Spaces:
Sleeping
Sleeping
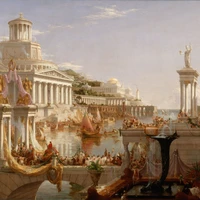
trying to move json content type to None, to disable that check entirely (might be the bug cause)
134d4df
import discord | |
import aiohttp | |
import ast | |
import os | |
import threading | |
intents = discord.Intents.default() | |
intents.message_content = True | |
bot = discord.Bot(intents = intents) | |
token = os.environ.get('TOKEN_DISCORD') | |
class Like_Dislike(discord.ui.View): | |
async def like_button(self, button, interaction): | |
await interaction.response.send_message("You liked the response") | |
async def dislike_button(self, button, interaction): | |
await interaction.response.send_message("You disliked the response") | |
async def on_ready(): | |
print(f"{bot.user} is ready and online!") | |
async def help(ctx: discord.ApplicationContext): | |
await ctx.respond("Hello! FURY Bot responds to all your messages\ | |
\n1)Inside Forum channel and\ | |
\n2)Those that tag the bot.") | |
async def llm_output(question: str) -> tuple[str, str]: | |
URL_LLM = 'https://robinroy03-fury-engine.hf.space' | |
obj = { | |
"query": question, | |
"llm": "llama3-70b-8192", | |
"knn": 3, | |
"stream": False | |
} | |
async with aiohttp.ClientSession() as session: | |
async with session.post(URL_LLM + "/api/generate", json=obj) as response: | |
if response.status == 500: | |
return "Error 500" | |
response_json = await response.json(content_type=None) | |
return response_json['response'], response_json['references'] | |
async def on_message(message): | |
""" | |
Returns llm answer with the relevant context. | |
""" | |
if (message.author == bot.user) or not(bot.user.mentioned_in(message)): | |
return | |
print(message.content) | |
await message.reply(content="Your message was received, it'll take around 30 seconds for FURY to process an answer.") | |
question = message.content.replace("<@1243428204124045385>", "") | |
engine_response = await llm_output(question) | |
try: | |
llm_answer, references = engine_response | |
start = 0 | |
while llm_answer[start: start+1990] != "": | |
if (start == 0): | |
await message.reply(content=llm_answer[start: start+1990]) | |
else: | |
await message.reply(content=llm_answer[start: start+1990]) | |
start += 1990 | |
await message.reply(content=f"**References**\n{references}", view=Like_Dislike()) | |
except Exception as e: # TODO: make exception handling better | |
print(e) | |
await message.reply("An error occurred. Retry again. Try different prompts.") | |
def run_bot(): | |
bot.run(token) | |
# =========================================================================================================================================================== | |
from flask import Flask | |
app = Flask(__name__) | |
def home(): | |
return "The bot is online." | |
threading.Thread(target=run_bot).start() | |
app.run() | |