Spaces:
Runtime error
Runtime error
Upload app.py
Browse files
app.py
ADDED
@@ -0,0 +1,147 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
#!/usr/bin/env python
|
2 |
+
# coding: utf-8
|
3 |
+
|
4 |
+
# In[113]:
|
5 |
+
|
6 |
+
|
7 |
+
import gradio as gr
|
8 |
+
from transformers import pipeline
|
9 |
+
import torch
|
10 |
+
from scipy.special import softmax
|
11 |
+
from transformers import BertForSequenceClassification, BertTokenizer, BertConfig
|
12 |
+
|
13 |
+
|
14 |
+
# In[114]:
|
15 |
+
|
16 |
+
|
17 |
+
tokenizer = BertTokenizer.from_pretrained("indobenchmark/indobert-base-p1")
|
18 |
+
config = BertConfig.from_pretrained('indobenchmark/indobert-base-p1')
|
19 |
+
model = BertForSequenceClassification.from_pretrained('indobenchmark/indobert-base-p1', config = config)
|
20 |
+
|
21 |
+
|
22 |
+
# In[115]:
|
23 |
+
|
24 |
+
|
25 |
+
def preprocess(text):
|
26 |
+
new_text = []
|
27 |
+
for t in text.split(" "):
|
28 |
+
t = '@user' if t.startswith('@') and len(t) > 1 else t
|
29 |
+
t = 'http' if t.startswith('http') else t
|
30 |
+
new_text.append(t)
|
31 |
+
return " ".join(new_text)
|
32 |
+
|
33 |
+
|
34 |
+
# In[116]:
|
35 |
+
|
36 |
+
|
37 |
+
def sentiment_analysis(text):
|
38 |
+
text = preprocess(text)
|
39 |
+
encoded_input = tokenizer(text, return_tensors='pt')
|
40 |
+
output = model(**encoded_input)
|
41 |
+
scores_ = output[0][0].detach().numpy()
|
42 |
+
scores_ = softmax(scores_)
|
43 |
+
# Format output dict of scores
|
44 |
+
labels = ['Negative', 'Neutral', 'Positive']
|
45 |
+
scores = {l:float(s) for (l,s) in zip(labels, scores_) }
|
46 |
+
return scores
|
47 |
+
|
48 |
+
|
49 |
+
# In[117]:
|
50 |
+
|
51 |
+
|
52 |
+
example_sentence_1 = "aduh mahasiswa sombong kasih kartu kuning belajar usahlah politik selesai kuliah nya politik telat dasar mahasiswa"
|
53 |
+
example_sentence_2 = "lokasi strategis jalan sumatra bandung nya nyaman sofa lantai paella nya enak pas dimakan minum bir dingin appetiser nya enak enak"
|
54 |
+
example_sentence_3 = "pakai kartu kredit bca tidak untung malah rugi besar"
|
55 |
+
example_sentence_4 = "makanan beragam , harga makanan di food stall akan ditambahkan 10 % lagi di kasir , suasana ramai dan perlu perhatian untuk mendapatkan parkir dan tempat duduk"
|
56 |
+
examples = [[example_sentence_1], [example_sentence_2],
|
57 |
+
[example_sentence_3], [example_sentence_4]]
|
58 |
+
|
59 |
+
|
60 |
+
# In[118]:
|
61 |
+
|
62 |
+
|
63 |
+
markdownn = '''
|
64 |
+
## Sentiment Analysis IndoBERT by IndoNLU
|
65 |
+
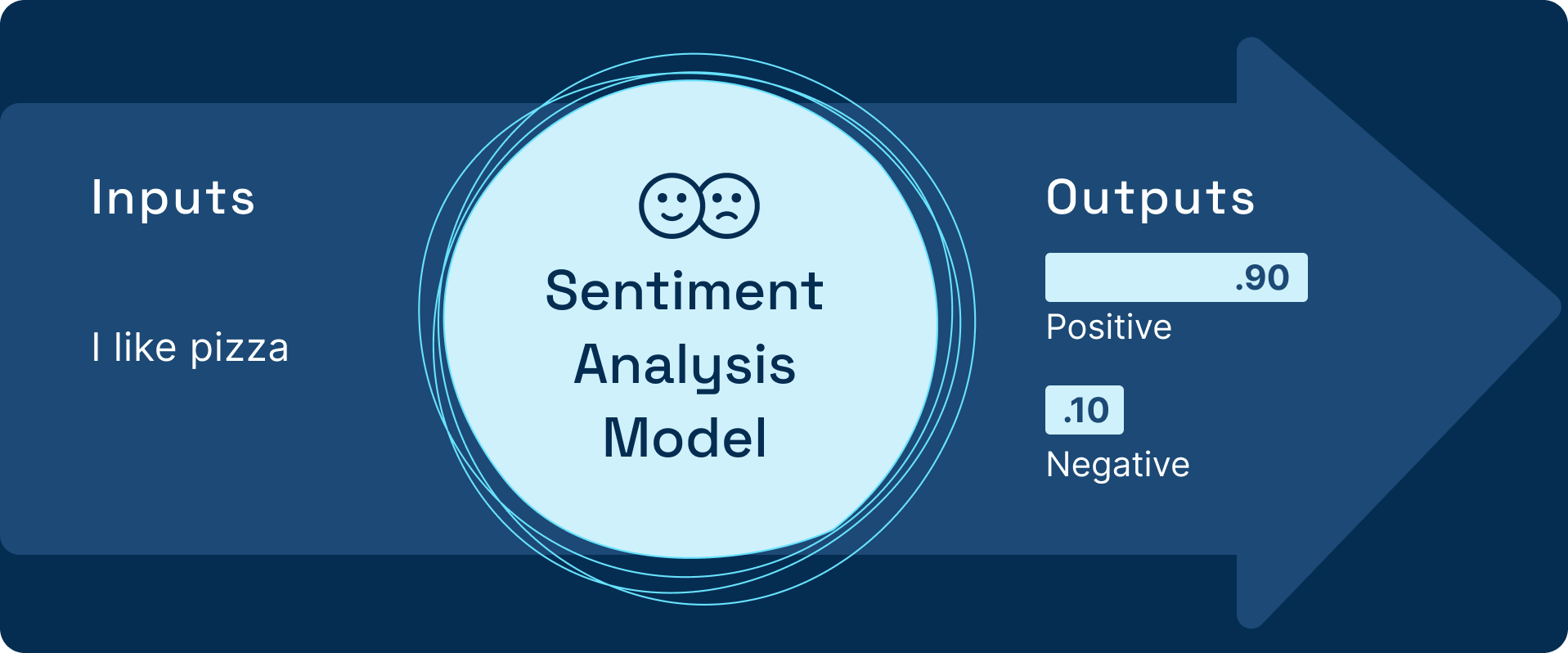
|
66 |
+
|
67 |
+
### Apa itu Sentimen Analisis?
|
68 |
+
Sentimen analisis atau sentiment analysis adalah proses untuk mengidentifikasi, mengekstrak,
|
69 |
+
dan memproses sentimen atau opini dari suatu teks, seperti artikel, review, tweet, atau status media sosial lainnya.
|
70 |
+
Tujuannya adalah untuk menentukan apakah sentimen yang diekspresikan dalam teks tersebut adalah positif, negatif, atau netral.
|
71 |
+
Sentimen analisis sering digunakan dalam berbagai industri, seperti bisnis, pemasaran, media sosial, dan politik untuk memahami opini atau persepsi publik tentang produk, merek, acara, atau isu tertentu.
|
72 |
+
|
73 |
+
### Tujuan Penggunaan
|
74 |
+
Analisis sentimen adalah teknik yang dapat digunakan dalam berbagai aplikasi. Berikut adalah beberapa contoh kasus penggunaan untuk analisis sentimen. Pemantauan media sosial: Analisis sentimen dapat digunakan untuk memantau sentimen postingan media sosial tentang perusahaan atau merek. Teknik dapat membantu perusahaan memahami bagaimana merek mereka dirasakan oleh publik dan mengidentifikasi area untuk perbaikan.
|
75 |
+
|
76 |
+
Secara keseluruhan, analisis sentimen adalah teknik serbaguna yang dapat digunakan di berbagai bidang untuk mendapatkan wawasan dari data tekstual.
|
77 |
+
|
78 |
+
#### Dokumentasi Pipeline
|
79 |
+
Here is sample code for a sentiment analysis pipeline:
|
80 |
+
```python
|
81 |
+
from deepsparse import Pipeline
|
82 |
+
tokenizer = BertTokenizer.from_pretrained("indobenchmark/indobert-base-p1")
|
83 |
+
config = BertConfig.from_pretrained('indobenchmark/indobert-base-p1')
|
84 |
+
model = BertForSequenceClassification.from_pretrained('indobenchmark/indobert-base-p1')
|
85 |
+
|
86 |
+
def preprocess(text):
|
87 |
+
new_text = []
|
88 |
+
for t in text.split(" "):
|
89 |
+
t = '@user' if t.startswith('@') and len(t) > 1 else t
|
90 |
+
t = 'http' if t.startswith('http') else t
|
91 |
+
new_text.append(t)
|
92 |
+
return " ".join(new_text)
|
93 |
+
|
94 |
+
def sentiment_analysis(text):
|
95 |
+
text = preprocess(text)
|
96 |
+
encoded_input = tokenizer(text, return_tensors='pt')
|
97 |
+
output = model(**encoded_input)
|
98 |
+
scores_ = output[0][0].detach().numpy()
|
99 |
+
scores_ = softmax(scores_)
|
100 |
+
# Format output dict of scores
|
101 |
+
labels = ['Negative', 'Neutral', 'Positive']
|
102 |
+
scores = {l:float(s) for (l,s) in zip(labels, scores_) }
|
103 |
+
return scores
|
104 |
+
```
|
105 |
+
'''
|
106 |
+
|
107 |
+
|
108 |
+
# In[119]:
|
109 |
+
|
110 |
+
|
111 |
+
with gr.Blocks() as demo:
|
112 |
+
|
113 |
+
|
114 |
+
with gr.Row():
|
115 |
+
with gr.Column():
|
116 |
+
gr.Markdown(markdownn)
|
117 |
+
|
118 |
+
with gr.Column():
|
119 |
+
gr.Markdown("""
|
120 |
+
### Sentiment analysis demo
|
121 |
+
by [Created by @Vrooh933 Production](https://www.linkedin.com/in/m-afif-rizky-a-a96048182)
|
122 |
+
""")
|
123 |
+
text = gr.Text(label="Text")
|
124 |
+
btn = gr.Button("Submit")
|
125 |
+
|
126 |
+
sparse_answers = gr.Label(label="label")
|
127 |
+
|
128 |
+
gr.Examples([[example_sentence_1], [example_sentence_2],
|
129 |
+
[example_sentence_3], [example_sentence_4]], inputs = [text])
|
130 |
+
|
131 |
+
|
132 |
+
btn.click(
|
133 |
+
sentiment_analysis,
|
134 |
+
inputs=[text],
|
135 |
+
outputs=[sparse_answers],
|
136 |
+
)
|
137 |
+
|
138 |
+
|
139 |
+
if __name__ == "__main__":
|
140 |
+
demo.launch()
|
141 |
+
|
142 |
+
|
143 |
+
# In[ ]:
|
144 |
+
|
145 |
+
|
146 |
+
|
147 |
+
|