
// ...
});
}
if (!newUser && loggedInUser.email && loggedInUser.password){
//console.log("submitting");
firebase.auth().signInWithEmailAndPassword(loggedInUser.email, loggedInUser.password)
.then(res =>{
const {displayName, email} = res.user;
const emailUser = {name: displayName, email: email};
// const newUserInfo = {...loggedInUser};
// newUserInfo.error = '';
// newUserInfo.success = true;
setLoggedInUser(emailUser);
updateUserName(loggedInUser.name);
// const signedInUser = {
// // isSignedIn: 'true',
// name: res.user.displayName,
// email: res.user.email
// };
// setLoggedInUser(signedInUser);
history.replace(from);
})
.catch(error => {
// Handle Errors here.
const newUserInfo = {...loggedInUser};
newUserInfo.error = error.message;
newUserInfo.success = false;
setLoggedInUser(newUserInfo);
// ...
});
}
event.preventDefault();
}
const updateUserName = name => {
const user = firebase.auth().currentUser;
user.updateProfile({
displayName: name
}).then(function() {
// Update successful.
}).catch(function(error) {
// An error happened.
});
}
const handleGoogleSignIn = () => {
var provider = new firebase.auth.GoogleAuthProvider();
firebase.auth().signInWithPopup(provider).then(function (result) {
const { displayName, email } = result.user;
const signedInUser = { name: displayName, email }
setLoggedInUser(signedInUser);
history.replace(from);
// ..
}).catch(function (error) {
const errorMessage = error.message;
console.log(errorMessage);
});
}
const handleFaceBookSignIn = () => {
var fbprovider = new firebase.auth.FacebookAuthProvider();
firebase.auth().signInWithPopup(fbprovider).then(function (result) {
const { displayName, email } = result.user;
const signedInuser = { displayName, email }
setLoggedInUser(signedInuser);
console.log(signedInuser);
history.replace(from);
// ...
}).catch(function (error) {
// Handle Errors here.
var errorCode = error.code;
var errorMessage = error.message;
// The email of the user's account used.
var email = error.email;
// The firebase.auth.AuthCredential type that was used.
var credential = error.credential;
// ...
});
}
return (
<div>
<div className="homeimage">
<div>
<Header></Header>
</div>
<div className="container">
<div className="row justify-content-center" style={{ marginTop: '90px', marginLeft: '290px', backgroundColor: 'white', border: '1px solid white', borderRadius: '20px', height: '440px', width: '500px' }}>
<div col-md-8>
<Form onSubmit={handleSubmit} style={{ width: '250px', color: 'gray' }}>
<Form.Group>
<Form.Check type="checkbox" onClick={() => setNewUser(!newUser)} label="Click for Sign up" />
</Form.Group>
<FormGroup>
{newUser && <Form.Control type="name" name="name" className="form-control" onBlur={handleBlur} placeholder="Your name" required />}
</FormGroup>
<FormGroup>
<Form.Label>Email address</Form.Label>
<Form.Control type="email" name="email" onBlur={handleBlur} placeholder="Username or Email" required />
</FormGroup>
<FormGroup>
<Form.Label>Password</Form.Label>
<Form.Control type="password" name="password" onBlur={handleBlur} placeholder="<PASSWORD>" required />
</FormGroup>
<br />
<input style={{ marginLeft: '75px', fontWeight: 'border', backgroundColor: '#ffc107' }} value="Submit" type="submit" />
</Form>
<br />
<button onClick={handleGoogleSignIn} style={{ borderRadius: '10px', marginLeft: '15px' }} >
Continue With Google
</button>
<br />
<br />
<button onClick={handleFaceBookSignIn} style={{ borderRadius: '10px', marginLeft: '15px' }}>
Continue With Facebook
</button>
</div>
</div>
</div>
</div>
</div>
);
};
export default Login;<file_sep>/src/Components/Destination/Destination.js
import React from 'react';
import { useParams } from 'react-router-dom';
import Header from '../Header/Header';
import fakedata from '../../fakedata/index'
const Destination = () => {
const { destinationid } = useParams();
const hotelinformation = fakedata.find(data => data.id === destinationid)
const { title, picture1, picture2, picture3,name1,name2,name3 } = hotelinformation;
console.log(hotelinformation);
return (
<div>
<div className="homeimage">
<Header></Header>
<div className="container">
<div className="row d-flex align-items-center">
<div className="col-md-7">
<div style={{ color: 'white' }}>
<p>252 stays Apr 13-17 3 guests</p>
<h4>{title}</h4>
</div>
{/* First room information */}
<div className="d-flex">
<div>
<img style={{ width: '200px',height:'130px' }} src={picture1} alt="" />
</div>
<div className="apartment" style={{ marginLeft: "35px" }}>
<h3 style={{ color: "white" }}>{name1} </h3>
<p style={{ color: "white" }}>
4 guests 2 bedrooms 2 beds 2 baths <br />
Wifi Air conditioning Kitchen <br />
Cancellation fexibility availiable <br />
<span > 4.9 (20) $52/ night $167 total</span>
</p>
</div>
</div>
{/* second room information */}
<div className="d-flex">
<div>
<img style={{ width: '200px',height:'130px' }} src={picture2} alt="" />
</div>
<div className="apartment" style={{ marginLeft: "35px" }}>
<h3 style={{ color: "white" }}>{name2} </h3>
<p style={{ color: "white" }}>
4 guests 2 bedrooms 2 beds 2 baths <br />
Wifi Air conditioning Kitchen <br />
Cancellation fexibility availiable <br />
<span > 4.9 (20) $52/ night $167 total</span>
</p>
</div>
</div>
{/*Third room information */}
<div className="d-flex">
<div>
<img style={{ width: '200px',height:'130px' }} src={picture3} alt="" />
</div>
<div className="apartment" style={{ marginLeft: "35px" }}>
<h3 style={{ color: "white" }}>{name3} </h3>
<p style={{ color: "white" }}>
4 guests 2 bedrooms 2 beds 2 baths <br />
Wifi Air conditioning Kitchen <br />
Cancellation fexibility availiable <br />
<span > 4.9 (20) $52/ night $167 total</span>
</p>
</div>
</div>
</div>
<div className="col-md-5">
<iframe
src="https://www.google.com/maps/embed?pb=!1m10!1m8!1m3!1d2766243.7879151287!2d89.97055095426357!3d24.142547837459787!3m2!1i1024!2i768!4f13.1!5e0!3m2!1sen!2sbd!4v1600294700894!5m2!1sen!2sbd"
width="470px" height="500px" frameborder="0" style={{ border: '0' }} allowfullscreen="" aria-hidden="false" tabindex="0">
</iframe>
</div>
</div>
</div>
</div>
</div>
);
};
export default Destination;<file_sep>/src/Components/Book/Book.js
import React from 'react';
import { Button, Form } from 'react-bootstrap';
import { Link, useHistory, useParams } from 'react-router-dom';
import fakedata from '../../fakedata/index'
import Header from '../Header/Header';
const Book = () => {
const history = useHistory();
const handleclick = (id) => {
const url = `/destination/${id}`;
history.push(url)
}
const { bookid } = useParams();
const bookData = fakedata.find(data => data.id === bookid)
const { name, id, description, origin, destination } = bookData;
return (
<div className="homeimage">
<div>
<Header></Header>
</div>
<div className="container">
<div className="row" style={{ color: 'white', marginTop: '100px' }}>
<div className="col-md-6">
<h1 >
{name}
</h1>
<br />
{description}
</div>
<div className="col-md-6 bg-white" style={{ border: '1px solid white', borderRadius: '5px' }}>
<Form>
<Form.Group>
<Form.Label style={{ color: 'gray' }}>Origin</Form.Label>
<Form.Control type="text" placeholder={origin} disabled />
</Form.Group>
<Form.Group >
<Form.Label style={{ color: 'gray' }}>Destination</Form.Label>
<Form.Control type="text" placeholder={destination} disabled />
</Form.Group>
<Form.Group >
<div className="d-flex justify-content-between">
<div>
<Form.Label style={{ color: 'gray' }}>From</Form.Label>
<Form.Control type="date" placeholder={destination} />
</div>
<div>
<Form.Label style={{ color: 'gray' }}>To</Form.Label>
<Form.Control type="date" placeholder={destination} />
</div>
</div>
</Form.Group>
</Form>
<div className="d-flex justify-content-center">
{/* <Link to ={`/destination/${id}`}>Confirm Now</Link> */}
<Button onClick={() => handleclick(id)} variant="warning"> Confirm Now </Button>
</div>
</div>
</div>
</div>
</div>
);
};
export default Book;<file_sep>/src/Components/Header/Header.js
import React, { useContext } from 'react';
import { Button, Form, FormControl, Nav, Navbar } from 'react-bootstrap';
import { UserContext } from '../../App';
import Logo from '../../Image/Logo.png'
import './Header.css'
const Header = () => {
const [loggedInUser, setLoggedInUser] = useContext(UserContext);
return (
<div>
<Navbar bg="none">
<Navbar.Brand href="#home" style={{ marginLeft: '140px' }}>
<img style={{ width: '100px', color: 'white', filter: 'contrast(0%) brightness(500%)' }}
src={Logo}
alt="React Bootstrap logo"
/>
</Navbar.Brand >
<Form inline style={{ marginLeft: '70px', weight: '70px' }}>
<FormControl type="text" placeholder="Search Your Destination" className="mr-sm-2" />
</Form>
<Nav className="nav" >
<Nav.Link to="/news" style={{ marginLeft: '45px', color: 'white', fontWeight: '600', fontFamily: 'Montserrat' }} href="#home">News</Nav.Link>
<Nav.Link to="/destination" style={{ marginLeft: '50px', color: 'white', fontWeight: '600', fontFamily: 'Montserrat' }} href="#features">Destination</Nav.Link>
<Nav.Link to="/blog" style={{ marginLeft: '50px', color: 'white', fontWeight: '600', fontFamily: 'Montserrat' }} href="#pricing">Blog</Nav.Link>
<Nav.Link to="/contact" style={{ marginLeft: '50px', color: 'white', fontWeight: '600', fontFamily: 'Montserrat' }} href="#features">Contact</Nav.Link>
<li>
<Button style={{ marginLeft: '50px', width: '80px', height: '40px', borderRadius: '10px', fontWeight: '600' }} href="/login" variant="warning" >
Login </Button>
</li>
</Nav>
</Navbar>
</div>
);
};
export default Header;<file_sep>/README.md
## Travel Lover
Features of this project :
1. Any Travel lover person can book his/her choice place which is shown in homepage
2. To book hotel or any place he/she first should be logged using gmail.
3. He can see how many hotels there are in that place through this application
[Live Site](https://travel-lover-fe8f6.web.app/home) [github](https://github.com/Toushiat96/travel-lover)
<file_sep>/src/Components/Home/Home.js
import React from 'react';
import Header from '../Header/Header';
import HomeInformation from '../HomeInformation/HomeInformation';
import fakedata from '../../fakedata/index';
import './Home.css'
const Home = () => {
return (
<div className="homeimage">
<div>
<Header></Header>
</div>
<div className="carousel">
{
fakedata.map( data => <HomeInformation data ={data}></HomeInformation>)
}
</div>
</div>
);
};
export default Home;<file_sep>/src/Components/HomeInformation/HomeInformation.js
import { Card, CardActionArea, CardActions, CardContent, CardMedia, makeStyles, Typography } from '@material-ui/core';
import React from 'react';
import { Button, Carousel } from 'react-bootstrap';
import { Link, useHistory } from 'react-router-dom';
import './HomeInformation.css'
const useStyles = makeStyles({
root: {
maxWidth: 345,
},
media: {
height: 140,
},
});
const HomeInformation = (props) => {
const {img,id,name,description} = props.data;
const classes = useStyles();
const history = useHistory();
const handleclick = (id) =>{
const url = `/book/${id}`;
history.push(url)
}
return (
<Card className={classes.root } className="card-container">
<CardActionArea>
<CardMedia
className={classes.media}
image={img}
title="Contemplative Reptile"
/>
<CardActions>
{/* <Link to={`/book/${id}`}>Booking Now</Link> */}
<Button onClick={ () => handleclick(id) } variant="warning"> Booking Now </Button>
</CardActions>
<CardContent>
<Typography gutterBottom variant="h5" component="h2">
{name}
</Typography>
<Typography variant="body2" color="textSecondary" component="p">
{description}
</Typography>
</CardContent>
</CardActionArea>
</Card>
);
};
export default HomeInformation;<file_sep>/src/fakedata/index.js
var data =
[
{
img: 'https://i.ibb.co/yYB6hBc/Rectangle-1.png',
picture1:"https://i.ibb.co/sV8fFLD/Rectangle-26.png",
picture2:"https://i.ibb.co/x6y14Mf/Rectangle-27.png",
picture3:"https://i.ibb.co/cDFKfd5/Rectangle-28.png",
name1:"Hotel Sea Crown",
name2 :"Sayeman Beach Resort",
name3:"Prime Park Hotel",
title:"Stay in Sajekvely",
id:'1',
origin: 'Dhaka',
destination: "Cox's Bazar",
name:"<NAME>",
description:"Cox's Bazar sea beach is the longest sea beach in the world, 120 km long, having no 2nd instance. The wavy water of Bay of Bengal touches the beach throughout this 120 km.For Bangladeshi's it doesn't get much better than Cox's Bazar, the country's most popular beach town than the other one 'Kuakata beach town. It's sort of a Cancun of the east. It's choc-a-bloc with massive well-architectured concrete structures, affluent 5 & 3 star hotels, catering largely to the country's elite and overseas tourists. The beach is only a bit crowded in tourist season, October to March, especially near the hotel-motel zone, but remains virgin during the rest of the year, April to September, when it's better to take a trip there.",
},
{
img:'https://i.ibb.co/RTkdXyX/Sreemongol.png',
picture1:"https://i.ibb.co/WFsdjWW/sreemangal-inn.jpg",
picture2:"https://i.ibb.co/TqMr2KX/1.jpg",
picture3:"https://i.ibb.co/F73JscJ/images.jpg",
name1:"Sreemangal Inn Hotel & Restaurant",
name2:"Sreemangal Resort",
name3:"<NAME>",
title:"Stay in Sreemangal",
id:'2',
origin: 'Dhaka',
destination: 'Sreemangal',
name:"SREEMANGAL",
description:"It is said the name Sreemangal (or Srimangal) is named after <NAME> and <NAME>; two brothers who settled on the banks of the Hail Haor. A copper plate of Raja Marundanath from the 11th century was found in Kalapur. During an excavation at Lamua, an ancient statue of Ananta Narayan was dug out. In 1454, the Nirmai Shiva Bari was built and still stands today. Srimangal thana was established in 1912. The central town later became a pourashava in 1935. In 1963, two peasants were killed by police officers which kicked off the Balishira peasant riots."
},
{
img:'https://i.ibb.co/f9wQGRK/sundorbon.png',
picture1:"https://i.ibb.co/1sh3Rh7/sd-1.jpg",
picture2:"https://i.ibb.co/ZW7b39r/imag-1.jpg",
picture3:"https://i.ibb.co/X8Jn29x/download.jpg",
name1:"Royal Sundarban Wild Resort",
name2:"Sundarban Royal Eco Resort",
name3:"Sunderban Tiger Camp- Wildlife Resort",
title:"Stay in Sundarban",
id:'3',
origin: 'Dhaka',
destination: 'Sundarban',
name:"SUNDARBANS",
description:"The Sundarbans is a mangrove area in the delta formed by the confluence of the Ganges, Brahmaputra and Meghna Rivers in the Bay of Bengal. It spans from the Hooghly River in India's state of West Bengal to the Baleswar River in Bangladesh. It comprises closed and open mangrove forests, agriculturally used land, mudflats and barren land, and is intersected by multiple tidal streams and channels. Four protected areas in the Sundarbans are enlisted as UNESCO World Heritage Sites, viz. Sundarbans National Park, Sundarbans West, Sundarbans South and Sundarbans East Wildlife Sanctuaries."
},
{
img:'https://i.ibb.co/dmNqGhs/Sajek.png',
picture1:"https://i.ibb.co/c6s3fHh/sah.jpg",
picture2:"https://i.ibb.co/gSSVqxH/sajek1.jpg",
picture3:"https://i.ibb.co/H7J9BXf/sajek2.jpg",
name1:"<NAME>",
name2:"<NAME>, S<NAME>",
name3:"<NAME> Sajek",
title:"Stay in Sajekvely",
id:'4',
origin: 'Dhaka',
destination: 'Sajekvely',
name:"SAJEKVELY",
description:"The name of Sajek Valley came from the Sajek River that originates from Karnafuli river. The Sajek river works as a border between Bangladesh and India.Sajek is a union located in the north of Chittagong Hill Tracts. It's under Baghaichori Upazila in Rangamati hill district, it is situated 67 kilometres (42 mi) north-east from Khagrachhari town and 95 kilometres (59 mi) north from Rangamati city. The border of Bangladesh and Mizoram of India is 8 kilometres (5.0 mi) east from Sajek"
}
];
export default data; | 068197d3671d63ff8d8fcd53cf8ea0c9c52cbf58 | [
"JavaScript",
"Markdown"
] | 8 | JavaScript | Toushiat96/travel-lover | be2140bcca6330177c5eb3a4ea762586620c7c52 | 2076183fb21d55446e00717ec6ef4d9dfb5af425 | |
refs/heads/master | <repo_name>wimvanhaaren/backbaseCodeChallenge<file_sep>/README.md
# Case backend developer for Backbase
**<NAME>**
<EMAIL>
+31 6 44 11 68 35
### Coding Challenge
We are asking you to program a Java web application that runs a game of 6-stone Kalah.
This web application should enable to let 2 human players play the game; there is no AI required. It doesn't need a fancy web interface or front end, it can also be a service endpoint.
#### About the game
Each of the two players has six pits in front of him/her. To the right of the six pits, each player has a larger pit, his Kalah or house. At the start of the game, six stones are put In each pit.
The player who begins picks up all the stones in any of their own pits, and sows the stones on to the right, one in each of the following pits, including his own Kalah. No stones are put in the opponent's' Kalah. If the players last stone lands in his own Kalah, he gets another turn. This can be repeated any number of times before it's the other player's turn.
when the last stone lands in an own empty pit, the player captures this stone and all stones in the opposite pit (the other players' pit) and puts them in his own Kalah.
The game is over as soon as one of the sides run out of stones. The player who still has stones in his/her pits keeps them and puts them in his/hers Kalah. The winner of the game is the player who has the most stones in his Kalah.
### Starting the application
Assuming maven is installed, from the root folder of the project enter the following command:
```
mvn spring-boot:run
```
Then open your web browser and navigate to <http://localhost:8080>
<file_sep>/src/main/java/it/jdev/kalah/domain/model/Game.java
package it.jdev.kalah.domain.model;
import java.util.UUID;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Data;
@Data
public class Game {
@JsonProperty(value = "gameId")
private UUID id;
private Player player1;
private Player player2;
private Player currentPlayer;
private boolean gameOver = false;
private Player winner;
@JsonIgnore
private Board board;
public void start() {
this.id = UUID.randomUUID();
this.board = new Board();
this.board.initialize();
this.currentPlayer = player1;
}
public void move(int pit, Player player) {
checkPlayersTurn(player);
checkPlayersPit(pit, player);
Pit lastPit = board.moveStones(pit);
if (!(lastPit instanceof KalahPit)) {
// switch to other player but not if last stone ended in his kalah pit...
currentPlayer = (player.equals(player1) ? player2 : player1);
}
if (gameOver()) {
this.gameOver = true;
this.board.moveRemainingStonesToKalahPits();
andTheWinnerIs();
}
}
private void andTheWinnerIs() {
int stonesPlayer1 = board.getPits()[6].getNumberOfStones();
int stonesPlayer2 = board.getPits()[13].getNumberOfStones();
if (stonesPlayer1 > stonesPlayer2) {
winner = player1;
} else {
winner = player2;
}
}
private void checkPlayersTurn(Player player) {
if (! currentPlayer.equals(player)) {
throw new IllegalMoveException("It is not this player's turn.");
}
}
private void checkPlayersPit(int pit, Player player) {
if ( (player.equals(player1) && pit > 6 ) ||
(player.equals(player2) && pit < 7)) {
throw new IllegalMoveException("Player is not allowed to use other player's pit.");
}
}
private boolean gameOver() {
Pit[] pits = board.getPits();
int count = 0;
for (int i=0; i< 6; i++) {
count += pits[i].getNumberOfStones();
}
if (count == 0) {
return true;
}
count = 0;
for (int i=7; i< 13; i++) {
count += pits[i].getNumberOfStones();
}
if (count == 0) {
return true;
}
return false;
}
}
<file_sep>/src/main/java/it/jdev/kalah/domain/model/RegularPit.java
package it.jdev.kalah.domain.model;
import lombok.Data;
import lombok.EqualsAndHashCode;
@Data
@EqualsAndHashCode(callSuper = true)
public class RegularPit extends Pit {
public RegularPit() {}
public RegularPit(int stones) {
super.numberOfStones = stones;
}
}
<file_sep>/src/main/resources/static/js/kalah.js
angular.module('kalahApp', []).controller('GameController', ['$http', function($http) {
var game = this;
game.status = '';
game.players = [ {id:1, name:'player 1'}, {id:1, name:'player 2'} ];
game.board = {};
game.board.pits = [];
game.start = function() {
$http.post('/kalah', { 'player1':game.players[0], 'player2':game.players[1]})
.then(function successCallback(response) {
game.gameId = response.data.gameId;
game.status = 'STARTED';
game.currentPlayer = response.data.currentPlayer;
updateBoard();
}, function errorCallback(response) {
game.status = 'ERROR';
});
};
var updateBoard = function() {
$http.get('/kalah/'+game.gameId+"/board")
.then(function successCallback(response) {
game.board = response.data;
game.status = 'PLAYING';
},
function errorCallback(res) {
game.status = 'ERROR';
});
}
game.move = function(index) {
$http.post("/kalah/"+game.gameId+"/board/"+index, game.currentPlayer)
.then(function successCallback(response) {
game.currentPlayer = response.data.currentPlayer;
if (response.data.gameOver === true) {
game.status = 'AND THE WINNER IS ' + response.data.winner.name + '!!!';
alert('We have a winner!\nCongratulations ' + response.data.winner.name);
} else {
updateBoard();
}
}, function errorCallback(response) {
game.status = 'ERROR';
});
}
}]);
<file_sep>/src/test/java/it/jdev/kalah/GameSetupTest.java
package it.jdev.kalah;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertTrue;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.post;
import static org.springframework.test.web.servlet.result.MockMvcResultHandlers.print;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.UUID;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.WebMvcTest;
import org.springframework.http.MediaType;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.MvcResult;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import it.jdev.kalah.api.GameController;
import it.jdev.kalah.domain.model.Board;
import it.jdev.kalah.domain.model.Game;
import it.jdev.kalah.domain.model.KalahPit;
import it.jdev.kalah.domain.model.Pit;
import it.jdev.kalah.domain.model.Player;
import it.jdev.kalah.domain.model.RegularPit;
@RunWith(SpringRunner.class)
@WebMvcTest(GameController.class)
public class GameSetupTest {
@Autowired
private MockMvc mockMvc;
@Test
public void newGame() throws Exception {
Game game = startNewGame();
assertNotNull(game.getId());
}
private Game startNewGame() throws JsonProcessingException, Exception, UnsupportedEncodingException, IOException,
JsonParseException, JsonMappingException {
Game game = new Game();
game.setPlayer1(new Player(1, "David"));
game.setPlayer2(new Player(2, "Neal"));
ObjectMapper objectMapper = new ObjectMapper();
String gameJson = objectMapper.writeValueAsString(game);
MvcResult mvcResult = this.mockMvc
.perform(post("/kalah").contentType(MediaType.APPLICATION_JSON_UTF8).content(gameJson))
.andDo(print())
.andExpect(status().isCreated())
.andReturn();
String response = mvcResult.getResponse().getContentAsString();
Game result = objectMapper.readValue(response, Game.class);
return result;
}
@Test
public void getBoard() throws Exception {
Game game = startNewGame();
Board board = getBoard(game.getId());
assertNotNull(board);
Pit[] pits = board.getPits();
assertEquals(14, pits.length);
for (int i=0; i<14; i++) {
Pit p = pits[i];
if (i == 6 || i == 13) {
assertTrue(p instanceof KalahPit);
assertEquals(0, p.getNumberOfStones());
} else {
assertTrue(p instanceof RegularPit);
assertEquals(6, p.getNumberOfStones());
}
}
}
private Board getBoard(UUID gameId) throws Exception {
MvcResult mvcResult = this.mockMvc.perform(get("/kalah/" + gameId + "/board")
.accept(MediaType.APPLICATION_JSON_UTF8))
.andExpect(status().isOk())
.andDo(print())
.andReturn();
String response = mvcResult.getResponse().getContentAsString();
ObjectMapper objectMapper = new ObjectMapper();
Board board = objectMapper.readValue(response, Board.class);
return board;
}
}
<file_sep>/src/main/java/it/jdev/kalah/domain/model/KalahPit.java
package it.jdev.kalah.domain.model;
import lombok.Data;
import lombok.EqualsAndHashCode;
@Data
@EqualsAndHashCode(callSuper = true)
public class KalahPit extends Pit {
public KalahPit() {}
}
| f44fb0ac9c17d4480938ae99c56e63c8a91304dc | [
"Markdown",
"Java",
"JavaScript"
] | 6 | Markdown | wimvanhaaren/backbaseCodeChallenge | 66c9ac6e57b8b23cb6923e70da5b8583ae0d98f9 | b81ac9675435c0276f39a68675b6cf354aeef726 | |
refs/heads/master | <repo_name>deekshati/GetADoc-Flask<file_sep>/app/models.py
from app import db, login
from werkzeug.security import generate_password_hash, check_password_hash
from flask_login import UserMixin
from datetime import datetime
@login.user_loader
def load_user(id):
if(id[0] == 'P'):
return Patient.query.get(id)
else:
return Doctor.query.get(id)
class Patient(UserMixin, db.Model):
id = db.Column(db.String(120), primary_key=True)
full_name = db.Column(db.String(64), index=True)
city = db.Column(db.String(20))
email = db.Column(db.String(120), index=True, unique=True)
password_hash = db.Column(db.String(120))
appointments = db.relationship('Appointment', backref='patient', lazy='dynamic')
def __repr__(self):
return '<Patient {}>'.format(self.full_name)
def set_password(self, password):
self.password_hash = generate_password_hash(password)
def check_password(self, password):
return check_password_hash(self.password_hash, password)
class Doctor(UserMixin, db.Model):
id = db.Column(db.String(120), primary_key=True)
full_name = db.Column(db.String(64), index=True)
city = db.Column(db.String(20))
qual = db.Column(db.String(20))
fees = db.Column(db.Integer)
phone = db.Column(db.Integer)
address = db.Column(db.String(120))
email = db.Column(db.String(120), index=True, unique=True)
password_hash = db.Column(db.String(120))
appointments = db.relationship('Appointment', backref='doctor', lazy='dynamic')
def __repr__(self):
return '<Doctor {}>'.format(self.full_name)
def set_password(self, password):
self.password_hash = generate_password_hash(password)
def check_password(self, password):
return check_password_hash(self.password_hash, password)
class Appointment(db.Model):
id = db.Column(db.Integer, primary_key=True)
requested_date = db.Column(db.Date)
appointment_date = db.Column(db.Date)
appointment_time = db.Column(db.Time)
doctor_id = db.Column(db.String(120), db.ForeignKey('doctor.id'))
patient_id = db.Column(db.String(120), db.ForeignKey('patient.id'))
reject_msg = db.Column(db.String(120))
status = db.Column(db.Integer)<file_sep>/app/forms.py
from flask_wtf import FlaskForm
from wtforms import StringField, PasswordField, BooleanField, SubmitField, SelectField, IntegerField, TextField
from wtforms.fields.html5 import DateField, TimeField, DateTimeField
from wtforms.validators import ValidationError, DataRequired, Email, Length, Optional
from app.models import Doctor, Patient
class LoginForm(FlaskForm):
choice = SelectField('Are you a Patient or Doctor?', choices=['Patient', 'Doctor'])
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('<PASSWORD>', validators=[DataRequired()])
remember_me = BooleanField('Remember me')
submit = SubmitField('Log In')
class DoctorRegister(FlaskForm):
name = StringField('Full Name', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('<PASSWORD>', validators=[DataRequired()])
city = StringField('City', validators=[DataRequired()])
phone = IntegerField('Phone No.', validators=[DataRequired()])
address = StringField('Address', validators=[DataRequired()])
qual = StringField('Qualifications', validators=[DataRequired()])
fees = IntegerField('Fees per Person', validators=[DataRequired()])
submit = SubmitField('Register')
def validate_email(self, email):
doctor = Doctor.query.filter_by(email=email.data).first()
if doctor is not None:
raise ValidationError('Email is already Registered!!')
class PatientRegister(FlaskForm):
name = StringField('Full Name', validators=[DataRequired()])
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('<PASSWORD>', validators=[DataRequired()])
city = StringField('City', validators=[DataRequired()])
submit = SubmitField('Register')
def validate_email(self, email):
patient = Patient.query.filter_by(email=email.data).first()
if patient is not None:
raise ValidationError('Email is already Registered!!')
class AppointmentForm(FlaskForm):
doctor_id = StringField('Doctor ID', validators=[Optional()])
patient_id = StringField('Patient ID', validators=[Optional()])
patient_name = StringField('Patient Name', validators=[DataRequired()])
mobile = IntegerField('Mobile Number', validators=[DataRequired()])
date = DateField('Enter Appointment Date', validators=[DataRequired()])
submit = SubmitField('Submit Request Form', validators=[DataRequired()])
class confirmAppointment(FlaskForm):
appoint_date = DateField("Appointment Date", validators=[DataRequired()])
appoint_time = TimeField("Appointment Time", validators=[DataRequired()])
submit = SubmitField("Confirm Appointment")
class rejectAppointment(FlaskForm):
rejectMessage = TextField('Reject Message', validators=[DataRequired()])
submit = SubmitField('Reject Appointment')
<file_sep>/getadoc.py
from app import app, db
from app.models import Patient, Doctor, Appointment
@app.shell_context_processor
def make_shell_context():
return {'db': db, 'Patient': Patient, 'Doctor': Doctor, 'Appointment': Appointment}<file_sep>/migrations/versions/cbe32a1e2540_doctor_patients_table.py
"""Doctor & Patients table
Revision ID: cbe32a1e2540
Revises:
Create Date: 2020-08-24 19:11:23.284040
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = '<KEY>'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.create_table('doctor',
sa.Column('id', sa.String(length=120), nullable=False),
sa.Column('full_name', sa.String(length=64), nullable=True),
sa.Column('city', sa.String(length=20), nullable=True),
sa.Column('qual', sa.String(length=20), nullable=True),
sa.Column('fees', sa.Integer(), nullable=True),
sa.Column('phone', sa.Integer(), nullable=True),
sa.Column('address', sa.String(length=120), nullable=True),
sa.Column('email', sa.String(length=120), nullable=True),
sa.Column('password_hash', sa.String(length=120), nullable=True),
sa.PrimaryKeyConstraint('id')
)
op.create_index(op.f('ix_doctor_email'), 'doctor', ['email'], unique=True)
op.create_index(op.f('ix_doctor_full_name'), 'doctor', ['full_name'], unique=False)
op.create_table('patient',
sa.Column('id', sa.String(length=120), nullable=False),
sa.Column('full_name', sa.String(length=64), nullable=True),
sa.Column('city', sa.String(length=20), nullable=True),
sa.Column('email', sa.String(length=120), nullable=True),
sa.Column('password_hash', sa.String(length=120), nullable=True),
sa.PrimaryKeyConstraint('id')
)
op.create_index(op.f('ix_patient_email'), 'patient', ['email'], unique=True)
op.create_index(op.f('ix_patient_full_name'), 'patient', ['full_name'], unique=False)
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.drop_index(op.f('ix_patient_full_name'), table_name='patient')
op.drop_index(op.f('ix_patient_email'), table_name='patient')
op.drop_table('patient')
op.drop_index(op.f('ix_doctor_full_name'), table_name='doctor')
op.drop_index(op.f('ix_doctor_email'), table_name='doctor')
op.drop_table('doctor')
# ### end Alembic commands ###
<file_sep>/app/routes.py
from secrets import token_hex
from flask import render_template, url_for, redirect, flash, request
from app import app, db
from flask_login import current_user, login_user, logout_user, login_required
from app.forms import LoginForm, DoctorRegister, PatientRegister, AppointmentForm, confirmAppointment, rejectAppointment
from app.models import Patient, Doctor, Appointment
from werkzeug.urls import url_parse
from datetime import datetime
@app.route('/')
def home():
date = datetime.utcnow()
return render_template('home.html', date=date)
@app.route('/about')
@login_required
def about():
return render_template('about.html')
@app.route('/finddoctor')
@login_required
def finddoctor():
doctors = Doctor.query.filter_by(city=current_user.city).all()
return render_template('doctorlist.html', doclist=doctors)
@app.route('/book/<Did>', methods=['GET', 'POST'])
@login_required
def book(Did):
app = Appointment(doctor_id=Did, patient_id=current_user.id)
form = AppointmentForm(obj=app)
if form.validate_on_submit():
appoint = Appointment(requested_date=form.date.data, doctor_id=form.doctor_id.data, patient_id=form.patient_id.data, status=0)
db.session.add(appoint)
db.session.commit()
flash('Congratulations, your appointment is successfully booked!')
return redirect(url_for('home'))
return render_template('bookdoctor.html', form=form)
@app.route('/myappointments')
@login_required
def myappointments():
if(current_user.id[0] == 'P'):
pending_data = Appointment.query.filter_by(patient_id=current_user.id, status=0).all()
confirmed_data = Appointment.query.filter_by(patient_id=current_user.id, status=1).all()
rejected_data = Appointment.query.filter_by(patient_id=current_user.id, status=-1).all()
return render_template('pat_appointment.html', confirm=confirmed_data, pending=pending_data, reject=rejected_data)
else:
pending_data = Appointment.query.filter_by(doctor_id=current_user.id, status=0).all()
confirmed_data = Appointment.query.filter_by(doctor_id=current_user.id, status=1).all()
#print(pending_data)
return render_template('doc_appointment.html', confirm=confirmed_data, pending=pending_data)
@app.route('/confirmappointment/<aid>', methods=['GET', 'POST'])
@login_required
def confirmappointment(aid):
app = Appointment.query.filter_by(id=aid).first()
if(current_user.id[0] == 'P'):
return redirect(url_for('home'))
form = confirmAppointment()
if form.validate_on_submit():
app.appointment_date = form.appoint_date.data
app.appointment_time = form.appoint_time.data
#print(app.appointment_date, app.appointment_time)
app.status = 1
db.session.commit()
return redirect(url_for('myappointments'))
return render_template('confirm.html', form=form, request = app.requested_date)
@app.route('/rejectappointment/<aid>', methods=['GET', 'POST'])
@login_required
def rejectappointment(aid):
app = Appointment.query.filter_by(id=aid).first()
if(current_user.id[0] == 'P'):
return redirect(url_for('home'))
form = rejectAppointment()
if form.validate_on_submit():
app.reject_msg = form.rejectMessage.data
app.status = -1
db.session.commit()
return redirect(url_for('myappointments'))
return render_template('reject.html', form=form)
@app.route('/login', methods=['GET', 'POST'])
def login():
if current_user.is_authenticated:
return redirect(url_for('home'))
form = LoginForm()
if form.validate_on_submit():
if(form.choice.data == 'Patient'):
daba = Patient
else:
daba = Doctor
user = daba.query.filter_by(email=form.email.data).first()
if user is None or not user.check_password(form.password.data):
flash('Invalid Username or Password')
return redirect(url_for('login'))
login_user(user, remember=form.remember_me.data)
next_page = request.args.get('next')
if not next_page or url_parse(next_page).netloc != '':
next_page = url_for('home')
return redirect(next_page)
return render_template('login.html', form=form)
@app.route('/register/<choice>', methods=['GET', 'POST'])
def register(choice):
if current_user.is_authenticated:
return redirect(url_for('home'))
idd = token_hex(16)
if(choice=='doctor'):
idd = 'D'+idd
form = DoctorRegister()
if form.validate_on_submit():
user = Doctor(id = idd, full_name=form.name.data, email=form.email.data, city=form.city.data, phone=form.phone.data, address=form.address.data, qual=form.qual.data, fees=form.fees.data)
user.set_password(form.password.data)
db.session.add(user)
db.session.commit()
flash('Congratulations, you are now a registered user!')
return redirect(url_for('login'))
else:
idd = 'P'+idd
form = PatientRegister()
if form.validate_on_submit():
user = Patient(id=idd, full_name=form.name.data, email=form.email.data, city=form.city.data)
user.set_password(form.password.data)
db.session.add(user)
db.session.commit()
flash('Congratulations, you are now a registered user!')
return redirect(url_for('login'))
return render_template('register.html', choice=choice, form=form)
@app.route('/logout')
def logout():
logout_user()
return redirect(url_for('home')) | b46c84327f5825898b31c371963f49aca4822f57 | [
"Python"
] | 5 | Python | deekshati/GetADoc-Flask | 5e12f230ddab1bb7ac3209d0d190bfff4e371ea2 | 738f032f1fff06db45d14628d0a9d4a9e6a47bdc | |
refs/heads/main | <repo_name>Trendyol-Front-End-Bootcamp/trendyol-frontend-bootcamp-js-201-202<file_sep>/5-promise-api/8-resolve.js
const fetch = require("node-fetch");
let cache = new Map();
const getUserName = () => {
if (cache.has("name")) {
return Promise.resolve(cache.get("name"));
}
return fetch(`https://api.github.com/users/enginustun`)
.then((res) => res.json())
.then((user) => {
cache.set("name", `from cache: ${user.name}`);
return user.name;
});
};
getUserName().then((name) => {
console.log({ name });
getUserName().then((name) => {
console.log({ name });
});
});
<file_sep>/4-promise/4-promise-finally.js
function getUserComments(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve([
{ id: 1, content: "hello world!" },
{ id: 2, content: "hello bootcamp!" },
]);
}, 1500);
});
}
function getUser(id) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ id: 1, name: "engin" });
}, 1000);
});
}
function setLoading(isLoading) {
if (isLoading) {
console.log("comments are loading...");
} else {
console.log("comments are loaded");
}
}
setLoading(true);
getUser(1).then((user) => {
getUserComments(user.id)
.then((comments) => {
console.log(comments);
})
.catch(() => {})
.finally(() => setLoading(false));
});
<file_sep>/3-callbacks/1-settimeout.js
function anotherFunc() {
console.log("<NAME>");
}
function logWithDelay() {
anotherFunc();
}
setTimeout(logWithDelay, 2000);
console.log("<NAME>");
/**
* callstack callback queue web api / cpp api
*
*
*
*
*
*
*
*
*
*
*/
<file_sep>/1-try-catch/5-works-sync-2.js
setTimeout(function () {
try {
noSuchVariable; // try...catch handles the error!
} catch {
console.log("error is caught here!");
}
}, 1000);
<file_sep>/5-promise-api/1-all.js
Promise.all([
new Promise((resolve) => setTimeout(() => resolve(1), 3000)), // 1
new Promise((resolve) => setTimeout(() => resolve(2), 2000)), // 2
new Promise((resolve) => setTimeout(() => resolve(3), 1000)), // 3
]).then(console.log); // 1,2,3 when promises are ready: each promise contributes an array member
<file_sep>/1-try-catch/6-error-object.js
try {
lalala; // error, variable is not defined!
} catch (err) {
console.log(err.name); // ReferenceError
console.log(err.message); // lalala is not defined
console.log(err.stack); // ReferenceError: lalala is not defined at (...call stack)
// Can also show an error as a whole
// The error is converted to string as "name: message"
console.log(err); // ReferenceError: lalala is not defined
}
<file_sep>/6-microtasks/1-microtasks.js
let promise = Promise.resolve();
setTimeout(() => {
console.log("I am finished first?");
}, 0);
promise.then(() => console.log("promise done!"));
setTimeout(() => {
console.log("I am finished last");
}, 0);
console.log("hello bootcamp 1");
console.log("hello bootcamp 2");
console.log("hello bootcamp 3");
console.log("hello bootcamp 4");
console.log("hello bootcamp 5");
// setTimeout(() => {
// console.log("Middle log");
// }, 0);
console.log("hello bootcamp 6");
console.log("hello bootcamp 7");
console.log("hello bootcamp 8");
console.log("hello bootcamp 9");
console.log("hello bootcamp 10");
for (let i = 0; i < 1000000000; i++) {
const x = Math.random();
}
<file_sep>/7-async-await/1-async-await.js
async function f() {
return 1;
}
f().then(console.log); // 1
<file_sep>/1-try-catch/3-runtime-error.js
try {
{{{{{{{
} catch (err) {
alert("The engine can't understand this code, it's invalid");
}<file_sep>/8-generators/2-generators-2.js
function* generateSequence() {
yield 1;
yield 2;
yield 3;
}
let it = generateSequence();
let one = it.next();
console.log(one, it.next(), it.next(), it.next()); // {value: 1, done: false}
// for (const value of generateSequence()) {
// console.log(value);
// }
<file_sep>/4-promise/3-promise-error.js
function getUserComments(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error("Boyle user olmaz olsun"));
resolve([
{ id: 1, content: "hello world!" },
{ id: 2, content: "hello bootcamp!" },
]);
}, 1500);
});
}
function getUser(id) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ id: 1, name: "engin" });
}, 1000);
});
}
getUser(1).then((user) => {
getUserComments(user.id)
.then((comments) => {
console.log(comments);
})
.catch((err) => {
console.log(err);
});
});
setTimeout(() => {
console.log("cok gec calisacagim");
}, 5000);
<file_sep>/1-try-catch/12-rethrow-2.js
function readData() {
let json = '{ "age": 30 }';
try {
// ...
blabla(); // error!
} catch (err) {
// ...
if (!(err instanceof SyntaxError)) {
throw err; // rethrow (don't know how to deal with it)
}
} finally {
console.log("Finally");
}
}
try {
readData();
} catch (err) {
console.log("External catch got: " + err); // caught it!
}
<file_sep>/1-try-catch/11-rethrow.js
let json = '{ "age": 30 }'; // incomplete data
try {
let user = JSON.parse(json);
if (!user.age) {
throw new SyntaxError("Incomplete data: no name");
}
blabla(); // unexpected error
console.log(user.name);
} catch (err) {
if (err instanceof SyntaxError) {
console.log("JSON Error: " + err.message);
} else {
throw err; // rethrow (*)
}
}
<file_sep>/7-async-await/7-async-await-error-handling.js
const fetch = require("node-fetch");
async function f() {
try {
let response = await fetch("/no-user-here");
let user = await response.json();
} catch (err) {
// catches errors both in fetch and response.json
console.log(err);
}
}
f();
// // top-level caselerinde
// async function f2() {
// let response = await fetch("/no-user-here");
// }
// // f2() becomes a rejected promise
// f2().catch(console.log); // TypeError: failed to fetch // (*)
<file_sep>/7-async-await/6-async-await-class-methods.js
class Waiter {
async wait() {
return await Promise.resolve("I am waiting");
}
}
new Waiter().wait().then(console.log); // 1 (this is the same as (result => console.log(result)))
<file_sep>/4-promise/1-promise.js
const logAfterSomeTime = (ms) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Zamani geldiginde calisacagim");
}, ms);
});
};
logAfterSomeTime(2000).then((message) => console.log(message));
console.log("<NAME>");
<file_sep>/1-try-catch/15-global-error-handling.js
// window.addEventListener('error', function(event) { ... })
process.on("uncaughtException", function (err) {
console.log("Caught exception: ", err.name, err.message, err.stack);
});
setTimeout(function () {
console.log("This will still run.");
}, 1000);
// Intentionally cause an exception, but don't catch it.
nonexistentFunc();
console.log("This will not run.");
<file_sep>/5-promise-api/2-all-2.js
const fetch = require("node-fetch");
let urls = [
"https://api.github.com/users/enginustun",
"https://api.github.com/users/remy",
"https://api.github.com/users/jeresig",
];
// map every url to the promise of the fetch
let requests = urls.map((url) => fetch(url));
// Promise.all waits until all jobs are resolved
Promise.all(requests).then((responses) =>
responses.forEach((response) =>
console.log(`${response.url}: ${response.status}`)
)
);
<file_sep>/8-generators/4-generators-4.js
let range = {
from: 1,
to: 5,
*[Symbol.iterator]() {
// a shorthand for [Symbol.iterator]: function*()
for (let value = this.from; value <= this.to; value++) {
yield value;
}
},
};
console.log(range, [...range]);
for (const val of range) {
console.log(val);
}
<file_sep>/4-promise/9-promise-error-handling-3.js
// rethrowing
// the execution: catch -> catch
new Promise((resolve, reject) => {
throw new Error("Whoops!");
})
.catch(function (error) {
// (*)
if (error instanceof URIError) {
// handle it
} else {
console.log("Can't handle such error");
throw error; // throwing this or another error jumps to the next catch
}
})
.then(function () {
/* doesn't run here */
})
.catch((error) => {
// (**)
console.log(`The unknown error has occurred: ${error}`);
// don't return anything => execution goes the normal way
});
<file_sep>/1-try-catch/8-json-parse-error.js
let json = "{ bad json }";
try {
let user = JSON.parse(json); // <-- when an error occurs...
console.log(user.name); // doesn't work
} catch (err) {
// ...the execution jumps here
console.log(
"Our apologies, the data has errors, we'll try to request it one more time."
);
console.log(err.name);
console.log(err.message);
}
<file_sep>/1-try-catch/1-no-error.js
try {
console.log("Start of try runs"); // (1) <--
// ...no errors here
console.log("End of try runs"); // (2) <--
} catch (err) {
console.log("Catch is ignored, because there are no errors"); // (3)
}
<file_sep>/1-try-catch/7-json-parse.js
let json = '{"name":"Engin", "age": 30}'; // data from the server
let user = JSON.parse(json); // convert the text representation to JS object
// now user is an object with properties from the string
console.log(user.name); // Engin
console.log(user.age); // 30
<file_sep>/4-promise/8-promise-error-handling-2.js
new Promise((resolve, reject) => {
resolve("ok");
})
.then((result) => {
throw new Error("Whoops!"); // rejects the promise
})
.catch(console.log); // Error: Whoops!
new Promise((resolve, reject) => {
resolve("ok");
})
.then((result) => {
blabla(); // no such function
})
.catch(console.log); // ReferenceError: blabla is not defined
<file_sep>/1-try-catch/9-throw-own-error.js
// no name in data
let json = '{ "age": 30 }'; // incomplete data
try {
let user = JSON.parse(json); // <-- no errors
console.log(user.name); // no name!
} catch (err) {
console.log("doesn't execute");
}
<file_sep>/8-generators/5-generators-5.js
var standard_input = process.stdin;
function* gen() {
// Pass a question to the outer code and wait for an answer
let result = yield "2 + 2 = ?";
while (true) {
console.log({ result });
if (result === 4) {
return "<NAME>!";
} else {
result = yield "Patladin";
}
}
}
let generator = gen();
let question = generator.next().value; // <-- yield returns the value
console.log(question);
standard_input.on("data", function (data) {
// User input exit.
if (data === "exit\n") {
process.exit();
} else {
let result = generator.next(+data); // --> pass the result into the generator
console.log(result);
if (result.value === "<NAME>!") {
process.exit();
}
}
});
<file_sep>/4-promise/2-promise-2.js
function getUserComments(userId) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve([
{ id: 1, content: "hello world!" },
{ id: 2, content: "hello bootcamp!" },
]);
}, 1500);
});
}
function getUser(id) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve({ id: 1, name: "engin" });
}, 1000);
});
}
getUser(1).then((user) => {
getUserComments(user.id).then((comments) => {
console.log(comments);
});
});
| 5f5724622c9d2c19fb9640fe3af99beff5e4ff08 | [
"JavaScript"
] | 27 | JavaScript | Trendyol-Front-End-Bootcamp/trendyol-frontend-bootcamp-js-201-202 | 23d9d99b1bbc3685f7078d368e6eb7928e7a0953 | 5deee87e6a9c0ccc8ab370b94331aa86980c4d2f | |
refs/heads/master | <file_sep>require './model/length'
require './model/weight'
public
def method_missing(method, *args, &block)
val = method[-1] == 's' ? method : "#{method}s" # Поддержка единственного и множественного наименования метода
condition(val.to_sym)
end
private
# Условие вывода результата
def condition(method)
if Length::STANDARTS.include?(method)
Length.new(self, method)
elsif Weight::STANDARTS.include?(method)
Weight.new(self, method)
else
raise NoMethodError, "undefined method #{method}"
end
end
<file_sep>class Weight < Length
# Конвертирующие коэффициенты
STANDARTS = {
kilograms: 1,
gramms: 1000,
pounds: 2.205,
ounces: 35.2739619
}
end<file_sep>class Length
require './model/error'
# Конвертирующие коэффициенты
STANDARTS = {
meters: 1,
miles: 0.000621371192,
inches: 39.3700787,
centimeters: 100
}
# Инициализация переменных
def initialize(num, type)
@num = num
@type = type
end
# Конвертация одной велечины в другую
def in(val)
standarts = self.class::STANDARTS # выбор констант в зависимости от класса
error(@type, val) unless standarts.include?(val) # вывод ошибки
@num * ( standarts[val] / standarts[@type] )
end
# Ошибка конвертации разных типов велечин
def error(type, value)
raise TypeMismatch, "impossible to convert #{type} per #{value}"
end
end<file_sep>class TypeMismatch < Exception; end | 2c78adc2763577e817bd08b03354472bedb17901 | [
"Ruby"
] | 4 | Ruby | akiba88/test1 | 8e446acea82e1e4b51095deb5a34705eea7e1d33 | f049985511f7abf3f25aa45cd1e1f5f193f803e4 | |
refs/heads/master | <repo_name>ninjaja/task3_composite<file_sep>/src/com/htp/matrizaev/constant/Constant.java
package com.htp.matrizaev.constant;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Constant {
public static final String FILENAME = "./input/input.txt";
public static final String FILETESTNAME = "input/test.txt";
public static final Logger LOGGER = LogManager.getLogger();
public static final String PARAGRAPH_DELIMITER = "(?sm)^[^\\s]+.*?\\.\\s*$";
public static final String SENTENCE_DELIMITER = "(?:[^!?.]|\\.(?=\\d))+[!?.]";
public static final String LEXEME_DELIMITER = "\\s";
public static final String SYMBOL_DELIMITER = "\\w";
}
<file_sep>/src/com/htp/matrizaev/reader/TextReader.java
package com.htp.matrizaev.reader;
import com.htp.matrizaev.exception.TextReaderException;
import org.apache.logging.log4j.*;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.stream.Stream;
import static com.htp.matrizaev.constant.Constant.LOGGER;
public class TextReader {
public static String readText(String filePath) throws TextReaderException {
if (filePath == null || filePath.isEmpty() || !new File(filePath).exists()) {
throw new TextReaderException("Invalid file path or file does not exist");
}
StringBuilder builder = new StringBuilder();
try (Stream<String> stream = Files.lines(Paths.get(filePath), StandardCharsets.UTF_8)) {
stream.forEach(s -> builder.append(s).append("\n"));
} catch (IOException e) {
throw new TextReaderException("Cannot read the file", e);
}
LOGGER.log(Level.INFO, "File has been read");
return builder.toString();
}
}
<file_sep>/src/com/htp/matrizaev/parser/TextParser.java
package com.htp.matrizaev.parser;
import com.htp.matrizaev.entity.Component;
public class TextParser implements MainParser {
private ParagraphParser paragraphParser;
private Component textComposite;
public TextParser() {
SymbolParser symbolParser = new SymbolParser();
LexemeParser lexemeParser = new LexemeParser(symbolParser);
SentenceParser sentenceParser = new SentenceParser(lexemeParser);
this.paragraphParser = new ParagraphParser(sentenceParser);
}
public Component getTextComposite() {
return textComposite;
}
@Override
public void parse(Component textComposite, String text) {
if (paragraphParser !=null){
paragraphParser.parse(textComposite, text);
}
this.textComposite = textComposite;
}
}
<file_sep>/src/com/htp/matrizaev/entity/Leaf.java
package com.htp.matrizaev.entity;
public class Leaf implements Component {
private char leaf;
private ComponentType componentType;
public Leaf(char leaf, ComponentType componentType) {
this.leaf = leaf;
this.componentType = componentType;
}
@Override
public void addComponent(Component component) {
}
@Override
public ComponentType defineType() {
return componentType;
}
@Override
public String toString() {
return String.valueOf(leaf);
}
}
<file_sep>/src/com/htp/matrizaev/composer/Composer.java
package com.htp.matrizaev.composer;
import java.util.List;
public class Composer {
List<String> listToCompose;
public String composeText(List<String> list) {
StringBuilder builder = new StringBuilder();
for (int i = 0; i < list.size(); i++) {
builder.append(list.get(i));
}
return builder.toString();
}
}
<file_sep>/src/com/htp/matrizaev/parser/MainParser.java
package com.htp.matrizaev.parser;
import com.htp.matrizaev.entity.Component;
public interface MainParser {
void parse(Component component, String text);
}
<file_sep>/src/com/htp/matrizaev/test/Main.java
package com.htp.matrizaev.test;
import com.htp.matrizaev.entity.Component;
import com.htp.matrizaev.entity.ComponentType;
import com.htp.matrizaev.entity.Composite;
import com.htp.matrizaev.exception.TextReaderException;
import com.htp.matrizaev.parser.TextParser;
import com.htp.matrizaev.reader.TextReader;
import static com.htp.matrizaev.constant.Constant.FILENAME;
public class Main {
public static void main(String[] args) throws TextReaderException {
String text = TextReader.readText(FILENAME);
Component composite = new Composite(ComponentType.TEXT);
TextParser textParser = new TextParser();
textParser.parse(composite, text);
composite = textParser.getTextComposite();
String result = composite.toString();
System.out.println(result);
}
}
<file_sep>/test/com/htp/matrizaev/entity/CompositeTest.java
package com.htp.matrizaev.entity;
import com.htp.matrizaev.exception.TextReaderException;
import com.htp.matrizaev.parser.TextParser;
import com.htp.matrizaev.reader.TextReader;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import static com.htp.matrizaev.constant.Constant.FILETESTNAME;
import static org.testng.Assert.*;
public class CompositeTest {
private TextParser textParser;
private Component textComposite;
private String text;
@BeforeTest
public void setUp() {
textParser = new TextParser();
textComposite = new Composite(ComponentType.TEXT);
}
@AfterTest
public void tearDown() {
textParser = null;
textComposite = null;
text = null;
}
@Test
public void test() {
String expected = " Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
String actual = null;
try {
text = TextReader.readText(FILETESTNAME);
textParser.parse(textComposite, text);
textComposite = textParser.getTextComposite();
actual = textComposite.toString();
} catch (TextReaderException e) {
fail();
}
Assert.assertEquals(actual, expected);
}
}
| 59046a747751f0d488867638403994889e12f237 | [
"Java"
] | 8 | Java | ninjaja/task3_composite | b28bb278f55ec03f2b695ae2abd2a2e7912c583f | ca4a152f94631f96508f13608d808a7887f4cd99 | |
refs/heads/master | <file_sep>from . import db
class Demand(db.Model):
_tablename_ = 'demand'
id = db.Column(db.INTEGER, primary_key=True)
address = db.Column(db.VARCHAR(20))
channel = db.Column(db.INTEGER)
model = db.Column(db.VARCHAR(20))
datetime = db.Column(db.DATETIME)
demand_min = db.Column(db.FLOAT(precision='20,2'))
demand_quarter = db.Column(db.FLOAT(precision='20,2'))
R_value = db.Column(db.FLOAT(precision='20,2'))
S_value = db.Column(db.FLOAT(precision='20,2'))
T_value = db.Column(db.FLOAT(precision='20,2'))
Total_value = db.Column(db.FLOAT(precision='20,2'))
def __init__(self, address, channel, model, datetime,
demand_min, demand_quarter, R_value, S_value,
T_value, Total_value):
self.address = address
self.channel = channel
self.model = model
self.datetime = datetime
self.demand_min = demand_min
self.demand_quarter = demand_quarter
self.R_value = R_value
self.S_value = S_value
self.T_value = T_value
self.Total_value = Total_value
<file_sep># DAE
測試上傳至GitHub
<file_sep>function randNum(){
return ((Math.floor( Math.random()* (1+40-20) ) ) + 20)* 1200;
}
function randNum2(){
return ((Math.floor( Math.random()* (1+40-20) ) ) + 20) * 500;
}
function randNum3(){
return ((Math.floor( Math.random()* (1+40-20) ) ) + 20) * 300;
}
function randNum4(){
return ((Math.floor( Math.random()* (1+40-20) ) ) + 20) * 100;
}
$(document).ready(function(){
/* ---------- Event ---------- */
var ldata1 = [], ldata2 = [], ldata3 = [], ldata4 = [], ldata5 = [];
var d1 = [];
for (var i = 1; i <= 96; i += 1)
d1.push([i, parseInt(Math.random() * 5)]);
var d2 = [];
for (var i = 1; i <= 24; i += 1)
d2.push([i, 10 + parseInt(Math.random() * 5)]);
var d3 = [];
for (var i = 1; i <= 1; i += 1)
d3.push([i, 200 + parseInt(Math.random() * 80)]);
ldata1.push(d1);
ldata1.push(d2);
ldata1.push(d3);
var d1 = [];
for (var i = 1; i <= 96; i += 1)
d1.push([i, parseInt(Math.random() * 5)]);
var d2 = [];
for (var i = 1; i <= 24; i += 1)
d2.push([i, 10 + parseInt(Math.random() * 5)]);
var d3 = [];
for (var i = 1; i <= 1; i += 1)
d3.push([i, 200 + parseInt(Math.random() * 80)]);
ldata2.push(d1);
ldata2.push(d2);
ldata2.push(d3);
var d1 = [];
for (var i = 1; i <= 96; i += 1)
d1.push([i, parseInt(Math.random() * 5)]);
var d2 = [];
for (var i = 1; i <= 24; i += 1)
d2.push([i, 10 + parseInt(Math.random() * 5)]);
var d3 = [];
for (var i = 1; i <= 1; i += 1)
d3.push([i, 200 + parseInt(Math.random() * 80)]);
ldata3.push(d1);
ldata3.push(d2);
ldata3.push(d3);
var d1 = [];
for (var i = 1; i <= 96; i += 1)
d1.push([i, parseInt(Math.random() * 5)]);
var d2 = [];
for (var i = 1; i <= 24; i += 1)
d2.push([i, 10 + parseInt(Math.random() * 5)]);
var d3 = [];
for (var i = 1; i <= 1; i += 1)
d3.push([i, 200 + parseInt(Math.random() * 80)]);
ldata4.push(d1);
ldata4.push(d2);
ldata4.push(d3);
var d1 = [];
for (var i = 1; i <= 96; i += 1)
d1.push([i, parseInt(Math.random() * 5)]);
var d2 = [];
for (var i = 1; i <= 24; i += 1)
d2.push([i, 10 + parseInt(Math.random() * 5)]);
var d3 = [];
for (var i = 1; i <= 1; i += 1)
d3.push([i, 200 + parseInt(Math.random() * 80)]);
ldata5.push(d1);
ldata5.push(d2);
ldata5.push(d3);
$("#stack_btn").click(function(){
var d = [];
if($("#stackchart").length)
{
if(stack_check_box_1.checked){
d = ldata1;
}
if(stack_check_box_2.checked){
d = ldata2;
}
if(stack_check_box_3.checked){
d = ldata3;
}
if(stack_check_box_4.checked){
d = ldata4;
}
if(stack_check_box_5.checked){
d = ldata5;
}
var stack = 0, bars = true, lines = false, steps = false;
function plotWithOptions(d) {
$.plot($("#stackchart"), [ d ], {
series: {
stack: stack,
bars: { show: true, barWidth: 0.6 },
},
colors: ["#FA5833", "#2FABE9", "#FABB3D"]
});
}
plotWithOptions(d[0]);
$(".graphControls input").click(function (e) {
e.preventDefault();
bars = $(this).val().indexOf("15分鐘") != -1;
lines = $(this).val().indexOf("1小時") != -1;
steps = $(this).val().indexOf("1天") != -1;
week = $(this).val().indexOf("1周") != -1;
//console.log(bars, lines, steps);
if(bars)
plotWithOptions(d[0]);
else if(lines)
plotWithOptions(d[1]);
else
plotWithOptions(d[2]);
});
}
});
var rd1 = [], rd2 = [], rd3 = [], rd4 = [], rd5 = [];
for (var i = 1; i < 25; i += 1){
rd1.push([i, 3 + Math.random()*5]);
rd2.push([i, 3 + Math.random()*5]);
rd3.push([i, 3 + Math.random()*5]);
rd4.push([i, 3 + Math.random()*5]);
rd5.push([i, 3 + Math.random()*5]);
}
$("#lines_btn").click(function(){
if(check_box_1.checked){
var d1 = rd1;
}
if(check_box_2.checked){
var d2 = rd2;
}
if(check_box_3.checked){
var d3 = rd3;
}
if(check_box_4.checked){
var d4 = rd4;
}
if(check_box_5.checked){
var d5 = rd5;
}
if($("#sincos").length)
{
var plot = $.plot($("#sincos"),
[ { data: d1, label: "電表一"}, { data: d2, label: "電表二" }, { data: d3, label: "電表三" }, { data: d4, label: "電表四" }, { data: d5, label: "電表五" } ], {
series: {
lines: { show: true,
lineWidth: 2,
},
points: { show: true },
shadowSize: 2
},
grid: { hoverable: true,
clickable: true,
tickColor: "#dddddd",
borderWidth: 0
},
//yaxis: { min: -1.2, max: 1.2 },
colors: ["#FA5833", "#2FABE9", "yellow", "green"]
});
function showTooltip(x, y, contents) {
$('<div id="tooltip">' + contents + '</div>').css( {
position: 'absolute',
display: 'none',
top: y + 5,
left: x + 5,
border: '1px solid #fdd',
padding: '2px',
'background-color': '#dfeffc',
opacity: 0.80
}).appendTo("body").fadeIn(200);
}
var previousPoint = null;
$("#sincos").bind("plothover", function (event, pos, item) {
$("#x").text(pos.x.toFixed(2));
$("#y").text(pos.y.toFixed(2));
if (item) {
if (previousPoint != item.dataIndex) {
previousPoint = item.dataIndex;
$("#tooltip").remove();
var x = item.datapoint[0].toFixed(2),
y = item.datapoint[1].toFixed(2);
showTooltip(item.pageX, item.pageY,
item.series.label + " of " + x + " = " + y);
}
}
else {
$("#tooltip").remove();
previousPoint = null;
}
});
}
});
/* ---------- Chart with points ---------- */
/* ---------- Stack chart ---------- */
/* ---------- Donut chart ---------- */
if($("#osChart").length)
{
$.plot($("#osChart"), data,
{
series: {
pie: {
innerRadius: 0.6,
show: true
}
},
legend: {
show: true
},
colors: ["#FA5833", "#2FABE9", "#FABB3D", "#78CD51"]
});
}
/* ---------- Pie chart ---------- */
var data = [
{ label: "Internet Explorer", data: 12},
{ label: "Mobile", data: 27},
{ label: "Safari", data: 85},
{ label: "Opera", data: 64},
{ label: "Firefox", data: 90},
{ label: "Chrome", data: 112}
];
if($("#piechart").length)
{
$.plot($("#piechart"), data,
{
series: {
pie: {
show: true
}
},
grid: {
hoverable: true,
clickable: true
},
legend: {
show: false
},
colors: ["#FA5833", "#2FABE9", "#FABB3D", "#78CD51"]
});
function pieHover(event, pos, obj)
{
if (!obj)
return;
percent = parseFloat(obj.series.percent).toFixed(2);
$("#hover").html('<span style="font-weight: bold; color: '+obj.series.color+'">'+obj.series.label+' ('+percent+'%)</span>');
}
$("#piechart").bind("plothover", pieHover);
}
/* ---------- Donut chart ---------- */
if($("#donutchart").length)
{
$.plot($("#donutchart"), data,
{
series: {
pie: {
innerRadius: 0.5,
show: true
}
},
legend: {
show: false
},
colors: ["#FA5833", "#2FABE9", "#FABB3D", "#78CD51"]
});
}
// we use an inline data source in the example, usually data would
// be fetched from a server
var data = [], totalPoints = 300;
function getRandomData() {
if (data.length > 0)
data = data.slice(1);
// do a random walk
while (data.length < totalPoints) {
var prev = data.length > 0 ? data[data.length - 1] : 50;
var y = prev + Math.random() * 10 - 5;
if (y < 0)
y = 0;
if (y > 100)
y = 100;
data.push(y);
}
// zip the generated y values with the x values
var res = [];
for (var i = 0; i < data.length; ++i)
res.push([i, data[i]])
return res;
}
// setup control widget
var updateInterval = 30;
$("#updateInterval").val(updateInterval).change(function () {
var v = $(this).val();
if (v && !isNaN(+v)) {
updateInterval = +v;
if (updateInterval < 1)
updateInterval = 1;
if (updateInterval > 2000)
updateInterval = 2000;
$(this).val("" + updateInterval);
}
});
if($("#realtimechart").length)
{
var options = {
series: { shadowSize: 1 },
lines: { fill: true, fillColor: { colors: [ { opacity: 1 }, { opacity: 0.1 } ] }},
yaxis: { min: 0, max: 100 },
xaxis: { show: false },
colors: ["#F4A506"],
grid: { tickColor: "#dddddd",
borderWidth: 0
},
};
var plot = $.plot($("#realtimechart"), [ getRandomData() ], options);
function update() {
plot.setData([ getRandomData() ]);
// since the axes don't change, we don't need to call plot.setupGrid()
plot.draw();
setTimeout(update, updateInterval);
}
update();
}
});<file_sep>$(function() {
$("#dialog_div").dialog({
autoOpen: false,
show: "blind",
hide: "explode",
dialogClass: "dlg-no-close",
width: 400,
height:350,
buttons: {
"Ok": function() {
$(this).dialog("close");
$("#value").empty();
$("#cost").empty();
$( "#count_btn").unbind( "click" );
},
"Cancel": function() {
$(this).dialog("close");
$("#value").empty();
$("#cost").empty();
$( "#count_btn").unbind( "click" );
}
}
});
$("#refresh").click(function() {
location.reload();
});
$(".info-box.red-bg").click(function() {
$("#dialog_div").dialog("open");
$("#count_btn").click(function() {
$("#value").empty();
$("#cost").empty();
$.ajax({
url:"assets/php/query_count.php",
type: "GET",
data: {
houlddeviceindex: 'SMB350-4-B CH1 R',
sDate: $('#datetimepicker1').val(),
eDate: $('#datetimepicker2').val()
},
dataType: "json",
success: function(response) {
var money = $("#money").val();
$("#value").append(response+" KWH");
$("#cost").append(response*money+" 元");
}
});
});
});
$(".info-box.green-bg").click(function() {
$("#dialog_div").dialog("open");
$("#count_btn").click(function() {
$("#value").empty();
$("#cost").empty();
$.ajax({
url:"assets/php/query_count.php",
type: "GET",
data: {
houlddeviceindex: 'SMB350-4-B CH1 S',
sDate: $('#datetimepicker1').val(),
eDate: $('#datetimepicker2').val()
},
dataType: "json",
success: function(response) {
var money = $("#money").val();
$("#value").append(response+" KWH");
$("#cost").append(response*money+" 元");
}
});
});
});
$(".info-box.blue-bg").click(function() {
$("#dialog_div").dialog("open");
$("#count_btn").click(function() {
$("#value").empty();
$("#cost").empty();
$.ajax({
url:"assets/php/query_count.php",
type: "GET",
data: {
houlddeviceindex: 'SMB350-4-B CH1 T',
sDate: $('#datetimepicker1').val(),
eDate: $('#datetimepicker2').val()
},
dataType: "json",
success: function(response) {
var money = $("#money").val();
$("#value").append(response+" KWH");
$("#cost").append(response*money+" 元");
}
});
});
});
$(".info-box.magenta-bg").click(function() {
$("#dialog_div").dialog("open");
$("#count_btn").click(function() {
$("#value").empty();
$("#cost").empty();
$.ajax({
url:"assets/php/query_count.php",
type: "GET",
data: {
houlddeviceindex: 'SMB350-4-B CH2 R',
sDate: $('#datetimepicker1').val(),
eDate: $('#datetimepicker2').val()
},
dataType: "json",
success: function(response) {
var money = $("#money").val();
$("#value").append(response+" KWH");
$("#cost").append(response*money+" 元");
}
});
});
});
$(".info-box.yellow-bg").click(function() {
$("#dialog_div").dialog("open");
$("#count_btn").click(function() {
$("#value").empty();
$("#cost").empty();
$.ajax({
url:"assets/php/query_count.php",
type: "GET",
data: {
houlddeviceindex: 'PM210',
sDate: $('#datetimepicker1').val(),
eDate: $('#datetimepicker2').val()
},
dataType: "json",
success: function(response) {
var money = $("#money").val();
$("#value").append(response+" KWH");
$("#cost").append(response*money+" 元");
}
});
});
});
$.ajax({
url:"assets/php/query.php",
type: "GET",
data: {
houlddeviceindex: ['SMB350-4-B CH1 R','SMB350-4-B CH1 S','SMB350-4-B CH1 T','SMB350-4-B CH2 R', 'PM210']
},
dataType: "json",
success: function(response) {
remove();
$("#updatetime").append(getDateTime());
for(var i=1;i<6;i++){
var index = i-1;
if(response[index])
$("#dev"+i).append(response[index]);
else
$("#dev"+i).append(0);
}
$("#connect").empty();
$("#connect").append("通訊良好");
}
});
function getDateTime(){
var currentTime = new Date();
var currentDate = new Date();
var hours = currentTime.getHours();
var minutes = currentTime.getMinutes();
var day = currentDate.getDate();
var month = currentDate.getMonth();
var year = currentDate.getFullYear();
if (minutes < 10)
minutes = "0" + minutes;
if (month < 10)
month = "0" + month;
if (day < 10)
day = "0" + day;
var time = year + "-" + month + "-" + day + " " + hours + ":" + minutes;
return time;
}
function remove(){
$("#dev1").empty();
$("#dev2").empty();
$("#dev3").empty();
$("#dev4").empty();
}
});<file_sep>import logging
from logging.handlers import RotatingFileHandler
class BasicConfig(object):
SECRET_KEY = '421d7fa062a76ca25669e91923a3c79f'
#SQLALCHEMY_COMMIT_ON_TEARDOWN = True
SQLALCHEMY_TRACK_MODIFICATIONS = True
SQLALCHEMY_RECORD_QUERIES = True
@staticmethod
def init_app(app):
_handler = RotatingFileHandler('app.log',
maxBytes=10000,
backupCount=1)
_handler.setLevel(logging.WARNING)
app.logger.addHandler(_handler)
class DevelopmentConfig(BasicConfig):
DEBUG = True
SQLALCHEMY_DATABASE_URI = 'mysql://root:kDd414o6@localhost/dae'
SQLALCHEMY_ECHO = True
# SQLALCHEMY_DATABASE_URI = os.environ.get('DEV_DATABASE_URL') or \
# 'sqlite:///' + os.path.join(basedir, 'data-dev.sqlite')
class TestingConfig(BasicConfig):
pass
class ProductionConfig(BasicConfig):
pass
config = {
'development': DevelopmentConfig,
'testing': TestingConfig,
'production': ProductionConfig,
'default': DevelopmentConfig
}
<file_sep>"""initial migration
Revision ID: fce4c7d2f0f6
Revises:
Create Date: 2017-06-07 13:19:58.722466
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = 'fce<KEY>'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.create_table('demand',
sa.Column('id', sa.INTEGER(), nullable=False),
sa.Column('address', sa.VARCHAR(length=20), nullable=True),
sa.Column('channel', sa.INTEGER(), nullable=True),
sa.Column('model', sa.VARCHAR(length=20), nullable=True),
sa.Column('datetime', sa.DATETIME(), nullable=True),
sa.Column('demand_min', sa.FLOAT(precision='20,2'), nullable=True),
sa.Column('demand_quarter', sa.FLOAT(precision='20,2'), nullable=True),
sa.Column('R_value', sa.FLOAT(precision='20,2'), nullable=True),
sa.Column('S_value', sa.FLOAT(precision='20,2'), nullable=True),
sa.Column('T_value', sa.FLOAT(precision='20,2'), nullable=True),
sa.Column('Total_value', sa.FLOAT(precision='20,2'), nullable=True),
sa.PrimaryKeyConstraint('id')
)
# ### end Alembic commands ###
def downgrade():
# ### commands auto generated by Alembic - please adjust! ###
op.drop_table('demand')
# ### end Alembic commands ###
<file_sep>/*每日最大需量*/
function daily_maxDemand(data) {
var chart = AmCharts.makeChart("daily_maxDemand", {
"type": "serial",
"theme": "light",
"dataProvider": data,
"legend": {
// "equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"valueAxes": [{
"gridColor": "#FFFFFF",
"gridAlpha": 0.2,
"dashLength": 0
}],
"gridAboveGraphs": true,
"startDuration": 1,
"graphs": [{
"balloonText": "[[category]]: <b>[[value]]</b>kW",
"fillAlphas": 0.8,
"lineAlpha": 0.2,
"type": "column",
"title": "最高需量",
"legendValueText": "[[value]] Watt",
"valueField": "peak_demand"
}],
"chartCursor": {
"categoryBalloonEnabled": false,
"cursorAlpha": 0,
"zoomable": false
},
"categoryField": "time",
"categoryAxis": {
"gridPosition": "start",
"gridAlpha": 0,
"tickPosition": "start",
"tickLength": 20
},
});
}
//每日最大需輛區間
function daily_demand(data) {
var chart = AmCharts.makeChart("daily_maxDemand_period", {
"type": "serial",
//圖例
"mouseWheelZoomEnabled": false,
"dataDateFormat": "YYYY-MM-DD JJ:NN:SS",
"legend": {
// "equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
//資料
"dataProvider": data["demands"],
"valueAxes": [
//Demand值軸
{
"id": "demandAxis",
"gridColor": "#FFFFFF",
"gridAlpha": 0.5,
"position": "left",
"title": "kW",
"minimum": 0
},
],
"gridAboveGraphs": true,
"graphs": [{
"id": "g1",
"balloonText": "<span style='font-size:12px; font-family:Microsoft JhengHei;'> 時間:[[category]]<br><b>需量:[[value]]kW</b>[[additional]]</span>",
"bullet": "round",
"bulletsize": 0.5,
"bulletBorderThickness": 1.5,
"bulletBorderAlpha": 0.5,
"bulletColor": "#FFFFFF",
"useLineColorForBulletBorder": true,
"balloon": {
"drop": false
},
"hideBulletsCount": 24,
"legendValueText": "[[value]] kW",
"title": "每10秒功率",
"labelPosition": "right",
"valueField": "Value",
"valueAxis": "demandAxis",
"lineColor": " #1f77b4",
"lineThickness": 2.5
}, ],
"chartScrollbar": {
"graph": "g1",
"autoGridCount": true,
"scrollbarHeight": 40,
"color": "#000000"
},
"chartCursor": {
"categoryBalloonEnabled": false,
"categoryBalloonDateFormat": "MMM DD,JJ:NN:SS",
"cursorPosition": "mouse",
"cursorColor": "#FFFFFF",
"cursorAlpha": 0
},
"valueScrollbar": {
"autoGridCount": true,
"color": "#000000",
"scrollbarHeight": 50
},
"categoryField": "time",
"categoryAxis": {
"minPeriod": "10ss",
"parseDates": true
},
});
}
/*該月前三大需量*/
function Demand_high(data) {
var chart = AmCharts.makeChart("Demand_high", {
"type": "serial",
"theme": "light",
"dataProvider": data,
"legend": {
// "equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"valueAxes": [{
"gridColor": "#FFFFFF",
"gridAlpha": 0.2,
"dashLength": 0
}],
"gridAboveGraphs": true,
"startDuration": 1,
"graphs": [{
"balloonText": "[[category]]: <b>[[value]]</b>kW",
"fillAlphas": 0.8,
"lineAlpha": 0.2,
"type": "column",
"title": "最高需量",
"legendValueText": "[[value]] Watt",
"valueField": "peak_demand"
}],
"chartCursor": {
"categoryBalloonEnabled": false,
"cursorAlpha": 0,
"zoomable": false
},
"categoryField": "time",
"categoryAxis": {
"gridPosition": "start",
"gridAlpha": 0,
"tickPosition": "start",
"tickLength": 20,
},
});
}
/*用電折線圖*/
function power(divs, data) {
for (var i in divs) {
if (data[i] !== undefined) {
console.log(i + " " + divs[i] + "\n" + data[i]["demands"])
var chart = AmCharts.makeChart(divs[i], {
"type": "serial",
//圖例
"mouseWheelZoomEnabled": false,
"dataDateFormat": "YYYY-MM-DD JJ:NN:SS",
"legend": {
// "equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
//資料
"dataProvider": data[i]["demands"],
"valueAxes": [
//Demand值軸
{
"id": "demandAxis",
"gridColor": "#FFFFFF",
"gridAlpha": 0.5,
"position": "left",
"title": "kW",
"minimum": 0
},
],
"gridAboveGraphs": true,
"graphs": [{
"id": "g1",
"balloonText": "<span style='font-size:12px; font-family:Microsoft JhengHei;'> 時間:[[category]]<br><b>需量:[[value]]kW</b>[[additional]]</span>",
"bullet": "round",
"bulletsize": 0.5,
"bulletBorderThickness": 1.5,
"bulletBorderAlpha": 0.5,
"bulletColor": "#FFFFFF",
"useLineColorForBulletBorder": true,
"balloon": {
"drop": false
},
"hideBulletsCount": 24,
"legendValueText": "[[value]] kW",
"title": "每10秒功率",
"labelPosition": "right",
"valueField": "Value",
"valueAxis": "demandAxis",
"lineColor": " #1f77b4",
"lineThickness": 2.5
}, ],
"chartScrollbar": {
"graph": "g1",
"autoGridCount": true,
"scrollbarHeight": 40,
"color": "#000000"
},
"chartCursor": {
"categoryBalloonEnabled": false,
"categoryBalloonDateFormat": "MMM DD,JJ:NN:SS",
"cursorPosition": "mouse",
"cursorColor": "#FFFFFF",
"cursorAlpha": 0
},
"valueScrollbar": {
"autoGridCount": true,
"color": "#000000",
"scrollbarHeight": 50
},
"categoryField": "time",
"categoryAxis": {
"minPeriod": "10ss",
"parseDates": true
},
});
}
}
}<file_sep>function startTime(type) {
var today = new Date(); //定義日期對象
var yyyy = today.getFullYear(); //通過日期對象的getFullYear()方法返回年
var MM = today.getMonth() + 1; //通過日期對象的getMonth()方法返回年
var dd = today.getDate(); //通過日期對象的getDate()方法返回年
var hh = today.getHours(); //通過日期對象的getHours方法返回小時
var mm = today.getMinutes(); //通過日期對象的getMinutes方法返回分?
var ss = today.getSeconds(); //通過日期對象的getSeconds方法返回秒
// 如果分?或小時的值小於10,則在其值前加0,比如如果時間是下午3點20分9秒的話,則顯示15:20:09
MM = checkTime(MM);
dd = checkTime(dd);
mm = checkTime(mm);
ss = checkTime(ss);
var day; //用於保存星期(getDay()方法得到星期編號)
if (today.getDay() == 0) day = "星期日";
if (today.getDay() == 1) day = "星期一";
if (today.getDay() == 2) day = "星期二";
if (today.getDay() == 3) day = "星期三";
if (today.getDay() == 4) day = "星期四";
if (today.getDay() == 5) day = "星期五";
if (today.getDay() == 6) day = "星期六";
switch (type) {
case '1':
document.getElementById('nowDateTimeSpan-demend').innerHTML = yyyy + "-" + MM + "-" + dd + " " + hh + ":" + mm + ":" + ss + " " + day;
setTimeout('startTime("1")', 1000); //每一秒中重新加載startTime()方法
break;
case '2':
return yyyy + "-" + MM + "-" + dd + " " + hh + ":" + mm + ":" + ss;
}
}
function checkTime(i) {
if (i < 10)
i = "0" + i;
return i;
} // checkTime function
//set the button
var check = 0;
var index_year = 0;
var index_month = 0;
var index_day = 0;
$(document).ready(function () {
$("a.list-group-item").click(function () {
$(this).siblings().removeClass('active');
$(this).addClass('active');
});
//dialog show
$("button.btn.btn-info.col-md-12").click(function () {
$("#dialog_model").dialog({
dialogClass: "dlg-no-close",
width: 1000,
height: 750,
resizeStop: function () {
$(this).height($(this).parent().height() - $(this).prev('.ui-dialog-titlebar').height() - $(this).prev('.ui-dialog-buttonpane').height() - 34);
$(this).width($(this).prev('.ui-dialog-titlebar').width() + 2);
// $("#Demand_high, #Demand_high_Interval, #Demand").height($(this).height() - $(".modal-header").height() - $(".dateframe").height() - $("#myTab").height() - 34);
},
open: function () {
// $("#Demand_high, #Demand_high_Interval, #Demand").height($(this).height() - $(".modal-header").height() - $(".dateframe").height() - $("#myTab").height() - 34);
$(document).ready(function () {
realtime_demand();
$('#demand_page').show();
$('#setting_page').hide();
//open 即插入需量值
// $("#real_demand > span").append("456");
});
},
});
});
$("#Demand_Setting").click(function () {
$("#page-wrapper").dialog({
title: "需量反應設定",
dialogClass: "dlg-no-close",
width: 800,
height: 700,
resizeStop: function () {
$(this).height($(this).parent().height() - $(this).prev('.ui-dialog-titlebar').height() - $(this).prev('.ui-dialog-buttonpane').height() - 34);
$(this).width($(this).prev('.ui-dialog-titlebar').width() + 2);
// $("#Demand_high, #Demand_high_Interval, #Demand").height($(this).height() - $(".modal-header").height() - $(".dateframe").height() - $("#myTab").height() - 34);
},
open: function () {
// $("#Demand_high, #Demand_high_Interval, #Demand").height($(this).height() - $(".modal-header").height() - $(".dateframe").height() - $("#myTab").height() - 34);
$(document).ready(function () {
realtime_demand();
$('#demandsetting_page').show();
$('#groupdown_setting').hide();
//open 即插入需量值
// $("#real_demand > span").append("456");
});
},
});
});
$('#datetime').datepicker({
changeMonth: true,
changeYear: true,
dateFormat: 'yy-MM-dd',
maxDate: 0,
minDate: "-23m",
onClose: function (dateText, inst) {
$(this).datepicker('setDate', new Date(inst.selectedYear, inst.selectedMonth, inst.selectedDay));
}
});
$('#demandControl').click(function (e) {
$('#demand_page').hide();
$('#setting_page').show();
});
$('#returnpage').click(function (e) {
$('#setting_page').hide();
$('#demand_page').show();
});
$('#groupdown').click(function (e) {
$('#demandsetting_page').hide();
$('#groupdown_setting').show();
});
$('#group_returnpage').click(function (e) {
$('#groupdown_setting').hide();
$('#demandsetting_page').show();
});
});
// }
//Demans_Setting
$(function () {
$("#demand-range").slider({
range: true,
min: 0,
max: 100,
step: 5,
values: [45, 70],
slide: function (event, ui) {
$("#demand_bottom").val(ui.values[0]);
$("#demand_top").val(ui.values[1]);
}
});
$("#demand_bottom").val($("#demand-range").slider("values", 0));
$("#demand_top").val($("#demand-range").slider("values", 1));
});
$(function () {
$("#demand-max").slider({
range: "min",
min: 500,
max: 1500,
step: 10,
value: 1000,
slide: function (event, ui) {
$("#max").val(ui.value);
}
});
$("#max").val($("#demand-max").slider("value"));
});
$(function () {
var spinner = $("#gap1").spinner();
});
$(function () {
var spinner = $("#delay").spinner();
});
$(function () {
var spinner = $("#gap2").spinner();
});<file_sep>//////////////////////////////////////純每15分鐘平均用電需量//未使用
function barchart2(data) {
//console.log(data);
var chart = AmCharts.makeChart("chartdiv2", {
"theme": "light",
"type": "serial",
"startDuration": 2,
"dataProvider": data,
"graphs": [{
"balloonText": "[[category]]: <b>[[value]]</b>kW",
"fillColorsField": "color",
"fillAlphas": 1,
"lineAlpha": 0.1,
"type": "column",
"valueField": "Average_demand"
}],
"depth3D": 20,
"angle": 30,
"chartCursor": {
"categoryBalloonEnabled": false,
"cursorAlpha": 0,
"zoomable": false
},
"categoryField": "Time",
"categoryAxis": {
"gridPosition": "start",
"labelRotation": 90
},
"categorxAxis": {
"gridPosition": "start",
"labelRotation": 0
},
"export": {
"enabled": true
}
});
}<file_sep>alembic==0.9.1
click==6.7
Flask==0.12.1
Flask-Migrate==2.0.3
Flask-Script==2.0.5
Flask-SQLAlchemy==2.2
itsdangerous==0.24
Jinja2==2.9.6
Mako==1.0.6
MarkupSafe==1.0
mysqlclient==1.3.10
python-editor==1.0.3
requests==2.13.0
SQLAlchemy==1.1.9
Werkzeug==0.12.1
<file_sep>$(document).ready(function () {
// $.ajax({
// type: "GET", //傳值方式有分 post & get
// url: "/api/v1.0/", //第一筆用電折線圖
// dataType: 'json',
// data: {
// //傳送2017/06取得資料格式為該月第一筆最大需量的270筆(每10秒)如2017-06-10 12:15-12:45
// },
// success: function (meter_count) {
// meter(meter_count);
// },
// error: function () {
// alert("no data");
// }
// });
$('#load').click(function () {
//該月每日最大需量
if ($("#datetime").val() == "") {
alert("請選擇日期");
return;
}
var spinner_daily_maxDemand = show_spinner($("#daily_maxDemand"));
$.ajax({
type: "GET", //傳值方式有分 post & get
url: "/api/v1.0/peak_period_everyday_in_month", //第一筆用電折線圖
dataType: 'json',
data: {
"address": "0",
"channel": "0",
"datetime": $("#datetime").val()
//傳送2017/06取得資料格式為該月第一筆最大需量的270筆(每10秒)如2017-06-10 12:15-12:45
},
success: function (response) {
daily_maxDemand(response);
remove_spinner(spinner_daily_maxDemand);
},
error: function () {
alert("no data");
}
});
//每日最大需量區間
var spinner_daily_maxDemand_period = show_spinner($("#daily_maxDemand_period"));
$.ajax({
type: "GET", //傳值方式有分 post & get
url: "/api/v1.0/peak_period_in_day", //第一筆用電折線圖
dataType: 'json',
data: {
"address": "0",
"channel": "0",
"datetime": $("#datetime").val()
//傳送2017/06取得資料格式為該月第一筆最大需量的270筆(每10秒)如2017-06-10 12:15-12:45
},
success: function (response) {
daily_demand(response);
remove_spinner(spinner_daily_maxDemand_period);
},
error: function () {
alert("no data");
}
});
//該月前三高需量
var spinner_Demand_high = show_spinner($("#Demand_high"));
$.ajax({
type: "GET", //傳值方式有分 post & get
url: "/api/v1.0/peak_periods_in_month", //第一筆用電折線圖
dataType: 'json',
data: {
"address": "0",
"channel": "0",
"datetime": $("#datetime").val()
//傳送2017/06取得資料格式為該月第一筆最大需量的270筆(每10秒)如2017-06-10 12:15-12:45
},
success: function (response) {
Demand_high(response);
remove_spinner(spinner_Demand_high);
},
error: function (response) {
alert("no data");
}
});
Demand_high();
//最高需量折線圖
$(".col-sm-12> nav > ul > li > a").click(function () {
var data_select = $(this).text();
if (data_select = "最高需量用電折線圖") {
first_graph();
//second_graph();
//third_graph();
}
});
});
});
// function meter(meterdata) {
// for (var meter in meterdata) {
// $("#showmeter").append('<div class="info-box red-bg">\
// <div class="count">' +meter["name"]+ meter["channel"] + '/' + meter["address"] + '</div>\
// <button type="button" class="btn btn-info col-md-12" style="border-radius:10px;">\
// <div style="font-size: 18px ; font-family:Microsoft JhengHei;">用電資訊</div>\
// </button>\
// </div>');
// }
// }
function first_graph() {
var spinner_first_graph = show_spinner($("#first_graph"));
var spinner_second_graph = show_spinner($("#second_graph"));
var spinner_third_graph = show_spinner($("#third_graph"));
$.ajax({
type: "GET", //傳值方式有分 post & get
url: "/api/v1.0/peak_periods_in_month_interval", //第一筆用電折線圖
dataType: 'json',
data: {
"address": "0",
"channel": "0",
"datetime": $("#datetime").val() //傳送2017/06取得資料格式為該月第一筆最大需量的270筆(每10秒)如2017-06-10 12:15-12:45
},
success: function (response) {
power(["first_graph", "second_graph", "third_graph"], response);
remove_spinner(spinner_first_graph);
remove_spinner(spinner_second_graph);
remove_spinner(spinner_third_graph);
},
error: function () {
alert("no data");
}
});
}
function show_spinner(div) {
$(div).empty();
return $('<div class="spinner"> \
<div class="rect1"></div> \
<div class="rect2"></div> \
<div class="rect3"></div> \
<div class="rect4"></div> \
<div class="rect5"></div> \
</div>').appendTo(div);
}
function remove_spinner(spinner) {
spinner.remove();
}
var speed = "10000";
var first = 0;
var pause = "60000";
setInterval(realtime_demand, pause);
function realtime_demand() {
$.ajax({
type: "GET", //傳值方式有分 post & get
url: "/api/v1.0/demand_min", //第二筆用電折線圖
dataType: 'json',
success: function (response) {
$('#real_demand > #total >span ').remove();
$('#real_demand ').animate({}, speed).show(function () {
total = '<span>' + response["demand_min"] + ' </span>';
$('#real_demand > #total').append(total);
});
},
error: function () {
alert("no data");
}
});
}<file_sep>import datetime
import calendar
import time
def get_interval_of_day(today):
today = datetime.datetime.strptime(today, '%Y-%m-%d')
return (datetime.datetime.combine(today, datetime.time.min),
datetime.datetime.combine(today, datetime.time.max))
def get_interval_of_month(today):
today = datetime.datetime.strptime(today, '%Y-%m-%d')
_, last_day_num = calendar.monthrange(today.year, today.month)
first_date = datetime.date(today.year, today.month, 1)
last_date = datetime.date(today.year, today.month, last_day_num)
return (datetime.datetime.combine(first_date, datetime.time.min),
datetime.datetime.combine(last_date, datetime.time.max))
<file_sep>from flask import jsonify, request
from sqlalchemy import func, and_, or_, between, exists
from . import api
from datetime import timedelta
from decimal import Decimal
import datetime
import math
import json
from .. import db
from ..models import Demand
from . import helper
@api.route('/demand', methods=['POST', 'GET'])
def demand_post():
if request.method == 'POST':
if request.is_json:
req_json = request.get_json()
print(type(req_json))
insert_data = Demand(
req_json['address'],
req_json['channel'],
req_json['model'],
req_json['datetime'],
req_json['demand_min']['value'],
req_json['demand_quarter']['value'],
req_json['instantaneous_power'][0]['value'],
req_json['instantaneous_power'][1]['value'],
req_json['instantaneous_power'][2]['value'],
req_json['instantaneous_power'][3]['value'])
db.session.add(insert_data)
db.session.commit()
return jsonify({"message": "feedback success"}), 201
'''
@api.route('/demand2', methods=['POST', 'GET'])
def demand_post():
if request.method == 'POST':
print(request.is_json)
if request.is_json:
print("json received.")
req_json = request.get_json()
print(req_json['instantaneous_power'][0]['tag'])
insert_data = Demand(
req_json['address'],
req_json['channel'],
req_json['model'],
req_json['datetime'],
req_json['demand_min']['value'],
req_json['demand_quarter']['value'],
req_json['instantaneous_power'][0]['value'],
req_json['instantaneous_power'][1]['value'],
req_json['instantaneous_power'][2]['value'],
req_json['instantaneous_power'][3]['value'])
db.session.add(insert_data)
db.session.commit()
return jsonify({"message": "feedback success"}), 201
'''
@api.route('/demand_min')
def demand_min():
# Get the identification of the device
req_address = request.args.get('address', default="0", type=str)
req_channel = request.args.get('channel', default="0", type=str)
# Try get data of the latest instantaneous power
power_data = db.session.query(Demand.datetime, Demand.demand_min).filter(
Demand.address == req_address,
Demand.channel == req_channel).order_by(Demand.datetime.desc()).first()
# Pack the result
data_output = {}
if power_data:
data_output['time'] = power_data.datetime.strftime("%Y-%m-%d %H:%M:%S")
data_output['demand_min'] = power_data.demand_min
return jsonify(data_output), 201
@api.route('/peak_period_in_day')
def get_peak_period_in_day():
# Get the identification of the device, and the time to check
req_address = request.args.get('address', default="0", type=str)
req_channel = request.args.get('channel', default="0", type=str)
req_datetime = request.args.get('datetime',
default=datetime.datetime.now().strftime("%Y-%m-%d"),
type=str)
req_interval = helper.get_interval_of_day(req_datetime)
# Try get data of the latest instantaneous power
power_data = db.session.query(Demand.datetime, Demand.demand_quarter).filter(
Demand.address == req_address,
Demand.channel == req_channel,
func.SECOND(Demand.datetime) == 0,
Demand.datetime.between(
*req_interval)).order_by(Demand.demand_quarter.desc()).first()
data_output = {}
final_data_output = {}
if power_data:
data_output['time'] = power_data.datetime.strftime("%Y-%m-%d %H:%M:%S")
data_output['peak_demand'] = power_data.demand_quarter
final_data_output['peak'] = data_output
date = power_data.datetime
interval_start = date - timedelta(minutes=30)
interval_end = date + timedelta(minutes=15)
interval_power_data = db.session.query(Demand.datetime, Demand.Total_value).filter(
Demand.address == req_address,
Demand.channel == req_channel,
Demand.datetime.between(interval_start, interval_end))
# Pack the result
final_data_output['demands'] = []
for data in interval_power_data:
final_data_output['demands'].append({'time': data.datetime.strftime("%Y-%m-%d %H:%M:%S"),
'Value': data.Total_value})
return jsonify(final_data_output), 201
@api.route('/peak_period_everyday_in_month')
def get_peak_period_everyday_in_month():
# Get the identification of the device, and the time to check
req_address = request.args.get('address', default="0", type=str)
req_channel = request.args.get('channel', default="0", type=str)
req_datetime = request.args.get('datetime',
default=datetime.datetime.now().strftime("%Y-%m-%d"),
type=str)
req_interval = helper.get_interval_of_month(req_datetime)
power_data = []
data_output = []
date = req_interval[0]
# Try get data of the latest instantaneous power
for i in range(req_interval[0].day, req_interval[1].day):
interval_start = req_interval[0] + timedelta(hours=24 * i - 24)
interval_end = req_interval[0] + timedelta(hours=24 * i)
power_data = db.session.query(Demand.datetime, Demand.demand_quarter).filter(
Demand.address == req_address,
Demand.channel == req_channel,
func.DAY(Demand.datetime) == interval_start.day,
Demand.datetime.between(
interval_start, interval_end)).order_by(Demand.demand_quarter.desc()).first()
# Pack the result
if(power_data):
data_output.append({'time': power_data.datetime.strftime("%Y-%m-%d %H:%M:%S"),
'peak_demand': power_data.demand_quarter})
return jsonify(data_output), 201
@api.route('/peak_periods_in_month')
def get_peak_periods_in_month():
# Get the identification of the device, and the time to check
req_address = request.args.get('address', default="0", type=str)
req_channel = request.args.get('channel', default="0", type=str)
req_datetime = request.args.get('datetime',
default=datetime.datetime.now().strftime("%Y-%m-%d"),
type=str)
req_interval = helper.get_interval_of_month(req_datetime)
# Try get data of the latest instantaneous power
power_data = db.session.query(Demand.datetime, Demand.demand_quarter).filter(
Demand.address == req_address,
Demand.channel == req_channel,
or_(
func.MINUTE(Demand.datetime) == 0,
func.MINUTE(Demand.datetime) == 15,
func.MINUTE(Demand.datetime) == 30,
func.MINUTE(Demand.datetime) == 45,
func.MINUTE(Demand.datetime) == 60),
func.SECOND(Demand.datetime) == 0,
Demand.datetime.between(
*req_interval)).order_by(Demand.demand_quarter.desc()).order_by(Demand.datetime).limit(3)
# Pack the result
data_output = []
if power_data:
for idx in range(power_data.count()):
data_output.append(
{'peak_demand': power_data[idx].demand_quarter,
'time': power_data[idx].datetime.strftime("%Y-%m-%d %H:%M:%S")})
return jsonify(data_output), 201
@api.route('/peak_periods_in_month_interval')
def get_peak_periods_in_month_interval():
# Get the identification of the device, and the time to check
req_address = request.args.get('address', default="0", type=str)
req_channel = request.args.get('channel', default="0", type=str)
req_datetime = request.args.get('datetime',
default=datetime.datetime.now().strftime("%Y-%m-%d"),
type=str)
req_interval = helper.get_interval_of_month(req_datetime)
# Try get data of the latest instantaneous power
power_data = db.session.query(Demand.datetime, Demand.demand_quarter).filter(
Demand.address == req_address,
Demand.channel == req_channel,
or_(
func.MINUTE(Demand.datetime) == 0,
func.MINUTE(Demand.datetime) == 15,
func.MINUTE(Demand.datetime) == 30,
func.MINUTE(Demand.datetime) == 45,
func.MINUTE(Demand.datetime) == 60),
func.SECOND(Demand.datetime) == 0,
Demand.datetime.between(
*req_interval)).order_by(Demand.demand_quarter.desc()).order_by(Demand.datetime).limit(3)
# Pack the result
final_data_output = []
if power_data:
for idx in range(power_data.count()):
data_output = {}
data_output['peak'] = {'peak_demand': power_data[idx].demand_quarter,
'time': power_data[idx].datetime.strftime("%Y-%m-%d %H:%M:%S")}
date = power_data[idx].datetime
interval_start = date - timedelta(minutes=30)
interval_end = date + timedelta(minutes=15)
interval_power_data = db.session.query(Demand.datetime, Demand.Total_value).filter(
Demand.address == req_address,
Demand.channel == req_channel,
Demand.datetime.between(interval_start, interval_end))
data_output['demands'] = []
for data in interval_power_data:
data_output['demands'].append(
{'time': data.datetime.strftime("%Y-%m-%d %H:%M:%S"),
'Value': data.Total_value})
final_data_output.append(data_output)
return jsonify(final_data_output), 201
<file_sep>/////////////////////////////////////////C3.js圖 未使用
function linebarchart() {
var chart = c3.generate({
bindto: '#linebarchart',
data: {
x: 'Time',
xFormat: '%H:%M:%S', // how the date is parsed
json: [{
Time: "00:15:00",
Peak_count: '12',
Average_Demand: '200',
Workday_count: '20',
Workday_Demand: '400'
},
{
Time: "00:30:00",
Peak_count: '15',
Average_Demand: '100',
Workday_count: '30',
Workday_Demand: '400'
},
{
Time: "00:45:00",
Peak_count: '20',
Average_Demand: '300',
Workday_count: '20',
Workday_Demand: '500'
},
{
Time: "01:00:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "01:15:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "01:30:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "01:45:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "02:00:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "02:15:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
},
{
Time: "02:30:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "02:45:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "03:00:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "03:15:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "03:30:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "03:45:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}, {
Time: "04:00:00",
Peak_count: '28',
Average_Demand: '400',
Workday_count: '5',
Workday_Demand: '500'
}
],
keys: {
// x: 'name', // it's possible to specify 'x' when category axis
x: 'Time',
value: ['Peak_count', 'Average_Demand', 'Workday_count', 'Workday_Demand']
},
axes: {
Average_Demand: 'y2',
Workday_Demand: 'y2'
},
types: {
Peak_count: 'bar',
Workday_count: 'bar'
}
},
bar: {
width: {
ratio: 0.9 // this makes bar width 50% of length between ticks
}
// or
//width: 100 // this makes bar width 100px
},
title: {
text: 'Peak_count-Demand Diagram'
},
tooltip: {
grouped: false // Default true
},
axis: {
x: {
label: {
text: 'Time',
position: 'outer-center'
},
type: 'timeseries',
tick: {
format: ' %H:%M:%S' // how the date is displayed
}
},
y: {
show: true,
label: {
text: 'Peak_count',
position: 'outer-middle'
},
},
y2: {
show: true,
label: {
text: 'kW',
position: 'outer-middle'
}
}
}
});
}<file_sep>from flask import render_template
from . import main
@main.route('/')
def index():
return render_template('index.html')
@main.route('/chart-flot')
def chart_flot():
return render_template('chart-flot.html')
@main.route('/chart-other')
def chart_other():
return render_template('chart-other.html')
@main.route('/demand-analysis')
def demand_analysis():
return render_template('demand-analysis.html')
| af7bcea5dcf0433d760465f27283b4c13bd52efa | [
"Markdown",
"Python",
"JavaScript",
"Text"
] | 15 | Python | GaryTsai/DAE | 37a95b44f46e7637f9e5a3c1199a0b3870bb621c | ab325ce631c92e6467f1583b79be0b076455de5b | |
refs/heads/master | <repo_name>tbulubas/patterns-examples<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step2/TurboEngine.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step2;
public class TurboEngine {
public TurboEngine(int i) {
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Prototype/step2/AbstractVan.java
package com.tboptimus.patterns.patternsexamples.Creational.Prototype.step2;
public abstract class AbstractVan extends AbstractVehicle {
public AbstractVan(Engine engine) {
super(engine);
}
public abstract Engine getEngine();
public abstract Colour getColour();
public abstract void paint();
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/CarWindows.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public class CarWindows implements Windows, Car {
@Override
public String getWindowParts() {
return getAutoType() + getType();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Prototype/step1/Engine.java
package com.tboptimus.patterns.patternsexamples.Creational.Prototype.step1;
public class Engine {
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step2/VehicleFactory.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step2;
public abstract class VehicleFactory {
public enum DrivingStyle {
ECONOMICAL,
MIDRANGE,
POWERFUL
}
public Vehicle build(DrivingStyle style, Vehicle.Colour colour) {
Vehicle v = selectVehicle(style);
v.paint(colour);
return v;
}
// This is the "factory method"
protected abstract Vehicle selectVehicle(DrivingStyle style);
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/basic/CarBuilder.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder.basic;
public class CarBuilder extends VehicleBuilder {
private AbstractCar carInProgress;
public CarBuilder(AbstractCar car) {
this.carInProgress = car;
}
public void buildBody() {
System.out.println("body");
}
public void buildBoot() {
System.out.println("boot");
}
public void buildChassis() {
System.out.println("chassis");
}
public void buildPassengerArea() {
System.out.println("passarea");
}
public void buildWindows() {
System.out.println("windows");
}
@Override
public Vehicle getVehicle() {
return carInProgress;
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/Chassis.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public interface Chassis {
default String getType() {
return "Chassis";
}
String getChassisParts();
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step3/Pickup.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step3;
public class Pickup extends AbstractVan {
public Pickup(StandardEngine standardEngine) {
}
@Override
public String getEngine() {
return null;
}
@Override
public String getColour() {
return null;
}
@Override
public void paint(Colour colour) {
}
@Override
public String toString() {
return "Pickup{} " + super.toString();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/basic/AbstractVan.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder.basic;
public class AbstractVan extends Vehicle {
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/basic/AbstractCar.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder.basic;
public class AbstractCar extends Vehicle {
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/StandardEngine.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder;
import com.tboptimus.patterns.patternsexamples.Creational.Builder.basic.Vehicle;
public class StandardEngine extends Vehicle {
public StandardEngine(int i) {
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step3/TurboEngine.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step3;
public class TurboEngine {
public TurboEngine(int i) {
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step2/Sport.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step2;
public class Sport extends AbstractCar {
public Sport(TurboEngine turboEngine) {
}
@Override
public String getEngine() {
return null;
}
@Override
public String getColour() {
return null;
}
@Override
public void paint(Vehicle.Colour colour) {
}
@Override
public String toString() {
return "Sport{} " + super.toString();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Prototype/step2/Engine.java
package com.tboptimus.patterns.patternsexamples.Creational.Prototype.step2;
public class Engine {
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step1/BoxVan.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step1;
public class BoxVan extends AbstractVan {
@Override
public String getEngine() {
return null;
}
@Override
public String getColour() {
return null;
}
@Override
public void paint() {
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Singleton/BillPughSingleton.java
package com.tboptimus.patterns.patternsexamples.Creational.Singleton;
public class BillPughSingleton {
private int count;
public synchronized int getNextSerial() {
return SingletonHelper.INSTANCE.count++;
}
private BillPughSingleton() {
}
private static class SingletonHelper {
private static final BillPughSingleton INSTANCE = new BillPughSingleton();
}
public static BillPughSingleton getInstance() {
return SingletonHelper.INSTANCE;
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/CarChassis.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public class CarChassis implements Chassis, Car{
@Override
public String getChassisParts() {
return getAutoType() + getType();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Singleton/Client.java
package com.tboptimus.patterns.patternsexamples.Creational.Singleton;
public class Client {
public static void main(String [] args) {
System.out.println("Using traditional singleton");
SerialNumberGeneratorTraditional generator = SerialNumberGeneratorTraditional.getInstance();
System.out.println("next serial: " + generator.getNextSerial());
System.out.println("next serial: " + generator.getNextSerial());
System.out.println("next serial: " + generator.getNextSerial());
System.out.println("Using enum singleton");
System.out.println("next vehicle: " + SerialNumberGenerator.INSTANCE.getNextSerial());
System.out.println("next vehicle: " + SerialNumberGenerator.INSTANCE.getNextSerial());
System.out.println("next engine: " + SerialNumberGenerator.INSTANCE.getNextSerial());
System.out.println("Using enum singleton");
System.out.println("next vehicle: " + SerialNumberGeneratorMulti.VEHICLE.getNextSerial());
System.out.println("next vehicle: " + SerialNumberGeneratorMulti.VEHICLE.getNextSerial());
System.out.println("next engine: " + SerialNumberGeneratorMulti.ENGINE.getNextSerial());
System.out.println("next vehicle: " + SerialNumberGeneratorMulti.VEHICLE.getNextSerial());
System.out.println("next engine: " + SerialNumberGeneratorMulti.ENGINE.getNextSerial());
System.out.println("Using static block singleton");
StaticBlockSingleton staticBlockSingleton = StaticBlockSingleton.getInstance();
System.out.println("next engine: " + staticBlockSingleton.getNextSerial());
System.out.println("next engine: " + staticBlockSingleton.getNextSerial());
System.out.println("next engine: " + staticBlockSingleton.getNextSerial());
System.out.println("Using private inner static class singleton");
BillPughSingleton billPughSingleton = BillPughSingleton.getInstance();
System.out.println("next engine: " + billPughSingleton.getNextSerial());
System.out.println("next engine: " + billPughSingleton.getNextSerial());
System.out.println("next engine: " + billPughSingleton.getNextSerial());
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step3/AbstractVehicle.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step3;
public abstract class AbstractVehicle implements Vehicle {
public abstract String getEngine();
public abstract String getColour();
public abstract void paint(Colour colour);
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/PatternsExamplesApplication.java
package com.tboptimus.patterns.patternsexamples;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class PatternsExamplesApplication {
public static void main(String[] args) {
SpringApplication.run(PatternsExamplesApplication.class, args);
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/FactoryMethod/step3/Coupe.java
package com.tboptimus.patterns.patternsexamples.Creational.FactoryMethod.step3;
public class Coupe extends AbstractCar {
public Coupe(StandardEngine standardEngine) {
}
@Override
public String getEngine() {
return null;
}
@Override
public String getColour() {
return null;
}
@Override
public void paint(Colour colour) {
}
@Override
public String toString() {
return "Coupe{} " + super.toString();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Singleton/SerialNumberGenerator.java
package com.tboptimus.patterns.patternsexamples.Creational.Singleton;
public enum SerialNumberGenerator {
INSTANCE;
private int count;
public synchronized int getNextSerial() {
return ++count;
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/basic/Vehicle.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder.basic;
public abstract class Vehicle {
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/VanWindows.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public class VanWindows implements Windows, Van {
@Override
public String getWindowParts() {
return getAutoType() + getType();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Builder/Saloon2.java
package com.tboptimus.patterns.patternsexamples.Creational.Builder;
import com.tboptimus.patterns.patternsexamples.Creational.Builder.basic.AbstractVan;
public class Saloon2 extends AbstractVan {
public Saloon2(StandardEngine standardEngine) {
super();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/Body.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public interface Body {
default String getType() {
return "Body";
}
String getBodyParts();
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/base/VanChassis.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base;
public class VanChassis implements Chassis, Van {
@Override
public String getChassisParts() {
return getAutoType() + getType();
}
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/AbstractFactory/withFactory/AbstractVehicleFactory.java
package com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.withFactory;
import com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base.Body;
import com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base.Chassis;
import com.tboptimus.patterns.patternsexamples.Creational.AbstractFactory.base.Windows;
public abstract class AbstractVehicleFactory {
public abstract Body createBody();
public abstract Chassis createChassis();
public abstract Windows createWindows();
}
<file_sep>/src/main/java/com/tboptimus/patterns/patternsexamples/Creational/Prototype/step2/StandardEngine.java
package com.tboptimus.patterns.patternsexamples.Creational.Prototype.step2;
public class StandardEngine extends Engine {
public StandardEngine(int i) {
super(
);
}
}
<file_sep>/README.md
Based on:
**<NAME>. Java Design Pattern Essentials**
Ability First Limited. Kindle Edition.
| a8720d46a651ec2c1a7d08410a67d88ce886dc84 | [
"Markdown",
"Java"
] | 30 | Java | tbulubas/patterns-examples | 7e5376d042883ce6651089e3efb40c63e15bd67b | 29eca47354ebe068096d60f4855dc1217d5b8c9d | |
refs/heads/master | <file_sep># danfe-simplificada
Visualizador de DANFE Simplificada (Documento Auxiliar Da Nota Fiscal Eletrônica) em html.
## Preparação
### Pré-requisitos
NodeJS 8.x
### Instalação
NPM
```
npm i danfe-simplificada
```
---------------------------------------------------------------------
Yarn
```
yarn add danfe-simplificada
```
### Exemplos
Utilizando template padrão
```
const Danfe = require('danfe-simplificada')
var danfe = Danfe.fromXML('conteudo XML', 'url logo')
console.log(danfe.toHtml())
```
----------------------------------------------------------------------
Utilizando template customizado
```
const Danfe = require('danfe-simplificada')
var danfe = Danfe.fromXML('conteudo XML', 'url logo')
console.log(danfe.toHtml('caminho-template.hbs'))
```
## Especificações
### Funções
* Criar representação do DANFE em html baseado somente em um arquivo XML existente.
* Criar a representação somente no formato retrato.
### Arquitetura
* Usa [template engine handlebars](https://github.com/wycats/handlebars.js) para gerar o html.
## Testes
```
npm run test
```
### Codificação
[standardjs](https://standardjs.com/rules.html)
## Licença
[MIT](https://github.com/mateusjose/danfe-simplificada/blob/master/LICENSE)
## Colaboradores
[<NAME>](https://github.com/mateustozoni)
[<NAME>](https://github.com/lucaslacroix)
<file_sep>const handlebars = require('handlebars')
const NFe = require('djf-nfe')
const TEMPLATE_DANFE = __dirname + '/template-danfe.hbs'
const fs = require('fs')
const path = require('path')
/**
* Retorna <valor> especificado com máscara do CPF.
*
* @param {string} valor
* @return {string}
*/
function mascaraCPF (valor) {
var retorno
var grupo01 = valor.substring(0, 3)
retorno = grupo01
var grupo02 = valor.substring(3, 6)
if (grupo02 !== '') {
retorno += '.' + grupo02
}
var grupo03 = valor.substring(6, 9)
if (grupo03 !== '') {
retorno += '.' + grupo03
}
var grupo04 = valor.substring(9)
if (grupo04 !== '') {
retorno += '-' + grupo04
}
return retorno
}
/**
* Retorna <valor> especificado com máscara do CNPJ.
*
* @param {string} valor
* @return {string}
*/
function mascaraCNPJ (valor) {
var retorno
var grupo01 = valor.substring(0, 2)
retorno = grupo01
var grupo02 = valor.substring(2, 5)
if (grupo02 !== '') {
retorno += '.' + grupo02
}
var grupo03 = valor.substring(5, 8)
if (grupo03 !== '') {
retorno += '.' + grupo03
}
var grupo04 = valor.substring(8, 12)
if (grupo04 !== '') {
retorno += '/' + grupo04
}
var grupo05 = valor.substring(12)
if (grupo05 !== '') {
retorno += '-' + grupo05
}
return retorno
}
/**
* Retorna <numero> especificado formatado de acordo com seu tipo (cpf ou cnpj).
*
* @param {string} numero
* @return {string}
*/
function formataInscricaoNacional (numero) {
if (numero) {
if (numero.length === 11) {
return mascaraCPF(numero)
}
if (numero.length === 14) {
return mascaraCNPJ(numero)
}
}
return numero
}
/**
* Formata data de acordo com <dt> esoecificado.
* <dt> é no formato UTC, YYYY-MM-DDThh:mm:ssTZD (https://www.w3.org/TR/NOTE-datetime)
*
* @param {string} dt
* @return {string}
*/
function formataData (dt) {
dt = dt ? dt.toString() : ''
if (!dt) { return '' }
if (dt && dt.length === 10) {
dt += 'T00:00:00+00:00'
}
var [data, hora] = dt.split('T')
var [hora, utc] = hora.split(/[-+]/)
var [ano, mes, dia] = data.split('-')
var [hora, min, seg] = hora.split(':')
var [utchora, utcmin] = utc ? utc.split(':') : ['', '']
return dia.padStart(2, '0') + '/' + mes.toString().padStart(2, '0') + '/' + ano
}
function formataHora (dt) {
if (dt) {
var data = new Date(dt)
return data.getHours().toString().padStart(2, '0') + ':' + (data.getMinutes().toString().padStart(2, '0')) + ':' + data.getSeconds().toString().padStart(2, '0')
}
return ''
}
/**
* Retorna o valor formatado em moeda de acordo com <numero> e <decimais> especificados.
*
* @param {number} numero
* @param {number} decimais
* @return {string}
*/
function formataMoeda (numero, decimais) {
decimais = decimais || 4
var symbol = ''
var decimal = ','
var thousand = '.'
var negative = numero < 0 ? '-' : ''
var i = parseInt(numero = Math.abs(+numero || 0).toFixed(decimais), 10) + ''
var j = 0
decimais = !isNaN(decimais = Math.abs(decimais)) ? decimais : 2
symbol = symbol !== undefined ? symbol : '$'
thousand = thousand || ','
decimal = decimal || '.'
j = (j = i.length) > 3 ? j % 3 : 0
return symbol + negative + (j ? i.substr(0, j) + thousand : '') + i.substr(j).replace(/(\d{3})(?=\d)/g, '$1' + thousand) + (decimais ? decimal + Math.abs(numero - i).toFixed(decimais).slice(2) : '')
};
/**
* Retorna objeto representando os dados da <entidade> especificada.
*
* @param {Object} entidade djf-nfe
* @return {Object}
*/
function dadosEntidade (entidade) {
if (entidade) {
return {
nome: entidade.nome(),
fantasia: entidade.fantasia(),
ie: entidade.inscricaoEstadual(),
ie_st: entidade.inscricaoEstadualST(),
inscricao_municipal: entidade.inscricaoMunicipal(),
inscricao_nacional: formataInscricaoNacional(entidade.inscricaoNacional()),
telefone: entidade.telefone()
}
}
return {}
}
/**
* Retorna objeto representando os dados do <endereco> especificado.
*
* @param {Object} endereco djf-nfe
* @return {Object}
*/
function endereco (endereco) {
if (endereco) {
return {
endereco: endereco.logradouro(),
numero: endereco.numero(),
complemento: endereco.complemento(),
bairro: endereco.bairro(),
municipio: endereco.municipio(),
cep: endereco.cep(),
uf: endereco.uf()
}
}
return {}
}
/**
* Retorna a <cahve> da NFE formata.
* Formatação: grupos de 4 números separados por espaço.
* @param {string} chave
* @return {string}
*/
function formataChave (chave) {
var out = ''
if (chave && chave.length === 44) {
for (var i = 0; i < chave.split('').length; i++) {
if (i % 4 === 0) {
out += ' ' + chave.charAt(i)
} else {
out += chave.charAt(i)
}
}
return out
}
return chave
}
/**
* Retorna array de objetos com os dados dos itens de acordo com <nfe> especificado.
*
* @param {<object>} nfe djf-nfe
* @return {array}
*/
function itens (nfe) {
var itens = []
var nrItens = nfe.nrItens()
for (var i = 1; i <= nrItens; i++) {
var row = nfe.item(i)
var item = {
codigo: row.codigo(),
descricao: row.descricao(),
ncm: row.ncm(),
cst: row.origem() + '' + row.cst(),
cfop: row.cfop(),
unidade: row.unidadeComercial(),
quantidade: formataMoeda(row.quantidadeComercial()),
valor: formataMoeda(row.valorUnitario()),
desconto: formataMoeda(row.valorDesconto()),
total: formataMoeda(row.valorProdutos()),
base_calculo: formataMoeda(row.baseCalculoIcms()),
icms: formataMoeda(row.valorIcms()),
ipi: formataMoeda(row.valorIPI()),
porcentagem_icms: formataMoeda(row.porcetagemIcms(), 2),
porcentagem_ipi: formataMoeda(row.porcentagemIPI(), 2)
}
itens.push(item)
}
return itens
}
/**
* Retorna array de objetos com os dados das duplicatas de acordo com <nfe> especificado
*
* @param {object} nfe djf-nfe
* @return {array}
*/
function duplicatas (nfe) {
var dups = []
if (nfe.cobranca() && nfe.cobranca().nrDuplicatas() > 0) {
var quant = nfe.cobranca().nrDuplicatas()
for (var i = 1; i <= quant; i++) {
var dup = nfe.cobranca().duplicata(i)
dups.push({
numero: dup.numeroDuplicata(),
vencimento: formataData(dup.vencimentoDuplicata()),
valor: formataMoeda(dup.valorDuplicata(), 2)
})
}
}
return dups
}
/**
* Retorna os dados da observação de acordo com <nfe> especificado.
*
* @param {object} nfe djf-nfe
* @return {string}
*/
function observacoes (nfe) {
var quant = nfe.nrObservacoes()
var result = ''
for (var i = 1; i <= quant; i++) {
result += '\n' + nfe.observacao(i).texto()
}
return result
}
/**
* Retorna o template html do Danfe preenchido com os dados em <data> especificado.
* Retorna vazio se não gerado.
* @param {object} data
* @param {string} logo
* @return {string}
*/
function renderHtml (data, logo = "", customTemplate) {
if (!data) {
return ''
}
var pathToTemplate =
customTemplate &&
customTemplate.includes('.hbs') &&
fs.existsSync(customTemplate)
? customTemplate
: TEMPLATE_DANFE
var template = fs.readFileSync(pathToTemplate, 'utf8')
return handlebars.compile(template)({...data, emitente: {...data.emitente, logo}})
}
/**
* Retorna objeto com os dados do template de acordo com <nfe> especificado.
*
* @param {object} nfe djf-nfe
* @return {object}
*/
function getTemplateData (nfe) {
if (!nfe) {
return null
}
var data = {
operacao: nfe.tipoOperacao(),
natureza: nfe.naturezaOperacao(),
numero: nfe.nrNota(),
serie: nfe.serie(),
chave: formataChave(nfe.chave()),
protocolo: nfe.protocolo(),
data_protocolo: formataData(nfe.dataHoraRecebimento()) + ' ' + formataHora(nfe.dataHoraRecebimento()),
destinatario: Object.assign(dadosEntidade(nfe.destinatario()), endereco(nfe.destinatario())),
emitente: Object.assign(dadosEntidade(nfe.emitente()), endereco(nfe.emitente())),
data_emissao: formataData(nfe.dataEmissao()),
data_saida: formataData(nfe.dataEntradaSaida()),
base_calculo_icms: formataMoeda(nfe.total().baseCalculoIcms(), 2),
imposto_icms: formataMoeda(nfe.total().valorIcms(), 2),
base_calculo_icms_st: formataMoeda(nfe.total().baseCalculoIcmsST(), 2),
imposto_icms_st: formataMoeda(nfe.total().valorIcmsST(), 2),
imposto_tributos: formataMoeda(nfe.total().valorTotalTributos(), 2),
total_produtos: formataMoeda(nfe.total().valorProdutos(), 2),
total_frete: formataMoeda(nfe.total().valorFrete(), 2),
total_seguro: formataMoeda(nfe.total().valorSeguro(), 2),
total_desconto: formataMoeda(nfe.total().valorDesconto(), 2),
total_despesas: formataMoeda(nfe.total().valorOutrasDespesas(), 2),
total_ipi: formataMoeda(nfe.total().valorIPI(), 2),
total_nota: formataMoeda(nfe.total().valorNota(), 2),
transportador: Object.assign(dadosEntidade(nfe.transportador()), endereco(nfe.transportador())),
informacoes_fisco: nfe.informacoesFisco(),
informacoes_complementares: nfe.informacoesComplementares(),
observacao: observacoes(nfe),
modalidade_frete: nfe.modalidadeFrete(),
modalidade_frete_texto: nfe.modalidadeFreteTexto(),
itens: itens(nfe),
duplicatas: duplicatas(nfe)
}
if (nfe.transporte().volume()) {
let volume = nfe.transporte().volume()
data.volume_quantidade = formataMoeda(volume.quantidadeVolumes())
data.volume_especie = volume.especie()
data.volume_marca = volume.marca()
data.volume_numeracao = volume.numeracao()
data.volume_pesoBruto = formataMoeda(volume.pesoBruto())
data.volume_pesoLiquido = formataMoeda(volume.pesoLiquido())
}
if (nfe.transporte().veiculo()) {
data.veiculo_placa = nfe.transporte().veiculo().placa()
data.veiculo_placa_uf = nfe.transporte().veiculo().uf()
data.veiculo_antt = nfe.transporte().veiculo().antt()
}
if (nfe.servico()) {
data.total_servico = formataMoeda(nfe.servico().valorTotalServicoNaoIncidente())
data.total_issqn = formataMoeda(nfe.servico().valorTotalISS())
data.base_calculo_issqn = formataMoeda(nfe.servico().baseCalculo())
}
return data
}
/**
* Retorna modelo Danfe de acordo com objeto <nfe> especificado.
*
* @param {<type>} nfe djf-nfe
* @param {string} logo
* @return {Object} { description_of_the_return_value }
*/
function model (nfe, logo = "") {
return {
/**
* @param {string} customTemplate Caminho para um template de NF customizado em .hbs (handlebars)
* @returns {string} Retorna um html em string
*/
toHtml: (customTemplate = null) =>
renderHtml(getTemplateData(nfe), logo, customTemplate)
}
}
/**
* Retorna modelo Danfe de acordo com objeto <nfe> especificado.
*
* @param {object} nfe djf-nfe
* @return {<object>}
*/
module.exports.fromNFe = function (nfe, logo = "") {
if (!nfe || typeof nfe.nrNota !== 'function') {
return model(null)
}
return model(nfe, logo)
}
/**
* Retorna modelo Danfe de acordo com <xml> especificado.
*
* @param {string} xml
* @param {string} logo
* @return {<object>}
*/
module.exports.fromXML = function (xml, logo = "") {
if (!xml || typeof xml !== 'string') {
return model(null)
}
return model(NFe(xml), logo)
}
/**
* Retorna modelo Danfe de acordo com <filePath> especificado.
*
* @param {string} filePath
* @param {string} logo
* @return {<object>}
*/
module.exports.fromFile = function (filePath, logo = "") {
var content = ''
if (!filePath || typeof filePath !== 'string') {
return model(null)
}
try {
content = fs.readFileSync(filePath, 'utf8')
} catch (err) {
throw new Error('File not found: ' + filePath + ' => ' + err.message)
}
return module.exports.fromXML(content, logo)
}
| 874f1ac4a8376e45bc70d1368bc47fe7244b314b | [
"Markdown",
"JavaScript"
] | 2 | Markdown | mateustozoni/danfe-simplificada | 5950cf5a82e3551454dce6263a5b9edb8628d5fc | d6ea55fe9a4fc84a94ab53069de91c473e80428c | |
refs/heads/master | <file_sep># Mapping Data
Mymapping <- read.csv(file.choose())
YearsMymapping <- Mymapping[ , c('Year.of.publication')]
hist(YearsMymapping, main = "Publications over the years", xlab = "Years", ylab = "Density", xlim=c(2000,2020), ylim=c(0,0.7), prob=T, cex.axis=1.5, cex.main=2, cex.lab=1.5, cex.sub=1.5)
box(bty="l")
#NFV Data
NFVIEEE <- read.csv(file.choose())
NFVACM <- read.csv(file.choose())
NFVElsevier <- read.csv(file.choose())
NFVSpringer <- read.csv(file.choose())
YearsNFVIEEE <- NFVIEEE[ , c('Publication_Year')]
YearsNFVACM <- NFVACM[ , c('year')]
YearsNFVElsevier <- unlist(c(NFVElsevier))
YearsNFVSpringer <- unlist(c(NFVSpringer))
YearsNFV <- c(YearsNFVIEEE, YearsNFVACM,YearsNFVElsevier,YearsNFVSpringer)
lines(density(YearsNFV[YearsNFV < 2020],na.rm=T), col="red", lwd=2)
legend("topleft",legend="NFV", cex=2, lty=1, col="red", lwd=2, bty="n")
#SDN Data
SDNIEEE <- read.csv(file.choose())
SDNACM <- read.csv(file.choose())
SDNElsevier <- read.csv(file.choose())
SDNSpringer <- read.csv(file.choose())
YearsSDNIEEE <- SDNIEEE[ , c('Publication_Year')]
YearsSDNACM <- SDNACM[ , c('year')]
YearsSDNElsevier <- unlist(c(SDNElsevier))
YearsSDNSpringer <- SDNSpringer[ , c('Publication.Year')]
YearsSDN <- c(YearsSDNIEEE, YearsSDNACM,YearsSDNElsevier,YearsSDNSpringer)
lines(density(YearsSDN[YearsSDN < 2020],na.rm=T), col="blue", lwd=2)
legend("topleft",legend="SDN", cex=2, lty=1, col="blue", lwd=2, bty="n")
#Cloud Data
CloudIEEE <- read.csv(file.choose())
CloudACM <- read.csv(file.choose())
CloudElsevier <- read.csv(file.choose())
CloudSpringer <- read.csv(file.choose())
YearsCloudIEEE <- CloudIEEE[ , c('Publication_Year')]
YearsCloudIEEE <- YearsCloudIEEE[-141]
YearsCloudACM <- CloudACM[ , c('year')]
YearsCloudElsevier <- unlist(c(CloudElsevier))
YearsCloudSpringer <- CloudSpringer[ , c('Publication.Year')]
YearsCloud <- c(YearsCloudIEEE,YearsCloudACM, YearsCloudElsevier, YearsCloudSpringer)
lines(density(YearsCloud,na.rm=T), col="green", lwd=2)
legend("topleft", legend="Cloud Computing", cex=2, lty=1, col="green", lwd=2, bty="n")
#Virtualization Data
VirtIEEE <- read.csv(file.choose())
VirtACM <- read.csv(file.choose())
VirtElsevier <- read.csv(file.choose())
VirtSpringer <- read.csv(file.choose())
YearsVirtIEEE <- VirtIEEE[ , c('Publication_Year')]
YearsVirtACM <- VirtACM[ , c('year')]
YearsVirtElsevier <- unlist(c(VirtElsevier))
YearsVirtSpringer <- VirtSpringer[ , c('Publication.Year')]
YearsVirt <- c(YearsVirtIEEE, YearsVirtACM,YearsVirtElsevier,YearsVirtSpringer)
lines(density(YearsVirt[YearsVirt < 2020],na.rm=T), col="orange", lwd=2)
legend("topleft", legend="Virtualization", cex=2, lty=1, col="orange", lwd=2, bty="n")
#DTN Data
DTNIEEE <- read.csv(file.choose())
DTNACM <- read.csv(file.choose())
DTNElsevier <- read.csv(file.choose())
DTNSpringer <- read.csv(file.choose())
YearsDTNIEEE <- DTNIEEE[ , c('Publication_Year')]
YearsDTNACM <- DTNACM[ , c('year')]
YearsDTNElsevier <- unlist(c(DTNElsevier))
YearsDTNSpringer <- DTNSpringer[ , c('Publication.Year')]
YearsDTN <- c(YearsDTNIEEE, YearsDTNACM,YearsDTNElsevier,YearsDTNSpringer)
lines(density(YearsDTN[YearsDTN < 2020],na.rm=T), col="purple", lwd=2)
legend("topleft", legend="DTN", cex = 2, lty=1, col="purple", lwd=2, bty="n")
#Grid Data
GridIEEE <- read.csv(file.choose())
GridACM <- read.csv(file.choose())
GridElsevier <- read.csv(file.choose())
GridSpringer <- read.csv(file.choose())
YearsGridIEEE <- GridIEEE[ , c('Publication_Year')]
YearsGridACM <- GridACM[ , c('year')]
YearsGridElsevier <- unlist(c(GridElsevier))
YearsGridSpringer <- GridSpringer[ , c('Publication.Year')]
YearsGrid <- c(YearsGridIEEE, YearsGridACM,YearsGridElsevier,YearsGridSpringer)
lines(density(YearsGrid[YearsGrid < 2020],na.rm=T), col="brown", lwd=2)
legend("topleft", legend="Grid Computing", cex=2, lty=1, col="brown", lwd=2, bty="n")
#P2P Data
P2PIEEE <- read.csv(file.choose())
P2PACM <- read.csv(file.choose())
P2PElsevier <- read.csv(file.choose())
P2PSpringer <- read.csv(file.choose())
YearsP2PIEEE <- P2PIEEE[ , c('Publication_Year')]
YearsP2PACM <- P2PACM[ , c('year')]
YearsP2PElsevier <- unlist(c(P2PElsevier))
YearsP2PSpringer <- P2PSpringer[ , c('Publication.Year')]
YearsP2P <- c(YearsP2PIEEE, YearsP2PACM,YearsP2PElsevier,YearsP2PSpringer)
lines(density(YearsP2P[YearsP2P < 2020],na.rm=T), col="cyan", lwd=2)
legend("topleft", legend="Peer-to-Peer", cex=2, lty=1, col="cyan", lwd=2, bty="n")
<file_sep>library(ggplot2)
#Gráfico de Barras Empilhados
#Management
Management <- read.csv(file.choose(), sep = ';')
Management <- as.data.frame(Management)
colnames(Management) <- c("Years", "Topics", "Quantity")
ggplot(data = Management, aes(x= Years, y= Quantity, fill= Topics)) +
geom_bar(stat = "identity") +
scale_fill_brewer(palette = 18) +
scale_x_continuous(breaks = seq(2010,2019,1)) +
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
axis.text = element_text(size = 17),
axis.title = element_text(size=20),
legend.text = element_text(size=17),
legend.title = element_text(size=17)
)
#Orchestration
Orchestration <- read.csv(file.choose(), sep = ';')
Orchestration <- as.data.frame(Orchestration)
colnames(Orchestration) <- c("Years", "Topics", "Quantity")
ggplot(data = Orchestration, aes(x= Years, y= Quantity, fill= Topics)) +
geom_bar(stat = "identity") +
scale_fill_brewer(palette = 10) +
scale_x_continuous(breaks = seq(2010,2019,1)) +
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
axis.text = element_text(size = 17),
axis.title = element_text(size=20),
legend.text = element_text(size=17),
legend.title = element_text(size=17)
)
#5G
FiveG <- read.csv(file.choose(), sep = ';')
FiveG <- as.data.frame(FiveG)
colnames(FiveG) <- c("Years", "Topics", "Quantity")
ggplot(data = FiveG, aes(x= Years, y= Quantity, fill= Topics)) +
geom_bar(stat = "identity") +
scale_fill_brewer(palette = 11) +
scale_x_continuous(breaks = seq(2010,2019,1)) +
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
axis.text = element_text(size = 17),
axis.title = element_text(size=20),
legend.text = element_text(size=17),
legend.title = element_text(size=17)
)
#Architecture
Architecture <- read.csv(file.choose(), sep = ';')
Architecture <- as.data.frame(Architecture)
colnames(Architecture) <- c("Years", "Topics", "Quantity")
ggplot(data = Architecture, aes(x= Years, y= Quantity, fill= Topics)) +
geom_bar(stat = "identity") +
scale_fill_brewer(palette = 8) +
scale_x_continuous(breaks = seq(2010,2019,1)) +
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
axis.text = element_text(size = 17),
axis.title = element_text(size=20),
legend.text = element_text(size=17),
legend.title = element_text(size=17)
)
#BillingPrice
BillingPrice <- read.csv(file.choose(), sep = ';')
BillingPrice <- as.data.frame(BillingPrice)
colnames(BillingPrice) <- c("Years", "Topics", "Quantity")
ggplot(data = BillingPrice, aes(x= Years, y= Quantity, fill= Topics)) +
geom_bar(stat = "identity") +
scale_fill_brewer(palette = 12) +
scale_x_continuous(breaks = seq(2010,2019,1)) +
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
axis.text = element_text(size = 17),
axis.title = element_text(size=20),
legend.text = element_text(size=17),
legend.title = element_text(size=17)
)
<file_sep>library(ggplot2)
library(dplyr)
library(scales)
library(plotly)
#Gráfico de Bolha
data <- read.csv(file.choose(), sep =';')
data$Value <- sub(",", ".",data$Value)
data$radius <- sqrt(as.numeric(data$Value)/pi)
colnames(data) <- c("TF", "RF", "Value", "Radius")
data$RF <- tools::toTitleCase(as.vector(data$RF))
data$RF <- as.character(data$RF)
data$RF <- factor(data$RF, levels=unique(data$RF))
data$RF <- factor(data$RF, levels=c("Evaluation Research","Opinion Papers","Personal Experience Papers","Solution Proposal","Philosophical Papers","Validation Research","Total (Technological Facet)"))
data$TF <- as.character(data$TF)
data$TF <- factor(data$TF, levels=unique(data$TF))
data$TF <- factor(data$TF, levels=c("Total (Research Facet)","5G","Architecture","Management","Orchestration","Pricing Model"))
ggplot(data,aes(RF,TF))+
geom_point(aes(size=as.numeric(data$Radius)*25, colour =factor(data$RF)), shape=20, alpha = 0.4)+
geom_text(aes(label = paste0(data$Value,"%")),size=5.5)+
scale_size_identity()+
annotate("label", x="Total (Technological Facet)", y="Total (Research Facet)", label="100%")+
annotate("label", x="Evaluation Research", y="Total (Research Facet)", label="2,54%")+
annotate("label", x="Solution Proposal", y="Total (Research Facet)", label="51,54%")+
annotate("label", x="Validation Research", y="Total (Research Facet)", label="38,59%")+
annotate("label", x="Philosophical Papers", y="Total (Research Facet)", label="4,54%")+
annotate("label", x="Personal Experience Papers", y="Total (Research Facet)", label="0,73%")+
annotate("label", x="Opinion Papers", y="Total (Research Facet)", label="2,05%")+
annotate("label", x="Total (Technological Facet)", y="Management", label="45,04%")+
annotate("label", x="Total (Technological Facet)", y="Orchestration", label="7,77%")+
annotate("label", x="Total (Technological Facet)", y="5G", label="32,49%")+
annotate("label", x="Total (Technological Facet)", y="Architecture", label="12,16%")+
annotate("label", x="Total (Technological Facet)", y="Pricing Model", label="2,56%")+
theme(panel.grid.major=element_line(linetype=2,color="gray90"),
axis.text.x = element_text(angle=30, hjust = 1, colour = "black", size = rel(1.5)),
axis.text.y = element_text(colour = "black", size = rel(1.5)),
panel.background = element_rect(fill = "white", colour = "black", size = 1),
plot.margin = margin(2, 2, 0, 0, "cm"),
axis.title.x=element_blank(),
axis.title.y=element_blank(),
legend.position = "none"
)
| f76d2107cd3e53e417e8da7a3684f3dd1a4b00d9 | [
"R"
] | 3 | R | leandrocalmeida/R-Scripts | 79852ed4a6a9d37dde87ed7102c96463309d946f | 3df1a757e0b96b3269622b1a28ca5b8f21b795b0 | |
refs/heads/main | <repo_name>robmoraes/essentia-teste-fullstack<file_sep>/frontend/src/store/admin/security/users/actions.js
import Vue from 'vue'
const USERS_ROUTE = '/security/users'
export function list (context) {
const p = new Promise((resolve, reject) => {
return Vue.prototype.$axios
.get(`${USERS_ROUTE}`)
.then(res => resolve(res.data))
.catch(err => reject(err.response))
})
return p
}
export function insert (context, payload) {
const p = new Promise((resolve, reject) => {
return Vue.prototype.$axios
.post(`${USERS_ROUTE}`, payload, {
headers: {
'Content-Type': 'multipart/form-data'
}
})
.then(res => resolve(res.data))
.catch(err => reject(err.response))
})
return p
}
export function show (context, id) {
const p = new Promise((resolve, reject) => {
return Vue.prototype.$axios
.get(`${USERS_ROUTE}/${id}`)
.then(res => resolve(res.data))
.catch(err => reject(err.response))
})
return p
}
export function update (context, payload) {
const formData = new FormData()
formData.append('name', payload.name)
formData.append('email', payload.email)
formData.append('phone', payload.phone)
formData.append('photo', payload.photo)
formData.append('photo_upload', payload.photo_upload)
formData.append('password', payload.password)
const p = new Promise((resolve, reject) => {
return Vue.prototype.$axios
.post(`${USERS_ROUTE}/${payload.id}`, formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
})
.then(res => resolve(res.data))
.catch(err => reject(err.response))
})
return p
}
export function destroy (context, id) {
const p = new Promise((resolve, reject) => {
return Vue.prototype.$axios
.delete(`${USERS_ROUTE}/${id}`)
.then(res => resolve(res.data))
.catch(err => reject(err.response))
})
return p
}
<file_sep>/backend/routes/api.php
<?php
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| API Routes
|--------------------------------------------------------------------------
|
| Here is where you can register API routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
// Authentication
Route::post('/login', 'Api\AuthController@login');
// Access by permission
Route::middleware('auth:api')->get('/user', 'Api\Security\UserController@getAuthUser');
Route::middleware('auth:api')->apiResource('/security/users', 'Api\Security\UserController');
Route::middleware('auth:api')->apiResource('/security/permissions', 'Api\Security\PermissionController');
Route::middleware('auth:api')->apiResource('/security/roles', 'Api\Security\RoleController');
Route::middleware('auth:api')->post('/security/users/{user}', 'Api\Security\UserController@update');
<file_sep>/frontend/src/store/admin/security/permissions/actions.js
import Vue from 'vue'
const PERMISSIONS_ROUTE = 'security/permissions'
export function setList (context, obj) {
const p = new Promise(function (resolve, reject) {
return Vue.prototype.$axios
.get(PERMISSIONS_ROUTE)
.then(res => {
const payload = res.data
context.commit('SET_PERMISSIONS', payload)
resolve(res)
})
.catch(err => {
reject(err.response)
})
})
return p
}
export function post (context, payload) {
const p = new Promise(function (resolve, reject) {
return Vue.prototype.$axios
.post(PERMISSIONS_ROUTE, payload)
.then(res => {
resolve(res)
})
.catch(err => {
reject(err.response)
})
})
return p
}
export function put (context, payload) {
const p = new Promise(function (resolve, reject) {
return Vue.prototype.$axios
.put(`${PERMISSIONS_ROUTE}/${payload.id}`, payload)
.then(res => {
resolve(res)
})
.catch(err => {
reject(err.response)
})
})
return p
}
export function destroy (context, payload) {
const p = new Promise(function (resolve, reject) {
return Vue.prototype.$axios
.delete(`${PERMISSIONS_ROUTE}/${payload}`)
.then(res => {
resolve(res)
})
.catch(err => {
reject(err.response)
})
})
return p
}
<file_sep>/README.md
# essentia-teste-fullstack
Teste para vaga de desenvolvedor Full stack da Essentia Group
## Visão Geral
Este é um teste para processo seletivo de full stack, as camadas de backend e frontend foram desenvolvidas em projetos separados, para realizar a instalação de cada uma, é necessário seguir as instruções que estão nas pastas
- /backend
- /frontend
## Back-end
Para o backend foi usado PHP/Laravel com banco de dados sqlite3, foi construída uma API para prover recursos de front-end. Foi implementado sistema de autenticação com oauth2.
## Front-end
Para o front foi usado Vue2/Quasar.dev com o qual foi construída uma SPA para montar as telas, o framework implementa Material Design 2 e possui um robusto pacote de componentes além de uma estrutura de pastas que garante a organização do código e adoção de boas práticas de desenvolvimento.
## Instalação
Acesse cada uma das pastas para ver as instruções de instalação de cada ambiente.
## Playground
Para facilitar a demonstração do sistema rodando, distribuí uma versão no link abaixo:
[Playground do Teste da Essentia](https://essentia.seemann.com.br)
- Login: <EMAIL>
- Senha: <PASSWORD>
<file_sep>/frontend/README.md
# essentia-teste-fulltack Frontend
Projeto de frontend para processo de seleção da empresa Essentia
## Visão Geral
Este é um teste para processo seletivo de full stack, as camadas de backend e frontend foram desenvolvidas em projetos separados, para realizar a instalação de cada uma, é necessário seguir as instruções que estão nas pastas
- /backend
- /frontend
Estas são as instruções para instalação da camada de Front-end.
## Requisitos
- Quasar 1.15
- Node >=10
- NPM >=5
A instalação do quasar pode ser guiada no seguinte link (atenção as recomendações sobre Node e NPM):
- [https://quasar.dev/quasar-cli/installation](https://quasar.dev/quasar-cli/installation)
## Download do projeto
```bash
$ git clone https://github.com/robmoraes/essentia-teste-fullstack.git
```
## Instalação das dependências
Acessar a pasta /frontend:
```bash
cd /pasta/do/projeto/frontend
npm install
```
Após instalar, algumas alterações no arquivo "/quasar.conf.js" podem ser necessárias:
```js
build: {
env: {
API: ctx.dev
? 'http://localhost:8000/api' // Informar URL da API
: 'http://localhost:8000/api' // Seria a URL da API de produção
},
// Caso a aplicação frontend deva rodar na rede,
// deve ser configurado devServer:
devServer: {
https: false,
host: '10.0.0.190', // ip para acesso na rede
port: 8080, // porta -- o acesso ficará http://10.0.0.190:8080 por exemplo
open: true // opens browser window automatically
},
```
### Rodar a aplicação no modo de Desenvolvimento (hot-code reloading, error reporting, etc.)
Depois de instalado com npm, e configurado o arquivo "quasar.conf.js", a aplicação pode ser executada com o comando abaixo:
```bash
cd /pasta/do/projeto/frontend
quasar dev
```
E já pode ser acessado pela URL configurada que é exibida após a conclusão do comando quasar dev.
As credênciais de acesso podem ser obtidas após a instalação da aplicação backend.
## Degustação
Mas como o processo de instalação é relativamente longo, preparei um ambiente de degustação para que possam avaliar. Para isso, publiquei o build do frontend na estrutura de views do laravel e fiz um pequeno ajuste nas rotas do laravel (/routes/web.php) para resolver as uris do front. Sendo assim a API e o Front rodam sob mesmo domínio, mas a construção foi completamente desacoplada.
- [Playground do desafio](https://essentia.seemann.com.br)
Lembrando:
- e-mail: <EMAIL>
- senha: <PASSWORD>
### Build da aplicação para produção
```bash
quasar build
```
<file_sep>/frontend/src/boot/axios.js
import axios from 'axios'
import qs from 'qs'
import auth from '../services/auth'
const axiosInstance = axios.create({
paramsSerializer: params => {
return qs.stringify(params, { arrayFormat: 'repeat' })
},
// baseURL: 'http://essentia.local/api'
baseURL: process.env.API
})
const a = auth.get()
if (a) {
if (a.check) {
axiosInstance.defaults.headers.common.Authorization = `${a.token.token_type} ${a.token.access_token}`
}
}
export default ({ Vue }) => {
Vue.prototype.$axios = axiosInstance
}
export { axiosInstance }
<file_sep>/frontend/src/router/routes.js
const routes = [
{
path: '/',
component: () => import('layouts/DesktopLayout.vue'),
children: [
{ path: '', component: () => import('pages/Index.vue') },
{ path: 'admin/security/permissions', component: () => import('pages/admin/security/Permissions.vue') },
{ path: 'admin/security/roles', component: () => import('pages/admin/security/roles/List.vue') },
{ path: 'admin/security/roles/create', component: () => import('pages/admin/security/roles/Create.vue') },
{ path: 'admin/security/roles/:id/edit', component: () => import('pages/admin/security/roles/Edit.vue') },
{ path: 'admin/security/users', component: () => import('pages/admin/security/users/List.vue') },
{ path: 'admin/security/users/:id/edit', component: () => import('pages/admin/security/users/Edit.vue') },
{ path: 'admin/security/users/create', component: () => import('pages/admin/security/users/Create.vue') }
],
meta: { requiresAuth: true }
},
{
path: '/login',
component: () => import('layouts/LoginLayout.vue'),
meta: { requiresAuth: false }
},
// Always leave this as last one,
// but you can also remove it
{
path: '*',
component: () => import('pages/Error404.vue')
}
]
export default routes
<file_sep>/frontend/src/store/auth/mutations.js
export function updateAuthState (state, payload) {
state.auth = payload
sessionStorage.setItem('auth', JSON.stringify(payload))
}
<file_sep>/frontend/src/store/auth/getters.js
// export function check (state) {
// }
<file_sep>/frontend/src/services/auth.js
const auth = {
get: function () {
return JSON.parse(sessionStorage.getItem('auth'))
}
}
export default auth
<file_sep>/frontend/src/store/auth/actions.js
import Vue from 'vue'
// import { axiosInstance } from 'boot/axios'
const LOGIN_ROUTE = '/login'
export function login (context, credentials) {
const p = new Promise(function (resolve, reject) {
return Vue.prototype.$axios
.post(LOGIN_ROUTE, credentials)
.then(response => {
const payload = {
user: response.data.user,
token: response.data.token,
check: true
}
context.commit('updateAuthState', payload)
Vue.prototype.$axios.defaults.headers.common.Authorization =
`${payload.token.token_type} ${payload.token.access_token}`
resolve(response)
})
.catch(error => {
console.log(error)
reject(error)
})
})
return p
}
export function logout (context) {
Vue.prototype.$axios.defaults.headers.common.Authorization = ''
context.commit('updateAuthState', {
user: null,
token: null,
check: false
})
}
<file_sep>/frontend/src/store/admin/security/permissions/mutations.js
export function SET_PERMISSIONS (state, payload) {
state.list = payload
}
| a3b4dca4a6c8e88867f791dbcf4473c3166d4d5c | [
"JavaScript",
"Markdown",
"PHP"
] | 12 | JavaScript | robmoraes/essentia-teste-fullstack | b98316b87259d108d6548d9a17000681752ae1c0 | eca5e98402e9d67c059148a8862e273b6c07cd75 | |
refs/heads/master | <file_sep><?php
$semester = 'Fall 2016';
$problems = array();
$account = user_load('1342');
// Courses with section 2xx are online.
$query = db_select('node', 'n');
$query->leftJoin('field_data_field_course_section', 's', 's.entity_id=n.nid');
$query->leftJoin('field_data_field_semester', 'y', 'y.entity_id=n.nid');
$nids = $query->fields('n', array('nid'))
->condition('n.type', 'course_section')
->condition('s.field_course_section_value', '2%', 'LIKE')
->condition('y.field_semester_value', $semester)
->execute()
->fetchCol();
drush_print(count($nids));
foreach ($nids as $nid) {
$success = flag('flag', 'course_online', $nid, $account);
if (!$success) {
$problems[] = $nid;
}
drush_print($nid);
}
drush_print('problems:');
foreach ($problems as $problem) {
drush_print($problem);
}
<file_sep><?php
$semester = 'Fall 2016';
// Prefixes and numbers in each that satisfy the language req.
$classes = array(
'AIS' => array(101,102,201,202),
'CHI' => array(101,102,201,202),
'CLA' => array(101,102,151,152,201,202,251,252),
'CSD' => array(220,320,420),
'FR' => array(101,102,106,201,202,204),
'GER' => array(101,102,201,202),
'HJS' => array(101,102,201,202),
'ITA' => array(101,102,201,202),
'JPN' => array(101,102,201,202),
'RUS' => array(101,102,201,202),
'SPA' => array(101,102,103,141,142,201,202,203,205,210,211,215,241,242),
);
$problems = array();
$account = user_load('1342');
foreach ($classes as $prefix => $numbers) {
$query = db_select('node', 'n');
$query->leftJoin('field_data_field_course_prefix', 'p', 'p.entity_id=n.nid');
$query->leftJoin('field_data_field_course_number', 'c', 'c.entity_id=n.nid');
$query->leftJoin('field_data_field_semester', 'y', 'y.entity_id=n.nid');
$nids = $query->fields('n', array('nid'))
->condition('n.type', 'course_section')
->condition('p.field_course_prefix_value', $prefix)
->condition('c.field_course_number_value', $numbers, 'IN')
->condition('y.field_semester_value', $semester)
->execute()
->fetchCol();
drush_print(implode(', ', $nids));
foreach ($nids as $nid) {
$success = flag('flag', 'courses_language', $nid, $account);
if (!$success) {
$problems[] = $line . ' ::: ' . $nid;
}
drush_print(implode(' - ', array($prefix, $course, $nid)));
}
}
foreach ($problems as $problem) {
drush_print($problem);
}
<file_sep># Flag Examples
This code sample shows scripts that are run after the A&S course catalog importer to set various flags used in the catalog interface.
###flag_uk_core
The most important script splits data from a csv string containing UK Core requirement codes along with the course numbers that satisfy them. Each course is then flagged appropriately.
###Other Flag Files
The other files each set flags based on a pattern (e.g. flag_online finds courses with section number 2xx, which are always online at UK). Like the UK Core script, they perform a query on the database to find the courses that should be flagged.
<file_sep><?php
$semester = 'Fall 2016';
/*
* The list of course prefix+numbers that satisfy each
* UK Core requirement. Provided as 2-col excel from admin.
*/
$raw = "ACR,A-S 102
ACR,CME 455
ACR,A-S 103
ACR,A-S 200
ACR,A-S 380
ACR,EE 101
ACR,MNG 592
ACR,ME 411
ACR,A-S 130
ACR,A-S 280
ACR,TA 120
ACR,TAD 140
ACR,A-E 120
ACR,TA 110
ACR,UKC 300
ACR,UKC 100
ACR,UKC 101
ACR,A-S 245
ACR,TA 370
ACR,A-S 270
ACR,MUS 123
ACR,ENG 107
ACR,MUS 200
ACR,PLS 240
ACR,GEO 109
CC1,CIS 110
CC1,WRD 110
CC2,CIS 111
CC2,WRD 111
CC2,UKC 150
CCC,HIS 108
CCC,HIS 109
CCC,PHI 130
CCC,PS 101
CCC,PHI 335
CCC,HIS 261
CCC,APP 200
CCC,GEO 320
CCC,SOC 235
CCC,EPE 301
CCC,GEN 100
CCC,ANT 221
CCC,AAS 261
CCC,AAS 235
CCC,COM 315
CCC,SOC 360
CCC,CLD 360
CCC,GEO 220
CCC,GWS 301
CCC,A-H 360
CCC,UKC 180
CCC,UKC 380
CCC,GEO 221
CCC,ENG 191
CCC,UKC 181
CCC,COM 312
CCC,GRN 250
GDY,ANT 241
GDY,HIS 105
GDY,HIS 202
GDY,HIS 203
GDY,HIS 208
GDY,HIS 296
GDY,LAS 201
GDY,PS 210
GDY,GER 361
GDY,GEO 222
GDY,PHI 343
GDY,ANT 160
GDY,ANT 242
GDY,ANT 321
GDY,GEO 160
GDY,GEO 260
GDY,MUS 330
GDY,SOC 380
GDY,HIS 206
GDY,JPN 321
GDY,JPN 320
GDY,A-H 104
GDY,RUS 370
GDY,RUS 271
GDY,SAG 201
GDY,ANT 311
GDY,CLD 380
GDY,GEO 162
GDY,GEO 255
GDY,CHI 331
GDY,HIS 208
GDY,ANT 225
GDY,HIS 121
GDY,ANT 329
GDY,GWS 302
GDY,GER 342
GDY,GEO 164
GDY,RUS 125
GDY,ANT 222
GDY,GEO 163
GDY,GEO 261
GDY,PLS 103
GDY,SOC 180
GDY,A-H 311
GDY,ENG 181
GDY,EGR 240
GDY,HIS 122
HUM,CLA 229
HUM,CLA 230
HUM,ENG 264
HUM,ENG 281
HUM,HIS 104
HUM,HIS 105
HUM,HIS 202
HUM,HIS 203
HUM,HIS 229
HUM,HIS 230
HUM,PHI 100
HUM,A-H 105
HUM,A-H 106
HUM,MUS 100
HUM,A-H 334
HUM,AAS 264
HUM,CLA 135
HUM,GER 103
HUM,ARC 314
HUM,ENG 230
HUM,ENG 234
HUM,FR 103
HUM,RUS 270
HUM,SPA 371
HUM,SPA 372
HUM,GWS 201
HUM,TA 171
HUM,TA 271
HUM,TA 273
HUM,TA 274
HUM,CHI 330
HUM,CHI 331
HUM,HIS 121
HUM,GER 105
HUM,EGR 201
HUM,RUS 125
HUM,ID 161
HUM,ID 162
HUM,A-H 101
HUM,UKC 310
HUM,MCL 100
HUM,ENG 191
HUM,CLA 191
NPM,AST 191
NPM,BIO 102
NPM,BIO 103
NPM,CHE 101
NPM,PHY 211
NPM,PHY 231
NPM,PHY 241
NPM,CHE 105
NPM,ENT 110
NPM,GEO 130
NPM,PLS 104
NPM,EES 110
NPM,EES 120
NPM,ANT 230
NPM,EES 150
NPM,ARC 333
NPM,PHY 120
NPM,CHE 111
NPM,GEO 135
NPM,UKC 121
NPM,ABT 120
QFO,MA 113
QFO,MA 123
QFO,PHI 120
QFO,MA 111
QFO,MA 137
QFO,EES 151
QFO,EES 185
SIR,PSY 215
SIR,PSY 216
SIR,BAE 202
SIR,STA 210
SSC,COM 101
SSC,SOC 101
SSC,PSY 100
SSC,PS 235
SSC,ECO 101
SSC,GEO 172
SSC,ANT 101
SSC,CPH 201
SSC,CLD 102
SSC,GWS 200
SSC,ANT 102
SSC,UKC 130
SSC,UKC 131
SSC,COM 311
SSC,COM 313
SSC,COM 314 ";
$core = array(
'ACR' => 'arts_creativity',
'CC1' => 'composition_communications_i',
'CC2' => 'composition_communication_ii',
'CCC' => 'ccc',
'GDY' => 'global_dynamics',
'HUM' => 'courses_humanities',
'NPM' => 'courses_natural_sciences',
'QFO' => 'quantitative_foundations',
'SIR' => 'statistical_inferential_reasonin',
'SSC' => 'courses_social_sciences',
);
$data = explode("\n", $raw);
unset($raw);
$problems = array();
$account = user_load('1342');
foreach ($data as $line) {
drush_print($line);
$parts = explode(',', $line);
$flag = $core[$parts[0]];
$course_parts = explode(' ', trim($parts[1]));
$prefix = $course_parts[0];
$course = $course_parts[1];
$query = db_select('node', 'n');
$query->leftJoin('field_data_field_course_prefix', 'p', 'p.entity_id=n.nid');
$query->leftJoin('field_data_field_course_number', 'c', 'c.entity_id=n.nid');
$query->leftJoin('field_data_field_semester', 'y', 'y.entity_id=n.nid');
$nids = $query->fields('n', array('nid'))
->condition('n.type', 'course_section')
->condition('p.field_course_prefix_value', $prefix)
->condition('c.field_course_number_value', $course)
->condition('y.field_semester_value', $semester)
->execute()
->fetchCol();
drush_print(implode(', ', $nids));
foreach ($nids as $nid) {
$success = flag('flag', $flag, $nid, $account);
if (!$success) {
$problems[] = $line . ' ::: ' . $nid;
}
drush_print(implode(' - ', array($prefix, $course, $nid)));
}
}
foreach ($problems as $problem) {
drush_print($problem);
}
<file_sep><?php
/* If this needs to be rerun, it needs the value changed for field_course_number_value to 3%, 4%, 5%, etc... */
$semester = 'Fall 2016';
$problems = array();
$account = user_load('1342');
// Exclude 6xx and 7xx from the search (undergrad classes only)
foreach(array('6', '7') as $num) {
$query = db_select('node', 'n');
$query->leftJoin('field_data_field_course_number', 'c', 'c.entity_id=n.nid');
$query->leftJoin('field_data_field_semester', 'y', 'y.entity_id=n.nid');
$nids = $query->fields('n', array('nid'))
->condition('n.type', 'course_section')
->condition('c.field_course_number_value', $num . '%', 'LIKE')
->condition('y.field_semester_value', $semester)
->execute()
->fetchCol();
drush_print(count($nids));
foreach ($nids as $nid) {
$success = flag('unflag', 'courses_exclude', $nid, $account);
if (!$success) {
$problems[] = $nid;
}
drush_print($nid);
}
}
drush_print('problems:');
foreach ($problems as $problem) {
drush_print($problem);
}
| 415ab58185680507f274345932328f420b7cb533 | [
"Markdown",
"PHP"
] | 5 | PHP | scotthorn/flag_examples | 88f5a85e69279d4ca7fb6c5cfab5dc36ee70d55a | c1ccac27d789533c0460ff86da8f048a43a297f2 | |
refs/heads/master | <file_sep># express-graphql-sample
This is a personal sample project to get more familiarity with node (express) as well as graphql.
<file_sep>const express = require("express");
const app = express();
const PORT = process.env.PORT || 5000;
app.use(require("./controllers/routes"));
app.listen(PORT, () => {
console.log(
`Server listening on port ${PORT}. \nRunning GraphQL API server on localhost:${PORT}/graphql`
);
});
| 6a928924f6a3fbe3872fbb2643857ed1b3cc1699 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | salehmoh/express-graphql-sample | 9d799eceda9db70eee44d7c6941185bf82fc2672 | 11ca8be2c7c502a5040c9a2e1d9df6f8ab66944e | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml.Serialization;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.Graphics;
namespace TileMapEditor
{
class Editor : GraphicsDeviceControl
{
ContentManager content;
SpriteBatch spriteBatch;
Map map;
int layerNumber;
public List<Image> Selector;
string[] selectorPath = { "Graphics/SelectorT1", "Graphics/SelectorT2", "Graphics/SelectorB1", "Graphics/SelectorB2" };
public event EventHandler OnInitialize;
public Editor()
{
map = new Map();
layerNumber = 0;
Selector = new List<Image>();
for (int i = 0; i < 4; i++)
{
Selector.Add(new Image());
}
}
public Layer CurrentLayer
{
get { return map.Layer[layerNumber]; }
}
protected override void Initialize()
{
spriteBatch = new SpriteBatch(GraphicsDevice);
content = new ContentManager(Services, "Content");
for (int i = 0; i < 4; i++)
{
Selector[i].Path = selectorPath[i];
Selector[i].Initialize(content);
}
XmlManager<Map> mapLoader = new XmlManager<Map>();
map = mapLoader.Load("Load/Map1.xml");
map.Initialize(content);
if (OnInitialize != null)
{
OnInitialize(this, null);
}
}
protected override void Draw()
{
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin();
map.Draw(spriteBatch);
spriteBatch.End();
}
}
}
<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml.Serialization;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.Graphics;
namespace MonoFort
{
public class Map
{
[XmlElement("Layer")]
public List<Layer> Layers;
public Vector2 TileDimensions;
public Map()
{
Layers = new List<Layer>();
TileDimensions = Vector2.Zero;
}
public void LoadContent()
{
foreach (var layer in Layers)
{
layer.LoadContent(TileDimensions);
}
}
public void UnloadContent()
{
foreach (var layer in Layers)
{
layer.UnloadContent();
}
}
public void Update(GameTime gameTime, ref Player player)
{
foreach (var layer in Layers)
{
layer.Update(gameTime, ref player);
}
}
public void Draw(SpriteBatch spriteBatch, string drawType)
{
foreach (var layer in Layers)
{
layer.Draw(spriteBatch, drawType);
}
}
}
}
<file_sep>BOX OF EVIL (by Studio Evil)
All the contents of the Box of Evil are provided as freeware. Use them as you see fit.
A link to our website would be nice: www.studioevil.com
| c6f894e2b6bd4fba7f1be03b40a780396cab8142 | [
"C#",
"Text"
] | 3 | C# | mkniffen/MonoFort | 1c28858a09c0d53f6f713eb4f847e20f18ccb0a0 | a0cf55b51f0bf2c7ef9b95d45d84589712456d0b | |
refs/heads/master | <file_sep>#!/bin/bash
# 检测Node进程是否存在
ps aux | grep -v grep | grep node && exit 0
exit 1
<file_sep>#!/bin/bash
source ~/.bashrc
echo "{ \"appid\": \"$APPID\", \"secret\": \"$SECRET\" }" > yourconfig.json
agenthub start yourconfig.json
# 递归创建工作目录
# mkdir -p $BASE_PATH/$APP_NAME
# 切换到工作目录
cd $BASE_PATH/$APP_NAME
# 如果tnpm不存在,则安装tnpm
# npm install @tencent/tnpm -g --registry=http://r.tnpm.oa.com --proxy=http://r.tnpm.oa.com:80 --verbose
# 安装npm依赖包
npm install
# touch $LOG_PATH/nohub.log
# 启动服务,是否开启调试模式
if [ $DEBUG ];then
ENABLE_NODE_LOG=YES $EXEC_FILE --inspect=0.0.0.0:9229 app.js
else
ENABLE_NODE_LOG=YES $EXEC_FILE app.js
fi
| 06544dc893c93d05b54eb2b94d04cdf219898e5f | [
"Shell"
] | 2 | Shell | fringe/docker-script | 94bb485045002a34e73fac2fb1a649672a752e00 | 8d9522b57cdb7a9a13fe7d020ac13e17943ce308 | |
refs/heads/main | <file_sep># Developing-Data-Products-Assignment
Final project, developing data products
An app to calculate area of a rectangle or square.
<file_sep>library(shiny)
shinyServer(
function(input, output) {
output$length <- renderText({as.numeric(input$length)})
output$breadth <- renderText({as.numeric(input$length)})
output$area <- renderText({
# if (input$goButton == 0) "Please enter a number"
# else if (input$goButton >= 1) numberGuessed(as.numeric(input$guess), number)
output$area <- renderPrint ({as.numeric(input$length) * as.numeric(input$breadth)})
})
}
)
<file_sep>library(shiny)
shinyUI( pageWithSidebar(
headerPanel("Rectangle Area Calculation"),
sidebarPanel(
textInput (inputId = 'length', 'Enter Length', value = '0'),
textInput (inputId = 'breadth', 'Enter Width', value = '0')
),
mainPanel(
h4('The Area of your rectangle is'),
verbatimTextOutput("area")
)
)
)
| ad36006160670ebbdcfdcf4dd0942f1a6f2977ba | [
"Markdown",
"R"
] | 3 | Markdown | singulritarian7/Developing-Data-Products-Assignment | 66709754d9109b71fd41e44c561d318f60565248 | 9995a54636caf7cc8d3035d5c1dba5303914362e | |
refs/heads/master | <file_sep>package com.shawllvey.hspring4.event;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
/**
* Created by carpenshaw on 2017/3/19.
*/
public class Main {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(EventConfig.class);
DemoPublisher demoPublisher = context.getBean(DemoPublisher.class);
demoPublisher.publishDemoEvent("Hello demo");
context.close();
}
}
<file_sep>package com.shawllvey.hspring4.prepost;
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
/**
* 使用JSR250形式的Bean
*
* Created by carpenshaw on 2017/3/19.
*/
public class JSR250WayService {
@PostConstruct // 在构造函数执行完之后执行
public void init() {
System.out.println("JSR250WayService init");
}
@PreDestroy // 在Bean销毁之前执行
public void destroy() {
System.out.println("JSR250WayService destroy");
}
public JSR250WayService() {
System.out.println("JSR250WayService constructor");
}
}
<file_sep>package com.shawllvey.hspring4.condition;
/**
* Created by carpenshaw on 2017/3/20.
*/
public interface ListService {
String showListCmd();
}
<file_sep>package com.shawllvey.hspring4.profile;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Profile;
/**
* Profile为在不同环境下使用不同的配置提供了支持
*
* Created by carpenshaw on 2017/3/19.
*/
@Configuration
public class ProfileConfig {
@Bean
@Profile("dev")
DemoBean devDemoBean() {
return new DemoBean("devDemoBean");
}
@Bean
@Profile("prod")
DemoBean prodDemoBean() {
return new DemoBean("prodDemoBean");
}
}
<file_sep>book.name=hspring4
book.author=shawllvey<file_sep>package com.shawllvey.hspring4.el;
import org.apache.commons.io.IOUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.PropertySource;
import org.springframework.context.support.PropertySourcesPlaceholderConfigurer;
import org.springframework.core.env.Environment;
import org.springframework.core.io.Resource;
import java.io.IOException;
/**
* 演示配置类
* Spring EL-Spring表达式语言,支持在xml和注解中使用表达式
*
* Created by carpenshaw on 2017/3/19.
*/
@Configuration
@ComponentScan("com.shawllvey.hspring4.el")
@PropertySource("classpath:com/shawllvey/hspring4/el/test.properties") // 注入配置properties
public class ElConfig {
@Value("I Love You") // 注入普通字符串
private String normal;
@Value("#{systemProperties['os.name']}") // 注入操作系统属性
private String osName;
@Value("#{T(java.lang.Math).random()*100.0}") // 注入表达式结果
private double randomNumber;
@Value("#{demoService.another}") // 注入其它Bean属性
private String fromAnother;
@Value("classpath:com/shawllvey/hspring4/el/test.txt") // 注入文件资源
private Resource testFile;
@Value("http://www.baidu.com") // 注入网址资源
private Resource testUrl;
@Value("${book.name}") // 注入配置项,需要与@PropertySource配合
private String bookName;
@Autowired
private Environment environment; // 注入的properties还可以从environment中获得
@Bean // 当使用@Value注入properties的值时,需要此Bean(实际并不一定需要,Spring会自动生成此Bean?)
public static PropertySourcesPlaceholderConfigurer propertyConfigure() {
return new PropertySourcesPlaceholderConfigurer();
}
public void outputResource() {
System.out.println(this.normal);
System.out.println(this.osName);
System.out.println(this.randomNumber);
System.out.println(this.fromAnother);
try {
System.out.println(IOUtils.toString(testFile.getInputStream()));
System.out.println(IOUtils.toString(testUrl.getInputStream()));
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(bookName);
System.out.println(environment.getProperty("book.author"));
System.out.println(environment);
}
}
<file_sep>package com.shawllvey.hspring4.scope;
import org.springframework.stereotype.Service;
/**
* 编写Singleton的Bean
*
* Created by carpenshaw on 2017/3/16.
*/
@Service
//@Scope("singleton") 默认
public class DemoSingletonService {
}
<file_sep>package com.shawllvey.hspring4.annotation;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import java.lang.annotation.*;
/**
* 组合注解
*
* Created by carpenshaw on 2017/3/20.
*/
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Configuration // 组合@Configuration注解
@ComponentScan // 组合@ComponentScan注解
public @interface WiselyConfiguration {
String[] value() default {}; // 覆盖value参数
}
<file_sep>package com.shawllvey.hspring4.aop;
import org.springframework.stereotype.Service;
/**
* 编写使用方法规则的被拦截器类
*
* Created by carpenshaw on 2017/3/16.
*/
@Service
public class DemoMethodService {
public void add() {}
}
<file_sep>package com.shawllvey.hspring4.event;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Component;
/**
* 事件发布类
*
* Created by carpenshaw on 2017/3/19.
*/
@Component
public class DemoPublisher {
@Autowired
ApplicationContext context; // 注入ApplicationContext来发布事件
public void publishDemoEvent(String msg) {
// 使用applicationContext的publishEvent方法来发布
context.publishEvent(new DemoEvent(this, msg));
}
}
<file_sep>package com.shawllvey.hspring4.di;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* 功能类的Bean
*
* Created by carpenshaw on 2017/3/16.
*/
@Service // 声明当前类是Spring管理的一个Bean
public class UseFunctionService {
// 使用@Autowired注解将FunctionService的实体Bean注入到UserFunctionService中,让UserFunctionService具备FunctionService的功能
// 体现了一种“组合”的理念
@Autowired
FunctionService functionService;
public String sayHello(String word) {
return functionService.sayHello(word);
}
}
<file_sep>package com.shawllvey.hspring4.condition;
/**
* Created by carpenshaw on 2017/3/20.
*/
public class LinuxListService implements ListService {
@Override
public String showListCmd() {
return "ls";
}
}
| b0b1b660c9e6c6042563509f4e858055d938e62c | [
"Java",
"INI"
] | 12 | Java | shawllvey/hspring4 | c6a7b6ccbddb7ba6661fdbe732daa8430b9dc856 | abfa6ca74fc45625f5d6c2381e3390d705d0de84 | |
refs/heads/master | <file_sep>import { Component, Input, Output, EventEmitter } from '@angular/core';
import { User } from '../../models/user.model';
@Component({
selector: 'ng-e-app-content',
templateUrl: './app-content.component.html',
styleUrls: ['./app-content.component.scss']
})
export class AppContentComponent {
user: User = {
firstName: 'Ahsan',
lastName: 'Ayaz'
};
@Input()
isLoggedIn = false;
@Output()
loginNotificator = new EventEmitter();
/**
* @author <NAME>
* @desc Logs the user in
*/
login() {
this.setLogin(true);
}
/**
* @author <NAME>
* @desc Logs the user out
*/
logout() {
this.setLogin(false);
}
/**
* @author <NAME>
* @desc handle the internal and external status of login
* @param value status to be set (representing logged or not)
*/
setLogin(value: boolean) {
this.isLoggedIn = value;
this.loginNotificator.emit(value);
}
}
<file_sep>import { Component } from '@angular/core';
@Component({
selector: 'ng-e-home',
templateUrl: './home.component.html',
styleUrls: ['./home.component.scss']
})
export class HomeComponent {
logged: Boolean = false;
/**
* @author <NAME>
* @desc handle the status of user
* @param value status to be set (representing logged or not)
*/
setLogin (logged) {
this.logged = logged;
}
}
<file_sep>import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { HttpClientModule } from '@angular/common/http';
import { UsersListRoutingModule } from './users-list-routing.module';
import { ListComponent } from './list/list.component';
import { UsersService } from './users.service';
import { UserDetailComponent } from './user-detail/user-detail.component';
@NgModule({
imports: [
CommonModule,
UsersListRoutingModule,
HttpClientModule
],
providers: [UsersService],
declarations: [
ListComponent,
UserDetailComponent
]
})
export class UsersListModule { }
<file_sep>import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { UsersService } from '../users.service';
import { randomuser } from '../../core/models/randomuser.model';
@Component({
selector: 'ng-e-list',
templateUrl: './list.component.html',
styleUrls: ['./list.component.scss']
})
export class ListComponent implements OnInit {
usersList: Array<randomuser.Result>;
constructor(
private usersService: UsersService,
private router: Router
) { }
ngOnInit() {
this.usersService
.getUsers()
.subscribe((response: randomuser.Result[]) => {
this.usersList = response;
});
}
viewDetail(id: number) {
this.router.navigate(['users-list/' + id]);
}
goBack() {
this.router.navigate(['landing']);
}
}
<file_sep>import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { of } from 'rxjs/observable/of';
import 'rxjs/add/operator/map';
import { randomuser } from '../core/models/randomuser.model';
@Injectable()
export class UsersService {
usersList;
NRESULTS: Number = 20;
ENDPOINT = {
baseUrl: 'https://randomuser.me',
relativeUrl: '/api',
queryParams: {
results: String(this.NRESULTS)
}
};
constructor(private http: HttpClient) { }
getUsers() {
return this.usersList
? of(this.usersList)
: this.http
.get(
this.ENDPOINT.baseUrl + this.ENDPOINT.relativeUrl,
{ params: this.ENDPOINT.queryParams }
)
.map((result: randomuser.RootObject) => {
this.usersList = result.results;
console.log('Service stored users list: ', this.usersList);
return this.usersList;
});
}
getUser(id: number = 0) {
if (this.usersList) {
return (id >= 0 && id < this.NRESULTS)
? of(this.usersList[id])
: of(null);
} else {
return this.getUsers().map(() => this.usersList[id]);
}
}
}
| 5f6d4d6debf0a488c33e5bcd0dcabdc0dbc4c88a | [
"TypeScript"
] | 5 | TypeScript | Ricard/ng-exercise | 587c16765003216ff7709b0e0ce1a490a84a9254 | 6d0a1c0dbd86791d0a2e043ee248fbf123f954d2 | |
refs/heads/master | <repo_name>mrjgreen/formbuilder<file_sep>/lib/Fieldset/Element/Checkbox.php
<?php namespace Fieldset\Element;
class Checkbox extends \Fieldset\Element {
/**
* Create a form input
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function draw($field, $value = null, array $attributes = array()) {
if (is_array($field)) {
$attributes = $field;
} else {
$attributes['name'] = (string) $field;
}
array_key_exists('value', $attributes) or $attributes['value'] = 1;
if($value == $attributes['value']){
$attributes['checked'] = 'checked';
}
else{
unset($attributes['checked']);
}
$attributes['type'] = empty($attributes['type']) ? 'text' : $attributes['type'];
return '<input type="hidden" value="0" name="'.$attributes['name'].'"><input ' . $this->attrToString($attributes) . '>';
}
}
<file_sep>/lib/Fieldset/ElementInterface.php
<?php
namespace Fieldset;
interface ElementInterface {
public function getName();
public function populate($data);
public function getRules();
public function setErrors($errors);
}
<file_sep>/lib/Fieldset/Element.php
<?php
namespace Fieldset;
/**
* Input Element Class
*
* Helper for creating input elements with support for creating dynamic form objects.
*
*/
class Element implements ElementInterface, \ArrayAccess {
/**
* @var string Name of this field
*/
protected $_name = '';
/**
* @var string Field type for form generation, false to prevent it showing
*/
protected $_type = 'text';
/**
* @var string Field label for validation errors and form label generation
*/
protected $_label = '';
/**
* @var mixed (Default) value of this field
*/
protected $_value;
/**
* @var array Rules for validation
*/
protected $_rules = array();
/**
* @var array Errors for validation
*/
protected $_errors = array();
/**
* @var array Attributes for form generation
*/
protected $_attributes = array();
/**
* @var array Options, only available for select, radio & checkbox types
*/
protected $_options = array();
/**
* @var string Template for form building
*/
protected $_defaultTemplate = '{label}{element}{error}';
/**
* @var string Template for form building
*/
protected $_defaultErrorTemplate = '<p class="error">{message}</p>';
/**
* @var string Template for form building
*/
protected $_defaultLabelClass = '';
/**
*
* @var string
*/
protected $_labelClass;
/**
* @var string Template for form building
*/
protected $_template;
/**
* @var string Template for form building
*/
protected $_errorTemplate;
/**
*
* @param array $settings
*/
public function __construct(array $settings = array()) {
foreach ($settings as $key => $setting) {
$this->set($key, $setting);
}
}
/**
*
* @param type $name
*/
public function setName($name) {
$this->_name = $name;
return $this;
}
/**
*
* @return type
*/
public function getName() {
return preg_replace('/\[.*\]$/', '', $this->_name);
}
/**
*
* @param type $value
*/
public function setValue($value) {
$this->_value = $value;
return $this;
}
/**
*
* @return type
*/
public function getValue() {
return $this->_value;
}
/**
*
* @param type $type
*/
public function setType($type) {
$this->_type = $type;
return $this;
}
/**
*
* @return type
*/
public function getType() {
return $this->_type;
}
/**
*
* @param type $options
*/
public function setOptions($options) {
$this->_options = $options;
return $this;
}
/**
*
* @return type
*/
public function getOptions() {
return $this->_options;
}
/**
*
* @param type $label
*/
public function setLabel($label) {
$this->_label = $label;
return $this;
}
/**
*
* @return type
*/
public function getLabel() {
return $this->_label;
}
/**
* if the elements are in array notation form ie something[34], we can set the index to be a value of our choice
* @param mixed $id
*/
public function setOrdinal($id) {
$this->_name = preg_replace('/\[.*\]$/', '[' . $id . ']', $this->_name);
return $this;
}
/**
* if the elements are in array notation form ie something[34], we can get the index in this case, 34
* @return mixed $id
*/
public function getOrdinal() {
$array = array();
if (preg_match('/\[(.*)\]$/', $this->_name, $array) === 1) {
return $array[1];
}
return null;
}
/**
*
* @param type $name
* @param type $value
*/
public function setAttribute($name, $value) {
$this->_attributes[$name] = $value;
return $this;
}
/**
*
* @param type $name
* @param type $value
*/
public function appendAttribute($name, $value) {
$this->_attributes[$name] .= ' ' . $value;
return $this;
}
/**
*
* @param type $name
* @return type
*/
public function getAttribute($name) {
return isset($this->_attributes[$name]) ? $this->_attributes[$name] : false;
}
/**
*
* @param type $attr
*/
public function setAttributes($attr) {
$this->_attributes = $attr;
return $this;
}
/**
*
* @return type
*/
public function getAttributes() {
return $this->_attributes;
}
/**
*
* @param type $rules
*/
public function setRules($rules) {
$this->_rules = $rules;
return $this;
}
/**
*
* @return type
*/
public function getRules() {
return $this->_rules;
}
public function setLabelClass($class) {
$this->_labelClass = $class;
return $this;
}
/**
*
* @param type $template
*/
public function setTemplate($template) {
$this->_template = $template;
return $this;
}
/**
*
* @return type
*/
public function getTemplate() {
return $this->_template;
}
/**
*
* @param type $template
*/
public function setErrorTemplate($template) {
$this->_errorTemplate = $template;
return $this;
}
/**
*
* @return type
*/
public function getErrorTemplate() {
return $this->_errorTemplate;
}
/**
*
* @return array
*/
public function setErrors($errors) {
$this->_errors = $errors->get($this->getName());
return $this;
}
/**
*
* @param type $name
* @param type $error
*/
public function addError($name, $error) {
$this->_errors[$name] = $error;
return $this;
}
public function populate($data) {
if (is_array($data)) {
$name = $this->getName();
if (!isset($data[$name])) {
return $this;
}
$data = $data[$name];
}
$this->_value = $data;
return $this;
}
/**
*
* @param type $template
*/
public function setDefaultTemplate($template) {
$this->_defaultTemplate = $template;
}
/**
*
* @param type $template
*/
public function setDefaultErrorTemplate($template) {
$this->_defaultErrorTemplate = $template;
}
/**
*
* @param type $template
*/
public function setDefaultLabelClass($class) {
$this->_defaultLabelClass = $class;
}
public function hasErrors(){
return count($this->_errors);
}
/**
* Place the element and label into the template and return the HTML
* @return string HTML label and element inside the specified template
*/
public function build() {
$template = $this->_template === null ? $this->_defaultTemplate : $this->_template;
$search = array('{label}','{label_raw}','{element}', '{error}','{value}','{error-class}');
$replace = array(
$this->_buildLabel(),
$this->_label,
$this->_buildElement(),
$this->_buildError(),
$this->_value,
$this->hasErrors() ? 'error' : ''
);
return str_replace($search, $replace, $template);
}
public function _buildError() {
$str = '';
$template = $this->_errorTemplate === null ? $this->_defaultErrorTemplate : $this->_errorTemplate;
foreach ($this->_errors as $error) {
$str .= str_replace('{message}', $error, $template);
break; //#GOTCHA only show one error message at a time
}
return $str;
}
protected function _rulesClass() {
$str = '';
foreach ($this->_rules as $rulename) {
if (is_array($rulename)) {
$class = array_shift($rulename);
$class.= '[' . implode(',', $rulename) . ']';
} else {
$class = $rulename;
}
$str .= ' ' . $class;
}
return $str;
}
/**
*
* @return string The HTML element ready for print
*/
protected function _buildElement() {
if (!isset($this->_attributes['class'])) {
$this->_attributes['class'] = '';
}
if($validate = $this->_rulesClass()){
isset($this->_attributes['data-validate']) or $this->_attributes['data-validate'] = '';
$this->_attributes['data-validate'] .= $validate;
}
if ($this instanceof Element\Select || $this instanceof Element\MultiRadio) { //special select element parameter format due to the additional "options" parameter
return $this->draw($this->_name, $this->_value, $this->_options, $this->_attributes);
}
$this->_attributes['type'] = $this->_type;
return $this->draw($this->_name, $this->_value, $this->_attributes);
}
public static function forge(array $settings) {
isset($settings['type']) or $settings['type'] = 'text';
$class = __NAMESPACE__ . '\\Element\\' . ucwords($settings['type']);
if (@class_exists($class)) { //quietly for loaders that MAKE NOISE!
return new $class($settings);
}
return new Element\Input($settings);
//throw new \Exception("Element $class could not be loaded.");
}
/**
* The HTML label element ready for print
* @return string
*/
protected function _buildLabel() {
return $this->_label ? $this->label($this->_label) : '';
}
/**
* Magic serializer
* @return string
*/
public function __toString() {
return $this->build();
}
/**
* Create a label field
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function label($label) {
if (is_array($label)) {
$attributes = $label;
$label = $attributes['label'];
}
$attributes = array();
isset($this->_attributes['id']) and $attributes['for'] = $this->_attributes['id'];
$attributes['class'] = isset($this->_labelClass) ? $this->_labelClass : $this->_defaultLabelClass;
return '<label ' . $this->attrToString($attributes) . '>' . $label . '</label>';
}
/**
* Prep Value
*
* Prepares the value for display in the form
*
* @param string
* @return string
*/
public function prep_value($value) {
return str_replace(array("'", '"'), array("'", """), htmlspecialchars($value));
}
/**
* Attr to String
*
* Wraps the global attributes function and does some form specific work
*
* @param array $attr
* @return string
*/
public function attrToString($attr) {
$string = "";
foreach ($attr as $k => $v) {
$v !== null and $string .= is_numeric($k) ? $v : sprintf($k . '="%s" ', $v);
}
return $string;
}
public function set($key, $setting) {
$method = 'set' . ucwords($key);
if (method_exists($this, $method)) {
$this->$method($setting);
} else {
$this->setAttribute($key, $setting);
}
}
public function get($key) {
$method = 'get' . ucwords($key);
if (method_exists($this, $method)) {
return $this->$method();
} elseif (isset($this->_attributes[$key])) {
return $this->_attributes[$key];
}
return null;
}
public function __get($name) {
return $this->get($name);
}
public function __set($name, $value) {
return $this->set($name, $value);
}
public function offsetSet($offset, $value) {
is_null($offset) or $this->set($offset, $value);
}
public function offsetExists($offset) {
$val = $this->get($offset);
return isset($val);
}
public function offsetUnset($offset) {
$this->set($offset, null);
}
public function offsetGet($offset) {
return $this->get($offset);
}
}<file_sep>/lib/Fieldset/Element/Multiradio.php
<?php
namespace Fieldset\Element;
class Multiradio extends Input {
public function draw($field, $value = null, array $options = array(), array $attributes = array()) {
$inputs = '<div class="radio-group">';
if (is_array($field)) {
$this->_attributes = $field;
} else {
$this->_attributes = $attributes;
$this->_attributes['name'] = (string) $field;
$this->_attributes['value'] = (string) $value;
}
$this->_attributes['type'] = 'radio';
$i = 0;
foreach ($options as $label => $option) {
$inputs .= '<div class="radio-element">';
$attributes = $this->_attributes;
$attributes['label'] = $option;
$attributes['value'] = $label;
$attributes['template'] = isset($attributes['elementTemplate'])?$attributes['elementTemplate']:'{element} {error}{label}';
$attributes['id'] = $field.'-'.$i++;
$attributes['checked'] = ($value == $label ? 'checked' : null);
$attributes['labelClass'] = ($value == $label ? 'active' : null);
unset($attributes['help'],$attributes['elementTemplate']);
$inputs .= \Fieldset\Element::forge($attributes);
$inputs .= '</div>';
}
return $inputs.'</div>';
}
}<file_sep>/lib/Fieldset/Element/Heading.php
<?php
namespace Fieldset\Element;
class Heading extends \Fieldset\Element {
public function draw($field, $values = null, array $attributes = array()) {
if (is_array($field)) {
$attributes = $field;
}
unset($attributes['value']);
isset($attributes['class']) or $attributes['class'] = '';
$attributes['class'] .= ' cman-heading';
return '<h2 ' . $this->attrToString($attributes) . '>'.$this->label.'</h2>';
}
}<file_sep>/lib/Fieldset/Element/Plain.php
<?php
namespace Fieldset\Element;
class Plain extends \Fieldset\Element {
public function draw($field, $values = null, array $attributes = array()) {
return $values;
}
}<file_sep>/lib/Fieldset/Element/Select.php
<?php
namespace Fieldset\Element;
class Select extends \Fieldset\Element {
/**
* Select
*
* Generates a html select element based on the given parameters
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string selected value(s)
* @param array array of options and option groups
* @param array
* @return string
*/
public function draw($field, $values = null, array $options = array(), array $attributes = array())
{
if (is_array($field))
{
$attributes = $field;
if ( ! isset($attributes['selected']))
{
$attributes['selected'] = ! isset($attributes['value']) ? null : $attributes['value'];
}
}
else
{
$attributes['name'] = (string) $field;
$attributes['selected'] = $values;
$attributes['options'] = $options;
}
unset($attributes['value']);
if ( ! isset($attributes['options']) || ! is_array($attributes['options']))
{
throw new \InvalidArgumentException(sprintf('Select element "%s" is either missing the "options" or "options" is not array.', $attributes['name']));
}
// Get the options then unset them from the array
$options = $attributes['options'];
unset($attributes['options']);
// Get the selected options then unset it from the array
// and make sure they're all strings to avoid type conversions
$selected = ! isset($attributes['selected']) ? array() : array_map(function($a) { return (string) $a; }, array_values((array) $attributes['selected']));
unset($attributes['selected']);
// workaround to access the current object context in the closure
$current_obj =& $this;
// closure to recusively process the options array
$listoptions = function (array $options, $selected, $level = 1) use (&$listoptions, &$current_obj, &$attributes)
{
$input = PHP_EOL;
foreach ($options as $key => $val)
{
if (is_array($val))
{
$optgroup = $listoptions($val, $selected, $level + 1);
$optgroup .= str_repeat("\t", $level);
$input .= str_repeat("\t", $level).'<optgroup label="'.$key.'" style="text-indent:'.(10*($level-1)).'px;">'. $optgroup.'</optgroup>'.PHP_EOL;
}
else
{
$opt_attr = array('value' => $key, 'style' => 'text-indent: '.(10*($level-1)).'px;');
(in_array((string)$key, $selected, true)) && $opt_attr[] = 'selected';
$input .= str_repeat("\t", $level);
$opt_attr['value'] = (empty($attributes['dont_prep'])) ?
$current_obj->prep_value($opt_attr['value']) : $opt_attr['value'];
$input .= '<option ' . $current_obj->attrToString($opt_attr) . '>' . $val . '</option>' . PHP_EOL;
}
}
unset($attributes['dont_prep']);
return $input;
};
// generate the select options list
$input = $listoptions($options, $selected).str_repeat("\t", 0);
return '<select ' . $this->attrToString($attributes) . '>' . $input . '</select>';
}
}
<file_sep>/lib/Fieldset/Element/Button.php
<?php namespace Fieldset\Element;
class Button extends Fieldset\Element {
/**
* Create a button
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function draw($field, $value = null, array $attributes = array()) {
if (is_array($field)) {
$attributes = $field;
$value = isset($attributes['value']) ? $attributes['value'] : $value;
} else {
$attributes['name'] = (string) $field;
$value = isset($value) ? $value : $attributes['name'];
}
$attributes['type'] = 'submit';
return '<button ' . $this->attrToString($attributes) . '>' . $value . '</button>';
}
}
<file_sep>/lib/Fieldset/Element/Multicheckbox.php
<?php
#NOT WORKING!
namespace Jg\Fieldset\Element;
class MultiCheckbox extends \Jg\Fieldset\Element {
/**
* Select
*
* Generates a html select element based on the given parameters
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string selected value(s)
* @param array array of options and option groups
* @param array
* @return string
*/
public function draw($field, $values = null, array $options = array(), array $attributes = array())
{
unset($attributes['array']);
$inputs = '';
if (is_array($field)) {
$this->_attributes = $field;
} else {
$this->_attributes = $attributes;
$this->_attributes['name'] = (string) $field;
$this->_attributes['value'] = (string) $values;
}
$this->_type = 'checkbox';
foreach ($options as $label => $option) {
$this->_label = $label;
$this->_value = $option;
$this->_attributes['selected'] = $values == $option ? 'selected' : false;
$inputs .= $this->build();
}
return $inputs;
}
}
<file_sep>/lib/Fieldset/Element/Imagepicker.php
<?php
namespace Fieldset\Element;
class Imagepicker extends \Fieldset\Element {
/**
* Create a form input
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function draw($field, $value = null, array $attributes = array()) {
$tag = $value ? basename($value) : "No Image Selected";
$src = $value ?: "http://placehold.it/250x150";
return
'<input type="hidden" name="'.$field.'" value="'.$value.'">' .
'<span class="imagepicker-tag">'.$tag.'</span> <a href="#" class="clearImage">(Clear Image)</a>' .
'<img src="'.$src.'@maxWidth-225" class="imagepicker" >';
}
}
<file_sep>/lib/Fieldset/Element/Textarea.php
<?php
namespace Fieldset\Element;
class Textarea extends \Fieldset\Element {
/**
* Create a textarea field
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function draw($field, $value = null, array $attributes = array()) {
if (is_array($field)) {
$attributes = $field;
} else {
$attributes['name'] = (string) $field;
$attributes['value'] = (string) $value;
}
$value = empty($attributes['value']) ? '' : $attributes['value'];
unset($attributes['value']);
if (empty($attributes['dont_prep'])) {
$value = $this->prep_value($value);
unset($attributes['dont_prep']);
}
return '<textarea ' . $this->attrToString($attributes) . '>' . $value . '</textarea>';
}
}
<file_sep>/lib/Fieldset/Element/Input.php
<?php
namespace Fieldset\Element;
class Input extends \Fieldset\Element {
/**
* Valid types for input tags (including HTML5)
*/
protected $_valid_inputs = array(
'button', 'checkbox', 'color', 'date', 'datetime',
'datetime-local', 'email', 'file', 'hidden', 'image',
'month', 'number', 'password', 'radio', 'range',
'reset', 'search', 'submit', 'tel', 'text', 'time',
'url', 'week'
);
/**
* Create a form input
*
* @param string|array either fieldname or full attributes array (when array other params are ignored)
* @param string
* @param array
* @return string
*/
public function draw($field, $value = null, array $attributes = array()) {
if (is_array($field)) {
$attributes = $field;
array_key_exists('value', $attributes) or $attributes['value'] = '';
} else {
$attributes['name'] = (string) $field;
$attributes['value'] = (string) $value;
}
$attributes['type'] = empty($attributes['type']) ? 'text' : $attributes['type'];
if (!in_array($attributes['type'], $this->_valid_inputs)) {
throw new \Exception(sprintf('"%s" is not a valid input type.', $attributes['type']));
}
if (empty($attributes['dont_prep'])) {
$attributes['value'] = $this->prep_value($attributes['value']);
unset($attributes['dont_prep']);
}
return '<input ' . $this->attrToString($attributes) . '>';
}
}
| e54fde0ccff381002b4a899065be0053b6fa6d7e | [
"PHP"
] | 12 | PHP | mrjgreen/formbuilder | e7784ee805d821b0380a18371ae37ec7067a22a0 | b7f5f7e56fab22fea8b84902a462ccb2a87e4b0f | |
refs/heads/master | <repo_name>alexanderson1993/react-lazy-presentation<file_sep>/src/pages/page2.js
import React from "react";
export default () => {
return (
<>
<h1>Dynamic `import()`</h1>
<ul>
<li>Webpack & Browserify keyword for code splitting</li>
</ul>
</>
);
};
<file_sep>/src/pages/page6.js
import React from "react";
export default () => (
<>
<div
style={{
display: "flex",
justifyContent: "space-around",
alignItems: "center"
}}
>
<img
style={{ width: "50%" }}
src={require("../images/before.png")}
alt="before"
/>
<img
style={{ width: "50%" }}
src={require("../images/after.png")}
alt="after"
/>
</div>
</>
);
<file_sep>/src/pages/page7.js
import React from "react";
export default () => (
<>
<h1>Other Considerations</h1>
<ul>
<li>Route-based code splitting with React Router or Reach Router</li>
<li>
Dynamic Preload with <code>onMouseEnter</code>
</li>
<li>Include Error boundaries to catch 404s and request errors</li>
<li>
Imported modules <strong>must</strong> be default exports
</li>
</ul>
</>
);
<file_sep>/src/pages/page4.js
import React from "react";
import Highlight from "react-highlight";
export default () => (
<>
<h1>{`<Suspense>`}</h1>
<p>Loading fallback handler</p>
<Highlight className="javascript">
{`const SuspendedComp = () => (
<Suspense fallback={<Loading />}>
<MyComponent />
</Suspense>
)`}
</Highlight>
<p>Put it somewhere in the component tree</p>
</>
);
<file_sep>/src/pages/page8.js
import React from "react";
export default () => (
<>
<h1 style={{ fontSize: "100px" }}>
<span role="img" aria-label="wave">
👋
</span>
</h1>
<ul>
<li>
<a href="https://reactjs.org/docs/code-splitting.html">
https://reactjs.org/docs/code-splitting.html
</a>
</li>
<li>
<a href="https://twitter.com/ralex1993">
https://twitter.com/ralex1993
</a>
</li>
<li>
<a href="https://github.com/alexanderson1993">
https://github.com/alexanderson1993
</a>
</li>
</ul>
</>
);
<file_sep>/src/index.js
import React, { lazy, Suspense } from "react";
import ReactDOM from "react-dom";
import { Router, Link } from "@reach/router";
import Spinner from "react-spinkit";
import ErrorBoundary from "./ErrorBoundary";
import "./styles.css";
import "highlight.js/styles/monokai.css";
const pages = Array(8)
.fill(0)
.map((_, i) => lazy(() => import(`./pages/page${i + 1}.js`)));
const NavLink = props => (
<Link
{...props}
getProps={({ isCurrent }) => ({ className: isCurrent ? "active" : "" })}
/>
);
function App() {
const Page1 = pages[0];
return (
<div className="container">
<div className="nav">
<NavLink to="/">About Me</NavLink>
<NavLink to="/page2">import()</NavLink>
<NavLink to="/page3">React.lazy</NavLink>
<NavLink to="/page4">{`<Suspense>`}</NavLink>
<NavLink to="/page5" onMouseEnter={() => import(`./pages/page6.js`)}>
All Together
</NavLink>
<NavLink to="/page6">Comparison</NavLink>
<NavLink to="/page7">Considerations</NavLink>
<NavLink to="/page8">Thanks</NavLink>
</div>
<div className="page">
<ErrorBoundary>
<Suspense
fallback={
<Spinner
name="folding-cube"
color="purple"
fadeIn="none"
className="big-spinner"
/>
}
>
<Router>
<Page1 path="/" />
{pages.map((Page, i) => (
<Page key={`page-${i}`} path={`/page${i + 1}`} />
))}
</Router>
</Suspense>
</ErrorBoundary>
</div>
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
<file_sep>/src/pages/page3.js
import React from "react";
import Highlight from "react-highlight";
export default () => {
return (
<>
<h1>React.lazy</h1>
<p>Special Wrapper for `import()`</p>
<Highlight className="javascript">
{`const MyComponent = React.lazy(() => import('./myComponent.js'))`}
</Highlight>
<p />
</>
);
};
<file_sep>/src/pages/page5.js
import React from "react";
import Highlight from "react-highlight";
export default () => (
<>
<h1>All Together</h1>
<Highlight className="javascript">
{`const MyComponent = React.lazy(() => import('./myComponent.js'))
const SuspendedComponent = () => (
<ErrorBoundary>
<Suspense fallback={<Loading />}>
<MyComponent /> {/* Only loaded when rendered.*/}
</Suspense>
</ErrorBoundary
)`}
</Highlight>
</>
);
| 5d417c7cc0c9ad21000bdf8609f6a1f84a41e9f2 | [
"JavaScript"
] | 8 | JavaScript | alexanderson1993/react-lazy-presentation | 4a169af7d712142ff77159831000d3225938f95f | d5840d10ff025af83f4846faaaa2adcd210628fe | |
refs/heads/master | <file_sep>//
// CommentsButton.swift
// VKDemo
//
// Created by <NAME> on 20/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import QuartzCore
@IBDesignable
class CommentsButton: UIControl {
private let plashkaFillColor = UIColor.white
private let fillColor = UIColor.clear
private let strokeColor = UIColor(white: 0.7, alpha: 1.0)
private let borderStrokeColor = #colorLiteral(red: 0.6666666667, green: 0.6666666667, blue: 0.6666666667, alpha: 0.5)
private lazy var commentsIconLayer: CAShapeLayer = {
let commentsPath = UIBezierPath()
commentsPath.move(to: CGPoint(x: 30.29, y: 28.78))
commentsPath.addCurve(to: CGPoint(x: 30.57, y: 29.42), controlPoint1: CGPoint(x: 30.38, y: 29.01), controlPoint2: CGPoint(x: 30.48, y: 29.22))
commentsPath.addCurve(to: CGPoint(x: 30.92, y: 29.97), controlPoint1: CGPoint(x: 30.67, y: 29.61), controlPoint2: CGPoint(x: 30.79, y: 29.79))
commentsPath.addCurve(to: CGPoint(x: 31.24, y: 30.39), controlPoint1: CGPoint(x: 31.06, y: 30.15), controlPoint2: CGPoint(x: 31.16, y: 30.28))
commentsPath.addCurve(to: CGPoint(x: 31.6, y: 30.81), controlPoint1: CGPoint(x: 31.31, y: 30.49), controlPoint2: CGPoint(x: 31.43, y: 30.63))
commentsPath.addCurve(to: CGPoint(x: 31.92, y: 31.17), controlPoint1: CGPoint(x: 31.77, y: 30.99), controlPoint2: CGPoint(x: 31.88, y: 31.11))
commentsPath.addCurve(to: CGPoint(x: 31.98, y: 31.23), controlPoint1: CGPoint(x: 31.93, y: 31.18), controlPoint2: CGPoint(x: 31.95, y: 31.2))
commentsPath.addCurve(to: CGPoint(x: 32.04, y: 31.3), controlPoint1: CGPoint(x: 32.01, y: 31.27), controlPoint2: CGPoint(x: 32.03, y: 31.29))
commentsPath.addCurve(to: CGPoint(x: 32.1, y: 31.38), controlPoint1: CGPoint(x: 32.05, y: 31.32), controlPoint2: CGPoint(x: 32.07, y: 31.34))
commentsPath.addCurve(to: CGPoint(x: 32.14, y: 31.46), controlPoint1: CGPoint(x: 32.12, y: 31.41), controlPoint2: CGPoint(x: 32.13, y: 31.44))
commentsPath.addLine(to: CGPoint(x: 32.18, y: 31.53))
commentsPath.addLine(to: CGPoint(x: 32.21, y: 31.61))
commentsPath.addLine(to: CGPoint(x: 32.21, y: 31.71))
commentsPath.addLine(to: CGPoint(x: 32.2, y: 31.8))
commentsPath.addCurve(to: CGPoint(x: 32.02, y: 32.12), controlPoint1: CGPoint(x: 32.17, y: 31.93), controlPoint2: CGPoint(x: 32.11, y: 32.04))
commentsPath.addCurve(to: CGPoint(x: 31.71, y: 32.22), controlPoint1: CGPoint(x: 31.93, y: 32.19), controlPoint2: CGPoint(x: 31.82, y: 32.23))
commentsPath.addCurve(to: CGPoint(x: 30.51, y: 31.99), controlPoint1: CGPoint(x: 31.25, y: 32.15), controlPoint2: CGPoint(x: 30.85, y: 32.07))
commentsPath.addCurve(to: CGPoint(x: 26.63, y: 30.15), controlPoint1: CGPoint(x: 29.08, y: 31.6), controlPoint2: CGPoint(x: 27.79, y: 30.99))
commentsPath.addCurve(to: CGPoint(x: 24.18, y: 30.38), controlPoint1: CGPoint(x: 25.8, y: 30.3), controlPoint2: CGPoint(x: 24.98, y: 30.38))
commentsPath.addCurve(to: CGPoint(x: 17.59, y: 28.48), controlPoint1: CGPoint(x: 21.66, y: 30.38), controlPoint2: CGPoint(x: 19.46, y: 29.75))
commentsPath.addCurve(to: CGPoint(x: 18.82, y: 28.54), controlPoint1: CGPoint(x: 18.13, y: 28.52), controlPoint2: CGPoint(x: 18.54, y: 28.54))
commentsPath.addCurve(to: CGPoint(x: 23.13, y: 27.89), controlPoint1: CGPoint(x: 20.32, y: 28.54), controlPoint2: CGPoint(x: 21.76, y: 28.33))
commentsPath.addCurve(to: CGPoint(x: 26.82, y: 26.04), controlPoint1: CGPoint(x: 24.51, y: 27.46), controlPoint2: CGPoint(x: 25.74, y: 26.85))
commentsPath.addCurve(to: CGPoint(x: 29.49, y: 23), controlPoint1: CGPoint(x: 27.98, y: 25.16), controlPoint2: CGPoint(x: 28.87, y: 24.15))
commentsPath.addCurve(to: CGPoint(x: 30.43, y: 19.35), controlPoint1: CGPoint(x: 30.12, y: 21.85), controlPoint2: CGPoint(x: 30.43, y: 20.63))
commentsPath.addCurve(to: CGPoint(x: 30.11, y: 17.17), controlPoint1: CGPoint(x: 30.43, y: 18.61), controlPoint2: CGPoint(x: 30.32, y: 17.89))
commentsPath.addCurve(to: CGPoint(x: 32.95, y: 19.72), controlPoint1: CGPoint(x: 31.31, y: 17.85), controlPoint2: CGPoint(x: 32.26, y: 18.7))
commentsPath.addCurve(to: CGPoint(x: 34, y: 23.03), controlPoint1: CGPoint(x: 33.65, y: 20.75), controlPoint2: CGPoint(x: 34, y: 21.85))
commentsPath.addCurve(to: CGPoint(x: 33.01, y: 26.25), controlPoint1: CGPoint(x: 34, y: 24.18), controlPoint2: CGPoint(x: 33.67, y: 25.25))
commentsPath.addCurve(to: CGPoint(x: 30.29, y: 28.78), controlPoint1: CGPoint(x: 32.35, y: 27.25), controlPoint2: CGPoint(x: 31.44, y: 28.1))
commentsPath.close()
commentsPath.move(to: CGPoint(x: 18.82, y: 12))
commentsPath.addCurve(to: CGPoint(x: 23.75, y: 12.98), controlPoint1: CGPoint(x: 20.6, y: 12), controlPoint2: CGPoint(x: 22.24, y: 12.33))
commentsPath.addCurve(to: CGPoint(x: 27.33, y: 15.66), controlPoint1: CGPoint(x: 25.26, y: 13.64), controlPoint2: CGPoint(x: 26.46, y: 14.53))
commentsPath.addCurve(to: CGPoint(x: 28.64, y: 19.35), controlPoint1: CGPoint(x: 28.21, y: 16.79), controlPoint2: CGPoint(x: 28.64, y: 18.02))
commentsPath.addCurve(to: CGPoint(x: 27.33, y: 23.04), controlPoint1: CGPoint(x: 28.64, y: 20.68), controlPoint2: CGPoint(x: 28.21, y: 21.91))
commentsPath.addCurve(to: CGPoint(x: 23.75, y: 25.72), controlPoint1: CGPoint(x: 26.46, y: 24.17), controlPoint2: CGPoint(x: 25.26, y: 25.06))
commentsPath.addCurve(to: CGPoint(x: 18.82, y: 26.7), controlPoint1: CGPoint(x: 22.24, y: 26.37), controlPoint2: CGPoint(x: 20.6, y: 26.7))
commentsPath.addCurve(to: CGPoint(x: 16.37, y: 26.47), controlPoint1: CGPoint(x: 18.02, y: 26.7), controlPoint2: CGPoint(x: 17.2, y: 26.63))
commentsPath.addCurve(to: CGPoint(x: 12.49, y: 28.31), controlPoint1: CGPoint(x: 15.21, y: 27.32), controlPoint2: CGPoint(x: 13.92, y: 27.93))
commentsPath.addCurve(to: CGPoint(x: 11.29, y: 28.54), controlPoint1: CGPoint(x: 12.15, y: 28.4), controlPoint2: CGPoint(x: 11.75, y: 28.47))
commentsPath.addLine(to: CGPoint(x: 11.25, y: 28.54))
commentsPath.addCurve(to: CGPoint(x: 10.96, y: 28.43), controlPoint1: CGPoint(x: 11.14, y: 28.54), controlPoint2: CGPoint(x: 11.05, y: 28.5))
commentsPath.addCurve(to: CGPoint(x: 10.8, y: 28.12), controlPoint1: CGPoint(x: 10.87, y: 28.35), controlPoint2: CGPoint(x: 10.82, y: 28.25))
commentsPath.addCurve(to: CGPoint(x: 10.79, y: 28.03), controlPoint1: CGPoint(x: 10.79, y: 28.1), controlPoint2: CGPoint(x: 10.79, y: 28.06))
commentsPath.addCurve(to: CGPoint(x: 10.79, y: 27.94), controlPoint1: CGPoint(x: 10.79, y: 28), controlPoint2: CGPoint(x: 10.79, y: 27.97))
commentsPath.addCurve(to: CGPoint(x: 10.82, y: 27.85), controlPoint1: CGPoint(x: 10.8, y: 27.91), controlPoint2: CGPoint(x: 10.81, y: 27.88))
commentsPath.addLine(to: CGPoint(x: 10.86, y: 27.78))
commentsPath.addLine(to: CGPoint(x: 10.9, y: 27.7))
commentsPath.addLine(to: CGPoint(x: 10.96, y: 27.63))
commentsPath.addLine(to: CGPoint(x: 11.02, y: 27.56))
commentsPath.addLine(to: CGPoint(x: 11.08, y: 27.49))
commentsPath.addCurve(to: CGPoint(x: 11.4, y: 27.13), controlPoint1: CGPoint(x: 11.12, y: 27.43), controlPoint2: CGPoint(x: 11.23, y: 27.32))
commentsPath.addCurve(to: CGPoint(x: 11.76, y: 26.71), controlPoint1: CGPoint(x: 11.57, y: 26.95), controlPoint2: CGPoint(x: 11.69, y: 26.81))
commentsPath.addCurve(to: CGPoint(x: 12.08, y: 26.29), controlPoint1: CGPoint(x: 11.84, y: 26.61), controlPoint2: CGPoint(x: 11.94, y: 26.47))
commentsPath.addCurve(to: CGPoint(x: 12.43, y: 25.74), controlPoint1: CGPoint(x: 12.21, y: 26.12), controlPoint2: CGPoint(x: 12.33, y: 25.93))
commentsPath.addCurve(to: CGPoint(x: 12.71, y: 25.11), controlPoint1: CGPoint(x: 12.52, y: 25.55), controlPoint2: CGPoint(x: 12.62, y: 25.34))
commentsPath.addCurve(to: CGPoint(x: 9.99, y: 22.57), controlPoint1: CGPoint(x: 11.56, y: 24.42), controlPoint2: CGPoint(x: 10.65, y: 23.57))
commentsPath.addCurve(to: CGPoint(x: 9, y: 19.35), controlPoint1: CGPoint(x: 9.33, y: 21.56), controlPoint2: CGPoint(x: 9, y: 20.49))
commentsPath.addCurve(to: CGPoint(x: 10.31, y: 15.66), controlPoint1: CGPoint(x: 9, y: 18.02), controlPoint2: CGPoint(x: 9.44, y: 16.79))
commentsPath.addCurve(to: CGPoint(x: 13.89, y: 12.98), controlPoint1: CGPoint(x: 11.19, y: 14.53), controlPoint2: CGPoint(x: 12.38, y: 13.64))
commentsPath.addCurve(to: CGPoint(x: 18.82, y: 12), controlPoint1: CGPoint(x: 15.4, y: 12.33), controlPoint2: CGPoint(x: 17.05, y: 12))
commentsPath.close()
commentsPath.move(to: CGPoint(x: 18.82, y: 13.84))
commentsPath.addCurve(to: CGPoint(x: 14.83, y: 14.58), controlPoint1: CGPoint(x: 17.4, y: 13.84), controlPoint2: CGPoint(x: 16.07, y: 14.09))
commentsPath.addCurve(to: CGPoint(x: 11.88, y: 16.61), controlPoint1: CGPoint(x: 13.59, y: 15.08), controlPoint2: CGPoint(x: 12.61, y: 15.76))
commentsPath.addCurve(to: CGPoint(x: 10.79, y: 19.35), controlPoint1: CGPoint(x: 11.15, y: 17.46), controlPoint2: CGPoint(x: 10.79, y: 18.37))
commentsPath.addCurve(to: CGPoint(x: 11.52, y: 21.62), controlPoint1: CGPoint(x: 10.79, y: 20.14), controlPoint2: CGPoint(x: 11.03, y: 20.89))
commentsPath.addCurve(to: CGPoint(x: 13.6, y: 23.52), controlPoint1: CGPoint(x: 12.02, y: 22.35), controlPoint2: CGPoint(x: 12.71, y: 22.98))
commentsPath.addLine(to: CGPoint(x: 14.96, y: 24.32))
commentsPath.addLine(to: CGPoint(x: 14.47, y: 25.53))
commentsPath.addCurve(to: CGPoint(x: 15.33, y: 24.97), controlPoint1: CGPoint(x: 14.79, y: 25.33), controlPoint2: CGPoint(x: 15.07, y: 25.15))
commentsPath.addLine(to: CGPoint(x: 15.95, y: 24.52))
commentsPath.addLine(to: CGPoint(x: 16.69, y: 24.66))
commentsPath.addCurve(to: CGPoint(x: 18.82, y: 24.86), controlPoint1: CGPoint(x: 17.41, y: 24.8), controlPoint2: CGPoint(x: 18.12, y: 24.86))
commentsPath.addCurve(to: CGPoint(x: 22.81, y: 24.12), controlPoint1: CGPoint(x: 20.24, y: 24.86), controlPoint2: CGPoint(x: 21.57, y: 24.62))
commentsPath.addCurve(to: CGPoint(x: 25.76, y: 22.09), controlPoint1: CGPoint(x: 24.05, y: 23.62), controlPoint2: CGPoint(x: 25.03, y: 22.95))
commentsPath.addCurve(to: CGPoint(x: 26.86, y: 19.35), controlPoint1: CGPoint(x: 26.49, y: 21.24), controlPoint2: CGPoint(x: 26.86, y: 20.33))
commentsPath.addCurve(to: CGPoint(x: 25.76, y: 16.61), controlPoint1: CGPoint(x: 26.86, y: 18.37), controlPoint2: CGPoint(x: 26.49, y: 17.46))
commentsPath.addCurve(to: CGPoint(x: 22.81, y: 14.58), controlPoint1: CGPoint(x: 25.03, y: 15.76), controlPoint2: CGPoint(x: 24.05, y: 15.08))
commentsPath.addCurve(to: CGPoint(x: 18.82, y: 13.84), controlPoint1: CGPoint(x: 21.57, y: 14.09), controlPoint2: CGPoint(x: 20.24, y: 13.84))
commentsPath.close()
strokeColor.setStroke()
commentsPath.lineWidth = 1
commentsPath.stroke()
let layer = CAShapeLayer()
layer.path = commentsPath.cgPath
layer.fillColor = fillColor.cgColor
layer.strokeColor = strokeColor.cgColor
layer.lineWidth = 1
return layer
}()
private lazy var borderLayer: CAShapeLayer = {
let layer = CAShapeLayer()
let rectanglePath = UIBezierPath(roundedRect: CGRect(x: 1, y: 1, width: 93, height: 42), cornerRadius: 21)
fillColor.setStroke()
rectanglePath.lineWidth = 0.5
rectanglePath.lineCapStyle = .round
rectanglePath.lineJoinStyle = .round
rectanglePath.stroke()
layer.path = rectanglePath.cgPath
layer.strokeColor = borderStrokeColor.cgColor
layer.fillColor = plashkaFillColor.cgColor
layer.lineWidth = 0.5
return layer
}()
private lazy var countLabel: UILabel = {
let label = UILabel()
label.textColor = UIColor(white: 0.3, alpha: 1.0)
label.text = "123"
label.frame = CGRect(x: 42, y: 11, width: 41, height: 21)
label.font = UIFont.systemFont(ofSize: 13.0)
return label
}()
private func addLayers() {
[borderLayer, commentsIconLayer].forEach { layer.addSublayer($0) }
addSubview(countLabel)
}
private func removeLayersIfNeeded() {
layer.sublayers?.forEach { $0.removeFromSuperlayer() }
}
private func configureLayers() {
removeLayersIfNeeded()
addLayers()
for subLayer in [
borderLayer,
commentsIconLayer
] {
subLayer.frame = bounds
subLayer.setNeedsDisplay()
}
}
override init(frame: CGRect) {
super.init(frame: frame)
addLayers()
backgroundColor = .clear
}
required init?(coder: NSCoder) {
super.init(coder: coder)
addLayers()
backgroundColor = .clear
}
public func setup(commentsCount: Int) {
countLabel.text = "\(commentsCount)"
}
}
<file_sep>//
// ViperRouterProtocol.swift
// Vanilla
//
// Created by <NAME> on 6/8/17.
// Copyright © 2017 BrightBox. All rights reserved.
//
import Foundation
public protocol ViperRouterProtocol {
var transitionHandler: ViperModuleTransitionHandler! { get set }
}
<file_sep>//
// FeedRouterInput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import Foundation
protocol FeedRouterInput {
}
<file_sep>//
// ConversationsResponse.swift
// VKDemo
//
// Created by <NAME> on 01.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct ConversationsResponse: Codable {
let count: Int
let items: [ConversationDto]
}
struct ConversationDto: Codable {
let peer: PeerDto
let inRead: Int
let outRead: Int
let unreadCount: Int
let important: BoolInt
let unanswered: BoolInt
let pushSettings: PushSettingsDto
let canWrite: CanWriteDto
let chatSettings: ChatSettingsDto
private enum CodingKeys: String, CodingKey {
case peer
case inRead = "in_read"
case outRead = "out_read"
case unreadCount = "unread_count"
case important
case unanswered
case pushSettings = "push_settings"
case canWrite = "can_write"
case chatSettings = "chat_settings"
}
}
struct PeerDto: Codable {
let id: Int
let type: String
let localId: Int
private enum CodingKeys: String, CodingKey {
case id
case type
case localId = "local_id"
}
}
struct PushSettingsDto: Codable {
let disabledUntil: Int
let disabledForever: BoolInt
let noSound: BoolInt
private enum CodingKeys: String, CodingKey {
case disabledUntil = "disabled_until"
case disabledForever = "disabled_forever"
case noSound = "no_sound"
}
}
struct CanWriteDto: Codable {
let allowed: BoolInt
let reason: Int
}
struct ChatSettingsDto: Codable {
let membersCount: Int
let title: String
let pinnedMessage: PinnedMessageDto
let state: String
let photo: ChatPhotoDto
let activeIds: [Int]
let isGroupChannel: BoolInt
private enum CodingKeys: String, CodingKey {
case membersCount = "members_count"
case title
case pinnedMessage = "pinned_message"
case state
case photo
case activeIds = "active_ids"
case isGroupChannel = "is_group_channel"
}
}
struct PinnedMessageDto: Codable {
let id: Int
let date: Int
let fromId: Int
let text: String
let attachments: String // TODO: Struct
let geo: GeoDto
}
struct ChatPhotoDto: Codable {
let photo50: String?
let photo100: String?
let photo200: String?
private enum CodingKeys: String, CodingKey {
case photo50 = "photo_50"
case photo100 = "photo_100"
case photo200 = "photo_200"
}
}
struct GeoDto: Codable {
let type: String
let coordinates: String
let place: GeoPlaceDto
}
struct GeoPlaceDto: Codable {
let id: Int
let title: String
let latitude: Double
let longitude: Double
let created: Int
let iconUrl: String
let country: String
let city: String
private enum CodingKeys: String, CodingKey {
case id
case title
case latitude
case longitude
case created
case iconUrl = "icon"
case country
case city
}
}
<file_sep>//
// FeedTextView.swift
// VKDemo
//
// Created by <NAME> on 07.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class FeedTextView: UITextView {
override init(frame: CGRect, textContainer: NSTextContainer?) {
super.init(frame: frame, textContainer: textContainer)
font = UIFont.systemFont(ofSize: 14.0)
isScrollEnabled = false
}
required init?(coder: NSCoder) {
super.init(coder: coder)
font = UIFont.systemFont(ofSize: 14.0)
isScrollEnabled = false
}
}
<file_sep>//
// FeedResponse.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct FeedResponse: Codable {
let items: [FeedItemDto]
let profiles: [UserDto]?
// TODO:
// Поле profiles содержит массив объектов пользователей (UserDto) с дополнительными полями:
// photo — адрес фотографии пользователя размером 50x50px;
// photo_medium_rec — адрес фотографии пользователя раз мером 100x100px;
// screen_name — короткий адрес страницы пользователя (например, andrew или id6492).
let groups: [GroupDto]?
let nextFrom: String
private enum CodingKeys: String, CodingKey {
case items
case profiles
case groups
case nextFrom = "next_from"
}
}
<file_sep>//
// DI.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
class DI {
private static let shared = DI()
static var container: DIContainerProtocol {
return shared.container
}
private let container: DIContainerProtocol
init() {
container = DIContainer()
}
}
<file_sep>//
// MessagesParser.swift
// VKDemo
//
// Created by <NAME> on 01.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct ConversationItem {
// let item: FeedItemDto
// let profiles: [UserDto]?
// let groups: [GroupDto]?
}
protocol IMessagesParser {
func parse(conversationsResponse: ConversationsResponse) -> [ConversationItem]
}
class MessagesParser: IMessagesParser {
func parse(conversationsResponse: ConversationsResponse) -> [ConversationItem] {
// var feedItems = [FeedItem]()
// let profiles = feedResponse.profiles ?? []
// let groups = feedResponse.groups ?? []
//
// feedResponse.items.forEach { feedItemDto in
// let copyHistoryOwnerIds: [Int]
// if let copyHistory = feedItemDto.copyHistory {
// copyHistoryOwnerIds = copyHistory.map { $0.ownerId }
// } else {
// copyHistoryOwnerIds = [Int]()
// }
//
// let copyHistoryFromIds: [Int]
// if let copyHistory = feedItemDto.copyHistory {
// copyHistoryFromIds = copyHistory.map { $0.fromId }
// } else {
// copyHistoryFromIds = [Int]()
// }
//
// var optionalProfileAndGroupIds = [Int]()
// if let signerId = feedItemDto.signerId {
// optionalProfileAndGroupIds.append(signerId)
// }
//
// let distinctProfileAndGroupIds = Set(
// copyHistoryOwnerIds
// + copyHistoryFromIds
// + optionalProfileAndGroupIds
// + [feedItemDto.sourceId]
// )
//
// let distinctProfileIds = distinctProfileAndGroupIds
// .filter { $0 > 0 }
//
// let distinctGroupIds = distinctProfileAndGroupIds
// .filter { $0 < 0 }
// .map { abs($0) }
//
// let profilesForCurrentFeedItem = profiles.filter { distinctProfileIds.contains($0.id) }
// let groupsForCurrentFeedItem = groups.filter { distinctGroupIds.contains($0.id) }
//
// let feedItem = FeedItem(item: feedItemDto,
// profiles: profilesForCurrentFeedItem,
// groups: groupsForCurrentFeedItem)
// feedItems.append(feedItem)
// }
//
// return feedItems
return [ConversationItem]()
}
}
<file_sep>//
// FeedTarget.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
import Alamofire
enum FeedTarget {
case getFeed(count: Int, startFrom: String?)
case addLike(feedItem: FeedItem)
case deleteLike(feedItem: FeedItem)
}
extension FeedTarget: TargetType {
private var sessionManager: IUserSessionManager {
return DI.container.core.sessionManager
}
var baseURL: URL {
return URL(string: "https://api.vk.com/method/")!
}
var path: String {
switch self {
case .getFeed:
return "newsfeed.get"
case .addLike:
return "likes.add"
case .deleteLike:
return "likes.delete"
}
}
var method: HTTPMethod {
switch self {
case .getFeed:
return .get
case .addLike:
return .post
case .deleteLike:
return .post
}
}
var sampleData: Data {
return Data()
}
var task: Task {
switch self {
case .getFeed(let count, let startFrom):
let startFromString = startFrom ?? ""
return .requestParameters(parameters: ["v": 5.95,
"access_token": sessionManager.getAccessToken() ?? "",
"filters" : "post,photo",
"count": count,
"start_from": startFromString],
encoding: URLEncoding.queryString)
case .addLike(let feedItem):
let feedType = feedItem.item.type
let ownerId = feedItem.item.sourceId
let itemId = (feedItem.item.postId != nil) ? String(describing: feedItem.item.postId!) : ""
let standardParams = ["v": 5.103,
"access_token": sessionManager.getAccessToken() ?? "",
"type": feedType,
"owner_id": ownerId,
"item_id": itemId] as [String: Any]
return .requestParameters(parameters: standardParams,
encoding: URLEncoding.httpBody)
case .deleteLike(let feedItem):
let feedType = feedItem.item.type
let ownerId = feedItem.item.sourceId
let itemId = (feedItem.item.postId != nil) ? String(describing: feedItem.item.postId!) : ""
let standardParams = ["v": 5.103,
"access_token": sessionManager.getAccessToken() ?? "",
"type": feedType,
"owner_id": ownerId,
"item_id": itemId] as [String: Any]
return .requestParameters(parameters: standardParams,
encoding: URLEncoding.httpBody)
}
}
var headers: [String : String]? {
switch self {
default:
return nil
}
}
}
extension FeedTarget: VKAccessTokenAuthorizable {
var authorizationType: VKAuthorizationType {
return .oauth
}
}
<file_sep>//
// MainTabBarCoordinator.swift
// VKDemo
//
// Created by <NAME> on 08/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
protocol IMainTabBarCoordinator {
func start()
}
class MainTabBarCoordinator {
}
extension MainTabBarCoordinator: IMainTabBarCoordinator {
func start() {
guard let window = (UIApplication.shared.delegate as? AppDelegate)?.window else {
return
}
let tabBarController = UITabBarController()
let feedVC = FeedRouter().createModule()
let chatVC = ChatRouter().createModule()
feedVC.tabBarItem = UITabBarItem(tabBarSystemItem: .recents, tag: 0)
chatVC.tabBarItem = UITabBarItem(tabBarSystemItem: .contacts, tag: 1)
let controllers = [feedVC, chatVC]
tabBarController.viewControllers = controllers.map { UINavigationController(rootViewController: $0)}
window.rootViewController = tabBarController
}
}
<file_sep>//
// VkLogoView.swift
// VKDemo
//
// Created by <NAME> on 13/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
private let fillColor = UIColor(red: 1.000, green: 1.000, blue: 1.000, alpha: 1.000)
private let blueMain = UIColor(red: 0.294, green: 0.471, blue: 0.706, alpha: 1.000)
enum VkLogoViewShape {
case rect
case roundedRect
case circle
case star
}
enum VkLogoViewState {
case normal
case loading
case success
case fail
}
class VkLogoView: UIView {
private var currentShape: VkLogoViewShape
private var currentState: VkLogoViewState
private let vkLayer = CAShapeLayer()
private let backgroundLayer = CAShapeLayer()
init(radius: CGFloat, currentShape: VkLogoViewShape?, currentState: VkLogoViewState?) {
self.currentShape = currentShape ?? .roundedRect
self.currentState = currentState ?? .normal
super.init(frame: CGRect(origin: .zero, size: CGSize(width: 2*radius, height: 2*radius)))
commonInit()
}
private func commonInit() {
backgroundColor = .clear
}
// Сами буквы VK
private func getVkPath(frame: CGRect) -> UIBezierPath {
let vkPath = UIBezierPath()
vkPath.move(to: CGPoint(x: frame.minX + 0.80737 * frame.width, y: frame.minY + 0.71355 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.73445 * frame.width, y: frame.minY + 0.71355 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.64906 * frame.width, y: frame.minY + 0.64193 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.70665 * frame.width, y: frame.minY + 0.71355 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.69849 * frame.width, y: frame.minY + 0.69136 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.57644 * frame.width, y: frame.minY + 0.59481 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.60579 * frame.width, y: frame.minY + 0.60021 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.58725 * frame.width, y: frame.minY + 0.59481 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.61980 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.56175 * frame.width, y: frame.minY + 0.59481 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.59896 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.68518 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.50529 * frame.width, y: frame.minY + 0.71355 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.70312 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.55164 * frame.width, y: frame.minY + 0.71355 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.28289 * frame.width, y: frame.minY + 0.58013 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.42803 * frame.width, y: frame.minY + 0.71355 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.34315 * frame.width, y: frame.minY + 0.66664 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.16779 * frame.width, y: frame.minY + 0.33833 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.19251 * frame.width, y: frame.minY + 0.45344 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.16779 * frame.width, y: frame.minY + 0.35764 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.19279 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.16779 * frame.width, y: frame.minY + 0.32752 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.17195 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.26571 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.29834 * frame.width, y: frame.minY + 0.34606 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.28434 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.29134 * frame.width, y: frame.minY + 0.32587 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.41885 * frame.width, y: frame.minY + 0.54074 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.33399 * frame.width, y: frame.minY + 0.44994 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.39414 * frame.width, y: frame.minY + 0.54074 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.43238 * frame.width, y: frame.minY + 0.51291 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.42812 * frame.width, y: frame.minY + 0.54074 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.43238 * frame.width, y: frame.minY + 0.53645 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.43238 * frame.width, y: frame.minY + 0.40554 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.40339 * frame.width, y: frame.minY + 0.33447 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.42966 * frame.width, y: frame.minY + 0.35611 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.40339 * frame.width, y: frame.minY + 0.35205 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.42196 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.40339 * frame.width, y: frame.minY + 0.32632 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.41036 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.53653 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.34451 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.55199 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.32597 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.48897 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.56871 * frame.width, y: frame.minY + 0.50983 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.55736 * frame.width, y: frame.minY + 0.50441 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.56408 * frame.width, y: frame.minY + 0.50983 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.60270 * frame.width, y: frame.minY + 0.48742 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.57798 * frame.width, y: frame.minY + 0.50983 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.58571 * frame.width, y: frame.minY + 0.50441 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.69231 * frame.width, y: frame.minY + 0.33833 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.65523 * frame.width, y: frame.minY + 0.42871 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.69231 * frame.width, y: frame.minY + 0.33833 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.72403 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.69695 * frame.width, y: frame.minY + 0.32752 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.70550 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addLine(to: CGPoint(x: frame.minX + 0.79695 * frame.width, y: frame.minY + 0.31770 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.81900 * frame.width, y: frame.minY + 0.34451 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.81900 * frame.width, y: frame.minY + 0.31770 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.82364 * frame.width, y: frame.minY + 0.32907 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.72089 * frame.width, y: frame.minY + 0.51214 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.80974 * frame.width, y: frame.minY + 0.38700 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.72089 * frame.width, y: frame.minY + 0.51214 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.72089 * frame.width, y: frame.minY + 0.54459 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.71316 * frame.width, y: frame.minY + 0.52451 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.71008 * frame.width, y: frame.minY + 0.53069 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.77110 * frame.width, y: frame.minY + 0.59712 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.72862 * frame.width, y: frame.minY + 0.55541 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.75411 * frame.width, y: frame.minY + 0.57703 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.83261 * frame.width, y: frame.minY + 0.68257 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.80243 * frame.width, y: frame.minY + 0.63235 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.82603 * frame.width, y: frame.minY + 0.66208 * frame.height))
vkPath.addCurve(to: CGPoint(x: frame.minX + 0.80737 * frame.width, y: frame.minY + 0.71355 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.83862 * frame.width, y: frame.minY + 0.70312 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.82820 * frame.width, y: frame.minY + 0.71355 * frame.height))
vkPath.close()
return vkPath
}
// Фон
private func getBackgroundPath(frame: CGRect) -> UIBezierPath {
let backgroundPath = UIBezierPath()
backgroundPath.move(to: CGPoint(x: frame.minX + 0.65333 * frame.width, y: frame.minY + 1.00000 * frame.height))
backgroundPath.addLine(to: CGPoint(x: frame.minX + 0.34667 * frame.width, y: frame.minY + 1.00000 * frame.height))
backgroundPath.addCurve(to: CGPoint(x: frame.minX + 0.00000 * frame.width, y: frame.minY + 0.65333 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.06667 * frame.width, y: frame.minY + 1.00000 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.00000 * frame.width, y: frame.minY + 0.93333 * frame.height))
backgroundPath.addLine(to: CGPoint(x: frame.minX + 0.00000 * frame.width, y: frame.minY + 0.34666 * frame.height))
backgroundPath.addCurve(to: CGPoint(x: frame.minX + 0.34667 * frame.width, y: frame.minY + 0.00000 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.00000 * frame.width, y: frame.minY + 0.06667 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.06667 * frame.width, y: frame.minY + 0.00000 * frame.height))
backgroundPath.addLine(to: CGPoint(x: frame.minX + 0.65333 * frame.width, y: frame.minY + 0.00000 * frame.height))
backgroundPath.addCurve(to: CGPoint(x: frame.minX + 1.00000 * frame.width, y: frame.minY + 0.34666 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.93333 * frame.width, y: frame.minY + 0.00000 * frame.height), controlPoint2: CGPoint(x: frame.minX + 1.00000 * frame.width, y: frame.minY + 0.06667 * frame.height))
backgroundPath.addLine(to: CGPoint(x: frame.minX + 1.00000 * frame.width, y: frame.minY + 0.65333 * frame.height))
backgroundPath.addCurve(to: CGPoint(x: frame.minX + 0.65333 * frame.width, y: frame.minY + 1.00000 * frame.height), controlPoint1: CGPoint(x: frame.minX + 1.00000 * frame.width, y: frame.minY + 0.93333 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.93333 * frame.width, y: frame.minY + 1.00000 * frame.height))
fillColor.setFill()
backgroundPath.fill()
backgroundPath.usesEvenOddFillRule = true
backgroundPath.close()
return backgroundPath
}
private func getApplePath(frame: CGRect) -> UIBezierPath {
let bezierPath = UIBezierPath()
bezierPath.move(to: CGPoint(x: frame.minX + 0.62723 * frame.width, y: frame.minY + 0.51575 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.63882 * frame.width, y: frame.minY + 0.46806 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.62723 * frame.width, y: frame.minY + 0.49667 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.63212 * frame.width, y: frame.minY + 0.48070 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.67766 * frame.width, y: frame.minY + 0.42550 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.65323 * frame.width, y: frame.minY + 0.44021 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.67612 * frame.width, y: frame.minY + 0.42631 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.59226 * frame.width, y: frame.minY + 0.37806 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.65040 * frame.width, y: frame.minY + 0.38426 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.60795 * frame.width, y: frame.minY + 0.37885 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.50351 * frame.width, y: frame.minY + 0.40000 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.55625 * frame.width, y: frame.minY + 0.37446 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.52178 * frame.width, y: frame.minY + 0.40000 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.42635 * frame.width, y: frame.minY + 0.37911 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.48523 * frame.width, y: frame.minY + 0.40000 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.45644 * frame.width, y: frame.minY + 0.37885 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.32989 * frame.width, y: frame.minY + 0.43892 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.38698 * frame.width, y: frame.minY + 0.38012 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.35046 * frame.width, y: frame.minY + 0.40284 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.31600 * frame.width, y: frame.minY + 0.47476 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.32345 * frame.width, y: frame.minY + 0.45001 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.31908 * frame.width, y: frame.minY + 0.46214 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.35973 * frame.width, y: frame.minY + 0.67842 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.29851 * frame.width, y: frame.minY + 0.54361 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.32602 * frame.width, y: frame.minY + 0.62843 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.43304 * frame.width, y: frame.minY + 0.73850 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.37902 * frame.width, y: frame.minY + 0.70705 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.40268 * frame.width, y: frame.minY + 0.73981 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.50943 * frame.width, y: frame.minY + 0.71915 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.46262 * frame.width, y: frame.minY + 0.73748 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.47369 * frame.width, y: frame.minY + 0.71915 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.58634 * frame.width, y: frame.minY + 0.73774 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.54518 * frame.width, y: frame.minY + 0.71915 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.55497 * frame.width, y: frame.minY + 0.73850 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.65785 * frame.width, y: frame.minY + 0.67973 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.61823 * frame.width, y: frame.minY + 0.73748 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.63829 * frame.width, y: frame.minY + 0.70834 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.69000 * frame.width, y: frame.minY + 0.61218 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.68022 * frame.width, y: frame.minY + 0.64597 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.68925 * frame.width, y: frame.minY + 0.61374 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.62723 * frame.width, y: frame.minY + 0.51575 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.68925 * frame.width, y: frame.minY + 0.61168 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.62802 * frame.width, y: frame.minY + 0.58769 * frame.height))
bezierPath.close()
bezierPath.move(to: CGPoint(x: frame.minX + 0.56885 * frame.width, y: frame.minY + 0.33889 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.59329 * frame.width, y: frame.minY + 0.26333 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.58531 * frame.width, y: frame.minY + 0.31902 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.59587 * frame.width, y: frame.minY + 0.29091 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.52434 * frame.width, y: frame.minY + 0.29918 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.56962 * frame.width, y: frame.minY + 0.26410 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.54107 * frame.width, y: frame.minY + 0.27883 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.49991 * frame.width, y: frame.minY + 0.37293 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.50943 * frame.width, y: frame.minY + 0.31723 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.49630 * frame.width, y: frame.minY + 0.34534 * frame.height))
bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.56885 * frame.width, y: frame.minY + 0.33914 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.52564 * frame.width, y: frame.minY + 0.37472 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.55264 * frame.width, y: frame.minY + 0.35924 * frame.height))
bezierPath.addLine(to: CGPoint(x: frame.minX + 0.56885 * frame.width, y: frame.minY + 0.33889 * frame.height))
bezierPath.close()
bezierPath.usesEvenOddFillRule = true
blueMain.setFill()
bezierPath.fill()
blueMain.setStroke()
bezierPath.lineWidth = 2.31
bezierPath.stroke()
return bezierPath
}
private func getDotPath(frame: CGRect) -> UIBezierPath {
func fastFloor(_ x: CGFloat) -> CGFloat { return floor(x) }
//// Oval Drawing
let ovalPath = UIBezierPath(ovalIn: CGRect(x: frame.minX + fastFloor(frame.width * 0.44667 + 0.5), y: frame.minY + fastFloor(frame.height * 0.44667 + 0.5), width: fastFloor(frame.width * 0.55333 + 0.5) - fastFloor(frame.width * 0.44667 + 0.5), height: fastFloor(frame.height * 0.55333 + 0.5) - fastFloor(frame.height * 0.44667 + 0.5)))
blueMain.setFill()
ovalPath.fill()
blueMain.setStroke()
ovalPath.lineWidth = 10
ovalPath.stroke()
return ovalPath
}
private func getBlankPath(frame: CGRect) -> UIBezierPath {
func fastFloor(_ x: CGFloat) -> CGFloat { return floor(x) }
return UIBezierPath(rect: CGRect(x: frame.midX, y: frame.midY, width: 0, height: 0) )
}
required init?(coder aDecoder: NSCoder) {
self.currentShape = .roundedRect
self.currentState = .normal
super.init(coder: aDecoder)
commonInit()
}
override func prepareForInterfaceBuilder() {
super.prepareForInterfaceBuilder()
commonInit()
}
override func draw(_ rect: CGRect) {
let backgroundPath = getBackgroundPath(frame: rect)
backgroundLayer.path = backgroundPath.cgPath
backgroundLayer.fillColor = UIColor.white.cgColor
layer.addSublayer(backgroundLayer)
let vkPath = getVkPath(frame: rect)
vkLayer.path = vkPath.cgPath
vkLayer.fillColor = blueMain.cgColor
layer.addSublayer(vkLayer)
}
func showAnimation() {
// CATransaction.begin()
let animation: CABasicAnimation = CABasicAnimation(keyPath: "path")
animation.delegate = self
animation.fromValue = vkLayer.path
animation.toValue = getBlankPath(frame: bounds).cgPath
animation.duration = 0.75
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseIn)
animation.fillMode = kCAFillModeForwards
animation.autoreverses = false
vkLayer.add(animation, forKey: "pathAnimation")
// CATransaction.commit()
self.vkLayer.path = self.getBlankPath(frame: self.bounds).cgPath
}
}
extension VkLogoView: CAAnimationDelegate {
func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
let animatio: CABasicAnimation = CABasicAnimation(keyPath: "path")
// animatio.delegate = self
animatio.fromValue = self.getBlankPath(frame: self.bounds).cgPath
animatio.toValue = self.getApplePath(frame: self.bounds).cgPath
animatio.duration = 0.75
animatio.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseOut)
animatio.fillMode = kCAFillModeForwards
animatio.autoreverses = false
self.vkLayer.add(animatio, forKey: "pathAnimation")
self.vkLayer.path = self.getApplePath(frame: self.bounds).cgPath
}
}
<file_sep>//
// IFeedManager.swift
// VKDemo
//
// Created by <NAME> on 05/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol IFeedManager {
func getFeed(count: Int,
startFrom: String?,
success: @escaping ([FeedItem], String) -> Void,
failure: @escaping FailureClosure)
func addLike(for feedItem: FeedItem,
success: @escaping (FeedItem) -> Void,
failure: @escaping FailureClosure)
func deleteLike(from feedItem: FeedItem,
success: @escaping (FeedItem) -> Void,
failure: @escaping FailureClosure)
func add(feedLikeUpdateHandler: @escaping (_ feedItem: FeedItem) -> Void)
}
extension IFeedManager {
func getFeed(count: Int,
startFrom: String? = nil,
success: @escaping ([FeedItem], String) -> Void,
failure: @escaping FailureClosure) {
getFeed(count: count,
startFrom: nil,
success: success,
failure: failure)
}
}
<file_sep>//
// PresentationContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol PresentationContainerProtocol {
}
class PresentationContainer: PresentationContainerProtocol {
}
<file_sep>//
// DaoContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol DaoContainerProtocol {
var sessionDao: ISessionDao { get }
}
class DaoContainer {
private let serviceContainer = ServiceContainer()
init() {
let sessionDao = SessionDao()
serviceContainer.add(services: sessionDao)
}
}
extension DaoContainer: DaoContainerProtocol {
var sessionDao: ISessionDao {
return serviceContainer.get(service: SessionDao.self)!
}
}
<file_sep>//
// WikiPageDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct WikiPageDto: Codable {
let id: Int
let groupId: Int
let creatorId: Int
let title: String
let currentUserCanEdit: BoolInt
let currentUserCanEditAccess: BoolInt
let readRights: WikiPageAccessRights
let editRights: WikiPageAccessRights
let edited: Int
let created: Int
let editorId: Int
let viewsCount: Int
let parent: String?
let parent2: String?
let source: String?
let html: String?
let viewUrlString: String
enum WikiPageAccessRights: Int, Codable {
case groupManagersOnly = 0
case groupMembersOnly = 1
case everybody = 2
}
private enum CodingKeys: String, CodingKey {
case id
case groupId = "group_id"
case creatorId = "creator_id"
case title
case currentUserCanEdit = "current_user_can_edit"
case currentUserCanEditAccess = "current_user_can_edit_access"
case readRights = "who_can_view"
case editRights = "who_can_edit"
case edited
case created
case editorId = "editor_id"
case viewsCount = "views"
case parent
case parent2
case source
case html
case viewUrlString = "view_url"
}
}
<file_sep>//
// RepliesButton.swift
// VKDemo
//
// Created by <NAME> on 20/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import QuartzCore
@IBDesignable
class RepostsButton: UIControl {
private let plashkaFillColor = UIColor.white
private let fillColor = UIColor.clear
private let strokeColor = UIColor(white: 0.7, alpha: 1.0)
private let borderStrokeColor = #colorLiteral(red: 0.6666666667, green: 0.6666666667, blue: 0.6666666667, alpha: 0.5)
private lazy var repliesIconLayer: CAShapeLayer = {
let repliesIconPath = UIBezierPath()
repliesIconPath.move(to: CGPoint(x: 15.88, y: 17.62))
repliesIconPath.addCurve(to: CGPoint(x: 11.5, y: 22), controlPoint1: CGPoint(x: 13.46, y: 17.62), controlPoint2: CGPoint(x: 11.5, y: 19.58))
repliesIconPath.addCurve(to: CGPoint(x: 15.88, y: 26.38), controlPoint1: CGPoint(x: 11.5, y: 24.42), controlPoint2: CGPoint(x: 13.46, y: 26.38))
repliesIconPath.addCurve(to: CGPoint(x: 20.25, y: 22), controlPoint1: CGPoint(x: 18.29, y: 26.38), controlPoint2: CGPoint(x: 20.25, y: 24.42))
repliesIconPath.addCurve(to: CGPoint(x: 15.88, y: 17.62), controlPoint1: CGPoint(x: 20.25, y: 19.58), controlPoint2: CGPoint(x: 18.29, y: 17.62))
repliesIconPath.close()
repliesIconPath.move(to: CGPoint(x: 21.63, y: 19.92))
repliesIconPath.addLine(to: CGPoint(x: 26.43, y: 17.36))
repliesIconPath.addCurve(to: CGPoint(x: 29, y: 18.5), controlPoint1: CGPoint(x: 27.07, y: 18.06), controlPoint2: CGPoint(x: 27.98, y: 18.5))
repliesIconPath.addCurve(to: CGPoint(x: 32.5, y: 15), controlPoint1: CGPoint(x: 30.93, y: 18.5), controlPoint2: CGPoint(x: 32.5, y: 16.93))
repliesIconPath.addCurve(to: CGPoint(x: 29, y: 11.5), controlPoint1: CGPoint(x: 32.5, y: 13.07), controlPoint2: CGPoint(x: 30.93, y: 11.5))
repliesIconPath.addCurve(to: CGPoint(x: 25.5, y: 15), controlPoint1: CGPoint(x: 27.07, y: 11.5), controlPoint2: CGPoint(x: 25.5, y: 13.07))
repliesIconPath.addCurve(to: CGPoint(x: 25.61, y: 15.82), controlPoint1: CGPoint(x: 25.5, y: 15.28), controlPoint2: CGPoint(x: 25.54, y: 15.55))
repliesIconPath.addLine(to: CGPoint(x: 20.8, y: 18.38))
repliesIconPath.addCurve(to: CGPoint(x: 21.63, y: 19.92), controlPoint1: CGPoint(x: 21.15, y: 18.85), controlPoint2: CGPoint(x: 21.43, y: 19.37))
repliesIconPath.close()
repliesIconPath.move(to: CGPoint(x: 29, y: 25.5))
repliesIconPath.addCurve(to: CGPoint(x: 26.43, y: 26.64), controlPoint1: CGPoint(x: 27.98, y: 25.5), controlPoint2: CGPoint(x: 27.07, y: 25.94))
repliesIconPath.addLine(to: CGPoint(x: 21.63, y: 24.08))
repliesIconPath.addCurve(to: CGPoint(x: 20.8, y: 25.62), controlPoint1: CGPoint(x: 21.43, y: 24.63), controlPoint2: CGPoint(x: 21.15, y: 25.15))
repliesIconPath.addLine(to: CGPoint(x: 25.61, y: 28.18))
repliesIconPath.addCurve(to: CGPoint(x: 25.5, y: 29), controlPoint1: CGPoint(x: 25.54, y: 28.45), controlPoint2: CGPoint(x: 25.5, y: 28.72))
repliesIconPath.addCurve(to: CGPoint(x: 29, y: 32.5), controlPoint1: CGPoint(x: 25.5, y: 30.93), controlPoint2: CGPoint(x: 27.07, y: 32.5))
repliesIconPath.addCurve(to: CGPoint(x: 32.5, y: 29), controlPoint1: CGPoint(x: 30.93, y: 32.5), controlPoint2: CGPoint(x: 32.5, y: 30.93))
repliesIconPath.addCurve(to: CGPoint(x: 29, y: 25.5), controlPoint1: CGPoint(x: 32.5, y: 27.07), controlPoint2: CGPoint(x: 30.93, y: 25.5))
repliesIconPath.close()
strokeColor.setStroke()
repliesIconPath.stroke()
let layer = CAShapeLayer()
layer.path = repliesIconPath.cgPath
layer.fillColor = fillColor.cgColor
layer.strokeColor = strokeColor.cgColor
layer.lineWidth = 1.0
return layer
}()
private lazy var borderLayer: CAShapeLayer = {
let layer = CAShapeLayer()
let rectanglePath = UIBezierPath(roundedRect: CGRect(x: 1, y: 1, width: 93, height: 42), cornerRadius: 21)
fillColor.setStroke()
rectanglePath.lineWidth = 0.5
rectanglePath.lineCapStyle = .round
rectanglePath.lineJoinStyle = .round
rectanglePath.stroke()
layer.path = rectanglePath.cgPath
layer.strokeColor = borderStrokeColor.cgColor
layer.fillColor = plashkaFillColor.cgColor
layer.lineWidth = 0.5
return layer
}()
private lazy var countLabel: UILabel = {
let label = UILabel()
label.textColor = UIColor(white: 0.3, alpha: 1.0)
label.text = "123"
label.frame = CGRect(x: 42, y: 11, width: 41, height: 21)
label.font = UIFont.systemFont(ofSize: 13.0)
return label
}()
private func addLayers() {
[borderLayer, repliesIconLayer].forEach { layer.addSublayer($0) }
addSubview(countLabel)
}
private func removeLayersIfNeeded() {
layer.sublayers?.forEach { $0.removeFromSuperlayer() }
}
private func configureLayers() {
removeLayersIfNeeded()
addLayers()
for subLayer in [
borderLayer,
repliesIconLayer
] {
subLayer.frame = bounds
subLayer.setNeedsDisplay()
}
}
override init(frame: CGRect) {
super.init(frame: frame)
addLayers()
backgroundColor = .clear
}
required init?(coder: NSCoder) {
super.init(coder: coder)
addLayers()
backgroundColor = .clear
}
public func setup(repliesCount: Int) {
countLabel.text = "\(repliesCount)"
}
}
<file_sep>platform :ios, '12.0'
supports_swift_versions '>= 4.2'
use_frameworks!
source 'https://cdn.cocoapods.org/'
target 'VKDemo' do
pod 'RxSwift'
pod 'RxCocoa'
pod 'RxAlamofire'
pod 'Moya'
pod 'Reachability'
pod 'Moya/RxSwift'
pod 'RxWebKit'
pod 'SkeletonView'
end
<file_sep>import Foundation
import UIKit
import QuartzCore
protocol LikeButtonDelegate: class {
func didLike(likeButton: LikeButton?)
func didUnlike(likeButton: LikeButton?)
}
enum LikeState {
case liked
case notLiked
case inProgress
}
@IBDesignable
class LikeButton: UIControl {
private weak var delegate: LikeButtonDelegate?
private var likeState: LikeState = LikeState.notLiked
private var isIdle: Bool {
get {
return (likeState == .inProgress) ? false : true
}
}
private let fillColor = #colorLiteral(red: 0.6666666667, green: 0.6666666667, blue: 0.6666666667, alpha: 0.5)
private let heartStrokeColor = UIColor(white: 0.7, alpha: 1.0)
private let borderStrokeColor = #colorLiteral(red: 0.6666666667, green: 0.6666666667, blue: 0.6666666667, alpha: 0.5)
private let likedFillColor = #colorLiteral(red: 0.9960784314, green: 0.4901960784, blue: 0.6980392157, alpha: 1)
private let notLikedFillColor = #colorLiteral(red: 1, green: 1, blue: 1, alpha: 1)
private func showInProgress(_ show: Bool = true) {
if show {
inProgressLayer.isHidden = false
let pulseAnimation = CASpringAnimation(keyPath: "transform.scale")
pulseAnimation.duration = 0.45
pulseAnimation.fromValue = 1.0
pulseAnimation.toValue = 1.15
pulseAnimation.autoreverses = true
pulseAnimation.repeatCount = 1
pulseAnimation.initialVelocity = 0.5
pulseAnimation.damping = 0.4
let animationGroup = CAAnimationGroup()
animationGroup.duration = 1.1
animationGroup.repeatCount = .greatestFiniteMagnitude
animationGroup.animations = [pulseAnimation]
inProgressLayer.add(animationGroup, forKey: "pulse")
} else {
inProgressLayer.isHidden = true
inProgressLayer.removeAnimation(forKey: "pulse")
}
}
private func animateTo(_ state: LikeState) {
switch state {
case .inProgress:
showInProgress(true)
break
case .liked:
showInProgress(false)
let fromPath =
UIBezierPath(ovalIn: CGRect(x: 14.25, y: 13.25, width: 13, height: 13))
let toPath = UIBezierPath(cgPath: Utils.pathForCircleThatContains(rect: self.frame))
let animation = CABasicAnimation(keyPath: "path")
activeBackgroundLayer.mask = borderLayer2
animation.fromValue = fromPath.cgPath
animation.toValue = toPath.cgPath
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseOut)
animation.duration = 0.6
activeBackgroundLayer.add(animation, forKey: "pathAnimation")
activeBackgroundLayer.path = toPath.cgPath
borderLayer.fillColor = UIColor.clear.cgColor
borderLayer.setNeedsLayout()
break
case .notLiked:
showInProgress(false)
let fromPath = UIBezierPath(cgPath: Utils.pathForCircleThatContains(rect: self.frame))
let toPath =
UIBezierPath(ovalIn: CGRect(x: 14.25, y: 13.25, width: 13, height: 13))
let animation = CABasicAnimation(keyPath: "path")
animation.fromValue = fromPath
animation.toValue = toPath
animation.timingFunction = CAMediaTimingFunction(name: kCAMediaTimingFunctionEaseIn)
animation.duration = 0.6
activeBackgroundLayer.add(animation, forKey: "pathAnimation")
activeBackgroundLayer.path = toPath.cgPath
borderLayer.fillColor = UIColor.clear.cgColor
borderLayer.setNeedsLayout()
break
}
}
private func goTo(_ state: LikeState) {
switch state {
case .inProgress:
showInProgress(true)
break
case .liked:
showInProgress(false)
let activeBackgroundPath = UIBezierPath(cgPath: Utils.pathForCircleThatContains(rect: self.frame))
activeBackgroundLayer.mask = borderLayer2
activeBackgroundLayer.path = activeBackgroundPath.cgPath
borderLayer.fillColor = UIColor.clear.cgColor
borderLayer.setNeedsLayout()
break
case .notLiked:
showInProgress(false)
let activeBackgroundPath = UIBezierPath(ovalIn: CGRect(x: 14.25, y: 13.25, width: 13, height: 13))
activeBackgroundLayer.path = activeBackgroundPath.cgPath
borderLayer.fillColor = UIColor.clear.cgColor
borderLayer.setNeedsLayout()
break
}
}
private lazy var heartLayer: CAShapeLayer = {
let heartPath = UIBezierPath()
heartPath.move(to: CGPoint(x: 21.5, y: 32))
heartPath.addLine(to: CGPoint(x: 20.03, y: 30.49))
heartPath.addCurve(to: CGPoint(x: 11, y: 17.3), controlPoint1: CGPoint(x: 14.57, y: 25.21), controlPoint2: CGPoint(x: 11, y: 21.66))
heartPath.addCurve(to: CGPoint(x: 16.77, y: 11), controlPoint1: CGPoint(x: 11, y: 13.75), controlPoint2: CGPoint(x: 13.52, y: 11))
heartPath.addCurve(to: CGPoint(x: 21.5, y: 13.41), controlPoint1: CGPoint(x: 18.56, y: 11), controlPoint2: CGPoint(x: 20.34, y: 11.92))
heartPath.addCurve(to: CGPoint(x: 26.22, y: 11), controlPoint1: CGPoint(x: 22.66, y: 11.92), controlPoint2: CGPoint(x: 24.44, y: 11))
heartPath.addCurve(to: CGPoint(x: 32, y: 17.3), controlPoint1: CGPoint(x: 29.48, y: 11), controlPoint2: CGPoint(x: 32, y: 13.75))
heartPath.addCurve(to: CGPoint(x: 22.97, y: 30.49), controlPoint1: CGPoint(x: 32, y: 21.66), controlPoint2: CGPoint(x: 28.43, y: 25.21))
heartPath.addLine(to: CGPoint(x: 21.5, y: 32))
heartPath.close()
heartPath.lineCapStyle = .round
let layer = CAShapeLayer()
layer.path = heartPath.cgPath
layer.fillColor = UIColor.white.cgColor
layer.strokeColor = heartStrokeColor.cgColor
layer.lineWidth = 1
return layer
}()
private lazy var borderLayer: CAShapeLayer = {
let layer = CAShapeLayer()
let rectanglePath = UIBezierPath(roundedRect: CGRect(x: 1, y: 1, width: 93, height: 42), cornerRadius: 21)
rectanglePath.lineCapStyle = .round
rectanglePath.lineJoinStyle = .round
UIColor.clear.setFill()
rectanglePath.fill()
layer.path = rectanglePath.cgPath
layer.strokeColor = borderStrokeColor.cgColor
layer.fillColor = UIColor.white.cgColor
layer.lineWidth = 0.5
layer.backgroundColor = UIColor.clear.cgColor
return layer
}()
private lazy var borderLayer2: CAShapeLayer = {
let layer = CAShapeLayer()
let rectanglePath = UIBezierPath(roundedRect: CGRect(x: 1.5, y: 1.5, width: 92, height: 41), cornerRadius: 20.5)
rectanglePath.lineCapStyle = .round
rectanglePath.lineJoinStyle = .round
UIColor.clear.setFill()
rectanglePath.fill()
layer.path = rectanglePath.cgPath
layer.strokeColor = borderStrokeColor.cgColor
layer.fillColor = UIColor.white.cgColor
layer.lineWidth = 0
layer.backgroundColor = UIColor.clear.cgColor
return layer
}()
private lazy var inProgressLayer: CALayer = {
let inProgressLayer = CALayer()
inProgressLayer.backgroundColor = likedFillColor.cgColor
inProgressLayer.frame = CGRect(x: 0.0, y: 0.0, width: 40.0, height: 40.0)
let outerHeartLayer = CAShapeLayer()
let outerHeartPath = UIBezierPath()
outerHeartPath.move(to: CGPoint(x: 21.5, y: 33))
outerHeartPath.addLine(to: CGPoint(x: 19.89, y: 31.34))
outerHeartPath.addCurve(to: CGPoint(x: 10, y: 16.91), controlPoint1: CGPoint(x: 13.91, y: 25.57), controlPoint2: CGPoint(x: 10, y: 21.68))
outerHeartPath.addCurve(to: CGPoint(x: 16.32, y: 10), controlPoint1: CGPoint(x: 10, y: 13.01), controlPoint2: CGPoint(x: 12.76, y: 10))
outerHeartPath.addCurve(to: CGPoint(x: 21.5, y: 12.64), controlPoint1: CGPoint(x: 18.28, y: 10), controlPoint2: CGPoint(x: 20.23, y: 11))
outerHeartPath.addCurve(to: CGPoint(x: 26.67, y: 10), controlPoint1: CGPoint(x: 22.77, y: 11), controlPoint2: CGPoint(x: 24.72, y: 10))
outerHeartPath.addCurve(to: CGPoint(x: 33, y: 16.91), controlPoint1: CGPoint(x: 30.24, y: 10), controlPoint2: CGPoint(x: 33, y: 13.01))
outerHeartPath.addCurve(to: CGPoint(x: 23.11, y: 31.34), controlPoint1: CGPoint(x: 33, y: 21.68), controlPoint2: CGPoint(x: 29.09, y: 25.57))
outerHeartPath.addLine(to: CGPoint(x: 21.5, y: 33))
outerHeartPath.close()
outerHeartPath.lineWidth = 0.1
outerHeartPath.lineCapStyle = .round
outerHeartLayer.path = outerHeartPath.cgPath
inProgressLayer.mask = outerHeartLayer
inProgressLayer.isHidden = true
return inProgressLayer
}()
private lazy var activeBackgroundLayer: CAShapeLayer = {
let layer = CAShapeLayer()
layer.path = UIBezierPath(ovalIn: CGRect(x: 14.25, y: 13.25, width: 13, height: 13)).cgPath
layer.fillColor = likedFillColor.cgColor
layer.strokeColor = likedFillColor.cgColor
layer.backgroundColor = likedFillColor.cgColor
return layer
}()
private lazy var countLabel: UILabel = {
let label = UILabel()
label.textColor = UIColor(white: 0.3, alpha: 1.0)
label.frame = CGRect(x: 42, y: 11, width: 41, height: 21)
label.font = UIFont.systemFont(ofSize: 13.0)
return label
}()
private func addLayers() {
[activeBackgroundLayer,
borderLayer,
borderLayer2,
inProgressLayer,
heartLayer].forEach { layer.addSublayer($0) }
addSubview(countLabel)
}
private func removeLayersIfNeeded() {
layer.sublayers?.forEach { $0.removeFromSuperlayer() }
}
private func configureLayers() {
removeLayersIfNeeded()
borderLayer.backgroundColor = UIColor.clear.cgColor
addLayers()
}
override init(frame: CGRect) {
super.init(frame: frame)
addLayers()
backgroundColor = .clear
addTarget(self, action: #selector(buttonPressed), for: .touchUpInside)
}
required init?(coder: NSCoder) {
super.init(coder: coder)
addLayers()
backgroundColor = .clear
addTarget(self,
action: #selector(buttonPressed),
for: .touchUpInside)
}
func setup(likesCount: Int,
state: LikeState,
animated: Bool,
delegate: LikeButtonDelegate? = nil) {
self.likeState = state
self.delegate = delegate
countLabel.text = "\(likesCount)"
if animated {
animateTo(state)
} else {
goTo(state)
}
}
private func getNextStateOnButtonPressed() -> LikeState {
switch likeState {
case .inProgress:
return .inProgress
case .liked:
return .notLiked
case .notLiked:
return .inProgress
}
}
@objc private func buttonPressed() {
guard isIdle else { return }
let nextState = getNextStateOnButtonPressed()
animateTo(nextState)
switch nextState {
case .liked:
break
case .notLiked:
delegate?.didUnlike(likeButton: self)
case .inProgress:
delegate?.didLike(likeButton: self)
break
}
}
}
<file_sep>//
// FeedViewInput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
protocol FeedViewInput: AnyObject {
func setup(feedItems: [FeedItem])
func addNewFeedItems(feedItems: [FeedItem])
func updateFeedAfterLike(feedItem: FeedItem)
}
<file_sep>//
// BaseCoordinator.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
public enum RequestError: Error {
case error(code: HTTPStatusCode)
case mappingError
case badResponse
case unauthorized
case noInternetConnection
case other(error: CustomRequestError)
case vkError(error: VkError)
}
public struct ErrorDto: Codable {
let error: VkError
}
public struct VkError: Codable {
let errorCode: Int
let errorMsg: String
private enum CodingKeys: String, CodingKey {
case errorCode = "error_code"
case errorMsg = "error_msg"
}
}
public protocol CustomRequestError {
var title: String { get }
var description: String? { get }
}
public struct TextRequestError: CustomRequestError {
public let title: String
public let description: String?
public init(title: String, description: String? = nil) {
self.title = title
self.description = description
}
}
<file_sep>//
// UIView+Constraints.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
extension UIView {
func addSubviewOnBorders(_ subview: UIView) {
self.addSubview(subview)
subview.translatesAutoresizingMaskIntoConstraints = false
self.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "H:|[subview]|", options: [], metrics: nil, views: ["subview": subview]))
self.addConstraints(NSLayoutConstraint.constraints(withVisualFormat: "V:|[subview]|", options: [], metrics: nil, views: ["subview": subview]))
}
func addSubviewOnCenter(_ subview: UIView) {
self.addSubview(subview)
subview.translatesAutoresizingMaskIntoConstraints = false
self.addConstraints([NSLayoutConstraint(item: subview, attribute: .centerX, relatedBy: .equal, toItem: self, attribute: .centerX, multiplier: 1, constant: 0)])
self.addConstraints([NSLayoutConstraint(item: subview, attribute: .centerY, relatedBy: .equal, toItem: self, attribute: .centerY, multiplier: 1, constant: 0)])
}
}
<file_sep>//
// ChatViewController.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import UIKit
class ChatViewController: BaseViewController, ViperModuleTransitionHandler {
var presenter: ChatViewOutput?
override func viewDidLoad() {
super.viewDidLoad()
presenter?.viewLoaded()
}
}
extension ChatViewController: ChatViewInput {
func setup() {
}
}
<file_sep>//
// AuthViewModel.swift
// VKDemo
//
// Created by <NAME> on 13/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import RxSwift
class AuthViewModel {
// Input
let showSignInScreen: AnyObserver<Void>
// Output
let authorize: Observable<Void>
init() {
let _showSignInScreen = PublishSubject<Void>()
self.showSignInScreen = _showSignInScreen.asObserver()
self.authorize = _showSignInScreen.asObservable()
}
}
<file_sep>//
// UIBezierPath+MyPaths.swift
// VKDemo
//
// Created by <NAME> on 15/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
private let fillColor = #colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1)
private let blueMain = UIColor(red: 0.294, green: 0.471, blue: 0.706, alpha: 1.000)
//extension UIBezierPath {
// static func heartPath(frame: CGRect, fillColor: UIColor = fillColor, strokeColor: UIColor = blueMain) -> UIBezierPath {
// /*
// let bezierPath = UIBezierPath()
// bezierPath.move(to: CGPoint(x: frame.minX + 0.50000 * frame.width, y: frame.minY + 0.98298 * frame.height))
// bezierPath.addLine(to: CGPoint(x: frame.minX + 0.43192 * frame.width, y: frame.minY + 0.90900 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.01373 * frame.width, y: frame.minY + 0.30128 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.17906 * frame.width, y: frame.minY + 0.66591 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.01373 * frame.width, y: frame.minY + 0.50209 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.28118 * frame.width, y: frame.minY + 0.01064 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.01373 * frame.width, y: frame.minY + 0.13747 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.13043 * frame.width, y: frame.minY + 0.01064 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.50000 * frame.width, y: frame.minY + 0.12161 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.36384 * frame.width, y: frame.minY + 0.01064 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.44651 * frame.width, y: frame.minY + 0.05291 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.71882 * frame.width, y: frame.minY + 0.01064 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.55349 * frame.width, y: frame.minY + 0.05291 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.63616 * frame.width, y: frame.minY + 0.01064 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.98627 * frame.width, y: frame.minY + 0.30128 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.86957 * frame.width, y: frame.minY + 0.01064 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.98627 * frame.width, y: frame.minY + 0.13747 * frame.height))
// bezierPath.addCurve(to: CGPoint(x: frame.minX + 0.56808 * frame.width, y: frame.minY + 0.90900 * frame.height), controlPoint1: CGPoint(x: frame.minX + 0.98627 * frame.width, y: frame.minY + 0.50209 * frame.height), controlPoint2: CGPoint(x: frame.minX + 0.82094 * frame.width, y: frame.minY + 0.66591 * frame.height))
// bezierPath.addLine(to: CGPoint(x: frame.minX + 0.50000 * frame.width, y: frame.minY + 0.98298 * frame.height))
//
// bezierPath.close()
// fillColor.setFill()
// bezierPath.fill()
// strokeColor.setStroke()
// bezierPath.lineWidth = 8
// bezierPath.stroke()
// */
//
// }
//}
<file_sep>//
// UIView+Init.swift
// VKDemo
//
// Created by <NAME> on 05.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
extension UIView {
static func loadFromXib() -> UIView? {
let bundle = Bundle(for: self)
let nib = UINib(nibName: String(describing: self), bundle: bundle)
let view = nib.instantiate(withOwner: self, options: nil).first as? UIView
return view
}
}
<file_sep>//
// NoteDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct NoteDto: Codable {
let id: Int
let ownerId: Int
let title: String
let text: String
let date: Int
let commentsCount: Int
let readComments: Int?
let viewUrlString: String
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case title
case text
case date
case commentsCount = "comments"
case readComments = "read_comments"
case viewUrlString = "view_url"
}
}
<file_sep>//
// WelcomeCoordinator.swift
// VKDemo
//
// Created by <NAME> on 08/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import RxSwift
import RxCocoa
import RxWebKit
import WebKit
import SafariServices
class WelcomeCoordinator: BaseCoordinator<Void> {
private let window: UIWindow
private let _authorizedSubject: PublishSubject<Void>
private let authorizedObservable: Observable<Void>
init(window: UIWindow) {
self.window = window
_authorizedSubject = PublishSubject<Void>()
authorizedObservable = _authorizedSubject.asObservable()
super.init()
}
override func start() -> Observable<Void> {
let authVc = AuthViewController.loadFromXib()
let authVm = AuthViewModel()
let navigationController = UINavigationController(rootViewController: authVc)
authVc.viewModel = authVm
window.rootViewController = navigationController
window.makeKeyAndVisible()
authVm.authorize
.subscribe(onNext:{ [weak self] in
guard let `self` = self,
let rootVC = self.window.rootViewController else { return }
_ = self.showSignInVC(on: rootVC)
.filter({ signInResult -> Bool in
return signInResult == .success
})
.subscribe({ _ in
self._authorizedSubject.onNext(Void())
})
})
.disposed(by: disposeBag)
return authorizedObservable
}
private func showSignInVC(on rootViewController: UIViewController) -> Observable<SignInCoordinationResult> {
let signInCoordinator = SignInCoordinator(rootViewController: rootViewController)
return coordinate(to: signInCoordinator)
}
}
<file_sep>//
// PollDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct PollDto: Codable {
let id: Int
let ownerId: Int
let created: Int
let question: String
let votesCount: Int
let answers: [PossibleAnswer]
let isAnonymous: Bool
let isMultiple: Bool
let answerIds: [Int]
let endDate: Int
let isClosed: Bool
let isBoard: Bool
let canEdit: Bool
let canVote: Bool
let canReport: Bool
let canShare: Bool
let authorId: Int
let photo: PhotoDto?
let background: PollBackground?
let friends: [FriendIdDto]?
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case created
case question
case votesCount = "votes"
case answers
case isAnonymous = "anonymous"
case isMultiple = "multiple"
case answerIds = "answer_ids"
case endDate = "end_date"
case isClosed = "closed"
case isBoard = "is_board"
case canEdit = "can_edit"
case canVote = "can_vote"
case canReport = "can_report"
case canShare = "can_share"
case authorId = "author_id"
case photo
case background
case friends
}
}
struct PossibleAnswer: Codable {
let id: Int
let text: String
let votes: Int
let rate: Double
}
struct FriendIdDto: Codable {
let id: Int
}
struct PollBackground: Codable {
let id: Int
let type: PollBackgroundType
let angle: Int?
let color: String
let width: Int?
let height: Int?
let images: [PhotoDto]?
let points: [PollBackgroundPoint]?
enum PollBackgroundType: String, Codable {
case gradient = "gradient"
case tile = "tile"
}
struct PollBackgroundPoint: Codable {
let position: Double
let color: String
}
}
<file_sep>//
// ChatRouterInput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import Foundation
protocol ChatRouterInput {
}
<file_sep>//
// AppCoordinator.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import RxSwift
protocol IAppCoordinator {
func start() -> Observable<Void>
}
class AppCoordinator: BaseCoordinator<Void>, IAppCoordinator {
private var window: UIWindow {
guard let window = (UIApplication.shared.delegate as? AppDelegate)?.window else {
fatalError("No UIWindow found!")
}
return window
}
private lazy var mainCoordinator = DI.container.coordinator.mainTabBarCoordinator
override func start() -> Observable<Void> {
// Если нет пользака
let welcomeCoordinator = WelcomeCoordinator(window: window)
let observable = coordinate(to: welcomeCoordinator)
// Вернуть когда понадобится авторизация
// _ = observable.subscribe({ event in
// let mainCoordinator = MainTabBarCoordinator()
// mainCoordinator.start()
// })
// Вернуть когда НЕ НУЖНА авторизация
let mainCoordinator = MainTabBarCoordinator()
mainCoordinator.start()
return .never()
// Если есть пользак, сразу переход в MainTabBarCoordinator,
// который не реактивный
}
}
<file_sep>//
// PlayerView.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import AVKit
import AVFoundation
protocol PlayerViewDelegate: class {
func videoTapped(videoUrl: String, formTime: CMTime?)
}
class PlayerView: UIView {
private var playerLayer: AVPlayerLayer?
private var url: String?
private weak var delegate: PlayerViewDelegate?
private var statusObservation: NSKeyValueObservation?
private let loadingView = UIView()
private let activityIndicator = UIActivityIndicatorView(frame: CGRect.zero)
override func awakeFromNib() {
super.awakeFromNib()
self.backgroundColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)
let player = AVPlayer()
player.isMuted = true
if #available(iOS 12.0, *) {
player.preventsDisplaySleepDuringVideoPlayback = false
}
let playerLayer = AVPlayerLayer(player: player)
self.playerLayer = playerLayer
self.layer.addSublayer(playerLayer)
playerLayer.frame = self.bounds
let videoButton = UIButton()
videoButton.addTarget(self, action: #selector(videoButtonTapped), for: .touchUpInside)
self.addSubviewOnBorders(videoButton)
loadingView.addSubviewOnCenter(activityIndicator)
activityIndicator.startAnimating()
self.addSubviewOnBorders(loadingView)
}
override func layoutSubviews() {
super.layoutSubviews()
playerLayer?.frame = self.bounds
}
func playVideo(urlString: String, delegate: PlayerViewDelegate) {
if playerLayer?.player?.currentItem != nil,
url == urlString {
playerLayer?.player?.play()
return
}
guard let encodedUrlString = urlString.addingPercentEncoding(withAllowedCharacters: .urlFragmentAllowed),
let videoURL = URL(string: encodedUrlString) else {
return
}
self.url = urlString
self.delegate = delegate
let videoPlayerItem = AVPlayerItem(url: videoURL)
NotificationCenter.default.removeObserver(self)
NotificationCenter.default.addObserver(forName: .AVPlayerItemDidPlayToEndTime,
object: videoPlayerItem,
queue: .main) { [weak self] _ in
self?.playerLayer?.player?.seek(to: kCMTimeZero)
self?.playerLayer?.player?.play()
}
playerLayer?.player?.replaceCurrentItem(with: videoPlayerItem)
playerLayer?.player?.seek(to: kCMTimeZero)
playerLayer?.player?.play()
showLoading()
statusObservation?.invalidate()
statusObservation = videoPlayerItem.observe(\.status, options: [.new]) { [weak self] (_, _) in
if videoPlayerItem.status == .readyToPlay {
self?.loadingView.isHidden = true
}
}
}
func showLoading() {
loadingView.isHidden = false
activityIndicator.startAnimating()
}
func stopPlaying() {
playerLayer?.player?.pause()
}
@objc func videoButtonTapped() {
guard let url = url else {
return
}
delegate?.videoTapped(videoUrl: url, formTime: playerLayer?.player?.currentTime())
}
deinit {
playerLayer?.player?.replaceCurrentItem(with: nil)
playerLayer?.removeFromSuperlayer()
statusObservation?.invalidate()
statusObservation = nil
NotificationCenter.default.removeObserver(self)
}
}
<file_sep>//
// SessionDao.swift
// VKDemo
//
// Created by <NAME> on 31/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol ISessionDao {
func saveAccessToken(_ accessToken: String?)
func getAccessToken() -> String?
}
class SessionDao {
private lazy var userDefaults = UserDefaults.standard
private lazy var accessTokenKey = "accessToken"
}
extension SessionDao: ISessionDao {
func saveAccessToken(_ accessToken: String?) {
userDefaults.set(accessToken, forKey: accessTokenKey)
}
func getAccessToken() -> String? {
return userDefaults.object(forKey: accessTokenKey) as? String
}
}
<file_sep>//
// FeedManager.swift
// VKDemo
//
// Created by <NAME> on 05/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
class FeedManager {
private let feedProvider: MoyaProvider<FeedTarget>
private let feedParser: IFeedParser
private let onFeedLikeUpdate = Events<FeedItem>()
init(feedProvider: MoyaProvider<FeedTarget>,
feedParser: IFeedParser) {
self.feedProvider = feedProvider
self.feedParser = feedParser
}
}
extension FeedManager: IFeedManager {
func addLike(for feedItem: FeedItem, success: @escaping (FeedItem) -> Void, failure: @escaping FailureClosure) {
_ = feedProvider.request(.addLike(feedItem: feedItem), success: { [weak self, feedItem] (likesCountResponse: LikesCountResponse) in
var feedItem = feedItem
feedItem.item.likes?.count = likesCountResponse.likesCount
feedItem.item.likes?.userLikes = BoolInt.true
self?.onFeedLikeUpdate.raise(feedItem)
success(feedItem)
}, failure: { error in
failure(error)
})
}
func deleteLike(from feedItem: FeedItem,
success: @escaping (FeedItem) -> Void,
failure: @escaping FailureClosure) {
_ = feedProvider.request(.deleteLike(feedItem: feedItem), success: { [weak self, feedItem] (likesCountResponse: LikesCountResponse) in
var feedItem = feedItem
feedItem.item.likes?.count = likesCountResponse.likesCount
feedItem.item.likes?.userLikes = BoolInt.false
self?.onFeedLikeUpdate.raise(feedItem)
success(feedItem)
}, failure: { error in
failure(error)
})
}
func getFeed(count: Int,
startFrom: String?,
success: @escaping ([FeedItem], String) -> Void,
failure: @escaping FailureClosure) {
_ = feedProvider.request(.getFeed(count: count, startFrom: startFrom),
success: { (feedResponse: FeedResponse) in
let feedItems = self.feedParser.parse(feedResponse: feedResponse)
success(feedItems, feedResponse.nextFrom)
}, failure: { error in
failure(error)
})
}
func add(feedLikeUpdateHandler: @escaping (_ feedItem: FeedItem) -> Void) {
onFeedLikeUpdate.addHandler(feedLikeUpdateHandler)
}
}
<file_sep>//
// Events.swift
// VKDemo
//
// Created by <NAME> on 08.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
class Events<T> {
typealias EventHandler = (T) -> Void
private var eventHandlers = [EventHandler]()
func addHandler(_ handler: @escaping EventHandler) {
eventHandlers.append(handler)
}
func raise(_ data: T) {
for handler in eventHandlers {
handler(data)
}
}
func clearHandlers() {
eventHandlers.removeAll()
}
}
<file_sep>//
// FeedViewController.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import UIKit
class FeedViewController: BaseViewController, ViperModuleTransitionHandler {
var presenter: FeedViewOutput?
@IBOutlet var dataSource: FeedTableViewDataSource!
override func viewDidLoad() {
super.viewDidLoad()
setupAppearance()
if let output = presenter as? (FeedViewOutput & SocialButtonsDelegate){
dataSource.output = output
}
DispatchQueue.main.asyncAfter(deadline: .now() + 2.5) {
self.presenter?.viewLoaded()
}
}
private func setupAppearance() {
navigationController?.navigationBar.prefersLargeTitles = true
}
}
extension FeedViewController: FeedViewInput {
func setup(feedItems: [FeedItem]) {
dataSource.setup(with: feedItems)
}
func addNewFeedItems(feedItems: [FeedItem]) {
dataSource.addNew(feedItems: feedItems)
}
func updateFeedAfterLike(feedItem: FeedItem) {
dataSource.updateFeedItem(feedItem: feedItem)
}
}
extension FeedViewController: UITableViewDelegate {
}
<file_sep>//
// BoolInt.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
enum BoolInt: Int, Codable {
case `true` = 1
case `false` = 0
}
extension BoolInt {
func asBool() -> Bool {
return self == .true
}
}
<file_sep>//
// ViperModuleOutput.swift
// Vanilla
//
// Created by <NAME> on 11/05/2017.
// Copyright © 2017 BrightBox. All rights reserved.
//
import Foundation
public protocol ViperModuleOutputProtocol: class {
}
<file_sep>//
// MoyaProvider+Mapping.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
import Alamofire
public typealias FailureClosure = (RequestError) -> Void
extension MoyaProvider {
open func request<T: Codable>(_ target: Target,
callbackQueue: DispatchQueue? = .none,
progress: ProgressBlock? = .none,
success: @escaping (T) -> Void,
failure: @escaping FailureClosure) -> Cancellable {
return request(target, callbackQueue: callbackQueue, progress: progress) { result in
switch result {
case let .success(moyaResponse):
do {
_ = try moyaResponse.filterSuccessfulStatusCodes()
}
catch _ {
// Неправильный статус код
failure(.error(code: HTTPStatusCode(rawValue: moyaResponse.statusCode) ?? .badRequest))
}
let jsonDecoder = JSONDecoder()
jsonDecoder.dateDecodingStrategy = .secondsSince1970
do {
let mappedData = try jsonDecoder.decode(MappedResponse<T>.self, from: moyaResponse.data)
// Все прошло хорошо, размапилось и не было VkError
success(mappedData.response)
} catch let error1 {
do {
let errorMappedData = try jsonDecoder.decode(ErrorDto.self, from: moyaResponse.data)
// Пришел VkError
failure(.vkError(error: errorMappedData.error))
return
} catch {
// Ошибка маппинга
guard let decodingError = error1 as? DecodingError else {
failure(.mappingError)
return
}
switch decodingError {
case .typeMismatch(let type, let context):
print(type)
print(context)
break
case .dataCorrupted(let context):
print("dataCorrupted, context:")
print(context)
break
case .keyNotFound(let codingKey, let context):
let a = context.codingPath
let b = context.debugDescription
let c = context.underlyingError
let d = codingKey
print("keyNotFound")
break
case .valueNotFound(let type, let context):
print("valueNotFound")
break
}
failure(.mappingError)
}
}
case .failure(_):
// Сюда не должен попадать
fatalError("FATAL ERROR!!!")
}
}
}
}
// Не используется
extension MoyaProvider {
// TODO: удалить или привести к нормальному виду!
// Идея: нам может понадобиться response object
open func request1<T: Codable>(_ target: Target,
callbackQueue: DispatchQueue? = .none,
progress: ProgressBlock? = .none,
success: @escaping (RequestResponse<T>) -> Void,
failure: @escaping FailureClosure) -> Cancellable {
return request(target, callbackQueue: callbackQueue, progress: progress) { result in
switch result {
case let .success(moyaResponse):
do {
let decoder = JSONDecoder()
let filteredResponse = try moyaResponse.filterSuccessfulStatusCodes()
let json = try filteredResponse.mapJSON() // type Any
let mappedData = try decoder.decode(T.self, from: filteredResponse.data)
print("JSON: \(json)")
print("Mapped data: \(mappedData)")
// Do something with your json.
}
catch let error {
// Here we get either statusCode error or jsonMapping error.
// TODO: handle the error == best. comment. ever.
print(error)
}
case .failure(let error):
print("case .failure(error):")
print(error)
}
}
}
}
// TODO:
//extension Bool {
// override public init(from decoder: Decoder) throws {
// do {
// let container = try decoder.singleValueContainer()
// self = try container.decode(Bool.self)
// } catch {
// assertionFailure("ERROR: \(error)")
// // TODO: automatically send a report about a corrupted data
// self = false
// }
// }
//}
extension Bool {
init(_ int: Int) {
self = (int == 1)
}
}
<file_sep>//
// AudioDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct AudioDto: Codable {
let id: Int
let ownerId: Int
let artist: String
let title: String
let duration: Int
let urlString: String
let lyricsId: Int?
let albumId: Int?
let genreId: Int?
let date: Int
let noSearch: Int?
let isHq: Bool?
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case artist
case title
case duration
case urlString = "url"
case lyricsId = "lyrics_id"
case albumId = "album_id"
case genreId = "genre_id"
case date
case noSearch = "no_search"
case isHq = "is_hq"
}
}
<file_sep>//
// FeedRouter.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import UIKit
class FeedRouter {
private weak var transitionHandler: ViperModuleTransitionHandler?
func createModule() -> UIViewController {
let vc = FeedViewController.loadFromXib()
transitionHandler = vc
let presenter = FeedPresenter(view: vc,
router: self,
feedManager: DI.container.data.feedManager)
vc.presenter = presenter
vc.title = "Feed"
vc.view.backgroundColor = UIColor.orange
return vc
}
func openModule(with transitionHandler: ViperModuleTransitionHandler, transitionStyle: TransitionStyle) {
transitionHandler.openModule(vc: createModule(), style: transitionStyle)
}
}
extension FeedRouter: FeedRouterInput {
}
<file_sep>//
// ViperViewController.swift
// Vanilla
//
// ViperViewController is essentially a mere UIViewController
// which hides boilerplate ModuleViewWithOutput protocol code
// and overrides prepare(for segue) func.
//
// This is a base class for UIViewController instances which
// implement VIPER and it should not be edited.
//
// Created by <NAME> on 6/5/17.
// Copyright © 2017 BrightBox. All rights reserved.
//
/// IMPORTANT:
/// THIS .swift FILE SHOULD NOT BE CHANGED OR UPDATED!
/// ANY MODIFICATIONS CAN LEAD TO LOSS OF INTEGRITY!
import UIKit
public protocol ModuleViewWithOutput {
var presenter: ViperModuleInputProtocol { get }
}
public protocol ViperViewControllerProtocol: ModuleViewWithOutput {
associatedtype OutputType
var output: OutputType! { get set }
}
open class ViperViewController: UIViewController {
open override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
handleSegue(segue, sender: sender)
}
}
open class ViperTableViewController: UITableViewController {
open override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
handleSegue(segue, sender: sender)
}
}
open class ViperCollectionViewController: UICollectionViewController {
open override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
self.handleSegue(segue, sender: sender)
}
}
class ViperNavigationController: UINavigationController {
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
handleSegue(segue, sender: sender)
}
}
public extension ViperViewControllerProtocol where Self: UIViewController {
var presenter: ViperModuleInputProtocol {
guard let output = output as? ViperModuleInputProtocol else {
fatalError("Presenter should conform 'ViperModuleInputProtocol'")
}
return output
}
}
extension ViperViewController: ViperModuleTransitionHandler {}
extension ViperNavigationController: ViperModuleTransitionHandler {}
extension ViperTableViewController: ViperModuleTransitionHandler {}
extension ViperCollectionViewController: ViperModuleTransitionHandler {}
<file_sep>//
// GroupDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct GroupDto: Codable {
let id: Int
let name: String
let screenName: String
let accessType: GroupAccessType
let deactivated: String?
let isAdmin: BoolInt?
let adminLevel: GroupAdminLevelType?
let isMember: BoolInt?
let isAdvertiser: BoolInt?
let invitedBy: Int?
let type: GroupType
let photo50: String
let photo100: String
let photo200: String
private enum CodingKeys: String, CodingKey {
case id
case name
case screenName = "screen_name"
case accessType = "is_closed"
case deactivated
case isAdmin = "is_admin"
case adminLevel = "admin_level"
case isMember = "is_member"
case isAdvertiser = "is_advertiser"
case invitedBy = "invited_by"
case type
case photo50 = "photo_50"
case photo100 = "photo_100"
case photo200 = "photo_200"
}
enum GroupAccessType: Int, Codable {
case open = 0
case closed = 1
case `private` = 2
}
enum GroupAdminLevelType: Int, Codable {
case moderator
case editor
case admin
}
enum GroupType: String, Codable {
case group
case page
case event
}
}
<file_sep>//
// SignInViewModel.swift
// VKDemo
//
// Created by <NAME> on 27/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import RxSwift
import RxCocoa
import WebKit
class SignInViewModel {
private let sessionManager: UserSessionManager
// Input
let decidePolicyNavigationActionObserver: AnyObserver<URL>
// Output
let authorized: Observable<SignInCoordinationResult>
init(sessionManager: UserSessionManager) {
let _authorized = PublishSubject<SignInCoordinationResult>()
authorized = _authorized.asObservable()
decidePolicyNavigationActionObserver = AnyObserver(eventHandler: { event in
switch event {
case .next(let url):
guard let parametersSubstring = url.absoluteString.split(separator: "#").last else { return }
let parametersComponents = parametersSubstring.split(separator: "&")
var parametersDict = [String: String]()
parametersComponents.forEach({ substring in
let keyValueArray = substring.split(separator: "=")
parametersDict[String(keyValueArray[0])] = String(keyValueArray[1])
})
sessionManager.saveAccessToken(parametersDict["access_token"])
_authorized.onNext(.success)
break
default:
break
}
})
self.sessionManager = sessionManager
}
}
<file_sep>import PlaygroundSupport
import Foundation
import UIKit
@IBDesignable
class LikeButton: UIControl {
private let fillColor = UIColor(red: 0.000, green: 0.000, blue: 0.000, alpha: 1.000)
private let likedFillColor = #colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1)
private let notLikedFillColor = #colorLiteral(red: 1, green: 1, blue: 1, alpha: 1)
private let blueMain = UIColor(red: 0.294, green: 0.471, blue: 0.706, alpha: 1.000)
override var frame: CGRect {
didSet {
configureAppearance()
}
}
override func layoutSublayers(of layer: CALayer) {
super.layoutSublayers(of: layer)
for layer in [
borderLayer,
heartLayer
] {
layer.bounds = bounds
layer.position = center
}
configureAppearance()
}
private lazy var heartLayer: CAShapeLayer = {
let bezierPath = UIBezierPath()
bezierPath.move(to: CGPoint(x: 50, y: 81))
bezierPath.addLine(to: CGPoint(x: 45.66, y: 76.97))
bezierPath.addCurve(to: CGPoint(x: 19, y: 41.81), controlPoint1: CGPoint(x: 29.54, y: 62.91), controlPoint2: CGPoint(x: 19, y: 53.43))
bezierPath.addCurve(to: CGPoint(x: 36.05, y: 25), controlPoint1: CGPoint(x: 19, y: 32.34), controlPoint2: CGPoint(x: 26.44, y: 25))
bezierPath.addCurve(to: CGPoint(x: 50, y: 31.42), controlPoint1: CGPoint(x: 41.32, y: 25), controlPoint2: CGPoint(x: 46.59, y: 27.45))
bezierPath.addCurve(to: CGPoint(x: 63.95, y: 25), controlPoint1: CGPoint(x: 53.41, y: 27.45), controlPoint2: CGPoint(x: 58.68, y: 25))
bezierPath.addCurve(to: CGPoint(x: 81, y: 41.81), controlPoint1: CGPoint(x: 73.56, y: 25), controlPoint2: CGPoint(x: 81, y: 32.34))
bezierPath.addCurve(to: CGPoint(x: 54.34, y: 76.97), controlPoint1: CGPoint(x: 81, y: 53.43), controlPoint2: CGPoint(x: 70.46, y: 62.91))
bezierPath.addLine(to: CGPoint(x: 50, y: 81))
bezierPath.close()
UIColor.white.setFill()
bezierPath.fill()
UIColor.lightGray.setStroke()
bezierPath.lineWidth = 6.5
bezierPath.miterLimit = 4
bezierPath.stroke()
let layer = CAShapeLayer()
layer.path = bezierPath.cgPath
layer.fillColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0).cgColor
layer.strokeColor = #colorLiteral(red: 0.6000000238, green: 0.6000000238, blue: 0.6000000238, alpha: 1).cgColor
return layer
}()
private lazy var borderLayer: CAShapeLayer = {
let layer = CAShapeLayer()
let rectanglePath = UIBezierPath(roundedRect: CGRect(x: 4, y: 7, width: 201.5, height: 91.5), cornerRadius: 39)
fillColor.setStroke()
rectanglePath.lineWidth = 3
rectanglePath.lineCapStyle = .round
rectanglePath.lineJoinStyle = .round
rectanglePath.stroke()
layer.path = rectanglePath.cgPath
layer.strokeColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0).cgColor
layer.fillColor = #colorLiteral(red: 0.501960814, green: 0.501960814, blue: 0.501960814, alpha: 1).cgColor
return layer
}()
override func prepareForInterfaceBuilder() {
super.prepareForInterfaceBuilder()
configureAppearance()
}
private func configureAppearance() {
configureLayers()
configOther()
}
private func configureLayers() {
layer.addSublayer(borderLayer)
layer.addSublayer(heartLayer)
}
private func configOther() {
}
override init(frame: CGRect) {
super.init(frame: frame)
configureAppearance()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
backgroundColor = .clear
configureAppearance()
}
}
let likeButton = LikeButton(frame: CGRect(x: 0, y: 0, width: 210, height: 105))
PlaygroundPage.current.liveView = likeButton
<file_sep>//
// UserSessionManager.swift
// VKDemo
//
// Created by <NAME> on 28/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
class UserSessionManager {
private let sessionDao: ISessionDao
private var accessToken: String?
init(sessionDao: ISessionDao) {
self.sessionDao = sessionDao
}
}
extension UserSessionManager: IUserSessionManager {
func clearSession() {
accessToken = nil
sessionDao.saveAccessToken(nil)
}
func getAccessToken() -> String? {
return accessToken ?? sessionDao.getAccessToken()
}
func saveAccessToken(_ accessToken: String?) {
self.accessToken = accessToken
sessionDao.saveAccessToken(accessToken)
}
}
<file_sep>import UIKit
var str = "Hello, playground"
//// Present the view controller in the Live View window
//PlaygroundPage.current.liveView = button
<file_sep>//
// BaseCoordinator.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import RxSwift
class BaseCoordinator<ResultType> {
typealias CoordinationResult = ResultType
let disposeBag = DisposeBag()
private let identifier = UUID()
private var childCoordinators: [UUID: Any] = [UUID: Any]()
private func store<T>(coordinator: BaseCoordinator<T>) {
childCoordinators[coordinator.identifier] = coordinator
}
private func free<T>(coordinator: BaseCoordinator<T>) {
childCoordinators[coordinator.identifier] = nil
}
func coordinate<T>(to coordinator: BaseCoordinator<T>) -> Observable<T> {
store(coordinator: coordinator)
return coordinator.start()
.do(onNext: { [weak self] _ in
self?.free(coordinator: coordinator)
})
}
func start() -> Observable<ResultType> {
fatalError("Should be implemneted in concrete implementation!")
}
}
<file_sep>//
// FeedTableViewDataSource.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class FeedTableViewDataSource: NSObject {
weak var output: (FeedViewOutput & SocialButtonsDelegate)?
private let cellName = FeedTableViewCell.cellName
private var feedCellModels = [FeedCellModel]()
@IBOutlet weak var tableView: UITableView! {
didSet {
initialSetup()
}
}
private lazy var templateFeedTextView: UITextView = {
let textView = FeedTextView(frame: CGRect(x: 0.0,
y: 0.0,
width: tableView.frame.size.width - 32.0,
height: 0.0))
return textView
}()
private func templateFeedTextViewHeight(text: String) -> Double {
guard text.count > 0 else {
return 0.0
}
templateFeedTextView.text = text
let fixedWidth = templateFeedTextView.frame.size.width
let textViewSize = templateFeedTextView.sizeThatFits(CGSize(width: fixedWidth, height: CGFloat.greatestFiniteMagnitude))
return Double(textViewSize.height)
}
func setup(with feedItems: [FeedItem]) {
feedCellModels = feedItems.map({ FeedCellModel(feedItem: $0) })
tableView.reloadData()
tableView.refreshControl?.endRefreshing()
}
func addNew(feedItems: [FeedItem]) {
let newFeedCellModels = feedItems.map({ FeedCellModel(feedItem: $0) })
let startIndex = feedCellModels.count
feedCellModels += newFeedCellModels
let endIndex = feedCellModels.count - 1
let newIndexPaths = Array(startIndex...endIndex).map { IndexPath(item: $0, section: 0)
}
tableView.insertRows(at: newIndexPaths, with: .none)
}
func updateFeedItem(feedItem: FeedItem) {
guard let indexOfCellModelToUpdate = feedCellModels.firstIndex (where: {
$0.feedItem.item.postId == feedItem.item.postId
}) else { return }
let updatedFeedCellModel = FeedCellModel.init(feedItem: feedItem)
feedCellModels[indexOfCellModelToUpdate] = updatedFeedCellModel
}
}
// MARK: - Private functions
extension FeedTableViewDataSource {
private func initialSetup() {
tableView.register(UINib(nibName: cellName, bundle: nil), forCellReuseIdentifier: cellName)
tableView.dataSource = self
tableView.delegate = self
tableView.estimatedRowHeight = 300.0
tableView.tableFooterView = FeedLoadMoreFooterView.loadFromXib()
let refreshControl = UIRefreshControl(frame: .zero)
refreshControl.addTarget(self,
action: #selector(didPulledRefresh(_:)),
for: .valueChanged)
tableView.refreshControl = refreshControl
}
@objc private func didPulledRefresh(_: UIRefreshControl) {
output?.didRefresh()
}
}
// MARK: - UITableViewDataSource, UITableViewDelegate
extension FeedTableViewDataSource: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, viewForHeaderInSection section: Int) -> UIView? {
guard feedCellModels.count == 0,
let cell = tableView.dequeueReusableCell(withIdentifier: cellName) as? FeedTableViewCell else { return nil }
cell.showSkeleton()
return cell
}
func tableView(_ tableView: UITableView, heightForHeaderInSection section: Int) -> CGFloat {
guard feedCellModels.count == 0 else { return CGFloat(0) }
return CGFloat(300.0)
}
func tableView(_ tableView: UITableView, willDisplay cell: UITableViewCell, forRowAt indexPath: IndexPath) {
if indexPath.row == (feedCellModels.count - 1) {
DispatchQueue.main.asyncAfter(deadline: .now() + 2.2) {
self.output?.didScrollForMore()
}
}
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return feedCellModels.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
guard let cell = tableView.dequeueReusableCell(withIdentifier: cellName, for: indexPath) as? FeedTableViewCell else {
return UITableViewCell()
}
let feedCellModel = feedCellModels[indexPath.row]
// TODO: output as! SocialButtonsDelegate переделать
if let outputAsSocialButtonsDelegate = output as? SocialButtonsDelegate {
}
cell.setup(feedCellModel: feedCellModel, delegate: output as! SocialButtonsDelegate)
return cell
}
func tableView(_ tableView: UITableView, heightForRowAt indexPath: IndexPath) -> CGFloat {
// 82+169+44+52
if indexPath.row >= feedCellModels.count { return 347.0 }
let feedCellModel = feedCellModels[indexPath.row]
let textViewHeight = templateFeedTextViewHeight(text: feedCellModel.feedItem.item.text ?? "")
let collectionViewHeigh = collectionViewHeight(for: feedCellModel.feedItem.item.attachments ?? [AttachmentDto]())
// ceil(Double(feedCellModel.photos.count) / 2.0) * 125.0 + 10
let headerViewHeight = 82.0
let socialButtonsViewHeight = 44.0
let sumMarginsHeight = 50.5 + 2.0 * 0.5
let overallHeight = headerViewHeight +
textViewHeight +
Double(collectionViewHeigh) +
socialButtonsViewHeight +
sumMarginsHeight
return CGFloat(overallHeight + 200.0)
}
func collectionViewHeight(for attachments: [AttachmentDto]) -> CGFloat {
if (attachments.count == 1),
let photo = attachments.first?.photo,
photo.sizes.count > 0 {
let photoSizes = photo.sizes
let photoSizesOrderArray = ["z", "y", "x", "w"]
let properPhotoSize = photoSizes.sorted(by: {
guard let index0 = photoSizesOrderArray.index(of: $0.type) else { return false }
guard let index1 = photoSizesOrderArray.index(of: $1.type) else { return true }
return index0 <= index1
}).first
let width = tableView.frame.width - 32.0
let height = CGFloat(properPhotoSize?.height ?? 0) / CGFloat(properPhotoSize?.width ?? 1) * width
return height
}
let height = CGFloat(120.0)
let photosCount = attachments.filter({
$0.type == .photo
}).count
return ceil(CGFloat(photosCount) / 2.0) * height
}
}
<file_sep>//
// AttachmentPhotoCollectionViewCell.swift
// VKDemo
//
// Created by <NAME> on 29/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class AttachmentPhotoCollectionViewCell: UICollectionViewCell {
static let cellName: String = String(describing: AttachmentPhotoCollectionViewCell.self)
var photoDto: PhotoDto?
var photoUrlString: String?
let photoSizesOrderArray = ["z", "y", "x", "w"]
@IBOutlet weak var imageView: UIImageView!
func setup(photoDto: PhotoDto) {
self.photoDto = photoDto
photoUrlString?.append("")
guard let properSizedPhotoDto = photoDto.sizes.correctSize() else { return }
// sorted(by: {
// guard let index0 = photoSizesOrderArray.index(of: $0.type) else { return false }
// guard let index1 = photoSizesOrderArray.index(of: $1.type) else { return true }
// return index0 <= index1
// }).first else {
// return
// }
imageView.image = nil
properSizedPhotoDto.url.getAsyncImageFromUrl(completion: { [weak self] image, urlStrig in
guard urlStrig == properSizedPhotoDto.url else { return }
self?.imageView.image = image
})
}
}
<file_sep>//
// UserDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct UserDto: Codable {
let id: Int
let firstName: String
let lastName: String
let isClosed: Bool
let canAccessClosed: Bool
let sex: Sex
let screenName: String
let photo50: String
let photo100: String
let online: BoolInt
enum Sex: Int, Codable {
case unknown = 0
case male
case female
}
private enum CodingKeys: String, CodingKey {
case id
case firstName = "first_name"
case lastName = "last_name"
case isClosed = "is_closed"
case canAccessClosed = "can_access_closed"
case sex
case screenName = "screen_name"
case photo50 = "photo_50"
case photo100 = "photo_100"
case online
}
}
<file_sep>//
// VkEnterButton.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class VkEnterButton: UIButton {
private let activeBgColor = UIColor(red: 60/255, green: 100/255, blue: 160/255, alpha: 1.0)
private let highlightedBgColor = UIColor(red: 62/255, green: 90/255, blue: 140/255, alpha: 1.0)
private let disabledBgColor = UIColor(red: 105/255, green: 145/255, blue: 205/255, alpha: 1.0)
private let activeFontColor = UIColor(red: 250/255, green: 250/255, blue: 250/255, alpha: 1.0)
private let highlightedFontColor = UIColor(red: 156/255, green: 176/255, blue: 212/255, alpha: 1.0)
private let disabledFontColor = UIColor(red: 170/255, green: 170/255, blue: 190/255, alpha: 1.0)
override init(frame: CGRect) {
super.init(frame: frame)
commonInit()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
commonInit()
}
private func commonInit() {
setTitle("Войти", for: .normal)
setBackgroundColor(color: activeBgColor, forState: .normal)
setBackgroundColor(color: highlightedBgColor, forState: .highlighted)
setBackgroundColor(color: disabledBgColor, forState: .disabled)
setTitleColor(activeFontColor, for: .normal)
setTitleColor(disabledFontColor, for: .disabled)
setTitleColor(highlightedFontColor, for: .highlighted)
layer.cornerRadius = 15.0
}
}
<file_sep>//
// AuthViewController.swift
// VKDemo
//
// Created by <NAME> on 13/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import RxSwift
import RxCocoa
class AuthViewController: UIViewController {
@IBOutlet weak var vkLogoView: VkLogoView!
@IBOutlet weak var logoViewCenterYConstraint: NSLayoutConstraint!
@IBOutlet weak var logoViewTopConstraint: NSLayoutConstraint!
@IBOutlet weak var enterButton: VkEnterButton!
@IBOutlet weak var emailTextField: UITextField!
@IBOutlet weak var passwordTextField: UITextField!
var viewModel: AuthViewModel!
private let disposeBag = DisposeBag()
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
setupBindings()
startWelcomeAnimation()
}
private func setupBindings() {
enterButton.rx
.tap
.bind(to: viewModel.showSignInScreen)
.disposed(by: disposeBag)
emailTextField.rx
.controlEvent(.editingDidBegin)
.do(onNext: { [weak self] in
self?.emailTextField.endEditing(true)
})
.bind(to: viewModel.showSignInScreen)
.disposed(by: disposeBag)
passwordTextField.rx
.controlEvent(.editingDidBegin)
.do(onNext: { [weak self] in
self?.passwordTextField.endEditing(true)
})
.bind(to: viewModel.showSignInScreen)
.disposed(by: disposeBag)
}
private func startWelcomeAnimation() {
logoViewCenterYConstraint.isActive = false
logoViewTopConstraint.isActive = true
UIView.animate(withDuration: 1.0, animations: {
self.vkLogoView.transform = CGAffineTransform(scaleX: 0.5, y: 0.5)
self.view.layoutIfNeeded()
}) { completed in
self.enterButton.isEnabled = true
}
}
}
<file_sep>//
// VideoDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct VideoDto: Codable {
let id: Int
let ownerId: Int
let title: String
let description: String
let duration: Int
let photo130: String
let photo320: String
let photo640: String?
let photo800: String?
let photo1280: String?
let firstFrame130: String?
let firstFrame320: String?
let firstFrame640: String?
let firstFrame800: String?
let firstFrame1280: String?
let date: Int
let addingDate: Int?
let views: Int
let comments: Int
let player: String?
let platform: String?
let canEdit: Bool?
let canAdd: BoolInt?
let isPrivate: Bool?
let accessKey: String
let processing: Bool?
let live: BoolInt?
let upcoming: BoolInt?
let isFavorite: Bool
let canComment: BoolInt?
let canRepost: BoolInt?
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case title
case description
case duration
case photo130 = "photo_130"
case photo320 = "photo_320"
case photo640 = "photo_640"
case photo800 = "photo_800"
case photo1280 = "photo_1280"
case firstFrame130 = "first_frame_130"
case firstFrame320 = "first_frame_320"
case firstFrame640 = "first_frame_640"
case firstFrame800 = "first_frame_800"
case firstFrame1280 = "first_frame_1280"
case date
case addingDate = "adding_date"
case views
case comments
case player
case platform
case canEdit = "can_edit"
case canAdd = "can_add"
case isPrivate = "is_private"
case accessKey = "access_key"
case processing
case live
case upcoming
case isFavorite = "is_favorite"
case canRepost = "can_repost"
case canComment = "can_comment"
}
}
struct VideoListDto: Codable {
let count: Int
let items: [VideoDto]
}
<file_sep>//
// BaseViewController.swift
// Vanilla
//
// Created by <NAME> on 5/21/18.
// Copyright © 2018 BrightBox. All rights reserved.
//
import UIKit
class BaseViewController: UIViewController {
var statusBarStyle: UIStatusBarStyle = .default {
didSet {
setNeedsStatusBarAppearanceUpdate()
}
}
override var preferredStatusBarStyle: UIStatusBarStyle {
return statusBarStyle
}
override func viewDidLoad() {
super.viewDidLoad()
hideKeyboardOnTappingAround()
view.backgroundColor = UIColor.white
}
func setNavBarStyle(_ style: BarStyle) {
navigationItem.backBarButtonItem?.title = ""
switch style {
case .standard:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
statusBarStyle = .default
setLargeTitlesEnabled(false)
setSeparatorHidden(false)
case .standardNoSeparator:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
statusBarStyle = .default
setLargeTitlesEnabled(false)
setSeparatorHidden(true)
case .translucent:
navigationController?.isNavigationBarHidden = true
statusBarStyle = .lightContent
case .transparent:
navigationController?.navigationBar.isTranslucent = true
navigationController?.navigationBar.backgroundColor = .clear
navigationController?.navigationBar.barTintColor = .clear
statusBarStyle = .lightContent
setSeparatorHidden(true)
case .hidden:
navigationController?.isNavigationBarHidden = true
case .standardLargeTitles:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
statusBarStyle = .default
setLargeTitlesEnabled(true)
setSeparatorHidden(false)
}
navigationController?.navigationBar.isExclusiveTouch = true
}
private func setLargeTitlesEnabled(_ enabled: Bool) {
guard #available(iOS 11.0, *) else {
return
}
if navigationController?.navigationBar.prefersLargeTitles == true {
navigationItem.largeTitleDisplayMode = enabled ? .automatic : .never
} else if enabled {
navigationController?.navigationBar.prefersLargeTitles = true
}
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
setNavBarStyle(.standard)
}
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
view.setInCallSafeFrame()
}
deinit {
NotificationCenter.default.removeObserver(self)
}
}
// MARK: - Hide Keyboard When View Tapped
extension BaseViewController {
private func hideKeyboardOnTappingAround() {
let tap: UITapGestureRecognizer = UITapGestureRecognizer(target: self,
action: #selector(hideKeyboard))
tap.cancelsTouchesInView = false
view.addGestureRecognizer(tap)
}
@objc private func hideKeyboard() {
view.endEditing(true)
}
}
// MARK: - Keyboard Show/Hide Observers (Subclassing)
extension BaseViewController {
open func addKeyboardWillShowObserver(selector: Selector) {
NotificationCenter.default.addObserver(self,
selector: selector,
name: .UIKeyboardWillShow,
object: nil)
}
open func addKeyboardWillHideObserver(selector: Selector) {
NotificationCenter.default.addObserver(self,
selector: selector,
name: .UIKeyboardWillHide,
object: nil)
}
open func addKeyboardDidHideObserver(selector: Selector) {
NotificationCenter.default.addObserver(self,
selector: selector,
name: .UIKeyboardDidHide,
object: nil)
}
}
<file_sep>//
// UIViewController+Init.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
extension UIViewController {
static func loadFromXib(bundle: Bundle? = nil, overriddenXib: String? = nil) -> Self {
return self.init(nibName: overriddenXib ?? String(describing: self), bundle: bundle)
}
}
<file_sep>//
// FeedPresenter.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import UIKit
class FeedPresenter {
private weak var view: FeedViewInput?
private let router: FeedRouterInput
private let feedManager: IFeedManager
var feedItems = [FeedItem]()
var nextFrom: String?
init(view: FeedViewInput,
router: FeedRouterInput,
feedManager: IFeedManager) {
self.view = view
self.router = router
self.feedManager = feedManager
}
private func loadFeed(take: Int,
startFrom: String?,
completion: (() -> Void)? = nil) {
feedManager.getFeed(count: take, startFrom: startFrom, success: { [weak self] feedItems, nextFrom in
self?.nextFrom = nextFrom
let isDownloadingFromBeginning = (startFrom == nil)
if isDownloadingFromBeginning {
self?.feedItems = feedItems
self?.view?.setup(feedItems: feedItems)
} else {
self?.feedItems += feedItems
self?.view?.addNewFeedItems(feedItems: feedItems)
completion?()
}
}) { [weak self] error in
completion?()
}
}
private func likeFeed(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void)) {
feedManager.addLike(for: feedItem,
success: { [weak self] feedItem in
completion(feedItem)
}) { [weak self] error in
}
}
private func unlikeFeed(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void)) {
feedManager.deleteLike(from: feedItem, success: { feedItem in
completion(feedItem)
}) { error in
}
}
}
extension FeedPresenter: FeedViewOutput {
func didRefresh() {
loadFeed(take: 15, startFrom: nil)
}
func viewLoaded() {
feedManager.add(feedLikeUpdateHandler: { [weak self] feedItem in
guard let strongSelf = self else { return }
strongSelf.feedItems.updateWithFeedItem(feedItem)
strongSelf.view?.updateFeedAfterLike(feedItem: feedItem)
})
loadFeed(take: 15, startFrom: nil, completion: nil)
}
func didScrollForMore() {
loadFeed(take: 15, startFrom: nextFrom, completion: nil)
}
}
extension FeedPresenter: SocialButtonsDelegate {
func didLike(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void)) {
likeFeed(feedItem: feedItem, completion: completion)
}
func didUnlike(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void)) {
unlikeFeed(feedItem: feedItem, completion: completion)
}
}
<file_sep>//
// FeedItemCellViewModel.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct FeedCellModel {
let sourceIconUrl: String
let sourceName: String
let dateAdded: Date
let likes: LikesCountDto?
let comments: CommentsCountDto?
let reposts: RepostCountDto?
let photos: [PhotoDto]
let videos: [VideoDto]
let audios: [AudioDto]
let docs: [DocDto]
let links: [LinkDto]
let notes: [NoteDto]
let polls: [PollDto]
let wikiPages: [WikiPageDto]
let marketItems: [MarketItem]
let marketAlbums: [MarketAlbum]
let stickers: [Sticker]
let podcasts: [PodcastDto]
var feedItem: FeedItem
// Для большой картинки подходят:
// w, z, y, x, m, s
// Для превью картинки, если картинка 1:
// w, z, y, x, m, s
init(feedItem: FeedItem) {
self.feedItem = feedItem
// Для шапки: sourceName, sourceIconUrl
// dateAdded
if feedItem.item.sourceId > 0 {
// Post napisan user'om
guard let sourceUserDto = feedItem.profiles?
.filter({ $0.id == feedItem.item.sourceId })
.first else {
fatalError("No source user found!")
}
sourceName = "\(sourceUserDto.lastName) \(sourceUserDto.firstName)"
sourceIconUrl = sourceUserDto.photo100
} else {
// Post napisan group'oy
let sourceGroupId = -1 * feedItem.item.sourceId
guard let sourceGroupDto = feedItem.groups?
.filter({ $0.id == sourceGroupId })
.first else {
fatalError("No source user found!")
}
sourceName = sourceGroupDto.name
sourceIconUrl = sourceGroupDto.photo100
}
dateAdded = feedItem.item.date
// ************************* //
// Attachments //
self.photos = feedItem.item.attachments?.compactMap({ $0.photo }) ?? [PhotoDto]()
self.videos = feedItem.item.attachments?.compactMap({ $0.video }) ?? [VideoDto]()
self.audios = feedItem.item.attachments?.compactMap({ $0.audio }) ?? [AudioDto]()
self.docs = feedItem.item.attachments?.compactMap({ $0.doc }) ?? [DocDto]()
self.links = feedItem.item.attachments?.compactMap({ $0.link }) ?? [LinkDto]()
self.notes = feedItem.item.attachments?.compactMap({ $0.note }) ?? [NoteDto]()
self.polls = feedItem.item.attachments?.compactMap({ $0.poll }) ?? [PollDto]()
self.wikiPages = feedItem.item.attachments?.compactMap({ $0.wikiPage }) ?? [WikiPageDto]()
self.marketItems = feedItem.item.attachments?.compactMap({ $0.marketItem }) ?? [MarketItem]()
self.marketAlbums = feedItem.item.attachments?.compactMap({ $0.marketAlbum }) ?? [MarketAlbum]()
self.stickers = feedItem.item.attachments?.compactMap({ $0.sticker }) ?? [Sticker]()
self.podcasts = feedItem.item.attachments?.compactMap({ $0.podcast }) ?? [PodcastDto]()
self.likes = feedItem.item.likes
self.comments = feedItem.item.comments
self.reposts = feedItem.item.reposts
// ****************** //
// Likes, shares, views and info for these buttons
// Mojno vzat' iz feedItem, mojno perenesti yavno
// TODO: podumat'!
// ****************** //
}
}
<file_sep>//
// CircleImageView.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class CircleImageView: UIImageView {
override func layoutSubviews() {
super.layoutSubviews()
layer.cornerRadius = bounds.size.height / 2.0
}
}
<file_sep>//
// DataContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
protocol DataContainerProtocol {
var feedManager: IFeedManager { get }
var messagesManager: IMessagesManager { get }
}
class DataContainer {
private let serviceContainer = ServiceContainer()
private let core: CoreContainerProtocol
private let parser: ParserContainerProtocol
init(core: CoreContainerProtocol,
parser: ParserContainerProtocol) {
self.core = core
self.parser = parser
let authPlugin = VKAccessTokenPlugin(userSessionManager: core.sessionManager)
let loggerPlugin = NetworkLoggerPlugin(verbose: true)
let feedProvider = MoyaProvider<FeedTarget>(plugins: [authPlugin, loggerPlugin])
serviceContainer.add(services: feedProvider)
let feedManager = FeedManager(feedProvider: feedProvider,
feedParser: parser.feedParser)
serviceContainer.add(services: feedManager)
let messagesProvider = MoyaProvider<MessagesTarget>(plugins: [authPlugin, loggerPlugin])
serviceContainer.add(services: messagesProvider)
let messagesManager = MessagesManager(messagesProvider: messagesProvider,
messagesParser: parser.messagesParser)
serviceContainer.add(services: messagesManager)
}
}
extension DataContainer: DataContainerProtocol {
var messagesManager: IMessagesManager {
serviceContainer.get(service: MessagesManager.self)!
}
var feedManager: IFeedManager {
return serviceContainer.get(service: FeedManager.self)!
}
}
<file_sep>//
// ImageLoadingCache.swift
// Vanilla
//
// Created by <NAME> on 15.03.18.
// Copyright © 2018 BrightBox. All rights reserved.
//
import Foundation
class ImageLoadingCache {
static let sharedCache: NSCache = { () -> NSCache<AnyObject, AnyObject> in
let cache = NSCache<AnyObject, AnyObject>()
cache.name = "ImageLoadingCache"
cache.countLimit = 200
cache.totalCostLimit = 10*1024*1024
return cache
}()
}
<file_sep>//
// FeedViewOutput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
protocol FeedViewOutput: class {
func viewLoaded()
func didRefresh()
func didScrollForMore()
}
<file_sep>//
// FeedParser.swift
// VKDemo
//
// Created by <NAME> on 22/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct FeedItem {
var item: FeedItemDto
let profiles: [UserDto]?
let groups: [GroupDto]?
}
extension Array where Element == FeedItem {
mutating func updateWithFeedItem(_ feedItem: FeedItem) {
guard let index = firstIndex (where: { $0.item.postId == feedItem.item.postId }) else { return }
self[index] = feedItem
}
}
protocol IFeedParser {
func parse(feedResponse: FeedResponse) -> [FeedItem]
}
class FeedParser: IFeedParser {
func parse(feedResponse: FeedResponse) -> [FeedItem] {
var feedItems = [FeedItem]()
let profiles = feedResponse.profiles ?? []
let groups = feedResponse.groups ?? []
feedResponse.items.forEach { feedItemDto in
let copyHistoryOwnerIds: [Int]
if let copyHistory = feedItemDto.copyHistory {
copyHistoryOwnerIds = copyHistory.map { $0.ownerId }
} else {
copyHistoryOwnerIds = [Int]()
}
let copyHistoryFromIds: [Int]
if let copyHistory = feedItemDto.copyHistory {
copyHistoryFromIds = copyHistory.map { $0.fromId }
} else {
copyHistoryFromIds = [Int]()
}
var optionalProfileAndGroupIds = [Int]()
if let signerId = feedItemDto.signerId {
optionalProfileAndGroupIds.append(signerId)
}
let distinctProfileAndGroupIds = Set(
copyHistoryOwnerIds
+ copyHistoryFromIds
+ optionalProfileAndGroupIds
+ [feedItemDto.sourceId]
)
let distinctProfileIds = distinctProfileAndGroupIds
.filter { $0 > 0 }
let distinctGroupIds = distinctProfileAndGroupIds
.filter { $0 < 0 }
.map { abs($0) }
let profilesForCurrentFeedItem = profiles.filter { distinctProfileIds.contains($0.id) }
let groupsForCurrentFeedItem = groups.filter { distinctGroupIds.contains($0.id) }
let feedItem = FeedItem(item: feedItemDto,
profiles: profilesForCurrentFeedItem,
groups: groupsForCurrentFeedItem)
feedItems.append(feedItem)
}
return feedItems
}
}
<file_sep>//
// ChatViewOutput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
protocol ChatViewOutput {
func viewLoaded()
}
<file_sep>//
// AttachmentDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct AttachmentDto: Codable {
let type: AttachmentType
let photo: PhotoDto?
let video: VideoDto?
let audio: AudioDto?
let doc: DocDto?
let link: LinkDto?
let note: NoteDto?
let poll: PollDto?
let wikiPage: WikiPageDto?
let marketItem: MarketItem?
let marketAlbum: MarketAlbum?
let sticker: Sticker?
let podcast: PodcastDto?
// case album = "album" TODO:доделать
// case photosList = "photos_list" Массив из строк, содержащих идентификаторы фотографий
private enum CodingKeys: String, CodingKey {
case type
case photo
case video
case audio
case doc
case link
case note
case poll
case wikiPage = "page"
case marketItem
case marketAlbum
case sticker
case podcast
}
}
enum AttachmentType: String, Codable {
case photo = "photo"
case video = "video"
case audio = "audio"
case doc = "doc"
case link = "link"
case note = "note"
case poll = "poll"
case page = "page"
case album = "album"
case photosList = "photos_list"
case market = "market"
case marketAlbum = "market_album"
case prettyCards = "pretty_cards"
case podcast = "podcast"
}
<file_sep>//
// LinkDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct LinkDto: Codable {
let urlString: String
let title: String
let caption: String?
let description: String
let photo: PhotoDto?
let product: Product?
let button: ButtonDto?
let previewPage: String?
let previewUrl: String?
private enum CodingKeys: String, CodingKey {
case urlString = "url"
case title
case caption
case description
case photo
case product
case button
case previewPage = "preview_page"
case previewUrl = "preview_url"
}
}
struct ButtonDto: Codable {
let title: String
let action: ButtonActionDto
}
struct ButtonActionDto: Codable {
let type: String
let urlString: String
private enum CodingKeys: String, CodingKey {
case type
case urlString = "url"
}
}
<file_sep>//
// ChatRouter.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
import UIKit
class ChatRouter {
private weak var transitionHandler: ViperModuleTransitionHandler?
func createModule() -> UIViewController {
let vc = ChatViewController.loadFromXib()
transitionHandler = vc
let presenter = ChatPresenter(view: vc,
router: self,
messagesManager: DI.container.data.messagesManager)
vc.presenter = presenter
vc.title = "Chat"
vc.view.backgroundColor = UIColor.blue
return vc
}
func openModule(with transitionHandler: ViperModuleTransitionHandler, transitionStyle: TransitionStyle) {
transitionHandler.openModule(vc: createModule(), style: transitionStyle)
}
}
extension ChatRouter: ChatRouterInput {
}
<file_sep>//
// AppDelegate.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import RxSwift
@UIApplicationMain class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
let disposeBag = DisposeBag()
private let appCoordinator = DI.container.coordinator.appCoordinator
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
setupAppearance()
_ = appCoordinator.start()
return true
}
func applicationWillResignActive(_ application: UIApplication) {
}
func applicationDidEnterBackground(_ application: UIApplication) {
}
func applicationWillEnterForeground(_ application: UIApplication) {
}
func applicationDidBecomeActive(_ application: UIApplication) {
}
func applicationWillTerminate(_ application: UIApplication) {
}
private func setupAppearance() {
UINavigationBar.appearance().isTranslucent = false
UINavigationBar.appearance().barTintColor = UIColor.blueMain
UINavigationBar.appearance().tintColor = .white
UINavigationBar.appearance().shadowImage = UIImage()
UINavigationBar.appearance().setBackgroundImage(UIImage(), for: .default)
}
}
<file_sep>//
// PodcastDto.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct PodcastDto: Codable {
let artist: String
let id: Int
let ownerId: Int
let title: String
let duration: Int
let trackCode: String
let url: String
let date: Int
let lyricsId: Int
let noSearch: BoolInt
let isExplicit: BoolInt?
let podcastInfo: PodcastInfoDto
private enum CodingKeys: String, CodingKey {
case artist
case id
case ownerId = "owner_id"
case title
case duration
case trackCode = "track_code"
case url
case date
case lyricsId = "lyrics_id"
case noSearch = "no_search"
case isExplicit = "is_explicit"
case podcastInfo = "podcast_info"
}
}
struct PodcastInfoDto: Codable {
let cover: PodcastInfoCoverDto
let plays: Int
let position: Int?
let isFavorite: Bool?
let description: String
private enum CodingKeys: String, CodingKey {
case cover
case plays
case position
case isFavorite = "is_favorite"
case description
}
}
struct PodcastInfoCoverDto: Codable {
let sizes: [PhotoSizeCopy]
}
<file_sep>//
// BaseCoordinator.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Alamofire
public enum DateError: Error {
case invalidDate
}
public enum Result<Value> {
case success(Value)
case failure(RequestError)
public var value: Value? {
switch self {
case .success(let value):
return value
case .failure:
return nil
}
}
public var error: Error? {
switch self {
case .success:
return nil
case .failure(let error):
return error
}
}
}
public struct NoData: Codable {}
public struct RequestResponse<T: Codable> {
public private(set) var data: Data?
public private(set) var mappedData: T!
public private(set) var response: HTTPURLResponse?
public var code: HTTPStatusCode {
return response != nil ? HTTPStatusCode(HTTPResponse: response)! : .internalServerError
}
public private(set) var error: Error?
public private(set) var result: Result<T>
init(data: Data?, response: URLResponse?, error: Error?, successCodes: Range<Int>? = nil) {
let decoder = JSONDecoder()
// decoder.dateDecodingStrategy = .custom({ (decoder) -> Date in
// let container = try decoder.singleValueContainer()
// let dateStr = try container.decode(String.self)
//
// if let date = Date.create(from: dateStr, format: "yyyy-MM-dd") {
// return date
// }
// if let date = Date.create(from: dateStr, format: "yyyy-MM-dd'T'HH:mm") {
// return date
// }
// if let date = Date.create(from: dateStr, format: "yyyy-MM-dd'T'HH:mm:ss") {
// return date
// }
// if let date = Date.create(from: dateStr, format: "yyyy-MM-dd'T'HH:mm:ss.SSSZ") {
// return date
// }
// if let date = Date.create(from: dateStr, format: "yyyy-MM-dd'T'HH:mm:ssZ") {
// return date
// }
// throw DateError.invalidDate
// })
self.response = response as? HTTPURLResponse
self.data = data
self.error = error
let httpCode = HTTPStatusCode(HTTPResponse: self.response) ?? .internalServerError
guard httpCode != .unauthorized else {
result = .failure(RequestError.unauthorized)
return
}
guard (error as? URLError)?.code != .notConnectedToInternet else {
result = .failure(RequestError.noInternetConnection)
return
}
guard let code = self.response?.statusCode, 200...299 ~= code else {
self.result = .failure(RequestError.error(code: httpCode))
return
}
guard let data = data else {
result = .failure(RequestError.error(code: httpCode))
return
}
if let noData = NoData() as? T {
self.result = .success(noData)
return
}
do {
self.mappedData = try decoder.decode(T.self, from: data)
self.result = .success(mappedData)
} catch let error {
print(error.localizedDescription)
self.error = error
result = .failure(RequestError.error(code: httpCode))
}
}
}
<file_sep>//
// FeedTableViewCell.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import SkeletonView
protocol SocialButtonsDelegate: class {
func didLike(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void))
func didUnlike(feedItem: FeedItem, completion: @escaping ((FeedItem) -> Void))
}
class FeedTableViewCell: UITableViewCell {
static let cellName: String = String(describing: FeedTableViewCell.self)
@IBOutlet weak var sourceIconImageView: UIImageView!
@IBOutlet weak var sourceNameLabel: UILabel!
@IBOutlet weak var dateAddedLabel: UILabel!
@IBOutlet weak var textView: UITextView!
@IBOutlet weak var likeButton: LikeButton!
@IBOutlet weak var commentsButton: CommentsButton!
@IBOutlet weak var repostsButton: RepostsButton!
@IBOutlet weak var previewCollectionView: UICollectionView! {
didSet {
previewCollectionViewDataSource.collectionView = previewCollectionView
}
}
private lazy var previewCollectionViewDataSource = { AttachmentsCollectionViewDataSource()
}()
private var cellModel: FeedCellModel?
private weak var delegate: SocialButtonsDelegate?
@IBOutlet weak var textViewHeightConstraint: NSLayoutConstraint!
@IBOutlet weak var collectionViewHeightConstraint: NSLayoutConstraint!
func setup(feedCellModel: FeedCellModel, delegate: SocialButtonsDelegate? = nil) {
self.cellModel = feedCellModel
self.delegate = delegate
let feedText = feedCellModel.feedItem.item.text
let feedTextLength = feedText?.count ?? 0
textView.text = feedText
sourceNameLabel.text = feedCellModel.sourceName
dateAddedLabel.text = "Date added"
let likesCount = feedCellModel.likes?.count ?? 0
let userLiked = (feedCellModel.likes?.userLikes == BoolInt.true) ? true : false
let commentsCount = feedCellModel.comments?.count ?? 0
let repostsCount = feedCellModel.reposts?.count ?? 0
likeButton.setup(likesCount: likesCount,
state: userLiked ? .liked : .notLiked,
animated: false,
delegate: self)
commentsButton.setup(commentsCount: commentsCount)
repostsButton.setup(repliesCount: repostsCount)
feedCellModel.sourceIconUrl.getAsyncImageFromUrl { [weak self] image, url in
guard let strongSelf = self,
let cellModel = strongSelf.cellModel,
cellModel.sourceIconUrl == url,
let image = image,
let strongImageView = strongSelf.sourceIconImageView else {
return
}
UIView.transition(with: strongImageView,
duration: 0.3,
options: .transitionCrossDissolve,
animations: {
strongImageView.image = image
}, completion: nil)
}
if feedTextLength > 0 {
let fixedWidth = textView.frame.size.width
let newSize = textView.sizeThatFits(CGSize(width: fixedWidth, height: CGFloat.greatestFiniteMagnitude))
textViewHeightConstraint.constant = newSize.height
} else {
textViewHeightConstraint.constant = 0.0
}
collectionViewHeightConstraint.constant = previewCollectionViewDataSource.collectionViewHeight(for: cellModel?.feedItem.item.attachments ?? [AttachmentDto]()) + 200.0
previewCollectionViewDataSource.setup(attachments: cellModel?.feedItem.item.attachments ?? [])
}
func showSkeleton() {
textView.showAnimatedGradientSkeleton()
sourceIconImageView.showAnimatedGradientSkeleton()
sourceNameLabel.showAnimatedGradientSkeleton()
dateAddedLabel.showAnimatedGradientSkeleton()
likeButton.showAnimatedGradientSkeleton()
commentsButton.showAnimatedGradientSkeleton()
repostsButton.showAnimatedGradientSkeleton()
previewCollectionView.showAnimatedGradientSkeleton()
}
func hideSkeleton() {
textView.hideSkeleton()
sourceIconImageView.hideSkeleton()
sourceNameLabel.hideSkeleton()
dateAddedLabel.hideSkeleton()
likeButton.hideSkeleton()
commentsButton.hideSkeleton()
repostsButton.hideSkeleton()
previewCollectionView.hideSkeleton()
}
private func hideCollectionView() {
collectionViewHeightConstraint.constant = 0.0
}
}
extension FeedTableViewCell: LikeButtonDelegate {
func didLike(likeButton: LikeButton?) {
guard let feedItem = cellModel?.feedItem else { return }
delegate?.didLike(feedItem: feedItem, completion: { [weak self] updatedFeedItem in
guard self?.cellModel?.feedItem.item.postId == updatedFeedItem.item.postId else { return }
self?.cellModel?.feedItem = updatedFeedItem
DispatchQueue.main.asyncAfter(deadline: .now() + 1.5) {
likeButton?.setup(likesCount: updatedFeedItem.item.likes?.count ?? 0,
state: .liked,
animated: true,
delegate: self)
}
})
}
func didUnlike(likeButton: LikeButton?) {
guard let feedItem = cellModel?.feedItem else { return }
delegate?.didUnlike(feedItem: feedItem, completion: { [weak self] updatedFeedItem in
guard self?.cellModel?.feedItem.item.sourceId == updatedFeedItem.item.sourceId else { return }
self?.cellModel?.feedItem = updatedFeedItem
likeButton?.setup(likesCount: updatedFeedItem.item.likes?.count ?? 0,
state: .notLiked,
animated: true,
delegate: self)
})
}
}
<file_sep>//
// AppCoordinator.swift
// VKDemo
//
// Created by <NAME> on 24/04/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import RxSwift
class AppCoordinator: BaseCoordinator<Void> {
private let window: UIWindow
init(window: UIWindow) {
self.window = window
super.init()
}
override func start() -> Observable<Void> {
let welcomeCoordinator = WelcomeCoordinator(window: window)
let observable = coordinate(to: welcomeCoordinator).share()
_ = observable.subscribe({ event in
})
return observable
}
}
<file_sep>//
// FeedItemDto.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct FeedItemDto: Codable {
let type: FeedType
let sourceId: Int
let date: Date
let postId: Int?
let postType: String? // TODO: enum
let text: String?
let markedAsAds: BoolInt?
let attachments: [AttachmentDto]?
let postSource: FeedPostSource?
let comments: CommentsCountDto?
var likes: LikesCountDto?
let reposts: RepostCountDto?
let views: ViewsCountDto?
let isFavorite: Bool?
let photos: PhotosListDto?
let friends: FriendsDto?
let signerId: Int?
let video: VideoListDto?
let copyHistory: [CopyHistory]? // Если есть, то это репост
let copyOwnerId: Int?
let copyPostId: Int?
let copyPostDate: Date?
let canEdit: Bool?
let canDelete: Bool?
private enum CodingKeys: String, CodingKey {
case sourceId = "source_id"
case text
case postType = "post_type"
case markedAsAds = "marked_as_ads"
case postSource = "post_source"
case isFavorite = "is_favorite"
case postId = "post_id"
case signerId = "signer_id"
case copyHistory = "copy_history"
case type
case date
case attachments
case comments
case likes
case reposts
case views
case photos
case friends
case video
case copyOwnerId = "copy_owner_id" //Не факт, что приходит вообще
case copyPostId = "copy_post_id" //Не факт, что приходит вообще
case copyPostDate = "copy_post_date" //Не факт, что приходит вообще
case canEdit = "can_edit"
case canDelete = "can_delete"
}
}
enum FeedType: String, Codable {
case post = "post"
case photo = "photo"
case photoTag = "photo_tag"
case wallPhoto = "wall_photo"
case friend = "friend"
case note = "note"
case audio = "audio"
case video = "video"
}
struct FeedPostSource: Codable {
let type: String
let platform: String?
}
struct CommentsCountDto: Codable {
let count: Int
let canPost: BoolInt?
let groupsCanPost: Bool?
private enum CodingKeys: String, CodingKey {
case count
case canPost = "can_post"
case groupsCanPost = "groups_can_post"
}
}
struct LikesCountDto: Codable {
var count: Int
var userLikes: BoolInt
let canLike: BoolInt?
let canPublish: BoolInt?
private enum CodingKeys: String, CodingKey {
case count
case userLikes = "user_likes"
case canLike = "can_like"
case canPublish = "can_publish"
}
}
struct RepostCountDto: Codable {
let count: Int
let userReposted: BoolInt
private enum CodingKeys: String, CodingKey {
case count
case userReposted = "user_reposted"
}
}
struct ViewsCountDto: Codable {
let count: Int
}
struct FriendsDto: Codable {
let count: Int
let items: [UserIdDto]
}
struct UserIdDto: Codable {
let userId: Int
private enum CodingKeys: String, CodingKey {
case userId = "user_id"
}
}
struct CopyHistory: Codable {
let id: Int
let ownerId: Int
let fromId: Int
let date: Date
let postType: String? // TODO: enum
let text: String
let attachments: [AttachmentDto]?
let postSource: FeedPostSource
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case fromId = "from_id"
case date
case postType = "post_type"
case text
case attachments
case postSource = "post_source"
}
}
struct MarketItem: Codable {
}
struct MarketAlbum: Codable {
}
struct Sticker: Codable {
}
struct Product: Codable {
let price: Price
}
struct Price: Codable {
let amount: Int
let currency: Currency
let text: String
}
struct Currency: Codable {
let id: Int
let name: String
}
<file_sep>//
// SignInCoordinator.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import UIKit
import RxSwift
import RxCocoa
import RxWebKit
import WebKit
import SafariServices
enum SignInCoordinationResult {
case success
case fail
}
class SignInCoordinator: BaseCoordinator<SignInCoordinationResult> {
private let rootViewController: UIViewController
// Input
private var didAuthorized: AnyObserver<SignInCoordinationResult>?
// Output
private var authorized: Observable<SignInCoordinationResult>?
init(rootViewController: UIViewController) {
self.rootViewController = rootViewController
super.init()
}
override func start() -> Observable<SignInCoordinationResult> {
let signInVC = SignInViewController()
let signInVM = SignInViewModel(sessionManager: DI.container.core.sessionManager)
signInVC.viewModel = signInVM
guard let navC = rootViewController as? UINavigationController else {
fatalError("123!!!")
}
navC.pushViewController(signInVC, animated: true)
return signInVM.authorized
}
}
<file_sep>//
// UIImageView+Loading.swift
// Vanilla
//
// Created by <NAME> on 28.06.17.
// Copyright © 2017 BrightBox. All rights reserved.
//
import UIKit
extension UIImageView {
public func imageFromUrl(urlString: String) {
self.imageFromUrl(urlString: urlString, heightCConstraint: nil)
}
public func imageFromUrl(urlString: String, heightCConstraint: NSLayoutConstraint?) {
if let data = ImageLoadingCache.sharedCache.object(forKey: urlString as AnyObject) as? Data {
self.image = UIImage(data: data)
} else {
let sessionConfig = URLSessionConfiguration.default
let url = URL(string: urlString)
let session = URLSession(configuration: sessionConfig)
let request = URLRequest(url: url!)
updateHeightConstreint(heightCConstraint: heightCConstraint)
let dataTask = session.dataTask(with: request) { responseData, _, error in
if let error = error {
print(error)
} else {
if let data = responseData {
ImageLoadingCache.sharedCache.setObject(data as AnyObject, forKey: urlString as AnyObject)
DispatchQueue.main.async {
self.image = UIImage(data: data)
self.updateHeightConstreint(heightCConstraint: heightCConstraint)
}
} else {
print("no data")
}
}
}
dataTask.resume()
}
}
private func updateHeightConstreint(heightCConstraint: NSLayoutConstraint?) {
guard let heightCConstraint = heightCConstraint, let image = self.image else {
return
}
let width = image.size.width
let height = image.size.height
heightCConstraint.constant = height*self.frame.size.width/width
}
}
extension String {
public func imageFromUrlAsync(_ completion: ((UIImage?) -> Void)?) {
guard let url = URL(string: self) else {
return
}
if let data = ImageLoadingCache.sharedCache.object(forKey: self as AnyObject) as? Data {
async_main {
completion?(UIImage(data: data))
}
return
}
let sessionConfig = URLSessionConfiguration.default
let session = URLSession(configuration: sessionConfig)
let request = URLRequest(url: url)
let dataTask = session.dataTask(with: request) { responseData, _, error in
if let error = error {
print(error)
} else {
async_main {
if let data = responseData {
ImageLoadingCache.sharedCache.setObject(data as AnyObject, forKey: self as AnyObject)
completion?(UIImage(data: data))
} else {
completion?(nil)
print("no data")
}
}
}
}
dataTask.resume()
}
public func getImageFromCache() -> UIImage? {
if let data = ImageLoadingCache.sharedCache.object(forKey: self as AnyObject) as? Data {
return UIImage(data: data)
} else {
return nil
}
}
public func getAsyncImageFromUrl(completion: @escaping (UIImage?, String) -> Void) {//
async {
if let data = ImageLoadingCache.sharedCache.object(forKey: self as AnyObject) as? Data {
async_main {
completion(UIImage(data: data), self)
}
return
}
self.forceLoadImageFromUrl(completion: completion)
}
}
func forceLoadImageFromUrl(completion: @escaping (UIImage?, String) -> Void) {
let sessionConfig = URLSessionConfiguration.default
let url = URL(string: self)
let session = URLSession(configuration: sessionConfig)
let request = URLRequest(url: url!)
let dataTask = session.dataTask(with: request) { responseData, _, error in
if let error = error {
print(error)
} else {
async_main {
if let data = responseData {
ImageLoadingCache.sharedCache.setObject(data as AnyObject, forKey: self as AnyObject)
completion(UIImage(data: data), self)
} else {
completion(nil, self)
print("no data")
}
}
}
}
dataTask.resume()
}
public func dataFromUrlSync(timeout: Int = 10) -> Data! {
let sessionConfig = URLSessionConfiguration.default
let url = URL(string: self)
let session = URLSession(configuration: sessionConfig)
let request = URLRequest(url: url!)
var asyncData: Data!
let sema = DispatchSemaphore(value: 0)
let dataTask = session.dataTask(with: request) { responseData, _, error in
if let error = error {
print(error)
} else {
if let data = responseData {
asyncData = data
} else {
print("no data")
}
}
sema.signal()
}
dataTask.resume()
_ = sema.wait(timeout: .now() + Double(timeout))
return asyncData
}
public func imageFromUrlSync(timeout: Int = 20) -> UIImage! {
if let data = ImageLoadingCache.sharedCache.object(forKey: self as AnyObject) as? Data {
return UIImage(data: data)
}
let sessionConfig = URLSessionConfiguration.default
let url = URL(string: self)
let session = URLSession(configuration: sessionConfig)
let request = URLRequest(url: url!)
var syncImage: UIImage!
let sema = DispatchSemaphore(value: 0)
let dataTask = session.dataTask(with: request) { responseData, response, error in
if let error = error {
if let response = response as? HTTPURLResponse {
print(response.description)
print(response.allHeaderFields)
print(response.statusCode)
}
print(error)
} else {
if let data = responseData {
ImageLoadingCache.sharedCache.setObject(data as AnyObject, forKey: self as AnyObject)
syncImage = UIImage(data: data)
} else {
print("no data")
}
}
}
dataTask.resume()
_ = sema.wait(timeout: .now() + .seconds(timeout))
return syncImage
}
}
<file_sep>//
// FeedLoadMoreFooterView.swift
// VKDemo
//
// Created by <NAME> on 05.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class FeedLoadMoreFooterView: UIView {
@IBOutlet weak var loadingLabel: UILabel!
@IBOutlet weak var copyLoadingLabel: UILabel!
@IBOutlet weak var borderView: UIView! {
didSet {
borderView.layer.cornerRadius = borderView.frame.size.height / 2.0
borderView.layer.borderColor = UIColor.blueMain.withAlphaComponent(0.6).cgColor
borderView.layer.borderWidth = CGFloat(1)
}
}
override func didMoveToSuperview() {
startAnimating()
}
lazy var gradientLayer: CAGradientLayer = {
let gradientLayer = CAGradientLayer()
gradientLayer.colors = [UIColor.clear.cgColor, UIColor.white.cgColor, UIColor.clear.cgColor]
gradientLayer.locations = [0, 0.5, 1]
gradientLayer.frame = copyLoadingLabel.frame
let angle = 45 * CGFloat.pi / 180
gradientLayer.transform = CATransform3DMakeRotation(angle, 0, 0, 1)
return gradientLayer
}()
private func startAnimating() {
let animation = CABasicAnimation(keyPath: "transform.translation.x")
animation.duration = 3
animation.fromValue = -2*loadingLabel.frame.width
animation.toValue = loadingLabel.frame.width
animation.repeatCount = Float.infinity
gradientLayer.add(animation, forKey: "transform.x")
}
override func awakeFromNib() {
super.awakeFromNib()
loadingLabel.layer.mask = gradientLayer
}
override init(frame: CGRect) {
fatalError("Not for initializng in code")
}
required init?(coder: NSCoder) {
super.init(coder: coder)
}
}
<file_sep>//
// MessagesManager.swift
// VKDemo
//
// Created by <NAME> on 01.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
class MessagesManager {
private let messagesProvider: MoyaProvider<MessagesTarget>
private let messagesParser: IMessagesParser
init(messagesProvider: MoyaProvider<MessagesTarget>,
messagesParser: IMessagesParser) {
self.messagesProvider = messagesProvider
self.messagesParser = messagesParser
}
}
extension MessagesManager: IMessagesManager {
func getConversations(success: @escaping ([FeedItem]) -> Void,
failure: @escaping FailureClosure) {
_ = messagesProvider.request(.getConversations, success: { (conversationsResponse: ConversationsResponse) in
print("HOORAY!!!!!!! CONVERSATIONS ARE OK")
print(conversationsResponse)
}, failure: { error in
print("OMG! SO BAD! CONVERSATIONS FAILED !! ERROR:")
print(error)
})
}
}
<file_sep>//
// MessagesTarget.swift
// VKDemo
//
// Created by <NAME> on 01.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Moya
import Alamofire
enum MessagesTarget {
case getConversations
}
extension MessagesTarget: TargetType {
private var sessionManager: IUserSessionManager {
return DI.container.core.sessionManager
}
var baseURL: URL {
return URL(string: "https://api.vk.com/method/")!
}
var path: String {
switch self {
case .getConversations:
return "messages.getConversations"
}
}
var method: HTTPMethod {
switch self {
default:
return .get
}
}
var sampleData: Data {
return Data()
}
var task: Task {
switch self {
case .getConversations:
return .requestParameters(parameters: ["v": 5.108, "access_token": sessionManager.getAccessToken() ?? "", "count" : "200"], encoding: URLEncoding.queryString)
default:
return .requestPlain
}
}
var headers: [String : String]? {
switch self {
default:
return nil
}
}
}
extension MessagesTarget: VKAccessTokenAuthorizable {
var authorizationType: VKAuthorizationType {
return .oauth
}
}
<file_sep>//
// UIButton+BackgroundColor.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
extension UIButton {
func setBackgroundColor(color: UIColor, forState: UIControl.State) {
self.clipsToBounds = true
UIGraphicsBeginImageContext(CGSize(width: 1, height: 1))
if let context = UIGraphicsGetCurrentContext() {
context.setFillColor(color.cgColor)
context.fill(CGRect(x: 0, y: 0, width: 1, height: 1))
let colorImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
self.setBackgroundImage(colorImage, for: forState)
}
}
}
<file_sep>//
// DocDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct DocDto: Codable {
let id: Int
let ownerId: Int
let title: String
let size: Int
let ext: String
let url: String
let date: Int
let type: Int
let preview: DocPreview
private enum CodingKeys: String, CodingKey {
case id
case ownerId = "owner_id"
case title
case size
case ext
case url
case date
case type
case preview
}
}
struct DocPreview: Codable {
let photo: DocPreviewPhoto?
let audioMessage: DocPreviewAudio?
let video: DocPreviewVideo?
private enum CodingKeys: String, CodingKey {
case photo
case audioMessage = "audio_message"
case video
}
}
struct DocPreviewPhoto: Codable {
let sizes: [DocPhotoSizeCopy]
}
struct DocPreviewVideo: Codable {
let src: String
let width: Int
let height: Int
let fileSize: Int
private enum CodingKeys: String, CodingKey {
case src
case width
case height
case fileSize = "file_size"
}
}
struct DocPhotoSizeCopy: Codable {
let src: String
let width: Int
let height: Int
let type: String
}
struct DocPreviewAudio: Codable {
let duration: Int
let waveform: [Int]
let linkOgg: String
let linkMp3: String
private enum CodingKeys: String, CodingKey {
case duration
case waveform
case linkOgg = "link_ogg"
case linkMp3 = "link_mp3"
}
}
<file_sep>//
// VKAccessTokenPlugin.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import Result
import Moya
public protocol VKAccessTokenAuthorizable {
var authorizationType: VKAuthorizationType { get }
}
public enum VKAuthorizationType {
case none
case oauth
}
public struct VKAccessTokenPlugin: PluginType {
let userSessionManager: IUserSessionManager
init(userSessionManager: IUserSessionManager) {
self.userSessionManager = userSessionManager
}
public func prepare(_ request: URLRequest, target: TargetType) -> URLRequest {
// guard let authorizable = target as? VKAccessTokenAuthorizable else {
// return request
// }
//
// var changedRequest = request
//
// switch authorizable.authorizationType {
// case .oauth:
// guard let accessToken = userSessionManager.getAccessToken() else {
// break
// }
//// changedRequest.setValue(accessToken, forHTTPHeaderField: "access_token")
//// changedRequest.allHTTPHeaderFields?["access_token"] = accessToken
// let accessTokenPathComponent = "access_token=\(accessToken)"
// changedRequest.url?.appendPathComponent(accessTokenPathComponent)
//
// default:
// break
// }
//
// return changedRequest
return request
}
public func willSend(_ request: RequestType, target: TargetType) {
print(request)
}
}
<file_sep>//
// ViperModuleTransitionHandler.swift
// Vanilla
//
// Created by <NAME> on 10/05/2017.
// Copyright © 2017 BrightBox. All rights reserved.
//
import UIKit
public enum TransitionStyle {
case push
case modal
case fade
}
public typealias ConfigurationBlock = (ViperModuleInputProtocol) -> Void
public protocol ViperModuleTransitionHandler: class {
func openModule(segueIdentifier: String, configurationBlock: @escaping ConfigurationBlock)
func openModule(segueIdentifier: String, source: ViperModuleOutputProtocol)
func openModule(segueIdentifier: String)
func openModule(vc: UIViewController, style: TransitionStyle, completion: (() -> Swift.Void)?)
func openModule(transitionHandler: ViperModuleTransitionHandler, style: TransitionStyle)
func closeModule()
func popModule()
func popToRootViewController()
func swapAndPopToRootViewController(vc: UIViewController)
func swapToViewController(vc: UIViewController)
}
extension ViperModuleTransitionHandler {
func openModule(vc: UIViewController, style: TransitionStyle, completion: (() -> Swift.Void)? = nil) {
self.openModule(vc: vc, style: style, completion: completion)
}
}
public extension ViperModuleTransitionHandler where Self: UIViewController {
func openModule(segueIdentifier: String, configurationBlock: @escaping ConfigurationBlock) {
performSegue(withIdentifier: segueIdentifier, sender: configurationBlock)
}
func openModule(segueIdentifier: String, source: ViperModuleOutputProtocol) {
performSegue(withIdentifier: segueIdentifier, sender: source)
}
func openModule(segueIdentifier: String) {
performSegue(withIdentifier: segueIdentifier, sender: nil)
}
func openModule(vc: UIViewController, style: TransitionStyle, completion: (() -> Swift.Void)? = nil) {
switch style {
case .modal:
self.present(vc, animated: true, completion: completion)
case .push:
self.navigationController?.pushViewController(vc, animated: true)
defer {
completion?()
}
case .fade:
vc.modalTransitionStyle = .crossDissolve
if let nc = self.navigationController {
nc.present(vc, animated: true, completion: completion)
} else {
self.present(vc, animated: true, completion: completion)
}
}
}
func openModule(transitionHandler: ViperModuleTransitionHandler, style: TransitionStyle) {
guard let vc = transitionHandler as? UIViewController else {
return
}
switch style {
case .modal:
self.present(vc, animated: true, completion: nil)
case .push:
self.navigationController?.pushViewController(vc, animated: true)
case .fade:
vc.modalTransitionStyle = .crossDissolve
self.present(vc, animated: true, completion: nil)
}
}
//TODO: Подумать как сохранять TransitionStyle и уходить с экрана соответственно
func closeModule() {
if presentingViewController != nil {
dismiss(animated: true, completion: nil)
} else {
let animated = presentedViewController == nil
navigationController?.popToRootViewController(animated: animated)
}
}
func popModule() {
self.navigationController?.popViewController(animated: true)
}
func popToRootViewController() {
self.navigationController?.popToRootViewController(animated: true)
}
func swapAndPopToRootViewController(vc: UIViewController) {
self.navigationController?.viewControllers = [vc]
self.navigationController?.popToRootViewController(animated: true)
}
func swapToViewController(vc: UIViewController) {
CATransaction.begin()
CATransaction.setCompletionBlock {
if let count = self.navigationController?.viewControllers.count,
count > 1 {
self.navigationController?.viewControllers.remove(at: count - 2)
}
}
self.navigationController?.pushViewController(vc, animated: true)
CATransaction.commit()
}
func handleSegue(_ segue: UIStoryboardSegue, sender: Any?) {
if sender == nil {
return
}
guard let configurationHolder = segue.destination as? ModuleViewWithOutput else {
fatalError("Destination should conform 'ModuleViewWithOutput'")
}
if let source = sender as? ViperModuleOutputProtocol {
configurationHolder.presenter.output = source
} else if let configure = sender as? ConfigurationBlock {
configure(configurationHolder.presenter)
}
}
}
<file_sep>//
// UIViewController+Style.swift
// Vanilla
//
// Created by Alexander on 06.08.2018.
// Copyright © 2018 BrightBox. All rights reserved.
//
import UIKit
enum BarStyle {
case standard
case standardNoSeparator
case translucent // With gradient
case transparent
case hidden
case standardLargeTitles
}
extension UIViewController {
@available(*, deprecated, message: "Use setNavBarStyle(_ style: BarStyle) from BaseViewController superclass instead")
func setBarStyle(_ style: BarStyle) {
navigationItem.backBarButtonItem?.title = ""
switch style {
case .standard:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
setSeparatorHidden(false)
setLargeTitlesEnabled(false)
case .standardNoSeparator:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
setSeparatorHidden(true)
setLargeTitlesEnabled(false)
case .translucent:
navigationController?.isNavigationBarHidden = true
case .transparent:
navigationController?.navigationBar.isTranslucent = true
navigationController?.navigationBar.backgroundColor = .clear
navigationController?.navigationBar.barTintColor = .clear
setSeparatorHidden(true)
case .hidden:
navigationController?.isNavigationBarHidden = true
case .standardLargeTitles:
navigationController?.isNavigationBarHidden = false
navigationController?.navigationBar.backgroundColor = UIColor.blueMain
navigationController?.navigationBar.barTintColor = UIColor.blueMain
setSeparatorHidden(false)
setLargeTitlesEnabled(true)
}
navigationController?.navigationBar.isExclusiveTouch = true
}
private func setLargeTitlesEnabled(_ enabled: Bool) {
guard #available(iOS 11.0, *) else {
return
}
if navigationController?.navigationBar.prefersLargeTitles == true {
navigationItem.largeTitleDisplayMode = enabled ? .automatic : .never
} else if enabled {
navigationController?.navigationBar.prefersLargeTitles = true
}
}
func setBarTitle(_ title: String?) {
navigationItem.title = title
}
func setSeparatorHidden(_ hidden: Bool) {
if #available(iOS 11.0, *) {
navigationController?.navigationBar.shadowImage = (hidden ? UIImage() : nil)
} else {
navigationController?.navigationBar.shadowImage = (hidden ? UIImage() : nil)
navigationController?.navigationBar.setBackgroundImage((hidden ? UIImage() : nil), for: .default)
}
}
}
<file_sep>//
// CoordinatorContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol CoordinatorContainerProtocol {
var appCoordinator: IAppCoordinator { get }
var mainTabBarCoordinator: IMainTabBarCoordinator { get }
}
class CoordinatorContainer {
private let serviceContainer = ServiceContainer()
init() {
let mainTabBarCoordinator = MainTabBarCoordinator()
serviceContainer.add(services: mainTabBarCoordinator)
let appCoordinator = AppCoordinator()
serviceContainer.add(services: appCoordinator)
}
}
extension CoordinatorContainer: CoordinatorContainerProtocol {
var mainTabBarCoordinator: IMainTabBarCoordinator {
return serviceContainer.get(service: MainTabBarCoordinator.self)!
}
var appCoordinator: IAppCoordinator {
return serviceContainer.get(service: AppCoordinator.self)!
}
}
<file_sep>//
// DIContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol DIContainerProtocol {
var core: CoreContainerProtocol { get }
var data: DataContainerProtocol { get }
var dao: DaoContainerProtocol { get }
var presentation: PresentationContainerProtocol { get }
var parser: ParserContainerProtocol { get }
var config: ConfigContainerProtocol { get }
var coordinator: CoordinatorContainerProtocol { get }
}
class DIContainer: DIContainerProtocol {
internal var core: CoreContainerProtocol
internal var data: DataContainerProtocol
internal var dao: DaoContainerProtocol
internal var presentation: PresentationContainerProtocol
internal var parser: ParserContainerProtocol
internal var config: ConfigContainerProtocol
var coordinator: CoordinatorContainerProtocol
init() {
self.parser = ParserContainer()
self.config = ConfigContainer()
self.dao = DaoContainer()
self.core = CoreContainer(dao: self.dao)
self.presentation = PresentationContainer()
self.data = DataContainer(core: self.core,
parser: self.parser)
self.coordinator = CoordinatorContainer()
}
}
<file_sep>//
// ViperModuleInput.swift
// Vanilla
//
// Created by <NAME> on 11/05/2017.
// Copyright © 2017 BrightBox. All rights reserved.
//
import Foundation
public protocol ViperModuleInputProtocol: class {
var output: ViperModuleOutputProtocol! { get set }
}
<file_sep>import PlaygroundSupport
import UIKit
var str = "Hello, playground"
#colorLiteral(red: 0, green: 0.4784313725, blue: 1, alpha: 1)
//// Present the view controller in the Live View window
class LikeButton: UIButton {
//// Color Declarations
private let fillColor = #colorLiteral(red: 0.8078431487, green: 0.02745098062, blue: 0.3333333433, alpha: 1)
private let blueMain = UIColor(red: 0.294, green: 0.471, blue: 0.706, alpha: 1.000)
private lazy var heartLayer: CAShapeLayer = {
let layer = CAShapeLayer()
func fastFloor(_ x: CGFloat) -> CGFloat { return floor(x) }
//// Subframes
let group: CGRect = CGRect(x: frame.minX + 72, y: frame.minY + fastFloor((frame.height - 56) * 0.10784 + 0.5), width: fastFloor((frame.width - 72) * 0.74149 + 0.5), height: frame.height - 56 - fastFloor((frame.height - 56) * 0.10784 + 0.5))
let bezierPath = UIBezierPath()
bezierPath.move(to: CGPoint(x: group.minX + 0.27104 * group.width, y: group.minY + 1.00000 * group.height))
bezierPath.addLine(to: CGPoint(x: group.minX + 0.23309 * group.width, y: group.minY + 0.92797 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.00000 * group.width, y: group.minY + 0.30023 * group.height), controlPoint1: CGPoint(x: group.minX + 0.09215 * group.width, y: group.minY + 0.67688 * group.height), controlPoint2: CGPoint(x: group.minX + 0.00000 * group.width, y: group.minY + 0.50766 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.14907 * group.width, y: group.minY + 0.00000 * group.height), controlPoint1: CGPoint(x: group.minX + 0.00000 * group.width, y: group.minY + 0.13101 * group.height), controlPoint2: CGPoint(x: group.minX + 0.06505 * group.width, y: group.minY + 0.00000 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.27104 * group.width, y: group.minY + 0.11463 * group.height), controlPoint1: CGPoint(x: group.minX + 0.19515 * group.width, y: group.minY + 0.00000 * group.height), controlPoint2: CGPoint(x: group.minX + 0.24122 * group.width, y: group.minY + 0.04367 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.39301 * group.width, y: group.minY + 0.00000 * group.height), controlPoint1: CGPoint(x: group.minX + 0.30085 * group.width, y: group.minY + 0.04367 * group.height), controlPoint2: CGPoint(x: group.minX + 0.34693 * group.width, y: group.minY + 0.00000 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.54208 * group.width, y: group.minY + 0.30023 * group.height), controlPoint1: CGPoint(x: group.minX + 0.47703 * group.width, y: group.minY + 0.00000 * group.height), controlPoint2: CGPoint(x: group.minX + 0.54208 * group.width, y: group.minY + 0.13101 * group.height))
bezierPath.addCurve(to: CGPoint(x: group.minX + 0.30898 * group.width, y: group.minY + 0.92797 * group.height), controlPoint1: CGPoint(x: group.minX + 0.54208 * group.width, y: group.minY + 0.50766 * group.height), controlPoint2: CGPoint(x: group.minX + 0.44992 * group.width, y: group.minY + 0.67688 * group.height))
bezierPath.addLine(to: CGPoint(x: group.minX + 0.27104 * group.width, y: group.minY + 1.00000 * group.height))
bezierPath.close()
UIColor.white.setFill()
bezierPath.fill()
UIColor.lightGray.setStroke()
bezierPath.lineWidth = 6.5
bezierPath.miterLimit = 4
bezierPath.stroke()
layer.path = bezierPath.cgPath
return layer
}()
override init(frame: CGRect) {
super.init(frame: frame)
configureLayers()
}
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
configureLayers()
}
private func configureLayers() {
backgroundColor = #colorLiteral(red: 1.0, green: 1.0, blue: 1.0, alpha: 1.0)
layer.addSublayer(heartLayer)
}
}
let likeButton = LikeButton(frame: CGRect(x: 0, y: 0, width: 500, height: 500))
PlaygroundPage.current.liveView = likeButton
<file_sep>//
// ParseContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol ParserContainerProtocol {
var feedParser: IFeedParser { get }
var messagesParser: IMessagesParser { get }
}
class ParserContainer: ParserContainerProtocol {
var feedParser: IFeedParser {
return FeedParser()
}
var messagesParser: IMessagesParser {
return MessagesParser()
}
}
<file_sep>//
// SignInViewController.swift
// VKDemo
//
// Created by <NAME> on 27/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
import RxSwift
import RxCocoa
import WebKit
import RxWebKit
class SignInViewController: UIViewController {
var viewModel: SignInViewModel!
private let disposeBag = DisposeBag()
@IBOutlet weak var webView: WKWebView!
override func viewDidLoad() {
super.viewDidLoad()
guard let url = URL(string: "https://oauth.vk.com/authorize?client_id=5513701&display=mobile&redirect_uri=https://oauth.vk.com/blank.html&scope=friends,photos,status,notes,messages,wall,groups,notifications,stats,messages&response_type=token&v=5.95&state=123456") else {
return
}
webView.load(URLRequest(url: url))
webView.rx
.decidePolicyNavigationAction
.asObservable()
.do(onNext: { webView, action, handler in
handler(.allow)
}, onError: { _ in
print("22")
}, onCompleted: {
print("11")
})
.filter({ arg -> Bool in
let (_, action, _) = arg
guard let url = action.request.url else {
return false
}
return url.absoluteString.contains("#")
})
.flatMap({ _, action, handler -> Observable<URL> in
return Observable.just(action.request.url!)
})
.bind(to: viewModel.decidePolicyNavigationActionObserver)
.disposed(by: disposeBag)
}
}
<file_sep>//
// ChatPresenter.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
class ChatPresenter {
private weak var view: ChatViewInput?
private let router: ChatRouterInput
private let messagesManager: IMessagesManager
init(view: ChatViewInput,
router: ChatRouterInput,
messagesManager: IMessagesManager) {
self.view = view
self.router = router
self.messagesManager = messagesManager
}
}
extension ChatPresenter: ChatViewOutput {
func viewLoaded() {
}
}
<file_sep>//
// PhotoDto.swift
// VKDemo
//
// Created by <NAME> on 12/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct PhotoDto: Codable {
let id: Int
let albumId: Int
let ownerId: Int
let userId: Int?
let text: String
let date: Int
let sizes: [PhotoSizeCopy]
private enum CodingKeys: String, CodingKey {
case id
case albumId = "album_id"
case ownerId = "owner_id"
case userId = "user_id"
case text
case date
case sizes
}
}
extension Array where Element == PhotoSizeCopy {
func correctSize() -> PhotoSizeCopy? {
return [self.first(where: { $0.type == "z" }),
self.first(where: { $0.type == "y" }),
self.first(where: { $0.type == "x" }),
self.first(where: { $0.type == "w" })]
.compactMap({$0})
.first
}
}
struct PhotoSizeCopy: Codable {
let type: String
let url: String
let width: Int
let height: Int
}
struct PhotosListDto: Codable {
let count: Int
let items: [ExtendedPhotoDto]
}
struct ExtendedPhotoDto: Codable {
let id: Int
let albumId: Int
let ownerId: Int
let userId: Int?
let text: String
let date: Int
let sizes: [PhotoSizeCopy]
let accessKey: String
let likes: LikesCountDto
let reposts: RepostCountDto
let comments: CommentsCountDto
let canComment: BoolInt
let canRepost: BoolInt
private enum CodingKeys: String, CodingKey {
case id
case albumId = "album_id"
case ownerId = "owner_id"
case userId = "user_id"
case text
case date
case sizes
case accessKey = "access_key"
case likes
case reposts
case comments
case canComment = "can_comment"
case canRepost = "can_repost"
}
}
<file_sep>//
// ChatViewInput.swift
// VKDemo
//
// Created by AntonKrylovBrightBox on 10/06/2019.
// Copyright © 2019 BrightBox. All rights reserved.
//
protocol ChatViewInput: AnyObject {
func setup()
}
<file_sep>//
// ServiceContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
class ServiceContainer {
private lazy var container = [String: Any]()
func add(services: Any...) {
for service in services {
container["\(type(of: service))"] = service
}
}
func get<T>(service: T.Type) -> T? {
return container["\(T.self)"] as? T
}
}
<file_sep>//
// IUserSessionManager.swift
// VKDemo
//
// Created by <NAME> on 28/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//f
import Foundation
protocol IUserSessionManager {
func clearSession()
func getAccessToken() -> String?
func saveAccessToken(_ accessToken: String?)
}
<file_sep>//
// ConfigContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol ConfigContainerProtocol {
}
class ConfigContainer: ConfigContainerProtocol {
}
<file_sep>//
// IMessagesManager.swift
// VKDemo
//
// Created by <NAME> on 01.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol IMessagesManager {
func getConversations(success: @escaping ([FeedItem]) -> Void,
failure: @escaping FailureClosure)
}
<file_sep>//
// AttachmentVideoCollectionViewCell.swift
// VKDemo
//
// Created by <NAME> on 28/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class AttachmentVideoCollectionViewCell: UICollectionViewCell {
static let cellName: String = String(describing: AttachmentVideoCollectionViewCell.self)
var videoDto: VideoDto?
var photoUrlString: String?
@IBOutlet weak var imageView: UIImageView!
func setup(videoDto: VideoDto) {
self.videoDto = videoDto
guard let appropriatePhotoUrlString = [videoDto.photo1280,
videoDto.photo800,
videoDto.photo640,
videoDto.photo320]
.compactMap({$0})
.first
else {
imageView.image = nil
photoUrlString = nil
return
}
photoUrlString = appropriatePhotoUrlString
appropriatePhotoUrlString.getAsyncImageFromUrl { [weak self] image, url in
guard let strongSelf = self,
let photoUrlString = strongSelf.photoUrlString,
photoUrlString == url,
let image = image,
let strongImageView = strongSelf.imageView else {
return
}
UIView.transition(with: strongImageView,
duration: 0.3,
options: .transitionCrossDissolve,
animations: {
strongImageView.image = image
}, completion: nil)
}
}
}
<file_sep>//
// MappedResponse.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct MappedResponse<T: Codable>: Codable {
let response: T
}
<file_sep>//
// ViperModuleInitializer.swift
// Vanilla
//
// Created by <NAME> on 12/7/17.
// Copyright © 2017 BrightBox. All rights reserved.
//
import Foundation
import UIKit
//TODO: remove ViperModuleInitializer protocol after deleting storyboards
public protocol ViperModuleInitializer: class {
var viewController: UIViewController? { get }
}
public protocol ViperInitializer: class {
func createModule(values: [Any])
}
public extension ViperModuleInitializer where Self: NSObject {
public static func assemble(values: Any...) -> Self {
let instance = Self.init()
if let instance = instance as? ViperInitializer {
instance.createModule(values: values)
} else {
instance.awakeFromNib()
}
return instance
}
public var viewController: UIViewController? {
return value(forKey: "viewController") as? UIViewController
}
}
<file_sep>//
// CoreContainer.swift
// VKDemo
//
// Created by <NAME> on 15/05/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
protocol CoreContainerProtocol {
var sessionManager: UserSessionManager { get }
}
class CoreContainer: CoreContainerProtocol {
private let serviceContainer = ServiceContainer()
let dao: DaoContainerProtocol
init(dao: DaoContainerProtocol) {
self.dao = dao
let sessionManager = UserSessionManager(sessionDao: dao.sessionDao)
serviceContainer.add(services: sessionManager)
}
var sessionManager: UserSessionManager {
return serviceContainer.get(service: UserSessionManager.self)!
}
}
<file_sep>//
// UIColor+Factory.swift
// VKDemo
//
// Created by <NAME> on 10/06/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit.UIColor
extension UIColor {
static var blueMain: UIColor {
return UIColor(named: "blueMain")!
}
static var redMain: UIColor {
return UIColor(named: "redMain")!
}
}
<file_sep>//
// UIView+InCallStatusBar.swift
// Vanilla
//
// Created by <NAME> on 14.08.2018.
// Copyright © 2018 BrightBox. All rights reserved.
//
import UIKit
extension UIView {
func setInCallSafeFrame() {
self.autoresizingMask = [.flexibleHeight, .flexibleTopMargin]
}
}
<file_sep>//
// LikesCountResponse.swift
// VKDemo
//
// Created by <NAME> on 07.11.2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
struct LikesCountResponse: Codable {
let likesCount: Int
private enum CodingKeys: String, CodingKey {
case likesCount = "likes"
}
}
<file_sep>//
// AttachmentsCollectionViewDataSource.swift
// VKDemo
//
// Created by <NAME> on 25/08/2019.
// Copyright © 2019 <NAME>. All rights reserved.
//
import Foundation
import UIKit
class AttachmentsCollectionViewDataSource: NSObject {
weak var output: FeedViewOutput?
var attachments: [AttachmentDto]?
weak var collectionView: UICollectionView!{
didSet {
initialSetup()
}
}
func generateLayout() -> UICollectionViewLayout {
// We have three row styles
// Style 1: 'Full'
// A full width photo
// Style 2: 'Main with pair'
// A 2/3 width photo with two 1/3 width photos stacked vertically
// Style 3: 'Triplet'
// Three 1/3 width photos stacked horizontally
// Full
// let fullPhotoItem = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalWidth(3/2)))
// fullPhotoItem.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
//
// // Main with pair
// let mainItem = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(2/3),
// heightDimension: .fractionalHeight(1.0)))
// mainItem.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
//
// let pairItem = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalHeight(0.5)))
// pairItem.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
// let trailingGroup = NSCollectionLayoutGroup.vertical(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1/3),
// heightDimension: .fractionalHeight(1.0)),
// subitem: pairItem,
// count: 2)
//
// let mainWithPairGroup = NSCollectionLayoutGroup.horizontal(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalWidth(4/9)),
// subitems: [mainItem, trailingGroup])
//
// // Triplet
// let tripletItem1 = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1/3.35),
// heightDimension: .fractionalHeight(1.0)))
//
// let tripletItem2 = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.35/3.35),
// heightDimension: .fractionalHeight(1.0)))
//
// let tripletItem3 = NSCollectionLayoutItem(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1/3.35),
// heightDimension: .fractionalHeight(1.0)))
// tripletItem1.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
// tripletItem2.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
// tripletItem3.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
//
// let tripletGroup = NSCollectionLayoutGroup.horizontal(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalWidth(2/3)),
// subitems: [tripletItem1, tripletItem2, tripletItem3])
//
// // Reversed main with pair
// let mainWithPairReversedGroup = NSCollectionLayoutGroup.horizontal(
// layoutSize: NSCollectionLayoutSize(
// widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalWidth(4/9)),
// subitems: [trailingGroup, mainItem])
guard let photoAttachments = attachments?.filter({ $0.photo != nil }),
photoAttachments.count > 0 else {
// let layoutGroup = NSCollectionLayoutGroup(layoutSize: NSCollectionLayoutSize(widthDimension: .fractionalWidth(1.0),
// heightDimension: .fractionalHeight(1.0)))
// let section = NSCollectionLayoutSection(group: layoutGroup)
// let layout = UICollectionViewCompositionalLayout(section: section)
return UICollectionViewLayout()
}
var subgroups = [NSCollectionLayoutGroup]()
var k = 0
for _ in 1...3 {
if k > photoAttachments.count - 1 { break }
let j = Int.random(in: 1...4)
let photo1 = (k < photoAttachments.count) ? photoAttachments[k].photo : photoAttachments.first?.photo!
let photo2 = (k < photoAttachments.count - 1) ? photoAttachments[k+1].photo : photoAttachments.first?.photo!
let photo3 = (k < photoAttachments.count - 2) ? photoAttachments[k+2].photo : photoAttachments.first?.photo!
switch j {
case 1:
subgroups.append(generateSingleLayoutGroup(photoDto: photo1!))
k += 1
case 2:
subgroups.append(generateMainWithPairLayoutGroup(photoDto1: photo1!, photoDto2: photo2!, photoDto3: photo3!))
k += 3
case 3:
subgroups.append(generateTripletLayoutGroup(photoDto1: photo1!, photoDto2: photo2!, photoDto3: photo3!))
k += 3
case 4:
break
default:
break
}
}
let photo1 = (k < photoAttachments.count) ? photoAttachments[k].photo : photoAttachments.first?.photo!
if subgroups.count == 0 { subgroups.append(generateSingleLayoutGroup(photoDto: photo1!)) }
let nestedGroup = NSCollectionLayoutGroup.vertical(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalHeight(1.0)),
subitems: subgroups)
// [fullPhotoItem, mainWithPairGroup, tripletGroup, mainWithPairReversedGroup])
let section = NSCollectionLayoutSection(group: nestedGroup)
let layout = UICollectionViewCompositionalLayout(section: section)
return layout
}
func generateSingleLayoutGroup(photoDto: PhotoDto) -> NSCollectionLayoutGroup {
let width = collectionView.frame.width
let photo = photoDto
let photoSize = photo.sizes.correctSize()
let photoRatio = CGFloat(photoSize?.height ?? 0) / CGFloat(photoSize?.width ?? 1)
let photoHeight = photoRatio * width
// Full
let fullPhotoItem = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalWidth(3/2)))
fullPhotoItem.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
let layoutGroup = NSCollectionLayoutGroup.horizontal(layoutSize: NSCollectionLayoutSize(widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalWidth(photoRatio)),
subitem: fullPhotoItem,
count: 1)
return layoutGroup
}
func generateMainWithPairLayoutGroup(photoDto1: PhotoDto, photoDto2: PhotoDto, photoDto3: PhotoDto) -> NSCollectionLayoutGroup {
let width1 = collectionView.frame.width * 2.0 / 3.0
let photo1 = photoDto1
let photo1Size = photo1.sizes.correctSize()
let photo1Ratio = CGFloat(photo1Size?.height ?? 0) / CGFloat(photo1Size?.width ?? 1)
let photo1Height = photo1Ratio * width1
let width2 = collectionView.frame.width / 3.0
let photo2 = photoDto2
let photo2Size = photo2.sizes.correctSize()
let photo2Ratio = CGFloat(photo2Size?.height ?? 0) / CGFloat(photo2Size?.width ?? 1)
let photo2Height = photo2Ratio * width2
let width3 = collectionView.frame.width / 3.0
let photo3 = photoDto3
let photo3Size = photo2.sizes.correctSize()
let photo3Ratio = CGFloat(photo3Size?.height ?? 0) / CGFloat(photo3Size?.width ?? 1)
let photo3Height = photo3Ratio * width3
// photo1Height < maxHeight
// photo2Height 20% diff with photo3Height
let mainItem = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(photo1Ratio),
heightDimension: .fractionalHeight(1.0)))
mainItem.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
let pairItem1 = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalHeight(photo2Ratio))) // меняем
pairItem1.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
let pairItem2 = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalHeight(photo3Ratio))) // меняем
pairItem2.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
let trailingGroup = NSCollectionLayoutGroup.vertical(layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0 - photo1Ratio),
heightDimension: .fractionalHeight(1.0)), subitems: [pairItem1, pairItem2])
let mainWithPairGroup = NSCollectionLayoutGroup.horizontal(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .fractionalWidth(4/9)),
subitems: [mainItem, trailingGroup])
return mainWithPairGroup
}
func generateTripletLayoutGroup(photoDto1: PhotoDto, photoDto2: PhotoDto, photoDto3: PhotoDto) -> NSCollectionLayoutGroup {
let width1 = collectionView.frame.width / 3.0
let photo1 = photoDto1
let photo1Size = photo1.sizes.correctSize()
let photo1Ratio = CGFloat(photo1Size?.height ?? 0) / CGFloat(photo1Size?.width ?? 1)
let photo1Height = photo1Ratio * width1
let width2 = collectionView.frame.width / 3.0
let photo2 = photoDto2
let photo2Size = photo2.sizes.correctSize()
let photo2Ratio = CGFloat(photo2Size?.height ?? 0) / CGFloat(photo2Size?.width ?? 1)
let photo2Height = photo2Ratio * width2
let width3 = collectionView.frame.width / 3.0
let photo3 = photoDto3
let photo3Size = photo3.sizes.correctSize()
let photo3Ratio = CGFloat(photo3Size?.height ?? 0) / CGFloat(photo3Size?.width ?? 1)
let photo3Height = photo3Ratio * width3
// photo1Height < maxHeight photo2Height < maxHeight photo3Height < maxHeight
// photo2Height 20% diff with photo3Height and photo1Height
// Triplet
let tripletItem1 = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalHeight(photo1Ratio),
heightDimension: .fractionalHeight(1.0)))
let tripletItem2 = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalHeight(photo2Ratio),
heightDimension: .fractionalHeight(1.0)))
let tripletItem3 = NSCollectionLayoutItem(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalHeight(photo3Ratio),
heightDimension: .fractionalHeight(1.0)))
tripletItem1.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
tripletItem2.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
tripletItem3.contentInsets = NSDirectionalEdgeInsets(top: 2, leading: 2, bottom: 2, trailing: 2)
let tripletGroup = NSCollectionLayoutGroup.horizontal(
layoutSize: NSCollectionLayoutSize(
widthDimension: .fractionalWidth(1.0),
heightDimension: .absolute(CGFloat(300))),
subitems: [tripletItem1, tripletItem2, tripletItem3])
return tripletGroup
UIApplication().applicationState
}
func setup(attachments: [AttachmentDto]) {
self.attachments = attachments
collectionView.reloadData()
collectionView.setCollectionViewLayout(generateLayout(), animated: false)
}
func collectionViewHeight(for attachments: [AttachmentDto]) -> CGFloat {
if (attachments.count == 1),
let photo = attachments.first?.photo,
photo.sizes.count > 0 {
let photoSizes = photo.sizes
let photoSizesOrderArray = ["z", "y", "x", "w"]
let properPhotoSize = photoSizes.correctSize()
// photoSizes.sorted(by: {
// guard let index0 = photoSizesOrderArray.index(of: $0.type) else { return false }
// guard let index1 = photoSizesOrderArray.index(of: $1.type) else { return true }
// return index0 <= index1
// }).first
let width = collectionView.frame.width
let height = CGFloat(properPhotoSize?.height ?? 0) / CGFloat(properPhotoSize?.width ?? 1) * width
return height
}
let height = CGFloat(120.0)
let photosCount = attachments.filter({
$0.type == .photo
}).count
return ceil(CGFloat(photosCount) / 2.0) * height
}
func createLayoutForPhotos(_ photos:[PhotoDto]) -> [CompositionalLayout] {
for i in 4...1 {
for j in 4...0 {
for k in 4...0 {
guard i + j + k == photos.count,
(j != 0) || (k == 0) else {
continue
}
let row1Photos = Array(photos[0..<i])
let row2Photos = Array(photos[i..<j+i])
let row3Photos = Array(photos.dropFirst(j+i))
createRowsLayout(row1: row1Photos,
row2: row2Photos,
row3: row3Photos)
}
}
}
return [CompositionalLayout]()
}
func createRowsLayout(row1: [PhotoDto], row2: [PhotoDto]?, row3: [PhotoDto]?) {
}
}
struct CompositionalLayout {
var rows: [RowLayout]
}
struct RowLayout {
var cells: Any
}
// MARK: - Private functions
extension AttachmentsCollectionViewDataSource {
private func initialSetup() {
collectionView.delegate = self
collectionView.dataSource = self
collectionView.register(UINib(nibName: AttachmentVideoCollectionViewCell.cellName,
bundle: nil),
forCellWithReuseIdentifier: AttachmentVideoCollectionViewCell.cellName)
collectionView.register(UINib(nibName: AttachmentPhotoCollectionViewCell.cellName,
bundle: nil),
forCellWithReuseIdentifier: AttachmentPhotoCollectionViewCell.cellName)
}
}
// MARK: - UICollectionViewDelegate
extension AttachmentsCollectionViewDataSource: UICollectionViewDelegate {
}
// MARK: - UICollectionViewDataSource
extension AttachmentsCollectionViewDataSource: UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
let photoAttachments = attachments?.filter({ $0.type == .photo }) ?? []
var strings = [String]()
for _ in 0..<15 {
strings.append("")
}
for i in 0..<photoAttachments.count {
guard let photoDto = photoAttachments[i].photo else {
return 0
}
strings[0] += "\(i+1) фото\t\t"
strings[1] += "\(photoDto.sizes.count) размеров\t"
var j = 2
photoDto.sizes.forEach {
let wxh = "\($0.width)x\($0.height)"
let aspRatio = ((CGFloat($0.width)/CGFloat($0.height))*100).rounded() / 100.0
strings[j] += "\(wxh) \(aspRatio)\t\t"
j += 1
}
let urlString = "\(photoDto.sizes.last!.url)\t"
strings[j] += urlString
}
if photoAttachments.count == 10 {
print("101010101010")
}
print("\n\n\n************Photo info*************")
for i in 0..<15 {
print(strings[i])
}
return photoAttachments.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let photoAttachments = attachments?.filter({ $0.type == .photo }) ?? []
let attachment = photoAttachments[indexPath.row]
switch attachment.type {
case .photo:
guard let cell = collectionView.dequeueReusableCell(withReuseIdentifier: AttachmentPhotoCollectionViewCell.cellName, for: indexPath) as? AttachmentPhotoCollectionViewCell,
let photoDto = attachment.photo else {
return UICollectionViewCell()
}
cell.setup(photoDto: photoDto)
return cell
case .video:
guard let cell = collectionView.dequeueReusableCell(withReuseIdentifier: AttachmentVideoCollectionViewCell.cellName, for: indexPath) as? AttachmentVideoCollectionViewCell,
let videoDto = attachment.video else {
return UICollectionViewCell()
}
cell.setup(videoDto: videoDto)
return cell
case .market:
return UICollectionViewCell()
case .marketAlbum:
return UICollectionViewCell()
case .prettyCards:
return UICollectionViewCell()
case .podcast:
return UICollectionViewCell()
case .audio:
return UICollectionViewCell()
case .doc:
return UICollectionViewCell()
case .link:
return UICollectionViewCell()
case .note:
return UICollectionViewCell()
case .poll:
return UICollectionViewCell()
case .page:
return UICollectionViewCell()
case .album:
return UICollectionViewCell()
case .photosList:
return UICollectionViewCell()
}
}
}
// MARK: - UICollectionViewDelegateFlowLayout
extension AttachmentsCollectionViewDataSource: UICollectionViewDelegateFlowLayout {
// func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
//
// if (attachments?.count == 1),
// let photo = attachments?.first?.photo,
// photo.sizes.count > 0 {
// let photoSizes = photo.sizes
// let photoSizesOrderArray = ["z", "y", "x", "w"]
// let properPhotoSize = photoSizes.sorted(by: {
// guard let index0 = photoSizesOrderArray.index(of: $0.type) else { return false }
// guard let index1 = photoSizesOrderArray.index(of: $1.type) else { return true }
// return index0 <= index1
// }).first
// let width = collectionView.frame.width
// let height = CGFloat(properPhotoSize?.height ?? 0) / CGFloat(properPhotoSize?.width ?? 1) * width
//
// return CGSize(width: width, height: height)
// }
//
// let width = collectionView.bounds.width / 2.0 - 5.0
// let height = CGFloat(120.0)
//
// return CGSize(width: width, height: height)
// }
}
| 4c878469331b84470c15ecd1f30306a746eb7e61 | [
"Swift",
"Ruby"
] | 103 | Swift | anton0701/VKDemo | ee7cba2a348904c7eaabbfc9751b3d5d348e3f52 | f6c864518e614cd0397eeb7f4ca1a1a29a4e78b0 | |
refs/heads/master | <repo_name>spaceshipsamurai/samurai-home<file_sep>/scripts/app/home/HomeCtrl.js
angular.module('ssHome').controller('HomeCtrl', function($scope, ssYoutubeFeed) {
ssYoutubeFeed.getRecent(3).then(function(videos) {
$scope.videos = videos;
});
$scope.contacts = [
{
id: 1694010657,
name: '<NAME>',
title: 'Alliance Exec.'
},
{
id: 92988604,
name: '<NAME>',
title: '2nd in command'
},
{
id: 92940230,
name: '<NAME>',
title: 'Diplomacy Director'
},
{
id: 92507729,
name: '<NAME>',
title: 'IT Director'
}
];
$scope.corporations = [
{
id: 98041262,
name: 'Community against Justice'
},
{
id: 851230678,
name: 'The New Era'
},
{
id: 555073675,
name: 'The Green Cross'
},
{
id: 692190945,
name: '<NAME>'
},
{
id: 98097817,
name: 'Concordiat'
},
{
id: 98189045,
name: '<NAME>'
}
];
});
<file_sep>/scripts/app/ssHome.js
angular.module('ssHome', ['ngRoute']);
angular.module('ssHome').config(['$locationProvider', '$routeProvider', function($locationProvider, $routeProvider) {
$locationProvider.html5Mode(true);
$routeProvider.when('/', {
templateUrl: '/templates/index.html',
controller: 'HomeCtrl'
});
}]);
<file_sep>/scripts/app/home/ssYoutubeFeed.js
angular.module('ssHome').factory('ssYoutubeFeed', function($http, $q) {
return {
getRecent: function(count) {
var dfd = $q.defer();
var feed = 'http://gdata.youtube.com/feeds/api/videos?author=SpaceshipSamurai&v=2&alt=json&max-results='+ count +'&orderby=published';
$http.get(feed).then(function(response){
var videos = new Array();
if(response.data) {
var entries = response.data.feed.entry;
entries.forEach(function(entry) {
videos.push({
id: entry['media$group']['yt$videoid']['$t'],
title: entry['title']['$t'],
desc: entry['media$group']['media$description']['$t'],
thumbnail: entry['media$group']['media$thumbnail'][0]['url'],
published: new Date(entry['published']['$t']).toLocaleDateString("en-US")
})
});
}
console.log(videos)
dfd.resolve(videos);
});
return dfd.promise;
}
}
}); | 13ddcf41c5776befadae39c28cecf07044c7d10a | [
"JavaScript"
] | 3 | JavaScript | spaceshipsamurai/samurai-home | 0222034b07bfc6b85111336401ce916ddf4977f9 | a92ad9c0c4bd6fa79cf63a0453ac0cc135dd2dd7 | |
refs/heads/master | <repo_name>Ochkalov/squest<file_sep>/js/data/quest.js
export const initialGame = {
level: 0,
lives: 3,
time:0
};
export const setLives = (game, lives) => {
if ( lives < 0 ) {
throw new RangeError(`Can't set negative lives`);
}
game = Object.assign({}, game);
game.lives = lives;
return game;
};
export const getLevel = (num, quest) => quest[`level-${num}`];
export const nextLevel = (state, quest) => {
const next = state.level + 1;
if (!getLevel(next, quest)) {
throw new RangeError(`Can't find level ${next}`);
}
state = Object.assign({}, state);
state.level = next;
return state;
};
const LEFT = `LEFT`;
const RIGHT = `RIGHT`;
const JUMP = `JUMP`;
const ONE = `1`;
const TWO = `2`;
const THREE = `3`;
const FOUR = `4`;
export const Action = {
LEFT, RIGHT, JUMP, ONE, TWO, THREE, FOUR
};
export const Result = {
DIE: `die`,
NOOP: `noop`,
NEXT: `next`,
WIN: `win`
};<file_sep>/js/welcome/welcome.js
import {changeView} from '../util';
import WelcomeView from './welcome-view';
import App from '../main';
export default class Welcome {
constructor() {
this.view = new WelcomeView();
}
init() {
App.showWelcome();
changeView(this.view);
this.view.onStart = () => {
App.showGame();
};
}
}<file_sep>/js/main.js
import {changeView} from './util';
import Game from './game/game';
import Model from './model';
import questAdapter from './data/quest-adapter';
import SplashScreen from './splash-screen/splash-screen';
import Welcome from './welcome/welcome';
const ControllerID = {
WELCOME: ``,
GAME: `game`,
WIN: `die`,
DIE: `win`,
};
const getControllerIDFromHash = (hash) => hash.replace(`#`, ``);
class App {
constructor() {
const preloaderRemove = this.showPreloader();
this.model = new class extends Model {
get urlRead() {
return `https://intensive-ecmascript-server-srmhvdwcks.now.sh/text-quest/quest`;
}
get urlWrite() {
return `true`;
}
}();
this.model.load(questAdapter)
.then((data) => this.setup(data))
.then(preloaderRemove)
.then(() => this.changeController(getControllerIDFromHash(location.hash)))
.catch(window.console.error);
}
setup(data) {
this.routes = {
[ControllerID.WELCOME]: new Welcome(),
[ControllerID.GAME]: new Game(this.model, data)
};
window.onhashchange = () => {
this.changeController(getControllerIDFromHash(location.hash));
};
}
changeController(route = ``) {
this.routes[route].init();
}
showPreloader() {
const splashScreen = new SplashScreen();
changeView(splashScreen);
splashScreen.start();
return () => splashScreen.hide();
}
showWelcome() {
location.hash = ControllerID.WELCOME;
}
showGame() {
location.hash = ControllerID.GAME;
}
}
export default new App();
<file_sep>/Readme.md
# Проект «Квест»
# Для запуска: npm start
| 9e07b7fc3c19bc96b8f75437b3115f080b7fd401 | [
"JavaScript",
"Markdown"
] | 4 | JavaScript | Ochkalov/squest | ba0f166546ebeb04968ce8895e293eb0c405f9a2 | 7bf6f2faced4fcb2597b2747bb95f0269162c666 | |
refs/heads/master | <file_sep>from django.urls import path
from basicapp import views
urlpatterns=[
path('',views.signup_view,name='signup')
]
<file_sep>from django import forms
from .models import SignUp
class FormName(forms.Form):
name = forms.CharField()
email = forms.EmailField()
text = forms.CharField(widget=forms.Textarea)
class SignupForm(forms.ModelForm):
class Meta:
model = SignUp
fields = ('__all__')
| 0c4a3e6dc61d548528dce2f48e564d20cef21c26 | [
"Python"
] | 2 | Python | pravinc22/D_level_three | 71f1e41e73d89424cb480536757df64d4caded1b | 83e229a87d09fbe62fd3aacca2c869776b9f1034 | |
refs/heads/main | <file_sep># -*- coding: utf-8 -*-
from vectorizers.bert_vectorizer import BERTVectorizer
from vectorizers import tokenizationK
from models.bert_nlu_model import BertNLUModel
from utils import flatten
import argparse
import os
import pickle
import tensorflow as tf
# read command-line parameters
parser = argparse.ArgumentParser('Evaluating the Joint BERT / ALBERT NLU model')
parser.add_argument('--model', '-m', help = 'Path to BERT / ALBERT NLU model', type = str, required = True)
parser.add_argument('--type', '-tp', help = 'bert or albert', type = str, default = 'bert', required = False)
VALID_TYPES = ['bert', 'albert']
args = parser.parse_args()
load_folder_path = args.model
type_ = args.type
# this line is to disable gpu
os.environ["CUDA_VISIBLE_DEVICES"]="-1"
config = tf.ConfigProto(intra_op_parallelism_threads=1,
inter_op_parallelism_threads=1,
allow_soft_placement=True,
device_count = {'CPU': 1})
sess = tf.compat.v1.Session(config=config)
if type_ == 'bert':
bert_model_hub_path = './bert-module'
is_bert = True
elif type_ == 'albert':
bert_model_hub_path = 'https://tfhub.dev/google/albert_base/1'
is_bert = False
else:
raise ValueError('type must be one of these values: %s' % str(VALID_TYPES))
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
# loading models
print('Loading models ...')
if not os.path.exists(load_folder_path):
print('Folder `%s` not exist' % load_folder_path)
with open(os.path.join(load_folder_path, 'intents_label_encoder.pkl'), 'rb') as handle:
intents_label_encoder = pickle.load(handle)
intents_num = len(intents_label_encoder.classes_)
model = BertNLUModel.load(load_folder_path, sess)
vocab_file = "./bert-module/assets/vocab.korean.rawtext.list"
tokenizer = tokenizationK.FullTokenizer(vocab_file=vocab_file)
while True:
print('\nEnter your sentence: ')
try:
input_text = input().strip()
except:
continue
if input_text == 'quit':
break
tokens = tokenizer.tokenize(input_text)
tokens = [' '.join(tokens)]
data_input_ids, data_input_mask, data_segment_ids = bert_vectorizer.transform(tokens)
first_inferred_intent, first_inferred_intent_score, _, _ = model.predict_intent([data_input_ids, data_input_mask, data_segment_ids], intents_label_encoder)
print(first_inferred_intent)
print(first_inferred_intent_score)
tf.compat.v1.reset_default_graph()
<file_sep>import re
import os
import sys
import json
import logging
import numpy as np
import pandas as pd
import tensorflow as tf
import tensorflow_hub as hub
from tensorflow import keras
from tensorflow.keras.callbacks import ReduceLROnPlateau, ModelCheckpoint
from sklearn.model_selection import train_test_split
from modeling import BertModel, BertConfig
# get TF logger
log = logging.getLogger('tensorflow')
log.handlers = []
def build_module_fn(config_path, vocab_path, do_lower_case=True):
def bert_module_fn(is_training):
"""Spec function for a token embedding module."""
input_ids = tf.placeholder(shape=[None, None], dtype=tf.int32, name="input_ids")
input_mask = tf.placeholder(shape=[None, None], dtype=tf.int32, name="input_mask")
token_type = tf.placeholder(shape=[None, None], dtype=tf.int32, name="segment_ids")
config = BertConfig.from_json_file(config_path)
model = BertModel(config=config, is_training=is_training,
input_ids=input_ids, input_mask=input_mask, token_type_ids=token_type)
model.input_to_output()
seq_output = model.get_all_encoder_layers()[-1]
pool_output = model.get_pooled_output()
config_file = tf.constant(value=config_path, dtype=tf.string, name="config_file")
vocab_file = tf.constant(value=vocab_path, dtype=tf.string, name="vocab_file")
lower_case = tf.constant(do_lower_case)
tf.add_to_collection(tf.GraphKeys.ASSET_FILEPATHS, config_file)
tf.add_to_collection(tf.GraphKeys.ASSET_FILEPATHS, vocab_file)
input_map = {"input_ids": input_ids,
"input_mask": input_mask,
"segment_ids": token_type}
output_map = {"pooled_output": pool_output,
"sequence_output": seq_output}
output_info_map = {"vocab_file": vocab_file,
"do_lower_case": lower_case}
hub.add_signature(name="tokens", inputs=input_map, outputs=output_map)
hub.add_signature(name="tokenization_info", inputs={}, outputs=output_info_map)
return bert_module_fn
MODEL_DIR = "004_bert_eojeol_tensorflow"
config_path = "{}/bert_config.json".format(MODEL_DIR)
vocab_path = "{}/vocab.korean.rawtext.list".format(MODEL_DIR)
tags_and_args = []
for is_training in (True, False):
tags = set()
if is_training:
tags.add("train")
tags_and_args.append((tags, dict(is_training=is_training)))
module_fn = build_module_fn(config_path, vocab_path)
spec = hub.create_module_spec(module_fn, tags_and_args=tags_and_args)
spec.export("bert-module",
checkpoint_path="{}/model.ckpt-56000".format(MODEL_DIR))
print('complete')
<file_sep>import os, re
import pandas as pd
import numpy as np
from selenium import webdriver
import time
class WebUtil :
def __init__(self) :
self.category_dict = {'치킨':5, '피자&양식':6,
'중국집':7, '한식':8, '일식&돈까스':9,
'족발&보쌈':10, '야식':11,
'분식':12, '카페&디저트':13 }
def open_url(self, chromedriver_path, url) :
self.driver = webdriver.Chrome(chromedriver_path)
self.driver.maximize_window()
self.driver.get(url)
def set_location(self, location) :
print(location +'으로 위치 설정 하는중...')
self.driver.find_element_by_css_selector('#search > div > form > input').click()
time.sleep(2)
self.driver.find_element_by_css_selector('#button_search_address > button.btn-search-location-cancel.btn-search-location.btn.btn-default > span').click()
time.sleep(2)
self.driver.find_element_by_css_selector('#search > div > form > input').send_keys(location)
self.driver.find_element_by_css_selector('#button_search_address > button.btn.btn-default.ico-pick').click()
time.sleep(2)
self.driver.find_element_by_css_selector('#search > div > form > ul > li:nth-child(3) > a').click()
time.sleep(2)
print(location+'으로 위치 설정 완료!')
def go_to_category(self, category) :
print(category+' 카테고리 페이지 로드중...')
self.driver.find_element_by_xpath('//*[@id="category"]/ul/li[{}]/span'.format(self.category_dict.get(category))).click()
time.sleep(2)
print(category+' 카테고리 페이지 로드 완료!')
def scroll_bottom(self) :
self.driver.execute_script("window.scrollTo(0, document.body.scrollHeight)")
def stretch_category_store_page(self) :
print("카테고리 가게 펼치는 중...")
prev_height = self.driver.execute_script("return document.body.scrollHeight")
while True :
self.scroll_bottom()
time.sleep(2)
curr_height = self.driver.execute_script("return document.body.scrollHeight")
if curr_height == prev_height:
break
prev_height = curr_height
print("카테고리 가게 펼치기 완료!")
def get_restaurant_list(self) :
print("가게 리스트 만들기 시작...")
restaurant_list = []
restaurants = self.driver.find_elements_by_css_selector('#content > div > div:nth-child(4) > div > div.restaurant-list > div.col-sm-6.contract')
for restaurant in restaurants:
review_cnt = restaurant.find_element_by_css_selector('div > table > tbody > tr > td:nth-child(2) > div > div.stars > span:nth-child(2)').text
try :
if int(review_cnt.replace('리뷰 ', '')) >= 10 :
restaurant_list.append(restaurant.find_element_by_css_selector('div > table > tbody > tr > td:nth-child(2) > div > div.restaurant-name.ng-binding').text)
except ValueError as e :
pass
self.restaurant_list = list(set(restaurant_list))
print("가게 리스트 만들기 성공!")
def search_restaurant(self, restaurant_name):
print("가게 검색중...")
self.driver.find_element_by_xpath('//*[@id="category"]/ul/li[1]/a').click()
self.driver.find_element_by_xpath('//*[@id="category"]/ul/li[15]/form/div/input').send_keys(restaurant_name)
self.driver.find_element_by_xpath('//*[@id="category_search_button"]').click()
time.sleep(5)
print("가게 검색 성공!")
def go_to_restaurant(self):
print("검색한 가게 가는 중...")
self.driver.find_element_by_xpath('//*[@id="content"]/div/div[5]/div/div/div/div/table/tbody/tr/td[2]/div/div[1]').click()
time.sleep(5)
print("검색한 가게 갔음!")
def go_to_cleanreview(self):
print("클린리뷰 가는 중")
self.driver.find_element_by_xpath('//*[@id="content"]/div[2]/div[1]/ul/li[2]/a').click()
time.sleep(2)
print("클린리뷰 갔음!")
def click_more_review(self):
self.driver.find_element_by_class_name('btn-more').click()
time.sleep(2)
def stretch_review_page(self):
review_count = int(self.driver.find_element_by_xpath('//*[@id="content"]/div[2]/div[1]/ul/li[2]/a/span').text)
#click_count = min(int(review_count/10), 10)
click_count = int((review_count/10))
print('모든 리뷰 불러오기 시작...')
for _ in range(click_count):
self.scroll_bottom()
self.click_more_review()
self.scroll_bottom()
print('모든 리뷰 불러오기 완료!')
def get_all_review_elements(self):
print('리뷰 모든 요소 가져오는 중...')
self.reviews = self.driver.find_elements_by_css_selector('#review > li.list-group-item.star-point.ng-scope')
print('리뷰 모든 요소 가져옴!')
def go_back_page(self):
print('페이지 돌아가기중...')
self.driver.execute_script("window.history.go(-1)")
time.sleep(5)
print('페이지 돌아가기 완료!'+'\n')
def Crawl_Main(self, gu: str, file_path: str, encoding: str, chromedriver_path: str, url: str) :
self.open_url(chromedriver_path, url)
dong_list = Location_setting(gu)
for dong in dong_list :
location = gu + " " + dong
self.set_location(location)
for key in self.category_dict :
self.go_to_category(key)
self.stretch_category_store_page()
self.get_restaurant_list()
for restaurant_name in self.restaurant_list :
self.search_restaurant(restaurant_name)
self.go_to_restaurant()
self.go_to_cleanreview()
self.stretch_review_page()
self.get_all_review_elements()
store = Store()
self.review_Dataframe = store.make_review_dataframe(self.reviews, self.driver, key, location)
write_dataframe_to_csv(file_path, restaurant_name, self.review_Dataframe, encoding)
self.go_back_page()
class Store :
def __init__(self) :
self.review_Dataframe = pd.DataFrame(columns=['Category','Location','Restaurant','UserID','Menu','Review',
'Total','Taste','Quantity','Delivery','Date'])
def make_review_dataframe(self, reviews, driver, category, location) :
print("리뷰 데이터프레임 만드는 중...")
for review in reviews :
# 가-힣, 공백 이외의 리뷰는 제외
review_txt = review.find_element_by_css_selector('p').text
re_review_txt = re.sub('[^가-힣 ]', '', review_txt)
if len(re_review_txt) >= 12 :
self.review_Dataframe.loc[len(self.review_Dataframe)] = {
'Category': category,
'Location': location,
'Restaurant': driver.find_element_by_class_name('restaurant-name').text,
'UserID': review.find_element_by_css_selector('span.review-id.ng-binding').text,
'Menu': review.find_element_by_css_selector('div.order-items.default.ng-binding').text,
'Review': re_review_txt,
'Total': str(len(review.find_elements_by_css_selector('div > span.total > span.full.ng-scope'))),
'Taste': review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(3)').text,
'Quantity': review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(6)').text,
'Delivery': review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(9)').text,
'Date': review.find_element_by_css_selector('div:nth-child(1) > span.review-time.ng-binding').text,
}
print("리뷰 데이터프레임 완성!")
return self.review_Dataframe
def Location_setting(gu) :
if gu == '송파구' :
dong_list = ['가락동','거여동','마천동','문정동','방이동','삼전동','석촌동','송파동','오금동','오륜동','잠실동','장지동','풍납동']
return dong_list
elif gu == '강남구' :
dong_list = ['논현동','삼성동','청담동','개포동','대치동','신사동','역삼동','압구정동','세곡동','자곡동','율현동','일원동','수서동','도곡동']
return dong_list
elif gu == '서초구' :
dong_list = ['방배동','양재동','우면동','원지동','잠원동','반포동','서초동','내곡동','염곡동','신원동']
return dong_list
def write_dataframe_to_csv(file_path, restaurant_name, review_Dataframe, encoding) :
print("csv파일 생성중...")
review_Dataframe.to_csv(f"{file_path}{restaurant_name}.csv", encoding = encoding)
print("csv파일 생성 완료!")
if __name__ == "__main__" :
gu = "강남구"
file_path = "C:/sklee/Academy/미디어젠 프로젝트/Crawl_test/"
encoding = "UTF-8-sig"
chromedriver_path = "C:/Users/samsung/Downloads/chromedriver.exe"
url = "https://www.yogiyo.co.kr/mobile/#/"
Crawl = WebUtil()
Crawl.Crawl_Main(gu, file_path, encoding, chromedriver_path, url)
##############################################################################
"""
file_path에는 csv파일이 생성될 폴더 경로
chromedirver_path에는 chromedriver의 위치 경로
Location_setting에는 형식에 맞춰 '구'와 '동'을 기입한 뒤에
gu에 검색할 '구'를 써넣고 실행시키면 됩니다.
리뷰수 10개 이상인 가게만
가-힣과 공백 이외의 텍스트는 제외
"""
<file_sep>import pandas as pd
from selenium import webdriver
import time
from tqdm import tqdm
from tqdm import trange
# 크롬 드라이버로 크롬 켜기
driver = webdriver.Chrome("./data/chromedriver.exe")
# 창 최소화모드에서는 카테고리가 보이지 않기에 창 최대화
driver.maximize_window()
# 접속할 웹 사이트 주소(요기요 홈페이지)
url = "https://www.yogiyo.co.kr/mobile/#/"
driver.get(url)
# 검색할 주소(나중에 구+동으로 나눠야 함)
location = "화성시 능동"
# 시간을 2초로 줌
interval = 2
# 주소 입력해서 페이지 넘어감
# 입력창 클릭 -> 입력창에 써져있는거 지우기 클릭 -> 로케이션 값 보내기 -> 검색버튼 클릭 -> 첫번째 검색결과 클릭
print(location +'으로 위치 설정 하는중...')
driver.find_element_by_css_selector('#search > div > form > input').click()
driver.find_element_by_css_selector('#button_search_address > button.btn-search-location-cancel.btn-search-location.btn.btn-default > span').click()
driver.find_element_by_css_selector('#search > div > form > input').send_keys(location)
driver.find_element_by_css_selector('#button_search_address > button.btn.btn-default.ico-pick').click()
time.sleep(interval)
driver.find_element_by_css_selector('#search > div > form > ul > li:nth-child(3) > a').click()
time.sleep(interval)
print(location+'으로 위치 설정 완료!')
#요기요 카테고리 페이지의 Element Number Dictionary 정의
#카테고리의 xpath 값이 카테고리 별로 다르기에 숫자를 다르게 넣어주려고 이렇게 정의한 것
food_dict = { '치킨':5, '피자&양식':6,
'중국집':7, '한식':8, '일식&돈까스':9,
'족발&보쌈':10, '야식':11,
'분식':12, '카페&디저트':13 }
for category in food_dict:
print(category+' 카테고리 페이지 로드중...')
# food_dict.get(category) -> category라는 key에 대응되는 value를 리턴
# .format() 이건 문자열 포매팅을 한 것이다.
# food_dict.get() 이건 괄호 안에 들어가는 key에 대한 value를 리턴하는 것이다.
# 그리고 문자열 안의 {} 안에 그 value(여기선 숫자)가 들어가게 되는 것이다.
driver.find_element_by_xpath('//*[@id="category"]/ul/li[{}]/span'.format(food_dict.get(category))).click()
time.sleep(interval)
print(category+' 카테고리 페이지 로드 완료!')
# 현재 문서 높이를 가져와서 저장
prev_height = driver.execute_script("return document.body.scrollHeight")
# 반복 수행
while True:
# 스크롤을 가장 아래로 내림
driver.execute_script("window.scrollTo(0, document.body.scrollHeight)")
# 페이지 로딩 대기
time.sleep(interval)
# 현재 문서 높이를 가져와서 저장
curr_height = driver.execute_script("return document.body.scrollHeight")
# 전에 저장한 문서 높이와 현재 저장한 문서 높이가 같으면 while문 빠져나감
if curr_height == prev_height:
break
prev_height = curr_height
print("스크롤 완료")
# 리스트 객체 만들고
restaurant_list=[]
# 우리동네플러스나 슈퍼레드위크추천이 아닌 요기요등록음식점 부분으로 설정하는 것
restaurants = driver.find_elements_by_css_selector('#content > div > div:nth-child(4) > div > div.restaurant-list > div.col-sm-6.contract')
# restaurants에 저장된 elements에서 loop를 돌리며 (각각의 가게들을 순회하며)
for restaurant in restaurants:
restaurant_list.append(restaurant.find_element_by_css_selector('div > table > tbody > tr > td:nth-child(2) > div > div.restaurant-name.ng-binding').text)
# 일단 실험해보는거니까 리스트에서 첫번째것만 빼서 전달
for restaurant_name in restaurant_list:
# 주소 설정창 말고 음식점 검색 버튼 클릭
driver.find_element_by_xpath('//*[@id="category"]/ul/li[1]/a').click()
# 음식점 검색 입력창에 가게 이름 전달
driver.find_element_by_xpath('//*[@id="category"]/ul/li[15]/form/div/input').send_keys(restaurant_name)
# 음식점 검색 버튼 클릭
driver.find_element_by_xpath('//*[@id="category_search_button"]').click()
time.sleep(interval)
# 첫번째 나오는 음식점 클릭
driver.find_element_by_css_selector('#content > div > div:nth-child(5) > div > div > div:nth-child(1) > div').click()
time.sleep(interval)
# 클린리뷰 클릭
driver.find_element_by_xpath('//*[@id="content"]/div[2]/div[1]/ul/li[2]/a').click()
time.sleep(interval)
# 클린리뷰 옆에 있는 리뷰 숫자가 써져있는데 그걸 보여주는 요소의 text를 가져와서 정수형으로 변환
review_count = int(driver.find_element_by_xpath('//*[@id="content"]/div[2]/div[1]/ul/li[2]/a/span').text)
# 요기요 리뷰는 더보기를 눌렀을 때 10개를 로드하니까 몇번 더보기를 누를지 정해야하니까 10으로 나누어줌
click_count = int((review_count/10))
# 리뷰 페이지를 모두 펼치는 for문 시작
print('모든 리뷰 불러오는 중...')
for _ in trange(click_count):
try:
# 스크롤을 가장 아래로 내림
driver.execute_script("window.scrollTo(0,document.body.scrollHeight);")
# 더보기 클릭
driver.find_element_by_class_name('btn-more').click()
# 2초 쉼
time.sleep(2)
except Exception as e:
print(e)
print('페이지 돌아가기중...')
driver.execute_script("window.history.go(-1)")
time.sleep(interval)
print('페이지 돌아가기 완료!\n')
continue
# 스크롤을 가장 아래로 내림
driver.execute_script("window.scrollTo(0,document.body.scrollHeight);")
print('모든 리뷰 불러오기 완료!')
### 리뷰 크롤링
# 리뷰 가져와서 revies객체에 할당
reviews = driver.find_elements_by_css_selector('#review > li.list-group-item.star-point.ng-scope')
# 데이터프레임 객체 생성 및 열에 이름 부여
print('리뷰 저장하는 중...')
df = pd.DataFrame(columns=['Category','Location','Restaurant','UserID','Menu','Review','Date','Total','Taste','Quantity','Delivery'])
for review in tqdm(reviews):
try:
df.loc[len(df)] = {
'Category':category,
'Location':location,
'Restaurant':driver.find_element_by_class_name('restaurant-name').text,
'UserID':review.find_element_by_css_selector('span.review-id.ng-binding').text,
'Menu':review.find_element_by_css_selector('div.order-items.default.ng-binding').text,
'Review':review.find_element_by_css_selector('p').text,
'Date':review.find_element_by_css_selector('div:nth-child(1) > span.review-time.ng-binding').text,
'Total':str(len(review.find_elements_by_css_selector('div > span.total > span.full.ng-scope'))),
'Taste':review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(3)').text,
'Quantity':review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(6)').text,
'Delivery':review.find_element_by_css_selector('div:nth-child(2) > div > span.category > span:nth-child(9)').text,
}
except Exception as e:
print('리뷰 페이지 에러')
print(e)
print('페이지 돌아가기중...')
driver.execute_script("window.history.go(-1)")
time.sleep(interval)
print('페이지 돌아가기 완료!\n')
continue
try:
df.to_csv('./data/yogiyo/' + df.Restaurant[0] + '.csv')
print('리뷰 저장하는 완료!')
except:
pass
print('페이지 돌아가기중...')
driver.execute_script("window.history.go(-1)")
time.sleep(interval)
print('페이지 돌아가기 완료!\n')
<file_sep># -*- coding: utf-8 -*-
from readers.data_reader import Reader
from vectorizers.bert_vectorizer import BERTVectorizer
from vectorizers import tokenizationK as tk
from models.bert_nlu_model import BertNLUModel
from utils import flatten
import argparse
import os
import pickle
import tensorflow as tf
from sklearn import metrics
# read command-line parameters
parser = argparse.ArgumentParser('Evaluating the BERT / ALBERT NLU model')
parser.add_argument('--model', '-m', help = 'Path to BERT / ALBERT NLU model', type = str, required = True)
parser.add_argument('--data', '-d', help = 'Path to data', type = str, required = True)
parser.add_argument('--type', '-tp', help = 'bert or albert', type = str, default = 'bert', required = False)
VALID_TYPES = ['bert', 'albert']
args = parser.parse_args()
load_folder_path = args.model
data_folder_path = args.data
type_ = args.type
# this line is to disable gpu
os.environ['CUDA_VISIBLE_DEVICES']='-1'
config = tf.ConfigProto(intra_op_parallelism_threads=8,
inter_op_parallelism_threads=0,
allow_soft_placement=True,
device_count = {'CPU': 8})
sess = tf.Session(config=config)
if type_ == 'bert':
bert_model_hub_path = './bert-module'
is_bert = True
elif type_ == 'albert':
bert_model_hub_path = 'https://tfhub.dev/google/albert_base/1'
is_bert = False
else:
raise ValueError('type must be one of these values: %s' % str(VALID_TYPES))
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
# loading models
print('Loading models ...')
if not os.path.exists(load_folder_path):
print('Folder `%s` not exist' % load_folder_path)
with open(os.path.join(load_folder_path, 'intents_label_encoder.pkl'), 'rb') as handle:
intents_label_encoder = pickle.load(handle)
intents_num = len(intents_label_encoder.classes_)
model = BertNLUModel.load(load_folder_path, sess)
tokenizer = tk.FullTokenizer('./bert-module/assets/vocab.korean.rawtext.list')
data_text_arr, data_intents = Reader.read(data_folder_path)
data_input_ids, data_input_mask, data_segment_ids = bert_vectorizer.transform(data_text_arr)
def get_results(input_ids, input_mask, segment_ids, intents, intents_label_encoder):
first_inferred_intent, first_inferred_intent_score, _, _ = model.predict_intent([data_input_ids, data_input_mask, data_segment_ids], intents_label_encoder)
acc = metrics.accuracy_score(intents, first_inferred_intent)
intent_incorrect = ''
for i, sent in enumerate(input_ids):
if intents[i] != first_inferred_intent[i]:
tokens = tokenizer.convert_ids_to_tokens(input_ids[i])
intent_incorrect += ('sent {}\n'.format(tokens))
intent_incorrect += ('pred: {}\n'.format(first_inferred_intent[i].strip()))
intent_incorrect += ('score: {}\n'.format(first_inferred_intent_score[i]))
intent_incorrect += ('ansr: {}\n'.format(intents[i].strip()))
intent_incorrect += '\n'
return acc, intent_incorrect
print('==== Evaluation ====')
acc, intent_incorrect = get_results(data_input_ids, data_input_mask, data_segment_ids,
data_intents, intents_label_encoder)
# 테스트 결과를 모델 디렉토리의 하위 디렉토리 'test_results'에 저장해 준다.
result_path = os.path.join(load_folder_path, 'test_results')
if not os.path.isdir(result_path):
os.mkdir(result_path)
with open(os.path.join(result_path, 'intent_incorrect.txt'), 'w') as f:
f.write(intent_incorrect)
with open(os.path.join(result_path, 'test_total.txt'), 'w') as f:
f.write('Intent accuracy = {}\n'.format(acc))
tf.compat.v1.reset_default_graph()
<file_sep># -*- coding: utf-8 -*-
import tensorflow as tf
import os
from tensorflow.keras import backend as K
import tensorflow_hub as hub
import json
class KorBertLayer(tf.keras.layers.Layer):
def __init__(self, bert_path="./bert-module", n_tune_layers=10,
pooling="cls", tune_embeddings=True, trainable=True, **kwargs):
self.trainable = trainable
self.n_tune_layers = n_tune_layers
self.tune_embeddings = tune_embeddings
self.pooling = pooling
self.bert_path = bert_path
self.output_size = 768
self.var_per_encoder = 16
if self.pooling not in ["cls", "mean", None]:
raise NameError(
f"Undefined pooling type (must be either 'cls', 'mean', or None, but is {self.pooling}"
)
super(KorBertLayer, self).__init__(**kwargs)
print('init ok')
def build(self, input_shape):
self.bert = hub.Module(self.build_abspath(self.bert_path),
trainable=self.trainable, name=f"{self.name}_module")
# Remove unused layers
trainable_vars = self.bert.variables
if self.pooling == 'cls':
trainable_vars = [var for var in trainable_vars if not "/cls/" in var.name]
trainable_layers = ["pooler/dense"]
elif self.pooling == 'mean':
trainable_vars = [var for var in trainable_vars
if not "/cls/" in var.name and not "/pooler/" in var.name
]
trainable_layers = []
else:
raise NameError(
f"Undefined pooling type (must be either first or mean, but is {self.pooling}"
)
# append word_embedding to trainable_layers
trainable_layers.append('bert/embeddings/word_embeddings:0')
# Select how many layers to fine tune
for i in range(self.n_tune_layers):
trainable_layers.append(f"encoder/layer_{str(11 - i)}")
# Update trainable vars to contain only the specified layers
trainable_vars = [
var
for var in trainable_vars
if any([l in var.name for l in trainable_layers])
]
# Add to trainable weights
for var in trainable_vars:
self._trainable_weights.append(var)
for var in self.bert.variables:
if var not in self._trainable_weights:
self._non_trainable_weights.append(var)
super(KorBertLayer, self).build(input_shape)
def build_abspath(self, path):
if path.startswith("https://") or path.startswith("gs://"):
return path
else:
return os.path.abspath(path)
def call(self, inputs):
inputs = [K.cast(x, dtype="int32") for x in inputs]
input_ids, input_mask, segment_ids= inputs
bert_inputs = dict(
input_ids=input_ids, input_mask=input_mask, segment_ids=segment_ids
)
result = self.bert(inputs=bert_inputs, signature='tokens', as_dict=True)
print('call ok')
return result['pooled_output']
def compute_output_shape(self, input_shape):
return (input_shape[0], input_shape[1], self.output_size)
def get_config(self):
config = super().get_config().copy()
config.update({
'n_tune_layers': self.n_tune_layers,
'trainable': self.trainable,
'output_size': self.output_size,
'pooling': self.pooling,
'bert_path': self.bert_path,
})
return config
<file_sep># -*- coding: utf-8 -*-
import tensorflow as tf
import numpy as np
import sys
from vectorizers import tokenizationK as tk
class BERTVectorizer:
def __init__(self, is_bert,
bert_model_hub_path="./bert-module"):
print('initializing!')
self.is_bert = is_bert
print('is_bert :', self.is_bert)
self.bert_model_hub_path = bert_model_hub_path
print('bert_model_hub_path :', self.bert_model_hub_path)
self.tokenizer = tk.FullTokenizer('./bert-module/assets/vocab.korean.rawtext.list')
print('initialized!')
def transform(self, text_arr):
print('transform started')
input_ids = []
input_mask = []
segment_ids = []
# print('text arr :', text_arr)
for text in text_arr:
ids, mask, seg_ids= self.__vectorize(text.strip())
# print('text :', text)
# print('ids :', ids)
# print('mask :', mask)
# print('seg_ids :', seg_ids)
# print('valid pos :', valid_pos)
input_ids.append(ids)
input_mask.append(mask)
segment_ids.append(seg_ids)
input_ids = tf.keras.preprocessing.sequence.pad_sequences(input_ids, padding='post')
input_mask = tf.keras.preprocessing.sequence.pad_sequences(input_mask, padding='post')
segment_ids = tf.keras.preprocessing.sequence.pad_sequences(segment_ids, padding='post')
return input_ids, input_mask, segment_ids
def __vectorize(self, text: str):
# str -> tokens list
tokens = text.split() # whitespace tokenizer
# insert "[CLS]"
tokens.insert(0, '[CLS]')
# insert "[SEP]"
tokens.append('[SEP]')
segment_ids = [0] * len(tokens)
input_ids = self.tokenizer.convert_tokens_to_ids(tokens)
input_mask = [1] * len(input_ids)
return input_ids, input_mask, segment_ids
<file_sep># flask_app/server.py
import datetime
import re
from flask_ngrok import run_with_ngrok
from flask import Flask, request, jsonify, render_template, session, url_for, redirect
from flask_dropzone import Dropzone
import time
from urllib.parse import unquote
import os
import uuid
import secrets
from run_intent_classify import sentiment_predict, csv_predict
from settings import *
import requests
import pdb
from input_module import read_file
app = Flask(__name__)
# colab에서의 서빙을 위해 flask_ngrok 모듈의 run_with_ngrok 함수를 사용합니다.
run_with_ngrok(app)
UPLOAD_FOLDER = os.path.join(app.root_path,'upload_files')
app.config['SEND_FILE_MAX_AGE_DEFAULT'] = 0
app.config['DROPZONE_UPLOAD_MULTIPLE'] = True
app.config['DROPZONE_ALLOWED_FILE_TYPE'] = 'text'
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
app.config.update(
SECRET_KEY=secrets.token_urlsafe(32),
SESSION_COOKIE_NAME='InteractiveTransformer-WebSession'
)
dropzone = Dropzone(app)
def delay_func(func):
def inner(*args, **kwargs):
returned_value = func(*args, **kwargs)
time.sleep(0)
return returned_value
return inner
# 실행 시작
@app.route("/")
def index():
# 처음 보여지는 화면입니다.
return render_template("index.html")
@app.route('/_evaluate_helper')
def evaluate_helper():
eval_text = request.args.get("evaluate_data", "", type=str).strip()
eval_text = re.sub("%20", "", eval_text)
prediction = sentiment_predict(eval_text)
if prediction and eval_text:
return jsonify(result=render_template('live_eval.html', predict=[prediction[0]], eval_text=[eval_text], intent=[prediction[1]]))
return jsonify(result="")
@app.route('/_evaluate_helper_file', methods=['POST'])
def evaluate_helper_file():
if 'file' not in request.files:
print('No file part')
else:
file = request.files.get('file')
print(file)
input_df = read_file(file)
prediction = csv_predict(input_df)
return jsonify(result=render_template("result_file.html", predict=[prediction]))
if __name__ == '__main__':
#app.run(host="0.0.0.0", debug=True, port=PORT)
app.run()
<file_sep># -*- coding: utf-8 -*-
from readers.data_reader import Reader
from vectorizers.bert_vectorizer import BERTVectorizer
from models.bert_nlu_model import BertNLUModel
import argparse
from sklearn.preprocessing import LabelEncoder
import numpy as np
import os
import pickle
import tensorflow as tf
from tensorflow import keras
# read command-line parameters
parser = argparse.ArgumentParser('Training the BERT NLU model')
parser.add_argument('--train', '-t', help = 'Path to training data', type = str, required = True)
parser.add_argument('--val', '-v', help = 'Path to validation data', type = str, default = "", required = False)
parser.add_argument('--save', '-s', help = 'Folder path to save the trained model', type = str, required = True)
parser.add_argument('--epochs', '-e', help = 'Number of epochs', type = int, default = 5, required = False)
parser.add_argument('--batch', '-bs', help = 'Batch size', type = int, default = 64, required = False)
parser.add_argument('--type', '-tp', help = 'bert or albert', type = str, default = 'bert', required = False)
parser.add_argument('--model', '-mo', help = 'load model', type = str, default = "", required = False)
VALID_TYPES = ['bert', 'albert']
args = parser.parse_args()
train_data_folder_path = args.train
val_data_folder_path = args.val
save_folder_path = args.save
epochs = args.epochs
batch_size = args.batch
type_ = args.type
model_path = args.model
# # this line is to disable gpu
# os.environ["CUDA_VISIBLE_DEVICES"]="-1"
tf.compat.v1.random.set_random_seed(7)
config = tf.ConfigProto(intra_op_parallelism_threads=8,
inter_op_parallelism_threads=0,
allow_soft_placement=True,
device_count = {'CPU': 8})
sess = tf.compat.v1.Session(config=config)
if type_ == 'bert':
bert_model_hub_path = "./bert-module" # to use KorBert by Etri
is_bert = True
elif type_ == 'albert':
bert_model_hub_path = 'https://tfhub.dev/google/albert_base/1'
is_bert = False
else:
raise ValueError('type must be one of these values: %s' % str(VALID_TYPES))
#이 부분을 고쳐서 Database를 이용해서 데이터를 읽어올 수 있음
print('read data ...')
train_text_arr, train_intents = Reader.read(train_data_folder_path)
print('train_text_arr[0:2] :', train_text_arr[0:2])
print('train_intents[0:2] :', train_intents[0:2])
print('vectorize data ...')
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
# now bert model hub path exists --> already tokenized dataset
# bert vectorizer MUST NOT tokenize input !!!
print('bert vectorizer started ...') #valid pos removed
train_input_ids, train_input_mask, train_segment_ids = bert_vectorizer.transform(train_text_arr)
print('encode labels ...')
intents_label_encoder = LabelEncoder()
train_intents = intents_label_encoder.fit_transform(train_intents).astype(np.int32)
intents_num = len(intents_label_encoder.classes_)
print('intents num :', intents_num)
if model_path == "":
model = BertNLUModel(intents_num, bert_model_hub_path, sess, num_bert_fine_tune_layers=10, is_bert=is_bert)
else:
model = BertNLUModel.load(load_folder_path, sess)
print('train input shape :', train_input_ids.shape, train_input_ids[0:2])
print('train_input_mask :', train_input_mask.shape, train_input_mask[0:2])
print('train_segment_ids :', train_segment_ids.shape, train_segment_ids[0:2])
print('train_intents :', train_intents.shape, train_intents[0:2])
if not os.path.exists(save_folder_path):
os.makedirs(save_folder_path)
print('Folder `%s` created' % save_folder_path)
checkpoint_cb = keras.callbacks.ModelCheckpoint(save_folder_path+"/my_yogiyo_model.h5", save_best_only=True)
early_stopping_cb = keras.callbacks.EarlyStopping(patience=5, restore_best_weights=True)
if val_data_folder_path:
print('preparing validation data')
val_text_arr, val_intents = Reader.read(val_data_folder_path)
val_input_ids, val_input_mask, val_segment_ids = bert_vectorizer.transform(val_text_arr)
val_intents = intents_label_encoder.transform(val_intents).astype(np.int32)
print('training model ...')
model.fit([train_input_ids, train_input_mask, train_segment_ids], train_intents,
validation_data=([val_input_ids, val_input_mask, val_segment_ids], val_intents),
epochs=epochs, batch_size=batch_size, callbacks=[checkpoint_cb, early_stopping_cb])
else:
print('training model ...')
model.fit([train_input_ids, train_input_mask, train_segment_ids], train_intents,
validation_data=None,
epochs=epochs, batch_size=batch_size, callbacks=[checkpoint_cb, early_stopping_cb])
### saving
print('Saving ..')
model.save(save_folder_path)
with open(os.path.join(save_folder_path, 'intents_label_encoder.pkl'), 'wb') as handle:
pickle.dump(intents_label_encoder, handle, protocol=pickle.HIGHEST_PROTOCOL)
tf.compat.v1.reset_default_graph()
<file_sep>Flask==1.1.1
tensorflow_gpu==1.15
tensorflow_hub==0.7.0
flask_dropzone
flask-ngrok
konlpy
h5py==2.10.0
<file_sep>import pandas as pd
def read_file(file):
input_df = pd.read_csv(file, header=None)
return input_df<file_sep># -*- coding: utf-8 -*-
from vectorizers.bert_vectorizer import BERTVectorizer
from vectorizers import tokenizationK
from models.bert_nlu_model import BertNLUModel
from utils import flatten
import argparse
import os
import pickle
import numpy as np
import tensorflow as tf
import math
def sentiment_predict(input_sentence):
# read command-line parameters
load_folder_path = "yogiyo_model"
type_ = "bert"
# this line is to disable gpu
#os.environ["CUDA_VISIBLE_DEVICES"]="-1"
config = tf.ConfigProto(intra_op_parallelism_threads=1,
inter_op_parallelism_threads=1,
allow_soft_placement=True,)
sess = tf.compat.v1.Session(config=config)
bert_model_hub_path = './bert-module'
is_bert = True
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
with open(os.path.join(load_folder_path, 'intents_label_encoder.pkl'), 'rb') as handle:
intents_label_encoder = pickle.load(handle)
intents_num = len(intents_label_encoder.classes_)
model = BertNLUModel.load(load_folder_path, sess)
vocab_file = "./bert-module/assets/vocab.korean.rawtext.list"
tokenizer = tokenizationK.FullTokenizer(vocab_file=vocab_file)
tokens = tokenizer.tokenize(input_sentence)
tokens = [' '.join(tokens)]
data_input_ids, data_input_mask, data_segment_ids = bert_vectorizer.transform(tokens)
first_inferred_intent, first_inferred_intent_score, _, _ = model.predict_intent([data_input_ids, data_input_mask, data_segment_ids], intents_label_encoder)
intent = str(first_inferred_intent[0])
score = float(first_inferred_intent_score[0]) * 100
tf.compat.v1.reset_default_graph()
string = ''
rating = int(float(intent))
for i in range(1, 6):
if i > rating:
string +='☆'
else:
string += '★'
answer_text = "{:.2f}% 확률로 [{} : {}점] 입니다.".format(score, string, intent)
return answer_text, intent
def csv_predict(input_df):
# read command-line parameters
load_folder_path = "yogiyo_model"
type_ = "bert"
# this line is to disable gpu
os.environ["CUDA_VISIBLE_DEVICES"]="-1"
config = tf.ConfigProto(intra_op_parallelism_threads=1,
inter_op_parallelism_threads=1,
allow_soft_placement=True,)
sess = tf.compat.v1.Session(config=config)
bert_model_hub_path = './bert-module'
is_bert = True
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
with open(os.path.join(load_folder_path, 'intents_label_encoder.pkl'), 'rb') as handle:
intents_label_encoder = pickle.load(handle)
intents_num = len(intents_label_encoder.classes_)
model = BertNLUModel.load(load_folder_path, sess)
vocab_file = "./bert-module/assets/vocab.korean.rawtext.list"
tokenizer = tokenizationK.FullTokenizer(vocab_file=vocab_file)
score_list = []
for i,input_sentence in input_df[0].items():
print(f'{i}번째 데이터:{input_sentence}')
tokens = tokenizer.tokenize(input_sentence)
tokens = [' '.join(tokens)]
data_input_ids, data_input_mask, data_segment_ids = bert_vectorizer.transform(tokens)
first_inferred_intent, first_inferred_intent_score, _, _ = model.predict_intent([data_input_ids, data_input_mask, data_segment_ids], intents_label_encoder)
# intent = str(first_inferred_intent[0])
intent = float(str(first_inferred_intent[0]))
score_list.append(intent)
score = float(first_inferred_intent_score[0]) * 100
mean_score = np.mean(score_list)
tf.compat.v1.reset_default_graph()
# answer_text = "{:.2f}% 확률로 {}점입니다.".format(score, intent)
print('평점의 평균:',mean_score)
return round(mean_score, 1), math.floor(mean_score)
if __name__ == "__main__":
print(sentiment_predict("최악입니다."))
<file_sep># -*- coding: utf-8 -*-
import os, re
import argparse
from vectorizers.tokenizationK import FullTokenizer
import pdb
# 상황에 따라서 다른 tokenizer를 사용해도 된다.
tokenizer = FullTokenizer(vocab_file="./bert-module/assets/vocab.korean.rawtext.list")
multi_spaces = re.compile(r"\s+")
def process_file(file_path, output_dir):
"""
단방향 데이터가 있는 file_path을 argument로 주면 가공을 한 이후에
output_dir 아래에 2개의 파일(seq.in, label)을 저장해 주는 함수.
output_dir의 경우 만약 존재하지 않는다면
"""
if not os.path.isdir(output_dir):
os.mkdir(output_dir)
data = open(file_path).read().splitlines()
# line별로 process를 해준 뒤,
processed_data = [process_line(line, tokenizer) for line in data]
intentions = list(map(lambda x: x[0], processed_data))
tokens = list(map(lambda x: x[1], processed_data))
# seq_in : 토큰들로만 이루어진 파일
intention_file = os.path.join(output_dir, "label")
seq_in = os.path.join(output_dir, "seq.in")
with open(intention_file, "w") as f:
f.write("\n".join(intentions) + "\n")
with open(seq_in, "w") as f:
f.write("\n".join(tokens)+ "\n")
def process_line(line, tokenizer):
"""
데이터를 라인별로 처리해 주는 함수이다.
"""
intention, sentence = line.split("\t")
tokens = ""
for word in sentence.split():
word_tokens = " ".join(tokenizer.tokenize(word))
tokens += word_tokens + " "
tokens = multi_spaces.sub(" ", tokens.strip())
return intention, tokens
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument('--input', '-i', help = '단방향 데이터 형식의 텍스트 파일', type = str, required = True)
parser.add_argument('--output', '-o', help = 'Path to data', type = str, required = True)
args = parser.parse_args()
file_path = args.input
output_dir = args.output
process_file(file_path, output_dir)
<file_sep># -*- coding: utf-8 -*-
import tensorflow as tf
from tensorflow.keras import backend as K
import tensorflow_hub as hub
class AlbertLayer(tf.keras.layers.Layer):
def __init__(self, fine_tune=True, pooling='first',
albert_path="https://tfhub.dev/google/albert_base/1",
**kwargs,):
self.fine_tune = fine_tune
self.output_size = 768
self.pooling = pooling
self.albert_path = albert_path
if self.pooling not in ['first', 'mean']:
raise NameError(
f"Undefined pooling type (must be either first or mean, but is {self.pooling}"
)
super(AlbertLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.albert = hub.Module(
self.albert_path, trainable=self.fine_tune, name=f"{self.name}_module"
)
if self.fine_tune:
# Remove unused layers
trainable_vars = self.albert.variables
if self.pooling == 'first':
trainable_vars = [var for var in trainable_vars if not "/cls/" in var.name]
trainable_layers = ["pooler/dense"]
elif self.pooling == 'mean':
trainable_vars = [var for var in trainable_vars
if not "/cls/" in var.name and not "/pooler/" in var.name
]
trainable_layers = []
else:
raise NameError(
f"Undefined pooling type (must be either first or mean, but is {self.pooling}"
)
# Select how many layers to fine tune
trainable_layers.append("encoder/transformer/group_0")
# Update trainable vars to contain only the specified layers
trainable_vars = [
var
for var in trainable_vars
if any([l in var.name for l in trainable_layers])
]
# Add to trainable weights
for var in trainable_vars:
self._trainable_weights.append(var)
for var in self.albert.variables:
if var not in self._trainable_weights:
self._non_trainable_weights.append(var)
super(AlbertLayer, self).build(input_shape)
def call(self, inputs):
inputs = [K.cast(x, dtype="int32") for x in inputs]
input_ids, input_mask, segment_ids, valid_positions = inputs
bert_inputs = dict(
input_ids=input_ids, input_mask=input_mask, segment_ids=segment_ids
)
result = self.albert(inputs=bert_inputs, signature='tokens', as_dict=True)
return result['pooled_output']
def compute_output_shape(self, input_shape):
return (input_shape[0], input_shape[1], self.output_size)
def get_config(self):
config = super().get_config().copy()
config.update({
'fine_tune': self.fine_tune,
'output_size': self.output_size,
'pooling': self.pooling,
'albert_path': self.albert_path,
})
return config
<file_sep>from readers.data_reader import Reader
from vectorizers import tokenizationK as tk
import argparse
import os
import pdb
# read command-line parameters
parser = argparse.ArgumentParser('Get the vectors from tokens')
parser.add_argument('--data', '-d', help = 'Path to data', type = str, required = True)
parser.add_argument('--tsv', '-t', help = 'Path to {meta,vecs}.tsv', type = str, required = True)
args = parser.parse_args()
data_folder_path = args.data
tsv_folder_path = args.tsv
# read tokenized data from ${data_folder_path}
with open(os.path.join(data_folder_path, 'seq.in'), encoding='utf-8') as f:
text_arr = f.read().splitlines()
# read tsvs from ${tsv_folder_path}
with open(os.path.join(tsv_folder_path, 'vecs.tsv'), encoding='utf-8') as f:
vecs = f.read().splitlines()
with open(os.path.join(tsv_folder_path, 'meta.tsv'), encoding='utf-8') as f:
meta = f.read().splitlines()
# tokenizer
tokenizer = tk.FullTokenizer('./bert-module/assets/vocab.korean.rawtext.list')
# get all token ids that is used in the data
print('collecting token_ids from tokenized data...')
token_id_set = set()
for text in text_arr:
token_id_set.update(tokenizer.convert_tokens_to_ids(text.split()))
# token_ids = sorted(map(lambda x: x+1, token_id_set)) # add 1 since id starts from 0..?
token_ids = sorted(token_id_set)
# get vecs and meta from
vecs_appeared = [vecs[i] for i in token_ids]
meta_appeared = [meta[i] for i in token_ids]
# save
print('saving vecs and meta that appeared in the dataset')
with open(os.path.join(data_folder_path, 'vecs_appeared.tsv'), 'w') as f:
f.write('\n'.join(vecs_appeared))
with open(os.path.join(data_folder_path, 'meta_appeared.tsv'), 'w') as f:
f.write('\n'.join(meta_appeared))
<file_sep># -*- coding: utf-8 -*-
from vectorizers.bert_vectorizer import BERTVectorizer
from vectorizers import tokenizationK
from models.bert_nlu_model import BertNLUModel
from utils import flatten
import argparse
import os
import pickle
import tensorflow as tf
import pdb
# read command-line parameters
parser = argparse.ArgumentParser('Evaluating the Joint BERT / ALBERT NLU model')
parser.add_argument('--model', '-m', help = 'Path to BERT / ALBERT NLU model', type = str, required = True)
parser.add_argument('--type', '-tp', help = 'bert or albert', type = str, default = 'bert', required = False)
VALID_TYPES = ['bert', 'albert']
args = parser.parse_args()
load_folder_path = args.model
type_ = args.type
# this line is to disable gpu
os.environ["CUDA_VISIBLE_DEVICES"]="-1"
config = tf.ConfigProto(intra_op_parallelism_threads=1,
inter_op_parallelism_threads=1,
allow_soft_placement=True,
device_count = {'CPU': 1})
sess = tf.compat.v1.Session(config=config)
if type_ == 'bert':
bert_model_hub_path = './bert-module'
is_bert = True
elif type_ == 'albert':
bert_model_hub_path = 'https://tfhub.dev/google/albert_base/1'
is_bert = False
else:
raise ValueError('type must be one of these values: %s' % str(VALID_TYPES))
bert_vectorizer = BERTVectorizer(is_bert, bert_model_hub_path)
# loading models
print('Loading models ...')
if not os.path.exists(load_folder_path):
print('Folder `%s` not exist' % load_folder_path)
with open(os.path.join(load_folder_path, 'intents_label_encoder.pkl'), 'rb') as handle:
intents_label_encoder = pickle.load(handle)
intents_num = len(intents_label_encoder.classes_)
model = BertNLUModel.load(load_folder_path, sess)
vocab_file = "./bert-module/assets/vocab.korean.rawtext.list"
tokenizer = tokenizationK.FullTokenizer(vocab_file=vocab_file)
kobert_layer = model.model.layers[3]
# token_embedding = kobert_layer.weights[0]
token_embedding = kobert_layer.weights[0].eval(session=sess)
# pdb.set_trace()
out_m_path = os.path.join(load_folder_path, 'meta.tsv')
out_v_path = os.path.join(load_folder_path, 'vecs.tsv')
# make out_m(meta.tsv) first
print(f'creating {out_m_path} out of {vocab_file}')
os.system(f"sed 1,2d {vocab_file} | cut -f 1 > {out_m_path}")
out_v = open(out_v_path, 'w', encoding='utf-8')
print(f'creating {out_v_path}')
for token_emb in token_embedding:
# pdb.set_trace()
out_v.write('\t'.join([str(x) for x in token_emb]) + "\n")
<file_sep># koBERT-yogiyo-reviews-classfication
## 1. 프로젝트 기획
- 현 별점 시스템의 문제점 의식
- 리뷰 내용-별점 불일치가 많아 별점만으로는 식당에 대한 긍정/부정 판단이 어려움
- 쿠폰 및 리뷰 이벤트로 인한 이벤트성 리뷰와 의미 없는 댓글로 매장에 대한 정확한 평가가 어려움
- 리뷰 데이터에 대한 평점을 KorBERT 모델을 이용하여 계산하는 시스템
## 2. ETRI koBERT(pre trained)
- https://aiopen.etri.re.kr/service_dataset.php 에서 협약서 작성 및 tensorflow 버전 다운로드
## 3. 데이터 수집 및 전처리
- pre processing
- Merge_Regex: 크롤링된 파일 다루기
- Make_Sample: 재라벨링한 파일 다루기
- 카테고리별 식당 리뷰 무작위 수집 (Selenium을 사용하여 리뷰 크롤링 진행)
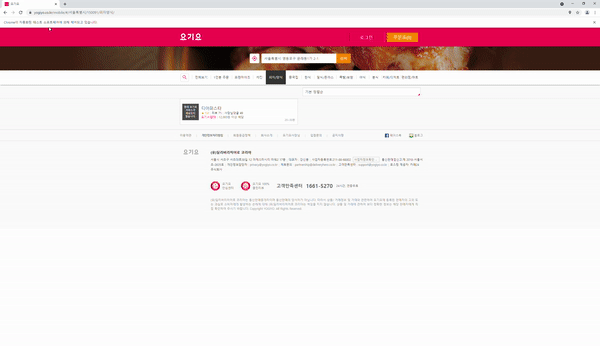
- 수집된 데이터에 대해 한글, 공백 제외한 모든 숫자/특수문자/모음과 자음만 있는 글자 제거
- 맞춤법 교정
- 리뷰 최소 길이 : 12음절
- 성능 개선을 위해 문장당 긍부정 비율에 기반한 기준 수립후 재라벨링
- 수집된 리뷰 코멘트를 30797 vocabs로 토큰화
- KorBERT 모델을 파인튜닝 할 수 있게 label과 input sequence로 나누어 줌 data.txt -> label, seq.in
- seq.in은 KorBERT가 pre-train된 대로 tokenization 과정을 거쳐 생성됨
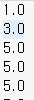

## 4. 파인튜닝 및 서비스
- 생성된 label, seq.in을 통해 파인튜닝 진행
- Flask를 이용해 웹 서버를 구동하고, 개별 리뷰에 대한 평점과
csv파일 업로드를 한 후 개선된 평점 확인 가능
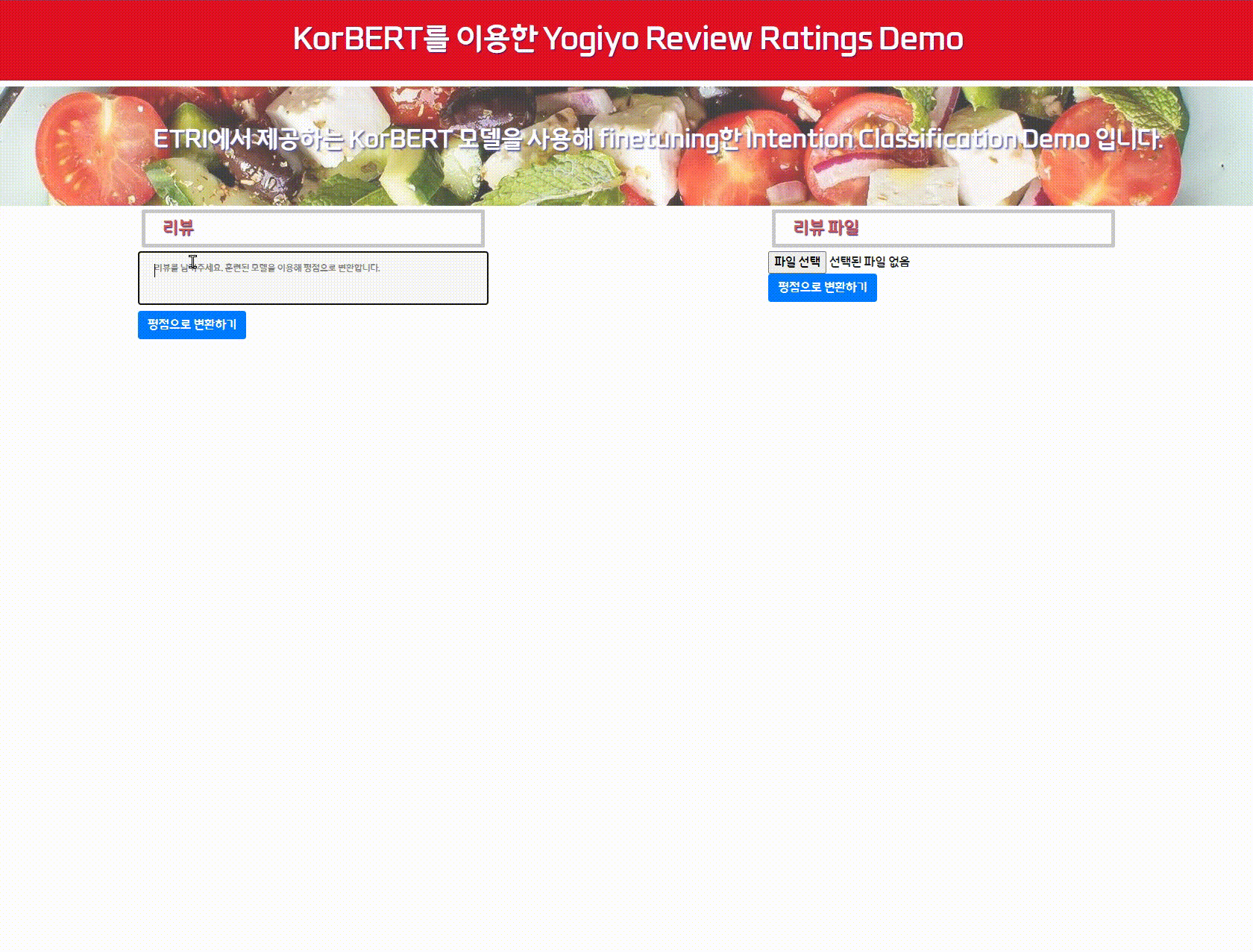
## 5. 결론
- BERT 모델은 동일한 단어의 문맥적 의미를 파악하여 표현
- 대용량 코퍼스 기반으로 사전학습된 모델을 가지고 수행하고자 하는 작업에 따라
미세조정을 하여 다양하게 활용가능하다.
- 다른 모델들과 성능비교시 정확도 또한 더 우수하다.

<file_sep>$(function() {
$('button#evaluate').on('click', function () {
$.getJSON($SCRIPT_ROOT + '/_evaluate_helper', {
evaluate_data: $('#evaluate-data').val()
}, function (data) {
$("#senti-result").prepend(data.result);
$("#evaluate-input").show();
$("#evaluate-data").val("");
});
return false;
});
});
$('#evaluate_csv').on('click', function () {
var url = $('#fileForm').attr('action');
var form_data = new FormData();
console.log($('#file').prop('files')[0]);
form_data.append('file', $('#file').prop('files')[0]);
$.ajax({
type: 'POST',
url: url,
data: form_data,
contentType: false,
processData: false,
success: function (data) {
$("#csv-result").prepend(data.result);
}
});
});
$('textarea').each(function () {
this.setAttribute('style', 'height:' + (this.scrollHeight) + 'px;overflow-y:hidden;');
}).on('input', function () {
this.style.height = 'auto';
this.style.height = (this.scrollHeight) + 'px';
});
$('a#toggle-history').on('click', function () {
var hist_toggle = $('#toggle-history');
hist_toggle.text() === "(hide)" ? hist_toggle.text("(show)") : hist_toggle.text("(hide)");
$("#history").toggle();
});
<file_sep># -*- coding: utf-8 -*-
import tensorflow as tf
from tensorflow.python.keras import backend as K
from tensorflow.python.keras.models import Model
from tensorflow.python.keras.layers import Input, Dense, Multiply, TimeDistributed
from models.base_nlu_model import BaseNLUModel
from layers.korbert_layer import KorBertLayer
import numpy as np
import os
import json
class BertNLUModel(BaseNLUModel):
def __init__(self, intents_num, bert_hub_path, sess, num_bert_fine_tune_layers=10,
is_bert=True, is_training=True):
self.intents_num = intents_num
self.bert_hub_path = bert_hub_path
self.num_bert_fine_tune_layers = num_bert_fine_tune_layers
self.is_bert = is_bert
self.is_training = is_training
self.model_params = {
'intents_num': intents_num,
'bert_hub_path': bert_hub_path,
'num_bert_fine_tune_layers': num_bert_fine_tune_layers,
'is_bert': is_bert
}
self.build_model()
self.compile_model()
self.initialize_vars(sess)
def compile_model(self):
optimizer = tf.keras.optimizers.Adam(lr=5e-5)#0.001)
losses = {
'intent_classifier': 'sparse_categorical_crossentropy',
}
metrics = {'intent_classifier': 'acc'}
self.model.compile(optimizer=optimizer, loss=losses, metrics=metrics)
self.model.summary()
def build_model(self):
in_id = Input(shape=(None,), dtype=tf.int32, name='input_ids')
in_mask = Input(shape=(None,), dtype=tf.int32, name='input_masks')
in_segment = Input(shape=(None,), dtype=tf.int32, name='segment_ids')
bert_inputs = [in_id, in_mask, in_segment] # tf.keras layers
print('bert inputs :', bert_inputs)
bert_pooled_output = KorBertLayer(
n_tune_layers=self.num_bert_fine_tune_layers,
pooling='mean', name='KorBertLayer')(bert_inputs)
# fine-tuning layer
intents_fc = Dense(self.intents_num, activation='softmax', name='intent_classifier')(bert_pooled_output)
self.model = Model(inputs=bert_inputs, outputs=intents_fc)
def fit(self, X, Y, validation_data=None, epochs=5, batch_size=64, callbacks=None):
"""
X: batch of [input_ids, input_mask, segment_ids, valid_positions]
"""
X = (X[0], X[1], X[2])
if validation_data is not None:
X_val, Y_val = validation_data
validation_data = ((X_val[0], X_val[1], X_val[2]), Y_val)
history = self.model.fit(X, Y, validation_data=validation_data,
epochs=epochs, batch_size=batch_size, callbacks=callbacks)
print(history.history)
self.visualize_metric(history.history, 'loss')
self.visualize_metric(history.history, 'acc')
def initialize_vars(self, sess):
sess.run(tf.compat.v1.local_variables_initializer())
sess.run(tf.compat.v1.global_variables_initializer())
K.set_session(sess)
def predict_intent(self, x, intent_vectorizer, remove_start_end=True):
# x = (x[0], x[1], x[2])
y_intent = self.predict(x)
# print('y_intent :', y_intent)
first_intents_score = np.array([np.max(y_intent[i]) for i in range(y_intent.shape[0])])
first_intents = np.array([intent_vectorizer.inverse_transform([np.argmax(y_intent[i])])[0] for i in range(y_intent.shape[0])])
second_intents_score = np.array([np.sort(y_intent[i])[-2] for i in range(y_intent.shape[0])])
second_intents = np.array([intent_vectorizer.inverse_transform([np.argsort(y_intent[i])[-2]])[0] for i in range(y_intent.shape[0])])
return first_intents, first_intents_score, second_intents, second_intents_score
def save(self, model_path):
with open(os.path.join(model_path, 'params.json'), 'w') as json_file:
json.dump(self.model_params, json_file)
self.model.save(os.path.join(model_path, 'bert_nlu_model.h5'))
def load(load_folder_path, sess): # load for inference or model evaluation
with open(os.path.join(load_folder_path, 'params.json'), 'r') as json_file:
model_params = json.load(json_file)
intents_num = model_params['intents_num']
bert_hub_path = model_params['bert_hub_path']
num_bert_fine_tune_layers = model_params['num_bert_fine_tune_layers']
is_bert = model_params['is_bert']
new_model = BertNLUModel(intents_num, bert_hub_path, sess, num_bert_fine_tune_layers, is_bert, is_training=False)
new_model.model.load_weights(os.path.join(load_folder_path,'bert_nlu_model.h5'))
return new_model
<file_sep># -*- coding: utf-8 -*-
from tensorflow.keras.models import load_model
import matplotlib.pyplot as plt
import numpy as np
class BaseNLUModel:
def __init__(self):
self.model = None
def visualize_metric(self, history_dic, metric_name):
plt.plot(history_dic[metric_name])
legend = ['train']
if 'val_' + metric_name in history_dic:
plt.plot(history_dic['val_' + metric_name])
legend.append('test')
plt.title('model ' + metric_name)
plt.ylabel(metric_name)
plt.xlabel('epoch')
plt.legend(legend, loc='upper left')
plt.savefig('train_log.png')
def predict(self, x):
return self.model.predict(x)
def save(self, model_path):
self.model.save(model_path)
def load(model_path, custom_objects=None):
new_model = BaseNLUModel()
new_model.model = load_model(model_path, custom_objects=custom_objects)
return new_model
def predict_intent(self, x, intents_label_encoder):
if len(x.shape) == 1:
x = x[np.newaxis, ...]
y = self.predict(x)
intents = np.array([intents_label_encoder.inverse_transform([np.argmax(y[i])])[0] for i in range(y.shape[0])])
return intents, slots
| efa6c4fbfbdf3479fe526e76bff6629db267e6ae | [
"Markdown",
"Python",
"Text",
"JavaScript"
] | 20 | Python | jinyoungsul/koBERT-yogiyo-reviews-classfication | ab483b0702753b12d40d44bec80b2c19828a1d7a | a8f4147bfb41a3ede67a23ef1263c36a5d8b7e08 | |
refs/heads/master | <repo_name>vikjogit/openmicevent1<file_sep>/app/src/main/java/com/vikjo/openmicevent1/CreateEventActivity.java
package com.vikjo.openmicevent1;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.google.android.gms.tasks.OnCompleteListener;
import com.google.android.gms.tasks.Task;
import com.google.firebase.auth.AuthResult;
import com.google.firebase.auth.FirebaseAuth;
import com.google.firebase.database.FirebaseDatabase;
public class CreateEventActivity extends AppCompatActivity implements View.OnClickListener {
private EditText mEventName;
private EditText mBusinessName;
private EditText mEventDate;
private EditText mEventTime;
private EditText mEventLocation;
private String databaseeventname;
private Button Btncreateevent;
private FirebaseAuth mAuth;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_create_event);
mEventName = (EditText)findViewById(R.id.editEventName);
mBusinessName = (EditText)findViewById(R.id.editCompanyName);
mEventDate = (EditText)findViewById(R.id.editEventDate);
mEventTime = (EditText)findViewById(R.id.editEventTime);
mEventLocation = (EditText)findViewById(R.id.editEventLocation);
mAuth = FirebaseAuth.getInstance();
findViewById(R.id.CreateEventButton).setOnClickListener(this);
}
private void CreateTheEvent(){
final String eventname = mEventName.getText().toString();
final String businessname = mBusinessName.getText().toString();
final String eventdate = mEventDate.getText().toString();
final String eventtime = mEventTime.getText().toString();
final String eventlocation = mEventLocation.getText().toString();
if(eventname.isEmpty()) {
mEventName.setError("Event Name required");
mEventName.requestFocus();
return;
}
else if(businessname.isEmpty()) {
mBusinessName.setError("Add your Business Name");
mBusinessName.requestFocus();
return;
}
else if(eventdate.isEmpty()) {
mEventDate.setError("Add the date of the event");
mEventDate.requestFocus();
return;
}
else if(eventtime.isEmpty()) {
mEventTime.setError("Add the time for the event");
mEventTime.requestFocus();
return;
}
else if(eventlocation.isEmpty()) {
mEventLocation.setError("Add Location of the event");
mEventLocation.requestFocus();
return;
}
//we will store additional fields in firebase database
Event event = new Event(eventname,businessname,eventdate,eventtime,eventlocation);
FirebaseDatabase.getInstance().getReference("Events")
.child(FirebaseAuth.getInstance().getCurrentUser().getUid())
.setValue(event).addOnCompleteListener(new OnCompleteListener<Void>() {
@Override
public void onComplete(@NonNull Task<Void> task) {
if(task.isSuccessful()){
Toast.makeText(CreateEventActivity.this,"Event Created Succesfully",Toast.LENGTH_SHORT).show();
//FirebaseDatabase.getInstance().getReference("Events")
// .child(FirebaseAuth.getInstance().getCurrentUser().getUid())
// .setValue()
Intent i = (new Intent(CreateEventActivity.this, BusinessHome.class));
finish();
startActivity(i);
}
else{
Toast.makeText(CreateEventActivity.this,"Event was not created, Try Again",Toast.LENGTH_SHORT).show();
}
}
});
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.CreateEventButton:
CreateTheEvent();
}
}
}
<file_sep>/README.md
# openmicevent1
This app is an open mic event management app. An open mic is an event hosted by pubs and similar venues to gain new customers and audiences by providing them with entertainment.
When a business creates an open mic event, an artist is supposed to contact the business and let them know that he wants to perform there. This gives him/her a chance to showcase
their talents in a comfortable space.
Basically, an artist gets a chance to perform in front of an audience without spending time to build one while the business gains new customers who come to see the performance
This app helps to bridge the gap between open mic venues and artists. Once you open the app, you can sign up as a business or an artist.
If you are an artist:
You are shown what events are available for you to join, you can join them accordingly
If you're a business:
You need to create an event using the app and then the event will be visible to the artist
This app looks to make open mics a convinient affair for both artists as well as businesses.
<file_sep>/settings.gradle
include ':app'
rootProject.name='openmicevent1'
<file_sep>/app/src/main/java/com/vikjo/openmicevent1/MyArtistEvent.java
package com.vikjo.openmicevent1;
public class MyArtistEvent {
String eventforparticipants;
public MyArtistEvent(String eventforparticipants) {
this.eventforparticipants = eventforparticipants;
}
public void setEventforparticipants(String eventforparticipants) {
this.eventforparticipants = eventforparticipants;
}
public MyArtistEvent(){
}
public String getEventforparticipants() {
return eventforparticipants;
}
}
| 894cfa445a8e55ed09dd423c6f3aa7d0d6264f3b | [
"Markdown",
"Java",
"Gradle"
] | 4 | Java | vikjogit/openmicevent1 | 63f5170f0cfee0cea14d419a833e27436db3b846 | cb13c77b53357b71e0fde07663b21f71c369e2cb | |
refs/heads/master | <repo_name>s1ckn3zz1337/stocard-be-challenge<file_sep>/test/integratiom/cities.test.js
const request = require('supertest');
const nock = require('nock');
const app = require('../../src');
const { configApp: { openWeather: { appId } } } = require('../../src/const');
const {
ids: { cityId },
values: { cities: { cityLng, cityLat } },
expectedResponses: { cities: { cityListExpected, cityIdWeatherExpected, cityIdExpected } },
rawResponses: { openWeather: { openWeatherCityIdResponse, openWeatherCityListResponse } },
} = require('../dummy/data');
describe('test cities route', () => {
afterAll((done) => {
app.close(done);
});
describe('test GET /cities route', () => {
it('should return 200 and expected body on succes if query is valid', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/find?lat=${cityLat}&lon=${cityLng}&appid=${appId}`)
.reply(200, openWeatherCityListResponse);
await request(app)
.get(`/cities?lat=${cityLat}&lng=${cityLng}`)
.expect('Content-Type', /json/)
.expect(200, cityListExpected);
});
it('should return 400 if lat param is missing', async () => {
await request(app)
.get('/cities')
.expect('Content-Type', /json/)
.expect(400, {
code: 'BadRequestError',
message: 'lat/lng required',
});
});
it('should return 400 if lng param is missing', async () => {
await request(app)
.get('/cities')
.expect('Content-Type', /json/)
.expect(400, {
code: 'BadRequestError',
message: 'lat/lng required',
});
});
it('should return 204 on no result', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/find?lat=${cityLat}&lon=${cityLng}&appid=${appId}`)
.reply(200, { list: [] });
await request(app)
.get(`/cities?lat=${cityLat}&lng=${cityLng}`)
.expect(204, {});
});
it('should return 500 on error', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/find?lat=${cityLat}&lon=${cityLng}&appid=${appId}`)
.reply(500, {});
await request(app)
.get(`/cities?lat=${cityLat}&lng=${cityLng}`)
.expect(500, {
code: 'InternalServerError',
message: 'Hoops looks like something went wrong :(',
});
});
});
describe('test GET /cities/{city_id} route', () => {
it('should return right body and http 200 on success', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(200, openWeatherCityIdResponse);
await request(app)
.get(`/cities/${cityId}`)
.expect('Content-Type', /json/)
.expect(200, cityIdExpected);
});
it('should return 404 if city not found', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(404, {});
await request(app)
.get(`/cities/${cityId}`)
.expect('Content-Type', /json/)
.expect(404, {
code: 'NotFoundError',
message: 'not found',
});
});
it('should return 500 on error', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(500, {});
await request(app)
.get(`/cities/${cityId}`)
.expect('Content-Type', /json/)
.expect(500, {
code: 'InternalServerError',
message: 'Hoops looks like something went wrong :(',
});
});
});
describe('test GET /cities/{city_id}/weather route', () => {
it('should return http 200 and right body on success', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(200, openWeatherCityIdResponse);
await request(app)
.get(`/cities/${cityId}/weather`)
.expect('Content-Type', /json/)
.expect(200, cityIdWeatherExpected);
});
it('should return http 404 on not found', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(404, {});
await request(app)
.get(`/cities/${cityId}/weather`)
.expect('Content-Type', /json/)
.expect(404, {
code: 'NotFoundError',
message: 'not found',
});
});
it('should return http 500 on error', async () => {
nock('https://api.openweathermap.org/')
.get(`/data/2.5/weather?id=${cityId}&appid=${appId}`)
.reply(500, {});
await request(app)
.get(`/cities/${cityId}/weather`)
.expect('Content-Type', /json/)
.expect(500, {
code: 'InternalServerError',
message: 'Hoops looks like something went wrong :(',
});
});
});
});
<file_sep>/src/route/index.js
const routeCities = require('./cities.route');
module.exports = {
routeCities,
};
<file_sep>/src/model/City.js
module.exports = (
{
externalApiOpenWeather:
{
getCitiesByLngLat,
getWeatherByCityId,
},
ModelWeather,
restifyErrors: { NotFoundError },
},
) => class City {
static async listAllByLngLat(lng, lat) {
const results = [];
try {
const { list } = await getCitiesByLngLat(lng, lat);
list.forEach(({ id, coord, name }) => {
const city = new City({
id, name, lat: coord.lat, lng: coord.lon,
});
results.push(city);
});
return results;
} catch (err) {
console.error('error parsing cities');
throw err;
}
}
static async getById(id) {
try {
const cityData = await getWeatherByCityId(id);
const weather = ModelWeather.parseFromCityData({ cityId: id, ...cityData });
return new City({
id: cityData.id,
name: cityData.name,
weather,
lng: cityData.coord.lon,
lat: cityData.coord.lat,
});
} catch (err) {
if (err.response.status === NotFoundError.prototype.statusCode) {
throw new NotFoundError('not found');
}
throw err;
}
}
constructor({
id, name, lat, lng,
weather,
}) {
this.attributes = {
id, name, lat, lng,
};
this.currentWeather = weather;
}
toDetailedJSON() {
return this.attributes;
}
toJSON() {
return { id: this.attributes.id, name: this.attributes.name };
}
};
<file_sep>/src/external/index.js
const externalApiOpenWeather = require('./openWeather');
module.exports = {
externalApiOpenWeather,
};
<file_sep>/src/route/cities.route.js
module.exports = ({ validate, cities }) => ([
{
location: '/cities',
method: 'get',
middlewares: [
validate,
cities.getCityByLanLng,
],
},
{
location: '/cities/:city_id',
method: 'get',
middlewares: [
validate,
cities.getCityById,
],
},
{
location: '/cities/:city_id/weather',
method: 'get',
middlewares: [
validate,
cities.getWeatherByCityId,
],
},
]);
<file_sep>/src/model/index.js
const ModelCity = require('./City');
const ModelWeather = require('./Weather');
module.exports = {
ModelCity,
ModelWeather,
};
<file_sep>/src/external/openWeather.js
module.exports = ({ axios, configApp: { openWeather: { appId } } }) => {
const { get } = axios.create({
baseURL: 'https://api.openweathermap.org/data/2.5/',
});
return {
/**
* https://openweathermap.org/current#cycle
* @param lng length °
* @param lat lat °
* @returns {Promise<*>} resolves with raw weather data
*/
getCitiesByLngLat: async (lng, lat) => {
try {
const { data } = await get('/find', {
params: {
lat,
lon: lng,
appid: appId,
},
});
return data;
} catch (err) {
console.error('could not load city by lng lat');
throw (err);
}
},
/**
* https://openweathermap.org/current#cityid
* @param id cityId
* @returns {*}
*/
getWeatherByCityId: async (id) => {
try {
const { data } = await get('/weather', {
params: {
id,
appid: appId,
},
});
return data;
} catch (err) {
console.error('could not load weather by city id');
throw err;
}
},
};
};
<file_sep>/src/model/Weather.js
/* eslint-disable camelcase */
module.exports = () => class Weather {
static parseFromCityData({
id, weather, sys: { sunrise, sunset }, main: {
temp, pressure, humidity, temp_min, temp_max,
}, clouds: { all }, wind: { speed },
}) {
return new Weather({
cityId: id,
weatherData: {
type: weather[0].main,
type_description: weather[0].description,
sunrise: sunrise * 1000,
sunset: sunset * 1000,
temp,
temp_min,
temp_max,
clouds_percent: all,
wind_speed: speed,
pressure,
humidity,
},
});
}
constructor({
cityId, weatherData: {
type,
type_description,
sunrise,
sunset,
temp,
temp_min,
temp_max,
pressure,
humidity,
clouds_percent,
wind_speed,
},
}) {
this.cityId = cityId;
this.attributes = {
type,
type_description,
sunrise,
sunset,
temp,
temp_min,
temp_max,
pressure,
humidity,
clouds_percent,
wind_speed,
};
}
toJSON() {
const attributes = { ...this.attributes };
attributes.sunset = new Date(this.attributes.sunset).toISOString();
attributes.sunrise = new Date(this.attributes.sunrise).toISOString();
return attributes;
}
};
<file_sep>/src/middleware/validate.js
/* eslint-disable no-case-declarations */
module.exports = ({ Joi, restifyErrors: { BadRequestError } }) => async (req, res, next) => {
switch (true) {
case /\/cities\/\d*\/weather/.test(req.url):
break;
case /\/cities\/\d*/.test(req.url):
break;
case /\/cities\?\w/.test(req.url) || /\/cities\/*/.test(req.url):
const citiesShema = Joi.object({
lng: Joi.number().required(),
lat: Joi.number().required(),
});
const { error } = citiesShema.validate(req.query);
if (error) {
throw new BadRequestError('lat/lng required');
}
break;
default:
throw Error('not found');
}
return next();
};
<file_sep>/src/const/index.js
const configApp = require('./configApp');
module.exports = {
configApp,
};
<file_sep>/src/middleware/cities.js
module.exports = ({ ModelCity }) => ({
async getCityById(req, res, next) {
const city = await ModelCity.getById(req.params.city_id);
res.send(city.toDetailedJSON());
return next();
},
async getCityByLanLng(req, res, next) {
const { lat, lng } = req.query;
const cities = (await ModelCity.listAllByLngLat(lng, lat)).map((city) => city.toJSON());
if (cities.length === 0) {
res.send(204, []);
} else {
res.send(cities);
}
return next();
},
async getWeatherByCityId(req, res, next) {
const city = await ModelCity.getById(req.params.city_id);
const weather = city.currentWeather;
res.send(weather.toJSON());
return next();
},
});
<file_sep>/README.md
# stocard-be-challenge
## First steps
* install all dependencies and run app in hot refresh mode
```
yarn
yarn dev
```
## Architecture
* uses awilix container for dependency injections
* uses axios for request/response
* all dependencies are registered at server start
* create routes and add them dynamically to server
* each route contains multiple middlewares
* each middleware does one simple task
* outbound API implementations are located in ./external
* domain models are located in domains
## Testing
* jest with supertest
```
yarn test
```
## Linting
* eslint with air-bnb-base
```
yarn lint
```
##Commiting
* code quality tools running as precommit such as lintting, duplicate, tests and outdate deps
```
yarn commit
```
## Open tasks
* add proper logging (debug log every function, error log not catched errors, json output, context for loggers)
* improve func naming
* improve error handling (add error codes, own error class et)
* add swagger doc
* create server from swagger doc
* add more JSDoc
* create Dockerfile
* create docker-compose files
* create K8S files | 2999773737ccbc3d2edc6c8fa4195c914d540b39 | [
"JavaScript",
"Markdown"
] | 12 | JavaScript | s1ckn3zz1337/stocard-be-challenge | 7b6598ae79db8854af63c7db21c36d685aafd569 | c557eb726589b2a36a9432dce5135941e60484f8 | |
refs/heads/master | <file_sep># JS20151
Project
package com.socket;
import java.io.*;
import java.net.*;
import java.util.Date;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
import com.gui.ChatFrame;
public class SocketClient implements Runnable{
public int port;
public String serverAddr;
public Socket socket;
public ChatFrame ui;
public ObjectInputStream In;
public ObjectOutputStream Out;
public History hist;
public SocketClient(ChatFrame frame) throws IOException{
ui = frame; this.serverAddr = ui.serverAddr; this.port = ui.port;
socket = new Socket(InetAddress.getByName(serverAddr), port);
Out = new ObjectOutputStream(socket.getOutputStream());
Out.flush();
In = new ObjectInputStream(socket.getInputStream());
hist = ui.hist;
}
@Override
public void run() {
boolean keepRunning = true;
while(keepRunning){
try {
Message msg = (Message) In.readObject();
System.out.println("Incoming : "+msg.toString());
if(msg.type.equals("message")){
if(msg.recipient.equals(ui.username)){
ui.jTextArea1.append("["+msg.sender +" > Me] : " + msg.content + "\n");
}
else{
ui.jTextArea1.append("["+ msg.sender +" > "+ msg.recipient +"] : " + msg.content + "\n");
}
if(!msg.content.equals(".bye") && !msg.sender.equals(ui.username)){
String msgTime = (new Date()).toString();
try{
hist.addMessage(msg, msgTime);
DefaultTableModel table = (DefaultTableModel) ui.historyFrame.jTable1.getModel();
table.addRow(new Object[]{msg.sender, msg.content, "Me", msgTime});
}
catch(Exception ex){}
}
}
else if(msg.type.equals("login")){
if(msg.content.equals("TRUE")){
ui.jButton2.setEnabled(false); ui.jButton3.setEnabled(false);
ui.jButton4.setEnabled(true); ui.jButton5.setEnabled(true);
ui.jTextArea1.append("[SERVER > Me] : Login Successful\n");
ui.jTextField3.setEnabled(false); ui.jPasswordField1.setEnabled(false);
}
else{
ui.jTextArea1.append("[SERVER > Me] : Login Failed\n");
}
}
else if(msg.type.equals("test")){
ui.jButton1.setEnabled(false);
ui.jButton2.setEnabled(true); ui.jButton3.setEnabled(true);
ui.jTextField3.setEnabled(true); ui.jPasswordField1.setEnabled(true);
ui.jTextField1.setEditable(false); ui.jTextField2.setEditable(false);
ui.jButton7.setEnabled(true);
}
else if(msg.type.equals("newuser")){
if(!msg.content.equals(ui.username)){
boolean exists = false;
for(int i = 0; i < ui.model.getSize(); i++){
if(ui.model.getElementAt(i).equals(msg.content)){
exists = true; break;
}
}
if(!exists){ ui.model.addElement(msg.content); }
}
}
else if(msg.type.equals("signup")){
if(msg.content.equals("TRUE")){
ui.jButton2.setEnabled(false); ui.jButton3.setEnabled(false);
ui.jButton4.setEnabled(true); ui.jButton5.setEnabled(true);
ui.jTextArea1.append("[SERVER > Me] : Singup Successful\n");
}
else{
ui.jTextArea1.append("[SERVER > Me] : Signup Failed\n");
}
}
else if(msg.type.equals("signout")){
if(msg.content.equals(ui.username)){
ui.jTextArea1.append("["+ msg.sender +" > Me] : Bye\n");
ui.jButton1.setEnabled(true); ui.jButton4.setEnabled(false);
ui.jTextField1.setEditable(true); ui.jTextField2.setEditable(true);
for(int i = 1; i < ui.model.size(); i++){
ui.model.removeElementAt(i);
}
ui.clientThread.stop();
}
else{
ui.model.removeElement(msg.content);
ui.jTextArea1.append("["+ msg.sender +" > All] : "+ msg.content +" has signed out\n");
}
}
else if(msg.type.equals("upload_req")){
if(JOptionPane.showConfirmDialog(ui, ("Accept '"+msg.content+"' from "+msg.sender+" ?")) == 0){
JFileChooser jf = new JFileChooser();
jf.setSelectedFile(new File(msg.content));
int returnVal = jf.showSaveDialog(ui);
String saveTo = jf.getSelectedFile().getPath();
if(saveTo != null && returnVal == JFileChooser.APPROVE_OPTION){
Download dwn = new Download(saveTo, ui);
Thread t = new Thread(dwn);
t.start();
send(new Message("upload_res", (""+InetAddress.getLocalHost().getHostAddress()), (""+dwn.port), msg.sender));
send(new Message("upload_res", ui.username, (""+dwn.port), msg.sender));
}
else{
send(new Message("upload_res", ui.username, "NO", msg.sender));
}
}
else{
send(new Message("upload_res", ui.username, "NO", msg.sender));
}
}
else if(msg.type.equals("upload_res")){
if(!msg.content.equals("NO")){
int port = Integer.parseInt(msg.content);
String addr = msg.sender;
ui.jButton5.setEnabled(false); ui.jButton6.setEnabled(false);
Upload upl = new Upload(addr, port, ui.file, ui);
Thread t = new Thread(upl);
t.start();
}
else{
ui.jTextArea1.append("[SERVER > Me] : "+msg.sender+" rejected file request\n");
}
}
else{
ui.jTextArea1.append("[SERVER > Me] : Unknown message type\n");
}
}
catch(Exception ex) {
keepRunning = false;
ui.jTextArea1.append("[Application > Me] : Connection Failure\n");
ui.jButton1.setEnabled(true); ui.jTextField1.setEditable(true); ui.jTextField2.setEditable(true);
ui.jButton4.setEnabled(false); ui.jButton5.setEnabled(false); ui.jButton5.setEnabled(false);
for(int i = 1; i < ui.model.size(); i++){
ui.model.removeElementAt(i);
}
ui.clientThread.stop();
System.out.println("Exception SocketClient run()");
ex.printStackTrace();
}
}
}
public void send(Message msg){
try {
Out.writeObject(msg);
Out.flush();
System.out.println("Outgoing : "+msg.toString());
if(msg.type.equals("message") && !msg.content.equals(".bye")){
String msgTime = (new Date()).toString();
try{
hist.addMessage(msg, msgTime);
DefaultTableModel table = (DefaultTableModel) ui.historyFrame.jTable1.getModel();
table.addRow(new Object[]{"Me", msg.content, msg.recipient, msgTime});
}
catch(Exception ex){}
}
}
catch (IOException ex) {
System.out.println("Exception SocketClient send()");
}
}
public void closeThread(Thread t){
t = null;
}
}
<file_sep>package chatClient.com.socket;
import java.io.*;
import java.net.*;
import java.util.Date;
import javax.swing.JFileChooser;
import javax.swing.JOptionPane;
import javax.swing.table.DefaultTableModel;
import chatClient.com.gui.clientFrame;
public class SocketClient implements Runnable{
public int port;
public String serverAddr;
public Socket socket;
public clientFrame gui;
public ObjectInputStream In;
public ObjectOutputStream Out;
public History hist;
public SocketClient(clientFrame frame) throws IOException{
gui = frame; this.serverAddr = gui.serverAddr; this.port = gui.port;
socket = new Socket(InetAddress.getByName(serverAddr), port);
Out = new ObjectOutputStream(socket.getOutputStream());
Out.flush();
In = new ObjectInputStream(socket.getInputStream());
hist = gui.hist;
}
@SuppressWarnings({ "unchecked", "deprecation" })
@Override
public void run() {
boolean keepRunning = true;
while(keepRunning){
try {
Message msg = (Message) In.readObject();
System.out.println("Incoming : "+msg.toString());
if(msg.type.equals("message")){
if(msg.recipient.equals(gui.username)){
gui.jTextArea1.append("["+msg.sender +" > Me] : " + msg.content + "\n");
}
else{
gui.jTextArea1.append("["+ msg.sender +" > "+ msg.recipient +"] : " + msg.content + "\n");
}
if(!msg.content.equals(".bye") && !msg.sender.equals(gui.username)){
String msgTime = (new Date()).toString();
try{
hist.addMessage(msg, msgTime);
DefaultTableModel table = (DefaultTableModel) gui.historyFrame.jTable1.getModel();
table.addRow(new Object[]{msg.sender, msg.content, "Me", msgTime});
}
catch(Exception ex){}
}
}
else if(msg.type.equals("login")){
if(msg.content.equals("TRUE")){
gui.jButton2.setEnabled(false); gui.jButton3.setEnabled(false);
gui.jButton4.setEnabled(true); gui.jButton5.setEnabled(true);
gui.jTextArea1.append("[SERVER > Me] : Login Successful\n");
gui.jTextField3.setEnabled(false); gui.jPasswordField1.setEnabled(false);
}
else{
gui.jTextArea1.append("[SERVER > Me] : Login Failed\n");
}
}
else if(msg.type.equals("test")){
gui.jButton1.setEnabled(false);
gui.jButton2.setEnabled(true); gui.jButton3.setEnabled(true);
gui.jTextField3.setEnabled(true); gui.jPasswordField1.setEnabled(true);
gui.jTextField1.setEditable(false); gui.jTextField2.setEditable(false);
gui.jButton7.setEnabled(true);
}
else if(msg.type.equals("newuser")){
if(!msg.content.equals(gui.username)){
boolean exists = false;
for(int i = 0; i < gui.model.getSize(); i++){
if(gui.model.getElementAt(i).equals(msg.content)){
exists = true; break;
}
}
if(!exists){ gui.model.addElement(msg.content); }
}
}
else if(msg.type.equals("signup")){
if(msg.content.equals("TRUE")){
gui.jButton2.setEnabled(false); gui.jButton3.setEnabled(false);
gui.jButton4.setEnabled(true); gui.jButton5.setEnabled(true);
gui.jTextArea1.append("[SERVER > Me] : Singup Successful\n");
}
else{
gui.jTextArea1.append("[SERVER > Me] : Signup Failed\n");
}
}
else if(msg.type.equals("signout")){
if(msg.content.equals(gui.username)){
gui.jTextArea1.append("["+ msg.sender +" > Me] : Bye\n");
gui.jButton1.setEnabled(true); gui.jButton4.setEnabled(false);
gui.jTextField1.setEditable(true); gui.jTextField2.setEditable(true);
for(int i = 1; i < gui.model.size(); i++){
gui.model.removeElementAt(i);
}
gui.clientThread.stop();
}
else{
gui.model.removeElement(msg.content);
gui.jTextArea1.append("["+ msg.sender +" > All] : "+ msg.content +" has signed out\n");
}
}
else if(msg.type.equals("upload_req")){
if(JOptionPane.showConfirmDialog(gui, ("Accept '"+msg.content+"' from "+msg.sender+" ?")) == 0){
JFileChooser jf = new JFileChooser();
jf.setSelectedFile(new File(msg.content));
int returnVal = jf.showSaveDialog(gui);
String saveTo = jf.getSelectedFile().getPath();
if(saveTo != null && returnVal == JFileChooser.APPROVE_OPTION){
Download dwn = new Download(saveTo, gui);
Thread t = new Thread(dwn);
t.start();
send(new Message("upload_res", gui.username, (""+dwn.port), msg.sender));
}
else{
send(new Message("upload_res", gui.username, "NO", msg.sender));
}
}
else{
send(new Message("upload_res", gui.username, "NO", msg.sender));
}
}
else if(msg.type.equals("upload_res")){
if(!msg.content.equals("NO")){
int port = Integer.parseInt(msg.content);
String addr = msg.sender;
gui.jButton5.setEnabled(false); gui.jButton6.setEnabled(false);
Upload upl = new Upload(addr, port, gui.file, gui);
Thread t = new Thread(upl);
t.start();
}
else{
gui.jTextArea1.append(" [SERVER > Me] : "+msg.sender+" rejected file request\n ");
}
}
else{
gui.jTextArea1.append("[SERVER > Me] : Unknown message type\n");
}
}
catch(Exception ex) {
keepRunning = false;
gui.jTextArea1.append("[Application > Me] : Connection Failure\n");
gui.jButton1.setEnabled(true); gui.jTextField1.setEditable(true); gui.jTextField2.setEditable(true);
gui.jButton4.setEnabled(false); gui.jButton5.setEnabled(false); gui.jButton5.setEnabled(false);
for(int i = 1; i < gui.model.size(); i++){
gui.model.removeElementAt(i);
}
gui.clientThread.stop();
System.out.println("Exception SocketClient run()");
ex.printStackTrace();
}
}
}
public void send(Message msg){
try {
Out.writeObject(msg);
Out.flush();
System.out.println("Outgoing : "+msg.toString());
if(msg.type.equals("message") && !msg.content.equals(".bye")){
String msgTime = (new Date()).toString();
try{
hist.addMessage(msg, msgTime);
DefaultTableModel table = (DefaultTableModel) gui.historyFrame.jTable1.getModel();
table.addRow(new Object[]{"Me", msg.content, msg.recipient, msgTime});
}
catch(Exception ex){}
}
}
catch (IOException ex) {
System.out.println(" Exception SocketClient send() ");
}
}
public void closeThread(Thread t){
t = null;
}
}
| 9ba0727d2823b023e4984e292b5d5247983cd2af | [
"Markdown",
"Java"
] | 2 | Markdown | Duong201093/JS20151 | ca2b390db74cfefbc7a87f59653fea4ad81ed066 | e789c400ff103b0dd9ed5ffa9d0be1f2697e1e93 | |
refs/heads/master | <file_sep>package com.example.zhang.abercrombiedemo.Activity;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.design.widget.FloatingActionButton;
import android.support.design.widget.Snackbar;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.support.design.widget.NavigationView;
import android.support.v4.view.GravityCompat;
import android.support.v4.widget.DrawerLayout;
import android.support.v7.app.ActionBarDrawerToggle;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.Toolbar;
import android.view.Menu;
import android.view.MenuItem;
import android.view.ViewGroup;
import android.widget.Toast;
import com.android.volley.AuthFailureError;
import com.android.volley.NetworkError;
import com.android.volley.NoConnectionError;
import com.android.volley.ParseError;
import com.android.volley.Request;
import com.android.volley.RequestQueue;
import com.android.volley.Response;
import com.android.volley.ServerError;
import com.android.volley.TimeoutError;
import com.android.volley.VolleyError;
import com.android.volley.toolbox.JsonArrayRequest;
import com.example.zhang.abercrombiedemo.AppController.AppController;
import com.example.zhang.abercrombiedemo.Items.ProductItem;
import com.example.zhang.abercrombiedemo.ProductAdapter.ProductAdapter;
import com.example.zhang.abercrombiedemo.R;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
/**
* Activity for show recyclerView
*
* This activity is used to display items in RecyclerView by using volley library
*
* @author <NAME>
* @version 1.0
* @since 2016-10-31
*/
public class MainActivity extends AppCompatActivity
implements NavigationView.OnNavigationItemSelectedListener {
String url = "https://www.abercrombie.com/anf/nativeapp/qa/codetest/codeTest_exploreData.json";
ArrayList<ProductItem> itemList = new ArrayList<>();
ProductAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
ActionBarDrawerToggle toggle = new ActionBarDrawerToggle(
this, drawer, toolbar, R.string.navigation_drawer_open, R.string.navigation_drawer_close);
drawer.setDrawerListener(toggle);
toggle.syncState();
NavigationView navigationView = (NavigationView) findViewById(R.id.nav_view);
navigationView.setNavigationItemSelectedListener(this);
// put item in recyclerView
RecyclerView recyclerView = (RecyclerView)findViewById(R.id.itemHolder);
LinearLayoutManager linearLayoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(linearLayoutManager);
adapter = new ProductAdapter(itemList, this);
recyclerView.setAdapter(adapter);
makeRequest(url);
}
/**
* Method make network request using volley, load data in ArrayList
*@param url The string for making JsonArray request
*/
public void makeRequest(String url)
{
JsonArrayRequest jsonArrayRequest = new JsonArrayRequest(url, new Response.Listener<JSONArray>() {
@Override
public void onResponse(JSONArray response)
{
try {
for (int i = 0; i < response.length(); i++)
{
JSONObject josonObject = response.getJSONObject(i);
ProductItem productItem = new ProductItem();
productItem.setBackgroundImage(josonObject.getString("backgroundImage"));
productItem.setTitle(josonObject.getString("title"));
//check wether element exist, if not, assign empty
if(josonObject.has("topDescription"))
{
productItem.setTopDescription(josonObject.getString("topDescription"));
}
else
{
productItem.setTopDescription("");
}
if(josonObject.has("promoMessage"))
{
productItem.setPromoMessage(josonObject.getString("promoMessage"));
}
else
{
productItem.setPromoMessage("");
}
if(josonObject.has("bottomDescription"))
{
productItem.setBottomDescription(josonObject.getString("bottomDescription"));
}
else
{
productItem.setBottomDescription("");
}
if (josonObject.has("content"))
{
JSONArray jsonContent = josonObject.getJSONArray("content");
for(int j=0; j<jsonContent.length();j++)
{
JSONObject contentObject = jsonContent.getJSONObject(j);
if(j==0)
{
productItem.setSelectButton_one(contentObject.getString("title"));
productItem.setGetSelectButton_two("");
productItem.setButton_one_link(contentObject.getString("target"));
}
else
{
productItem.setGetSelectButton_two(contentObject.getString("title"));
productItem.setButton_two_link(contentObject.getString("target"));
}
}
}
else
{
productItem.setSelectButton_one("");
productItem.setGetSelectButton_two("");
}
itemList.add(productItem);
}
}
catch (JSONException e) {
e.printStackTrace();
}
adapter.notifyDataSetChanged();
}
},new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
String message = null;
//network status check
if (error instanceof NetworkError) {
message = "Cannot connect to Internet...Please check your connection!";
} else if (error instanceof ServerError) {
message = "The server could not be found. Please try again after some time!!";
} else if (error instanceof AuthFailureError) {
message = "Cannot connect to Internet...Please check your connection!";
} else if (error instanceof ParseError) {
message = "Parsing error! Please try again after some time!!";
} else if (error instanceof NoConnectionError) {
message = "Cannot connect to Internet...Please check your connection!";
} else if (error instanceof TimeoutError) {
message = "Connection TimeOut! Please check your internet connection.";
}
}
});
AppController.getInstance().addToRequestQueue(jsonArrayRequest);
}
@Override
public void onBackPressed() {
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
if (drawer.isDrawerOpen(GravityCompat.START)) {
drawer.closeDrawer(GravityCompat.START);
} else {
super.onBackPressed();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
@SuppressWarnings("StatementWithEmptyBody")
@Override
public boolean onNavigationItemSelected(MenuItem item) {
// Handle navigation view item clicks here.
int id = item.getItemId();
if (id == R.id.nav_shop) {
// Handle the navigation action
} else if (id == R.id.save) {
} else if (id == R.id.nav_massage) {
} else if (id == R.id.nav_myAccount) {
}
DrawerLayout drawer = (DrawerLayout) findViewById(R.id.drawer_layout);
drawer.closeDrawer(GravityCompat.START);
return true;
}
}
<file_sep>package com.example.zhang.abercrombiedemo.Activity;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import com.example.zhang.abercrombiedemo.R;
/**
* Activity for show WebView
*
* This activity is used to display WebView from previews RecyclerView
*
* @author <NAME>
* @version 1.0
* @since 2016-10-31
*/
public class WebViewActivity extends AppCompatActivity {
WebView webView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_web_view);
getSupportActionBar().hide();
Intent intent = getIntent();
String url = intent.getStringExtra("webView");
webView = (WebView) findViewById(R.id.web);
webView.setWebViewClient(new WebViewClient());
webView.getSettings().setJavaScriptEnabled(true);
webView.loadUrl(url);
}
}
| ebe0be487e95aaaf0781905db2ad9ccd2e411a14 | [
"Java"
] | 2 | Java | zhanglianyu0911/RecyclerViewDemo_AF | a278c213743ed3e8b24eeaee422e69fe281696eb | 81dbd1ac423e3b8706ea112bc4cbfa529dd36816 | |
refs/heads/master | <file_sep>'use strict';
var app = angular.module('ToDoList', ['ngTable']);<file_sep>'use strict';
global.__base = __dirname + '/';
require('dotenv').config();
require(`${__base}app`);<file_sep>'use strict';
const express = require('express'),
bodyParser = require('body-parser'),
Bluebird = require('bluebird'),
http = require('http'),
app = express(),
server = http.createServer(app),
fs = Bluebird.promisifyAll(require('fs'));
app.set('view engine', 'pug');
app.set('views', `${__base}app/views`);
app.use(bodyParser.json({'extended': true}));
app.use('/static', express.static(`${__base}public`));
app.get('/', (req, res) => {
res.render('index');
});
app.get('/public/lodash/lodash.js', (req, res) => {
fs.readFileAsync(`${__base}node_modules/lodash/lodash.js`)
.then(data => res.send(data))
.catch(e => res.status(500).send(e));
});
require(`${__base}app/server/router`)(app);
app.listen(process.env.PORT, () => {
console.log(`Todo application up and running on port ${process.env.PORT}`);
});
<file_sep># Aplicação 2 - To-do list
## Getting started:
```bash
$ git clone <EMAIL>:desoares1975/to-do-list.git
```
### After clonning on the same folder:
```bash
$ cd to-do-list
```
```bash
$ npm install
```
Save the file in the main folder .env.sample as .env
```bash
$cp .env.sample .env
```
## Starting application
```bash
$ node index.js
```
<file_sep>'use strict';
const todos = require(`${__base}app/server/router/todos`);
module.exports = app => {
app.post('/todo', todos.create);
app.get('/todo', todos.list);
app.get('/todo/:_id', todos.read);
app.put('/todo/:_id', todos.update);
app.delete('/todo/:_id', todos.delete);
}; | 7fa72370784ea86f0e0d29c5d2f5da85de42a9df | [
"JavaScript",
"Markdown"
] | 5 | JavaScript | desoares1975/to-do-list | e255dcfca72aea2940af4e84042eb45853334d43 | d151a589d46f6385e90b8d17d24c0553706a0109 | |
refs/heads/master | <repo_name>ccoley/js3DGraphics<file_sep>/Tuple3.js
/**
* Created with IntelliJ IDEA.
* User: Chris
* Date: 1/21/14
* Time: 5:38 PM
*/
function Tuple3(x, y, z) {
this.x = x !== undefined ? x : 0;
this.y = y !== undefined ? y : 0;
this.z = z !== undefined ? z : 0;
}
Tuple3.prototype.set = function(x, y, z) {
this.x = x;
this.y = y;
this.z = z;
};
//Tuple3.prototype.copy = function(tuple) {
// this.x = tuple.x;
// this.y = tuple.y;
// this.z = tuple.z;
//};
Tuple3.prototype.scale = function(scaleFactor) {
this.x *= scaleFactor;
this.y *= scaleFactor;
this.z *= scaleFactor;
};
Tuple3.prototype.toString = function() {
return "[" + this.x + "," + this.y + "," + this.z + "]";
};<file_sep>/README.md
js3DGraphics
================
This is a 3D graphics engine written in JavaScript.
I am following along with the tutorial located [here](http://gamedevelopment.tutsplus.com/series/lets-build-a-3d-graphics-software-engine--gamedev-12718).
<file_sep>/Point.js
/**
* Created with IntelliJ IDEA.
* User: Chris
* Date: 1/21/14
* Time: 5:01 PM
*/
// Point extends Tuple3
function Point(x, y, z) {
Tuple3.call(this, x, y, z);
}
// inherit Tuple3
Point.prototype = new Tuple3();
// correct the constructor pointer because it points to Tuple3
Point.prototype.constructor = Point;
/** Point Operations */
Point.prototype.addVector = function(vector) {
this.x += vector.x;
this.y += vector.y;
this.z += vector.z;
};
Point.prototype.subtractVector = function(vector) {
this.x -= vector.x;
this.y -= vector.y;
this.z -= vector.z;
};
Point.prototype.addScaledVector = function(scale, vector) {
this.x += scale * vector.x;
this.y += scale * vector.y;
this.z += scale * vector.z;
};
/** Point Functions */
Point.prototype.distance = function(point) {
return Math.sqrt((this.x - point.x)*(this.x - point.x) + (this.y - point.y)*(this.y - point.y) + (this.z - point.z)*(this.z - point.z));
};
Point.prototype.distanceSquared = function(point) {
return (this.x - point.x)*(this.x - point.x) + (this.y - point.y)*(this.y - point.y) + (this.z - point.z)*(this.z - point.z);
};
Point.prototype.drawPoint = function() {
console.log("Point: " + this.toString());
};<file_sep>/Vector.js
/**
* Created with IntelliJ IDEA.
* User: Chris
* Date: 1/21/14
* Time: 5:01 PM
*/
// Vector extends Tuple3
function Vector(x, y, z) {
Tuple3.call(this, x, y, z);
}
// inherit Tuple3
Vector.prototype = new Tuple3();
// correct the constructor pointer because it points to Tuple3
Vector.prototype.constructor = Vector;
/** Vector Operations */
Vector.prototype.addVector = function(vector) {
this.x += vector.x;
this.y += vector.y;
this.z += vector.z;
};
Vector.prototype.subtractVector = function(vector) {
this.x -= vector.x;
this.y -= vector.y;
this.z -= vector.z;
};
Vector.prototype.addScaledVector = function(scale, vector) {
this.x += scale * vector.x;
this.y += scale * vector.y;
this.z += scale * vector.z;
};
Vector.prototype.makeFromPointSubtraction = function(point1, point2) {
this.x = point1.x - point2.x;
this.y = point1.y - point2.y;
this.z = point1.z - point2.z;
};
Vector.prototype.cross = function(vector1, vector2) {
this.x = vector1.y * vector2.z - vector1.z * vector2.y;
this.y = vector1.z * vector2.x - vector1.x * vector2.z;
this.x = vector1.x * vector2.y - vector1.y * vector2.x;
};
/*
* This method will normalize this Vector so that its length is 1.0. If the
* length of the Vector is 0 or 1, no action is taken.
*/
Vector.prototype.normalize = function() {
var lsqr = this.x * this.x + this.y * this.y + this.z * this.z;
if (lsqr != 1 && lsqr != 0) {
var dist = Math.sqrt(lsqr);
this.x /= dist;
this.y /= dist;
this.z /= dist;
}
};
/** Vector Functions */
Vector.prototype.dot = function(vector) {
return this.x * vector.x + this.y * vector.y + this.z * vector.z;
};
Vector.prototype.magnitude = function() {
return Math.sqrt(this.x * this.x + this.y * this.y + this.z * this.z);
};
Vector.prototype.magnitudeSquared = function() {
return this.x * this.x + this.y * this.y + this.z * this.z;
};<file_sep>/main.js
/**
* Created with IntelliJ IDEA.
* User: Chris
* Date: 1/21/14
* Time: 8:24 PM
*/
function main() {
var point1 = new Point(1,2,1);
var point2 = new Point(0,4,4);
var vector1 = new Vector(2,0,0);
var vector2;
point1.drawPoint(); // should display (1,2,1)
point2.drawPoint(); // should display (0,4,4)
vector2 = new Vector();
vector2.makeFromPointSubtraction(point1, point2);
vector1.addVector(vector2);
point1.addVector(vector1);
point1.drawPoint(); // should display (4,0,-2)
point2.subtractVector(vector2);
point2.drawPoint(); // should display (-1,6,7)
} | 877a3e879d1e7c7d234037c34773d70db9927e11 | [
"JavaScript",
"Markdown"
] | 5 | JavaScript | ccoley/js3DGraphics | c26f0137e7487e655267a9152ae5742ebec1b84e | 9e7ddab475f52b6f7b44f4e88f2366d8735dcfe8 | |
refs/heads/master | <repo_name>armstj7/CAS757_AutomatedAdvagrafAdjustment<file_sep>/Test/File.swift
//
// File.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-12.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
func getNow() -> String {
let date = Date()
let formatter = DateFormatter()
formatter.dateFormat = "MMM d, yyyy"
let now = formatter.string(from: date)
return now
}
func getNowTimestamp() -> String {
let date = Date()
let formatter = DateFormatter()
formatter.dateFormat = "MMM d, h:mm a"
let now = formatter.string(from: date)
return now
}
<file_sep>/Test/PatientListCell.swift
//
// PatientListCell.swift
// Test
//
// Created by <NAME> on 2018-11-26.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class PatientListCell: UITableViewCell {
@IBOutlet weak var patientNameLabel: UILabel!
@IBOutlet weak var patientDOBLabel: UILabel!
func setPatient(_ patient: Patient){
patientNameLabel.text = patient.getName()
patientDOBLabel.text = patient.DOB
}
}
<file_sep>/Test/PatientListScreenViewController.swift
//
// PatientListScreenViewController.swift
// Test
//
// Created by <NAME> on 2018-11-26.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
import FirebaseDatabase
class PatientListScreenViewController: UIViewController {
@IBOutlet weak var patientTableView: UITableView!
@IBOutlet weak var patientSearchBar: UISearchBar!
var patients: [Patient] = []
var allPatients: [Patient] = []
var searching:Bool = false
var filtering:Bool = false
var ref:DatabaseReference?
var databaseHandle:DatabaseHandle?
var postData: [String] = []
override func viewDidLoad() {
super.viewDidLoad()
createPatientArray()
/*
var tempPatients: [Patient] = []
let patient1 = Patient(firstName: "Harry", lastName: "Potter", DOB: "June 05, 1980")
let patient2 = Patient(firstName: "Hermione", lastName: "Granger", DOB: "Sept 19, 1979")
let patient3 = Patient(firstName: "Ron", lastName: "Weasley", DOB: "Mar 1, 1980")
let patient4 = Patient(firstName: "Albus", lastName: "Dumbledore", DOB: "August 8, 1881")
let patient5 = Patient(firstName: "Minerva", lastName: "McGonagall", DOB: "c")
tempPatients.append(patient1)
tempPatients.append(patient2)
tempPatients.append(patient3)
tempPatients.append(patient4)
tempPatients.append(patient5)
self.allPatients = tempPatients
self.patients = tempPatients
*/
}
func createPatientArray(){
/*
let patient1 = Patient(firstName: "Harry", lastName: "Potter", DOB: "June 05, 1980")
let patient2 = Patient(firstName: "Hermione", lastName: "Granger", DOB: "Sept 19, 1979")
let patient3 = Patient(firstName: "Ron", lastName: "Weasley", DOB: "Mar 1, 1980")
let patient4 = Patient(firstName: "Albus", lastName: "Dumbledore", DOB: "August 8, 1881")
let patient5 = Patient(firstName: "Minerva", lastName: "McGonagall", DOB: "Oct 04, 1935")
tempPatients.append(patient1)
tempPatients.append(patient2)
tempPatients.append(patient3)
tempPatients.append(patient4)
tempPatients.append(patient5)
*/
ref = Database.database().reference()
//get all patient data
databaseHandle = ref?.child("Patients").observe(.value, with: {(snapshot) in
var tempPatients: [Patient] = []
for pat in snapshot.children.allObjects as! [DataSnapshot] {
//getting values
let patObject = pat.value as? [String: AnyObject]
let tempPatient = setPatient(patObject: patObject!)
tempPatients.append(tempPatient)
}
self.allPatients = tempPatients
self.patients = tempPatients
DispatchQueue.main.async {
self.patientTableView.reloadData()
}
})
}
func filterPatientArray(allPatients: [Patient]) -> [Patient] {
var tempPatients: [Patient] = []
for patIndex in 0 ..< allPatients.count {
if(allPatients[patIndex].getAlert()){
tempPatients.append(allPatients[patIndex])
}
}
/*
let patient1 = Patient(firstName: "Harry", lastName: "Potter", DOB: "June 05, 1980")
let patient2 = Patient(firstName: "Hermione", lastName: "Granger", DOB: "Sept 19, 1979")
let patient3 = Patient(firstName: "Ron", lastName: "Weasley", DOB: "Mar 1, 1980")
tempPatients.append(patient1)
tempPatients.append(patient2)
tempPatients.append(patient3)
*/
return tempPatients
}
@IBAction func switchViewAction(_ sender: UISegmentedControl) {
switch sender.selectedSegmentIndex {
case 0:
filtering = false
patients = allPatients
if searching {
patients = performPatientSearch()
}
UIView.transition(with: patientTableView, duration: 0.35, options: .transitionCrossDissolve , animations: {() -> Void in self.patientTableView.reloadData()}, completion: nil)
//patientTableView.reloadData()
default:
filtering = true
patients = filterPatientArray(allPatients: allPatients)
if searching {
patients = performPatientSearch()
}
UIView.transition(with: patientTableView, duration: 0.35, options: .transitionCrossDissolve , animations: {() -> Void in self.patientTableView.reloadData()}, completion: nil)
//patientTableView.reloadData()
}
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "PatientSegue" {
let destVC = segue.destination as! TabBarController
destVC.patient = sender as? Patient
}
}
}
extension PatientListScreenViewController: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return patients.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let patient = patients[(indexPath as NSIndexPath).row]
let cell = tableView.dequeueReusableCell(withIdentifier: "PatientListCell") as! PatientListCell
cell.setPatient(patient)
return cell
}
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let patient = patients[(indexPath as NSIndexPath).row]
performSegue(withIdentifier: "PatientSegue", sender: patient)
}
}
extension PatientListScreenViewController: UISearchBarDelegate {
func searchBar(_ searchBar: UISearchBar, textDidChange searchText: String) {
searching = true
patients = performPatientSearch()
patientTableView.reloadData()
}
func searchBarCancelButtonClicked(_ searchBar: UISearchBar) {
searching = false
searchBar.text = ""
if filtering {
patients = filterPatientArray(allPatients: allPatients)
} else {
patients = allPatients
}
patientTableView.reloadData()
}
func performPatientSearch() -> [Patient] {
var searchPatients: [Patient] = []
if filtering {
patients = filterPatientArray(allPatients: allPatients)
} else {
patients = allPatients
}
if(patientSearchBar.text!.isEmpty){
searchPatients = patients
} else {
for rows in 0 ..< patients.count {
if(patients[rows].getName().lowercased().contains(patientSearchBar.text!.lowercased())){
searchPatients.append(patients[rows])
}
}
}
return searchPatients
}
}
<file_sep>/Test/PhysNotifScreen.swift
//
// PhysNotifScreen.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
import FirebaseDatabase
class PhysNotifScreen: UIViewController {
@IBOutlet weak var notifTableView: UITableView!
var ref:DatabaseReference?
var databaseHandle:DatabaseHandle?
var notifs: [Notification] = []
var patient:Patient?
override func viewDidLoad() {
super.viewDidLoad()
createNotifArray()
//notifs = createNotifArray()
// Do any additional setup after loading the view.
}
func createNotifArray(){
ref = Database.database().reference()
databaseHandle = ref?.child("Notifications").child("1").observe(.value, with: {(snapshot) in
var tempNotifs: [Notification] = []
for notif in snapshot.children.allObjects as! [DataSnapshot] {
//getting values
let notifObject = notif.value as? [String: AnyObject]
print(notifObject)
if let doseObject = notifObject?["doseType"] as? [String: AnyObject] {
let notifText = doseObject["text"]
let patientID = doseObject["patientID"]
let dose = doseObject["dose"]
let tempNotif = Notification(notifStr: notifText as! String, type: "dose", patientID: patientID as! String)
tempNotif.setDose(dose: Float(dose as! String)!)
tempNotifs.append(tempNotif)
}
if let rangeObject = notifObject?["rangeType"] as? [String: AnyObject] {
let notifText = rangeObject["text"]
let patientID = rangeObject["patientID"]
let rangeLow = rangeObject["rangeLow"]
let rangeHigh = rangeObject["rangeHigh"]
let tempNotif = Notification(notifStr: notifText as! String, type: "range", patientID: patientID as! String)
tempNotif.setRange(rangeLow: Float(rangeLow as! String)!, rangeHigh: Float(rangeHigh as! String)!)
tempNotifs.append(tempNotif)
}
}
self.notifs = tempNotifs
DispatchQueue.main.async {
self.notifTableView.reloadData()
}
//return tempNotifs
})
}
func removeNotif(index:Int) {
ref = Database.database().reference()
ref?.child("Notifications").child("1").child(notifs[index].patientID).removeValue()
}
func updateValue(index:Int, attribute:String, value:String){
ref = Database.database().reference()
ref?.child("Patients").child(notifs[index].patientID).updateChildValues([attribute: value])
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "NotifSegue" {
let destVC = segue.destination as! TabBarController
destVC.patient = sender as? Patient
}
}
}
extension PhysNotifScreen: PhysNotifCellDelegate {
func didTapAccept(_ cellIndex: Int){
updateValue(index: cellIndex, attribute:"lastAdvagrafDose", value: String(notifs[cellIndex].dose))
updateValue(index: cellIndex, attribute: "alert", value: "false")
removeNotif(index: cellIndex)
var ref:DatabaseReference?
var tempString = "<NAME>"
tempString += " changed the dose to "
tempString += String(String(notifs[cellIndex].dose)) + "mg"
tempString += " on " + getNow()
let history = ["text": tempString]
ref = Database.database().reference().child("History").child(notifs[cellIndex].patientID).childByAutoId()
ref?.setValue(history)
notifs.remove(at: cellIndex)
notifTableView.reloadData()
}
func didTapDecline(_ cellIndex: Int){
updateValue(index: cellIndex, attribute: "alert", value: "false")
removeNotif(index: cellIndex)
var ref:DatabaseReference?
var tempString = "<NAME>"
tempString += " declined changing the dose"
tempString += " on " + getNow()
let history = ["text": tempString]
ref = Database.database().reference().child("History").child(notifs[cellIndex].patientID).childByAutoId()
ref?.setValue(history)
notifs.remove(at: cellIndex)
notifTableView.reloadData()
}
func didTapSeePat(_ cellIndex: Int) {
ref = Database.database().reference()
ref?.child("Patients").child(notifs[cellIndex].patientID).observeSingleEvent(of: .value, with: {(snapshot) in
let patObject = snapshot.value as? [String: AnyObject]
let tempPatient = setPatient(patObject: patObject!)
self.patient = tempPatient
DispatchQueue.main.async {
self.performSegue(withIdentifier: "NotifSegue", sender: self.patient)
}
})
}
}
extension PhysNotifScreen: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return notifs.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let notif = notifs[(indexPath as NSIndexPath).row]
let cell = tableView.dequeueReusableCell(withIdentifier: "PhysNotifCell") as! PhysNotifCell
cell.setNotif(notif, cellIndex: (indexPath as NSIndexPath).row)
cell.delegate = self
return cell
}
}
<file_sep>/Test/EnterResultVC.swift
//
// EnterResultVC.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-05.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class EnterResultVC: UIViewController {
@IBOutlet weak var resultTextField: UITextField!
var patient:Patient?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func submitTapped(_ sender: Any) {
if(resultTextField.text != ""){
if let result = Float(resultTextField.text!) {
patient?.addBloodResult(result, entryDate: getNow())
_ = self.navigationController?.popViewController(animated: true)
}
}
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
<file_sep>/Test/PhysNotifCell.swift
//
// PhysNotifCell.swift
// Test
//
// Created by <NAME> on 2018-11-26.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
protocol PhysNotifCellDelegate {
func didTapAccept(_ cellIndex: Int)
func didTapDecline(_ cellIndex: Int)
func didTapSeePat(_ cellIndex: Int)
}
class PhysNotifCell: UITableViewCell {
@IBOutlet weak var notifText: UILabel!
@IBOutlet weak var acceptNotif: UIButton!
@IBOutlet weak var declineNotif: UIButton!
@IBOutlet weak var seePatient: UIButton!
var delegate: PhysNotifCellDelegate?
var notif: Notification!
var index:Int = 0
func setNotif(_ notification: Notification, cellIndex: Int){
notif = notification
self.index = cellIndex
notifText.text = notification.notifStr
}
@IBAction func acceptTapped(_ sender: AnyObject) {
delegate?.didTapAccept(index)
}
@IBAction func declineTapped(_ sender: AnyObject) {
delegate?.didTapDecline(index)
}
//update patient values
@IBAction func seePatientTapped(_ sender: AnyObject) {
delegate?.didTapSeePat(index)
}
//add to history
//update notification variable
//remove notification cell
}
<file_sep>/Test/Clinic.swift
//
// Clinic.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Clinic {
var clinicName:String
var nurses:[Nurse] = []
var doctors:[Doctor] = []
init(clinicName:String){
self.clinicName = clinicName
}
func addNurse(_ firstName: String, lastName: String, employeeID: Int){
let nurse = Nurse(firstName: firstName, lastName: lastName, employeeID: employeeID)
self.nurses.append(nurse)
}
func addDoctor(_ firstName: String, lastName: String, employeeID: Int){
let doctor = Doctor(firstName: firstName, lastName: lastName, employeeID: employeeID)
self.doctors.append(doctor)
}
}
<file_sep>/Test/PatHistCell.swift
//
// PatHistCell.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class PatHistCell: UITableViewCell {
@IBOutlet weak var patHistLabel: UILabel!
func setHist(_ history:History){
patHistLabel.text = history.histStr
}
}
<file_sep>/Test/setPatient.swift
//
// setPatient.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-12.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
func setPatient(patObject:[String: AnyObject]) -> Patient {
let patFirstName = patObject["firstName"]
let patLastName = patObject["lastName"]
let patDOB = patObject["DOB"]
let patAlert = patObject["alert"]
let patientID = patObject["userID"]
let patDoctorID = patObject["doctorID"]
let patDoctorName = patObject["doctorName"]
//let patientID = patObject?["userID"]
let tempPatient = Patient(firstName: patFirstName as! String, lastName: patLastName as! String, DOB: patDOB as! String, doctorID: patDoctorID as! String, doctorName: patDoctorName as! String)
tempPatient.setPatientID(patientID as! String)
tempPatient.setAlert(state: Bool(patAlert as! String)!)
if let patMRN = patObject["MRN"] as? String {
tempPatient.setMRN(patMRN)
}
if let patTransDate = patObject["transDate"] as? String {
tempPatient.setTransDate(patTransDate)
}
if let patlastBloodResult = patObject["lastBloodResult"] as? String {
if let patDateBloodResult = patObject["dateOfBloodResult"] as? String {
tempPatient.setBloodResult(Float(patlastBloodResult)!, entryDate: patDateBloodResult)
}
}
if let patBloodLow = patObject["bloodRangeLow"] as? String {
if let patBloodHigh = patObject["bloodRangeHigh"] as? String {
tempPatient.setBloodWorkRange(Float(patBloodLow)!, bloodWorkHigher: Float(patBloodHigh)!)
}
}
//THIS DATE IS WRONG, FIX ME!!!!!!!!!!
if let patLastAdvagrafDose = patObject["lastAdvagrafDose"] as? String {
tempPatient.setAdvagrafDose(Float(patLastAdvagrafDose)!, entryDate: "")
}
return tempPatient
}
<file_sep>/Test/Patient.swift
//
// Patient.swift
// Test
//
// Created by <NAME> on 2018-11-26.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
/*
import Foundation
class Patient {
var name: String
var DOB: String
init(name:String, DOB:String){
self.name = name
self.DOB = DOB
}
}
*/
import UIKit
import FirebaseDatabase
class Patient:Person{
var DOB:String
var MRN:String = ""
var transplantDate:String = ""
var doctorID:String
var doctorName:String
var bloodWorkLower:Float = 0
var bloodWorkHigher:Float = 0
var patientID = ""
var alert:Bool = false
var advagrafDoses:[Dose] = []
var bloodWorkResults:[BloodWorkResult] = []
var historyEvents:[HistoryEvent] = []
var doctorNotifcations:[DoctorNotification] = []
init(firstName: String, lastName:String, DOB: String, doctorID:String, doctorName:String){
self.DOB = DOB
self.doctorID = doctorID
self.doctorName = doctorName
super.init()
self.setName(firstName, lastName: lastName)
}
func setMRN(_ MRN: String){
self.MRN = MRN
}
func setPatientID(_ patientID: String){
self.patientID = patientID
}
func setTransDate(_ transplantDate: String){
self.transplantDate = transplantDate
}
func setBloodWorkRange(_ bloodWorkLower:Float, bloodWorkHigher: Float){
self.bloodWorkLower = bloodWorkLower
self.bloodWorkHigher = bloodWorkHigher
}
func setBloodWorkRange(_ bloodWorkBoundary:Float, high:Int){
if(high==0){
self.bloodWorkLower = bloodWorkBoundary
}else{
self.bloodWorkHigher = bloodWorkBoundary
}
}
func setAdvagrafDose(_ doseInMG:Float, entryDate:String){
let advagrafDose = Dose(doseInMG: doseInMG, entryDate: entryDate)
self.advagrafDoses.insert(advagrafDose, at: 0)
}
func addAdvagrafDose(_ doseInMG:Float, entryDate:String){
var ref:DatabaseReference?
let advagrafDose = Dose(doseInMG: doseInMG, entryDate: entryDate)
self.advagrafDoses.insert(advagrafDose, at: 0)
self.updateValue(attribute:"lastAdvagrafDose", value: String(doseInMG))
self.updateValue(attribute: "alert", value: "false")
ref = Database.database().reference()
ref?.child("Notifications").child(self.doctorID).child(self.patientID).removeValue()
self.addHistoryEvent(doctorName, action: "changed dose", entryDate: getNowTimestamp())
}
func declineDoseChange(entryDate:String){
var ref:DatabaseReference?
self.updateValue(attribute: "alert", value: "false")
ref = Database.database().reference()
ref?.child("Notifications").child(self.doctorID).child(self.patientID).removeValue()
self.addHistoryEvent(doctorName, action: "declined dose", entryDate: getNowTimestamp())
}
func setBloodResult(_ result:Float, entryDate:String){
let bloodWorkResult = BloodWorkResult(result: result, entryDate: entryDate)
self.bloodWorkResults.insert(bloodWorkResult, at: 0)
}
func addBloodResult(_ result:Float, entryDate:String){
let bloodWorkResult = BloodWorkResult(result: result, entryDate: entryDate)
self.bloodWorkResults.insert(bloodWorkResult, at: 0)
self.updateValue(attribute: "lastBloodResult", value: String(result))
self.updateValue(attribute: "dateOfBloodResult", value: entryDate)
self.addHistoryEvent(doctorName, action: "changed result", entryDate: getNowTimestamp())
if !self.inRange() {
self.updateValue(attribute: "alert", value: "true")
self.addDoseChangeNotif("The app", dateSuggested: getNow(), newDose: self.suggestedDose())
self.addHistoryEvent("The app", action: "suggested dose", entryDate: getNowTimestamp())
}
}
func addHistoryEvent(_ actor:String, action:String, entryDate:String){
var ref:DatabaseReference?
//create text
var tempString = actor
switch action {
case "suggested dose":
tempString += " suggested changing the dose to "
tempString += String(self.suggestedDose()) + "mg"
break
case "changed dose":
tempString += " changed the dose to "
tempString += String(self.advagrafDoses[0].doseInMG) + "mg"
break
case "declined dose":
tempString += " declined changing the dose"
break
case "changed range":
tempString += " changed the blood test range to "
tempString += String(self.bloodWorkLower) + "-"
tempString += String(self.bloodWorkHigher) + "ng/L"
break
case "changed result":
tempString += " entered the new blood test result of "
tempString += String(self.bloodWorkResults[0].result) + "ng/L"
break
default:
break
}
tempString += " on " + getNow()
let history = ["text": tempString]
ref = Database.database().reference().child("History").child(self.patientID).childByAutoId()
ref?.setValue(history)
}
func addDoseChangeNotif(_ suggester:String, dateSuggested:String, newDose:Float){
var ref:DatabaseReference?
//create text
var tempString = suggester
tempString += " suggested changing the Advagraf dose for "
tempString += String(self.getName())
tempString += " from " + String(self.advagrafDoses[0].doseInMG)
tempString += " to " + String(newDose)
tempString += " on " + getNow()
let notification = [
"text": tempString,
"dose": String(newDose),
"patientID": self.patientID
]
ref = Database.database().reference().child("Notifications").child(self.doctorID).child(self.patientID).child("doseType")
ref?.setValue(notification)
}
func addRangeUpdateNotif(_ suggester:String, suggestType:Character, dateSuggested:String, newRangeLow:Float, newRangeHigh:Float){
let doctorNotification = DoctorNotification(suggester: suggester, suggestType: suggestType, dateSuggested: dateSuggested, newRangeLow: newRangeLow, newRangeHigh: newRangeHigh)
self.doctorNotifcations.insert(doctorNotification, at: 0)
}
func inRange() -> Bool {
if(bloodWorkResults.isEmpty){
return true
} else {
if(bloodWorkResults[0].result>=bloodWorkLower && bloodWorkResults[0].result<=bloodWorkHigher){
return true
} else {
return false
}
}
}
func setAlert(state:Bool){
self.alert = state
}
func getAlert() -> Bool {
return self.alert
}
func suggestedDose() -> Float {
let midRange = (bloodWorkLower + bloodWorkHigher)/2
let adjustment:Float = (midRange/bloodWorkResults[0].result)
var suggestedDose = round(adjustment*advagrafDoses[0].doseInMG*2)/2
if (suggestedDose>25){
suggestedDose = 25
}
return suggestedDose
}
func hasDoctorNotification() -> Bool {
if (doctorNotifcations.isEmpty) {
return false
} else {
for rows in 0 ..< doctorNotifcations.count {
if(!doctorNotifcations[rows].approved){
return true
}
}
return false
}
}
//change to returning only indices??
func getDoctorNotification() -> [DoctorNotification] {
var tempNotification:[DoctorNotification] = []
if !(doctorNotifcations.isEmpty) {
for rows in 0 ..< doctorNotifcations.count {
if(!doctorNotifcations[rows].approved){
tempNotification.append(doctorNotifcations[rows])
}
}
}
return tempNotification
}
func getPatientHistory() -> [String] {
var tempStringArr:[String] = []
for rows in 0..<historyEvents.count{
tempStringArr.append(historyEvents[rows].getHistory())
}
return tempStringArr
}
func updateValue(attribute:String, value:String){
var ref:DatabaseReference?
ref = Database.database().reference()
ref?.child("Patients").child(self.patientID).updateChildValues([attribute: value])
}
}
class Dose {
var doseInMG:Float
var entryDate:String
init(doseInMG:Float, entryDate:String){
self.doseInMG = doseInMG
self.entryDate = entryDate
}
}
class BloodWorkResult {
var result:Float
var entryDate:String
init(result:Float, entryDate:String){
self.result = result
self.entryDate = entryDate
}
}
class HistoryEvent {
var actor:String
var actorType:Character
var action:String
var entryDate:String
init(actor:String, actorType:Character, action:String, entryDate:String){
self.actor = actor
self.actorType = actorType
self.action = action
self.entryDate = entryDate
}
func getHistory () -> String {
return (actor + " " + action + " on " + entryDate)
}
}
class DoctorNotification {
var approved:Bool = false
var suggester:String
var suggestType:Character
var dateSuggested:String
var rangeChange:Bool = false
var newRangeLow:Float = 0
var newRangeHigh:Float = 0
var doseChange:Bool = false
var newDose:Float = 0
init(suggester:String, suggestType:Character, dateSuggested:String, newRangeLow:Float, newRangeHigh:Float){
self.suggester = suggester
self.suggestType = suggestType
self.dateSuggested = dateSuggested
self.rangeChange = true
self.newRangeLow = newRangeLow
self.newRangeHigh = newRangeHigh
}
init(suggester:String, suggestType:Character, dateSuggested:String, newDose:Float){
self.suggester = suggester
self.suggestType = suggestType
self.dateSuggested = dateSuggested
self.doseChange = true
self.newDose = newDose
}
func getNotification (_ patient:Patient) -> String {
var tempString:String = ""
tempString += suggester + " "
if(doseChange){
tempString += "changed the Advagraf dose for "
tempString += patient.getName()
tempString += " from " + (newRangeLow).description
tempString += " to " + (newRangeHigh).description
} else if (rangeChange) {
}
tempString += "on " + dateSuggested
return tempString
}
}
<file_sep>/Test/LoginScreen.swift
//
// LoginScreen.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
import FirebaseDatabase
class LoginScreen: UIViewController {
@IBOutlet weak var usernameTextField: UITextField!
@IBOutlet weak var passwordTextField: UITextField!
//var ref:DatabaseReference?
override func viewDidLoad() {
super.viewDidLoad()
//ref = Database.database().reference()
//ref?.child("Patients")
//.setValue(["username": username])
/*
let postRef = ref?.child("Patients").childByAutoId()
let patient = [
"firstName":"Ginny",
"lastName":"Weasley",
"DOB":"-",
"MRN":"9876543214RT",
"transDate":"Nov 9, 2018",
"lastBloodResult": "2",
"dateOfBloodResult": "Nov 26, 2018",
"bloodRangeLow": "3",
"bloodRangeHigh": "6",
"lastAdvagrafDose": "9",
"doctorID": "2",
"doctorName": "-",
//"doctorName": "<NAME>",
"userID": postRef?.key]
postRef?.setValue(patient)
*/
// Do any additional setup after loading the view.
}
@IBAction func loginTapped(_ sender: AnyObject) {
// let username = usernameTextField.text
//let password = passwordTextField.text
}
}
<file_sep>/Test/Doctor.swift
//
// Doctor.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Doctor:Provider{
var patients:[Patient] = []
init(firstName: String, lastName: String, employeeID: Int){
super.init()
self.setName(firstName, lastName: lastName)
self.setID(employeeID)
}
func addPatient(_ firstName: String, lastName: String, DOB: String, MRN: String, transplantDate: String, bloodWorkLower:Float, bloodWorkHigher: Float){
/*let patient = Patient(firstName: firstName, lastName: lastName, DOB: DOB, doctorID: String(self.employeeID))
patient.setMRN(MRN)
patient.setTransDate(transplantDate)
patient.setBloodWorkRange(bloodWorkLower, bloodWorkHigher: bloodWorkHigher)
self.patients.append(patient)*/
}
}
<file_sep>/Test/PatientDetailScreen.swift
//
// PatientDetailScreen.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
import FirebaseDatabase
class PatientDetailScreen: UIViewController {
@IBOutlet weak var patientNameLabel: UILabel!
@IBOutlet weak var patientDOBLabel: UILabel!
@IBOutlet weak var patientMRNLabel: UILabel!
@IBOutlet weak var patientDateofTransLabel: UILabel!
@IBOutlet weak var resultDateLabel: UILabel!
@IBOutlet weak var resultCurrentLabel: UILabel!
@IBOutlet weak var resultRangeLabel: UILabel!
@IBOutlet weak var advagrafDoseLabel: UILabel!
@IBOutlet weak var doseAlertLabel: UILabel!
@IBOutlet weak var doseSuggestionLabel: UILabel!
@IBOutlet weak var acceptDoseButton: UIButton!
@IBOutlet weak var declineDoseButton: UIButton!
var patient: Patient?
var ref:DatabaseReference?
var databaseHandle:DatabaseHandle?
override func viewDidLoad() {
super.viewDidLoad()
let tabBar = tabBarController as! TabBarController
self.patient = tabBar.patient
updateView()
//self.patient = setPatient(patObje)(tabBar.patient?.patientID)
// Do any additional setup after loading the view.
}
func updateView(){
ref = Database.database().reference()
ref?.child("Patients").child((self.patient?.patientID)!).observe(.value, with: {(snapshot) in
let patObject = snapshot.value as? [String: AnyObject]
let tempPatient = setPatient(patObject: patObject!)
self.patient = tempPatient
DispatchQueue.main.async {
self.setUI()
}
})
}
func setUI(){
patientNameLabel.text = patient?.getName()
patientDOBLabel.text = "Date of Birth: " + patient!.DOB
patientMRNLabel.text = "OHIP: " + patient!.MRN
patientDateofTransLabel.text = "Date of Transplant: " + patient!.transplantDate
if(!patient!.bloodWorkResults.isEmpty){
resultDateLabel.text = patient!.bloodWorkResults[0].entryDate
resultCurrentLabel.text = (NSString(format: "%.1f",patient!.bloodWorkResults[0].result) as String) + "ng/mL"
} else {
resultDateLabel.text = ""
resultCurrentLabel.text = "__ ng/L"
}
if(patient!.bloodWorkLower==0 && patient!.bloodWorkHigher==0){
resultRangeLabel.text = "__ - __ ng/L"
} else {
resultRangeLabel.text = (NSString(format: "%.1f",patient!.bloodWorkLower) as String) + " - " + (NSString(format: "%.1f",patient!.bloodWorkHigher) as String) + "ng/mL"
}
if(!patient!.advagrafDoses.isEmpty){
advagrafDoseLabel.text = (NSString(format: "%.1f",patient!.advagrafDoses[0].doseInMG) as String) + "mg"
} else {
advagrafDoseLabel.text = "__ mg"
}
if(!((patient?.getAlert())!)){
self.hideAlert()
} else {
self.showAlert()
}
}
@IBAction func acceptTapped(_ sender: Any) {
patient?.addAdvagrafDose((patient?.suggestedDose())!, entryDate: getNow())
updateView()
}
@IBAction func declineTapped(_ sender: Any) {
patient?.declineDoseChange(entryDate: getNow())
updateView()
}
@IBAction func enterResultTapped(_ sender: Any) {
self.performSegue(withIdentifier: "ResultSegue", sender: self.patient)
}
@IBAction func updateRangeTapped(_ sender: Any) {
self.performSegue(withIdentifier: "RangeSegue", sender: self.patient)
}
@IBAction func adjustDoseTapped(_ sender: Any) {
self.performSegue(withIdentifier: "DoseSegue", sender: self.patient)
}
func hideAlert(){
acceptDoseButton.isHidden = true
declineDoseButton.isHidden = true
doseAlertLabel.text = ""
doseSuggestionLabel.text = ""
}
func showAlert(){
acceptDoseButton.isHidden = false
declineDoseButton.isHidden = false
doseAlertLabel.text = "ALERT!"
doseSuggestionLabel.text = "Suggestion: Change dose to " + (NSString(format: "%.1f",(patient?.suggestedDose())!) as String) + "mg"
}
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
if segue.identifier == "ResultSegue" {
let destVC = segue.destination as! EnterResultVC
destVC.patient = sender as? Patient
} else if segue.identifier == "DoseSegue" {
let destVC = segue.destination as! EnterDoseVC
destVC.patient = sender as? Patient
} else if segue.identifier == "RangeSegue" {
let destVC = segue.destination as! EnterRangeVC
destVC.patient = sender as? Patient
}
}
}
<file_sep>/Test/History.swift
//
// History.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class History {
var histStr: String
init(histStr:String){
self.histStr = histStr
}
}<file_sep>/Test/EnterRangeVC.swift
//
// EnterRangeVC.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-11.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class EnterRangeVC: UIViewController {
var patient:Patient?
@IBOutlet weak var rangeLowTF: UITextField!
@IBOutlet weak var rangeHighTF: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func submitTapped(_ sender: Any) {
var updated = false
if(rangeLowTF.text != ""){
if let rangeLow = Float(rangeLowTF.text!) {
patient?.updateValue(attribute: "bloodRangeLow", value: String(rangeLow))
patient?.setBloodWorkRange(rangeLow, high: 0)
updated = true
}
}
if(rangeHighTF.text != ""){
if let rangeHigh = Float(rangeHighTF.text!) {
patient?.updateValue(attribute: "bloodRangeHigh", value: String(rangeHigh))
patient?.setBloodWorkRange(rangeHigh, high: 1)
updated = true
}
}
if(updated){
patient?.addHistoryEvent((patient?.doctorName)!, action: "changed range", entryDate: getNowTimestamp())
_ = self.navigationController?.popViewController(animated: true)
}
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
<file_sep>/Test/Nurse.swift
//
// Nurse.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Nurse:Provider{
init(firstName: String, lastName: String, employeeID: Int){
super.init()
self.setName(firstName, lastName: lastName)
self.setID(employeeID)
}
}<file_sep>/Test/Provider.swift
//
// Provider.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Provider:Person{
var employeeID:Int = 0
func setID(_ employeeID:Int){
self.employeeID = employeeID
}
}
<file_sep>/Test/Notification.swift
//
// Notification.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Notification{
var notifStr: String
var type:String
var patientID:String
var dose:Float = 0
var rangeLow:Float = 0
var rangeHigh:Float = 0
init(notifStr:String, type:String, patientID:String){
self.notifStr = notifStr
self.type = type
self.patientID = patientID
}
func setDose(dose:Float){
self.dose = dose
}
func setRange(rangeLow:Float, rangeHigh:Float){
self.rangeLow = rangeLow
self.rangeHigh = rangeHigh
}
func acceptNotif(patient: Patient){
//update patient values
if(type=="dose"){
patient.addAdvagrafDose(dose, entryDate: "")
} else {
patient.setBloodWorkRange(rangeLow, bloodWorkHigher: rangeHigh)
}
//remove warning
//add to patient history
}
func declineNotif(){
//remove warning
//add to patient history
}
func addNotif(patient: Patient, rangeLow:Float, rangeHigh:Float){
//create text
//write to database
}
func addNotif(patient: Patient, dose:Float){
//create text
//write to database
}
}
<file_sep>/Test/PatientHistoryScreen.swift
//
// PatientHistoryScreen.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
import FirebaseDatabase
class PatientHistoryScreen: UIViewController {
@IBOutlet weak var patHistTableView: UITableView!
@IBOutlet weak var patientDOBLabel: UILabel!
@IBOutlet weak var patientNameLabel: UILabel!
@IBOutlet weak var patientMRNLabel: UILabel!
@IBOutlet weak var patientDateofTransLabel: UILabel!
var ref:DatabaseReference?
var databaseHandle:DatabaseHandle?
var histories: [History] = []
var patient:Patient!
override func viewDidLoad() {
super.viewDidLoad()
let tabBar = tabBarController as! TabBarController
self.patient = tabBar.patient
setUI()
createHistArray()
// Do any additional setup after loading the view.
}
func setUI(){
patientNameLabel.text = patient?.getName()
patientDOBLabel.text = "Date of Birth: " + patient!.DOB
patientMRNLabel.text = "OHIP: " + patient!.MRN
patientDateofTransLabel.text = "Date of Transplant: " + patient!.transplantDate
}
func createHistArray() {
ref = Database.database().reference()
ref?.child("History").child(patient.patientID).observe(.value, with: { (snapshot) in
var tempHists: [History] = []
for history in snapshot.children.allObjects as! [DataSnapshot] {
//getting values
let historyObject = history.value as? [String: AnyObject]
let text = historyObject?["text"]
let tempHist = History(histStr: text as! String)
tempHists.append(tempHist)
}
self.histories = tempHists
DispatchQueue.main.async {
self.patHistTableView.reloadData()
}
})
}
}
extension PatientHistoryScreen: UITableViewDataSource, UITableViewDelegate {
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return histories.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let history = histories[(indexPath as NSIndexPath).row]
let cell = tableView.dequeueReusableCell(withIdentifier: "PatHistCell") as! PatHistCell
cell.setHist(history)
return cell
}
}
<file_sep>/Test/PatientStatsScreen.swift
//
// PatientStatsScreen.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-06.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class PatientStatsScreen: UIViewController {
@IBOutlet weak var patientNameLabel: UILabel!
@IBOutlet weak var patientDOBLabel: UILabel!
@IBOutlet weak var patientMRNLabel: UILabel!
@IBOutlet weak var patientDateofTransLabel: UILabel!
var patient:Patient!
override func viewDidLoad() {
super.viewDidLoad()
let tabBar = tabBarController as! TabBarController
self.patient = tabBar.patient
setUI()
// Do any additional setup after loading the view.
}
func setUI(){
patientNameLabel.text = patient?.getName()
patientDOBLabel.text = "Date of Birth: " + patient!.DOB
patientMRNLabel.text = "OHIP: " + patient!.MRN
patientDateofTransLabel.text = "Date of Transplant: " + patient!.transplantDate
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
<file_sep>/Test/TabBarController.swift
//
// TabBarController.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class TabBarController: UITabBarController {
var patient:Patient?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
}
<file_sep>/Test/EnterDoseVC.swift
//
// EnterDoseVC.swift
// AdvagrafApp
//
// Created by <NAME> on 2018-12-11.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import UIKit
class EnterDoseVC: UIViewController {
var patient:Patient?
@IBOutlet weak var doseTextField: UITextField!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func submitTapped(_ sender: Any) {
if(doseTextField.text != ""){
if let dose = Float(doseTextField.text!) {
/*let date = Date()
let formatter = DateFormatter()
formatter.dateFormat = "MMM d, yyyy"
let now = formatter.string(from: date)*/
patient?.addAdvagrafDose(dose, entryDate: getNow())
_ = self.navigationController?.popViewController(animated: true)
}
}
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
<file_sep>/Test/Person.swift
//
// Person.swift
// Test
//
// Created by <NAME> on 2018-11-27.
// Copyright © 2018 CAS757_Group6. All rights reserved.
//
import Foundation
class Person{
var firstName:String = ""
var lastName:String = ""
init(){
}
func setName(_ firstName: String, lastName: String){
self.firstName = firstName
self.lastName = lastName
}
func getName() -> String {
return (firstName + " " + lastName)
}
}
| 9aaeeb2b3925c24d847bf060be06e3ce5c37eef6 | [
"Swift"
] | 23 | Swift | armstj7/CAS757_AutomatedAdvagrafAdjustment | dbd037f6d5fcc2d057a33981994a0c655c0cda1b | 6366d567b2e26f658a600076f5f5e2c3908be4b5 | |
refs/heads/master | <file_sep>#include<iostream.h>
#include<conio.h>
#include<math.h>
/**
*@author:<NAME>
*http://in.linkedin.com/meetdagod
*print a circle without using graphic.h
*save text as circle.cpp
*run it in Turbo C++ or CMD or any other C++ compiler
*/
void main()
{clrscr();
cout<<"Program to draw an empty circle."<<endl;
cout<<"------------------------------"<<endl;
cout<<"Input desired diameter:";
int a=0;
cin>>a;
if(a%2!=0){a=a+1;}
cout<<"Enter character to print.:";
char ch;
cin>>ch;
cout<<"--------------------"<<endl;
char circle[100][100];
int b=a/2;
double y=0;
double z=0;
int r=0;
int Y=0;
int Z=0;
for(int s=0;s<=a;s++)
{for(int t=0;t<=a;t++)
{circle[s][t]=32;
}
}
circle[b][b]=79;
double m=0;
for(int i=0;i<a;i++) //calcualtes circle coordinates by equation to circle
{
m=abs(i*i-2*b*i);
y=(b+sqrt(m));
z=(b-sqrt(m));
Y=floor(y+0.5);
Z=floor(z+0.5);
circle[i][Y]=ch;
circle[i][Z]=ch;
}
for(int p=0;p<=a;p++)
{for(int q=0;q<=a;q++)
{cout<<circle[p][q]<<" "; //add or remove spaces to correct shape!
}cout<<endl;
}getch();
}
<file_sep>#include<iostream.h>
#include<conio.h>
#include<dos.h>
#include<stdlib.h>
#include<stdio.h>
#include<process.h>
#include<string.h>
#include<iomanip.h>
char game[30][90]={' '};
enum option{off,on};
enum level{beginner=1,mediocre,advanced};
struct player
{
char name[80];
option sound;
option roof;
level speed;
level difficulty;
unsigned int score;
int locat;
player()
{strcpy(name,"Pilot");
sound =on;
roof=off;
speed=mediocre;
difficulty =mediocre;
locat =15;
score =0;
}
void reset()
{strcpy(name,"Pilot");
roof=off;
sound =on;
speed=mediocre;
difficulty =mediocre;
locat =15;
score =0;
}
};
struct obstruction
{unsigned int size;
unsigned int start;
int pos;
obstruction()
{size=0;
start=0;
pos=80;
}
void reset()
{do
{size=random(16);
}while(size<4);
//size=random(12); //use random(12) instead
start=random(24-size);
pos=80;
}
};
void menu(int& mode)
{
nosound();
mode=1;
char ch='e';
clrscr();
cout<<"Helicopter\n"
<<"(P)lay\n"
<<"(A)bout\n"
<<"(E)xit\n"
<<"\tHit appropriate Key: ";
ch=getche();
switch(ch)
{case 'P':
case 'p':clrscr();
cout<<"Mode\n"
<<"(1) Player\n"
<<"(2) Player\n"
<<"\tHit appropriate Key: ";
do
{
mode=getch();
}while(mode!=49&&mode!=50);
mode-=48;
cout<<mode<<endl;
// cin>>mode;
break;
case 'a':
case 'A':clrscr();
cout<<"Details\n"
<<"Welcome to Helicopter!!\n"
<<"This game has been designed as a part of my Computer Applications Project.\n"
<<"In the game you are the pilot of a quadricopter in Pandora and you have to save your crew from the flying rocks. Go ahead try your luck...\n"
<<"The controls are as follows:/n"
<<"Use arrow Keys for Navigation [Up] key for moving your copter upwards and [Down] key for maving it downwards\n"
<<"Simple ain't it? For those of you who can't handle the difficulty.. just type in your name as {dIWANK} or {Diwank}.\n";
getch();
menu(mode);
break;
case 'e':
case 'E':exit(1);
break;
default:menu(mode);
}
}
void printer(player pl1)
{clrscr();
for(int i=0;i<24;i++)
{for(int j=0;j<80;j++)
{cout<<game[i][j];
}
}
cout<<pl1.name<<"\tScore: "<<pl1.score;
}
void options(player& pl1)
{char ch='\0';int s,d,p,h;
char option[2][15]={"Off","On"};
char level[3][25]={"Beginner","Mediocre","Advanced"};
clrscr();
cout<<"\nOptions\n"
<<"Enter name: ";
gets(pl1.name);
clrscr();
do{clrscr();
s=pl1.sound;
d=pl1.difficulty;
p=pl1.speed;
h=pl1.roof;
cout<<"\nSettings: "<<pl1.name;
cout<<"\nS(p)eed:\t"<<level[p-1];
cout<<"\n(D)ifficulty: "<<level[d-1]
<<"\n(S)ound:\t"<<option[s]
<<"\n(H)it Roof:\t"<<option[h]
<<"\n\tHit appropriate Key: ";
ch=getche();
switch(ch)
{case 's':
case 'S':pl1.sound=(!pl1.sound);
break;
case 'h':
case 'H':pl1.roof=(!pl1.roof);
break;
case 'p':
case 'P':pl1.speed=(pl1.speed==3)?1:pl1.speed+1;
break;
case 'd':
case 'D':pl1.difficulty=(pl1.difficulty==3)?1:pl1.difficulty+1;
break;
case 'e':
case 'E':exit(0);
}
}while(ch!='\r');
}
void obstructor(obstruction obs1)
{char ch='*';
obs1.pos=(obs1.pos>=79)?79:obs1.pos;
for(int i=obs1.start;(i<(obs1.start+obs1.size))&&obs1.size>0;i++)
{
if(obs1.pos>=0)
{game[i][obs1.pos]=ch;
game[i][obs1.pos+1]=' ';
game[i][obs1.pos-1]=ch;
}
else{game[i][obs1.pos]=' ';
game[i][obs1.pos+1]=' ';
}
}
}
void play(player& pl1)
{
int i=1;
char key=' ';
obstruction obs1,obs2,tmp;
obs1.reset();
do{
do{delay(3360/(pl1.speed*80)); //check speed
for(int w=0;w<26;w++){for(int x=0;x<82;x++){game[w][x]=' ';}}
game[pl1.locat][0]='#';
obstructor(obs1);
obstructor(obs2);
printer(pl1);
if(pl1.sound)
{sound(120*(random(3)+1+(i%3)));}
if(obs1.pos==(80-(10*(6-pl1.difficulty))))
{obs2.reset();}
else if(obs1.pos==1)
{tmp=obs1;
obs1=obs2;
obs2=obs1;
obs2.reset();
}
obs1.pos--;obs2.pos--;
i+=(!strcmp(pl1.name,"Diwank"))?10:1;
pl1.score=i/10;
}while(kbhit()==0&&game[pl1.locat+1][0]==' '&&game[pl1.locat-1][0]==' ');
if(kbhit()!=0||!strcmp(pl1.name,"dIWANK"))
{
key=getch();
if(key==0)
{key=getch();
if(key==72)pl1.locat--;
else if(key==80)pl1.locat++;
if(!pl1.roof)
{pl1.locat=(pl1.locat<1)?22:pl1.locat;
pl1.locat=(pl1.locat>22)?1:pl1.locat;
}
for(int j=0;j<24;j++){game[j][0]=' ';}
}
else if(key=='e'||key=='E')exit(0);}
}while(key!='\r'&&game[pl1.locat+1][0]==' '&&game[pl1.locat-1][0]==' ');
}
void main()
{
randomize();
textmode(C80);
char ch;
int mode=1;
player pl1,pl2,tmp;
do{
menu(mode);
for(int i=1;i<=mode;i++)
{options(pl1);
for(int w=0;w<26;w++){for(int x=0;x<82;x++){game[w][x]=' ';}}
play(pl1);
//if(mode==2)
tmp=pl1;
pl1=pl2;pl1.reset();
pl2=tmp;
}
cout<<"\nScoreboard\n"
<<pl2.name<<setw(10)<<pl2.score<<endl;
if(mode==2)
{cout<<pl1.name<<setw(10)<<pl1.score<<endl;
cout<<((pl2.score>pl1.score)?pl2.name:pl1.name)<<" Wins!\n";
}
cout<<"\nHit n to exit: ";
cin>>ch;
//ch=getche();
}while(ch!='n'||ch!='N');
nosound();
}<file_sep>//Begin- Includes
#include<fstream.h>
#include<conio.h>
#include<string.h>
#include<ctype.h>
#include<time.h>
#include<stdlib.h>
#include<stdio.h>
#include<graphics.h>
#include<dos.h>
//#include"mouse.h"
//End- Includes
//Begin- Types
class colorC
{
unsigned int R,G,B;
char hex[6];
int hextoint(char);
unsigned int red();
unsigned int blue();
unsigned int green();
public:
colorC();
colorC(char []);
void setColor(char []);
unsigned int getColor();
char* hexValue();
};
int colorC::hextoint(char a)
{
unsigned int tmp;
if(isdigit(a)) tmp=(a-48);
else if(isalpha(a)) tmp=(tolower(a)-87);
else tmp=0;
return tmp;
}
colorC::colorC()
{
R=255,G=255,B=255;
strcpy(hex,"ffffff");
}
colorC::colorC(char temp[])
{
strcpy(hex,temp);
R=hextoint(hex[0])*16+hextoint(hex[1]);
G=hextoint(hex[2])*16+hextoint(hex[3]);
B=hextoint(hex[4])*16+hextoint(hex[5]);
}
void colorC::setColor(char temp[])
{
strcpy(hex,temp);
R=hextoint(hex[0])*16+hextoint(hex[1]);
G=hextoint(hex[2])*16+hextoint(hex[3]);
B=hextoint(hex[4])*16+hextoint(hex[5]);
}
unsigned int colorC::red()
{
return R;
}
unsigned int colorC::blue()
{
return B;
}
unsigned int colorC::green()
{
return G;
}
char* colorC::hexValue()
{
return hex;
}
unsigned int colorC::getColor()
{
float r=R/255;
float g=G/255;
float b=B/255;
int c;
if(r==1&&g==0&&b==0) c=RED;
else if(r==0&&g==1&&b==0) c=GREEN;
else if(r==0&&g==0&&b==1) c=BLUE;
else if(r==1&&g==1&&b==0) c=YELLOW;
else if(r==1&&g==0&&b==1) c=MAGENTA;
else if(r==0&&g==1&&b==1) c=CYAN;
else if(r==1&&g==1&&b==1) c=WHITE;
else if(r==0&&g==0&&b==0) c=BLACK;
else if(r==g&&g==b&&b>=0.5&&b<1) c=LIGHTGRAY;
else if(r==g&&g==b&&b<=0.5&&b>0) c=DARKGRAY;
else if(r<1&&r>=0.5&&g==0&&b==0) c=LIGHTRED;
else if(g<1&&g>=0.5&&r==0&&b==0) c=LIGHTGREEN;
else if(b<1&&b>=0.5&&g==0&&r==0) c=LIGHTBLUE;
else if(r<1&&r>=0.5&&g==0&&b<1&&b>=0.5) c=LIGHTMAGENTA;
else if(g<1&&g>=0.5&&r==0&&b<1&&b>=0.5) c=LIGHTCYAN;
else if(r<1&&r>=0.5&&g<=0.5&&g>0&&b<=0.5&&b>0) c=BROWN;
else c=WHITE;
return c;
}
//-------------------------------------------//
class fontC
{
unsigned int s;
char f[24];
public:
colorC color;
fontC();
fontC(colorC , unsigned int, char []);
void setFontColor(char []);
void setFontSize(unsigned int);
void setFontFace(char []);
unsigned int size();
char* face();
};
fontC::fontC()
{
colorC c1("ffffff");
color=c1;
s=4;
strcpy(f,"serif");
}
fontC::fontC(colorC c1, unsigned int s1, char f1[24])
{
strcpy(f,f1);
color=c1;
//s=(s1%8);
s=s1;
}
void fontC::setFontColor(char c1[])
{
color.setColor(c1);
}
void fontC::setFontSize(unsigned int s1)
{
//s=(s1%8);
s=s1;
}
void fontC::setFontFace(char f1[24])
{
strcpy(f,f1);
}
unsigned int fontC::size()
{
return s;
}
char* fontC::face()
{
return f;
}
//-------------------------------------------//
class borderC
{
unsigned int s;
public:
colorC color;
borderC();
borderC(unsigned int , colorC );
void setBorderWidth(unsigned int);
void setBorderColor(char []);
unsigned int size();
};
borderC::borderC()
{
s=1;
colorC c1("ffffff");
color=c1;
}
borderC::borderC(unsigned int s1, colorC c1)
{
s=s1;
color=c1;
}
void borderC::setBorderWidth(unsigned int s1)
{
s=s1;
}
void borderC::setBorderColor(char c1[])
{
color.setColor(c1);
}
unsigned int borderC::size()
{
return s;
}
//-------------------------------------------//
class opC
{
unsigned int r;
unsigned int c;
public:
opC();
opC(unsigned int);
void incChild(unsigned int);
void incRank(int);
unsigned int children();
unsigned int rank();
};
opC::opC()
{
r=0;
c=0;
}
opC::opC(unsigned int r1)
{
c=0;
r=r1;
}
void opC::incChild(unsigned int c1=1)
{
c+=c1;
}
void opC::incRank(int r1=1)
{
r+=r1;
}
unsigned int opC::children()
{
return c;
}
unsigned int opC::rank()
{
return r;
}
//-------------------------------------------//
class metaC
{
char i[20];
char h[60];
public:
metaC();
metaC(char [],char []);
void setId(char []);
void setHref(char []);
char* id();
char* href();
};
metaC::metaC()
{
i[0]='\0';
h[0]='\0';
}
metaC::metaC(char i1[],char h1[])
{
strcpy(i,i1);
strcpy(h,h1);
}
char* metaC::id()
{
return i;
}
char* metaC::href()
{
return h;
}
void metaC::setId(char i1[])
{
strcpy(i,i1);
}
void metaC::setHref(char h1[])
{
strcpy(h,h1);
}
//-------------------------------------------//
class generalC
{
char n[20];
char s;
public:
generalC();
generalC(char []);
char* name();
void setName(char []);
void setSupported(char);
unsigned int isSupported();
};
generalC::generalC()
{
n[0]='\0';
s='N';
}
generalC::generalC(char n1[])
{
strcpy(n,n1);
s='Y';
}
char* generalC::name()
{
return n;
}
void generalC::setName(char n1[])
{
strcpy(n,n1);
s='y';
}
void generalC::setSupported(char s1='Y')
{
s=s1;
}
unsigned int generalC::isSupported()
{
unsigned int flag=0;
if(s=='y'||s=='Y')
{
flag=1;
}
return flag;
}
//-------------------------------------------//
class contentC
{
char tmp[20];
fstream file;
public:
contentC();
char* name();
~contentC();
void insert(char []);
void insertChar(char);
void clear();
char* read();
void reset(unsigned int);
char readChar();
};
contentC::contentC()
{
unsigned int r= random(9000)+1000;
char s[20];
itoa(r,s,10);
strcpy(tmp,s);
strcat(tmp,".txt");
file.open(tmp,ios::in|ios::out);
}
char* contentC::name()
{
return tmp;
}
contentC::~contentC()
{
file.close();
unlink(tmp);
}
void contentC::insert(char line[80])
{
file<<line;
}
void contentC::insertChar(char ch)
{
file<<ch;
}
void contentC::clear()
{
file.clear();
}
char* contentC::read()
{
char line[80];
file.getline(line,80);
return line;
}
void contentC::reset(unsigned int ctr=0)
{
file.seekp(ctr);
}
char contentC::readChar()
{
char ch;
file.get(ch);
return ch;
}
//End- Types
//Begin- Elements
class style
{
protected:
unsigned int h,w,t,l;
public:
colorC color,background;
fontC font;
borderC border;
unsigned int height();
unsigned int width();
unsigned int top();
unsigned int left();
void setHeight(unsigned int);
void setWidth(unsigned int);
void setTop(unsigned int);
void setLeft(unsigned int);
};
void style::setHeight(unsigned int h1)
{
h=h1;
}
void style::setWidth(unsigned int w1)
{
w=w1;
}
void style::setTop(unsigned int t1)
{
t=t1;
}
void style::setLeft(unsigned int l1)
{
l=l1;
}
unsigned int style::height()
{
return h;
}
unsigned int style::width()
{
return w;
}
unsigned int style::top()
{
return t;
}
unsigned int style::left()
{
return l;
}
//-------------------------------------------//
class element: public style
{
char s;
public:
element *link; //for q
generalC general; //name and support
metaC meta; //id & href
opC op; //rank & child
contentC content;
void setStyled(char);
void displayProp();
unsigned int isStyled();
//element();
};
void element::setStyled(char s1)
{
s=s1;
}
unsigned int element::isStyled()
{
unsigned int flag=0;
if(s=='y'||s=='Y')
{
flag=1;
}
return flag;
}
void element::displayProp()
{
cout<<"---------------------------------\n"
<<"PROPERTIES - "<<general.name()<<endl
<<"---------------------------------\n"
<<"Pointer: "<<link<<endl
<<"Name: "<<general.name()<<endl
<<"Support: "<<(general.isSupported())//?'Y':'N'<<endl
<<"ID: "<<meta.id()<<endl
<<"href: "<<meta.href()<<endl
<<"Style: "<<(isStyled())//?'Y':'N'<<endl
<<"---------------------------------\n"
<<"\tHeight: "<<height()<<endl
<<"\tWidth: "<<width()<<endl
<<"\tTop: "<<top()<<endl
<<"\tLeft: "<<left()<<endl
<<"\tColor: #"<<color.hexValue()<<endl
<<"\tBackground Color: #"<<background.hexValue()<<endl
<<"\tFont Face: "<<font.face()<<endl
<<"\tFont Size: "<<font.size()<<endl
<<"\tFont Color: #"<<font.color.hexValue()<<endl
<<"\tBorder Size: "<<border.size()<<endl
<<"\tBorder Color: #"<<border.color.hexValue()<<endl
<<"---------------------------------\n";
}
//-------------------------------------------//
class queueC
{
element *Front, *Rear;
public:
element* insert();
void removeLast();
unsigned int count();
element* process();
void test();
queueC();
~queueC();
};
element* queueC::insert()
{
element *T=new element;
//Read element properties into T
T->link=NULL;
if(Rear==NULL)
{
Front=T;
Rear=T;
}
else
{
Rear->link=T;
Rear=T;
}
return Rear;
}
void queueC::removeLast()
{
if(Front!=NULL)
{
element *T=Front;
Front=Front->link;
delete T;
if(Front==NULL) Rear=NULL;
}
}
unsigned int queueC::count()
{
unsigned int ctr;
element *T=Front;
while(T!=NULL)
{
ctr++;
T=T->link;
}
return ctr;
}
element* queueC::process()
{
element* tmp;
if(Front!=NULL)
{
element *T=Front;
Front=Front->link;
tmp=T;
//Process element
delete T;
if(Front==NULL) Rear=NULL;
}
return tmp;
}
queueC::queueC()
{
Front=NULL;
Rear=NULL;
}
queueC::~queueC()
{
while(Front!=NULL)
{
element *T=Front;
Front=Front->link;
delete T;
}
}
void queueC::test()
{
element *T=Front;
while(T!=NULL)
{
T->displayProp();
T=T->link;
}
}
//End- Elements
//Begin- Parser
char statusMessage[180];
void setGeneral(element*, char []); //correct
unsigned int isSupported(char []); //correct
void readContent(fstream &, element*); //correct
char* getToken(fstream &, char, unsigned int); //correct
void parse(char [], queueC &); //correct
void readElement(fstream &, element*); //correct
void readStyle(fstream &, element*); //correct
unsigned int isSupported(char name[])
{
unsigned int flag=0,i;
char list[15][80]={"html","head","body","title","br","h1","h2","h3","h4","h5","h6","span","p","a","div"};
for(i=0;i<3;i++)
{
if(!strcmpi(name,list[i])) flag=1; //Not Supported. Unstyled.
}
for(i=3;i<5;i++)
{
if(!strcmpi(name,list[i])) flag=2; //Supported. Unstyled.
}
for(i=5;i<15;i++)
{
if(!strcmpi(name,list[i])) flag=3; //Supported. Styled.
}
return flag;
}
void setGeneral(element* T, char tmp[])
{
T->general.setName(tmp);
switch(isSupported(tmp))
{
case 1:
T->general.setSupported('N');
T->setStyled('N');
break;
case 2:
T->general.setSupported('Y');
T->setStyled('N');
break;
case 3:
T->general.setSupported('Y');
T->setStyled('Y');
break;
default:
break;
}
}
void readContent(fstream &file, element* T)
{
char c='\0';
while(c!='<')
{
file.get(c);
if(c!='<')
T->content.insertChar(c);
else
file.seekg(-sizeof(c));
}
}
char* getToken(fstream &file, char c1, unsigned int s=0)
{
char tmp[100];
char c;
int i=-1;
for(int ctr=1;ctr<=s;ctr++) file.seekg(sizeof(c));
while(c!=c1&&c!='>')
{
file.get(c);
if(c!=c1&&c!='>')
{
++i;
tmp[i]=c;
}
else if(c=='>')
file.seekg(-sizeof(c));
}
tmp[i]='\0';
return tmp;
}
void readElement(fstream &file, element* T)
{
if(T==NULL)
{
return;
}
char tmp[100]; //read token
char c='\0'; //read char
strcpy(tmp,getToken(file,' ')); //read name
setGeneral(T,tmp); //read gen prop
while(c!='>')
{
file.get(c);
if(c=='>')
{
file.seekg(-sizeof(c));
return;
}
strcpy(tmp,getToken(file,' '));
if(strcmpi(tmp,"id=\"")==0)
{
T->meta.setId(getToken(file,'\"'));
}
else if(strcmpi(tmp,"href=\"")==0)
{
T->meta.setHref(getToken(file,'\"'));
}
else if(strcmpi(tmp,"style=\"")==0)
{
readStyle(file,T);
}
}
}
void readStyle(fstream &file, element* T)
{
int a;
char tmp[100];
while(c!='\"')
{
file.get(c);
if(c=='\"')
{
return;
//file.seekg(-sizeof(c));
}
strcpy(tmp,getToken(file,':'));
if(strcmpi(tmp,"height")==0)
{
a=atoi(getToken(file,';'));
T->setHeight(a);
}
else if(strcmpi(tmp,"width")==0)
{
a=atoi(getToken(file,';'));
T->setWidth(a);
}
else if(strcmpi(tmp,"top")==0)
{
a=atoi(getToken(file,';'));
T->setTop(a);
}
else if(strcmpi(tmp,"left")==0)
{
a=atoi(getToken(file,';'));
T->setLeft(a);
}
else if(strcmpi(tmp,"color")==0)
{
T->color.setColor(getToken(file,';',1));
}
else if(strcmpi(tmp,"background")==0)
{
T->background.setColor(getToken(file,';',1));
}
else if(strcmpi(tmp,"border-color")==0)
{
T->border.setBorderColor(getToken(file,';',1));
}
else if(strcmpi(tmp,"border-width")==0)
{
a=atoi(getToken(file,';'));
T->border.setBorderWidth(a);
}
else if(strcmpi(tmp,"font-size")==0)
{
a=atoi(getToken(file,';'));
T->font.setFontSize(a);
}
else if(strcmpi(tmp,"font-face")==0)
{
T->font.setFontFace(getToken(file,';'));
}
else if(strcmpi(tmp,"font-color")==0)
{
T->font.setFontColor(getToken(file,';',1));
}
}
}
void parse(char filename[], queueC &q)
{
//<types>
int flag=-1, rank=0; //counters
char c; //char read
char tmp[100]; //store tokens
element *T=NULL; //element pointer
fstream file; //html file
//</types>
//<fopen>
int i=0,j=0;
char ext[4]; //Read File Extension
while(filename[i]!='.')++i;
++i;
while(filename[i]!='\0'&&j<4)
{
ext[j]=tmp[i];
j++;
i++;
}
if(strcmpi(ext,"html")!=0||strcmpi(ext,"htm")!=0)
{
//cout<<"problem\n";
strcpy(statusMessage,"Invalid File");
return;
}
file.open(filename,ios::in);
if(file.good()==0) //To check
{
//cout<<"problem\n";
strcpy(statusMessage,"Invalid File");
return;
}
//</fopen>
while(!file.eof())
{
file.get(c);
switch(c)
{
case '<':
file.get(c);
if(c=='/')
{
flag=0;
getToken(file,'>');
break;
}
else
{
file.seekg(-sizeof(c));
flag=1;
rank++;
T=q.insert();
readElement(file,T);
T->op.incRank(rank-(T->op.rank()));
}
break;
case '>':
if(flag==1&&T!=NULL)
{
readContent(file,T);
}
else if(flag==0)
{
rank--;
}
break;
default:
file.get(c);
break;
}
}
}
//End- Parser
//Begin- Printer
unsigned int xmax,ymax;
void displayChrome();
void welcome();
void farewell();
void splashScreen();
void printer(queueC q);
void splashScreen()
{
clrscr();
cout<<"About:SPUTNIK!\n";
delay(5000);
clrscr();
gotoxy(11,12);
cout<<"SPUTNIK 0.0.1b\n";
delay(5000);
clrscr();
}
void welcome()
{
setcolor(1);
setbkcolor(11);
setfillstyle(0,1);
clearviewport();
rectangle(10,10,630,470);
settextstyle(0,0,3);
outtextxy(140,360,"Please fasten your seatbelts!");
outtextxy(140,360,"SPUTNIK 0.0.1b");
settextstyle(0,0,1);
outtextxy(80, 450, "Developers: <NAME>, <NAME>, <NAME>");
}
void farewell()
{
setcolor(1);
setbkcolor(11);
setfillstyle(0,1);
clearviewport();
rectangle(10,10,630,470);
settextstyle(0,0,3);
outtextxy(140,360,"Mind the parachute!");
outtextxy(140,360,"SPUTNIK 0.0.1b");
settextstyle(0,0,1);
outtextxy(80, 450, "Developers: <NAME>, <NAME>, <NAME>");
}
void displayChrome()
{
setcolor(1);
setbkcolor(11);
setfillstyle(0,1);
clearviewport();
rectangle(10,10,630,470);
rectangle(60,280,120,310);
rectangle(520,280,580,310);
outtextxy(140,360,"Ship's on sail, Cap'n!");
}
//End- Printer
//Begin- Main
void main()
{
clrscr();
/*
TESTS
colorC c("ffffff");
cout<<c.red()<<c.blue()<<c.green();
contentC content;
content.insertChar('a');
content.reset();
charch=content.readChar();
cout<<ch<<endl;
queueC queue;
parse("sample.htm",queue);
queue.test();
circle(330,180,100);
rectangle (10,30,500,400);
ellipse(100,150,0,360,100,50);
arc(120,160,300,90,70);
line(100,50,100,400);
getch();
*/
splashScreen();
int gd=DETECT, gm, exit=0, mx, my, click;
initgraph(&gd, &gm, "c:/tc/bgi ");
welcome();
displayChrome();
mouseshow();
do
{
mouseposi(mx,my,click);
if(click==1)
{
delay(500);
if(((mx>520)&&(mx<580))&&((my>280)&&(my<310)))
{
exit=1;
}
//if(((mx>60)&&(mx<120))&&((my>280)&&(my<310)))
//{
//Do something!
//}
}
}
while(exit==0);
farewell();
closegraph();
restorecrtmode();
getch();
}
//End- Main<file_sep># CPP makes sad
**Turbo C++** is to blame here. _(It's what they asked me to use at school. Sigh.)_
* * *
## Projects
### Semyorka
* HTML Parser written in Turbo C++
* Semyorka was the name of the rocket that carried **Sputnik** to space
* It won me an award though. :P
### Sputnik
* The C++ based browser I had hoped to write atop **Semyorka**.
* You don't see it here because I gave up after struggling with inline css.
### Helicopter
* The Pointless command line C++ game.
* **Statutory Warning** : Highly addictive. You may also experience withdrawal symptoms.
### Circle
* My first serious attempt at writing a C++ program.
* Draws some circles on the screen.
* It appears here for emotional reasons. Please bear with me. Sob.
* * *
## Thanks
<NAME>, <NAME> and <NAME> for working with me on Semyorka.
Mr. <NAME> for his help and debugging skills.
Ditto for Mr. <NAME>.
You can write to me at: *<EMAIL>*
* * *
## License
Copyright (c) 2012 <NAME>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
"Software"), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
| 6e33a142af6278b57c4a5b0898fb6d933da29546 | [
"Markdown",
"C++"
] | 4 | C++ | creatorrr/CPP-is-Sad | bea2a54c3456f6831b256bda9f0edf0315d93686 | 10a578fb327444580a5f54e793c3edd5a436a557 | |
refs/heads/master | <repo_name>moak/dumbo<file_sep>/app/www/js/services/tournamentQueue.js
angular.module('starter')
.factory('TournamentQueue', function($http, $q, $log) {
var httpOptions = function () {
return {headers: {'Content-Type': 'application/json'}};
};
var TournamentQueue = function() {
};
TournamentQueue.prototype.fetch = function() {
var deferred = $q.defer();
$http.get('http://localhost:8080/api/tournaments/fetch')
.success(function(data) {
console.log(data);
deferred.resolve(data);
})
.error(function(err, code) {
deferred.reject(err);
$log.error(err, code);
});
return deferred.promise;
};
return TournamentQueue;
});
<file_sep>/app/www/js/controllers/profileCtrl.js
angular.module('starter.profileCtrl', [])
.controller('ProfileCtrl', function($scope, $ionicModal, $timeout, ngFB ) {
ngFB.api({
path: '/me',
params: {fields: 'id,name'}
}).then(
function (user) {
$scope.user = user;
},
function (error) {
alert('Facebook error: ' + error.error_description);
});
});
<file_sep>/app/www/js/services/Auth.js
angular.module('starter')
.factory('Auth', function($http, $q, $log, $window) {
return {
isAuth : function() {
return $window.localStorage['token'] !== undefined;
},
getToken : function() {
return $window.localStorage['token'];
},
logout : function() {
return $window.localStorage['token'] = undefined;
},
getUser : function() {
var user = {
id : $window.localStorage['id'],
token : $window.localStorage['token'],
name : $window.localStorage['name']
};
return user;
}
};
});
<file_sep>/app/www/js/controllers/loginFBCtrl.js
angular.module('starter.loginFBCtrl', [])
.controller('LoginFBCtrl', function($scope, $ionicModal, $timeout, ngFB, $window , $location, $state, $ionicHistory, ngFB, Player ) {
$ionicHistory.nextViewOptions({
disableBack: true
});
$scope.fbLogin = function(){
ngFB.login({scope: 'email,publish_actions'}).then(
function (response) {
if (response.status === 'connected') {
ngFB.api({
path: '/me',
params: {fields: 'id,name'}
}).then(function (user) {
var player = new Player(user.id, response.authResponse.accessToken, user.name );
player.save();
$state.go('app.tournaments')
},
function (error) {
alert('Facebook error: ' + error.error_description);
});
} else {
alert('Facebook login failed');
}
});
};
});
<file_sep>/app/www/js/services/tournament.js
angular.module('starter')
.factory('Tournament', function($http, $q, $log) {
var Tournament = function(name) {
this.name = name;
};
var httpOptions = function () {
return {headers: {'Content-Type': 'application/json'}};
};
Tournament.prototype.create = function(name) {
var deferred = $q.defer();
var body = {
name : this.name
};
$http.post('http://localhost:8080/api/tournament/create', body, httpOptions())
.success(function(data) {
deferred.resolve(data);
})
.error(function(err, code) {
deferred.reject(err);
$log.error(err, code);
});
return deferred.promise;
};
Tournament.prototype.fetch = function(id) {
var deferred = $q.defer();
var url = 'http://localhost:8080/api/tournaments/' + id + '/fetch';
$http.get(url)
.success(function(data) {
deferred.resolve(data);
})
.error(function(err, code) {
deferred.reject(err);
$log.error(err, code);
});
return deferred.promise;
};
return Tournament;
});
<file_sep>/app/www/js/services/player.js
angular.module('starter')
.factory('Player', function($http, $q, $log, $window) {
var Player = function(id,token,name) {
this.id = id;
this.token = token;
this.name = name;
};
var httpOptions = function () {
return {headers: {'Content-Type': 'application/json'}};
};
Player.prototype.save = function() {
$window.localStorage['id'] = this.id;
$window.localStorage['token'] = this.token;
$window.localStorage['name'] = this.name;
};
Player.prototype.getPlayer = function() {
return new Player(this.id, this.token, this.name);
};
Player.prototype.register = function(idTournament) {
console.log(this);
var deferred = $q.defer();
var body = {
name : this.name,
token : this.token,
id : this.id
};
console.log(body);
$http.post('http://localhost:8080/api/tournaments/' + idTournament + '/register', body, httpOptions())
.success(function(data) {
deferred.resolve(data);
})
.error(function(err, code) {
console.log(err);
deferred.reject(err);
$log.error(err, code);
});
return deferred.promise;
};
return Player;
});
<file_sep>/app/www/js/controllers/createCtrl.js
angular.module('starter.createCtrl', [])
.controller('CreateCtrl', function($state , $scope, $ionicModal, $timeout, Tournament, $ionicHistory) {
$ionicHistory.nextViewOptions({
disableBack: true
});
$scope.create = function(name) {
var t = new Tournament(name);
t.create(name).then(function(res){
$state.go('app.tournaments');
});
};
});
<file_sep>/app/www/js/controllers/tournamentCtrl.js
angular.module('starter.tournamentCtrl', [])
.controller('TournamentCtrl', function($scope, $ionicModal, $timeout, Tournament, Player, $stateParams, Auth ) {
var t = new Tournament();
t.fetch($stateParams.id).then(function(result){
console.log(result);
$scope.tournament = result;
});
$scope.register = function() {
var player = Auth.getUser();
var p = new Player(player.id, player.token, player.name);
p.register($stateParams.id).then(function(res){
console.log(res);
});
};
});
<file_sep>/api/server.js
var express = require('express'); // call express
var app = express(); // define our app using express
var bodyParser = require('body-parser');
var mongoose = require('mongoose');
var Tournament = require("./models/tournament");
// configure app to use bodyParser()
// this will let us get the data from a POST
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
app.all('*', function(req, res, next) {
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "X-Requested-With");
res.header('Access-Control-Allow-Headers', 'Content-Type');
next();
});
var port = process.env.PORT || 8080; // set our port
// ROUTES FOR OUR API
// =============================================================================
var router = express.Router(); // get an instance of the express Router
// test route to make sure everything is working (accessed at GET http://localhost:8080/api)
router.get('/', function(req, res) {
res.json({ message: 'hooray! welcome to our api!' });
});
// create a tournament
router.post('/tournament/create', function(req, res) {
var name = req.body.name;
if(!name) {
return res.status(404).send("Name missing");
}
Tournament.count({name : name}, function (err, count){
if( count > 0 ){
return res.status(404).send("Tournament exsists");
}
else {
var t = new Tournament({name: name});
t.save(function (err) {
if (err) {
return err;
}
else {
return res.json(t);
}
});
}
});
});
router.post('/tournaments/:id/register', function(req,res){
var _id = mongoose.Types.ObjectId.fromString(req.params.id);
var query = {"_id": _id};
var options = {upsert: true};
var player = { id : req.body.id, token : req.body.token, name : req.body.name };
console.log(player);
Tournament.findOneAndUpdate(req.params.id, {$push: { players: player }}, options, function(err, tournamentsDB) {
console.log(err);
console.log(tournamentsDB);
if (err) {
console.log('got an error');
return res.status(404).send(err);
}
if (!tournamentsDB) {
return res.status(404).send("Tournament doest not exsist");
}
else {
return res.json(tournamentsDB);
}
});
});
router.get('/tournaments/:id/fetch', function(req,res){
var _id = mongoose.Types.ObjectId.fromString(req.params.id);
Tournament.findById(_id, function(err,tournamentsDB) {
if (!tournamentsDB)
return res.status(404).send("Tournament doest not exsist");
else {
return res.json(tournamentsDB);
}
});
});
router.get('/tournaments/fetch', function(req,res){
Tournament.find({}, function(err,tournamentsDB) {
if (!tournamentsDB)
return res.status(404).send("Tournament doest not exsist");
else {
return res.json(tournamentsDB);
}
});
});
router.get('/tournaments', function(req,res){
Tournament.find().all(function(tournaments) {
res.json(tournaments);
});
});
// more routes for our API will happen here
// REGISTER OUR ROUTES -------------------------------
// all of our routes will be prefixed with /api
app.use('/api', router);
// START THE SERVER
// =============================================================================
app.listen(port);
console.log('Magic happens on port ' + port);
<file_sep>/api/models/tournament.js
var mongoose = require("mongoose");
//connect to database
var db = mongoose.connect('mongodb://localhost/dumbo');
var playerSchema = new mongoose.Schema({
name: String,
date: { type: Date, default: Date.now },
});
//create schema for blog post
var tournamentSchema = new mongoose.Schema({
name: String,
author: String,
//players: [playerSchema],
players : [],
created: { type: Date, default: Date.now }
});
//compile schema to model
module.exports = db.model('tournament', tournamentSchema);
| e9174170726dfa024ec3a0444a7d2710528b443f | [
"JavaScript"
] | 10 | JavaScript | moak/dumbo | 7426964f74f385137b8fb6f84a9b0feb53a73120 | 7580e557a87d80e6f46f050df262ceb06b13a89f | |
refs/heads/master | <repo_name>k-dominik/ilastik-publish-packages<file_sep>/FLYEM_README.md
FlyEM Publish Packages
======================
For most of the packages FlyEM depends on, developers should obtain them via the [conda-forge][2] project.
But some of the packages we use are not hosted on conda-forge.
These fall into three categories:
1. Packages which we (FlyEM) are actively developing
2. Packages which we are not the original authors of, but which conda-forge does not currently host
3. Packages which conda-forge hosts, but with a version or build configuration we can't use.
In those cases, we must build the packages ourselves, and host them on [our own channel ("flyem-forge")][3] in the Anaconda cloud.
This repo contains a script to fetch and build such packages. Before building each one, the script checks to see if it has already been built and uploaded to our channel, in which case the build can be skipped. For more details, see the docstrings in `build-recipes.py`.
Usage
-----
```bash
cd flyem-publish-packages
# Activate the root conda environment. Must be Python 3.6
source activate root
# Make sure you have a very recent version of conda-build
conda install --override-channels -c defaults conda-build
# Build/upload all FlyEM dependencies
./build-recipes.py flyem-recipe-specs.yaml
```
To add a new package, simply add a new item to `flyem-recipe-specs.yaml`.
[1]: https://github.com/ilastik/ilastik-publish-packages
[2]: https://conda-forge.org
[3]: https://anaconda.org/flyem-forge
<file_sep>/build-recipes.py
#!/usr/bin/env python
# PYTHON_ARGCOMPLETE_OK
import os
from os.path import basename, splitext, abspath, exists, dirname, normpath
from pathlib import Path
import sys
import argparse
import subprocess
import platform
import json
import tempfile
import yaml
import textwrap
import datetime
try:
import argcomplete
from argcomplete.completers import FilesCompleter
ENABLE_TAB_COMPLETION = True
except:
# See --help text for instructions.
ENABLE_TAB_COMPLETION = False
# See _init_globals(), below
CONDA_PLATFORM = None
PLATFORM_STR = None
BUILD_PKG_DIR = None
# Disable git pager for log messages, etc.
os.environ['GIT_PAGER'] = ''
def parse_cmdline_args():
"""
Parse the user's command-lines, with support for tab-completion.
"""
bashrc = '~/.bashrc'
if os.path.exists(os.path.expanduser('~/.bash_profile')):
bashrc = '~/.bash_profile'
prog_name = sys.argv[0]
if prog_name[0] not in ('.', '/'):
prog_name = './' + prog_name
help_epilog = textwrap.dedent(f"""\
--------------------------------------------------------------------
To activate command-line tab-completion, run the following commands:
conda install argcomplete
echo 'eval "$(register-python-argcomplete {prog_name} -s bash)"' >> {bashrc}
...and run this script directly as "{prog_name}", not "python {prog_name}"
--------------------------------------------------------------------
""")
parser = argparse.ArgumentParser(
epilog=help_epilog, formatter_class=argparse.RawDescriptionHelpFormatter)
parser.add_argument('-l', '--list', action='store_true',
help='List the recipe names in the specs file')
specs_path_arg = parser.add_argument(
'recipe_specs_path', help='Path to a recipe specs YAML file')
selection_arg = parser.add_argument(
'selected_recipes', nargs='*', help='Which recipes to process (Default: process all)')
parser.add_argument(
'--start-from', default='', help='Recipe name to start from building recipe specs in YAML file.')
if ENABLE_TAB_COMPLETION:
def complete_recipe_selection(prefix, action, parser, parsed_args):
specs_file_contents = yaml.load(
open(parsed_args.recipe_specs_path, 'r'))
recipe_specs = specs_file_contents["recipe-specs"]
names = (spec['name'] for spec in recipe_specs)
return filter(lambda name: name.startswith(prefix), names)
specs_path_arg.completer = FilesCompleter(
('.yml', '.yaml'), directories=False)
selection_arg.completer = complete_recipe_selection
argcomplete.autocomplete(parser)
args = parser.parse_args()
return args
def main():
start_time = datetime.datetime.now()
args = parse_cmdline_args()
_init_globals()
specs_file_contents = yaml.load(open(args.recipe_specs_path, 'r'))
# Read the 'shared-config' section
shared_config = specs_file_contents["shared-config"]
expected_shared_config_keys = [
'pinned-versions', 'source-channels', 'destination-channel', 'repo-cache-dir']
assert set(shared_config.keys()) == set(expected_shared_config_keys), \
f"shared-config section is missing expected keys or has too many. Expected: {expected_shared_config_keys}"
# make path to config file absolute:
pin_specs_path = os.path.abspath(shared_config['pinned-versions'])
# Convenience member
shared_config['source-channel-string'] = ' '.join(
[f'-c {ch}' for ch in shared_config['source-channels']])
# Overwrite repo-cache-dir with an absolute path
# Path is given relative to the specs file directory.
if not shared_config['repo-cache-dir'].startswith('/'):
specs_dir = Path(dirname(abspath(args.recipe_specs_path)))
shared_config['repo-cache-dir'] = Path(
normpath(specs_dir / shared_config['repo-cache-dir']))
os.makedirs(shared_config['repo-cache-dir'], exist_ok=True)
selected_recipe_specs = get_selected_specs(
args,
specs_file_contents["recipe-specs"])
if args.list:
print_recipe_list(selected_recipe_specs)
sys.exit(0)
result = {
'found': [],
'built': [],
'start_time': start_time.isoformat(timespec='seconds'),
'args': vars(args)
}
script_path = os.path.abspath(os.path.split(__file__)[0])
result_file = os.path.join(script_path, f"{start_time.strftime('%Y%m%d-%H%M%S')}_build_out.yaml")
for spec in selected_recipe_specs:
status = build_and_upload_recipe(spec, shared_config, pin_specs_path)
for k, v in status.items():
result[k].append(v)
with open(result_file, 'w') as f:
yaml.dump(result, f, default_flow_style=False)
end_time = datetime.datetime.now()
result['end_time'] = end_time.isoformat(timespec='seconds')
result['duration'] = str(end_time - start_time)
with open(result_file, 'w') as f:
yaml.dump(result, f, default_flow_style=False)
print("--------")
print(f"DONE, Result written to {result_file}")
print("--------")
print("Summary:")
print(yaml.dump(result, default_flow_style=False))
def _init_globals():
"""
We initialize globals in this function, AFTER argcomplete is used.
(Using subprocess interferes with argcomplete.)
"""
global CONDA_PLATFORM
global BUILD_PKG_DIR
global PLATFORM_STR
CONDA_PLATFORM = {'Darwin': 'osx-64',
'Linux': 'linux-64',
'Windows': 'win-64'}[platform.system()]
PLATFORM_STR = CONDA_PLATFORM.replace('-64', '')
# There's probably some proper way to obtain BUILD_PKG_DIR
# via the conda.config python API, but I can't figure it out.
CONDA_ROOT = Path(subprocess.check_output(
'conda info --root', shell=True).rstrip().decode())
BUILD_PKG_DIR = CONDA_ROOT / 'conda-bld'
def print_recipe_list(recipe_specs):
max_name = max(len(spec['name']) for spec in recipe_specs)
for spec in recipe_specs:
print(
f"{spec['name']: <{max_name}} : {spec['recipe-repo']} ({spec['tag']})")
def get_selected_specs(args, full_recipe_specs):
"""
If the user gave a list of specific recipes to process,
select them from the given recipe specs.
Args:
start_from (str): Name of the recipe from from full_recipe_specs to
start from. If not '', then the recipe should be in full_recipe_specs.
selected_recipes (list): List of recipe names to build
full_recipe_specs (list): List of recipes, usually loaded from yaml
Returns:
list: list of recipes to build
"""
available_recipe_names = [spec['name'] for spec in full_recipe_specs]
if args.start_from != '':
if args.start_from not in available_recipe_names:
sys.exit(
f"'start-from' parameter invalid: {args.start_from} not found in full_recipe_specs."
)
full_recipe_specs = full_recipe_specs[available_recipe_names.index(args.start_from)::]
if not args.selected_recipes:
return full_recipe_specs
invalid_names = set(args.selected_recipes) - set(available_recipe_names)
if invalid_names:
sys.exit("Invalid selection: The following recipes are not listed"
f" in {args.recipe_specs_path}: {', '.join(invalid_names)}")
# Remove non-selected recipes
filtered_specs = list(
filter(lambda spec: spec['name'] in args.selected_recipes, full_recipe_specs))
filtered_names = [spec['name'] for spec in filtered_specs]
if filtered_names != args.selected_recipes:
print(
f"WARNING: Your recipe list was not given in the same order as in {args.recipe_specs_path}.")
print(
f" They will be processed in the following order: {', '.join(filtered_names)}")
return filtered_specs
def build_and_upload_recipe(recipe_spec, shared_config, variant_config):
"""
Given a recipe-spec dictionary, build and upload the recipe if
it doesn't already exist on ilastik-forge.
More specifically:
1. Clone the recipe repo to our cache directory (if necessary)
2. Check out the tag (with submodules, if any)
3. Render the recipe's meta.yaml ('conda render')
4. Query the 'ilastik-forge' channel for the exact package.
5. If the package doesn't exist on ilastik-forge channel yet, build it and upload it.
A recipe-spec is a dict with the following keys:
- name -- The package name
- recipe-repo -- A URL to the git repo that contains the package recipe.
- recipe-subdir -- The name of the recipe directory within the git repo
- tag -- Which tag/branch/commit of the recipe-repo to use.
- environment (optional) -- Extra environment variables to define before building the recipe
- conda-build-flags (optional) -- Extra arguments to pass to conda build for this package
- build-on (optional) -- A list of operating systems on which the package should be built. Available are: osx, win, linux
"""
# Extract spec fields
package_name = recipe_spec['name']
recipe_repo = recipe_spec['recipe-repo']
tag = recipe_spec['tag']
recipe_subdir = recipe_spec['recipe-subdir']
conda_build_flags = recipe_spec.get('conda-build-flags', '')
print("-------------------------------------------")
print(f"Processing {package_name}")
# check whether we need to build the package on this OS at all
if 'build-on' in recipe_spec:
platforms_to_build_on = recipe_spec['build-on']
assert isinstance(platforms_to_build_on, list)
assert(all(o in ['win', 'osx', 'linux']
for o in platforms_to_build_on))
else:
platforms_to_build_on = ['win', 'osx', 'linux']
if PLATFORM_STR not in platforms_to_build_on:
print(
f"Not building {package_name} on platform {PLATFORM_STR}, only builds on {platforms_to_build_on}")
return {}
# configure build environment
build_environment = dict(**os.environ)
if 'environment' in recipe_spec:
for key in recipe_spec['environment'].keys():
recipe_spec['environment'][key] = str(
recipe_spec['environment'][key])
build_environment.update(recipe_spec['environment'])
os.chdir(shared_config['repo-cache-dir'])
repo_dir = checkout_recipe_repo(recipe_repo, tag)
# All subsequent work takes place within the recipe repo
os.chdir(repo_dir)
# Render
recipe_version, recipe_build_string = get_rendered_version(
package_name, recipe_subdir, build_environment, shared_config, variant_config)
print(
f"Recipe version is: {package_name}-{recipe_version}-{recipe_build_string}")
# Check our channel. Did we already upload this version?
package_info = {
'pakage_name': package_name,
'recipe_version': recipe_version,
'recipe_build_string': recipe_build_string
}
if check_already_exists(package_name, recipe_version, recipe_build_string, shared_config):
print(
f"Found {package_name}-{recipe_version}-{recipe_build_string} on {shared_config['destination-channel']}, skipping build.")
ret_dict = {'found': package_info}
else:
# Not on our channel. Build and upload.
build_recipe(package_name, recipe_subdir, conda_build_flags,
build_environment, shared_config, variant_config)
upload_package(package_name, recipe_version,
recipe_build_string, shared_config)
ret_dict = {'built': package_info}
return ret_dict
def checkout_recipe_repo(recipe_repo, tag):
"""
Checkout the given repository and tag.
Clone it first if necessary, and update any submodules it has.
"""
try:
repo_name = splitext(basename(recipe_repo))[0]
cwd = abspath(os.getcwd())
if not exists(repo_name):
# assuming url of the form github.com/remote-name/myrepo[.git]
remote_name = recipe_repo.split('/')[-2]
subprocess.check_call(
f"git clone -o {remote_name} {recipe_repo}", shell=True)
os.chdir(repo_name)
else:
# The repo is already cloned in the cache,
# but which remote do we want to fetch from?
os.chdir(repo_name)
remote_output = subprocess.check_output(
"git remote -v", shell=True).decode('utf-8').strip()
remotes = {}
for line in remote_output.split('\n'):
name, url, role = line.split()
remotes[url] = name
if recipe_repo in remotes:
remote_name = remotes[recipe_repo]
else:
# Repo existed locally, but was missing the desired remote.
# Add it.
remote_name = recipe_repo.split('/')[-2]
subprocess.check_call(
f"git remote add {remote_name} {recipe_repo}", shell=True)
subprocess.check_call(f"git fetch {remote_name}", shell=True)
print(f"Checking out {tag} of {repo_name} into {cwd}...")
subprocess.check_call(f"git checkout {tag}", shell=True)
subprocess.check_call(
f"git pull --ff-only {remote_name} {tag}", shell=True)
subprocess.check_call(
f"git submodule update --init --recursive", shell=True)
except subprocess.CalledProcessError:
raise RuntimeError(f"Failed to clone or update the repository: {recipe_repo}\n"
"Double-check the repo url, or delete your repo cache and try again.")
print(f"Recipe checked out at tag: {tag}")
print("Most recent commit:")
subprocess.call("git log -n1", shell=True)
os.chdir(cwd)
return repo_name
def get_rendered_version(package_name, recipe_subdir, build_environment, shared_config, variant_config):
"""
Use 'conda render' to process a recipe's meta.yaml (processes jinja templates and selectors).
Returns the version and build string from the rendered file.
Returns
tuple: recipe_version, recipe_build_string
"""
print(f"Rendering recipe in {recipe_subdir}...")
temp_meta_file = tempfile.NamedTemporaryFile(delete=False)
temp_meta_file.close()
render_cmd = (f"conda render"
f" -m {variant_config}"
f" {recipe_subdir}"
f" {shared_config['source-channel-string']}"
f" --file {temp_meta_file.name}")
print('\t' + render_cmd)
rendered_meta_text = subprocess.check_output(
render_cmd, env=build_environment, shell=True).decode()
meta = yaml.load(open(temp_meta_file.name, 'r'))
os.remove(temp_meta_file.name)
if meta['package']['name'] != package_name:
raise RuntimeError(
f"Recipe for package '{package_name}' has unexpected name: '{meta['package']['name']}'")
render_cmd += " --output"
rendered_filename = subprocess.check_output(
render_cmd, env=build_environment, shell=True).decode()
build_string_with_hash = rendered_filename.split('-')[-1].split('.tar.bz2')[0]
return meta['package']['version'], build_string_with_hash
def check_already_exists(package_name, recipe_version, recipe_build_string, shared_config):
"""
Check if the given package already exists on anaconda.org in the
ilastik-forge channel with the given version and build string.
"""
print(f"Searching channel: {shared_config['destination-channel']}")
search_cmd = f"conda search --json --full-name --override-channels --channel={shared_config['destination-channel']} {package_name}"
print('\t' + search_cmd)
try:
search_results_text = subprocess.check_output(
search_cmd, shell=True).decode()
except Exception:
# In certain scenarios, the search can crash.
# In such cases, the package wasn't there anyway, so return False
return False
search_results = json.loads(search_results_text)
if package_name not in search_results:
return False
for result in search_results[package_name]:
if result['build'] == recipe_build_string and result['version'] == recipe_version:
print("Found package!")
return True
return False
def build_recipe(package_name, recipe_subdir, build_flags, build_environment, shared_config, variant_config):
"""
Build the recipe.
"""
print(f"Building {package_name}")
build_cmd = (f"conda build {build_flags}"
f" -m {variant_config}"
f" {shared_config['source-channel-string']}"
f" {recipe_subdir}")
print('\t' + build_cmd)
try:
subprocess.check_call(build_cmd, env=build_environment, shell=True)
except subprocess.CalledProcessError as ex:
sys.exit(f"Failed to build package: {package_name}")
def upload_package(package_name, recipe_version, recipe_build_string, shared_config):
"""
Upload the package to the ilastik-forge channel.
"""
pkg_file_name = f"{package_name}-{recipe_version}-{recipe_build_string}.tar.bz2"
pkg_file_path = BUILD_PKG_DIR / CONDA_PLATFORM / pkg_file_name
if not os.path.exists(pkg_file_path):
# Maybe it's a noarch package?
pkg_file_path = BUILD_PKG_DIR / 'noarch' / pkg_file_name
if not os.path.exists(pkg_file_path):
raise RuntimeError(f"Can't find built package: {pkg_file_name}")
upload_cmd = f"anaconda upload -u {shared_config['destination-channel']} {pkg_file_path}"
print(f"Uploading {pkg_file_name}")
print(upload_cmd)
subprocess.check_call(upload_cmd, shell=True)
if __name__ == "__main__":
# import os
# from os.path import dirname
# os.chdir(dirname(__file__))
# sys.argv.append('recipe-specs.yaml')
sys.exit(main())
<file_sep>/README.md
# ilastik-publish-packages
Scripts to update our published conda packages when our recipes have changed.
By <NAME>, <NAME>, in 2017
## Introduction:
ilastik has quite a lot of dependencies, which we handle by using the _conda package manager_.
Conda can retrieve packages from different channels, and most of our dependencies we take from the `conda-forge` channel (see also https://conda-forge.org).
Some of ilastik's dependencies are developed by us, and for some external packages we require special builds or patches, hence we need to build those conda packages ourselves.
The build process is automated by the scripts in this repository.
## Prerequisites:
### Linux:
We build all Linux packages in the conda-forge docker image to be compatible, and compile with GCC 4.8.
To start an interactive session in this barebones linux, execute
```
docker run -i -t condaforge/linux-anvil
```
If you want to mount some local folders into the docker container, e.g. to install the commercial solvers there, append `-v localfolder:mountfolder` for every folder you want to make accessible.
Inside the docker container, you might want to install some necessities:
```sh
yum install vim
yum install java-1.8.0-openjdk-headless.x86_64 unzip # (java and unzip needed for CPLEX installer)
conda install cmake
conda install conda-build --override-channels -c defaults # to get the latest conda-build
```
### MacOS:
* Install XCode (freely available on the AppStore)
* Install the MacOS 10.9 SDK to build for older OS versions than your one from https://github.com/devernay/xcodelegacy by following their instructions.
* Install [Miniconda](https://repo.continuum.io/miniconda/Miniconda3-latest-MacOSX-x86_64.sh) if you don't have it yet.
* Install CMake, e.g. by `conda install cmake` or through `brew`.
* Install `conda-build` (otherwise `conda render` won't work): `conda install conda-build`
* Install the anaconda client for uploading packages to our `ilastik-forge` channel: `conda install anaconda-client` in your conda root environment
### Windows:
* Install the Visual Studio 2015 build tools (which contain the VC14 compiler) from http://landinghub.visualstudio.com/visual-cpp-build-tools
- select _custom_ installation and select the Win 10 SDK
* Install Miniconda Python 3 64-bit and let it add conda to the environment vars
- If you do not do this, the `activate` step below will fail. Then you'll have to add `C:\Users\YOURUSERNAME\Miniconda3\Scripts` to `PATH` via the control panel.
* Open a command prompt by executing: `C:\Program Files (x86)\Microsoft Visual C++ Build Tools\Visual C++ 2015 x64 Native Build Tools CMD`
* Install some prerequisites in conda's root environment:
```sh
activate root
conda install git cmake pyyaml conda-build anaconda-client m2-patch
```
### Solvers:
To build the tracking and multicut C++ packages with all features (listed in `ilastik-dependencies` at the bottom), you need to install the ILP solvers Gurobi and CPLEX on your development machine, and **adjust the paths to the solvers in the `ilastik-recipe-specs.yaml` file**:
* **CPLEX**
* Linux Docker Container:
* start a clean shell for cplex installation: env -i bash --noprofile --norc
* install cplex (e.g. `/opt/cplex`)
```
bash cplex_studio12.7.1.linux-x86-64.bin
```
* quit the clean shell (exit)
* macOS (requires CPLEX version 12.7 or newer, the ones before weren't built with clang):
```
bash cplex_studio12.7.1.osx.bin
```
* **Gurobi:**
* Extract/Install Gurobi on the machine
* If you do not have that yet, obtain a licence for the build machine (needs to be done for each new docker container!) and run `grbgetkey ...` as described on their website.
## Building packages:
Run `conda install anaconda-client`. You need to be logged in to your https://anaconda.org account by running `anaconda login`. Then you can build the packages by running the commands below:
```bash
git clone https://github.com/ilastik/ilastik-publish-packages
cd ilastik-publish-packages
source activate root # needs to have latest conda-build and anaconda, and being logged into an anaconda account
# on Linux and Windows:
python build-recipes.py ilastik-recipe-specs.yaml
# on Mac:
MACOSX_DEPLOYMENT_TARGET=10.9 python build-recipes.py ilastik-recipe-specs.yaml
```
The `build-recipes.py` script parses the packages from `ilastik-recipe-specs.yaml`, and for each package checks whether that version is already available on the `ilastik-forge` channel. If that is not the case, it will build the package and upload it to `ilastik-forge`. By default, the script **assumes you have both solvers** and wants to build all packages. If you do not have CPLEX or Gurobi, comment out the sections near the end that have `cplex` or `gurobi` in their name, as well as the `ilastik-dependencies` package as described below.
### Configuration:
If you want to change which packages are built, _e.g._ to build **without solvers** edit the ilastik-recipe-specs.yaml file. There, you can comment or change the sections specific to respective packages.
It is a YAML file with the following format:
```yaml
recipe-specs:
- name: PACKAGE_NAME
recipe-repo: PACKAGE_RECIPE_GIT_URL
tag: RECIPE_GIT_TAG_OR_BRANCH
recipe-subdir: RECIPE_DIR_IN_GIT_REPO
# Optional specs
environment:
MY_VAR: VALUE
conda-build-flags: STRING_THAT_WILL_BE_APPENDED_TO_CONDA_BUILD
# by default a package is built on all 3 platforms, you can restrict that by specifying the following
build-on:
- linux
- win
- osx
- name: NEXT_PACKAGE
...
```
| 665990de1fc017d2dce49e16b614d69029484372 | [
"Markdown",
"Python"
] | 3 | Markdown | k-dominik/ilastik-publish-packages | 30c464ace420096bddf220b4882a030cbfcd0e96 | 0a6c845634aff5f64c06a6d17b039c8cf2cac687 | |
refs/heads/master | <repo_name>whanata/pacman_no_ui<file_sep>/Pacman/Player.java
/**
* Player Class
*
* @author Whanata
* @version 20:41 25/03/2014
*/
public class Player
{
//Player's fields
private int x;
private int y;
private int collectedDots;
private boolean life;
//Constructor for objects of class Player
Player(int initialX,int initialY)
{
collectedDots = 0;
this.x = initialX;
this.y = initialY;
this.life = true;
}
public boolean alive()
{
return this.life;
}
public void died()
{
this.life = false;
}
//Player changing position
public void changePosition(int changeX,int changeY)
{
this.x += changeX;
this.y += changeY;
}
//Player's x position
public int getX()
{
return this.x;
}
//Player's y position
public int getY()
{
return this.y;
}
public String end()
{
if (this.life)
{
return "You win!";
}
else
{
return "You lose!";
}
}
//Increase collectedDots by 1
public void collectDot()
{
collectedDots++;
}
public boolean allDots()
{
return (collectedDots == 3);
}
public String dots()
{
int i;
String star = "";
for (i = 0; i < collectedDots; i++)
{
star += "*";
}
return star;
}
//Return to string
public String toString()
{
String dots = "Player[" + this.dots() + "](";
if (this.life)
{
return dots + this.x + "," + this.y + ")";
}
else
{
return dots + "-,-)";
}
}
}
<file_sep>/Pacman/Enemy.java
/**
* Write a description of class Enemy here.
*
* @author (your name)
* @version (a version number or a date)
*/
public class Enemy
{
private int x;
private int y;
private String direction;
Enemy(int initialX,int initialY)
{
x = initialX;
y = initialY;
direction = "neutral";
}
public int getX()
{
return x;
}
public int getY()
{
return y;
}
public void movement()
{
if (direction == "right")
{
this.x++;
}
else if (direction == "left")
{
this.x--;
}
else if (direction == "down")
{
this.y++;
}
else if (direction == "up")
{
this.y--;
}
}
public int distance(int player, int enemy)
{
return player - enemy;
}
public void decision(Player player)
{
int playerX = player.getX();
int playerY = player.getY();
int xDistance = distance(playerX,this.x);
int yDistance = distance(playerY,this.y);
boolean rightDistance = this.absDecision(xDistance - 1,xDistance);
boolean leftDistance = this.absDecision(xDistance + 1,xDistance);
boolean downDistance = this.absDecision(yDistance - 1,yDistance);
boolean upDistance = this.absDecision(yDistance + 1,yDistance);
if (direction == "right" && rightDistance || direction == "left" && leftDistance || direction == "down" && downDistance || direction == "up" && upDistance || direction == "neutral")
{
this.decisionMaking(xDistance,yDistance);
}
this.movement();
if (this.check(playerX,playerY))
{
player.died();
}
}
public boolean check(int playerX,int playerY)
{
return distance(playerX,this.x) == 0 && distance(playerY,this.y) == 0;
}
public boolean absDecision(int a,int b)
{
return Math.abs(a) > Math.abs(b);
}
public void decisionMaking(int xDistance,int yDistance)
{
if (this.absDecision(xDistance,yDistance))
{
if (xDistance > 0)
{
direction = "right";
}
else
{
direction = "left";
}
}
else if (this.absDecision(yDistance,xDistance))
{
if (yDistance > 0)
{
direction = "down";
}
else
{
direction = "up";
}
}
else
{
direction = "neutral";
}
}
public String toString()
{
return "Enemy(" + this.x + "," + this.y + ")";
}
}
<file_sep>/README.md
pacman_no_ui
============
<file_sep>/Pacman/Dot.java
/**
* Class Dot
*
* @author Whanata
* @version 20:40 25/03/2014
*/
public class Dot
{
// Dot's field
private int x;
private int y;
private boolean exists;
//Constructor for objects of Class Dot
Dot(int initialX,int initialY)
{
this.exists = true;
this.x = initialX;
this.y = initialY;
}
//The dot will disappear
public void disappear()
{
this.exists = false;
}
public void check(Player player)
{
if (player.getX() == this.x && player.getY() == this.y && this.getExists())
{
player.collectDot();
this.disappear();
}
}
//Dot's state
public boolean getExists()
{
return this.exists;
}
//Return to string
public String toString()
{
if (exists)
{
return "Dot(" + this.x + "," + this.y +")";
}
else
{
return "Dot(-,-)";
}
}
}
<file_sep>/Pacman/Game.java
import java.util.*;
/**
* Class Game
*
* @author Whanata
* @version 20:40 25/03/2014
*/
public class Game
{
//Game's fields
private Player player;
private Dot dot1;
private Dot dot2;
private Dot dot3;
private Exit exit;
private Enemy enemy;
private boolean advanced;
//Constructor for objects of class Game
Game(int xPosition,int yPosition)
{
// initialise instance variables
player = new Player(xPosition,yPosition);
dot1 = new Dot(1,1);
dot2 = new Dot(2,2);
dot3 = new Dot(3,3);
exit = new Exit(4,4);
enemy = new Enemy(5,5);
if (xPosition == 5)
{
this.advanced = true;
}
else
{
this.advanced = false;
}
}
public void advanceMode()
{
enemy.decision(player);
}
public void start()
{
do
{
System.out.println(this);
this.input();
if (this.advanced)
{
this.advanceMode();
}
}
while (exit.check(player) && player.alive());
System.out.println(this);
System.out.println(player.end());
}
public void input()
{
Scanner keyboard = new Scanner(System.in);
System.out.print("Move (l/r/u/d): ");
String movement = keyboard.nextLine();
switch (movement.charAt(0))
{
case 'l': move(-1,0);
break;
case 'r': move(1,0);
break;
case 'u': move(0,-1);
break;
case 'd': move(0,1);
break;
default: System.out.println("Invalid move");
break;
}
}
//Player moves around by adding dx and dy to original position, Checks if player is on dot
public void move(int dx,int dy)
{
player.changePosition(dx,dy);
dot1.check(player);
dot2.check(player);
dot3.check(player);
exit.check(player);
}
//Return to string
public String toString()
{
String normal = player + " " + dot1 + " " + dot2 + " " + dot3 + " " + exit;
if (this.advanced)
{
return normal + " " + enemy;
}
else
{
return normal;
}
}
}
| e9c97a9d8815040997f932a176199257b6416cf9 | [
"Markdown",
"Java"
] | 5 | Java | whanata/pacman_no_ui | a28c3ab209799cf9282c03cab9016a809e380cae | 3cf4fc6b0ba5847209f34e71fff0a7e8ef513c7f | |
refs/heads/master | <file_sep>var img;
function setup(){
img = loadImage('assets/IMG_9682.jpg')
createCanvas(windowWidth, windowHeight)
background(0,0,255)
}
function draw(){
image(img,0,0,windowWidth, windowHeight)
rect(40,30,60,60)
ellipse(700,100,70,70)
textAlign(CENTER);
textSize(40);
text("Camino", windowWidth/2, 300);
textSize(12);
text("Those who come to the Camino with a heavy heart leave with a lighter load. Don't cry because it's over, smile because it happened. As in any journey, it's not what you take with you, but what you leave behind. Listen to your heart and it will show you the way.", windowWidth/2, 400);
}
| 88a3e19a635a37d22e8359aaef92ae01a594bcd2 | [
"JavaScript"
] | 1 | JavaScript | sydneymcauliffe/CAMINOO | bfad3dfabc1319cbe4d3a74a32c691684331592e | 011e88222854d2de262f35f46a8a2a9c85b5efe1 | |
refs/heads/master | <repo_name>rasl/memirror<file_sep>/README.md
# memirror
create replicated directory in memory
<file_sep>/memirror.sh
#!/bin/sh
MOUNT_POINT=~/work/memory
SRC=~/work/b2bcenter
DST=~/work/memory
DST_FULL=~/work/memory/b2bcenter/
SIZE_IN_MB=4000
PROG=$0
PROG_DIR=$(dirname $0)
usage()
{
cat <<USAGE
This program replicate dir to memory partition
Usage
$PROG <cmd>
cmd:
enable, disable, start_auto_sync, stop_auto_sync, manual_sync
USAGE
}
enable()
{
# init disk &&
# sync from SRC to DST
$PROG_DIR/memindisk/mount-ram.sh $MOUNT_POINT $SIZE_IN_MB && \
rsync -ah --exclude ".fseventsd" --delete $SRC $DST
if [ $? -ne 0 ]; then
echo "disk not created and files does not synced"
exit $?
fi
echo "disk created"
echo "mount point $MOUNT_POINT"
echo "folders synced $SRC<->$DST"
}
disable()
{
# unmount
$PROG_DIR/memindisk/umount-ram.sh $MOUNT_POINT
if [ $? -ne 0 ]; then
echo "disk does not unmount"
exit $?
fi
echo "disk unmounted"
}
manual_sync()
{
rsync -ah --exclude ".fseventsd" --delete $DST_FULL $SRC
if [ $? -ne 0 ]; then
echo "folders not synced $DST_FULL<->$SRC"
exit $?
fi
echo "folders synced $DST_FULL<->$SRC"
}
start_auto_sync()
{
# todo
echo "start_auto_sync"
}
stop_auto_sync()
{
# todo
echo "stop_auto_sync"
}
if [ $# -eq 0 ]; then
usage
fi
for i in "$@"; do
case $i in
enable)
enable
shift
;;
disable)
disable
shift
;;
start_auto_sync)
start_auto_sync
shift
;;
stop_auto_sync)
stop_auto_sync
shift
;;
manual_sync)
manual_sync
shift
;;
*)
usage
exit 1
;;
esac
done
| bb659c8267e5648794dec1b01fe83b63aa0effd4 | [
"Markdown",
"Shell"
] | 2 | Markdown | rasl/memirror | 1cd06d173cc0ec859a764fca89c8e6bc4dd3a400 | d7f2b7fe70672098c1824a6900784903fbd0b2b6 | |
refs/heads/master | <repo_name>tenato/webservice-client-api<file_sep>/src/main/java/fr/foop/ws/tools/configurators/CxfClientBuilderConfigurator.java
package fr.foop.ws.tools.configurators;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Optional;
import com.google.common.base.Splitter;
import com.google.common.collect.Iterables;
import fr.foop.ws.CxfClientBuilder;
public interface CxfClientBuilderConfigurator {
public final static Logger LOGGER = LoggerFactory.getLogger(CxfClientBuilderConfigurator.class);
public static abstract class BasicBuilderConfigurator implements
CxfClientBuilderConfigurator {
@Override
public CxfClientBuilder configure(CxfClientBuilder configured,
Optional<String> propValue) {
LOGGER.debug("autoconfigure : {} -> {}", this.getClass().getName(), propValue.toString());
if (propValue.isPresent()) {
return ensurePresentConfigured(configured, propValue.get());
}
return configured;
}
protected abstract CxfClientBuilder ensurePresentConfigured(
final CxfClientBuilder configured, final String propValue);
}
public final static CxfClientBuilderConfigurator NOOP_CONFIGURATOR = new CxfClientBuilderConfigurator() {
@Override
public CxfClientBuilder configure(CxfClientBuilder configured,
Optional<String> propValue) {
return configured;
}
};
public final static CxfClientBuilderConfigurator ENDPOINT_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withEndpoint(propValue);
}
};
public final static CxfClientBuilderConfigurator INLOGGER_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withInLogger(propValue);
}
};
public final static CxfClientBuilderConfigurator OUTLOGGER_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withOutLogger(propValue);
}
};
public final static CxfClientBuilderConfigurator LOGGER_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withLogger(propValue);
}
};
public final static CxfClientBuilderConfigurator WSSE_USER_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withWsseUser(propValue);
}
};
public final static CxfClientBuilderConfigurator WSSE_PWD_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withWssePwd(propValue);
}
};
public final static CxfClientBuilderConfigurator CONNECTION_TIMEOUT_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withConnectionTimeout(Long.valueOf(propValue));
}
};
public final static CxfClientBuilderConfigurator RECEIVE_TIMEOUT_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withReceiveTimeout(Long.valueOf(propValue));
}
};
public final static CxfClientBuilderConfigurator MOCKED_PORT_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withMockedPort(propValue);
}
};
public final static CxfClientBuilderConfigurator USE_MOCK_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
final boolean useMock = Boolean.valueOf(propValue);
if(useMock) {
return configured.enableMocking();
}
else {
return configured.disableMocking();
}
}
};
public final static CxfClientBuilderConfigurator SERVERS_CONFIGURATOR = new BasicBuilderConfigurator() {
@Override
protected CxfClientBuilder ensurePresentConfigured(
CxfClientBuilder configured, String propValue) {
return configured.withServers(Iterables.toArray(Splitter.on(",")
.trimResults().omitEmptyStrings().split(propValue),
String.class));
}
};
public CxfClientBuilder configure(final CxfClientBuilder configured,
final Optional<String> propValue);
}
<file_sep>/README.md
# WebService Client API
An API to easily built webservice client, based on CXF.
## Quick start Guide
The easiest way is to clone the existing example project. Although you can start from scratch by creating a maven simple jar project.
+ Clone the example project :
`git clone https://github.com/cedricbou/webservice-client-example.git <<your project name here>>`
+ (Optional) Remove the .git folder to "ungit" the project :
`cd <<your project name here>>`
`rm -rf .git`
+ Open the pom.xml file :
1. Modify the groupId, artifactId, version with yours.
2. Change description and name to something describing the client you are creating.
3. Update the `<wsdl>` tag in the cxf plugin with the URL to your WSDL.
+ Clean and generate source
`mvn clean generate-sources`
+ The project will contain compilation error, we need to adapt the Client class :
1. Open the CRMClient class, rename it to whatever you need, and change the package as well to something you like.
2. Adapt the CxfClient extended class with generic types corresponding to the Port generated by CXF, and the service manager class generated by CXF :
`public class CRMClient extends CxfClient<_WebServicePort_, _WebServiceManager_>`
3. In the `newPort` method, calls the manager method to instanciate your port interface, the manager is passed in parameter.
4. In the `checkIfPortUp` method, implements a health check. If an exception is thrown the service is considered unhealthy.
## Configuration DSL
### Basic features
```java
builder()
.withWsseCredentials(username, password) // Configure username and password using the WSSE standard, as PasswordText.
.withConnectionTimeout(1000) // Set the timeout in millis on socket connection
.withReceiveTimeout(3000) // Set the timeout in millis to wait for data.
.withEndpoint("http://....") // Define the endpoint to use.
.withInLogger(logger) // The SLF4J logger to use for payload sent to the server, the level is INFO.
.withOutLogger(logger) // The SLF4J logger to use for payload received from the server, the level is INFO.
.withLogger(logger) // Shortcut for withInLogger(logger).withOutLogger(logger)
```
### Endpoint and servers
During service instanciation, the API endpoint failover. For an endpoint,
you can defined several target servers address.
When instanciating the service, for each server, it will attempt to configure a client. It uses the `checkIfPortUp` method to check if the server is available. If everything is fine, it will use this port, otherwise, it would try the next server. If nothing works, it returns `null`.
For this to work, you need to write your endpoint with the special `{{server}}` tag :
```java
builder()
.withEndpoint("http://{{server}}/mywebservice")
```
Then you'll configure the server list to use :
```java
builder()
.withEndpoint("http://{{server}}/mywebservice")
.withServers("localhost:8080", "10.10.10.10:6541");
```
### Loading from properties
```java
Properties props = new Properties();
props.load(...);
builder()
.withProperties("com.myprops", props)
.withServers("localhost:8080", "10.10.10.10:6541");
```
## The Client class
To build the client, use the `build` method :
```java
final CRMClient client = CRMClient.builder()
.withReceiveTimeout(2000)
.withLogger("com.myservice.Trames")
.build(CRMClient.class); // The port is instanciated at this stage.
final CRMServicePT port = client.service(); // Return the port instanciated above.
// Now you can call the web services.
port.getAll();
port.create(new CustomerType(...));
```
You need to pass the Client class. This is used to automatically cast to the right type in the return.
<file_sep>/src/main/java/fr/foop/ws/tools/WebServicePortConfigurer.java
package fr.foop.ws.tools;
import java.util.HashMap;
import java.util.Map;
import javax.xml.ws.BindingProvider;
import org.apache.cxf.endpoint.Client;
import org.apache.cxf.endpoint.Endpoint;
import org.apache.cxf.frontend.ClientProxy;
import org.apache.cxf.interceptor.LoggingInInterceptor;
import org.apache.cxf.interceptor.LoggingOutInterceptor;
import org.apache.cxf.transport.http.HTTPConduit;
import org.apache.cxf.transports.http.configuration.HTTPClientPolicy;
import org.apache.cxf.ws.security.wss4j.WSS4JOutInterceptor;
import org.apache.ws.security.WSConstants;
import org.apache.ws.security.handler.WSHandlerConstants;
import org.slf4j.Logger;
public class WebServicePortConfigurer<Port> {
private final Client client;
private final HTTPConduit http;
private final BindingProvider provider;
private final Endpoint cxfEndpoint;
public WebServicePortConfigurer(final Port port) {
this.client = ClientProxy.getClient(port);
this.http = (HTTPConduit) client.getConduit();
this.provider = (BindingProvider) port;
this.cxfEndpoint = client.getEndpoint();
}
public void configureEndpoint(final String endpoint, final String server) {
final String concreteEndpoint = endpoint.replaceFirst(
"\\{\\{server\\}\\}", server);
provider.getRequestContext().put(
BindingProvider.ENDPOINT_ADDRESS_PROPERTY, concreteEndpoint);
}
public void configureWsse(final String wsseUser, final String wssePwd) {
Map<String, Object> outProps = new HashMap<String, Object>();
outProps.put(WSHandlerConstants.ACTION,
WSHandlerConstants.USERNAME_TOKEN);
outProps.put(WSHandlerConstants.USER, wsseUser);
outProps.put(WSHandlerConstants.PASSWORD_TYPE, WSConstants.PW_TEXT);
outProps.put(WSHandlerConstants.PW_CALLBACK_REF,
new ClientPasswordCallback(wsseUser, wssePwd));
WSS4JOutInterceptor wssOut = new WSS4JOutInterceptor(outProps);
cxfEndpoint.getOutInterceptors().add(wssOut);
}
public void configureInLogger(final Logger logger) {
cxfEndpoint.getInInterceptors().add(new LoggingInInterceptor() {
@Override
protected void log(java.util.logging.Logger arg0, String arg1) {
logger.info(arg1);
}
});
}
public void configureOutLogger(final Logger logger) {
cxfEndpoint.getOutInterceptors().add(new LoggingOutInterceptor() {
@Override
protected void log(java.util.logging.Logger arg0, String arg1) {
logger.info(arg1);
}
});
}
public void configureTimeouts(final long connectionTimeout, final long receiveTimeout) {
final HTTPClientPolicy httpClientPolicy = new HTTPClientPolicy();
httpClientPolicy.setConnectionTimeout(connectionTimeout);
httpClientPolicy.setAllowChunking(false);
httpClientPolicy.setReceiveTimeout(receiveTimeout);
http.setClient(httpClientPolicy);
}
}
<file_sep>/src/main/java/fr/foop/ws/CxfClient.java
package fr.foop.ws;
import java.lang.reflect.InvocationTargetException;
import java.net.URL;
import org.apache.cxf.frontend.ClientProxy;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import fr.foop.ws.tools.WebServicePortConfigurer;
public abstract class CxfClient<Port, ServiceManager> {
private static final Logger LOGGER = LoggerFactory
.getLogger(CxfClient.class);
private final CxfClientBuilder config;
private final Port port;
private final Class<ServiceManager> smClazz;
private final String endpoint;
protected CxfClient(final CxfClientBuilder config,
final Class<ServiceManager> smClazz) {
this.config = config;
this.smClazz = smClazz;
this.endpoint = detectEndpoint();
this.port = enableMockIfRequired();
}
@SuppressWarnings("unchecked")
private Port enableMockIfRequired() {
if(config.useMock && config.mockedPort.isPresent()) {
return (Port)config.mockedPort.get();
}
else {
return electPort();
}
}
public CxfClientBuilder config() {
return config;
}
public abstract void checkIfPortUp(final Port port) throws Exception;
public abstract Port newPort(final ServiceManager serviceManager);
private String detectEndpoint() {
if (config.endpoint.isPresent()) {
return config.endpoint.get();
} else {
return ClientProxy.getClient(newPort(newDefaultServiceManager()))
.getEndpoint().getEndpointInfo().getAddress();
}
}
private ServiceManager newNoEndpointServiceManager() {
try {
return smClazz.getConstructor(URL.class).newInstance((URL) null);
} catch (InstantiationException | IllegalAccessException
| IllegalArgumentException | InvocationTargetException
| NoSuchMethodException | SecurityException e) {
throw new RuntimeException(
"failed to instanciate new service manager", e);
}
}
private ServiceManager newDefaultServiceManager() {
try {
return smClazz.newInstance();
} catch (InstantiationException | IllegalAccessException
| IllegalArgumentException | SecurityException e) {
throw new RuntimeException(
"failed to instanciate new service manager", e);
}
}
/**
* Instanciate a configured web service port the provided server.
* @param server
* @return
*/
private Port instanciateForServer(final String server) {
final Port port = newPort(newNoEndpointServiceManager());
final WebServicePortConfigurer<Port> configurer = new WebServicePortConfigurer<Port>(port);
configurer.configureEndpoint(endpoint, server);
if(config.inLogger.isPresent()) {
configurer.configureInLogger(config.inLogger.get());
}
if(config.outLogger.isPresent()) {
configurer.configureOutLogger(config.outLogger.get());
}
if(config.wsseUser.isPresent() && config.wssePwd.isPresent()) {
configurer.configureWsse(config.wsseUser.get(), config.wssePwd.get());
}
configurer.configureTimeouts(config.connectionTimeout, config.receiveTimeout);
return port;
}
/**
* Try to find a working Web Service Port for the given server list.
* @return
*/
private Port electPort() {
if (config.servers.size() == 0) {
return instanciateForServer("");
} else {
int i = 0;
while (i < config.servers.size()) {
final Port port = instanciateForServer(config.servers.get(i++));
try {
checkIfPortUp(port);
return port;
} catch (Exception e) {
LOGGER.warn(
"Failed to instanciate Service [{}] : {}",
config.endpoint + " <-- "
+ config.servers.get(i - 1), e.getMessage());
LOGGER.debug("Failed to instanciate Service", e);
}
}
}
return null;
}
public Port service() {
return port;
}
}
<file_sep>/src/main/java/fr/foop/ws/CxfClientBuilder.java
package fr.foop.ws;
import java.lang.reflect.InvocationTargetException;
import java.util.Properties;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.base.Joiner;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import fr.foop.ws.tools.configurators.CxfClientBuilderConfigurator;
import fr.foop.ws.tools.configurators.PropertyMeta;
public class CxfClientBuilder {
public final Optional<String> endpoint;
public final Optional<String> wsseUser;
public final Optional<String> wssePwd;
public final long connectionTimeout;
public final long receiveTimeout;
public final ImmutableList<String> servers;
public final Optional<Logger> inLogger;
public final Optional<Logger> outLogger;
public final Optional<Object> mockedPort;
public final boolean useMock;
private final static String[] propNames = new String[] { "endpoint",
"wsseUser", "wssePwd", "connectionTimeout", "receiveTimeout",
"inLogger", "outLogger", "logger", "mockedPort", "useMock", "servers" };
private final static ImmutableMap<String, PropertyMeta> propMetas = ImmutableMap
.<String, PropertyMeta> builder()
.put("endpoint",
new PropertyMeta(
"The web service endpoint, can contain the variable {{server}}, in this case it would be replaced by one of the server defined in servers.",
CxfClientBuilderConfigurator.ENDPOINT_CONFIGURATOR))
.put("wsseUser",
new PropertyMeta(
"The user to pass as WSSE user credential", CxfClientBuilderConfigurator.WSSE_USER_CONFIGURATOR))
.put("wssePwd",
new PropertyMeta(
"The password to pass as the WSSE password credential (only PasswordText supported for now)",
CxfClientBuilderConfigurator.WSSE_PWD_CONFIGURATOR))
.put("connectionTimeout",
new PropertyMeta(
"the time in millis before timeout during connection",
CxfClientBuilderConfigurator.CONNECTION_TIMEOUT_CONFIGURATOR))
.put("receiveTimeout",
new PropertyMeta(
"The time in millis to wait before timeout while not receiving data",
CxfClientBuilderConfigurator.RECEIVE_TIMEOUT_CONFIGURATOR))
.put("inLogger",
new PropertyMeta(
"The logger name to use for client request sent to the server (seen from server side)",
CxfClientBuilderConfigurator.INLOGGER_CONFIGURATOR))
.put("outLogger",
new PropertyMeta(
"The logger name to log server response sent to the client (seen from server side)",
CxfClientBuilderConfigurator.OUTLOGGER_CONFIGURATOR))
.put("logger",
new PropertyMeta(
"The logger name to use for both in/out logger. It will override in/out logger settings",
CxfClientBuilderConfigurator.LOGGER_CONFIGURATOR))
.put("mockedPort",
new PropertyMeta(
"The mocked object to use as port, it must implements the CXF generated port interface",
CxfClientBuilderConfigurator.MOCKED_PORT_CONFIGURATOR))
.put("useMock",
new PropertyMeta(
"A flag to enable or disable the use of the mocked port object",
CxfClientBuilderConfigurator.USE_MOCK_CONFIGURATOR))
.put("servers",
new PropertyMeta(
"Comma separated list of server to use in the endpoint, they will be tried turn by turn at service initialisation",
CxfClientBuilderConfigurator.SERVERS_CONFIGURATOR))
.build();
public CxfClientBuilder() {
this(Optional.<String> absent(), Optional.<String> absent(), Optional
.<String> absent(), 1000, 3000, Optional.<Logger> absent(),
Optional.<Logger> absent(), Optional.<Object> absent(), false, ImmutableList.<String> of());
}
public CxfClientBuilder(final String endPoint, final String... servers) {
this(Optional.of(endPoint), Optional.<String> absent(), Optional
.<String> absent(), 1000, 3000, Optional.<Logger> absent(),
Optional.<Logger> absent(), Optional.<Object> absent(), false, ImmutableList.copyOf(servers));
}
public CxfClientBuilder(final Optional<String> endpoint,
final Optional<String> username, final Optional<String> password,
final long connectionTimeout, final long receiveTimeout,
final Optional<Logger> inLogger, final Optional<Logger> outLogger,
final Optional<Object> mockedPort,
final boolean useMock,
final ImmutableList<String> servers) {
this.endpoint = endpoint;
this.wsseUser = username;
this.wssePwd = <PASSWORD>;
this.connectionTimeout = connectionTimeout;
this.receiveTimeout = receiveTimeout;
this.outLogger = outLogger;
this.inLogger = inLogger;
this.mockedPort = mockedPort;
this.useMock = useMock;
this.servers = ImmutableList.<String> copyOf(servers);
}
public CxfClientBuilder withEndpoint(final String endpoint) {
return new CxfClientBuilder(Optional.of(endpoint), wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withReceiveTimeout(final long receiveTimeout) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withConnectionTimeout(final long connectionTimeout) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withWsseUser(final String username) {
return new CxfClientBuilder(endpoint, Optional.fromNullable(username),
wssePwd, connectionTimeout, receiveTimeout, inLogger,
outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withWssePwd(final String password) {
return new CxfClientBuilder(endpoint, wsseUser,
Optional.fromNullable(password), connectionTimeout,
receiveTimeout, inLogger, outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withWsseCredentials(final String username,
final String password) {
return this.withWsseUser(username).withWssePwd(password);
}
public CxfClientBuilder withInLogger(final Logger logger) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout,
Optional.fromNullable(logger), outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withInLogger(final String loggerName) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, Optional.of(LoggerFactory
.getLogger(loggerName)), outLogger, mockedPort, useMock, servers);
}
public CxfClientBuilder withOutLogger(final Logger logger) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger,
Optional.fromNullable(logger), mockedPort, useMock, servers);
}
public CxfClientBuilder withOutLogger(final String loggerName) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger,
Optional.of(LoggerFactory.getLogger(loggerName)), mockedPort, useMock, servers);
}
public CxfClientBuilder withLogger(final Logger logger) {
return this.withInLogger(logger).withOutLogger(logger);
}
public CxfClientBuilder withLogger(final String loggerName) {
return this.withInLogger(loggerName).withOutLogger(loggerName);
}
public CxfClientBuilder withInOutLogger(final Logger logger) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout,
Optional.fromNullable(logger), Optional.fromNullable(logger),
mockedPort, useMock, servers);
}
public CxfClientBuilder withServers(final String... servers) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, useMock,
ImmutableList.copyOf(servers));
}
public CxfClientBuilder withProperties(final String rootKey,
final Properties props) {
CxfClientBuilder configured = this;
final boolean rootKeyEndsWithDot = rootKey.endsWith(".");
for (final String property : propNames) {
final String rootedProperty = Joiner.on(rootKeyEndsWithDot?"":".").join(rootKey, property);
configured = Optional
.fromNullable(propMetas.get(property).configurator)
.or(CxfClientBuilderConfigurator.NOOP_CONFIGURATOR)
.configure(configured,
Optional.fromNullable((String) props.get(rootedProperty)));
}
return configured;
}
public <Port> CxfClientBuilder withMockedPort(final Port port) {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, Optional.<Object>of((Object)port), useMock,
servers);
}
public <Port> CxfClientBuilder withMockedPort(final Class<Port> portClass) {
try {
return withMockedPort(portClass.newInstance());
} catch (InstantiationException | IllegalAccessException e) {
throw new RuntimeException("unable to instianciate mocked port class : " + portClass.getName(), e);
}
}
public CxfClientBuilder enableMocking() {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, true,
servers);
}
public CxfClientBuilder disableMocking() {
return new CxfClientBuilder(endpoint, wsseUser, wssePwd,
connectionTimeout, receiveTimeout, inLogger, outLogger, mockedPort, false,
servers);
}
public CxfClientBuilder withMockedPort(final String portClassName) {
try {
return withMockedPort(Class.forName(portClassName));
} catch (ClassNotFoundException e) {
throw new RuntimeException("unable to find class for named mocked port : " + portClassName, e);
}
}
public <Port, Client extends CxfClient<Port, ?>> Client build(
Class<Client> clazz) {
try {
return clazz.getConstructor(CxfClientBuilder.class).newInstance(
this);
} catch (InstantiationException | IllegalAccessException
| IllegalArgumentException | InvocationTargetException
| NoSuchMethodException | SecurityException e) {
throw new IllegalArgumentException(
"failed to build CxfClient with class : "
+ clazz.toString(), e);
}
}
} | 45bc7f40f6ea602812bf6939dc3c2e66a534fd77 | [
"Markdown",
"Java"
] | 5 | Java | tenato/webservice-client-api | 5e96af81feefba83dc02ef79c57640ff1d316417 | 9ee9a2c459290f9fbab07603093f9e17c747a1cd | |
refs/heads/master | <file_sep>angular.module("waitStaffCalc", [])
.controller("WaitStaffCalcController", function(){
this.data={
basePrice : 0.0,
taxRate : 0.0,
tipPercentage : 0.0,
subTotal : 0.0,
tip : 0.0,
total : 0.0,
tipTotal : 0.0,
mealCount : 0.0,
avgTip : 0.0
};
this.submit = function(){
this.data.subTotal = this.data.basePrice + this.data.basePrice*(this.data.taxRate/100);
this.data.tip = this.data.subTotal * (this.data.tipPercentage/100);
this.data.tipTotal += this.data.tip;
this.data.mealCount++;
this.data.avgTip = this.data.tipTotal/this.data.mealCount;
this.data.total = this.data.subTotal + this.data.tip;
};
this.cancel = function(){
this.data.basePrice = 0.0;
this.data.taxRate = 0.0;
this.data.tipPercentage = 0.0;
};
this.reset = function(){
//I could do this.data="" but I wanted the default values as placeholders.
//Anyway to do this without recreating the object?
this.data={
basePrice : 0.0,
taxRate : 0.0,
tipPercentage : 0.0,
subTotal : 0.0,
tip : 0.0,
total : 0.0,
tipTotal : 0.0,
mealCount : 0.0,
avgTip : 0.0
};
};
});
| 7512c1774c6b807c64383c7291208a9c11830976 | [
"JavaScript"
] | 1 | JavaScript | tanviraslam/WaitstaffCalculator | b7d4f6b5821c7818a6137f95fb24db31d4858af9 | e419251c5dc28b0fb17fad55b9c0c4b78929749c | |
refs/heads/master | <repo_name>evanJensengit/problems<file_sep>/interviewProblems/main.cpp
//
// main.cpp
// interviewProblems
//
// Created by <NAME> on 8/22/20.
// Copyright © 2020 <NAME>. All rights reserved.
//
#include <iostream>
#include "problems.h"
using namespace std;
int main() {
// insert code here...
problems a;
int num = 2147;
int reversedNum = a.reverse(num);
// cout << reverse(num) << endl;
cout << reversedNum << endl;
int num2 = 2147;
int b = num2 % 10;
//cout << b << endl;
}
<file_sep>/README.md
# problems
practice problems
<file_sep>/reverseInt.cpp
int problems::reverse (int num) {
if (num > 2147483648 || num < -2147483648 ) {
return 0;
}
string reversed = "";
int div = 10;
int oneDigitLess, numAtEnd;
bool isNegative = false;
if (num < 0) {
isNegative = true;
}
for (int i = 0; i < 10; i++) {
oneDigitLess = num / div;
numAtEnd = num % div;
num = oneDigitLess;
if (isNegative && i < 1) {
numAtEnd *= -1;
num *= -1;
}
cout << num << endl;
reversed += to_string(numAtEnd);
if (oneDigitLess == 0) {
break;
}
}
cout << reversed << endl;
bool isNonZero = false;
int length = reversed.length();
for (int i = 0; i< length; i++) {
// cout << reversed << endl;
if (!isNonZero) {
if (reversed.at(i) == '0') {
reversed.erase(0, 1);
i--;
length--;
}
else
isNonZero = true;
}
}
try {
num = stoi(reversed);
}
catch (const exception& e) {
cout << "exception caught " << e.what() << endl;
return 0;
}
if (isNegative) {
num *= -1;
}
//cout << num << endl;
return num;
}
<file_sep>/twoSum.cpp
//
// main.cpp
// TwoSumProblem
//
// Created by <NAME> on 8/5/20.
// Copyright © 2020 <NAME>. All rights reserved.
//
using namespace std;
#include <iostream>
#include <vector>
vector<int> twoSum(vector<int>& nums, int target) {
bool solutionNotFound = true;
int indexBase = 0;
int baseAndCurrentSum;
int currentElement;
int currentElementBase;
while (solutionNotFound) {
for (int i = indexBase; i < nums.size(); i++) {
baseAndCurrentSum = nums[i] + nums[indexBase];
//cout << baseAndCurrentSum << endl;
if (baseAndCurrentSum == target) {
solutionNotFound = false;
currentElementBase = nums[indexBase];
currentElement = nums[i];
nums.erase(nums.begin(), nums.end());
nums.push_back (currentElement);
nums.push_back (currentElementBase);
break;
}
}
if (indexBase == nums.size()) {
break;
}
indexBase++;
}
return nums;
}
int main(int argc, const char * argv[]) {
vector<int> myInts {12, 14, 22, 7, 8, 13 };
for (auto i = myInts.begin(); i != myInts.end(); ++i) {
cout << *i << endl;
}
int target = 21;
myInts = twoSum(myInts, target);
cout << "New vector after twoSum: " << endl;
for (auto i = myInts.begin(); i != myInts.end(); ++i) {
cout << *i << endl;
}
// cout << myInts.size();
// for (int i = 0; i <= 20; i++) {
// myInts.push_back(i);
// }
// for (int i = 0; i <= 20; i++) {
// cout << myInts[i] << endl;
// }
}
<file_sep>/interviewProblems/problems.h
//
// reverseInteger.hpp
// interviewProblems
//
// Created by <NAME> on 8/22/20.
// Copyright © 2020 <NAME>. All rights reserved.
//
#ifndef problems_h
#define problems_h
using namespace std;
#include <iostream>
#include<string>
#include <stdio.h>
#include <vector>
class problems {
public:
void start();
int reverse (int);
};
#endif /* reverseInteger_hpp */
<file_sep>/interviewProblems/problems.cpp
//
// reverseInteger.cpp
// interviewProblems
//
// Created by <NAME> on 8/22/20.
// Copyright © 2020 <NAME>. All rights reserved.
//
#include "problems.h"
int problems::reverse (int num) {
string reversed = "";
int div = 10;
int oneDigitLess, numAtEnd;
bool isNegative = false;
if (num < 0) {
isNegative = true;
num *= -1;
}
for (int i = 0; i < 10; i++) {
oneDigitLess = num / div;
numAtEnd = num % div;
num = oneDigitLess;
// cout << num << endl;
reversed += to_string(numAtEnd);
if (oneDigitLess == 0 ) {
break;
}
}
bool isNonZero = false;
int length = reversed.length();
for (int i = 0; i< length; i++) {
// cout << reversed << endl;
if (!isNonZero) {
if (reversed.at(i) == '0') {
reversed.erase(0, 1);
i--;
length--;
}
else
isNonZero = true;
}
}
num = stoi(reversed);
if (isNegative) {
num *= -1;
}
//cout << num << endl;
return num;
}
| 3df5a878c42ccd1f0a4cd512d72d54025d99102a | [
"Markdown",
"C++"
] | 6 | C++ | evanJensengit/problems | b71f2c60847dda524930035d1ca332fbe5ce5387 | 601d874329fdf572bf3e11e3cd07e14149847467 | |
refs/heads/master | <file_sep><?php
// Initialize the session
session_start();
// Check if the user is logged in, if not then redirect him to login page
if(!isset($_SESSION["loggedin"]) || $_SESSION["loggedin"] !== true){
header("location: login.php");
exit;
}
?>
<!doctype html>
<html>
<head>
<style>
.button3 {width: 80%;}
</style>
<meta charset="utf-8">
<title>Golfzilla</title>
<link rel="stylesheet" href= "stylesheet.css">
</head>
<br><img src="golfzillalogo.png" alt="Logo"><br>
<br>
<br>
<h1>Welcome to Golfzilla!</h1> <br>
<input type = "submit" class="button button3" onclick="document.location = 'golfzilla2.php'" name = "start" value = "Start Round"></button><br>
<button class="button button3" onclick="document.location = 'pastscores.php'">View Past Scores</button>
<p>
<button onclick="document.location = 'reset-password.php'">Reset Your Password</button>
<button onclick="document.location = 'logout.php'">Sign Out of Your Account</button>
</p>
<div class="container">
<p id="response"></p>
</div>
</body>
</html>
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "golfzilla";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if(!isset($_GET["start"])){
$sql = "INSERT INTO roundinfo(id, tdate) VALUES (1, NOW());";
}
$conn->close();
?><file_sep><!doctype html>
<html>
<head>
<style>
.button3 {width: 80%;}
</style>
<meta charset="utf-8">
<title>Golfzilla</title>
<link rel="stylesheet" href= "stylesheet.css">
</head>
<body>
<img src="golfzillalogo.png" alt="Logo"><br>
<p>Welcome to Golfzilla!</p>
<h2><label for="fname">Score:</label></h2>
<div class="container">
<form method = "GET" action = "golfzilla2.php" >
<p>
<input type = "submit" name = "strokes" value = 1 id = 1></button>
<input type = "submit" name = "strokes" value = 2 id = 2></button>
<input type = "submit" name = "strokes" value = 3 id = 3></button>
<input type = "submit" name = "strokes" value = 4 id = 4></button>
<input type = "submit" name = "strokes" value = 5 id = 5></button>
<input type = "submit" name = "strokes" value = 6 id = 6></button>
<input type = "submit" name = "strokes" value = 7 id = 7></button>
<input type = "submit" name = "strokes" value = 8 id = 8></button>
<input type = "submit" name = "strokes" value = 9 id = 9></button>
<input type = "submit" name = "strokes" value = 10 id = 10></button>
</p>
</form>
</div>
<button class="button button3" onclick="document.location = 'endofround.php'">End Round</button>
<br>
<button class="button button3" onclick="document.location = 'golfzilla.php'">Go back to Home Page</button>
</body>
</html>
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "golfzilla";
$conn = new mysqli($servername, $username, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$hole = 1;
if(!isset($_POST["strokes"])){
$strokes = $_GET['strokes'];
$sql = "INSERT INTO score(id, rid, strokes) VALUES (1,1, '$strokes');";
if ($conn->query($sql)){
echo "<p> Score Recorded </p>";
}
}
$conn->close();
?>
<file_sep><?php
// Initialize the session
session_start();
// Check if the user is logged in, if not then redirect him to login page
if(!isset($_SESSION["loggedin"]) || $_SESSION["loggedin"] !== true){
header("location: login.php");
exit;
}
?>
<!doctype html>
<html>
<head>
<style>
.button3 {width: 80%;}
</style>
<meta charset="utf-8">
<title>Golfzilla</title>
<link rel="stylesheet" href= "stylesheet.css">
</head>
<br><img src="golfzillalogo.png" alt="Logo"><br>
<br>
<br>
<h1>Your Scorecard!</h1> <br>
<table>
<tr>
<th>1</th>
<th>2</th>
<th>3</th>
<th>4</th>
<th>5</th>
<th>6</th>
<th>7</th>
<th>8</th>
<th>9</th>
<th>10</th>
<th>11</th>
<th>12</th>
<th>13</th>
<th>14</th>
<th>15</th>
<th>16</th>
<th>17</th>
<th>18</th>
</tr>
<tr>
<td>4</td>
<td>4</td>
<td>3</td>
<td>5</td>
<td>7</td>
<td>3</td>
<td>5</td>
<td>4</td>
<td>4</td>
<td>5</td>
<td>4</td>
<td>3</td>
<td>5</td>
<td>6</td>
<td>4</td>
<td>5</td>
<td>3</td>
<td>4</td>
</tr>
</table>
<br>
<button class="button button3" onclick="document.location = 'pastScores.php'">View Past Scores</button> <br>
<button class="button button3" onclick="document.location = 'golfzilla.php'">Go back to Home Page</button>
<p>
<button onclick="document.location = 'logout.php'">Sign Out of Your Account</button>
</p>
<div class="container">
<p id="response"></p>
</div>
</body>
</html>
<file_sep><!doctype html>
<html>
<head>
<style>
.button3 {width: 50%;}
</style>
<meta charset="utf-8">
<title>Scores</title>
<link rel="stylesheet" href= "stylesheet.css">
</head>
<img src="golfzillalogo.png" alt="Logo"><br>
<p>Your Scores</p> <br>
<h3>2020-06-02</h3>
<table>
<tr>
<th>1</th>
<th>2</th>
<th>3</th>
<th>4</th>
<th>5</th>
<th>6</th>
<th>7</th>
<th>8</th>
<th>9</th>
<th>10</th>
<th>11</th>
<th>12</th>
<th>13</th>
<th>14</th>
<th>15</th>
<th>16</th>
<th>17</th>
<th>18</th>
</tr>
<tr>
<td>5</td>
<td>8</td>
<td>3</td>
<td>5</td>
<td>7</td>
<td>3</td>
<td>4</td>
<td>9</td>
<td>4</td>
<td>3</td>
<td>4</td>
<td>3</td>
<td>5</td>
<td>6</td>
<td>4</td>
<td>6</td>
<td>4</td>
<td>3</td>
</tr>
</table>
</div>
<br>
<?php
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "golfzilla";
$conn = new mysqli($servername, $username, $password, $dbname);
// Check connection: No need for else. It will quit if the connection thorws an error.
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$sql = "SELECT * FROM scores";
$result = $conn->query($sql);
if ($result->num_rows > 0){
echo "<table><tr><th>1</th><th>2</th><th>3</th><th>4</th><th>5</th><th>6</th><th>7</th><th>8</th><th>9</th> <th>10</th><th>11</th><th>12</th><th>13</th><th>14</th><th>15</th><th>16</th> <th>17</th><th>18</th></tr>";
while($row = $result->fetch_assoc()) {
echo "<tr><td>" .$row["strokes"];
}
echo "</table>";
} else {
echo "0 results";
}
$conn->close();
?>
<button class="button button3" onclick="document.location = 'golfzilla.php'">Go back to Home Page</button>
<p>
<button onclick="document.location = 'logout.php'">Sign Out of Your Account</button>
</p>
<div class="container">
<p id="response"></p>
</div>
</body>
</html> | dd18b7fda3093764d99159d9f40063ceab234b30 | [
"PHP"
] | 4 | PHP | mackenzie08/Golfzilla | b5f2420a7890d9b9f9de030bc4ccd6b3483fb2d3 | df1def2bfbb9affceb4a7f89e86d17cd281a4b45 | |
refs/heads/main | <file_sep>$('#ham, #menu-back').click(function(e) {
e.preventDefault();
if($('#menu').hasClass('in')) {
resetMenu();
} else {
var overlayHeight = $('body').height() + 'px';
$('#menu').addClass('in');
$('#menu').css({'height': overlayHeight });
$('#nav-overlay').css({'height': overlayHeight, 'display': 'block' });
}
});
$('#nav-overlay').click(function() {
resetMenu();
});
$(window).resize(function() {
var width = $(this).width();
if(width > 768) {
resetMenu();
$('#menu').css({'height':'auto'});
}
});
function resetMenu() {
$('#menu').removeClass('in');
$('#nav-overlay').css({'display':'none'});
setTimeout(function() {
$('#menu').css({'height':'auto'});
}, 300);
}<file_sep>theme = "digitalservices"
languageCode = "en-us"
title = "San Francisco Digital Services"
MetaDataFormat = "toml"
baseURL = "/"
<file_sep>+++
type = "default"
+++
<!-- Header -->
<div id="background-image" style="background-image:url(/assets/sf_city_hall-bg.jpg);"></div>
<div id="home" class="main">
<h1 class= "title">San Francisco Digital Services</h1>
<h3>Making services for everyone</h3>
<!-- <br/><br/><br/>
<a class="btn-custom" href="/#contact">Read the Full Report (PDF)</a> -->
</div>
<!-- Header End -->
<!-- About -->
<div id="about">
<div class="container">
<!-- <h2 class="sub-heading">About</h2><div class="separator center"></div> -->
<div class="about-box">
<div class="about-body col-xs-12 col-sm-10 col-sm-offset-1 col-md-6 col-md-offset-3">
<p>San Francisco Digital Services works with other City departments to improve public services. We use technology to make it easier for people to get things done.</p>
<p>We're working on critical issues like <a href="https://housing.sfgov.org/" target="_blank"> affordable housing</a>, <a href="https://businessportal.sfgov.org/" target="_blank">small businesses</a>, <a href="https://immigrants.sfgov.org/" target="_blank">legal aid for immigrants</a>, and more. Our team is also rebuilding the city’s website from the ground up. This means taking a service-led approach so that anyone using the site can get what they need.</p>
<p>Our work goes beyond websites. We’re re-thinking how public services are designed, by understanding what our users need and building with an agile approach.</p>
<!--<p><a href="ourwork.html">Read more about our work »</a></p>-->
<br/><br/><br/>
<a id="btn-cdso" class="btn-custom2 center" href="/joinus.html">Join us</a>
<br/><br/><br/><br/>
</div>
</div>
</div>
</div>
<!-- About End -->
<!-- <div id="separator-image" style="background-image:url('assets/bg-icons.png');"></div>
-->
<!-- Values -->
<div id="separator-image">
<div class="container">
<div class="col-xs-12 col-md-4 quote-photo">
<img src="/assets/Carmen.jpg" alt="<NAME>, City Administrator">
</div>
<div class="col-xs-12 col-md-8 carmen-quote">
<p class="separator-quote-2">San Francisco Digital Services is part of the San Francisco City Administrator's office, led by City Administrator <NAME>.
</p>
<p class="separator-quote-2"><NAME> was the elected Assessor for the City and County of San Francisco. Prior to becoming Assessor, <NAME> was an elected representative on the San Francisco Board of Supervisors from 2007 to 2013. She served as Deputy Director of the Mayor’s Office of Public Policy and Finance from 2004 to 2007. Ms. Chu has over 16 years of governmental management and finance experience with the City and County of San Francisco.</p>
<p class="separator-quote-2">
She believes good government works for all people. A financial steward and proven leader, she stands for transparency, accountability and fairness.</p>
</div>
</div>
</div>
<!-- Values End -->
<!-- Services -->
<div id="top3" class="container">
<div class="row">
<div class="col-xs-12 col-md-4 blog">
<a href="https://medium.com/san-francisco-digital-service" target="_blank"><img src="/assets/icons/blog.svg" /></a>
<h3>Our Blog</h3>
<p>Read our latest updates.<br/>
<a href="https://medium.com/san-francisco-digital-service" target="_blank">Visit our blog »</a>
</p>
</div>
<!--blog-->
<div class="col-xs-12 col-md-4 team">
<a href="/joinus.html"><img src="/assets/icons/team.svg" /></a>
<h3>Join us</h3>
<p>We're technologists brought together by our love of San Francisco.<br/>
<a href="/joinus.html">Join our team »</a>
</p>
</div>
<!--team-->
<div class="col-xs-12 col-md-4 participate">
<a href="https://www.surveymonkey.com/r/ResidentsImprovingSanFranciscoCityServices?source=sfds_website8" target="_blank"><img src="/assets/icons/participate.svg" /></a>
<h3>Participate</h3>
<p>If you're an SF resident, sign up to hear about user testing opportunities.<br/>
<a href="https://www.surveymonkey.com/r/ResidentsImprovingSanFranciscoCityServices?source=sfds_website" target="_blank">Sign up for research »</a>
</p>
</div>
<!--team-->
</div>
<!--row-->
</div>
<!--container-->
<!-- Services End -->
{{% subscribeform %}}
<file_sep>#!/bin/bash
set -eo pipefail
static_branch=$CIRCLE_BRANCH-static-ci
cd ~/hugo
echo "Run hugo to build static site"
HUGO_ENV=production hugo -v
git config --global user.email $GH_EMAIL
git config --global user.name $GH_NAME
git fetch && git checkout -b origin/$static_branch $static_branch || git checkout -b $static_branch
mkdir -p ../tmp/.circleci && cp -a .circleci/. ../tmp/.circleci/. # copy circleci config to tmp dir
git rm -rf . # remove everything
mv public/* . # move hugo generated files into root of branch dir
cp -a ../tmp/.circleci . # copy circleci config back to prevent triggering build on ignored branches
git add -A
# this is necessary because a separate manual deploy (which serves the gh-pages branch) happens on a city server
# because the domain digitalservices.sfgov.org is in use internally
if [ $CIRCLE_BRANCH == $SOURCE_BRANCH ]; then
# main branch, force push gh-pages
git commit -m "build $CIRCLE_SHA1 to gh-pages"
git push -f origin $static_branch:gh-pages
fi
<file_sep>$('#ham, #menu-back').click(function(e) {
e.preventDefault();
if($('#menu').hasClass('in')) {
resetMenu();
} else {
var overlayHeight = $('body').height() + 'px';
$('#menu').addClass('in');
$('#menu').css({'height': overlayHeight });
$('#nav-overlay').css({'height': overlayHeight, 'display': 'block' });
}
});
$('#nav-overlay').click(function() {
resetMenu();
});
$(window).resize(function() {
var width = $(this).width();
if(width > 768) {
resetMenu();
$('#menu').css({'height':'auto'});
}
});
function resetMenu() {
$('#menu').removeClass('in');
$('#nav-overlay').css({'display':'none'});
setTimeout(function() {
$('#menu').css({'height':'auto'});
}, 300);
}
(function() {
$('#skip-link').click(function() {
$(window).scrollTop($('.main').position().top - $('.navbar-brand.logo img').height());
$('.main').attr('tabindex', '-1');
$('.main').focus();
});
$('#skip-link').on('focus', function() {
$(this).addClass('skip-link-active');
var minLeftPos = $('#skip-link').position().left + $('#skip-link').width();
var logoLeftPos = $('.navbar-brand.logo').position().left;
if(logoLeftPos < minLeftPos) $('.navbar-brand.logo').css({'margin-left': (parseFloat($('.navbar-brand.logo').css('margin-left').replace('px','')) + $(this).width()) + 'px'});
});
$('#skip-link').on('blur', function() {
$(this).removeClass('skip-link-active');
$('.navbar-brand.logo').attr('style', '');
});
})();<file_sep>[](https://circleci.com/gh/SFDigitalServices/sfdigitalservices)
# Build locally
## Get Hugo
### via homebrew:
```
$ brew install hugo
```
### or binary install:
Pick the appropriate binary from here:
[https://github.com/gohugoio/hugo/releases](https://github.com/gohugoio/hugo/releases)
## Generate site
```
$ hugo
```
## Serve it up
```
$ hugo server -w
```
Hit [http://localhost:1313](http://localhost:1313) to verify that the site was generated correctly
## Create a new page
Creating a new page can be as simple as creating a file (xyz.html) in the `/content` folder. Or via the command line (at the root directory of this repo):
```
$ hugo new xyz.html
```
These new pages will follow the layout template in `/themes/digitalservices/_default/single.html`
# How to add team members to website
* When a new person joins the team, get their profile photo in .jpg or .png formats
* Add their profile photo to the `themes/static/assets/staff-imgs` folder, name it like this: `firstname.jpg` or `firstname.png`
* Follow the instructions above to build the site locally and preview changes.
* Edit the `content/ourteam.html` file, add team members in `<div id="staff">` by adding a new `<li class="col-xs-12 col-md-6 col-lg-4">` block
* Make sure that their name, job title and photo are correct
* Submit a PR
* Once your PR has been approved by a reviewer, who will also send you a preview of your changes (in the form of a Pantheon link), check your preview then merge your PR. If you are not happy with the changes, commit to the same branch, which will generate another Pantheon preview.
* Merge your PR
* Reach out to someone with server access (currently, that would be @skinnylatte, @aekong and @henryjiang-sfgov) to deploy with the following commands
As of August 2021, you no longer have sudo access to this server. This readme file is being updated to remove all mention of using sudo for git fetch.
```
$ cd /opt/rh/httpd24/root/var/www/html/digitalservices/public
$ git fetch --all
$ git reset --hard origin/gh-pages
```
## Notes
* Never ever edit files in /public directly (this is hugo generated)
* This repo is a very basic site, with very few (what hugo calls) content types
* Refer to Hugo documentation at [https://gohugo.io/documentation/](https://gohugo.io/documentation/)
<file_sep>---
aliases:
- /joinus.html
type: jobs
roles:
- title: Program Manager, Strategic Initiatives
url: https://careers.sf.gov/role/?id=3743990000627536
- title: Senior QA Engineer
url: https://jobs.smartrecruiters.com/ni/CityAndCountyOfSanFrancisco1/070dc0d7-d018-4f52-85fc-34756d7ca78c-senior-quality-assurance-engineer-digital-services-1043-
- title: Digital Translation Project Manager
url: https://jobs.smartrecruiters.com/ni/CityAndCountyOfSanFrancisco1/213d1773-015a-4f46-a3f7-611df281cc9d-digital-translations-project-manager-digital-services-9976-1053-
- title: Analytics Engineer, DataSF
url: https://jobs.smartrecruiters.com/CityAndCountyOfSanFrancisco1/3743990000587056-analytics-engineer-datasf-1042-
- title: Product Manager for Platforms and Infrastructure
url: https://jobs.smartrecruiters.com/CityAndCountyOfSanFrancisco1/743999801085935-product-manager-for-platforms-infrastructure-digital-services-9976-1053-
- title: Senior Service Designer
url: https://jobs.smartrecruiters.com/CityAndCountyOfSanFrancisco1/743999807919460-senior-service-designer-digital-services-9976-1054-
- title: Bloomberg Data Program Manager, DataSF
url: https://jobs.smartrecruiters.com/CityAndCountyOfSanFrancisco1/743999811945241-bloomberg-data-program-manager-datasf-1054-
- title: Bloomberg Data Engineer, DataSF
url: https://jobs.smartrecruiters.com/CityAndCountyOfSanFrancisco1/743999812423990-bloomberg-data-engineer-datasf-1043-
---
{{% subscribeform %}}
<file_sep><!doctype html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
<title>Thank you for submitting your application</title>
<style>
/* -------------------------------------
GLOBAL RESETS
------------------------------------- */
/*All the styling goes here*/
img {
border: none;
-ms-interpolation-mode: bicubic;
max-width: 100%;
}
body {
background-color: #f6f6f6;
font-family: sans-serif;
-webkit-font-smoothing: antialiased;
font-size: 14px;
line-height: 1.4;
margin: 0;
padding: 0;
-ms-text-size-adjust: 100%;
-webkit-text-size-adjust: 100%;
}
table {
border-collapse: separate;
mso-table-lspace: 0pt;
mso-table-rspace: 0pt;
width: 100%; }
table td {
font-family: sans-serif;
font-size: 14px;
vertical-align: top;
}
/* -------------------------------------
BODY & CONTAINER
------------------------------------- */
.body {
background-color: #f6f6f6;
width: 100%;
}
/* Set a max-width, and make it display as block so it will automatically stretch to that width, but will also shrink down on a phone or something */
.container {
display: block;
Margin: 0 auto !important;
/* makes it centered */
max-width: 580px;
padding: 10px;
width: 580px;
}
/* This should also be a block element, so that it will fill 100% of the .container */
.content {
box-sizing: border-box;
display: block;
Margin: 0 auto;
max-width: 580px;
padding: 10px;
}
/* -------------------------------------
HEADER, FOOTER, MAIN
------------------------------------- */
.main {
background: #ffffff;
border-radius: 3px;
width: 100%;
}
.wrapper {
box-sizing: border-box;
padding: 20px;
}
.content-block {
padding-bottom: 10px;
padding-top: 10px;
}
.footer {
clear: both;
Margin-top: 10px;
text-align: center;
width: 100%;
}
.footer td,
.footer p,
.footer span,
.footer a {
color: #999999;
font-size: 12px;
text-align: center;
}
/* -------------------------------------
TYPOGRAPHY
------------------------------------- */
h1,
h2,
h3,
h4 {
color: #000000;
font-family: sans-serif;
font-weight: 400;
line-height: 1.4;
margin: 0;
margin-bottom: 30px;
}
h1 {
font-size: 35px;
font-weight: 300;
text-align: center;
text-transform: capitalize;
}
p,
ul,
ol {
font-family: sans-serif;
font-size: 14px;
font-weight: normal;
margin: 0;
margin-bottom: 15px;
}
p li,
ul li,
ol li {
list-style-position: inside;
margin-left: 5px;
}
a {
color: #3498db;
text-decoration: underline;
}
/* -------------------------------------
BUTTONS
------------------------------------- */
.btn {
box-sizing: border-box;
background-color: #3498db;
border: solid 1px #3498db;
border-radius: 5px;
box-sizing: border-box;
color: #ffffff;
cursor: pointer;
display: inline-block;
font-size: 14px;
font-weight: bold;
margin: 0;
padding: 12px 25px;
text-decoration: none;
text-transform: capitalize;
}
/* -------------------------------------
OTHER STYLES THAT MIGHT BE USEFUL
------------------------------------- */
.last {
margin-bottom: 0;
}
.first {
margin-top: 0;
}
.align-center {
text-align: center;
}
.align-right {
text-align: right;
}
.align-left {
text-align: left;
}
.clear {
clear: both;
}
.mt0 {
margin-top: 0;
}
.mb0 {
margin-bottom: 0;
}
.preheader {
color: transparent;
display: none;
height: 0;
max-height: 0;
max-width: 0;
opacity: 0;
overflow: hidden;
mso-hide: all;
visibility: hidden;
width: 0;
}
.powered-by a {
text-decoration: none;
}
hr {
border: 0;
border-bottom: 1px solid #f6f6f6;
Margin: 20px 0;
}
/* -------------------------------------
RESPONSIVE AND MOBILE FRIENDLY STYLES
------------------------------------- */
@media only screen and (max-width: 620px) {
table[class=body] h1 {
font-size: 28px !important;
margin-bottom: 10px !important;
}
table[class=body] p,
table[class=body] ul,
table[class=body] ol,
table[class=body] td,
table[class=body] span,
table[class=body] a {
font-size: 16px !important;
}
table[class=body] .wrapper,
table[class=body] .article {
padding: 10px !important;
}
table[class=body] .content {
padding: 0 !important;
}
table[class=body] .container {
padding: 0 !important;
width: 100% !important;
}
table[class=body] .main {
border-left-width: 0 !important;
border-radius: 0 !important;
border-right-width: 0 !important;
}
table[class=body] .btn table {
width: 100% !important;
}
table[class=body] .btn a {
width: 100% !important;
}
table[class=body] .img-responsive {
height: auto !important;
max-width: 100% !important;
width: auto !important;
}
}
/* -------------------------------------
PRESERVE THESE STYLES IN THE HEAD
------------------------------------- */
@media all {
.ExternalClass {
width: 100%;
}
.ExternalClass,
.ExternalClass p,
.ExternalClass span,
.ExternalClass font,
.ExternalClass td,
.ExternalClass div {
line-height: 100%;
}
.apple-link a {
color: inherit !important;
font-family: inherit !important;
font-size: inherit !important;
font-weight: inherit !important;
line-height: inherit !important;
text-decoration: none !important;
}
.btn-primary table td:hover {
background-color: #34495e !important;
}
.btn-primary a:hover {
background-color: #34495e !important;
border-color: #34495e !important;
}
}
</style>
</head>
<body class="">
<table role="presentation" border="0" cellpadding="0" cellspacing="0" class="body">
<tr>
<td> </td>
<td class="container">
<div class="content">
<table role="presentation" class="main">
<tr>
<td class="wrapper">
<table role="presentation" border="0" cellpadding="0" cellspacing="0">
<tr>
<td>
<h2>Thank you for submitting your application</h2>
<p>Dear {{ data.name }},</p>
{% if data.outdoorspace.sidewalk %}
<p>You are registered to use sidewalk space at your location address.</p>
<p>You may start using this space in 2 business days. We will contact you if we find issues with your application, proposed location, or insurance documents.</p>
<p>Print <a href="https://sfdsoewd.blob.core.usgovcloudapi.net/permits/ProvisionalPermit-SidewalkSeatingOrRetail.pdf" target="_blank">this PDF of your permit</a> to display. This provisional permit covers sidewalk seating or retail use only. We will also email you a legally binding permit later this week. This temporary permit is valid until December 31, 2020.</p>
<p>You must provide and install diverters around your outdoor space before you operate.</p>
{% endif %}
{% if data.outdoorspace.parkingLane %}
<p>We will review your application for the parking lane. You may not use the parking lane now. We will contact you with next steps within 3 business days.</p>
{% endif %}
{% if data.outdoorspace.sidewalk or data.curbspace == "socialDistancingSpaceForYourCustomersToStandInLine" or data.curbspace == "seatingOrDining" or data.curbspace == "retailUse" %}
<p>You must follow these rules:</p>
<ul>
<li>Follow physical distancing protocols</li>
<li>Display a copy of the permit during business hours</li>
<li>Comply with the Americans with Disabilities Act (ADA) and the SF Better Streets Plan</li>
<li>Keep curb ramps, doors, driveways, fire escapes, or Fire Department connections free of obstructions</li>
<li>Keep furniture in the approved area</li>
<li>Don’t place or store food trays or carts on the sidewalk or parking lane</li>
<li>Keep the parking lane and sidewalk clean of trash, debris, and food waste at all times</li>
<li>Don’t obstruct the sidewalk next to a bus stop or blue curb zone</li>
</ul>
{% endif %}
{% if data.outdoorspace.sidewalk %}
<p>Additional sidewalk rules</p>
<ul>
<li>Make and maintain a straight, clear travel path at least 6 feet (2 yards) wide across your entire sidewalk</li>
<li>Provide and use approved diverters</li>
<li>Keep furniture and diverters clear and free of advertising</li>
<li>Bring in furniture and diverters when you close every day</li>
</ul>
{% endif %}
{% if data.curbspace == "seatingOrDining" %}
<p>Additional parking lane seating rules</p>
<ul>
<li>Add approved traffic barriers</li>
<li>Provide an ADA accessible table and route if another is not available</li>
</ul>
{% endif %}
<p>We will visit your business location to inspect your approved area. We may revoke your permit.</p>
<p><a href="https://sf.gov/information/what-expect-after-you-apply-use-sidewalk-or-parking-lane" target="_blank">What to expect after you apply</a></p>
<p>Email us at <a href="mailto:<EMAIL>"><EMAIL><a/></p>
<p>To track the progress of your application, please use the Shared Spaces tracker: <a href="https://sf.gov/shared-spaces-tracker" target="_blank">https://sf.gov/shared-spaces-tracker</a></p>
</td>
</tr>
</table>
</td>
</tr>
</table>
<div class="footer">
</div>
</div>
</td>
<td> </td>
</tr>
</table>
</body>
</html> | 2a46b6cf9250730c24a7ef0b39ad84f42a30fded | [
"HTML",
"JavaScript",
"TOML",
"Markdown",
"Shell"
] | 8 | JavaScript | SFDigitalServices/sfdigitalservices | 911c8a01d71db57a75c75f64e6245d03a00bd6d3 | ba062d5e6273894983ab4f7a5a1d2e6e6b5aa5ba | |
refs/heads/master | <file_sep>package com.danisable.appdatapersistence
import android.graphics.Color
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import com.danisable.appdatapersistence.utils.Utils
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//PUBLICA 1 PRIVADA 0 COMPRATIDA 2
//Private: 0
//Public: 1
//Share:2
swPer.setOnCheckedChangeListener{ buttonView, isChecked ->
if(isChecked){
Utils.setPreferencesAppData(this, Utils.PREF_DARK_MODE,true)
/*val sharedPreferences: SharedPreferences = getSharedPreferences("PREF_USER", 0)
val editor = sharedPreferences.edit()
editor.putBoolean("isDark",true)
editor.apply()*/
changeBackgroundColor(Color.BLACK)
}else{
Utils.setPreferencesAppData(this, Utils.PREF_DARK_MODE,false)
/*val sharedPreferences: SharedPreferences = getSharedPreferences("PREF_USER", 0)
val editor = sharedPreferences.edit()
editor.putBoolean("isDark",false)
editor.apply()*/
changeBackgroundColor(Color.WHITE)
}
val result: String? = Utils.getPreferencesAppData(this, Utils.PREF_DARK_MODE)
Log.e("TAG",result.toString())
tvText.text = "PREF_DARK_MODE: $result"
}
}
override fun onStart() {
super.onStart()
val result: String? = Utils.getPreferencesAppData(this, Utils.PREF_DARK_MODE)
Log.e("TAG",result.toString())
if (result=="true") {
changeBackgroundColor(Color.BLACK)
}else{
changeBackgroundColor(Color.WHITE)
}
}
private fun changeBackgroundColor(color: Int){
viewLayout.setBackgroundColor(color)
if(color==Color.BLACK) {
tvText.setTextColor(Color.WHITE)
}else{
tvText.setTextColor(Color.BLACK)
}
}
/*private fun getSharedPreferences(): Boolean {
val sharePreferences: SharedPreferences = getSharedPreferences("PREF_USER",0)
return sharePreferences.getBoolean("isDark",false)
}*/
}<file_sep>package com.danisable.appdatapersistence.utils
import android.content.Context
import android.content.SharedPreferences
object Utils {
const val PREF_DARK_MODE = "darkMode"
const val PREF_USER = "pref_user"
private const val PREF_DEFAULT = ""
fun setPreferencesAppData(context: Context, key: String, value: Boolean){
val sharedPreferences: SharedPreferences = context.getSharedPreferences(PREF_USER, 0)
val editor = sharedPreferences.edit()
editor.putString(key,value.toString())
editor.apply()
}
fun getPreferencesAppData(context: Context, key: String): String? {
val sharePreferences: SharedPreferences = context.getSharedPreferences(PREF_USER,0)
return sharePreferences.getString(key, PREF_USER)
}
} | 731e7f1e33fd0cb905202a178aa950b79607637f | [
"Kotlin"
] | 2 | Kotlin | JoseVQuintero/AppDataPersistence | 5a93db8828676705f1d38051fd9e974de6877272 | aa205663b15b7a10a8a10cbfa4edd8c9f35e05ea | |
refs/heads/master | <file_sep>import openpyxl as op
col1 = "Apples"
col2 = "2"
wb = op.load_workbook("file.xlsx")
ws = wb.active
print(ws)
ws.append([col1, col2])
wb.save("file.xlsx")
wb.close()
<file_sep>from bs4 import BeautifulSoup
import os
import datetime
import getpass, os, imaplib, email
import re
import mysql.connector
def filterString(str):
str= str.strip()
return re.sub("[\n\r' ]*","",str)
# method to get the content from a given string
def getContentFromString(mailbody):
# msg_key = mailbody.values()[mailbody.keys().index("Message-ID")]
# print(msg_key)
name=''
email=''
phone=''
message=''
url=''
emailDate=''
emailTo=''
emailFrom=''
tempDate = mailbody.values()[mailbody.keys().index("Date")]
emailDate = datetime.datetime.strptime(tempDate, "%a, %d %b %Y %H:%M:%S %z").strftime('%Y-%m-%d %H:%M:%S')
emailTo = mailbody.values()[mailbody.keys().index("To")]
# print(emailTo)
emailFrom = mailbody.values()[mailbody.keys().index("From")]
# print(emailFrom)
splitstring = mailbody.keys()[-1]+": "+mailbody.values()[-1]
content_str= str(mailbody).split(splitstring)[1]
content_html = BeautifulSoup(content_str,"html.parser")
content = (content_html.findAll("td"))
# print(content_html)
for i in range(0,len(content)):
content_value = content[i].text
if(content_value == "Name:"):
name = content[i+1].text
elif(content_value == "Email:"):
email = content[i+1].text
elif(content_value == "Phone:"):
phone = content[i+1].text
elif(content_value == "Message:"):
message = content[i+1].text
elif(content_value == "URL:"):
url = content[i+1].text;
# print(name)
# print(email)
# print(phone)
# print(message)
# print(url)
sql = (
"INSERT INTO leads1 (name, email, phone, message, url,emaildate,emailto,emailfrom) VALUES ('"
+ filterString(name)
+ "','"
+ filterString(email)
+ "','"
+ filterString(phone)
+ "','"
+ filterString(message)
+ "','"
+ filterString(url)
+ "','"
+ filterString(str(emailDate))
+ "','"
+ filterString(emailTo)
+ "','"
+ filterString(emailFrom)
+ "')"
)
print(sql)
try:
if(url!=''):
sqlcursor.execute(sql)
mydb.commit()
else:
print(content)
with open("emptyurls2.csv","a+")as f:
f.write(str(content_str)+",\n")
f.close()
# exit()
except Exception as e:
print(e)
exit()
# getting messages from the mail
def getMessages():
typ, messages = conn.search(None, '(FROM "MHB" SUBJECT "%s")' % subject)
for i in messages[0].split():
type1, message = conn.fetch(i, "RFC822")
m = email.message_from_bytes(message[0][1])
try:
status = getContentFromString(m)
# input()
except Exception as e:
print("in excepion")
print(e)
# ===================================================
# creating the mysql db connetion to record contents of mails
mydb = mysql.connector.connect(
host="localhost", user="root", passwd="", database="manipalgmail"
)
sqlcursor = mydb.cursor(dictionary=True)
# ======================================================
# credentials of server
servername = "imap.gmail.com"
usernm = "<EMAIL>"
passwd = "<PASSWORD>"
subject = "Manipal"
# establishing connection using imap
conn = imaplib.IMAP4_SSL(servername)
conn.login(usernm, passwd)
conn.select("Inbox")
getMessages()
| 0c449b3ade936e14c239c37fa57dea2c504906f6 | [
"Python"
] | 2 | Python | AbhishekMultiplier/ImportantDocs | 86f0503b1bd83723f1370fe9dbf8a172f87c3e17 | 87685db73b6de8f7707451f15526f9cd5db18e94 | |
refs/heads/master | <file_sep>---
layout: post
title: java源码-ThreadPoolExecutor
date: 2017-08-04 16:21:00
categories:
- java
tags:
- java
- 源码
- 并发
---
我们通过源码来瞅瞅java的线程池是如何实现的。
<br/>
<br/>
<br/>
<br/>
<br/>
<br/>
<br/>
### 简单例子
先通过一个简单的例子来看看吧。
```java
public class TestThreadPoolExecutor {
public static void main(String[] args) {
ExecutorService executor = new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
executor.execute(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
System.out.println("sssssss");
}
});
executor.shutdown();
}
}
```
* 实例化一个executor,调用execute(Runnable)方法
* 调用shutdown()方法。
* 下面我们就通过execute(Runnable)方法进入开始了解```ThreadPoolExecutor```
在了解方法前,我们先来了解下```ThreadPoolExecutor```中的一些重要的参数
* clt:高三位记录当前线程池状态,低29位记录当前线程数量
* corePoolSize:线程池核心线程数量,allowCoreThreadTimeOut(默认为false)为false且当前线程数量小于corePoolSize时,现在不会死亡。
* maximunPoolSize:线程池最大线程数量;
* keepAliveTime:线程执行玩当前任务后活着的状态;
```java
private final AtomicInteger ctl = new AtomicInteger(ctlOf(RUNNING, 0));
//29
private static final int COUNT_BITS = Integer.SIZE - 3;
////00011111111111111111111111111111
private static final int CAPACITY = (1 << COUNT_BITS) - 1;
// runState is stored in the high-order bits
//11100000000000000000000000000000
private static final int RUNNING = -1 << COUNT_BITS;
//0
private static final int SHUTDOWN = 0 << COUNT_BITS;
//00100000000000000000000000000000
private static final int STOP = 1 << COUNT_BITS;
//01000000000000000000000000000000
private static final int TIDYING = 2 << COUNT_BITS;
//01100000000000000000000000000000
private static final int TERMINATED = 3 << COUNT_BITS;
// Packing and unpacking ctl
//获取ctl高三位,状态
private static int runStateOf(int c) { return c & ~CAPACITY; }
//获取线程池数量
private static int workerCountOf(int c) { return c & CAPACITY; }
private static int ctlOf(int rs, int wc) { return rs | wc; }
```
### execute(Runnable)方法
```java
public void execute(Runnable command) {
if (command == null)
throw new NullPointerException();
/*
* Proceed in 3 steps:
*
* 1. If fewer than corePoolSize threads are running, try to
* start a new thread with the given command as its first
* task. The call to addWorker atomically checks runState and
* workerCount, and so prevents false alarms that would add
* threads when it shouldn't, by returning false.
*
* 2. If a task can be successfully queued, then we still need
* to double-check whether we should have added a thread
* (because existing ones died since last checking) or that
* the pool shut down since entry into this method. So we
* recheck state and if necessary roll back the enqueuing if
* stopped, or start a new thread if there are none.
*
* 3. If we cannot queue task, then we try to add a new
* thread. If it fails, we know we are shut down or saturated
* and so reject the task.
*/
//得到ctl
int c = ctl.get();
//判断当前的worker数量是否还是小于自定义的线程池容量 (1)步
if (workerCountOf(c) < corePoolSize) {
//把传入的线程添加到worker中
if (addWorker(command, true))
return;
//添加不成功,得到当前的ctl(因为可能有外因导致了当前线程状态的改变,才会导致addWorker失败,所以需要重新获得ctl)
c = ctl.get();
}
//当前线程池是否运行中 && 把command添加到线程队列的队尾 (2)步
if (isRunning(c) && workQueue.offer(command)) {
int recheck = ctl.get();
if (! isRunning(recheck) && remove(command))
reject(command);
else if (workerCountOf(recheck) == 0)
//如果当前线程池中没有线程 调用addWorker方法
addWorker(null, false);
}
// (3)步
else if (!addWorker(command, false))
reject(command);
}
```
* 主要分三步
- 判断当前线程池中线程数量是否小于```corePoolSize```,小于则添加新的线程,添加成功直接返回
- 判断当前是否运行中&向队列中添加线程,判断当前线程数量是否为0,为0则向线程池中添加线程
- 如果向队列中添加线程失败,然后就尝试向线程池中添加一个新的线程,失败则调用```RejectedExecutionHandler```的方法,默认是抛出一个```RejectedExecutionException```异常
### addWorker(Runnable, boolean)
* Runnable:线程对象
* boolean:false:表示当前线程数量不能大于```maximumPoolSize```;true:表示当前线程数量不能大于```corePoolSize```
```java
private boolean addWorker(Runnable firstTask, boolean core) {
retry:
for (;;) {
int c = ctl.get();
int rs = runStateOf(c);
// Check if queue empty only if necessary.
if (rs >= SHUTDOWN &&
! (rs == SHUTDOWN &&
firstTask == null &&
! workQueue.isEmpty()))
return false;
for (;;) {
int wc = workerCountOf(c);
//当前线程数量大于CAPACITY(线程池中所能添加最大值2^29-1)
//或者线程数量大于自定义的线程最大量,也返回false
if (wc >= CAPACITY ||
wc >= (core ? corePoolSize : maximumPoolSize))
return false;
//线程数量加一
if (compareAndIncrementWorkerCount(c))
break retry;
c = ctl.get(); // Re-read ctl
//线程数量新增失败,如果当前线程状态已经改变,则需要重新判断当前线程的状态
//如果线程状态没变,则说明线程数量变了,只需要重新新增线程数量即可
if (runStateOf(c) != rs)
continue retry;
// else CAS failed due to workerCount change; retry inner loop
}
}
boolean workerStarted = false;
boolean workerAdded = false;
Worker w = null;
try {
// 封装到worker中
w = new Worker(firstTask);
final Thread t = w.thread;
if (t != null) {
final ReentrantLock mainLock = this.mainLock;
//获取锁
mainLock.lock();
try {
// Recheck while holding lock.
// Back out on ThreadFactory failure or if
// shut down before lock acquired.
int rs = runStateOf(ctl.get());
//判断线程池当前是否是RUNNING状态
//亦或线程池状态是SHUTDOWN&传入的线程是null
if (rs < SHUTDOWN ||
(rs == SHUTDOWN && firstTask == null)) {
//判断当前线程是否已经运行
if (t.isAlive()) // precheck that t is startable
throw new IllegalThreadStateException();
//把worker添加到workers中
workers.add(w);
int s = workers.size();
//设置largestPoolSize
if (s > largestPoolSize)
largestPoolSize = s;
workerAdded = true;
}
} finally {
mainLock.unlock();
}
if (workerAdded) {
//运行线程
t.start();
workerStarted = true;
}
}
} finally {
if (! workerStarted)
addWorkerFailed(w);
}
return workerStarted;
}
```
* 先根据线程池的状态,把线程池数量```workercount```数量+1
* 把线程封装到```Worker```类中,然后添加到```workers```集合中
* 如果添加成功,则运行线程
* 看到这里注意到,这个运行的线程是```worker.thread```,那跟我们传入的```firstTask```有什么关系呢
### Worker
```java
private final class Worker
extends AbstractQueuedSynchronizer
implements Runnable
{
/**
* This class will never be serialized, but we provide a
* serialVersionUID to suppress a javac warning.
*/
private static final long serialVersionUID = 6138294804551838833L;
/** Thread this worker is running in. Null if factory fails. */
final Thread thread;
/** Initial task to run. Possibly null. */
Runnable firstTask;
/** Per-thread task counter */
volatile long completedTasks;
/**
* Creates with given first task and thread from ThreadFactory.
* @param firstTask the first task (null if none)
*/
Worker(Runnable firstTask) {
setState(-1); // inhibit interrupts until runWorker
this.firstTask = firstTask;
this.thread = getThreadFactory().newThread(this);
}
/** Delegates main run loop to outer runWorker */
public void run() {
runWorker(this);
}
// Lock methods
//
// The value 0 represents the unlocked state.
// The value 1 represents the locked state.
protected boolean isHeldExclusively() {
return getState() != 0;
}
protected boolean tryAcquire(int unused) {
if (compareAndSetState(0, 1)) {
setExclusiveOwnerThread(Thread.currentThread());
return true;
}
return false;
}
protected boolean tryRelease(int unused) {
setExclusiveOwnerThread(null);
setState(0);
return true;
}
public void lock() { acquire(1); }
public boolean tryLock() { return tryAcquire(1); }
public void unlock() { release(1); }
public boolean isLocked() { return isHeldExclusively(); }
void interruptIfStarted() {
Thread t;
if (getState() >= 0 && (t = thread) != null && !t.isInterrupted()) {
try {
t.interrupt();
} catch (SecurityException ignore) {
}
}
}
}
```
* 很明显可以看到,worker.thread是线程工厂生成的,传入的是worker本身这个Runnable对象
* 所以```t.start()```运行的是worker中的run()方法,也就是```runWorker(this)```方法
### runWorker(this)
```java
final void runWorker(Worker w) {
Thread wt = Thread.currentThread();
Runnable task = w.firstTask;
w.firstTask = null; // 辅助gc
w.unlock(); // allow interrupts
boolean completedAbruptly = true;
try {
// 如果线程task不为空,说明是新增加的线程
// 当然task为空不代表,这个线程就一定不是新添加的线程
// 如果task为空,则通过getTask()方法尝试获取task
while (task != null || (task = getTask()) != null) {
w.lock();
// If pool is stopping, ensure thread is interrupted;
// if not, ensure thread is not interrupted. This
// requires a recheck in second case to deal with
// shutdownNow race while clearing interrupt
// 如果线程正在停止或已经停止,则interrupt当前线程
if ((runStateAtLeast(ctl.get(), STOP) ||
(Thread.interrupted() &&
runStateAtLeast(ctl.get(), STOP))) &&
!wt.isInterrupted())
wt.interrupt();
try {
beforeExecute(wt, task);
Throwable thrown = null;
try {
// 运行线程,也就是firstTask,也就是传入的线程
task.run();
} catch (RuntimeException x) {
thrown = x; throw x;
} catch (Error x) {
thrown = x; throw x;
} catch (Throwable x) {
thrown = x; throw new Error(x);
} finally {
afterExecute(task, thrown);
}
} finally {
task = null;
w.completedTasks++;
w.unlock();
}
}
completedAbruptly = false;
} finally {
processWorkerExit(w, completedAbruptly);
}
}
```
* 如果传入的```Worker```的firstTask不为空,则直接运行这个task
* 如果为空,则通过```getTask()```方法获取task。
* 然后里面会有两个钩子方法```beforeExecute```和```afterExecute```方法
### getTask()
```java
private Runnable getTask() {
boolean timedOut = false; // Did the last poll() time out?
for (;;) {
int c = ctl.get();
int rs = runStateOf(c);
// Check if queue empty only if necessary.
//判断当前线程是否shutdown
if (rs >= SHUTDOWN && (rs >= STOP || workQueue.isEmpty())) {
decrementWorkerCount();
return null;
}
int wc = workerCountOf(c);
// Are workers subject to culling?
// 判断从队列中获取task时使用poll还是take
boolean timed = allowCoreThreadTimeOut || wc > corePoolSize;
// 线程数量大于maximumPoolSize || 已经获取超时过
// 线程队列为空
if ((wc > maximumPoolSize || (timed && timedOut))
&& (wc > 1 || workQueue.isEmpty())) {
// 线程数量减一,返回null,关闭当前线程
if (compareAndDecrementWorkerCount(c))
return null;
continue;
}
try {
//获取线程
Runnable r = timed ?
workQueue.poll(keepAliveTime, TimeUnit.NANOSECONDS) :
workQueue.take();
if (r != null)
return r;
timedOut = true;
} catch (InterruptedException retry) {
timedOut = false;
}
}
}
```
* 这个方法的作用其实就是从线程队列中获取线程。
### 总结
* 看到这里,线程池的总体流程就出来了。
1. 通过入口execute调用方法
2. 判断当前线程池中的总线程数量是否小于```corePoolSize```,小于则直接添加一个线程worker1;大于则把runnable添加到队列中,如果添加到队列失败,则会想线程池中添加非核心线程,但是线程数量不能大于```maximumPoolSize```和```CAPACITY```
3. 如果worker1运行完firstTask,则会继续从线程池中获取task。获取线程时,使用take还是poll根据```allowCoreThreadTimeOut```和```wc > corePoolSize```来确定,超时时间根据```keepAliveTime```来确定
4. 如果线程队列为空,并且已经超时,则关闭当前worker1。如果```timed```为false的话,则worker1不会关闭。
### Executors.newCachedThreadPool()
```java
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<Runnable>());
}
public ThreadPoolExecutor(int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue) {
this(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue,
Executors.defaultThreadFactory(), defaultHandler);
}
```
* corePoolSize:0 核心线程为0,表示当线程队列为空时,所有的线程都会关闭
* maximumPoolSize:Integer.MAX_VALUE 最大的线程数量,其实```CAPACITY```比这个还小
* keepAliveTime:60 线程从队列中获取task的超时时间,超时后关闭当前线程
* 线程队列采用```SynchronousQueue```
- SynchronousQueue有个特点:没有数据缓冲,生产者线程对其的插入操作put必须等待消费者的移除操作take。
- 所以第一次execute(Runnable)调用时,会运行到(3)步;如果运行完firstTask,在60秒内又调用execute(Runnable),则会运行(2)步
### Executors.newFixedThreadPool(int nThreads)
```java
public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
```
* corePoolSize:nThreads,核心线程数为设置值
* maximumPoolSize:nThreads,最大线程数也为设置值,maximumPoolSize与corePoolSize一样大,所以```timed```永远都是false,除非主动的设置```allowCoreThreadTimeOut```的值,所以getTask()时队列获取task时采用的是take(),获取不到会一直阻塞;而且线程也不会自动关闭。
* keepAliveTime:0,
* 线程队列采用```LinkedBlockingQueue```
<file_sep>---
layout: post
title: docker简单mysql主备
date: 2019-12-10 23:00:00
categories:
- mysql
- docker
tags:
- docker
- mysql
---
以下例子的mysql版本为5.7
docker官网下载docker for mac然后直接安装。下载地址自行百度\google
#### 拉取mysql5.7
```
docker pull mysql:5.7
```
#### 配置mysql的master、slave的my.cnf
##### 配置目录
* master
* conf
* my.cnf
* logs
* data
* slave
* conf
* my.cnf
* logs
* data
##### my.cnf
master的my.cnf
```
[mysqld]
#skip-grant-tables
character_set_server=utf8
server_id=1
log_bin=mysql-bin
```
slave的my.cnf
```
[mysqld]
#skip-grant-tables
character_set_server=utf8
server_id=2
log_bin=mysql-slave-bin
relay_log=edu-mysql-relay-bin
```
#### 启动mysql
```
//master目录下
docker run -p 3306:3306 --name mysql_master -v $PWD/conf/my.cnf:/etc/mysql/my.cnf -v $PWD/logs:/logs -v $PWD/data:/mysql_data -e MYSQL_ROOT_PASSWORD=<PASSWORD> -d mysql:5.7
//slave目录下
docker run -p 3307:3306 --name mysql_slave -v $PWD/conf/my.cnf:/etc/mysql/my.cnf -v $PWD/logs:/logs -v $PWD/data:/mysql_data -e MYSQL_ROOT_PASSWORD=<PASSWORD> -d mysql:5.7
```
#### master配置
新建一个主备账户,并且记录当前master的status
```
grant replication slave on *.* to 'testSlave'@'%' identified by 'testSlave';
flush privileges;
show master status;
+------------------+----------+--------------+------------------+-------------------+
| File | Position | Binlog_Do_DB | Binlog_Ignore_DB | Executed_Gtid_Set |
+------------------+----------+--------------+------------------+-------------------+
| mysql-bin.000003 | 594 | | | |
+------------------+----------+--------------+------------------+-------------------+
//记录File和Positoin
```
#### slave配置
获取master容器的ip,假如是:172.17.0.2
```
docker inspect mysql_master | grep IPAddress
```
配置slave
```
CHANGE MASTER TO
MASTER_HOST='172.17.0.2',
MASTER_USER='testSlave',
MASTER_PASSWORD='<PASSWORD>',
MASTER_LOG_FILE='mysql-bin.000003',
MASTER_LOG_POS=594;
start slave;
```
查看slave的status
```
show slave status\G;
```
如下显示,则表示配置成功
```
Slave_IO_State: Waiting for master to send event
.
.
.
Slave_IO_Running: Yes
Slave_SQL_Running: Yes
.
.
.
```
#### 测试
进入master容器,新建数据库
```
docker exec -it mysql_master bash;
mysql -uroot -p123456;
create database canal_sync;
```
查看slave容器内的数据库,可以看到已经有了canal_sync数据库
<file_sep>---
layout: post
title: spring源码-spring初始化时bean的生成
date: 2017-09-05 16:21:00
categories:
- java
- spring
tags:
- spring
- java
- 源码
---
在spring中,我们可以通过xml或注解的方式去配置一个bean,配置好后,在spring启动的时候,spring会根据配置的属性去实例化这个bean,有可能是单例的,也有可能是多例的。
这里不会对spring如何收集bean属性做过多的说明,前面有一篇博文已经对xml的bean属性解析做过说明了,这里主要想要聊得还是spring在启动的时候,是如何生成一个个的bean对象的。
### getBean方法
* 通过跟踪spring的源码,会发现bean对象都是通过```AbstractBeanFactory.getBean(参数)```方法来生成的。
* ```AbstractBeanFactory.getBean(参数)```方法实际上会去调用```doGetBean(参数)```方法。
### doGetBean方法
```java
protected <T> T doGetBean(
final String name, final Class<T> requiredType, final Object[] args, boolean typeCheckOnly)
throws BeansException {
//获取实际的beanName,去除beanFactory的&,根据alias获取实际的beanId
final String beanName = transformedBeanName(name);
Object bean;
// Eagerly check singleton cache for manually registered singletons.
//获取单例对象
Object sharedInstance = getSingleton(beanName);
if (sharedInstance != null && args == null) {
if (logger.isDebugEnabled()) {
if (isSingletonCurrentlyInCreation(beanName)) {
logger.debug("Returning eagerly cached instance of singleton bean '" + beanName +
"' that is not fully initialized yet - a consequence of a circular reference");
}
else {
logger.debug("Returning cached instance of singleton bean '" + beanName + "'");
}
}
//当前类是factoryBean对象,则通过其生成对象
//如果是普通对象,则直接返回
bean = getObjectForBeanInstance(sharedInstance, name, beanName, null);
}
else {
// Fail if we're already creating this bean instance:
// We're assumably within a circular reference.
//判断多例类型的对象是否正在创建,显然可以看出,非单例如果有循环依赖的话,会直接抛出异常
if (isPrototypeCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(beanName);
}
// Check if bean definition exists in this factory.
//获取父的beanFactory,如果存在,而且当前没有beanName的定义,则从父的beanFactory中获取对象
BeanFactory parentBeanFactory = getParentBeanFactory();
if (parentBeanFactory != null && !containsBeanDefinition(beanName)) {
// Not found -> check parent.
String nameToLookup = originalBeanName(name);
if (args != null) {
// Delegation to parent with explicit args.
return (T) parentBeanFactory.getBean(nameToLookup, args);
}
else {
// No args -> delegate to standard getBean method.
return parentBeanFactory.getBean(nameToLookup, requiredType);
}
}
//标记bean的创建
if (!typeCheckOnly) {
markBeanAsCreated(beanName);
}
try {
//获取beanDefinition
final RootBeanDefinition mbd = getMergedLocalBeanDefinition(beanName);
//检查当前要创建对象是否是abstract
checkMergedBeanDefinition(mbd, beanName, args);
// Guarantee initialization of beans that the current bean depends on.
String[] dependsOn = mbd.getDependsOn();
//为depend-on依赖创建对象,递归调用getBean
if (dependsOn != null) {
for (String dep : dependsOn) {
if (isDependent(beanName, dep)) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Circular depends-on relationship between '" + beanName + "' and '" + dep + "'");
}
registerDependentBean(dep, beanName);
getBean(dep);
}
}
// Create bean instance.
//单例的方式创建对象
if (mbd.isSingleton()) {
sharedInstance = getSingleton(beanName, new ObjectFactory<Object>() {
@Override
public Object getObject() throws BeansException {
try {
return createBean(beanName, mbd, args);
}
catch (BeansException ex) {
// Explicitly remove instance from singleton cache: It might have been put there
// eagerly by the creation process, to allow for circular reference resolution.
// Also remove any beans that received a temporary reference to the bean.
destroySingleton(beanName);
throw ex;
}
}
});
bean = getObjectForBeanInstance(sharedInstance, name, beanName, mbd);
}
//多例的方式创建对象
else if (mbd.isPrototype()) {
// It's a prototype -> create a new instance.
Object prototypeInstance = null;
try {
beforePrototypeCreation(beanName);
prototypeInstance = createBean(beanName, mbd, args);
}
finally {
afterPrototypeCreation(beanName);
}
bean = getObjectForBeanInstance(prototypeInstance, name, beanName, mbd);
}
//其他方式创建对象
else {
String scopeName = mbd.getScope();
final Scope scope = this.scopes.get(scopeName);
if (scope == null) {
throw new IllegalStateException("No Scope registered for scope name '" + scopeName + "'");
}
try {
Object scopedInstance = scope.get(beanName, new ObjectFactory<Object>() {
@Override
public Object getObject() throws BeansException {
beforePrototypeCreation(beanName);
try {
return createBean(beanName, mbd, args);
}
finally {
afterPrototypeCreation(beanName);
}
}
});
bean = getObjectForBeanInstance(scopedInstance, name, beanName, mbd);
}
catch (IllegalStateException ex) {
throw new BeanCreationException(beanName,
"Scope '" + scopeName + "' is not active for the current thread; consider " +
"defining a scoped proxy for this bean if you intend to refer to it from a singleton",
ex);
}
}
}
catch (BeansException ex) {
cleanupAfterBeanCreationFailure(beanName);
throw ex;
}
}
// Check if required type matches the type of the actual bean instance.
if (requiredType != null && bean != null && !requiredType.isAssignableFrom(bean.getClass())) {
try {
return getTypeConverter().convertIfNecessary(bean, requiredType);
}
catch (TypeMismatchException ex) {
if (logger.isDebugEnabled()) {
logger.debug("Failed to convert bean '" + name + "' to required type '" +
ClassUtils.getQualifiedName(requiredType) + "'", ex);
}
throw new BeanNotOfRequiredTypeException(name, requiredType, bean.getClass());
}
}
return (T) bean;
}
```
1. 根据传入的beanName获取实际的name
* factoryBean如果是以&开头,则去除它
* 如果传入的是一个alias,则会返回其实际的beanId
2. 根据beanName获取单例对象(此对象有可能是提早暴露出来的对象),如果对象存在,则返回它。
3. 会判断当前对象是否正以非单例的方式创建,这里说明如果有循环依赖存在,且循环依赖的对象还是多例的,则会抛出异常
4. 获取父beanFactory
5. 标记对象正在创建
6. 根据对象scope进行对象的实际创建工作
#### transformedBeanName(name)
```java
protected String transformedBeanName(String name) {
return canonicalName(BeanFactoryUtils.transformedBeanName(name));
}
//去除开始的&符号
public static String transformedBeanName(String name) {
Assert.notNull(name, "'name' must not be null");
String beanName = name;
while (beanName.startsWith(BeanFactory.FACTORY_BEAN_PREFIX)) {
beanName = beanName.substring(BeanFactory.FACTORY_BEAN_PREFIX.length());
}
return beanName;
}
//根据别名获取beanid
public String canonicalName(String name) {
String canonicalName = name;
String resolvedName;
do {
resolvedName = (String)this.aliasMap.get(canonicalName);
if(resolvedName != null) {
canonicalName = resolvedName;
}
} while(resolvedName != null);
return canonicalName;
}
```
1. 如果beanName是以&开头,则去除&,有&表示```getBean()```获取factoryBean对象,并不是获取factoryBean生成的对象
2. 如果传入的是alias,则获取其实际的beanId
#### getSingleton(beanName)
```java
public Object getSingleton(String beanName) {
return getSingleton(beanName, true);
}
//allowEarlyReference表示是否可以获取过早暴露(还未创建完成)的单例对象
protected Object getSingleton(String beanName, boolean allowEarlyReference) {
Object singletonObject = this.singletonObjects.get(beanName);
if (singletonObject == null && isSingletonCurrentlyInCreation(beanName)) {
synchronized (this.singletonObjects) {
singletonObject = this.earlySingletonObjects.get(beanName);
if (singletonObject == null && allowEarlyReference) {
ObjectFactory<?> singletonFactory = this.singletonFactories.get(beanName);
if (singletonFactory != null) {
singletonObject = singletonFactory.getObject();
this.earlySingletonObjects.put(beanName, singletonObject);
this.singletonFactories.remove(beanName);
}
}
}
}
return (singletonObject != NULL_OBJECT ? singletonObject : null);
}
```
1. 如果单例对象已经创建成功的话,这个方法会返回一个单例对象
2. 这个方法对于解决单例对象的循环依赖起到了很好的作用,具体在这就不讨论了,有兴趣的可以看看我下面贴出的链接!!
#### getObjectForBeanInstance
```java
protected Object getObjectForBeanInstance(
Object beanInstance, String name, String beanName, RootBeanDefinition mbd) {
// Don't let calling code try to dereference the factory if the bean isn't a factory.
//判断以&开头 且不是factorybean
if (BeanFactoryUtils.isFactoryDereference(name) && !(beanInstance instanceof FactoryBean)) {
throw new BeanIsNotAFactoryException(transformedBeanName(name), beanInstance.getClass());
}
// Now we have the bean instance, which may be a normal bean or a FactoryBean.
// If it's a FactoryBean, we use it to create a bean instance, unless the
// caller actually wants a reference to the factory.
//不是beanFactory对象亦或以&开头的对象 直接返回
if (!(beanInstance instanceof FactoryBean) || BeanFactoryUtils.isFactoryDereference(name)) {
return beanInstance;
}
Object object = null;
if (mbd == null) {
//从缓存中获取
object = getCachedObjectForFactoryBean(beanName);
}
if (object == null) {
// Return bean instance from factory.
FactoryBean<?> factory = (FactoryBean<?>) beanInstance;
// Caches object obtained from FactoryBean if it is a singleton.
if (mbd == null && containsBeanDefinition(beanName)) {
//合并属性
mbd = getMergedLocalBeanDefinition(beanName);
}
boolean synthetic = (mbd != null && mbd.isSynthetic());
//通过factoryBean生成对象并放入缓存
object = getObjectFromFactoryBean(factory, beanName, !synthetic);
}
return object;
}
protected Object getObjectFromFactoryBean(FactoryBean<?> factory, String beanName, boolean shouldPostProcess) {
if (factory.isSingleton() && containsSingleton(beanName)) {
//单例模式下factoryBean生成对象逻辑
synchronized (getSingletonMutex()) {
Object object = this.factoryBeanObjectCache.get(beanName);
if (object == null) {
//通过factory生成对象
object = doGetObjectFromFactoryBean(factory, beanName);
// Only post-process and store if not put there already during getObject() call above
// (e.g. because of circular reference processing triggered by custom getBean calls)
Object alreadyThere = this.factoryBeanObjectCache.get(beanName);
if (alreadyThere != null) {
object = alreadyThere;
}
else {
if (object != null && shouldPostProcess) {
try {
//调用beanProcess的after处理方法
object = postProcessObjectFromFactoryBean(object, beanName);
}
catch (Throwable ex) {
throw new BeanCreationException(beanName,
"Post-processing of FactoryBean's singleton object failed", ex);
}
}
this.factoryBeanObjectCache.put(beanName, (object != null ? object : NULL_OBJECT));
}
}
return (object != NULL_OBJECT ? object : null);
}
}
else {
Object object = doGetObjectFromFactoryBean(factory, beanName);
if (object != null && shouldPostProcess) {
try {
object = postProcessObjectFromFactoryBean(object, beanName);
}
catch (Throwable ex) {
throw new BeanCreationException(beanName, "Post-processing of FactoryBean's object failed", ex);
}
}
return object;
}
}
private Object doGetObjectFromFactoryBean(final FactoryBean<?> factory, final String beanName)
throws BeanCreationException {
Object object;
try {
if (System.getSecurityManager() != null) {
AccessControlContext acc = getAccessControlContext();
try {
object = AccessController.doPrivileged(new PrivilegedExceptionAction<Object>() {
@Override
public Object run() throws Exception {
return factory.getObject();
}
}, acc);
}
catch (PrivilegedActionException pae) {
throw pae.getException();
}
}
else {
object = factory.getObject();//通过factoryBean生成对象
}
}
catch (FactoryBeanNotInitializedException ex) {
throw new BeanCurrentlyInCreationException(beanName, ex.toString());
}
catch (Throwable ex) {
throw new BeanCreationException(beanName, "FactoryBean threw exception on object creation", ex);
}
// Do not accept a null value for a FactoryBean that's not fully
// initialized yet: Many FactoryBeans just return null then.
if (object == null && isSingletonCurrentlyInCreation(beanName)) {
throw new BeanCurrentlyInCreationException(
beanName, "FactoryBean which is currently in creation returned null from getObject");
}
return object;
}
```
1. 如果是factoryBean且beanName不以&开头,则通过factoryBean生成对象,并且返回。
2. 如果是普通bean则直接返回
#### 获取单例对象 getSingleton(String beanName, ObjectFactory<?> singletonFactory)
```java
public Object getSingleton(String beanName, ObjectFactory<?> singletonFactory) {
Assert.notNull(beanName, "'beanName' must not be null");
synchronized (this.singletonObjects) {
//如果单例对象已经存在则,直接返回
Object singletonObject = this.singletonObjects.get(beanName);
if (singletonObject == null) {
//判断当前单例是否正在创建
if (this.singletonsCurrentlyInDestruction) {
throw new BeanCreationNotAllowedException(beanName,
"Singleton bean creation not allowed while singletons of this factory are in destruction " +
"(Do not request a bean from a BeanFactory in a destroy method implementation!)");
}
if (logger.isDebugEnabled()) {
logger.debug("Creating shared instance of singleton bean '" + beanName + "'");
}
//向集合singletonsCurrentlyInCreation中添加值
beforeSingletonCreation(beanName);
boolean newSingleton = false;
boolean recordSuppressedExceptions = (this.suppressedExceptions == null);
if (recordSuppressedExceptions) {
this.suppressedExceptions = new LinkedHashSet<Exception>();
}
try {
//通过ObjectFactory获取单例对象,实际的处理方法是上层的createBean(beanName, ex1, args)方法
singletonObject = singletonFactory.getObject();
newSingleton = true;
}
catch (IllegalStateException ex) {
// Has the singleton object implicitly appeared in the meantime ->
// if yes, proceed with it since the exception indicates that state.
singletonObject = this.singletonObjects.get(beanName);
if (singletonObject == null) {
throw ex;
}
}
catch (BeanCreationException ex) {
if (recordSuppressedExceptions) {
for (Exception suppressedException : this.suppressedExceptions) {
ex.addRelatedCause(suppressedException);
}
}
throw ex;
}
finally {
if (recordSuppressedExceptions) {
this.suppressedExceptions = null;
}
afterSingletonCreation(beanName);
}
if (newSingleton) {
//把生成的对象添加到缓存中
addSingleton(beanName, singletonObject);
}
}
return (singletonObject != NULL_OBJECT ? singletonObject : null);
}
}
```
1. 首先从缓存中获取单例对象,存在则直接返回
2. 调用ObjectFactory的方法获取单例对象
3. 把获取的单例对象添加到缓存中
### 正式创建bean对象 createBean(String beanName, RootBeanDefinition mbd, Object[] args)
```java
protected Object createBean(String beanName, RootBeanDefinition mbd, Object[] args) throws BeanCreationException {
if (logger.isDebugEnabled()) {
logger.debug("Creating instance of bean '" + beanName + "'");
}
RootBeanDefinition mbdToUse = mbd;
// Make sure bean class is actually resolved at this point, and
// clone the bean definition in case of a dynamically resolved Class
// which cannot be stored in the shared merged bean definition.
Class<?> resolvedClass = resolveBeanClass(mbd, beanName);
if (resolvedClass != null && !mbd.hasBeanClass() && mbd.getBeanClassName() != null) {
mbdToUse = new RootBeanDefinition(mbd);
mbdToUse.setBeanClass(resolvedClass);
}
// Prepare method overrides.
try {
//主要标记一下有没有方法增强
mbdToUse.prepareMethodOverrides();
}
catch (BeanDefinitionValidationException ex) {
throw new BeanDefinitionStoreException(mbdToUse.getResourceDescription(),
beanName, "Validation of method overrides failed", ex);
}
try {
// Give BeanPostProcessors a chance to return a proxy instead of the target bean instance.
//给beanPostProcessors一个机会返回一个代理类
//内部会调用InstantiationAwareBeanPostProcessor类的方法
//aop处理类实现了此接口
Object bean = resolveBeforeInstantiation(beanName, mbdToUse);
if (bean != null) {
return bean;
}
}
catch (Throwable ex) {
throw new BeanCreationException(mbdToUse.getResourceDescription(), beanName,
"BeanPostProcessor before instantiation of bean failed", ex);
}
Object beanInstance = doCreateBean(beanName, mbdToUse, args);
if (logger.isDebugEnabled()) {
logger.debug("Finished creating instance of bean '" + beanName + "'");
}
return beanInstance;
}
```
1. 保证beanName能正确的解析成class
2. 标记一下当前方法有没有增强方法
3. 给beanPostProcessors一个机会,一旦其返回一个对象,则直接使用这个对象;其调用的是```InstantiationAwareBeanPostProcessor```接口类的方法;而aop的处理器则是实现的这个接口的子类```SmartInstantiationAwareBeanPostProcessor```。
4. 如果beanPostProcessors没有返回对象,则走正常的创建bean的流程,把创建的工作交给```doCreateBean(beanName, mbdToUse, args)```方法
#### doCreateBean(beanName, mbdToUse, args)
```java
protected Object doCreateBean(final String beanName, final RootBeanDefinition mbd, final Object[] args)
throws BeanCreationException {
// Instantiate the bean.
BeanWrapper instanceWrapper = null;
if (mbd.isSingleton()) {
instanceWrapper = this.factoryBeanInstanceCache.remove(beanName);
}
if (instanceWrapper == null) {
//创建bean的包装类
instanceWrapper = createBeanInstance(beanName, mbd, args);
}
final Object bean = (instanceWrapper != null ? instanceWrapper.getWrappedInstance() : null);
Class<?> beanType = (instanceWrapper != null ? instanceWrapper.getWrappedClass() : null);
// Allow post-processors to modify the merged bean definition.
synchronized (mbd.postProcessingLock) {
if (!mbd.postProcessed) {
try {
//调用MergedBeanDefinitionPostProcessor处理器
applyMergedBeanDefinitionPostProcessors(mbd, beanType, beanName);
}
catch (Throwable ex) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Post-processing of merged bean definition failed", ex);
}
mbd.postProcessed = true;
}
}
// Eagerly cache singletons to be able to resolve circular references
// even when triggered by lifecycle interfaces like BeanFactoryAware.
//判断是不是需要提早暴露对象,主要用于处理循环依赖
boolean earlySingletonExposure = (mbd.isSingleton() && this.allowCircularReferences &&
isSingletonCurrentlyInCreation(beanName));
if (earlySingletonExposure) {
if (logger.isDebugEnabled()) {
logger.debug("Eagerly caching bean '" + beanName +
"' to allow for resolving potential circular references");
}
addSingletonFactory(beanName, new ObjectFactory<Object>() {
@Override
public Object getObject() throws BeansException {
return getEarlyBeanReference(beanName, mbd, bean);
}
});
}
// Initialize the bean instance.
Object exposedObject = bean;
try {
//填装属性
populateBean(beanName, mbd, instanceWrapper);
if (exposedObject != null) {
//初始化对象
exposedObject = initializeBean(beanName, exposedObject, mbd);
}
}
catch (Throwable ex) {
if (ex instanceof BeanCreationException && beanName.equals(((BeanCreationException) ex).getBeanName())) {
throw (BeanCreationException) ex;
}
else {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Initialization of bean failed", ex);
}
}
//这主要做一个循环依赖的检测,检测循环依赖的对象是否相同
if (earlySingletonExposure) {
Object earlySingletonReference = getSingleton(beanName, false);
if (earlySingletonReference != null) {
if (exposedObject == bean) {
exposedObject = earlySingletonReference;
}
else if (!this.allowRawInjectionDespiteWrapping && hasDependentBean(beanName)) {
String[] dependentBeans = getDependentBeans(beanName);
Set<String> actualDependentBeans = new LinkedHashSet<String>(dependentBeans.length);
for (String dependentBean : dependentBeans) {
if (!removeSingletonIfCreatedForTypeCheckOnly(dependentBean)) {
actualDependentBeans.add(dependentBean);
}
}
if (!actualDependentBeans.isEmpty()) {
throw new BeanCurrentlyInCreationException(beanName,
"Bean with name '" + beanName + "' has been injected into other beans [" +
StringUtils.collectionToCommaDelimitedString(actualDependentBeans) +
"] in its raw version as part of a circular reference, but has eventually been " +
"wrapped. This means that said other beans do not use the final version of the " +
"bean. This is often the result of over-eager type matching - consider using " +
"'getBeanNamesOfType' with the 'allowEagerInit' flag turned off, for example.");
}
}
}
}
// Register bean as disposable.
try {
registerDisposableBeanIfNecessary(beanName, bean, mbd);
}
catch (BeanDefinitionValidationException ex) {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Invalid destruction signature", ex);
}
return exposedObject;
}
```
1. 生成bean的包装类对象
2. 调用MergedBeanDefinitionPostProcessor处理器的postProcessMergedBeanDefinition方法
3. 单例模式下提早的暴露当前对象,用于处理循环依赖问题
4. 填装属性,如果内部属性也是一个对象,则会递归调用getBean,所以有可能会存在循环依赖的问题
5. 单例模式下做一个循环依赖对象的检测,检测循环依赖的对象是否相同
#### createBeanInstance
```java
protected BeanWrapper createBeanInstance(String beanName, RootBeanDefinition mbd, Object[] args) {
// Make sure bean class is actually resolved at this point.
Class<?> beanClass = resolveBeanClass(mbd, beanName);
if (beanClass != null && !Modifier.isPublic(beanClass.getModifiers()) && !mbd.isNonPublicAccessAllowed()) {
throw new BeanCreationException(mbd.getResourceDescription(), beanName,
"Bean class isn't public, and non-public access not allowed: " + beanClass.getName());
}
if (mbd.getFactoryMethodName() != null) {
return instantiateUsingFactoryMethod(beanName, mbd, args);
}
// Shortcut when re-creating the same bean...
boolean resolved = false;
boolean autowireNecessary = false;
if (args == null) {
synchronized (mbd.constructorArgumentLock) {
if (mbd.resolvedConstructorOrFactoryMethod != null) {
resolved = true;
autowireNecessary = mbd.constructorArgumentsResolved;
}
}
}
if (resolved) {
if (autowireNecessary) {
return autowireConstructor(beanName, mbd, null, null);
}
else {
return instantiateBean(beanName, mbd);
}
}
// Need to determine the constructor...
Constructor<?>[] ctors = determineConstructorsFromBeanPostProcessors(beanClass, beanName);
if (ctors != null ||
mbd.getResolvedAutowireMode() == RootBeanDefinition.AUTOWIRE_CONSTRUCTOR ||
mbd.hasConstructorArgumentValues() || !ObjectUtils.isEmpty(args)) {
return autowireConstructor(beanName, mbd, ctors, args);
}
// No special handling: simply use no-arg constructor.
return instantiateBean(beanName, mbd);
}
```
1. 如果是factoryBean则委托```instantiateUsingFactoryMethod(beanName, mbd, args)```方法来创建对象
2. 如果构造方法上有autowire注解,则委托```autowireConstructor(beanName, mbd, null, null)```方法来创建对象
3. 最后使用```instantiateBean(beanName, mbd)```来创建对象,即constructor.newInstance();
#### addSingletonFactory
```java
protected void addSingletonFactory(String beanName, ObjectFactory<?> singletonFactory) {
Assert.notNull(singletonFactory, "Singleton factory must not be null");
synchronized (this.singletonObjects) {
if (!this.singletonObjects.containsKey(beanName)) {
this.singletonFactories.put(beanName, singletonFactory);
this.earlySingletonObjects.remove(beanName);
this.registeredSingletons.add(beanName);
}
}
}
```
1. 内部主要有两个中间集合```singletonFactories```:用于存储beanName和ObjectFactory关系。```earlySingletonObjects```:用于存储提早暴露出来的对象。两个集合在单例对象正式生成后都会被清空。
2. 此方法主要用于解决单例对象的循环依赖
#### populateBean
```java
protected void populateBean(String beanName, RootBeanDefinition mbd, BeanWrapper bw) {
PropertyValues pvs = mbd.getPropertyValues();
if (bw == null) {
if (!pvs.isEmpty()) {
throw new BeanCreationException(
mbd.getResourceDescription(), beanName, "Cannot apply property values to null instance");
}
else {
// Skip property population phase for null instance.
return;
}
}
// Give any InstantiationAwareBeanPostProcessors the opportunity to modify the
// state of the bean before properties are set. This can be used, for example,
// to support styles of field injection.
boolean continueWithPropertyPopulation = true;
if (!mbd.isSynthetic() && hasInstantiationAwareBeanPostProcessors()) {
for (BeanPostProcessor bp : getBeanPostProcessors()) {
if (bp instanceof InstantiationAwareBeanPostProcessor) {
InstantiationAwareBeanPostProcessor ibp = (InstantiationAwareBeanPostProcessor) bp;
if (!ibp.postProcessAfterInstantiation(bw.getWrappedInstance(), beanName)) {
continueWithPropertyPopulation = false;
break;
}
}
}
}
if (!continueWithPropertyPopulation) {
return;
}
if (mbd.getResolvedAutowireMode() == RootBeanDefinition.AUTOWIRE_BY_NAME ||
mbd.getResolvedAutowireMode() == RootBeanDefinition.AUTOWIRE_BY_TYPE) {
MutablePropertyValues newPvs = new MutablePropertyValues(pvs);
// Add property values based on autowire by name if applicable.
if (mbd.getResolvedAutowireMode() == RootBeanDefinition.AUTOWIRE_BY_NAME) {
autowireByName(beanName, mbd, bw, newPvs);
}
// Add property values based on autowire by type if applicable.
if (mbd.getResolvedAutowireMode() == RootBeanDefinition.AUTOWIRE_BY_TYPE) {
autowireByType(beanName, mbd, bw, newPvs);
}
pvs = newPvs;
}
boolean hasInstAwareBpps = hasInstantiationAwareBeanPostProcessors();
boolean needsDepCheck = (mbd.getDependencyCheck() != RootBeanDefinition.DEPENDENCY_CHECK_NONE);
if (hasInstAwareBpps || needsDepCheck) {
PropertyDescriptor[] filteredPds = filterPropertyDescriptorsForDependencyCheck(bw, mbd.allowCaching);
if (hasInstAwareBpps) {
for (BeanPostProcessor bp : getBeanPostProcessors()) {
if (bp instanceof InstantiationAwareBeanPostProcessor) {
InstantiationAwareBeanPostProcessor ibp = (InstantiationAwareBeanPostProcessor) bp;
pvs = ibp.postProcessPropertyValues(pvs, filteredPds, bw.getWrappedInstance(), beanName);
if (pvs == null) {
return;
}
}
}
}
if (needsDepCheck) {
checkDependencies(beanName, mbd, filteredPds, pvs);
}
}
applyPropertyValues(beanName, mbd, bw, pvs);
}
```
1. 先调用InstantiationAwareBeanPostProcessor的postProcessAfterInstantiation方法,如果返回false,则跳过属性的自动填充
2. 对象的填充会递归调用getBean方法
#### initializeBean
```java
protected Object initializeBean(final String beanName, final Object bean, RootBeanDefinition mbd) {
if (System.getSecurityManager() != null) {
AccessController.doPrivileged(new PrivilegedAction<Object>() {
@Override
public Object run() {
invokeAwareMethods(beanName, bean);
return null;
}
}, getAccessControlContext());
}
else {
//设置aware属性
invokeAwareMethods(beanName, bean);
}
Object wrappedBean = bean;
if (mbd == null || !mbd.isSynthetic()) {
//调用beanProcessor.postProcessBeforeInitialization(result, beanName);
wrappedBean = applyBeanPostProcessorsBeforeInitialization(wrappedBean, beanName);
}
try {
//调用初始化方法
invokeInitMethods(beanName, wrappedBean, mbd);
}
catch (Throwable ex) {
throw new BeanCreationException(
(mbd != null ? mbd.getResourceDescription() : null),
beanName, "Invocation of init method failed", ex);
}
if (mbd == null || !mbd.isSynthetic()) {
//调用beanProcessor.postProcessAfterInitialization(result, beanName);
wrappedBean = applyBeanPostProcessorsAfterInitialization(wrappedBean, beanName);
}
return wrappedBean;
}
private void invokeAwareMethods(final String beanName, final Object bean) {
if (bean instanceof Aware) {
if (bean instanceof BeanNameAware) {
((BeanNameAware) bean).setBeanName(beanName);
}
if (bean instanceof BeanClassLoaderAware) {
((BeanClassLoaderAware) bean).setBeanClassLoader(getBeanClassLoader());
}
if (bean instanceof BeanFactoryAware) {
((BeanFactoryAware) bean).setBeanFactory(AbstractAutowireCapableBeanFactory.this);
}
}
}
protected void invokeInitMethods(String beanName, final Object bean, RootBeanDefinition mbd)
throws Throwable {
boolean isInitializingBean = (bean instanceof InitializingBean);
if (isInitializingBean && (mbd == null || !mbd.isExternallyManagedInitMethod("afterPropertiesSet"))) {
if (logger.isDebugEnabled()) {
logger.debug("Invoking afterPropertiesSet() on bean with name '" + beanName + "'");
}
if (System.getSecurityManager() != null) {
try {
AccessController.doPrivileged(new PrivilegedExceptionAction<Object>() {
@Override
public Object run() throws Exception {
((InitializingBean) bean).afterPropertiesSet();
return null;
}
}, getAccessControlContext());
}
catch (PrivilegedActionException pae) {
throw pae.getException();
}
}
else {
//调用afterPropertiesSet方法
((InitializingBean) bean).afterPropertiesSet();
}
}
if (mbd != null) {
//调用init-method方法
String initMethodName = mbd.getInitMethodName();
if (initMethodName != null && !(isInitializingBean && "afterPropertiesSet".equals(initMethodName)) &&
!mbd.isExternallyManagedInitMethod(initMethodName)) {
invokeCustomInitMethod(beanName, bean, mbd);
}
}
}
```
1. 为Aware类自动装配属性
2. 调用初始化的方法
### 总结
这里主要针对的是spring bean对象的初始化,关于方法的增强,比如aop会通过beanPostProcessor生成代理类等待这里都没有细说。
spring循环依赖的问题可以参考下面的链接:[Spring中循环引用的处理](https://www.iflym.com/index.php/code/201208280001.html"Spring中循环引用的处理")
参考:
1. [i flym 博客](https://www.iflym.com/"i flym 博客")
2. [Spring源码深度解析 郝佳]
<file_sep>---
layout: post
title: spring的event事件
date: 2017-08-04 16:21:00
categories:
- java
- spring
tags:
- spring
- java
---
先来看个简单的spring事件监听的例子
```java
@Component
public class TestListener implements ApplicationListener<TestEvent> {
@Override
public void onApplicationEvent(TestEvent event) {
// TODO Auto-generated method stub
String name = event.getName() ;
System.out.println("name= " + name);
}
}
public class TestEvent extends ApplicationEvent {
private String name ;
public TestEvent(String source) {
super(source);
this.name = source ;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
*
*/
private static final long serialVersionUID = 1L;
}
public class TestMain {
public static void main(String[] args) {
ApplicationContext ctx = new ClassPathXmlApplicationContext("spring/dispatcherServlet-servlet.xml");
ctx.publishEvent(new TestEvent("111111"));
}
}
```
运行结果:<br />
> name= 111111
* 调用了```ApplicationContext.publishEvent()```方法后,```listener```中的方法就会被自动调用。
* 那么具体是怎么调用的呢?我们进入```ApplicationContext.publishEvent()```方法看看是怎么实现的
```java
@Override
public void publishEvent(ApplicationEvent event) {
Assert.notNull(event, "Event must not be null");
if (logger.isTraceEnabled()) {
logger.trace("Publishing event in " + getDisplayName() + ": " + event);
}
getApplicationEventMulticaster().multicastEvent(event);
if (this.parent != null) {
this.parent.publishEvent(event);
}
}
```
* 实际上```ApplicationContext```类中并没有```publishEvent```方法,这个方法是在其父类中```AbstractApplicationContext```实现的
* 实际上处理是委托给```SimpleApplicationEventMulticaster```的```multicastEvent```方法
```java
@Override
public void multicastEvent(final ApplicationEvent event) {
for (final ApplicationListener<?> listener : getApplicationListeners(event)) {
Executor executor = getTaskExecutor();
if (executor != null) {
executor.execute(new Runnable() {
@Override
public void run() {
invokeListener(listener, event);
}
});
}
else {
invokeListener(listener, event);
}
}
}
@SuppressWarnings({"unchecked", "rawtypes"})
protected void invokeListener(ApplicationListener listener, ApplicationEvent event) {
ErrorHandler errorHandler = getErrorHandler();
if (errorHandler != null) {
try {
listener.onApplicationEvent(event);
}
catch (Throwable err) {
errorHandler.handleError(err);
}
}
else {
listener.onApplicationEvent(event);
}
}
```
* 先获取所有event的的listener,如果listener实现了ordered接口,spring会先排序。
* 然后遍历listener,循环调用listener的onApplicationEvent方法。
* 这是一个非常典型的观察者模式。
- ```ApplicationEvent```:发布事件实体
- ```ApplicationListener```:监听事件
- ```ApplicationContext.publishEvent()```方法:发布事件
<br/>
***
<br/>
* 细心的朋友能看到在```multicastEvent```方法中调用的时候分两种情况,一个是同异步调用,但是需要一个```executor```类,一中是同步调用。
* 那么这个```executor```在什么时候设置的呢?
* 看过spring初始化源码的朋友都清楚spring会调用```AbstractApplicationContext```的```refresh()```方法
* ```refresh()```方法内会调用一个```initApplicationEventMulticaster```方法。代码如下:
```java
@Override
public void refresh() throws BeansException, IllegalStateException {
synchronized (this.startupShutdownMonitor) {
// Prepare this context for refreshing.
prepareRefresh();
// Tell the subclass to refresh the internal bean factory.
ConfigurableListableBeanFactory beanFactory = obtainFreshBeanFactory();
// Prepare the bean factory for use in this context.
prepareBeanFactory(beanFactory);
try {
// Allows post-processing of the bean factory in context subclasses.
postProcessBeanFactory(beanFactory);
// Invoke factory processors registered as beans in the context.
invokeBeanFactoryPostProcessors(beanFactory);
// Register bean processors that intercept bean creation.
registerBeanPostProcessors(beanFactory);
// Initialize message source for this context.
initMessageSource();
// 这里我们只需要关注这个方法
initApplicationEventMulticaster();
// Initialize other special beans in specific context subclasses.
onRefresh();
// Check for listener beans and register them.
registerListeners();
// Instantiate all remaining (non-lazy-init) singletons.
finishBeanFactoryInitialization(beanFactory);
// Last step: publish corresponding event.
finishRefresh();
}
catch (BeansException ex) {
logger.warn("Exception encountered during context initialization - cancelling refresh attempt", ex);
// Destroy already created singletons to avoid dangling resources.
destroyBeans();
// Reset 'active' flag.
cancelRefresh(ex);
// Propagate exception to caller.
throw ex;
}
}
}
protected void initApplicationEventMulticaster() {
ConfigurableListableBeanFactory beanFactory = getBeanFactory();
if (beanFactory.containsLocalBean(APPLICATION_EVENT_MULTICASTER_BEAN_NAME)) {
this.applicationEventMulticaster =
beanFactory.getBean(APPLICATION_EVENT_MULTICASTER_BEAN_NAME, ApplicationEventMulticaster.class);
if (logger.isDebugEnabled()) {
logger.debug("Using ApplicationEventMulticaster [" + this.applicationEventMulticaster + "]");
}
}
else {
this.applicationEventMulticaster = new SimpleApplicationEventMulticaster(beanFactory);
beanFactory.registerSingleton(APPLICATION_EVENT_MULTICASTER_BEAN_NAME, this.applicationEventMulticaster);
if (logger.isDebugEnabled()) {
logger.debug("Unable to locate ApplicationEventMulticaster with name '" +
APPLICATION_EVENT_MULTICASTER_BEAN_NAME +
"': using default [" + this.applicationEventMulticaster + "]");
}
}
}
public static final String APPLICATION_EVENT_MULTICASTER_BEAN_NAME = "applicationEventMulticaster";
```
* 首先会去判断是否已经有了名称为```applicationEventMulticaster```的自定义对象。
* 如果有则使用自定义的,没有则使用系统默认的```SimpleApplicationEventMulticaster```类。
* 那么现在就很清楚了,如果我们要设置```executor```,可以实现自己的```SimpleApplicationEventMulticaster```,如下:
```java
@Component("applicationEventMulticaster")
public class MySimpleApplicationEventMulticaster extends SimpleApplicationEventMulticaster {
public MySimpleApplicationEventMulticaster() {
setTaskExecutor(Executors.newCachedThreadPool());
}
}
```
* 这样spring在使用事件监听的时候,就会采用异步的方式去处理。
* 这里有个问题需要注意的:
- 如果有多个listener监听同一个event,并实现了ordered(排序)接口;处理顺序在前面的listener抛出异常的话,如果当前采用的是异步方式,那么后面的listener还会调用;如果采用的是同步方式,那么后面的listener就不会调用了。
- 代码如下:
```java
@Component("applicationEventMulticaster")
public class MySimpleApplicationEventMulticaster extends SimpleApplicationEventMulticaster {
public MySimpleApplicationEventMulticaster() {
setTaskExecutor(Executors.newCachedThreadPool());
}
}
@Component
public class TestListener implements ApplicationListener<TestEvent>, Ordered {
@Override
public void onApplicationEvent(TestEvent event) {
// TODO Auto-generated method stub
String name = event.getName() ;
System.out.println("name= " + name);
throw new RuntimeException("RuntimeException") ;
}
@Override
public int getOrder() {
// TODO Auto-generated method stub
return 1;
}
}
@Component
public class TestListener2 implements ApplicationListener<TestEvent>, Ordered {
@Override
public int getOrder() {
// TODO Auto-generated method stub
return 2;
}
@Override
public void onApplicationEvent(TestEvent event) {
// TODO Auto-generated method stub
String name = event.getName() ;
System.out.println("name2= " + name);
}
}
```
* 最终运行结果如下:<br/>
>name= 111111<br/>
>name2= 111111<br/>
>Exception in thread "pool-1-thread-1" java.lang.RuntimeException: RuntimeException<br/>
> at com.wgq.spring.source.event.TestListener.onApplicationEvent(TestListener.java:15)<br/>
> at com.wgq.spring.source.event.TestListener.onApplicationEvent(TestListener.java:1)<br/>
> at org.springframework.context.event.SimpleApplicationEventMulticaster.invokeListener(SimpleApplicationEventMulticaster.java:151)<br/>
> at org.springframework.context.event.SimpleApplicationEventMulticaster$1.run(SimpleApplicationEventMulticaster.java:123)<br/>
> at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142)<br/>
> at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617)<br/>
> at java.lang.Thread.run(Thread.java:745)<br/>
<br/>
***
<br/>
```SimpleApplicationEventMulticaster```类中还有一个```errorHandler```的属性,如果我们想处理listener抛出的异常的话,那么可以在实现自己的```MySimpleApplicationEventMulticaster```的时候,同理的也设置一个```errorHandler```属性即可。
<file_sep>---
layout: post
title: 初识dubbo
date: 2017-09-11 15:21:00
categories:
- rpc
tags:
- dubbo
- rpc
---
<p>dubbo是一个分布式、高性能、透明化的RPC服务框架,提供服务自动注册、自动发现等高效的服务治理方案。</p>
官网地址:[Dubbo](http://dubbo.io/"Dubbo")
<p>核心包括:</p>
* 远程通讯: 提供对多种基于长连接的NIO框架抽象封装,包括多种线程模型,序列化,以及“请求-响应”模式的信息交换方式。
* 集群容错: 提供基于接口方法的透明远程过程调用,包括多协议支持,以及软负载均衡,失败容错,地址路由,动态配置等集群支持
* 自动发现: 基于注册中心目录服务,使服务消费方能动态的查找服务提供方,使地址透明,使服务提供方可以平滑增加或减少机器。
<p>Dubbo能做什么?</p>
* 透明化的远程方法调用,就像调用本地方法一样调用远程方法,只需简单配置,没有任何API侵入。
* 软负载均衡及容错机制,可在内网替代F5等硬件负载均衡器,降低成本,减少单点。
* 服务自动注册与发现,不再需要写死服务提供方地址,注册中心基于接口名查询服务提供者的IP地址,并且能够平滑添加或删除服务提供者。
<p>Dubbo提供了四种注册中心:redis、zookeeper、Multicast、simple。redis和zookeeper可用于生产;simple则想当于新建了一个项目作为注册中心,如果想要已集群的方式运行需要自行扩展;Multicast一般用于测试。</p>
* 如果使用redis作为注册中心的话,redis是通过心跳检测的方式检测脏数据,所以对服务器会有一定的压力
* zookeeper有临时节点和watch机制,相比redis的话,对服务器压力更小,个人认为更适合作为注册中心。
### 单台机器搭建zookeeper伪集群
1. 首先,zookeeper官网下载zookeeper最新版本[下载地址](http://www.apache.org/dyn/closer.cgi/zookeeper/"下载地址")
2. 分别解压到server0、server1、server2三个目录下(zookeeper集群内节点一般推荐奇数个,这里3个),然后分别新建data、logs目录,并在data目录下新建myid文件,结构如下:<br />
```
.
├── server0
│ ├── data
│ ├── logs
│ └── zookeeper-3.4.9
├── server1
│ ├── data
│ ├── logs
│ └── zookeeper-3.4.9
└── server2
├── data
├── logs
└── zookeeper-3.4.9
```
3. 三个server的/zookeeper/conf/目录下分别新建zoo.cfg,内容如下:<br />
```
#server0/zookeeper/conf/zoo.cfg
tickTime=2000
initLimit=10
syncLimit=5
dataDir=/home/wgq/zkGroup/server0/data
dataLogDir=/home/wgq/zkGroup/server0/logs
clientPort=2181
server.0=127.0.0.1:2888:3888
server.1=127.0.0.1:2889:3889
server.2=127.0.0.1:2890:3890
#server1/zookeeper/conf/zoo.cfg
tickTime=2000
initLimit=10
syncLimit=5
dataDir=/home/wgq/zkGroup/server1/data
dataLogDir=/home/wgq/zkGroup/server1/logs
clientPort=2182
server.0=127.0.0.1:2888:3888
server.1=127.0.0.1:2889:3889
server.2=127.0.0.1:2890:3890
#server2/zookeeper/conf/zoo.cfg
tickTime=2000
initLimit=10
syncLimit=5
dataDir=/home/wgq/zkGroup/server2/data
dataLogDir=/home/wgq/zkGroup/server2/logs
clientPort=2183
server.0=127.0.0.1:2888:3888
server.1=127.0.0.1:2889:3889
server.2=127.0.0.1:2890:3890
#如果是部署在不同机器上的话,配置文件可能是这样的
tickTime=2000
initLimit=10
syncLimit=5
dataDir=/home/wgq/zkGroup/server/data
dataLogDir=/home/wgq/zkGroup/server/logs
clientPort=2181
server.0=192.168.1.1:2888:3888
server.1=192.168.1.2:2888:3888
server.2=192.168.1.3:2888:3888
```
* clientPort表示zookeeper节点的端口,如果部署在不同的机器上可以相同
* server.myid 这里的myid就是data目录下myid文件的值,server0/data/myid=0,对应server.0
* server.0=127.0.0.1:2888:3888,3888表示推举lead时候需要使用的端口,需要同2888不一样
4. 分别调用zkServer.sh start启动服务,启动后可通过zkServer.sh status观看服务状态,如下表示没有问题。
```
[wgq@localhost zookeeper-3.4.9]$ ./bin/zkServer.sh status
ZooKeeper JMX enabled by default
Using config: /home/wgq/zkGroup/server0/zookeeper-3.4.9/bin/../conf/zoo.cfg
Mode: follower
```
### 服务提供者-provider
* 通过spring-boot新建一个maven项目,pom.xml、dubbo等关键配置和类如下
```xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>mydubbo</groupId>
<artifactId>com.wgq.dubbo.product</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>1.4.7.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test </artifactId>
<version>1.4.7.RELEASE</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.5.3</version>
<exclusions>
<exclusion>
<artifactId>spring</artifactId>
<groupId>org.springframework</groupId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.8</version>
</dependency>
<dependency>
<groupId>com.101tec</groupId>
<artifactId>zkclient</artifactId>
<version>0.3</version>
</dependency>
<dependency>
<groupId>cglib</groupId>
<artifactId>cglib</artifactId>
<version>2.2.2</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<fork>true</fork>
<mainClass>com.wgq.dubbo.product.AppClass</mainClass>
</configuration>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
```
* application.properties
```
server.port=8080
```
* dubbo.properties
```
#服务名称
dubbo.application.name=mydubbo
##dubbo zookeeper config
dubbo.registry.address=zookeeper://192.168.110.65:2181?backup=192.168.110.65:2182,192.168.110.65:2183
dubbo.registry.client=zkclient
dubbo.registry.group=dubbo
##dubbo 协议
dubbo.service.protocol=dubbo
dubbo.protocol.dubbo.port=20880
## 关闭服务启动时检查
dubbo.consumer.check=false
```
* application-dubbo.xml
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<dubbo:provider delay="-1" />
<dubbo:service interface="com.wgq.dubbo.product.service.HelloWordService" ref="helloWordService"></dubbo:service>
</beans>
```
* spring-boot程序入口AppClass
```java
package com.wgq.dubbo.product;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ImportResource;
@SpringBootApplication
@ImportResource(
"classpath:application-main.xml"
)
public class AppClass {
public static void main(String[] args) {
SpringApplication.run(AppClass.class, args) ;
}
}
```
* 因为dubbo的接口一般都是独立的项目,打成一个jar包提供给服务端和消费端分别使用的,但是这里我们为了测试方便,紧紧是分别在服务端和消费端新建了两个包和类名相同的接口,接口如下:
```java
//
package com.wgq.dubbo.product.service;
public interface HelloWordService {
String helloWord() ;
}
//HelloWordServiceImpl
package com.wgq.dubbo.product.service.impl;
import com.wgq.dubbo.product.service.HelloWordService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Service;
@Service
public class HelloWordServiceImpl implements HelloWordService {
private static Logger LOGGER = LoggerFactory.getLogger(HelloWordServiceImpl.class) ;
public String helloWord() {
return "product1" ;
}
}
```
### 服务消费者-consumer
* 服务消费者的pom.xml文件的依赖和插件同服务提供者是一样的。
* dubbo.properties
```
dubbo.application.name=mydubbo
##dubbo zookeeper config
dubbo.registry.address=zookeeper://192.168.110.65:2181?backup=192.168.110.65:2182,192.168.110.65:2183
dubbo.registry.client=zkclient
dubbo.registry.group=dubbo
```
* application.properties
```
server.port=8084
```
* AppClass
```
@SpringBootApplication
@ImportResource(
"classpath:application-main.xml"
)
public class AppClass {
public static void main(String[] args) {
SpringApplication.run(AppClass.class, args) ;
}
}
```
* application-dubbo.xml
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<dubbo:consumer></dubbo:consumer>
<dubbo:reference interface="com.wgq.dubbo.product.service.HelloWordService" id="helloWordService"></dubbo:reference>
</beans>
```
* ConsumerController
```
package com.wgq.dubbo.consumer.controller;
import com.wgq.dubbo.product.service.HelloWordService;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.annotation.Resource;
@Controller
@RequestMapping("/consumer")
public class ConsumerController {
@Resource(name = "helloWordService")
private HelloWordService helloWordServiceImpl ;
@RequestMapping("hello")
@ResponseBody
public String helloWord() {
return helloWordServiceImpl.helloWord();
}
}
```
* 和服务提供者包名类名相同的接口
```
package com.wgq.dubbo.product.service;
public interface HelloWordService {
String helloWord() ;
}
```
* 整体上消费者和提供者不同的地方在于xml配置文件。
### dubbo-admin dubbo的管理控制台
* 上github下载dubbo的源码,地址如下:https://github.com/alibaba/dubbo
* 修改dubbo-admin项目下WEB-INF/dubbo.properties(dubbo\dubbo-admin\src\main\webapp\WEB-INF\dubbo.properties)内注册地址:
```
#其实主要就是修改注册中心地址
dubbo.registry.address=zookeeper://192.168.110.65:2181?backup=192.168.110.65:2182,192.168.110.65:2183
dubbo.admin.root.password=<PASSWORD>
dubbo.admin.guest.password=<PASSWORD>
```
* 然后通过maven打包项目 mvn package,生成dubbo-admin-2.5.4.war的war包,放入tomcat中运行,结果如下图:

### 测试
* 这里提供三个服务的提供者,一个服务的消费者用于测试
#### 运行服务提供者
* 其实就是修改server.port和dubbo协议的端口后,生成三个不同的jar,分别运行。(当然这里为了调用清晰,还需要修改serverImpl返回值)
* 进入到项目目录下,运行mvn clean package命令,生成jar包
* java -jar xxxxx.jar 分别运行三个服务
#### 运行消费者
* 直接运行消费者的AppClass的main方法,然后在浏览器输入测试地址:localhost:8084/consumer/hello/
* 可以很清楚的看到返回值product1,product2,product3都会出现
<file_sep>---
title: java源码--AbstractQueuedSynchronizer
date: 2017-07-27 23:48:00
categories:
- java
tags:
- 并发
- java
- 源码
---
### 通过AQS实现一个简单的Lock
java并发包下有个非常重要的类```AbstractQueuedSynchronizer```,```ReentrantLock```、```ReentrantReadWriteLock```、```CountDownLatch```、```Semaphore```都是通过```AbstractQueuedSynchronizer```(以下简称AQS)来实现的;既然AQS的用处这么大,那么这里就让我们通过阅读AQS的源码来了解一下它到底提供了一个什么样的功能。
AQS的源码中有下面的一段注释的代码,
```java
class Mutex implements Lock, java.io.Serializable {
// Our internal helper class
private static class Sync extends AbstractQueuedSynchronizer {
// Reports whether in locked state
protected boolean isHeldExclusively() {
return getState() == 1;
}
// Acquires the lock if state is zero
public boolean tryAcquire(int acquires) {
assert acquires == 1; // Otherwise unused
if (compareAndSetState(0, 1)) {
setExclusiveOwnerThread(Thread.currentThread());
return true;
}
return false;
}
// Releases the lock by setting state to zero
protected boolean tryRelease(int releases) {
assert releases == 1; // Otherwise unused
if (getState() == 0) throw new IllegalMonitorStateException();
setExclusiveOwnerThread(null);
setState(0);
return true;
}
// Provides a Condition
Condition newCondition() { return new ConditionObject(); }
// Deserializes properly
private void readObject(ObjectInputStream s)
throws IOException, ClassNotFoundException {
s.defaultReadObject();
setState(0); // reset to unlocked state
}
}
// The sync object does all the hard work. We just forward to it.
private final Sync sync = new Sync();
public void lock() { sync.acquire(1); }
public boolean tryLock() { return sync.tryAcquire(1); }
public void unlock() { sync.release(1); }
public Condition newCondition() { return sync.newCondition(); }
public boolean isLocked() { return sync.isHeldExclusively(); }
public boolean hasQueuedThreads() { return sync.hasQueuedThreads(); }
public void lockInterruptibly() throws InterruptedException {
sync.acquireInterruptibly(1);
}
public boolean tryLock(long timeout, TimeUnit unit)
throws InterruptedException {
return sync.tryAcquireNanos(1, unit.toNanos(timeout));
}
}}
```
* 这段代码的意思就是通过AQS来简单的实现一个Lock,这个锁只有两个状态0、1,0:已释放锁,1:获取锁,并且这个锁是不可重入的。
* 从这个例子上来看,实现一个这样一个锁,主要依赖的是AQS的```acquire```和```release```方法。
* 那么下面我们就通过```acquire```和```release```方法这两个方法去认识AQS到底做了些啥
### AQS的acquire方法
```java
public final void acquire(int arg) {
//Node.EXCLUSIVE代表独占锁
if (!tryAcquire(arg) &&
acquireQueued(addWaiter(Node.EXCLUSIVE), arg))
selfInterrupt();
}
```
* 调用```tryAcquire(int)```方法,成功则方法执行成功,返回void
* ```tryAcquire(int)```尝试获取锁失败,则调用```acquireQueued```方法。
* ```acquireQueued```方法内会先调用```addWaiter```方法,那么我们先看看```addWaiter```方法
#### addWaiter
```java
private Node addWaiter(Node mode) {
//根据当前线程new一个新的node节点
Node node = new Node(Thread.currentThread(), mode);
// Try the fast path of enq; backup to full enq on failure
//获取尾节点
Node pred = tail;
if (pred != null) {
//设置当前节点的prev为原尾节点
node.prev = pred;
//通过cas方法把当前节点设置为尾节点,并把原尾节点的next设置为当前节点
if (compareAndSetTail(pred, node)) {
pred.next = node;
return node; //放回当前节点
}
}
enq(node);
return node;
}
//尾节点为空
private Node enq(final Node node) {
for (;;) {
Node t = tail;
//重新判断当前尾节点是否为空
//为空表示当前队列为空
if (t == null) { // Must initialize
//通过cas设置当前head节点,并把tail赋值为head
if (compareAndSetHead(new Node()))
tail = head;
} else {
node.prev = t;
if (compareAndSetTail(t, node)) {
t.next = node;
return t;
}
}
}
}
```
* 根据当前线程初始化一个node节点
* 如果当前链表的尾节点为null,表面当前链接还未初始化,那么初始化链接,设置tail=head=new node()
* 如果当前链表的尾节点不为null,则把当前节点放到链表的末尾
* 替换tail和head值都是通过cas操作来保证线程安全
#### acquireQueued
```java
private final boolean acquireQueued(final Node node, int arg) {
boolean failed = true;
try {
//线程是否interrupted
boolean interrupted = false;
for (;;) {
//当前节点的上一个节点
final Node p = node.predecessor();
//如果当前节点是头节点,并且尝试获取锁
if (p == head && tryAcquire(arg)) {
//获取锁成功,设置头节点。
setHead(node);
p.next = null; // help GC
failed = false;
return interrupted;
}
if (shouldParkAfterFailedAcquire(p, node) &&
parkAndCheckInterrupt())
interrupted = true;
}
} finally {
if (failed)
cancelAcquire(node);
}
}
//获取锁失败后,当前线程需要阻塞等待
private static boolean shouldParkAfterFailedAcquire(Node pred, Node node) {
int ws = pred.waitStatus;
//判断上一个节点的状态是否是 SIGNAL
if (ws == Node.SIGNAL)
/*
* This node has already set status asking a release
* to signal it, so it can safely park.
*/
return true;
if (ws > 0) {
// 表示当前正在处理的线程已经cancelled
/*
* Predecessor was cancelled. Skip over predecessors and
* indicate retry.
*/
do {
node.prev = pred = pred.prev;
} while (pred.waitStatus > 0);
pred.next = node;
} else {
//node的waitStatus 初始状态都是 0,这里需要替换成SIGNAL,表示后面有节点正在等待唤醒
//或者在共享模式下,head节点的有可能会设置成PROPAGATE状态
/*
* waitStatus must be 0 or PROPAGATE. Indicate that we
* need a signal, but don't park yet. Caller will need to
* retry to make sure it cannot acquire before parking.
*/
compareAndSetWaitStatus(pred, ws, Node.SIGNAL);
}
return false;
}
//阻塞当前线程,并且当前线程是否interrupted
private final boolean parkAndCheckInterrupt() {
LockSupport.park(this);
return Thread.interrupted();
}
```
* 首先判断上一个节点是否是head节点,如果是,则尝试获取资源,获取成功则直接返回。
* 尝试获取资源失败,则去判断prev节点的状态。
* SIGNAL:阻塞当前线程,等待唤醒。0:表示初始状态。>0:表示已取消
* 然后一直循环直到获取资源成功。
#### cancelAcquire方法
当获取资源失败时,在finally里面会调用cancelAcquire(Node node)方法。
```java
private void cancelAcquire(Node node) {
// Ignore if node doesn't exist
if (node == null)
return;
node.thread = null;
// Skip cancelled predecessors
//获取当前节点的prev节点,并跳过waitStatus=CANCELLED的节点
Node pred = node.prev;
while (pred.waitStatus > 0)
node.prev = pred = pred.prev;
// predNext is the apparent node to unsplice. CASes below will
// fail if not, in which case, we lost race vs another cancel
// or signal, so no further action is necessary.
Node predNext = pred.next;
// Can use unconditional write instead of CAS here.
// After this atomic step, other Nodes can skip past us.
// Before, we are free of interference from other threads.
//设置当前节点为CANCELLED
node.waitStatus = Node.CANCELLED;
// If we are the tail, remove ourselves.
// 如果是当前节点是tail节点,把pred节点设置为tail节点,并且pred节点的next节点为空
if (node == tail && compareAndSetTail(node, pred)) {
compareAndSetNext(pred, predNext, null);
} else {
// If successor needs signal, try to set pred's next-link
// so it will get one. Otherwise wake it up to propagate.
int ws;
if (pred != head &&
((ws = pred.waitStatus) == Node.SIGNAL ||
(ws <= 0 && compareAndSetWaitStatus(pred, ws, Node.SIGNAL))) &&
pred.thread != null) {
Node next = node.next;
if (next != null && next.waitStatus <= 0)
//pred节点不是head节点
//pred节点的状态是 SIGNAL
//pred的thread不为空
//next节点不为空并且next节点状态不为CANCELLED
//则替换pred的next节点为当前节点的next节点
compareAndSetNext(pred, predNext, next);
} else {
//直接尝试唤醒 实际上唤醒的是从链表末尾的第一个 waitStatus<=0的节点
unparkSuccessor(node);
}
node.next = node; // help GC
}
}
```
* 主要的作用就是获取资源失败后删除当前添加的节点。
### AQS的链表节点Node
上面我们了解了acquire方法后,发现内部是通过一个链表来实现功能的,所以这里我们先来了解一下这个队列的Node
```java
static final class Node {
/** Marker to indicate a node is waiting in shared mode */
static final Node SHARED = new Node();
/** Marker to indicate a node is waiting in exclusive mode */
static final Node EXCLUSIVE = null;
/** waitStatus value to indicate thread has cancelled */
static final int CANCELLED = 1;
/** waitStatus value to indicate successor's thread needs unparking */
static final int SIGNAL = -1;
/** waitStatus value to indicate thread is waiting on condition */
static final int CONDITION = -2;
/**
* waitStatus value to indicate the next acquireShared should
* unconditionally propagate
*/
static final int PROPAGATE = -3;
/**
* Status field, taking on only the values:
* SIGNAL: The successor of this node is (or will soon be)
* blocked (via park), so the current node must
* unpark its successor when it releases or
* cancels. To avoid races, acquire methods must
* first indicate they need a signal,
* then retry the atomic acquire, and then,
* on failure, block.
* CANCELLED: This node is cancelled due to timeout or interrupt.
* Nodes never leave this state. In particular,
* a thread with cancelled node never again blocks.
* CONDITION: This node is currently on a condition queue.
* It will not be used as a sync queue node
* until transferred, at which time the status
* will be set to 0. (Use of this value here has
* nothing to do with the other uses of the
* field, but simplifies mechanics.)
* PROPAGATE: A releaseShared should be propagated to other
* nodes. This is set (for head node only) in
* doReleaseShared to ensure propagation
* continues, even if other operations have
* since intervened.
* 0: None of the above
*
* The values are arranged numerically to simplify use.
* Non-negative values mean that a node doesn't need to
* signal. So, most code doesn't need to check for particular
* values, just for sign.
*
* The field is initialized to 0 for normal sync nodes, and
* CONDITION for condition nodes. It is modified using CAS
* (or when possible, unconditional volatile writes).
*/
volatile int waitStatus;
/**
* Link to predecessor node that current node/thread relies on
* for checking waitStatus. Assigned during enqueuing, and nulled
* out (for sake of GC) only upon dequeuing. Also, upon
* cancellation of a predecessor, we short-circuit while
* finding a non-cancelled one, which will always exist
* because the head node is never cancelled: A node becomes
* head only as a result of successful acquire. A
* cancelled thread never succeeds in acquiring, and a thread only
* cancels itself, not any other node.
*/
volatile Node prev;
/**
* Link to the successor node that the current node/thread
* unparks upon release. Assigned during enqueuing, adjusted
* when bypassing cancelled predecessors, and nulled out (for
* sake of GC) when dequeued. The enq operation does not
* assign next field of a predecessor until after attachment,
* so seeing a null next field does not necessarily mean that
* node is at end of queue. However, if a next field appears
* to be null, we can scan prev's from the tail to
* double-check. The next field of cancelled nodes is set to
* point to the node itself instead of null, to make life
* easier for isOnSyncQueue.
*/
volatile Node next;
/**
* The thread that enqueued this node. Initialized on
* construction and nulled out after use.
*/
volatile Thread thread;
/**
* Link to next node waiting on condition, or the special
* value SHARED. Because condition queues are accessed only
* when holding in exclusive mode, we just need a simple
* linked queue to hold nodes while they are waiting on
* conditions. They are then transferred to the queue to
* re-acquire. And because conditions can only be exclusive,
* we save a field by using special value to indicate shared
* mode.
*/
Node nextWaiter;
/**
* Returns true if node is waiting in shared mode.
*/
final boolean isShared() {
return nextWaiter == SHARED;
}
/**
* Returns previous node, or throws NullPointerException if null.
* Use when predecessor cannot be null. The null check could
* be elided, but is present to help the VM.
*
* @return the predecessor of this node
*/
final Node predecessor() throws NullPointerException {
Node p = prev;
if (p == null)
throw new NullPointerException();
else
return p;
}
Node() { // Used to establish initial head or SHARED marker
}
Node(Thread thread, Node mode) { // Used by addWaiter
this.nextWaiter = mode;
this.thread = thread;
}
Node(Thread thread, int waitStatus) { // Used by Condition
this.waitStatus = waitStatus;
this.thread = thread;
}
}
```
| waitStatus | 说明 |
| :----: | :----: |
| SIGNAL | 表示当前节点的next节点正在等待唤醒 |
| CANCELLED | 表示当前节点已取消获取锁 |
| CONDITION | 表示当前节点在condition队列上 |
| PROPAGATE | |
| 0 | 一个非codition的Node节点初始状态 |
* nextWaiter :SHARED 表示当前节点是共享,EXCLUSIVE 表示当前是独占
### AQS的release方法
```java
public final boolean release(int arg) {
//尝试释放资源
if (tryRelease(arg)) {
Node h = head;
//head节点waitStatus=0 表示后面没有其他节点
if (h != null && h.waitStatus != 0)
//唤醒下一个节点
unparkSuccessor(h);
return true;
}
return false;
}
/**
* Wakes up node's successor, if one exists.
*
* @param node the node
*/
private void unparkSuccessor(Node node) {
/*
* If status is negative (i.e., possibly needing signal) try
* to clear in anticipation of signalling. It is OK if this
* fails or if status is changed by waiting thread.
*/
int ws = node.waitStatus;
//重置waitStatus为0
if (ws < 0)
compareAndSetWaitStatus(node, ws, 0);
/*
* Thread to unpark is held in successor, which is normally
* just the next node. But if cancelled or apparently null,
* traverse backwards from tail to find the actual
* non-cancelled successor.
*/
//获取next节点
Node s = node.next;
//如果next节点为空或者next节点为取消状态
//则从后往前遍历队列获取非node节点并且 waitStatus<=0的那个节点
if (s == null || s.waitStatus > 0) {
s = null;
for (Node t = tail; t != null && t != node; t = t.prev)
if (t.waitStatus <= 0)
s = t;
}
//直接唤醒
if (s != null)
LockSupport.unpark(s.thread);
}
```
* 尝试释放资源,成功则根据当前head节点去唤醒下一个节点
* 唤醒过程中会先把当前节点的waitStatus设置为0,这边设置为0后,acquire时便不会去park阻塞next节点了。
* 失败则直接返回false
### AQS的acquireInterruptibly
```java
public final void acquireInterruptibly(int arg)
throws InterruptedException {
if (Thread.interrupted())
throw new InterruptedException();
if (!tryAcquire(arg))
doAcquireInterruptibly(arg);
}
private void doAcquireInterruptibly(int arg)
throws InterruptedException {
final Node node = addWaiter(Node.EXCLUSIVE);
boolean failed = true;
try {
for (;;) {
final Node p = node.predecessor();
if (p == head && tryAcquire(arg)) {
setHead(node);
p.next = null; // help GC
failed = false;
return;
}
if (shouldParkAfterFailedAcquire(p, node) &&
parkAndCheckInterrupt())
throw new InterruptedException();
}
} finally {
if (failed)
cancelAcquire(node);
}
}
```
* 如果仔细的看完过上面的acquire方法的同学,这里应该就能一目了然了。
* 这个方法跟acquire方法不同的地方在于,如果线程已经中断,则直接抛出InterruptedException。
### AQS的独占模式总结
* 尝试获取资源,获取失败,把当前线程封装成EXCLUSIVE类型Node添加到队列。
* 如果pred不是head节点或者再次尝试获取资源失败,则会把当前对象从线程调度上取下,并自旋一直尝试获取资源
* 当head节点调用了```release```方法时,会唤醒head的next节点,如果next节点是CANCELLED状态或者为null,则会并从tail节点往前遍历队列,知道获取一个非CANCELLED节点,并唤醒他。
### AQS的acquireShared方法
AQS的共享模式
```java
public final void acquireShared(int arg) {
if (tryAcquireShared(arg) < 0)
doAcquireShared(arg);
}
```
* ```tryAcquireShared```会返回一个int值,>0: 表示可以唤醒next的share节点,<0: 表示获取失败,=0:表示获取成功,但是不可唤醒next节点
* 返回值小于0,加入到同步队列中。
#### AQS的doAcquireShared
```java
/**
* Acquires in shared uninterruptible mode.
* @param arg the acquire argument
*/
private void doAcquireShared(int arg) {
//添加节点,SHARED模式
final Node node = addWaiter(Node.SHARED);
boolean failed = true;
try {
boolean interrupted = false;
for (;;) {
final Node p = node.predecessor();
//判断pred节点是否为head节点
if (p == head) {
//尝试获取共享资源
int r = tryAcquireShared(arg);
if (r >= 0) {
//设置head节点,r>0时,next节点是share节点,则唤醒他
setHeadAndPropagate(node, r);
p.next = null; // help GC
if (interrupted)
selfInterrupt();
failed = false;
return;
}
}
if (shouldParkAfterFailedAcquire(p, node) &&
parkAndCheckInterrupt())
interrupted = true;
}
} finally {
if (failed)
cancelAcquire(node);
}
}
```
* 把当前节点封装为SHARED节点添加到队列尾部
* 当前节点的pred节点是head节点,则尝试获取共享资源
* 获取共享资源成功后,返回值>0,则重新设置节点并唤醒后置的share节点。
#### AQS的setHeadAndPropagate节点
```java
private void setHeadAndPropagate(Node node, int propagate) {
Node h = head; // Record old head for check below
//设置头节点
setHead(node);
/*
* Try to signal next queued node if:
* Propagation was indicated by caller,
* or was recorded (as h.waitStatus either before
* or after setHead) by a previous operation
* (note: this uses sign-check of waitStatus because
* PROPAGATE status may transition to SIGNAL.)
* and
* The next node is waiting in shared mode,
* or we don't know, because it appears null
*
* The conservatism in both of these checks may cause
* unnecessary wake-ups, but only when there are multiple
* racing acquires/releases, so most need signals now or soon
* anyway.
*/
if (propagate > 0 || h == null || h.waitStatus < 0 ||
(h = head) == null || h.waitStatus < 0) {
Node s = node.next;
if (s == null || s.isShared())
doReleaseShared();
}
}
```
* 先设置head节点
* 返回值```propagate```>0时,如果next节点为空,或者next节点是SHARED节点,则唤醒next节点
#### AQS的doReleaseShared
```java
/**
* Release action for shared mode -- signals successor and ensures
* propagation. (Note: For exclusive mode, release just amounts
* to calling unparkSuccessor of head if it needs signal.)
*/
private void doReleaseShared() {
/*
* Ensure that a release propagates, even if there are other
* in-progress acquires/releases. This proceeds in the usual
* way of trying to unparkSuccessor of head if it needs
* signal. But if it does not, status is set to PROPAGATE to
* ensure that upon release, propagation continues.
* Additionally, we must loop in case a new node is added
* while we are doing this. Also, unlike other uses of
* unparkSuccessor, we need to know if CAS to reset status
* fails, if so rechecking.
*/
for (;;) {
Node h = head;
//head节点不为空,head节点不等于tail节点
if (h != null && h != tail) {
int ws = h.waitStatus;
//head节点的状态为SIGNAL直接唤醒后置节点
if (ws == Node.SIGNAL) {
if (!compareAndSetWaitStatus(h, Node.SIGNAL, 0))
continue; // loop to recheck cases
unparkSuccessor(h);
}
//head节点状态为0,状态设置为PROPAGATE
//两种情况,一种是head节点没有next节点,则状态还是初始的0
//一种是另外一个线程把head节点状态更新为0
else if (ws == 0 &&
!compareAndSetWaitStatus(h, 0, Node.PROPAGATE))
continue; // loop on failed CAS
}
if (h == head) // loop if head changed
break;
}
}
```
* head节点状态为SIGNAL,则直接唤醒后一个节点。
* head节点状态为0,则设置为PROPAGATE状态。
### AQS的releaseShared
共享模式释放资源
```java
public final boolean releaseShared(int arg) {
if (tryReleaseShared(arg)) {
doReleaseShared();
return true;
}
return false;
}
```
* ```tryReleaseShared```返回true的时候表示所有的共享资源全部被释放
* 调用```doReleaseShared```方法。
* ```doReleaseShared```方法上面有说过,这个时候会唤醒下一个节点,这个时候无论下一个节点是独占节点还是SHARED节点
### AQS共享模式总结
* 获取资源时,根据```tryAcquireShared```返回值,
+ 小于0 获取资源失败,则初始化一个SHARED模式Node添加到队列末尾
+ 等于0 获取资源成功,但是不唤醒next的SHARED节点
+ 大于0 获取资源成功,如果next节点是SHARED节点,则直接唤醒。
* 释放资源时,```tryReleaseShared```只有释放所有的资源时才会返回true,这个时候会唤醒下一个资源。
### AQS的ConditionObject
这个我们首先得先了解一下ConditionObject的用法
```java
package test.wgq;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.ReentrantLock;
public class ThreadConditionTest {
private static ReentrantLock lock = new ReentrantLock() ;
private static Condition condition = lock.newCondition() ;
public static void main(String[] args) throws Exception {
Thread thread = new Thread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
try {
lock.lock();
System.out.println("线程1获取到锁");
System.out.println("线程1等待condition信号");
condition.await();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
System.out.println("线程1获取到condition信号");
lock.unlock();
}
}) ;
thread.start();
Thread.currentThread().sleep(1000l);
Thread thread1 = new Thread(new Runnable() {
@Override
public void run() {
// TODO Auto-generated method stub
lock.lock();
System.out.println("线程2获取到锁");
condition.signal();
System.out.println("线程2发送信号量");
lock.unlock();
}
}) ;
thread1.start();
}
}
```
运行结果:
>线程1获取到锁<br/>
>线程1等待condition信号<br/>
>线程2获取到锁<br/>
>线程2发送信号量<br/>
>线程1获取到condition信号<br/>
* 调用顺序 线程一获取锁 -> 线程一codition.await -> 线程二获取到锁 -> 线程二codition.signal -> 线程二释放锁 -> 线程一释放锁
* 上面了解了codition的用法,那么下面通过源码看看await和signal是怎么实现的。
#### await
```java
public final void await() throws InterruptedException {
if (Thread.interrupted())
throw new InterruptedException();
//向Codition队列上添加一个codition节点
Node node = addConditionWaiter();
//释放当前资源
int savedState = fullyRelease(node);
int interruptMode = 0;
//判断当前节点是否在同步队列上
//不在则一直自旋直到调用signal方法
while (!isOnSyncQueue(node)) {
LockSupport.park(this);
if ((interruptMode = checkInterruptWhileWaiting(node)) != 0)
break;
}
//重新把当前节点添加到同步队列上
if (acquireQueued(node, savedState) && interruptMode != THROW_IE)
interruptMode = REINTERRUPT;
if (node.nextWaiter != null) // clean up if cancelled
unlinkCancelledWaiters();
if (interruptMode != 0)
reportInterruptAfterWait(interruptMode);
}
private Node addConditionWaiter() {
Node t = lastWaiter;
// If lastWaiter is cancelled, clean out.
if (t != null && t.waitStatus != Node.CONDITION) {
unlinkCancelledWaiters();
t = lastWaiter;
}
Node node = new Node(Thread.currentThread(), Node.CONDITION);
if (t == null)
firstWaiter = node;
else
t.nextWaiter = node;
lastWaiter = node;
return node;
}
final boolean isOnSyncQueue(Node node) {
if (node.waitStatus == Node.CONDITION || node.prev == null)
return false;
if (node.next != null) // If has successor, it must be on queue
return true;
/*
* node.prev can be non-null, but not yet on queue because
* the CAS to place it on queue can fail. So we have to
* traverse from tail to make sure it actually made it. It
* will always be near the tail in calls to this method, and
* unless the CAS failed (which is unlikely), it will be
* there, so we hardly ever traverse much.
*/
return findNodeFromTail(node);
}
```
* 首先先把当前线程的锁释放掉。
* 然后等待其他线程signal发送信号:判断节点是否在同步队列上,如果在了,则重新调用acquireQueued,获取到锁,继续执行
#### signal
```java
public final void signal() {
if (!isHeldExclusively())
throw new IllegalMonitorStateException();
Node first = firstWaiter;
if (first != null)
doSignal(first);
}
private void doSignal(Node first) {
do {
if ( (firstWaiter = first.nextWaiter) == null)
lastWaiter = null;
first.nextWaiter = null;
} while (!transferForSignal(first) &&
(first = firstWaiter) != null);
}
final boolean transferForSignal(Node node) {
/*
* If cannot change waitStatus, the node has been cancelled.
*/
if (!compareAndSetWaitStatus(node, Node.CONDITION, 0))
return false;
/*
* Splice onto queue and try to set waitStatus of predecessor to
* indicate that thread is (probably) waiting. If cancelled or
* attempt to set waitStatus fails, wake up to resync (in which
* case the waitStatus can be transiently and harmlessly wrong).
*/
Node p = enq(node);
int ws = p.waitStatus;
if (ws > 0 || !compareAndSetWaitStatus(p, ws, Node.SIGNAL))
LockSupport.unpark(node.thread);
return true;
}
```
* 获取到codition队列的first节点
* 把first节点的状态从CONDITION替换成0
* 把first节点添加到同步队列尾部,并获取到原tail节点p
#### codition总结
* 线程1调用reentrantLock.lock时,线程被加入到AQS的等待队列中。
* 线程1调用await方法被调用时,该线程从AQS中移除,对应操作是锁的释放。
* 接着马上被加入到Condition的等待队列中,以为着该线程需要signal信号。
* 线程2,因为线程1释放锁的关系,被唤醒,并判断可以获取锁,于是线程2获取锁,并被加入到AQS的等待队列中。
* 线程2调用signal方法,这个时候Condition的等待队列中只有线程1一个节点,于是它被取出来,并被加入到AQS的等待队列中。 注意,这个时候,线程1 并没有被唤醒。
* signal方法执行完毕,线程2调用reentrantLock.unLock()方法,释放锁。这个时候因为AQS中只有线程1,于是,AQS释放锁后按从头到尾的顺序唤醒线程时,线程1被唤醒,于是线程1回复执行。
* 直到释放所整个过程执行完毕
<file_sep>---
title: id生成
date: 2017-07-23 16:00:00
categories:
- java
tags:
- java
---
美团点评上有一片关于id生成的文章写的很好,大家可以参考一下:
[Leaf——美团点评分布式ID生成系统](https://tech.meituan.com/MT_Leaf.html "Leaf——美团点评分布式ID生成系统")
这里要说的主要还是我们公司当前内部自己的生成id的实现;其实现上来说其实有些类似美团点评的```Leaf-segment```实现。
下面具体的说明一下是怎么实现的。
公司内部的应用一般都有2个以上的节点,每个节点下部署的代码是一样的,获取id时,调用:getNextSeq("tbl_account_history_id", 20);
每个节点的在获取id时,采用```synchronized```关键字保证当前节点应用下的数据安全。
#### 获取id ```getNextSeq```
```java
public synchronized String getNextSeq(String sequenceName, int width) throws Exception{
SequencePool pool = seqMap.get(sequenceName);
if(seqMap.get(sequenceName) == null || pool.isEmpty()){
pool = refillPool(sequenceName);
seqMap.put(sequenceName, pool);
}
return formatSequence(String.valueOf(pool.next()),width);
}
```
上面一段代码主要干了两件事情:
* 从seqMap中获取到SequencePool对象,如果SequencePool对象不存在或者为空则重新初始化一个SequencePool对象,放入到seqMap中。
* 通过SequencePool格式化返回的id字符串
```SequencePool```<br />
```java
private static class SequencePool{
private long low;
private long high;
public SequencePool(long low, long high){
this.low = low;
this.high = high;
}
public long next() {
return low++;
}
public boolean isEmpty(){
return low > high;
}
}
```
#### 初始化pool ```refillPool```
```java
private SequencePool refillPool(String sequenceName) throws Exception{
int allotment = 100 ;
long nextSeq = batchSequenceDao.getNextSeq(sequenceName, allotment);
return new SequencePool(nextSeq, nextSeq + allotment -1);
}
//batchSequenceDao.getNextSeq
public long getNextSeq(String key, int allotment) throws Exception{
HashMap<String, Object> parameters = new HashMap<String, Object>();
parameters.put("sequenceName", key);
parameters.put("allotment", allotment);
Long result = (Long)sqlMapClient.queryForObject("batch_sequence.getNextSequence", parameters);
return result;
}
```
初始化的时候干了啥呢?
* 先通过数据库函数batch_sequence.getNextSequence获取到id。
* 然后初始化SequencePool,很明显可以看到pool里的high=low+100(不要纠结99还是100哈)。
那么这个数据库函数又干了啥呢?继续往下看
#### 更新数据库并返回id
```sql
--表
CREATE TABLE common.sequence_center
(
sequence_name character varying(32) COLLATE pg_catalog."default" NOT NULL,
next_val numeric(18),
CONSTRAINT sequence_center_pkey PRIMARY KEY (sequence_name)
)
--函数
CREATE OR REPLACE FUNCTION common.batch_sequence(
p_sequence_name character varying,
allotment integer)
RETURNS numeric
LANGUAGE 'plpgsql'
COST 100.0
VOLATILE NOT LEAKPROOF
AS $function$
declare
begin_sequence numeric(18);
cur_val numeric(18);
result numeric(18);
begin
select next_val into cur_val from common.sequence_center where sequence_name=p_sequence_name for update;
if cur_val is null
then
begin
cur_val := allotment + 1;
insert into common.sequence_center(sequence_name,next_val) values (p_sequence_name, cur_val);
exception when unique_violation then
select common.batch_sequence(p_sequence_name, allotment) into result;
return result;
end;
else
cur_val := allotment + cur_val;
update common.sequence_center set next_val=cur_val where sequence_name=p_sequence_name;
end if;
result := cur_val - allotment;
return result;
end;
```
* sequence_name:序列名称
* next_val:表示<next_val的值都已被应用节点缓存.
这个函数主要的作用是根据传入的id序列号```sequence_name```和缓存id的长度```allotment```来更新common.sequence_center表内的值
* 1、 首先根据主键sequence_name查询出当前列
* 2、 如果当前列存在next_val的值就等于next_val+allotment,更新common.sequence_center表,返回原始的next_val。
* 3、 如果当前列不存在,说明是第一次获取id,直接插入一条数据next_val=allotment+1,因为当数据不存在时使用select...for update加排它锁是无效的,所以会有可能有两个节点同时调用函数插入数据的情况存在,那么第二个插入的必定失败,失败则重新调用这个函数,这个时候则select...for update排它锁生效,然后重复步骤2。
#### 总结
上面看完了各个步骤的实现后,id生成的业务逻辑总结如下:
* 每个应用节点内部使用```synchronized```保证应用内部不会出现多线程的问题
* 根据```sequence_name```获取id时,先从当前应用节点的缓存中获取,没有或者```SequencePool```中的low > high,则需要更新数据库,并返回当前最新的一段数据,然后缓存起来,缓存的id的数量为```allotment```大小。
* 根据```sequence_name```更新数据库id时,使用select...for update排它锁来保证应用多个节点下的数据安全。
<file_sep>---
layout: post
title: 简单算法-java实现
date: 2017-07-23 15:00:00
categories:
- java
tags:
- 算法
- java
---
算法的稳定性:假定在待排序的记录序列中,存在多个具有相同的关键字的记录,若经过排序,这些记录的相对次序保持不变,即在原序列中,ri=rj,且ri在rj之前,而在排序后的序列中,ri仍在rj之前,则称这种排序算法是稳定的;否则称为不稳定的。
### 冒泡排序
\\( o(n^2) \\) 稳定
```java
public class BubbleSort {
/**
* 冒泡排序
* 比较相邻的元素,如果第一个比第二个大/第一个比第二个小,则交换他们
* 排序一趟后,最后一个应该是最大的或者最小的
* 重复上面的步骤,除了最后一个
* @param args
*/
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,4,33,2,3,4,32,543,63,76} ;
int temp ;
for(int i=0;i<a.length-1;i++) {
for(int j=0;j<a.length-1-i;j++) {
if(a[j] > a[j+1]) {
temp = a[j] ;
a[j] = a[j+1] ;
a[j+1] = temp ;
}
System.out.print(a[j] + " ");
}
System.out.println("");
}
}
}
```
### 快速排序
\\( o(nlogn) \\) 不稳定
```java
public class QuickSort {
/**
* 快速排序思想
* 选取数组第一个数字作为中轴
* 和最后一个比,比他大则交换;交换后再和最前面那个比,比他小则交换。
* 这样循环,一趟排序完成后,左边的都是比中轴小的,右边的都是比中轴大的。
* 然后左右两边分别递归排序。
* @param a
* @param low
* @param high
* @return
*/
public static int getMiddle(int[] a, int low, int high) {
int temp = a[low] ;
while(low < high) {
while(low < high && a[high] >= temp) {
high-- ;
}
a[low] = a[high] ;
while(low < high && a[low] <= temp) {
low++ ;
}
a[high] = a[low] ;
}
a[low] = temp ;
return low ;
}
public static void quickSort(int[] a, int low, int high) {
if(low < high) {
int middle = getMiddle(a, low, high) ;
quickSort(a, middle + 1, high) ;
quickSort(a, low, middle - 1) ;
}
}
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,33,2,3,4,32,543,63,76} ;
long startTime = System.currentTimeMillis() ;
quickSort(a, 0, a.length - 1) ;
long endTime = System.currentTimeMillis() ;
System.out.println("排序时间:" + (startTime - endTime));
for(int i=0;i<a.length - 1;i++) {
System.out.print(a[i] + " ");
}
}
}
```
### 选择排序
\\( o(n^2) \\) 不稳定
```java
public class SelectSort {
/**
* 选择排序
* 选择数组中最小元素与第一个置换,选择数组剩余元素中最小元素与第二个置换,以此类推...
* @param a
*/
public static void selectSort(int[] a) {
int size = a.length ;
int temp = 0 ;
for(int i=0;i < size;i++) {
int k = i ;
for(int j=i;j<size;j++) {
if(a[j] < a[k]) {
k = j ;
}
}
temp = a[i] ;
a[i] = a[k] ;
a[k] = temp ;
}
}
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,33,2,3,4,32,543,63,76} ;
long startTime = System.currentTimeMillis() ;
selectSort(a) ;
long endTime = System.currentTimeMillis() ;
System.out.println("排序时间:" + (startTime - endTime));
for(int i=0;i<a.length - 1;i++) {
System.out.print(a[i] + " ");
}
}
}
```
### 插入排序
\\( o(n^2) \\) 稳定
```java
public class InsertSort {
/**
* 插入排序
* 从第一个元素开始,改元素可以认为已经被排序
* 取出下一个元素,与有序的元素从后往前比较
* 如果当前元素小于有序的元素,则把有序元素移到下一位。
* 直到当前元素大于有序的元素,则把当前元素放入有序的元素中。
* @param a
*/
public static void insetSort(int[] a) {
int temp ;
int j = 0;
for(int i=1;i<a.length;i++) {
temp = a[i] ;
for(j=i;j > 0 && temp < a[j-1];j--) {
a[j] = a[j-1] ;
}
a[j] = temp ;
}
}
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,33,2,3,4,32,543,63,76} ;
long startTime = System.currentTimeMillis() ;
insetSort(a) ;
long endTime = System.currentTimeMillis() ;
System.out.println("排序时间:" + (startTime - endTime));
for(int i=0;i<a.length - 1;i++) {
System.out.print(a[i] + " ");
}
}
}
```
### 希尔排序
\\( o(n^2) \\) 不稳定
```java
public class ShellSort {
/**
* 希尔排序
* 根据需求,如果你想要结果从大到小排列,它会首先将数组进行分组,然后将较大值移到前面,较小值移到后面,
* 最后将整个数组进行插入排序,这样比起一开始就用插入排序减少了数据交换和移动的次数,可以说希尔排序是加强版的插入排序
* @param a
*/
public static void shellSort(int[] a) {
int size = a.length ;
int j,temp ;
for(int ic=size/2; ic>0 ; ic = ic/2) {//就相当于在插入排序外面套了一层这个遍历
for(int i=ic;i<a.length;i++) {
temp = a[i] ;
for(j=i;j >= ic && temp < a[j - ic];j = j - ic) {
a[j] = a[j-ic] ;
}
a[j] = temp ;
}
}
}
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,33,2,3,4,32,543,63,76} ;
long startTime = System.currentTimeMillis() ;
shellSort(a) ;
long endTime = System.currentTimeMillis() ;
System.out.println("排序时间:" + (startTime - endTime));
for(int i=0;i<a.length;i++) {
System.out.print(a[i] + " ");
}
}
}
```
### 归并排序
\\( o(nlogn) \\) 稳定
```java
/**
* 归并排序
* 将两个或两个以上的有序表合成一个新的有序表。
* 把待排序的序列分成若干子序列,每个子序列都是有序的,然后再把有序的子序列合并为整体有序的序列。
* 时间复杂度0(nlogn)
* 稳定排序
* @author wangguangqiao
*
*/
public class MergeSort {
public static void mergeSort(int[] a, int low, int high) {
int mid = (low + high) / 2 ;
if(low < high) {
mergeSort(a, low, mid) ;
mergeSort(a, mid + 1, high) ;
merge(a, low, mid, high) ;
}
}
/**
* 讲数组中 low 到 high的位置进行排序
* @param a 待排序数组
* @param low 待排序开始位置
* @param mid 待排序中间位置
* @param high 待排序结束位置
*/
public static void merge(int[] a, int low, int mid, int high) {
int[] temp = new int[high - low + 1] ;
int i = low ;
int j = mid + 1 ;
int k = 0 ;
//将两个有序队列中较小的移到新数组
//总会有一个数组会有剩余的元素
while(i <= mid && j <= high) {
if(a[i] < a[j]) {
temp[k++] = a[i++] ;
}else {
temp[k++] = a[j++] ;
}
}
//将剩余的元素加入到数组
while(i <= mid) {
temp[k++] = a[i++] ;
}
//将剩余的元素加入到数组
while(j <= high) {
temp[k++] = a[j++] ;
}
for(int t = 0;t<temp.length;t++) {
a[t + low] = temp[t] ;
}
}
public static void main(String[] args) {
int[] a = {88,23,32,44,12,45,33,2,3,4,32,543,63,76} ;
long startTime = System.currentTimeMillis() ;
mergeSort(a, 0, a.length - 1) ;
long endTime = System.currentTimeMillis() ;
System.out.println("排序时间:" + (startTime - endTime));
for(int i=0;i<a.length;i++) {
System.out.print(a[i] + " ");
}
}
}
```
### 堆排序
利用大根堆的特性,先建堆,建堆后把根节点和最后一个值交换。然后把1-(n-1)重新建堆,然后把根节点同n-1的值交换,以此类推。<file_sep>---
layout: post
title: xxl-job源码解析一
date: 2018-04-10 16:04:00
categories:
- quartz
tags:
- quartz
- xxl-job
---
XXL-JOB是一个轻量级分布式任务调度框架,其核心设计目标是开发迅速、学习简单、轻量级、易扩展。现已开放源代码并接入多家公司线上产品线,开箱即用。
主要特性如下:
* 1、简单:支持通过Web页面对任务进行CRUD操作,操作简单,一分钟上手;
* 2、动态:支持动态修改任务状态、暂停/恢复任务,以及终止运行中任务,即时生效;
* 3、调度中心HA(中心式):调度采用中心式设计,“调度中心”基于集群Quartz实现并支持集群部署,可保证调度中心HA;
* 4、执行器HA(分布式):任务分布式执行,任务"执行器"支持集群部署,可保证任务执行HA;
* 5、注册中心: 执行器会周期性自动注册任务, 调度中心将会自动发现注册的任务并触发执行。同时,也支持手动录入执行器地址;
* 6、弹性扩容缩容:一旦有新执行器机器上线或者下线,下次调度时将会重新分配任务;
* 7、路由策略:执行器集群部署时提供丰富的路由策略,包括:第一个、最后一个、轮询、随机、一致性HASH、最不经常使用、最近最久未使用、故障转移、忙碌转移等;
* 8、故障转移:任务路由策略选择"故障转移"情况下,如果执行器集群中某一台机器故障,将会自动Failover切换到一台正常的执行器发送调度请求。
* 9、失败处理策略;调度失败时的处理策略,策略包括:失败告警(默认)、失败重试;
* 10、失败重试:调度中心调度失败且启用"失败重试"策略时,将会自动重试一次;执行器执行失败且回调失败重试状态时,也将会自动重试一次;
* 11、阻塞处理策略:调度过于密集执行器来不及处理时的处理策略,策略包括:单机串行(默认)、丢弃后续调度、覆盖之前调度;
* 12、分片广播任务:执行器集群部署时,任务路由策略选择"分片广播"情况下,一次任务调度将会广播触发集群中所有执行器执行一次任务,可根据分片参数开发分片任务;
* 13、动态分片:分片广播任务以执行器为维度进行分片,支持动态扩容执行器集群从而动态增加分片数量,协同进行业务处理;在进行大数据量业务操作时可显著提升任务处理能力和速度。
* 14、事件触发:除了"Cron方式"和"任务依赖方式"触发任务执行之外,支持基于事件的触发任务方式。调度中心提供触发任务单次执行的API服务,可根据业务事件灵活触发。
* 15、任务进度监控:支持实时监控任务进度;
* 16、Rolling实时日志:支持在线查看调度结果,并且支持以Rolling方式实时查看执行器输出的完整的执行日志;
* 17、GLUE:提供Web IDE,支持在线开发任务逻辑代码,动态发布,实时编译生效,省略部署上线的过程。支持30个版本的历史版本回溯。
* 18、脚本任务:支持以GLUE模式开发和运行脚本任务,包括Shell、Python、NodeJS等类型脚本;
* 19、任务依赖:支持配置子任务依赖,当父任务执行结束且执行成功后将会主动触发一次子任务的执行, 多个子任务用逗号分隔;
* 20、一致性:“调度中心”通过DB锁保证集群分布式调度的一致性, 一次任务调度只会触发一次执行;
* 21、自定义任务参数:支持在线配置调度任务入参,即时生效;
* 22、调度线程池:调度系统多线程触发调度运行,确保调度精确执行,不被堵塞;
* 23、数据加密:调度中心和执行器之间的通讯进行数据加密,提升调度信息安全性;
* 24、邮件报警:任务失败时支持邮件报警,支持配置多邮件地址群发报警邮件;
* 25、推送maven中央仓库: 将会把最新稳定版推送到maven中央仓库, 方便用户接入和使用;
* 26、运行报表:支持实时查看运行数据,如任务数量、调度次数、执行器数量等;以及调度报表,如调度日期分布图,调度成功分布图等;
* 27、全异步:系统底层实现全部异步化,针对密集调度进行流量削峰,理论上支持任意时长任务的运行;
* 28、国际化:调度中心支持国际化设置,提供中文、英文两种可选语言,默认为中文;
官方的地址为:[XXL-JOB](http://www.xuxueli.com/xxl-job/"XXL-JOB")
XXL-JOB依托于spring quartz集群的实现,对于quartz集群有想了解的朋友可以先看看美团点评的这篇文章:[Quartz应用与集群原理分析](https://tech.meituan.com/mt-crm-quartz.html"Quartz应用与集群原理分析")
* XXL-JOB主要分为两个部分,一个是任务的调度中心,一个是任务的执行器,调度中心和执行器会公用一套核心的代码,执行器为具体的任务系统。
* 下面我们就通过一个任务具体执行的过程,先来通读一遍代码
* 调度中心新建任务
* 调度中心触发任务,通过http发送请求到执行器,获取调用结果
* 执行器具体的去执行任务,记录日志,执行完后通过http请求通知调度中心执行结果
* 调度中心查询执行结果
### 调度中心新建任务
```java
/**
* index controller
* @author xuxueli 2015-12-19 16:13:16
*/
@Controller
@RequestMapping("/jobinfo")
public class JobInfoController {
@Resource
private XxlJobGroupDao xxlJobGroupDao;
@Resource
private XxlJobService xxlJobService;
...........
//添加任务的入口
@RequestMapping("/add")
@ResponseBodyadd(XxlJobInfo jobInfo)
public ReturnT<String> {
return xxlJobService.add(jobInfo);
}
...........
}
/**
* core job action for xxl-job
* @author xuxueli 2016-5-28 15:30:33
*/
@Service
public class XxlJobServiceImpl implements XxlJobService {
private static Logger logger = LoggerFactory.getLogger(XxlJobServiceImpl.class);
@Resource
private XxlJobGroupDao xxlJobGroupDao;
@Resource
private XxlJobInfoDao xxlJobInfoDao;
@Resource
public XxlJobLogDao xxlJobLogDao;
@Resource
private XxlJobLogGlueDao xxlJobLogGlueDao;
.............................
@Override
public ReturnT<String> add(XxlJobInfo jobInfo) {
// valid
.....................
// add in db 保存到数据库
xxlJobInfoDao.save(jobInfo);
if (jobInfo.getId() < 1) {
return new ReturnT<String>(ReturnT.FAIL_CODE, (I18nUtil.getString("jobinfo_field_add")+I18nUtil.getString("system_fail")) );
}
// add in quartz
String qz_group = String.valueOf(jobInfo.getJobGroup());
String qz_name = String.valueOf(jobInfo.getId());
try {
//添加任务到quartz集群
XxlJobDynamicScheduler.addJob(qz_name, qz_group, jobInfo.getJobCron());
//XxlJobDynamicScheduler.pauseJob(qz_name, qz_group);
return ReturnT.SUCCESS;
} catch (SchedulerException e) {
logger.error(e.getMessage(), e);
try {
xxlJobInfoDao.delete(jobInfo.getId());
XxlJobDynamicScheduler.removeJob(qz_name, qz_group);
} catch (SchedulerException e1) {
logger.error(e.getMessage(), e1);
}
return new ReturnT<String>(ReturnT.FAIL_CODE, (I18nUtil.getString("jobinfo_field_add")+I18nUtil.getString("system_fail"))+":" + e.getMessage());
}
}
............................
}
```
* 上面的代码可以看出,调度中心的任务管理类主要是```XxlJobDynamicScheduler```,通过```XxlJobDynamicScheduler.addJob```方法添加任务到quartz集群中去
* xxlJobDynamicScheduler类在配置文件中有配置
```xml
//spring 实现的scheduler工厂类
<bean id="quartzScheduler" lazy-init="false" class="org.springframework.scheduling.quartz.SchedulerFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="autoStartup" value="true" /> <!--自动启动 -->
<property name="startupDelay" value="20" /> <!--延时启动,应用启动成功后在启动 -->
<property name="overwriteExistingJobs" value="true" /> <!--覆盖DB中JOB:true、以数据库中已经存在的为准:false -->
<property name="applicationContextSchedulerContextKey" value="applicationContextKey" />
<property name="configLocation" value="classpath:quartz.properties"/>
</bean>
//xxl-job封装的scheduler工具类
<bean id="xxlJobDynamicScheduler" class="com.xxl.job.admin.core.schedule.XxlJobDynamicScheduler" init-method="init" destroy-method="destroy" >
<!-- (轻易不要变更“调度器名称”, 任务创建时会绑定该“调度器名称”) -->
<property name="scheduler" ref="quartzScheduler"/>
<property name="accessToken" value="${xxl.job.accessToken}" />
</bean>
```
下面看看xxlJobDynamicScheduler类
```java
/**
* base quartz scheduler util
* @author xuxueli 2015-12-19 16:13:53
*/
public final class XxlJobDynamicScheduler implements ApplicationContextAware {
private static final Logger logger = LoggerFactory.getLogger(XxlJobDynamicScheduler.class);
// ---------------------- param ----------------------
// scheduler
private static Scheduler scheduler;
public void setScheduler(Scheduler scheduler) {
XxlJobDynamicScheduler.scheduler = scheduler;
}
// accessToken
private static String accessToken;
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
// dao
public static XxlJobLogDao xxlJobLogDao;
public static XxlJobInfoDao xxlJobInfoDao;
public static XxlJobRegistryDao xxlJobRegistryDao;
public static XxlJobGroupDao xxlJobGroupDao;
public static AdminBiz adminBiz;
// ---------------------- applicationContext ----------------------
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
XxlJobDynamicScheduler.xxlJobLogDao = applicationContext.getBean(XxlJobLogDao.class);
XxlJobDynamicScheduler.xxlJobInfoDao = applicationContext.getBean(XxlJobInfoDao.class);
XxlJobDynamicScheduler.xxlJobRegistryDao = applicationContext.getBean(XxlJobRegistryDao.class);
XxlJobDynamicScheduler.xxlJobGroupDao = applicationContext.getBean(XxlJobGroupDao.class);
XxlJobDynamicScheduler.adminBiz = applicationContext.getBean(AdminBiz.class);
}
// ---------------------- init + destroy ----------------------
public void init() throws Exception {
// admin registry monitor run 辅助线程
JobRegistryMonitorHelper.getInstance().start();
// admin monitor runJob 辅助线程
JobFailMonitorHelper.getInstance().start();
// admin-server(spring-mvc) 用于callback
NetComServerFactory.putService(AdminBiz.class, XxlJobDynamicScheduler.adminBiz);
NetComServerFactory.setAccessToken(accessToken);
// valid
Assert.notNull(scheduler, "quartz scheduler is null");
logger.info(">>>>>>>>> init xxl-job admin success.");
}
public void destroy(){
// admin registry stop
JobRegistryMonitorHelper.getInstance().toStop();
// admin monitor stop
JobFailMonitorHelper.getInstance().toStop();
}
// ---------------------- executor-client ----------------------
private static ConcurrentHashMap<String, ExecutorBiz> executorBizRepository = new ConcurrentHashMap<String, ExecutorBiz>();
public static ExecutorBiz getExecutorBiz(String address) throws Exception {
// valid
if (address==null || address.trim().length()==0) {
return null;
}
// load-cache
address = address.trim();
ExecutorBiz executorBiz = executorBizRepository.get(address);
if (executorBiz != null) {
return executorBiz;
}
// set-cache NetComClientProxy主要用于rpc的调用
executorBiz = (ExecutorBiz) new NetComClientProxy(ExecutorBiz.class, address, accessToken).getObject();
executorBizRepository.put(address, executorBiz);
return executorBiz;
}
// ---------------------- schedule util ----------------------
........................................
/**
* addJob
*
* @param jobName
* @param jobGroup
* @param cronExpression
* @return
* @throws SchedulerException
*/
public static boolean addJob(String jobName, String jobGroup, String cronExpression) throws SchedulerException {
// TriggerKey : name + group
TriggerKey triggerKey = TriggerKey.triggerKey(jobName, jobGroup);
JobKey jobKey = new JobKey(jobName, jobGroup);
// TriggerKey valid if_exists
if (checkExists(jobName, jobGroup)) {
logger.info(">>>>>>>>> addJob fail, job already exist, jobGroup:{}, jobName:{}", jobGroup, jobName);
return false;
}
// CronTrigger : TriggerKey + cronExpression // withMisfireHandlingInstructionDoNothing 忽略掉调度终止过程中忽略的调度
CronScheduleBuilder cronScheduleBuilder = CronScheduleBuilder.cronSchedule(cronExpression).withMisfireHandlingInstructionDoNothing();
CronTrigger cronTrigger = TriggerBuilder.newTrigger().withIdentity(triggerKey).withSchedule(cronScheduleBuilder).build();
// JobDetail : jobClass
Class<? extends Job> jobClass_ = RemoteHttpJobBean.class; // Class.forName(jobInfo.getJobClass());
JobDetail jobDetail = JobBuilder.newJob(jobClass_).withIdentity(jobKey).build();
/*if (jobInfo.getJobData()!=null) {
JobDataMap jobDataMap = jobDetail.getJobDataMap();
jobDataMap.putAll(JacksonUtil.readValue(jobInfo.getJobData(), Map.class));
// JobExecutionContext context.getMergedJobDataMap().get("mailGuid");
}*/
// schedule : jobDetail + cronTrigger
Date date = scheduler.scheduleJob(jobDetail, cronTrigger);
logger.info(">>>>>>>>>>> addJob success, jobDetail:{}, cronTrigger:{}, date:{}", jobDetail, cronTrigger, date);
return true;
}
..............................
}
```
* 代码太长,这里我们主要关注两点,init()和addJob(String jobName, String jobGroup, String cronExpression)方法
* init()内会初始化两个线程,JobRegistryMonitorHelper和JobFailMonitorHelper
* JobRegistryMonitorHelper用于监控执行器的注册
* JobFailMonitorHelper用于监控任务执行结果
* init()还会初始化NetComServerFactory类,此类主要的作用在于对AdminBizImpl做了一层代理,实际调用的方法还是AdminBizImpl的方法
* getExecutorBiz()主要为每个地址生成一个```ExecutorBiz```的代理类,此代理类主要的作用是发送http请求,这里不做过多赘述
* addJob()其实就是向quartz内添加一个job
* 此类中还有其他的比如暂停任务,重新运行任务等其他方法这里就不过多介绍。
### 调度中心触发任务
* 调度中心按照cron表达式新建好任务之后,就是等待quartz定时的去执行任务了。
* 细心的朋友可能发现了,addJob()内向quartz集群中添加的job固定为```RemoteHttpJobBean```。
```java
//@DisallowConcurrentExecution
public class RemoteHttpJobBean extends QuartzJobBean {
private static Logger logger = LoggerFactory.getLogger(RemoteHttpJobBean.class);
@Override
protected void executeInternal(JobExecutionContext context)
throws JobExecutionException {
// load jobId
JobKey jobKey = context.getTrigger().getJobKey();
Integer jobId = Integer.valueOf(jobKey.getName());
// trigger
XxlJobTrigger.trigger(jobId);
}
}
```
* 可以看到,定时任务启动后,调用```RemoteHttpJobBean```的方法,其实已经代理给了```XxlJobTrigger```类了
```java
/**
* xxl-job trigger
* Created by xuxueli on 17/7/13.
*/
public class XxlJobTrigger {
private static Logger logger = LoggerFactory.getLogger(XxlJobTrigger.class);
/**
* trigger job
*
* @param jobId
*/
public static void trigger(int jobId) {
// load data
XxlJobInfo jobInfo = XxlJobDynamicScheduler.xxlJobInfoDao.loadById(jobId); // job info
if (jobInfo == null) {
logger.warn(">>>>>>>>>>>> trigger fail, jobId invalid,jobId={}", jobId);
return;
}
XxlJobGroup group = XxlJobDynamicScheduler.xxlJobGroupDao.load(jobInfo.getJobGroup()); // group info
//阻塞策略
ExecutorBlockStrategyEnum blockStrategy = ExecutorBlockStrategyEnum.match(jobInfo.getExecutorBlockStrategy(), ExecutorBlockStrategyEnum.SERIAL_EXECUTION); // block strategy
//失败策略
ExecutorFailStrategyEnum failStrategy = ExecutorFailStrategyEnum.match(jobInfo.getExecutorFailStrategy(), ExecutorFailStrategyEnum.FAIL_ALARM); // fail strategy
//路由策略
ExecutorRouteStrategyEnum executorRouteStrategyEnum = ExecutorRouteStrategyEnum.match(jobInfo.getExecutorRouteStrategy(), null); // route strategy
ArrayList<String> addressList = (ArrayList<String>) group.getRegistryList();
// broadcast
if (ExecutorRouteStrategyEnum.SHARDING_BROADCAST == executorRouteStrategyEnum && CollectionUtils.isNotEmpty(addressList)) {
//数据分片先略过
...........
} else {
// 1、save log-id
XxlJobLog jobLog = new XxlJobLog();
jobLog.setJobGroup(jobInfo.getJobGroup());
jobLog.setJobId(jobInfo.getId());
XxlJobDynamicScheduler.xxlJobLogDao.save(jobLog);
logger.debug(">>>>>>>>>>> xxl-job trigger start, jobId:{}", jobLog.getId());
// 2、prepare trigger-info
//jobLog.setExecutorAddress(executorAddress);
jobLog.setGlueType(jobInfo.getGlueType());
jobLog.setExecutorHandler(jobInfo.getExecutorHandler());
jobLog.setExecutorParam(jobInfo.getExecutorParam());
jobLog.setTriggerTime(new Date());
ReturnT<String> triggerResult = new ReturnT<String>(null);
StringBuffer triggerMsgSb = new StringBuffer();
triggerMsgSb.append(I18nUtil.getString("jobconf_trigger_admin_adress")).append(":").append(IpUtil.getIp());
triggerMsgSb.append("<br>").append(I18nUtil.getString("jobconf_trigger_exe_regtype")).append(":")
.append( (group.getAddressType() == 0)?I18nUtil.getString("jobgroup_field_addressType_0"):I18nUtil.getString("jobgroup_field_addressType_1") );
triggerMsgSb.append("<br>").append(I18nUtil.getString("jobconf_trigger_exe_regaddress")).append(":").append(group.getRegistryList());
triggerMsgSb.append("<br>").append(I18nUtil.getString("jobinfo_field_executorRouteStrategy")).append(":").append(executorRouteStrategyEnum.getTitle());
triggerMsgSb.append("<br>").append(I18nUtil.getString("jobinfo_field_executorBlockStrategy")).append(":").append(blockStrategy.getTitle());
triggerMsgSb.append("<br>").append(I18nUtil.getString("jobinfo_field_executorFailStrategy")).append(":").append(failStrategy.getTitle());
// 3、trigger-valid 验证是否有可用的执行器
if (triggerResult.getCode()==ReturnT.SUCCESS_CODE && CollectionUtils.isEmpty(addressList)) {
triggerResult.setCode(ReturnT.FAIL_CODE);
triggerMsgSb.append("<br>----------------------<br>").append(I18nUtil.getString("jobconf_trigger_address_empty"));
}
if (triggerResult.getCode() == ReturnT.SUCCESS_CODE) {
// 4.1、trigger-param 准备参数
TriggerParam triggerParam = new TriggerParam();
triggerParam.setJobId(jobInfo.getId());
triggerParam.setExecutorHandler(jobInfo.getExecutorHandler());
triggerParam.setExecutorParams(jobInfo.getExecutorParam());
triggerParam.setExecutorBlockStrategy(jobInfo.getExecutorBlockStrategy());
triggerParam.setLogId(jobLog.getId());
triggerParam.setLogDateTim(jobLog.getTriggerTime().getTime());
triggerParam.setGlueType(jobInfo.getGlueType());
triggerParam.setGlueSource(jobInfo.getGlueSource());
triggerParam.setGlueUpdatetime(jobInfo.getGlueUpdatetime().getTime());
triggerParam.setBroadcastIndex(0);
triggerParam.setBroadcastTotal(1);
// 4.2、trigger-run (route run / trigger remote executor)
//根据路由策略获取address然后去执行
triggerResult = executorRouteStrategyEnum.getRouter().routeRun(triggerParam, addressList);
triggerMsgSb.append("<br><br><span style=\"color:#00c0ef;\" > >>>>>>>>>>>"+ I18nUtil.getString("jobconf_trigger_run") +"<<<<<<<<<<< </span><br>").append(triggerResult.getMsg());
// 4.3、trigger (fail retry)
//如果设置了失败重试的策略,如果调用不成功,会再调用一次
if (triggerResult.getCode()!=ReturnT.SUCCESS_CODE && failStrategy == ExecutorFailStrategyEnum.FAIL_RETRY) {
triggerResult = executorRouteStrategyEnum.getRouter().routeRun(triggerParam, addressList);
triggerMsgSb.append("<br><br><span style=\"color:#F39C12;\" > >>>>>>>>>>>"+ I18nUtil.getString("jobconf_trigger_fail_retry") +"<<<<<<<<<<< </span><br>").append(triggerResult.getMsg());
}
}
// 5、save trigger-info
jobLog.setExecutorAddress(triggerResult.getContent());
jobLog.setTriggerCode(triggerResult.getCode());
jobLog.setTriggerMsg(triggerMsgSb.toString());
XxlJobDynamicScheduler.xxlJobLogDao.updateTriggerInfo(jobLog);
// 6、monitor trigger
//添加任务执行失败监控
JobFailMonitorHelper.monitor(jobLog.getId());
logger.debug(">>>>>>>>>>> xxl-job trigger end, jobId:{}", jobLog.getId());
}
}
/**
* run executor
* @param triggerParam
* @param address
* @return ReturnT.content: final address
*/
public static ReturnT<String> runExecutor(TriggerParam triggerParam, String address){
ReturnT<String> runResult = null;
try {
//获取ExecutorBiz,然后去执行
//获取到的是个代理类
//实际上就是个http请求
ExecutorBiz executorBiz = XxlJobDynamicScheduler.getExecutorBiz(address);
runResult = executorBiz.run(triggerParam);
} catch (Exception e) {
logger.error(">>>>>>>>>>> xxl-job trigger error, please check if the executor[{}] is running.", address, e);
runResult = new ReturnT<String>(ReturnT.FAIL_CODE, ""+e );
}
StringBuffer runResultSB = new StringBuffer(I18nUtil.getString("jobconf_trigger_run") + ":");
runResultSB.append("<br>address:").append(address);
runResultSB.append("<br>code:").append(runResult.getCode());
runResultSB.append("<br>msg:").append(runResult.getMsg());
runResult.setMsg(runResultSB.toString());
runResult.setContent(address);
return runResult;
}
}
```
* 先获取到任务中配置的阻塞、失败重试(只针对调用)、路由策略。
* 添加trigger调用日志
* 准备传递参数
* 验证是否有可用的执行器
* 根据路由策略去调用执行,实际上是通过```NetComClientProxy```工厂类获取代理生成```ExecutorBiz```的去执行的
* 调用失败如果设置了失败重试则会再调用一次
* 把当前任务添加到任务执行结果监控线程中去
* 各种执行策略可以自己单独去看或者后面会单独拉出一篇文章来讲解
```java
public class NetComClientProxy implements FactoryBean<Object> {
private static final Logger logger = LoggerFactory.getLogger(NetComClientProxy.class);
// ---------------------- config ----------------------
private Class<?> iface;
private String serverAddress;
private String accessToken;
private JettyClient client = new JettyClient();
public NetComClientProxy(Class<?> iface, String serverAddress, String accessToken) {
this.iface = iface;
this.serverAddress = serverAddress;
this.accessToken = accessToken;
}
@Override
public Object getObject() throws Exception {
return Proxy.newProxyInstance(Thread.currentThread()
.getContextClassLoader(), new Class[] { iface },
new InvocationHandler() {
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
// filter method like "Object.toString()"
if (Object.class.getName().equals(method.getDeclaringClass().getName())) {
logger.error(">>>>>>>>>>> xxl-rpc proxy class-method not support [{}.{}]", method.getDeclaringClass().getName(), method.getName());
throw new RuntimeException("xxl-rpc proxy class-method not support");
}
// request
RpcRequest request = new RpcRequest();
request.setServerAddress(serverAddress);
request.setCreateMillisTime(System.currentTimeMillis());
request.setAccessToken(accessToken);
request.setClassName(method.getDeclaringClass().getName());
request.setMethodName(method.getName());
request.setParameterTypes(method.getParameterTypes());
request.setParameters(args);
// send
RpcResponse response = client.send(request);
// valid response
if (response == null) {
logger.error(">>>>>>>>>>> xxl-rpc netty response not found.");
throw new Exception(">>>>>>>>>>> xxl-rpc netty response not found.");
}
if (response.isError()) {
throw new RuntimeException(response.getError());
} else {
return response.getResult();
}
}
});
}
@Override
public Class<?> getObjectType() {
return iface;
}
@Override
public boolean isSingleton() {
return false;
}
}
public class JettyClient {
private static Logger logger = LoggerFactory.getLogger(JettyClient.class);
public RpcResponse send(RpcRequest request) throws Exception {
try {
// serialize request
byte[] requestBytes = HessianSerializer.serialize(request);
// reqURL
String reqURL = request.getServerAddress();
if (reqURL!=null && reqURL.toLowerCase().indexOf("http")==-1) {
reqURL = "http://" + request.getServerAddress() + "/"; // IP:PORT, need parse to url
}
// remote invoke
byte[] responseBytes = HttpClientUtil.postRequest(reqURL, requestBytes);
if (responseBytes == null || responseBytes.length==0) {
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("RpcResponse byte[] is null");
return rpcResponse;
}
// deserialize response
RpcResponse rpcResponse = (RpcResponse) HessianSerializer.deserialize(responseBytes, RpcResponse.class);
return rpcResponse;
} catch (Exception e) {
logger.error(e.getMessage(), e);
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("Client-error:" + e.getMessage());
return rpcResponse;
}
}
}
```
* NetComClientProxy生产的ExecutorBiz类,实际上就是一个http请求的方法
* http请求的序列化采用的hessian
### 总结前两步的调用链,调度中心->执行器
1. XxlJobDynamicScheduler.addJob()添加任务到quartz
2. RemoteHttpJobBean.executeInternal()定时触发任务执行
3. XxlJobTrigger.trigger()
4. XxlJobDynamicScheduler.getExecutorBiz() -> NetComClientProxy.getObject()获取代理的ExecutorBiz
5. ExecutorBiz.run() -> JettyClient.send()通过http请求调用执行器
6. 此处忽略了各种执行策略
### 执行器启动
执行器启动的时候,需要初始化```XxlJobExecutor```类,此类在初始化的时候,会进行一系列的准备。
```java
/**
* Created by xuxueli on 2016/3/2 21:14.
*/
public class XxlJobExecutor implements ApplicationContextAware {
private static final Logger logger = LoggerFactory.getLogger(XxlJobExecutor.class);
// ---------------------- param ----------------------
private String adminAddresses;
private String appName;
private String ip;
private int port;
private String accessToken;
private String logPath;
private int logRetentionDays;
public void setAdminAddresses(String adminAddresses) {
this.adminAddresses = adminAddresses;
}
public void setAppName(String appName) {
this.appName = appName;
}
public void setIp(String ip) {
this.ip = ip;
}
public void setPort(int port) {
this.port = port;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public void setLogPath(String logPath) {
this.logPath = logPath;
}
public void setLogRetentionDays(int logRetentionDays) {
this.logRetentionDays = logRetentionDays;
}
// ---------------------- applicationContext ----------------------
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext applicationContext) throws BeansException {
this.applicationContext = applicationContext;
}
public static ApplicationContext getApplicationContext() {
return applicationContext;
}
// ---------------------- start + stop ----------------------
public void start() throws Exception {
// init admin-client 初始化admin-http-client
initAdminBizList(adminAddresses, accessToken);
// init executor-jobHandlerRepository 初始化jobHandler
initJobHandlerRepository(applicationContext);
// init logpath 初始化XxlJobLog
XxlJobFileAppender.initLogPath(logPath);
// init executor-server 初始化内嵌的jetty服务器
initExecutorServer(port, ip, appName, accessToken);
// init JobLogFileCleanThread 初始化定时清理日志线程
JobLogFileCleanThread.getInstance().start(logRetentionDays);
}
public void destroy(){
// destory JobThreadRepository
if (JobThreadRepository.size() > 0) {
for (Map.Entry<Integer, JobThread> item: JobThreadRepository.entrySet()) {
removeJobThread(item.getKey(), "Web容器销毁终止");
}
JobThreadRepository.clear();
}
// destory executor-server
stopExecutorServer();
// destory JobLogFileCleanThread
JobLogFileCleanThread.getInstance().toStop();
}
// ---------------------- admin-client ----------------------
private static List<AdminBiz> adminBizList;
private static void initAdminBizList(String adminAddresses, String accessToken) throws Exception {
if (adminAddresses!=null && adminAddresses.trim().length()>0) {
for (String address: adminAddresses.trim().split(",")) {
if (address!=null && address.trim().length()>0) {
String addressUrl = address.concat(AdminBiz.MAPPING);
AdminBiz adminBiz = (AdminBiz) new NetComClientProxy(AdminBiz.class, addressUrl, accessToken).getObject();
if (adminBizList == null) {
adminBizList = new ArrayList<AdminBiz>();
}
adminBizList.add(adminBiz);
}
}
}
}
public static List<AdminBiz> getAdminBizList(){
return adminBizList;
}
// ---------------------- executor-server(jetty) ----------------------
private NetComServerFactory serverFactory = new NetComServerFactory();
private void initExecutorServer(int port, String ip, String appName, String accessToken) throws Exception {
// valid param
port = port>0?port: NetUtil.findAvailablePort(9999);
// start server
NetComServerFactory.putService(ExecutorBiz.class, new ExecutorBizImpl()); // rpc-service, base on jetty
NetComServerFactory.setAccessToken(accessToken);
serverFactory.start(port, ip, appName); // jetty + registry
}
private void stopExecutorServer() {
serverFactory.destroy(); // jetty + registry + callback
}
// ---------------------- job handler repository ----------------------
private static ConcurrentHashMap<String, IJobHandler> jobHandlerRepository = new ConcurrentHashMap<String, IJobHandler>();
public static IJobHandler registJobHandler(String name, IJobHandler jobHandler){
logger.info(">>>>>>>>>>> xxl-job register jobhandler success, name:{}, jobHandler:{}", name, jobHandler);
return jobHandlerRepository.put(name, jobHandler);
}
public static IJobHandler loadJobHandler(String name){
return jobHandlerRepository.get(name);
}
private static void initJobHandlerRepository(ApplicationContext applicationContext){
if (applicationContext == null) {
return;
}
// init job handler action
Map<String, Object> serviceBeanMap = applicationContext.getBeansWithAnnotation(JobHandler.class);
if (serviceBeanMap!=null && serviceBeanMap.size()>0) {
for (Object serviceBean : serviceBeanMap.values()) {
if (serviceBean instanceof IJobHandler){
String name = serviceBean.getClass().getAnnotation(JobHandler.class).value();
IJobHandler handler = (IJobHandler) serviceBean;
if (loadJobHandler(name) != null) {
throw new RuntimeException("xxl-job jobhandler naming conflicts.");
}
registJobHandler(name, handler);
}
}
}
}
// ---------------------- job thread repository ----------------------
private static ConcurrentHashMap<Integer, JobThread> JobThreadRepository = new ConcurrentHashMap<Integer, JobThread>();
public static JobThread registJobThread(int jobId, IJobHandler handler, String removeOldReason){
JobThread newJobThread = new JobThread(jobId, handler);
newJobThread.start();
logger.info(">>>>>>>>>>> xxl-job regist JobThread success, jobId:{}, handler:{}", new Object[]{jobId, handler});
JobThread oldJobThread = JobThreadRepository.put(jobId, newJobThread); // putIfAbsent | oh my god, map's put method return the old value!!!
if (oldJobThread != null) {
oldJobThread.toStop(removeOldReason);
oldJobThread.interrupt();
}
return newJobThread;
}
public static void removeJobThread(int jobId, String removeOldReason){
JobThread oldJobThread = JobThreadRepository.remove(jobId);
if (oldJobThread != null) {
oldJobThread.toStop(removeOldReason);
oldJobThread.interrupt();
}
}
public static JobThread loadJobThread(int jobId){
JobThread jobThread = JobThreadRepository.get(jobId);
return jobThread;
}
}
```
* 主要关注XxlJobExecutor的init方法
* 初始化AdminBiz的类集合,通过工厂类生产的代理类,生成的方法雷同前面的代理ExecutorBiz类,实际上也是一个NetComClientProxy.getObject(),http请求的地址为"调用中心地址+/api"
* 初始化jobHandler,也就是实际的任务执行类
* 初始化XxlJobLog,通过此log记录日志,日志记录到执行器服务器的文件内。
* 初始化执行器内嵌的jetty服务器,端口号默认9999;初始化内嵌服务器时,还会启动一个执行器自动注册到调度中心的线程。
* 初始化自动清除XxlJobLog生产的日志文件的线程
#### 初始化AdminBiz类的集合
前面有讲过,再调度中心调用执行器的过程中,是通过NetComClientProxy工厂类生产的代理ExecutorBiz类,此生成的类实际上就是封装了一个http请求;这里出书画生成的AdminBiz类也是如此,实际上就是封装了一个http请求
#### 初始化jobHandler
jobHandler在xxl-job中,就是执行器的实际任务执行类,例子如下:
```java
@JobHandler(value="demoJobHandler")
@Component
public class DemoJobHandler extends IJobHandler {
@Override
public ReturnT<String> execute(String param) throws Exception {
XxlJobLogger.log("XXL-JOB, Hello World.");
for (int i = 0; i < 5; i++) {
XxlJobLogger.log("beat at:" + i);
TimeUnit.SECONDS.sleep(2);
}
return SUCCESS;
}
}
```
* demoJobHandler在调度中心中添加任务的时候需要用到
#### 初始化XxlJobLog
```java
/**
* store trigger log in each log-file
* @author xuxueli 2016-3-12 19:25:12
*/
public class XxlJobFileAppender {
private static Logger logger = LoggerFactory.getLogger(XxlJobFileAppender.class);
// for JobThread (support log for child thread of job handler)
//public static ThreadLocal<String> contextHolder = new ThreadLocal<String>();
public static final InheritableThreadLocal<String> contextHolder = new InheritableThreadLocal<String>();
/**
* log base path
*
* strut like:
* ---/
* ---/gluesource/
* ---/gluesource/10_1514171108000.js
* ---/gluesource/10_1514171108000.js
* ---/2017-12-25/
* ---/2017-12-25/639.log
* ---/2017-12-25/821.log
*
*/
private static String logBasePath = "/data/applogs/xxl-job/jobhandler";
private static String glueSrcPath = logBasePath.concat("/gluesource");
public static void initLogPath(String logPath){
// init
if (logPath!=null && logPath.trim().length()>0) {
logBasePath = logPath;
}
// mk base dir
File logPathDir = new File(logBasePath);
if (!logPathDir.exists()) {
logPathDir.mkdirs();
}
logBasePath = logPathDir.getPath();
// mk glue dir
File glueBaseDir = new File(logPathDir, "gluesource");
if (!glueBaseDir.exists()) {
glueBaseDir.mkdirs();
}
glueSrcPath = glueBaseDir.getPath();
}
........
}
```
* 主要是初始化日志存放的路径和glue过来的代码存放路径
#### 初始化jetty服务器
```java
public class JettyServer {
private static final Logger logger = LoggerFactory.getLogger(JettyServer.class);
private Server server;
private Thread thread;
public void start(final int port, final String ip, final String appName) throws Exception {
thread = new Thread(new Runnable() {
@Override
public void run() {
// The Server
server = new Server(new ExecutorThreadPool()); // 非阻塞
// HTTP connector
ServerConnector connector = new ServerConnector(server);
if (ip!=null && ip.trim().length()>0) {
connector.setHost(ip); // The network interface this connector binds to as an IP address or a hostname. If null or 0.0.0.0, then bind to all interfaces.
}
connector.setPort(port);
server.setConnectors(new Connector[]{connector});
// Set a handler
HandlerCollection handlerc =new HandlerCollection();
handlerc.setHandlers(new Handler[]{new JettyServerHandler()});
server.setHandler(handlerc);
try {
// Start server 启动内嵌服务器
server.start();
logger.info(">>>>>>>>>>> xxl-job jetty server start success at port:{}.", port);
// Start Registry-Server 自动注册线程
ExecutorRegistryThread.getInstance().start(port, ip, appName);
// Start Callback-Server 任务执行结束异步回调线程
TriggerCallbackThread.getInstance().start();
server.join(); // block until thread stopped
logger.info(">>>>>>>>>>> xxl-rpc server join success, netcon={}, port={}", JettyServer.class.getName(), port);
} catch (Exception e) {
logger.error(e.getMessage(), e);
} finally {
//destroy();
}
}
});
thread.setDaemon(true); // daemon, service jvm, user thread leave >>> daemon leave >>> jvm leave
thread.start();
}
......
}
```
* 启动内嵌的jetty服务器,设置JettyServerHandler,当接受到调用请求后,会调用此类
* 启动自动注册到调度中心线程
* 启动任务执行结束异步回调线程
### 执行器执行任务
执行器收到请求后,调用```JettyServerHandler```的handle方法
```java
/**
* jetty handler
* @author xuxueli 2015-11-19 22:32:36
*/
public class JettyServerHandler extends AbstractHandler {
private static Logger logger = LoggerFactory.getLogger(JettyServerHandler.class);
@Override
public void handle(String target, Request baseRequest, HttpServletRequest request, HttpServletResponse response) throws IOException, ServletException {
// invoke
RpcResponse rpcResponse = doInvoke(request);
// serialize response
byte[] responseBytes = HessianSerializer.serialize(rpcResponse);
response.setContentType("text/html;charset=utf-8");
response.setStatus(HttpServletResponse.SC_OK);
baseRequest.setHandled(true);
OutputStream out = response.getOutputStream();
out.write(responseBytes);
out.flush();
}
private RpcResponse doInvoke(HttpServletRequest request) {
try {
// deserialize request
byte[] requestBytes = HttpClientUtil.readBytes(request);
if (requestBytes == null || requestBytes.length==0) {
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("RpcRequest byte[] is null");
return rpcResponse;
}
//hession 反序列化
RpcRequest rpcRequest = (RpcRequest) HessianSerializer.deserialize(requestBytes, RpcRequest.class);
// invoke 实际调用
RpcResponse rpcResponse = NetComServerFactory.invokeService(rpcRequest, null);
return rpcResponse;
} catch (Exception e) {
logger.error(e.getMessage(), e);
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("Server-error:" + e.getMessage());
return rpcResponse;
}
}
}
public class NetComServerFactory {
private static final Logger logger = LoggerFactory.getLogger(NetComServerFactory.class);
// ---------------------- server start ----------------------
JettyServer server = new JettyServer();
public void start(int port, String ip, String appName) throws Exception {
server.start(port, ip, appName);
}
// ---------------------- server destroy ----------------------
public void destroy(){
server.destroy();
}
// ---------------------- server instance ----------------------
/**
* init local rpc service map
*/
private static Map<String, Object> serviceMap = new HashMap<String, Object>();
private static String accessToken;
public static void putService(Class<?> iface, Object serviceBean){
serviceMap.put(iface.getName(), serviceBean);
}
public static void setAccessToken(String accessToken) {
NetComServerFactory.accessToken = accessToken;
}
public static RpcResponse invokeService(RpcRequest request, Object serviceBean) {
if (serviceBean==null) {
serviceBean = serviceMap.get(request.getClassName());
}
if (serviceBean == null) {
// TODO
}
RpcResponse response = new RpcResponse();
if (System.currentTimeMillis() - request.getCreateMillisTime() > 180000) {
response.setResult(new ReturnT<String>(ReturnT.FAIL_CODE, "The timestamp difference between admin and executor exceeds the limit."));
return response;
}
if (accessToken!=null && accessToken.trim().length()>0 && !accessToken.trim().equals(request.getAccessToken())) {
response.setResult(new ReturnT<String>(ReturnT.FAIL_CODE, "The access token[" + request.getAccessToken() + "] is wrong."));
return response;
}
try {
Class<?> serviceClass = serviceBean.getClass();
//获取调度中心调用的方法
String methodName = request.getMethodName();
Class<?>[] parameterTypes = request.getParameterTypes();
Object[] parameters = request.getParameters();
FastClass serviceFastClass = FastClass.create(serviceClass);
FastMethod serviceFastMethod = serviceFastClass.getMethod(methodName, parameterTypes);
//去实际调用方法
Object result = serviceFastMethod.invoke(serviceBean, parameters);
response.setResult(result);
} catch (Throwable t) {
t.printStackTrace();
response.setError(t.getMessage());
}
return response;
}
}
```
* 首先通过hession反序列化获取调度中心传过来的数据,内包含serviceBean和调用方法
* serviceMap.get(request.getClassName());获取的一般就是ExecutorBizImpl类,在XxlJobExecutor初始化的时候会添加进serviceMap中
* 根据传过来的调用方法名称(run()方法),通过反射调用
下面来看看ExecutorBizImpl的run方法
```java
@Override
public ReturnT<String> run(TriggerParam triggerParam) {
// load old:jobHandler + jobThread
JobThread jobThread = XxlJobExecutor.loadJobThread(triggerParam.getJobId());
IJobHandler jobHandler = jobThread!=null?jobThread.getHandler():null;
String removeOldReason = null;
// valid:jobHandler + jobThread
GlueTypeEnum glueTypeEnum = GlueTypeEnum.match(triggerParam.getGlueType());
if (GlueTypeEnum.BEAN == glueTypeEnum) {
// new jobhandler
IJobHandler newJobHandler = XxlJobExecutor.loadJobHandler(triggerParam.getExecutorHandler());
// valid old jobThread
if (jobThread!=null && jobHandler != newJobHandler) {
// change handler, need kill old thread
removeOldReason = "更换JobHandler或更换任务模式,终止旧任务线程";
jobThread = null;
jobHandler = null;
}
// valid handler
if (jobHandler == null) {
jobHandler = newJobHandler;
if (jobHandler == null) {
return new ReturnT<String>(ReturnT.FAIL_CODE, "job handler [" + triggerParam.getExecutorHandler() + "] not found.");
}
}
} else if (GlueTypeEnum.GLUE_GROOVY == glueTypeEnum) {
// valid old jobThread
if (jobThread != null &&
!(jobThread.getHandler() instanceof GlueJobHandler
&& ((GlueJobHandler) jobThread.getHandler()).getGlueUpdatetime()==triggerParam.getGlueUpdatetime() )) {
// change handler or gluesource updated, need kill old thread
removeOldReason = "更新任务逻辑或更换任务模式,终止旧任务线程";
jobThread = null;
jobHandler = null;
}
// valid handler
if (jobHandler == null) {
try {
IJobHandler originJobHandler = GlueFactory.getInstance().loadNewInstance(triggerParam.getGlueSource());
jobHandler = new GlueJobHandler(originJobHandler, triggerParam.getGlueUpdatetime());
} catch (Exception e) {
logger.error(e.getMessage(), e);
return new ReturnT<String>(ReturnT.FAIL_CODE, e.getMessage());
}
}
} else if (glueTypeEnum!=null && glueTypeEnum.isScript()) {
// valid old jobThread
if (jobThread != null &&
!(jobThread.getHandler() instanceof ScriptJobHandler
&& ((ScriptJobHandler) jobThread.getHandler()).getGlueUpdatetime()==triggerParam.getGlueUpdatetime() )) {
// change script or gluesource updated, need kill old thread
removeOldReason = "更新任务逻辑或更换任务模式,终止旧任务线程";
jobThread = null;
jobHandler = null;
}
// valid handler
if (jobHandler == null) {
jobHandler = new ScriptJobHandler(triggerParam.getJobId(), triggerParam.getGlueUpdatetime(), triggerParam.getGlueSource(), GlueTypeEnum.match(triggerParam.getGlueType()));
}
} else {
return new ReturnT<String>(ReturnT.FAIL_CODE, "glueType[" + triggerParam.getGlueType() + "] is not valid.");
}
// executor block strategy
if (jobThread != null) {
ExecutorBlockStrategyEnum blockStrategy = ExecutorBlockStrategyEnum.match(triggerParam.getExecutorBlockStrategy(), null);
if (ExecutorBlockStrategyEnum.DISCARD_LATER == blockStrategy) {
// discard when running
if (jobThread.isRunningOrHasQueue()) {
return new ReturnT<String>(ReturnT.FAIL_CODE, "阻塞处理策略-生效:"+ExecutorBlockStrategyEnum.DISCARD_LATER.getTitle());
}
} else if (ExecutorBlockStrategyEnum.COVER_EARLY == blockStrategy) {
// kill running jobThread
if (jobThread.isRunningOrHasQueue()) {
removeOldReason = "阻塞处理策略-生效:" + ExecutorBlockStrategyEnum.COVER_EARLY.getTitle();
jobThread = null;
}
} else {
// just queue trigger
}
}
// replace thread (new or exists invalid)
if (jobThread == null) {
jobThread = XxlJobExecutor.registJobThread(triggerParam.getJobId(), jobHandler, removeOldReason);
}
// push data to queue
ReturnT<String> pushResult = jobThread.pushTriggerQueue(triggerParam);
return pushResult;
}
```
* 根据调度中心配置的任务执行方法,分为三种:bean、glue、脚本(shell,python等),这里先不讨论他们具体的实现方式,主要讨论bean的方式
* 先去缓存中获取jobThread,一个jobId一般只会有一个线程(再不考虑堵塞策略和线程生命周期的情况下)
* 如果jobThread已经存在,并且老的jobHandler和新的jobHandler不一致,则会把jobHandler设置为新的,并且重新生成一个新的jobThread代替老的
* 如果jobThread不存在,则会生成一个新的jobThread,用于执行任务
* 把任务推送到jobThread队列中
* 注意:这里有一点要注意的地方是,阻塞策略是调度中心传递到执行来实现的。
- 阻塞策略的覆盖策略,如果任务已经开始执行,则要等待此任务执行完
```java
/**
* handler thread
* @author xuxueli 2016-1-16 19:52:47
*/
public class JobThread extends Thread{
private static Logger logger = LoggerFactory.getLogger(JobThread.class);
private int jobId;
private IJobHandler handler;
private LinkedBlockingQueue<TriggerParam> triggerQueue;
private ConcurrentHashSet<Integer> triggerLogIdSet; // avoid repeat trigger for the same TRIGGER_LOG_ID
private boolean toStop = false;
private String stopReason;
private boolean running = false; // if running job
private int idleTimes = 0; // idel times
public JobThread(int jobId, IJobHandler handler) {
this.jobId = jobId;
this.handler = handler;
this.triggerQueue = new LinkedBlockingQueue<TriggerParam>();
this.triggerLogIdSet = new ConcurrentHashSet<Integer>();
}
public IJobHandler getHandler() {
return handler;
}
/**
* new trigger to queue
*
* @param triggerParam
* @return
*/
public ReturnT<String> pushTriggerQueue(TriggerParam triggerParam) {
// avoid repeat
if (triggerLogIdSet.contains(triggerParam.getLogId())) {
logger.info(">>>>>>>>>>> repeate trigger job, logId:{}", triggerParam.getLogId());
return new ReturnT<String>(ReturnT.FAIL_CODE, "repeate trigger job, logId:" + triggerParam.getLogId());
}
triggerLogIdSet.add(triggerParam.getLogId());
triggerQueue.add(triggerParam);
return ReturnT.SUCCESS;
}
/**
* kill job thread
*
* @param stopReason
*/
public void toStop(String stopReason) {
/**
* Thread.interrupt只支持终止线程的阻塞状态(wait、join、sleep),
* 在阻塞出抛出InterruptedException异常,但是并不会终止运行的线程本身;
* 所以需要注意,此处彻底销毁本线程,需要通过共享变量方式;
*/
this.toStop = true;
this.stopReason = stopReason;
}
/**
* is running job
* @return
*/
public boolean isRunningOrHasQueue() {
return running || triggerQueue.size()>0;
}
@Override
public void run() {
// init
try {
handler.init();
} catch (Throwable e) {
logger.error(e.getMessage(), e);
}
// execute
while(!toStop){
running = false;
idleTimes++;
TriggerParam triggerParam = null;
ReturnT<String> executeResult = null;
try {
// to check toStop signal, we need cycle, so wo cannot use queue.take(), instand of poll(timeout)
triggerParam = triggerQueue.poll(3L, TimeUnit.SECONDS);
if (triggerParam!=null) {
running = true;
idleTimes = 0;
triggerLogIdSet.remove(triggerParam.getLogId());
// log filename, like "logPath/yyyy-MM-dd/9999.log"
String logFileName = XxlJobFileAppender.makeLogFileName(new Date(triggerParam.getLogDateTim()), triggerParam.getLogId());
XxlJobFileAppender.contextHolder.set(logFileName);
ShardingUtil.setShardingVo(new ShardingUtil.ShardingVO(triggerParam.getBroadcastIndex(), triggerParam.getBroadcastTotal()));
// execute
XxlJobLogger.log("<br>----------- xxl-job job execute start -----------<br>----------- Param:" + triggerParam.getExecutorParams());
executeResult = handler.execute(triggerParam.getExecutorParams());
if (executeResult == null) {
executeResult = IJobHandler.FAIL;
}
XxlJobLogger.log("<br>----------- xxl-job job execute end(finish) -----------<br>----------- ReturnT:" + executeResult);
} else {
if (idleTimes > 30) {
XxlJobExecutor.removeJobThread(jobId, "excutor idel times over limit.");
}
}
} catch (Throwable e) {
if (toStop) {
XxlJobLogger.log("<br>----------- JobThread toStop, stopReason:" + stopReason);
}
StringWriter stringWriter = new StringWriter();
e.printStackTrace(new PrintWriter(stringWriter));
String errorMsg = stringWriter.toString();
executeResult = new ReturnT<String>(ReturnT.FAIL_CODE, errorMsg);
XxlJobLogger.log("<br>----------- JobThread Exception:" + errorMsg + "<br>----------- xxl-job job execute end(error) -----------");
} finally {
if(triggerParam != null) {
// callback handler info
if (!toStop) {
// commonm
TriggerCallbackThread.pushCallBack(new HandleCallbackParam(triggerParam.getLogId(), executeResult));
} else {
// is killed
ReturnT<String> stopResult = new ReturnT<String>(ReturnT.FAIL_CODE, stopReason + " [业务运行中,被强制终止]");
TriggerCallbackThread.pushCallBack(new HandleCallbackParam(triggerParam.getLogId(), stopResult));
}
}
}
}
// callback trigger request in queue
while(triggerQueue !=null && triggerQueue.size()>0){
TriggerParam triggerParam = triggerQueue.poll();
if (triggerParam!=null) {
// is killed
ReturnT<String> stopResult = new ReturnT<String>(ReturnT.FAIL_CODE, stopReason + " [任务尚未执行,在调度队列中被终止]");
TriggerCallbackThread.pushCallBack(new HandleCallbackParam(triggerParam.getLogId(), stopResult));
}
}
// destroy
try {
handler.destroy();
} catch (Throwable e) {
logger.error(e.getMessage(), e);
}
logger.info(">>>>>>>>>>> xxl-job JobThread stoped, hashCode:{}", Thread.currentThread());
}
}
```
* 基本就是从队列中取出任务,获取jobHandler,然后执行
* 执行玩之后,会把任务执行结果添加到TriggerCallbackThread线程中
```java
/**
* Created by xuxueli on 16/7/22.
*/
public class TriggerCallbackThread {
private static Logger logger = LoggerFactory.getLogger(TriggerCallbackThread.class);
private static TriggerCallbackThread instance = new TriggerCallbackThread();
public static TriggerCallbackThread getInstance(){
return instance;
}
/**
* job results callback queue
*/
private LinkedBlockingQueue<HandleCallbackParam> callBackQueue = new LinkedBlockingQueue<HandleCallbackParam>();
public static void pushCallBack(HandleCallbackParam callback){
getInstance().callBackQueue.add(callback);
logger.debug(">>>>>>>>>>> xxl-job, push callback request, logId:{}", callback.getLogId());
}
/**
* callback thread
*/
private Thread triggerCallbackThread;
private volatile boolean toStop = false;
public void start() {
// valid
if (XxlJobExecutor.getAdminBizList() == null) {
logger.warn(">>>>>>>>>>> xxl-job, executor callback config fail, adminAddresses is null.");
return;
}
triggerCallbackThread = new Thread(new Runnable() {
@Override
public void run() {
// normal callback
while(!toStop){
try {
HandleCallbackParam callback = getInstance().callBackQueue.take();
if (callback != null) {
// callback list param
List<HandleCallbackParam> callbackParamList = new ArrayList<HandleCallbackParam>();
int drainToNum = getInstance().callBackQueue.drainTo(callbackParamList);
callbackParamList.add(callback);
// callback, will retry if error
if (callbackParamList!=null && callbackParamList.size()>0) {
doCallback(callbackParamList);
}
}
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
}
// last callback
try {
List<HandleCallbackParam> callbackParamList = new ArrayList<HandleCallbackParam>();
int drainToNum = getInstance().callBackQueue.drainTo(callbackParamList);
if (callbackParamList!=null && callbackParamList.size()>0) {
doCallback(callbackParamList);
}
} catch (Exception e) {
logger.error(e.getMessage(), e);
}
logger.info(">>>>>>>>>>> xxl-job, executor callback thread destory.");
}
});
triggerCallbackThread.setDaemon(true);
triggerCallbackThread.start();
}
public void toStop(){
toStop = true;
// interrupt and wait
triggerCallbackThread.interrupt();
try {
triggerCallbackThread.join();
} catch (InterruptedException e) {
logger.error(e.getMessage(), e);
}
}
/**
* do callback, will retry if error
* @param callbackParamList
*/
private void doCallback(List<HandleCallbackParam> callbackParamList){
// callback, will retry if error
for (AdminBiz adminBiz: XxlJobExecutor.getAdminBizList()) {
try {
ReturnT<String> callbackResult = adminBiz.callback(callbackParamList);
if (callbackResult!=null && ReturnT.SUCCESS_CODE == callbackResult.getCode()) {
callbackResult = ReturnT.SUCCESS;
logger.info(">>>>>>>>>>> xxl-job callback success, callbackParamList:{}, callbackResult:{}", new Object[]{callbackParamList, callbackResult});
break;
} else {
logger.info(">>>>>>>>>>> xxl-job callback fail, callbackParamList:{}, callbackResult:{}", new Object[]{callbackParamList, callbackResult});
}
} catch (Exception e) {
logger.error(">>>>>>>>>>> xxl-job callback error, callbackParamList:{}", callbackParamList, e);
//getInstance().callBackQueue.addAll(callbackParamList);
}
}
}
}
```
* 先从队列中取出任务执行结果
* 通过admin-http-client(前面初始化的AdminBiz代理类)逐个调用,直至成功,如果全部失败不会重试(被注释掉了,不知道为什么)
调度中心对执行器开放的接口
```java
@Controller
public class JobApiController {
private static Logger logger = LoggerFactory.getLogger(JobApiController.class);
private RpcResponse doInvoke(HttpServletRequest request) {
try {
// deserialize request
byte[] requestBytes = HttpClientUtil.readBytes(request);
if (requestBytes == null || requestBytes.length==0) {
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("RpcRequest byte[] is null");
return rpcResponse;
}
RpcRequest rpcRequest = (RpcRequest) HessianSerializer.deserialize(requestBytes, RpcRequest.class);
// invoke
RpcResponse rpcResponse = NetComServerFactory.invokeService(rpcRequest, null);
return rpcResponse;
} catch (Exception e) {
logger.error(e.getMessage(), e);
RpcResponse rpcResponse = new RpcResponse();
rpcResponse.setError("Server-error:" + e.getMessage());
return rpcResponse;
}
}
@RequestMapping(AdminBiz.MAPPING)
@PermessionLimit(limit=false)
public void api(HttpServletRequest request, HttpServletResponse response) throws IOException {
// invoke
RpcResponse rpcResponse = doInvoke(request);
// serialize response
byte[] responseBytes = HessianSerializer.serialize(rpcResponse);
response.setContentType("text/html;charset=utf-8");
response.setStatus(HttpServletResponse.SC_OK);
//baseRequest.setHandled(true);
OutputStream out = response.getOutputStream();
out.write(responseBytes);
out.flush();
}
}
```
* 执行器注册和执行器结果的异步回调都是统一调用此接口
* 然后去实际的调用AdminBizImpl类中的具体方法,下面是AdminBizImpl
```java
public class AdminBizImpl implements AdminBiz {
private static Logger logger = LoggerFactory.getLogger(AdminBizImpl.class);
@Resource
public XxlJobLogDao xxlJobLogDao;
@Resource
private XxlJobInfoDao xxlJobInfoDao;
@Resource
private XxlJobRegistryDao xxlJobRegistryDao;
@Resource
private XxlJobService xxlJobService;
@Override
public ReturnT<String> callback(List<HandleCallbackParam> callbackParamList) {
for (HandleCallbackParam handleCallbackParam: callbackParamList) {
ReturnT<String> callbackResult = callback(handleCallbackParam);
logger.info(">>>>>>>>> JobApiController.callback {}, handleCallbackParam={}, callbackResult={}",
(callbackResult.getCode()==IJobHandler.SUCCESS.getCode()?"success":"fail"), handleCallbackParam, callbackResult);
}
return ReturnT.SUCCESS;
}
private ReturnT<String> callback(HandleCallbackParam handleCallbackParam) {
// valid log item
XxlJobLog log = xxlJobLogDao.load(handleCallbackParam.getLogId());
if (log == null) {
return new ReturnT<String>(ReturnT.FAIL_CODE, "log item not found.");
}
if (log.getHandleCode() > 0) {
return new ReturnT<String>(ReturnT.FAIL_CODE, "log repeate callback."); // avoid repeat callback, trigger child job etc
}
// trigger success, to trigger child job
String callbackMsg = null;
if (IJobHandler.SUCCESS.getCode() == handleCallbackParam.getExecuteResult().getCode()) {
XxlJobInfo xxlJobInfo = xxlJobInfoDao.loadById(log.getJobId());
if (xxlJobInfo!=null && StringUtils.isNotBlank(xxlJobInfo.getChildJobId())) {
callbackMsg = "<br><br><span style=\"color:#00c0ef;\" > >>>>>>>>>>>"+ I18nUtil.getString("jobconf_trigger_child_run") +"<<<<<<<<<<< </span><br>";
String[] childJobIds = xxlJobInfo.getChildJobId().split(",");
for (int i = 0; i < childJobIds.length; i++) {
int childJobId = (StringUtils.isNotBlank(childJobIds[i]) && StringUtils.isNumeric(childJobIds[i]))?Integer.valueOf(childJobIds[i]):-1;
if (childJobId > 0) {
ReturnT<String> triggerChildResult = xxlJobService.triggerJob(childJobId);
// add msg
callbackMsg += MessageFormat.format(I18nUtil.getString("jobconf_callback_child_msg1"),
(i+1),
childJobIds.length,
childJobIds[i],
(triggerChildResult.getCode()==ReturnT.SUCCESS_CODE?I18nUtil.getString("system_success"):I18nUtil.getString("system_fail")),
triggerChildResult.getMsg());
} else {
callbackMsg += MessageFormat.format(I18nUtil.getString("jobconf_callback_child_msg2"),
(i+1),
childJobIds.length,
childJobIds[i]);
}
}
}
} else if (IJobHandler.FAIL_RETRY.getCode() == handleCallbackParam.getExecuteResult().getCode()){
ReturnT<String> retryTriggerResult = xxlJobService.triggerJob(log.getJobId());
callbackMsg = "<br><br><span style=\"color:#F39C12;\" > >>>>>>>>>>>"+ I18nUtil.getString("jobconf_exe_fail_retry") +"<<<<<<<<<<< </span><br>";
callbackMsg += MessageFormat.format(I18nUtil.getString("jobconf_callback_msg1"),
(retryTriggerResult.getCode()==ReturnT.SUCCESS_CODE?I18nUtil.getString("system_success"):I18nUtil.getString("system_fail")), retryTriggerResult.getMsg());
}
// handle msg
StringBuffer handleMsg = new StringBuffer();
if (log.getHandleMsg()!=null) {
handleMsg.append(log.getHandleMsg()).append("<br>");
}
if (handleCallbackParam.getExecuteResult().getMsg() != null) {
handleMsg.append(handleCallbackParam.getExecuteResult().getMsg());
}
if (callbackMsg != null) {
handleMsg.append(callbackMsg);
}
// success, save log
log.setHandleTime(new Date());
log.setHandleCode(handleCallbackParam.getExecuteResult().getCode());
log.setHandleMsg(handleMsg.toString());
xxlJobLogDao.updateHandleInfo(log);
return ReturnT.SUCCESS;
}
@Override
public ReturnT<String> registry(RegistryParam registryParam) {
int ret = xxlJobRegistryDao.registryUpdate(registryParam.getRegistGroup(), registryParam.getRegistryKey(), registryParam.getRegistryValue());
if (ret < 1) {
xxlJobRegistryDao.registrySave(registryParam.getRegistGroup(), registryParam.getRegistryKey(), registryParam.getRegistryValue());
}
return ReturnT.SUCCESS;
}
@Override
public ReturnT<String> registryRemove(RegistryParam registryParam) {
xxlJobRegistryDao.registryDelete(registryParam.getRegistGroup(), registryParam.getRegistryKey(), registryParam.getRegistryValue());
return ReturnT.SUCCESS;
}
@Override
public ReturnT<String> triggerJob(int jobId) {
return xxlJobService.triggerJob(jobId);
}
}
```
* 至此,一个完成的流程就完成了,其他的比如路由策略,各种线程,xxl日志等等,后面有时间会用一篇单独的文章来书写。
<file_sep>---
layout: post
title: java的spi
date: 2017-09-13 15:21:00
categories:
- java
tags:
- java
---
<p>最近在看dubbo的源码时看到了其spi(Service Provider Interface)的实现,想要了解dubbo的spi的实现的话,就不得不要先了解一下java原生提供的spi实现了。</p>
<p>dubbo在其官网上是这样评价他们的spi机制的:</p>
Dubbo改进了JDK标准的SPI的以下问题:
* JDK标准的SPI会一次性实例化扩展点所有实现,如果有扩展实现初始化很耗时,但如果没用上也加载,会很浪费资源。
* 如果扩展点加载失败,连扩展点的名称都拿不到了。比如:JDK标准的ScriptEngine,通过 getName() 获取脚本类型的名称,但如果RubyScriptEngine因为所依赖的jruby.jar不存在,导致RubyScriptEngine类加载失败,这个失败原因被吃掉了,和ruby对应不起来,当用户执行ruby脚本时,会报不支持ruby,而不是真正失败的原因。
* 增加了对扩展点IoC和AOP的支持,一个扩展点可以直接setter注入其它扩展点。
<p>接下来就是对java原生提供的spi机制的详细说明了,dubbo的留到下次再说</p>
#### spi功能实现
* java原生的spi相对来说还是比较简单的,主要通过java.util.ServiceLoader这个类来实现功能
* 首先定义一个interface
```java
package com.wgq.spi.test;
public interface ISearch {
void search() ;
}
```
* 接口的实现
```java
package com.wgq.spi.test;
public class SearchBean implements ISearch {
public void search() {
System.out.println("spi search");
}
}
```
* 然后在resources配置文件下新建文件夹META-INF/services/*,*表示接口名称的全称,所以这里*=com.wgq.spi.test.ISearch
* 在文件META-INF/services/com.wgq.spi.test.ISearch内添加接口的实现类全称,如果有多个实现,则一行一个
```
####com.wgq.spi.test.ISearch文件
com.wgq.spi.test.SearchBean
```
* 然后通过java.util.ServiceLoader调用接口
```java
public static void main(String[] args) {
ServiceLoader<ISearch> serviceLoader = ServiceLoader.load(ISearch.class) ;
Iterator<ISearch> iterator = serviceLoader.iterator() ;
while (iterator.hasNext()) {
ISearch search = iterator.next() ;
search.search();
}
}
```
* 到这里一个简单的java原生spi功能就演示完毕了。
#### ServiceLoader源码阅读
```java
package java.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.URL;
import java.security.AccessController;
import java.security.AccessControlContext;
import java.security.PrivilegedAction;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
/**
* A simple service-provider loading facility.
*
* <p> A <i>service</i> is a well-known set of interfaces and (usually
* abstract) classes. A <i>service provider</i> is a specific implementation
* of a service. The classes in a provider typically implement the interfaces
* and subclass the classes defined in the service itself. Service providers
* can be installed in an implementation of the Java platform in the form of
* extensions, that is, jar files placed into any of the usual extension
* directories. Providers can also be made available by adding them to the
* application's class path or by some other platform-specific means.
*
* <p> For the purpose of loading, a service is represented by a single type,
* that is, a single interface or abstract class. (A concrete class can be
* used, but this is not recommended.) A provider of a given service contains
* one or more concrete classes that extend this <i>service type</i> with data
* and code specific to the provider. The <i>provider class</i> is typically
* not the entire provider itself but rather a proxy which contains enough
* information to decide whether the provider is able to satisfy a particular
* request together with code that can create the actual provider on demand.
* The details of provider classes tend to be highly service-specific; no
* single class or interface could possibly unify them, so no such type is
* defined here. The only requirement enforced by this facility is that
* provider classes must have a zero-argument constructor so that they can be
* instantiated during loading.
*
* <p><a name="format"> A service provider is identified by placing a
* <i>provider-configuration file</i> in the resource directory
* <tt>META-INF/services</tt>.</a> The file's name is the fully-qualified <a
* href="../lang/ClassLoader.html#name">binary name</a> of the service's type.
* The file contains a list of fully-qualified binary names of concrete
* provider classes, one per line. Space and tab characters surrounding each
* name, as well as blank lines, are ignored. The comment character is
* <tt>'#'</tt> (<tt>'\u0023'</tt>,
* <font style="font-size:smaller;">NUMBER SIGN</font>); on
* each line all characters following the first comment character are ignored.
* The file must be encoded in UTF-8.
*
* <p> If a particular concrete provider class is named in more than one
* configuration file, or is named in the same configuration file more than
* once, then the duplicates are ignored. The configuration file naming a
* particular provider need not be in the same jar file or other distribution
* unit as the provider itself. The provider must be accessible from the same
* class loader that was initially queried to locate the configuration file;
* note that this is not necessarily the class loader from which the file was
* actually loaded.
*
* <p> Providers are located and instantiated lazily, that is, on demand. A
* service loader maintains a cache of the providers that have been loaded so
* far. Each invocation of the {@link #iterator iterator} method returns an
* iterator that first yields all of the elements of the cache, in
* instantiation order, and then lazily locates and instantiates any remaining
* providers, adding each one to the cache in turn. The cache can be cleared
* via the {@link #reload reload} method.
*
* <p> Service loaders always execute in the security context of the caller.
* Trusted system code should typically invoke the methods in this class, and
* the methods of the iterators which they return, from within a privileged
* security context.
*
* <p> Instances of this class are not safe for use by multiple concurrent
* threads.
*
* <p> Unless otherwise specified, passing a <tt>null</tt> argument to any
* method in this class will cause a {@link NullPointerException} to be thrown.
*
*
* <p><span style="font-weight: bold; padding-right: 1em">Example</span>
* Suppose we have a service type <tt>com.example.CodecSet</tt> which is
* intended to represent sets of encoder/decoder pairs for some protocol. In
* this case it is an abstract class with two abstract methods:
*
* <blockquote><pre>
* public abstract Encoder getEncoder(String encodingName);
* public abstract Decoder getDecoder(String encodingName);</pre></blockquote>
*
* Each method returns an appropriate object or <tt>null</tt> if the provider
* does not support the given encoding. Typical providers support more than
* one encoding.
*
* <p> If <tt>com.example.impl.StandardCodecs</tt> is an implementation of the
* <tt>CodecSet</tt> service then its jar file also contains a file named
*
* <blockquote><pre>
* META-INF/services/com.example.CodecSet</pre></blockquote>
*
* <p> This file contains the single line:
*
* <blockquote><pre>
* com.example.impl.StandardCodecs # Standard codecs</pre></blockquote>
*
* <p> The <tt>CodecSet</tt> class creates and saves a single service instance
* at initialization:
*
* <blockquote><pre>
* private static ServiceLoader<CodecSet> codecSetLoader
* = ServiceLoader.load(CodecSet.class);</pre></blockquote>
*
* <p> To locate an encoder for a given encoding name it defines a static
* factory method which iterates through the known and available providers,
* returning only when it has located a suitable encoder or has run out of
* providers.
*
* <blockquote><pre>
* public static Encoder getEncoder(String encodingName) {
* for (CodecSet cp : codecSetLoader) {
* Encoder enc = cp.getEncoder(encodingName);
* if (enc != null)
* return enc;
* }
* return null;
* }</pre></blockquote>
*
* <p> A <tt>getDecoder</tt> method is defined similarly.
*
*
* <p><span style="font-weight: bold; padding-right: 1em">Usage Note</span> If
* the class path of a class loader that is used for provider loading includes
* remote network URLs then those URLs will be dereferenced in the process of
* searching for provider-configuration files.
*
* <p> This activity is normal, although it may cause puzzling entries to be
* created in web-server logs. If a web server is not configured correctly,
* however, then this activity may cause the provider-loading algorithm to fail
* spuriously.
*
* <p> A web server should return an HTTP 404 (Not Found) response when a
* requested resource does not exist. Sometimes, however, web servers are
* erroneously configured to return an HTTP 200 (OK) response along with a
* helpful HTML error page in such cases. This will cause a {@link
* ServiceConfigurationError} to be thrown when this class attempts to parse
* the HTML page as a provider-configuration file. The best solution to this
* problem is to fix the misconfigured web server to return the correct
* response code (HTTP 404) along with the HTML error page.
*
* @param <S>
* The type of the service to be loaded by this loader
*
* @author <NAME>
* @since 1.6
*/
public final class ServiceLoader<S>
implements Iterable<S>
{
private static final String PREFIX = "META-INF/services/";
// The class or interface representing the service being loaded
private final Class<S> service;
// The class loader used to locate, load, and instantiate providers
private final ClassLoader loader;
// The access control context taken when the ServiceLoader is created
private final AccessControlContext acc;
// Cached providers, in instantiation order
private LinkedHashMap<String,S> providers = new LinkedHashMap<>();
// The current lazy-lookup iterator
private LazyIterator lookupIterator;
/**
* Clear this loader's provider cache so that all providers will be
* reloaded.
*
* <p> After invoking this method, subsequent invocations of the {@link
* #iterator() iterator} method will lazily look up and instantiate
* providers from scratch, just as is done by a newly-created loader.
*
* <p> This method is intended for use in situations in which new providers
* can be installed into a running Java virtual machine.
*/
public void reload() {
providers.clear();
lookupIterator = new LazyIterator(service, loader);
}
private ServiceLoader(Class<S> svc, ClassLoader cl) {
service = Objects.requireNonNull(svc, "Service interface cannot be null");
loader = (cl == null) ? ClassLoader.getSystemClassLoader() : cl;
acc = (System.getSecurityManager() != null) ? AccessController.getContext() : null;
reload();
}
private static void fail(Class<?> service, String msg, Throwable cause)
throws ServiceConfigurationError
{
throw new ServiceConfigurationError(service.getName() + ": " + msg,
cause);
}
private static void fail(Class<?> service, String msg)
throws ServiceConfigurationError
{
throw new ServiceConfigurationError(service.getName() + ": " + msg);
}
private static void fail(Class<?> service, URL u, int line, String msg)
throws ServiceConfigurationError
{
fail(service, u + ":" + line + ": " + msg);
}
// Parse a single line from the given configuration file, adding the name
// on the line to the names list.
//
private int parseLine(Class<?> service, URL u, BufferedReader r, int lc,
List<String> names)
throws IOException, ServiceConfigurationError
{
String ln = r.readLine();
if (ln == null) {
return -1;
}
int ci = ln.indexOf('#');
if (ci >= 0) ln = ln.substring(0, ci);
ln = ln.trim();
int n = ln.length();
if (n != 0) {
if ((ln.indexOf(' ') >= 0) || (ln.indexOf('\t') >= 0))
fail(service, u, lc, "Illegal configuration-file syntax");
int cp = ln.codePointAt(0);
if (!Character.isJavaIdentifierStart(cp))
fail(service, u, lc, "Illegal provider-class name: " + ln);
for (int i = Character.charCount(cp); i < n; i += Character.charCount(cp)) {
cp = ln.codePointAt(i);
if (!Character.isJavaIdentifierPart(cp) && (cp != '.'))
fail(service, u, lc, "Illegal provider-class name: " + ln);
}
if (!providers.containsKey(ln) && !names.contains(ln))
names.add(ln);
}
return lc + 1;
}
// Parse the content of the given URL as a provider-configuration file.
//
// @param service
// The service type for which providers are being sought;
// used to construct error detail strings
//
// @param u
// The URL naming the configuration file to be parsed
//
// @return A (possibly empty) iterator that will yield the provider-class
// names in the given configuration file that are not yet members
// of the returned set
//
// @throws ServiceConfigurationError
// If an I/O error occurs while reading from the given URL, or
// if a configuration-file format error is detected
//
private Iterator<String> parse(Class<?> service, URL u)
throws ServiceConfigurationError
{
InputStream in = null;
BufferedReader r = null;
ArrayList<String> names = new ArrayList<>();
try {
in = u.openStream();
r = new BufferedReader(new InputStreamReader(in, "utf-8"));
int lc = 1;
while ((lc = parseLine(service, u, r, lc, names)) >= 0);
} catch (IOException x) {
fail(service, "Error reading configuration file", x);
} finally {
try {
if (r != null) r.close();
if (in != null) in.close();
} catch (IOException y) {
fail(service, "Error closing configuration file", y);
}
}
return names.iterator();
}
// Private inner class implementing fully-lazy provider lookup
//
private class LazyIterator
implements Iterator<S>
{
Class<S> service;
ClassLoader loader;
Enumeration<URL> configs = null;
Iterator<String> pending = null;
String nextName = null;
private LazyIterator(Class<S> service, ClassLoader loader) {
this.service = service;
this.loader = loader;
}
private boolean hasNextService() {
if (nextName != null) {
return true;
}
if (configs == null) {
try {
String fullName = PREFIX + service.getName();
if (loader == null)
configs = ClassLoader.getSystemResources(fullName);
else
configs = loader.getResources(fullName);
} catch (IOException x) {
fail(service, "Error locating configuration files", x);
}
}
while ((pending == null) || !pending.hasNext()) {
if (!configs.hasMoreElements()) {
return false;
}
pending = parse(service, configs.nextElement());
}
nextName = pending.next();
return true;
}
private S nextService() {
if (!hasNextService())
throw new NoSuchElementException();
String cn = nextName;
nextName = null;
Class<?> c = null;
try {
c = Class.forName(cn, false, loader);
} catch (ClassNotFoundException x) {
fail(service,
"Provider " + cn + " not found");
}
if (!service.isAssignableFrom(c)) {
fail(service,
"Provider " + cn + " not a subtype");
}
try {
S p = service.cast(c.newInstance());
providers.put(cn, p);
return p;
} catch (Throwable x) {
fail(service,
"Provider " + cn + " could not be instantiated",
x);
}
throw new Error(); // This cannot happen
}
public boolean hasNext() {
if (acc == null) {
return hasNextService();
} else {
PrivilegedAction<Boolean> action = new PrivilegedAction<Boolean>() {
public Boolean run() { return hasNextService(); }
};
return AccessController.doPrivileged(action, acc);
}
}
public S next() {
if (acc == null) {
return nextService();
} else {
PrivilegedAction<S> action = new PrivilegedAction<S>() {
public S run() { return nextService(); }
};
return AccessController.doPrivileged(action, acc);
}
}
public void remove() {
throw new UnsupportedOperationException();
}
}
/**
* Lazily loads the available providers of this loader's service.
*
* <p> The iterator returned by this method first yields all of the
* elements of the provider cache, in instantiation order. It then lazily
* loads and instantiates any remaining providers, adding each one to the
* cache in turn.
*
* <p> To achieve laziness the actual work of parsing the available
* provider-configuration files and instantiating providers must be done by
* the iterator itself. Its {@link java.util.Iterator#hasNext hasNext} and
* {@link java.util.Iterator#next next} methods can therefore throw a
* {@link ServiceConfigurationError} if a provider-configuration file
* violates the specified format, or if it names a provider class that
* cannot be found and instantiated, or if the result of instantiating the
* class is not assignable to the service type, or if any other kind of
* exception or error is thrown as the next provider is located and
* instantiated. To write robust code it is only necessary to catch {@link
* ServiceConfigurationError} when using a service iterator.
*
* <p> If such an error is thrown then subsequent invocations of the
* iterator will make a best effort to locate and instantiate the next
* available provider, but in general such recovery cannot be guaranteed.
*
* <blockquote style="font-size: smaller; line-height: 1.2"><span
* style="padding-right: 1em; font-weight: bold">Design Note</span>
* Throwing an error in these cases may seem extreme. The rationale for
* this behavior is that a malformed provider-configuration file, like a
* malformed class file, indicates a serious problem with the way the Java
* virtual machine is configured or is being used. As such it is
* preferable to throw an error rather than try to recover or, even worse,
* fail silently.</blockquote>
*
* <p> The iterator returned by this method does not support removal.
* Invoking its {@link java.util.Iterator#remove() remove} method will
* cause an {@link UnsupportedOperationException} to be thrown.
*
* @implNote When adding providers to the cache, the {@link #iterator
* Iterator} processes resources in the order that the {@link
* java.lang.ClassLoader#getResources(java.lang.String)
* ClassLoader.getResources(String)} method finds the service configuration
* files.
*
* @return An iterator that lazily loads providers for this loader's
* service
*/
public Iterator<S> iterator() {
return new Iterator<S>() {
Iterator<Map.Entry<String,S>> knownProviders
= providers.entrySet().iterator();
public boolean hasNext() {
if (knownProviders.hasNext())
return true;
return lookupIterator.hasNext();
}
public S next() {
if (knownProviders.hasNext())
return knownProviders.next().getValue();
return lookupIterator.next();
}
public void remove() {
throw new UnsupportedOperationException();
}
};
}
/**
* Creates a new service loader for the given service type and class
* loader.
*
* @param <S> the class of the service type
*
* @param service
* The interface or abstract class representing the service
*
* @param loader
* The class loader to be used to load provider-configuration files
* and provider classes, or <tt>null</tt> if the system class
* loader (or, failing that, the bootstrap class loader) is to be
* used
*
* @return A new service loader
*/
public static <S> ServiceLoader<S> load(Class<S> service,
ClassLoader loader)
{
return new ServiceLoader<>(service, loader);
}
/**
* Creates a new service loader for the given service type, using the
* current thread's {@linkplain java.lang.Thread#getContextClassLoader
* context class loader}.
*
* <p> An invocation of this convenience method of the form
*
* <blockquote><pre>
* ServiceLoader.load(<i>service</i>)</pre></blockquote>
*
* is equivalent to
*
* <blockquote><pre>
* ServiceLoader.load(<i>service</i>,
* Thread.currentThread().getContextClassLoader())</pre></blockquote>
*
* @param <S> the class of the service type
*
* @param service
* The interface or abstract class representing the service
*
* @return A new service loader
*/
public static <S> ServiceLoader<S> load(Class<S> service) {
ClassLoader cl = Thread.currentThread().getContextClassLoader();
return ServiceLoader.load(service, cl);
}
/**
* Creates a new service loader for the given service type, using the
* extension class loader.
*
* <p> This convenience method simply locates the extension class loader,
* call it <tt><i>extClassLoader</i></tt>, and then returns
*
* <blockquote><pre>
* ServiceLoader.load(<i>service</i>, <i>extClassLoader</i>)</pre></blockquote>
*
* <p> If the extension class loader cannot be found then the system class
* loader is used; if there is no system class loader then the bootstrap
* class loader is used.
*
* <p> This method is intended for use when only installed providers are
* desired. The resulting service will only find and load providers that
* have been installed into the current Java virtual machine; providers on
* the application's class path will be ignored.
*
* @param <S> the class of the service type
*
* @param service
* The interface or abstract class representing the service
*
* @return A new service loader
*/
public static <S> ServiceLoader<S> loadInstalled(Class<S> service) {
ClassLoader cl = ClassLoader.getSystemClassLoader();
ClassLoader prev = null;
while (cl != null) {
prev = cl;
cl = cl.getParent();
}
return ServiceLoader.load(service, prev);
}
/**
* Returns a string describing this service.
*
* @return A descriptive string
*/
public String toString() {
return "java.util.ServiceLoader[" + service.getName() + "]";
}
}
```
* ServiceLoader.load(Class)方法其实就是通过传入的Class对象new一个ServiceLoader对象出来
* 在new对象时,会生成一个LazyIterator的内部类对象
* 然后通过LazyIterator这个对象迭代生成配置文件中的对象。
<file_sep>---
layout: post
title: dubbo的扩展点机制-spi
date: 2017-09-25 18:21:00
categories:
- spi
tags:
- dubbo
- spi
---
Dubbo改进了JDK标准的SPI的以下问题:
* JDK标准的SPI会一次性实例化扩展点所有实现,如果有扩展实现初始化很耗时,但如果没用上也加载,会很浪费资源。
* 如果扩展点加载失败,连扩展点的名称都拿不到了。比如:JDK标准的ScriptEngine,通过 getName() 获取脚本类型的名称,但如果RubyScriptEngine因为所依赖的jruby.jar不存在,导致RubyScriptEngine类加载失败,这个失败原因被吃掉了,和ruby对应不起来,当用户执行ruby脚本时,会报不支持ruby,而不是真正失败的原因。
* 增加了对扩展点IoC和AOP的支持,一个扩展点可以直接setter注入其它扩展点。
<p>Dubbo的spi实现类是com.alibaba.dubbo.common.extension.ExtensionLoader。</p>
<p>Dubbo会为每个接口都生成一个ExtensionLoader对象,并放入到缓存中。</p>
```java
public static <T> ExtensionLoader<T> getExtensionLoader(Class<T> type) {
if (type == null)
throw new IllegalArgumentException("Extension type == null");
if(!type.isInterface()) {
throw new IllegalArgumentException("Extension type(" + type + ") is not interface!");
}
if(!withExtensionAnnotation(type)) {
throw new IllegalArgumentException("Extension type(" + type +
") is not extension, because WITHOUT @" + SPI.class.getSimpleName() + " Annotation!");
}
//先从缓存中获取ExtensionLoader
ExtensionLoader<T> loader = (ExtensionLoader<T>) EXTENSION_LOADERS.get(type);
if (loader == null) {
//缓存中不存在,则new一个ExtensionLoader对象并放入缓存中
EXTENSION_LOADERS.putIfAbsent(type, new ExtensionLoader<T>(type));
loader = (ExtensionLoader<T>) EXTENSION_LOADERS.get(type);
}
return loader;
}
private ExtensionLoader(Class<?> type) {
this.type = type;
objectFactory = (type == ExtensionFactory.class ? null : ExtensionLoader.getExtensionLoader(ExtensionFactory.class).getAdaptiveExtension());
}
```
* 根据class类型获取到ExtensionLoader对象后,一般有三个方法获取扩展点对象
* getAdaptiveExtension(): 获取适配的扩展点。
* getExtension(String name):根据key获取扩展点
* getActivateExtension(URL url, String[] values, String group):获取激活状态的扩展点
* 在这三个方法中,都会调用到一个方法,getExtensionClasses(),这个方法的主要作用就是加载配置文件,然后根据配置把数据放入到缓存中。
#### getExtensionClasses
先来看看getExtensionClasses方法,了解一下Dubbo读取的配置文件有哪些,又是如何读取配置文件中的内容的。
```java
private Map<String, Class<?>> getExtensionClasses() {
//holder缓存中获取
Map<String, Class<?>> classes = cachedClasses.get();
//double check
if (classes == null) {
synchronized (cachedClasses) {
classes = cachedClasses.get();
if (classes == null) {
//缓存中没有,加载扩展点
classes = loadExtensionClasses();
cachedClasses.set(classes);
}
}
}
return classes;
}
private Map<String, Class<?>> loadExtensionClasses() {
//根据spi注解中设置的name,设置当前接口的默认扩展点
final SPI defaultAnnotation = type.getAnnotation(SPI.class);
if(defaultAnnotation != null) {
String value = defaultAnnotation.value();
if(value != null && (value = value.trim()).length() > 0) {
String[] names = NAME_SEPARATOR.split(value);
if(names.length > 1) {
throw new IllegalStateException("more than 1 default extension name on extension " + type.getName()
+ ": " + Arrays.toString(names));
}
if(names.length == 1) cachedDefaultName = names[0];
}
}
Map<String, Class<?>> extensionClasses = new HashMap<String, Class<?>>();
//加载 META-INF/dubbo/internal/ 下的文件
loadFile(extensionClasses, DUBBO_INTERNAL_DIRECTORY);
//加载 META-INF/dubbo/ 下的文件
loadFile(extensionClasses, DUBBO_DIRECTORY);
//加载 META-INF/services/ 下的文件
loadFile(extensionClasses, SERVICES_DIRECTORY);
return extensionClasses;
}
private void loadFile(Map<String, Class<?>> extensionClasses, String dir) {
//根据目录和接口的全名 获取到文件路径
String fileName = dir + type.getName();
try {
Enumeration<java.net.URL> urls;
ClassLoader classLoader = findClassLoader();
if (classLoader != null) {
urls = classLoader.getResources(fileName);
} else {
urls = ClassLoader.getSystemResources(fileName);
}
if (urls != null) {
while (urls.hasMoreElements()) {
java.net.URL url = urls.nextElement();
try {
//读入文件到reader
BufferedReader reader = new BufferedReader(new InputStreamReader(url.openStream(), "utf-8"));
try {
String line = null;
//按行获取
while ((line = reader.readLine()) != null) {
final int ci = line.indexOf('#');
if (ci >= 0) line = line.substring(0, ci);
line = line.trim();
if (line.length() > 0) {
try {
String name = null;
int i = line.indexOf('=');
if (i > 0) {
//扩展点name
name = line.substring(0, i).trim();
//扩展点的类
line = line.substring(i + 1).trim();
}
if (line.length() > 0) {
//获取到扩展点class
Class<?> clazz = Class.forName(line, true, classLoader);
if (! type.isAssignableFrom(clazz)) {
throw new IllegalStateException("Error when load extension class(interface: " +
type + ", class line: " + clazz.getName() + "), class "
+ clazz.getName() + "is not subtype of interface.");
}
if (clazz.isAnnotationPresent(Adaptive.class)) { //判断是否有Adaptive注解
if(cachedAdaptiveClass == null) {
cachedAdaptiveClass = clazz; //如果有则设置cachedAdaptiveClass属性
} else if (! cachedAdaptiveClass.equals(clazz)) {
throw new IllegalStateException("More than 1 adaptive class found: "
+ cachedAdaptiveClass.getClass().getName()
+ ", " + clazz.getClass().getName());
}
} else {
try {
//判断是否是一个wrapper包装类,包装类会有一个传入一个当前扩展点对象的构造函数
clazz.getConstructor(type);
Set<Class<?>> wrappers = cachedWrapperClasses;
if (wrappers == null) {
cachedWrapperClasses = new ConcurrentHashSet<Class<?>>();
wrappers = cachedWrapperClasses;
}
wrappers.add(clazz);
} catch (NoSuchMethodException e) {
//没有这个构造函数,说明是普通的扩展点类
clazz.getConstructor();
if (name == null || name.length() == 0) {
name = findAnnotationName(clazz);
if (name == null || name.length() == 0) {
if (clazz.getSimpleName().length() > type.getSimpleName().length()
&& clazz.getSimpleName().endsWith(type.getSimpleName())) {
name = clazz.getSimpleName().substring(0, clazz.getSimpleName().length() - type.getSimpleName().length()).toLowerCase();
} else {
throw new IllegalStateException("No such extension name for the class " + clazz.getName() + " in the config " + url);
}
}
}
String[] names = NAME_SEPARATOR.split(name);
if (names != null && names.length > 0) {
//判断是否有activate注解,加入到缓存中
Activate activate = clazz.getAnnotation(Activate.class);
if (activate != null) {
cachedActivates.put(names[0], activate);
}
for (String n : names) {
if (! cachedNames.containsKey(clazz)) {
//放入扩展点name缓存
cachedNames.put(clazz, n);
}
Class<?> c = extensionC lasses.get(n);
if (c == null) {
//放入扩展点缓存
extensionClasses.put(n, clazz);
} else if (c != clazz) {
throw new IllegalStateException("Duplicate extension " + type.getName() + " name " + n + " on " + c.getName() + " and " + clazz.getName());
}
}
}
}
}
}
} catch (Throwable t) {
IllegalStateException e = new IllegalStateException("Failed to load extension class(interface: " + type + ", class line: " + line + ") in " + url + ", cause: " + t.getMessage(), t);
exceptions.put(line, e);
}
}
} // end of while read lines
} finally {
reader.close();
}
} catch (Throwable t) {
logger.error("Exception when load extension class(interface: " +
type + ", class file: " + url + ") in " + url, t);
}
} // end of while urls
}
} catch (Throwable t) {
logger.error("Exception when load extension class(interface: " +
type + ", description file: " + fileName + ").", t);
}
}
```
* 先从holder类中获取扩展点class集合,获取不到才会去加载一遍
* 加载扩展点前会先根据spi注解内设置的name,设置默认的扩展点
* 然后会去三个文件夹下加载扩展点,分别是:```META-INF/dubbo/internal/```,```META-INF/dubbo/```,```META-INF/services/```
#### getAdaptiveExtension
```java
public T getAdaptiveExtension() {
//getExtensionClasses时设置
Object instance = cachedAdaptiveInstance.get();
if (instance == null) {
if(createAdaptiveInstanceError == null) {
synchronized (cachedAdaptiveInstance) {
instance = cachedAdaptiveInstance.get();
if (instance == null) {
try {
instance = createAdaptiveExtension();
cachedAdaptiveInstance.set(instance);
} catch (Throwable t) {
createAdaptiveInstanceError = t;
throw new IllegalStateException("fail to create adaptive instance: " + t.toString(), t);
}
}
}
}
else {
throw new IllegalStateException("fail to create adaptive instance: " + createAdaptiveInstanceError.toString(), createAdaptiveInstanceError);
}
}
return (T) instance;
}
private T createAdaptiveExtension() {
try {
return injectExtension((T) getAdaptiveExtensionClass().newInstance());
} catch (Exception e) {
throw new IllegalStateException("Can not create adaptive extenstion " + type + ", cause: " + e.getMessage(), e);
}
}
private Class<?> getAdaptiveExtensionClass() {
//加载扩展点
getExtensionClasses();
if (cachedAdaptiveClass != null) {
return cachedAdaptiveClass;
}
return cachedAdaptiveClass = createAdaptiveExtensionClass();
}
private Class<?> createAdaptiveExtensionClass() {
//组装扩展点的类代码
String code = createAdaptiveExtensionClassCode();
ClassLoader classLoader = findClassLoader();
//获取编译器,默认javassist
com.alibaba.dubbo.common.compiler.Compiler compiler = ExtensionLoader.getExtensionLoader(com.alibaba.dubbo.common.compiler.Compiler.class).getAdaptiveExtension();
return compiler.compile(code, classLoader);
}
private String createAdaptiveExtensionClassCode() {
StringBuilder codeBuidler = new StringBuilder();
Method[] methods = type.getMethods();
boolean hasAdaptiveAnnotation = false;
for(Method m : methods) {
if(m.isAnnotationPresent(Adaptive.class)) {
hasAdaptiveAnnotation = true;
break;
}
}
// 完全没有Adaptive方法,则不需要生成Adaptive类
if(! hasAdaptiveAnnotation)
throw new IllegalStateException("No adaptive method on extension " + type.getName() + ", refuse to create the adaptive class!");
codeBuidler.append("package " + type.getPackage().getName() + ";");
codeBuidler.append("\nimport " + ExtensionLoader.class.getName() + ";");
codeBuidler.append("\npublic class " + type.getSimpleName() + "$Adpative" + " implements " + type.getCanonicalName() + " {");
for (Method method : methods) {
Class<?> rt = method.getReturnType();
Class<?>[] pts = method.getParameterTypes();
Class<?>[] ets = method.getExceptionTypes();
Adaptive adaptiveAnnotation = method.getAnnotation(Adaptive.class);
StringBuilder code = new StringBuilder(512);
if (adaptiveAnnotation == null) {
code.append("throw new UnsupportedOperationException(\"method ")
.append(method.toString()).append(" of interface ")
.append(type.getName()).append(" is not adaptive method!\");");
} else {
int urlTypeIndex = -1;
for (int i = 0; i < pts.length; ++i) {
if (pts[i].equals(URL.class)) {
urlTypeIndex = i;
break;
}
}
// 有类型为URL的参数
if (urlTypeIndex != -1) {
// Null Point check
String s = String.format("\nif (arg%d == null) throw new IllegalArgumentException(\"url == null\");",
urlTypeIndex);
code.append(s);
s = String.format("\n%s url = arg%d;", URL.class.getName(), urlTypeIndex);
code.append(s);
}
// 参数没有URL类型
else {
String attribMethod = null;
// 找到参数的URL属性
LBL_PTS:
for (int i = 0; i < pts.length; ++i) {
Method[] ms = pts[i].getMethods();
for (Method m : ms) {
String name = m.getName();
if ((name.startsWith("get") || name.length() > 3)
&& Modifier.isPublic(m.getModifiers())
&& !Modifier.isStatic(m.getModifiers())
&& m.getParameterTypes().length == 0
&& m.getReturnType() == URL.class) {
urlTypeIndex = i;
attribMethod = name;
break LBL_PTS;
}
}
}
if(attribMethod == null) {
throw new IllegalStateException("fail to create adative class for interface " + type.getName()
+ ": not found url parameter or url attribute in parameters of method " + method.getName());
}
// Null point check
String s = String.format("\nif (arg%d == null) throw new IllegalArgumentException(\"%s argument == null\");",
urlTypeIndex, pts[urlTypeIndex].getName());
code.append(s);
s = String.format("\nif (arg%d.%s() == null) throw new IllegalArgumentException(\"%s argument %s() == null\");",
urlTypeIndex, attribMethod, pts[urlTypeIndex].getName(), attribMethod);
code.append(s);
s = String.format("%s url = arg%d.%s();",URL.class.getName(), urlTypeIndex, attribMethod);
code.append(s);
}
String[] value = adaptiveAnnotation.value();
// 没有设置Key,则使用“扩展点接口名的点分隔 作为Key
if(value.length == 0) {
char[] charArray = type.getSimpleName().toCharArray();
StringBuilder sb = new StringBuilder(128);
for (int i = 0; i < charArray.length; i++) {
if(Character.isUpperCase(charArray[i])) {
if(i != 0) {
sb.append(".");
}
sb.append(Character.toLowerCase(charArray[i]));
}
else {
sb.append(charArray[i]);
}
}
value = new String[] {sb.toString()};
}
boolean hasInvocation = false;
for (int i = 0; i < pts.length; ++i) {
if (pts[i].getName().equals("com.alibaba.dubbo.rpc.Invocation")) {
// Null Point check
String s = String.format("\nif (arg%d == null) throw new IllegalArgumentException(\"invocation == null\");", i);
code.append(s);
s = String.format("\nString methodName = arg%d.getMethodName();", i);
code.append(s);
hasInvocation = true;
break;
}
}
String defaultExtName = cachedDefaultName;
String getNameCode = null;
for (int i = value.length - 1; i >= 0; --i) {
if(i == value.length - 1) {
if(null != defaultExtName) {
if(!"protocol".equals(value[i]))
if (hasInvocation)
getNameCode = String.format("url.getMethodParameter(methodName, \"%s\", \"%s\")", value[i], defaultExtName);
else
getNameCode = String.format("url.getParameter(\"%s\", \"%s\")", value[i], defaultExtName);
else
getNameCode = String.format("( url.getProtocol() == null ? \"%s\" : url.getProtocol() )", defaultExtName);
}
else {
if(!"protocol".equals(value[i]))
if (hasInvocation)
getNameCode = String.format("url.getMethodParameter(methodName, \"%s\", \"%s\")", value[i], defaultExtName);
else
getNameCode = String.format("url.getParameter(\"%s\")", value[i]);
else
getNameCode = "url.getProtocol()";
}
}
else {
if(!"protocol".equals(value[i]))
if (hasInvocation)
getNameCode = String.format("url.getMethodParameter(methodName, \"%s\", \"%s\")", value[i], defaultExtName);
else
getNameCode = String.format("url.getParameter(\"%s\", %s)", value[i], getNameCode);
else
getNameCode = String.format("url.getProtocol() == null ? (%s) : url.getProtocol()", getNameCode);
}
}
code.append("\nString extName = ").append(getNameCode).append(";");
// check extName == null?
String s = String.format("\nif(extName == null) " +
"throw new IllegalStateException(\"Fail to get extension(%s) name from url(\" + url.toString() + \") use keys(%s)\");",
type.getName(), Arrays.toString(value));
code.append(s);
s = String.format("\n%s extension = (%<s)%s.getExtensionLoader(%s.class).getExtension(extName);",
type.getName(), ExtensionLoader.class.getSimpleName(), type.getName());
code.append(s);
// return statement
if (!rt.equals(void.class)) {
code.append("\nreturn ");
}
s = String.format("extension.%s(", method.getName());
code.append(s);
for (int i = 0; i < pts.length; i++) {
if (i != 0)
code.append(", ");
code.append("arg").append(i);
}
code.append(");");
}
codeBuidler.append("\npublic " + rt.getCanonicalName() + " " + method.getName() + "(");
for (int i = 0; i < pts.length; i ++) {
if (i > 0) {
codeBuidler.append(", ");
}
codeBuidler.append(pts[i].getCanonicalName());
codeBuidler.append(" ");
codeBuidler.append("arg" + i);
}
codeBuidler.append(")");
if (ets.length > 0) {
codeBuidler.append(" throws ");
for (int i = 0; i < ets.length; i ++) {
if (i > 0) {
codeBuidler.append(", ");
}
codeBuidler.append(pts[i].getCanonicalName());
}
}
codeBuidler.append(" {");
codeBuidler.append(code.toString());
codeBuidler.append("\n}");
}
codeBuidler.append("\n}");
if (logger.isDebugEnabled()) {
logger.debug(codeBuidler.toString());
}
return codeBuidler.toString();
}
```
* 先从holder缓存中获取当前接口的Adaptive类
* 如果为空,则尝试加载一下扩展点,如果还没有默认的Adaptive类
* 则会自动生成一个Adaptive类。
* 如下就是Protocol接口和自动生成的Adaptive类
```java
/*
* Copyright 1999-2011 Alibaba Group.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.alibaba.dubbo.rpc;
import com.alibaba.dubbo.common.URL;
import com.alibaba.dubbo.common.extension.Adaptive;
import com.alibaba.dubbo.common.extension.SPI;
/**
* Protocol. (API/SPI, Singleton, ThreadSafe)
*
* @author william.liangf
*/
@SPI("dubbo")
public interface Protocol {
/**
* 获取缺省端口,当用户没有配置端口时使用。
*
* @return 缺省端口
*/
int getDefaultPort();
/**
* 暴露远程服务:<br>
* 1. 协议在接收请求时,应记录请求来源方地址信息:RpcContext.getContext().setRemoteAddress();<br>
* 2. export()必须是幂等的,也就是暴露同一个URL的Invoker两次,和暴露一次没有区别。<br>
* 3. export()传入的Invoker由框架实现并传入,协议不需要关心。<br>
*
* @param <T> 服务的类型
* @param invoker 服务的执行体
* @return exporter 暴露服务的引用,用于取消暴露
* @throws RpcException 当暴露服务出错时抛出,比如端口已占用
*/
@Adaptive
<T> Exporter<T> export(Invoker<T> invoker) throws RpcException;
/**
* 引用远程服务:<br>
* 1. 当用户调用refer()所返回的Invoker对象的invoke()方法时,协议需相应执行同URL远端export()传入的Invoker对象的invoke()方法。<br>
* 2. refer()返回的Invoker由协议实现,协议通常需要在此Invoker中发送远程请求。<br>
* 3. 当url中有设置check=false时,连接失败不能抛出异常,并内部自动恢复。<br>
*
* @param <T> 服务的类型
* @param type 服务的类型
* @param url 远程服务的URL地址
* @return invoker 服务的本地代理
* @throws RpcException 当连接服务提供方失败时抛出
*/
@Adaptive
<T> Invoker<T> refer(Class<T> type, URL url) throws RpcException;
/**
* 释放协议:<br>
* 1. 取消该协议所有已经暴露和引用的服务。<br>
* 2. 释放协议所占用的所有资源,比如连接和端口。<br>
* 3. 协议在释放后,依然能暴露和引用新的服务。<br>
*/
void destroy();
}
//自动生成的代码
package com.alibaba.dubbo.rpc;
import com.alibaba.dubbo.common.extension.ExtensionLoader;
public class Protocol$Adpative implements com.alibaba.dubbo.rpc.Protocol {
public void destroy() {throw new UnsupportedOperationException("method public abstract void com.alibaba.dubbo.rpc.Protocol.destroy() of interface com.alibaba.dubbo.rpc.Protocol is not adaptive method!");
}
public int getDefaultPort() {throw new UnsupportedOperationException("method public abstract int com.alibaba.dubbo.rpc.Protocol.getDefaultPort() of interface com.alibaba.dubbo.rpc.Protocol is not adaptive method!");
}
public com.alibaba.dubbo.rpc.Exporter export(com.alibaba.dubbo.rpc.Invoker arg0) throws com.alibaba.dubbo.rpc.Invoker {
if (arg0 == null) throw new IllegalArgumentException("com.alibaba.dubbo.rpc.Invoker argument == null");
if (arg0.getUrl() == null) throw new IllegalArgumentException("com.alibaba.dubbo.rpc.Invoker argument getUrl() == null");com.alibaba.dubbo.common.URL url = arg0.getUrl();
String extName = ( url.getProtocol() == null ? "dubbo" : url.getProtocol() );
if(extName == null) throw new IllegalStateException("Fail to get extension(com.alibaba.dubbo.rpc.Protocol) name from url(" + url.toString() + ") use keys([protocol])");
com.alibaba.dubbo.rpc.Protocol extension = (com.alibaba.dubbo.rpc.Protocol)ExtensionLoader.getExtensionLoader(com.alibaba.dubbo.rpc.Protocol.class).getExtension(extName);
return extension.export(arg0);
}
public com.alibaba.dubbo.rpc.Invoker refer(java.lang.Class arg0, com.alibaba.dubbo.common.URL arg1) throws java.lang.Class {
if (arg1 == null) throw new IllegalArgumentException("url == null");
com.alibaba.dubbo.common.URL url = arg1;
String extName = ( url.getProtocol() == null ? "dubbo" : url.getProtocol() );
if(extName == null) throw new IllegalStateException("Fail to get extension(com.alibaba.dubbo.rpc.Protocol) name from url(" + url.toString() + ") use keys([protocol])");
com.alibaba.dubbo.rpc.Protocol extension = (com.alibaba.dubbo.rpc.Protocol)ExtensionLoader.getExtensionLoader(com.alibaba.dubbo.rpc.Protocol.class).getExtension(extName);
return extension.refer(arg0, arg1);
}
}
```
#### getExtension(String name)
通过扩展点名称获取扩展点对象
```java
public T getExtension(String name) {
if (name == null || name.length() == 0)
throw new IllegalArgumentException("Extension name == null");
if ("true".equals(name)) {
return getDefaultExtension();
}
Holder<Object> holder = cachedInstances.get(name);
if (holder == null) {
cachedInstances.putIfAbsent(name, new Holder<Object>());
holder = cachedInstances.get(name);
}
Object instance = holder.get();
if (instance == null) {
synchronized (holder) {
instance = holder.get();
if (instance == null) {
//创建
instance = createExtension(name);
holder.set(instance);
}
}
}
return (T) instance;
}
private T createExtension(String name) {
//加载扩展点
Class<?> clazz = getExtensionClasses().get(name);
if (clazz == null) {
throw findException(name);
}
try {
T instance = (T) EXTENSION_INSTANCES.get(clazz);
if (instance == null) {
EXTENSION_INSTANCES.putIfAbsent(clazz, (T) clazz.newInstance());
instance = (T) EXTENSION_INSTANCES.get(clazz);
}
injectExtension(instance);
Set<Class<?>> wrapperClasses = cachedWrapperClasses;
//想当于aop,实现wrapper类的扩展功能
if (wrapperClasses != null && wrapperClasses.size() > 0) {
for (Class<?> wrapperClass : wrapperClasses) {
instance = injectExtension((T) wrapperClass.getConstructor(type).newInstance(instance));
}
}
//返回扩展类
return instance;
} catch (Throwable t) {
throw new IllegalStateException("Extension instance(name: " + name + ", class: " +
type + ") could not be instantiated: " + t.getMessage(), t);
}
}
```
* 先从holder缓存中获取扩展点对象
* 缓存中不存在,则创建扩展点对象,如果存在wapper类,则会通过wapper封装扩展点对象,所以最后返回的是wapper对象
<file_sep>source 'https://ruby.taobao.org/'
gem 'github-pages', group: :jekyll_plugins
#gem 'jekyll-admin', group: :jekyll_plugins
<file_sep>---
layout: post
title: docker简单mysql主备
date: 2022-03-15 23:00:00
categories:
- mysql
tags:
- mysql
---
以下例子的mysql版本为5.7
讨论对象主要针对X锁和gap锁,且只讨论rc和rr级别下的锁,最后给结论;
#### 表信息
| 字段名称 | 类型 | 是否可为空 | 其他 |
| :------: | :------: | :-------: | :----: |
| id | bigint | N | 主键 |
| user_name | varchar(50) | N | 名称,普通索引 |
| real_name | varchar(50) | N | 真实姓名 |
| age | int | N | 年龄 |
具体的建表语句如下
```sql
CREATE TABLE `user_info` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`user_name` varchar(50) NOT NULL DEFAULT '',
`real_name` varchar(50) NOT NULL DEFAULT '',
`age` int(11) NOT NULL,
`create_time` datetime DEFAULT NULL,
`update_time` datetime DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `idx_user_name` (`user_name`)
)
```
插入一些默认数据:
```sql
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(1, '小王', '王X', 12, now(), now());
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(2, '大王', '王XX', 22, now(), now());
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(5, '老王', '王XXX', 52, now(), now());
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(10, '小李', '李X', 12, now(), now());
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(20, '大李', '李XX', 22, now(), now());
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(30, '老李', '李XXX', 52, now(), now());
```
#### 给不存在的数据加锁
##### rr隔离级别
###### 事物1(select ... for update) + 事物2(select ... for update)
事物1执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物2执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物1执行:
```sql
commit;
```
事物2执行:
```sql
commit;
```
可以正常运行
###### 事物1(select ... for update + insert) + 事物2(select ... for update)
事物1执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物2执行:
```sql
begin ;
select * from payment_detail where id = 4 for update ;
```
事物1执行:
```sql
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(3, '老天', '天下第一', 99999, now(), now());
commit;
```
此时事物1进入等待,等带事物2 ``` select ... from update ```产生的gap锁;
事物2执行:
```sql
commit;
```
* 因为事物2执行的id = 3;出于已存在数据(2,5)之间,gap锁会把(2,5)之间的空隙加上锁,不让插入;
* 因为事物1也执行了``` select ... for update ```语句,且条件一样,也侧面验证了,gap锁不是排他锁;
* 也就是上面``` 事物1(select ... for update) + 事物2(select ... for update) ```可以正常执行的原因;
###### gap锁导致的死锁
事物1执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物2执行:
```sql
begin ;
select * from payment_detail where id = 4 for update ;
```
事物1执行:
```sql
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(3, '老天', '天下第一', 99999, now(), now());
commit;
```
事物2执行:
```sql
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(4, '小天', '天下第二', 9999, now(), now());
commit;
```
此时事物1在等待事物2的gap锁释放;事物2在等待事物1的gap锁释放,会产生死锁;
###### 事物1(select ... for update) + 事物2(select ... for update) 列无索引
事物1执行:
```sql
begin ;
select * from user_info where age = 13 for update ;
```
事物2执行:
```sql
begin ;
select * from user_info where age = 14 for update ;
```
事物2无法顺利执行,在等待事物1释放锁
事物1执行:
```sql
commit;
```
事物2顺利执行
* 此处事物1的``` select ... for update ```因为age字段没有索引,且数据不存在,直接锁全表,导致事物2无法顺利执行;
* 即使事物2的age改成12,数据库中存在,也是无法获取到锁的;因为事物1已经锁的全表。
###### 事物1(select ... for update) + 事物2(insert) 列无索引
事物1执行:
```sql
begin ;
select * from user_info where age = 13 for update ;
```
事物2执行:
```sql
begin ;
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(4, '小四', '十四是十四', 14, now(), now());
```
* 和上面一样的结果,insert无法插入到数据,必须等待事物1释放表锁
##### rc隔离级别
###### 事物1(select ... for update) + 事物2(select ... for update)
事物1执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物2执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物1执行:
```sql
commit;
```
事物2执行:
```sql
commit;
```
可以正常运行
###### 事物1(select ... for update + insert) + 事物2(select ... for update)
事物1执行:
```sql
begin ;
select * from payment_detail where id = 3 for update ;
```
事物2执行:
```sql
begin ;
select * from payment_detail where id = 4 for update ;
```
事物1执行:
```sql
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(3, '老天', '天下第一', 99999, now(), now());
commit;
```
事物2执行:
```sql
commit;
```
* 正常运行;rc隔离级别没有gap锁,不会锁insert;
###### 事物1(select ... for update) + 事物2(select ... for update) 列无索引
事物1执行:
```sql
begin ;
select * from user_info where age = 13 for update ;
```
事物2执行:
```sql
begin ;
select * from user_info where age = 14 for update ;
select * from user_info where age = 12 for update ;
```
可以顺利执行。
###### 事物1(select ... for update) + 事物2(insert) 列无索引
事物1执行:
```sql
begin ;
select * from user_info where age = 13 for update ;
```
事物2执行:
```sql
begin ;
insert into user_info(id,user_name, real_name, age,create_time,update_time)
values(4, '小四', '十四是十四', 14, now(), now());
```
可以顺利执行
##### 总结
* 不管是rc的隔离级别还是rr的隔离级别,即使在有索引的情况下,``` select ... for update ```在所查询的数据不存在的时候都不能很好的获取到排他锁,在rr的隔离级别下能获取到gap锁防止插入而已,并不能让任务的并发执行变成顺序执行。反而有可能导致死锁
* 别给无索引的列加锁,rr级别会锁全表;但是rc在操作的时候并不会;猜测是会先锁表中的所有数据,但是mysql发现其中的数据不满足条件,又把锁给释放掉了。
#### 给存在的数据加锁
##### rr隔离级别
#### 范围加锁
##### rr隔离级别
<file_sep>---
layout: post
title: spring源码解析--默认标签
date: 2017-08-03 15:18:00
categories:
- java
- spring
tags:
- spring
- java
- 源码
---
前面的文章中,我们有了解过spring的自定义标签的使用和解析过程,相比于自定义标签,默认标签的解析就要复杂的多,下面就让我们开始吧。

通过上面的时序图,我们发现自定义标签和默认标签在解析前面的步骤都是一样的,只是在后面调用的方法不一样。默认标签是:```parseDefaultElement```方法,自定义标签是```parseCustomElement```方法,前面我们已经讲过```parseCustomElement```方法了。
### parseCustomElement
```java
private void parseDefaultElement(Element ele, BeanDefinitionParserDelegate delegate) {
// 解析import标签
if (delegate.nodeNameEquals(ele, IMPORT_ELEMENT)) {
importBeanDefinitionResource(ele);
}
// 解析alias标签
else if (delegate.nodeNameEquals(ele, ALIAS_ELEMENT)) {
processAliasRegistration(ele);
}
// 解析bean标签
else if (delegate.nodeNameEquals(ele, BEAN_ELEMENT)) {
processBeanDefinition(ele, delegate);
}
// 递归解析beans标签
else if (delegate.nodeNameEquals(ele, NESTED_BEANS_ELEMENT)) {
// recurse
doRegisterBeanDefinitions(ele);
}
}
```
* 对默认标签的解析,主要委托给四个private方法进行,分别对import,alias,bean,beans标签进行解析。
* 这里我们先看对bean标签的解析,因为bean标签解析了解了,其他几个标签也就差不多了。
### bean标签解析
```java
/**
* Process the given bean element, parsing the bean definition
* and registering it with the registry.
*/
protected void processBeanDefinition(Element ele, BeanDefinitionParserDelegate delegate) {
//解析bean标签
BeanDefinitionHolder bdHolder = delegate.parseBeanDefinitionElement(ele);
if (bdHolder != null) {
//如果子标签有自定义标签则解析他
bdHolder = delegate.decorateBeanDefinitionIfRequired(ele, bdHolder);
try {
// Register the final decorated instance.
//注册bean
BeanDefinitionReaderUtils.registerBeanDefinition(bdHolder, getReaderContext().getRegistry());
}
catch (BeanDefinitionStoreException ex) {
getReaderContext().error("Failed to register bean definition with name '" +
bdHolder.getBeanName() + "'", ele, ex);
}
// Send registration event.
//发送注册bean事件
getReaderContext().fireComponentRegistered(new BeanComponentDefinition(bdHolder));
}
}
```
* 解析bean标签,解析的属性存放在```AbstractBeanDefinition```内
* 如果子标签有自定义的标签则解析他
* 注册bean
#### 解析bean标签:parseBeanDefinitionElement
```java
/**
* Parses the supplied {@code <bean>} element. May return {@code null}
* if there were errors during parse. Errors are reported to the
* {@link org.springframework.beans.factory.parsing.ProblemReporter}.
*/
public BeanDefinitionHolder parseBeanDefinitionElement(Element ele) {
return parseBeanDefinitionElement(ele, null);
}
/**
* Parses the supplied {@code <bean>} element. May return {@code null}
* if there were errors during parse. Errors are reported to the
* {@link org.springframework.beans.factory.parsing.ProblemReporter}.
*/
public BeanDefinitionHolder parseBeanDefinitionElement(Element ele, BeanDefinition containingBean) {
// bean标签的id属性
String id = ele.getAttribute(ID_ATTRIBUTE);
// bean标签的name属性
String nameAttr = ele.getAttribute(NAME_ATTRIBUTE);
List<String> aliases = new ArrayList<String>();
if (StringUtils.hasLength(nameAttr)) {
String[] nameArr = StringUtils.tokenizeToStringArray(nameAttr, MULTI_VALUE_ATTRIBUTE_DELIMITERS);
aliases.addAll(Arrays.asList(nameArr));
}
String beanName = id;
if (!StringUtils.hasText(beanName) && !aliases.isEmpty()) {
beanName = aliases.remove(0);
if (logger.isDebugEnabled()) {
logger.debug("No XML 'id' specified - using '" + beanName +
"' as bean name and " + aliases + " as aliases");
}
}
//检查beanName是否唯一
if (containingBean == null) {
checkNameUniqueness(beanName, aliases, ele);
}
//解析bean
AbstractBeanDefinition beanDefinition = parseBeanDefinitionElement(ele, beanName, containingBean);
if (beanDefinition != null) {
if (!StringUtils.hasText(beanName)) {
try {
if (containingBean != null) {
beanName = BeanDefinitionReaderUtils.generateBeanName(
beanDefinition, this.readerContext.getRegistry(), true);
}
else {
beanName = this.readerContext.generateBeanName(beanDefinition);
// Register an alias for the plain bean class name, if still possible,
// if the generator returned the class name plus a suffix.
// This is expected for Spring 1.2/2.0 backwards compatibility.
String beanClassName = beanDefinition.getBeanClassName();
if (beanClassName != null &&
beanName.startsWith(beanClassName) && beanName.length() > beanClassName.length() &&
!this.readerContext.getRegistry().isBeanNameInUse(beanClassName)) {
aliases.add(beanClassName);
}
}
if (logger.isDebugEnabled()) {
logger.debug("Neither XML 'id' nor 'name' specified - " +
"using generated bean name [" + beanName + "]");
}
}
catch (Exception ex) {
error(ex.getMessage(), ele);
return null;
}
}
String[] aliasesArray = StringUtils.toStringArray(aliases);
return new BeanDefinitionHolder(beanDefinition, beanName, aliasesArray);
}
return null;
}
```
* 根据id,name属性,确定beanName,并保证beanName的唯一
* 然后委托给```parseBeanDefinitionElement(ele, beanName, containingBean)```方法
```java
public AbstractBeanDefinition parseBeanDefinitionElement(
Element ele, String beanName, BeanDefinition containingBean) {
this.parseState.push(new BeanEntry(beanName));
String className = null;
if (ele.hasAttribute(CLASS_ATTRIBUTE)) {
className = ele.getAttribute(CLASS_ATTRIBUTE).trim();
}
try {
String parent = null;
if (ele.hasAttribute(PARENT_ATTRIBUTE)) {
parent = ele.getAttribute(PARENT_ATTRIBUTE);
}
AbstractBeanDefinition bd = createBeanDefinition(className, parent);
parseBeanDefinitionAttributes(ele, beanName, containingBean, bd);
bd.setDescription(DomUtils.getChildElementValueByTagName(ele, DESCRIPTION_ELEMENT));
parseMetaElements(ele, bd);
parseLookupOverrideSubElements(ele, bd.getMethodOverrides());
parseReplacedMethodSubElements(ele, bd.getMethodOverrides());
parseConstructorArgElements(ele, bd);
parsePropertyElements(ele, bd);
parseQualifierElements(ele, bd);
bd.setResource(this.readerContext.getResource());
bd.setSource(extractSource(ele));
return bd;
}
catch (ClassNotFoundException ex) {
error("Bean class [" + className + "] not found", ele, ex);
}
catch (NoClassDefFoundError err) {
error("Class that bean class [" + className + "] depends on not found", ele, err);
}
catch (Throwable ex) {
error("Unexpected failure during bean definition parsing", ele, ex);
}
finally {
this.parseState.pop();
}
return null;
}
```
* 先获取className,parent等属性,然后新建一个AbstractBeanDefinition对象。
* 解析bean标签上的属性
* 然后分别对bean的一些子节点进行解析
- description标签
- meta标签
- lookup-method标签
- replaced-method标签
- constructor-arg标签
- property标签
- qualifier标签
#### 解析bean标签上的属性
```java
public AbstractBeanDefinition parseBeanDefinitionAttributes(Element ele, String beanName,
BeanDefinition containingBean, AbstractBeanDefinition bd) {
// 判断singleton节点
if (ele.hasAttribute(SINGLETON_ATTRIBUTE)) {
error("Old 1.x 'singleton' attribute in use - upgrade to 'scope' declaration", ele);
}
// scope属性
else if (ele.hasAttribute(SCOPE_ATTRIBUTE)) {
bd.setScope(ele.getAttribute(SCOPE_ATTRIBUTE));
}
// 继承父bean的scope属性
else if (containingBean != null) {
// Take default from containing bean in case of an inner bean definition.
bd.setScope(containingBean.getScope());
}
// abstract属性
if (ele.hasAttribute(ABSTRACT_ATTRIBUTE)) {
bd.setAbstract(TRUE_VALUE.equals(ele.getAttribute(ABSTRACT_ATTRIBUTE)));
}
// lazy-init属性
String lazyInit = ele.getAttribute(LAZY_INIT_ATTRIBUTE);
if (DEFAULT_VALUE.equals(lazyInit)) {
lazyInit = this.defaults.getLazyInit();
}
bd.setLazyInit(TRUE_VALUE.equals(lazyInit));
// autowire属性
String autowire = ele.getAttribute(AUTOWIRE_ATTRIBUTE);
bd.setAutowireMode(getAutowireMode(autowire));
// dependency-check属性
String dependencyCheck = ele.getAttribute(DEPENDENCY_CHECK_ATTRIBUTE);
bd.setDependencyCheck(getDependencyCheck(dependencyCheck));
// depends-on属性
if (ele.hasAttribute(DEPENDS_ON_ATTRIBUTE)) {
String dependsOn = ele.getAttribute(DEPENDS_ON_ATTRIBUTE);
bd.setDependsOn(StringUtils.tokenizeToStringArray(dependsOn, MULTI_VALUE_ATTRIBUTE_DELIMITERS));
}
// autowire-candidate属性
String autowireCandidate = ele.getAttribute(AUTOWIRE_CANDIDATE_ATTRIBUTE);
if ("".equals(autowireCandidate) || DEFAULT_VALUE.equals(autowireCandidate)) {
String candidatePattern = this.defaults.getAutowireCandidates();
if (candidatePattern != null) {
String[] patterns = StringUtils.commaDelimitedListToStringArray(candidatePattern);
bd.setAutowireCandidate(PatternMatchUtils.simpleMatch(patterns, beanName));
}
}
else {
bd.setAutowireCandidate(TRUE_VALUE.equals(autowireCandidate));
}
// primary属性
if (ele.hasAttribute(PRIMARY_ATTRIBUTE)) {
bd.setPrimary(TRUE_VALUE.equals(ele.getAttribute(PRIMARY_ATTRIBUTE)));
}
// init-method属性
if (ele.hasAttribute(INIT_METHOD_ATTRIBUTE)) {
String initMethodName = ele.getAttribute(INIT_METHOD_ATTRIBUTE);
if (!"".equals(initMethodName)) {
bd.setInitMethodName(initMethodName);
}
}
else {
if (this.defaults.getInitMethod() != null) {
bd.setInitMethodName(this.defaults.getInitMethod());
bd.setEnforceInitMethod(false);
}
}
// destroy-method属性
if (ele.hasAttribute(DESTROY_METHOD_ATTRIBUTE)) {
String destroyMethodName = ele.getAttribute(DESTROY_METHOD_ATTRIBUTE);
if (!"".equals(destroyMethodName)) {
bd.setDestroyMethodName(destroyMethodName);
}
}
else {
if (this.defaults.getDestroyMethod() != null) {
bd.setDestroyMethodName(this.defaults.getDestroyMethod());
bd.setEnforceDestroyMethod(false);
}
}
// factory-method属性
if (ele.hasAttribute(FACTORY_METHOD_ATTRIBUTE)) {
bd.setFactoryMethodName(ele.getAttribute(FACTORY_METHOD_ATTRIBUTE));
}
// factory-bean属性
if (ele.hasAttribute(FACTORY_BEAN_ATTRIBUTE)) {
bd.setFactoryBeanName(ele.getAttribute(FACTORY_BEAN_ATTRIBUTE));
}
return bd;
}
```
* this.defaults缓存的是root节点上的参数。
* 包含的属性如下:
- scope属性
- abstract属性
- lazy-init属性
- autowire属性
- dependency-check属性
- depends-on属性
- autowire-candidate属性
- primary属性
- init-method属性
- destroy-method属性
- factory-method属性
- factory-bean属性
* 这些属性被保存在```AbstractBeanDefinition```对象内
#### meta标签
```java
public void parseMetaElements(Element ele, BeanMetadataAttributeAccessor attributeAccessor) {
NodeList nl = ele.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
//判断子节点是否默认标签&子节点的标签名称是否是meta标签
if (isCandidateElement(node) && nodeNameEquals(node, META_ELEMENT)) {
Element metaElement = (Element) node;
String key = metaElement.getAttribute(KEY_ATTRIBUTE);
String value = metaElement.getAttribute(VALUE_ATTRIBUTE);
BeanMetadataAttribute attribute = new BeanMetadataAttribute(key, value);
attribute.setSource(extractSource(metaElement));
attributeAccessor.addMetadataAttribute(attribute);
}
}
}
```
* 先判断子节点内是否有meta标签
* 封装到```AbstractBeanDefinition```的一个名称为```attributes```的```LinkedHashMap```内
#### lookup-method标签和replaced-method标签
```java
/**
* Parse lookup-override sub-elements of the given bean element.
*/
public void parseLookupOverrideSubElements(Element beanEle, MethodOverrides overrides) {
NodeList nl = beanEle.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
// 判断子标签是否有lookup-method标签
if (isCandidateElement(node) && nodeNameEquals(node, LOOKUP_METHOD_ELEMENT)) {
Element ele = (Element) node;
String methodName = ele.getAttribute(NAME_ATTRIBUTE);
String beanRef = ele.getAttribute(BEAN_ELEMENT);
LookupOverride override = new LookupOverride(methodName, beanRef);
override.setSource(extractSource(ele));
overrides.addOverride(override);
}
}
}
/**
* Parse replaced-method sub-elements of the given bean element.
*/
public void parseReplacedMethodSubElements(Element beanEle, MethodOverrides overrides) {
NodeList nl = beanEle.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
//判断子标签是否有replaced-method标签
if (isCandidateElement(node) && nodeNameEquals(node, REPLACED_METHOD_ELEMENT)) {
Element replacedMethodEle = (Element) node;
String name = replacedMethodEle.getAttribute(NAME_ATTRIBUTE);
String callback = replacedMethodEle.getAttribute(REPLACER_ATTRIBUTE);
ReplaceOverride replaceOverride = new ReplaceOverride(name, callback);
// Look for arg-type match elements.
List<Element> argTypeEles = DomUtils.getChildElementsByTagName(replacedMethodEle, ARG_TYPE_ELEMENT);
for (Element argTypeEle : argTypeEles) {
String match = argTypeEle.getAttribute(ARG_TYPE_MATCH_ATTRIBUTE);
match = (StringUtils.hasText(match) ? match : DomUtils.getTextValue(argTypeEle));
if (StringUtils.hasText(match)) {
replaceOverride.addTypeIdentifier(match);
}
}
replaceOverride.setSource(extractSource(replacedMethodEle));
overrides.addOverride(replaceOverride);
}
}
}
```
* lookup-method标签封装成```LookupOverride```,replaced-method标签封装成```ReplaceOverride```
* 都添加到```MethodOverrides```的一个名称为```overrides```的```HashSet```内
#### constructor-arg标签
```java
/**
* Parse constructor-arg sub-elements of the given bean element.
*/
public void parseConstructorArgElements(Element beanEle, BeanDefinition bd) {
NodeList nl = beanEle.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
if (isCandidateElement(node) && nodeNameEquals(node, CONSTRUCTOR_ARG_ELEMENT)) {
parseConstructorArgElement((Element) node, bd);
}
}
}
/**
* Parse a constructor-arg element.
*/
public void parseConstructorArgElement(Element ele, BeanDefinition bd) {
//index属性
String indexAttr = ele.getAttribute(INDEX_ATTRIBUTE);
//type属性
String typeAttr = ele.getAttribute(TYPE_ATTRIBUTE);
//name属性
String nameAttr = ele.getAttribute(NAME_ATTRIBUTE);
//判断是否有index属性
if (StringUtils.hasLength(indexAttr)) {
try {
int index = Integer.parseInt(indexAttr);
if (index < 0) {
error("'index' cannot be lower than 0", ele);
}
else {
try {
this.parseState.push(new ConstructorArgumentEntry(index));
//获取value,也有可能是一个ref对象
Object value = parsePropertyValue(ele, bd, null);
ConstructorArgumentValues.ValueHolder valueHolder = new ConstructorArgumentValues.ValueHolder(value);
if (StringUtils.hasLength(typeAttr)) {
valueHolder.setType(typeAttr);
}
if (StringUtils.hasLength(nameAttr)) {
valueHolder.setName(nameAttr);
}
valueHolder.setSource(extractSource(ele));
if (bd.getConstructorArgumentValues().hasIndexedArgumentValue(index)) {
error("Ambiguous constructor-arg entries for index " + index, ele);
}
else {
bd.getConstructorArgumentValues().addIndexedArgumentValue(index, valueHolder);
}
}
finally {
this.parseState.pop();
}
}
}
catch (NumberFormatException ex) {
error("Attribute 'index' of tag 'constructor-arg' must be an integer", ele);
}
}
else {
try {
this.parseState.push(new ConstructorArgumentEntry());
Object value = parsePropertyValue(ele, bd, null);
ConstructorArgumentValues.ValueHolder valueHolder = new ConstructorArgumentValues.ValueHolder(value);
if (StringUtils.hasLength(typeAttr)) {
valueHolder.setType(typeAttr);
}
if (StringUtils.hasLength(nameAttr)) {
valueHolder.setName(nameAttr);
}
valueHolder.setSource(extractSource(ele));
bd.getConstructorArgumentValues().addGenericArgumentValue(valueHolder);
}
finally {
this.parseState.pop();
}
}
}
public Object parsePropertyValue(Element ele, BeanDefinition bd, String propertyName) {
String elementName = (propertyName != null) ?
"<property> element for property '" + propertyName + "'" :
"<constructor-arg> element";
// Should only have one child element: ref, value, list, etc.
//只能拥有一个子节点
NodeList nl = ele.getChildNodes();
Element subElement = null;
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
if (node instanceof Element && !nodeNameEquals(node, DESCRIPTION_ELEMENT) &&
!nodeNameEquals(node, META_ELEMENT)) {
// Child element is what we're looking for.
if (subElement != null) {
error(elementName + " must not contain more than one sub-element", ele);
}
else {
subElement = (Element) node;
}
}
}
boolean hasRefAttribute = ele.hasAttribute(REF_ATTRIBUTE);
boolean hasValueAttribute = ele.hasAttribute(VALUE_ATTRIBUTE);
if ((hasRefAttribute && hasValueAttribute) ||
((hasRefAttribute || hasValueAttribute) && subElement != null)) {
error(elementName +
" is only allowed to contain either 'ref' attribute OR 'value' attribute OR sub-element", ele);
}
if (hasRefAttribute) {
//解析ref属性
String refName = ele.getAttribute(REF_ATTRIBUTE);
if (!StringUtils.hasText(refName)) {
error(elementName + " contains empty 'ref' attribute", ele);
}
RuntimeBeanReference ref = new RuntimeBeanReference(refName);
ref.setSource(extractSource(ele));
return ref;
}
else if (hasValueAttribute) {
//解析value属性
TypedStringValue valueHolder = new TypedStringValue(ele.getAttribute(VALUE_ATTRIBUTE));
valueHolder.setSource(extractSource(ele));
return valueHolder;
}
else if (subElement != null) {
//如果有子节点,解析子节点
return parsePropertySubElement(subElement, bd);
}
else {
// Neither child element nor "ref" or "value" attribute found.
error(elementName + " must specify a ref or value", ele);
return null;
}
}
```
* 看上去代码好像很长,但是读进去的话业务逻辑也不是很复杂
* index属性不能有两个相同的值。
* constructor-arg标签的属性会封装到```constructorArgumentValues```属性内
* constructor-arg标签只能有一个子节点,ref,value或者其他。
#### property标签
```java
/**
* Parse property sub-elements of the given bean element.
*/
public void parsePropertyElements(Element beanEle, BeanDefinition bd) {
NodeList nl = beanEle.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
if (isCandidateElement(node) && nodeNameEquals(node, PROPERTY_ELEMENT)) {
parsePropertyElement((Element) node, bd);
}
}
}
/**
* Parse a property element.
*/
public void parsePropertyElement(Element ele, BeanDefinition bd) {
String propertyName = ele.getAttribute(NAME_ATTRIBUTE);
if (!StringUtils.hasLength(propertyName)) {
error("Tag 'property' must have a 'name' attribute", ele);
return;
}
this.parseState.push(new PropertyEntry(propertyName));
try {
if (bd.getPropertyValues().contains(propertyName)) {
error("Multiple 'property' definitions for property '" + propertyName + "'", ele);
return;
}
Object val = parsePropertyValue(ele, bd, propertyName);
PropertyValue pv = new PropertyValue(propertyName, val);
parseMetaElements(ele, pv);
pv.setSource(extractSource(ele));
bd.getPropertyValues().addPropertyValue(pv);
}
finally {
this.parseState.pop();
}
}
```
* 只能有一个propertyName,多个相同的会报错
* 解析property标签下的meta子标签
#### qualifier标签
```java
/**
* Parse qualifier sub-elements of the given bean element.
*/
public void parseQualifierElements(Element beanEle, AbstractBeanDefinition bd) {
NodeList nl = beanEle.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
if (isCandidateElement(node) && nodeNameEquals(node, QUALIFIER_ELEMENT)) {
parseQualifierElement((Element) node, bd);
}
}
}
/**
* Parse a qualifier element.
*/
public void parseQualifierElement(Element ele, AbstractBeanDefinition bd) {
String typeName = ele.getAttribute(TYPE_ATTRIBUTE);
if (!StringUtils.hasLength(typeName)) {
error("Tag 'qualifier' must have a 'type' attribute", ele);
return;
}
this.parseState.push(new QualifierEntry(typeName));
try {
AutowireCandidateQualifier qualifier = new AutowireCandidateQualifier(typeName);
qualifier.setSource(extractSource(ele));
String value = ele.getAttribute(VALUE_ATTRIBUTE);
if (StringUtils.hasLength(value)) {
qualifier.setAttribute(AutowireCandidateQualifier.VALUE_KEY, value);
}
NodeList nl = ele.getChildNodes();
for (int i = 0; i < nl.getLength(); i++) {
Node node = nl.item(i);
if (isCandidateElement(node) && nodeNameEquals(node, QUALIFIER_ATTRIBUTE_ELEMENT)) {
Element attributeEle = (Element) node;
String attributeName = attributeEle.getAttribute(KEY_ATTRIBUTE);
String attributeValue = attributeEle.getAttribute(VALUE_ATTRIBUTE);
if (StringUtils.hasLength(attributeName) && StringUtils.hasLength(attributeValue)) {
BeanMetadataAttribute attribute = new BeanMetadataAttribute(attributeName, attributeValue);
attribute.setSource(extractSource(attributeEle));
qualifier.addMetadataAttribute(attribute);
}
else {
error("Qualifier 'attribute' tag must have a 'name' and 'value'", attributeEle);
return;
}
}
}
bd.addQualifier(qualifier);
}
finally {
this.parseState.pop();
}
}
```
#### delegate.decorateBeanDefinitionIfRequired 解析子标签的自定义标签
```java
public BeanDefinitionHolder decorateBeanDefinitionIfRequired(Element ele, BeanDefinitionHolder definitionHolder) {
return decorateBeanDefinitionIfRequired(ele, definitionHolder, null);
}
public BeanDefinitionHolder decorateBeanDefinitionIfRequired(
Element ele, BeanDefinitionHolder definitionHolder, BeanDefinition containingBd) {
BeanDefinitionHolder finalDefinition = definitionHolder;
// Decorate based on custom attributes first.
NamedNodeMap attributes = ele.getAttributes();
for (int i = 0; i < attributes.getLength(); i++) {
Node node = attributes.item(i);
finalDefinition = decorateIfRequired(node, finalDefinition, containingBd);
}
// Decorate based on custom nested elements.
NodeList children = ele.getChildNodes();
for (int i = 0; i < children.getLength(); i++) {
Node node = children.item(i);
if (node.getNodeType() == Node.ELEMENT_NODE) {
finalDefinition = decorateIfRequired(node, finalDefinition, containingBd);
}
}
return finalDefinition;
}
public BeanDefinitionHolder decorateIfRequired(
Node node, BeanDefinitionHolder originalDef, BeanDefinition containingBd) {
String namespaceUri = getNamespaceURI(node);
//非默认标签
if (!isDefaultNamespace(namespaceUri)) {
NamespaceHandler handler = this.readerContext.getNamespaceHandlerResolver().resolve(namespaceUri);
if (handler != null) {
return handler.decorate(node, originalDef, new ParserContext(this.readerContext, this, containingBd));
}
else if (namespaceUri != null && namespaceUri.startsWith("http://www.springframework.org/")) {
error("Unable to locate Spring NamespaceHandler for XML schema namespace [" + namespaceUri + "]", node);
}
else {
// A custom namespace, not to be handled by Spring - maybe "xml:...".
if (logger.isDebugEnabled()) {
logger.debug("No Spring NamespaceHandler found for XML schema namespace [" + namespaceUri + "]");
}
}
}
return originalDef;
}
```
* 看过前面我写的自定义标签的应该都能看懂上面的代码。
* 对自定义标签,根据命名空间获取处理器来进行处理
#### 注册解析的bean
```java
public static void registerBeanDefinition(
BeanDefinitionHolder definitionHolder, BeanDefinitionRegistry registry)
throws BeanDefinitionStoreException {
// Register bean definition under primary name.
String beanName = definitionHolder.getBeanName();
//注册bean
registry.registerBeanDefinition(beanName, definitionHolder.getBeanDefinition());
// Register aliases for bean name, if any.
String[] aliases = definitionHolder.getAliases();
if (aliases != null) {
for (String alias : aliases) {
//注册别名
registry.registerAlias(beanName, alias);
}
}
}
public void registerBeanDefinition(String beanName, BeanDefinition beanDefinition)
throws BeanDefinitionStoreException {
Assert.hasText(beanName, "Bean name must not be empty");
Assert.notNull(beanDefinition, "BeanDefinition must not be null");
if (beanDefinition instanceof AbstractBeanDefinition) {
try {
// 注册前的校验
((AbstractBeanDefinition) beanDefinition).validate();
}
catch (BeanDefinitionValidationException ex) {
throw new BeanDefinitionStoreException(beanDefinition.getResourceDescription(), beanName,
"Validation of bean definition failed", ex);
}
}
BeanDefinition oldBeanDefinition;
// 根据beanName获取已注册的beanDetinition
oldBeanDefinition = this.beanDefinitionMap.get(beanName);
if (oldBeanDefinition != null) {
// 如果beanDetinition已被注册且不允许覆盖,则抛出异常
if (!this.allowBeanDefinitionOverriding) {
throw new BeanDefinitionStoreException(beanDefinition.getResourceDescription(), beanName,
"Cannot register bean definition [" + beanDefinition + "] for bean '" + beanName +
"': There is already [" + oldBeanDefinition + "] bound.");
}
else if (oldBeanDefinition.getRole() < beanDefinition.getRole()) {
// e.g. was ROLE_APPLICATION, now overriding with ROLE_SUPPORT or ROLE_INFRASTRUCTURE
if (this.logger.isWarnEnabled()) {
this.logger.warn("Overriding user-defined bean definition for bean '" + beanName +
" with a framework-generated bean definition ': replacing [" +
oldBeanDefinition + "] with [" + beanDefinition + "]");
}
}
else {
if (this.logger.isInfoEnabled()) {
this.logger.info("Overriding bean definition for bean '" + beanName +
"': replacing [" + oldBeanDefinition + "] with [" + beanDefinition + "]");
}
}
}
else {
// 记录beanName
this.beanDefinitionNames.add(beanName);
this.manualSingletonNames.remove(beanName);
this.frozenBeanDefinitionNames = null;
}
// 注册beanDetinition
this.beanDefinitionMap.put(beanName, beanDefinition);
if (oldBeanDefinition != null || containsSingleton(beanName)) {
resetBeanDefinition(beanName);
}
}
```
* 注册beandefinition也只是把beanName作为key,beanDefinition作为value放到一个map中
#### 注册别名
```java
@Override
public void registerAlias(String name, String alias) {
Assert.hasText(name, "'name' must not be empty");
Assert.hasText(alias, "'alias' must not be empty");
if (alias.equals(name)) {
//如果别名和beanName相同,直接remove掉
this.aliasMap.remove(alias);
}
else {
if (!allowAliasOverriding()) {
//如果不允许别名覆盖,并且别名已经被注册,则抛出异常
String registeredName = this.aliasMap.get(alias);
if (registeredName != null && !registeredName.equals(name)) {
throw new IllegalStateException("Cannot register alias '" + alias + "' for name '" +
name + "': It is already registered for name '" + registeredName + "'.");
}
}
//检测循环别名设置 A->B->C->B->A
checkForAliasCircle(name, alias);
//注册别名
this.aliasMap.put(alias, name);
}
}
/**
* Determine the raw name, resolving aliases to canonical names.
* @param name the user-specified name
* @return the transformed name
*/
public String canonicalName(String name) {
String canonicalName = name;
// Handle aliasing...
String resolvedName;
do {
resolvedName = this.aliasMap.get(canonicalName);
if (resolvedName != null) {
canonicalName = resolvedName;
}
}
while (resolvedName != null);
return canonicalName;
}
/**
* Check whether the given name points back to given alias as an alias
* in the other direction, catching a circular reference upfront and
* throwing a corresponding IllegalStateException.
* @param name the candidate name
* @param alias the candidate alias
* @see #registerAlias
*/
protected void checkForAliasCircle(String name, String alias) {
if (alias.equals(canonicalName(name))) {
throw new IllegalStateException("Cannot register alias '" + alias +
"' for name '" + name + "': Circular reference - '" +
name + "' is a direct or indirect alias for '" + alias + "' already");
}
}
```
* 注册别名的时候,会判断是否有别名的循环设置。有的话会抛出异常。
### import标签
```java
protected void importBeanDefinitionResource(Element ele) {
String location = ele.getAttribute(RESOURCE_ATTRIBUTE);
if (!StringUtils.hasText(location)) {
getReaderContext().error("Resource location must not be empty", ele);
return;
}
// Resolve system properties: e.g. "${user.dir}"
location = getReaderContext().getEnvironment().resolveRequiredPlaceholders(location);
Set<Resource> actualResources = new LinkedHashSet<Resource>(4);
// Discover whether the location is an absolute or relative URI
boolean absoluteLocation = false;
try {
// 判断是否绝对地址
absoluteLocation = ResourcePatternUtils.isUrl(location) || ResourceUtils.toURI(location).isAbsolute();
}
catch (URISyntaxException ex) {
// cannot convert to an URI, considering the location relative
// unless it is the well-known Spring prefix "classpath*:"
}
// Absolute or relative?
if (absoluteLocation) {
try {
int importCount = getReaderContext().getReader().loadBeanDefinitions(location, actualResources);
if (logger.isDebugEnabled()) {
logger.debug("Imported " + importCount + " bean definitions from URL location [" + location + "]");
}
}
catch (BeanDefinitionStoreException ex) {
getReaderContext().error(
"Failed to import bean definitions from URL location [" + location + "]", ele, ex);
}
}
else {
// No URL -> considering resource location as relative to the current file.
try {
int importCount;
Resource relativeResource = getReaderContext().getResource().createRelative(location);
if (relativeResource.exists()) {
importCount = getReaderContext().getReader().loadBeanDefinitions(relativeResource);
actualResources.add(relativeResource);
}
else {
String baseLocation = getReaderContext().getResource().getURL().toString();
importCount = getReaderContext().getReader().loadBeanDefinitions(
StringUtils.applyRelativePath(baseLocation, location), actualResources);
}
if (logger.isDebugEnabled()) {
logger.debug("Imported " + importCount + " bean definitions from relative location [" + location + "]");
}
}
catch (IOException ex) {
getReaderContext().error("Failed to resolve current resource location", ele, ex);
}
catch (BeanDefinitionStoreException ex) {
getReaderContext().error("Failed to import bean definitions from relative location [" + location + "]",
ele, ex);
}
}
Resource[] actResArray = actualResources.toArray(new Resource[actualResources.size()]);
// 发送event事件
getReaderContext().fireImportProcessed(location, actResArray, extractSource(ele));
}
```
* import标签的解析,首先解析文件的地址,转换为绝对地址,然后递归调用```loadBeanDefinitions```方法。
* 最后发送event事件
### alias标签
```java
/**
* Process the given alias element, registering the alias with the registry.
*/
protected void processAliasRegistration(Element ele) {
String name = ele.getAttribute(NAME_ATTRIBUTE);
String alias = ele.getAttribute(ALIAS_ATTRIBUTE);
boolean valid = true;
if (!StringUtils.hasText(name)) {
getReaderContext().error("Name must not be empty", ele);
valid = false;
}
if (!StringUtils.hasText(alias)) {
getReaderContext().error("Alias must not be empty", ele);
valid = false;
}
if (valid) {
try {
getReaderContext().getRegistry().registerAlias(name, alias);
}
catch (Exception ex) {
getReaderContext().error("Failed to register alias '" + alias +
"' for bean with name '" + name + "'", ele, ex);
}
getReaderContext().fireAliasRegistered(name, alias, extractSource(ele));
}
}
```
* alias标签的注册同bean标签解析,最后注册别名调用的方法是同一个。
### beans标签
```java
/**
* Register each bean definition within the given root {@code <beans/>} element.
*/
protected void doRegisterBeanDefinitions(Element root) {
// Any nested <beans> elements will cause recursion in this method. In
// order to propagate and preserve <beans> default-* attributes correctly,
// keep track of the current (parent) delegate, which may be null. Create
// the new (child) delegate with a reference to the parent for fallback purposes,
// then ultimately reset this.delegate back to its original (parent) reference.
// this behavior emulates a stack of delegates without actually necessitating one.
BeanDefinitionParserDelegate parent = this.delegate;
this.delegate = createDelegate(getReaderContext(), root, parent);
if (this.delegate.isDefaultNamespace(root)) {
String profileSpec = root.getAttribute(PROFILE_ATTRIBUTE);
if (StringUtils.hasText(profileSpec)) {
String[] specifiedProfiles = StringUtils.tokenizeToStringArray(
profileSpec, BeanDefinitionParserDelegate.MULTI_VALUE_ATTRIBUTE_DELIMITERS);
if (!getReaderContext().getEnvironment().acceptsProfiles(specifiedProfiles)) {
return;
}
}
}
preProcessXml(root);
parseBeanDefinitions(root, this.delegate);
postProcessXml(root);
this.delegate = parent;
}
```
* 递归调用解析。
<file_sep>---
layout: post
title: dubbo自定义标签解析
date: 2017-09-14 11:00:00
categories:
- dubbo
tags:
- dubbo
- spring
---
<p>dubbo依赖spring容器,想要先了解dubbo的实现机制和原理的话,那么首先就需要先了解dubbo的自定义标签是如何去解析的,是如何把参数转化成beenDefinition的。</p>
<p>前面已经对spring的自定义标签做过详细的讲解,这里就不做过多的介绍了,直接进入主题吧</p>
### DubboNamespaceHandler
```java
public class DubboNamespaceHandler extends NamespaceHandlerSupport {
static {
Version.checkDuplicate(DubboNamespaceHandler.class);
}
public void init() {
registerBeanDefinitionParser("application", new DubboBeanDefinitionParser(ApplicationConfig.class, true));
registerBeanDefinitionParser("module", new DubboBeanDefinitionParser(ModuleConfig.class, true));
registerBeanDefinitionParser("registry", new DubboBeanDefinitionParser(RegistryConfig.class, true));
registerBeanDefinitionParser("monitor", new DubboBeanDefinitionParser(MonitorConfig.class, true));
registerBeanDefinitionParser("provider", new DubboBeanDefinitionParser(ProviderConfig.class, true));
registerBeanDefinitionParser("consumer", new DubboBeanDefinitionParser(ConsumerConfig.class, true));
registerBeanDefinitionParser("protocol", new DubboBeanDefinitionParser(ProtocolConfig.class, true));
registerBeanDefinitionParser("service", new DubboBeanDefinitionParser(ServiceBean.class, true));
registerBeanDefinitionParser("reference", new DubboBeanDefinitionParser(ReferenceBean.class, false));
registerBeanDefinitionParser("annotation", new DubboBeanDefinitionParser(AnnotationBean.class, true));
}
}
```
* dubbo标签的解析都是交给```DubboBeanDefinitionParser```这个类来进行的。
* ```DubboBeanDefinitionParser```会传入两个参数,第一个Class类型的表示的是这个beanDefinition的beanClass,也就是后面生成bean对象的类型;第二个表示,如果当前标签没有传入id字段,是否自动生成id字段
### DubboBeanDefinitionParser
* DubboBeanDefinitionParser继承了BeanDefinitionParser类,并重写了其parse方法
* 这个parse方法有点长,因为dubbo的所有标签都是通过其来解析。
```java
public BeanDefinition parse(Element element, ParserContext parserContext) {
return parse(element, parserContext, beanClass, required);
}
@SuppressWarnings("unchecked")
private static BeanDefinition parse(Element element, ParserContext parserContext, Class<?> beanClass, boolean required) {
RootBeanDefinition beanDefinition = new RootBeanDefinition();
beanDefinition.setBeanClass(beanClass);
beanDefinition.setLazyInit(false);
String id = element.getAttribute("id");
//没有自定义id,则根据条件自动生成id
if ((id == null || id.length() == 0) && required) {
String generatedBeanName = element.getAttribute("name");
if (generatedBeanName == null || generatedBeanName.length() == 0) {
if (ProtocolConfig.class.equals(beanClass)) { //ProtocolConfig.class默认id为dubbo
generatedBeanName = "dubbo";
} else {
generatedBeanName = element.getAttribute("interface"); //其他的则从interface节点获取
}
}
if (generatedBeanName == null || generatedBeanName.length() == 0) { //如果还为空,则获取beanClass的全名称
generatedBeanName = beanClass.getName();
}
id = generatedBeanName;
int counter = 2;
while(parserContext.getRegistry().containsBeanDefinition(id)) { //确定id的最终名称。
id = generatedBeanName + (counter ++);
}
}
//注册beanDefinition
if (id != null && id.length() > 0) {
if (parserContext.getRegistry().containsBeanDefinition(id)) {
throw new IllegalStateException("Duplicate spring bean id " + id);
}
parserContext.getRegistry().registerBeanDefinition(id, beanDefinition);
beanDefinition.getPropertyValues().addPropertyValue("id", id);
}
//解析ProtocolConfig.class时,找出其他beanDefinition中依赖当前protocol的地方,把当前对象注入进去
if (ProtocolConfig.class.equals(beanClass)) {
for (String name : parserContext.getRegistry().getBeanDefinitionNames()) {
BeanDefinition definition = parserContext.getRegistry().getBeanDefinition(name);
PropertyValue property = definition.getPropertyValues().getPropertyValue("protocol");
if (property != null) {
Object value = property.getValue();
if (value instanceof ProtocolConfig && id.equals(((ProtocolConfig) value).getName())) {
definition.getPropertyValues().addPropertyValue("protocol", new RuntimeBeanReference(id));
}
}
}
} else if (ServiceBean.class.equals(beanClass)) {
//解析ServiceBean.class时,节点上有class属性的解析
String className = element.getAttribute("class");
if(className != null && className.length() > 0) {
RootBeanDefinition classDefinition = new RootBeanDefinition();
classDefinition.setBeanClass(ReflectUtils.forName(className));
classDefinition.setLazyInit(false);
parseProperties(element.getChildNodes(), classDefinition);
beanDefinition.getPropertyValues().addPropertyValue("ref", new BeanDefinitionHolder(classDefinition, id + "Impl"));
}
} else if (ProviderConfig.class.equals(beanClass)) {
//解析ProviderConfig.class时,如果有<dubbo:service>子节点,则解析它
parseNested(element, parserContext, ServiceBean.class, true, "service", "provider", id, beanDefinition);
} else if (ConsumerConfig.class.equals(beanClass)) {
//解析ConsumerConfig.class时,如果有<dubbo:reference>子节点,则解析它
parseNested(element, parserContext, ReferenceBean.class, false, "reference", "consumer", id, beanDefinition);
}
Set<String> props = new HashSet<String>();
ManagedMap parameters = null;
for (Method setter : beanClass.getMethods()) {
String name = setter.getName();
//找set方法,比如setName
if (name.length() > 3 && name.startsWith("set")
&& Modifier.isPublic(setter.getModifiers())
&& setter.getParameterTypes().length == 1) {
Class<?> type = setter.getParameterTypes()[0];
//得到setName后面的name
String property = StringUtils.camelToSplitName(name.substring(3, 4).toLowerCase() + name.substring(4), "-");
props.add(property);
Method getter = null;
//获取set方法对应的get方法,如果没有可能是boolean属性,则判断是否有isName方法
try {
getter = beanClass.getMethod("get" + name.substring(3), new Class<?>[0]);
} catch (NoSuchMethodException e) {
try {
getter = beanClass.getMethod("is" + name.substring(3), new Class<?>[0]);
} catch (NoSuchMethodException e2) {
}
}
//get方法不存在,或者get方法不是public,或者get返回值类型与set的类型不同,则跳过当前set方法
if (getter == null
|| ! Modifier.isPublic(getter.getModifiers())
|| ! type.equals(getter.getReturnType())) {
continue;
}
if ("parameters".equals(property)) {
//当前方法是setParameters方法
parameters = parseParameters(element.getChildNodes(), beanDefinition);
} else if ("methods".equals(property)) {
//当前方法是setMethods方法
parseMethods(id, element.getChildNodes(), beanDefinition, parserContext);
} else if ("arguments".equals(property)) {
//当前方法是setArguments方法
parseArguments(id, element.getChildNodes(), beanDefinition, parserContext);
} else {
//其他set方法
//获取set的属性在标签中设置的值
String value = element.getAttribute(property);
if (value != null) {
value = value.trim();
if (value.length() > 0) {
if ("registry".equals(property) && RegistryConfig.NO_AVAILABLE.equalsIgnoreCase(value)) {
RegistryConfig registryConfig = new RegistryConfig();
registryConfig.setAddress(RegistryConfig.NO_AVAILABLE);
beanDefinition.getPropertyValues().addPropertyValue(property, registryConfig);
} else if ("registry".equals(property) && value.indexOf(',') != -1) {
//解析多个registry地址
parseMultiRef("registries", value, beanDefinition, parserContext);
} else if ("provider".equals(property) && value.indexOf(',') != -1) {
//解析多个provider属性
parseMultiRef("providers", value, beanDefinition, parserContext);
} else if ("protocol".equals(property) && value.indexOf(',') != -1) {
//解析多个protocol属性
parseMultiRef("protocols", value, beanDefinition, parserContext);
} else {
Object reference;
if (isPrimitive(type)) {
if ("async".equals(property) && "false".equals(value)
|| "timeout".equals(property) && "0".equals(value)
|| "delay".equals(property) && "0".equals(value)
|| "version".equals(property) && "0.0.0".equals(value)
|| "stat".equals(property) && "-1".equals(value)
|| "reliable".equals(property) && "false".equals(value)) {
// 兼容旧版本xsd中的default值
value = null;
}
reference = value;
} else if ("protocol".equals(property)
&& ExtensionLoader.getExtensionLoader(Protocol.class).hasExtension(value)
&& (! parserContext.getRegistry().containsBeanDefinition(value)
|| ! ProtocolConfig.class.getName().equals(parserContext.getRegistry().getBeanDefinition(value).getBeanClassName()))) {
if ("dubbo:provider".equals(element.getTagName())) {
logger.warn("Recommended replace <dubbo:provider protocol=\"" + value + "\" ... /> to <dubbo:protocol name=\"" + value + "\" ... />");
}
// 兼容旧版本配置
ProtocolConfig protocol = new ProtocolConfig(); //感觉像占个位置,等待protocolConfig标签初始化时,再设置
protocol.setName(value);
reference = protocol;
} else if ("monitor".equals(property)
&& (! parserContext.getRegistry().containsBeanDefinition(value)
|| ! MonitorConfig.class.getName().equals(parserContext.getRegistry().getBeanDefinition(value).getBeanClassName()))) {
// 兼容旧版本配置
reference = convertMonitor(value);
} else if ("onreturn".equals(property)) {
//设置return钩子方法
int index = value.lastIndexOf(".");
String returnRef = value.substring(0, index);
String returnMethod = value.substring(index + 1);
reference = new RuntimeBeanReference(returnRef);
beanDefinition.getPropertyValues().addPropertyValue("onreturnMethod", returnMethod);
} else if ("onthrow".equals(property)) {
//设置throw钩子方法
int index = value.lastIndexOf(".");
String throwRef = value.substring(0, index);
String throwMethod = value.substring(index + 1);
reference = new RuntimeBeanReference(throwRef);
beanDefinition.getPropertyValues().addPropertyValue("onthrowMethod", throwMethod);
} else {
if ("ref".equals(property) && parserContext.getRegistry().containsBeanDefinition(value)) {
BeanDefinition refBean = parserContext.getRegistry().getBeanDefinition(value);
if (! refBean.isSingleton()) {
throw new IllegalStateException("The exported service ref " + value + " must be singleton! Please set the " + value + " bean scope to singleton, eg: <bean id=\"" + value+ "\" scope=\"singleton\" ...>");
}
}
reference = new RuntimeBeanReference(value);
}
beanDefinition.getPropertyValues().addPropertyValue(property, reference);
}
}
}
}
}
}
//处理标签上未处理的属性
NamedNodeMap attributes = element.getAttributes();
int len = attributes.getLength();
for (int i = 0; i < len; i++) {
Node node = attributes.item(i);
String name = node.getLocalName();
if (! props.contains(name)) {
if (parameters == null) {
parameters = new ManagedMap();
}
String value = node.getNodeValue();
parameters.put(name, new TypedStringValue(value, String.class));
}
}
if (parameters != null) {
beanDefinition.getPropertyValues().addPropertyValue("parameters", parameters);
}
return beanDefinition;
}
```
* 先获取标签的id,如果id不存在,则会根据规则自动生成一个id,然后注册当前beanDefinition
* 根据beanClass的不同做一些特定的工作
- 解析ProtocolConfig.class时,找出其他beanDefinition中依赖当前protocol的地方,把当前对象注入进去
- 解析ServiceBean.class时,节点上有class属性会转化成ref属性
- 解析ProviderConfig.class时,如果有<dubbo:service>子节点,则解析它
- 解析ConsumerConfig.class时,如果有<dubbo:reference>子节点,则解析它
* 根据beanClass的set方法到标签属性上寻找对应的值,并设置到beanDefinition中去
- 设置前需要判断是否有get方法或者is方法,get或者is方法是否public,且set方法的参数类型是否和get方法的返回类型相同
- 单个属性有多个值得解析,registry、provider、protocol
- 对旧版本的兼容处理
- 设置onreturn和onthrow钩子方法
- 然后才是其他所有的属性
* 最后会遍历节点的所有属性,找到未处理的属性,然后处理它。
#### parseNested方法
* parseNested(element, parserContext, ServiceBean.class, true, "service", "provider", id, beanDefinition);
* parseNested(element, parserContext, ReferenceBean.class, false, "reference", "consumer", id, beanDefinition);
```java
private static void parseNested(Element element, ParserContext parserContext, Class<?> beanClass, boolean required, String tag, String property, String ref, BeanDefinition beanDefinition) {
NodeList nodeList = element.getChildNodes();
if (nodeList != null && nodeList.getLength() > 0) {
boolean first = true;
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node instanceof Element) {
if (tag.equals(node.getNodeName())
|| tag.equals(node.getLocalName())) {
if (first) {
first = false;
String isDefault = element.getAttribute("default");
if (isDefault == null || isDefault.length() == 0) {
beanDefinition.getPropertyValues().addPropertyValue("default", "false");
}
}
//递归调用解析子标签
BeanDefinition subDefinition = parse((Element) node, parserContext, beanClass, required);
if (subDefinition != null && ref != null && ref.length() > 0) {
//子标签添加属性
subDefinition.getPropertyValues().addPropertyValue(property, new RuntimeBeanReference(ref));
}
}
}
}
}
}
```
* <dubbo:provider>节点下有<dubbo:service>或者<dubbo:consumer>节点下有<dubbo:reference>节点时,才会调用当前方法
* 递归调用parse解析子节点,并把父节点注入到子节点中去
#### parseParameters
* 解析beanClass的setParameters方法时调用方法
```java
private static ManagedMap parseParameters(NodeList nodeList, RootBeanDefinition beanDefinition) {
if (nodeList != null && nodeList.getLength() > 0) {
ManagedMap parameters = null;
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node instanceof Element) {
if ("parameter".equals(node.getNodeName())
|| "parameter".equals(node.getLocalName())) {
if (parameters == null) {
parameters = new ManagedMap();
}
String key = ((Element) node).getAttribute("key");
String value = ((Element) node).getAttribute("value");
boolean hide = "true".equals(((Element) node).getAttribute("hide"));
if (hide) {
key = Constants.HIDE_KEY_PREFIX + key;
}
parameters.put(key, new TypedStringValue(value, String.class));
}
}
}
return parameters;
}
return null;
}
```
* 子节点是否有<dubbo:parameter>节点,如果有则解析它并添加到parameters集合中去
#### parseMethods
* 解析beanClass的setMethods方法时调用的方法
```java
private static void parseMethods(String id, NodeList nodeList, RootBeanDefinition beanDefinition,
ParserContext parserContext) {
if (nodeList != null && nodeList.getLength() > 0) {
ManagedList methods = null;
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node instanceof Element) {
Element element = (Element) node;
if ("method".equals(node.getNodeName()) || "method".equals(node.getLocalName())) {
String methodName = element.getAttribute("name");
if (methodName == null || methodName.length() == 0) {
throw new IllegalStateException("<dubbo:method> name attribute == null");
}
if (methods == null) {
methods = new ManagedList();
}
BeanDefinition methodBeanDefinition = parse(((Element) node),
parserContext, MethodConfig.class, false);
String name = id + "." + methodName;
BeanDefinitionHolder methodBeanDefinitionHolder = new BeanDefinitionHolder(
methodBeanDefinition, name);
methods.add(methodBeanDefinitionHolder);
}
}
}
if (methods != null) {
beanDefinition.getPropertyValues().addPropertyValue("methods", methods);
}
}
}
```
* 解析method子节点。
#### parseArguments
```java
private static void parseArguments(String id, NodeList nodeList, RootBeanDefinition beanDefinition,
ParserContext parserContext) {
if (nodeList != null && nodeList.getLength() > 0) {
ManagedList arguments = null;
for (int i = 0; i < nodeList.getLength(); i++) {
Node node = nodeList.item(i);
if (node instanceof Element) {
Element element = (Element) node;
if ("argument".equals(node.getNodeName()) || "argument".equals(node.getLocalName())) {
String argumentIndex = element.getAttribute("index");
if (arguments == null) {
arguments = new ManagedList();
}
BeanDefinition argumentBeanDefinition = parse(((Element) node),
parserContext, ArgumentConfig.class, false);
String name = id + "." + argumentIndex;
BeanDefinitionHolder argumentBeanDefinitionHolder = new BeanDefinitionHolder(
argumentBeanDefinition, name);
arguments.add(argumentBeanDefinitionHolder);
}
}
}
if (arguments != null) {
beanDefinition.getPropertyValues().addPropertyValue("arguments", arguments);
}
}
}
```
* 解析argument子节点
#### parseMultiRef
```java
private static void parseMultiRef(String property, String value, RootBeanDefinition beanDefinition,
ParserContext parserContext) {
String[] values = value.split("\\s*[,]+\\s*");
ManagedList list = null;
for (int i = 0; i < values.length; i++) {
String v = values[i];
if (v != null && v.length() > 0) {
if (list == null) {
list = new ManagedList();
}
list.add(new RuntimeBeanReference(v));
}
}
beanDefinition.getPropertyValues().addPropertyValue(property, list);
}
```
* 总结:dubbo标签解析时并不会去读取dubbo.properties内的属性值。
<file_sep>---
layout: post
title: spring源码解析--自定义标签
date: 2017-07-25 15:48:00
categories:
- java
- spring
tags:
- spring
- java
- 源码
---
spring初始化的时候,是如何解析自定义标签的呢?先看看下面的的时序图。

最终spring是交给```BeanDefinitionParserDelegate```类的```parseCustomElement(Element ele, BeanDefinition containingBd)```方法去解析的
下面看看```parseCustomElement(Element ele, BeanDefinition containingBd)```方法
```java
public BeanDefinition parseCustomElement(Element ele, BeanDefinition containingBd) {
//获取命名空间 http://www.wgq.com/schema/user
String namespaceUri = getNamespaceURI(ele);
//根据命名空间获取 NamespaceHandler
NamespaceHandler handler = this.readerContext.getNamespaceHandlerResolver().resolve(namespaceUri);
if (handler == null) {
error("Unable to locate Spring NamespaceHandler for XML schema namespace [" + namespaceUri + "]", ele);
return null;
}
return handler.parse(ele, new ParserContext(this.readerContext, this, containingBd));
}
```
* 获取命名空间直接调用的是```org.w3c.dom.Node```自带的方法获取
* 然后便是根据命名空间获取```NamespaceHandler```了,跟踪```resolve```方法
### resolve方法
```java
public NamespaceHandler resolve(String namespaceUri) {
//获取handler缓存集合
Map<String, Object> handlerMappings = getHandlerMappings();
Object handlerOrClassName = handlerMappings.get(namespaceUri);
if (handlerOrClassName == null) {
return null;
}
//根据缓存获取hanlder已初始化直接返回
else if (handlerOrClassName instanceof NamespaceHandler) {
return (NamespaceHandler) handlerOrClassName;
}
//未初始化则进行初始化,并替换缓存中的值为初始化后的对象
else {
String className = (String) handlerOrClassName;
try {
Class<?> handlerClass = ClassUtils.forName(className, this.classLoader);
if (!NamespaceHandler.class.isAssignableFrom(handlerClass)) {
throw new FatalBeanException("Class [" + className + "] for namespace [" + namespaceUri +
"] does not implement the [" + NamespaceHandler.class.getName() + "] interface");
}
NamespaceHandler namespaceHandler = (NamespaceHandler) BeanUtils.instantiateClass(handlerClass);
namespaceHandler.init();
handlerMappings.put(namespaceUri, namespaceHandler);
return namespaceHandler;
}
catch (ClassNotFoundException ex) {
throw new FatalBeanException("NamespaceHandler class [" + className + "] for namespace [" +
namespaceUri + "] not found", ex);
}
catch (LinkageError err) {
throw new FatalBeanException("Invalid NamespaceHandler class [" + className + "] for namespace [" +
namespaceUri + "]: problem with handler class file or dependent class", err);
}
}
}
```
观看上面的源码,主要分成以下几步:
* 获取```handlerMappings```缓存集合
* 从缓存中获取handler,如果已初始化则直接返回,未初始化则进行初始化工作。
我们先看看```getHandlerMappings()```干了些什么
#### getHandlerMappings()
```java
private Map<String, Object> getHandlerMappings() {
if (this.handlerMappings == null) {
synchronized (this) {
if (this.handlerMappings == null) {
try {
Properties mappings =
PropertiesLoaderUtils.loadAllProperties(this.handlerMappingsLocation, this.classLoader);
if (logger.isDebugEnabled()) {
logger.debug("Loaded NamespaceHandler mappings: " + mappings);
}
Map<String, Object> handlerMappings = new ConcurrentHashMap<String, Object>(mappings.size());
CollectionUtils.mergePropertiesIntoMap(mappings, handlerMappings);
this.handlerMappings = handlerMappings;
}
catch (IOException ex) {
throw new IllegalStateException(
"Unable to load NamespaceHandler mappings from location [" + this.handlerMappingsLocation + "]", ex);
}
}
}
}
return this.handlerMappings;
}
```
* 从上面的代码可以看出,主要是从```handlerMappingsLocation```这个地址下获取键值然后缓存下来
* 那么```handlerMappingsLocation```值是什么呢?看下面这张图

* 可以很清楚的看到this.handlerMappingsLocation=META-INF/spring.handlers。
```java
##spring.handlers
http\://www.wgq.com/schema/user=com.wgq.spring.source.namespacehandler.MyNamespaceHandler
```
* 看完getHandlerMappings我们在看看```namespaceHandler.init()```初始化的时候做了啥
#### namespaceHandler.init()
```java
@Override
public void init() {
// TODO Auto-generated method stub
registerBeanDefinitionParser("user", new UserBeanDefintionParser());
}
protected final void registerBeanDefinitionParser(String elementName, BeanDefinitionParser parser) {
this.parsers.put(elementName, parser);
}
```
* 可以很清楚的看到,在handler初始化的时候会把我们自定义的```UserBeanDefintionParser```注册进去
### handler.parse
看完了resolve方法,下面来看看handler.parse这个方法,其调用的是```NamespaceHandlerSupport```里面的parse方法
```java
@Override
public BeanDefinition parse(Element element, ParserContext parserContext) {
return findParserForElement(element, parserContext).parse(element, parserContext);
}
private BeanDefinitionParser findParserForElement(Element element, ParserContext parserContext) {
String localName = parserContext.getDelegate().getLocalName(element);
BeanDefinitionParser parser = this.parsers.get(localName);
if (parser == null) {
parserContext.getReaderContext().fatal(
"Cannot locate BeanDefinitionParser for element [" + localName + "]", element);
}
return parser;
}
```
* 根据```localName```找到```BeanDefinitionParser```,例:localName="user"。
* ```this.parsers```在前面提到过,通过```namespaceHandler.init()```的方法把自定义的BeanDefinitionParser注册进去
* 然后直接调用```AbstractBeanDefinitionParser```下parse方法
一般来说我们的自定义BeanDefinitionParser的类会实现```AbstractSingleBeanDefinitionParser```,比如:
```java
package com.wgq.spring.source.parser;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.xml.AbstractSingleBeanDefinitionParser;
import org.w3c.dom.Element;
import com.wgq.spring.source.pojo.User;
public class UserBeanDefintionParser extends AbstractSingleBeanDefinitionParser {
protected Class getBeanClass(Element element) {
return User.class;
}
protected void doParse(Element element, BeanDefinitionBuilder bean) {
String userName = element.getAttribute("username");
String email = element.getAttribute("email");
bean.addPropertyValue("username", userName);
bean.addPropertyValue("email", email);
}
}
```
* 上面我们说直接调用的是```AbstractBeanDefinitionParser```下```parse```方法,那为什么这里继承的是```AbstractSingleBeanDefinitionParser```类呢?先看看```AbstractBeanDefinitionParser```下```parse```方法
```java
//AbstractBeanDefinitionParser
@Override
public final BeanDefinition parse(Element element, ParserContext parserContext) {
AbstractBeanDefinition definition = parseInternal(element, parserContext);
if (definition != null && !parserContext.isNested()) {
try {
String id = resolveId(element, definition, parserContext);
if (!StringUtils.hasText(id)) {
parserContext.getReaderContext().error(
"Id is required for element '" + parserContext.getDelegate().getLocalName(element)
+ "' when used as a top-level tag", element);
}
String[] aliases = null;
if (shouldParseNameAsAliases()) {
String name = element.getAttribute(NAME_ATTRIBUTE);
if (StringUtils.hasLength(name)) {
aliases = StringUtils.trimArrayElements(StringUtils.commaDelimitedListToStringArray(name));
}
}
BeanDefinitionHolder holder = new BeanDefinitionHolder(definition, id, aliases);
registerBeanDefinition(holder, parserContext.getRegistry());
if (shouldFireEvents()) {
BeanComponentDefinition componentDefinition = new BeanComponentDefinition(holder);
postProcessComponentDefinition(componentDefinition);
parserContext.registerComponent(componentDefinition);
}
}
catch (BeanDefinitionStoreException ex) {
parserContext.getReaderContext().error(ex.getMessage(), element);
return null;
}
}
return definition;
}
//AbstractSingleBeanDefinitionParser
protected final AbstractBeanDefinition parseInternal(Element element, ParserContext parserContext) {
BeanDefinitionBuilder builder = BeanDefinitionBuilder.genericBeanDefinition();
String parentName = getParentName(element);
if (parentName != null) {
builder.getRawBeanDefinition().setParentName(parentName);
}
Class<?> beanClass = getBeanClass(element);
if (beanClass != null) {
builder.getRawBeanDefinition().setBeanClass(beanClass);
}
else {
String beanClassName = getBeanClassName(element);
if (beanClassName != null) {
builder.getRawBeanDefinition().setBeanClassName(beanClassName);
}
}
builder.getRawBeanDefinition().setSource(parserContext.extractSource(element));
if (parserContext.isNested()) {
// Inner bean definition must receive same scope as containing bean.
builder.setScope(parserContext.getContainingBeanDefinition().getScope());
}
if (parserContext.isDefaultLazyInit()) {
// Default-lazy-init applies to custom bean definitions as well.
builder.setLazyInit(true);
}
doParse(element, parserContext, builder);
return builder.getBeanDefinition();
}
```
* ```parse```方法会调用其子类```AbstractSingleBeanDefinitionParser```的```parseInternal```方法,在```parseInternal```方法内,会调用```doParse```方法(也就是我们自定义的方法)
* 当然您也可以直接继承```AbstractBeanDefinitionParser```类,去重写其的```parse```方法,spring内开启aop注解功能的自定义标签就是这样的。
### aop自定义标签的实现
```java
public class AopNamespaceHandler extends NamespaceHandlerSupport {
/**
* Register the {@link BeanDefinitionParser BeanDefinitionParsers} for the
* '{@code config}', '{@code spring-configured}', '{@code aspectj-autoproxy}'
* and '{@code scoped-proxy}' tags.
*/
@Override
public void init() {
// In 2.0 XSD as well as in 2.1 XSD.
registerBeanDefinitionParser("config", new ConfigBeanDefinitionParser());
registerBeanDefinitionParser("aspectj-autoproxy", new AspectJAutoProxyBeanDefinitionParser());
registerBeanDefinitionDecorator("scoped-proxy", new ScopedProxyBeanDefinitionDecorator());
// Only in 2.0 XSD: moved to context namespace as of 2.1
registerBeanDefinitionParser("spring-configured", new SpringConfiguredBeanDefinitionParser());
}
}
class AspectJAutoProxyBeanDefinitionParser implements BeanDefinitionParser {
@Override
public BeanDefinition parse(Element element, ParserContext parserContext) {
AopNamespaceUtils.registerAspectJAnnotationAutoProxyCreatorIfNecessary(parserContext, element);
extendBeanDefinition(element, parserContext);
return null;
}
private void extendBeanDefinition(Element element, ParserContext parserContext) {
BeanDefinition beanDef =
parserContext.getRegistry().getBeanDefinition(AopConfigUtils.AUTO_PROXY_CREATOR_BEAN_NAME);
if (element.hasChildNodes()) {
addIncludePatterns(element, parserContext, beanDef);
}
}
private void addIncludePatterns(Element element, ParserContext parserContext, BeanDefinition beanDef) {
ManagedList<TypedStringValue> includePatterns = new ManagedList<TypedStringValue>();
NodeList childNodes = element.getChildNodes();
for (int i = 0; i < childNodes.getLength(); i++) {
Node node = childNodes.item(i);
if (node instanceof Element) {
Element includeElement = (Element) node;
TypedStringValue valueHolder = new TypedStringValue(includeElement.getAttribute("name"));
valueHolder.setSource(parserContext.extractSource(includeElement));
includePatterns.add(valueHolder);
}
}
if (!includePatterns.isEmpty()) {
includePatterns.setSource(parserContext.extractSource(element));
beanDef.getPropertyValues().add("includePatterns", includePatterns);
}
}
}
//aop标签的spring.schemas
http\://www.springframework.org/schema/aop/spring-aop-2.0.xsd=org/springframework/aop/config/spring-aop-2.0.xsd
http\://www.springframework.org/schema/aop/spring-aop-2.5.xsd=org/springframework/aop/config/spring-aop-2.5.xsd
http\://www.springframework.org/schema/aop/spring-aop-3.0.xsd=org/springframework/aop/config/spring-aop-3.0.xsd
http\://www.springframework.org/schema/aop/spring-aop-3.1.xsd=org/springframework/aop/config/spring-aop-3.1.xsd
http\://www.springframework.org/schema/aop/spring-aop-3.2.xsd=org/springframework/aop/config/spring-aop-3.2.xsd
http\://www.springframework.org/schema/aop/spring-aop-4.0.xsd=org/springframework/aop/config/spring-aop-4.0.xsd
http\://www.springframework.org/schema/aop/spring-aop-4.1.xsd=org/springframework/aop/config/spring-aop-4.1.xsd
http\://www.springframework.org/schema/aop/spring-aop.xsd=org/springframework/aop/config/spring-aop-4.1.xsd
//aop标签的spring.handlers
http\://www.springframework.org/schema/aop=org.springframework.aop.config.AopNamespaceHandler
```
### 自定义标签实现(例子)
```MyNamespaceHandler```<br />
```java
package com.wgq.spring.source.namespacehandler;
import org.springframework.beans.factory.xml.NamespaceHandlerSupport;
import com.wgq.spring.source.parser.UserBeanDefintionParser;
public class MyNamespaceHandler extends NamespaceHandlerSupport {
@Override
public void init() {
// TODO Auto-generated method stub
registerBeanDefinitionParser("user", new UserBeanDefintionParser());
}
}
```
```UserBeanDefintionParser```<br />
```java
package com.wgq.spring.source.parser;
import org.springframework.beans.factory.support.BeanDefinitionBuilder;
import org.springframework.beans.factory.xml.AbstractSingleBeanDefinitionParser;
import org.w3c.dom.Element;
import com.wgq.spring.source.pojo.User;
public class UserBeanDefintionParser extends AbstractSingleBeanDefinitionParser {
protected Class getBeanClass(Element element) {
return User.class;
}
protected void doParse(Element element, BeanDefinitionBuilder bean) {
String userName = element.getAttribute("username");
String email = element.getAttribute("email");
bean.addPropertyValue("username", userName);
bean.addPropertyValue("email", email);
}
}
```
```spring.handlers```<br />
```java
http\://www.wgq.com/schema/user=com.wgq.spring.source.namespacehandler.MyNamespaceHandler
```
```spring.schemas```<br />
```java
http\://www.wgq.com/schema/user.xsd=META-INF/spring-test.xsd
```
```spring-test.xsd```<br />
```xml
<?xml version="1.0" encoding="UTF-8"?>
<schema xmlns="http://www.w3.org/2001/XMLSchema" targetNamespace="http://www.wgq.com/schema/user"
xmlns:tns="http://www.wgq.com/schema/user" elementFormDefault="qualified">
<element name="user">
<complexType>
<attribute name="id" type="string" />
<attribute name="username" type="string" />
<attribute name="password" type="<PASSWORD>" />
<attribute name="email" type="string" />
</complexType>
</element>
</schema>
```
```dispatcherServlet-custom-tag.xml```<br />
```xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:myname="http://www.wgq.com/schema/user"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.wgq.com/schema/user
http://www.wgq.com/schema/user.xsd">
<myname:user id="user" username="1111" email="sss"></myname:user>
</beans>
``` | d9c71f26a3f1557812a8fab6ccff37f5dae78d19 | [
"Markdown",
"Ruby"
] | 16 | Markdown | joyswang/joyswang.github.io | 3dec888acecd7859314998b76459aa40c7772741 | 62594802e58728169b05e1117e87edbe187d77e2 | |
refs/heads/master | <file_sep>package biz.lungo.rentcars;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.database.Cursor;
import android.os.Bundle;
import android.text.InputType;
import android.view.LayoutInflater;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.View.OnTouchListener;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.DatePicker;
import android.widget.EditText;
import android.widget.RadioGroup;
import android.widget.RelativeLayout;
import android.widget.Spinner;
import android.widget.Toast;
public class AddOrderFragment extends FrontFragment implements OnTouchListener, OnClickListener {
private static final int START = 0;
private static final int END = 1;
EditText fieldName, fieldDateStart, fieldDateEnd;
Button buttonAdd;
Spinner spinnerCars;
private String result;
DatePicker dp;
ArrayAdapter<CharSequence> adapter;
RadioGroup radioGroupVip;
public AddOrderFragment(int color, int resource) {
super(color, resource);
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
RelativeLayout rl = new RelativeLayout(getActivity());
rl = (RelativeLayout) RelativeLayout.inflate(getActivity(), super.resource, null);
rl.setBackgroundColor(super.mColorRes);
spinnerCars = (Spinner) rl.findViewById(R.id.spinnerCars);
fieldName = (EditText) rl.findViewById(R.id.editTextName);
fieldDateStart = (EditText) rl.findViewById(R.id.editTextDateStart);
fieldDateEnd = (EditText) rl.findViewById(R.id.editTextDateEnd);
radioGroupVip = (RadioGroup) rl.findViewById(R.id.radioGroup1);
fieldDateStart.setClickable(true);
fieldDateStart.setInputType(InputType.TYPE_NULL);
fieldDateStart.setOnTouchListener(this);
fieldDateEnd.setClickable(true);
fieldDateEnd.setInputType(InputType.TYPE_NULL);
fieldDateEnd.setOnTouchListener(this);
buttonAdd = (Button) rl.findViewById(R.id.buttonAdd);
buttonAdd.setOnClickListener(this);
String array_cars[] = fillArrayCars();
adapter = new ArrayAdapter<CharSequence>(getActivity(), android.R.layout.simple_spinner_dropdown_item);
adapter.addAll(array_cars);
spinnerCars.setAdapter(adapter);
return rl;
}
private String[] fillArrayCars() {
String statementSelect = "SELECT * FROM cars;";
Cursor c = MainActivity.db.rawQuery(statementSelect, null);
int rowCount = c.getCount();
String result[] = new String[rowCount];
if(c.moveToFirst()){
for (int i = 0; i < rowCount; i++){
result[i] = c.getString(1);
c.moveToNext();
}
}
return result;
}
@Override
public boolean onTouch(View v, MotionEvent e) {
if (e.getAction() == MotionEvent.ACTION_DOWN){
switch (v.getId()){
case R.id.editTextDateStart:
showDateDialog(START, getResources().getString(R.string.calendar_start));
break;
case R.id.editTextDateEnd:
showDateDialog(END, getResources().getString(R.string.calendar_end));
break;
}
}
return false;
}
private void showDateDialog(int state, String title) {
final int curstate = state;
dp = new DatePicker(getActivity());
dp.setCalendarViewShown(false);
AlertDialog.Builder ab = new AlertDialog.Builder(getActivity());
ab.setView(dp);
ab.setCancelable(false);
ab.setTitle(title);
ab.setPositiveButton(android.R.string.ok, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
result = ((dp.getDayOfMonth() < 10)?("0" + dp.getDayOfMonth()):dp.getDayOfMonth()) + "."
+ (((dp.getMonth() + 1) < 10)?("0" + (dp.getMonth() + 1)):(dp.getMonth() + 1)) + "."
+ dp.getYear();
if (curstate == START)
fieldDateStart.setText(result);
else if (curstate == END)
fieldDateEnd.setText(result);
}
});
ab.setNegativeButton(android.R.string.cancel, null);
ab.show();
}
@Override
public void onClick(View v) {
String carModel = spinnerCars.getSelectedItem().toString();
Cursor cursor = MainActivity.db.rawQuery("SELECT * FROM cars WHERE _model = '" + carModel + "';", null);
cursor.moveToFirst();
String carLicensePlate = cursor.getString(2);
String clientName = fieldName.getText().toString();
long startDate = getDate(START);
long endDate = getDate(END);
int isVip = isVip();
String queryAdd = "INSERT INTO orders(_car_model, _license_plate, _client_name, _start_date, _end_date, _is_vip) VALUES('"
+ spinnerCars.getSelectedItem().toString() + "', '"
+ carLicensePlate + "', '"
+ clientName + "', "
+ startDate + ", "
+ endDate + ", "
+ isVip + ");";
MainActivity.db.execSQL(queryAdd);
getDBContent();
}
private void getDBContent() {
String statementSelect = "SELECT * FROM orders;";
StringBuilder sb = new StringBuilder();
Cursor c = MainActivity.db.rawQuery(statementSelect, null);
int columns = c.getColumnCount();
if (c.moveToFirst()){
while(!c.isAfterLast()){
for (int i = 0; i < columns; i++){
sb.append(c.getString(i) + " ");
}
sb.append("\n");
c.moveToNext();
}
}
Toast.makeText(getActivity(), sb.toString(), Toast.LENGTH_SHORT).show();
}
private int isVip() {
int result = 0;
if (radioGroupVip.getCheckedRadioButtonId() == R.id.radio0){
result = 1;
}
return result;
}
private long getDate(int i){
SimpleDateFormat sdf = new SimpleDateFormat("dd.mm.yyyy");
Date date = null;
switch(i){
case START:
try {
date = sdf.parse(fieldDateStart.getText().toString());
} catch (ParseException e) {
e.printStackTrace();
}
break;
case END:
try {
date = sdf.parse(fieldDateEnd.getText().toString());
} catch (ParseException e) {
e.printStackTrace();
}
break;
}
return date.getTime();
}
}
| 6e6a598b26ef0a5a8578d771f54d3caa8bffc2cb | [
"Java"
] | 1 | Java | Higmans/RentCars | 1510960af1d7441a3de40da87c7c760a8fb1ee77 | 7b689eab96c1ed7ede6126093686dd225077fc17 | |
refs/heads/master | <repo_name>Aditya170894/test-dumbways-21-kloter-2<file_sep>/dwbike/detail.php
<?php
require 'functions.php';
$datas = query("SELECT * FROM `cycle` WHERE id = {$_GET["id"]}");
$data = $datas[0];
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="<KEY>" crossorigin="anonymous">
<title>DW Bike</title>
<style>
*{
padding: 0;
margin: 0;
}
a {
text-decoration: none;
}
.container {
height: 100%;
}
p {
color: #ffffff;
}
.d-flex {
color: #ffffff;
}
.image-author img {
height: 200px;
width: 120px;
object-fit: cover;
}
.card-list {
padding: 20px;
}
.image-author {
width: 120px;
}
.card-title {
font-size: 30px;
font-weight: bold;
}
.card {
height: 700px;
}
.body-img {
width: 50%;
height: 350px;
object-fit: cover;
}
.card-text {
height: 100px;
}
.btn-body {
background-color: #292938;
color: blue;
}
</style>
</head>
<body style="background-color:#000000">
<div class="container">
<div class="d-flex flex-row my-5 justify-content-between">
<div>
<div class="card-body">
<h5 class="card-title" style="color: #ffffff;"><?=$data["name"]?></h5>
<img class="body-img my-5" src=<?=$data["image"]?> alt="...">
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/umd/popper.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
</body>
</html><file_sep>/dwbike/index.php
<?php
require 'functions.php';
$datas = query("SELECT * FROM `cycle`");
if (isset($_POST["submit-post"])) {
insertPost($_POST);
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="<KEY>" crossorigin="anonymous">
<title>DW Bike</title>
<style>
*{
padding: 0;
margin: 0;
}
a {
text-decoration: none;
}
.container {
height: 100%;
}
p {
color: #ffffff;
}
.d-flex {
color: #ffffff;
}
.image-author img {
height: 120px;
width: 120px;
object-fit: cover;
}
.card-list {
padding: 20px;
}
.image-author {
width: 120px;
}
.card {
height: 300px;
width: 180px;
}
.body-img {
width: 900px;
height: 350px;
object-fit: cover;
}
.card-text {
height: 100px;
}
.btn-body {
background-color: grey;
color: blue;
}
</style>
</head>
<body style="background-color:#000000">
<div class="container">
<div class="d-flex flex-row my-5 justify-content-between">
<h3>DW Bike</h3>
<button type="button" data-bs-toggle="modal" data-bs-target="#modalPost" class="btn btn-warning btn-post">My Order</button>
</div>
<?php $i = 1; ?>
<?php foreach ($datas as $data) : ?>
<div class="card mb-3 mt-3 p-2 d-flex" style="background-color: #292929;">
<div class="d-flex flex-row card-list">
<div class="col-md-4 image-author text-center">
<img src=<?= $data['image']; ?> alt="...">
<div><?=$data["name"]?></div>
<div>stock: <?=$data["stock"]?></div>
<div class="text-center">
<a href=<?="./detail.php?id={$data["id"]}";?> >
<button class="btn btn-body" type="button">Buy</button>
</a>
</div>
</div>
<div>
</div>
</div>
</div>
<?php $i++; ?>
<?php endforeach; ?>
<!-- Modal Post -->
<div class="modal fade" id="modalPost" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<form class="modal-content" method="post">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">My Order</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text" id="basic-addon1">Nama Sepeda</span>
</div>
<input type="text" class="form-control" placeholder="<NAME>" aria-label="Username" aria-describedby="basic-addon1" name="name" id="name">
</div>
</div>
<div class="modal-body">
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text" id="basic-addon1">Address</span>
</div>
<input type="text" class="form-control" placeholder="Address" aria-label="Username" aria-describedby="basic-addon1" name="address" id="address">
</div>
</div>
<div class="modal-body">
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text" id="basic-addon1">image</span>
</div>
<input type="text" class="form-control" placeholder="image" aria-label="Username" aria-describedby="basic-addon1" name="image" id="image">
</div>
</div>
<div class="modal-body">
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text" id="basic-addon1">Akreditasi</span>
</div>
<select name="school_level" id="school_level">
<option value="A">A</option>
<option value="B">B</option>
<option value="C">C</option>
</select>
</div>
</div>
<div class="modal-body">
<div class="input-group mb-3">
<div class="input-group-prepend">
<span class="input-group-text" id="basic-addon1">Status Sekolah</span>
</div>
<select name="status_school" id="status_school">
<option value="Negeri">Negeri</option>
<option value="Swasta">Swasta</option>
</select>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary" name="submit-post">Save changes</button>
</div>
</form>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="<KEY>" crossorigin="anonymous"></script>
</body>
</html><file_sep>/2.js
const array1 = ['apa', 'saya', 'anda', 'kamu', 'hallo'];
const array2 = [
'apa yang anda lakukan?',
'selamat pagi',
'kamu ternyata cantik juga ya',
'yukk belajar javascript',
'kamu itu adalah kebanggaan saya',
'music hari ini yang akan di putar oleh gabut FM apa ya?'];
function filterKoneversiArr(array1, array2, vocal) {
let countArray1 = {};
const newArray = array2.map((item) => {
return item.split(' ').map((str) => {
let newStr = str;
array1.forEach(element => {
if (countArray1[element] === undefined) {
countArray1[element] = 0;
}
if (element === str) {
newStr = str.replace(/a|i|u|o|e/g, vocal);
countArray1[element] += 1
}
});
return newStr
}).join(' ')
})
array1.forEach(letter => {
console.log('Kata ' + letter + ' muncul sebanyak: ' + countArray1[letter]);
});
console.log(newArray);
console.log(countArray1);
return newArray;
}
filterKoneversiArr(array1, array2, 'i');<file_sep>/dw_bike.sql
-- phpMyAdmin SQL Dump
-- version 4.0.4
-- http://www.phpmyadmin.net
--
-- Host: 127.0.0.1
-- Generation Time: Feb 06, 2021 at 03:58 PM
-- Server version: 5.5.32
-- PHP Version: 5.4.16
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8 */;
--
-- Database: `dw_bike`
--
CREATE DATABASE IF NOT EXISTS `dw_bike` DEFAULT CHARACTER SET latin1 COLLATE latin1_swedish_ci;
USE `dw_bike`;
-- --------------------------------------------------------
--
-- Table structure for table `cycle`
--
CREATE TABLE IF NOT EXISTS `cycle` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`price` varchar(50) NOT NULL,
`stock` int(11) NOT NULL,
`image` varchar(300) NOT NULL,
`id_merk` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=8 ;
--
-- Dumping data for table `cycle`
--
INSERT INTO `cycle` (`id`, `name`, `price`, `stock`, `image`, `id_merk`) VALUES
(6, 'M6E 2020', '35000000', 5, 'https://s0.bukalapak.com/img/58283534962/large/data.jpeg.webp', 0),
(7, 'M6L 2021 BNIB', '44000000', 15, 'https://s3.bukalapak.com/img/32924155672/large/data.png.webp', 0);
-- --------------------------------------------------------
--
-- Table structure for table `merk`
--
CREATE TABLE IF NOT EXISTS `merk` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(20) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=5 ;
--
-- Dumping data for table `merk`
--
INSERT INTO `merk` (`id`, `name`) VALUES
(3, 'BROMPTON'),
(4, 'HIMO');
-- --------------------------------------------------------
--
-- Table structure for table `user`
--
CREATE TABLE IF NOT EXISTS `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`email` varchar(100) NOT NULL,
`password` varchar(100) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=5 ;
--
-- Dumping data for table `user`
--
INSERT INTO `user` (`id`, `name`, `email`, `password`) VALUES
(1, 'komar', '<EMAIL>', '<PASSWORD>'),
(2, 'udin', '<EMAIL>', '<PASSWORD>'),
(3, 'kurapika', '<EMAIL>', ''),
(4, 'gon', '<EMAIL>', '<PASSWORD>');
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/4a.sql
SELECT * FROM `cycle`
SELECT `id`, `name`, `price`, `stock`, `image`, `id_merk` FROM `cycle` WHERE id_merk = "BROMPTON"
UPDATE `cycle` SET `name`="M6E 2020",`price`="35000000",`stock`="5",`image`="https://s0.bukalapak.com/img/58283534962/large/data.jpeg.webp" WHERE id_merk = "BROMPTON"
SELECT * FROM `cycle` WHERE stock = 15
SELECT * FROM `cycle` WHERE id_merk = "BROMPTON" | 5b23518469eefff9f24771b2f23fc9754954515d | [
"JavaScript",
"SQL",
"PHP"
] | 5 | PHP | Aditya170894/test-dumbways-21-kloter-2 | 1327550d8834fc5af40cf3f9c09558c14e0eb13a | d5b683f9410745514fadd23c08b93423e3293eac | |
refs/heads/master | <file_sep>export class LoginUser {
mail : string;
password :string;
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { Task } from "../../model/task";
import { Router } from "@angular/router";
import { FormGroup, FormBuilder, Validators, FormControl } from '@angular/forms';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-create-task',
templateUrl: './create-task.component.html',
styleUrls: ['./create-task.component.scss']
})
export class CreateTaskComponent implements OnInit {
userTask: Task = new Task();
taskForm: FormGroup;
constructor(
private formBuilder: FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.taskForm = this.formBuilder.group({
'name': new FormControl(this.userTask.name, [Validators.required]),
'description': new FormControl(this.userTask.description, [Validators.required])
})
}
create() {
console.log(this.userTask);
this.http.post('createtask', this.userTask).subscribe(
(response: any) => {
if (response.ststusCode == 200) {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 })
} else {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 })
}
}
)
}
}
<file_sep>import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { LoginComponent } from "./component/login/login.component";
import { DashboardComponent } from "./component/dashboard/dashboard.component";
import { AddMemberComponent } from "./component/add-member/add-member.component";
import { ViewListComponent } from "./component/view-list/view-list.component";
import { BackgroundComponent } from "./component/background/background.component";
import { TLDashboardComponent } from "./component/tldashboard/tldashboard.component";
import { DdDashboardComponent } from "./component/dd-dashboard/dd-dashboard.component";
import { ResetPasswordComponent } from "./component/reset-password/reset-password.component";
import { CreateProjectComponent } from "./component/create-project/create-project.component";
import { ViewProjectsComponent } from "./component/view-projects/view-projects.component";
import { ViewTaskComponent } from "./component/view-task/view-task.component";
import { CreateTaskComponent } from "./component/create-task/create-task.component";
import { ViewMemberDetailComponent } from "./component/view-member-detail/view-member-detail.component";
import { ViewTlProjectComponent } from "./component/view-tl-project/view-tl-project.component";
import { AddTaskToProjectComponent } from "./component/add-task-to-project/add-task-to-project.component";
import { ViewDdTaskComponent } from "./component/view-dd-task/view-dd-task.component";
const routes: Routes = [
{ path: '', component: LoginComponent },
{
path: 'pmdashboard', component: DashboardComponent, children: [
{
path: '', component: BackgroundComponent
}, {
path: 'add', component: AddMemberComponent
},
{
path: 'list', component: ViewListComponent
},
{
path: 'create', component: CreateProjectComponent
},
{
path: 'view', component: ViewProjectsComponent
},
{
path: 'task', component: ViewTaskComponent
},
{
path: 'createtask', component: CreateTaskComponent
},
]
},
{
path: 'tldashboard', component: TLDashboardComponent, children: [
{
path: 'list', component: ViewMemberDetailComponent
},
{
path: 'viewProject', component: ViewTlProjectComponent
},
{
path: 'addTask', component: AddTaskToProjectComponent
},
]
},
{
path: 'dddashboard', component: DdDashboardComponent, children: [
{
path: 'viewTask', component: ViewDdTaskComponent
},
]
},
{ path: 'reset', component: ResetPasswordComponent },
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
<file_sep>import { Component, OnInit, Inject } from '@angular/core';
import { FormGroup, FormBuilder, FormControl, Validators } from "@angular/forms";
import { Task } from "../../model/task";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MAT_DIALOG_DATA } from '@angular/material/dialog';
import { DataServiceService } from "src/app/service/data-service.service";
@Component({
selector: 'app-dialog-edit-task',
templateUrl: './dialog-edit-task.component.html',
styleUrls: ['./dialog-edit-task.component.scss']
})
export class DialogEditTaskComponent implements OnInit {
taskList: Task = new Task();
addForm: FormGroup;
message: any;
// name : new FormControl(this.)
constructor(
@Inject(MAT_DIALOG_DATA) public data: any,
private formBuilder: FormBuilder,
private http: HttpServerService,
private snackBar: MatSnackBar,
private service: DataServiceService,
) { }
ngOnInit() {
this.service.currentMessage.subscribe(
(message: any) => {
this.message = message;
this.taskList.name = this.data.element.name;
this.taskList.description = this.data.element.description;
this.addForm = this.formBuilder.group(
{
'name': new FormControl(this.taskList.name, [Validators.required]),
'description': new FormControl(this.taskList.description, [Validators.required]),
}
)
}
)
}
updateTask() {
this.http.put('updatetask/' + this.data.element.tid, this.taskList).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.service.changeMessage(response.statusMessage);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
}else{
this.service.changeMessage(response.statusMessage);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
}
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { Project } from "../../model/project";
import { Router } from "@angular/router";
import { FormGroup, FormBuilder, Validators, FormControl } from '@angular/forms';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-create-project',
templateUrl: './create-project.component.html',
styleUrls: ['./create-project.component.scss']
})
export class CreateProjectComponent implements OnInit {
userProject: Project = new Project();
projForm: FormGroup;
constructor(
private formBuilder: FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.projForm = this.formBuilder.group({
'name': new FormControl(this.userProject.name, [Validators.required]),
'description': new FormControl(this.userProject.description, [Validators.required])
})
}
create() {
console.log(this.userProject);
this.http.post('createProject', this.userProject).subscribe(
(response: any) => {
if (response.ststusCode == 200) {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 })
} else {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 })
}
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { Router } from "@angular/router";
@Component({
selector: 'app-tldashboard',
templateUrl: './tldashboard.component.html',
styleUrls: ['./tldashboard.component.scss']
})
export class TLDashboardComponent implements OnInit {
constructor(
private router : Router ,
) { }
ngOnInit() {
}
logout(){
localStorage.clear();
this.router.navigate(['/']);
}
reset(){
this.router.navigate(['/reset'])
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, FormControl, Validators } from "@angular/forms";
import { Router } from "@angular/router";
import { AddMember } from "../../model/add-member";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-add-member',
templateUrl: './add-member.component.html',
styleUrls: ['./add-member.component.scss']
})
export class AddMemberComponent implements OnInit {
member: AddMember = new AddMember();
addForm: FormGroup;
constructor(
private formBuilder: FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.addForm = this.formBuilder.group(
{
'name': new FormControl(this.member.name, [Validators.required]),
'desg': new FormControl(this.member.desg, [Validators.required]),
'mobile': new FormControl(this.member.mobileNo, [Validators.required]),
'email': new FormControl(this.member.mail, [Validators.required]),
'password': new FormControl(<PASSWORD>, [Validators.required, Validators.minLength(6)]),
}
)
}
register() {
console.log(this.member);
this.http.post('register', this.member).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
} else {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
}
}
)
}
}
<file_sep>export class ResetPasswordModel {
password : string;
conformPassword : string;
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { Router } from "@angular/router";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MatDialog } from "@angular/material/dialog";
@Component({
selector: 'app-add-task-to-project',
templateUrl: './add-task-to-project.component.html',
styleUrls: ['./add-task-to-project.component.scss']
})
export class AddTaskToProjectComponent implements OnInit {
displayedColumns: string[] = ['id', 'name', 'description', 'asign task'];
projectInfo: any;
mail: string;
data: any;
taskList: any;
constructor(
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
private dialog: MatDialog,
) { }
ngOnInit() {
this.getData();
}
getData() {
this.mail = localStorage.getItem('mail');
this.http.get('getProject?mail=' + this.mail).subscribe(
(response: any) => {
this.projectInfo = response;
}
)
}
onClick(items) {
this.http.put('asigntask?pid=' +this.data.pid + '&tid=' + items.tid ,null).subscribe(
(response : any) =>{
if(response.statusCode == 200){
this.snackBar.open(response.statusMessage , 'close' ,{duration:3000});
}else{
this.snackBar.open(response.statusMessage , 'close' ,{duration:3000});
}
}
)
}
send(element) {
this.data = element;
this.http.get('getalltasks').subscribe(
(response : any) => {
this.taskList = response;
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MatDialog } from "@angular/material/dialog";
import { DialodBoxComponent } from '../dialod-box/dialod-box.component';
import { DataServiceService } from "src/app/service/data-service.service";
@Component({
selector: 'app-view-list',
templateUrl: './view-list.component.html',
styleUrls: ['./view-list.component.scss']
})
export class ViewListComponent implements OnInit {
displayedColumns: string[] = ['id', 'name', 'mail', 'mobileNo', 'designation', 'asign project' ,'delete' , 'edit'];
userInfo: any;
id: number;
projects: any;
data: any;
message : any;
constructor(
private http: HttpServerService,
private snackBar: MatSnackBar,
private dialog: MatDialog,
private service : DataServiceService,
) { }
ngOnInit() {
this.service.currentMessage.subscribe(
( message : any)=>{
this.message = message;
this.getData();
}
)
}
getData() {
this.http.get('usersinfo').subscribe(
(response: any) => {
this.userInfo = response;
}
)
}
edit(items) {
this.dialog.open(DialodBoxComponent, {
data: { items }
});
}
delete(items) {
this.http.delete("deleteUser/" + items.id).subscribe(
(response: any) => {
if (response.status == 200) {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}else{
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}
}
)
}
// asignProjects(items) {
// this.dialog.open(DialogBoxAsignProjectsComponent, {
// data: { items }
// });
// }
getProjects(element) {
this.data = element;
this.http.get('getAllProjects').subscribe(
(response: any) => {
this.projects = response;
console.log("hi ",this.projects);
}
)
}
onClick(items) {
this.http.put('assignProject?pid=' + items.pid + '&eid=' + this.data.id, null).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
} else {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}
}
)
}
}
<file_sep>export class AddMember {
name : string;
desg :string;
mobileNo : string;
mail : string;
password :string;
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators, FormControl } from '@angular/forms';
import { ResetPasswordModel } from "../../model/reset-password-model";
import { Router } from "@angular/router";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-reset-password',
templateUrl: './reset-password.component.html',
styleUrls: ['./reset-password.component.scss']
})
export class ResetPasswordComponent implements OnInit {
passwordModel : ResetPasswordModel = new ResetPasswordModel();
passwordForm : FormGroup ;
status : string;
token = localStorage.getItem('token');
constructor(
private formBulder :FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.passwordForm = this.formBulder.group({
'password': new FormControl(this.passwordModel.password, [Validators.required, Validators.minLength(6)]),
'conformPassword': new FormControl(this.passwordModel.conformPassword, [Validators.required, Validators.minLength(6)]),
})
}
reset(){
console.log(this.token);
console.log(this.passwordModel);
this.status = localStorage.getItem('code');
if(this.passwordModel.password == this.passwordModel.conformPassword){
this.http.putWithToken('resetpassword' , this.passwordModel).subscribe(
(response: any) =>{
if(response.statusCode == 205){
console.log("hi");
if(this.status == '201' || this.status == '204'){
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/dddashboard']);
}else if (this.status == '202') {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/tldashboard']);
}else if (this.status == '203') {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/pmdashboard']);
}else {
console.log(response);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
}
}
}
)
}
}
skip(){
this.status = localStorage.getItem('code');
if(this.status == '201' || this.status == '204'){
this.router.navigate(['/dddashboard']);
}else if (this.status == '202') {
this.router.navigate(['/tldashboard']);
}else if (this.status == '203') {
this.router.navigate(['/pmdashboard']);
}else {
this.snackBar.open(" sorry spik is failed ", 'close', { duration: 3000 });
}
}
}
<file_sep>import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { DdDashboardComponent } from './dd-dashboard.component';
describe('DdDashboardComponent', () => {
let component: DdDashboardComponent;
let fixture: ComponentFixture<DdDashboardComponent>;
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ DdDashboardComponent ]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(DdDashboardComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
<file_sep>import { Component, OnInit } from '@angular/core';
import { Router } from "@angular/router";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MatDialog } from "@angular/material/dialog";
import { DialogEditTaskComponent } from '../dialog-edit-task/dialog-edit-task.component';
@Component({
selector: 'app-view-tl-project',
templateUrl: './view-tl-project.component.html',
styleUrls: ['./view-tl-project.component.scss']
})
export class ViewTlProjectComponent implements OnInit {
projectList: any;
mail: string;
taskList: any;
project : any ;
displayedColumns: string[] = ['id', 'name', 'description','edit' , 'delete'];
constructor(
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
private dialog : MatDialog,
) { }
ngOnInit() {
this.getData();
}
getData() {
this.mail = localStorage.getItem('mail');
this.http.get('getProject?mail=' + this.mail).subscribe(
(response: any) => {
console.log(" hellow ", response);
this.projectList = response;
}
)
}
getProjectTask(items){
this.project = items;
this.http.get('getProjectTask?pid='+items.pid).subscribe(
(response : any) =>{
this.taskList = response;
}
)
}
editTask(element){
this.dialog.open(DialogEditTaskComponent ,{
data : {element}
});
}
deleteTask(element){
this.http.delete('removeTaskfromProj?pid='+this.project.pid+'&tid='+element.tid).subscribe(
(response : any) =>{
this.snackBar.open(response.statusMessage , 'close' ,{duration : 3000});
}
)
}
}
<file_sep>import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { ViewMemberDetailComponent } from './view-member-detail.component';
describe('ViewMemberDetailComponent', () => {
let component: ViewMemberDetailComponent;
let fixture: ComponentFixture<ViewMemberDetailComponent>;
beforeEach(async(() => {
TestBed.configureTestingModule({
declarations: [ ViewMemberDetailComponent ]
})
.compileComponents();
}));
beforeEach(() => {
fixture = TestBed.createComponent(ViewMemberDetailComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
});
<file_sep>import { Component, OnInit } from '@angular/core';
import { Router } from "@angular/router";
@Component({
selector: 'app-dd-dashboard',
templateUrl: './dd-dashboard.component.html',
styleUrls: ['./dd-dashboard.component.scss']
})
export class DdDashboardComponent implements OnInit {
constructor(
private router : Router,
) { }
ngOnInit() {
}
logout(){
localStorage.clear();
this.router.navigate(['/']);
}
reset(){
this.router.navigate(['/reset'])
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material";
import { DataServiceService } from "../../service/data-service.service";
@Component({
selector: 'app-view-member-detail',
templateUrl: './view-member-detail.component.html',
styleUrls: ['./view-member-detail.component.scss']
})
export class ViewMemberDetailComponent implements OnInit {
userInfo: any;
mail: string;
userData: any;
data: any;
taskList: any;
projectList: any;
project: any;
message:string;
displayedColumns: string[] = ['id', 'name', 'mail', 'mobileNo', 'designation', 'asign task'];
constructor(
private http: HttpServerService,
private snackBar: MatSnackBar,
private service :DataServiceService,
) { }
ngOnInit() {
this.getData();
this.service.currentMessage.subscribe(message => this.message = message);
}
getUsers(items) {
this.project = items;
this.mail = localStorage.getItem('mail');
this.http.get('getOneProjects?pid='+items.pid).subscribe(
(response: any) => {
this.userInfo = response.userModel;
console.log(" usdser ", this.userInfo);
// for (let index = 0; index < this.userInfo.length; index++) {
// const element = this.userInfo[index];
// this.userData = element.userModel
// }
}
)
}
// getTask(element) {
// this.data = element;
// this.http.get('getalltasks').subscribe(
// (response: any) => {
// this.taskList = response;
// }
// )
// }
onClick(items) {
this.http.put('asigntaskToUser?eid=' + this.data.id + '&tid=' + items.tid, null).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
} else {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
}
}
)
}
getData() {
this.mail = localStorage.getItem('mail');
this.http.get('getProject?mail=' + this.mail).subscribe(
(response: any) => {
console.log(" hellow ", response);
this.projectList = response;
}
)
}
getProjectTask(element) {
this.data = element;
this.http.get('getProjectTask?pid=' + this.project.pid).subscribe(
(response: any) => {
this.taskList = response;
}
)
}
onEvent(event) {
event.stopPropagation();
}
}
<file_sep>import { ResetPasswordModel } from './reset-password-model';
describe('ResetPasswordModel', () => {
it('should create an instance', () => {
expect(new ResetPasswordModel()).toBeTruthy();
});
});
<file_sep>import { Component, OnInit } from '@angular/core';
import { FormGroup, FormBuilder, Validators, FormControl } from '@angular/forms';
import { LoginUser } from "../../model/login-user";
import { Router } from "@angular/router";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-login',
templateUrl: './login.component.html',
styleUrls: ['./login.component.scss']
})
export class LoginComponent implements OnInit {
user: LoginUser = new LoginUser();
loginForm: FormGroup;
token: string;
constructor(
private formBuilder: FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.loginForm = this.formBuilder.group(
{
'mail': new FormControl(this.user.mail, [Validators.required]),
'password': new FormControl(this.user.password, [Validators.required, Validators.minLength(6)]),
}
)
}
navigate() {
console.log(this.user.mail);
console.log(this.user.password);
console.log(this.user)
this.token = localStorage.getItem('token');
this.http.post('login', this.user).subscribe(
(response: any) => {
if (response.statusCode == 201 || response.statusCode == 204) {
localStorage.setItem('mail', this.user.mail);
localStorage.setItem('password', <PASSWORD>);
localStorage.setItem('token', response.token);
localStorage.setItem('code', response.statusCode);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/dddashboard']);
} else if (response.statusCode == 202) {
localStorage.setItem('mail', this.user.mail);
localStorage.setItem('password', <PASSWORD>);
localStorage.setItem('token', response.token);
localStorage.setItem('code', response.statusCode);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/tldashboard']);
} else if (response.statusCode == 203) {
localStorage.setItem('mail', this.user.mail);
localStorage.setItem('password', <PASSWORD>);
localStorage.setItem('token', response.token);
localStorage.setItem('code', response.statusCode);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.router.navigate(['/pmdashboard']);
} else {
console.log(response);
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
}
}
)
}
}
<file_sep>import { AddMember } from './add-member';
describe('AddMember', () => {
it('should create an instance', () => {
expect(new AddMember()).toBeTruthy();
});
});
<file_sep>import { Component, OnInit } from '@angular/core';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { DataServiceService } from "../../service/data-service.service";
@Component({
selector: 'app-view-dd-task',
templateUrl: './view-dd-task.component.html',
styleUrls: ['./view-dd-task.component.scss']
})
export class ViewDdTaskComponent implements OnInit {
taskList: any;
displayedColumns: string[] = ['id', 'name', 'status', 'Description', 'change status'];
mail: string;
message: string;
constructor(
private http: HttpServerService,
private snackBar: MatSnackBar,
private data: DataServiceService,
) { }
ngOnInit() {
this.data.currentMessage.subscribe(
message => {
this.message = message;
this.getTask();
})
}
getTask() {
this.mail = localStorage.getItem('mail');
this.http.get('getUserTask?mail=' + this.mail).subscribe(
(response: any) => {
this.taskList = response;
}
)
}
onClick(element) {
this.http.put('changeStatus?tid=' + element.tid, null).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.data.changeMessage(response.statusMessage);
} else {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.data.changeMessage(response.statusMessage);
}
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MatDialog } from "@angular/material/dialog";
import { DialogEditTaskComponent } from '../dialog-edit-task/dialog-edit-task.component';
@Component({
selector: 'app-view-projects',
templateUrl: './view-projects.component.html',
styleUrls: ['./view-projects.component.scss']
})
export class ViewProjectsComponent implements OnInit {
projectList : any;
taskList : any ;
displayedColumns: string[] = ['id', 'name', 'description','edit' , 'delete'];
project : any ;
constructor(
private http: HttpServerService,
private snackBar: MatSnackBar,
private dialog : MatDialog,
) { }
ngOnInit() {
this.getProjects();
}
getProjects(){
this.http.get('getAllProjects').subscribe(
(response : any) =>{
this.projectList = response;
}
)
}
editTask(element){
this.dialog.open(DialogEditTaskComponent ,{
data : {element}
});
}
deleteTask(element){
this.http.delete('removeTaskfromProj?pid='+this.project.pid+'&tid='+element.tid).subscribe(
(response : any) =>{
this.snackBar.open(response.statusMessage , 'close' ,{duration : 3000});
}
)
}
getProjectTask(items){
this.project = items ;
this.http.get('getProjectTask?pid='+items.pid).subscribe(
(response : any) =>{
this.taskList = response;
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MatDialog } from "@angular/material/dialog";
import { DialogEditTaskComponent } from '../dialog-edit-task/dialog-edit-task.component';
import { DataServiceService } from "src/app/service/data-service.service";
@Component({
selector: 'app-view-task',
templateUrl: './view-task.component.html',
styleUrls: ['./view-task.component.scss']
})
export class ViewTaskComponent implements OnInit {
taskList: any;
displayedColumns: string[] = ['id', 'name', 'Description', 'asignTaskToProj', 'edit', 'delete'];
projects: any;
data: any;
message : any;
constructor(
private http: HttpServerService,
private snackBar: MatSnackBar,
private dialog: MatDialog,
private service : DataServiceService,
) { }
ngOnInit() {
this.service.currentMessage.subscribe(
( message : any)=>{
this.message = message;
this.getTask();
}
)
}
getTask() {
this.http.get('getalltasks').subscribe(
(response: any) => {
this.taskList = response;
}
)
}
editTask(element) {
this.dialog.open(DialogEditTaskComponent, {
data: { element }
});
}
deleteTask(element) {
console.log(element);
this.http.delete('deletetask/' + element.tid).subscribe(
(response: any) => {
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}
)
}
asignTask(element) {
this.data = element;
this.http.get('getAllProjects').subscribe(
(response: any) => {
this.projects = response;
}
)
}
onClick(items) {
this.http.put('asigntask?pid=' +items.pid + '&tid=' + this.data.tid,null).subscribe(
(response : any)=>{
if(response.statusCode == 200){
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}else{
this.snackBar.open(response.statusMessage, 'close', { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}
}
)
}
}
<file_sep>import { Component, OnInit ,Inject} from '@angular/core';
import { FormGroup, FormBuilder, FormControl, Validators } from "@angular/forms";
import { Router } from "@angular/router";
import { Project } from "../../model/project";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MAT_DIALOG_DATA} from '@angular/material/dialog';
@Component({
selector: 'app-dialog-edit-projects',
templateUrl: './dialog-edit-projects.component.html',
styleUrls: ['./dialog-edit-projects.component.scss']
})
export class DialogEditProjectsComponent implements OnInit {
project : Project = new Project();
addForm : FormGroup;
constructor(
@Inject(MAT_DIALOG_DATA) public data : any,
private formBuilder: FormBuilder,
private router: Router,
private http: HttpServerService,
private snackBar: MatSnackBar,
) { }
ngOnInit() {
this.addForm = this.formBuilder.group(
{
'name': new FormControl(this.project.name, [Validators.required]),
'description': new FormControl(this.project.description, [Validators.required]),
}
)
}
updateProject(){
this.http.put('updateProj/'+this.data.items.pid,this.project).subscribe(
(response : any) =>{
if(response.statusCode == 200){
this.snackBar.open(response.statusMessage , 'close' ,{duration :3000});
}
}
)
}
}
<file_sep>import { BrowserModule } from '@angular/platform-browser';
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { LoginComponent } from './component/login/login.component';
import { FlexLayoutModule } from "@angular/flex-layout";
import { MatCardModule } from '@angular/material/card';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { ReactiveFormsModule } from '@angular/forms';
import { MatFormFieldModule } from '@angular/material/form-field';
import {
MatIconModule, MatButtonModule, MatInputModule, MatToolbarModule,
MatSidenavModule, MatListModule, MatDividerModule, MatExpansionModule,
MatTableModule
} from '@angular/material';
import { DashboardComponent } from './component/dashboard/dashboard.component';
import { AddMemberComponent } from './component/add-member/add-member.component';
import { ViewListComponent } from './component/view-list/view-list.component';
import { BackgroundComponent } from './component/background/background.component';
import { TLDashboardComponent } from './component/tldashboard/tldashboard.component';
import { DdDashboardComponent } from './component/dd-dashboard/dd-dashboard.component';
import { HttpClientModule } from '@angular/common/http';
import { MatSnackBarModule } from '@angular/material/snack-bar';
import { ResetPasswordComponent } from './component/reset-password/reset-password.component';
import { CreateProjectComponent } from './component/create-project/create-project.component';
import { ViewProjectsComponent } from './component/view-projects/view-projects.component';
import { ViewTaskComponent } from './component/view-task/view-task.component';
import { MatDialogModule } from '@angular/material/dialog';
import { DialodBoxComponent } from './component/dialod-box/dialod-box.component';
import { DialogEditProjectsComponent } from './component/dialog-edit-projects/dialog-edit-projects.component';
import { DialogEditTaskComponent } from './component/dialog-edit-task/dialog-edit-task.component';
import { CreateTaskComponent } from './component/create-task/create-task.component';
import { ViewMemberDetailComponent } from './component/view-member-detail/view-member-detail.component';
import { ViewTlProjectComponent } from './component/view-tl-project/view-tl-project.component';
import { AddTaskToProjectComponent } from './component/add-task-to-project/add-task-to-project.component';
import {MatMenuModule} from '@angular/material/menu';
import { ViewDdTaskComponent } from "./component/view-dd-task/view-dd-task.component";
@NgModule({
declarations: [
AppComponent,
LoginComponent,
DashboardComponent,
AddMemberComponent,
ViewListComponent,
BackgroundComponent,
TLDashboardComponent,
DdDashboardComponent,
ResetPasswordComponent,
CreateProjectComponent,
ViewProjectsComponent,
ViewTaskComponent,
DialodBoxComponent,
DialogEditProjectsComponent,
DialogEditTaskComponent,
CreateTaskComponent,
ViewMemberDetailComponent,
ViewTlProjectComponent,
AddTaskToProjectComponent,
ViewDdTaskComponent,
],
entryComponents: [DialodBoxComponent,
DialogEditProjectsComponent,
DialogEditTaskComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
HttpClientModule,
FlexLayoutModule,
MatCardModule,
BrowserAnimationsModule,
ReactiveFormsModule,
MatFormFieldModule,
MatIconModule,
MatToolbarModule,
MatButtonModule,
MatInputModule,
MatSidenavModule,
MatListModule,
MatDividerModule,
MatSnackBarModule,
MatExpansionModule,
MatDialogModule,
MatTableModule,
MatMenuModule,
],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class AppModule { }
<file_sep>import { Component, OnInit ,Inject } from '@angular/core';
import { FormGroup, FormBuilder, FormControl, Validators } from "@angular/forms";
import { AddMember } from "../../model/add-member";
import { HttpServerService } from "../../service/http-server.service";
import { MatSnackBar } from "@angular/material/snack-bar";
import { MAT_DIALOG_DATA} from '@angular/material/dialog';
import { DataServiceService } from "src/app/service/data-service.service";
@Component({
selector: 'app-dialod-box',
templateUrl: './dialod-box.component.html',
styleUrls: ['./dialod-box.component.scss']
})
export class DialodBoxComponent implements OnInit {
member: AddMember = new AddMember();
addForm: FormGroup;
message : any;
constructor(
@Inject(MAT_DIALOG_DATA) public data : any,
private formBuilder: FormBuilder,
private http: HttpServerService,
private snackBar: MatSnackBar,
private service : DataServiceService,
) { }
ngOnInit() {
this.service.currentMessage.subscribe(
( message : any)=>{
this.message = message;
this.addForm = this.formBuilder.group(
{
'name': new FormControl(this.member.name, [Validators.required]),
'desg': new FormControl(this.member.desg, [Validators.required]),
'mobile': new FormControl(this.member.mobileNo, [Validators.required]),
}
)
}
)
}
update() {
console.log(this.member);
this.http.put('updateUser/'+this.data.items.id, this.member).subscribe(
(response: any) => {
if (response.statusCode == 200) {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
} else {
this.snackBar.open(response.statusMessage, "close", { duration: 3000 });
this.service.changeMessage(response.statusMessage);
}
}
)
}
}
| 9b61b561a2376099ac849af1a1f19de0f66451b1 | [
"TypeScript"
] | 26 | TypeScript | Lalit340/FrontEndAngular | f482aa1315f4d3a618ebf093fe23f670f4b9b807 | 8ee6ba62ce536c9b5749ebf8427b94345673b1d4 | |
refs/heads/master | <file_sep># Estudos-JavaScript
JavaScript | HTML5 | Digital Innovation One
<file_sep>function botao() {
var nome
var validar
nome=prompt("Qual seu nome?");
if(nome=="Gabriel"){
validar= true
//alert("Parabéns "+nome+", você ganhou 1 milhão de reais");
document.getElementById("premio").innerHTML = "Parabéns "+ nome +", você ganhou a oportunidade de conhecer uma plataforma de ensino de tecnologia a distância, clique aqui para conhecer"
console.log(document.getElementById("premio"))
}else {
validar=false
//alert("Seu nome não está no banco de dados");
document.getElementById("premio").innerHTML = "Desculpe "+ nome +", mas seu nome não está no banco de dados"
console.log(document.getElementById("premio"))
}
}
botao(validar)
alert(nome)
function redirecionar() {
//window.open("https://digitalinnovation.one/");
window.location.href = "https://digitalinnovation.one/";
}
function trocar(elemento){
elemento.innerHTML="Parabéns"
//document.getElementById("mouse").innerHTML = "Parabéns";
//alert ("trocar texto");
}
function voltar (elemento) {
elemento.innerHTML= "Passe o mouse aqui";
//document.getElementById("mouse").innerHTML = "Passe o mouse aqui";
}
function load() {
alert("Carregamento completo")
}
function Change (elemento) {
var questão
if (elemento.value==1) {
questão= true
console.log("Correto")
} else {
questão= false
console.log("errado")
}
}
Change(questão)
alert(value)
/*
function soma (n1,n2){
return (n1+n2);
}
*/
/*
var validacao
function validaNome (nome){
validacao;
alert(nome);
if(nome=="Gabriel"){
validacao=true;
alert("Você ganhou um prêmio");
}else{
validacao=false;
alert("Você não está autorizado");
}
return validacao
}
var nome = prompt ("Quem é você?");
validaNome(nome)
alert(validacao)
*/
/*
var d= new Date ();
alert(d.getMinutes());
alert(d.getHours());
alert(d.getDate());
alert(d.getMonth()+1);
alert(d)
*/
/*
var count;
for(count=0; count<=3; count++){
alert (count);
};
*/
/*
var count= 0
while (count<=10) {
alert (count);
count++
}
*/
//var idade = prompt ("Qual sua idade?")
//if (idade>=18) {
//if (idade>18) {
//alert("maior de idade")
//}
//if (idade=18) {
//alert ("Você possui 18 anos")
//}
//} else {
//alert ("menor de idade")
//}
//var legumes = [{nome:"cenoura", cor:"laranja",}, {nome:"batata", cor:"amarela",}]
//console.log(legumes[0])
//alert(legumes[0])
//var lista = ["cenoura", "beterraba", "batata"]
//lista.push("mandioca");
//lista.pop();
//console.log(lista[0]);
//console.log(lista.toString()[0]);
//console.log(lista.join(" | "))
//var nome = "<NAME>";
//var n1 = 16
//var n2 = 2
//var frase = "Bem vindo ao site da SMG Soluções"
//alert (nome + " tem " + idade + " anos.");
//alert (idade+idade2)
//console.log (nome);
//console.log (n1*n2)
//console.log (frase.toLowerCase()) | 4459ff9e392ff7ab56d841d7ec4b6f32d0055102 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | Gabriel-Avelino/Estudos-JavaScript | b92bcd5ba987ddf85659abfea435647babae6c2e | 2fdc061a41f5b00901cfa74c27d0721142c951f2 | |
refs/heads/master | <file_sep># Software-Defined-Networking
1) stateful_firewall.py is the main firewall program that blacklists the IP's based on counter and time thresholds. For more information, refer to the presentation attached.
2) stateless_firewall.py module can be used along with the main firewall module to block the IP's that you want to block even before the (If the malicious IP list is known beforehand)
3) learning_l2.py is the learning module is responsible for learning(discovering) the entire network
Note: stateful_firewall.py module runs along with any other compatible modules.
For more information on usage, please refer to the attached presentation.
Execution of the module:
Run the stateful_firewall.py module along with other modules. You can use the learning_l2.py for learning the network(or any other module which is available in market) and stateless_firewall.py module(to block malicious IP's that are known beforehand)
Eg: sudo ~/pox/pox.py learning_l2 stateful_firewall stateless_firewall
<file_sep>#POX and general imports
from pox.core import core
import pox.openflow.libopenflow_01 as of
from pox.lib.util import dpid_to_str
from pox.lib.util import str_to_bool
from pox.lib.packet.ethernet import ethernet, ETHER_BROADCAST
from pox.lib.packet.ethernet import ethernet, ETHER_BROADCAST
from pox.lib.packet.ipv4 import ipv4
from pox.lib.packet.arp import arp
import time
"""
Firewall module that detects and prevents intrusion
***It can be used along with other modules***
Requirements: Environment should contain fake IP's(IP's that are not used) to detect suspicious IP's
When a IP scanner tries to scan fake IP in our network, we record the IP address as suspicious
and keep track of those IP's. If a suspicious IP tries to flood the network above threshold limit in specified
amount of time, then it is blacklisted(Firewall rule is added to the switch)
Algorithm in brief:
For each packet do the following:
1)look whether it is ARP request or other IPV4 packet
2)If dst ip is one of the fake ip's, then record the source address
a)if src address is not in suspicious list, make a record and start a timer
b)if src address is in suspicious list, increse the counter
c)If the counter of a suspicious list exceeds the threshold, blacklist the IP by installing a flow.
d)Check the entire suspicious list to delete the one's for which timer is exceeded.
e)Also delete the flow which is injected by other modules for suspicious ip
"""
#log purpose
log = core.getLogger()
# the switch on which this dynamic firewall should be applied
FIREWALL_SWITCH = 1
THRESHOLD = 10
# the IP's that are being used by the switch
used_IP = ["10.0.0.1", "10.0.0.2", "10.0.0.3", "10.0.0.4"]
fake_IP = ["10.0.0.5", "10.0.0.6","10.0.0.7", "10.0.0.8","10.0.0.9","10.0.0.10","10.0.0.11","10.0.0.12",\
"10.0.0.13","10.0.0.14","10.0.0.15","10.0.0.16","10.0.0.17","10.0.0.18","10.0.0.19","10.0.0.20"]
#suspicious IP's that are flodding the network
suspicious_IP_list = {}
suspicious_IP_time = {}
#add a rule to block a suspicious ip(doesn't deal with ARP flows)
def AddRule(event,packet):
#installing flow to block IP that is reponsible for flooding
log.info("Adding rules to blacklist %s",packet.next.srcip)
msg = of.ofp_flow_mod()
msg.idle_timeout = 300
msg.priority = 30
msg.match.dl_type = 0x0800
msg.match.nw_src = packet.next.srcip
msg.buffer_id = None
event.connection.send(msg)
#by default if no action is specified, then the packet is rejected
#add a rule to block a suspicious ip(deals with ARP requests)
def AddRule_ARP(event,packet):
#installing flow to block IP that is reponsible for flooding
log.info("Adding rules to blacklist %s",packet.next.protosrc)
msg = of.ofp_flow_mod()
msg.idle_timeout = 300
msg.priority = 30
msg.match.dl_type = 0x0806
msg.match.nw_src = packet.next.protosrc
msg.buffer_id = None
event.connection.send(msg)
#by default if no action is specified, then the packet ss rejected
#Timeout check of suspicious IPs
def check_timeout(suspicious_IP_list,suspicious_IP_time):
#check the timer corresponding to each suspicious IP. If timer exceeds threshold, delete it.
for key in suspicious_IP_time:
if time.time()-suspicious_IP_time[key] > 1000: #threshold time of 1000 sec..
#deleting src from suspicious list as the time exceeded the limit
del suspicious_IP_list[key]
del suspicious_IP_time[key]
#delete a flow
def Delrule(packet,event):
#deleting the flow installed for fake IP's by other modules.
#log.info("Deleting a flow corresponding to a fake IP")
msg = of.ofp_flow_mod()
msg.match = of.ofp_match.from_packet(packet, event.port)
msg.idle_timeout = 10
msg.hard_timeout = 30
msg.command = of.OFPFC_DELETE
event.connection.send(msg)
def _handle_PacketIn (event):
global FIREWALL_SWITCH
global used_IP
global used_PORT
global suspicious_src_list
global THRESHOLD
global suspicious_IP_time
packet = event.parsed
#install firewall rules only on the a particular switch
if event.connection.dpid != FIREWALL_SWITCH:
return
#check whether ARP
if isinstance(packet.next, arp):
#ignore if the packet is originated from our own source ip's(internal)
if packet.next.protosrc in used_IP or packet.next.protosrc in fake_IP:
return
if not packet.next.protodst in used_IP:
if not packet.next.protosrc in suspicious_IP_list:
suspicious_IP_list[packet.next.protosrc] = 1
log.info("A suspicious source %s is trying to attack. Monitoring it's activity", packet.next.protosrc)
suspicious_IP_time[packet.next.protosrc] = time.time()
else:
suspicious_IP_list[packet.next.protosrc] = suspicious_IP_list[packet.next.protosrc]+1
if suspicious_IP_list[packet.next.protosrc] > THRESHOLD:
AddRule_ARP(event,packet)
del suspicious_IP_list[packet.next.protosrc]
del suspicious_IP_time[packet.next.protosrc]
check_timeout(suspicious_IP_list,suspicious_IP_time)
Delrule(packet,event)
#checking whether l3
if isinstance(packet.next, ipv4):
#ignore if the packet is originated from our own source ip's(internal)
if packet.next.srcip in used_IP or packet.next.srcip in fake_IP:
return
#log.info("IP Packet %s ===> %s", packet.next.srcip,packet.next.dstip)
if not packet.next.dstip in used_IP:
if not packet.next.srcip in suspicious_IP_list:
suspicious_IP_list[packet.next.srcip] = 1
log.info("A suspicious source %s is trying to attack. Monitoring it's activity", packet.next.srcip)
suspicious_IP_time[packet.next.srcip] = time.time()
else:
suspicious_IP_list[packet.next.srcip] = suspicious_IP_list[packet.next.srcip]+1
#log.info("Increased the counter for %s",packet.next.srcip)
if suspicious_IP_list[packet.next.srcip] > THRESHOLD :
AddRule(event,packet)
#deleting IP from suspicious list
del suspicious_IP_list[packet.next.srcip]
del suspicious_IP_time[packet.next.srcip]
#also check if the timeout has exceeded for a particular ip. If so delete it from suspicious list
check_timeout(suspicious_IP_list,suspicious_IP_time)
#l2_learning must have installed a flow for forwarding to fake IP. Delete those flows
Delrule(packet,event)
#main function that starts the module
def launch ():
core.openflow.addListenerByName("PacketIn", _handle_PacketIn)
| 2a42cee79a7791264896f1fc1f9b998f0a9b9eba | [
"Markdown",
"Python"
] | 2 | Markdown | sandprddy/Software-Defined-Networking | 05b36c3922f4e62014fdb69b521ab108906c27ed | f42c0fb239350136501d70dc1142c4a16db6e3d4 | |
refs/heads/master | <repo_name>fr830/opcua-cmake<file_sep>/server/CMakeLists.txt
include_directories(${MAPPINGSDIR}/include )
include_directories(${COREDIR}/include )
include_directories(${CLIENTDIR}/include )
include_directories(${SERVERDIR})
include_directories(${SERVERDIR}/binary_protocol/include )
include_directories(${SERVERDIR}/endpoint_services/include )
include_directories(${SERVERDIR}/services_registry/include )
include_directories(${SERVERDIR}/address_space/include )
include_directories(${SERVERDIR}/tcp_server/include )
include_directories(${SERVERDIR}/builtin_server/include )
include_directories(${SERVERDIR}/xml_address_space_loader/include )
include_directories(${SERVERDIR}/standard_namespace/include )
include_directories(${SERVERDIR}/server/include)
get_property(inc_dirs DIRECTORY PROPERTY INCLUDE_DIRECTORIES)
set(TMPSRC ${SERVERDIR}/services_registry/src)
add_library(services_registry_addon SHARED
${TMPSRC}/services_registry_impl.cpp
${TMPSRC}/services_registry_factory.cpp
${TMPSRC}/services_registry_module.cpp
)
target_link_libraries(services_registry_addon opcuabinary opcuacommon)
set(TMPSRC ${SERVERDIR}/endpoint_services/src)
add_library(endpoints_services_addon SHARED
${TMPSRC}/endpoints_parameters.cpp
${TMPSRC}/endpoints_services_addon.cpp ${TMPSRC}/endpoints_services_module.cpp
)
target_link_libraries(endpoints_services_addon opcuabinary)
set(TMPSRC ${SERVERDIR}/binary_protocol/src)
add_library(opcua_protocol SHARED
${TMPSRC}/opc_tcp_processor.cpp
${TMPSRC}/opcua_protocol_addon.cpp
${TMPSRC}/opcua_protocol_module.cpp
)
target_link_libraries(opcua_protocol opcuacommon endpoints_services_addon xml2)
set(TMPSRC ${SERVERDIR}/standard_namespace/src)
add_library(standard_ns_addon SHARED
${TMPSRC}/standard_namespace_addon.cpp
${TMPSRC}/standard_namespace.cpp ${TMPSRC}/standard_namespace_module.cpp
)
target_link_libraries(standard_ns_addon opcuabinary)
set(TMPSRC ${SERVERDIR}/address_space/src)
add_library(address_space_addon SHARED
${TMPSRC}/address_space_addon.cpp ${TMPSRC}/address_space_internal.cpp ${TMPSRC}/address_space_module.cpp
)
target_link_libraries(address_space_addon opcuabinary opcuacommon ${Boost_LIBRARIES})
set(TMPSRC ${SERVERDIR}/xml_address_space_loader/src)
add_library(xml_address_space_loader_addon SHARED
${TMPSRC}/xml_address_space_addon.cpp ${TMPSRC}/xml_address_space_loader.cpp ${TMPSRC}/xml_address_space_module.cpp
)
target_link_libraries(xml_address_space_loader_addon opcuacommon opcuabinary xml2)
set(TMPSRC ${SERVERDIR}/tcp_server/src)
add_library(opcua_tcp_server SHARED
${TMPSRC}/tcp_server_addon_impl.cpp
${TMPSRC}/tcp_server_factory.cpp
${TMPSRC}/tcp_server.cpp
${TMPSRC}/tcp_server_module.cpp
)
target_link_libraries(opcua_tcp_server opcuacommon)
set(TMPSRC ${SERVERDIR}/server/src)
add_library(opcuaserver SHARED
${TMPSRC}/opcuaserver.cpp
)
target_link_libraries(opcuaserver opcuabinary opcuacommon standard_ns_addon opcua_protocol address_space_addon services_registry_addon opcua_tcp_server endpoints_services_addon)
#command line server
set(TMPSRC ${SERVERDIR}/exe/src)
add_executable(server
${TMPSRC}/daemon.cpp ${TMPSRC}/server_main.cpp ${TMPSRC}/server_options.cpp
)
target_link_libraries(server opcuabinary opcuacommon ${Boost_LIBRARIES} )
#example server
add_executable(example
${TMPSRC}/daemon.cpp ${TMPSRC}/example.cpp
)
target_link_libraries(example opcuaserver opcuabinary opcuacommon ${Boost_LIBRARIES} )
<file_sep>/mappings/CMakeLists.txt
include_directories(${MAPPINGSDIR}/include)
file(GLOB_RECURSE HEADERS ${MAPPINGSDIR}/include/*.h )
#the OPCUA lib
add_library(opcuabinary SHARED
${MAPPINGSDIR}/src/binary_attribute.cpp
${MAPPINGSDIR}/src/binary_node_management.cpp
${MAPPINGSDIR}/src/binary_endpoints.cpp
${MAPPINGSDIR}/src/binary_raw_size.cpp
${MAPPINGSDIR}/src/binary_session.cpp
${MAPPINGSDIR}/src/binary_variant.cpp
${MAPPINGSDIR}/src/monitored_items.cpp
${MAPPINGSDIR}/src/subscriptions.cpp
${MAPPINGSDIR}/src/binary_data_value.cpp
${MAPPINGSDIR}/src/binary_messages.cpp
${MAPPINGSDIR}/src/binary_stream.cpp
${MAPPINGSDIR}/src/binary_view.cpp
${MAPPINGSDIR}/src/nodeid.cpp
${MAPPINGSDIR}/src/types.cpp
${MAPPINGSDIR}/src/input_from_buffer.cpp
${MAPPINGSDIR}/src/string_utils.cpp
${HEADERS}
)
if(BUILD_TESTS)
set(MAPPINGSTESTDIR ${MAPPINGSDIR}/tests)
include_directories(${MAPPINGSTESTDIR})
add_executable(opcuabinary_tests EXCLUDE_FROM_ALL
${MAPPINGSTESTDIR}/binary_deserialize.cpp
${MAPPINGSTESTDIR}/binary_serialize_variant.cpp
${MAPPINGSTESTDIR}/binary_serialize.cpp
${MAPPINGSTESTDIR}/binary_serialize_attribute.cpp
${MAPPINGSTESTDIR}/binary_serialize_data_value.cpp
${MAPPINGSTESTDIR}/binary_serialize_endpoints.cpp
${MAPPINGSTESTDIR}/binary_serialize_monitored_items.cpp
${MAPPINGSTESTDIR}/binary_serialize_session.cpp
${MAPPINGSTESTDIR}/binary_serialize_view.cpp
${MAPPINGSTESTDIR}/binary_subscriptions.cpp
${MAPPINGSTESTDIR}/binary_node_management.cpp
${MAPPINGSTESTDIR}/message_id.cpp
${MAPPINGSTESTDIR}/node_id.cpp
${MAPPINGSTESTDIR}/reference_id.cpp
${MAPPINGSTESTDIR}/test_input_from_buffer.cpp
${MAPPINGSTESTDIR}/utils.cpp
${MAPPINGSTESTDIR}/common.h
)
target_link_libraries(opcuabinary_tests ${GTEST_BOTH_LIBRARIES} opcuabinary pthread)
endif(BUILD_TESTS)
<file_sep>/start_server.sh
set +x
build/server/server --config server/config/ --log-file=server.log
<file_sep>/CMakeLists.txt
cmake_minimum_required (VERSION 2.6)
project (FreeOpcUa)
option(CLANG "Build using Clang++" ON)
option(BUILD_PYTHON "Build Python bindings" ON)
option(BUILD_MAPPINGS "Build Mappings" ON)
option(BUILD_CORE "Build Core" ON)
option(BUILD_CLIENT "Build Client" ON)
option(BUILD_SERVER "Build Server" ON)
option(BUILD_TESTS "Build Tests Target" ON)
set(MAPPINGSDIR ${CMAKE_SOURCE_DIR}/reps/opcua-mappings)
set(COREDIR ${CMAKE_SOURCE_DIR}/reps/opcua-core)
set(CLIENTDIR ${CMAKE_SOURCE_DIR}/reps/opcua-client)
set(SERVERDIR ${CMAKE_SOURCE_DIR}/reps/opcua-server)
set(PYTHONDIR ${CMAKE_SOURCE_DIR}/reps/opcua-python)
if(NOT WIN32)
set(CMAKE_CXX_FLAGS "-std=c++0x -ggdb -Wall ${CMAKE_CXX_FLAGS_DEBUG} " )
if(CLANG)
set(CMAKE_CXX_COMPILER "clang++")
set(CMAKE_SHARED_LINKER_FLAGS "--no-undefined" )
endif()
endif(NOT WIN32)
find_package( Boost COMPONENTS system program_options filesystem thread REQUIRED )
include_directories( ${Boost_INCLUDE_DIR} )
message(STATUS "Boost INCLUDE DIR IS: " ${Boost_INCLUDE_DIR})
find_package(LibXml2 REQUIRED)
message(STATUS "LibXML2 INCLUDE DIR IS: " ${LIBXML2_INCLUDE_DIR})
include_directories( ${LIBXML2_INCLUDE_DIR} )
if(BUILD_TESTS)
find_package(GTest)
if(GTEST_FOUND)
MESSAGE(STATUS "GTest found, building tests")
enable_testing()
include_directories(${GTEST_INCLUDE_DIRS})
else()
MESSAGE(STATUS "GTest NOT found, Not building tests")
set(BUILD_TESTS OFF)
endif()
endif()
if(BUILD_MAPPINGS)
message(STATUS "Building Mappings")
add_subdirectory (mappings)
if(BUILD_TESTS)
add_test("OPC-UAMappings" ${PROJECT_BINARY_DIR}/mappings/opcuabinary_tests )
set(TEST_MAPPINGS_DEPEND opcuabinary_tests)
endif(BUILD_TESTS)
endif(BUILD_MAPPINGS)
if(BUILD_CORE)
message(STATUS "Building Core")
add_subdirectory (core)
if(BUILD_TESTS)
add_test("OPC-UACore" ${PROJECT_BINARY_DIR}/core/opcuacore_tests )
set(TEST_CORE_DEPEND opcuacore_tests)
endif(BUILD_TESTS)
endif(BUILD_CORE)
if(BUILD_CLIENT)
message(STATUS "Building Client")
add_subdirectory (client)
if(BUILD_TESTS)
endif(BUILD_TESTS)
endif(BUILD_CLIENT)
if(BUILD_SERVER)
message(STATUS "Building Server")
add_subdirectory (server)
if(BUILD_TESTS)
endif(BUILD_TESTS)
endif(BUILD_SERVER)
if(BUILD_PYTHON)
message(STATUS "Building Python")
add_subdirectory (python)
if(BUILD_TESTS)
add_test("PythonTests" python3 ${PYTHONDIR}/tests/test_highlevel.py)
#find_package(PythonInterp REQUIRED ) #cmake only find python2 ?!?!?
#add_test("PythonTests" ${PYTHON_EXECUTABLE} ${PYTHONDIR}/tests/test_highlevel.py)
endif(BUILD_TESTS)
endif(BUILD_PYTHON)
if(BUILD_TESTS)
add_custom_target(check COMMAND ${CMAKE_CTEST_COMMAND} --output-on-failure DEPENDS ${TEST_MAPPINGS_DEPEND} ${OPCUA_CORE_DEPEND})
endif()
<file_sep>/core/CMakeLists.txt
include_directories(${MAPPINGSDIR}/include )
include_directories(${COREDIR}/include )
file(GLOB_RECURSE HEADERS ${COREDIR}/include/*.h )
set(TMPSRC ${COREDIR}/src/)
add_library(opcuacommon SHARED
${TMPSRC}/socket_channel.cpp
${TMPSRC}/common/application.cpp
${TMPSRC}/common/common_errors.cpp
${TMPSRC}/common/exception.cpp
${TMPSRC}/common/object_id.cpp
${TMPSRC}/common/thread.cpp
${TMPSRC}/common/value.cpp
${TMPSRC}/node.cpp
${TMPSRC}/common/addons_core/addon_manager.cpp
${TMPSRC}/common/addons_core/config_file.cpp
${TMPSRC}/common/addons_core/dynamic_addon_factory.cpp
${TMPSRC}/common/addons_core/dynamic_library.cpp
${TMPSRC}/common/addons_core/errors_addon_manager.cpp
${TMPSRC}/opcua_errors.cpp
${HEADERS}
)
target_link_libraries(opcuacommon opcuabinary ${Boost_LIBRARIES} dl xml2)
if(BUILD_TESTS)
set(CORETESTDIR ${COREDIR}/tests)
include_directories(${CORETESTDIR})
add_executable(opcuacore_tests EXCLUDE_FROM_ALL
${CORETESTDIR}/common/error_test.cpp
${CORETESTDIR}/common/main.cpp
${CORETESTDIR}/common/value_test.cpp
)
target_link_libraries(opcuacore_tests ${GTEST_BOTH_LIBRARIES} opcuabinary opcuacommon pthread cppunit)
endif(BUILD_TESTS)
<file_sep>/python/CMakeLists.txt
SET(PYTHONFOUND FALSE)
FIND_PACKAGE( Boost COMPONENTS python-py34 )
IF(Boost_PYTHON-PY34_FOUND)
SET(Python_ADDITIONAL_VERSIONS 3.4) #HACK UNTIL CORRENT CMAKE SUPPORT
FIND_PACKAGE(PythonLibs 3.4)
IF(PYTHONLIBS_FOUND)
MESSAGE(STATUS "Compiling Python module with Python 3.4")
SET(PYTHONFOUND TRUE)
ENDIF()
ENDIF()
IF(NOT PYTHONFOUND)
FIND_PACKAGE( Boost COMPONENTS python )
IF(Boost_PYTHON_FOUND)
FIND_PACKAGE(PythonLibs 2.7)
IF(PYTHONLIBS_FOUND)
MESSAGE("Compiling Python module")
SET(PYTHONFOUND TRUE)
ENDIF()
ENDIF()
ENDIF()
IF(NOT PYTHONFOUND)
MESSAGE(STATUS "Python boost or lib not found: Not building python module")
RETURN()
ENDIF()
INCLUDE_DIRECTORIES( ${BOOST_INCLUDE_DIR} )
INCLUDE_DIRECTORIES( ${PYTHON_INCLUDE_DIR} )
include_directories(${CMAKE_SOURCE_DIR}/mappings/include )
include_directories(${CMAKE_SOURCE_DIR}/core/include )
include_directories(${CMAKE_SOURCE_DIR}/client/include )
include_directories(${CMAKE_SOURCE_DIR}/server )
include_directories(${CMAKE_SOURCE_DIR}/server/binary_protocol/include )
include_directories(${CMAKE_SOURCE_DIR}/server/endpoint_services/include )
include_directories(${CMAKE_SOURCE_DIR}/server/services_registry/include )
include_directories(${CMAKE_SOURCE_DIR}/server/address_space/include )
include_directories(${CMAKE_SOURCE_DIR}/server/tcp_server/include )
include_directories(${CMAKE_SOURCE_DIR}/server/builtin_server/include )
include_directories(${CMAKE_SOURCE_DIR}/server/server/include )
include_directories(${CMAKE_SOURCE_DIR}/server/xml_address_space_loader/include )
add_definitions(-DMODULE_NAME=opcua)
ADD_LIBRARY(opcua SHARED src/module.cpp)
set_target_properties(opcua PROPERTIES PREFIX "")
TARGET_LINK_LIBRARIES(opcua opcuaserver opcuabinary opcuacommon opcua_client ${Boost_LIBRARIES} ${PYTHON_LIBRARIES})
<file_sep>/example_server.sh
set +x
build/server/example_server --config server/config/ --log-file=server.log
<file_sep>/client/CMakeLists.txt
include_directories(${MAPPINGSDIR}/include )
include_directories(${COREDIR}/include )
include_directories(${CLIENTDIR}/include )
#the OPCUA lib
add_library(opc_tcp_client SHARED
${CLIENTDIR}/src/binary_computer_addon.cpp
${CLIENTDIR}/src/binary_connection.cpp
${CLIENTDIR}/src/binary_computer.cpp
${CLIENTDIR}/src/binary_secure_channel.cpp
${CLIENTDIR}/src/computer.cpp
${CLIENTDIR}/src/errors.cpp
)
target_link_libraries(opc_tcp_client opcuacommon opcuabinary)
add_library(opcua_client SHARED ${CLIENTDIR}/src/client.cpp )
target_link_libraries(opcua_client opcuacommon opcuabinary opc_tcp_client)
add_executable(client ${CLIENTDIR}/src/opcua_main.cpp ${CLIENTDIR}/src/opcua_options.cpp)
target_link_libraries(client opcuacommon opcuabinary)
add_executable(example_client ${CLIENTDIR}/src/example_main.cpp )
target_link_libraries(example_client opcua_client )
| db600a75e3815970d005ba2a23e4bd344f618b01 | [
"CMake",
"Shell"
] | 8 | CMake | fr830/opcua-cmake | 89e9545de13bbebc44cb44b2c415ca1ed42d1721 | e545f2b13f79f6c2344d71c87ef4bc3aa8831f6e | |
refs/heads/main | <repo_name>POOPYFARTS-CODE/AutoShoPer<file_sep>/README.md
# AutoShoPer
Auto Shopper Is A sneaker Bot developed by Lonewolf Discord -> 𝓡𝓮𝓰𝓮𝔁#7777
It Have Some Dependency
from selenium import webdriver
from selenium import *
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
This Bot Is Easy To Handle And Manage It Can Have Some Errors And Bugs So Pull requests are welcome....
WEBSITES IT WILL SUPPORT ARE....
1)Nike
2)Adidas
3)Supreme
<file_sep>/[FINAL]supreme.py
from selenium import webdriver
from selenium import *
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
import time
print('''
░█████╗░ ██████╗░░█████╗░████████╗ ████╗██████╗░██╗░░░██╗
██╔══██╗ ██╔══██╗██╔══██╗╚══██╔══╝ ██╔═╝██╔══██╗╚██╗░██╔╝
███████║ ██████╦╝██║░░██║░░░██║░░░ ██║░░██████╦╝░╚████╔╝░
██╔══██║ ██╔══██╗██║░░██║░░░██║░░░ ██║░░██╔══██╗░░╚██╔╝░░
██║░░██║ ██████╦╝╚█████╔╝░░░██║░░░ ████╗██████╦╝░░░██║░░░
╚═╝░░╚═╝ ╚═════╝░░╚════╝░░░░╚═╝░░░ ╚═══╝╚═════╝░░░░╚═╝░░░
██╗░░░░░░█████╗░███╗░░██╗███████╗░██╗░░░░░░░██╗░█████╗░██╗░░░░░███████╗████╗
██║░░░░░██╔══██╗████╗░██║██╔════╝░██║░░██╗░░██║██╔══██╗██║░░░░░██╔════╝╚═██║
██║░░░░░██║░░██║██╔██╗██║█████╗░░░╚██╗████╗██╔╝██║░░██║██║░░░░░█████╗░░░░██║
██║░░░░░██║░░██║██║╚████║██╔══╝░░░░████╔═████║░██║░░██║██║░░░░░██╔══╝░░░░██║
███████╗╚█████╔╝██║░╚███║███████╗░░╚██╔╝░╚██╔╝░╚█████╔╝███████╗██║░░░░░████║
╚══════╝░╚════╝░╚═╝░░╚══╝╚══════╝░░░╚═╝░░░╚═╝░░░╚════╝░╚══════╝╚═╝░░░░░╚═══╝
''')
link = input('Enter Your Link[Format: https://example.com/us/product_name/product_code.html]:')
print('''
█░░ █▀█ ▄▀█ █▀▄ █ █▄░█ █▀▀ ▄▀█ █░█ ▄▀█ █ █░░ ▄▀█ █▄▄ █░░ █▀▀ █▀ █ ▀█ █▀▀ █▀ ░ ░ ░ ░
█▄▄ █▄█ █▀█ █▄▀ █ █░▀█ █▄█ █▀█ ▀▄▀ █▀█ █ █▄▄ █▀█ █▄█ █▄▄ ██▄ ▄█ █ █▄ ██▄ ▄█ ▄ ▄ ▄ ▄''')
def checkStock():
try:
options = webdriver.ChromeOptions()# options for driver
options.add_argument('--ignore-certificate-errors')#argumen added
options.add_argument('--ignore-ssl-errors')#argumen added
driver = webdriver.Chrome("E:/chromedriver_win32 (3)/chromedriver.exe", chrome_options= options )#initiaizing driver
driver.get(link)#userapp
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.CLASS_NAME, 'swatch')))#wait till element specified is located
username = driver.find_element_by_class_name('swatch')#sizes element parent
options = username.find_elements_by_tag_name('label')#element conatins sizes
optionList = []#sizes array
for option in options:
optionList.append(option.get_attribute('innerHTML'))
for sizes in range(len(optionList)-2):
print("Size "+ str(optionList[sizes][:20]) + " for is available.")
finally:
driver.quit()
def selectSize():
input_user = input('Please Select your Size:')
return(input_user)
def final_stage():
size_ = selectSize()
driver = webdriver.Chrome("E:/chromedriver_win32 (3)/chromedriver.exe" )
driver.get(link)
element1 = driver.find_element_by_xpath("//*[text()="+size_+"]")
element1.click()
time.sleep(10)
# element2 = driver.find_element_by_xpath("//SPAN[@class='gl-cta__content'][text()='Checkout']")
# element2.click()
# time.sleep(15)
checkStock()
selectSize()
final_stage()
# * ,MMM8&&&. *
# MMMM88&&&&& .
# MMMM88&&&&&&&
# * MMM88&&&&&&&&
# MMM88&&&&&&&&
# 'MMM88&&&&&&'
# 'MMM8&&&' *
# |\___/|
# ) ( . '
# =\ /=
# )===( *
# / \
# | |
# / \
# \ /
# _/\_/\_/\__ _/_/\_/\_/\_/\_/\_/\_/\_/\_/\_
# | | | |( ( | | | | | | | | | |
# | | | | ) ) | | | | | | | | | |
# | | | |(_( | | | | | | | | | |
# | | | | | | | | | | | | | | |
# | | | | | | | | | | | | | | |<file_sep>/[FINAL]adidas.py
from selenium import webdriver
from selenium import *
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
from selenium.webdriver.common.action_chains import ActionChains
import time
print('''
░█████╗░ ██████╗░░█████╗░████████╗ ████╗██████╗░██╗░░░██╗
██╔══██╗ ██╔══██╗██╔══██╗╚══██╔══╝ ██╔═╝██╔══██╗╚██╗░██╔╝
███████║ ██████╦╝██║░░██║░░░██║░░░ ██║░░██████╦╝░╚████╔╝░
██╔══██║ ██╔══██╗██║░░██║░░░██║░░░ ██║░░██╔══██╗░░╚██╔╝░░
██║░░██║ ██████╦╝╚█████╔╝░░░██║░░░ ████╗██████╦╝░░░██║░░░
╚═╝░░╚═╝ ╚═════╝░░╚════╝░░░░╚═╝░░░ ╚═══╝╚═════╝░░░░╚═╝░░░
██╗░░░░░░█████╗░███╗░░██╗███████╗░██╗░░░░░░░██╗░█████╗░██╗░░░░░███████╗████╗
██║░░░░░██╔══██╗████╗░██║██╔════╝░██║░░██╗░░██║██╔══██╗██║░░░░░██╔════╝╚═██║
██║░░░░░██║░░██║██╔██╗██║█████╗░░░╚██╗████╗██╔╝██║░░██║██║░░░░░█████╗░░░░██║
██║░░░░░██║░░██║██║╚████║██╔══╝░░░░████╔═████║░██║░░██║██║░░░░░██╔══╝░░░░██║
███████╗╚█████╔╝██║░╚███║███████╗░░╚██╔╝░╚██╔╝░╚█████╔╝███████╗██║░░░░░████║
╚══════╝░╚════╝░╚═╝░░╚══╝╚══════╝░░░╚═╝░░░╚═╝░░░╚════╝░╚══════╝╚═╝░░░░░╚═══╝
''')
link = input('Enter Your Link[Format: https://example.com/us/product_name/product_code.html]:')
print('''
█░░ █▀█ ▄▀█ █▀▄ █ █▄░█ █▀▀ ▄▀█ █░█ ▄▀█ █ █░░ ▄▀█ █▄▄ █░░ █▀▀ █▀ █ ▀█ █▀▀ █▀ ░ ░ ░ ░
█▄▄ █▄█ █▀█ █▄▀ █ █░▀█ █▄█ █▀█ ▀▄▀ █▀█ █ █▄▄ █▀█ █▄█ █▄▄ ██▄ ▄█ █ █▄ ██▄ ▄█ ▄ ▄ ▄ ▄''')
def checkStock():
try:
options = webdriver.ChromeOptions()# options for driver
options.add_argument('--ignore-certificate-errors')#argumen added
options.add_argument('--ignore-ssl-errors')#argumen added
driver = webdriver.Chrome("E:/chromedriver_win32 (3)/chromedriver.exe", chrome_options= options )#initiaizing driver
driver.get(link)#userapp
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.CLASS_NAME, 'buy-section___yYtjH')))#wait till element specified is located
username = driver.find_element_by_class_name('buy-section___yYtjH')#sizes element parent
options = username.find_elements_by_tag_name('span')#element conatins sizes
optionList = []#sizes array
for option in options:
optionList.append(option.get_attribute('innerHTML'))
for sizes in range(len(optionList)-2):
print("Size "+ optionList[sizes] + " for is available.")
finally:
driver.quit()
def selectSize():
input_user = int(input('Please Select your Size:'))
base = 530 #this is base
size = (input_user -4)*10
final_size = base + size
# final_link = input_user + final_size
return(final_size)
def linkWithSize():
model_number = input('Enter Model Number[Example https://www.adidas.com/us/ultraboost-20-shoes/--->FZ0174<---.html] :')
size_to_be_selected = selectSize()
str_fina_one = '?forceSelSize='
br_link = str(link)+str(str_fina_one)+str(model_number)+'_'+str(size_to_be_selected)
return(br_link)
def bus():
lol_link = linkWithSize()
return(lol_link)
def final_stage():
link_ = bus()
driver = webdriver.Chrome("E:/chromedriver_win32 (3)/chromedriver.exe" )
driver.get(link_)
element1 = driver.find_element_by_xpath("(//SPAN[@class='gl-cta__content'][text()='Add To Bag'][text()='Add To Bag'])[5]")
element1.click()
time.sleep(10)
element2 = driver.find_element_by_xpath("//SPAN[@class='gl-cta__content'][text()='Checkout']")
element2.click()
time.sleep(15)
checkStock()
selectSize()
linkWithSize()
bus()
final_stage()
# * ,MMM8&&&. *
# MMMM88&&&&& .
# MMMM88&&&&&&&
# * MMM88&&&&&&&&
# MMM88&&&&&&&&
# 'MMM88&&&&&&'
# 'MMM8&&&' *
# |\___/|
# ) ( . '
# =\ /=
# )===( *
# / \
# | |
# / \
# \ /
# _/\_/\_/\__ _/_/\_/\_/\_/\_/\_/\_/\_/\_/\_
# | | | |( ( | | | | | | | | | |
# | | | | ) ) | | | | | | | | | |
# | | | |(_( | | | | | | | | | |
# | | | | | | | | | | | | | | |
# | | | | | | | | | | | | | | | | 01f4218d0bd516a474d1c74a01010ebe19e9d810 | [
"Markdown",
"Python"
] | 3 | Markdown | POOPYFARTS-CODE/AutoShoPer | c441437dae37043733e54cf20c5643c73df156ac | 34c0d3dd57998fdfc5526c07cd15a50920e29ca6 | |
refs/heads/master | <file_sep># Essay_scoring
This repo has the implementation of the Intelligent Text Rater (ITR) with the proposed novel approach offeature vector generation of text essays which significantly reduces the required training data size.
<file_sep>from nltk import word_tokenize, pos_tag
from nltk.corpus import wordnet as wn
from sklearn import linear_model
from sklearn.linear_model import Ridge
from nltk.corpus import stopwords
from sklearn.externals import joblib
import pickle
from sklearn.cluster import KMeans
import numpy as np
import pandas as pd
import re
def penn_to_wn(tag):
if tag.startswith('N'):
return 'n'
if tag.startswith('V'):
return 'v'
if tag.startswith('J'):
return 'a'
if tag.startswith('R'):
return 'r'
return None
def tagged_to_synset(word, tag):
wn_tag = penn_to_wn(tag)
if wn_tag is None:
return None
try:
return wn.synsets(word, wn_tag)[0]
except:
return None
def sentence_similarity(synset,sentence):
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(sentence)
filtered_sentence = [w for w in word_tokens if not w in stop_words]
sentence = pos_tag(filtered_sentence)
synsets = [tagged_to_synset(*tagged_word) for tagged_word in sentence]
synsets = [ss for ss in synsets if ss]
score, count = 0.0, 0
fin = []
for i in synset:
a = [i.path_similarity(ss) for ss in synsets]
a = [ss for ss in a if ss]
if(len(a) == 0):
best_score = 0.0
else:
best_score = max(a)
score += best_score
count += 1
fin.append(best_score)
score /= count
return fin
path ='training_set_rel3.tsv'
record = pd.DataFrame.from_csv(path, sep='\t', header=0)
record = record[['essay_set', 'essay','domain1_score']]
record = record[(record['domain1_score'] == 12)&(record['essay_set'] == 1)]
bEssay = record['essay'].tolist()
mSynset = []
for i in bEssay:
dum = re.sub('\W+',' ',i)
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(dum)
filtered_sentence = [w for w in word_tokens if not w in stop_words]
s = pos_tag(filtered_sentence)
synset = [tagged_to_synset(*tagged_word) for tagged_word in s]
synset = [ss for ss in synset if ss]
for j in synset:
mSynset.append(j)
print ("master synset created")
print (len(mSynset))
path ='training_set_rel3.tsv'
record = pd.DataFrame.from_csv(path, sep='\t', header=0)
record = record[['essay_set', 'essay','domain1_score']]
record = record[record['essay_set'] == 1]
record = record[['essay','domain1_score']]
msk = np.random.rand(len(record)) < 0.7
train = record[msk]
test = record[~msk]
essays_train = train['essay'].tolist()
essays_test = test['essay'].tolist()
scores_train = train['domain1_score'].tolist()
scores_test = test['domain1_score'].tolist()
mat1 = {}
mat = []
for i in range(0,len(essays_train)):
fin = sentence_similarity(mSynset,essays_train[i])
mat1[i] = {v: k for v,k in enumerate(fin)}
mat1[i]['score'] = scores_train[i]
print (scores_train[i])
mat.append(fin)
df = pd.DataFrame(mat1)
df = df.transpose()
#df.drop('Unnamed: 0',inplace=True)
df.to_csv('hclust30Lasso.csv',sep=',')
print ("clust done")
reg = linear_model.Ridge(alpha = .5)
reg.fit(mat, scores_train )
print ("training done")
#s = pickle.dumps(reg)
joblib.dump(reg, 'Regression30Lassoo.pkl')
#reg = joblib.load('Regression10.pkl')
# test_essay = essays_test[0:10]
# for i in range(0,len(test_essay)):
# fin_predict = sentence_similarity(mSynset,test_essay[i])
# predicted = reg.predict([fin_predict])
# print ("predicted score : " + str(predicted[0]))
# print ("actual score : " + scores_test[i])
# print ("")
# mat = np.array(mat)
# kmeans = KMeans(n_clusters=3,random_state=0).fit(mat)
# print (kmeans.labels_)
# ----- ADD REGRESSION CODE HERE ----- #<file_sep>from nltk import word_tokenize, pos_tag
from nltk.corpus import wordnet as wn
from nltk.corpus import stopwords
from sklearn.cluster import KMeans
import numpy as np
import pandas as pd
import re
def penn_to_wn(tag):
if tag.startswith('N'):
return 'n'
if tag.startswith('V'):
return 'v'
if tag.startswith('J'):
return 'a'
if tag.startswith('R'):
return 'r'
return None
def tagged_to_synset(word, tag):
wn_tag = penn_to_wn(tag)
if wn_tag is None:
return None
try:
return wn.synsets(word, wn_tag)[0]
except:
return None
def sentence_similarity(synset,sentence):
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(sentence)
filtered_sentence = [w for w in word_tokens if not w in stop_words]
sentence = pos_tag(filtered_sentence)
synsets = [tagged_to_synset(*tagged_word) for tagged_word in sentence]
synsets = [ss for ss in synsets if ss]
score, count = 0.0, 0
fin = []
for i in synset:
a = [i.path_similarity(ss) for ss in synsets]
a = [ss for ss in a if ss]
if(len(a) == 0):
best_score = 0.0
else:
best_score = max(a)
score += best_score
count += 1
fin.append(best_score)
score /= count
return fin,score
path ='training_set_rel3.tsv'
record = pd.DataFrame.from_csv(path, sep='\t', header=0)
record = record[['essay_set', 'essay','domain1_score']]
record = record[(record['domain1_score'] == 12)&(record['essay_set'] == 1)]
bEssay = record['essay'].tolist()
mSynset = []
for i in bEssay:
dum = re.sub('\W+',' ',i)
stop_words = set(stopwords.words('english'))
word_tokens = word_tokenize(dum)
filtered_sentence = [w for w in word_tokens if not w in stop_words]
s = pos_tag(filtered_sentence)
synset = [tagged_to_synset(*tagged_word) for tagged_word in s]
synset = [ss for ss in synset if ss]
for j in synset:
mSynset.append(j)
print ("master synset created")
print (len(mSynset))
path ='training_set_rel3.tsv'
record = pd.DataFrame.from_csv(path, sep='\t', header=0)
record = record[['essay_set', 'essay','domain1_score']]
record = record[record['essay_set'] == 1]
record = record[['essay','domain1_score']]
msk = np.random.rand(len(record)) < 0.7
train = record[msk]
test = record[~msk]
essays = train['essay'].tolist()
scores = train['domain1_score'].tolist()
mat1 = {}
mat = []
for i in range(0,len(essays)):
fin = sentence_similarity(mSynset,essays[i])
mat1[i] = {v: k for v,k in enumerate(fin)}
mat1[i]['score'] = scores[i]
mat.append(fin)
df = pd.DataFrame(mat1)
df = df.transpose()
df.drop('Unnamed: 0',inplace=True)
df.to_csv('hclust.csv',sep=',')
# mat = np.array(mat)
# kmeans = KMeans(n_clusters=3,random_state=0).fit(mat)
# print (kmeans.labels_)
# ----- ADD REGRESSION CODE HERE ----- # | 7ddb840539531568a63a0fa85876ffee14dcf68b | [
"Markdown",
"Python"
] | 3 | Markdown | Anup-Deshmukh/Essay_scoring | 7d2e37d47f21d1da53bf759ea526b74f0c035a48 | ac718bba0521e8c7cfb18e03aae1d963cafa5e66 | |
refs/heads/master | <file_sep>require 'lotus/generators/abstract'
require 'lotus/utils/string'
module Lotus
module Generators
# @since 0.5.0
# @api private
class Mailer < Abstract
# @since 0.5.0
# @api private
TXT_FORMAT = '.txt'.freeze
# @since 0.5.0
# @api private
HTML_FORMAT = '.html'.freeze
# @since 0.5.0
# @api private
DEFAULT_ENGINE = 'erb'.freeze
# @since 0.5.0
# @api private
DEFAULT_FROM = "'<from>'".freeze
# @since 0.5.0
# @api private
DEFAULT_TO = "'<to>'".freeze
# @since 0.5.0
# @api private
DEFAULT_SUBJECT = "'Hello'".freeze
# @since 0.5.0
# @api private
def initialize(command)
super
@mailer_name = Utils::String.new(name).classify
cli.class.source_root(source)
end
# @since 0.5.0
# @api private
def start
assert_mailer!
opts = {
mailer: @mailer_name,
from: DEFAULT_FROM,
to: DEFAULT_TO,
subject: DEFAULT_SUBJECT,
}
templates = {
'mailer_spec.rb.tt' => _mailer_spec_path,
'mailer.rb.tt' => _mailer_path,
'template.txt.tt' => _txt_template_path,
'template.html.tt' => _html_template_path,
}
templates.each do |src, dst|
cli.template(source.join(src), target.join(dst), opts)
end
end
# @since 0.5.0
# @api private
def assert_mailer!
if @mailer_name.nil? || @mailer_name.empty?
raise Lotus::Commands::Generate::Error.new("Missing mailer name")
end
end
# @since 0.5.0
# @api private
def _mailer_path
core_root.join('mailers', "#{ name }.rb").to_s
end
# @since 0.5.0
# @api private
def _mailer_spec_path
spec_root.join(::File.basename(Dir.getwd), 'mailers', "#{ name }_spec.rb")
end
# @since 0.5.0
# @api private
def _txt_template_path
__template_path(TXT_FORMAT)
end
# @since 0.5.0
# @api private
def _html_template_path
__template_path(HTML_FORMAT)
end
# @since 0.5.0
# @api private
def __template_path(format)
core_root.join('mailers', 'templates', "#{ name }#{ format }.#{ DEFAULT_ENGINE }")
end
# @since 0.5.0
# @api private
def name
Utils::String.new(app_name || super).underscore
end
end
end
end
<file_sep>module Lotus
module Commands
class Routes
def initialize(environment)
@environment = environment
@environment.require_application_environment
end
def start
puts app.routes.inspector.to_s
end
private
def app
if @environment.container?
Lotus::Container.new
else
Lotus::Application.applications.first.new
end
end
end
end
end
| c3e78e7a429f5681c85adfe8e849e46145f176b0 | [
"Ruby"
] | 2 | Ruby | DEIGirls/lotus | fc4e48098b0ebf7bc9d1165562a406d8a1d0054d | a7dbdf4446400db4d82fe2ac510f62fe22381d68 | |
refs/heads/master | <repo_name>rovkit/Organet<file_sep>/queue.h
/*
* IncFile1.h
*
* Created: 22.05.2015 14:05:09
* Author: Viktor
*/
#ifndef INCFILE1_H_
#define INCFILE1_H_
//#include <stdio.h>
#define MAX_SIZE 32 // Define maximum length of the queue
#include <stdint.h>
typedef struct{
uint16_t head, tail; // Declare global indices to head and tail of queue
char theQueue[MAX_SIZE]; // The queue
}queue;
// List Function Prototypes
void initQueue(queue *q); // Initialize the queue
void clearQueue(queue *q); // Remove all items from the queue
char enqueue(queue *q,char ch); // Enter an item in the queue
char dequeue(queue *q); // Remove an item from the queue
char isEmpty(queue *q); // Return true if queue is empty
char isFull(queue *q); // Return true if queue is full
#endif /* INCFILE1_H_ */<file_sep>/comhelper.h
#ifndef COMHELPER_H_
#define COMHELPER_H_
#include "SoftUart2.h"
#include "queue.h"
/*##############################################################
This is the comhelper. It manages the incoming bytes from the uart_port and returns them as messages to the organet client.
So somehow a layer between the actual software and the uart library. Used fot controlling the uart library.
Todo:
-port four pins have to be changed
-every port should get its own debug led.
-at uart init there is a comment: not using port d for anything else. this is not true anymore with multiple com lines.
-rewrite the print char method.
-the timer interrupt has to be 4 times faster.
################################################################
*/
//here, as a macro the transmission (tx) and receiving (rx) pins are set as macros.
#define PORTONERX PIND2;
#define PORTONETX PIND3;
#define PORTTWORX PIND4;
#define PORTTWOTX PIND5;
#define PORTTHREERX PIND6;
#define PORTTHREETX PIND7;
#define PORTFOURRX PIND0; //probably not a good idea. has to be changed
#define PORTFOURTX PIND1;
//not yet used. maybe not needed after all.
typedef struct{
uint8_t txpin;
uint8_t rxpin;
unsigned char name;
unsigned char incoming_chars_buffer[32];
unsigned char outgoin_chars_buffer[32];
} comhelper;
//this as well is not needed. maybe not after all.
//comhelper comhelpers[4];
//a very basic message, with receipient and sender and a short routestack to save the past relay stations.
typedef struct{
char receipient;
char sender;
unsigned char routestack[5];
}message;
queue incoming_port[4];
void com_helper_init(); //initializes all four connection ports, with the pins set on the macros.
char com_helper_send_message(message,comhelper); //not implemented yet.
char com_helper_is_msg(queue *q); //check if chars in buffer represent message
char com_helper_parse_msg(queue *q);
#endif /* COMHELPER_H_ */
<file_sep>/comhelper.c
/*
* OrganetP.c
*
* Created: 24.02.2015 10:07:45
* Author: Viktor
*/
#include "comhelper.h"
void com_helper_init(){
/************************************************************************/
/* This method initializes all four connection lines. */
/************************************************************************/
initQueue(&incoming_port[0]);
initQueue(&incoming_port[1]);
initQueue(&incoming_port[2]);
initQueue(&incoming_port[3]);
port_rotator = 1; //this couldnt be done in the header file
port[0].uartrx = PORTONERX; //load the pins for each line.
port[0].uarttx = PORTONETX;
port[0].port_number = 1;
port[1].uartrx = PORTTWORX;
port[1].uarttx = PORTTWOTX;
port[1].port_number = 2;
port[2].uartrx = PORTTHREERX;
port[2].uarttx = PORTTHREETX;
port[2].port_number = 3;
port[3].uartrx = PORTFOURRX; //right now, this pin is still wrong.
port[3].uarttx = PORTFOURTX;
port[3].port_number = 4;
for(int i = 0;i<4;i++){
switch (i){ //init each com line
case 1:
uart_init(&port[0]);
break;
case 2:
uart_init(&port[1]);
break;
case 3:
uart_init(&port[2]);
break;
case 4:
uart_init(&port[3]);
break;
}
}
}
char com_helper_send_message(message msg,comhelper cmhlpr)
{
return 0;
}
char com_helper_is_msg(queue *q)
{
return 0;
}
char com_helper_parse_msg(queue *q)
{
}<file_sep>/SoftUart2.c
/*
* SoftUart.c
*
* Created: 22.02.2015 22:19:11
* Author: Viktor
*/
#include "SoftUart2.h"
////////////////////////////////////////////////////////////////////////////////////////////
//
// Software uart routines
//
// we need two serial lines, and there's only one usart on the M32
// so we implement another in software
//
// we set up a clock with a timeout of 883 cycles - four times baud rate at 2400 baud -
// and use it to sample an input line and clock an output line
// we also use it to maintain a 20mS tick for timouts and such
//
///////////////////////////////////////////////////////////////////////////////////////////
//this is called periodically, then our periodical routine is called to read/write on the current port, which is afterwards incremented.
ISR(TIMER1_COMPA_vect)
{
//DEBUG
switch (port_rotator){
case 1:
do_what_needs_to_be_done(&port[0]);
break;
case 2:
do_what_needs_to_be_done(&port[1]);
break;
case 3:
do_what_needs_to_be_done(&port[2]);
break;
case 4:
do_what_needs_to_be_done(&port[3]);
break;
}
if(port_rotator == 4){ // <= rotate the port.
port_rotator = 1;
}
else{
port_rotator +=1;
}
}
//this is the main method for reading/writing
//probably doesnt work right now, as interrupt has to be 4 times faster.
void do_what_needs_to_be_done(uart_port *curr_port){
if(curr_port->yeah){ //First of all, the debug led is lit if yeah==1.
//has to be improved to all ports
DDRB |= _BV(PINB3);
PORTB |= _BV(PINB3);
}
else{
PORTB &= ~_BV(PINB3);
}
// the interrupt signal on a compare for the timer 1
// we arrive here four times per baud rate
// we first send the output signal, if there's anything to send,
// since it needs to be somewhere close to accurate...
// then we read the incoming signal, if there is one,
// and finally we update the nearly-real-time clock
// (we never display the clock but it timestamps the output data)
if (curr_port->uart_status & _BV(txbusy))
{
// we're transmitting something
// increment the tick - we only do things every four ticks
curr_port->uart_txtick++;
if (curr_port->uart_txtick == 4)
{
// okay, we've work to do
curr_port->uart_txtick = 0;
// is it the start bit?
if (curr_port->uart_txbit == 0)
{
// yes...
PORTD &= ~(_BV(curr_port->uarttx)); // clear the start bit output
curr_port->uart_txbit++;
}
else
{
if (curr_port->uart_txbit != 9)
{
// deal with the data bits
if (curr_port->uart_txd & 1){// low bit set?
PORTD |= _BV(curr_port->uarttx); // then set the data stream bit
}
else{
PORTD &= ~(_BV(curr_port->uarttx)); //Here the tx pin is set as low. This doesnt work properly.
//_delay_us(5);
//PORTD &= ~(_BV(curr_port->uarttx)); //maybe we can set it low twice?
}
// or clear, as required
curr_port->uart_txbit++; // increment the bit count
// and shift the data right
curr_port->uart_txd /= 2;
}
else
{
// deal with the stop bit (stop bit is allways on high.
PORTD |= _BV(curr_port->uarttx);
curr_port->uart_txbit++;
}
}
// and finally, if txbit is more than 9, we're done
if (curr_port->uart_txbit > 9)
{
curr_port->uart_txbit = 0; // clear the bit counter
curr_port->uart_status &= ~(_BV(txbusy)); // and the busy status
}
}
}
// we may be receiving something
// if we're *not* yet receiving, we check every clock tick to see if the input
// line has gone into a stop bit
// if it has, we wait for half a bit period and then sample every four ticks
// to put together the rx data
if ((curr_port->uart_status & _BV(rxbusy)) == 0)
{
// check to see if there's a start
if ((PIND & _BV(curr_port->uartrx)) == 0)
{
// we found a start bit!
// set the tick count to 2, so we get the sample near the middle of the bit
curr_port->uart_rxtick = 2;
// and flag that we're now busy
curr_port->uart_status |= _BV(rxbusy);
// we're expecting the start bit now...
curr_port->uart_rxbit = 0;
// and carry on with life
}
}
else
{
// we're receiving something
// inc the tick count
curr_port->uart_rxtick++;
if (curr_port->uart_rxtick == 4)
{
// we only sample when the tick = 0
curr_port->uart_rxtick = 0;
// what we do depends on the bit count
// 0 = start,
// 1-8 = data
// 9 = stop
if (curr_port->uart_rxbit == 0)
{
// start bit
// it had better be 0 or it was a line glitch
if ((PIND & _BV(curr_port->uartrx)) == 0)
{
// it's a real start bit (probably) so deal with it
// next bit will be data
curr_port->uart_rxbit ++;
curr_port->uart_rxd = 0;
}
else
{
// bad start bit, flag us back as not busy
curr_port->uart_status |= ~(_BV(rxbusy));
}
}
else
{
if (curr_port->uart_rxbit < 9)
{
// data bit
// if the data bit is a one, we add the bit value to the rxd value
if (PIND & _BV(curr_port->uartrx))
{
curr_port->uart_rxd += uart_bitpattern[curr_port->uart_rxbit];
}
curr_port->uart_rxbit ++;
}
else
{
if (curr_port->uart_rxbit == 9)
{
// stop bit
// we're going to assume it's a valid bit, though we could check for
// framing error here, and simply use this bit to wait for the first
// stop bit period
curr_port->uart_rxbit++;
}
else
{
// finished, ready to start again
curr_port->uart_status &= ~(_BV(rxbusy));
// store the data into the buffer
curr_port->buffer2[curr_port->next_write_2++] = curr_port->uart_rxd;
if(curr_port->uart_rxd == 0xF0){
}
if (curr_port->next_write_2 == BUFFERSIZE)
curr_port->next_write_2 = 0;
}
}
}
}
}
}
//will be executed multiple times during startup, but who cares.
void uart_init (uart_port *curr_port)
{
curr_port->next_write_2 = 0; // set up the buffer pointers
curr_port->next_read_2 = 0;
curr_port->yeah = 0; //set up debug char
// we wake up the timer, preset the clock and uart variables, and enable the ocr1a interrupt
TCCR1B = 0; //resetting TCCR1B.
TCCR1B |= _BV(CS10); //clock enabled, prescaling = 1
TCCR1B |= _BV(WGM12); // ctc mode is enabled
TCCR1A = 0; //nothing special is used.
OCR1AH = 0x03; //for four connections at the same time: has to be D0
OCR1AL = 0x41; //The compare A value is set, right now it is the value it used to be for only one port, has to be four times higher.
OCR1AH = 0x00;
OCR1AL = 0xD0;
//OCR1AH = 0x01; //bullshit numbers
//OCR1AL = 0x59;
TIMSK1 |= _BV(OCIE1A); //Timer/Counter Compare Match is enabled.
curr_port->uart_status = 0; // nothing happening either tx or rx
//Here, the ports are set and the pullups configured. This implementation worked with one port, maybe changes have to be made so that we only edit "our" pin as a initializing port.
DDRD = _BV(curr_port->uarttx); // and set the input and output pins
PORTD |= _BV(curr_port->uarttx); // we're not using port d for anything else and
PORTD |= _BV(curr_port->uartrx); // the usart overrides the pin directions anyway, here the pull up resistor on the rx port is enabled.
}
void uart_out_char (char ch, uart_port *curr_port)
{
// output the character to the software serial port, waiting until the output
// buffer is available
while ((curr_port->uart_status & _BV(txbusy)) == 1); // wait...
{
// once the system isn't busy...
// load the register
curr_port->uart_txd = ch;
// and tell it there's something to send
curr_port->uart_status |= _BV(txbusy);
}
}
void uart_print (char * text)
{
// print a string of characters to the soft uart
//for (int q=0; q<strlen(text); q++)
//ser2_out(text[q]);
}
//checks if a char is waiting to be read.
char uart_ischar (uart_port *curr_port)
{
// returns true if there is a character available from the soft uart
// false if there is nothing waiting
// call ser2_in after this to retrieve the data
if (curr_port->next_read_2 != curr_port->next_write_2)
return 1;
else
return 0;
}
//reads this char. Argument in both methods: a port struct, this struct contains the buffer object where the char is read from (if it exists).
char uart_get_char (uart_port *curr_port)
{
// return a character from the soft uart
// do *not* call without first testing with ser2_ischar as it modifies the
// next_read pointer
char ch;
ch = curr_port->buffer2[curr_port->next_read_2++];
if (curr_port->next_read_2 == BUFFERSIZE)
curr_port->next_read_2 = 0;
return ch;
}
<file_sep>/README.md
# Organet
This is heavily work in progress. As of the current state, only the concept of the organet and a fully working SoftwareUART class exist. The SoftwareUART Class can send and receive bytes, in the future it is to be encapsulated by the comhelper component and the comhelper to be used by the organet class.
<file_sep>/organet.c
/*
* CFile1.c
*
* Created: 24.02.2015 23:32:03
* Author: Viktor
*/
#include "organet.h"
#include <util/delay.h>
int main(){
com_helper_init(); //initializes all four ports by calling the uart_init method with each port struct as an argument.
sei();
while(1){
uart_out_char(0xAA,&port[0]);
_delay_ms(50);
if(uart_ischar(&port[0])){ //checks if the is a char to be read on port 1
//activate debug led.
char x = uart_get_char(&port[0]);
if(x == 0x04){
port[0].yeah = 1;
}
}
}
}
void check_for_new_chars(){
if(uart_ischar(&port[0])){
enqueue(&incoming_port[0], uart_get_char(&port[0]));
if(com_helper_is_msg(&incoming_port[0])){
com_helper_parse_msg(&incoming_port[0]);
}
}
if(uart_ischar(&port[1])){
enqueue(&incoming_port[1], uart_get_char(&port[1]));
if(com_helper_is_msg(&incoming_port[1])){
com_helper_parse_msg(&incoming_port[1]);
}
}
if(uart_ischar(&port[2])){
enqueue(&incoming_port[0], uart_get_char(&port[2]));
if(com_helper_is_msg(&incoming_port[2])){
com_helper_parse_msg(&incoming_port[2]);
}
}
if(uart_ischar(&port[3])){
enqueue(&incoming_port[0], uart_get_char(&port[3]));
if(com_helper_is_msg(&incoming_port[3])){
com_helper_parse_msg(&incoming_port[3]);
}
}
}
void parse_message(){
//check if message is for me
//if nessesary send a response message
//if not for me, send the message on the other 3 ports.
}
void send_message(){
//on which ports?
//add message to cue
}
void manage_transmissions(){
//is uart currently sending a byte?
//yes: return
//no: is a message currently being sent?
//yes: uart_send next byte of message
//no: take next message from message cue, send first byte.
}<file_sep>/SoftUart2.h
/*
* SoftUart2.h
*
* Created: 24.02.2015 09:57:33
* Author: Viktor
*/
#ifndef SOFTUART_H_
#define SOFTUART_H_
#define F_CPU 16000000UL
#include <avr/io.h>
#include <avr/interrupt.h>
#include <util/delay.h>
/*
################################################################################
This is a full duplex software Uart library.
IMPORTANT: set port_rotator to 1 when doing first init.
Todo: Output buffer
#############################################################################
*/
#define BUFFERSIZE 32
#define txbusy 0 // set if a byte is in transmission
#define rxbusy 1 // set if a byte is being received
#define rxframe 2
static uint8_t uart_bitpattern[9] = {0,1,2,4,8,16,32,64,128}; //this is used to know the actual value of a incoming bit in the final char. Read only, so no need to put it in every uart_port object.
volatile char port_rotator; // this variable determines on which port the read/send method will be executed at the moment.
// it is incremented/set to 0 after each interrupt. The interrupt is called by a timer periodically.
//this is the uart_port struct. A port represents a physical uart port, made of two pins on the arduino.
//The set of uart_port attributes are where the state of a port is saved, the method for reading exists only once, but the port which is given as an argument changes.
//Therefore, depending on which uart_port object is given to the read/write method, the according port is used.
//Addition: A uart_message consist of more than just one bit, so the bits have to be saved for each port. This happens in the buffer2 of every uart_port object.
typedef struct {
char port_number; //The port number as an actual number.
volatile uint8_t yeah; //if true, this port lights the debug led.
volatile uint8_t uart_rxd; // the byte being received
volatile uint8_t uart_txd; // the byte being transmitted
volatile uint8_t uart_txtick; // tick count for the transmitter
volatile uint8_t uart_rxtick; // tick count for the receiver
volatile uint8_t uart_txbit; // bit count for tx
volatile uint8_t uart_rxbit; // bit count for rx
volatile uint8_t uart_status; // uart status
volatile uint8_t next_read_2;
volatile uint8_t next_write_2; // the pointers to the receive2 buffer
volatile uint8_t uartrx; //the pin used by the port to receive something.
volatile uint8_t uarttx; //the pin used by the port to send something.
volatile unsigned char buffer2[BUFFERSIZE]; // circular buffer for receiver 2
}uart_port;
//this is our port array:
//it has positions 0-3 althoug the port numbers are 1-4. attention!
//in this port array, all four port objects are saved.
uart_port port[4];
//######################################################################
//
// METHODS
//
//######################################################################
void uart_init (uart_port *curr_port); //Used for initialization, argument is a port object, in which for example tha pins to use are specified. where specified? at com_helper_init().
char uart_ischar (uart_port *curr_port); //checks if there is a new char to be read.
char uart_get_char (uart_port *curr_port); // gets this char, which is to be read.
void uart_out_char (char ch, uart_port *curr_port); // prints a char via the software Uart. Currently the main routine waits until its printed, in the future abuffer will be implemented
void uart_print (char * text); //prints a whole string, doesnt work currently
void do_what_needs_to_be_done(uart_port *curr_port);
#endif /* SOFTUART_H_ */
<file_sep>/organet.h
/*
* IncFile1.h
*
* Created: 25.02.2015 01:05:51
* Author: Viktor
*/
#ifndef ORGANET_H_
#define ORGANET_H_
#include "comhelper.h"
void check_for_new_chars ();
void parse_message();
void send_message();
void manage_transmissions();
#endif /* ORGANET_H_ */<file_sep>/Debug/makedep.mk
################################################################################
# Automatically-generated file. Do not edit or delete the file
################################################################################
queue.c
organet.c
comhelper.c
SoftUart2.c
<file_sep>/queue.c
/*
* CFile1.c
*
* Created: 22.05.2015 14:05:19
* Author: Viktor
*/
#include "queue.h"
// Declare these as static so no code outside of this source
// can access them.
//--------------------------------------------
// Function: InitQueue()
// Purpose: Initialize queue to empty.
// Returns: void
//--------------------------------------------
void initQueue(queue *q)
{
q->head = q->tail = -1;
}
//--------------------------------------------
// Function: ClearQueue()
// Purpose: Remove all items from the queue
// Returns: void
//--------------------------------------------
void clearQueue(queue *q)
{
q->head = q->tail = -1; // Reset indices to start over
}
//--------------------------------------------
// Function: Enqueue()
// Purpose: Enqueue an item into the queue.
// Returns: TRUE if enqueue was successful
// or FALSE if the enqueue failed.
// Note: We let head and tail continuing
// increasing and use [head % MAX_SIZE]
// and [tail % MAX_SIZE] to get the real
// indices. This automatically handles
// wrap-around when the end of the array
// is reached.
//--------------------------------------------
char enqueue(queue *q,char ch)
{
// Check to see if the Queue is full
if(isFull(q)) return 0;
// Increment tail index
q->tail++;
// Add the item to the Queue
q->theQueue[q->tail % MAX_SIZE] = ch;
return 1;
}
//--------------------------------------------
// Function: Dequeue()
// Purpose: Dequeue an item from the Queue.
// Returns: TRUE if dequeue was successful
// or FALSE if the dequeue failed.
//--------------------------------------------
char dequeue(queue *q)
{
char ch;
// Check for empty Queue
if(isEmpty(q)) return '\0'; // Return null character if queue is empty
else
{
q->head++;
ch = q->theQueue[q->head % MAX_SIZE]; // Get character to return
return ch; // Return popped character
}
}
//--------------------------------------------
// Function: isEmpty()
// Purpose: Return true if the queue is empty
// Returns: TRUE if empty, otherwise FALSE
// Note: C has no boolean data type so we use
// the defined int values for TRUE and FALSE
// instead.
//--------------------------------------------
char isEmpty(queue *q)
{
return (q->head == q->tail);
}
//--------------------------------------------
// Function: isFull()
// Purpose: Return true if the queue is full.
// Returns: TRUE if full, otherwise FALSE
// Note: C has no boolean data type so we use
// the defined int values for TRUE and FALSE
// instead.
//--------------------------------------------
char isFull(queue *q)
{
// Queue is full if tail has wrapped around
// to location of the head. See note in
// Enqueue() function.
return ((q->tail - MAX_SIZE) == q->head);
} | 97a3847578382d2bac2c80504868da275b756fbd | [
"Markdown",
"C",
"Makefile"
] | 10 | C | rovkit/Organet | d9532ee263bfa15d6204c77c3791a177a32602db | 58b17d89ca83069a89643da7658e90c2c3eca1ca | |
refs/heads/master | <repo_name>masalennon/vimrc<file_sep>/.zshrc
# 色設定
source ~/.zplug/init.zsh
# ダブルクォーテーションで囲うと良い
zplug "zsh-users/zsh-history-substring-search"
zplug "sorin-ionescu/prezto"
zplug "b4b4r07/gomi", as:command, use:"bin"
zplug load --verbose
# コマンドも管理する
# グロブを受け付ける(ブレースやワイルドカードなど)
zplug "Jxck/dotfiles", as:command, use:"bin/{histuniq,color}"
# frozen タグが設定されているとアップデートされない
zplug "k4rthik/git-cal", as:command, frozen:1
# GitHub Releases からインストールする
# また、コマンドは rename-to でリネームできる
zplug "junegunn/fzf-bin", \
from:gh-r, \
as:command, \
rename-to:fzf, \
use:"*darwin*amd64*"
# oh-my-zsh をサービスと見なして、
# そこからインストールする
zplug "plugins/git", from:oh-my-zsh
# if タグが true のときのみインストールされる
zplug "lib/clipboard", from:oh-my-zsh, if:"[[ $OSTYPE == *darwin* ]]"
# インストール・アップデート後に実行されるフック
# この場合は以下のような設定が別途必要
# ZPLUG_SUDO_PASSWORD="********"
zplug "jhawthorn/fzy", \
as:command, \
rename-to:fzy, \
hook-build:"make && sudo make install"
# リビジョンロック機能を持つ
zplug "mollifier/anyframe", at:4c23cb60
zplug "b4b4r07/enhancd", use:init.sh
# ノート: 読み込み順序を遅らせるなら defer タグを使いましょう
# 読み込み順序を設定する
# 例: "zsh-syntax-highlighting" は compinit の前に読み込まれる必要がある
# (2 以上は compinit 後に読み込まれるようになる)
zplug "zsh-users/zsh-syntax-highlighting", defer:2
# ローカルプラグインも読み込める
zplug "~/.zsh", from:local
# 未インストール項目をインストールする
if ! zplug check --verbose; then
printf "Install? [y/N]: "
if read -q; then
echo; zplug install
fi
fi
# コマンドをリンクして、PATH に追加し、プラグインは読み込む
zplug load --verbose
# プラグインを読み込み、コマンドにパスを通す
zplug load --verbose
autoload -U colors; colors
# もしかして機能
setopt correct
zplug "junegunn/fzf-bin", \
as:command, \
rename-to:"fzf", \
from:gh-r, \
on: zplug "b4b4r07/enhancd", use:enhancd.sh
function is_exists() { type "$1" >/dev/null 2>&1; return $?; }
function is_osx() { [[ $OSTYPE == darwin* ]]; }
function is_screen_running() { [ ! -z "$STY" ]; }
function is_tmux_runnning() { [ ! -z "$TMUX" ]; }
function is_screen_or_tmux_running() { is_screen_running || is_tmux_runnning; }
function shell_has_started_interactively() { [ ! -z "$PS1" ]; }
function is_ssh_running() { [ ! -z "$SSH_CONECTION" ]; }
function tmux_automatically_attach_session()
{
if is_screen_or_tmux_running; then
! is_exists 'tmux' && return 1
if is_tmux_runnning; then
echo " "
echo "${fg_bold[red]} _____ __ __ _ ___ __ ${reset_color}"
echo "${fg_bold[red]}|_ _| \/ | | | \ \/ / ${reset_color}"
echo "${fg_bold[red]} | | | |\/| | | | |\ / ${reset_color}"
echo "${fg_bold[red]} | | | | | | |_| |/ \ ${reset_color}"
echo "${fg_bold[red]} |_| |_| |_|\___//_/\_\ ${reset_color}"
echo " "
elif is_screen_running; then
echo "This is on screen."
fi
else
if shell_has_started_interactively && ! is_ssh_running; then
if ! is_exists 'tmux'; then
echo 'Error: tmux command not found' 2>&1
return 1
fi
if tmux has-session >/dev/null 2>&1 && tmux list-sessions | grep -qE '.*]$'; then
# detached session exists
tmux list-sessions
echo -n "Tmux: attach? (y/N/num) "
read
if [[ "$REPLY" =~ ^[Yy]$ ]] || [[ "$REPLY" == '' ]]; then
tmux attach-session
if [ $? -eq 0 ]; then
echo "$(tmux -V) attached session"
return 0
fi
elif [[ "$REPLY" =~ ^[0-9]+$ ]]; then
tmux attach -t "$REPLY"
if [ $? -eq 0 ]; then
echo "$(tmux -V) attached session"
return 0
fi
fi
fi
if is_osx && is_exists 'reattach-to-user-namespace'; then
# on OS X force tmux's default command
# to spawn a shell in the user's namespace
tmux_config=$(cat $HOME/.tmux.conf <(echo 'set-option -g default-command "reattach-to-user-namespace -l $SHELL"'))
tmux -f <(echo "$tmux_config") new-session && echo "$(tmux -V) created new session supported OS X"
else
tmux new-session && echo "tmux created new session"
fi
fi
fi
}
tmux_automatically_attach_session
bindkey -M vicmd 'k' history-substring-search-up
bindkey -M vicmd 'j' history-substring-search-down
bindkey '^[[A' history-substring-search-up
bindkey '^[[B' history-substring-search-down
alias -g C="| color"
| 61a76d1c6a277862e3e1a291ea5fa094ab5e3d57 | [
"Shell"
] | 1 | Shell | masalennon/vimrc | a514db9c41651f69acc21640d146ff108a5290b5 | 4f2e8462e8f12ded9ebb2ab6c9b5742b378a10ef | |
refs/heads/master | <file_sep>DROP DATABASE IF EXISTS employee_trackerDB;
CREATE DATABASE employee_trackerDB;
USE employee_trackerDB;
CREATE TABLE department(
id INT AUTO_INCREMENT NOT NULL,
name VARCHAR(30),
PRIMARY KEY (id)
);
CREATE TABLE role(
id INT AUTO_INCREMENT NOT NULL,
title VARCHAR(30),
salary DECIMAL,
department_id INTEGER,
constraint fk_department foreign key (department_id) references department(id),
PRIMARY KEY (id)
);
CREATE TABLE employee(
id INT AUTO_INCREMENT NOT NULL,
first_name VARCHAR(30),
last_name VARCHAR(30),
role_id INTEGER,
constraint fk_role foreign key (role_id) references role(id),
manager_id INTEGER,
constraint fk_manager foreign key (manager_id) references employee(id),
PRIMARY KEY (id)
);
INSERT INTO department (id, name)
VALUES (1, "Marketing");
<file_sep>
USE employee_trackerDB;
INSERT INTO department (name)
VALUES
("Marketing"),
("Human Resources"),
("Engineering"),
("Finance"),
("Legal"),
("Sales");
INSERT INTO role (title, salary, department_id)
VALUES
("Manager", 60000, 2),
("Intern", 10000, 1),
("Sales Lead", 100000, 6),
("Salesperson", 80000, 6),
("Lead Engineer", 150000, 3),
("Software Engineer", 120000, 3),
("Accountant", 125000, 4),
("Legal Team Lead", 250000, 5),
("Lawyer", 190000, 5),
("Software Engineer", 120000, 3);
INSERT INTO employee (first_name, last_name, role_id, manager_id)
VALUES
("Bob", "Smith", 1, null),
("George", "Glass", 2, 1);
<file_sep>const mysql = require("mysql");
const inquirer = require("inquirer");
const cTable = require('console.table');
var connection = mysql.createConnection({
host: "localhost",
// Your port; if not 3306
port: 3306,
// Your username
user: "root",
// Your password
password: "<PASSWORD>",
database: "employee_trackerDB"
});
connection.connect(function(err) {
if (err) throw err;
console.log("connected as id " + connection.threadId + "\n");
addRole();
});
function viewDepartment()
{
console.log("Selecting all department...\n");
connection.query("SELECT * FROM department", function(err, res) {
if (err) throw err;
// Log all results of the SELECT statement
console.table(res);
// for(var i=0; i<res.length;i++)
// {
// console.log(res[i].name);
// }
}
);
}
function viewRoles()
{
console.log("Selecting all roles...\n");
connection.query("SELECT * FROM role", function(err, res) {
if (err) throw err;
// Log all results of the SELECT statement
console.table(res);
// for(var i=0; i<res.length;i++)
// {
// console.log(res[i].title);
// console.log(res[i].salary);
// console.log(res[i].department_id);
// }
});
}
function viewEmployees(){
{
console.log("Selecting all employees...\n");
connection.query("SELECT * FROM employee", function(err, res) {
if (err) throw err;
// Log all results of the SELECT statement
console.table(res);
// for(var i=0; i<res.length;i++)
// {
// console.log(res[i].first_name);
// console.log(res[i].last_name);
// console.log(res[i].role_id);
// console.log(res[i].manager_id);
// }
});
}
}
function addDepartment(){
//1. get user input
inquirer
.prompt([
{
type: "choice",
name: "deptName",
message: "What department name would you like to add?",
},
])
.then((data) => {
console.log(data)
console.log("Inserting a new product...\n");
var query = connection.query(
"INSERT INTO department SET ?",
{
name: data.deptName,
},
function(err, res) {
if (err) throw err;
console.log(res.affectedRows + " product inserted!\n");
// Call updateProduct AFTER the INSERT completes
console.log("department updated")
}
);
// logs the actual query being run
console.log(query.sql);
});
//2. add to database
}
//this function will add an action to the database
const addItems = () => {
inquirer
.prompt([
{
type: "list",
name: "action",
message: "What would you like to do?",
choices: ["View all employees","View all employees by department","View all employees by manager", "Add employee", "Remove employee", "Update employee role",
"Update employee manager"]
},
])
.then((data) => {
connection.query(
"INSERT INTO items SET ?",
{
name: data.item,
importance: data.importance,
},
(err) => {
if (err) throw err;
console.log(`Item ${data.item} has been added!!!`);
viewItems();
}
);
});
};
async function addRole(){
//1. get user input
// var department= await viewDepartment();
// console.log(department)
// mangerID= department.map(
// ({id, name}) =>
// ({name:name, value:id})
// );
//console.log("department: "+mangerID)
inquirer
.prompt([
{
type: "choice",
name: "title",
message: "What title would you like to add?",
},
{
type: "choice",
name: "salary",
message: "What salary would you like to add?",
},
{
type: "choice",
name: "department_id",
message: "What department id would you like to add?",
},
])
.then((data) => {
console.log(data)
console.log("Inserting a new role...\n");
var query = connection.query(
"INSERT INTO role SET ?",
{
title: data.title,
salary: data.salary,
department_id: data.department_id,
},
function(err, res) {
if (err) throw err;
console.log(res.affectedRows + " product inserted!\n");
// Call updateProduct AFTER the INSERT completes
console.log("department updated")
}
);
// logs the actual query being run
console.log(query.sql);
});
}
function addEmployee(){}
function updateEmployeeRole(){}
// function createProduct() {
// console.log("Inserting a new product...\n");
// var query = connection.query(
// "INSERT INTO products SET ?",
// {
// flavor: "Rocky Road",
// price: 3.0,
// quantity: 50
// },
// function(err, res) {
// if (err) throw err;
// console.log(res.affectedRows + " product inserted!\n");
// // Call updateProduct AFTER the INSERT completes
// updateProduct();
// }
// );
// // logs the actual query being run
// console.log(query.sql);
// }
// function updateProduct() {
// console.log("Updating all Rocky Road quantities...\n");
// var query = connection.query(
// "UPDATE products SET ? WHERE ?",
// [
// {
// quantity: 100
// },
// {
// flavor: "Rocky Road"
// }
// ],
// function(err, res) {
// if (err) throw err;
// console.log(res.affectedRows + " products updated!\n");
// // Call deleteProduct AFTER the UPDATE completes
// deleteProduct();
// }
// );
// // logs the actual query being run
// console.log(query.sql);
// }
// function readProducts() {
// console.log("Selecting all products...\n");
// connection.query("SELECT * FROM products", function(err, res) {
// if (err) throw err;
// // Log all results of the SELECT statement
// console.log(res);
// connection.end();
// });
// }
<file_sep># employee-tracker
A small CMS to make it easy for non-developers to view and interact with database content. This one is for managing a company's employees. It uses node, inquirer, and MySQL
| 1e0bd14a72d6aae5b0fca7e9f12b03c3fa9f1c92 | [
"JavaScript",
"SQL",
"Markdown"
] | 4 | SQL | amandalmorris31/employee-tracker | af492eab8da590c3642956adac324b01252f707c | f503475568977b2b6f87334da5a6f48a4c81b70f | |
refs/heads/master | <repo_name>DrewKjellWCTC/bookWebApp<file_sep>/src/main/java/edu/wctc/adk/distjava/bookwebapp/model/MySQLDBAccessor.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package edu.wctc.adk.distjava.bookwebapp.model;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.StringJoiner;
import java.util.stream.Collectors;
/**
*
* @author drew
*/
public class MySQLDBAccessor implements DBAccessor {
private Connection conn;
// Consider creating custom exception
@Override
public void openConnection(String driverClass, String url, String userName, String pwd)
throws ClassNotFoundException, SQLException{
// Needs null check validation
Class.forName(driverClass);
conn = DriverManager.getConnection(url, userName, pwd);
}
@Override
public int insertRecord(String table, Map<String,Object> colInfo) throws SQLException{
int recordCount = -1;
StringJoiner sj = new StringJoiner(",", "(", ")");
for(String key : colInfo.keySet())
{
sj.add(key);
}
String sql = "INSERT into " + table + " " + sj.toString();
sj = new StringJoiner(",", "(", ")");
for(Object val : colInfo.values())
{
sj.add("?");
}
sql += " VALUES " + sj.toString();
PreparedStatement stmt = conn.prepareStatement(sql);
List<String> keys = new ArrayList(colInfo.keySet());
for(int i = 0; i< keys.size(); i++)
{
stmt.setObject(i+1, colInfo.get(keys.get(i)));
}
recordCount = stmt.executeUpdate();
return recordCount;
}
@Override
public int deleteById(Object dataId, String table, String colName) throws SQLException
{
int recordCount = -1;
String sql = "DELETE FROM " + table + " WHERE " + colName + " = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setObject(1, dataId);
recordCount = stmt.executeUpdate();
return recordCount;
}
@Override
public int updateById(Object dataId, String table, String colName, Map<String,Object> colInfo) throws SQLException
{
int recordCount = -1;
String sql = "UPDATE " + table + " SET ";
StringJoiner sj = new StringJoiner(",");
for(String key : colInfo.keySet())
{
sj.add(" " + key + " = ?");
}
sql += sj.toString();
sql += " WHERE " + colName + " = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
int colNum = 0;
for (Object val : colInfo.values())
{
stmt.setObject(colNum + 1, val);
colNum++;
}
stmt.setObject(colNum + 1, dataId);
recordCount = stmt.executeUpdate();
return recordCount;
}
@Override
public List<Map<String, Object>> findRecordsFor(String tableName, int maxRecords)
throws SQLException{
String sql = "";
if (maxRecords > 0)
sql = "SELECT * FROM " + tableName + " LIMIT " + maxRecords;
else
sql = "SELECT * FROM " + tableName;
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql);
List<Map<String,Object>> results = new ArrayList<>();
ResultSetMetaData rsmd = rs.getMetaData();
// Get column count from meta data
// Knowing # of columns using getInt() and column number
int colCount = rsmd.getColumnCount();
Map<String,Object> record = null;
while (rs.next())
{
// Linked hash map maintains the order of the keys
// Also faster for looping over the contents
// Normal hash map does not maintain the order
record = new LinkedHashMap<>();
for (int colNo = 1; colNo <= colCount; colNo++)
{
// Store each column for each row as a map
String colName = rsmd.getColumnName(colNo);
Object data = rs.getObject(colNo);
record.put(colName, data);
}
results.add(record);
}
return results;
}
@Override
public void closeConnection() throws SQLException{
if (conn != null)
conn.close();
}
public static void main(String[] args) throws Exception{
DBAccessor db = new MySQLDBAccessor();
db.openConnection("com.mysql.jdbc.Driver",
"jdbc:mysql://localhost:3306/book",
"root", "root");
Map<String, Object> colInfo = new LinkedHashMap<>();
colInfo.put("author_name", "<NAME>");
colInfo.put("date_added", new Date());
db.insertRecord("author", colInfo);
List<Map<String,Object>> records = db.findRecordsFor("author", 10);
for(Map<String,Object> record : records)
{
System.out.println(record);
}
colInfo.clear();
colInfo.put("author_name", "<NAME>");
colInfo.put("date_added", new Date());
db.updateById(3, "author", "author_id", colInfo);
records = db.findRecordsFor("author", 10);
for(Map<String,Object> record : records)
{
System.out.println(record);
}
db.closeConnection();
}
}
<file_sep>/src/main/java/edu/wctc/adk/distjava/bookwebapp/model/AuthorDao.java
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package edu.wctc.adk.distjava.bookwebapp.model;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
/**
*
* @author drew
*/
public class AuthorDao implements IAuthorDao {
private DBAccessor db;
private String driverClass;
private String url;
private String userName;
private String pwd;
public AuthorDao(DBAccessor db, String driverClass, String url, String userName, String pwd) {
this.db = db;
this.driverClass = driverClass;
this.url = url;
this.userName = userName;
this.pwd = pwd;
}
@Override
public DBAccessor getDb() {
return db;
}
@Override
public void setDb(DBAccessor db) {
this.db = db;
}
@Override
public List<Author> getAuthorList(String tableName, int maxRecords)
throws ClassNotFoundException, SQLException{
List<Author> authorList = new ArrayList<>();
db.openConnection(driverClass, url, userName, pwd);
List<Map<String,Object>> rawData = db.findRecordsFor(tableName, maxRecords);
db.closeConnection();
// Translate from rawData to authorList
for(Map<String,Object> record : rawData)
{
Author author = new Author();
// Don't need null check as author id is a PK
author.setAuthorId((Integer)record.get("author_id"));
Object objAuthorName = record.get("author_name");
if (objAuthorName != null)
author.setAuthorName(objAuthorName.toString());
else
author.setAuthorName("");
// can use one line.
String name = objAuthorName != null ? objAuthorName.toString() : "";
author.setAuthorName(name);
Object objDate = record.get("date_added");
Date dateAdded = objDate != null ? (Date)objDate : null;
author.setDateAdded(dateAdded);
authorList.add(author);
}
return authorList;
}
@Override
public int addAuthor(String table, Author newAuth) throws SQLException, ClassNotFoundException{
db.openConnection(driverClass, url, userName, pwd);
// Map author object to Map for insert
Map<String,Object> colInfo = new HashMap<>();
colInfo.put("author_name", newAuth.getAuthorName());
colInfo.put("date_added", newAuth.getDateAdded());
int affectedRecords = db.insertRecord(table, colInfo);
db.closeConnection();
return affectedRecords;
}
@Override
public int removeAuthor(String table, Author remAuth) throws ClassNotFoundException, SQLException{
db.openConnection(driverClass, url, userName, pwd);
int affected = db.deleteById(remAuth.getAuthorId(), table, "author_id");
db.closeConnection();
return affected;
}
@Override
public int updateAuthor(String table, Author updAuth) throws SQLException, ClassNotFoundException{
db.openConnection(driverClass, url, userName, pwd);
Map<String,Object> colInfo = new HashMap<>();
colInfo.put("author_name", updAuth.getAuthorName());
if (updAuth.getDateAdded() != null)
colInfo.put("date_added", updAuth.getDateAdded());
int affected = db.updateById(updAuth.getAuthorId(), table, "author_id", colInfo);
db.closeConnection();
return affected;
}
@Override
public String getDriverClass() {
return driverClass;
}
@Override
public void setDriverClass(String driverClass) {
this.driverClass = driverClass;
}
@Override
public String getUrl() {
return url;
}
@Override
public void setUrl(String url) {
this.url = url;
}
@Override
public String getUserName() {
return userName;
}
@Override
public void setUserName(String userName) {
this.userName = userName;
}
@Override
public String getPwd() {
return pwd;
}
@Override
public void setPwd(String pwd) {
this.pwd = pwd;
}
public static void main(String[] args) throws Exception {
IAuthorDao test;
test = new AuthorDao(new MySQLDBAccessor(),
"com.mysql.jdbc.Driver",
"jdbc:mysql://localhost:3306/book", "root", "root");
Author tmp = new Author();
tmp.setAuthorName("<NAME>");
tmp.setDateAdded(new Date());
test.addAuthor("author", tmp);
tmp.setAuthorId(11);
tmp.setAuthorName("DAO Updated");
System.out.println(test.updateAuthor("author", tmp));
List<Author> results = test.getAuthorList("author", 100);
for (Author row : results)
{
System.out.println(row);
}
System.out.println(test.removeAuthor("author", tmp));
}
}
| baecb72f87231c8b958f44a7b5bdf407d4769729 | [
"Java"
] | 2 | Java | DrewKjellWCTC/bookWebApp | dc0e6af52b7b556f33ddd0a9ecf8e1bdb3c78bff | 6ea7325a49a1eebdad2e49eed7f7c24eedf07a95 | |
refs/heads/master | <file_sep>#include <iostream>
#include <string>
#include <ctime>
#include <cstdlib>
#include <fstream>
#include <ctype.h>
using namespace std;
//structs---------------------------------------------------------------------=
struct Salesperson {
string name;
string passchar;
};
struct Pizza {
string nameOfCustomer;
string pizzaKind;
string size;
string status;
//int priceOfOnePiece;
int numberOfPieces;
//char coupon;
int couponSize;
string specialOrder;
string topping;
string dessert;
int paid;
};
//global Vars-------------------------------------------------------------=
struct Pizza Pizzas[1000];
int index=0;
string menu[10] = {"Berry","Arugula","Prosciutto","Macaroni","Cheese","Cantaloupe","Sweet Ricotta","Chicken Alfredo","null","null"};
int prices[10] = {15,24,56,35,74,83,96,25,0,0};
int numberMenu=8;
//functions Prototypes-----------------------------------------------------=
void newPizza();
void checkStatus();
void changeStatus();
void generateCoupon();
bool isValid(string coupon);
void history();
void printToFile();
int captureCouponSize(string CouponID);
void showPizzaMenu();
//---------------------------------------------------------=
int main(){
bool isManager=false;
int choice;
Salesperson Salespersons[1];
cout<<"\n\t=========Welcome to the Pizza Ordering System=========\n\t";
cout<<"\n\t\t=========The Login Window=========\n\t";
cout<<"\n\t\t\tName : ";
getline(cin,Salespersons[0].name);
cout<<"\n\t\t\tPassChar : ";
getline(cin,Salespersons[0].passchar);
if(Salespersons[0].passchar=="M"){
isManager=true;
cout<<"\n\t\t\tWelcome Back Manager!!\n";
goto MainScreen;
}
while(1){
MainScreen:
cout<<"\n\t\t=========Welcome to the Pizza Ordering System=========\n\t";
cout<<"\n\t\t\t=========The Menu Window=========\n\t";
cout<<"\n\t\t|| [1]Sumbit a new order ||";
cout<<"\n\t\t|| [2]Change the status of an order ||";
cout<<"\n\t\t|| [3]Check the status of an order ||";
cout<<"\n\t\t|| [4]Generate a coupon ||";
if(isManager){
cout<<"\n\t\t|| [5]History ||";
cout<<"\n\t\t|| [6]Print the History to an external file ||";
}
cout<<"\n\t\t|| [0]EXIT ||";
while(1){
cout<<"\n\t\t\tYour Choice : ";
cin>>choice;
if(choice>=0&&choice<=6){
break;
}else{
continue;
}
}
switch(choice){
case 0:
cout<<"\n\t\t\t=============";
break;
case 1:
newPizza();
//break;
goto MainScreen;
case 2:
changeStatus();
//break;
goto MainScreen;
case 3:
checkStatus();
//break;
goto MainScreen;
case 4:
generateCoupon();
//break;
goto MainScreen;
case 5:
history();
//break;
goto MainScreen;
case 6:
printToFile();
goto MainScreen;
}
if(choice==0){
break;
}
}
return 0;
}
//-------------------------------------------------------------------------=
void newPizza(){
string specialOrder;
bool isSpecialOrder=false;
string couponID;
string isFinal;
string newOrder;
char wantDessert;
int ofMenu;
string hasCoupon;
for(int i=index;i<1000;i++){
/*
===Tried to implement the time of creation===
time_t now = time(0);
string* tm=ctime(&now);
strcpy(tm,Pizzas[i].systemTime);
*/
while(1){
cout<<"\n\t\tIs this a special order(Y|N)?";
cin>>specialOrder;
if(specialOrder=="Y"||specialOrder=="N"){
break;
}else{
cout<<"\n\t\t\tInvalid Input";
continue;
}
}
if(specialOrder=="Y"){
Pizzas[i].specialOrder="Yes";
}else if(specialOrder=="N"){
Pizzas[i].specialOrder="No";
}
cout<<"\n\t\tName of the Customer : ";
cin>>Pizzas[i].nameOfCustomer;
cout<<"\n\t\tWe have : ";
showPizzaMenu();
cout<<"\n\t\tYour choice : ";
cin>>ofMenu;
Pizzas[i].pizzaKind=menu[ofMenu-1];
cout<<"\n\t\tSmall-Medium-Big";
cout<<"\n\t\tPizza Size : ";
cin>>Pizzas[i].size;
if(specialOrder=="Y"){
cout<<"\n\t\t Available : 1,2,3,4,5";
cout<<"\n\t\t please choose the topping you want : ";
cin>>Pizzas[i].topping;
cout<<"\n\t\tDo you want any dessert(Y for Yes)?";
cin>>wantDessert;
if(wantDessert=='Y'){
cout<<"\n\t\t Available : 1,2,3,4,5";
cout<<"\n\t\tplease choose the dessert you want : ";
cin>>Pizzas[i].dessert;
}
}
nospecial:
cout<<"\n\t\tNumber of pieces : ";
cin>>Pizzas[i].numberOfPieces;
//cout<<"\n\t\tPrice of one piece : ";
//cin>>Pizzas[i].priceOfOnePiece;
while(1){
cout<<"\n\t\tAvaliablity of Coupons(Y|N) : ";
cin>>hasCoupon;
if(hasCoupon=="Y"||hasCoupon=="N"){
break;
}else{
cout<<"\n\t\tEnter Either \"Y\" or \"N\"!!";
continue;
}
while(1){
cout<<"\n\t\tCoupon ID : ";
cin>>couponID;
if(isValid(couponID)==false){
cout<<"\n\t\tInvalid Coupon ID!!";
continue;
}else{
break;
}
}
}
cout<<"\n\t\tPreparing-Finished-Delivered\n";
cout<<"\n\t\tOrder Status : ";
//cout<<"\t\t";
cin>>Pizzas[i].status;
Pizzas[i].paid=prices[ofMenu-1]*Pizzas[i].numberOfPieces-captureCouponSize(couponID);
cout<<"\n\t\tThis Customer Gotta pay : "<<Pizzas[i].paid;
while(1){
cout<<"\n\t\tIs this FINAL(Y|N)?";
cin>>isFinal;
if(isFinal=="Y"||isFinal=="N"){
break;
}else{
cout<<"\n\t\tEnter Either \"Y\" or \"N\"!!";
continue;
}
}
if(isFinal=="Y"){
cout<<"\n\t\tYou Just Sumbitted a new Order!!";
while(1){
cout<<"\n\t\tDo you want to sumbit another order(Y|N)?";
cin>>newOrder;
if(newOrder=="Y"||newOrder=="N"){
break;
}else{
cout<<"\n\t\tEnter Either \"Y\" or \"N\"!!";
continue;
}
}
if(newOrder=="Y"){
index++;
continue;
}else if(newOrder=="N"){
index++;
break;
}
}else if(isFinal=="N"){
index--;
continue;
}
}
}
//----------------------------------------------------------------------=
void checkStatus(){
string customerName;
int anotherChecking=1;
while(!anotherChecking==0){
cout<<"\n\t\tPlease Enter the name of the customer : ";
cin>>customerName;
for(int i =0;i<1000;i++){
if(Pizzas[i].nameOfCustomer==customerName){
cout<<"\n\t\tName : "<<Pizzas[i].nameOfCustomer<<"\n\t\tStatus of the order : "<<Pizzas[i].status;
break;
}else{
cout<<"\n\t\tThere is not any customers with that name in the data!!";
break;
}
}
while(1){
cout<<"\n\t\tDo you want to check another order(0 to return)?";
cin>>anotherChecking;
if(anotherChecking==0){
break;
}else{
cout<<"\n\t\tYou can only enter \"0\"!";
continue;
}
}
}
//TODO: sensitivity for duplicate names
}
//-------------------------------------------------------------------------=
void changeStatus(){
string customerName;
string newStatus;
int anotherChange=1;
while(!anotherChange==0){
cout<<"\n\t\tPlease Enter the name of the customer : ";
cin>>customerName;
for(int i =0;i<1000;i++){
if(Pizzas[i].nameOfCustomer==customerName){
cout<<"\n\t\tPreparing-Finished-Delivered";
cout<<"\n\t\tNew Status : ";
cin>>newStatus;
Pizzas[i].status=newStatus;
break;
}else{
cout<<"\n\t\tThere is not any customers with that name in the data!!";
break;
}
} while(1){
cout<<"\n\t\tDo you want to change another Status(0 to return)?";
cin>>anotherChange;
if(anotherChange==0){
break;
}else{
cout<<"\n\t\tYou can only enter \"0\"!";
continue;
}
}
}
//TODO: sensitivity for duplicate names
}
//------------------------------------------------------------------------------=
void history(){
int toreturn=1;
cout<<"\n\t\tThe History : ";
while(toreturn==1){
for(int i =0;i<index;i++){
cout<<"\n"<<"Customer name : "<<Pizzas[i].nameOfCustomer<<" || Pizza Kind : "<<Pizzas[i].pizzaKind<<" || Size : "<<Pizzas[i].size<<" || Status : "<<Pizzas[i].status<<" || Paid : "<<Pizzas[i].paid;
//TODO: Displaying if the customer had a special order or not
}
while(1){
cout<<"\n\t\tTo return Enter 0 : ";
cin>>toreturn;
if(toreturn==0){
break;
}else{
cout<<"\n\t\tYou can only enter \"0\"!";
continue;
}
}
}
}
//-------------------------------------------------------------------------------=
void generateCoupon(){
int sizeOfCoupon;
string couponID;
while(1){
cout<<"\n\t\tEnter the Size of the coupon to be generated : ";
cin>>sizeOfCoupon;
if(isdigit(sizeOfCoupon)){
break;
}else{
cout<<"\n\t\tPlease enter a proper size!";
continue;
}
}
srand((unsigned)time(0));
int randomnum = rand()%1000;
couponID="Pi-"+to_string(randomnum)+'.'+to_string(sizeOfCoupon)+'z';
cout<<"\n\t\tcoupon ID : "<<couponID;
//TODO: Add ability to generate more than one coupon
}
//----------------------------------------------------------------------------------=
bool isValid(string couponID){
bool isValid=false;
if(couponID.find("-")==2&&couponID.find(".")==6&&couponID.find("z")==9){
isValid=true;
}
return isValid;
}
//-------------------------------------------------------------------------------------=
void printToFile(){
ofstream history;
history.open("history.txt");
for(int i =0;i<index;i++){
history<<"\n"<<"Customer name : "<<Pizzas[i].nameOfCustomer<<" || Pizza Kind : "<<Pizzas[i].pizzaKind<<" || Size : "<<Pizzas[i].size<<" || Status : "<<Pizzas[i].status<<" || Paid : "<<Pizzas[i].paid;
}
history.close();
cout<<"Done Printing To File";
}
//imp TODO: names with spaces in the newPizza function
//------------------------------------------------------------------------------------------=
int captureCouponSize(string couponID){
int couponSize;
couponSize = atoi(couponID.substr(couponID.find(".")+1,couponID.find("z")).c_str());
return couponSize;
}
//---------------------------------------------------------------------------------------=
void showPizzaMenu(){
for(int i =0;i<10;i++){
if(i%2==0&&menu[i]!="null"){
cout<<"\n\t\t\t>> "<<"["<<i+1<<"]"<<menu[i]<<" - ";
}else if(i%2!=0&&menu[i]!="null"){
cout<<"["<<i+1<<"]"<<menu[i];
}
}
} | e8e7d893f62373535b7e43c25178257ea4322d9b | [
"C++"
] | 1 | C++ | ahmdaeyz/Academic-Projects | 079b5ed5d22896c9133e893302ba5d3a4df8ddf0 | 2c3a08bc5310794a2f37beb18ec9dc2e3e4c8466 | |
refs/heads/netush | <repo_name>Netabutensky/Vlogy-Project<file_sep>/vlogy-front-end/src/App.js
import React, { Component } from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import axios from 'axios';
import './App.css';
import Landing from './Components/Landing';
class App extends Component {
constructor() {
super()
this.state = {
MovieData: [],
data: [],
UserData: {},
allData: []
}
}
updateUser = (data) =>{
// console.log(data)
axios.put('http://localhost:5000/updateUser', data)
.then( (response) => {
console.log(response)
this.myData()
})
}
myData = async () => {
let data = await axios.get('http://localhost:5000/users')
let allData = data.data
this.setState({
allData
})
}
newUser = async (user) => {
axios.post('http://localhost:5000/newUser', user)
this.myData()
console.log(this.state.data)
}
UserExict = async(login) => {
let res = await axios.get(`http://localhost:5000/username/${login.username}/password/${login.password}`)
if (res.data[0] === undefined) {
console.log('User not found')
} else {
let UserData = res.data[0]
localStorage.setItem("username", UserData.username)
localStorage.setItem("password", <PASSWORD>)
this.setState({
UserData
}, function () {
console.log(this.state.UserData)
})
}
return res.data[0]
}
componentDidMount = async () => {
this.myData()
}
deleteuser=()=>{
this.setState({
UserData:{}
})}
render() {
return (
<Router>
<div className="App" >
<Landing updateUser={this.updateUser} allData={this.state.allData} newUser={this.newUser} deleteuser={this.deleteuser} UserData={this.state.UserData} UserExict={this.UserExict} newUser={this.newUser} />
</div>
</Router>
);
}
}
export default App;
<file_sep>/vlogy-front-end/src/Components/UserSearch.js
import React, { Component } from 'react'
import axios from 'axios'
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import UserPage from './UserPage';
class UserSearch extends Component {
constructor(props) {
super(props)
this.state = {
comment: '',
follow: this.chekFollowStatus(),
}
}
chekFollowStatus = async() =>{
let username = localStorage.getItem("username")
let password = <PASSWORD>("password")
let user = await axios.get(`http://localhost:5000/username/${username}/password/${password}`)
let i = this.props.followers.findIndex(d => d == user.data[0]._id)
if(this.props.followers.findIndex(d => d == user.data[0]._id) > -1){
this.setState({
follow : true
})
}
else{
this.setState({
follow : false
})
}
return true
}
componentDidMount = () =>{
this.chekFollowStatus()
}
followbutton = (f) => {
if (this.state.follow == true) {
alert('UNFRIENDED')
this.setState({
follow: !this.state.follow
})
} else {
this.setState({
follow: !this.state.follow
})
}
let data ={
follwer: localStorage.getItem("username"),
user: this.props.username
}
this.props.follow(data)
}
render() {
console.log(this.props.followers)
console.log(this.props.following)
let urls = `/user/${this.props.username}`
// console.log(this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(p => p.profilePic))
return (
<div>
<div class="card">
<div class="container">
<h4><b>{this.props.username}</b></h4>
{this.state.follow ? <div onClick={this.followbutton}><i class="fas fa-user-minus"></i>
</div> : <div value={this.props.username} onClick={this.followbutton} > <i class="fas fa-user-plus"></i>
</div>}
{this.props.allData.filter(u => u.username == this.props.username).map(f => f.profilePic === undefined ?
<div> {this.props.username === localStorage.getItem("username") ?
<a href='/userprofile' ><i class="fas fa-user-alt"> </i> </a> :
<a href={urls} ><i class="fas fa-user-alt"> </i> </a>}</div> :
<div> {this.props.username === localStorage.getItem("username") ?
<a href='/userprofile'> <img className="usernameimg" src={f.profilePic} /> </a> :
<a href={urls}> <img className="usernameimg" src={f.profilePic} /> </a>}</div>
)}
<p>following:{this.props.following.length}</p>
<p>followers:{this.props.followers.length}</p>
</div>
</div>
</div>
)
}
}
export default UserSearch<file_sep>/vlogy-front-end/src/Components/FeedComment.js
import React, { Component } from 'react';
class FeedComment extends Component {
delete = () => {
let data = {
username: this.props.vid.user.username,
videoId: this.props.vid.id,
comment: this.props.c
}
// console.log(data)
this.props.deleteComment(data)
}
render() {
let comment = this.props.c
return (<div >
<span> <div class="dialogbox">
<div class="body">
{localStorage.getItem("username") === comment.user ? <span onClick={this.delete}><i class="fas fa-trash"></i></span>:null}
<span class="tip tip-left"></span>
{localStorage.getItem("username") === comment.user ? <h6>Me:</h6> : <h6>{comment.user}:</h6>}
{/* <div onClick={this.delete}><i class="fas fa-trash-alt"></i></div> */}
<div class="message">
<span>{comment.text}</span>
</div>
</div>
</div> </span>
</div>)
}
}
export default FeedComment<file_sep>/vlogy-back-end/server.js
const express = require('express');
const bodyParser = require('body-parser');
const methodOverride = require('method-override');
const router = require('./Routes/Router')
const app = express();
// Middleware
app.use(bodyParser.json());
app.use(methodOverride('_method'));
app.use(function (req, res, next) {
res.header('Access-Control-Allow-Origin', '*')
res.header('Access-Control-Allow-Methods', 'GET,PUT,POST,DELETE,OPTIONS')
res.header('Access-Control-Allow-Headers', 'Content-Type, Authorization, Content-Length, X-Requested-With')
next()
})
app.use('/', router)
const port = 5000;
app.listen(port, () => console.log(`Server started on port ${port}`));<file_sep>/vlogy-front-end/src/Components/Feed.js
import React, { Component } from 'react'
import App from '../App'
import axios from 'axios'
import FeedVideo from './FeedVideo'
import { Link } from 'react-router-dom';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import Draggable, { DraggableCore } from 'react-draggable';
import GridFeed from './GridFeed'
import Feedsecond from './Feedsecond';
class Feed extends Component {
constructor() {
super()
this.state = {
file: React.createRef(),
data: [],
UserData: {},
showupload: false
}
}
handleUploadFile = () => {
const data = new FormData();
let username = localStorage.getItem("username")
data.append('file', this.state.file.current.files[0]);
axios.post('http://localhost:5000/upload', data).then((response) => {
axios({
method: 'put',
url: 'http://localhost:5000/uploadVideo',
data: {
filename: response.data,
username: username
}
}).then((response) => {
this.getFeed()
this.setState({
showupload: false,
file: React.createRef()
})
})
})
}
exit = () => {
alert(`${localStorage.getItem("username")} you are Loging-Out`)
localStorage.clear()
}
showupload = () => {
this.setState({
showupload: !this.state.showupload
})
}
getFeed = async () => {
let videos = await axios.get('http://localhost:5000/feed')
console.log(videos)
let feed = videos.data.filter(o => o.user.username !== localStorage.getItem("username"))
this.setState({ data: feed })
}
componentDidMount = async () => {
this.getFeed()
}
handleinput = (e) => {
this.setState({ file: e.target.value })
}
comment = (data) => {
axios.put('http://localhost:5000/addComment', data)
.then((response) => {
console.log(response)
this.getFeed()
})
}
deleteComment = (data) => {
axios({
method: 'delete',
url: 'http://localhost:5000/comment',
data: data
}).then((response) => {
this.getFeed()
})
}
likeVid = (data) => {
axios.put('http://localhost:5000/like', data)
.then((response) => {
this.getFeed()
})
}
scrolltop=()=>{
window.scrollTo(0, 0)
}
render() {
let scroll=this.scrolltop
return (
<Router>
<div className='feed'>
<span className="myfeed">Feed</span>
<div className="grid"> <a href='/gridfeed' ><i class="fas fa-th-large"></i></a></div>
<a href='/' >
<div onClick={this.exit} className="logOut"><i class="fas fa-walking"></i>
<i class="fas fa-door-open"></i>
</div>
</a>
<div className='input'>
<div className="scrolltop" onClick={scroll} ><i class="fas fa-angle-double-up"></i></div>
{this.state.showupload ?
<div><div onClick={this.showupload} > <li class="fas fa-video"></li></div>
<Draggable>
<div className="uploadcontainer2">
<input className="inputupload" type='file' ref={this.state.file} />
<button className="uploadbutton" onClick={this.handleUploadFile} >upload</button>
</div></Draggable> </div> :
<div onClick={this.showupload} ><li class="fas fa-video"></li></div>}
<div>
{this.state.data.map(v => <FeedVideo users={v.likes.users} deleteComment={this.deleteComment} likeVid={this.likeVid} vid={v} UserData={this.state.UserData} date={v.user.uploads[0].date[0]} comment={this.comment} />)}
</div>
</div>
</div>
</Router>
)
}
}
export default Feed;
<file_sep>/vlogy-front-end/src/Components/Landing.js
import React, { Component } from 'react';
import { Link } from 'react-router-dom';
import Login from './Login';
import Signup from './Signup'
import { BrowserRouter as Router, Route } from 'react-router-dom';
import UserProfile from './UserProfile';
import UserSearch from './UserSearch';
import Feed from './Feed'
import UserPage from './UserPage';
import GridFeed from './GridFeed';
import axios from 'axios';
import Feedsecond from './Feedsecond'
class Landing extends Component {
constructor() {
super()
this.state = {
searchPut: '',
allData: []
}
}
myData = async () => {
let data = await axios.get('http://localhost:5000/users')
let allData = data.data
this.setState({
allData
})
}
componentDidMount = () =>{
this.myData()
}
followUser = (data) =>{
axios({
method: 'put',
url: 'http://localhost:5000/follow',
data: {
user: data.user,
follwer: data.follwer
}
}).then((res) => {
this.myData()
})
}
searchPutChange = (event) => {
const input = event.target.value
this.setState({
searchPut: input,
})
}
render() {
let userPic = this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(p => p.profilePic)
let data = this.state.allData
let searchdata = data.filter(r => r.username.toLowerCase().includes(this.state.searchPut))
return (
<div >
<div>
<ul>
{localStorage.getItem("username") === 'undefined'? <li><div><a class="fas fa-home" href="/"></a></div></li>:<li><a class="fas fa-home" href="/feed"></a></li>}
{localStorage.getItem("username") === 'undefined'? null:<li><a href="/userprofile"><img className="usernameimg4" src={userPic} /></a></li>}
{localStorage.getItem("username") === 'undefined' ? null : <a href="/usersearch"><button className="inputcon" type="submit"><i class="fa fa-search"></i></button></a>}
{localStorage.getItem("username") === 'undefined' ? null : <input value={this.state.searchPut} onChange={this.searchPutChange} className="searchcontainer" type="text" placeholder="Search.." name="search" />}
<div className="vlogy">Vlogy</div>
</ul>
</div>
<Router>
<Route path="/feed" exact render={() => <Feed />} />
<Route path="/" exact render={() => <Login UserData={this.props.UserData} UserExict={this.props.UserExict} />} />
<Route path="/signup" exact render={() => <Signup newUser={this.props.newUser} />} />
<Route path="/gridfeed" exact render={() => <Feedsecond />}/>
<Route path="/usersearch" exact render={() =>
this.state.searchPut === '' ?
data.map(r => <UserSearch follow ={this.followUser} allData={this.state.allData} username={r.username} following={r.following} followers={r.followers} />) :
searchdata.map(r => <UserSearch follow ={this.followUser} updateUser={this.props.updateUser} allData={this.state.allData} username={r.username} following={r.following} followers={r.followers} />)
} />
<Route path='/userprofile' exact render={() => <UserProfile updateUser={this.props.updateUser} allData={this.state.allData} updateprofile={this.props.updateprofile} deleteuser={this.props.deleteuser} UserExict={this.props.UserExict} UserData={this.props.UserData} />} />
<Route path='/user/:username' exact render={({ match }) => <UserPage updateUser={this.props.updateUser} match={match} UserData={this.props.UserData} />} />
</Router>
</div>
)
}
}
export default Landing
<file_sep>/vlogy-front-end/src/Components/Feedsecond.js
import React, { Component } from 'react'
import App from '../App'
import axios from 'axios'
import FeedVideo from './FeedVideo'
import { Link } from 'react-router-dom';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import Draggable, { DraggableCore } from 'react-draggable';
import GridFeed from './GridFeed';
import InfiniteScroll from 'react-infinite-scroller';
class Feed extends Component {
constructor() {
super()
this.state = {
data: []
}
}
getFeed = async () => {
let videos = await axios.get('http://localhost:5000/feed')
let feed = videos.data.filter(o => o.user.username !== localStorage.getItem("username"))
console.log(feed)
this.setState({ data: feed })
}
componentDidMount = async () => {
this.getFeed()
}
render() {
return (
<div>
<div className='feed'>
<span className="myfeed">Feed</span>
<div className="grid"> <a href='/feed' ><i class="fas fa-th-large"></i></a></div>
</div>
{/* <InfiniteScroll
pageStart={2}
loadMore= {this.state.data.map(v => <GridFeed deleteComment={this.deleteComment} likeVid={this.likeVid} vid={v} UserData={this.state.UserData} date={v.user.uploads[0].date[0]} comment={this.comment} />)}
hasMore={true || false}
loader={<div className="loader" key={3}>Loading ...</div>}
>
{this.state.data.map(v => <GridFeed deleteComment={this.deleteComment} likeVid={this.likeVid} vid={v} UserData={this.state.UserData} date={v.user.uploads[0].date[0]} comment={this.comment} />)}
</InfiniteScroll> */}
{this.state.data.map(v => <GridFeed deleteComment={this.deleteComment} likeVid={this.likeVid} vid={v} UserData={this.state.UserData} date={v.user.uploads[0].date[0]} comment={this.comment} />)}
</div>
)
}
}
export default Feed;
<file_sep>/vlogy-front-end/src/Components/UserPage.js
import React, { Component } from 'react'
import axios from 'axios'
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import VideoUserP from './VideoUserP'
import Feed from './Feed';
import FeedVideo from './FeedVideo'
class UserPage extends Component {
constructor() {
super()
this.state = {
userdata: {}
}
}
getUser = async () => {
let username = this.props.match.params.username
let res = await axios.get(`http://localhost:5000/user/${username}`)
this.setState({
userdata: res.data
})
}
componentDidMount = async () => {
this.getUser()
}
likeVid = (data) =>{
axios.put('http://localhost:5000/like', data)
.then( (response) => {
this.getUser()
})
}
comment = (data) => {
console.log(data)
axios.put('http://localhost:5000/addComment', data)
.then((response) => {
console.log(response)
this.getUser()
})
}
deleteComment = (data) =>{
axios({
method: 'delete',
url: 'http://localhost:5000/comment',
data: data
}).then((response) => {
this.getUser()
})
}
deleteuser = () => {
localStorage.username = { 'username': localStorage.getItem("username") };
localStorage.username = undefined
}
render() {
return (
<div className='userprofile'>
<div>
<div className='usernameprofile'> <img className="usernameimg" src={this.state.userdata.profilePic} /> <div > </div>
<a href='/' > <div onClick={this.deleteuser} className="logOut"><i class="fas fa-walking"></i>
<i class="fas fa-door-open"></i>
</div></a>
<form >
<label for="fname">About {this.state.userdata.username}</label>
<div type="text" className="aboutmyself" name="fname" >{this.state.userdata.about} </div>
</form>
<div >
{!this.state.userdata.uploads ? null : this.state.userdata.uploads.map(d =><div className="vidcontainer" >
<VideoUserP deleteComment={this.deleteComment} data={this.state.userdata.uploads} comment ={this.comment} likeVid={this.likeVid} d={d} username={this.state.userdata.username} />
</div> )}
</div>
</div>
</div>
</div>
)
}
}
export default UserPage<file_sep>/vlogy-back-end/Routes/CreateFeed.js
const timeSort = function(vidosarr1, vidosarr2){
let i = 0
let newArr = []
while(i < vidosarr1.length || i < vidosarr2.length){
if(vidosarr1[i] === undefined && vidosarr2[i] !== undefined){
newArr.push(vidosarr2[i])
}
else if(vidosarr2[i] == undefined && vidosarr2[i] !== undefined){
newArr.push(vidosarr2[i])
}
else{
vidosarr1[i].date > vidosarr2[i].date ? newArr.push(vidosarr1[i]) : newArr.push(vidosarr2[i])
vidosarr1[i].date < vidosarr2[i].date ? newArr.push(vidosarr1[i]) : newArr.push(vidosarr2[i])
}
}
return newArr
}
const likeSort = function(vidosarr){
let likeArr = []
for(let vid of vidosarr){
likeArr.push(vid.likes)
}
likeArr.sort(function(a, b){return b - a})
let newArr = []
for(let i = 0; i < likeArr.length; i++){
for(let vid of vidosarr){
if(vid.likes == likeArr[i])
newArr.push(vid)
break
}
}
return newArr
}
const createFeed = function(vidosarr1, vidosarr2){
let sortedByDate = timeSort(vidosarr1, vidosarr2)
let lastday = new Date(), lastweek = new Date(), lastmonth = new Date()
lastday.setDate(lastday.getDate()-1)
lastweek.setDate(lastday.getDate() -7)
lastmonth.setMonth(lastday.getMonth() -1)
let lastDayVideos = []
let lastWeekVideos = []
let lastMonthVideos = []
let others = []
for(let vid of sortedByDate){
if(vid.date > lastday){
lastDayVideos.push(vid)
}
else if(vid.date > lastweek){
lastWeekVideos.push(vid)
}
else if(vid.date > lastmonth){
lastMonthVideos.push(vid)
}
else{
others.push(vid)
}
}
let Feed = []
Feed.concat(likeSort(lastDayVideos))
Feed.concat(likeSort(lastWeekVideos))
Feed.concat(likeSort(lastMonthVideos))
Feed.concat(likeSort(others))
return Feed
}
module.exports = createFeed<file_sep>/vlogy-front-end/src/Components/UserProfile.js
import React, { Component } from 'react';
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
import axios from 'axios';
import feed from './Feed'
import Login from './Login';
import Video from './Video';
class UserProfile extends Component {
constructor() {
super()
this.state = {
username: '',
password: '',
about: '',
showlogout: false,
UserData: {uploads : []},
img: '',
editimg: false,
editingtwo: false,
file: React.createRef(),
showupload: false,
edit:false
}
}
edit=()=>{
this.setState({
edit:!this.state.edit
})
}
updateUser = () => {
let datas = this.props.updateUser({ data: this.state.img, prop: 'profilePic', username: localStorage.getItem("username") })
console.log(datas)
}
updateabout = () => {
let datas = this.props.updateUser({ data: this.state.about, prop: 'about', username: localStorage.getItem("username") })
console.log(datas)
}
handleeditimg = () => {
this.setState({
img: '',
editimg: !this.state.editimg,
})
}
handleeditimgtwo = () => {
this.setState({
editingtwo: !this.state.editingtwo,
})
}
showupload = () => {
this.setState({
showupload: !this.state.showupload,
})
}
handleUserName = (u) => {
let username = u.target.value
this.setState({
username
})
}
handleimg = (u) => {
let img = u.target.value
this.setState({
img
}, function () {
this.updateUser()
})
}
handleshow = () => {
this.setState({
showlogout: !this.state.showlogout
})
}
handlePassword = (p) => {
let password = p.target.value
this.setState({
password
})
}
UserExict = async () => {
let UserData = await this.props.UserExict({ username: this.state.username, password: <PASSWORD> })
console.log('hello')
console.log(UserData)
}
handleAbout = (v) => {
let about = v.target.value
this.setState({
about
}, function () {
this.updateabout()
})
}
exit = () => {
alert(`${localStorage.getItem("username")} you are Loging-Out`)
localStorage.clear()
this.deleteuser()
}
getUser = async () => {
let username = localStorage.getItem('username')
let password = localStorage.getItem('password')
let user = await axios.get(`http://localhost:5000/username/${username}/password/${password}`)
this.setState({ UserData: user.data[0] })
}
deleteVideo = (videoId) => {
let username = localStorage.getItem("username")
axios({
method: 'delete',
url: `http://localhost:5000/files/${videoId}/${username}`,
}).then((response) => {
console.log(response)
this.getUser()
})
}
componentDidMount = () => {
this.getUser()
}
handleUploadFile = () => {
const data = new FormData();
let username = localStorage.getItem("username")
data.append('file', this.state.file.current.files[0]);
axios.post('http://localhost:5000/upload', data).then((response) => {
axios({
method: 'put',
url: 'http://localhost:5000/uploadVideo',
data: {
filename: response.data,
username: username
}
}).then((response) => {
this.setState({
file: React.createRef(),
showupload: false
})
this.getUser()
})
})
}
deleteuser = () => {
localStorage.username = { 'username': localStorage.getItem("username") };
localStorage.username = undefined
}
likeVid = (data) =>{
axios.put('http://localhost:5000/like', data)
.then( (response) => {
this.getUser()
})
}
comment = (data) => {
axios.put('http://localhost:5000/addComment', data)
.then((response) => {
console.log(response)
this.getUser()
})
}
deleteComment = (data) =>{
axios({
method: 'delete',
url: 'http://localhost:5000/comment',
data: data
}).then((response) => {
this.getUser()
})
}
render() {
// console.log(this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(r => r.about))
let userPic = this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(p => p.profilePic)
console.log(userPic)
// console.log(this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(p => p.following)[0])
return (
< Router >
<div className='userprofile'>
<div>
{this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(p => p.profilePic)[0] === undefined ?
<div><input className="imginput" value={this.state.img} onChange={this.handleimg} placeholder="put image url" /> <div className='usernameprofile'> <i class="fas fa-user-alt"> </i>
{localStorage.getItem("username")}</div> </div>
:
<div className='usernameprofile'> <img className="usernameimg" src={userPic} /> <div onClick={this.handleeditimg} ><i class="fas fa-user-edit"></i></div> {localStorage.getItem("username")} </div>}
<div>{this.state.editimg ? <input className="imginput" value={this.state.img} onChange={this.handleimg} placeholder="put image url" /> : null}</div>
<div onClick={this.edit}><i class="far fa-edit"></i></div>
{/* {this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(f => <div className="followersprofile"><div>Following: {f.following} </div><div>Followers: {f.followers}</div></div>)} */}
<a href='/' > <div onClick={this.deleteuser} className="logOut"><i class="fas fa-walking"></i>
<i class="fas fa-door-open"></i>
</div></a>
{this.state.showupload ?
<div><div onClick={this.showupload} ><li class="fas fa-video"></li></div>
<div className="uploadcontainer2">
<input className="inputupload" type='file' ref={this.state.file} />
<button className="uploadbutton" onClick={this.handleUploadFile} >upload</button>
</div></div> :
<div onClick={this.showupload} ><li class="fas fa-video"></li></div>}
<form >
<label for="fname">About MySelf</label>
{this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(r => r.about) === undefined || this.state.editingtwo ? <input value={this.props.UserData.about} onChange={this.handleAbout} type="text" className="aboutmyself" name="fname" /> :
<div type="text" className="aboutmyself" name="fname">{this.props.allData.filter(u => u.username == localStorage.getItem("username")).map(r => r.about)}<span> {this.state.edit?<i onClick={this.handleeditimgtwo} className="fas fa-pencil-alt"></i>:null} </span></div> }
</form>
<div>
{this.state.UserData.uploads.map(d =>
this.state.edit?
<div className="video-container">
<div onClick={this.deleteVideo}><i class="fas fa-trash"></i>
</div>
<Video likeVid={this.likeVid} userdata={this.state.UserData} d={d} deleteComment={this.deleteComment} comment={this.comment} />
</div>:
<div className="video-container">
<Video likeVid={this.likeVid} userdata={this.state.UserData} d={d} deleteComment={this.deleteComment} comment={this.comment} />
</div>
)}
</div>
</div>
</div>
</Router >
);
}
}
export default UserProfile;
<file_sep>/vlogy-front-end/src/Components/VideoUserP.js
import React, { Component } from 'react';
import { Link } from 'react-router-dom';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import CommentPage from './CommentPage';
import axios from 'axios';
class VideoUserP extends Component {
constructor() {
super()
this.state = {
like: 0,
likes: false,
timeclick: 0,
comment: "",
showcomments: false,
red: false
}
}
showcomments = () => {
this.setState({
showcomments: !this.state.showcomments
})
}
postLike = () => {
if (this.state.like == 0) {
this.setState({
likes: true,
like: this.props.d.likes + 1,
}, function () {
this.updatelikes()
})
}
else if (this.state.like < 2) {
this.setState({
like: this.props.d.like == 0
})
}
else if (this.state.like > 1) {
this.setState({
likes: true,
like: this.props.d.likes - 1,
}, function () {
this.updatelikes()
})
}
}
updateUserVideo = (data) => {
axios.put('http://localhost:5000/like', data)
// .then( (response) => {
// this.myData()
// })
}
like = () => {
let vid = this.props.d
let data = {
username: localStorage.getItem("username"),
videoId: vid.videoId,
uploader: this.props.username
}
this.props.likeVid(data)
this.setState({
red: !this.state.red
})
}
handleInput = (e) => {
this.setState({
comment: e.target.value,
})
}
commentfunction = () => {
let data = {
username: localStorage.getItem("username"),
videoId: this.props.d.videoId,
comment: this.state.comment,
uploader: this.props.username
}
this.props.comment(data)
this.setState({
comment: ""
})
}
render() {
console.log(this.props.d.comments)
let d = this.props.d;
// let liked= d.likes.users.map(r=> r === localStorage.getItem("username") )
return (
<div >
<div class="card7">
<div className="container" >
<video className="videoss" width="355" height="300" controls>
<source src={`http://localhost:5000/video/${this.props.d.videoId}`} />
</video>
<div>
<i onClick={this.showcomments} class="far fa-comment-dots"></i>
{/* {d.likes.users.map(r=> r === localStorage.getItem("username") ?
<div><i id="mylikes" onClick={this.like} class="fab fa-gratipay" id= "red" ></i><span className="numlikes">{d.likes.num}</span> </div>:
null)} */}
<i id="mylikes" onClick={this.like} class="fab fa-gratipay" id={this.state.red ? "red" : "b"} ></i><span className="numlikes">{d.likes.num}</span>
</div>
{this.state.showcomments ? <div className="dugma"><div class="dialogbox7">
{this.props.d.comments.map(c => <CommentPage deleteComment={this.props.deleteComment} comment={c} user={this.props.username} vidId={this.props.d.videoId} />)}
</div> <span><input className="commentsss8" type='text' value={this.state.comment} onChange={this.handleInput} placeholder='comment' /> <span onClick={this.commentfunction}><i class="fas fa-plus"></i>
</span></span></div> : null}
</div>
</div>
</div>
)
}
}
export default VideoUserP
| 3990f19d57874a6509a18d27e05d22da2aa097e9 | [
"JavaScript"
] | 11 | JavaScript | Netabutensky/Vlogy-Project | 442a0e52bded413144c24cfc11822b1aa50c6bc6 | d2ea8c69c6ca0dbfa917db9881036fc5def775b3 | |
refs/heads/master | <file_sep>import org.apache.log4j.Logger;
/**
* Created by dvalo on 23.10.2016.
* Data class for coordinate with 2 values longtitude and latitude
*/
public class Coordinate {
static Logger log = Logger.getLogger(Coordinate.class.getName());
private double x = 0;
private double y = 0;
//cordinatesPlaintText = [-122.48369693756104, 37.83381888486939]
public Coordinate(String cordinatesPlaintText) {
cordinatesPlaintText = cordinatesPlaintText.replaceAll("\\[","").replaceAll("]","");
String[] coordinates = cordinatesPlaintText.split(",");
if(coordinates.length==2) {
this.x = Double.parseDouble(coordinates[0]);
this.y = Double.parseDouble(coordinates[1]);
}else{
log.info("Coordinates does not have 2 values: "+cordinatesPlaintText);
}
}
/**
*
* @return coordinates in format: [-122.48369693756104, 37.83381888486939], where [x,y]
*/
public String getCoordinate(){
String coordinate = "";
coordinate ="["+x+","+y+"]";
return coordinate;
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
}
<file_sep># General course assignment
Build a map-based application, which lets the user see geo-based data on a map and filter/search through it in a meaningfull way. Specify the details and build it in your language of choice. The application should have 3 components:
1. Custom-styled background map, ideally built with [mapbox](http://mapbox.com). Hard-core mode: you can also serve the map tiles yourself using [mapnik](http://mapnik.org/) or similar tool.
2. Local server with [PostGIS](http://postgis.net/) and an API layer that exposes data in a [geojson format](http://geojson.org/).
3. The user-facing application (web, android, ios, your choice..) which calls the API and lets the user see and navigate in the map and shows the geodata. You can (and should) use existing components, such as the Mapbox SDK, or [Leaflet](http://leafletjs.com/).
# Application description
- Zobrazovanie parkov a pláží na území Slovenska podľa mesta v ktorom sa nachádzajú
- Zobrazovanie parkov a pláží na území Slovenska podľa mesta v ktorom sa nachádzajú alebo výber mesta kurzorom alebo aktuálnou GPS polohou
- Zobrazovanie parkov a pláží na území Slovenska podľa mesta v ktorom sa nachádzajú, podľa ich rozlohy
- Zobrazovanie parkov a pláží na území Slovenska ak sa okolo nich nachádzajú bary do špecifikovanej vzdialenosti - zobrazenie aj barov
- Zobrazovanie parkov a pláží na území Slovenska podľa toho, či sa v nich nachádza občerstvenie alebo wc
# Môj projekt (Hybridná aplikácia Web+Android+IOS)
- Map-based hybridná aplikácia
založená na Apache-Cordova, ktorá komunikuje s backendom, realizovaným v Jave pomocou frameworku Spark,využívaného na REST-ové volania. Front-end je vytvorený pomocou frameworku apache-cordova, ktorý nám umožnuje vybuildovať aplikáciu pre rôzne platformy(screenshots/platforma-android.png,screenshots/platforma-ios.png,screenshots/platforma-web.png,). Hybridnú aplikáciu sme zvolili, kvôli multiplaformovosti a jednoduchému prístupu k natívnym komponentom ako je GPS, ktorú využívame. Môžeme vyhľadať všetky pláže a parky v meste v ktorom sa práve nachádzame. Toto získame práve pomocou GPS.
Front-end aplikáciu som vyexportoval ako webovú aplikáciu, spustiteľnú v ľubovoľnom prehliadači(Ja som používal Chrome) a zdrojáky priložil k odovzdaniu, pretože celý projekt zahrňujúci všetky platformy mal okolo 120MB. Projekt som uložil na googledrive, odkiaľ je možné si ho stiahnuť ().
Zdrojové kódy webovej aplikácie, spustiteľnej v prehliadači som vložil do tohto repozitára do zložky front-end.
Projekt sa spúšťa:
-na back-ende pomocou triedy /src/java/Main.java
-na front-ende pomocou súboru /front_end/index.html a logika k nemu je v súbore /scripts/index.js
- Databáza
Dáta sme získali z voľne dostupných datasetov, importovali ich vo formáte shapeFile pomocou postGis importera.
S dátami administratívnych území bol problém, pretože geom hodota nebola správna a bolo potrebné celý shapeFile
skonvertovať pomocou konvertera: (https://zbgis.skgeodesy.sk/zbgisconvert/). Využívali sme nasledujúce tabuľky:
- gis_osm_pois_free_1 -wc,bary
- gis_osm_pois_a_free_1 -obcerstvenie
- Parky -parky
- gis_osm_natural_a_free_1 -pláže
- hranice_obce_6 -mesta
- Back-end Pomocou fraeworku Spark pracujeme s REStovými volaniami.
Selecty som chcel pôvodne robiť pomocou MyBatis frameworku (http://www.mybatis.org/mybatis-3/) s využitím mapovača ale potom som sa rozhodol urobiť generátor selectov a kvôli tomu som ho nemohol použiť pretože musím selecty postupne vyskladávať podľa používateľových vstupov.
1. Zachytíme request.
2. Po zachytení requestu zoberieme argumenty a vytvoríme požadovaný select.
3. Bolo viacero zadefinovaných scenárov ako využívať rozlohu parkov, vzdialenosť barov a toho čo sa nachádza v parkoch, ale tie obmedzovali používateľa, preto som nevytvoril len natvrdo zadefinované selecty ale som vytvoril generátor na selecty, ktorý kombinuje rôzne možnosti, ktoré si používateľ môže zvoliť a tak využil všetky prvy rôznych scenárov naplno.
4. Ukážka vygenerovaného jednoduchého a zložitejšieho selectu:
SELECT obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS parky,park.name AS parkName
FROM Parky AS park,hranice_obce_6 AS obce
WHERE obce.nm2 LIKE '%'
AND ST_WITHIN(park.geom, obce.geom)
SELECT wc.name AS wcName,ST_AsGeoJSON(wc.geom) AS wcka,obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city,ST_Area(park.geom::geography) AS rozloha, ST_AsGeoJSON(park.geom) AS parky,park.name AS parkName,obcer.name AS obcerName ,obcer.fclass AS obcerClass,ST_AsGeoJSON(obcer.geom) AS obcerstvenie,Obar.name AS obarName ,Obar.fclass AS obarClass,ST_AsGeoJSON(Obar.geom) AS obar
FROM gis_osm_pois_a_free_1 AS obcer, gis_osm_pois_free_1 AS Obar,Parky AS park,hranice_obce_6 AS obce,gis_osm_pois_free_1 AS wc
WHERE obce.nm2 LIKE '%'
AND wc.fclass='toilet'
AND ST_WITHIN(wc.geom, park.geom)
AND ST_WITHIN(park.geom, obce.geom)
AND ST_Area(park.geom::geography) < 5600000
AND ST_Area(park.geom::geography) > 0
AND (obcer.fclass='theatre' OR obcer.fclass='fast_food' OR obcer.fclass='restaurant' OR obcer.fclass='cafe' OR obcer.fclass='bar' OR obcer.fclass='playground' OR obcer.fclass='attraction' OR obcer.fclass='theme_park')
AND ST_WITHIN(obcer.geom, park.geom)
AND (Obar.fclass='pub' OR Obar.fclass='bar' OR Obar.fclass='nightclub' OR Obar.fclass='cafe' OR Obar.fclass='nightclub')
AND ST_WITHIN(Obar.geom, obce.geom)
AND ST_DISTANCE(park.geom::geography,Obar.geom::geography)<500
//-- Another select generator with coordinates(dlzka,sirka) as input --
String select = "" +
"SELECT "+
showWc + showDistance + showObcerstvenie + showRozloha +
"obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS parky,park.name AS parkName "+
"FROM " +
fromWc + fromDistance + fromObcerstvenie+
"Parky AS park,hranice_obce_6 AS obce "+
"WHERE ST_WITHIN(ST_MakePoint("+dlzka+","+sirka+"),obce.geom) "+
whereWc+
"AND ST_WITHIN(park.geom, obce.geom)"+
whereRozloha+
whereObcerstvenie+
whereDistance;
5. Odpoveď z databázy spracujeme a vytvoríme novú odpoveď ako GeoJSON, ktorý rovno na front-ende vložíme do mapbox API volania.
6. Bolo potrebné vytvoriť konvertej GeoJson-u z databázy na GeoJson, ktorý príjma mapbox API.
- Frontend
1. Overíme vstupy - či sú hodnoty zadané
2. Vytvoríme volanie na back-end
3. Po prijatí refreshneme mapu, naplníme tabuľku prijatými dátami a ukážeme notifikáciu o prijatí dát
4. Dáta so zobrazia pomocou niektorej z vytvorených vrstiev:
a. Mesto(city)
b. Park(park)
c. Plaz(beach)
d. Obcerstvenie(obcerstvenie)
e. Bary a wc(points)
- Frontend
1. Overíme vstupy - či sú hodnoty zadané
2. Vytvoríme volanie na back-end
3. Po prijatí refreshneme mapu, naplníme tabuľku prijatými dátami a ukážeme notifikáciu o prijatí dát
4. Dáta so zobrazia pomocou niektorej z vytvorených vrstiev:
a. Mesto(city)
b. Park(park)
c. Plaz(beach)
d. Obcerstvenie(obcerstvenie)
e. Bary a wc(points)
## Screenshots
- Záber zobrazujúci bočný panel obsahujúci filter + vyhľadanie oblasti pláže + tabuľu všetkých zaujímavých bodov/oblastí + okolitých barov + detail na bar
(screenshots/screensho-detail.PNG)
- Záber zobrazujúci bočný panel obsahujúci filter + vyhľadanie oblasti parku a všetkých občerstvení/wc, ktoré sa v ňom nachádzajú + tabuľu všetkých zaujímavých bodov/oblastí + detail na zaujímavý bod + okolité bary
(screenshots/screenshot-park_s_obcerstvenim_aj_wc_aj_okolitymi_barmi.PNG)
- Záber zobrazujúci bočný panel obsahujúci filter + tabuľu všetkých zaujímavých bodov/oblastí + vyhľadanie oblasti parku podľa kurzora na mape a notifikáciu o prijatých výsledkoch
(screenshots/screenshot-vyhladanie_podla_kurzora-notifikacia.PNG)
##Implementovanie zaujimave funkcie
- Možnosť kliknutia na body aj polygóny na mape- zobrazenie detailov, realizované pop-upom
- Hľadanie podľa GPS polohy
- Zobrazenie všetkých zaujímavých bodov v tabuľke, pričom po kliknutí na daný bod v tabuľke sa mapa vycentruje na tento bod
- Notifikácia o to, že hľadanie výsledkov bolo dokončené
- Možnosť výberu mesta pomocou pohybovateľného kurzora
- Responzívne otvárateľný filter
## Zdroje dát
- [Vektorové dáta administratívnych území Slovenska] http://wiki.freemap.sk/HraniceAdministrativnychUzemi - bolo otrebné prekonvertovať geom dáta, pomocou konvertera na stránke
- [Zaujimave body a ostatne data] http://wiki.freemap.sk/OpenStreetMap
- [Uploadnute shp súbory] https://drive.google.com/open?id=0B1H7sUvssn2MdEVJU2h1Q1E5SDg
- [Pozadie] https://wallpaperscraft.com/image/park_autumn_foliage_benches_trees_99110_1920x1080.jpg
## Použité nástroje
- Intelij IDEA 2016 - Back-end
- Visual Studio 2015/Apache-Cordova - Front-end
- Postgres/Postgis - Databáza
## Použité tehnológie
- [Spark] http://sparkjava.com/ (v2.3)
- [Mapbox](http://mapbox.com) (v2.2.2)
- [PostgreSQL](http://www.postgresql.org/) (v9.6)
- [PostGIS](http://postgis.net/) (v2.2.4)
- [Apache/Cordova] Hybridna aplikacia
- [Html/CSS/JS] Bootsrtap, Front-end
- [Java] Back-end (v1.8)
- [PNotify] Notifikacie https://sciactive.com/pnotify/
- [Simple Sidebar] Bočný bar na filter http://startbootstrap.com/template-overviews/simple-sidebar/
<file_sep>import org.apache.log4j.Logger;
import org.json.JSONArray;
import org.json.JSONObject;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Exchanger;
/**
* Created by dvalo on 23.10.2016.
* Class that keeps method for processing response from database. Convert result to objects.
*/
public class PostgisResponseProcessing {
static Logger log = Logger.getLogger(PostgisResponseProcessing.class.getName());
/**
* Parse response from query and generate new Json for MapBox
* @param response Response from query to database
* @return GeoJson for MapBox
*/
public static String processPostgisResponse( ResultSet response){
String geoJson = "";
List<GeoGeometry> responseObjects = new ArrayList<GeoGeometry>();
Map<String,GeoGeometry> responseObjectsAnyDuplicities = new HashMap<String,GeoGeometry>();
try {
String type ="";
//we have to parse response to objects
log.info("Processing response : ");
while ( response.next() ) {
JSONObject st_asGeoJson = new JSONObject(response.getString("city"));
String cityName = null;
String parkName = null;
String obcerName = null;
String wckaName = null;
String obarName = null;
String plazName = null;
try{
cityName = response.getString("cityName");
processResponse(response,"city",cityName,"",GeoGeometry.MY_TYPE_CITY,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
try{
parkName = response.getString("parkName");
processResponse(response,"parky",parkName,"",GeoGeometry.MY_TYPE_PARK,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
try{
obcerName = response.getString("obcerName");
processResponse(response,"obcerstvenie",obcerName,"",GeoGeometry.MY_TYPE_OBCERSTVENIE,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
try{
wckaName = response.getString("wcName");
processResponse(response,"wcka",wckaName,"",GeoGeometry.MY_TYPE_WC,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
try{
obarName = response.getString("obarName");
processResponse(response,"obar",obarName,"",GeoGeometry.MY_TYPE_OUTSIDE_BAR,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
try{
plazName = response.getString("plazName");
processResponse(response,"plaze",plazName,"",GeoGeometry.MY_TYPE_BEACH,responseObjects,responseObjectsAnyDuplicities);
}catch (Exception e){
}
}
//we generate geoJson for mapbox according type
geoJson = GeoJsonMapboxFactory.generateMapBoxJson(responseObjects);
response.close();
} catch (SQLException e) {
e.printStackTrace();
}
return geoJson;
}
/**
* Method fill hashmap and list with new Geo object, and method prevent duplicities
*
* @param response
* @param columnGeomName
* @param name
* @param description
* @param myType
* @param responseObjects
* @param responseObjectsAnyDuplicities
*/
private static void processResponse(ResultSet response,String columnGeomName,String name,String description, String myType,List<GeoGeometry> responseObjects,Map<String,GeoGeometry> responseObjectsAnyDuplicities){
try {
JSONObject parky = new JSONObject(response.getString(columnGeomName));
String type = parky.getString("type");
String coordinatesPark = parky.getJSONArray("coordinates").toString();
GeoGeometry geoGeometryPark = null;
if(!responseObjectsAnyDuplicities.containsKey(coordinatesPark)) {
switch (type) {
case GeoPoint.TYPE_POSTGRES:
geoGeometryPark = processPointResponse(parky, name, description, myType);
break;
case GeoMultiLineString.TYPE_POSTGRES:
geoGeometryPark = processMultiStringResponse(parky, name, description, myType);
break;
case GeoPolygon.TYPE_POSTGRES:
geoGeometryPark = processPolygonResponse(parky, name, description, myType);
break;
}
if (geoGeometryPark != null) {
responseObjects.add(geoGeometryPark);
responseObjectsAnyDuplicities.put(coordinatesPark,geoGeometryPark);
}
}
} catch (SQLException e) {
e.printStackTrace();
}
}
private static GeoPoint processPointResponse(JSONObject st_asGeoJson,String name,String description,String myType){
GeoPoint geoPoint = null;
JSONArray coordinateArray = st_asGeoJson.getJSONArray("coordinates");
geoPoint = new GeoPoint(coordinateArray.toString(),name,description,myType);
return geoPoint;
}
private static GeoMultiLineString processMultiStringResponse(JSONObject st_asGeoJson,String name,String description,String myType){
GeoMultiLineString geoMultiLineString = null;
JSONArray coordinateArray = st_asGeoJson.getJSONArray("coordinates");
geoMultiLineString = new GeoMultiLineString(coordinateArray.toString(),name,description,myType);
return geoMultiLineString;
}
private static GeoPolygon processPolygonResponse(JSONObject st_asGeoJson,String name,String description,String myType){
GeoPolygon geoPolygon = null;
String type = st_asGeoJson.getString("type");
JSONArray coordinateArray = st_asGeoJson.getJSONArray("coordinates");
geoPolygon = new GeoPolygon(coordinateArray.toString(),name,description,myType);
return geoPolygon;
}
}
<file_sep>/**
* Created by dvalo on 27.11.2016.
* Class for dynamic generating selects according user inputs
*/
public abstract class SelectFactory {
private static final String SHOW_WC ="wc.name AS wcName,ST_AsGeoJSON(wc.geom) AS wcka,";
private static final String FROM_WC ="gis_osm_pois_free_1 AS wc,";
private static final String WHERE_WC ="AND wc.fclass='toilet' " +
"AND ST_WITHIN(wc.geom, park.geom) ";
private static final String SHOW_OBCERSTVENIE ="obcer.name AS obcerName ,obcer.fclass AS obcerClass,ST_AsGeoJSON(obcer.geom) AS obcerstvenie,";
private static final String FROM_OBCERSTVENIE ="gis_osm_pois_a_free_1 AS obcer,";
private static final String WHERE_OBCERSTVENIE ="AND (obcer.fclass='theatre' OR obcer.fclass='fast_food' OR obcer.fclass='restaurant' OR obcer.fclass='cafe' OR obcer.fclass='bar' OR obcer.fclass='playground' OR obcer.fclass='attraction' OR obcer.fclass='theme_park') " +
"AND ST_WITHIN(obcer.geom, park.geom) ";
private static final String SHOW_DISTANCE ="Obar.name AS obarName ,Obar.fclass AS obarClass,ST_AsGeoJSON(Obar.geom) AS obar,";
private static final String FROM_DISTANCE ="gis_osm_pois_free_1 AS Obar,";
private static String getWHERE_DISTANCE(String reqInputVzdialenost){
return "AND (Obar.fclass='pub' OR Obar.fclass='bar' OR Obar.fclass='nightclub' OR Obar.fclass='cafe' OR Obar.fclass='nightclub') " +
"AND ST_WITHIN(Obar.geom, obce.geom) " +
"AND ST_DWITHIN(park.geom::geography,Obar.geom::geography,"+ reqInputVzdialenost +")";
}
private static final String SHOW_ROZLOHA ="";
private static final String FROM_ROZLOHA ="";
private static String getWHERE_ROZLOHA(String reqInputMaxRozloha,String reqInputMinRozloha){
return "AND ST_Area(park.geom::geography) < " + reqInputMaxRozloha + " " +
"AND ST_Area(park.geom::geography) > " + reqInputMinRozloha + " ";
}
/*
Create select from template. This method create selects for parks.
Method create selects for request with specified city.
*/
public static String getSelectTemplate(
boolean wc,boolean obcerstvenie,boolean rozloha,boolean distance, String placeName, String reqInputVzdialenost,String reqInputMaxRozloha,String reqInputMinRozloha
){
/*
Skontrolujeme ci nedostavame suradnice namiesto miesta
*/
if(placeName.contains("MyPoint")){
return getSelectTemplateFromCircle( wc, obcerstvenie, rozloha, distance, placeName, reqInputVzdialenost, reqInputMaxRozloha, reqInputMinRozloha);
}
String showWc = "";
String fromWc = "";
String whereWc ="";
String showObcerstvenie = "";
String fromObcerstvenie = "";
String whereObcerstvenie ="";
String showDistance = "";
String fromDistance = "";
String whereDistance ="";
String showRozloha = "";
String fromRozloha = "";
String whereRozloha ="";
if(wc){
showWc = SHOW_WC;
fromWc =FROM_WC;
whereWc = WHERE_WC;
}
if(obcerstvenie){
showObcerstvenie = SHOW_OBCERSTVENIE;
fromObcerstvenie = FROM_OBCERSTVENIE;
whereObcerstvenie = WHERE_OBCERSTVENIE;
}
if(distance){
showDistance = SHOW_DISTANCE;
fromDistance = FROM_DISTANCE;
whereDistance = getWHERE_DISTANCE(reqInputVzdialenost);
}
if(rozloha){
showRozloha = SHOW_ROZLOHA;
fromRozloha =FROM_ROZLOHA;
whereRozloha = getWHERE_ROZLOHA(reqInputMaxRozloha,reqInputMinRozloha);
}
//-------Generate select
String select = "" +
"SELECT "+
showWc + showDistance + showObcerstvenie + showRozloha +
"obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS parky,park.name AS parkName "+
"FROM " +
fromWc + fromDistance + fromObcerstvenie+
"Parky AS park,hranice_obce_6 AS obce "+
"WHERE obce.nm2 LIKE '"+placeName+"%' "+
whereWc+
"AND ST_WITHIN(park.geom, obce.geom)"+
whereRozloha+
whereObcerstvenie+
whereDistance;
return select;
}
/*
Create select from template. This method create selects for beach.
Method create selects for request with specified city.
*/
public static String getSelectTemplateForBeach(
boolean wc,boolean obcerstvenie,boolean rozloha,boolean distance, String placeName, String reqInputVzdialenost,String reqInputMaxRozloha,String reqInputMinRozloha
){
/*
Skontrolujeme ci nedostavame suradnice namiesto miesta
*/
if(placeName.contains("MyPoint")){
return getSelectTemplateForBeachFromCircle( wc, obcerstvenie, rozloha, distance, placeName, reqInputVzdialenost, reqInputMaxRozloha, reqInputMinRozloha);
}
String showWc = "";
String fromWc = "";
String whereWc ="";
String showObcerstvenie = "";
String fromObcerstvenie = "";
String whereObcerstvenie ="";
String showDistance = "";
String fromDistance = "";
String whereDistance ="";
String showRozloha = "";
String fromRozloha = "";
String whereRozloha ="";
if(wc){
showWc = SHOW_WC;
fromWc =FROM_WC;
whereWc = WHERE_WC;
}
if(obcerstvenie){
showObcerstvenie = SHOW_OBCERSTVENIE;
fromObcerstvenie = FROM_OBCERSTVENIE;
whereObcerstvenie = WHERE_OBCERSTVENIE;
}
if(distance){
showDistance = SHOW_DISTANCE;
fromDistance = FROM_DISTANCE;
whereDistance = getWHERE_DISTANCE(reqInputVzdialenost);
}
if(rozloha){
showRozloha = SHOW_ROZLOHA;
fromRozloha =FROM_ROZLOHA;
whereRozloha = getWHERE_ROZLOHA(reqInputMaxRozloha,reqInputMinRozloha);
}
//-------Generate select
String select = "" +
"SELECT "+
showWc + showDistance + showObcerstvenie + showRozloha +
"obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS plaze,park.name AS plazName "+
"FROM " +
fromWc + fromDistance + fromObcerstvenie+
"gis_osm_natural_a_free_1 AS park,hranice_obce_6 AS obce "+
"WHERE obce.nm2 LIKE '"+placeName+"%' "+
"AND park.fclass='beach'"+
whereWc+
"AND ST_WITHIN(park.geom, obce.geom)"+
whereRozloha+
whereObcerstvenie+
whereDistance;
return select;
}
/*
Create select from template. This method create selects for parks.
Method create selects for request with coordinates(dlzka,sirka) as input.
For example, from current GPS location or cursor on the map.
*/
public static String getSelectTemplateFromCircle(
boolean wc,boolean obcerstvenie,boolean rozloha,boolean distance, String placeName, String reqInputVzdialenost,String reqInputMaxRozloha,String reqInputMinRozloha
){
String dlzka ="0";
String sirka= "0";
placeName = placeName.replaceAll("MyPoint","");
dlzka = placeName.split(",")[0];
sirka = placeName.split(",")[1];
String showWc = "";
String fromWc = "";
String whereWc ="";
String showObcerstvenie = "";
String fromObcerstvenie = "";
String whereObcerstvenie ="";
String showDistance = "";
String fromDistance = "";
String whereDistance ="";
String showRozloha = "";
String fromRozloha = "";
String whereRozloha ="";
if(wc){
showWc = SHOW_WC;
fromWc =FROM_WC;
whereWc = WHERE_WC;
}
if(obcerstvenie){
showObcerstvenie = SHOW_OBCERSTVENIE;
fromObcerstvenie = FROM_OBCERSTVENIE;
whereObcerstvenie = WHERE_OBCERSTVENIE;
}
if(distance){
showDistance = SHOW_DISTANCE;
fromDistance = FROM_DISTANCE;
whereDistance = getWHERE_DISTANCE(reqInputVzdialenost);
}
if(rozloha){
showRozloha = SHOW_ROZLOHA;
fromRozloha =FROM_ROZLOHA;
whereRozloha = getWHERE_ROZLOHA(reqInputMaxRozloha,reqInputMinRozloha);
}
//-------Generate select
String select = "" +
"SELECT "+
showWc + showDistance + showObcerstvenie + showRozloha +
"obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS parky,park.name AS parkName "+
"FROM " +
fromWc + fromDistance + fromObcerstvenie+
"Parky AS park,hranice_obce_6 AS obce "+
"WHERE ST_WITHIN(ST_MakePoint("+dlzka+","+sirka+"),obce.geom) "+
whereWc+
"AND ST_WITHIN(park.geom, obce.geom)"+
whereRozloha+
whereObcerstvenie+
whereDistance;
return select;
}
/*
Create select from template. This method create selects for beach.
Method create selects for request with coordinates(dlzka,sirka) as input.
For example, from current GPS location or cursor on the map.
*/
public static String getSelectTemplateForBeachFromCircle(
boolean wc,boolean obcerstvenie,boolean rozloha,boolean distance, String placeName, String reqInputVzdialenost,String reqInputMaxRozloha,String reqInputMinRozloha
){
String dlzka ="0";
String sirka= "0";
placeName = placeName.replaceAll("MyPoint","");
dlzka = placeName.split(",")[0];
sirka = placeName.split(",")[1];
String showWc = "";
String fromWc = "";
String whereWc ="";
String showObcerstvenie = "";
String fromObcerstvenie = "";
String whereObcerstvenie ="";
String showDistance = "";
String fromDistance = "";
String whereDistance ="";
String showRozloha = "";
String fromRozloha = "";
String whereRozloha ="";
if(wc){
showWc = SHOW_WC;
fromWc =FROM_WC;
whereWc = WHERE_WC;
}
if(obcerstvenie){
showObcerstvenie = SHOW_OBCERSTVENIE;
fromObcerstvenie = FROM_OBCERSTVENIE;
whereObcerstvenie = WHERE_OBCERSTVENIE;
}
if(distance){
showDistance = SHOW_DISTANCE;
fromDistance = FROM_DISTANCE;
whereDistance = getWHERE_DISTANCE(reqInputVzdialenost);
}
if(rozloha){
showRozloha = SHOW_ROZLOHA;
fromRozloha =FROM_ROZLOHA;
whereRozloha = getWHERE_ROZLOHA(reqInputMaxRozloha,reqInputMinRozloha);
}
//-------Generate select
String select = "" +
"SELECT "+
showWc + showDistance + showObcerstvenie + showRozloha +
"obce.nm2 AS cityName,ST_AsGeoJSON(obce.geom) AS city, ST_AsGeoJSON(park.geom) AS plaze,park.name AS plazName "+
"FROM " +
fromWc + fromDistance + fromObcerstvenie+
"gis_osm_natural_a_free_1 AS park,hranice_obce_6 AS obce "+
"WHERE ST_WITHIN(ST_MakePoint("+dlzka+","+sirka+"),obce.geom) "+
"AND park.fclass='beach'"+
whereWc+
"AND ST_WITHIN(park.geom, obce.geom)"+
whereRozloha+
whereObcerstvenie+
whereDistance;
return select;
}
}
<file_sep>import org.apache.log4j.Logger;
import java.util.ArrayList;
import java.util.List;
/**
* Created by dvalo on 23.10.2016.
* Class for geometry Point, keeps attributes like name,description,myType(city,park,..),icon,coordinatesList
*/
public class GeoPolygon implements GeoGeometry{
public static final String TYPE_POSTGRES = "MultiPolygon";
public static final String TYPE_MAPBOX = "Polygon";
private String name = "\"\"";
private String description = "\"\"";
private String myType = "\"\""; //this can be park/beach whatever
private String icon = "\"\"";
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = "\""+icon+"\"";
}
private List<Coordinate> coordinatesList = new ArrayList<Coordinate>();
static Logger log = Logger.getLogger(GeoPolygon.class.getName());
public String getName() {
return name;
}
public void setName(String name) {
if(name != null) this.name = "\""+name+"\"";
}
public String getDescription() {
String rtrn = " <h4>Title: "+name.replaceAll("\"","")+
" </h4><p>Description: "+description.replaceAll("\"","")+
" Druh: "+myType.replaceAll("\"","")+"</p>";
return "\""+rtrn+"\"";
}
public void setDescription(String description) {
this.description = "\""+description+"\"";
}
public String getMyType() {
return myType;
}
public void setMyType(String myType) {
this.myType = "\""+myType+"\"";
switch (myType){
case(GeoGeometry.MY_TYPE_PARK):
setIcon(GeoGeometry.MY_TYPE_ICON_PARK);
break;
case(GeoGeometry.MY_TYPE_WC):
setIcon(GeoGeometry.MY_TYPE_ICON_WC);
break;
case(GeoGeometry.MY_TYPE_BEACH):
//setIcon(GeoGeometry.MY_TYPE_ICON_);
break;
}
}
public GeoPolygon(String coordinatesPlainText,String name,String description,String myType) {
setName(name);
setDescription(description);
setMyType(myType);
coordinatesPlainText = coordinatesPlainText.substring(2,coordinatesPlainText.length()-2);
//log.info("Polygon Plain Text: "+coordinatesPlainText);
String[] coordinates = coordinatesPlainText.split("],");
for(String coord: coordinates){
Coordinate coordinate = new Coordinate(coord);
addCoordinate(coordinate);
}
}
@Override
public String generateGeoJsonMapbox() {
//generate geometry
String geoJsonGeometry = "";
geoJsonGeometry = "{"+
TYPE_TEXT+": "+"\""+ TYPE_MAPBOX +"\","+
COORDINATES_TEXT+": [[";
StringBuilder builder =new StringBuilder();
for(int i=0;i<coordinatesList.size();i++){
builder.append( coordinatesList.get(i).getCoordinate());
if(i!=coordinatesList.size()-1)builder.append(",");
}
geoJsonGeometry+=builder.toString();
geoJsonGeometry += "]]"+
"}";
//generate properties
String geoJsonProperties = "";
geoJsonProperties = PROPERTIES_TEXT+": {"+
TITLE_TEXT+": "+name+","+
NAME_TEXT+": "+getDescription()+","+
TITLE_DECRIPTION+": "+getDescription()+","+
MY_TYPE_TEXT+": "+myType+","+
ICON_TEXT+": "+icon+
"},";
//generate rest of polygon
String geoJson = "{"+
GeoGeometry.TYPE_TEXT+": "+GeoGeometry.TYPE_FEATURE+","
+geoJsonProperties
+GeoGeometry.GEOMETRY_TEXT+": "
+geoJsonGeometry
+"}";
return geoJson;
}
public List<Coordinate> getCoordinatesList() {
return coordinatesList;
}
public void setCoordinatesList(List<Coordinate> coordinatesList) {
this.coordinatesList = coordinatesList;
}
public void addCoordinate(Coordinate coordinate) {
this.coordinatesList.add(coordinate);
}
public void removeCoordinate(Coordinate coordinate) {
this.coordinatesList.remove(coordinate);
}
@Override
public String getPostgresType() {
return TYPE_POSTGRES;
}
@Override
public String getMapBoxType() {
return TYPE_MAPBOX;
}
}
<file_sep>import org.apache.log4j.Logger;
/**
* Created by dvalo on 22.10.2016.
* Class for geometry Point, keeps attributes like name,description,myType(city,park,..),title,coordinate
*/
public class GeoPoint implements GeoGeometry{
static Logger log = Logger.getLogger(GeoPoint.class.getName());
public static final String TYPE_POSTGRES = "Point";
public static final String TYPE_MAPBOX = "Point";
private String name = "\"\"";
private String description = "\"\"";
private String myType = "\"\""; //this can be park/beach whatever
private String title = "\"\"";
private String icon = "\"\"";
private Coordinate coordinate;
public GeoPoint(String coordinates,String name,String description,String myType) {
setName(name);
setDescription(description);
setMyType(myType);
this.coordinate = new Coordinate(coordinates);
}
public String getName() {
return name;
}
public void setName(String name) {
if(name != null){
name=name.replaceAll("\"","");
this.name = "\""+name+"\"";
}
}
public String getDescription() {
String rtrn = " <h4>Title: "+name.replaceAll("\"","")+
" </h4><p>Description: "+description.replaceAll("\"","")+"</br>"+
" Druh: "+myType.replaceAll("\"","")+"</br>"+
" GPS coordinates: "+coordinate.getX()+" "+coordinate.getY()+"</br>"+
"</p>";
return "\""+rtrn+"\"";
}
public void setDescription(String description) {
this.description = "\""+description+"\"";
}
public String getMyType() {
return myType;
}
public void setMyType(String myType) {
this.myType = "\""+myType+"\"";
switch (myType){
case(GeoGeometry.MY_TYPE_PARK):
setIcon(GeoGeometry.MY_TYPE_ICON_PARK);
break;
case(GeoGeometry.MY_TYPE_WC):
setIcon(GeoGeometry.MY_TYPE_ICON_WC);
break;
case(GeoGeometry.MY_TYPE_BEACH):
//setIcon(GeoGeometry.MY_TYPE_ICON_);
break;
}
}
public String getPostgresType() {
return TYPE_POSTGRES;
}
@Override
public String getMapBoxType() {
return TYPE_MAPBOX;
}
public Coordinate getCoordinate() {
return coordinate;
}
public void setCoordinate(Coordinate coordinate) {
this.coordinate = coordinate;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = "\""+icon+"\"";
}
@Override
public String generateGeoJsonMapbox() {
String geoJson = "";
geoJson = "{"+
TYPE_TEXT+": "+TYPE_FEATURE+","+
GEOMETRY_TEXT+": {"+
TYPE_TEXT+": "+"\""+ TYPE_MAPBOX +"\","+
COORDINATES_TEXT+": "+coordinate.getCoordinate()+
"},"+
PROPERTIES_TEXT+": {"+
TITLE_TEXT+": "+name+","+
TITLE_DECRIPTION+": "+getDescription()+","+
ICON_TEXT+": "+icon+","+
MARKER_SYMBOL_TEXT+": "+icon+","+
MY_TYPE_TEXT+": "+myType+
"}"+
"}";
return geoJson;
}
}
<file_sep>import org.apache.log4j.Logger;
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.util.UUID;
import static spark.Spark.port;
/**
* Created by dvalo on 21.10.2016.
* Main class for application. We are setting port for Spark framework here.
*/
public class Main {
static Logger logger = Logger.getLogger(Main.class.getName());
public static void main(String[] args) {
port(4567);
String id;
try {
id = InetAddress.getLocalHost().getHostAddress();
} catch (UnknownHostException e1) {
id = UUID.randomUUID().toString();
e1.printStackTrace();
}
try {
logger.info("Starting mapnode on port 4567 with " + id);
new PostgreSQLJDBC();
new MapApiController();
} catch(Exception e) {
e.printStackTrace();
logger.fatal("Cannot initialize mapnode, exiting...");
return;
}
}
}
| dc5417d28a9e628b3ba657ea45987d80411365c9 | [
"Markdown",
"Java"
] | 7 | Java | valocky/PDT | 5cbc2681c2e47e0fb862345a586332bfc0a15214 | 887f35d266c9c7fc9c7005b6c9d2b326fbd78e5c | |
refs/heads/main | <repo_name>Tamara570/DSA-recursion<file_sep>/logic.js
// 1. Counting Sheep
// Write a recursive function that counts how many sheep jump over the fence. Your program should take a number as input. That number should be the number of sheep you have. The function should display the number along with the message "Another sheep jumps over the fence" until no more sheep are left.
const sheep = (num) => {
//base case
if (num === 0) {
return 'All sheep jumped over the fence';
}
console.log(`${num} Another sheep jumps over the fence`);
return sheep(num - 1);
};
console.log(sheep(3));
// 2. Power Calculator
// Write a function called powerCalculator() that takes two parameters, an integer as a base, and another integer as an exponent. The function returns the value of the base raised to the power of the exponent. Use only exponents greater than or equal to 0 (positive numbers)
const powerCalculator = (num, power) => {
// base case
if (power < 0) {
return "exponent should be '>= 0'";
}
// base case part 2
if (power === 0) {
return 1;
}
const current = num;
const rest = powerCalculator(num, power - 1);
return current * rest;
};
console.log(powerCalculator(10, 3));
// 3. Reverse String
// Write a function that reverses a string. Take a string as input, reverse the string, and return the new string.
const reverse = (str) => {
// base case
if (str === '') {
return '';
}
const current = str[0];
const rest = str.slice(1);
return reverse(rest) + current;
};
console.log(reverse('sheep'));
// 4. nth Triangular Number
// Calculate the nth triangular number. A triangular number counts the objects that can form an equilateral triangle. The nth triangular number is the number of dots composing a triangle with n dots on a side, and is equal to the sum of the n natural numbers from 1 to n. This is the Triangular Number Sequence: 1, 3, 6, 10, 15, 21, 28, 36, 45.
const nth = (n) => {
// base case
if (n === 1) {
return 1;
}
// recursion
return n + nth(n - 1);
};
console.log(nth(4)); //10
// 5. String Splitter
// Write a recursive function that splits a string based on a separator (similar to String.prototype.split). Don't use JS array's split function to solve this problem. Input: 02/20/2020 Output: ["02", "20", "2020"]
const split = (str) => {};
// 6. Fibonacci
// Write a recursive function that prints the Fibonacci sequence of a given number. The Fibonacci sequence is a series of numbers in which each number is the sum of the 2 preceding numbers. For example, the 7th Fibonacci number in a Fibonacci sequence is 13. The sequence looks as follows: 1, 1, 2, 3, 5, 8, 13.
const fib = (num) => {
// base case
if (num <= 0) {
return 0;
} else if (num === 1) {
return 1;
}
// recursion
return fib(num - 1) + fib(num - 2);
};
console.log(fib(7)); // 13
// 7. Factorial
// Write a recursive function that finds the factorial of a given number. The factorial of a number can be found by multiplying that number by each number between itself and 1. For example, the factorial of 5 is 5 * 4 * 3 * 2 * 1 = 120.
const factorial = (num) => {
// base case
if (num === 1) {
return 1;
}
// recursion
return num * factorial(num - 1);
};
| e15f0d905dd859a8ac57b6784fdb3198785a1a99 | [
"JavaScript"
] | 1 | JavaScript | Tamara570/DSA-recursion | ea536bcf7d09270dfb11622f90f7fa137e3c5acb | 1746468605c5ab7c5f31782fb5793a0b8f1ea133 | |
refs/heads/master | <repo_name>rickertl/FSND_movie-page<file_sep>/entertainment_center.py
# importing fresh_tomatoes to get access to open_movies_page function
import fresh_tomatoes
# importing media to get access to Movie() class
import media
# listing of movie objects/instances for Movie() class
spiderman = media.Movie(
"Spider-Man",
"A high school student who turns to crimefighting after developing " +
"spider-like super powers.",
"http://upload.wikimedia.org/wikipedia/en/f/f3/Spider-Man2002Poster.jpg",
"https://www.youtube.com/watch?v=O7zvehDxttM",
"http://www.rottentomatoes.com/m/spiderman/")
spiderman2 = media.Movie(
"Spider-Man 2",
"Set two years after the events of Spider-Man, the film focuses on " +
"<NAME> struggling to manage both his personal life and his " +
"duties as Spider-Man, while Dr. <NAME> (Doctor Octopus) becomes" +
" diabolical following a failed experiment and his wife's death.",
"http://upload.wikimedia.org/wikipedia/en/0/02/Spider-Man_2_Poster.jpg",
"https://www.youtube.com/watch?v=nbp3Ra3Yp74",
"http://www.rottentomatoes.com/m/spiderman_2/")
spiderman3 = media.Movie(
"Spider-Man 3",
"Set months after the events of Spider-Man 2, <NAME> has become " +
"a cultural phenomenon as Spider-Man, while <NAME> continues " +
"her Broadway career.",
"http://upload.wikimedia.org/wikipedia/en/thumb/7/7a/Spider-Man_3%2C_" +
"International_Poster.jpg/220px-Spider-Man_3%2C_International_Poster.jpg",
"https://www.youtube.com/watch?v=wPosLpgMtTY",
"http://www.rottentomatoes.com/m/spiderman_3/")
iron_man = media.Movie(
"Iron Man",
"<NAME>, an industrialist and master engineer, builds a powered " +
"exoskeleton and becomes the technologically advanced superhero Iron Man.",
"http://upload.wikimedia.org/wikipedia/en/7/70/Ironmanposter.JPG",
"https://youtu.be/7H0yo-lDuk0",
"http://www.rottentomatoes.com/m/iron_man/")
iron_man2 = media.Movie(
"Iron Man 2",
"Six months after the events of Iron Man, <NAME> is resisting calls" +
" by the United States government to hand over the Iron Man technology " +
"while also combating his declining health from the arc reactor in his " +
"chest.",
"http://upload.wikimedia.org/wikipedia/en/e/ed/Iron_Man_2_poster.jpg",
"https://www.youtube.com/watch?v=FNQowwwwYa0",
"http://www.rottentomatoes.com/m/iron_man_2/")
iron_man3 = media.Movie(
"Iron Man 3",
"<NAME> tries to recover from posttraumatic stress disorder caused " +
"by the events of The Avengers, while investigating a terrorist " +
"organization led by the mysterious Mandarin.",
"http://upload.wikimedia.org/wikipedia/en/d/d5/Iron_Man_3_theatrical_" +
"poster.jpg",
"https://youtu.be/RsxsAN_bsvQ",
"http://www.rottentomatoes.com/m/iron_man_3/")
# creating an array of movies
movies = [spiderman, spiderman2, spiderman3, iron_man, iron_man2, iron_man3]
# passing movies array as arguement to open_movies_page function
fresh_tomatoes.open_movies_page(movies)
<file_sep>/README.txt
##Overview##
Server-side code to store a list of my favorite movies,
including box art imagery, movie trailer URL, and link
to Rotten Tomatoes page.
Code will generate a static web page allowing visitors
to browse movies, watch the trailers, and visit Rotten
Tomatoes page.
##Requirements##
1) Make sure these files are in the same directory
- entertainment_center.py
- fresh_tomatoes.py
- media.py
2) Have Python 2.7.9 Shell installed
##How To##
1) Open IDLE app
2) Open 'entertainment_center.py'
3) Goto and select 'Run > Run Module'
4) fresh_tomatoes.html will open in your browser
5) Browse my favorite movies
6) To watch trailers, click on movie box art image
7) To view Rotten Tomatoes page, click link
<file_sep>/media.py
# importing webbrowser module to use for playing youtube video
import webbrowser
class Movie():
# creating/initializing space in memory for movie details data
""" This class provides a way store movie related information """
def __init__(
self, movie_title, movie_storyline, poster_image, trailer_youtube,
rotten_page):
self.title = movie_title
self.storyline = movie_storyline
self.poster_image_url = poster_image
self.trailer_youtube_url = trailer_youtube
self.rotten = rotten_page
# calling webbrowser.open and creating/initializing space in memory to
# link to youtube trailer
def show_trailer(self):
webbrowser.open(self.trailer_youtube_url)
| 963d52d739500a06db8811f47d422f03ad91740f | [
"Python",
"Text"
] | 3 | Python | rickertl/FSND_movie-page | eb832f0652a19e4f534771335d49ee6ca404ce45 | d1b8393e6046ef735016592149d563c7902dcfea | |
refs/heads/master | <repo_name>JohannesFischer/grunt-starter-kit<file_sep>/assets/source/js/script.js
// Put JS code here
console.info('Add your JS code to "assets/source/js"');
| f23bdef20716f600f230fcdfd7ee64f00a1c652a | [
"JavaScript"
] | 1 | JavaScript | JohannesFischer/grunt-starter-kit | 2dc3f25488f6fdde7b7e36196773f5ff9f4244b4 | e5fb86158ddb6e43222e1565a9a642f0243c1ce0 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using SCI_PLT.Models;
using System.Diagnostics;
namespace SCI_PLT.Controllers
{
public class MaterialAuxController : Controller
{
private bd_sci_pltEntities4 db = new bd_sci_pltEntities4();
//
// GET: /MaterialAux/
public ActionResult Index()
{
return View(db.material_aux.ToList());
}
//
// GET: /MaterialAux/Details/5
public ActionResult Details(int id = 0)
{
material_aux material_aux = db.material_aux.Find(id);
if (material_aux == null)
{
return HttpNotFound();
}
return View(material_aux);
}
//
// GET: /MaterialAux/Create
public ActionResult Create()
{
return View();
}
//
// POST: /MaterialAux/Create
[HttpPost]
public ActionResult Create(material_aux material_aux)
{
if (ModelState.IsValid)
{
int c = db.material_aux.Count();
material_aux.mat_aux_cod = c;
db.material_aux.Add(material_aux);
Debug.WriteLine("Entreeeeeeeeeeeeeeeeeeeeeeee");
Debug.WriteLine(c);
//Debug.WriteLine("Entreeeeeeeeeeeeeeeeeeeeeeee22222222222");
db.SaveChanges();
return RedirectToAction("Index");
}
return View(material_aux);
}
//
// GET: /MaterialAux/Edit/5
public ActionResult Edit(int id = 0)
{
material_aux material_aux = db.material_aux.Find(id);
if (material_aux == null)
{
return HttpNotFound();
}
return View(material_aux);
}
//
// POST: /MaterialAux/Edit/5
[HttpPost]
public ActionResult Edit(material_aux material_aux)
{
if (ModelState.IsValid)
{
db.Entry(material_aux).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
return View(material_aux);
}
//
// GET: /MaterialAux/Delete/5
public ActionResult Delete(int id = 0)
{
material_aux material_aux = db.material_aux.Find(id);
if (material_aux == null)
{
return HttpNotFound();
}
return View(material_aux);
}
//
// POST: /MaterialAux/Delete/5
[HttpPost, ActionName("Delete")]
public ActionResult DeleteConfirmed(int id)
{
material_aux material_aux = db.material_aux.Find(id);
db.material_aux.Remove(material_aux);
db.SaveChanges();
return RedirectToAction("Index");
}
protected override void Dispose(bool disposing)
{
db.Dispose();
base.Dispose(disposing);
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using SCI_PLT.Models;
namespace SCI_PLT.Controllers
{
public class MaterialController : Controller
{
bd_sci_pltEntities4 entidad = new bd_sci_pltEntities4();
//
// GET: /Material/
public ActionResult Index()
{
var listaMateriales = entidad.material;
return View(listaMateriales.ToList());
}
//
// GET: /Material/Create
public ActionResult Create() {
return View();
}
//
// POST: /Material/Create
[HttpPost]
public ActionResult Create(material material)
{
if (ModelState.IsValid) {
int c = entidad.material_aux.Count();
material.mat_aux_cod = c;
entidad.material.Add(material);
entidad.SaveChanges();
return RedirectToAction("Index");
}
return View(material);
}
public ActionResult ListadoMaterial()
{
var listaMateriales = entidad.material;
return View(listaMateriales.ToList());
}
public ActionResult BuscarMaterial(string descripcion)
{
var objeto = from i in entidad.material where i.mat_descripcion == descripcion select i;
return View(objeto.ToList());
}
public ActionResult BuscarMaterialconMatAux()
{
var objeto = from i in entidad.material
join c in entidad.material_aux
on i.mat_cod equals c.mat_aux_cod
select new bdMaterial
{
id = i.mat_cod,
descripcion = i.mat_descripcion,
existencia = i.mat_existencia
};
return View(objeto.ToList());
}
}
}
<file_sep><h1>SCI_PLT</h1>
- Descripción: SCI_PLT es un sistema de control de inventarios para una planta de producción. Elaborado con ASP .NET MVC 4 Web Application
| 4173406376864298a658f06208eff120a1de538d | [
"Markdown",
"C#"
] | 3 | C# | oggs22/SCI_PLT | 726ee7db0f384bd702af26cbd2bcf30190f81a48 | 57796c6f43140f60d717915e33a022d942ba9013 | |
refs/heads/master | <repo_name>Ala-de/movie-app<file_sep>/src/films.js
import { getDefaultNormalizer } from '@testing-library/dom';
import React, {useState,useEffect}from 'react'
import {Card,Button } from 'react-bootstrap'
import './film.css'
import Rater from 'react-rater'
import 'react-rater/lib/react-rater.css'
function Film ({input,getFavorie}) {
const [film, setfilm ]= useState([])
const getfilm = ()=>{
fetch('film.json').then(
response => response.json()).then
(film => setfilm(film));
}
useEffect(()=> {getfilm()
},[])
console.log(film)
return(
film.filter((al)=>{
if(input===""){
return al
}
else if (al.Title.toLowerCase().includes(input.toLowerCase())){
return al
}
}).map(el=>
<div className= "navbarrr">
<div className="crd">
<div className='cwart'>
<Card style={{ width: '18rem' }}>
<Card.Img variant="top" src={el.Images} />
<Card.Body>
<Card.Title>{el.Title}</Card.Title>
<Card.Text>
{el.Plot}
</Card.Text>
<Card.Text>
<Rater total={10} rating={el.imdbRating} />
</Card.Text>
<Button variant="primary" className="bbb" >watch</Button>
<Button variant="primary" className="bbb" onClick={()=>{getFavorie(el)}}>favrie</Button>
</Card.Body>
</Card>
</div>
</div>
</div>
)
)
}
export default Film
<file_sep>/src/Admin.js
import React,{useEffect} from 'react'
import {Form,Button,Modal, Card } from 'react-bootstrap'
import Update from './Update'
import {useState} from 'react';
import axios from 'axios';
import './styleadmin.css'
const Admin = () => {
// **********get movies************
const [movie, setMovie] = useState([])
const getMovie = ()=> {axios.get('http://localhost:3004/posts')
.then((response )=> {setMovie(response.data) }) }
useEffect(()=> {getMovie() },[])
const [input,setInput]=useState(
{
title:"",
desc:""
}
);
//handleChage
const handleChange = e => {
const { name, value } = e.target;
//e.target.name=e.target.value
setInput({
...input,
[name]: value,
});
};
// delete function//
// DELETE request using axios with error handling
const deleteData=(id)=> {
axios.delete(`http://localhost:3004/posts/${id}`)
.then((response) => console.log(response) )
.catch((err) => console.log(err) )
}
//post data
const handlesubmit = (e) => {
e.preventDefault();
axios
.post("http://localhost:3004/posts",input)
.then((response) => console.log(response))
.catch((error) => console.log(error));
}
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
return(
<>
<div className='container md-2'>
<Button className="buton" variant="primary" onClick={handleShow}>
Add Film
</Button>
<Modal show={show} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Modal heading</Modal.Title>
</Modal.Header>
<Modal.Body>
<Form onSubmit={handlesubmit}>
<Form.Group controlId="formBasicEmail">
<Form.Label>Title</Form.Label>
<Form.Control type="text" placeholder="title" name='title' onChange={handleChange}/>
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>description</Form.Label>
<Form.Control type="text" placeholder="description" name='desc' onChange={handleChange} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label> Rating</Form.Label>
<Form.Control type="text" placeholder="rating" name='rating' onChange={handleChange} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>image</Form.Label>
<Form.Control type="text" placeholder="image" name='image' onChange={handleChange} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>type</Form.Label>
<Form.Control type="text" placeholder="image" name='type' onChange={handleChange} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>year</Form.Label>
<Form.Control type="text" placeholder="image" name='year' onChange={handleChange} />
</Form.Group>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button type='submit' variant="primary" onClick={handleClose}>
Save Changes
</Button>
</Form>
</Modal.Body>
</Modal>
</div>
{ movie.map(el=>
<div className="crd d-flex justify-content-around">
<div className='cwart'>
<Card style={{ width: '18rem' }}>
<Card.Img variant="top" src={el.image} />
<Card.Body>
<Card.Title>{el.title}</Card.Title>
<Card.Text>
{el.desc}
</Card.Text>
<Card.Text>
{el.rating}<img src="./star.png" className="star"/>
</Card.Text>
<Update el={el}/>
<Button className="buttonn" variant="primary" onClick={()=>deleteData(el.id)} >
Supprission
</Button>
</Card.Body>
</Card>
</div>
</div>
)}
</>
)
}
export default Admin
<file_sep>/src/Update.js
import React from 'react'
import axios from 'axios';
import {useState} from 'react';
import {Form,Button,Modal, Card }from 'react-bootstrap'
export default function Update({el}) {
const [show, setShow] = useState(false);
const handleClose = () => setShow(false);
const handleShow = () => setShow(true);
//EDit======================================================================
// edit//
const [edit, setEdit]=useState({
title: el.title,
desc: el.Plot,
rating: el.Rated,
image: el.Poster,
type: el.Genre,
year: el.Year
})
const handleEdit = e => {
const {name, value} = e.target
setEdit({...edit, [name]:value})
}
const Edit = async (id) => {
console.log(edit)
await axios.put(`http://localhost:3004/posts/${id}`, edit)
.then((response) => {console.log(response.data)
setEdit(response.data)})
.catch((error) => console.log(error));
}
return (
<div>
<Button className="buton" variant="primary" onClick={handleShow}>
edit
</Button>
<Modal show={show} onHide={handleClose}>
<Modal.Header closeButton>
<Modal.Title>Modal heading</Modal.Title>
</Modal.Header>
<Modal.Body>
<Form>
<Form.Group controlId="formBasicEmail">
<Form.Label>Title</Form.Label>
<Form.Control type="text" placeholder="title" defaultValue={el.title} name='title' onChange={handleEdit}/>
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>description</Form.Label>
<Form.Control type="text" placeholder="description" defaultValue={el.Plot} name='desc' onChange={handleEdit} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label> Rating</Form.Label>
<Form.Control type="text" placeholder="rating" defaultValue={el.Rated} name='rating' onChange={handleEdit} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>image</Form.Label>
<Form.Control type="text" placeholder="image" defaultValue={el.Poster} name='image' onChange={handleEdit} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>type</Form.Label>
<Form.Control type="text" placeholder="image" defaultValue={el.Genre} name='type' onChange={handleEdit} />
</Form.Group>
<Form.Group controlId="formBasicPassword">
<Form.Label>year</Form.Label>
<Form.Control type="text" placeholder="image" defaultValue={el.Year} name='year' onChange={handleEdit} />
</Form.Group>
<Button variant="secondary" onClick={handleClose}>
Close
</Button>
<Button variant="primary" onClick={()=>Edit(el.id)}>
Save Changes
</Button>
</Form>
</Modal.Body>
</Modal>
</div>
)
}
<file_sep>/src/navgation.js
import React from 'react'
import {Navbar,Nav,Form,FormControl } from 'react-bootstrap';
import {Link, Route, Router} from 'react-router-dom'
import './film.css'
export default function Navgation({handelchange}) {
return (
<div>
<Navbar className="azaz" expand="lg">
<Navbar.Brand href="#home">MOVIES</Navbar.Brand>
<Navbar.Toggle aria-controls="basic-navbar-nav" />
<Navbar.Collapse id="basic-navbar-nav">
<Nav className="links">
<Link to="/"><li>Home</li></Link>
<Link to="/"><li>Films</li></Link>
<Link to="/favorie"><li>Favorie</li></Link>
<Link to="/Contact"><li>contact</li></Link>
</Nav>
<Form inline className="limine ml-auto">
<FormControl type="text" placeholder="Search" className="mr-sm-2" onChange={handelchange} />
</Form>
</Navbar.Collapse>
</Navbar>
</div>
)
}
<file_sep>/src/App.js
import './App.css';
import Navgation from'./navgation'
import 'bootstrap/dist/css/bootstrap.min.css';
import Footer from'./footer'
import Apropos from './apropo'
import Favorie from './favorie'
import { BrowserRouter, Route } from 'react-router-dom';
import Home from './home'
import Contact from './contact'
import Admin from './Admin'
import {useState} from "react"
import Film from './films';
function App() {
const [favorie,setFavorie]=useState([])
const getFavorie=(el)=>{
favorie.push(el);
console.log (favorie);
};
return (
<div className="App">
<BrowserRouter>
<Navgation/>
<Route exact path="/" component={Home} />
<Route path="/favorie" component={Favorie} favorie={favorie} />
<Route path ="/a propos" component={Apropos}/>
<Route path ="/Contact" component={Contact}/>
<Route path="/Admin"component={Admin}/>
<Route path="/films" component={Film} getFavorie={getFavorie}/>
<Footer/>
</BrowserRouter>
</div>
);
}
export default App;
<file_sep>/src/favorie.js
import React from 'react'
import Navgation from './navgation'
import {Button,Card} from 'react-bootstrap'
import Rater from 'react-rater'
import 'react-rater/lib/react-rater.css'
const Favorie = ({favorie}) => {
return (
<div className='cwart'>
{favorie.map((el)=>
<Card style={{ width: '18rem' }}>
<Card.Img variant="top" src={el.Images} />
<Card.Body>
<Card.Title>{el.Title}</Card.Title>
<Card.Text>
{el.Plot}
</Card.Text>
<Card.Text>
<Rater total={10} rating={el.imdbRating} />
</Card.Text>
<Button variant="primary" className="bbb">watch</Button>
</Card.Body>
</Card>
) }
</div>
)
}
export default Favorie<file_sep>/src/home.js
import {React,useState} from 'react'
import Film from './films'
import './film.css'
import Navgation from './navgation'
import { Container } from "react-bootstrap";
function Home() {
const [input,setInput]=useState("")
const handelchange=(e)=>{
setInput(e.target.value)
}
return (
<div>
<Container className="d-flex flex-wrap">
<Film input={input}/>
</Container>
</div>
)
}
export default Home<file_sep>/src/footer.js
import React from 'react'
import './film.css'
export default function Footer() {
return (
<div>
<footer class="text-footer" >
<div class="container p-4"></div>
<div class="text-center p-3">
© 2021 Copyright:
<a class="text-white" href="https://mdbootstrap.com/">Movie.TN:</a>
</div>
</footer>
</div>
)
}
| cc0a81907361d530689fef7fdc819047a25e45e8 | [
"JavaScript"
] | 8 | JavaScript | Ala-de/movie-app | 3775b5c4b1427ecb97906a4cccbbf8fa84e31fe8 | e71590baa0f6da30c2331c45aa4b30b6f8086b6f | |
refs/heads/master | <file_sep>using Core;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.RazorPages;
using Microsoft.Extensions.Options;
namespace App.Pages
{
public class IndexModel : PageModel
{
[BindProperty]
public string MapboxToken { get; set; }
private readonly IOptions<AppSettings> _options;
public IndexModel(IOptions<AppSettings> options)
{
this._options = options;
}
public void OnGet()
{
this.MapboxToken = this._options.Value.MapboxToken;
}
}
}
<file_sep>using Core;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.DependencyInjection.Extensions;
using Microsoft.Extensions.Hosting;
using Core.Data.Service.GoogleServices;
namespace App
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
// configure strongly typed settings objects
var section = Configuration.GetSection("AppSettings");
// services.Configure<AppSettings>(section);
var appSettings = section.Get<AppSettings>();
// INFO: must have, let IOptions<T> knows and worked out
services.Configure<AppSettings>(section);
services.AddRazorPages();
services.AddCors(c =>
{
c.AddPolicy("AllowOrigin", options => options
.AllowAnyOrigin()
.AllowAnyHeader()
.AllowAnyMethod()
);
});
// ref: https://github.com/aspnet/AspNetCore/issues/9348
services.AddRouting(options => options.SuppressCheckForUnhandledSecurityMetadata = true);
services.TryAddSingleton<IHttpContextAccessor, HttpContextAccessor>();
services.AddHttpClient();
services.AddTransient<Place, Place>();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
endpoints.MapControllers();
});
app.UseCors(options => options.AllowAnyOrigin());
AppSettings.WebRootPath = env.WebRootPath;
AppSettings.ContentRootPath = env.ContentRootPath;
}
}
}
<file_sep>using Core.Data.GoogleServices;
using Microsoft.Extensions.Options;
using System;
using System.Net.Http;
using System.Text.Json;
using System.Threading.Tasks;
namespace Core.Data.Service.GoogleServices
{
public class Place
{
private readonly IOptions<AppSettings> _options;
private readonly IHttpClientFactory _httpClientFactory;
public Place(IOptions<AppSettings> options, IHttpClientFactory httpClientFactory)
{
this._options = options;
this._httpClientFactory = httpClientFactory;
}
public async Task<GooglePlaceData> Nearbysearch(double lng, double lat, string name, string lang)
{
return await Nearbysearch(lng, lat, name, 1000, "airport", lang);
}
public async Task<GooglePlaceData> Nearbysearch(double lng, double lat, string name, int radius, string type, string lang)
{
var api = string.Format(
this._options.Value?.GooglePlaceApi.Nearbysearch,
this._options.Value?.GoogleApiToken,
lat,
lng,
radius,
name,
type,
lang);
var jsonStr = await this._httpClientFactory.CreateClient().GetStringAsync(api);
var data = JsonSerializer.Deserialize<GooglePlaceData>(jsonStr);
return data;
}
public async Task<GooglePlaceData2> NearbySearch(string name)
{
var api = string.Format(
this._options.Value?.GooglePlaceApi.FindFromText,
this._options.Value?.GoogleApiToken,
name);
var jsonStr = await this._httpClientFactory.CreateClient().GetStringAsync(api);
try
{
var jn = jsonStr.Replace("\n", "");
var temp = JsonSerializer.Deserialize<GooglePlaceData2>(jn);
if (temp != null)
{
return temp;
}
else
{
return new GooglePlaceData2 { };
}
}
catch (Exception)
{
throw;
}
}
public async Task<GooglePlaceDetailData> Detail(string placeid, string lang)
{
var api = string.Format(this._options.Value?.GooglePlaceApi.Detail, this._options.Value?.GoogleApiToken, placeid, lang);
var jsonStr = await this._httpClientFactory.CreateClient().GetStringAsync(api);
var data = JsonSerializer.Deserialize<GooglePlaceDetailData>(jsonStr);
return data;
}
public async Task<string> Photo(string photoreference, int maxWidth)
{
var api = string.Format(this._options.Value?.GooglePlaceApi.Photo, this._options.Value?.GoogleApiToken, photoreference, maxWidth);
var strm = await this._httpClientFactory.CreateClient().GetStreamAsync(api);
var base64Str = Convert.ToBase64String(StreamToBytes(strm));
return base64Str;
}
private static byte[] StreamToBytes(System.IO.Stream stream)
{
using var memoryStream = new System.IO.MemoryStream();
stream.CopyTo(memoryStream);
return memoryStream.ToArray();
}
}
}
<file_sep>using System.Reflection;
namespace Core
{
public class AppSettings
{
public string MapboxToken { get; set; }
public string GoogleApiToken { get; set; }
public GooglePlaceApi GooglePlaceApi { get; set; }
public static string WebRootPath { get; set; }
public static string ContentRootPath { get; set; }
public static string Version
{
get
{
return typeof(AppSettings)
.GetTypeInfo()
.Assembly
.GetCustomAttribute<AssemblyInformationalVersionAttribute>()
.InformationalVersion;
}
}
public static string OSDescription
{
get
{
return System.Runtime.InteropServices.RuntimeInformation.OSDescription;
}
}
}
public class GooglePlaceApi
{
public string Nearbysearch { get; set; }
public string FindFromText { get; set; }
public string Detail { get; set; }
public string Photo { get; set; }
}
}
<file_sep>using System.Collections.Generic;
namespace Core.Data.GoogleServices
{
public class GooglePlaceData2
{
public IEnumerable<TextPlaceData> candidates { get; set; }
public string status { get; set; }
}
public class TextPlaceData
{
public string formatted_address { get; set; }
public Geometry geometry { get; set; }
public string name { get; set; }
public OpeningHours opening_hours { get; set; }
public IEnumerable<Photo> photos { get; set; }
public double rating { get; set; }
}
public class OpeningHours
{
public bool open_now { get; set; }
}
}
<file_sep>using Core.Data.GoogleServices;
using Microsoft.AspNetCore.Mvc;
using System.Linq;
using System.Threading.Tasks;
namespace Core.Data.Api
{
[Route("api/g")]
[ApiController]
public class GoogleController : ControllerBase
{
private readonly Service.GoogleServices.Place _placeService;
public GoogleController(Service.GoogleServices.Place placeService)
{
this._placeService = placeService;
}
[HttpGet("nearby/{lng}/{lat}/{name}/{lang?}")]
public async Task<ActionResult<GooglePlaceData>> GetNearby(double lng, double lat, string name, string lang)
{
var ret = await this._placeService.Nearbysearch(lng, lat, name, lang);
// return new JsonResult(ret); // same results by using Ok(ret);
return Ok(ret);
}
[HttpGet("find/{name}")]
public async Task<ActionResult<GooglePlaceData2>> FindFromText(string name)
{
var ret = await this._placeService.NearbySearch(name);
return Ok(ret);
}
[HttpGet("detail/{placeid}/{lang?}")]
public async Task<ActionResult<GooglePlaceDetailData>> GetDetail(string placeid, string lang)
{
var ret = await this._placeService.Detail(placeid, lang);
return Ok(ret);
}
[HttpGet("r/{lng}/{lat}/{name}/{width}/{lang?}")]
public async Task<IActionResult> GetMixedUp(double lng, double lat, string name, int width, string lang)
{
var nearby = await this._placeService.Nearbysearch(lng, lat, name, lang);
if (nearby == null || nearby.status != "OK" || !nearby.results.Any())
{
return Ok(new
{
name = string.Empty,
photo = string.Empty,
formattedAddress = string.Empty,
phoneNumber = string.Empty,
website = string.Empty
});
}
if (!nearby.results.Any())
{
return Ok(new
{
name = string.Empty,
photo = string.Empty,
formattedAddress = string.Empty,
phoneNumber = string.Empty,
website = string.Empty
});
}
else
{
var result = nearby.results.FirstOrDefault();
var detail = await this._placeService.Detail(result.place_id, lang);
var detailResult = detail?.result;
var photos = result.photos;
var photo = string.Empty;
if (photos != null && photos.Any())
{
photo = await this._placeService.Photo(photos.FirstOrDefault().photo_reference, width);
}
return Ok(new
{
detailResult.name,
photo,
formattedAddress = detailResult?.formatted_address,
phoneNumber = detailResult?.international_phone_number,
detailResult?.website
});
}
}
[HttpGet("r/{name}")]
public async Task<IActionResult> GetMixedUp(string name)
{
var detail = await this._placeService.NearbySearch(name);
if (detail != null && detail.status == "OK")
{
if (detail.candidates.Any())
{
var candidate = detail.candidates.FirstOrDefault();
var photos = candidate.photos;
var photoBase64 = "";
if (photos.Any())
{
var photo = candidate.photos.FirstOrDefault();
photoBase64 = await this._placeService.Photo(photo.photo_reference, photo.width);
}
return Ok(new
{
candidate.name,
photo = photoBase64,
formattedAddress = candidate.formatted_address,
website = candidate.photos.Any() ? candidate.photos.FirstOrDefault().html_attributions.FirstOrDefault() : string.Empty
});
}
return Ok(new
{
name = string.Empty,
photo = string.Empty,
formattedAddress = string.Empty,
website = string.Empty
});
}
else
{
return Ok(new
{
name = string.Empty,
photo = string.Empty,
formattedAddress = string.Empty,
website = string.Empty
});
}
}
}
}
<file_sep>using System.Collections.Generic;
namespace Core.Data
{
/// <summary>
/// feature data use for mapbox
/// </summary>
public class FeatureCollection
{
public string Type { get; set; } = "FeatureCollection";
public IEnumerable<FeaturePoint> Features { get; set; }
}
public class FeaturePoint
{
public string Type { get; set; } = "Feature";
public Geometry Geometry { get; set; }
public Property Properties { get; set; }
}
public class Geometry
{
public Geometry(double longitude, double latitude)
{
this.Coordinates = new[] { longitude, latitude };
}
public string Type { get; set; } = "Point";
public double[] Coordinates { get; set; }
}
public class Property
{
public string Name { get; set; }
}
}
<file_sep>namespace Core.Data
{
/// <summary>
/// main data: scenery from excel file
/// </summary>
public class Scenery
{
public string Name { get; set; }
public Coordinates Coordinates { get; set; }
}
public class Coordinates
{
public double Longitude { get; set; }
public double Latidue { get; set; }
}
}
<file_sep>using Core.Data.Srvice;
using Microsoft.AspNetCore.Cors;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace Core.Data.Api
{
[Route("api/[controller]")]
[ApiController]
public class SceneriesController : ControllerBase
{
[ResponseCache(Duration = 3600, Location = ResponseCacheLocation.Client)]
[HttpGet]
[EnableCors("AllowOrigin")]
public async Task<ActionResult<IEnumerable<FeatureCollection>>> Get()
// public async Task<IActionResult> Get()
{
var sceneries = await Task.FromResult(FileHandlerService.XlsHandler());
var result = new FeatureCollection
{
Features = sceneries.Select(p => new FeaturePoint
{
Geometry = new Geometry(p.Coordinates.Longitude, p.Coordinates.Latidue),
Properties = new Property
{
Name = p.Name
}
})
};
return Ok(result);
}
}
}
<file_sep>namespace Core.Data.GoogleServices
{
public class GooglePlaceDetailData
{
public string[] html_attributions { get; set; }
public Dresult result { get; set; }
public string status { get; set; }
}
public class Dresult
{
// only grab info needed
public string adr_address { get; set; }
public string formatted_address { get; set; }
public string formatted_phone_number { get; set; }
public string international_phone_number { get; set; }
public string name { get; set; }
public string url { get; set; }
public string vicinity { get; set; }
public string website { get; set; }
}
}
<file_sep>using ExcelDataReader;
using System;
using System.Collections.Generic;
using System.IO;
namespace Core.Data.Srvice
{
public class FileHandlerService
{
public static IEnumerable<Scenery> XlsHandler()
{
System.Text.Encoding.RegisterProvider(System.Text.CodePagesEncodingProvider.Instance);
try
{
// remember, it's bad for unit test, bad smells: too much dependency there
var data = new List<Scenery>();
var xls = Path.Combine(AppSettings.ContentRootPath, "sceneries.xlsx");
using var stream = File.Open(xls, FileMode.Open, FileAccess.Read);
using var reader = ExcelReaderFactory.CreateReader(stream);
do
{
while (reader.Read())
{
// in the excel file, 0 is name column, 4 is coordinates column
if (reader.GetString(0) != null && reader.GetString(4) != null)
{
var name = Convert.ToString(reader.GetString(0));
var coordinatesString = Convert.ToString(reader.GetString(4));
var spliter = coordinatesString.Split(",");
if (spliter.Length == 2)
{
double.TryParse(spliter[0], out var y); // latitude
double.TryParse(spliter[1], out var x); // longitude
if (x != 0 && y != 0)
{
data.Add(new Scenery
{
Name = name,
Coordinates = new Coordinates
{
Longitude = x,
Latidue = y
}
});
}
}
}
}
} while (reader.NextResult());
return data;
}
catch(Exception)
{
throw;
}
}
}
}
<file_sep>let lang = '';
function rendermap(loc) {
if (!loc || loc === null) { return; }
mapboxgl.accessToken = document.getElementById('mapboxtoken').value;
const map = new mapboxgl.Map({
container: 'map',
style: 'mapbox://styles/mapbox/streets-v11',
center: [ loc.coords.longitude, loc.coords.latitude ],
zoom: 7 // starting zoom
});
const nav = new mapboxgl.NavigationControl();
map.addControl(nav, 'bottom-right');
map.on('load', () => {
mapcallback(map);
});
}
function mapcallback(map) {
map.addSource('sceneries', {
type: 'geojson',
data: `${apiRoot}/sceneries`,
cluster: true, // Enable clustering
clusterRadius: 50, // Radius of each cluster when clustering points
clusterMaxZoom: 6 // Max zoom to cluster points on
});
map.addLayer({
id: 'sceneries',
type: 'circle',
source: 'sceneries',
filter: ['!has', 'point_count'],
paint: {
'circle-color': '#a890f0',
'circle-radius': 5,
'circle-stroke-width': 1,
'circle-stroke-color': '#fff'
}
});
map.on('mouseenter', 'sceneries', (data) => {
map.getCanvas().style.cursor = 'pointer';
const name = data.features[0].properties.name;
$('#card').modal('toggle');
const api = `/api/g/r/${name}`;
fetch(api)
.then(resp => {
if (resp.status === 200) {
return resp.json();
}
return {};
})
.then(data => {
setSceneryProperties(data);
})
.catch(error => {
console.log(error);
document.getElementById('scenery-image').src = 'https://via.placeholder.com/400x200?text=Error+while+loading+data';
});
});
map.on('mouseleave', 'sceneries', () => {
map.getCanvas().style.cursor = '';
closeInfoCard();
});
var placesAutocomplete = places({
container: document.querySelector('#where-to-go'),
type: 'city'
});
placesAutocomplete.on('change', e => {
map.flyTo({
center: [e.suggestion.latlng.lng, e.suggestion.latlng.lat],
zoom: 9
});
});
}
function setSceneryProperties(data) {
if (!data || data === null) { return; }
if (data.photo && data.photo !== null) {
document.getElementById('scenery-image').src = `data:image/png;base64,${data.photo}`;
}
else {
document.getElementById('scenery-image').src = 'https://via.placeholder.com/500x300?text=No+Image+Found';
}
document.getElementById('name').innerText = data.name || '';
document.getElementById('address').innerText = data.formattedAddress || '';
document.getElementById('website').innerHTML = data.website || '';
}
function closeInfoCard() {
document.getElementById('scenery-image').src = 'https://via.placeholder.com/500x300?text=loading';
document.getElementById('name').innerText = '';
document.getElementById('address').innerText = '';
document.getElementById('website').innerHTML = '';
$('#card').modal('toggle');
}
function getBaseUri(isApi = false) {
const loc = window.location;
const protocol = loc.protocol;
const host = loc.host;
if (isApi) {
return `${protocol}//${host}/api`;
}
return `${protocol}//${host}`;
}
const apiRoot = getBaseUri(true);
(() => {
if (navigator.geolocation) {
// we get geolocation from browsers, to make map centered upon current location.
navigator.geolocation.getCurrentPosition(rendermap);
}
// what if, geolocation did NOT support by browsers, or premission denied by users !?
})();
| ce74db04fbdcc7aea62805b779e77e47106b3cbf | [
"JavaScript",
"C#"
] | 12 | C# | mattyyzac/tp_mapbox_demo | 14b165b57254b8d9fe85673f7566e8001478ddbb | 03f1cc4a96e6d13fc151045754b73f442d08b3d0 | |
refs/heads/master | <file_sep>import io
import unittest
import unittest.mock
from p01 import print_depth
class TestProblem01(unittest.TestCase):
@unittest.mock.patch('sys.stdout', new_callable=io.StringIO)
def assert_stdout(self, data, expected_output, mock_stdout):
print_depth(data)
self.assertEqual(mock_stdout.getvalue(), expected_output)
def test01(self):
a = {
"key1": 1,
"key2": 2,
"key3": {
"key4": 3
}
}
self.assert_stdout(a, 'key1 1\nkey2 1\nkey3 1\nkey4 2\n')
def test02(self):
a = {
"key1": 1,
"key2": {
"key3": 1,
"key4": {
"key5": 4
}
}
}
self.assert_stdout(a, "key1 1\nkey2 1\nkey3 2\nkey4 2\nkey5 3\n")
if __name__ == '__main__':
unittest.main()
<file_sep>import unittest
from p03 import *
class TestProblem03(unittest.TestCase):
def test_01(self):
self.assertEqual(lca(n6, n7), 3)
def test_02(self):
self.assertEqual(lca(n3, n7), 3)
def test_03(self):
self.assertEqual(lca(n1, n2), 1)
def test_04(self):
self.assertEqual(lca(n1, n7), 1)
def test_05(self):
self.assertEqual(lca(n8, n5), 2)
def test_06(self):
self.assertEqual(lca(n8, n8), 8)
def test_07(self):
self.assertEqual(lca(n3, n7), 3)
def test_08(self):
self.assertEqual(lca(n1, n1), 1)
if __name__ == '__main__':
unittest.main()
<file_sep>import io
import unittest
import unittest.mock
from p02 import print_depth, person_b
class TestProblem02(unittest.TestCase):
@unittest.mock.patch('sys.stdout', new_callable=io.StringIO)
def assert_stdout(self, data, expected_output, mock_stdout):
print_depth(data)
self.assertEqual(mock_stdout.getvalue(), expected_output)
def test_01(self):
a = {
"key1": 1,
"key2": {
"key3": 1,
"key4": {
"key5": 4,
"user": person_b,
"key6": {
"key7": 4
}
}
}
}
self.assert_stdout(a, 'key1 1\nkey2 1\nkey3 2\nkey4 2\nkey5 3\nuser: 3\nfirst_name: 4\nlast_name: 4\n'
+ 'father: 4\nfirst_name: 5\nlast_name: 5\nfather: 5\nkey6 3\nkey7 4\n')
def test_02(self):
a = {
"key1": 1,
"key2": {
"key3": 1,
"key4": {
"key5": 4
}
}
}
self.assert_stdout(a, 'key1 1\nkey2 1\nkey3 2\nkey4 2\nkey5 3\n')
def test_03(self):
a = {
"key1": 1,
"key2": 2,
"key3": {
"key4": 3
}
}
self.assert_stdout(a, 'key1 1\nkey2 1\nkey3 1\nkey4 2\n')
if __name__ == '__main__':
unittest.main()
<file_sep>'''
Time and Memory complexity analysis
-->Time complexity<--
* every step program prints one element until the total number of keys,
so total costs of the program as follows: 1+2+3+.....+n
Hence time complexity of this program is linear or O(n)
-->Space/Memory<--
* The recursive calls of this program does not save its state to the Stack, But in order to create
Dictionary it costs linear space which is proportional to number of keys
So we can consider the Space complexity of this program is O(n)
'''
class Person(object):
def __init__(self, first_name, last_name, father):
self.first_name = first_name
self.last_name = last_name
self.father = father
person_a = Person("User", None, None)
person_b = Person("User", "2", person_a)
test = {
"key1": {
"key2": {
"key3": 1
}
},
"key4": {
"key5": {
"key6": 2
}
},
"key7": {
"key8": {
"key9": 3
}
}
}
a = {
"key1": 1,
"key2": {
"key3": 1,
"key4": {
"key5": 4,
"user1": person_b,
"key6": {
"key7": 4,
}
}
}
}
# Print function when it's an instance of Person
def print_for_person(k, depth):
print(str(k)+': '+str(depth))
# Print function when it's an instance of dictionary
def print_for_dict(k, depth):
print(str(k)+' '+str(depth))
# Driver function
def print_depth(data, depth=1):
# If value of a key is Instance of Person
if isinstance(data, Person):
for k, v in vars(data).items():
# Handling all of the properties of Person instance
if isinstance(v, Person):
print_for_person(k, depth)
print_depth(v, depth+1)
# Handling None property of Person instance
else:
print_for_person(k, depth)
# When value of a key is Instance of dictionary
else:
for k, v in data.items():
# A value of dictionary can be instance of either dict or Person
# When value of a key is instance of dict
if isinstance(v, dict):
print_for_dict(k, depth)
print_depth(v, depth+1)
# When value of a key is instance of Person
elif isinstance(v, Person):
print_for_person(k, depth)
print_depth(v, depth+1)
# If value of a key is not another dict or Person instance
else:
print_for_dict(k, depth)
# print_depth(person_b)
<file_sep>'''
Time and Memory complexity analysis
-->Time complexity<--
* every step program prints one element until the total number of keys,
so total costs of the program as follows: 1+2+3+.....+n
Hence time complexity of this program is linear or O(n)
-->Space/Memory<--
* The recursive calls of this program does not save its state to the Stack, But in order to create
Dictionary it costs linear space which is proportional to number of keys
So we can consider the Space complexity of this program is O(n)
'''
def print_depth(data, depth=1):
for key in data:
print(key, depth)
if isinstance(data[key], dict):
print_depth(data[key], depth+1)
# print_depth(a)
<file_sep>'''
Time and Memory complexity analysis
-->Time complexity<--
* For constructing the binary tree total cost is numbers of nodes "n"
so overall time complexity O(n)
* For getting the paths to root node of two given nodes is equal to the numbers of
nodes to the root, In worst case it will be height of the tree O(h)+O(h)
* and selecting least common node between two Stacks cost would be in worst case O(h), where
h height of the tree
-> Overall time complexity in worst case is O(n)
-->Space/Memory<--
* For constructing the binary tree total Space complexity in worst case in O(n)
where n is number of nodes of the tree
* and Space complexity of storing values to Stack is propotional to height of the tree
in worst case so we can consider O(h)
-> Overall Space complexity of this problem is O(n)
'''
from collections import deque
class Node:
def __init__(self, value, parent):
self.value = value
self.parent = parent
# This function returns all of the nodes from target node to the root node
def pathToRoot(Node):
paths = deque()
currentNode = Node
while currentNode.parent:
paths.append(currentNode.value)
currentNode = currentNode.parent
paths.append(currentNode.value)
return paths
def lca(node1, node2):
# Getting paths of two given nodes
paths_of_node1 = pathToRoot(node1)
paths_of_node2 = pathToRoot(node2)
# Finding out the least common value between two paths
lca_of_two = None
while paths_of_node1 and paths_of_node2:
pop1 = paths_of_node1.pop()
pop2 = paths_of_node2.pop()
if pop1 == pop2:
lca_of_two = pop1
else:
break
return lca_of_two
# inserting nodes
n1 = Node(1, None)
n2 = Node(2, n1)
n3 = Node(3, n1)
n4 = Node(4, n2)
n5 = Node(5, n2)
n6 = Node(6, n3)
n7 = Node(7, n3)
n8 = Node(8, n4)
n9 = Node(9, n4)
# result = lca(n3, n7)
# print(result)
<file_sep># Instruction for Unittesting
## For testing Task 01
$ python3 -m unittest tests/test_p01.py
## For testing Task 02
$ python3 -m unittest tests/test_p02.py
## For testing Task 03
$ python3 -m unittest tests/test_p03.py
| e44f8ee3f4ec20d9601a126091d44dcc958a54af | [
"Markdown",
"Python"
] | 7 | Python | aip777/assessment | 2a6e3a9c1197b92151bff75bed894157b80dd702 | 263f1f6cf8197b95ef5da2e836583383fa64bced | |
refs/heads/master | <file_sep><!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>深入理解 KVO | 张不坏的博客</title>
<meta name="description" content="Just For Fun">
<link rel="icon" href="/favicon.ico">
<link rel="preload" href="/assets/css/0.styles.37bfab3d.css" as="style"><link rel="preload" href="/assets/js/app.50bca889.js" as="script"><link rel="preload" href="/assets/js/5.081d1701.js" as="script"><link rel="preload" href="/assets/js/107.5af47fd0.js" as="script"><link rel="prefetch" href="/assets/js/10.7bb33f06.js"><link rel="prefetch" href="/assets/js/100.014ff06c.js"><link rel="prefetch" href="/assets/js/101.28f11de0.js"><link rel="prefetch" href="/assets/js/102.be9d2e87.js"><link rel="prefetch" href="/assets/js/103.a1210d81.js"><link rel="prefetch" href="/assets/js/104.7101a956.js"><link rel="prefetch" href="/assets/js/105.833e6f80.js"><link rel="prefetch" href="/assets/js/106.978e1fc0.js"><link rel="prefetch" href="/assets/js/108.efc3ce89.js"><link rel="prefetch" href="/assets/js/109.a69d6b5a.js"><link rel="prefetch" href="/assets/js/11.2de4afc9.js"><link rel="prefetch" href="/assets/js/110.30984b63.js"><link rel="prefetch" href="/assets/js/12.bfc099bd.js"><link rel="prefetch" href="/assets/js/13.20253e0d.js"><link rel="prefetch" href="/assets/js/14.67131b1c.js"><link rel="prefetch" href="/assets/js/15.1af26cbd.js"><link rel="prefetch" href="/assets/js/16.4b261ee0.js"><link rel="prefetch" href="/assets/js/17.1216332f.js"><link rel="prefetch" href="/assets/js/18.c0159773.js"><link rel="prefetch" href="/assets/js/19.0f007f87.js"><link rel="prefetch" href="/assets/js/2.b4633a05.js"><link rel="prefetch" href="/assets/js/20.4b295001.js"><link rel="prefetch" href="/assets/js/21.0c46767c.js"><link rel="prefetch" href="/assets/js/22.a5e065ea.js"><link rel="prefetch" href="/assets/js/23.f43a6a7e.js"><link rel="prefetch" href="/assets/js/24.245f4f15.js"><link rel="prefetch" href="/assets/js/25.618f74a1.js"><link rel="prefetch" href="/assets/js/26.274a606b.js"><link rel="prefetch" href="/assets/js/27.c2d8fe18.js"><link rel="prefetch" href="/assets/js/28.5c522d2a.js"><link rel="prefetch" href="/assets/js/29.c90fdb1a.js"><link rel="prefetch" href="/assets/js/3.9babd8f1.js"><link rel="prefetch" href="/assets/js/30.1ccbdebc.js"><link rel="prefetch" href="/assets/js/31.acf3eca6.js"><link rel="prefetch" href="/assets/js/32.ccfdc859.js"><link rel="prefetch" href="/assets/js/33.9b262756.js"><link rel="prefetch" href="/assets/js/34.c59a4044.js"><link rel="prefetch" href="/assets/js/35.2b10fefb.js"><link rel="prefetch" href="/assets/js/36.2daeeb7b.js"><link rel="prefetch" href="/assets/js/37.d649866c.js"><link rel="prefetch" href="/assets/js/38.aba1ac95.js"><link rel="prefetch" href="/assets/js/39.58a95fd1.js"><link rel="prefetch" href="/assets/js/4.a7413ce2.js"><link rel="prefetch" href="/assets/js/40.8ef4d374.js"><link rel="prefetch" href="/assets/js/41.5799de7a.js"><link rel="prefetch" href="/assets/js/42.b7ee7489.js"><link rel="prefetch" href="/assets/js/43.28a65d64.js"><link rel="prefetch" href="/assets/js/44.90f92ea2.js"><link rel="prefetch" href="/assets/js/45.30b683fd.js"><link rel="prefetch" href="/assets/js/46.f57ccc19.js"><link rel="prefetch" href="/assets/js/47.7a82bd74.js"><link rel="prefetch" href="/assets/js/48.72503020.js"><link rel="prefetch" href="/assets/js/49.3a4ba077.js"><link rel="prefetch" href="/assets/js/50.0c3297f3.js"><link rel="prefetch" href="/assets/js/51.e9ba9363.js"><link rel="prefetch" href="/assets/js/52.473ee9ff.js"><link rel="prefetch" href="/assets/js/53.166d6e7a.js"><link rel="prefetch" href="/assets/js/54.78af3662.js"><link rel="prefetch" href="/assets/js/55.f0d54751.js"><link rel="prefetch" href="/assets/js/56.5de81531.js"><link rel="prefetch" href="/assets/js/57.6e18322f.js"><link rel="prefetch" href="/assets/js/58.1fccc879.js"><link rel="prefetch" href="/assets/js/59.773775e1.js"><link rel="prefetch" href="/assets/js/6.0c9cc532.js"><link rel="prefetch" href="/assets/js/60.0d665185.js"><link rel="prefetch" href="/assets/js/61.d9ae36dc.js"><link rel="prefetch" href="/assets/js/62.fb5e3b65.js"><link rel="prefetch" href="/assets/js/63.5ace8fda.js"><link rel="prefetch" href="/assets/js/64.d44fb0af.js"><link rel="prefetch" href="/assets/js/65.ed8fe56f.js"><link rel="prefetch" href="/assets/js/66.809078da.js"><link rel="prefetch" href="/assets/js/67.2489499e.js"><link rel="prefetch" href="/assets/js/68.e3ee952d.js"><link rel="prefetch" href="/assets/js/69.071411f8.js"><link rel="prefetch" href="/assets/js/7.8188415c.js"><link rel="prefetch" href="/assets/js/70.be8269cf.js"><link rel="prefetch" href="/assets/js/71.a320347a.js"><link rel="prefetch" href="/assets/js/72.f4fda48b.js"><link rel="prefetch" href="/assets/js/73.0f9f9284.js"><link rel="prefetch" href="/assets/js/74.b4028d07.js"><link rel="prefetch" href="/assets/js/75.6d63415f.js"><link rel="prefetch" href="/assets/js/76.d5b4df24.js"><link rel="prefetch" href="/assets/js/77.62b794e1.js"><link rel="prefetch" href="/assets/js/78.63e767ab.js"><link rel="prefetch" href="/assets/js/79.45056905.js"><link rel="prefetch" href="/assets/js/8.20d7cb0f.js"><link rel="prefetch" href="/assets/js/80.e06c5521.js"><link rel="prefetch" href="/assets/js/81.bc82bd01.js"><link rel="prefetch" href="/assets/js/82.4aeb6081.js"><link rel="prefetch" href="/assets/js/83.3ed6146f.js"><link rel="prefetch" href="/assets/js/84.f2aff9f4.js"><link rel="prefetch" href="/assets/js/85.2b8f4e50.js"><link rel="prefetch" href="/assets/js/86.27aea1da.js"><link rel="prefetch" href="/assets/js/87.7f5dc71e.js"><link rel="prefetch" href="/assets/js/88.9ca6511c.js"><link rel="prefetch" href="/assets/js/89.e8f54ad1.js"><link rel="prefetch" href="/assets/js/9.ee6c43f7.js"><link rel="prefetch" href="/assets/js/90.9abac718.js"><link rel="prefetch" href="/assets/js/91.9d8f5f36.js"><link rel="prefetch" href="/assets/js/92.2277b907.js"><link rel="prefetch" href="/assets/js/93.efca2f57.js"><link rel="prefetch" href="/assets/js/94.e9cc0386.js"><link rel="prefetch" href="/assets/js/95.fa3326f7.js"><link rel="prefetch" href="/assets/js/96.82bafc57.js"><link rel="prefetch" href="/assets/js/97.da22d13e.js"><link rel="prefetch" href="/assets/js/98.d745e5ec.js"><link rel="prefetch" href="/assets/js/99.79a6f693.js">
<link rel="stylesheet" href="/assets/css/0.styles.37bfab3d.css">
</head>
<body>
<div id="app" data-server-rendered="true"><div class="theme-container"><div class="navbar"><div class="navbar-content"><div class="slogan"><NAME></div> <div class="links"><span class="link-item"><a href="/">首页</a></span> <span class="link-item"><a href="/category/iOS/">iOS</a></span> <span class="link-item"><a href="/category/other/">其他</a></span></div></div></div> <div class="content-header"><div class="post-title">深入理解 KVO</div> <div class="post-info">2015-04-29 • iOS</div></div> <div class="content content__default"><p>程序设计语言中有各种各样的设计模式(pattern)和与此对应的反设计模式(anti-pattern),譬如 singleton、factory、observer、MVC 等等。对于基于 Objective-C 的 iOS 开发而言,有些设计模式几乎已经成为开发环境的一部分,譬如 MVC,自打我们设计第一个页面开始就已经开始与之打交道了;KVO,即 Key-Value Observing(根据我的理解它属于 observer 设计模式)也一样,只是它已经成为 Objective-C 事实标准了,作为一个 iOS 开发者,必须对它有相当的了解。</p> <p>之前对 KVO 的了解仅限于使用层面,没有去想过它是如何实现的,更没有想过它会存在一些坑;甚至在刚接触它时,会尽可能创造机会使用它,譬如监听<code>UITextField#text</code>值的变化;但近几天接触了 Objective-C 的 Runtime 相关的知识,从 Runtime 层面了解到了 KVO 的实现原理(即 KVO 的消息转发机制),也通过阅读各位大神的博客了解到了它的坑。</p> <p>本文首先分析 KVO 和 Runtime 的关系,阐述 KVO 的实现原理;然后结合大神们的博客整理 KVO 存在的坑以及避免掉坑的正确使用姿势。</p> <h2 id="kvo-和-runtime"><a href="#kvo-和-runtime" class="header-anchor">#</a> KVO 和 Runtime</h2> <p>关于 KVO,即 Key-Value Observing,官方文档《Key-Value Observing Programming Guide》里的介绍比较简短明了:</p> <blockquote><p>Key-value observing is a mechanism that allows objects to be notified of changes to specified properties of other objects.</p></blockquote> <p><strong>KVO 的实现</strong></p> <p>KVO 的实现也依赖于 Objective-C 的 Runtime,官方文档《Key-Value Observing Programming Guide》中在《<a href="https://developer.apple.com/library/prerelease/content/documentation/Cocoa/Conceptual/KeyValueObserving/Articles/KVOImplementation.html" target="_blank" rel="noopener noreferrer">Key-Value Observing Implementation Details<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>》部分简单提到它的实现:</p> <blockquote><p>Automatic key-value observing is implemented using a technique called isa-swizzling.
The isa pointer, as the name suggests, points to the object's class which maintains a dispatch table. This dispatch table essentially contains pointers to the methods the class implements, among other data.
When an observer is registered for an attribute of an object the isa pointer of the observed object is modified, pointing to an intermediate class rather than at the true class. As a result the value of the isa pointer does not necessarily reflect the actual class of the instance.
You should never rely on the isa pointer to determine class membership. Instead, you should use the class method to determine the class of an object instance.</p></blockquote> <p>简单概述下 KVO 的实现:
当你观察一个对象(称该对象为<strong>被观察对象</strong>)时,一个新的类会动态被创建。这个类继承自<strong>被观察对象</strong>所对应类的,并重写该被观察属性的 setter 方法;针对 setter 方法的重写无非是在赋值语句前后加上相应的通知(或曰方法调用);最后,把被观察对象的<code>isa</code>指针(<code>isa</code>指针告诉 Runtime 系统这个对象的类是什么)指向这个新创建的中间类,对象就神奇变成了新创建类的实例。</p> <p>根据文档的描述,虽然被观察对象的<code>isa</code>指针被修改了,但是调用其<code>class</code>方法得到的类信息仍然是它之前所继承类的类信息,而不是这个新创建类的类信息。</p> <p>补充:下面对<code>isa</code>指针和类方法<code>class</code>作以更多的说明。</p> <p><code>isa</code>指针和类方法<code>class</code>的返回值都是<code>Class</code>类型,如下:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token keyword">@interface</span> NSObject <span class="token operator"><</span>NSObject<span class="token operator">></span> <span class="token punctuation">{</span>
Class isa OBJC_ISA_AVAILABILITY<span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token operator">+</span> <span class="token punctuation">(</span>Class<span class="token punctuation">)</span>class<span class="token punctuation">;</span>
</code></pre></div><p>根据我的理解,一般情况下,<code>isa</code>指针和<code>class</code>方法返回值都是一样的;但 KVO 底层实现时,动态创建的类只是重写了被观察属性的 setter 方法,并未重写类方法<code>class</code>,因此向被观察者发送<code>class</code>消息实际上仍然调用的是被观察者原先类的类方法<code>+(Class)class</code>,得到的类型信息当然是原先类的类信息,根据我的猜测,<code>isKindOfClass:</code>和<code>isMemberOfClass:</code>与<code>class</code>方法紧密相关。</p> <p>国外的大神 <NAME> 早在 2009 年就做了关于 KVO 的实现细节的探究,更多详细参考<a href="https://www.mikeash.com/pyblog/friday-qa-2009-01-23.html" target="_blank" rel="noopener noreferrer">这里<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>。</p> <h2 id="kvo-的槽点"><a href="#kvo-的槽点" class="header-anchor">#</a> KVO 的槽点</h2> <p>AFNetworking 作者 <NAME> 在<a href="http://nshipster.com/key-value-observing/" target="_blank" rel="noopener noreferrer">Key-Value Observing<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>中说:</p> <blockquote><p>Ask anyone who's been around the NSBlock a few times: Key-Value Observing has the worst API in all of Cocoa.</p></blockquote> <p>另一位不认识的大神在<a href="http://khanlou.com/2013/12/kvo-considered-harmful/" target="_blank" rel="noopener noreferrer">KVO Considered Harmful<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>中也写道:</p> <blockquote><p>KVO, or key-value observing, is a pattern that Cocoa provides for us for subscribing to changes to the properties of other objects. It’s hands down the most poorly designed API in all of Cocoa, and even when implemented perfectly, it’s still an incredibly dangerous tool to use, reserved only for when no other technique will suffice.</p></blockquote> <p>总之,两位大神都认为 KVO 的 API 非常差劲!</p> <p>其中<a href="http://khanlou.com/2013/12/kvo-considered-harmful/" target="_blank" rel="noopener noreferrer">KVO Considered Harmful<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>中对 KVO 的槽点有比较详细的阐述,这一部分内容就取材于此。</p> <p>为了更好说明这些槽点,假设一个应用场景:<code>ZWTableViewController</code>继承自<code>UITableViewController</code>,它现在需要做一件事情,即监测<code>self.tableView.contentSize</code>的变化,现采用典型的方式(即 KVO)处理这么个需求。</p> <p><strong>所有的 observe 处理都放在一个方法里</strong></p> <p>实现上述「监测<code>self.tableView.contentSize</code>的变化」的需求,最基本处理方式是:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token comment">// register observer</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>viewDidLoad <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">super</span> viewDidLoad<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">[</span>_tableView addObserver<span class="token punctuation">:</span><span class="token keyword">self</span> forKeyPath<span class="token punctuation">:</span><span class="token string">@"contentSize"</span> options<span class="token punctuation">:</span><span class="token number">0</span> context<span class="token punctuation">:</span><span class="token constant">NULL</span><span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token comment">/* ... */</span>
<span class="token punctuation">}</span>
<span class="token comment">// 处理observe</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>observeValueForKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
ofObject<span class="token punctuation">:</span><span class="token punctuation">(</span>id<span class="token punctuation">)</span>object
change<span class="token punctuation">:</span><span class="token punctuation">(</span>NSDictionary <span class="token operator">*</span><span class="token punctuation">)</span>change
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">self</span> configureView<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
</code></pre></div><p>但考虑到<code>observeValueForKeyPath:ofObject:change:context:</code>中可能会很多其他的 observe 事务,所以<code>observeValueForKeyPath:ofObject:change:context:</code>更好的逻辑是:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>observeValueForKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
ofObject<span class="token punctuation">:</span><span class="token punctuation">(</span>id<span class="token punctuation">)</span>object
change<span class="token punctuation">:</span><span class="token punctuation">(</span>NSDictionary <span class="token operator">*</span><span class="token punctuation">)</span>change
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context <span class="token punctuation">{</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>object <span class="token operator">==</span> _tableView <span class="token operator">&&</span> <span class="token punctuation">[</span>keyPath isEqualToString<span class="token punctuation">:</span><span class="token string">@"contentSize"</span><span class="token punctuation">]</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">self</span> configureView<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre></div><p>但如果 KVO 处理的事情种类多且繁杂,这会造成<code>observeValueForKeyPath:ofObject:change:context:</code>代码特别长,极不优雅。</p> <p><strong>严重依赖于 string</strong></p> <p>KVO 严重依赖 string,换句话说,KVO 中的 keyPath 必须是<code>NSString</code>这个事实使得编译器没办法在编译阶段将错误的 keyPath 给找出来;譬如很容易将「contentSize」写成「contentsize」;</p> <p><strong>需要自己处理 superclass 的 observe 事务</strong></p> <p>对于 Objective-C,很多时候 Runtime 系统都会自动帮助处理 superclass 的方法。譬如对于<code>dealloc</code>,假设类<code>Father</code>继承自<code>NSObject</code>,而类<code>Son</code>继承自<code>Father</code>,创建一个<code>Son</code>的实例<code>aSon</code>,在<code>aSon</code>被释放的时候,Runtime 会先调用<code>Son#dealloc</code>,之后会自动调用<code>Father#dealloc</code>,而无需在<code>Son#dealloc</code>中显式执行<code>[super dealloc];</code>。但 KVO 不会这样,所以为了保证父类(父类可能也会自己 observe 事务要处理)的 observe 事务也能被处理,上述<code>observeValueForKeyPath:ofObject:change:context:</code>代码得改成这样:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>observeValueForKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
ofObject<span class="token punctuation">:</span><span class="token punctuation">(</span>id<span class="token punctuation">)</span>object
change<span class="token punctuation">:</span><span class="token punctuation">(</span>NSDictionary <span class="token operator">*</span><span class="token punctuation">)</span>change
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context <span class="token punctuation">{</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>object <span class="token operator">==</span> _tableView <span class="token operator">&&</span> <span class="token punctuation">[</span>keyPath isEqualToString<span class="token punctuation">:</span><span class="token string">@"contentSize"</span><span class="token punctuation">]</span><span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">self</span> configureView<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span> <span class="token keyword">else</span> <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">super</span> observeValueForKeyPath<span class="token punctuation">:</span>keyPath ofObject<span class="token punctuation">:</span>object change<span class="token punctuation">:</span>change context<span class="token punctuation">:</span>context<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre></div><p><strong>多次相同的 remove observer 会导致 crash</strong></p> <p>写过 KVO 代码的人都知道,对同一个对象执行两次 remove observer 操作会导致程序 crash。</p> <p>在同一个文件中执行两次相同的 remove observer 属于粗心,比较容易 debug 出来;但是跨文件执行两次相同的 remove observer 就不是那么容易发现了。</p> <p>我们一般会在<code>dealloc</code>中进行 remove observer 操作(这也是 Apple 所推荐的)。</p> <p>譬如,假设上述的<code>ZWTableViewController</code>的父类<code>UITableViewController</code>也对<code>tableView.contentSize</code>注册了相同的监听;那么<code>UITableViewController#dealloc</code>中常常会写出如下这样的代码:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token punctuation">[</span>_tableView removeObserver<span class="token punctuation">:</span><span class="token keyword">self</span> forKeyPath<span class="token punctuation">:</span><span class="token string">@"contentSize"</span> context<span class="token punctuation">:</span><span class="token constant">NULL</span><span class="token punctuation">]</span><span class="token punctuation">;</span>
</code></pre></div><p>按照一般习惯,<code>ZWTableViewController#dealloc</code>也会有相同的处理;那么当<code>ZWTableViewController</code>对象被释放时,<code>ZWTableViewController</code>的<code>dealloc</code>和其父类<code>UITableViewController</code>的<code>dealloc</code>都被调用,这样会导致相同的 remove observer 被执行两次,自然会导致 crash。</p> <p><a href="http://khanlou.com/2013/12/kvo-considered-harmful/" target="_blank" rel="noopener noreferrer">KVO Considered Harmful<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>中还有很多其他的槽点,<a href="https://www.mikeash.com/pyblog/key-value-observing-done-right.html" target="_blank" rel="noopener noreferrer">Key-Value Observing Done Right<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>也描述了一些,这里就不多说了,更多信息还是建议看原文。</p> <p>不过好在上述的槽点「严重依赖于 string」和「多次相同的 remove observer 会导致 crash」有比较好的解决方案,如下会讲到。</p> <h2 id="使用-kvo"><a href="#使用-kvo" class="header-anchor">#</a> 使用 KVO</h2> <p>这一部分将阐述 KVO 的使用方法。</p> <h3 id="订阅"><a href="#订阅" class="header-anchor">#</a> 订阅</h3> <p>KVO 中与订阅相关的 API 只有一个:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>addObserver<span class="token punctuation">:</span><span class="token punctuation">(</span>NSObject <span class="token operator">*</span><span class="token punctuation">)</span>observer
forKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
options<span class="token punctuation">:</span><span class="token punctuation">(</span>NSKeyValueObservingOptions<span class="token punctuation">)</span>options
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context<span class="token punctuation">;</span>
</code></pre></div><p>对于这四个参数:</p> <ul><li><strong>observer</strong>: The object to register for KVO notifications. The observer must implement the key-value observing method <code>observeValueForKeyPath:ofObject:change:context:</code>.</li> <li><strong>keyPath</strong>: The key path, relative to the receiver, of the property to observe. This value must not be nil.</li> <li><strong>options</strong>: A combination of the NSKeyValueObservingOptions values that specifies what is included in observation notifications. For possible values, see <code>NSKeyValueObservingOptions</code>.</li> <li><strong>context</strong>: Arbitrary data that is passed to observer in <code>observeValueForKeyPath:ofObject:change:context:</code>.</li></ul> <p>大神们认为这个 API 丑陋的重要原因是因为后面两个参数:options 和 context。</p> <p>下面来对这两个参数进行详细介绍。</p> <p>首先是<strong>options</strong>。options 可选值是一个<code>NSKeyValueObservingOptions</code>枚举值,到目前为止,一共包括四个值,在介绍这四个值各自表示的意思之前,先得有一个概念,即 KVO 响应方法有一个<code>NSDictionary</code>类型参数 change(下面<strong>响应</strong>中可以看到),这个字典中会有一个与被监听属性相关的值,譬如被改变之前的值、新值等,<code>NSDictionary</code>中有啥值由订阅时的 options 值决定,options 可取值如下:</p> <ul><li><code>NSKeyValueObservingOptionNew</code>: 指示 change 字典中包含新属性值;</li> <li><code>NSKeyValueObservingOptionOld</code>: 指示 change 字典中包含旧属性值;</li> <li><code>NSKeyValueObservingOptionInitial</code>: 相对复杂一些,NSKeyValueObserving.h 文件中有详细说明,此处略过;</li> <li><code>NSKeyValueObservingOptionPrior</code>: 相对复杂一些,NSKeyValueObserving.h 文件中有详细说明,此处略过;</li></ul> <p>现在细想,options 这个参数也忒复杂了,难怪大神们觉得这个 API 丑陋(不过我等小民之前从未想过这个问题,=_=,没办法,Apple 是个大帝国,我只是其中一个跪舔的小屁民)。</p> <p>不过更糟心的是下面的 context 参数。</p> <p>options 信息量稍大,但其实蛮好理解的,然而对于 context,在写这篇博客之前,一直不知道 context 参数有啥用(也没在意)。</p> <p>context 作用大了去了,在上面的<strong>KVO 的槽点</strong>中提到一个槽点「多次相同的 remove observer 会导致 crash」。导致「多次调用相同的 remove observer」一个很重要的原因是我们经常在 add observer 时为 context 参数赋值<code>NULL</code>,关于如何使用 context 参数,下面会提到。</p> <h3 id="响应"><a href="#响应" class="header-anchor">#</a> 响应</h3> <p>iOS 的 UI 交互(譬如<code>UIButton</code>的一次点击)有一个非常不错的消息转发机制 -- Target-Action 模型,简单来说,为指定的 event 指定 target 和 action 处理方法。</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code>UIButton <span class="token operator">*</span>button <span class="token operator">=</span> <span class="token punctuation">[</span>UIButton new<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">[</span>button addTarget<span class="token punctuation">:</span><span class="token keyword">self</span> action<span class="token punctuation">:</span><span class="token keyword">@selector</span><span class="token punctuation">(</span>buttonDidClicked<span class="token punctuation">:</span><span class="token punctuation">)</span> forControlEvents<span class="token punctuation">:</span>UIControlEventTouchUpInside<span class="token punctuation">]</span><span class="token punctuation">;</span>
</code></pre></div><p>这种 target-action 模型逻辑非常清晰。作为对比,KVO 的响应处理就非常糟糕了,所有的响应都对应是同一个方法<code>observeValueForKeyPath:ofObject:change:context:</code>,其原型如下:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>observeValueForKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
ofObject<span class="token punctuation">:</span><span class="token punctuation">(</span>id<span class="token punctuation">)</span>object
change<span class="token punctuation">:</span><span class="token punctuation">(</span>NSDictionary <span class="token operator">*</span><span class="token punctuation">)</span>change
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context<span class="token punctuation">;</span>
</code></pre></div><p>除了<code>NSDictionary</code>类型参数 change 之外,其余几个参数都能在<code>addObserver:forKeyPath:options:context:</code>找到对应。</p> <p>change 参数上文已经讲过了,这里不多说了。下面将针对「严重依赖于 string」和「多次相同的 remove observer 会导致 crash」这两个槽点对 keyPath 和 context 参数进行阐述。</p> <p><strong>keyPath</strong>。keyPath 的类型是<code>NSString</code>,这导致了我们使用了错误的 keyPath 而不自知,譬如将<code>@"contentSize"</code>错误写成<code>@"contentsize"</code>,一个更好的方法是不直接使用<code>@"xxxoo"</code>,而是积极使用<code>NSStringFromSelector(SEL aSelector)</code>方法,即改<code>@"contentSize"</code>为<code>NSStringFromSelector(@selector(contentSize))</code>。</p> <p><strong>context</strong>。对于 context,上文已经提到一种场景:假如父类(设为<code>ClassA</code>)和子类(设为<code>ClassB</code>)都监听了同一个对象肿么办?是<code>ClassB</code>处理呢还是交给父类<code>ClassA</code>的<code>observeValueForKeyPath:ofObject:change:context:</code>处理呢?更复杂一点,如果子类的子类(设为<code>ClassC</code>)也监听了同一个对象,当<code>ClassB</code>接收到<code>ClassC</code>的<code>[super observeValueForKeyPath:keyPath ofObject:object change:change context:context];</code>消息时又该如何处理呢?</p> <p>这么一想,KVO 的 API 还真的是设计非常糟糕。一般来说,比较靠谱的做法是自己的屁股自己擦。<code>ClassB</code>的 observe 事务在<code>ClassB</code>中处理,怎么知道是自己的事务还是<code>ClassC</code>传上来的事务呢?用 context 参数判断!</p> <p>在 add observer 时为 context 参数设置一个独一无二的值即可,在 responding 处理时对这个 context 值进行检验。如此就解决了问题,但这需要靠用户(各个层级类的程序员用户)自觉遵守。</p> <h3 id="取消订阅"><a href="#取消订阅" class="header-anchor">#</a> 取消订阅</h3> <p>和<strong>订阅</strong>以及<strong>响应</strong>不同,取消订阅有两个方法:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>removeObserver<span class="token punctuation">:</span><span class="token punctuation">(</span>NSObject <span class="token operator">*</span><span class="token punctuation">)</span>observer forKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context<span class="token punctuation">;</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>removeObserver<span class="token punctuation">:</span><span class="token punctuation">(</span>NSObject <span class="token operator">*</span><span class="token punctuation">)</span>observer forKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath<span class="token punctuation">;</span>
</code></pre></div><p>个人觉得应该尽可能使用第一个方法,保持「订阅-响应-取消订阅」的一致性嘛,养成好习惯!</p> <p>此外,为了避免取消订阅时造成的 crash,可以把取消订阅代码放在<code>@try-@catch</code>语句中,如下是一个比较全面的的 KVO 使用示例:</p> <div class="language-objectivec extra-class"><pre class="language-objectivec"><code><span class="token keyword">static</span> <span class="token keyword">void</span> <span class="token operator">*</span> zwContentSize <span class="token operator">=</span> <span class="token operator">&</span>zwContentSize<span class="token punctuation">;</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>viewDidLoad <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">super</span> viewDidLoad<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token comment">// 1. subscribe</span>
<span class="token punctuation">[</span>_tableView addObserver<span class="token punctuation">:</span><span class="token keyword">self</span>
forKeyPath<span class="token punctuation">:</span><span class="token function">NSStringFromSelector</span><span class="token punctuation">(</span><span class="token keyword">@selector</span><span class="token punctuation">(</span>contentSize<span class="token punctuation">)</span><span class="token punctuation">)</span>
options<span class="token punctuation">:</span>NSKeyValueObservingOptionNew
context<span class="token punctuation">:</span>zwContentSize<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token comment">// 2. responding</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>observeValueForKeyPath<span class="token punctuation">:</span><span class="token punctuation">(</span>NSString <span class="token operator">*</span><span class="token punctuation">)</span>keyPath
ofObject<span class="token punctuation">:</span><span class="token punctuation">(</span>id<span class="token punctuation">)</span>object
change<span class="token punctuation">:</span><span class="token punctuation">(</span>NSDictionary <span class="token operator">*</span><span class="token punctuation">)</span>change
context<span class="token punctuation">:</span><span class="token punctuation">(</span><span class="token keyword">void</span> <span class="token operator">*</span><span class="token punctuation">)</span>context <span class="token punctuation">{</span>
<span class="token keyword">if</span> <span class="token punctuation">(</span>context <span class="token operator">==</span> zwContentSize<span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token comment">// configure view</span>
<span class="token punctuation">}</span> <span class="token keyword">else</span> <span class="token punctuation">{</span>
<span class="token punctuation">[</span><span class="token keyword">super</span> observeValueForKeyPath<span class="token punctuation">:</span>keyPath ofObject<span class="token punctuation">:</span>object change<span class="token punctuation">:</span>change context<span class="token punctuation">:</span>context<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
<span class="token operator">-</span> <span class="token punctuation">(</span><span class="token keyword">void</span><span class="token punctuation">)</span>dealloc <span class="token punctuation">{</span>
<span class="token keyword">@try</span> <span class="token punctuation">{</span>
<span class="token comment">// 3. unsubscribe</span>
<span class="token punctuation">[</span>_tableView removeObserver<span class="token punctuation">:</span><span class="token keyword">self</span>
forKeyPath<span class="token punctuation">:</span><span class="token function">NSStringFromSelector</span><span class="token punctuation">(</span><span class="token keyword">@selector</span><span class="token punctuation">(</span>contentSize<span class="token punctuation">)</span><span class="token punctuation">)</span>
context<span class="token punctuation">:</span>zwContentSize<span class="token punctuation">]</span><span class="token punctuation">;</span>
<span class="token punctuation">}</span>
<span class="token keyword">@catch</span> <span class="token punctuation">(</span>NSException <span class="token operator">*</span>exception<span class="token punctuation">)</span> <span class="token punctuation">{</span>
<span class="token punctuation">}</span>
<span class="token punctuation">}</span>
</code></pre></div><p>总之,KVO 很强大,但也挺坑,使用它要养成好习惯,避免入坑!</p> <h2 id="本文参考"><a href="#本文参考" class="header-anchor">#</a> 本文参考</h2> <ul><li>《Key-Value Observing Programming Guide》</li> <li><a href="http://tech.glowing.com/cn/implement-kvo/" target="_blank" rel="noopener noreferrer">如何自己动手实现 KVO<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="https://www.mikeash.com/pyblog/friday-qa-2009-01-23.html" target="_blank" rel="noopener noreferrer">KVO Implementation<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="https://www.mikeash.com/pyblog/friday-qa-2010-11-6-creating-classes-at-runtime-in-objective-c.html" target="_blank" rel="noopener noreferrer">Creating Classes at Runtime in Objective-C<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="https://www.mikeash.com/pyblog/key-value-observing-done-right.html" target="_blank" rel="noopener noreferrer">Key-Value Observing Done Right<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="http://khanlou.com/2013/12/kvo-considered-harmful/" target="_blank" rel="noopener noreferrer">KVO Considered Harmful<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="http://nshipster.com/key-value-observing/" target="_blank" rel="noopener noreferrer">Key-Value Observing<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li> <li><a href="http://objccn.io/issue-7-3/" target="_blank" rel="noopener noreferrer">KVC 和 KVO<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></li></ul></div></div><div class="global-ui"></div></div>
<script src="/assets/js/app.50bca889.js" defer></script><script src="/assets/js/5.081d1701.js" defer></script><script src="/assets/js/107.5af47fd0.js" defer></script>
</body>
</html>
<file_sep><!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>NSError 分析 | 张不坏的博客</title>
<meta name="description" content="我的第 1 篇博客">
<link rel="icon" href="/favicon.ico">
<link rel="preload" href="/assets/css/0.styles.37bfab3d.css" as="style"><link rel="preload" href="/assets/js/app.50bca889.js" as="script"><link rel="preload" href="/assets/js/5.081d1701.js" as="script"><link rel="preload" href="/assets/js/87.7f5dc71e.js" as="script"><link rel="prefetch" href="/assets/js/10.7bb33f06.js"><link rel="prefetch" href="/assets/js/100.014ff06c.js"><link rel="prefetch" href="/assets/js/101.28f11de0.js"><link rel="prefetch" href="/assets/js/102.be9d2e87.js"><link rel="prefetch" href="/assets/js/103.a1210d81.js"><link rel="prefetch" href="/assets/js/104.7101a956.js"><link rel="prefetch" href="/assets/js/105.833e6f80.js"><link rel="prefetch" href="/assets/js/106.978e1fc0.js"><link rel="prefetch" href="/assets/js/107.5af47fd0.js"><link rel="prefetch" href="/assets/js/108.efc3ce89.js"><link rel="prefetch" href="/assets/js/109.a69d6b5a.js"><link rel="prefetch" href="/assets/js/11.2de4afc9.js"><link rel="prefetch" href="/assets/js/110.30984b63.js"><link rel="prefetch" href="/assets/js/12.bfc099bd.js"><link rel="prefetch" href="/assets/js/13.20253e0d.js"><link rel="prefetch" href="/assets/js/14.67131b1c.js"><link rel="prefetch" href="/assets/js/15.1af26cbd.js"><link rel="prefetch" href="/assets/js/16.4b261ee0.js"><link rel="prefetch" href="/assets/js/17.1216332f.js"><link rel="prefetch" href="/assets/js/18.c0159773.js"><link rel="prefetch" href="/assets/js/19.0f007f87.js"><link rel="prefetch" href="/assets/js/2.b4633a05.js"><link rel="prefetch" href="/assets/js/20.4b295001.js"><link rel="prefetch" href="/assets/js/21.0c46767c.js"><link rel="prefetch" href="/assets/js/22.a5e065ea.js"><link rel="prefetch" href="/assets/js/23.f43a6a7e.js"><link rel="prefetch" href="/assets/js/24.245f4f15.js"><link rel="prefetch" href="/assets/js/25.618f74a1.js"><link rel="prefetch" href="/assets/js/26.274a606b.js"><link rel="prefetch" href="/assets/js/27.c2d8fe18.js"><link rel="prefetch" href="/assets/js/28.5c522d2a.js"><link rel="prefetch" href="/assets/js/29.c90fdb1a.js"><link rel="prefetch" href="/assets/js/3.9babd8f1.js"><link rel="prefetch" href="/assets/js/30.1ccbdebc.js"><link rel="prefetch" href="/assets/js/31.acf3eca6.js"><link rel="prefetch" href="/assets/js/32.ccfdc859.js"><link rel="prefetch" href="/assets/js/33.9b262756.js"><link rel="prefetch" href="/assets/js/34.c59a4044.js"><link rel="prefetch" href="/assets/js/35.2b10fefb.js"><link rel="prefetch" href="/assets/js/36.2daeeb7b.js"><link rel="prefetch" href="/assets/js/37.d649866c.js"><link rel="prefetch" href="/assets/js/38.aba1ac95.js"><link rel="prefetch" href="/assets/js/39.58a95fd1.js"><link rel="prefetch" href="/assets/js/4.a7413ce2.js"><link rel="prefetch" href="/assets/js/40.8ef4d374.js"><link rel="prefetch" href="/assets/js/41.5799de7a.js"><link rel="prefetch" href="/assets/js/42.b7ee7489.js"><link rel="prefetch" href="/assets/js/43.28a65d64.js"><link rel="prefetch" href="/assets/js/44.90f92ea2.js"><link rel="prefetch" href="/assets/js/45.30b683fd.js"><link rel="prefetch" href="/assets/js/46.f57ccc19.js"><link rel="prefetch" href="/assets/js/47.7a82bd74.js"><link rel="prefetch" href="/assets/js/48.72503020.js"><link rel="prefetch" href="/assets/js/49.3a4ba077.js"><link rel="prefetch" href="/assets/js/50.0c3297f3.js"><link rel="prefetch" href="/assets/js/51.e9ba9363.js"><link rel="prefetch" href="/assets/js/52.473ee9ff.js"><link rel="prefetch" href="/assets/js/53.166d6e7a.js"><link rel="prefetch" href="/assets/js/54.78af3662.js"><link rel="prefetch" href="/assets/js/55.f0d54751.js"><link rel="prefetch" href="/assets/js/56.5de81531.js"><link rel="prefetch" href="/assets/js/57.6e18322f.js"><link rel="prefetch" href="/assets/js/58.1fccc879.js"><link rel="prefetch" href="/assets/js/59.773775e1.js"><link rel="prefetch" href="/assets/js/6.0c9cc532.js"><link rel="prefetch" href="/assets/js/60.0d665185.js"><link rel="prefetch" href="/assets/js/61.d9ae36dc.js"><link rel="prefetch" href="/assets/js/62.fb5e3b65.js"><link rel="prefetch" href="/assets/js/63.5ace8fda.js"><link rel="prefetch" href="/assets/js/64.d44fb0af.js"><link rel="prefetch" href="/assets/js/65.ed8fe56f.js"><link rel="prefetch" href="/assets/js/66.809078da.js"><link rel="prefetch" href="/assets/js/67.2489499e.js"><link rel="prefetch" href="/assets/js/68.e3ee952d.js"><link rel="prefetch" href="/assets/js/69.071411f8.js"><link rel="prefetch" href="/assets/js/7.8188415c.js"><link rel="prefetch" href="/assets/js/70.be8269cf.js"><link rel="prefetch" href="/assets/js/71.a320347a.js"><link rel="prefetch" href="/assets/js/72.f4fda48b.js"><link rel="prefetch" href="/assets/js/73.0f9f9284.js"><link rel="prefetch" href="/assets/js/74.b4028d07.js"><link rel="prefetch" href="/assets/js/75.6d63415f.js"><link rel="prefetch" href="/assets/js/76.d5b4df24.js"><link rel="prefetch" href="/assets/js/77.62b794e1.js"><link rel="prefetch" href="/assets/js/78.63e767ab.js"><link rel="prefetch" href="/assets/js/79.45056905.js"><link rel="prefetch" href="/assets/js/8.20d7cb0f.js"><link rel="prefetch" href="/assets/js/80.e06c5521.js"><link rel="prefetch" href="/assets/js/81.bc82bd01.js"><link rel="prefetch" href="/assets/js/82.4aeb6081.js"><link rel="prefetch" href="/assets/js/83.3ed6146f.js"><link rel="prefetch" href="/assets/js/84.f2aff9f4.js"><link rel="prefetch" href="/assets/js/85.2b8f4e50.js"><link rel="prefetch" href="/assets/js/86.27aea1da.js"><link rel="prefetch" href="/assets/js/88.9ca6511c.js"><link rel="prefetch" href="/assets/js/89.e8f54ad1.js"><link rel="prefetch" href="/assets/js/9.ee6c43f7.js"><link rel="prefetch" href="/assets/js/90.9abac718.js"><link rel="prefetch" href="/assets/js/91.9d8f5f36.js"><link rel="prefetch" href="/assets/js/92.2277b907.js"><link rel="prefetch" href="/assets/js/93.efca2f57.js"><link rel="prefetch" href="/assets/js/94.e9cc0386.js"><link rel="prefetch" href="/assets/js/95.fa3326f7.js"><link rel="prefetch" href="/assets/js/96.82bafc57.js"><link rel="prefetch" href="/assets/js/97.da22d13e.js"><link rel="prefetch" href="/assets/js/98.d745e5ec.js"><link rel="prefetch" href="/assets/js/99.79a6f693.js">
<link rel="stylesheet" href="/assets/css/0.styles.37bfab3d.css">
</head>
<body>
<div id="app" data-server-rendered="true"><div class="theme-container"><div class="navbar"><div class="navbar-content"><div class="slogan"><NAME></div> <div class="links"><span class="link-item"><a href="/">首页</a></span> <span class="link-item"><a href="/category/iOS/">iOS</a></span> <span class="link-item"><a href="/category/other/">其他</a></span></div></div></div> <div class="content-header"><div class="post-title">NSError 分析</div> <div class="post-info">2015-01-19 • iOS</div></div> <div class="content content__default"><h2 id="写在前面"><a href="#写在前面" class="header-anchor">#</a> 写在前面</h2> <p>在 iOS 开发中,<code>NSError</code>的使用非常常见,使用也比较简单,也正因为简单,所以对这一部分知识不甚注重。但是近期在做 app 底层网络封装时发现了一些问题。我使用的网络框架是 AFNetworking,AFNetworking 常常会返回一些错误信息,有时需要将这些错误信息告诉用户,通常做法是将<code>NSError#localizedDescription</code>以弹框的形式原原本本展现给用户(譬如“网络不畅”之类的),但是这样非常不友好,一是这些信息往往是英文,二是这些信息过于冗长,不够简洁。所以自然想到了对这些 error 信息进行包装。这就迫使我不得不去了解更多关于 NSError 相关的信息,本文着重叙述 Error Domain 和 Error Code。</p> <h2 id="error-domain"><a href="#error-domain" class="header-anchor">#</a> Error Domain</h2> <p>首先,error domain 是一个字符串。因为历史原因,在 OS X 中将 errors 分为不同的 domains。譬如,对于 Carbon 框架的 error,归于 OSStatus domain(<code>NSOSStatusErrorDomain</code>),对于 POSIX error,归于<code>NSPOSIXErrorDomain</code>,而对于我们的 iOS 开发,一般使用<code>NSCocoaErrorDomain</code>。NSError.h 定义了四个 domain,如下:</p> <div class="language-c extra-class"><pre class="language-c"><code><span class="token comment">// Predefined domain for errors from most AppKit and Foundation APIs.</span>
FOUNDATION_EXPORT NSString <span class="token operator">*</span><span class="token keyword">const</span> NSCocoaErrorDomain<span class="token punctuation">;</span>
<span class="token comment">// Other predefined domains; value of "code" will correspond to preexisting values in these domains.</span>
FOUNDATION_EXPORT NSString <span class="token operator">*</span><span class="token keyword">const</span> NSPOSIXErrorDomain<span class="token punctuation">;</span>
FOUNDATION_EXPORT NSString <span class="token operator">*</span><span class="token keyword">const</span> NSOSStatusErrorDomain<span class="token punctuation">;</span>
FOUNDATION_EXPORT NSString <span class="token operator">*</span><span class="token keyword">const</span> NSMachErrorDomain<span class="token punctuation">;</span>
</code></pre></div><p>除了上述的四个 domain 之外,不同的 framework 甚至一些 classes group(相关的几个 classes)也定义了自己的 domain,譬如对于 Web Kit framework,定义了<code>WebKitErrorDomain</code>,而更常见的,URL 相关的 classes 定义了<code>NSURLErrorDomain</code>。</p> <p>Domains 非常有用,特别当程序非常复杂庞大时,官方文档是这么说的:</p> <blockquote><p>Domains serve several useful purposes. They give Cocoa programs a way to identify the OS X subsystem that is detecting an error. They also help to prevent collisions between error codes from different subsystems with the same numeric value. In addition, domains allow for a causal relationship between error codes based on the layering of subsystems; for example, an error in the NSOSStatusErrorDomain may have an underlying error in the NSMachErrorDomain.</p></blockquote> <p>用户也可以为自己的 framework 或者 app 定义自己的 domain,官方推荐的 domain 命名规则是:
<code>com.company.framework_or_app.ErrorDomain</code>。</p> <h2 id="error-code"><a href="#error-code" class="header-anchor">#</a> Error Code</h2> <p>Error Code 的类型是 signed integer。Error Code 指定了特殊的错误。这个信息对于程序开发来说极为有用。比如访问 URL 资源 timeout 错误对应的是<code>NSURLErrorTimedOut</code>(<code>-1001</code>)。</p> <p>那么如何知道各个 error code 对应什么样的值呢?iOS 开发中常用的 error code 所对应的头文件如下:</p> <ul><li>Foundation/FoundationErrors.h - Generic Foundation error codes</li> <li>CoreData/CoreDataErrors.h - Core Data error codes</li> <li>Foundation/NSURLError.h - URL error codes</li></ul> <p>以 Foundation/NSURLError.h 为例,其中的 URLError Code 值从<code>NSURLErrorDataLengthExceedsMaximum</code>到<code>NSURLErrorCancelled</code>,二者分别对应<code>-1103</code>和<code>-999</code>。如果对所有网络 error 笼统处理,这两个值可以为我所用。</p> <h2 id="the-user-info-dictionary"><a href="#the-user-info-dictionary" class="header-anchor">#</a> The User Info Dictionary</h2> <p>Every NSError object has a “user info” dictionary to hold error information beyond domain and code.</p> <p>User info 可以包含很多自定义信息,最常用的或许是 localized error information。访问 localized error information 有两种方式,其一是访问 NSError 的 localizedDescription 属性,其二是访问<code>NSError#userInfo</code>的<code>NSLocalizedDescriptionKey</code>域。</p> <p>关于 user info dictionary,比较常见,这里不多讲了,更多内容参考《Error Handling Programming Guide》。</p></div></div><div class="global-ui"></div></div>
<script src="/assets/js/app.50bca889.js" defer></script><script src="/assets/js/5.081d1701.js" defer></script><script src="/assets/js/87.7f5dc71e.js" defer></script>
</body>
</html>
<file_sep><!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>Cookie & Session | 张不坏的博客</title>
<meta name="description" content="Just For Fun">
<link rel="icon" href="/favicon.ico">
<link rel="preload" href="/assets/css/0.styles.37bfab3d.css" as="style"><link rel="preload" href="/assets/js/app.50bca889.js" as="script"><link rel="preload" href="/assets/js/5.081d1701.js" as="script"><link rel="preload" href="/assets/js/57.6e18322f.js" as="script"><link rel="preload" href="/assets/js/4.a7413ce2.js" as="script"><link rel="prefetch" href="/assets/js/10.7bb33f06.js"><link rel="prefetch" href="/assets/js/100.014ff06c.js"><link rel="prefetch" href="/assets/js/101.28f11de0.js"><link rel="prefetch" href="/assets/js/102.be9d2e87.js"><link rel="prefetch" href="/assets/js/103.a1210d81.js"><link rel="prefetch" href="/assets/js/104.7101a956.js"><link rel="prefetch" href="/assets/js/105.833e6f80.js"><link rel="prefetch" href="/assets/js/106.978e1fc0.js"><link rel="prefetch" href="/assets/js/107.5af47fd0.js"><link rel="prefetch" href="/assets/js/108.efc3ce89.js"><link rel="prefetch" href="/assets/js/109.a69d6b5a.js"><link rel="prefetch" href="/assets/js/11.2de4afc9.js"><link rel="prefetch" href="/assets/js/110.30984b63.js"><link rel="prefetch" href="/assets/js/12.bfc099bd.js"><link rel="prefetch" href="/assets/js/13.20253e0d.js"><link rel="prefetch" href="/assets/js/14.67131b1c.js"><link rel="prefetch" href="/assets/js/15.1af26cbd.js"><link rel="prefetch" href="/assets/js/16.4b261ee0.js"><link rel="prefetch" href="/assets/js/17.1216332f.js"><link rel="prefetch" href="/assets/js/18.c0159773.js"><link rel="prefetch" href="/assets/js/19.0f007f87.js"><link rel="prefetch" href="/assets/js/2.b4633a05.js"><link rel="prefetch" href="/assets/js/20.4b295001.js"><link rel="prefetch" href="/assets/js/21.0c46767c.js"><link rel="prefetch" href="/assets/js/22.a5e065ea.js"><link rel="prefetch" href="/assets/js/23.f43a6a7e.js"><link rel="prefetch" href="/assets/js/24.245f4f15.js"><link rel="prefetch" href="/assets/js/25.618f74a1.js"><link rel="prefetch" href="/assets/js/26.274a606b.js"><link rel="prefetch" href="/assets/js/27.c2d8fe18.js"><link rel="prefetch" href="/assets/js/28.5c522d2a.js"><link rel="prefetch" href="/assets/js/29.c90fdb1a.js"><link rel="prefetch" href="/assets/js/3.9babd8f1.js"><link rel="prefetch" href="/assets/js/30.1ccbdebc.js"><link rel="prefetch" href="/assets/js/31.acf3eca6.js"><link rel="prefetch" href="/assets/js/32.ccfdc859.js"><link rel="prefetch" href="/assets/js/33.9b262756.js"><link rel="prefetch" href="/assets/js/34.c59a4044.js"><link rel="prefetch" href="/assets/js/35.2b10fefb.js"><link rel="prefetch" href="/assets/js/36.2daeeb7b.js"><link rel="prefetch" href="/assets/js/37.d649866c.js"><link rel="prefetch" href="/assets/js/38.aba1ac95.js"><link rel="prefetch" href="/assets/js/39.58a95fd1.js"><link rel="prefetch" href="/assets/js/40.8ef4d374.js"><link rel="prefetch" href="/assets/js/41.5799de7a.js"><link rel="prefetch" href="/assets/js/42.b7ee7489.js"><link rel="prefetch" href="/assets/js/43.28a65d64.js"><link rel="prefetch" href="/assets/js/44.90f92ea2.js"><link rel="prefetch" href="/assets/js/45.30b683fd.js"><link rel="prefetch" href="/assets/js/46.f57ccc19.js"><link rel="prefetch" href="/assets/js/47.7a82bd74.js"><link rel="prefetch" href="/assets/js/48.72503020.js"><link rel="prefetch" href="/assets/js/49.3a4ba077.js"><link rel="prefetch" href="/assets/js/50.0c3297f3.js"><link rel="prefetch" href="/assets/js/51.e9ba9363.js"><link rel="prefetch" href="/assets/js/52.473ee9ff.js"><link rel="prefetch" href="/assets/js/53.166d6e7a.js"><link rel="prefetch" href="/assets/js/54.78af3662.js"><link rel="prefetch" href="/assets/js/55.f0d54751.js"><link rel="prefetch" href="/assets/js/56.5de81531.js"><link rel="prefetch" href="/assets/js/58.1fccc879.js"><link rel="prefetch" href="/assets/js/59.773775e1.js"><link rel="prefetch" href="/assets/js/6.0c9cc532.js"><link rel="prefetch" href="/assets/js/60.0d665185.js"><link rel="prefetch" href="/assets/js/61.d9ae36dc.js"><link rel="prefetch" href="/assets/js/62.fb5e3b65.js"><link rel="prefetch" href="/assets/js/63.5ace8fda.js"><link rel="prefetch" href="/assets/js/64.d44fb0af.js"><link rel="prefetch" href="/assets/js/65.ed8fe56f.js"><link rel="prefetch" href="/assets/js/66.809078da.js"><link rel="prefetch" href="/assets/js/67.2489499e.js"><link rel="prefetch" href="/assets/js/68.e3ee952d.js"><link rel="prefetch" href="/assets/js/69.071411f8.js"><link rel="prefetch" href="/assets/js/7.8188415c.js"><link rel="prefetch" href="/assets/js/70.be8269cf.js"><link rel="prefetch" href="/assets/js/71.a320347a.js"><link rel="prefetch" href="/assets/js/72.f4fda48b.js"><link rel="prefetch" href="/assets/js/73.0f9f9284.js"><link rel="prefetch" href="/assets/js/74.b4028d07.js"><link rel="prefetch" href="/assets/js/75.6d63415f.js"><link rel="prefetch" href="/assets/js/76.d5b4df24.js"><link rel="prefetch" href="/assets/js/77.62b794e1.js"><link rel="prefetch" href="/assets/js/78.63e767ab.js"><link rel="prefetch" href="/assets/js/79.45056905.js"><link rel="prefetch" href="/assets/js/8.20d7cb0f.js"><link rel="prefetch" href="/assets/js/80.e06c5521.js"><link rel="prefetch" href="/assets/js/81.bc82bd01.js"><link rel="prefetch" href="/assets/js/82.4aeb6081.js"><link rel="prefetch" href="/assets/js/83.3ed6146f.js"><link rel="prefetch" href="/assets/js/84.f2aff9f4.js"><link rel="prefetch" href="/assets/js/85.2b8f4e50.js"><link rel="prefetch" href="/assets/js/86.27aea1da.js"><link rel="prefetch" href="/assets/js/87.7f5dc71e.js"><link rel="prefetch" href="/assets/js/88.9ca6511c.js"><link rel="prefetch" href="/assets/js/89.e8f54ad1.js"><link rel="prefetch" href="/assets/js/9.ee6c43f7.js"><link rel="prefetch" href="/assets/js/90.9abac718.js"><link rel="prefetch" href="/assets/js/91.9d8f5f36.js"><link rel="prefetch" href="/assets/js/92.2277b907.js"><link rel="prefetch" href="/assets/js/93.efca2f57.js"><link rel="prefetch" href="/assets/js/94.e9cc0386.js"><link rel="prefetch" href="/assets/js/95.fa3326f7.js"><link rel="prefetch" href="/assets/js/96.82bafc57.js"><link rel="prefetch" href="/assets/js/97.da22d13e.js"><link rel="prefetch" href="/assets/js/98.d745e5ec.js"><link rel="prefetch" href="/assets/js/99.79a6f693.js">
<link rel="stylesheet" href="/assets/css/0.styles.37bfab3d.css">
</head>
<body>
<div id="app" data-server-rendered="true"><div class="theme-container"><div class="navbar"><div class="navbar-content"><div class="slogan"><NAME></div> <div class="links"><span class="link-item"><a href="/">首页</a></span> <span class="link-item"><a href="/category/iOS/">iOS</a></span> <span class="link-item"><a href="/category/other/">其他</a></span></div></div></div> <div class="content-header"><div class="post-title">Cookie & Session</div> <div class="post-info">2018-05-20</div></div> <div class="content content__default"><p>最近写 Web 服务,过程中梳理了一下 Cookie 和 Session 的知识,完成度勉强可以,于是以博客的方式进行展示。</p> <p>HTTP 请求都是无状态的,但 Web 应用通常都需要记录一些状态信息,譬如知道发起请求的人是谁。Cookie 和 Session 正是用来弥补用户状态追踪的两种机制;它们的区别是啥呢?博文<a href="https://justsee.iteye.com/blog/1570652" target="_blank" rel="noopener noreferrer">Session 机制详解<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>里举了几个非常精彩的例子,直接摘抄如下:</p> <div class="custom-block tip"><p class="custom-block-title">🌰</p> <p>让我们用几个例子来描述一下 cookie 和 session 机制之间的区别与联系。笔者曾经常去的一家咖啡店有喝5杯咖啡免费赠一杯咖啡的优惠,然而一次性消费5杯咖啡的机会微乎其微,这时就需要某种方式来纪录某位顾客的消费数量。想象一下其实也无外乎下面的几种方案:</p> <p>1、该店的店员很厉害,能记住每位顾客的消费数量,只要顾客一走进咖啡店,店员就知道该怎么对待了。这种做法就是协议本身支持状态。</p> <p>2、发给顾客一张卡片,上面记录着消费的数量,一般还有个有效期限。每次消费时,如果顾客出示这张卡片,则此次消费就会与以前或以后的消费相联系起来。这种做法就是在客户端保持状态。</p> <p>3、发给顾客一张会员卡,除了卡号之外什么信息也不纪录,每次消费时,如果顾客出示该卡片,则店员在店里的纪录本上找到这个卡号对应的纪录添加一些消费信息。这种做法就是在服务器端保持状态。</p> <p>由于HTTP协议是无状态的,而出于种种考虑也不希望使之成为有状态的,因此,后面两种方案就成为现实的选择。具体来说cookie机制采用的是在客户端保持状态的方案,而session机制采用的是在服务器端保持状态的方案。同时我们也看到,由于采用服务器端保持状态的方案在客户端也需要保存一个标识,所以session机制可能需要借助于cookie机制来达到保存标识的目的,但实际上它还有其他选择。</p></div> <h1 id="cookie"><a href="#cookie" class="header-anchor">#</a> Cookie</h1> <h2 id="相关首部"><a href="#相关首部" class="header-anchor">#</a> 相关首部</h2> <p>站在 HTTP 协议的角度来看,HTTP 协议中有两个与 Cookie 直接相关首部:</p> <ul><li>Set-Cookie,由 response 携带,指示 client 存储 cookie 到本地</li> <li>Cookie,由 request 携带,将本地 cookie 传给 server</li></ul> <p>Server 发送 response 时,通过 Set-Cookie 首部,告诉客户端(譬如浏览器)将 cookie 内容给存储起来。该字段包括如下常用属性用来指导「客户端如何存储」:</p> <ul><li>Domain:cookie 适用的域名,若不指定,则默认为创建 cookie 的服务器的域名</li> <li>Path:cookie 适用的 path</li> <li>Expires:到期时间,若不指定,则浏览器关闭时即删除</li> <li>Secure:指示是否仅当在 HTTPS 链接上才传输</li> <li>HttpOnly:加以限制,使得 cookie 不能被 JavaScript 脚本访问(意味着不能 JS 不能通过<code>document.cookie</code>的方式访问 cookie)</li></ul> <p>这几个常用属性都不难理解,其中 Domain 和 Path 限制了 cookie 的使用范围,浏览器在发起 request 的时候,会根据这两个属性将符合使用范围的 cookie 发给 server;Secure 指示是否在 HTTPS 环境下才能传输。</p> <p>事实上,Cookie 还有更多其他属性,只是不怎么常用;之前的博客<a href="/post/http-draft.html">HTTP 碎片信息</a>对 Cookie 的发展史和字段详情有更详细的描述。</p> <p>下图是使用 Charles 抓包的一个 Set-Cookie 信息样例:</p> <div class="custom-image-wrapper" data-v-339c7bd5><img src="/image/charles-set-cookie.png" srcset="/image/charles-set-cookie.png 2x" data-v-339c7bd5></div> <p>客户端在发起 request 请求时,会根据 Domain 和 Path 将符合规则的本地 Cookie 内容给找出来发送给 server,内容存储在 Cookie 首部中,如下图:</p> <div class="custom-image-wrapper" data-v-339c7bd5><img src="/image/charles-cookie.png" srcset="/image/charles-cookie.png 2x" data-v-339c7bd5></div> <h2 id="持久-cookie-会话-cookie"><a href="#持久-cookie-会话-cookie" class="header-anchor">#</a> 持久 Cookie & 会话 Cookie</h2> <p>从存活时长来看,Cookie 通常被笼统划分为两种类型:会话 cookie、持久 cookie。前者是一种临时 cookie,会话结束(一般是浏览器被关闭)时,cookie 就被删除了。持久 cookie 的生存时间更长一些,它们存储在硬盘上,应用程序退出时,仍然会保留它们。</p> <p>简单来说,当设置了 Discard,或者没有设置 Expires 或 Max-Age,就意味着这个 cookie 是一个会话 cookie。</p> <h1 id="session"><a href="#session" class="header-anchor">#</a> Session</h1> <p>关于 Cookie 和 Session 的对比,一种常见的说法是:</p> <ul><li>Cookie:在客户端保存用户状态</li> <li>Session:在服务端保存用户状态</li></ul> <p>在我看来,第一句没问题,第二句不是很准确。为啥不准确呢?Cookie 是一种事实上的标准,但 Session 不是,它只是一种比较常见的概念,没有所谓的实现标准;换句话说,不同的服务框架,Session 的实现机制可能是不一样的,它可能被保存在服务端,也可能保存在客户端。</p> <h2 id="session-的实现机制"><a href="#session-的实现机制" class="header-anchor">#</a> Session 的实现机制</h2> <p>这一部分讲述 Session 的常见实现机制。据我所知,从存储的角度看,session 的常见实现机制有两种。</p> <p>第一种姿势:session 信息存储在服务端。这种实现机制中,服务端负责生成和管理 session 信息,每个 session 会有一个 session id,server 返回 response 给 client 时会让后者以 cookie 的形式存储 session id,后续发起 request 请求时,都会在 HTTP 报文的 Cookie 首部中携带 session id,server 根据 id 找到对应的 session 信息。</p> <p>第二种姿势:session 信息存储在客户端。这种实现机制中,服务端负责生成 session 信息,但不存储,在 response 中,通过 Set-Cookie 首部让 client 把 session 信息(一般都进行了加密)存在 cookie 中,后续 client 发起 request 请求时,server 从 Cookie 首部取出 session 信息进行解密读取,以及进行各种操作,然后再通过 Set-Cookie 回写到 client。</p> <p>这两种姿势有何优劣?如下谈谈个人的理解。</p> <p>从客户端角度看,第一种姿势 client 承担的任务更轻一些,毕竟只需管理 session id,第二种姿势负责管理的信息更重一些:整个 session 信息,虽然可能是加密压缩的。当 session 信息比较大时,负载无疑更重。</p> <p>第一种姿势更常见,好处貌似更明显;为啥还有第二种姿势存在呢?</p> <p>对于轻量级的应用,以线程或协程为粒度处理 request 的服务框架(譬如 Java、Go 生态),一般都采用第一种。而笔者实践过的 Egg.js 框架,提供的默认 session 实现机制是第二种。我认为很重要的原因是 Node 无法提供线程服务,为了提高服务端响应速度,通常开启多个 node 进程,进程之间共享数据却是个麻烦,把 session 数据放在客户端能规避这个麻烦,服务端基本上无需在管理 session 上费心,因为 session 已然是 request 的一部分。</p> <p>然而,对于大型分布式应用,一般都采用第一种姿势,在分布式场景下,管理 session 是一个重要的议题,而 Redis 在其中扮演者重要角色,本文就不展开描述了。</p></div></div><div class="global-ui"></div></div>
<script src="/assets/js/app.50bca889.js" defer></script><script src="/assets/js/5.081d1701.js" defer></script><script src="/assets/js/57.6e18322f.js" defer></script><script src="/assets/js/4.a7413ce2.js" defer></script>
</body>
</html>
<file_sep><!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width,initial-scale=1">
<title>HTTPS 碎片信息 | 张不坏的博客</title>
<meta name="description" content="Just For Fun">
<link rel="icon" href="/favicon.ico">
<link rel="preload" href="/assets/css/0.styles.37bfab3d.css" as="style"><link rel="preload" href="/assets/js/app.50bca889.js" as="script"><link rel="preload" href="/assets/js/5.081d1701.js" as="script"><link rel="preload" href="/assets/js/72.f4fda48b.js" as="script"><link rel="preload" href="/assets/js/4.a7413ce2.js" as="script"><link rel="prefetch" href="/assets/js/10.7bb33f06.js"><link rel="prefetch" href="/assets/js/100.014ff06c.js"><link rel="prefetch" href="/assets/js/101.28f11de0.js"><link rel="prefetch" href="/assets/js/102.be9d2e87.js"><link rel="prefetch" href="/assets/js/103.a1210d81.js"><link rel="prefetch" href="/assets/js/104.7101a956.js"><link rel="prefetch" href="/assets/js/105.833e6f80.js"><link rel="prefetch" href="/assets/js/106.978e1fc0.js"><link rel="prefetch" href="/assets/js/107.5af47fd0.js"><link rel="prefetch" href="/assets/js/108.efc3ce89.js"><link rel="prefetch" href="/assets/js/109.a69d6b5a.js"><link rel="prefetch" href="/assets/js/11.2de4afc9.js"><link rel="prefetch" href="/assets/js/110.30984b63.js"><link rel="prefetch" href="/assets/js/12.bfc099bd.js"><link rel="prefetch" href="/assets/js/13.20253e0d.js"><link rel="prefetch" href="/assets/js/14.67131b1c.js"><link rel="prefetch" href="/assets/js/15.1af26cbd.js"><link rel="prefetch" href="/assets/js/16.4b261ee0.js"><link rel="prefetch" href="/assets/js/17.1216332f.js"><link rel="prefetch" href="/assets/js/18.c0159773.js"><link rel="prefetch" href="/assets/js/19.0f007f87.js"><link rel="prefetch" href="/assets/js/2.b4633a05.js"><link rel="prefetch" href="/assets/js/20.4b295001.js"><link rel="prefetch" href="/assets/js/21.0c46767c.js"><link rel="prefetch" href="/assets/js/22.a5e065ea.js"><link rel="prefetch" href="/assets/js/23.f43a6a7e.js"><link rel="prefetch" href="/assets/js/24.245f4f15.js"><link rel="prefetch" href="/assets/js/25.618f74a1.js"><link rel="prefetch" href="/assets/js/26.274a606b.js"><link rel="prefetch" href="/assets/js/27.c2d8fe18.js"><link rel="prefetch" href="/assets/js/28.5c522d2a.js"><link rel="prefetch" href="/assets/js/29.c90fdb1a.js"><link rel="prefetch" href="/assets/js/3.9babd8f1.js"><link rel="prefetch" href="/assets/js/30.1ccbdebc.js"><link rel="prefetch" href="/assets/js/31.acf3eca6.js"><link rel="prefetch" href="/assets/js/32.ccfdc859.js"><link rel="prefetch" href="/assets/js/33.9b262756.js"><link rel="prefetch" href="/assets/js/34.c59a4044.js"><link rel="prefetch" href="/assets/js/35.2b10fefb.js"><link rel="prefetch" href="/assets/js/36.2daeeb7b.js"><link rel="prefetch" href="/assets/js/37.d649866c.js"><link rel="prefetch" href="/assets/js/38.aba1ac95.js"><link rel="prefetch" href="/assets/js/39.58a95fd1.js"><link rel="prefetch" href="/assets/js/40.8ef4d374.js"><link rel="prefetch" href="/assets/js/41.5799de7a.js"><link rel="prefetch" href="/assets/js/42.b7ee7489.js"><link rel="prefetch" href="/assets/js/43.28a65d64.js"><link rel="prefetch" href="/assets/js/44.90f92ea2.js"><link rel="prefetch" href="/assets/js/45.30b683fd.js"><link rel="prefetch" href="/assets/js/46.f57ccc19.js"><link rel="prefetch" href="/assets/js/47.7a82bd74.js"><link rel="prefetch" href="/assets/js/48.72503020.js"><link rel="prefetch" href="/assets/js/49.3a4ba077.js"><link rel="prefetch" href="/assets/js/50.0c3297f3.js"><link rel="prefetch" href="/assets/js/51.e9ba9363.js"><link rel="prefetch" href="/assets/js/52.473ee9ff.js"><link rel="prefetch" href="/assets/js/53.166d6e7a.js"><link rel="prefetch" href="/assets/js/54.78af3662.js"><link rel="prefetch" href="/assets/js/55.f0d54751.js"><link rel="prefetch" href="/assets/js/56.5de81531.js"><link rel="prefetch" href="/assets/js/57.6e18322f.js"><link rel="prefetch" href="/assets/js/58.1fccc879.js"><link rel="prefetch" href="/assets/js/59.773775e1.js"><link rel="prefetch" href="/assets/js/6.0c9cc532.js"><link rel="prefetch" href="/assets/js/60.0d665185.js"><link rel="prefetch" href="/assets/js/61.d9ae36dc.js"><link rel="prefetch" href="/assets/js/62.fb5e3b65.js"><link rel="prefetch" href="/assets/js/63.5ace8fda.js"><link rel="prefetch" href="/assets/js/64.d44fb0af.js"><link rel="prefetch" href="/assets/js/65.ed8fe56f.js"><link rel="prefetch" href="/assets/js/66.809078da.js"><link rel="prefetch" href="/assets/js/67.2489499e.js"><link rel="prefetch" href="/assets/js/68.e3ee952d.js"><link rel="prefetch" href="/assets/js/69.071411f8.js"><link rel="prefetch" href="/assets/js/7.8188415c.js"><link rel="prefetch" href="/assets/js/70.be8269cf.js"><link rel="prefetch" href="/assets/js/71.a320347a.js"><link rel="prefetch" href="/assets/js/73.0f9f9284.js"><link rel="prefetch" href="/assets/js/74.b4028d07.js"><link rel="prefetch" href="/assets/js/75.6d63415f.js"><link rel="prefetch" href="/assets/js/76.d5b4df24.js"><link rel="prefetch" href="/assets/js/77.62b794e1.js"><link rel="prefetch" href="/assets/js/78.63e767ab.js"><link rel="prefetch" href="/assets/js/79.45056905.js"><link rel="prefetch" href="/assets/js/8.20d7cb0f.js"><link rel="prefetch" href="/assets/js/80.e06c5521.js"><link rel="prefetch" href="/assets/js/81.bc82bd01.js"><link rel="prefetch" href="/assets/js/82.4aeb6081.js"><link rel="prefetch" href="/assets/js/83.3ed6146f.js"><link rel="prefetch" href="/assets/js/84.f2aff9f4.js"><link rel="prefetch" href="/assets/js/85.2b8f4e50.js"><link rel="prefetch" href="/assets/js/86.27aea1da.js"><link rel="prefetch" href="/assets/js/87.7f5dc71e.js"><link rel="prefetch" href="/assets/js/88.9ca6511c.js"><link rel="prefetch" href="/assets/js/89.e8f54ad1.js"><link rel="prefetch" href="/assets/js/9.ee6c43f7.js"><link rel="prefetch" href="/assets/js/90.9abac718.js"><link rel="prefetch" href="/assets/js/91.9d8f5f36.js"><link rel="prefetch" href="/assets/js/92.2277b907.js"><link rel="prefetch" href="/assets/js/93.efca2f57.js"><link rel="prefetch" href="/assets/js/94.e9cc0386.js"><link rel="prefetch" href="/assets/js/95.fa3326f7.js"><link rel="prefetch" href="/assets/js/96.82bafc57.js"><link rel="prefetch" href="/assets/js/97.da22d13e.js"><link rel="prefetch" href="/assets/js/98.d745e5ec.js"><link rel="prefetch" href="/assets/js/99.79a6f693.js">
<link rel="stylesheet" href="/assets/css/0.styles.37bfab3d.css">
</head>
<body>
<div id="app" data-server-rendered="true"><div class="theme-container"><div class="navbar"><div class="navbar-content"><div class="slogan"><NAME></div> <div class="links"><span class="link-item"><a href="/">首页</a></span> <span class="link-item"><a href="/category/iOS/">iOS</a></span> <span class="link-item"><a href="/category/other/">其他</a></span></div></div></div> <div class="content-header"><div class="post-title">HTTPS 碎片信息</div> <div class="post-info">2017-02-26</div></div> <div class="content content__default"><p>本文是我了解 HTTPS 过程中记录的一些碎片信息。</p> <h2 id="基本概念"><a href="#基本概念" class="header-anchor">#</a> 基本概念</h2> <ul><li>对称密钥加密系统:编/解码使用相同密钥的算法</li> <li>不对称密钥加密系统:编/解码使用不同密钥的算法</li> <li>公开密钥加密系统:一种能够使百万计算机便携地发送机密报文的系统</li> <li>数字签名:用来验证报文未被伪造或篡改的校验和</li> <li>数字证书:由一个可信的组织验证和签发的识别信息</li></ul> <h3 id="对称密钥加密技术"><a href="#对称密钥加密技术" class="header-anchor">#</a> 对称密钥加密技术</h3> <p>保持密钥的机密状态是很重要的。在很多情况下,编/解码算法都是众所周知的,密钥是唯一保密的东西。</p> <p>好的加密算法会迫使攻击者尝遍每一个可能的密钥,才能破解代码。</p> <p>可用密钥值的数量取决于密钥的位数,以及可能的密钥中有多少位是有效的。有些加密技术中只有部分密钥是有效的。</p> <p><strong>枚举攻击</strong>:用暴力去尝试所有的密钥值称为枚举攻击(enumeration attack)。</p> <p>对称密钥加密技术的痛点之一:发送者和接收者在互相对话之前,一定要有一个共享的保密密钥。如果有 N 个节点,每个节点都要和其他所有 N-1 个节点进行安全对话,总共大概会有 N*N 个保密密钥,这几乎无法管理。</p> <p>常见的对称加密算法有 DES、3DES、AES、Blowfish、IDEA、RC5、RC6。</p> <h3 id="公开密钥加密技术"><a href="#公开密钥加密技术" class="header-anchor">#</a> 公开密钥加密技术</h3> <p>公开密钥加密技术没有为每对主机使用单独的加密/解密密钥,而是使用了两个非对称密钥:一个用来对主机报文编码,另一个用来对主机报文解码。</p> <p>编码密钥是众所周知的,但是解码密钥只能为报文的目标主机所持有。</p> <p>P.S: 公开密钥加密技术似乎只适合多对一通信。</p> <p>公开密钥加密一般都是非对称的,即加密密钥和解密密钥不同。共同挑战是,要确保即便有人拥有了下面线索,也无法计算出保密的私有密钥:</p> <ul><li>公开密钥(是公有的,所有人都可以获得)</li> <li>一小片拦截下来的密文(可通过对网络的嗅探获取)</li> <li>一条报文与之相关的密文(对任意一段文本运行加密器就可以得到)</li></ul> <p><strong>RSA</strong></p> <p>RSA 是一个满足了所有上述条件的公开密钥加密系统,破解 RSA 系统的私密钥匙的困难程度相当于对一个极大的数进行质因数分解,这是计算机科学中最困难的问题之一。</p> <p>关于更多 RSA 的内容,参考:<a href="http://www.ruanyifeng.com/blog/2013/06/rsa_algorithm_part_one.html" target="_blank" rel="noopener noreferrer">RSA 算法原理(一)<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>、<a href="http://www.ruanyifeng.com/blog/2013/07/rsa_algorithm_part_two.html" target="_blank" rel="noopener noreferrer">RSA 算法原理(二)<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>。</p> <h3 id="数字签名"><a href="#数字签名" class="header-anchor">#</a> 数字签名</h3> <p>数字签名是附加在报文上的特殊加密校验码,即所谓的校验和。</p> <p>数字签名算法的输入是啥呢?报文消息?No!数字签名算法对消息的长度和格式是有要求的,要求数据满足一定的条件。所以不能直接使用报文消息作为输入。一般策略是是对报文消息先进行哈希,得到固定长度的哈希值,然后在对哈希值进行签名。如下图:</p> <div class="custom-image-wrapper" data-v-339c7bd5><img src="/image/[email protected]" srcset="/image/[email protected] 2x" data-v-339c7bd5></div> <ul><li>节点 A 将变长报文进行哈希,生成定长的消息摘要</li> <li>节点 A 对摘要应用了一个签名函数,该函数会将用户的私有密钥作为参数,输出校验和</li> <li>签名被附着在报文的末尾,一并发送给节点 B</li> <li>接收端 B 对签名进行逆向处理(使用公开密钥),将得到的消息摘要与自己计算得到的消息摘要进行对比,进而判断报文是否被篡改</li></ul> <p>显然,签名一般是在非对称公开加密技术基础上建立的,签名作者持有私钥,签名接收者持有公钥。</p> <p>综上,签名的作用有两点:</p> <ul><li>证明是作者编写了这条报文,因为只有作者持有的密钥才能生成校验和</li> <li>签名可以防止报文被篡改,攻击者若拦截报文,然后对之进行修改,然而校验和无法匹配,这会在接收者那里被识破</li></ul> <p>P.S: 消息摘要是哈希算法通过哈希处理得到的,哈希算法的安全性从很大程度上决定了数字签名的安全性,所谓哈希算法的安全性,在这里指的是,如果消息报文被篡改了,那么篡改后的摘要和篡改前的摘要必须不同。最近 Google 宣布实现了对著名的哈希算法 SHA-1 的碰撞,详见<a href="https://www.zhihu.com/question/56234281/answer/148349930" target="_blank" rel="noopener noreferrer">这里<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>。</p> <h3 id="数字证书"><a href="#数字证书" class="header-anchor">#</a> 数字证书</h3> <p>数字证书是由证书颁发机构签发的,像身份证一样,不易伪造。包括如下内容:</p> <ul><li>证书序列号</li> <li>证书签名算法</li> <li>证书颁发者</li> <li>有效期</li> <li>公开密钥</li> <li>证书签发机构的数字签名</li> <li>等等</li></ul> <p>P.S: 特别需要注意的是 <em>证书签发机构的数字签名</em>,浏览器收到某个 Web 服务器的证书时,会对签名颁发机构的签名进行检查,一般来说,浏览器事先就已经预安装了很多签名颁发机构的证书(含有公开密钥),因此可以对签名进行验证。如果浏览器对签名颁发机构一无所知,浏览器就无法确定是否应该信任该签名颁发机构,它通常会向用户显示一个对话框,看看用户是否相信这个签名发布者,这种签名发布者往往是本地的 IT 部门或者软件厂商。</p> <p>P.S: 大多数证书都遵循 X.509 v3 标准。</p> <p>基于 X.509 证书的签名有好几种,包括:</p> <ul><li>Web 服务器证书</li> <li>客户端电子邮件证书</li> <li>软件代码签名证书</li> <li>证书颁发机构证书</li></ul> <h2 id="http-的缺点"><a href="#http-的缺点" class="header-anchor">#</a> HTTP 的缺点</h2> <p>HTTP 主要有三大不足:</p> <ul><li>通信使用明文(不加密),内容可能会被窃听</li> <li>不验证通信方的身份,有可能遭到伪装</li> <li>无法证明报文的完整性,报文可能被篡改</li></ul> <p>或者参考阮一峰大神的博客<a href="http://www.ruanyifeng.com/blog/2014/02/ssl_tls.html" target="_blank" rel="noopener noreferrer">SSL/TLS 协议运行机制<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>的概述的说法,简称为三大风险:</p> <ul><li>窃听风险(eavesdropping)</li> <li>冒充风险(pretending)</li> <li>篡改风险(tampering)</li></ul> <p>本文的目的是就是详细阐述这三大问题,以便以后更好地理解 HTTPS。</p> <h3 id="窃听风险与加密"><a href="#窃听风险与加密" class="header-anchor">#</a> 窃听风险与加密</h3> <p>一个重要的事实是:TCP/IP 是可能被窃听的网络。TCP/IP 协议簇的工作机制,通信内容在所有的通信线路上都有可能遭到窥视:</p> <div class="custom-image-wrapper" data-v-339c7bd5><img src="/image/[email protected]" srcset="/image/[email protected] 2x" data-v-339c7bd5></div> <p>所谓互联网,是由能联通到全世界的网络设备组成的,无论哪里的 server 和 client 通信,此线路上的网络设备(包括电缆、计算机、路由器、交换机等)都不可能是个人的私有物,因此根本无法避免某个环节的报文会被窥探。</p> <p>窃听报文并非难事,只要收集互联网上流动的数据包即可,然后对它们进行解析。</p> <h3 id="通过加密防止报文被窃听"><a href="#通过加密防止报文被窃听" class="header-anchor">#</a> 通过加密防止报文被窃听</h3> <p>在应对报文被窃听的对策中,最为普遍的做法是加密技术,加密并不是个陌生的概念,两个重要问题:被加密的对象是啥?采用哪种加密方式?</p> <p><strong>对内容进行加密</strong></p> <p>对内容本身进行加密是最容易想到的,加密后的内容,即便报文被捕获,没有解密秘钥,拿到的也是一堆乱码。此处的 <em>内容</em> 指的是啥?众所周知,HTTP message 有三大组成部分:起始行、首部、报文主体。对内容加密的这种方式,被加密对象是哪一种呢?</p> <p>一般来说,不会对起始行和首部进行加密。为啥呢?根据我的理解,一方面是意义不大,另一方面首部和起始行信息不光是给接收端看的,也是给沿路中的各种代理看的,加密后的首部和起始行叫人家怎么看?因此,加密对象一般是报文主体或者部分报文主体(敏感内容)。</p> <p>诚然,为了做到有效的内容加密,前提是要求 client 和 server 同时具备加密和解密机制。</p> <p><strong>内容加密的相关算法</strong></p> <p>另一个问题,对内容加密的方式中,一般采用何种加密呢?加密算法太多了,这里就不展开了...</p> <p><strong>对内容加密的不足</strong></p> <p>只要加密算法足够安全,并且密钥被保护得当,对内容加密能够避免报文主体的敏感内容被监听,但是仍然无法避免内容被篡改。</p> <p>这个也容易理解,在传输过程中,窃听者虽然读不懂报文的内容的意义,但是仍然可以修改报文内容,譬如把报文的主体内容给清掉,进而对接收者进行误导。</p> <p><strong>对信道加密</strong></p> <p>相对于内容加密,<em>信道加密</em> 这个概念比较生僻一些,内容是比较容易理解的,那么,何为 <em>信道</em> 呢?</p> <p>我们知道,网络协议是一层层的,HTTP 建立在 TCP 的基础上,TCP 建立在 IP 的基础上。对于 HTTP 协议来说,所谓信道,其实是 TCP 层(类似,TCP 报文的信道是 IP 层),如果 TCP 层的通信是安全的,那么我们谈论 HTTP 加密似乎就没啥意义了。因此,所谓信道加密,是指 HTTP 所依赖的更底层协议被加密。</p> <p>这里所指的信道加密和 SSL/TLS 有关,搁在后面再讲。</p> <p>P.S: 对 <em>信道</em> 和 <em>信道加密</em> 的阐述,只是我的理解,可能是错的。</p> <h3 id="冒充风险与身份认证"><a href="#冒充风险与身份认证" class="header-anchor">#</a> 冒充风险与身份认证</h3> <p>另一个重要的事实是:任何人都可以发起请求。</p> <p>HTTP 协议并没有要求对通信方进行确认,server 只要接收到 request,就会给出一个相应的 response,因此会存在如下隐患:</p> <ul><li>无法保证 request 发送到了目标 server,有可能接收者是伪装的 server</li> <li>无法保证 response 是被正确的 client 接收,有可能接收者是伪装的 client</li> <li>无法确定正在通信的对方是否具备访问权限,因为某些 Web 服务器上保存着重要的信息,只想发给具备某种特定权限的 client</li> <li>无法避免无意义的 request 涌入,既无法阻止海量请求下的 DoS 攻击(Denial of Service,拒绝服务攻击)</li></ul> <p><strong>通过证书验证身份</strong></p> <p>身份认证可以解决这个问题,SSL/TLS 提供了这种服务,详见之后的博客。</p> <h3 id="篡改风险与完整性保护"><a href="#篡改风险与完整性保护" class="header-anchor">#</a> 篡改风险与完整性保护</h3> <p>上文已经提到,通过内容加密方式,可以避免报文敏感信息被窃听,但是无法避免报文被篡改。譬如,request 或 response 在传输途中,遭受攻击者拦截并篡改内容,这种攻击行为被称为中间人攻击(Man-In-The-Middle attack,MITM attack)。</p> <p>什么是完整性保护呢?所谓完整性并不是指报文没有受损,而是指报文的准确度;换句话说,如果无法证明报文的完整性,那么意味着无法判断报文信息是否准确。</p> <p>与窃听风险、冒充风险不同,篡改风险的应对措施比较多,根据《HTTP 权威指南》的说法,至少包括:基本认证、摘要认证,以及数字签名。</p> <p>在 HTTP 应用中,一种常见的策略是使用散列值校验,简单来说,发送方使用散列值算法(最常见的有 MD5、SHA-1,又被称为 <em>摘要算法</em> ),对消息进行哈希,得到固定长度的哈希值(是谓 <em>摘要</em> ),将该哈希值与消息一起传给对方,对方在收到 message 后,也使用约定的散列值算法对报文进行哈希,再与发送方的哈希值进行对比,如果不一致,则说明报文已被篡改,不安全。</p> <p>然而,这是会存在问题的,一方面是 MD5、SHA-1 不再安全,另一方面摘要本身也是可以被篡改的。</p> <p>更好的做法是,对摘要本身也进行保护,这个过程一般被称为数字签名,上文已经对数字签名有所阐述,这里就不再赘述。</p> <h2 id="初步了解-ssl-tls"><a href="#初步了解-ssl-tls" class="header-anchor">#</a> 初步了解 SSL/TLS</h2> <p>HTTPS(Hypertext Transfer Protocol Secure)被称为 <em>HTTP over TLS</em> 或 <em>HTTP over SSL</em> 或 <em>HTTP Secure</em> 。它并不是应用层的一种新协议,只是 HTTP 通信接口部分用 SSL 或 TLS 协议代替而已。通常,HTTP 直接和 TCP 通信,当使用 SSL/TLS 时,则演变为先和 SSL/TLS 通信,再由 SSL/TLS 和 TCP 通信,简单来说,HTTPS 在 HTTP 和 TCP 之间隔了一层 SSL/TLS(通常被称为安全层)。</p> <p>上文谈到了 HTTP 存在的三个重大毛病,SSL/TLS 解决了三个毛病的哪一个呢?答案是:所有。</p> <p>因此,此处引用《图解 HTTP》对 HTTPS 的总结:</p> <blockquote><p>HTTPS = HTTP + 加密 + 认证 + 完整性保护。</p></blockquote> <p>简单来说,建立在 SSL/TLS 协议之上,HTTP 具备加密、认证和完整性保护这些特点。本文旨在理清 SSL/TLS 是如何实现加密、认证和完整性保护的。</p> <p>首先一个必要的任务是搞清楚 SSL 和 TLS 的关系。SSL 是网景公司推出的,3.0 版本后,被 IETF 标准化,写入 RFC,是谓 TLS。下表是对它们的说明:</p> <table><thead><tr><th style="text-align:left;">版本</th> <th style="text-align:left;">发布时间</th> <th style="text-align:left;">RFC</th> <th style="text-align:left;">说明</th></tr></thead> <tbody><tr><td style="text-align:left;">SSL 1.0</td> <td style="text-align:left;">无</td> <td style="text-align:left;">无</td> <td style="text-align:left;">从未公开过,因为存在严重的安全漏洞</td></tr> <tr><td style="text-align:left;">SSL 2.0</td> <td style="text-align:left;">1995 年 2 月</td> <td style="text-align:left;">无</td> <td style="text-align:left;">因为存在数个严重的安全漏洞而被摈弃</td></tr> <tr><td style="text-align:left;">SSL 3.0</td> <td style="text-align:left;">1996 年</td> <td style="text-align:left;">无(后来作为历史文献被添加到 RFC 中,即<a href="https://tools.ietf.org/html/rfc6101" target="_blank" rel="noopener noreferrer">RFC6101<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>)</td> <td style="text-align:left;">2014 年 10 月,Google 发现在 SSL 3.0 中发现设计缺陷,建议禁用此一协议,后续 Microsoft、Mozilla 跟进</td></tr> <tr><td style="text-align:left;">TLS 1.0</td> <td style="text-align:left;">1999 年 1 月</td> <td style="text-align:left;"><a href="https://tools.ietf.org/html/rfc2246" target="_blank" rel="noopener noreferrer">RFC2246<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></td> <td style="text-align:left;">从技术上讲,TLS 1.0 与 SSL 3.0 的差异非常微小</td></tr> <tr><td style="text-align:left;">TLS 1.1</td> <td style="text-align:left;">2006 年 4 月</td> <td style="text-align:left;"><a href="https://tools.ietf.org/html/rfc4346" target="_blank" rel="noopener noreferrer">RFC4346<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></td> <td style="text-align:left;"></td></tr> <tr><td style="text-align:left;">TLS 1.2</td> <td style="text-align:left;">2008 年 8 月</td> <td style="text-align:left;"><a href="https://tools.ietf.org/html/rfc5246" target="_blank" rel="noopener noreferrer">RFC5246<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></td> <td style="text-align:left;">现在主流</td></tr> <tr><td style="text-align:left;">TLS 1.3</td> <td style="text-align:left;">草案阶段</td> <td style="text-align:left;"></td> <td style="text-align:left;"></td></tr></tbody></table> <p>可见,SSL 已是历史了;但是大家仍然习惯使用术语 SSL 指代 SSL 或 TLS。</p> <p>对于 SSL/TLS,最重要的内容莫过于其握手过程的分析,以后专门开辟博客阐述吧。</p> <h2 id="各种劫持与攻击"><a href="#各种劫持与攻击" class="header-anchor">#</a> 各种劫持与攻击</h2> <p>经常看到或者听到一些与安全相关的名词,譬如 DNS 劫持、HTTP 劫持、DDoS 攻击等,这一部分旨在对这些名词进行简单概述。</p> <h3 id="http-报文劫持"><a href="#http-报文劫持" class="header-anchor">#</a> HTTP 报文劫持</h3> <p>所谓 HTTP 报文劫持,指的是 HTTP 报文在 server 和 client 的传输过程中被修改的现象,这是运营商(ISP)比较喜欢做的事情,凑不要脸!HTTP 劫持主要发生在 Web 网页中,故而又被称为网页注入。简单来说,运营商常常在 server 发往 client 的 HTTP 报文中插入一段代码,这段代码通常与广告信息有关。相当于你让某个商店邮寄一包东西到你家,你收到的包裹里却有运营商附加的一坨屎。</p> <p><strong>HTTPS 能解决 HTTP 报文劫持的问题吗?</strong></p> <p>显然可以,SSL 的完整性保护确保了这件事情不会发生。</p> <h3 id="dns-劫持"><a href="#dns-劫持" class="header-anchor">#</a> DNS 劫持</h3> <p>DNS(域名系统)的作用是把网络地址对应到能够识别的 IP 地址,以便设备能够进一步通信,传递网址和内容等。DNS 劫持的实质是把 client 的域名劫持指向到非正常的 IP 地址。</p> <p>P.S: DNS 劫持是流量劫持的一种,又称 <em>域名劫持</em> ,或称 <em>DNS 钓鱼攻击</em> 。</p> <p>P.P.S: 除了 DNS 劫持,流量劫持还包括哪些呢?</p> <p><strong>HTTPS 能解决 DNS 劫持的问题吗?</strong></p> <p>答案是一般可以,但没有什么事情是绝对的。Server 与 client 建立 HTTPS 通信的前提是 server 持有受信任的合法证书;换句话说,如果被劫持到的目标 server 搞到一张假的受信任的证书,又或者它自己签发一个证书,并且 client 信任了该证书,那么 DNS 攻击依然存在。</p> <p>总之,HTTPS 下的 DNS 攻击门槛非常高,要么搞定 CA 机构,要么让用户信任自签发的证书。从另外一个角度来看,作为用户,我们不应该轻易相信别人自签发的证书,根据我的理解,这一点在网页端的问题要比移动客户端严重得多。</p> <p>P.S: <a href="https://www.zhihu.com/question/22795329" target="_blank" rel="noopener noreferrer">知乎<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>的这个话题下有些比较好的说明。</p> <p>P.S: DNS 劫持也常常发生在路由器中,譬如被人诟病的小米路由器。</p> <h3 id="运营商-路由器缓存造成的劫持"><a href="#运营商-路由器缓存造成的劫持" class="header-anchor">#</a> 运营商/路由器缓存造成的劫持</h3> <p>上述的 HTTP 报文劫持和 DNS 劫持都被统称为网络劫持,除了这两种,还有一种常见的劫持:运营商或者路由器无脑的缓存。</p> <p>点评大神周辉我司内部分享时举了一个相关例子:</p> <div class="custom-image-wrapper" data-v-339c7bd5><img src="/image/[email protected]" srcset="/image/[email protected] 2x" data-v-339c7bd5></div> <p>如上图所示,不同用户居然获取到同一个用户的个人信息,这几个用户连接的都是上海地铁上的花生 wifi,最后定位到的原因是花生 wifi 服务商对 HTTP response 进行了缓存,缓存的 key 自然是 HTTP request,但是在对 request 处理时居然将参数给去掉了...</p> <h3 id="ddos-攻击"><a href="#ddos-攻击" class="header-anchor">#</a> DDoS 攻击</h3> <p>这种攻击比较简单了,一般情况下的 SSL/TLS 通信模式是单向认证,并不能解决 DDoS 问题...</p> <h2 id="https-与实践"><a href="#https-与实践" class="header-anchor">#</a> HTTPS 与实践</h2> <p>本文记录我的一些 HTTPS 实践经历或体会。</p> <p>P.S: 然而,到目前为止,其实没啥近距离的 HTTPS 实践机会...</p> <h3 id="ats"><a href="#ats" class="header-anchor">#</a> ATS</h3> <p>ATS 并不等价于<em>所有 App 的 HTTP API 都得使用 HTTPS</em> ,它比后者要求得更多,<a href="https://developer.apple.com/library/content/documentation/General/Reference/InfoPlistKeyReference/Articles/CocoaKeys.html#//apple_ref/doc/uid/TP40009251-SW57" target="_blank" rel="noopener noreferrer">这里<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a>有详细说明。简单来说:</p> <ul><li>默认情况下,server 的 TLS 版本得是 1.2+</li> <li>对此加密算法得是 AES-128 或者 AES-256 级别的</li> <li>等等</li></ul> <p>处理业务时,遇到一个 case:外网 https://api.guanaitong.com 的 web 页面在我们 app 内不能访问,经查,发现是 server 的 TLS 版本过低(TLS 1.0),给他们的 server 反馈后,已解决...可惜当时没留下截图,一个难得的 case。</p> <p><strong>如何知道 server 支持的 TLS 的版本呢?</strong></p> <p>可以使用 openssl 工具,譬如<code>openssl s_client -connect api.guanaitong.com:443 -tls1_2</code>,参考自:<a href="https://serverfault.com/questions/638691/how-can-i-verify-if-tls-1-2-is-supported-on-a-remote-web-server-from-the-rhel-ce" target="_blank" rel="noopener noreferrer">How can I verify if TLS 1.2 is supported on a remote Web server from the RHEL/CentOS shell?<svg xmlns="http://www.w3.org/2000/svg" aria-hidden="true" x="0px" y="0px" viewBox="0 0 100 100" width="15" height="15" class="icon outbound"><path fill="currentColor" d="M18.8,85.1h56l0,0c2.2,0,4-1.8,4-4v-32h-8v28h-48v-48h28v-8h-32l0,0c-2.2,0-4,1.8-4,4v56C14.8,83.3,16.6,85.1,18.8,85.1z"></path> <polygon fill="currentColor" points="45.7,48.7 51.3,54.3 77.2,28.5 77.2,37.2 85.2,37.2 85.2,14.9 62.8,14.9 62.8,22.9 71.5,22.9"></polygon></svg></a></p> <p>P.S: macOS 默认已安装 openssl 工具,但是版本比较老(低于 v1.0),使用<code>-tls1_2</code>选项会有问题,需要升级...</p> <p><strong>如何知道 server 对 ATS 的支持情况呢?</strong></p> <p>使用另一个工具 nscurl,譬如:</p> <div class="language-sh extra-class"><pre class="language-sh"><code>nscurl --ats-diagnostics --verbose https://api.guanaitong.com
</code></pre></div></div></div><div class="global-ui"></div></div>
<script src="/assets/js/app.50bca889.js" defer></script><script src="/assets/js/5.081d1701.js" defer></script><script src="/assets/js/72.f4fda48b.js" defer></script><script src="/assets/js/4.a7413ce2.js" defer></script>
</body>
</html>
<file_sep>(window.webpackJsonp=window.webpackJsonp||[]).push([[110],{6:function(n,w,o){}}]); | e6f0c4e08d2008b5d46313b0932ea49ac6d297e7 | [
"JavaScript",
"HTML"
] | 5 | HTML | sadjason/sadjason.github.io | b91bfbce3f3d79cd2ff0877bdd18d93dd2a6c861 | 4b61b5e876e0361c23625f6b058bb74e379550be | |
refs/heads/master | <repo_name>DarwinGonzalez/JPA-example<file_sep>/src/es/uv/prnr/p2/ProjectService.java
package es.uv.prnr.p2;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.persistence.*;
public class ProjectService {
EntityManagerFactory emf;
EntityManager em;
public ProjectService() {
this.emf = Persistence.createEntityManagerFactory("acmeEmployees");
this.em = emf.createEntityManager();
}
/**
* Busca un departamento
* @param id identificador del departamento
* @return entidad con el deparamenteo encontrado
*/
public Department getDepartmentById (String id) {
return em.find(Department.class, id);
}
/**
* Asciende a un empleado a manager. Utilizar una estrateg�a de herencia adecuada
* en employee. Tened en cuenta que NO puede haber dos entidades con el mismo id
* por lo que habr� que eliminar el empleado original en algun momento.
* @param employeeId
* @param bonus
* @return
*/
public Manager promoteToManager(int employeeId, long bonus) {
em.getTransaction().begin();
Employee employee = em.find(Employee.class, employeeId);
Manager man = new Manager(employee, bonus);
em.remove(employee);
em.persist(man);
em.getTransaction().commit();
return man;
}
/**
* Crea un nuevo proyecto en el area de Big Data que comienza en la fecha actual y que finaliza
* en 3 a�os.
* @param name
* @param d departamento asignado al proyecto
* @param m manager que asignado al proyecto
* @param budget
* @return el proyecto creado
*/
public Project createBigDataProject(String name, Department d, Manager m, BigDecimal budget) {
em.getTransaction().begin();
Project project = new Project(name, d, m,budget,LocalDate.now(),LocalDate.now().plusYears(3),"BigData");
em.persist(project);
em.getTransaction().commit();
return project;
}
/**
* Crea un equipo de proyecto. Se debera implementa el m�todo addEmployee de
* Project para incluir los empleados
* @param p proyecto al cual asignar el equipo
* @param startId identificador a partir del cual se asignan empleado
* @param endId identificador final de empleados. Se asume que start id < endId
*/
public void assignTeam (Project p, int startId, int endId) {
em.getTransaction().begin();
for(int i = startId; i <= endId; i++) {
if(em.find(Employee.class, i) != null) {
p.addEmployee(em.find(Employee.class, i));
}
}
em.merge(p);
em.getTransaction().commit();
}
/**
* Genera un conjunto de horas inicial para cada empleado. El m�todo asigna para cada
* mes de duraci�n del proyecto, un n�mero entre 10-165 de horas a cada empleado.
* @param projectId
* @return total de horas generadas para el proyecto
*/
public int assignInitialHours (int projectId) {
em.getTransaction().begin();
int totalHours = 0;
Project p = em.find(Project.class, projectId);
LocalDate start = p.getStartDate();
while (start.isBefore(p.getEndDate())) {
for (Employee e: p.getEmployees()) {
int hours = new Random().nextInt(165) + 10;
totalHours += hours;
p.addHours(e, start.getMonthValue(), start.getYear(), hours);
}
start = start.plusMonths(1);
}
em.persist(p);
em.getTransaction().commit();
return totalHours;
}
/**
* Busca si un empleado se encuentra asignado en el proyecto utilizando la
* namedQuery Project.findEmployee
* @param projectId
* @param firstName
* @param lastName
* @return cierto si se encuentra asignado al proyecto
*/
public boolean employeeInProject (int projectId, String firstName, String lastName){
Query consulta= em.createNamedQuery("Project.findEmployee", Integer.class);
consulta.setParameter("idProyecto", projectId);
consulta.setParameter("nombre", firstName);
consulta.setParameter("apellido", lastName);
@SuppressWarnings("unchecked")
List<Integer> lista= consulta.getResultList();
if (lista.size() == 0) {
return false;
} else {
return true;
}
}
/**
* Devuelve los meses con mayor n�mero de horas de un a�o determinado
* utilizando la namedQuery Project.getTopMonths
* @param projectId
* @param year a�o a seleccionar
* @param rank n�mero de meses a mostrar, se asume que rank <= 12
* @return una lista de objetos mes,hora ordenados de mayor a menor
*/
public List getTopHourMonths(int projectId, int year, int rank) {
// Query consulta= em.createNamedQuery("Project.getTopMonths");
// consulta.setParameter("idProyecto", projectId);
// consulta.setParameter("año", year);
// consulta.setMaxResults(rank);
return em.createNamedQuery("Project.getTopMonths").setParameter("idProyecto", projectId).setParameter("año", year).setMaxResults(rank).getResultList();
}
/**
* Devuelve para cada par mes-a�o el presupuesto teniendo en cuenta el
* coste/hora de los empleados asociado utilizando la namedQuery Project.getMonthlyBudget
* que realiza una consulta nativa
* @param projectId
* @return una colecci�n de objetos MonthlyBudget
*/
public List<MonthlyBudget> getMonthlyBudget (int projectId){
return em.createNamedQuery("Project.getMonthlyBudget", MonthlyBudget.class).setParameter("idProyecto", projectId)
.getResultList();
}
}
<file_sep>/src/es/uv/prnr/p2/Project.java
package es.uv.prnr.p2;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javax.persistence.*;
//TODO JPQL de Ejercicio3 employeeInProject
@NamedQuery(name = "Project.findEmployee",
query = "select e.id from Employee e inner join e.assignedTo aT where e.firstName like :nombre and e.last_name like :apellido and aT.id = :idProyecto")
//TODO JPQL de Ejercicio3 getTopHoursMonth
@NamedQuery(
name="Project.getTopMonths",
query="select ph.month, max(ph.hours) as top from ProjectHours ph WHERE ph.year = :año and ph.project.id = :idProyecto group by ph.month order by top"
)
@NamedNativeQuery(name = "Project.getMonthlyBudget", query = "select mh.year, mh.month, sum(round(((s.salary/1650)*mh.hours),0)) as amount from monthly_hours mh "
+ " inner join employees e on e.emp_no = mh.fk_employee"
+ " inner join salaries s on s.emp_no = mh.fk_employee and s.to_date = '9999-01-01'"
+ " where mh.fk_project = :idProyecto" + " group by mh.month, mh.year"
+ " order by mh.year, mh.month", resultSetMapping = "MonthBudgetMapping")
// Mapeo del ResultSet para la consulta anterior
@SqlResultSetMapping(name = "MonthBudgetMapping", classes = {
@ConstructorResult(targetClass = MonthlyBudget.class, columns = { @ColumnResult(name = "year"),
@ColumnResult(name = "month"), @ColumnResult(name = "amount", type = Float.class) }) })
@Entity
@Table(name="project")
public class Project {
@Id @Column(name="id")
private int id;
@Column(name = "name", unique = false,
nullable = false, length = 45)
private String name;
@ManyToOne
@JoinColumn(name="fk_department")
private Department department;
@Column(name = "budget", unique = false,
nullable = false, length = 10, scale = 2)
private BigDecimal budget;
@Column(name="start_date")
private LocalDate startDate;
@Column(name="end_date")
private LocalDate endDate;
@Column(name="area")
private String area;
@ManyToOne
@JoinColumn(name="fk_manager")
private Manager manager;
@ManyToMany
@JoinTable(
name="project_team",
joinColumns=@JoinColumn(name="project_id",
referencedColumnName="id"),
inverseJoinColumns=@JoinColumn(name="employee_id",
referencedColumnName="emp_no")
)
private Set<Employee> team = new HashSet<Employee>(0);
// @OneToMany(fetch=FetchType.LAZY)
// @JoinColumn(name="fk_employee")
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.MERGE)
@JoinColumn(name = "fk_project", updatable = false)
private List<ProjectHours> hours = new ArrayList<ProjectHours>();
public Project() {
}
public Project(String name, Department department, Manager manager, BigDecimal budget, LocalDate startDate, LocalDate endDate, String area) {
this.name = name;
this.department = department;
this.manager = manager;
this.budget = budget;
this.startDate = startDate;
this.endDate = endDate;
this.area = area;
}
/**
* Relaciona el proyecto con el empleado e
* @param e
*/
public void addEmployee(Employee e) {
this.team.add(e);
}
/**
* A�ade un numero de horas al empleado e para un mes-a�o concreto
* @param e
* @param month
* @param year
* @param hours
*/
public void addHours(Employee e, int month, int year, int hours) {
ProjectHours ph = new ProjectHours(month, year, hours, e, this);
this.hours.add(ph);
}
public int getId() {
return id;
}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name = name;
}
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public Department getDepartment() {
return this.department;
}
public void setDepartment(Department Department) {
this.department = Department;
}
public BigDecimal getBudget() {
return this.budget;
}
public void setBudget(BigDecimal budget) {
this.budget = budget;
}
public String getArea() {
return this.area;
}
public void setArea(String area) {
this.area = area;
}
public Manager getManager() {
return manager;
}
public Set<Employee> getEmployees() {
return this.team;
}
public List<ProjectHours> getHours(){
return this.hours;
}
public void print () {
System.out.println("Project " + this.name + " from department " + this.department.getDeptName() );
System.out.print("Managed by ");
this.manager.print();
System.out.println("Project Team");
System.out.println(this.team.toString());
}
}
| b41516e9c7fc9b9c08004776a248956a51036c84 | [
"Java"
] | 2 | Java | DarwinGonzalez/JPA-example | 9794551983b29b66cadacdf52961a72b9c77eb2f | 25063cb43d19bc9c2b23df23928f6edeb9f956fb | |
refs/heads/main | <file_sep>using Microsoft.EntityFrameworkCore;
namespace Giansar.Demo.TodoApi.Models
{
public class TodoContext : DbContext
{
public TodoContext(DbContextOptions<TodoContext> options) : base(options)
{
}
public DbSet<TodoItem> TodoItems { get; set; }
//protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder) => optionsBuilder.UseNpgsql("Host=localhost;Database=demo_todo_api;Username=demo_db;Password=<PASSWORD>");
}
} | 1f4978424872bf320130354ef06eb337cc21bc6e | [
"C#"
] | 1 | C# | giansar/demo-dotnet-todo-api | 09160e5a0d1270d4dcc030b93bd53b6ceae14e3a | dce7d4bae6b765d7b32a3cfb84ebcaec18a32839 | |
refs/heads/master | <repo_name>boer0924/BoerOps<file_sep>/app/services/roles.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
from . import Base
from app.models import Role
class RoleService(Base):
__model__ = Role
roles = RoleService()<file_sep>/boerops_dev.sql
-- MySQL dump 10.13 Distrib 5.7.18, for Linux (x86_64)
--
-- Host: localhost Database: boerops_dev
-- ------------------------------------------------------
-- Server version 5.7.18-0ubuntu0.16.04.1
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8 */;
/*!40103 SET @OLD_TIME_ZONE=@@TIME_ZONE */;
/*!40103 SET TIME_ZONE='+00:00' */;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
--
-- Table structure for table `deploys`
--
DROP TABLE IF EXISTS `deploys`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `deploys` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`project_id` int(10) unsigned NOT NULL,
`user_id` int(10) unsigned NOT NULL,
`version` varchar(64) COLLATE utf8mb4_bin NOT NULL COMMENT 'commit id',
`mode` tinyint(1) unsigned DEFAULT '0' COMMENT '0-test30; 1-test31; 2-prod; 3-rollback',
`status` tinyint(1) unsigned DEFAULT '0' COMMENT '0-input; 1-test; 2-test_result; 3-prod; 4-prod_result; 5release',
`comment` text COLLATE utf8mb4_bin COMMENT 'release note',
`result` tinyint(1) unsigned DEFAULT NULL COMMENT '0-success; 1-failed',
`created_at` datetime NOT NULL,
`updated_at` datetime NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='发布部署';
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `hosts`
--
DROP TABLE IF EXISTS `hosts`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `hosts` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`hostname` varchar(32) COLLATE utf8mb4_bin NOT NULL,
`ip_address` varchar(32) COLLATE utf8mb4_bin NOT NULL,
`ssh_port` int(8) unsigned NOT NULL DEFAULT '22',
`username` varchar(32) COLLATE utf8mb4_bin NOT NULL,
`password` varchar(64) COLLATE utf8mb4_bin DEFAULT NULL,
`ssh_method` tinyint(1) DEFAULT '0' COMMENT '0-password;1-public key',
`environ` tinyint(1) DEFAULT '0' COMMENT '0-30test;1-31test;2-prod',
`created_at` datetime NOT NULL,
`updated_at` datetime NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `ip_address` (`ip_address`)
) ENGINE=InnoDB AUTO_INCREMENT=9 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='主机资产表';
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `projects`
--
DROP TABLE IF EXISTS `projects`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `projects` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(64) COLLATE utf8mb4_bin NOT NULL,
`repo_url` varchar(128) COLLATE utf8mb4_bin NOT NULL COMMENT 'git repo url',
`checkout_dir` varchar(128) COLLATE utf8mb4_bin NOT NULL COMMENT '检出目录',
`compile_dir` varchar(128) COLLATE utf8mb4_bin NOT NULL COMMENT '编译/打包/发布目录',
`compile_cmd` text COLLATE utf8mb4_bin COMMENT '编译命令',
`playbook_path` varchar(128) COLLATE utf8mb4_bin NOT NULL COMMENT 'ansible playbook yaml文件',
`created_at` datetime NOT NULL,
`updated_at` datetime NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `name` (`name`)
) ENGINE=InnoDB AUTO_INCREMENT=11 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='项目信息表';
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `rel_host_project`
--
DROP TABLE IF EXISTS `rel_host_project`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `rel_host_project` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`host_id` int(10) unsigned NOT NULL,
`project_id` int(10) unsigned NOT NULL,
`created_at` datetime NOT NULL COMMENT 'create time',
`updated_at` datetime NOT NULL COMMENT 'update time',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=32 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='主机、项目关联';
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `roles`
--
DROP TABLE IF EXISTS `roles`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `roles` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(32) COLLATE utf8mb4_bin NOT NULL,
`created_at` datetime NOT NULL,
`updated_at` datetime NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `name` (`name`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='用户角色';
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `users`
--
DROP TABLE IF EXISTS `users`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `users` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(64) COLLATE utf8mb4_bin NOT NULL,
`password_hash` varchar(128) COLLATE utf8mb4_bin NOT NULL,
`name` varchar(64) COLLATE utf8mb4_bin DEFAULT NULL COMMENT '姓名',
`role_id` int(10) unsigned NOT NULL,
`email` varchar(64) COLLATE utf8mb4_bin DEFAULT NULL,
`phone` varchar(16) COLLATE utf8mb4_bin DEFAULT NULL,
`apikey` varchar(64) COLLATE utf8mb4_bin DEFAULT NULL,
`created_at` datetime NOT NULL COMMENT 'create time',
`updated_at` datetime NOT NULL COMMENT 'update time',
PRIMARY KEY (`id`),
UNIQUE KEY `username` (`username`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='用户信息';
/*!40101 SET character_set_client = @saved_cs_client */;
/*!40103 SET TIME_ZONE=@OLD_TIME_ZONE */;
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
-- Dump completed on 2017-06-28 18:27:38
<file_sep>/app/static/js/projects/index.js
layui.use(['element', 'layer', 'util', 'form', 'layedit', 'laypage', 'upload'], function () {
var $ = layui.jquery;
var element = layui.element();
var layer = layui.layer;
var util = layui.util;
var form = layui.form();
var layedit = layui.layedit;
var laypage = layui.laypage;
// 文件上传
layui.upload({
url: '/projects/uploads',
type: 'file',
ext: 'yaml|yml|txt',
success: function (res, input) {
// console.log(res.filepath);
$('#playbook').val(res.filepath);
}
});
// 添加项目表单提交
form.on('submit(addProject)', function(data) {
// layer.msg(JSON.stringify(data.field));
$.ajax({
type: 'POST',
url: '/projects/',
data: data.field,
dataType: 'json',
// contentType: false,
processData: true,
success: function (data, txtStatus, jqXHR) {
if (data.code === 200) {
layer.msg(data.msg, {
icon: 1,
time: 2000,
anim: 0,
shade: [0.6, '#c2c2c2']
},
function () {
location.href="/projects/";
});
} else {
layer.msg(data.msg, {
icon: 2,
time: 2000,
anim: 6,
shade: [0.6, '#c2c2c2']
});
}
}
});
return false;
});
// 封装数据
var hosts = [];
form.on('checkbox(chk-hosts)', function (data) {
hosts.push(data.value);
});
// 执行绑定
form.on('submit(bindHost)', function(data) {
data.field.hosts = hosts;
// layer.msg(JSON.stringify(data.field));
$.ajax({
type: 'POST',
url: '/projects/bind',
data: data.field,
dataType: 'json',
// contentType: false,
processData: true,
success: function (data, txtStatus, jqXHR) {
if (data.code === 200) {
layer.msg(data.msg, {
icon: 1,
time: 2000,
anim: 0,
shade: [0.6, '#c2c2c2']
},
function () {
location.href="/projects/";
});
} else {
layer.msg(data.msg, {
icon: 2,
time: 2000,
anim: 6,
shade: [0.6, '#c2c2c2']
});
}
}
});
return false;
});
// 添加项目弹出层
$('#add-project-btn').on('click', function () {
layer.open({
type: 1,
title: '添加项目',
skin: 'layui-layer-molv',
content: $('#add-project-ctx'),
area: ['680px', '520px'],
// area: 'auto',
// maxWidth: '900px',
closeBtn: 1,
resize: true
// shadeClose: true,
});
});
// 修改项目
$(document).on('click', '#update-project-btn', function () {
layer.open({
type: 1,
title: '编辑项目',
skin: 'layui-layer-molv',
content: $('#add-project-ctx'),
area: ['680px', '520px'],
// area: 'auto',
// maxWidth: '900px',
closeBtn: 1,
resize: true
// shadeClose: true,
});
return false;
});
// 绑定主机
$('table').on('click', '#bind-host-btn', function (e) {
var e = window.event || e;
var id = $(e.target).parent().parent().parent().find('td:eq(0)').find('input').val();
$('#project_id').val(id);
layer.open({
type: 1,
title: '绑定主机',
skin: 'layui-layer-molv',
content: $('#bind-host-ctx'),
area: ['520px', '360px'],
// area: 'auto',
// maxWidth: '900px',
closeBtn: 1,
resize: true
// shadeClose: true,
});
return false;
});
});<file_sep>/api.py
#!/usr/bin/env python
import os
import sys
from collections import namedtuple
from ansible.parsing.dataloader import DataLoader
from ansible.vars import VariableManager
from ansible.inventory import Inventory
from ansible.executor.playbook_executor import PlaybookExecutor
variable_manager = VariableManager()
loader = DataLoader()
inventory = Inventory(loader=loader, variable_manager=variable_manager, host_list='/home/slotlocker/hosts2')
playbook_path = '/home/slotlocker/ls.yml'
if not os.path.exists(playbook_path):
print '[INFO] The playbook does not exist'
sys.exit()
Options = namedtuple('Options', ['listtags', 'listtasks', 'listhosts', 'syntax', 'connection','module_path', 'forks', 'remote_user', 'private_key_file', 'ssh_common_args', 'ssh_extra_args', 'sftp_extra_args', 'scp_extra_args', 'become', 'become_method', 'become_user', 'verbosity', 'check'])
options = Options(listtags=False, listtasks=False, listhosts=False, syntax=False, connection='ssh', module_path=None, forks=100, remote_user='slotlocker', private_key_file=None, ssh_common_args=None, ssh_extra_args=None, sftp_extra_args=None, scp_extra_args=None, become=True, become_method=None, become_user='root', verbosity=None, check=False)
variable_manager.extra_vars = {'hosts': 'mywebserver'} # This can accomodate various other command line arguments.`
passwords = {}
pbex = PlaybookExecutor(playbooks=[playbook_path], inventory=inventory, variable_manager=variable_manager, loader=loader, options=options, passwords=passwords)
results = pbex.run()
# ad-hoc
#!/usr/bin/env python
import json
from collections import namedtuple
from ansible.parsing.dataloader import DataLoader
from ansible.vars import VariableManager
from ansible.inventory import Inventory
from ansible.playbook.play import Play
from ansible.executor.task_queue_manager import TaskQueueManager
from ansible.plugins.callback import CallbackBase
class ResultCallback(CallbackBase):
"""A sample callback plugin used for performing an action as results come in
If you want to collect all results into a single object for processing at
the end of the execution, look into utilizing the ``json`` callback plugin
or writing your own custom callback plugin
"""
def v2_runner_on_ok(self, result, **kwargs):
"""Print a json representation of the result
This method could store the result in an instance attribute for retrieval later
"""
host = result._host
print json.dumps({host.name: result._result}, indent=4)
Options = namedtuple('Options', ['connection', 'module_path', 'forks', 'become', 'become_method', 'become_user', 'check'])
# initialize needed objects
variable_manager = VariableManager()
loader = DataLoader()
options = Options(connection='local', module_path='/path/to/mymodules', forks=100, become=None, become_method=None, become_user=None, check=False)
passwords = dict(vault_pass='<PASSWORD>')
# Instantiate our ResultCallback for handling results as they come in
results_callback = ResultCallback()
# create inventory and pass to var manager
inventory = Inventory(loader=loader, variable_manager=variable_manager, host_list='localhost')
variable_manager.set_inventory(inventory)
# create play with tasks
play_source = dict(
name = "Ansible Play",
hosts = 'localhost',
gather_facts = 'no',
tasks = [
dict(action=dict(module='shell', args='ls'), register='shell_out'),
dict(action=dict(module='debug', args=dict(msg='{{shell_out.stdout}}')))
]
)
play = Play().load(play_source, variable_manager=variable_manager, loader=loader)
# actually run it
tqm = None
try:
tqm = TaskQueueManager(
inventory=inventory,
variable_manager=variable_manager,
loader=loader,
options=options,
passwords=passwords,
stdout_callback=results_callback, # Use our custom callback instead of the ``default`` callback plugin
)
result = tqm.run(play)
finally:
if tqm is not None:
tqm.cleanup()
# ipython shell
# from app.models import User, Role, db
# admin_role = Role(name='admin')
# admin_user = User(username='boer', password='123', name='海博', job='Ops', role=admin_role)
# from app import create_app
# app = create_app('dev')
# with app.app_context():
# db.session.add(admin_role)
# db.session.add(admin_user)
# db.session.commit()
pydelo
<EMAIL>:meanstrong/pydelo.git
/tmp/pydelo_chk
/tmp/pydelo_pkg
echo "***begin compile***"
touch /tmp/pydelo_pkg/compile_test.txt
echo "***end compile***"
cd /tmp/pydelo_pkg
rm -f /tmp/pydelo.tar.gz
tar -zcf /tmp/pydelo.tar.gz *<file_sep>/app/services/hosts.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
from . import Base
from app.models import Host
class HostService(Base):
__model__ = Host
hosts = HostService()<file_sep>/runserver.py
from app import create_app
import socket
if __name__ == '__main__':
app = create_app('dev')
if socket.gethostname() not in ['Boer-PC', 'boer-PC']:
app = create_app('prod')
app.run(host='0.0.0.0')
<file_sep>/requirements.txt
Flask
Flask-Login
Flask-Mail
Flask-SQLAlchemy
PyMySQL
requests
ansible<file_sep>/app/projects/__init__.py
from flask import Blueprint
projects = Blueprint('projects', __name__, url_prefix='/projects')
from . import views<file_sep>/app/services/projects.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
from . import Base
from app.models import Project
from .hosts import hosts
class ProjectService(Base):
__model__ = Project
projs = ProjectService()
# C:\Users\Public\Documents\Hyper-V\Virtual Hard Disks\MobyLinuxVM.vhdx <file_sep>/Dockerfile
FROM python:3.5
ADD ./requirements.txt /tmp/requirements.txt
RUN pip install -r /tmp/requirements.txt && pip install ipython<file_sep>/app/utils/uploads.py
# -*- coding: utf-8 -*-
"""文件上传"""
import os
from flask import request, current_app, jsonify
from werkzeug.utils import secure_filename
# UPLOAD_FOLDER = os.path.join(os.path.dirname(current_app.root_path), 'playbook')
# UPLOAD_FOLDER = os.path.join(os.path.dirname(current_app.root_path), 'playbook')
ALLOWED_EXTENSIONS = set(['yml', 'yaml', 'txt'])
def allowed_file(filename):
return '.' in filename and filename.rsplit('.', 1)[1] in ALLOWED_EXTENSIONS
def gen_file_name(filename):
flag = 1
while os.path.exists(os.path.join(UPLOAD_FOLDER, filename)):
name, ext = os.path.splitext(filename)
filename = '%s_%s%s' % (name, str(flag), ext)
flag += 1
return filename
def upload_file(UPLOAD_FOLDER):
if request.method == 'POST':
file = request.files.get('playbook')
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
uploaded_file_path = os.path.join(UPLOAD_FOLDER, filename)
if not os.path.exists(os.path.dirname(uploaded_file_path)):
try:
os.makedirs(os.path.dirname(uploaded_file_path))
except OSError as e:
return jsonify(rc=0)
file.save(uploaded_file_path)
return jsonify(rc=1, filepath=uploaded_file_path)
else:
return jsonify(rc=0)
<file_sep>/app/projects/views.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
from . import projects
from app.utils.uploads import upload_file
from app.utils.remoteshell import MyRunner
from app.utils.helper import get_dynamic_inventory, login_required
from app.services.projects import projs
from app.services.hosts import hosts
from app.services.deploys import deploys
from flask import render_template, request, jsonify, current_app, g
import os
@projects.route('/uploads', methods=['GET', 'POST'])
@login_required
def uploads():
UPLOAD_FOLDER = os.path.join(os.path.dirname(current_app.root_path), 'playbook')
return upload_file(UPLOAD_FOLDER)
@projects.route('/bind', methods=['POST'])
@login_required
def binds():
_project_id = request.form.get('project_id')
_hosts = request.form.getlist('hosts[]')
proj = projs.get(_project_id)
for host in set(_hosts):
proj.hosts.append(hosts.get(int(host)))
projs.save(proj)
return jsonify(code=200, msg='绑定成功')
@projects.route('/', methods=['GET', 'POST'])
@login_required
def index():
if request.method == 'POST':
_id = request.form.get('id')
fields = request.form.to_dict()
fields.pop('playbook')
# 修改操作
if _id is not None:
proj = projs.first(id=_id)
if not proj:
return jsonify(code=404, msg='记录不存在')
try:
projs.update(proj, **fields)
except Exception as e:
return jsonify(code=500, msg='修改失败')
return jsonify(code=200, msg='修改成功')
# 添加操作
try:
projs.create(**fields)
except Exception as e:
print(e)
return jsonify(code=500, msg='添加失败')
return jsonify(code=200, msg='添加成功')
_projects = projs.all()
_hosts = hosts.all()
return render_template('projects/index.html', projects=_projects, hosts=_hosts)
@projects.route('/hosts', methods=['GET', 'POST'])
@login_required
def project_hosts():
if request.method == 'POST':
_id = request.form.get('id')
# 修改操作
if _id is not None:
host = hosts.first(id=_id)
if not host:
return jsonify(code=404, msg='记录不存在')
try:
hosts.update(host, **request.form.to_dict())
except Exception as e:
return jsonify(code=500, msg='修改失败')
return jsonify(code=200, msg='修改成功')
# 添加操作
try:
hosts.create(**request.form.to_dict())
except Exception as e:
print(e)
return jsonify(code=500, msg='添加失败')
return jsonify(code=200, msg='添加成功')
_hosts = hosts.all()
return render_template('projects/hosts.html', hosts=_hosts)
@projects.route('/deploy', methods=['GET', 'POST'])
@login_required
def deploy_step_first():
if request.method == 'POST':
fields = request.form.to_dict()
project_name = fields.get('name')
version = fields.get('version')
environ = fields.get('environ')
proj = projs.first(name=project_name.strip())
if not proj:
return jsonify(rc=400, msg='项目不存在')
if int(environ) == 2:
num_deploy = deploys.count(project_id=proj.id, status=3)
if int(num_deploy) >= 1:
return jsonify(code=500, msg='有其他任务在上线中...')
deploys.create(
project_id=proj.id,
user_id=g.user.id,
version=version,
mode=environ,
status=3)
else:
num_deploy = deploys.count(project_id=proj.id, status=0)
if int(num_deploy) >= 1:
return jsonify(code=500, msg='有其他任务在提测中...')
deploys.create(
project_id=proj.id,
user_id=g.user.id,
version=version,
mode=environ)
results = deploys.deploy_task(proj.id, int(environ))
print(results)
return jsonify(code=200, msg='job done', status=results)
return render_template('projects/deploy.html')
@projects.route('/deploy/second', methods=['GET', 'POST'])
@login_required
def deploy_step_second():
if request.method == 'POST':
results = deploys.deploy_task(proj.id)
return jsonify(code=200, msg='job done', status=status)
@projects.route('/rollback')
def rollback():
return render_template('projects/rollback.html')
<file_sep>/app/auth/views.py
# -*- coding: utf-8 -*-
import datetime
import json
from . import auth
from flask import render_template, redirect, url_for, request, jsonify, g, make_response, current_app
import jwt
from app.services.roles import roles
from app.services.users import users
from app.utils.helper import login_required, permission_required, write_required
from app import redis
@auth.route('/login', methods=['GET', 'POST'])
def login():
redirect_uri = request.args.get('redirect_uri') or '/dashboard'
if request.method == 'POST':
username = request.form.get('username')
password = request.form.get('password')
redirect_uri = request.form.get('redirect_uri')
_u = users.first(username=username)
if _u and _u.verify_password(password):
# 获取缓存,动态设置config
role_map = redis.get('roles').decode('utf-8')
current_app.config['roles'] = json.loads(role_map)
# 处理登录、生成token
resp = jsonify(code=200, msg='登录成功', redirect_uri=redirect_uri)
payload = dict(
exp = datetime.datetime.utcnow() + datetime.timedelta(hours=8),
rid = _u.role_id,
uid = _u.id
)
token = jwt.encode(payload, current_app.config['SECRET_KEY'], algorithm='HS256')
resp.set_cookie('token', token)
return resp
else:
return jsonify(code=500, msg='用户名或密码错误')
return render_template('auth/login.html', redirect_uri=redirect_uri)
@auth.route('/users', methods=['GET', 'POST'])
@login_required
@permission_required(3)
def user():
if request.method == 'POST':
users.create(**request.form.to_dict())
return jsonify(code=200, msg='添加成功')
_users = users.all()
_roles = roles.all()
return render_template('auth/users.html', users=_users, roles=_roles)
@auth.route('/groups', methods=['GET', 'POST'])
@login_required
@permission_required(3, alls=True)
def group():
if request.method == 'POST':
roles.create(**request.form.to_dict())
# 每次添加角色,更新redis roles
all_roles = roles.all()
all_role_ids = map(lambda x: x.id, all_roles)
all_role_names = map(lambda x: x.name, all_roles)
role_map = dict(zip(all_role_names, all_role_ids))
redis.set('roles', json.dumps(role_map))
return jsonify(code=200, msg='添加成功')
_roles = roles.all()
return render_template('auth/groups.html', roles=_roles)
@auth.route('/logout')
@login_required
def logout():
resp = make_response(redirect(url_for('.login')))
resp.set_cookie('token', '', expires=0)
return resp
@auth.route('/reset', methods=['POST'])
@login_required
def reset_password():
if request.method == 'POST':
current_pass = request.form.get('current_pwd')
new_pass = request.form.get('new_pwd')
if g.user and g.user.verify_password(current_pass):
g.user.password = <PASSWORD>
users.session_commit()
return jsonify(code=200, msg='修改成功')
else:
return jsonify(code=403, msg='权限不足')
@auth.route('/test')
def test_for_someting():
print(current_app.config['roles'].get('测试'))
return ''
@auth.errorhandler(403)
def access_forbidden(e):
return render_template('auth/403.html'), 403<file_sep>/app/main/views.py
# -*- coding: utf-8 -*-
from . import main
from flask import render_template, redirect
from app.utils.helper import login_required
from app.services.deploys import deploys
@main.route('/')
def index():
return redirect('/dashboard')
@main.route('/dashboard')
@login_required
def dashboard():
u_ds = deploys.find(status=5)
return render_template('main/index.html')<file_sep>/app/utils/sendemail.py
# -*- coding: utf-8 -*-
import os
import datetime
import shutil
import subprocess
from subprocess import Popen, PIPE
import json
from threading import Thread
from flask import Flask, request, render_template
from flask_mail import Mail, Message
app = Flask(__name__)
mail = Mail(app)
app.config['MAIL_SERVER'] = 'mail.heclouds.com'
app.config['MAIL_PORT'] = 25
app.config['MAIL_USERNAME'] = 'boer'
app.config['MAIL_PASSWORD'] = '<PASSWORD>'
app.config['CHECKOUT_DIR'] = '/data/deployment/gitrepos'
app.config['DEPLOY_DIR'] = '/data/deployment/deploydir'
app.config['PROJECTS_DIR'] = '/data/deployment/projects'
def update_repo(repo_path, repo_url, commit_id):
git_path = os.path.join(repo_path, '.git')
if os.path.exists(git_path) and os.path.isdir(git_path):
cmd = 'cd %s && git reset --hard origin/master && git pull -q' % repo_path
rc = subprocess.check_call(cmd, shell=True, cwd=repo_path)
else:
if os.path.exists(os.path.dirname(repo_path)) and os.path.isdir(os.path.dirname(repo_path)):
shutil.rmtree(os.path.dirname(repo_path))
else:
os.makedirs(os.path.dirname(repo_path))
cmd = 'git clone -q %s' % repo_url
rc = subprocess.check_call(cmd.split(), cwd=os.path.dirname(repo_path))
# 指定commit_id
cmd = 'git reset -q --hard %s' % commit_id
rc = subprocess.check_call(cmd.split(), cwd=repo_path)
def rsync_local(src, dest, excludes=[]):
excludes.append('.git')
exclude_args = ''
for e in excludes:
exclude_args = exclude_args + ' --exclude %s' % e
cmd = 'rsync -qa --delete %s %s%s %s%s' % (exclude_args, src, os.sep, dest, os.sep)
rc = subprocess.check_call(cmd.split())
def chk_and_set_exe(src_path):
if not os.access(src_path, os.X_OK):
os.chmod(src_path, 755)
def exec_script(script_file):
if os.path.exists(script_file) and os.path.isfile(script_file):
chk_and_set_exe(script_file)
outputs = subprocess.check_output(script_file, shell=True)
# p = Popen(script_file, stdout=PIPE)
# c_pid = p.pid
# outputs = p.communicate()[0]
# rc = p.poll()
#if rc == 0:
# p.terminate()
#else:
# p.kill()
return outputs
# 同步邮件
def send_sync_email(sender, to, cc, subject, template, **kwargs):
msg = Message(subject, sender=sender, recipients=to, cc=cc)
# msg.body = render_template(template + '.txt', **kwargs)
msg.html = render_template(template + '.html', **kwargs)
mail.send(msg)
# 异步发邮件
def send_async_email(app, msg):
with app.app_context():
mail.send(msg)
def send_email(sender, to, cc, subject, template, **kwargs):
msg = Message(subject, sender=sender, recipients=to, cc=cc)
# msg.body = render_template(template + '.txt', **kwargs)
msg.html = render_template(template + '.html', **kwargs)
thr = Thread(target=send_async_email, args=[app, msg])
thr.start()
return thr
@app.route('/sendmail')
def sendmail():
user_email_map = {
'zhanghaibo': '<EMAIL>',
'mazhijie': '<EMAIL>',
'jieyuanfei': '<EMAIL>',
'weizhengdong': '<EMAIL>',
'tanjiang': '<EMAIL>',
'chenbo': '<EMAIL>'
}
send_email('<EMAIL>', ['<EMAIL>', '<EMAIL>', '<EMAIL>'], ['<EMAIL>', '<EMAIL>'], 'Just a test', 'deploy')
# msg = Message('Hello', sender="<EMAIL>", recipients=['<EMAIL>'])
# msg.body = "testing"
# mail.send(msg)
return 'done'
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
results = json.loads(request.data)
noteable_type = results['object_attributes']['noteable_type']
# Triggered when a new comment is made on commits, merge requests, issues, and code snippets.
if noteable_type != 'Commit':
return '', 404
# user 相关
name = results['user']['name']
username = results['user']['username']
if username != 'zhanghaibo':
return '', 403
# repository 相关
repo_name = results['repository']['name']
repo_url = results['repository']['url']
repo_path = git_path = os.path.join(app.config['CHECKOUT_DIR'], repo_name, repo_name)
# commit 相关
commit_id = results['commit']['id']
print('---commit_id---', commit_id)
commit_msg = results['commit']['message'].strip('\n')
# object_attributes 相关
try:
notes = results['object_attributes']['note']
if not notes.startswith('```json') and not notes.endswith('```'):
return ''
notes = notes.lstrip('```json').rstrip('```').replace('\r\n', '')
notes = json.loads(notes)
except Exception as e:
print('<-debug->', e)
# send_email()
return ''
deploy_type = notes.get('deploy_type')
recipients = notes.get('recipients')
carbon_copy = notes.get('carbon_copy')
functions = notes.get('functions')
update_repo(repo_path, repo_url, commit_id)
rsync_local(repo_path, os.path.join(app.config['DEPLOY_DIR'], repo_name, repo_name))
script_file = ''
subject = ''
if deploy_type == 'weekfix':
script_file = os.path.join(app.config['PROJECTS_DIR'], repo_name + '_weekfix', 'script/local_after.sh')
subject = datetime.datetime.strftime(datetime.date.today(), '%Y/%m/%d') + 'weekfix测试'
elif deploy_type == 'hotfix':
pass
elif deploy_type == 'feature':
pass
elif deploy_type == 'prod':
pass
else:
print('滚蛋吧')
outputs = exec_script(script_file)
print(outputs)
print(type(outputs))
send_sync_email('<EMAIL>', recipients, carbon_copy, subject, 'deploy', functions=functions, outputs=outputs)
return ''
return '', 405
if __name__ == '__main__':
app.run(host='172.19.3.23', port=8080)
# {
# "deploy_type": "weekfix/feature/hotfix/prod",
# "recipients": ["mazhijie", "limao", "dengdeng"],
# "carbon_copy": ["bmwlee", "limao"],
# "functions": [
# {
# "name": "功能点一",
# "content": "balabala~"
# },
# {
# "name": "功能点二",
# "content": "balabala~"
# }
# ]
# }
# onenet_v3
# onenet_ee
# forum_v2
# passport
# phpcorelib
# groupservice
# admin_onenetv3
# campaignmap<file_sep>/app/services/users.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
from . import Base
from app.models import User
class UserService(Base):
__model__ = User
users = UserService()<file_sep>/app/models.py
from app import db, login_manager
from werkzeug.security import generate_password_hash, check_password_hash
from flask_login import UserMixin
class Permission(db.Model):
__tablename__ = 'permissions'
id = db.Column(db.Integer, primary_key=True)
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
class Role(db.Model):
__tablename__ = 'roles'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64), unique=True)
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
users = db.relationship('User', backref='role', lazy="dynamic")
def __repr__(self):
return '<%s %r>' % (self.__class__.__name__, self.name)
class User(UserMixin, db.Model):
__tablename__ = 'users'
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(64), unique=True)
password_hash = db.Column(db.String(64))
name = db.Column(db.String(64))
# job = db.Column(db.String(64))
email = db.Column(db.String(64))
phone = db.Column(db.String(64))
apikey = db.Column(db.String(64))
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
role_id = db.Column(db.Integer, db.ForeignKey('roles.id'))
@property
def password(self):
raise AttributeError('password is not a readable attribute')
@password.setter
def password(self, pwd):
self.password_hash = generate_password_hash(pwd, salt_length=16)
def verify_password(self, pwd):
return check_password_hash(self.password_hash, pwd)
def __repr__(self):
return '<%s %r>' % (self.__class__.__name__, self.username)
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
rel_host_project = db.Table(
"rel_host_project",
db.Column("id", db.Integer, primary_key=True),
db.Column("host_id", db.Integer, db.ForeignKey("hosts.id")),
db.Column("project_id", db.Integer, db.ForeignKey("projects.id")),
db.Column("created_at", db.DateTime, default=db.func.now()),
db.Column("updated_at", db.DateTime, default=db.func.now(), onupdate=db.func.now()),
)
class Project(db.Model):
__tablename__ = 'projects'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64), unique=True)
repo_url = db.Column(db.String(128))
checkout_dir = db.Column(db.String(128))
compile_dir = db.Column(db.String(128))
compile_cmd = db.Column(db.String(512))
playbook_path = db.Column(db.String(128))
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
hosts = db.relationship("Host", secondary=rel_host_project,
backref=db.backref("projects", lazy="dynamic"))
def __repr__(self):
return '<%s %r>' % (self.__class__.__name__, self.name)
class Host(db.Model):
__tablename__ = 'hosts'
id = db.Column(db.Integer, primary_key=True)
hostname = db.Column(db.String(64))
ip_address = db.Column(db.String(64), unique=True)
ssh_port = db.Column(db.Integer)
username = db.Column(db.String(32))
password = db.Column(db.String(128))
ssh_method = db.Column(db.Integer)
environ = db.Column(db.Integer)
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
def __repr__(self):
return '<%s %r>' % (self.__class__.__name__, self.ip_address)
class Deploy(db.Model):
__tablename__ = 'deploys'
id = db.Column(db.Integer, primary_key=True)
project_id = db.Column(db.Integer, db.ForeignKey('projects.id'))
user_id = db.Column(db.Integer, db.ForeignKey('users.id'))
version = db.Column(db.String(64))
mode = db.Column(db.Integer)
status = db.Column(db.Integer, default=0)
comment = db.Column(db.Text)
result = db.Column(db.Integer)
created_at = db.Column(db.DateTime, default=db.func.now())
updated_at = db.Column(db.DateTime, default=db.func.now(), onupdate=db.func.now())
user = db.relationship('User',
backref=db.backref("deploys", lazy="dynamic"))
project = db.relationship('Project',
backref=db.backref("deploys", lazy="dynamic"))
def __repr__(self):
return '<%s %r>' % (self.__class__.__name__, self.version)<file_sep>/app/utils/helper.py
# -*- coding: utf-8 -*-
from functools import wraps
from flask import g, request, redirect, url_for, json, abort, jsonify, current_app
import jwt
from app.services.users import users
from app.services.roles import roles
__all__ = [
'get_dynamic_inventory', 'login_required', 'permission_required',
'write_required'
]
def get_dynamic_inventory(proj, environ):
return {
proj.name: {
'hosts': [{
'hostname': h.ip_address,
'port': h.ssh_port,
'username': h.username,
'password': h.<PASSWORD>
} for h in proj.hosts if h.environ == int(environ)],
'vars': {
'ansible_user': 'boer',
'ansible_become': True,
'ansible_become_method': 'sudo',
'ansible_become_user': 'root',
'ansible_become_pass': '<PASSWORD>'
}
}
}
def login_required(f):
@wraps(f)
def decorated_function(*args, **kwargs):
token = request.cookies.get('token')
if not token:
return redirect(url_for('auth.login', redirect_uri=request.path))
try:
token = jwt.decode(token, current_app.config['SECRET_KEY'])
g.user = users.get(token['uid'])
g.role = roles.get(token['rid'])
except jwt.InvalidTokenError as e:
return redirect(url_for('auth.login', redirect_uri=request.path))
return f(*args, **kwargs)
return decorated_function
def permission_required(*roles, alls=False):
# http://flask.pocoo.org/snippets/98/
def wrapper(f):
@wraps(f)
def wrapped(*args, **kwargs):
if g.role.id in roles:
if alls:
abort(403)
if request.method == 'POST':
return jsonify(code=405, msg='权限不足')
return f(*args, **kwargs)
return wrapped
return wrapper
def write_required(f):
@wraps(f)
def wrapped(*args, **kwargs):
if request.method == 'POST':
return jsonify(code=405, msg='权限不足')
return f(*args, **kwargs)
return wrapped<file_sep>/app/services/deploys.py
#!/usr/local/env python
# -*- coding: utf-8 -*-
import os
import subprocess
from flask import g
from . import Base
from app.models import Deploy
from app.utils.remoteshell import MyRunner
from app.utils.helper import get_dynamic_inventory
class DeployService(Base):
__model__ = Deploy
def deploy_task(self, project_id, environ):
c_d = self.first(project_id=project_id, status=0) if int(environ) != 2 else self.first(project_id=project_id, status=3)
if not c_d:
return
# 检出代码库
cmd = 'git clone -q %s %s' % (c_d.project.repo_url, c_d.project.checkout_dir)
git_path = os.path.join(c_d.project.checkout_dir, '.git')
if os.path.exists(git_path) and os.path.isdir(git_path):
cmd = 'cd %s && git fetch --all -fq' % c_d.project.checkout_dir
rc = subprocess.call(cmd, shell=True)
# 指定版本
cmd = 'cd %s && git reset -q --hard %s' % (c_d.project.checkout_dir, c_d.version.strip())
rc = subprocess.call(cmd, shell=True)
# 同步到编译/打包目录
cmd = 'rsync -qa --delete --exclude .git %s%s %s%s' % (c_d.project.checkout_dir, os.sep, c_d.project.compile_dir, os.sep)
rc = subprocess.call(cmd, shell=True)
# 执行用户自定义命令(权限,fis编译,打包)
cmds = c_d.project.compile_cmd.split('\n')
cmds = ' && '.join(cmds)
rc = subprocess.call(cmds, shell=True)
# 获取ansible动态Inventory
resource = get_dynamic_inventory(c_d.project, environ)
host_lists = [h.ip_address for h in c_d.project.hosts if h.environ == int(environ)]
# 执行ansible playbook
runner = MyRunner(resource)
runner.run_playbook(host_lists, c_d.project.playbook_path)
if int(environ) == 2:
self.update(c_d, status=4)
else:
self.update(c_d, status=1)
# return runner.get_playbook_result()
return self.get(id=c_d.id).status
def rollback(self, deploy):
pass
deploys = DeployService()<file_sep>/app/static/js/auth/users.js
layui.use(['element', 'layer', 'util', 'form', 'layedit', 'laypage'], function () {
var $ = layui.jquery;
var element = layui.element();
var layer = layui.layer;
var util = layui.util;
var form = layui.form();
var layedit = layui.layedit;
var laypage = layui.laypage;
// 表单提交
form.on('submit(addUser)', function(data) {
// layer.msg(JSON.stringify(data.field));
$.ajax({
type: 'POST',
url: '/users',
data: data.field,
dataType: 'json',
processData: true,
success: function (data, txtStatus, jqXHR) {
if (data.code === 200) {
layer.msg(data.msg, {
icon: 1,
time: 2000,
anim: 0,
shade: [0.6, '#c2c2c2']
},
function () {
location.href="/users";
});
} else {
layer.msg(data.msg, {
icon: 2,
time: 2000,
anim: 6,
shade: [0.6, '#c2c2c2']
});
}
}
});
return false;
});
// 添加主机弹出层
$('#add-user-btn').on('click', function () {
layer.open({
type: 1,
title: '添加用户',
skin: 'layui-layer-molv',
content: $('#add-user-ctx'),
area: ['600px', '480px'],
// area: 'auto',
// maxWidth: '900px',
closeBtn: 1,
resize: true
// shadeClose: true,
});
});
}); | 06f606e481112021c2d6bfcd2a0a8fcfd37d5711 | [
"SQL",
"JavaScript",
"Python",
"Text",
"Dockerfile"
] | 20 | Python | boer0924/BoerOps | a5451d4cdbc276cd56acd3d11b8f269d77183820 | fe919e39042b3f8edadf86c032ed0e175c5751d7 | |
refs/heads/master | <file_sep>import { Component, OnInit } from '@angular/core';
import { Injectable } from '@angular/core';
import { Http } from '@angular/http';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-school-result',
templateUrl: './school-result.component.html',
styleUrls: ['./school-result.component.css']
})
export class SchoolResultComponent implements OnInit {
data;
studentResults: any;
studentName: String;
total = [];
totalMarks: number;
students = [];
max:number =0;
maxMarks: any;
constructor(private http: HttpClient) {
}
getStudentResults() {
return this.http.get('../assets/data.json')
.toPromise().then(res => {
return res
}
)
}
ngOnInit() {
this.getStudentResults().then(
res => {
this.studentResults = res;
if (this.studentResults) {
let result;
for (let i = 0; i < this.studentResults.length; i++) {
this.totalMarks = parseInt(this.studentResults[i].marks.English) + parseInt(this.studentResults[i].marks.Maths) + parseInt(this.studentResults[i].marks.Science);
if (this.totalMarks >this.max) {
this.max=this.totalMarks;
this.maxMarks=this.totalMarks;
}
if (this.studentResults[i].marks.English < 20 || this.studentResults[i].marks.Maths < 20 || this.studentResults[i].marks.Science < 20) {
result = "fail";
}
else {
result = "pass";
}
this.students.push({ 'rollNumber': this.studentResults[i].rollNumber, 'total': this.totalMarks, 'result': result, 'student': this.studentResults[i] });
}
for (let i = 0; i < this.students.length; i++) {
if(this.maxMarks == this.students[i].total){
this.students[i].result = "topper";
}
}
console.log(this.students);
console.log(this.maxMarks);
// var sorted = this.students.sort();
// console.log("Returned string is : " + sorted );
}
}
)
}
}
<file_sep>import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-school-form',
templateUrl: './school-form.component.html',
styleUrls: ['./school-form.component.css']
})
export class SchoolFormComponent implements OnInit {
firstName: string;
lastname: string;
class: string;
percentage: string;
yop: number;
submitBtn :boolean = false;
constructor() { }
onKeyUpValidation(){
if(this.firstName && this.lastname && this.class && this.percentage && this.yop){
if(this.firstName.length > 1 && this.firstName.length <= 20 && this.lastname.length > 1 && this.lastname.length <= 20 && this.class.length > 1 && this.percentage.length > 1 && this.yop < 2018 && this.yop > 1980){
this.submitBtn = true;
}
else{
this.submitBtn = false;
}
}
}
ngOnInit() {
}
submit(){
alert("Vaild");
}
}
| 2ce4c2c903a6eb1838be51402bf4b0c668bf8486 | [
"TypeScript"
] | 2 | TypeScript | gadeajaykumar/Techolution-Angular | 9627e9855738bd62e812faa7a87ce778a1eb3553 | 8abb210afb038ecd291b521b97d3ceb91e2010ad | |
refs/heads/master | <repo_name>elko-dev/github-generator<file_sep>/todos/create.ts
'use strict'
import * as uuid from 'uuid'
module.exports.create = (event, context, callback) => {
const timestamp = new Date().getTime()
const data = JSON.parse(event.body)
if (typeof data.text !== 'string') {
console.error('Validation Failed')
callback(new Error('Couldn\'t create the todo item.'))
return
}
// create a response
const response = {
statusCode: 200,
body: JSON.stringify({ hello: "world" })
}
callback(null, response)
}
<file_sep>/tests/hello.test.ts
import { } from 'ts-jest';
describe('My First Test', () => {
it('hello test', () => {
expect("hellow").toEqual("hello");
});
});<file_sep>/integration/run.sh
#!/bin/bash
set -e
SCRIPT_DIR="$(cd "$(dirname "${BASH_SOURCE[0]}")" && pwd)"
COLLECTION=$1
STACK=$2
ENV=$3
LOCAL="local"
STAGE="stage"
DEV="dev"
POSTMAN_ENV="github_generator_$ENV.postman_environment.json"
if [ "$ENV" != "$LOCAL" ] && [ "$ENV" != "$STAGE" ] && [ "$ENV" != "$DEV" ]
then
echo "$ENV is not a valid environment, must be either $LOCAL, $DEV or $STAGE"
exit 1
else
echo "Running $ENV tests"
fi
function run_postman {
newman run "$SCRIPT_DIR/$COLLECTION" -e "$SCRIPT_DIR/$POSTMAN_ENV"
}
function setup_url {
##define variables
MACOS="Mac"
NEWURL=`aws cloudformation describe-stacks \
--stack-name $STACK \
--query "Stacks[0].[Outputs[? starts_with(OutputKey, 'ServiceEndpoint')]][0][*].{OutputValue:OutputValue}" \
--output text`
echo $NEWURL
##Check OS
unameOut="$(uname -s)"
case "${unameOut}" in
Linux*) machine=Linux;;
Darwin*) machine=Mac;;
CYGWIN*) machine=Cygwin;;
MINGW*) machine=MinGw;;
*) machine="UNKNOWN:${unameOut}"
esac
if [ "$machine" == "$MACOS" ]
then
echo "You are on device with MAC OS, running sed with '' "
sed -i '' "s#{{URL}}#$NEWURL#g" "$SCRIPT_DIR/$COLLECTION"
else
echo "You are not on a mac, running sed normally"
sed -i "s#{{URL}}#$NEWURL#g" "$SCRIPT_DIR/$COLLECTION"
fi
}
setup_url
run_postman | 20513718f729d48809f914755b46e4ee0d57711a | [
"TypeScript",
"Shell"
] | 3 | TypeScript | elko-dev/github-generator | f40b876f56185c8a4a6f01ee971a17befae06483 | e3bb28886041bb0d64d1bff6d17ac2a3a80e6785 | |
refs/heads/master | <repo_name>pibahamondes/projet-INF442<file_sep>/Graph.cpp
#include <set>
#include <utility>
class Graph{
private:
std::set<int> vertex;
std::set<std::pair<int, int> > edges;
public:
void ajoutev(int sommet){
vert.insert(sommet);
}
void ajoutee(int a, int b){
somm.insert(std::make_pair(a, b));
}
};
int main(){
Graph g;
for(int i=0; i<5; i++){
g.ajoutev(i);
g.ajoutes(i, i);
}
return 0;
}
| 8a954b9939e935ab9e5a71d6359e7dd5a698b0b6 | [
"C++"
] | 1 | C++ | pibahamondes/projet-INF442 | 658edc2f466276191dec532797384c7c3f90797f | acb351a613ca7d0e634185da4dc63bd76daae715 | |
refs/heads/master | <repo_name>glowingmoth/AlphabetForKids<file_sep>/src/Gemfile
# frozen_string_literal: true
source "https://rubygems.org"
git_source(:github) {|repo_name| "https://github.com/#{repo_name}" }
# gem "rails"
#gem "ruby2d", "~> 0.9.4"
#gem "ascii-image", "~> 0.1.5"
#gem "rmagick", "~> 2.16"
gem "colorize", "~> 0.8.1"
<file_sep>/docs/HelpFile.md
# Instructions - Alphabet for Kids
1. Download current repository
2. Install Ruby.
https://www.ruby-lang.org/en/downloads/
3. In the terminal navigate to the src directory within the downloaded repository type: ./play.sh and hit Enter. Make sure to maximize the terminal window if text Title screen looks broken.
4. User will be presented with menu screen containing the following options
- **Sight Words** [Press 1 & hit Enter]
- **Missing Letter** [Press 2 & hit Enter]
- **Exit** [Press 3 & hit Enter]
4. When the parent enters Sight Words it will enter a mode of allowing a the child to press a single **[letter]** that displays the **[letter]** pressed and a word it's for. Pressing the **[3]** key will exit back to the menu.
5. When the parent enters Missing Letter mode the child will be able to press a **[letter]** and and the app will display the **[letter]** and the word with a random missing letter. The child will have 3 attempts to guess the letter. After **[3]** attempts it will let the child choose another letter for a new word. Pressing the **[3]** key will exit back to the menu.
6. If the parent presses **[3]** and **[Enter]** they will exit the program.
## Known Issues:
There is an issue with the key presses when in the sight words or missing letter modes.
holding the key down or pressing it before the ascii art disappears will cause the app to work through every key press, causing a back log.
The work around/temp fix If you don't want to wait for each press to be executed is to press ctrl + c. This will exit the app, then you can ./play.sh to run it again.
<file_sep>/src/tests.rb
require 'test/unit'
require_relative './ascii_art'
require 'colorize'
# Nested hash holding both alphabet categories
$words_hash = {
'A' => {animal: 'Ant', object: 'Apple'},
'B' => {animal: 'Bird', object: 'Bus'},
'C' => {animal: 'Cat', object: 'Castle'},
'D' => {animal: 'Dog', object: 'Drums'},
'E' => {animal: 'Elephant', object: 'Earmuffs'},
'F' => {animal: 'Fish', object: 'Flowers'},
'G' => {animal: 'Goat', object: 'Guitar'},
'H' => {animal: 'Horse', object: 'Helicopter'},
'I' => {animal: 'Impala', object: 'Igloo'},
'J' => {animal: 'Jellyfish', object: 'Jeep'},
'K' => {animal: 'Kangaroo', object: 'Key'},
'L' => {animal: 'Lion', object: 'Ladder'},
'M' => {animal: 'Mouse', object: 'Moon'},
'N' => {animal: 'Numbat', object: 'Nest'},
'O' => {animal: 'Octopus', object: 'Orange'},
'P' => {animal: 'Pig', object: 'Planet'},
'Q' => {animal: 'Quail', object: 'Queen'},
'R' => {animal: 'Rabbit', object: 'Ring'},
'S' => {animal: 'Snake', object: 'Scissors'},
'T' => {animal: 'Turtle', object: 'Tree'},
'U' => {animal: 'Urchin', object: 'Umbrella'},
'V' => {animal: 'Vulture', object: 'Violin'},
'W' => {animal: 'Whale', object: 'Watch'},
'X' => {animal: 'Xerus', object: 'X-ray'},
'Y' => {animal: 'Yak', object: 'Yoyo'},
'Z' => {animal: 'Zebra', object: 'Zip'}
}
class AppTest < Test::Unit::TestCase
def test_hash_value
assert_equal($words_hash['N'][:animal],'Numbat')
end
def test_apple
assert_equal( apple, "
,*/.
(& .((((((((###%%%.
%.,////((((###########.
% ********/((((((//(#
(%%##%###%(/((#%#//****/(#(#(/*,(
(%%#%%%#%*,**(##%#********/(/,.
%&%%%%#/**/#%#/*,,,......,#%%%%##
%&&&&&&%##(##((/,,,,,,,,,,.,,*%%%%%%.
&&&&&&&%&%%%%%%%%#((###%%%%%%#%%&%%&%%*
&&&&&&&&&&&&&&&%&&&%&&&%&%%%&&%%%%%%%%%%
/@@&&&&&&&&&&&&&&&&&&&&&&%&%&&%&%%#%%%%%%*
%&&&&&&&&&&&&&&&&&&%&&&&&&&&&&%%%%###%%%%*
#&&&&&&&&&&&&&&&&&&&&&&&&&&&&&&%%%%%%%%%%
&&&%%%&&&&%&&&&&&&&%&%&&&%&%%%%%%%%%%#(#
,((##%%%%#%&&&&&&&&&%%%&%%%%%%#%#%##((/
*((###%%%%%%%%&%&&%%%%%%%%%%#####((/*
*(##%%%%%%%#%%#%#%%%%%%%#%(####((/*
.%%%%%%%%%%%%%%%%%%%%%######(#(/
/%&&&&&&&&&%%%%#%%%%%%###((//,
%%%&&&&&&&&%%%%%%%(##(##((*.
##%%&&&&&&&&%%&%%&&&%%#/,.
.,,***///((((/*,....".colorize(:red))
end
end
<file_sep>/src/alphabet_for_kids.rb
require 'tty-cursor'
require 'colorize'
require_relative 'ascii_art'
#Title Screen
def title
system('clear')
puts ""
puts ""
puts "
____ _ ___ _ _ ____ ___ ____ ___ ____ ____ ____ _ _ _ ___ ____
|__| | |__] |__| |__| |__] |___ | |___ | | |__/ |_/ | | \\ [__
| | |___ | | | | | |__] |___ | | |__| | \\ |\\_ | |__/ ___]".colorize(:cyan)
puts ""
puts ""
puts " Choose an option and press Enter:"
puts ""
puts " 1 = Sight Words".colorize(:light_blue)
puts " 2 = Missing Letter".colorize(:light_red)
puts " 3 = Exit".colorize(:green)
end
#This is for fetching the letter pressed and randomly choosing from either category
def missing(letter, image)
#Random number to choose either category
category = rand(1..2)
if category == 1
category = :animal
elsif category == 2
category = :object
end
#This prints a message if any key pressed other than a letter or Exit [3]
unless $words_hash.key?(letter)
puts "no match"
else
selected_word = $words_hash[letter][category]
arry_m_letter = selected_word.split("")
num = arry_m_letter.length
num -= 1
# the random index number for removing a letter
fn = rand(0..num)
# returns the letter at the random index and puts in m_l
$m_l = arry_m_letter[fn]
# deletes the letter at the random index
arry_m_letter.delete_at(fn)
# replace the deleted letter with an empty space
arry_m_letter.insert(fn,"_")
# convert back into a string
new_word = arry_m_letter.join("").capitalize
sleep(2)
puts ""
puts ""
puts ""
puts " #{letter} is for #{new_word}"
puts ""
puts ""
puts image[letter][category]
end
#sleep(1)
end
#This fetches the key pressed and randomly removes a letter from the word
def is_for(letter, image)
#Random number to choose either category
category = rand(1..2)
if category == 1
category = :animal
elsif category == 2
category = :object
end
#This prints a message if any key pressed other than a letter or exit [3]
unless $words_hash.key?(letter)
puts "no match"
else
# system("clear")
# sleep(1)
# rabbit
# sleep(2)
puts ""
puts ""
puts " #{letter} is for #{$words_hash[letter][category]}"
puts ""
puts ""
puts image[letter][category]
end
return
end
# Nested hash holding both alphabet categories
$words_hash = {
'A' => {animal: 'Ant', object: 'Apple'},
'B' => {animal: 'Bird', object: 'Bus'},
'C' => {animal: 'Cat', object: 'Castle'},
'D' => {animal: 'Dog', object: 'Drums'},
'E' => {animal: 'Elephant', object: 'Earmuffs'},
'F' => {animal: 'Fish', object: 'Flowers'},
'G' => {animal: 'Goat', object: 'Guitar'},
'H' => {animal: 'Horse', object: 'Helicopter'},
'I' => {animal: 'Impala', object: 'Igloo'},
'J' => {animal: 'Jellyfish', object: 'Jeep'},
'K' => {animal: 'Kangaroo', object: 'Key'},
'L' => {animal: 'Lion', object: 'Ladder'},
'M' => {animal: 'Mouse', object: 'Moon'},
'N' => {animal: 'Numbat', object: 'Nest'},
'O' => {animal: 'Octopus', object: 'Orange'},
'P' => {animal: 'Pig', object: 'Planet'},
'Q' => {animal: 'Quail', object: 'Queen'},
'R' => {animal: 'Rabbit', object: 'Ring'},
'S' => {animal: 'Snake', object: 'Scissors'},
'T' => {animal: 'Turtle', object: 'Tree'},
'U' => {animal: 'Urchin', object: 'Umbrella'},
'V' => {animal: 'Vulture', object: 'Violin'},
'W' => {animal: 'Whale', object: 'Watch'},
'X' => {animal: 'Xerus', object: 'X-ray'},
'Y' => {animal: 'Yak', object: 'Yoyo'},
'Z' => {animal: 'Zebra', object: 'Zip'}
}
# Ascii art hash
image = {}
image["A"] = {}
image["A"][:object] = apple
image["A"][:animal] = ant
image["B"]= {}
image["B"][:object]= bus
image["B"][:animal] = bird
image["C"] = {}
image["C"][:object] = castle
image["C"][:animal] = cat
image["D"] = {}
image["D"][:object] = drums
image["D"][:animal] = dog
image["E"] = {}
image["E"][:object] = earmuffs
image["E"][:animal] = elephant
image["F"] = {}
image["F"][:object] = flowers
image["F"][:animal] = fish
image["G"] = {}
image["G"][:object] = guitar
image["G"][:animal] = goat
image["H"] = {}
image["H"][:object] = helicopter
image["H"][:animal] = horse
image["I"] = {}
image["I"][:object] = igloo
image["I"][:animal] = impala
image["J"] = {}
image["J"][:object] = jeep
image["J"][:animal] = jellyfish
image["K"] = {}
image["K"][:object] = key
image["K"][:animal] = kangaroo
image["L"] = {}
image["L"][:object] = ladder
image["L"][:animal] = lion
image["M"] = {}
image["M"][:object] = moon
image["M"][:animal] = mouse
image["N"] = {}
image["N"][:object] = nest
image["N"][:animal] = numbat
image["O"] = {}
image["O"][:object] = orange
image["O"][:animal] = octopus
image["P"] = {}
image["P"][:object] = planet
image["P"][:animal] = pig
image["Q"] = {}
image["Q"][:object] = queen
image["Q"][:animal] = quail
image["R"] = {}
image["R"][:object] = ring
image["R"][:animal] = rabbit
image["S"] = {}
image["S"][:object] = scissors
image["S"][:animal] = snake
image["T"] = {}
image["T"][:object] = tree
image["T"][:animal] = turtle
image["U"] = {}
image["U"][:object] = umbrella
image["U"][:animal] = urchin
image["V"] = {}
image["V"][:object] = violin
image["V"][:animal] = vulture
image["W"] = {}
image["W"][:object] = watch
image["W"][:animal] = whale
image["X"] = {}
image["X"][:object] = xray
image["X"][:animal] = xerus
image["Y"] = {}
image["Y"][:object] = yoyo
image["Y"][:animal] = yak
image["Z"] = {}
image["Z"][:object] = zip
image["Z"][:animal] = zebra
#Main menu loop
while true
#Run the title screen method
title
options = gets.chomp
case options
when '1'
#Loop for Sight Words option
while true
system('clear')
puts ""
puts ""
puts "(Press 3 to Exit) Press a letter to begin! "
#Get key press without having to press enter key after
begin
system('stty raw -echo') #This terminal/system command wil
key = STDIN.getc #Using I/O to get key press and convert to string
system("stty -raw echo")
if key == "3"
break
end
letter = key.upcase
#Method for grabbing letter pressed by user
is_for(letter, image)
sleep(4)
ensure
system("stty -raw echo")
end
end
when '2'
#Loop for the Missing Letter option
while true
system('clear')
puts ""
puts ""
puts "(Press 3 to Exit) Guess the missing letter!"
begin
system('stty raw -echo') #This terminal/system command wil
first_key = STDIN.getc #Using I/O to get key press and convert to string
system('stty -raw echo') #This terminal/system command wil
if first_key == "3"
break
end
correct_letter_attempts = 0
while correct_letter_attempts <= 3
game_letter = first_key.upcase
#Method for grabbing letter pressed by user
#is_for(letter)
missing(game_letter, image)
begin
system('stty raw -echo') #This terminal/system command wil
answer_key = STDIN.getc #Using I/O to get key press and convert to string
system('stty -raw echo') #This terminal/system command wil
if answer_key == $m_l
system('clear')
puts ""
puts ""
puts " Correct!".colorize(:light_blue)
sleep(1)
break
elsif answer_key != $m_l
system('clear')
puts ""
puts ""
puts " Try Again".colorize(:light_red)
sleep(0.5)
break
elsif answer_key == '3'
break
end
correct_letter_attempts += 1
ensure
system("stty -raw echo")
end
end
ensure
system("stty -raw echo")
end
end
# Exiting the App
when '3'
system('clear')
puts ""
puts ""
puts " Thank you for using ALPHABET for KIDS!".colorize(:cyan)
puts ""
puts ""
sleep(1)
exit
end
end
<file_sep>/README.md
### R4 - **Provide a link to your source control repository**
https://github.com/glowingmoth/AlphabetForKids
### R5 - **Statement of Purpose and Scope**
Being a father of two young children I'm aware that early education is vital for their development.
- The app is for learning the alphabet and basic spelling.
- The target audience is for the ages between 5-8, however this may vary slightly as each child is different.
- Learning that is fun and that can engage is always better and in the case of children, this app was no exception.
- The app will have a main menu where the child (or parent) can choose between 1 of two options. Choosing any letter on the keyboard will allow them to see a word from either category of animals or objects. There is a slightly more advanced options where instead of showing a complete word relating to the chosen letter, it will remove a random letter from the word and give them the options to guess what letter is missing.
### R6 - **Features**
- **Archive of words** - The app has 52 words for children to learn through 2 categories available ie. animals and objects. Essentially these are sight words and when presented repeatedly with the letter that is pressed the child will be able to see how the first letter is at the beginning and related to the word.
- **Guidance** - The app is designed for children and so has to be as simple as possible. To accomplish this, the app will not require the child to do anything after it receives input, instead the app will go back to the menu and allow them to press another letter.
- **Missing Letters** - For children needing slightly more challenging learning, there is the ability to choose the missing letter option of the app. The child presses any letter on the keyboard and this time instead of showing the complete word it will show the word from either category ie, animals or objects and removes a random letter.
If the child where to press the same letter again (after being back at the start of the missing letter option) a different letter would be missing. This means the **missing letter isn't hard coded** and the child can't get the letter right purely by memory.
- **Ascii images/art** - Images are the universal language and so they're a MUST when trying to create a fun learning experience for children. The MVP is purely text based however the implementation of images/ascii would greatly enhance the boring environment that is the terminal, especially in the context of a child user. There are 52 words and to accompany these words, 52 different pieces of ascii art
- **Animations** - The next step up from images in the same category would be animations. Due to the nature of the terminal this feature will be a bonus and implemented last, if there is still enough time. Otherwise this can be added after the due date.
### R7 - **User Interaction & Experience**
- **How to Interact** Dealing with an age (in the case of no parent to supervise) that is still learning to read, things need to be shown visually with images/pictures or be intuitive. For this reason the child will essentially learn how to interact by pressing keys on the key board.
- **Interaction** By pressing any letter on the key board the child can choose whatever letter they like and see a word and art relating to that letter.
- **Errors** will be handled in a simplified way from the child's perspective, however still informing a parent of the wrong key or letter being pressed. The app will simply ignore any keys pressed that are not letters or numbers in the case of the main menu loop.
### R9 - **Implementation Plan**
https://github.com/glowingmoth/AlphabetForKids/blob/master/docs/ControlFlow_AlphabetKids.png
- With the idea in mind I needed to get a clear image of the program logic and so created a flow chart to flesh out any unforeseen issues. This proved to be very useful as I actually recognized several issue immediately once I could see them. The flowchart proved invaluable through the early to mid stages of development.
https://github.com/glowingmoth/AlphabetForKids/blob/master/docs/Trello_7.png
- Having never used any project management system such as Agile and Trello there was some learning and experience to gain. But I can really see the benefit now. My use in the future, now that I have some experience, will be to add more check lists, as I wasn't aware of them and kept creating new cards instead of adding a check list to what was already there.
- From this point , with my limited understanding or perhaps brain jammed full of information from the past to weeks of the bootcamp I decided to go with a hash to store the alphabet, words and categories as I still lacked in understand all the features of using classes and inheritance.
- To store the ascii art and call them later I placed the strings inside methods which may not have been the best choice. I separated the files and had the rest of the source code ie. methods with main while loops, hashes etc in the main.rb file (now renamed).
- In conclusion, I truly did my best to incorporate everything I had learnt from my instructors. Whilst not following best practices/coding conventions everywhere I still managed to clean up as much of the source code with what damage had all ready been done. If I were to attempt this again, I would most definitely plan and flesh out on paper how to structure the code.
<file_sep>/src/play.sh
bundle install
ruby ../src/alphabet_for_kids.rb | 8da7f1cc31785d303f06ba290136be86f6599638 | [
"Markdown",
"Ruby",
"Shell"
] | 6 | Ruby | glowingmoth/AlphabetForKids | a55084df8c814b83052f8cf4353af471078297af | 52ac98152e087a3d667d0d123a0b4ad338b3072c | |
refs/heads/master | <repo_name>josemariaallegue/Laboratorio_II_TP<file_sep>/TP-01/Entidades/MiCalculadora/Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using Entidades;
namespace MiCalculadora
{
public partial class LaCalculadora : Form
{
public LaCalculadora()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
this.MaximizeBox = false;
this.MinimizeBox = false;
}
private void btnLimpiar_Click(object sender, EventArgs e)
{
this.Limpiar();
}
private void btnOperar_Click(object sender, EventArgs e)
{
lblResultado.Text = LaCalculadora.Operar(txtNumero1.Text, txtNumero2.Text, cmbOperador.Text).ToString();
//aplicacion de formatos a los textbox y comobox para hacer que la calculadora sea
//mas agradable visualmente
if (txtNumero1.Text == "")
{
txtNumero1.Text = "0";
}
if (txtNumero2.Text == "")
{
txtNumero2.Text = "0";
}
if (cmbOperador.Text != "+" && cmbOperador.Text != "-" && cmbOperador.Text != "/" && cmbOperador.Text != "*")
{
cmbOperador.Text = "+";
}
}
private void btnCerrar_Click(object sender, EventArgs e)
{
this.Close();
}
private void btnConvertirADecimal_Click(object sender, EventArgs e)
{
Numero numero = new Numero();
lblResultado.Text = numero.BinarioDecimal(lblResultado.Text);
}
private void btnConvertirABinario_Click(object sender, EventArgs e)
{
Numero numero = new Numero();
lblResultado.Text = numero.DecimalBinario(lblResultado.Text);
}
/// <summary>
/// Limpia todos los textbox y combobox
/// </summary>
private void Limpiar()
{
this.txtNumero1.Clear();
this.txtNumero2.Clear();
this.cmbOperador.Text = "";
this.lblResultado.Text = "";
}
/// <summary>
/// Calcula el resultado a partir de los numeros y operadores ingresados
/// </summary>
/// <param name="numero1"> Primer numero a operar </param>
/// <param name="numero2"> Segundo numero a operar </param>
/// <param name="operador"> El string que determinar la operacion </param>
/// <returns> El resultado de la operacion </returns>
private static double Operar(string numero1, string numero2, string operador)
{
double retorno;
Calculadora calculadora = new Calculadora();
Numero num1 = new Numero(numero1);
Numero num2 = new Numero(numero2);
retorno = calculadora.Operar(num1,num2, operador);
return retorno;
}
}
}
<file_sep>/TP-01/Entidades/Entidades/Calculadora.cs
using System;
namespace Entidades
{
public class Calculadora
{
/// <summary>
/// Suma
/// </summary>
/// <param name="num1"> Primer numero a operar </param>
/// <param name="num2"> Segundo numero a operar</param>
/// <param name="operador"> Determina que cuenta se va a realizar </param>
/// <returns> Devuelve el resultado de la operacion entre los dos numeros </returns>
public double Operar(Numero num1, Numero num2, string operador)
{
double retorno = 0;
switch (Calculadora.ValidarOperador(operador))
{
case "+":
retorno = num1 + num2;
break;
case "-":
retorno = num1 - num2;
break;
case "/":
retorno = num1 / num2;
break;
case "*":
retorno = num1 * num2;
break;
}
return retorno;
}
/// <summary>
/// Valida que operador sea +, - , / o *
/// </summary>
/// <param name="operador"> El string a comprobar </param>
/// <returns> Si es distinto a alguna de las 4 opciones devuelve + si no el correspondiente</returns>
private static string ValidarOperador(string operador)
{
string retorno = "+";
if (operador == "-" || operador == "*" || operador == "/")
{
retorno = operador;
}
return retorno;
}
}
}
<file_sep>/TP-01/Entidades/Entidades/Numero.cs
using System;
using System.Collections.Generic;
using System.Text;
namespace Entidades
{
public class Numero
{
private double numero;
private string SetNumero
{
set
{
this.numero = this.ValidarNumero(value);
}
}
public Numero()
{
}
public Numero(double numero)
{
this.numero = numero;
}
public Numero(string numero)
{
this.SetNumero = numero;
}
/// <summary>
/// Convierte un string a double
/// </summary>
/// <param name="strNumero"> La cadena a convertir </param>
/// <returns> El numero en formato double </returns>
private double ValidarNumero(string strNumero)
{
double retorno = 0;
if (double.TryParse(strNumero, out double auxiliarRetorno))
{
retorno = auxiliarRetorno;
}
return retorno;
}
/// <summary>
/// Convierte un numero binario en formato string a un decimal en formato string
/// </summary>
/// <param name="binario"> El binario a convertir </param>
/// <returns> Un string que contiene el numero en decimal </returns>
public string BinarioDecimal(string binario)
{
double retorno = 0;
bool flag = true;
if (binario.Length > 1)
{
if (binario[0] == '-')
{
binario = binario.Substring(3, binario.Length - 3);
}
else
{
binario = binario.Substring(2, binario.Length - 2);
}
}
for (int j = 0; j <= binario.Length - 1; j++)
{
if (binario[j].ToString() != "0" && binario[j].ToString() != "1")
{
flag = false;
break;
}
}
if (flag)
{
for (int i = 1; i <= binario.Length; i++)
{
retorno += double.Parse(binario[i - 1].ToString()) * (double)Math.Pow(2, binario.Length - i);
}
}
else
{
return "Valor inválido";
}
return retorno.ToString();
}
/// <summary>
/// Convierte un decimal en formato double a binario
/// </summary>
/// <param name="numero"> Numero a convertir a binario </param>
/// <returns> El numero binario en formato string </returns>
public string DecimalBinario(double numero)
{
string retorno = "";
int numeroEntero = (int)numero;
if (numeroEntero < 0)
{
numeroEntero = numeroEntero * -1;
}
while (numeroEntero > 0)
{
retorno = (numeroEntero % 2).ToString() + retorno;
numeroEntero = numeroEntero / 2;
}
if (numero >= 0)
{
retorno = "0b" + retorno;
}
else
{
retorno = "-0b" + retorno;
}
return retorno.ToString();
}
/// <summary>
/// Convierte un decimal en formato string a binario
/// </summary>
/// <param name="numero"> Numero a convertir a bianario </param>
/// <returns> El numero binario en formato string </returns>
public string DecimalBinario(string numero)
{
string retorno = "";
if (double.TryParse(numero, out double auxiliar))
{
retorno = this.DecimalBinario(auxiliar);
}
return retorno;
}
/// <summary>
/// Permite que al sumar dos objetos numero no haga falta llamar a sus variables
/// </summary>
/// <param name="n1"> Primer numero a operar </param>
/// <param name="n2"> Segundo numero a operar </param>
/// <returns> El resultado de la operacion </returns>
public static double operator +(Numero n1, Numero n2)
{
return n1.numero + n2.numero;
}
/// <summary>
/// Permite que al restar dos objetos numero no haga falta llamar a sus variables
/// </summary>
/// <param name="n1"> Primer numero a operar </param>
/// <param name="n2"> Segundo numero a operar </param>
/// <returns> El resultado de la operacion </returns>
public static double operator -(Numero n1, Numero n2)
{
return n1.numero - n2.numero;
}
/// <summary>
/// Permite que al dividir dos objetos numero no haga falta llamar a sus variables
/// </summary>
/// <param name="n1"> Primer numero a operar </param>
/// <param name="n2"> Segundo numero a operar </param>
/// <returns> El resultado de la operacion </returns>
public static double operator /(Numero n1, Numero n2)
{
return n1.numero / n2.numero;
}
/// <summary>
/// Permite que al multiplicar dos objetos numero no haga falta llamar a sus variables
/// </summary>
/// <param name="n1"> Primer numero a operar </param>
/// <param name="n2"> Segundo numero a operar </param>
/// <returns> El resultado de la operacion </returns>
public static double operator *(Numero n1, Numero n2)
{
return n1.numero * n2.numero;
}
}
}
| eac131b3590340fb9ad5379b71b69135d1a78122 | [
"C#"
] | 3 | C# | josemariaallegue/Laboratorio_II_TP | 3204428ad1604bb243ec6131345e6d67a254c66b | 34e704a0bcdc90f517782a4fd201c85084ab703e | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
import sys
from nacl.public import PrivateKey, PublicKey, SealedBox
from nacl.encoding import Base64Encoder
def write_file(file_name, data, base64 = False):
if base64:
data = Base64Encoder.encode(data).decode('ascii')
f = open(file_name, 'w')
f.write(data)
f.close()
def read_file(file_name, base64 = False):
f = open(file_name, 'r')
data = f.read()
if base64:
data = Base64Encoder.decode(data)
f.close()
return data
def generate_keypair():
key_pair = PrivateKey.generate()
secret_key_bin = key_pair._private_key
public_key_bin = key_pair.public_key._public_key
write_file('secret_key.txt', secret_key_bin, True)
write_file('public_key.txt', public_key_bin, True)
def encrypt():
decrypted_utf8 = read_file('decrypted.txt')
decrypted_bin = bytes(decrypted_utf8, 'utf-8')
public_key_bin = read_file('public_key.txt', True)
public_key = PublicKey(public_key_bin)
sealed_box = SealedBox(public_key)
encrypted_bin = sealed_box.encrypt(decrypted_bin)
write_file("encrypted.txt", encrypted_bin, True)
def decrypt():
encrypted_bin = read_file('encrypted.txt', True)
secret_key_bin = read_file('secret_key.txt', True)
secret_key = PrivateKey(secret_key_bin)
sealed_box = SealedBox(secret_key)
decrypted_bin = sealed_box.decrypt(encrypted_bin)
decrypted_utf8 = decrypted_bin.decode('utf-8')
write_file("decrypted.txt", decrypted_utf8)
# Depending on script argument, generate keypair, encrypt, or decrypt
def execute_command(command):
switcher = {
'g': generate_keypair,
'e': encrypt,
'd': decrypt
}
func = switcher.get(command)
func()
if len(sys.argv) > 1:
execute_command(sys.argv[1])
<file_sep># Example of libsodium in Python
The scripts in this repo show how to:
* generate a public and secret key
* encrypt a sealed box via a public key
* decrypt a sealed box via a secret key
For portability, all keys and encrypted data are stored in base64.
## Required modules
* [PyNaCl](https://github.com/pyca/pynacl)
## Examples in other languages
See the [libsodium_examples](https://github.com/users/tpronk/projects/1) project.
| 80e425ccffc6f91e2010b3929fc189149087bf73 | [
"Markdown",
"Python"
] | 2 | Python | tpronk/libsodium_example_python | cfa53c617add7fb90ea53016c17ba9c13510fb36 | 48f422bef25d80eba258a3ec4d8825bd4af975b8 | |
refs/heads/master | <file_sep>#!/bin/bash
QT_VERSION=4.8
QT_FOLDER=Qt-$QT_VERSION
# Tip: change this to local/shared mirror
QT_URL=git://gitorious.org/~lfranchi/qt/lfranchi-qt.git
COMPILE_JOBS=10
# Step 1: Download Qt source tarball
# Note: only if it does not exist yet in the current directory
echo "Cloning Qt $QT_VERSION from Gitorious. Please wait..."
if [ ! -d $QT_FOLDER ]
then
git clone $QT_URL $QT_FOLDER
git checkout -b phantomjs origin/phantomjs
else
cd $QT_FOLDER
git checkout -f
git clean -xdf
git checkout phantomjs
cd ..
fi
cd $QT_FOLDER
git pull
#
# if [ ! -f $QT_TARBALL ]
# then
# echo "Downloading Qt $QT_VERSION from Nokia. Please wait..."
# if ! curl -C - -O -S $QT_URL
# then
# echo
# echo "Fatal error: fail to download from $QT_URL !"
# exit 1
# fi
# fi
# Step 2: Extract Qt source
# [ -d $QT_FOLDER ] && rm -rf $QT_FOLDER
# echo "Extracting Qt $QT_VERSION source tarball..."
# echo
# tar xzf $QT_TARBALL
# mv qt-everywhere-opensource-src-$QT_VERSION Qt-$QT_VERSION
# Step 3: Build Qt
# cd $QT_FOLDER
patch -p1 < ../qapplication_skip_qtmenu.patch
echo "Building Qt $QT_VERSION. Please wait..."
echo
./configure -opensource -confirm-license -release -no-exceptions -no-stl -no-xmlpatterns -no-phonon -no-qt3support -no-opengl -no-declarative -qt-libpng -qt-libjpeg -no-libmng -no-libtiff -D QT_NO_STYLE_CDE -D QT_NO_STYLE_CLEANLOOKS -D QT_NO_STYLE_MOTIF -D QT_NO_STYLE_PLASTIQUE -prefix $PWD -nomake demos -nomake examples -nomake tools -qpa
make -j$COMPILE_JOBS
cd ..
#
# # Extra step: copy JavaScriptCore/release, needed for jscore static lib
# mkdir ../JavaScriptCore
# cp -rp $QT_FOLDER/src/3rdparty/webkit/JavaScriptCore/release ../JavaScriptCore/
# Step 4: Build PhantomJS
echo "Building PhantomJS. Please wait..."
echo
cd ..
[ -f Makefile ] && make distclean
deploy/$QT_FOLDER/bin/qmake
make -j$COMPILE_JOBS
# Step 5: Prepare for deployment
echo "Compressing PhantomJS executable..."
echo
strip bin/phantomjs
if [ `command -v upx` ]; then
upx -9 bin/phantomjs
else
echo "You don't have UPX. Consider installing it to reduce the executable size."
fi
<file_sep>/*
This file is part of the PhantomJS project from Ofi Labs.
Copyright (C) 2011 <NAME> <<EMAIL>>
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the <organization> nor the
names of its contributors may be used to endorse or promote products
derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
ARE DISCLAIMED. IN NO EVENT SHALL <COPYRIGHT HOLDER> BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
#include "mimesniffer.h"
#define CHECK_START(magic, type) if (checkStart(data, magic)) { return type; }
#define CHECK_CONTAIN(magic, type) if (ldata.contains(magic) != NULL) { return type; }
static bool checkStart(const QString data, const QString magic)
{
for (int i = 0; i < magic.length(); ++i)
{
if (magic[i] == '.')
{
continue;
}
if (data[i] != magic[i])
{
return false;
}
}
return true;
}
QString mimeSniff(const QByteArray &data)
{
// Only check the first 512 bytes.
QByteArray ldata = data.left(512).toLower();
// HTML Tags
// XML processing directive. Although this is not an HTML mime type,
// we sniff for this in the HTML phase because text/xml is just as powerful as HTML.
CHECK_CONTAIN("<?xml", "text/xml") // # Mozilla
// DOCTYPEs
CHECK_CONTAIN("<!DOCTYPE html", "text/html") // # HTML5 spec
// Sniffable tags, ordered by how often they occur in sniffable documents.
CHECK_CONTAIN("<script", "text/html") // HTML5 spec, Mozilla
CHECK_CONTAIN("<html", "text/html") // HTML5 spec, Mozilla
CHECK_CONTAIN("<!--", "text/html")
CHECK_CONTAIN("<head", "text/html") // HTML5 spec, Mozilla
CHECK_CONTAIN("<iframe", "text/html") // Mozilla
CHECK_CONTAIN("<h1", "text/html") // Mozilla
CHECK_CONTAIN("<div", "text/html") // Mozilla
CHECK_CONTAIN("<font", "text/html") // Mozilla
CHECK_CONTAIN("<table", "text/html") // Mozilla
CHECK_CONTAIN("<a", "text/html") // Mozilla
CHECK_CONTAIN("<style", "text/html") // Mozilla
CHECK_CONTAIN("<title", "text/html") // Mozilla
CHECK_CONTAIN("<b", "text/html") // Mozilla
CHECK_CONTAIN("<body", "text/html") // Mozilla
CHECK_CONTAIN("<br", "text/html")
CHECK_CONTAIN("<p", "text/html") // Mozilla
// XML Tags
// We want to be very conservative in interpreting text/xml content as
// XHTML -- we just want to sniff enough to make things pass.
// So we match explicitly on this, and don't match other ways of writing
// it in semantically-equivalent ways.
CHECK_CONTAIN("<html xmlns=\"http://www.w3.org/1999/xhtml\"", "application/xhtml+xml")
CHECK_CONTAIN("<feed", "application/atom+xml")
CHECK_CONTAIN("<rss", "application/rss+xml") // UTF-8
// Images
// Source: HTML 5 specification
CHECK_START("GIF87a", "image/gif")
CHECK_START("GIF89a", "image/gif")
CHECK_START("\x89PNG\x0D\x0A\x1A\x0A", "image/png")
CHECK_START("\xFF\xD8\xFF", "image/jpeg")
CHECK_START("BM", "image/bmp")
// Source: Chrome
CHECK_START("I I", "image/tiff")
CHECK_START("II*", "image/tiff")
CHECK_START("MM\x00*", "image/tiff")
CHECK_START("RIFF....WEBPVP8 ", "image/webp") // The space at the end is no error
// General magic numbers
// Source: HTML 5 specification
CHECK_START("%PDF-", "application/pdf")
CHECK_START("%!PS-Adobe-", "application/postscript")
// Source: Mozilla
CHECK_START("#!", "text/plain") // Script
CHECK_START("%!", "text/plain") // Script, similar to PS
CHECK_START("From", "text/plain")
CHECK_START(">From", "text/plain")
// Source: Chrome
CHECK_START("\x1F\x8B\x08", "application/x-gzip")
CHECK_START("PK\x03\x04", "application/zip")
CHECK_START("Rar!\x1A\x07\x00", "application/x-rar-compressed")
CHECK_START("\xD7\xCD\xC6\x9A", "application/x-msmetafile")
CHECK_START("MZ", "application/octet-stream") // Exe
// Audio
// Source: Chrome
CHECK_START("\x2E\x52\x4D\x46", "audio/x-pn-realaudio")
CHECK_START("ID3", "audio/mpeg")
// Video
// Source: Chrome
CHECK_START("\x30\x26\xB2\x75\x8E\x66\xCF\x11\xA6\xD9\x00\xAA\x00\x62\xCE\x6C", "video/x-ms-asf")
CHECK_START("\x1A\x45\xDF\xA3", "video/webm")
// FLash
CHECK_START("CWS", "application/x-shockwave-flash")
// Byte order marks
CHECK_CONTAIN("\xFE\xFF", "text/plain") // UTF-16BE
CHECK_CONTAIN("\xFF\xFE", "text/plain") // UTF-16LE
CHECK_CONTAIN("\xEF\xBB\xBF", "text/plain") // UTF-8
// Whether a given byte looks like it might be part of binary content.
// Source: HTML5 specification
bool magic_byte_looks_binary[256] = {
1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, // 0x00 - 0x0F
1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, // 0x10 - 0x1F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x20 - 0x2F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x30 - 0x3F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x40 - 0x4F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x50 - 0x5F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x60 - 0x6F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x70 - 0x7F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x80 - 0x8F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0x90 - 0x9F
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0xA0 - 0xAF
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0xB0 - 0xBF
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0xC0 - 0xCF
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0xD0 - 0xDF
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // 0xE0 - 0xEF
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 // 0xF0 - 0xFF
};
for (int i = 0; i < data.length(); ++i)
{
if (magic_byte_looks_binary[(int)data.at(i)])
{
return "application/octet-stream";
}
}
// fall back to text/plain
return "text/plain";
}
bool mimeIsText(QString mime)
{
return (mime == "text/plain");
}
bool mimeIsHtml(QString mime)
{
return ((mime == "text/html") ||
(mime == "text/xml"));
}
bool mimeIsXml(QString mime)
{
return ((mime == "application/xhtml+xml") ||
(mime == "application/atom+xml") ||
(mime == "application/rss+xml"));
}
bool mimeIsImage(QString mime)
{
return ((mime == "image/gif") ||
(mime == "image/png") ||
(mime == "image/jpeg") ||
(mime == "image/bmp") ||
(mime == "image/tiff") ||
(mime == "image/webp"));
}
bool mimeIsAudio(QString mime)
{
return ((mime == "udio/x-pn-realaudio") ||
(mime == "audio/mpeg"));
}
bool mimeIsVideo(QString mime)
{
return ((mime == "video/x-ms-asf") ||
(mime == "video/webm"));
}
| 455cafd8c4c53b887032cc0087f98f272c88cbdf | [
"C++",
"Shell"
] | 2 | Shell | lfranchi/phantomjs | 2549f6d25565b9ac6e9c9547eff9c1c6d9728d67 | 25c49b8ae8d3b952efbaa0ad71db9ed14a094f88 | |
refs/heads/master | <repo_name>ncalderon/sf-web-client<file_sep>/src/test/java/com/calderon/sf/web/rest/AccountTransactionResourceIntTest.java
package com.calderon.sf.web.rest;
import com.calderon.sf.SfWebClientApp;
import com.calderon.sf.domain.AccountTransaction;
import com.calderon.sf.domain.FinanceAccount;
import com.calderon.sf.repository.AccountTransactionRepository;
import com.calderon.sf.web.rest.errors.ExceptionTranslator;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.MockitoAnnotations;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.web.PageableHandlerMethodArgumentResolver;
import org.springframework.http.MediaType;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.transaction.annotation.Transactional;
import javax.persistence.EntityManager;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.time.ZoneId;
import java.util.List;
import static org.assertj.core.api.Assertions.assertThat;
import static org.hamcrest.Matchers.hasItem;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
import com.calderon.sf.domain.enumeration.TranType;
import com.calderon.sf.domain.enumeration.PaymentMethod;
/**
* Test class for the AccountTransactionResource REST controller.
*
* @see AccountTransactionResource
*/
@RunWith(SpringRunner.class)
@SpringBootTest(classes = SfWebClientApp.class)
public class AccountTransactionResourceIntTest {
private static final TranType DEFAULT_TRAN_TYPE = TranType.EXPENSE;
private static final TranType UPDATED_TRAN_TYPE = TranType.INCOME;
private static final String DEFAULT_TRAN_NUMBER = "AAAAAAAAAA";
private static final String UPDATED_TRAN_NUMBER = "BBBBBBBBBB";
private static final String DEFAULT_REFERENCE_NUMBER = "AAAAAAAAAA";
private static final String UPDATED_REFERENCE_NUMBER = "BBBBBBBBBB";
private static final LocalDate DEFAULT_POST_DATE = LocalDate.ofEpochDay(0L);
private static final LocalDate UPDATED_POST_DATE = LocalDate.now(ZoneId.systemDefault());
private static final String DEFAULT_DESCRIPTION = "AAAAAAAAAA";
private static final String UPDATED_DESCRIPTION = "BBBBBBBBBB";
private static final BigDecimal DEFAULT_AMOUNT = new BigDecimal(1);
private static final BigDecimal UPDATED_AMOUNT = new BigDecimal(2);
private static final PaymentMethod DEFAULT_PAYMENT_METHOD = PaymentMethod.UNSPECIFIED;
private static final PaymentMethod UPDATED_PAYMENT_METHOD = PaymentMethod.CASH;
@Autowired
private AccountTransactionRepository accountTransactionRepository;
@Autowired
private MappingJackson2HttpMessageConverter jacksonMessageConverter;
@Autowired
private PageableHandlerMethodArgumentResolver pageableArgumentResolver;
@Autowired
private ExceptionTranslator exceptionTranslator;
@Autowired
private EntityManager em;
private MockMvc restAccountTransactionMockMvc;
private AccountTransaction accountTransaction;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
final AccountTransactionResource accountTransactionResource = new AccountTransactionResource(accountTransactionRepository);
this.restAccountTransactionMockMvc = MockMvcBuilders.standaloneSetup(accountTransactionResource)
.setCustomArgumentResolvers(pageableArgumentResolver)
.setControllerAdvice(exceptionTranslator)
.setMessageConverters(jacksonMessageConverter).build();
}
/**
* Create an entity for this test.
*
* This is a static method, as tests for other entities might also need it,
* if they test an entity which requires the current entity.
*/
public static AccountTransaction createEntity(EntityManager em) {
AccountTransaction accountTransaction = new AccountTransaction()
.tranType(DEFAULT_TRAN_TYPE)
.tranNumber(DEFAULT_TRAN_NUMBER)
.referenceNumber(DEFAULT_REFERENCE_NUMBER)
.postDate(DEFAULT_POST_DATE)
.description(DEFAULT_DESCRIPTION)
.amount(DEFAULT_AMOUNT)
.paymentMethod(DEFAULT_PAYMENT_METHOD);
// Add required entity
FinanceAccount financeAccount = FinanceAccountResourceIntTest.createEntity(em);
em.persist(financeAccount);
em.flush();
accountTransaction.setFinanceAccount(financeAccount);
return accountTransaction;
}
@Before
public void initTest() {
accountTransaction = createEntity(em);
}
@Test
@Transactional
public void createAccountTransaction() throws Exception {
int databaseSizeBeforeCreate = accountTransactionRepository.findAll().size();
// Create the AccountTransaction
restAccountTransactionMockMvc.perform(post("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isCreated());
// Validate the AccountTransaction in the database
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeCreate + 1);
AccountTransaction testAccountTransaction = accountTransactionList.get(accountTransactionList.size() - 1);
assertThat(testAccountTransaction.getTranType()).isEqualTo(DEFAULT_TRAN_TYPE);
assertThat(testAccountTransaction.getTranNumber()).isEqualTo(DEFAULT_TRAN_NUMBER);
assertThat(testAccountTransaction.getReferenceNumber()).isEqualTo(DEFAULT_REFERENCE_NUMBER);
assertThat(testAccountTransaction.getPostDate()).isEqualTo(DEFAULT_POST_DATE);
assertThat(testAccountTransaction.getDescription()).isEqualTo(DEFAULT_DESCRIPTION);
assertThat(testAccountTransaction.getAmount()).isEqualTo(DEFAULT_AMOUNT);
assertThat(testAccountTransaction.getPaymentMethod()).isEqualTo(DEFAULT_PAYMENT_METHOD);
}
@Test
@Transactional
public void createAccountTransactionWithExistingId() throws Exception {
int databaseSizeBeforeCreate = accountTransactionRepository.findAll().size();
// Create the AccountTransaction with an existing ID
accountTransaction.setId(1L);
// An entity with an existing ID cannot be created, so this API call must fail
restAccountTransactionMockMvc.perform(post("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isBadRequest());
// Validate the AccountTransaction in the database
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void checkTranTypeIsRequired() throws Exception {
int databaseSizeBeforeTest = accountTransactionRepository.findAll().size();
// set the field null
accountTransaction.setTranType(null);
// Create the AccountTransaction, which fails.
restAccountTransactionMockMvc.perform(post("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isBadRequest());
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void checkPostDateIsRequired() throws Exception {
int databaseSizeBeforeTest = accountTransactionRepository.findAll().size();
// set the field null
accountTransaction.setPostDate(null);
// Create the AccountTransaction, which fails.
restAccountTransactionMockMvc.perform(post("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isBadRequest());
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void checkAmountIsRequired() throws Exception {
int databaseSizeBeforeTest = accountTransactionRepository.findAll().size();
// set the field null
accountTransaction.setAmount(null);
// Create the AccountTransaction, which fails.
restAccountTransactionMockMvc.perform(post("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isBadRequest());
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void getAllAccountTransactions() throws Exception {
// Initialize the database
accountTransactionRepository.saveAndFlush(accountTransaction);
// Get all the accountTransactionList
restAccountTransactionMockMvc.perform(get("/api/account-transactions?sort=id,desc"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.[*].id").value(hasItem(accountTransaction.getId().intValue())))
.andExpect(jsonPath("$.[*].tranType").value(hasItem(DEFAULT_TRAN_TYPE.toString())))
.andExpect(jsonPath("$.[*].tranNumber").value(hasItem(DEFAULT_TRAN_NUMBER.toString())))
.andExpect(jsonPath("$.[*].referenceNumber").value(hasItem(DEFAULT_REFERENCE_NUMBER.toString())))
.andExpect(jsonPath("$.[*].postDate").value(hasItem(DEFAULT_POST_DATE.toString())))
.andExpect(jsonPath("$.[*].description").value(hasItem(DEFAULT_DESCRIPTION.toString())))
.andExpect(jsonPath("$.[*].amount").value(hasItem(DEFAULT_AMOUNT.intValue())))
.andExpect(jsonPath("$.[*].paymentMethod").value(hasItem(DEFAULT_PAYMENT_METHOD.toString())));
}
@Test
@Transactional
public void getAccountTransaction() throws Exception {
// Initialize the database
accountTransactionRepository.saveAndFlush(accountTransaction);
// Get the accountTransaction
restAccountTransactionMockMvc.perform(get("/api/account-transactions/{id}", accountTransaction.getId()))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.id").value(accountTransaction.getId().intValue()))
.andExpect(jsonPath("$.tranType").value(DEFAULT_TRAN_TYPE.toString()))
.andExpect(jsonPath("$.tranNumber").value(DEFAULT_TRAN_NUMBER.toString()))
.andExpect(jsonPath("$.referenceNumber").value(DEFAULT_REFERENCE_NUMBER.toString()))
.andExpect(jsonPath("$.postDate").value(DEFAULT_POST_DATE.toString()))
.andExpect(jsonPath("$.description").value(DEFAULT_DESCRIPTION.toString()))
.andExpect(jsonPath("$.amount").value(DEFAULT_AMOUNT.intValue()))
.andExpect(jsonPath("$.paymentMethod").value(DEFAULT_PAYMENT_METHOD.toString()));
}
@Test
@Transactional
public void getNonExistingAccountTransaction() throws Exception {
// Get the accountTransaction
restAccountTransactionMockMvc.perform(get("/api/account-transactions/{id}", Long.MAX_VALUE))
.andExpect(status().isNotFound());
}
@Test
@Transactional
public void updateAccountTransaction() throws Exception {
// Initialize the database
accountTransactionRepository.saveAndFlush(accountTransaction);
int databaseSizeBeforeUpdate = accountTransactionRepository.findAll().size();
// Update the accountTransaction
AccountTransaction updatedAccountTransaction = accountTransactionRepository.findOne(accountTransaction.getId());
updatedAccountTransaction
.tranType(UPDATED_TRAN_TYPE)
.tranNumber(UPDATED_TRAN_NUMBER)
.referenceNumber(UPDATED_REFERENCE_NUMBER)
.postDate(UPDATED_POST_DATE)
.description(UPDATED_DESCRIPTION)
.amount(UPDATED_AMOUNT)
.paymentMethod(UPDATED_PAYMENT_METHOD);
restAccountTransactionMockMvc.perform(put("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(updatedAccountTransaction)))
.andExpect(status().isOk());
// Validate the AccountTransaction in the database
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeUpdate);
AccountTransaction testAccountTransaction = accountTransactionList.get(accountTransactionList.size() - 1);
assertThat(testAccountTransaction.getTranType()).isEqualTo(UPDATED_TRAN_TYPE);
assertThat(testAccountTransaction.getTranNumber()).isEqualTo(UPDATED_TRAN_NUMBER);
assertThat(testAccountTransaction.getReferenceNumber()).isEqualTo(UPDATED_REFERENCE_NUMBER);
assertThat(testAccountTransaction.getPostDate()).isEqualTo(UPDATED_POST_DATE);
assertThat(testAccountTransaction.getDescription()).isEqualTo(UPDATED_DESCRIPTION);
assertThat(testAccountTransaction.getAmount()).isEqualTo(UPDATED_AMOUNT);
assertThat(testAccountTransaction.getPaymentMethod()).isEqualTo(UPDATED_PAYMENT_METHOD);
}
@Test
@Transactional
public void updateNonExistingAccountTransaction() throws Exception {
int databaseSizeBeforeUpdate = accountTransactionRepository.findAll().size();
// Create the AccountTransaction
// If the entity doesn't have an ID, it will be created instead of just being updated
restAccountTransactionMockMvc.perform(put("/api/account-transactions")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(accountTransaction)))
.andExpect(status().isCreated());
// Validate the AccountTransaction in the database
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeUpdate + 1);
}
@Test
@Transactional
public void deleteAccountTransaction() throws Exception {
// Initialize the database
accountTransactionRepository.saveAndFlush(accountTransaction);
int databaseSizeBeforeDelete = accountTransactionRepository.findAll().size();
// Get the accountTransaction
restAccountTransactionMockMvc.perform(delete("/api/account-transactions/{id}", accountTransaction.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().isOk());
// Validate the database is empty
List<AccountTransaction> accountTransactionList = accountTransactionRepository.findAll();
assertThat(accountTransactionList).hasSize(databaseSizeBeforeDelete - 1);
}
@Test
@Transactional
public void equalsVerifier() throws Exception {
TestUtil.equalsVerifier(AccountTransaction.class);
AccountTransaction accountTransaction1 = new AccountTransaction();
accountTransaction1.setId(1L);
AccountTransaction accountTransaction2 = new AccountTransaction();
accountTransaction2.setId(accountTransaction1.getId());
assertThat(accountTransaction1).isEqualTo(accountTransaction2);
accountTransaction2.setId(2L);
assertThat(accountTransaction1).isNotEqualTo(accountTransaction2);
accountTransaction1.setId(null);
assertThat(accountTransaction1).isNotEqualTo(accountTransaction2);
}
}
<file_sep>/src/main/webapp/app/entities/finance-account/finance-account.module.ts
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { RouterModule } from '@angular/router';
import { SfWebClientSharedModule } from '../../shared';
import { SfWebClientAdminModule } from '../../admin/admin.module';
import {
FinanceAccountService,
FinanceAccountPopupService,
FinanceAccountComponent,
FinanceAccountDetailComponent,
FinanceAccountDialogComponent,
FinanceAccountPopupComponent,
FinanceAccountDeletePopupComponent,
FinanceAccountDeleteDialogComponent,
financeAccountRoute,
financeAccountPopupRoute,
FinanceAccountResolvePagingParams,
} from './';
const ENTITY_STATES = [
...financeAccountRoute,
...financeAccountPopupRoute,
];
@NgModule({
imports: [
SfWebClientSharedModule,
SfWebClientAdminModule,
RouterModule.forRoot(ENTITY_STATES, { useHash: true })
],
declarations: [
FinanceAccountComponent,
FinanceAccountDetailComponent,
FinanceAccountDialogComponent,
FinanceAccountDeleteDialogComponent,
FinanceAccountPopupComponent,
FinanceAccountDeletePopupComponent,
],
entryComponents: [
FinanceAccountComponent,
FinanceAccountDialogComponent,
FinanceAccountPopupComponent,
FinanceAccountDeleteDialogComponent,
FinanceAccountDeletePopupComponent,
],
providers: [
FinanceAccountService,
FinanceAccountPopupService,
FinanceAccountResolvePagingParams,
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientFinanceAccountModule {}
<file_sep>/src/main/java/com/calderon/sf/service/dto/package-info.java
/**
* Data Transfer Objects.
*/
package com.calderon.sf.service.dto;
<file_sep>/src/main/webapp/app/sf-entities/finance-account/index.ts
export * from './finance-account.model';
export * from './finance-account-popup.service';
export * from './finance-account.service';
export * from './finance-account-dialog.component';
export * from './finance-account-delete-dialog.component';
export * from './finance-account-detail.component';
export * from './finance-account.component';
export * from './finance-account.route';
export * from './transaction/transaction.module'
<file_sep>/src/main/webapp/app/app.module.ts
import './vendor.ts';
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import {BrowserAnimationsModule} from '@angular/platform-browser/animations';
import { Ng2Webstorage } from 'ng2-webstorage';
import { SfWebClientSharedModule, UserRouteAccessService } from './shared';
import { SfWebClientHomeModule } from './home/home.module';
import { SfWebClientAdminModule } from './admin/admin.module';
import { SfWebClientAccountModule } from './account/account.module';
/*import { SfWebClientEntityModule } from './entities/entity.module';*/
import { SfWebClientEntityModule as AppSfWebClientEntityModule } from './sf-entities/entity.module';
import { customHttpProvider } from './blocks/interceptor/http.provider';
import { PaginationConfig } from './blocks/config/uib-pagination.config';
// jhipster-needle-angular-add-module-import JHipster will add new module here
import {
JhiMainComponent,
LayoutRoutingModule,
NavbarComponent,
FooterComponent,
ProfileService,
PageRibbonComponent,
ActiveMenuDirective,
ErrorComponent
} from './layouts';
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
LayoutRoutingModule,
Ng2Webstorage.forRoot({ prefix: 'jhi', separator: '-'}),
SfWebClientSharedModule,
SfWebClientHomeModule,
SfWebClientAdminModule,
SfWebClientAccountModule,
/*SfWebClientEntityModule,*/
// jhipster-needle-angular-add-module JHipster will add new module here
AppSfWebClientEntityModule
],
declarations: [
JhiMainComponent,
NavbarComponent,
ErrorComponent,
PageRibbonComponent,
ActiveMenuDirective,
FooterComponent
],
providers: [
ProfileService,
customHttpProvider(),
PaginationConfig,
UserRouteAccessService
],
bootstrap: [ JhiMainComponent ]
})
export class SfWebClientAppModule {}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/tran-category-dialog.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Response } from '@angular/http';
import { Observable } from 'rxjs/Rx';
import { NgbActiveModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { JhiEventManager, JhiAlertService } from 'ng-jhipster';
import { TranCategory } from './tran-category.model';
import { TranCategoryPopupService } from './tran-category-popup.service';
import { TranCategoryService } from './tran-category.service';
import { User, UserService } from '../../shared';
import { TranCategoryRegex, TranCategoryRegexService } from '../tran-category-regex';
import { ResponseWrapper } from '../../shared';
@Component({
selector: 'jhi-tran-category-dialog',
templateUrl: './tran-category-dialog.component.html'
})
export class TranCategoryDialogComponent implements OnInit {
tranCategory: TranCategory;
isSaving: boolean;
users: User[];
trancategoryregexes: TranCategoryRegex[];
constructor(
public activeModal: NgbActiveModal,
private alertService: JhiAlertService,
private tranCategoryService: TranCategoryService,
private userService: UserService,
private tranCategoryRegexService: TranCategoryRegexService,
private eventManager: JhiEventManager
) {
}
ngOnInit() {
this.isSaving = false;
this.userService.query()
.subscribe((res: ResponseWrapper) => { this.users = res.json; }, (res: ResponseWrapper) => this.onError(res.json));
this.tranCategoryRegexService.query()
.subscribe((res: ResponseWrapper) => { this.trancategoryregexes = res.json; }, (res: ResponseWrapper) => this.onError(res.json));
}
clear() {
this.activeModal.dismiss('cancel');
}
save() {
this.isSaving = true;
if (this.tranCategory.id !== undefined) {
this.subscribeToSaveResponse(
this.tranCategoryService.update(this.tranCategory));
} else {
this.subscribeToSaveResponse(
this.tranCategoryService.create(this.tranCategory));
}
}
private subscribeToSaveResponse(result: Observable<TranCategory>) {
result.subscribe((res: TranCategory) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private onSaveSuccess(result: TranCategory) {
this.eventManager.broadcast({ name: 'tranCategoryListModification', content: 'OK'});
this.isSaving = false;
this.activeModal.dismiss(result);
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
trackUserById(index: number, item: User) {
return item.id;
}
trackTranCategoryRegexById(index: number, item: TranCategoryRegex) {
return item.id;
}
}
@Component({
selector: 'jhi-tran-category-popup',
template: ''
})
export class TranCategoryPopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(
private route: ActivatedRoute,
private tranCategoryPopupService: TranCategoryPopupService
) {}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
if ( params['id'] ) {
this.tranCategoryPopupService
.open(TranCategoryDialogComponent as Component, params['id']);
} else {
this.tranCategoryPopupService
.open(TranCategoryDialogComponent as Component);
}
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/sf-entities/account-transaction/account-transaction.service.ts
import {Injectable} from '@angular/core';
import {Http, Response, URLSearchParams} from '@angular/http';
import {Observable} from 'rxjs/Rx';
import {SERVER_API_URL} from '../../app.constants';
import {AccountTransaction} from './account-transaction.model';
import {createRequestOption, ResponseWrapper} from '../../shared';
import {JhiDateUtils} from 'ng-jhipster';
@Injectable()
export class AccountTransactionService {
private resourceUrl = SERVER_API_URL + 'api/account-transactions';
constructor(private http: Http, private dateUtils: JhiDateUtils) {
}
create(accountTransaction: AccountTransaction): Observable<AccountTransaction> {
const copy = this.convert(accountTransaction);
return this.http.post(this.resourceUrl, copy).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
createBulk(transactions: AccountTransaction[]): Observable<AccountTransaction[]> {
const copy = this.convertArray(transactions);
return this.http.post(this.resourceUrl + "/bulk", copy).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
update(accountTransaction: AccountTransaction): Observable<AccountTransaction> {
const copy = this.convert(accountTransaction);
return this.http.put(this.resourceUrl, copy).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
find(id: number): Observable<AccountTransaction> {
return this.http.get(`${this.resourceUrl}/${id}`).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
query(req?: any): Observable<ResponseWrapper> {
const options = createRequestOption(req);
return this.http.get(this.resourceUrl, options)
.map((res: Response) => this.convertResponse(res));
}
delete(id: number): Observable<Response> {
return this.http.delete(`${this.resourceUrl}/${id}`);
}
private convertResponse(res: Response): ResponseWrapper {
const jsonResponse = res.json();
for (let i = 0; i < jsonResponse.length; i++) {
this.convertItemFromServer(jsonResponse[i]);
}
return new ResponseWrapper(res.headers, jsonResponse, res.status);
}
private convertItemsFromServer(accountTransactions: AccountTransaction[]) {
for (let tran of accountTransactions) {
this.convertItemFromServer(tran);
}
}
private convertItemFromServer(entity: any) {
entity.postDate = this.dateUtils
.convertLocalDateFromServer(entity.postDate);
}
private convert(accountTransaction: AccountTransaction): AccountTransaction {
const copy: AccountTransaction = Object.assign({}, accountTransaction);
copy.postDate = this.dateUtils.convertLocalDateToServer(accountTransaction.postDate);
return copy;
}
private convertArray(accountTransactions: AccountTransaction[]): AccountTransaction[] {
const copyTrans: AccountTransaction[] = [];
for (let tran of accountTransactions) {
copyTrans.push(this.convert(tran));
}
return copyTrans;
}
findTransactionsByAccounts(accountsId: number[]): Observable<AccountTransaction[]> {
return this.findTransactionsByAccountsAndYear(accountsId, 0);
}
findTransactionsByAccountsAndYear(accountsId: number[], year: number): Observable<AccountTransaction[]> {
const options = createRequestOption();
const params: URLSearchParams = new URLSearchParams();
params.set('accountsId', <any>accountsId);
params.set('year', year + '');
options.params = params;
return this.http.get(`${this.resourceUrl}/findByAccountsIdAndYear`, options).map((res: Response) => {
const jsonResponse = res.json();
this.convertTransactionsFromServer(jsonResponse);
return jsonResponse;
});
}
private convertTransactionsFromServer(accountTransactions: AccountTransaction[]) {
for (let tran of accountTransactions) {
this.convertTransactionFromServer(tran);
}
}
private convertTransactionFromServer(entity: any) {
entity.postDate = this.dateUtils
.convertLocalDateFromServer(entity.postDate);
}
}
<file_sep>/src/main/java/com/calderon/sf/web/rest/BankResource.java
package com.calderon.sf.web.rest;
import com.calderon.sf.domain.Bank;
import com.calderon.sf.repository.BankRepository;
import com.calderon.sf.web.rest.util.HeaderUtil;
import com.calderon.sf.web.rest.util.PaginationUtil;
import com.codahale.metrics.annotation.Timed;
import io.github.jhipster.web.util.ResponseUtil;
import io.swagger.annotations.ApiParam;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.List;
import java.util.Optional;
/**
* REST controller for managing Bank.
*/
@RestController
@RequestMapping("/api")
public class BankResource {
private final Logger log = LoggerFactory.getLogger(BankResource.class);
private static final String ENTITY_NAME = "bank";
private final BankRepository bankRepository;
public BankResource(BankRepository bankRepository) {
this.bankRepository = bankRepository;
}
/**
* POST /banks : Create a new bank.
*
* @param bank the bank to create
* @return the ResponseEntity with status 201 (Created) and with body the new bank, or with status 400 (Bad Request) if the bank has already an ID
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PostMapping("/banks")
@Timed
public ResponseEntity<Bank> createBank(@Valid @RequestBody Bank bank) throws URISyntaxException {
log.debug("REST request to save Bank : {}", bank);
if (bank.getId() != null) {
return ResponseEntity.badRequest().headers(HeaderUtil.createFailureAlert(ENTITY_NAME, "idexists", "A new bank cannot already have an ID")).body(null);
}
Bank result = bankRepository.save(bank);
return ResponseEntity.created(new URI("/api/banks/" + result.getId()))
.headers(HeaderUtil.createEntityCreationAlert(ENTITY_NAME, result.getId().toString()))
.body(result);
}
/**
* PUT /banks : Updates an existing bank.
*
* @param bank the bank to update
* @return the ResponseEntity with status 200 (OK) and with body the updated bank,
* or with status 400 (Bad Request) if the bank is not valid,
* or with status 500 (Internal Server Error) if the bank couldn't be updated
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PutMapping("/banks")
@Timed
public ResponseEntity<Bank> updateBank(@Valid @RequestBody Bank bank) throws URISyntaxException {
log.debug("REST request to update Bank : {}", bank);
if (bank.getId() == null) {
return createBank(bank);
}
Bank result = bankRepository.save(bank);
return ResponseEntity.ok()
.headers(HeaderUtil.createEntityUpdateAlert(ENTITY_NAME, bank.getId().toString()))
.body(result);
}
/**
* GET /banks : get all the banks.
*
* @param pageable the pagination information
* @return the ResponseEntity with status 200 (OK) and the list of banks in body
*/
@GetMapping("/banks")
@Timed
public ResponseEntity<List<Bank>> getAllBanks(@ApiParam Pageable pageable) {
log.debug("REST request to get a page of Banks");
Page<Bank> page = bankRepository.findAll(pageable);
HttpHeaders headers = PaginationUtil.generatePaginationHttpHeaders(page, "/api/banks");
return new ResponseEntity<>(page.getContent(), headers, HttpStatus.OK);
}
/**
* GET /banks/:id : get the "id" bank.
*
* @param id the id of the bank to retrieve
* @return the ResponseEntity with status 200 (OK) and with body the bank, or with status 404 (Not Found)
*/
@GetMapping("/banks/{id}")
@Timed
public ResponseEntity<Bank> getBank(@PathVariable Long id) {
log.debug("REST request to get Bank : {}", id);
Bank bank = bankRepository.findOne(id);
return ResponseUtil.wrapOrNotFound(Optional.ofNullable(bank));
}
/**
* DELETE /banks/:id : delete the "id" bank.
*
* @param id the id of the bank to delete
* @return the ResponseEntity with status 200 (OK)
*/
@DeleteMapping("/banks/{id}")
@Timed
public ResponseEntity<Void> deleteBank(@PathVariable Long id) {
log.debug("REST request to delete Bank : {}", id);
bankRepository.delete(id);
return ResponseEntity.ok().headers(HeaderUtil.createEntityDeletionAlert(ENTITY_NAME, id.toString())).build();
}
}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/transaction-dialog/transaction-dialog.component.ts
import {Component, OnDestroy, OnInit} from '@angular/core';
import {ActivatedRoute} from '@angular/router';
import {TransactionPopupService} from '../transaction-popup.service';
import {AccountTransaction} from '../../../account-transaction/account-transaction.model';
import {User} from '../../../../shared/user/user.model';
import {TranCategory} from '../../../tran-category/tran-category.model';
import {NgbActiveModal} from '@ng-bootstrap/ng-bootstrap';
import {JhiAlertService, JhiEventManager} from 'ng-jhipster';
import {AccountTransactionService} from '../../../account-transaction/account-transaction.service';
import {FinanceAccountService} from '../../finance-account.service';
import {TranCategoryService} from '../../../tran-category/tran-category.service';
import {LoggerService} from '../../../../shared/logger/logger.service';
import {ResponseWrapper} from '../../../../shared/model/response-wrapper.model';
import {Observable} from 'rxjs/Observable';
import {TranType} from '../../../../sf-entities/account-transaction/account-transaction.model';
import {FinanceAccount} from '../../finance-account.model';
@Component({
selector: 'jhi-transaction-dialog',
templateUrl: './transaction-dialog.component.html'
})
export class TransactionDialogComponent implements OnInit {
accountId: number;
currentAccount: FinanceAccount;
transaction: AccountTransaction;
isSaving: boolean;
categoryTypeaheadValue: string;
categories: TranCategory[] = [];
postDateDp: any;
isDetailsCollapsed = true;
constructor(private activatedRoute: ActivatedRoute,
public activeModal: NgbActiveModal,
private alertService: JhiAlertService,
private tranService: AccountTransactionService,
private accountService: FinanceAccountService,
private tranCategoryService: TranCategoryService,
private eventManager: JhiEventManager,
private logger: LoggerService) {
}
ngOnInit() {
this.activatedRoute.children.find((value) => {
if (value.outlet === 'popup') {
value.params.subscribe((params) => {
this.accountId = params['accountId'];
this.load();
});
return true;
}
});
/*this.activatedRoute.params.subscribe((params) => {
this.accountId = params['accountId'];
this.load();
});*/
}
load() {
this.isSaving = false;
this.accountService.find(this.accountId)
.subscribe((account) => {
this.currentAccount = account;
this.transaction.financeAccount = account;
}, (res: ResponseWrapper) => this.onError(res.json));
this.tranCategoryService.query()
.subscribe((res: ResponseWrapper) => {
this.categories = res.json;
}, (res: ResponseWrapper) => this.onError(res.json));
}
clear() {
this.activeModal.dismiss('cancel');
}
save() {
this.isSaving = true;
if (this.transaction.id !== undefined) {
this.subscribeToSaveResponse(
this.tranService.update(this.transaction));
} else {
this.subscribeToSaveResponse(
this.tranService.create(this.transaction));
}
}
private subscribeToSaveResponse(result: Observable<AccountTransaction>) {
result.subscribe((res: AccountTransaction) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private onSaveSuccess(result: AccountTransaction) {
this.eventManager.broadcast({name: 'transactionListModification', content: 'OK'});
this.isSaving = false;
this.activeModal.dismiss(result);
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
trackUserById(index: number, item: User) {
return item.id;
}
trackTranCategoryById(index: number, item: TranCategory) {
return item.id;
}
getDetailsCollapseClass() {
return {
'form-row': !this.isDetailsCollapsed,
'collapse': this.isDetailsCollapsed
};
}
getTranIncomeClass() {
return {
'btn': true,
'btn-secondary': this.transaction.tranType == <TranType><any>'EXPENSE',
'btn-success': this.transaction.tranType == <TranType><any>'INCOME'
};
}
getTranExpenseClass() {
return {
'btn': true,
'btn-danger': this.transaction.tranType == <TranType><any>'EXPENSE',
'btn-secondary': this.transaction.tranType == <TranType><any>'INCOME'
};
}
onTypeaheadSelect(event: any) {
this.transaction.tranCategory = event.item;
}
}
@Component({
selector: 'jhi-transaction-popup',
template: ''
})
export class TransactionPopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(private route: ActivatedRoute,
private accountTransactionPopupService: TransactionPopupService) {
}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
if (params['id']) {
this.accountTransactionPopupService
.open(TransactionDialogComponent as Component, params['id']);
} else {
this.accountTransactionPopupService
.open(TransactionDialogComponent as Component);
}
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/entities/finance-account/finance-account-detail.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Subscription } from 'rxjs/Rx';
import { JhiEventManager } from 'ng-jhipster';
import { FinanceAccount } from './finance-account.model';
import { FinanceAccountService } from './finance-account.service';
@Component({
selector: 'jhi-finance-account-detail',
templateUrl: './finance-account-detail.component.html'
})
export class FinanceAccountDetailComponent implements OnInit, OnDestroy {
financeAccount: FinanceAccount;
private subscription: Subscription;
private eventSubscriber: Subscription;
constructor(
private eventManager: JhiEventManager,
private financeAccountService: FinanceAccountService,
private route: ActivatedRoute
) {
}
ngOnInit() {
this.subscription = this.route.params.subscribe((params) => {
this.load(params['id']);
});
this.registerChangeInFinanceAccounts();
}
load(id) {
this.financeAccountService.find(id).subscribe((financeAccount) => {
this.financeAccount = financeAccount;
});
}
previousState() {
window.history.back();
}
ngOnDestroy() {
this.subscription.unsubscribe();
this.eventManager.destroy(this.eventSubscriber);
}
registerChangeInFinanceAccounts() {
this.eventSubscriber = this.eventManager.subscribe(
'financeAccountListModification',
(response) => this.load(this.financeAccount.id)
);
}
}
<file_sep>/src/test/javascript/e2e/entities/tran-category.spec.ts
import { browser, element, by, $ } from 'protractor';
import { NavBarPage } from './../page-objects/jhi-page-objects';
const path = require('path');
describe('TranCategory e2e test', () => {
let navBarPage: NavBarPage;
let tranCategoryDialogPage: TranCategoryDialogPage;
let tranCategoryComponentsPage: TranCategoryComponentsPage;
const fileToUpload = '../../../../main/webapp/content/images/logo-jhipster.png';
const absolutePath = path.resolve(__dirname, fileToUpload);
beforeAll(() => {
browser.get('/');
browser.waitForAngular();
navBarPage = new NavBarPage();
navBarPage.getSignInPage().autoSignInUsing('admin', 'admin');
browser.waitForAngular();
});
it('should load TranCategories', () => {
navBarPage.goToEntity('tran-category');
tranCategoryComponentsPage = new TranCategoryComponentsPage();
expect(tranCategoryComponentsPage.getTitle()).toMatch(/sfWebClientApp.tranCategory.home.title/);
});
it('should load create TranCategory dialog', () => {
tranCategoryComponentsPage.clickOnCreateButton();
tranCategoryDialogPage = new TranCategoryDialogPage();
expect(tranCategoryDialogPage.getModalTitle()).toMatch(/sfWebClientApp.tranCategory.home.createOrEditLabel/);
tranCategoryDialogPage.close();
});
it('should create and save TranCategories', () => {
tranCategoryComponentsPage.clickOnCreateButton();
tranCategoryDialogPage.setNameInput('name');
expect(tranCategoryDialogPage.getNameInput()).toMatch('name');
tranCategoryDialogPage.setDescriptionInput('description');
expect(tranCategoryDialogPage.getDescriptionInput()).toMatch('description');
tranCategoryDialogPage.userSelectLastOption();
tranCategoryDialogPage.tranCategoryRegexSelectLastOption();
tranCategoryDialogPage.save();
expect(tranCategoryDialogPage.getSaveButton().isPresent()).toBeFalsy();
});
afterAll(() => {
navBarPage.autoSignOut();
});
});
export class TranCategoryComponentsPage {
createButton = element(by.css('.jh-create-entity'));
title = element.all(by.css('jhi-tran-category div h2 span')).first();
clickOnCreateButton() {
return this.createButton.click();
}
getTitle() {
return this.title.getAttribute('jhiTranslate');
}
}
export class TranCategoryDialogPage {
modalTitle = element(by.css('h4#myTranCategoryLabel'));
saveButton = element(by.css('.modal-footer .btn.btn-primary'));
closeButton = element(by.css('button.close'));
nameInput = element(by.css('input#field_name'));
descriptionInput = element(by.css('input#field_description'));
userSelect = element(by.css('select#field_user'));
tranCategoryRegexSelect = element(by.css('select#field_tranCategoryRegex'));
getModalTitle() {
return this.modalTitle.getAttribute('jhiTranslate');
}
setNameInput = function (name) {
this.nameInput.sendKeys(name);
}
getNameInput = function () {
return this.nameInput.getAttribute('value');
}
setDescriptionInput = function (description) {
this.descriptionInput.sendKeys(description);
}
getDescriptionInput = function () {
return this.descriptionInput.getAttribute('value');
}
userSelectLastOption = function () {
this.userSelect.all(by.tagName('option')).last().click();
}
userSelectOption = function (option) {
this.userSelect.sendKeys(option);
}
getUserSelect = function () {
return this.userSelect;
}
getUserSelectedOption = function () {
return this.userSelect.element(by.css('option:checked')).getText();
}
tranCategoryRegexSelectLastOption = function () {
this.tranCategoryRegexSelect.all(by.tagName('option')).last().click();
}
tranCategoryRegexSelectOption = function (option) {
this.tranCategoryRegexSelect.sendKeys(option);
}
getTranCategoryRegexSelect = function () {
return this.tranCategoryRegexSelect;
}
getTranCategoryRegexSelectedOption = function () {
return this.tranCategoryRegexSelect.element(by.css('option:checked')).getText();
}
save() {
this.saveButton.click();
}
close() {
this.closeButton.click();
}
getSaveButton() {
return this.saveButton;
}
}
<file_sep>/src/main/java/com/calderon/sf/service/FinanceService.java
package com.calderon.sf.service;
import com.calderon.sf.domain.AccountTransaction;
import com.calderon.sf.domain.FinanceAccount;
import com.calderon.sf.domain.enumeration.PaymentMethod;
import com.calderon.sf.domain.enumeration.TranType;
import com.calderon.sf.repository.AccountTransactionRepository;
import com.calderon.sf.repository.FinanceAccountRepository;
import com.querydsl.core.types.Predicate;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
@Service
public class FinanceService {
private AccountTransactionRepository tranRepository;
private FinanceAccountRepository accountRepository;
public FinanceService(AccountTransactionRepository tranRepository, FinanceAccountRepository accountRepository) {
this.tranRepository = tranRepository;
this.accountRepository = accountRepository;
}
public List<AccountTransaction> findTransactionBy(Long accountId){
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_Id(accountId);
}
public Page<AccountTransaction> findTransactionBy(Long accountId, Pageable page){
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_Id(accountId, page);
}
public Page<AccountTransaction> findTransactionBy(Predicate predicate, Pageable page){
return tranRepository.findAll(predicate, page);
}
/*public Page<AccountTransaction> findTransactionBy(Long accountId, Pageable page, Predicate predicate){
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_Id(accountId, page, predicate);
}*/
public Page<AccountTransaction> findTransactionBy(FinanceAccount account, Pageable pageable){
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_Id(account.getId(), pageable);
}
public List<AccountTransaction> findTransactionBy(Long accountId, LocalDate start, LocalDate end) {
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_IdAndPostDateGreaterThanEqualAndPostDateLessThanEqual(accountId, start, end);
}
public List<AccountTransaction> findTransactionBy(LocalDate start, LocalDate end) {
return tranRepository.findByUserIsCurrentUserAndPostDateGreaterThanEqualAndPostDateLessThanEqual(start, end);
}
public List<AccountTransaction> findTransactionBy(long[] accountId, LocalDate start, LocalDate end) {
return tranRepository.findByUserIsCurrentUserAndFinanceAccount_IdIsInAndPostDateGreaterThanEqualAndPostDateLessThanEqual(accountId, start, end);
}
@Transactional
public AccountTransaction saveTransaction(AccountTransaction tran){
FinanceAccount account = tran.getFinanceAccount();
account.setBalance(calcNewBalance(account.getBalance(), tran.getAmount(), tran.getTranType()== TranType.INCOME));
AccountTransaction transaction = tranRepository.save(tran);
accountRepository.save(account);
return transaction;
}
private BigDecimal calcNewBalance(BigDecimal currentBalance, BigDecimal tranValue, boolean isIncomeType){
if (isIncomeType)
return currentBalance.add(tranValue);
else
return currentBalance.subtract(tranValue);
}
@Transactional
public List<AccountTransaction> saveTransactions(List<AccountTransaction> transactions) {
FinanceAccount account = transactions.get(0).getFinanceAccount();
transactions.stream().forEach(tran -> {
account.setBalance(calcNewBalance(account.getBalance(), tran.getAmount(), tran.getTranType()== TranType.INCOME));
});
List<AccountTransaction> transactionPersited = tranRepository.save(transactions);
accountRepository.save(account);
return transactionPersited;
}
@Transactional
public void deleteTransaction(Long id) {
AccountTransaction tran = tranRepository.getOne(id);
FinanceAccount account = tran.getFinanceAccount();
account.setBalance(calcNewBalance(account.getBalance(), tran.getAmount(), tran.getTranType()== TranType.INCOME));
tranRepository.delete(id);
accountRepository.save(account);
}
public FinanceAccount saveAccount(FinanceAccount account) {
return saveAccount(account, true);
}
public FinanceAccount saveAccount(FinanceAccount account, boolean createDefaultTrans){
FinanceAccount persistedAccount = accountRepository.save(account);
if(createDefaultTrans)
saveTransactions(createDefaultTransactions(persistedAccount));
return persistedAccount;
}
public List<FinanceAccount> saveAccounts(List<FinanceAccount> accounts){
return saveAccounts(accounts, true);
}
public List<FinanceAccount> saveAccounts(List<FinanceAccount> accounts, boolean createDefaultTrans){
List<FinanceAccount> persistedAccounts = accountRepository.save(accounts);
persistedAccounts.forEach(account -> {
if(createDefaultTrans)
saveTransactions(createDefaultTransactions(account));
});
return persistedAccounts;
}
private List<AccountTransaction> createDefaultTransactions(FinanceAccount account){
List<AccountTransaction> transactions = new ArrayList<>();
transactions.add(new AccountTransaction().amount(new BigDecimal(0)).description("Initial Balance").paymentMethod(PaymentMethod.UNSPECIFIED).postDate(LocalDate.now()).tranType(TranType.INCOME).user(account.getUser()).financeAccount(account));
return transactions;
}
public Page<FinanceAccount> findAccounts(Pageable pageable) {
return accountRepository.findAll(pageable);
}
public FinanceAccount findAccount(Long id) {
return accountRepository.findOne(id);
}
public void deleteAccount(Long id) {
accountRepository.delete(id);
}
public Page<FinanceAccount> findAccountsByCurrentUserAndActive(Pageable pageable) {
return accountRepository.findByUserIsCurrentUserAndAccountStatusIsActive(pageable);
}
}
<file_sep>/src/test/java/com/calderon/sf/web/rest/TranCategoryResourceIntTest.java
package com.calderon.sf.web.rest;
import com.calderon.sf.SfWebClientApp;
import com.calderon.sf.domain.TranCategory;
import com.calderon.sf.repository.TranCategoryRepository;
import com.calderon.sf.web.rest.errors.ExceptionTranslator;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.MockitoAnnotations;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.web.PageableHandlerMethodArgumentResolver;
import org.springframework.http.MediaType;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.transaction.annotation.Transactional;
import javax.persistence.EntityManager;
import java.util.List;
import static org.assertj.core.api.Assertions.assertThat;
import static org.hamcrest.Matchers.hasItem;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
/**
* Test class for the TranCategoryResource REST controller.
*
* @see TranCategoryResource
*/
@RunWith(SpringRunner.class)
@SpringBootTest(classes = SfWebClientApp.class)
public class TranCategoryResourceIntTest {
private static final String DEFAULT_NAME = "AAAAAAAAAA";
private static final String UPDATED_NAME = "BBBBBBBBBB";
private static final String DEFAULT_DESCRIPTION = "AAAAAAAAAA";
private static final String UPDATED_DESCRIPTION = "BBBBBBBBBB";
@Autowired
private TranCategoryRepository tranCategoryRepository;
@Autowired
private MappingJackson2HttpMessageConverter jacksonMessageConverter;
@Autowired
private PageableHandlerMethodArgumentResolver pageableArgumentResolver;
@Autowired
private ExceptionTranslator exceptionTranslator;
@Autowired
private EntityManager em;
private MockMvc restTranCategoryMockMvc;
private TranCategory tranCategory;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
final TranCategoryResource tranCategoryResource = new TranCategoryResource(tranCategoryRepository);
this.restTranCategoryMockMvc = MockMvcBuilders.standaloneSetup(tranCategoryResource)
.setCustomArgumentResolvers(pageableArgumentResolver)
.setControllerAdvice(exceptionTranslator)
.setMessageConverters(jacksonMessageConverter).build();
}
/**
* Create an entity for this test.
*
* This is a static method, as tests for other entities might also need it,
* if they test an entity which requires the current entity.
*/
public static TranCategory createEntity(EntityManager em) {
TranCategory tranCategory = new TranCategory()
.name(DEFAULT_NAME)
.description(DEFAULT_DESCRIPTION);
return tranCategory;
}
@Before
public void initTest() {
tranCategory = createEntity(em);
}
@Test
@Transactional
public void createTranCategory() throws Exception {
int databaseSizeBeforeCreate = tranCategoryRepository.findAll().size();
// Create the TranCategory
restTranCategoryMockMvc.perform(post("/api/tran-categories")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(tranCategory)))
.andExpect(status().isCreated());
// Validate the TranCategory in the database
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeCreate + 1);
TranCategory testTranCategory = tranCategoryList.get(tranCategoryList.size() - 1);
assertThat(testTranCategory.getName()).isEqualTo(DEFAULT_NAME);
assertThat(testTranCategory.getDescription()).isEqualTo(DEFAULT_DESCRIPTION);
}
@Test
@Transactional
public void createTranCategoryWithExistingId() throws Exception {
int databaseSizeBeforeCreate = tranCategoryRepository.findAll().size();
// Create the TranCategory with an existing ID
tranCategory.setId(1L);
// An entity with an existing ID cannot be created, so this API call must fail
restTranCategoryMockMvc.perform(post("/api/tran-categories")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(tranCategory)))
.andExpect(status().isBadRequest());
// Validate the TranCategory in the database
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void checkNameIsRequired() throws Exception {
int databaseSizeBeforeTest = tranCategoryRepository.findAll().size();
// set the field null
tranCategory.setName(null);
// Create the TranCategory, which fails.
restTranCategoryMockMvc.perform(post("/api/tran-categories")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(tranCategory)))
.andExpect(status().isBadRequest());
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void getAllTranCategories() throws Exception {
// Initialize the database
tranCategoryRepository.saveAndFlush(tranCategory);
// Get all the tranCategoryList
restTranCategoryMockMvc.perform(get("/api/tran-categories?sort=id,desc"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.[*].id").value(hasItem(tranCategory.getId().intValue())))
.andExpect(jsonPath("$.[*].name").value(hasItem(DEFAULT_NAME.toString())))
.andExpect(jsonPath("$.[*].description").value(hasItem(DEFAULT_DESCRIPTION.toString())));
}
@Test
@Transactional
public void getTranCategory() throws Exception {
// Initialize the database
tranCategoryRepository.saveAndFlush(tranCategory);
// Get the tranCategory
restTranCategoryMockMvc.perform(get("/api/tran-categories/{id}", tranCategory.getId()))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.id").value(tranCategory.getId().intValue()))
.andExpect(jsonPath("$.name").value(DEFAULT_NAME.toString()))
.andExpect(jsonPath("$.description").value(DEFAULT_DESCRIPTION.toString()));
}
@Test
@Transactional
public void getNonExistingTranCategory() throws Exception {
// Get the tranCategory
restTranCategoryMockMvc.perform(get("/api/tran-categories/{id}", Long.MAX_VALUE))
.andExpect(status().isNotFound());
}
@Test
@Transactional
public void updateTranCategory() throws Exception {
// Initialize the database
tranCategoryRepository.saveAndFlush(tranCategory);
int databaseSizeBeforeUpdate = tranCategoryRepository.findAll().size();
// Update the tranCategory
TranCategory updatedTranCategory = tranCategoryRepository.findOne(tranCategory.getId());
updatedTranCategory
.name(UPDATED_NAME)
.description(UPDATED_DESCRIPTION);
restTranCategoryMockMvc.perform(put("/api/tran-categories")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(updatedTranCategory)))
.andExpect(status().isOk());
// Validate the TranCategory in the database
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeUpdate);
TranCategory testTranCategory = tranCategoryList.get(tranCategoryList.size() - 1);
assertThat(testTranCategory.getName()).isEqualTo(UPDATED_NAME);
assertThat(testTranCategory.getDescription()).isEqualTo(UPDATED_DESCRIPTION);
}
@Test
@Transactional
public void updateNonExistingTranCategory() throws Exception {
int databaseSizeBeforeUpdate = tranCategoryRepository.findAll().size();
// Create the TranCategory
// If the entity doesn't have an ID, it will be created instead of just being updated
restTranCategoryMockMvc.perform(put("/api/tran-categories")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(tranCategory)))
.andExpect(status().isCreated());
// Validate the TranCategory in the database
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeUpdate + 1);
}
@Test
@Transactional
public void deleteTranCategory() throws Exception {
// Initialize the database
tranCategoryRepository.saveAndFlush(tranCategory);
int databaseSizeBeforeDelete = tranCategoryRepository.findAll().size();
// Get the tranCategory
restTranCategoryMockMvc.perform(delete("/api/tran-categories/{id}", tranCategory.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().isOk());
// Validate the database is empty
List<TranCategory> tranCategoryList = tranCategoryRepository.findAll();
assertThat(tranCategoryList).hasSize(databaseSizeBeforeDelete - 1);
}
@Test
@Transactional
public void equalsVerifier() throws Exception {
TestUtil.equalsVerifier(TranCategory.class);
TranCategory tranCategory1 = new TranCategory();
tranCategory1.setId(1L);
TranCategory tranCategory2 = new TranCategory();
tranCategory2.setId(tranCategory1.getId());
assertThat(tranCategory1).isEqualTo(tranCategory2);
tranCategory2.setId(2L);
assertThat(tranCategory1).isNotEqualTo(tranCategory2);
tranCategory1.setId(null);
assertThat(tranCategory1).isNotEqualTo(tranCategory2);
}
}
<file_sep>/src/main/webapp/app/shared/charts/sf-charts.module.ts
import {CUSTOM_ELEMENTS_SCHEMA, NgModule} from '@angular/core';
import {LineChartComponent} from './line-chart/line-chart.component';
import {ChartsModule} from 'ng2-charts';
import {SfWebClientSharedModule} from '../shared.module';
import {BarChartComponent} from './bar-chart/bar-chart.component';
@NgModule({
imports: [
ChartsModule, SfWebClientSharedModule
],
declarations: [LineChartComponent, BarChartComponent],
exports: [LineChartComponent, BarChartComponent],
providers: [],
entryComponents: [],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfChartsModule {
}
<file_sep>/src/main/webapp/app/shared/charts/tran-chart-data.ts
import {AccountTransaction, TranType} from '../../sf-entities/account-transaction/account-transaction.model';
export class TranChartData {
constructor(private _transactions: AccountTransaction[]) {
}
set transactions(value: AccountTransaction[]) {
this._transactions = value;
}
getChartData(year: number): Array<any> {
let arr = [];
let expenseDataObj = { data: [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1], label: 'EXPENSES', backgroundColor: 'rgb(255, 99, 132)',
borderColor: 'rgb(255, 99, 132)'};
let incomesDataObj = { data: [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1], label: 'INCOMES'
, backgroundColor: 'rgb(54, 162, 235)',
borderColor: 'rgb(54, 162, 235)'};
for(const tran of this._transactions){
if (tran.postDate.getFullYear() !== year) {
continue;
}
const month = tran.postDate.getMonth();
let currentValExp = expenseDataObj.data[month];
currentValExp = currentValExp === -1 ? 0 : currentValExp;
let currentValInc = incomesDataObj.data[month];
currentValInc = currentValInc === -1 ? 0 : currentValInc;
if (<string><any>tran.tranType === 'EXPENSE') {
currentValExp = tran.amount + currentValExp;
}else {
currentValInc = tran.amount + currentValInc;
}
expenseDataObj.data[month] = currentValExp;
incomesDataObj.data[month] = currentValInc;
/*let label = new DecimalPipe('USD').transform(acc, '2.2-2');*/
}
expenseDataObj.data = expenseDataObj.data.filter((value) => {
return value !== -1;
});
incomesDataObj.data = incomesDataObj.data.filter((value) => {
return value !== -1;
});
arr.push(expenseDataObj);
arr.push(incomesDataObj);
return arr;
}
getChartLabels(year: number) {
const arr = [];
const months = {
'Jan' : false,
'Feb' : false,
'Mar' : false,
'Apr' : false,
'May' : false,
'Jun' : false,
'Jul' : false,
'Aug' : false,
'Sep': false,
'Oct' : false,
'Nov' : false,
'Dec' : false
};
const chartLabels = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'];
for (const tran of this._transactions) {
if (tran.postDate.getFullYear() !== year) {
continue;
}
const month = tran.postDate.getMonth();
months[chartLabels[month]] = true;
}
for (const val in months) {
if (months[val] === true) {
arr.push(val);
}
}
return arr;
}
}
<file_sep>/src/main/java/com/calderon/sf/domain/TranCategory.java
package com.calderon.sf.domain;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
import javax.persistence.*;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import java.io.Serializable;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
/**
* A TranCategory.
*/
@Entity
@Table(name = "tran_category")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class TranCategory extends AbstractAuditingEntity implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@NotNull
@Size(min = 2, max = 64)
@Column(name = "name", length = 64, nullable = false)
private String name;
@Size(max = 256)
@Column(name = "description", length = 256)
private String description;
@OneToMany(mappedBy = "tranCategory")
@JsonIgnore
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
private Set<AccountTransaction> transactions = new HashSet<>();
@ManyToOne
private User user;
@ManyToOne
private TranCategoryRegex tranCategoryRegex;
// jhipster-needle-entity-add-field - Jhipster will add fields here, do not remove
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public TranCategory name(String name) {
this.name = name;
return this;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public TranCategory description(String description) {
this.description = description;
return this;
}
public void setDescription(String description) {
this.description = description;
}
public Set<AccountTransaction> getTransactions() {
return transactions;
}
public TranCategory transactions(Set<AccountTransaction> accountTransactions) {
this.transactions = accountTransactions;
return this;
}
public TranCategory addTransaction(AccountTransaction accountTransaction) {
this.transactions.add(accountTransaction);
accountTransaction.setTranCategory(this);
return this;
}
public TranCategory removeTransaction(AccountTransaction accountTransaction) {
this.transactions.remove(accountTransaction);
accountTransaction.setTranCategory(null);
return this;
}
public void setTransactions(Set<AccountTransaction> accountTransactions) {
this.transactions = accountTransactions;
}
public User getUser() {
return user;
}
public TranCategory user(User user) {
this.user = user;
return this;
}
public void setUser(User user) {
this.user = user;
}
public TranCategoryRegex getTranCategoryRegex() {
return tranCategoryRegex;
}
public TranCategory tranCategoryRegex(TranCategoryRegex tranCategoryRegex) {
this.tranCategoryRegex = tranCategoryRegex;
return this;
}
public void setTranCategoryRegex(TranCategoryRegex tranCategoryRegex) {
this.tranCategoryRegex = tranCategoryRegex;
}
// jhipster-needle-entity-add-getters-setters - Jhipster will add getters and setters here, do not remove
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TranCategory tranCategory = (TranCategory) o;
if (tranCategory.getId() == null || getId() == null) {
return false;
}
return Objects.equals(getId(), tranCategory.getId());
}
@Override
public int hashCode() {
return Objects.hashCode(getId());
}
@Override
public String toString() {
return "TranCategory{" +
"id=" + getId() +
", name='" + getName() + "'" +
", description='" + getDescription() + "'" +
"}";
}
}
<file_sep>/src/main/webapp/app/shared/search/search-options.ts
export interface SearchOptions {
cssClass: string[];
placeHolder: string;
}
<file_sep>/src/main/java/com/calderon/sf/repository/BankRepository.java
package com.calderon.sf.repository;
import com.calderon.sf.domain.Bank;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
/**
* Spring Data JPA repository for the Bank entity.
*/
@SuppressWarnings("unused")
@Repository
public interface BankRepository extends JpaRepository<Bank, Long> {
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category-regex/tran-category-regex-dialog.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Response } from '@angular/http';
import { Observable } from 'rxjs/Rx';
import { NgbActiveModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { JhiEventManager, JhiAlertService } from 'ng-jhipster';
import { TranCategoryRegex } from './tran-category-regex.model';
import { TranCategoryRegexPopupService } from './tran-category-regex-popup.service';
import { TranCategoryRegexService } from './tran-category-regex.service';
import { User, UserService } from '../../shared';
import { ResponseWrapper } from '../../shared';
@Component({
selector: 'jhi-tran-category-regex-dialog',
templateUrl: './tran-category-regex-dialog.component.html'
})
export class TranCategoryRegexDialogComponent implements OnInit {
tranCategoryRegex: TranCategoryRegex;
isSaving: boolean;
users: User[];
constructor(
public activeModal: NgbActiveModal,
private alertService: JhiAlertService,
private tranCategoryRegexService: TranCategoryRegexService,
private userService: UserService,
private eventManager: JhiEventManager
) {
}
ngOnInit() {
this.isSaving = false;
this.userService.query()
.subscribe((res: ResponseWrapper) => { this.users = res.json; }, (res: ResponseWrapper) => this.onError(res.json));
}
clear() {
this.activeModal.dismiss('cancel');
}
save() {
this.isSaving = true;
if (this.tranCategoryRegex.id !== undefined) {
this.subscribeToSaveResponse(
this.tranCategoryRegexService.update(this.tranCategoryRegex));
} else {
this.subscribeToSaveResponse(
this.tranCategoryRegexService.create(this.tranCategoryRegex));
}
}
private subscribeToSaveResponse(result: Observable<TranCategoryRegex>) {
result.subscribe((res: TranCategoryRegex) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private onSaveSuccess(result: TranCategoryRegex) {
this.eventManager.broadcast({ name: 'tranCategoryRegexListModification', content: 'OK'});
this.isSaving = false;
this.activeModal.dismiss(result);
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
trackUserById(index: number, item: User) {
return item.id;
}
}
@Component({
selector: 'jhi-tran-category-regex-popup',
template: ''
})
export class TranCategoryRegexPopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(
private route: ActivatedRoute,
private tranCategoryRegexPopupService: TranCategoryRegexPopupService
) {}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
if ( params['id'] ) {
this.tranCategoryRegexPopupService
.open(TranCategoryRegexDialogComponent as Component, params['id']);
} else {
this.tranCategoryRegexPopupService
.open(TranCategoryRegexDialogComponent as Component);
}
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/tran-category-popup.service.ts
import { Injectable, Component } from '@angular/core';
import { Router } from '@angular/router';
import { NgbModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { TranCategory } from './tran-category.model';
import { TranCategoryService } from './tran-category.service';
import {Principal} from '../../shared/auth/principal.service';
import {DatetimeService} from '../../shared/datetime/datetime.service';
import {LoggerService} from '../../shared/logger/logger.service';
import {User} from '../../shared/user/user.model';
@Injectable()
export class TranCategoryPopupService {
private ngbModalRef: NgbModalRef;
private currentUser: User;
constructor(
private modalService: NgbModal,
private router: Router,
private tranCategoryService: TranCategoryService,
private principal: Principal,
private logger: LoggerService,
private dateTimeService: DatetimeService
) {
this.ngbModalRef = null;
}
newCategory(): TranCategory{
const category: TranCategory = new TranCategory();
this.principal.identity().then((user) => {
this.currentUser = user;
category.user = user;
});
return category;
}
open(component: Component, id?: number | any): Promise<NgbModalRef> {
return new Promise<NgbModalRef>((resolve, reject) => {
const isOpen = this.ngbModalRef !== null;
if (isOpen) {
resolve(this.ngbModalRef);
}
if (id) {
this.tranCategoryService.find(id).subscribe((tranCategory) => {
this.ngbModalRef = this.tranCategoryModalRef(component, tranCategory);
resolve(this.ngbModalRef);
});
} else {
// setTimeout used as a workaround for getting ExpressionChangedAfterItHasBeenCheckedError
setTimeout(() => {
this.ngbModalRef = this.tranCategoryModalRef(component, this.newCategory());
resolve(this.ngbModalRef);
}, 0);
}
});
}
tranCategoryModalRef(component: Component, tranCategory: TranCategory): NgbModalRef {
const modalRef = this.modalService.open(component, { size: 'lg', backdrop: 'static'});
modalRef.componentInstance.tranCategory = tranCategory;
modalRef.result.then((result) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
}, (reason) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
});
return modalRef;
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category-regex/tran-category-regex-detail.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Subscription } from 'rxjs/Rx';
import { JhiEventManager } from 'ng-jhipster';
import { TranCategoryRegex } from './tran-category-regex.model';
import { TranCategoryRegexService } from './tran-category-regex.service';
@Component({
selector: 'jhi-tran-category-regex-detail',
templateUrl: './tran-category-regex-detail.component.html'
})
export class TranCategoryRegexDetailComponent implements OnInit, OnDestroy {
tranCategoryRegex: TranCategoryRegex;
private subscription: Subscription;
private eventSubscriber: Subscription;
constructor(
private eventManager: JhiEventManager,
private tranCategoryRegexService: TranCategoryRegexService,
private route: ActivatedRoute
) {
}
ngOnInit() {
this.subscription = this.route.params.subscribe((params) => {
this.load(params['id']);
});
this.registerChangeInTranCategoryRegexes();
}
load(id) {
this.tranCategoryRegexService.find(id).subscribe((tranCategoryRegex) => {
this.tranCategoryRegex = tranCategoryRegex;
});
}
previousState() {
window.history.back();
}
ngOnDestroy() {
this.subscription.unsubscribe();
this.eventManager.destroy(this.eventSubscriber);
}
registerChangeInTranCategoryRegexes() {
this.eventSubscriber = this.eventManager.subscribe(
'tranCategoryRegexListModification',
(response) => this.load(this.tranCategoryRegex.id)
);
}
}
<file_sep>/src/main/webapp/app/shared/datetime/datetime.service.ts
import {Injectable} from '@angular/core';
@Injectable()
export class DatetimeService {
constructor() {
}
now(): string {
const date: Date = new Date();
return date.toString();
}
nowLocalDate(): any {
const date: Date = new Date();
return {
year: date.getFullYear()
, month: date.getMonth()+1
, day: date.getDate()
};
}
convertToObjDate(date: any): any {
return {
year: date.getFullYear()
, month: date.getMonth()+1
, day: date.getDate()
};
}
}
<file_sep>/src/main/webapp/app/shared/animation/enter-leave-animation.ts
import { trigger, state, style, animate, transition, stagger, query} from '@angular/animations';
export const ENTER_LEAVE_ANIMATION = [
trigger('in-out', [
state('in', style({
transform: 'translateX(0)',
opacity: 1
})),
transition('void => *', [
style({
transform: 'translateX(-100%)',
opacity: 1
}),
animate('0.4s ease-in')
]),
transition('* => void', [
style({
transform: 'translateX(100%)',
opacity: 0
}),
animate('0.2s 0.5s ease-out')
])
])
];
<file_sep>/src/test/java/com/calderon/sf/web/rest/BankResourceIntTest.java
package com.calderon.sf.web.rest;
import com.calderon.sf.SfWebClientApp;
import com.calderon.sf.domain.Bank;
import com.calderon.sf.repository.BankRepository;
import com.calderon.sf.web.rest.errors.ExceptionTranslator;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.MockitoAnnotations;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.web.PageableHandlerMethodArgumentResolver;
import org.springframework.http.MediaType;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.test.context.junit4.SpringRunner;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.setup.MockMvcBuilders;
import org.springframework.transaction.annotation.Transactional;
import javax.persistence.EntityManager;
import java.util.List;
import static org.assertj.core.api.Assertions.assertThat;
import static org.hamcrest.Matchers.hasItem;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
/**
* Test class for the BankResource REST controller.
*
* @see BankResource
*/
@RunWith(SpringRunner.class)
@SpringBootTest(classes = SfWebClientApp.class)
public class BankResourceIntTest {
private static final String DEFAULT_NAME = "AAAAAAAAAA";
private static final String UPDATED_NAME = "BBBBBBBBBB";
private static final String DEFAULT_DESCRIPTION = "AAAAAAAAAA";
private static final String UPDATED_DESCRIPTION = "BBBBBBBBBB";
@Autowired
private BankRepository bankRepository;
@Autowired
private MappingJackson2HttpMessageConverter jacksonMessageConverter;
@Autowired
private PageableHandlerMethodArgumentResolver pageableArgumentResolver;
@Autowired
private ExceptionTranslator exceptionTranslator;
@Autowired
private EntityManager em;
private MockMvc restBankMockMvc;
private Bank bank;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
final BankResource bankResource = new BankResource(bankRepository);
this.restBankMockMvc = MockMvcBuilders.standaloneSetup(bankResource)
.setCustomArgumentResolvers(pageableArgumentResolver)
.setControllerAdvice(exceptionTranslator)
.setMessageConverters(jacksonMessageConverter).build();
}
/**
* Create an entity for this test.
*
* This is a static method, as tests for other entities might also need it,
* if they test an entity which requires the current entity.
*/
public static Bank createEntity(EntityManager em) {
Bank bank = new Bank()
.name(DEFAULT_NAME)
.description(DEFAULT_DESCRIPTION);
return bank;
}
@Before
public void initTest() {
bank = createEntity(em);
}
@Test
@Transactional
public void createBank() throws Exception {
int databaseSizeBeforeCreate = bankRepository.findAll().size();
// Create the Bank
restBankMockMvc.perform(post("/api/banks")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(bank)))
.andExpect(status().isCreated());
// Validate the Bank in the database
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeCreate + 1);
Bank testBank = bankList.get(bankList.size() - 1);
assertThat(testBank.getName()).isEqualTo(DEFAULT_NAME);
assertThat(testBank.getDescription()).isEqualTo(DEFAULT_DESCRIPTION);
}
@Test
@Transactional
public void createBankWithExistingId() throws Exception {
int databaseSizeBeforeCreate = bankRepository.findAll().size();
// Create the Bank with an existing ID
bank.setId(1L);
// An entity with an existing ID cannot be created, so this API call must fail
restBankMockMvc.perform(post("/api/banks")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(bank)))
.andExpect(status().isBadRequest());
// Validate the Bank in the database
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeCreate);
}
@Test
@Transactional
public void checkNameIsRequired() throws Exception {
int databaseSizeBeforeTest = bankRepository.findAll().size();
// set the field null
bank.setName(null);
// Create the Bank, which fails.
restBankMockMvc.perform(post("/api/banks")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(bank)))
.andExpect(status().isBadRequest());
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeTest);
}
@Test
@Transactional
public void getAllBanks() throws Exception {
// Initialize the database
bankRepository.saveAndFlush(bank);
// Get all the bankList
restBankMockMvc.perform(get("/api/banks?sort=id,desc"))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.[*].id").value(hasItem(bank.getId().intValue())))
.andExpect(jsonPath("$.[*].name").value(hasItem(DEFAULT_NAME.toString())))
.andExpect(jsonPath("$.[*].description").value(hasItem(DEFAULT_DESCRIPTION.toString())));
}
@Test
@Transactional
public void getBank() throws Exception {
// Initialize the database
bankRepository.saveAndFlush(bank);
// Get the bank
restBankMockMvc.perform(get("/api/banks/{id}", bank.getId()))
.andExpect(status().isOk())
.andExpect(content().contentType(MediaType.APPLICATION_JSON_UTF8_VALUE))
.andExpect(jsonPath("$.id").value(bank.getId().intValue()))
.andExpect(jsonPath("$.name").value(DEFAULT_NAME.toString()))
.andExpect(jsonPath("$.description").value(DEFAULT_DESCRIPTION.toString()));
}
@Test
@Transactional
public void getNonExistingBank() throws Exception {
// Get the bank
restBankMockMvc.perform(get("/api/banks/{id}", Long.MAX_VALUE))
.andExpect(status().isNotFound());
}
@Test
@Transactional
public void updateBank() throws Exception {
// Initialize the database
bankRepository.saveAndFlush(bank);
int databaseSizeBeforeUpdate = bankRepository.findAll().size();
// Update the bank
Bank updatedBank = bankRepository.findOne(bank.getId());
updatedBank
.name(UPDATED_NAME)
.description(UPDATED_DESCRIPTION);
restBankMockMvc.perform(put("/api/banks")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(updatedBank)))
.andExpect(status().isOk());
// Validate the Bank in the database
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeUpdate);
Bank testBank = bankList.get(bankList.size() - 1);
assertThat(testBank.getName()).isEqualTo(UPDATED_NAME);
assertThat(testBank.getDescription()).isEqualTo(UPDATED_DESCRIPTION);
}
@Test
@Transactional
public void updateNonExistingBank() throws Exception {
int databaseSizeBeforeUpdate = bankRepository.findAll().size();
// Create the Bank
// If the entity doesn't have an ID, it will be created instead of just being updated
restBankMockMvc.perform(put("/api/banks")
.contentType(TestUtil.APPLICATION_JSON_UTF8)
.content(TestUtil.convertObjectToJsonBytes(bank)))
.andExpect(status().isCreated());
// Validate the Bank in the database
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeUpdate + 1);
}
@Test
@Transactional
public void deleteBank() throws Exception {
// Initialize the database
bankRepository.saveAndFlush(bank);
int databaseSizeBeforeDelete = bankRepository.findAll().size();
// Get the bank
restBankMockMvc.perform(delete("/api/banks/{id}", bank.getId())
.accept(TestUtil.APPLICATION_JSON_UTF8))
.andExpect(status().isOk());
// Validate the database is empty
List<Bank> bankList = bankRepository.findAll();
assertThat(bankList).hasSize(databaseSizeBeforeDelete - 1);
}
@Test
@Transactional
public void equalsVerifier() throws Exception {
TestUtil.equalsVerifier(Bank.class);
Bank bank1 = new Bank();
bank1.setId(1L);
Bank bank2 = new Bank();
bank2.setId(bank1.getId());
assertThat(bank1).isEqualTo(bank2);
bank2.setId(2L);
assertThat(bank1).isNotEqualTo(bank2);
bank1.setId(null);
assertThat(bank1).isNotEqualTo(bank2);
}
}
<file_sep>/src/main/java/com/calderon/sf/domain/predicates/TransactionCriteria.java
package com.calderon.sf.domain.predicates;
import com.fasterxml.jackson.annotation.JsonInclude;
import org.json.JSONObject;
import java.math.BigDecimal;
import java.time.LocalDate;
@JsonInclude(JsonInclude.Include.NON_NULL)
public class TransactionCriteria {
private boolean active;
private LocalDate startDate;
private LocalDate endDate;
private BigDecimal startAmount;
private BigDecimal endAmount;
private String desc;
public TransactionCriteria(JSONObject json) {
this.active = json.optBoolean("active", false);
this.desc = json.optString("desc", "");
String startDate = json.optString("startDate", "");
if (!startDate.isEmpty()){
this.startDate = LocalDate.parse(startDate);
}
String endDate = json.optString("endDate", "");
if (!endDate.isEmpty()){
this.endDate = LocalDate.parse(endDate);
}
String startAmount = json.optString("startAmount", "");
if (!startAmount.isEmpty()){
this.startAmount = new BigDecimal(startAmount);
}
String endAmount = json.optString("endAmount", "");
if (!endAmount.isEmpty()){
this.endAmount = new BigDecimal(endAmount);
}
}
public boolean isActive() {
return active;
}
public void setActive(boolean active) {
this.active = active;
}
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public BigDecimal getStartAmount() {
return startAmount;
}
public void setStartAmount(BigDecimal startAmount) {
this.startAmount = startAmount;
}
public BigDecimal getEndAmount() {
return endAmount;
}
public void setEndAmount(BigDecimal endAmount) {
this.endAmount = endAmount;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
/*private String desc;
public boolean isActive() {
return active;
}
public void setActive(boolean active) {
this.active = active;
}
public LocalDate getStartDate() {
return startDate;
}
public void setStartDate(LocalDate startDate) {
this.startDate = startDate;
}
public LocalDate getEndDate() {
return endDate;
}
public void setEndDate(LocalDate endDate) {
this.endDate = endDate;
}
public BigDecimal getStartAmount() {
return startAmount;
}
public void setStartAmount(BigDecimal startAmount) {
this.startAmount = startAmount;
}
public BigDecimal getEndAmount() {
return endAmount;
}
public void setEndAmount(BigDecimal endAmount) {
this.endAmount = endAmount;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public TransactionCriteria active(boolean active) {
this.active = active;
return this;
}
public TransactionCriteria startDate(LocalDate startDate) {
this.startDate = startDate;
return this;
}
public TransactionCriteria endDate(LocalDate endDate) {
this.endDate = endDate;
return this;
}
public TransactionCriteria startAmount(BigDecimal startAmount) {
this.startAmount = startAmount;
return this;
}
public TransactionCriteria endAmount(BigDecimal endAmount) {
this.endAmount = endAmount;
return this;
}
public TransactionCriteria desc(String desc) {
this.desc = desc;
return this;
}*/
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category-regex/tran-category-regex.module.ts
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { RouterModule } from '@angular/router';
import { SfWebClientSharedModule } from '../../shared';
import { SfWebClientAdminModule } from '../../admin/admin.module';
import {
TranCategoryRegexService,
TranCategoryRegexPopupService,
TranCategoryRegexComponent,
TranCategoryRegexDetailComponent,
TranCategoryRegexDialogComponent,
TranCategoryRegexPopupComponent,
TranCategoryRegexDeletePopupComponent,
TranCategoryRegexDeleteDialogComponent,
tranCategoryRegexRoute,
tranCategoryRegexPopupRoute,
TranCategoryRegexResolvePagingParams,
} from './';
const ENTITY_STATES = [
...tranCategoryRegexRoute,
...tranCategoryRegexPopupRoute,
];
@NgModule({
imports: [
SfWebClientSharedModule,
SfWebClientAdminModule,
RouterModule.forRoot(ENTITY_STATES, { useHash: true })
],
declarations: [
TranCategoryRegexComponent,
TranCategoryRegexDetailComponent,
TranCategoryRegexDialogComponent,
TranCategoryRegexDeleteDialogComponent,
TranCategoryRegexPopupComponent,
TranCategoryRegexDeletePopupComponent,
],
entryComponents: [
TranCategoryRegexComponent,
TranCategoryRegexDialogComponent,
TranCategoryRegexPopupComponent,
TranCategoryRegexDeleteDialogComponent,
TranCategoryRegexDeletePopupComponent,
],
providers: [
TranCategoryRegexService,
TranCategoryRegexPopupService,
TranCategoryRegexResolvePagingParams,
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientTranCategoryRegexModule {}
<file_sep>/src/main/webapp/app/entities/tran-category/tran-category.route.ts
import { Injectable } from '@angular/core';
import { Resolve, ActivatedRouteSnapshot, RouterStateSnapshot, Routes, CanActivate } from '@angular/router';
import { UserRouteAccessService } from '../../shared';
import { JhiPaginationUtil } from 'ng-jhipster';
import { TranCategoryComponent } from './tran-category.component';
import { TranCategoryDetailComponent } from './tran-category-detail.component';
import { TranCategoryPopupComponent } from './tran-category-dialog.component';
import { TranCategoryDeletePopupComponent } from './tran-category-delete-dialog.component';
@Injectable()
export class TranCategoryResolvePagingParams implements Resolve<any> {
constructor(private paginationUtil: JhiPaginationUtil) {}
resolve(route: ActivatedRouteSnapshot, state: RouterStateSnapshot) {
const page = route.queryParams['page'] ? route.queryParams['page'] : '1';
const sort = route.queryParams['sort'] ? route.queryParams['sort'] : 'id,asc';
return {
page: this.paginationUtil.parsePage(page),
predicate: this.paginationUtil.parsePredicate(sort),
ascending: this.paginationUtil.parseAscending(sort)
};
}
}
export const tranCategoryRoute: Routes = [
{
path: 'tran-category',
component: TranCategoryComponent,
resolve: {
'pagingParams': TranCategoryResolvePagingParams
},
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategory.home.title'
},
canActivate: [UserRouteAccessService]
}, {
path: 'tran-category/:id',
component: TranCategoryDetailComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategory.home.title'
},
canActivate: [UserRouteAccessService]
}
];
export const tranCategoryPopupRoute: Routes = [
{
path: 'tran-category-new',
component: TranCategoryPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategory.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'tran-category/:id/edit',
component: TranCategoryPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategory.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'tran-category/:id/delete',
component: TranCategoryDeletePopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategory.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
}
];
<file_sep>/src/main/webapp/app/shared/search/searcher.ts
import {Observable} from "rxjs/Observable";
export class Searcher {
onSearch(term: string): Observable<any[]>{
return Observable.of<any[]>([]);
}
}
<file_sep>/src/main/java/com/calderon/sf/web/rest/TranCategoryResource.java
package com.calderon.sf.web.rest;
import com.calderon.sf.domain.TranCategory;
import com.calderon.sf.repository.TranCategoryRepository;
import com.calderon.sf.web.rest.util.HeaderUtil;
import com.calderon.sf.web.rest.util.PaginationUtil;
import com.codahale.metrics.annotation.Timed;
import io.github.jhipster.web.util.ResponseUtil;
import io.swagger.annotations.ApiParam;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.List;
import java.util.Optional;
/**
* REST controller for managing TranCategory.
*/
@RestController
@RequestMapping("/api")
public class TranCategoryResource {
private final Logger log = LoggerFactory.getLogger(TranCategoryResource.class);
private static final String ENTITY_NAME = "tranCategory";
private final TranCategoryRepository tranCategoryRepository;
public TranCategoryResource(TranCategoryRepository tranCategoryRepository) {
this.tranCategoryRepository = tranCategoryRepository;
}
/**
* POST /tran-categories : Create a new tranCategory.
*
* @param tranCategory the tranCategory to create
* @return the ResponseEntity with status 201 (Created) and with body the new tranCategory, or with status 400 (Bad Request) if the tranCategory has already an ID
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PostMapping("/tran-categories")
@Timed
public ResponseEntity<TranCategory> createTranCategory(@Valid @RequestBody TranCategory tranCategory) throws URISyntaxException {
log.debug("REST request to save TranCategory : {}", tranCategory);
if (tranCategory.getId() != null) {
return ResponseEntity.badRequest().headers(HeaderUtil.createFailureAlert(ENTITY_NAME, "idexists", "A new tranCategory cannot already have an ID")).body(null);
}
TranCategory result = tranCategoryRepository.save(tranCategory);
return ResponseEntity.created(new URI("/api/tran-categories/" + result.getId()))
.headers(HeaderUtil.createEntityCreationAlert(ENTITY_NAME, result.getId().toString()))
.body(result);
}
/**
* PUT /tran-categories : Updates an existing tranCategory.
*
* @param tranCategory the tranCategory to update
* @return the ResponseEntity with status 200 (OK) and with body the updated tranCategory,
* or with status 400 (Bad Request) if the tranCategory is not valid,
* or with status 500 (Internal Server Error) if the tranCategory couldn't be updated
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PutMapping("/tran-categories")
@Timed
public ResponseEntity<TranCategory> updateTranCategory(@Valid @RequestBody TranCategory tranCategory) throws URISyntaxException {
log.debug("REST request to update TranCategory : {}", tranCategory);
if (tranCategory.getId() == null) {
return createTranCategory(tranCategory);
}
TranCategory result = tranCategoryRepository.save(tranCategory);
return ResponseEntity.ok()
.headers(HeaderUtil.createEntityUpdateAlert(ENTITY_NAME, tranCategory.getId().toString()))
.body(result);
}
/**
* GET /tran-categories : get all the tranCategories.
*
* @param pageable the pagination information
* @return the ResponseEntity with status 200 (OK) and the list of tranCategories in body
*/
@GetMapping("/tran-categories")
@Timed
public ResponseEntity<List<TranCategory>> getAllTranCategories(@ApiParam Pageable pageable) {
log.debug("REST request to get a page of TranCategories");
/*Page<TranCategory> page = tranCategoryRepository.findAll(pageable);*/
Page<TranCategory> page = tranCategoryRepository.findByUserIsCurrentUser(pageable);
HttpHeaders headers = PaginationUtil.generatePaginationHttpHeaders(page, "/api/tran-categories");
return new ResponseEntity<>(page.getContent(), headers, HttpStatus.OK);
}
/**
* GET /tran-categories/:id : get the "id" tranCategory.
*
* @param id the id of the tranCategory to retrieve
* @return the ResponseEntity with status 200 (OK) and with body the tranCategory, or with status 404 (Not Found)
*/
@GetMapping("/tran-categories/{id}")
@Timed
public ResponseEntity<TranCategory> getTranCategory(@PathVariable Long id) {
log.debug("REST request to get TranCategory : {}", id);
TranCategory tranCategory = tranCategoryRepository.findOne(id);
return ResponseUtil.wrapOrNotFound(Optional.ofNullable(tranCategory));
}
/**
* DELETE /tran-categories/:id : delete the "id" tranCategory.
*
* @param id the id of the tranCategory to delete
* @return the ResponseEntity with status 200 (OK)
*/
@DeleteMapping("/tran-categories/{id}")
@Timed
public ResponseEntity<Void> deleteTranCategory(@PathVariable Long id) {
log.debug("REST request to delete TranCategory : {}", id);
tranCategoryRepository.delete(id);
return ResponseEntity.ok().headers(HeaderUtil.createEntityDeletionAlert(ENTITY_NAME, id.toString())).build();
}
}
<file_sep>/src/main/java/com/calderon/sf/service/TranFileReaderService.java
package com.calderon.sf.service;
import com.calderon.sf.domain.AccountTransaction;
import com.calderon.sf.domain.Bank;
import com.calderon.sf.reader.CsvReader;
import com.calderon.sf.reader.Reader;
import com.calderon.sf.service.mapper.TransactionMapper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.nio.file.Path;
import java.util.List;
@Service
public class TranFileReaderService {
private final Logger log = LoggerFactory.getLogger(TranFileReaderService.class);
private TransactionMapper tranMapper;
@Autowired
public void setTranMapper(TransactionMapper tranMapper) {
this.tranMapper = tranMapper;
}
public List<AccountTransaction> read(Bank bank, Path from){
return tranMapper.TransactionToAccountTransaction(Readers.of(Integer.parseInt(bank.getId().toString()), from).getTransactions());
}
private static class Readers {
public static Reader of(int bankId, Path from){
switch (bankId){
case 1:
return new CsvReader(from);
default:
return new CsvReader(from);
}
}
}
}
<file_sep>/src/main/webapp/app/dashboard/dashboard.component.ts
import {Component, OnInit} from '@angular/core';
import {FinanceAccount} from '../sf-entities/finance-account/finance-account.model';
import {TranChartData} from '../shared/charts/tran-chart-data';
import {AccountTransactionService} from '../sf-entities/account-transaction/account-transaction.service';
import {FinanceAccountService} from '../sf-entities/finance-account/finance-account.service';
import {LoggerService} from '../shared/logger/logger.service';
import {Principal} from '../shared/auth/principal.service';
import {JhiAlertService} from 'ng-jhipster';
import {AccountTransaction} from '../sf-entities/account-transaction/account-transaction.model';
import {ResponseWrapper} from '../shared/model/response-wrapper.model';
import {ENTER_LEAVE_ANIMATION} from '../shared/animation/enter-leave-animation';
@Component({
selector: 'jhi-dashboard',
templateUrl: './dashboard.component.html',
styles: [],
animations: ENTER_LEAVE_ANIMATION
})
export class DashboardComponent implements OnInit {
accChecked: number[] = [];
currentUser: any;
accounts: FinanceAccount[];
year: number = new Date().getFullYear();
tranYears: number[] = [];
tranChartData: TranChartData = new TranChartData([]);
transactions: AccountTransaction[];
// chart
chartData: Array<any> = [
{data: [], label: 'EXPENSES'},
{data: [], label: 'INCOMES'}
];
chartLabels: Array<any> = [];
constructor(private tranService: AccountTransactionService,
private accountService: FinanceAccountService,
private logger: LoggerService,
private principal: Principal,
private alertService: JhiAlertService
) {
}
ngOnInit() {
this.principal.identity().then((user) => {
this.currentUser = user;
});
this.load();
}
isAuthenticated() {
return this.principal.isAuthenticated();
}
private load() {
this.tranYears.push(this.year);
this.accountService.query()
.subscribe((res: ResponseWrapper) => {
this.accounts = res.json;
const allAcc = new FinanceAccount();
allAcc.id = -1;
allAcc.name = 'ALL';
this.accounts.unshift(allAcc);
for (let account of this.accounts) {
this.accChecked.push(account.id);
}
this.loadTransactions()
}, (res: ResponseWrapper) => this.onError(res.json));
}
onRefresh() {
this.loadTransactions();
}
private loadTransactions() {
const accoundsId = this.accChecked.filter((value) => {
return value !== -1;
});
this.tranService.findTransactionsByAccountsAndYear(accoundsId, this.year)
.subscribe((transactions: AccountTransaction[]) => {
this.transactions = transactions;
this.refreshChart();
});
}
refreshChart() {
this.tranChartData.transactions = this.transactions;
this.chartLabels = this.tranChartData.getChartLabels(this.year);
this.chartData = this.tranChartData.getChartData(this.year);
}
// events
public chartClicked(e: any): void {
//console.log(e);
}
public chartHovered(e: any): void {
//console.log(e);
}
private onError(error) {
this.alertService.error(error.message, null, null);
}
isChecked(id: number): boolean {
return -1 !== this.accChecked.indexOf(id)
}
onChangeAcc(checked: boolean, acc: FinanceAccount) {
if (checked) {
if (acc.id === -1) {
for (let account of this.accounts) {
this.accChecked.push(account.id);
}
}else {
this.accChecked.push(acc.id);
}
} else {
if (acc.id === -1) {
this.accChecked = [];
} else {
this.accChecked = this.accChecked.filter((value, index, array) => {
return value !== acc.id;
});
}
}
}
}
<file_sep>/src/main/webapp/app/entities/entity.module.ts
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { SfWebClientBankModule } from './bank/bank.module';
import { SfWebClientTranCategoryModule } from './tran-category/tran-category.module';
import { SfWebClientTranCategoryRegexModule } from './tran-category-regex/tran-category-regex.module';
import { SfWebClientAccountTransactionModule } from './account-transaction/account-transaction.module';
import { SfWebClientFinanceAccountModule } from './finance-account/finance-account.module';
/* jhipster-needle-add-entity-module-import - JHipster will add entity modules imports here */
@NgModule({
imports: [
SfWebClientBankModule,
SfWebClientTranCategoryModule,
SfWebClientTranCategoryRegexModule,
SfWebClientAccountTransactionModule,
SfWebClientFinanceAccountModule,
/* jhipster-needle-add-entity-module - JHipster will add entity modules here */
],
declarations: [],
entryComponents: [],
providers: [],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientEntityModule {}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/transaction.component.ts
import {Component, Input, OnDestroy, OnInit} from '@angular/core';
import {AccountTransaction} from '../../account-transaction/account-transaction.model';
import {Subscription} from 'rxjs/Subscription';
import {JhiAlertService, JhiEventManager, JhiPaginationUtil, JhiParseLinks} from 'ng-jhipster';
import {ActivatedRoute, Router} from '@angular/router';
import {PaginationConfig} from '../../../blocks/config/uib-pagination.config';
import {ITEMS_PER_PAGE} from '../../../shared/constants/pagination.constants';
import {ResponseWrapper} from '../../../shared/model/response-wrapper.model';
import {FinanceAccount} from '../finance-account.model';
import {FinanceAccountService} from '../finance-account.service';
import {LoggerService} from '../../../shared/logger/logger.service';
import {ENTER_LEAVE_ANIMATION} from '../../../shared/animation/enter-leave-animation';
@Component({
selector: 'jhi-transaction',
templateUrl: './transaction.component.html',
animations: ENTER_LEAVE_ANIMATION
})
export class TransactionComponent implements OnInit, OnDestroy {
accountId: number;
@Input()
currentAccount: FinanceAccount;
transactions: AccountTransaction[];
error: any;
success: any;
eventSubscriber: Subscription;
routeData: any;
links: any;
totalItems: any;
queryCount: any;
itemsPerPage: any;
page: any;
predicate: any;
previousPage: any;
reverse: any;
private subscription: Subscription;
hidePagingDiv = false;
/****** filter ******/
dateRange: Date[];
filterObj = {
description: '',
amount: 0,
operator: 1
};
constructor(private accountService: FinanceAccountService,
private parseLinks: JhiParseLinks,
private alertService: JhiAlertService,
private activatedRoute: ActivatedRoute,
private router: Router,
private eventManager: JhiEventManager,
private paginationUtil: JhiPaginationUtil,
private paginationConfig: PaginationConfig,
private logger: LoggerService) {
this.itemsPerPage = ITEMS_PER_PAGE;
this.routeData = this.activatedRoute.data.subscribe((data) => {
this.page = data['pagingParams'].page;
this.previousPage = data['pagingParams'].page;
this.reverse = data['pagingParams'].ascending;
this.predicate = data['pagingParams'].predicate;
});
}
loadAll() {
if (!this.currentAccount) {
this.accountService.find(this.accountId)
.subscribe((account) => {
this.currentAccount = account;
}, (res: ResponseWrapper) => this.onError(res.json));
}
this.accountService.queryTransactions(this.accountId, {
page: this.page - 1,
size: this.itemsPerPage,
sort: this.sort()
}).subscribe(
(res: ResponseWrapper) => this.onSuccess(res.json, res.headers),
(res: ResponseWrapper) => this.onError(res.json)
);
}
loadPage(page: number) {
if (page !== this.previousPage) {
this.previousPage = page;
this.transition();
}
}
transition() {
this.router.navigate(['./finance-account', this.accountId], {
queryParams:
{
page: this.page,
size: this.itemsPerPage,
sort: this.predicate + ',' + (this.reverse ? 'asc' : 'desc')
}
});
this.loadAll();
}
clear() {
this.page = 0;
this.router.navigate(['./finance-account/' + this.accountId, {
page: this.page,
sort: this.predicate + ',' + (this.reverse ? 'asc' : 'desc')
}]);
this.loadAll();
}
ngOnInit() {
this.subscription = this.activatedRoute.params.subscribe((params) => {
this.accountId = params['id'];
});
this.predicate = 'createdDate';
this.reverse = false;
this.loadAll();
this.registerChangeInAccountTransactions();
}
ngOnDestroy() {
this.eventManager.destroy(this.eventSubscriber);
}
trackId(index: number, item: AccountTransaction) {
return item.id;
}
registerChangeInAccountTransactions() {
this.eventSubscriber = this.eventManager.subscribe('transactionListModification', (response) => {
if(!response.data)
this.loadAll();
if(response.data.action === "transactionDeleted"){
this.transactions = this.transactions.filter(value => {
return value.id != response.data.item.id;
});
}
});
}
sort() {
const result = [this.predicate + ',' + (this.reverse ? 'asc' : 'desc')];
if (this.predicate !== 'id') {
result.push('id');
}
return result;
}
private onSuccess(data, headers) {
this.links = this.parseLinks.parse(headers.get('link'));
this.totalItems = headers.get('X-Total-Count');
this.queryCount = this.totalItems;
// this.page = pagingParams.page;
this.transactions = data;
}
private onError(error) {
this.alertService.error(error.message, null, null);
}
onFilterClick() {
this.logger.info("****** On Filter click *****");
this.accountService.queryTransactionsBy(this.accountId, {
page: this.page - 1,
size: this.itemsPerPage,
sort: this.sort()
}, this.mapToTranCriteria(this.filterObj)).subscribe(
(res: ResponseWrapper) => this.onSuccess(res.json, res.headers),
(res: ResponseWrapper) => this.onError(res.json)
);
}
mapToTranCriteria(filterObj: any): any{
let tranCriteria = {};
this.filterObj["dateRange"] = this.dateRange;
if (filterObj.dateRange && filterObj.dateRange.length>0){
tranCriteria["startDate"]=filterObj.dateRange[0];
tranCriteria["endDate"]=filterObj.dateRange[1];
}
if (filterObj.description.length>0){
tranCriteria["desc"]=filterObj.description;
}
if (filterObj.amount != null){
if(filterObj.operator>0){
tranCriteria["startAmount"]=filterObj.amount;
}
else {
tranCriteria["endAmount"]=filterObj.amount;
}
}
return tranCriteria;
}
onDateRangeShown(): any{
this.hidePagingDiv = true;
}
onDateRangeHidden(): any{
this.hidePagingDiv = false;
}
}
<file_sep>/src/main/webapp/app/entities/finance-account/finance-account.route.ts
import { Injectable } from '@angular/core';
import { Resolve, ActivatedRouteSnapshot, RouterStateSnapshot, Routes, CanActivate } from '@angular/router';
import { UserRouteAccessService } from '../../shared';
import { JhiPaginationUtil } from 'ng-jhipster';
import { FinanceAccountComponent } from './finance-account.component';
import { FinanceAccountDetailComponent } from './finance-account-detail.component';
import { FinanceAccountPopupComponent } from './finance-account-dialog.component';
import { FinanceAccountDeletePopupComponent } from './finance-account-delete-dialog.component';
@Injectable()
export class FinanceAccountResolvePagingParams implements Resolve<any> {
constructor(private paginationUtil: JhiPaginationUtil) {}
resolve(route: ActivatedRouteSnapshot, state: RouterStateSnapshot) {
const page = route.queryParams['page'] ? route.queryParams['page'] : '1';
const sort = route.queryParams['sort'] ? route.queryParams['sort'] : 'id,asc';
return {
page: this.paginationUtil.parsePage(page),
predicate: this.paginationUtil.parsePredicate(sort),
ascending: this.paginationUtil.parseAscending(sort)
};
}
}
export const financeAccountRoute: Routes = [
{
path: 'finance-account',
component: FinanceAccountComponent,
resolve: {
'pagingParams': FinanceAccountResolvePagingParams
},
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.financeAccount.home.title'
},
canActivate: [UserRouteAccessService]
}, {
path: 'finance-account/:id',
component: FinanceAccountDetailComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.financeAccount.home.title'
},
canActivate: [UserRouteAccessService]
}
];
export const financeAccountPopupRoute: Routes = [
{
path: 'finance-account-new',
component: FinanceAccountPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.financeAccount.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'finance-account/:id/edit',
component: FinanceAccountPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.financeAccount.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'finance-account/:id/delete',
component: FinanceAccountDeletePopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.financeAccount.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
}
];
<file_sep>/src/main/webapp/app/sf-entities/account-transaction/account-transaction.model.ts
import { BaseEntity, User } from './../../shared';
export const enum TranType {
'EXPENSE',
'INCOME'
}
export const enum PaymentMethod {
'UNSPECIFIED',
'CASH',
'CHECK',
'CREDIT_CARD',
'DEBIT',
'ELECTRONIC_TRANSFER',
'OTHER'
}
export class AccountTransaction implements BaseEntity {
constructor(
public id?: number,
public tranType?: TranType,
public tranNumber?: string,
public referenceNumber?: string,
public postDate?: any,
public createdDate?: any,
public description?: string,
public amount?: number,
public paymentMethod?: PaymentMethod,
public user?: User,
public financeAccount?: BaseEntity,
public tranCategory?: BaseEntity,
) {
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category-regex/index.ts
export * from './tran-category-regex.model';
export * from './tran-category-regex-popup.service';
export * from './tran-category-regex.service';
export * from './tran-category-regex-dialog.component';
export * from './tran-category-regex-delete-dialog.component';
export * from './tran-category-regex-detail.component';
export * from './tran-category-regex.component';
export * from './tran-category-regex.route';
<file_sep>/src/main/webapp/app/shared/search/search.directive.ts
import {Directive, ElementRef, HostListener, Input, Output} from '@angular/core';
import {Searcher} from "./searcher";
import {Subject} from "rxjs/Subject";
import {Observable} from "rxjs/Observable";
@Directive({
selector: '[jhiSearch]'
})
export class SearchDirective {
@Input() searcher: Searcher;
@Input() items: Observable<any[]>;
private searchTerms = new Subject<string>();
constructor(private el: ElementRef) {
this.searchTerms
.debounceTime(300) // wait 300ms after each keystroke before considering the term
.distinctUntilChanged()
.subscribe((term: string) => this.searcher.onSearch(term), (res: Response) => Observable.of<any[]>([]));
}
@HostListener("keyup") onKeyUp(){
this.searchTerms.next(this.el.nativeElement.value);
//this.searcher.onSearch(this.el.nativeElement.value);
}
}
<file_sep>/src/main/java/com/calderon/sf/config/audit/package-info.java
/**
* Audit specific code.
*/
package com.calderon.sf.config.audit;
<file_sep>/src/main/webapp/app/entities/tran-category-regex/tran-category-regex.route.ts
import { Injectable } from '@angular/core';
import { Resolve, ActivatedRouteSnapshot, RouterStateSnapshot, Routes, CanActivate } from '@angular/router';
import { UserRouteAccessService } from '../../shared';
import { JhiPaginationUtil } from 'ng-jhipster';
import { TranCategoryRegexComponent } from './tran-category-regex.component';
import { TranCategoryRegexDetailComponent } from './tran-category-regex-detail.component';
import { TranCategoryRegexPopupComponent } from './tran-category-regex-dialog.component';
import { TranCategoryRegexDeletePopupComponent } from './tran-category-regex-delete-dialog.component';
@Injectable()
export class TranCategoryRegexResolvePagingParams implements Resolve<any> {
constructor(private paginationUtil: JhiPaginationUtil) {}
resolve(route: ActivatedRouteSnapshot, state: RouterStateSnapshot) {
const page = route.queryParams['page'] ? route.queryParams['page'] : '1';
const sort = route.queryParams['sort'] ? route.queryParams['sort'] : 'id,asc';
return {
page: this.paginationUtil.parsePage(page),
predicate: this.paginationUtil.parsePredicate(sort),
ascending: this.paginationUtil.parseAscending(sort)
};
}
}
export const tranCategoryRegexRoute: Routes = [
{
path: 'tran-category-regex',
component: TranCategoryRegexComponent,
resolve: {
'pagingParams': TranCategoryRegexResolvePagingParams
},
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategoryRegex.home.title'
},
canActivate: [UserRouteAccessService]
}, {
path: 'tran-category-regex/:id',
component: TranCategoryRegexDetailComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategoryRegex.home.title'
},
canActivate: [UserRouteAccessService]
}
];
export const tranCategoryRegexPopupRoute: Routes = [
{
path: 'tran-category-regex-new',
component: TranCategoryRegexPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategoryRegex.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'tran-category-regex/:id/edit',
component: TranCategoryRegexPopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategoryRegex.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
},
{
path: 'tran-category-regex/:id/delete',
component: TranCategoryRegexDeletePopupComponent,
data: {
authorities: ['ROLE_USER'],
pageTitle: 'sfWebClientApp.tranCategoryRegex.home.title'
},
canActivate: [UserRouteAccessService],
outlet: 'popup'
}
];
<file_sep>/src/main/webapp/app/dashboard/dashboard.module.ts
import {CUSTOM_ELEMENTS_SCHEMA, NgModule} from '@angular/core';
import {SfChartsModule} from '../shared/charts/sf-charts.module';
import {DashboardComponent} from './dashboard.component';
import {SfWebClientSharedModule} from '../shared/shared.module';
@NgModule({
imports: [
SfWebClientSharedModule,
/*RouterModule.forRoot([ DASHBOARD_ROUTE ], { useHash: true }),*/
SfChartsModule
],
declarations: [DashboardComponent],
exports: [DashboardComponent],
entryComponents: [],
providers: [],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class DashboardModule {
}
<file_sep>/src/main/webapp/app/shared/charts/line-chart/line-chart.component.ts
import {Component, Input, OnInit} from '@angular/core';
import {DecimalPipe} from '@angular/common';
@Component({
selector: 'jhi-line-chart',
templateUrl: './line-chart.component.html',
styles: []
})
export class LineChartComponent implements OnInit {
@Input()
chartLabels: Array<any> = [];
@Input()
// chart
chartData: Array<any> = [
{data: [], label: 'EXPENSES'},
{data: [], label: 'INCOMES'}
];
chartOptions: any = {
responsive: true,
title: {
display: false,
text: 'Line Chart'
}
,
hover: {
mode: 'nearest',
intersect: true
},
scales: {
xAxes: [{
display: true,
scaleLabel: {
display: false,
labelString: 'Month'
}
}],
yAxes: [{
display: true,
scaleLabel: {
display: false,
labelString: 'Value'
},
ticks: {
// Include a dollar sign in the ticks
callback: (value: any, index: any, values: any) => {
return this.formatTick(value, index, values);
}
}
}]
},
tooltips: {
mode: 'index',
intersect: false,
callbacks: {
label: (value: any, data: any) => {
return this.formatTooltip(value, data);
}
}
}
/*tooltipTemplate: '<%if (label){%><%=label %>: <%}%><%= value + \' %\' %>',
// String - Template string for multiple tooltips
multiTooltipTemplate: '<%= value + \' %\' %>'*/
};
chartLegend = true;
chartType = 'line';
chartColors = [
/*{
backgroundColor: 'rgb(255, 99, 132)',
borderColor: 'rgb(255, 99, 132)'
},
{
backgroundColor: 'rgb(54, 162, 235)',
borderColor: 'rgb(54, 162, 235)'
}*/
];
constructor() {
}
ngOnInit() {
}
formatTick(value: any, index: any, values: any): any {
return new DecimalPipe('USD').transform(value, '2.2-2');
;
}
formatTooltip(value: any, values: any): any {
return new DecimalPipe('USD').transform(value.yLabel, '2.2-2');
;
}
// events
chartClicked(e: any): void {
console.log(e);
}
chartHovered(e: any): void {
console.log(e);
}
}
<file_sep>/src/main/webapp/app/entities/account-transaction/account-transaction.module.ts
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { RouterModule } from '@angular/router';
import { SfWebClientSharedModule } from '../../shared';
import { SfWebClientAdminModule } from '../../admin/admin.module';
import {
AccountTransactionService,
AccountTransactionPopupService,
AccountTransactionComponent,
AccountTransactionDetailComponent,
AccountTransactionDialogComponent,
AccountTransactionPopupComponent,
AccountTransactionDeletePopupComponent,
AccountTransactionDeleteDialogComponent,
accountTransactionRoute,
accountTransactionPopupRoute,
AccountTransactionResolvePagingParams,
} from './';
const ENTITY_STATES = [
...accountTransactionRoute,
...accountTransactionPopupRoute,
];
@NgModule({
imports: [
SfWebClientSharedModule,
SfWebClientAdminModule,
RouterModule.forRoot(ENTITY_STATES, { useHash: true })
],
declarations: [
AccountTransactionComponent,
AccountTransactionDetailComponent,
AccountTransactionDialogComponent,
AccountTransactionDeleteDialogComponent,
AccountTransactionPopupComponent,
AccountTransactionDeletePopupComponent,
],
entryComponents: [
AccountTransactionComponent,
AccountTransactionDialogComponent,
AccountTransactionPopupComponent,
AccountTransactionDeleteDialogComponent,
AccountTransactionDeletePopupComponent,
],
providers: [
AccountTransactionService,
AccountTransactionPopupService,
AccountTransactionResolvePagingParams,
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientAccountTransactionModule {}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/index.ts
export * from './transaction-popup.service';
export * from './transaction-dialog/transaction-dialog.component';
export * from './transaction-delete-dialog/transaction-delete-dialog.component';
export * from './transaction.component';
export * from './tran-upload/tran-upload.component';
export * from './transaction.route';
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/tran-upload/tran-upload.component.ts
import {Component, OnDestroy, OnInit} from '@angular/core';
import {Searcher} from '../../../../shared/search/searcher';
import {FileItem, FileUploader, ParsedResponseHeaders} from 'ng2-file-upload';
import {FinanceAccount} from '../../finance-account.model';
import {TranCategory} from '../../../tran-category/tran-category.model';
import {AccountTransaction} from '../../../account-transaction/account-transaction.model';
import {Observable} from 'rxjs/Observable';
import {Bank} from '../../../bank/bank.model';
import {User} from '../../../../shared/user/user.model';
import {LoggerService} from '../../../../shared/logger/logger.service';
import {JhiAlertService, JhiDateUtils, JhiEventManager} from 'ng-jhipster';
import {FinanceAccountService} from '../../finance-account.service';
import {TranCategoryService} from '../../../tran-category/tran-category.service';
import {AccountTransactionService} from '../../../account-transaction/account-transaction.service';
import {BankService} from '../../../bank/bank.service';
import {ActivatedRoute} from '@angular/router';
import {AuthServerProvider} from '../../../../shared/auth/auth-jwt.service';
import {Principal} from '../../../../shared/auth/principal.service';
import {ResponseWrapper} from '../../../../shared/model/response-wrapper.model';
import {ENTER_LEAVE_ANIMATION} from '../../../../shared/animation/enter-leave-animation';
@Component({
selector: 'jhi-tran-upload',
templateUrl: './tran-upload.component.html',
styles: [],
animations: ENTER_LEAVE_ANIMATION
})
export class TranUploadComponent implements OnInit, OnDestroy {
searcher: Searcher;
/*component property*/
hasBaseDropZoneOver = false;
isSaving: boolean;
uploader: FileUploader;
/*dropdown data*/
categories: TranCategory[];
categoryValues: string[];
/*model*/
accountId: number;
transactions: AccountTransaction[] = [];
transactionsObservables: Observable<AccountTransaction[]> = Observable.of([]);
account: FinanceAccount;
bank: Bank;
banks: Bank[] = [];
currentUser: User;
fileInput: any;
constructor(private logger: LoggerService,
private alertService: JhiAlertService,
private activatedRoute: ActivatedRoute,
private accountService: FinanceAccountService,
private tranCategoryService: TranCategoryService,
private transactionService: AccountTransactionService,
private bankService: BankService,
private eventManager: JhiEventManager,
private authServerProvider: AuthServerProvider,
private principal: Principal,
private dateUtils: JhiDateUtils) {
}
// TODO: Remove this when we're done
get diagnostic() {
return JSON.stringify(this.transactions);
}
ngOnInit() {
this.activatedRoute.params.subscribe((params) => {
this.accountId = params['accountId'];
this.load();
});
}
load() {
this.logger.log('***Loading***');
this.isSaving = false;
this.searcher = new Searcher();
this.searcher.onSearch = (term: string) => {
return this.OnSearch(term.toLocaleLowerCase());
}
this.uploader = new FileUploader(
{
url: 'api/account-transactions/upload',
authToken: 'Bearer ' + this.authServerProvider.getToken()
/*allowedFileType: ['xls'],
allowedMimeType: ['text/csv']*/
});
this.uploader.onCompleteItem = (item: FileItem, response: string, status: number, headers: ParsedResponseHeaders) => {
this.onCompleteUpload(item, response, status, headers);
};
this.uploader.onBuildItemForm = (fileItem: FileItem, form: any) => {
this.onBuildItemForm(fileItem, form);
};
this.principal.identity().then((user) => {
this.currentUser = user;
});
this.accountService.find(this.accountId)
.subscribe((account) => {
this.account = account;
}, (res: ResponseWrapper) => this.onError(res.json));
this.tranCategoryService.query()
.subscribe((res: ResponseWrapper) => {
this.categories = res.json;
}, (res: ResponseWrapper) => this.onError(res.json));
this.bankService.query().subscribe((res: ResponseWrapper) => {
this.banks = res.json;
this.bank = this.banks.length > 0 ? this.banks[0] : this.bank;
}, (res: ResponseWrapper) =>
this.onError(res.json)
);
}
private OnSearch(term: string): Observable<any[]> {
this.logger.info('***** OnSearch ******');
this.logger.info(term);
this.transactionsObservables = Observable.of(this.transactions);
if (term.length <= 0) {
return this.transactionsObservables;
}
this.transactionsObservables = this.transactionsObservables.map((trans) =>
trans.filter((tran) =>
(<AccountTransaction>tran).description.toLocaleLowerCase().match(term) ||
((<AccountTransaction>tran).amount + '').toLocaleLowerCase().match(term)
)
);
return this.transactionsObservables;
}
private onBuildItemForm(fileItem: FileItem, form: any): any {
this.logger.info('******onBuildItemForm*****');
form.append('bank', this.bank.id);
}
private onCompleteUpload(item: FileItem, response: string, status: number, headers: ParsedResponseHeaders): any {
this.logger.info('******* Response from upload file endpoint *******');
this.logger.info(JSON.parse(response));
this.transactions = <AccountTransaction[]>JSON.parse(response);
this.setCategoryValues(this.transactions);
this.transactionsObservables = Observable.of(this.transactions);
this.uploader.clearQueue();
this.fileInput = null;
return void 0;
}
private setCategoryValues(transactions: AccountTransaction[]) {
this.categoryValues = [];
for (const tran of transactions) {
this.categoryValues.push(tran.tranCategory ? tran.tranCategory['name'] : '');
}
}
upload() {
this.logger.info('Uploading');
this.uploader.uploadItem(<FileItem>this.uploader.getNotUploadedItems()[0]);
/*this.uploader.component = this;*/
}
remove(index: number, item: AccountTransaction) {
this.transactions.splice(index, 1);
}
trackBankById(index: number, item: Bank) {
return item.id;
}
trackAccountById(index: number, item: FinanceAccount) {
return item.id;
}
trackTranCategoryById(index: number, item: TranCategory) {
return item.id;
}
trackId(index: number, item: AccountTransaction) {
return item.tranNumber;
}
onChangeCategory() {
this.logger.info(this.categoryValues);
}
onTypeaheadSelect(index: number, transaction: AccountTransaction, event: any) {
/*this.logger.info('***** on select typeahead category');
this.logger.info(index);
this.logger.info(transaction);
this.logger.info(event);*/
transaction.tranCategory = event.item;
}
goBack() {
this.logger.log('***Back***');
this.clear();
window.history.back();
}
clear() {
this.logger.log('***Clear***');
this.transactions = [];
this.account = null;
this.uploader.clearQueue();
this.fileInput = null;
}
private onSaveSuccess(result: AccountTransaction[]) {
this.eventManager.broadcast({name: 'accountTransactionListModification', content: 'OK'});
this.isSaving = false;
this.goBack();
}
private subscribeToSaveResponse(result: Observable<AccountTransaction[]>) {
result.subscribe((res: AccountTransaction[]) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private prepareTransactionsForServer(): AccountTransaction[] {
const copyTransactions: AccountTransaction[] = [];
for (const tran of this.transactions) {
const copy: AccountTransaction = Object.assign({}, tran);
copy.id = null;
copy.financeAccount = this.account;
copy.user = this.currentUser;
const postDate: Date = this.dateUtils.convertLocalDateFromServer(copy.postDate);
copy.postDate = {
year: postDate.getFullYear(),
month: postDate.getMonth() + 1,
day: postDate.getDate()
};
copyTransactions.push(copy);
}
return copyTransactions;
}
save() {
this.isSaving = true;
this.logger.log('***Saving***');
this.subscribeToSaveResponse(this.transactionService.createBulk(this.prepareTransactionsForServer()));
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
ngOnDestroy() {
}
}
<file_sep>/src/main/webapp/app/sf-entities/account-transaction/transaction-upload/transaction-upload.component.ts
import {Component, OnDestroy, OnInit} from '@angular/core';
import {LoggerService} from '../../../shared/logger/logger.service';
import {FileItem, FileUploader, ParsedResponseHeaders} from 'ng2-file-upload';
import {FinanceAccount} from '../../finance-account/finance-account.model';
import {AccountTransaction} from '../account-transaction.model';
import {JhiAlertService, JhiDateUtils, JhiEventManager} from 'ng-jhipster';
import {FinanceAccountService} from '../../finance-account/finance-account.service';
import {ActivatedRoute} from '@angular/router';
import {AuthServerProvider} from '../../../shared/auth/auth-jwt.service';
import {ResponseWrapper} from '../../../shared/model/response-wrapper.model';
import {AccountTransactionService} from '../account-transaction.service';
import {TranCategoryService} from '../../tran-category/tran-category.service';
import {TranCategory} from '../../tran-category/tran-category.model';
import {Observable} from 'rxjs/Observable';
import {User} from '../../../shared/user/user.model';
import {Principal} from '../../../shared/auth/principal.service';
import {Bank} from '../../bank/bank.model';
import {BankService} from '../../bank/bank.service';
import {Searcher} from '../../../shared/search/searcher';
@Component({
selector: 'jhi-transaction-upload',
templateUrl: './transaction-upload.component.html',
styles: []
})
export class TransactionUploadComponent implements OnInit, OnDestroy {
searcher: Searcher;
/*component property*/
hasBaseDropZoneOver = false;
isSaving: boolean;
uploader: FileUploader;
/*dropdown data*/
accounts: FinanceAccount[];
categories: TranCategory[];
/*model*/
transactions: AccountTransaction[] = [];
transactionsObservables: Observable<AccountTransaction[]> = Observable.of([]);
account: FinanceAccount;
bank: Bank;
banks: Bank[] = [];
currentUser: User;
fileInput: any;
constructor(private logger: LoggerService,
private alertService: JhiAlertService,
private accountService: FinanceAccountService,
private tranCategoryService: TranCategoryService,
private transactionService: AccountTransactionService,
private bankService: BankService,
private eventManager: JhiEventManager,
private route: ActivatedRoute,
private authServerProvider: AuthServerProvider,
private principal: Principal,
private dateUtils: JhiDateUtils) {
}
// TODO: Remove this when we're done
get diagnostic() {
return JSON.stringify(this.transactions);
}
ngOnInit() {
this.load();
}
load() {
this.logger.log('***Loading***');
this.isSaving = false;
this.searcher = new Searcher();
this.searcher.onSearch = (term: string) => {
return this.OnSearch(term.toLocaleLowerCase());
};
this.uploader = new FileUploader(
{
url: 'api/account-transactions/upload',
authToken: 'Bearer ' + this.authServerProvider.getToken()
/*allowedFileType: ['xls'],
allowedMimeType: ['text/csv']*/
});
this.uploader.onCompleteItem = (item: FileItem, response: string, status: number, headers: ParsedResponseHeaders) => {
this.onCompleteUpload(item, response, status, headers);
};
this.uploader.onBuildItemForm = (fileItem: FileItem, form: any) => {
this.onBuildItemForm(fileItem, form);
};
this.principal.identity().then((user) => {
this.currentUser = user;
});
this.accountService.query()
.subscribe((res: ResponseWrapper) => {
this.accounts = res.json;
this.account = this.accounts.length > 0 ? this.accounts[0] : this.account;
}, (res: ResponseWrapper) => this.onError(res.json));
this.tranCategoryService.query()
.subscribe((res: ResponseWrapper) => {
this.categories = res.json;
}, (res: ResponseWrapper) => this.onError(res.json));
this.bankService.query().subscribe((res: ResponseWrapper) => {
this.banks = res.json;
this.bank = this.banks.length > 0 ? this.banks[0] : this.bank;
}, (res: ResponseWrapper) =>
this.onError(res.json)
);
}
private OnSearch(term: string): Observable<any[]> {
this.logger.info("***** OnSearch ******");
this.logger.info(term);
this.transactionsObservables = Observable.of(this.transactions);
if (term.length <= 0) {
return this.transactionsObservables;
}
this.transactionsObservables = this.transactionsObservables.map(trans =>
trans.filter(tran =>
(<AccountTransaction>tran).description.toLocaleLowerCase().match(term) ||
((<AccountTransaction>tran).amount + "").toLocaleLowerCase().match(term)
)
);
return this.transactionsObservables;
}
private onBuildItemForm(fileItem: FileItem, form: any): any {
this.logger.info("******onBuildItemForm*****");
form.append("bank", this.bank.id);
}
private onCompleteUpload(item: FileItem, response: string, status: number, headers: ParsedResponseHeaders): any {
this.logger.info("******* Response from upload file endpoint *******");
this.logger.info(JSON.parse(response));
this.transactions = <AccountTransaction[]>JSON.parse(response);
this.transactionsObservables = Observable.of(this.transactions);
this.uploader.clearQueue();
this.fileInput = null;
return void 0;
}
upload() {
this.logger.info("Uploading");
this.uploader.uploadItem(<FileItem>this.uploader.getNotUploadedItems()[0]);
/*this.uploader.component = this;*/
}
remove(index: number, item: AccountTransaction) {
this.transactions.splice(index, 1);
}
trackBankById(index: number, item: Bank) {
return item.id;
}
trackAccountById(index: number, item: FinanceAccount) {
return item.id;
}
trackTranCategoryById(index: number, item: TranCategory) {
return item.id;
}
trackId(index: number, item: AccountTransaction) {
return item.id;
}
goBack() {
this.logger.log('***Back***');
this.clear();
window.history.back();
}
clear() {
this.logger.log('***Clear***');
this.transactions = [];
this.account = null;
this.uploader.clearQueue();
this.fileInput = null;
}
private onSaveSuccess(result: AccountTransaction[]) {
this.eventManager.broadcast({name: 'accountTransactionListModification', content: 'OK'});
this.isSaving = false;
this.goBack();
}
private subscribeToSaveResponse(result: Observable<AccountTransaction[]>) {
result.subscribe((res: AccountTransaction[]) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private prepareTransactions(): AccountTransaction[] {
const copyTransactions: AccountTransaction[] = [];
for (let tran of this.transactions) {
const copy: AccountTransaction = Object.assign({}, tran);
copy.id = null;
copy.financeAccount = this.account;
copy.user = this.currentUser;
let postDate: Date = this.dateUtils.convertLocalDateFromServer(copy.postDate);
copy.postDate = {
year: postDate.getFullYear(),
month: postDate.getMonth() + 1,
day: postDate.getDate()
};
copyTransactions.push(copy);
}
return copyTransactions;
}
save() {
this.isSaving = true;
this.logger.log('***Saving***');
this.subscribeToSaveResponse(this.transactionService.createBulk(this.prepareTransactions()));
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
ngOnDestroy() {
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/tran-category-delete-dialog.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { NgbActiveModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { JhiEventManager } from 'ng-jhipster';
import { TranCategory } from './tran-category.model';
import { TranCategoryPopupService } from './tran-category-popup.service';
import { TranCategoryService } from './tran-category.service';
@Component({
selector: 'jhi-tran-category-delete-dialog',
templateUrl: './tran-category-delete-dialog.component.html'
})
export class TranCategoryDeleteDialogComponent {
tranCategory: TranCategory;
constructor(
private tranCategoryService: TranCategoryService,
public activeModal: NgbActiveModal,
private eventManager: JhiEventManager
) {
}
clear() {
this.activeModal.dismiss('cancel');
}
confirmDelete(id: number) {
this.tranCategoryService.delete(id).subscribe((response) => {
this.eventManager.broadcast({
name: 'tranCategoryListModification',
content: 'Deleted an tranCategory'
});
this.activeModal.dismiss(true);
});
}
}
@Component({
selector: 'jhi-tran-category-delete-popup',
template: ''
})
export class TranCategoryDeletePopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(
private route: ActivatedRoute,
private tranCategoryPopupService: TranCategoryPopupService
) {}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
this.tranCategoryPopupService
.open(TranCategoryDeleteDialogComponent as Component, params['id']);
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/entities/finance-account/finance-account.model.ts
import { BaseEntity, User } from './../../shared';
export const enum AccountStatus {
'INACTIVE',
'ACTIVE'
}
export class FinanceAccount implements BaseEntity {
constructor(
public id?: number,
public accountStatus?: AccountStatus,
public accountNumber?: string,
public name?: string,
public description?: string,
public balance?: number,
public isCreditCard?: boolean,
public dueDate?: any,
public closingDate?: any,
public accountTransactions?: BaseEntity[],
public user?: User,
) {
this.isCreditCard = false;
}
}
<file_sep>/src/main/webapp/app/sf-entities/account-transaction/account-transaction-dialog.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Response } from '@angular/http';
import { Observable } from 'rxjs/Rx';
import { NgbActiveModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { JhiEventManager, JhiAlertService } from 'ng-jhipster';
import {AccountTransaction, TranType} from './account-transaction.model';
import { AccountTransactionPopupService } from './account-transaction-popup.service';
import { AccountTransactionService } from './account-transaction.service';
import { User, UserService } from '../../shared';
import { FinanceAccount, FinanceAccountService } from '../finance-account';
import { TranCategory, TranCategoryService } from '../tran-category';
import { ResponseWrapper } from '../../shared';
import {Principal} from '../../shared/auth/principal.service';
import {LoggerService} from '../../shared/logger/logger.service';
@Component({
selector: 'jhi-account-transaction-dialog',
templateUrl: './account-transaction-dialog.component.html'
})
export class AccountTransactionDialogComponent implements OnInit {
accountTransaction: AccountTransaction;
isSaving: boolean;
currentUser: User;
users: User[];
financeaccounts: FinanceAccount[] = [];
trancategories: TranCategory[] = [];
postDateDp: any;
isDetailsCollapsed: boolean = true;
constructor(
public activeModal: NgbActiveModal,
private alertService: JhiAlertService,
private accountTransactionService: AccountTransactionService,
private userService: UserService,
private financeAccountService: FinanceAccountService,
private tranCategoryService: TranCategoryService,
private eventManager: JhiEventManager,
private principal: Principal,
private logger: LoggerService
) {
}
ngOnInit() {
this.load();
}
load() {
this.isSaving = false;
this.userService.query().subscribe((res: ResponseWrapper) =>
{
this.users = res.json;
}, (res: ResponseWrapper) => this.onError(res.json));
this.financeAccountService.query()
.subscribe((res: ResponseWrapper) => {
this.financeaccounts = res.json;
if(!this.accountTransaction.id)
this.accountTransaction.financeAccount = this.financeaccounts.length > 0? this.financeaccounts[0]:this.accountTransaction.financeAccount;
}, (res: ResponseWrapper) => this.onError(res.json));
this.tranCategoryService.query()
.subscribe((res: ResponseWrapper) =>
{
this.trancategories = res.json;
if(!this.accountTransaction.id)
this.accountTransaction.tranCategory = this.trancategories.length > 0? this.trancategories[0]:this.accountTransaction.tranCategory;
}, (res: ResponseWrapper) => this.onError(res.json));
this.loadDefaults();
}
loadDefaults() {
}
clear() {
this.activeModal.dismiss('cancel');
}
save() {
this.isSaving = true;
/*this.accountTransaction.user = this.currentUser;*/
if (this.accountTransaction.id !== undefined) {
this.subscribeToSaveResponse(
this.accountTransactionService.update(this.accountTransaction));
} else {
this.subscribeToSaveResponse(
this.accountTransactionService.create(this.accountTransaction));
}
}
private subscribeToSaveResponse(result: Observable<AccountTransaction>) {
result.subscribe((res: AccountTransaction) =>
this.onSaveSuccess(res), (res: Response) => this.onSaveError());
}
private onSaveSuccess(result: AccountTransaction) {
this.eventManager.broadcast({ name: 'accountTransactionListModification', content: 'OK'});
this.isSaving = false;
this.activeModal.dismiss(result);
}
private onSaveError() {
this.isSaving = false;
}
private onError(error: any) {
this.alertService.error(error.message, null, null);
}
trackUserById(index: number, item: User) {
return item.id;
}
trackFinanceAccountById(index: number, item: FinanceAccount) {
return item.id;
}
trackTranCategoryById(index: number, item: TranCategory) {
return item.id;
}
getDetailsCollapseClass() {
return {
'form-row': !this.isDetailsCollapsed,
'collapse': this.isDetailsCollapsed
};
}
getTranIncomeClass() {
return {
'btn': true,
'btn-secondary': this.accountTransaction.tranType == <TranType><any>'EXPENSE',
'btn-success': this.accountTransaction.tranType == <TranType><any>'INCOME'
};
}
getTranExpenseClass() {
return {
'btn': true,
'btn-danger': this.accountTransaction.tranType == <TranType><any>'EXPENSE',
'btn-secondary': this.accountTransaction.tranType == <TranType><any>'INCOME'
};
}
}
@Component({
selector: 'jhi-account-transaction-popup',
template: ''
})
export class AccountTransactionPopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(
private route: ActivatedRoute,
private accountTransactionPopupService: AccountTransactionPopupService
) {}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
if ( params['id'] ) {
this.accountTransactionPopupService
.open(AccountTransactionDialogComponent as Component, params['id']);
} else {
this.accountTransactionPopupService
.open(AccountTransactionDialogComponent as Component);
}
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/entities/tran-category/tran-category.module.ts
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { RouterModule } from '@angular/router';
import { SfWebClientSharedModule } from '../../shared';
import { SfWebClientAdminModule } from '../../admin/admin.module';
import {
TranCategoryService,
TranCategoryPopupService,
TranCategoryComponent,
TranCategoryDetailComponent,
TranCategoryDialogComponent,
TranCategoryPopupComponent,
TranCategoryDeletePopupComponent,
TranCategoryDeleteDialogComponent,
tranCategoryRoute,
tranCategoryPopupRoute,
TranCategoryResolvePagingParams,
} from './';
const ENTITY_STATES = [
...tranCategoryRoute,
...tranCategoryPopupRoute,
];
@NgModule({
imports: [
SfWebClientSharedModule,
SfWebClientAdminModule,
RouterModule.forRoot(ENTITY_STATES, { useHash: true })
],
declarations: [
TranCategoryComponent,
TranCategoryDetailComponent,
TranCategoryDialogComponent,
TranCategoryDeleteDialogComponent,
TranCategoryPopupComponent,
TranCategoryDeletePopupComponent,
],
entryComponents: [
TranCategoryComponent,
TranCategoryDialogComponent,
TranCategoryPopupComponent,
TranCategoryDeleteDialogComponent,
TranCategoryDeletePopupComponent,
],
providers: [
TranCategoryService,
TranCategoryPopupService,
TranCategoryResolvePagingParams,
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientTranCategoryModule {}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/transaction-popup.service.ts
import { Injectable, Component } from '@angular/core';
import { Router } from '@angular/router';
import { NgbModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import {User} from '../../../shared/user/user.model';
import {AccountTransactionService} from '../../account-transaction/account-transaction.service';
import {Principal} from '../../../shared/auth/principal.service';
import {LoggerService} from '../../../shared/logger/logger.service';
import {DatetimeService} from '../../../shared/datetime/datetime.service';
import {AccountTransaction, PaymentMethod, TranType} from '../../account-transaction/account-transaction.model';
@Injectable()
export class TransactionPopupService {
private ngbModalRef: NgbModalRef;
private currentUser: User;
constructor(
private modalService: NgbModal,
private router: Router,
private accountTransactionService: AccountTransactionService,
private principal: Principal,
private logger: LoggerService,
private dateTimeService: DatetimeService
) {
this.ngbModalRef = null;
}
open(component: Component, id?: number | any): Promise<NgbModalRef> {
return new Promise<NgbModalRef>((resolve, reject) => {
const isOpen = this.ngbModalRef !== null;
if (isOpen) {
resolve(this.ngbModalRef);
}
if (id) {
this.accountTransactionService.find(id).subscribe((accountTransaction) => {
if (accountTransaction.postDate) {
accountTransaction.postDate = {
year: accountTransaction.postDate.getFullYear(),
month: accountTransaction.postDate.getMonth() + 1,
day: accountTransaction.postDate.getDate()
};
}
this.logger.info(accountTransaction);
this.ngbModalRef = this.accountTransactionModalRef(component, accountTransaction);
resolve(this.ngbModalRef);
});
} else {
// setTimeout used as a workaround for getting ExpressionChangedAfterItHasBeenCheckedError
setTimeout(() => {
this.ngbModalRef = this.accountTransactionModalRef(component, this.newAccountTransaction());
resolve(this.ngbModalRef);
}, 0);
}
});
}
newAccountTransaction() {
const tran: AccountTransaction = new AccountTransaction();
tran.tranType = <TranType><any>'EXPENSE';
tran.paymentMethod = <PaymentMethod><any>'UNSPECIFIED';
tran.postDate = this.dateTimeService.nowLocalDate();
this.principal.identity().then((user) => {
this.currentUser = user;
tran.user = user;
});
this.logger.info(tran);
return tran;
}
accountTransactionModalRef(component: Component, accountTransaction: AccountTransaction): NgbModalRef {
const modalRef = this.modalService.open(component, { size: 'lg', backdrop: 'static'});
modalRef.componentInstance.transaction = accountTransaction;
modalRef.result.then((result) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
}, (reason) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
});
return modalRef;
}
}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/transaction/transaction.module.ts
import {
TransactionPopupService,
TransactionComponent,
TransactionDialogComponent,
TransactionPopupComponent,
TransactionDeletePopupComponent,
TransactionDeleteDialogComponent,
TranUploadComponent,
transactionRoute,
transactionPopupRoute,
TransactionResolvePagingParams
} from './';
import {SfWebClientSharedModule} from '../../../shared/shared.module';
import {SfWebClientAdminModule} from '../../../admin/admin.module';
import {RouterModule} from '@angular/router';
import {FileUploadModule} from 'ng2-file-upload';
import {SearchModule} from '../../../shared/search/search.module';
import {AccountTransactionService} from '../../account-transaction/account-transaction.service';
import {CUSTOM_ELEMENTS_SCHEMA, NgModule} from '@angular/core';
import {TypeaheadModule} from 'ngx-bootstrap/typeahead';
import {BsDatepickerModule} from "ngx-bootstrap/datepicker";
const ENTITY_STATES = [
...transactionRoute,
...transactionPopupRoute,
];
@NgModule({
imports: [
SfWebClientSharedModule,
SfWebClientAdminModule,
RouterModule.forRoot(ENTITY_STATES, { useHash: true, enableTracing: true }),
FileUploadModule,
SearchModule,
TypeaheadModule.forRoot(),
BsDatepickerModule.forRoot()
],
declarations: [
TransactionComponent,
TransactionDialogComponent,
TransactionDeleteDialogComponent,
TransactionPopupComponent,
TransactionDeletePopupComponent,
TranUploadComponent
],
providers: [
AccountTransactionService,
TransactionPopupService,
TransactionResolvePagingParams,
],
entryComponents: [
TranUploadComponent,
TransactionDialogComponent,
TransactionDeleteDialogComponent,
TransactionPopupComponent,
TransactionDeletePopupComponent
],
exports: [
TransactionComponent
],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class SfWebClientTransactionModule {}
<file_sep>/src/main/java/com/calderon/sf/domain/package-info.java
/**
* JPA domain objects.
*/
package com.calderon.sf.domain;
<file_sep>/src/main/webapp/app/entities/account-transaction/index.ts
export * from './account-transaction.model';
export * from './account-transaction-popup.service';
export * from './account-transaction.service';
export * from './account-transaction-dialog.component';
export * from './account-transaction-delete-dialog.component';
export * from './account-transaction-detail.component';
export * from './account-transaction.component';
export * from './account-transaction.route';
<file_sep>/src/main/webapp/app/entities/account-transaction/account-transaction-popup.service.ts
import { Injectable, Component } from '@angular/core';
import { Router } from '@angular/router';
import { NgbModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { AccountTransaction } from './account-transaction.model';
import { AccountTransactionService } from './account-transaction.service';
@Injectable()
export class AccountTransactionPopupService {
private ngbModalRef: NgbModalRef;
constructor(
private modalService: NgbModal,
private router: Router,
private accountTransactionService: AccountTransactionService
) {
this.ngbModalRef = null;
}
open(component: Component, id?: number | any): Promise<NgbModalRef> {
return new Promise<NgbModalRef>((resolve, reject) => {
const isOpen = this.ngbModalRef !== null;
if (isOpen) {
resolve(this.ngbModalRef);
}
if (id) {
this.accountTransactionService.find(id).subscribe((accountTransaction) => {
if (accountTransaction.postDate) {
accountTransaction.postDate = {
year: accountTransaction.postDate.getFullYear(),
month: accountTransaction.postDate.getMonth() + 1,
day: accountTransaction.postDate.getDate()
};
}
this.ngbModalRef = this.accountTransactionModalRef(component, accountTransaction);
resolve(this.ngbModalRef);
});
} else {
// setTimeout used as a workaround for getting ExpressionChangedAfterItHasBeenCheckedError
setTimeout(() => {
this.ngbModalRef = this.accountTransactionModalRef(component, new AccountTransaction());
resolve(this.ngbModalRef);
}, 0);
}
});
}
accountTransactionModalRef(component: Component, accountTransaction: AccountTransaction): NgbModalRef {
const modalRef = this.modalService.open(component, { size: 'lg', backdrop: 'static'});
modalRef.componentInstance.accountTransaction = accountTransaction;
modalRef.result.then((result) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
}, (reason) => {
this.router.navigate([{ outlets: { popup: null }}], { replaceUrl: true });
this.ngbModalRef = null;
});
return modalRef;
}
}
<file_sep>/src/main/java/com/calderon/sf/web/rest/TranCategoryRegexResource.java
package com.calderon.sf.web.rest;
import com.calderon.sf.domain.TranCategoryRegex;
import com.calderon.sf.repository.TranCategoryRegexRepository;
import com.calderon.sf.web.rest.util.HeaderUtil;
import com.calderon.sf.web.rest.util.PaginationUtil;
import com.codahale.metrics.annotation.Timed;
import io.github.jhipster.web.util.ResponseUtil;
import io.swagger.annotations.ApiParam;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.validation.Valid;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.List;
import java.util.Optional;
/**
* REST controller for managing TranCategoryRegex.
*/
@RestController
@RequestMapping("/api")
public class TranCategoryRegexResource {
private final Logger log = LoggerFactory.getLogger(TranCategoryRegexResource.class);
private static final String ENTITY_NAME = "tranCategoryRegex";
private final TranCategoryRegexRepository tranCategoryRegexRepository;
public TranCategoryRegexResource(TranCategoryRegexRepository tranCategoryRegexRepository) {
this.tranCategoryRegexRepository = tranCategoryRegexRepository;
}
/**
* POST /tran-category-regexes : Create a new tranCategoryRegex.
*
* @param tranCategoryRegex the tranCategoryRegex to create
* @return the ResponseEntity with status 201 (Created) and with body the new tranCategoryRegex, or with status 400 (Bad Request) if the tranCategoryRegex has already an ID
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PostMapping("/tran-category-regexes")
@Timed
public ResponseEntity<TranCategoryRegex> createTranCategoryRegex(@Valid @RequestBody TranCategoryRegex tranCategoryRegex) throws URISyntaxException {
log.debug("REST request to save TranCategoryRegex : {}", tranCategoryRegex);
if (tranCategoryRegex.getId() != null) {
return ResponseEntity.badRequest().headers(HeaderUtil.createFailureAlert(ENTITY_NAME, "idexists", "A new tranCategoryRegex cannot already have an ID")).body(null);
}
TranCategoryRegex result = tranCategoryRegexRepository.save(tranCategoryRegex);
return ResponseEntity.created(new URI("/api/tran-category-regexes/" + result.getId()))
.headers(HeaderUtil.createEntityCreationAlert(ENTITY_NAME, result.getId().toString()))
.body(result);
}
/**
* PUT /tran-category-regexes : Updates an existing tranCategoryRegex.
*
* @param tranCategoryRegex the tranCategoryRegex to update
* @return the ResponseEntity with status 200 (OK) and with body the updated tranCategoryRegex,
* or with status 400 (Bad Request) if the tranCategoryRegex is not valid,
* or with status 500 (Internal Server Error) if the tranCategoryRegex couldn't be updated
* @throws URISyntaxException if the Location URI syntax is incorrect
*/
@PutMapping("/tran-category-regexes")
@Timed
public ResponseEntity<TranCategoryRegex> updateTranCategoryRegex(@Valid @RequestBody TranCategoryRegex tranCategoryRegex) throws URISyntaxException {
log.debug("REST request to update TranCategoryRegex : {}", tranCategoryRegex);
if (tranCategoryRegex.getId() == null) {
return createTranCategoryRegex(tranCategoryRegex);
}
TranCategoryRegex result = tranCategoryRegexRepository.save(tranCategoryRegex);
return ResponseEntity.ok()
.headers(HeaderUtil.createEntityUpdateAlert(ENTITY_NAME, tranCategoryRegex.getId().toString()))
.body(result);
}
/**
* GET /tran-category-regexes : get all the tranCategoryRegexes.
*
* @param pageable the pagination information
* @return the ResponseEntity with status 200 (OK) and the list of tranCategoryRegexes in body
*/
@GetMapping("/tran-category-regexes")
@Timed
public ResponseEntity<List<TranCategoryRegex>> getAllTranCategoryRegexes(@ApiParam Pageable pageable) {
log.debug("REST request to get a page of TranCategoryRegexes");
/*Page<TranCategoryRegex> page = tranCategoryRegexRepository.findAll(pageable);*/
Page<TranCategoryRegex> page = tranCategoryRegexRepository.findByUserIsCurrentUser(pageable);
HttpHeaders headers = PaginationUtil.generatePaginationHttpHeaders(page, "/api/tran-category-regexes");
return new ResponseEntity<>(page.getContent(), headers, HttpStatus.OK);
}
/**
* GET /tran-category-regexes/:id : get the "id" tranCategoryRegex.
*
* @param id the id of the tranCategoryRegex to retrieve
* @return the ResponseEntity with status 200 (OK) and with body the tranCategoryRegex, or with status 404 (Not Found)
*/
@GetMapping("/tran-category-regexes/{id}")
@Timed
public ResponseEntity<TranCategoryRegex> getTranCategoryRegex(@PathVariable Long id) {
log.debug("REST request to get TranCategoryRegex : {}", id);
TranCategoryRegex tranCategoryRegex = tranCategoryRegexRepository.findOne(id);
return ResponseUtil.wrapOrNotFound(Optional.ofNullable(tranCategoryRegex));
}
/**
* DELETE /tran-category-regexes/:id : delete the "id" tranCategoryRegex.
*
* @param id the id of the tranCategoryRegex to delete
* @return the ResponseEntity with status 200 (OK)
*/
@DeleteMapping("/tran-category-regexes/{id}")
@Timed
public ResponseEntity<Void> deleteTranCategoryRegex(@PathVariable Long id) {
log.debug("REST request to delete TranCategoryRegex : {}", id);
tranCategoryRegexRepository.delete(id);
return ResponseEntity.ok().headers(HeaderUtil.createEntityDeletionAlert(ENTITY_NAME, id.toString())).build();
}
}
<file_sep>/src/main/java/com/calderon/sf/domain/enumeration/AccountStatus.java
package com.calderon.sf.domain.enumeration;
/**
* The AccountStatus enumeration.
*/
public enum AccountStatus {
INACTIVE, ACTIVE
}
<file_sep>/src/main/java/com/calderon/sf/domain/enumeration/AccountType.java
package com.calderon.sf.domain.enumeration;
/**
* The AccountType enumeration.
*/
public enum AccountType {
GENERIC, CHECKING_ACCOUNT, SAVING_ACCOUNT, CREDIT_CARD
}
<file_sep>/src/main/webapp/app/shared/logger/logger.service.ts
import {Injectable} from '@angular/core';
@Injectable()
export class LoggerService {
constructor() {
}
public log(msg: any) {
console.log(msg);
}
info(msg: any){
console.info(msg);
}
error(msg: any) {
console.error(msg);
}
warn(msg: any) {
console.warn(msg);
}
}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/finance-account-delete-dialog.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { NgbActiveModal, NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import { JhiEventManager } from 'ng-jhipster';
import { FinanceAccount } from './finance-account.model';
import { FinanceAccountPopupService } from './finance-account-popup.service';
import { FinanceAccountService } from './finance-account.service';
@Component({
selector: 'jhi-finance-account-delete-dialog',
templateUrl: './finance-account-delete-dialog.component.html'
})
export class FinanceAccountDeleteDialogComponent {
financeAccount: FinanceAccount;
constructor(
private financeAccountService: FinanceAccountService,
public activeModal: NgbActiveModal,
private eventManager: JhiEventManager
) {
}
clear() {
this.activeModal.dismiss('cancel');
}
confirmDelete(id: number) {
this.financeAccountService.delete(id).subscribe((response) => {
this.eventManager.broadcast({
name: 'financeAccountListModification',
content: 'Deleted an financeAccount'
});
this.activeModal.dismiss(true);
});
}
}
@Component({
selector: 'jhi-finance-account-delete-popup',
template: ''
})
export class FinanceAccountDeletePopupComponent implements OnInit, OnDestroy {
routeSub: any;
constructor(
private route: ActivatedRoute,
private financeAccountPopupService: FinanceAccountPopupService
) {}
ngOnInit() {
this.routeSub = this.route.params.subscribe((params) => {
this.financeAccountPopupService
.open(FinanceAccountDeleteDialogComponent as Component, params['id']);
});
}
ngOnDestroy() {
this.routeSub.unsubscribe();
}
}
<file_sep>/src/main/webapp/app/sf-entities/finance-account/finance-account.service.ts
import {Injectable} from '@angular/core';
import {Http, Response} from '@angular/http';
import {Observable} from 'rxjs/Rx';
import {SERVER_API_URL} from '../../app.constants';
import {JhiDateUtils} from 'ng-jhipster';
import {FinanceAccount} from './finance-account.model';
import {createRequestOption, ResponseWrapper} from '../../shared';
import {AccountTransaction} from '../account-transaction/account-transaction.model';
import {HttpObserve} from '@angular/common/http/src/client';
import {HttpParams} from '@angular/common/http';
import {createQueryRequestOption} from "../../shared/model/request-util";
import {DatetimeService} from "../../shared/datetime/datetime.service";
@Injectable()
export class FinanceAccountService {
private resourceUrl = SERVER_API_URL + 'api/finance-accounts';
constructor(private http: Http, private dateUtils: JhiDateUtils, private dateTimeService: DatetimeService) {
}
create(financeAccount: FinanceAccount): Observable<FinanceAccount> {
const copy = this.convert(financeAccount);
return this.http.post(this.resourceUrl, copy).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
update(financeAccount: FinanceAccount): Observable<FinanceAccount> {
const copy = this.convert(financeAccount);
return this.http.put(this.resourceUrl, copy).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
find(id: number): Observable<FinanceAccount> {
return this.http.get(`${this.resourceUrl}/${id}`).map((res: Response) => {
const jsonResponse = res.json();
this.convertItemFromServer(jsonResponse);
return jsonResponse;
});
}
findTransactions(id: number): Observable<AccountTransaction[]> {
return this.findTransactionsByYear(id, 0);
}
findTransactionsByYear(id: number, year: number): Observable<AccountTransaction[]> {
return this.http.get(`${this.resourceUrl}/${id}/transactionsByYear`, {
params: new HttpParams().set('year', year + '')
}).map((res: Response) => {
const jsonResponse = res.json();
this.convertTransactionsFromServer(jsonResponse);
return jsonResponse;
});
}
query(req?: any): Observable<ResponseWrapper> {
const options = createRequestOption(req);
return this.http.get(this.resourceUrl, options)
.map((res: Response) => this.convertResponse(res));
}
queryTransactions(id: number, req?: any): Observable<ResponseWrapper> {
const options = createQueryRequestOption(req);
return this.http.get(`${this.resourceUrl}/${id}/transactions`, options)
.map((res: Response) => this.convertResponse(res));
}
queryTransactionsBy(id: number, req?: any, criteria?: any): Observable<ResponseWrapper> {
const options = createQueryRequestOption(req, this.convertCriteria(criteria));
return this.http.get(`${this.resourceUrl}/${id}/transactions`, options)
.map((res: Response) => this.convertResponse(res));
}
delete(id: number): Observable<Response> {
return this.http.delete(`${this.resourceUrl}/${id}`);
}
private convertTransactionsFromServer(accountTransactions: AccountTransaction[]) {
for (let tran of accountTransactions) {
this.convertTransactionFromServer(tran);
}
}
private convertTransactionFromServer(entity: any) {
entity.postDate = this.dateUtils
.convertLocalDateFromServer(entity.postDate);
}
private convertResponse(res: Response): ResponseWrapper {
const jsonResponse = res.json();
for (let i = 0; i < jsonResponse.length; i++) {
this.convertItemFromServer(jsonResponse[i]);
}
return new ResponseWrapper(res.headers, jsonResponse, res.status);
}
private convertTranResponse(res: Response): ResponseWrapper {
const jsonResponse = res.json();
for (let i = 0; i < jsonResponse.length; i++) {
this.convertItemFromServer(jsonResponse[i]);
}
return new ResponseWrapper(res.headers, jsonResponse, res.status);
}
private convertTranFromServer(entity: any) {
entity.postDate = this.dateUtils
.convertLocalDateFromServer(entity.postDate);
entity.createdDate = this.dateUtils
.convertLocalDateFromServer(entity.createdDate);
}
private convertItemFromServer(entity: any) {
entity.dueDate = this.dateUtils
.convertLocalDateFromServer(entity.dueDate);
entity.closingDate = this.dateUtils
.convertLocalDateFromServer(entity.closingDate);
}
private convert(financeAccount: FinanceAccount): FinanceAccount {
const copy: FinanceAccount = Object.assign({}, financeAccount);
copy.dueDate = this.dateUtils
.convertLocalDateToServer(financeAccount.dueDate);
copy.closingDate = this.dateUtils
.convertLocalDateToServer(financeAccount.closingDate);
return copy;
}
private convertCriteria(criteria: any): any {
const copy: any = Object.assign({}, criteria);
if(copy.startDate)
copy.startDate = this.dateUtils
.convertLocalDateToServer(this.dateTimeService.convertToObjDate(criteria.startDate));
if(copy.endDate)
copy.endDate = this.dateUtils
.convertLocalDateToServer(this.dateTimeService.convertToObjDate(criteria.endDate));
return copy;
}
}
<file_sep>/src/main/webapp/app/shared/search/search.component.ts
import {Component, OnInit} from "@angular/core";
import {Observable} from "rxjs/Observable";
import {Subject} from "rxjs/Subject";
@Component({
selector: 'jhi-search',
templateUrl: './search.component.html',
providers: []
})
export class SearchComponent implements OnInit {
items: Observable<any[]>;
private searchTerms = new Subject<string>();
constructor() {}
// Push a search term into the observable stream.
search(term: string): void {
this.searchTerms.next(term);
}
ngOnInit(): void {
this.items = this.searchTerms
.debounceTime(300) // wait 300ms after each keystroke before considering the term
.distinctUntilChanged() // ignore if next search term is same as previous
.switchMap(term => term // switch to new observable each time the term changes
// return the http search observable
? this.searchAysnc(term)
// or the observable of empty heroes if there was no search term
: Observable.of<any[]>([]))
.catch(error => {
// TODO: add real error handling
console.log(error);
return Observable.of<any[]>([]);
});
}
private searchAysnc(term: string): Observable<any[]> {
return Observable.of<any[]>([]);
}
}
<file_sep>/src/main/webapp/app/shared/model/request-util.ts
import { URLSearchParams, BaseRequestOptions } from '@angular/http';
export const createRequestOption = (req?: any): BaseRequestOptions => {
const options: BaseRequestOptions = new BaseRequestOptions();
if (req) {
const params: URLSearchParams = new URLSearchParams();
params.set('page', req.page);
params.set('size', req.size);
if (req.sort) {
params.paramsMap.set('sort', req.sort);
}
params.set('query', req.query);
options.params = params;
}
return options;
};
export const createQueryRequestOption = (req?: any, criteria?: any): BaseRequestOptions => {
const options: BaseRequestOptions = new BaseRequestOptions();
if (req) {
const urlParams: URLSearchParams = new URLSearchParams();
const params = {};
params['page'] = req.page;
params['size'] = req.size;
if (req.sort) {
params['sort'] = req.sort;
}
params['query'] = req.query;
urlParams.set("pageable", JSON.stringify(params));
if(criteria){
criteria["active"]=true;
urlParams.set("criteria", JSON.stringify(criteria));
}
else
//urlParams.set("criteria", JSON.stringify({"active": false, "startDate":"", "endDate":"","desc":"","startAmount":"","endAmount":""}));
urlParams.set("criteria", JSON.stringify({"active": false}));
options.params = urlParams;
}
return options;
};
<file_sep>/src/test/javascript/e2e/entities/bank.spec.ts
import { browser, element, by, $ } from 'protractor';
import { NavBarPage } from './../page-objects/jhi-page-objects';
const path = require('path');
describe('Bank e2e test', () => {
let navBarPage: NavBarPage;
let bankDialogPage: BankDialogPage;
let bankComponentsPage: BankComponentsPage;
const fileToUpload = '../../../../main/webapp/content/images/logo-jhipster.png';
const absolutePath = path.resolve(__dirname, fileToUpload);
beforeAll(() => {
browser.get('/');
browser.waitForAngular();
navBarPage = new NavBarPage();
navBarPage.getSignInPage().autoSignInUsing('admin', 'admin');
browser.waitForAngular();
});
it('should load Banks', () => {
navBarPage.goToEntity('bank');
bankComponentsPage = new BankComponentsPage();
expect(bankComponentsPage.getTitle()).toMatch(/sfWebClientApp.bank.home.title/);
});
it('should load create Bank dialog', () => {
bankComponentsPage.clickOnCreateButton();
bankDialogPage = new BankDialogPage();
expect(bankDialogPage.getModalTitle()).toMatch(/sfWebClientApp.bank.home.createOrEditLabel/);
bankDialogPage.close();
});
it('should create and save Banks', () => {
bankComponentsPage.clickOnCreateButton();
bankDialogPage.setNameInput('name');
expect(bankDialogPage.getNameInput()).toMatch('name');
bankDialogPage.setDescriptionInput('description');
expect(bankDialogPage.getDescriptionInput()).toMatch('description');
bankDialogPage.save();
expect(bankDialogPage.getSaveButton().isPresent()).toBeFalsy();
});
afterAll(() => {
navBarPage.autoSignOut();
});
});
export class BankComponentsPage {
createButton = element(by.css('.jh-create-entity'));
title = element.all(by.css('jhi-bank div h2 span')).first();
clickOnCreateButton() {
return this.createButton.click();
}
getTitle() {
return this.title.getAttribute('jhiTranslate');
}
}
export class BankDialogPage {
modalTitle = element(by.css('h4#myBankLabel'));
saveButton = element(by.css('.modal-footer .btn.btn-primary'));
closeButton = element(by.css('button.close'));
nameInput = element(by.css('input#field_name'));
descriptionInput = element(by.css('input#field_description'));
getModalTitle() {
return this.modalTitle.getAttribute('jhiTranslate');
}
setNameInput = function (name) {
this.nameInput.sendKeys(name);
}
getNameInput = function () {
return this.nameInput.getAttribute('value');
}
setDescriptionInput = function (description) {
this.descriptionInput.sendKeys(description);
}
getDescriptionInput = function () {
return this.descriptionInput.getAttribute('value');
}
save() {
this.saveButton.click();
}
close() {
this.closeButton.click();
}
getSaveButton() {
return this.saveButton;
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/index.ts
export * from './tran-category.model';
export * from './tran-category-popup.service';
export * from './tran-category.service';
export * from './tran-category-dialog.component';
export * from './tran-category-delete-dialog.component';
export * from './tran-category-detail.component';
export * from './tran-category.component';
export * from './tran-category.route';
<file_sep>/src/main/webapp/app/shared/search/search.module.ts
import {NgModule} from '@angular/core';
import {SearchDirective} from './search.directive';
@NgModule({
imports: [],
declarations: [SearchDirective],
exports: [
SearchDirective
]
})
export class SearchModule {
}
<file_sep>/src/test/javascript/e2e/entities/tran-category-regex.spec.ts
import { browser, element, by, $ } from 'protractor';
import { NavBarPage } from './../page-objects/jhi-page-objects';
const path = require('path');
describe('TranCategoryRegex e2e test', () => {
let navBarPage: NavBarPage;
let tranCategoryRegexDialogPage: TranCategoryRegexDialogPage;
let tranCategoryRegexComponentsPage: TranCategoryRegexComponentsPage;
const fileToUpload = '../../../../main/webapp/content/images/logo-jhipster.png';
const absolutePath = path.resolve(__dirname, fileToUpload);
beforeAll(() => {
browser.get('/');
browser.waitForAngular();
navBarPage = new NavBarPage();
navBarPage.getSignInPage().autoSignInUsing('admin', 'admin');
browser.waitForAngular();
});
it('should load TranCategoryRegexes', () => {
navBarPage.goToEntity('tran-category-regex');
tranCategoryRegexComponentsPage = new TranCategoryRegexComponentsPage();
expect(tranCategoryRegexComponentsPage.getTitle()).toMatch(/sfWebClientApp.tranCategoryRegex.home.title/);
});
it('should load create TranCategoryRegex dialog', () => {
tranCategoryRegexComponentsPage.clickOnCreateButton();
tranCategoryRegexDialogPage = new TranCategoryRegexDialogPage();
expect(tranCategoryRegexDialogPage.getModalTitle()).toMatch(/sfWebClientApp.tranCategoryRegex.home.createOrEditLabel/);
tranCategoryRegexDialogPage.close();
});
it('should create and save TranCategoryRegexes', () => {
tranCategoryRegexComponentsPage.clickOnCreateButton();
tranCategoryRegexDialogPage.setNameInput('name');
expect(tranCategoryRegexDialogPage.getNameInput()).toMatch('name');
tranCategoryRegexDialogPage.setDescriptionInput('description');
expect(tranCategoryRegexDialogPage.getDescriptionInput()).toMatch('description');
tranCategoryRegexDialogPage.setRegexInput('regex');
expect(tranCategoryRegexDialogPage.getRegexInput()).toMatch('regex');
tranCategoryRegexDialogPage.userSelectLastOption();
tranCategoryRegexDialogPage.save();
expect(tranCategoryRegexDialogPage.getSaveButton().isPresent()).toBeFalsy();
});
afterAll(() => {
navBarPage.autoSignOut();
});
});
export class TranCategoryRegexComponentsPage {
createButton = element(by.css('.jh-create-entity'));
title = element.all(by.css('jhi-tran-category-regex div h2 span')).first();
clickOnCreateButton() {
return this.createButton.click();
}
getTitle() {
return this.title.getAttribute('jhiTranslate');
}
}
export class TranCategoryRegexDialogPage {
modalTitle = element(by.css('h4#myTranCategoryRegexLabel'));
saveButton = element(by.css('.modal-footer .btn.btn-primary'));
closeButton = element(by.css('button.close'));
nameInput = element(by.css('input#field_name'));
descriptionInput = element(by.css('input#field_description'));
regexInput = element(by.css('input#field_regex'));
userSelect = element(by.css('select#field_user'));
getModalTitle() {
return this.modalTitle.getAttribute('jhiTranslate');
}
setNameInput = function (name) {
this.nameInput.sendKeys(name);
}
getNameInput = function () {
return this.nameInput.getAttribute('value');
}
setDescriptionInput = function (description) {
this.descriptionInput.sendKeys(description);
}
getDescriptionInput = function () {
return this.descriptionInput.getAttribute('value');
}
setRegexInput = function (regex) {
this.regexInput.sendKeys(regex);
}
getRegexInput = function () {
return this.regexInput.getAttribute('value');
}
userSelectLastOption = function () {
this.userSelect.all(by.tagName('option')).last().click();
}
userSelectOption = function (option) {
this.userSelect.sendKeys(option);
}
getUserSelect = function () {
return this.userSelect;
}
getUserSelectedOption = function () {
return this.userSelect.element(by.css('option:checked')).getText();
}
save() {
this.saveButton.click();
}
close() {
this.closeButton.click();
}
getSaveButton() {
return this.saveButton;
}
}
<file_sep>/src/test/javascript/e2e/entities/account-transaction.spec.ts
import { browser, element, by, $ } from 'protractor';
import { NavBarPage } from './../page-objects/jhi-page-objects';
const path = require('path');
describe('AccountTransaction e2e test', () => {
let navBarPage: NavBarPage;
let accountTransactionDialogPage: AccountTransactionDialogPage;
let accountTransactionComponentsPage: AccountTransactionComponentsPage;
const fileToUpload = '../../../../main/webapp/content/images/logo-jhipster.png';
const absolutePath = path.resolve(__dirname, fileToUpload);
beforeAll(() => {
browser.get('/');
browser.waitForAngular();
navBarPage = new NavBarPage();
navBarPage.getSignInPage().autoSignInUsing('admin', 'admin');
browser.waitForAngular();
});
it('should load AccountTransactions', () => {
navBarPage.goToEntity('account-transaction');
accountTransactionComponentsPage = new AccountTransactionComponentsPage();
expect(accountTransactionComponentsPage.getTitle()).toMatch(/sfWebClientApp.accountTransaction.home.title/);
});
it('should load create AccountTransaction dialog', () => {
accountTransactionComponentsPage.clickOnCreateButton();
accountTransactionDialogPage = new AccountTransactionDialogPage();
expect(accountTransactionDialogPage.getModalTitle()).toMatch(/sfWebClientApp.accountTransaction.home.createOrEditLabel/);
accountTransactionDialogPage.close();
});
/* it('should create and save AccountTransactions', () => {
accountTransactionComponentsPage.clickOnCreateButton();
accountTransactionDialogPage.tranTypeSelectLastOption();
accountTransactionDialogPage.setTranNumberInput('tranNumber');
expect(accountTransactionDialogPage.getTranNumberInput()).toMatch('tranNumber');
accountTransactionDialogPage.setReferenceNumberInput('referenceNumber');
expect(accountTransactionDialogPage.getReferenceNumberInput()).toMatch('referenceNumber');
accountTransactionDialogPage.setPostDateInput('2000-12-31');
expect(accountTransactionDialogPage.getPostDateInput()).toMatch('2000-12-31');
accountTransactionDialogPage.setDescriptionInput('description');
expect(accountTransactionDialogPage.getDescriptionInput()).toMatch('description');
accountTransactionDialogPage.setAmountInput('5');
expect(accountTransactionDialogPage.getAmountInput()).toMatch('5');
accountTransactionDialogPage.paymentMethodSelectLastOption();
accountTransactionDialogPage.userSelectLastOption();
accountTransactionDialogPage.financeAccountSelectLastOption();
accountTransactionDialogPage.tranCategorySelectLastOption();
accountTransactionDialogPage.save();
expect(accountTransactionDialogPage.getSaveButton().isPresent()).toBeFalsy();
}); */
afterAll(() => {
navBarPage.autoSignOut();
});
});
export class AccountTransactionComponentsPage {
createButton = element(by.css('.jh-create-entity'));
title = element.all(by.css('jhi-account-transaction div h2 span')).first();
clickOnCreateButton() {
return this.createButton.click();
}
getTitle() {
return this.title.getAttribute('jhiTranslate');
}
}
export class AccountTransactionDialogPage {
modalTitle = element(by.css('h4#myAccountTransactionLabel'));
saveButton = element(by.css('.modal-footer .btn.btn-primary'));
closeButton = element(by.css('button.close'));
tranTypeSelect = element(by.css('select#field_tranType'));
tranNumberInput = element(by.css('input#field_tranNumber'));
referenceNumberInput = element(by.css('input#field_referenceNumber'));
postDateInput = element(by.css('input#field_postDate'));
descriptionInput = element(by.css('input#field_description'));
amountInput = element(by.css('input#field_amount'));
paymentMethodSelect = element(by.css('select#field_paymentMethod'));
userSelect = element(by.css('select#field_user'));
financeAccountSelect = element(by.css('select#field_financeAccount'));
tranCategorySelect = element(by.css('select#field_tranCategory'));
getModalTitle() {
return this.modalTitle.getAttribute('jhiTranslate');
}
setTranTypeSelect = function (tranType) {
this.tranTypeSelect.sendKeys(tranType);
}
getTranTypeSelect = function () {
return this.tranTypeSelect.element(by.css('option:checked')).getText();
}
tranTypeSelectLastOption = function () {
this.tranTypeSelect.all(by.tagName('option')).last().click();
}
setTranNumberInput = function (tranNumber) {
this.tranNumberInput.sendKeys(tranNumber);
}
getTranNumberInput = function () {
return this.tranNumberInput.getAttribute('value');
}
setReferenceNumberInput = function (referenceNumber) {
this.referenceNumberInput.sendKeys(referenceNumber);
}
getReferenceNumberInput = function () {
return this.referenceNumberInput.getAttribute('value');
}
setPostDateInput = function (postDate) {
this.postDateInput.sendKeys(postDate);
}
getPostDateInput = function () {
return this.postDateInput.getAttribute('value');
}
setDescriptionInput = function (description) {
this.descriptionInput.sendKeys(description);
}
getDescriptionInput = function () {
return this.descriptionInput.getAttribute('value');
}
setAmountInput = function (amount) {
this.amountInput.sendKeys(amount);
}
getAmountInput = function () {
return this.amountInput.getAttribute('value');
}
setPaymentMethodSelect = function (paymentMethod) {
this.paymentMethodSelect.sendKeys(paymentMethod);
}
getPaymentMethodSelect = function () {
return this.paymentMethodSelect.element(by.css('option:checked')).getText();
}
paymentMethodSelectLastOption = function () {
this.paymentMethodSelect.all(by.tagName('option')).last().click();
}
userSelectLastOption = function () {
this.userSelect.all(by.tagName('option')).last().click();
}
userSelectOption = function (option) {
this.userSelect.sendKeys(option);
}
getUserSelect = function () {
return this.userSelect;
}
getUserSelectedOption = function () {
return this.userSelect.element(by.css('option:checked')).getText();
}
financeAccountSelectLastOption = function () {
this.financeAccountSelect.all(by.tagName('option')).last().click();
}
financeAccountSelectOption = function (option) {
this.financeAccountSelect.sendKeys(option);
}
getFinanceAccountSelect = function () {
return this.financeAccountSelect;
}
getFinanceAccountSelectedOption = function () {
return this.financeAccountSelect.element(by.css('option:checked')).getText();
}
tranCategorySelectLastOption = function () {
this.tranCategorySelect.all(by.tagName('option')).last().click();
}
tranCategorySelectOption = function (option) {
this.tranCategorySelect.sendKeys(option);
}
getTranCategorySelect = function () {
return this.tranCategorySelect;
}
getTranCategorySelectedOption = function () {
return this.tranCategorySelect.element(by.css('option:checked')).getText();
}
save() {
this.saveButton.click();
}
close() {
this.closeButton.click();
}
getSaveButton() {
return this.saveButton;
}
}
<file_sep>/src/main/java/com/calderon/sf/domain/AccountTransaction.java
package com.calderon.sf.domain;
import com.calderon.sf.domain.enumeration.PaymentMethod;
import com.calderon.sf.domain.enumeration.TranType;
import org.hibernate.annotations.Cache;
import org.hibernate.annotations.CacheConcurrencyStrategy;
import javax.persistence.*;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import java.io.Serializable;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.util.Objects;
/**
* A AccountTransaction.
*/
@Entity
@Table(name = "account_transaction")
@Cache(usage = CacheConcurrencyStrategy.NONSTRICT_READ_WRITE)
public class AccountTransaction extends AbstractAuditingEntity implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@NotNull
@Enumerated(EnumType.STRING)
@Column(name = "tran_type", nullable = false)
private TranType tranType;
@Column(name = "tran_number")
private String tranNumber;
@Column(name = "reference_number")
private String referenceNumber;
@NotNull
@Column(name = "post_date", nullable = false)
private LocalDate postDate;
@Size(max = 256, min = 2)
@Column(name = "description", length = 256, nullable = false)
private String description;
@NotNull
@Column(name = "amount", precision=10, scale=2, nullable = false)
private BigDecimal amount;
@Enumerated(EnumType.STRING)
@Column(name = "payment_method")
private PaymentMethod paymentMethod;
@ManyToOne
private User user;
@ManyToOne(optional = false)
@NotNull
private FinanceAccount financeAccount;
@ManyToOne
private TranCategory tranCategory;
// jhipster-needle-entity-add-field - Jhipster will add fields here, do not remove
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public TranType getTranType() {
return tranType;
}
public AccountTransaction tranType(TranType tranType) {
this.tranType = tranType;
return this;
}
public void setTranType(TranType tranType) {
this.tranType = tranType;
}
public String getTranNumber() {
return tranNumber;
}
public AccountTransaction tranNumber(String tranNumber) {
this.tranNumber = tranNumber;
return this;
}
public void setTranNumber(String tranNumber) {
this.tranNumber = tranNumber;
}
public String getReferenceNumber() {
return referenceNumber;
}
public AccountTransaction referenceNumber(String referenceNumber) {
this.referenceNumber = referenceNumber;
return this;
}
public void setReferenceNumber(String referenceNumber) {
this.referenceNumber = referenceNumber;
}
public LocalDate getPostDate() {
return postDate;
}
public AccountTransaction postDate(LocalDate postDate) {
this.postDate = postDate;
return this;
}
public void setPostDate(LocalDate postDate) {
this.postDate = postDate;
}
public String getDescription() {
return description;
}
public AccountTransaction description(String description) {
this.description = description;
return this;
}
public void setDescription(String description) {
this.description = description;
}
public BigDecimal getAmount() {
return amount;
}
public AccountTransaction amount(BigDecimal amount) {
this.amount = amount;
return this;
}
public void setAmount(BigDecimal amount) {
this.amount = amount;
}
public PaymentMethod getPaymentMethod() {
return paymentMethod;
}
public AccountTransaction paymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public User getUser() {
return user;
}
public AccountTransaction user(User user) {
this.user = user;
return this;
}
public void setUser(User user) {
this.user = user;
}
public FinanceAccount getFinanceAccount() {
return financeAccount;
}
public AccountTransaction financeAccount(FinanceAccount financeAccount) {
this.financeAccount = financeAccount;
return this;
}
public void setFinanceAccount(FinanceAccount financeAccount) {
this.financeAccount = financeAccount;
}
public TranCategory getTranCategory() {
return tranCategory;
}
public AccountTransaction tranCategory(TranCategory tranCategory) {
this.tranCategory = tranCategory;
return this;
}
public void setTranCategory(TranCategory tranCategory) {
this.tranCategory = tranCategory;
}
// jhipster-needle-entity-add-getters-setters - Jhipster will add getters and setters here, do not remove
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
AccountTransaction accountTransaction = (AccountTransaction) o;
if (accountTransaction.getId() == null || getId() == null) {
return false;
}
return Objects.equals(getId(), accountTransaction.getId());
}
@Override
public int hashCode() {
return Objects.hashCode(getId());
}
@Override
public String toString() {
return "AccountTransaction{" +
"id=" + getId() +
", tranType='" + getTranType() + "'" +
", tranNumber='" + getTranNumber() + "'" +
", referenceNumber='" + getReferenceNumber() + "'" +
", postDate='" + getPostDate() + "'" +
", description='" + getDescription() + "'" +
", amount='" + getAmount() + "'" +
", paymentMethod='" + getPaymentMethod() + "'" +
"}";
}
}
<file_sep>/src/test/javascript/e2e/entities/finance-account.spec.ts
import { browser, element, by, $ } from 'protractor';
import { NavBarPage } from './../page-objects/jhi-page-objects';
const path = require('path');
describe('FinanceAccount e2e test', () => {
let navBarPage: NavBarPage;
let financeAccountDialogPage: FinanceAccountDialogPage;
let financeAccountComponentsPage: FinanceAccountComponentsPage;
const fileToUpload = '../../../../main/webapp/content/images/logo-jhipster.png';
const absolutePath = path.resolve(__dirname, fileToUpload);
beforeAll(() => {
browser.get('/');
browser.waitForAngular();
navBarPage = new NavBarPage();
navBarPage.getSignInPage().autoSignInUsing('admin', 'admin');
browser.waitForAngular();
});
it('should load FinanceAccounts', () => {
navBarPage.goToEntity('finance-account');
financeAccountComponentsPage = new FinanceAccountComponentsPage();
expect(financeAccountComponentsPage.getTitle()).toMatch(/sfWebClientApp.financeAccount.home.title/);
});
it('should load create FinanceAccount dialog', () => {
financeAccountComponentsPage.clickOnCreateButton();
financeAccountDialogPage = new FinanceAccountDialogPage();
expect(financeAccountDialogPage.getModalTitle()).toMatch(/sfWebClientApp.financeAccount.home.createOrEditLabel/);
financeAccountDialogPage.close();
});
it('should create and save FinanceAccounts', () => {
financeAccountComponentsPage.clickOnCreateButton();
financeAccountDialogPage.accountStatusSelectLastOption();
financeAccountDialogPage.setAccountNumberInput('accountNumber');
expect(financeAccountDialogPage.getAccountNumberInput()).toMatch('accountNumber');
financeAccountDialogPage.setNameInput('name');
expect(financeAccountDialogPage.getNameInput()).toMatch('name');
financeAccountDialogPage.setDescriptionInput('description');
expect(financeAccountDialogPage.getDescriptionInput()).toMatch('description');
financeAccountDialogPage.setBalanceInput('5');
expect(financeAccountDialogPage.getBalanceInput()).toMatch('5');
financeAccountDialogPage.getIsCreditCardInput().isSelected().then(function (selected) {
if (selected) {
financeAccountDialogPage.getIsCreditCardInput().click();
expect(financeAccountDialogPage.getIsCreditCardInput().isSelected()).toBeFalsy();
} else {
financeAccountDialogPage.getIsCreditCardInput().click();
expect(financeAccountDialogPage.getIsCreditCardInput().isSelected()).toBeTruthy();
}
});
financeAccountDialogPage.setDueDateInput('2000-12-31');
expect(financeAccountDialogPage.getDueDateInput()).toMatch('2000-12-31');
financeAccountDialogPage.setClosingDateInput('2000-12-31');
expect(financeAccountDialogPage.getClosingDateInput()).toMatch('2000-12-31');
financeAccountDialogPage.userSelectLastOption();
financeAccountDialogPage.save();
expect(financeAccountDialogPage.getSaveButton().isPresent()).toBeFalsy();
});
afterAll(() => {
navBarPage.autoSignOut();
});
});
export class FinanceAccountComponentsPage {
createButton = element(by.css('.jh-create-entity'));
title = element.all(by.css('jhi-finance-account div h2 span')).first();
clickOnCreateButton() {
return this.createButton.click();
}
getTitle() {
return this.title.getAttribute('jhiTranslate');
}
}
export class FinanceAccountDialogPage {
modalTitle = element(by.css('h4#myFinanceAccountLabel'));
saveButton = element(by.css('.modal-footer .btn.btn-primary'));
closeButton = element(by.css('button.close'));
accountStatusSelect = element(by.css('select#field_accountStatus'));
accountNumberInput = element(by.css('input#field_accountNumber'));
nameInput = element(by.css('input#field_name'));
descriptionInput = element(by.css('input#field_description'));
balanceInput = element(by.css('input#field_balance'));
isCreditCardInput = element(by.css('input#field_isCreditCard'));
dueDateInput = element(by.css('input#field_dueDate'));
closingDateInput = element(by.css('input#field_closingDate'));
userSelect = element(by.css('select#field_user'));
getModalTitle() {
return this.modalTitle.getAttribute('jhiTranslate');
}
setAccountStatusSelect = function (accountStatus) {
this.accountStatusSelect.sendKeys(accountStatus);
}
getAccountStatusSelect = function () {
return this.accountStatusSelect.element(by.css('option:checked')).getText();
}
accountStatusSelectLastOption = function () {
this.accountStatusSelect.all(by.tagName('option')).last().click();
}
setAccountNumberInput = function (accountNumber) {
this.accountNumberInput.sendKeys(accountNumber);
}
getAccountNumberInput = function () {
return this.accountNumberInput.getAttribute('value');
}
setNameInput = function (name) {
this.nameInput.sendKeys(name);
}
getNameInput = function () {
return this.nameInput.getAttribute('value');
}
setDescriptionInput = function (description) {
this.descriptionInput.sendKeys(description);
}
getDescriptionInput = function () {
return this.descriptionInput.getAttribute('value');
}
setBalanceInput = function (balance) {
this.balanceInput.sendKeys(balance);
}
getBalanceInput = function () {
return this.balanceInput.getAttribute('value');
}
getIsCreditCardInput = function () {
return this.isCreditCardInput;
}
setDueDateInput = function (dueDate) {
this.dueDateInput.sendKeys(dueDate);
}
getDueDateInput = function () {
return this.dueDateInput.getAttribute('value');
}
setClosingDateInput = function (closingDate) {
this.closingDateInput.sendKeys(closingDate);
}
getClosingDateInput = function () {
return this.closingDateInput.getAttribute('value');
}
userSelectLastOption = function () {
this.userSelect.all(by.tagName('option')).last().click();
}
userSelectOption = function (option) {
this.userSelect.sendKeys(option);
}
getUserSelect = function () {
return this.userSelect;
}
getUserSelectedOption = function () {
return this.userSelect.element(by.css('option:checked')).getText();
}
save() {
this.saveButton.click();
}
close() {
this.closeButton.click();
}
getSaveButton() {
return this.saveButton;
}
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/tran-category-detail.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { Subscription } from 'rxjs/Rx';
import { JhiEventManager } from 'ng-jhipster';
import { TranCategory } from './tran-category.model';
import { TranCategoryService } from './tran-category.service';
@Component({
selector: 'jhi-tran-category-detail',
templateUrl: './tran-category-detail.component.html'
})
export class TranCategoryDetailComponent implements OnInit, OnDestroy {
tranCategory: TranCategory;
private subscription: Subscription;
private eventSubscriber: Subscription;
constructor(
private eventManager: JhiEventManager,
private tranCategoryService: TranCategoryService,
private route: ActivatedRoute
) {
}
ngOnInit() {
this.subscription = this.route.params.subscribe((params) => {
this.load(params['id']);
});
this.registerChangeInTranCategories();
}
load(id) {
this.tranCategoryService.find(id).subscribe((tranCategory) => {
this.tranCategory = tranCategory;
});
}
previousState() {
window.history.back();
}
ngOnDestroy() {
this.subscription.unsubscribe();
this.eventManager.destroy(this.eventSubscriber);
}
registerChangeInTranCategories() {
this.eventSubscriber = this.eventManager.subscribe(
'tranCategoryListModification',
(response) => this.load(this.tranCategory.id)
);
}
}
<file_sep>/src/main/webapp/app/account/activate/activate.component.ts
import {Component, OnDestroy, OnInit} from '@angular/core';
import { NgbModalRef } from '@ng-bootstrap/ng-bootstrap';
import {ActivatedRoute, Router} from '@angular/router';
import { ActivateService } from './activate.service';
import { LoginModalService } from '../../shared';
import {Principal} from "../../shared/auth/principal.service";
import {Subscription} from "rxjs/Subscription";
import {JhiEventManager} from "ng-jhipster";
@Component({
selector: 'jhi-activate',
templateUrl: './activate.component.html'
})
export class ActivateComponent implements OnInit, OnDestroy {
error: string;
success: string;
modalRef: NgbModalRef;
eventSubscriber: Subscription;
constructor(
private eventManager: JhiEventManager,
private activateService: ActivateService,
private loginModalService: LoginModalService,
private route: ActivatedRoute,
private router: Router,
private principal: Principal,
) {
}
ngOnInit() {
this.onAuthentication();
this.route.queryParams.subscribe((params) => {
this.activateService.get(params['key']).subscribe(() => {
this.error = null;
this.success = 'OK';
}, () => {
this.success = null;
this.error = 'ERROR';
});
});
this.registerOnUserLogin();
}
login() {
this.modalRef = this.loginModalService.open();
}
onAuthentication() {
if(this.principal.isAuthenticated())
this.router.navigate(['/']);
}
registerOnUserLogin() {
this.eventSubscriber = this.eventManager.subscribe('authenticationSuccess', (content) => {
this.onAuthentication();
});
}
ngOnDestroy(): void {
this.eventManager.destroy(this.eventSubscriber);
}
}
<file_sep>/src/main/java/com/calderon/sf/repository/TranCategoryRegexRepository.java
package com.calderon.sf.repository;
import com.calderon.sf.domain.TranCategoryRegex;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Query;
import org.springframework.stereotype.Repository;
import java.util.List;
/**
* Spring Data JPA repository for the TranCategoryRegex entity.
*/
@SuppressWarnings("unused")
@Repository
public interface TranCategoryRegexRepository extends JpaRepository<TranCategoryRegex, Long> {
@Query("select tran_category_regex from TranCategoryRegex tran_category_regex where tran_category_regex.user.login = ?#{principal.username}")
List<TranCategoryRegex> findByUserIsCurrentUser();
@Query("select tran_category_regex from TranCategoryRegex tran_category_regex where tran_category_regex.user.login = ?#{principal.username}")
Page<TranCategoryRegex> findByUserIsCurrentUser(Pageable pageable);
}
<file_sep>/src/main/webapp/app/sf-entities/tran-category/tran-category.model.ts
import { BaseEntity, User } from './../../shared';
export class TranCategory implements BaseEntity {
constructor(
public id?: number,
public name?: string,
public description?: string,
public transactions?: BaseEntity[],
public user?: User,
public tranCategoryRegex?: BaseEntity,
) {
}
}
<file_sep>/src/main/java/com/calderon/sf/domain/projections/Transaction.java
package com.calderon.sf.domain.projections;
import java.math.BigDecimal;
import java.time.LocalDate;
public interface Transaction {
String getDescription();
BigDecimal getAmount();
LocalDate getPostDate();
}
| 3bdc0a0705a1a509601ea2abff1972f7ee17cd9e | [
"Java",
"TypeScript"
] | 74 | Java | ncalderon/sf-web-client | d957e2ffddc4c41fabebffa80c851ed68cf4e522 | 6bd743a0cd99387176f35814d348077516bfe90d | |
refs/heads/master | <file_sep>#include "foo.h"
int foo_add(int x, int y) {
return x + y;
}
void foo_hello() {
std::cout << "hello from foo\n";
}
<file_sep>#ifndef FOO_H
#define FOO_H
#include <iostream>
int foo_add(int x, int y);
void foo_hello();
#endif
<file_sep>bazel C++ example
build with:
bazel build //src/utils/bar:bar<file_sep>//#include "src/include/libfoo/foo.h" // works
#include "foo.h"
int main() {
foo_hello();
return foo_add(0, 0);
}
| bd1a49d3770fff6b2377bb3433088915447742fb | [
"Markdown",
"C++"
] | 4 | C++ | nitingupta910/bazel_cpp_example | 9fbfd72602a5335aaf9dfaa7f15b0037b51a8dd8 | e471e84dd300b57bc7becc008147fcccab22fd5e | |
refs/heads/master | <file_sep>### Florida Facts Quiz App project
[Test your knowledge of the Sunshine State!](https://orndorfftyler.github.io/quiz-app/)
This is a simple 5 question quiz that keeps track of the user's current question and score.
This exercise helped me get familiar with jQuery and get used to breaking up a program into many smaller specialized functions.
I also used wireframing for the first time in this project. Before writing the JS allowing the user to navigate the quiz, I wrote some basic HMTL/CSS describing each different screen that would be needed. This basic wireframe is in a separate repo called quiz-app-wireframe.
Built with HTML, CSS, JavaScript, jQuery.
<file_sep>/**
*
* Technical requirements:
*
* Your app should include a render() function, that regenerates the view each time the store is updated.
* See your course material and access support for more details.
*
* NO additional HTML elements should be added to the index.html file.
*
* You may add attributes (classes, ids, etc) to the existing HTML elements, or link stylesheets or additional scripts if necessary
*
* SEE BELOW FOR THE CATEGORIES OF THE TYPES OF FUNCTIONS YOU WILL BE CREATING
*
*/
/********** TEMPLATE GENERATION FUNCTIONS **********/
// These functions return HTML templates
/********** RENDER FUNCTION(S) **********/
// This function conditionally replaces the contents of the <main> tag based on the state of the store
/********** EVENT HANDLER FUNCTIONS **********/
// These functions handle events (submit, click, etc)
const store = {
// 5 or more questions are required
questions: [
{
question: 'In what year did Florida become a state?',
answers: [
'1845',
'1869',
'1902',
'1801'
],
correctAnswer: '1845'
},
{
question: 'What is the most populous city in Florida?',
answers: [
'Tallahassee',
'Miami',
'Jacksonville',
'Orlando'
],
correctAnswer: 'Jacksonville'
},
{
question: 'Excluding barrier islands, how many miles of coastline does Florida have?',
answers: [
'1350',
'958',
'576',
'215'
],
correctAnswer: '1350'
},
{
question: 'What is the largest National Park in Florida?',
answers: [
'Alafia River State Park',
'Everglades National Park',
'Big Lagoon State Park',
'Grayton Beach State Park'
],
correctAnswer: 'Everglades National Park'
},
{
question: 'What is the largest freshwater lake in Florida?',
answers: [
'Lake Eustis',
'Lake Istokpoga',
'Lake Okeechobee',
'Crescent Lake'
],
correctAnswer: 'Lake Okeechobee'
}
],
quizStarted: false,
questionNumber: 0,
score: 0,
//potential values of view: start, question, check, final
//(changed when submit button clicked)
view: 'start'
};
function scoreKeeper() { //used by multiple render functions
let num = store.questionNumber + 1;
if (store.view == 'start') {
num = store.questionNumber;
}
let score = store.score;
return '\
<h1>Florida Quiz</h1>\
<div class="mobile">\
<p>Score '+ score +'/5</p>\
<p>Question '+ num +'/5</p>\
</div>\
';
}
//---------------------------startRender() and helper functions
function startBuilder() {
return '\
<form>\
<h2>Want to test your knowledge of Florida?</h2>\
<button id="start" type="submit">Begin</button>\
</form>\
'
}
function startRender() {
let score = scoreKeeper();
$('header').html(score);
let startMain = startBuilder();
$('main').html(startMain);
}
//---------------------------end of startRender() and helper functions
//---------------------------questionRender() and helper functions
function qGrabber() {
let num = store.questionNumber;
let q = store.questions[num].question;
let a = store.questions[num].answers; // a will be an array of 4 strings
return [q,a];
}
function qBuilder() {
let qa = qGrabber();
return '\
<form>\
<h2>' + qa[0] + '</h2>\
<div>\
<input id="a1" name="q" type="radio" value="Answer1">\
<label for="a1">' + qa[1][0] + '</label>\
</div>\
<div>\
<input id="b1" name="q" type="radio" value="Answer2">\
<label for="b1">' + qa[1][1] + '</label>\
</div>\
<div>\
<input id="c1" name="q" type="radio" value="Answer3">\
<label for="c1">' + qa[1][2] + '</label>\
</div>\
<div>\
<input id="d1" name="q" type="radio" value="Answer4">\
<label for="d1">' + qa[1][3] + '</label>\
</div>\
<button id="question" type="submit">Submit</button>\
</form>\
';
}
function questionRender() {
let score = scoreKeeper();
$('header').html(score);
let question = qBuilder();
$('main').html(question);
}
//---------------------------end of questionRender() and helper functions
//---------------------------checkRender() and helper functions
function correct() {
return '\
<form>\
<h2>Correct!</h2>\
<p>Good job!</p>\
<button id="check" type="submit">Next</button>\
</form>\
';
}
function incorrect(rightAnswer) {
return '\
<form>\
<h2>Incorrect</h2>\
<p>Correct answer was '+ rightAnswer +'</p>\
<button id="check" type="submit">Next</button>\
</form>\
';
}
function checkRender(state, rightAnswer) {
let score = scoreKeeper();
$('header').html(score);
if (state) {
let congrats = correct();
$('main').html(congrats);
} else {
let niceTry = incorrect(rightAnswer);
$('main').html(niceTry);
}
}
//---------------------------end of checkRender() and helper functions
//---------------------------finalRender() and helper functions
function finalScore() {
let score = store.score;
let final = '\
<h2>Final Score: '+ score +'/5</h2>\
';
if (score >= 5) {
return pass(final);
} else {
return fail(final);
}
}
function pass(final) {
return '\
<form>\
'+ final +'\
<p>Congrats! Your knowledge of Florida is vast.</p>\
<button id="final" type="submit">Again</button>\
</form>\
';
}
function fail(final) {
return '\
<form>\
'+ final +'\
<p>Not perfect, but good attempt!</p>\
<button id="final" type="submit">Again</button>\
</form>\
';
}
function finalRender() {
let score = scoreKeeper();
$('header').html(score);
let finalMain = finalScore();
$('main').html(finalMain);
}
//---------------------------end of finalRender() and helper functions
//---------------------------render() function
function render(state, rightAnswer) {
if (store.view == 'start') {
startRender();
}
if (store.view == 'question') {
questionRender();
}
if (store.view == 'check') {
checkRender(state, rightAnswer);
}
if (store.view == 'final') {
finalRender();
}
}
//---------------------------event handler functions and helper functions
function startButtonClicked() {
$('main').on('click', '#start', function(event) {
event.preventDefault();
store.view = 'question';
render();
});
};
function qGrader(answer, qNum) {
let correct = store.questions[qNum].correctAnswer;
if (answer == correct) {
store.score ++;
return [true, correct];
} else {
return [false, correct];
}
}
function questionButtonClicked() {
$('main').on('click', '#question', function(event) {
event.preventDefault();
let answer = $("input[name='q']:checked").siblings('label').text();
if (answer != "") {
let state = qGrader(answer, store.questionNumber);
store.view = 'check';
render(state[0], state[1]);
}
});
};
function questionOrFinalView() {
if (store.questionNumber >= 4) {
store.view = 'final';
} else {
store.questionNumber ++;
store.view = 'question';
console.log('q to:'+ store.questionNumber);
}
}
function checkButtonClicked() {
$('main').on('click', '#check', function(event) {
event.preventDefault();
questionOrFinalView();
render();
});
}
function finalButtonClicked() {
$('main').on('click', '#final', function(event) {
event.preventDefault();
store.questionNumber = 0;
store.score = 0;
store.view = 'start';
render();
});
}
function handleQuizApp() {
render();
startButtonClicked();
questionButtonClicked();
checkButtonClicked();
finalButtonClicked();
}
$(handleQuizApp);
| 147c4bd2992342645cfe4e6ac43300350c2f1e9e | [
"Markdown",
"JavaScript"
] | 2 | Markdown | orndorfftyler/quiz-app | 88cc3c4853ebc7f9c6acd501c350cf702248f5be | 1907cc1c3b316571a52a150a7cf366aabfce0c12 | |
refs/heads/master | <repo_name>yantze/fs-lockfile<file_sep>/index.js
const fs = require('fs')
const path = require('path')
const { promisify } = require('util')
const fsp = {
readFile : promisify(fs.readFile),
writeFile : promisify(fs.writeFile),
}
const lockMap = new Map()
function generateLock() {
const lock = {
resolve: null,
obtain: null,
}
const promise = new Promise(resolve => lock.resolve = resolve)
lock.obtain = () => promise
return lock
}
async function obtainReadLock(fp) {
let lockMeta = lockMap.get(fp)
if (!lockMeta) {
lockMeta = {
filePath: fp,
readCount: 0,
writeQueue: [],
readLock: null,
}
lockMap.set(fp, lockMeta)
}
// 循环等待最后一个写锁
while(lockMeta.writeQueue.length) {
await lockMeta.writeQueue[lockMeta.writeQueue.length - 1].obtain()
}
if (!lockMeta.readLock) {
lockMeta.readLock = generateLock()
}
++lockMeta.readCount
return lockMeta
}
async function obtainWriteLock(fp) {
let lockMeta = lockMap.get(fp)
if (!lockMeta) {
lockMeta = {
filePath: fp,
readCount: 0,
writeQueue: [],
readLock: null,
}
lockMap.set(fp, lockMeta)
}
let prevLock = null
const lockNum = lockMeta.writeQueue.length
if (lockNum >= 1) {
prevLock = lockMeta.writeQueue[lockNum-1]
}
// 先放进队列中,让其它请求都能获取这个队列
lockMeta.writeQueue.push(generateLock())
// 检查当前是否有读锁
if (lockMeta.readLock) {
await lockMeta.readLock.obtain()
}
if (prevLock) {
// 等待最后一个锁释放
await prevLock.obtain()
}
return lockMeta
}
async function releaseReadLock(lockMeta) {
if (lockMeta.readLock) {
--lockMeta.readCount
} else {
throw new Error('No read lock found')
}
if (lockMeta.readCount === 0) {
lockMeta.readLock.resolve()
lockMeta.readLock = null
}
if (lockMeta.readCount === 0 && lockMeta.writeQueue.length === 0) {
lockMap.delete(lockMeta.filePath)
}
}
async function releaseWriteLock(lockMeta) {
if (lockMeta.writeQueue.length) {
lockMeta.writeQueue[0].resolve()
} else {
throw new Error('No write lock.')
}
lockMeta.writeQueue.shift()
if (lockMeta.readCount === 0 && lockMeta.writeQueue.length === 0) {
lockMap.delete(lockMeta.filePath)
}
}
async function read(fp) {
if (!path.isAbsolute(fp)) throw new Error('Must be an absolute path.')
const lockMeta = await obtainReadLock(fp)
try {
return await fsp.readFile(fp)
} finally {
await releaseReadLock(lockMeta)
}
}
async function write(fp, content) {
if (!path.isAbsolute(fp)) throw new Error('Must be an absolute path.')
if (content === undefined || content === null) throw new Error('Content can not be undefined or null.')
const lockMeta = await obtainWriteLock(fp)
try {
return await fsp.writeFile(fp, content)
} finally {
await releaseWriteLock(lockMeta)
}
}
module.exports = {
read,
write,
obtainReadLock,
obtainWriteLock,
releaseReadLock,
releaseWriteLock,
}
<file_sep>/README.md
# fs-lockfile
Managing the reading and writing of files in the application.
Only one action can manipulate file in the mean time.
No Dependencies.
## Installation
```
npm i --save fs-lockfile
```
## Method of fs-lockfile
- **read(filePath): Promise**
- **write(filePath, content): Promise**
- **obtainReadLock(filePath): Object**
- **obtainWriteLock(filePath): Object**
- **releaseReadLock(lockMeta): undefined**
- **releaseWriteLock(lockMeta): undefined**
## Usage
**Example:**
```js
const path = require('path')
const { read, write } = require('fs-lockfile')
async function demo(filePath) {
await write(filePath, 'test data')
const content = await read(filePath)
console.log('File content:', content.toString())
}
const filePath = path.join(__dirname, './test.txt')
demo(filePath)
```
Another example:
```js
async function read(fp) {
if (!path.isAbsolute(fp)) throw new Error('Must be an absolute path.')
const lockMeta = await obtainReadLock(fp)
try {
return await fsp.readFile(fp)
} catch (readError) {
throw readError
} finally {
await releaseReadLock(lockMeta)
}
}
```
## LISENCE
MIT
| ef22c5aeed04c545d6b64a14e47a6dfcf65cb624 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | yantze/fs-lockfile | 297c3767a5b4e4a776e42a82e79e16d1cb8694d7 | be8eebf0a771467bf0a06f887baee812bcfe3f57 | |
refs/heads/master | <repo_name>matpalm/dead-simple-ga<file_sep>/member.rb
class Array
def randomize
clear
GENE_SIZE.times { self << random_gene_value }
self
end
def random_gene_value
(rand()*10).to_i
end
def fitness
inject { |a,v| a+v }
end
def mutate
point_wise_mutation
inversion_mutation
end
def point_wise_mutation
return unless rand() < 0.01
self[rand(size)] = random_gene_value
end
def inversion_mutation
return unless rand() < 0.01
from, to = rand(size), rand(size)
from, to = to, from if from > to
splice = self.slice(from,to-from).reverse
(from...to).each { |idx| self[idx] = splice.shift }
end
def crossover_with other
self.zip(other).collect { |p| rand()>0.5 ? p[0] : p[1]}
end
end
def random_member
[].randomize
end
<file_sep>/main.rb
GENE_SIZE = 30
POPN_SIZE = 20
require 'member'
require 'population'
p = Population.new
5000.times { p.epoch }
<file_sep>/population.rb
class Population
def initialize
@members = []
POPN_SIZE.times { @members << random_member }
end
def epoch
calculate_fitness
breed_next_gen
mutate_members
#inject_random_member
output_members
end
def calculate_fitness
@fitness = @members.collect {|m| m.fitness }
@total_fitness = @fitness.inject{|a,v| a+v}
puts "@fitness=#{@fitness.inspect} @total_fitness=#{@total_fitness}"
end
def breed_next_gen
next_gen_members = []
(POPN_SIZE/2).times do
parent1 = select_member
parent2 = select_member
next_gen_members << parent1.crossover_with(parent2)
next_gen_members << parent2.crossover_with(parent1)
end
@members = next_gen_members
end
def select_member
selection_value = rand(@total_fitness)
@fitness.each_with_index do |v,i|
selection_value -= v
return @members[i] if selection_value<=0
end
raise "fail!"
end
def mutate_members
@members.each {|m| m.mutate }
end
def inject_random_member
@members.first.randomize
end
def output_members
@members.each {|m| puts m.inspect }
end
end
| addc61961b0b020c75c1ffdc30234a414f693c93 | [
"Ruby"
] | 3 | Ruby | matpalm/dead-simple-ga | dbcec3d7d1f6797f95389dcb299d2b2ee3807613 | 4c91d978e244e568fac6bb107cf06fb375deab05 | |
refs/heads/master | <repo_name>lhx295801268/marraySetApp<file_sep>/ProductionCreateTest/ProductionCreateTest/model/Config/YCNoticationNameConfig.h
//
// YCNoticationNameConfig.h
// ProductionCreateTest
//
// Created by MDJ on 2018/9/26.
// Copyright © 2018年 TsunamiLi. All rights reserved.
//
#ifndef YCNoticationNameConfig_h
#define YCNoticationNameConfig_h
#endif /* YCNoticationNameConfig_h */
<file_sep>/ProductionCreateTest/ProductionCreateTest/model/Config/YCPathConfig.h
//
// YCPathConfig.h
// ProductionCreateTest
//
// Created by MDJ on 2018/9/26.
// Copyright © 2018年 TsunamiLi. All rights reserved.
//
#ifndef YCPathConfig_h
//url地址集合
#define YCDefRootUrl @"https://www.baidu.com"
//document 文件夹下的文件
#define YCDefFamilyObjFilePath @"familyObjListFile"
#define YCPathConfig_h
#endif /* YCPathConfig_h */
<file_sep>/ProductionCreateTest/Podfile
target 'ProductionCreateTest' do
# 自动布局
pod 'PureLayout', '~> 3.1.2'
# 监听
pod 'ReactiveObjC', '~> 3.1.0'
# 多图片网络w缓存
pod 'SDWebImage', '~> 4.4.2'
# 网络监测
pod 'Reachability', '~> 3.2'
# 本地数据库
pod 'FMDB', '~> 2.7.5'
# 网络请求组件
pod 'AFNetworking', '~> 3.2.1'
# 数据解析
pod 'YYModel', '~> 1.0.4'
# 上下拉刷新
pod 'MJRefresh', '~> 3.1.15.7'
# tableView collectionview空白页面展示
pod 'DZNEmptyDataSet', '~> 1.8.0'
# 自定义布局yytext yylabel
pod 'YYText', '~> 1.0.7'
end
<file_sep>/ProductionCreateTest/ProductionCreateTest/model/Obj/YCTypeDefObj.h
//
// YCTypeDefObj.h
// ProductionCreateTest
//
// Created by TsunamiLi on 2018/9/28.
// Copyright © 2018年 TsunamiLi. All rights reserved.
//
#define YCDefScreenWidth ([UIScreen mainScreen].bounds.size.width)
#define YCDefScreenHeight ([UIScreen mainScreen].bounds.size.height)
#define YCDefImageWithName(name) ((name == nil || name.length <= 0) ? (nil) : ([UIImage imageNamed:imageName]))
typedef void (^ONE_PARAM_BLOCK)(id __nullable param);
typedef void (^TWO_PARAM_BLOCK)(id __nullable param1, id __nullable param2);
typedef NS_ENUM(NSInteger, YCHttpRequestMethod) {
kYCHttpRequestMethod4Post = 0,
kYCHttpRequestMethod4Get,
kYCHttpRequestMethod4Delete
};
<file_sep>/README.md
# marraySetApp
创建于2018-9-27
| ff021e388b9f3a490a15379efe47b24e37798f68 | [
"Markdown",
"C",
"Ruby"
] | 5 | C | lhx295801268/marraySetApp | 0949d805df27ac0893e41d941dd47c1652fffc7a | 65e0c0e636c376de0650217c24002430958e9b42 | |
refs/heads/master | <file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerInput : MonoBehaviour
{
public string inputPostfix;
public float AxisMoveX { get; private set; }
public float AxisMoveY { get; private set; }
public bool Punch { get; private set; }
// Update is called once per frame
void Update()
{
AxisMoveX = Input.GetAxisRaw("Horizontal_"+ inputPostfix);
AxisMoveY = Input.GetAxisRaw("Vertical_" + inputPostfix);
Punch = Input.GetButtonDown("Fire_" + inputPostfix);
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[System.Serializable]
public struct PlayerSettingContainer
{
private int id;
public PlayerController playerController;
public HeadquarterController headquarter;
public UIHealthManager uiHealthHeadquarterController;
public UIHealthManager uiHealthPlayerController;
internal void SetPlayerId(int id)
{
this.id = id;
playerController.SetPlayerId(id);
headquarter.SetPlayerId(id);
uiHealthPlayerController.SetHealthManager(playerController);
uiHealthHeadquarterController.SetHealthManager(headquarter);
}
public int GetId()
{
return id;
}
}
<file_sep>using System;
public interface IHealthManager
{
event Action<int,int> OnHealthChange;
event Action<int> OnHealtZero;
int GetMaxHealth();
int GetHealth();
void ModifyHealt(int modifier);
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameController : MonoBehaviour
{
public static GameController INSTANCE { get; private set; }
private void Awake()
{
if (INSTANCE == null)
{
INSTANCE = this;
}
else
{
Destroy(this);
}
}
private void Start()
{
foreach(PlayerSettingContainer container in PlayerSettingsManager.INSTANCE.playersContainers)
{
container.headquarter.OnHealtZero += OnPlayerLoose;
}
}
private void OnPlayerLoose(int playerId)
{
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HeadquarterController : MonoBehaviour, IHealthManager
{
public event Action<int,int> OnHealthChange;
public event Action<int> OnHealtZero;
public int maxHealth;
private int health;
private int playerId;
private void Awake()
{
health = maxHealth;
}
public int GetPlayerId()
{
return playerId;
}
public int GetHealth()
{
return health;
}
public int GetMaxHealth()
{
return maxHealth;
}
public void ModifyHealt(int modifier)
{
health += modifier;
if (OnHealthChange != null)
OnHealthChange(playerId,modifier);
if (health <= 0)
{
if (OnHealtZero != null)
OnHealtZero(playerId);
}
else
{
if (health > maxHealth)
health = maxHealth;
}
}
internal void SetPlayerId(int playerId)
{
this.playerId = playerId;
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMain : MonoBehaviour
{
private int id;
public PlayerController PlayerController { get; private set; }
private void Awake()
{
PlayerController = GetComponent<PlayerController>();
}
internal void SetPlayerId(int id)
{
this.id = id;
}
public int GetId()
{
return id;
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerController : MonoBehaviour, IHealthManager
{
public event Action<int, int> OnHealthChange;
public event Action<int> OnHealtZero;
public float movementSpeed;
public int maxEnergy;
public float stunTime;
public Vector2 FacingVector { get; private set; }
private int playerId;
private PlayerInput playerInput;
private Rigidbody2D mRigidbody2D;
private Vector2 currentVelocity;
private int energy;
private bool canPunch;
private void Awake()
{
playerInput = GetComponent<PlayerInput>();
mRigidbody2D = GetComponent<Rigidbody2D>();
currentVelocity = mRigidbody2D.velocity;
FacingVector = transform.right;
energy = maxEnergy;
canPunch = true;
}
void Update()
{
if (energy > 0)
{
currentVelocity.x = playerInput.AxisMoveX;
currentVelocity.y = playerInput.AxisMoveY;
Flip();
currentVelocity.Normalize();
mRigidbody2D.velocity = movementSpeed * currentVelocity;
if (canPunch && playerInput.Punch)
{
Debug.Log("punch " + playerInput.inputPostfix);
}
}
}
void FixedUpdate()
{
Facing();
}
public int GetHealth()
{
return energy;
}
public int GetMaxHealth()
{
return maxEnergy;
}
public void ModifyHealt(int modifier)
{
energy += modifier;
if (OnHealthChange != null)
OnHealthChange(playerId, modifier);
if (energy <= 0)
{
if (OnHealtZero != null)
OnHealtZero(playerId);
}
else
{
if (energy > maxEnergy)
energy = maxEnergy;
}
}
private void Flip()
{
if ((currentVelocity.x * transform.right.x) < 0)
{
transform.Rotate(0, 180, 0);
}
if ((currentVelocity.y * transform.up.y) < 0)
{
transform.Rotate(180, 0, 0);
}
}
private void Facing()
{
if (currentVelocity.x != 0 || currentVelocity.y != 0)
{
FacingVector = (currentVelocity.x * Vector2.right) + (currentVelocity.y * Vector2.up);
}
FacingVector.Normalize();
Debug.DrawRay(transform.position, FacingVector * 3, Color.red);
}
internal void SetPlayerId(int id)
{
this.playerId = id;
}
public int GetId()
{
return this.playerId;
}
}
<file_sep>using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class UIHealthManager : MonoBehaviour
{
private IHealthManager healthManager;
public Slider sliderHealth;
public void SetHealthManager(IHealthManager healthManager)
{
if (this.healthManager!=null)
{
this.healthManager.OnHealthChange -= OnHealthChange;
this.healthManager.OnHealtZero -= OnHealthZero;
}
this.healthManager = healthManager;
this.healthManager.OnHealthChange += OnHealthChange;
this.healthManager.OnHealtZero += OnHealthZero;
sliderHealth.minValue = 0;
sliderHealth.maxValue = this.healthManager.GetMaxHealth();
sliderHealth.value = this.healthManager.GetHealth();
}
private void OnHealthChange(int playerId, int modify)
{
sliderHealth.value = sliderHealth.value + modify;
}
private void OnHealthZero(int playerId)
{
}
}
| d8536bbf42c52dca3dcf3fe24de5cb040b0e73c5 | [
"C#"
] | 8 | C# | kevinesaa/planticas | 95c07a9ec2964f7262a9cef7a0ffb8a501ca0287 | 4485e81b2b063e685faec1290940f75b8a0df028 | |
refs/heads/main | <repo_name>Ivan-Jimenez/facial-gesture-detection-demo<file_sep>/face-gesture-detection-demo/FacialGestureCameraView.swift
//
// FacialGestureCameraView.swift
// face-gesture-detection-demo
//
// Created by <NAME> on 17/12/20.
//
import UIKit
import AVFoundation
import FirebaseMLVision
class FacialGestureCameraView: UIView {
private var restingFace: Bool = true
private lazy var vision: Vision = {
return Vision.vision()
}()
private lazy var options: VisionFaceDetectorOptions = {
let option = VisionFaceDetectorOptions()
option.performanceMode = .accurate
option.landmarkMode = .none
option.classificationMode = .all
option.isTrackingEnabled = false
option.contourMode = .none
return option
}()
private lazy var videoDataOutput: AVCaptureVideoDataOutput = {
let videoOutput = AVCaptureVideoDataOutput()
videoOutput.alwaysDiscardsLateVideoFrames = true
videoOutput.setSampleBufferDelegate(self, queue: videoDataOutputQueue)
videoOutput.connection(with: .video)?.isEnabled = true
return videoOutput
}()
private let videoDataOutputQueue: DispatchQueue = DispatchQueue(label: "Constants.videoDataOutputQueue")
private lazy var session: AVCaptureSession = {
return AVCaptureSession()
}()
private lazy var previewLayer: AVCaptureVideoPreviewLayer = {
let layer = AVCaptureVideoPreviewLayer(session: session)
layer.videoGravity = .resizeAspectFill
return layer
}()
private let captureDevice: AVCaptureDevice? = AVCaptureDevice.default(.builtInWideAngleCamera, for: .video, position: .front)
public var leftNodThreshold: CGFloat = 20.0
public var rightNodThreshold: CGFloat = -4
public var smileProbability: CGFloat = 0.8
public var openEyeMaxProbability: CGFloat = 0.95
public var openEyeMinProbability: CGFloat = 0.1
public weak var delegate: FacialGestureCameraViewDelegate?
}
// MARK: - Logic
extension FacialGestureCameraView {
private func detectFacesOnDevice(in image: VisionImage, width: CGFloat, height: CGFloat) {
let faceDetector = vision.faceDetector(options: options)
faceDetector.process(image, completion: { features, error in
if let error = error {
print(error.localizedDescription)
return
}
guard error == nil, let features = features, !features.isEmpty else { return }
if let face = features.first {
let leftEyeOpenProbability = face.leftEyeOpenProbability
let rightEyeOpenProbability = face.rightEyeOpenProbability
// left head node
if face.headEulerAngleZ > self.leftNodThreshold {
if self.restingFace {
self.restingFace = false
self.delegate?.nodLeftDetected?()
}
} else if face.headEulerAngleZ < self.rightNodThreshold {
// right head tilt
if self.restingFace {
self.restingFace = false
self.delegate?.nodRightDetected?()
}
} else if leftEyeOpenProbability > self.openEyeMaxProbability && rightEyeOpenProbability < self.openEyeMinProbability {
// right eye blink
if self.restingFace {
self.restingFace = false
self.delegate?.rightEyeBlinkDetected?()
}
} else if rightEyeOpenProbability > self.openEyeMaxProbability && leftEyeOpenProbability < self.openEyeMinProbability {
// left eye blink
if self.restingFace {
self.restingFace = false
self.delegate?.leftEyeBlinkDetected?()
}
} else if face.smilingProbability > self.smileProbability {
// smile detected
if self.restingFace {
self.restingFace = false
self.delegate?.smileDetected?()
}
} else if leftEyeOpenProbability < self.openEyeMinProbability && rightEyeOpenProbability < self.openEyeMinProbability {
// full/both eye blink
if self.restingFace {
self.restingFace = false
self.delegate?.doubleEyeBlinkDetected?()
}
} else {
// face got reseted
self.restingFace = true
}
}
})
}
}
// MARK: - Handlers
extension FacialGestureCameraView {
func beginSession() {
guard let deviceCapture = captureDevice else { return }
guard let deviceInput = try? AVCaptureDeviceInput(device: deviceCapture) else { return }
if session.canAddInput(deviceInput) {
session.addInput(deviceInput)
}
if session.canAddOutput(videoDataOutput) {
session.addOutput(videoDataOutput)
}
layer.masksToBounds = true
layer.addSublayer(previewLayer)
previewLayer.frame = bounds
session.startRunning()
}
func stopSession() {
session.stopRunning()
}
}
// MARK: - AVCaptureVideoDataOutputSampleBufferDelegate
extension FacialGestureCameraView: AVCaptureVideoDataOutputSampleBufferDelegate {
func captureOutput(_ output: AVCaptureOutput, didOutput sampleBuffer: CMSampleBuffer, from connection: AVCaptureConnection) {
guard let imageBuffer = CMSampleBufferGetImageBuffer(sampleBuffer) else {
print("Failed to get image buffer from sample buffer.")
return
}
let visionImage = VisionImage(buffer: sampleBuffer)
let metadata = VisionImageMetadata()
let visionOrientation = visionImageOrientation(from: imageOrientation())
metadata.orientation = visionOrientation
visionImage.metadata = metadata
let imageWidth = CGFloat(CVPixelBufferGetWidth(imageBuffer))
let imageHeight = CGFloat(CVPixelBufferGetHeight(imageBuffer))
DispatchQueue.global().async {
self.detectFacesOnDevice(in: visionImage, width: imageWidth, height: imageHeight)
}
}
}
// MARK: - Util Functions
extension FacialGestureCameraView {
private func visionImageOrientation(from imageOrientation: UIImage.Orientation) -> VisionDetectorImageOrientation {
switch imageOrientation {
case .up: return .topLeft
case .down: return .bottomRight
case .left: return .leftBottom
case .right: return .topRight
case .upMirrored: return .topRight
case .downMirrored: return .bottomLeft
case .leftMirrored: return .leftTop
case .rightMirrored: return .rightBottom
@unknown default:
fatalError()
}
}
private func imageOrientation(fromDevicePosition devicePosition: AVCaptureDevice.Position = .front) -> UIImage.Orientation {
var deviceOrientation = UIDevice.current.orientation
if deviceOrientation == .faceDown || deviceOrientation == .faceUp || deviceOrientation == .unknown {
deviceOrientation = currentUIOrientation()
}
switch deviceOrientation {
case .portrait: return devicePosition == .front ? .leftMirrored : .right
case .landscapeLeft: return devicePosition == .front ? .downMirrored : .up
case .portraitUpsideDown: return devicePosition == .front ? .rightMirrored : .left
case .landscapeRight: return devicePosition == .front ? .upMirrored : .down
case .faceDown, .faceUp, .unknown: return .up
@unknown default:
fatalError()
}
}
private func currentUIOrientation() -> UIDeviceOrientation {
let deviceOrientation = { () -> UIDeviceOrientation in
switch UIApplication.shared.windows.first?.windowScene?.interfaceOrientation {
case .landscapeLeft: return .landscapeRight
case .landscapeRight: return .landscapeLeft
case .portraitUpsideDown: return .portraitUpsideDown
case .portrait, .unknown, .none: return .portrait
@unknown default:
fatalError()
}
}
guard Thread.isMainThread else {
var currentOrientation: UIDeviceOrientation = .portrait
DispatchQueue.main.async {
currentOrientation = deviceOrientation()
}
return currentOrientation
}
return deviceOrientation()
}
}
// MARK: - Delegate
@objc public protocol FacialGestureCameraViewDelegate: class {
@objc optional func doubleEyeBlinkDetected()
@objc optional func smileDetected()
@objc optional func nodLeftDetected()
@objc optional func nodRightDetected()
@objc optional func leftEyeBlinkDetected()
@objc optional func rightEyeBlinkDetected()
}
<file_sep>/face-gesture-detection-demo/ViewController.swift
//
// ViewController.swift
// face-gesture-detection-demo
//
// Created by <NAME> on 17/12/20.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var cameraView: FacialGestureCameraView!
override func viewDidLoad() {
super.viewDidLoad()
addCameraViewDelegate()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
startGestureDetection()
}
override func viewDidDisappear(_ animated: Bool) {
super.viewDidDisappear(animated)
stopGestureDetection()
}
}
extension ViewController: FacialGestureCameraViewDelegate {
func doubleEyeBlinkDetected() {
print("Double Eye Blink Detected")
}
func smileDetected() {
print("Smile Detected")
}
func nodLeftDetected() {
print("Nod Left Detected")
}
func nodRightDetected() {
print("Nod Right Detected")
}
func leftEyeBlinkDetected() {
print("Left Eye Blink Detected")
}
func rightEyeBlinkDetected() {
print("Right Eye Blink Detected")
}
}
extension ViewController {
private func addCameraViewDelegate() {
cameraView.delegate = self
}
private func startGestureDetection() {
cameraView.beginSession()
}
private func stopGestureDetection() {
cameraView.stopSession()
}
}
| 0d46e3bb86d5e94e571ef853d626b2526fe94f34 | [
"Swift"
] | 2 | Swift | Ivan-Jimenez/facial-gesture-detection-demo | a59c4237a97ad476f3f61144e923854b5caa045b | c522088d549e7de765717584935ef20712308fc5 | |
refs/heads/master | <file_sep>package org.uma.jmetal.util.experiment.util;
import org.uma.jmetal.problem.DoubleProblem;
import org.uma.jmetal.solution.Solution;
import org.uma.jmetal.solution.impl.DefaultDoubleSolution;
import org.uma.jmetal.util.extremevalues.impl.SolutionListExtremeValues;
import org.uma.jmetal.util.naming.DescribedEntity;
/**
* Created by ajnebro on 23/12/15.
*/
public interface TaggedSolution<T> extends Solution<T> {
public String getTag() ;
}
| 2319bcf94a9591cd37acc95d5631fcffd2570a8e | [
"Java"
] | 1 | Java | Navieclipse/jMetal | c9851ff633fa53de9357c606e35e8034d64b475b | 1d02fff4b44845f6f58564e13c107a87c012028f | |
refs/heads/master | <repo_name>oHa510/ioBroker.alpha2<file_sep>/main.js
/**
*
* Moehlenhoff Alpha2 Adapter
*
*
* file io-package.json comments:
*
*
*/
/* jshint -W097 */// jshint strict:false
/*jslint node: true */
'use strict';
// you have to require the utils module and call adapter function
const utils = require('@iobroker/adapter-core'); // Get common adapter utils
// you have to call the adapter function and pass a options object
// name has to be set and has to be equal to adapters folder name and main file name excluding extension
// adapter will be restarted automatically every time as the configuration changed, e.g system.adapter.template.0
const adapter = new utils.Adapter('alpha2');
const request = require('request');
const parser = require('xml2js').parseString;
const http = require('http');
// define adapter wide vars
var device_id;
/*Variable declaration, since ES6 there are let to declare variables. Let has a more clearer definition where
it is available then var.The variable is available inside a block and it's childs, but not outside.
You can define the same variable name inside a child without produce a conflict with the variable of the parent block.*/
let variable = 1234;
// is called when adapter shuts down - callback has to be called under any circumstances!
adapter.on('unload', function (callback) {
try {
adapter.log.info('cleaned everything up...');
callback();
} catch (e) {
callback();
}
});
// is called if a subscribed object changes
adapter.on('objectChange', function (id, obj) {
// Warning, obj can be null if it was deleted
adapter.log.debug('objectChange ' + id + ' ' + JSON.stringify(obj));
});
// is called if a subscribed state changes
adapter.on('stateChange', function (id, state) {
// Warning, state can be null if it was deleted
//adapter.log.info('stateChange ' + id + ' ' + JSON.stringify(state));
// you can use the ack flag to detect if it is status (true) or command (false)
if (state && !state.ack) {
adapter.log.debug('ack is not set!');
adapter.log.debug('Value: ' + state.val);
adapter.log.debug('id: ' + id);
// save value in var
var new_val = state.val;
// Set HEATAREA values
for (var i = 1; i < 13; i++) //Alle möglichen HEATAREA's abprüfen..
{
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.T_TARGET') {var heatarea = i; var new_target = 'T_TARGET'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.T_HEAT_DAY') {var heatarea = i; var new_target = 'T_HEAT_DAY'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.T_HEAT_NIGHT') {var heatarea = i; var new_target = 'T_HEAT_NIGHT'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.T_COOL_DAY') {var heatarea = i; var new_target = 'T_COOL_DAY'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.T_COOL_NIGHT') {var heatarea = i; var new_target = 'T_COOL_NIGHT'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.HEATAREA_MODE') {var heatarea = i; var new_target = 'HEATAREA_MODE'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.PROGRAM_WEEK') {var heatarea = i; var new_target = 'PROGRAM_WEEK'; var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.PROGRAM_WEEKEND') {var heatarea = i;var new_target = 'PROGRAM_WEEKEND';var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
if (id == adapter.namespace + '.' + 'HEATAREA.' + i + '.ISLOCKED') {var heatarea = i;var new_target = 'ISLOCKED';var xml_construct = '<HEATAREA nr="'+ heatarea +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></HEATAREA>';}
}
if (id == adapter.namespace + '.' + 'DEVICE.T_HEAT_VACATION') {var new_target = 'T_HEAT_VACATION';var xml_construct = '<'+ new_target +'>'+ new_val +'</'+ new_target +'>';}
if (id == adapter.namespace + '.' + 'DEVICE.LOCK_MODE') {var new_target = 'LOCK_MODE';var xml_construct = '<'+ new_target +'>'+ new_val +'</'+ new_target +'>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.1.END') {var nr = '1'; var shift = '1';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.1.START') {var nr = '1'; var shift = '1';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.2.END') {var nr = '1'; var shift = '2';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.2.START') {var nr = '1'; var shift = '2';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.3.END') {var nr = '1'; var shift = '3';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.3.START') {var nr = '1'; var shift = '3';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.4.END') {var nr = '1'; var shift = '4';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.1.4.START') {var nr = '1'; var shift = '4';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.1.END') {var nr = '2'; var shift = '1';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.1.START') {var nr = '2'; var shift = '1';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.2.END') {var nr = '2'; var shift = '2';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.2.START') {var nr = '2'; var shift = '2';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.3.END') {var nr = '2'; var shift = '3';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.3.START') {var nr = '2'; var shift = '3';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.4.END') {var nr = '2'; var shift = '4';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.2.4.START') {var nr = '2'; var shift = '4';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.1.END') {var nr = '3'; var shift = '1';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.1.START') {var nr = '3'; var shift = '1';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.2.END') {var nr = '3'; var shift = '2';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.2.START') {var nr = '3'; var shift = '2';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.3.END') {var nr = '3'; var shift = '3';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.3.START') {var nr = '3'; var shift = '3';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.4.END') {var nr = '3'; var shift = '4';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.3.4.START') {var nr = '3'; var shift = '4';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.1.END') {var nr = '4'; var shift = '1';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.1.START') {var nr = '4'; var shift = '1';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.2.END') {var nr = '4'; var shift = '2';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.2.START') {var nr = '4'; var shift = '2';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.3.END') {var nr = '41'; var shift = '3';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.3.START') {var nr = '4'; var shift = '3';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.4.END') {var nr = '4'; var shift = '4';var new_target = 'END';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'PROGRAM.4.4.START') {var nr = '4'; var shift = '4';var new_target = 'START';var xml_construct = '<PROGRAM><SHIFT_PROGRAM nr="'+ nr +'" shiftingtime="'+ shift +'"><'+ new_target +'>'+ new_val +'</'+ new_target +'></SHIFT_PROGRAM></PROGRAM>';}
if (id == adapter.namespace + '.' + 'VACATION.START_DATE') {var new_target = 'START_DATE';var xml_construct = '<VACATION><'+ new_target +'>'+ new_val +'</'+ new_target +'></VACATION>';}
if (id == adapter.namespace + '.' + 'VACATION.END_DATE') {var new_target = 'END_DATE';var xml_construct = '<VACATION><'+ new_target +'>'+ new_val +'</'+ new_target +'></VACATION>';}
// Post DATA to DEVICE
var data = '<?xml version="1.0" encoding="UTF-8"?><Devices><Device><ID>'+ device_id +'</ID>'+ xml_construct +'</Device></Devices>';
httpPost(data);
}
});
// Some message was sent to adapter instance over message box. Used by email, pushover, text2speech, ...
adapter.on('message', function (obj) {
if (typeof obj === 'object' && obj.message) {
if (obj.command === 'send') {
// e.g. send email or pushover or whatever
console.log('send command');
// Send response in callback if required
if (obj.callback) adapter.sendTo(obj.from, obj.command, 'Message received', obj.callback);
}
}
});
// is called when databases are connected and adapter received configuration.
// start here!
adapter.on('ready', function () {
main();
});
// Post Data to XML-API
function httpPost(data) {
// URL, die abgefragt, bzw. gesendet werden soll:
var options = {
host: adapter.config.host,
path: '/data/changes.xml',
method: 'POST' // in der Regel: "GET"
};
var req = http.request(options, function(res) {
adapter.log.debug("http Status: " + res.statusCode);
adapter.log.debug('HEADERS: ' + JSON.stringify(res.headers), (res.statusCode != 200 ? "warn" : "info")); // Header (R�ckmeldung vom Webserver)
if (res.statusCode == 200) {
setTimeout(getXMLcyclic,5000);
}
});
req.on('error', function(e) { // Fehler abfangen
adapter.log.info('ERROR: ' + e.message,"warn");
});
adapter.log.debug("Data to request body: " + data);
// write data to request body
(data ? req.write(data) : adapter.log.info("Daten: keine Daten angegeben"));
req.end();
}
// Get XML Data from API
function getXMLcyclic() {
request('http://'+adapter.config.host+'/data/static.xml', function (error, response, body) {
if (!error && response.statusCode == 200) {
getTemp(body);
} else {
adapter.log.info("Fehler beim Herunterladen: " + error, 'error');
}
});
}
function getTemp(xml) {
parser(xml, {explicitArray: false, mergeAttrs: true, explicitRoot: false}, function(err, obj) {
if(err) adapter.log.info('Fehler XML-Parsen: ' + err, 'error');
else {
adapter.log.debug("XMLcyclic: " + JSON.stringify(obj));
adapter.setState(adapter.namespace + '.' + 'DEVICE.ID', {val: obj.Device.ID, ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.NAME', {val: obj.Device.NAME, ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.TYPE', {val: obj.Device.TYPE, ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.DATETIME', {val: obj.Device.DATETIME, ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.TIMEZONE', {val: obj.Device.TIMEZONE, ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.NTPSYNC', {val: Boolean(Number(obj.Device.NTPTIMESYNC)), ack: true});
adapter.setState(adapter.namespace + '.' + 'DEVICE.T_HEAT_VACATION', {val: parseFloat(obj.Device.T_HEAT_VACATION), ack: true});
adapter.setState(adapter.namespace + '.' + 'VACATION.STATE', {val: Boolean(Number(obj.Device.VACATION.VACATION_STATE)), ack: true});
adapter.setState(adapter.namespace + '.' + 'VACATION.START_DATE', {val: obj.Device.VACATION.START_DATE, ack: true});
adapter.setState(adapter.namespace + '.' + 'VACATION.START_TIME', {val: obj.Device.VACATION.START_TIME, ack: true});
adapter.setState(adapter.namespace + '.' + 'VACATION.END_DATE', {val: obj.Device.VACATION.END_DATE, ack: true});
adapter.setState(adapter.namespace + '.' + 'VACATION.END_TIME', {val: obj.Device.VACATION.END_TIME, ack: true});
adapter.log.debug("Program lenght 0: " + obj.Device.PROGRAM.SHIFT_PROGRAM.length);
// Fill PROGRAM objects with variable XML array lenght
if (obj.Device.PROGRAM.SHIFT_PROGRAM.length < 16) {
for (var i = 1; i < 17; i++) {
adapter.setState(adapter.namespace + '.' + 'PROGRAM.'+ [i] +'.'+ [i] +'.START', {val: null, ack: true});
adapter.setState(adapter.namespace + '.' + 'PROGRAM.'+ [i] +'.'+ [i] +'.END', {val: null, ack: true});
}
}
for (var i = 0; i < obj.Device.PROGRAM.SHIFT_PROGRAM.length; i++) {
adapter.log.debug("--- for loop position: " + i);
adapter.log.debug("Program NR: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].nr);
adapter.log.debug("Shiftingtime: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].shiftingtime);
adapter.log.debug("Start: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].START);
adapter.log.debug("End: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].END);
adapter.log.debug("PROGRAM: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].nr +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].shiftingtime +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].START);
adapter.log.debug("PROGRAM: " + obj.Device.PROGRAM.SHIFT_PROGRAM[i].nr +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].shiftingtime +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].END);
adapter.setState(adapter.namespace + '.' + 'PROGRAM.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].nr +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].shiftingtime +'.START', {val: obj.Device.PROGRAM.SHIFT_PROGRAM[i].START , ack: true});
adapter.setState(adapter.namespace + '.' + 'PROGRAM.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].nr +'.'+ obj.Device.PROGRAM.SHIFT_PROGRAM[i].shiftingtime +'.END', {val: obj.Device.PROGRAM.SHIFT_PROGRAM[i].END , ack: true});
}
for (var i = 0; i < obj.Device.HEATAREA.length; i++) {
var HeatareaNr = obj.Device.HEATAREA[i].nr; //Nummer der Heatarea auslesen
//adapter.log.info(JSON.stringify(obj.Device.HEATAREA));
//adapter.log.info(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.');
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.HEATAREA_NAME', {val: obj.Device.HEATAREA[i].HEATAREA_NAME, ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.HEATAREA_MODE', {val: obj.Device.HEATAREA[i].HEATAREA_MODE, ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_ACTUAL', {val: parseFloat(obj.Device.HEATAREA[i].T_ACTUAL), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_TARGET', {val: parseFloat(obj.Device.HEATAREA[i].T_TARGET), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.PROGRAM_WEEK', {val: obj.Device.HEATAREA[i].PROGRAM_WEEK, ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.PROGRAM_WEEKEND', {val: obj.Device.HEATAREA[i].PROGRAM_WEEKEND, ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_HEAT_DAY', {val: parseFloat(obj.Device.HEATAREA[i].T_HEAT_DAY), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_HEAT_NIGHT', {val: parseFloat(obj.Device.HEATAREA[i].T_HEAT_NIGHT), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_COOL_DAY', {val: parseFloat(obj.Device.HEATAREA[i].T_COOL_DAY), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.T_COOL_NIGHT', {val: parseFloat(obj.Device.HEATAREA[i].T_COOL_NIGHT), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATAREA.'+ [HeatareaNr] +'.ISLOCKED', {val: obj.Device.HEATAREA[i].ISLOCKED, ack: true});
}
for (var i = 0; i < obj.Device.HEATCTRL.length; i++) {
var HeatctrlNr = obj.Device.HEATCTRL[i].nr; //Nummer des Heatctrl auslesen
//adapter.log.info(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.');
adapter.setState(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.INUSE', {val: Boolean(Number(obj.Device.HEATCTRL[i].INUSE)), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.HEATAREA_NR', {val: obj.Device.HEATCTRL[i].HEATAREA_NR, ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.ACTOR', {val: Boolean(Number(obj.Device.HEATCTRL[i].ACTOR)), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.HEATCTRL_STATE', {val: Number(obj.Device.HEATCTRL[i].HEATCTRL_STATE), ack: true});
adapter.setState(adapter.namespace + '.' + 'HEATCTRL.'+ [HeatctrlNr] +'.ACTOR_PERCENT', {val: Number(obj.Device.HEATCTRL[i].ACTOR_PERCENT), ack: true});
}
for (var i = 0; i < obj.Device.IODEVICE.length; i++) {
var IODeviceNr = obj.Device.IODEVICE[i].nr; //Nummer des Iodevice auslesen
//adapter.log.info(JSON.stringify(obj.Device.IODEVICE));
//adapter.log.info(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.');
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.IODEVICE_TYPE', {val: obj.Device.IODEVICE[i].IODEVICE_TYPE, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.IODEVICE_ID', {val: obj.Device.IODEVICE[i].IODEVICE_ID, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.IODEVICE_STATE', {val: obj.Device.IODEVICE[i].IODEVICE_STATE, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.IODEVICE_COMERROR', {val: obj.Device.IODEVICE[i].IODEVICE_COMERROR, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.HEATAREA_NR', {val: obj.Device.IODEVICE[i].HEATAREA_NR, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.SIGNALSTRENGTH', {val: obj.Device.IODEVICE[i].SIGNALSTRENGTH, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.BATTERY', {val: obj.Device.IODEVICE[i].BATTERY, ack: true});
adapter.setState(adapter.namespace + '.' + 'IODEVICE.'+ [IODeviceNr] +'.ISON', {val: obj.Device.IODEVICE[i].ISON, ack: true});
}
// fill global vals
device_id = obj.Device.ID;
}
});
}
function main() {
// The adapters config (in the instance object everything under the attribute "native") is accessible via
// adapter.config:
/*
* Interval
* example
* setInterval(pifaceread, adapter.config.piinterval);
*/
setInterval(getXMLcyclic, adapter.config.polltime);
adapter.log.info('config host: ' + adapter.config.host);
adapter.log.info('config polltime: ' + adapter.config.polltime);
/**
*
* For every state in the system there has to be also an object of type state
*
* Here a simple template for a boolean variable named "testVariable"
*
* Because every adapter instance uses its own unique namespace variable names can't collide with other adapters variables
*
*/
// in this template all states changes inside the adapters namespace are subscribed
adapter.subscribeStates('*');
getXMLcyclic();
/**
* setState examples
*
* you will notice that each setState will cause the stateChange event to fire (because of above subscribeStates cmd)
*
*/
// the variable testVariable is set to true as command (ack=false)
// adapter.setState('testVariable', ack: true});
// same thing, but the value is flagged "ack"
// ack should be always set to true if the value is received from or acknowledged from the target system
// adapter.setState('testVariable', {val: true, ack: true});
// same thing, but the state is deleted after 30s (getState will return null afterwards)
// adapter.setState('testVariable', {val: true, ack: true, expire: 30});
// examples for the checkPassword/checkGroup functions
// adapter.checkPassword('admin', '<PASSWORD>', function (res) {
// console.log('check user admin pw ioboker: ' + res);
// });
// adapter.checkGroup('admin', 'admin', function (res) {
// console.log('check group user admin group admin: ' + res);
// });
}
| 0552f660411689b98f709ca2b69334aba0e95388 | [
"JavaScript"
] | 1 | JavaScript | oHa510/ioBroker.alpha2 | 21cb01d811a748792db32ff29abd566bd421acb1 | 544df6d1fca437df0b85b573432d252f9441fbd6 | |
refs/heads/master | <repo_name>THZs/DataTablesSrc<file_sep>/test/api/core/on().js
// todo tests
// - Check it exists and is a function
// - Able to add a single event
// - Able to listen for multiple events with a single call
// - Event handlers are correctly triggered
// - The callback function is passed at least the event object as the first parameter (exact parameters depend upon the event)
// - returns API instance
describe( "core- on()", function() {
dt.libs( {
js: [ 'jquery', 'datatables' ],
css: [ 'datatables' ]
} );
describe("Check the defaults", function () {
dt.html( 'basic' );
it("Default should be null", function () {
});
});
});
| 92549f70486dbade9cbeb44c5a1dda4c76ad1d85 | [
"JavaScript"
] | 1 | JavaScript | THZs/DataTablesSrc | 5806dc045acccae2f309a103321c2758279161e2 | c04a7d9f27240c5822a48ce59a7eb0c86b228e45 | |
refs/heads/master | <repo_name>JingwenX/data-structure-algorithm<file_sep>/warm-up/lint_no627_longestPalindrome.py
#LINT NO 627 Longest Palindrome
#5 min
#time used: 25min
# abAbAbabazzz
class Solution:
"""
@param s: a string which consists of lowercase or uppercase letters
@return: the length of the longest palindromes that can be built
"""
def longestPalindrome(self, s):
"""
Given a string which consists of lowercase or uppercase letters, find the length of the longest palindromes that can be built with those letters.
Sol:
this can be finished in O(n). We just loop over and check if any letter comes in double, and then
make a list of all singles, pop it out when meets a double.
O(52n)
Worst case: abcdefg....ABCDEFG...abcdefg...ABCDEFG
Cannot be determined until you meet the very last letter
"""
if not s:
return 0
#init i and list
i = 0
singles = []
while i < len(s):
count_in_singles = singles.count(s[i])
if count_in_singles > 0:
singles.pop(singles.index(s[i]))
else:
singles.append(s[i])
i += 1
if len(singles) > 0:
len_longest_palindrome = len(s) - len(singles) + 1
else:
len_longest_palindrome = len(s)
return len_longest_palindrome
# accepted on second submission
# problem with first submission: used list.index(), which returns value error when encounter no value in list.
# problem with second submission: if "aaaa", the length calculation is wrong. <file_sep>/README.md
# data-structure-algorithm
Practice my data structure and algorithm skills. Mainly using Leetcode and Lintcode
<file_sep>/5binary_tree_and_tree_based_dfs/lint_no596minimum_subtree_v1.py
"""
596. Minimum Subtree
====
A solution using only divide and conquer
====
Description
https://www.lintcode.com/problem/minimum-subtree/description
Given a binary tree, find the subtree with minimum sum. Return the root of the subtree.
LintCode will print the subtree which root is your return node.
It's guaranteed that there is only one subtree with minimum sum and the given binary tree is not an empty tree.
Have you met this question in a real interview?
Example
Example 1:
Input:
1
/ \
-5 2
/ / \
0 2 -4 -5
Output:1
Example 2:
Input:
1
Output:1
"""
"""
Definition of TreeNode:
class TreeNode:
def __init__(self, val):
self.val = val
self.left, self.right = None, None
"""
#v0.9
class Solution:
"""
@param root: the root of binary tree
@return: the root of the minimum subtree
"""
def findSubtree(self, root):
# write your code here
# set up global variable
self.min_weight = sys.maxsize
self.min_root = None
self.helper(root)
return self.min_root
def helper(self, root):
"""
@return: the weight of the subtree
"""
if root is None:
return 0
left_weight = self.helper(root.left)
right_weight = self.helper(root.right)
root_weight = left_weight + right_weight + root.val
# compare with the current min to update †he current min_weight
if root_weight < self.min_weight:
self.min_weight = root_weight
self.min_root = root
return root_weight
#v1.0
class Solution:
def findSubtree(self, root):
min_subtree, min_weight, _ = None, sys.maxsize, 0
min_subtree, min_weight, _ = self.helper(root)
return min_subtree
def helper(self, root):
"""
@return: current minimum subtree root, current minimum subtree weight, current subtree weight.
"""
if root is None:
return None, sys.maxsize, 0
# recursion function that return three parameter.
# the key is, that the return level of the three need to be the same.
min_subtree_l, min_subtree_weight_l, subtree_weight_l = self.helper(root.left)
min_subtree_r, min_subtree_weight_r, subtree_weight_r = self.helper(root.right)
root_subtree_weight = subtree_weight_l + subtree_weight_r + root.val
"""
last method: v0.9
we are using divide and conquer to get the sum of the subtree.
Our helper function calculate the sum of the left, sum of the right, and return the sum of the root subtree.
This method: v1.0
we are using divide and conquer to get the minimum of the subtree.
Our helper function calculate the min , and return the min of left subtree, right subtree, and root subtree.
"""
# if left is the min, return the left
cur_min_subtree_weight = min(min_subtree_weight_l, min_subtree_weight_r, root_subtree_weight)
if min_subtree_weight_l == cur_min_subtree_weight:
return min_subtree_l, cur_min_subtree_weight, root_subtree_weight
if min_subtree_weight_r == cur_min_subtree_weight:
return min_subtree_r, cur_min_subtree_weight, root_subtree_weight
if root_subtree_weight == cur_min_subtree_weight:
return root, cur_min_subtree_weight, root_subtree_weight
#v1.1 linghu optimization
class Solution:
def findSubtree(self, root):
# jw/o: here you don't have to init the parameter.
# min_subtree, min_weight, _ = None, sys.maxsize, 0
minimum, subtree, sum = self.helper(root)
return subtree
def helper(self, root):
# jw/b: remember to have a aligned return type, if you return three things.
if root is None:
return sys.maxsize, None, 0 # jw: using sys.maxsize, for the default value of calculating the min
# jw/o: variable naming. please make it as simple.
# minimum_l: minimum weight of the subtree in the left
# subtree_l: minimum subtree in the left
# sum_l: sum of the left subtree
# comment: use minimum and sum could include the meaning of weight.
left_minimum, left_subtree, left_sum = self.helper(root.left)
right_minimum, right_subtree, right_sum = self.helper(root.right)
sum = left_sum + right_sum + root.val
if left_minimum == min(left_minimum, right_minimum, sum):
return left_minimum, left_subtree, sum
if right_minimum == min(left_minimum, right_minimum, sum):
return right_minimum, right_subtree, sum
# jw/O: After the two ifs, you don't need a third if, instead you can just return.
# if root_subtree_weight == cur_min_subtree_weight:
# return root, cur_min_subtree_weight, root_subtree_weight
return sum, root, sum
<file_sep>/5binary_tree_and_tree_based_dfs/lint_no596.minimum_subtree.py
"""
596. Minimum Subtree
Description
https://www.lintcode.com/problem/minimum-subtree/description
Given a binary tree, find the subtree with minimum sum. Return the root of the subtree.
LintCode will print the subtree which root is your return node.
It's guaranteed that there is only one subtree with minimum sum and the given binary tree is not an empty tree.
Have you met this question in a real interview?
Example
Example 1:
Input:
1
/ \
-5 2
/ \ / \
0 2 -4 -5
Output:1
Example 2:
Input:
1
Output:1
"""
"""
Definition of TreeNode:
class TreeNode:
def __init__(self, val):
self.val = val
self.left, self.right = None, None
"""
class Solution:
"""
@param root: the root of binary tree
@return: the root of the minimum subtree
"""
def findSubtree(self, root):
# write your code here
# set up global variable
self.min_weight = sys.maxsize
self.min_root = None
self.helper(root)
return self.min_root
def helper(self, root):
"""
@return: the weight of the subtree
"""
if root is None:
return 0
left_weight = self.helper(root.left)
right_weight = self.helper(root.right)
root_weight = left_weight + right_weight + root.val
# compare with the current min to update †he current min_weight
if root_weight < self.min_weight:
self.min_weight = root_weight
self.min_root = root
return root_weight<file_sep>/warm-up/lint_no13_ImplementingStrstr.py
class Solution:
"""
@param source:
@param target:
@return: return the index
Example 1:
Input: source = "source" ,target = "target"
Output: -1
Explanation:
If the source does not contain the target content, return - 1.
Example 2:
Input:source = "abcdabcdefg" ,target = "bcd"
Output: 1
Explanation:
If the source contains the target content, return the location where the target first appeared in the source.
test 1:
Input: source = "source", target = "sourcez"
test 2:
Input: source = "source", target = "source"
first submission:
cannot pass
Input: source = "tartarget", target = "target"
expected: 3
real: -1
"""
def strStr(self, source, target):
# Write your code here
# thinking: one pointer, when encounter something starting with target, then index starting from that index, until the last one is comfirmed to be align. If encounter something diff, restart the i.
i_source = 0
i_target = 0
first_ocr_index = -1
if not target:
return 0
#while 3 < 9 - 6 + 1; source[3] == target[0], first_ocr_index = 3.
while i_source < len(source) - len(target) + 1:
print(i_source)
if source[i_source] == target[0]:
first_ocr_index = i_source
#check if the later ones are align
while i_target < len(target):
if i_target == len(target) - 1:
return first_ocr_index
elif source[i_source] == target[i_target]:
i_target += 1
i_source += 1
#corner case: when new i_source cannot keep aligning, but could possibly start a new alignment.
elif source[i_source] == target[0]:
first_ocr_index = i_source
continue
else:
first_ocr_index = -1
i_source += 1
break
else:
i_source += 1
return first_ocr_index
# this one has not pass the test yet...
<file_sep>/python_common_methos.py
str.lower
#convert to lower case
len(str)
#check length
str[1]
#extract letter
str.isalnum
#check if is alphanumeric
0 to len(str) -1
#rmb the -1
list.index(ele)
#The method index() returns the lowest index in list that obj appears.
"""
list.index(x[, start[, end]])
Return zero-based index in the list of the first item whose value is equal to x.
!!!!Raises a ValueError if there is no such item!!!!
The optional arguments start and end are interpreted as in the slice notation and are used to limit the search to a particular subsequence of the list. The returned index is computed relative to the beginning of the full sequence rather than the start argument.
"""
list.count(ele)
#to find some element to see if that is in a list, you can use this one instead.
palindrome problem:
usually odd and even are different cases. Consider them seperately.
<file_sep>/5binary_tree_and_tree_based_dfs/lint_no632_find_maximum_node.py
#lint_no632 find maximum node. Not maximum subtree!!
"""
Definition of TreeNode:
class TreeNode:
def __init__(self, val):
self.val = val
self.left, self.right = None, None
"""
class Solution:
"""
@param: root: the root of tree
@return: the max node
jw/o: this method is totally divide and conquer
"""
def maxNode(self, root):
# write your code here
max_node, _ = self.helper(root)
return max_node
def helper(self, root):
"""
compare left_max_node, right_max_node and root, return the max_node and maximum
@return max_node, maximum
"""
if root is None:
# jw/b: remember to use lowest possible number in order to calculate a max
# return None, 0
return None, -sys.maxsize - 1
left_max_node, left_maximum = self.helper(root.left)
right_max_node, right_maximum = self.helper(root.right)
maximum = max(left_maximum, right_maximum, root.val)
if left_maximum == maximum:
return left_max_node, left_maximum
if right_maximum == maximum:
return right_max_node, right_maximum
return root, root.val | 36cc9c52840680bab07c2af011a06344c40b2af0 | [
"Markdown",
"Python"
] | 7 | Python | JingwenX/data-structure-algorithm | 86a400775655d7c50b0e4bd9592700a8fdbfb8da | e5f807102dab11dcd152926585499b94c7403f0b | |
refs/heads/master | <file_sep># rabbitmq-demo5-topics
Demo 5 Topics
- Receiving messages based on a pattern (topics)
<file_sep>using RabbitMQ.Client;
using RabbitMQ.Client.Events;
using System;
using System.Text;
using System.Threading;
namespace ReceiveLogs
{
class ReceiveLogs
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
Console.WriteLine("Write the log types you want to receive:");
Console.WriteLine("The options are: system.info, application.warning and system.error");
Console.WriteLine("Try combination with * and # to bind to desired topic");
Console.WriteLine("Ex.: '*.info' , 'system.*'");
var input = Console.ReadLine();
var severities = input.Split(' ');
var factory = new ConnectionFactory()
{
HostName = "192.168.15.33",
UserName = "renatocolaco",
Password = "<PASSWORD>"
};
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
// This tells RabbitMQ not to give more than one message to a worker at a time
// prefetchCount: 1
channel.ExchangeDeclare(exchange: "topic_logs", type: "topic");
var queueName = channel.QueueDeclare().QueueName;
foreach (var sev in severities)
{
channel.QueueBind(queue: queueName, exchange: "topic_logs", routingKey: sev);
}
var consumer = new EventingBasicConsumer(channel);
consumer.Received += (model, ea) => {
var body = ea.Body;
var message = Encoding.UTF8.GetString(body);
Console.WriteLine(" [x] message received {0}", message);
Thread.Sleep(4000);
Console.WriteLine(" [#] Done");
};
channel.BasicConsume(
queue: queueName,
autoAck: true,
consumer: consumer
);
Console.WriteLine(" Press [enter] to exit.");
Console.ReadLine();
}
}
}
}
<file_sep>using RabbitMQ.Client;
using System;
using System.Text;
using System.Timers;
using System.Security.Cryptography;
namespace EmitLog
{
class EmitLog
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
SetTimer();
Console.ReadKey();
}
private static void SetTimer()
{
// Create a timer with a two second interval.
var messageTimer = new Timer(2000);
// Hook up the Elapsed event for the timer.
messageTimer.Elapsed += OnTimedEvent;
messageTimer.AutoReset = true;
messageTimer.Enabled = true;
}
private static void OnTimedEvent(Object source, ElapsedEventArgs e)
{
var factory = new ConnectionFactory()
{
HostName = "192.168.15.33",
UserName = "renatocolaco",
Password = "<PASSWORD>"
};
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.ExchangeDeclare(exchange: "topic_logs", type: "topic");
var message = GetMessage();
string severity = GetSeverity();
var body = Encoding.UTF8.GetBytes($"[{severity}] {message}");
channel.BasicPublish(exchange: "topic_logs",
routingKey: severity,
basicProperties: null,
body: body);
}
Console.WriteLine("A new message published to the queue at {0:HH:mm:ss.fff}",
e.SignalTime);
}
/// <summary>
/// Gets a random severity
/// </summary>
/// <returns></returns>
private static string GetSeverity()
{
var severityArr = new string[] {
"system.info",
"system.warning",
"system.error",
"application.info",
"application.warning",
"application.error"
};
using (var randomNumberGenerator = RandomNumberGenerator.Create())
{
var randomNumber = new byte[1];
randomNumberGenerator.GetBytes(randomNumber);
var rest = randomNumber[0] % severityArr.Length;
return severityArr[rest];
}
}
private static string GetMessage()
{
return $"Hello World! {DateTime.Now:HH:mm:ss}";
}
}
}
| 8fa9fd98dfd98aca2d01923f7fe896faeaf79db8 | [
"Markdown",
"C#"
] | 3 | Markdown | renatoinline/rabbitmq-demo5-topics | 1a6ada929e2abbb5f118274ee3c116311dbb3a4e | fa129a0a3e82f60ab03052a38d082bd4a3b71414 | |
refs/heads/master | <file_sep>CampusPathfinder
================
This is a path-finding tool to help you find the shortest distance walking path on the Clayton State University Campus. It was created with the javascript game framework Phaser using an EasyStar.js plugin.
Demo: [http://boggsman.com/class/pathfinder](http://boggsman.com/class/pathfinder/)
Using
================
It is written in Javascript. Drop it in a folder on your favorite web server and enjoy.
Customizing
================
It is easily customizable with a different map image and tilemap. I created the tilemap using the Tiled map editor. Take a look at the files in the /assets folder and also Map.js.
<file_sep>
var game = new Phaser.Game(1024, 768, Phaser.CANVAS, 'mapContainer', { preload: preload, create: create, update: update, render: render });
function preload() {
game.load.tilemap('csu', 'assets/csu.json', null, Phaser.Tilemap.TILED_JSON);
game.load.image('background', 'assets/csumap.png');
game.load.image('tiles', 'assets/tiles.png');
}
var map;
var tileset;
var layer;
var pathfinder
function create() {
background = game.add.tileSprite(0, 0, 1024, 768, "background");
map = game.add.tilemap('csu');
map.addTilesetImage('tiles', 'tiles');
layer = map.createLayer('layer1');
layer.resizeWorld();
var walkables = [2]; //walkable tiles, look in the csu.json file
pathfinder = game.plugins.add(Phaser.Plugin.PathFinderPlugin);
pathfinder.setGrid(map.layers[0].data, walkables);
}
function getFormData() {
var fromXY = document.getElementById('from').value;
var toXY = document.getElementById('to').value;
if (fromXY == toXY) {
alert('To: and From: must be different.');
}
else {
var fromArry = fromXY.split(",");
var toArry = toXY.split(",");
drawPath(parseInt(fromArry[0]), parseInt(fromArry[1]), parseInt(toArry[0]), parseInt(toArry[1]));
}
}
function drawPath(fromTilex, fromTiley, toTilex, toTiley) {
pathfinder.setCallbackFunction(function(path) {
path = path || [];
for(var i = 0, ilen = path.length; i < ilen; i++) {
map.putTile(1, path[i].x, path[i].y); //mark each tile in the path as walkable
}
});
pathfinder.preparePathCalculation([fromTilex,fromTiley], [toTilex,toTiley]);
pathfinder.calculatePath();
}
function update() {
}
function render() {
}
| 665c2a503398324029bf1361261124122665cf11 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | boggsman/CampusPathfinder | 59bafbd0072d26b1b670455d3b0c4d69232d318e | e7a3066da0298a8a308d8fbcf9cf4be8f0981ae8 | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
import argparse
import pickle
import numpy as np
import keras.utils.np_utils as np_utils
import dataset_io
import nn
def extract_misclassified(image_properties, predictions, labels):
'''Given a list of image properties and the class probabilities, extract the image properties and
class probabilities of the misclassified samples.
image_properties: list type, content can be anything
predictions: numpy array of shape (samples, classes)
labels: numpy array of shape (samples, classes), labels in one-hot encoding
returns wrong_image_properties: the part of image_properties that belongs to misclassified images
returns wrong_predictions: the part of predictions that belongs to misclassified images'''
pred_labels = np.argmax(predictions, axis=1) # from one-hot encoding to a class id
labels = np.argmax(labels, axis=1) # same
mistakes = pred_labels != labels # check which images are misclassified
wrong_image_properties = [prop for prop, m in zip(image_properties, mistakes) if m]
wrong_predictions = predictions[mistakes]
return wrong_image_properties, wrong_predictions
#~ Parse parameters
parser = argparse.ArgumentParser()
parser.add_argument('weights', help='Path weights in xyz.w file')
parser.add_argument('layout', help='Path network layout specification')
parser.add_argument('data', help='Path to csv file that lists input images')
parser.add_argument('path', help='Location for storing the predictions')
parser.add_argument('-b', '--batchsize', help='Size of the batches to be learned on [default 16]', type=int, default=16)
parser.add_argument('-d', '--datalimit', help='Maximum number of data points to read from DATA [if missing, read all]', type=int, default=None)
parser.add_argument('-m', '--misclassified', help='Only store predictions for missclassified images', action='store_true')
args = parser.parse_args()
#~ Load model
print('Loading model from {0}'.format(args.layout))
layout = nn.load_layout(args.layout)
model, optimizer = nn.build_model_to_layout(layout)
#~ Load weights
print('Loading weights from {0}'.format(args.weights))
model.load_weights(args.weights)
#~ Load data
input_shape = layout[0][1]['input_shape']
resolution = input_shape[1:]
print('Loading data from {0} and rescaling it to {1}x{2}'.format(args.data, resolution[0], resolution[1]))
x_test, y_test, num_classes, image_properties = dataset_io.read_data(args.data, resolution, args.datalimit, return_image_properties=True)
y_test = np_utils.to_categorical(y_test, num_classes)
#~ Create predictions
print('Predicting labels for {0} samples at resolution {1}x{2} in batches of size {3}'.format(x_test.shape[0], resolution[0], resolution[1], args.batchsize))
predictions = model.predict_proba(x_test, batch_size=args.batchsize)
#~ If desired, reduce output to the misclassified images
if args.misclassified:
print('Reducing output to misclassified samples')
sample_count = len(image_properties)
image_properties, predictions = extract_misclassified(image_properties, predictions, y_test)
print('\tMisclassified images: {0} out of {1}'.format(len(image_properties), sample_count))
#~ Store preditions on disk
print('Storing samples to {0}'.format(args.path))
with open(args.path, 'wb') as out_file:
pickle.dump((image_properties, predictions), out_file, pickle.HIGHEST_PROTOCOL)
print('Done')
<file_sep># -*- coding: utf-8 -*-
'''Take a network layout and weights, and test its performance on a given data set.
Print score and accuracy.
User can specify batchsize, number of samples to consider (randomly chosen), and keras verbosity'''
import argparse
import theano
theano.config.openmp = True
import keras.utils.np_utils as np_utils
import nn
import dataset_io
#~ Parse parameters
parser = argparse.ArgumentParser()
parser.add_argument('weights', help='Path weights in xyz.w file')
parser.add_argument('layout', help='Path network layout specification')
parser.add_argument('path', help='Path to csv file that lists input images')
parser.add_argument('-b', '--batchsize', help='Size of the batches to be learned on [default 16]', type=int, default=16)
parser.add_argument('-d', '--datalimit', help='Maximum number of data points to read from PATH [if missing, read all]', type=int, default=None)
parser.add_argument('-v', '--verbosity', help='Set the verbosity level of keras (valid values: 0, 1, 2)', type=int, default=1)
args = parser.parse_args()
#~ Load model
print('Loading model from {0}'.format(args.layout))
layout = nn.load_layout(args.layout)
model, optimizer = nn.build_model_to_layout(layout)
#~ Load weights
print('Loading weights from {0}'.format(args.weights))
model.load_weights(args.weights)
#~ Load data
input_shape = layout[0][1]['input_shape']
resolution = input_shape[1:]
print('Loading data from {0} and rescaling it to {1}x{2}'.format(args.path, resolution[0], resolution[1]))
x_test, y_test, num_classes = dataset_io.read_data(args.path, resolution, args.datalimit)
y_test = np_utils.to_categorical(y_test, num_classes)
#~ Test the model
print('Testing on {0} samples at resolution {1}x{2} in batches of size {3}'.format(x_test.shape[0], resolution[0], resolution[1], args.batchsize))
score = model.evaluate(x_test, y_test, batch_size=args.batchsize, show_accuracy=True, verbose=args.verbosity)
print('Test score:', score[0])
print('Test accuracy:', score[1])
print('Done')<file_sep>import theano
import numpy as np
class stepwise_tanh_op(theano.Op):
__props__ = ()
def make_node(self, x):
# check that the theano version has support for __props__.
# This next line looks like it has a typo,
# but it's actually a way to detect the theano version
# is sufficiently recent to support the use of __props__.
assert hasattr(self, '_props'), "Your version of theano is too old to support __props__."
x = theano.tensor.as_tensor_variable(x)
return theano.Apply(self, [x], [x.type()])
def perform(self, node, inputs, output_storage):
x = inputs[0]
z = output_storage[0]
y = x.copy()
a = 1
y[y > a] = a
y[y < -a] = -a
z[0] = y
def infer_shape(self, node, i0_shapes):
return i0_shapes
def grad(self, inputs, output_grads):
g = np.ones(inputs[0].shape)
a = 1
g[inputs[0] > a] = 0
g[inputs[0] < -a] = 0
return [g[0]]
#return [output_grads[0]]
def R_op(self, inputs, eval_points):
# R_op can receive None as eval_points.
# That mean there is no diferientiable path through that input
# If this imply that you cannot compute some outputs,
# return None for those.
if eval_points[0] is None:
return eval_points
return self.grad(inputs, eval_points)
def create():
x = theano.tensor.matrix()
return theano.function([x], stepwise_tanh_op()(x))
if __name__ == "__main__":
stepwise_tanh = create()
inp = np.random.rand(5, 4) * 4 - 2
out = stepwise_tanh(inp)
#assert numpy.allclose(inp * 2, out)
print(inp)
print(out)
<file_sep>import os
def search_folder(path):
list = ""
for subdir, dirs, files in os.walk(path):
for file in files:
if file[-4:] == ".csv":
list += subdir + "/" + file + "\n"
return list
path = "data/GTSRB_TEST/"
list = search_folder(path)[:-1]
with open("csv_list_test.txt", "w") as list_file:
list_file.write(list)
<file_sep>#!/bin/bash
# Path settings
weights_path="weights/"
results_path="results/"
# Will get path to layout, dataset and out_path for a weight path in $1.
# They are stored in layout, dataset and out_path, respectively
generate_paths() {
layout_hint="${1%.w}"
layout_hint=$(echo "$layout_hint" | sed -e "s/[0-9]*$//")
layout_hint="${layout_hint}layout.txt"
layout=$(cat "$layout_hint")
dataset_hint=$(dirname $1)
dataset_hint="${dataset_hint}/test_dataset.txt"
dataset=$(cat "$dataset_hint")
out_path="${1##$weights_path}"
out_path="${out_path%.w}"
out_path="${results_path}${out_path}.txt"
}
# Stage 1: Evaluate all weights in $weights_path, and write the output to
# $results_path, keeping the folder structure.
weights=$(find $weights_path -iname "*.w" | sort) # The list of weights to evaluate
for w in $weights ; do
generate_paths $w
# If output already exists, don't re-compute it
if [ -f $out_path ] ; then
echo "Results for $w exist, skipping"
continue
else
echo "Evaluating $w"
fi
# Create output folders if they don't exist
out_dir="$(dirname $out_path)"
mkdir -p "$out_dir"
nice -n 19 python src/test.py $w $layout $dataset > $out_path
if [ "$?" -ne "0" ] ; then
echo "Test failed, deleting $out_path"
rm "$out_path"
fi
done
# Stage 2: Agglomerate the resulting values in a single file per configuration
# that is gnuplot-readable
results=$(find $results_path -iname "*.txt" | sort) # The list of results to agglomerate
# Remove old files
for result in $results ; do
out_file="${result%.txt}"
out_file=$(echo "$out_file" | sed -e "s/[0-9]*$//")
out_file="${out_file}result.dat"
rm -f "$out_file"
#echo "# epoch accuracy" > "$out_file"
echo "# epoch accuracy" > "$out_file"
done
# Create new ones with fresh values
for result in $results ; do
# The file to store agglomerated results in
out_file="${result%.txt}"
out_file=$(echo "$out_file" | sed -e "s/[0-9]*$//")
out_file="${out_file}result.dat"
# Epoch number
epoch=$(basename "$result")
epoch="${epoch%.w}"
epoch=$(echo "$epoch" | sed 's/[^0-9]//g')
# Accuracy
accuracy_line=$(tail -n 2 "$result" | head -n 1)
accuracy=${accuracy_line##"('Test accuracy:', "}
accuracy=${accuracy%%)}
# Write to file
line="$epoch $accuracy"
echo "$line" >> "$out_file"
done
<file_sep># -*- coding: utf-8 -*-
'''Will train a neural network with a given layout on a data set. The user can opt to load weights
before and store them after training. Furthermore, number of epochs, batchsize, maximum number
of training samples to consider, and keras verbosity can be specified. The user can also chose to
apply slight affine transformations of the input images before each epoch.
For loading and saving weights, specify paths without a filename extension. Two files will be
created / read from, a .w file containing the weights, and a .t file containing the training
parameters like learning rate.'''
import argparse
import pickle
import theano
theano.config.openmp = True
import keras.utils.np_utils as np_utils
import nn
import dataset_io
import distortions
#~ Parse arguments
parser = argparse.ArgumentParser()
parser.add_argument('layout', help='Path network layout specification')
parser.add_argument('path', help='Path to csv file that lists input images')
parser.add_argument('-e', '--epochs', help='Numper of epochs to train for [default 1]', type=int, default=1)
parser.add_argument('-b', '--batchsize', help='Size of the batches to be learned on [default 16]', type=int, default=16)
parser.add_argument('-d', '--datalimit', help='Maximum number of data points to read from PATH [if missing, read all]', type=int, default=None)
parser.add_argument('-m', '--morph', help='Morph training data between epochs', action='store_true')
parser.add_argument('-l', '--load-status', help='Basename of the files to load status from')
parser.add_argument('-s', '--store-status', help='Basename of the files to store status in')
parser.add_argument('-v', '--verbosity', help='Set the verbosity level of keras (valid values: 0, 1, 2)', type=int, default=1)
args = parser.parse_args()
#~ Load model
print('Loading model from {0}'.format(args.layout))
layout = nn.load_layout(args.layout)
model, optimizer = nn.build_model_to_layout(layout)
#~ Load status
if args.load_status:
print('Loading status from {0}'.format(args.load_status))
#~ weights
weight_filename = args.load_status + ".w"
model.load_weights(weight_filename)
#~ training parameters
try:
train_filename = args.load_status + ".t"
with open(train_filename, 'rb') as train_file:
optimizer_state = pickle.load(train_file)
optimizer.set_state(optimizer_state)
except IOError:
print('\t{0} not found: using initial parameters'.format(train_filename))
#~ Load data
input_shape = layout[0][1]['input_shape']
resolution = input_shape[1:]
print('Loading data from {0} and rescaling it to {1}x{2}'.format(args.path, resolution[0], resolution[1]))
x_train, y_train, num_classes = dataset_io.read_data(args.path, resolution, args.datalimit)
y_train = np_utils.to_categorical(y_train, num_classes)
#~ Create distortions callback
if args.morph:
distcall = distortions.Distortions(x_train, x_train.shape[0])
callbacks = [distcall]
print('Distortions will be applied to training data between epochs')
else:
callbacks = []
#~ Train the model
print('Training on {0} samples in batches of size {1} for {2} epochs'.format(x_train.shape[0], args.batchsize, args.epochs))
model.fit(x_train, y_train, nb_epoch=args.epochs, callbacks=callbacks, batch_size=args.batchsize, show_accuracy=True, verbose=args.verbosity)
#~ Store status
if args.store_status:
print('Storing status to {0}'.format(args.store_status))
#~ weights
weight_filename = args.store_status + ".w"
model.save_weights(weight_filename, overwrite=True)
#~ training parameters
train_filename = args.store_status + ".t"
optimizer_state = optimizer.get_state()
with open(train_filename, 'wb') as train_file:
pickle.dump(optimizer_state, train_file, pickle.HIGHEST_PROTOCOL)
print('Done')
<file_sep># -*- coding: utf-8 -*-
import PIL.Image as pil
import PIL.ImageOps as pilops
import numpy as np
import time
import random
import csv
def create_image_list(filename):
'''This method takes the path to a .txt file, which contains the paths to the csv files (one per row), which contain the information about the images, which are to be loaded. The csv files contain the filename (with the path relative to the csv file), the width of the image, the height of the image, the coordinates X1, Y1, X2, Y2 of the bounding box (X1 and Y1 are inclusive, X2 and Y2 exclusive) and the class id of the image. Semicolons are used as seperators.'''
# open file which contains the csv list
with open(filename, "r") as f:
# read csv paths from file and save them in a list
csv_paths = f.readlines()
# create list, which saves a dictionary for each image
image_list = []
# iterate over csv files
for csv_path in csv_paths:
# remove possible '\n' at the end of the path
csv_path = csv_path.strip()
# open csv file to read images
with open(csv_path) as csv_file:
# create csv reader
csv_reader = csv.reader(csv_file, delimiter=";")
# skip first line, which contains the headlines
next(csv_reader)
# save path to the folder, which contains the images
folder_path = csv_path[:csv_path.rfind('/')] + "/"
# iterate over images
for image_row in csv_reader:
# build path to image
image_path = folder_path + image_row[0]
# append image to image list
image_list.append({'path': image_path, 'corners': (int(image_row[3]), int(image_row[4]), int(image_row[5]), int(image_row[6])), 'label': image_row[7]})
# shuffle images
random.shuffle(image_list)
return image_list
def count_classes(image_list):
classes = set()
for image in image_list:
classes.add(image['label'])
return len(classes)
def read_data(filename, resolution, d=None, normalize=True, autocontrast=True, return_image_properties=False):
'''This method takes the path to a .txt file containing the paths to the csv files, which save information about the images. For a more detailed description of those files cf. method create_image_list(). In addition, this method takes the 'resolution' to which the images are to be scaled, a parameter 'd' which determines how many images are to be processed, a boolean parameter 'normalize' which controls whether the images shall be normalized to the interval [0,1] and a boolean parameter 'autocontrast' which controls whether a PIL intern method shall be used to increase the contrast of the images. The boolean parameter 'return_image_properties' can be set to 'True' in order to return the image list created by the create_image_list method called within this method.'''
# create image list
image_list = create_image_list(filename)
# count classes
num_classes = count_classes(image_list)
# check whether there is a limit for the images to be loaded
num_images = d if d is not None else len(image_list)
# create empty arrays with appropriate size
X = np.empty((num_images, 3, resolution[0], resolution[1]), dtype=float)
y = np.empty((num_images))
# iterate over images
for idx, image in enumerate(image_list):
if idx >= num_images:
break
# open the image
im = pil.open(image['path'])
# crop image
im = im.crop(image['corners'])
# autocontrast
if autocontrast:
im = pilops.autocontrast(im)
# resize image to desired size
im = im.resize(resolution)
# save image as array within the result array
X[idx] = np.transpose(np.asarray(im), [2,0,1])
# save label
y[idx] = image['label']
# normalize images to range [0,1] if desired
if normalize:
X /= 255.0
if return_image_properties:
return X, y, num_classes, image_list
else:
return X, y, num_classes
if __name__ == "__main__":
size = (48,48)
csv_filename = "data/csv_list_train.txt"
start_time = time.time()
X_train, y_train = read_data(csv_filename, size, 1000, True, True)
end_time = time.time()
print("Execution time: " + str(end_time - start_time) + "s")
<file_sep># -*- coding: utf-8 -*-
import numpy as np
import keras.callbacks
import scipy.ndimage.interpolation as trans
class Distortions(keras.callbacks.Callback):
def __init__(self, x, number_of_images):
self.original_x = np.copy(x)
self.x = x
self.resolution = x.shape[2:4]
self.number_of_images = number_of_images
def cut_image(self, image):
# ATTENTION! It is assumed that the images are square!
# image has a smaller resolution than desired
if image.shape[1] < self.resolution[0]:
# initialize result image
res = np.empty((3,self.resolution[0],self.resolution[1]))
# calculate boundaries
left = (self.resolution[0] - image.shape[1]) // 2
top = (self.resolution[1] - image.shape[2]) // 2
right = left + image.shape[1]
bottom = top + image.shape[2]
res[:,left:right,top:bottom] = image
# interpolate empty pixels (left, right, above, below distorted image)
res[:,:left,top:bottom] = image[:,0,:].reshape(3,1,-1)
res[:,right:,top:bottom] = image[:,-1,:].reshape(3,1,-1)
res[:,left:right,:top] = image[:,:,0].reshape(3,-1,1)
res[:,left:right,bottom:] = image[:,:,-1].reshape(3,-1,1)
# interpolate empty pixels (topleft, topright, bottomleft, bottomright corner)
res[:,:left,:top] = trans.zoom(image[:,0,0].reshape(3,1,1), zoom=[1,left,top])
res[:,right:,:top] = trans.zoom(image[:,-1,0].reshape(3,1,1), zoom=[1,self.resolution[0]-right,top])
res[:,:left,bottom:] = trans.zoom(image[:,0,-1].reshape(3,1,1), zoom=[1, left, self.resolution[1]-bottom])
res[:,right:,bottom:] = trans.zoom(image[:,-1,-1].reshape(3,1,1), zoom=[1, self.resolution[0]-right, self.resolution[1]-bottom])
return res
# image has a larger resolution than desired
else:
# calculate boundaries
left = (image.shape[1] - self.resolution[0]) // 2
top = (image.shape[2] - self.resolution[1]) // 2
right = left + self.resolution[0]
bottom = top + self.resolution[1]
# return result image
return image[:,left:right,top:bottom]
def on_epoch_begin(self, epoch, logs={}):
# create random values for shift, rotation and scaling
rotate_angles = np.random.uniform(-5., 5., (self.number_of_images))
scale_factors = np.random.uniform(0.9, 1.1, (self.number_of_images))
shift_values = np.random.uniform(- 0.1 * self.x.shape[1], 0.1 * self.x.shape[2], (self.number_of_images))
# iterate over all images and transform them
for img_id in range(self.number_of_images):
# shift
img = trans.shift(self.original_x[img_id], [0, shift_values[img_id], shift_values[img_id]], mode="nearest")
# rotate
img = trans.rotate(img, axes=(1,2), angle=rotate_angles[img_id], reshape=False, mode="nearest")
# scale
img = trans.zoom(img, zoom=[1,scale_factors[img_id],scale_factors[img_id]], mode="nearest")
self.x[img_id] = self.cut_image(img)
<file_sep>#!/bin/bash
# To use this, set ADDITIONAL_LIBS to the folder containing the required
# libaries and source this file.
# This script will append to the LD_LIBRARY_PATH environment variable so that
# the libraries located in ADDITIONAL_LIBS will be found by ld when running
# the program. Libraries that need to be found in ADDITIONAL_LIBS if they are
# not found in the regular system paths include:
# libopenblas.so
# libopenblas.so.0
# libhdf5_serial.so.10
# libhdf5_serial_hl.so.10
# libhdf5_serial_hl.so.10.0.1
ADDITIONAL_LIBS=/path/to/libraries
export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:$ADDITIONAL_LIBS
echo "LD_LIBRARY_PATH=$LD_LIBRARY_PATH"
<file_sep>import os
import random
import numpy as np
# variable definitions
inriapath = "data/INRIA"
nb_testimg = 37
# initialize strings which contain the content of the csv file
csv_str_train = "Filename;Width;Height;Roi.X1;Roi.Y1;Roi.X2;Roi.Y2;ClassId"
csv_str_test = "Filename;Width;Height;Roi.X1;Roi.Y1;Roi.X2;Roi.Y2;ClassId"
# determine which images shall be used as test images
test_images = np.empty((15,nb_testimg))
for id, row in enumerate(test_images):
test_images[id] = random.sample(range(186), nb_testimg)
test_images = test_images.astype(np.int16)
# iterate over files
for path, subdirs, files in os.walk(inriapath):
# check whether we are in folder 'deFace' and skip it
if not path.endswith("deFace"):
# iterate over file
for idx, f in enumerate(files):
# check whether file is an image
if f.endswith(".jpg"):
obj_id = int(path[-2:])
for i in range(nb_testimg):
if idx == test_images[int(obj_id) - 1, i]:
csv_str_test += "\nPersonne" + str(obj_id).zfill(2) + "/" + f + ";384;288;0;0;384;288;" + str(obj_id - 1)
break
else:
csv_str_train += "\nPersonne" + str(obj_id).zfill(2) + "/" + f + ";384;288;0;0;384;288;" + str(obj_id - 1)
with open("data/INRIA/INRIA_TRAIN.csv", "w") as text_file:
text_file.write(csv_str_train)
with open("data/INRIA/INRIA_TEST.csv", "w") as text_file:
text_file.write(csv_str_test)
<file_sep># study-project-gtsrb
A study project of using convolutional neural networks on the GTSRB dataset.
# Performance
On 6 Intel Xeon X7542 CPUs the implementation currently processes 18432 images
in 15000 seconds. That means it trains about 1,22 images per second.
<file_sep>import argparse
import sys
import os
sys.path.insert(1, os.path.join(sys.path[0], '../src'))
import nn
parser = argparse.ArgumentParser()
parser.add_argument('source_layout', metavar='source-layout', help='Path source network layout specification')
parser.add_argument('source_weights', metavar='source-weights', help='Path sourcce network weights')
parser.add_argument('target_layout', metavar='target-layout', help='Path target network layout specification')
parser.add_argument('target_weights', metavar='target-weights', help='Path target network weights')
parser.add_argument('layerspec', help='Which layers to copy. Format: a-b-...-z where a-z are 0-based layer numbers')
args = parser.parse_args()
#~ Load source model
print('Loading source model from {0}'.format(args.source_layout))
source_layout = nn.load_layout(args.source_layout)
source_model, source_optimizer = nn.build_model_to_layout(source_layout)
#~ Load source weights
print('\tLoading source weights from {0}'.format(args.source_weights))
source_model.load_weights(args.source_weights)
#~ Load target model
print('Loading target model from {0}'.format(args.target_layout))
target_layout = nn.load_layout(args.target_layout)
target_model, target_optimizer = nn.build_model_to_layout(target_layout)
#~ Load target weights
if os.path.isfile(args.target_weights):
print('\tLoading target weights from {0}'.format(args.target_weights))
target_model.load_weights(args.target_weights)
else:
print('\tNot loading target weights. Initialization values will be used where not overwritten.')
layers = args.layerspec.split('-')
layers = [int(l) for l in layers]
#~ Copy layer weights
for layer_idx in layers:
print('Copying weights on layer {0}'.format(layer_idx))
weights = source_model.layers[layer_idx].get_weights()
target_model.layers[layer_idx].set_weights(weights)
#~ Store target weights
print('Storing target weights to {0}'.format(args.target_weights))
target_model.save_weights(args.target_weights, overwrite=True)
<file_sep>import os
import random
import numpy as np
# variable definitions
coilpath = "data/COIL100"
nb_testimg = 14
# initialize strings which contain the content of the csv file
csv_str_train = "Filename;Width;Height;Roi.X1;Roi.Y1;Roi.X2;Roi.Y2;ClassId"
csv_str_test = "Filename;Width;Height;Roi.X1;Roi.Y1;Roi.X2;Roi.Y2;ClassId"
# determine which images shall be used as test images
test_images = np.empty((100,nb_testimg))
for id, row in enumerate(test_images):
test_images[id] = random.sample(range(72), nb_testimg)
test_images = test_images.astype(np.int16) * 5
# iterate over files
for subdir, dirs, files in os.walk(coilpath):
for f in files:
# check whether file is an image
if f[-4:] == ".png":
# extract object id and degree from file name
obj_id, degree = f[3:-4].split('__', 1)
# append image to respective csv file
for i in range(nb_testimg):
if int(degree) == test_images[int(obj_id) - 1, i]:
csv_str_test += "\nobj" + obj_id + "__" + degree + ".png;128;128;0;0;128;128;" + str(int(obj_id) - 1)
break
else:
csv_str_train += "\nobj" + obj_id + "__" + degree + ".png;128;128;0;0;128;128;" + str(int(obj_id) - 1)
with open("data/COIL100/COIL_TRAIN.csv", "w") as text_file:
text_file.write(csv_str_train)
with open("data/COIL100/COIL_TEST.csv", "w") as text_file:
text_file.write(csv_str_test)
<file_sep># -*- coding: utf-8 -*-
import argparse
import json
import theano
theano.config.openmp = True
import keras.models as models
import keras.layers.core as core_layers
import keras.layers.convolutional as conv_layers
import keras.optimizers as optimizers
import stepwise_tanh_op as stanh
def build_model_to_layout(layout, momentum=0.0, nesterov=False):
model = models.Sequential()
#stepwise_tanh = stanh.create()
x = theano.tensor.matrix()
stepwise_tanh = theano.function([x], stanh.stepwise_tanh_op()(x))
for ltype, lspec in layout:
try:
if lspec['activation'] == 'stepwise_tanh':
lspec['activation'] = stepwise_tanh
except KeyError:
pass
if ltype == 'conv2D':
layer = conv_layers.Convolution2D(**lspec)
elif ltype == 'maxpool2D':
layer = conv_layers.MaxPooling2D(**lspec)
elif ltype == 'flatten':
layer = core_layers.Flatten()
elif ltype == 'dense':
layer = core_layers.Dense(**lspec)
else:
raise NotImplementedError
model.add(layer)
sgd = optimizers.SGD(lr=0.1, decay=1e-6, momentum=momentum, nesterov=nesterov) # TODO parameters
model.compile(loss='categorical_crossentropy', optimizer=sgd)
return model, sgd
def get_gtsrb_layout(input_shape, num_classes):
'''Builds a keras CNN layout according to one column of the Multi-CNN architecture described in
Multi-column deep neural network for traffic sign classification
(<NAME> ∗ , <NAME>, <NAME>, <NAME>)'''
layout = []
layout.append( ('conv2D', {'nb_filter': 100, 'nb_row': 7, 'nb_col': 7, 'init': 'uniform', 'activation': 'tanh', 'input_shape': input_shape}) )
layout.append( ('maxpool2D', {'pool_size': (2,2)}) )
layout.append( ('conv2D', {'nb_filter': 150, 'nb_row': 4, 'nb_col': 4, 'init': 'uniform', 'activation': 'tanh'}) )
layout.append( ('maxpool2D', {'pool_size': (2,2)}) )
layout.append( ('conv2D', {'nb_filter': 250, 'nb_row': 4, 'nb_col': 4, 'init': 'uniform', 'activation': 'tanh'}) )
layout.append( ('maxpool2D', {'pool_size': (2,2)}) )
layout.append( ('flatten', {}) )
layout.append( ('dense', {'output_dim': 300, 'init': 'uniform', 'activation': 'tanh'}) )
layout.append( ('dense', {'output_dim': num_classes, 'init': 'uniform', 'activation': 'softmax'}) )
return layout
def load_layout(path):
with open(path) as in_file:
layout = json.load(in_file)
return layout
def store_layout(layout, path):
with open(path, 'w') as out_file:
json.dump(layout, out_file, indent=2, sort_keys=True)
if __name__ == '__main__':
#~ Parse arguments
parser = argparse.ArgumentParser()
parser.add_argument('path', help='Folder to store known layouts in')
args = parser.parse_args()
#~ Make sure path ends in a /
if args.path[-1] != '/':
args.path += '/'
#~ Generate list of models to store
layouts = []
layouts.append( ('gtsrb', get_gtsrb_layout, {'input_shape': (3, 48, 48), 'num_classes': 43}) )
#~ Store models to disk
for name, function, kwargs in layouts:
path = args.path + name + '.l'
store_layout(function(**kwargs), path)
<file_sep># -*- coding: utf-8 -*-
import argparse
import sys
import os
import numpy as np
import PIL.Image as pil_img
sys.path.insert(1, os.path.join(sys.path[0], '../src'))
import nn
def visualize_filters(model, path, filename):
# add "/" to path, if it does not end on this character
if path[-1] != "/":
path += "/"
# iterate over layers
for layer_id,layer in enumerate(model.layers):
# ignore all layers which are not convolutional layers
if layer.get_config()['name'] != "Convolution2D":
continue
# iterate over outputs
for weight_matrices in layer.get_weights():
# ignore 1-dimensional filters
if len(weight_matrices.shape) > 1:
# get number of output maps, number of filters per input map and the x and y dimensions of the filters
num_outputs, num_filters, xdim, ydim = weight_matrices.shape
# calculate the size of the filter collection image and create it
height = num_filters * (xdim + 1) + 1
width = num_outputs * (ydim + 1) + 1
filter_collection = pil_img.new("L", (width, height), "white")
# iterate over the output maps of the next layer
for i_id, inputs in enumerate(weight_matrices):
# iterate over the filters of the maps on the current layer
for f_id, filter in enumerate(inputs):
# determine position of the filter in the filter collection
xpos = i_id * (xdim+1) + 1
ypos = f_id * (ydim+1) + 1
# rescale the grayvalues to visualize them
filter -= np.min(filter)
filter *= 255./np.max(filter)
im_filter = pil_img.fromarray(filter)
# paste the filter into the filter collection
filter_collection.paste(im_filter, (xpos, ypos))
# save the filter collection of layer 'id'
filter_collection.save(path + "weights_on_layer_" + str(layer_id) + "_" + filename + ".png")
if __name__ == "__main__":
#~ Parse parameters
parser = argparse.ArgumentParser()
parser.add_argument('weights', help='Path to the weights which are to be loaded')
parser.add_argument('layout', help='Path network layout specification')
parser.add_argument('-s', '--savepath', help='Path to save location of the visualized filters', default='./')
parser.add_argument('-v', '--verbose', help='Determine whether the programm shall print information in the terminal or not', action="store_true")
parser.add_argument('-n', '--filename', help='Pass a string which is appended to the created image files.', default='')
args = parser.parse_args()
# Load model
print('Loading model from {0}'.format(args.layout))
layout = nn.load_layout(args.layout)
model, optimizer = nn.build_model_to_layout(layout)
#~ Load weights
print('Loading weights from \"{0}\"'.format(args.weights))
model.load_weights(args.weights)
# visualize filters
print('Generating visualizations and storing to {0}'.format(args.savepath))
visualize_filters(model, args.savepath, args.filename)
print('Done')
<file_sep># -*- coding: utf-8 -*-
'''If executed, this program will display images, together with the specified number of most likely
candidates and their probabilities. For the candidates, class representatives are used that reside
inside a specified class representative folder and are named classCLASSID.ppm'''
import argparse
import pickle
import PIL.Image as pil_img
import PIL.ImageOps as pil_img_ops
import numpy as np
import matplotlib.pyplot as ppt
class Image_Loader():
'''Provides methods to load an image from a given path and to load an image according to a class
id, from a folder containing class representatives which has to be passed to __init__.
All images will be provided in the resolution passed to __init__.'''
def __init__(self, resolution, class_representative_path):
'''resolution: 2-tuple of x- and y-resolution
class_representative_path: path to a folder of class representative images named class$.ppm where $ is the class id'''
self.resolution = resolution
self.class_representative_path = class_representative_path
def get_image(self, path):
'''load an image and rescale it to the resolution specified in __init__
path: path to the image file
returns: the image'''
image = pil_img.open(path)
image = image.resize(self.resolution)
return image
def get_class_representative(self, class_id):
'''load image that represents a certain class in the resolution specified in __init__
class_id: id of the class
returns: representative image'''
path = self.class_representative_path.format(class_id)
return self.get_image(path)
def visualize(image_info, class_ids, probabilites, image_loader):
'''Displays an image, the most likely candidates and their probabilities
image_info: iterable that contains dictionaries, where the key "path" provides the path to an image as a string
class_ids: numpy array of shape (number of samples, number of candidates), contains candidate class ids
probabilites: numpy array of shape (number of samples, number of candidates), contains candidate class probabilities
image_loader: Instance of Image_Loader
'''
#~ Iterate over sample images with their respective candidates and their probabilities
for i, c, p in zip(image_info, class_ids, probabilites):
fig, axes = ppt.subplots(1, c.size + 1, figsize=(12, 6), subplot_kw={'xticks': [], 'yticks': []})
#~ Load and display the image
image = image_loader.get_image(i['path'])
axes[0].imshow(image, interpolation='none')
axes[0].set_title('Input', y=-0.15)
#~ Load and display representatives of the candidate classes and their probabilities
for idx, (class_id, probability) in enumerate(zip(c,p)):
class_representative = image_loader.get_class_representative(class_id)
axes[idx+1].imshow(class_representative, interpolation='none')
axes[idx+1].set_title('{0:.4f}'.format(probability), y=-0.15)
ppt.show()
def get_candidates(probabilities, num_candidates=3):
'''Transform the class probabilites into the num_candidates most likely class ids and their probabilites
probabilites: numpy array of shape (samples, classes)
num_candidates: integer, the number of candidates to extract
returns class_ids: numpy array of shape (samples, num_candidates), the ids of the most likely classes
returns winner_probabilities: numpy array of shape (samples, num_candidates), the corresponding probabilites to class_ids'''
num_samples = probabilities.shape[0]
#~ Generate the ids of the most likely classes (the most likely one should be at the lowest index)
class_ids = np.argsort(probabilities, axis=1)
class_ids = class_ids[:,-num_candidates:] # Take the num_candidates most likely classes
class_ids = class_ids[:,::-1] # Put them in descending order
# Also generate probabilities of the num_candidates most likely classes
row_indices = np.arange(num_samples).reshape((num_samples, 1))
winner_probabilities = probabilities[row_indices, class_ids]
return class_ids, winner_probabilities
if __name__ == '__main__':
#~ Parse parameters
parser = argparse.ArgumentParser()
parser.add_argument('predictions', help='Location of the stored predictions')
parser.add_argument('representatives', help='Path to the directory containing class representatives')
parser.add_argument('-c', '--candidates', help='Number of candidates to show for each test image [default 3]', type=int, default=3)
parser.add_argument('-d', '--datalimit', help='Maximum number of data points to read from DATA [if missing, read all]', type=int, default=None)
args = parser.parse_args()
#~ Read data, a list of the sample image properties (including the path), and the class probabilites calculated for them
#~ Data should be a pickle dump of (image_info, probabilities) akin to the one produced by predict.py
#~ image_info is a list of {'path': path_as_string} dictionaries (may contain other key-value pairs)
#~ probabilities is a (number_of_samples, number_of_classes) numpy array.
with open(args.predictions, 'rb') as in_file:
image_info, probabilities = pickle.load(in_file)
#~ Limit the amount of data if specified per parameter
if args.datalimit:
image_info = image_info[:args.datalimit]
probabilities = probabilities[:args.datalimit,...]
#~ Get class ids and their probabilities of the most likely classes
class_ids, winner_probabilities = get_candidates(probabilities, num_candidates=args.candidates)
#~ Actual visualization
if args.representatives[-1] != '/':
args.representatives += '/'
class_representative_path_spec = args.representatives + 'class{0}.ppm'
image_loader = Image_Loader((48, 48), class_representative_path_spec)
visualize(image_info, class_ids, winner_probabilities, image_loader)
<file_sep>import sys
import os
for i in range(31,61):
os.system("python src/train.py layouts/inria_no_filter_train.l data/csv_list_train_inria.txt -m -l weights/inria/gtsrb_filters_" + str(i - 1) + " -s weights/inria/gtsrb_filters_" + str(i))
| 4e7fe7ed82c86a856c645a3d70a5d24e114bcc75 | [
"Markdown",
"Python",
"Shell"
] | 17 | Python | chris-code/study-project-gtsrb | 7fbdf1348373aa2ee4b692fa2f49494e98e7067d | 8f1fc60b6b0feef7babac9018fccb1ab38303dda | |
refs/heads/master | <file_sep>const express = require("express");
const bodyParser = require("body-parser");
const mongoose = require("mongoose");
const _ = require("lodash");
const app = express();
app.set('view engine', 'ejs');
app.use(bodyParser.urlencoded({extended: true}));
app.use(express.static("public"));
//create new database
mongoose.connect("mongodb+srv://admin-dixon:<EMAIL>.apw55.mongodb.net/usersDB", {useNewUrlParser: true});
const userSchema = new mongoose.Schema ({
firstName: String,
email: String,
mobile: String
});
const User = mongoose.model('Fruit', userSchema);
app.post('/src/app', function(req, res){
const { firstName, email, mobile } = req.body;
// storedata on database
User.insertOne({ firstName, email, mobile }, function (err, users){
users.save();
}
});
});
app.listen(3000, function() {
console.log("Server started on port 3000");
});
<file_sep>import { Component } from '@angular/core';
import { FormControl, Validators } from '@angular/forms';
import { User } from 'src/app/entity/user';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-name-editor',
templateUrl: './name-editor.component.html',
styleUrls: ['./name-editor.component.css']
})
export class NameEditorComponent {
firstName = new FormControl('', Validators.required);
email = new FormControl('', Validators.email);
mobile = new FormControl('', Validators.minLength(10));
// updateName() {
// this.firstName.setValue('JohnDoe');
// }
// updateEmail() {
// this.email.setValue('');
// }
// updateMobile() {
// this.mobile.setValue('012-345-6789'); }
user = new User('Thabo', '<EMAIL>', '0123456789');
constructor(private http: HttpClient) {
}
public show: boolean = true;
public showmail: boolean = false;
public showmobile: boolean = false;
public showstart: boolean = false;
public buttonName: any = 'Show';
toggle() {
// hide form element onclick
this.show = !this.show;
this.showmail = !this.showmail;
// post to node
this.http.post('mongodb+srv://admin-dixon:[email protected]/usersDB', this.user)
// success message to user
alert("success!");
}
toggleEmail() {
// hide form element onclick
this.showmail = !this.showmail;
this.showmobile = !this.showmobile;
// post to node
this.http.post('mongodb+srv://admin-dixon:[email protected]/usersDB', this.user)
// success message to user
alert("success!");
}
toggleNumber() {
// hide form element onclick
this.showmobile = !this.showmobile;
this.showstart = !this.showstart;
// post to node
this.http.post('mongodb+srv://admin-dixon:<EMAIL>/usersDB', this.user)
// success message to user
alert("success!");
}
toggleProfile() {
// hide form element onclick
this.showstart = !this.showstart;
// Show initial element form element onclick
this.show = !this.show;
}
}
| 57e035eb350d8daf118ada23ba06a79e14022783 | [
"JavaScript",
"TypeScript"
] | 2 | JavaScript | RailwayGunDora/appdocker | 3a0a78bf5d1fb39c8f20b383c56c31362b7e73a6 | dfec58c56eea5eb55069ff4e7d360659d6f64ab8 | |
refs/heads/master | <file_sep>package com.jbk.sopan.AdminLTE;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import com.aventstack.extentreports.utils.FileUtil;
public class Utilty {
static WebDriver driver;
public static String Scrrenshort(WebDriver driver) {
TakesScreenshot ts=(TakesScreenshot) driver;
File src=ts.getScreenshotAs(OutputType.FILE);
String des=System.getProperty("user.dir"+"/scrrenshort"+System.currentTimeMillis());
File destination=new File(des);
try {
FileUtils.copyFile(src, destination);
} catch (IOException e) {
System.out.println("capture failes"+e.getMessage());
}
return des;
}
}
<file_sep> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.jbk.sopan</groupId>
<artifactId>AdminLTE</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>AdminLTE</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<!-- https://mvnrepository.com/artifact/javax.mail/mail -->
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.activation/activation -->
<dependency>
<groupId>javax.activation</groupId>
<artifactId>activation</artifactId>
<version>1.1.1</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>3.17</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>2.53.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.7</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.github.yev/screenshot -->
<dependency>
<groupId>com.github.yev</groupId>
<artifactId>screenshot</artifactId>
<version>0.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>3.0.1-FINAL</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.aventstack/extentreports -->
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>3.0.2</version>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.testng/testng -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>6.9.10</version>
</dependency>
<!-- https://mvnrepository.com/artifact/log4j/log4j -->
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
</dependencies>
</project>
<file_sep>package com.jbk.scrrenshort;
public class demo1 {
}
<file_sep>package com.jbk.scrrenshort;
import org.apache.log4j.Logger;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class GenerateLog {
public void demo() {
Logger log =Logger.getLogger(GenerateLog.class);
System.setProperty("webdriver.chrome.driver", "E:\\softwae\\chromedriver_win32\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
log.info("launching browser");
driver.get("http://www.google.com");
log.info("browser launch");
String title=driver.getTitle();
log.info("title is"+title);
if(title.equals("Googel"))
{
log.info("title is correct");
}
else {
log.info("title is not correct");
}
driver.quit();
log.info("browser is closed");
}
}
<file_sep>package com.cookies;
import java.util.Iterator;
import java.util.Set;
import org.openqa.selenium.Cookie;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class cookiesDemo {
@Test
public void demo() {
System.setProperty("webdriver.chrome.driver", "E:\\softwae\\chromedriver_win32\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://www.flipkart.com/");
driver.manage().window().maximize();
Set<Cookie> cook=driver.manage().getCookies();
Cookie coo=new Cookie("uname", "kiran");
driver.manage().getCookieNamed("coo");
for(Cookie cooki:cook)
{
System.out.println("Cookies details");
System.out.println("cookies name" +cooki.getName());
System.out.println("cookies name" +cooki.getValue());
System.out.println("cookies name" +cooki.getDomain());
System.out.println("cookies name" +cooki.getPath());
System.out.println("cookies name" +cooki.getClass());
System.out.println("cookies name" +cooki.getExpiry());
}
driver.close();
}
}
| c0a570969c5dee626e8e3afc016cca4b317040d3 | [
"Java",
"Maven POM"
] | 5 | Java | sopan1234/demo | 826f99a74dec2452ff303094c95c30457e531568 | 7286c0f272620f3af613a96b2f44fc83e68a8c4d | |
refs/heads/master | <repo_name>catlizard/Heos-Bamboozler<file_sep>/readme.md
$ npm/yarn install
$ npm/yarn start
<file_sep>/Heos-Bamboozler/app.js
// This file is required by the index.html file and will
// be executed in the renderer process for that window.
// All of the Node.js APIs are available in this process.
const { app, BrowserWindow } = require('electron');
const heos = require('heos-api');
const { volume_level, play_state } = heos.constants;
let volumeSlider, vol, playing;
window.$ = window.jQuery = require('jquery');
window.onload = function () {
var canvas = document.getElementById('ultraCanvas');
volumeSlider = document.getElementById('volumeRange');
vol = document.getElementById('currentVolume');
getInitialVol();
getCurrentlyPlaying();
checkPlayState();
setInterval(function() {
getCurrentlyPlaying()
}, 5000);
var calcVolume = debounce(() => {
setVolume(volumeSlider.value);
vol.innerText = volumeSlider.value;
}, 50);
$(volumeSlider).on('input', calcVolume);
$('#bamboozleTheme').click(function() {
if($(this).is(':checked')) {
$('html').addClass('bamboozle');
$('#volumeRange').addClass('super-bamboozle');
} else {
$('html').removeClass('bamboozle');
$('#volumeRange').removeClass('super-bamboozle');
}
});
$('.btn-prev').on('click', function() {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.play_previous(
connection,
player.pid,
)
).then(bamboozle => {
getCurrentlyPlaying();
})
);
});
$('.btn-pause').on('click', function () {
var _this = this;
var newState;
playing = !playing;
if(playing) {
newState = play_state.play;
} else {
newState = play_state.pause;
}
switch (newState) {
case 'play':
$(_this).html('<i class="fa fa-pause"></i>')
playing = true;
break;
default:
$(_this).html('<i class="fa fa-play"></i>')
playing = false;
break;
}
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.set_play_state(
connection,
player.pid,
newState
)
).then(bamboozle => {
getCurrentlyPlaying();
})
);
});
$('.btn-next').on('click', function () {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.play_next(
connection,
player.pid,
)
).then(bamboozle => {
getCurrentlyPlaying();
})
);
});
}
function debounce(func, wait, immediate) {
var timeout;
return function () {
var context = this, args = arguments;
var later = function () {
timeout = null;
if (!immediate) func.apply(context, args);
};
var callNow = immediate && !timeout;
clearTimeout(timeout);
timeout = setTimeout(later, wait);
if (callNow) func.apply(context, args);
};
};
function checkPlayState() {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.get_play_state(
connection,
player.pid,
)
)
.then(playState => {
switch (playState.message.state) {
case 'play':
$('.btn-pause').html('<i class="fa fa-pause"></i>')
playing = true;
break;
default:
$('.btn-pause').html('<i class="fa fa-play"></i>')
playing = false;
break;
}
})
);
}
function getCurrentlyPlaying() {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.get_now_playing_media(
connection,
player.pid,
null
)
).then(media => {
$('.now-playing').html(media.payload.artist + ' – ' + media.payload.song );
})
);
}
function getInitialVol() {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.get_volume(
connection,
player.pid
)
).then(volume => {
setDisplayVolume(volume.message.level);
})
);
}
function setDisplayVolume(volume) {
vol.innerText = volume;
volumeSlider.value = volume;
}
function setVolume(vol) {
heos.discoverAndCreateConnection()
.then(connection =>
heos.commands.player.get_players(connection)
.then(players => players.payload[0])
.then(player =>
heos.commands.player.set_volume(
connection,
player.pid,
volume_level(vol)
)
)
)
} | 5d2ba41cfa90c843adb6383d7f37919d2bc92d9f | [
"Markdown",
"JavaScript"
] | 2 | Markdown | catlizard/Heos-Bamboozler | 0a5402bc4fbab2964ed6d718dbe5a6aa63771468 | 582e343f8550839c8132698c7d25657f6e7350a8 |