Update Main Models/Vbai-1.1 Dementia/README.md
Browse files- Main Models/Vbai-1.1 Dementia/README.md +186 -184
Main Models/Vbai-1.1 Dementia/README.md
CHANGED
@@ -1,185 +1,187 @@
|
|
1 |
-
# Vbai-1.1 Dementia (11178564 parametre)
|
2 |
-
|
3 |
-
## "Vbai-1.1 Dementia" modeli, bir önceki modele göre daha fazla veriyle eğitilmiş olup üzerinde ince ayar yapılmış versiyonudur.
|
4 |
-
|
5 |
-
## -----------------------------------------------------------------------------------
|
6 |
-
|
7 |
-
# Vbai-1.1 Dementia (11178564 parameters)
|
8 |
-
|
9 |
-
## The "Vbai-1.1 Dementia" model is a fine-tuned version of the previous model, trained with more data.
|
10 |
-
|
11 |
-
|
12 |
-
|
13 |
-
|
14 |
-
|
15 |
-
|
16 |
-
|
17 |
-
|
18 |
-
import
|
19 |
-
import
|
20 |
-
|
21 |
-
|
22 |
-
|
23 |
-
|
24 |
-
|
25 |
-
|
26 |
-
model
|
27 |
-
model.
|
28 |
-
model =
|
29 |
-
model.
|
30 |
-
|
31 |
-
|
32 |
-
|
33 |
-
|
34 |
-
|
35 |
-
transforms.
|
36 |
-
|
37 |
-
|
38 |
-
|
39 |
-
|
40 |
-
|
41 |
-
|
42 |
-
|
43 |
-
|
44 |
-
|
45 |
-
|
46 |
-
|
47 |
-
|
48 |
-
|
49 |
-
|
50 |
-
|
51 |
-
|
52 |
-
|
53 |
-
|
54 |
-
plt.
|
55 |
-
plt.
|
56 |
-
|
57 |
-
|
58 |
-
|
59 |
-
|
60 |
-
|
61 |
-
|
62 |
-
|
63 |
-
|
64 |
-
|
65 |
-
|
66 |
-
|
67 |
-
|
68 |
-
|
69 |
-
import
|
70 |
-
|
71 |
-
|
72 |
-
import
|
73 |
-
from
|
74 |
-
|
75 |
-
from PyQt5.
|
76 |
-
|
77 |
-
|
78 |
-
|
79 |
-
|
80 |
-
|
81 |
-
|
82 |
-
transforms.
|
83 |
-
|
84 |
-
|
85 |
-
|
86 |
-
|
87 |
-
|
88 |
-
|
89 |
-
|
90 |
-
|
91 |
-
|
92 |
-
|
93 |
-
self.
|
94 |
-
|
95 |
-
|
96 |
-
|
97 |
-
|
98 |
-
self.
|
99 |
-
|
100 |
-
self.
|
101 |
-
|
102 |
-
|
103 |
-
self.
|
104 |
-
|
105 |
-
|
106 |
-
self.
|
107 |
-
|
108 |
-
self.predictButton
|
109 |
-
|
110 |
-
self.
|
111 |
-
|
112 |
-
|
113 |
-
self.
|
114 |
-
|
115 |
-
|
116 |
-
|
117 |
-
|
118 |
-
layout
|
119 |
-
layout.addWidget(self.
|
120 |
-
layout.addWidget(self.
|
121 |
-
layout.addWidget(self.
|
122 |
-
|
123 |
-
self.
|
124 |
-
|
125 |
-
|
126 |
-
|
127 |
-
|
128 |
-
|
129 |
-
|
130 |
-
|
131 |
-
|
132 |
-
self.model
|
133 |
-
self.model.
|
134 |
-
self.model =
|
135 |
-
self.model.
|
136 |
-
self.
|
137 |
-
|
138 |
-
|
139 |
-
|
140 |
-
|
141 |
-
|
142 |
-
|
143 |
-
|
144 |
-
|
145 |
-
|
146 |
-
self.
|
147 |
-
|
148 |
-
|
149 |
-
|
150 |
-
|
151 |
-
|
152 |
-
|
153 |
-
|
154 |
-
|
155 |
-
|
156 |
-
|
157 |
-
|
158 |
-
|
159 |
-
|
160 |
-
|
161 |
-
|
162 |
-
|
163 |
-
|
164 |
-
|
165 |
-
|
166 |
-
|
167 |
-
|
168 |
-
|
169 |
-
|
170 |
-
|
171 |
-
|
172 |
-
|
173 |
-
|
174 |
-
|
175 |
-
|
176 |
-
|
177 |
-
|
178 |
-
|
179 |
-
|
180 |
-
|
181 |
-
|
182 |
-
|
183 |
-
|
184 |
-
|
|
|
|
|
185 |
```
|
|
|
1 |
+
# Vbai-1.1 Dementia (11178564 parametre)
|
2 |
+
|
3 |
+
## "Vbai-1.1 Dementia" modeli, bir önceki modele göre daha fazla veriyle eğitilmiş olup üzerinde ince ayar yapılmış versiyonudur.
|
4 |
+
|
5 |
+
## -----------------------------------------------------------------------------------
|
6 |
+
|
7 |
+
# Vbai-1.1 Dementia (11178564 parameters)
|
8 |
+
|
9 |
+
## The "Vbai-1.1 Dementia" model is a fine-tuned version of the previous model, trained with more data.
|
10 |
+
|
11 |
+
[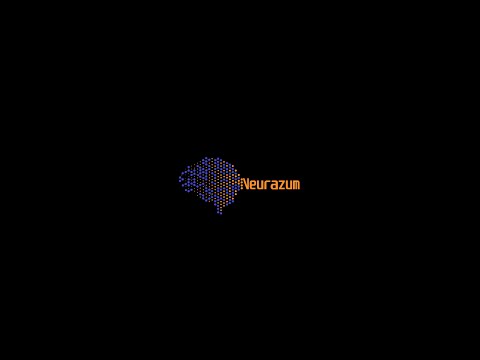](https://youtu.be/wDfsFwusGQU)
|
12 |
+
|
13 |
+
# Kullanım / Usage
|
14 |
+
|
15 |
+
```python
|
16 |
+
import torch
|
17 |
+
import torch.nn as nn
|
18 |
+
from torchvision import transforms, models
|
19 |
+
from PIL import Image
|
20 |
+
import matplotlib.pyplot as plt
|
21 |
+
import os
|
22 |
+
from torchsummary import summary
|
23 |
+
|
24 |
+
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
|
25 |
+
|
26 |
+
model = models.resnet18(pretrained=False)
|
27 |
+
num_ftrs = model.fc.in_features
|
28 |
+
model.fc = nn.Linear(num_ftrs, 4)
|
29 |
+
model.load_state_dict(torch.load('Vbai-1.1 Dementia/path'))
|
30 |
+
model = model.to(device)
|
31 |
+
model.eval()
|
32 |
+
summary(model, (3, 224, 224))
|
33 |
+
|
34 |
+
transform = transforms.Compose([
|
35 |
+
transforms.Resize((224, 224)),
|
36 |
+
transforms.ToTensor(),
|
37 |
+
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
|
38 |
+
])
|
39 |
+
|
40 |
+
class_names = ['No Dementia', 'Mild Dementia', 'Avarage Dementia', 'Very Mild Dementia']
|
41 |
+
|
42 |
+
def predict(image_path, model, transform):
|
43 |
+
image = Image.open(image_path).convert('RGB')
|
44 |
+
image = transform(image).unsqueeze(0).to(device)
|
45 |
+
model.eval()
|
46 |
+
with torch.no_grad():
|
47 |
+
outputs = model(image)
|
48 |
+
probs = torch.nn.functional.softmax(outputs, dim=1)
|
49 |
+
_, preds = torch.max(outputs, 1)
|
50 |
+
return preds.item(), probs[0][preds.item()].item()
|
51 |
+
|
52 |
+
def show_image_with_prediction(image_path, prediction, confidence, class_names):
|
53 |
+
image = Image.open(image_path)
|
54 |
+
plt.imshow(image)
|
55 |
+
plt.title(f"Prediction: {class_names[prediction]} (%{confidence * 100:.2f})")
|
56 |
+
plt.axis('off')
|
57 |
+
plt.show()
|
58 |
+
|
59 |
+
test_image_path = 'image-path'
|
60 |
+
prediction, confidence = predict(test_image_path, model, transform)
|
61 |
+
print(f'Prediction: {class_names[prediction]} (%{confidence * 100})')
|
62 |
+
|
63 |
+
show_image_with_prediction(test_image_path, prediction, confidence, class_names)
|
64 |
+
```
|
65 |
+
|
66 |
+
# Uygulama / As App
|
67 |
+
|
68 |
+
```python
|
69 |
+
import sys
|
70 |
+
import torch
|
71 |
+
import torch.nn as nn
|
72 |
+
from torchvision import transforms, models
|
73 |
+
from PIL import Image
|
74 |
+
import matplotlib.pyplot as plt
|
75 |
+
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QFileDialog, QVBoxLayout, QMessageBox
|
76 |
+
from PyQt5.QtGui import QPixmap, QIcon
|
77 |
+
from PyQt5.QtCore import Qt
|
78 |
+
|
79 |
+
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
|
80 |
+
|
81 |
+
transform = transforms.Compose([
|
82 |
+
transforms.Resize((224, 224)),
|
83 |
+
transforms.ToTensor(),
|
84 |
+
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
|
85 |
+
])
|
86 |
+
|
87 |
+
class_names = ['No Dementia', 'Mild Dementia', 'Avarage Dementia', 'Very Mild Dementia']
|
88 |
+
|
89 |
+
|
90 |
+
class DementiaApp(QWidget):
|
91 |
+
def __init__(self):
|
92 |
+
super().__init__()
|
93 |
+
self.initUI()
|
94 |
+
self.model = None
|
95 |
+
self.image_path = None
|
96 |
+
|
97 |
+
def initUI(self):
|
98 |
+
self.setWindowTitle('Prediction App by Neurazum')
|
99 |
+
self.setWindowIcon(QIcon('C:/Users/eyupi/PycharmProjects/Neurazum/NeurAI/Assets/neurazumicon.ico'))
|
100 |
+
self.setGeometry(2500, 300, 400, 200)
|
101 |
+
|
102 |
+
self.loadModelButton = QPushButton('Upload Model', self)
|
103 |
+
self.loadModelButton.clicked.connect(self.loadModel)
|
104 |
+
|
105 |
+
self.loadImageButton = QPushButton('Upload Image', self)
|
106 |
+
self.loadImageButton.clicked.connect(self.loadImage)
|
107 |
+
|
108 |
+
self.predictButton = QPushButton('Make a Prediction', self)
|
109 |
+
self.predictButton.clicked.connect(self.predict)
|
110 |
+
self.predictButton.setEnabled(False)
|
111 |
+
|
112 |
+
self.resultLabel = QLabel('', self)
|
113 |
+
self.resultLabel.setAlignment(Qt.AlignCenter)
|
114 |
+
|
115 |
+
self.imageLabel = QLabel('', self)
|
116 |
+
self.imageLabel.setAlignment(Qt.AlignCenter)
|
117 |
+
|
118 |
+
layout = QVBoxLayout()
|
119 |
+
layout.addWidget(self.loadModelButton)
|
120 |
+
layout.addWidget(self.loadImageButton)
|
121 |
+
layout.addWidget(self.imageLabel)
|
122 |
+
layout.addWidget(self.predictButton)
|
123 |
+
layout.addWidget(self.resultLabel)
|
124 |
+
|
125 |
+
self.setLayout(layout)
|
126 |
+
|
127 |
+
def loadModel(self):
|
128 |
+
options = QFileDialog.Options()
|
129 |
+
fileName, _ = QFileDialog.getOpenFileName(self, "Choose Model Path", "",
|
130 |
+
"PyTorch Model Files (*.pt);;All Files (*)", options=options)
|
131 |
+
if fileName:
|
132 |
+
self.model = models.resnet18(pretrained=False)
|
133 |
+
num_ftrs = self.model.fc.in_features
|
134 |
+
self.model.fc = nn.Linear(num_ftrs, 4)
|
135 |
+
self.model.load_state_dict(torch.load(fileName, map_location=device))
|
136 |
+
self.model = self.model.to(device)
|
137 |
+
self.model.eval()
|
138 |
+
self.predictButton.setEnabled(True)
|
139 |
+
QMessageBox.information(self, "Model Uploaded", "Model successfully uploaded!")
|
140 |
+
|
141 |
+
def loadImage(self):
|
142 |
+
options = QFileDialog.Options()
|
143 |
+
fileName, _ = QFileDialog.getOpenFileName(self, "Choose Image File", "",
|
144 |
+
"Image Files (*.jpg *.jpeg *.png);;All Files (*)", options=options)
|
145 |
+
if fileName:
|
146 |
+
self.image_path = fileName
|
147 |
+
pixmap = QPixmap(self.image_path)
|
148 |
+
self.imageLabel.setPixmap(pixmap.scaled(224, 224, Qt.KeepAspectRatio))
|
149 |
+
|
150 |
+
def predict(self):
|
151 |
+
if self.model and self.image_path:
|
152 |
+
prediction, confidence = self.predictImage(self.image_path, self.model, transform)
|
153 |
+
self.resultLabel.setText(f'Prediction: {class_names[prediction]} (%{confidence * 100:.2f})')
|
154 |
+
else:
|
155 |
+
QMessageBox.warning(self, "Missing Information", "Model and picture must be uploaded.")
|
156 |
+
|
157 |
+
def predictImage(self, image_path, model, transform):
|
158 |
+
image = Image.open(image_path).convert('RGB')
|
159 |
+
image = transform(image).unsqueeze(0).to(device)
|
160 |
+
model.eval()
|
161 |
+
with torch.no_grad():
|
162 |
+
outputs = model(image)
|
163 |
+
probs = torch.nn.functional.softmax(outputs, dim=1)
|
164 |
+
_, preds = torch.max(outputs, 1)
|
165 |
+
return preds.item(), probs[0][preds.item()].item()
|
166 |
+
|
167 |
+
|
168 |
+
if __name__ == '__main__':
|
169 |
+
app = QApplication(sys.argv)
|
170 |
+
ex = DementiaApp()
|
171 |
+
ex.show()
|
172 |
+
sys.exit(app.exec_())
|
173 |
+
```
|
174 |
+
|
175 |
+
# Python Sürümü / Python Version
|
176 |
+
|
177 |
+
### 3.9 <=> 3.13
|
178 |
+
|
179 |
+
# Modüller / Modules
|
180 |
+
|
181 |
+
```bash
|
182 |
+
matplotlib==3.8.0
|
183 |
+
Pillow==10.0.1
|
184 |
+
torch==2.3.1
|
185 |
+
torchsummary==1.5.1
|
186 |
+
torchvision==0.18.1
|
187 |
```
|