id
int64 5
1.93M
| title
stringlengths 0
128
| description
stringlengths 0
25.5k
| collection_id
int64 0
28.1k
| published_timestamp
timestamp[s] | canonical_url
stringlengths 14
581
| tag_list
stringlengths 0
120
| body_markdown
stringlengths 0
716k
| user_username
stringlengths 2
30
|
---|---|---|---|---|---|---|---|---|
1,912,383 | Deep Dive into Chromium: A Comprehensive Analysis from Architecture Design to Core Code | 1. Introduction Since its inception by Google in 2008, the Chromium project has become the... | 0 | 2024-07-05T07:52:34 | https://dev.to/happyer/deep-dive-into-chromium-a-comprehensive-analysis-from-architecture-design-to-core-code-4jdg | webdev, web, website, development | ## 1. Introduction
Since its inception by Google in 2008, the Chromium project has become the cornerstone of modern browser technology. The initial goal was to create a fast, stable, and secure browser that supports modern web technologies. Over time, Chromium has not only provided the foundation for Google Chrome but also for browsers like Microsoft Edge, Brave, and Vivaldi. This article will delve into Chromium's architecture and core components, analyzing its source code to reveal the mechanisms behind its high performance and security.
## 2. History
The Chromium project originated in 2008 when Google decided to develop a new browser. The project aimed to create a fast, stable, and secure browser that supports modern web technologies. The initial version of Chromium was released in September 2008, and the first commercial version based on Chromium, Google Chrome, was released in December 2008.
Over time, Chromium has evolved into a community-driven project, attracting developers from around the world. Today, Chromium not only supports Google Chrome but also serves as the foundation for browsers like Microsoft Edge, Brave, and Vivaldi.
## 3. Architecture
Chromium's architecture can be divided into several main parts:
### 3.1. Browser Process
The browser process is the core component of Chromium, responsible for managing the overall operation of the browser. Its main responsibilities include:
- Creating and managing browser windows.
- Handling user input, such as clicks, scrolls, and keyboard input.
- Managing other processes, such as renderer processes, plugin processes, and network processes.
- Handling bookmarks, history, and other browser settings.
- Interacting with the operating system to integrate the browser into the OS.
The browser process is closely related to the user interface (UI) thread, ensuring that the browser can respond to user actions and run smoothly.
### 3.2. Renderer Process
The renderer process is responsible for converting web content into visual images. Each tab has a separate renderer process, which helps improve the browser's security and stability. The main responsibilities of the renderer process include:
- Parsing HTML documents and constructing the DOM tree.
- Parsing CSS styles, calculating layouts, and drawing styles.
- Executing JavaScript code, responding to user actions, and updating the DOM tree.
- Displaying the rendered images on the screen.
The renderer process is closely related to the main thread, which handles DOM operations, CSS style calculations, and JavaScript execution. To improve performance, Chromium can also use multiple worker threads to handle tasks such as image decoding and text layout.
### 3.3. Plugin Process
The plugin process is responsible for managing browser plugins, such as Flash and PDF readers. The main responsibilities of the plugin process include:
- Loading and initializing plugins.
- Handling interactions between plugins and web pages.
- Managing the lifecycle and resources of plugins.
While the plugin process allows the browser to extend its functionality, it also introduces security risks. Therefore, modern browsers have gradually reduced their reliance on plugins, opting instead for native web technologies based on HTML5, CSS3, and JavaScript.
### 3.4. GPU Process
The GPU process handles graphics rendering, particularly using hardware acceleration to improve page rendering performance. The main responsibilities of the GPU process include:
- Managing GPU resources, such as textures and buffers.
- Assigning rendering tasks to GPU hardware.
- Handling graphics drawing requests from renderer processes.
- Collaborating with the browser process and other processes to ensure that graphics are correctly displayed on the screen.
The GPU process enables Chromium to achieve high-performance graphics rendering on various devices, including desktop computers, laptops, tablets, and smartphones.
### 3.5. Network Process
The network process handles network requests, such as HTTP requests and DNS queries. The main responsibilities of the network process include:
- Parsing URLs to determine the type of requested resources.
- Establishing connections with servers, sending requests, and receiving responses.
- Caching and managing network resources to improve page load speed.
- Handling proxy settings and security policies, such as HTTPS connections and cross-origin access control.
The network process allows Chromium to efficiently handle network requests, ensuring the fast loading of web content. Additionally, the network process is responsible for implementing the browser's security policies, protecting users from network attacks.
## 4. Core Components
Chromium's core components form the foundation of the browser, working together to provide high-performance, secure, and scalable browser functionality. The core components of Chromium include:
### 4.1. Blink
**Blink** is Chromium's rendering engine, responsible for parsing HTML, CSS, and JavaScript to generate the visual representation of web pages. Blink is based on the WebKit project but later became an independent project. The main responsibilities of Blink include:
- Parsing HTML documents and constructing the DOM tree.
- Parsing CSS styles, calculating layouts, and drawing styles.
- Executing JavaScript code, responding to user actions, and updating the DOM tree.
- Displaying the rendered images on the screen.
Blink employs various optimization techniques to improve rendering performance, such as compositing layers, hardware acceleration, and lazy loading. Additionally, Blink supports web standards like CSS Grid, Flexbox, and Web Components, enabling developers to create modern, responsive web pages.
### 4.2. V8
**V8** is a high-performance JavaScript engine used to execute JavaScript code in web pages. V8 employs Just-In-Time (JIT) compilation to convert JavaScript code into native machine code, thereby improving execution speed. The main features of V8 include:
- Fast execution speed: V8 uses a JIT compiler to compile JavaScript code into native machine code, improving execution speed.
- Memory optimization: V8 uses a garbage collection mechanism to manage memory, reducing memory leaks and fragmentation.
- Isolation and sandboxing: V8 supports isolation and sandboxing techniques to enhance the browser's security and stability.
V8's performance advantages enable Chromium to smoothly run complex web applications, such as online games, real-time communication, and big data visualization.
### 4.3. Chromium Content API
The **Chromium Content API** defines the interface between the browser and renderer processes, allowing developers to easily extend Chromium's functionality. The Content API provides a set of C++ classes and methods for handling tasks such as network requests, file I/O, and GPU rendering. Through the Content API, developers can create custom browser extensions, plugins, or embedded browser instances.
The design of the Chromium Content API considers security and stability, enabling developers to create high-performance, secure browser applications on different operating systems and devices.
These core components collectively form the foundation of the Chromium project, enabling Chromium to provide high-performance, secure, and scalable browser functionality across multiple platforms.
## 5. Chromium Source Code Analysis
First, ensure you have installed the Chromium source code. To obtain the source code, visit the [Chromium Git repository](https://chromium.googlesource.com/chromium/src/) and follow the instructions to clone it.
### 5.1. Main Function (main.cc)
The entry point of Chromium is the `main.cc` file. In this file, we can see the `main()` function, which is the starting point of the Chromium application.
```cpp
int main(int argc, char** argv) {
// ...
return content::ContentMain(MakeChromeMainDelegate());
}
```
The `main()` function calls `content::ContentMain()`, which is the actual entry point of the Chromium application. The `MakeChromeMainDelegate()` function creates a `ChromeMainDelegate` instance, responsible for initializing various components of Chromium.
### 5.2. Initialization (chrome_main_delegate.cc)
The `ChromeMainDelegate` class is the controller of the Chromium initialization process. In the `chrome_main_delegate.cc` file, we can see the `Create()` function creating a `ChromeMainDelegate` instance.
```cpp
std::unique_ptr<content::MainDelegate> Create() {
return std::make_unique<ChromeMainDelegate>();
}
```
The `ChromeMainDelegate` class overrides several key virtual functions, such as `PreEarlyInitialization()` and `PostEarlyInitialization()`, which are called at different stages of Chromium's initialization to ensure the correct initialization of various components.
### 5.3. Creating the Browser Process (browser_process.cc)
In the `browser_process.cc` file, we can see the implementation of the `BrowserProcessImpl` class. This class is responsible for managing various components of the browser process, such as `PrefService` and `ExtensionService`.
```cpp
BrowserProcessImpl::BrowserProcessImpl() {
// ...
}
```
The constructor of the `BrowserProcessImpl` class initializes the various components required by the browser process. For example, it creates a `PrefService` instance to manage browser settings and an `ExtensionService` instance to manage browser extensions.
### 5.4. Creating the Renderer Process (render_process_impl.cc)
In the `render_process_impl.cc` file, we can see the implementation of the `RenderProcessImpl` class. This class is responsible for managing various components of the renderer process, such as `RenderThreadImpl` and `RendererNetworkService`.
```cpp
RenderProcessImpl::RenderProcessImpl() {
// ...
}
```
The constructor of the `RenderProcessImpl` class initializes the various components required by the renderer process. For example, it creates a `RenderThreadImpl` instance to handle inter-thread communication in the renderer process and a `RendererNetworkService` instance to handle network requests.
### 5.5. Creating the Plugin Process (plugin_process.cc)
In the `plugin_process.cc` file, we can see the implementation of the `PluginProcess` class. This class is responsible for managing various components of the plugin process, such as `PluginService` and `PluginProcessHost`.
```cpp
PluginProcess::PluginProcess() {
// ...
}
```
The constructor of the `PluginProcess` class initializes the various components required by the plugin process. For example, it creates a `PluginService` instance to manage browser plugins and a `PluginProcessHost` instance to handle communication between the plugin process and the browser process.
Through the above code analysis, we can see the complexity and modularity of the Chromium project. The various components of Chromium collaborate through well-designed interfaces and classes to achieve high-performance, secure, and scalable browser functionality.
## 6. Conclusion
The Chromium project, through its modular architecture and efficient core components, achieves high-performance, secure, and scalable browser functionality. The browser process, renderer process, plugin process, GPU process, and network process each play their roles, ensuring the smooth operation of the browser. Core components such as the Blink rendering engine and the V8 JavaScript engine provide excellent performance and security through various optimization techniques and just-in-time compilation. By analyzing the Chromium source code, we can gain a deeper understanding of its complexity and modular design, which enable Chromium to provide a consistent user experience across multiple platforms. The success of the Chromium project lies not only in its technical implementation but also in its openness and community-driven development model, allowing it to continuously adapt to and lead the development of web technologies.
## 7. Codia AI's products
Codia AI has rich experience in multimodal, image processing, development, and AI.
1.[**Codia AI Figma to code:HTML, CSS, React, Vue, iOS, Android, Flutter, Tailwind, Web, Native,...**](https://codia.ai/s/YBF9)
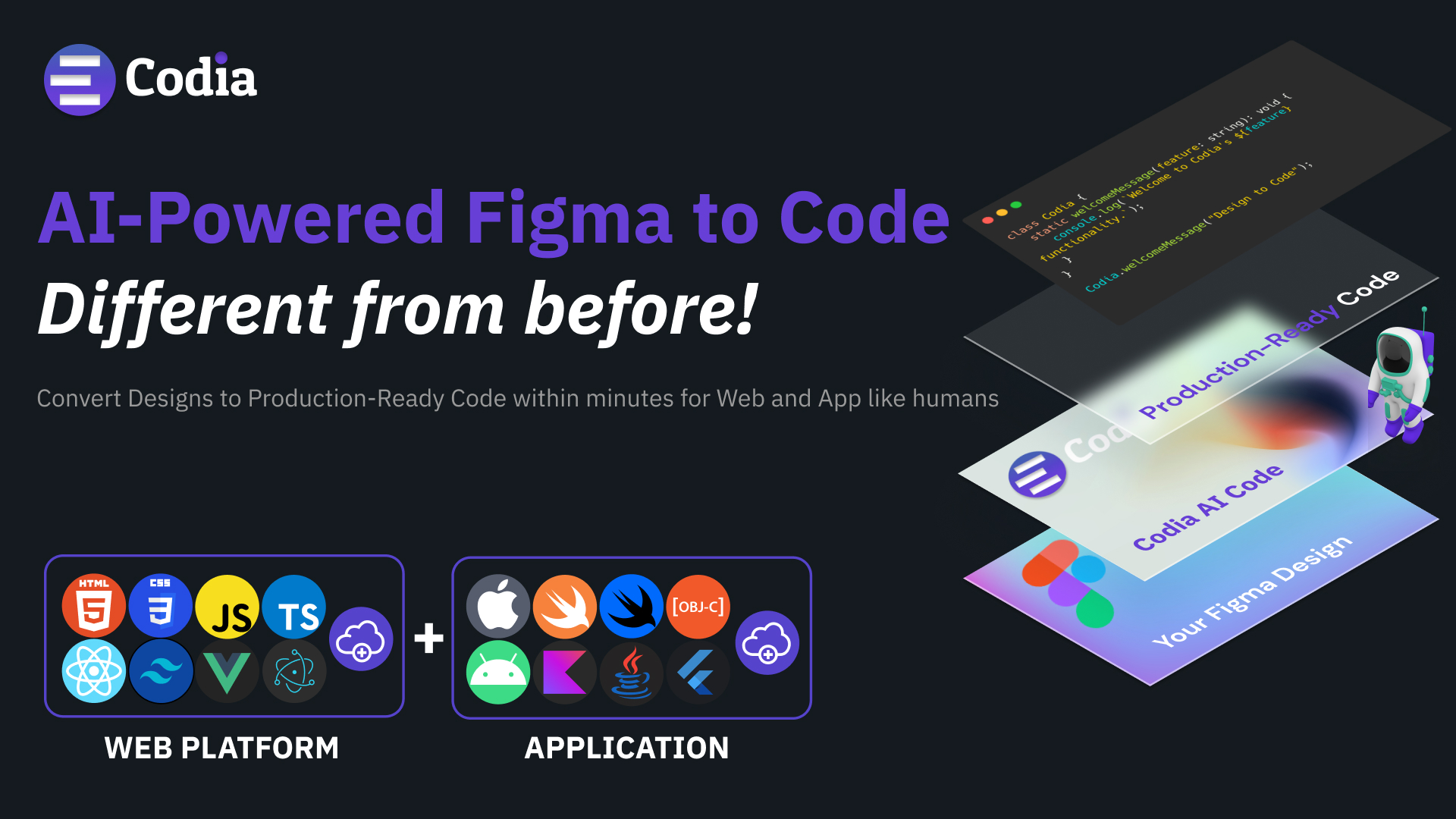
2.[**Codia AI DesignGen: Prompt to UI for Website, Landing Page, Blog**](https://codia.ai/t/pNFx)
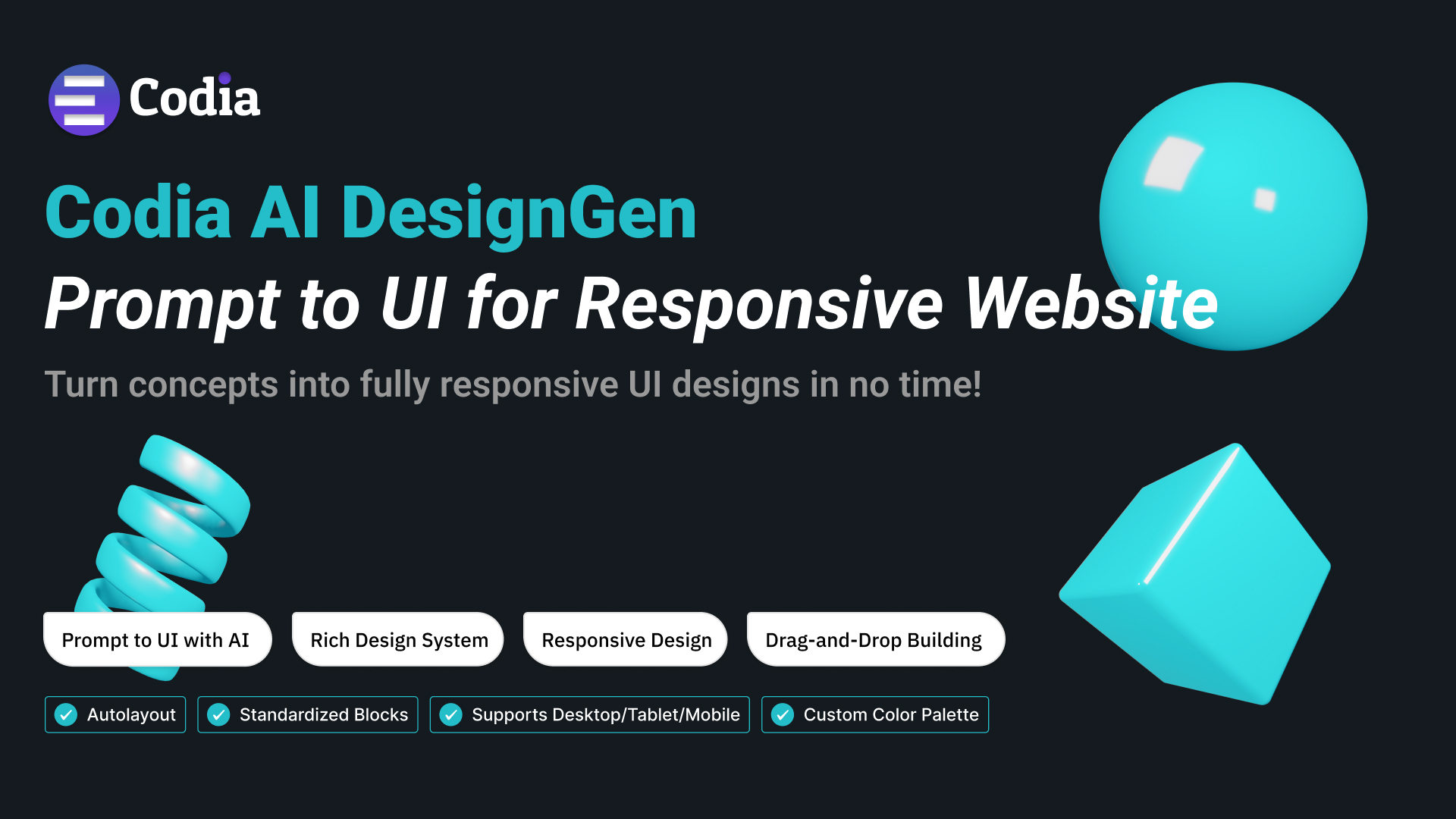
3.[**Codia AI Design: Screenshot to Editable Figma Design**](https://codia.ai/d/5ZFb)
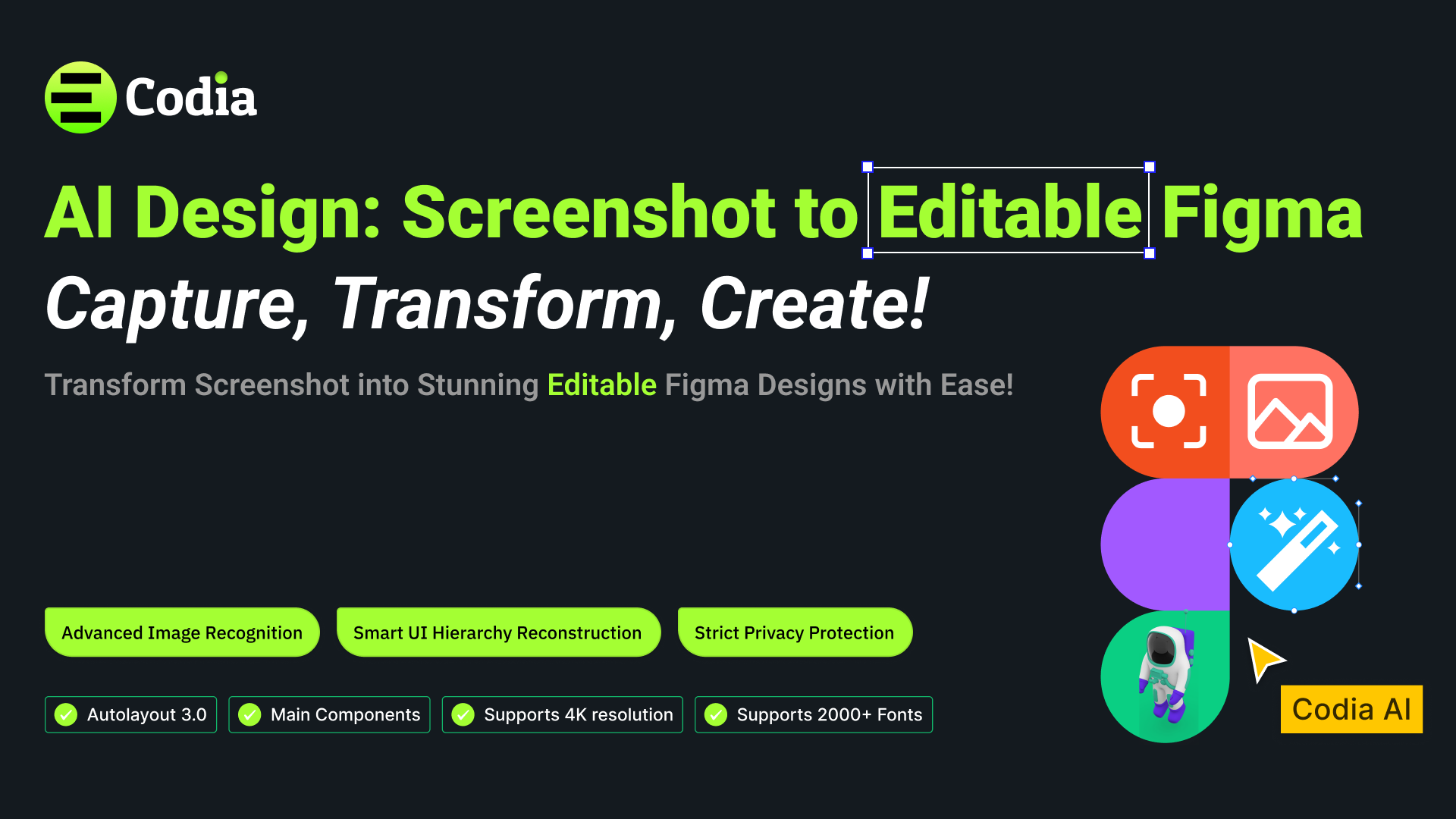
4.[**Codia AI VectorMagic: Image to Full-Color Vector/PNG to SVG**](https://codia.ai/v/bqFJ)
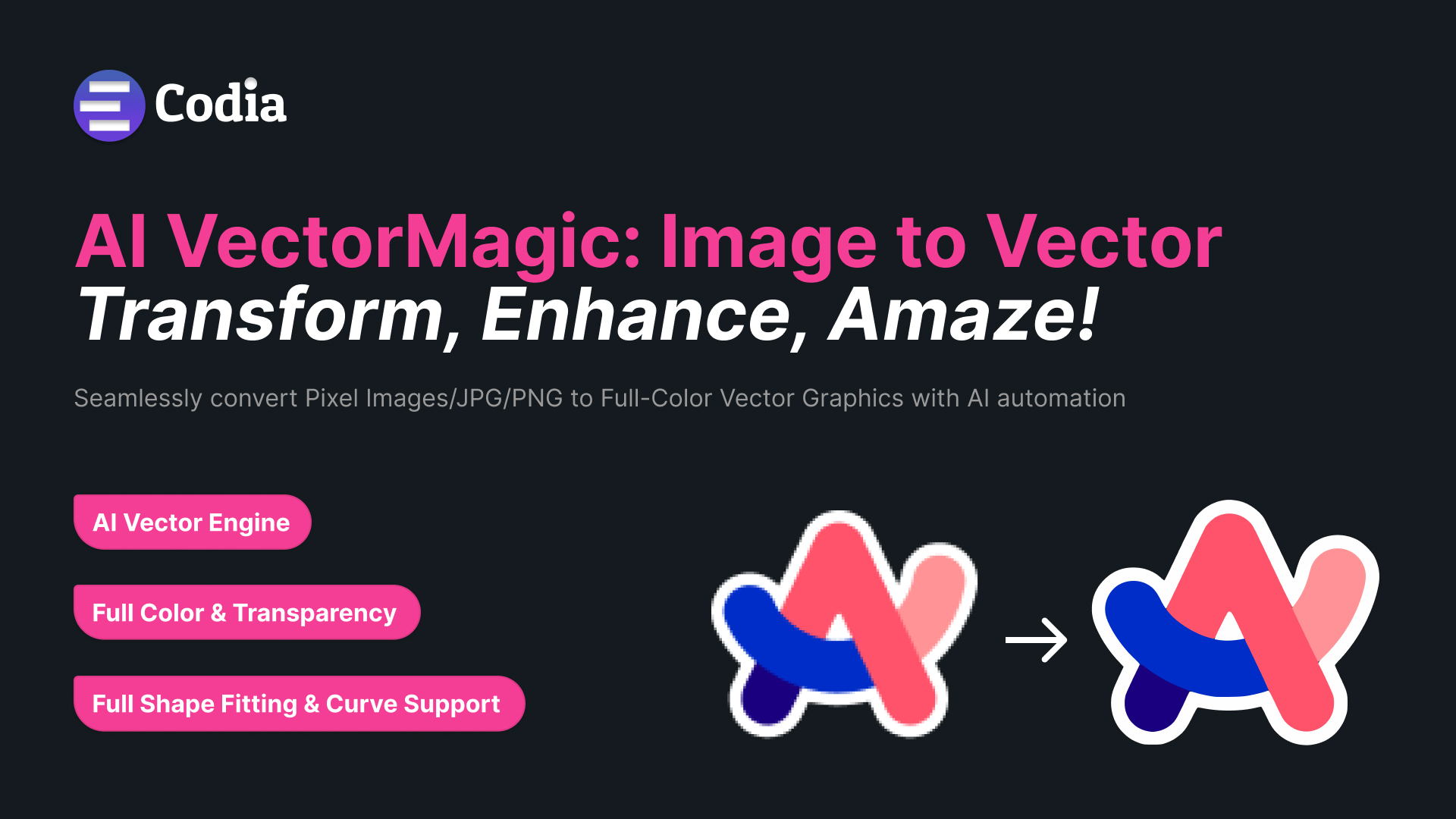
| happyer |
1,912,381 | 10 Detailed Artificial Intelligence Case Studies 2024 | Artificial Intelligence isn’t a thing of science fiction anymore. It is in every industry, seeking to... | 0 | 2024-07-05T07:39:52 | https://dev.to/bosctechlabs/10-detailed-artificial-intelligence-case-studies-2024-2d9f | flutter | **[Artificial Intelligence](https://bosctechlabs.com/ai-artificial-intelligence-use-cases-and-benefits-in-mobile-app-development-2024/)** isn’t a thing of science fiction anymore. It is in every industry, seeking to revolutionize things and bring out top-level efficiency at everything- from process to manufacturing.
Not long ago, AI wasn’t considered at the level of human consciousness. But with time, and extensive research, trial and error of AI models, and testing of AI at larger stages have brought about an era where AI is everywhere.
You might also be an AI enthusiast or looking to build your AI model. Well, once you’ve decided what AI model, you’ll build to address a problem, the only thing left for you to do is engage yourself with Java developers. You’d need to leverage the best Java development services around you to build the best quality AI programs and models!
With all the buzz around AI, it is easy to get lost in chaos. And for this exact reason, we’ve compiled top 10 detailed artificial intelligence case studies in the year 2024 to get an insight into how exactly AI is helping people map out new courses in business, innovation, and healthcare, all in real time.
**10 Detailed AI Case Studies [2024] **
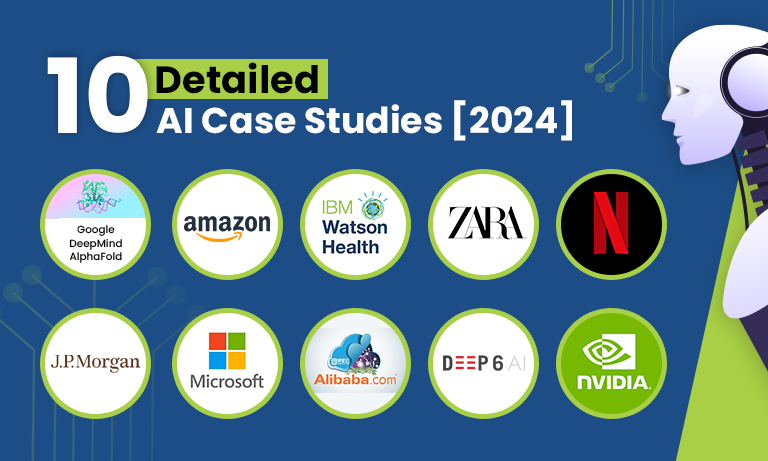
**
1. Google DeepMind AlphaFold
**
**Challenge:** Proteins have unique and complex 3D structures made of amino acid sequences that aren’t easy to determine. There are about 200 million proteins that exist in the world. It is quite crucial to understand protein structures to understand and decode things like diseases and patterns on a molecular level- but biological structural complexity is a hurdle.
**AI Solution:** AlphaFold, developed by **Google DeepMind**, is trained on the vast datasets of already-known protein structures. It works by assessing the distances and angles between amino acids to predict protein folding patterns; thus, reducing the time factor required concerning computational biology.
**Impact: **
- Acceleration in drug discovery and molecular studies
- Revolutionized the field of computational biology for researchers.
**2. Amazon: Revolutionizing Supply Chain Management**
**Challenge:** Managing a global supply chain is no simple feat. From predicting product demands, managing the inventory, and streamlining shipping and delivery, to managing blocks in the supply chain. The main challenge for Amazon was to meet customer demands with reduced prices while managing its massive inventory.
**Solution:** Amazon is currently employing AI algorithms for predictive inventory management. It works by assessing changes in the market, buying trends, and more to predict product demand. The best part about this AI is that it can adjust to real-time market changes.
**Impact:**
- Reduction in operational costs worldwide
- Time-efficient deliveries, suited to customer satisfaction.
**3. IBM Watson Health**
**Challenge:** In the Healthcare Industry, one of the biggest challenges is the vast amount of patient data, reports, registrations, appointments, diagnoses, etc. Analyzing and organizing this data will be useful to predict disease progression as well as personalize treatment plans.
**Solution:** IBM Watson uses cognitive computing to analyze large volumes of medical records, patient data, research papers, and more. By using NLP to process medical jargon and present useful structured data to help healthcare professionals in treating patients.
**Impact:**
- Efficiency and accuracy in patient diagnoses
- Laying the foundations for personalized healthcare
**4. Zara: Retail Fashion With AI**
**Challenges:** The fashion industry is quite fast-paced, and trends change within weeks! At such a pace, it becomes quite important to manage inventory, keep track of trends, and more. The challenge is to deliver on changing trends and avoid overstocking.
**Solution:** Zara now uses AI algorithms to analyze their sales data, current fashion trends, sift through customer preferences, and more. This helps in understanding customer preferences and stocking popular items quickly, as well as reducing unsold products at outlets.
**Impact:**
- Enhanced customer satisfaction by aligning products with trends
- Optimized inventory helps to increase sales and profits.
**5. Netflix: Personalized Entertainment**
**Challenge:** While we may not realize it, the streaming industry has become far more vicious. Personalized entertainment is perhaps one of the best ways to retain existing customers. Here’s where AI comes in.
**Solution:** Netflix employs AI to drive recommendations suited to ratings, individual viewing habits, and preferences. This tactic helps in keeping users engaged for a longer period, thus enhancing the overall viewing experience.
**Impact:**
**Increasing subscription rates as more users onboard on the platform.**
**Higher user engagement due to personalized preferences.**
**6. JP Morgan: Legal Documents Analysis With AI**
**Challenge:** Legal document analysis is a time-consuming and exhaustive process and may also be prone to errors. JP Morgan, with its extensive list of clientele, couldn’t afford to waste time over this. So, they chose the easier and possibly more efficient route- AI.
**Solution:** COIN, short for Contract Intelligence, is an AI employed currently by JP Morgan that analyzes and interprets legal data accurately and efficiently. It uses NLP to extract relevant information from documents, significantly cutting down on the time required for document review.
**Impact:**
- Drastic time reduction in legal document analysis
- Reduced human error in contract interpretation.
**7. Microsoft: AI Accessibility**
**Challenges:** Disabled people often face challenges in using technology. Microsoft resorted to creating AI tools to address this issue- particularly for people with visual, hearing, or cognitive disabilities.
**Solution:** Seeing AI, by Microsoft, helps visually impaired people to realize their surroundings by resorting to vividly describing them. The range of tools that Microsoft created for this issue included voice recognition applications, visual assistance, cognitive support, and more.
**Impact:**
- Improved technological accessibility, and subsequently, more independence for disabled people.
- Focus on more inclusive technology development.
**8. Alibaba’s City Brain: Revolutionizing Urban Traffic Management**
**Challenges:** Traffic congestion in many urban cities has spiked after the pandemic. And while this may seem miniscule, it has many environmental effects as well as leads to movement issues in the city. Efficient traffic management is always the need of the hour.
**Solution:** City Brain, developed by AliBaba, leverages AI to assess real-time traffic data from GPS systems, traffic cameras, and sensors. This data is then processed and used to predict traffic patterns and optimize the timing of traffic lights, thus reducing congestion.
**Impact:**
- Drastic reduction in travel time for commuters.
- Efficient overall traffic congestion reduction.
**9. Deep 6 AI: For Accelerated Clinical Trials**
**Challenges:** Recruiting suitable patients for clinical trials is quite a slow and wearisome process, and this slow approach often negatively affects medical research. The process involves going through humongous amounts of patient data and assessing their history to determine if they can be recruited for clinical trials.
If AI is leveraged for the same, it can help go through the vast amounts of patient data to identify the eligible candidates for the same in a time-efficient manner.
**Solution:** Deep 6 AI can sift through extensive medical data to locate potential trial participants depending on the given criteria. The AI can go through doctor’s notes, and vast amounts of structured and unstructured data, to find prospective matches for clinical trials, thus revolutionizing the world of healthcare clinical trials.
**Impact:**
- Efficient and better recruitment of potential candidates suited for clinical trials.
- Assisting the world of medical research and healthcare by leveraging AI to quicken up processes.
**10. NVIDIA: Using AI To Evolve Gaming Graphics**
**Challenges:** The gaming industry is rapidly evolving, but so are the desires of the people who wish for more realism and performance games. Gaming graphics enhancement is challenging, but who is better than AI to give gamers what they want?
**Solutions:** NVIDIA’s graphic processing technologies, driven by AI (like Deep Learning Super Sampling (DLSS)) can provide extremely detailed and highly realistic gaming graphics. These technologies use AI to render graphics much more efficiently and do not compromise the games’ visual appeal either.
As of today, they’ve set out to create new standards in gaming graphics, keeping the spirit of gamers alive and raging.
**Impact:**
- Better industry standards for gaming.
- Highly engaging and realistic gaming experience, thus elevating gamers’ experiences.
**Conclusion**
The above case studies are barely a glimpse into the vast world of how AI can engage and revolutionize various industries. The key lies in identifying a conflict, a challenge, or a problem. The solution usually lies in tackling the said challenge from different perspectives, and figuring out how AI can make it more efficient, swifter, or cheaper.
Who knows, in the future, you might have your own AI tool that enhances the experiences of thousands of people in healthcare, gaming, or entertainment. Explore the possibilities with Bosc Tech Labs’ **[custom software development services](https://bosctechlabs.com/services/custom-software-development-company/)**. Bosc Tech Labs specializes in creating innovative and tailor-made software solutions designed to meet the unique needs of various industries, ensuring your vision is brought to life with cutting-edge technology and expertise.
And since we already know that Java is perfect for the stages of building AI, you might also want to be on the lookout for awesome Java development services to assist you with it! Contact Generative AI development company **[Bosc Tech Labs](https://bosctechlabs.com/)** for more Details. | bosctechlabs |
1,912,378 | Where to Add Your Additional JavaScript in Elementor Pro? | Ever wanted to add some custom functionality to your Elementor Pro-designed website? JavaScript is... | 0 | 2024-07-05T07:36:10 | https://dev.to/rashedulhridoy/where-to-add-your-additional-javascript-in-elementor-pro-21na | webdev, javascript |
Ever wanted to add some custom functionality to your Elementor Pro-designed website? JavaScript is your friend! But where exactly do you put that code to make it work its magic?
Elementor Pro offers two main ways to add JavaScript:
**1. Using the Custom HTML Widget (Free):**
This is a great option for simple scripts or those specific to a particular page element. Here's how:
- **Edit the Page in Elementor Pro:** Open the page you want to add the script to and enter the Elementor editor.
- **Drag and Drop the HTML Widget:** Find the "HTML" widget in the Elementor panel on the left and drag it to the desired location on your page.
- **Paste Your JavaScript Code:** Click the edit button on the HTML widget and paste your JavaScript code wrapped in `<script>` tags within the editor.
- **Update and Check:** Save your changes and preview the page to ensure the script functions correctly.
**2. Leveraging Elementor Pro's Custom Code Feature (Pro):**
This method is ideal for site-wide scripts or those that need to be loaded in specific areas like the header or footer. Here's the process:
- **Navigate to Elementor > Custom Code:** In your WordPress dashboard, go to Elementor and then "Custom Code."
- **Create a New Code Snippet:** Click "Add New" and give your code a descriptive title.
- **Select Location and Priority:** Choose where you want the script to load (header, footer, etc.) and set its priority (lower number means higher priority).
- **Paste Your JavaScript:** Add your JavaScript code within the designated area.
- **Publish and Test:** Publish your changes and test your website to ensure the script functions as intended.
**Remember:**
- Always ensure your JavaScript code is properly formatted and free of errors.
- For complex functionality, consider consulting a developer to ensure optimal implementation.
By following these steps, you can easily add your custom JavaScript to your Elementor Pro website and unlock new levels of interactivity and functionality!
| rashedulhridoy |
1,912,377 | Leading Study Abroad Consultant in Kolkata: YES Germany | YES Germany Overview YES Germany is a leading study abroad consultancy that has... | 0 | 2024-07-05T07:35:37 | https://dev.to/narwatharsh152/leading-study-abroad-consultant-in-kolkata-yes-germany-1d8i | studyabroad, kolkata, education, consultant | ## **YES Germany Overview**
YES Germany is a leading study abroad consultancy that has established a strong presence in Kolkata, India. With over a decade of experience, the company has carved out a reputation as one of the most trusted and reliable overseas education consultants in the region.
## **Best Study Abroad Consultant in Kolkata**
According to a recent industry report, YES Germany has emerged as the **#1 [study abroad consultant in Kolkata](https://www.yesgermany.com/study-abroad-consultant-in-kolkata/)**, outpacing its competitors through its comprehensive suite of services and unwavering commitment to student success. The company's expert team of counselors, with their in-depth knowledge of the German education system, have been instrumental in guiding countless students towards their academic and career goals.
## **Services Offered in Kolkata**
YES Germany's services in Kolkata encompass the entire study abroad journey, from initial counseling and university selection to visa assistance and pre-departure preparation. Some of their key offerings include:
- **Comprehensive Counseling:** Helping students choose the right academic program and university based on their interests, qualifications, and career aspirations.
- **Admission Support:** Providing end-to-end assistance with the application process, including SOP and LOR preparation.
- **Visa Guidance:** Offering step-by-step support throughout the visa application process, ensuring a smooth and hassle-free experience.
- **Language Training:** Offering German language courses to help students develop the necessary linguistic skills for their studies.
- **Accommodation and Arrival Assistance:** Helping students secure suitable accommodation and providing on-ground support upon arrival in Germany.
## **Study Abroad and Overseas Education Consultant Market Size**
The study abroad and overseas education consultant market has witnessed significant growth in recent years. According to a report by the Federation of Indian Chambers of Commerce and Industry (FICCI), the Indian study abroad market is expected to reach $80 billion by 2024, growing at a CAGR of 18%.
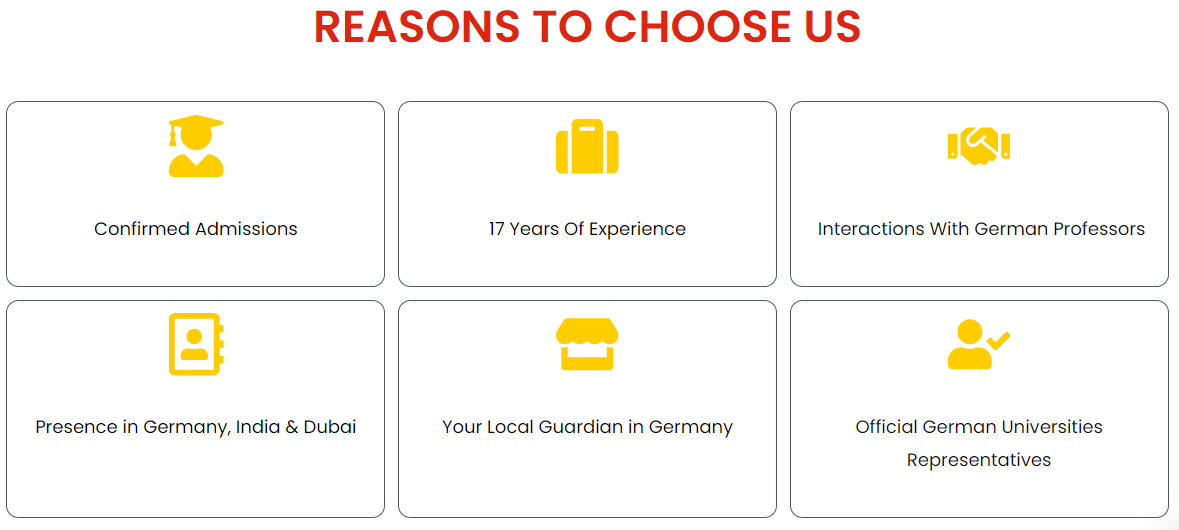
**For More Info Call Us On: +91 8070606070**
## **Most Trusted Consultant for Public University Admission**
YES Germany has established strong partnerships with numerous public universities in Germany, making them the go-to choice for students seeking admission to these prestigious institutions. Their deep understanding of the admission criteria and their ability to navigate the complex application process have earned them the trust of both students and universities alike.
## **Study Abroad Market Trends in Kolkata**
The demand for study abroad opportunities in Kolkata has been on the rise, driven by factors such as the growing aspirations of the city's youth, the increasing affordability of overseas education, and the perceived value of a global degree. The COVID-19 pandemic has also accelerated the shift towards online and hybrid learning models, further fueling the growth of the study abroad consultancy market.
**Click Here to Know More About: [Study Abroad Consultant in Kolkata](https://www.yesgermany.com/study-abroad-consultant-in-kolkata/)**
## **Study Abroad Industry Challenges and Opportunities in Kolkata**
While the study abroad industry in Kolkata presents numerous opportunities, it also faces its fair share of challenges. These include intense competition, the need for specialized expertise, and the evolving regulatory landscape. However, with its comprehensive services, strong industry partnerships, and a dedicated team of experts, YES Germany is well-positioned to navigate these challenges and capitalize on the growing demand for overseas education.
## **Overseas Education Consultants Industry Outlook Kolkata**
The outlook for the overseas education consultants industry in Kolkata remains positive, with the market expected to continue its upward trajectory in the coming years. As more students in Kolkata seek to expand their horizons and pursue higher education abroad, the demand for reliable and experienced study abroad consultants like YES Germany is likely to increase. The company's commitment to innovation, customer satisfaction, and industry leadership will be key to its continued success in this dynamic market. | narwatharsh152 |
1,912,376 | Warum? | Bin mir nicht sicher, was da bringt, da ich mein Wissen in die Dokumentation meiner Firma integriere.... | 0 | 2024-07-05T07:34:24 | https://dev.to/dubrau/warum-4c5a | barrierefreiheit, cobol | Bin mir nicht sicher, was da bringt, da ich mein Wissen in die Dokumentation meiner Firma integriere. Ich brauche keine extra Zettel, Ablagen oder private Ordner, um mich zu organisieren. | dubrau |
1,912,375 | Leveraging AI in Workday Testing: Ensuring Quality and Efficiency | AI-powered technology is permeating every area of the Cloud-based ERP industry. We’ve already seen... | 0 | 2024-07-05T07:33:54 | https://www.opkey.com/blog/leveraging-ai-in-workday-testing-ensuring-quality-and-efficiency | ai, in, workday, testing | 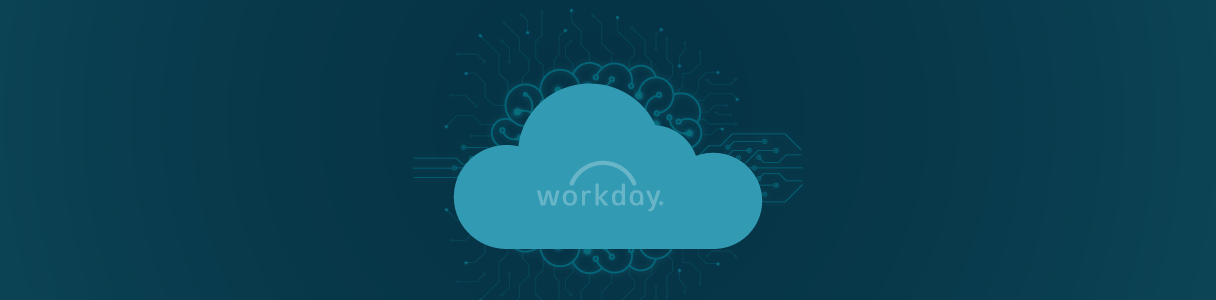
AI-powered technology is permeating every area of the Cloud-based ERP industry. We’ve already seen how AI enables individuals, and enterprises to increase productivity, make better decisions quickly, and drive innovation. It's no surprise that Workday has emerged as a pioneer in utilizing AI to manage their workforce and financial processes.
This blog explores two aspects of AI and Workday:
1. How AI is changing Workday’s internal processes, features, and functionalities.
2.AI-powered test automation innovations that are helping customers test Workday better. Are you looking to increase efficiency, strengthen compliance, and get a better return on your Workday AI investment? A Workday AI testing tool ensures that your business processes do not break with new enhancements and updates. Opkey is an official Workday testing partner, and built out specifically for Workday testing needs.
Let’s dive in!
**How AI Is Changing Workday Itself**
Machine Learning and Artificial Intelligence (AI) influence is improving the Workday experience by providing intelligent solutions for end users.
Here are different ways that Workday itself is elevating performance with Generative AI capabilities.
**Advanced data analytics**: Workday's platform now includes AI-powered data analytics capabilities, allowing organizations to obtain deeper insights into their workforce and financial data.
**Improving the employee experience**: Workday Generative AI capabilities go beyond back-office activities and improve the overall employee experience. Employees can use Workday AI-powered chatbots and virtual assistants to access information, submit requests, and receive personalized recommendations.
**Customizing training and development for the workforce**: Workday users can leverage AI to develop a trained and adaptable workforce. This targeted approach ensures that employees receive the proper training at the right time, allowing them to advance and thrive within the organization.
**Intelligent risk management**: Workday uses generative AI capabilities to assist customers simplify risk management duties, minimize business process errors, and improve overall reporting and compliance accuracy.
**Turning to Testing: Why Is AI Enabled Testing Now Necessary**?
According to Statista, the global software testing industry is anticipated to reach $50.4 billion by 2027. This emphasizes the importance of software testing in the development and deployment of software applications.
Testing remains one of the most time-consuming and costly efforts in software development. Augmenting AI in testing processes can dramatically improve efficiency and efficacy, resulting in better outcomes. AI-powered testing can easily identify defects, and even predict future issues and vulnerabilities, and thus provide deeper insights into system performance, eventually saving time and money.
**Why Do You Need Workday AI Testing**?
As we've seen, Workday has implemented advanced AI features into its platform, emphasizing the need for AI-enabled testing. Workday AI automated testing assures that features are reliable, effective, and secure. By using AI in testing, Workday users can make sure that their AI-driven features deliver accurate and valuable results while also maintaining high performance and customer satisfaction.
- Workday's AI features, like predictive analytics and automated decision-making, are complex and require advanced AI testing to ensure their correctness and reliability.
- Workday ERP systems handle massive volumes of HR, payroll, and financial data. AI testing solutions can efficiently handle and validate big datasets, which is critical when evaluating AI features that need considerable data processing.
- Workday ERP is susceptible to biannual major releases and minor changes throughout the year. Artificial intelligence testing automates repetitive and time-consuming testing procedures, such as regression tests, which are required with each new update or release.
- By automating big portions of the testing process, AI lowers the need for considerable manual testing, resulting in cost savings.
**How Opkey Utilizes AI to Enhance Testing, Compliance, and Security for Workday Customers**
Workday's ongoing AI advances transform various business activities. This is excellent, but it means you must test your Workday on a frequent basis to ensure everything is running properly. Let’s discuss Opkey’s AI-powered testing features that help Workday clients streamline their testing procedures.
**AI-powered Impact Analysis**: Opkey's AI-powered Impact Analysis feature provides smart analytics of the changes within two Workday releases. Instead of testing all the test cases customers only need to test those that are highly risky. Thus, eliminating hours of effort and struggle. Additionally, it provides detailed analytical reports to see changes across the releases that affect your Workday application.
**AI-powered Self-Healing**: Workday application is prone to frequent changes. Opkey's AI-powered Self-Healing feature uses AI algorithms to detect and automatically resolve issues and changes that would otherwise require (direct) human intervention. One of the major advantages of Self-Healing is that it allows for easier refactoring and maintenance.
**AI-powered Test Builder**: It is now possible to generate test cases even before the code has been written or built. Opkey uses AI algorithms to analyze and identify various graphical elements such as buttons, fields, and menus. Based on these analytics, it develops test cases that mimic user interactions. Additionally, during test execution, the Opkey AI engine simulates user interactions like clicks, inputs, and selections. It then confirms that the program responds appropriately to these activities and notifies any faults or defects. Making test case generation and execution easy for users.
**Wilfred, Opkey’s Chatbot**: Wilfred helps you generate, execute, and maintain tests with Natural Language Processing (NLP). Opkey has a proprietary ERP-specific language model that means Wilfred is fast, accurate, and customized to your business needs.
**Test Discovery**: Opkey’s Test Discovery engine accesses an ERP software’s event and configuration logs to extract relevant data. By using AI-analyzed ERP data, teams can confidently know which tests they are currently running, gain complete process transparency, pinpoint inefficiencies, and find the gaps in their processes. By comparing the tests they are running with the processes, QA teams can remove uncertainty about which tests must be executed. With this knowledge, QA teams can prioritize important test cases, increase test coverage, and enhance overall testing efforts.
As Workday keeps evolving its platform with complex AI features, the use of AI-enabled testing solutions such as Opkey becomes vital. Opkey not only streamlines and automates testing, but also ensures that its AI features are resilient, accurate, and secure. 1,200+ pre-built Workday tests also speed up testing and onboarding. | johnste39558689 |
1,912,374 | Automating User and Group Management on Linux with a Bash Script | Introduction Managing users and groups on a Linux system can be daunting, especially when... | 0 | 2024-07-05T07:31:20 | https://dev.to/hellowale/automating-user-and-group-management-on-linux-with-a-bash-script-2dm5 | bash, devops | ## Introduction
Managing users and groups on a Linux system can be daunting, especially when onboarding new employees. As a SysOps engineer, automating these tasks can save time and reduce errors. This article explores a bash script called create_users.sh that automates user creation, password management, and group assignments. This script reads from an input file and logs all actions, ensuring a smooth and auditable process.
## Script Features
Reading Input File: Parses a text file containing usernames and group names.
User Creation: Creates users with personal groups if they don't already exist.
Password Management: Generates and assigns random passwords to users.
Group Assignment: Adds users to specified groups.
Logging: Records all actions to a log file for auditing purposes.
## The Script
Below is the create_users.sh script. Save this script to your server and make it executable.
```
#!/bin/bash
# Function to read and parse the input file
read_input_file() {
local filename="$1"
while IFS=';' read -r user groups; do
users+=("$(echo "$user" | xargs)")
group_list+=("$(echo "$groups" | tr -d '[:space:]')")
done < "$filename"
}
# Function to create a user with its group
create_user_with_group() {
local username="$1"
if id "$username" &>/dev/null; then
echo "User $username already exists." | tee -a "$log_file"
else
groupadd "$username"
useradd -m -g "$username" -s /bin/bash "$username"
echo "Created user $username with personal group $username." | tee -a "$log_file"
fi
}
# Function to set a password for the user
set_user_password() {
local username="$1"
local password=$(openssl rand -base64 12)
echo "$username:$password" | chpasswd
echo "$username,$password" >> "$password_file"
echo "Password for $username set and stored." | tee -a "$log_file"
}
# Function to add users to additional groups
add_user_to_groups() {
local username="$1"
IFS=',' read -r -a groups <<< "$2"
for group in "${groups[@]}"; do
if ! getent group "$group" &>/dev/null; then
groupadd "$group"
echo "Group $group created." | tee -a "$log_file"
fi
usermod -aG "$group" "$username"
echo "Added $username to group $group." | tee -a "$log_file"
done
}
# Check for an input file argument
if [[ $# -ne 1 ]]; then
echo "Usage: $0 <input_file>"
exit 1
fi
# Initialize variables
input_file="$1"
log_file="/var/log/user_management.log"
password_file="/var/secure/user_passwords.txt"
declare -a users
declare -a group_list
# Create log and password files if they do not exist
mkdir -p /var/log /var/secure
touch "$log_file"
touch "$password_file"
chmod 600 "$password_file"
# Read input file
read_input_file "$input_file"
# Process each user
for ((i = 0; i < ${#users[@]}; i++)); do
username="${users[i]}"
user_groups="${group_list[i]}"
if [[ "$username" == "" ]]; then
continue # Skip empty usernames
fi
create_user_with_group "$username"
set_user_password "$username"
add_user_to_groups "$username" "$user_groups"
done
echo "User creation and group assignment completed." | tee -a "$log_file"
```
## How to Use the Script
- Prepare the Input File: Create a file named users.txt with the following format:
```
light;sudo,dev,www-data
idimma;sudo
mayowa;dev,www-data
```
- Reading the Input File
The script begins by defining a function to read and parse the input file. Each line in the file contains a username and a list of groups separated by a semicolon (;).
```
# Function to read and parse the input file
read_input_file() {
local filename="$1"
while IFS=';' read -r user groups; do
users+=("$(echo "$user" | xargs)")
group_list+=("$(echo "$groups" | tr -d '[:space:]')")
done < "$filename"
}
```
- Creating a User with a Personal Group
Next, we define a function to create a user and their group. If the user already exists, the script logs a message and skips the creation.
```
# Function to create a user with its group
create_user_with_group() {
local username="$1"
if id "$username" &>/dev/null; then
echo "User $username already exists." | tee -a "$log_file"
else
groupadd "$username"
useradd -m -g "$username" -s /bin/bash "$username"
echo "Created user $username with personal group $username." | tee -a "$log_file"
fi
}
```
- Setting a Password for the User
This function generates a random password for the user, sets it, and stores the password in a secure file.
```
# Function to set a password for the user
set_user_password() {
local username="$1"
local password=$(openssl rand -base64 12)
echo "$username:$password" | chpasswd
echo "$username,$password" >> "$password_file"
echo "Password for $username set and stored." | tee -a "$log_file"
}
```
- Adding the User to Additional Groups
The following function adds the user to the specified groups. If a group does not exist, it is created.
```
# Function to add users to additional groups
add_user_to_groups() {
local username="$1"
IFS=',' read -r -a groups <<< "$2"
for group in "${groups[@]}"; do
if ! getent group "$group" &>/dev/null; then
groupadd "$group"
echo "Group $group created." | tee -a "$log_file"
fi
usermod -aG "$group" "$username"
echo "Added $username to group $group." | tee -a "$log_file"
done
}
```
- Main Script Execution
The main part of the script checks for the input file argument initializes variables, creates log and password files if they don't exist, and processes each user in the input file.
```
# Check for an input file argument
if [[ $# -ne 1 ]]; then
echo "Usage: $0 <input_file>"
exit 1
fi
# Initialize variables
input_file="$1"
log_file="/var/log/user_management.log"
password_file="/var/secure/user_passwords.txt"
declare -a users
declare -a group_list
# Create log and password files if they do not exist
mkdir -p /var/log /var/secure
touch "$log_file"
touch "$password_file"
chmod 600 "$password_file"
# Read input file
read_input_file "$input_file"
# Process each user
for ((i = 0; i < ${#users[@]}; i++)); do
username="${users[i]}"
user_groups="${group_list[i]}"
if [[ "$username" == "" ]]; then
continue # Skip empty usernames
fi
create_user_with_group "$username"
set_user_password "$username"
add_user_to_groups "$username" "$user_groups"
done
echo "User creation and group assignment completed." | tee -a "$log_file"
```
Run the Script: Execute the script with the input file as an argument:
```
./create_users.sh users.txt
```
Check Logs and Passwords: Review the log file at /var/log/user_management.log for actions taken and find user passwords in /var/secure/user_passwords.txt.
## Benefits of Automation
- Efficiency: Automates repetitive tasks, freeing time for more critical activities.
- Consistency: Ensures that user and group configurations are applied uniformly.
- Security: Randomly generated passwords enhance security, and storing them securely minimizes risks.
- Auditing: Detailed logging helps in tracking changes and troubleshooting.
## Learn More
If you're interested in advancing your career in tech, consider joining the HNG Internship program by visiting [HNG Internship](https://hng.tech/internship) or [HNG Premium](https://hng.tech/premium). It's an excellent opportunity to gain hands-on experience and learn from industry professionals.
For those looking to hire top tech talent, [HNG Hire](https://hng.tech/hire) connects you with skilled developers who have undergone rigorous training.
## Conclusion
Automating user and group management tasks with a bash script can significantly improve efficiency and security in a Linux environment. By following the steps outlined in this article, you can streamline your onboarding process and ensure proper user management.
| hellowale |
1,912,373 | How I Plan to Make Money Using ChatGPT (And Other AI Tools). | Probably 2023 is going to be the year that many entrepreneurs and freelancers will make a lot of... | 0 | 2024-07-05T07:30:41 | https://dev.to/manojgohel/how-i-plan-to-make-money-using-chatgpt-and-other-ai-tools-2pme | python, programming, webdev, beginners | Probably 2023 is going to be the year that many entrepreneurs and freelancers will make a lot of money thanks to Artificial Intelligence (AI).
I don’t want to miss that opportunity, so over the past weeks, I’ve been exploring different ways to make money using [ChatGPT](https://medium.com/geekculture/i-spent-14-days-testing-chatgpt-here-are-3-ways-it-can-improve-your-everyday-life-30852a349ad1) and [other AI tools](https://frank-andrade.medium.com/6-ai-tools-that-will-make-your-life-easier-a1b71d15cbff).
I found different options, but I only listed here those that I consider worth a try.
## 1\. Creating a Product or Service and Power It with AI
There are many products and services that are powered by AI, but guess what? Most of them never developed the AI technology used in their products.
They only integrated others’ AI technology into their products by connecting to their APIs.
OpenAI, the developer of ChatGPT, has an API that has been used by companies such as Duolingo and GitHub. Duolingo uses OpenAI’s GPT-3 to provide French grammar corrections on its app, while GitHub uses OpenAI’s Codex to help programmers write code faster with less work.
All of this is possible through an API! (you can learn how to use OpenAI API [in this article I wrote](https://frank-andrade.medium.com/a-simple-guide-to-openai-api-with-python-3bb4ed9a4b0a))
Another example is Jasper. Jasper is an AI content generator that tailors the generic language created by OpenAI’s technology to specific use cases. We can say that Jasper is the “middleman” between you and Open AI.
But why would anyone choose a middleman over OpenAI’s technology?
* They optimize the outputs to be more relevant to their customers.
* They use an interface that makes AI much more accessible for those who don’t have the resources/expertise to access the technology themselves.
But OpenAI isn’t the only tech you can use. There are other options.
For example, the app Lensa uses Stable Diffusion’s open-source image generator to turn selfies into different styles of artwork. They charge a fee for this and they only act as a middleman between users and Stable Diffusion technology.
To sum it up: Pick a niche. Develop a product or service. Power it with AI.
## 2\. Teaching
There are many people that are unfamiliar with artificial intelligence, but they will need to familiarize themselves with AI and learn how to use AI tools sooner or later.
That’s an opportunity to educate them through blogs, video tutorials, or complete paid courses.
To give you an example, very few people used to watch [my YouTube videos](https://www.youtube.com/@ThePyCoach) before ChatGPT was released. My videos averaged 8k views, but only in December I got hundreds of thousands of views simply because I was teaching others how to use ChatGPT.
And, believe me, I’m not the only content creator who leveraged this tool.
This shows how even a small YouTuber like me can grow their channel by talking about a hot topic like AI. If you ever thought about creating online content, pick an AI tool and teach others how to make the most of it through blog posts or YouTube videos.
You’ll learn twice and make money. What else can you ask for?
## 3\. Writing
Probably many people will use AI to try to make money blogging or writing books, but here’s the problem: most are either new to AI or writing.
I’ve been writing articles and using AI technology for some years and I think 2023 is a good opportunity for those willing to learn how to use AI to enhance their writing.
Why? Some writers who are new to AI will ignore AI tools and complain about how useless they are, while those new to writing might use AI tools the wrong way.
Here’s how I’d use AI to make money writing (if I could start over).
Say I want to start blogging, I’d pick topics I love talking about and use AI tools to overcome the common problems we face when writing.
* You lack ideas for your article title? ChatGPT can generate them for you
* You lack the inspiration to complete your article? Jasper can help finish your draft
* You lack the vocabulary to express your ideas? Quillbot can paraphrase sentences and you can control how much vocabulary change you want.
* You want unique pictures for your article? Midjourney can create AI art for you
Rather than a lazy solution to a problem, you should think of AI as a tool that can help you become a better writer. We only need to pick the right AI tool for a specific task and master it.
In case you want to write a book (like me), the AI tools I listed above can also help you.
## 4\. Building and selling apps, or APIs
This is something you can do if you at least have good knowledge of one programming language.
As I showed in some of my [YouTube videos](https://youtu.be/WYiU0NuyQwk), you can build complete scripts from scratch with ChatGPT, but sometimes you might need to make some tweaks to make it work as you expect.
The same happens with APIs. We can build an API using ChatGPT. Here’s a simple example I found on YouTube.
> Generate code for python flask API server that take GET request with url as string, converts it to QR code image and sends it back as an API responde
The problem is creating an API people want to buy.
This means we need to spend time analyzing niches that don’t have too much competition, generate ideas for our API, and only then use ChatGPT as a code assistant to convert our ideas into reality. Once we build an API, we can sell it on sites like RapidAPI.
If you enjoy reading stories like these and want to support me | manojgohel |
1,912,372 | CQRS and Mediator pattern | CQRS- Command Query Responsibility Segregation CQRS stands for Command Query... | 0 | 2024-07-05T07:29:52 | https://dev.to/shashanksaini203/cqrs-and-mediator-pattern-159k | dotnet, cqrs, mediatr, restapi | ## CQRS- Command Query Responsibility Segregation
CQRS stands for **Command Query Responsibility Segregation**. Its a design pattern in software architecture that separates the operations that update data- **COMMANDS**, from the operations that read data - **QUERIES**.
This separation can improve performance, scalability, and security of an application.
• **Commands**: Operations that change the state of the application. For example, creating a new user, updating an order, or deleting a record. Commands are often validated before execution and might involve complex business logic.
• **Queries**: Operations that read data without modifying it. For example, fetching user details, retrieving a list of orders, or getting the status of a specific record. Queries are typically optimized for read performance and can be cached for faster retrieval.
• **Segregation**: By separating commands from queries, you can optimize and scale each side independently. For instance, you might want to use different databases or models for reads and writes. This can also lead to a more secure system, as read and write permissions can be managed separately.
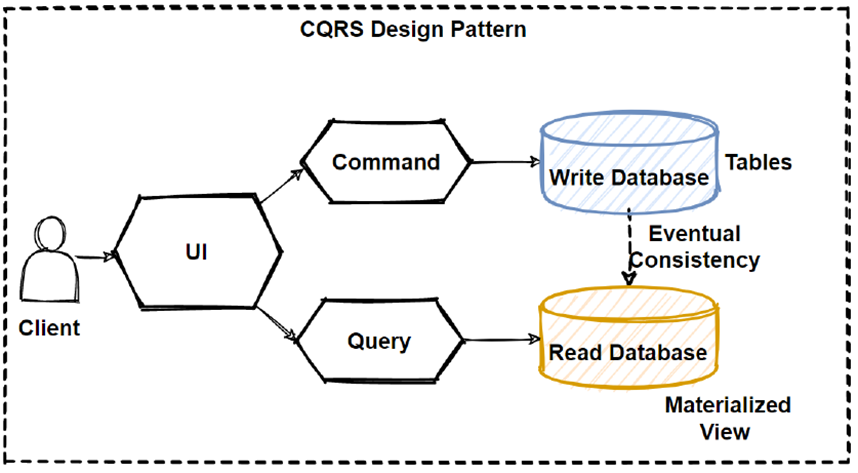
Benefits of CQRS:
• **Scalability**: You can scale read and write operations independently based on their specific load and performance requirements.
• **Optimized Performance**: By tailoring the data model for reading and writing separately, you can optimize each for its specific use case.
• **Simplified Business Logic**: Commands can be handled in a way that ensures complex business rules are applied correctly, while queries can be optimized for fast data retrieval.
• **Improved Security**: With clear segregation, you can apply different security measures to read and write operations.
CQRS is often used in conjunction with the Mediator pattern. This can be implemented using the MediatR library.
## Mediator Design Pattern -
Mediator design pattern is used to handle communication between different components or objects in a system. It promotes loose coupling by ensuring that objects do not communicate directly with each other but instead through a **mediator**. This can simplify the dependencies between objects and make the system easier to maintain and extend.
Implementation of mediator pattern in .NET is popular through the use of the **MediatR** library. It provides a simple in-process messaging library that helps implement the mediator pattern.
Key Components of MediatR -
• **Requests**: These are the messages that are sent through the mediator. It can be a Command or a Query(explained above)
• **Handlers**: These are the components that handle the requests. Each request has a corresponding handler that contains the logic to process the request. We usually have separate handlers for Commands and Queries.
• **Mediator**: The mediator component routes the requests to the appropriate handlers. MediatR provides the IMediator interface to send requests and publish notifications.
Checkout the repository for understanding this through code - https://github.com/ShashankSaini203/RestApiPlayground
Feel free to reach out. Connect me at [LinkedIn](https://www.linkedin.com/in/shashanksaini203) | shashanksaini203 |
1,912,371 | I Reduced Our CSS Size by 20% Using This Tailwind Hack - You Won't Believe What Happened Next at @Parentune! | At our @Parentune Gurugram headquarter, we heavily focus on SEO and increasing our user base through... | 0 | 2024-07-05T07:28:13 | https://dev.to/slimpython/i-reduced-our-css-size-by-20-using-this-tailwind-hack-you-wont-believe-what-happened-next-at-parentune-3cd8 | css, react, webdev | At our @Parentune Gurugram headquarter, we heavily focus on SEO and increasing our user base through organic channels, we realized our site’s CSS was bloated with unused styles, negatively impacting our SEO. We turned to [PageSpeed Insights](https://pagespeed.web.dev/) to understand our site’s performance issues and confirmed the problem using the DevTools Coverage tab.
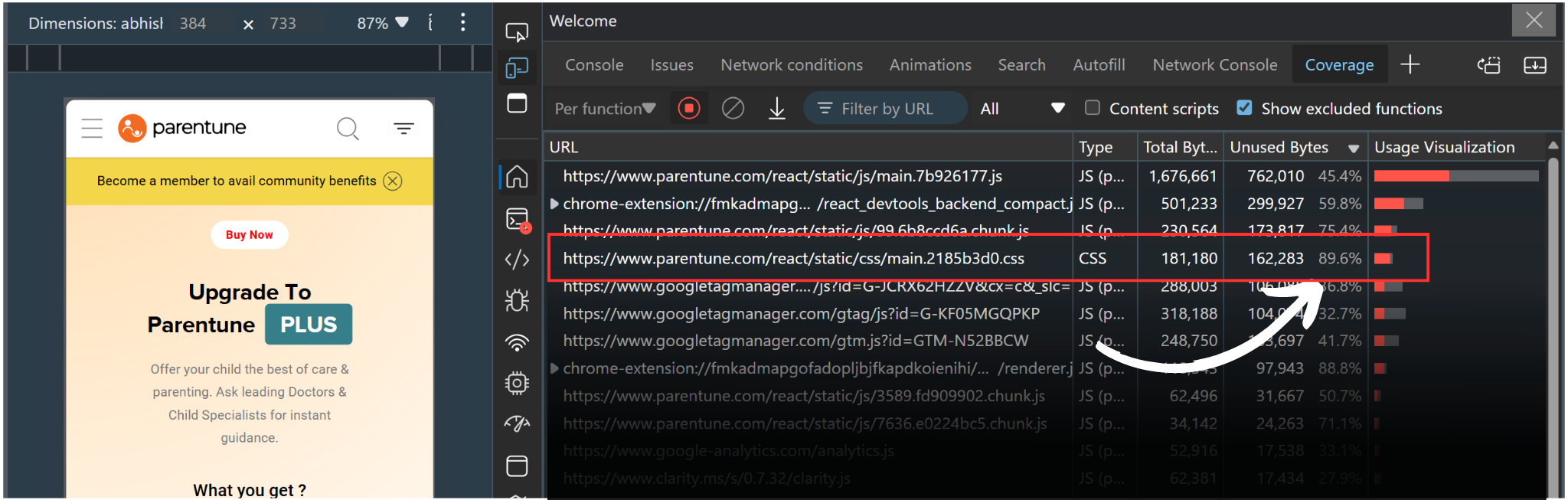
**Here’s how we tackled it:**
1. **Consulted Tailwind Docs:** We started by thoroughly reading the [Tailwind CSS optimization guide](https://tailwindcss.com/docs/optimizing-for-production). This provided us with the necessary steps to streamline our CSS for production.
2. **Modified Package.json:** We updated our `package.json` file to automate the CSS build process. Here’s the relevant snippet:
```json
"build:css": "npx tailwindcss -o src/build.css --minify",
"build": "npm run build:css && react-scripts build",
```
3. **Updated index.tsx:** To ensure we used the optimized CSS, we modified our `index.tsx`:
```typescript
// import './index.css';
import './build.css';
```
4. **Moved Fonts to CDN:** We shifted our heavy fonts to a CDN and served them asynchronously to further enhance performance.
**The Results:**
Since implementing these changes, we’ve noticed significant improvements in our SEO scores and overall site performance. We are confident these enhancements will continue to drive better SEO results.
Optimizing your CSS can seem daunting, but with the right tools and steps, it can lead to substantial performance gains. Give it a try and watch your site speed and SEO improve!
> Feel free to reach out for any kind of help, open for change😊
| slimpython |
1,912,370 | The Role of Cloud Computing in Custom Website Development Services | In the dynamic landscape of custom website development services, staying abreast of technological... | 0 | 2024-07-05T07:27:15 | https://dev.to/hooria_khan_d825e94f48402/the-role-of-cloud-computing-in-custom-website-development-services-3j9d | In the dynamic landscape of custom website development services, staying abreast of technological advancements is crucial for delivering innovative solutions that meet the evolving needs of businesses and users alike. Cloud computing is one such transformative technology that has reshaped how websites are built and deployed. This blog explores the pivotal role of cloud computing in empowering software development companies to deliver [top-tier custom website solutions](url=https://algoace.com/website-development/) efficiently and effectively.
## Understanding Cloud Computing in Website Development
Cloud computing refers to the delivery of computing services—including servers, storage, databases, networking, software, and analytics—over the internet (the cloud). Instead of owning and maintaining physical hardware and infrastructure, businesses can access these resources on-demand from cloud service providers like Amazon Web Services (AWS), Microsoft Azure, or Google Cloud Platform.
### Importance of Cloud Computing for Custom Website Development Services
For [software development companies](url=https://algoace.com/) specializing in top-tier custom website solutions, leveraging cloud computing offers numerous strategic advantages that enhance flexibility, scalability, security, and performance:
#### 1. Flexibility and Scalability
Cloud computing provides unparalleled flexibility in scaling resources based on demand. Whether you're developing a simple business website or a complex e-commerce platform, cloud infrastructure allows developers to provision and deploy computing resources (such as virtual machines, databases, and storage) quickly and efficiently.
This flexibility is particularly advantageous for businesses experiencing fluctuating traffic patterns, seasonal spikes, or rapid growth. Instead of investing in costly hardware upgrades or over-provisioning resources, cloud services enable software development companies to scale resources up or down dynamically, ensuring optimal performance and cost-efficiency.
#### 2. Cost Efficiency and Resource Optimization
Cloud computing follows a pay-as-you-go pricing model, where businesses only pay for the resources they consume. This eliminates the upfront capital expenditures associated with purchasing and maintaining physical infrastructure, such as servers and data centers.
Moreover, cloud providers offer a range of pricing plans and discounts for long-term usage, allowing businesses to optimize costs further. By leveraging cloud services, software development companies can allocate resources more efficiently, invest savings into innovation, and deliver competitive pricing for top-tier custom website development services.
#### 3. Enhanced Collaboration and Remote Work Capabilities
Cloud computing facilitates seamless collaboration among distributed teams and remote workforces—a growing trend in today's digital economy. Cloud-based development environments enable developers to access shared code repositories, collaborate on projects in real-time, and deploy updates instantly from anywhere with an internet connection.
This agility in collaboration not only improves productivity and teamwork but also accelerates time-to-market for custom website projects. Whether team members are located across different offices or working remotely, cloud computing fosters a cohesive and efficient development workflow.
#### 4. Improved Security and Data Protection
Security is a paramount concern for businesses entrusted with sensitive data and transactions. Cloud service providers invest heavily in state-of-the-art security measures, including data encryption, multi-factor authentication, and regular security audits, to protect client information and ensure regulatory compliance.
By leveraging cloud infrastructure, software development companies can enhance data security and resilience against cyber threats. Cloud providers offer robust disaster recovery solutions, automated backups, and geographic redundancy to mitigate risks and ensure business continuity. This proactive approach to security instills confidence among clients seeking top-tier custom website development services, reinforcing trust and compliance with industry standards.
#### 5. Rapid Deployment and Scalable Architecture
Cloud computing enables rapid deployment of custom websites and applications through automated provisioning and configuration management tools. Developers can leverage Infrastructure as Code (IaC) principles to define and deploy cloud resources using code, ensuring consistency and reproducibility across development, testing, and production environments.
Moreover, cloud-native architectures support microservices and containerization technologies (such as Docker and Kubernetes), enabling modular and scalable application designs. This modular approach simplifies updates, enhances fault tolerance, and facilitates continuous integration and deployment (CI/CD) pipelines, accelerating the delivery of new features and improvements to clients' custom websites.
## Implementing Cloud Computing in Your Custom Website Development Strategy
Integrating cloud computing into your custom website development strategy requires careful planning, expertise in cloud architecture, and collaboration with a reputable cloud service provider. Here's a step-by-step approach to maximize the benefits of cloud computing for your next project:
### 1. Assessing Project Requirements and Workloads
Evaluate your client's business objectives, scalability requirements, and compliance considerations. Determine the types of workloads (e.g., web hosting, databases, content delivery) that can benefit from cloud services.
### 2. Choosing the Right Cloud Service Provider
Select a cloud service provider (e.g., AWS, Azure, Google Cloud) that aligns with your project's technical requirements, budget constraints, and geographic preferences. Consider factors such as service reliability, global infrastructure, security certifications, and pricing models.
### 3. Designing Scalable and Resilient Architectures
Architect scalable and resilient cloud-native solutions using best practices such as serverless computing, auto-scaling, and load balancing. Design fault-tolerant architectures that prioritize high availability and performance optimization.
### 4. Implementing DevOps Practices
Adopt DevOps practices and tools to streamline development, testing, and deployment processes in cloud environments. Implement CI/CD pipelines to automate build, test, and release workflows, ensuring rapid iteration and continuous improvement.
### 5. Monitoring, Optimization, and Cost Management
Implement robust monitoring and logging solutions to track application performance, detect anomalies, and optimize resource utilization. Use cloud-native tools for cost management and budget allocation to control expenses and maximize ROI.
## Conclusion
In conclusion, cloud computing has revolutionized custom website development services by offering unparalleled flexibility, scalability, security, and cost efficiency. From enabling rapid deployment and fostering collaboration to enhancing data protection and supporting scalable architectures, cloud computing empowers software development companies to deliver top-tier custom website solutions that exceed client expectations.
As a software development company committed to excellence in custom website development services, integrating cloud computing into your workflow can differentiate your offerings, drive innovation, and accelerate business growth. Embrace the transformative power of cloud computing and position your business as a leader in delivering agile, scalable, and secure custom website solutions tailored to client success.
For businesses seeking top-tier custom website development services and partnering with a trusted provider proficient in cloud computing, our expertise ensures seamless integration and exceptional outcomes. Contact us today to discover how we can elevate your digital strategy, optimize your online presence, and achieve business excellence through cloud-powered custom website solutions. | hooria_khan_d825e94f48402 |
|
1,912,369 | Mobile Car Washing Market Economic and Environmental Benefits Driving Adoption | Mobile Car Washing Market Introduction & Size Analysis: The global market for mobile car washing... | 0 | 2024-07-05T07:27:03 | https://dev.to/ganesh_dukare_34ce028bb7b/mobile-car-washing-market-economic-and-environmental-benefits-driving-adoption-kpe | Mobile Car Washing Market Introduction & Size Analysis:
The global market for mobile car washing is poised for substantial growth, projected to expand at an impressive 8.8% compound annual growth rate (CAGR) to reach approximately US$ 21.7 billion by 2033, up from US$ 9.3 billion in 2024.
The [Mobile car wash services market](https://www.persistencemarketresearch.com/market-research/mobile-car-washing-market.asp) offer numerous advantages over traditional drive-through options, including convenience, cost savings, water conservation, and reduced environmental impact. Customers can have their vehicles cleaned at home or work, eliminating the need to search for a service location, thereby saving time and fuel costs.
Compared to traditional car washes, mobile services typically use less than half the water, with some employing water recycling systems, further enhancing their eco-friendliness. Entry into this market is relatively accessible, with moderate startup costs, allowing entrepreneurs to start with basic services like hand washing and vacuuming before expanding their offerings as they build a client base.
As consumer preferences shift towards convenience and environmental sustainability, the mobile car washing market is well-positioned for growth. Technological advancements, including automation and AI, promise increased operational efficiency and scalability, enabling providers to serve more customers efficiently.
The mobile car washing market is witnessing significant expansion globally, driven by top manufacturers who are innovating and expanding their services to meet growing consumer demand for convenient and eco-friendly vehicle cleaning solutions.
The mobile car washing market is experiencing increasing adoption driven by compelling economic and environmental benefits. Here’s an exploration of how these factors are influencing the industry’s growth:
Economic Benefits
Cost Savings for Consumers: Mobile car washing offers cost-effective solutions compared to traditional car wash facilities. By eliminating the need for physical infrastructure and optimizing operational efficiency, mobile operators can often provide competitive pricing, saving customers money on vehicle maintenance.
Business Efficiency: For fleet owners and businesses, mobile car washing reduces operational downtime and increases productivity. Vehicles can be cleaned on-site, minimizing disruptions and allowing employees to focus on core activities, ultimately improving operational efficiency.
Low Barrier to Entry for Entrepreneurs: Starting a mobile car wash business typically requires lower initial investment compared to establishing a fixed-location car wash. This accessibility encourages entrepreneurship and fosters market competition, benefiting consumers through improved service offerings and pricing options.
Environmental Benefits
Water Conservation: Mobile car wash services use significantly less water per vehicle compared to traditional car washes. Advanced technologies such as water recycling systems further minimize water consumption, addressing environmental concerns and promoting sustainable practices.
Reduced Chemical Usage: Eco-friendly cleaning products are commonly used in mobile car washing, reducing the environmental impact of chemical runoff into waterways. Biodegradable products and non-toxic cleaning agents contribute to cleaner ecosystems and support environmental conservation efforts.
Lower Carbon Footprint: Mobile car washing reduces vehicle travel to and from car wash facilities, thereby lowering carbon emissions associated with transportation. By operating on-site, mobile services contribute to local air quality improvements and mitigate traffic congestion.
Consumer Preferences and Industry Growth
Shift Towards Convenience: Increasing urbanization and busy lifestyles have intensified consumer demand for convenient services. Mobile car washing meets this demand by offering on-demand cleaning at customers’ preferred locations, such as homes or workplaces, saving time and enhancing convenience.
Alignment with Sustainable Practices: As environmental awareness grows, consumers are increasingly choosing service providers that prioritize sustainability. Mobile car wash businesses that adopt eco-friendly practices and communicate their environmental commitment effectively are well-positioned to attract and retain environmentally conscious clientele.
Future Outlook
The economic and environmental benefits driving the adoption of mobile car washing services underscore its growth potential in the automotive service sector. As industry players innovate with technology, expand service offerings, and enhance environmental stewardship, the market is expected to continue expanding, meeting evolving consumer expectations for cost-effective, convenient, and sustainable vehicle cleaning solutions.
Conclusion
The mobile car washing market is propelled by compelling economic advantages such as cost savings and business efficiency, alongside significant environmental benefits including water conservation and reduced chemical usage. By aligning with consumer preferences for convenience and sustainability, mobile car wash businesses are poised for sustained growth and market leadership, contributing to a cleaner, more efficient automotive service industry.
| ganesh_dukare_34ce028bb7b |
|
1,912,368 | How can you implement a conditional login mechanism between LDAP and local Database using Spring Boot. | Implementing a conditional login mechanism in Spring Boot that seamlessly switches between LDAP... | 0 | 2024-07-05T07:25:57 | https://dev.to/kailashnirmal/how-can-you-implement-a-conditional-login-mechanism-between-ldap-and-local-database-using-spring-boot-5glm | webdev, springboot, ldap, postgressql | Implementing a conditional login mechanism in Spring Boot that seamlessly switches between LDAP authentication and local database authentication based on the availability of the LDAP server is a crucial aspect of ensuring uninterrupted access to your application.
By incorporating this dynamic authentication approach, you can enhance the reliability and resilience of your system, providing users with a seamless login experience even during LDAP server downtime.
To implement a conditional login mechanism that checks whether the LDAP server is available and, based on that, either authenticate using the LDAP server or fall back to a local PostgreSQL database, you will need to:
1. **Check LDAP server availability**: Implement a mechanism to check if the LDAP server is reachable.
2. **Attempt LDAP Authentication**: If the LDAP server is available, authenticate using LDAP.
3. **Fallback to Local Authentication**: If the LDAP server is not reachable, authenticate using credentials stored in the local PostgreSQL database.
_Here are the Step-by-Step Implementation_ :
## 1. Add Dependencies :
Here I am considering the Maven as build tool.Ensure you have the following dependencies in your
pom.xml
file:
`<dependencies><!-- Spring Boot and Web --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-web</artifactId></dependency><!-- Spring Boot and Data JPA --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-data-jpa</artifactId></dependency><!-- PostgreSQL Driver --><dependency><groupId>org.postgresql</groupId><artifactId>postgresql</artifactId><scope>runtime</scope></dependency><!-- Spring Boot LDAP --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-ldap</artifactId></dependency><!-- Spring Boot Actuator (Optional) --><dependency><groupId>org.springframework.boot</groupId><artifactId>spring-boot-starter-actuator</artifactId></dependency></dependencies>`
##
## 2. Configure Application Properties
Set the necessary properties in application.properties:
```
# LDAP Server Configuration
ldap.url=ldap://url:389
ldap.admin.dn=cn=admin,dc=urdc,dc=urdc
ldap.admin.password=ldappassword
ldap.search.base=dc=cspurdc,dc=csp
# PostgreSQL Configuration
spring.datasource.url=jdbc:postgresql://localhost:5432/yourdb
spring.datasource.username=yourusername
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=org.postgresql.Driver
spring.jpa.hibernate.ddl-auto=update
# User Authentication Configuration
user.samaccountname=username
user.password=ldappassword
```
##
## 3. Create User Entity and Repository for PostgreSQL
Define a simple User entity and repository for the PostgreSQL database. **User.java**
```
package com.example.ldapauth.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String username;
private String password;
// Getters and Setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
```
**UserRepository.java**
```
package com.example.ldapauth.repository;
import com.example.ldapauth.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
### 4. Service for Local Authentication
Add a service to handle local database authentication.
**LocalAuthService.java**
```
package com.example.ldapauth.service;
import com.example.ldapauth.entity.User;
import com.example.ldapauth.repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class LocalAuthService {
@Autowired
private UserRepository userRepository;
public boolean authenticate(String username, String password) {
User user = userRepository.findByUsername(username);
return user != null && user.getPassword().equals(password);
}
}
```
## 5. Modify LdapService to Include Health Check:
Add a method to check the availability of the LDAP server.
**LdapService.java**
```
package com.example.ldapauth.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.ldap.support.LdapUtils;
import org.springframework.stereotype.Service;
import javax.naming.Context;
import javax.naming.NamingEnumeration;
import javax.naming.NamingException;
import javax.naming.directory.*;
import java.util.Hashtable;
@Service
public class LdapService {
@Autowired
private DirContext ldapContext;
@Value("${ldap.search.base}")
private String searchBase;
@Value("${user.samaccountname}")
private String sAMAccountName;
@Value("${user.password}")
private String userPassword;
public boolean isLdapAvailable() {
try {
// Try a no-op search to check server availability
NamingEnumeration<SearchResult> results = ldapContext.search("", "(objectClass=*)", new SearchControls());
return results != null; // If this does not throw an exception, LDAP is available
} catch (NamingException e) {
return false; // LDAP is not available
}
}
public String getUserDn(String username) {
try {
String searchFilter = "(sAMAccountName=" + username + ")";
SearchControls searchControls = new SearchControls();
searchControls.setSearchScope(SearchControls.SUBTREE_SCOPE);
NamingEnumeration<SearchResult> results = ldapContext.search(searchBase, searchFilter, searchControls);
if (results.hasMore()) {
SearchResult searchResult = results.next();
return searchResult.getNameInNamespace();
}
} catch (NamingException e) {
e.printStackTrace();
}
return null;
}
public boolean authenticateUser(String userDn, String password) {
if (userDn == null) {
System.out.println("User authentication failed: User DN not found.");
return false;
}
try {
Hashtable<String, String> env = new Hashtable<>();
env.put(Context.INITIAL_CONTEXT_FACTORY, "com.sun.jndi.ldap.LdapCtxFactory");
env.put(Context.PROVIDER_URL, ldapContext.getEnvironment().get(Context.PROVIDER_URL).toString());
env.put(Context.SECURITY_AUTHENTICATION, "simple");
env.put(Context.SECURITY_PRINCIPAL, userDn);
env.put(Context.SECURITY_CREDENTIALS, password);
DirContext ctx = new InitialDirContext(env);
ctx.close();
System.out.println("User authentication successful.");
return true;
} catch (NamingException e) {
System.out.println("User authentication failed: " + e.getMessage());
return false;
}
}
}
```
## 6. Modify Controller to Implement Conditional Login
**LdapController.java**
```
package com.example.ldapauth.controller;
import com.example.ldapauth.service.LdapService;
import com.example.ldapauth.service.LocalAuthService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class LdapController {
@Autowired
private LdapService ldapService;
@Autowired
private LocalAuthService localAuthService;
@PostMapping("/login")
public String performLogin(@RequestParam String username, @RequestParam String password) {
if (ldapService.isLdapAvailable()) {
String userDn = ldapService.getUserDn(username);
boolean isAuthenticated = ldapService.authenticateUser(userDn, password);
if (isAuthenticated) {
return "LDAP authentication successful!";
}
}
boolean isLocalAuthenticated = localAuthService.authenticate(username, password);
if (isLocalAuthenticated) {
return "Local authentication successful!";
}
return "Authentication failed!";
}
}
```
## Explanation:
1. Check LDAP Availability:
isLdapAvailable method in LdapService performs a dummy LDAP
search to check if the LDAP server is available.
2. Conditional Login Logic:
In LdapController, the /login endpoint checks LDAP
availability.
If LDAP is available, it tries to authenticate the user with
LDAP.
If LDAP authentication fails or LDAP is not available, it
falls back to local PostgreSQL database authentication using
LocalAuthService.
3. Local Authentication:
If LDAP is unavailable or the user fails LDAP authentication,
it checks the local PostgreSQL database for user credentials
using UserRepository.
4. REST Controller:
The @PostMapping on /login endpoint handles login requests and
applies the conditional logic for authentication.
This setup provides a robust mechanism to switch between LDAP and local authentication based on the availability of the LDAP server.
I hope this was helpful guys. Please note that you have to modify few things based on your requirements and logic. I have given here overall idea only.
Happy Coding :)
Thanks,
JavaCharter
Kailash Nirmal
Written on : 5th July 2024. | kailashnirmal |
1,912,366 | For playing | So, I've been exploring different Australian online casinos lately, and let me tell you, the perks... | 0 | 2024-07-05T07:24:11 | https://dev.to/adam_braun_a2cf762f8caabb/for-playing-j69 | playing | So, I've been exploring different Australian online casinos lately, and let me tell you, the perks are incredible. Besides the usual games, some sites offer loyalty programs that give you points just for playing – and you can redeem them for cash or bonuses later on. It's like getting rewarded for having fun! Plus, the mobile experience is top-notch. I can play my favorite games on the go without any glitches. If you're looking for a great time and some sweet rewards, definitely give these [https://play-au-casino.com/](https://play-au-casino.com/) sites a shot. | adam_braun_a2cf762f8caabb |
1,912,356 | 了解业务流程分析(BPA)和有效的流程设计 | A post by 宏天软件BPM&低代码平台 | 0 | 2024-07-05T07:14:37 | https://dev.to/hotentbpm/liao-jie-ye-wu-liu-cheng-fen-xi-bpahe-you-xiao-de-liu-cheng-she-ji-6ci | hotentbpm |
||
1,912,364 | How Secure Are Blockchain Networks Against Hacking And Other Cyber Threats? | Introduction The decentralized structure of blockchain networks, along with encryption protocols,... | 0 | 2024-07-05T07:21:12 | https://dev.to/capsey/how-secure-are-blockchain-networks-against-hacking-and-other-cyber-threats-jh1 | blockchain, cryptocurrency, bitcoin | **Introduction**
The decentralized structure of blockchain networks, along with encryption protocols, provides robust protection over cyber-attacks and hacking. Tailor-proof payments and resilience against unauthorized access are guaranteed by blockchain, that distributes information among a network of nodes and uses consensual techniques like Proof of Work or Proof of Stake. Smart contracts ensure visible and irreversible processes, and the use of cryptography protects data integrity. The ongoing enhancement of safeguards and procedures is necessary in light of the weaknesses in smart contract technology and the possibility of 51% attacks, regardless of their strengths.
This blog discusses the core safety features of blockchain networks and assesses how resistant they are to cyberattacks and hacking.
**What Is Blockchain ? and Its Importance**
Blockchain is a decentralized database system that safely records payments. Through saving data throughout a network of computers, it offers safety by rendering it almost impossible to change or hack without the approval of the whole network.
**Importance:**Nowadays, businesses need safety in blockchain due to the safeguards private information and builds confidence between participants without the need for middlemen like banks or authorities.
**Comparing Blockchain Security with Traditional Systems**
**Overview of Blockchain Security Features:** Key security elements of the blockchain system include consensus methods for transactional validation, data protection via encrypted hashing, and decentralization—the ability to operate independently of a single institution.
**Comparison with Traditional Systems:**The integrity of blockchain is not like that of conventional systems that are centralized. Because blockchain distributes information over an internet rather than centralized frameworks that depend on one entity, it is more difficult to attack or corrupt.
**Emerging Cyber Threats in Blockchain Security**
Cyber attacks such as 51% attacks, in which an organization gains authority over most of a blockchain's processing capacity, are prevalent and have the ability to manipulate payments. The act of using the exact same digital currency more than once is known as double-spends. Automatic contract vulnerabilities in code are holes that can be utilized.
Instances from the real world included attacks such as the Ethereum Decentralized Autonomous Organizations (DAOs) hack, in which the smart contract's weakness enabled fifty million dollars to be siphoned off. These events demonstrate how crucial blockchain safety has become.
**Security Measures in Blockchain Networks**
**Encryption and Cryptography:**Information is safeguarded by cryptography and cryptography processes, which jumble it up so that only those with permission may decipher it. This guarantees the privacy and security of data on blockchain-based systems.
**Consensus Mechanisms:** Safety on networks is maintained through consensus techniques like Proof of Work and Proof of Stake, which validate operations and stop fraud. They make it possible for users of the blockchain to concur on a transaction's legitimacy without depending on a governing body.
**Smart Contract Audits:** Finding and addressing these issues requires smart contract audits. It lessens the possibility of flaws or faults that might be taken advantage of by bad actors and helps guarantee that smart contracts work as planned.
**Challenges and Controversies**
The continuing debate is on striking a balance between blockchain networks' capacity to process massive volumes of operations (scalability) and their ability to provide robust defenses against intrusions and other threats (safety). Administrative Issues: These are problems pertaining to laws and regulations that have an impact on the applications and security of blockchain-based technologies. The design and maintenance of blockchain-based systems might be influenced by legal difficulties.
**Practical Tips for Businesses**
Companies should implement a number of doable strategies to improve [blockchain development services](https://bidbits.org/blockchain-development-company) and protection. Start by selecting a platform for blockchain technology that is trustworthy, safe, and has a lot of safeguarding capabilities. Verify thoroughly that suppliers of services follow strict security guidelines. Detects and remediate problems by conducting routine security audits and assessments. Employee education is essential; teach staff members how to identify malware, create safe passwords, and protect secret keys, among additional best practices for security on the blockchain. Frequently update software and use multiple authentication methods to guard against emerging risks. These actions can help firms greatly strengthen their blockchain safety measures.
| capsey |
1,912,363 | Learn AI Online Free: Top Courses to Kickstart Your Artificial Intelligence Journey | Top Free AI Courses Online How to Learn Artificial Intelligence for Free with... | 0 | 2024-07-05T07:21:04 | https://dev.to/educatinol_courses_806c29/learn-ai-online-free-top-courses-to-kickstart-your-artificial-intelligence-journey-4807 | education | Top Free AI Courses Online How to Learn Artificial Intelligence for Free with Certificates
Navigating the myriad options for learning of AI can be overwhelming, especially when trying to find resources that align with your specific needs. To determine the best pathway for mastering artificial intelligence, consider the following pivotal criteria:
1. Gratuitous Learning: You seek a course that incurs no cost.
2. Expedited Completion: You desire a swift educational journey.
3. Reputed Institution: You prefer accreditation from a prestigious university.
Premier Complimentary Online AI Courses
Even for neophytes, it is essential to recognize that AI courses vary widely, concentrating on distinct facets of the domain. Based on your predilections, select the appropriate AI course for you.
Diploma in Artificial Intelligence
This all-encompassing program is tailored for novices. Diploma in Artificial Intelligence encapsulates foundational concepts requisite for grasping artificial intelligence. The curriculum is concise, enabling completion within a fortnight, while simultaneously imparting advanced AI applications.
Checkout Diploma in Artificial Intelligence : https://bit.ly/45QvL5e
Why Opt for This Course: Ideal for those desiring to comprehend AI fundamentals applicable across multiple fields.
Basics of Artificial Intelligence: Learning Models
Basics of Artificial Intelligence: Learnings Models is Crafted by Cambridge International Qualifications, UK, this certification course delves into various AI learning paradigms. Subjects covered include Deep Learning, Probabilistic Models, and Fuzzy Logic.
Checkout Basics of Artificial Intelligence: Learning Models : https://bit.ly/4csXhIn
Why Opt for This Course: Highly beneficial for AI researchers, developers, or professionals dealing with extensive datasets.
Basics of Artificial Intelligence
Basics of Artificial Intelligence Is Another introductory course from Cambridge International Qualifications, UK, this program investigates the evolution of AI and future prospects. The material is designed for completion in a mere 6 hours.
Checkout Basics of Artificial Intelligence : https://bit.ly/4csXhIn
Why Opt for This Course: One of the finest introductory courses for beginners seeking a rapid overview of AI.
Basics of Agents & Environments in AI
Basics of Agents and Environments in AI This course elucidates the fundamentals of AI with a focus on intelligent agents. It encompasses various environments where AI is utilized and includes the renowned Turing Test for evaluating an agent's intelligence.
Checkout Basics of Agents & Environments in AI : https://bit.ly/3VZjhns
Why Opt for This Course: Suitable for those wanting a concise course emphasizing agents and environments in AI.
Why Individuals in Botswana Should Undertake These Courses :
Employment Opportunities: Proficiency in AI opens doors to lucrative job opportunities, both domestically and internationally, addressing unemployment issues.
Technological Advancement: AI expertise can propel Botswana towards technological leadership within Africa, attracting foreign investments and partnerships.
Problem-Solving Acumen: AI can be harnessed to tackle local challenges such as wildlife conservation, climate change, and resource management. With AI expertise, Botswanans can develop bespoke solutions tailored to their specific needs.
Conclusion
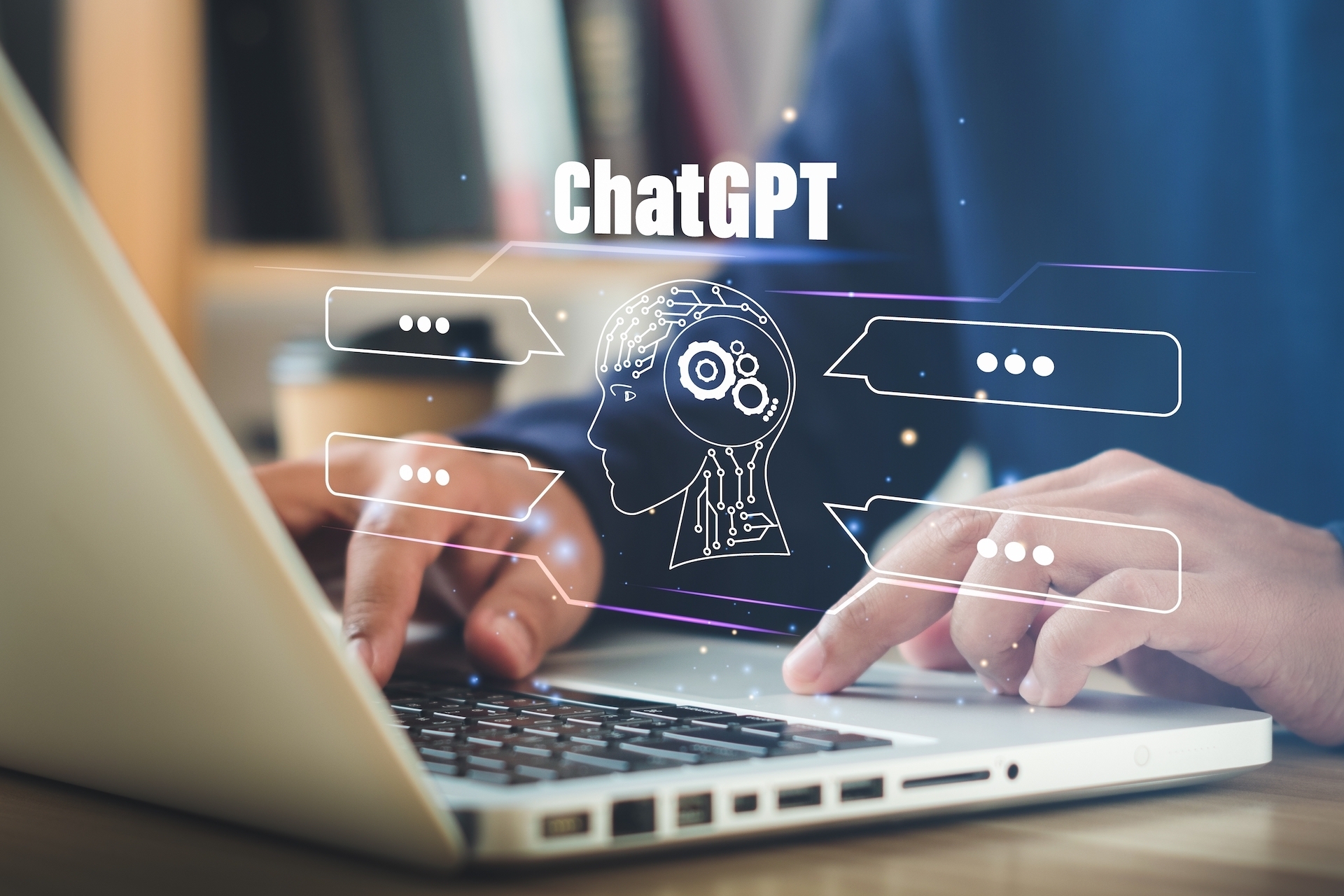
Checkout Uniathena Courses : https://bit.ly/3zDrC8w
These courses represent some of the finest avenues for learning AI online without cost, particularly for beginners. All courses are available through UniAthena and come with certificates, enhancing your skill set and bolstering your resume for the job market.
| educatinol_courses_806c29 |
1,912,362 | How to Create and Optimize a Personal Blog with WordPress and EdgeOne Acceleration? | So far, blogging remains a platform that allows anyone, from individuals to large enterprises, to... | 0 | 2024-07-05T07:18:29 | https://dev.to/chuck7chen/how-to-create-and-optimize-a-personal-blog-with-wordpress-and-edgeone-acceleration-9fc |
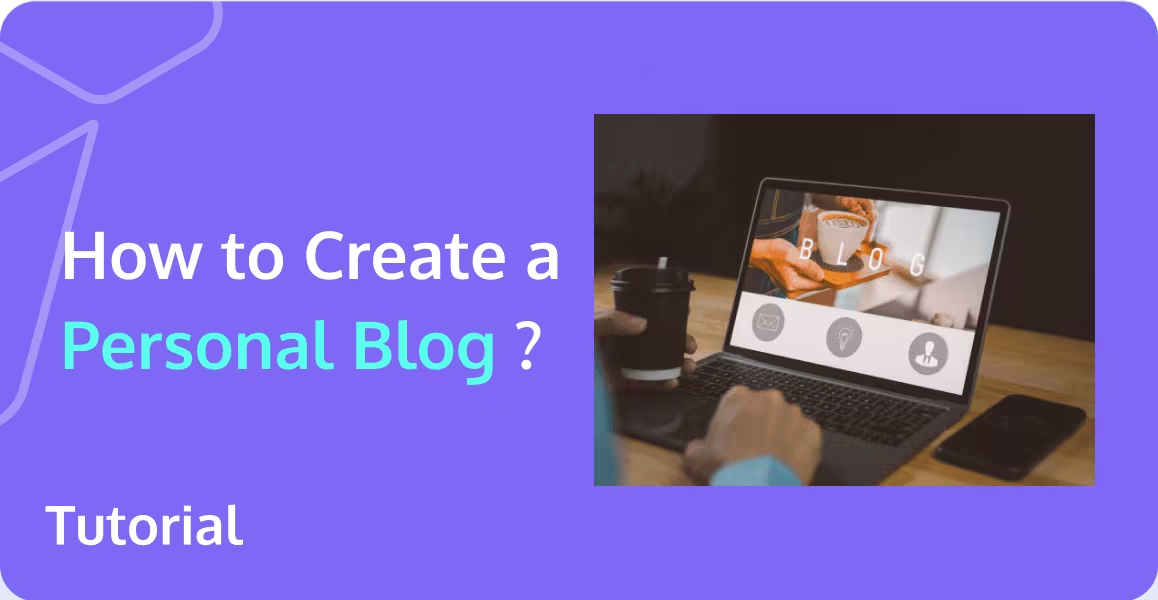
So far, blogging remains a platform that allows anyone, from individuals to large enterprises, to reach a large number of potential customers at a meager cost. You can use a blog to write and record your daily thoughts, share your stories, engage with others, or build a community. As your blog's traffic increases, you can even earn income through your blog content. This article will help interested individuals set up their own independent personal blog.
Understanding Key Web Terminology
Considering that some friends may not be familiar with the concepts involved, let's start with a brief explanation:
When we want to access a website, the text we enter in the browser, such as "www.tencentcloud.com" is called a domain name. It is a user-friendly and memorable address that identifies a computer's electronic location for various services like websites, email, FTP, etc.
Now, what happens after we enter a domain name?
The browser doesn't know which server to contact for the domain "www.tencentcloud.com". Instead, it relies on the DNS (Domain Name System) server configured on our computer to provide an answer.
The DNS server holds the record of the server's IP address associated with "www.tencentcloud.com". An IP address is a unique identifier for a computer, and the DNS server provides this IP address to the browser.
The browser uses the IP address obtained from the DNS server to send a request to the server and retrieve the requested data. The server then sends the data back to our browser.
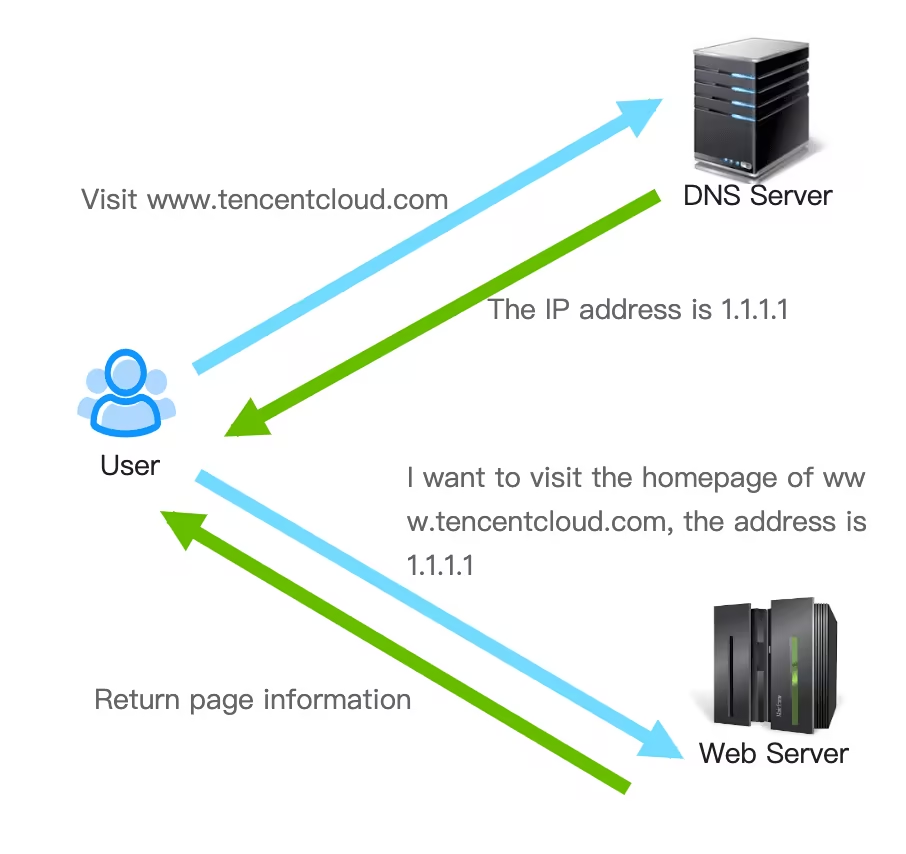
## Creating Your Personal Blog
WordPress is a blogging platform developed using PHP language. Users can set up their own websites on servers that support PHP and MySQL databases. Approximately 34% of websites worldwide use WordPress. Additionally, WordPress offers nearly 50,000 extension plugins and 5,000 theme templates, allowing users to create communities or even online stores.
### Preparation
To build a personal blog, we will need:
1. Domain Name (Can be registered on platforms like Tencent Cloud )
2. Hosting (Web server server to store website files, images, etc. For example, Tencent Cloud offers Lighthouse, a web hosting service)
3. WordPress Software (Download from cn.wordpress.org, or use the WordPress image available on Tencent Cloud servers. Refer to Tencent Cloud's CVM product documentation for more details.)
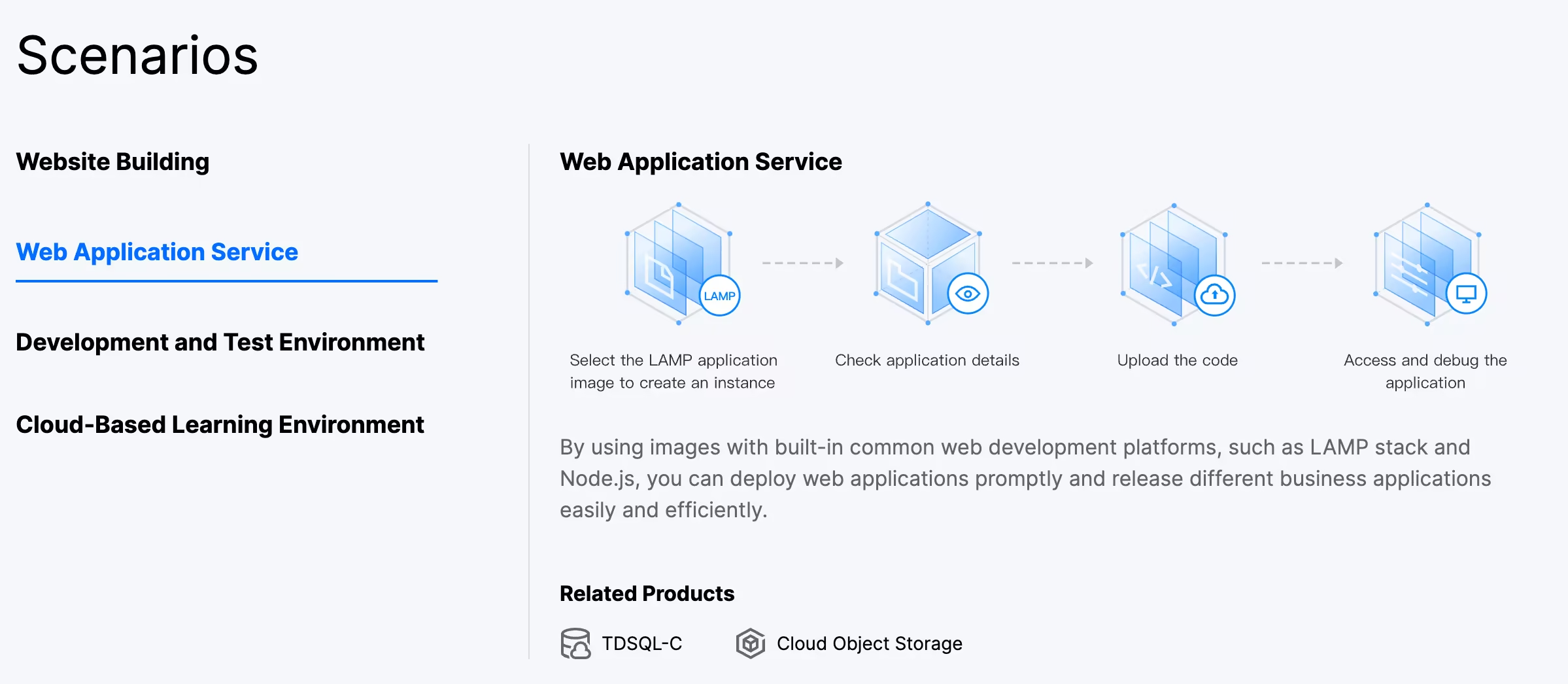
### Specific Steps
#### 1. Purchase a Domain Name
You can register a domain name on platforms like Tencent Cloud.
#### 2. Purchase a Server
You can purchase a CVM (Cloud Virtual Machine) server on Tencent Cloud.
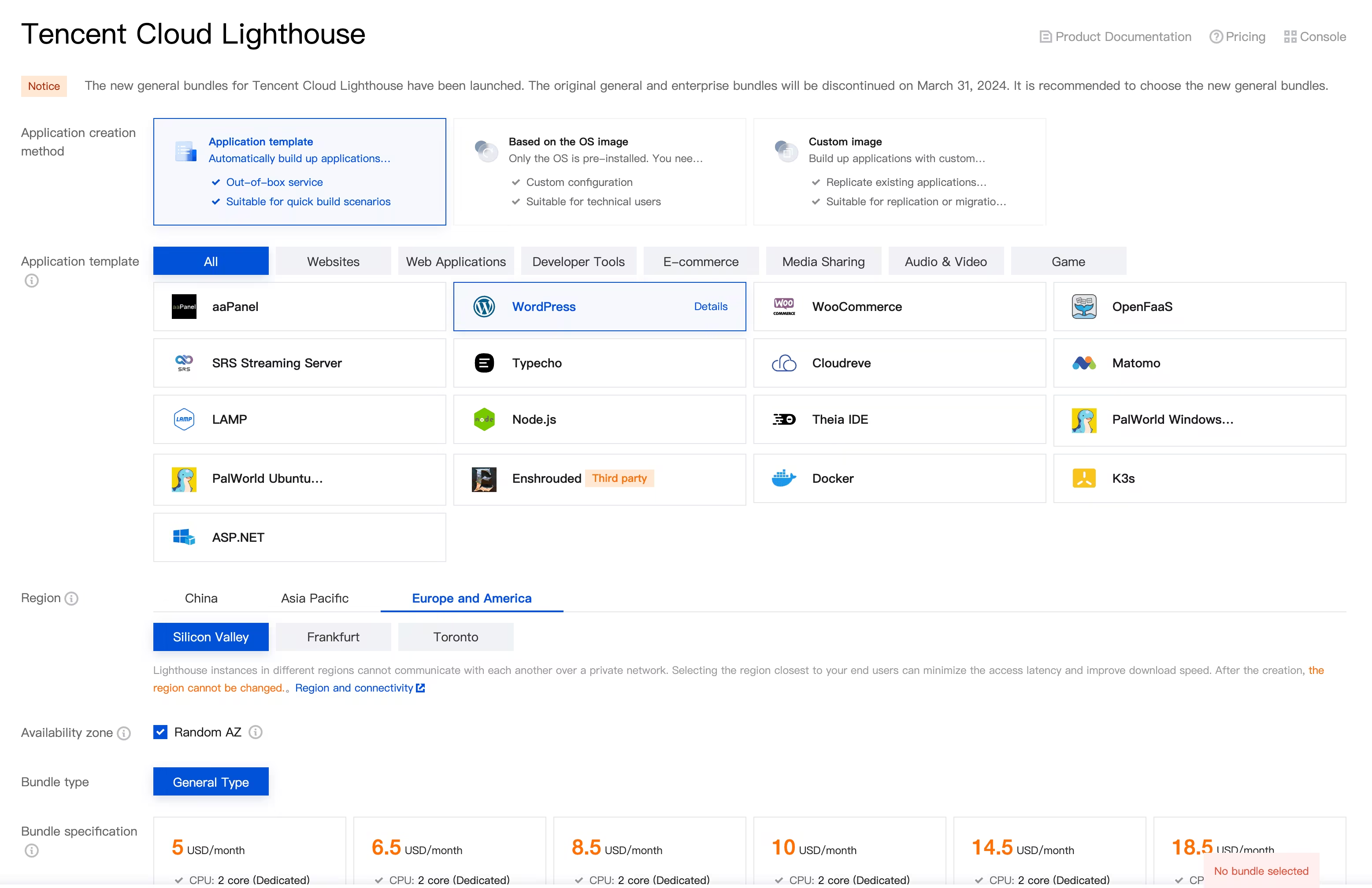
#### 3. Domain Name Resolution
Domain name resolution involves recording the association between the registered domain name and the IP address of the hosting server on the DNS server. This allows the DNS server to provide the corresponding server IP address to the browser when a user accesses the domain name.
To perform domain name resolution, go to the domain name service provider and find the domain name resolution entry. Add DNS records with the host records as "www" and "@" respectively, and the record value as the public IP address of the hosting server.
Once the domain name resolution is complete, our domain name will be accessible.
#### 4. Log in to the WordPress Admin Dashboard
On the main page of the blog, click on the login option and enter the username and password obtained from the provided credentials to access the admin dashboard.
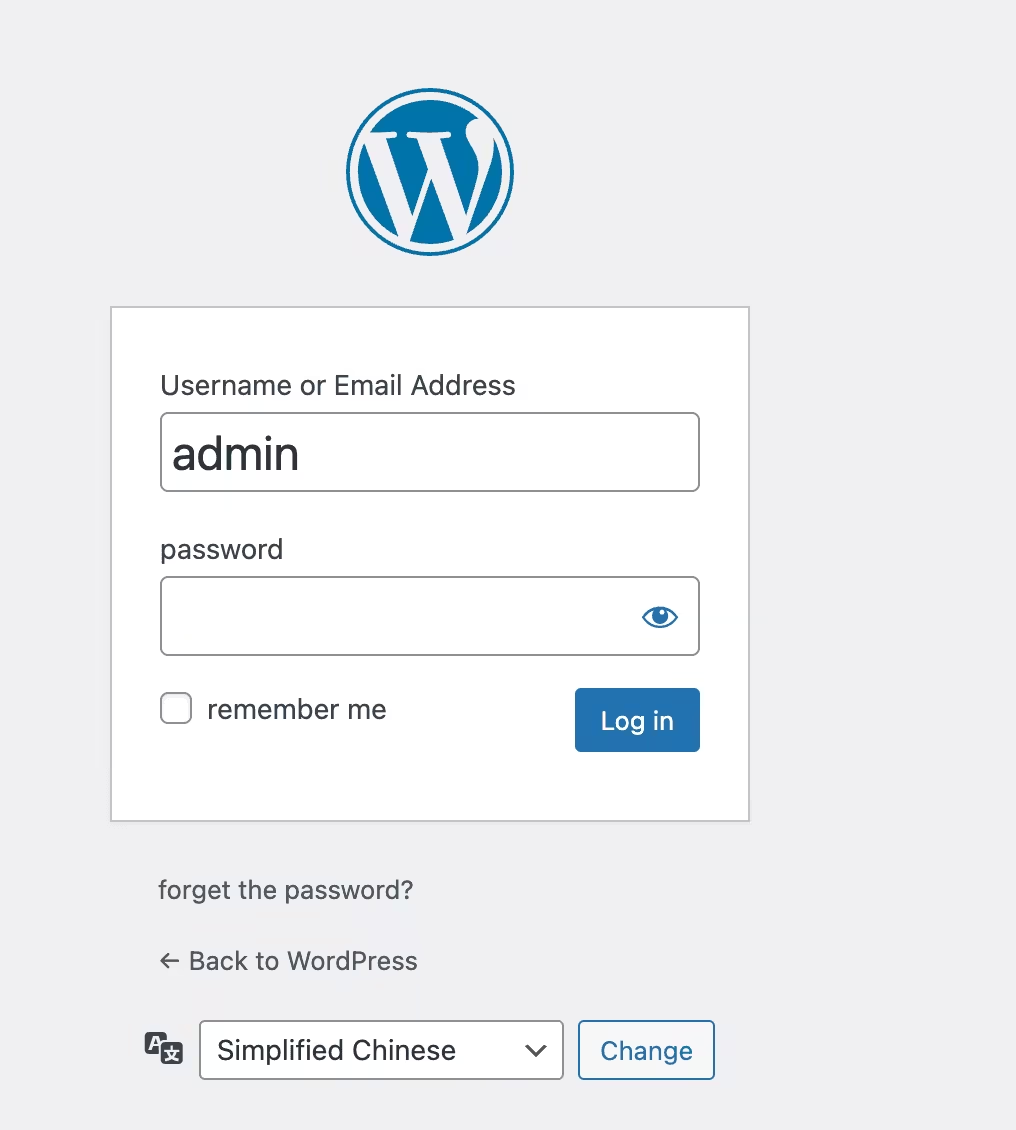
The admin dashboard address is YourHostingIP/wp-admin.
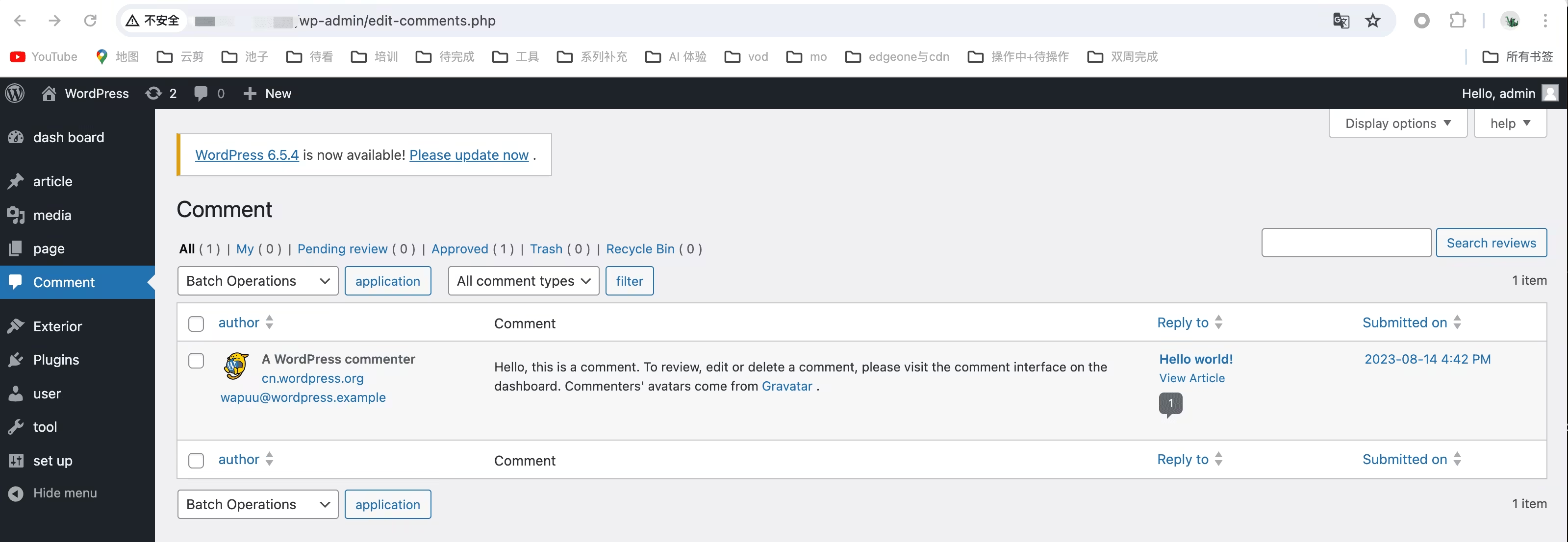
At this point, your WordPress blog website is successfully set up. You can now write articles, publish posts, and perform various operations such as managing comments on your blog.
## Blog Optimization
### 1. [EdgeOne](https://edgeone.ai/) Acceleration Principle
Although the blog website is successfully set up, when there is a significant physical distance between users and the server, multiple network hops are required during the access process, resulting in high and unstable latency. Additionally, having a large number of image files on the blog can significantly impact the loading speed. In such cases, we can use [EdgeOne](https://edgeone.ai/) to accelerate the domain name and improve the user's browsing experience. Let's take a look at [how EdgeOne accelerates domain names](https://edgeone.ai/document/56724).
[EdgeOne](https://edgeone.ai/) adds a new network architecture layer to the existing internet network, consisting of high-performance acceleration nodes distributed globally. These nodes store business content based on certain caching strategies. When a user requests specific content, the request is routed to the nearest acceleration node, which quickly responds to the request, effectively reducing user access latency and improving availability. In simpler terms, you can think of [EdgeOne](https://edgeone.ai/) acceleration nodes as warehouses located worldwide. When a user in Singapore places an order, it is shipped from the warehouse in Singapore, and when a user in China places an order, it is shipped from the warehouse in China (these are the nearest acceleration nodes). This strategy ensures that users receive their goods faster.
### 2. Configuring [EdgeOne](https://edgeone.ai/)
Taking Tencent Cloud's [EdgeOne](https://edgeone.ai/) as an example, you need to register a Tencent Cloud account and activate the service in the [Tencent EdgeOne Console](https://console.tencentcloud.com/edgeone). After activating [EdgeOne](https://edgeone.ai/), the configuration involves two steps: domain name integration and CNAME configuration.
First, you need to configure the **domain name integration**. In the console, enter the domain name you want to accelerate, which is the domain name of your blog platform.
IP/Domain Name: Used to integrate a single origin server. You can enter a single IP or domain name as the origin server.
Object Storage Source: Used to add Tencent Cloud COS (Cloud Object Storage) or AWS S3-compatible authenticated object storage buckets as origin servers. If the storage bucket has public read/write access, you can also use the IP/Domain Name origin server type directly.
Origin Server Group: If there are multiple origin servers, you can add them by configuring an origin server group.
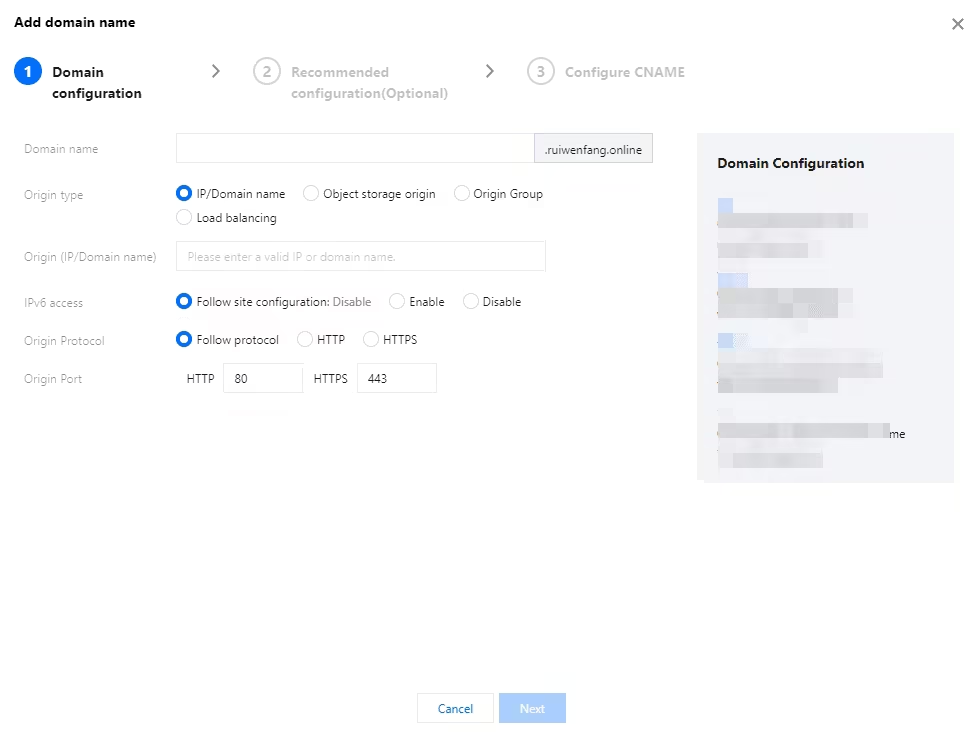
After you add the domain name, [EdgeOne](https://edgeone.ai/) provides you with recommended configurations for different business scenarios to ensure that your business runs securely and smoothly. You can select a recommended configuration as needed and click Next to deploy the configuration, or click Skip.
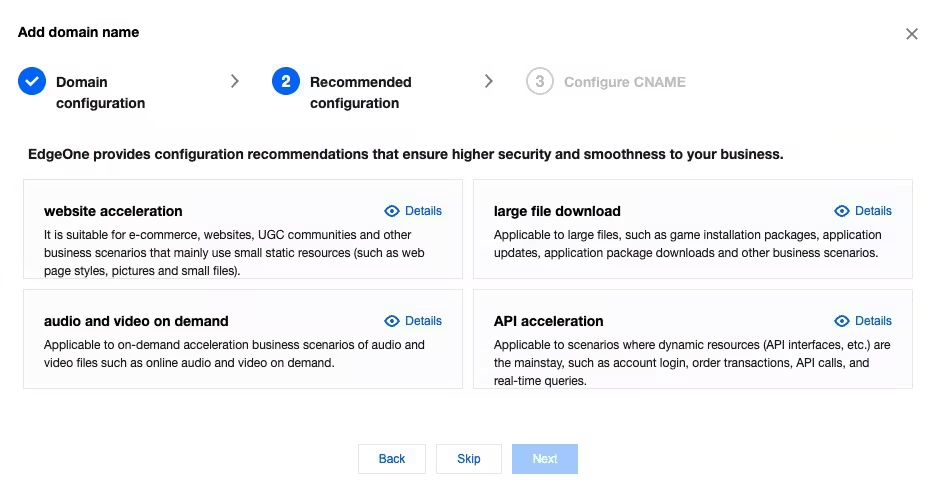
After completing the configuration, it will appear as shown in the following image:
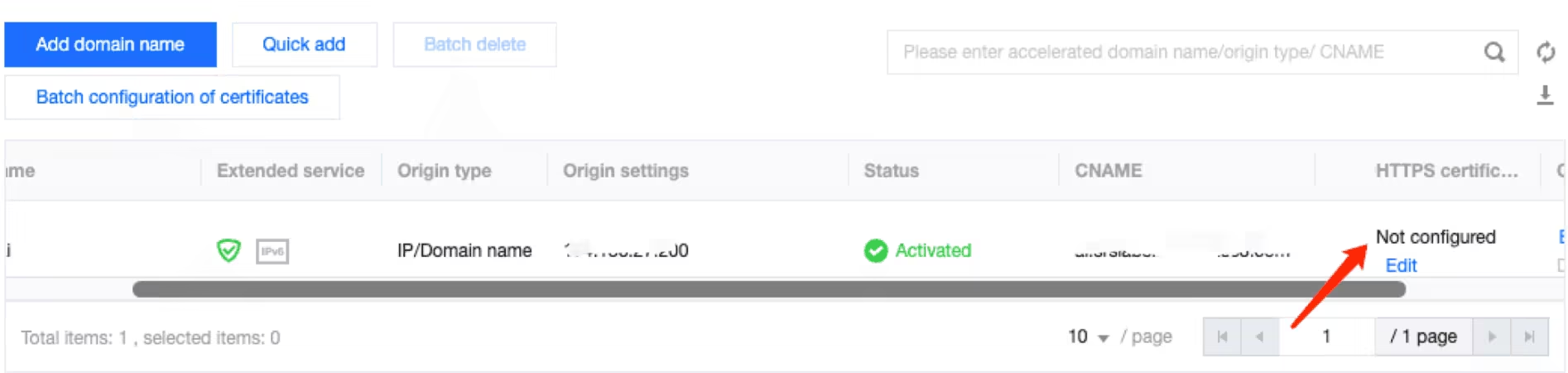
For a more detailed configuration process, refer to [Site Acceleration](https://test.edgeone.ai/blog/details/accelerate-site).
Next, let's discuss how to configure the CNAME.
After adding the domain name, [EdgeOne](https://edgeone.ai/) will provide you with a CNAME that points to the [EdgeOne](https://edgeone.ai/) node.
The steps to configure CNAME are similar to the process of domain name resolution in setting up the blog website. In the domain name service provider, find the domain name resolution entry and add a record. As shown in the image, add the domain name prefix in the host record, set the record type as CNAME, enter the CNAME domain name in the record value, and click **Confirm** to complete the CNAME configuration.

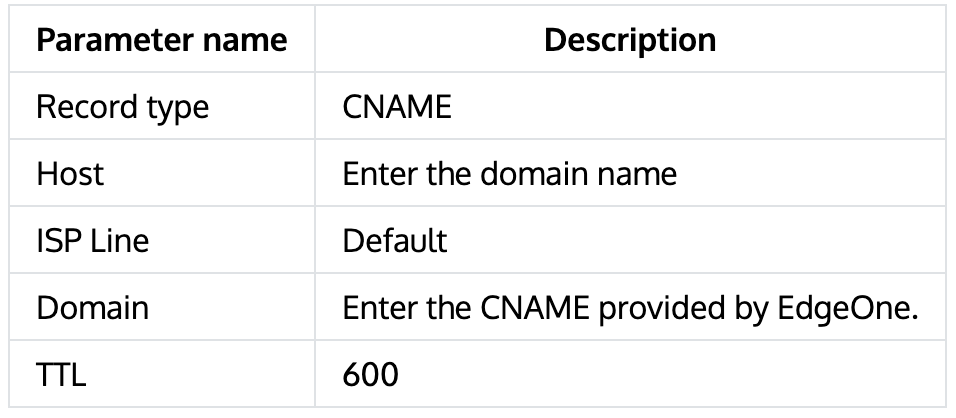
Once the CNAME configuration is complete, go back to the domain name management page and check the corresponding domain name. The prompt for CNAME not being configured should disappear. At this point, the [EdgeOne](https://edgeone.ai/) acceleration for that domain name is configured.

The above steps cover the entire process from setting up a personal blog to configuring [EdgeOne](https://edgeone.ai/). | chuck7chen |
|
1,912,360 | Find the Best Software Development Company in Calicut. | If you're on the lookout for [best software development company in Calicut, Kerala. look no further... | 0 | 2024-07-05T07:16:42 | https://dev.to/wis_branding_84cec990b812/find-the-best-software-development-company-in-calicut-15jj | softwaredevelopment, softwarecompany, software |
If you're on the lookout for [best software development company in Calicut, Kerala. look no further than Wisbato. Renowned for its innovative solutions and customer-first approach, Wisbato has earned its reputation as the best software company in the region.
**Why Wisbato Stands Out**
1. Expertise and Experience
Wisbato boasts a team of highly skilled professionals proficient in various programming languages and cutting-edge technologies. With years of experience under their belt, they have successfully delivered a wide range of projects, from simple applications to complex enterprise solutions.
2. Client-Centric Approach
At Wisbato, the client is always at the forefront. They take the time to understand your unique needs and tailor their solutions accordingly. Their commitment to delivering high-quality, customized software ensures that your project not only meets but exceeds expectations.
3. Robust Portfolio
Wisbato's impressive portfolio is a testament to their capabilities. They have worked across various industries, providing innovative solutions that drive business success. Whether it's web development, mobile app development, or software design, Wisbato has done it all with excellence.
4. Continuous Support
The relationship with Wisbato doesn't end with project delivery. They offer ongoing support and maintenance to ensure your software remains up-to-date and functions smoothly. Their dedicated support team is always ready to assist with any issues or updates.
5. Cutting-Edge Technology
Staying ahead in the tech game is crucial, and Wisbato excels at it. They leverage the latest technologies and methodologies to deliver solutions that are not only current but also future-proof. This forward-thinking approach ensures your software stays relevant in the rapidly evolving tech landscape.
**What Clients Say**
Client testimonials speak volumes about Wisbato's reliability and excellence. Many have praised their professional approach, timely delivery, and exceptional quality of work. This positive feedback underscores why Wisbato is the go-to choice for [software company in Calicut.](https://www.wisbato.com/)
**Conclusion**
Choosing the right software development company can make a significant difference in the success of your project. With Wisbato, you're not just getting a service provider; you're partnering with a team dedicated to driving your business forward. Their expertise, client-centric approach, robust portfolio, and continuous support make them the best [software development company in Calicut](https://www.wisbato.com/), Kerala.
| wis_branding_84cec990b812 |
1,912,359 | ☁️ The Future is Cloud: Embracing Cloud Computing in the IT Industry 🚀 | Cloud computing is transforming the IT landscape, offering unparalleled scalability, flexibility, and... | 0 | 2024-07-05T07:16:11 | https://dev.to/m_hussain/the-future-is-cloud-embracing-cloud-computing-in-the-it-industry-3f5m |
Cloud computing is transforming the IT landscape, offering unparalleled scalability, flexibility, and cost savings. As businesses adapt to this digital revolution, here are some key benefits and trends to watch:
Scalability: Easily scale your resources up or down based on demand, ensuring optimal performance without over-provisioning.
Flexibility: Access your data and applications from anywhere, at any time, fostering remote work and collaboration.
Cost Savings: Reduce infrastructure costs by paying only for the resources you use, eliminating the need for expensive on-premises hardware. | m_hussain |
|
1,912,355 | Responsive Column List of Reviews | Sleek and adaptable column list to display reviews. Perfect for any website, this item showcases a... | 0 | 2024-07-05T07:12:01 | https://dev.to/dmtlo/responsive-column-list-of-reviews-448a | codepen, webdev, css, html | Sleek and adaptable column list to display reviews. Perfect for any website, this item showcases a design that automatically adjusts to different screen sizes, ensuring an optimal viewing experience on desktops, tablets, and mobile devices. Featuring clean HTML and CSS code, this example helps you organize user reviews in a visually appealing and accessible manner.
**Check out this Pen I made!**
{% codepen https://codepen.io/dmtlo/pen/poXzaoa %} | dmtlo |
1,912,353 | Cotton Candy Vending Machines: Spinning Delightful Treats in Seconds | Vending Machine Makes Cotton Candy Fun And Easy You know how you go to a carnival or fair and they... | 0 | 2024-07-05T07:09:55 | https://dev.to/ted_slunaisy_edbc0713b6/cotton-candy-vending-machines-spinning-delightful-treats-in-seconds-4a3 | design | Vending Machine Makes Cotton Candy Fun And Easy
You know how you go to a carnival or fair and they have that machine spinning out fluffy cotton candy? A scrumptious indulgence, poised to vanish within the blink of an eye. Well what if I told you could have access to this sweet nectar whenever your heart desires? Cotton candy vending machines are now available to let you do just this! These incredible devices make cotton candy even more fun to enjoy since they sit right on your countertop and you can eat a deliciously sweet snack exactly when you want one. Keep reading and join our in-depth exploration of the amazing world cotton candy vending machines including what makes them awesome, how they secure your safety, a walk-though on using one in 3 simple steps and more importantly learn about their production quality receive some insight into maintenance requirements as well as where to find such novelties.
Cotton Candy Vending Machines Are Amazing
When it comes to cotton candy vending machines, they are a bit like wizards who make the sweet confection in virtually no time at all. I mean honestly can you even imagine how nice it feels seeing this huge poofy, sweet cloud appear the instant! These commercial popcorn machine can also be found in locations that have high traffic, like malls, arcades or amusement park due to their super fast speed. They also happen to be as user-friendly as can be. You can make yourself some luscious cotton candy by following a few basic instructions.
How to remain safe with vending machines
How can you be safe when it comes to meals We should be grateful for cotton candy vending machines because of the special safety features they come with to give you peace as snacking on them. For example, a safety guard surrounds the whirling bowl to keep idle fingers at a safe distance when it is running. Moreover, the heating element is strategically off to one side thereby eliminating near misses from hot surfaces.
Using A Cotton Candy Vending Machine
It's as simple to use a vending machine of cotton candy, it is literally like stealing the money. First of all, get to understand about the instructions given. So here is what you can do, just get the machine plugged and let it heat itself to a level where its ready for use. Then you should pour in your sugar mix, turn on the commercial popcorn popper machine and be mesmerized as it turns that sugary concoction into delicious cotton candy right before your eyes. Afterwards, you can collect your treat on a stick or in cone to bite into its fluffy sweetness.
Enjoy Delicious Cotton Candy Every Time
One of the most important aspects of cotton candy is consistency, and these vending machines provide exactly that every time. Everything they prepare is accomplished with scientifically accurate sugar blends and their signature spinning bowl - creating the perfect consistency for every serving of cotton candy. In addition, it has an adaptable alternation to its spinning speed that enables you to modify the texture and denseness of your cotton candy as how do like.
Maintaining Vending Machines
Cotton candy vending machines must be properly managed and maintained to ensure that it keeps producing the perfect treats. Fortunately, the majority of places that offer these devices will have a standardised service plan which includes cleaning and regular maintenance. Taking the initiative this way will keep your machines running smoothly and enable you to continue indulging in that sweet treat distraction from life as per usual.
Where To Locate Cotton Candy Vending Machines
These fantastic machines are popular in high traffic areas like airports, malls and theme parks. These treats are the life of carnivals, fairs and weddings because they provide immediate happiness Additionally, cotton candy vending machines can both add unique eye-catching vibrancy to retail spaces and at the same time offer a lucrative revenue stream aside from its functional benefits.
So in conclusion, one of the most recognisable old school sweet treats can be obtain through a vending machine. Not only are they beautiful additions for any setting with their speedy production, safety-first attitude and high-quality final good popcorn machine product; Our machines have a wonderfully classic appeal that can't be beaten! I figure the vending machines here in busy town are for people who like variety (not me, no way) or others who have something special to celebrate (also - not interested because true happiness is when cotton candy settles permanently somewhere warm and comfortable inside of you). | ted_slunaisy_edbc0713b6 |
1,912,350 | Hỗ trợ luận văn thạc sỹ | Với 15 năm kinh nghiệm thực tiễn viết luận văn thạc sỹ, đội ngũ chuyên gia có trình độ và chuyên môn... | 0 | 2024-07-05T07:03:21 | https://dev.to/hotroluanvanthacsi/ho-tro-luan-van-thac-sy-k1c | Với 15 năm kinh nghiệm thực tiễn viết luận văn thạc sỹ, đội ngũ chuyên gia có trình độ và chuyên môn cao, các bài viết luận văn của chúng tôi cam kết nội dung mới 100%, trình bày theo đúng tiêu chuẩn mà khách hàng yêu cầu về [hỗ trợ luận văn thạc sỹ](https://hotroluanvanthacsi.com/). | hotroluanvanthacsi |
|
1,912,349 | Is JavaScript Really as Insecure as They Say? | That's something I have heard sometimes over internet discussions. Let's see the truth to it.... | 0 | 2024-07-05T07:03:20 | https://dev.to/nikl/is-javascript-really-as-insecure-as-they-say-mi8 | javascript, node, react, discuss | That's something I have heard sometimes over internet discussions. Let's see the truth to it.
JavaScript, especially through frameworks like React and Node/Express, has a lingering reputation for being "insecure" compared to other web technologies like .NET or Laravel. But is this reputation deserved, or is it just another case of tech snobbery?
#### 1. **Client-Side Exposure**
Yes, JavaScript runs in the browser, making its code accessible to anyone with basic developer tools. This visibility makes some developers sweat bullets, imagining hordes of hackers cracking open their precious code. But let's get real: client-side code visibility is not a death sentence. If you're storing sensitive data or performing critical operations on the client side, you're doing it wrong. Period.
The truth is, the client-side nature of JavaScript is only a problem if you treat your front end like Fort Knox. Sensitive operations should always happen server-side, whether you're using JavaScript, Python, or good old COBOL.
#### 2. **Server-Side JavaScript**
With Node.js, JavaScript stepped into the server-side arena, and guess what? It’s holding its own just fine. Critics argue that server-side JavaScript isn't as robust as .NET or Java, but let's not forget that security isn't about the language; it's about the implementation. Node.js, when configured properly, can be as secure as any other server-side technology.
Anyone who claims otherwise is either stuck in the past or hasn't bothered to learn about the modern capabilities of Node.js. If you can’t handle the heat, stay out of the codebase.
#### 3. **Framework Flexibility**
Frameworks like Next.js offer the flexibility to mix server-side and client-side code, which can be a double-edged sword. Some developers accidentally expose server-only code to the client, but let’s face it, that’s not the framework's fault. That’s like blaming the hammer for missing the nail.
Accidents happen, but with proper knowledge and vigilance, these can be avoided. Blaming the tool for the user's mistakes is a cop-out.
#### 4. **Dependency Hell**
JavaScript's rich ecosystem, particularly through npm, is both a blessing and a curse. Sure, there are a million packages, but with great power comes great responsibility. Lazy developers who fail to audit their dependencies can introduce vulnerabilities into their applications. But this isn't a problem unique to JavaScript; it’s just more visible because of its popularity.
Every ecosystem has its dark corners. The difference is, JavaScript’s are just more popular—and more scrutinized.
#### 5. **Security Practices**
Security boils down to practices, not languages. You can write insecure code in any language if you don’t follow best practices. React and Express are designed with security in mind, but they can't fix lazy or uninformed coding. Using eval recklessly or failing to sanitize inputs isn't JavaScript’s fault—it’s yours.
Blaming JavaScript for security flaws is like blaming your car for running out of gas because you didn’t fill it up.
#### 6. **Developer Inexperience: A Silent Threat**
JavaScript is often the first language many new developers learn, leading to a proliferation of poorly secured apps. This influx of green developers contributes to the perception of JavaScript’s insecurity. But let's be fair: every language has its fair share of noobs. It’s just that JavaScript’s low entry barrier makes it a common starting point.
Blaming JavaScript for newbie mistakes is like blaming a pencil for misspelled words.
So? Is it that?
So next time someone dismisses JavaScript as "insecure," ask them this: Is it the language, or is it you? | nikl |
1,911,839 | Builder Design Pattern | O padrão de design Builder é utilizado para construir objetos complexos de forma incremental,... | 0 | 2024-07-04T18:42:09 | https://dev.to/rflpazini/builder-design-pattern-2hm3 | go, coding, softwaredevelopment, designpatterns | O padrão de design Builder é utilizado para construir objetos complexos de forma incremental, permitindo a criação de diferentes representações de um objeto utilizando o mesmo processo de construção. Neste artigo, vamos explorar como implementar o padrão Builder em Golang, entender seus benefícios e analisar um exemplo prático de uso.
## O que é o Builder?
O padrão Builder separa a construção de um objeto complexo da sua representação, permitindo que o mesmo processo de construção possa criar diferentes representações. Isso é especialmente útil quando um objeto precisa ser criado em várias etapas ou com várias configurações possíveis.
## Benefícios do Builder
* Separação de Construção e Representação: Permite que a construção de um objeto seja separada da sua representação final.
* Construção Incremental: Permite a construção de objetos complexos de maneira incremental e passo a passo.
* Reutilização de Código: Facilita a reutilização de código ao definir passos de construção comuns que podem ser combinados de várias maneiras.
## Implementando um Builder
Para implementar nosso Builder, vamos imaginar um objeto complexo onde será necessário inicializar vários campos e até outros objetos agrupados. Que tal uma Casa? Onde teremos dois tipos de construção, uma convencional onde será usado concreto e tijolos, e uma segunda de madeira.
### 1 - Definindo a Estrutura
Primeiro, precisamos definir a estrutura do objeto que queremos construir. Como dito antes, vamos construir uma casa. Dentro dessa Struct colocaremos o que é necessário para criar uma.
```go
// house.go
package main
type House struct {
Foundation string
Structure string
Roof string
Interior string
}
```
### 2 - Definindo a Interface do Builder
Ainda no mesmo arquivo, vamos definir a interface do nosso Builder que especifica os métodos necessários para construir as diferentes partes da `House`.
```go
//house.go
package main
type House struct {
Foundation string
Structure string
Roof string
Interior string
}
type HouseBuilder interface {
SetFoundation()
SetStructure()
SetRoof()
SetInterior()
GetHouse() House
}
```
### 3 - Implementando concretamente o Builder
Vamos criar dois arquivos novos, `concreteHouse` e `woodHouse`. Eles serão a implementação de uma classe concreta que siga a interface `HouseBuilder`.
```go
//concreteHouse.go
package main
type ConcreteHouseBuilder struct {
house House
}
func (b *ConcreteHouseBuilder) SetFoundation() {
b.house.Foundation = "Concrete, brick, and stone"
}
func (b *ConcreteHouseBuilder) SetStructure() {
b.house.Structure = "Wood and brick"
}
func (b *ConcreteHouseBuilder) SetRoof() {
b.house.Roof = "Concrete and reinforced steel"
}
func (b *ConcreteHouseBuilder) SetInterior() {
b.house.Interior = "Gypsum board, plywood, and paint"
}
func (b *ConcreteHouseBuilder) GetHouse() House {
return b.house
}
```
```go
//woodHouse.go
package main
type WoodHouseBuilder struct {
house House
}
func (b *WoodHouseBuilder) SetFoundation() {
b.house.Foundation = "Wooden piles"
}
func (b *WoodHouseBuilder) SetStructure() {
b.house.Structure = "Wooden frame"
}
func (b *WoodHouseBuilder) SetRoof() {
b.house.Roof = "Wooden shingles"
}
func (b *WoodHouseBuilder) SetInterior() {
b.house.Interior = "Wooden panels and paint"
}
func (b *WoodHouseBuilder) GetHouse() House {
return b.house
}
```
### 4 - Definindo o `Director`
O `Director` é uma classe que gerencia a construção de um objeto, garantindo que as etapas de construção sejam chamadas na ordem correta. Ele não sabe nada sobre os detalhes das implementações específicas do Builder, apenas chama os métodos do Builder em uma sequência lógica para criar o produto final.
```go
//director.go
package main
type Director struct {
builder HouseBuilder
}
func (d *Director) Build() {
d.builder.SetFoundation()
d.builder.SetStructure()
d.builder.SetRoof()
d.builder.SetInterior()
}
func (d *Director) SetBuilder(b HouseBuilder) {
d.builder = b
}
```
### 5 - Utilizando o Builder
Finalmente, vamos utilizar o `Director` e os `Builders` concretos para construir diferentes tipos de casas.
```go
//main.go
package main
import (
"fmt"
)
func main() {
cb := &builder.ConcreteHouseBuilder{}
director := builder.Director{Builder: cb}
director.Build()
concreteHouse := cb.GetHouse()
fmt.Println("Concrete House")
fmt.Println("Foundation:", concreteHouse.Foundation)
fmt.Println("Structure:", concreteHouse.Structure)
fmt.Println("Roof:", concreteHouse.Roof)
fmt.Println("Interior:", concreteHouse.Interior)
fmt.Println("-------------------------------------------")
wb := &builder.WoodHouseBuilder{}
director.SetBuilder(wb)
director.Build()
woodHouse := wb.GetHouse()
fmt.Println("Wood House")
fmt.Println("Foundation:", woodHouse.Foundation)
fmt.Println("Structure:", woodHouse.Structure)
fmt.Println("Roof:", woodHouse.Roof)
fmt.Println("Interior:", woodHouse.Interior)
}
```
### Resumindo
1. Struct `House`: Representa o produto final que estamos construindo.
2. Interface `HouseBuilder`: Define os métodos para construir as diferentes partes da casa.
3. Implementações Concretas (`ConcreteHouseBuilder` e `WoodHouseBuilder`): Implementam a interface `HouseBuilder` e definem as etapas de construção específicas.
4. `Director`: Gerencia o processo de construção, garantindo que as etapas sejam chamadas na ordem correta.
5. Função `main`: Demonstra o uso do padrão Builder para construir diferentes tipos de casas, chamando o Director para gerenciar o processo e obtendo o produto final.
## Conclusão
O padrão Builder é uma ferramenta para construir objetos complexos de maneira incremental e flexível. Em Golang, a implementação deste padrão é direta e eficaz, permitindo a criação de sistemas modulares e fáceis de manter. Com o uso de interfaces e classes concretas, podemos centralizar a lógica de construção e simplificar a evolução do código à medida que novos requisitos surgem.
| rflpazini |
1,912,348 | Cracking the Code of Global Expansion: Your Ultimate Guide to International Consulting Services | Cracking the Code of Global Expansion: Your Ultimate Guide to International Consulting Services Are... | 0 | 2024-07-05T07:03:06 | https://dev.to/international_consulting/cracking-the-code-of-global-expansion-your-ultimate-guide-to-international-consulting-services-ndi | Cracking the Code of Global Expansion: Your Ultimate Guide to International Consulting Services
Are you dreaming of expanding your business beyond borders but feeling overwhelmed by the complexities of international markets? Don't worry, you're not alone. Many companies, from ambitious startups to established corporations, face similar challenges when venturing into new territories. That's where international consulting services come in, your trusty compass in the vast landscape of global expansion.
What are International Consulting Services?
Think of international consulting firms as your seasoned Sherpas, guiding you through the treacherous terrains of global business. They offer specialized services, from market research and entry strategies to regulatory compliance and cultural adaptation. Their expertise can differentiate between a successful international venture and a costly misstep.
Why Do You Need International Consulting Services?
In-Depth Market Knowledge: International consultants have their fingers on the pulse of global markets. They understand different regions' unique challenges and opportunities, helping you make informed decisions.
Tailored Strategies: There's no one-size-fits-all approach to international expansion. Consultants develop customized strategies that align with your business goals and specific market conditions.
Regulatory Compliance: Navigating a foreign market's legal and regulatory landscape can be a nightmare. Consultants ensure you stay on the right side of the law, avoiding costly fines and penalties.
Cultural Sensitivity: Understanding and respecting cultural differences is crucial for building successful business relationships overseas. Consultants help you bridge the cultural gap, ensuring smooth communication and collaboration.
Risk Mitigation: International business has inherent risks, such as political instability, economic fluctuations, and supply chain disruptions. Consultants help you identify and mitigate these risks, protecting your investments.
Operational Efficiency: Expanding internationally can be complex and resource-intensive. Consultants streamline your operations, optimize processes, and help you achieve maximum efficiency.
How to Choose the Right International Consulting Firm
Selecting the right consulting partner is as important as expanding internationally. Consider the following factors:
Expertise: Choose a firm with a proven track record in your industry and target markets.
Global Reach: Look for a firm with a worldwide network of offices and resources, ensuring comprehensive support wherever you expand.
Cultural Fit: Ensure the firm's values and work culture align with your own for a seamless collaboration.
Client-Centric Approach: Partner with a firm that prioritizes your business goals and tailors solutions to your needs.
The Bottom Line
Expanding your business internationally can be a daunting but rewarding endeavor. With the right international consulting partner by your side, you can confidently navigate the complexities, mitigate risks, and unlock the full potential of global markets. Remember, knowledge is power in international business, and the right consultants can be the key to unlocking your global success.
Are you ready to take your business to the next level? Contact us today to explore how we can help you achieve your global ambitions.[](https://int-consultants.com/)[](https://maps.app.goo.gl/Wp3w5cxjYfB7XAdUA)
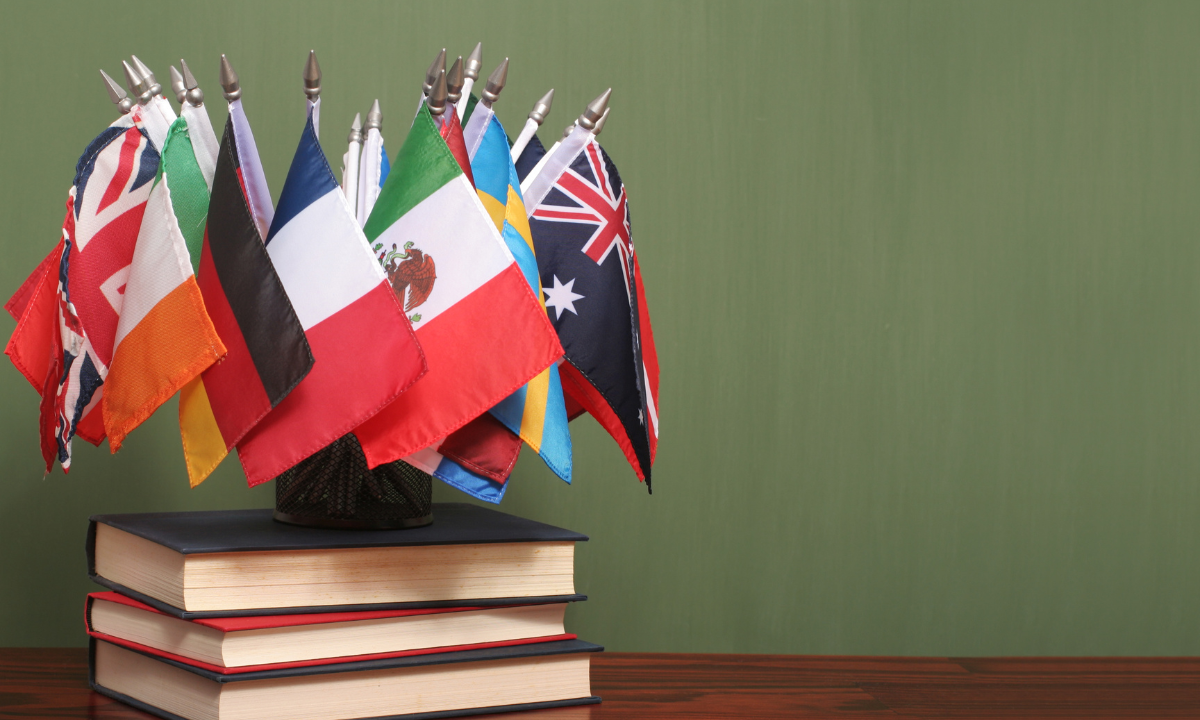
| international_consulting |
|
1,907,360 | Keep launching | You just launched your developer tool on Product Hunt. Bravo! There's two more things to keep the... | 27,917 | 2024-07-05T07:01:00 | https://dev.to/fmerian/keep-launching-4p93 | startup, developer, marketing, devjournal | You just launched your developer tool on Product Hunt. Bravo!
There's two more things to keep the momentum going:
1. Maximize efforts and,
2. Keep launching.
## Maximize efforts
With the assets you've worked on for your Product Hunt launch, you can maximize efforts by launching on more places, like Hacker News and Dev Hunt, an open-source alternative for dev-first products.
## Launch early, launch often
Launching on Product Hunt isn't a one-time opportunity. Launch early, launch often.
### You can pre-launch your product.
When submitting a new product on Product Hunt, you can mark it as pre-launch.
### You can launch multiple times.
Take Stripe. The company launched [68 times](https://www.producthunt.com/products/stripe/launches) on Product Hunt in the last 10 years. Supabase launched [12 times](https://www.producthunt.com/products/supabase/launches) in the last 4 years. Raycast has launched [11 products](https://www.producthunt.com/products/raycast/launches) since 2020.
**Launching multiple times creates a tailwind.**
— [Repost this](https://twitter.com/fmerian/status/1676554020348100610)
Luis Guzmán, Head of Marketing at n8n (launched [5 times](https://www.producthunt.com/products/n8n-io/launches) since 2019), sums it up perfectly:
> **Every follower we collected from past launches receives a notification about recent launches. This helps get more exposure.**
> — [Luis Guzmán](https://www.linkedin.com/in/guzmanluis/), Head of Marketing, n8n
So, keep launching. | fmerian |
1,912,347 | Taizhou Chengyan Houseware Co., Ltd: Your Trusted Source for Houseware | Here is the Best to Know Mould Manufactory_products offered by Taizhou Chengyan'_s houseware Taizhou... | 0 | 2024-07-05T06:59:40 | https://dev.to/ted_slunaisy_edbc0713b6/taizhou-chengyan-houseware-co-ltd-your-trusted-source-for-houseware-2037 | design | Here is the Best to Know Mould Manufactory_products offered by Taizhou Chengyan'_s houseware
Taizhou Chengyan Houseware Co., Ltd is a well-known company which produces good + quality home products and make your life easier with smart housewares. Comprising items like kitchen utensils, bathroom accessories and intelligent storage solutions designed to suit any lifestyle. All products are constructed with quality materials that will last and perform overtime, providing you peace of mind in your home.
Reliable And Attractive Housewares By Taizhou Chengyan
The recipient is Taizhou Chengyan, a company whose priority isn't just utility but style. They focus on developing houseware items that both looks great and are functional.axis of living)prepare gourmet food. It is essential for them to express themselves based on that, and so they offer an array of Desktop Storage designs as well colors which will suitably complement your home decor. Taizhou Chengyan is worth it regardless of whether you are searching for a gift or making an investment in your future.
The Solutions Smart Housewares from Taizhou Chengyan Organize Your Life
But Chengyan gets far beyond products only, they provide solutions that use your everyday and also enable you arrange at home better. With innovative kitchen helpers to practical bathroom solutions, they offer products that make your life easier. To ensure that your home feels clutter-free and clean, whether you are living in a tiny apartment or an expansive house (or something in-between), they have designed solutions for every corner of your home.
High-Quality Houseware from Taizhou Chengyan for Upgrading Home
Look no further than Taizhou Chengyan to fulfill your premium houseware needs if you want to upgrade the living space. Their catalog ranges from basic kitchen utilities to fashionable bathroom add-ons - all of which are intricately Bathroom Storage Series designed using the highest-grade materials. Whether it is sophistication that you wish to add in your bathroom or efficiency in your kitchen, Taizhou Chengyan has a wide range of options for both.
Pour That Molten Glas with Taizhou Chengyan Premium Houseware
Fed up with houseware items that let you down every time? Youfield, Taizhou Chengyan to provide you with premium enjoyment range of household Storage products designed for comfortable living. Stackable food storage containers to help with meal prep, or bathroom caddies that you can use for anything from shower essentials, keeping them close but out of the way. And thanks to the dedication towards practicality and quality, keeping tidy home is breeze -all of this with Taizhou Chengyan.
Conclusion
To sum up, is that Taizhou Chengyan Houseware Co., Ltd is your one-stop shop for tough and trendy houseware products. Their clever design solutions are for every nook and cranny of your house, so you can get over the room reno in a snap. Vanities to send out your things, cooking area basics-- you call it, Chengyan has actually gotten cleaned up! They are experts in making sure you can get everything houseware related from the same location, allowing for a relaxing and carefree calm to envelope your life. | ted_slunaisy_edbc0713b6 |
1,912,346 | The Invaluable Advantages of System Integration Testing (SIT) | Ensuring smooth integration between different components is crucial in the complex world of software... | 0 | 2024-07-05T06:59:14 | https://elephantsands.com/the-invaluable-advantages-of-system-integration-testing-sit/ | system, integration, testing | 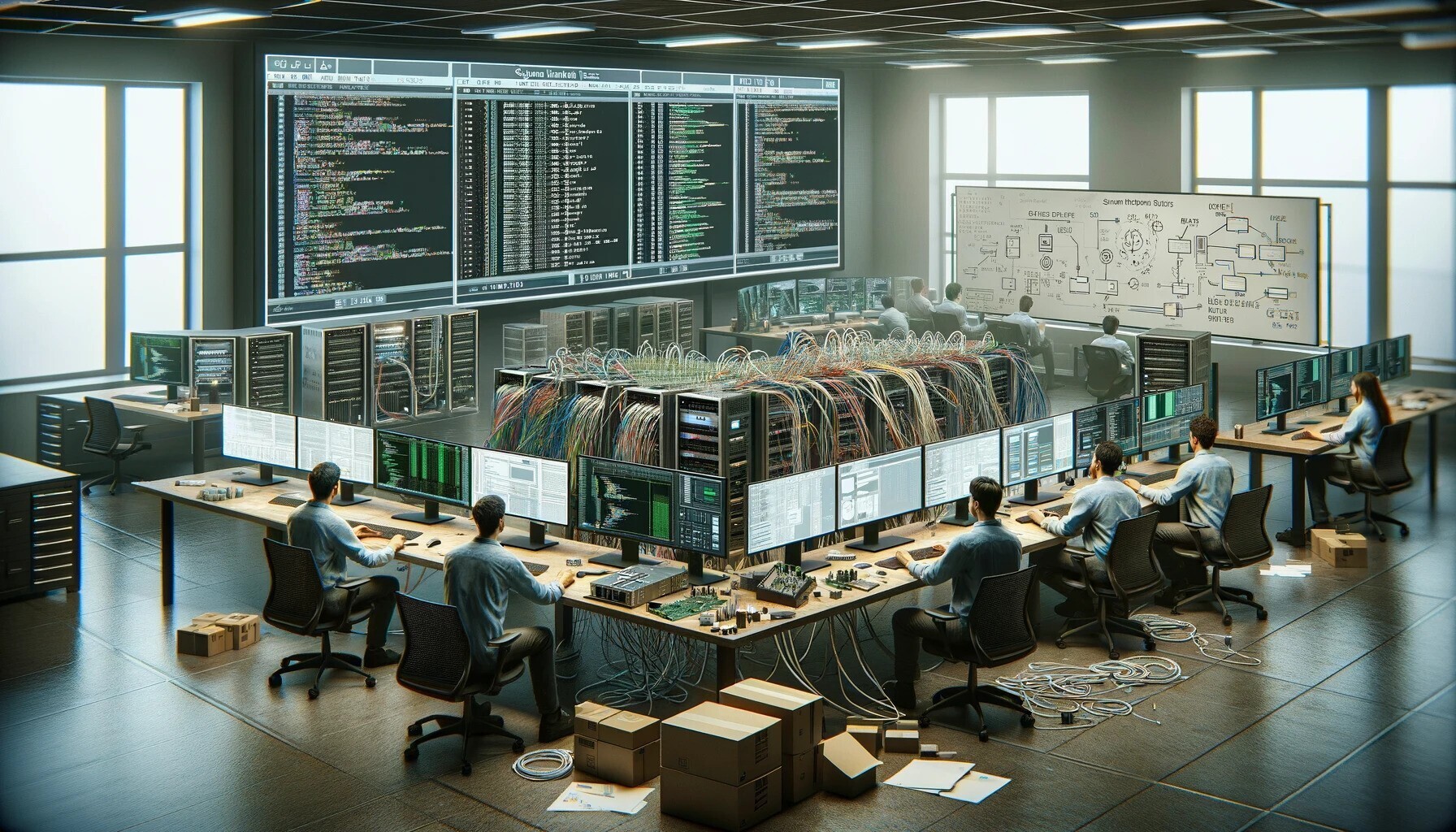
Ensuring smooth integration between different components is crucial in the complex world of software development that exists today. This is where SIT testing excels, providing a wealth of priceless advantages that improve software systems’ quality, effectiveness, and dependability. Let’s examine the top five benefits that render SIT a crucial procedure.
1. **Unveiling Integration Defects Early**
The capacity of SIT to identify integration flaws early on is one of its main benefits. The likelihood of integration problems grows as software systems get more intricate, involving numerous interconnected components and interfaces. SIT proactively finds these flaws before they worsen and end up requiring more time and resources to fix. Through the simulation of real-world scenarios and testing of the smooth integration of different modules, SIT helps developers identify and address problems early on.
2. **Ensuring End-to-End System Functionality**
SIT assesses the overall functionality of the system rather than just testing individual parts separately. By using a comprehensive approach, it is ensured that all parts function together and exchange data and information in an accurate and efficient manner. SIT confirms the software satisfies the requirements and provides the intended functionality by examining the proper data in addition to process flow throughout the integrated system. SIT also aids in locating possible bottlenecks, performance concerns, or compatibility issues that might develop from the interaction of various components.
3. **Fostering Collaboration and Communication**
SIT encourages cross-functional teams working on software development to collaborate and communicate with one another. Developers, testers, and subject matter experts must collaborate closely as various modules in addition to components are integrated. By creating a shared knowledge of the needs, expectations, and behavior of the system, SIT makes this collaboration easier. Team members can gain a better understanding of the complex dependencies and interactions between components by sharing knowledge and communicating on a regular basis.
4. **Mitigating Risks and Reducing Costs**
SIT is essential for risk mitigation and cost reduction because it finds and fixes integration defects early in the development lifecycle. Resolving problems later on, like after deployment or during user acceptance testing, can be much more costly and time-consuming. SIT serves as a preventative measure, identifying possible issues before they worsen, necessitating more thorough rework or expensive system failures. Moreover, SIT reduces the risks involved in integrating components created by various teams or vendors.
5. **Facilitating Continuous Integration and Delivery**
Continuous Integration along with Delivery (CI/CD) practices are now critical to delivering software quickly in addition to reliably in today’s agile development environments. Through the validation of the integration of updates and code changes from multiple sources, SIT is essential to the success of CI/CD processes. Teams are able to promptly detect integration problems brought about by fresh code commits or updates by routinely running SIT as part of the CI/CD pipeline.
**Final Thought**
System Integration Testing is a vital practice that offers numerous benefits to software development teams as well as organizations. With Opkey, organizations can streamline System Integration Testing (SIT) for seamless connectivity across applications and platforms. Opkey’s no-code integration platform enables comprehensive SIT, validating data synchronization and process workflows between disparate systems. Businesses can ensure their ecosystem operates as a cohesive whole with Opkey’s automated SIT capabilities, minimizing disruptions and maximizing operational efficiency. | rohitbhandari102 |
1,912,345 | Develop Full Stack Flight Booking System | Creating a full-stack flight booking system with Next.js for the front end and NestJS for the back... | 0 | 2024-07-05T06:58:06 | https://dev.to/nadim_ch0wdhury/develop-full-stack-flight-booking-system-o98 | Creating a full-stack flight booking system with Next.js for the front end and NestJS for the back end is a substantial project. Here are some key features and functionalities that such a system could include:
### User Features
1. **User Registration and Authentication:**
- Sign up, login, and logout.
- Password recovery/reset.
- Social media login (Google, Facebook, etc.).
2. **User Profile:**
- View and edit profile information.
- Upload profile picture.
3. **Flight Search and Booking:**
- Search flights by destination, date, and other criteria.
- View flight details (airline, departure/arrival time, duration, etc.).
- Filter and sort search results.
- Book selected flights.
- View and manage bookings.
- Receive booking confirmations via email.
4. **Payment Integration:**
- Integration with payment gateways (PayPal, Stripe, etc.).
- Secure payment processing.
- View payment history.
5. **Notifications:**
- Email and SMS notifications for booking confirmations, flight status updates, etc.
6. **Reviews and Ratings:**
- Rate and review airlines and flights.
- View reviews and ratings from other users.
### Admin Features
1. **Dashboard:**
- Overview of bookings, users, flights, and revenue.
2. **Flight Management:**
- Add, edit, and delete flights.
- Manage flight schedules and availability.
3. **User Management:**
- View and manage users.
- Assign roles (e.g., admin, customer support).
4. **Booking Management:**
- View and manage all bookings.
- Cancel or modify bookings.
5. **Reporting and Analytics:**
- Generate reports on bookings, revenue, user activity, etc.
- Analyze data to make informed decisions.
### Functionalities
1. **API Integration:**
- Integrate with third-party APIs for real-time flight data (e.g., Amadeus, Skyscanner).
2. **Security:**
- Implement authentication and authorization (JWT, OAuth).
- Secure data transmission (HTTPS).
3. **Performance Optimization:**
- Optimize database queries.
- Implement caching mechanisms.
- Use CDN for static assets.
4. **Scalability:**
- Design for horizontal scalability.
- Use microservices architecture if needed.
5. **Testing:**
- Unit and integration tests for back-end (NestJS).
- End-to-end tests for the front end (Next.js).
6. **Documentation:**
- API documentation (Swagger).
- User manuals and help sections.
### Optional Advanced Features
1. **Multi-language Support:**
- Provide interface and notifications in multiple languages.
2. **Loyalty Program:**
- Implement a points or rewards system for frequent flyers.
3. **Customer Support:**
- Live chat or chatbot integration.
- Ticketing system for support requests.
4. **Mobile App:**
- Develop a companion mobile app (using React Native or Flutter).
5. **Dynamic Pricing:**
- Implement algorithms for dynamic pricing based on demand, season, etc.
By incorporating these features and functionalities, you'll have a comprehensive flight booking system that caters to both users and administrators effectively.
To implement user registration and authentication features in a NestJS backend, you'll need to set up a few key components, including modules, controllers, services, and guards for handling authentication and authorization. Below is a step-by-step guide with sample code for implementing these features:
### 1. Setting Up the Project
First, create a new NestJS project if you haven't already:
```bash
nest new flight-booking-backend
cd flight-booking-backend
```
### 2. Install Required Packages
You'll need some additional packages for authentication:
```bash
npm install @nestjs/jwt @nestjs/passport passport passport-jwt bcryptjs
```
### 3. Create Auth Module
Generate the authentication module and related components:
```bash
nest generate module auth
nest generate service auth
nest generate controller auth
```
### 4. User Entity
Create a user entity to represent users in the database. Assuming you are using TypeORM, it would look something like this:
```typescript
// src/user/user.entity.ts
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ unique: true })
email: string;
@Column()
password: string;
@Column({ nullable: true })
googleId: string;
@Column({ nullable: true })
facebookId: string;
}
```
### 5. Auth Service
Implement the authentication logic in the AuthService:
```typescript
// src/auth/auth.service.ts
import { Injectable, UnauthorizedException } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import * as bcrypt from 'bcryptjs';
import { User } from '../user/user.entity';
import { JwtPayload } from './jwt-payload.interface';
import { AuthCredentialsDto } from './dto/auth-credentials.dto';
@Injectable()
export class AuthService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
private jwtService: JwtService,
) {}
async signUp(authCredentialsDto: AuthCredentialsDto): Promise<void> {
const { email, password } = authCredentialsDto;
const salt = await bcrypt.genSalt();
const hashedPassword = await bcrypt.hash(password, salt);
const user = this.userRepository.create({ email, password: hashedPassword });
await this.userRepository.save(user);
}
async validateUser(email: string, pass: string): Promise<any> {
const user = await this.userRepository.findOne({ email });
if (user && (await bcrypt.compare(pass, user.password))) {
const { password, ...result } = user;
return result;
}
return null;
}
async login(user: any) {
const payload: JwtPayload = { email: user.email, sub: user.id };
return {
access_token: this.jwtService.sign(payload),
};
}
}
```
### 6. Auth Controller
Create the controller to handle authentication requests:
```typescript
// src/auth/auth.controller.ts
import { Controller, Post, Body, UseGuards, Request } from '@nestjs/common';
import { AuthService } from './auth.service';
import { AuthCredentialsDto } from './dto/auth-credentials.dto';
import { JwtAuthGuard } from './jwt-auth.guard';
@Controller('auth')
export class AuthController {
constructor(private authService: AuthService) {}
@Post('/signup')
signUp(@Body() authCredentialsDto: AuthCredentialsDto): Promise<void> {
return this.authService.signUp(authCredentialsDto);
}
@Post('/login')
async login(@Body() authCredentialsDto: AuthCredentialsDto) {
const user = await this.authService.validateUser(authCredentialsDto.email, authCredentialsDto.password);
if (!user) {
throw new UnauthorizedException();
}
return this.authService.login(user);
}
}
```
### 7. DTOs
Define the DTOs (Data Transfer Objects) for authentication:
```typescript
// src/auth/dto/auth-credentials.dto.ts
export class AuthCredentialsDto {
email: string;
password: string;
}
```
### 8. JWT Strategy
Configure the JWT strategy for handling authentication:
```typescript
// src/auth/jwt.strategy.ts
import { Strategy, ExtractJwt } from 'passport-jwt';
import { PassportStrategy } from '@nestjs/passport';
import { Injectable, UnauthorizedException } from '@nestjs/common';
import { JwtPayload } from './jwt-payload.interface';
import { AuthService } from './auth.service';
import { ConfigService } from '@nestjs/config';
@Injectable()
export class JwtStrategy extends PassportStrategy(Strategy) {
constructor(
private authService: AuthService,
private configService: ConfigService,
) {
super({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
ignoreExpiration: false,
secretOrKey: configService.get<string>('JWT_SECRET'),
});
}
async validate(payload: JwtPayload) {
const user = await this.authService.validateUser(payload.email, null);
if (!user) {
throw new UnauthorizedException();
}
return user;
}
}
```
### 9. JWT Auth Guard
Create a guard to protect routes:
```typescript
// src/auth/jwt-auth.guard.ts
import { Injectable, ExecutionContext } from '@nestjs/common';
import { AuthGuard } from '@nestjs/passport';
@Injectable()
export class JwtAuthGuard extends AuthGuard('jwt') {
canActivate(context: ExecutionContext) {
return super.canActivate(context);
}
}
```
### 10. Auth Module Configuration
Configure the AuthModule to include necessary imports and providers:
```typescript
// src/auth/auth.module.ts
import { Module } from '@nestjs/common';
import { JwtModule } from '@nestjs/jwt';
import { PassportModule } from '@nestjs/passport';
import { TypeOrmModule } from '@nestjs/typeorm';
import { User } from '../user/user.entity';
import { AuthService } from './auth.service';
import { AuthController } from './auth.controller';
import { JwtStrategy } from './jwt.strategy';
import { ConfigModule, ConfigService } from '@nestjs/config';
@Module({
imports: [
ConfigModule,
TypeOrmModule.forFeature([User]),
PassportModule,
JwtModule.registerAsync({
imports: [ConfigModule],
inject: [ConfigService],
useFactory: async (configService: ConfigService) => ({
secret: configService.get<string>('JWT_SECRET'),
signOptions: { expiresIn: '60m' },
}),
}),
],
providers: [AuthService, JwtStrategy],
controllers: [AuthController],
})
export class AuthModule {}
```
### 11. Environment Configuration
Finally, add the JWT secret to your environment variables:
```bash
# .env
JWT_SECRET=your_jwt_secret_key
```
And configure TypeORM to connect to your database:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { AuthModule } from './auth/auth.module';
import { ConfigModule } from '@nestjs/config';
import { User } from './user/user.entity';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User],
synchronize: true,
}),
AuthModule,
],
})
export class AppModule {}
```
This setup should provide a robust foundation for user registration, login, and JWT-based authentication. You can expand on this by adding social login functionality, password reset, and other features as needed.
To implement user profile management, including viewing and editing profile information and uploading a profile picture, you'll need to extend the functionality of your existing NestJS application. Here's how to do it step by step:
### 1. Update User Entity
First, update the `User` entity to include fields for profile information and profile picture:
```typescript
// src/user/user.entity.ts
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column({ unique: true })
email: string;
@Column()
password: string;
@Column({ nullable: true })
firstName: string;
@Column({ nullable: true })
lastName: string;
@Column({ nullable: true })
profilePicture: string;
@Column({ nullable: true })
googleId: string;
@Column({ nullable: true })
facebookId: string;
}
```
### 2. Create User Service
Implement methods in the UserService to handle profile operations:
```typescript
// src/user/user.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import { UpdateProfileDto } from './dto/update-profile.dto';
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async getProfile(userId: number): Promise<User> {
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new NotFoundException(`User with ID ${userId} not found`);
}
return user;
}
async updateProfile(userId: number, updateProfileDto: UpdateProfileDto): Promise<User> {
await this.userRepository.update(userId, updateProfileDto);
return this.getProfile(userId);
}
async updateProfilePicture(userId: number, profilePicture: string): Promise<User> {
await this.userRepository.update(userId, { profilePicture });
return this.getProfile(userId);
}
}
```
### 3. Create DTOs
Define the DTOs for updating profile information:
```typescript
// src/user/dto/update-profile.dto.ts
export class UpdateProfileDto {
firstName?: string;
lastName?: string;
profilePicture?: string;
}
```
### 4. Create User Controller
Implement the UserController to handle profile-related requests:
```typescript
// src/user/user.controller.ts
import { Controller, Get, Patch, Body, Param, UseGuards, UploadedFile, UseInterceptors } from '@nestjs/common';
import { UserService } from './user.service';
import { UpdateProfileDto } from './dto/update-profile.dto';
import { JwtAuthGuard } from '../auth/jwt-auth.guard';
import { FileInterceptor } from '@nestjs/platform-express';
import { diskStorage } from 'multer';
import { extname } from 'path';
@Controller('user')
@UseGuards(JwtAuthGuard)
export class UserController {
constructor(private userService: UserService) {}
@Get(':id')
getProfile(@Param('id') id: string) {
return this.userService.getProfile(+id);
}
@Patch(':id')
updateProfile(@Param('id') id: string, @Body() updateProfileDto: UpdateProfileDto) {
return this.userService.updateProfile(+id, updateProfileDto);
}
@Patch(':id/profile-picture')
@UseInterceptors(
FileInterceptor('file', {
storage: diskStorage({
destination: './uploads/profile-pictures',
filename: (req, file, cb) => {
const uniqueSuffix = Date.now() + '-' + Math.round(Math.random() * 1e9);
const ext = extname(file.originalname);
cb(null, `${file.fieldname}-${uniqueSuffix}${ext}`);
},
}),
}),
)
uploadProfilePicture(@Param('id') id: string, @UploadedFile() file: Express.Multer.File) {
const profilePicture = `/uploads/profile-pictures/${file.filename}`;
return this.userService.updateProfilePicture(+id, profilePicture);
}
}
```
### 5. Update App Module
Ensure that the UserModule is imported in your AppModule and configure the `ServeStaticModule` for serving uploaded files:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { AuthModule } from './auth/auth.module';
import { ConfigModule } from '@nestjs/config';
import { UserModule } from './user/user.module';
import { User } from './user/user.entity';
import { ServeStaticModule } from '@nestjs/serve-static';
import { join } from 'path';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User],
synchronize: true,
}),
ServeStaticModule.forRoot({
rootPath: join(__dirname, '..', 'uploads'),
serveRoot: '/uploads',
}),
AuthModule,
UserModule,
],
})
export class AppModule {}
```
### 6. Create User Module
Finally, create the UserModule to tie everything together:
```typescript
// src/user/user.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { UserService } from './user.service';
import { UserController } from './user.controller';
import { User } from './user.entity';
@Module({
imports: [TypeOrmModule.forFeature([User])],
providers: [UserService],
controllers: [UserController],
exports: [UserService],
})
export class UserModule {}
```
With this setup, you have created endpoints for viewing and editing user profile information and uploading a profile picture. The profile pictures are saved to the `./uploads/profile-pictures` directory and served statically via the `/uploads` URL path.
To implement flight search and booking functionality in the backend using NestJS, we'll need to define entities, services, controllers, and DTOs. Here's a step-by-step guide:
### 1. Define Flight and Booking Entities
First, define the `Flight` and `Booking` entities:
```typescript
// src/flight/flight.entity.ts
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class Flight {
@PrimaryGeneratedColumn()
id: number;
@Column()
airline: string;
@Column()
from: string;
@Column()
to: string;
@Column()
departureTime: Date;
@Column()
arrivalTime: Date;
@Column('decimal')
price: number;
@Column()
duration: string;
}
```
```typescript
// src/booking/booking.entity.ts
import { Entity, Column, PrimaryGeneratedColumn, ManyToOne, JoinColumn } from 'typeorm';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Entity()
export class Booking {
@PrimaryGeneratedColumn()
id: number;
@ManyToOne(() => User, user => user.bookings)
@JoinColumn({ name: 'userId' })
user: User;
@ManyToOne(() => Flight)
@JoinColumn({ name: 'flightId' })
flight: Flight;
@Column()
bookingDate: Date;
@Column()
status: string;
}
```
### 2. Create DTOs
Define DTOs for creating bookings and searching flights:
```typescript
// src/flight/dto/search-flight.dto.ts
export class SearchFlightDto {
from: string;
to: string;
departureDate: Date;
returnDate?: Date;
}
// src/booking/dto/create-booking.dto.ts
export class CreateBookingDto {
flightId: number;
userId: number;
}
```
### 3. Create Services
Implement services for flight search and booking operations:
```typescript
// src/flight/flight.service.ts
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Flight } from './flight.entity';
import { SearchFlightDto } from './dto/search-flight.dto';
@Injectable()
export class FlightService {
constructor(
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
) {}
async searchFlights(searchFlightDto: SearchFlightDto): Promise<Flight[]> {
const { from, to, departureDate, returnDate } = searchFlightDto;
const query = this.flightRepository.createQueryBuilder('flight')
.where('flight.from = :from', { from })
.andWhere('flight.to = :to', { to })
.andWhere('DATE(flight.departureTime) = :departureDate', { departureDate });
if (returnDate) {
query.andWhere('DATE(flight.arrivalTime) = :returnDate', { returnDate });
}
return query.getMany();
}
async getFlightById(id: number): Promise<Flight> {
return this.flightRepository.findOne(id);
}
}
```
```typescript
// src/booking/booking.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Booking } from './booking.entity';
import { CreateBookingDto } from './dto/create-booking.dto';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Injectable()
export class BookingService {
constructor(
@InjectRepository(Booking)
private bookingRepository: Repository<Booking>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
) {}
async createBooking(createBookingDto: CreateBookingDto): Promise<Booking> {
const { flightId, userId } = createBookingDto;
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new NotFoundException(`User with ID ${userId} not found`);
}
const flight = await this.flightRepository.findOne(flightId);
if (!flight) {
throw new NotFoundException(`Flight with ID ${flightId} not found`);
}
const booking = this.bookingRepository.create({
user,
flight,
bookingDate: new Date(),
status: 'CONFIRMED',
});
await this.bookingRepository.save(booking);
// Add email confirmation logic here
return booking;
}
async getBookingsByUserId(userId: number): Promise<Booking[]> {
return this.bookingRepository.find({
where: { user: { id: userId } },
relations: ['flight'],
});
}
}
```
### 4. Create Controllers
Implement controllers to handle flight search and booking requests:
```typescript
// src/flight/flight.controller.ts
import { Controller, Get, Query, Param } from '@nestjs/common';
import { FlightService } from './flight.service';
import { SearchFlightDto } from './dto/search-flight.dto';
@Controller('flights')
export class FlightController {
constructor(private flightService: FlightService) {}
@Get()
searchFlights(@Query() searchFlightDto: SearchFlightDto) {
return this.flightService.searchFlights(searchFlightDto);
}
@Get(':id')
getFlightById(@Param('id') id: number) {
return this.flightService.getFlightById(id);
}
}
```
```typescript
// src/booking/booking.controller.ts
import { Controller, Post, Body, Get, Param, UseGuards } from '@nestjs/common';
import { BookingService } from './booking.service';
import { CreateBookingDto } from './dto/create-booking.dto';
import { JwtAuthGuard } from '../auth/jwt-auth.guard';
@Controller('bookings')
@UseGuards(JwtAuthGuard)
export class BookingController {
constructor(private bookingService: BookingService) {}
@Post()
createBooking(@Body() createBookingDto: CreateBookingDto) {
return this.bookingService.createBooking(createBookingDto);
}
@Get(':userId')
getBookingsByUserId(@Param('userId') userId: number) {
return this.bookingService.getBookingsByUserId(userId);
}
}
```
### 5. Update App Module
Ensure that the `FlightModule` and `BookingModule` are imported in your AppModule:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { ConfigModule } from '@nestjs/config';
import { AuthModule } from './auth/auth.module';
import { UserModule } from './user/user.module';
import { FlightModule } from './flight/flight.module';
import { BookingModule } from './booking/booking.module';
import { User } from './user/user.entity';
import { Flight } from './flight/flight.entity';
import { Booking } from './booking/booking.entity';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User, Flight, Booking],
synchronize: true,
}),
AuthModule,
UserModule,
FlightModule,
BookingModule,
],
})
export class AppModule {}
```
### 6. Create Flight and Booking Modules
Create the Flight and Booking modules to tie everything together:
```typescript
// src/flight/flight.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { FlightService } from './flight.service';
import { FlightController } from './flight.controller';
import { Flight } from './flight.entity';
@Module({
imports: [TypeOrmModule.forFeature([Flight])],
providers: [FlightService],
controllers: [FlightController],
exports: [FlightService],
})
export class FlightModule {}
```
```typescript
// src/booking/booking.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { BookingService } from './booking.service';
import { BookingController } from './booking.controller';
import { Booking } from './booking.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Module({
imports: [TypeOrmModule.forFeature([Booking, User, Flight])],
providers: [BookingService],
controllers: [BookingController],
exports: [BookingService],
})
export class BookingModule {}
```
### 7. Email Confirmation (Optional)
For email confirmation, you can use a package like `nodemailer`. Here’s an example of how to integrate it:
```typescript
// src/booking/booking.service.ts (inside createBooking method)
import * as nodemailer from 'nodemailer';
async function sendBookingConfirmation(email: string, booking: Booking) {
const transporter = nodemailer.createTransport({
host: 'smtp.example.com',
port: 587,
secure: false,
auth: {
user: '[email protected]',
pass: 'your_email_password',
},
});
const mailOptions = {
from: '[email protected]',
to: email,
subject:
'Booking Confirmation',
text: `Your booking is confirmed. Booking details: ${JSON.stringify(booking)}`,
};
await transporter.sendMail(mailOptions);
}
// Call this function after saving the booking
await this.bookingRepository.save(booking);
await sendBookingConfirmation(user.email, booking);
```
This setup should provide a comprehensive backend for flight search and booking functionalities. You can expand on this by adding more features like filtering, sorting, and better error handling as needed.
To integrate payment processing in a NestJS backend, you can use popular payment gateways like Stripe or PayPal. Below is an example of integrating Stripe for payment processing, secure payments, and viewing payment history.
### 1. Install Required Packages
First, install the Stripe package:
```bash
npm install @nestjs/common @nestjs/config stripe @nestjs/stripe
```
### 2. Configure Stripe
Add your Stripe API keys to your environment variables:
```bash
# .env
STRIPE_SECRET_KEY=your_stripe_secret_key
STRIPE_PUBLISHABLE_KEY=your_stripe_publishable_key
```
### 3. Create Payment Module
Generate the payment module and related components:
```bash
nest generate module payment
nest generate service payment
nest generate controller payment
```
### 4. Create Payment Entity
Define a `Payment` entity to store payment information:
```typescript
// src/payment/payment.entity.ts
import { Entity, Column, PrimaryGeneratedColumn, ManyToOne, JoinColumn } from 'typeorm';
import { User } from '../user/user.entity';
import { Booking } from '../booking/booking.entity';
@Entity()
export class Payment {
@PrimaryGeneratedColumn()
id: number;
@Column()
stripePaymentId: string;
@Column()
amount: number;
@Column()
currency: string;
@Column()
status: string;
@ManyToOne(() => User)
@JoinColumn({ name: 'userId' })
user: User;
@ManyToOne(() => Booking)
@JoinColumn({ name: 'bookingId' })
booking: Booking;
@Column()
createdAt: Date;
}
```
### 5. Create Payment Service
Implement methods in the PaymentService to handle payment processing and viewing payment history:
```typescript
// src/payment/payment.service.ts
import { Injectable, BadRequestException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Payment } from './payment.entity';
import { User } from '../user/user.entity';
import { Booking } from '../booking/booking.entity';
import { ConfigService } from '@nestjs/config';
import Stripe from 'stripe';
@Injectable()
export class PaymentService {
private stripe: Stripe;
constructor(
@InjectRepository(Payment)
private paymentRepository: Repository<Payment>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Booking)
private bookingRepository: Repository<Booking>,
private configService: ConfigService,
) {
this.stripe = new Stripe(this.configService.get<string>('STRIPE_SECRET_KEY'), {
apiVersion: '2020-08-27',
});
}
async processPayment(userId: number, bookingId: number, token: string): Promise<Payment> {
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new BadRequestException(`User with ID ${userId} not found`);
}
const booking = await this.bookingRepository.findOne(bookingId);
if (!booking) {
throw new BadRequestException(`Booking with ID ${bookingId} not found`);
}
const amount = 1000; // Amount in cents
const currency = 'usd';
const charge = await this.stripe.charges.create({
amount,
currency,
source: token,
description: `Payment for booking ${bookingId}`,
receipt_email: user.email,
});
const payment = this.paymentRepository.create({
stripePaymentId: charge.id,
amount,
currency,
status: charge.status,
user,
booking,
createdAt: new Date(),
});
return this.paymentRepository.save(payment);
}
async getPaymentHistory(userId: number): Promise<Payment[]> {
return this.paymentRepository.find({
where: { user: { id: userId } },
relations: ['booking'],
order: { createdAt: 'DESC' },
});
}
}
```
### 6. Create Payment Controller
Implement the PaymentController to handle payment-related requests:
```typescript
// src/payment/payment.controller.ts
import { Controller, Post, Body, Get, Param, UseGuards } from '@nestjs/common';
import { PaymentService } from './payment.service';
import { JwtAuthGuard } from '../auth/jwt-auth.guard';
@Controller('payments')
@UseGuards(JwtAuthGuard)
export class PaymentController {
constructor(private paymentService: PaymentService) {}
@Post('process')
async processPayment(@Body('userId') userId: number, @Body('bookingId') bookingId: number, @Body('token') token: string) {
return this.paymentService.processPayment(userId, bookingId, token);
}
@Get('history/:userId')
async getPaymentHistory(@Param('userId') userId: number) {
return this.paymentService.getPaymentHistory(userId);
}
}
```
### 7. Update App Module
Ensure that the `PaymentModule` is imported in your AppModule:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { ConfigModule } from '@nestjs/config';
import { AuthModule } from './auth/auth.module';
import { UserModule } from './user/user.module';
import { FlightModule } from './flight/flight.module';
import { BookingModule } from './booking/booking.module';
import { PaymentModule } from './payment/payment.module';
import { User } from './user/user.entity';
import { Flight } from './flight/flight.entity';
import { Booking } from './booking/booking.entity';
import { Payment } from './payment/payment.entity';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User, Flight, Booking, Payment],
synchronize: true,
}),
AuthModule,
UserModule,
FlightModule,
BookingModule,
PaymentModule,
],
})
export class AppModule {}
```
### 8. Create Payment Module
Create the PaymentModule to tie everything together:
```typescript
// src/payment/payment.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { PaymentService } from './payment.service';
import { PaymentController } from './payment.controller';
import { Payment } from './payment.entity';
import { User } from '../user/user.entity';
import { Booking } from '../booking/booking.entity';
import { ConfigModule } from '@nestjs/config';
@Module({
imports: [TypeOrmModule.forFeature([Payment, User, Booking]), ConfigModule],
providers: [PaymentService],
controllers: [PaymentController],
exports: [PaymentService],
})
export class PaymentModule {}
```
### 9. Stripe Payment Processing Frontend Integration (Optional)
For the frontend, you would typically use Stripe.js to collect payment details and generate a payment token, which is then sent to your backend API to process the payment. Here’s a brief example of how you might collect the token:
```html
<!-- Add this script to your HTML -->
<script src="https://js.stripe.com/v3/"></script>
```
```javascript
// Example frontend code to get the Stripe token
const stripe = Stripe('your_stripe_publishable_key');
const handlePayment = async () => {
const { token } = await stripe.createToken(cardElement); // cardElement is a reference to the card input field
// Send token to your backend
const response = await fetch('/payments/process', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
userId: 1,
bookingId: 1,
token: token.id,
}),
});
const result = await response.json();
console.log(result);
};
```
With this setup, you should have a fully functional payment processing system integrated with Stripe, along with secure payment handling and payment history viewing capabilities in your NestJS backend.
To implement email and SMS notifications for booking confirmations, flight status updates, and other notifications in a NestJS backend, we need to integrate with email and SMS service providers. We'll use Nodemailer for email notifications and Twilio for SMS notifications.
### 1. Install Required Packages
First, install the necessary packages:
```bash
npm install @nestjs/common @nestjs/config nodemailer twilio
```
### 2. Configure Environment Variables
Add your Twilio and email SMTP service credentials to your environment variables:
```bash
# .env
SMTP_HOST=smtp.example.com
SMTP_PORT=587
[email protected]
SMTP_PASS=your_email_password
TWILIO_ACCOUNT_SID=your_twilio_account_sid
TWILIO_AUTH_TOKEN=your_twilio_auth_token
TWILIO_PHONE_NUMBER=your_twilio_phone_number
```
### 3. Create Notification Module
Generate the notification module and related components:
```bash
nest generate module notification
nest generate service notification
nest generate controller notification
```
### 4. Create Notification Service
Implement methods in the NotificationService to handle email and SMS notifications:
```typescript
// src/notification/notification.service.ts
import { Injectable } from '@nestjs/common';
import { ConfigService } from '@nestjs/config';
import * as nodemailer from 'nodemailer';
import { Twilio } from 'twilio';
@Injectable()
export class NotificationService {
private transporter: nodemailer.Transporter;
private twilioClient: Twilio;
constructor(private configService: ConfigService) {
this.transporter = nodemailer.createTransport({
host: this.configService.get<string>('SMTP_HOST'),
port: this.configService.get<number>('SMTP_PORT'),
secure: false,
auth: {
user: this.configService.get<string>('SMTP_USER'),
pass: this.configService.get<string>('SMTP_PASS'),
},
});
this.twilioClient = new Twilio(
this.configService.get<string>('TWILIO_ACCOUNT_SID'),
this.configService.get<string>('TWILIO_AUTH_TOKEN'),
);
}
async sendEmail(to: string, subject: string, text: string, html?: string) {
const mailOptions = {
from: this.configService.get<string>('SMTP_USER'),
to,
subject,
text,
html,
};
await this.transporter.sendMail(mailOptions);
}
async sendSms(to: string, body: string) {
await this.twilioClient.messages.create({
body,
from: this.configService.get<string>('TWILIO_PHONE_NUMBER'),
to,
});
}
async sendBookingConfirmationEmail(userEmail: string, bookingDetails: any) {
const subject = 'Booking Confirmation';
const text = `Your booking is confirmed. Booking details: ${JSON.stringify(bookingDetails)}`;
await this.sendEmail(userEmail, subject, text);
}
async sendBookingConfirmationSms(userPhone: string, bookingDetails: any) {
const body = `Your booking is confirmed. Booking details: ${JSON.stringify(bookingDetails)}`;
await this.sendSms(userPhone, body);
}
// Add more methods for other types of notifications as needed
}
```
### 5. Integrate Notification Service in Booking Service
Update the `BookingService` to send notifications upon booking confirmation:
```typescript
// src/booking/booking.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Booking } from './booking.entity';
import { CreateBookingDto } from './dto/create-booking.dto';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
import { NotificationService } from '../notification/notification.service';
@Injectable()
export class BookingService {
constructor(
@InjectRepository(Booking)
private bookingRepository: Repository<Booking>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
private notificationService: NotificationService,
) {}
async createBooking(createBookingDto: CreateBookingDto): Promise<Booking> {
const { flightId, userId } = createBookingDto;
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new NotFoundException(`User with ID ${userId} not found`);
}
const flight = await this.flightRepository.findOne(flightId);
if (!flight) {
throw new NotFoundException(`Flight with ID ${flightId} not found`);
}
const booking = this.bookingRepository.create({
user,
flight,
bookingDate: new Date(),
status: 'CONFIRMED',
});
await this.bookingRepository.save(booking);
// Send notifications
await this.notificationService.sendBookingConfirmationEmail(user.email, booking);
if (user.phone) {
await this.notificationService.sendBookingConfirmationSms(user.phone, booking);
}
return booking;
}
async getBookingsByUserId(userId: number): Promise<Booking[]> {
return this.bookingRepository.find({
where: { user: { id: userId } },
relations: ['flight'],
});
}
}
```
### 6. Update App Module
Ensure that the `NotificationModule` is imported in your AppModule:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { ConfigModule } from '@nestjs/config';
import { AuthModule } from './auth/auth.module';
import { UserModule } from './user/user.module';
import { FlightModule } from './flight/flight.module';
import { BookingModule } from './booking/booking.module';
import { PaymentModule } from './payment/payment.module';
import { NotificationModule } from './notification/notification.module';
import { User } from './user/user.entity';
import { Flight } from './flight/flight.entity';
import { Booking } from './booking/booking.entity';
import { Payment } from './payment/payment.entity';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User, Flight, Booking, Payment],
synchronize: true,
}),
AuthModule,
UserModule,
FlightModule,
BookingModule,
PaymentModule,
NotificationModule,
],
})
export class AppModule {}
```
### 7. Create Notification Module
Create the NotificationModule to tie everything together:
```typescript
// src/notification/notification.module.ts
import { Module } from '@nestjs/common';
import { ConfigModule } from '@nestjs/config';
import { NotificationService } from './notification.service';
@Module({
imports: [ConfigModule],
providers: [NotificationService],
exports: [NotificationService],
})
export class NotificationModule {}
```
This setup should provide a robust notification system that sends email and SMS notifications for booking confirmations and other updates. You can expand this by adding more notification types and integrating with additional email/SMS providers if needed.
To implement a reviews and ratings feature for airlines and flights in your NestJS backend, we need to create entities for reviews and ratings, services to handle the business logic, and controllers to expose the endpoints. Here’s how you can do it:
### 1. Create Review and Rating Entities
Define the `Review` and `Rating` entities. These will store the reviews and ratings given by users to flights.
```typescript
// src/review/review.entity.ts
import { Entity, Column, PrimaryGeneratedColumn, ManyToOne, JoinColumn, CreateDateColumn } from 'typeorm';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Entity()
export class Review {
@PrimaryGeneratedColumn()
id: number;
@Column()
content: string;
@CreateDateColumn()
createdAt: Date;
@ManyToOne(() => User)
@JoinColumn({ name: 'userId' })
user: User;
@ManyToOne(() => Flight)
@JoinColumn({ name: 'flightId' })
flight: Flight;
}
// src/rating/rating.entity.ts
import { Entity, Column, PrimaryGeneratedColumn, ManyToOne, JoinColumn, CreateDateColumn } from 'typeorm';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Entity()
export class Rating {
@PrimaryGeneratedColumn()
id: number;
@Column()
score: number;
@CreateDateColumn()
createdAt: Date;
@ManyToOne(() => User)
@JoinColumn({ name: 'userId' })
user: User;
@ManyToOne(() => Flight)
@JoinColumn({ name: 'flightId' })
flight: Flight;
}
```
### 2. Create Review and Rating Modules
Generate the review and rating modules and services:
```bash
nest generate module review
nest generate service review
nest generate controller review
nest generate module rating
nest generate service rating
nest generate controller rating
```
### 3. Create Review Service
Implement methods in the `ReviewService` to handle adding and viewing reviews:
```typescript
// src/review/review.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Review } from './review.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
import { CreateReviewDto } from './dto/create-review.dto';
@Injectable()
export class ReviewService {
constructor(
@InjectRepository(Review)
private reviewRepository: Repository<Review>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
) {}
async addReview(createReviewDto: CreateReviewDto): Promise<Review> {
const { userId, flightId, content } = createReviewDto;
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new NotFoundException(`User with ID ${userId} not found`);
}
const flight = await this.flightRepository.findOne(flightId);
if (!flight) {
throw new NotFoundException(`Flight with ID ${flightId} not found`);
}
const review = this.reviewRepository.create({
content,
user,
flight,
createdAt: new Date(),
});
return this.reviewRepository.save(review);
}
async getReviewsByFlight(flightId: number): Promise<Review[]> {
return this.reviewRepository.find({
where: { flight: { id: flightId } },
relations: ['user'],
order: { createdAt: 'DESC' },
});
}
}
```
### 4. Create Rating Service
Implement methods in the `RatingService` to handle adding and viewing ratings:
```typescript
// src/rating/rating.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Rating } from './rating.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
import { CreateRatingDto } from './dto/create-rating.dto';
@Injectable()
export class RatingService {
constructor(
@InjectRepository(Rating)
private ratingRepository: Repository<Rating>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
) {}
async addRating(createRatingDto: CreateRatingDto): Promise<Rating> {
const { userId, flightId, score } = createRatingDto;
const user = await this.userRepository.findOne(userId);
if (!user) {
throw new NotFoundException(`User with ID ${userId} not found`);
}
const flight = await this.flightRepository.findOne(flightId);
if (!flight) {
throw new NotFoundException(`Flight with ID ${flightId} not found`);
}
const rating = this.ratingRepository.create({
score,
user,
flight,
createdAt: new Date(),
});
return this.ratingRepository.save(rating);
}
async getRatingsByFlight(flightId: number): Promise<Rating[]> {
return this.ratingRepository.find({
where: { flight: { id: flightId } },
relations: ['user'],
order: { createdAt: 'DESC' },
});
}
}
```
### 5. Create Review and Rating DTOs
Define DTOs for creating reviews and ratings:
```typescript
// src/review/dto/create-review.dto.ts
export class CreateReviewDto {
userId: number;
flightId: number;
content: string;
}
// src/rating/dto/create-rating.dto.ts
export class CreateRatingDto {
userId: number;
flightId: number;
score: number;
}
```
### 6. Create Review and Rating Controllers
Implement the `ReviewController` and `RatingController` to expose the endpoints:
```typescript
// src/review/review.controller.ts
import { Controller, Post, Body, Get, Param, UseGuards } from '@nestjs/common';
import { ReviewService } from './review.service';
import { CreateReviewDto } from './dto/create-review.dto';
import { JwtAuthGuard } from '../auth/jwt-auth.guard';
@Controller('reviews')
@UseGuards(JwtAuthGuard)
export class ReviewController {
constructor(private reviewService: ReviewService) {}
@Post()
async addReview(@Body() createReviewDto: CreateReviewDto) {
return this.reviewService.addReview(createReviewDto);
}
@Get('flight/:flightId')
async getReviewsByFlight(@Param('flightId') flightId: number) {
return this.reviewService.getReviewsByFlight(flightId);
}
}
// src/rating/rating.controller.ts
import { Controller, Post, Body, Get, Param, UseGuards } from '@nestjs/common';
import { RatingService } from './rating.service';
import { CreateRatingDto } from './dto/create-rating.dto';
import { JwtAuthGuard } from '../auth/jwt-auth.guard';
@Controller('ratings')
@UseGuards(JwtAuthGuard)
export class RatingController {
constructor(private ratingService: RatingService) {}
@Post()
async addRating(@Body() createRatingDto: CreateRatingDto) {
return this.ratingService.addRating(createRatingDto);
}
@Get('flight/:flightId')
async getRatingsByFlight(@Param('flightId') flightId: number) {
return this.ratingService.getRatingsByFlight(flightId);
}
}
```
### 7. Update App Module
Ensure that the `ReviewModule` and `RatingModule` are imported in your AppModule:
```typescript
// src/app.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { ConfigModule } from '@nestjs/config';
import { AuthModule } from './auth/auth.module';
import { UserModule } from './user/user.module';
import { FlightModule } from './flight/flight.module';
import { BookingModule } from './booking/booking.module';
import { PaymentModule } from './payment/payment.module';
import { NotificationModule } from './notification/notification.module';
import { ReviewModule } from './review/review.module';
import { RatingModule } from './rating/rating.module';
import { User } from './user/user.entity';
import { Flight } from './flight/flight.entity';
import { Booking } from './booking/booking.entity';
import { Payment } from './payment/payment.entity';
import { Review } from './review/review.entity';
import { Rating } from './rating/rating.entity';
@Module({
imports: [
ConfigModule.forRoot(),
TypeOrmModule.forRoot({
type: 'postgres',
host: process.env.DATABASE_HOST,
port: parseInt(process.env.DATABASE_PORT, 10),
username: process.env.DATABASE_USERNAME,
password: process.env.DATABASE_PASSWORD,
database: process.env.DATABASE_NAME,
entities: [User, Flight, Booking, Payment, Review, Rating],
synchronize: true,
}),
AuthModule,
UserModule,
FlightModule,
BookingModule,
PaymentModule,
NotificationModule,
ReviewModule,
RatingModule,
],
})
export class AppModule {}
```
### 8. Create Review and Rating Modules
Create the ReviewModule and RatingModule to tie everything together:
```typescript
// src/review
/review.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { ReviewService } from './review.service';
import { ReviewController } from './review.controller';
import { Review } from './review.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Module({
imports: [TypeOrmModule.forFeature([Review, User, Flight])],
providers: [ReviewService],
controllers: [ReviewController],
})
export class ReviewModule {}
// src/rating/rating.module.ts
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { RatingService } from './rating.service';
import { RatingController } from './rating.controller';
import { Rating } from './rating.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
@Module({
imports: [TypeOrmModule.forFeature([Rating, User, Flight])],
providers: [RatingService],
controllers: [RatingController],
})
export class RatingModule {}
```
This setup should provide a comprehensive review and rating system for flights. You can expand this by adding more fields, validation, and additional features as needed.
To implement admin features such as a dashboard, flight management, and user management in your NestJS backend, you need to create the necessary entities, services, controllers, and guards for authorization. Here’s how you can do it:
### 1. Install Required Packages
Make sure you have the necessary packages installed:
```bash
npm install @nestjs/common @nestjs/config @nestjs/typeorm @nestjs/jwt bcryptjs
```
### 2. Create Admin Guard
Create a guard to protect routes that only admins should access:
```typescript
// src/auth/roles.guard.ts
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { Reflector } from '@nestjs/core';
import { JwtService } from '@nestjs/jwt';
@Injectable()
export class RolesGuard implements CanActivate {
constructor(private reflector: Reflector, private jwtService: JwtService) {}
canActivate(context: ExecutionContext): boolean {
const requiredRoles = this.reflector.getAllAndOverride<string[]>('roles', [
context.getHandler(),
context.getClass(),
]);
if (!requiredRoles) {
return true;
}
const request = context.switchToHttp().getRequest();
const token = request.headers.authorization?.split(' ')[1];
if (!token) {
return false;
}
const user = this.jwtService.decode(token);
return requiredRoles.some((role) => user['roles']?.includes(role));
}
}
```
Add a decorator to set roles on routes:
```typescript
// src/auth/roles.decorator.ts
import { SetMetadata } from '@nestjs/common';
export const Roles = (...roles: string[]) => SetMetadata('roles', roles);
```
### 3. Create Dashboard Service
Implement methods in the `DashboardService` to fetch booking, user, flight, and revenue data:
```typescript
// src/admin/dashboard.service.ts
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Booking } from '../booking/booking.entity';
import { User } from '../user/user.entity';
import { Flight } from '../flight/flight.entity';
import { Payment } from '../payment/payment.entity';
@Injectable()
export class DashboardService {
constructor(
@InjectRepository(Booking)
private bookingRepository: Repository<Booking>,
@InjectRepository(User)
private userRepository: Repository<User>,
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
@InjectRepository(Payment)
private paymentRepository: Repository<Payment>,
) {}
async getOverview() {
const totalBookings = await this.bookingRepository.count();
const totalUsers = await this.userRepository.count();
const totalFlights = await this.flightRepository.count();
const totalRevenue = await this.paymentRepository
.createQueryBuilder('payment')
.select('SUM(payment.amount)', 'sum')
.getRawOne();
return {
totalBookings,
totalUsers,
totalFlights,
totalRevenue: totalRevenue.sum || 0,
};
}
}
```
### 4. Create Flight Management Service
Implement methods in the `FlightService` to handle adding, editing, and deleting flights:
```typescript
// src/flight/flight.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Flight } from './flight.entity';
import { CreateFlightDto } from './dto/create-flight.dto';
import { UpdateFlightDto } from './dto/update-flight.dto';
@Injectable()
export class FlightService {
constructor(
@InjectRepository(Flight)
private flightRepository: Repository<Flight>,
) {}
async createFlight(createFlightDto: CreateFlightDto): Promise<Flight> {
const flight = this.flightRepository.create(createFlightDto);
return this.flightRepository.save(flight);
}
async updateFlight(id: number, updateFlightDto: UpdateFlightDto): Promise<Flight> {
const flight = await this.flightRepository.preload({
id,
...updateFlightDto,
});
if (!flight) {
throw new NotFoundException(`Flight with ID ${id} not found`);
}
return this.flightRepository.save(flight);
}
async deleteFlight(id: number): Promise<void> {
const result = await this.flightRepository.delete(id);
if (result.affected === 0) {
throw new NotFoundException(`Flight with ID ${id} not found`);
}
}
async getFlights(): Promise<Flight[]> {
return this.flightRepository.find();
}
}
```
### 5. Create User Management Service
Implement methods in the `UserService` to handle viewing and managing users, and assigning roles:
```typescript
// src/user/user.service.ts
import { Injectable, NotFoundException } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { User } from './user.entity';
import { UpdateUserDto } from './dto/update-user.dto';
@Injectable()
export class UserService {
constructor(
@InjectRepository(User)
private userRepository: Repository<User>,
) {}
async getUsers(): Promise<User[]> {
return this.userRepository.find();
}
async updateUser(id: number, updateUserDto: UpdateUserDto): Promise<User> {
const user = await this.userRepository.preload({
id,
...updateUserDto,
});
if (!user) {
throw new NotFoundException(`User with ID ${id} not found`);
}
return this.userRepository.save(user);
}
async deleteUser(id: number): Promise<void> {
const result = await this.userRepository.delete(id);
if (result.affected === 0) {
throw new NotFoundException(`User with ID ${id} not found`);
}
}
}
```
### 6. Create DTOs
Define DTOs for creating and updating flights and users:
```typescript
// src/flight/dto/create-flight.dto.ts
export class CreateFlightDto {
airline: string;
departure: string;
arrival: string;
departureTime: Date;
arrivalTime: Date;
price: number;
availability: number;
}
// src/flight/dto/update-flight.dto.ts
import { PartialType } from '@nestjs/mapped-types';
import { CreateFlightDto } from './create-flight.dto';
export class UpdateFlightDto extends PartialType(CreateFlightDto) {}
// src/user/dto/update-user.dto.ts
export class UpdateUserDto {
username?: string;
email?: string;
roles?: string[];
}
```
### 7. Create Admin Controllers
Implement the `AdminController` to expose the endpoints for the dashboard, flight management, and user management:
```typescript
// src/admin/admin.controller.ts
import { Controller, Get, Post, Put, Delete, Body, Param, UseGuards } from '@nestjs/common';
import { DashboardService } from './dashboard.service';
import { FlightService } from '../flight/flight.service';
import { UserService } from '../user/user.service';
import { CreateFlightDto } from '../flight/dto/create-flight.dto';
import { UpdateFlightDto } from '../flight/dto/update-flight.dto';
import { UpdateUserDto } from '../user/dto/update-user.dto';
import { RolesGuard } from '../auth/roles.guard';
import { Roles } from '../auth/roles.decorator';
@Controller('admin')
@UseGuards(RolesGuard)
@Roles('admin')
export class AdminController {
constructor(
private dashboardService: DashboardService,
private flightService: FlightService,
private userService: UserService,
) {}
@Get('dashboard')
async getDashboard() {
return this.dashboardService.getOverview();
}
@Get('flights')
async getFlights() {
return this.flightService.getFlights();
}
@Post('flights')
async createFlight(@Body() createFlightDto: CreateFlightDto) {
return this.flightService.createFlight(createFlightDto);
}
@Put('flights/:id')
async updateFlight(@Param('id') id: number, @Body() updateFlightDto: UpdateFlightDto) {
return this.flightService.updateFlight(id, updateFlightDto);
}
@Delete('flights/:id')
async deleteFlight(@Param('id') id: number) {
return this.flightService.deleteFlight(id);
}
@Get('users')
async getUsers() {
return this.userService.getUsers();
}
@Put('users/:id')
async updateUser(@Param('id') id: number, @Body() updateUserDto: UpdateUserDto) {
return this.userService.updateUser(id, updateUserDto);
}
@Delete('users/:id')
async deleteUser(@Param('id') id: number) {
return this.userService.deleteUser(id);
}
}
```
### 8. Update App Module
Ensure that the `AdminModule`, `FlightModule`, and `UserModule` are imported in your AppModule:
Creating a backend for a full-stack flight booking system using NestJS involves several components. Below is a step-by-step guide with code snippets for each feature you listed. This guide assumes you have a basic understanding of NestJS and TypeScript.
1. **Initialize NestJS Project**
First, create a new NestJS project:
```bash
nest new flight-booking-backend
cd flight-booking-backend
```
2. **Install Required Packages**
Install the necessary packages:
```bash
npm install @nestjs/typeorm typeorm mysql @nestjs/passport passport passport-local passport-jwt bcryptjs
npm install @nestjs/jwt
```
3. **Set Up Database Connection**
Configure TypeORM in your `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { User } from './users/user.entity';
import { Flight } from './flights/flight.entity';
import { Booking } from './bookings/booking.entity';
@Module({
imports: [
TypeOrmModule.forRoot({
type: 'mysql',
host: 'localhost',
port: 3306,
username: 'root',
password: 'password',
database: 'flight_booking',
entities: [User, Flight, Booking],
synchronize: true,
}),
TypeOrmModule.forFeature([User, Flight, Booking]),
],
})
export class AppModule {}
```
4. **User Registration and Authentication**
Create a user entity and authentication module.
**user.entity.ts**
```typescript
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
username: string;
@Column()
email: string;
@Column()
password: string;
@Column({ default: 'user' })
role: string;
}
```
**auth.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { JwtModule } from '@nestjs/jwt';
import { PassportModule } from '@nestjs/passport';
import { AuthService } from './auth.service';
import { JwtStrategy } from './jwt.strategy';
import { UsersModule } from '../users/users.module';
@Module({
imports: [
PassportModule,
JwtModule.register({
secret: 'secretKey',
signOptions: { expiresIn: '1h' },
}),
UsersModule,
],
providers: [AuthService, JwtStrategy],
exports: [AuthService],
})
export class AuthModule {}
```
**auth.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
import * as bcrypt from 'bcryptjs';
import { UsersService } from '../users/users.service';
@Injectable()
export class AuthService {
constructor(
private readonly usersService: UsersService,
private readonly jwtService: JwtService,
) {}
async validateUser(username: string, password: string): Promise<any> {
const user = await this.usersService.findOne(username);
if (user && bcrypt.compareSync(password, user.password)) {
const { password, ...result } = user;
return result;
}
return null;
}
async login(user: any) {
const payload = { username: user.username, sub: user.id, role: user.role };
return {
access_token: this.jwtService.sign(payload),
};
}
async register(user: any) {
const hashedPassword = bcrypt.hashSync(user.password, 8);
return this.usersService.create({ ...user, password: hashedPassword });
}
}
```
**jwt.strategy.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PassportStrategy } from '@nestjs/passport';
import { ExtractJwt, Strategy } from 'passport-jwt';
@Injectable()
export class JwtStrategy extends PassportStrategy(Strategy) {
constructor() {
super({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
ignoreExpiration: false,
secretOrKey: 'secretKey',
});
}
async validate(payload: any) {
return { userId: payload.sub, username: payload.username, role: payload.role };
}
}
```
5. **Flight Search and Booking**
Create flight and booking entities and their respective modules and services.
**flight.entity.ts**
```typescript
import { Entity, Column, PrimaryGeneratedColumn } from 'typeorm';
@Entity()
export class Flight {
@PrimaryGeneratedColumn()
id: number;
@Column()
airline: string;
@Column()
departureTime: Date;
@Column()
arrivalTime: Date;
@Column()
from: string;
@Column()
to: string;
@Column()
price: number;
@Column()
seatsAvailable: number;
}
```
**flight.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { Flight } from './flight.entity';
import { FlightService } from './flight.service';
import { FlightController } from './flight.controller';
@Module({
imports: [TypeOrmModule.forFeature([Flight])],
providers: [FlightService],
controllers: [FlightController],
})
export class FlightModule {}
```
**flight.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Flight } from './flight.entity';
@Injectable()
export class FlightService {
constructor(
@InjectRepository(Flight)
private readonly flightRepository: Repository<Flight>,
) {}
findAll(): Promise<Flight[]> {
return this.flightRepository.find();
}
findOne(id: number): Promise<Flight> {
return this.flightRepository.findOne(id);
}
create(flight: Flight): Promise<Flight> {
return this.flightRepository.save(flight);
}
update(id: number, flight: Flight): Promise<any> {
return this.flightRepository.update(id, flight);
}
delete(id: number): Promise<any> {
return this.flightRepository.delete(id);
}
}
```
**flight.controller.ts**
```typescript
import { Controller, Get, Post, Put, Delete, Body, Param } from '@nestjs/common';
import { FlightService } from './flight.service';
import { Flight } from './flight.entity';
@Controller('flights')
export class FlightController {
constructor(private readonly flightService: FlightService) {}
@Get()
findAll(): Promise<Flight[]> {
return this.flightService.findAll();
}
@Get(':id')
findOne(@Param('id') id: number): Promise<Flight> {
return this.flightService.findOne(id);
}
@Post()
create(@Body() flight: Flight): Promise<Flight> {
return this.flightService.create(flight);
}
@Put(':id')
update(@Param('id') id: number, @Body() flight: Flight): Promise<any> {
return this.flightService.update(id, flight);
}
@Delete(':id')
delete(@Param('id') id: number): Promise<any> {
return this.flightService.delete(id);
}
}
```
**booking.entity.ts**
```typescript
import { Entity, Column, PrimaryGeneratedColumn, ManyToOne } from 'typeorm';
import { User } from '../users/user.entity';
import { Flight } from '../flights/flight.entity';
@Entity()
export class Booking {
@PrimaryGeneratedColumn()
id: number;
@ManyToOne(() => User)
user: User;
@ManyToOne(() => Flight)
flight: Flight;
@Column()
status: string;
@Column()
bookingDate: Date;
}
```
**booking.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { TypeOrmModule } from '@nestjs/typeorm';
import { Booking } from './booking.entity';
import { BookingService } from './booking.service';
import { BookingController } from './booking.controller';
@Module({
imports: [TypeOrmModule.forFeature([Booking])],
providers: [BookingService],
controllers: [BookingController],
})
export class BookingModule {}
```
**booking.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { InjectRepository } from '@nestjs/typeorm';
import { Repository } from 'typeorm';
import { Booking } from './booking.entity';
@Injectable()
export class BookingService {
constructor(
@InjectRepository(Booking)
private readonly bookingRepository: Repository<Booking>,
) {}
findAll(): Promise<Booking[]> {
return this.bookingRepository.find({ relations: ['user', 'flight'] });
}
findOne(id: number): Promise<Booking> {
return this.bookingRepository.findOne(id, { relations: ['user', 'flight'] });
}
create(booking: Booking): Promise<Booking> {
return this.bookingRepository.save(booking);
}
update
(id: number, booking: Booking): Promise<any> {
return this.bookingRepository.update(id, booking);
}
delete(id: number): Promise<any> {
return this.bookingRepository.delete(id);
}
}
```
**booking.controller.ts**
```typescript
import { Controller, Get, Post, Put, Delete, Body, Param } from '@nestjs/common';
import { BookingService } from './booking.service';
import { Booking } from './booking.entity';
@Controller('bookings')
export class BookingController {
constructor(private readonly bookingService: BookingService) {}
@Get()
findAll(): Promise<Booking[]> {
return this.bookingService.findAll();
}
@Get(':id')
findOne(@Param('id') id: number): Promise<Booking> {
return this.bookingService.findOne(id);
}
@Post()
create(@Body() booking: Booking): Promise<Booking> {
return this.bookingService.create(booking);
}
@Put(':id')
update(@Param('id') id: number, @Body() booking: Booking): Promise<any> {
return this.bookingService.update(id, booking);
}
@Delete(':id')
delete(@Param('id') id: number): Promise<any> {
return this.bookingService.delete(id);
}
}
```
6. **Admin Features**
For admin features, you can create specific controllers and services that will be accessible only to users with admin roles. You can use the `@Roles` decorator to restrict access.
**roles.guard.ts**
```typescript
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { Reflector } from '@nestjs/core';
@Injectable()
export class RolesGuard implements CanActivate {
constructor(private reflector: Reflector) {}
canActivate(context: ExecutionContext): boolean {
const roles = this.reflector.get<string[]>('roles', context.getHandler());
if (!roles) {
return true;
}
const request = context.switchToHttp().getRequest();
const user = request.user;
return roles.some(role => role === user.role);
}
}
```
**roles.decorator.ts**
```typescript
import { SetMetadata } from '@nestjs/common';
export const Roles = (...roles: string[]) => SetMetadata('roles', roles);
```
**flight.controller.ts (Admin)**
```typescript
import { Controller, Get, Post, Put, Delete, Body, Param, UseGuards } from '@nestjs/common';
import { FlightService } from './flight.service';
import { Flight } from './flight.entity';
import { Roles } from '../auth/roles.decorator';
import { RolesGuard } from '../auth/roles.guard';
import { AuthGuard } from '@nestjs/passport';
@Controller('admin/flights')
@UseGuards(AuthGuard('jwt'), RolesGuard)
@Roles('admin')
export class AdminFlightController {
constructor(private readonly flightService: FlightService) {}
@Get()
findAll(): Promise<Flight[]> {
return this.flightService.findAll();
}
@Get(':id')
findOne(@Param('id') id: number): Promise<Flight> {
return this.flightService.findOne(id);
}
@Post()
create(@Body() flight: Flight): Promise<Flight> {
return this.flightService.create(flight);
}
@Put(':id')
update(@Param('id') id: number, @Body() flight: Flight): Promise<any> {
return this.flightService.update(id, flight);
}
@Delete(':id')
delete(@Param('id') id: number): Promise<any> {
return this.flightService.delete(id);
}
}
```
This is a comprehensive setup for the backend of a flight booking system. You can expand and refine it according to your specific requirements, such as adding more sophisticated search and filter options, integrating third-party APIs for real-time flight data, or implementing payment gateways.
Creating the frontend for a user registration and authentication system using Next.js involves several components. Below is a step-by-step guide with code snippets for each feature you listed. This guide assumes you have a basic understanding of Next.js, React, and Apollo Client.
1. **Initialize Next.js Project**
First, create a new Next.js project:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
```
2. **Install Required Packages**
Install the necessary packages:
```bash
npm install @apollo/client graphql next-auth @mantine/core @mantine/hooks @mantine/dropzone
```
3. **Set Up Apollo Client**
Create an `ApolloClient` instance in a file called `apollo-client.js`:
**apollo-client.js**
```javascript
import { ApolloClient, InMemoryCache, createHttpLink } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
const httpLink = createHttpLink({
uri: 'http://localhost:4000/graphql',
});
const authLink = setContext((_, { headers }) => {
const token = localStorage.getItem('token');
return {
headers: {
...headers,
authorization: token ? `Bearer ${token}` : '',
},
};
});
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache(),
});
export default client;
```
4. **User Registration and Authentication**
Create the necessary GraphQL queries and mutations:
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const LOGIN_USER = gql`
mutation LoginUser($username: String!, $password: String!) {
login(username: $username, password: $password) {
access_token
}
}
`;
export const REGISTER_USER = gql`
mutation RegisterUser($username: String!, $email: String!, $password: String!) {
register(username: $username, email: $email, password: $password) {
id
username
email
}
}
`;
```
Create a login page:
**pages/login.js**
```javascript
import { useState } from 'react';
import { useMutation } from '@apollo/client';
import { LOGIN_USER } from '../graphql/queries';
import { useRouter } from 'next/router';
export default function Login() {
const [username, setUsername] = useState('');
const [password, setPassword] = useState('');
const [login, { data, loading, error }] = useMutation(LOGIN_USER);
const router = useRouter();
const handleSubmit = async (e) => {
e.preventDefault();
try {
const { data } = await login({ variables: { username, password } });
localStorage.setItem('token', data.login.access_token);
router.push('/');
} catch (error) {
console.error('Login failed', error);
}
};
return (
<div>
<h1>Login</h1>
<form onSubmit={handleSubmit}>
<div>
<label>Username</label>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
</div>
<div>
<label>Password</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</div>
<button type="submit" disabled={loading}>
{loading ? 'Logging in...' : 'Login'}
</button>
{error && <p>Error: {error.message}</p>}
</form>
</div>
);
}
```
Create a registration page:
**pages/register.js**
```javascript
import { useState } from 'react';
import { useMutation } from '@apollo/client';
import { REGISTER_USER } from '../graphql/queries';
import { useRouter } from 'next/router';
export default function Register() {
const [username, setUsername] = useState('');
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [register, { data, loading, error }] = useMutation(REGISTER_USER);
const router = useRouter();
const handleSubmit = async (e) => {
e.preventDefault();
try {
await register({ variables: { username, email, password } });
router.push('/login');
} catch (error) {
console.error('Registration failed', error);
}
};
return (
<div>
<h1>Register</h1>
<form onSubmit={handleSubmit}>
<div>
<label>Username</label>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
</div>
<div>
<label>Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<div>
<label>Password</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</div>
<button type="submit" disabled={loading}>
{loading ? 'Registering...' : 'Register'}
</button>
{error && <p>Error: {error.message}</p>}
</form>
</div>
);
}
```
5. **User Profile**
Create a profile page to view and edit profile information:
**pages/profile.js**
```javascript
import { useQuery, useMutation } from '@apollo/client';
import { useState } from 'react';
import { GET_USER_PROFILE, UPDATE_USER_PROFILE } from '../graphql/queries';
import { useRouter } from 'next/router';
export default function Profile() {
const { data, loading, error } = useQuery(GET_USER_PROFILE);
const [updateProfile] = useMutation(UPDATE_USER_PROFILE);
const [username, setUsername] = useState('');
const [email, setEmail] = useState('');
const router = useRouter();
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const handleSubmit = async (e) => {
e.preventDefault();
try {
await updateProfile({ variables: { username, email } });
router.push('/');
} catch (error) {
console.error('Update failed', error);
}
};
return (
<div>
<h1>Profile</h1>
<form onSubmit={handleSubmit}>
<div>
<label>Username</label>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
</div>
<div>
<label>Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
</div>
<button type="submit">Update</button>
</form>
</div>
);
}
```
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const GET_USER_PROFILE = gql`
query GetUserProfile {
userProfile {
id
username
email
}
}
`;
export const UPDATE_USER_PROFILE = gql`
mutation UpdateUserProfile($username: String!, $email: String!) {
updateUserProfile(username: $username, email: $email) {
id
username
email
}
}
`;
```
6. **Upload Profile Picture**
Use Mantine for the file upload component. Install Mantine and set up the file upload:
```bash
npm install @mantine/core @mantine/dropzone
```
Create a file upload component:
**components/ProfilePictureUpload.js**
```javascript
import { useState } from 'react';
import { Dropzone, IMAGE_MIME_TYPE } from '@mantine/dropzone';
import { useMutation } from '@apollo/client';
import { UPLOAD_PROFILE_PICTURE } from '../graphql/queries';
export default function ProfilePictureUpload() {
const [files, setFiles] = useState([]);
const [uploadProfilePicture] = useMutation(UPLOAD_PROFILE_PICTURE);
const handleDrop = async (acceptedFiles) => {
setFiles(acceptedFiles);
const formData = new FormData();
formData.append('file', acceptedFiles[0]);
try {
await uploadProfilePicture({ variables: { file: acceptedFiles[0] } });
} catch (error) {
console.error('Upload failed', error);
}
};
return (
<Dropzone
onDrop={handleDrop}
accept={IMAGE_MIME_TYPE}
multiple={false}
>
{(status) => (
<div>
<p>Drag images here or click to select files</p>
</div>
)}
</Dropzone>
);
}
```
**graphql/queries.js**
```javascript
import
{ gql } from '@apollo/client';
export const UPLOAD_PROFILE_PICTURE = gql`
mutation UploadProfilePicture($file: Upload!) {
uploadProfilePicture(file: $file) {
url
}
}
`;
```
7. **Integrate with Pages**
Add the `ProfilePictureUpload` component to your profile page:
**pages/profile.js**
```javascript
import ProfilePictureUpload from '../components/ProfilePictureUpload';
export default function Profile() {
// ...existing code
return (
<div>
<h1>Profile</h1>
<ProfilePictureUpload />
<form onSubmit={handleSubmit}>
{/* ...existing code */}
</form>
</div>
);
}
```
8. **Social Media Login**
Set up social media login using `next-auth`:
```bash
npm install next-auth
```
Configure `next-auth` in a file called `[...nextauth].js` in the `pages/api/auth` directory:
**pages/api/auth/[...nextauth].js**
```javascript
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
Providers.Facebook({
clientId: process.env.FACEBOOK_CLIENT_ID,
clientSecret: process.env.FACEBOOK_CLIENT_SECRET,
}),
],
callbacks: {
async jwt(token, user) {
if (user) {
token.id = user.id;
}
return token;
},
async session(session, token) {
session.user.id = token.id;
return session;
},
},
});
```
Create environment variables for your social media client IDs and secrets:
**.env.local**
```plaintext
GOOGLE_CLIENT_ID=your-google-client-id
GOOGLE_CLIENT_SECRET=your-google-client-secret
FACEBOOK_CLIENT_ID=your-facebook-client-id
FACEBOOK_CLIENT_SECRET=your-facebook-client-secret
```
Add social media login buttons to your login page:
**pages/login.js**
```javascript
import { signIn } from 'next-auth/client';
export default function Login() {
// ...existing code
return (
<div>
<h1>Login</h1>
<form onSubmit={handleSubmit}>
{/* ...existing code */}
</form>
<button onClick={() => signIn('google')}>Login with Google</button>
<button onClick={() => signIn('facebook')}>Login with Facebook</button>
</div>
);
}
```
This should cover the main features for user registration, authentication, profile management, and social media login for your flight booking system's frontend using Next.js.
To implement flight search, booking, and management features on the frontend using Next.js, you need to set up several components and pages. Below is a step-by-step guide to help you build these features.
### 1. Initialize Next.js Project and Install Dependencies
If you haven't already, create a Next.js project and install the necessary dependencies:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
npm install @apollo/client graphql react-hook-form date-fns
```
### 2. Set Up Apollo Client
Create an `ApolloClient` instance to interact with your GraphQL backend.
**apollo-client.js**
```javascript
import { ApolloClient, InMemoryCache, createHttpLink } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
const httpLink = createHttpLink({
uri: 'http://localhost:4000/graphql',
});
const authLink = setContext((_, { headers }) => {
const token = localStorage.getItem('token');
return {
headers: {
...headers,
authorization: token ? `Bearer ${token}` : '',
},
};
});
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache(),
});
export default client;
```
### 3. GraphQL Queries and Mutations
Define the necessary queries and mutations for flight search, booking, and user bookings.
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const SEARCH_FLIGHTS = gql`
query SearchFlights($from: String!, $to: String!, $departureDate: String!) {
searchFlights(from: $from, to: $to, departureDate: $departureDate) {
id
airline
from
to
departureTime
arrivalTime
duration
price
}
}
`;
export const BOOK_FLIGHT = gql`
mutation BookFlight($flightId: ID!, $userId: ID!) {
bookFlight(flightId: $flightId, userId: $userId) {
id
flight {
id
airline
from
to
departureTime
arrivalTime
duration
}
user {
id
username
}
bookingTime
}
}
`;
export const GET_USER_BOOKINGS = gql`
query GetUserBookings($userId: ID!) {
userBookings(userId: $userId) {
id
flight {
id
airline
from
to
departureTime
arrivalTime
duration
}
bookingTime
}
}
`;
```
### 4. Flight Search Component
Create a flight search component to search for flights by destination, date, and other criteria.
**components/FlightSearch.js**
```javascript
import { useState } from 'react';
import { useForm } from 'react-hook-form';
import { useLazyQuery } from '@apollo/client';
import { SEARCH_FLIGHTS } from '../graphql/queries';
import { format } from 'date-fns';
export default function FlightSearch({ onFlightsFound }) {
const { register, handleSubmit } = useForm();
const [searchFlights, { data, loading, error }] = useLazyQuery(SEARCH_FLIGHTS);
const onSubmit = async (formData) => {
const { from, to, departureDate } = formData;
await searchFlights({
variables: {
from,
to,
departureDate: format(new Date(departureDate), 'yyyy-MM-dd'),
},
});
if (data) {
onFlightsFound(data.searchFlights);
}
};
return (
<div>
<h2>Search Flights</h2>
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>From:</label>
<input type="text" {...register('from')} required />
</div>
<div>
<label>To:</label>
<input type="text" {...register('to')} required />
</div>
<div>
<label>Departure Date:</label>
<input type="date" {...register('departureDate')} required />
</div>
<button type="submit" disabled={loading}>
{loading ? 'Searching...' : 'Search'}
</button>
{error && <p>Error: {error.message}</p>}
</form>
</div>
);
}
```
### 5. Flight List Component
Create a component to display the list of searched flights and allow users to book a flight.
**components/FlightList.js**
```javascript
import { useMutation } from '@apollo/client';
import { BOOK_FLIGHT } from '../graphql/queries';
export default function FlightList({ flights, userId }) {
const [bookFlight] = useMutation(BOOK_FLIGHT);
const handleBook = async (flightId) => {
try {
await bookFlight({ variables: { flightId, userId } });
alert('Flight booked successfully!');
} catch (error) {
console.error('Booking failed', error);
}
};
return (
<div>
<h2>Available Flights</h2>
{flights.map((flight) => (
<div key={flight.id}>
<p>Airline: {flight.airline}</p>
<p>From: {flight.from}</p>
<p>To: {flight.to}</p>
<p>Departure: {flight.departureTime}</p>
<p>Arrival: {flight.arrivalTime}</p>
<p>Duration: {flight.duration}</p>
<p>Price: ${flight.price}</p>
<button onClick={() => handleBook(flight.id)}>Book Flight</button>
</div>
))}
</div>
);
}
```
### 6. Bookings Page
Create a page to view and manage user bookings.
**pages/bookings.js**
```javascript
import { useQuery } from '@apollo/client';
import { GET_USER_BOOKINGS } from '../graphql/queries';
import { useEffect, useState } from 'react';
export default function Bookings({ userId }) {
const { data, loading, error } = useQuery(GET_USER_BOOKINGS, {
variables: { userId },
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h2>Your Bookings</h2>
{data.userBookings.map((booking) => (
<div key={booking.id}>
<p>Airline: {booking.flight.airline}</p>
<p>From: {booking.flight.from}</p>
<p>To: {booking.flight.to}</p>
<p>Departure: {booking.flight.departureTime}</p>
<p>Arrival: {booking.flight.arrivalTime}</p>
<p>Duration: {booking.flight.duration}</p>
<p>Booking Time: {booking.bookingTime}</p>
</div>
))}
</div>
);
}
```
### 7. Home Page
Integrate the flight search and flight list components into the home page.
**pages/index.js**
```javascript
import { useState } from 'react';
import FlightSearch from '../components/FlightSearch';
import FlightList from '../components/FlightList';
import { useRouter } from 'next/router';
export default function Home() {
const [flights, setFlights] = useState([]);
const router = useRouter();
const userId = 'current-user-id'; // Replace with the actual logged-in user ID
const handleFlightsFound = (foundFlights) => {
setFlights(foundFlights);
};
return (
<div>
<h1>Flight Booking System</h1>
<FlightSearch onFlightsFound={handleFlightsFound} />
{flights.length > 0 && <FlightList flights={flights} userId={userId} />}
<button onClick={() => router.push('/bookings')}>View Bookings</button>
</div>
);
}
```
### 8. Setup Email Notifications (Optional)
To handle email notifications for booking confirmations, you would typically implement this feature on the backend. You can trigger an email notification when a booking is created using a service like SendGrid, Nodemailer, or any other email service.
### Final Steps
Ensure that your backend GraphQL API supports all the necessary queries and mutations for flights and bookings. This setup provides a robust foundation for your flight booking system's frontend. You can further enhance it with features like filtering, sorting, and more advanced error handling as needed.
Integrating payment gateways like Stripe into your Next.js application involves setting up payment forms, handling secure payment processing, and displaying payment history. Below is a step-by-step guide to implement these features on the frontend.
### 1. Initialize Project and Install Dependencies
If you haven't already, create a Next.js project and install the necessary dependencies:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
npm install @stripe/stripe-js @stripe/react-stripe-js @apollo/client graphql react-hook-form date-fns
```
### 2. Set Up Apollo Client
If you haven't already, set up Apollo Client as shown in the previous sections.
### 3. Create Payment Form Component
Create a payment form component using Stripe's React components.
**components/CheckoutForm.js**
```javascript
import { useStripe, useElements, CardElement } from '@stripe/react-stripe-js';
import { useState } from 'react';
import { useMutation } from '@apollo/client';
import { CREATE_PAYMENT_INTENT, SAVE_PAYMENT } from '../graphql/queries';
export default function CheckoutForm({ bookingId }) {
const stripe = useStripe();
const elements = useElements();
const [createPaymentIntent] = useMutation(CREATE_PAYMENT_INTENT);
const [savePayment] = useMutation(SAVE_PAYMENT);
const [loading, setLoading] = useState(false);
const [error, setError] = useState(null);
const handleSubmit = async (event) => {
event.preventDefault();
setLoading(true);
try {
const { data } = await createPaymentIntent({ variables: { bookingId } });
const clientSecret = data.createPaymentIntent.clientSecret;
const cardElement = elements.getElement(CardElement);
const paymentResult = await stripe.confirmCardPayment(clientSecret, {
payment_method: { card: cardElement },
});
if (paymentResult.error) {
setError(`Payment failed: ${paymentResult.error.message}`);
} else if (paymentResult.paymentIntent.status === 'succeeded') {
await savePayment({ variables: { bookingId, paymentIntentId: paymentResult.paymentIntent.id } });
alert('Payment successful!');
}
} catch (error) {
setError(`Payment failed: ${error.message}`);
}
setLoading(false);
};
return (
<form onSubmit={handleSubmit}>
<CardElement />
<button type="submit" disabled={!stripe || loading}>
{loading ? 'Processing...' : 'Pay'}
</button>
{error && <div>{error}</div>}
</form>
);
}
```
### 4. GraphQL Queries and Mutations
Define the necessary queries and mutations for creating payment intents and saving payments.
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const CREATE_PAYMENT_INTENT = gql`
mutation CreatePaymentIntent($bookingId: ID!) {
createPaymentIntent(bookingId: $bookingId) {
clientSecret
}
}
`;
export const SAVE_PAYMENT = gql`
mutation SavePayment($bookingId: ID!, $paymentIntentId: String!) {
savePayment(bookingId: $bookingId, paymentIntentId: $paymentIntentId) {
id
booking {
id
flight {
airline
from
to
}
}
amount
currency
status
}
}
`;
export const GET_PAYMENT_HISTORY = gql`
query GetPaymentHistory($userId: ID!) {
paymentHistory(userId: $userId) {
id
amount
currency
status
createdAt
}
}
`;
```
### 5. Payment Page
Create a page to handle payment processing.
**pages/payment.js**
```javascript
import { loadStripe } from '@stripe/stripe-js';
import { Elements } from '@stripe/react-stripe-js';
import CheckoutForm from '../components/CheckoutForm';
import { useRouter } from 'next/router';
const stripePromise = loadStripe(process.env.NEXT_PUBLIC_STRIPE_PUBLISHABLE_KEY);
export default function Payment() {
const router = useRouter();
const { bookingId } = router.query;
return (
<div>
<h1>Complete Your Payment</h1>
<Elements stripe={stripePromise}>
<CheckoutForm bookingId={bookingId} />
</Elements>
</div>
);
}
```
### 6. View Payment History
Create a page to view the user's payment history.
**pages/payment-history.js**
```javascript
import { useQuery } from '@apollo/client';
import { GET_PAYMENT_HISTORY } from '../graphql/queries';
import { useRouter } from 'next/router';
export default function PaymentHistory({ userId }) {
const { data, loading, error } = useQuery(GET_PAYMENT_HISTORY, { variables: { userId } });
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h2>Payment History</h2>
{data.paymentHistory.map((payment) => (
<div key={payment.id}>
<p>Amount: {payment.amount} {payment.currency}</p>
<p>Status: {payment.status}</p>
<p>Date: {new Date(payment.createdAt).toLocaleString()}</p>
</div>
))}
</div>
);
}
```
### 7. Environment Variables
Ensure you have your Stripe publishable key set in your environment variables.
**.env.local**
```plaintext
NEXT_PUBLIC_STRIPE_PUBLISHABLE_KEY=your-stripe-publishable-key
```
### 8. Backend Integration (Necessary for Full Implementation)
While this guide focuses on the frontend, note that you must implement the corresponding backend logic in your NestJS application to handle:
1. Creating payment intents using Stripe.
2. Saving payment information after successful payments.
3. Providing payment history data via GraphQL queries.
### Final Steps
1. Ensure your backend is properly set up to handle Stripe payments and is accessible to your frontend.
2. Test the entire flow from searching flights, booking, making payments, and viewing payment history.
This setup should provide a robust foundation for integrating payment gateways into your flight booking system's frontend using Next.js.
### Email and SMS Notifications
To handle notifications, we will integrate with services like SendGrid for emails and Twilio for SMS notifications. We'll create components and hooks to send these notifications when needed. The actual sending logic will typically be handled by your backend, but we can trigger these notifications from the frontend.
### Reviews and Ratings
We'll create components to rate and review airlines and flights, and display reviews and ratings from other users.
### Step-by-Step Implementation
### 1. Initialize Project and Install Dependencies
If you haven't already, create a Next.js project and install the necessary dependencies:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
npm install @apollo/client graphql react-hook-form date-fns
```
### 2. Set Up Apollo Client
Set up Apollo Client as shown in the previous sections.
### 3. GraphQL Queries and Mutations
Define the necessary queries and mutations for sending notifications, creating reviews, and fetching reviews.
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const SEND_NOTIFICATION = gql`
mutation SendNotification($type: String!, $to: String!, $message: String!) {
sendNotification(type: $type, to: $to, message: $message) {
success
message
}
}
`;
export const CREATE_REVIEW = gql`
mutation CreateReview($flightId: ID!, $rating: Int!, $comment: String!) {
createReview(flightId: $flightId, rating: $rating, comment: $comment) {
id
flightId
rating
comment
user {
id
username
}
}
}
`;
export const GET_REVIEWS = gql`
query GetReviews($flightId: ID!) {
reviews(flightId: $flightId) {
id
rating
comment
user {
id
username
}
}
}
`;
```
### 4. Notification Hook
Create a hook to send notifications.
**hooks/useNotification.js**
```javascript
import { useMutation } from '@apollo/client';
import { SEND_NOTIFICATION } from '../graphql/queries';
export const useNotification = () => {
const [sendNotification, { loading, error }] = useMutation(SEND_NOTIFICATION);
const notify = async (type, to, message) => {
try {
const response = await sendNotification({ variables: { type, to, message } });
return response.data.sendNotification;
} catch (error) {
console.error('Notification error:', error);
throw new Error('Failed to send notification');
}
};
return { notify, loading, error };
};
```
### 5. Notification Component
Create a component to send notifications.
**components/Notification.js**
```javascript
import { useForm } from 'react-hook-form';
import { useNotification } from '../hooks/useNotification';
export default function Notification() {
const { register, handleSubmit } = useForm();
const { notify, loading, error } = useNotification();
const onSubmit = async (formData) => {
const { type, to, message } = formData;
try {
await notify(type, to, message);
alert('Notification sent successfully!');
} catch (error) {
alert('Failed to send notification');
}
};
return (
<div>
<h2>Send Notification</h2>
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>Type (email/sms):</label>
<input type="text" {...register('type')} required />
</div>
<div>
<label>To:</label>
<input type="text" {...register('to')} required />
</div>
<div>
<label>Message:</label>
<textarea {...register('message')} required />
</div>
<button type="submit" disabled={loading}>
{loading ? 'Sending...' : 'Send'}
</button>
{error && <p>Error: {error.message}</p>}
</form>
</div>
);
}
```
### 6. Review and Rating Components
Create components to handle reviews and ratings.
**components/ReviewForm.js**
```javascript
import { useForm } from 'react-hook-form';
import { useMutation } from '@apollo/client';
import { CREATE_REVIEW } from '../graphql/queries';
export default function ReviewForm({ flightId, onReviewSubmitted }) {
const { register, handleSubmit } = useForm();
const [createReview, { loading, error }] = useMutation(CREATE_REVIEW);
const onSubmit = async (formData) => {
const { rating, comment } = formData;
try {
const response = await createReview({ variables: { flightId, rating: parseInt(rating), comment } });
onReviewSubmitted(response.data.createReview);
} catch (error) {
console.error('Review submission error:', error);
}
};
return (
<div>
<h2>Submit a Review</h2>
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>Rating (1-5):</label>
<input type="number" {...register('rating')} min="1" max="5" required />
</div>
<div>
<label>Comment:</label>
<textarea {...register('comment')} required />
</div>
<button type="submit" disabled={loading}>
{loading ? 'Submitting...' : 'Submit'}
</button>
{error && <p>Error: {error.message}</p>}
</form>
</div>
);
}
```
**components/Reviews.js**
```javascript
import { useQuery } from '@apollo/client';
import { GET_REVIEWS } from '../graphql/queries';
export default function Reviews({ flightId }) {
const { data, loading, error } = useQuery(GET_REVIEWS, { variables: { flightId } });
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
return (
<div>
<h2>Reviews</h2>
{data.reviews.length > 0 ? (
data.reviews.map((review) => (
<div key={review.id}>
<p>Rating: {review.rating}</p>
<p>Comment: {review.comment}</p>
<p>User: {review.user.username}</p>
</div>
))
) : (
<p>No reviews yet.</p>
)}
</div>
);
}
```
### 7. Integrate Components into Pages
**pages/flight/[id].js**
```javascript
import { useRouter } from 'next/router';
import { useState } from 'react';
import ReviewForm from '../../components/ReviewForm';
import Reviews from '../../components/Reviews';
export default function FlightDetails() {
const router = useRouter();
const { id } = router.query;
const [reviews, setReviews] = useState([]);
const handleReviewSubmitted = (newReview) => {
setReviews((prevReviews) => [...prevReviews, newReview]);
};
return (
<div>
<h1>Flight Details</h1>
<p>Flight ID: {id}</p>
{/* Render flight details here */}
<ReviewForm flightId={id} onReviewSubmitted={handleReviewSubmitted} />
<Reviews flightId={id} reviews={reviews} />
</div>
);
}
```
**pages/send-notification.js**
```javascript
import Notification from '../components/Notification';
export default function SendNotificationPage() {
return (
<div>
<h1>Send Notification</h1>
<Notification />
</div>
);
}
```
### Final Steps
1. Ensure your backend is properly set up to handle notifications and reviews.
2. Test the entire flow for sending notifications, submitting reviews, and viewing reviews.
3. Enhance the UI/UX as needed and handle edge cases and error scenarios gracefully.
This setup provides a robust foundation for handling notifications and reviews/ratings in your flight booking system's frontend using Next.js.
To implement the admin features for your flight booking system, we'll create components for the dashboard, flight management (adding, editing, and deleting flights), and managing flight schedules and availability. We'll use Apollo Client for GraphQL queries and mutations to interact with the backend.
### 1. Initialize Project and Install Dependencies
If you haven't already, create a Next.js project and install the necessary dependencies:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
npm install @apollo/client graphql react-hook-form
```
### 2. Set Up Apollo Client
Set up Apollo Client as shown in the previous sections.
### 3. GraphQL Queries and Mutations
Define the necessary queries and mutations for managing flights and getting the dashboard overview.
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const GET_DASHBOARD_OVERVIEW = gql`
query GetDashboardOverview {
dashboardOverview {
bookingsCount
usersCount
flightsCount
revenue
}
}
`;
export const GET_FLIGHTS = gql`
query GetFlights {
flights {
id
airline
from
to
departureTime
arrivalTime
availableSeats
}
}
`;
export const CREATE_FLIGHT = gql`
mutation CreateFlight($input: FlightInput!) {
createFlight(input: $input) {
id
airline
from
to
departureTime
arrivalTime
availableSeats
}
}
`;
export const UPDATE_FLIGHT = gql`
mutation UpdateFlight($id: ID!, $input: FlightInput!) {
updateFlight(id: $id, input: $input) {
id
airline
from
to
departureTime
arrivalTime
availableSeats
}
}
`;
export const DELETE_FLIGHT = gql`
mutation DeleteFlight($id: ID!) {
deleteFlight(id: $id) {
success
}
}
`;
```
### 4. Dashboard Component
Create a component to display the dashboard overview.
**components/Dashboard.js**
```javascript
import { useQuery } from '@apollo/client';
import { GET_DASHBOARD_OVERVIEW } from '../graphql/queries';
export default function Dashboard() {
const { data, loading, error } = useQuery(GET_DASHBOARD_OVERVIEW);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const { bookingsCount, usersCount, flightsCount, revenue } = data.dashboardOverview;
return (
<div>
<h1>Admin Dashboard</h1>
<div>
<h3>Overview</h3>
<p>Bookings: {bookingsCount}</p>
<p>Users: {usersCount}</p>
<p>Flights: {flightsCount}</p>
<p>Revenue: ${revenue}</p>
</div>
</div>
);
}
```
### 5. Flight Management Components
Create components to manage flights: adding, editing, and deleting flights.
**components/FlightForm.js**
```javascript
import { useForm } from 'react-hook-form';
import { useMutation } from '@apollo/client';
import { CREATE_FLIGHT, UPDATE_FLIGHT } from '../graphql/queries';
export default function FlightForm({ flight, onCompleted }) {
const { register, handleSubmit, reset } = useForm({
defaultValues: flight || { airline: '', from: '', to: '', departureTime: '', arrivalTime: '', availableSeats: 0 },
});
const [createFlight] = useMutation(CREATE_FLIGHT, { onCompleted });
const [updateFlight] = useMutation(UPDATE_FLIGHT, { onCompleted });
const onSubmit = async (formData) => {
if (flight) {
await updateFlight({ variables: { id: flight.id, input: formData } });
} else {
await createFlight({ variables: { input: formData } });
}
reset();
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>Airline:</label>
<input type="text" {...register('airline')} required />
</div>
<div>
<label>From:</label>
<input type="text" {...register('from')} required />
</div>
<div>
<label>To:</label>
<input type="text" {...register('to')} required />
</div>
<div>
<label>Departure Time:</label>
<input type="datetime-local" {...register('departureTime')} required />
</div>
<div>
<label>Arrival Time:</label>
<input type="datetime-local" {...register('arrivalTime')} required />
</div>
<div>
<label>Available Seats:</label>
<input type="number" {...register('availableSeats')} required />
</div>
<button type="submit">{flight ? 'Update' : 'Add'} Flight</button>
</form>
);
}
```
**components/FlightList.js**
```javascript
import { useQuery, useMutation } from '@apollo/client';
import { GET_FLIGHTS, DELETE_FLIGHT } from '../graphql/queries';
export default function FlightList({ onEdit }) {
const { data, loading, error } = useQuery(GET_FLIGHTS);
const [deleteFlight] = useMutation(DELETE_FLIGHT, {
refetchQueries: [{ query: GET_FLIGHTS }],
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const handleDelete = async (id) => {
await deleteFlight({ variables: { id } });
};
return (
<div>
<h2>Flights</h2>
<ul>
{data.flights.map((flight) => (
<li key={flight.id}>
<div>
<p>Airline: {flight.airline}</p>
<p>From: {flight.from}</p>
<p>To: {flight.to}</p>
<p>Departure Time: {new Date(flight.departureTime).toLocaleString()}</p>
<p>Arrival Time: {new Date(flight.arrivalTime).toLocaleString()}</p>
<p>Available Seats: {flight.availableSeats}</p>
<button onClick={() => onEdit(flight)}>Edit</button>
<button onClick={() => handleDelete(flight.id)}>Delete</button>
</div>
</li>
))}
</ul>
</div>
);
}
```
### 6. Integrate Components into Pages
**pages/admin/dashboard.js**
```javascript
import Dashboard from '../../components/Dashboard';
export default function AdminDashboardPage() {
return (
<div>
<Dashboard />
</div>
);
}
```
**pages/admin/flights.js**
```javascript
import { useState } from 'react';
import FlightForm from '../../components/FlightForm';
import FlightList from '../../components/FlightList';
export default function AdminFlightsPage() {
const [selectedFlight, setSelectedFlight] = useState(null);
const handleEdit = (flight) => {
setSelectedFlight(flight);
};
const handleFormCompleted = () => {
setSelectedFlight(null);
};
return (
<div>
<h1>Manage Flights</h1>
<FlightForm flight={selectedFlight} onCompleted={handleFormCompleted} />
<FlightList onEdit={handleEdit} />
</div>
);
}
```
### Final Steps
1. Ensure your backend is properly set up to handle flight management and provide dashboard data.
2. Test the entire flow for adding, editing, deleting flights, and viewing the dashboard.
3. Enhance the UI/UX as needed and handle edge cases and error scenarios gracefully.
This setup provides a robust foundation for managing flights and viewing dashboard data in your flight booking system's frontend using Next.js.
To implement the user management, booking management, and reporting and analytics features for your flight booking system, we'll create components for each feature and integrate them into your Next.js application. We'll use Apollo Client for GraphQL queries and mutations to interact with the backend.
### 1. Initialize Project and Install Dependencies
If you haven't already, create a Next.js project and install the necessary dependencies:
```bash
npx create-next-app@latest flight-booking-frontend
cd flight-booking-frontend
npm install @apollo/client graphql react-hook-form
```
### 2. Set Up Apollo Client
Set up Apollo Client as shown in the previous sections.
### 3. GraphQL Queries and Mutations
Define the necessary queries and mutations for managing users, bookings, and generating reports.
**graphql/queries.js**
```javascript
import { gql } from '@apollo/client';
export const GET_USERS = gql`
query GetUsers {
users {
id
username
email
role
}
}
`;
export const UPDATE_USER_ROLE = gql`
mutation UpdateUserRole($id: ID!, $role: String!) {
updateUserRole(id: $id, role: $role) {
id
username
email
role
}
}
`;
export const GET_BOOKINGS = gql`
query GetBookings {
bookings {
id
flight {
id
airline
from
to
}
user {
id
username
}
status
createdAt
}
}
`;
export const UPDATE_BOOKING_STATUS = gql`
mutation UpdateBookingStatus($id: ID!, $status: String!) {
updateBookingStatus(id: $id, status: $status) {
id
status
}
}
`;
export const GET_REPORTS = gql`
query GetReports {
reports {
bookingsCount
revenue
userActivity
}
}
`;
```
### 4. User Management Components
Create components to view and manage users and assign roles.
**components/UserList.js**
```javascript
import { useQuery, useMutation } from '@apollo/client';
import { GET_USERS, UPDATE_USER_ROLE } from '../graphql/queries';
import { useForm } from 'react-hook-form';
export default function UserList() {
const { data, loading, error } = useQuery(GET_USERS);
const [updateUserRole] = useMutation(UPDATE_USER_ROLE, {
refetchQueries: [{ query: GET_USERS }],
});
const { register, handleSubmit } = useForm();
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const onSubmit = async (formData) => {
await updateUserRole({ variables: { id: formData.id, role: formData.role } });
};
return (
<div>
<h2>User Management</h2>
<ul>
{data.users.map((user) => (
<li key={user.id}>
<p>Username: {user.username}</p>
<p>Email: {user.email}</p>
<p>Role: {user.role}</p>
<form onSubmit={handleSubmit(onSubmit)}>
<input type="hidden" value={user.id} {...register('id')} />
<select {...register('role')}>
<option value="admin">Admin</option>
<option value="customer_support">Customer Support</option>
<option value="user">User</option>
</select>
<button type="submit">Update Role</button>
</form>
</li>
))}
</ul>
</div>
);
}
```
### 5. Booking Management Components
Create components to view and manage bookings.
**components/BookingList.js**
```javascript
import { useQuery, useMutation } from '@apollo/client';
import { GET_BOOKINGS, UPDATE_BOOKING_STATUS } from '../graphql/queries';
import { useForm } from 'react-hook-form';
export default function BookingList() {
const { data, loading, error } = useQuery(GET_BOOKINGS);
const [updateBookingStatus] = useMutation(UPDATE_BOOKING_STATUS, {
refetchQueries: [{ query: GET_BOOKINGS }],
});
const { register, handleSubmit } = useForm();
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const onSubmit = async (formData) => {
await updateBookingStatus({ variables: { id: formData.id, status: formData.status } });
};
return (
<div>
<h2>Booking Management</h2>
<ul>
{data.bookings.map((booking) => (
<li key={booking.id}>
<p>Flight: {booking.flight.airline} from {booking.flight.from} to {booking.flight.to}</p>
<p>User: {booking.user.username}</p>
<p>Status: {booking.status}</p>
<form onSubmit={handleSubmit(onSubmit)}>
<input type="hidden" value={booking.id} {...register('id')} />
<select {...register('status')}>
<option value="confirmed">Confirmed</option>
<option value="cancelled">Cancelled</option>
</select>
<button type="submit">Update Status</button>
</form>
</li>
))}
</ul>
</div>
);
}
```
### 6. Reporting and Analytics Components
Create components to generate reports and display analytics.
**components/Reports.js**
```javascript
import { useQuery } from '@apollo/client';
import { GET_REPORTS } from '../graphql/queries';
export default function Reports() {
const { data, loading, error } = useQuery(GET_REPORTS);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: {error.message}</p>;
const { bookingsCount, revenue, userActivity } = data.reports;
return (
<div>
<h2>Reports and Analytics</h2>
<div>
<p>Bookings Count: {bookingsCount}</p>
<p>Revenue: ${revenue}</p>
<p>User Activity: {userActivity}</p>
</div>
</div>
);
}
```
### 7. Integrate Components into Pages
**pages/admin/users.js**
```javascript
import UserList from '../../components/UserList';
export default function AdminUsersPage() {
return (
<div>
<h1>Manage Users</h1>
<UserList />
</div>
);
}
```
**pages/admin/bookings.js**
```javascript
import BookingList from '../../components/BookingList';
export default function AdminBookingsPage() {
return (
<div>
<h1>Manage Bookings</h1>
<BookingList />
</div>
);
}
```
**pages/admin/reports.js**
```javascript
import Reports from '../../components/Reports';
export default function AdminReportsPage() {
return (
<div>
<h1>Reports and Analytics</h1>
<Reports />
</div>
);
}
```
### Final Steps
1. Ensure your backend is properly set up to handle user management, booking management, and generating reports.
2. Test the entire flow for viewing and managing users, bookings, and generating reports.
3. Enhance the UI/UX as needed and handle edge cases and error scenarios gracefully.
This setup provides a robust foundation for managing users, bookings, and generating reports in your flight booking system's frontend using Next.js.
Disclaimer: This content is generated by AI. | nadim_ch0wdhury |
|
1,912,344 | Continuous Testing: Ensuring Quality in the Fast-Paced World of Software Development | In the dynamic realm of software development, delivering high-quality software quickly is a critical... | 0 | 2024-07-05T06:55:48 | https://dev.to/keploy/continuous-testing-ensuring-quality-in-the-fast-paced-world-of-software-development-a9i |
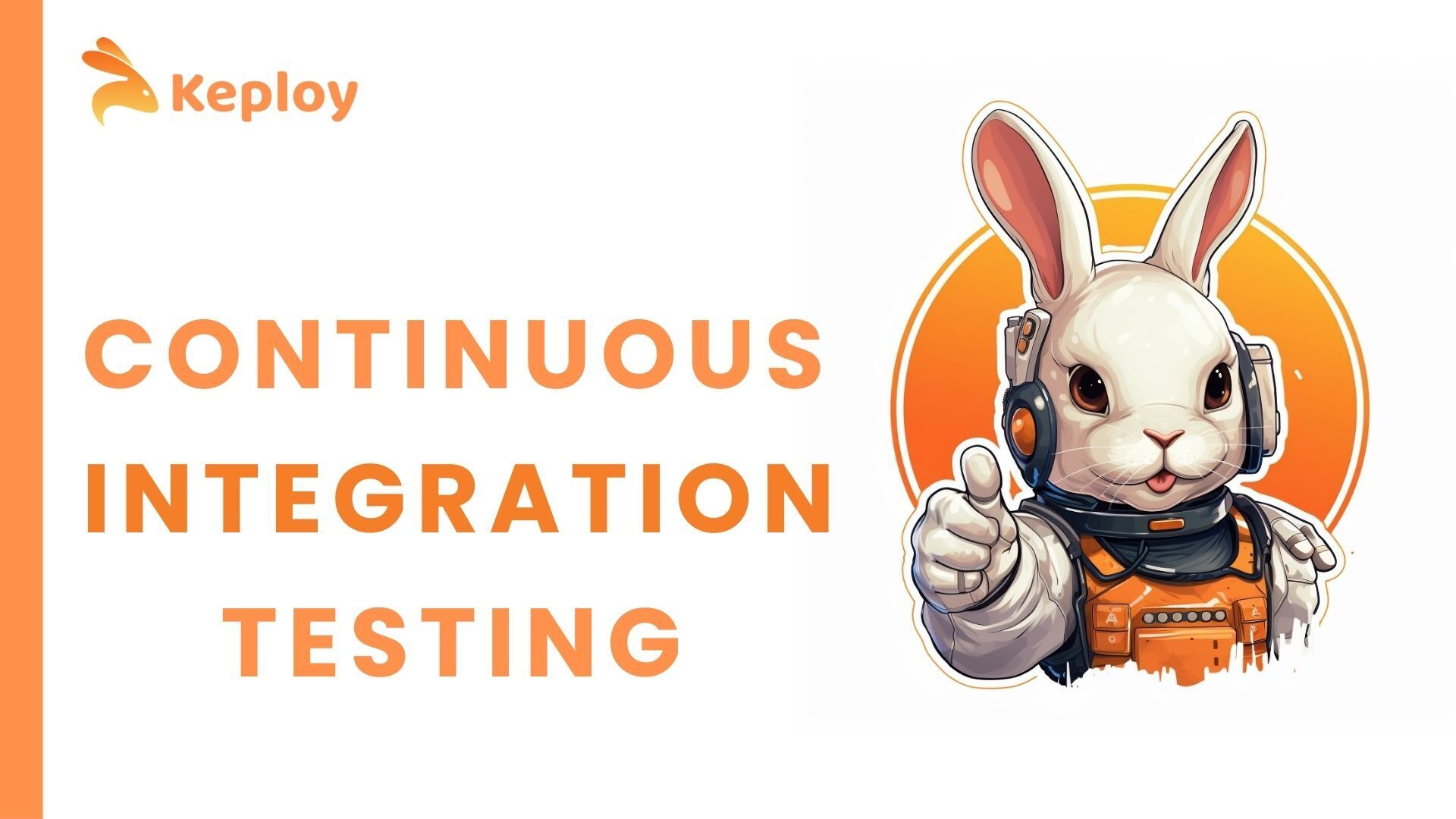
In the dynamic realm of software development, delivering high-quality software quickly is a critical requirement. Continuous testing has become a fundamental practice, ensuring that applications are rigorously tested throughout their lifecycle. This article delves into the essence of [continuous testing](https://keploy.io/blog/community/understand-the-role-of-continuous-testing-in-ci-cd), its benefits, the methodologies involved, and the tools that facilitate this practice.
What is Continuous Testing?
Continuous testing is the practice of executing automated tests as part of the software delivery pipeline to obtain immediate feedback on the business risks associated with a software release candidate. It is integrated into the Continuous Integration (CI) and Continuous Deployment (CD) pipelines, ensuring that tests are run automatically whenever code changes are made. This approach contrasts with traditional testing, which is often performed at the end of the development cycle.
Core Principles of Continuous Testing
1. Automation: Automation is the foundation of continuous testing. Automated tests run repeatedly and consistently, ensuring rapid feedback and enabling quick identification of defects. Automation tools like Selenium, JUnit, and TestNG are widely used to create and manage these tests.
2. Shift-Left Testing: The shift-left approach moves testing activities earlier in the development cycle. By identifying and addressing defects early, teams can avoid the compounding impact of issues that surface later. This approach enhances software quality and accelerates development.
3. Comprehensive Test Coverage: Continuous testing involves various types of tests, including unit tests, integration tests, system tests, performance tests, and security tests. This comprehensive coverage ensures that all aspects of the application are thoroughly tested.
4. Integration with CI/CD: Continuous testing is seamlessly integrated with CI/CD pipelines. Automated tests run whenever code changes are committed, providing immediate feedback and ensuring that new code integrates smoothly with existing functionality.
Benefits of Continuous Testing
1. Early Detection of Defects: Continuous testing facilitates early detection of defects, allowing developers to resolve issues promptly. This reduces the cost and complexity of fixes, as defects are addressed before they propagate further into the development cycle.
2. Improved Code Quality: By ensuring that new code is continuously tested against existing functionality, continuous testing promotes high code quality. It prevents regressions and maintains the stability of the software.
3. Faster Time to Market: Continuous testing streamlines the development process, enabling faster releases. Automated tests and immediate feedback loops make development more efficient, allowing teams to deliver features and updates quickly.
4. Enhanced Collaboration: Continuous testing fosters better collaboration between development and QA teams. By integrating testing into the development process, both teams work together towards delivering high-quality software.
5. Increased Confidence: Continuous testing provides greater confidence in the software’s reliability. Automated tests run consistently, ensuring that any changes do not introduce new issues, and the software remains robust.
Implementing Continuous Testing
1. Automate Early and Often: Start by automating unit tests, which validate individual components in isolation. Tools like JUnit, TestNG, and NUnit are ideal for this purpose.
2. Expand Test Coverage: As development progresses, expand automation to include integration tests, system tests, and end-to-end tests. Integration tests validate the interaction between components, while system tests assess the entire application’s functionality.
3. Incorporate Performance and Security Testing: Performance and security are critical aspects of any application. Incorporate performance tests to evaluate how the application performs under load and stress. Security tests help identify vulnerabilities and ensure the application is secure.
4. Use CI/CD Tools: Leverage CI/CD tools like Jenkins, Travis CI, CircleCI, and GitLab CI/CD to automate integration and deployment processes. These tools facilitate continuous testing by running automated tests whenever code is committed or deployed.
5. Monitor and Analyze Results: Continuous testing generates a wealth of data. Use monitoring and analysis tools to track test results, identify trends, and pinpoint areas that need improvement. Tools like Grafana, Kibana, and Splunk can help visualize and analyze test data.
Challenges in Continuous Testing
While continuous testing offers numerous benefits, it also presents certain challenges that teams must address:
1. Test Maintenance: Automated tests require regular maintenance to ensure they remain accurate and effective. As the application evolves, tests may need updates to reflect changes in functionality.
2. Flaky Tests: Flaky tests produce inconsistent results and can undermine confidence in the testing process. Identifying and resolving the root causes of flaky tests is crucial for maintaining test reliability.
3. Integration Complexity: Integrating continuous testing into existing development workflows can be complex. It requires careful planning and coordination between development, QA, and operations teams.
4. Resource Management: Continuous testing can be resource-intensive, especially when running a large number of tests. Efficient resource management and optimization are essential to ensure tests run smoothly without impacting other processes.
Best Practices for Continuous Testing
1. Prioritize Test Automation: Focus on automating high-value tests that provide significant coverage and quick feedback. Prioritize critical functionalities and frequently used features.
2. Implement Test-Driven Development (TDD): TDD is a development practice where tests are written before the code. This approach ensures that the code meets the requirements from the outset and promotes better design.
3. Use Mocking and Stubbing: Mocking and stubbing simulate external dependencies in tests. They help isolate the components being tested and ensure tests run reliably and quickly.
4. Parallelize Tests: Running tests in parallel can significantly reduce the time required for testing. CI/CD tools often support parallel test execution, enabling faster feedback.
5. Foster a Culture of Quality: Continuous testing should be a shared responsibility across development and QA teams. Foster a culture where quality is a priority, and everyone is committed to maintaining high standards.
Conclusion
Continuous testing is essential for modern software development, enabling teams to deliver high-quality software rapidly and reliably. By integrating testing into every stage of the development lifecycle, continuous testing ensures that defects are detected and resolved early, code quality is maintained, and releases are accelerated. While it presents certain challenges, the benefits of continuous testing far outweigh the difficulties. By embracing automation, leveraging CI/CD tools, and fostering a culture of quality, teams can achieve the full potential of continuous testing and deliver exceptional software products. | keploy |
|
1,912,343 | How to Build Your Own Distributed KV Storage System Using the etcd Raft Library | Introduction raftexample is an example provided by etcd that demonstrates the use of the... | 0 | 2024-07-05T06:55:45 | https://dev.to/justlorain/how-to-build-your-own-distributed-kv-storage-system-using-the-etcd-raft-library-2j69 | webdev, tutorial, database, go | ## Introduction
[raftexample](https://github.com/etcd-io/etcd/tree/main/contrib/raftexample) is an example provided by etcd that demonstrates the use of the etcd raft consensus algorithm library. raftexample ultimately implements a distributed key-value storage service that provides a REST API.
This article will read and analyze the code of raftexample, hoping to help readers better understand how to use the etcd raft library and the implementation logic of the raft library.
## Architecture
The architecture of raftexample is very simple, with the main files as follows:
- **main.go:** Responsible for organizing the interaction between the raft module, the httpapi module, and the kvstore module;
- **raft.go:** Responsible for interacting with the raft library, including submitting proposals, receiving RPC messages that need to be sent, and performing network transmission, etc.;
- **httpapi.go:** Responsible for providing the REST API, serving as the entry point for user requests;
- **kvstore.go:** Responsible for persistently storing committed log entries, equivalent to the state machine in the raft protocol.
## The Processing Flow of a Write Request
A write request arrives in the `ServeHTTP` method of the httpapi module via an HTTP PUT request.
```shell
curl -L http://127.0.0.1:12380/key -XPUT -d value
```
After matching the HTTP request method via `switch`, it enters the PUT method processing flow:
- Read the content from the HTTP request body (i.e., the value);
- Construct a proposal through the `Propose` method of the kvstore module (adding a key-value pair with key as key and value as value);
- Since there is no data to return, respond to the client with 204 StatusNoContent;
> **The proposal is submitted to the raft algorithm library through the `Propose` method provided by the raft algorithm library.**
>
> **The content of a proposal can be adding a new key-value pair, updating an existing key-value pair, etc.**
```go
// httpapi.go
v, err := io.ReadAll(r.Body)
if err != nil {
log.Printf("Failed to read on PUT (%v)\n", err)
http.Error(w, "Failed on PUT", http.StatusBadRequest)
return
}
h.store.Propose(key, string(v))
w.WriteHeader(http.StatusNoContent)
```
Next, let's look into the `Propose` method of the kvstore module to see how a proposal is constructed and processed.
In the `Propose` method, we first encode the key-value pair to be written using gob, and then pass the encoded content to `proposeC`, a channel responsible for transmitting proposals constructed by the kvstore module to the raft module.
```go
// kvstore.go
func (s *kvstore) Propose(k string, v string) {
var buf strings.Builder
if err := gob.NewEncoder(&buf).Encode(kv{k, v}); err != nil {
log.Fatal(err)
}
s.proposeC <- buf.String()
}
```
The proposal constructed by kvstore and passed to `proposeC` is received and processed by the `serveChannels` method in the raft module.
After confirming that `proposeC` has not been closed, the raft module submits the proposal to the raft algorithm library for processing using the `Propose` method provided by the raft algorithm library.
```go
// raft.go
select {
case prop, ok := <-rc.proposeC:
if !ok {
rc.proposeC = nil
} else {
rc.node.Propose(context.TODO(), []byte(prop))
}
```
After a proposal is submitted, it follows the raft algorithm process. The proposal will eventually be forwarded to the leader node (if the current node is not the leader and you allow followers to forward proposals, controlled by the `DisableProposalForwarding` configuration). The leader will add the proposal as a log entry to its raft log and synchronize it with other follower nodes. After being deemed committed, it will be applied to the state machine and the result will be returned to the user.
However, since the etcd raft library itself does not handle communication between nodes, appending to the raft log, applying to the state machine, etc., the raft library only prepares the data required for these operations. The actual operations must be performed by us.
Therefore, we need to receive this data from the raft library and process it accordingly based on its type. The `Ready` method returns a read-only channel through which we can receive the data that needs to be processed.
> **It should be noted that the received data includes multiple fields, such as snapshots to be applied, log entries to be appended to the raft log, messages to be transmitted over the network, etc.**
Continuing with our write request example (leader node), after receiving the corresponding data, we need to persistently save snapshots, `HardState`, and `Entries` to handle issues caused by server crashes (e.g., a follower voting for multiple candidates). `HardState` and `Entries` together comprise the `Persistent state on all servers` as mentioned in the paper. After persistently saving them, we can apply the snapshot and append to the raft log.
Since we are currently the leader node, the raft library will return `MsgApp` type messages to us (corresponding to `AppendEntries` RPC in the paper). We need to send these messages to the follower nodes. Here, we use the rafthttp provided by etcd for node communication and send the messages to follower nodes using the `Send` method.
```go
// raft.go
case rd := <-rc.node.Ready():
if !raft.IsEmptySnap(rd.Snapshot) {
rc.saveSnap(rd.Snapshot)
}
rc.wal.Save(rd.HardState, rd.Entries)
if !raft.IsEmptySnap(rd.Snapshot) {
rc.raftStorage.ApplySnapshot(rd.Snapshot)
rc.publishSnapshot(rd.Snapshot)
}
rc.raftStorage.Append(rd.Entries)
rc.transport.Send(rc.processMessages(rd.Messages))
applyDoneC, ok := rc.publishEntries(rc.entriesToApply(rd.CommittedEntries))
if !ok {
rc.stop()
return
}
rc.maybeTriggerSnapshot(applyDoneC)
rc.node.Advance()
```
Next, we use the `publishEntries` method to apply the committed raft log entries to the state machine. As mentioned earlier, in raftexample, the kvstore module acts as the state machine. In the `publishEntries` method, we pass the log entries that need to be applied to the state machine to `commitC`. Similar to the earlier `proposeC`, `commitC` is responsible for transmitting the log entries that the raft module has deemed committed to the kvstore module for application to the state machine.
```go
// raft.go
rc.commitC <- &commit{data, applyDoneC}
```
In the `readCommits` method of the kvstore module, messages read from `commitC` are gob-decoded to retrieve the original key-value pairs, which are then stored in a map structure within the kvstore module.
```go
// kvstore.go
for commit := range commitC {
...
for _, data := range commit.data {
var dataKv kv
dec := gob.NewDecoder(bytes.NewBufferString(data))
if err := dec.Decode(&dataKv); err != nil {
log.Fatalf("raftexample: could not decode message (%v)", err)
}
s.mu.Lock()
s.kvStore[dataKv.Key] = dataKv.Val
s.mu.Unlock()
}
close(commit.applyDoneC)
}
```
Returning to the raft module, we use the `Advance` method to notify the raft library that we have finished processing the data read from the `Ready` channel and are ready to process the next batch of data.
Earlier, on the leader node, we sent `MsgApp` type messages to the follower nodes using the `Send` method. The follower node's rafthttp listens on the corresponding port to receive requests and return responses. Whether it's a request received by a follower node or a response received by a leader node, it will be submitted to the raft library for processing through the `Step` method.
> **`raftNode` implements the `Raft` interface in rafthttp, and the `Process` method of the `Raft` interface is called to handle the received request content (such as `MsgApp` messages).**
```go
// raft.go
func (rc *raftNode) Process(ctx context.Context, m raftpb.Message) error {
return rc.node.Step(ctx, m)
}
```
The above describes the complete processing flow of a write request in raftexample.
## Summary
This concludes the content of this article. By outlining the structure of raftexample and detailing the processing flow of a write request, I hope to help you better understand how to use the etcd raft library to build your own distributed KV storage service.
If there are any mistakes or issues, please feel free to comment or message me directly. Thank you.
## References
- https://github.com/etcd-io/etcd/tree/main/contrib/raftexample
- https://github.com/etcd-io/raft
- https://raft.github.io/raft.pdf | justlorain |
1,899,740 | Introduction to CSS Frameworks | Introduction to CSS Frameworks CSS frameworks are pre-prepared libraries that are supposed... | 0 | 2024-07-05T06:54:24 | https://dev.to/hillaryprosper_wahua_bce/introduction-to-css-frameworks-1g4b | beginners, webdev, css, frontend | # Introduction to CSS Frameworks
CSS frameworks are pre-prepared libraries that are supposed to be used as the starting design for any website or web application. They are set up to include CSS styles and guidelines that standardize and make web development much easier. Most frameworks come with pre-written classes and components of common web elements such as grids, buttons, forms, and navigation menus. This way, a developer can easily structure and design web projects without much effort.
Using a CSS framework offers several advantages that streamline the web development process and improve the quality of the end product:
- Rapid Development: CSS frameworks provide pre-written, ready-to-use code that is reusable for the most common UI elements one might come across—buttons, forms, and navigation bars. It thus speeds up the development process and allows developers to put together a website in less time.
- Consistency: Frameworks offer stylized standards and components used for designing the entire site. Consistency in design maintains coherent user experience and branding across the website.
- Responsive Design: Most of the CSS frameworks come with a built-in support to make your web designs responsive. This indicates that a website can inherently look great on any device and in any screen size automatically—possibly using grid systems, responsive utilities, and media query integrations. Cross-Browser Compatibility: CSS frameworks are built to feature the same functionality and look in each browser and device. It reduces the need for extra fixes and optimizations based on the special browsers.
- Code Quality: Frameworks should encourage development with modularity, separation of concerns, and organization of code best practices for the development of CSS. In this way, one could achieve a cleaner, more maintainable code base written with less effort, which would then also become much easier to debug and extend.
- Community Support: Common CSS frameworks like Bootstrap, Tailwind CSS have a massive community of developers contributing to plugins, extensions, and documentation continuously in the ecosystem. They are invaluable resources that provide useful tutorials for the developer working with the framework. Customizability: While it is true that CSS frameworks come with their own styles and components out of the box, that does not at all mean that they are highly unchangeable. In fact, they are very easy to tweak and customize for a specific project and brand.
## Getting Started with Bootstrap
Bootstrap, by Twitter engineers Mark Otto and Jacob Thornton, was first released in early August 2011 for internal usage at Twitter; later, it turned open source in August 2011. It has subsequently evolved to be one of the most popular CSS frameworks for responsive and mobile-first website development.
**Key Features:**
- Responsive grid system for layout design.
- Pre-styled UI components like buttons, forms, and navigation bars.
- Built-in JavaScript plugins for interactive elements.
- Extensive documentation and community support.
### Setting Up Bootstrap in a Project
Using Bootstrap via `CDN`
Add the following `<link>` tag to the <head> section of your HTML file
```
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" rel="stylesheet">
```
This will load the Bootstrap CSS file from a Content Delivery Network (CDN) into your project.
Installing Bootstrap via `npm`
If you're using `npm` as your package manager, you can install Bootstrap by running the following command in your project directory:
```
npm install bootstrap
```
Once installed, you can import Bootstrap into your project's JavaScript or CSS files as needed.
```
import 'bootstrap/dist/css/bootstrap.min.css';
```
**Basic Structure of a Bootstrap Project**
Here's a basic structure for a Bootstrap project using `npm`:
```
project-root/
│
├── node_modules/ # Folder containing npm packages (automatically generated)
│
├── src/ # Source code directory
│ ├── css/ # CSS files
│ │ └── styles.css # Your custom CSS files
│ │
│ ├── js/ # JavaScript files
│ │ └── script.js # Your custom JavaScript files
│ │
│ └── index.html # Main HTML file
│
├── package.json # npm package configuration file
│
└── webpack.config.js # Webpack configuration file (if using Webpack)
```
Here's a basic structure for a Bootstrap project using `CDM`:
```
project/
│
├── css/
│ ├── bootstrap.min.css // Bootstrap CSS file
│ └── custom.css // Custom styles (optional)
│
├── js/
│ ├── bootstrap.min.js // Bootstrap JavaScript file
│ └── custom.js // Custom scripts (optional)
│
├── img/
│ └── ... // Images used in the project
│
│
└── index.html // Main HTML file
```
### Bootstrap Components and Utilities
**Layout and Grid System**
Understanding the Bootstrap Grid
- Bootstrap utilizes a responsive, mobile-first grid system based on a 12-column layout.
- Columns are wrapped in a `.container` for fixed-width layouts or .container-fluid for full-width layouts.
- Columns are defined using classes such as `.col`, `.col-sm`, `.col-md`, etc. specifying the number of columns to occupy at different breakpoints.
**Creating Responsive Layouts**
Different column classes can be mixed and combined with varied breakpoints to design a responsive layout.
For example, `<div class="col-12 col-md-6 col-lg-4">` creates a column with sizes 12 for small screens, 6 for medium screens, and 4 for large screens.
**Common Components**
Navbar:
The Bootstrap navbar component provides navigation links and branding in a horizontal bar.
It can be customized with various options like color schemes, positioning, and responsiveness.
```html
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">Navbar</a>
<button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item active">
<a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a>
</li>
<!-- Add more navigation links here -->
</ul>
</div>
</nav>
```
Buttons:
Bootstrap provides pre-styled buttons in various styles and sizes.
Buttons can have different contextual variations like primary, secondary, success, etc.
Example:
```html
<button type="button" class="btn btn-primary">Primary</button>
```
Forms:
Bootstrap offers styles for form elements like input fields, checkboxes, radio buttons, etc.
Forms can be styled with grid classes for layout control.
Example :
```html
<form>
<div class="form-group">
<label for="exampleInputEmail1">Email address</label>
<input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp">
<small id="emailHelp" class="form-text text-muted">We'll never share your email with anyone else.</small>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
```
Modals:
Bootstrap modals are dialog boxes that float over the main content.
They can contain any HTML content and are commonly used for notifications, alerts, or interactive forms.
Example:
```html
<div class="modal fade" id="exampleModal" tabindex="-1" role="dialog" aria-labelledby="exampleModalLabel" aria-hidden="true">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Modal title</h5>
<button type="button" class="close" data-dismiss="modal" aria-label="Close">
<span aria-hidden="true">×</span>
</button>
</div>
<div class="modal-body">
<!-- Modal content goes here -->
</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button>
<button type="button" class="btn btn-primary">Save changes</button>
</div>
</div>
</div>
</div>
```
### Utility Classes
**Spacing**:
Bootstrap provides utility classes for adding margin and padding to elements.
Classes like m-1, p-2, mt-3, etc., allow developers to add margin and padding in various sizes and directions.
Example
```html
<div class="mt-3 mb-2 p-4">Content with margin and padding</div>
```
**Typography**
Bootstrap includes utility classes for typography, such as text alignment, font weights, and text transformations.
Classes like text-center, font-weight-bold, text-uppercase, etc., can be used to style text elements.
Example
```html
<p class="text-center font-weight-bold">Centered and bold text</p>
```
Colors:
Bootstrap offers utility classes for applying background and text colors.
Classes like bg-primary, text-success, bg-dark, etc., allow developers to quickly apply colors to elements.
Example:
```html
<div class="bg-primary text-light p-3">Primary background color with light text</div>
```
Bootstrap's components and utilities provide a convenient and consistent way to design and build responsive websites with minimal effort. Developers can leverage these features to create visually appealing and user-friendly web applications.
## Getting Started with Tailwind CSS
Tailwind CSS is a utility-first CSS framework created by Adam Wathan and Steve Schoger, first released in 2017. Unlike traditional CSS frameworks that provide pre-designed components, Tailwind offers a set of utility classes that enable developers to build custom designs directly in their HTML. This approach allows for highly customizable and flexible designs, promoting a design system approach where styles are composed by applying utility classes directly to HTML elements.
### Setting Up Tailwind CSS in a Project
Using Tailwind CSS via`CDN`
To quickly get started with Tailwind CSS, you can use the `CDN` link in your HTML file:
```
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/tailwind.min.css" rel="stylesheet">
```
This method is ideal for prototyping or smaller projects where you don't need extensive customization.
Installing Tailwind CSS via` npm`
For larger projects or when you need to customize Tailwind, it's best to install it via `npm`. Follow these steps:
Run the following command in your project directory:
```
npm install -D tailwindcss
```
Create Configuration File:
Generate a `tailwind.config.js` file to customize your Tailwind setup:
```
npx tailwindcss init
```
Configure Your Template Paths:
In your tailwind.config.js file, specify the paths to all of your template files:
```js
module.exports = {
content: [
"./src/**/*.{html,js}",
],
theme: {
extend: {},
},
plugins: [],
}
```
Add Tailwind Directives to Your CSS:
Create a CSS file (e.g., `styles.css`) and add the following directives:
```css
@tailwind base;
@tailwind components;
@tailwind utilities;
```
Build Your CSS:
Configure your build process to include Tailwind. Here's an example using PostCSS:
```
npm install -D postcss postcss-cli autoprefixer
```
Create a postcss.config.js file:
```js
module.exports = {
plugins: [
require('tailwindcss'),
require('autoprefixer'),
]
}
```
Build your CSS
```
npx postcss styles.css -o output.css
```
Basic Structure of a Tailwind CSS Project
A basic Tailwind CSS project structure looks like this:
```
project/
│
├── src/
│ ├── index.html // Main HTML file
│ └── styles.css // Tailwind directives
│
├── dist/
│ └── output.css // Compiled CSS file
│
├── node_modules/ // Installed npm packages
│
├── tailwind.config.js // Tailwind configuration file
│
├── postcss.config.js // PostCSS configuration file
│
└── package.json // Project metadata and dependencies
```
#### Tailwind CSS Concepts and Utilities
- Utility-First Approach
The utility-first approach in Tailwind CSS means that instead of writing custom CSS, you use pre-defined utility classes to style your HTML elements. Each utility class does one specific thing, such as setting a margin, padding, font size, or color. This approach encourages a highly composable and flexible design system.
```html
<div class="p-4 m-2 bg-blue-500 text-white">
Hello, Tailwind CSS!
</div>
```
In this example, `p-4` sets padding, `m-2` sets margin, `bg-blue-500` sets the background color, and `text-white` sets the text color.
- Customizing Tailwind Configuration
Tailwind is highly customizable via its configuration file (`tailwind.config.js`). You can extend the default theme, add new utility classes, and configure plugins.
```javascript
// tailwind.config.js
module.exports = {
content: [
"./src/**/*.{html,js}",
],
theme: {
extend: {
colors: {
customBlue: '#1e40af',
},
spacing: {
'128': '32rem',
},
},
},
plugins: [],
}
```
In this example, a custom blue color and a new spacing value are added to the default theme.
- Common Utilities
- Flexbox and Grid
Tailwind provides utility classes for flexbox and CSS grid to create complex layouts.
Flexbox
```html
<div class="flex justify-center items-center h-screen">
<div class="p-6 bg-gray-200">
Centered Content
</div>
</div>
```
In this example, flex creates a flex container, justify-center centers the content horizontally, and items-center centers it vertically.
Grid:
```html
<div class="grid grid-cols-3 gap-4">
<div class="bg-gray-200 p-4">1</div>
<div class="bg-gray-200 p-4">2</div>
<div class="bg-gray-200 p-4">3</div>
</div>
```
In this example, `grid` creates a grid container, `grid-cols-3` defines a three-column layout, and `gap-4` adds spacing between the grid items.
- Spacing and Sizing
Tailwind's spacing utilities handle margin, padding, and width/height.
```html
<div class="m-4 p-4 w-64 h-32 bg-gray-200">
Box with margin, padding, width, and height
</div>
```
In this example, `m-4` adds margin, `p-4` adds padding, `w-64` sets the width, and `h-32` sets the height.
#### Typography and Colors.
Tailwind provides extensive typography and color utilities.
Typography
```html
<p class="text-lg font-semibold text-gray-800">
Large, semibold, gray text
</p>
```
In this example, `text-lg` sets the font size, `font-semibold` sets the font weight, and `text-gray-800` sets the text color.
Colors:
```html
<div class="bg-red-500 text-white p-4">
Red background with white text
</div>
```
In this example, bg-red-500 sets the background color, and text-white sets the text color.
- Creating Reusable Components with Tailwind
Reusable components in Tailwind can be created by combining utility classes into custom class names using @apply in your CSS files.
```css
.btn {
@apply px-4 py-2 bg-blue-500 text-white rounded;
}
.btn-primary {
@apply bg-blue-600 hover:bg-blue-700;
}
```
In this example, `@apply` combines multiple utility classes into a custom class. The `.btn `class includes padding, background color, text color, and border-radius `.btn-primary `extends it with additional styles.
#### Advantages of CSS Frameworks
- **Rapid Development**:
- Time-Saving: Pre-designed components and grid systems accelerate development.
- Consistency: Ensures a consistent look and feel across the entire project.
- **Responsive Desig**n:
- Built-In Responsiveness: Most frameworks include responsive design principles, making it easier to create mobile-friendly sites.
- Cross-Browser Compatibility: Frameworks are tested across various browsers, reducing compatibility issues.
- **Customization and Flexibility**:
- Customizable Components: Allows for the customization of predefined styles and components.
- Themeable: Easily apply different themes or modify existing ones to suit project needs.
#### Potential Drawbacks and Limitations
- Overhead and Performance:
- File Size: Frameworks can be bulky, adding unnecessary code that might not be used.
- Performance Issues: Can slow down page load times if not optimized properly.
- Learning Curve:
- Initial Learning: Time and effort are required to learn the framework’s structure and classes.
- Customization Complexity: Customizing beyond basic modifications can become complex and time-consuming.
- When to Use and When to Avoid
- When to Use:
- Prototyping: For quick prototyping and getting a project off the ground rapidly.
- Standard Projects: When building standard websites or applications with common requirements.
- Team Projects: In projects with multiple developers to ensure consistency and faster collaboration.
- Beginner Projects: For beginners who can benefit from structured, ready-to-use components and best practices.
- When to Avoid:
- Highly Customized Projects: When a unique, bespoke design is required that might not fit within the framework's constraints.
- Performance-Critical Applications: When performance is a critical factor, and every byte of CSS and JS counts.
- Minimalist Projects: For very simple projects where a framework would add unnecessary complexity and overhead.
- Experimental Designs: When experimenting with new design trends or techniques that aren’t well-supported by existing frameworks.
### Conclusion
Using CSS frameworks can greatly enhance the efficiency, consistency, and quality of web development projects. They offer a range of pre-designed components, utilities, and responsive design features that simplify the process of creating professional, cross-browser compatible websites. However, it's essential to weigh their advantages against potential drawbacks, such as performance overhead and customization complexity, to determine if they are suitable for your specific project needs.
**Additional Resources and Tutorials**
To further enhance your skills and understanding of CSS frameworks, explore the following resources:
Bootstrap:
- [Bootstrap Documentation](https://getbootstrap.com/docs/4.1/getting-started/introduction/)
- [Bootstrap 4 Tutorial by W3Schools](https://www.w3schools.com/bootstrap4/)
- [Bootstrap Components](https://getbootstrap.com/docs/5.0/customize/components/)
Tailwind CSS:
- [Tailwind CSS Documentation](https://tailwindcss.com/docs/installation)
- [Tailwind CSS Tutorial by Traversy Media](https://www.youtube.com/watch?v=UBOj6rqRUME)
- [Building with Tailwind CSS](https://www.youtube.com/watch?v=21HuwjmuS7A&list=PL7CcGwsqRpSM3w9BT_21tUU8JN2SnyckR&index=2)
| hillaryprosper_wahua_bce |
1,912,341 | How to Scan a Barcode in C# | How to Scan a Barcode in C Create or Open Visual Studio Project Install IronBarcode... | 0 | 2024-07-05T06:52:29 | https://dev.to/mhamzap10/how-to-scan-a-barcode-in-c-ced | webdev, barcode, csharp, dotnet | ###How to Scan a Barcode in C#
1. Create or Open Visual Studio Project
2. Install IronBarcode Library
3. Pass Input Image File Path to BarcodeReader.Read() Method
4. Loop Through Extracted Results to Get Barcode Value
In today’s digital age, [barcodes](https://en.wikipedia.org/wiki/Barcode) are ubiquitous, used across various industries for tracking products, managing inventory, and facilitating transactions. Developing a barcode scanner application in C# is a valuable skill, particularly with the robust capabilities provided by the [IronBarcode](https://ironsoftware.com/csharp/barcode/) library. This article will guide you through building a barcode scanner in C# using IronBarcode, covering installation, basic usage, advanced features, and best practices.
##What is IronBarcode:
[IronBarcode](https://ironsoftware.com/csharp/barcode/) is a comprehensive C# library designed for [barcode generation](https://ironsoftware.com/csharp/barcode/tutorials/csharp-barcode-image-generator/) and [reading](https://ironsoftware.com/csharp/barcode/tutorials/reading-barcodes/), offering a robust set of features and capabilities that make it a versatile tool for developers. It supports a wide range of [barcode formats](https://ironsoftware.com/csharp/barcode/troubleshooting/support-barcode-formats/), including QR codes, Code 128, Code 39, and EAN, and can handle [multiple barcodes](https://ironsoftware.com/csharp/barcode/how-to/read-multiple-barcodes/) in a single image. IronBarcode allows for barcode reading from images, PDFs, and live video feeds, making it suitable for various applications such as inventory management, point-of-sale systems, and document processing. It also enables [barcode customization](https://ironsoftware.com/csharp/barcode/how-to/customize-barcode-style/), including color, size, and text annotations. With its ease of integration and extensive documentation, IronBarcode is ideal for both simple and complex barcode-related tasks in diverse industries.
##Getting Started with IronBarcode
###Installation
To begin, you need to install the IronBarcode library. This can be easily done via NuGet Package Manager in Visual Studio. Open the NuGet Package Manager Console and run the following command:
```
Install-Package Barcode
```
Alternatively, you can install it via the NuGet Package Manager GUI by searching for "IronBarcode".
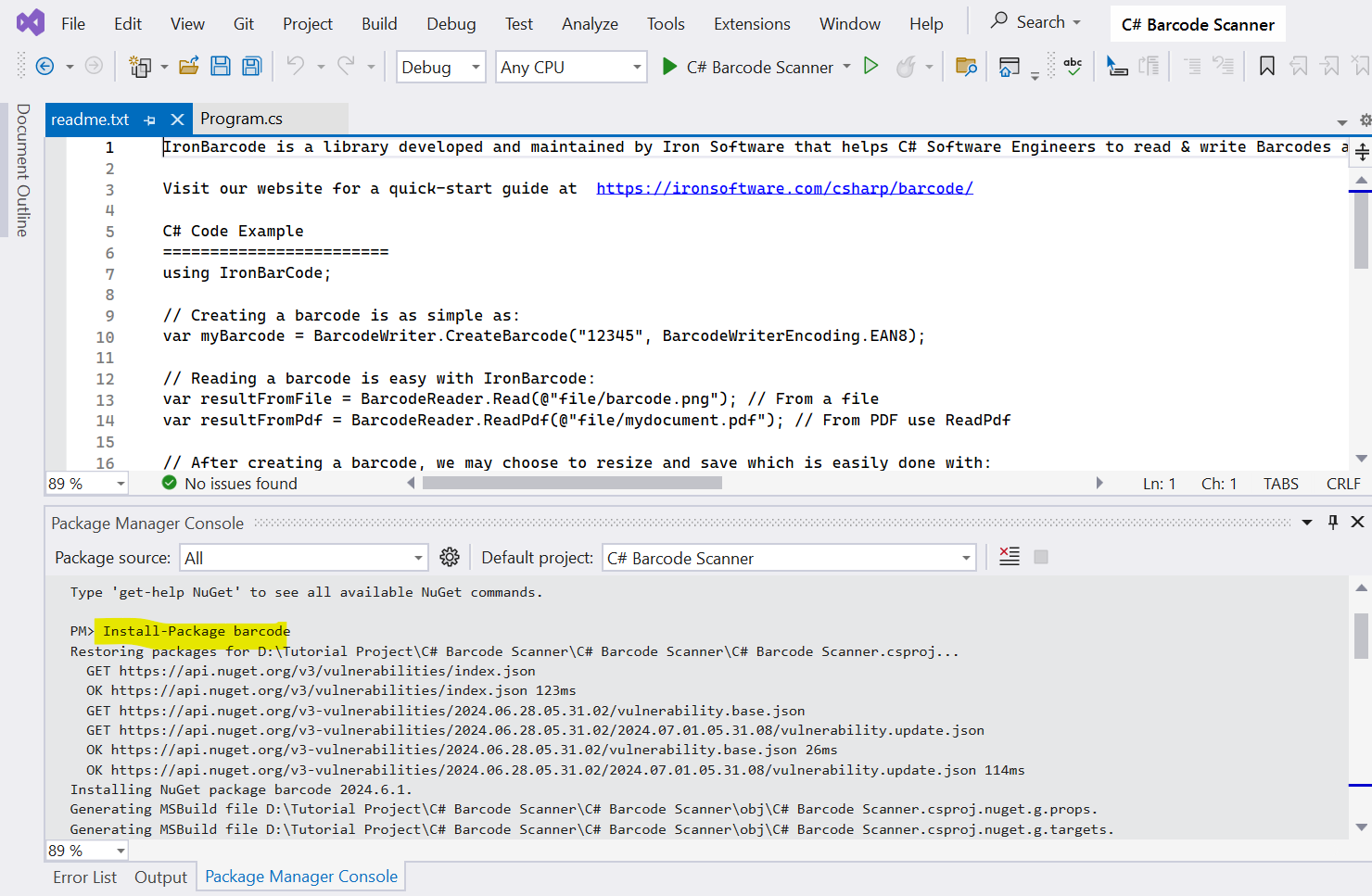
##Basic Barcode Scanning
###Reading Barcodes from Images
IronBarcode makes it straightforward to read barcodes from images. Here’s a simple example of how to read a barcode from an image file:
```
var barcodeResult = BarcodeReader.Read(@"barcode.png");
foreach (var barcode in barcodeResult)
{
Console.WriteLine("Barcode Value: " + barcode.Text);
Console.WriteLine("Barcode Format: " + barcode.BarcodeType);
}
```
The above code uses IronBarcode to read barcodes from an image file named "barcode.png". The BarcodeReader.Read method reads the barcodes from the specified image. It then iterates through each detected barcode in the barcodeResult collection, printing its value and format to the console for each barcode found.
The Barcode Image used in this example is as:
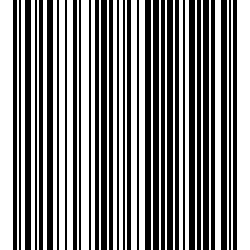
The Output generated from the above code is as:
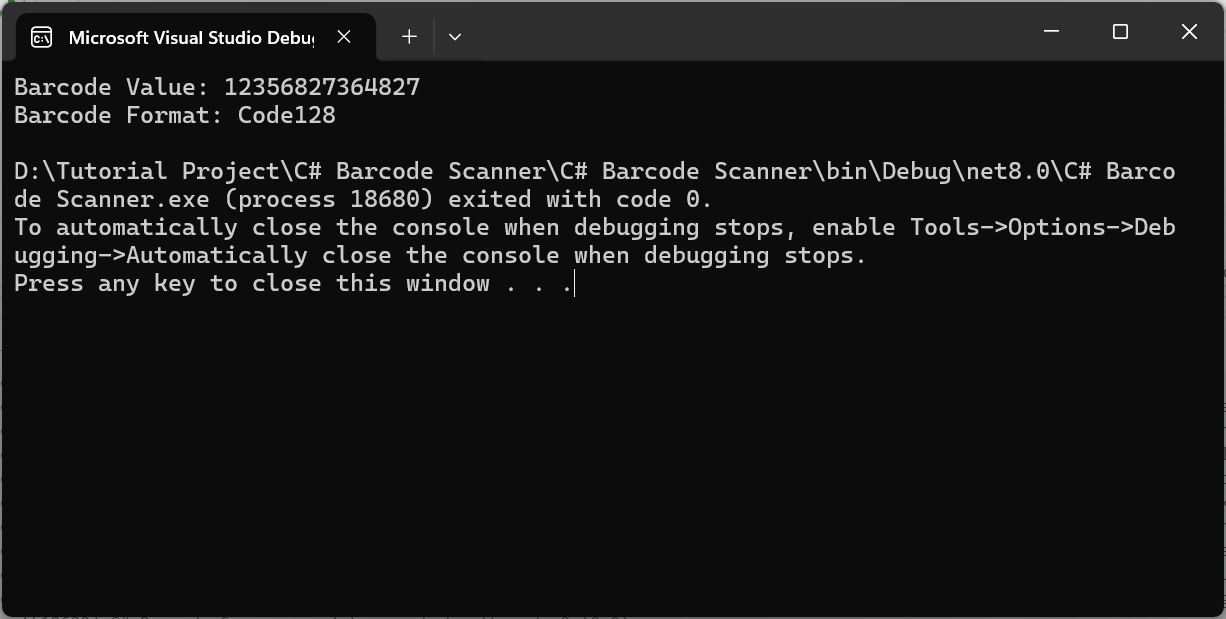
###Reading Barcode from PDF Files:
IronBarcode makes it straightforward to read barcodes from PDF file. Here’s a simple example of how to read a barcode from a pdf file:
```
var barcodeResult = BarcodeReader.ReadPdf(@"barcode.pdf");
foreach (var barcode in barcodeResult)
{
Console.WriteLine("Barcode Value: " + barcode.Text);
Console.WriteLine("Barcode Format: " + barcode.BarcodeType);
}
```
This code snippet uses IronBarcode to read barcodes from a PDF file named "barcode.pdf" using the BarcodeReader.ReadPdf method. It iterates through each detected barcode in the barcodeResult collection, printing the barcode's value and format to the console for each barcode found.
The PDF file used in this example is as:
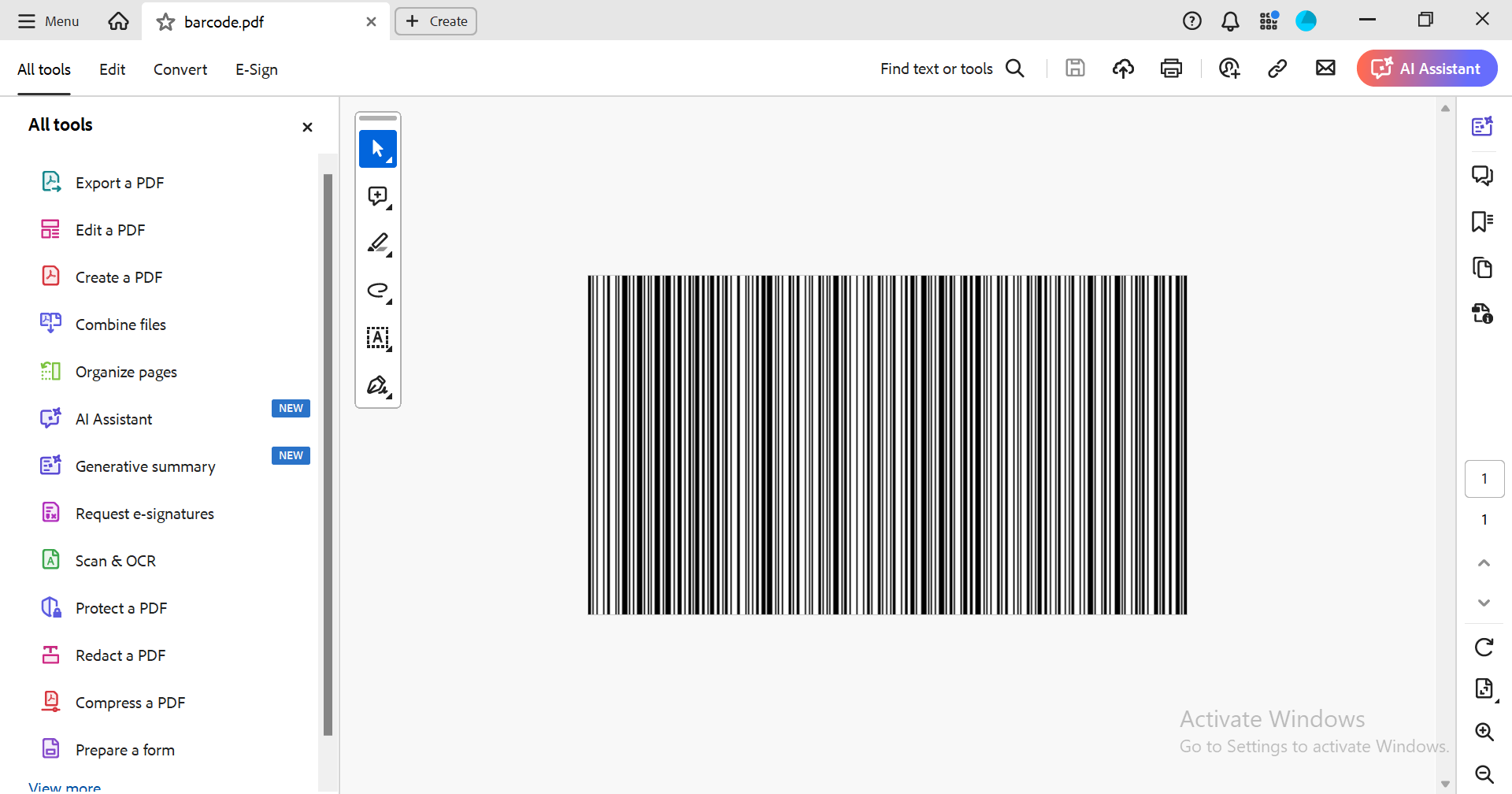
The output generated is as:
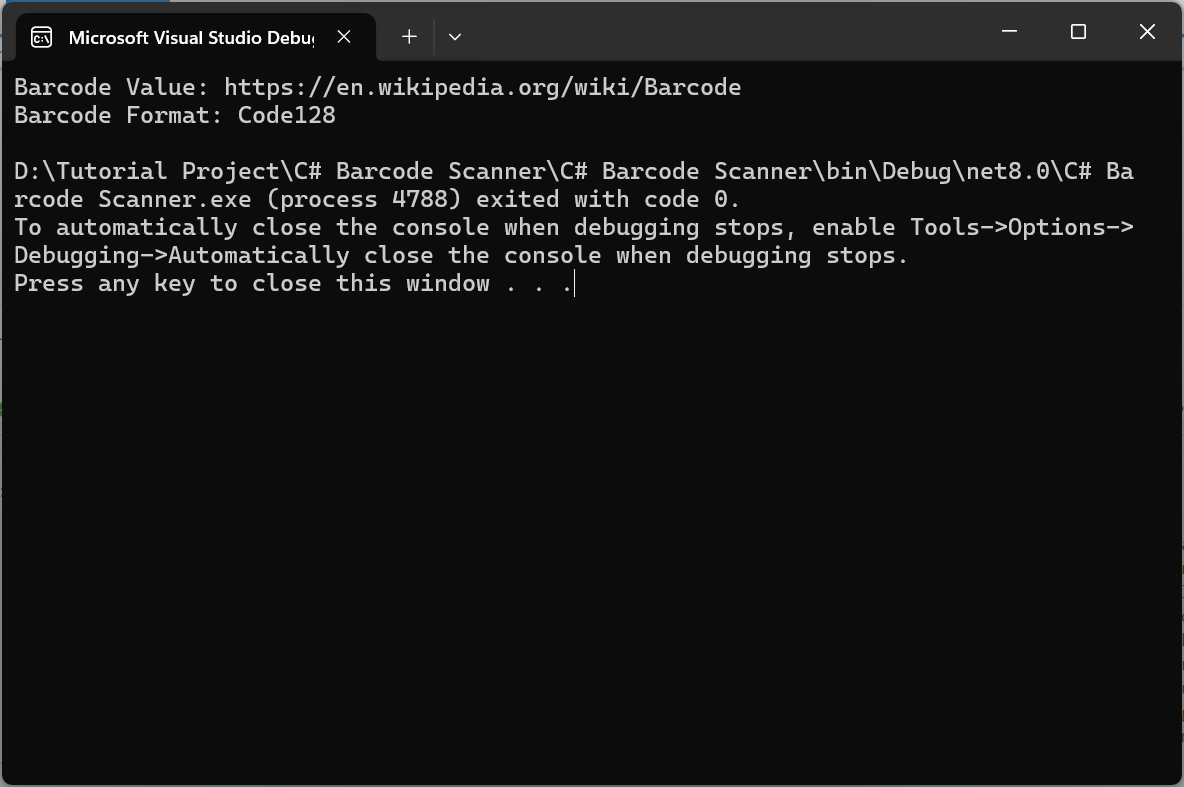
##Advanced Features
###Multiple Barcode Formats
IronBarcode supports a wide range of barcode formats, including QR code, Code 128, Code 39, EAN, and more. You can specify the type of barcode you want to read from barcode images:
```
BarcodeReaderOptions options = new BarcodeReaderOptions();
options.ExpectBarcodeTypes = BarcodeEncoding.EAN13;
options.Speed = ReadingSpeed.Balanced;
var barcodeResult = BarcodeReader.Read(@"barcode_EAN13.png", options);
foreach (var barcode in barcodeResult)
{
Console.WriteLine("Barcode Value: " + barcode.Text);
Console.WriteLine("Barcode Format: " + barcode.BarcodeType);
}
```
This code snippet configures IronBarcode to read EAN-13 barcodes from an image file named "barcode_EAN13.png" using specific options. It first creates a BarcodeReaderOptions object, setting the expected barcode type to EAN-13 and the reading speed to balanced. You may set the reading speed to ReadingSpeed.Faster for faster barcode scanning. The BarcodeReader.Read method then reads the barcodes from the specified image using these options. It automatically managed the speed. Finally, it iterates through each detected barcode in the barcodeResult collection, printing the barcode's value and format to the console for each barcode found.
Barcode Image used in this example is as:
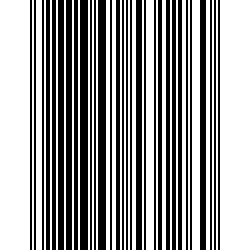
The Output of the above code is:
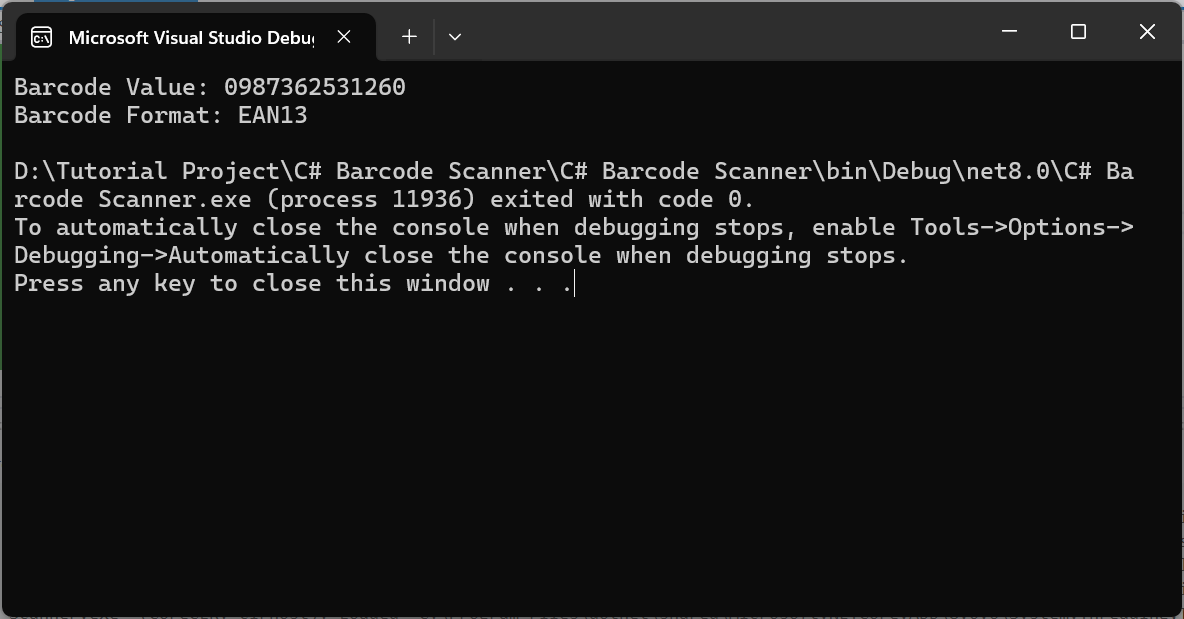
###Handling Multiple Barcodes in a Single Image
In cases where you have [multiple barcodes](https://ironsoftware.com/csharp/barcode/how-to/read-multiple-barcodes/) in a single image, IronBarcode can read multiple barcodes:
```
BarcodeReaderOptions options = new BarcodeReaderOptions();
options.ExpectBarcodeTypes = BarcodeEncoding.AllOneDimensional;
options.Speed = ReadingSpeed.Balanced;
options.ExpectMultipleBarcodes = true;
var barcodeResult = BarcodeReader.Read(@"multiple_barcode.jpg", options);
foreach (var barcode in barcodeResult)
{
Console.WriteLine("----------------------------------------");
Console.WriteLine("Barcode Value: " + barcode.Text);
Console.WriteLine("Barcode Format: " + barcode.BarcodeType);
Console.WriteLine("----------------------------------------");
}
```
The above code snippet configures IronBarcode to read multiple one-dimensional barcodes from an image file named "multiple_barcode.png" using specific options. First, it creates a BarcodeReaderOptions object and sets the ExpectBarcodeTypes property to all one-dimensional barcode types. It also sets the reading speed to balanced and enables the ExpectMultipleBarcodes property to true, allowing the reading of multiple barcodes from a single image. The BarcodeReader.Read method then reads the barcodes from the specified image using these options. The code iterates through each detected barcode in the barcodeResult collection and prints each barcode's value and format to the console, surrounded by separators for clarity.
The Input Image is as:
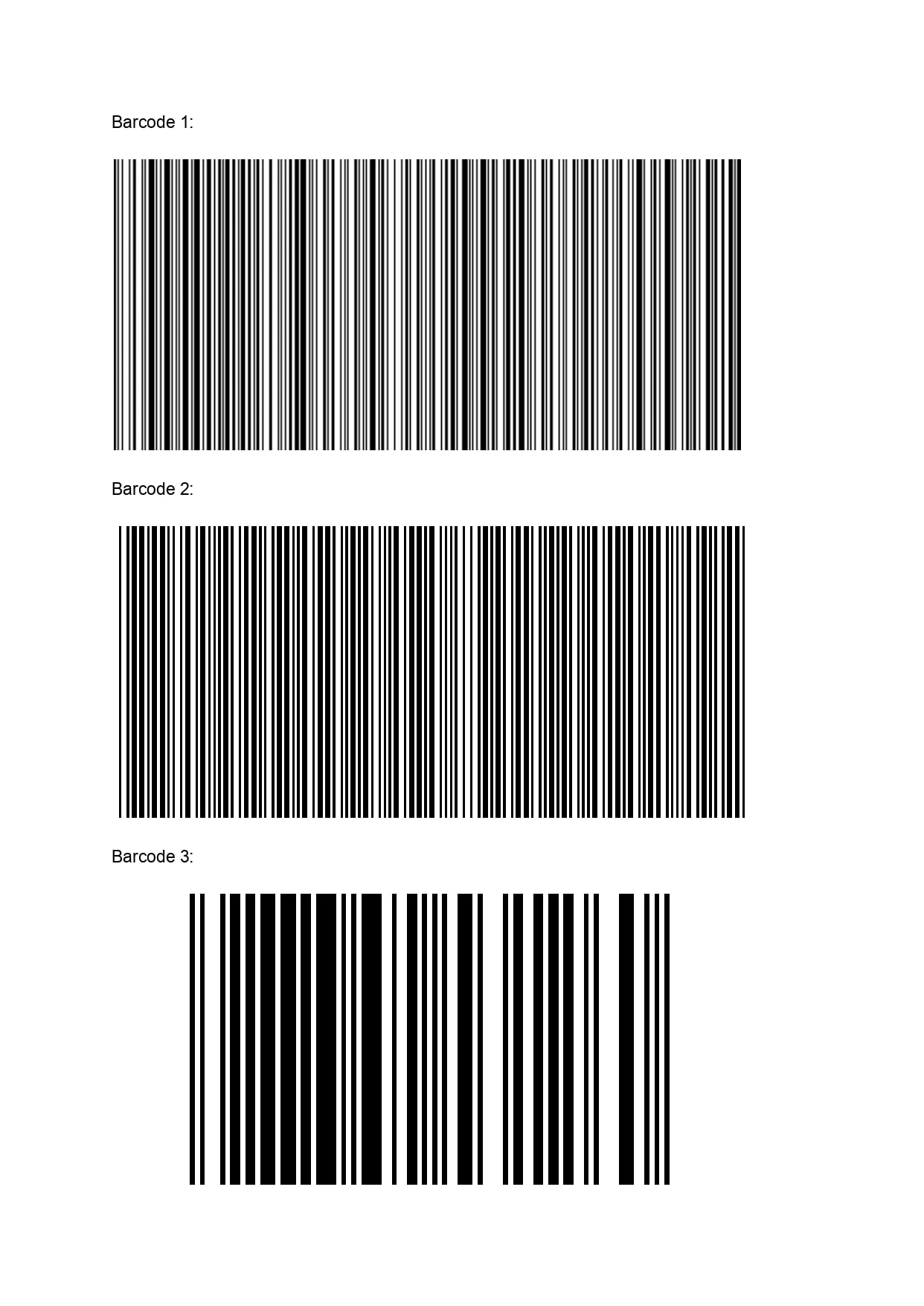
The Output of the code is as:
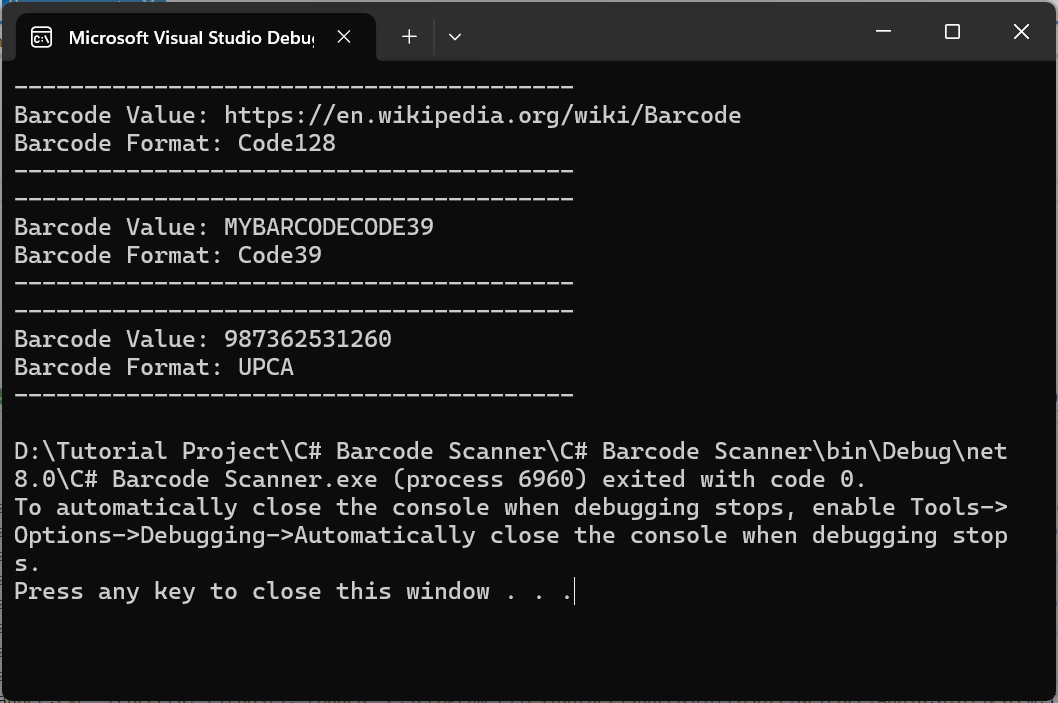
##Generating Barcodes
IronBarcode is not only limited to reading barcodes; it also provides functionalities to generate them. Here’s an example of how to create a simple barcode:
```
var barcode = BarcodeWriter.CreateBarcode("My_Barcode_Code128", BarcodeEncoding.Code128);
barcode.SaveAsPng("barcode_128.png");
```
This code snippet uses the IronBarcode library to generate a barcode. It creates a Code 128 barcode with the text "My_Barcode_Code128" using the BarcodeWriter.CreateBarcode method. After generating the barcode, it saves the barcode as a PNG image file named "barcode_128.png" using the SaveAsPng method.
The output Image is as:
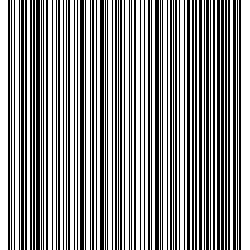
##Conclusion:
In conclusion, building a barcode scanner application in C# using IronBarcode offers developers a robust solution with extensive capabilities for barcode generation and reading. IronBarcode, as a versatile .NET library, supports a wide array of barcode formats including QR codes, Code 128, and EAN, making it suitable for diverse applications across industries such as inventory management and retail. Its ability to handle multiple barcodes from various sources, including images, PDFs, and live video feeds, underscores its adaptability in different scenarios. Moreover, IronBarcode's intuitive integration into Windows Application and its automatic management of barcode generation and reading processes simplify development tasks, enhancing efficiency and productivity.
For developers interested in exploring IronBarcode, it offers a [free trial](https://ironsoftware.com/csharp/barcode/#trial-license) to test its functionalities before opting for a [commercial license](https://ironsoftware.com/csharp/barcode/licensing/). This trial period allows developers to evaluate its suitability for their projects, ensuring compatibility and performance across multiple images and multiple documents. Whether you are building a simple barcode scanner or integrating complex barcode functionalities into enterprise applications, IronBarcode provides the tools necessary to streamline development and deliver robust barcode solutions. | mhamzap10 |
1,912,340 | How the Best Exam Dumps Websites Ensure Your Exam Success | Unlike traditional study guides or textbooks, exam dumps offer a direct peek into the real exam... | 0 | 2024-07-05T06:51:19 | https://dev.to/dedge1945/how-the-best-exam-dumps-websites-ensure-your-exam-success-5898 | webdev, javascript, beginners, programming | Unlike traditional study guides or textbooks, exam dumps offer a direct peek into the real exam questions. While study guides provide comprehensive coverage of the exam <a href="https://pass2dumps.com/">best exam dumps websites</a> topics, exam dumps are more focused, often featuring exact questions from past exams.
The Pros and Cons of Using Exam Dumps
Advantages
1. Real Exam Questions: Access to actual exam questions helps in understanding the exam's structure and difficulty level.
2. Time-Saving: Quickly identify areas where you need improvement.
3. Focused Preparation: Concentrate on the most relevant topics.
Potential Risks
1. Outdated Information: Dumps may not reflect the most recent exam updates.
2. Over-Reliance: Solely relying <a href="https://pass2dumps.com/">best exam dumps websites free</a> on dumps can lead to inadequate understanding of the subject.
3. Ethical Concerns: The use of dumps might violate exam policies or ethical standards.
Click Here For More Info>>>>>>> https://pass2dumps.com/ | dedge1945 |
1,912,339 | Exploring the Scope of Laravel Development Services and Hiring Laravel Experts | PHP has developed a long way, and today there are many frameworks, such as Symfony, Yii,... | 0 | 2024-07-05T06:50:21 | https://dev.to/techpulzz/exploring-the-scope-of-laravel-development-services-and-hiring-laravel-experts-26ad | laravel, php, website, api |
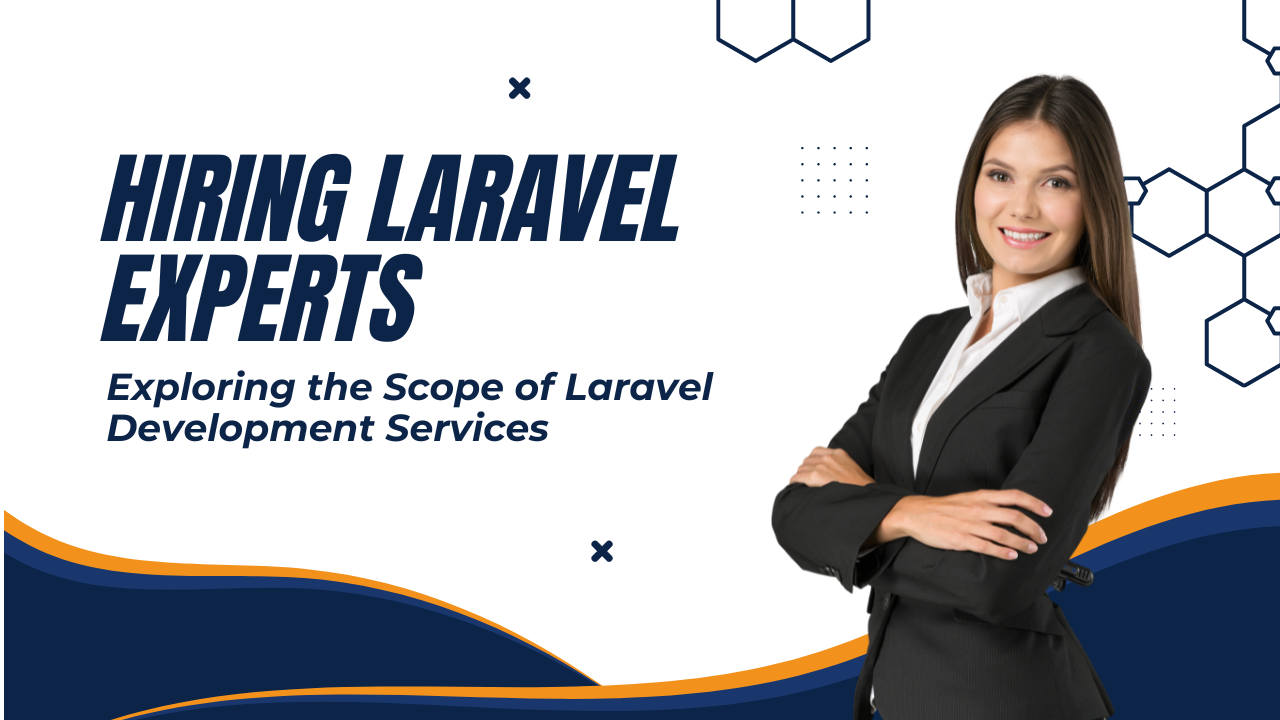
PHP has developed a long way, and today there are many frameworks, such as Symfony, Yii, CodeIgniter, and Phalcon, that can be used to make strong apps. When it comes to these, Laravel has always been one of the best MVC-based PHP platforms.
Developers prefer Laravel because it has an excellent layout and programming that is easy to understand. "Yes, we additionally enjoy stunning code, but this particular statement is not ours" is a common public statement from Laravel. The Laravel community also has stunning code.
If you want high-quality [Laravel development services](https://arhamwebworks.com/service/laravel-development/), you have to hire experienced Laravel experts who have the skills and knowledge to do a great job.
In this blog, I will discuss the advantages of hiring Laravel experts and the beginner level of Laravel developers. They can help you to use Laravel's powerful features and functionalities to create scalable and reliable web applications.
## What is Laravel?
Laravel is an open-source PHP web application framework that has increasing popularity. It has become the preferred choice for developers developing complex software systems and web applications for various types of businesses. There are a lot of opportunities open for experienced Laravel developers.
Laravel, having a Model-View-Controller (MVC) framework, provides a solid foundation for developers to build modern web applications. It is also known for having powerful features such as routing, Livewire Integration, Blade Templating Engine, database migration, and Integrated Authentication and Security. Laravel is easy to learn, use, and deploy, making it an ideal choice for startups, small businesses, and large enterprises.
## Top Features of Laravel Framework
**Routing:** Laravel offers a powerful routing system that enables developers to define clean and expressive routes for their applications.
**Templating Engine:** Laravel includes a lightweight templating engine called Blade, which makes it easy to create reusable templates.
**Authentication:** Laravel simplifies user authentication and authorization with its built-in system.
**Artisan CLI:** Laravel's robust command-line interface (CLI), Artisan, provides a variety of developer-friendly commands.
**Security:** Laravel prioritizes security with features like encryption, CSRF protection, and secure password hashing.
**Testing:** Laravel supports automated testing with PHPUnit, ensuring that applications function correctly.
**Database Migration:** Laravel's migration system allows developers to manage database schema changes using code.
**Eloquent ORM:** Laravel's Object-Relational Mapping (ORM), known as Eloquent, offers a straightforward and sophisticated way to interact with databases.
**Blade Components and Slots:** Blade's templating engine includes the ability to define reusable components and slots, simplifying complex UI development.
**Queues:** Laravel provides an intuitive API for working with job queues, improving application performance by offloading time-consuming tasks.
The demand for Laravel developers is high due to the increasing number of web-based applications across sectors such as healthcare, education, e-commerce, banking, finance, and entertainment. The rise of digital transformation and cloud-based technologies has further fueled the need for skilled Laravel experts who can build scalable, secure, and high-performance web applications.
Laravel has an active [community of developers](https://laravel.io/) and contributors regularly enhances its development, documentation, and support. This community is growing, with numerous meet-ups, conferences, and workshops being organized. These events offer developers excellent opportunities to network, learn, and collaborate, keeping them updated with the latest trends and technologies in Laravel development.
## Job Opportunities for Laravel Developers
Laravel developers can explore various career options, including web developers, software engineers, full-stack developers, PHP developers, front-end developers, back-end developers, and Laravel developers. Salaries for Laravel developers vary based on experience, skills, and location, with senior-level positions commanding higher salaries. For current salary information, you can refer to resources like [Upwork](https://www.upwork.com/hire/laravel-developer/).
In conclusion, the scope for Laravel developers is extensive due to the increasing demand for high-quality web applications and software solutions. Laravel offers a robust foundation for building modern web applications and benefits from a supportive and active community. The growth of digital transformation and cloud-based technologies continues to drive the demand for skilled Laravel developers capable of creating scalable, secure, and high-performance web applications. With the right skills and experience, Laravel developers can look forward to a wide range of career opportunities and competitive salaries.
| techpulzz |
1,912,338 | Intravenous Solutions Market: Top Trends and Innovations Shaping the Future | The global Intravenous Solutions Market, valued at US$ 10.9 billion in 2021, is poised for... | 0 | 2024-07-05T06:46:13 | https://dev.to/swara_353df25d291824ff9ee/intravenous-solutions-market-top-trends-and-innovations-shaping-the-future-58op | 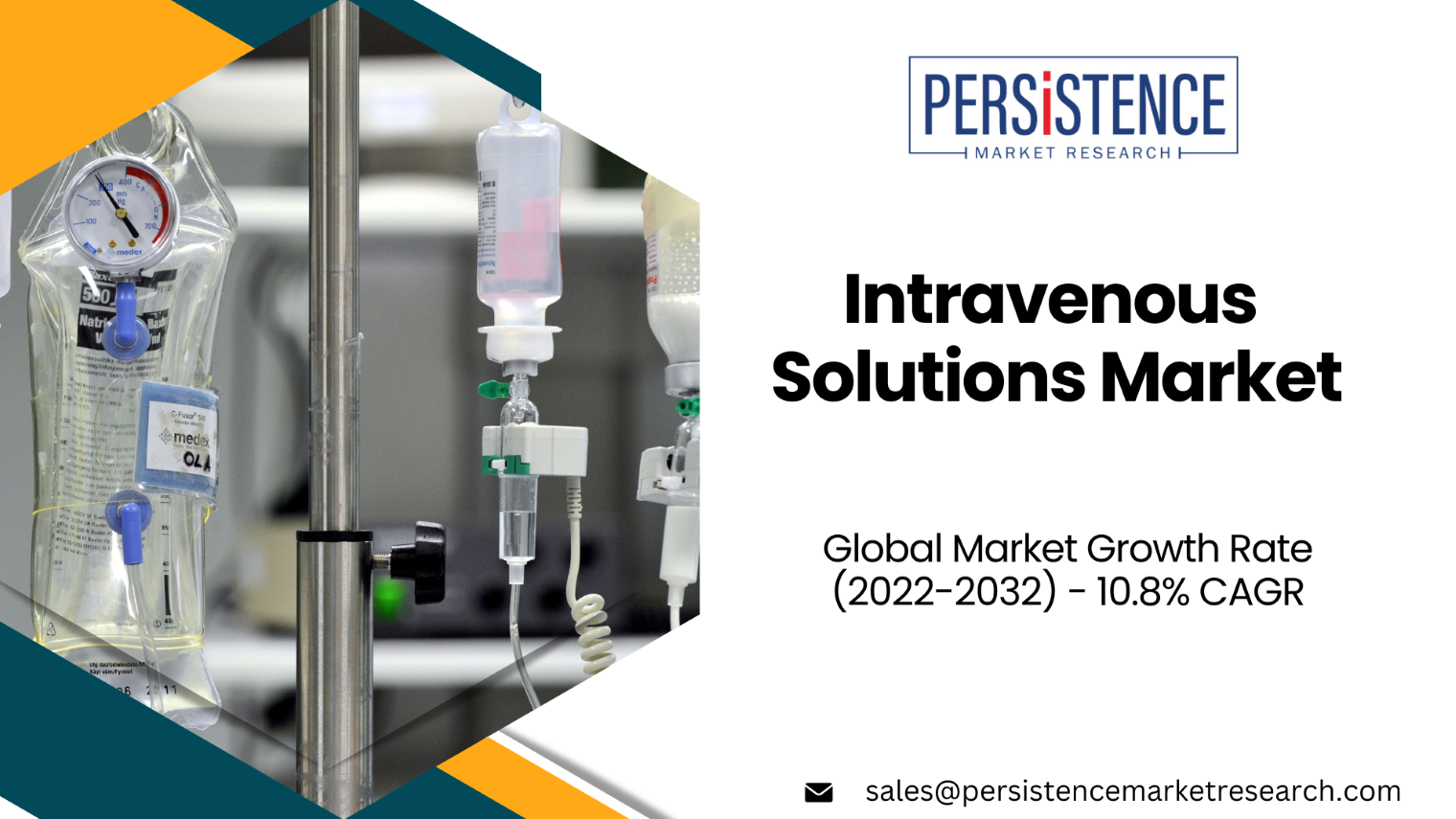
The global [Intravenous Solutions Market](https://www.persistencemarketresearch.com/market-research/intravenous-solutions-market.asp), valued at US$ 10.9 billion in 2021, is poised for substantial growth with a projected CAGR of 10.8% through 2032, reaching around US$ 33.6 billion. This growth is driven by increasing demand due to sedentary lifestyles affecting 39% of the global population, particularly among aging demographics with chronic conditions like cancer and heart disease. The United States, expected to lead consumption with significant growth opportunities, underscores the market's expansion. Major players like Fresenius Kabi and Baxter International are pivotal in meeting escalating global healthcare needs, especially in intensive care settings where IV therapy plays a critical role in patient care and recovery.
**Key Trends**
The intravenous (IV) solutions market is undergoing rapid transformation, driven by technological advancements, changing healthcare landscapes, and evolving patient needs. As the demand for efficient and effective IV therapies grows, several key trends and innovations are shaping the future of this critical healthcare sector.
**Technological Advancements in IV Delivery Systems**
Technological innovations in IV delivery systems are revolutionizing patient care. Smart infusion pumps equipped with advanced software and connectivity features are enhancing the precision and safety of IV therapy. These pumps enable healthcare providers to monitor infusion rates, detect errors, and adjust treatment plans in real-time, thereby reducing medication errors and improving patient outcomes.
**Shift Towards Personalized Medicine**
There is a notable shift towards personalized medicine in the IV solutions market. Advances in genomic research and biomarker identification are enabling healthcare providers to tailor IV therapies to individual patient profiles. Personalized IV solutions not only improve treatment efficacy but also minimize adverse reactions, leading to better patient compliance and satisfaction.
**Expansion of Home Healthcare Services**
The expansion of home healthcare services is significantly influencing the IV solutions market. Patients are increasingly opting for IV therapies administered in the comfort of their homes, driven by convenience, cost-effectiveness, and the desire for independence. This trend is prompting manufacturers to develop portable and user-friendly IV solutions that meet the stringent safety and efficacy standards required for home-based care.
**Focus on Patient Safety and Infection Control**
Patient safety remains a paramount concern in the IV solutions market. Manufacturers are investing in innovative technologies and materials to minimize the risk of contamination and infections associated with IV therapy. Antimicrobial coatings, sterile packaging, and closed-system transfer devices (CSTDs) are among the advancements aimed at enhancing product safety and protecting patient health.
**Sustainable Practices and Eco-friendly Solutions**
There is a growing emphasis on sustainability within the healthcare industry, including the IV solutions market. Manufacturers are increasingly adopting eco-friendly practices and materials in product development and packaging. From biodegradable materials to energy-efficient manufacturing processes, sustainability initiatives are gaining traction as healthcare providers and consumers prioritize environmental responsibility.
**Integration of Artificial Intelligence (AI) and Data Analytics**
The integration of artificial intelligence (AI) and data analytics is revolutionizing healthcare delivery, including IV therapy. AI-powered algorithms analyze patient data in real-time, enabling healthcare providers to optimize IV treatment protocols, predict patient responses, and mitigate risks. Data-driven insights enhance treatment precision, reduce healthcare costs, and improve overall patient care outcomes.
**Regulatory Advancements and Compliance**
Stringent regulatory standards continue to shape the landscape of the IV solutions market. Regulatory bodies worldwide are updating guidelines to ensure the safety, efficacy, and quality of IV products. Manufacturers are focusing on regulatory compliance, clinical validation, and post-market surveillance to meet evolving regulatory requirements and maintain market competitiveness.
**Collaborative Partnerships and Strategic Alliances**
Collaborative partnerships and strategic alliances are fostering innovation and market growth in the IV solutions sector. Industry players are forming alliances with research institutions, healthcare providers, and technology firms to co-develop next-generation IV therapies and delivery systems. These partnerships accelerate product innovation, expand market reach, and address unmet medical needs more effectively.
**Future Outlook**
Looking ahead, the future of the intravenous solutions market is promising, driven by continuous innovation, expanding healthcare access, and the increasing prevalence of chronic diseases globally. As technology continues to advance and healthcare delivery models evolve, the demand for safer, more efficient, and personalized IV solutions will continue to grow. Manufacturers and healthcare providers are poised to capitalize on these trends by leveraging cutting-edge technologies, embracing sustainable practices, and fostering collaborative partnerships to shape the future of IV therapy.
**Conclusion**
The intravenous solutions market is at a pivotal juncture, characterized by transformative trends and innovations that are redefining patient care. From advanced IV delivery systems and personalized medicine to sustainable practices and regulatory advancements, stakeholders across the healthcare continuum are driving progress and improving outcomes. As the market evolves, embracing innovation, ensuring patient safety, and meeting regulatory requirements will be crucial for unlocking new opportunities and delivering value in the global healthcare landscape.
```
| swara_353df25d291824ff9ee |
|
1,912,337 | Revolutionizing Sales with AI: The Role of AI Content Management Systems | In the fast-evolving landscape of sales and marketing, staying ahead requires leveraging cutting-edge... | 0 | 2024-07-05T06:43:24 | https://dev.to/luke_warner_f8e11a728b516/revolutionizing-sales-with-ai-the-role-of-ai-content-management-systems-4m1m | ai, contentmanagement | In the fast-evolving landscape of sales and marketing, staying ahead requires leveraging cutting-edge technologies. Among these, Artificial Intelligence (AI) has emerged as a game-changer, particularly through AI Content Management Systems (CMS). These systems are transforming how businesses manage, optimize, and personalize their content to drive sales and enhance customer engagement.
Understanding AI Content Management Systems
**[AI Content Management System](https://salesier.com/solution/sales-training-coaching.html)** integrate advanced AI algorithms with traditional content management functionalities. They enable businesses to automate content creation, curation, distribution, and performance analysis. By harnessing machine learning and natural language processing, AI CMS platforms can:
Automate Content Creation: AI can generate content such as blog posts, product descriptions, and social media updates based on predefined parameters and data inputs. This not only saves time but also ensures consistency and relevance.
Enhance Content Curation: AI algorithms analyze vast amounts of data to identify trends, customer preferences, and market demands. This helps in curating content that resonates with target audiences, increasing engagement and conversion rates.
Optimize Content Distribution: AI CMS systems use predictive analytics to determine the most effective channels, timing, and formats for content distribution. This targeted approach maximizes reach and impact while minimizing resource wastage.
Personalize Customer Experiences: Through AI-powered insights, businesses can deliver personalized content recommendations and experiences. This level of customization strengthens customer relationships and improves satisfaction levels.
Benefits of AI Content Management Systems
Implementing an AI CMS offers numerous advantages that directly impact sales performance and operational efficiency:
Improved Content Quality: AI-driven content creation ensures higher quality outputs with minimal human intervention, reducing errors and enhancing relevance.
Enhanced Efficiency: Automation of repetitive tasks frees up valuable human resources to focus on strategic initiatives and creative endeavors.
Real-time Insights: AI analytics provide real-time performance metrics and actionable insights, enabling agile decision-making and continuous optimization of content strategies.
Scalability: AI CMS platforms can scale operations seamlessly, accommodating growing content volumes and expanding customer bases without compromising efficiency.
Case Studies: Real-world Applications
1. Retail Industry
Major retail chains use AI CMS to dynamically adjust product descriptions and promotional content based on customer behavior and market trends. This not only boosts sales but also enhances customer satisfaction by delivering relevant and timely information.
2. Media and Publishing
Publishing houses utilize AI to automate content creation for news articles and digital publications. AI algorithms analyze reader preferences to tailor content recommendations, driving subscription rates and ad revenues.
3. E-commerce Platforms
Leading e-commerce platforms leverage AI CMS to personalize product recommendations and optimize search engine visibility. This results in increased conversions and higher average order values through targeted marketing campaigns.
Future Trends and Considerations
Looking ahead, the evolution of AI CMS is set to continue, with advancements in natural language understanding, image and video processing, and predictive analytics. Businesses must adapt by:
Investing in AI Talent: Developing in-house expertise or partnering with AI service providers to leverage the full potential of AI CMS.
Ensuring Data Security: Implementing robust data privacy measures to protect customer information and comply with regulatory standards.
Embracing Innovation: Continuously exploring new AI applications and integrating emerging technologies to maintain a competitive edge.
Conclusion
AI Content Management Systems represent a paradigm shift in how businesses create, manage, and optimize content to drive sales and foster meaningful customer relationships. By harnessing the power of AI, organizations can unlock new levels of efficiency, creativity, and profitability in an increasingly digital marketplace. Embracing AI CMS isn't just about keeping pace—it's about leading the charge towards a more intelligent and customer-centric future of sales and marketing.
In essence, integrating AI Content Management Systems isn't just a choice for businesses—it's a strategic imperative for those looking to thrive in the era of digital transformation.
By incorporating AI content management systems into their operations, businesses can optimize their content strategy to meet the needs of their target audience more effectively | luke_warner_f8e11a728b516 |
1,912,336 | Creating dynamic Search Bar using CSS Translate property | What is Translate Property in CSS? CSS translate property moves an element along the X and Y axes.... | 0 | 2024-07-05T06:43:16 | https://dev.to/code_passion/creating-dynamic-search-bar-using-css-translate-property-59a5 | html, css, webdesign, webdev | **What is Translate Property in CSS?**
[CSS translate property](https://skillivo.in/css-translate-property-guide/) moves an element along the X and Y axes. Unlike other positioning attributes, such as position, translate does not disrupt the document’s natural flow, making it excellent for producing smooth animations and transitions.
**What is transform property in CSS**
Visually appealing and dynamic user interfaces have become the norm in the ever-changing web development landscape. CSS (Cascading Style Sheets) is a core technology for styling and layout that stands out among the many tools available to developers. The transform property in CSS emerges as a strong technique for controlling the display of items on a web page.([Read more](https://skillivo.in/css-translate-property-guide/) example of translate property)
**Syntax:**
```
selector {
transform: transform-function;
}
```
The term selector refers to the element to which the transformation will be applied, whereas transform-function describes the type of transformation to be done.
** Let’s explore some of the most commonly used Translate functions**
**Creating dynamic Search Bars using CSS Translate property:**
Forms are essential for user engagement on the web. Forms serve as the gateway to involvement, whether it’s logging into a favourite website, signing up for a subscription, or completing a purchase.However, creating forms that are both functional and aesthetically beautiful can be difficult. This is where CSS classes like.form-group come in handy, providing an organised approach to form design. ([Read more](https://skillivo.in/css-translate-property-guide/) example)
output:

**HTML:**
```
<!DOCTYPE html>
<html>
<head>
<meta charset='utf-8'>
<meta http-equiv='X-UA-Compatible' content='IE=edge'>
<title>Page Title</title>
<meta name='viewport' content='width=device-width, initial-scale=1'>
</head>
<body>
<div class="form-group">
<input type="text" placeholder=" " id="name" required>
<label for="name">Your Name</label>
</div>
</body>
</html>
```
**CSS:**
```
.form-group {
position: relative;
margin-bottom: 15px;
max-width: 900px;
margin-top: 50px;
}
.form-group input {
width: 100%;
padding: 10px;
font-size: 16px;
border: 1px solid #ccc;
border-radius: 5px;
outline: none;
border-radius: 20px;
}
.form-group label {
position: absolute;
top: 10px;
left: 10px;
font-size: 17px;
color: #999;
pointer-events: none;
transition: all 0.3s;
}
.form-group input:focus + label,
.form-group input:not(:placeholder-shown) + label {
top: -20px;
left: 10px;
font-size: 16px;
color: #ee3646;
transform: translateY(-10px);
}
```
1. The.form-group class serves as the basis for our enchantment. It creates a structural layout for our form elements, ensuring they are well-organized and visually consistent. Our form groups are elegantly positioned and styled using properties such as position: relative, margin, and max-width.
2. The.form-group input and.form-group label selectors are where the magic happens. These selectors apply certain styles to our input fields and labels, making them into interactive elements.
3. Input fields in the.form-group inherit styles that stretch to the entire width of the container, with padding for comfort and a slight border to establish limits. The outline: none declaration maintains a clean design, while border-radius adds beauty with rounded edges.
**Conclusion**
In the ever-changing world of web development, learning CSS properties like as translate is critical for developing engaging and flexible user experiences. | code_passion |
1,912,335 | How to Develop an Eventbrite-like App for Android and iOS? | Creating an event management and ticketing app like Eventbrite involves careful planning, design, and... | 0 | 2024-07-05T06:43:03 | https://dev.to/gauravkanabar/how-to-develop-an-eventbrite-like-app-for-android-and-ios-4meg | androidapp, iosapp, eventbritelikeapp, eventmanagementplatform | Creating an event management and ticketing app like Eventbrite involves careful planning, design, and development. This guide will walk you through the key steps in the process and provide a coding snippet to help you get started with user registration using Firebase Authentication.
## Key Features to Include
There are a few [must-have features](https://www.pinoybisnes.com/business-tools/must-have-features-for-an-event-management-platform/) to include in your event management and ticketing system, these include:
**User Registration and Profiles:** Allow users to sign up and manage their profiles.
**Event Creation and Management:** Enable users to create, edit, and manage events.
**Ticket Sales and Distribution:** Facilitate ticket purchasing and distribution.
**Payment Integration:** Integrate secure payment gateways.
**Search and Filter:** Provide advanced search and filtering options for events.
**Social Media Integration:** Allow sharing of events on social media platforms.
**Notifications:** Implement push notifications for event updates and reminders.
**Reviews and Ratings:** Enable users to review and rate events.
**Customer Support:** Provide in-app customer support features.
## Technology Stack
Front-end: React Native or Flutter for cross-platform development.
Back-end: Node.js, Python (Django), or Ruby on Rails.
Database: PostgreSQL, MySQL, or MongoDB.
Cloud Services: AWS, Google Cloud, or Azure.
## Step-by-Step Guide to Development
Below is a comprehensive step-by-step guide to developing an [Eventbrite clone](https://www.alphansotech.com/eventbrite-clone) for Android and iOS, detailing each phase from planning to deployment and maintenance.
## Planning and Research
Start with market research to understand the needs and preferences of your target audience. Identify the unique selling points that will differentiate your app from competitors.
## Design
Create wireframes and prototypes to visualize the user flow. Design a user-friendly interface (UI) that is consistent across both Android and iOS platforms.
## Development
Set up the development environment and begin implementing core features. Here's a coding snippet to help you get started with user registration using Firebase Authentication in a React Native app.
## iOS (Swift) - Event Management and Ticketing System
## User Registration with Firebase
Add Firebase to your iOS project by following the instructions in the Firebase documentation.
**_Podfile:_**
```
# Uncomment the next line to define a global platform for your project
platform :ios, '10.0'
target 'YourApp' do
# Comment the next line if you're not using Swift and don't want to use dynamic frameworks
use_frameworks!
# Pods for YourApp
pod 'Firebase/Core'
pod 'Firebase/Auth'
end
```
**AppDelegate.swift:**
```
import UIKit
import Firebase
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
FirebaseApp.configure()
return true
}
}
```
**RegisterViewController.swift:**
```
import UIKit
import FirebaseAuth
class RegisterViewController: UIViewController {
@IBOutlet weak var emailTextField: UITextField!
@IBOutlet weak var passwordTextField: UITextField!
@IBOutlet weak var registerButton: UIButton!
@IBOutlet weak var errorLabel: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
errorLabel.isHidden = true
}
@IBAction func registerTapped(_ sender: UIButton) {
guard let email = emailTextField.text, !email.isEmpty,
let password = passwordTextField.text, !password.isEmpty else {
errorLabel.text = "Please fill in all fields"
errorLabel.isHidden = false
return
}
Auth.auth().createUser(withEmail: email, password: password) { (authResult, error) in
if let error = error {
self.errorLabel.text = error.localizedDescription
self.errorLabel.isHidden = false
} else {
self.errorLabel.text = "User registered successfully!"
self.errorLabel.isHidden = false
// Navigate to another screen or perform other actions
}
}
}
}
```
## Event Creation
**Event.swift:**
```
import Foundation
struct Event {
var id: String
var title: String
var description: String
var date: Date
var location: String
}
```
**CreateEventViewController.swift:**
```
import UIKit
class CreateEventViewController: UIViewController {
@IBOutlet weak var titleTextField: UITextField!
@IBOutlet weak var descriptionTextField: UITextField!
@IBOutlet weak var datePicker: UIDatePicker!
@IBOutlet weak var locationTextField: UITextField!
@IBOutlet weak var createButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func createEventTapped(_ sender: UIButton) {
guard let title = titleTextField.text, !title.isEmpty,
let description = descriptionTextField.text, !description.isEmpty,
let location = locationTextField.text, !location.isEmpty else {
// Show error message
return
}
let event = Event(id: UUID().uuidString, title: title, description: description, date: datePicker.date, location: location)
// Save event to database
saveEvent(event)
}
func saveEvent(_ event: Event) {
// Implement database saving logic here
// For example, save to Firebase Firestore
}
}
```
## Android (Kotlin) - Event Management and Ticketing System
## User Registration with Firebase
Add Firebase to your Android project by following the instructions in the Firebase documentation.
**build.gradle (Project):**
```
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.google.gms:google-services:4.3.10'
}
}
```
**build.gradle (App):**
```
plugins {
id 'com.android.application'
id 'kotlin-android'
id 'com.google.gms.google-services'
}
dependencies {
implementation platform('com.google.firebase:firebase-bom:31.0.2')
implementation 'com.google.firebase:firebase-auth-ktx'
}
```
**RegisterActivity.kt:**
```
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.TextView
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.google.firebase.auth.FirebaseAuth
class RegisterActivity : AppCompatActivity() {
private lateinit var auth: FirebaseAuth
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_register)
auth = FirebaseAuth.getInstance()
val emailEditText = findViewById<EditText>(R.id.emailEditText)
val passwordEditText = findViewById<EditText>(R.id.passwordEditText)
val registerButton = findViewById<Button>(R.id.registerButton)
val errorTextView = findViewById<TextView>(R.id.errorTextView)
registerButton.setOnClickListener {
val email = emailEditText.text.toString()
val password = passwordEditText.text.toString()
if (email.isEmpty() || password.isEmpty()) {
errorTextView.text = "Please fill in all fields"
} else {
auth.createUserWithEmailAndPassword(email, password)
.addOnCompleteListener(this) { task ->
if (task.isSuccessful) {
Toast.makeText(baseContext, "User registered successfully!", Toast.LENGTH_SHORT).show()
// Navigate to another screen or perform other actions
} else {
errorTextView.text = task.exception?.message
}
}
}
}
}
}
```
## Event Creation
**Event.kt:**
```
data class Event(
val id: String,
val title: String,
val description: String,
val date: Long,
val location: String
)
```
**CreateEventActivity.kt:**
```
import android.os.Bundle
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
import com.google.firebase.firestore.FirebaseFirestore
import java.util.*
class CreateEventActivity : AppCompatActivity() {
private lateinit var firestore: FirebaseFirestore
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_create_event)
firestore = FirebaseFirestore.getInstance()
val titleEditText = findViewById<EditText>(R.id.titleEditText)
val descriptionEditText = findViewById<EditText>(R.id.descriptionEditText)
val dateEditText = findViewById<EditText>(R.id.dateEditText)
val locationEditText = findViewById<EditText>(R.id.locationEditText)
val createButton = findViewById<Button>(R.id.createButton)
createButton.setOnClickListener {
val title = titleEditText.text.toString()
val description = descriptionEditText.text.toString()
val date = dateEditText.text.toString().toLongOrNull()
val location = locationEditText.text.toString()
if (title.isEmpty() || description.isEmpty() || date == null || location.isEmpty()) {
Toast.makeText(this, "Please fill in all fields", Toast.LENGTH_SHORT).show()
} else {
val event = Event(UUID.randomUUID().toString(), title, description, date, location)
saveEvent(event)
}
}
}
private fun saveEvent(event: Event) {
firestore.collection("events").add(event)
.addOnSuccessListener {
Toast.makeText(this, "Event created successfully!", Toast.LENGTH_SHORT).show()
// Navigate to another screen or perform other actions
}
.addOnFailureListener { e ->
Toast.makeText(this, "Error creating event: ${e.message}", Toast.LENGTH_SHORT).show()
}
}
}
```
Both the iOS and Android snippets provide a basic framework for user registration and event creation. In a production app, you would expand these examples to include more features, error handling, and UI enhancements. Additionally, ensure you follow [best practices](https://rakeebkhandurani.livepositively.com/eventbrite-mobile-app-development-best-practices-and-pitfalls/) for security, performance, and user experience in your final app.
## Testing and Quality Assurance
Perform extensive testing on different devices and screen sizes. Ensure the app is secure, performs well, and is user-friendly.
## Deployment
Prepare for launch by creating developer accounts on Google Play Store and Apple App Store. Submit the app and address any feedback during the review process.
## Marketing and Promotion
Execute a marketing strategy to promote your app. Use social media, influencers, and other digital marketing channels to attract users.
## Maintenance and Updates
Regularly update the app to fix bugs and add new features. Gather user feedback to make continuous improvements.
## Conclusion
Developing an Eventbrite-like app is a complex but rewarding process. By following these steps and focusing on user needs, you can create a successful event management app. The provided coding snippet is a starting point for implementing user registration, an essential feature of your app.
Stay tuned for more detailed guides and coding examples to help you build a fully functional event management app!
| gauravkanabar |
1,912,334 | Install google fonts using terminal. | Repository https://github.com/nureon22/gfont I created a python project to install google fonts... | 0 | 2024-07-05T06:42:20 | https://dev.to/nureon22/my-first-python-project-in-github-3o53 | python, cli, fonts | Repository https://github.com/nureon22/gfont
I created a python project to install google fonts directly from terminal. It also allows packing woff2 fonts to use in websites as locally hosted fonts. It works on Linux and macOS. Windows is not supported yet because I don't know to register fonts on Windows. | nureon22 |
1,912,333 | Master These 10 Essential DSA Concepts to Supercharge Your Coding Skills | Data Structures and Algorithms (DSA) form the foundation of computer science and software... | 0 | 2024-07-05T06:42:05 | https://dev.to/futuristicgeeks/master-these-10-essential-dsa-concepts-to-supercharge-your-coding-skills-340l | webdev, dsa, algorithms, beginners | Data Structures and Algorithms (DSA) form the foundation of computer science and software engineering. Mastering these concepts is crucial for efficient problem-solving and optimizing performance. Whether you are preparing for coding interviews, working on projects, or simply looking to improve your coding skills, understanding these core DSA concepts is essential. This article will cover the top 10 must-know DSA concepts for every developer, complete with detailed explanations and interactive examples to help solidify your understanding.
## 1. Arrays and Lists
Arrays and lists are the most fundamental data structures in programming. They store collections of elements in a linear order. Arrays have a fixed size, while lists (such as linked lists) can dynamically grow and shrink.
**Arrays**
An array is a collection of elements identified by index or key. It is a basic data structure that allows you to store multiple items of the same type together.
**Linked Lists**
A linked list is a linear data structure where each element is a separate object, and elements are linked using pointers. A node in a linked list contains data and a reference (or pointer) to the next node in the sequence.
## 2. Stacks and Queues
Stacks and queues are linear data structures that follow specific order principles. Stacks follow the Last In First Out (LIFO) principle, while queues follow the First In First Out (FIFO) principle.
**Stacks**
A stack is a collection of elements that supports two main operations: push (adding an element to the top of the stack) and pop (removing the top element).
**Queues**
A queue is a collection of elements that supports two main operations: enqueue (adding an element to the end of the queue) and dequeue (removing the front element).
## 3. Trees
Trees are hierarchical data structures with a root node and child nodes. The most common type is the binary tree, where each node has at most two children. Trees are used to represent hierarchical relationships, such as files in a directory.
**Binary Trees**
A binary tree is a tree data structure in which each node has at most two children, referred to as the left child and the right child.
## 4. Graphs
Graphs are a collection of nodes (vertices) connected by edges. They can represent various real-world structures like social networks, maps, and more. Graphs can be directed or undirected.
**Adjacency List**
An adjacency list is a way to represent a graph as a collection of lists. Each list corresponds to a node in the graph and contains a list of its adjacent nodes.
## 5. Hash Tables
Hash tables (or hash maps) store key-value pairs and provide fast lookup, insertion, and deletion operations. They use a hash function to map keys to indexes in an array, allowing for quick access to values.
## 6. Sorting Algorithms
Sorting algorithms arrange elements in a particular order. Common sorting algorithms include Bubble Sort, Merge Sort, Quick Sort, and Insertion Sort. Sorting is a fundamental operation that can significantly affect the efficiency of other algorithms.
**Quick Sort**
Quick Sort is an efficient, comparison-based sorting algorithm. It uses a divide-and-conquer strategy to sort elements.
## 7. Searching Algorithms
Searching algorithms find an element within a data structure. Common searching algorithms include Linear Search and Binary Search. Efficient searching is crucial for performance in many applications.
**Binary Search**
Binary Search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing the search interval in half.
## 8. Dynamic Programming
Dynamic Programming (DP) is a method for solving complex problems by breaking them down into simpler subproblems. It is applicable when the subproblems are overlapping and can be optimized by storing the results of subproblems to avoid redundant computations.
**Fibonacci Sequence**
The Fibonacci sequence is a classic example of dynamic programming. It involves storing previously computed values to avoid redundant calculations.
## 9. Recursion
Recursion is a process where a function calls itself directly or indirectly. It is a powerful tool for solving problems that can be broken down into smaller, similar problems. Recursive solutions are often cleaner and more intuitive.
**Factorial Calculation**
Factorial of a number is a classic example of recursion, where the function repeatedly calls itself with decremented values.
## 10. Breadth-First Search (BFS) and Depth-First Search (DFS)
BFS and DFS are fundamental algorithms for traversing or searching tree or graph data structures. BFS explores all neighbors at the present depth level before moving on to nodes at the next depth level. DFS explores as far as possible along each branch before backtracking.
**Breadth-First Search (BFS)**
BFS uses a queue to keep track of the next location to visit.
** Depth-First Search (DFS)**
DFS uses a stack to keep track of the next location to visit. It can be implemented using recursion or iteration.
Example
[Click here to Read the complete article with examples.](https://futuristicgeeks.com/master-these-10-essential-dsa-concepts-to-supercharge-your-coding-skills/)
| futuristicgeeks |
1,912,332 | Beyond ChatGPT: How to Maximize Its Use with Interactive Graph Models | In the fast changing field of artificial intelligence (AI), OpenAI's ChatGPT has emerged as a... | 0 | 2024-07-05T06:41:26 | https://dev.to/nim12/beyond-chatgpt-how-to-maximize-its-use-with-interactive-graph-models-135g | chatgpt, graphmodels, genai, apacheage | In the fast changing field of artificial intelligence (AI), OpenAI's ChatGPT has emerged as a powerful tool for natural language processing and comprehension. To fully realize its potential across multiple domains, merging ChatGPT with interactive graph models can considerably improve its capabilities. This integration not only broadens the scope of information retrieval and context management, but it also enables ChatGPT to provide more intelligent and contextually aware responses.
## 1. Understanding Interactive Graph Models
Before delving into the synergies between ChatGPT and interactive graph models, it's important to understand what these models are:
1. **Graph-Based Knowledge Representation:** Interactive graph models use graph structures to represent data, with nodes representing entities (for example, objects or concepts) and edges denoting relationships between them. This framework is extremely customizable and scalable, making it excellent for documenting complicated relationships and situations.
2. **Graph Neural Networks (GNNs):** These specialized networks excel at handling graph-structured data. GNNs may perform tasks like node categorization, link prediction, and graph-level prediction by aggregating data from surrounding nodes.
## 2. Enhancing ChatGPT with Graph Models
Integrating ChatGPT with interactive graph models opens several characteristics that improve its utility and efficacy in various applications:
**1. Knowledge enrichment and contextual understanding**:
- **Dynamic Knowledge Retrieval:** ChatGPT can obtain relevant information in real time from knowledge graphs in response to user questions. This capability ensures that responses are both accurate and contextually rich.
- **Context Management:** Graph architectures can preserve the context of ongoing interactions. Nodes can represent previous interactions, current subjects of conversation, or user preferences, allowing ChatGPT to create logical and tailored responses across long talks.
**2. Multi-modal Integration:**
- **Text-Image Interaction:** Graph models can connect textual descriptions to corresponding images, allowing ChatGPT to produce responses based on visual context. This integration is especially valuable in applications that require image description, visual search, and multimedia content development.
- **Text-Code Interaction:** For programming and technical support applications, integrating ChatGPT with graph-based representations of code snippets and programming concepts improves the system's capacity to deliver accurate code suggestions, explanations, and troubleshooting advice.
**3. Enhanced reasoning and inference:**
- **Graph-Based Reasoning:** Using graph neural networks, ChatGPT can execute complex reasoning tasks. It traverses structured graph data to infer associations, make logical conclusions, and handle complicated questions.
- **Probabilistic Reasoning:** Graphical models that work with ChatGPT can manage uncertainty and probabilistic reasoning. This competence is critical in decision-making situations where probabilistic consequences must be evaluated.
**4. Real-time Updates and Feedback Loops:**
- **Adaptive Learning:** Interactive graph models enable real-time modifications based on user input and external data sources. This guarantees that ChatGPT's knowledge base is current and useful.
- **Feedback Integration:** Adding user feedback to graph models enhances the accuracy and relevance of ChatGPT responses over time. Changing node weights or edges depending on user interactions improves the learning and adaption processes.
## Practical applications
Integrating ChatGPT with interactive graph models offers up a plethora of practical applications across numerous areas.
- **Individualized Assistants:** Create intelligent virtual assistants that can understand complex user queries, get individualized information from knowledge graphs, and tailor responses to ongoing interactions.
- **Educational Tools:** Create interactive learning environments in which ChatGPT assists students by explaining, answering questions based on graph-based knowledge, and giving tailored learning paths.
- **Customer Support:** Use ChatGPT in customer support systems where interactive graph models store customer profiles, service history, and product information. This configuration allows ChatGPT to provide targeted support and troubleshooting assistance.
## Implementation Considerations:
To maximize the use of ChatGPT with interactive graph models, consider the following implementation factors:
- **Scalability:** Ensure that graph-based systems can manage enormous amounts of data and interactions while maintaining performance.
- **Design** a seamless interface between ChatGPT and graph models to ensure smooth data flow and effective information retrieval.
- **Ethical Considerations:** When incorporating user-specific information into graph models, consider privacy problems and ensure ethical data use.
The combination of ChatGPT with interactive graph models provides a substantial improvement in AI-powered capabilities. ChatGPT goes beyond basic natural language understanding by using graph-based knowledge representation to provide intelligent, context-aware responses across a wide range of applications. As artificial intelligence advances, combining ChatGPT with interactive graph models has the potential to open up new horizons in tailored user experiences, informed decision-making, and adaptive learning systems.
| nim12 |
1,912,329 | Gantt Project Planners in Software Development: Powerful Tools in Action | Project teams involved in software development need to organize, track, and adjust their plans and... | 0 | 2024-07-05T06:38:53 | https://dev.to/thomasy0ung/gantt-project-planners-in-software-development-powerful-tools-in-action-233c | projectmanagement, ganttchart, planning, projects | Project teams involved in software development need to organize, track, and adjust their plans and work schedules in real time. They often apply an online Gantt chart to do it without headaches.
This diagram stands out for its ability to visually represent project timelines, allocate resources, and track progress. Therefore, Gantt project planners in software development continue to gain popularity and trust.
Let’s define the pros and cons of modern Gantt chart tools popular in the software development sphere and other professional fields and explore some bright examples of such platforms.
## What is an online Gantt chart project planner?
> An online Gantt project planner is a project management tool based on an online Gantt diagram that provides a visual timeline for project tasks.
It helps teams schedule, coordinate, and track project activities, ensuring that everyone is on the same page. This visual representation makes it easier to manage complex projects and ensure timely delivery.
While some developers still believe that the success of their project depends solely on coding, more and more teams rely on professional planning to ensure the effective accomplishment of any project.
Derek Holt, the author of Forbes, [thinks](https://www.forbes.com/sites/forbesbusinesscouncil/2024/04/18/the-future-of-software-development-is-upon-us/) that _"writing code is only part of the broader set of processes required to deliver great software. Organizations that embrace a modern approach to planning, product-centric thinking, test automation, automated code scanning, and more will be poised to further differentiate themselves in an AI world"_.
This statement seems true, especially if considering planning as the starting point of the project management process. And when it comes to high-quality planning, a Gantt project planner is just the best assistant.
It is worth noting here that these tools are widely used in many industries and professional fields, not just in the IT sector.
For instance, you may find the effective implementation of a Gantt chart construction software like [this](https://ganttpro.com/gantt-project-planner-for-construction/).
High-demand project planning solutions also include a Gantt chart architecture [tool](https://ganttpro.com/gantt-project-planner-for-architecture/) or a related platform for manufacturing projects. Event spheres and game development also require reliable work planners. Consequently, a good [example](https://ganttpro.com/gantt-project-planner-for-game-development/) of a Gantt project planner for game development is also highly valuable.
## What are the benefits of using Gantt project planners in software development?
Software development teams can use a Gantt diagram regardless of the complexity of their project.
By visualizing project timelines, deadlines, and milestones, they can gain a clear understanding of the [project scope](https://www.project-management-skills.com/project-management-scope.html) and progress. It allows them to set realistic goals, allocate resources, and track the development process from start to finish.
Let’s dive deeper into the key advantages of online Gantt project planners.
- **Advanced project visualization**. Modern Gantt diagram planners provide a clear visual representation of project timelines, making it easier to see task durations, dependencies, and deadlines.
- **Improved resource management**. Online planners with Gantt charts allow project managers to allocate resources efficiently, ensuring that team members are neither overburdened nor underutilized.
- **Enhanced team coordination**. Gantt charts help team members understand their roles and responsibilities by displaying the entire project in one view. It fosters better collaboration.
- **Simplified monitoring**. With Gantt charts, tracking progress becomes straightforward. Managers can easily see which tasks are on schedule and which are lagging.
- **Effective risk control**. Identifying potential bottlenecks and risks early in a project timeline helps mitigate issues before they escalate.
- **Enriched communication**. Most Gantt project planners are excellent tools for communicating project status to stakeholders. They provide a clear overview without overwhelming people with details.
## What are the possible disadvantages of Gantt project planners in software development?
There is no tool without drawbacks and bottlenecks.
Below you’ll find some evident cons of Gantt chart planning solutions.
- **Complexity for large projects**. Some project planners offer a Gantt chart that is too simple and limited in its functions. You can even build it in Excel, but it is unlikely to be suitable for complex multi-level projects.
- **Time-consuming updates**. Sometimes regularly updating a Gantt chart to reflect changes can be time-consuming and may require dedicated effort.
- **Complex dependency management**. Managing dependencies within a Gantt chart can be challenging, especially in projects with numerous interdependent tasks. Therefore, it’s better to choose a professional Gantt chart maker with robust features.
Fortunately, the advantages outweigh the disadvantages. However, it’s better to be aware of all of them.
Now let’s consider some reliable tools with the Gantt planning functionality.
## Examples of robust Gantt project planners used in software development projects
The world of project planning tools evolves and identifies new competitive solutions, including those for use in software development projects.
Here are 3 trustworthy Gantt project planners you can consider for your future work.
### 1. GanttPRO
This comprehensive Gantt chart-based project management tool comes with a competitive set of features. It is known for professional scheduling capabilities, robust task management, resource location, progress tracking, collaboration, and integration features. Software development teams that use this Gantt project planner get everything they need to evolve and succeed in the competitive environment.
### 2. Asana
The tool is known for its user-friendly interface and simple yet demanded features. Asana offers a Gantt diagram view through its timeline functionality. It looks appropriate for teams looking for a straightforward yet effective project management solution.
### 3. Trello
This platform will satisfy software development teams that only begin their PM journey. Therefore, Trello is a frequent choice among startups. While primarily a Kanban-based tool, Trello offers the Gantt chart functionality through power-ups like [BigPicture](https://bigpicture.one/news/). It is perfect if you need a flexible and customizable project management planner.
#### Apply professional Gantt project planners to lead your software development projects to success
Gantt project planners play a pivotal role in software development by providing a clear visual timeline, enhancing team coordination, and improving resource management. While they offer numerous benefits, it is essential to be aware of their potential drawbacks.
By selecting the right Gantt chart tool and using it effectively, software development teams can significantly boost their productivity and project success rates.
| thomasy0ung |
1,912,323 | Why Choose AIRI Consultancy Services for Your Digital Needs? | Now it is almost mandatory for any company to have a sufficient presence on the World Wide Web.... | 0 | 2024-07-05T06:34:15 | https://dev.to/gillywork/why-choose-airi-consultancy-services-for-your-digital-needs-ng5 | Now it is almost mandatory for any company to have a sufficient presence on the World Wide Web. Whether you are in Jaipur, Jodhpur or any part of Rajasthan, then your premier choice is the AIRI Consultancy Services that offers all end to end digital services. But, here is the endow and why should you consider choosing AIRI Consultancy Services for the digital need? It is now time to explore the main aspect which is the specialists and the services they provide that includes [website development in Jaipur](https://acspvtltd.com/), digital marketing and SEO.
Website Development in Jaipur
The website of a business organization is the contact point of the world of digital. Website design Company in Jaipur We provide implausible, magnificent and efficient web designs that attract visitors and fulfil the requirements of the business. The team of efficient and professional web developers apply all the modern trends and technologies to make your website unique among the others. Based on your needs that might vary from a basic informative website to a sophisticated Internet store, AIRI’s developers are capable of doing excellent work.
Jaipur based Best Digital Marketing Company
Organic marketing essential for communication with your audience and attracting traffic to your website. Being a [top digital marketing agency in Jaipur ](https://acspvtltd.com/)– based AIRI Consultancy Services provides services to help increase your online presence. The services range from SEO and social media marketing, content writing, and PPC campaigns with an emphasis on your objectives. This way, every marketing effort made will go through approximate data analysis, thus, maximizing the return on investment.
Web developers in Jaipur know about the platform.
Jaipur Web Development team of talented and trustworthy Web developers that aim at fulfilling your dream at AIRI Consultancy Services. They know that each enterprise is different and that it is impossible to approach the web development of such companies mechanically. While offering personalized design of the project, including the choice of the site’s layout and colour range, AIRI’s web developers also ensure that the site will run smoothly and meet all the technical requirements. They pay a lot of attention to the aspect of ‘Responsive Web Design’ which guarantees the proper working of sites in different devices.
Best website design company in Jaipur
The phenomenon of web design is considered as an essential ingredient of any website. In the websites designed by AIRI Consultancy Services – one of the top [website design companies in Jaipur](https://acspvtltd.com/), Reliability, Attractiveness/Beauty, and Efficiency goes hand in hand. Their designers sit with you, listen to what you intend to achieve for your organisation and make sure the website you come up with is unique to the brand and captures the target customers’ attention. Consider the use of colours, fonts, arrangement of the content, and many other factors that improve the user experience and result in a sale.
Best SEO Company in Jodhpur
All these uses can be achieved through search engine optimization better known as SEO – very critical in managing rankings for websites. AIRI Consultancy Services is a professional SEO company in Jodhpur that uses modern SEO strategies to increase the rank and traffic. Their SEO specialists identify the right keywords, internal and external link structures and they always keep an eye on your competitors. By doing so, the business can find specialized help in ways to climb the search engine ladder and increase the site’s visibility.
A leading Digital Marketing Company in Vaishali Nagar
Present in Vaishali Nagar, which is a very active region, AIRI Consultancy Services is also an experienced digital marketing company in Vaishali Nagar. Indeed, their services in digital marketing are highly extensive, and they have adopted a strategy of tailoring their services to suit the needs of different companies in this region. AIRI provides solutions on how to work with local SEO, Google My Business, and numerous special targeting social media marketing. Their activities focus on getting visitors to their websites, achieving customer interest, and making sales.
Conclusion
Welcome to AIRI Consultancy Services our company will provide you all what you need in the digital world. Be it web design in Jaipur service or digital marketing services or even SEO services, their team of professionals can help you create excellent solutions. When you select us, that is AIRI, you make an investment to a company whose main goal is to ensure it brings the best out of your internet visibility. You can reach out to AIRI Consultancy Services now to help you soar higher in the digital world for your business.
https://acspvtltd.com/
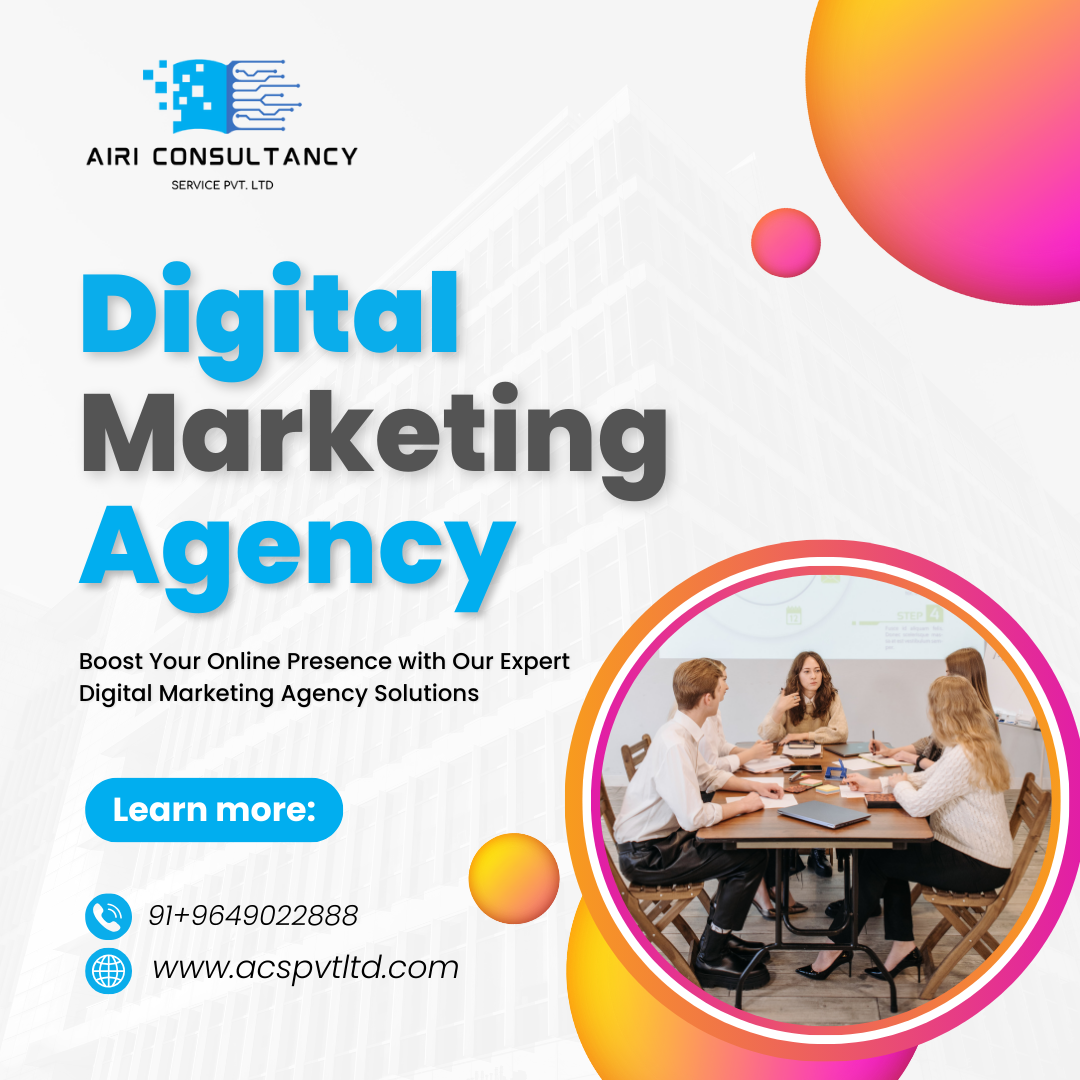 | gillywork |
|
1,912,328 | The Allure of Jewelry Canada: A Deep Dive into Canada's Jewelry Industry | In the vast landscape of the jewelry world, Canada stands out not only for its breathtaking... | 0 | 2024-07-05T06:38:31 | https://dev.to/vineeta_rana_0bf9c747f488/the-allure-of-jewelry-canada-a-deep-dive-into-canadas-jewelry-industry-3b8d | luxury, jewelry, bridaljewelry, tutorial | In the vast landscape of the jewelry world, Canada stands out not only for its breathtaking landscapes but also for its rich and diverse jewelry industry. From traditional indigenous designs to contemporary masterpieces, [Canadian jewelry](https://vinnis.ca/) reflects the country's cultural tapestry and its deep-rooted connection to nature. In this comprehensive guide, we delve into the intricacies of Jewelry Canada, exploring its history, craftsmanship, market trends, and the future of the industry.
1. A Glittering Legacy: The History of Canadian Jewelry
1.1 Indigenous Roots: Honoring Tradition and Heritage
The history of Canadian jewelry dates back centuries, with indigenous peoples crafting intricate adornments long before the arrival of European settlers. These early pieces served not only as decorative ornaments but also as symbols of cultural identity and spiritual significance.
1.2 Colonial Influence: European Artistry Meets Canadian Craftsmanship
With the arrival of European settlers, Canadian jewelry began to evolve, blending traditional indigenous techniques with European styles. Artisans across the country embraced new materials and techniques, creating a unique fusion of cultural influences.
1.3 Modern Era: Innovation and Creativity in Canadian Jewelry
In the 20th and 21st centuries, Canadian jewelry experienced a renaissance, with artisans pushing the boundaries of design and craftsmanship. From avant-garde creations to sustainable practices, the modern jewelry landscape in Canada is as diverse as it is dynamic.
2. The Art of Canadian Jewelry Making: Craftsmanship and Techniques
2.1 Traditional Methods: Handcrafted Treasures
Many Canadian jewelry artisans still rely on traditional techniques passed down through generations. From hand-forging metals to intricate stone setting, these time-honored methods imbue each piece with a sense of authenticity and craftsmanship.
2.2 Innovative Approaches: Embracing Technology
While traditional craftsmanship remains integral to Canadian jewelry making, many artisans are also embracing technology to push the boundaries of design and innovation. Computer-aided design (CAD) and 3D printing are revolutionizing the way jewelry is conceptualized and produced, allowing for greater precision and creativity.
3. Gems of the Great White North: Canadian Gemstones and Minerals
3.1 Diamonds: Canada's Shining Contribution to the World
Canada is renowned for its high-quality diamonds, with mines in the Northwest Territories and Ontario producing some of the world's most sought-after gems. Known for their exceptional clarity and brilliance, Canadian diamonds have become synonymous with luxury and quality.
3.2 Other Precious Gemstones: A Wealth of Natural Beauty
In addition to diamonds, Canada is home to a wealth of other precious gemstones, including sapphires, emeralds, and rubies. These gems are prized for their unique colors and exceptional quality, making them highly sought after by jewelry enthusiasts around the world.
4. The Business of Jewelry: Market Trends and Opportunities
4.1 Domestic Market: A Growing Appetite for Canadian Jewelry
The demand for Canadian-made jewelry is on the rise, fueled by a growing appreciation for locally sourced and ethically produced goods. Canadian consumers are increasingly seeking out jewelry that tells a story and reflects their values, driving demand for artisanal and sustainably sourced pieces.
4.2 International Trade: Exporting Canadian Craftsmanship
Canada's reputation as a hub for quality craftsmanship has made its jewelry products highly sought after in international markets. From traditional indigenous designs to contemporary luxury brands, Canadian jewelry enjoys a global reputation for excellence and innovation.
5. Navigating the Future: Trends and Innovations in Canadian Jewelry
5.1 Sustainability: A Growing Focus on Ethical Practices
As consumers become more conscious of the environmental and social impact of their purchases, sustainability is emerging as a key trend in the jewelry industry. Canadian artisans are leading the way, embracing recycled materials and ethical sourcing practices to create jewelry that is both beautiful and environmentally responsible.
5.2 Customization: Personalized Pieces for Every Taste
With advances in technology, customization has become increasingly popular in the jewelry industry. Canadian artisans are leveraging CAD software and 3D printing technology to create bespoke pieces that cater to individual tastes and preferences, offering customers a truly unique and personalized experience.
6. Conclusion: Celebrating the Beauty of Jewelry Canada
From its rich cultural heritage to its commitment to innovation and sustainability, Jewelry Canada offers a captivating glimpse into the diverse and dynamic world of Canadian jewelry. Whether you're drawn to the timeless elegance of indigenous designs or the cutting-edge creativity of modern artisans, there's something for everyone to discover and admire in Canada's vibrant jewelry industry.
[Source Link](https://vinnis.ca/)
| vineeta_rana_0bf9c747f488 |
1,912,327 | Benefits of Laser Cutting in Coimbatore | Masters of LASER Cutting and Fabrication Since 1980 Universal Machine Works, established in 1980, has... | 0 | 2024-07-05T06:38:03 | https://dev.to/universalmachineworks/benefits-of-laser-cutting-in-coimbatore-12fn | lasercutting, lasercuttingincoimbatore, universalmachineworks | **Masters of LASER Cutting and Fabrication Since 1980**[](https://www.universalmachineworks.com/content/Unleash-the-Power-of-Laser-Cutting-in-Coimbatore/)
[Universal Machine Works](https://www.universalmachineworks.com/), established in 1980, has evolved as a leading player in the precision engineering and fabrication industry. Specializing in LASER cutting, sheet metal, and fabricated components, the company has consistently met the diverse needs of clients across various sectors such as AGRO MACHINERY, AUTOMOBILES, PUMPS & VALVES, ELECTRIC EQUIPMENT, among others. With a commitment to excellence, innovation, and customer satisfaction, Universal Machine Works has become synonymous with precision, reliability, and timely delivery.
Empowering Precision with Cutting-Edge Technology and Versatile Machinery
| universalmachineworks |
1,912,326 | Supercharge Your Knowledge Base: Turning Your Developer Community into Content Creators | Learn how to leverage user-generated content to build a powerful knowledge base for your... | 0 | 2024-07-05T06:37:56 | https://dev.to/swati1267/supercharge-your-knowledge-base-turning-your-developer-community-into-content-creators-338o |
## Learn how to leverage user-generated content to build a powerful knowledge base for your DevTool. This guide provides actionable strategies to empower your community and scale support efficiently.
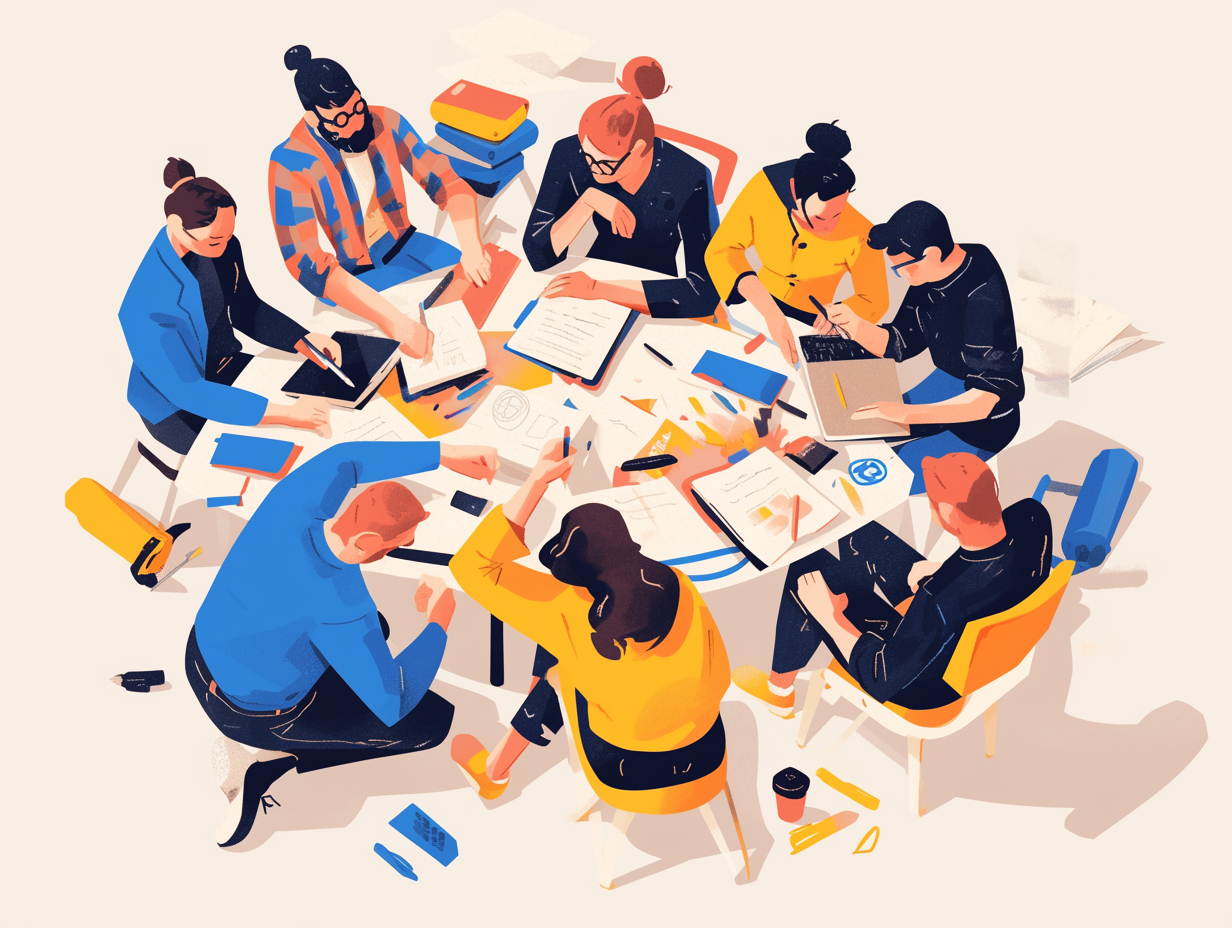
As your DevTool gains traction and your community grows, your support team might start feeling like they're drowning in a sea of questions. How do you keep up with the demand for answers without sacrificing the quality of support or burning out your team?
The answer lies within your community itself! Tapping into user-generated content (UGC) can be a game-changer, transforming your knowledge base into a thriving hub of information and collaboration.
**Why User-Generated Content Is a Support Superhero**
Think of your community as a team of expert problem-solvers who are already using your product every day. They're encountering issues, finding workarounds, and discovering clever tips and tricks. Their collective wisdom is a goldmine of knowledge just waiting to be tapped.
Here's why UGC is essential for scaling your knowledge base:
- **Authenticity and Trust**: Developers trust information from their peers who are experiencing the same challenges and using the same tools.
- **Diversity of Perspectives**: Your community brings a wide range of experiences and use cases to the table, ensuring your knowledge base covers various scenarios.
- **Increased Engagement**: When users see their contributions valued and shared,they feel more connected to your community and are more likely to continue contributing.
- **Reduced Support Burden**: Empowering your community to help itself means fewer tickets for your support team, allowing them to focus on more complex issues.
- **Constant Updates**: UGC keeps your knowledge base fresh and relevant as your product evolves and new use cases emerge.
**How to Encourage and Capture User-Generated Content**
1. **Create a Welcoming Environment**:
- Foster a Culture of Sharing: Encourage users to ask questions, share solutions, and offer feedback.
- Recognize and Reward: Highlight valuable contributions through shout-outs,badges, or even swag.
- Make it Easy: Provide clear guidelines and simple tools for users to submit their content.
2. **Incentivize Participation**:
- Contests and Challenges: Run contests for the best tutorial or solution to a common problem.
- Gamification: Award points or badges for helpful contributions.
- Exclusive Access: Offer early access to new features or beta programs to active contributors.
3. **Curate and Organize**:
- Review and Edit: Ensure content is accurate, well-formatted, and aligned with your brand voice.
- Tag and Categorize: Make it easy for users to find relevant content by organizing it into clear categories and using appropriate tags.
- Integrate with Your Knowledge Base: Make UGC easily accessible alongside your official documentation.
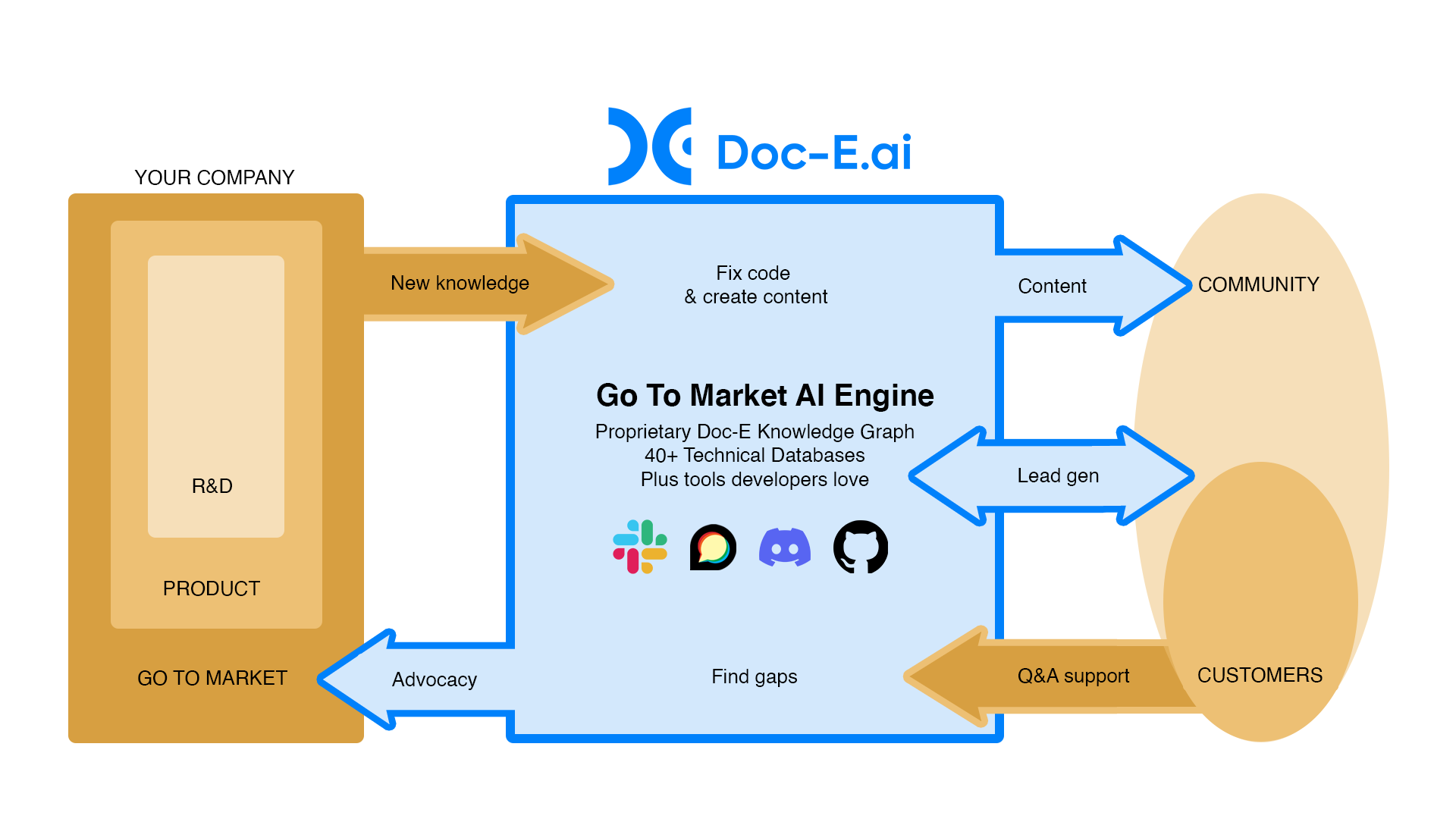
Doc-E.ai - The Developer Engagement Platform
**Doc-E.ai: Your UGC Power Tool**
Doc-E.ai is a developer engagement platform that supercharges your ability to leverage user-generated content:
- **Identify Valuable Insights**: Doc-E.ai analyzes community discussions and support interactions to surface the most helpful answers, solutions, and tutorials.
- **Automate Content Creation**: It can automatically turn these interactions into knowledge base articles, FAQs, or even blog posts, saving you time and effort.
- **Measure Impact**: Track how UGC performs in terms of views, engagement, and user feedback. This helps you understand what resonates with your audience and refine your content strategy.
**Real-World Examples**
- **Stack Overflow**: This platform thrives on user-generated content, with millions of developers contributing questions, answers, and code snippets.
- **Hashnode**: This developer blogging platform encourages users to share their knowledge and insights, fostering a collaborative learning environment.
- **Open Source Projects**: Many open-source projects rely on community contributions to improve documentation, write tutorials, and fix bugs.
**Conclusion**
By embracing user-generated content, you're not just building a knowledge base; you're building a community of empowered developers who are invested in your product's success. This collaborative approach leads to a richer, more relevant knowledge base, reduced support burden, and increased developer satisfaction.
**Ready to unlock the power of your community?**
Try Doc-E.ai for free today!
| swati1267 |
|
1,912,325 | ?? vs || in JavaScript: The little-known difference | At first glance, it might seem like you can interchange ?? (Nullish Coalescing Operator) and ||... | 0 | 2024-07-05T06:36:03 | https://dev.to/safdarali/-vs-in-javascript-the-little-known-difference-18a6 | jsoperators, webdev, javascript, programming | At first glance, it might seem like you can interchange ?? (Nullish Coalescing Operator) and || (Logical OR) in JavaScript. However, they are fundamentally different in how they handle truthy and falsy values.
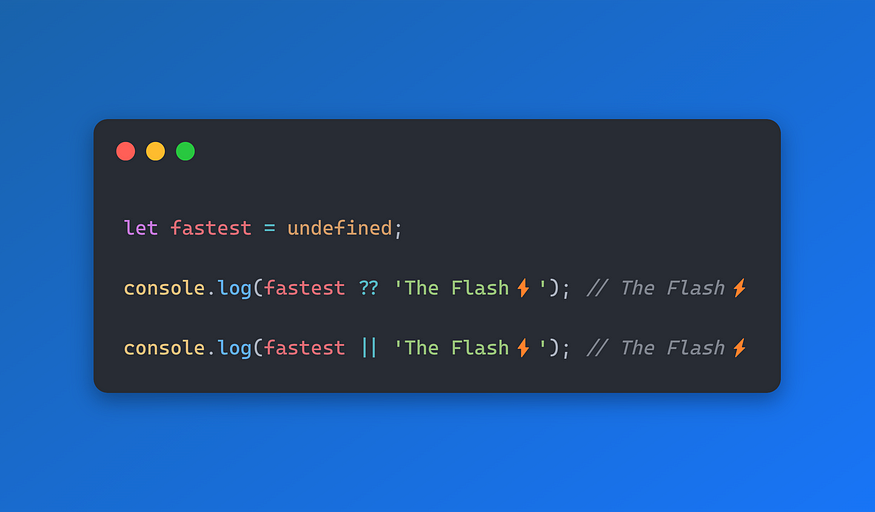
## Falsy Values in JavaScript
Falsy values become **false** in a Boolean context:
- **0
- undefined
- null
- NaN
- false
- '' (empty string)**
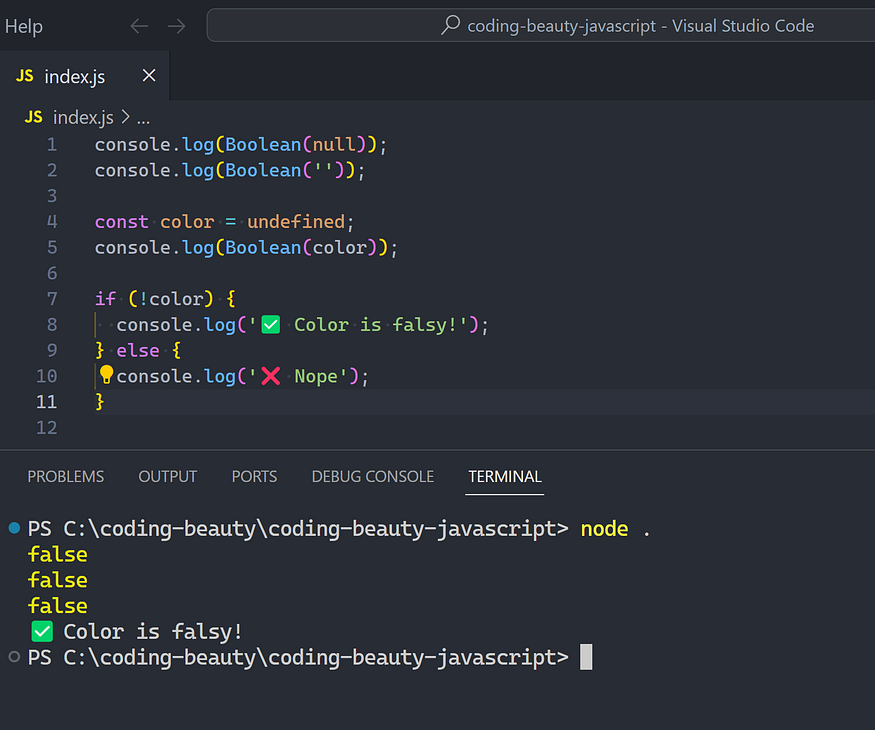
## Truthy Values
Everything else.
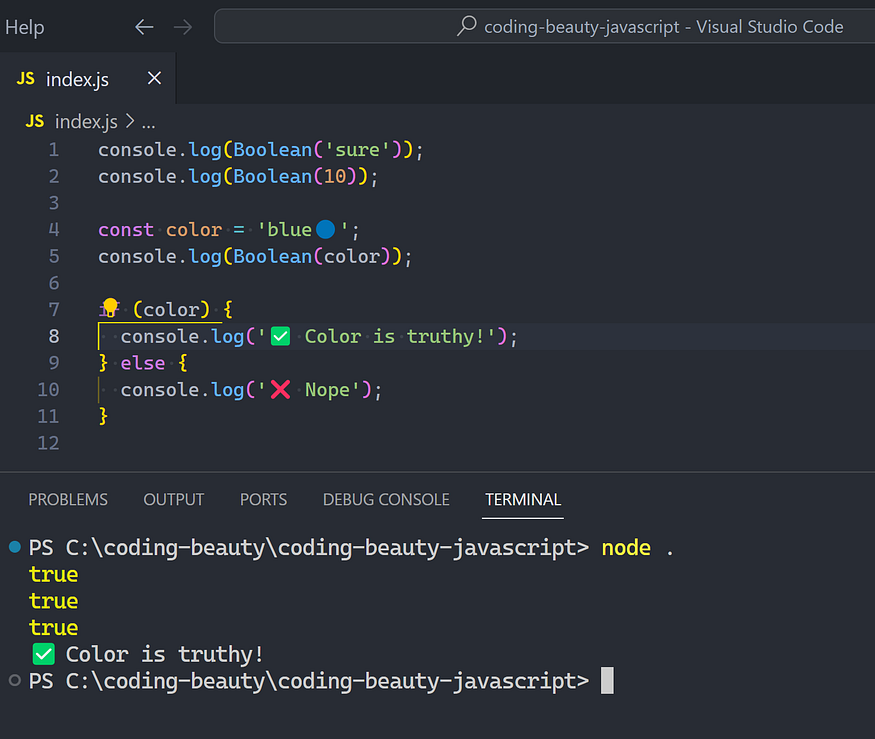
## The Difference Explained
- **|| (Logical OR):** Returns the first truthy value. If the first operand is falsy, it returns the second operand.
```
let x = 0 || "default"; // "default"
```
Here, 0 is falsy, so "default" is returned.
- **?? (Nullish Coalescing):** Returns the right-hand operand if the left-hand operand is null or undefined. It ignores other falsy values.
```
let x = 0 ?? "default"; // 0
```
Here, 0 is not null or undefined, so 0 is returned.
## Use Cases
- Use || when you want to provide a fallback for any falsy value.
- Use ?? when you only want to provide a fallback for null or undefined.
Understanding this difference helps avoid bugs and ensures your code behaves as expected.
That's all for today.
And also, share your favourite web dev resources to help the beginners here!
Connect with me:@ [LinkedIn ](https://www.linkedin.com/in/safdarali25/)and checkout my [Portfolio](https://safdarali.vercel.app/).
Explore my [YouTube ](https://www.youtube.com/@safdarali_?sub_confirmation=1)Channel! If you find it useful.
Please give my [GitHub ](https://github.com/Safdar-Ali-India) Projects a star ⭐️
Thanks for 24956! 🤗 | safdarali |
1,912,324 | The Rise of 3D Printing in Mold Making for Plastic and Silicone Products | One of the more exciting recent uses is in 3D printed molds. Traditionally mold making processes were... | 0 | 2024-07-05T06:34:23 | https://dev.to/gloria_jchavarriausi_fb/the-rise-of-3d-printing-in-mold-making-for-plastic-and-silicone-products-3j04 | design | One of the more exciting recent uses is in 3D printed molds. Traditionally mold making processes were labor-intensive, time consuming and costly. Making molds was a complex and detail-oriented process that demanded the hands of specialists from specific fields, such practices often cost companies both time as well money. In 3D printing fashion, though, the entire process adapted to that single technique has had a seismic shift as well - making almost everything easier and less expensive.
Benefits of 3D Printing for Manufacturing Molds
Fundamentally, 3D printing works by building up a three-dimensional object from the model of the digital data layer-by-layer. In the field of mold making for plastic and silicone products, this technology has many advantages. A key selling point is the speed at which 3D printing can create molds 3D printing can cure a mold in only hours, depending on intricacy, while traditional methods may take several weeks or even months to produce the same plastic injection product.
A major benefit also is the accuracy obtained in 3D Printed Molds. The technology has the ability to produce molds that are highly intricate and involved, more complex than what can be created by traditional mold making methods. Moreover, 3D printing enables the molds of different thickness wall angles and complex shapes which cannot be possible with traditional methods too.
The revolutionized world of mold making with 3D Printing
3D printing, although it is a relatively recent technology, already influences the mold making industry and modifies its landscape. The introduction of 3D printing has enabled more accurate and precise mold production which opened up a whole new world in design. New designs can be intricate and complex, beyond what was possible for designers using traditional mold making methods.
One more area in which 3D printing is anticipated to change the mold making industry by allowing it for little and medium enterprises. Making molds used to be the domain of specialised manufacturers with all their machinery and know how. But with 3D printing, companies can now create molds in-house to dramatically decrease the time and cost necessary for parts and prototypes production.
Advantages of adopting 3D printing in mold making:
The benefits of preparing plastic or silicone product molds through 3D printing can indeed be very attractive. When lead times, costs and complexity can be reduced companies will benefit from having more flexible tools in the manufacturing process. This assures that the quality of mold is maintained at a good level as manufacturing molds externally are one way to increase risk for production delays or defects. In addition to this, 3D printing greatly reduces the time required for injection moulder product development or prototyping, allowing companies have products on the market sooner.
SustainabilityAnother significant benefit of utilizing 3D printing is that it can produce the molds in a different way. By contrast, conventional mold making involves milling away metal from a block until shape of molten material is formed and like any machining process there can produce large waste that 3D printing eliminates by using the purpose desired amount when building the mold.
Growing Importance of 3D Printing in Mold Making
Now the 3D printing is growing, a trend that has meant to claiming remarkable ground on several fronts - with many prospects in terms of plastic and silicone mold manufacturing. Additive manufacturing is already used in a range of industries, from aerospace to healthcare. As the need for custom, high performing parts continues to grow 3D printing is transforming into a compelling asset the businesses seeking complex or individual components.
This is why most of the people now use 3d printing services from china to make molds for plastic and silicone mold plastic injection products. Businesses gain the flexibility and agility required to be competitive by significantly reducing lead times, costs, complexity. In the future, 3D printing will continue defining new paths in technology, and we might still not understand where all this is heading. As the possibilities for AR in business only seem to expand, companies hoping to keep up with innovation must jump on board or risk being left behind. | gloria_jchavarriausi_fb |
1,912,322 | How to Use an Active Button After Click in Tailwind CSS | To use an active button state after clicking it in Tailwind CSS, you can utilize the active utility... | 0 | 2024-07-05T06:34:14 | https://larainfo.com/blogs/how-to-use-an-active-button-after-click-in-tailwind-css/ | html, tailwindcss, webdev | To use an active button state after clicking it in Tailwind CSS, you can utilize the active utility class. The active class is applied when the button is clicked or activated
```html
<button class="rounded bg-blue-500 px-4 py-2 text-white hover:bg-blue-700 active:bg-blue-800">
Click me
</button>
```

bg-blue-500 sets the initial background to blue. hover:bg-blue-700 changes it to a darker blue on hover. text-white makes the text white. font-bold makes the text bold. py-2 px-4 adds vertical (0.5rem) and horizontal (1rem) padding. rounded gives the button rounded corners. `active:bg-blue-800` changes the background to an even darker blue when clicked, providing visual feedback.
Create a button that becomes active after clicking using the Tailwind CSS classes active:bg-blue-800, along with `focus:outline-none` and focus:ring `focus:border-blue-300`.
```html
<button class="bg-blue-500 hover:bg-blue-700 text-white py-2 px-4 rounded focus:outline-none focus:ring focus:border-blue-300 active:bg-blue-800">
Active Button
</button>
```

Active Button with Gradient and Icon in Tailwind CSS, Outlined Active Button.
```html
<div>
<button class="rounded border border-blue-500 px-4 py-2 font-semibold text-blue-500 hover:bg-blue-500 hover:text-white focus:border-blue-300 focus:outline-none focus:ring">Outlined Active Button</button>
</div>
<div>
<button class="rounded bg-blue-500 px-4 py-2 text-white shadow-md hover:bg-blue-700 focus:border-blue-300 focus:outline-none focus:ring active:bg-blue-800">Primary Button</button>
</div>
<div>
<button class="rounded bg-gradient-to-r from-purple-400 to-blue-500 px-4 py-2 text-white hover:from-purple-500 hover:to-blue-600 focus:border-blue-300 focus:outline-none focus:ring active:bg-blue-800">
<svg xmlns="http://www.w3.org/2000/svg" class="inline-block h-5 w-5" viewBox="0 0 20 20" fill="currentColor">
<path fill-rule="evenodd" d="M10 2a1 1 0 00-1 1v5H5a1 1 0 100 2h4v5a1 1 0 102 0v-5h4a1 1 0 100-2h-4V3a1 1 0 00-1-1z" clip-rule="evenodd" />
</svg>
Button with Icon
</button>
</div>
```
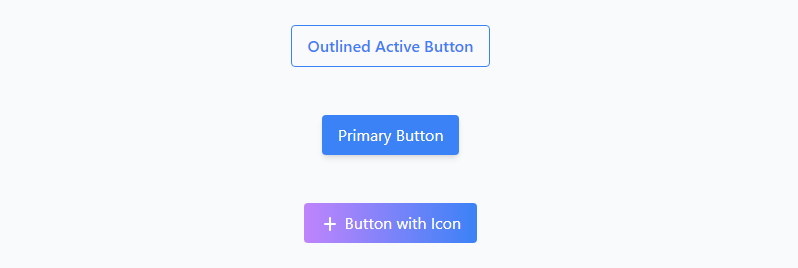 | saim_ansari |
1,912,318 | How I send a personal message (incl. follow ups) to 100's of sign-ups with this $5 tool | As Formbricks matures, I’m less involved in building the product and instead focus on selling... | 0 | 2024-07-05T06:31:02 | https://dev.to/jobenjada/how-i-send-a-personal-message-incl-follow-ups-to-100s-of-sign-ups-with-this-5-tool-32dl | opensource, webdev, tutorial | _As Formbricks matures, I’m less involved in building the product and instead focus on selling it._
With hundreds of people signing up every week, we’re fortunate to have a good starting point.
Obviously, every sign-up is an opportunity: Someone found us, was intrigued enough to sign up and test the product. We want to leverage that. Not every sign-up will buy, but every sign-up can provide valuable feedback or insights.
However, without a good process, the follow-ups quickly occupied a good chunk of my workday…
## How to Follow-Up: Delegate or Automate?
I ran into a bunch of problems:
- The sign-ups piled up in our list of new users when I was working on other things.
- Our product analytics tool doesn’t let me tick off/mark sign-ups in the people view.
- I didn’t want to set up a custom Slack notifier for every sign-up because it would make Slack notifications redundant. But I also kept forgetting to follow up within 24h…
- Each follow up took too long to be effective.
So in short: I struggled to **reach out on time** and **personalized**. And the leads went stale…
Since customer research and communication are key for a successful product development, _I didn’t want to delegate_.
And since personalized messages work much better, _I didn’t want to fully automate_.
So I looked for a better process and tooling - and found Vocus.
### The $5 Tool That Makes It All Possible
The new process I set out to design needed to tick the following boxes:
- Filter out personal email addresses from the sign-up list.
- Let me reach out within 24h after sign up.
- Reach out with a personalized message.
- Auto-follow up twice (a lot of sales happen after the 2nd and 3rd follow-up).
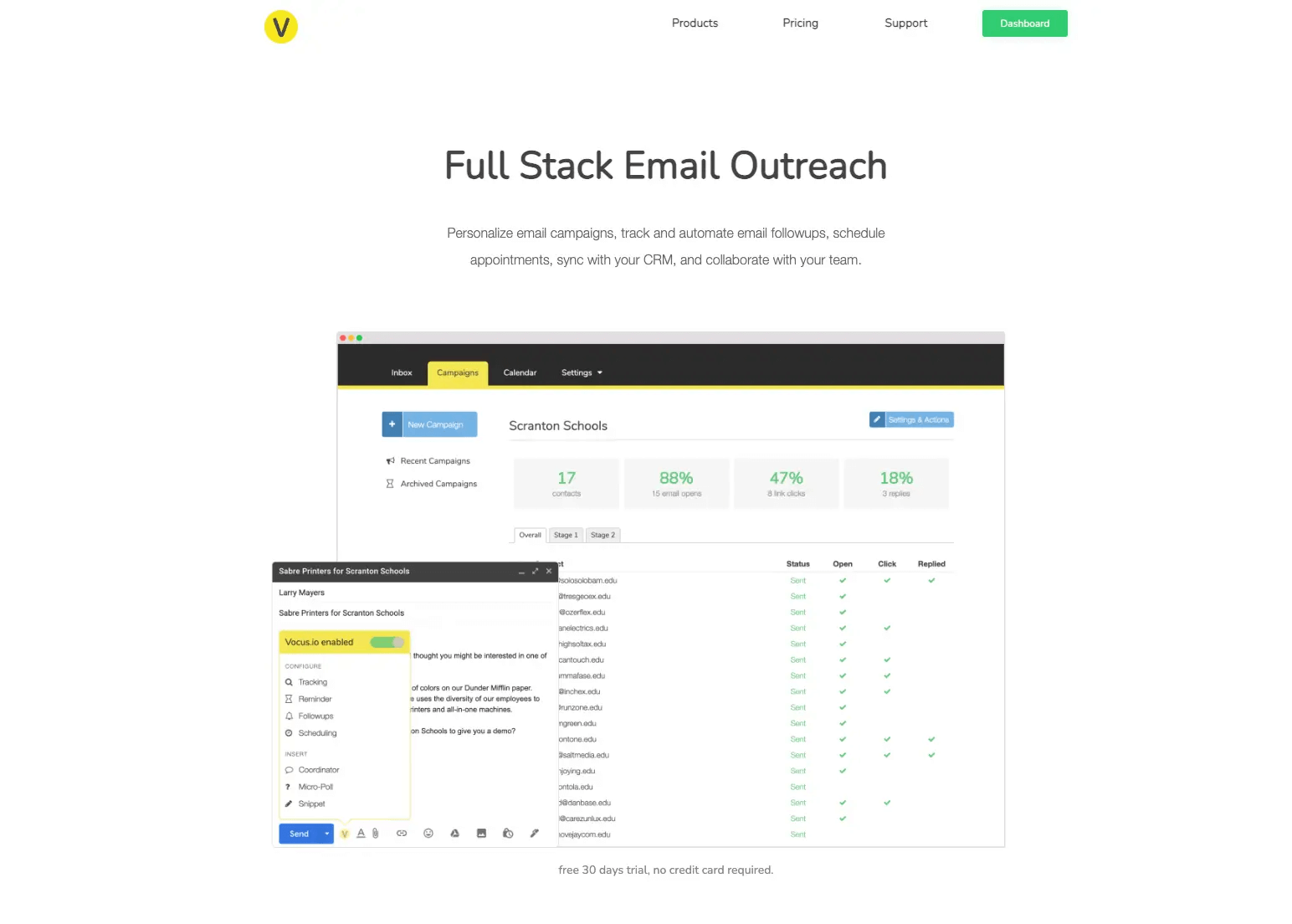
Vocus.io is a simple tool that does exactly what I need (and a tiny bit more). But even the $5 plan is enough for now because it packs the two things I need: **Email templates with placeholders** (they call this Snippets) and **automatic follow-ups 😍.** And it comes at a really fair price!
## Setting Up Semi-Automated Follow-Ups
Before we look at how to set up Vocus and Formbricks, here is the process in a nutshell:
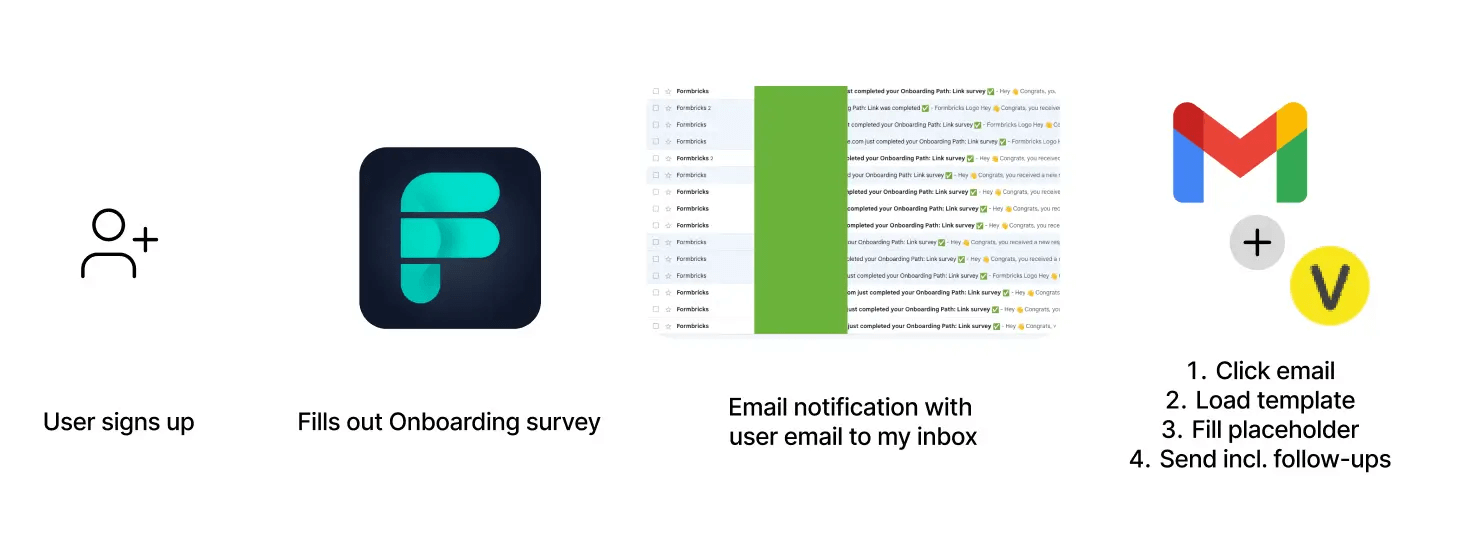
Alrighty, let’s dive into the details!
### Setting Up Vocus.io
Create a Snippet to send out as the initial email:
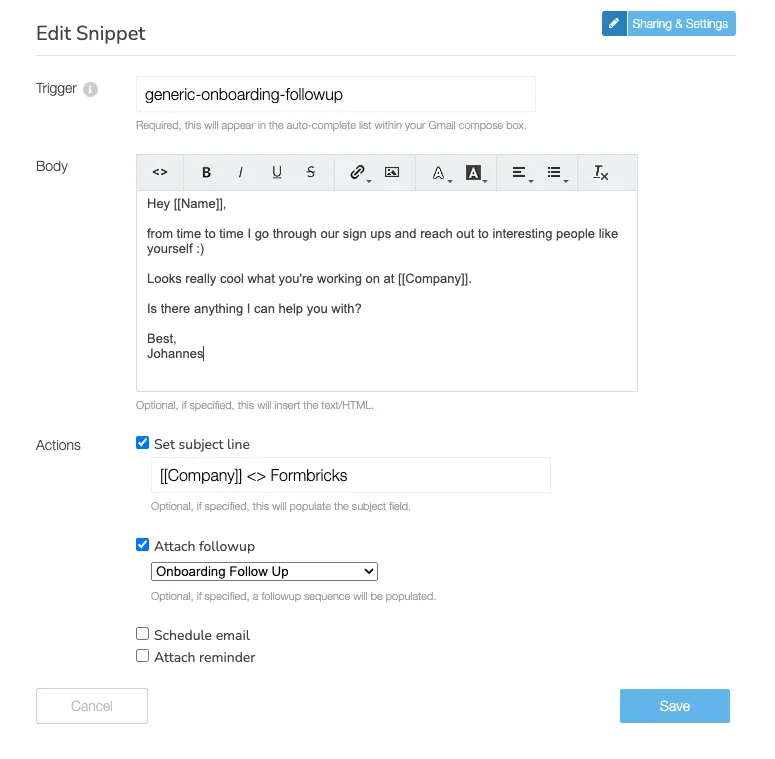
It’s important to keep it short (I’m likely too wordy already) and generic enough so that you keep the option to send it even without further personalization. Here is my current message before personalization:
> Hey [[Name]],
> from time to time I go through our sign ups and reach out to interesting people like yourself :)
> Looks really cool what you're working on at [[Company]].
> Is there anything I can help you with?
> Best, Johannes
Secondly, we set up the template for the follow-ups:
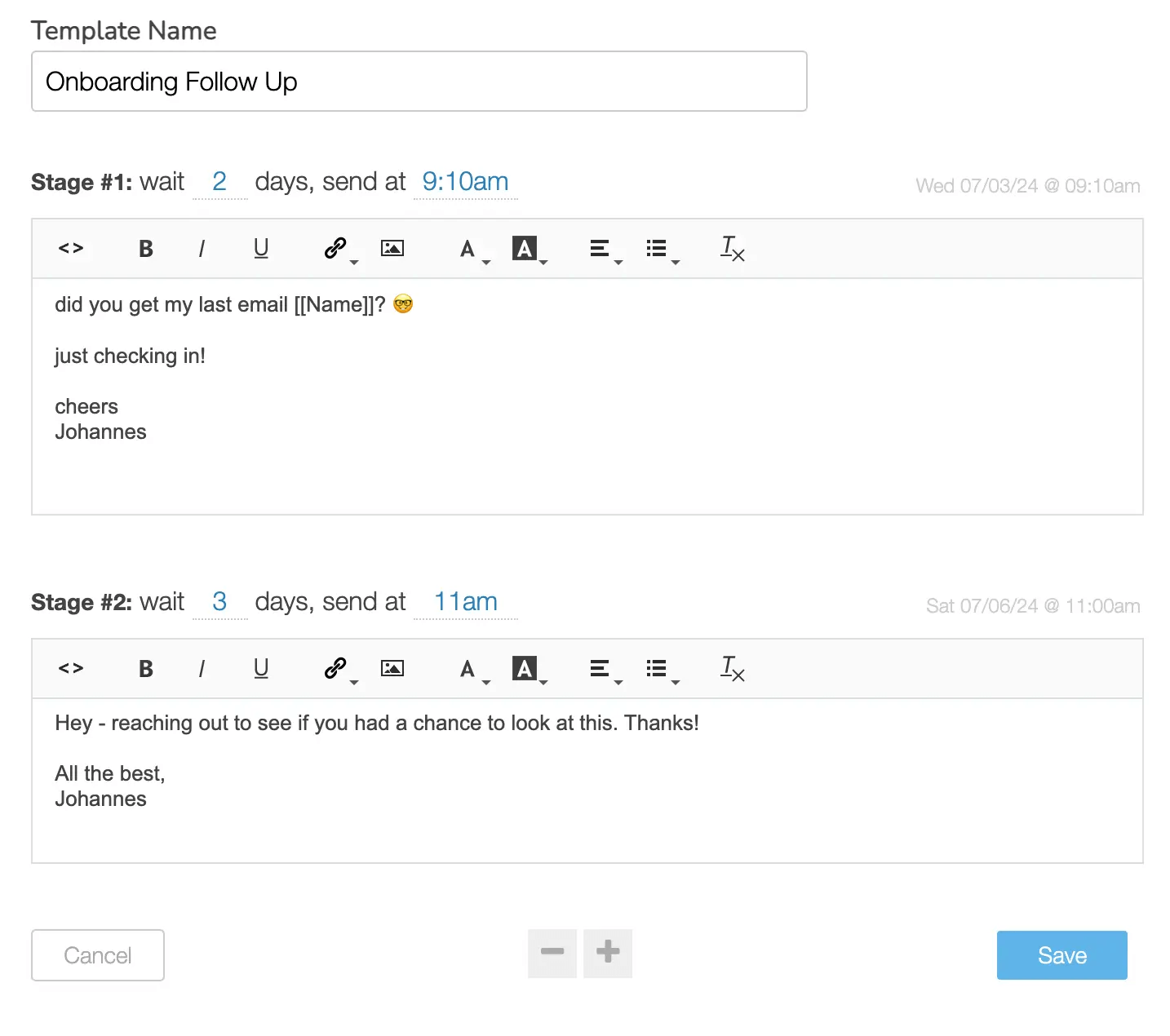
Once you hit Save, you have to **attach the Follow-Up to the Snippet** we created above.
Once Vocus is set up, let's have a look at Formbricks.
## Setting Up Formbricks
How and where you embed Formbricks for your onboarding survey is out of scope for this article.
The only important thing is that you do two things:
1. Identify the user (with Formbricks possible for both [link surveys embedded via iframe](https://formbricks.com/docs/link-surveys/user-identification) as well as [native surveys](https://formbricks.com/docs/app-surveys/user-identification)).
2. Activate email notifications for the Onboarding survey:

Aight, we’re good to go!
## The Process in Action
1) When a user [signs up for Formbricks](https://formbricks.com/signup), they are asked to fill out the Onboarding survey:
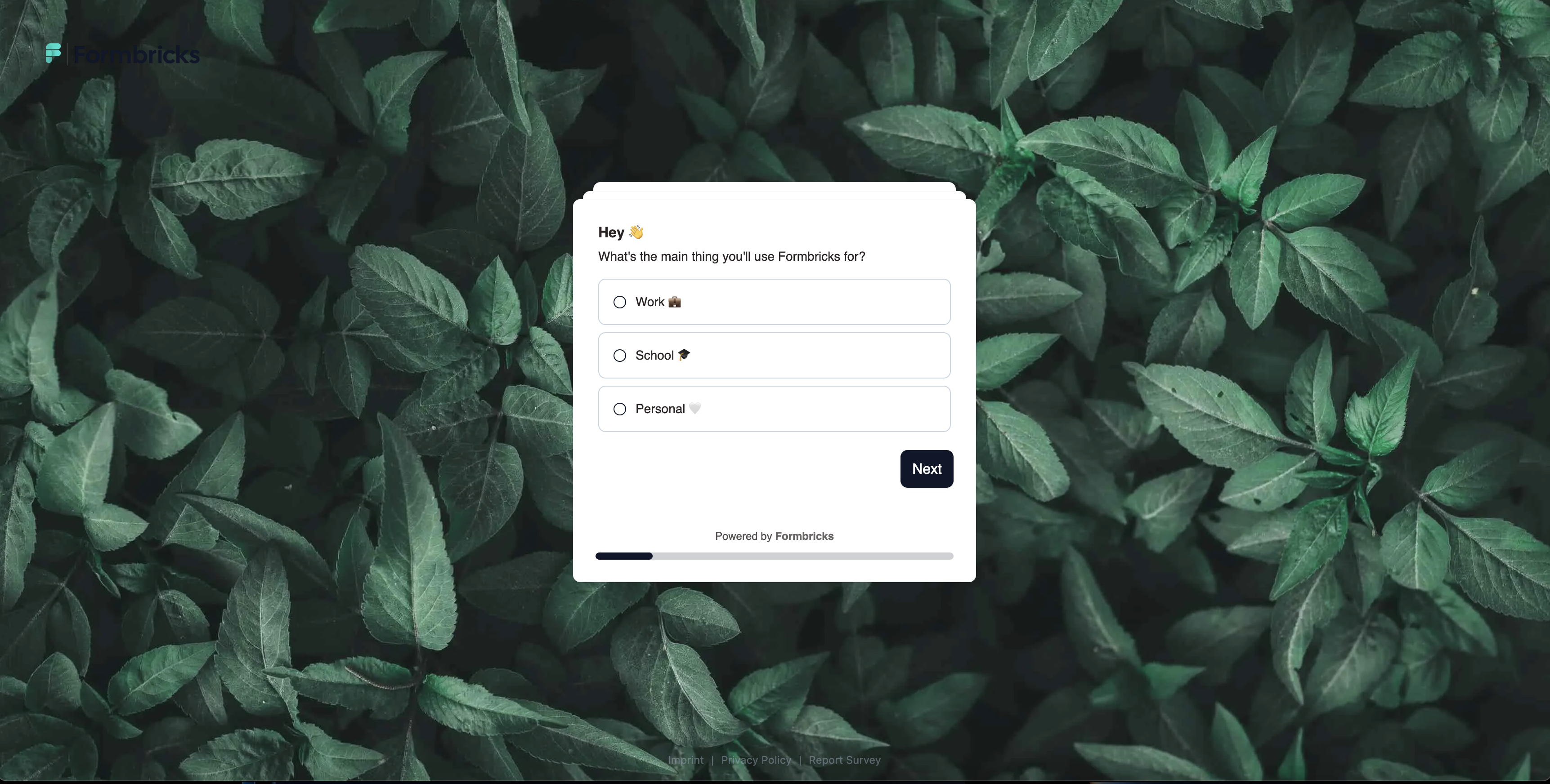
2) Because we set up both user identification and email notifications, I get an email as soon as someone signs up. Before the email hits my inbox, I filter out all personal sign-ups which are unlikely to buy an Enterprise plan from us:

3) The Formbricks email notification contains both the email of the new sign-up and the content of the Onboarding survey:
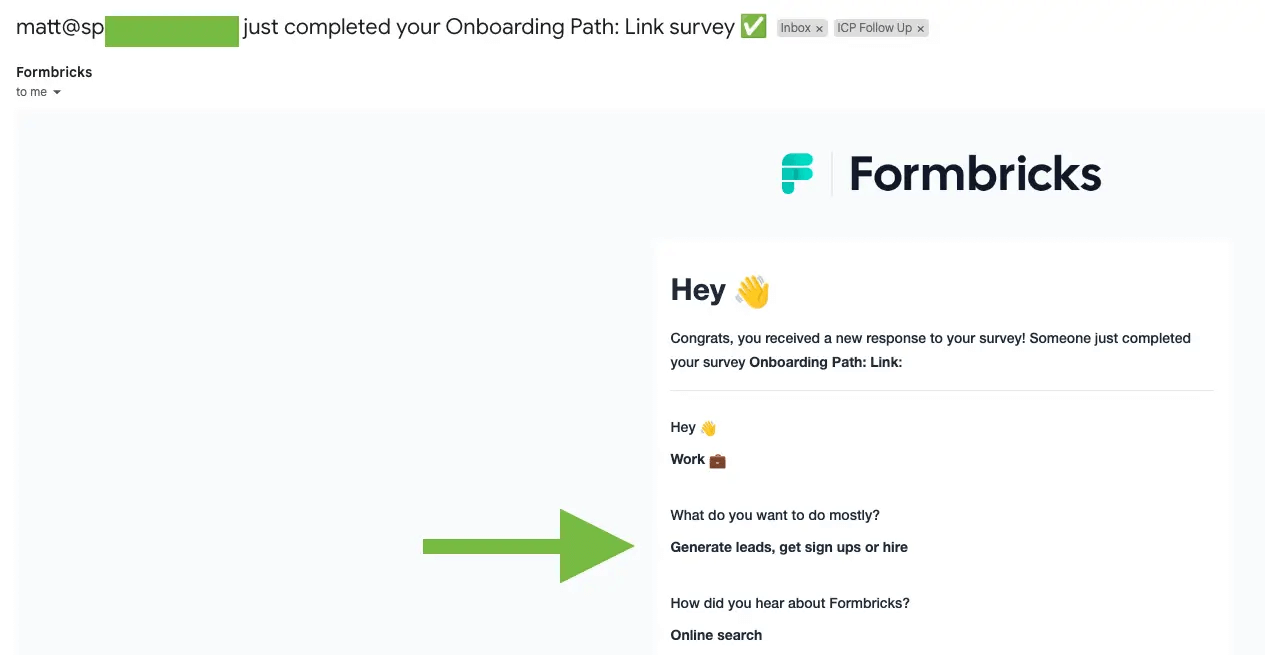
I look at the domain and website to understand what the company is working on. For promising leads, I try to find the person on LinkedIn. Together with the info from the onboarding survey, I have enough to personalize my outreach message.
4) I then draft an email to the user and type `/` into the body of the email. Vocus opens and lets me pick a template by typing the name of it. In this case, I have a “generic-onboarding-followup” template in both English and German:
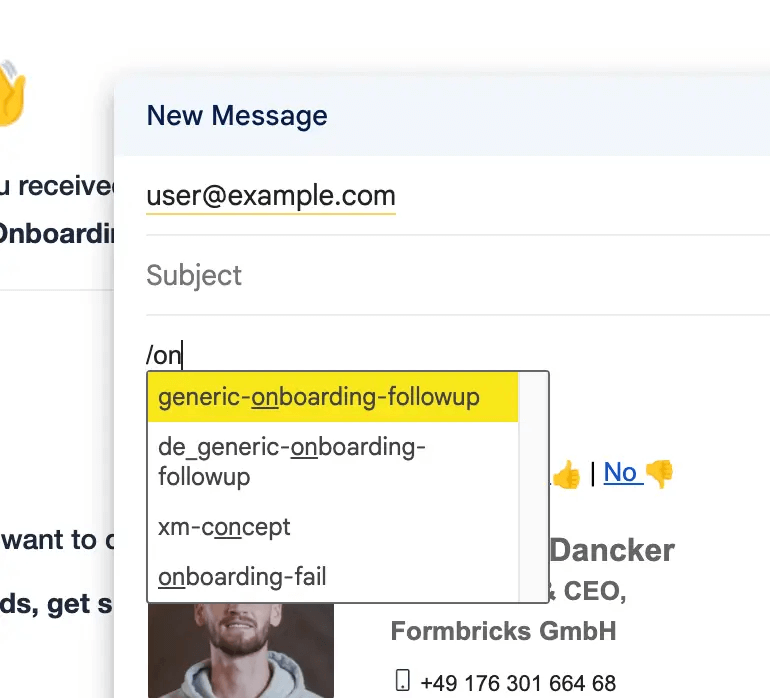
Now Vocus asks me to fill in the placeholders I created in the template:
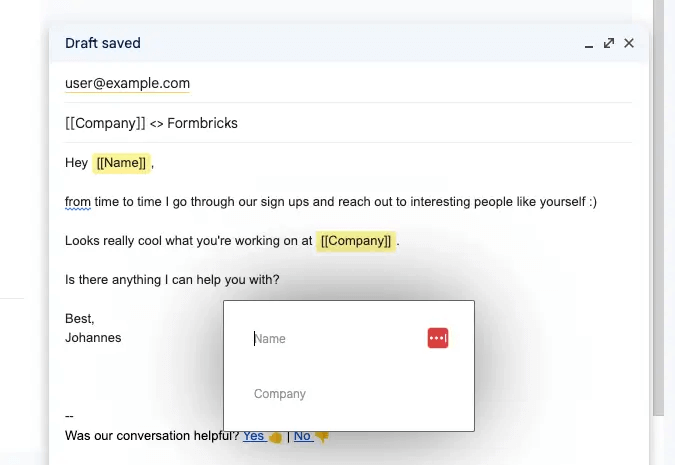
It fills the values in both the template and the follow-ups:
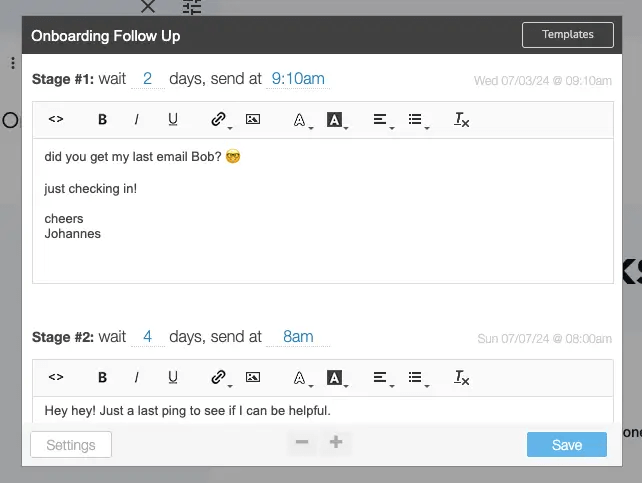
I really like that I can update the text or just go with the default. It lets me personalize the messages including follow-ups all in one step. I can set and forget until people reply.
5) After hitting “Save” to automatically send the follow-ups after 2 and 4 days, I can personalize my initial message. In my experience, it’s not super important what exactly you write. The main goal is to give the recipient the impression that the email is not automated:
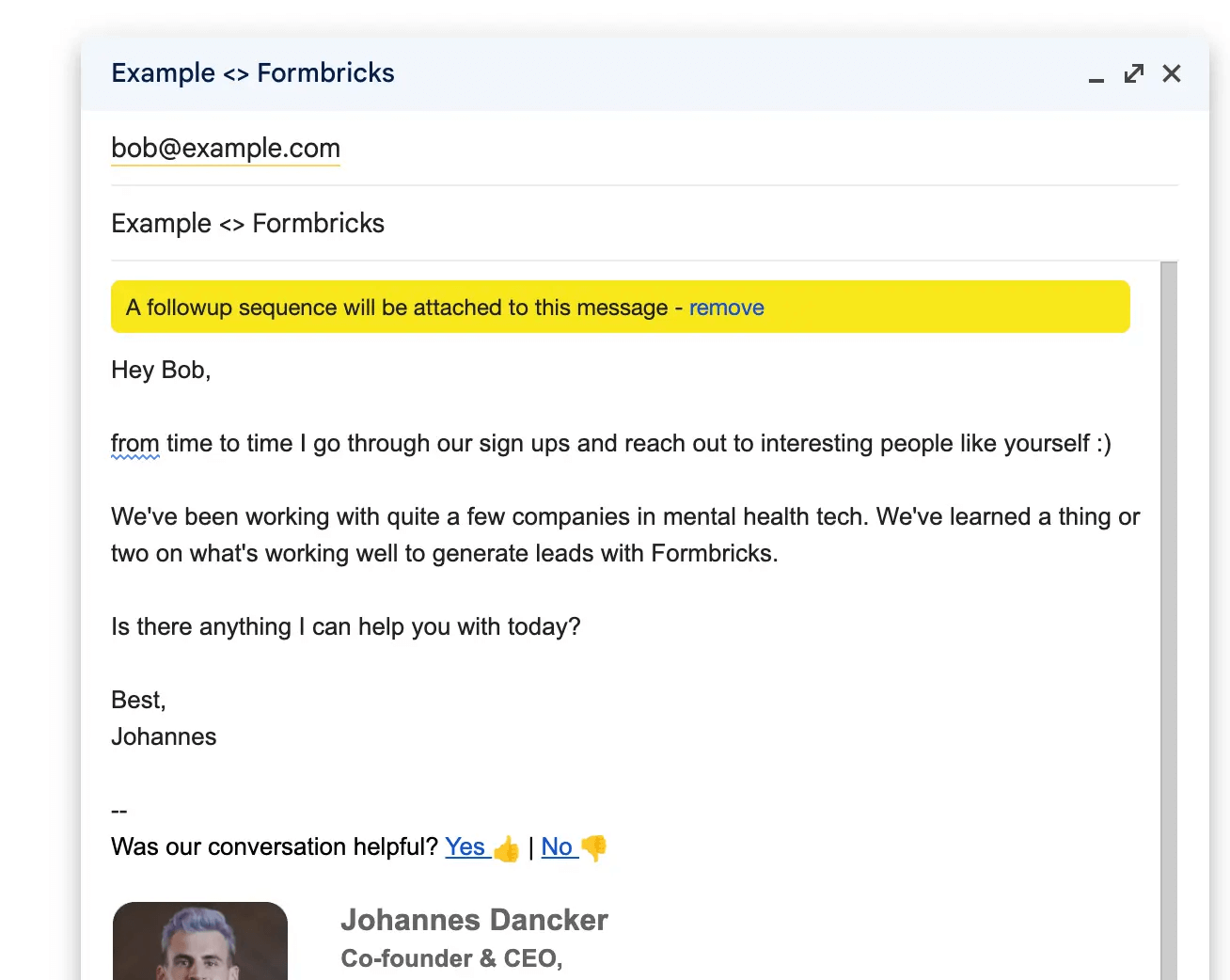
### That’s It!
For me, this is the perfect balance of keeping control over personalization and automating away the tedious stuff (templating, follow-ups, mental load of having to think of doing a daily task in different tools).
It’s simple, cheap, and effective.
Have a look at both [Vocus.io](https://vocus.io) and the [Formbricks Onboarding Survey](https://formbricks.com/onboarding-segmentation).
### Keep shipping 🚢
| jobenjada |
1,902,223 | Choosing Your Readability & Maintainability Path | Ugh, we've all been there. You open some code, see a jumble of weird symbols, and think: "What in the... | 25,953 | 2024-07-05T06:30:00 | https://dev.to/jaloplo/choosing-your-readability-maintainability-path-4abj | softwareengineering, softwaredevelopment | Ugh, we've all been there. You open some code, see a jumble of weird symbols, and think: "What in the world is this?!" Even a simple to-do list app code can feel like a secret message!
But what if you could see how the code works? What if you could see different ways to build the same app, each a bit different? This article is your code detective kit!
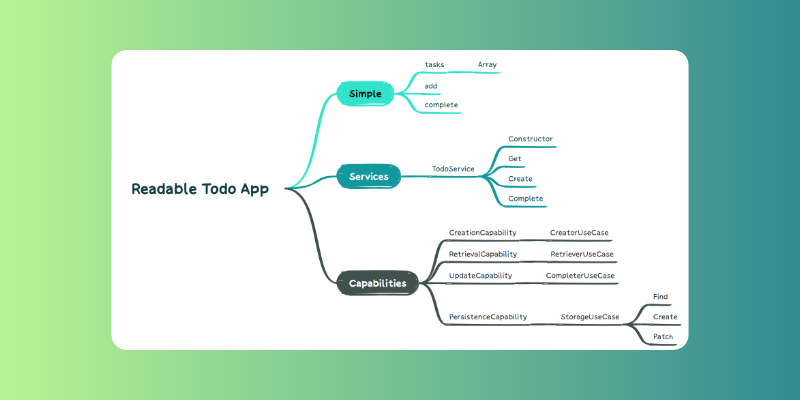
We'll explore three ways to build a basic to-do list app. By looking at the code itself, you'll get a feel for how easy it is to read and change the code. There's no one "perfect" way – it's all about finding what works best for you. So, grab a drink, turn on your code detective brain, and get ready to see how different coding styles tackle the same task! Maybe you'll even find a favorite way to build your own apps in the future!
## Code in One File: Friend or Foe?
This chapter dives into a unique approach: all the code in one file! This code only includes the functions it absolutely needs to work. Here's the code itself:
```TypeScript
type Task = {
title: string;
done: boolean;
};
type Collection = Task[];
const tasks: Collection = [];
const add: (title: string) => Task = (title) => {
const task = {
title: title,
done: false
};
tasks.push(task);
return task;
};
const complete: (title: string) => Task = (title) => {
let taskIndex = tasks.findIndex(t => t.title === title);
tasks[taskIndex].done = true;
return tasks[taskIndex];
};
add('Task 1');
add('Task 2');
add('Task 3');
complete('Task 1');
complete('Task 3');
console.log(tasks);
```
At first glance, it might seem a bit jumbled. But don't worry! With a closer look (or two!), you'll see how it all works together. This approach makes future changes and updates seem pretty straightforward, as long as the logic stays clear.
Think of it like a simple to-do list on a single piece of paper. Easy to understand, easy to update with new tasks or mark things done. But imagine having multiple to-do lists scattered around – that's when things can get messy! Adding a feature like marking all tasks as completed might be simple here, but keeping things organized with multiple lists could become tricky.
**So, when is this single-file approach a good friend to have?** It shines for smaller projects or prototypes. When the codebase is compact and the logic is clear, this approach can be a great time-saver. Everything is in one place, making it easy to find what you need and make quick edits. But remember, just like a to-do list that gets too long, a single code file can become overwhelming as your project grows.
## Code on the Case: Split Up for Success
This chapter explores a different approach. Here, the code is spread out across multiple files, making it easier to grasp at a quick glance. Imagine a well-organized to-do list with each task on its own sticky note. Much easier to understand, right?
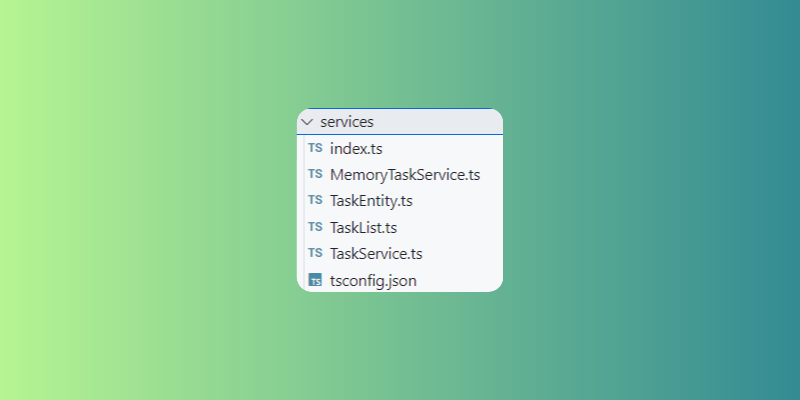
For example, we have a special code part named `TaskEntity` that represents each individual task we manage. The goal here isn't to become a code master – it's about understanding what happens in the main code file, which in this case is called `index.js`. Here's a peek at the code:
```TypeScript
import MemoryTaskService from './MemoryTaskService';
const service = MemoryTaskService();
service.add('Task 1');
service.add('Task 2');
service.add('Task 3');
service.complete('Task 1');
service.complete('Task 3');
const allTasks = service.get();
console.log(allTasks);
```
See the difference? This code is much easier to read and follow, even for our first-time code detectives. We can clearly see how three tasks are created and two are marked as completed. Plus, it's easy to spot where we might add new features.
Think of it like adding a new category to your to-do list. We already know where to go (the service) to implement marking all tasks as complete. But adding a whole new "list" (another set of tasks) isn't as clear from this one file. That's where the other code files come in – they hold the details behind the scenes!
**When is this multi-file approach a champion?** It's a great choice for larger projects or when the logic gets complex. By splitting the code into smaller, focused files, it becomes easier to understand, maintain, and modify. Imagine a massive to-do list – breaking it down into categories (like separate files) makes it much less overwhelming!
## Cracking the Code Case: Power Up with Domains!
This chapter dives into the final approach. Here, the code is split into multiple files, just like in the last case. But this time, it uses the hexagonal architecture with a twist of *capabilities*. Understanding the file structure might require some extra detective work at first, but the explanation will make it all clear.
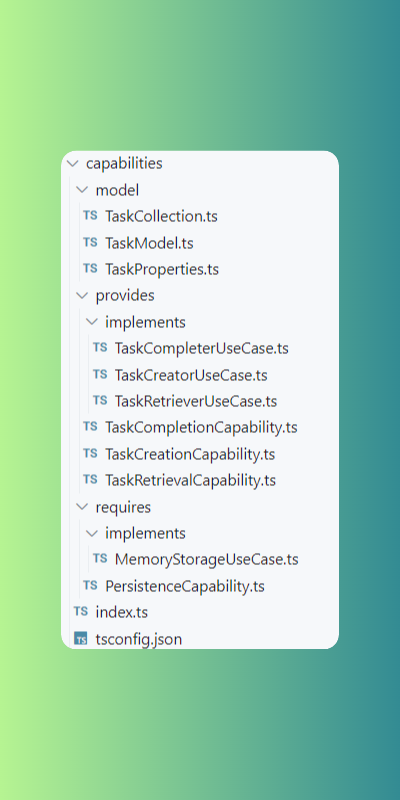
Similar to the previous chapter, we'll focus on the main code file, `index.js`, to see what's happening:
```TypeScript
import MemoryStorageUseCase from "./requires/implements/MemoryStorageUseCase";
import TaskCompleterUseCase from "./provides/implements/TaskCompleterUseCase";
import TaskCreatorUseCase from "./provides/implements/TaskCreatorUseCase";
import TaskRetrieverUseCase from "./provides/implements/TaskRetrieverUseCase";
const storage = MemoryStorageUseCase();
const taskCreator = TaskCreatorUseCase(storage);
const taskRetriever = TaskRetrieverUseCase(storage);
const taskCompleter = TaskCompleterUseCase(storage);
taskCreator.create('Task 1');
taskCreator.create('Task 2');
taskCreator.create('Task 3');
taskCompleter.update('Task 1');
taskCompleter.update('Task 3');
const tasks = taskRetriever.retrieve();
console.log(tasks);
```
Don't get discouraged if it seems like there's more code here compared to the last case. There's a hidden benefit to this complexity! While it might have a few more lines, this approach offers superpowers for future changes.
Imagine your to-do list on steroids. This approach lets you break it down into specific areas (domains) with clear capabilities (like adding tasks or marking them complete). This makes it easier for different people to work on different parts without stepping on each other's toes. Think of it as separate to-do lists for different categories, each with its own set of rules.
Let's say you want to add a new feature like marking all tasks as completed. With this approach, a single code detective can focus on that specific task (capability) without affecting anything else. They can even use a separate *storage* for testing purposes to make sure it works perfectly.
But what if you want a whole new list entirely? The code might not give away all the details at first glance, but the underlying structure allows for easy creation of new domains with their own capabilities. This gives you ultimate flexibility as your to-do list (or project) grows and evolves.
**When is this capabilities-based approach most beneficial?** It shines for complex projects. By clearly separating concerns and capabilities, this approach makes it easier to understand, maintain, and scale your codebase. Think of it as the ultimate organizational tool for keeping your ever-growing to-do list under control!
## Conclusion
Congratulations! You've cracked the case and explored three unique approaches to building a basic task management application. We've seen how a single file can be quick for small projects, while multiple files offer better organization for larger ones. Finally, the capabilities-based approach on top of hexagonal architecture provides ultimate power and flexibility for complex projects.
There's no single *right* answer here. The best approach depends on the size and complexity of your project, as well as your personal preferences. This article has equipped you with the knowledge to choose the path that best suits your coding needs. So, grab your favorite beverage and get ready to build amazing things!
## References
- *Github repository: [https://github.com/jaloplo/me-readable-todo-app](https://github.com/jaloplo/me-readable-todo-app)*
- *Simplifying Hexagonal Architecture: Using Capabilities and Requirements for Better Code: [https://dev.to/jaloplo/simplifying-hexagonal-architecture-using-capabilities-and-requirements-for-better-code-5aei](https://dev.to/jaloplo/simplifying-hexagonal-architecture-using-capabilities-and-requirements-for-better-code-5aei)* | jaloplo |
1,912,321 | iSEE Lab Stores 500M+ Files on JuiceFS Replacing NFS | Sun Yat-sen University is one of the top universities in China. Its Intelligence Science and System... | 0 | 2024-07-05T06:29:58 | https://dev.to/daswu/isee-lab-stores-500m-files-on-juicefs-replacing-nfs-3ag0 | ai | [Sun Yat-sen University](https://en.wikipedia.org/wiki/Sun_Yat-sen_University) is one of the top universities in China. Its Intelligence Science and System (iSEE) Lab focuses on human identification, activity recognition, and other related topics in visual surveillance. For dealing with large-scale vision data, we’re interested in large-scale machine learning algorithms such as online learning, clustering, fast search, cloud computing, and deep learning.
During deep learning tasks, we needed to handle massive small file reads.** In high-concurrency read and write scenarios, our network file system (NFS) setup showed poor performance. We often faced node freezes during peak periods. In addition, NFS had single point of failure issues**. If that node failed, data on the server was completely inaccessible. Scalability was also challenging. Each new data node required multiple mounts across all compute nodes. Small data volumes of the new node did not effectively alleviate read or write pressures.
To address these issues, we chose [JuiceFS](https://juicefs.com/docs/community/introduction/), an open-source distributed file system. Integrated with TiKV, JuiceFS successfully manages over 500 million files. **The solution markedly enhanced performance and system stability in high-concurrency scenarios. It ensures continuous operation of compute nodes during deep learning training, while effectively mitigating single point of failure concerns.**
JuiceFS is easy to learn and operate. It doesn’t require dedicated storage administrators for maintenance. This significantly reduces the operational burden for cluster management teams primarily composed of students in the AI field.
In this article, we’ll deep dive into our storage requirements in deep learning scenarios, why we chose JuiceFS, and how we built a storage system based on JuiceFS.
## Storage requirements in deep learning scenarios
Our lab's cluster primarily supports deep learning training and method validation. During training, we faced four read and write requirements:
- During the training process, a large number of dataset files needed to be read.
- At the initial stage of model training, we needed to load a Conda environment, which involved reading many library files of different sizes.
- Throughout the training process, as model parameters were adjusted, we frequently wrote model parameter switch files of varying sizes. These files ranged from tens of megabytes to several gigabytes, depending on the model size.
- We also needed to record training logs (for each training session), which mainly involved frequent writing of small amounts of data.
Based on these needs, we expected the storage system to:
- **Prioritize stability in high-concurrency read and write environments**, with performance improvements built upon stability.
- **Eliminate single points of failure**, ensuring that no node failure would disrupt cluster training processes.
- **Feature user-friendly operational characteristics**. Given that our team mainly focused on AI deep learning and we had limited storage expertise, we needed an easy-to-operate storage system that had low maintenance frequency.
- **Provide a POSIX interface to minimize learning costs and reduce code modifications**. This was crucial, because we used a PyTorch framework in model training.
### Current hardware configuration
Our hardware consists of three types of devices:
- Data nodes: About 3 to 4 nodes, each equipped with a lot of mechanical hard drives in RAID 6 arrays, totaling nearly 700 TB storage capacity.
- Compute nodes: Equipped with GPUs for intensive compute tasks, supplemented with 1 to 3 SSD cache disks per node (each about 800 GB), since JuiceFS has caching capabilities.
- TiKV nodes: Three nodes serving as metadata engines, each with about 2 TB data disk capacity and 512 GB memory. According to current usage, each node uses 300+ GB of memory when handling 500 to 600 million files.
### Challenges with NFS storage
Initially, when there were not many compute nodes, we built standalone file systems on data nodes and mounted these file systems onto the directory of compute nodes via NFS mounts for data sharing. This approach simplified operations but as the number of nodes increased, we encountered significant performance degradation with our NFS-based storage system.
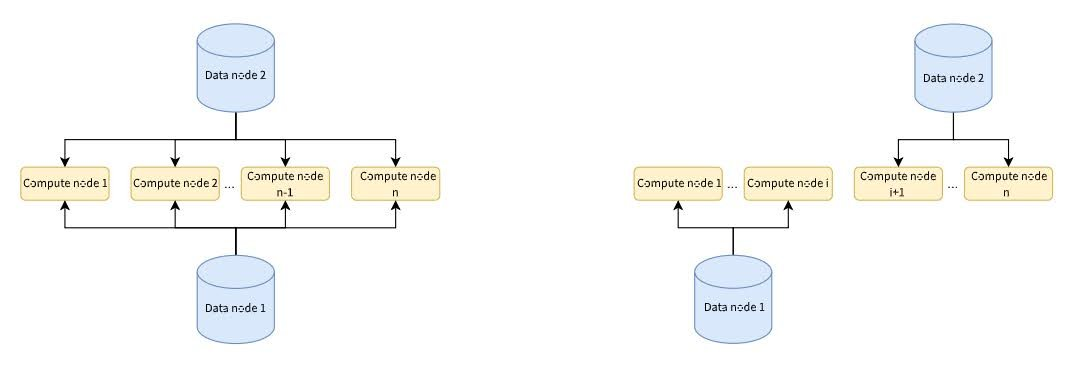
During peak periods of high concurrency and training, the system frequently experienced severe slowdowns or even freezes. This severely impacted our work efficiency. To mitigate this issue, we tried to divide the cluster into two smaller clusters to reduce data pressure on each node. However, this temporary measure did not bring significant improvements and introduced new challenges such as limited data interchangeability between different clusters.
Overall, **our NFS storage solution demonstrated poor read performance and high risk of system crashes, especially in scenarios involving reading numerous small files**. In addition, the entire system lacked a caching mechanism. But deep learning dataset training needed frequent reads. This exacerbated read and write pressures. Therefore, we sought a more efficient and stable storage solution to address these challenges.
## Why we chose JuiceFS
We chose JuiceFS due to the following reasons:
- **POSIX compatibility**: JuiceFS supports POSIX interfaces. This ensures a seamless migration experience without disruption to users when switching systems.
- **Cache feature**: JuiceFS’ caching feature is crucial for training deep learning models. Although the cache may not be directly hit during the first round of data loading, as training progresses, subsequent rounds can use the cache almost 100%. This significantly improves the data reading speed during the training process.
- **Trash**: JuiceFS trash allows data recovery. The one-day trash we set up allows accidentally deleted files to be recovered within one day. In practical applications, it has helped users recover accidentally deleted data in a timely manner.
- **Specific file management commands**: JuiceFS offers a set of unique file management commands that are not only user-friendly but also more efficient compared to traditional file systems.
- **Operational excellence**: Since our lab does not have full-time personnel responsible for storage, we expect a storage system that should be easy to use and have low operation frequency, and JuiceFS exactly meets these needs. It provides rich documentation resources, allowing us to quickly find solutions when getting started and solving problems. JuiceFS can automatically back up metadata and provides an extra layer of protection for data. This enhanced our sense of security. The Prometheus and Grafana monitoring functionalities that come with JuiceFS allow us to easily view the status of the entire system on the web page, including key information such as the growth of the number of files, file usage, and the size of the entire system. This provides us with timely system monitoring and management convenience.
## Building a JuiceFS-based storage system
Our JuiceFS setup uses TiKV as the metadata engine and SeaweedFS for object storage.
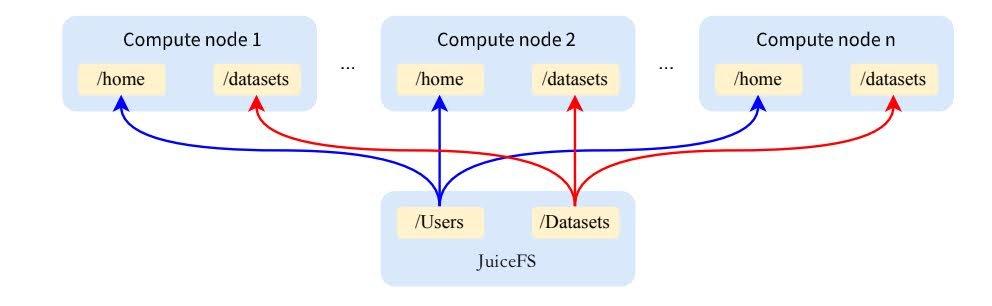
JuiceFS is divided into two directories for mounting:
- One directory stores user files. The independent mounting method allows us to flexibly adjust the mounting parameters to meet the specific needs of different directories. In terms of user file directories, we mainly use the default mount parameters to ensure stability and compatibility.
- The other directory is for the dataset. Given its almost read-only nature, we specifically increased the expiration time of the metadata cache. This can hit the metadata cache multiple times during multiple reads, thereby avoiding repeated access to the original data and significantly improving the speed of data access. Based on this change, we chose to mount the dataset and user files in two different directories.
In addition, we’ve implemented practical optimizations. Considering that the main task of the compute node is to process computing tasks rather than background tasks, **we equipped an idle node with a large memory specifically for processing background tasks, such as backing up metadata. As the number of files increases, the memory requirements for backup metadata also gradually increase. Therefore, this allocation method not only ensures the performance of the compute nodes, but also meets the resource requirements of background tasks**.
### Metadata engine selection: Redis vs. TiKV
Initially, we employed two metadata engines: Redis and TiKV. During the early stages when data volume was small, in the range of tens of millions of data records, we chose Redis. At that time, because we were not familiar with these software solutions, we referred to relevant documents and chose Redis for its ease of adoption, high performance, and abundant resources. However, as the number of files rapidly increased, Redis' performance significantly degraded.
Specifically, we set up Redis database (RDB) persistence for Redis. When the memory usage increased, Redis was almost constantly in RDB backup state. This led to a significant decline in performance. In addition, we adopted sentinel mode at the time and enabled primary-secondary replication to increase availability, but this also caused problems. Because it was asynchronous replication, it was difficult to guarantee the data consistency of the secondary node after the primary node went down.
In addition, we learned that the client did not read metadata from the Redis secondary node, but mainly relied on the primary node for read and write operations. Therefore, as the number of files increased, the performance of Redis further degraded.
We then considered and tested TiKV as an alternative metadata engine. Judging from the official documentation, the performance of TiKV was second only to Redis. In actual use, the user experience is not much different from Redis. TiKV's performance is quite stable when the data volume reaches 500 to 600 million files.
Another advantage of TiKV is its ability to load balance and redundant storage. We use a three-node configuration, each node has multiple copies. This ensures data security and availability. For the operation and maintenance team, these features greatly reduce the workload and improve the stability and reliability of the system.
### Metadata migration from Redis to TiKV
We migrated the system around January this year and have been using TiKV stably for nearly half a year without any downtime or any major problems.
In the early stages of the migration, because Redis could not support the metadata load of 500 to 600 million files, we decided to use the export and import method to implement the migration. Specifically, we used specific commands to export the metadata in Redis into a unified JSON file, and planned to load it into TiKV through the `load` command.
However, during the migration process, we encountered a challenge. We noticed that a user's directory encountered a failure during export due to excessive file depth or other reasons. To solve this problem, we took an innovative approach. We exported all directories except the problematic directory separately and manually opened and joined these JSON files to reconstruct the complete metadata structure.
When we manually processed these files, we found that the metadata JSON files had a clear structure and the join operation was simple. Its nested structure was basically consistent with the directory structure, which allowed us to process metadata efficiently. Finally, we successfully imported these files into TiKV and completed the migration process.
## Why choose SeaweedFS for object storage?
Overall, we followed the main components and basic features recommended in the official SeaweedFS documentation without going into too many novel or advanced features. In terms of specific deployment, we use the CPU node as the core and run the master server on it. The data nodes are distributed and run on different bare metals, and each node runs the volume service to handle data storage. In addition, we run the filer server on the same CPU node, which provides the S3 service API for JuiceFS and is responsible for connecting with JuiceFS. Judging from the current operating conditions, the load on the CPU node is not heavy, and the main data reading, writing and processing tasks are distributed on each worker node.
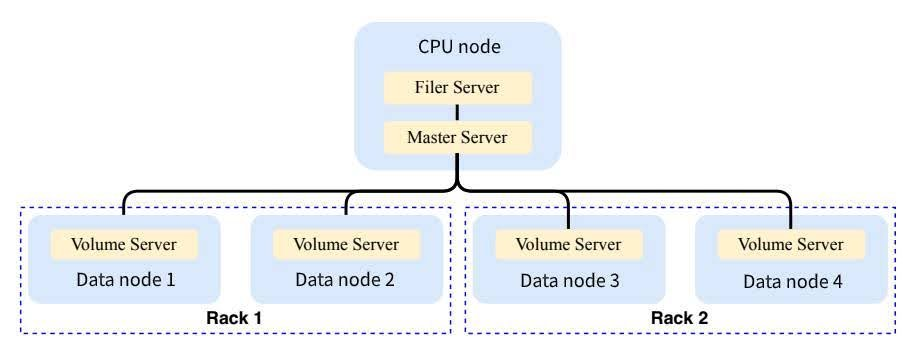
Regarding data redundancy and backup, we used SeaweedFS' redundancy features extensively. Logically, we divided data nodes into two racks. When writing data, it’s simultaneously written to both Rack 0 and Rack 1 to ensure dual backups. Data is considered successfully written only when it’s successfully written to both racks. While this strategy logically reduces our disk capacity by half (as each piece of data is stored twice), it ensures high availability and data safety. Even if a node in one rack fails or goes offline, it does not impact overall read, write operations, or data safety.
## Challenges and solutions
When we used JuiceFS, we encountered the following challenges.
### Client abnormal exits
We faced issues with clients unexpectedly exiting. Upon analysis, we identified these exits were caused by out-of-memory (OOM) errors. As the original data grew, specific nodes without the `--no-bgjob` option enabled experienced high memory consumption tasks (primarily automatic metadata backups). This led to insufficient remaining memory for backing up original data. This triggered OOM errors and client exits. To resolve this issue, we added the `--no-bgjob` option across all compute nodes and used idle data nodes specifically for background task processing.
### Slow initial read of large files
During our initial use phase, we observed significantly slower read speeds than the gigabit network bandwidth limit particularly when accessing large files for the first time. Upon deeper investigation, we found this was due to incorrect configuration of JuiceFS command parameters during performance testing.
We did not specify the `--storage s3` option. As a result, the performance was local disk performance by default rather than actual object storage performance. This misunderstanding led to a misjudgment of object storage performance. Through further inspection, we found performance bottlenecks in the SeaweedFS Filer metadata engine, primarily due to the use of a single mechanical disk at the underlying level. Thus, we considered optimizing this aspect to enhance performance.
### Slow dataset decompression (large volume of small file writes)
We occasionally needed to decompress datasets in daily use. This involved writing a large number of small files. We found this process to be significantly slower compared to local decompression. The JuiceFS team suggested using the write-back acceleration feature. This feature allows for immediate returns after a file is written, while the background uploads the data to object storage. We plan to implement this recommendation in the future to optimize decompression performance.
If you have any questions for this article, feel free to join [JuiceFS discussions on GitHub](https://github.com/juicedata/juicefs/discussions) and their [community on Slack](https://juicefs.slack.com/ssb/redirect).
| daswu |
1,912,317 | Mastering Binary Search in JavaScript: A Comprehensive Guide for Beginners | Table of Contents Introduction What is Binary Search? How Binary Search Works Pseudo-code... | 0 | 2024-07-05T06:27:42 | https://dev.to/pr4san/mastering-binary-search-in-javascript-a-comprehensive-guide-for-beginners-2m6i | webdev, javascript, algorithms, beginners |
## Table of Contents
1. [Introduction](#introduction)
2. [What is Binary Search?](#what-is-binary-search)
3. [How Binary Search Works](#how-binary-search-works)
4. [Pseudo-code for Binary Search](#pseudo-code-for-binary-search)
5. [Implementing Binary Search in JavaScript](#implementing-binary-search-in-javascript)
6. [Original Examples](#original-examples)
- [Example 1: Finding a Number in a Sorted Array](#example-1-finding-a-number-in-a-sorted-array)
- [Example 2: Finding a Book in a Library](#example-2-finding-a-book-in-a-library)
- [Example 3: Guessing a Number Game](#example-3-guessing-a-number-game)
7. [Time Complexity of Binary Search](#time-complexity-of-binary-search)
8. [When to Use Binary Search](#when-to-use-binary-search)
9. [Common Pitfalls and How to Avoid Them](#common-pitfalls-and-how-to-avoid-them)
10. [Conclusion](#conclusion)
## Introduction
Binary search is a fundamental algorithm in computer science that efficiently locates a target value within a sorted array. It's like a high-tech version of the "higher or lower" guessing game. In this blog post, we'll dive deep into binary search, exploring its concepts, implementation in JavaScript, and real-world applications.
## What is Binary Search?
Binary search is a search algorithm that finds the position of a target value within a sorted array. It works by repeatedly dividing the search interval in half. If the value of the search key is less than the item in the middle of the interval, narrow the interval to the lower half. Otherwise, narrow it to the upper half. Repeatedly check until the value is found or the interval is empty.
## How Binary Search Works
Imagine you're looking for a specific page in a book:
1. You open the book in the middle.
2. If the page number you want is lower, you look in the first half of the book.
3. If it's higher, you look in the second half.
4. You repeat this process, halving the search area each time until you find your page.
That's essentially how binary search works!
## Pseudo-code for Binary Search
Here's a simple pseudo-code representation of the binary search algorithm:
```
function binarySearch(array, target):
left = 0
right = length of array - 1
while left <= right:
middle = (left + right) / 2
if array[middle] == target:
return middle
else if array[middle] < target:
left = middle + 1
else:
right = middle - 1
return -1 // Target not found
```
## Implementing Binary Search in JavaScript
Now, let's implement this algorithm in JavaScript:
```javascript
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid; // Target found, return its index
} else if (arr[mid] < target) {
left = mid + 1; // Target is in the right half
} else {
right = mid - 1; // Target is in the left half
}
}
return -1; // Target not found
}
```
## Original Examples
Let's explore some original examples to better understand how binary search works in different scenarios.
### Example 1: Finding a Number in a Sorted Array
Let's start with a simple example of finding a number in a sorted array.
```javascript
const sortedNumbers = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47];
function findNumber(numbers, target) {
const result = binarySearch(numbers, target);
if (result !== -1) {
console.log(`The number ${target} is found at index ${result}.`);
} else {
console.log(`The number ${target} is not in the array.`);
}
}
findNumber(sortedNumbers, 23); // Output: The number 23 is found at index 8.
findNumber(sortedNumbers, 25); // Output: The number 25 is not in the array.
```
In this example, we use binary search to quickly find a number in a sorted array of prime numbers.
### Example 2: Finding a Book in a Library
Let's create a more real-world example: finding a book in a sorted library catalog.
```javascript
class Book {
constructor(title, isbn) {
this.title = title;
this.isbn = isbn;
}
}
const libraryCatalog = [
new Book("Algorithms", "9780262033848"),
new Book("Clean Code", "9780132350884"),
new Book("Design Patterns", "9780201633610"),
new Book("JavaScript: The Good Parts", "9780596517748"),
new Book("You Don't Know JS", "9781491924464")
];
function findBook(catalog, targetIsbn) {
const compare = (book, target) => {
if (book.isbn === target) return 0;
return book.isbn < target ? -1 : 1;
};
let left = 0;
let right = catalog.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
const comparison = compare(catalog[mid], targetIsbn);
if (comparison === 0) {
return catalog[mid];
} else if (comparison < 0) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return null;
}
const searchIsbn = "9780596517748";
const foundBook = findBook(libraryCatalog, searchIsbn);
if (foundBook) {
console.log(`Book found: ${foundBook.title} (ISBN: ${foundBook.isbn})`);
} else {
console.log(`No book found with ISBN ${searchIsbn}`);
}
```
In this example, we use binary search to find a book in a sorted library catalog using its ISBN. This demonstrates how binary search can be applied to more complex data structures.
### Example 3: Guessing a Number Game
Let's implement a number guessing game that uses binary search to guess the player's number efficiently.
```javascript
function guessingGame() {
const min = 1;
const max = 100;
let guesses = 0;
console.log("Think of a number between 1 and 100, and I'll guess it!");
function makeGuess(low, high) {
if (low > high) {
console.log("You must have changed your number! Let's start over.");
return;
}
const guess = Math.floor((low + high) / 2);
guesses++;
console.log(`Is your number ${guess}? (Type 'h' for higher, 'l' for lower, or 'c' for correct)`);
// In a real implementation, we'd get user input here.
// For this example, let's assume the number is 73.
const userNumber = 73;
if (guess === userNumber) {
console.log(`I guessed your number ${guess} in ${guesses} guesses!`);
} else if (guess < userNumber) {
console.log("You said it's higher. I'll guess again.");
makeGuess(guess + 1, high);
} else {
console.log("You said it's lower. I'll guess again.");
makeGuess(low, guess - 1);
}
}
makeGuess(min, max);
}
guessingGame();
```
This example demonstrates how binary search can be used in an interactive scenario, efficiently guessing a number between 1 and 100 in no more than 7 guesses.
## Time Complexity of Binary Search
One of the key advantages of binary search is its efficiency. The time complexity of binary search is O(log n), where n is the number of elements in the array. This means that even for very large datasets, binary search can find the target quickly.
For example:
- In an array of 1,000,000 elements, binary search will take at most 20 comparisons.
- Doubling the size of the array only adds one extra comparison in the worst case.
This logarithmic time complexity makes binary search significantly faster than linear search (O(n)) for large datasets.
## When to Use Binary Search
Binary search is ideal when:
1. You have a sorted collection of elements.
2. You need to repeatedly search for elements in this collection.
3. The collection is large enough that the efficiency gain matters.
4. The collection allows for random access (like arrays).
It's particularly useful in scenarios like:
- Searching in large databases
- Implementing autocomplete features
- Finding entries in a phone book or dictionary
- Debugging by pinpointing where in a large codebase a problem occurs
## Common Pitfalls and How to Avoid Them
1. **Unsorted Array**: Binary search only works on sorted arrays. Always ensure your array is sorted before applying binary search.
2. **Integer Overflow**: In languages prone to integer overflow, calculating the middle index as `(left + right) / 2` can cause issues for very large arrays. A safer alternative is `left + (right - left) / 2`.
3. **Off-by-One Errors**: Be careful with your comparisons and index adjustments. It's easy to accidentally exclude the correct element by being off by one.
4. **Infinite Loops**: Ensure that your search space is actually shrinking in each iteration. If not, you might end up in an infinite loop.
5. **Forgetting Edge Cases**: Don't forget to handle edge cases like an empty array or when the target is not in the array.
## Conclusion
Binary search is a powerful algorithm that dramatically improves search efficiency in sorted datasets. By repeatedly dividing the search interval in half, it achieves logarithmic time complexity, making it invaluable for working with large datasets.
As you've seen through our examples, binary search can be applied to various scenarios beyond simple number searches. Whether you're developing a search function for a large database, creating a game, or optimizing any search process on sorted data, binary search is a crucial tool in your programming toolkit.
Remember, the key to mastering binary search is practice. Try implementing it in different scenarios, and soon you'll find yourself reaching for this efficient algorithm whenever you need to search through sorted data.
Happy coding, and may your searches always be logarithmic! | pr4san |
1,912,320 | Unlock the Power and the Benefits of Vy6ys: A Guide to Success | In today's fast-paced world, finding a competitive edge is essential for success. Enter Vy6ys, a... | 0 | 2024-07-05T06:26:07 | https://dev.to/loloy5544/unlock-the-power-and-the-benefits-of-vy6ys-a-guide-to-success-1a0p | vy6ys, benefits, greencric, power | In today's fast-paced world, finding a competitive edge is essential for success. Enter Vy6ys, a revolutionary solution designed to transform how individuals and businesses achieve their goals. This guide explores the power and benefits of Vy6ys, offering insights into how it can drive your success.
## What is Vy6ys?
**[Vy6ys](https://greencric.com/vy6ys/)** is a cutting-edge platform that integrates advanced technology with user-friendly features to enhance productivity, streamline operations, and boost overall performance. Whether you're a solo entrepreneur, a small business owner, or part of a large corporation, Vy6ys offers tailored solutions to meet your specific needs.
## The Power of Vy6ys
Advanced Analytics: Vy6ys leverages state-of-the-art analytics to provide deep insights into your business processes. By analyzing data patterns, Vy6ys helps you make informed decisions, optimize workflows, and identify growth opportunities.
Seamless Integration: One of the standout features of Vy6ys is its ability to integrate seamlessly with existing systems. This means you can easily incorporate Vy6ys into your current operations without disrupting your workflow, ensuring a smooth transition and immediate benefits.
User-Friendly Interface: Vy6ys is designed with the user in mind. Its intuitive interface ensures that even those with limited technical expertise can navigate and utilize its features effectively. This ease of use accelerates adoption and maximizes productivity from day one.
Customization: Every business is unique, and Vy6ys recognizes this by offering customizable solutions. Whether you need specific modules, tailored dashboards, or personalized reports, Vy6ys can be configured to suit your exact requirements.
## The Benefits of Vy6ys
Increased Efficiency: By automating repetitive tasks and providing real-time insights, Vy6ys helps you save time and resources. This increased efficiency allows you to focus on strategic initiatives that drive growth and innovation.
Enhanced Collaboration: Vy6ys facilitates better communication and collaboration among team members. Its integrated tools enable seamless sharing of information, fostering a collaborative environment that enhances teamwork and productivity.
Scalability: As your business grows, Vy6ys grows with you. Its scalable architecture ensures that it can handle increased workloads and more complex processes, making it a future-proof solution for businesses of all sizes.
Cost Savings: By optimizing processes and improving efficiency, Vy6ys helps reduce operational costs. The insights provided by Vy6ys can also identify areas where expenses can be cut, further contributing to your bottom line.
Improved Customer Experience: With Vy6ys, you can deliver a superior customer experience. Its analytics tools help you understand customer behavior and preferences, allowing you to tailor your offerings and provide personalized service that meets their needs.
## How to Get Started with Vy6ys
Assess Your Needs: Begin by evaluating your current processes and identifying areas where Vy6ys can add value. This will help you determine which features and modules are most relevant to your business.
Request a Demo: Seeing Vy6ys in action is the best way to understand its capabilities. Schedule a demo to explore its features and see how it can benefit your organization.
Implement and Train: Once you've decided to adopt Vy6ys, the implementation process is straightforward. Vy6ys offers comprehensive training to ensure your team can effectively use the platform and maximize its potential.
Monitor and Optimize: After implementation, continuously monitor the performance of Vy6ys and look for ways to optimize its use. Regularly reviewing analytics and feedback will help you refine processes and achieve even greater success.
## **Conclusion**
**[Vy6ys](https://greencric.com/)** is more than just a tool; it's a comprehensive solution designed to propel your business towards success. By unlocking the power and benefits of Vy6ys, you can transform your operations, enhance productivity, and achieve your goals with greater efficiency and effectiveness. Embrace the future of business optimization with Vy6ys and set yourself on the path to sustained success.
| loloy5544 |
1,912,319 | Revolutionizing Architectural Projects with Design Visualization & 3D Rendering Services | In the fast-evolving Architecture, Engineering, and Construction (AEC) industry, visualization plays... | 0 | 2024-07-05T06:25:50 | https://dev.to/pavan_sai_7/revolutionizing-architectural-projects-with-design-visualization-3d-rendering-services-2f5l | architecture, design | In the fast-evolving Architecture, Engineering, and Construction (AEC) industry, visualization plays a pivotal role in the design process. The ability to see and understand a project's intricacies before it is built can significantly impact its success. Architectural Design Visualization and 3D Rendering Services are transforming how architects and designers approach projects, providing tools that enhance precision, creativity, and client communication. This blog explores the transformative impact of these services on architectural projects, detailing their benefits, applications, and future trends.
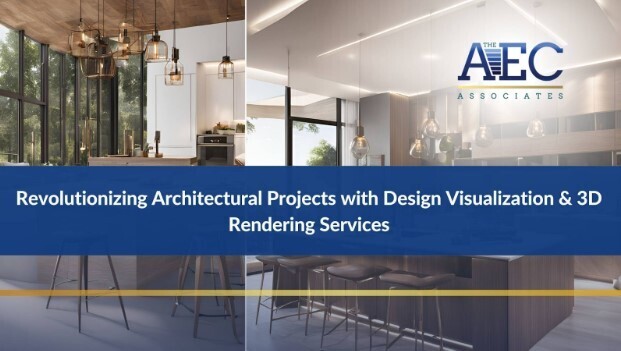
**Understanding Design Visualization & 3D Rendering**
**Definition and Scope of Design Visualization & 3D Rendering**
**[Design Visualization and 3D Rendering](https://theaecassociates.weebly.com/blog/visualizing-hospitality-elegance-architectural-design-visualization-and-3d-rendering-services-for-luxury-hotels-and-resorts)** involve creating detailed visual representations of architectural projects using advanced software tools. These services include photorealistic images, walkthroughs, and virtual tours that bring architectural concepts to life. They enable stakeholders to experience and interact with the design in a virtual environment, providing a comprehensive understanding of the project's scope and potential.
**Key Components**
- Photorealistic Images: High-quality images that depict the project with realistic lighting, textures, and materials.
- Walkthroughs: Animated sequences that guide viewers through the virtual space, simulating a real-life experience.
- Virtual Tours: Interactive experiences that allow users to explore the design from various angles and perspectives.
**Role of Software Tools**
Software tools like 3ds Max, V-Ray, and SketchUp are instrumental in creating detailed visualizations. These tools offer advanced features for modeling, texturing, lighting, and rendering, enabling architects to produce highly realistic and detailed representations of their designs.
**Benefits of Design Visualization & 3D Rendering Services**
**Enhanced Client Communication**
One of the most significant benefits of **[design visualization and 3D rendering services](https://theaecassociates.com/blog/transforming-educational-institutes-the-power-of-architectural-design-visualization-3d-rendering-services/)** is improved client communication. These services provide clients with a clear, realistic view of the project, facilitating better decision-making and faster approvals. Clients can visualize the end product, ask informed questions, and provide precise feedback, ensuring that the final design aligns with their expectations.
**Improved Design Accuracy**
3D rendering helps visualize complex design elements and spatial relationships, allowing architects to identify and address potential issues early in the design process. This proactive approach reduces the likelihood of errors and rework, enhancing the overall accuracy of the project.
**Marketing and Presentation**
High-quality visualizations are powerful tools for marketing and presentations. They create impactful visual content that can be used in promotional materials, investor pitches, and stakeholder meetings. Stunning visuals capture attention, convey the project's value, and help secure funding and approvals.
**Sector-Specific Applications**
**Residential Projects**
In residential architecture, detailed visualizations of interior and exterior spaces enhance client engagement and satisfaction. Clients can see their future homes in realistic detail, making it easier to visualize customizations and design elements. This clarity helps ensure that the final design meets their needs and preferences.
**Commercial Buildings**
Commercial projects often involve complex layouts and space planning. 3D rendering services provide detailed visualizations that address these complexities, ensuring compliance with commercial standards and regulations. Accurate visualizations help stakeholders understand the design's functionality and aesthetic appeal, leading to better planning and execution.
**Healthcare Facilities**
Healthcare facilities have unique requirements that must comply with stringent regulations and standards. Visualization and 3D rendering services help create functional and compliant medical facilities by providing detailed and realistic representations of the design. These visualizations ensure that all standards are met and that the facility is designed for optimal efficiency and patient care.
**Educational Institutions**
In educational architecture, accurate visualizations are essential for creating effective learning environments. 3D renderings help incorporate modern educational facility requirements, such as flexible learning spaces, accessibility features, and sustainable design elements. These visualizations ensure that the design supports the educational goals and enhances the learning experience.
**Implementing Visualization & 3D Rendering in Projects**
**Initial Design Phase**
The implementation of visualization and 3D rendering services begins with creating conceptual visualizations. These initial renderings set the design direction and provide a foundation for further development. They help communicate the design concept to clients and stakeholders, ensuring alignment from the project's outset.
**Detailed Rendering Phase**
In the detailed rendering phase, high-quality 3D models and renderings are developed. These detailed visualizations provide a comprehensive view of the project, showcasing all design elements with precision and clarity. They are used for in-depth reviews and presentations, facilitating better decision-making and approvals.
**Review and Feedback**
The visualization process is iterative, involving multiple rounds of review and feedback. Clients and stakeholders provide input on the visualizations, which are then refined to address their comments and concerns. This collaborative approach ensures that the final design meets all requirements and expectations.
**Challenges and Solutions in Visualization & 3D Rendering**
**Managing Large Projects**
Handling large and complex projects can be challenging in terms of visualization. Techniques such as dividing the project into manageable sections and using advanced rendering tools can help manage these complexities effectively, ensuring that all elements are accurately represented.
**Ensuring Realism**
Achieving photorealism in renderings requires advanced techniques and attention to detail. Using high-quality textures, accurate lighting, and realistic materials are essential for creating lifelike images. Continuous learning and adopting new rendering technologies can enhance the realism of visualizations.
**Balancing Costs and Quality**
Creating high-quality visualizations can be costly, but strategies for balancing costs and quality include optimizing rendering settings, using efficient workflows, and leveraging cloud-based rendering solutions. These approaches help produce high-quality visualizations within budget constraints.
**Future Trends in Design Visualization & 3D Rendering**
**Advancements in Rendering Technology**
Rendering technology continues to evolve, with new features and capabilities emerging regularly. Advancements such as real-time rendering, AI-driven enhancements, and improved rendering engines are making it possible to create more detailed and realistic visualizations faster and more efficiently.
**Integration with VR/AR**
The integration of 3D rendering with Virtual Reality (VR) and Augmented Reality (AR) is providing immersive design experiences. These technologies allow clients and stakeholders to interact with the design in new ways, offering deeper insights and better decision-making.
**Sustainable Visualization Practices**
Sustainability is becoming a priority in architectural design, and visualization practices are evolving to support this trend. Incorporating eco-friendly elements in visualizations, such as green roofs, energy-efficient systems, and sustainable materials, helps promote sustainable design practices and educate clients about the benefits of green architecture.
**Conclusion**
**[Architectural Design Visualization and 3D Rendering Services](https://theaecassociates.com/blog/architectural-design-visualization-3d-rendering-services-visualizing-future-cities/)** are revolutionizing the AEC industry by enhancing precision, creativity, and client communication. These services provide clear and realistic representations of architectural designs, facilitating better decision-making and project outcomes. As the industry continues to evolve, adopting these advanced tools and methodologies will be essential for achieving successful architectural projects. Embracing visualization and 3D rendering services can help architects deliver innovative, efficient, and client-focused designs. | pavan_sai_7 |
1,912,316 | First Coast Foot and Ankle Clinic | At First Coast Foot & Ankle Clinic, we are ready to help get you moving again. We believe healthy... | 0 | 2024-07-05T06:24:19 | https://dev.to/firstcoast0011/first-coast-foot-and-ankle-clinic-23ff | At First Coast Foot & Ankle Clinic, we are ready to help get you moving again. We believe healthy feet are the key to a happy, active lifestyle. Our specialty and focus is the medical and surgical treatment of the foot and ankle. Utilizing the latest techniques and technology, we provide our patient's the most advanced, comprehensive podiatric care available in a calming, informative environment.
In today's medical environment it is easy for a patient to seem lost. At First Coast Foot & Ankle Clinic we pride ourselves by treating the patient with the utmost respect, compassion, and understanding.
We realize that patient education is a vital part of the healing process and should not be overlooked. It is important for us to provide the most accurate information and treatment options so the patient can make an educated and informed decision about his/her health care.
Website link: https://firstcoastfootclinic.com/
GMB Link: https://g.page/r/CVMCEoXtFAkSEBM
Phone: 1 904-739-9129
Location: 8075 Gate Pkwy W STE 301, Jacksonville, FL 32216, United States
| firstcoast0011 |
|
1,912,315 | Deep Groove Ball Bearings: Providing Longevity and Reliability in Machinery | Deep Groove Ball Bearings are very important for the smooth operation of different types of... | 0 | 2024-07-05T06:22:33 | https://dev.to/luella_dreahsi_1059caea/deep-groove-ball-bearings-providing-longevity-and-reliability-in-machinery-49n1 | design | Deep Groove Ball Bearings are very important for the smooth operation of different types of machinery. Made from high quality component in small circular shape to exclude the friction between various components of machine processes by delivering a smooth long lasting movement. You may find them across a wide range of machines like motors, pumps and power tools among others to enable the equipment to function effectively.
But one of the remarkable attributes is this type, which has a high yield for days without end. Designed to resist wear, they save time and money on regular repairs and replacements. This is why you can be confident that if a machine comes with a Deep Groove Ball Bearing, it will function correctly for years to come.
Advancements in Deep Groove Ball Bearing Technology
Due to advancements in technology, Deep Groove Ball Bearings have only gotten better over time: more durable and long-lasting. The manufacturers have developed these Bearings with strategic designs; they use superior quality materials and incorporate advanced technologies in an attempt to make their tapered roller bearing products more reliable. Similarly, more studies are going toward improved security features so that no sudden incidents happen reducing your machine running ability too.
At the end of it all, keeping people and machines safe continues to be a top measure in designing any machinery application. A safety design which is suitable for high-speed operation protection; The other significant feature of Deep Groove Ball Bearings are the ease that they allow in installation. For this purpose, safety equipment such as seals, shields or cages are used which prevent escaping of contaminants and protect the outside environment (water/dust) to enter into machinery for maintaining safe operational surroundings.
How To Make The Best Use Of Deep Groove Ball Bearings
Deep Groove Ball Bearings are easy to install in your machine. It's important to choose the right specification and size that matches with your project needs. Take care with the installation and make sure they are not damaged, so that we can use them to their full potential. Additionally, correct treatment is a crucial phase in maintaining their surface finishes fine on the way to steer clear of rust and contamination encumbrances.
Correct locating and alignmentare very important when mounting Deep Groove Ball Bearings, so the manufacturerinstructions should be followed inprecision.CodeAnalysis This can lead to higher vibrations, unplanned friction and damage of the tapered bearing machine. Thus, it is important to follow the authorized instructions for installation if one wishes to ensure in keeping functionality and reliability of the Bearings.
Deep Groove Ball Bearing Quality Assurance and Maintenance
Going for premium grade Deep Groove Ball Bearings from reliable manufacturers assures quality and durability with every purchase. These tests check the Bearings for effectiveness and durability, only after which they are able to meet standards set by respected manufacturers. Moreover, these companies also offer technical support along with training and can help you to optimize the performance of the Bearings.
Deep Groove Ball Bearings can become less effective with continuous use, contamination or damage over time. Frequent examination and maintenance ensures longer life of those components while retaining high level functions. Unusual sounds, excessive vibration or machine failure simply means that you need to call a professional help immediately for them to remove Drums and fix broken Bearings.
Deep Groove Ball Bearings Applications
Among the most widely used of rolling bearings, Deep Groove Ball Bearing serve a broad spectrum of machinery and products employing such in everything from individual gadgets to significant industrial equipment as well as cars. ATEX adaptable to different load configurations such as Light-Duty (LD)/and Heavy Duty(HD) offering a flexible solution for diverse machinery setups. At the end of the day, choosing tapered roller bearings that fits for your kit will ensure efficient and accurate but also safe operation.
To sum up, the deep groove ball bearing is an essential part that can help machines to achieve smooth and safe operation. The strength and their unique composition increasing them make essential for the better performance of machines. Prioritizing the replacement of quality Bearings, proper maintenance and inspection regularly as well for right selection of bearings according to your needs allows you to maintain your machines longevity. | luella_dreahsi_1059caea |
1,912,314 | Wellhealth How To Build Muscle Tag | Introduction Wellhealth How To Build Muscle Tag Wellhealth How To Build Muscle Tag is more... | 0 | 2024-07-05T06:21:22 | https://dev.to/loloy5544/wellhealth-how-to-build-muscle-tag-27f8 | wellhealth, muscle, fitness, gym | ## Introduction Wellhealth How To Build Muscle Tag
**[Wellhealth How To Build Muscle Tag](https://cyberpulseltd.co.uk/wellhealth-how-to-build-muscle-tag/)** is more than just lifting weights—it's a comprehensive approach that includes proper nutrition, consistent training, and adequate rest. Whether you’re a beginner or looking to refine your approach, this guide from Wellhealth will provide you with essential tips and strategies to maximize your muscle-building potential.
## 1. Set Clear Goals
Before diving into any muscle-building program, it's crucial to set clear, realistic goals. Whether you aim to gain a specific amount of muscle mass, increase strength, or improve overall fitness, having a defined target helps keep you motivated and focused.
## 2. Optimize Your Diet
Nutrition plays a critical role in muscle growth. Ensure your diet includes:
Protein: Aim for at least 1.2 to 2.2 grams of protein per kilogram of body weight. Great sources include lean meats, fish, eggs, dairy, legumes, and plant-based proteins.
Carbohydrates: These are essential for fueling your workouts and aiding recovery. Opt for complex carbs like whole grains, fruits, and vegetables.
Fats: Healthy fats are important for hormone production and overall health. Include sources like avocados, nuts, seeds, and olive oil.
Hydration: Drink plenty of water to stay hydrated, especially during intense workouts.
## 3. Strength Training Principles
To build muscle, incorporate the following principles into your training routine:
Progressive Overload: Gradually increase the weight, frequency, or intensity of your workouts to continually challenge your muscles.
Compound Exercises: Focus on exercises that work multiple muscle groups at once, such as squats, deadlifts, bench presses, and pull-ups.
Consistency: Stick to a regular workout schedule. Aim for at least 3-4 strength training sessions per week.
Rest and Recovery: Muscles grow during rest periods, so ensure you get adequate sleep and rest days between intense workouts.
## 4. Supplement Smartly
While whole foods should be your primary source of nutrients, certain supplements can support muscle growth:
Protein Powder: Convenient for meeting protein goals, especially post-workout.
Creatine: Enhances strength and performance.
Branched-Chain Amino Acids (BCAAs): May reduce muscle soreness and improve recovery.
## 5. Track Your Progress
Monitoring your progress helps you stay on track and make necessary adjustments. Keep a workout journal, take regular measurements, and consider periodic body composition analyses.
## 6. Avoid Common Mistakes
Overtraining: More isn’t always better. Overtraining can lead to injuries and hinder progress.
Poor Form: Using improper technique can result in injuries. Consider working with a trainer to learn correct form.
Neglecting Nutrition: Training hard without proper nutrition won't yield optimal results.
## 7. Stay Motivated
Maintaining motivation is key to long-term success. Set mini-goals, celebrate small victories, and consider joining a community or finding a workout partner for support and accountability.
## Conclusion
Building muscle is a journey that requires dedication, consistency, and a holistic approach. By setting clear goals, optimizing your diet, following effective training principles, and staying motivated, you can achieve impressive muscle gains and improve your overall health and fitness. Remember, every small step counts toward your larger goal. Keep pushing, stay focused, and enjoy the process of becoming a stronger, healthier you.
For more tips regarding this, stay tuned to **[CyberPulseLTD](https://cyberpulseltd.co.uk/)**
| loloy5544 |
1,912,300 | Node js Rest API | Creating a REST API using Node.js in the MERN stack involves several steps, including setting up... | 0 | 2024-07-05T06:19:05 | https://dev.to/thirdearnest123/node-js-rest-api-3dkb | node, restapi, mern |
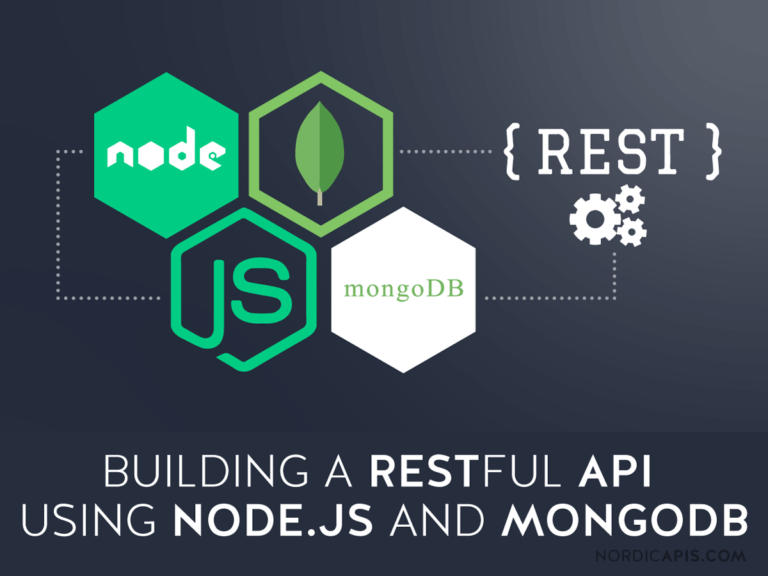Creating a REST API using Node.js in the MERN stack involves several steps, including setting up MongoDB, creating the backend with Express, and using tools like Postman to test the API. Here's a detailed guide to help you through the process:
### 1. Set Up Your Environment
#### Install Node.js and npm
Download and install Node.js from [nodejs.org](https://nodejs.org/). npm is included with Node.js.
#### Install MongoDB
Download and install MongoDB from [mongodb.com](https://www.mongodb.com/try/download/community).
### 2. Create a New Project
1. **Initialize a new Node.js project:**
```sh
mkdir mern-rest-api
cd mern-rest-api
npm init -y
```
2. **Install dependencies:**
```sh
npm install express mongoose body-parser cors
npm install --save-dev nodemon
```
3. **Install additional tools:**
```sh
npm install express-validator
```
### 3. Set Up MongoDB
1. **Start MongoDB:**
- On Windows: Run `mongod` in your terminal.
- On Mac: You can use `brew services start mongodb-community`.
2. **Create a new database and collection:**
- Use MongoDB Compass or the MongoDB shell to create a new database (e.g., `mern_db`) and a collection (e.g., `users`).
### 4. Create the Express Server
1. **Create the directory structure:**
```sh
mkdir src
cd src
mkdir config controllers models routes
touch server.js
```
2. **Set up the `server.js` file:**
```javascript
const express = require('express');
const mongoose = require('mongoose');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
// Middleware
app.use(bodyParser.json());
app.use(cors());
// MongoDB connection
mongoose.connect('mongodb://localhost:27017/mern_db', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
mongoose.connection.once('open', () => {
console.log('Connected to MongoDB');
});
// Routes
const users = require('./routes/users');
app.use('/api/users', users);
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
```
### 5. Create Models
1. **Create `models/User.js`:**
```javascript
const mongoose = require('mongoose');
const UserSchema = new mongoose.Schema({
name: {
type: String,
required: true,
},
email: {
type: String,
required: true,
unique: true,
},
password: {
type: String,
required: true,
},
});
module.exports = mongoose.model('User', UserSchema);
```
### 6. Create Controllers
1. **Create `controllers/userController.js`:**
```javascript
const User = require('../models/User');
const { body, validationResult } = require('express-validator');
// Get all users
exports.getUsers = async (req, res) => {
try {
const users = await User.find();
res.json(users);
} catch (err) {
res.status(500).json({ message: err.message });
}
};
// Create a new user
exports.createUser = [
body('name').notEmpty().withMessage('Name is required'),
body('email').isEmail().withMessage('Email is not valid'),
body('password').isLength({ min: 6 }).withMessage('Password must be at least 6 characters long'),
async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const { name, email, password } = req.body;
try {
const user = new User({ name, email, password });
await user.save();
res.status(201).json(user);
} catch (err) {
res.status(500).json({ message: err.message });
}
},
];
```
### 7. Create Routes
1. **Create `routes/users.js`:**
```javascript
const express = require('express');
const router = express.Router();
const userController = require('../controllers/userController');
// Get all users
router.get('/', userController.getUsers);
// Create a new user
router.post('/', userController.createUser);
module.exports = router;
```
### 8. Test the API with Postman
1. **Start the server:**
```sh
nodemon src/server.js
```
2. **Open Postman and create a new request:**
- **GET** `http://localhost:5000/api/users` to fetch all users.
- **POST** `http://localhost:5000/api/users` to create a new user. In the body, use the following JSON format:
```json
{
"name": "thirdearnest123",
"email": "[email protected]",
"password": "password123"
}
```
### Useful Resources
- [Node.js](https://nodejs.org/)
- [Express.js](https://expressjs.com/)
- [MongoDB](https://www.mongodb.com/)
- [Postman](https://www.postman.com/)
- [Mongoose](https://mongoosejs.com/)
- [Express Validator](https://express-validator.github.io/docs/)
By following these steps, you will have a basic REST API setup using the MERN stack. You can further expand this by adding more features, authentication, and front-end integration. | thirdearnest123 |
1,912,312 | 7 Standard Reasons Why Test Automation Fails [With Solution] | If test automation promises to deliver fast, efficient, consistent, and reliable testing, why don’t... | 0 | 2024-07-05T06:18:59 | https://dev.to/morrismoses149/7-standard-reasons-why-test-automation-fails-with-solution-3ca9 | testautomationfails, testgrid | If test automation promises to deliver fast, efficient, consistent, and reliable testing, why don’t more companies take advantage?
Less than 25% of QA teams are using test automation, and why are so many companies still sticking with manual testing even when they have an automated testing tool available?
According to CA Technologies’ report, Test Automation Trends, the number one reason for test automation failure is that there isn’t enough testing expertise in the organization.
Test automation provides numerous benefits to software development projects, such as reducing manual QA time and raising code quality by catching bugs early.
Yet, many teams still don’t automate their tests—and it’s not because they lack the money or time to do so. Indeed, there are several other reasons why test automation fails, so these are the most common ones. Here are some additional reasons why test automation fails in organizations.
## Standard Reasons Why Test Automation Fails
### 1. Unrealistic Expectations
Development teams have come to believe that test automation is a one-size-fits-all solution is the most common reason why test automation fails. Developers, who may not be accustomed to thinking like testers, are sold on test automation with unrealistic expectations.
For example, they may assume it will eliminate all manual testing or that it will drastically reduce test cycle times. This leads to disappointment when expectations aren’t met and leaves them questioning whether automated tests provide any value.
### 2. “One Size Fits All” Mindset
One of the leading causes of the failures of test automation is that test automation does not provide a one size fits all solution as it depends on various parameters like requirements, the type of tests, and the number of tests that need to be automated.
You’ll need to be patient and willing to grow along with continuous improvement for automated testing to work efficiently.
### 3. Improper Management
There are plenty of reasons why your software projects might not be automated, but poor management is likely near or at the top of that list and responsible for test automation failure.
When management doesn’t value test automation—or worse, does not prioritize it above other activities—there will always be a long list of pressing tasks that will get done before anyone gets around to Automation.
And once you do begin to automate, you can expect resistance from everyone on your team who isn’t quite sure how it fits into their workflow. So make sure you have support from upper management and don’t be afraid to help them understand why it’s important in term of avoiding failures of test automation. After all, if you have time for another meeting about defects or issues with code quality, there’s time for test automation!
It is naïve to think that Automation will fix everything. This issue is complicated because only half of the team members know how automation testing is performed.
Each team member should have the necessary skills to perform their role effectively, as Automation is a team effort rather than something one person can handle independently.
### 4. Ignoring Manual Testing Completely
Humans aren’t always consistent. The same test can be executed multiple times by different people and get vastly different results.
For example, one person might be in a good mood on a particular day and interpret things more favourably than another. Even if all users execute tests with 100% accuracy, a manual tester is still limited to only being able to test one thing at a time—and they can only do that once every 24 hours!
Most teams find it faster and cheaper to automate tests instead of solely relying on manual testing during peak hours.
If you want your tests to run fast and frequently, consider automating them. Also, remember: even better than Automation alone is having both humans and machines work toward an accurate final result!
### 5. Unclarity on When to Use Automation
Some organizations that have embraced test automation haven’t given up manual testing; they’ve just automated only a small set of tests. Even though automating those tests may make sense (perhaps there are high levels of re-testing or manual testing is slowing down development), there may be no strategy for transitioning from primarily manual to primarily automated.
This confusion leads some teams to abandon test automation altogether because it’s not meeting their goals or doesn’t align with their plans to reduce headcount. If you want to convince your organization that you need to automate more tests than you already do, then be sure everyone understands how test automation fits your long-term strategy.
Read more: [When to use Automation Testing for Better Results](https://testgrid.io/blog/no-code-automation-testing-when-to-use/)?
### 6. Improper Staff Selection and Resource Planning
Resources that are not adequately trained to use a specific test automation tool or technology can create severe issues with the tests and execution of those tests resulting in test automation failure.
You need to have tools for your staff that are easier for them to use, and you need to ensure you have enough of these
skilled resources on staff to handle all your automation needs. When choosing a test automation tool or technology, look at your existing team’s skillsets, what they already know, and any training they may need.
If you don’t pick a tool with an easy learning curve, it will be a lot harder to convince others in your organization that they should give it a try. It also doesn’t help if none of them has any experience using it.
### 7. Not Giving Attention to Test Reports
Why does test automation fail? It’s simply an answer maybe you’re not giving attention to testing reports.
Automated testing does many checks at once, making failure more likely, so it’s important to sift through test reports.
If you fail to look at the test reports carefully and attend to the test results, you might overlook the primary defects, wasting time, resources, and efforts.
Some tests succeed, and some fail, so it is necessary to analyze the causes of failures to solve problems before they even occur.
This blog is originally published at [TestGrid](https://testgrid.io/blog/why-test-automation-fails/) | morrismoses149 |
1,912,311 | Develop Full Stack Event Management System | Creating a full-stack event management system with Next.js, NestJS, and Tailwind CSS involves several... | 0 | 2024-07-05T06:15:49 | https://dev.to/nadim_ch0wdhury/develop-full-stack-event-management-system-45f9 | Creating a full-stack event management system with Next.js, NestJS, and Tailwind CSS involves several key features and functionalities. Here's an outline of the features, the architecture, and the documentation to guide you through the development process:
## Features and Functionalities
### 1. User Authentication
- **Sign Up**: Users can create an account.
- **Login**: Users can log in to their account.
- **Password Reset**: Users can reset their password.
### 2. User Roles
- **Admin**: Can manage events, users, and system settings.
- **Organizer**: Can create and manage their own events.
- **Attendee**: Can view and register for events.
### 3. Event Management
- **Create Event**: Organizers can create events with details like title, description, date, time, venue, and ticket information.
- **Edit Event**: Organizers can edit their events.
- **Delete Event**: Organizers can delete their events.
- **View Event**: Users can view event details.
- **Search Events**: Users can search for events by various criteria (e.g., date, location, type).
### 4. Ticket Management
- **Create Tickets**: Organizers can create different types of tickets for an event.
- **Purchase Tickets**: Attendees can purchase tickets.
- **View Tickets**: Attendees can view their purchased tickets.
### 5. Notification System
- **Email Notifications**: Users receive email notifications for important actions (e.g., event creation, ticket purchase).
- **In-App Notifications**: Real-time notifications within the app.
### 6. Payment Integration
- **Payment Gateway**: Integrate with a payment gateway for ticket purchases.
### 7. Dashboard
- **Admin Dashboard**: Overview of system metrics, user management, event management.
- **Organizer Dashboard**: Overview of their events, ticket sales, attendee list.
- **Attendee Dashboard**: Overview of registered events and purchased tickets.
### 8. Analytics
- **Event Analytics**: Insights into event performance (e.g., ticket sales, attendee demographics).
- **User Analytics**: Insights into user behavior and engagement.
## Architecture and Tech Stack
### Frontend
- **Next.js**: For server-side rendering and frontend development.
- **Tailwind CSS**: For styling the frontend components.
- **React**: Core library for building UI components.
### Backend
- **NestJS**: For building the server-side application with TypeScript.
- **PostgreSQL**: For the database.
- **Prisma**: ORM for database interactions.
- **GraphQL**: API for communication between frontend and backend.
### Deployment
- **Vercel**: For deploying the Next.js application.
- **Heroku**: For deploying the NestJS backend.
- **Docker**: For containerizing the applications.
## Documentation
### 1. Setting Up the Development Environment
#### Prerequisites
- Node.js
- npm or yarn
- PostgreSQL
- Docker
#### Frontend (Next.js + Tailwind CSS)
1. Initialize Next.js project:
```bash
npx create-next-app@latest event-management-frontend
cd event-management-frontend
```
2. Install Tailwind CSS:
```bash
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
```
3. Configure `tailwind.config.js`:
```javascript
module.exports = {
content: [
"./pages/**/*.{js,ts,jsx,tsx}",
"./components/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
```
4. Add Tailwind directives to `styles/globals.css`:
```css
@tailwind base;
@tailwind components;
@tailwind utilities;
```
#### Backend (NestJS + Prisma)
1. Initialize NestJS project:
```bash
npm i -g @nestjs/cli
nest new event-management-backend
cd event-management-backend
```
2. Install Prisma and PostgreSQL client:
```bash
npm install @nestjs/graphql @nestjs/apollo graphql apollo-server-express
npm install @prisma/client
npm install -D prisma
```
3. Initialize Prisma:
```bash
npx prisma init
```
4. Configure `prisma/schema.prisma` for PostgreSQL:
```prisma
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
email String @unique
password String
role String
events Event[]
tickets Ticket[]
}
model Event {
id Int @id @default(autoincrement())
title String
description String
date DateTime
organizer User @relation(fields: [organizerId], references: [id])
organizerId Int
tickets Ticket[]
}
model Ticket {
id Int @id @default(autoincrement())
type String
price Float
event Event @relation(fields: [eventId], references: [id])
eventId Int
attendee User @relation(fields: [attendeeId], references: [id])
attendeeId Int
}
```
5. Run Prisma migrations:
```bash
npx prisma migrate dev --name init
```
### 2. Building the Features
#### User Authentication
- Implement signup, login, and password reset in NestJS.
- Create corresponding frontend pages in Next.js.
#### Event Management
- Create APIs for creating, editing, deleting, and viewing events in NestJS.
- Develop frontend components and pages for event management in Next.js.
#### Ticket Management
- Develop APIs for ticket creation, purchase, and viewing in NestJS.
- Create frontend components and pages for ticket management in Next.js.
#### Notification System
- Implement email notifications using a service like SendGrid.
- Develop in-app notifications using WebSockets.
#### Payment Integration
- Integrate with a payment gateway like Stripe or PayPal in NestJS.
- Implement payment workflows in the frontend.
#### Dashboard
- Build admin, organizer, and attendee dashboards with necessary metrics and features.
#### Analytics
- Use a library like Chart.js to display analytics in the frontend.
- Implement backend logic for aggregating and providing analytics data.
## Deployment
### Frontend
1. Deploy the Next.js application on Vercel.
2. Connect your GitHub repository to Vercel for automatic deployments.
### Backend
1. Create a Dockerfile for the NestJS application.
2. Deploy the NestJS application on Heroku using Docker.
This documentation provides a comprehensive guide to developing a full-stack event management system. Feel free to ask for more detailed information or specific code examples for any part of the process.
Sure! Here’s how you can implement the frontend for user authentication (Sign Up, Login, Password Reset) using Next.js and Tailwind CSS.
### Project Structure
```
event-management-frontend
├── components
│ ├── Auth
│ │ ├── LoginForm.js
│ │ ├── SignupForm.js
│ │ ├── PasswordResetForm.js
├── pages
│ ├── auth
│ │ ├── login.js
│ │ ├── signup.js
│ │ ├── reset-password.js
│ ├── index.js
└── styles
└── globals.css
```
### 1. Sign Up
#### `components/Auth/SignupForm.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const SignupForm = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [confirmPassword, setConfirmPassword] = useState('');
const [error, setError] = useState('');
const router = useRouter();
const handleSignup = async (e) => {
e.preventDefault();
if (password !== confirmPassword) {
setError('Passwords do not match');
return;
}
try {
await axios.post('/api/auth/signup', { email, password });
router.push('/auth/login');
} catch (err) {
setError(err.response.data.message);
}
};
return (
<div className="max-w-md mx-auto mt-10">
<h1 className="text-2xl font-bold mb-6">Sign Up</h1>
<form onSubmit={handleSignup}>
<div className="mb-4">
<label className="block text-gray-700">Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Password</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Confirm Password</label>
<input
type="password"
value={confirmPassword}
onChange={(e) => setConfirmPassword(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
{error && <p className="text-red-500">{error}</p>}
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Sign Up
</button>
</form>
</div>
);
};
export default SignupForm;
```
#### `pages/auth/signup.js`
```javascript
import SignupForm from '../../components/Auth/SignupForm';
const SignupPage = () => {
return (
<div>
<SignupForm />
</div>
);
};
export default SignupPage;
```
### 2. Login
#### `components/Auth/LoginForm.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const LoginForm = () => {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [error, setError] = useState('');
const router = useRouter();
const handleLogin = async (e) => {
e.preventDefault();
try {
await axios.post('/api/auth/login', { email, password });
router.push('/');
} catch (err) {
setError(err.response.data.message);
}
};
return (
<div className="max-w-md mx-auto mt-10">
<h1 className="text-2xl font-bold mb-6">Login</h1>
<form onSubmit={handleLogin}>
<div className="mb-4">
<label className="block text-gray-700">Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Password</label>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
{error && <p className="text-red-500">{error}</p>}
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Login
</button>
</form>
</div>
);
};
export default LoginForm;
```
#### `pages/auth/login.js`
```javascript
import LoginForm from '../../components/Auth/LoginForm';
const LoginPage = () => {
return (
<div>
<LoginForm />
</div>
);
};
export default LoginPage;
```
### 3. Password Reset
#### `components/Auth/PasswordResetForm.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
const PasswordResetForm = () => {
const [email, setEmail] = useState('');
const [message, setMessage] = useState('');
const [error, setError] = useState('');
const handlePasswordReset = async (e) => {
e.preventDefault();
try {
await axios.post('/api/auth/reset-password', { email });
setMessage('A password reset link has been sent to your email');
} catch (err) {
setError(err.response.data.message);
}
};
return (
<div className="max-w-md mx-auto mt-10">
<h1 className="text-2xl font-bold mb-6">Reset Password</h1>
<form onSubmit={handlePasswordReset}>
<div className="mb-4">
<label className="block text-gray-700">Email</label>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
{message && <p className="text-green-500">{message}</p>}
{error && <p className="text-red-500">{error}</p>}
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Reset Password
</button>
</form>
</div>
);
};
export default PasswordResetForm;
```
#### `pages/auth/reset-password.js`
```javascript
import PasswordResetForm from '../../components/Auth/PasswordResetForm';
const PasswordResetPage = () => {
return (
<div>
<PasswordResetForm />
</div>
);
};
export default PasswordResetPage;
```
### Styles
Make sure you have Tailwind CSS properly configured in your `globals.css` as mentioned in the initial setup.
### API Endpoints
The above forms make POST requests to `/api/auth/signup`, `/api/auth/login`, and `/api/auth/reset-password`. You need to implement these endpoints in your backend (NestJS) to handle these requests.
This setup should give you a fully functioning frontend for user authentication in your event management system.
To implement user roles (Admin, Organizer, and Attendee) in the frontend using Next.js and Tailwind CSS, you'll need to create different components and pages for each role. Here's an example setup for each role's dashboard and basic functionality.
### Project Structure
```
event-management-frontend
├── components
│ ├── Admin
│ │ ├── AdminDashboard.js
│ │ ├── ManageEvents.js
│ │ ├── ManageUsers.js
│ ├── Organizer
│ │ ├── OrganizerDashboard.js
│ │ ├── CreateEvent.js
│ │ ├── ManageOwnEvents.js
│ ├── Attendee
│ │ ├── AttendeeDashboard.js
│ │ ├── ViewEvents.js
│ │ ├── RegisterEvent.js
├── pages
│ ├── admin
│ │ ├── index.js
│ │ ├── manage-events.js
│ │ ├── manage-users.js
│ ├── organizer
│ │ ├── index.js
│ │ ├── create-event.js
│ │ ├── manage-events.js
│ ├── attendee
│ │ ├── index.js
│ │ ├── view-events.js
│ │ ├── register-event.js
└── styles
└── globals.css
```
### 1. Admin
#### `components/Admin/AdminDashboard.js`
```javascript
const AdminDashboard = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Admin Dashboard</h1>
<div className="flex space-x-4">
<a href="/admin/manage-events" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">Manage Events</a>
<a href="/admin/manage-users" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">Manage Users</a>
</div>
</div>
);
};
export default AdminDashboard;
```
#### `components/Admin/ManageEvents.js`
```javascript
const ManageEvents = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Manage Events</h1>
{/* Add functionality to list and manage all events */}
</div>
);
};
export default ManageEvents;
```
#### `components/Admin/ManageUsers.js`
```javascript
const ManageUsers = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Manage Users</h1>
{/* Add functionality to list and manage all users */}
</div>
);
};
export default ManageUsers;
```
#### `pages/admin/index.js`
```javascript
import AdminDashboard from '../../components/Admin/AdminDashboard';
const AdminPage = () => {
return (
<div>
<AdminDashboard />
</div>
);
};
export default AdminPage;
```
#### `pages/admin/manage-events.js`
```javascript
import ManageEvents from '../../components/Admin/ManageEvents';
const ManageEventsPage = () => {
return (
<div>
<ManageEvents />
</div>
);
};
export default ManageEventsPage;
```
#### `pages/admin/manage-users.js`
```javascript
import ManageUsers from '../../components/Admin/ManageUsers';
const ManageUsersPage = () => {
return (
<div>
<ManageUsers />
</div>
);
};
export default ManageUsersPage;
```
### 2. Organizer
#### `components/Organizer/OrganizerDashboard.js`
```javascript
const OrganizerDashboard = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Organizer Dashboard</h1>
<div className="flex space-x-4">
<a href="/organizer/create-event" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">Create Event</a>
<a href="/organizer/manage-events" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">Manage Events</a>
</div>
</div>
);
};
export default OrganizerDashboard;
```
#### `components/Organizer/CreateEvent.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
const CreateEvent = () => {
const [title, setTitle] = useState('');
const [description, setDescription] = useState('');
const [date, setDate] = useState('');
const [time, setTime] = useState('');
const [venue, setVenue] = useState('');
const handleCreateEvent = async (e) => {
e.preventDefault();
try {
await axios.post('/api/events', { title, description, date, time, venue });
alert('Event created successfully');
} catch (err) {
console.error(err);
alert('Error creating event');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Create Event</h1>
<form onSubmit={handleCreateEvent}>
<div className="mb-4">
<label className="block text-gray-700">Title</label>
<input
type="text"
value={title}
onChange={(e) => setTitle(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Description</label>
<textarea
value={description}
onChange={(e) => setDescription(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
></textarea>
</div>
<div className="mb-4">
<label className="block text-gray-700">Date</label>
<input
type="date"
value={date}
onChange={(e) => setDate(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Time</label>
<input
type="time"
value={time}
onChange={(e) => setTime(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Venue</label>
<input
type="text"
value={venue}
onChange={(e) => setVenue(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Create Event
</button>
</form>
</div>
);
};
export default CreateEvent;
```
#### `components/Organizer/ManageOwnEvents.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const ManageOwnEvents = () => {
const [events, setEvents] = useState([]);
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/organizer/events');
setEvents(response.data);
};
fetchEvents();
}, []);
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Manage Your Events</h1>
<div>
{events.map((event) => (
<div key={event.id} className="mb-4 p-4 border rounded">
<h2 className="text-2xl font-bold">{event.title}</h2>
<p>{event.description}</p>
<p>{new Date(event.date).toLocaleDateString()} {event.time}</p>
<p>{event.venue}</p>
{/* Add buttons for editing and deleting the event */}
</div>
))}
</div>
</div>
);
};
export default ManageOwnEvents;
```
#### `pages/organizer/index.js`
```javascript
import OrganizerDashboard from '../../components/Organizer/OrganizerDashboard';
const OrganizerPage = () => {
return (
<div>
<OrganizerDashboard />
</div>
);
};
export default OrganizerPage;
```
#### `pages/organizer/create-event.js`
```javascript
import CreateEvent from '../../components/Organizer/CreateEvent';
const CreateEventPage = () => {
return (
<div>
<CreateEvent />
</div>
);
};
export default CreateEventPage;
```
#### `pages/organizer/manage-events.js`
```javascript
import ManageOwnEvents from '../../components/Organizer/ManageOwnEvents';
const ManageOwnEventsPage = () => {
return (
<div>
<ManageOwnEvents />
</div>
);
};
export default ManageOwnEventsPage;
```
### 3. Attendee
#### `components/Attendee/AttendeeDashboard.js`
```javascript
const AttendeeDashboard = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Attendee Dashboard</h1>
<div className="flex space-x-4">
<a href="/attendee/view-events" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">View Events</a>
<a href="/attendee/register-event" className="bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700">Register for Event</a>
</div>
</div>
);
};
export default AttendeeDashboard;
```
#### `components/Attendee/ViewEvents.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const ViewEvents = () => {
const [events, setEvents] = useState([]);
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/events');
setEvents(response.data);
};
fetchEvents();
}, []);
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">View Events</h1>
<div>
{events.map((event) => (
<div key={event.id} className="mb-4 p-4 border rounded">
<h2 className="text-2xl font-bold">{event.title}</h2>
<p>{event.description}</p>
<p>{new Date(event.date).toLocaleDateString()} {event.time}</p>
<p>{event.venue}</p>
</div>
))}
</div>
</div>
);
};
export default ViewEvents;
```
#### `components/Attendee/RegisterEvent.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
const RegisterEvent = () => {
const [eventId, setEventId] = useState('');
const handleRegister = async (e) => {
e.preventDefault();
try {
await axios.post(`/api/events/${eventId}/register`);
alert('Successfully registered for the event');
} catch (err) {
console.error(err);
alert('Error registering for the event');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Register for Event</h1>
<form onSubmit={handleRegister}>
<div className="mb-4">
<label className="block text-gray-700">Event ID</label>
<input
type="text"
value={eventId}
onChange={(e) => setEventId(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Register
</button>
</form>
</div>
);
};
export default RegisterEvent;
```
#### `pages/attendee/index.js`
```javascript
import AttendeeDashboard from '../../components/Attendee/AttendeeDashboard';
const AttendeePage = () => {
return (
<div>
<AttendeeDashboard />
</div>
);
};
export default AttendeePage;
```
#### `pages/attendee/view-events.js`
```javascript
import ViewEvents from '../../components/Attendee/ViewEvents';
const ViewEventsPage = () => {
return (
<div>
<ViewEvents />
</div>
);
};
export default ViewEventsPage;
```
#### `pages/attendee/register-event.js`
```javascript
import RegisterEvent from '../../components/Attendee/RegisterEvent';
const RegisterEventPage = () => {
return (
<div>
<RegisterEvent />
</div>
);
};
export default RegisterEventPage;
```
### Navigation and Authorization
You should also implement navigation and role-based authorization checks to ensure users only access the pages they are allowed to. This can be achieved using a combination of custom hooks, context providers, and middleware.
### Styles
Make sure Tailwind CSS is properly configured in your `globals.css`.
With these components and pages, you can build a user role-based frontend for your event management system. The functionality for each role can be expanded as needed.
To implement event management features in the frontend using Next.js and Tailwind CSS, you'll need components and pages for creating, editing, deleting, viewing, and searching events. Below is an example setup for these functionalities.
### Project Structure
```
event-management-frontend
├── components
│ ├── Organizer
│ │ ├── CreateEvent.js
│ │ ├── EditEvent.js
│ │ ├── DeleteEvent.js
│ │ ├── ManageOwnEvents.js
│ ├── Event
│ │ ├── EventDetails.js
│ │ ├── SearchEvents.js
├── pages
│ ├── organizer
│ │ ├── create-event.js
│ │ ├── edit-event.js
│ │ ├── manage-events.js
│ ├── events
│ │ ├── [id].js
│ │ ├── search.js
└── styles
└── globals.css
```
### 1. Create Event
#### `components/Organizer/CreateEvent.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const CreateEvent = () => {
const [title, setTitle] = useState('');
const [description, setDescription] = useState('');
const [date, setDate] = useState('');
const [time, setTime] = useState('');
const [venue, setVenue] = useState('');
const [ticketInfo, setTicketInfo] = useState('');
const router = useRouter();
const handleCreateEvent = async (e) => {
e.preventDefault();
try {
await axios.post('/api/events', { title, description, date, time, venue, ticketInfo });
router.push('/organizer/manage-events');
} catch (err) {
console.error(err);
alert('Error creating event');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Create Event</h1>
<form onSubmit={handleCreateEvent}>
<div className="mb-4">
<label className="block text-gray-700">Title</label>
<input
type="text"
value={title}
onChange={(e) => setTitle(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Description</label>
<textarea
value={description}
onChange={(e) => setDescription(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
></textarea>
</div>
<div className="mb-4">
<label className="block text-gray-700">Date</label>
<input
type="date"
value={date}
onChange={(e) => setDate(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Time</label>
<input
type="time"
value={time}
onChange={(e) => setTime(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Venue</label>
<input
type="text"
value={venue}
onChange={(e) => setVenue(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Ticket Information</label>
<input
type="text"
value={ticketInfo}
onChange={(e) => setTicketInfo(e.target.value)}
className="w-full px-3 py-2 border rounded"
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Create Event
</button>
</form>
</div>
);
};
export default CreateEvent;
```
#### `pages/organizer/create-event.js`
```javascript
import CreateEvent from '../../components/Organizer/CreateEvent';
const CreateEventPage = () => {
return (
<div>
<CreateEvent />
</div>
);
};
export default CreateEventPage;
```
### 2. Edit Event
#### `components/Organizer/EditEvent.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
import { useRouter } from 'next/router';
const EditEvent = () => {
const router = useRouter();
const { id } = router.query;
const [event, setEvent] = useState(null);
const [title, setTitle] = useState('');
const [description, setDescription] = useState('');
const [date, setDate] = useState('');
const [time, setTime] = useState('');
const [venue, setVenue] = useState('');
const [ticketInfo, setTicketInfo] = useState('');
useEffect(() => {
if (id) {
axios.get(`/api/events/${id}`)
.then(response => {
const eventData = response.data;
setEvent(eventData);
setTitle(eventData.title);
setDescription(eventData.description);
setDate(eventData.date);
setTime(eventData.time);
setVenue(eventData.venue);
setTicketInfo(eventData.ticketInfo);
})
.catch(error => console.error('Error fetching event:', error));
}
}, [id]);
const handleEditEvent = async (e) => {
e.preventDefault();
try {
await axios.put(`/api/events/${id}`, { title, description, date, time, venue, ticketInfo });
router.push('/organizer/manage-events');
} catch (err) {
console.error(err);
alert('Error editing event');
}
};
if (!event) return <div>Loading...</div>;
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Edit Event</h1>
<form onSubmit={handleEditEvent}>
<div className="mb-4">
<label className="block text-gray-700">Title</label>
<input
type="text"
value={title}
onChange={(e) => setTitle(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Description</label>
<textarea
value={description}
onChange={(e) => setDescription(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
></textarea>
</div>
<div className="mb-4">
<label className="block text-gray-700">Date</label>
<input
type="date"
value={date}
onChange={(e) => setDate(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Time</label>
<input
type="time"
value={time}
onChange={(e) => setTime(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Venue</label>
<input
type="text"
value={venue}
onChange={(e) => setVenue(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Ticket Information</label>
<input
type="text"
value={ticketInfo}
onChange={(e) => setTicketInfo(e.target.value)}
className="w-full px-3 py-2 border rounded"
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Edit Event
</button>
</form>
</div>
);
};
export default EditEvent;
```
#### `pages/organizer/edit-event.js`
```javascript
import EditEvent from '../../components/Organizer/EditEvent';
const EditEventPage = () => {
return (
<div>
<EditEvent
/>
</div>
);
};
export default EditEventPage;
```
### 3. Delete Event
#### `components/Organizer/DeleteEvent.js`
```javascript
import axios from 'axios';
import { useRouter } from 'next/router';
const DeleteEvent = ({ eventId }) => {
const router = useRouter();
const handleDeleteEvent = async () => {
try {
await axios.delete(`/api/events/${eventId}`);
router.push('/organizer/manage-events');
} catch (err) {
console.error(err);
alert('Error deleting event');
}
};
return (
<button
onClick={handleDeleteEvent}
className="bg-red-500 text-white py-2 px-4 rounded hover:bg-red-700"
>
Delete Event
</button>
);
};
export default DeleteEvent;
```
### 4. Manage Own Events
#### `components/Organizer/ManageOwnEvents.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import Link from 'next/link';
const ManageOwnEvents = () => {
const [events, setEvents] = useState([]);
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/events/mine');
setEvents(response.data);
};
fetchEvents();
}, []);
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Manage My Events</h1>
<div>
{events.map((event) => (
<div key={event.id} className="mb-4 p-4 border rounded">
<h2 className="text-2xl font-bold">{event.title}</h2>
<p>{event.description}</p>
<p>{new Date(event.date).toLocaleDateString()} {event.time}</p>
<p>{event.venue}</p>
<div className="flex space-x-4 mt-4">
<Link href={`/organizer/edit-event?id=${event.id}`}>
<a className="bg-green-500 text-white py-2 px-4 rounded hover:bg-green-700">Edit</a>
</Link>
<DeleteEvent eventId={event.id} />
</div>
</div>
))}
</div>
</div>
);
};
export default ManageOwnEvents;
```
### 5. View Event
#### `components/Event/EventDetails.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const EventDetails = () => {
const router = useRouter();
const { id } = router.query;
const [event, setEvent] = useState(null);
useEffect(() => {
if (id) {
axios.get(`/api/events/${id}`)
.then(response => {
setEvent(response.data);
})
.catch(error => console.error('Error fetching event:', error));
}
}, [id]);
if (!event) return <div>Loading...</div>;
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">{event.title}</h1>
<p className="mb-4">{event.description}</p>
<p className="mb-2">Date: {new Date(event.date).toLocaleDateString()}</p>
<p className="mb-2">Time: {event.time}</p>
<p className="mb-2">Venue: {event.venue}</p>
<p className="mb-2">Tickets: {event.ticketInfo}</p>
</div>
);
};
export default EventDetails;
```
#### `pages/events/[id].js`
```javascript
import EventDetails from '../../components/Event/EventDetails';
const EventDetailsPage = () => {
return (
<div>
<EventDetails />
</div>
);
};
export default EventDetailsPage;
```
### 6. Search Events
#### `components/Event/SearchEvents.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
const SearchEvents = () => {
const [query, setQuery] = useState('');
const [events, setEvents] = useState([]);
const handleSearch = async (e) => {
e.preventDefault();
try {
const response = await axios.get(`/api/events/search?query=${query}`);
setEvents(response.data);
} catch (err) {
console.error(err);
alert('Error searching events');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Search Events</h1>
<form onSubmit={handleSearch} className="mb-6">
<input
type="text"
value={query}
onChange={(e) => setQuery(e.target.value)}
className="w-full px-3 py-2 border rounded mb-4"
placeholder="Search by title, date, location..."
required
/>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Search
</button>
</form>
<div>
{events.map((event) => (
<div key={event.id} className="mb-4 p-4 border rounded">
<h2 className="text-2xl font-bold">{event.title}</h2>
<p>{event.description}</p>
<p>{new Date(event.date).toLocaleDateString()} {event.time}</p>
<p>{event.venue}</p>
</div>
))}
</div>
</div>
);
};
export default SearchEvents;
```
#### `pages/events/search.js`
```javascript
import SearchEvents from '../../components/Event/SearchEvents';
const SearchEventsPage = () => {
return (
<div>
<SearchEvents />
</div>
);
};
export default SearchEventsPage;
```
### Navigation and Authorization
Ensure you have navigation links set up and proper authorization checks for each role to access specific pages.
### Styles
Make sure Tailwind CSS is properly configured in your `globals.css`.
With these components and pages, you can build a comprehensive event management system frontend using Next.js and Tailwind CSS.
To implement ticket management features in the frontend using Next.js and Tailwind CSS, you need components and pages for creating tickets, purchasing tickets, and viewing purchased tickets. Below is the example setup for these functionalities.
### Project Structure
```
event-management-frontend
├── components
│ ├── Organizer
│ │ ├── CreateTickets.js
│ ├── Attendee
│ │ ├── PurchaseTickets.js
│ │ ├── ViewTickets.js
├── pages
│ ├── organizer
│ │ ├── create-tickets.js
│ ├── attendee
│ │ ├── purchase-tickets.js
│ │ ├── view-tickets.js
└── styles
└── globals.css
```
### 1. Create Tickets
#### `components/Organizer/CreateTickets.js`
```javascript
import { useState } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const CreateTickets = () => {
const [eventId, setEventId] = useState('');
const [ticketType, setTicketType] = useState('');
const [price, setPrice] = useState('');
const [quantity, setQuantity] = useState('');
const router = useRouter();
const handleCreateTickets = async (e) => {
e.preventDefault();
try {
await axios.post(`/api/events/${eventId}/tickets`, { ticketType, price, quantity });
router.push('/organizer/manage-events');
} catch (err) {
console.error(err);
alert('Error creating tickets');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Create Tickets</h1>
<form onSubmit={handleCreateTickets}>
<div className="mb-4">
<label className="block text-gray-700">Event ID</label>
<input
type="text"
value={eventId}
onChange={(e) => setEventId(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Ticket Type</label>
<input
type="text"
value={ticketType}
onChange={(e) => setTicketType(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Price</label>
<input
type="number"
value={price}
onChange={(e) => setPrice(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<div className="mb-4">
<label className="block text-gray-700">Quantity</label>
<input
type="number"
value={quantity}
onChange={(e) => setQuantity(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Create Tickets
</button>
</form>
</div>
);
};
export default CreateTickets;
```
#### `pages/organizer/create-tickets.js`
```javascript
import CreateTickets from '../../components/Organizer/CreateTickets';
const CreateTicketsPage = () => {
return (
<div>
<CreateTickets />
</div>
);
};
export default CreateTicketsPage;
```
### 2. Purchase Tickets
#### `components/Attendee/PurchaseTickets.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const PurchaseTickets = () => {
const [events, setEvents] = useState([]);
const [selectedEvent, setSelectedEvent] = useState('');
const [tickets, setTickets] = useState([]);
const [selectedTicket, setSelectedTicket] = useState('');
const [quantity, setQuantity] = useState(1);
const router = useRouter();
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/events');
setEvents(response.data);
};
fetchEvents();
}, []);
useEffect(() => {
if (selectedEvent) {
const fetchTickets = async () => {
const response = await axios.get(`/api/events/${selectedEvent}/tickets`);
setTickets(response.data);
};
fetchTickets();
}
}, [selectedEvent]);
const handlePurchase = async (e) => {
e.preventDefault();
try {
await axios.post(`/api/tickets/purchase`, { eventId: selectedEvent, ticketId: selectedTicket, quantity });
alert('Successfully purchased tickets');
router.push('/attendee/view-tickets');
} catch (err) {
console.error(err);
alert('Error purchasing tickets');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Purchase Tickets</h1>
<form onSubmit={handlePurchase}>
<div className="mb-4">
<label className="block text-gray-700">Select Event</label>
<select
value={selectedEvent}
onChange={(e) => setSelectedEvent(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Event</option>
{events.map(event => (
<option key={event.id} value={event.id}>{event.title}</option>
))}
</select>
</div>
{selectedEvent && (
<>
<div className="mb-4">
<label className="block text-gray-700">Select Ticket</label>
<select
value={selectedTicket}
onChange={(e) => setSelectedTicket(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Ticket</option>
{tickets.map(ticket => (
<option key={ticket.id} value={ticket.id}>{ticket.ticketType} - ${ticket.price}</option>
))}
</select>
</div>
<div className="mb-4">
<label className="block text-gray-700">Quantity</label>
<input
type="number"
value={quantity}
onChange={(e) => setQuantity(e.target.value)}
className="w-full px-3 py-2 border rounded"
min="1"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Purchase Tickets
</button>
</>
)}
</form>
</div>
);
};
export default PurchaseTickets;
```
#### `pages/attendee/purchase-tickets.js`
```javascript
import PurchaseTickets from '../../components/Attendee/PurchaseTickets';
const PurchaseTicketsPage = () => {
return (
<div>
<PurchaseTickets />
</div>
);
};
export default PurchaseTicketsPage;
```
### 3. View Tickets
#### `components/Attendee/ViewTickets.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const ViewTickets = () => {
const [tickets, setTickets] = useState([]);
useEffect(() => {
const fetchTickets = async () => {
const response = await axios.get('/api/tickets');
setTickets(response.data);
};
fetchTickets();
}, []);
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">My Tickets</h1>
<div>
{tickets.map((ticket) => (
<div key={ticket.id} className="mb-4 p-4 border rounded">
<h2 className="text-2xl font-bold">{ticket.event.title}</h2>
<p>Type: {ticket.ticketType}</p>
<p>Price: ${ticket.price}</p>
<p>Quantity: {ticket.quantity}</p>
<p>Date: {new Date(ticket.event.date).toLocaleDateString()}</p>
<p>Time: {ticket.event.time}</p>
<p>Venue: {ticket.event.venue}</p>
</div>
))}
</div>
</div>
);
};
export default ViewTickets;
```
#### `pages/attendee/view-tickets.js`
```javascript
import ViewTickets from '../../components/Attendee/ViewTickets';
const ViewTicketsPage = () => {
return (
<div>
<ViewTickets />
</div>
);
};
export default ViewTicketsPage;
```
### Navigation and Authorization
Ensure you have navigation links set up and proper authorization checks
for each role to access specific pages.
### Styles
Make sure Tailwind CSS is properly configured in your `globals.css`.
With these components and pages, you can build a comprehensive ticket management system frontend using Next.js and Tailwind CSS.
To implement a notification system in the frontend using Next.js and Tailwind CSS, you'll need components for displaying in-app notifications and mechanisms to trigger email notifications (typically handled by the backend). Below is an example setup for in-app notifications and placeholders for triggering email notifications.
### Project Structure
```
event-management-frontend
├── components
│ ├── Notifications
│ │ ├── InAppNotifications.js
├── pages
│ ├── notifications
│ │ ├── index.js
└── styles
└── globals.css
```
### 1. In-App Notifications
#### `components/Notifications/InAppNotifications.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const InAppNotifications = () => {
const [notifications, setNotifications] = useState([]);
useEffect(() => {
const fetchNotifications = async () => {
const response = await axios.get('/api/notifications');
setNotifications(response.data);
};
fetchNotifications();
}, []);
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Notifications</h1>
<div>
{notifications.map((notification) => (
<div key={notification.id} className="mb-4 p-4 border rounded bg-gray-100">
<h2 className="text-2xl font-bold">{notification.title}</h2>
<p>{notification.message}</p>
<p className="text-sm text-gray-500">{new Date(notification.createdAt).toLocaleString()}</p>
</div>
))}
</div>
</div>
);
};
export default InAppNotifications;
```
#### `pages/notifications/index.js`
```javascript
import InAppNotifications from '../../components/Notifications/InAppNotifications';
const NotificationsPage = () => {
return (
<div>
<InAppNotifications />
</div>
);
};
export default NotificationsPage;
```
### Triggering Email Notifications
Email notifications are typically handled by the backend. Here's an example of how you might trigger email notifications from the frontend by making API calls to your backend.
### Example: Triggering Email Notification on Ticket Purchase
#### `components/Attendee/PurchaseTickets.js` (Modified)
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
const PurchaseTickets = () => {
const [events, setEvents] = useState([]);
const [selectedEvent, setSelectedEvent] = useState('');
const [tickets, setTickets] = useState([]);
const [selectedTicket, setSelectedTicket] = useState('');
const [quantity, setQuantity] = useState(1);
const router = useRouter();
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/events');
setEvents(response.data);
};
fetchEvents();
}, []);
useEffect(() => {
if (selectedEvent) {
const fetchTickets = async () => {
const response = await axios.get(`/api/events/${selectedEvent}/tickets`);
setTickets(response.data);
};
fetchTickets();
}
}, [selectedEvent]);
const handlePurchase = async (e) => {
e.preventDefault();
try {
await axios.post(`/api/tickets/purchase`, { eventId: selectedEvent, ticketId: selectedTicket, quantity });
alert('Successfully purchased tickets');
// Trigger email notification
await axios.post(`/api/notifications/email`, {
type: 'TICKET_PURCHASE',
userId: 'current_user_id', // Replace with actual user ID
eventId: selectedEvent,
ticketId: selectedTicket,
});
router.push('/attendee/view-tickets');
} catch (err) {
console.error(err);
alert('Error purchasing tickets');
}
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Purchase Tickets</h1>
<form onSubmit={handlePurchase}>
<div className="mb-4">
<label className="block text-gray-700">Select Event</label>
<select
value={selectedEvent}
onChange={(e) => setSelectedEvent(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Event</option>
{events.map(event => (
<option key={event.id} value={event.id}>{event.title}</option>
))}
</select>
</div>
{selectedEvent && (
<>
<div className="mb-4">
<label className="block text-gray-700">Select Ticket</label>
<select
value={selectedTicket}
onChange={(e) => setSelectedTicket(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Ticket</option>
{tickets.map(ticket => (
<option key={ticket.id} value={ticket.id}>{ticket.ticketType} - ${ticket.price}</option>
))}
</select>
</div>
<div className="mb-4">
<label className="block text-gray-700">Quantity</label>
<input
type="number"
value={quantity}
onChange={(e) => setQuantity(e.target.value)}
className="w-full px-3 py-2 border rounded"
min="1"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Purchase Tickets
</button>
</>
)}
</form>
</div>
);
};
export default PurchaseTickets;
```
### Summary
- **In-App Notifications:** The `InAppNotifications` component displays real-time notifications. The notifications are fetched from the backend using an API call.
- **Email Notifications:** Email notifications are triggered by making an API call to the backend whenever an important action occurs (e.g., ticket purchase).
Make sure to implement the necessary backend logic to handle these API endpoints and send emails.
To integrate a payment gateway in your frontend for ticket purchases, you can use popular options like Stripe. Below is an example of how to integrate Stripe into your Next.js application for handling ticket purchases.
### Project Structure
```
event-management-frontend
├── components
│ ├── Attendee
│ │ ├── PurchaseTickets.js
│ │ ├── CheckoutForm.js
├── pages
│ ├── attendee
│ │ ├── purchase-tickets.js
│ │ ├── success.js
└── styles
└── globals.css
```
### 1. Setting Up Stripe
First, install the necessary Stripe packages:
```bash
npm install @stripe/stripe-js @stripe/react-stripe-js
```
### 2. Checkout Form Component
#### `components/Attendee/CheckoutForm.js`
```javascript
import { CardElement, useStripe, useElements } from '@stripe/react-stripe-js';
import axios from 'axios';
import { useRouter } from 'next/router';
import { useState } from 'react';
const CheckoutForm = ({ eventId, ticketId, quantity }) => {
const stripe = useStripe();
const elements = useElements();
const router = useRouter();
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
const handleSubmit = async (event) => {
event.preventDefault();
setLoading(true);
if (!stripe || !elements) {
return;
}
const cardElement = elements.getElement(CardElement);
try {
const { data: clientSecret } = await axios.post('/api/create-payment-intent', {
eventId,
ticketId,
quantity,
});
const { error: stripeError, paymentIntent } = await stripe.confirmCardPayment(clientSecret, {
payment_method: {
card: cardElement,
billing_details: {
name: 'Test User',
},
},
});
if (stripeError) {
setError(`Payment failed: ${stripeError.message}`);
setLoading(false);
return;
}
if (paymentIntent.status === 'succeeded') {
alert('Payment successful');
router.push('/attendee/success');
}
} catch (error) {
setError(`Payment failed: ${error.message}`);
} finally {
setLoading(false);
}
};
return (
<form onSubmit={handleSubmit} className="w-full max-w-lg mx-auto p-6">
<CardElement className="border p-4 rounded mb-4" />
{error && <div className="text-red-500 mb-4">{error}</div>}
<button
type="submit"
disabled={!stripe || loading}
className={`w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700 ${loading && 'opacity-50 cursor-not-allowed'}`}
>
{loading ? 'Processing...' : 'Pay Now'}
</button>
</form>
);
};
export default CheckoutForm;
```
### 3. Purchase Tickets Component
#### `components/Attendee/PurchaseTickets.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { useRouter } from 'next/router';
import { Elements } from '@stripe/react-stripe-js';
import { loadStripe } from '@stripe/stripe-js';
import CheckoutForm from './CheckoutForm';
const stripePromise = loadStripe('your-publishable-key-from-stripe');
const PurchaseTickets = () => {
const [events, setEvents] = useState([]);
const [selectedEvent, setSelectedEvent] = useState('');
const [tickets, setTickets] = useState([]);
const [selectedTicket, setSelectedTicket] = useState('');
const [quantity, setQuantity] = useState(1);
const [checkout, setCheckout] = useState(false);
const router = useRouter();
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/events');
setEvents(response.data);
};
fetchEvents();
}, []);
useEffect(() => {
if (selectedEvent) {
const fetchTickets = async () => {
const response = await axios.get(`/api/events/${selectedEvent}/tickets`);
setTickets(response.data);
};
fetchTickets();
}
}, [selectedEvent]);
const handlePurchase = async (e) => {
e.preventDefault();
setCheckout(true);
};
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Purchase Tickets</h1>
<form onSubmit={handlePurchase}>
<div className="mb-4">
<label className="block text-gray-700">Select Event</label>
<select
value={selectedEvent}
onChange={(e) => setSelectedEvent(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Event</option>
{events.map(event => (
<option key={event.id} value={event.id}>{event.title}</option>
))}
</select>
</div>
{selectedEvent && (
<>
<div className="mb-4">
<label className="block text-gray-700">Select Ticket</label>
<select
value={selectedTicket}
onChange={(e) => setSelectedTicket(e.target.value)}
className="w-full px-3 py-2 border rounded"
required
>
<option value="">Select Ticket</option>
{tickets.map(ticket => (
<option key={ticket.id} value={ticket.id}>{ticket.ticketType} - ${ticket.price}</option>
))}
</select>
</div>
<div className="mb-4">
<label className="block text-gray-700">Quantity</label>
<input
type="number"
value={quantity}
onChange={(e) => setQuantity(e.target.value)}
className="w-full px-3 py-2 border rounded"
min="1"
required
/>
</div>
<button
type="submit"
className="w-full bg-blue-500 text-white py-2 px-4 rounded hover:bg-blue-700"
>
Proceed to Checkout
</button>
</>
)}
</form>
{checkout && (
<Elements stripe={stripePromise}>
<CheckoutForm eventId={selectedEvent} ticketId={selectedTicket} quantity={quantity} />
</Elements>
)}
</div>
);
};
export default PurchaseTickets;
```
#### `pages/attendee/purchase-tickets.js`
```javascript
import PurchaseTickets from '../../components/Attendee/PurchaseTickets';
const PurchaseTicketsPage = () => {
return (
<div>
<PurchaseTickets />
</div>
);
};
export default PurchaseTicketsPage;
```
### 4. Success Page
#### `pages/attendee/success.js`
```javascript
const SuccessPage = () => {
return (
<div className="max-w-4xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Payment Successful</h1>
<p className="mb-4">Thank you for your purchase. You will receive an email confirmation shortly.</p>
<a href="/attendee/view-tickets" className="text-blue-500 hover:underline">View Your Tickets</a>
</div>
);
};
export default SuccessPage;
```
### Summary
- **Checkout Form:** The `CheckoutForm` component handles the Stripe payment process.
- **Purchase Tickets:** The `PurchaseTickets` component handles the ticket selection and triggers the checkout process.
- **Success Page:** A simple page to display after successful payment.
This setup integrates Stripe into your Next.js application for handling payments. Ensure you have the necessary backend setup to create payment intents and handle webhook events from Stripe for a complete integration.
To create dashboards for Admin, Organizer, and Attendee roles in your Next.js application using Tailwind CSS, you will need to set up the structure and components for each dashboard. Each dashboard will have different views and functionalities according to the role.
### Project Structure
```
event-management-frontend
├── components
│ ├── Admin
│ │ ├── AdminDashboard.js
│ ├── Organizer
│ │ ├── OrganizerDashboard.js
│ ├── Attendee
│ │ ├── AttendeeDashboard.js
├── pages
│ ├── admin
│ │ ├── dashboard.js
│ ├── organizer
│ │ ├── dashboard.js
│ ├── attendee
│ │ ├── dashboard.js
└── styles
└── globals.css
```
### 1. Admin Dashboard
#### `components/Admin/AdminDashboard.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const AdminDashboard = () => {
const [metrics, setMetrics] = useState({});
const [users, setUsers] = useState([]);
const [events, setEvents] = useState([]);
useEffect(() => {
const fetchMetrics = async () => {
const response = await axios.get('/api/admin/metrics');
setMetrics(response.data);
};
const fetchUsers = async () => {
const response = await axios.get('/api/admin/users');
setUsers(response.data);
};
const fetchEvents = async () => {
const response = await axios.get('/api/admin/events');
setEvents(response.data);
};
fetchMetrics();
fetchUsers();
fetchEvents();
}, []);
return (
<div className="max-w-7xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Admin Dashboard</h1>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">System Metrics</h2>
<div className="grid grid-cols-3 gap-6">
<div className="bg-white p-6 rounded shadow">
<h3 className="text-xl font-bold">Total Users</h3>
<p className="text-3xl">{metrics.totalUsers}</p>
</div>
<div className="bg-white p-6 rounded shadow">
<h3 className="text-xl font-bold">Total Events</h3>
<p className="text-3xl">{metrics.totalEvents}</p>
</div>
<div className="bg-white p-6 rounded shadow">
<h3 className="text-xl font-bold">Total Tickets Sold</h3>
<p className="text-3xl">{metrics.totalTicketsSold}</p>
</div>
</div>
</div>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">User Management</h2>
<table className="min-w-full bg-white border">
<thead>
<tr>
<th className="border px-4 py-2">ID</th>
<th className="border px-4 py-2">Name</th>
<th className="border px-4 py-2">Email</th>
<th className="border px-4 py-2">Role</th>
</tr>
</thead>
<tbody>
{users.map(user => (
<tr key={user.id}>
<td className="border px-4 py-2">{user.id}</td>
<td className="border px-4 py-2">{user.name}</td>
<td className="border px-4 py-2">{user.email}</td>
<td className="border px-4 py-2">{user.role}</td>
</tr>
))}
</tbody>
</table>
</div>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Event Management</h2>
<table className="min-w-full bg-white border">
<thead>
<tr>
<th className="border px-4 py-2">ID</th>
<th className="border px-4 py-2">Title</th>
<th className="border px-4 py-2">Organizer</th>
<th className="border px-4 py-2">Date</th>
</tr>
</thead>
<tbody>
{events.map(event => (
<tr key={event.id}>
<td className="border px-4 py-2">{event.id}</td>
<td className="border px-4 py-2">{event.title}</td>
<td className="border px-4 py-2">{event.organizer}</td>
<td className="border px-4 py-2">{new Date(event.date).toLocaleDateString()}</td>
</tr>
))}
</tbody>
</table>
</div>
</div>
);
};
export default AdminDashboard;
```
#### `pages/admin/dashboard.js`
```javascript
import AdminDashboard from '../../components/Admin/AdminDashboard';
const AdminDashboardPage = () => {
return (
<div>
<AdminDashboard />
</div>
);
};
export default AdminDashboardPage;
```
### 2. Organizer Dashboard
#### `components/Organizer/OrganizerDashboard.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const OrganizerDashboard = () => {
const [events, setEvents] = useState([]);
const [ticketSales, setTicketSales] = useState([]);
useEffect(() => {
const fetchEvents = async () => {
const response = await axios.get('/api/organizer/events');
setEvents(response.data);
};
const fetchTicketSales = async () => {
const response = await axios.get('/api/organizer/ticket-sales');
setTicketSales(response.data);
};
fetchEvents();
fetchTicketSales();
}, []);
return (
<div className="max-w-7xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Organizer Dashboard</h1>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Your Events</h2>
<table className="min-w-full bg-white border">
<thead>
<tr>
<th className="border px-4 py-2">ID</th>
<th className="border px-4 py-2">Title</th>
<th className="border px-4 py-2">Date</th>
<th className="border px-4 py-2">Venue</th>
<th className="border px-4 py-2">Tickets Sold</th>
</tr>
</thead>
<tbody>
{events.map(event => (
<tr key={event.id}>
<td className="border px-4 py-2">{event.id}</td>
<td className="border px-4 py-2">{event.title}</td>
<td className="border px-4 py-2">{new Date(event.date).toLocaleDateString()}</td>
<td className="border px-4 py-2">{event.venue}</td>
<td className="border px-4 py-2">{ticketSales[event.id]}</td>
</tr>
))}
</tbody>
</table>
</div>
</div>
);
};
export default OrganizerDashboard;
```
#### `pages/organizer/dashboard.js`
```javascript
import OrganizerDashboard from '../../components/Organizer/OrganizerDashboard';
const OrganizerDashboardPage = () => {
return (
<div>
<OrganizerDashboard />
</div>
);
};
export default OrganizerDashboardPage;
```
### 3. Attendee Dashboard
#### `components/Attendee/AttendeeDashboard.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
const AttendeeDashboard = () => {
const [registeredEvents, setRegisteredEvents] = useState([]);
const [purchasedTickets, setPurchasedTickets] = useState([]);
useEffect(() => {
const fetchRegisteredEvents = async () => {
const response = await axios.get('/api/attendee/registered-events');
setRegisteredEvents(response.data);
};
const fetchPurchasedTickets = async () => {
const response = await axios.get('/api/attendee/purchased-tickets');
setPurchasedTickets(response.data);
};
fetchRegisteredEvents();
fetchPurchasedTickets();
}, []);
return (
<div className="max-w-7xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Attendee Dashboard</h1>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Registered Events</h2>
<table className="min-w-full bg-white border">
<thead>
<tr>
<th className="border
px-4 py-2">ID</th>
<th className="border px-4 py-2">Title</th>
<th className="border px-4 py-2">Date</th>
<th className="border px-4 py-2">Venue</th>
</tr>
</thead>
<tbody>
{registeredEvents.map(event => (
<tr key={event.id}>
<td className="border px-4 py-2">{event.id}</td>
<td className="border px-4 py-2">{event.title}</td>
<td className="border px-4 py-2">{new Date(event.date).toLocaleDateString()}</td>
<td className="border px-4 py-2">{event.venue}</td>
</tr>
))}
</tbody>
</table>
</div>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Purchased Tickets</h2>
<table className="min-w-full bg-white border">
<thead>
<tr>
<th className="border px-4 py-2">Ticket ID</th>
<th className="border px-4 py-2">Event</th>
<th className="border px-4 py-2">Quantity</th>
<th className="border px-4 py-2">Price</th>
</tr>
</thead>
<tbody>
{purchasedTickets.map(ticket => (
<tr key={ticket.id}>
<td className="border px-4 py-2">{ticket.id}</td>
<td className="border px-4 py-2">{ticket.eventTitle}</td>
<td className="border px-4 py-2">{ticket.quantity}</td>
<td className="border px-4 py-2">${ticket.price}</td>
</tr>
))}
</tbody>
</table>
</div>
</div>
);
};
export default AttendeeDashboard;
```
#### `pages/attendee/dashboard.js`
```javascript
import AttendeeDashboard from '../../components/Attendee/AttendeeDashboard';
const AttendeeDashboardPage = () => {
return (
<div>
<AttendeeDashboard />
</div>
);
};
export default AttendeeDashboardPage;
```
### Summary
- **Admin Dashboard:** Displays system metrics, user management, and event management.
- **Organizer Dashboard:** Shows organizer's events and ticket sales.
- **Attendee Dashboard:** Shows registered events and purchased tickets.
Each dashboard fetches data from the backend using Axios and displays it in tables. Tailwind CSS is used for styling. Make sure your backend APIs are set up to provide the necessary data for each dashboard.
To implement analytics for events and users in your Next.js application, you can use charting libraries such as Chart.js or Recharts. For this example, we'll use Recharts, a popular charting library for React, to display event performance and user engagement insights.
### Project Structure
```
event-management-frontend
├── components
│ ├── Analytics
│ │ ├── EventAnalytics.js
│ │ ├── UserAnalytics.js
├── pages
│ ├── analytics
│ │ ├── event.js
│ │ ├── user.js
└── styles
└── globals.css
```
### 1. Install Recharts
First, install Recharts:
```bash
npm install recharts
```
### 2. Event Analytics Component
#### `components/Analytics/EventAnalytics.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { BarChart, Bar, XAxis, YAxis, Tooltip, CartesianGrid, ResponsiveContainer } from 'recharts';
const EventAnalytics = () => {
const [ticketSales, setTicketSales] = useState([]);
const [attendeeDemographics, setAttendeeDemographics] = useState([]);
useEffect(() => {
const fetchTicketSales = async () => {
const response = await axios.get('/api/analytics/ticket-sales');
setTicketSales(response.data);
};
const fetchAttendeeDemographics = async () => {
const response = await axios.get('/api/analytics/attendee-demographics');
setAttendeeDemographics(response.data);
};
fetchTicketSales();
fetchAttendeeDemographics();
}, []);
return (
<div className="max-w-7xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">Event Analytics</h1>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Ticket Sales</h2>
<ResponsiveContainer width="100%" height={400}>
<BarChart data={ticketSales}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="event" />
<YAxis />
<Tooltip />
<Bar dataKey="sales" fill="#8884d8" />
</BarChart>
</ResponsiveContainer>
</div>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">Attendee Demographics</h2>
<ResponsiveContainer width="100%" height={400}>
<BarChart data={attendeeDemographics}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="ageGroup" />
<YAxis />
<Tooltip />
<Bar dataKey="attendees" fill="#82ca9d" />
</BarChart>
</ResponsiveContainer>
</div>
</div>
);
};
export default EventAnalytics;
```
### 3. User Analytics Component
#### `components/Analytics/UserAnalytics.js`
```javascript
import { useState, useEffect } from 'react';
import axios from 'axios';
import { LineChart, Line, XAxis, YAxis, Tooltip, CartesianGrid, ResponsiveContainer } from 'recharts';
const UserAnalytics = () => {
const [userBehavior, setUserBehavior] = useState([]);
const [userEngagement, setUserEngagement] = useState([]);
useEffect(() => {
const fetchUserBehavior = async () => {
const response = await axios.get('/api/analytics/user-behavior');
setUserBehavior(response.data);
};
const fetchUserEngagement = async () => {
const response = await axios.get('/api/analytics/user-engagement');
setUserEngagement(response.data);
};
fetchUserBehavior();
fetchUserEngagement();
}, []);
return (
<div className="max-w-7xl mx-auto mt-10">
<h1 className="text-3xl font-bold mb-6">User Analytics</h1>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">User Behavior</h2>
<ResponsiveContainer width="100%" height={400}>
<LineChart data={userBehavior}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="date" />
<YAxis />
<Tooltip />
<Line type="monotone" dataKey="activeUsers" stroke="#8884d8" />
</LineChart>
</ResponsiveContainer>
</div>
<div className="mb-6">
<h2 className="text-2xl font-bold mb-4">User Engagement</h2>
<ResponsiveContainer width="100%" height={400}>
<LineChart data={userEngagement}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="date" />
<YAxis />
<Tooltip />
<Line type="monotone" dataKey="engagement" stroke="#82ca9d" />
</LineChart>
</ResponsiveContainer>
</div>
</div>
);
};
export default UserAnalytics;
```
### 4. Analytics Pages
#### `pages/analytics/event.js`
```javascript
import EventAnalytics from '../../components/Analytics/EventAnalytics';
const EventAnalyticsPage = () => {
return (
<div>
<EventAnalytics />
</div>
);
};
export default EventAnalyticsPage;
```
#### `pages/analytics/user.js`
```javascript
import UserAnalytics from '../../components/Analytics/UserAnalytics';
const UserAnalyticsPage = () => {
return (
<div>
<UserAnalytics />
</div>
);
};
export default UserAnalyticsPage;
```
### Summary
- **Event Analytics:** Displays insights into event performance using bar charts to show ticket sales and attendee demographics.
- **User Analytics:** Shows insights into user behavior and engagement using line charts.
Each component fetches data from the backend using Axios and displays it using Recharts. Tailwind CSS is used for styling. Ensure your backend APIs are set up to provide the necessary data for each analytic view.
Here is a detailed setup for the backend of your full-stack event management system using NestJS, Prisma, PostgreSQL, and GraphQL. Let's start with the basic setup for user authentication.
### Backend Setup with NestJS
1. **Initialize a NestJS Project**
```bash
npm i -g @nestjs/cli
nest new event-management-backend
```
2. **Install Required Dependencies**
```bash
cd event-management-backend
npm install @nestjs/graphql @nestjs/apollo apollo-server-express graphql
npm install @prisma/client
npm install bcryptjs
npm install @nestjs/jwt passport-jwt @nestjs/passport passport
npm install class-validator class-transformer
```
3. **Set Up Prisma**
```bash
npx prisma init
```
Update your `prisma/schema.prisma` file:
```prisma
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
generator client {
provider = "prisma-client-js"
}
model User {
id Int @id @default(autoincrement())
email String @unique
password String
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
```
Update your `.env` file with your PostgreSQL connection string:
```
DATABASE_URL="postgresql://username:password@localhost:5432/mydb"
```
Run the Prisma migration:
```bash
npx prisma migrate dev --name init
```
4. **Configure Prisma in NestJS**
Create `prisma.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { PrismaService } from './prisma.service';
@Module({
providers: [PrismaService],
exports: [PrismaService],
})
export class PrismaModule {}
```
Create `prisma.service.ts`:
```typescript
import { Injectable, OnModuleInit, INestApplication } from '@nestjs/common';
import { PrismaClient } from '@prisma/client';
@Injectable()
export class PrismaService extends PrismaClient implements OnModuleInit {
async onModuleInit() {
await this.$connect();
}
async enableShutdownHooks(app: INestApplication) {
this.$on('beforeExit', async () => {
await app.close();
});
}
}
```
5. **Setup Authentication Module**
Create `auth.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthService } from './auth.service';
import { AuthResolver } from './auth.resolver';
import { JwtModule } from '@nestjs/jwt';
import { PassportModule } from '@nestjs/passport';
import { PrismaModule } from '../prisma/prisma.module';
import { JwtStrategy } from './jwt.strategy';
@Module({
imports: [
PrismaModule,
PassportModule,
JwtModule.register({
secret: 'your_secret_key', // use a better secret in production
signOptions: { expiresIn: '60m' },
}),
],
providers: [AuthService, AuthResolver, JwtStrategy],
})
export class AuthModule {}
```
Create `auth.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
import { PrismaService } from '../prisma/prisma.service';
import * as bcrypt from 'bcryptjs';
@Injectable()
export class AuthService {
constructor(
private readonly prisma: PrismaService,
private readonly jwtService: JwtService,
) {}
async validateUser(email: string, password: string): Promise<any> {
const user = await this.prisma.user.findUnique({ where: { email } });
if (user && (await bcrypt.compare(password, user.password))) {
const { password, ...result } = user;
return result;
}
return null;
}
async login(user: any) {
const payload = { email: user.email, sub: user.id };
return {
access_token: this.jwtService.sign(payload),
};
}
async signup(email: string, password: string) {
const hashedPassword = await bcrypt.hash(password, 10);
const user = await this.prisma.user.create({
data: {
email,
password: hashedPassword,
},
});
return user;
}
}
```
Create `auth.resolver.ts`:
```typescript
import { Resolver, Mutation, Args } from '@nestjs/graphql';
import { AuthService } from './auth.service';
import { AuthResponse } from './dto/auth-response';
import { UserInput } from './dto/user-input';
@Resolver()
export class AuthResolver {
constructor(private readonly authService: AuthService) {}
@Mutation(() => AuthResponse)
async login(@Args('userInput') userInput: UserInput) {
const user = await this.authService.validateUser(userInput.email, userInput.password);
if (!user) {
throw new Error('Invalid credentials');
}
return this.authService.login(user);
}
@Mutation(() => AuthResponse)
async signup(@Args('userInput') userInput: UserInput) {
const user = await this.authService.signup(userInput.email, userInput.password);
return this.authService.login(user);
}
}
```
Create DTOs (`dto/auth-response.ts` and `dto/user-input.ts`):
```typescript
// auth-response.ts
import { Field, ObjectType } from '@nestjs/graphql';
@ObjectType()
export class AuthResponse {
@Field()
access_token: string;
}
```
```typescript
// user-input.ts
import { InputType, Field } from '@nestjs/graphql';
@InputType()
export class UserInput {
@Field()
email: string;
@Field()
password: string;
}
```
6. **Setup JWT Strategy**
Create `jwt.strategy.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import { PassportStrategy } from '@nestjs/passport';
import { ExtractJwt, Strategy } from 'passport-jwt';
import { JwtPayload } from './jwt-payload.interface';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class JwtStrategy extends PassportStrategy(Strategy) {
constructor(private readonly prisma: PrismaService) {
super({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
ignoreExpiration: false,
secretOrKey: 'your_secret_key', // use a better secret in production
});
}
async validate(payload: JwtPayload) {
const user = await this.prisma.user.findUnique({ where: { id: payload.sub } });
if (!user) {
throw new UnauthorizedException();
}
return user;
}
}
```
Create `jwt-payload.interface.ts`:
```typescript
export interface JwtPayload {
email: string;
sub: number;
}
```
7. **Add GraphQL Module**
Configure `graphql.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { GraphQLModule } from '@nestjs/graphql';
import { ApolloDriver, ApolloDriverConfig } from '@nestjs/apollo';
import { join } from 'path';
@Module({
imports: [
GraphQLModule.forRoot<ApolloDriverConfig>({
driver: ApolloDriver,
autoSchemaFile: join(process.cwd(), 'src/schema.gql'),
sortSchema: true,
}),
],
})
export class GraphqlModule {}
```
8. **Integrate All Modules in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test the GraphQL API**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the authentication queries and mutations:
```graphql
mutation {
signup(userInput: {email: "[email protected]", password: "password"}) {
access_token
}
}
mutation {
login(userInput: {email: "[email protected]", password: "password"}) {
access_token
}
}
```
This setup provides the foundational authentication system. You can now build upon this by adding other features like event management, ticket management, notifications, and more.
Sure, let's extend the backend to support user roles and permissions. We'll add role-based access control to our authentication system. Here’s how we can achieve that:
1. **Update Prisma Schema for Roles**
Add roles and related fields to `prisma/schema.prisma`:
```prisma
model User {
id Int @id @default(autoincrement())
email String @unique
password String
role Role @default(ATTENDEE)
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
enum Role {
ADMIN
ORGANIZER
ATTENDEE
}
```
Run the Prisma migration:
```bash
npx prisma migrate dev --name add-roles
```
2. **Update User Registration to Assign Roles**
Update `auth.service.ts` to assign roles during signup:
```typescript
import { Injectable } from '@nestjs/common';
import { JwtService } from '@nestjs/jwt';
import { PrismaService } from '../prisma/prisma.service';
import * as bcrypt from 'bcryptjs';
import { Role } from '@prisma/client';
@Injectable()
export class AuthService {
constructor(
private readonly prisma: PrismaService,
private readonly jwtService: JwtService,
) {}
async validateUser(email: string, password: string): Promise<any> {
const user = await this.prisma.user.findUnique({ where: { email } });
if (user && (await bcrypt.compare(password, user.password))) {
const { password, ...result } = user;
return result;
}
return null;
}
async login(user: any) {
const payload = { email: user.email, sub: user.id, role: user.role };
return {
access_token: this.jwtService.sign(payload),
};
}
async signup(email: string, password: string, role: Role) {
const hashedPassword = await bcrypt.hash(password, 10);
const user = await this.prisma.user.create({
data: {
email,
password: hashedPassword,
role,
},
});
return user;
}
}
```
Update `auth.resolver.ts` to accept role during signup:
```typescript
import { Resolver, Mutation, Args } from '@nestjs/graphql';
import { AuthService } from './auth.service';
import { AuthResponse } from './dto/auth-response';
import { UserInput } from './dto/user-input';
import { Role } from '@prisma/client';
@Resolver()
export class AuthResolver {
constructor(private readonly authService: AuthService) {}
@Mutation(() => AuthResponse)
async login(@Args('userInput') userInput: UserInput) {
const user = await this.authService.validateUser(userInput.email, userInput.password);
if (!user) {
throw new Error('Invalid credentials');
}
return this.authService.login(user);
}
@Mutation(() => AuthResponse)
async signup(@Args('userInput') userInput: UserInput, @Args('role') role: Role) {
const user = await this.authService.signup(userInput.email, userInput.password, role);
return this.authService.login(user);
}
}
```
3. **Create Guard for Role-Based Authorization**
Create `roles.guard.ts`:
```typescript
import { Injectable, CanActivate, ExecutionContext } from '@nestjs/common';
import { Reflector } from '@nestjs/core';
import { Role } from '@prisma/client';
@Injectable()
export class RolesGuard implements CanActivate {
constructor(private reflector: Reflector) {}
canActivate(context: ExecutionContext): boolean {
const requiredRoles = this.reflector.get<Role[]>('roles', context.getHandler());
if (!requiredRoles) {
return true;
}
const { user } = context.switchToHttp().getRequest();
return requiredRoles.some((role) => user.role?.includes(role));
}
}
```
Create `roles.decorator.ts`:
```typescript
import { SetMetadata } from '@nestjs/common';
import { Role } from '@prisma/client';
export const Roles = (...roles: Role[]) => SetMetadata('roles', roles);
```
4. **Protect Routes with Role-Based Guards**
Update event-related resolvers and services to use the role-based guard:
Example for event management (`events.module.ts`, `events.service.ts`, `events.resolver.ts`):
Create `events.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { EventsService } from './events.service';
import { EventsResolver } from './events.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [EventsService, EventsResolver],
})
export class EventsModule {}
```
Create `events.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class EventsService {
constructor(private readonly prisma: PrismaService) {}
// Add methods for event creation, deletion, etc.
}
```
Create `events.resolver.ts`:
```typescript
import { Resolver, Mutation, Args, Query } from '@nestjs/graphql';
import { EventsService } from './events.service';
import { UseGuards } from '@nestjs/common';
import { RolesGuard } from '../auth/roles.guard';
import { Roles } from '../auth/roles.decorator';
import { Role } from '@prisma/client';
@Resolver()
export class EventsResolver {
constructor(private readonly eventsService: EventsService) {}
@Mutation(() => Boolean)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async createEvent(
@Args('title') title: string,
@Args('description') description: string,
// Add other event fields
) {
// Call service method to create event
return true;
}
@Mutation(() => Boolean)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async deleteEvent(@Args('id') id: number) {
// Call service method to delete event
return true;
}
@Query(() => [Event])
async events() {
// Call service method to get events
return [];
}
}
```
5. **Integrate Event Module in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Role-Based Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the role-based mutations and queries:
```graphql
mutation {
signup(userInput: {email: "[email protected]", password: "password"}, role: ORGANIZER) {
access_token
}
}
mutation {
login(userInput: {email: "[email protected]", password: "password"}) {
access_token
}
}
mutation {
createEvent(title: "Event Title", description: "Event Description") {
success
}
}
```
This setup provides a robust foundation for role-based access control in your event management system. You can now add more roles and permissions as needed, ensuring each user has access to only the appropriate features and functionalities.
Let's extend our backend to include event management functionality. We'll implement the ability for organizers to create, edit, and delete events, and for users to view and search for events. We'll start by updating the Prisma schema and then proceed to the NestJS service and resolver layers.
### Update Prisma Schema for Events
Update `prisma/schema.prisma` to include the `Event` model:
```prisma
model Event {
id Int @id @default(autoincrement())
title String
description String
date DateTime
time String
venue String
tickets Ticket[]
organizer User @relation(fields: [organizerId], references: [id])
organizerId Int
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
model Ticket {
id Int @id @default(autoincrement())
type String
price Float
quantity Int
event Event @relation(fields: [eventId], references: [id])
eventId Int
}
model User {
id Int @id @default(autoincrement())
email String @unique
password String
role Role @default(ATTENDEE)
events Event[]
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
enum Role {
ADMIN
ORGANIZER
ATTENDEE
}
```
Run the Prisma migration:
```bash
npx prisma migrate dev --name add-events
```
### Create Event Module in NestJS
1. **Create Event DTOs**
Create `dto/create-event.input.ts`:
```typescript
import { InputType, Field } from '@nestjs/graphql';
@InputType()
export class CreateEventInput {
@Field()
title: string;
@Field()
description: string;
@Field()
date: Date;
@Field()
time: string;
@Field()
venue: string;
@Field(() => [CreateTicketInput])
tickets: CreateTicketInput[];
}
```
Create `dto/create-ticket.input.ts`:
```typescript
import { InputType, Field } from '@nestjs/graphql';
@InputType()
export class CreateTicketInput {
@Field()
type: string;
@Field()
price: number;
@Field()
quantity: number;
}
```
Create `dto/update-event.input.ts`:
```typescript
import { InputType, Field, PartialType } from '@nestjs/graphql';
import { CreateEventInput } from './create-event.input';
@InputType()
export class UpdateEventInput extends PartialType(CreateEventInput) {
@Field()
id: number;
}
```
2. **Create Event Service**
Create `events.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
import { CreateEventInput } from './dto/create-event.input';
import { UpdateEventInput } from './dto/update-event.input';
@Injectable()
export class EventsService {
constructor(private readonly prisma: PrismaService) {}
async createEvent(createEventInput: CreateEventInput, organizerId: number) {
const { tickets, ...eventData } = createEventInput;
const event = await this.prisma.event.create({
data: {
...eventData,
organizer: { connect: { id: organizerId } },
tickets: {
create: tickets,
},
},
});
return event;
}
async updateEvent(updateEventInput: UpdateEventInput) {
const { id, tickets, ...eventData } = updateEventInput;
const event = await this.prisma.event.update({
where: { id },
data: {
...eventData,
tickets: {
deleteMany: { eventId: id },
create: tickets,
},
},
});
return event;
}
async deleteEvent(id: number) {
await this.prisma.event.delete({ where: { id } });
return true;
}
async getEvent(id: number) {
return this.prisma.event.findUnique({ where: { id } });
}
async getEvents() {
return this.prisma.event.findMany();
}
async searchEvents(searchTerm: string) {
return this.prisma.event.findMany({
where: {
OR: [
{ title: { contains: searchTerm, mode: 'insensitive' } },
{ description: { contains: searchTerm, mode: 'insensitive' } },
{ venue: { contains: searchTerm, mode: 'insensitive' } },
],
},
});
}
}
```
3. **Create Event Resolver**
Create `events.resolver.ts`:
```typescript
import { Resolver, Query, Mutation, Args } from '@nestjs/graphql';
import { EventsService } from './events.service';
import { CreateEventInput } from './dto/create-event.input';
import { UpdateEventInput } from './dto/update-event.input';
import { Event } from '@prisma/client';
import { UseGuards } from '@nestjs/common';
import { RolesGuard } from '../auth/roles.guard';
import { Roles } from '../auth/roles.decorator';
import { Role } from '@prisma/client';
@Resolver('Event')
export class EventsResolver {
constructor(private readonly eventsService: EventsService) {}
@Mutation(() => Event)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async createEvent(
@Args('createEventInput') createEventInput: CreateEventInput,
@Args('organizerId') organizerId: number,
) {
return this.eventsService.createEvent(createEventInput, organizerId);
}
@Mutation(() => Event)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async updateEvent(@Args('updateEventInput') updateEventInput: UpdateEventInput) {
return this.eventsService.updateEvent(updateEventInput);
}
@Mutation(() => Boolean)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async deleteEvent(@Args('id') id: number) {
return this.eventsService.deleteEvent(id);
}
@Query(() => Event)
async event(@Args('id') id: number) {
return this.eventsService.getEvent(id);
}
@Query(() => [Event])
async events() {
return this.eventsService.getEvents();
}
@Query(() => [Event])
async searchEvents(@Args('searchTerm') searchTerm: string) {
return this.eventsService.searchEvents(searchTerm);
}
}
```
4. **Create Event Module**
Create `events.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { EventsService } from './events.service';
import { EventsResolver } from './events.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [EventsService, EventsResolver],
})
export class EventsModule {}
```
5. **Integrate Event Module in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Event Management Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the event management queries and mutations:
```graphql
mutation {
createEvent(
createEventInput: {
title: "Sample Event"
description: "This is a sample event."
date: "2024-08-01T00:00:00.000Z"
time: "18:00"
venue: "Sample Venue"
tickets: [
{ type: "General", price: 100.0, quantity: 100 }
{ type: "VIP", price: 200.0, quantity: 50 }
]
}
organizerId: 1
) {
id
title
description
date
time
venue
tickets {
type
price
quantity
}
}
}
mutation {
updateEvent(
updateEventInput: {
id: 1
title: "Updated Event Title"
description: "Updated description."
date: "2024-08-02T00:00:00.000Z"
time: "19:00"
venue: "Updated Venue"
tickets: [
{ type: "General", price: 120.0, quantity: 100 }
{ type: "VIP", price: 250.0, quantity: 50 }
]
}
) {
id
title
description
date
time
venue
tickets {
type
price
quantity
}
}
}
mutation {
deleteEvent(id: 1)
}
query {
event(id: 1) {
id
title
description
date
time
venue
tickets {
type
price
quantity
}
}
}
query {
events {
id
title
description
date
time
venue
}
}
query {
searchEvents(searchTerm: "Sample") {
id
title
description
date
time
venue
}
}
```
This setup provides the necessary backend functionality for event management, allowing organizers to create, update, and delete events, and enabling users to view and search for events. You can further extend these functionalities as needed.
To add ticket management to our event management system, we'll extend our backend to include the functionality for creating tickets, purchasing tickets, and viewing purchased tickets. We'll update the Prisma schema to track ticket purchases, and then add the necessary service and resolver methods in NestJS.
### Update Prisma Schema for Ticket Purchases
Update `prisma/schema.prisma` to include the `TicketPurchase` model:
```prisma
model TicketPurchase {
id Int @id @default(autoincrement())
user User @relation(fields: [userId], references: [id])
userId Int
ticket Ticket @relation(fields: [ticketId], references: [id])
ticketId Int
quantity Int
totalPrice Float
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
model Ticket {
id Int @id @default(autoincrement())
type String
price Float
quantity Int
event Event @relation(fields: [eventId], references: [id])
eventId Int
purchases TicketPurchase[]
}
model Event {
id Int @id @default(autoincrement())
title String
description String
date DateTime
time String
venue String
tickets Ticket[]
organizer User @relation(fields: [organizerId], references: [id])
organizerId Int
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
model User {
id Int @id @default(autoincrement())
email String @unique
password String
role Role @default(ATTENDEE)
events Event[]
ticketPurchases TicketPurchase[]
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
enum Role {
ADMIN
ORGANIZER
ATTENDEE
}
```
Run the Prisma migration:
```bash
npx prisma migrate dev --name add-ticket-purchases
```
### Create Ticket Management Service
Create `tickets.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
import { CreateTicketInput } from './dto/create-ticket.input';
import { PurchaseTicketInput } from './dto/purchase-ticket.input';
@Injectable()
export class TicketsService {
constructor(private readonly prisma: PrismaService) {}
async createTicket(createTicketInput: CreateTicketInput, eventId: number) {
const ticket = await this.prisma.ticket.create({
data: {
...createTicketInput,
event: { connect: { id: eventId } },
},
});
return ticket;
}
async purchaseTicket(purchaseTicketInput: PurchaseTicketInput, userId: number) {
const { ticketId, quantity } = purchaseTicketInput;
const ticket = await this.prisma.ticket.findUnique({ where: { id: ticketId } });
if (!ticket || ticket.quantity < quantity) {
throw new Error('Insufficient ticket quantity');
}
const totalPrice = ticket.price * quantity;
const purchase = await this.prisma.ticketPurchase.create({
data: {
user: { connect: { id: userId } },
ticket: { connect: { id: ticketId } },
quantity,
totalPrice,
},
});
await this.prisma.ticket.update({
where: { id: ticketId },
data: {
quantity: ticket.quantity - quantity,
},
});
return purchase;
}
async getUserTickets(userId: number) {
return this.prisma.ticketPurchase.findMany({
where: { userId },
include: { ticket: true, event: true },
});
}
}
```
### Create Ticket Management Resolvers
Create `tickets.resolver.ts`:
```typescript
import { Resolver, Mutation, Args, Query } from '@nestjs/graphql';
import { TicketsService } from './tickets.service';
import { CreateTicketInput } from './dto/create-ticket.input';
import { PurchaseTicketInput } from './dto/purchase-ticket.input';
import { Ticket, TicketPurchase } from '@prisma/client';
import { UseGuards } from '@nestjs/common';
import { RolesGuard } from '../auth/roles.guard';
import { Roles } from '../auth/roles.decorator';
import { Role } from '@prisma/client';
@Resolver('Ticket')
export class TicketsResolver {
constructor(private readonly ticketsService: TicketsService) {}
@Mutation(() => Ticket)
@Roles(Role.ORGANIZER)
@UseGuards(RolesGuard)
async createTicket(
@Args('createTicketInput') createTicketInput: CreateTicketInput,
@Args('eventId') eventId: number,
) {
return this.ticketsService.createTicket(createTicketInput, eventId);
}
@Mutation(() => TicketPurchase)
@Roles(Role.ATTENDEE)
@UseGuards(RolesGuard)
async purchaseTicket(
@Args('purchaseTicketInput') purchaseTicketInput: PurchaseTicketInput,
@Args('userId') userId: number,
) {
return this.ticketsService.purchaseTicket(purchaseTicketInput, userId);
}
@Query(() => [TicketPurchase])
@Roles(Role.ATTENDEE)
@UseGuards(RolesGuard)
async userTickets(@Args('userId') userId: number) {
return this.ticketsService.getUserTickets(userId);
}
}
```
### Create Ticket Management DTOs
Create `dto/create-ticket.input.ts`:
```typescript
import { InputType, Field } from '@nestjs/graphql';
@InputType()
export class CreateTicketInput {
@Field()
type: string;
@Field()
price: number;
@Field()
quantity: number;
}
```
Create `dto/purchase-ticket.input.ts`:
```typescript
import { InputType, Field } from '@nestjs/graphql';
@InputType()
export class PurchaseTicketInput {
@Field()
ticketId: number;
@Field()
quantity: number;
}
```
### Create Ticket Module
Create `tickets.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { TicketsService } from './tickets.service';
import { TicketsResolver } from './tickets.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [TicketsService, TicketsResolver],
})
export class TicketsModule {}
```
### Integrate Ticket Module in App Module
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Ticket Management Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the ticket management queries and mutations:
```graphql
mutation {
createTicket(
createTicketInput: {
type: "General"
price: 100.0
quantity: 100
}
eventId: 1
) {
id
type
price
quantity
}
}
mutation {
purchaseTicket(
purchaseTicketInput: {
ticketId: 1
quantity: 2
}
userId: 1
) {
id
quantity
totalPrice
ticket {
type
price
}
}
}
query {
userTickets(userId: 1) {
id
quantity
totalPrice
ticket {
type
price
}
createdAt
}
}
```
This setup provides the necessary backend functionality for ticket management, allowing organizers to create tickets, attendees to purchase tickets, and users to view their purchased tickets. You can further extend these functionalities as needed.
To implement a notification system in the backend, we'll need to integrate both email notifications and in-app notifications. We'll use a service like SendGrid or Nodemailer for email notifications and a real-time mechanism like WebSockets for in-app notifications.
### Setting Up Email Notifications
1. **Install Nodemailer**
```bash
npm install nodemailer
```
2. **Create Email Service**
Create `email.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import * as nodemailer from 'nodemailer';
@Injectable()
export class EmailService {
private transporter;
constructor() {
this.transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: process.env.EMAIL_USER,
pass: process.env.EMAIL_PASS,
},
});
}
async sendMail(to: string, subject: string, text: string) {
const mailOptions = {
from: process.env.EMAIL_USER,
to,
subject,
text,
};
await this.transporter.sendMail(mailOptions);
}
}
```
3. **Update Environment Variables**
Add the following to your `.env` file:
```
[email protected]
EMAIL_PASS=your-email-password
```
4. **Integrate Email Service in Ticket Service**
Update `tickets.service.ts` to send an email notification after purchasing a ticket:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
import { CreateTicketInput } from './dto/create-ticket.input';
import { PurchaseTicketInput } from './dto/purchase-ticket.input';
import { EmailService } from '../email/email.service';
@Injectable()
export class TicketsService {
constructor(
private readonly prisma: PrismaService,
private readonly emailService: EmailService,
) {}
async createTicket(createTicketInput: CreateTicketInput, eventId: number) {
const ticket = await this.prisma.ticket.create({
data: {
...createTicketInput,
event: { connect: { id: eventId } },
},
});
return ticket;
}
async purchaseTicket(purchaseTicketInput: PurchaseTicketInput, userId: number) {
const { ticketId, quantity } = purchaseTicketInput;
const ticket = await this.prisma.ticket.findUnique({ where: { id: ticketId } });
if (!ticket || ticket.quantity < quantity) {
throw new Error('Insufficient ticket quantity');
}
const totalPrice = ticket.price * quantity;
const purchase = await this.prisma.ticketPurchase.create({
data: {
user: { connect: { id: userId } },
ticket: { connect: { id: ticketId } },
quantity,
totalPrice,
},
});
await this.prisma.ticket.update({
where: { id: ticketId },
data: {
quantity: ticket.quantity - quantity,
},
});
const user = await this.prisma.user.findUnique({ where: { id: userId } });
// Send email notification
await this.emailService.sendMail(
user.email,
'Ticket Purchase Confirmation',
`You have successfully purchased ${quantity} tickets for ${ticket.type} at ${ticket.event.title}. Total price: ${totalPrice}`,
);
return purchase;
}
async getUserTickets(userId: number) {
return this.prisma.ticketPurchase.findMany({
where: { userId },
include: { ticket: true, event: true },
});
}
}
```
5. **Create Email Module**
Create `email.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { EmailService } from './email.service';
@Module({
providers: [EmailService],
exports: [EmailService],
})
export class EmailModule {}
```
6. **Integrate Email Module in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
import { EmailModule } from './email/email.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
EmailModule,
],
})
export class AppModule {}
```
### Setting Up In-App Notifications
1. **Install WebSockets**
```bash
npm install @nestjs/websockets @nestjs/platform-socket.io
```
2. **Create Notification Gateway**
Create `notification.gateway.ts`:
```typescript
import {
WebSocketGateway,
WebSocketServer,
SubscribeMessage,
OnGatewayConnection,
OnGatewayDisconnect,
} from '@nestjs/websockets';
import { Server, Socket } from 'socket.io';
@WebSocketGateway()
export class NotificationGateway implements OnGatewayConnection, OnGatewayDisconnect {
@WebSocketServer() server: Server;
async handleConnection(client: Socket) {
console.log(`Client connected: ${client.id}`);
}
async handleDisconnect(client: Socket) {
console.log(`Client disconnected: ${client.id}`);
}
@SubscribeMessage('message')
async onMessage(client: Socket, message: string) {
console.log(message);
}
async sendNotification(event: string, data: any) {
this.server.emit(event, data);
}
}
```
3. **Integrate Notification Gateway in Ticket Service**
Update `tickets.service.ts` to send an in-app notification after purchasing a ticket:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
import { CreateTicketInput } from './dto/create-ticket.input';
import { PurchaseTicketInput } from './dto/purchase-ticket.input';
import { EmailService } from '../email/email.service';
import { NotificationGateway } from '../notification/notification.gateway';
@Injectable()
export class TicketsService {
constructor(
private readonly prisma: PrismaService,
private readonly emailService: EmailService,
private readonly notificationGateway: NotificationGateway,
) {}
async createTicket(createTicketInput: CreateTicketInput, eventId: number) {
const ticket = await this.prisma.ticket.create({
data: {
...createTicketInput,
event: { connect: { id: eventId } },
},
});
return ticket;
}
async purchaseTicket(purchaseTicketInput: PurchaseTicketInput, userId: number) {
const { ticketId, quantity } = purchaseTicketInput;
const ticket = await this.prisma.ticket.findUnique({ where: { id: ticketId } });
if (!ticket || ticket.quantity < quantity) {
throw new Error('Insufficient ticket quantity');
}
const totalPrice = ticket.price * quantity;
const purchase = await this.prisma.ticketPurchase.create({
data: {
user: { connect: { id: userId } },
ticket: { connect: { id: ticketId } },
quantity,
totalPrice,
},
});
await this.prisma.ticket.update({
where: { id: ticketId },
data: {
quantity: ticket.quantity - quantity,
},
});
const user = await this.prisma.user.findUnique({ where: { id: userId } });
// Send email notification
await this.emailService.sendMail(
user.email,
'Ticket Purchase Confirmation',
`You have successfully purchased ${quantity} tickets for ${ticket.type} at ${ticket.event.title}. Total price: ${totalPrice}`,
);
// Send in-app notification
await this.notificationGateway.sendNotification('ticketPurchased', {
userId,
message: `You have successfully purchased ${quantity} tickets for ${ticket.type} at ${ticket.event.title}.`,
});
return purchase;
}
async getUserTickets(userId: number) {
return this.prisma.ticketPurchase.findMany({
where: { userId },
include: { ticket: true, event: true },
});
}
}
```
4. **Create Notification Module**
Create `notification.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { NotificationGateway } from './notification.gateway';
@Module({
providers: [NotificationGateway],
exports: [NotificationGateway],
})
export class NotificationModule {}
```
5. **Integrate Notification Module in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
import { EmailModule } from './email/email.module';
import { NotificationModule } from './notification/notification.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
EmailModule,
NotificationModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Notification Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the ticket purchase mutation:
```graphql
mutation {
purchaseTicket(
purchaseTicketInput: {
ticketId: 1
quantity: 2
}
userId: 1
) {
id
quantity
totalPrice
ticket {
type
price
}
}
}
```
Check the console output for WebSocket connection messages and email inbox for the notification email.
This setup provides the necessary backend functionality for both email and in-app notifications. You can further extend these functionalities as needed.
To integrate a payment gateway for ticket purchases, we'll use Stripe. Stripe provides robust APIs for handling payments, subscriptions, and more. We'll integrate Stripe into our NestJS backend.
### Setting Up Stripe
1. **Install Stripe SDK**
```bash
npm install stripe @nestjs/stripe
```
2. **Create Stripe Service**
Create `stripe.service.ts`:
```typescript
import { Injectable } from '@nestjs/common';
import Stripe from 'stripe';
@Injectable()
export class StripeService {
private stripe: Stripe;
constructor() {
this.stripe = new Stripe(process.env.STRIPE_SECRET_KEY, {
apiVersion: '2020-08-27',
});
}
async createPaymentIntent(amount: number, currency: string) {
return await this.stripe.paymentIntents.create({
amount,
currency,
});
}
async confirmPaymentIntent(paymentIntentId: string) {
return await this.stripe.paymentIntents.confirm(paymentIntentId);
}
}
```
3. **Update Environment Variables**
Add the following to your `.env` file:
```
STRIPE_SECRET_KEY=your-stripe-secret-key
STRIPE_PUBLIC_KEY=your-stripe-public-key
```
4. **Create Payment DTOs**
Create `dto/create-payment.dto.ts`:
```typescript
export class CreatePaymentDto {
amount: number;
currency: string;
}
```
Create `dto/confirm-payment.dto.ts`:
```typescript
export class ConfirmPaymentDto {
paymentIntentId: string;
}
```
5. **Create Payment Resolver**
Create `payment.resolver.ts`:
```typescript
import { Resolver, Mutation, Args } from '@nestjs/graphql';
import { StripeService } from './stripe.service';
import { CreatePaymentDto } from './dto/create-payment.dto';
import { ConfirmPaymentDto } from './dto/confirm-payment.dto';
@Resolver()
export class PaymentResolver {
constructor(private readonly stripeService: StripeService) {}
@Mutation(() => String)
async createPaymentIntent(@Args('createPaymentDto') createPaymentDto: CreatePaymentDto) {
const paymentIntent = await this.stripeService.createPaymentIntent(
createPaymentDto.amount,
createPaymentDto.currency,
);
return paymentIntent.client_secret;
}
@Mutation(() => String)
async confirmPaymentIntent(@Args('confirmPaymentDto') confirmPaymentDto: ConfirmPaymentDto) {
const paymentIntent = await this.stripeService.confirmPaymentIntent(confirmPaymentDto.paymentIntentId);
return paymentIntent.status;
}
}
```
6. **Create Payment Module**
Create `payment.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { StripeService } from './stripe.service';
import { PaymentResolver } from './payment.resolver';
@Module({
providers: [StripeService, PaymentResolver],
})
export class PaymentModule {}
```
7. **Integrate Payment Module in App Module**
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
import { EmailModule } from './email/email.module';
import { NotificationModule } from './notification/notification.module';
import { PaymentModule } from './payment/payment.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
EmailModule,
NotificationModule,
PaymentModule,
],
})
export class AppModule {}
```
### Integrate Payment Flow in Ticket Service
Update `tickets.service.ts` to create a payment intent before confirming the ticket purchase:
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
import { CreateTicketInput } from './dto/create-ticket.input';
import { PurchaseTicketInput } from './dto/purchase-ticket.input';
import { EmailService } from '../email/email.service';
import { NotificationGateway } from '../notification/notification.gateway';
import { StripeService } from '../payment/stripe.service';
@Injectable()
export class TicketsService {
constructor(
private readonly prisma: PrismaService,
private readonly emailService: EmailService,
private readonly notificationGateway: NotificationGateway,
private readonly stripeService: StripeService,
) {}
async createTicket(createTicketInput: CreateTicketInput, eventId: number) {
const ticket = await this.prisma.ticket.create({
data: {
...createTicketInput,
event: { connect: { id: eventId } },
},
});
return ticket;
}
async purchaseTicket(purchaseTicketInput: PurchaseTicketInput, userId: number) {
const { ticketId, quantity, paymentIntentId } = purchaseTicketInput;
const ticket = await this.prisma.ticket.findUnique({ where: { id: ticketId } });
if (!ticket || ticket.quantity < quantity) {
throw new Error('Insufficient ticket quantity');
}
const totalPrice = ticket.price * quantity;
const paymentIntent = await this.stripeService.confirmPaymentIntent(paymentIntentId);
if (paymentIntent.status !== 'succeeded') {
throw new Error('Payment failed');
}
const purchase = await this.prisma.ticketPurchase.create({
data: {
user: { connect: { id: userId } },
ticket: { connect: { id: ticketId } },
quantity,
totalPrice,
},
});
await this.prisma.ticket.update({
where: { id: ticketId },
data: {
quantity: ticket.quantity - quantity,
},
});
const user = await this.prisma.user.findUnique({ where: { id: userId } });
// Send email notification
await this.emailService.sendMail(
user.email,
'Ticket Purchase Confirmation',
`You have successfully purchased ${quantity} tickets for ${ticket.type} at ${ticket.event.title}. Total price: ${totalPrice}`,
);
// Send in-app notification
await this.notificationGateway.sendNotification('ticketPurchased', {
userId,
message: `You have successfully purchased ${quantity} tickets for ${ticket.type} at ${ticket.event.title}.`,
});
return purchase;
}
async getUserTickets(userId: number) {
return this.prisma.ticketPurchase.findMany({
where: { userId },
include: { ticket: true, event: true },
});
}
}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Payment Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the payment flow:
1. Create a payment intent:
```graphql
mutation {
createPaymentIntent(createPaymentDto: { amount: 5000, currency: "usd" }) {
client_secret
}
}
```
2. Confirm the payment intent:
```graphql
mutation {
confirmPaymentIntent(confirmPaymentDto: { paymentIntentId: "pi_1JHYLR2eZvKYlo2C8kIfmQqN" }) {
status
}
}
```
3. Purchase a ticket:
```graphql
mutation {
purchaseTicket(
purchaseTicketInput: {
ticketId: 1
quantity: 2
paymentIntentId: "pi_1JHYLR2eZvKYlo2C8kIfmQqN"
}
userId: 1
) {
id
quantity
totalPrice
ticket {
type
price
}
}
}
```
This setup provides the necessary backend functionality for integrating a payment gateway (Stripe) for ticket purchases. You can further extend these functionalities as needed.
To create a dashboard for different user roles (Admin, Organizer, and Attendee), we will need to build appropriate resolvers and services to fetch and aggregate the required data. We'll create separate services and resolvers for each type of dashboard.
### Admin Dashboard
**admin-dashboard.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class AdminDashboardService {
constructor(private readonly prisma: PrismaService) {}
async getSystemMetrics() {
const userCount = await this.prisma.user.count();
const eventCount = await this.prisma.event.count();
const ticketSales = await this.prisma.ticketPurchase.count();
return { userCount, eventCount, ticketSales };
}
async getUsers() {
return this.prisma.user.findMany();
}
async getEvents() {
return this.prisma.event.findMany();
}
}
```
**admin-dashboard.resolver.ts**
```typescript
import { Resolver, Query } from '@nestjs/graphql';
import { AdminDashboardService } from './admin-dashboard.service';
@Resolver()
export class AdminDashboardResolver {
constructor(private readonly adminDashboardService: AdminDashboardService) {}
@Query(() => String)
async getSystemMetrics() {
return this.adminDashboardService.getSystemMetrics();
}
@Query(() => [User])
async getUsers() {
return this.adminDashboardService.getUsers();
}
@Query(() => [Event])
async getEvents() {
return this.adminDashboardService.getEvents();
}
}
```
**admin-dashboard.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { AdminDashboardService } from './admin-dashboard.service';
import { AdminDashboardResolver } from './admin-dashboard.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [AdminDashboardService, AdminDashboardResolver],
})
export class AdminDashboardModule {}
```
### Organizer Dashboard
**organizer-dashboard.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class OrganizerDashboardService {
constructor(private readonly prisma: PrismaService) {}
async getOrganizerEvents(organizerId: number) {
return this.prisma.event.findMany({
where: { organizerId },
include: {
tickets: true,
purchases: true,
},
});
}
async getEventAttendees(eventId: number) {
return this.prisma.ticketPurchase.findMany({
where: { eventId },
include: { user: true },
});
}
}
```
**organizer-dashboard.resolver.ts**
```typescript
import { Resolver, Query, Args, Int } from '@nestjs/graphql';
import { OrganizerDashboardService } from './organizer-dashboard.service';
@Resolver()
export class OrganizerDashboardResolver {
constructor(private readonly organizerDashboardService: OrganizerDashboardService) {}
@Query(() => [Event])
async getOrganizerEvents(@Args('organizerId', { type: () => Int }) organizerId: number) {
return this.organizerDashboardService.getOrganizerEvents(organizerId);
}
@Query(() => [TicketPurchase])
async getEventAttendees(@Args('eventId', { type: () => Int }) eventId: number) {
return this.organizerDashboardService.getEventAttendees(eventId);
}
}
```
**organizer-dashboard.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { OrganizerDashboardService } from './organizer-dashboard.service';
import { OrganizerDashboardResolver } from './organizer-dashboard.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [OrganizerDashboardService, OrganizerDashboardResolver],
})
export class OrganizerDashboardModule {}
```
### Attendee Dashboard
**attendee-dashboard.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class AttendeeDashboardService {
constructor(private readonly prisma: PrismaService) {}
async getUserEvents(userId: number) {
return this.prisma.ticketPurchase.findMany({
where: { userId },
include: { event: true, ticket: true },
});
}
}
```
**attendee-dashboard.resolver.ts**
```typescript
import { Resolver, Query, Args, Int } from '@nestjs/graphql';
import { AttendeeDashboardService } from './attendee-dashboard.service';
@Resolver()
export class AttendeeDashboardResolver {
constructor(private readonly attendeeDashboardService: AttendeeDashboardService) {}
@Query(() => [TicketPurchase])
async getUserEvents(@Args('userId', { type: () => Int }) userId: number) {
return this.attendeeDashboardService.getUserEvents(userId);
}
}
```
**attendee-dashboard.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { AttendeeDashboardService } from './attendee-dashboard.service';
import { AttendeeDashboardResolver } from './attendee-dashboard.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [AttendeeDashboardService, AttendeeDashboardResolver],
})
export class AttendeeDashboardModule {}
```
### Integrate Dashboard Modules in App Module
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
import { EmailModule } from './email/email.module';
import { NotificationModule } from './notification/notification.module';
import { PaymentModule } from './payment/payment.module';
import { AdminDashboardModule } from './admin-dashboard/admin-dashboard.module';
import { OrganizerDashboardModule } from './organizer-dashboard/organizer-dashboard.module';
import { AttendeeDashboardModule } from './attendee-dashboard/attendee-dashboard.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
EmailModule,
NotificationModule,
PaymentModule,
AdminDashboardModule,
OrganizerDashboardModule,
AttendeeDashboardModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Dashboard Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the dashboard queries:
- **Admin Dashboard**:
```graphql
query {
getSystemMetrics {
userCount
eventCount
ticketSales
}
getUsers {
id
email
role
}
getEvents {
id
title
date
organizer {
id
name
}
}
}
```
- **Organizer Dashboard**:
```graphql
query {
getOrganizerEvents(organizerId: 1) {
id
title
tickets {
id
type
price
quantity
}
purchases {
id
user {
id
email
}
quantity
totalPrice
}
}
getEventAttendees(eventId: 1) {
id
user {
id
email
}
quantity
totalPrice
}
}
```
- **Attendee Dashboard**:
```graphql
query {
getUserEvents(userId: 1) {
id
event {
id
title
date
}
ticket {
type
price
}
quantity
totalPrice
}
}
```
This setup provides the necessary backend functionality for the different dashboards (Admin, Organizer, and Attendee). You can further extend these functionalities as needed.
To implement analytics for event performance and user behavior, we'll create dedicated services and resolvers to handle these tasks. These services will fetch and process data from the database to provide insights.
### Event Analytics
**event-analytics.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class EventAnalyticsService {
constructor(private readonly prisma: PrismaService) {}
async getEventPerformance(eventId: number) {
const ticketSales = await this.prisma.ticketPurchase.aggregate({
_sum: { totalPrice: true },
_count: { id: true },
where: { eventId },
});
const attendeeDemographics = await this.prisma.user.findMany({
where: {
tickets: {
some: { eventId },
},
},
select: {
age: true,
gender: true,
location: true,
},
});
return { ticketSales, attendeeDemographics };
}
}
```
**event-analytics.resolver.ts**
```typescript
import { Resolver, Query, Args, Int } from '@nestjs/graphql';
import { EventAnalyticsService } from './event-analytics.service';
@Resolver()
export class EventAnalyticsResolver {
constructor(private readonly eventAnalyticsService: EventAnalyticsService) {}
@Query(() => String)
async getEventPerformance(@Args('eventId', { type: () => Int }) eventId: number) {
return this.eventAnalyticsService.getEventPerformance(eventId);
}
}
```
**event-analytics.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { EventAnalyticsService } from './event-analytics.service';
import { EventAnalyticsResolver } from './event-analytics.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [EventAnalyticsService, EventAnalyticsResolver],
})
export class EventAnalyticsModule {}
```
### User Analytics
**user-analytics.service.ts**
```typescript
import { Injectable } from '@nestjs/common';
import { PrismaService } from '../prisma/prisma.service';
@Injectable()
export class UserAnalyticsService {
constructor(private readonly prisma: PrismaService) {}
async getUserEngagement(userId: number) {
const eventsAttended = await this.prisma.ticketPurchase.count({
where: { userId },
});
const totalSpent = await this.prisma.ticketPurchase.aggregate({
_sum: { totalPrice: true },
where: { userId },
});
return { eventsAttended, totalSpent };
}
async getUserBehavior() {
const activeUsers = await this.prisma.user.findMany({
where: {
tickets: {
some: {},
},
},
select: {
id: true,
email: true,
tickets: {
select: { eventId: true },
},
},
});
const userDemographics = await this.prisma.user.groupBy({
by: ['age', 'gender', 'location'],
_count: { id: true },
});
return { activeUsers, userDemographics };
}
}
```
**user-analytics.resolver.ts**
```typescript
import { Resolver, Query, Args, Int } from '@nestjs/graphql';
import { UserAnalyticsService } from './user-analytics.service';
@Resolver()
export class UserAnalyticsResolver {
constructor(private readonly userAnalyticsService: UserAnalyticsService) {}
@Query(() => String)
async getUserEngagement(@Args('userId', { type: () => Int }) userId: number) {
return this.userAnalyticsService.getUserEngagement(userId);
}
@Query(() => String)
async getUserBehavior() {
return this.userAnalyticsService.getUserBehavior();
}
}
```
**user-analytics.module.ts**
```typescript
import { Module } from '@nestjs/common';
import { UserAnalyticsService } from './user-analytics.service';
import { UserAnalyticsResolver } from './user-analytics.resolver';
import { PrismaModule } from '../prisma/prisma.module';
@Module({
imports: [PrismaModule],
providers: [UserAnalyticsService, UserAnalyticsResolver],
})
export class UserAnalyticsModule {}
```
### Integrate Analytics Modules in App Module
Update `app.module.ts`:
```typescript
import { Module } from '@nestjs/common';
import { AuthModule } from './auth/auth.module';
import { PrismaModule } from './prisma/prisma.module';
import { GraphqlModule } from './graphql/graphql.module';
import { EventsModule } from './events/events.module';
import { TicketsModule } from './tickets/tickets.module';
import { EmailModule } from './email/email.module';
import { NotificationModule } from './notification/notification.module';
import { PaymentModule } from './payment/payment.module';
import { AdminDashboardModule } from './admin-dashboard/admin-dashboard.module';
import { OrganizerDashboardModule } from './organizer-dashboard/organizer-dashboard.module';
import { AttendeeDashboardModule } from './attendee-dashboard/attendee-dashboard.module';
import { EventAnalyticsModule } from './event-analytics/event-analytics.module';
import { UserAnalyticsModule } from './user-analytics/user-analytics.module';
@Module({
imports: [
AuthModule,
PrismaModule,
GraphqlModule,
EventsModule,
TicketsModule,
EmailModule,
NotificationModule,
PaymentModule,
AdminDashboardModule,
OrganizerDashboardModule,
AttendeeDashboardModule,
EventAnalyticsModule,
UserAnalyticsModule,
],
})
export class AppModule {}
```
### Running the Backend
1. **Start the NestJS Server**
```bash
npm run start:dev
```
2. **Test Analytics Functionality**
Access the GraphQL playground at `http://localhost:3000/graphql` and test the analytics queries:
- **Event Analytics**:
```graphql
query {
getEventPerformance(eventId: 1) {
ticketSales {
_sum {
totalPrice
}
_count {
id
}
}
attendeeDemographics {
age
gender
location
}
}
}
```
- **User Analytics**:
```graphql
query {
getUserEngagement(userId: 1) {
eventsAttended
totalSpent {
_sum {
totalPrice
}
}
}
getUserBehavior {
activeUsers {
id
email
tickets {
eventId
}
}
userDemographics {
age
gender
location
_count {
id
}
}
}
}
```
This setup provides the necessary backend functionality for event performance and user behavior analytics. You can further extend these functionalities as needed.
Disclaimer: This content is generated by AI. | nadim_ch0wdhury |
|
1,912,310 | Real-time Data Updates with Server-Sent Events (SSE) in Node.js | In this blog post, we'll explore how to use Server-Sent Events (SSE) to push real-time data from a... | 0 | 2024-07-05T06:15:46 | https://dev.to/franklinthaker/real-time-data-with-server-sent-events-sse-in-nodejs-4hba | javascript, pubsub, eventdriven, node | In this blog post, we'll explore how to use Server-Sent Events (SSE) to push real-time data from a server to clients. We'll create a simple example using Node.js and Express to demonstrate how SSE works.
## **What are Server-Sent Events (SSE)?**
Server-Sent Events (SSE) allow servers to push updates to the client over a single, long-lived HTTP connection. Unlike WebSockets, SSE is a unidirectional protocol where updates flow from server to client. This makes SSE ideal for live data feeds like news updates, stock prices, or notifications.
## **Creating the Server**
```
// app.js
const express = require("express");
const app = express();
const { v4 } = require("uuid");
let clients = [];
app.use(express.json());
app.use(express.static("./public"));
function sendDataToAllClients() {
const value_to_send_to_all_clients = Math.floor(Math.random() * 1000) + 1;
clients.forEach((client) =>
client.response.write("data: " + value_to_send_to_all_clients + "\n\n")
);
}
app.get("/subscribe", async (req, res) => {
const clients_id = v4();
const headers = {
"Content-Type": "text/event-stream",
"Cache-Control": "no-cache",
Connection: "keep-alive",
};
res.writeHead(200, headers);
clients.push({ id: clients_id, response: res });
// Close the connection when the client disconnects
req.on("close", () => {
clients = clients.filter((c) => c.id !== clients_id);
console.log(`${clients_id} Connection closed`);
res.end("OK");
});
});
app.get("/data", (req, res) => {
sendDataToAllClients();
res.send("Data sent to all subscribed clients.");
});
app.listen(80, () => {
console.log("Server is running on port 80");
});
```
## **Code Explanation**
- **Express Setup:** We create an Express app and set up JSON parsing and static file serving.
- **Client Management:** We maintain a list of connected clients.
- **SSE Headers:** In the /subscribe endpoint, we set the necessary headers to establish an SSE connection.
- **Send Data:** The **sendDataToAllClients** function sends random data to all subscribed clients.
- **Subscribe Endpoint:** Clients connect to this endpoint to receive real-time updates.
- **Data Endpoint:** This endpoint triggers the **sendDataToAllClients** function to send data.
## **Creating the Client**
Next, let's create a simple HTML page to subscribe to the server and display the real-time data.
```
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>SSE - Example (Server-Sent-Events)</title>
</head>
<body>
<div id="data"></div>
</body>
</html>
<script>
const subscription = new EventSource("/subscribe");
// Default events
subscription.addEventListener("open", () => {
console.log("Connection opened");
});
subscription.addEventListener("error", () => {
console.error("Subscription err'd");
subscription.close();
});
subscription.addEventListener("message", (event) => {
console.log("Receive message", event);
document.getElementById("data").innerHTML += `${event.data}<br>`;
});
</script>
```
## Code Explanation
- **EventSource:** We create a new **EventSource** object to subscribe to the /subscribe endpoint.
- **Event Listeners:** We set up listeners for open, error, and message events.
- **Display Data:** When a message is received, we append the data to a div.
## **Running the Example**
1. Start the server:
`node app.js`
2. Open your browser and navigate to http://localhost/subscribe. [Don't close it]
3. Now, open another tab & navigate to http://localhost/data and you should see random data prints onto the screen in other tab.
4. You can subscribe to multiple clients/tabs as you want & you can simply navigate to http://localhost/data & you would see the same data emits to all the subscribed clients.
## **Conclusion**
In this post, we've seen how to use Server-Sent Events (SSE) to push real-time updates from a server to connected clients using Node.js and Express. SSE is a simple yet powerful way to add real-time capabilities to your web applications without the complexity of WebSockets.
## **Warning⚠️:**
When not used over HTTP/2, SSE suffers from a **limitation** to the **maximum number of open connections**, which can be especially painful when opening multiple tabs, as the limit is per browser and is set to a very low number (6). The issue has been marked as "Won't fix" in [Chrome](https://bugs.chromium.org/p/chromium/issues/detail?id=275955) and [Firefox](https://bugzilla.mozilla.org/show_bug.cgi?id=906896). This limit is per browser + domain, which means that you can open 6 SSE connections across all of the tabs to `www.example1.com` and another 6 SSE connections to `www.example2.com` (per [Stackoverflow](https://stackoverflow.com/questions/5195452/websockets-vs-server-sent-events-eventsource/5326159)). When using HTTP/2, the maximum number of simultaneous HTTP streams is negotiated between the server and the client (defaults to 100).
Happy coding!
## **Resources:**
- https://developer.mozilla.org/en-US/docs/Web/API/Server-sent_events/Using_server-sent_events | franklinthaker |
1,911,613 | What Is React Native? – Intro To Top Mobile Framework | React Native: A Powerful Tool For Mobile App Development What is React Native? This is a... | 0 | 2024-07-05T06:15:33 | https://pagepro.co/blog/what-is-react-native/ | reactnative, mobile, leadership | ## React Native: A Powerful Tool For Mobile App Development
What is React Native? This is a question asked by many developers and business owers. According to Statista, there are over **7 billion smartphone users** in 2024. Meanwhile, the number of mobile internet users is reaching 5.4 billion. An average mobile user spends five and a half as much time on apps as on web.
These numbers are growing at a steady rate and there’s no sign that the tendency is about to reverse. This makes the [mobile app](https://pagepro.co/services/mobile-app-development) market a highly competitive place.
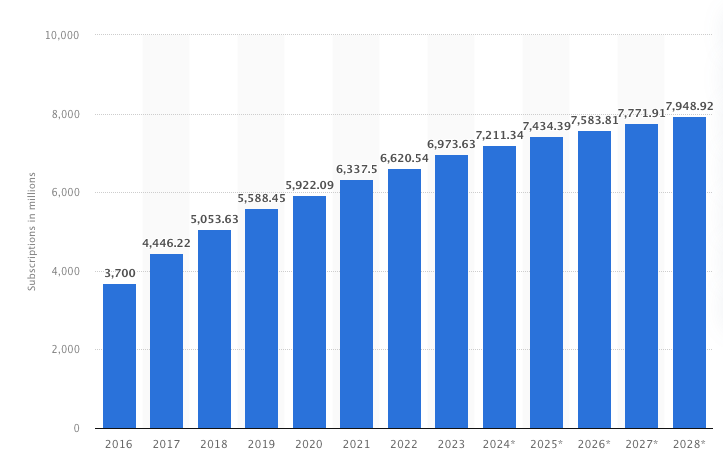
Source: Statista
Companies constantly challenge themselves to deliver better mobile experiences to their users. Among many mobile app development frameworks [React Native](https://pagepro.co/services/react-native-development) is **one of the most popular choices**.
In this comprehensive guide, we will explore:
- What is React Native
- What famous companies are using it
- When and why you should use it for your project
- What are its alternatives
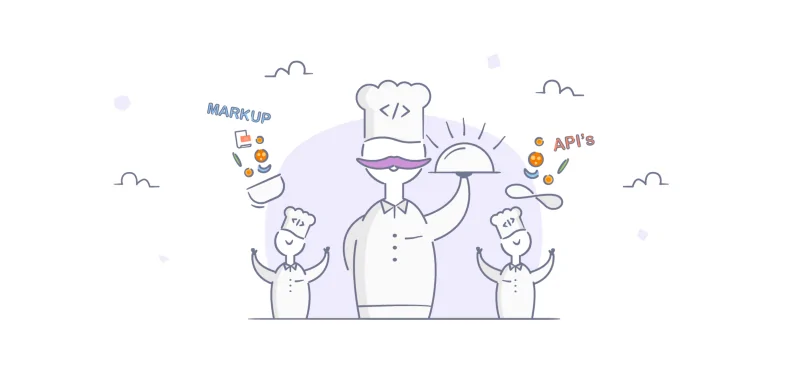
### WHAT IS REACT NATIVE
React Native is a JavaScript framework allowing **fast development of mobile apps** running on both Android and iOS. It was created upon React.js – a component-based framework for [building front-end web applications](https://pagepro.co/services/frontend-development) and user interfaces.
The work on the popular mobile app development framework started during an internal Facebook (Now Meta) hackathon in 2013. React Native was first released to a global audience at the React.js conference at the beginning of 2015.
In March of 2015, it became an open library on GitHub. Due to its **ability to develop UIs and native apps quickly** it took the mobile app world by a storm
React Native has proved **very successful**. The framework managed to beat the interest in classic native development and popularise the concept of cross-platform app development.
### What is cross-platform mobile development?
[Cross-platform mobile development](https://pagepro.co/services/mobile-app-development) is **cheaper and more convenient** alternative to native development. It allows you to create a mobile app for iOS and Android with **one codebase**.
React Native is considered to be one of the best cross-platform mobile development options. It lets you cut the time and costs of development nearly in half by producing apps that can run both on Android and iOS.
###React Native MVP
React Native is also a great idea for building the [Minimum Valuable Product (MVP)](https://pagepro.co/services/mvp-development) mostly because of its **super-fast and efficient development process**, as well as serving both iOS and Android on **one database**.
It delivers native-like experiences and adapts to unique needs with native modules. JavaScript expertise translates well, making it **ideal for launching your app and gathering user feedback fast**.
As an [experienced React Native app development company](https://pagepro.co/services/react-native-development), we’ve worked on many MVPs. For Payhip, a web-only platform, slow sales notifications and limited access to revenue data for sellers on their web platform were major hurdles to overcome.
Luckily, we provided them with a perfect solution – [a mobile app MVP built with React Native and Expo](https://pagepro.co/case-studies/payhip).
Watch our step-by step video on [how to create an MVP](https://www.youtube.com/watch?v=3cTCnopAvQA).
Learn more about [how to build your MVP](https://pagepro.co/blog/how-to-build-mvp/).
### How React Native works?
Just as in [React.js development](https://pagepro.co/services/reactjs-development), React Native applications are written with a combination of **JavaScript** and **JSX**, an **XML markdown language** for developing UIs which replace **HTML** and **CSS**. From JSX, the components are composed of native components. Dedicated to specific platforms, they can **create a fast and natural experience for app users**.
Then, it invokes the native rendering APIs in **Java, for Android, and Objective-C for iOS**. It also exposes JavaScript interfaces for platform APIs, which allows RN apps to access features like GPS location.
## What Are Popular React Native Apps (That Aren't Facebook)
Did you know React Native is used in some of your favourite apps? Let’s explore some examples of well-known companies that use this framework to create their mobile apps.
### Instagram
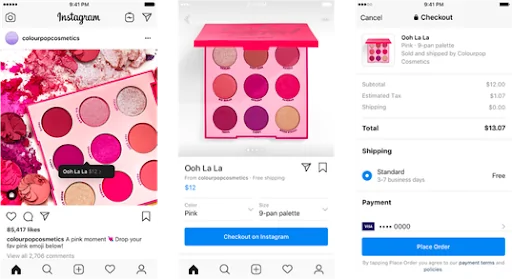
Source: TheQuint.com
In 2016 Instagram dev team began exploring the possibilities of React Native development. First, they tested the waters with a simple view of Push Notifications. When the results met their expectations, the company continued developing other parts of the app with the framework
This allowed Instagram’s devs to deliver features to both Android and iOS versions of the app much, much faster. Now, from **85% to 99%** of the code is shared between Android and iOS versions of the app.
### Tesla
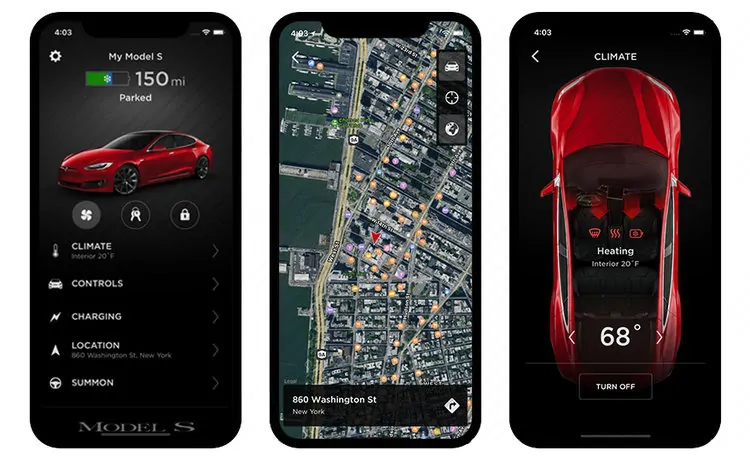
Source: SimplyTechnologies.net
Tesla leverages React Native to power their apps for the self-driving cars and Powerwall. This ensures a seamless and consistent user experience across both Android and iOS devices, allowing Tesla owners to control their vehicles’ features – lights, locks, roof, charger, and more – from **a single, familiar app**.
### Skype
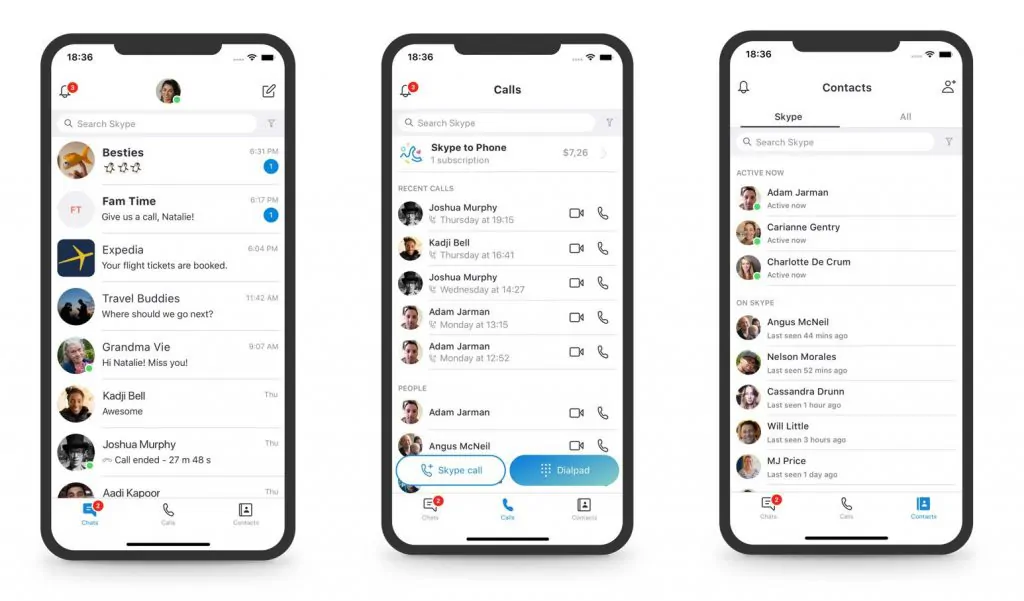
Source: Tabletowo.pl
Before switching to React Native, Skype native apps suffered from several issues, such as losing the speed of the app while sharing GIFs and other media.
In 2017 the company started working on a brand new application, which would be written completely in React Native, allowing the team to include new features.
Moreover, Microsoft decided to use React Native for the Windows desktop app, showing its **possibilities go beyond mobile app development**.
### Pinterest
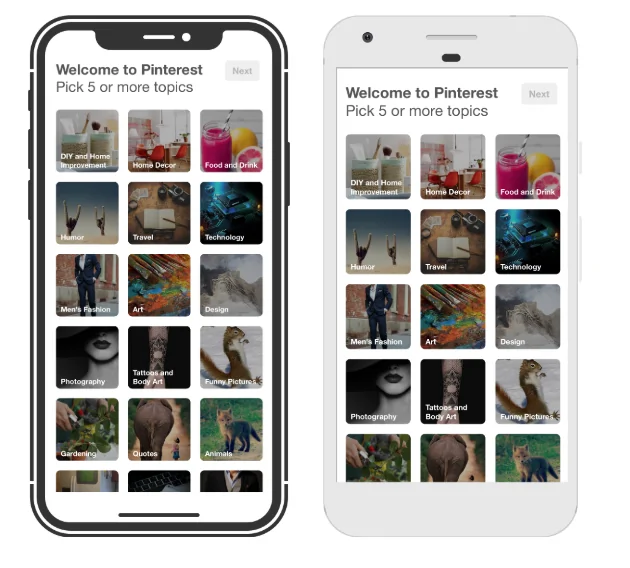
Source: Medium.com
Intrigued by React Native’s growth since 2015, a small Pinterest engineering team began exploring its potential last year to see if it could benefit their platform.
Internal testing kicked off with optimized prototypes focusing on a key onboarding screen – the Topic Picker – to assess React Native’s performance and iterate UI quickly.
The initial implementation on iOS took the Pinterest team [a record-breaking time of 10 days](https://medium.com/pinterest-engineering/supporting-react-native-at-pinterest-f8c2233f90e6).
### Bloomberg
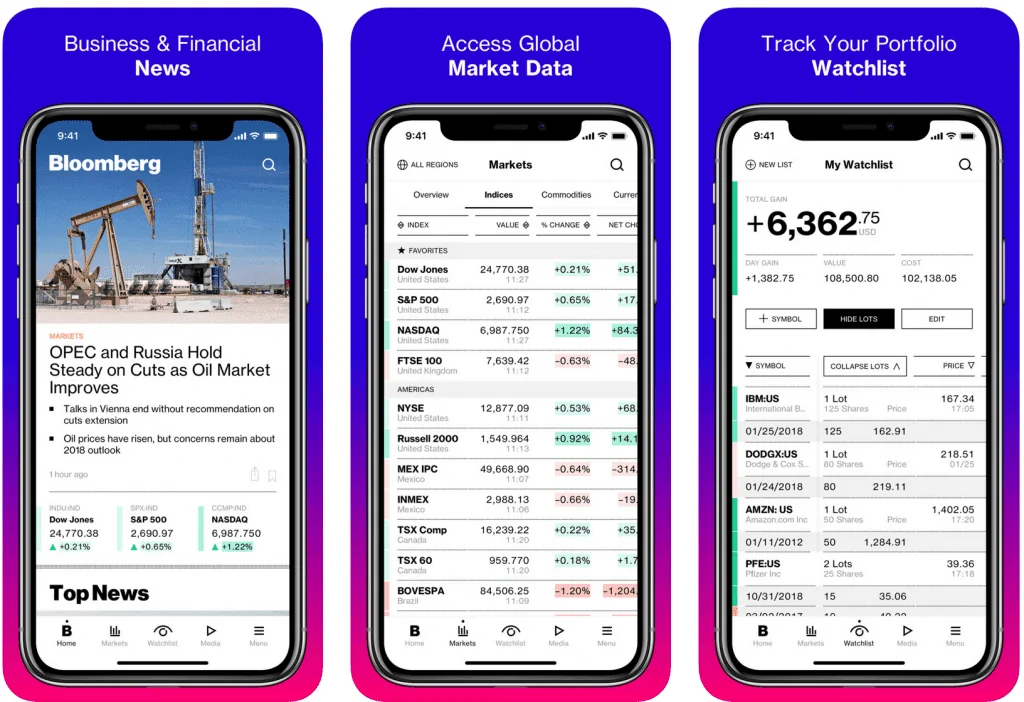
Source: Wiredelta.com
The development team at Bloomberg decided to move their entire product to cross-platform development technology. Before, the devs used to build their apps with native technologies like Java.
Their brand new consumer mobile app for both iOS and Android offers users a smooth, interactive experience letting them easily access personalised content across Bloomberg’s Media.
Another thing they loved about React Native is its automated code refreshments which speed up the process of the new feature release. [According to their website](https://www.bloomberg.com/company/stories/bloomberg-used-react-native-develop-new-consumer-app/), Bloomberg’s development took **only half the time they’d need otherwise** to create the app thanks to React Native.
### 113 more examples of React Native Apps
And that’s not all of it. **React Native is used by many Fortune 500 companies and startups**. Some other names would be **Walmart, Wix.com, Airbnb, Soundcloud Pulse**, and many others.
If you’d like to learn more about the possibilities provided by React Native [explore our list of over 113 real-world apps](https://pagepro.co/ebook/ebook-react-native-apps)! This list includes downloadable apps built with React Native, **including their ranking in the App Store**.
Whether you’re a seasoned developer or just getting started, these examples can inspire you and provide valuable insights.
## React Native Pros and Cons
Like any other framework, React Native has its [pros and cons](https://pagepro.co/blog/react-native-pros-and-cons/). Although the benefits radically outnumber the potential risks, let’s take a look at both.
## React Native Pros
### From A Business Owner's Perspective:
#### Time & Cost-saving
**Cross-platform mobile development:** React Native allows the use of the same code for developing both Android and iOS apps instead of native code for each.
Since you only need to write the code once, there’s no need to hire a bunch of engineers specialising in different languages – **all you need is a JavaScript developer** familiar with native UI libraries, APIs, and hybrid mobile app development.
**Cost-effective and time saving:** A single React Native team, reusable code, and a supported library mean **saving time and money**. It’s one of the biggest advantages of React Native for startups and young businesses who are careful about their budget.
Learn more about [how React Native can cut your development costs](https://pagepro.co/blog/how-react-native-can-cut-your-development-costs/).
**Live updates:** React Native solves the eternal iOS development problem – waiting for the App Store to approve any updates.
As long as your app has access to the server to check for a new version, you can publish [updates to your app](https://pagepro.co/blog/ota-updates-with-expo/) whenever you want, like in a web application.
**Extended code sharing:** With React Native you can share code not only between mobile platforms but also in a web browser. Just imagine, **all of your channels are covered by one shared codebase**. Isn’t it great?
Expand your knowledge of the capabilities of React Native on web platforms by reading our guide [React Native for Web](https://pagepro.co/blog/react-native-for-web/).
#### UX & Performance
**Great user experience:** A mobile application built with this framework results in a **highly responsive User Interface**, which guarantees a five-star user experience.
**Great performance:** By using a single codebase that translates to both Android and iOS, React Native allows developers to build apps that **work on both platforms while maintaining good performance**.
For example, [the Xbox team switched from Electron to React Native UWP](https://www.windowscentral.com/xbox-app-pc-gets-speed-boost-ditching-electron-react-native-uwp) for their Xbox app beta for Windows 10, which resulted in a better native experience on Windows, **decreased memory usage** (by over 50%), **increased performance**, and a **decrease in app installation size** – from nearly 300MB to 60MB.
**Stable future:** Meta (Facebook) is considered a **stable enterprise** and has stood by its framework, supporting and growing it. React Native is open source, meaning any react native developer can help improve it.
###From A Developer's Perspective:
**Pre-developed components:** React Native is widely **supported by a great community**, allowing you to find plenty of pre-made assets to utilise in your project, **shortening the release times**.
**Easy learning curve:** Many frameworks require you to learn a number of rules applicable only to that technology. React Native is **easily readable**, which is one of its greatest strengths.
**One ecosystem:** One of the advantages of cross-platform development is that a developer can build **a versatile application** with React Native without dealing with the ecosystem and language specifics of each OS.
**Third-party support:** **React Native is an open-source project**, so it comes with an open environment. You can integrate various third-party modules with ease.
**Great developer experience:** Real Native has a great development environment that will make building apps **easy and enjoyable**, from Chrome developer tools to [flexbox](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_flexible_box_layout/Basic_concepts_of_flexbox), among many other facilitations.
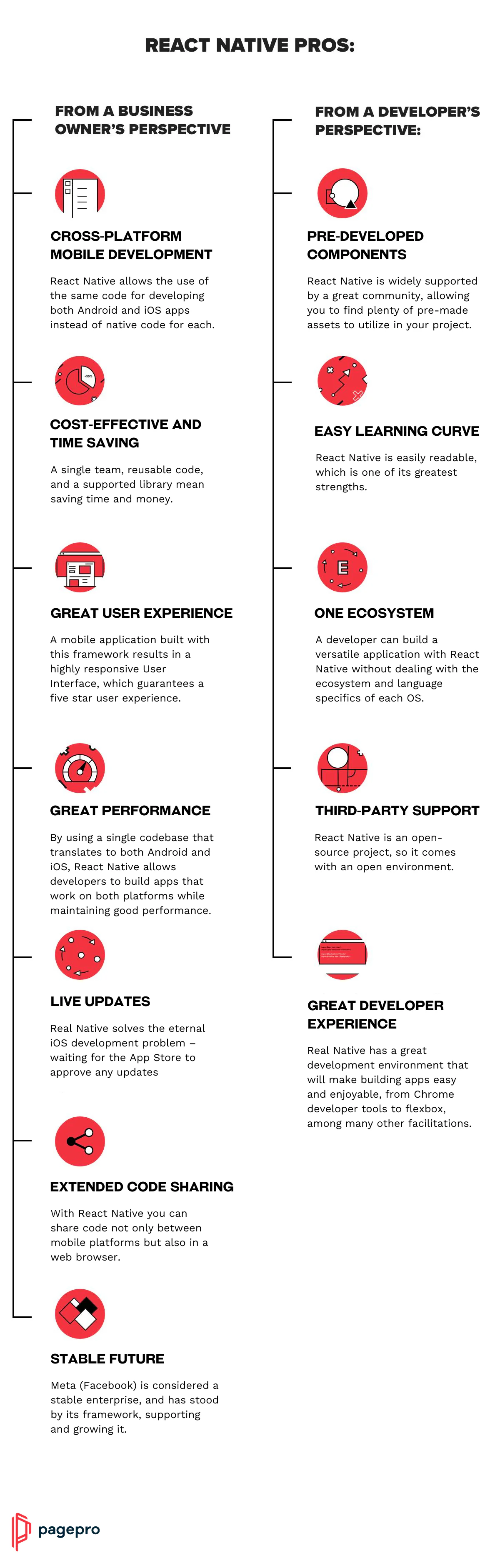
## React Native Cons
### From A Business Owner's Perspective:
**Increased app size:** While React Native offers faster development, its **apps can be larger** due to bundled JavaScript and external libraries for native features.
This **can affect downloads**, but code optimization and selective libraries can alleviate size concerns.
**You may still need help from a native developer:** The implementation of some more advanced features typical for native apps **may still require support from a dedicated Android and iOS developer**. This may be an issue for teams with limited project budgets.
###From A Developer's Perspective:
**Debugging:** As React Native brings another layer to your project, it **can make debugging harder** and more problematic.
**Configuration:** It’s possible that local library coordination inside a React Native app will **require plenty of configurations**, so consider allocating some extra time to account for that.
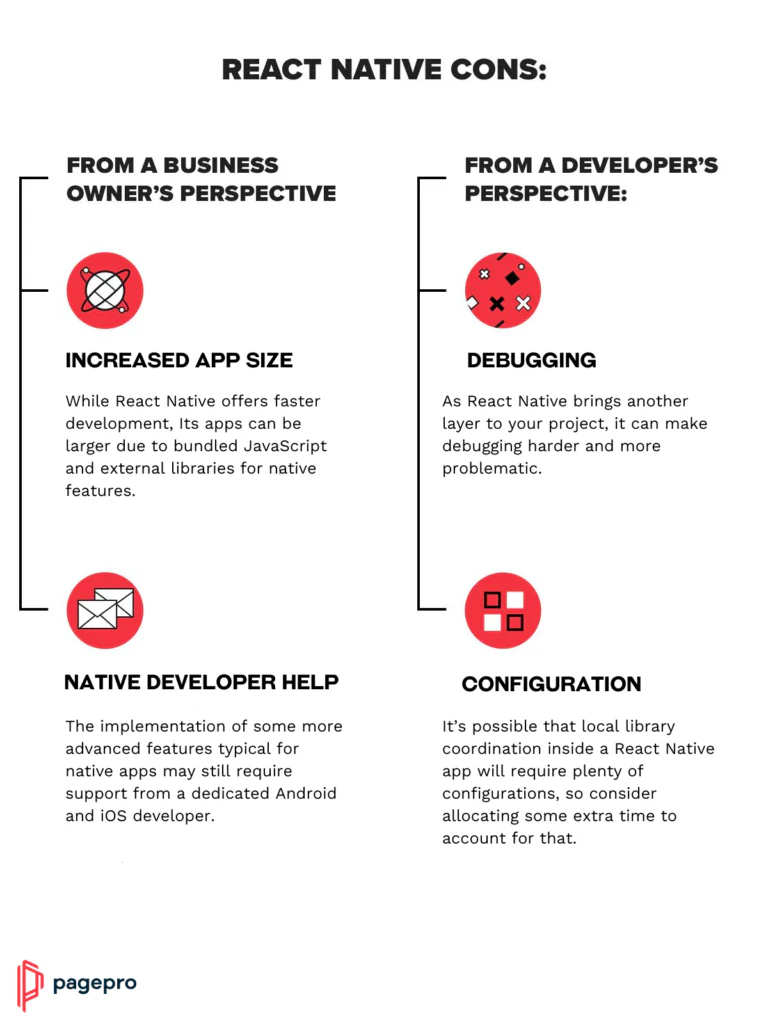
Despite potential **size considerations** and occasional native development needs, React Native’s benefits like faster development and live updates **make it a compelling choice**.
## When Should You Choose React Native For Your App?
To make the most of your development process, choosing the optimal technology is crucial. Here’s where React Native shines:
- When you want **a stable and fast solution** that performs just like a native app
- If you want to build an app that will work on various operating systems but **don’t want to support two or more separate development teams**
- When your app is all about the **User Interface**
- When you are currently working on a web app, but **consider creating a mobile app** someday in the future
##What Is The Future Of React Native?
With a thriving community of over **[2,600 contributors](https://github.com/facebook/react-native)**, React Native’s development is constantly moving forward and the best is yet to come!
In recent months, the team has provided frequent updates, including the release of **React Native 0.74** in April 2024. This release brought new, exciting features, which further improved the framework.
###React Native 0.74
**Yoga 3.0 Integration:** This improved the layout engine for more predictable styling and includes new properties like position: static and align-content: space-evenly.
It also enhanced the overall correctness of layouts and distributed Yoga’s JavaScript bindings as an ES Module, improving stability and integration with modern JavaScript projects.
**Bridgeless New Architecture:** Now set as the default when the New Architecture is enabled, this feature removes the need for the old bridge system that communicated between JavaScript and native modules.
This boosts performance by eliminating the serialisation and deserialization process.
**Batched onLayout Updates:** By reducing the number of re-renders required during layout changes by batching updates, this change allows for smoother performance and less computational overhead during rendering.
**Yarn 3 as Default Package Manager:** For new projects, Yarn 3 replaces Yarn Classic, providing faster dependency management and optimising how dependencies are stored, which can speed up project setup and updates.
**Android Minimum SDK Bump:** The minimum required SDK for Android has been updated to version 23 (Android 6.0), which can help in optimising compatibility and performance, along with reducing the overall app size on user devices.
**Removal of Deprecated PropTypes:** To streamline the framework and reduce memory overhead, React Native 0.74 has removed all built-in PropTypes that have been deprecated since React 15.5.
**API Changes to `PushNotificationIOS`:** As React Native prepares for the removal of this library in a future update, changes have been made to align with modern iOS notification frameworks and improve the handling and scheduling of notifications.
## What Are React Native Alternatives?
In this part of the guide, we will quickly present some of the most popular alternatives to React Native.
### Flutter
Flutter is a mobile development framework created by Google. It uses Dart programming language that supports most of the object-oriented concepts.
While it **doesn’t have a community or popularity the size of React Native’s**, it can provide **great performance**. Moreover, it has excellent build automation tooling. Flutter allows easy and painless setup on CI/CD servers, thanks to its **strong CLI tools**.
+ Great developer tools
+ Easy to learn Dart language
– Small community
Learn more about [React Native vs Flutter](https://pagepro.co/blog/react-native-vs-flutter-which-is-better-for-cross-platform-app/)
### Ionic
Ionic was created in 2013 as an open-source software development kit for hybrid mobile applications. Since then over 5 million apps were built using the framework.
It’s famous for **providing platform-specific UI elements** with its library of native components for Android and iOS. By using HTML, CSS, JavaScript, and Angular for app development it allows creating cross-platform mobile apps with a single codebase.
Ionic has a **large community** of active users who contribute to it on a regular basis.
+ Cordova native plugins
+ Active community
– Sometimes gets buggy
Learn more about [React Native Vs Ionic And Cordova](https://pagepro.co/blog/react-native-vs-ionic-and-cordova-comparison/)
### NativeScript
NativeScript is an open-source framework for building native apps.
Known for its **elasticity**, Native Scrip givies you plenty of choice. You can code a NativeScript app in JavaScript, TypeScript, Angular or go for Vue.js. You can make use of any components, classes and functions of the framework of your choice.
NativeScript has a **vibrant community** and **frequent releases** of new plugins that add new possibilities. What’s more, the NativeScript team released many great tools like [NativeScript Playground](https://preview.nativescript.org/), [NativeScript Marketplace](https://market.nativescript.org/), and [NativeScript Sidekick](https://github.com/ProgressNS/sidekick-feedback).
+ Works great with Vue.js
+ Also great with Angular
– Long startup times with Angular for Android
Learn more about [React Native Vs Nativescript](https://pagepro.co/blog/react-native-nativescript-comparison/)
## Summing Up
**[React Native](https://pagepro.co/blog/react-native-faq/) streamlines mobile app development**, allowing engineers to code once and deploy across iOS and Android. This means significant **cost savings** for businesses by reducing development time and resources needed for each platform.
Additionally, React Native’s efficient code sharing **fosters faster development cycles** and **easier maintenance**, further lowering costs.
React Native has a **rich library of pre-built components**, which speeds up the development process and ensures consistency. This is an advantage for companies aiming to deliver high-quality mobile apps efficiently, and without sacrificing user experience.
Thanks to its open-source nature, the React Native community is robust, offering **plenty of support** for cross-platform application development.
Popular companies like **Instagram and Pinterest** leverage React Native’s power to create **a feature-rich mobile experiences**. Consider it for your next versatile mobile app.
## Sources
[Statista - Number of smartphone mobile network subscriptions worldwide from 2016 to 2023, with forecasts from 2023 to 2028](https://www.statista.com/statistics/330695/number-of-smartphone-users-worldwide/)
[Statista - Number of internet and social media users worldwide as of April 2024](https://www.statista.com/statistics/617136/digital-population-worldwide/)
[SmartInsights - 2024 Mobile marketing statistics compilation](https://www.smartinsights.com/mobile-marketing/mobile-marketing-analytics/mobile-marketing-statistics/)
| itschrislojniewski |
1,912,309 | The Future of Generative AI: Possibilities, Challenges, and What Lies Ahead | The specter of AI domination has haunted us since "The Terminator" (or perhaps even earlier). The... | 0 | 2024-07-05T06:13:32 | https://dev.to/vikas_brilworks/the-future-of-generative-ai-possibilities-challenges-and-what-lies-ahead-1cam | generativeai | ##
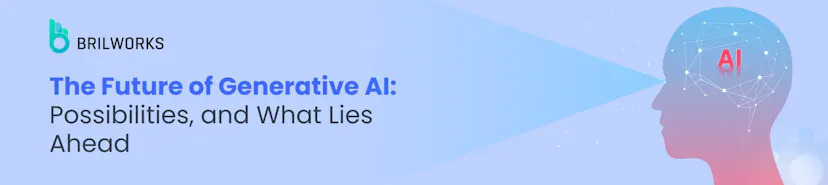
The specter of AI domination has haunted us since "The Terminator" (or perhaps even earlier). The evolution of AI goes back to the 1950s. Many of you may now know about its past, but what about its future?
Recent advancements in generative AI, including the development of Generative Adversarial Networks and transformers, have spurred a wave of innovation. These technologies work behind the scenes to help today’s model generate content on par with humans.
In the year 2022, ChatGPT took the internet by storm. It’s been more than a year since the ChatGPT launched and showed the potential of generative AI in business. Since then, business leaders have been busy finding use cases of generative AI in business and testing this technology across different operations.
Basic generative AI modals like ChatGPT and Bard are becoming more powerful and transforming into multimodal tools. The development of smaller LLMs is on the rise. Small businesses are rushing to integrate AI capabilities into their existing digital infrastructure.
Large organizations are moving beyond general-purpose AI applications by developing custom AI solutions. Gartner predicts that by 2027, the adoption of tailored GenAI models within large enterprises will grow from 1% to 50%.
Many business leaders who are unaware of this technology have begun experimenting with it for smaller use cases. This indicates that this technology will take center stage in the coming years, but with this power comes some ethical concerns that we cannot avoid.
In light of these developments, it is crucial to understand the future of generative AI. To truly understand it, we need to explore its ethical concerns, implementation challenges, limitations, and anticipated advancements.
It is not only affecting work related to facts and numbers but creativity and the artist's spirit as well, this is where AI-driven creativity comes into question. So, how will AI affect our daily lives? Should we run from it or adopt it? Questions and opinions are endless. Whatever the outcome is, it is definitely affecting our lives in one way or another. Let’s fast forward and see what the future holds for us and our friend AI.
## What is Generative AI Technology?
Generative AI is a technology that generates various kinds of content. The AI models can interpret human language and respond accordingly.
creates content, including images, text, audio, video, and other types of data. The AI models run on generative AI and can interpret human language, take text, video, audio, and other types of data, and provide results. They can generate original content.
## Here are the most popular applications of generative AI:
Marketing
You have a lot of customer data; generative AI can help. This technology excels at analyzing a massive amount of data. In your case, it could be customer data and preferences. AI can process this data at a blazing-fast speed and in record time, helping you create targeted marketing messages.
You can leverage generative AI for content creation as it can write compelling product descriptions, ad copy, social media content, and variations to test and optimize content and website landing pages for maximum conversion rates.
Note Taking
Generative AI can transcribe audio or video recordings of meetings and then summarize the key points, action items, and decisions taken.
Video generation
Tasks such as video editing and creating special effects are now much easier with the help of AI. The way AI technology is evolving, it will likely be able to generate entire videos from scratch, based on a script or storyboard. This could revolutionize the way videos are produced, making it faster and more affordable.
## Future of Generative AI Technology
## 1. Advancement of Multimodal AI
Multimodal AI is an ML (machine learning) Model that can process and understand information from various sources, not just one. These sources can be text, audio, images, or videos. The purpose of Multimodal AI is to enable machines to interpret and respond to information in a way that mimics human understanding across different channels.
Initially, early generative AI models such as ChatGPT were categorized as unimodal—they could only process one type of data input and generate outputs of the same type. Gradually, Multimodal AI is advancing. Updated versions of ChatGPT and Gemini now have the ability to process and generate outputs from images as well. Though still in the early stages, these developments are Advancing us toward AGI (Artificial General Intelligence).
Multimodal learning is unleashing new possibilities for AI By training on various data types (text, images, audio), AI models can now understand and respond using multiple formats. This means they can take in text and images, and generate outputs that combine text and visuals. Multimodal learning will increase the capabilities of applications of multimodal AI including:
Augmented Generative AI: Multimodal models like GPT-4 turbo, and Google Gemini, come with new possibilities that can improve user experience both at the input and output sides. Furthermore, Virtual assistants like Siri, Alexa, or Google Assistant will better understand voice commands and gestures.
Autonomous Vehicles: Self-driving cars depend significantly on multimodal AI. Equipped with multiple sensors capturing diverse data formats from their surroundings, these vehicles rely on multimodal learning to integrate and process this information efficiently, enabling them to make intelligent decisions in real-time.
However, Multimodal AI faces hurdles. The massive datasets needed, with their variety of formats (text, images, audio), pose challenges in storage, processing, and even data quality. Additionally, teaching AI nuance, like sarcasm in spoken language, and aligning data from different sources (e.g., ensuring a camera image aligns with a specific car's location data) require further development.
## Smaller LLMs
LLMs (Large Language Models) contain gigantic amounts of parameters to make applications of generative AI more accurate and reliable. for example, OpenAI added more and more parameters to their newer versions of ChatGPT. It started with GPT-2 with 1.2 billion and took a massive leap for GPT-3 with 175 billion and as for GPT-4, it is rumored to be more than 1 trillion.
These large language models require massive funds and server space to train and maintain energy-hungry models, which only some giant companies can do like OpenAI and Google or Microsoft. But many recent findings say otherwise.
For example, DeepMind's Chinchilla, an ensemble of large language models with 12 billion parameters, achieved comparable accuracy to GPT-3.5 on specific NLP datasets, despite GPT-3.5 having ten times more parameters. A research paper by Deepmind showed that training smaller models on more data gives better results. The “Quality over Quantity” approach has started to be adopted in the Generative AI technology world.
These smaller models will not only increase performance benchmarks but also help save energy. Sam Altman CEO of Open AI told the audience at an MIT event “I think we're at the end of the era where it's going to be these, like, giant, giant models,”
## 3. Impact on different industries
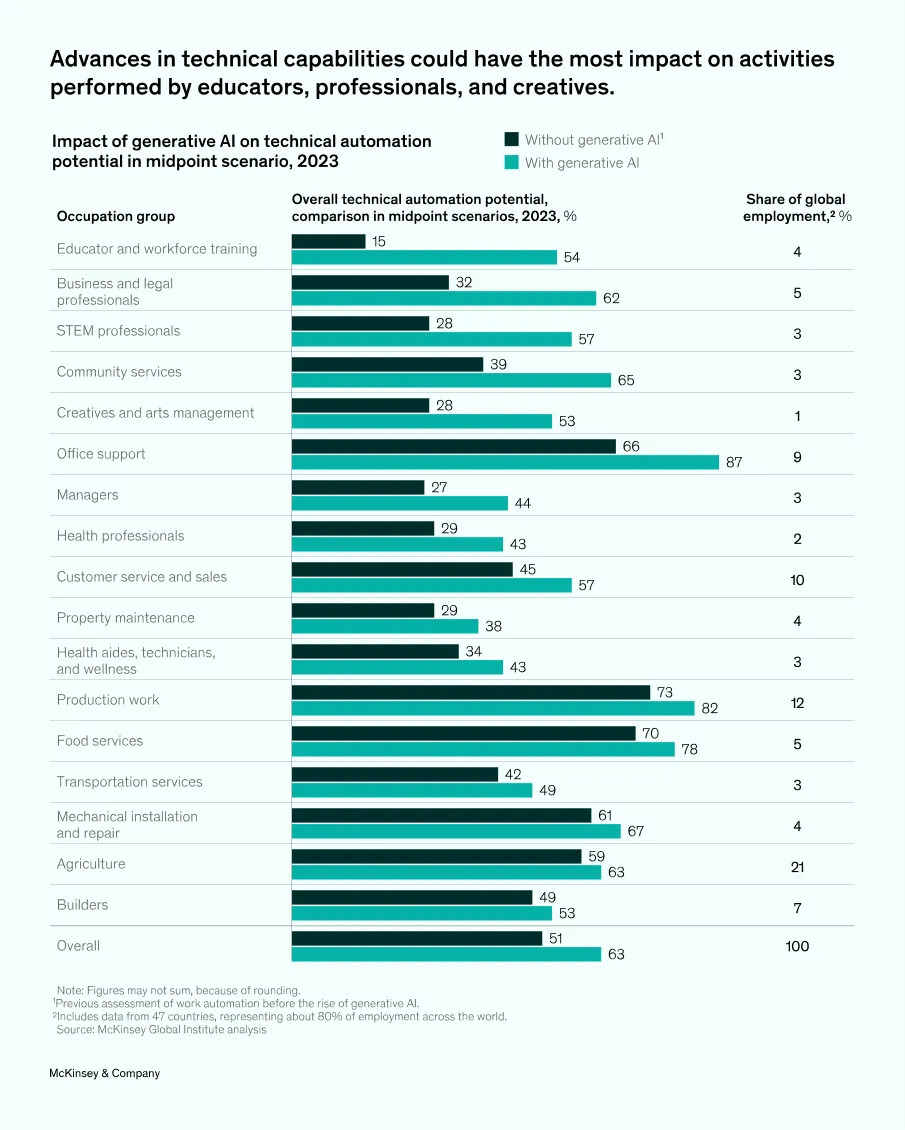
As AI becomes more accurate, efficient, and reliable businesses and individuals may become irresistible from implementing its use in their work. Different industries will start to adopt it as a tool to enhance their work productivity, cost efficiency, and time consumption.
Let’s look at how different industries will be impacted by the future gen AI.
## Healthcare
Generative AI will accelerate diagnosis for the patient as it will analyze medical data (like scans and test results) to identify potential diseases much earlier, potentially saving lives. AI analyzes patient medical history and genetic data to recommend personalized medications and therapies, leading to more effective treatment. AI can analyze vast datasets to accelerate the discovery of new drugs and even predict potential side effects, ensuring safety for human consumption.
## Finance
Generative AI presents a paradigm shift in data analysis, particularly within the financial sector. Its ability to process massive datasets in mere minutes promises to streamline cumbersome numerical tasks. Financial institutions will leverage this technology to generate comprehensive reports, potentially identifying lucrative investment opportunities that may have been previously overlooked. Furthermore, the implementation of AI chatbots within banks signifies a move towards enhanced customer service. These chatbots can efficiently handle account inquiries, and loan applications, and even offer basic financial planning assistance.
## Education
AI is set to change education by offering personalized learning experiences tailored to individual student needs. Through advanced algorithms, AI will analyze students' learning patterns and preferences, allowing for adaptive learning platforms that adjust content and pace accordingly. Virtual tutors powered by AI will provide real-time feedback and support, enhancing traditional teaching methods.
## Ethical Concerns
As with any powerful tool, AI comes with a double-edged sword. While it promises to revolutionize work, making tasks faster and less time-consuming, concerns about its responsible use are mounting. Threats such as deepfakes, which blur the line between reality and fabrication can be used to create life-like content from images to videos such power can be used to spread misinformation and manipulate the public.
Additionally, using AI for content creation can lead to some copyrighted legal battles, especially in the music industry where AI creates music that closely resembles the copyrighted music of the original artist.
Because of these Ethical concerns ongoing dialogue has been taken into account by the government. The current opacity of some AI models raises concerns about accountability and bias. Future AI development will prioritize explainability, allowing humans to understand the reasoning behind AI decisions. AI algorithms can perpetuate societal biases present in
their training data. The future prioritizes identifying and reducing bias in AI for fair treatment. Regulations will be built requiring AI systems to provide clear explanations for their outputs, empowering users to trust and assess the fairness of AI-driven decisions.
## Economic Potential
Generative AI has the potential to boost global GDP by trillions of dollars. Researchers at Mckinsey found that across 63 use cases suggest it could generate an annual value equivalent to $2.6 trillion to $4.4 trillion – eclipsing the entire GDP of the United Kingdom in 2021 ($3.1 trillion). This translates to a 15-40% boost in the overall impact of artificial intelligence.
In specific industries, Generative AI is projected to significantly enhance productivity. In retail and consumer packaged goods, it could boost annual revenues by 1.2% to 2.0%, equating to an additional $400 billion to $660 billion. Similarly, in banking, it is expected to increase productivity by 2.8% to 4.7%, adding $200 billion to $340 billion annually to industry revenues. Meanwhile, Generative AI could contribute from 2.6% to 4.5% of annual revenues in the pharmaceutical and medical-product sectors, translating from $60 billion to $110 billion annually.
## Conclusion
Generative AI holds immense promise for the future across various industries, promising exceptional levels of productivity, efficiency, and innovation. As this technology continues to advance, it will reshape how businesses operate, offering personalized solutions, automating complex tasks, and unleashing better opportunities for growth and development.
Adopting Generative AI represents not just a technological advancement, but a more liberating creative expression towards art. While challenges remain, like ensuring responsible development and addressing potential biases, the vast potential benefits outweigh them. In making sure that Generative AI technology not replacing humans but enhancing human capabilities, we can use this cutting-edge technology for the betterment of society. | vikas_brilworks |
1,912,308 | Mistakes to Steer Clear of During System Integration Testing | System integration testing is essential to the complex world of software development because it... | 0 | 2024-07-05T06:13:28 | https://www.newyorkcomputerhelp.com/mistakes-to-steer-clear-of-during-system-integration-testing/ | system, integration, testing | 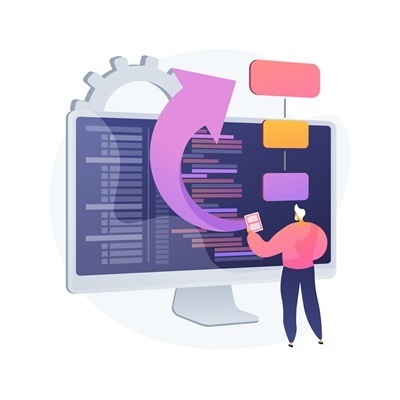
System integration testing is essential to the complex world of software development because it guarantees the smooth functioning and integration of different system components. But even the most thorough testing procedures can be compromised by typical errors that, if ignored, can have serious repercussions. When doing system integration testing in software testing, it is essential to be aware of these potential pitfalls and take proactive steps to avoid them in order to ensure the success of SIT endeavors.
**Neglecting Test Case Prioritization**
In SIT, one of the most frequent errors is not giving test cases the proper priority. Prioritizing the most important features and integration points first is crucial when there is limited time and resources available. Prioritizing test cases too low may cause users to miss high-risk areas, which can lead to expensive flaws and even system failures. Use a risk-based strategy when prioritizing test cases to steer clear of these pitfalls. Prioritize testing efforts by determining which parts in addition to integration points are most important to the overall operation of the system.
**Insufficient Test Data Management**
Inadequate management of test data can seriously impair SIT efficacy. Teams may encounter difficulties faithfully replicating real-world scenarios in the absence of a well-thought-out and thorough approach to test data, which could result in partial or inaccurate test results. Create a solid test data management plan right away to help with this problem. This should involve creating test data that is representative and realistic, protecting the privacy in addition to security of the data, and keeping a central repository for quick access to and upkeep of the data.
**Overlooking Non-Functional Requirements**
Functional testing is obviously important during SIT, but if non-functional requirements are ignored, there could be security risks, scalability problems, and less-than-ideal system performance. Performance, dependability, and security, as well as usability are examples of non-functional factors that are frequently disregarded or assigned a lower priority, leading to systems that may operate as intended but fall short of essential quality standards. Include non-functional testing in SIT strategy from the start to avoid making this error. Create thorough test cases that verify the system’s functionality under different load scenarios, gauge its defenses against possible security breaches and how accessible and user-friendly it is.
**Inadequate Test Environment Management**
The accuracy and dependability of SIT results can be significantly impacted by discrepancies between test environments. The validity of the testing efforts is jeopardized if the test environment is not a true reflection of the production environment, or if there are differences between test environments. Use strong test environment management techniques to reduce this risk. To guarantee uniformity throughout, clearly define policies and processes for establishing, and maintaining, in addition to keeping an eye on test environments. When it’s feasible, automate environment provisioning and configuration procedures to reduce human error and guarantee consistency.
**Insufficient Documentation and Traceability**
The efficacy of SIT and team member collaboration can be severely hampered by inadequate documentation and traceability. It becomes difficult to reproduce problems, comprehend the reasoning behind testing decisions and maintain consistency across testing cycles without thorough documentation of test cases, test data, and test results. Throughout the SIT process, give careful documentation top priority in order to avoid this trap. Provide clear and well-organized documentation of the test cases, test data, test environments, and test outcomes. Create a traceable relationship between test cases and system requirements to guarantee thorough coverage in addition to making impact analysis easier to conduct when changes take place.
**Conclusion**
In order to ensure that different system components are successfully integrated and functional, system integration testing is an essential part of software development. Organizations can expedite system integration testing (SIT) across various applications and systems by utilizing Opkey robust integration platform. Opkey makes it possible to thoroughly validate workflows and data synchronization, guaranteeing smooth communication between vital business solutions. Opkey ensures that the company’s ecosystem functions as a cohesive, integrated whole by automating SIT, which minimizes disruptions and maximizes operational efficiency. It also removes compatibility problems. | rohitbhandari102 |
1,912,307 | Building Flexible UIs: Leveraging React Native's Component Lifecycle Methods for Reusable Components | React Native's component-based architecture allows you to build modular and reusable UI elements. But... | 0 | 2024-07-05T06:11:54 | https://dev.to/epakconsultant/building-flexible-uis-leveraging-react-natives-component-lifecycle-methods-for-reusable-components-28e6 | react | React Native's component-based architecture allows you to build modular and reusable UI elements. But how do you ensure these components efficiently manage their state and behavior throughout their lifecycle? This article explores how React Native's component lifecycle methods empower you to develop reusable and adaptable components.
Understanding Component Lifecycle Methods:
React Native components go through various stages during their creation, update, and destruction. These stages are defined by lifecycle methods that provide hooks for you to execute specific logic at different points in the component's existence. Here are some key lifecycle methods to master:
componentDidMount: This method is invoked immediately after a component is mounted (inserted) into the UI. It's ideal for tasks like fetching data from an API, setting up subscriptions, or initializing third-party libraries.
componentDidUpdate(prevProps, prevState): This method is called whenever a component receives new props or its state changes. It allows you to react to these changes and update the UI accordingly. A common use case is comparing the previous props or state with the new ones to determine if an update is necessary.
componentWillUnmount: This method is called just before a component is removed from the UI. It's used for cleanup tasks like canceling subscriptions, clearing timers, or removing event listeners to prevent memory leaks and unexpected behavior.
[Jumpstart Your App Development Journey with React Native](https://www.amazon.com/dp/B0CRF8S8Z1)
Building Reusable Components with Lifecycle Methods:
Let's see how these lifecycle methods can be leveraged to create reusable components:
Fetching Data on Mount: Imagine a reusable "UserList" component that displays a list of users fetched from an API. You can utilize componentDidMount to fetch the user data and update the component's state upon mounting:
`JavaScript
import React, { useState, useEffect } from 'react';
const UserList = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/users');
const data = await response.json();
setUsers(data);
};
fetchData();
}, []);
// ... render logic using users data
return (
<View>
{/* Display the list of users */}
</View>
);
};
export default UserList;`
In this example, useEffect (a functional component alternative to componentDidMount) fetches data and updates the state within the component upon first render.
Handling Prop Changes: Let's consider a reusable "Button" component that can change its text and onPress behavior based on props. You can leverage componentDidUpdate to handle prop changes and update the button's appearance or functionality accordingly:
`JavaScript
const Button = ({ text, onPress }) => {
useEffect(() => {
// Handle potential side effects based on prop changes (optional)
}, [text, onPress]);
return (
<TouchableOpacity onPress={onPress}>
<Text>{text}</Text>
</TouchableOpacity>
);
};
export default Button;`
Here, useEffect with a dependency array containing text and onPress props ensures the button re-renders and updates its behavior whenever these props change.
Benefits of Utilizing Lifecycle Methods:
- Improved Code Organization: By encapsulating logic within lifecycle methods, you keep your components clean and well-organized. This promotes code readability and maintainability.
- Reusable Components: Lifecycle methods empower you to build components that can adapt to different situations based on props and state changes, leading to truly reusable UI elements.
- Performance Optimization: Conditional logic within lifecycle methods allows you to optimize performance by only updating the UI when necessary.
Beyond the Basics:
React Native offers additional lifecycle methods like shouldComponentUpdate for optimizing re-renders and getDerivedStateFromProps for managing derived state values based on props. As you delve deeper into React Native development, understanding these methods will further enhance your ability to create robust and efficient reusable components.
Conclusion:
By mastering React Native's component lifecycle methods, you can unlock the full potential of creating reusable and modular UI components. These components not only promote cleaner and more maintainable code but also empower you to build dynamic and adaptable user interfaces for your React Native mobile applications. So, leverage the power of lifecycle methods and empower your React Native development journey! | epakconsultant |
1,912,306 | The quotations for different application scenarios of LED display screens are different | At present, the quotations for LED display screens in different application scenarios are different.... | 0 | 2024-07-05T06:10:35 | https://dev.to/sostrondylan/the-quotations-for-different-application-scenarios-of-led-display-screens-are-different-5f8b | display, led, screen | At present, the quotations for [LED display screens](https://sostron.com/products/) in different application scenarios are different. Why is there such a difference? It is mainly related to the size, rental time, model parameters and other related factors of the LED display screen, and of course the entire level is also different.
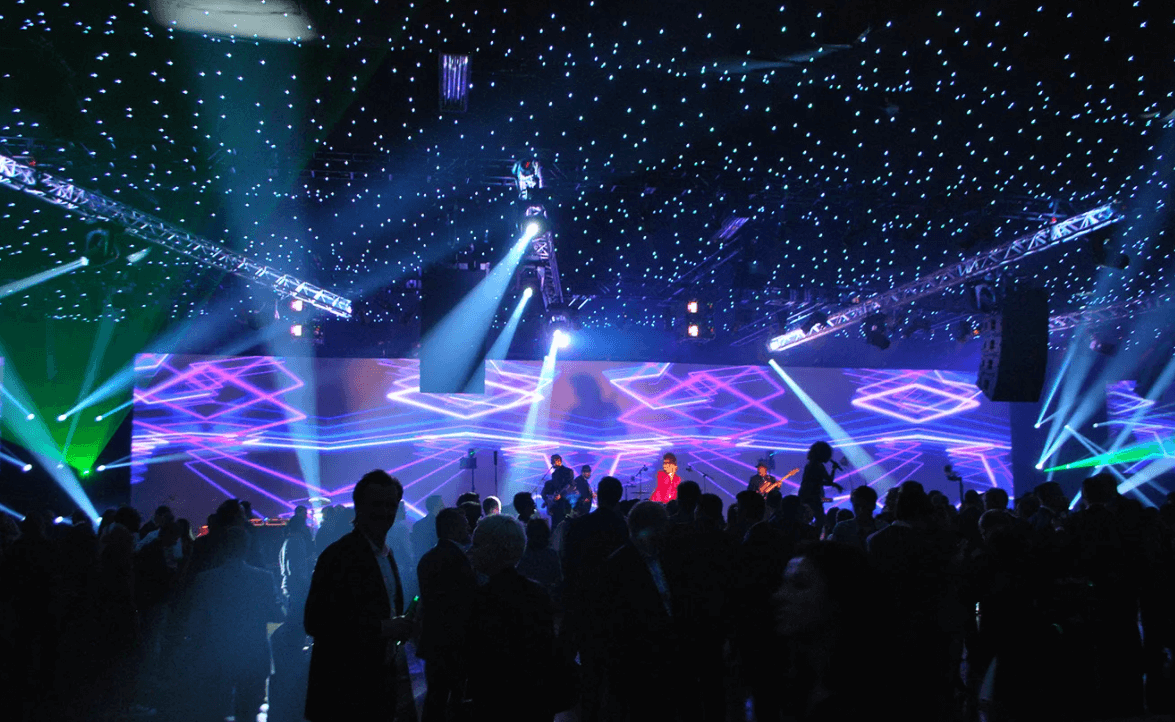
Factors affecting different application scenarios
1. Size and resolution
The larger the size of the LED display screen and the higher the resolution, the more LED lamp beads are required, and the production cost will naturally increase. In addition, ultra-high-definition displays usually use higher-quality LED lamp beads and control systems, and the price will also be higher. [Introduce you the working principle of LED lamp beads. ](https://sostron.com/the-working-principle-of-led-lamp-beads/)
2. Rental time
The length of time for renting an LED display screen is also an important factor affecting the price. Short-term rentals are usually more expensive because they require frequent installation and removal, while long-term rentals can enjoy certain discounts. [Provide you with a guide to calculating the rental price of LED display screens. ](https://sostron.com/led-screen-rental-price-calculation-guide/)
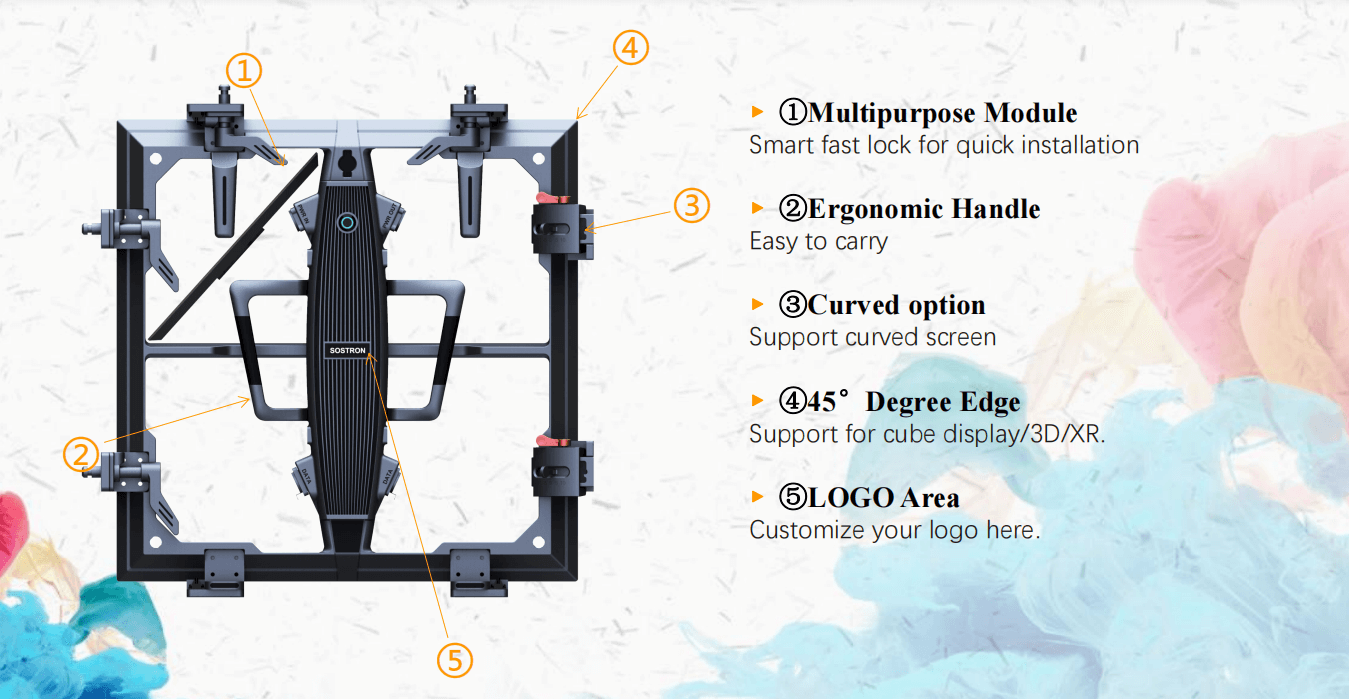
3. Model parameters
Different models of LED display screens differ in brightness, refresh rate, color performance, etc. High-end models usually have higher brightness, faster refresh rate and better color performance, so the price will be higher.
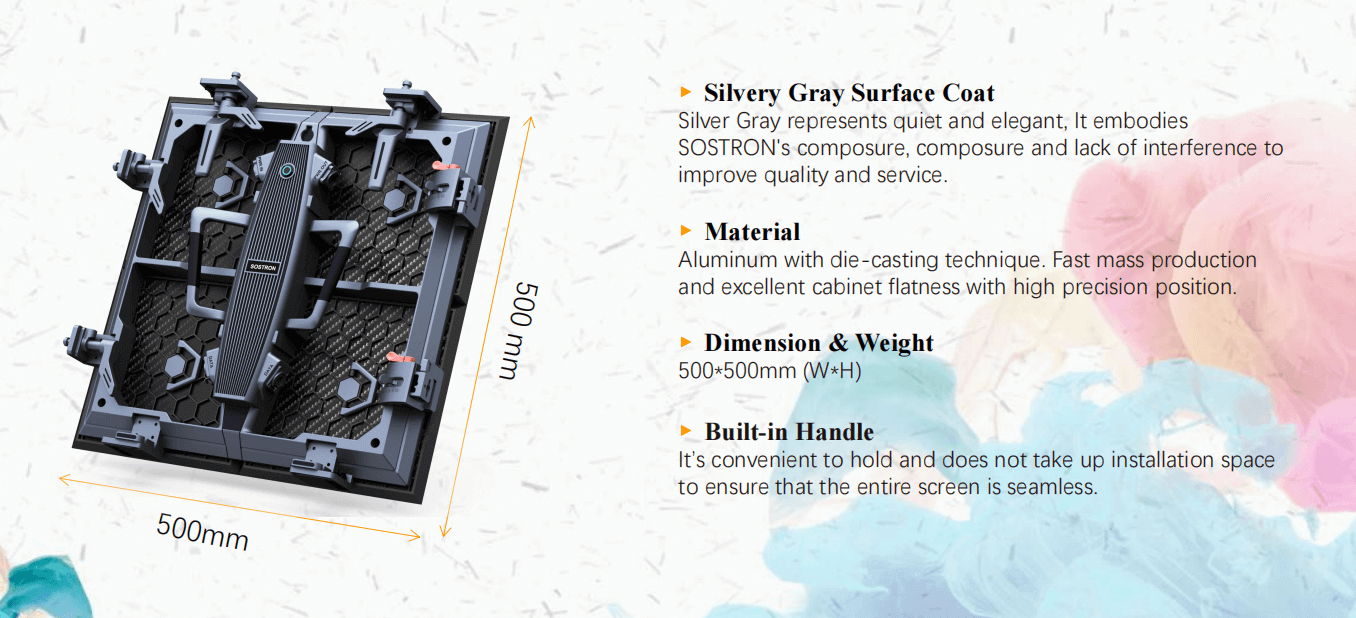
4. Installation environment
The installation environment of the LED display screen also affects the price. For example, outdoor LED display screens need to have functions such as waterproof, dustproof and windproof. The realization of these functions requires additional materials and technical support, and the price will increase accordingly. [Here are some installation methods of LED. ](https://sostron.com/some-ways-of-installing-led-display/)
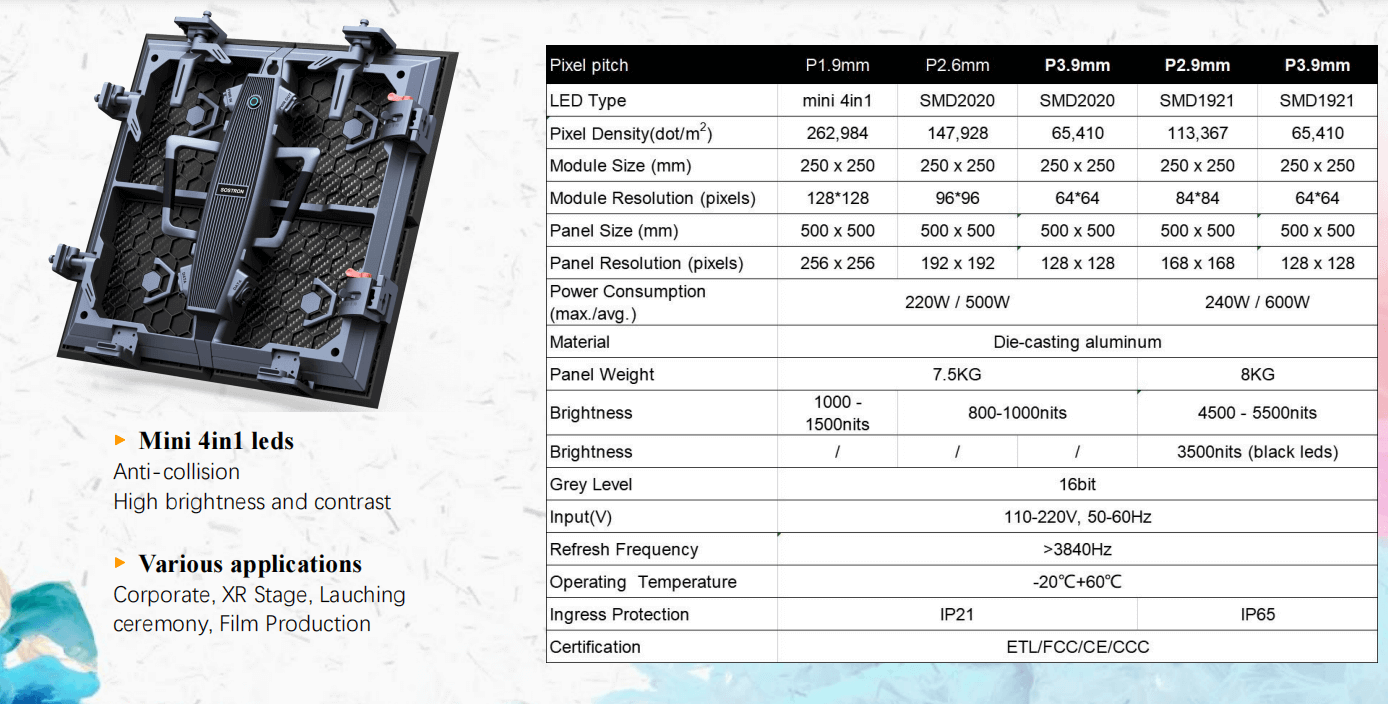
Features of LED display screens inside shopping malls
Main function
The LED display screen inside the shopping mall mainly plays the role of advertising screen, and at the same time, it must meet the design of the scene, create a good shopping atmosphere, and mobilize customer emotions.
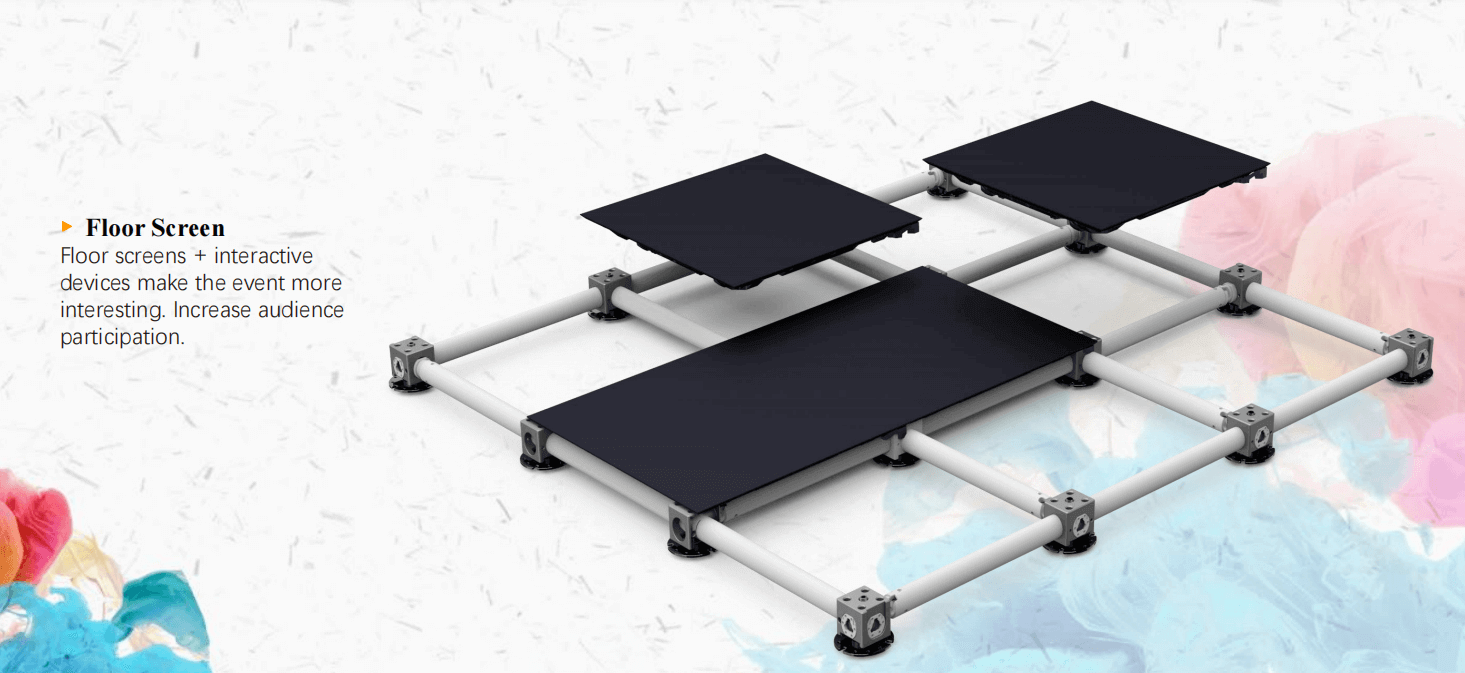
Features
Personalized design: coordinated and unified with the installation environment.
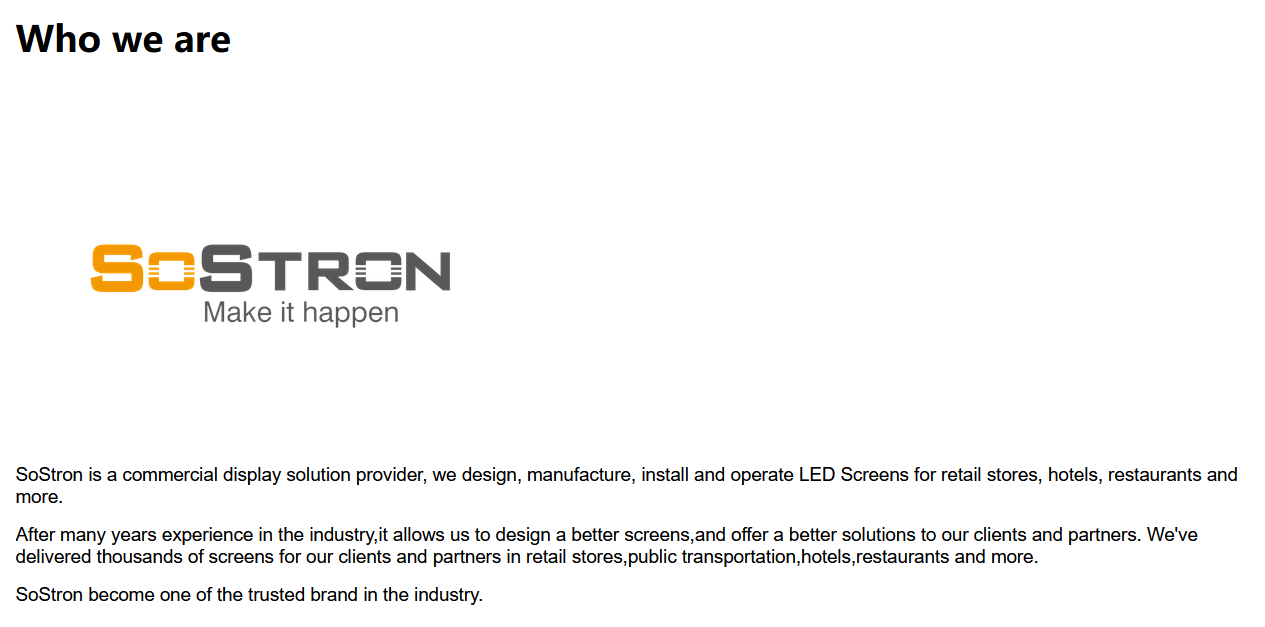
Insufficient maintenance space: usually installed in a location with limited space, the convenience of maintenance needs to be considered.
Complex and varied shapes: can be designed into various shapes according to needs to enhance visual appeal.
Different installation methods: choose different installation methods according to actual conditions, such as wall-mounted, embedded, etc.
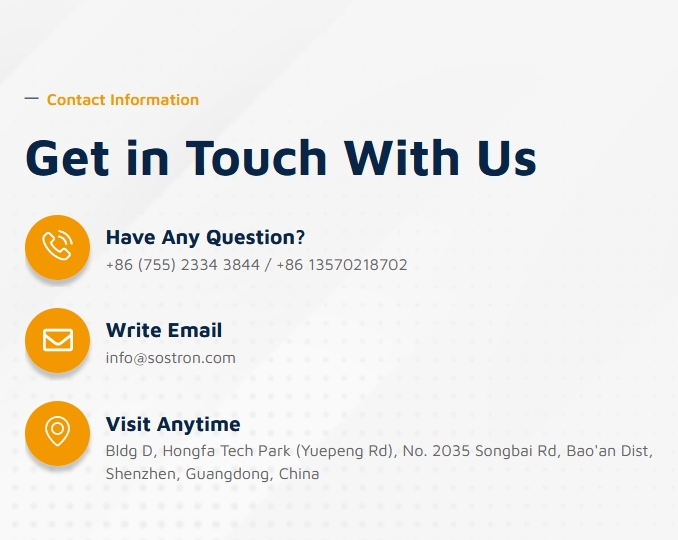
Solution
Manufacturable rental LED display: Choose a rental LED display that can be designed in a variety of shapes to meet different styling needs. [Here is the LED stage rental screen scheme. ](https://sostron.com/led-stage-rental-screen-scheme/)
Front-maintained ultra-thin display: The front-maintenance design is adopted to facilitate maintenance when there is insufficient maintenance space.
Small-size LED modules or cabinets: Choose ultra-light and thin, SMD technology indoor dedicated LED display with a dot pitch of ≤6mm.
Calculation of advertising costs
Advertising costs are usually calculated on a monthly, quarterly or half-year basis. Take a 15-second advertising film as an example, which is controlled at about 10 customers and played 320 times a day. For shopping malls with large traffic, the market quotation for a month is between 120,000 and 180,000 (different in different places). The price will also vary according to the area of the rental LED display. The larger the area, the higher the price. At the same time, good advertising benefits and an increase in the number of customers will also affect the final price. The playback material is usually provided by the advertiser, and of course the advertising company can also provide design services.
Matters needing attention when renting LED display screens in shopping malls
Refer to the advertising price of local hotel LED display screens: understand the local market situation and avoid quoting too high or too low.
Refer to the price of local print ads: compare with the price of print ads and reasonably formulate the advertising cost of LED display screens.
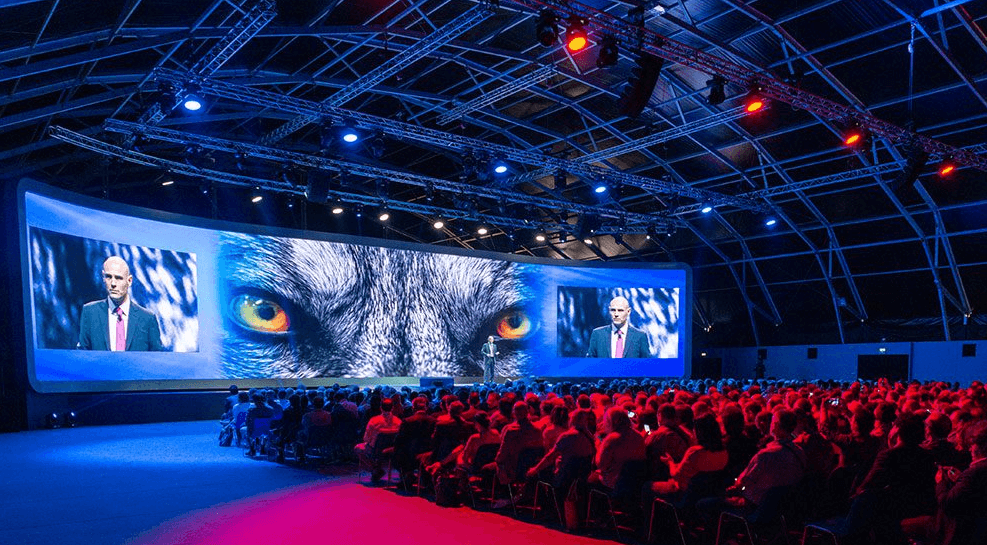
Consideration of installation environment
Brightness: LED display screens installed in ordinary indoor illumination environments should use indoor display screens; outdoor display screens should be used when installed outdoors. For environments with strong light such as open halls, eaves, outdoor awnings or sunny roofs, semi-outdoor display screens should be used. [Here are ten differences between indoor and outdoor LED walls. ](https://sostron.com/ten-differences-between-indoor-and-outdoor-led-walls/)
Through the above analysis, it can be seen that the price difference of LED display screens in different application scenarios is determined by many factors. Understanding these factors and reasonably choosing suitable LED display screens can better control costs and achieve the expected results.
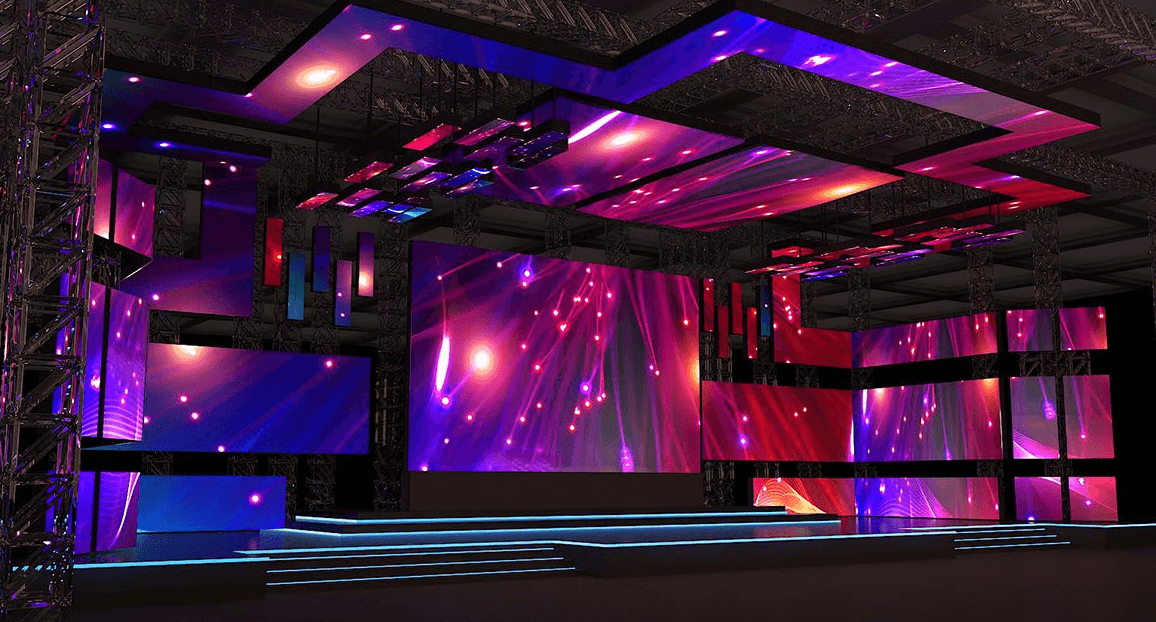
Thank you for watching. I hope we can solve your problems. Sostron is a professional [LED display manufacturer](https://sostron.com/about-us/). We provide all kinds of displays, display leasing and display solutions around the world. If you want to know: [LED transparent screen: visual revolution and technological breakthrough in the new era.](https://dev.to/sostrondylan/led-transparent-screen-visual-revolution-and-technological-breakthrough-in-the-new-era-5gh6) Please click read.
Follow me! Take you to know more about led display knowledge.
Contact us on WhatsApp:https://api.whatsapp.com/send?phone=+8613570218702&text=Hello | sostrondylan |
1,912,305 | Immerse Yourself in the Rich Tapestry of India: A Traveler’s Guide | Have you ever dreamt of being swept away by a kaleidoscope of cultures, breathtaking landscapes, and... | 0 | 2024-07-05T06:10:33 | https://dev.to/vacationinindia/immerse-yourself-in-the-rich-tapestry-of-india-a-travelers-guide-8pn | Have you ever dreamt of being swept away by a kaleidoscope of cultures, breathtaking landscapes, and rich history? Look no further than India, a land where ancient traditions and vibrant energy coexist in perfect harmony. This India Trip Package guide, crafted for the curious and adventurous traveler, is your gateway to an unforgettable experience.
Regions of Enchantment
India’s vastness offers something for everyone. Let’s explore some of the most captivating regions:
The Golden Triangle: Embark on a classic journey through Delhi, the bustling capital of India; Agra, home to the iconic Taj Mahal, a monument to eternal love; and Jaipur, the “Pink City,” famed for its rose-hued palaces and forts. Immerse yourself in the rich history of Mughal emperors and marvel at architectural wonders that stand as testaments to human ingenuity.
Spiritual Sojourn: For those seeking inner peace, India beckons. Explore the sacred city of Varanasi, where life unfolds along the banks of the holy Ganges River. Witness the mesmerizing bathing rituals and delve into the spiritual heart of Hinduism. Travel north to Rishikesh, the “Yoga Capital of the World,” and learn the ancient practice of yoga amidst the serenity of the Himalayas. Finally, pay your respects at McLeod Ganj, the residence of His Holiness the Dalai Lama, and experience the tranquility of Tibetan Buddhism.
Off the Beaten Path: Craving a unique adventure? Venture beyond the usual tourist trail. Kerala, nicknamed “God’s Own Country,” awaits with its tranquil backwaters, pristine beaches, and lush greenery. Glide through the emerald canals on a traditional houseboat, savoring the serenity of the palm-fringed landscapes. Goa, a former Portuguese colony, offers a taste of Europe on Indian soil. Explore its charming churches, sun-drenched beaches, and vibrant markets overflowing with local crafts. Darjeeling, a hill station nestled in the Himalayas, provides a welcome respite from the heat. Take in the breathtaking views of snow-capped peaks, explore the aromatic tea plantations, and experience the warmth of the local people.
Cultural Delights
India’s cultural tapestry is woven with vibrant threads of festivals, food, and art:
Festivals and Celebrations: Immerse yourself in the joyous spirit of India’s numerous festivals. Witness the dazzling illuminations of Diwali, the Festival of Lights, where homes and streets come alive with rows of diyas (oil lamps). Get swept up in the colorful chaos of Holi, the Festival of Colors, where revelers playfully throw colored powder at each other. Experience the grandeur of the Pushkar Camel Fair, a unique event where thousands of camels are adorned, showcased, and traded amidst a vibrant cultural spectacle.
Food and Flavors: Tantalize your taste buds with the vast culinary landscape of India. From the rich curries of the north to the delicate flavors of the south, Indian cuisine offers a symphony of spices and regional specialties. Explore the vibrant street food scene, where vendors tempt passersby with aromatic delights. Don’t forget to indulge in the explosion of flavors offered by vegetarian and vegan options, a true testament to India’s diverse culinary heritage.
Art and Architecture: India boasts a rich artistic legacy, evident in its magnificent architecture. Stand in awe of the white marble perfection of the Taj Mahal, a Mughal masterpiece symbolizing eternal love. Journey south to witness the intricate carvings and towering gopurams (gateway towers) of Dravidian temples. Cruise along the serene backwaters of Kerala and marvel at the architectural ingenuity of traditional houseboats. Every corner of India offers a glimpse into its artistic soul.
Experiences that Last a Lifetime
India Tourism Packages go beyond sightseeing. Here are some unforgettable experiences:
Yoga and Ayurveda: Enhance your well-being by learning yoga, an ancient Indian practice that combines physical postures, breathing exercises, and meditation. Explore Ayurveda, India’s traditional holistic healing system, and discover natural remedies for a healthier you.
Wildlife Watching: Embark on a thrilling jeep safari through India’s renowned national parks, such as Ranthambore and Kaziranga. Spot majestic tigers, one-horned rhinos, and a dazzling array of wildlife in their natural habitat. Capture breathtaking photographs and create memories that will last a lifetime.
Houseboat Cruises: Drift along the tranquil backwaters of Kerala on a traditional houseboat. Glide through palm-fringed canals, spotting local fishermen and lush green landscapes. Savor delicious meals prepared onboard and experience the serenity of this unique ecosystem.
Planning Your Indian Adventure
Now that you’re brimming with excitement, here are some essential tips to plan your perfect India Trip Package:
Best Time to Visit: India experiences a variety of seasons, from the monsoon’s refreshing showers to the cool winters and scorching summers. Research the best time to visit your chosen destinations to ensure a comfortable and enjoyable trip.
Prepare to be enchanted, inspired, and utterly captivated by India. This guide is your first step towards an adventure of a lifetime with India Tourism Packages designed to fulfill your wanderlust and leave you with unforgettable memories. | vacationinindia |
|
1,912,304 | What Makes Somerled Driving Courses the Best Choice for Montreal Drivers? | What Makes Somerled Driving Courses the Best Choice for Montreal Drivers? Choosing the right driving... | 0 | 2024-07-05T06:09:39 | https://dev.to/acs_40ce78f9e439578ffddbc/what-makes-somerled-driving-courses-the-best-choice-for-montreal-drivers-lg3 | What Makes Somerled Driving Courses the Best Choice for Montreal Drivers?
Choosing the right driving school is crucial for gaining the necessary skills and confidence to drive safely. Somerled driving courses, offered by a reputable Montreal driving school, are designed to meet the needs of both new and experienced drivers. Whether you're looking to obtain a Probationary Driver License in Montreal or need a refresher course, Somerled driving courses provide comprehensive training tailored to your specific needs.
Understanding Somerled Driving Courses
Somerled driving courses are renowned for their thorough curriculum and expert instruction. These courses are structured to provide a solid foundation in driving principles, road safety, and practical skills. The instructors at this Montreal driving school are highly experienced and dedicated to ensuring that each student gains the confidence and competence needed to drive safely. From beginner lessons to advanced driving techniques, Somerled driving courses cater to a wide range of learners.
SAAQ Driving Course in Somerled
The SAAQ driving course in Somerled is specifically designed to help students meet the requirements set by the Société de l'assurance automobile du Québec (SAAQ). This course covers all the essential aspects of driving, including traffic laws, defensive driving strategies, and practical road tests. By enrolling in the SAAQ driving course in Somerled, students can be assured of receiving high-quality instruction that prepares them thoroughly for the SAAQ examinations and road tests.
Obtaining a Probationary Driver License in Montreal
One of the key milestones for new drivers is obtaining a Probationary Montreal driving school. Somerled driving courses are tailored to help students achieve this goal. The comprehensive training includes both theoretical and practical components, ensuring that students are well-prepared for the challenges of the probationary period. The instructors focus on building strong foundational skills, emphasizing safe driving practices and adherence to traffic regulations.
Auto École Cote des Neiges: A Trusted Partner
Somerled driving courses are offered in collaboration with Auto École Cote des Neiges, another well-respected driving school in Montreal. This partnership enhances the training experience by combining the expertise and resources of both institutions. Students benefit from a diverse range of instructional techniques and a broader perspective on driving education. Auto École Cote des Neiges brings additional value to Somerled driving courses, ensuring a comprehensive and well-rounded learning experience.
Why Choose a Montreal Driving School?
Choosing a Montreal driving school like the one offering Somerled driving courses provides several advantages. Firstly, local driving schools are familiar with the specific traffic conditions, road layouts, and driving regulations unique to Montreal. This local knowledge is crucial for effective training. Secondly, a Montreal driving school ensures that the instruction is aligned with provincial requirements, such as those set by the SAAQ. Finally, the convenience of attending a driving school close to home reduces the stress of travel and allows for flexible scheduling.
Conclusion: Where to Begin Your Driving Journey?
In conclusion, Somerled driving courses offer a comprehensive, well-structured, and expert-led pathway to becoming a confident and competent driver. Whether you're aiming to obtain your Probationary Driver License in Montreal, need to complete the SAAQ driving course in Somerled, or are looking for quality instruction from a trusted Montreal driving school, Somerled driving courses provide the ideal solution. Partnering with Auto École Cote des Neiges further enhances the quality of training, ensuring that students receive the best possible driving education. Start your driving journey with Somerled driving courses and experience the benefits of top-tier driving instruction tailored to your needs.
VISIT - https://ecolesomerled.ca/
| acs_40ce78f9e439578ffddbc |
|
1,912,303 | Bridging the Divide: React Native vs. React Web Development | React, a popular JavaScript library, has taken the web development world by storm. But what if you... | 0 | 2024-07-05T06:09:00 | https://dev.to/epakconsultant/bridging-the-divide-react-native-vs-react-web-development-42hm | react | React, a popular JavaScript library, has taken the web development world by storm. But what if you want to leverage React's power to build mobile apps? Enter React Native, a framework that utilizes React principles for cross-platform mobile development. While both involve React, there are distinct differences to consider. Let's explore these key distinctions to help you choose the right tool for the job.
[Jumpstart Your App Development Journey with React Native](https://www.amazon.com/dp/B0CRF8S8Z1)
Target Platforms:
The most fundamental difference lies in the target platform. React web development focuses on creating user interfaces for web browsers, accessible on desktops, laptops, and tablets. React Native, on the other hand, targets mobile devices – iOS and Android. This translates to differences in the user experience and the underlying technologies used.
Development Environment:
React web development typically involves familiar web development tools like HTML, CSS, and JavaScript. Developers work within a web browser environment and utilize libraries like React to build interactive UI elements. In contrast, React Native development utilizes JavaScript primarily, with the code being compiled into native code for iOS and Android. While some knowledge of native platforms (Swift or Java) can be beneficial, it's not mandatory.
Performance and User Experience:
React web apps rely on the web browser's rendering engine, which can sometimes lead to performance limitations. React Native, however, leverages native UI components, resulting in a smoother and more responsive user experience that feels indistinguishable from native apps built with platform-specific languages.
Access to Native Features:
React web apps are primarily restricted to browser functionalities like making network requests or manipulating the DOM. React Native, with its bridge to native components, allows access to device-specific features like GPS, camera, or sensors. This empowers developers to create mobile apps that integrate seamlessly with the device's capabilities.
Learning Curve and Community Support:
If you're already familiar with web development concepts like HTML, CSS, and JavaScript, transitioning to React web development might be smoother. React Native, while utilizing JavaScript, requires an understanding of mobile development concepts and potentially some familiarity with native platforms (iOS or Android) for advanced functionalities. However, React Native benefits from a large and active community, offering extensive documentation, tutorials, and third-party libraries to ease the learning curve.
Choosing the Right Tool:
The decision between React web development and React Native depends on your project's specific needs. Here's a quick guide:
- For web-based applications: If your project is a web app, website, or single-page application accessible through a web browser, React web development is the ideal choice.
- For mobile applications: If you want to build a mobile app for iOS and/or Android, React Native is the way to go. You can leverage your React knowledge while creating native-looking mobile experiences.
- For hybrid applications: In some cases, you might consider a hybrid approach, where a single codebase using React Native can be used to create both a web app and a mobile app. However, this approach might have limitations in terms of performance and access to native features.
Conclusion
React and React Native, while sharing the same core principles, cater to different development needs. Understanding the distinctions between target platforms, development environment, performance, and feature accessibility will empower you to make an informed decision when choosing the best tool for your next project. So, whether you're building the next big web app or a feature-rich mobile experience, the React ecosystem offers a powerful and versatile toolkit to bring your ideas to life. | epakconsultant |
1,912,302 | Expanding Horizons: Exporting Superior Steel Pipes Worldwide | Steel Industry is pivotal for the global economy as it caters to construction, transportation and... | 0 | 2024-07-05T06:06:56 | https://dev.to/gloria_jchavarriausi_fb/expanding-horizons-exporting-superior-steel-pipes-worldwide-n59 | design | Steel Industry is pivotal for the global economy as it caters to construction, transportation and manufacturing. In fact, steel pipes of high quality are so much in demand throughout the world for use in industry and infrastructure projects. Exporting great steel pipes to many parts of the world is what a lot enterprises are doing. In this article, we analyze some of the highest exporting regions for steel pipes and reveal what manufacturers can do to increase their profits through tactical initiatives while also calculating the global political influences on industry - including technological innovations transforming it as well as strategies that could help domestic companies offset challenges faced from consumers in the rising markets.
The US is one of the main consumers of Steel Pipes that are manufactured in other countries and with their vast infrastructure need a continuous representation of high-quality steel pipes. Canada, Europe and Middle East are other primary destinations along with Asia The high-grade razor wire steel pipes are exported in large amounts to countries like Russia, Germany or the United Kingdom within Europe. The opportunities in the second segment can be seen very well in a part of Middle East countries such as Dub, Turkey and others are significant importer of oil & gas projects. Further, China, India, Japan and South Korea in the Asia continent are some of the key end markets for steel pipes offered from global suppliers.
For-profit enterprises need to: adopt strategic export strategies, so as to increase profits. Strategies to build strong relations with clients, on time superior quality steel pipes delivery. For companies to expand the customer base and reach new markets, they should diversify product offerings, target specific sectors with customized products or services, explore niche-markets opportunities like competitive pricing approaches, use innovative marketing models etc.
Where global politics are concerned, steel pipe export is no exception. Profitability can be affected by trade policies, as well as country embargoes. Companies can hedge these risks by expanding into new markets if they have a more diversified customer base. Navigating the ever-changing terrain of global politics requires a sound knowledge of worldwide political trends, and this also holds true for international trade.
Technology has changed the world and with it have arrived a number of innovations in steel pipe export. The production processes and efficiency are getting easier due to robotic technologies. Predictive maintenance is becoming smarter with AI and Machine Learning, leading to decreased downtime as productivity increases. In the world of complex pipe concertina wire design, 3D printing technology is now enabling reducation in lead time and cost for delivery. This technology can reduce the risk of fraudulent transactions and improve the transparency in supply chain operations.
Challenges of Growing Business in Emerging Markets Problems such as poor infrastructure, unstable political environments and incompatible cultures can throw up impediments to success. Developing local partnerships, investing in local talent and understanding of location-specific regulations and tapping into support from government as well financial institutions are key strategies for a successful expansion to emerging markets.
To sum up, the demand for steel pipe export is increasing rapidly with highly competitive companies all over the world. The major market areas where the export of steel pipes are done, include USA, Canada followed by Europe, Middle East and Asia. For companies in this industry, the need to maximize profits using best practices through strategic management while negotiating global politics and applying advanced rectangle tubing technology for growth is not lost on both old guards as well entry level contenders all want a share of what emerging markets have to offer. Businesses interested in joining the global steel pipe export industry must make use of strategic partnerships, technological advancements, and proper planning to increase their scope. | gloria_jchavarriausi_fb |
1,912,301 | Building Blocks of React Native Apps: Mastering View, Text, and Image Components | React Native empowers you to create mobile apps using JavaScript and React principles. But how do you... | 0 | 2024-07-05T06:06:10 | https://dev.to/epakconsultant/building-blocks-of-react-native-apps-mastering-view-text-and-image-components-14no | react | React Native empowers you to create mobile apps using JavaScript and React principles. But how do you translate UI ideas into actual on-screen elements? This article dives into three fundamental building blocks of any React Native app: View, Text, and Image components.
The Foundation: The View Component
The View component is the cornerstone of your React Native UI. It acts as a container for other UI elements and defines the overall layout of your app's screens. Think of it as a blank canvas on which you build your user interface. Here's a basic example of a View component:
`JavaScript
import React from 'react';
import { View } from 'react-native';
const App = () => {
return (
<View style={{ flex: 1, backgroundColor: 'lightblue' }}>
{/* Other UI components go here */}
</View>
);
};
export default App;`
In this example, we import the View component and use it to create a container that takes up the entire screen (flex: 1) and has a light blue background (backgroundColor: 'lightblue'). Everything else you render within this View component will become part of your screen's layout.
Styling Your Views:
React Native offers various ways to style your View components. You can define styles directly within the component using an inline style object, or you can create separate stylesheets and reference them within your component. Here's an example with inline styling:
`JavaScript
<View style={{ margin: 20, padding: 10, borderRadius: 10, backgroundColor: 'white' }}>
{/* Other UI components */}
</View>`
This code snippet adds margins, padding, rounded corners, and a white background to the View component.
Text: Displaying Content
The Text component, as the name suggests, is used to display text elements within your app. It allows you to define the text content, styling options like font size and color, and even handle text alignment. Here's an example of using the Text component:
`JavaScript
<Text style={{ fontSize: 24, fontWeight: 'bold', color: 'black' }}>
Welcome to React Native!
</Text>`
This code displays the text "Welcome to React Native!" with a font size of 24, bold weight, and black color.
Adding Visuals: The Image Component
The Image component allows you to integrate images into your React Native app. You can specify the image source by referencing a local file path or a remote URL. Additionally, you can control the image's size, aspect ratio, and how it resizes within its container. Here's an example of using the Image component:
`JavaScript
<Image
source={{ uri: 'https://placeimg.com/640/480/nature' }}
style={{ width: 200, height: 150, resizeMode: 'contain' }}
/>`
This code displays an image from a placeholder URL with a width of 200 pixels, a height of 150 pixels, and a resize mode set to "contain" which ensures the entire image is visible within the specified dimensions.
[Jumpstart Your App Development Journey with React Native](https://www.amazon.com/dp/B0CRF8S8Z1)
Combining the Essentials:
Now that you understand View, Text, and Image components, let's create a simple example that combines them:
`JavaScript
import React from 'react';
import { View, Text, Image } from 'react-native';
const App = () => {
return (
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Text style={{ fontSize: 32, marginBottom: 20 }}>React Native Example</Text>
<Image
source={{ uri: 'https://placeimg.com/320/240/tech' }}
style={{ width: 320, height: 240 }}
/>
</View>
);
};
export default App;`
This code creates a centered layout with the text "React Native Example" displayed above an image downloaded from a placeholder URL.
Beyond the Basics:
While View, Text, and Image are fundamental components, React Native offers a vast library of built-in components to handle various UI elements like buttons, scroll views, and input fields. By mastering these basic building blocks, you can embark on creating more complex and interactive mobile apps using React Native.
Remember, this is just the beginning! Explore the official React Native documentation to delve deeper into styling options, advanced layout techniques using Flexbox, and how to combine these components with others to build feature-rich mobile applications. | epakconsultant |
1,912,299 | Discover the Ultimate Delta 8 Vape Juice at SouthEast Vape | Experience the next level of vaping with our premium Delta 8 Vape Juice at SouthEast Vape. Crafted... | 0 | 2024-07-05T06:02:53 | https://dev.to/southeastvape/discover-the-ultimate-delta-8-vape-juice-at-southeast-vape-44j1 | Experience the next level of vaping with our premium Delta 8 Vape Juice at SouthEast Vape. Crafted for enthusiasts seeking a smooth and potent experience, our [Delta 8 Vape Juice](https://southeastvape.com/collections/delta-8) delivers a perfect balance of flavor and relaxation. Infused with high-quality Delta 8 THC, each puff offers a delightful sensation that enhances your vaping journey. Choose from a variety of tantalizing flavors that cater to every palate, ensuring a satisfying and enjoyable experience every time. Our Delta 8 Vape Juice is meticulously tested for purity and potency, guaranteeing a safe and exceptional product. Elevate your vape game with SouthEast Vape’s Delta 8 Vape Juice and indulge in the ultimate vaping pleasure. Shop now and discover why we are the go-to destination for Delta 8 enthusiasts! | southeastvape |
|
1,912,298 | Demystifying React Native: Core Concepts for Building Mobile Apps | React Native has emerged as a powerful tool for cross-platform mobile app development. It allows... | 0 | 2024-07-05T06:02:45 | https://dev.to/epakconsultant/demystifying-react-native-core-concepts-for-building-mobile-apps-2k7f | react | React Native has emerged as a powerful tool for cross-platform mobile app development. It allows developers to leverage JavaScript and React principles to create native-looking mobile apps for iOS and Android. But what lies beneath the hood of React Native? This article explores some core concepts that make it tick, including the virtual DOM and the bridge to native components.
Building Blocks: React Components
At its heart, React Native utilizes the concept of components. Just like in React for web development, components are reusable building blocks that encapsulate UI elements and their behavior. These components are written in JavaScript and define how the UI should look and interact. They can be nested within each other to create complex user interfaces.
The Virtual DOM: A Lightweight Representation
One of the key strengths of React Native is its use of a virtual DOM (Document Object Model). Unlike the traditional DOM used in web browsers, the virtual DOM is a lightweight in-memory representation of the actual UI. When a component's state or props change, React Native efficiently calculates the difference between the previous and the updated virtual DOM. This allows it to pinpoint the minimal changes needed in the actual UI, leading to a more performant and efficient update process.
JSX: Bridging the Gap Between JavaScript and UI
JSX (JavaScript XML) plays a crucial role in React Native development. It's a syntactic extension that allows writing HTML-like structures within JavaScript code. This makes it easier for developers to visualize the UI and define the component structure intuitively. However, it's important to remember that JSX is transformed into plain JavaScript functions before being executed by the JavaScript engine.
[Mastering ROS: Unleashing the Power of Robot Operating System for Next-Generation Robotics](https://www.amazon.com/dp/B0CTRJP3BZ)
The Bridge to Native Components: Connecting JavaScript to Native Power
React Native components themselves are not directly rendered on the device's screen. Here's where the bridge comes in. It acts as a communication channel between the JavaScript code running in React Native and the native platform (iOS or Android). When a React Native component needs to be displayed, the bridge translates the component's description (including its layout, style, and behavior) into calls to the platform's native UI components. This ensures that the app utilizes the native UI elements for a seamless and performant user experience.
[Beyond the Eraser: Advanced Background Removal Techniques with Channels in Photoshop](https://nocodeappdeveloper.blogspot.com/2024/07/beyond-eraser-advanced-background.html)
Benefits of the Bridge Approach
The bridge approach offers several advantages:
- Native Look and Feel: By leveraging native UI components, React Native apps inherit the look and feel of the underlying platform. This ensures a smooth user experience indistinguishable from native apps built with platform-specific languages like Swift or Java.
- Performance: Native UI components are typically optimized for the specific platform, leading to better performance and smoother rendering compared to purely web-based solutions.
- Access to Native Features: The bridge allows React Native apps to access native device functionalities like GPS, camera, or sensors. This empowers developers to create feature-rich mobile apps that integrate seamlessly with the device's capabilities.
Beyond the Basics: Additional Concepts
Understanding the virtual DOM and the bridge to native components is a solid foundation for React Native development. However, there are several other important concepts to explore, such as:
- State Management: Managing the state of your app's components is crucial and tools like Redux or MobX can be helpful in this regard.
- Navigation: Navigation libraries like React Navigation provide a structured way to handle transitions between different screens within your app.
- Styling: React Native offers various options for styling your components, including inline styles, stylesheets, and third-party libraries like Styled Components.
Conclusion
By understanding the core concepts like the virtual DOM and the bridge to native components, developers can leverage the power of React Native to build efficient, performant, and visually appealing mobile apps. With its growing ecosystem and active community, React Native continues to evolve, offering a compelling solution for cross-platform mobile development. So, delve deeper, explore these concepts, and embark on your journey of building amazing mobile apps with React Native!
| epakconsultant |
1,912,297 | Check colors in Angular Material theme builder | Now you can check all colors in the preview. Live on theme builder for angular material at... | 0 | 2024-07-05T05:56:28 | https://dev.to/ngmaterialdev/check-colors-in-angular-material-theme-builder-poi | angular, angularmaterial, webdev | ---
title: Check colors in Angular Material theme builder
published: true
description:
tags: angular,angularmaterial,webdevelopment
---
Now you can check all colors in the preview.
Live on theme builder for angular material at https://themes.angular-material.dev
{% embed https://dev.to/shhdharmen/colors-in-the-preview-are-live-on-theme-builder-for-angular-material-2lf0 %}
For now, it's only available for Material 3 previews.
Do try it out today
| shhdharmen |
1,912,295 | Colors in the preview are live on theme builder for angular material | A post by Dharmen Shah | 0 | 2024-07-05T05:54:52 | https://dev.to/shhdharmen/colors-in-the-preview-are-live-on-theme-builder-for-angular-material-2lf0 | angular, angularmaterial, webdev | ---
title: Colors in the preview are live on theme builder for angular material
published: true
description:
tags: angular,angularmaterial,webdevelopment
---
| shhdharmen |
1,912,296 | Exploring the Different Types of Heat Pumps and Their Applications | Heat pumps are amazing devices that may you need to just take heat from a area that is single... | 0 | 2024-07-05T05:54:00 | https://dev.to/gloria_jchavarriausi_fb/exploring-the-different-types-of-heat-pumps-and-their-applications-ji | design |
Heat pumps are amazing devices that may you need to just take heat from a area that is single transfer it to a different. What this signifies is into the cold weather they could cool your home during summer as well as heat it. There are numerous several kinds of heat pumps, and every has its advantages being own are own uses. , we'll explore the various types of temperature pumps and their applications
Advantages of Heat Pumps
Temperature pumps have many benefits over conventional heating and systems which are cooling. A few of these advantages consist of:
- power cost savings: temperature pumps are much more energy-efficient than old-fashioned heating and systems which are cooling they transfer temperature instead of build it. This implies you cash on your power bills they use less air source heatpumps electricity and will conserve
- green: Since heat pumps use less power, in addition they produce fewer greenhouse gasoline emissions, that is better when it comes to environment
- Versatility: Heat pumps is utilized both for heating and cooling, this means that you only need one system to achieve both
- Comfort: Heat pumps provide a far more temperature that is constant your property, unlike conventional heating systems which could result in cold spots
Innovation in Heat Pumps
Temperature pumps came an method that is easy is long they certainly were very first created. Today, you can find various types of heat pumps, each using their air source heatpump features that may be very own are unique. Several of the temperature that is most this is certainly innovative in the market consist of today:
- Geothermal temperature pumps: These temperature pumps utilize the consistent heat of this earth to heat and cool your home. They could be more expensive to set up initially but will save you profit to the run this can be long they have been really energy-efficient
- Air-source heat pumps: These heat pumps make use of the exterior atmosphere to heat up and cool your house. They are usually less costly to install than geothermal heat pumps, but they are additionally less efficient in extreme conditions
- Dual-fuel temperature pumps: These temperature pumps use both electricity and propane or propane to warm your home. They can be higher priced to set up, however they in many cases are more cost-effective in cold climates
Safety and Utilization Of Heat Pumps
Heat pumps are generally very safe to make use of, particularly contrasted to heating that is traditional that usage combustion to create heat. However, a safety may be found by you that is few you'll want to be familiar with whenever coming up with usage of a air to water heat pump:
- Ventilation: verify your heat pump is properly ventilated and that there clearly was air that is sufficient fresh the room to avoid carbon monoxide buildup
- upkeep: Regular maintenance is important to make sure your temperature pump is working properly and properly. This could easily include air that is changing and levels that are checking are refrigerant
- Installation: it is advisable to get heat pump installed by way of a expert to ensure it's properly wired and therefore the levels being refrigerant correct
Just how to Make Use Of Heat Pump
Employing a heat pump is simple, especially because so many models come with programmable thermostats that permit you to definitely set the heat for different times through the the full time that is full. To work very well with your temperature pump:
- Set the thermoregulator to your desired temperature
- await heat pump to begin up and commence heating or cooling your house
- enjoy temperatures that are consistent your house
Service and Quality of Heat Pumps
This is certainly certainly technical temperature pumps need regular service to keep them working properly like any device. You ought to get heat pump serviced at the minimum when a to make sure that its working properly year. When selecting a temperature pump, it is necessary to determine on a model this will be top-quality and from the brand that is reputable. Look for models which have SEER that is high (Seasonal Energy Efficiency Ratio) and reviews that are good many customers
Applications of Heat Pumps
Temperature pumps is utilized in several applications that are various. A number of the applications that are many may be common:
- Residential heating and cooling: Heat pumps are the ideal choice for cooling and heating domiciles, particularly in mild to moderate climates
- Commercial heating and cooling: Heat pumps may be used to heat up and cool commercial buildings, such as for example offices, schools, and hospitals
- kids' pool heating: temperature pumps allows you to heat swimming pools, helping you to enjoy your pool year-round
- Water heating: Heat pumps enable you to heat up water, that can be quite a more alternative that is energy-efficient water that is old-fashioned
Conclusion
Heat pumps are a choice this will be warming that is excellent cooling your building or property. They truly are energy-efficient, eco-friendly, and versatile. With several different types of heat pumps on the market, you need to buy a model that is correct for your needs and also to obtain it installed correctly and serviced. Regardless for you personally personally if you are trying to heat and cool your property, your swimming pool, or your water, there clearly was a heat pump you got that right
| gloria_jchavarriausi_fb |
1,912,294 | Fönsterrenovering i Haninge | När det gäller byggnadens skönhet, oavsett för vilket ändamål den är konstruerad, spelar dess fönster... | 0 | 2024-07-05T05:52:55 | https://dev.to/fonstria_marketing_2ac995/fonsterrenovering-i-haninge-4adk | digital | När det gäller byggnadens skönhet, oavsett för vilket ändamål den är konstruerad, spelar dess fönster en nyckelroll för att förbättra dess skönhet, klass och ventilation. Så, om du menar allvar med dessa specifika områden, kan du inte ta fönstret för givet. Oavsett om det gäller att sätta in nya [fönster](https://fonstria.se/) i en nybyggd byggnad eller fönsterbyte i en gammal så har vi kompetens inom båda.
[Fönsterrenovering i Haninge](https://fonstria.se/):
Om du sitter i Haninge och letar efter en bra fönsterrenoveringstjänsteleverantör som kan prissätta nya fönster effektivt. Vi har tillhandahållit tjänsterna under så lång tid med vårt team att vi har skapat en pålitlig relation med våra kunder eftersom vi ger dem pålitlig service, och vi prissätter nya fönster eller fönsterbyten till en mycket genuin pris där båda sidor är nöjda .
Fönsterrenovering i Djursholm:
Vi är här för att bara betjäna dig.Det har setts många gånger att kunden mycket väl vet att det finns ett behov av byte av ett fönster snarare än reparation, men de tvekar att dra nytta av den höga kostnaden för byte av fönster. Här tillhandahåller vi lösningen eftersom vi har många erfarna arbetare som ger en tydlig bild och den billigaste kostnaden, vilket räcker till fönsterrenoveringen.
Slutsats:
Kontakta oss bara för att förvandla din tillgång med ditt uppgraderade fönster. Vi är alltid tillgängliga för att ge dig våra tjänster.
https://fonstria.se/ | fonstria_marketing_2ac995 |
1,912,293 | How to Sequentially Fill and Submit Form Fields from CSV Using Chrome Extension and JavaScript | I'm developing a Chrome extension that uses a CSV file to populate and submit a form on a webpage.... | 0 | 2024-07-05T05:51:19 | https://dev.to/itscae/how-to-sequentially-fill-and-submit-form-fields-from-csv-using-chrome-extension-and-javascript-kda | javascript, extensions, csv | I'm developing a Chrome extension that uses a CSV file to populate and submit a form on a webpage. The goal is to enter data row-by-row from the CSV file into the form fields, wait for the form to be processed, submit the form, and then move to the next row.
Currently, my script processes all rows at once, causing the fields to be filled and submitted simultaneously. I need help to ensure that the form is filled and submitted sequentially for each row.
Here's my current approach:
a. Reading the CSV file using PapaParse.
b. Iterating over each row of the CSV data.
c. Each row contains a few fields of data
d. Using chrome.scripting.executeScript to fill the form fields and submit the form.
However, the script still processes all rows (including every field in every row) at once, instead of waiting for each submission to complete before moving to the next row. I tried to use setTimeout() to ensure the script waits before submitting, but that didn't work. It just processed all the rows (data) immediately and waited a few seconds before pressing the submit button. I also tried to use async functions, but it would always break; only one field would be submitted, none would be submitted, etc... I'm not sure how to do the logic I've stated above. There are no error messages, or build errors. I tried googling more about this issue and more about async functions, but I couldn't duplicate it for my project properly.
Here's my code below:
(i couldn't post it on the website, it was very buggy, apologies)
https://pastecode.io/s/3x2n46as | itscae |
1,912,291 | Best Invest in Commercial Property in hyderabad | Investing in Best Invest in Commercial Property in hyderabad means securing a high-quality Invest in... | 0 | 2024-07-05T05:47:07 | https://dev.to/kapildmseo1_01bae34b967eb/best-invest-in-commercial-property-in-hyderabad-3j51 | Investing in Best [Invest in Commercial Property](https://kapilinfraprojects.com/kapilinfraprojects/) in hyderabad means securing a high-quality Invest in commercial property with a minimum investment, enjoying regular and escalating rental income, and benefiting from significant capital appreciation. The added advantage of a long-term assured income makes it an exceptional opportunity for both novice and seasoned investors looking to enhance their portfolio with stable and profitable real estate assets. | kapildmseo1_01bae34b967eb |
|
1,912,290 | Tecpinion: Leading Sports Betting Software Development Company | Tecpinion is a premier sports betting software development company, dedicated to providing innovative... | 0 | 2024-07-05T05:44:24 | https://dev.to/kelly_william_e4f689ced84/tecpinion-leading-sports-betting-software-development-company-gf2 | sportsbettingdevelopement | Tecpinion is a premier [sports betting software development company](https://www.tecpinion.com/sports-betting-software-platform-development/
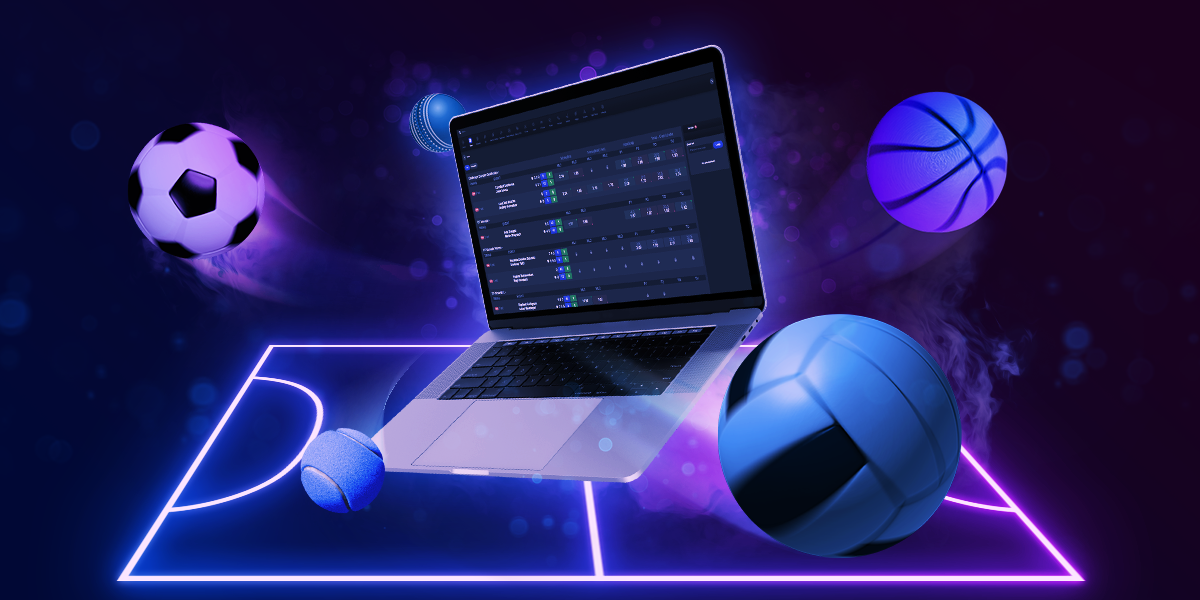), dedicated to providing innovative and customized solutions for the sports betting industry. With a team of experienced developers and industry experts, Tecpinion specializes in creating robust, scalable, and user-friendly betting platforms. Our cutting-edge technology and comprehensive services ensure that clients receive a competitive edge in the market. From intuitive user interfaces to secure payment integrations and real-time data analytics, Tecpinion delivers top-tier sports betting software tailored to meet the unique needs of each client. Choose Tecpinion to elevate your sports betting business to new heights. | kelly_william_e4f689ced84 |
1,912,289 | Understanding Dedicated Servers and Cloud Hosting: Scaling Your Website's Capacity | A dedicated server is a physical server leased by a single client, providing exclusive use of its... | 0 | 2024-07-05T05:43:06 | https://dev.to/leapswitch123/understanding-dedicated-servers-and-cloud-hosting-scaling-your-websites-capacity-14n6 | dedicatedservers, cloudhosting, webdev, website | A dedicated server is a physical server leased by a single client, providing exclusive use of its resources. This setup offers several advantages:
Performance and Control: Dedicated servers excel in providing consistent performance because all resources (CPU, RAM, storage, bandwidth) are dedicated solely to your website or application. This ensures reliable speed and responsiveness, crucial for high-traffic websites and applications that require stable performance.
**Scalability**: Scaling a dedicated server involves upgrading its hardware components, such as increasing CPU cores, RAM capacity, or adding more storage. This linear scaling model means you can expand your server's capacity as your traffic grows, but it requires proactive planning and potential downtime during upgrades.
**Use Cases**: Dedicated servers are ideal for resource-intensive applications that demand consistent performance and security, such as e-commerce platforms, large databases, or gaming servers. They provide a controlled environment where you have direct access to hardware configurations and can optimize performance based on specific requirements.
**Cloud Hosting**:
Cloud hosting operates on a network of virtual servers that draw resources from a pool of physical servers. This model offers distinct advantages:
Flexibility and Scalability: [Cloud hosting](https://leapswitch.com/vps/) allows for dynamic scaling of resources based on demand. Resources like CPU, RAM, and storage are allocated instantly as needed, accommodating sudden traffic spikes without manual intervention. This elasticity makes cloud hosting highly suitable for applications with unpredictable or fluctuating traffic patterns.
High Availability and Redundancy: Cloud infrastructure typically includes redundancy across multiple physical servers and data centers. This setup ensures high availability by distributing data and resources, minimizing downtime due to hardware failures or maintenance activities. Redundancy also enhances data security and disaster recovery capabilities.
Use Cases: Cloud hosting is well-suited for startups, SaaS (Software as a Service) applications, or any website expecting rapid growth or variable traffic volumes. It offers cost-efficiency as you pay for the resources you use, making it easier to scale resources up or down based on current needs.
Handling Increased Traffic:
Dedicated Servers: Scaling a [dedicated server](https://leapswitch.com/delhi-india/dedicated-servers/) to handle increased traffic involves upgrading hardware components. For example, you might upgrade to a server with more powerful CPUs, additional RAM, or higher bandwidth capacity. This approach provides predictable performance but requires upfront planning and potential downtime during upgrades.
Cloud Hosting: Cloud platforms automatically handle increased traffic by dynamically allocating more resources (CPU, RAM, storage) to your virtual servers. This scalability is seamless and immediate, ensuring your website remains responsive even during sudden traffic spikes. Since you pay for resources on-demand, cloud hosting is cost-effective for managing variable traffic patterns.
Choosing the Right Option:
Considerations: When deciding between dedicated servers and cloud hosting, consider factors such as your budget, technical expertise, scalability needs, and the predictability of your website’s traffic patterns.
Hybrid Approaches: Many businesses opt for hybrid solutions that combine dedicated servers for stable workloads with cloud services for handling unpredictable spikes in traffic. This approach offers flexibility, cost-efficiency, and the ability to customize resource allocation based on specific application needs.
Conclusion:
Choosing between dedicated servers and cloud hosting depends on your specific requirements for performance, scalability, budget, and operational control. Dedicated servers offer robust performance and direct hardware control but require upfront investment and manual scaling efforts. In contrast, cloud hosting provides flexibility, scalability, and cost-efficiency, making it suitable for dynamic or rapidly growing applications. Understanding these differences helps you make an informed decision to support your website's growth effectively while meeting performance demands. | leapswitch123 |
1,912,288 | Oracle Redwood: Redefining the User Experience | In when client experience is basic, Prophet declared at Prophet OpenWorld in September 2019 that it... | 0 | 2024-07-05T05:38:28 | https://www.jbsagolf.com/blogs/oracle-redwood-redefining-the-user-experience/ | oracle, redwood | 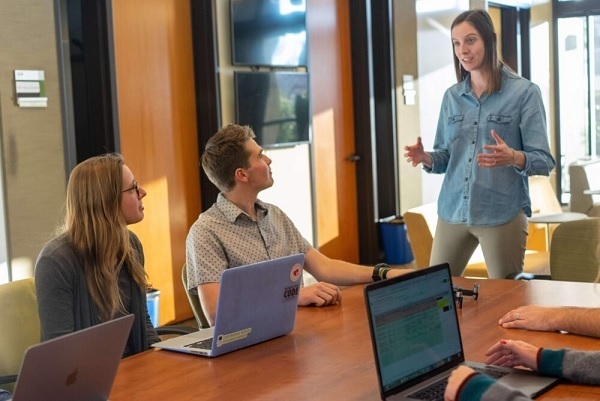
In when client experience is basic, Prophet declared at Prophet OpenWorld in September 2019 that it will be moving to the Redwood UI plan language. Bringing Prophet’s different items and administrations cutting-edge for clients is the point of this upgrade. With Redwood, Prophet means to upset how organizations associate with its expansive scope of uses and innovations. Be that as it may, what definitively is Oracle Redwood, also, what are the outcomes of carrying out it? Together, we can investigate the subtleties and find the testing information required.
**Preparing for the Transition**
Redwood gives a more regular and simple to-utilize interface, yet it likewise requires a lot of testing and arrangement. Similarly as with any critical programming update, it’s essential to ensure the current applications and work processes are viable with the new point of interaction. This could mean refreshing the testing techniques, reworking experiments, and guaranteeing that the staff is enough prepared on Redwood’s latest highlights and functionalities.
**Seamless Integration and Compatibility Testing**
It’s essential to completely test the momentum applications and frameworks’ similarity with Prophet Redwood to ensure a consistent exchange. A careful assessment of the cooperations between the new Redwood interface notwithstanding the ongoing programming biological system is expected at this basic stage. By leading top to bottom similarity appraisals, clients can recognize any similarity holes, incorporation issues, or clashes that could emerge during the change. Having this clever information, clients can proactively carry out any updates, patches, or workarounds expected to bring down chances and keep up with continuous tasks.
**User Acceptance Testing**
Changing to Prophet Redwood’s new UI requires end clients to team up with each other. Client agreeableness testing, or UAT, is significant to this cycle to recognize ease of use issues, check that the point of interaction fulfills client necessities and assumptions, and assemble priceless input for impending enhancements. Effectively remembering end clients for client acknowledgment testing (UAT) works with the distinguishing proof of potential trouble spots, work process improvement, and client experience upgrade.
**Performance Testing**
Prophet Redwood’s new point of interaction ought not be executed to the detriment of framework execution. Broad execution testing is expected to guarantee that the new UI keeps up with the planned speed, responsiveness, and in general application execution. Among the severe methods that might be applied in this cycle to survey framework conduct in unforgiving conditions and lay out execution benchmarks are pressure trying, load testing, and benchmarking. Exhaustive execution evaluations assist with recognizing expected bottlenecks and fix them, upgrade asset designation, and make the fundamental acclimations to convey a responsive and liquid client experience.
**Security Testing**
Powerful safety efforts should start things out when Prophet Redwood delivers its high level information representation as well as personalization highlights. Lead complete security testing, which ought to include entrance testing to mimic certifiable assault situations and weakness evaluations to recognize possible weaknesses. The clients can shield private information and stick to industry principles by being proactive in distinguishing and settling any security blemishes with the new connection point.
**Conclusion**
By being proactive about testing and readiness, the organizations can capitalize on this progressive upgrade of the client experience while ensuring a smooth change to Prophet Redwood. Opkey’s man-made intelligence fueled stage adjusts flawlessly to changes in the UI achieved by the natural Redwood interface, consequently decreasing support endeavors. Relapse testing is less important thanks to its self-mending abilities, which naturally fix broken test steps welcomed on by refreshes. It makes tests for new Redwood includes rapidly by recording and replaying activities. Opkey guides clients through mechanization testing by coordinating with the momentum devices to work with consistent work processes and by offering important Prophet skill. | rohitbhandari102 |
1,912,287 | Experience thrill at your nearest trampoline park| Maryland | Are you looking for trampoline parks near you in Maryland for a thrilling experience? Find all of... | 0 | 2024-07-05T05:33:27 | https://dev.to/funfull/experience-thrill-at-your-nearest-trampoline-park-maryland-1m18 | Are you looking for [trampoline parks near you in Maryland](https://funfull.com/fun-places-offer/maryland-amusement-parks) for a thrilling experience? Find all of them partnered with Funfull; all you have to do is search for "trampoline parks near me in Maryland" and find them on Funfull. [Funfull](https://funfull.com/) allows you to access the nearest trampoline parks for free and at discounted prices.
With a Funfull membership, you can access a variety of other amusement parks like bowling alleys, skating rinks, water parks, fun arcades, and others. Moreover, you can access Funfull's national-level partners like AMC, Cinemark, Regal, and Chuck-E-Cheese. Funfull also offers hundreds of fun places across seven markets (MD, DE, VA, IL, MO, PA, ID) in the USA. Keep having fun!
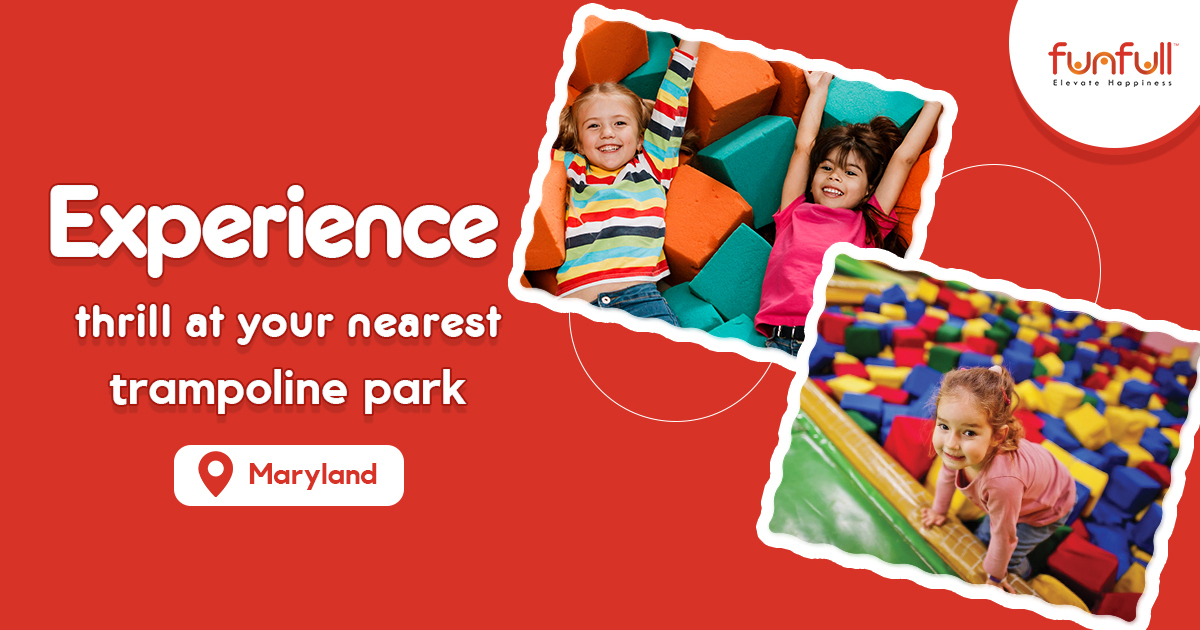
| funfull |
|
1,912,286 | Premium Hacks & Cheats By CHEATSERVICE | Explore premium hacks and cheats carefully chosen by CHEATSERVICE for top-tier gaming experiences.... | 0 | 2024-07-05T05:21:20 | https://dev.to/alexgrace012/premium-hacks-cheats-by-cheatservice-3e3b | Explore premium hacks and cheats carefully chosen by [CHEATSERVICE](https://cheatservice.co/) for top-tier gaming experiences. With elite offerings tested rigorously for outstanding performance and reliability, why settle for anything less? Benefit from undetectable cheats created by trusted developers prioritizing excellence over quantity. Safeguard your gaming environment with continuous updates, ensuring unmatched security. Experience around-the-clock live chat support for immediate assistance and expect prompt resolutions for seamless gameplay. Stay ahead with daily updates enhancing cheat performance while offering unbeatable pricing and a full compensation policy. Reveal the ultimate gaming experience with CHEATSERVICE's exceptional cheats and hacks.
**Key Takeaways**
- Rigorous testing and curation for premium quality.
- Undetectable cheats by anti-cheat systems.
- Dedicated support with 24/7 live chat assistance.
- Daily updates and maintenance for optimal performance.
- Competitive pricing without compromising excellence.
**Hand-Picked Quality Hacks**
The selection process for our [hand-picked quality hacks](https://cheatservice.co/our-hacks/) guarantees outstanding performance and reliability. Each hack undergoes rigorous testing and curation to ensure excellent quality.
Our approach prioritizes excellence over quantity, offering a select number of premium cheats that have been carefully vetted for best performance. Trusted developers design our hand-picked hacks, focusing on delivering cheats that meet the highest standards.
Customers can expect nothing less than exceptional performance and reliability from our exclusive selection of cheats, tailored to provide the most favorable results.
**Why Choose Us?**
For those seeking unparalleled quality and reliability in their cheats and hacks, our platform stands out as the best choice. With trusted developers boasting a proven track record of creating undetected cheats, users can rest assured that they're accessing premium tools for their gaming needs.
Our commitment to excellence is further exemplified by the availability of live chat customer support on the website, providing quick assistance whenever necessary.
Moreover, our dedication to staying ahead of detection is evident through daily updates and infrastructure improvements, ensuring that our hacks remain undetected.
Despite offering high-quality, we believe in providing affordable access to our services, with competitive pricing that offers great value for our users.
Additionally, by joining our supportive community on Discord, users can't only network and engage with like-minded individuals but also benefit from a collective knowledge base that enhances their overall gaming experience.
**Safety Measures**
To guarantee a secure gaming environment, our platform implements rigorous safety measures to protect users while utilizing our undetected cheats and hacks. Our hacks are designed to be undetectable by anti-cheat systems, ensuring that players can enjoy their gaming experience without the fear of being banned.
We prioritize security by continuously updating and maintaining our cheats to stay ahead of detection mechanisms employed by game developers.
All our cheats are created by trusted professionals within the hacking community, guaranteeing their reliability and safety for our users. With our hacker-to-hacker platform, users can securely purchase and utilize cheats without compromising their personal information or risking any security breaches.
Our industry-leading game hacks are tailored to provide a safe and enjoyable gaming experience for all our customers, maintaining the integrity of their gameplay while enhancing their overall enjoyment.
**Dedicated Support Team**
The dedicated support team at Premium Hacks & Cheats provides live chat assistance for immediate help with any hack-related concerns.
With quick response times, customers can rely on the team for prompt resolutions to their queries, ensuring a smooth experience.
This support system guarantees full compensation, including a 100% playtime guarantee and refunds for incomplete updates.
**Live Chat Assistance**
Our dedicated support team offers real-time live chat assistance for users seeking help with our premium hacks and cheats.
When you reach out to our support team, you can expect to receive prompt and expert guidance from our knowledgeable team members who specialize in hacking and game cheats.
Our live chat service guarantees quick response times, enabling efficient resolution of any concerns you may encounter while using our cheats.
Customer support is paramount to us, and we're committed to providing the best support experience for all our users.
Whether you require assistance with installation, troubleshooting, or have any other queries, our live chat assistance is available 24/7 to help you navigate through any challenges you may face.
Rest assured that our dedicated team is here to support you every step of the way, ensuring a smooth and seamless experience with our premium hacks and cheats.
**Quick Response Time**
With a focus on efficiency, the dedicated support team guarantees quick responses to user issues and queries. When you encounter any concerns or need assistance, our support team is committed to providing timely solutions.
Through our live chat service, immediate help is just a click away, ensuring that you can swiftly address any issues that may arise. We take pride in offering the best support experience by prioritizing quick and efficient responses to your inquiries, allowing you to continue enjoying our premium hacks and cheats without interruptions.
Our support team remains available on our website at all times, ready to assist you with any questions or problems you may encounter. Rest assured that you can rely on our dedicated support team to deliver prompt and helpful responses, ensuring a seamless experience with our services.
**Frequent Updates**
Frequent updates are a cornerstone of maintaining top-tier cheat systems. These updates involve daily maintenance tasks, ensuring cheats remain undetected and effective.
Additionally, strategies for avoiding detection are continuously enhanced to keep users protected.
**Daily Maintenance Tasks**
Regularly performing maintenance tasks is essential to guarantee the smooth operation and efficiency of our cheat system. These tasks encompass infrastructure improvements aimed at optimizing performance and ensuring seamless user experiences.
Daily updates play a pivotal role in staying ahead of detection methods, safeguarding the integrity of our cheats. Maintaining key parts of our system is paramount to its functionality, warranting meticulous attention to detail. Our ongoing enhancements are meticulously crafted to deliver the best possible user experience, reflecting our commitment to quality and innovation.
In the event of updates or maintenance causing any inconvenience, we provide full compensation for any lost time, prioritizing customer satisfaction and ensuring a seamless journey for our users. By prioritizing daily maintenance tasks and frequent updates, we reinforce our dedication to excellence and reliability in providing premium cheat services to our valued clientele.
**Detection Avoidance Strategies**
To maintain a competitive edge and ensure user security, the cheat system undergoes frequent updates to evade detection by anti-cheat systems.
Our hacks receive daily updates, striving to stay ahead of detection mechanisms. These updates not only focus on enhancing cheat performance but also include infrastructure improvements to fortify the security of our cheats.
Key components of our cheats undergo regular maintenance to address any potential vulnerabilities that could be exploited by anti-cheat systems. By continuously enhancing our cheat system, we aim to improve performance and evade detection effectively.
This proactive approach not only benefits our users by providing them with the best protection against detection but also ensures that their gameplay remains undisturbed.
Through these meticulous detection avoidance strategies, we aim to uphold the integrity of our cheat system and offer an unparalleled gaming experience to our users.
**Enhanced Cheat Performance**
Regularly updating our cheats guarantees they remain undetected and perform at their best in gameplay. With daily updates, our cheats stay ahead of detection, ensuring effectiveness while maintaining security.
Infrastructure improvements are continuously made to enhance cheat performance and reliability, optimizing your gaming experience. Our cheat system enhancements prioritize security, providing you with a seamless and efficient hacking experience. Maintenance of key parts is conducted regularly to address any potential issues and further enhance cheat performance.
Additionally, cheat update protection is in place to guarantee that your hacks are always up-to-date and ready to use whenever you need them. By prioritizing frequent updates and infrastructure enhancements, we aim to provide you with the best cheat performance possible, ensuring that your gaming remains undisturbed and enjoyable.
**Full Compensation Policy**
If a customer's key duration isn't met, our Full Compensation Policy guarantees they receive compensation for the lost time. This policy includes a 100% playtime guarantee for all our hacks, ensuring customers get the value they deserve.
In cases where an update completion is delayed, customers are eligible for a refund, demonstrating our commitment to reliable service. We go the extra mile by providing protection for hacks against updates, ensuring uninterrupted access for our users.
Our Full Compensation Policy is designed to prioritize customer satisfaction and offer peace of mind when using our [premium hacks](https://cheatservice.co/games/). By adhering to this policy, we aim to build trust with our customers and deliver on our promise of high-quality service.
Rest assured that with our Full Compensation Policy in place, you can confidently enjoy our hacks knowing that your satisfaction is our top priority.
**Cheapest Prices Offered**
Customers seeking high-quality cheats at unbeatable prices can rely on CHEATSERVICE's dedication to offering the most economical prices in the market. With our premium hacks and cheats, we aim to provide cost-effective solutions without compromising on excellence.
Our competitive pricing strategy guarantees that gamers can access exceptional cheats while staying within their budget. At CHEATSERVICE, we prioritize value for money, allowing customers to enjoy expertly curated cheats at unmatched prices.
Whether you're looking for a competitive edge or simply want to enhance your gaming experience, our budget-friendly options cater to all needs. By maintaining the lowest prices without sacrificing quality, we guarantee that players receive the best of both worlds.
Choose CHEATSERVICE for the most affordable prices on premium hacks and cheats, where affordability meets reliability seamlessly. Experience the thrill of gaming with our economical yet high-quality cheat options today.
| alexgrace012 |
|
1,912,284 | Build an Angular Video Chat App | Popular chat messaging apps like WhatsApp and Telegram offer real-time video calls, while video... | 0 | 2024-07-05T05:21:02 | https://getstream.io/blog/angular-video-chat-app/ | angular, javascript, css, html |
Popular chat messaging apps like WhatsApp and Telegram offer real-time video calls, while video conferencing apps like Zoom and [Google Meet](https://getstream.io/blog/zoom-clone-compose/) provide group chat during meetings. Chat apps with video calling and video conferencing apps with chat support focus on two similar communication needs.
This article guides you in building an Angular chat app with integrated video conferencing support. You can customize the final project to prioritize video conferencing with chat as a secondary feature.
## Prerequisites
The two main features of the sample project in this tutorial are chat messaging and video calling. For messaging functionality, we will use the [Stream Chat Angular SDK](https://getstream.io/chat/sdk/angular/). We will also power video calling support with the above SDK's companion [JavaScript Video SDK](https://getstream.io/video/sdk/javascript/). By integrating these two SDKs as in-apps, developers can build seamless communication experiences, allowing people to send and receive chat messages, livestream, video call, join, and leave live audio room conversations in their apps.
You can recreate this tutorial’s project with your favorite IDE, preferably [VS Code](https://code.visualstudio.com/).
## Run the Source Code
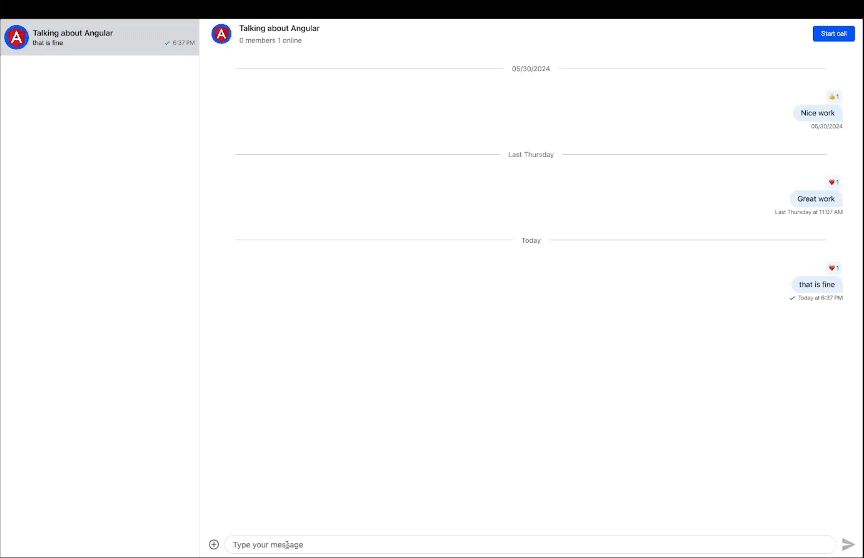
To test the sample project, you can clone and have a local version or create a GitHub Codespace for the app in this [repo](https://github.com/GetStream/angular-chat-video-demo).
## Start With the Chat Feature
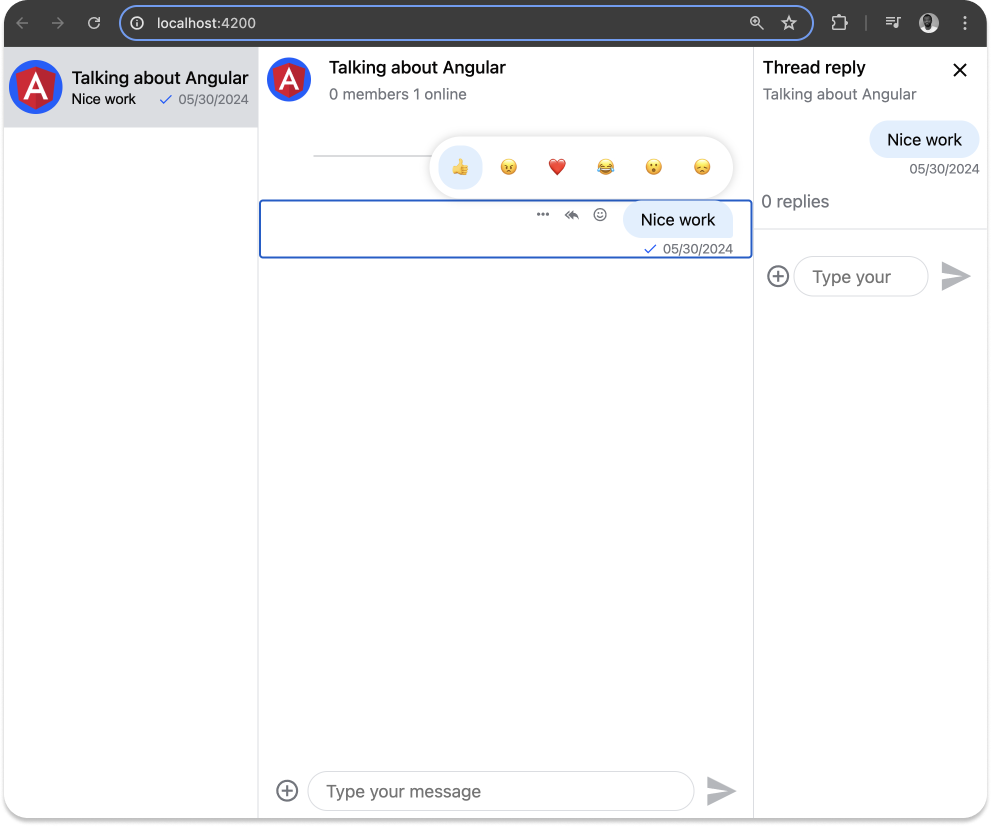
In this section, you will build the Angular chat UI with a look and feel similar to the image above. The leading chat features include instant messaging, media attachments, emojis, threads and replies, and [message interactions](https://getstream.io/chat/docs/sdk/angular/concepts/message-interactions/). Let's initiate a new Angular project and get started with the following steps.
### Create a New Angular Project
Creating a new Angular project requires the installation of [Node.js](https://nodejs.org/en). Open Terminal or your favorite command line tool and check the version of Node on your machine with the following:
`node -v`
If you don't have Node installed, click the link above to install the latest stable (LTS) version.
Execute the following command to install the [Angular CLI](https://www.npmjs.com/package/@angular/cli) globally on your machine.
`npm install -g @angular/cli`
**Note**: On macOS, you may be required to prepend `sudo` to the above command.
`sudo npm install -g @angular/cli`
With the Angular CLI installed, you can now create a new project with:
`ng new video-chat-angular --routing false --style css --ssr false`
where `video-chat-angular` is the project name. For styling, we use ***CSS* and also turn off routing. You can use whatever name you want for the project.
Open the app in your IDE and install the necessary dependencies as follows.
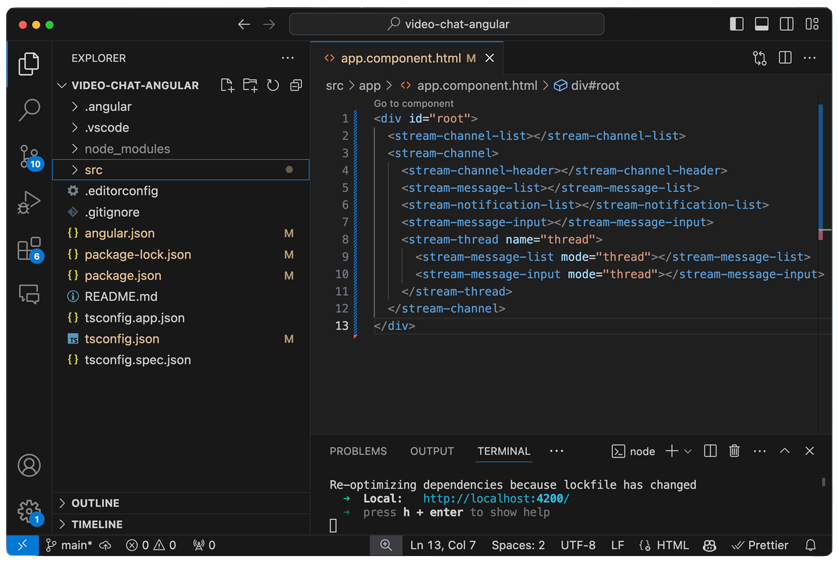
The example above shows the app opened in VS Code. Open the integrated terminal in VS Code and install Stream Chat Angular as a dependency with the following.
`npm install stream-chat-angular stream-chat @ngx-translate/core`
- `Stream-chat-angular`: [Stream Chat Angular](https://github.com/GetStream/stream-chat-angular) consists of reusable Angular chat components.
- `stream-chat`: The core Angular SDK without UI components. You can use this to build a completely custom chat messaging experience.
- `ngx-translate/core`: An internationalization (i18n) [library](https://github.com/ngx-translate/core) for Angular projects.
## Configure the Angular Chat SDK
Before we setup the Angular SDK, we should add the required imports in the project's `src/app/app.config.ts` and `src/app/app.component.ts`
Open the file `src/app/app.config.ts` and import the `TranslateModule` in `appConfig` with the following.
`providers: [importProvidersFrom(TranslateModule.forRoot())],`
Next, open `src/app/app.component.ts`, and import the `TranslateModule`, `StreamAutocompleteTextareaModule`, `StreamChatModule` in the `@Component` decorator.
`imports: [TranslateModule, StreamAutocompleteTextareaModule, StreamChatModule],`
The modified content of this file should look like this:
```typescript
import { Component, OnInit } from '@angular/core';
import { TranslateModule } from '@ngx-translate/core';
import {
ChatClientService,
ChannelService,
StreamI18nService,
StreamAutocompleteTextareaModule,
StreamChatModule,
} from 'stream-chat-angular';
// Video imports
@Component({
selector: 'app-root',
standalone: true,
imports: [TranslateModule, StreamAutocompleteTextareaModule, StreamChatModule],
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent implements OnInit {
constructor(
private chatService: ChatClientService,
private channelService: ChannelService,
private streamI18nService: StreamI18nService,
) {
const apiKey = 'REPLACE_WITH_YOUR_STREAM_APIKEY';
const userId = 'REPLACE_WITH_YOUR_STREAM_USERID';
const userToken = 'REPLACE_WITH_TOKEN';
this.chatService.init(apiKey, userId, userToken);
this.streamI18nService.setTranslation();
}
async ngOnInit() {
const channel = this.chatService.chatClient.channel('messaging', 'talking-about-angular', {
// add as many custom fields as you'd like
image: 'https://upload.wikimedia.org/wikipedia/commons/thumb/c/cf/Angular_full_color_logo.svg/2048px-Angular_full_color_logo.svg.png',
name: 'Talking about Angular',
});
await channel.create();
this.channelService.init({
type: 'messaging',
id: { $eq: 'talking-about-angular' },
});
}
}
```
In the code above, a valid user should be connected to the SDK's backend infrastructure to access the chat functionality. We are using a hard-coded user credential from our [Angular Chat Tutorial](https://getstream.io/chat/angular/tutorial/). To implement a chat functionality for a production app using this SDK, you should [sign up](https://getstream.io/try-for-free/) for a Dashboard account. On your Stream's Dashboard, you will find your API key to generate a token with our [User JWT Generator](https://getstream.io/chat/docs/react/token_generator/). After filling out the user credentials, we create a new channel and define a condition to populate it when the app runs.
In addition to the above configurations, the Stream Chat SDK uses TypeScript's concept [Allow Synthetic Default Imports](https://www.typescriptlang.org/tsconfig/#allowSyntheticDefaultImports) to write default imports in a more efficient way. Add the following to `tsconfig.json` in the project's root folder to turn it on.
`"allowSyntheticDefaultImports": true`
## Configure the Chat UI Structure and Style
In our generated Angular project, the UI structure is defined in `src/app/app.component.html`, while its styles are declared in `src/app/styles.css`. Replace the HTML content of `src/app/app.component.html` with the following to load the SDK's chat components such as [Channel](https://getstream.io/chat/docs/sdk/angular/components/ChannelComponent/), [Message List](https://getstream.io/chat/docs/sdk/angular/components/MessageComponent/), [Message Input](https://getstream.io/chat/docs/sdk/angular/components/MessageInputComponent/), and [Thread](https://getstream.io/chat/docs/sdk/angular/components/ThreadComponent/) when the app launches.
```html
<div id="root">
<stream-channel-list></stream-channel-list>
<stream-channel>
<stream-channel-header></stream-channel-header>
<stream-message-list></stream-message-list>
<stream-notification-list></stream-notification-list>
<stream-message-input></stream-message-input>
<stream-thread name="thread">
<stream-message-list mode="thread"></stream-message-list>
<stream-message-input mode="thread"></stream-message-input>
</stream-thread>
</stream-channel>
</div>
```
Let's define the following **CSS** styles in `src/app/styles.css`. The styles we specify here do not include that of the video calling integration. We will modify this file again in a later section to cover that.
```css
@import 'stream-chat-angular/src/assets/styles/v2/css/index.css';
html {
height: 100%;
}
body {
margin: 0;
height: 100%;
}
#root {
display: flex;
height: 100%;
stream-channel-list {
width: 30%;
}
stream-channel {
width: 100%;
}
stream-thread {
width: 45%;
}
}
```
## Test the Chat Functionality
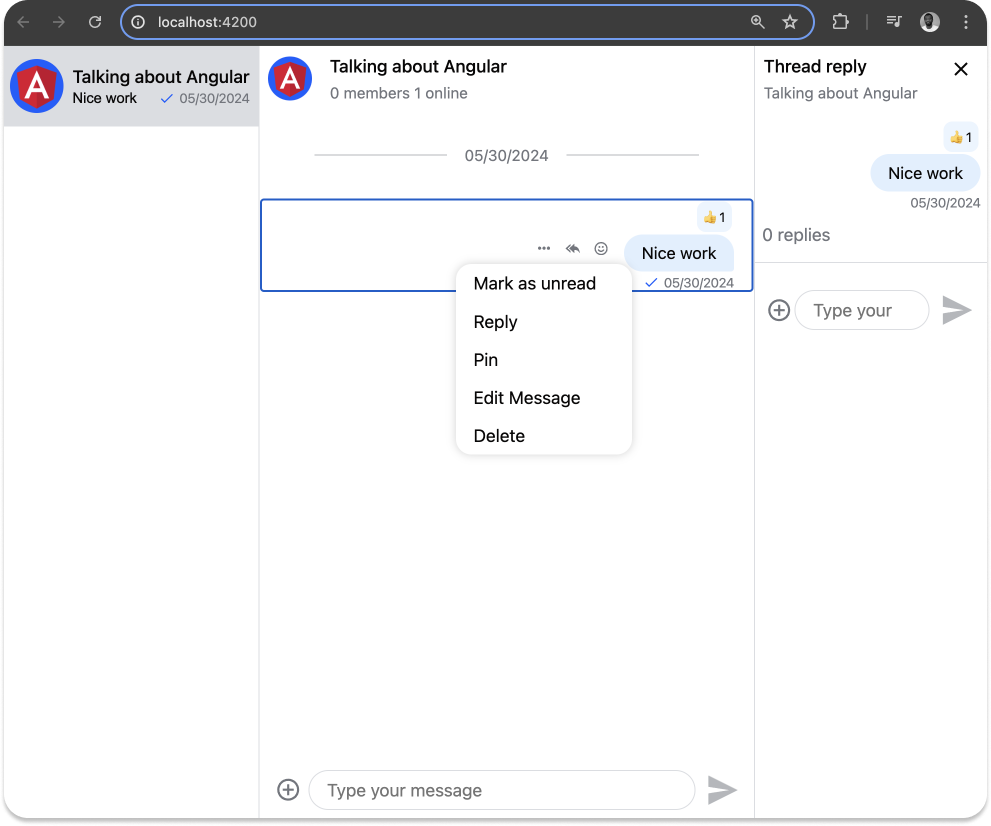
To start the development server in VS Code to run and test the chat version, launch the integrated Terminal and run:
`ng serve` or `npm start`
When the server runs successfully, you should see a screen similar to the one below.
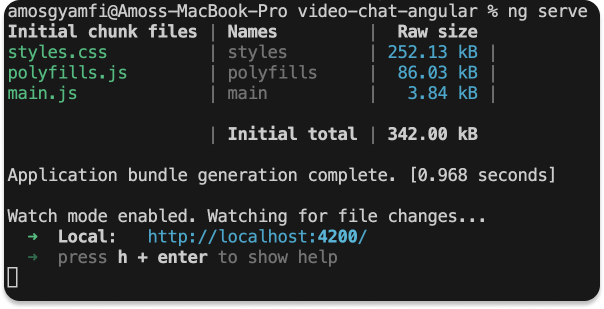
Open the link to the localhost http://localhost:4200/ in your preferred browser and start testing the chat interface.
For the rest of this tutorial, let's implement the ability to make video calls by clicking a button at the top-right of the messages list in the chat window.
## Integrate the Video Calling Functionality
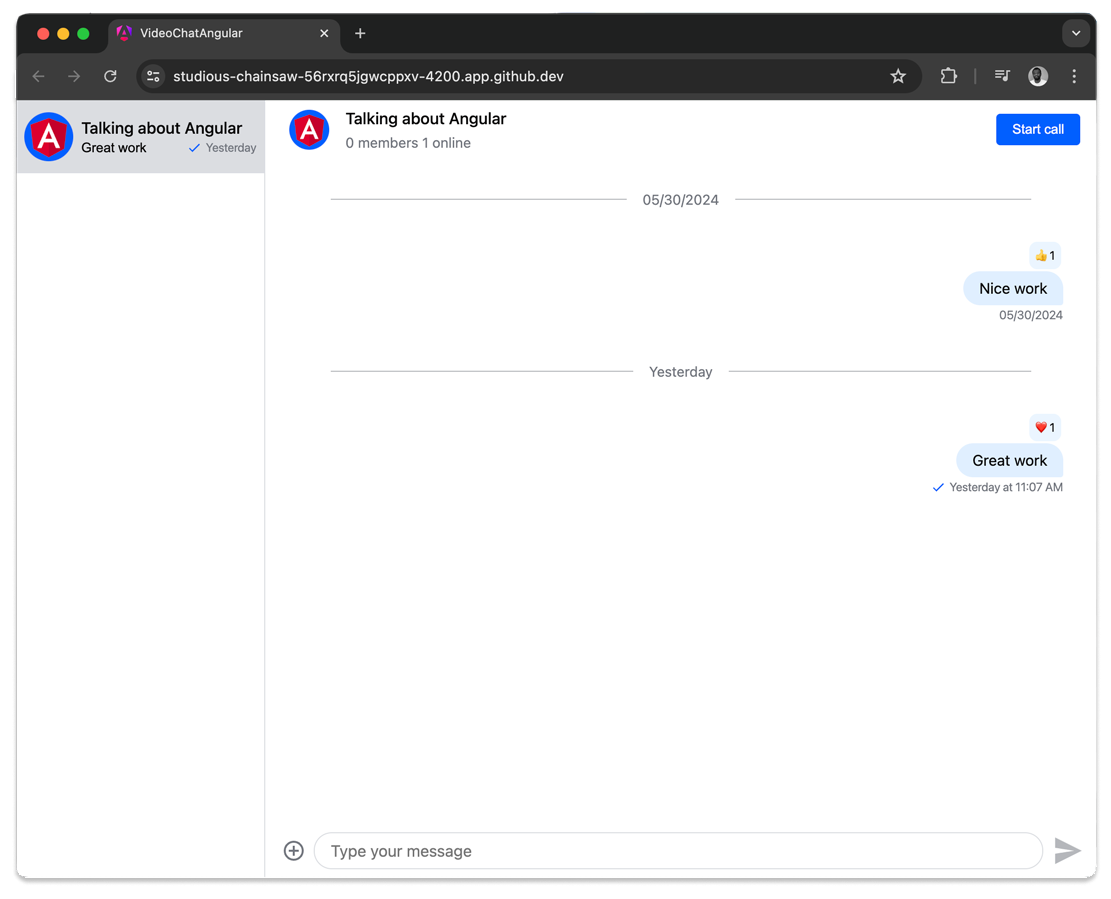
To allow users to initiate video calls from the chat UI, we will use Stream Video's [low-level JavaScript client](https://getstream.io/video/docs/javascript/), which developers can implement with any other SDK or platform.
You can test the app's video calling feature by creating a GitHub Codespace from the [final project](https://github.com/GetStream/angular-chat-video-demo). A Codespace allows you to run and test the app in the browser without cloning and installing it from the GitHub repo.
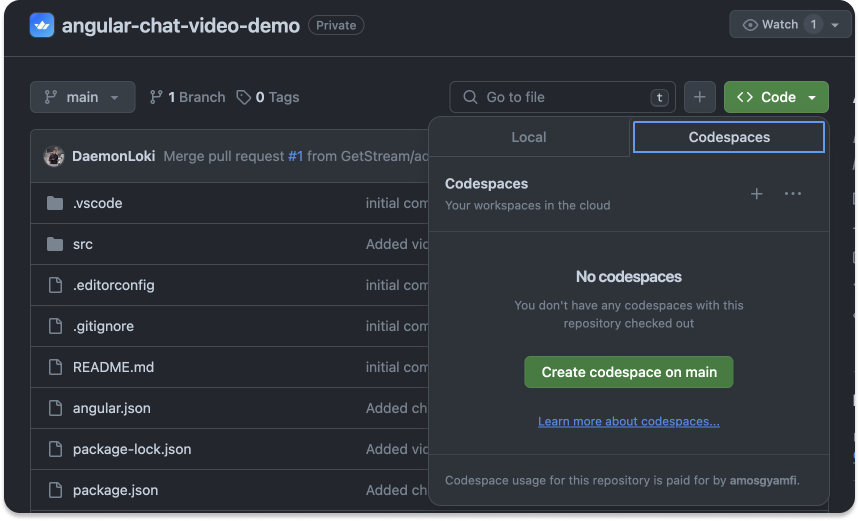
## Install and Configure the JavaScript Video SDK
To install the Video SDK, navigate to the project’s root folder in VS Code, open a new Terminal instance and run the following.
`npm i @stream-io/video-client`
Then start the development server with:
`npm start`
### Create a Video Calling Service
Ensure you are still in the project's `app` folder `/video-chat-angular/src/app`. Then, use the Angular CLI command below to generate a calling service.
`ng generate service calling`
After running the above, you will find two additional generated files When you expand the `app` directory to see its content.
- calling.service.spec.ts
- calling.service.ts
**Note**: The links above do not contain the original generated content. They have been modified to provide our calling service.
Let's open `calling.service.ts` and substitute what is in the file with the following.
```typescript
import { Injectable, computed, signal } from '@angular/core';
import { Call, StreamVideoClient, User } from '@stream-io/video-client';
@Injectable({
providedIn: 'root',
})
export class CallingService {
callId = signal<string | undefined>(undefined);
call = computed<Call | undefined>(() => {
const currentCallId = this.callId();
if (currentCallId !== undefined) {
const call = this.client.call('default', currentCallId);
call.join({ create: true }).then(async () => {
call.camera.enable();
call.microphone.enable();
});
return call;
} else {
return undefined;
}
});
client: StreamVideoClient;
constructor() {
const apiKey = '5cs7r9gv7sny';
const token =
'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyX2lkIjoiZmxvcmFsLW1lYWRvdy01In0.uASF95J3UqfS8zMtza0opjhtGk7r7xW0SJACsHXF0Do';
const user: User = { id: 'floral-meadow-5' };
this.client = new StreamVideoClient({ apiKey, token, user });
}
setCallId(callId: string | undefined) {
if (callId === undefined) {
this.call()?.leave();
}
this.callId.set(callId);
}
}
```
The service we created with the sample code above is designed to manage [video calls](https://getstream.io/video/sdk/javascript/tutorial/video-calling/), including [joining and creating calls](https://getstream.io/video/docs/javascript/guides/joining-and-creating-calls/) with video and audio enabled and leaving calls. We use a reactive programming pattern [Angular Signals](https://angular.dev/guide/signals) to manage state changes reactively. Check out our [YouTube tutorial](https://www.youtube.com/watch?v=lb_6vUfVAr8) to learn more about creating a video calling app with Angular Signals.
First, we import the JavaScript video SDK, create a video client instance, and initialize it with an API key and token. Check out our documentation's [Client and Authentication](https://getstream.io/video/docs/javascript/guides/client-auth/) section to learn more about user types and how to generate a user token for your projects.
Finally, we create and join a call if it has not been created using the `call.join` method. The `call.join` method also allows real-time audio and video transmission.
Next, open `calling.service.spec.ts` and replace the original code with the sample code below.
```typescript
import { TestBed } from '@angular/core/testing';
import { CallingService } from './calling.service';
describe('CallingService', () => {
let service: CallingService;
beforeEach(() => {
TestBed.configureTestingModule({});
service = TestBed.inject(CallingService);
});
it('should be created', () => {
expect(service).toBeTruthy();
});
});
```
The sample code above performs a basic unit test configuration for the `CallingService`. It checks to see if a calling service can be created or successfully initiated.
## How to Manage Audio/Video Calls
In this section, we should define a component to manage audio and video call-related functions such as toggling the microphone and camera on and off, accepting and leaving calls, and identifying participants with session IDs. Navigate to the app's `src/app` directory and create a new folder **call**.
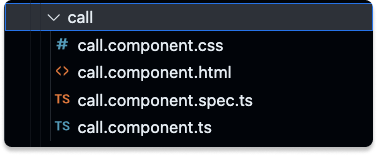
Add the following files inside the **call** folder and fill out each of their content by copying them from the [GitHub project’s](https://github.com/GetStream/angular-chat-video-demo) links below.
- [call.component.css](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/call/call.component.css)
- [call.component.html](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/call/call.component.html)
- [call.component.spec.ts](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/call/call.component.spec.ts)
- [call.component.ts](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/call/call.component.ts)
The files above provide the required UI and the logic for managing participants’ states and controlling microphone and camera states during audio/video calls.
## Display and Manage a Call Participant
In this section, we should define a component that sets up the necessary bindings for displaying a participant's video and playing audio in a video call. Let's create the following directory `src/app/participant`.
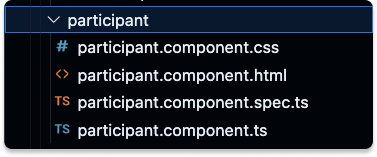
Add the participant's files in the image above in the **call** directory and replace each piece of content by copying it from its link to the GitHub project.
- [participant.component.css](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/participant/participant.component.css)
- [participant.component.html](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/participant/participant.component.html)
- [participant.component.spec.ts](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/participant/participant.component.spec.ts)
- [participant.component.ts](https://github.com/GetStream/angular-chat-video-demo/blob/main/src/app/participant/participant.component.ts)
## Add a Start Call Button to the Chat UI
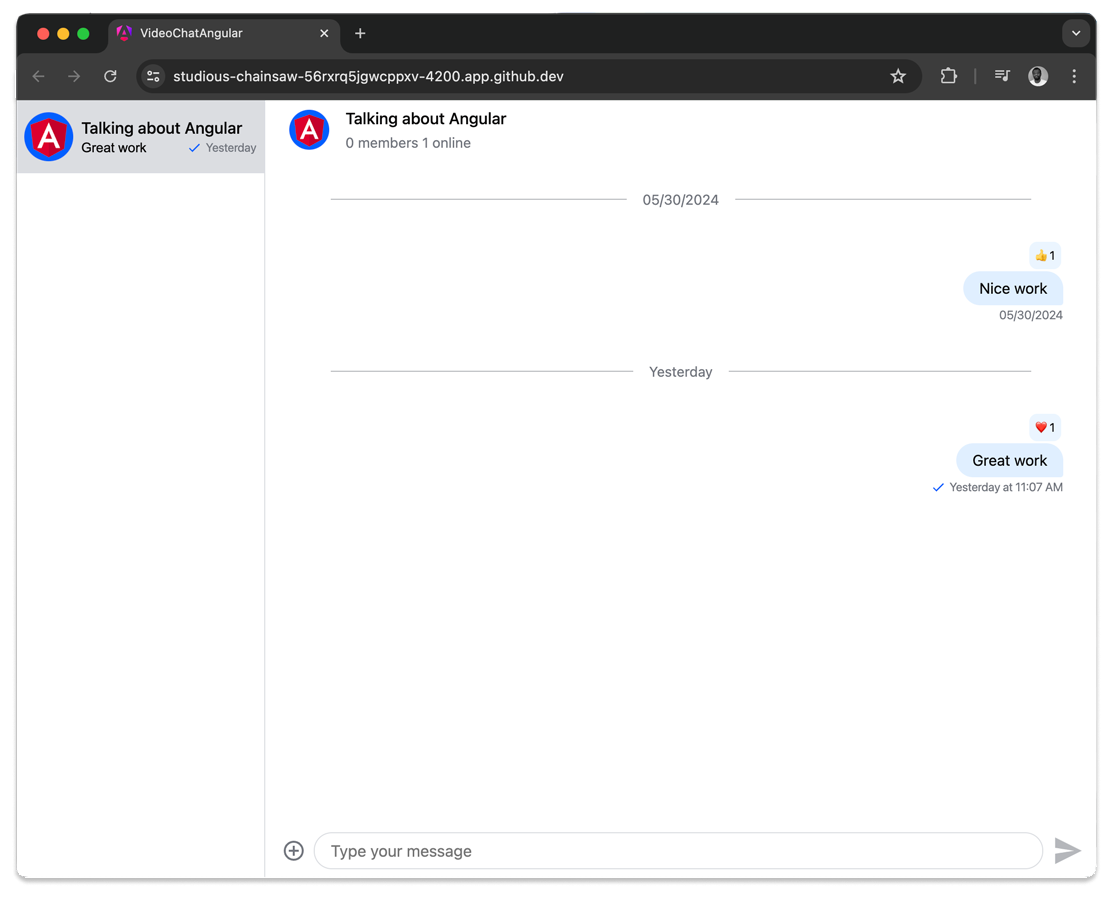
So far, the header of the chat UI's messages list consists of an avatar and the chat channel's title. Let's modify the message list’s header to add a **start call** button. We will also implement additional styles for the start button.
Open `style.css` located in the project's root folder and add this code snippet below the `@import`.
```css
:root {
--custom-blue: hsl(218, 100%, 50%);
--custom-blue-hover: hsl(218, 100%, 70%);
--custom-red: hsl(0, 80%, 60%);
--custom-red-hover: hsl(0, 80%, 50%);
}
```
The modified content of `style.css` is shown below.
```css
@import "stream-chat-angular/src/assets/styles/v2/css/index.css";
:root {
--custom-blue: hsl(218, 100%, 50%);
--custom-blue-hover: hsl(218, 100%, 70%);
--custom-red: hsl(0, 80%, 60%);
--custom-red-hover: hsl(0, 80%, 50%);
}
html {
height: 100%;
}
body {
margin: 0;
height: 100%;
}
#root {
display: flex;
height: 100%;
stream-channel-list {
width: 30%;
}
stream-channel {
width: 100%;
}
stream-thread {
width: 45%;
}
}
```
Locate `app.component.css`, an empty CSS file that comes with Angular project generation. Fill out its content with the following style snippet.
```css
.header-container {
display: flex;
align-items: center;
justify-content: space-between;
padding: 0 1rem;
}
button {
background: #005fff;
color: white;
padding: 0.5rem 1rem;
border-radius: 0.25rem;
border: none;
cursor: pointer;
}
```
Next, let's add the call service to the HTML structure of the chat UI.
```html
</ng-container>
<ng-container *ngIf="callingService.callId()">
<app-call [call]="callingService.call()!"></app-call>
</ng-container>
```
You must add the code snippet above to the `app.component.html` file in the `app` directory.
```html
<div id="root">
<stream-channel-list></stream-channel-list>
<ng-container *ngIf="!callingService.callId()">
<stream-channel>
<div class="header-container">
<stream-channel-header></stream-channel-header>
<button (click)="startCall()">Start call</button>
</div>
<stream-message-list></stream-message-list>
<stream-notification-list></stream-notification-list>
<stream-message-input></stream-message-input>
<stream-thread name="thread">
<stream-message-list mode="thread"></stream-message-list>
<stream-message-input mode="thread"></stream-message-input>
</stream-thread>
</stream-channel>
</ng-container>
<ng-container *ngIf="callingService.callId()">
<app-call [call]="callingService.call()!"></app-call>
</ng-container>
</div>
```
Finally, we should modify the `app.component.ts` file that was added in one of the chat sections to import the `CallingService` and `CallComponent` and add a `startCall` method. The modified sample code in `app.component.ts` should look like below.
```typescript
import { Component, OnInit } from '@angular/core';
import { TranslateModule } from '@ngx-translate/core';
import {
ChatClientService,
ChannelService,
StreamI18nService,
StreamAutocompleteTextareaModule,
StreamChatModule,
} from 'stream-chat-angular';
import { CallingService } from './calling.service';
import { CommonModule } from '@angular/common';
// Video imports
import { CallComponent } from './call/call.component';
@Component({
selector: 'app-root',
standalone: true,
imports: [
TranslateModule,
StreamAutocompleteTextareaModule,
StreamChatModule,
CommonModule,
CallComponent,
],
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent implements OnInit {
callingService: CallingService;
constructor(
private chatService: ChatClientService,
private channelService: ChannelService,
private streamI18nService: StreamI18nService,
callingService: CallingService
) {
this.callingService = callingService;
const apiKey = '5cs7r9gv7sny';
const userId = 'floral-meadow-5';
const userName = 'Floral Meadow';
const userToken =
'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyX2lkIjoiZmxvcmFsLW1lYWRvdy01In0.uASF95J3UqfS8zMtza0opjhtGk7r7xW0SJACsHXF0Do';
this.chatService.init(apiKey, userId, userToken);
this.streamI18nService.setTranslation();
}
async ngOnInit() {
const channel = this.chatService.chatClient.channel(
'messaging',
'talking-about-angular',
{
// add as many custom fields as you'd like
image:
'https://upload.wikimedia.org/wikipedia/commons/thumb/c/cf/Angular_full_color_logo.svg/2048px-Angular_full_color_logo.svg.png',
name: 'Talking about Angular',
}
);
await channel.create();
this.channelService.init({
type: 'messaging',
id: { $eq: 'talking-about-angular' },
});
}
startCall() {
const channelId = this.channelService.activeChannel?.id;
this.callingService.setCallId(channelId);
}
}
```
## Test the Integrated Chat and Video Calling Features
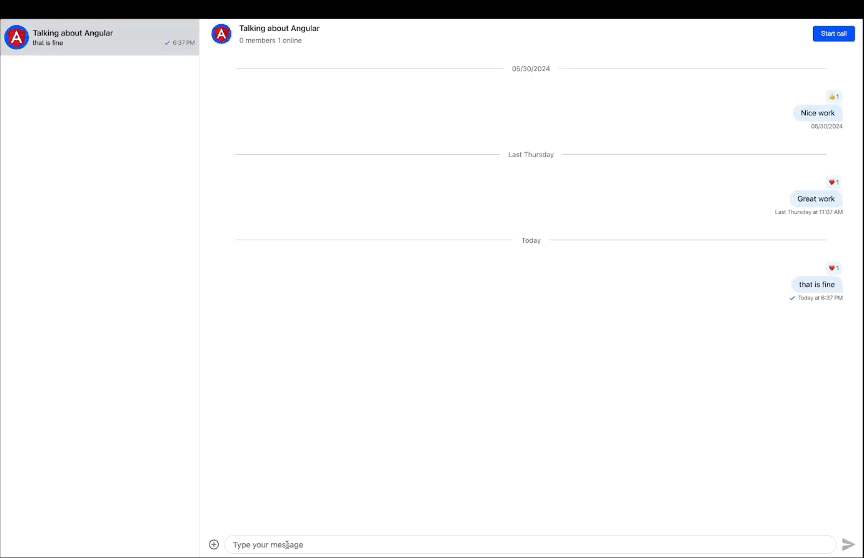
Start the development server again with:
`npm start`
The start call button you just implemented will appear at the top right of the message list's header. Clicking the button presents a live video of the local participant. On the live video or active call screen, there are buttons for performing standard call operations like switching the camera and microphone on and off and ending a call.
## Conclusion
Congratulations! 👏 You have followed all the steps to build a real-time and fully functioning Angular video chat application. The app allows users to send and receive rich text chats, add media as attachments, and connect with others through face-to-face video calling. Explore the related links of this tutorial to learn more about the advanced features and capabilities of the [Angular Chat](https://getstream.io/chat/sdk/angular/) and [JavaScript Video SDKs](https://getstream.io/video/sdk/javascript/).
| amosgyamfi |
1,912,250 | Buy Tapentadol 100mg Online with Fast & Secure Delivery | Buy Tapentadol 100mg Online with Fast & Secure Delivery Pain management is a crucial aspect of... | 0 | 2024-07-05T05:18:52 | https://dev.to/health_hub_daf376d3963572/buy-tapentadol-100mg-online-with-fast-secure-delivery-5nb | Buy Tapentadol 100mg Online with Fast & Secure Delivery
Pain management is a crucial aspect of modern medicine, and effective pain control is one of the toughest parts of treatments involving injury or trauma. Painkillers are arguably been the best-selling medications in America over the past few years. Both opioid and non-opioid medications have considerably increased their sales in US pharmacies. **Tapentadol** is one such opioid analgesic that has found its own space in both traditional and online pharmacies. For effective and instant pain relief, you can **Buy Tapentadol Online in USA with or without a Prescription**. However, it’s advised to purchase opioid medications with a recent medical prescription to avoid receiving counterfeit or expired products. In this article, we’ll provide some in-depth insights into how you should **[Buy Tapentadol 100mg online at Best Price in USA](https://tapentadolmeds.com/buy-tapentadol-online-with-best-price.php)** easily and quickly.
## Purchasing Pain Relief Online
When the pain strikes hard, with or without an underlying cause, it is difficult to manage it using a simple painkiller. This highlights the importance of opioid painkillers such as **Tapentadol 100 mg**. You can **[Buy Tapentadol without a Prescription](https://tapentadolmeds.com/about.php)** in emergencies from online pharmacy websites to avoid going to physical stores and waiting in long queues. The option to **[Buy tapentadol 100mg online in USA](https://tapentadolmeds.com/shop.php)** has increased its popularity among citizens due to its efficiency in primary pain management.
Convenience is the best key that opens online pharmacy stores wide to painkiller users. In the United States, most people prefer non-prescription online pharmacies to **Purchase Tapentadol** and other opioid painkillers due to the convenience and anonymity the process offers without compromising on transparency and product quality. All reputable online medical stores are licensed and registered with the US authorities to make the deal legal and secure in terms of personal and financial information.
## Tapentadol 100mg in Effective Pain Management
What is Tapentadol and How Does it Work? **Tapentadol 100 mg** is a centrally-acting opioid pain medication that belongs to the benzodiazepine class of drugs. It exhibits a dual mode of action; first, as an agonist of the mu-opioid receptor, and second, as a norepinephrine reuptake inhibitor. Physicians usually prescribe 200mg to 300mg as a daily tapentadol dose. These tablets will relieve pain within 30 minutes of ingestion, and their analgesic and sedative effects will last up to 4-6 hours. As sedation is a normal tapentadol side effect, you shouldn’t panic in such a situation.
## Tapentadol Uses and Dosage
Used in moderate to moderately severe pain conditions, **Tapentadol Tablets** do not rely on metabolic activity to produce their therapeutic effects. This property makes tapentadol pills highly recommended for patients who do not react well to non-opioid medicines for severe pain. **Tapentadol 100mg Tablets** or capsules are useful for both injury or surgery-caused acute pain and chronic pain caused by certain musculoskeletal conditions. You can also **[Buy Tapentadol 100mg Online](https://tapentadolmeds.com/)** for diabetes-related neuropathic pain, however, only after consulting a healthcare professional.
Immediate-release (IR) and extended-release (ER) forms of tapentadol are available in most US pharmacies. The former is used in moderate to severe acute pain, while the latter is used in severe chronic pain. Tapentadol IR is sold in 50mg, 75mg, and 100mg dosages, whereas the ER form is available in 50mg, 100mg, 150mg, and 200mg. This medication is listed by the FDA under category C-controlled substance, making it inappropriate and dangerous for certain age groups and pregnant women. So, be familiar with the indications and contraindications of the medication before you **buy tapentadol online**.
## Purchase tapentadol 100mg Online with Overnight and Fast Delivery
Despite the warnings due to tapentadol being a controlled substance, many users purchase it from online sources. The main reason why so many people prefer online purchases of tapentadol is the reduced and cheaper prices with fast and secure delivery. Almost every dosage and formulation of the medication is available in online pharmacies. Regardless of your location, their delivery services will reach you within hours.
Apart from the **100mg dosage**, you can **Buy Tapentadol 75mg online** when pain management requires a lower dosage. In case of an emergency during the night and you need **Tapentadol Tablets with Overnight delivery** and fast shipping, you can visit our website at **[https://tapentadol100mgonline.com/]** to order your preferred dosage of pain medication. It is better to **Buy Tapentadol 75mg online** if Tapentadol tablets are not available, as both medications are similar in controlling pain in adults.
## FAQs on Buy Tapentadol 100mg Online with Fast & Secure Delivery
**Q. Why is tapentadol 100mg costly?**
**Ans.** Tapentadol 100mg may be costly in traditional pharmacies. Purchase it from an online source to get it at a cheaper price and with discounts.
**Q. Are tapentadol tablets available in 150mg dosage?**
**Ans.** Yes, tapentadol extended-release tablets are available in 100mg dosage.
**Q. Does the tapentadol pill relieve pain faster?**
**Ans.** Yes, **Tapentadol 100mg Tablets** can relieve pain within 30 minutes.
| health_hub_daf376d3963572 |
|
1,911,184 | Create Convincing UX mockups using PowerPoint! | Ever wondered if there was just a tool for everything. Indeed, there is one, Microsoft PowerPoint.... | 0 | 2024-07-05T05:17:35 | https://dev.to/chandruchiku/eye-catching-ux-mockups-using-powerpoint-544a | mockup, powerpoint, ux, uxdesign | Ever wondered if there was just a tool for everything. Indeed, there is one, Microsoft PowerPoint. One tool for all your needs.
Imagine you are a product manager or team lead who wants to showcase a new idea or a UX wireframe to the team. Of course, if you have had completed a course in Figma or Adobe XD, it would be walk in the park. Otherwise, it would be plain old tools like draw.io, Visio etc. And they look like this.
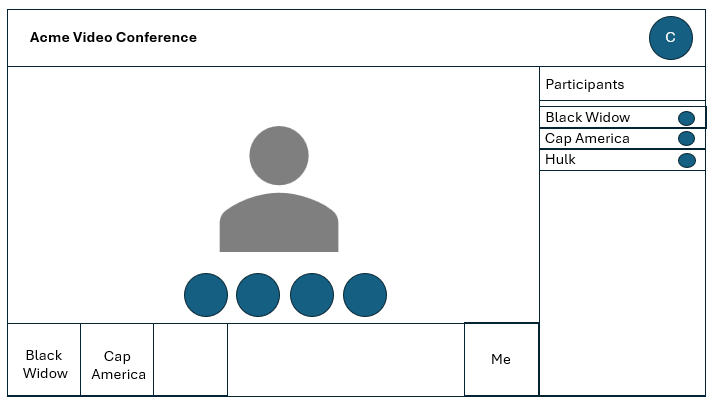
What if you could just leverage your existing skills and spend one time effort to create a common set of UX Components in PowerPoint and use them for your UX wireframe and prototyping and make your UX look way better to present and add some extra wow factor? And transform the same wireframe to this.
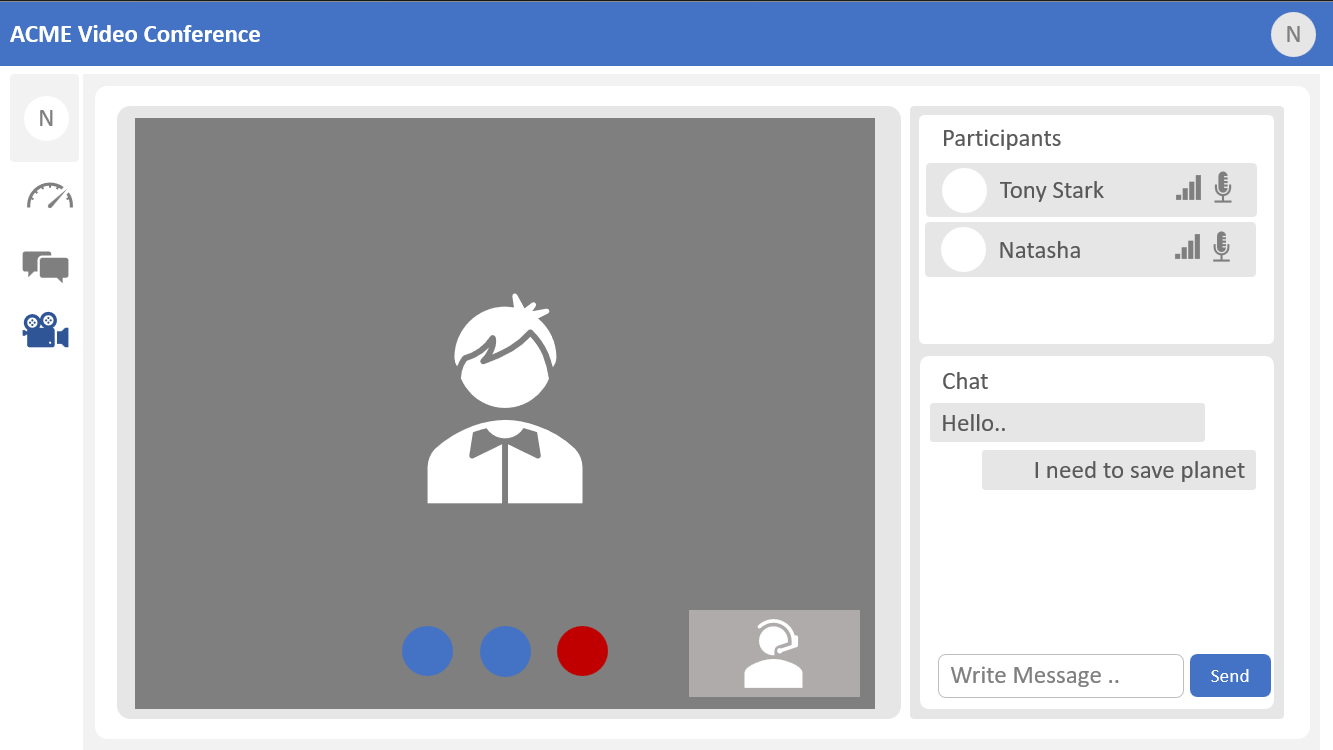
I understand that Figma/Adobe XD have their advantages and use cases which are beyond the scope of this article. What we are trying to do is just use existing PPT skills to create quick UX mockups without even talking to your UX team. For example, you are a small team and don't want to spend on expensive license or your UX person is on leave, and you urgently need a mockup.
**Let's get started:**
Our first step is to create a PowerPoint slide with the commonly used components (like button, text, slider, toggle), and couple of other slides as templates. Subsequently we can copy paste them to modify into mockup screens.
<!-- add image! -->
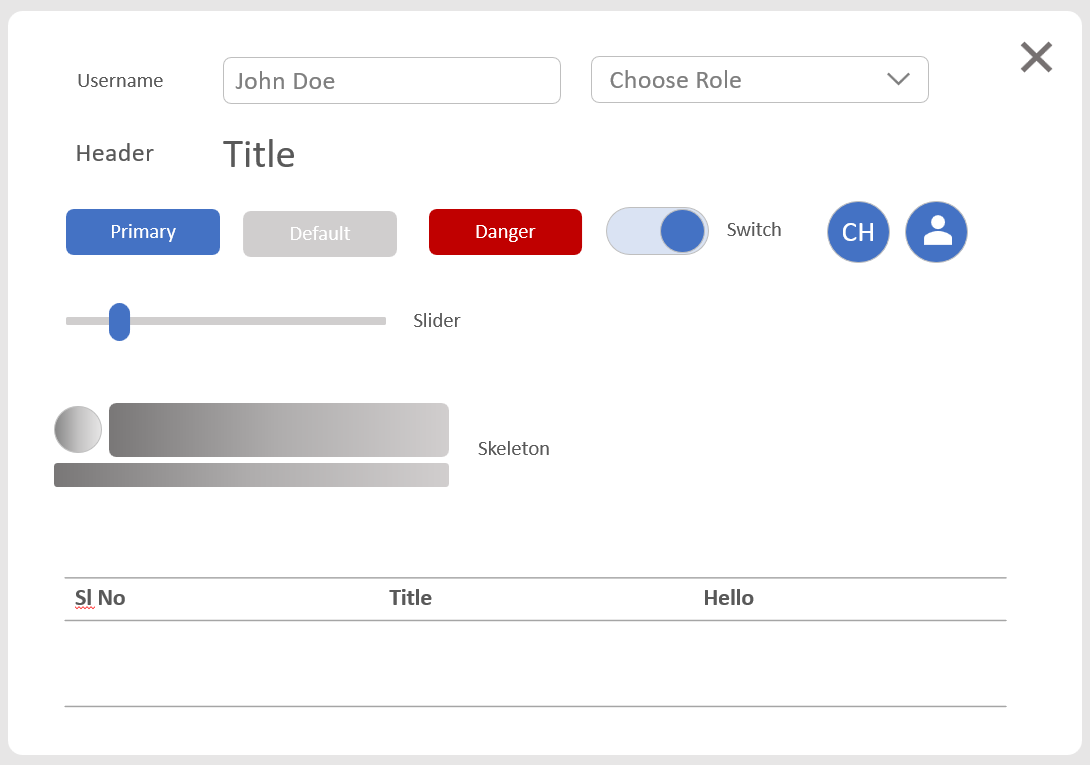
By the way PowerPoint has tons of vector icons to use for free.
A sample UX created in PowerPoint
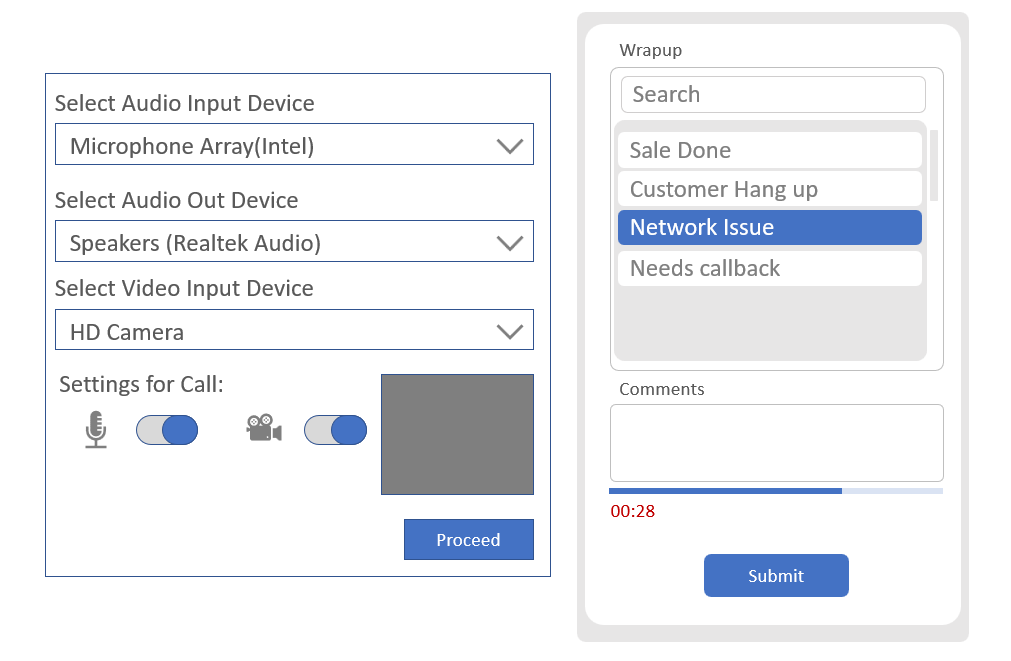
With those components ready and in place, next is just your idea and creativity to keep created UX mocks, wireframes and prototypes.
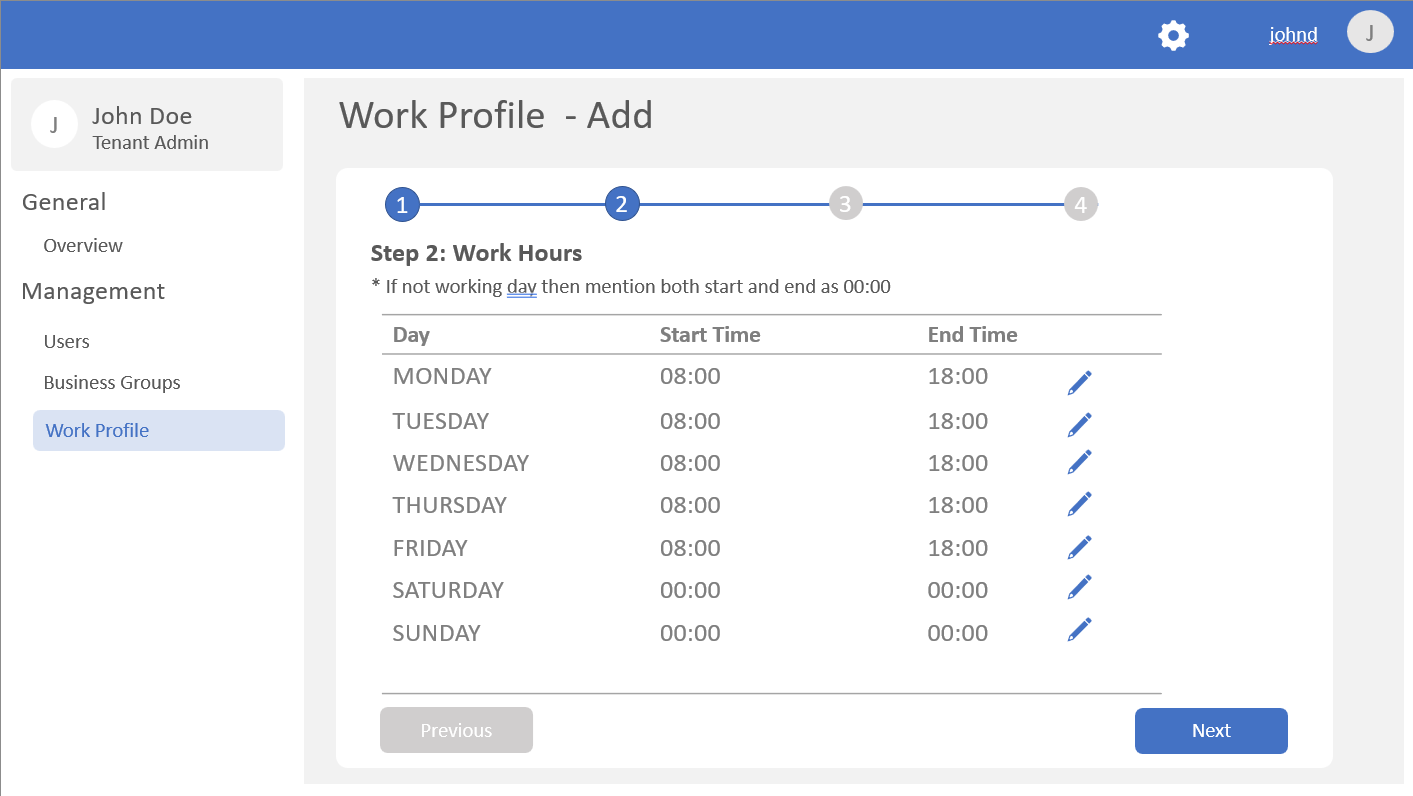
I have a sample PowerPoint slide created with some basic components available at this GitHub Location:
You can download and get an idea.
**Convert to prototype**
Now that we have the UX wireframes ready, why not make a working prototype. To achieve this, right click on button, navbar or links and add hyperlinks, finally select the destination slide you want the navigation to take you to.
Once all these steps are done, hit F5 to go to slideshow, present your working UX prototype with button clicks taking you to actual screens. How cool is that? | chandruchiku |
1,912,249 | Thinking in React: A Guide to Building User Interfaces. | (If you prefer to watch video: https://www.youtube.com/watch?v=RoRm6QVjkik) To utilize and harness... | 0 | 2024-07-05T05:14:33 | https://dev.to/itric/thinking-in-react-a-guide-to-building-user-interfaces-jp3 | learning, reactjsdevelopment, react | (If you prefer to watch video: https://www.youtube.com/watch?v=RoRm6QVjkik)
To utilize and harness the full power of React, it’s essential to adopt a specific mindset known as "Thinking in React". This approach involves breaking down the UI into components, managing state effectively, and ensuring a seamless data flow. In this article, we’ll explore the principles of Thinking in React and provide a step-by-step guide to building efficient and maintainable user interfaces with a practical example. And along the way we will map it to analogy for better understanding.
(code used in this article :https://github.com/code-blogger3/Youtube-demos/blob/main/src/App.jsx )
## Understanding the Core Principles
Before diving into the practical aspects of Thinking in React, it’s important to grasp the core principles that underpin this methodology:
1. **Component-Based Architecture**: React promotes the idea of building user interfaces using small, reusable components. Each component encapsulates a piece of the UI, making it easier to manage and maintain.
2. **Unidirectional Data Flow**: Data in React applications flows in one direction, from parent components to child components. This predictable data flow simplifies debugging and enhances the application’s stability.
3. **State Management**: React components can maintain their own state, which represents the dynamic parts of the UI. Understanding how to manage state effectively is crucial for building interactive applications.
## Step-by-Step Guide to Thinking in React
To illustrate the process of Thinking in React, let’s walk through the steps of building a simple searchable product data table.
### 1. Break the UI into a Component Hierarchy
The first step is to break down the UI into a component hierarchy. This involves identifying the distinct parts of the interface and mapping them to components. For our example, the product data table can be divided into the following components:
- **FilterableProductTable**: The main container component.
- **SearchBar**: A component for the search input.
- **ProductTable**: A component that displays the product data.
- **ProductCategoryRow**: A component for displaying product category headers.
- **ProductRow**: A component for displaying individual products.
Now let’s relate this step to an analogy.
Imagine you're a child again, surrounded by a sea of colorful Lego bricks. You have a vision in your mind—a towering castle with intricate details, bustling marketplaces, and brave knights. But how do you transform this vision into reality? Well, The answer lies in a step-by-step process, much like Thinking in React. Let’s see how building a complex Lego structure mirrors the process of developing a user interface with React.
## The Lego Metaphor: Building Block by Block
Breaking the UI into a component Hierarchy is similar to breaking Down the blueprint of the Castle.
### Breaking Down the Castle (Component Hierarchy)
When you start building your Lego castle, you don't just randomly stick pieces together. Instead, you think about the different parts of the castle: the towers, the walls, the gates, and the moat. In React, this is akin to breaking down your user interface into a component hierarchy.
- **The Castle**: This is your entire application, the big picture.
- **The Towers and Walls**: These represent the major sections of your app, like the header, main content, and footer.
- **The Gates and Moat**: These are smaller parts within those sections, like buttons, forms, and navigation links.
By breaking the castle into manageable parts, you can focus on building one section at a time, ensuring each piece fits perfectly with the others.
### 2. Build a Static Version in React
Once the component hierarchy is established, the next step is to build a static version of the UI in React. This involves creating the components and rendering them with static data. At this stage, focus on the layout and structure without adding any interactivity or state management.
This step corresponds to creating static structures of the castle.
### Creating Static Structures (Static Version in React)
Before adding the finishing touches and intricate details to your Lego castle, you first build a static version. You assemble the towers, walls, gates, and moat without any moving parts or decorations. In React, this is similar to creating a static version of your UI with components that don't yet have interactivity or dynamic behavior.
- **Lego Bricks**: These are your React components, each serving a specific purpose.
- **Building the Structure**: You put together the components to form the basic layout of your app.
This step helps you visualize the overall structure and ensures that all parts fit together seamlessly.
### 3. Identify the Minimal (But Complete) Representation of UI State
To add interactivity, it’s essential to identify the minimal set of mutable state that the application needs. For our product data table, the state can be categorized into two main types:
- **Search Query**: The text entered in the search bar.
- **Stock Only**: A boolean value indicating whether to show only products in stock.
Now this step maps to deciding which bricks can move in castle.
### Deciding Which Bricks Can Move (Identifying State)
Now that your static Lego castle is standing tall, you want to add some excitement—maybe a drawbridge that opens, knights that move, and flags that wave in the wind. To do this, you need to identify which parts of the castle should be dynamic. In React, this involves determining the minimal but complete set of state needed for your app.
- **Dynamic Lego Parts**: These are the movable pieces, like the drawbridge and knights.
- **UI State**: This is the state in React, such as the current page, user input, or whether a modal is open.
By identifying these dynamic parts, you can focus on making only the necessary pieces interactive, keeping your castle (and your code) efficient and manageable.
### 4. Identify Where Your State Should Live
Next, determine which components should own the state. According to the React documentation, state should be owned by the component that:
- Renders something based on that state.
- Needs to pass the state down to its child components.
For our example, the `FilterableProductTable` component is the best candidate to hold the state, as it renders both the `SearchBar` and the `ProductTable` and needs to pass the state to these child components.
This step overlaps to deciding who will control the drawbridge in the castle, where the state lives.
### Deciding Who Controls the Drawbridge (Where State Lives)
In your Lego castle, someone needs to control the drawbridge. Should it be the knight, the king, or perhaps a hidden mechanism? In React, this is like deciding which component should own the state.
- **Drawbridge Mechanism**: This represents the state in React.
- **Controller**: This is the component that manages the state, determining how and when it changes.
By placing the state in the appropriate component, you ensure a smooth and logical flow of information, making it easier to manage and update your app.
### 5. Add Inverse Data Flow
Finally, add inverse data flow to make the components interactive. This involves passing callback functions from the stateful parent component to the stateless child components. The child components call these functions to update the state in the parent component. For instance, the `SearchBar` component will receive a callback from `FilterableProductTable` to update the search query and stock-only state.
This step can be looked as allowing communication between knights.
### Communicating Between Knights (Adding Inverse Data Flow)
To make your Lego castle come alive, the knights need to communicate with each other—when the drawbridge is down, they charge out; when it's up, they retreat. In React, this involves adding inverse data flow, where child components send data back to their parent components.
- **Knight Communication**: This is like child components sending information back to their parents.
- **Parent Commands**: The parent component uses this information to update the state and trigger actions.
This two-way communication ensures that all parts of your castle (and your app) work together harmoniously, creating a dynamic and interactive experience.
## Practical Example: Building the Searchable Product Data Table
Let’s put these principles into practice by building the searchable product data table. Below is a simplified implementation in React:
```jsx
import React, { useState } from 'react';
// Sample product data
const PRODUCTS = [
{ category: "Sporting Goods", price: "$49.99", stocked: true, name: "Football" },
{ category: "Sporting Goods", price: "$9.99", stocked: true, name: "Baseball" },
{ category: "Sporting Goods", price: "$29.99", stocked: false, name: "Basketball" },
{ category: "Electronics", price: "$99.99", stocked: true, name: "iPod Touch" },
{ category: "Electronics", price: "$399.99", stocked: false, name: "iPhone 5" },
{ category: "Electronics", price: "$199.99", stocked: true, name: "Nexus 7" }
];
// FilterableProductTable Component
function FilterableProductTable() {
const [filterText, setFilterText] = useState('');
const [inStockOnly, setInStockOnly] = useState(false);
return (
<div>
<SearchBar
filterText={filterText}
inStockOnly={inStockOnly}
onFilterTextChange={setFilterText}
onInStockChange={setInStockOnly}
/>
<ProductTable
products={PRODUCTS}
filterText={filterText}
inStockOnly={inStockOnly}
/>
</div>
);
}
// SearchBar Component
function SearchBar({ filterText, inStockOnly, onFilterTextChange, onInStockChange }) {
return (
<form>
<input
type="text"
placeholder="Search..."
value={filterText}
onChange={(e) => onFilterTextChange(e.target.value)}
/>
<p>
<input
type="checkbox"
checked={inStockOnly}
onChange={(e) => onInStockChange(e.target.checked)}
/>
{' '}
Only show products in stock
</p>
</form>
);
}
// ProductTable Component
function ProductTable({ products, filterText, inStockOnly }) {
const rows = [];
let lastCategory = null;
products.forEach((product) => {
if (product.name.indexOf(filterText) === -1 || (!product.stocked && inStockOnly)) {
return;
}
if (product.category !== lastCategory) {
rows.push(
<ProductCategoryRow
category={product.category}
key={product.category}
/>
);
}
rows.push(
<ProductRow
product={product}
key={product.name}
/>
);
lastCategory = product.category;
});
return (
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
</tr>
</thead>
<tbody>{rows}</tbody>
</table>
);
}
// ProductCategoryRow Component
function ProductCategoryRow({ category }) {
return (
<tr>
<th colSpan="2">
{category}
</th>
</tr>
);
}
// ProductRow Component
function ProductRow({ product }) {
const name = product.stocked ? product.name : <span style={{ color: 'red' }}>{product.name}</span>;
return (
<tr>
<td>{name}</td>
<td>{product.price}</td>
</tr>
);
}
// Render the FilterableProductTable component
function App() {
return (
<div>
<h1>Product Table</h1>
<FilterableProductTable />
</div>
);
}
export default App;
```
Additional resource: https://elementarydot.blogspot.com/2024/07/thinking-in-react-relatable-scenarios.html
| itric |
1,912,248 | Benefits of Developing Pancakeswap clone script? | Introduction: PancakeSwap is a decentralized exchange (DEX) built on the Binance Smart Chain (BSC),... | 0 | 2024-07-05T05:11:13 | https://dev.to/sivaprasad_m_2ad653bf68aa/benefits-of-developing-pancakeswap-clone-script-146k | blockchain, cryptocurrency, web3 | **Introduction:**
PancakeSwap is a decentralized exchange (DEX) built on the Binance Smart Chain (BSC), It lets people exchange cryptocurrencies, add money to liquidity pools, and earn rewards by farming all while keeping fees low and processing transactions faster than many other blockchain platforms.
**Objective:**
PancakeSwap is a decentralized platform on the Binance Smart Chain for trading tokens, providing liquidity, and participating in yield farming. It is popular among users seeking affordable and effective DeFi options. The use of the CAKE token for governance and rewards enhances its attractiveness in the crypto community.
**How Does pancakeswap clone script works?**
When creating a PancakeSwap clone, the first step is to define what sets your platform apart from PancakeSwap. Next, decide whether to use a pre-made clone script from a trusted provider or build one yourself. Before launching, thoroughly check the smart contracts for any security issues through audits. Test the platform extensively to fix any bugs and ensure everything works smoothly. Once everything is ready, deploy your platform on the Binance Smart Chain.
**What is the importance of creating a PancakeSwap clone?**
Developing a PancakeSwap clone is crucial because it provides a quick and cost-effective way for developers to enter the DeFi market. By using a clone script, they can take advantage of proven technology and attract users more easily. Moreover, it encourages innovation by allowing developers to add unique features while benefiting from PancakeSwap's established success on the Binance Smart Chain. Overall, cloning PancakeSwap enables faster, cheaper, and more secure development of decentralized exchange platforms, promoting growth and diversity in DeFi.
**Features Of Pancakeswap Clone Script:**
The PancakeSwap clone has essential features such as an Automated Market Maker (AMM) protocol, which allows token trading without order books, and Liquidity Pools that enable users to earn rewards by providing liquidity. It also supports Yield Farming, where users can stake tokens to earn additional rewards. Furthermore, it includes an NFT Marketplace for buying, selling, and trading non-fungible tokens, as well as a Prediction feature that allows users to forecast cryptocurrency price movements and earn rewards. Additionally, it offers Profit Sharing, distributing a portion of the platform's revenue among token holders.
**Advantages of developing PancakeSwap clone:**
Using a clone script speeds up DeFi platform deployment, enabling quick responses to capture market opportunities. It is cost-effective, saving money compared to starting from scratch, and offers a customizable framework. Cloning a proven model reduces risks such as security issues and guarantees platform stability. Users trust familiar platforms, making it easier to attract an initial user base."
**Conclusion:**
"Beleaf Technologies recognizes the value of PancakeSwap clone script development for swiftly entering the DeFi market with a proven, cost-effective solution. By leveraging this approach, they aim to innovate while building on PancakeSwap's established success on the Binance Smart Chain. This strategic move positions Beleaf Technologies to contribute meaningfully to the decentralized finance ecosystem, driving adoption and fostering community trust in their platform with a secure and user-friendly platform that meets the evolving needs of cryptocurrency enthusiasts
| sivaprasad_m_2ad653bf68aa |
1,912,247 | Benefits of Partnering with a CakePHP Development Company/ TechnoProfiles | Digital transformation steps into the future with strategic partnering with a CakePHP development... | 0 | 2024-07-05T05:09:39 | https://dev.to/technoprofiles/benefits-of-partnering-with-a-cakephp-development-company-technoprofiles-2ppi | webdev, php, github, development |
Digital transformation steps into the future with strategic partnering with a CakePHP development company. Often, CakePHP has been referred to as a stronghold of robustness and flexibility. Whereby customized solutions are delivered on e-commerce development, sophisticated web applications, and so forth. You will be at the very top of cutting-edge technology. The empowers delivery of perfect user experience for scalable growth by partnering up with professional CakePHP developers. Harness limitless potential for CakePHP web development services in redefining your online presence. Execute the strategy, backed by unrivalled levels of innovation and precision in digital strategy execution.
**
## Unveiling CakePHP: A Framework for the Future
**
CakePHP is one of the powerful web development frameworks, flexible, extensible, and responsible for the innovation in productivity with almost all kinds of projects from e-commerce solutions to large web-based enterprise applications. CakePHP strongly puts a great deal of robust features at the disposal of the developers through a very streamlined development process.
**Powering E-commerce Excellence**
CakePHP is a master in e-commerce development and offers the best way of developing secure, scalable, and feature-rich online stores. Its built-in tools and libraries simplify complex tasks, ensuring integration of payment gateway systems, inventory management systems, and customer relationship management tools.
**Versatile Development Solutions**
Perfect for communities and university websites. CakePHP provides smooth way of work and adaptability which is unparalleled in the industry. With Model-View-Controller (MVC) programming, CakePHP is helping to encompass clean and structured coding. This also helps in quick test-driven development and easy maintenance.
**Global Reach with Local Expertise**
CakePHP development services in India provided a chance to rely on local area developers, much experienced in using the full potential of CakePHP to cater to worldwide standards, thereby helping businesses do the same. The service will just help the enterprises starting up or trying to scale up from the well-established straighten and, with CakePHP development service located in India, it brings opportunities for cost-effective solutions tailor-made to meet needs.
**Driving Innovation in Web Applications**
The advantages of CakePHP are its commitment to strong innovation with developers, an active community from different parts of the globe, proper community engagement, and driving steady improvements. A lot of plugins and extensions in the repository, helping to extend the functionalities of CakePHP.
**Choose CakePHP for Your Development Needs**
**[Expert CakePHP web development ](https://technoprofiles.com/cakephp-development-company)**that puts high performance, scalability, and the best degree of security above everything else. CakePHP lets developers build custom-fit solutions for entities both of today's needs and tomorrow's challenges. Take your web national with CakePHP and notice the difference notice the difference in speed, reliability, and innovation.
**
## Baking Success: Elevate Your Online Presence with CakePHP Development
**
In today's digital landscape, achieving online success hinges on choosing the right tools and partners. CakePHP emerges as a game-changer for businesses aiming to excel in e-commerce development, web applications, and beyond. Here’s why collaborating with a CakePHP development company can propel your digital aspirations
**Unleashing CakePHP Versatility**
CakePHP isn’t just a framework; it’s a versatile engine designed to streamline development processes across various digital fronts. Whether you’re venturing into e-commerce, crafting dynamic web apps, or enhancing your online presence. CakePHP robust MVC architecture ensures agile development, scalability, and efficient code management.
**Tailored Solutions for E-commerce Excellence**
For businesses diving into online retail, CakePHP offers tailored solutions that optimize everything from product management to secure payment gateways. Imagine seamless shopping experiences, intuitive interfaces, and backend systems that ensure smooth operations. With CakePHP, your e-commerce ambitions are backed by a framework that blends functionality with user-centric design.
**Harnessing Expertise with Global Reach**
Choosing CakePHP development services in India brings a strategic advantage. Indian developers are renowned for their expertise in CakePHP. It offers solutions that are economical without sacrificing quality. By partnering with Indian CakePHP experts, businesses gain access to a wealth of technical proficiency and strategic insights tailored to global and local market needs.
**Driving Innovation Through Collaboration
**
CakePHP thrives on community-driven innovation, constantly evolving with new updates, enhanced security features, and a vast library of plugins. This collaborative ethos ensures that businesses can adopt cutting-edge technologies and stay ahead in a competitive marketplace. With CakePHP, innovation isn’t just a latest update. It’s a cornerstone of sustainable digital growth.
**Choosing Your CakePHP Champion**
Selecting the right CakePHP development partner is crucial. Look for a company that not only boasts technical prowess but also aligns with your business goals and values. Whether you require seamless integrations, custom web applications and ongoing support. A trusted CakePHP partner can turn your digital vision into reality.
**
## Essential Ingredients: Exploring the Elements of CakePHP Development
**
CakePHP is not just a framework. it's a gateway to crafting exceptional web experiences that redefine digital landscapes. In this exploration, we uncover the essential components that make CakePHP the cornerstone of contemporary web development. It is especially true in the realm of e-commerce and beyond.
**1. CakePHP Development Services: Tailored Solutions for Every Digital Ambition**
CakePHP development services are more than just solutions; they are tailored digital masterpieces designed to elevate businesses across diverse sectors. Whether you're venturing into e-commerce, forging dynamic web applications, or creating intuitive website. CakePHP's versatile toolkit empowers developers to shape ideas into robust realities with precision and finesse.
**2. Expert CakePHP Development: Sculpting Technical Brilliance**
Harnessing the prowess of expert CakePHP developers is akin to sculpting a digital masterpiece. These skilled artisans bring not only technical expertise but also a deep-seated passion for innovation. Their adeptness in **[web development Services](https://technoprofiles.com/web-development-destiny-fullstack-mean-mern/)** ensures projects are not only functional but also scalable and future-ready. It ensuring a seamless journey from conception to deployment.
**3. CakePHP Web Development Services: Building Beyond Boundaries**
CakePHP web development services transcend conventional limitations, paving the way for boundless digital growth. Whether scaling existing infrastructures or architecting new ones. .CakePHP robust framework offers the flexibility and scalability needed to meet the most ambitious of digital endeavors. It's more than building. It’s crafting digital ecosystems that evolve and adapt alongside business aspirations.
**4. CakePHP Web Application Development: Powering Dynamics with Purpose**
At the heart of CakePHP lies its unparalleled ability to power dynamic web applications with purpose. Through its Model-View-Controller (MVC) architecture, CakePHP promotes seamless collaboration between functionality and design. It ensures applications not only perform optimally but also engage users intuitively. From feature-rich platforms to agile solutions. CakePHP empowers developers to innovate boldly and execute flawlessly.
**5. CakePHP Website Development: Crafting Experiences that Resonate**
CakePHP empowers developers to craft websites that transcend mere digital presence to become immersive experiences. With its rich ecosystem of plugins and libraries. CakePHP website development emphasizes user-centric design and unparalleled functionality. Whether optimizing for speed, security, or scalability. CakePHP ensures every interaction leaves a lasting impression, driving user engagement and business growth.
**6. CakePHP Programmers and Web Developers: Architects of Innovation**
In the realm of CakePHP, programmers and web developers aren't just creators. These architects of innovation. Their mastery in CakePHP application development navigates complexities with finesse, integrating cutting-edge technologies and industry insights seamlessly. This collaborative approach not only enhances project efficiency but also drives continuous improvement, ensuring solutions remain at the forefront of digital evolution.
**Finding the Perfect Slice: How to Select Your Ideal CakePHP Development Partner**
Choosing the perfect CakePHP development partner is crucial for your project's success. Much like finding the ideal slice of cake that satisfies your unique cravings. In today's digital landscape, where online presence is paramount, partnering with a seasoned CakePHP team can make all the difference. Here’s a comprehensive guide to help you navigate the process and find your ideal CakePHP development partner.
**1. Crafting Your Digital Recipe**
Start by defining your project’s flavour profile whether it’s setting up an **[innovative ecommerce platform](https://technoprofiles.com/e-commerce-management-system/)**, developing a robust web application, or enhancing your web presence. Clearly outlining your requirements. The foundation for finding a CakePHP partner capable of delivering a tailored solution.
**2. Mastery in CakePHP Artistry**
Look for a partner who excels in CakePHP development. Seek a team with a proven track record in crafting scalable solutions across diverse industries. Experience matters, so opt for a partner with expertise in CakePHP development services India and a portfolio showcasing their technical proficiency and creative flair.
**3. Innovation as the Secret Ingredient**
Beyond technical skills, prioritize innovation. Choose a partner who integrates cutting-edge technologies into their solutions and stays ahead of industry trends. A forward-thinking approach ensures your project remains relevant and competitive in the long term.
**4. Collaboration that’s a Perfect Blend**
Effective collaboration is key. Select a partner who values open communication and works closely with your team to understand your vision and goals. A collaborative approach fosters creativity and clarity.
**5. Refined by Client Raves**
Examine case studies and customer feedback before deciding. Insights from past projects can provide valuable perspectives on the partner’s reliability, service quality, and ability to deliver on promises.
**
## Sweet Rewards: Conclusion and Final Thoughts
**
A development with CakePHP opens the doorway toward new digital creation and growth. In this dynamic world of web development and e-commerce, choosing the **[perfect CakePHP development partner](https://technoprofiles.com/difference-between-cakephp-laravel-codeigniter/)** is not a choice but a strategic imperative. Through our journey so far in this paper. We have tried to deliberate how the robust framework that CakePHP enables businesses with bespoke solutions catering to various digital ambitions.
From building smooth e-commerce platforms to the orchestration of dynamic web applications. CakePHP stands high as a beacon when it comes to reliability and scalability. It allows not only code but also a canvas for agile development with ease of maintenance, fortified by a vibrant community powering innovation nonstop through updates and a far-reaching library of plugins.
While collaboration with a CakePHP development company is connected with an increase in technical knowledge, it aligns more with strategic business goals. From bootstrapping a startup to scaling an enterprise, availing of local expertise in CakePHP development services in India brings the most affordable deal without jeopardizing quality.
At the core of CakePHP is innovation, which places developers in a better position in the marketplace. CakePHP helps your business prepare for upcoming challenges and provides unmatched user experiences.
In the process, think about one important aspect: choosing the right champion in CakePHP. Seek a partner who is technically at the top of the class. Who champions collaboration, innovation, and unwavering client satisfaction.
| technoprofiles |
1,912,246 | Leetcode Day 4: Longest Common Prefix Explained | The problem is as follows: Write a function to find the longest common prefix string amongst an array... | 0 | 2024-07-05T05:08:18 | https://dev.to/simona-cancian/leetcode-day-4-longest-common-prefix-explained-62n | python, leetcode, beginners, codenewbie | The problem is as follows:
Write a function to find the longest common prefix string amongst an array of strings.
If there is no common prefix, return an empty string `""`.
Here is how I solved it:
- If there input list of strings is empty, it means that there is no common prefix to check so we return an empty string.
```
if not strs:
return ""
```
- Use the first string in the list as a reference for comparison
```
for i in range(len(strs[0])):
char = strs[0][i]
```
- For each character in the reference string, compare it with the corresponding character in all other strings. If any string has a different character at the same position or is shorter than current position `i`, it means that the common prefix ends before this character. Therefore, return the substring of the reference string up to this point.
```
for string in strs[1:]:
if i >= len(string) or string[i] != char:
return strs[0][:i]
```
- If loops completes without returning, it means that the reference string itself is the longest common prefix
```
return strs[0]
```
OR just use `os` standard library available in Python. It includes functions for file and directory manipulation, environment variables, process management etc.
The `os` module provides a way to interact with the operating system in a portable way.
Here is the completed solution:
```
import os
class Solution:
def longestCommonPrefix(self, strs: List[str]) -> str:
prefix = os.path.commonprefix(strs)
return prefix
```
| simona-cancian |
1,912,245 | Implementing JWT Authentication in Node.js | Page Content Introduction to JSON Web Token What is JSON Web Token Why use JSON Web... | 0 | 2024-07-05T04:52:53 | https://dev.to/mbugua70/implementing-jwt-authentication-in-nodejs-3m8g | webdev, backend, node, jsonwebtoken | ##Page Content
* [Introduction to JSON Web Token](#introduction-to-json-web-token)
* [What is JSON Web Token](#what-is-json-web-token)
* [Why use JSON Web Token](#why-use-json-web-token)
* [When You Should Use JSON Web Token](#when-you-should-use-json-web-token)
* [The Structure of JSON Web Token](#the-structure-of-json-web-token)
* [Setting Up JSON Web Token in a Node.js Application](#setting-up-json-web-token-in-a-node.js)
* [Using JSON Web Token with a Node.js Application](#using-json-web-token-with-a-node.js)
* [Securing Routes with JSON Web Token in a Node.js Application](#securing-routes-with-json-web-token-in-a-node.js-application)
* [Conclusion](#conclusion)
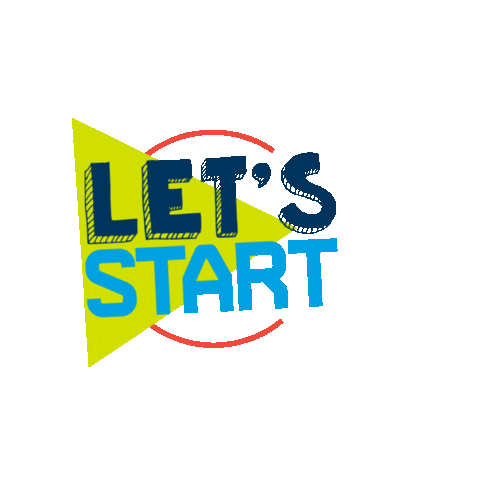
##Introduction to JSON Web Token
What's up, techies? 👋In this guide, we are going to explore the magic of JSON Web Tokens (JWT) and how they can make your authentication process secured. Buckle up,and get ready to implement robust authentication in your Node.js apps. Let's get started!
##What is JSON Web Token
JSON Web Token (JWT) is a compact and self-contained way to securely transmit information between parties as a JSON object. It is an open standard (RFC 7519) that ensures the information can be verified and trusted because it is digitally signed. JWTs can be signed using a secret (HMAC) or a public/private key pair (RSA or ECDSA).
##Why use JSON Web Token
JWT is stateless, meaning they don't require server-side session storage, making them highly scalable. They provide a secure way to transmit information and can be easily verified. JWT are also versatile, working well for authentication, authorization, and information exchange in web applications.
##When You Should Use JSON Web Token
**Authorization**:
- JWT are ideal for authorization. Once logged in, users can access routes, services, and resources with their JWT. Single Sign-On (SSO) commonly uses JWT due to its small overhead and cross-domain capabilities.
**Information Exchange**:
- JWT securely transmit information between parties. With digital signatures, you can verify the sender's identity and ensure the content has not been tampered with.
##The Structure of JSON Web Token
JWT consists of three parts separated by dots (.):
1. **Header**
- Specifies the token type (JWT) and signing algorithm (e.g., HS256). Encoded in Base64Url.
```
{
"alg": "HS256",
"typ": "JWT"
}
```
2. **Payload**
Contains claims about an entity (usually the user). There are three types of claims:
- _**Registered claims**_: Predefined, optional claims like iss (issuer), exp (expiration), sub (subject), and aud (audience).
- _**Public claims**_: Custom claims defined in the IANA JWT Registry or as a URI to avoid collisions.
- _**Private claims**_: Custom claims agreed upon by parties using the token.
```
{
"sub": "1234567890",
"name": "John Doe",
"admin": true
}
```
3.**Signature**
Verifies the token's integrity. Created using the encoded header, encoded payload, a secret, and the specified algorithm.
```
HMACSHA256(
base64UrlEncode(header) + "." +
base64UrlEncode(payload),
secret)
```
The resulting JWT looks like this: `xxxxx.yyyyy.zzzzz.` This format is compact and easily used in web environments.
##Setting Up JSON Web Token in a Node.js Application
Before we dive into implementing JSON Web Tokens (JWT) in your Node.js application, let's start with the basics, _installation_. To get started with JWT, you will need to install the jsonwebtoken package. This library will help you create, sign, and verify JWTs in your application.
**Installation**
First, you need to install the jsonwebtoken package using npm. Run the following command in your Node.js project directory
`npm install jsonwebtoken`
This will add the jsonwebtoken package to your project's dependencies.
**Next Steps**
Now that we have JWT installed, we are ready to implement it in our Node.js application. In the next section, we will cover how to use JSON Web Tokens for user authentication, including creating and verifying tokens using instance methods. Stay tuned!
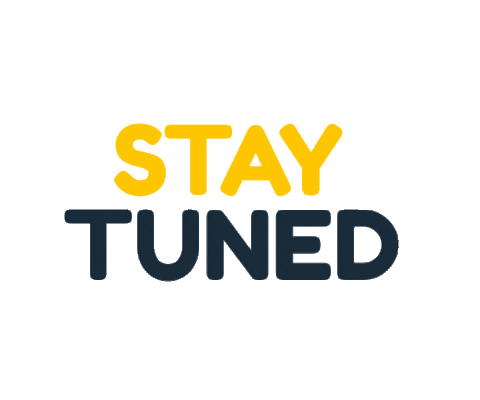
##Using JSON Web Token with a Node.js Application
I know this article is not about Mongoose, schemas, and models, but to effectively implement JSON Web Tokens (JWT) in our Node.js application, we will need to touch on these concepts briefly. Let's assume you have already set up your schema logic using Mongoose. For example:
```
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const UserSchema = new Schema({
name: {
type: String,
required: [true, "Please insert your name"],
minLength: [3, "Your name is too short"],
},
email: {
type: String,
required: [true, "Please insert your email"],
match: [
/^(([^<>()[\]\\.,;:\s@"]+(\.[^<>()[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/,
],
unique: true,
},
password: {
type: String,
required: [true, "Please insert password"],
minLength: [8, "Password is less than 8 character"],
},
});
```
Now, we will focus on creating a JWT using an instance method.
**Creating a JSON Web Token Using an Instance Method**
To start, we will create an instance method in our Mongoose model that will generate a JWT for a user. This instance method will be defined in the file where we have our model logic based on Mongoose.
```
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const jwt = require("jsonwebtoken");
const UserSchema = new Schema({
// your schema logic code
});
UserSchema.methods.createToken = function () {
return jwt.sign(
{ userId: this._id, userName: this.name },
process.env.SECRET,
{ expiresIn: process.env.JWT_LIFETIME }
);
};
```
**Explanation**
- We assume your schema for example my case UserSchema is already defined in your Mongoose model.
We add an instance method **createToken ** to the UserSchema. This method uses **jwt.sign** to create a token with the **user's** **ID** and **name ** as **payload**, a **secret key** from environment variables, and an **expiration time** also from environment variables.
This instance method can be called on any user document to generate a JWT, making it easy to handle user authentication in your application.
##Integrating JWT with Login and Registration
Now that we have the instance method to create JWTs, let us see how we can use it in the login and registration logic of our Node.js application. Here is how you can integrate the createToken method:
**User registration**
During user registration, once the user is successfully created, we can generate a JWT and send it back to the client.
```
const UserModel = require("../models/User");
const { StatusCodes } = require("http-status-codes");
const { BadRequestError, UnauthenticatedError } = require("../errors");
const register = async (req, res) => {
const { name, email, password } = req.body;
// Create a new user
const user = await UserModel.create({ ...req.body });
// Generate a JWT
const token = user.createToken();
// Respond with the user name and token
res.status(StatusCodes.CREATED).json({ user: { name: user.getName() }, token });
};
module.exports = {
register,
};
```
**User login**
For user login, after validating the user's credentials, we can generate a JWT and send it back to the client.
```
const UserModel = require("../models/User");
const { StatusCodes } = require("http-status-codes");
const { BadRequestError, UnauthenticatedError } = require("../errors");
const login = async (req, res) => {
const { email, password } = req.body;
// Validate the request
if (!email || !password) {
throw new BadRequestError("Please provide email and password");
}
// Find the user by email
const userLogin = await UserModel.findOne({ email });
// If user is not found
if (!userLogin) {
throw new UnauthenticatedError("Invalid credentials");
}
// Check if the password is correct
const isPasswordCorrect = await userLogin.comparePassword(password);
// If the password is incorrect
if (!isPasswordCorrect) {
throw new UnauthenticatedError("Invalid credentials");
}
// Generate a JWT
const token = userLogin.createToken();
// Respond with the user name and token
res.status(StatusCodes.OK).json({ user: { name: userLogin.getName() }, token });
};
module.exports = {
login,
};
```
In these examples, after a user registers or logs in successfully, we generate a JWT using the **createToken** instance method and send it back in the response. This token can then be used by the client to authenticate subsequent requests.
**Next Steps**
With the instance method in place and integrated into your login and registration logic, you can now secure your routes and verify tokens in your Node.js application. In the following section, we will cover how to use this token for securing routes. Stay tuned!
##Securing Routes with JSON Web Token in a Node.js Application
With our JWT creation logic in place, the next step is to secure our routes. To do this, we will create a file to store our authentication logic and use it as middleware in our application.
**Creating Middleware for Securing Routes**
We will create a middleware function that will verify the JWT in incoming requests. This middleware will check the authorization header, verify the token, and attach the user information to the request object if the token is valid.
```
const UserModel = require("../models/User");
const jwt = require("jsonwebtoken");
const { UnauthenticatedError } = require("../errors");
const auth = async (req, res, next) => {
const authHeader = req.headers.authorization;
if (!authHeader || !authHeader.startsWith("Bearer ")) {
throw new UnauthenticatedError("Authentication invalid");
}
const token = authHeader.split(" ")[1];
try {
const payload = jwt.verify(token, process.env.SECRET);
req.user = { userId: payload.userId };
next();
} catch (err) {
console.log(err);
throw new UnauthenticatedError("Authentication invalid");
}
};
module.exports = auth;
```
**Using the Middleware**
Now that we have our authentication middleware, we can use it to secure our routes in various ways. Here are three different ways to apply the middleware:
**1. Applying Middleware to Specific Routes**
```
const express = require('express');
const router = express.Router();
const auth = require('../middleware/auth');
const { getUserInfo } = require('../controllers/userController');
router.get('/user-info', auth, getUserInfo);
module.exports = router;
```
**2. Applying Middleware to All Routes in a Router**
```
const express = require('express');
const router = express.Router();
const auth = require('../middleware/auth');
const { getUserInfo, getJobs } = require('../controllers/userController');
router.use(auth);
router.get('/user-info', getUserInfo);
router.get('/jobs', getJobs);
module.exports = router;
```
**3. Applying Middleware in the Main File**
```
const express = require('express');
const app = express();
const auth = require('./middleware/auth');
const jobRoutes = require('./routes/jobRoutes');
app.use("/api/v1/jobs", auth, jobRoutes);
```
##Conclusion
In this article, we covered the basics of JSON Web Tokens (JWT) and how to use them in a Node.js application. We discussed what JWT is, why and when to use it, and how to implement it for user authentication. We also looked at securing routes using middleware in different ways. With these steps, you can enhance the security of your Node.js applications by ensuring only authenticated users can access protected resources.
For more detailed information on JSON Web Tokens, you can visit the [jsonwebtoken documentation](https://jwt.io/introduction).
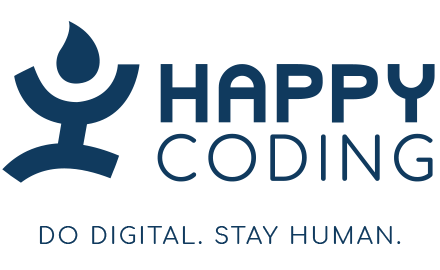
| mbugua70 |
1,912,244 | Exchange contact information: Mastering Business Cards — Subraa | A business card is more than just a convenient way to exchange contact information; it is a... | 0 | 2024-07-05T04:52:36 | https://dev.to/subraalogo/exchange-contact-information-mastering-business-cards-subraa-48d0 | businesscard, namecarddesign, propertyagentflyer |
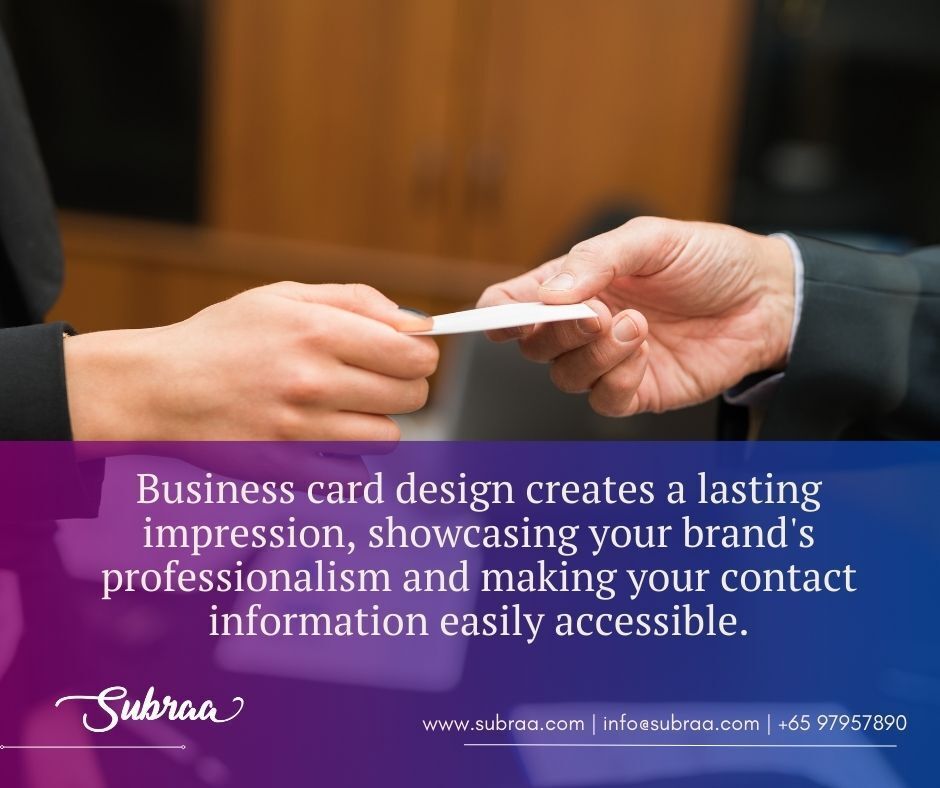
A [business card](https://www.subraa.com/name-card-design) is more than just a convenient way to exchange contact information; it is a critical marketing tool that can leave a lasting impression on potential clients, partners, and stakeholders. A well-designed business card should convey professionalism, credibility, and a clear message about your brand. Here are the essential elements that should be included in an effective [business card design](https://www.subraa.com/name-card-design):
1. Business Name and Logo
The name of your business should be prominently displayed on the card, as it is the primary identifier for your brand. Alongside the name, the logo acts as a visual representation of your business. The logo should be clear, professionally designed, and reflect the essence of your brand. Together, the business name and logo help in establishing brand recognition.
2. Your Name and Job Title
Including your name and job title personalizes the card and tells the recipient who they will be contacting. Your job title also gives context to your role within the company, whether you are the owner, manager, sales executive, or another key player. This information helps in building a personal connection and provides clarity on your position and authority.
3. Contact Information
Contact information is the core element of a business card design. It should include:
Phone Number: Preferably your direct line or mobile number to ensure direct communication.
Email Address: Use a professional email address associated with your business domain.
Website: Directing recipients to your website allows them to learn more about your business at their convenience.
4. Physical Address
If your business has a physical location, including the address can be beneficial. It indicates stability and a tangible presence, which can be reassuring to potential clients. For businesses that primarily operate online, this element may be less critical but can still add a level of
credibility.
5. Social Media Handles
In today’s digital age, social media presence is significant for most businesses. Including your social media handles in your business Card can drive engagement and allow recipients to connect with your brand on multiple platforms. Ensure these handles are current and actively managed to present a professional online image.
6. Tagline or Slogan
A tagline or slogan succinctly conveys what your business is about and what makes it unique. It should be short, memorable, and reflective of your brand’s value proposition. A good tagline can make your business card stand out and be more memorable.
7. Design and Layout
The overall design and layout of your business card are crucial for making a positive impression. This includes:
Typography: Use clean, legible fonts. Avoid overly decorative fonts that might be difficult to read.
Colors: Choose colors that align with your brand’s visual identity. Ensure there is sufficient contrast between the text and background for readability.
White Space: A cluttered card can be off-putting. Use white space effectively to ensure the card is easy to read and looks professional.
Material and Finish: The quality of the card stock and the finish (matte, glossy, embossed) can also impact the impression it leaves. High-quality materials and finishes can convey a sense of quality and attention to detail.
8. Additional Elements
Depending on your industry and business needs, additional elements such as QR codes, professional certifications, or a brief list of services can be included in your Business Card. QR codes can link directly to your website or portfolio, offering a convenient way for recipients to engage further with your brand.
In conclusion, a [name card](https://www.subraa.com/name-card-design) is a compact yet powerful marketing tool. By including essential elements such as the business name, logo, contact information, and a thoughtful design, you can create a [professional business card design](https://www.subraa.com/name-card-design) that effectively communicates your brand’s identity and professionalism. Remember, the goal is to make it easy for recipients to contact you and to leave a positive, lasting impression.
Design here: https://www.subraa.com/ | subraalogo |
1,912,243 | Pandas DataFrame Hist Method: Visualizing Data Distributions | The hist() method in the Pandas library allows us to create histograms, which are visual representations of the distribution of data. This method is used on a DataFrame object and calls the matplotlib.pyplot.hist() function on each series within the DataFrame, resulting in one histogram per column. | 27,675 | 2024-07-05T04:46:58 | https://dev.to/labex/pandas-dataframe-hist-method-visualizing-data-distributions-3png | pandas, coding, programming, tutorial |
## Introduction

This article covers the following tech skills:
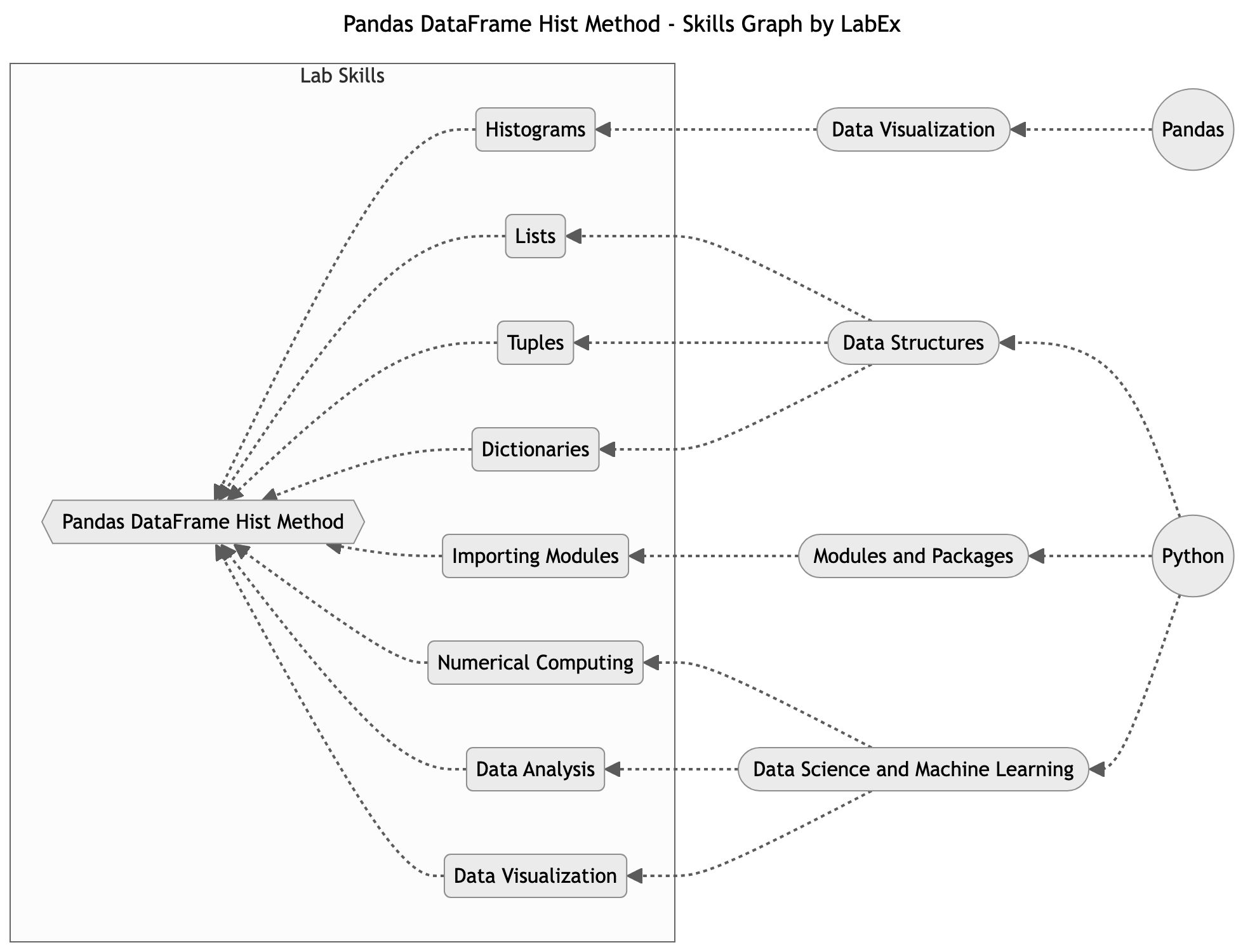
The `hist()` method in the Pandas library allows us to create histograms, which are visual representations of the distribution of data. This method is used on a DataFrame object and calls the `matplotlib.pyplot.hist()` function on each series within the DataFrame, resulting in one histogram per column.
### VM Tips
After the VM startup is done, click the top left corner to switch to the **Notebook** tab to access Jupyter Notebook for practice.
Sometimes, you may need to wait a few seconds for Jupyter Notebook to finish loading. The validation of operations cannot be automated because of limitations in Jupyter Notebook.
If you face issues during learning, feel free to ask Labby. Provide feedback after the session, and we will promptly resolve the problem for you.
## Import the necessary libraries
To use the `hist()` method, we need to import the required libraries, which are `pandas` and `matplotlib.pyplot`.
```python
import pandas as pd
import matplotlib.pyplot as plt
```
## Create a DataFrame
Next, we need to create a DataFrame object using the `pd.DataFrame()` method. We can pass a dictionary as an argument, where the keys represent the column names and the values represent the data.
```python
data = {'length': [1.5, 0.5, 1.2, 0.9, 3], 'width': [0.7, 0.2, 0.15, 0.2, 1.1]}
df = pd.DataFrame(data)
```
## Create a histogram
Now, we can use the `hist()` method on the DataFrame to create a histogram of each column.
```python
df.hist()
plt.show()
```
## Customize the histogram
We can customize the histogram by providing additional parameters to the `hist()` method. For example, we can specify the number of bins, the color of the histogram bars, and the title of the histogram.
```python
df.hist(bins=10, color='skyblue')
plt.title('Histogram')
plt.show()
```
## Summary
The `hist()` method in Pandas allows us to create histograms of the data within a DataFrame. By using this method, we can visualize the distribution of our data, which can be useful for data analysis and exploration. Additionally, we can customize the appearance of the histogram by providing additional parameters to the `hist()` method. Overall, the `hist()` method is a handy tool for analyzing and visualizing data in Pandas.
---
## Want to learn more?
- 🚀 Practice [Pandas DataFrame Hist Method](https://labex.io/tutorials/pandas-pandas-dataframe-hist-method-68633)
- 🌳 Learn the latest [Pandas Skill Trees](https://labex.io/skilltrees/pandas)
- 📖 Read More [Pandas Tutorials](https://labex.io/tutorials/category/pandas)
Join our [Discord](https://discord.gg/J6k3u69nU6) or tweet us [@WeAreLabEx](https://twitter.com/WeAreLabEx) ! 😄 | labby |
1,912,242 | How I Calculate Capacity for Systems Design - 2 | Part 1: Pre-requisites : How I Calculate Capacity for Systems Design - 1 Capacity... | 0 | 2024-07-05T04:45:51 | https://dev.to/zeeshanali0704/how-i-calculate-capacity-for-systems-design-399o | javascript, systemdesignwithzeeshanali | Part 1: [Pre-requisites : How I Calculate Capacity for Systems Design - 1](https://dev.to/zeeshanali0704/how-i-calculate-capacity-for-systems-design-1-25ob)
### Capacity Estimation Process Example: Twitter-like Application
**1. Traffic Estimation**
- **Monthly Active Users (MAU):** 500 million
- **Daily Active Users (DAU):** 100 million (assuming 1/5 of MAUs are active daily)
**2. Read and Write Requests**
To estimate read and write requests, assume:
- Each active user tweets 2 times per day.
- Each user reads 100 tweets per day (including their feed, replies, and notifications).
**Write Requests:**
- Each tweet is a write request.
- Additional write requests for likes, retweets, and replies. Assume 2 additional write requests per tweet.
**Calculations:**
- **Daily Write Requests:**
- Daily Write Requests = DAU × (Average Tweets per User + Additional Writes per Tweet)
- Daily Write Requests = 100 million × (2 + 2) = 400 million requests/day
- **Daily Read Requests:**
- Daily Read Requests = DAU × (Average Reads per User)
- Daily Read Requests = 100 million × 100 = 10 billion requests/day
- **Requests per Second (RPS):**
- There are 86,400 seconds in a day but we will consider 100,000 (100K) for simplifying calculations.
- Write RPS = Daily Write Requests / 100,000
- Write RPS = 400 million / 100K ≈ 4,000 writes per second
- Read RPS = Daily Read Requests / 100,000
- Read RPS = 10 billion / 100K ≈ 100,000 reads per second
**3. Storage Requirements**
Assume:
- Average size of a tweet: 300 bytes
- Retention period: 5 years (5 × 365 days)
- Additional storage for metadata (likes, retweets, etc.): 3 times the tweet size
**Calculations:**
- **Daily Storage for Tweets:**
- Daily Storage = Daily Write Requests × Average Tweet Size × 4
- Daily Storage = 400 million × 300 × 4 ≈ 400 Million × 1200Bytes ~ 480GB/Day ~ 500 GB/day
- **Annual Storage:**
- Annual Storage = Daily Storage × 365
- Annual Storage = 500 GB × 365 ≈ 182 TB
**Basic Formula**
If we assume a write request size of x KB and y million users:
` x KB × y Million users = xy GB;`
For example:
If each user writes 100 KB per day and there are 200 million users:
Storage per day = 100 KB × 200 M = 20000 KB X M ~ 20000 GB ~ 20 TB
**4. Bandwidth Requirements**
Assume:
- Average tweet size: 1,200 bytes (including metadata)
- Write RPS ≈ 4,000
- Read RPS ≈ 100,000
- Average read size: 500 bytes
**Calculations:**
- **Write Bandwidth:**
- Write Bandwidth (Mbps) = Write RPS × Size per Write
- Write Bandwidth = 4,000 × 1,200 ≈ 4.8 MB/second
- **Read Bandwidth:**
- Read Bandwidth (Mbps) = Read RPS × Size per Read
- Read Bandwidth = 100,000 × 500 ≈ 50 MB/second
- **Total Bandwidth:**
- Total Bandwidth = Write Bandwidth + Read Bandwidth
- Total Bandwidth ≈ 4.8 + 50 ≈ 54.8 MB/second
**5. RAM / Cache Estimation**
Assume:
- Cache the last 5 posts per user
- Average size of each post: 500 bytes
**Calculations:**
- **Cache Requirement:**
- 1 post = 500 bytes, so 5 posts = 2,500 bytes ≈ 3 KB
- Total cache for 100 million daily active users:
- Total Cache = DAU × Cache Size per User
- Total Cache = 100 million × 3 KB ≈ 300 GB
- **Number of Machines Required:**
- Assume each machine has 10 GB cache storage.
- Number of Machines = Total Cache / Cache per Machine
- Number of Machines = 300 GB / 10 GB ≈ 30 machines
**6. Latency Estimation**
Assume:
- Latency per request: 500 ms
- 1 second to serve 2 requests
- Write RPS ≈ 4,000
- Assume each server serves 100 requests/second.
**Calculations:**
- **Number of Servers Required:**
- Number of Servers = Write RPS / Requests per Server
- Number of Servers = 4,000 / 100 ≈ 40 servers
By following these calculations, you can estimate the capacity needed to handle traffic, storage, bandwidth, cache, and latency for your system.
Part 1: [Pre-requisites : How I Calculate Capacity for Systems Design - 1](https://dev.to/zeeshanali0704/how-i-calculate-capacity-for-systems-design-1-25ob)
More Details:
Get all articles related to system design
Hastag: SystemDesignWithZeeshanAli
Git: https://github.com/ZeeshanAli-0704/SystemDesignWithZeeshanAli
| zeeshanali0704 |
1,910,440 | Mastering npm: A Comprehensive Guide to Package Management | Ah, npm – the Node Package Manager. For web developers, it's like that quirky old friend who's... | 0 | 2024-07-05T04:44:09 | https://dev.to/chiragagg5k/mastering-npm-a-comprehensive-guide-to-package-management-3h0m | webdev, javascript, beginners, programming | Ah, npm – the Node Package Manager. For web developers, it's like that quirky old friend who's simultaneously invaluable and infuriating. Whether you're a newbie fumbling through your first `npm install` or a seasoned dev who can recite package versions in your sleep, npm is an inescapable part of the modern JavaScript ecosystem.
I've been on quite the journey with npm, from my early days of copy-pasting commands I barely understood, to now, where I can confidently say I've tamed this beast (most days, anyway). So, grab your favourite caffeinated beverage, and let's dive into the wild world of npm!
## Why Do We Even Need npm?
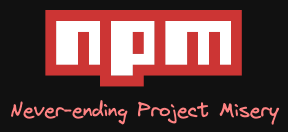
Picture this: You're building a web app, and you need a date picker. Sure, you *could* write one from scratch, accounting for leap years, time zones, and all those delightful edge cases. Or... you could type `npm install moment` and have a battle-tested solution at your fingertips in seconds.
That's the magic of npm. It's like having access to a vast library of code, written and maintained by developers worldwide. Need routing? Authentication? A library to validate email addresses? There's probably an npm package for that.
But npm isn't just about installing packages. It's a powerful tool for:
1. **Managing Dependencies**: Keep track of what your project needs and which versions.
2. **Script Running**: Standardize commands across your team (ever seen `npm run build`?).
3. **Version Control**: Ensure everyone on your team is using the same package versions.
4. **Publishing**: Share your own code with the world (or just your team).
In essence, npm is the glue that holds the JavaScript ecosystem together. It allows us to stand on the shoulders of giants and build amazing things without reinventing the wheel every time.
## But why just NPM?
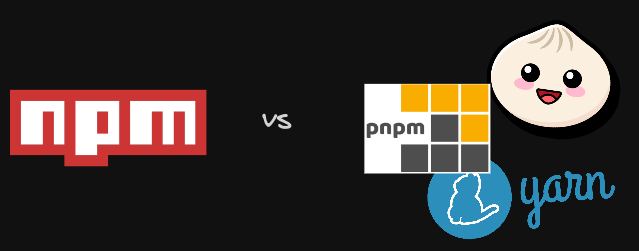
Of course NPM isn't alone, it has its own family! Sadly it isn't the most loved... but still, it's the good ol' reliable! If you want to be called a 10xengineer, you should probably switch to the alternatives. And the contenders are:
| | Pros | Cons |
|-----------------|------|------|
| npm | • Default for Node.js <br>• Massive package ecosystem | • Historically slower than alternatives <br>• node_modules can get large |
| Yarn | • Faster installation <br>• Offline mode | • Another tool to learn <br>• Occasional compatibility issues with npm |
| pnpm | • Efficient disk space usage <br>• Lightning-fast installations | • Different node_modules structure <br>• Less mainstream adoption |
| Bun | • Blazing fast performance <br>• All-in-one solution: runtime, transpiler, bundler | • Still in development <br>• Limited ecosystem compared to npm |
In contrast to popular belief, a 10x engineer like me is not using the freshly baked (pun intended) technology like bun! I still stick to pnpm. Why is that so you might ask? Well, it's a case specific to a Mac user like me, where Bun isn't very efficient with caching the files for repeated downloads. So it is less efficient for Macbook (or it was till the day I wrote this).
## But what are these files???
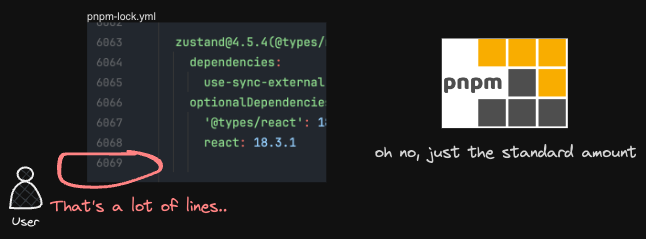
At the core of every JavaScript project, regardless of the package manager, lies the `package.json` file. This crucial manifest outlines project details and dependencies in a structured JSON format:
```json
{
"name": "my-awesome-project",
"version": "1.0.0",
"dependencies": { ... },
"devDependencies": { ... },
"scripts": { ... }
}
```
Complementing `package.json`, each package manager employs a unique lock file to ensure dependency consistency across environments. These files meticulously detail every dependency, including sub-dependencies and their exact versions:
- npm: package-lock.json
- Yarn: yarn.lock
- pnpm: pnpm-lock.yaml
- Bun: bun.lockb (in binary format)
If you've ever peeked inside these lock files, you've likely encountered a daunting wall of text or, in Bun's case, indecipherable binary data. Don't panic! These files aren't meant for human editing. They're the domain of your chosen package manager, automatically generated and updated to keep your project's dependency ecosystem in perfect harmony.
## Surviving the Dependency Management Nightmare
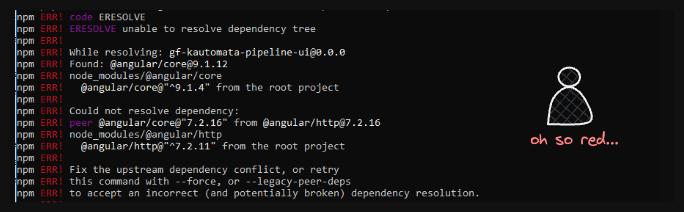
Picture this: It's 2 AM, and you're fueled by coffee and determination, trying to resurrect an old project. Suddenly, npm throws a fit. One package is outdated. No, wait—all of them are. And oh, joy! That innocent-looking major update just turned your project into a digital dumpster fire.
Welcome to dependency management hell, where "it works on my machine" goes to die.
While we can't completely exorcise these demons (it's part of the JavaScript circle of life), we can at least arm ourselves with some holy water. Let's explore two powerful tools to keep your sanity intact.
## 1. npm-check-updates: The Blunt Force Approach
First up is `npm-check-updates`, the sledgehammer of the update world. It doesn't care about your feelings or your project's delicate ecosystem. It has one job: update all the things.
```bash
npm install -g npm-check-updates # Install globally
ncu # List available updates (look before you leap)
ncu -u # Update everything and pray
```
## 2. npm-check: The Sophisticated Sibling
For those who prefer a more refined approach, meet `npm-check`. It's like having a personal assistant for your dependencies, complete with a monocle and a British accent.
```bash
npm install -g npm-check # Install globally
npm-check # Get a detailed report of your dependency situation
npm-check -u # Interactive update process, like a choose-your-own-adventure book
```
This tool doesn't just check for updates; it's also a snitch. It'll rat out those packages you installed and never used (we've all been there). Plus, it categorizes updates into patch, minor, and major groups, allowing you to update with the precision of a surgeon rather than the recklessness of a caffeinated developer at 2 AM.
## Conclusion
We've ventured through the npm universe, from decoding `package.json` to escaping dependency hell. Here's your survival kit:
1. Choose your package manager wisely - npm, Yarn, or pnpm each have their strengths.
2. Treat your `package.json` and lock files with respect - they're the backbone of your project.
3. Use tools like npm-check-updates and npm-check to keep dependencies in check.
4. Update regularly, but cautiously. Always read changelogs and run tests.
5. Remember, even seasoned devs sometimes get lost in dependency hell - you're not alone.
In the ever-changing JavaScript landscape, managing packages is more art than science. Stay curious, update wisely, and may your builds always be successful!
P.S. When all else fails, there's always `rm -rf node_modules && npm install`. It's the "turn it off and on again" of the npm world! | chiragagg5k |
1,912,241 | From Field to Blockchain Evolution of Agriculture in the Digital Age. | This article will examine how agriculture and blockchain technology can be leveraged. What is... | 0 | 2024-07-05T04:40:58 | https://dev.to/ukeziebenezer/from-field-to-blockchain-evolution-of-agriculture-in-the-digital-age-51a0 | blockchain, web3, agriculture |
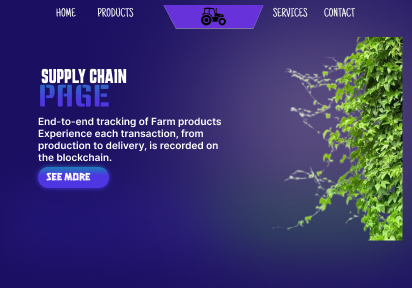
This article will examine how agriculture and blockchain technology can be leveraged.
What is Agriculture?
According to [Twinkl](https://www.twinkl.com), individuals can own and trade culture is the science of farming, it can transform soil for growing crops, rearing animals to provide food, wool, and other products, and harvesting grown crops as effectively as possible. You can also think of it as the practice of cultivating the soil, growing crops, and raising livestock to produce commodities essential for human life, including food, fiber, and raw materials.
Agriculture is vital for the global economy, providing commodities like grain, livestock, dairy, fiber, and raw materials for fuel. It supports livelihoods, habitats, and jobs, and contributes to building strong economies through trade.
**_What is Blockchain?_**
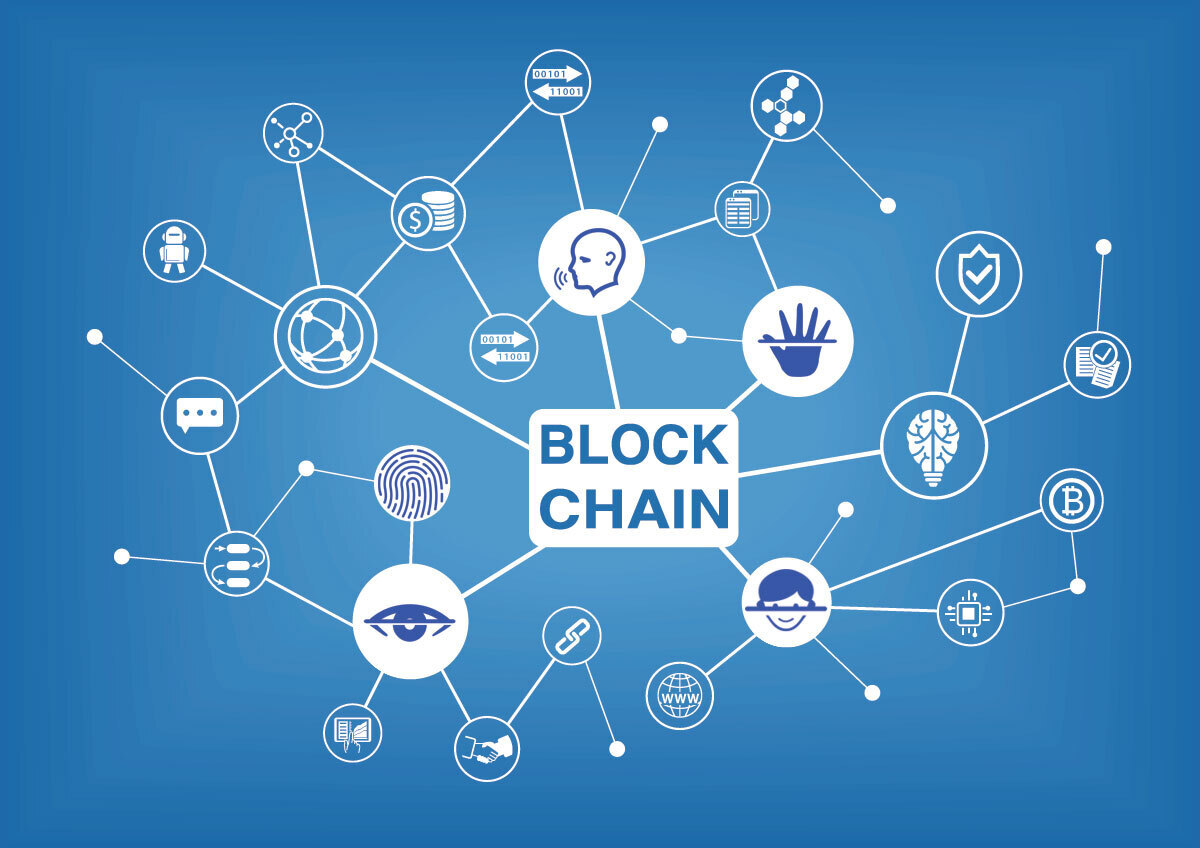
Blockchain is a distributed ledger technology that functions as a decentralized and highly secure database. A blockchain network consists of many nodes that contain a copy of the database. This makes it so a failure of one or more nodes does not affect the accessibility of the database.
Transactions that occur within the blockchain are recorded and added to the previous transaction block. The blocks are joined together using encryption to create a chain of transaction events. The encrypted chain makes it theoretically impossible to alter, interfere, or access the data contained within the blocks.
**
## Key Features of Blockchain
**
• **Decentralization**: Blockchain operates on a decentralized network, eliminating the need for a central authority. This structure ensures that the network is robust against failures and attacks, as there is no single point of failure. It also removes the need for third parties, reducing risks and costs associated with intermediaries.
• **Immutability**: Once data is recorded on the blockchain, it cannot be altered or deleted. This feature ensures the integrity and permanence of the information stored, making blockchain an ideal solution for creating transparent and tamper-proof ledgers.
•**Transparency**: Every transaction on the blockchain is visible to all participants in the network, fostering openness and accountability. This transparency helps in preventing fraudulent activities and ensures that all actions taken within the network are verifiable.
• **Security**: Blockchain leverages cryptographic techniques to secure transactions and data. The combination of public and private keys provides a secure method for authentication and authorization, ensuring that only authorized parties can perform transactions. Additionally, the decentralized nature of blockchain makes it resistant to hacking attempts.
• **Smart Contracts**: Blockchain enables the creation of smart contracts, which are self-executing contracts with the terms of the agreement directly written into lines of code. These contracts automatically execute when predefined conditions are met, streamlining processes and reducing the need for intermediaries.
**_What is Web3?_**
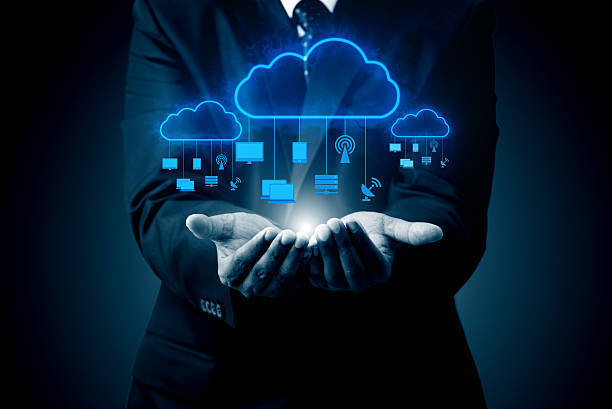
Web3 is a decentralized vision of the Internet that aspires to create an entirely new system of contracts and change the way that individuals and institutions reach agreements, represents a new phase of the Internet characterized by decentralization, leveraging blockchain technology to create a more user-centric and autonomous online environment.
**Key Features of Web3:**
• **Decentralization**: At the heart of Web3 is the principle of decentralization, moving away from centralized servers and databases towards a peer-to-peer network where data is stored across multiple nodes, making it more resilient and secure against censorship and failures.
• **Blockchain Technology**: Web3 relies heavily on blockchain technology, which provides a transparent and immutable ledger for transactions and agreements. This technology underpins the creation of cryptocurrencies, smart contracts, and decentralized applications (DApps).
• **User Control and Ownership**: In Web3, users have greater control over their personal data and digital assets. This is facilitated by technologies like blockchain, which enables the creation of digital assets and tokens that individuals can own and trade.
• **Smart Contracts** : These are self-executing contracts with the terms of the agreement directly written into lines of code. They run on blockchain networks, eliminating the need for intermediaries and reducing transaction costs.
• **Cryptocurrencies and Digital Assets**: Web3 introduces a variety of digital assets, including cryptocurrencies like Bitcoin and Ethereum, as well as non-fungible tokens (NFTs) and other forms of digital property that can be bought, sold, and traded on the blockchain.
**How Web3 Applications can transform the Agricultural Web3 Sector**
The world is fighting hunger globally, and in that situation loss of every crop should be something like extensive damage. With time, the world has upgraded its technology, and when everything is getting upgraded with time, why not merge technology with agriculture to make crop cultivation more accurate and productive? Web3 platform has great potential to take care of every sort of business, and that too with a completely decentralized format and ultimate security.
The work of the web3 platform is to make the platform completely decentralized and free from any third-party interference. Blockchain technology has brought a great revolution in the market with high-end solutions to protect and record data.
Web3 applications are robust technologies transforming the agricultural sector and making it more productive. Creation of your web3 application for farming, controlling crop cultivation process, monitoring soil, etc.
** What Makes Web3 A Place Of Benefit**
The creation of web3 applications for the agriculture sector can change the complete theory of the farming industry. Here are some reasons to consider a Web3 Application for the Agriculture Sector:
1. **Financial Support and Accessibility**
Web3 platforms enable farmers to access financial support without the need to rely on traditional banking systems. Through the use of crypto, farmers can borrow money quickly and efficiently, providing a valuable lifeline in situations where crops are affected by adverse weather or other unfortunate circumstances.
2. **Track and Trace**
Blockchain forms the backbone of Web3 applications, allowing for tamper-proof tracking of food origin, processing, and transportation. This fosters trust between consumers and producers. Imagine supermarket tomatoes with a QR code revealing the farm, growing methods, and carbon footprint.
3. **Decentralized Finance (DeFi)**
Cryptocurrencies and DeFi applications offer alternative financing options, bypassing traditional banks with their high fees and complex procedures. Farmers can secure loans or investments through DeFi platforms.
4. **Smart contracts and payments**
Web3 can enable farmers to use smart contracts, which are self-executing agreements that run on a blockchain, to manage their contracts with buyers, suppliers, insurers, and lenders. Smart contracts can reduce transaction costs, eliminate intermediaries, and enforce terms and conditions. For example, a smart contract can automatically release payment to a farmer once a product is delivered and verified, or trigger an insurance claim in case of a crop failure. Web3 can also enable farmers to access peer-to-peer payments and financing options, such as cryptocurrencies and decentralized lending platforms, that can reduce fees and barriers.
**Potential Blockchain Technology Benefits for Agriculture**
The blockchain is a ledger of accounts and transactions that are written and stored by all participants. It promises a reliable source of truth about the state of farms, inventories and contracts in agriculture, where the collection of such information is often incredibly costly.
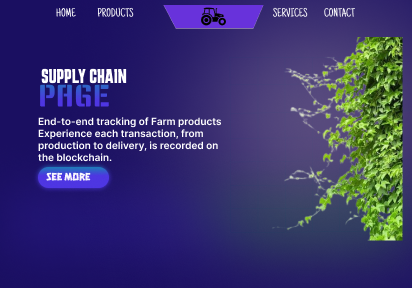
Technology can track the provenance of food and thus help create trustworthy food supply chains and build trust between producers and consumers. As a trusted way of storing data, it facilitates the use of data-driven technologies to make farming smarter. In addition, jointly used with smart contracts, it allows timely payments between stakeholders that can be triggered by data changes appearing in the blockchain.
Blockchain technology also offers a reliable approach to tracing transactions between anonymous participants. Fraud and malfunctions can thus be detected quickly. Moreover, problems can be reported in real time by incorporating smart contracts.
This helps address the challenge of tracking products in the wide-reaching supply chain due to the complexity of the agri-food system. The technology thus provides solutions to issues of food quality and safety, which are highly concerned by consumers, government, etc.
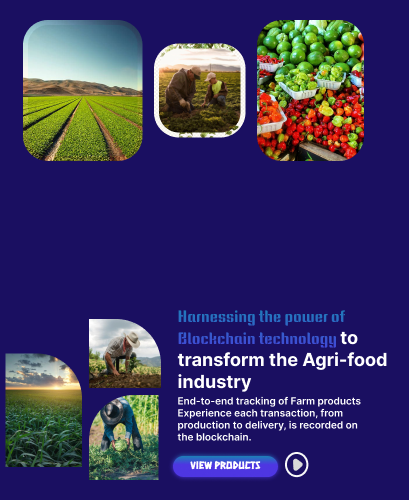
**APPLICATIONS OF BLOCKCHAIN IN THE AGRICULTURAL SECTOR**
1. **Agricultural Insurance**
Agricultural insurance schemes are traditionally a well-recognized tool to manage weather related risks. Farmers would have to pay an insurance premium before the cropping cycle begins and receive an insurance payout whenever they experience a loss on their farm.
The insurer has to bear all the insured risk and farmers can manage their financial exposure to weather extremes, i.e., financial losses caused by weather extremes. In addition, in case of weather threats that systemically affect all the insured farmers, the insurer can further hedge the systemic part of the risk with a reinsurance company.
2. **Smart Agriculture**
A key issue of establishing smart agriculture is developing a comprehensive security system that facilitates the use and management of data. Traditional ways manage data in a centralized fashion and are prone to inaccurate data, data distortion, and misuse as well as cyber-attacks.
For example, environmental monitoring data is generally managed by centralized government entities that have their interest. They can manipulate the decision-making related to data.
Blockchain technology serves to store data and information that various actors and stakeholders generate throughout the entire value-added process, from seed to sale, of producing an agricultural product. It ensures that the data and information are transparent to the involved actors and stakeholders and that all recorded data are immutable.
**> Blockchain technology provides proper solutions for many aspects of e-commerce problems**
a. **Information security**: Blockchain technology provides private key encryption which is a powerful tool that provides the authentication requirements, it can thus link the data of all aspects of planting and harvesting of agricultural products safely and unchangeable.
b. **Supply chain management**: Blockchain technology could enable supply chain management more efficiently than traditional monitoring mechanisms by lowering signaling costs for each entity. Every link in the supply chain – the producer, the place of origin, the shipping company, the destination, the multi-modal transport, the warehouse, and the final last mile – represents a “block” of information, with the advantage of visibility, aggregation, validation, automation and resiliency.
c. **Payment methods**: The blockchain provides a digital payment solution with zero rates. Furthermore, the application of cryptocurrency in the transaction of agricultural products will reduce transaction costs substantially.
d. **Consumer confidence**: Through the decentralized mechanism, the distributed accounting system of the blockchain is time-stamped, so that all information on the chain is transparent and unmodifiable. Consumers will be liberated from fakes and regain confidence in e-commerce.
e. **Reduce the cost of farmers**: Many agricultural products are produced by households. Due to the low transaction volume and small scale, traditional e-commerce is neither willing nor able to provide services for them, thus excluding these participants from the market. Blockchain technology can greatly reduce transaction costs and incorporate them into the market again.
**Conclusion**
Blockchain technology enables the traceability of information in the food supply chain and thus helps improve food safety. It provides a secure way of storing and managing data, which facilitates the development and use of data-driven innovations for smart farming and smart index-based agriculture insurance. In addition, it can reduce transaction costs, which will benefit farmers’ access to markets and generate new revenue streams. Despite enormous potential advantages, key limitations remain for applying blockchain technology in the agriculture and food sectors.
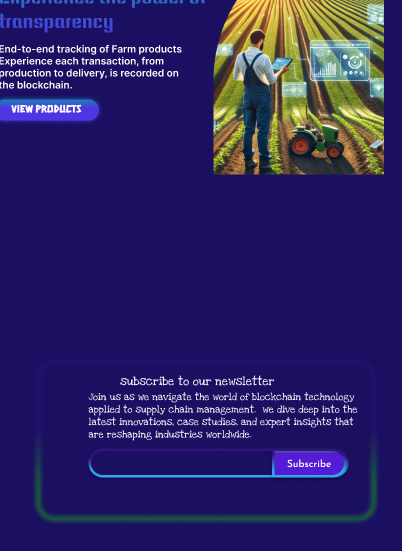
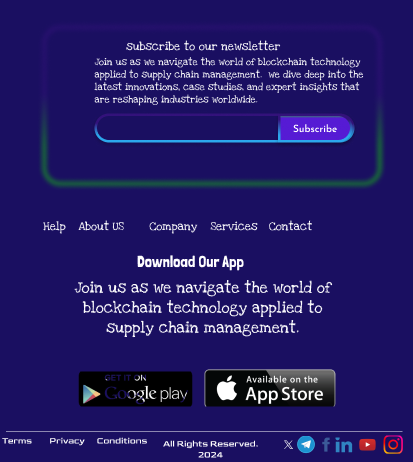
> Want to get more updates on Cybersecurity, Blockchain, and Web3 trends on other platforms?
> Let's Connect on
> [LinkedIn](https://www.linkedin.com/in/nwachukwu-ebenezer-542475217/): Connect with me to stay updated on tech-related insights. Let's network and collaborate on exciting projects.
> [X](https://x.com/UkeziEbenezer): Join me on X for tech news and engaging discussions. Follow me and let's share our thoughts in the X verse. Follow on X.
> [Portfolio Website](https://hashnode.com/@nwachukwu1): Explore my portfolio website to see a curated collection of my projects, learn more about my skills, and get in touch for potential collaborations.
| ukeziebenezer |