branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>Robotics-Team-5268/RobotCode2019<file_sep>/src/main/include/commands/MoveElevator.h
#pragma once
#include "CommandBase.h"
class MoveElevator : public CommandBase {
private:
double speed;
public:
MoveElevator(double spd);
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
};
<file_sep>/src/main/include/commands/Autonomous.h
#pragma once
#include <frc/commands/Command.h>
#include "CommandBase.h"
class Autonomous : public CommandBase {
public:
Autonomous(int selection);
};
<file_sep>/src/main/cpp/commands/DriveWithJoystick.cpp
#include "commands/DriveWithJoystick.h"
DriveWithJoystick::DriveWithJoystick() : CommandBase("DriveWithJoystick") {
Requires(drive.get());
}
void DriveWithJoystick::Initialize() {}
void DriveWithJoystick::Execute() {
drive->setMotors(
-CommandBase::oi->getDriverJoystick()->GetRawAxis(1) ,
-CommandBase::oi->getDriverJoystick()->GetRawAxis(5)
// 0.0,0.0
);
}
bool DriveWithJoystick::IsFinished() { return true; }
void DriveWithJoystick::End() {}
void DriveWithJoystick::Interrupted() {}
<file_sep>/src/main/include/subsystems/MB1013Ultrasonic.h
#pragma once
#include <frc/commands/Subsystem.h>
#include "RobotMap.h"
class MB1013Ultrasonic : public frc::Subsystem {
private:
frc::AnalogInput ultrasonic{ULTRASONIC};
public:
MB1013Ultrasonic();
double getVoltage();
};
/*
.29-.30 = 1 ft
.41-.42 = 1-1/2 ft
.58-.60 = 2 ft
.72-.72 = 2-1/2 ft
.86-.87 = 3 ft
1.09-1.10 = 4 ft
*/<file_sep>/src/main/cpp/commands/GrabberCommand.cpp
#include "commands/GrabberCommand.h"
#include "CommandBase.h"
GrabberCommand::GrabberCommand(std::string drct) {
// Use Requires() here to declare subsystem dependencies
// eg. Requires(Robot::chassis.get());
Requires(CommandBase::grabber.get());
direction = drct;
}
// Called just before this Command runs the first time
void GrabberCommand::Initialize() {}
// Called repeatedly when this Command is scheduled to run
void GrabberCommand::Execute() {
if(direction == "Output"){
CommandBase::grabber->GrabberOutput();
}
if(direction == "Intake"){
CommandBase::grabber->GrabberIntake();
}
}
// Make this return true when this Command no longer needs to run execute()
bool GrabberCommand::IsFinished() { return false; }
// Called once after isFinished returns true
void GrabberCommand::End() {
CommandBase::grabber->GrabberOff();
}
// Called when another command which requires one or more of the same
// subsystems is scheduled to run
void GrabberCommand::Interrupted() {}
<file_sep>/src/main/include/commands/Move.h
#pragma once
#include <frc/commands/Command.h>
#include <frc/Timer.h>
#include <frc/WPILib.h>
#include "CommandBase.h"
class Move : public CommandBase {
public:
Move(float tm, float spd);
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
float speed;
float seconds;
std::shared_ptr<frc::Timer> timer;
frc::PIDController* pid;
};
class MovePIDOutput: public frc::PIDOutput {
public:
MovePIDOutput(double sp);
virtual ~MovePIDOutput();
void PIDWrite(double a);
float speed;
};
<file_sep>/src/main/include/CommandBase.h
#pragma once
#include <memory>
#include <frc/commands/CommandGroup.h>
#include <string.h>
#include "subsystems/UDPReceiver.h"
#include "subsystems/Drive.h"
#include "subsystems/Sighting.h"
#include "subsystems/Encoders.h"
#include "subsystems/Elevator.h"
#include "subsystems/Grabber.h"
#include "subsystems/Arm.h"
#include "subsystems/Pneumatics.h"
#include "subsystems/MB1013Ultrasonic.h"
#include "OI.h"
class CommandBase : public frc::CommandGroup {
public:
CommandBase(const std::string &name);
CommandBase();
static void init();
static std::unique_ptr<Drive> drive;
static std::unique_ptr<Encoders> leftEncoder;
static std::unique_ptr<Encoders> rightEncoder;
static std::unique_ptr<Encoders> elevatorEncoder;
static std::unique_ptr<MB1013Ultrasonic> ultrasonic;
static std::unique_ptr<Elevator> elevator;
static std::unique_ptr<Grabber> grabber;
static std::unique_ptr<Pneumatics> pneumatics;
static std::unique_ptr<Arm> arm;
static std::unique_ptr<OI> oi;
static std::unique_ptr<UDPReceiver> udp;
static std::unique_ptr<Sighting> sight;
};
<file_sep>/src/main/cpp/commands/MovePneumatics.cpp
#include "commands/MovePneumatics.h"
#include "CommandBase.h"
MovePneumatics::MovePneumatics(frc::DoubleSolenoid::Value v) : CommandBase("MovePneumatics") {
Requires(CommandBase::pneumatics.get());
value = v;
}
void MovePneumatics::Initialize() {}
void MovePneumatics::Execute() {
if (value == frc::DoubleSolenoid::kForward) {
CommandBase::pneumatics->Forward();
} else if (value == frc::DoubleSolenoid::kReverse){
CommandBase::pneumatics->Reverse();
} else {
CommandBase::pneumatics->Off();
}
}
bool MovePneumatics::IsFinished() {
return true;
}
void MovePneumatics::End() {}
void MovePneumatics::Interrupted() {}
<file_sep>/src/main/include/subsystems/Drive.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/drive/DifferentialDrive.h>
#include <frc/Ultrasonic.h>
#include "RobotMap.h"
class Drive : public frc::Subsystem {
private:
frc::Talon speedControllerFL{DRIVE_SPEED_CONTROLLER_FL_CHANNEL};
frc::Talon speedControllerBL{DRIVE_SPEED_CONTROLLER_BL_CHANNEL};
frc::SpeedControllerGroup leftSC{speedControllerFL, speedControllerBL};
frc::Talon speedControllerFR{DRIVE_SPEED_CONTROLLER_FR_CHANNEL};
frc::Talon speedControllerBR{DRIVE_SPEED_CONTROLLER_BR_CHANNEL};
frc::SpeedControllerGroup rightSC{speedControllerFR, speedControllerBR};
frc::DifferentialDrive diffDrive{leftSC, rightSC};
float oldLeftSpeed, oldRightSpeed;
const float MAX_CHANGE = .05;
const float MAX_SPEED = 3000;//used to be 3000
float velocityToCommandSlope[4];
float velocityToCommandIntercept[4];
frc::AnalogGyro gyro{GYRO_ANALOG_PORT};
//std::ofstream fout;
public:
Drive();
void InitDefaultCommand() override;
virtual void setMotors(float leftSpeed, float rightSpeed);
virtual void safetyOff() {diffDrive.SetSafetyEnabled(false);}
virtual void takeInput();
frc::AnalogGyro* getGyro();
float getGyroAngle();
float getGyroRate();
void resetGyro();
void calibrateGyro();
void SetVelocity(float left, float right);
};<file_sep>/src/main/cpp/subsystems/REVDigitBoard.cpp
#include <frc/I2C.h>
#include <frc/Timer.h>
#include <frc/DigitalInput.h>
#include <frc/AnalogInput.h>
#include "Subsystems/RevDigitBoard.h"
#include <string.h>
#include <cctype>
REVDigitBoard::REVDigitBoard() : frc::Subsystem("REVDigitBoard")
, i2c( frc::I2C::kMXP, 0x70 )
, buttonA( 19 )
, buttonB( 20 )
, pot( 3 )
{
byte osc;
byte blink;
byte bright;
osc = (byte)0x21;
blink = (byte)0x81;
bright = (byte)0xEF;
i2c.WriteBulk(&osc, 1);
frc::Wait(.01);
i2c.WriteBulk(&bright, 1);
frc::Wait(.01);
i2c.WriteBulk(&blink, 1);
frc::Wait(.01);
charreg[0][0] = (byte)0b00111111; charreg[9][1] = (byte)0b00000000; //0
charmap['0'] = 0;
charreg[1][0] = (byte)0b00000110; charreg[0][1] = (byte)0b00000000; //1
charmap['1'] = 1;
charreg[2][0] = (byte)0b11011011; charreg[1][1] = (byte)0b00000000; //2
charmap['2'] = 2;
charreg[3][0] = (byte)0b11001111; charreg[2][1] = (byte)0b00000000; //3
charmap['3'] = 3;
charreg[4][0] = (byte)0b11100110; charreg[3][1] = (byte)0b00000000; //4
charmap['4'] = 4;
charreg[5][0] = (byte)0b11101101; charreg[4][1] = (byte)0b00000000; //5
charmap['5'] = 5;
charreg[6][0] = (byte)0b11111101; charreg[5][1] = (byte)0b00000000; //6
charmap['6'] = 6;
charreg[7][0] = (byte)0b00000111; charreg[6][1] = (byte)0b00000000; //7
charmap['7'] = 7;
charreg[8][0] = (byte)0b11111111; charreg[7][1] = (byte)0b00000000; //8
charmap['8'] = 8;
charreg[9][0] = (byte)0b11101111; charreg[8][1] = (byte)0b00000000; //9
charmap['9'] = 9;
charreg[10][0] = (byte)0b11110111; charreg[10][1] = (byte)0b00000000; //A
charmap['A'] = 10;
charreg[11][0] = (byte)0b10001111; charreg[11][1] = (byte)0b00010010; //B
charmap['B'] = 11;
charreg[12][0] = (byte)0b00111001; charreg[12][1] = (byte)0b00000000; //C
charmap['C'] = 12;
charreg[13][0] = (byte)0b00001111; charreg[13][1] = (byte)0b00010010; //D
charmap['D'] = 13;
charreg[14][0] = (byte)0b11111001; charreg[14][1] = (byte)0b00000000; //E
charmap['E'] = 14;
charreg[15][0] = (byte)0b11110001; charreg[15][1] = (byte)0b00000000; //F
charmap['F'] = 15;
charreg[16][0] = (byte)0b10111101; charreg[16][1] = (byte)0b00000000; //G
charmap['G'] = 16;
charreg[17][0] = (byte)0b11110110; charreg[17][1] = (byte)0b00000000; //H
charmap['H'] = 17;
charreg[18][0] = (byte)0b00001001; charreg[18][1] = (byte)0b00010010; //I
charmap['I'] = 18;
charreg[19][0] = (byte)0b00011110; charreg[19][1] = (byte)0b00000000; //J
charmap['J'] = 19;
charreg[20][0] = (byte)0b01110000; charreg[20][1] = (byte)0b00001100; //K
charmap['K'] = 20;
charreg[21][0] = (byte)0b00111000; charreg[21][1] = (byte)0b00000000; //L
charmap['L'] = 21;
charreg[22][0] = (byte)0b00110110; charreg[22][1] = (byte)0b00000101; //M
charmap['M'] = 22;
charreg[23][0] = (byte)0b00110110; charreg[23][1] = (byte)0b00001001; //N
charmap['N'] = 23;
charreg[24][0] = (byte)0b00111111; charreg[24][1] = (byte)0b00000000; //O
charmap['O'] = 24;
charreg[25][0] = (byte)0b11110011; charreg[25][1] = (byte)0b00000000; //P
charmap['P'] = 25;
charreg[26][0] = (byte)0b00111111; charreg[26][1] = (byte)0b00001000; //Q
charmap['Q'] = 26;
charreg[27][0] = (byte)0b11110011; charreg[27][1] = (byte)0b00001000; //R
charmap['R'] = 27;
charreg[28][0] = (byte)0b10001101; charreg[28][1] = (byte)0b00000001; //S
charmap['S'] = 28;
charreg[29][0] = (byte)0b00000001; charreg[29][1] = (byte)0b00010010; //T
charmap['T'] = 29;
charreg[30][0] = (byte)0b00111110; charreg[30][1] = (byte)0b00000000; //U
charmap['U'] = 30;
charreg[31][0] = (byte)0b00110000; charreg[31][1] = (byte)0b00100100; //V
charmap['V'] = 31;
charreg[32][0] = (byte)0b00110110; charreg[32][1] = (byte)0b00101000; //W
charmap['W'] = 32;
charreg[33][0] = (byte)0b00000000; charreg[33][1] = (byte)0b00101101; //X
charmap['X'] = 33;
charreg[34][0] = (byte)0b00000000; charreg[34][1] = (byte)0b00010101; //Y
charmap['Y'] = 34;
charreg[35][0] = (byte)0b00001001; charreg[35][1] = (byte)0b00100100; //Z
charmap['Z'] = 35;
charreg[36][0] = (byte)0b00000000; charreg[36][1] = (byte)0b00000000; //space
charmap[' '] = 36;
}
void REVDigitBoard::InitDefaultCommand() {}
void REVDigitBoard::display(std::string str) { // only displays first 4 chars
byte charz[4] = {36,36,36,36};
for(size_t i = 0; i < 4 && i < str.length(); i++) {
charz[i] = charmap[std::toupper(str[i])];
}
_display(charz);
}
void REVDigitBoard::display(double batt) { // optimized for battery voltage, needs a double like 12.34
byte charz[4] = {36,36,36,36};
// idk how to decimal point
int ten = (int)(batt/10);
int one = ((int)batt)%10;
int tenth = ((int)(batt*10)%10);
int hundredth = ((int)(batt*100)%10);
if (ten != 0) charz[0] = ten;
charz[1] = one;
charz[2] = tenth;
charz[3] = hundredth;
_display(charz);
}
void REVDigitBoard::clear() {
byte charz[4] = {36,36,36,36};
_display(charz);
}
bool REVDigitBoard::getButtonA() {
return !buttonA.Get();// Not symbol because digital input is true when open
}
bool REVDigitBoard::getButtonB() {
return !buttonB.Get();// Not symbol because digital input is true when open
}
double REVDigitBoard::getPot() {
return pot.GetVoltage();
}
////// not supposed to be publicly used..
void REVDigitBoard::_display( byte charz[4]) {
byte byte1[10];
byte1[0] = (byte)(0b00001111);
byte1[1] = 0; //(byte)(0b00001111);
byte1[2] = charreg[charz[3]][0];
byte1[3] = charreg[charz[3]][1];
byte1[4] = charreg[charz[2]][0];
byte1[5] = charreg[charz[2]][1];
byte1[6] = charreg[charz[1]][0];
byte1[7] = charreg[charz[1]][1];
byte1[8] = charreg[charz[0]][0];
byte1[9] = charreg[charz[0]][1];
//send the array to the board
i2c.WriteBulk(byte1, sizeof( byte1 ));
frc::Wait(0.01);
}
<file_sep>/src/main/cpp/commands/RampUpVelocityTest.cpp
#include "commands/RampUpVelocityTest.h"
RampUpVelocityTest::RampUpVelocityTest()
: fout("/home/lvuser/TestValue.csv"),
pdp()
{
driveCommand = 0.0;
count = 0;
fout << "Percent Power,Left Velocity,Right Velocity" << '\n';
}
void RampUpVelocityTest::Initialize() {}
void RampUpVelocityTest::Execute() {
if (count == 10) {
driveCommand += 0.02;
count = 0;
} else {
count++;
}
drive->setMotors(driveCommand, driveCommand);
double left = CommandBase::leftEncoder->getRate();
double right = CommandBase::rightEncoder->getRate();
fout << driveCommand << "," << left << "," << right << '\n';
}
bool RampUpVelocityTest::IsFinished() {
return (4 <= count && driveCommand >= 1.0);
}
void RampUpVelocityTest::End() {
fout.close();
}
void RampUpVelocityTest::Interrupted() {
fout.close();
}<file_sep>/src/main/include/subsystems/Encoders.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/WPILib.h>
class Encoders : public frc::Subsystem {
private:
public:
double getCount() const;
double getRaw() const;
double getDistance() const;
double getRate() const; //const keyword is placed afterwards so that the accessor method will be checked to make sure it doesn't modify variable values.
double getDirection() const;
double getStopped() const;
void reset() const;
Encoders(std::string shortName, unsigned int channelA, unsigned int channelB, bool reverseDirection);
private:
const double MAX_PERIOD = .1;
const double MIN_RATE = .05;
const double PULSEINCHES = .00920; //this is the appproximate value for the magnetic encoder. gear ratio for tike is 12.75 and 10.71 on the actual robot18
const double SAMPLES_TO_AVE = 7;
frc::Encoder *encoder;
};
<file_sep>/src/main/cpp/commands/DriveStraightDistance.cpp
#include <frc/WPILib.h>
#include "commands/DriveStraightDistance.h"
#include "subsystems/Encoders.h"
#include "commands/Velocity.h"
void DriveStraightDistance::End(){
velocity->done = true;
//velocity->End();
}
void DriveStraightDistance::Initialize(){
velocity = new Velocity();
//velocity->Initialize();
velocity->Start();
//AddSequential(velocity);
startingLeft = CommandBase::leftEncoder->getDistance();
startingRight = CommandBase::rightEncoder->getDistance();
}
void DriveStraightDistance::Execute(){
/*if (velocity == nullptr){
return;
}*/
double currentDistance = (CommandBase::leftEncoder->getDistance() + (CommandBase::rightEncoder->getDistance() * 7)) / 2;
double velocityPIDInput = 0;
if (currentDistance < ACCELERATION_DISTANCE) {
//This is when we start accelerating
velocityPIDInput = ACCELERATION * currentDistance;
if(velocityPIDInput < .1){ velocityPIDInput = .1;}
} else if (currentDistance >= ACCELERATION_DISTANCE && currentDistance < distance - DECELERATION_DISTANCE) {
//This is when we stay at a constant velocity
velocityPIDInput = MAX_VELOCITY;
} else {
//This is when we to start to deaccelerate
velocityPIDInput = -MAX_VELOCITY * ((currentDistance - distance)/DECELERATION_DISTANCE);
}
frc::SmartDashboard::PutNumber("Setpoint", velocityPIDInput);
velocity->SetSetpoint(velocityPIDInput);
//velocity->Execute();
}
bool DriveStraightDistance::IsFinished(){
if ((CommandBase::leftEncoder->getDistance() + (CommandBase::rightEncoder->getDistance() * 7)) / 2 > distance) {
return true;
}
return false;
}
DriveStraightDistance::DriveStraightDistance(double distance) : CommandBase("DriveStraightDistance") {
this->distance = distance;
frc::SmartDashboard::PutNumber("distance", distance);
}<file_sep>/src/main/cpp/commands/Move.cpp
#include "commands/Move.h"
Move::Move(float tm, float spd) : CommandBase() {
Requires(drive.get());
pid = nullptr;
seconds = tm;
speed = spd;
timer.reset(new frc::Timer());
}
void Move::Initialize() {}
void Move::Execute() {
if(pid) {
}
}
bool Move::IsFinished() {
return timer->HasPeriodPassed(seconds);
}
void Move::End() {}
void Move::Interrupted() {}
<file_sep>/src/main/cpp/subsystems/Elevator.cpp
#include "subsystems/Elevator.h"
#include "CommandBase.h"
Elevator::Elevator() : Subsystem("Elevator") {
}
void Elevator::InitDefaultCommand(){
}
void Elevator::MoveMotor(double speed) {
elevator_SC.Set(speed);
}<file_sep>/src/main/include/subsystems/Grabber.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/drive/DifferentialDrive.h>
#include "RobotMap.h"
//I want to make take and place item commands into one class so you will see a new command called GrabberCommand
//I left the two commands you made but if you want to get rid of them go ahead
//I feel that lower level things like what direction the wheels spin should be handled at the subsystem level
//which is why I made two methods in this class, one to handle input and another for output
//the third method is just called at the end of the GrabberCommand and sets the motors to 0 so they don't run indefinitly
class Grabber : public frc::Subsystem {
private:
frc::Talon speedControllerL{GRABBER_SPEED_CONTROLLER_L_CHANNEL};
frc::Talon speedControllerR{GRABBER_SPEED_CONTROLLER_R_CHANNEL};
frc::SpeedControllerGroup GrabberSCG{speedControllerL, speedControllerR};
public:
Grabber();
void GrabberIntake();
void GrabberOutput();
void GrabberOff();
};
<file_sep>/src/main/include/OI.h
#pragma once
#include "frc/WPILib.h"
class OI {
private:
std::shared_ptr<frc::Joystick> driverJoystick, mechanismsJoystick;
std::vector<frc::JoystickButton*> driverBtns, mechanismsBtns;
public:
std::shared_ptr<frc::Joystick> getDriverJoystick();
OI();
};
<file_sep>/src/main/cpp/commands/VelocityTest.cpp
#include "commands/VelocityTest.h"
VelocityTest::VelocityTest()
: fout("/home/lvuser/TestValue.csv"),
pdp()
{
driveCommand = 0.6;
count = 0;
fout << "Front Left,Front Right,Back Left,Back Right,LeftEncoderVelocity,RightEncoderVelocity" << '\n';
}
void VelocityTest::Initialize() {}
void VelocityTest::Execute() {
count++;
drive->SetVelocity(driveCommand, driveCommand);
double left = CommandBase::leftEncoder->getRate();
double right = CommandBase::rightEncoder->getRate();
fout << pdp.GetCurrent(1) << "," << pdp.GetCurrent(2) << "," << pdp.GetCurrent(0) << "," << pdp.GetCurrent(3) << "," << left << "," << right << '\n';
}
bool VelocityTest::IsFinished() {
return (200 <= count);
}
void VelocityTest::End() {
fout.close();
}
void VelocityTest::Interrupted() {}
<file_sep>/src/main/include/commands/GrabberCommand.h
#pragma once
#include <frc/commands/Command.h>
class GrabberCommand : public frc::Command {
private:
std::string direction;
public:
GrabberCommand(std::string drct);
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
};
<file_sep>/src/main/cpp/Robot.cpp
#include "Robot.h"
#include "subsystems/Drive.h"
#include "CommandBase.h"
#include <frc/commands/Scheduler.h>
#include <frc/smartdashboard/SmartDashboard.h>
std::unique_ptr<Autonomous> Robot::autonomousCommand;
AutonomousChooser Robot::AutoChooser;
void Robot::RobotInit() {
CommandBase::init();
CommandBase::drive->calibrateGyro();
CommandBase::drive->safetyOff();
}
void Robot::RobotPeriodic() {}
void Robot::DisabledInit() {}
void Robot::DisabledPeriodic() { frc::Scheduler::GetInstance()->Run(); }
void Robot::AutonomousInit() {
autonomousCommand.reset(new Autonomous(AutoChooser.AutonomousSelection()));
autonomousCommand->Start();
}
void Robot::AutonomousPeriodic() {
frc::Scheduler::GetInstance()->Run();
AddSmartDashboardItems();
}
void Robot::TeleopInit() {
//frc::SmartDashboard::PutNumber("breakpoint", 200);
CommandBase::drive->resetGyro();
CommandBase::drive->setMotors(0.0, 0.0);
}
void Robot::TeleopPeriodic() {
frc::Scheduler::GetInstance()->Run();
AddSmartDashboardItems();
}
void Robot::TestPeriodic() {}
void Robot::AddSmartDashboardItems() {
// frc::SmartDashboard::PutValue("Solenoid Value", CommandBase::pneumatics->getValue())
frc::SmartDashboard::PutNumber("Gyro Angle", CommandBase::drive->getGyroAngle());
frc::SmartDashboard::PutNumber("Gyro Rate", CommandBase::drive->getGyroRate());
frc::SmartDashboard::PutNumber("rightcount", CommandBase::rightEncoder->getCount());
frc::SmartDashboard::PutNumber("rightRaw Count", CommandBase::rightEncoder->getRaw());
frc::SmartDashboard::PutNumber("rightDistance", CommandBase::rightEncoder->getDistance()*7);
frc::SmartDashboard::PutNumber("rightRate", CommandBase::rightEncoder->getRate());
frc::SmartDashboard::PutBoolean("rightDirection", CommandBase::rightEncoder->getDirection());
frc::SmartDashboard::PutBoolean("rightStopped", CommandBase::rightEncoder->getStopped());
frc::SmartDashboard::PutNumber("leftcount", CommandBase::leftEncoder->getCount());
frc::SmartDashboard::PutNumber("leftRaw Count", CommandBase::leftEncoder->getRaw());
frc::SmartDashboard::PutNumber("leftDistance", CommandBase::leftEncoder->getDistance());
frc::SmartDashboard::PutNumber("leftRate", CommandBase::leftEncoder->getRate());
frc::SmartDashboard::PutBoolean("leftDirection", CommandBase::leftEncoder->getDirection());
frc::SmartDashboard::PutBoolean("leftStopped", CommandBase::leftEncoder->getStopped());
frc::SmartDashboard::PutNumber("Ultrasonic", CommandBase::ultrasonic->getVoltage());
frc::SmartDashboard::PutNumber("distance traveled with drive straight", (CommandBase::leftEncoder->getDistance() + (CommandBase::rightEncoder->getDistance() * 7)) / 2);
}
#ifndef RUNNING_FRC_TESTS
int main() { return frc::StartRobot<Robot>(); }
#endif
<file_sep>/src/main/include/commands/DriveWithJoystick.h
#pragma once
#include "CommandBase.h"
class DriveWithJoystick : public CommandBase {
public:
DriveWithJoystick();
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
};
<file_sep>/src/main/cpp/commands/MoveElevator.cpp
#include "commands/MoveElevator.h"
#include "subsystems/Elevator.h"
MoveElevator::MoveElevator(double spd) : CommandBase("MoveElevator") {
Requires(CommandBase::elevator.get());
speed = spd;
}
void MoveElevator::Initialize() {
}
void MoveElevator::Execute() {
CommandBase::elevator->MoveMotor(speed);
}
bool MoveElevator::IsFinished() {
return true;
}
void MoveElevator::End() {
CommandBase::elevator->MoveMotor(0.0);
}
void MoveElevator::Interrupted() {}
<file_sep>/src/main/include/commands/DriveStraightDistance.h
#ifndef DRIVE_STRAIGHT_DISTANCE_H
#define DRIVE_STRAIGHT_DISTANCE_H
#define MAX_VELOCITY .3
#define ACCELERATION_DISTANCE 20
#define DECELERATION_DISTANCE 47
#define ACCELERATION (MAX_VELOCITY / ACCELERATION_DISTANCE)
#define DECELERATION (MAX_VELOCITY / DECELERATION_DISTANCE)
#include "CommandBase.h"
#include "commands/Velocity.h"
class DriveStraightDistance : public CommandBase {
private:
double distance, startingRight, startingLeft;
Velocity* velocity;
public:
virtual void End();
virtual void Initialize();
virtual void Execute();
virtual bool IsFinished();
DriveStraightDistance(double distance);
};
#endif<file_sep>/src/main/cpp/subsystems/Arm.cpp
#include "subsystems/Arm.h"
Arm::Arm() : Subsystem("Arm") {
}
void Arm::MoveArm() {
arm_SC.Set(0.4);
}
int Arm::GetCount() {
return hallSensor.Get();
}<file_sep>/src/main/include/subsystems/Elevator.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/drive/DifferentialDrive.h>
#include "RobotMap.h"
class Elevator : public frc::Subsystem{
private:
frc::Talon elevator_SC{ELEVATOR_SPEED_CONTROLLER_CHANNEL};
public:
Elevator();
void InitDefaultCommand();
void MoveMotor(double speed);
};<file_sep>/src/main/cpp/subsystems/Grabber.cpp
#include "subsystems/Grabber.h"
Grabber::Grabber() : Subsystem("Grabber") {
//I have no idea if the inverts are correct here, if the intake/output go backwards then flip the trues to falses
speedControllerL.SetInverted(false);
speedControllerR.SetInverted(true);
}
void Grabber::GrabberIntake() {
GrabberSCG.Set(0.6);
}
void Grabber::GrabberOff() {
GrabberSCG.Set(0.0);
}
void Grabber::GrabberOutput() {
GrabberSCG.Set(-0.4);
}<file_sep>/src/main/include/commands/MovePneumatics.h
#pragma once
#include "CommandBase.h"
#include <frc/DoubleSolenoid.h>
class MovePneumatics : public CommandBase {
public:
MovePneumatics(frc::DoubleSolenoid::Value v);
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
private:
frc::DoubleSolenoid::Value value;
};
<file_sep>/src/main/include/RobotMap.h
#pragma once
#include <frc/drive/DifferentialDrive.h>
#include <frc/SpeedControllerGroup.h>
#include <frc/SpeedController.h>
#include <frc/WPILib.h>
#define DRIVE_SPEED_CONTROLLER_FL_CHANNEL 0
#define DRIVE_SPEED_CONTROLLER_FR_CHANNEL 1
#define DRIVE_SPEED_CONTROLLER_BL_CHANNEL 2
#define DRIVE_SPEED_CONTROLLER_BR_CHANNEL 3
#define SCFL_INVERTED false
#define SCBL_INVERTED false
#define SCFR_INVERTED false
#define SCBR_INVERTED false
#define GYRO_ANALOG_PORT 0
#define ULTRASONIC 1
#define ELEVATOR_SPEED_CONTROLLER_CHANNEL 5
#define GRABBER_SPEED_CONTROLLER_R_CHANNEL 6
#define GRABBER_SPEED_CONTROLLER_L_CHANNEL 7
#define ARM_SPEED_CONTROLLER_CHANNEL 8
#define HALL_SENSOR 6
#define PCM_PORT 0 //Pnuematics Control Module
#define DOUBLESOLENOID_FORWARD_CHANNEL 0
#define DOUBLESOLENOID_REVERSE_CHANNEL 1
#define TROLLEY_VICTOR_SPX_CAN_BUS_ID 0 //Needs to be changed later<file_sep>/src/main/cpp/subsystems/Drive.cpp
#include "subsystems/Drive.h"
#include "commands/DriveWithJoystick.h"
#include <frc/smartDashBoard/SmartDashBoard.h>
Drive::Drive() : Subsystem("Drive") {
oldLeftSpeed = 0.0;
oldRightSpeed = 0.0;
velocityToCommandIntercept[0] = -316.89;
velocityToCommandIntercept[1] = -378.00;
velocityToCommandIntercept[2] = -364.78;
velocityToCommandIntercept[3] = -331.27;
velocityToCommandSlope[0] = 1/3493.57;
velocityToCommandSlope[1] = 1/3493.90;
velocityToCommandSlope[2] = 1/3386.53;
velocityToCommandSlope[3] = 1/3744.83;
speedControllerFL.SetInverted(SCFL_INVERTED);
speedControllerBL.SetInverted(SCBL_INVERTED);
speedControllerFR.SetInverted(SCFR_INVERTED);
speedControllerBR.SetInverted(SCBR_INVERTED);
}
void Drive::InitDefaultCommand() {
SetDefaultCommand(new DriveWithJoystick());
}
void Drive::takeInput() {
float leftSpeed = -CommandBase::oi->getDriverJoystick()->GetRawAxis(1);
float rightSpeed = -CommandBase::oi->getDriverJoystick()->GetRawAxis(5);
if (leftSpeed > oldLeftSpeed + MAX_CHANGE) leftSpeed = oldLeftSpeed + MAX_CHANGE;
else if (leftSpeed < oldLeftSpeed - MAX_CHANGE) leftSpeed = oldLeftSpeed - MAX_CHANGE;
if (rightSpeed > oldRightSpeed + MAX_CHANGE) rightSpeed = oldRightSpeed + MAX_CHANGE;
else if (rightSpeed < oldRightSpeed - MAX_CHANGE) rightSpeed = oldRightSpeed - MAX_CHANGE;
if (leftSpeed >= .9 && rightSpeed >= .9) {
setMotors(1, 1);
} else {
setMotors(leftSpeed, rightSpeed);
}
oldLeftSpeed = leftSpeed;
oldRightSpeed = rightSpeed;
//fout << getGyroRate() << '\n';
}
void Drive::setMotors(float leftSpeed, float rightSpeed) {
diffDrive.TankDrive(leftSpeed, rightSpeed, false);
frc::SmartDashboard::PutNumber("Speed Controller FL", speedControllerFL.Get());
frc::SmartDashboard::PutNumber("Speed Controller FR", speedControllerFR.Get());
frc::SmartDashboard::PutNumber("Speed Controller BL", speedControllerBL.Get());
frc::SmartDashboard::PutNumber("Speed Controller BR", speedControllerBR.Get());
}
frc::AnalogGyro* Drive::getGyro() {
return &gyro;
}
float Drive::getGyroAngle() {
return gyro.GetAngle();
}
float Drive::getGyroRate() {
return gyro.GetRate();
}
void Drive::resetGyro() {
gyro.Reset();
}
void Drive::calibrateGyro() {
gyro.Calibrate();
}
void Drive::SetVelocity(float left, float right) {
float leftSpeed = left * MAX_SPEED;
float rightSpeed = right * MAX_SPEED;
if (leftSpeed < 0) {
leftSpeed = velocityToCommandSlope[0]*(leftSpeed + velocityToCommandIntercept[0]);
} else {
leftSpeed = velocityToCommandSlope[2]*(leftSpeed + velocityToCommandIntercept[2]);
}
if (rightSpeed < 0) {
rightSpeed = velocityToCommandSlope[1]*(rightSpeed + velocityToCommandIntercept[1]);
} else {
rightSpeed = velocityToCommandSlope[3]*(rightSpeed + velocityToCommandIntercept[3]);
}
setMotors(left, rightSpeed);
}<file_sep>/src/main/include/commands/LowerArm.h
#pragma once
#include "CommandBase.h"
class LowerArm : public CommandBase {
private:
const int STOP_POINT = 25;
public:
LowerArm();
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
};
<file_sep>/src/main/include/commands/VelocityTest.h
#pragma once
#include "CommandBase.h"
#include <frc/PowerDistributionPanel.h>
#include <iostream>
#include <fstream>
#include <string>
class VelocityTest : public CommandBase {
public:
VelocityTest();
void Initialize() override;
void Execute() override;
bool IsFinished() override;
void End() override;
void Interrupted() override;
private:
frc::PowerDistributionPanel pdp;
double driveCommand;
int count;
std::ofstream fout;
};
<file_sep>/src/main/cpp/CommandBase.cpp
#include "CommandBase.h"
#include "subsystems/Encoders.h"
#include "subsystems/Drive.h"
#include "subsystems/UDPReceiver.h"
#include "subsystems/Sighting.h"
#include "subsystems/Elevator.h"
#include <Subsystems/Encoders.h>
std::unique_ptr<Drive> CommandBase::drive;
std::unique_ptr<Encoders> CommandBase::leftEncoder;
std::unique_ptr<Encoders> CommandBase::rightEncoder;
std::unique_ptr<Encoders> CommandBase::elevatorEncoder;
std::unique_ptr<Elevator> CommandBase::elevator;
std::unique_ptr<Grabber> CommandBase::grabber;
std::unique_ptr<OI> CommandBase::oi;
std::unique_ptr<Arm> CommandBase::arm;
std::unique_ptr<Pneumatics> CommandBase::pneumatics;
std::unique_ptr<UDPReceiver> CommandBase::udp;
std::unique_ptr<Sighting> CommandBase::sight;
std::unique_ptr<MB1013Ultrasonic> CommandBase::ultrasonic;
CommandBase::CommandBase(const std::string &name) : frc::CommandGroup(name) {
}
CommandBase::CommandBase() : frc::CommandGroup() {
}
void CommandBase::init() {
drive.reset(new Drive());
udp.reset(new UDPReceiver());
sight.reset(new Sighting());
leftEncoder.reset(new Encoders("left", 3, 2, false));
rightEncoder.reset(new Encoders("right", 1, 0, false));
elevatorEncoder.reset(new Encoders("elevator", 4, 5, false));
grabber.reset(new Grabber());
arm.reset(new Arm());
pneumatics.reset(new Pneumatics());
ultrasonic.reset(new MB1013Ultrasonic());
elevator.reset(new Elevator());
// Keep at the end
oi.reset(new OI());
}<file_sep>/src/main/include/subsystems/REVDigitBoard.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/I2C.h>
#include <frc/Timer.h>
#include <frc/DigitalInput.h>
#include <frc/AnalogInput.h>
#include <string.h>
// this class controls the REV digit board that we use to choose our autonomous mode
//without having to redeploy every match.
class REVDigitBoard: public frc::Subsystem {
typedef unsigned char byte;
/*
* DOCUMENTATION::
*
* REVDigitBoard() : constructor
* void display(String str) : displays the first four characters of the string (only alpha (converted to uppercase), numbers, and spaces)
* void display(double batt) : displays a decimal number (like battery voltage) in the form of 12.34 (ten-one-decimal-tenth-hundredth)
* void clear() : clears the display
* boolean getButtonA() : button A on the board
* boolean getButtonB() : button B on the board
* double getPot() : potentiometer value
*/
public:
REVDigitBoard();
void InitDefaultCommand();
void clear();
bool getButtonA();
bool getButtonB();
double getPot();
void display(double batt);
void display(std::string str);
private:
void _display( byte charz[4]);
frc::I2C i2c;
frc::DigitalInput buttonA, buttonB;
frc::AnalogInput pot;
byte charreg[37][2];
byte charmap[256];
};
<file_sep>/src/main/cpp/subsystems/Pneumatics.cpp
#include "subsystems/Pneumatics.h"
Pneumatics::Pneumatics() : Subsystem("Pnuematics") {
}
void Pneumatics::Forward() {
doubleSolenoid.Set(frc::DoubleSolenoid::kForward);
}
void Pneumatics::Reverse() {
doubleSolenoid.Set(frc::DoubleSolenoid::kReverse);
}
void Pneumatics::Off() {
doubleSolenoid.Set(frc::DoubleSolenoid::kOff);
}<file_sep>/src/main/cpp/commands/Autonomous.cpp
#include "commands/Autonomous.h"
#include "commands/Move.h"
#include "commands/VelocityTest.h"
#include "commands/RampUpVelocityTest.h"
#include "commands/DriveStraightDistance.h"
Autonomous::Autonomous(int selection) : CommandBase("Autonomous") {
//std::string gameData;
//gameData = frc::DriverStation::GetInstance().GetGameSpecificMessage();
//AddSequential(new VelocityTest());
//AddSequential(new RampUpVelocityTest());
//AddSequential(new DriveStraightDistance(36));
//"Wes is Stupid and dumb" -Wes
}<file_sep>/src/main/include/commands/Velocity.h
#ifndef VELOCITY_H
#define VELOCITY_H
#include "CommandBase.h"
#include <frc/PIDController.h>
#include <frc/PIDOutput.h>
#include <frc/PIDSource.h>
//NOTICE: PLEASE READ: If encoders are not already required by the command you are calling this from,
//then you must add it to that command.
class VelocityPIDOutput: public frc::PIDOutput {
private:
double leftOutput , rightOutput ;
bool right;
public:
VelocityPIDOutput(bool right);
virtual ~VelocityPIDOutput();
virtual void PIDWrite(double output);
};
class VelocityPIDSource: public frc::PIDSource {
private:
bool right;
public:
VelocityPIDSource(bool right);
virtual ~VelocityPIDSource();
virtual double PIDGet();
};
class Velocity : public CommandBase {
private:
double setPointVelocity;
frc::PIDController * leftPID = nullptr, * rightPID = nullptr;
frc::PIDSource * rightPIDSource, * leftPIDSource;
frc::PIDOutput * rightPIDOutput, * leftPIDOutput;
public:
bool done;
Velocity();
virtual void End();
virtual void Initialize();
virtual void Execute();
virtual bool IsFinished();
virtual void Interrupted();
void SetSetpoint(double inputVelocity);
bool IsInterruptible() const;
};
#endif<file_sep>/src/main/cpp/OI.cpp
#include <frc/WPILib.h>
#include <frc/DoubleSolenoid.h>
#include "OI.h"
#include "frc/smartDashboard/SmartDashboard.h"
#include "Robot.h"
#include "commands/DriveStraightDistance.h"
#include "commands/LowerArm.h"
#include "commands/MoveElevator.h"
#include "commands/MovePneumatics.h"
OI::OI() {
driverJoystick.reset(new frc::Joystick(0));
mechanismsJoystick.reset(new frc::Joystick(1));
for (int i=1; i<=10; i++){
driverBtns.push_back(new frc::JoystickButton(driverJoystick.get(), i));
mechanismsBtns.push_back(new frc::JoystickButton(mechanismsJoystick.get(), i));
}
driverBtns[1]->WhenPressed(new DriveStraightDistance(100));
mechanismsBtns[1]->WhileHeld(new MoveElevator(0.6)); //This should be 'b' button on the XBox controller
mechanismsBtns[0]->WhileHeld(new MoveElevator(-0.6)); //This should be 'a' button on the XBox controller
mechanismsBtns[2]->WhileHeld(new MovePneumatics(frc::DoubleSolenoid::kForward)); //This should be 'x' button on the XBox controller
mechanismsBtns[2]->WhenReleased(new MovePneumatics(frc::DoubleSolenoid::kOff)); //This should be 'x' button on the XBox controller
mechanismsBtns[3]->WhileHeld(new MovePneumatics(frc::DoubleSolenoid::kReverse)); //This should be 'y' button on the XBox controller
mechanismsBtns[3]->WhenReleased(new MovePneumatics(frc::DoubleSolenoid::kOff)); //This should be 'y' button on the XBox controller
mechanismsBtns[5]->WhenPressed(new LowerArm()); //Ths should be 'RB' on the XBox controller
}
std::shared_ptr<frc::Joystick> OI::getDriverJoystick() {
return driverJoystick;
}
<file_sep>/src/main/cpp/subsystems/Encoders.cpp
#include <Subsystems/Encoders.h>
#include <frc/smartDashboard/SmartDashboard.h>
#include <String>
Encoders::Encoders(std::string shortName, unsigned int channelA, unsigned int channelB, bool reverseDirection) : frc::Subsystem("Encoders") {
encoder = new frc::Encoder(channelA, channelB, reverseDirection, frc::Encoder::EncodingType::k4X);
encoder->Reset();
encoder->SetMaxPeriod(MAX_PERIOD);
encoder->SetMinRate(MIN_RATE);
encoder->SetReverseDirection(false);
encoder->SetDistancePerPulse(PULSEINCHES);
encoder->SetSamplesToAverage(SAMPLES_TO_AVE);
frc::SmartDashboard::PutNumber(shortName + "count", encoder->Get());
frc::SmartDashboard::PutNumber(shortName + "Raw Count", encoder->GetRaw());
frc::SmartDashboard::PutNumber(shortName + "Distance", encoder->GetDistance());
frc::SmartDashboard::PutNumber(shortName + "Rate", encoder->GetRate());
frc::SmartDashboard::PutBoolean(shortName + "Direction", encoder->GetDirection());
frc::SmartDashboard::PutBoolean(shortName + "Stopped", encoder->GetStopped());
}
double Encoders::getCount() const {
return encoder->Get();
}
double Encoders::getRaw() const {
return encoder->GetRaw();
}
double Encoders::getDistance() const {
return encoder->GetDistance();
}
double Encoders::getRate() const {
return encoder->GetRate();
}
double Encoders::getDirection() const {
return encoder->GetDirection();
}
double Encoders::getStopped() const {
return encoder->GetStopped();
}
void Encoders::reset() const {
encoder->Reset();
}<file_sep>/src/main/cpp/commands/LowerArm.cpp
#include "commands/LowerArm.h"
LowerArm::LowerArm() :CommandBase("LowerArm") {
Requires(CommandBase::arm.get());
}
void LowerArm::Initialize() {}
void LowerArm::Execute() {
CommandBase::arm->MoveArm();
}
bool LowerArm::IsFinished() {
return CommandBase::arm->GetCount() <= STOP_POINT;
}
void LowerArm::End() {}
void LowerArm::Interrupted() {}
<file_sep>/src/main/cpp/subsystems/MB1013Ultrasonic.cpp
#include "subsystems/MB1013Ultrasonic.h"
MB1013Ultrasonic::MB1013Ultrasonic() : Subsystem("MB1013Ultrasonic") {}
double MB1013Ultrasonic::getVoltage() {
return ultrasonic.GetVoltage();
}<file_sep>/src/main/cpp/commands/Velocity.cpp
#include <frc/WPILib.h>
#include "CommandBase.h"
#include <frc/PIDController.h>
#include <frc/PIDOutput.h>
#include <frc/PIDSource.h>
#include "commands/Velocity.h"
Velocity::Velocity() : CommandBase("Velocity") {
done = false;
Requires(drive.get());
leftPIDOutput = new VelocityPIDOutput(false);
rightPIDOutput = new VelocityPIDOutput(true);
leftPIDSource = new VelocityPIDSource(false);
rightPIDSource = new VelocityPIDSource(true);
setPointVelocity = 0;
rightPID->SetSetpoint(0);
leftPID->SetSetpoint(0);
}
bool Velocity::IsInterruptible() const{
return true;
}
void Velocity::Interrupted() {
End();
}
void Velocity::End() {
leftPID->Disable();
rightPID->Disable();
delete leftPIDOutput;
delete leftPIDSource;
delete rightPIDOutput;
delete rightPIDSource;
delete leftPID;
delete rightPID;
drive->setMotors(0.0, 0.0);
}
void Velocity::Initialize() {
leftPID = new frc::PIDController(
frc::SmartDashboard::GetNumber("PLeftvelocity", .03),
frc::SmartDashboard::GetNumber("ILeftvelocity", .000),
frc::SmartDashboard::GetNumber("DLeftvelocity", .00),
frc::SmartDashboard::GetNumber("FLeftvelocity", 0),
leftPIDSource,
leftPIDOutput
);
rightPID = new frc::PIDController(
frc::SmartDashboard::GetNumber("PRightvelocity", .03),
frc::SmartDashboard::GetNumber("IRightvelocity", .000),
frc::SmartDashboard::GetNumber("DRightvelocity", .00),
frc::SmartDashboard::GetNumber("FRightvelocity", 0),
rightPIDSource,
rightPIDOutput
);
leftPID->SetInputRange(-352.0, 352.0);
leftPID->SetOutputRange(-1.0, 1.0);
rightPID->SetInputRange(-352.0, 352.0);
rightPID->SetOutputRange(-1.0, 1.0);
leftPID->Enable();
rightPID->Enable();
}
void Velocity::Execute() {
if(leftPID && rightPID){
frc::SmartDashboard::PutNumber("PLeftPIDvelocity", leftPID->GetP());
frc::SmartDashboard::PutNumber("ILeftPIDvelocity", leftPID->GetI());
frc::SmartDashboard::PutNumber("DLeftPIDvelocity", leftPID->GetD());
frc::SmartDashboard::PutNumber("FLeftPIDvelocity", leftPID->GetF());
frc::SmartDashboard::PutNumber("PRightPIDvelocity", rightPID->GetP());
frc::SmartDashboard::PutNumber("IRightPIDvelocity", rightPID->GetP());
frc::SmartDashboard::PutNumber("DRightPIDvelocity", rightPID->GetP());
frc::SmartDashboard::PutNumber("FRightPIDvelocity", rightPID->GetP());
}
//this is the hard coding way of avoiding the PID. if this is active then the line in PIDWrite should be commented or vice versa
CommandBase::drive->setMotors(0, 0);
}
void Velocity::SetSetpoint(double inputVelocity){
if(rightPID && leftPID){
rightPID->SetSetpoint(inputVelocity);
leftPID->SetSetpoint(inputVelocity);
}
}
//this is set to false by the DriveStraightDistance End() method
bool Velocity::IsFinished() {
return done;
}
VelocityPIDOutput::VelocityPIDOutput(bool right) {
this->right = right;
leftOutput = 0;
rightOutput = 0;
}
VelocityPIDOutput::~VelocityPIDOutput() {
}
void VelocityPIDOutput::PIDWrite(double output) {
if (right) {
rightOutput = output;
} else {
leftOutput = output;
}
//CommandBase::drive->setMotors(leftOutput, rightOutput);
}
VelocityPIDSource::VelocityPIDSource(bool right) {
this->right = right;
}
VelocityPIDSource::~VelocityPIDSource() {
}
double VelocityPIDSource::PIDGet() {
if (right) {
return (CommandBase::rightEncoder->getRate() * 7);
} else {
return CommandBase::leftEncoder->getRate();
}
}<file_sep>/src/main/include/Robot.h
#pragma once
#include <frc/TimedRobot.h>
#include <frc/commands/Command.h>
#include <frc/smartdashboard/SendableChooser.h>
#include "CommandBase.h"
#include "commands/Autonomous.h"
#include "AutonomousChooser.h"
class Robot : public frc::TimedRobot {
public:
static std::unique_ptr<Autonomous> autonomousCommand;
static AutonomousChooser AutoChooser;
private:
void RobotInit() override;
void RobotPeriodic() override;
void DisabledInit() override;
void DisabledPeriodic() override;
void AutonomousInit() override;
void AutonomousPeriodic() override;
void TeleopInit() override;
void TeleopPeriodic() override;
void TestPeriodic() override;
void AddSmartDashboardItems();
};
<file_sep>/src/main/include/subsystems/Arm.h
#pragma once
#include <frc/commands/Subsystem.h>
#include <frc/Counter.h>
#include "RobotMap.h"
class Arm : public frc::Subsystem {
private:
frc::Talon arm_SC{ARM_SPEED_CONTROLLER_CHANNEL};
frc::Counter hallSensor{HALL_SENSOR};
public:
Arm();
void MoveArm();
int GetCount();
};
| 9b699c29c1c9e4bd22d0489c53fbd0a166ddd4af | [
"C",
"C++"
] | 43 | C++ | Robotics-Team-5268/RobotCode2019 | c9047a171391b48a6b32748ebd4e1498d24697b5 | ba988f8f3aab9cdd827893e16e2f03abb0bdac1a | |
refs/heads/master | <file_sep>package db
import (
"os"
"path"
"testing"
"time"
)
var testDB *SQLiteDatastore
func TestMain(m *testing.M) {
setup()
retCode := m.Run()
teardown()
os.Exit(retCode)
}
func setup() {
os.MkdirAll(path.Join("./", "datastore"), os.ModePerm)
testDB, _ = Create("", "LetMeIn")
testDB.config.Init("LetMeIn")
testDB.config.Configure(time.Now())
}
func teardown() {
os.RemoveAll(path.Join("./", "datastore"))
}
func TestConfigDB_Create(t *testing.T) {
if _, err := os.Stat(path.Join("./", "datastore", "mainnet.db")); os.IsNotExist(err) {
t.Error("Failed to create database file")
}
}
func TestConfigDB_SignIn(t *testing.T) {
err := testDB.config.SignIn("woohoo!", "...", "...")
if err != nil {
t.Error(err)
}
}
func TestConfigDB_GetUsername(t *testing.T) {
un, err := testDB.config.GetUsername()
if err != nil {
t.Error(err)
return
}
if un != "woohoo!" {
t.Error("got bad username")
}
}
func TestConfigDB_GetTokens(t *testing.T) {
at, rt, err := testDB.config.GetTokens()
if err != nil {
t.Error(err)
return
}
if at != "..." {
t.Error("got bad access token")
return
}
if rt != "..." {
t.Error("got bad refresh token")
return
}
}
func TestConfigDB_SignOut(t *testing.T) {
err := testDB.config.SignOut()
if err != nil {
t.Error(err)
return
}
_, err = testDB.config.GetUsername()
if err == nil {
t.Error("signed out but username still present")
}
}
func TestConfigDB_GetCreationDate(t *testing.T) {
_, err := testDB.config.GetCreationDate()
if err != nil {
t.Error(err)
}
}
func TestConfigDB_GetSchemaVersion(t *testing.T) {
sv, err := testDB.config.GetSchemaVersion()
if err != nil {
t.Error(err)
}
if sv != schemaVersion {
t.Error("schema version mismatch")
}
}
func TestInterface(t *testing.T) {
if testDB.Config() != testDB.config {
t.Error("Config() return wrong value")
}
if testDB.Settings() != testDB.settings {
t.Error("Settings() return wrong value")
}
}
func TestConfigDB_IsEncrypted(t *testing.T) {
encrypted := testDB.Config().IsEncrypted()
if encrypted {
t.Error("IsEncrypted returned incorrectly")
}
}
<file_sep>// TODO: use lumberjack logger, not stdout, see #33
package main
import (
"errors"
"fmt"
"os"
"path/filepath"
"github.com/fatih/color"
"github.com/jessevdk/go-flags"
"github.com/mitchellh/go-homedir"
"github.com/op/go-logging"
"gopkg.in/abiosoft/ishell.v2"
"github.com/textileio/textile-go/cmd"
"github.com/textileio/textile-go/core"
)
type Opts struct {
Version bool `short:"v" long:"version" description:"print the version number and exit"`
DataDir string `short:"d" long:"datadir" description:"specify the data directory to be used"`
}
var Options Opts
var parser = flags.NewParser(&Options, flags.Default)
var shell *ishell.Shell
func main() {
// create a new shell
shell = ishell.New()
// handle version flag
if len(os.Args) > 1 && (os.Args[1] == "--version" || os.Args[1] == "-v") {
shell.Println(core.Version)
return
}
// handle data dir
var dataDir string
if len(os.Args) > 1 && (os.Args[1] == "--datadir" || os.Args[1] == "-d") {
if len(os.Args) < 3 {
shell.Println(errors.New("datadir option provided but missing value"))
return
}
dataDir = os.Args[2]
} else {
hd, err := homedir.Dir()
if err != nil {
shell.Println(errors.New("could not determine home directory"))
return
}
dataDir = filepath.Join(hd, ".textile")
}
// parse flags
if _, err := parser.Parse(); err != nil {
return
}
// handle interrupt
// TODO: shutdown on 'exit' command too
shell.Interrupt(func(c *ishell.Context, count int, input string) {
if count == 1 {
shell.Println("input Ctrl-C once more to exit")
return
}
shell.Println("interrupted")
shell.Printf("textile node shutting down...")
if core.Node.Online() {
core.Node.Stop()
}
shell.Printf("done\n")
os.Exit(1)
})
// add commands
shell.AddCmd(&ishell.Cmd{
Name: "start",
Help: "start the node",
Func: func(c *ishell.Context) {
if core.Node.Online() {
c.Println("already started")
return
}
if err := start(shell); err != nil {
c.Println(fmt.Errorf("start desktop node failed: %s", err))
return
}
c.Println("ok, started")
},
})
shell.AddCmd(&ishell.Cmd{
Name: "stop",
Help: "stop the node",
Func: func(c *ishell.Context) {
if !core.Node.Online() {
c.Println("already stopped")
return
}
if err := stop(); err != nil {
c.Println(fmt.Errorf("stop desktop node failed: %s", err))
return
}
c.Println("ok, stopped")
},
})
shell.AddCmd(&ishell.Cmd{
Name: "id",
Help: "show peer id",
Func: cmd.ShowId,
})
shell.AddCmd(&ishell.Cmd{
Name: "peers",
Help: "show connected peers (same as `ipfs swarm peers`)",
Func: cmd.SwarmPeers,
})
shell.AddCmd(&ishell.Cmd{
Name: "ping",
Help: "ping a peer (same as `ipfs ping`)",
Func: cmd.SwarmPing,
})
shell.AddCmd(&ishell.Cmd{
Name: "connect",
Help: "connect to a peer (same as `ipfs swarm connect`)",
Func: cmd.SwarmConnect,
})
{
photoCmd := &ishell.Cmd{
Name: "photo",
Help: "manage photos",
LongHelp: "Add, list, and get info about photos.",
}
photoCmd.AddCmd(&ishell.Cmd{
Name: "add",
Help: "add a new photo (default thread is \"#default\")",
Func: cmd.AddPhoto,
})
photoCmd.AddCmd(&ishell.Cmd{
Name: "share",
Help: "share a photo to a different thread",
Func: cmd.SharePhoto,
})
photoCmd.AddCmd(&ishell.Cmd{
Name: "get",
Help: "save a photo to a local file",
Func: cmd.GetPhoto,
})
photoCmd.AddCmd(&ishell.Cmd{
Name: "meta",
Help: "cat photo metadata",
Func: cmd.CatPhotoMetadata,
})
photoCmd.AddCmd(&ishell.Cmd{
Name: "ls",
Help: "list photos from a thread (defaults to \"#default\")",
Func: cmd.ListPhotos,
})
shell.AddCmd(photoCmd)
}
{
albumsCmd := &ishell.Cmd{
Name: "thread",
Help: "manage photo threads",
LongHelp: "Add, list, enable, disable, and get info about photo threads.",
}
albumsCmd.AddCmd(&ishell.Cmd{
Name: "add",
Help: "add a new thread",
Func: cmd.CreateAlbum,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "ls",
Help: "list threads",
Func: cmd.ListAlbums,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "enable",
Help: "enable a thread",
Func: cmd.EnableAlbum,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "disable",
Help: "disable a thread",
Func: cmd.DisableAlbum,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "mnemonic",
Help: "show mnemonic phrase",
Func: cmd.AlbumMnemonic,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "publish",
Help: "publish latest update",
Func: cmd.RepublishAlbum,
})
albumsCmd.AddCmd(&ishell.Cmd{
Name: "peers",
Help: "list peers",
Func: cmd.ListAlbumPeers,
})
shell.AddCmd(albumsCmd)
}
// create and start a desktop textile node
// TODO: darwin should use App. Support dir, not home dir
// TODO: make api url configuratable via an option flag
config := core.NodeConfig{
RepoPath: dataDir,
CentralApiURL: "https://api.textile.io",
IsMobile: false,
LogLevel: logging.DEBUG,
LogFiles: true,
}
node, err := core.NewNode(config)
if err != nil {
shell.Println(fmt.Errorf("create desktop node failed: %s", err))
return
}
core.Node = node
// auto start it
if err := start(shell); err != nil {
shell.Println(fmt.Errorf("start desktop node failed: %s", err))
}
// welcome
printSplashScreen(shell, core.Node.RepoPath)
// run shell
shell.Run()
}
func start(shell *ishell.Shell) error {
// start node
if err := core.Node.Start(); err != nil {
return err
}
// start garbage collection
// TODO: see method todo before enabling
//go startGarbageCollection()
// join existing rooms
for _, album := range core.Node.Datastore.Albums().GetAlbums("") {
cmd.JoinRoom(shell, album.Id)
}
return nil
}
func stop() error {
return core.Node.Stop()
}
// Start garbage collection
func startGarbageCollection() {
errc, err := core.Node.StartGarbageCollection()
if err != nil {
shell.Println(fmt.Errorf("auto gc error: %s", err))
return
}
for {
select {
case err, ok := <-errc:
if err != nil {
shell.Println(fmt.Errorf("auto gc error: %s", err))
}
if !ok {
shell.Println("auto gc stopped")
return
}
}
}
}
func printSplashScreen(shell *ishell.Shell, dataDir string) {
blue := color.New(color.FgBlue).SprintFunc()
banner :=
`
__ __ .__.__
_/ |_ ____ ___ ____/ |_|__| | ____
\ __\/ __ \\ \/ /\ __\ | | _/ __ \
| | \ ___/ > < | | | | |_\ ___/
|__| \___ >__/\_ \ |__| |__|____/\___ >
\/ \/ \/
`
shell.Println(blue(banner))
shell.Println("")
shell.Println("textile node v" + core.Version)
shell.Printf("using repo at %s\n", dataDir)
shell.Println("type `help` for available commands")
}
<file_sep>package repo
import (
"database/sql"
"time"
)
type Datastore interface {
Config() Config
Settings() ConfigurationStore
Photos() PhotoStore
Albums() AlbumStore
Ping() error
Close()
}
type Queryable interface {
BeginTransaction() (*sql.Tx, error)
PrepareQuery(string) (*sql.Stmt, error)
PrepareAndExecuteQuery(string, ...interface{}) (*sql.Rows, error)
ExecuteQuery(string, ...interface{}) (sql.Result, error)
}
type Config interface {
// Create the database and tables
Init(password string) error
// Configure the database
Configure(created time.Time) error
// Saves username, access token, and refresh token
SignIn(username string, at string, rt string) error
// Deletes username and jwt
SignOut() error
// Get username
GetUsername() (string, error)
// Retrieve JSON web tokens
GetTokens() (at string, rt string, err error)
// Returns the date the seed was created
GetCreationDate() (time.Time, error)
// Returns current schema version of the database
GetSchemaVersion() (string, error)
// Returns true if the database has failed to decrypt properly ex) wrong pw
IsEncrypted() bool
}
type ConfigurationStore interface {
Queryable
// Put settings to the database, overriding all fields
Put(settings SettingsData) error
// Update all non-nil fields
Update(settings SettingsData) error
// Return the settings object
Get() (SettingsData, error)
// Delete all settings data
Delete() error
}
type PhotoStore interface {
Queryable
// Put a new photo to the database
Put(set *PhotoSet) error
// Get a single photo set
GetPhoto(cid string) *PhotoSet
// A list of photos
GetPhotos(offsetId string, limit int, query string) []PhotoSet
// Delete a photo
DeletePhoto(cid string) error
}
type AlbumStore interface {
Queryable
// Put a new album to the database
Put(album *PhotoAlbum) error
// Get a single album
GetAlbum(id string) *PhotoAlbum
// Get a single album by name
GetAlbumByName(name string) *PhotoAlbum
// A list of albums
GetAlbums(query string) []PhotoAlbum
// Delete an album
DeleteAlbum(id string) error
DeleteAlbumByName(name string) error
}
<file_sep>package photos
import (
"bytes"
"image"
"image/gif"
"image/jpeg"
"image/png"
"os"
"path/filepath"
"strings"
"github.com/disintegration/imaging"
"github.com/rwcarlsen/goexif/exif"
)
// ImagePathWithoutExif makes a copy of image (jpg,png or gif) and applies
// all necessary operation to reverse its orientation to 1
// The result is a image with corrected orientation and without
// exif data.
func ImagePathWithoutExif(ifile *os.File) (*bytes.Buffer, error) {
var img image.Image
var err error
writer := &bytes.Buffer{}
filetype := strings.ToLower(filepath.Ext(ifile.Name()))
// deal with image
if filetype == ".jpg" || filetype == ".jpeg" {
img, err = jpeg.Decode(ifile)
if err != nil {
return nil, err
}
} else if filetype == ".png" {
img, err = png.Decode(ifile)
if err != nil {
return nil, err
}
} else if filetype == ".gif" {
img, err = gif.Decode(ifile)
if err != nil {
return nil, err
}
}
// deal with exif
ifile.Seek(0, 0)
x, err := exif.Decode(ifile)
if err != nil {
if x == nil {
// ignore - image exif data has been already stripped
}
log.Debugf("no exif data found for [%s]: %s", ifile.Name(), err.Error())
}
if x != nil {
orient, _ := x.Get(exif.Orientation)
if orient != nil {
log.Infof("%s had orientation %s", ifile.Name(), orient.String())
img = reverseOrientation(img, orient.String())
} else {
log.Errorf("%s had no orientation - implying 1", ifile.Name())
img = reverseOrientation(img, "1")
}
}
if filetype == ".jpg" || filetype == ".jpeg" {
err = jpeg.Encode(writer, img, nil)
if err != nil {
return nil, err
}
} else if filetype == ".png" {
err = png.Encode(writer, img)
if err != nil {
return nil, err
}
} else if filetype == ".gif" {
err = gif.Encode(writer, img, nil)
if err != nil {
return nil, err
}
}
return writer, nil
}
// reverseOrientation amply`s what ever operation is necessary to transform given orientation
// to the orientation 1
func reverseOrientation(img image.Image, o string) *image.NRGBA {
switch o {
case "1":
return imaging.Clone(img)
case "2":
return imaging.FlipV(img)
case "3":
return imaging.Rotate180(img)
case "4":
return imaging.Rotate180(imaging.FlipV(img))
case "5":
return imaging.Rotate270(imaging.FlipV(img))
case "6":
return imaging.Rotate270(img)
case "7":
return imaging.Rotate90(imaging.FlipV(img))
case "8":
return imaging.Rotate90(img)
}
log.Errorf("unknown orientation %s, expect 1-8", o)
return imaging.Clone(img)
}
<file_sep>package core_test
import (
"bytes"
"fmt"
"os"
"testing"
"time"
"github.com/op/go-logging"
"github.com/rwcarlsen/goexif/exif"
"github.com/segmentio/ksuid"
cmodels "github.com/textileio/textile-go/central/models"
. "github.com/textileio/textile-go/core"
util "github.com/textileio/textile-go/util/testing"
)
var node *TextileNode
var hash string
var centralReg = &cmodels.Registration{
Username: ksuid.New().String(),
Password: <PASSWORD>().String(),
Identity: &cmodels.Identity{
Type: cmodels.EmailAddress,
Value: ksuid.New().String() + "@textile.io",
},
Referral: "",
}
func TestNewNode(t *testing.T) {
os.RemoveAll("testdata/.ipfs")
config := NodeConfig{
RepoPath: "testdata/.ipfs",
CentralApiURL: util.CentralApiURL,
IsMobile: false,
LogLevel: logging.DEBUG,
LogFiles: false,
}
var err error
node, err = NewNode(config)
if err != nil {
t.Errorf("create node failed: %s", err)
}
}
func TestTextileNode_Start(t *testing.T) {
err := node.Start()
if err != nil {
t.Errorf("start node failed: %s", err)
}
}
func TestTextileNode_StartAgain(t *testing.T) {
err := node.Start()
if err != ErrNodeRunning {
t.Errorf("start node again reported wrong error: %s", err)
}
}
func TestTextileNode_StartGarbageCollection(t *testing.T) {
_, err := node.StartGarbageCollection()
if err != nil {
t.Errorf("start services failed: %s", err)
}
}
func TestTextileNode_Online(t *testing.T) {
if !node.Online() {
t.Errorf("should report online")
}
}
func TestTextileNode_SignUp(t *testing.T) {
_, ref, err := util.CreateReferral(util.RefKey, 1)
if err != nil {
t.Errorf("create referral for signup failed: %s", err)
return
}
if len(ref.RefCodes) == 0 {
t.Error("create referral for signup got no codes")
return
}
centralReg.Referral = ref.RefCodes[0]
err = node.SignUp(centralReg)
if err != nil {
t.Errorf("signup failed: %s", err)
return
}
}
func TestTextileNode_SignIn(t *testing.T) {
creds := &cmodels.Credentials{
Username: centralReg.Username,
Password: <PASSWORD>,
}
err := node.SignIn(creds)
if err != nil {
t.Errorf("signin failed: %s", err)
return
}
}
func TestTextileNode_IsSignedIn(t *testing.T) {
// TODO
}
func TestTextileNode_GetUsername(t *testing.T) {
// TODO
}
func TestTextileNode_GetAccessToken(t *testing.T) {
// TODO
}
func TestTextileNode_JoinRoom(t *testing.T) {
da := node.Datastore.Albums().GetAlbumByName("default")
if da == nil {
t.Error("default album not found")
return
}
go node.JoinRoom(da.Id, make(chan ThreadUpdate))
}
func TestTextileNode_LeaveRoom(t *testing.T) {
da := node.Datastore.Albums().GetAlbumByName("default")
if da == nil {
t.Error("default album not found")
return
}
time.Sleep(time.Second)
node.LeaveRoom(da.Id)
<-node.LeftRoomChs[da.Id]
}
func TestTextileNode_WaitForRoom(t *testing.T) {
// TODO
}
func TestTextileNode_CreateAlbum(t *testing.T) {
err := node.CreateAlbum("", "test")
if err != nil {
t.Errorf("create album failed: %s", err)
return
}
}
func TestTextileNode_AddPhoto(t *testing.T) {
caption := "i am not a crook"
mr, err := node.AddPhoto("testdata/image.jpg", "testdata/thumb.jpg", "default", caption)
if err != nil {
t.Errorf("add photo failed: %s", err)
return
}
if len(mr.Boundary) == 0 {
t.Errorf("add photo got bad hash")
}
hash = mr.Boundary
err = os.Remove("testdata/" + mr.Boundary)
if err != nil {
t.Errorf("error unlinking test multipart file: %s", err)
}
}
func TestTexileNode_ExifRemoved(t *testing.T) {
_, a, err := node.LoadPhotoAndAlbum(hash)
if err != nil {
t.Error(err.Error())
return
}
pb, err := node.GetFile(fmt.Sprintf("%s/photo", hash), a)
// create reader over photo bytes
pr := bytes.NewReader(pb)
// extract exif data from photo bytes
_, err = exif.Decode(pr)
if err == nil {
t.Errorf("exif not scrubbed: %s", err)
return
}
}
func TestTextileNode_SharePhoto(t *testing.T) {
caption := "a day that will live on in infamy"
mr, err := node.SharePhoto(hash, "test", caption)
if err != nil {
t.Errorf("share photo failed: %s", err)
return
}
if len(mr.Boundary) == 0 {
t.Errorf("share photo got bad hash")
}
}
func TestTextileNode_GetHashRequest(t *testing.T) {
// TODO
}
func TestTextileNode_GetPhotos(t *testing.T) {
list := node.GetPhotos("", -1, "default")
if len(list.Hashes) == 0 {
t.Errorf("get photos bad result")
}
}
func TestTextileNode_GetFile(t *testing.T) {
res, err := node.GetFile(hash+"/thumb", nil)
if err != nil {
t.Errorf("get photo failed: %s", err)
return
}
if len(res) == 0 {
t.Errorf("get photo bad result")
}
}
func TestTextileNode_GetFileBase64(t *testing.T) {
// TODO
}
func TestTextileNode_GetMetadata(t *testing.T) {
_, err := node.GetMetaData(hash, nil)
if err != nil {
t.Errorf("get metadata failed: %s", err)
return
}
}
func TestTextileNode_GetLastHash(t *testing.T) {
_, err := node.GetLastHash(hash, nil)
if err != nil {
t.Errorf("get last hash failed: %s", err)
return
}
}
func TestTextileNode_LoadPhotoAndAlbum(t *testing.T) {
// TODO
}
func TestTextileNode_UnmarshalPrivatePeerKey(t *testing.T) {
_, err := node.UnmarshalPrivatePeerKey()
if err != nil {
t.Errorf("unmarshal private peer key failed: %s", err)
return
}
}
func TestTextileNode_GetPublicPeerKeyString(t *testing.T) {
_, err := node.GetPublicPeerKeyString()
if err != nil {
t.Errorf("get peer public key as base 64 string failed: %s", err)
return
}
}
func TestTextileNode_PingPeer(t *testing.T) {
// TODO
}
func TestTextileNode_SignOut(t *testing.T) {
err := node.SignOut()
if err != nil {
t.Errorf("signout failed: %s", err)
return
}
}
func TestTextileNode_Stop(t *testing.T) {
err := node.Stop()
if err != nil {
t.Errorf("stop node failed: %s", err)
}
}
func TestTextileNode_OnlineAgain(t *testing.T) {
if node.Online() {
t.Errorf("should report offline")
}
}
// test signin in stopped state, should re-connect to db
func TestTextileNode_SignInAgain(t *testing.T) {
creds := &cmodels.Credentials{
Username: centralReg.Username,
Password: <PASSWORD>,
}
err := node.SignIn(creds)
if err != nil {
t.Errorf("signin failed: %s", err)
return
}
}
func Test_Teardown(t *testing.T) {
os.RemoveAll(node.RepoPath)
}
<file_sep>package cmd
import (
"bytes"
"encoding/json"
"errors"
"fmt"
"image"
_ "image/gif"
"image/jpeg"
_ "image/png"
"io/ioutil"
"os"
"path/filepath"
"github.com/disintegration/imaging"
"github.com/fatih/color"
"github.com/mitchellh/go-homedir"
"github.com/segmentio/ksuid"
"gopkg.in/abiosoft/ishell.v2"
"github.com/textileio/textile-go/core"
"github.com/textileio/textile-go/repo/photos"
)
func AddPhoto(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing photo path"))
return
}
// try to get path with home dir tilda
pp, err := homedir.Expand(c.Args[0])
if err != nil {
pp = c.Args[0]
}
// open the file
f, err := os.Open(pp)
if err != nil {
c.Err(err)
return
}
defer f.Close()
// try to create a thumbnail version
th, _, err := image.Decode(f)
if err != nil {
c.Err(err)
return
}
th = imaging.Resize(th, 300, 0, imaging.Lanczos)
thb := new(bytes.Buffer)
if err = jpeg.Encode(thb, th, nil); err != nil {
c.Err(err)
return
}
tp := filepath.Join(core.Node.RepoPath, "tmp", ksuid.New().String()+".jpg")
if err = ioutil.WriteFile(tp, thb.Bytes(), 0644); err != nil {
c.Err(err)
return
}
// parse album
album := "default"
if len(c.Args) > 1 {
album = c.Args[1]
}
c.Print("caption (optional): ")
caption := c.ReadLine()
// do the add
f.Seek(0, 0)
mr, err := core.Node.AddPhoto(pp, tp, album, caption)
if err != nil {
c.Err(err)
return
}
// clean up
if err = os.Remove(tp); err != nil {
c.Err(err)
return
}
if err = os.Remove(mr.PayloadPath); err != nil {
c.Err(err)
return
}
// show user root cid
cyan := color.New(color.FgCyan).SprintFunc()
c.Println(cyan("added " + mr.Boundary + " to thread " + album))
}
func SharePhoto(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing photo cid"))
return
}
if len(c.Args) == 1 {
c.Err(errors.New("missing destination thread name"))
return
}
cid := c.Args[0]
dest := c.Args[1]
c.Print("caption (optional): ")
caption := c.ReadLine()
mr, err := core.Node.SharePhoto(cid, dest, caption)
if err != nil {
c.Err(err)
return
}
green := color.New(color.FgHiGreen).SprintFunc()
c.Println(green("shared " + cid + " to thread " + dest + " (new cid: " + mr.Boundary + ")"))
}
func ListPhotos(c *ishell.Context) {
album := "default"
if len(c.Args) > 0 {
album = c.Args[0]
}
a := core.Node.Datastore.Albums().GetAlbumByName(album)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", album)))
return
}
sets := core.Node.Datastore.Photos().GetPhotos("", -1, "album='"+a.Id+"'")
if len(sets) == 0 {
c.Println(fmt.Sprintf("no photos found in: %s", album))
} else {
c.Println(fmt.Sprintf("found %v photos in: %s", len(sets), album))
}
magenta := color.New(color.FgHiMagenta).SprintFunc()
for _, s := range sets {
c.Println(magenta(fmt.Sprintf("cid: %s, name: %s%s", s.Cid, s.MetaData.Name, s.MetaData.Ext)))
}
}
func GetPhoto(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing photo cid"))
return
}
if len(c.Args) == 1 {
c.Err(errors.New("missing out directory"))
return
}
hash := c.Args[0]
// try to get path with home dir tilda
dest, err := homedir.Expand(c.Args[1])
if err != nil {
dest = c.Args[1]
}
set, a, err := core.Node.LoadPhotoAndAlbum(hash)
if err != nil {
c.Err(err)
return
}
pb, err := core.Node.GetFile(fmt.Sprintf("%s/photo", hash), a)
if err != nil {
c.Err(err)
return
}
path := filepath.Join(dest, set.MetaData.Name+set.MetaData.Ext)
if err := ioutil.WriteFile(path, pb, 0644); err != nil {
c.Err(err)
return
}
blue := color.New(color.FgHiBlue).SprintFunc()
c.Println(blue("wrote " + hash + " to " + path))
}
func CatPhotoMetadata(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing photo cid"))
return
}
hash := c.Args[0]
b, err := core.Node.GetFile(fmt.Sprintf("%s/meta", hash), nil)
if err != nil {
c.Err(err)
return
}
var md photos.Metadata
if err := json.Unmarshal(b, &md); err != nil {
c.Err(err)
return
}
jb, err := json.MarshalIndent(md, "", " ")
if err != nil {
c.Err(err)
return
}
black := color.New(color.FgHiBlack).SprintFunc()
c.Println(black(string(jb)))
}
<file_sep>package repo
import (
"github.com/textileio/textile-go/repo/photos"
libp2p "gx/ipfs/QmaPbCnUMBohSGo3KnxEa2bHqyJVVeEEcwtqJAYxerieBo/go-libp2p-crypto"
)
type SettingsData struct {
Version *string `json:"version"`
}
type PhotoSet struct {
Cid string `json:"cid"`
LastCid string `json:"last_cid"`
AlbumID string `json:"album_id"`
MetaData photos.Metadata `json:"metadata"`
Caption string `json:"caption,omitempty"`
IsLocal bool `json:"is_local"`
}
type PhotoAlbum struct {
Id string `json:"id"`
Key libp2p.PrivKey `json:"key"`
Mnemonic string `json:"mnemonic"`
Name string `json:"name"`
}
<file_sep>package db
import (
"database/sql"
"encoding/json"
"sync"
"testing"
"github.com/textileio/textile-go/repo"
)
var sdb repo.ConfigurationStore
var settings repo.SettingsData
func init() {
conn, _ := sql.Open("sqlite3", ":memory:")
initDatabaseTables(conn, "")
sdb = NewConfigurationStore(conn, new(sync.Mutex))
v := "/textile-go:0.1/"
settings = repo.SettingsData{
Version: &v,
}
}
func TestSettingsPut(t *testing.T) {
err := sdb.Put(settings)
if err != nil {
t.Error(err)
}
set := repo.SettingsData{}
stmt, err := sdb.PrepareQuery("select value from config where key=?")
defer stmt.Close()
var settingsBytes []byte
err = stmt.QueryRow("settings").Scan(&settingsBytes)
if err != nil {
t.Error(err)
}
err = json.Unmarshal(settingsBytes, &set)
if err != nil {
t.Error(err)
}
if *set.Version != "/textile-go:0.1/" {
t.Error("Settings put failed to put correct value")
}
}
func TestInvalidSettingsGet(t *testing.T) {
tx, err := sdb.BeginTransaction()
if err != nil {
t.Error(err)
}
stmt, _ := tx.Prepare("insert or replace into config(key, value) values(?,?)")
defer stmt.Close()
_, err = stmt.Exec("settings", string("Test fail"))
if err != nil {
tx.Rollback()
t.Error(err)
}
tx.Commit()
_, err = sdb.Get()
if err == nil {
t.Error("settings get didn't error with invalid data")
}
}
func TestSettingsGet(t *testing.T) {
err := sdb.Put(settings)
if err != nil {
t.Error(err)
}
set, err := sdb.Get()
if err != nil {
t.Error(err)
}
if *set.Version != "/textile-go:0.1/" {
t.Error("Settings put failed to put correct value")
}
}
func TestSettingsUpdate(t *testing.T) {
err := sdb.Put(settings)
if err != nil {
t.Error(err)
}
l := "/textile-go:0.2/"
setUpdt := repo.SettingsData{
Version: &l,
}
err = sdb.Update(setUpdt)
if err != nil {
t.Error(err)
}
set, err := sdb.Get()
if err != nil {
t.Error(err)
}
if *set.Version != "/textile-go:0.2/" {
t.Error("Settings update failed to put correct value")
}
}
<file_sep>package core
import (
"bytes"
"context"
"crypto/hmac"
"crypto/sha256"
"sort"
"strings"
"gx/ipfs/Qm<KEY>/go-ipfs/core"
"gx/ipfs/<KEY>/go-ipfs/core/corerepo"
iaddr "gx/ipfs/Qm<KEY>/go-ipfs-addr"
"gx/ipfs/<KEY>/go-ipfs-cmds"
ma "gx/ipfs/Qm<KEY>/go-multiaddr"
pstore "gx/ipfs/Qm<KEY>/go-libp2p-peerstore"
"gx/ipfs/Qm<KEY>/go-libp2p-peer"
libp2p "gx/ipfs/Q<KEY>/go-libp2p-crypto"
)
// start auto-garbage collection process
// TODO: investigate where this is gonna get datadir from
// TODO: it may use the env var IPFS_PATH, which we don't want
func runGC(ctx context.Context, node *core.IpfsNode) (<-chan error, error) {
errc := make(chan error)
go func() {
errc <- corerepo.PeriodicGC(ctx, node)
close(errc)
}()
log.Info("auto garbage collection started")
return errc, nil
}
// createMnemonic creates a new mnemonic phrase with given entropy
func createMnemonic(newEntropy func(int) ([]byte, error), newMnemonic func([]byte) (string, error)) (string, error) {
entropy, err := newEntropy(256)
if err != nil {
return "", err
}
mnemonic, err := newMnemonic(entropy)
if err != nil {
return "", err
}
return mnemonic, nil
}
// identityKeyFromSeed returns a new key identity from a seed
func identityKeyFromSeed(seed []byte, bits int) ([]byte, error) {
hm := hmac.New(sha256.New, []byte("sc<PASSWORD>"))
hm.Write(seed)
reader := bytes.NewReader(hm.Sum(nil))
sk, _, err := libp2p.GenerateKeyPairWithReader(libp2p.Ed25519, bits, reader)
if err != nil {
return nil, err
}
encodedKey, err := sk.Bytes()
if err != nil {
return nil, err
}
return encodedKey, nil
}
// printSwarmAddrs prints the addresses of the host
func printSwarmAddrs(node *core.IpfsNode) error {
if !node.OnlineMode() {
log.Info("swarm not listening, running in offline mode")
return nil
}
var lisAddrs []string
ifaceAddrs, err := node.PeerHost.Network().InterfaceListenAddresses()
if err != nil {
return err
}
for _, addr := range ifaceAddrs {
lisAddrs = append(lisAddrs, addr.String())
}
sort.Sort(sort.StringSlice(lisAddrs))
for _, addr := range lisAddrs {
log.Infof("swarm listening on %s\n", addr)
}
var addrs []string
for _, addr := range node.PeerHost.Addrs() {
addrs = append(addrs, addr.String())
}
sort.Sort(sort.StringSlice(addrs))
for _, addr := range addrs {
log.Infof("swarm announcing %s\n", addr)
}
return nil
}
// parsePeerParam takes a peer address string and returns p2p params
func parsePeerParam(text string) (ma.Multiaddr, peer.ID, error) {
// to be replaced with just multiaddr parsing, once ptp is a multiaddr protocol
idx := strings.LastIndex(text, "/")
if idx == -1 {
pid, err := peer.IDB58Decode(text)
if err != nil {
return nil, "", err
}
return nil, pid, nil
}
addrS := text[:idx]
peeridS := text[idx+1:]
var maddr ma.Multiaddr
var pid peer.ID
// make sure addrS parses as a multiaddr.
if len(addrS) > 0 {
var err error
maddr, err = ma.NewMultiaddr(addrS)
if err != nil {
return nil, "", err
}
}
// make sure idS parses as a peer.ID
var err error
pid, err = peer.IDB58Decode(peeridS)
if err != nil {
return nil, "", err
}
return maddr, pid, nil
}
// parseAddresses is a function that takes in a slice of string peer addresses
// (multiaddr + peerid) and returns slices of multiaddrs and peerids.
func parseAddresses(addrs []string) (iaddrs []iaddr.IPFSAddr, err error) {
iaddrs = make([]iaddr.IPFSAddr, len(addrs))
for i, saddr := range addrs {
iaddrs[i], err = iaddr.ParseString(saddr)
if err != nil {
return nil, cmds.ClientError("invalid peer address: " + err.Error())
}
}
return
}
// peersWithAddresses is a function that takes in a slice of string peer addresses
// (multiaddr + peerid) and returns a slice of properly constructed peers
func peersWithAddresses(addrs []string) (pis []pstore.PeerInfo, err error) {
iaddrs, err := parseAddresses(addrs)
if err != nil {
return nil, err
}
for _, a := range iaddrs {
pis = append(pis, pstore.PeerInfo{
ID: a.ID(),
Addrs: []ma.Multiaddr{a.Transport()},
})
}
return pis, nil
}
<file_sep>package db
import (
"database/sql"
"encoding/json"
"errors"
"sync"
"github.com/textileio/textile-go/repo"
)
var SettingsNotSetError error = errors.New("settings not set")
type SettingsDB struct {
modelStore
}
func NewConfigurationStore(db *sql.DB, lock *sync.Mutex) repo.ConfigurationStore {
return &SettingsDB{modelStore{db, lock}}
}
func (s *SettingsDB) Put(settings repo.SettingsData) error {
s.lock.Lock()
defer s.lock.Unlock()
tx, err := s.db.Begin()
if err != nil {
return err
}
b, err := json.MarshalIndent(&settings, "", " ")
if err != nil {
return err
}
stmt, err := tx.Prepare("insert or replace into config(key, value) values(?,?)")
if err != nil {
return err
}
defer stmt.Close()
_, err = stmt.Exec("settings", string(b))
if err != nil {
tx.Rollback()
return err
}
tx.Commit()
return nil
}
func (s *SettingsDB) Get() (repo.SettingsData, error) {
s.lock.Lock()
defer s.lock.Unlock()
settings := repo.SettingsData{}
stmt, err := s.db.Prepare("select value from config where key=?")
if err != nil {
return settings, err
}
defer stmt.Close()
var settingsBytes []byte
err = stmt.QueryRow("settings").Scan(&settingsBytes)
if err != nil {
return settings, SettingsNotSetError
}
err = json.Unmarshal(settingsBytes, &settings)
if err != nil {
return settings, err
}
return settings, nil
}
func (s *SettingsDB) Update(settings repo.SettingsData) error {
current, err := s.Get()
if err != nil {
return errors.New("not found")
}
if settings.Version == nil {
settings.Version = current.Version
}
err = s.Put(settings)
if err != nil {
return err
}
return nil
}
// Delete removes all settings from the database. It's a destructive action that should be used with care.
func (s *SettingsDB) Delete() error {
s.lock.Lock()
defer s.lock.Unlock()
stmt, err := s.db.Prepare("delete from config where key = ?")
if err != nil {
return err
}
defer stmt.Close()
_, err = stmt.Exec("settings")
return err
}
<file_sep>package photos
import (
"bytes"
"encoding/json"
"io"
"io/ioutil"
"os"
"path/filepath"
"strings"
"time"
"github.com/op/go-logging"
"github.com/rwcarlsen/goexif/exif"
"github.com/textileio/textile-go/net"
"gx/ipfs/Qm<KEY>/go-ipfs/core"
"gx/ipfs/Qm<KEY>/go-ipfs/core/coreunix"
uio "gx/ipfs/<KEY>/go-ipfs/unixfs/io"
libp2p "gx/ipfs/Q<KEY>/go-libp2p-crypto"
"gx/ipfs/Q<KEY>/go-cid"
ipld "gx/ipfs/Q<KEY>ES/go-ipld-format"
)
var log = logging.MustGetLogger("photos")
type Metadata struct {
// photo data
Name string `json:"name,omitempty"`
Ext string `json:"extension,omitempty"`
Created time.Time `json:"created,omitempty"`
Added time.Time `json:"added,omitempty"`
Latitude float64 `json:"latitude,omitempty"`
Longitude float64 `json:"longitude,omitempty"`
// user data
Username string `json:"username,omitempty"`
PeerID string `json:"peer_id,omitempty"`
}
// Add adds a photo, it's thumbnail, and it's metadata to ipfs
func Add(n *core.IpfsNode, pk libp2p.PubKey, p *os.File, t *os.File, lc string, un string, cap string) (*net.MultipartRequest, *Metadata, error) {
// path info
path := p.Name()
ext := strings.ToLower(filepath.Ext(path))
dname := filepath.Dir(t.Name())
// try to extract exif data
// TODO: get image size info
// TODO: break this up into one method with multi sub-methods for testing
var tm time.Time
x, err := exif.Decode(p)
if err == nil {
// time taken
tmTmp, err := x.DateTime()
if err == nil {
tm = tmTmp
}
}
// create a metadata file
md := &Metadata{
Name: strings.TrimSuffix(filepath.Base(path), ext),
Ext: ext,
Username: un,
PeerID: n.Identity.Pretty(),
Created: tm,
Added: time.Now(),
}
mdb, err := json.Marshal(md)
if err != nil {
return nil, nil, err
}
cmdb, err := net.Encrypt(pk, mdb)
if err != nil {
return nil, nil, err
}
// encrypt the last hash
clcb, err := net.Encrypt(pk, []byte(lc))
if err != nil {
return nil, nil, err
}
// encrypt the caption
ccapb, err := net.Encrypt(pk, []byte(cap))
if err != nil {
return nil, nil, err
}
// create an empty virtual directory
dirb := uio.NewDirectory(n.DAG)
// add the image
p.Seek(0, 0)
pr, err := ImagePathWithoutExif(p)
if err != nil {
return nil, nil, err
}
pb, err := getEncryptedReaderBytes(pr, pk)
if err != nil {
return nil, nil, err
}
err = addFileToDirectory(n, dirb, pb, "photo")
if err != nil {
return nil, nil, err
}
// add the thumbnail
tr, err := ImagePathWithoutExif(t)
if err != nil {
return nil, nil, err
}
tb, err := getEncryptedReaderBytes(tr, pk)
if err != nil {
return nil, nil, err
}
err = addFileToDirectory(n, dirb, tb, "thumb")
if err != nil {
return nil, nil, err
}
// add the metadata file
err = addFileToDirectory(n, dirb, cmdb, "meta")
if err != nil {
return nil, nil, err
}
// add caption
err = addFileToDirectory(n, dirb, ccapb, "caption")
if err != nil {
return nil, nil, err
}
// add last update's cid
err = addFileToDirectory(n, dirb, clcb, "last")
if err != nil {
return nil, nil, err
}
// pin it
dir, err := dirb.GetNode()
if err != nil {
return nil, nil, err
}
if err := pinPhoto(n, dir); err != nil {
return nil, nil, err
}
// create and init a new multipart request
mr := &net.MultipartRequest{}
mr.Init(dname, dir.Cid().Hash().B58String())
// add files
if err := mr.AddFile(pb, "photo"); err != nil {
return nil, nil, err
}
if err := mr.AddFile(tb, "thumb"); err != nil {
return nil, nil, err
}
if err := mr.AddFile(cmdb, "meta"); err != nil {
return nil, nil, err
}
if err := mr.AddFile(ccapb, "caption"); err != nil {
return nil, nil, err
}
if err := mr.AddFile(clcb, "last"); err != nil {
return nil, nil, err
}
// finish request
if err := mr.Finish(); err != nil {
return nil, nil, err
}
return mr, md, nil
}
// getEncryptedReaderBytes reads reader bytes and returns the encrypted result
func getEncryptedReaderBytes(r io.Reader, pk libp2p.PubKey) ([]byte, error) {
b, err := ioutil.ReadAll(r)
if err != nil {
return nil, err
}
return net.Encrypt(pk, b)
}
// addFileToDirectory adds bytes as file to a virtual directory (dag) structure
func addFileToDirectory(n *core.IpfsNode, dirb *uio.Directory, b []byte, fname string) error {
r := bytes.NewReader(b)
s, err := coreunix.Add(n, r)
if err != nil {
return err
}
c, err := cid.Decode(s)
if err != nil {
return err
}
node, err := n.DAG.Get(n.Context(), c)
if err != nil {
return err
}
if err := dirb.AddChild(n.Context(), fname, node); err != nil {
return err
}
return nil
}
// pinPhoto pins the entire photo set dag, minues the full res image
func pinPhoto(n *core.IpfsNode, dir ipld.Node) error {
// pin the top-level structure (recursive: false)
if err := n.Pinning.Pin(n.Context(), dir, false); err != nil {
return err
}
// pin thumbnail and metadata
for _, item := range dir.Links() {
if item.Name == "meta" || item.Name == "thumb" {
tnode, err := item.GetNode(n.Context(), n.DAG)
if err != nil {
return err
}
n.Pinning.Pin(n.Context(), tnode, false)
}
}
return n.Pinning.Flush()
}
<file_sep>package main
import (
"flag"
"fmt"
"github.com/asticode/go-astilectron"
"github.com/asticode/go-astilectron-bootstrap"
"github.com/asticode/go-astilog"
"github.com/op/go-logging"
"github.com/pkg/errors"
"github.com/textileio/textile-go/core"
)
var (
BuiltAt string
debug = flag.Bool("d", false, "enables the debug mode")
w *astilectron.Window
)
var textile *core.TextileNode
var gateway string
func main() {
AppName := "Textile"
flag.Parse()
astilog.FlagInit()
// create a desktop textile node
// TODO: on darwin, repo should live in Application Support
// TODO: make api url configurable somehow
config := core.NodeConfig{
RepoPath: "output/.ipfs",
CentralApiURL: "https://api.textile.io",
IsMobile: false,
LogLevel: logging.DEBUG,
LogFiles: true,
}
var err error
textile, err = core.NewNode(config)
if err != nil {
astilog.Errorf("create desktop node failed: %s", err)
return
}
// bring the node online and startup the gateway
err = textile.Start()
if err != nil {
astilog.Errorf("start desktop node failed: %s", err)
return
}
// save off the gateway address
gateway = fmt.Sprintf("http://localhost%s", textile.GatewayProxy.Addr)
// start garbage collection and gateway services
// TODO: see method todo before enabling
//errc, err := textile.StartGarbageCollection()
//if err != nil {
// astilog.Errorf("auto gc error: %s", err)
// return
//}
//go func() {
// for {
// select {
// case err, ok := <-errc:
// if err != nil {
// astilog.Errorf("auto gc error: %s", err)
// }
// if !ok {
// astilog.Info("auto gc stopped")
// return
// }
// }
// }
//}()
// run bootstrap
astilog.Debugf("Running app built at %s", BuiltAt)
if err := bootstrap.Run(bootstrap.Options{
Asset: Asset,
AstilectronOptions: astilectron.Options{
AppName: AppName,
AppIconDarwinPath: "resources/icon.icns",
AppIconDefaultPath: "resources/icon.png",
// TODO: Revisit: slightly dangerous because this will ignore _all_ certificate errors
ElectronSwitches: []string{"ignore-certificate-errors", "true"},
},
Debug: *debug,
Homepage: "index.html",
MessageHandler: handleMessages,
OnWait: start,
RestoreAssets: RestoreAssets,
WindowOptions: &astilectron.WindowOptions{
BackgroundColor: astilectron.PtrStr("#333333"),
Center: astilectron.PtrBool(true),
Height: astilectron.PtrInt(633),
Width: astilectron.PtrInt(1024),
},
}); err != nil {
astilog.Fatal(errors.Wrap(err, "running bootstrap failed"))
}
}
<file_sep>package db
import (
"database/sql"
"sync"
"github.com/textileio/textile-go/repo"
libp2p "gx/ipfs/QmaPbCnUMBohSGo3KnxEa2bHqyJVVeEEcwtqJAYxerieBo/go-libp2p-crypto"
)
type AlbumDB struct {
modelStore
}
func NewAlbumStore(db *sql.DB, lock *sync.Mutex) repo.AlbumStore {
return &AlbumDB{modelStore{db, lock}}
}
func (c *AlbumDB) Put(album *repo.PhotoAlbum) error {
c.lock.Lock()
defer c.lock.Unlock()
tx, err := c.db.Begin()
if err != nil {
return err
}
stm := `insert into albums(id, key, mnemonic, name) values(?,?,?,?)`
stmt, err := tx.Prepare(stm)
if err != nil {
log.Errorf("error in tx prepare: %s", err)
return err
}
skb, err := album.Key.Bytes()
if err != nil {
log.Errorf("error getting key bytes: %s", err)
return err
}
defer stmt.Close()
_, err = stmt.Exec(
album.Id,
skb,
album.Mnemonic,
album.Name,
)
if err != nil {
tx.Rollback()
log.Errorf("error in db exec: %s", err)
return err
}
tx.Commit()
return nil
}
func (c *AlbumDB) GetAlbum(id string) *repo.PhotoAlbum {
c.lock.Lock()
defer c.lock.Unlock()
ret := c.handleQuery("select * from albums where id='" + id + "';")
if len(ret) == 0 {
return nil
}
return &ret[0]
}
func (c *AlbumDB) GetAlbumByName(name string) *repo.PhotoAlbum {
c.lock.Lock()
defer c.lock.Unlock()
ret := c.handleQuery("select * from albums where name='" + name + "';")
if len(ret) == 0 {
return nil
}
return &ret[0]
}
func (c *AlbumDB) GetAlbums(query string) []repo.PhotoAlbum {
c.lock.Lock()
defer c.lock.Unlock()
q := ""
if query != "" {
q = " where " + query
}
return c.handleQuery("select * from albums" + q + ";")
}
func (c *AlbumDB) DeleteAlbum(id string) error {
c.lock.Lock()
defer c.lock.Unlock()
_, err := c.db.Exec("delete from albums where id=?", id)
return err
}
func (c *AlbumDB) DeleteAlbumByName(name string) error {
c.lock.Lock()
defer c.lock.Unlock()
_, err := c.db.Exec("delete from albums where name=?", name)
return err
}
func (c *AlbumDB) handleQuery(stm string) []repo.PhotoAlbum {
var ret []repo.PhotoAlbum
rows, err := c.db.Query(stm)
if err != nil {
log.Errorf("error in db query: %s", err)
return nil
}
for rows.Next() {
var id, mnemonic, name string
var key []byte
if err := rows.Scan(&id, &key, &mnemonic, &name); err != nil {
log.Errorf("error in db scan: %s", err)
continue
}
sk, err := libp2p.UnmarshalPrivateKey(key)
if err != nil {
log.Errorf("error unmarshaling private key: %s", err)
continue
}
album := repo.PhotoAlbum{
Id: id,
Key: sk,
Mnemonic: mnemonic,
Name: name,
}
ret = append(ret, album)
}
return ret
}
<file_sep>package cmd
import (
"github.com/fatih/color"
"gopkg.in/abiosoft/ishell.v2"
"github.com/textileio/textile-go/core"
"gx/<KEY>go-libp2p-peer"
)
func ShowId(c *ishell.Context) {
// peer id
psk, err := core.Node.UnmarshalPrivatePeerKey()
if err != nil {
c.Err(err)
return
}
pid, err := peer.IDFromPrivateKey(psk)
if err != nil {
c.Err(err)
return
}
// show user their id
red := color.New(color.FgRed).SprintFunc()
c.Println(red(pid.Pretty()))
}
<file_sep>package db
import (
"database/sql"
"sync"
"testing"
"time"
"github.com/textileio/textile-go/repo"
"github.com/textileio/textile-go/repo/photos"
)
var phdb repo.PhotoStore
func init() {
setupPhotoDB()
}
func setupPhotoDB() {
conn, _ := sql.Open("sqlite3", ":memory:")
initDatabaseTables(conn, "")
phdb = NewPhotoStore(conn, new(sync.Mutex))
}
func TestPhotoDB_Put(t *testing.T) {
err := phdb.Put(&repo.PhotoSet{
Cid: "Qmabc123",
LastCid: "",
AlbumID: "Qm",
MetaData: photos.Metadata{},
Caption: "tear down this wall",
IsLocal: false,
})
if err != nil {
t.Error(err)
}
stmt, err := phdb.PrepareQuery("select cid from photos where cid=?")
defer stmt.Close()
var cid string
err = stmt.QueryRow("Qmabc123").Scan(&cid)
if err != nil {
t.Error(err)
}
if cid != "Qmabc123" {
t.Errorf(`expected "Qmabc123" got %s`, cid)
}
}
func TestPhotoDB_GetPhoto(t *testing.T) {
setupPhotoDB()
err := phdb.Put(&repo.PhotoSet{
Cid: "Qmabc",
LastCid: "",
AlbumID: "Qm",
MetaData: photos.Metadata{
Added: time.Now(),
},
Caption: "one small step for man",
IsLocal: true,
})
if err != nil {
t.Error(err)
}
p := phdb.GetPhoto("Qmabc")
if p == nil {
t.Error("could not get photo")
return
}
}
func TestPhotoDB_GetPhotos(t *testing.T) {
setupPhotoDB()
err := phdb.Put(&repo.PhotoSet{
Cid: "Qm123",
LastCid: "",
AlbumID: "Qm",
MetaData: photos.Metadata{
Added: time.Now(),
},
Caption: "one giant leap for mankind",
IsLocal: true,
})
if err != nil {
t.Error(err)
}
time.Sleep(time.Second * 1)
err = phdb.Put(&repo.PhotoSet{
Cid: "Qm456",
LastCid: "Qm123",
AlbumID: "Qm",
MetaData: photos.Metadata{
Added: time.Now(),
},
IsLocal: false,
})
if err != nil {
t.Error(err)
}
ps := phdb.GetPhotos("", -1, "")
if len(ps) != 2 {
t.Error("returned incorrect number of photos")
return
}
limited := phdb.GetPhotos("", 1, "")
if len(limited) != 1 {
t.Error("returned incorrect number of photos")
return
}
offset := phdb.GetPhotos(limited[0].Cid, -1, "")
if len(offset) != 1 {
t.Error("returned incorrect number of photos")
return
}
filtered := phdb.GetPhotos("", -1, "local=1")
if len(filtered) != 1 {
t.Error("returned incorrect number of photos")
return
}
}
func TestPhotoDB_DeletePhoto(t *testing.T) {
setupPhotoDB()
err := phdb.Put(&repo.PhotoSet{
Cid: "Qm789",
LastCid: "",
AlbumID: "Qm",
MetaData: photos.Metadata{},
Caption: "",
IsLocal: true,
})
if err != nil {
t.Error(err)
}
ps := phdb.GetPhotos("", -1, "")
if len(ps) == 0 {
t.Error("returned incorrect number of photos")
return
}
err = phdb.DeletePhoto(ps[0].Cid)
if err != nil {
t.Error(err)
}
stmt, err := phdb.PrepareQuery("select cid from photos where cid=?")
defer stmt.Close()
var cid string
err = stmt.QueryRow(ps[0].Cid).Scan(&cid)
if err == nil {
t.Error("Delete failed")
}
}
<file_sep>package mobile
import (
"encoding/base64"
"encoding/json"
"errors"
"github.com/op/go-logging"
tcore "github.com/textileio/textile-go/core"
"github.com/textileio/textile-go/net"
"gx/<KEY>libp2p-peer"
libp2p "gx/<KEY>libp2p-crypto"
"github.com/textileio/textile-go/central/models"
)
var log = logging.MustGetLogger("mobile")
// Message is a generic go -> bridge message structure
type Event struct {
Name string `json:"name"`
Payload string `json:"payload"`
}
// newEvent transforms an event name and structured data in Event
func newEvent(name string, payload map[string]interface{}) *Event {
event := &Event{Name: name}
jsonb, err := json.Marshal(payload)
if err != nil {
log.Errorf("error creating event data json: %s", err)
}
event.Payload = string(jsonb)
return event
}
// Messenger is used to inform the bridge layer of new data waiting to be queried
type Messenger interface {
Notify(event *Event)
}
// Wrapper is the object exposed in the frameworks
type Wrapper struct {
RepoPath string
messenger Messenger
}
// NodeConfig is used to configure the mobile node
type NodeConfig struct {
RepoPath string
CentralApiURL string
LogLevel string
LogFiles bool
}
// NewNode is the mobile entry point for creating a node
// NOTE: logLevel is one of: CRITICAL ERROR WARNING NOTICE INFO DEBUG
func NewNode(config *NodeConfig, messenger Messenger) (*Wrapper, error) {
var m Mobile
return m.NewNode(config, messenger)
}
// Mobile is the name of the framework (must match package name)
type Mobile struct{}
// Create a gomobile compatible wrapper around TextileNode
func (m *Mobile) NewNode(config *NodeConfig, messenger Messenger) (*Wrapper, error) {
ll, err := logging.LogLevel(config.LogLevel)
if err != nil {
ll = logging.INFO
}
cconfig := tcore.NodeConfig{
RepoPath: config.RepoPath,
CentralApiURL: config.CentralApiURL,
IsMobile: true,
LogLevel: ll,
LogFiles: config.LogFiles,
}
node, err := tcore.NewNode(cconfig)
if err != nil {
return nil, err
}
tcore.Node = node
return &Wrapper{RepoPath: config.RepoPath, messenger: messenger}, nil
}
// Start the mobile node
func (w *Wrapper) Start() error {
if err := tcore.Node.Start(); err != nil {
if err == tcore.ErrNodeRunning {
return nil
}
return err
}
// join existing rooms
for _, album := range tcore.Node.Datastore.Albums().GetAlbums("") {
w.joinRoom(album.Id)
}
return nil
}
// Stop the mobile node
func (w *Wrapper) Stop() error {
if err := tcore.Node.Stop(); err != nil && err != tcore.ErrNodeNotRunning {
return err
}
return nil
}
// SignUpWithEmail creates an email based registration and calls core signup
func (w *Wrapper) SignUpWithEmail(username string, password string, email string, referral string) error {
// build registration
reg := &models.Registration{
Username: username,
Password: <PASSWORD>,
Identity: &models.Identity{
Type: models.EmailAddress,
Value: email,
},
Referral: referral,
}
// signup
return tcore.Node.SignUp(reg)
}
// SignIn build credentials and calls core SignIn
func (w *Wrapper) SignIn(username string, password string) error {
// build creds
creds := &models.Credentials{
Username: username,
Password: <PASSWORD>,
}
// signin
return tcore.Node.SignIn(creds)
}
// SignOut calls core SignOut
func (w *Wrapper) SignOut() error {
return tcore.Node.SignOut()
}
// IsSignedIn calls core IsSignedIn
func (w *Wrapper) IsSignedIn() bool {
si, _ := tcore.Node.IsSignedIn()
return si
}
// Update thread allows the mobile client to choose the 'all' thread to subscribe
func (w *Wrapper) UpdateThread(mnemonic string, name string) error {
if mnemonic == "" {
return errors.New("mnemonic must not be empty")
}
if err := tcore.Node.TouchDB(); err != nil {
return err
}
log.Debugf("deleting album if exists: %s", name)
err := tcore.Node.Datastore.Albums().DeleteAlbumByName(name)
if err != nil {
log.Errorf("error deleting album %s: %s", name, err)
return err
}
err = tcore.Node.CreateAlbum(mnemonic, name)
if err != nil {
log.Errorf("error creating album %s: %s", name, err)
return err
}
return nil
}
// GetUsername calls core GetUsername
func (w *Wrapper) GetUsername() (string, error) {
return tcore.Node.GetUsername()
}
// GetAccessToken calls core GetAccessToken
func (w *Wrapper) GetAccessToken() (string, error) {
return tcore.Node.GetAccessToken()
}
// GetGatewayPassword returns the current cookie value expected by the gateway
func (w *Wrapper) GetGatewayPassword() string {
return tcore.Node.GatewayPassword
}
// AddPhoto calls core AddPhoto
func (w *Wrapper) AddPhoto(path string, thumb string, thread string) (*net.MultipartRequest, error) {
return tcore.Node.AddPhoto(path, thumb, thread, "")
}
// SharePhoto calls core SharePhoto
func (w *Wrapper) SharePhoto(hash string, thread string, caption string) (*net.MultipartRequest, error) {
return tcore.Node.SharePhoto(hash, thread, caption)
}
// GetHashRequest calls core GetHashRequest
func (w *Wrapper) GetHashRequest(hash string) (string, error) {
request := tcore.Node.GetHashRequest(hash)
// gomobile does not allow slices. so, convert to json
jsonb, err := json.Marshal(request)
if err != nil {
log.Errorf("error marshaling json: %s", err)
return "", err
}
return string(jsonb), nil
}
// Get Photos returns core GetPhotos with json encoding
func (w *Wrapper) GetPhotos(offsetId string, limit int, thread string) (string, error) {
if tcore.Node.Online() {
go func() {
th := tcore.Node.Datastore.Albums().GetAlbumByName(thread)
if th != nil {
tcore.Node.RepublishLatestUpdate(th)
}
}()
}
list := tcore.Node.GetPhotos(offsetId, limit, thread)
if list == nil {
list = &tcore.PhotoList{
Hashes: make([]string, 0),
}
}
// gomobile does not allow slices. so, convert to json
jsonb, err := json.Marshal(list)
if err != nil {
log.Errorf("error marshaling json: %s", err)
return "", err
}
return string(jsonb), nil
}
// GetFileBase64 call core GetFileBase64
func (w *Wrapper) GetFileBase64(path string) (string, error) {
return tcore.Node.GetFileBase64(path)
}
// GetPeerID returns our peer id
func (w *Wrapper) GetPeerID() (string, error) {
if !tcore.Node.Online() {
return "", tcore.ErrNodeNotRunning
}
return tcore.Node.IpfsNode.Identity.Pretty(), nil
}
// PairDesktop publishes this nodes default album keys to a desktop node
// which is listening at it's own peer id
func (w *Wrapper) PairDesktop(pkb64 string) (string, error) {
if !tcore.Node.Online() {
return "", tcore.ErrNodeNotRunning
}
log.Info("pairing with desktop...")
pkb, err := base64.StdEncoding.DecodeString(pkb64)
if err != nil {
log.Errorf("error decoding string: %s: %s", pkb64, err)
return "", err
}
pk, err := libp2p.UnmarshalPublicKey(pkb)
if err != nil {
log.Errorf("error unmarshaling pub key: %s", err)
return "", err
}
// the phrase will be used by the desktop client to create
// the private key needed to decrypt photos
// we invite the desktop to _read and write_ to our default album
da := tcore.Node.Datastore.Albums().GetAlbumByName("default")
if da == nil {
err = errors.New("default album not found")
log.Error(err.Error())
return "", err
}
// encypt with the desktop's pub key
cph, err := net.Encrypt(pk, []byte(da.Mnemonic))
if err != nil {
log.Errorf("encrypt failed: %s", err)
return "", err
}
// get the topic to pair with from the pub key
peerID, err := peer.IDFromPublicKey(pk)
if err != nil {
log.Errorf("id from public key failed: %s", err)
return "", err
}
topic := peerID.Pretty()
// finally, publish the encrypted phrase
err = tcore.Node.Publish(topic, cph)
if err != nil {
log.Errorf("publish %s failed: %s", topic, err)
return "", err
}
log.Infof("published key phrase to desktop: %s", topic)
return topic, nil
}
// joinRoom and pass updates to messenger
func (w *Wrapper) joinRoom(id string) {
datac := make(chan tcore.ThreadUpdate)
go tcore.Node.JoinRoom(id, datac)
go func() {
for {
select {
case update, ok := <-datac:
if !ok {
return
}
w.messenger.Notify(newEvent("onThreadUpdate", map[string]interface{}{
"cid": update.Cid,
"thread": update.Thread,
"thread_id": update.ThreadID,
}))
}
}
}()
}
<file_sep>package db
import (
"database/sql"
"path"
"sync"
"time"
_ "github.com/mutecomm/go-sqlcipher"
"github.com/op/go-logging"
"github.com/textileio/textile-go/repo"
)
var log = logging.MustGetLogger("db")
const schemaVersion = "0"
type SQLiteDatastore struct {
config repo.Config
settings repo.ConfigurationStore
photos repo.PhotoStore
albums repo.AlbumStore
db *sql.DB
lock *sync.Mutex
}
func Create(repoPath, password string) (*SQLiteDatastore, error) {
var dbPath string
dbPath = path.Join(repoPath, "datastore", "mainnet.db")
conn, err := sql.Open("sqlite3", dbPath)
if err != nil {
return nil, err
}
if password != "" {
p := "pragma key='" + password + "';"
conn.Exec(p)
}
l := new(sync.Mutex)
sqliteDB := &SQLiteDatastore{
config: &ConfigDB{
db: conn,
lock: l,
path: dbPath,
},
settings: NewConfigurationStore(conn, l),
photos: NewPhotoStore(conn, l),
albums: NewAlbumStore(conn, l),
db: conn,
lock: l,
}
return sqliteDB, nil
}
func (d *SQLiteDatastore) Ping() error {
return d.db.Ping()
}
func (d *SQLiteDatastore) Close() {
d.db.Close()
}
func (d *SQLiteDatastore) Config() repo.Config {
return d.config
}
func (d *SQLiteDatastore) Settings() repo.ConfigurationStore {
return d.settings
}
func (d *SQLiteDatastore) Photos() repo.PhotoStore {
return d.photos
}
func (d *SQLiteDatastore) Albums() repo.AlbumStore {
return d.albums
}
func (d *SQLiteDatastore) Copy(dbPath string, password string) error {
d.lock.Lock()
defer d.lock.Unlock()
var cp string
stmt := "select name from sqlite_master where type='table'"
rows, err := d.db.Query(stmt)
if err != nil {
log.Errorf("error in copy: %s", err)
return err
}
var tables []string
for rows.Next() {
var name string
if err := rows.Scan(&name); err != nil {
return err
}
tables = append(tables, name)
}
if password == "" {
cp = `attach database '` + dbPath + `' as plaintext key '';`
for _, name := range tables {
cp = cp + "insert into plaintext." + name + " select * from main." + name + ";"
}
} else {
cp = `attach database '` + dbPath + `' as encrypted key '` + password + `';`
for _, name := range tables {
cp = cp + "insert into encrypted." + name + " select * from main." + name + ";"
}
}
_, err = d.db.Exec(cp)
if err != nil {
return err
}
return nil
}
func (d *SQLiteDatastore) InitTables(password string) error {
return initDatabaseTables(d.db, password)
}
func initDatabaseTables(db *sql.DB, password string) error {
var sqlStmt string
if password != "" {
sqlStmt = "PRAGMA key = '" + password + "';"
}
sqlStmt += `
PRAGMA user_version = 0;
create table config (key text primary key not null, value blob);
create table photos (cid text primary key not null, lastCid text, album text not null, name text not null, ext text not null, username text, peerId text, created integer, added integer not null, latitude real, longitude real, local integer not null, caption text);
create index index_album_added on photos (album, added);
create index index_album_local on photos (album, local);
create index index_album_username on photos (album, username);
create index index_album_peerid on photos (album, peerId);
create table albums (id text primary key not null, key blob not null, mnemonic text not null, name text not null);
create unique index index_name on albums (name);
`
_, err := db.Exec(sqlStmt)
if err != nil {
return err
}
return nil
}
type ConfigDB struct {
db *sql.DB
lock *sync.Mutex
path string
}
func (c *ConfigDB) Init(password string) error {
c.lock.Lock()
defer c.lock.Unlock()
return initDatabaseTables(c.db, password)
}
func (c *ConfigDB) Configure(created time.Time) error {
c.lock.Lock()
defer c.lock.Unlock()
tx, err := c.db.Begin()
if err != nil {
return err
}
stmt, err := tx.Prepare("insert or replace into config(key, value) values(?,?)")
if err != nil {
return err
}
defer stmt.Close()
_, err = stmt.Exec("created", created.Format(time.RFC3339))
if err != nil {
tx.Rollback()
return err
}
_, err = stmt.Exec("schema", schemaVersion)
if err != nil {
tx.Rollback()
return err
}
tx.Commit()
return nil
}
func (c *ConfigDB) SignIn(un string, at string, rt string) error {
c.lock.Lock()
defer c.lock.Unlock()
tx, err := c.db.Begin()
if err != nil {
return err
}
stmt, err := tx.Prepare("insert or replace into config(key, value) values(?,?)")
if err != nil {
return err
}
defer stmt.Close()
_, err = stmt.Exec("username", un)
if err != nil {
tx.Rollback()
return err
}
_, err = stmt.Exec("access", at)
if err != nil {
tx.Rollback()
return err
}
_, err = stmt.Exec("refresh", rt)
if err != nil {
tx.Rollback()
return err
}
tx.Commit()
return nil
}
func (c *ConfigDB) SignOut() error {
c.lock.Lock()
defer c.lock.Unlock()
stmt, err := c.db.Prepare("delete from config where key=?")
defer stmt.Close()
_, err = stmt.Exec("username")
if err != nil {
return err
}
_, err = stmt.Exec("access")
if err != nil {
return err
}
_, err = stmt.Exec("refresh")
if err != nil {
return err
}
return nil
}
func (c *ConfigDB) GetUsername() (string, error) {
c.lock.Lock()
defer c.lock.Unlock()
stmt, err := c.db.Prepare("select value from config where key=?")
defer stmt.Close()
var un string
err = stmt.QueryRow("username").Scan(&un)
if err != nil {
return "", err
}
return un, nil
}
func (c *ConfigDB) GetTokens() (at string, rt string, err error) {
c.lock.Lock()
defer c.lock.Unlock()
stmt, err := c.db.Prepare("select value from config where key=?")
defer stmt.Close()
err = stmt.QueryRow("access").Scan(&at)
if err != nil {
return "", "", err
}
err = stmt.QueryRow("refresh").Scan(&rt)
if err != nil {
return "", "", err
}
return at, rt, nil
}
func (c *ConfigDB) GetCreationDate() (time.Time, error) {
c.lock.Lock()
defer c.lock.Unlock()
var t time.Time
stmt, err := c.db.Prepare("select value from config where key=?")
if err != nil {
return t, err
}
defer stmt.Close()
var created []byte
err = stmt.QueryRow("created").Scan(&created)
if err != nil {
return t, err
}
return time.Parse(time.RFC3339, string(created))
}
func (c *ConfigDB) GetSchemaVersion() (string, error) {
c.lock.Lock()
defer c.lock.Unlock()
stmt, err := c.db.Prepare("select value from config where key=?")
defer stmt.Close()
var sv string
err = stmt.QueryRow("schema").Scan(&sv)
if err != nil {
return "", err
}
return sv, nil
}
func (c *ConfigDB) IsEncrypted() bool {
c.lock.Lock()
defer c.lock.Unlock()
pwdCheck := "select count(*) from sqlite_master;"
_, err := c.db.Exec(pwdCheck) // Fails if wrong password is entered
if err != nil {
return true
}
return false
}
<file_sep>package cmd
import (
"errors"
"fmt"
"sort"
"github.com/fatih/color"
"github.com/textileio/textile-go/core"
"gopkg.in/abiosoft/ishell.v2"
)
func ListAlbums(c *ishell.Context) {
rooms := core.Node.IpfsNode.Floodsub.GetTopics()
albums := core.Node.Datastore.Albums().GetAlbums("")
if len(albums) == 0 {
c.Println("no threads found")
} else {
c.Println(fmt.Sprintf("found %v threads", len(albums)))
}
blue := color.New(color.FgHiBlue).SprintFunc()
for _, a := range albums {
mem := "disabled"
for _, r := range rooms {
if r == a.Id {
mem = "enabled"
}
}
c.Println(blue(fmt.Sprintf("name: %s, id: %s, status: %s", a.Name, a.Id, mem)))
}
}
func CreateAlbum(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
c.Print("key pair mnemonic phrase (optional): ")
mnemonic := c.ReadLine()
if err := core.Node.CreateAlbum(mnemonic, name); err != nil {
c.Err(err)
return
}
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
JoinRoom(c, a.Id)
cyan := color.New(color.FgCyan).SprintFunc()
c.Println(cyan(fmt.Sprintf("created thread #%s", name)))
}
func EnableAlbum(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
if core.Node.LeftRoomChs[a.Id] != nil {
c.Printf("already enabled: %s\n", a.Id)
return
}
JoinRoom(c, a.Id)
c.Printf("ok, now enabled: %s\n", a.Id)
}
func DisableAlbum(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
if core.Node.LeftRoomChs[a.Id] == nil {
c.Printf("already disabled: %s\n", a.Id)
return
}
core.Node.LeaveRoom(a.Id)
<-core.Node.LeftRoomChs[a.Id]
c.Printf("ok, now disabled: %s\n", a.Id)
}
func AlbumMnemonic(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
green := color.New(color.FgGreen).SprintFunc()
c.Println(green(a.Mnemonic))
}
func RepublishAlbum(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
recent := core.Node.Datastore.Photos().GetPhotos("", 1, "album='"+a.Id+"' and local=1")
if len(recent) == 0 {
c.Println(fmt.Sprintf("no updates to publish in: %s", name))
return
}
latest := recent[0].Cid
// publish it
if err := core.Node.IpfsNode.Floodsub.Publish(a.Id, []byte(latest)); err != nil {
c.Err(fmt.Errorf("error re-publishing update: %s", err))
return
}
blue := color.New(color.FgHiBlue).SprintFunc()
c.Println(blue(fmt.Sprintf("published %s to %s", latest, a.Id)))
}
func ListAlbumPeers(c *ishell.Context) {
if len(c.Args) == 0 {
c.Err(errors.New("missing thread name"))
return
}
name := c.Args[0]
a := core.Node.Datastore.Albums().GetAlbumByName(name)
if a == nil {
c.Err(errors.New(fmt.Sprintf("could not find thread: %s", name)))
return
}
peers := core.Node.IpfsNode.Floodsub.ListPeers(a.Id)
var list []string
for _, peer := range peers {
list = append(list, peer.Pretty())
}
sort.Strings(list)
if len(list) == 0 {
c.Println(fmt.Sprintf("no peers found in: %s", name))
} else {
c.Println(fmt.Sprintf("found %v peers in: %s", len(list), name))
}
green := color.New(color.FgHiGreen).SprintFunc()
for _, peer := range list {
c.Println(green(peer))
}
}
func JoinRoom(shell ishell.Actions, id string) {
cyan := color.New(color.FgCyan).SprintFunc()
datac := make(chan core.ThreadUpdate)
go core.Node.JoinRoom(id, datac)
go func() {
for {
select {
case update, ok := <-datac:
if !ok {
return
}
msg := fmt.Sprintf("\nnew photo %s in %s thread", update.Cid, update.Thread)
shell.ShowPrompt(false)
shell.Printf(cyan(msg))
shell.ShowPrompt(true)
}
}
}()
}
<file_sep>package db
import (
"database/sql"
"sync"
"testing"
"github.com/textileio/textile-go/repo"
libp2p "gx/ipfs/QmaPbCnUMBohSGo3KnxEa2bHqyJVVeEEcwtqJAYxerieBo/go-libp2p-crypto"
)
var aldb repo.AlbumStore
func init() {
setupAlbumDB()
}
func setupAlbumDB() {
conn, _ := sql.Open("sqlite3", ":memory:")
initDatabaseTables(conn, "")
aldb = NewAlbumStore(conn, new(sync.Mutex))
}
func TestAlbumDB_Put(t *testing.T) {
priv, _, err := libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qmabc123",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err != nil {
t.Error(err)
}
stmt, err := aldb.PrepareQuery("select id from albums where id=?")
defer stmt.Close()
var id string
err = stmt.QueryRow("Qmabc123").Scan(&id)
if err != nil {
t.Error(err)
}
if id != "Qmabc123" {
t.Errorf(`expected "Qmabc123" got %s`, id)
}
}
func TestAlbumDB_GetAlbum(t *testing.T) {
setupAlbumDB()
priv, _, err := libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qmabc",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err != nil {
t.Error(err)
}
p := aldb.GetAlbum("Qmabc")
if p == nil {
t.Error("could not get album")
}
}
func TestAlbumDB_GetAlbumByName(t *testing.T) {
setupAlbumDB()
priv, _, err := libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qmabc",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qmabc2",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err == nil {
t.Error("unique constraint on name failed")
}
p := aldb.GetAlbumByName("boom")
if p == nil {
t.Error("could not get album")
}
}
func TestAlbumDB_GetAlbums(t *testing.T) {
setupAlbumDB()
priv, _, err := libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qm123",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err != nil {
t.Error(err)
}
priv, _, err = libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qm456",
Key: priv,
Mnemonic: "",
Name: "boom2",
})
if err != nil {
t.Error(err)
}
as := aldb.GetAlbums("")
if len(as) != 2 {
t.Error("returned incorrect number of albums")
return
}
filtered := aldb.GetAlbums("name='boom2'")
if len(filtered) != 1 {
t.Error("returned incorrect number of albums")
return
}
}
func TestAlbumDB_DeleteAlbum(t *testing.T) {
setupAlbumDB()
priv, _, err := libp2p.GenerateKeyPair(libp2p.Ed25519, 0)
if err != nil {
t.Error(err)
}
err = aldb.Put(&repo.PhotoAlbum{
Id: "Qm789",
Key: priv,
Mnemonic: "",
Name: "boom",
})
if err != nil {
t.Error(err)
}
as := aldb.GetAlbums("")
if len(as) == 0 {
t.Error("returned incorrect number of albums")
return
}
err = aldb.DeleteAlbum(as[0].Id)
if err != nil {
t.Error(err)
}
stmt, err := phdb.PrepareQuery("select id from albums where id=?")
defer stmt.Close()
var id string
err = stmt.QueryRow(as[0].Id).Scan(&id)
if err == nil {
t.Error("Delete failed")
}
}
<file_sep>package core
import (
"context"
"encoding/base64"
"encoding/json"
"errors"
"fmt"
"io"
"io/ioutil"
"net/http"
"os"
"path"
"path/filepath"
"strings"
"sync"
"time"
"github.com/op/go-logging"
"github.com/segmentio/ksuid"
"github.com/tyler-smith/go-bip39"
"gopkg.in/natefinch/lumberjack.v2"
cmodels "github.com/textileio/textile-go/central/models"
"github.com/textileio/textile-go/core/central"
"github.com/textileio/textile-go/net"
trepo "github.com/textileio/textile-go/repo"
tconfig "github.com/textileio/textile-go/repo/config"
"github.com/textileio/textile-go/repo/db"
"github.com/textileio/textile-go/repo/photos"
"github.com/textileio/textile-go/util"
utilmain "gx/ipfs/<KEY>/go-ipfs/cmd/ipfs/util"
oldcmds "gx/ipfs/<KEY>/go-ipfs/commands"
"gx/ipfs/<KEY>/go-ipfs/core"
"gx/ipfs/<KEY>/go-ipfs/core/coreapi"
"gx/ipfs/<KEY>/go-ipfs/core/coreapi/interface"
"gx/<KEY>go-ipfs/core/coreapi/interface/options"
"gx/<KEY>go-ipfs/repo/config"
"gx/<KEY>/repo/fsrepo"
"gx/<KEY>libp2p-floodsub"
"gx/<KEY>libp2p-swarm"
pstore "gx/<KEY>go-libp2p-peerstore"
"gx/<KEY>go-libp2p-peer"
libp2p "gx/<KEY>go-libp2p-crypto"
"gx/<KEY>libp2p-kad-dht"
)
const (
Version = "0.0.1"
)
var fileLogFormat = logging.MustStringFormatter(
`%{time:15:04:05.000} [%{shortfunc}] [%{level}] %{message}`,
)
var log = logging.MustGetLogger("core")
const roomRepublishInterval = time.Minute * 1
const pingTimeout = time.Second * 10
const pinTimeout = time.Minute * 1
const catTimeout = time.Second * 30
// Node is the single TextileNode instance
var Node *TextileNode
// ErrNodeRunning is an error for when node start is called on a running node
var ErrNodeRunning = errors.New("node is already running")
// ErrNodeNotRunning is an error for when node stop is called on a nil node
var ErrNodeNotRunning = errors.New("node is not running")
// TextileNode is the main node interface for textile functionality
type TextileNode struct {
// Context for issuing IPFS commands
Context oldcmds.Context
// IPFS node object
IpfsNode *core.IpfsNode
// The path to the openbazaar repo in the file system
RepoPath string
// Database for storing node specific data
Datastore trepo.Datastore
// Function to call for shutdown
Cancel context.CancelFunc
// The local decrypting gateway server
GatewayProxy *http.Server
// Map of hash passwords for the decrypting gateway server
HashPasses map[string]string
// The local password used to authenticate http gateway requests (username is TextileNode)
GatewayPassword string
// Signals for when we've left rooms
LeftRoomChs map[string]chan struct{}
// Signals for leaving rooms
leaveRoomChs map[string]chan struct{}
// IPFS configuration used to instantiate new ipfs nodes
ipfsConfig *core.BuildCfg
// Whether or not we're running on a mobile device
isMobile bool
// API URL of the central backup / recovery / pinning user service
centralUserAPI string
// Whether or not we've just inited, and never run, a "fresh" node :)
fresh bool
// Whether or no we're online
online bool
// Mutex for controlling lifecycle
mux sync.Mutex
// Captures stdout from ipfs packages
stdOutLogger *util.StdOutLogger
}
// PhotoList contains a list of photo hashes
type PhotoList struct {
Hashes []string `json:"hashes"`
}
// HashRequest represents a single-use gateway token
type HashRequest struct {
Token string `json:"token"`
Protocol string `json:"protocol"`
Host string `json:"host"`
}
// ThreadUpdate is used to notify listeners about updates in a thread
type ThreadUpdate struct {
Cid string `json:"cid"`
Thread string `json:"thread"`
ThreadID string `json:"thread_id"`
}
// NodeConfig is used to configure the node
type NodeConfig struct {
RepoPath string
CentralApiURL string
IsMobile bool
IsServer bool
LogLevel logging.Level
LogFiles bool
SwarmPort string
}
// NewNode creates a new TextileNode
func NewNode(config NodeConfig) (*TextileNode, error) {
// TODO: shouldn't need to manually remove these
repoLockFile := filepath.Join(config.RepoPath, fsrepo.LockFile)
os.Remove(repoLockFile)
dsLockFile := filepath.Join(config.RepoPath, "datastore", "LOCK")
os.Remove(dsLockFile)
// log handling
var backendFile *logging.LogBackend
if config.LogFiles {
w := &lumberjack.Logger{
Filename: path.Join(config.RepoPath, "logs", "textile.log"),
MaxSize: 10, // megabytes
MaxBackups: 3,
MaxAge: 30, // days
}
backendFile = logging.NewLogBackend(w, "", 0)
} else {
backendFile = logging.NewLogBackend(os.Stdout, "", 0)
}
backendFileFormatter := logging.NewBackendFormatter(backendFile, fileLogFormat)
logging.SetBackend(backendFileFormatter)
logging.SetLevel(config.LogLevel, "")
// capture stdout from ipfs packages
stdOutLogger, err := util.NewStdOutLogger(logging.MustGetLogger("ipfs"))
if err != nil {
log.Errorf("error creating stdout logger: %s", err)
return nil, err
}
// get database handle
sqliteDB, err := db.Create(config.RepoPath, "")
if err != nil {
return nil, err
}
// we may be running in an uninitialized state.
err = trepo.DoInit(config.RepoPath, config.IsMobile, sqliteDB.Config().Init, sqliteDB.Config().Configure)
if err != nil && err != trepo.ErrRepoExists {
return nil, err
}
// acquire the repo lock _before_ constructing a node. we need to make
// sure we are permitted to access the resources (datastore, etc.)
repo, err := fsrepo.Open(config.RepoPath)
if err != nil {
log.Errorf("error opening repo: %s", err)
return nil, err
}
// determine the best routing
var routingOption core.RoutingOption
if config.IsMobile {
routingOption = core.DHTClientOption
} else {
routingOption = core.DHTOption
}
// assemble node config
ncfg := &core.BuildCfg{
Repo: repo,
Permanent: true, // temporary way to signify that node is permanent
Online: true,
ExtraOpts: map[string]bool{
"pubsub": true,
"ipnsps": true,
"mplex": true,
},
Routing: routingOption,
}
// setup gateway
gwAddr, err := repo.GetConfigKey("Addresses.Gateway")
if err != nil {
log.Errorf("error getting ipfs config: %s", err)
return nil, err
}
gatewayProxy := &http.Server{
Addr: gwAddr.(string),
}
// if a specific swarm port was selected, set it in the config
if config.SwarmPort != "" {
log.Infof("using specified swarm port: %s", config.SwarmPort)
if err := tconfig.Update(repo, "Addresses.Swarm", []string{
fmt.Sprintf("/ip4/0.0.0.0/tcp/%s", config.SwarmPort),
fmt.Sprintf("/ip6/::/tcp/%s", config.SwarmPort),
}); err != nil {
return nil, err
}
}
// if this is a server node, apply the ipfs server profile
if config.IsServer {
if err := tconfig.Update(repo, "Addresses.NoAnnounce", tconfig.DefaultServerFilters); err != nil {
return nil, err
}
if err := tconfig.Update(repo, "Swarm.AddrFilters", tconfig.DefaultServerFilters); err != nil {
return nil, err
}
if err := tconfig.Update(repo, "Swarm.EnableRelayHop", true); err != nil {
return nil, err
}
if err := tconfig.Update(repo, "Discovery.MDNS.Enabled", false); err != nil {
return nil, err
}
log.Info("applied server profile")
}
// clean central api url
if len(config.CentralApiURL) > 0 {
ca := config.CentralApiURL
if ca[len(ca)-1:] == "/" {
ca = ca[0 : len(ca)-1]
}
config.CentralApiURL = ca
}
// finally, construct our node
node := &TextileNode{
RepoPath: config.RepoPath,
Datastore: sqliteDB,
GatewayProxy: gatewayProxy,
GatewayPassword: <PASSWORD>.New().String(),
HashPasses: make(map[string]string),
LeftRoomChs: make(map[string]chan struct{}),
leaveRoomChs: make(map[string]chan struct{}),
ipfsConfig: ncfg,
isMobile: config.IsMobile,
centralUserAPI: fmt.Sprintf("%s/api/v1/users", config.CentralApiURL),
fresh: true,
stdOutLogger: stdOutLogger,
}
// create default album
da := node.Datastore.Albums().GetAlbumByName("default")
if da == nil {
err = node.CreateAlbum("", "default")
if err != nil {
log.Errorf("error creating default album: %s", err)
return nil, err
}
}
return node, nil
}
// Start the node
func (t *TextileNode) Start() error {
t.mux.Lock()
defer t.mux.Unlock()
t.fresh = false
if t.Online() {
return ErrNodeRunning
}
log.Info("starting node...")
// start capturing
//t.stdOutLogger.Start()
// raise file descriptor limit
if err := utilmain.ManageFdLimit(); err != nil {
log.Errorf("setting file descriptor limit: %s", err)
}
// check db
if err := t.touchDB(); err != nil {
return err
}
// check repo
if t.ipfsConfig.Repo == nil {
log.Debug("re-opening repo...")
repo, err := fsrepo.Open(t.RepoPath)
if err != nil {
log.Errorf("error re-opening repo: %s", err)
return err
}
t.ipfsConfig.Repo = repo
}
// start the ipfs node
log.Debug("creating an ipfs node...")
cctx, cancel := context.WithCancel(context.Background())
t.Cancel = cancel
nd, err := core.NewNode(cctx, t.ipfsConfig)
if err != nil {
log.Errorf("error creating ipfs node: %s", err)
return err
}
nd.SetLocal(false)
// print swarm addresses
if err = printSwarmAddrs(nd); err != nil {
log.Errorf("failed to read listening addresses: %s", err)
}
// build the node
ctx := oldcmds.Context{}
ctx.Online = true
ctx.ConfigRoot = t.RepoPath
ctx.LoadConfig = func(path string) (*config.Config, error) {
return fsrepo.ConfigAt(t.RepoPath)
}
ctx.ConstructNode = func() (*core.IpfsNode, error) {
return nd, nil
}
t.Context = ctx
t.IpfsNode = nd
// construct decrypting http gateway
var gwpErrc <-chan error
gwpErrc, err = t.startGateway()
if err != nil {
log.Errorf("error starting decrypting gateway: %s", err)
return err
}
go func() {
for {
select {
case err, ok := <-gwpErrc:
if err != nil && err.Error() != "http: Server closed" {
log.Errorf("gateway error: %s", err)
}
if !ok {
log.Info("decrypting gateway was shutdown")
return
}
}
}
}()
// wait for dht to bootstrap
<-dht.DefaultBootstrapConfig.DoneChan
if !t.isMobile {
// every min, send out latest room updates
go t.startRepublishing()
}
// dunzo
if t.isMobile {
log.Info("mobile node is ready")
} else {
log.Info("desktop node is ready")
}
t.online = true
return nil
}
// Stop the node
func (t *TextileNode) Stop() error {
t.mux.Lock()
defer t.mux.Unlock()
if !t.Online() {
return ErrNodeNotRunning
}
log.Info("stopping node...")
// shutdown the gateway
cgCtx, cancelCGW := context.WithCancel(context.Background())
if err := t.GatewayProxy.Shutdown(cgCtx); err != nil {
log.Errorf("error shutting down gateway: %s", err)
return err
}
// close ipfs node command context
t.Context.Close()
// cancel textile node background context
t.Cancel()
// close the ipfs node
if err := t.IpfsNode.Close(); err != nil {
log.Errorf("error closing ipfs node: %s", err)
return err
}
// force the gateway closed if it's not already closed
cancelCGW()
// close db connection
t.Datastore.Close()
dsLockFile := filepath.Join(t.RepoPath, "datastore", "LOCK")
if err := os.Remove(dsLockFile); err != nil {
log.Errorf("error removing ds lock: %s", err)
}
// clean up node
t.IpfsNode = nil
t.ipfsConfig.Repo = nil
// stop capturing
//t.stdOutLogger.Stop()
t.online = false
return nil
}
func (t *TextileNode) Online() bool {
return t.fresh || t.online
}
// StartGarbageCollection starts auto garbage cleanup
// TODO: verify this is finding the correct repo, might be using IPFS_PATH
func (t *TextileNode) StartGarbageCollection() (<-chan error, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
if t.isMobile {
return nil, errors.New("services not available on mobile")
}
// repo blockstore GC
var gcErrc <-chan error
var err error
gcErrc, err = runGC(t.IpfsNode.Context(), t.IpfsNode)
if err != nil {
log.Errorf("error starting gc: %s", err)
return nil, err
}
return gcErrc, nil
}
// SignUp requests a new username and token from the central api and saves them locally
func (t *TextileNode) SignUp(reg *cmodels.Registration) error {
// check db
if err := t.touchDB(); err != nil {
return err
}
log.Debugf("signup: %s %s %s %s %s", reg.Username, "xxxxxx", reg.Identity.Type, reg.Identity.Value, reg.Referral)
// remote signup
res, err := central.SignUp(reg, t.centralUserAPI)
if err != nil {
log.Errorf("signup error: %s", err)
return err
}
if res.Error != nil {
log.Errorf("signup error from central: %s", *res.Error)
return errors.New(*res.Error)
}
// local signin
if err := t.Datastore.Config().SignIn(reg.Username, res.Session.AccessToken, res.Session.RefreshToken); err != nil {
log.Errorf("local signin error: %s", err)
return err
}
return nil
}
// SignIn requests a token with a username from the central api and saves them locally
func (t *TextileNode) SignIn(creds *cmodels.Credentials) error {
// check db
if err := t.touchDB(); err != nil {
return err
}
log.Debugf("signin: %s %s", creds.Username, "xxxxxx")
// remote signin
res, err := central.SignIn(creds, t.centralUserAPI)
if err != nil {
log.Errorf("signin error: %s", err)
return err
}
if res.Error != nil {
log.Errorf("signin error from central: %s", *res.Error)
return errors.New(*res.Error)
}
// local signin
if err := t.Datastore.Config().SignIn(creds.Username, res.Session.AccessToken, res.Session.RefreshToken); err != nil {
log.Errorf("local signin error: %s", err)
return err
}
return nil
}
// SignOut deletes the locally saved user info (username and tokens)
func (t *TextileNode) SignOut() error {
// check db
if err := t.touchDB(); err != nil {
return err
}
log.Debug("signing out...")
// remote is stateless, so we just ditch the local token
if err := t.Datastore.Config().SignOut(); err != nil {
log.Errorf("local signout error: %s", err)
return err
}
return nil
}
// IsSignedIn returns whether or not a user is signed in
func (t *TextileNode) IsSignedIn() (bool, error) {
// check db
if err := t.touchDB(); err != nil {
return false, err
}
_, err := t.Datastore.Config().GetUsername()
return err == nil, nil
}
// GetUsername returns the current user's username
func (t *TextileNode) GetUsername() (string, error) {
// check db
if err := t.touchDB(); err != nil {
return "", err
}
un, err := t.Datastore.Config().GetUsername()
if err != nil {
return "", err
}
return un, nil
}
// GetAccessToken returns the current access_token (jwt) for central
func (t *TextileNode) GetAccessToken() (string, error) {
// check db
if err := t.touchDB(); err != nil {
return "", err
}
at, _, err := t.Datastore.Config().GetTokens()
if err != nil {
return "", err
}
return at, nil
}
// JoinRoom with a given id
func (t *TextileNode) JoinRoom(id string, datac chan ThreadUpdate) {
if !t.Online() {
return
}
// create the subscription
sub, err := t.IpfsNode.Floodsub.Subscribe(id)
if err != nil {
log.Errorf("error creating subscription: %s", err)
return
}
log.Infof("joined room: %s\n", id)
t.leaveRoomChs[id] = make(chan struct{})
t.LeftRoomChs[id] = make(chan struct{})
api := coreapi.NewCoreAPI(t.IpfsNode)
ctx, cancel := context.WithCancel(context.Background())
leave := func() {
cancel()
close(t.LeftRoomChs[id])
delete(t.LeftRoomChs, id)
delete(t.leaveRoomChs, id)
log.Infof("left room: %s\n", sub.Topic())
}
defer func() {
defer func() {
if r := recover(); r != nil {
log.Errorf("room data channel already closed")
}
}()
close(datac)
}()
go func() {
for {
// unload new message
msg, err := sub.Next(ctx)
if err == io.EOF || err == context.Canceled {
log.Debugf("room subscription ended: %s", err)
return
} else if err != nil {
log.Debugf(err.Error())
return
}
// handle the update
go func(msg *floodsub.Message) {
if err = t.handleRoomUpdate(msg, id, api, datac); err != nil {
log.Errorf("error handling room update: %s", err)
}
}(msg)
}
}()
// block so we can shutdown with the leave room signal
for {
select {
case <-t.leaveRoomChs[id]:
leave()
return
case <-t.IpfsNode.Context().Done():
leave()
return
}
}
}
// LeaveRoom with a given id
func (t *TextileNode) LeaveRoom(id string) {
if !t.Online() {
return
}
if t.leaveRoomChs[id] == nil {
return
}
close(t.leaveRoomChs[id])
}
// WaitForRoom to join
func (t *TextileNode) WaitForRoom() {
if !t.Online() {
return
}
// we're in a lonesome state here, we can just sub to our own
// peer id and hope somebody sends us a priv key to join a room with
rid := t.IpfsNode.Identity.Pretty()
sub, err := t.IpfsNode.Floodsub.Subscribe(rid)
if err != nil {
log.Errorf("error creating subscription: %s", err)
return
}
log.Infof("waiting for room at own peer id: %s\n", rid)
ctx, cancel := context.WithCancel(context.Background())
cancelCh := make(chan struct{})
go func() {
for {
msg, err := sub.Next(ctx)
if err == io.EOF || err == context.Canceled {
log.Debugf("wait subscription ended: %s", err)
return
} else if err != nil {
log.Debugf(err.Error())
return
}
from := msg.GetFrom().Pretty()
log.Infof("got pairing request from: %s\n", from)
// get private peer key and decrypt the phrase
sk, err := t.UnmarshalPrivatePeerKey()
if err != nil {
log.Errorf("error unmarshaling priv peer key: %s", err)
return
}
p, err := net.Decrypt(sk, msg.GetData())
if err != nil {
log.Errorf("error decrypting msg data: %s", err)
return
}
ps := string(p)
log.Debugf("decrypted mnemonic phrase as: %s\n", ps)
// create a new album for the room
// TODO: let user name this or take phone's name, e.g., bob's iphone
// TODO: or auto name it, cause this means only one pairing can happen
t.CreateAlbum(ps, "mobile")
// we're done
close(cancelCh)
}
}()
for {
select {
case <-cancelCh:
cancel()
return
case <-t.IpfsNode.Context().Done():
cancel()
return
}
}
}
// CreateAlbum creates an album with a given name and mnemonic words
func (t *TextileNode) CreateAlbum(mnemonic string, name string) error {
// check db
if err := t.touchDB(); err != nil {
return err
}
log.Debugf("creating a new album: %s", name)
// use phrase if provided
if mnemonic == "" {
var err error
mnemonic, err = createMnemonic(bip39.NewEntropy, bip39.NewMnemonic)
if err != nil {
log.Errorf("error creating mnemonic: %s", err)
return err
}
log.Debugf("generating %v-bit Ed25519 keypair for: %s", trepo.NBitsForKeypair, name)
} else {
log.Debugf("regenerating Ed25519 keypair from mnemonic phrase for: %s", name)
}
// create the bip39 seed from the phrase
seed := bip39.NewSeed(mnemonic, "")
kb, err := identityKeyFromSeed(seed, trepo.NBitsForKeypair)
if err != nil {
log.Errorf("error creating identity from seed: %s", err)
return err
}
// convert to a libp2p crypto private key
sk, err := libp2p.UnmarshalPrivateKey(kb)
if err != nil {
log.Errorf("error unmarshaling private key: %s", err)
return err
}
// we need the resultant peer id to use as the album's id
id, err := peer.IDFromPrivateKey(sk)
if err != nil {
log.Errorf("error getting id from priv key: %s", err)
return err
}
// finally, create the album
album := &trepo.PhotoAlbum{
Id: id.Pretty(),
Key: sk,
Mnemonic: mnemonic,
Name: name,
}
return t.Datastore.Albums().Put(album)
}
// AddPhoto adds a photo and its thumbnail to an album
// TODO: Make this available offline
func (t *TextileNode) AddPhoto(path string, thumb string, album string, caption string) (*net.MultipartRequest, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
log.Debugf("adding photo %s to %s", path, album)
// read file from disk
p, err := os.Open(path)
if err != nil {
log.Errorf("error opening photo: %s", err)
return nil, err
}
defer p.Close()
th, err := os.Open(thumb)
if err != nil {
log.Errorf("error opening thumb: %s", err)
return nil, err
}
defer th.Close()
// get album private key
a := t.Datastore.Albums().GetAlbumByName(album)
if a == nil {
err = errors.New(fmt.Sprintf("could not find album: %s", album))
log.Error(err.Error())
return nil, err
}
// get last photo update which has local true
var lc string
recent := t.Datastore.Photos().GetPhotos("", 1, "album='"+a.Id+"' and local=1")
if len(recent) > 0 {
lc = recent[0].Cid
log.Debugf("found last hash: %s", lc)
}
// get username
un, err := t.Datastore.Config().GetUsername()
if err != nil {
log.Errorf("username not found (not signed in)")
un = ""
}
// add it
// TODO: clean up this nasty method signature
mr, md, err := photos.Add(t.IpfsNode, a.Key.GetPublic(), p, th, lc, un, caption)
if err != nil {
log.Errorf("error adding photo: %s", err)
return nil, err
}
// index
log.Debugf("indexing %s...", mr.Boundary)
set := &trepo.PhotoSet{
Cid: mr.Boundary,
LastCid: lc,
AlbumID: a.Id,
MetaData: *md,
Caption: caption,
IsLocal: true,
}
err = t.Datastore.Photos().Put(set)
if err != nil {
log.Errorf("error indexing photo: %s", err)
return nil, err
}
// publish
go func() {
err = t.Publish(a.Id, []byte(mr.Boundary))
if err != nil {
log.Errorf("error publishing photo update: %s", err)
return
}
log.Debugf("published update to %s", a.Id)
}()
return mr, nil
}
// SharePhoto re-encrypts a photo from an existing album and shares it into a different album
// TODO: Make this available offline
func (t *TextileNode) SharePhoto(hash string, album string, caption string) (*net.MultipartRequest, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
log.Debugf("sharing photo %s to %s...", hash, album)
// get the photo
set, a, err := t.LoadPhotoAndAlbum(hash)
if err != nil {
log.Error(err.Error())
return nil, err
}
// get dest album
na := t.Datastore.Albums().GetAlbumByName(album)
if na == nil {
return nil, errors.New(fmt.Sprintf("could not find album: %s", album))
}
// check if album is diff
if a.Id == na.Id {
return nil, errors.New(fmt.Sprintf("photo already in album: %s", album))
}
// get photo data
pb, err := t.GetFile(fmt.Sprintf("%s/photo", hash), a)
if err != nil {
return nil, err
}
tb, err := t.GetFile(fmt.Sprintf("%s/thumb", hash), a)
if err != nil {
return nil, err
}
// temp write to disk
ppath := filepath.Join(t.RepoPath, "tmp", set.MetaData.Name+set.MetaData.Ext)
tpath := filepath.Join(t.RepoPath, "tmp", "thumb_"+set.MetaData.Name+set.MetaData.Ext)
err = ioutil.WriteFile(ppath, pb, 0644)
if err != nil {
return nil, err
}
defer func() {
err := os.Remove(ppath)
if err != nil {
log.Errorf("error cleaning up shared photo path: %s", ppath)
}
}()
err = ioutil.WriteFile(tpath, tb, 0644)
if err != nil {
return nil, err
}
defer func() {
err = os.Remove(tpath)
if err != nil {
log.Errorf("error cleaning up shared thumb path: %s", tpath)
}
}()
return t.AddPhoto(ppath, tpath, album, caption)
}
// GetHashRequest returns a single-use token for requesting content via the gateway
func (t *TextileNode) GetHashRequest(hash string) HashRequest {
token := ksuid.New().String()
t.HashPasses[hash] = token
return HashRequest{
Token: token,
Protocol: "http",
Host: t.GatewayProxy.Addr,
}
}
// GetPhotos paginates photos from the datastore
func (t *TextileNode) GetPhotos(offsetId string, limit int, album string) *PhotoList {
// check db
if err := t.touchDB(); err != nil {
return nil
}
log.Debugf("getting photos: offsetId: %s, limit: %d, album: %s", offsetId, limit, album)
// query for available hashes
a := t.Datastore.Albums().GetAlbumByName(album)
if a == nil {
return &PhotoList{Hashes: make([]string, 0)}
}
list := t.Datastore.Photos().GetPhotos(offsetId, limit, "album='"+a.Id+"'")
// return json list of hashes
res := &PhotoList{
Hashes: make([]string, len(list)),
}
for i := range list {
res.Hashes[i] = list[i].Cid
}
log.Debugf("found %d photos in thread %s", len(list), album)
return res
}
// GetFile cats data from the ipfs node.
// e.g., Qm../thumb, Qm../photo, Qm../meta, Qm../caption
// Note: album is looked up if not present
// TODO: shouldn't this be available offline for local (pinned content)?
func (t *TextileNode) GetFile(path string, album *trepo.PhotoAlbum) ([]byte, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
// get bytes
cb, err := t.getDataAtPath(path)
if err != nil {
log.Errorf("error getting file data: %s", err)
return nil, err
}
// normalize path
ip, err := coreapi.ParsePath(path)
if err != nil {
log.Errorf("error parsing path: %s", err)
return nil, err
}
// parse root hash of path
tmp := strings.Split(ip.String(), "/")
if len(tmp) < 3 {
err := errors.New(fmt.Sprintf("bad path: %s", path))
log.Error(err.Error())
return nil, err
}
ci := tmp[2]
// look up key for decryption
if album == nil {
_, album, err = t.LoadPhotoAndAlbum(ci)
if err != nil {
log.Error(err.Error())
return nil, err
}
}
// finally, decrypt
b, err := net.Decrypt(album.Key, cb)
if err != nil {
log.Errorf("error decrypting file: %s", err)
return nil, err
}
return b, err
}
// GetFileBase64 returns data encoded as base64 under an ipfs path
func (t *TextileNode) GetFileBase64(path string) (string, error) {
b, err := t.GetFile(path, nil)
if err != nil {
return "error", err
}
return base64.StdEncoding.EncodeToString(b), nil
}
// GetMetaData returns metadata under a hash
// TODO: shouldn't this be available offline for local (pinned content)?
func (t *TextileNode) GetMetaData(hash string, album *trepo.PhotoAlbum) (*photos.Metadata, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
b, err := t.GetFile(fmt.Sprintf("%s/meta", hash), album)
if err != nil {
log.Errorf("error getting meta file with hash: %s: %s", hash, err)
return nil, err
}
var data *photos.Metadata
err = json.Unmarshal(b, &data)
if err != nil {
log.Errorf("error unmarshaling meta file with hash: %s: %s", hash, err)
return nil, err
}
return data, nil
}
func (t *TextileNode) TouchDB() error {
return t.touchDB()
}
// GetLastHash return the caption under a hash
// TODO: shouldn't this be available offline for local (pinned content)?
func (t *TextileNode) GetCaption(hash string, album *trepo.PhotoAlbum) (string, error) {
if !t.Online() {
return "", ErrNodeNotRunning
}
b, err := t.GetFile(fmt.Sprintf("%s/caption", hash), album)
if err != nil {
log.Errorf("error getting caption file with hash: %s: %s", hash, err)
return "", err
}
return string(b), nil
}
// GetLastHash return the last update's root cid under a hash
// TODO: shouldn't this be available offline for local (pinned content)?
func (t *TextileNode) GetLastHash(hash string, album *trepo.PhotoAlbum) (string, error) {
if !t.Online() {
return "", ErrNodeNotRunning
}
b, err := t.GetFile(fmt.Sprintf("%s/last", hash), album)
if err != nil {
log.Errorf("error getting last hash file with hash: %s: %s", hash, err)
return "", err
}
return string(b), nil
}
// LoadPhotoAndAlbum get the indexed photo and album for a hash
func (t *TextileNode) LoadPhotoAndAlbum(hash string) (*trepo.PhotoSet, *trepo.PhotoAlbum, error) {
if !t.Online() {
return nil, nil, ErrNodeNotRunning
}
ph := t.Datastore.Photos().GetPhoto(hash)
if ph == nil {
return nil, nil, errors.New(fmt.Sprintf("photo %s not found", hash))
}
album := t.Datastore.Albums().GetAlbum(ph.AlbumID)
if album == nil {
return nil, nil, errors.New(fmt.Sprintf("could not find album: %s", ph.AlbumID))
}
return ph, album, nil
}
// UnmarshalPrivatePeerKey returns a PrivKey instance from the base64 encoded ipfs peer key
func (t *TextileNode) UnmarshalPrivatePeerKey() (libp2p.PrivKey, error) {
if !t.Online() {
return nil, ErrNodeNotRunning
}
cfg, err := t.Context.GetConfig()
if err != nil {
return nil, err
}
skb, err := base64.StdEncoding.DecodeString(cfg.Identity.PrivKey)
if err != nil {
return nil, err
}
sk, err := libp2p.UnmarshalPrivateKey(skb)
if err != nil {
return nil, err
}
// check
id2, err := peer.IDFromPrivateKey(sk)
if err != nil {
return nil, err
}
if id2 != t.IpfsNode.Identity {
return nil, fmt.Errorf("private key in config does not match id: %s != %s", t.IpfsNode.Identity, id2)
}
return sk, nil
}
// GetPublicPeerKeyString returns the base64 encoded public ipfs peer key
func (t *TextileNode) GetPublicPeerKeyString() (string, error) {
sk, err := t.UnmarshalPrivatePeerKey()
if err != nil {
log.Errorf("error unmarshaling priv peer key: %s", err)
return "", err
}
pkb, err := sk.GetPublic().Bytes()
if err != nil {
log.Errorf("error getting pub key bytes: %s", err)
return "", err
}
return base64.StdEncoding.EncodeToString(pkb), nil
}
// Publish and ping
func (t *TextileNode) Publish(topic string, payload []byte) error {
if len(t.IpfsNode.PeerHost.Network().Peers()) == 0 {
return errors.New("no peers, aborting")
}
out, err := t.ConnectPeer([]string{fmt.Sprintf("/p2p-circuit/ipfs/%s", tconfig.RemoteRelayNode)})
if err != nil {
return err
}
for _, o := range out {
log.Debug(o)
}
return t.IpfsNode.Floodsub.Publish(topic, payload)
}
// ConnectPeer connect to another ipfs peer (i.e., ipfs swarm connect)
func (t *TextileNode) ConnectPeer(addrs []string) ([]string, error) {
if t.IpfsNode.PeerHost == nil {
return nil, errors.New("not online")
}
snet, ok := t.IpfsNode.PeerHost.Network().(*swarm.Network)
if !ok {
return nil, errors.New("peerhost network was not swarm")
}
swrm := snet.Swarm()
pis, err := peersWithAddresses(addrs)
if err != nil {
return nil, err
}
output := make([]string, len(pis))
for i, pi := range pis {
swrm.Backoff().Clear(pi.ID)
output[i] = "connect " + pi.ID.Pretty()
err := t.IpfsNode.PeerHost.Connect(t.IpfsNode.Context(), pi)
if err != nil {
return nil, fmt.Errorf("%s failure: %s", output[i], err)
}
output[i] += " success"
}
return output, nil
}
// PingPeer pings a peer num times, returning the result to out chan
func (t *TextileNode) PingPeer(addrs string, num int, out chan string) error {
if !t.Online() {
return ErrNodeNotRunning
}
addr, pid, err := parsePeerParam(addrs)
if addr != nil {
t.IpfsNode.Peerstore.AddAddr(pid, addr, pstore.TempAddrTTL) // temporary
}
if len(t.IpfsNode.Peerstore.Addrs(pid)) == 0 {
// Make sure we can find the node in question
log.Debugf("looking up peer: %s", pid.Pretty())
ctx, cancel := context.WithTimeout(t.IpfsNode.Context(), pingTimeout)
defer cancel()
p, err := t.IpfsNode.Routing.FindPeer(ctx, pid)
if err != nil {
err = fmt.Errorf("peer lookup error: %s", err)
log.Errorf(err.Error())
return err
}
t.IpfsNode.Peerstore.AddAddrs(p.ID, p.Addrs, pstore.TempAddrTTL)
}
ctx, cancel := context.WithTimeout(t.IpfsNode.Context(), pingTimeout*time.Duration(num))
defer cancel()
pings, err := t.IpfsNode.Ping.Ping(ctx, pid)
if err != nil {
log.Errorf("error pinging peer %s: %s", pid.Pretty(), err)
return err
}
var done bool
var total time.Duration
for i := 0; i < num && !done; i++ {
select {
case <-ctx.Done():
done = true
close(out)
break
case t, ok := <-pings:
if !ok {
done = true
close(out)
break
}
total += t
msg := fmt.Sprintf("ping %s completed after %f seconds", pid.Pretty(), t.Seconds())
select {
case out <- msg:
default:
}
log.Debug(msg)
time.Sleep(time.Second)
}
}
return nil
}
// RepublishLatestUpdate publishes latest in a single album
func (t *TextileNode) RepublishLatestUpdate(album *trepo.PhotoAlbum) {
// find latest local update
recent := t.Datastore.Photos().GetPhotos("", 1, "album='"+album.Id+"' and local=1")
var latest string
if len(recent) > 0 {
latest = recent[0].Cid
} else {
latest = "ping"
}
// publish it
log.Debugf("starting re-publish...")
if err := t.Publish(album.Id, []byte(latest)); err != nil {
log.Errorf("error re-publishing update: %s", err)
return
}
log.Debugf("re-published %s to %s", latest, album.Id)
}
// registerGatewayHandler registers a handler for the gateway
func (t *TextileNode) registerGatewayHandler() {
defer func() {
if recover() != nil {
log.Debug("gateway handler already registered")
}
}()
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
_, password, ok := r.BasicAuth()
log.Debugf("gateway request: %s", r.URL.RequestURI())
if ok == false {
w.Header().Set("WWW-Authenticate", `Basic realm="Restricted"`)
w.WriteHeader(401)
return
}
// parse root hash of path
tmp := strings.Split(r.URL.Path, "/")
if len(tmp) < 3 {
err := errors.New(fmt.Sprintf("bad path: %s", r.URL.Path))
log.Error(err.Error())
return
}
ci := strings.Join(tmp[2:], "/")
if password != t.HashPasses[ci] {
log.Debugf("wrong password: %s", ci, t.HashPasses[ci])
w.WriteHeader(401)
return
}
// invalidate previous password
// t.HashPasses[username] = ksuid.New().String()
b, err := t.GetFile(r.URL.Path, nil)
if err != nil {
log.Errorf("error decrypting path %s: %s", r.URL.Path, err)
w.WriteHeader(400)
return
}
w.Write(b)
})
}
// startGateway starts the secure HTTP gatway server
func (t *TextileNode) startGateway() (<-chan error, error) {
// try to register our handler
t.registerGatewayHandler()
// Start the HTTPS server in a goroutine
errc := make(chan error)
go func() {
errc <- t.GatewayProxy.ListenAndServe()
close(errc)
}()
log.Infof("decrypting gateway (readonly) server listening at %s\n", t.GatewayProxy.Addr)
return errc, nil
}
// startRepublishing continuously publishes the latest update in each thread
func (t *TextileNode) startRepublishing() {
// do it once right away
t.republishLatestUpdates()
// create a never-ending ticker
ticker := time.NewTicker(roomRepublishInterval)
defer func() {
ticker.Stop()
defer func() {
if recover() != nil {
log.Error("republishing ticker already stopped")
}
}()
}()
go func() {
for range ticker.C {
t.republishLatestUpdates()
}
}()
// we can stop when the node stops
for {
if !t.Online() {
return
}
select {
case <-t.IpfsNode.Context().Done():
log.Info("republishing stopped")
return
}
}
}
// republishLatestUpdates interates through albums, publishing each ones latest update
func (t *TextileNode) republishLatestUpdates() {
// do this for each album
albums := t.Datastore.Albums().GetAlbums("")
for _, album := range albums {
go func(a trepo.PhotoAlbum) {
t.RepublishLatestUpdate(&a)
}(album)
}
}
// handleRoomUpdate tries to recursively process an update sent to a thread
func (t *TextileNode) handleRoomUpdate(msg *floodsub.Message, aid string, api iface.CoreAPI, datac chan ThreadUpdate) error {
// unpack from
from := msg.GetFrom().Pretty()
if from == t.IpfsNode.Identity.Pretty() {
return nil
}
// unpack message data
data := string(msg.GetData())
if data == "ping" {
return nil
}
// determine if this is from a relay node
tmp := strings.Split(data, ":")
var hash string
if len(tmp) > 1 && tmp[0] == "relay" {
hash = tmp[1]
from = fmt.Sprintf("relay:%s", from)
} else {
hash = tmp[0]
}
log.Debugf("got update from %s in room %s", from, aid)
// recurse back in time starting at this hash
err := t.handleHash(hash, aid, api, datac)
if err != nil {
return err
}
return nil
}
// handleHash tries to process an update sent to a thread
func (t *TextileNode) handleHash(hash string, aid string, api iface.CoreAPI, datac chan ThreadUpdate) error {
// look up the album
a := t.Datastore.Albums().GetAlbum(aid)
if a == nil {
err := errors.New(fmt.Sprintf("could not find album with id: %s", aid))
return err
}
// first update?
if hash == "" {
log.Debugf("found genesis update, aborting")
return nil
}
log.Debugf("handling update: %s...", hash)
// check if we aleady have this hash
set := t.Datastore.Photos().GetPhoto(hash)
if set != nil {
log.Debugf("update %s exists, aborting", hash)
return nil
}
// pin the dag structure
log.Debugf("pinning %s...", hash)
if err := t.pinPath(hash, api, false); err != nil {
return err
}
// pin the thumbnail
log.Debugf("pinning %s/thumb...", hash)
if err := t.pinPath(fmt.Sprintf("%s/thumb", hash), api, false); err != nil {
return err
}
// pin the meta
log.Debugf("pinning %s/meta...", hash)
if err := t.pinPath(fmt.Sprintf("%s/meta", hash), api, false); err != nil {
return err
}
// pin the last hash
log.Debugf("pinning %s/last...", hash)
if err := t.pinPath(fmt.Sprintf("%s/last", hash), api, false); err != nil {
return err
}
// pin the caption (may not exist, ignore error)
log.Debugf("pinning %s/caption...", hash)
t.pinPath(fmt.Sprintf("%s/caption", hash), api, false)
// unpack data set
log.Debugf("unpacking %s...", hash)
md, err := t.GetMetaData(hash, a)
if err != nil {
return err
}
last, err := t.GetLastHash(hash, a)
if err != nil {
return err
}
caption, err := t.GetCaption(hash, a)
if err != nil {
caption = ""
}
// index
log.Debugf("indexing %s...", hash)
set = &trepo.PhotoSet{
Cid: hash,
LastCid: last,
AlbumID: aid,
MetaData: *md,
Caption: caption,
IsLocal: false,
}
err = t.Datastore.Photos().Put(set)
if err != nil {
return err
}
// don't block on the send since nobody might be listening
select {
case datac <- ThreadUpdate{Cid: hash, Thread: a.Name, ThreadID: a.Id}:
default:
}
defer func() {
if recover() != nil {
log.Error("update channel already closed")
}
}()
// check last hash
return t.handleHash(last, aid, api, datac)
}
// getDataAtPath cats any data under an ipfs path
func (t *TextileNode) getDataAtPath(path string) ([]byte, error) {
// convert string to an ipfs path
ip, err := coreapi.ParsePath(path)
if err != nil {
return nil, err
}
api := coreapi.NewCoreAPI(t.IpfsNode)
ctx, cancel := context.WithTimeout(t.IpfsNode.Context(), catTimeout)
defer cancel()
r, err := api.Unixfs().Cat(ctx, ip)
if err != nil {
return nil, err
}
defer r.Close()
defer func() {
if recover() != nil {
log.Debug("node stopped")
}
}()
return ioutil.ReadAll(r)
}
// pinPath takes an ipfs path string and pins it
func (t *TextileNode) pinPath(path string, api iface.CoreAPI, recursive bool) error {
ip, err := coreapi.ParsePath(path)
if err != nil {
log.Errorf("error pinning path: %s, recursive: %t: %s", path, recursive, err)
return err
}
ctx, cancel := context.WithTimeout(t.IpfsNode.Context(), pinTimeout)
defer cancel()
if err := api.Pin().Add(ctx, ip, options.Pin.Recursive(recursive)); err != nil {
return err
}
defer func() {
if recover() != nil {
log.Debug("node stopped")
}
}()
return nil
}
// touchDB ensures that we have a good db connection
func (t *TextileNode) touchDB() error {
if err := t.Datastore.Ping(); err != nil {
log.Debug("re-opening datastore...")
sqliteDB, err := db.Create(t.RepoPath, "")
if err != nil {
log.Errorf("error re-opening datastore: %s", err)
return err
}
t.Datastore = sqliteDB
}
return nil
}
<file_sep>package main
import (
"fmt"
"github.com/asticode/go-astilectron"
"github.com/asticode/go-astilog"
"github.com/textileio/textile-go/core"
)
var albumID string
func start(_ *astilectron.Astilectron, iw *astilectron.Window, _ *astilectron.Menu, _ *astilectron.Tray, _ *astilectron.Menu) error {
astilog.Info("TEXTILE STARTED")
astilog.Info("SENDING COOKIE INFO")
sendData(iw, "login.cookie", map[string]interface{}{
"name": "SessionId",
"value": textile.GatewayPassword,
"gateway": gateway,
})
// check if we're configured yet
a := textile.Datastore.Albums().GetAlbumByName("mobile")
if a != nil {
albumID = a.Id
// can join room
astilog.Info("FOUND MOBILE ALBUM")
// tell app we're ready and send initial html
sendData(iw, "sync.ready", map[string]interface{}{
"html": getPhotosHTML(),
})
} else {
// otherwise, start onboaring
astilog.Info("COULD NOT FIND MOBILE ALBUM")
astilog.Info("STARTING PAIRING")
go func() {
// sub to own peer id for pairing setup and wait
textile.WaitForRoom()
a := textile.Datastore.Albums().GetAlbumByName("mobile")
if a == nil {
astilog.Error("failed to create mobile album")
return
}
albumID = a.Id
// let the app know we're done pairing
sendMessage(iw, "onboard.complete")
// and that we're ready to go
sendData(iw, "sync.ready", map[string]interface{}{
"html": getPhotosHTML(),
})
}()
sendMessage(iw, "onboard.start")
}
return nil
}
func joinRoom(iw *astilectron.Window) error {
astilog.Info("STARTING SYNC")
datac := make(chan core.ThreadUpdate)
go textile.JoinRoom(albumID, datac)
for {
select {
case update, ok := <-datac:
if !ok {
return nil
}
sendData(iw, "sync.data", map[string]interface{}{
"update": update,
"gateway": gateway,
})
}
}
}
func getPhotosHTML() string {
var html string
for _, photo := range textile.Datastore.Photos().GetPhotos("", -1, "") {
ph := fmt.Sprintf("%s/ipfs/%s/photo", gateway, photo.Cid)
th := fmt.Sprintf("%s/ipfs/%s/thumb", gateway, photo.Cid)
md := fmt.Sprintf("%s/ipfs/%s/meta", gateway, photo.Cid)
img := fmt.Sprintf("<img src=\"%s\" />", th)
html += fmt.Sprintf("<div id=\"%s\" class=\"grid-item\" ondragstart=\"imageDragStart(event);\" draggable=\"true\" data-url=\"%s\" data-meta=\"%s\">%s</div>", photo.Cid, ph, md, img)
}
return html
}
func sendMessage(iw *astilectron.Window, name string) {
iw.SendMessage(map[string]string{"name": name})
}
func sendData(iw *astilectron.Window, name string, data map[string]interface{}) {
data["name"] = name
iw.SendMessage(data)
}
<file_sep>package db
import (
"database/sql"
"strconv"
"sync"
"time"
"github.com/textileio/textile-go/repo"
"github.com/textileio/textile-go/repo/photos"
)
type PhotoDB struct {
modelStore
}
func NewPhotoStore(db *sql.DB, lock *sync.Mutex) repo.PhotoStore {
return &PhotoDB{modelStore{db, lock}}
}
func (c *PhotoDB) Put(set *repo.PhotoSet) error {
c.lock.Lock()
defer c.lock.Unlock()
tx, err := c.db.Begin()
if err != nil {
return err
}
stm := `insert into photos(cid, lastCid, album, name, ext, username, peerId, created, added, latitude, longitude, local, caption) values(?,?,?,?,?,?,?,?,?,?,?,?,?)`
stmt, err := tx.Prepare(stm)
if err != nil {
log.Errorf("error in tx prepare: %s", err)
return err
}
localInt := 0
if set.IsLocal {
localInt = 1
}
defer stmt.Close()
_, err = stmt.Exec(
set.Cid,
set.LastCid,
set.AlbumID,
set.MetaData.Name,
set.MetaData.Ext,
set.MetaData.Username,
set.MetaData.PeerID,
int(set.MetaData.Created.Unix()),
int(set.MetaData.Added.Unix()),
set.MetaData.Latitude,
set.MetaData.Longitude,
localInt,
set.Caption,
)
if err != nil {
tx.Rollback()
log.Errorf("error in db exec: %s", err)
return err
}
tx.Commit()
return nil
}
func (c *PhotoDB) GetPhotos(offsetId string, limit int, query string) []repo.PhotoSet {
c.lock.Lock()
defer c.lock.Unlock()
var stm string
if offsetId != "" {
q := ""
if query != "" {
q = query + " and "
}
stm = "select * from photos where " + q + "added<(select added from photos where cid='" + offsetId + "') order by added desc limit " + strconv.Itoa(limit) + " ;"
} else {
q := ""
if query != "" {
q = "where " + query + " "
}
stm = "select * from photos " + q + "order by added desc limit " + strconv.Itoa(limit) + ";"
}
return c.handleQuery(stm)
}
func (c *PhotoDB) GetPhoto(cid string) *repo.PhotoSet {
c.lock.Lock()
defer c.lock.Unlock()
ret := c.handleQuery("select * from photos where cid='" + cid + "';")
if len(ret) == 0 {
return nil
}
return &ret[0]
}
func (c *PhotoDB) DeletePhoto(cid string) error {
c.lock.Lock()
defer c.lock.Unlock()
_, err := c.db.Exec("delete from photos where cid=?", cid)
return err
}
func (c *PhotoDB) handleQuery(stm string) []repo.PhotoSet {
var ret []repo.PhotoSet
rows, err := c.db.Query(stm)
if err != nil {
log.Errorf("error in db query: %s", err)
return nil
}
for rows.Next() {
var cid, lastCid, album, name, ext, username, peerId string
var createdInt, addedInt int
var latitude, longitude float64
var localInt int
var caption string
if err := rows.Scan(&cid, &lastCid, &album, &name, &ext, &username, &peerId, &createdInt, &addedInt, &latitude, &longitude, &localInt, &caption); err != nil {
log.Errorf("error in db scan: %s", err)
continue
}
created := time.Unix(int64(createdInt), 0)
added := time.Unix(int64(addedInt), 0)
local := false
if localInt == 1 {
local = true
}
photo := repo.PhotoSet{
Cid: cid,
LastCid: lastCid,
AlbumID: album,
MetaData: photos.Metadata{
Name: name,
Ext: ext,
Username: username,
PeerID: peerId,
Created: created,
Added: added,
Latitude: latitude,
Longitude: longitude,
},
Caption: caption,
IsLocal: local,
}
ret = append(ret, photo)
}
return ret
}
| aaef28f5be896e37813652f0e8c530f0880afc15 | [
"Go"
] | 22 | Go | lexrus/textile-go | b8b93fe1eacb85d22fc6b405711928bb33b2b285 | 950313e1e3052959cb34abd8d46e5025fc7abb0e | |
refs/heads/master | <repo_name>kdj1219/ess<file_sep>/app/controllers/UserController.php
<?php
class UserController extends BaseController {
/**
* 用户首页
*/
public function index()
{
$users = UserProcessor::getUserList();
return View::make('user.index', compact('users'));
}
/**
* 登入
*/
public function login()
{
if (Request::isMethod('get'))
{
return View::make('user.login');
}
elseif (Request::isMethod('post'))
{
$uname = Input::get('uname');
$pword = Input::get('pword');
$remember = intval(Input::get('remember'));
try
{
// Login credentials
$credentials = array(
'email' => $uname,
'password' => <PASSWORD>,
);
// Authenticate the user
$user = Sentry::authenticate($credentials, false);
if($user){
if($remember) Sentry::loginAndRemember($user);
return Redirect::to('/');
}
}
catch (Cartalyst\Sentry\Users\LoginRequiredException $e)
{
return Redirect::to('/login')
->with('message', 'Login field is required.');
}
catch (Cartalyst\Sentry\Users\PasswordRequiredException $e)
{
return Redirect::to('/login')
->with('message', 'Password field is required.');
}
catch (Cartalyst\Sentry\Users\WrongPasswordException $e)
{
return Redirect::to('/login')
->with('message', 'Wrong password, try again.');
}
catch (Cartalyst\Sentry\Users\UserNotFoundException $e)
{
return Redirect::to('/login')
->with('message', 'User was not found.');
}
catch (Cartalyst\Sentry\Users\UserNotActivatedException $e)
{
return Redirect::to('/login')
->with('message', 'User is not activated.');
}
// The following is only required if the throttling is enabled
catch (Cartalyst\Sentry\Throttling\UserSuspendedException $e)
{
return Redirect::to('/login')
->with('message', 'User is suspended.');
}
catch (Cartalyst\Sentry\Throttling\UserBannedException $e)
{
return Redirect::to('/login')
->with('message', 'User is banned.');
}
}
}
/**
* 登出
*/
public function logout()
{
Sentry::logout();
return Redirect::to('/login');
}
}
<file_sep>/app/models/SentenceProcessor.php
<?php
class SentenceProcessor {
/**
* 获取例句列表
*/
public static function getSentenceList($keywords='')
{
$user = Sentry::getUser();
if(empty($keywords)){
$sentences = Sentence::where('uid','=',$user->id)->orderBy('created_at','desc')->paginate(1);
}else{
$sentences = Sentence::where('uid','=',$user->id)->where('description', 'like', '%'.urldecode($keywords).'%')->orderBy('created_at','desc')->paginate(1);
}
return $sentences;
}
/**
* 通过例句id获取例句详细信息
*
* @param string $sentenceId 例句的id
* @return false
*/
public static function getDetailById($sentenceId=''){
$user = Sentry::getUser();
if(empty($user) || empty($sentenceId) || !is_numeric($sentenceId)){
return false;
}
$sentenceInfo = Sentence::where('uid','=',$user->id)->where('id','=',$sentenceId)->first();
if( empty($sentenceInfo) ) return false;
return $sentenceInfo;
}
/**
* 获取随机例句
*/
public static function getRandomSentence()
{
$user = Sentry::getUser();
$sentences = Sentence::where('uid','=',$user->id)->orderByRaw("RAND()")->first();
return $sentences;
}
/**
* 添加例句
*
* @param $newSentence 新的例句
* @param $description 新的例句的描述说明
*/
public static function addSentence($newSentence, $description){
$sentence = new Sentence;
$user = Sentry::getUser();
$sentence->sentence = $newSentence;
$sentence->description = $description;
$sentence->uid = $user->id;
$res = $sentence->save();
return $res;
}
/**
* 更新例句
*
* @param $sentenceId 例句的id
* @param $sentence 例句
* @param $description 例句的描述说明
*/
public static function updateSentence($sentenceId, $sentence, $description){
$user = Sentry::getUser();
$sentenceObj = Sentence::where('uid','=',$user->id)->find($sentenceId);
$sentenceObj->sentence = $sentence;
$sentenceObj->description = $description;
$res = $sentenceObj->save();
return $res;
}
/**
* 删除例句
*
* @param $sentenceId 例句ID
*
*/
public static function delSentence($sentenceId){
$res = Sentence::destroy($sentenceId);
return $res;
}
}
<file_sep>/app/models/User.php
<?php
class User extends Eloquent {
/**
* The database table used by the model.
*
* @var string
*/
protected $table = 'users';
protected $primaryKey = 'id';
/**
* The attributes excluded from the model's JSON form.
*
* @var array
*/
// protected $hidden = array('password', 'remember_token');
public function roles()
{
return $this->belongsToMany('Role','users_groups','user_id','group_id');
}
}
<file_sep>/app/controllers/WordController.php
<?php
class WordController extends BaseController {
/**
* 首页
*/
public function index()
{
$keywords = Input::get('key');
$words = WordProcessor::getWordList($keywords);
return View::make('word.index', compact('words'));
}
/**
* 添加词汇页面
*/
public function add(){
return View::make('word.add');
}
/**
* Ajax执行添加词汇
*/
public function doAdd(){
$word = Input::get('word');
$description = Input::get('description');
$res = array('stat'=>0,'msg'=>'');
if(''==$word || ''==$description){
$res['msg'] = 'Can not be empty.';
echo json_encode($res);
die;
}
$succ = WordProcessor::addWord($word, $description);
if($succ){
$res['stat'] = 1;
$res['msg'] = 'Add Successfully.';
}else{
$res['msg'] = 'Add Unsuccessfully.';
}
echo json_encode($res);
die;
}
/**
* Ajax执行删除词汇
*/
public function doDelete(){
$wordId = Input::get('word_id');
$res = array('stat'=>0,'msg'=>'');
if(''==$wordId || !is_numeric($wordId)){
$res['msg'] = 'Word Id is wrong.';
echo json_encode($res);
die;
}
$succ = WordProcessor::delWord($wordId);
if($succ){
$res['stat'] = 1;
$res['msg'] = 'Delete Successfully.';
}else{
$res['msg'] = 'Delete Unsuccessfully.';
}
echo json_encode($res);
die;
}
}
<file_sep>/app/libraries/paypal/lib/Api/RelatedResources.php
<?php
namespace PayPal\Api;
use PayPal\Common\PPModel;
use PayPal\Rest\ApiContext;
/**
* Class RelatedResources
*
* @property \PayPal\Api\Sale sale
* @property \PayPal\Api\Authorization authorization
* @property \PayPal\Api\Capture capture
* @property \PayPal\Api\Refund refund
*/
class RelatedResources extends PPModel
{
/**
* Set Sale
* A sale transaction
*
* @param \PayPal\Api\Sale $sale
*
* @return $this
*/
public function setSale($sale)
{
$this->sale = $sale;
return $this;
}
/**
* Get Sale
* A sale transaction
*
* @return \PayPal\Api\Sale
*/
public function getSale()
{
return $this->sale;
}
/**
* Set Authorization
* An authorization transaction
*
* @param \PayPal\Api\Authorization $authorization
*
* @return $this
*/
public function setAuthorization($authorization)
{
$this->authorization = $authorization;
return $this;
}
/**
* Get Authorization
* An authorization transaction
*
* @return \PayPal\Api\Authorization
*/
public function getAuthorization()
{
return $this->authorization;
}
/**
* Set Capture
* A capture transaction
*
* @param \PayPal\Api\Capture $capture
*
* @return $this
*/
public function setCapture($capture)
{
$this->capture = $capture;
return $this;
}
/**
* Get Capture
* A capture transaction
*
* @return \PayPal\Api\Capture
*/
public function getCapture()
{
return $this->capture;
}
/**
* Set Refund
* A refund transaction
*
* @param \PayPal\Api\Refund $refund
*
* @return $this
*/
public function setRefund($refund)
{
$this->refund = $refund;
return $this;
}
/**
* Get Refund
* A refund transaction
*
* @return \PayPal\Api\Refund
*/
public function getRefund()
{
return $this->refund;
}
/**
* Set Order
*
* @param \PayPal\Api\Order $order
*
* @return $this
*/
public function setOrder($order)
{
$this->order = $order;
return $this;
}
/**
* Get Order
*
* @return \PayPal\Api\Order
*/
public function getOrder()
{
return $this->order;
}
}
<file_sep>/app/database/seeds/WordTableSeeder.php
<?php
/**
* Created by PhpStorm.
* User: <NAME>
* Date: 2014/10/14
* Time: 14:47
*/
class WordTableSeeder extends Seeder {
public function run()
{
DB::table('words')->insert(
array(
'word' => 'cat',
'description' => '猫',
'created_at'=>date('Y-m-d H:i:s',time()),
'updated_at'=>date('Y-m-d H:i:s',time()),
)
);
}
} <file_sep>/config/mgt/config.php
<?php
/**
* config.php
*
* Descript Here
*
* @filename config.php
* @version v1.0
* @update 2013-3-28
* @author randy.hong
* @contact <EMAIL>
* @package chinascope
*/
//DB配置
define('OMS_DB_DRIVER', 'mysql');
define('OMS_DB_HOST', '192.168.250.200');
define('OMS_DB_PORT', '3306');
define('OMS_DB_NAME', 'ada_cam');
define('OMS_DB_USER', 'ada_user');
define('OMS_DB_PASS', '<PASSWORD>');
define('OMS_DB_CHARSET', 'utf8');
define('OMS_DB_DEBUG', TRUE);
define('BASE_DOMAIN', 'chinascope.net');
//redis缓存配置
define('REDIS_HOST', '192.168.250.200'); //redis host
define('REDIS_PORT', 6379);
//redis缓存配置
define('REDIS_HOST_SH', '192.168.250.200'); //redis host
define('REDIS_PORT_SH', 6379); //redis port
//MONGO ADA服务器配置
define('MONGO_ADA_HOST', '192.168.250.200');
define('MONGO_ADA_PORT', '27017');
define('MONGO_ADA_DB', 'ada');
//MONGO ADMIN服务器配置
define('MONGO_ADMIN_HOST', '192.168.250.200');
define('MONGO_ADMIN_PORT', '27017');
define('MONGO_ADMIN_DB', 'csf_admin');
//REST接口和JAVA借口地址配置
define('REST_SERVER', 'http://1172.16.31.10:18080'); //rest search service
//SMTP服务器配置
define('SMTP_HOST', 'mail.chinascopefinancial.com'); // SMTP URL
define('SMTP_PORT', 25); // SMTP PORT
define('SMTP_USER', '<EMAIL>'); // SMTP USER
define('SMTP_PASS', '<PASSWORD>'); // SMTP PASSWORD
define('MAIL_CHARSET', 'utf-8'); // CHARSET CONFIG
define('MAIL_SUPPORT', "<EMAIL>"); // support team email address
define('MAIL_SALES', "<EMAIL>"); // sales team email address
define('MAIL_TRIAL', "<EMAIL>"); // sales team email address
//程序环境变量
!defined('ENVIRONMENT') && define('ENVIRONMENT', 'production');<file_sep>/app/database/seeds/UserTableSeeder.php
<?php
/**
* Created by PhpStorm.
* User: <NAME>
* Date: 2014/10/14
* Time: 14:47
*/
class UserTableSeeder extends Seeder {
public function run()
{
try
{
// Create the group
$group = Sentry::createGroup(array(
'name' => 'Administrator',
'permissions' => array(
'admin' => 1,
'users' => 1,
),
));
}
catch (Cartalyst\Sentry\Groups\NameRequiredException $e)
{
echo 'Name field is required';
}
catch (Cartalyst\Sentry\Groups\GroupExistsException $e)
{
echo 'Group already exists';
}
try
{
// Create the user
$user = Sentry::createUser(array(
'email' => '<EMAIL>',
'password' => '<PASSWORD>',
'activated' => true,
'first_name' => 'Daniel',
'last_name' => 'Kong',
));
// Find the group using the group id
$adminGroup = Sentry::findGroupById(1);
// Assign the group to the user
$user->addGroup($adminGroup);
}
catch (Cartalyst\Sentry\Users\LoginRequiredException $e)
{
echo 'Login field is required.';
}
catch (Cartalyst\Sentry\Users\PasswordRequiredException $e)
{
echo 'Password field is required.';
}
catch (Cartalyst\Sentry\Users\UserExistsException $e)
{
echo 'User with this login already exists.';
}
catch (Cartalyst\Sentry\Groups\GroupNotFoundException $e)
{
echo 'Group was not found.';
}
}
} <file_sep>/config/www/config.php
<?php
/**
* config.php
*
* Descript Here
*
* @filename config.php
* @version v1.0
* @update 2013-3-28
* @author randy.hong
* @contact <EMAIL>
* @package chinascope
*/
//DB配置
define('OMS_DB_DRIVER', 'mysql');
define('OMS_DB_HOST', 'sg-com-csf-web-db.cwiif0vzcyt6.ap-southeast-1.rds.amazonaws.com');
define('OMS_DB_PORT', '3306');
define('OMS_DB_NAME', 'ada_cam');
define('OMS_DB_USER', 'website');
define('OMS_DB_PASS', '<PASSWORD>');
define('OMS_DB_CHARSET', 'utf8');
define('OMS_DB_DEBUG', TRUE);
define('BASE_DOMAIN', 'chinascopefinancial.com');
//redis缓存配置
define('REDIS_HOST', '10.142.123.144'); //redis host
define('REDIS_PORT', 6379);
//redis缓存配置
define('REDIS_HOST_SH', '172.16.17.32'); //redis host
define('REDIS_PORT_SH', 6379); //redis port
//MONGO ADA服务器配置
define('MONGO_ADA_HOST', '10.142.123.144');
define('MONGO_ADA_PORT', '27017');
define('MONGO_ADA_DB', 'ada');
//MONGO ADMIN服务器配置
define('MONGO_ADMIN_HOST', '10.134.105.228');
define('MONGO_ADMIN_PORT', '27017');
define('MONGO_ADMIN_DB', 'ada');
//REST接口和JAVA借口地址配置
define('REST_SERVER', 'http://10.134.213.90:18080');
//SMTP服务器配置
define('SMTP_HOST', 'mail.chinascopefinancial.com'); // SMTP URL
define('SMTP_PORT', 25); // SMTP PORT
define('SMTP_USER', '<EMAIL>'); // SMTP USER
define('SMTP_PASS', '<PASSWORD>'); // SMTP PASSWORD
define('MAIL_CHARSET', 'utf-8'); // CHARSET CONFIG
define('MAIL_SUPPORT', "<EMAIL>"); // support team email address
define('MAIL_SALES', "<EMAIL>"); // sales team email address
define('MAIL_TRIAL', "<EMAIL>"); // trial team email address
//程序环境变量
!defined('ENVIRONMENT') && define('ENVIRONMENT', 'production');<file_sep>/app/routes.php
<?php
Route::model('user', 'User');
Route::model('order', 'Order');
/*
|--------------------------------------------------------------------------
| Application Routes
|--------------------------------------------------------------------------
|
| Here is where you can register all of the routes for an application.
| It's a breeze. Simply tell Laravel the URIs it should respond to
| and give it the Closure to execute when that URI is requested.
|
*/
Route::match(array('get', 'post'), '/login', 'UserController@login');
Route::match(array('get', 'post'), 'refund/alipay/notify', 'RefundController@alipayNotify');
/**
* Sentry filter
*
* Checks if the user is logged in
*/
Route::filter('sentry', function()
{
if ( ! Sentry::check()) {
return Redirect::to('/login');
}
});
/**
* InGroup filter
*
* Check if the user belongs to a group
*/
Route::filter('inGroup', function($route, $request, $value)
{
try
{
$user = Sentry::getUser();
$group = Sentry::findGroupByName($value);
if( ! $user->inGroup($group))
{
throw new NotFoundException;
// return Response::make('Not Found', 404);
}
}
catch (Cartalyst\Sentry\Users\UserNotFoundException $e)
{
throw new NotFoundException;
// return Response::make('Not Found', 404);
}
catch (Cartalyst\Sentry\Groups\GroupNotFoundException $e)
{
throw new NotFoundException;
// return Response::make('Not Found', 404);
}
});
//against csrf attack
Route::when('*', 'csrf', array('post', 'put', 'delete'));
Route::group(array('before' => 'sentry|inGroup:Administrator'), function()
{
Route::get('/', function(){ return View::make('index'); });
Route::get('/logout', 'UserController@logout');
Route::get('user', 'UserController@index');
Route::get('word', 'WordController@index');
Route::get('word/add', 'WordController@add');
Route::post('word/add', 'WordController@doAdd');
Route::post('word/delete', 'WordController@doDelete');
Route::get('sentence', 'SentenceController@index');
Route::get('sentence/daily', 'SentenceController@daily');
Route::get('sentence/add', 'SentenceController@add');
Route::post('sentence/add', 'SentenceController@doAdd');
Route::get('sentence/edit/{sentence_id}', 'SentenceController@edit');
Route::post('sentence/edit', 'SentenceController@doEdit');
Route::post('sentence/delete', 'SentenceController@doDelete');
});
<file_sep>/gulpfile.js
//Load gulp and plug-ins
var gulp = require('gulp');
var del = require('del');
var path = require('path');
var runSequence = require('run-sequence');
var merge = require('merge-stream');
var $ = require('gulp-load-plugins')();
//Common tasks for sources
//step 3 Pre-compile Sass files
// sources/styles/*.scss -> public/styles/*.css
// echo "/.sass-cache" >> .gitignore
gulp.task('styles', function () {
var vendDeps = gulp.src('./sources/styles/vondors/**/*.css')
.pipe($.concat('vendor.css'))
.pipe($.minifyCss())
.pipe($.rename('vendor.min.css'))
.pipe(gulp.dest('public/assets/css'))
.pipe($.size({title: 'styles'}));
var mainDeps = gulp.src('./sources/styles/css/screen.css')
.pipe($.minifyCss())
.pipe($.rename('screen.min.css'))
.pipe(gulp.dest('public/assets/css'))
.pipe($.size({title: 'styles'}))
return merge(vendDeps, mainDeps);
});
//step 4 Check syntax and copy files
// sources/scripts/*.js -> public/scripts/*.js
gulp.task('scripts', function () {
var vendDeps = gulp.src(['./sources/scripts/vondors/**/*.js'])
.pipe($.jshint('.jshintrc'))
.pipe($.concat('vendor.js'))
.pipe($.uglify())
.pipe($.rename('vendor.min.js'))
.pipe(gulp.dest('public/assets/js'))
.pipe($.size({title: 'vendor-scripts'}));
var appDeps = gulp.src('./sources/scripts/app.js')
.pipe($.jshint('.jshintrc'))
.pipe($.uglify())
.pipe($.rename('app.min.js'))
.pipe(gulp.dest('public/assets/js'))
.pipe($.size({title: 'app-scripts'}));
return merge(vendDeps, appDeps);
});
//step 6 Copy fonts
//sources/fonts/**/*.* -> public/assets/fonts/
gulp.task('fonts', function () {
return gulp.src([
'sources/fonts/*.{otf,eot,svg,ttf,woff}',
'public/bower_components/**/fonts/**/*.{otf,eot,svg,ttf,woff}'
])
.pipe($.flatten())
.pipe(gulp.dest('public/assets/fonts'))
.pipe($.size({title: 'fonts'}));
});
/////// DEVELOPMENT ///////
// Clean
gulp.task('clean:develop', function (cb) {
del(['public/**/*.html', '!public/bower_components/**/*.html', '.sass-cache'], cb);
});
// Prepare for development
gulp.task('prepare', function (cb) {
runSequence(
'clean:develop',
['styles', 'scripts', 'fonts'],
cb);
});
// Watch
gulp.task('watch', ['prepare'], function () {
gulp.watch('sources/styles/vondors/**/*.css', ['styles']);
gulp.watch('sources/scripts/**/*.js', ['scripts']);
});
/////// BUILD ///////
// HTML
gulp.task('build', ['styles', 'scripts', 'fonts'], function () {
});
// Clean Cache
gulp.task('clean:cache', function (cb) {
return $.cache.clearAll(cb);
});
// Clean
gulp.task('clean', ['clean:develop', 'clean:cache'], function (cb) {
del([
'public/assets/css', 'public/assets/js',
'public/assets/fonts',
], cb);
});
// Clean temporary sources
gulp.task('clean:temporary', function (cb) {
del([
'.sass-cache'
], cb);
});
// Build
gulp.task('default', function (cb) {
runSequence('clean', 'build', 'clean:temporary', cb);
});
<file_sep>/app/controllers/SentenceController.php
<?php
class SentenceController extends BaseController {
/**
* 首页
*/
public function index()
{
$keywords = Input::get('key');
$sentences = SentenceProcessor::getSentenceList($keywords);
return View::make('sentence.index', compact('sentences','keywords'));
}
/**
* 每日一句
*/
public function daily()
{
$sentences = SentenceProcessor::getRandomSentence();
return View::make('sentence.daily', compact('sentences'));
}
/**
* 添加例句页面
*/
public function add(){
return View::make('sentence.add');
}
/**
* Ajax执行添加例句
*/
public function doAdd(){
$sentence = Input::get('sentence');
$description = Input::get('description');
$res = array('stat'=>0,'msg'=>'');
if(''==$sentence || ''==$description){
$res['msg'] = 'Can not be empty.';
echo json_encode($res);
die;
}
$succ = SentenceProcessor::addSentence($sentence, $description);
if($succ){
$res['stat'] = 1;
$res['msg'] = 'Add Successfully.';
}else{
$res['msg'] = 'Add Unsuccessfully.';
}
echo json_encode($res);
die;
}
/**
* Ajax执行删除例句
*/
public function doDelete(){
$sentenceId = Input::get('sentence_id');
$res = array('stat'=>0,'msg'=>'');
if(''==$sentenceId || !is_numeric($sentenceId)){
$res['msg'] = 'Word Id is wrong.';
echo json_encode($res);
die;
}
$succ = SentenceProcessor::delSentence($sentenceId);
if($succ){
$res['stat'] = 1;
$res['msg'] = 'Delete Successfully.';
}else{
$res['msg'] = 'Delete Unsuccessfully.';
}
echo json_encode($res);
die;
}
/**
* 编辑例句页面
*/
public function edit(){
$sentenceId = Route::input('sentence_id');
if(''==$sentenceId || !is_numeric($sentenceId)){
return Redirect::to('/sentence');
}
$sentenceDetail = SentenceProcessor::getDetailById($sentenceId);
return View::make('sentence.edit', compact('sentenceDetail'));
}
/**
* Ajax执行更新例句
*/
public function doEdit(){
$sentenceId = Input::get('sentence_id');
$sentence = Input::get('sentence');
$description = Input::get('description');
$res = array('stat'=>0,'msg'=>'');
if(''==$sentenceId || ''==$sentence || ''==$description){
$res['msg'] = 'Can not be empty.';
echo json_encode($res);
die;
}
$succ = SentenceProcessor::updateSentence($sentenceId, $sentence, $description);
if($succ){
$res['stat'] = 1;
$res['msg'] = 'Update Successfully.';
}else{
$res['msg'] = 'Update Unsuccessfully.';
}
echo json_encode($res);
die;
}
}
<file_sep>/app/models/UserProcessor.php
<?php
class UserProcessor {
/**
* 获取用户列表
*/
public static function getUserList()
{
$users = User::where('activated','1')->get();
foreach($users as $key=>$user){
$user->roles = implode(',', array_column($user->roles->toArray(),'name'));
}
return $users;
}
}
<file_sep>/sources/scripts/app.js
/**
* Created by <NAME> on 2014/10/19.
*/
$(document).ready(function(){
/**
* 点击单词添加按钮
*/
$('#add_btn').click(function(){
var word = $('#inputWord').val();
var description = $('#inputDescription').val();
if(word=='' || description==''){
new $.flavr('Can not be empty.');
return false;
}
//防止csrf攻击
ajaxCsrfSetup();
$.ajax({
type:'post',
url:app_url + '/word/add',
data:{word:word,description:description},
beforeSend: function(){
// Handle the beforeSend event
$("#main-container").mask("Loading...");
},
complete: function(){
// Handle the complete event
$("#main-container").unmask();
},
success:function(data){
if(typeof(JSON) !== 'object' || typeof(JSON.parse) !== 'function'){
new $.flavr('Something Wrong!');
return false;
}
var res = JSON.parse(data);
new $.flavr(res.msg);
$('#inputWord').val('');
$('#inputDescription').val('');
}
});
});
/**
* 点击单词删除按钮
*/
$('.word_del_btn').click(function(){
var word_id = $(this).attr('item_id');
var item = $(this).parents('tr');
if(word_id=='' || isNaN(word_id)){
new $.flavr('Word Id is wrong.');
return false;
}
new $.flavr({ content : 'Are you sure to delete it?',
dialog : 'confirm',
onConfirm : function(){
//防止csrf攻击
ajaxCsrfSetup();
$.ajax({
type:'post',
url:app_url + '/word/delete',
data:{word_id:word_id},
beforeSend: function(){
// Handle the beforeSend event
$("#main-container").mask("Loading...");
},
complete: function(){
// Handle the complete event
$("#main-container").unmask();
},
success:function(data){
if(typeof(JSON) !== 'object' || typeof(JSON.parse) !== 'function'){
new $.flavr('Something Wrong!');
return false;
}
var res = JSON.parse(data);
new $.flavr(res.msg);
item.remove();
}
});
},
onCancel : function(){}
});
});
/**
* 点击例句添加按钮
*/
$('#sentence_add_btn').click(function(){
var sentence = $('#sentenceWord').val();
var description = $('#sentenceDescription').val();
if(sentence=='' || description==''){
new $.flavr('Can not be empty.');
return false;
}
//防止csrf攻击
ajaxCsrfSetup();
$.ajax({
type:'post',
url:app_url + '/sentence/add',
data:{sentence:sentence,description:description},
beforeSend: function(){
// Handle the beforeSend event
$("#main-container").mask("Loading...");
},
complete: function(){
// Handle the complete event
$("#main-container").unmask();
},
success:function(data){
if(typeof(JSON) !== 'object' || typeof(JSON.parse) !== 'function'){
new $.flavr('Something Wrong!');
return false;
}
var res = JSON.parse(data);
new $.flavr(res.msg);
$('#sentenceWord').val('');
$('#sentenceDescription').val('');
}
});
});
/**
* 点击例句编辑按钮
*/
$('.sentence_edit_btn').click(function(){
var sentence_id = $(this).attr('item_id');
if(sentence_id=='' || isNaN(sentence_id)){
new $.flavr('Sentence Id is wrong.');
return false;
}
window.location.href = app_url + '/sentence/edit/' + sentence_id;
});
/**
* 点击例句更新按钮
*/
$('#sentence_update_btn').click(function(){
var sentence_id = $('#sentenceId').val();
var sentence = $('#sentenceWord').val();
var description = $('#sentenceDescription').val();
if(sentence_id=='' || sentence=='' || description==''){
new $.flavr('Can not be empty.');
return false;
}
//防止csrf攻击
ajaxCsrfSetup();
$.ajax({
type:'post',
url:app_url + '/sentence/edit',
data:{sentence_id:sentence_id,sentence:sentence,description:description},
beforeSend: function(){
// Handle the beforeSend event
$("#main-container").mask("Loading...");
},
complete: function(){
// Handle the complete event
$("#main-container").unmask();
},
success:function(data){
if(typeof(JSON) !== 'object' || typeof(JSON.parse) !== 'function'){
new $.flavr('Something Wrong!');
return false;
}
var res = JSON.parse(data);
new $.flavr(res.msg);
window.location.href = app_url + '/sentence/edit/' + sentence_id;
}
});
});
/**
* 点击例句删除按钮
*/
$('.sentence_del_btn').click(function(){
var sentence_id = $(this).attr('item_id');
var item = $(this).parents('tr');
if(sentence_id=='' || isNaN(sentence_id)){
new $.flavr('Sentence Id is wrong.');
return false;
}
new $.flavr({ content : 'Are you sure to delete it?',
dialog : 'confirm',
onConfirm : function(){
//防止csrf攻击
ajaxCsrfSetup();
$.ajax({
type:'post',
url:app_url + '/sentence/delete',
data:{sentence_id:sentence_id},
beforeSend: function(){
// Handle the beforeSend event
$("#main-container").mask("Loading...");
},
complete: function(){
// Handle the complete event
$("#main-container").unmask();
},
success:function(data){
if(typeof(JSON) !== 'object' || typeof(JSON.parse) !== 'function'){
new $.flavr('Something Wrong!');
return false;
}
var res = JSON.parse(data);
new $.flavr(res.msg);
window.location.reload();
}
});
},
onCancel : function(){}
});
});
/**
* 隐藏显示例句按钮
*/
$("#sentence_show_btn").click(function(){
var sentence_holder = $("#sentence_holder");
if(sentence_holder.hasClass('hidden')){
sentence_holder.removeClass('hidden');
$(this).html('点击我隐藏');
}else{
sentence_holder.addClass('hidden');
$(this).html('点击我显示');
}
});
/**
* 点击换一句按钮
*/
$("#sentence_random_btn").click(function(){
location.reload();
});
/**
* 点击搜索按钮
*/
$("#sentence_search_btn").click(function(){
var key = $.trim( $('#sentence_key').val() );
if(''!=key){
window.location.href = app_url + '/sentence?key='+encodeURIComponent(key);
}
return false;
});
$("#word_search_btn").click(function(){
var key = $.trim( $('#word_key').val() );
if(''!=key){
window.location.href = app_url + '/word?key='+encodeURIComponent(key);
}
return false;
});
//这个就是键盘触发的函数
var SubmitOrHidden = function(evt){
var btn;
if(1==$("#sentence_search_btn").length){
btn = $("#sentence_search_btn");
}else if(1==$("#word_search_btn").length){
btn = $("#word_search_btn");
}
evt = window.event || evt;
if(evt.keyCode==13){//如果取到的键值是回车
btn.click();
}
}
window.document.onkeydown=SubmitOrHidden;//当有键按下时执行函数
/**
* ajax against csrf setup
*/
var ajaxCsrfSetup = function() {
$.ajaxSetup({
headers: {
'X-CSRF-Token': $('meta[name="_token"]').attr('content')
}
});
}
});
<file_sep>/app/libraries/paypal/lib/Api/Order.php
<?php
namespace PayPal\Api;
use PayPal\Common\PPModel;
use PayPal\Rest\ApiContext;
/**
* Class Order
*
* @property string id
* @property string createTime
* @property string updateTime
* @property string state
* @property \PayPal\Api\Amount amount
* @property string parentPayment
* @property \PayPal\Api\Links links
* @property string reasonCode
*/
class Order extends PPModel
{
/**
* Set the identifier of the order transaction.
*
* @param string $id
*
* @return $this
*/
public function setId($id)
{
$this->id = $id;
return $this;
}
/**
* Get the identifier of the order transaction.
*
* @return string
*/
public function getId()
{
return $this->id;
}
/**
* Set the time the resource was created.
*
* @param string $create_time
*
* @return $this
*/
public function setCreateTime($create_time)
{
$this->create_time = $create_time;
return $this;
}
/**
* Get the time the resource was created.
*
* @return string
*/
public function getCreateTime()
{
return $this->create_time;
}
/**
* Set the time the resource was last updated.
*
* @param string $update_time
*
* @return $this
*/
public function setUpdateTime($update_time)
{
$this->update_time = $update_time;
return $this;
}
/**
* Get the time the resource was last updated.
*
* @return string
*/
public function getUpdateTime()
{
return $this->update_time;
}
/**
* Set the state of the order transaction.
*
* Allowed values are: `PENDING`, `COMPLETED`, `REFUNDED` or `PARTIALLY_REFUNDED`.
*
* @param string $state
*
* @return $this
*/
public function setState($state)
{
$this->state = $state;
return $this;
}
/**
* Get the state of the order transaction.
*
* Allowed values are: `PENDING`, `COMPLETED`, `REFUNDED` or `PARTIALLY_REFUNDED`.
*
* @return string
*/
public function getState()
{
return $this->state;
}
/**
* Set the amount being collected.
*
* @param \PayPal\Api\Amount $amount
*
* @return $this
*/
public function setAmount($amount)
{
$this->amount = $amount;
return $this;
}
/**
* Get the amount being collected.
*
* @return \PayPal\Api\Amount
*/
public function getAmount()
{
return $this->amount;
}
/**
* Set ID of the payment resource on which this transaction is based.
*
* @param string $parent_payment
*
* @return $this
*/
public function setParentPayment($parent_payment)
{
$this->parent_payment = $parent_payment;
return $this;
}
/**
* Get ID of the payment resource on which this transaction is based.
*
* @return string
*/
public function getParentPayment()
{
return $this->parent_payment;
}
/**
* Set Links
*
* @param \PayPal\Api\Links $links
*
* @return $this
*/
public function setLinks($links)
{
$this->links = $links;
return $this;
}
/**
* Get Links
*
* @return \PayPal\Api\Links
*/
public function getLinks()
{
return $this->links;
}
/**
* Reason code for the transaction state being Pending or Reversed. Assigned in response.
*
* Allowed values: `CHARGEBACK`, `GUARANTEE`, `BUYER_COMPLAINT`, `REFUND`,
* `UNCONFIRMED_SHIPPING_ADDRESS`,
* `ECHECK`, `INTERNATIONAL_WITHDRAWAL`,
* `RECEIVING_PREFERENCE_MANDATES_MANUAL_ACTION`, `PAYMENT_REVIEW`,
* `REGULATORY_REVIEW`, `UNILATERAL`, or `VERIFICATION_REQUIRED`
* (`ORDER` can also be set in the response).
*
* @param string $reason_code
*
* @return $this
*/
public function setReasonCode($reason_code)
{
$this->reason_code = $reason_code;
return $this;
}
/**
* Reason code for the transaction state being Pending or Reversed. Assigned in response.
*
* Allowed values: `CHARGEBACK`, `GUARANTEE`, `BUYER_COMPLAINT`, `REFUND`,
* `UNCONFIRMED_SHIPPING_ADDRESS`,
* `ECHECK`, `INTERNATIONAL_WITHDRAWAL`,
* `RECEIVING_PREFERENCE_MANDATES_MANUAL_ACTION`, `PAYMENT_REVIEW`,
* `REGULATORY_REVIEW`, `UNILATERAL`, or `VERIFICATION_REQUIRED`
* (`ORDER` can also be set in the response).
*
* @return string
*/
public function getReasonCode()
{
return $this->reason_code;
}
}
<file_sep>/app/models/WordProcessor.php
<?php
class WordProcessor {
/**
* 获取单词列表
*/
public static function getWordList($keywords='')
{
$user = Sentry::getUser();
if(empty($keywords)){
$words = Word::where('uid','=',$user->id)->orderBy('created_at','desc')->paginate(10);
}else{
$words = Word::where('uid','=',$user->id)->where('description', 'like', '%'.urldecode($keywords).'%')->orderBy('created_at','desc')->paginate(10);
}
foreach($words as $key=>$word){
if(is_mobile()){
$word->url = 'http://m.youdao.com/dict?le=eng&q='.$word->word;
}else{
$word->url = 'http://dict.youdao.com/search?q='.$word->word.'&keyfrom=dict.index';
}
$word->jq_url = 'http://dictionary.cambridge.org/dictionary/american-english/'.$word->word;
}
return $words;
}
/**
* 添加单词
*
* @param $newword 新的单词
* @param $description 添加新的单词
*/
public static function addWord($newword, $description){
$word = new Word;
$user = Sentry::getUser();
$word->word = $newword;
$word->description = $description;
$word->uid = $user->id;
$res = $word->save();
return $res;
}
/**
* 删除单词
*
* @param $wordId 单词ID
*
*/
public static function delWord($wordId){
$res = Word::destroy($wordId);
return $res;
}
}
<file_sep>/app/libraries/alipay/alipay_config.class.php
<?php
/* *
* 类名:AlipayConfig
* 功能:支付宝获取配置类
* 详细:获取alipay.config.php配置文件
* 版本:3.3
* 日期:2012-07-23
* 说明:
* 以下代码只是为了方便商户测试而提供的样例代码,商户可以根据自己网站的需要,按照技术文档编写,并非一定要使用该代码。
* 该代码仅供学习和研究支付宝接口使用,只是提供一个参考。
*/
class AlipayConfig {
private static $config;
/**
* 获取支付宝配置
* return 配置文件数组
*/
public static function get() {
if(self::$config===null){
//引入配置文件
require_once("alipay.config.php");
self::$config = $alipay_config;
}
return self::$config;
}
}
?> | 2b062715e75547d286e5ff0bca9e8470f6ec8909 | [
"JavaScript",
"PHP"
] | 17 | PHP | kdj1219/ess | 816fadd2a3ed89820ab124ef0f7b964cbcb55cfb | c49f14362a073c1a7cacfc55ebcbbdf7d9322f1e | |
refs/heads/master | <file_sep>#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
#include<pthread.h>
#include<semaphore.h>
int TA_Sleeping;
sem_t semTA;
sem_t chairs;
pthread_mutex_t Helpmutex = PTHREAD_MUTEX_INITIALIZER;
pthread_mutex_t TAcreated = PTHREAD_MUTEX_INITIALIZER;
pthread_mutex_t helping = PTHREAD_MUTEX_INITIALIZER;
void helpStudent(){
while(1){
int availableChairs;
sem_getvalue(&chairs,&availableChairs);
if(availableChairs == 3){TA_Sleeping = 1;printf("The TA is sleeping\n");}
sem_wait(&semTA);
sleep(2);
pthread_mutex_unlock(&helping);
}
}
void getHelp(long studentID){
if(TA_Sleeping){
TA_Sleeping = 0;
printf("Student %lu woke up the TA\n",studentID);
sem_post(&semTA);
pthread_mutex_unlock(&Helpmutex);
pthread_mutex_lock(&helping);
}
else{
int availableChairs;
sem_getvalue(&chairs,&availableChairs);
if(availableChairs == 3){
sem_wait(&chairs);
printf("Student %lu took a seat in chair 1\n",studentID);
pthread_mutex_unlock(&Helpmutex);
sem_post(&semTA);
pthread_mutex_lock(&helping);
printf("TA is Helping a student %lu\n", studentID);
sem_post(&chairs);
printf("Chair 1 is now open\n");
}
if(availableChairs == 2){
sem_wait(&chairs);
printf("Student %lu took a seat in chair 2\n",studentID);
pthread_mutex_unlock(&Helpmutex);
sem_post(&semTA);
pthread_mutex_lock(&helping);
printf("TA is Helping a student %lu\n", studentID);
sem_post(&chairs);
printf("Chair 2 is now open\n");
}
if(availableChairs == 1){
sem_wait(&chairs);
printf("Student %lu took a seat in chair 3\n",studentID);
pthread_mutex_unlock(&Helpmutex);
sem_post(&semTA);
pthread_mutex_lock(&helping);
printf("TA is Helping a student %lu\n", studentID);
sem_post(&chairs);
printf("Chair 3 is now open\n");
}
if(availableChairs == 0){ printf("Student %lu went back to programming\n",studentID);
pthread_mutex_unlock(&Helpmutex);}
}
}
void *initTA(){
sem_init(&semTA,0,0);
sem_init(&chairs,0,3);
TA_Sleeping = 1;
pthread_mutex_unlock(&TAcreated);
helpStudent();
}
void *initStudent(void *t){
pthread_mutex_lock(&Helpmutex);
long id = (long)t;
getHelp(id);
}
int main(int argc,char **argv){
//Make n student threads
int n = 6;
int ret;
//Make TA Thread
pthread_mutex_lock(&TAcreated);
pthread_t TA;
ret = pthread_create(&TA,NULL,initTA,NULL);
pthread_mutex_lock(&TAcreated);
long t;
pthread_t threads[n];
for(t = 0; t<n;t++){
ret = pthread_create(&threads[t],NULL,initStudent,(void *)t);
}
//Make threads wait for all to terminate before ending program
for(t = 0; t<n;t++){
pthread_join(threads[t],NULL);
}
pthread_join(TA,NULL);
return 0;
}
<file_sep>#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
int getInput(){
printf("Enter a Positive Number: ");
int num;
scanf("%d", &num);
if(num < 0){getInput();}
else{return num;}
}
void collatz(int num){
printf("%d", num);
printf(" ");
if(num > 1){
if(num%2 == 0){ num = num/2;}
else{num = (3*num)+1;}
collatz(num);
}
if(num == 1){printf("\n");}//Print a new line to make answer more readable
}
int main()
{
int pid;
pid=fork();
int number;
if(pid<0)
{
printf("\n Error ");
exit(1);
}
else if(pid==0)
{
//This is the child process so make it do all the work
number = getInput();
collatz(number);
exit(0);
}
else
{
//This is the parent process so make it wait for child to finish
wait();
exit(1);
}
}
<file_sep>#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <stdlib.h>
#define MAX_LINE 80
int main(void){
int shouldWait = 0;
int should_run = 1;
char **hist = malloc(10*sizeof(char*));
int commandcount = 0;
//allocate the history array
int k = 0;
while(k < 10){
hist[k] = malloc(MAX_LINE);
strcpy(hist[k],"0");
k++;
}
void addHistory(char* arg){
int i = 0;
while(i < 9){
if(strcmp(hist[i+1],"0")!= 0){strcpy(hist[i],hist[i+1]);}
i++;
}
strcpy(hist[9],arg);
commandcount++;
}
void history(){
int i = 0;
int count = 0;
while(i < 10){
if(strcmp(hist[i],"0")!= 0){
printf("%d ", commandcount-count);
printf("%s\n", hist[i]);
count++;
}
i++;
}
}
int runShell(){
char *saveprt;
char arg[MAX_LINE];
char *temp;
int argnum = 0;
int i;
char *tempargs[MAX_LINE/2 +1];
char **args;
pid_t pid;
shouldWait = 0;
printf("osh>");
fflush(stdout);
fflush(stdin);
fgets(arg,MAX_LINE,stdin);
strtok_r(arg,"\n",&saveprt);
if(strcmp(arg,"history")!= 0 && strcmp(arg,"\n")!= 0 && arg[0]!= '!'){addHistory(arg);}
if(arg[0] == '!'){ if(arg[1] == '!'){
if(strcmp(hist[9],"0")!= 0){strcpy(arg,hist[9]);}
else{printf("No commands in history\n");}}
else{
char *end;
int n = strtol(&arg[1],&end,10);
if(0 < n && n <= 10){
if(strcmp(hist[10-n],"0")!= 0){strcpy(arg,hist[10-n]);}
else{printf("No such command in history\n");}}
else{printf("No such command in history\n");}
}
}
temp = strtok_r(arg," ",&saveprt);
i = 0;
while(temp != NULL){
tempargs[i] = temp;
i++;
argnum++;
temp = strtok_r(NULL," ",&saveprt);
}
i = 0;
if(strcmp(tempargs[argnum-1],"&") == 0){
shouldWait = 1;
argnum --;
args = malloc(argnum*sizeof(char*));
while(i < argnum){
args[i] = malloc(MAX_LINE);
strcpy(args[i],tempargs[i]);
i++;
}
}
else{
args = malloc(argnum*sizeof(char*));
while(i < argnum){
args[i] = malloc(MAX_LINE);
strcpy(args[i],tempargs[i]);
i++;
}
}
if(strcmp(args[0],"exit")==0){return 0;}
else if(strcmp(args[0],"history")==0){history();free(args);runShell();}
else{
fflush(stdout);
int status;
pid = fork();
if(pid<0){printf("Error in fork"); return 1;}
else if(pid == 0){execvp(args[0],args);return 0;}
else if(shouldWait){wait(&status);free(args);runShell();}
else{free(args);runShell();}
}
}
int run = runShell();
free(hist);
return run;
}
<file_sep>#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
#include<pthread.h>
#define MAX_RESOURCES 100
int numThreads = 1;
int available_resources = MAX_RESOURCES;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t resc_thresh;
void waitForResources(int resc){
while(resc > available_resources){
sleep(.5);
}
}
//Part A of the code
int decrease_count(int count,long t){
if(available_resources < count){
printf("XXXXXXX Insufficient Resources for thread %lu\n",t);
printf("XXXXXXX Tried to claim %d resources\n", count);
printf("XXXXXXX Available: %d\n\n", available_resources);
return -1;
}
else{
available_resources -= count;
printf("Thread %lu claimed %d resources\n",t,count);
printf("Claimed: %d resources\n", count);
printf("Available: %d\n", available_resources);
return 0;
}
}
int increase_count(int count,long t){
available_resources += count;
printf("Thread %lu added %d resources\n",t,count);
printf("There are now %d resources available\n\n\n",available_resources);
return 0;
}
void *getResources(void *t){
long threadID = (long)t;
int resc = rand()%100;
if(resc > MAX_RESOURCES){
printf("Thread %lu attempted to claim more than max resources.\n",threadID);
}
else{
int success = decrease_count(resc,threadID);
if(success == 0){increase_count(resc,threadID);}
}
pthread_exit(0);
}
//This is the code where the monitor is implemented
//Part C of the code
void decrease_countMonitor(int resc,long t){
pthread_mutex_lock(&mutex);
if(available_resources < resc){
printf("Thread %lu WAITING to claim %d resources\n\n",t, resc);
pthread_cond_wait(&resc_thresh, &mutex);
}
available_resources -= resc;
printf("Thread %lu\n",t);
printf("Claimed: %d resources\n", resc);
printf("Available: %d\n\n\n", available_resources);
pthread_mutex_unlock(&mutex);
}
void increase_countMonitor(int resc, long t){
pthread_mutex_lock(&mutex);
available_resources += resc;
printf("Thread %lu added %d resources\n",t,resc);
printf("There are now %d resources available\n\n\n",available_resources);
pthread_cond_signal(&resc_thresh);
pthread_mutex_unlock(&mutex);
}
void *getResourcesMonitor(void *t){
long threadID = (long)t;
int resc = rand()%10+90;
if(resc > MAX_RESOURCES){
printf("Thread %lu attempted to claim more than max resources.\n",threadID);
}
else{
decrease_countMonitor(resc,threadID);
increase_countMonitor(resc,threadID);
}
pthread_exit(0);
}
int main(int argc, char *argv[])
{
numThreads = atoi(argv[1]);
printf("Enter A to see race condition or M to see the Monitor: ");
char condition[80];
fgets(condition,80,stdin);
printf("%s",condition);
//Make all the threads
pthread_cond_init(&resc_thresh,NULL);
pthread_t threads[numThreads];
int ret;
long t;
if(strcmp(condition,"A\n") == 0 || strcmp(condition,"a\n") == 0){
printf("RACE CONDITION:\n");
for(t = 0; t<numThreads;t++){
ret = pthread_create(&threads[t],NULL,getResources,(void *)t);
}
//Make threads wait for all to terminate before ending program
for(t = 0; t<numThreads;t++){
pthread_join(threads[t],NULL);
}
}
else{
if(strcmp(condition,"M\n") == 0 || strcmp(condition,"m\n") == 0){
printf("Monitor\n");
for(t = 0; t<numThreads;t++){
ret = pthread_create(&threads[t],NULL,getResourcesMonitor,(void *)t);
}
//Make threads wait for all to terminate before ending program
for(t = 0; t<numThreads;t++){
pthread_join(threads[t],NULL);
}
}
}
pthread_exit(NULL);
}
<file_sep>#include<stdio.h>
#include<string.h>
#include<unistd.h>
#include<stdlib.h>
long numAdds = 0;
int *page;
int *offset;
long *pageTable;
long* physical;
int pageFault = 1;
int tlbHits = 0;
long **tlb;
long checkTlb(int index){
int i;
for(i = 0;i < 16; i++){
if(tlb[i][0] == page[index]){;
return tlb[i][1];
}
}
return (long)2;
}
void addTlb(int index){
int i;
for(i = 1;i < 16; i++){
tlb[i-1][0] = tlb[i][0];
tlb[i-1][1] = tlb[i][1];
}
tlb[15][0] = page[index];
tlb[15][1] = pageTable[page[index]];
}
void getPhysical(long *logicals){
physical = malloc(sizeof(long)*1000);
int i;
long frame = 0;
for(i = 0;i<numAdds;i++){
int off = offset[i];
long isTlb = checkTlb(i);
if(isTlb == 2){
if(pageTable[page[i]] == 1){
pageTable[page[i]] = frame;
frame += 256;
pageFault++;
}
physical[i] = pageTable[page[i]] + off;
addTlb(i);
}
else{physical[i] = isTlb + off;tlbHits++;}
}
}
int isFreeFrame(){
int i;
for(i = 0;i < 128;i++){
if(pageTable[i] == 1){return i;}
}
return 0;
}
int findVictim(){
int i;
for(i = 0; i < 128; i++){
int intlb = 0;
int j;
for(j = 0; j < 16; j++){
if(tlb[j][0] == i){intlb = 1;}
}
if(!intlb){return i;}
}
}
void getPhysicalMod(long *logicals){
physical = malloc(sizeof(long)*1000);
int i;
long frame = 0;
for(i = 0;i<numAdds;i++){
int off = offset[i];
long isTlb = checkTlb(i);
if(isTlb == 2){
if(pageTable[page[i]] == 1){
pageTable[page[i]] = frame;
frame += 128;
pageFault++;
}
else{
int freeFrame = isFreeFrame();
//Use a free frame if available
if(freeFrame>0){
pageTable[freeFrame] = frame;
frame += 128;
pageFault++;
}
//If no free frame find first frame no in use by tlb and make it victim
else{
int victim = findVictim();
pageTable[victim] = frame;
frame += 128;
pageFault++;
}
}
physical[i] = pageTable[page[i]] + off;
addTlb(i);
}
else{physical[i] = isTlb + off;tlbHits++;}
}
}
void initPageTable(){
pageTable = malloc(256*sizeof(long));
int i;
pageTable[0] = 0;
for(i = 1; i < 256;i++){
pageTable[i] = 1;
}
tlb = malloc(sizeof(long)*16);
for(i = 0;i < 16;i++){
tlb[i] = malloc(2*sizeof(long));
tlb[i][0] = 256;
tlb[i][1] = 0;
}
}
void toDec(int *bin,int index){
int dec = 0;
int i;
int pow = 1;
for(i = 15;i >= 0;i--){
if(i >7){
if(bin[i] == 1){
dec += pow;
}
pow = pow*2;
}
if(i == 7){pow = 1; offset[index] = dec; dec = 0;}
if(i <= 7){
if(bin[i] == 1){
dec += pow;
}
pow = pow*2;
}
}
page[index] = dec;
}
void toBinary(long *adds){
int i;
page = malloc(sizeof(long)*numAdds);
offset = malloc(sizeof(long)*numAdds);
for( i = 0; i < numAdds; i++){
long num = adds[i];
int index = 15;
int b[] = {0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
while(num != 0){
b[index] = num%2;
num = num/2;
index -= 1;
}
toDec(b,i);
}
}
long * openFile(char* filename){
//Open file
FILE *fp;
fp = fopen(filename,"r");
long *addresses = malloc(sizeof(long)*1000);
if(fp==NULL){printf("File not found.\n");}
else{
int i = 0;
char ch;
char l[5];
int j = 0;
while((ch = fgetc(fp))!= EOF){
if(ch == '\n'){
j = 0;
addresses[i] = atoll(l);
numAdds++;
i++;
int k;
for(k = 0;k<5;k++){
l[k] = 0;
}
}
else{l[j] = ch;j++;}
}
}
return addresses;
}
int main(int argc,char *argv[]){
//Get Command Line Args
char* addresses = argv[1];
//Prompt for Mod
printf("Input '1' for Modifications and '0' for No Modifications: ");
char input[80];
fgets(input,80,stdin);
int mod = atoi(input);
if(mod == 1){mod = 1;}
//Open file and read addresses
long *logicals = openFile(addresses);
toBinary(logicals);
initPageTable();
if(mod){getPhysicalMod(logicals);}
else{getPhysical(logicals);}
//Print out logical addresses
int i;
for(i = 0; i < numAdds;i++){
printf("Virtual address: %lu ",logicals[i]);
printf("Physical address: %lu ",physical[i]);
printf("Value: %d",*((signed char *)&physical[i]));
printf("\n");
}
printf("Number of Translated Addresses = %lu\n",numAdds);
printf("Page Faults = %d\n",pageFault);
printf("Page Fault Rate = %g\n", (double)pageFault/(double)numAdds);
printf("TLB Hits = %d\n",tlbHits);
printf("TLB Hit Rate = %g\n", (double)tlbHits/(double)numAdds);
return 0;
}
<file_sep>#include<stdio.h>
#include<string.h>
#include<unistd.h>
#include<stdlib.h>
#include<pthread.h>
#include<semaphore.h>
#include<time.h>
//Initialize exit variable to quit threads
int shouldExit = 0;
//Define Semaphores and Mutexes
sem_t empty;
sem_t full;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;;
//Define Buffer
typedef int buffer_item;
#define BUFFER_SIZE 5
buffer_item buffer[BUFFER_SIZE];
void initBuffer(){
printf("Setting up buffer....\n");
sleep(0);
//buffer = (buffer_item [5]);
printf("Buffer Initialized\n");
}
int insert_item(buffer_item item,long id){
int success = 0;
//Insert item into buffer
//Wait for something to be consumed
printf("Producer thread %lu waiting for empty spot in buffer\n",id);
sem_wait(&empty);
pthread_mutex_lock(&mutex);
printf("Producer thread %lu has lock on empty spot in buffer\n",id);
int emptySpots = 0;
sem_getvalue(&empty,&emptySpots);
buffer[emptySpots] = item;
pthread_mutex_unlock(&mutex);
sem_post(&full);
success = 1;
if(success){return 1;}
else{return 0;}
}
int remove_item(buffer_item *item,long id){
int success = 0;
//Remove Item from buffer
//Wait for something to be produced
printf("Consumer thread %lu waiting for production to finish\n",id);
sem_wait(&full);
pthread_mutex_lock(&mutex);
printf("Consumer thread %lu has a lock on an item for consumption\n",id);
int fullSpots = 0;
sem_getvalue(&full,&fullSpots);
*item = buffer[BUFFER_SIZE - fullSpots - 1];
buffer[BUFFER_SIZE - fullSpots-1] = 0;
pthread_mutex_unlock(&mutex);
sem_post(&empty);
success = 1;
if(success){return 1;}
else{return 0;}
}
void *initProducer(void *t){
buffer_item item = 0;
long id = (long)t;
while(1){
if(shouldExit){pthread_exit(0);}
//Sleep random period of time
sleep(rand()%3);
//Genereate random number for item size
int temp = rand();
item = temp;
//Insert and item
if(insert_item(item,id)){printf("Production Successful for thread %lu\n\n\n",id);}
else{printf("Error in production on thread %lu\n",id);}
}
}
void *initConsumer(void *t){
buffer_item item = 0;
long id = (long)t;
while(1){
if(shouldExit){pthread_exit(0);}
//Sleep random period of time
sleep(rand()%3);
//Remove an item
if(remove_item(&item,id)){printf("Consumer consumed %d on thread %lu\n\n\n",item,id);}
else{printf("Error in consumption on thread %lu\n",id);}
}
}
int main(int argc,char *argv[]){
//Get Command Line Args
int sleepTime = atoi(argv[1]);
int pThreads = atoi(argv[2]);
int cThreads = atoi(argv[3]);
//Initialize Buffer
initBuffer();
//Initialize Semaphores and Mutexes
sem_init(&empty,0,5);
sem_init(&full,0,0);
pthread_mutex_init(&mutex,NULL);
//Make Consumer and Producer Threads
pthread_t consumerThreads[cThreads];
pthread_t producerThreads[pThreads];
long t;
int ret;
for(t = 0; t<cThreads;t++){
ret = pthread_create(&consumerThreads[t],NULL,initConsumer,(void *)t);
}
for(t = 0; t<pThreads;t++){
ret = pthread_create(&producerThreads[t],NULL,initProducer,(void *)t);
}
/*
//Join Threads at the end
for(t = 0; t<cThreads;t++){
pthread_join(consumerThreads[t],NULL);
}
for(t = 0; t<pThreads;t++){
pthread_join(producerThreads[t],NULL);
}*/
//Sleep before terminating
sleep(sleepTime);
shouldExit = 1;
return 0;
}
<file_sep>#include<stdio.h>
#include<unistd.h>
#include<stdlib.h>
#include<pthread.h>
#define MAX_RESOURCES 6
int numThreads = 10;
int resc = 6;
int available_resources = MAX_RESOURCES;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
int decrease_count(int count,long t){
if(available_resources < count){printf("Insufficient Resources for thread %lu\n",t);return -1;}
else{available_resources -= count; printf("Thread %lu claimed some resources\n",t); return 0;}
}
int increase_count(int count,long t){
available_resources += count;
return 0;
}
int decrease_countFixed(int count,long t){
if(available_resources < count){printf("Insufficient Resources for thread %lu\n",t);return -1;}
else{available_resources -= count; printf("Thread %lu claimed some resources\n",t); return 0;}
}
int increase_countFixed(int count,long t){
available_resources += count;
return 0;
}
void *getResources(void *t){
long threadID = (long)t;
if(resc > MAX_RESOURCES){printf("Thread %lu attempted to claim more than max resources.\n",threadID);}
else{
decrease_count(resc,threadID);
increase_count(resc,threadID);
}
pthread_exit(0);
}
void *getResourcesFixed(void *t){
long threadID = (long)t;
pthread_mutex_lock(&mutex);
if(resc > MAX_RESOURCES){printf("Thread %lu attempted to claim more than max resources.\n",threadID);}
else{
decrease_countFixed(resc,threadID);
increase_countFixed(resc,threadID);
}
pthread_mutex_unlock(&mutex);
pthread_exit(0);
}
int main()
{
//Make all the threads
pthread_t threads[numThreads];
int ret;
long t;
printf("RACE CONDITION:\n");
for(t = 0; t<numThreads;t++){
ret = pthread_create(&threads[t],NULL,getResources,(void *)t);
}
//Make threads wait for all to terminate before ending program
for(t = 0; t<numThreads;t++){
pthread_join(threads[t],NULL);
}
printf("FIXED RACE CONDITION\n");
for(t = 0; t<numThreads;t++){
ret = pthread_create(&threads[t],NULL,getResourcesFixed,(void *)t);
}
//Make threads wait for all to terminate before ending program
for(t = 0; t<numThreads;t++){
pthread_join(threads[t],NULL);
}
pthread_exit(NULL);
}
<file_sep>#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int *unsorted;
int *sorted;
int lines;
int half;
int odd;
int compare( const void* a, const void* b)
{
int int_a = * ( (int*) a );
int int_b = * ( (int*) b );
if ( int_a == int_b ) return 0;
else if ( int_a < int_b ) return -1;
else return 1;
}
void *sortFirstHalf(){
int i;
int temp1[half];
for(i = 0;i < half;i++){
memcpy(&temp1[i],&unsorted[i],sizeof(unsorted[i]));
}
qsort(temp1,half,sizeof(int),compare);
for(i = 0;i < half;i++){
memcpy(&unsorted[i],&temp1[i],sizeof(unsorted[i]));
}
pthread_exit(0);
}
void *sortSecondHalf(){
int i;
int temp2[half];
for(i = 0;i < half-odd;i++){
memcpy(&temp2[i],&unsorted[i+half],sizeof(unsorted[i+half]));
}
qsort(temp2,half,sizeof(int),compare);
for(i = odd;i < half;i++){
memcpy(&unsorted[i+half-odd],&temp2[i],sizeof(unsorted[i+half]));
}
pthread_exit(0);
}
void *merge(){
int i = 0;
int j = 0;
int k = half;
while(i < lines){
int a = 99999999;//Init to infinity as our "null" value
int b = 99999999;
if(j < half){a = unsorted[j];}
if(k < lines){b = unsorted[k];}
if(a <= b){j++; memcpy(&sorted[i],&a,sizeof(a));}
else{k++; memcpy(&sorted[i],&b,sizeof(b));}
i++;
}
pthread_exit(0);
}
int main(int argc,char **argv){
char buff[255];
//read in file as a list
//count lines
FILE *fp;
fp = fopen(argv[1],"r");
fscanf(fp,"%s",buff);
lines = 0;
while(fgets(buff,255,(FILE*)fp)){
lines++;
}
fclose(fp);
//Allocate array and read in ints
unsorted = (int*)calloc(lines, sizeof(int));
sorted = (int*)calloc(lines,sizeof(int));
fp = fopen(argv[1],"r");
int i;
for(i = 0; i < lines; i++){
fscanf(fp,"%d",&unsorted[i]);
}
//split array into 2 arrays
if(lines%2 == 0){half = lines/2; odd = 0;}
else{half = (lines+1)/2; odd = 1;}
//Make 2 thread and assign a half on unsorted to be sorted in each thread
//sort each list in its own sorting thread
pthread_t tid1; //identifier
pthread_attr_t attr1; //attributes
pthread_attr_init(&attr1); //get default attributes
pthread_create(&tid1,&attr1,sortFirstHalf,NULL);
pthread_t tid2; //identifier 2
pthread_attr_t attr2; //attributes 2
pthread_attr_init(&attr2); //get default attributes
pthread_create(&tid2,&attr2,sortSecondHalf,NULL);
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
//merge lists in a merge thread
for(i = 0; i < lines; i++){
//printf("%d\n",unsorted[i]);
}
pthread_t tid3; //identifier 2
pthread_attr_t attr3; //attributes 2
pthread_attr_init(&attr3); //get default attributes
pthread_create(&tid3,&attr3,merge,NULL);
pthread_join(tid3,NULL);
//merge lists in a merge thread
printf("Sorted Array: [");
for(i = 0; i < lines; i++){
if(i < lines-1){printf("%d,",sorted[i]);}
else{printf("%d]\n",sorted[i]);}
}
free(sorted);
free(unsorted);
return 0;
}
| f1a5417121f8e2888a6a3549c77fb7fdbb1781ee | [
"C"
] | 8 | C | amdonham/C | 70640b87d08702296b76440b80ce6bbdd2ad9df4 | c8225bfcff24dd591d20815a9553821a3d9f0401 | |
refs/heads/main | <repo_name>liuyandong1988/Network<file_sep>/README.md
# Network
记录学习神经网络的过程
## 1. 全连接网络
- 神经网络的结构:
- 激活函数;
- 损失函数;
- 优化器;
- 前向传播和反向传播:
- 求损失函数的梯度;
- 链式法则;
- 代码 一步一步搭建神经网络<file_sep>/Fully_connected/code/nn_pytorch.py
"""
使用深度学习框架完成性别判断问题
"""
import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
import matplotlib.pyplot as plt
class Net(nn.Module):
def __init__(self, input_dim=2, out_put_dim=1):
super(Net, self).__init__()
self.fc1 = nn.Linear(in_features=input_dim, out_features=2)
self.fc2 = nn.Linear(in_features=2, out_features=out_put_dim)
def forward(self, x):
x = F.relu(self.fc1(x))
outputs = self.fc2(x)
return outputs
# --- 实例化一个网络 --- #
my_net = Net()
print("Network:", my_net)
# parameters
for name, params in my_net.named_parameters():
print(name, params)
print('Total parameters:', sum(param.numel() for param in my_net.parameters()))
# --- optimizer --- #
optimizer = optim.Adam(params=my_net.parameters(), lr=0.01)
# --- data --- #
# Define dataset
data = np.array([
[-2, -1], # Alice
[25, 6], # Bob
[17, 4], # Charlie
[-15, -6], # Diana
])
all_y_trues = np.array([
1, # Alice
0, # Bob
0, # Charlie
1, # Diana
])
# --- train --- #
loss_list = list()
for i in range(1000):
# forward
sample_index = np.random.randint(0, 4)
x_train = data[sample_index]
y_label = all_y_trues[sample_index]
# numpy to tensor
x_train = torch.tensor(x_train).to(dtype=torch.float32)
y_label = torch.tensor(y_label).to(dtype=torch.float32).unsqueeze(dim=0)
y_pred = my_net(x_train)
# loss
loss = F.mse_loss(y_pred, y_label)
# grad zero
optimizer.zero_grad()
# back propagation
loss.backward()
# update weight
optimizer.step()
print('Epoch: %d Loss : %f'%(i, loss.item()))
loss_list.append(loss.item())
plt.plot(loss_list)
plt.show()
<file_sep>/Fully_connected/code/simple_network.py
"""
简单的两层神经网络
"""
import numpy as np
from simple_neural import Neuron
# ------------------------ #
# --- 神经网络 --- #
# ------------------------ #
class OurNeuralNetwork:
'''
A neural network with:
- 2 inputs
- a hidden layer with 2 neurons (h1, h2)
- an output layer with 1 neuron (o1)
Each neuron has the same weights and bias:
- w = [0, 1]
- b = 0
'''
def __init__(self):
weights = np.array([0, 1])
bias = 0
# The Neuron class here is from the previous section
self.h1 = Neuron(weights, bias)
self.h2 = Neuron(weights, bias)
self.o1 = Neuron(weights, bias)
def feedforward(self, x):
out_h1 = self.h1.feedforward(x)
out_h2 = self.h2.feedforward(x)
# The inputs for o1 are the outputs from h1 and h2
out_o1 = self.o1.feedforward(np.array([out_h1, out_h2]))
return out_o1
network = OurNeuralNetwork()
x = np.array([2, 3])
print(network.feedforward(x)) # 0.721632560951842
# ------------------------ #
# --- 损失函数 --- #
# ------------------------ #
def mse_loss(y_true, y_pred):
# y_true and y_pred are numpy arrays of the same length.
return ((y_true - y_pred) ** 2).mean()
y_true = np.array([1, 0, 0, 1])
y_pred = np.array([0, 0, 0, 0])
print(mse_loss(y_true, y_pred)) # 0.5 | 83c948498d43e9fd427aa0772d61248928c6c918 | [
"Markdown",
"Python"
] | 3 | Markdown | liuyandong1988/Network | 9beb045d8b0e9ce3bd5f3321f33b99a73e0da7a2 | ad930162e9b6e60fbbc299cc2d97f7cb1d12a9b2 | |
refs/heads/main | <file_sep>use crate::error::Result;
use sqlparser::ast::Statement;
pub fn tokenize_statement(statement: &str) -> Result<Statement> {
unimplemented!();
}
<file_sep>pub mod create;
| 91c99884602d857235860ccea9e58a919400f281 | [
"Rust"
] | 2 | Rust | kangxiaoning/rust_sqlite | 66bb9674bdf2ce90d65f45f5ccc969838c37d020 | 2423d55873708292c588cc9e4561d471ffa761cf | |
refs/heads/master | <repo_name>soda460/exploring_genetic_context<file_sep>/scripts/expl_gen_context.py
#!/usr/bin/env python
"""
Ce script cherche a identifier les genes a proximite d'un gene de resistance passe
en argument.
Usage : ./context.py (i)amr_gene (ii)genbank_folder_path (iii)number_of_genes_in_context
"""
__auteur__ = "<NAME>"
__date__ = "2019-09-17"
# Importation de modules standards
import sys
import os
from pathlib import Path
#from Bio.Graphics import GenomeDiagram
from Bio import SeqIO
from Bio.SeqFeature import SeqFeature, FeatureLocation
from io import StringIO
from collections import OrderedDict
#import pandas as pd
import numpy
import re
import csv
import glob
# Importation des modules locaux
from foreign_code import prepare_color_dict
from foreign_code import digest_features
from foreign_code import get_coord_gene
from geneCluster import geneCluster
def get_gbk_files(start_dir):
""" Given a directory, make a list of genbank files
This function will find all files recursively.
"""
gbk_files = []
for filename in Path(start_dir).glob('**/*.gbk'):
gbk_files.append(str(filename))
return gbk_files
def examine_gbk_file (gene, gbk_f):
""" Parse the content of a genbank file with BioPython SeqFeature module
Decouple the parsing with the SeqFeature engine and the function
that will check gene presence for a particular gene.
"""
hits = [] # a list that may contain features and feature_iterators for that particular file
# Get the gbk records with BioPython | many files have more than 1 record (ended by //) !
for i_gbk, gbk_rec in enumerate(SeqIO.parse(gbk_f, "gb"), start=1):
# If required print stuff to standard output
# s = "Handling GBK record no. " + repr(i_gbk) + ":" + gbk_rec.name # car i_gbk est un int
# print (s)
# Check in each record for the gene of interest
abc = check_gene_presence(gene, gbk_rec)
hits.extend(abc)
return (hits)
def check_gene_presence(gene, record):
""" Check the presence of the AMR gene given in argument of the script
This should be a gene referenced in th CARD database and annotated in an inference fied by PROKKA
This function return a 4-items list : an index, the feature, the AMR gene that have been found, and the entire record
"""
record_out= []
for i_feature, feature in enumerate(record.features):
if feature.type == 'CDS':
if 'inference' in feature.qualifiers:
for inf in feature.qualifiers['inference']:
if ("protein_fasta_protein_homolog_model" or
"protein_fasta_protein_knockout_model" or
"protein_fasta_protein_overexpression_model" or
"protein_fasta_protein_variant_model") in inf:
inf2 = inf.replace(" ", '') # To remove extra space converted from \n in genbank
b = inf2.split("|") # To get the gene name
my_current_gene = (b[3])
# Fill an array with feature and iterator if current gene is the one that we are looking for
if gene in my_current_gene:
record_out.append([i_feature, feature, my_current_gene, record])
return (record_out)
def get_metadata(f, out_list):
""" Get and print important information about the strain, molecule, WGS contig, locus where
the amr gene was found
"""
# This is related to the organization of files
# For now, this script will work with the following files organization:
# strains folders then molecule folder then gbk files /Res13-blabla-strain1/plasmid_476/Res13-blabla_plasmid_476.gbk
gbk_file_name = (f.split('/')[-1])
molecule_name = (f.split('/')[-2])
strain_name = (f.split('/')[-3])
meta = strain_name + "\t" + molecule_name + "\t" + gbk_file_name
# First of all, try to grab the amr gene name
# In item we should have [index, feature, AMR_gene, gbk_record_name (== wgs contig)]
for item in out_list:
feature_position_in_record = repr(item[0]) # un index
# Here we have a seqFeature object for our desired AMR gene
amr_found_feature = item[1]
if 'locus_tag' in amr_found_feature.qualifiers:
locus = amr_found_feature.qualifiers['locus_tag'][0]
amr_found = item[2]
gbk_record_name = item[3].name
# Now we are ready to not print this, the infos is returned anyway
#print (amr_found + "\t"
# + meta + "\t"
# + gbk_record_name + "\t"
# + locus + "\t"
# + feature_position_in_record + "\n")
# Return a list, not a tuple
return [amr_found, gbk_file_name, molecule_name, strain_name, gbk_record_name, locus]
def splice_record(index, record, features_range):
"""This function will try to get features around a given feature"""
# to print the entire record
# print(record.features[index])
nb_features = len(record.features) # number of features in the record
# Math pour savoir la plage de feature qui sera consideree
upper_bound = index + features_range
# if the index is too close from the end, the upper_bound will be nb_features minus 1 (0-indexed)
upper_bound = min(upper_bound, (nb_features - 1))
lower_bound = index - features_range
# if the index is too close from the start, the lower_bound will be 0
if (lower_bound <= 0):
lower_bound = 1 # !!! Because the first feature is usually source and it describes the whole molecule
# s = "Feature range will be " + repr(lower_bound) + ":" + repr(upper_bound)
# print(s)
'''
Voici les qualifiers disponibles
locus_tag
codon_start
inference
translation
transl_table
location n'en fait pas partie
Pour acceder au coordonnes en nucletotide du
print (record.features[index].location.start)
https://biopython.org/DIST/docs/api/Bio.SeqFeature.FeatureLocation-class.html
En somme pour acceder aux elements on a les methodes start, end, et strand
'''
'''Note that the start and end location numbering follow Python's scheme, thus a GenBank entry of 123..150 (one based counting)
becomes a location of [122:150] (zero based counting).'''
lower_pos = record.features[lower_bound].location.start
upper_pos = record.features[upper_bound].location.end
# s = "Original record will be spliced at positions " + repr(lower_pos) + ":" + repr(upper_pos)
# print(s)
sub_record = record[lower_pos:upper_pos]
return (sub_record)
def extract_gene_cluster (record):
"""This function will give gene cluster and orientations"""
# Use our own class geneCluster
a = geneCluster(record.name)
for feature in record.features:
if feature.type == 'CDS': # We use the CDS fields
flagGeneDraw = 'FALSE' # Initialize this 'Boolean'!
if 'inference' in feature.qualifiers:
for inf in feature.qualifiers['inference']: # In some cases, more than one inference tag are present in gbk file.
if "protein_fasta_protein_homolog_model" in inf:
inf2 = inf.replace(" ", '') # To remove extra space converted from \n in genbank
b = inf2.split("|") # To get the gene name,
my_gene = (b[3])
a.add(my_gene, feature.strand)
flagGeneDraw = 'TRUE'
break
if "ISfinder" in inf:
inf2 = inf.replace(" ", '') # To remove extra space converted from \n in genbank
b = inf2.split(":") # To get the gene name,
my_gene = (b[2])
a.add(my_gene, feature.strand)
flagGeneDraw = 'TRUE'
break
# Continue searching...
if flagGeneDraw == 'FALSE':
if 'locus_tag' in feature.qualifiers:
if 'gene' in feature.qualifiers:
my_gene = feature.qualifiers['gene'][0]
a.add(my_gene, feature.strand)
else:
my_gene= '?'
a.add(my_gene, feature.strand)
return (a)
def strange_sort(L):
"""This function sort a list of gene cluster objets according to ther size (nb genes)
"""
newlist = sorted(L, key=lambda x: x.nb_genes, reverse=True)
return newlist
def cluster_Gene_clusters(gene_cluster):
"""This function will try to classify a cluster into an appropriate entry in a dictionnary d
Pour que cette fn soit efficace, il faut 'envoyer' les gene clusters a regrouper en ordre
decroissant de taille, de maniere a ce que les plus gros regroupements se forment, puis que
ceux qui sont identiques ou plus petits mais compatibles s'y ajoutent.
"""
s = ""
s = gene_cluster.read()
# check if we have an entry for that particular gene cluster
if s in d:
d[s]['n'] += 1
d[s]['geneClusterObjects'].append(gene_cluster)
#print ("--> Incrementing an existing gene cluster")
return
# remember ; for all the following there is no entry
else:
# Try if the reverse complement of the cluster match an entry in the dict
reverse_gene_cluster = gene_cluster.reverse()
r = ""
r = reverse_gene_cluster.read()
if r in d:
print ('--> Adding a reverse gene cluster to an existing category yeah!')
# We count this cluster and add relative info in the entry of the 'unreversed' cluster
d[r]['n'] += 1
d[r]['geneClusterObjects'].append(gene_cluster)
return
# other investigations , check if the cluster is included in another cluster(larger)
else:
a = check_if_included_in_larger_gene_cluster(gene_cluster, 'normal')
if a:
print (" --> Including a gene cluster into an existing cluster of gene clusters")
return
b = check_if_included_in_larger_gene_cluster(reverse_gene_cluster, 'reverse')
if b:
print (" --> Including a reversed gene cluster into an existing cluster of gene clusters")
return
# All other cases
print ('--> Adding a new gene cluster')
d[s] = {}
d[s]['amr_found'] = gene_cluster.amr_found
d[s]['n'] = 1
d[s]['geneClusterObjects'] = [gene_cluster]
return
def check_if_included_in_larger_gene_cluster(gene_cluster, status):
reverse_gene_cluster = gene_cluster.reverse()
# boucler sur tous les clusters presents dans le dict d | un dictionnaire ordinaire
for key, value in d.items():
for sub_key, sub_value in value.items():
if sub_key == 'geneClusterObjects':
for cluster_in_dict in sub_value:
if gene_cluster.isin(cluster_in_dict):
# Check if the cluster examined is directly the prototype of a larger cluster
if cluster_in_dict.read() in d:
#print ('clus.read()' + '\t', end='')
#print (cluster_in_dict.read())
#print ('gene_cluster.read()' + '\t', end='')
#print (gene_cluster.read())
if status=='normal':
d[cluster_in_dict.read()]['n'] += 1
d[cluster_in_dict.read()]['geneClusterObjects'].append(gene_cluster)
d[cluster_in_dict.read()]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.read()]['geneClusterObjects']) #!new
return True
if status=='reversed':
d[cluster_in_dict.read()]['n'] += 1
d[cluster_in_dict.read()]['geneClusterObjects'].append(reverse_gene_cluster)
d[cluster_in_dict.read()]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.read()]['geneClusterObjects']) #!new
return True
else:
# The following situation occurs occassionnaly when the examined gene cluster is found to be included in another gene cluster
# (beacause we iterate on all members of all clusters of gene clusters) and that the representative of this group is reversed
# For example consider a cluster of gene clusters with the key abcde in dictionnay d
# The cluster could contain many representatives [abcde, abcde, abcde, edcba]
# If the examined gene cluster is edc it would be found to be included in edcab
# We therefore need to increase the counter of this group and add edc to the list of gene cluster objects
# If we try to write in d with the key edcba, we would get a key error because the cluster of gene clusters
# is labeled with abcde. This is why we will use the revese_read() fn to access the appropriate key.
if cluster_in_dict.reverse_read() in d:
if status=='normal':
d[cluster_in_dict.reverse_read()]['n'] += 1
d[cluster_in_dict.reverse_read()]['geneClusterObjects'].append(gene_cluster)
d[cluster_in_dict.reverse_read()]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.reverse_read()]['geneClusterObjects']) #!new
return True
if status=='reversed':
d[cluster_in_dict.reverse_read()]['n'] += 1
d[cluster_in_dict.reverse_read()]['geneClusterObjects'].append(reverse_gene_cluster)
d[cluster_in_dict.reverse_read()]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.reverse_read()]['geneClusterObjects']) #!new
return True
else:
# The following situation occurs occassionnaly when one cluster is found to included in another gene cluster
# which is not the representative of the cluster of gene clusters (because the latter is not the full length
# version of the group. To handle it, we use the parent attribute which is assigned when one cluster was found to be included
# in another one (see the Class geneCluster).
if status=='normal':
d[cluster_in_dict.parent]['n'] += 1
d[cluster_in_dict.parent]['geneClusterObjects'].append(gene_cluster)
d[cluster_in_dict.parent]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.parent]['geneClusterObjects']) #!new
return True
if status=='reversed':
d[cluster_in_dict.parent]['n'] += 1
d[cluster_in_dict.parent]['geneClusterObjects'].append(reverse_gene_cluster)
d[cluster_in_dict.parent]['geneClusterObjects'] = strange_sort(d[cluster_in_dict.parent]['geneClusterObjects']) #!new
return True
# Programme principal
if __name__ == "__main__":
""" The program will find gbk files and will produce one features list per gbk file"""
# Dict of gene clusters
#d = {}
d = OrderedDict()
# List of geneCluster objects
L = []
# Parsing arguments
amr_in = sys.argv[1] # There is a subtle diff between amr_in (ex. 'TEM' and amr_found (TEM-1 or TEM-212)
folder = sys.argv[2]
nb_genes_in_context = int(sys.argv[3])
gbk_files = get_gbk_files(folder)
for f in gbk_files:
hits = examine_gbk_file(amr_in, f)
'''
Note that the hits list is a list of lists, which contains distinct objects
[0], is the index of the amr_gene, i.e. the feature position of the amr_gene in the record
[1], is the feature of the amr_gene
[2], is the amr_gene (string)
[3], is the complete record
'''
if hits:
for my_hit in hits:
# Get the metadata
my_meta_stuff = []
my_meta_stuff = get_metadata(f, hits)
# Give intelligent variables names to metadata
amr_found = my_meta_stuff.pop(0)
gbk_file_name = my_meta_stuff.pop(0)
molecule_name = my_meta_stuff.pop(0)
strain_name = my_meta_stuff.pop(0)
gbk_record_name = my_meta_stuff.pop(0)
locus = my_meta_stuff.pop(0)
# The index of the amr_gene, i.e. the feature position of the amr_gene in the record
amr_index = my_hit[0]
# Correspond to the complete record
amr_record = my_hit[3]
# Splice the record where an AMR gene has been found
sub_rec = splice_record(amr_index, amr_record, nb_genes_in_context)
# Obtain a GeneCluster object from a subrecord
my_gene_cluster = extract_gene_cluster(sub_rec)
# Add metadata to our gene cluster object
my_gene_cluster.locus=locus
my_gene_cluster.amr_found=amr_found
my_gene_cluster.molecule_name=molecule_name
my_gene_cluster.strain_name=strain_name
# Simply put the GeneCluster object in a list
L.append(my_gene_cluster)
# Sort geneCluster objects according to their size
newlist = sorted(L, key=lambda x: x.nb_genes, reverse=True)
for c in newlist:
# print (c.read())
# Verification que mes objects sont bien en ordre descendants de taille
# print(c.nb_genes, c.name, c.cluster)
# This will modify d a dictionnary
# Here we cluster similar or identical gene clusters
print('Dealing with-->' + c.read() + '<--')
cluster_Gene_clusters(c)
# we have a cool dictionnay d and we want to sorted it by the reverse order of the frequency of clusters
# We can do the following, but this will give just a list with our desired sorted keys
# Used at line 505
sorted_keys = sorted(d, key=lambda x: (d[x]['n']), reverse=True)
# After some stackoverflow searches, I,ve found the magic command that allow to create an OrderedDict object
# that will remember the insertion order of the items. We want to create the keys in reverse order of ['n']
# which is the number of occurence of the clusters
d2 = OrderedDict(sorted(d.items(), key=lambda x: x[1]['n'], reverse=True))
# For output...
detailed='Gene found' + '\t' + 'Number of instances' + '\t' + 'Cluster' + '\t' + 'Strain name' + '\t' + 'Molecule' + '\t' + 'Locus' + '\t' + 'Nb genes in cluster' + '\n'
summary='Gene found' + '\t' + 'Number of instances' + '\t' + 'Cluster' + '\n'
# Passe au travers du dictio
for key, value in d2.items():
if isinstance(value, dict):
for sub_key, sub_value in value.items():
if sub_key == 'amr_found':
detailed += '\n' + sub_value + '\t'
if sub_key == 'n':
print('Regroupement de taille', sub_value)
detailed += repr(sub_value) + '\t' + key + '\n'
if sub_key == 'geneClusterObjects':
for clus in sub_value:
print(clus.read(), clus.name, clus.nb_genes, clus.strain_name)
detailed += '\t\t' + clus.read() + '\t' + clus.strain_name + '\t' + clus.molecule_name + '\t' + clus.locus + '\t' + repr(clus.nb_genes) + '\n'
with open('detailed.txt', 'w') as file:
file.write(detailed)
<file_sep>/scripts/geneCluster.py
#!/usr/bin/env python
# -*- coding: utf-8 -*-
class geneCluster:
"""Classe définissant une regroupement de genes caractérisé par :
- son nom
- ses genes
- leur orientation
- le nombre de genes"""
def __init__(self, name): # Notre méthode constructeur
self.name = name
self.nb_genes = 0 # Au depart il est vide
self.cluster = []
self.strand = []
self.amr_found = []
self.molecule_name = []
self.strain_name = []
self.locus = []
self.parent = "" # Pour garder la trace des inclusions dans d'autres gene clusters
def add(self, gene, strand):
"""Methode pour ajouter un gene a la fois """
self.nb_genes += 1
self.cluster.append(gene)
self.strand.append(strand)
def read(self):
"""Une methode pour lire le contenu du cluster"""
s = ""
#s = self.name + ": "
for i,j in zip(self.cluster, self.strand):
if (j == 1 or j == '+'):
s = s + '5\'-' + i + '-3\' '
if (j == -1 or j == '-'):
s = s + '3\'-' + i + '-5\' '
return(s)
def __eq__(self, other):
"""Override the default Equals behavior"""
if isinstance(other, self.__class__):
return self.cluster == other.cluster and self.strand == other.strand
return False
def __ne__(self, other):
"""Override the default Unequals behavior"""
if isinstance(other, self.__class__):
return self.cluster != other.cluster or self.strand != other.strand
return False
def isin(self, other):
"""Methode pour tester si un regroupement de gene est inclus dans un autre"""
l3=[] # a list to store list index :)
if isinstance(other, self.__class__):
"""Pour s'assurer que le parametre passe a la fn appartienne a la classe geneCluster"""
for i, val in enumerate(self.cluster):
if val in other.cluster:
# Les indices des element du deuxieme cluster (other) communs a ceux du premier gene cluster sont gardes
l3.append(other.cluster.index(val))
else:
return False
# Here we test if the indices are consecutive
if ( (l3[-1] - l3[0]) == (len(l3) - 1) ):
self.parent = other.read()
return True
else:
# Try again with the reversed small cluster
l3=[]
rev_small = self.reverse()
for i, val in enumerate(rev_small.cluster):
if val in other.cluster:
l3.append(other.cluster.index(val))
else:
return False
# Here we test if the indices are consecutive
if ( (l3[-1] - l3[0]) == (len(l3) - 1) ):
self.parent = other.read()
return True
else:
return False
def load(self, gene_list, strand_list):
"""Methode pour loader des genes from scratch a partir d'une liste"""
self.cluster = gene_list
self.strand = strand_list
def get_size(self):
"""Methode pour avoir le nombre de genes"""
return(self.nb_genes)
def reverse(self):
"""Methode pour inverser un regroupement de genes"""
r_cluster=[] # to hold the inverted cluster
r_strand=[] # to hold the new polarity
# use the reversed function to reverse the list
r_cluster = list(reversed(self.cluster))
r_strand = list(reversed(self.strand))
# polariyy need to be changed (reverse complement)
r_strand_2 = []
for i in r_strand:
if i == '+' or i == 1:
r_strand_2.append(-1)
if i == '-' or i == -1:
r_strand_2.append(1)
new = geneCluster(self.name + '_reversed')
# keep other atributes
new.nb_genes = self.nb_genes
new.amr_found =self.amr_found
new.molecule_name = self.molecule_name
new.strain_name = self.strain_name
new.locus = self.locus
new.parent = self.parent # Pour garder la trace des inclusions dans d'autres gene clusters
new.load(r_cluster, r_strand_2)
return(new)
def reverse_read(self):
"""Une methode pour lire le reverse complement du cluster"""
r_string=""
reverse_object = self.reverse()
r_string = reverse_object.read()
return (r_string)
| 33dc16e2f98a9cba82065a6d856c62bab987d02b | [
"Python"
] | 2 | Python | soda460/exploring_genetic_context | cd55fc473ec533b535ffbd9c4976ae1f2a00fdfb | 7f20d0e8622578c3c8b9679c805d60d98ba6e539 | |
refs/heads/master | <repo_name>BestJason1/text_04b<file_sep>/week.php
<?php
// $a=0;
// for ($i=0; $i <=100; $i++) {
// $a+=$i;
// }
// echo $a;
// echo array_sum(range(1,100));
// function sum($str,$count){
// if ($count>100) {
// echo $str;
// }else{
// $str+=$count;
// sum($str,$count+1);
// }
// }
// sum($str=0,$count=0);
// $n=6;
// for ($i=0; $i <6; $i++) {
// $n=$n*($n-1);
// echo $n;echo "<hr>";
// }
// echo $n;
// function ishuiwen($str){
// $len=strlen($str);
// $l=1;
// $k=intval($len/2)+1;
// for($j=0;$j<$k;$j++){
// if (substr($str,$j,1)!=substr($str,$len-$j-1,1))
// {
// $l=0;
// break;
// }
// }
// if ($l==1)
// {
// return 1;
// }
// else
// {
// return -1;
// }
// }
// $str=1231;
// echo ishuiwen($str);
class Person{
public $name;
public $age;
}
interface Walk{
}
class Student extends Person implements Walk{
}
$obj1=new Student();
$obj1->name="申奇龙";
$obj1->age=6;
$obj2=new Student();
$obj2->name="王波";
$obj2->age=7;
$obj3=new Student();
$obj3->name="迟方毅";
$obj3->age=8;
$obj4=new Student();
$obj4->name="段狗";
$obj4->age=9;
$obj5=new Student();
$obj5->name="小高";
$obj5->age=10;
$obj6=new Student();
$obj6->name="老cai";
$obj6->age=11;
$data[$obj1->name]=$obj1->age;
$data[$obj2->name]=$obj2->age;
$data[$obj3->name]=$obj3->age;
$data[$obj4->name]=$obj4->age;
$data[$obj5->name]=$obj5->age;
$data[$obj6->name]=$obj6->age;
// print_r($data);
print_r(four($data));
function four($data){
return max($data);
}
?>
| 26b43876e220cbe0413dbc97fcb17368876f3dae | [
"PHP"
] | 1 | PHP | BestJason1/text_04b | 34461a68249cb462b466c8c9a60e5bb5ee6e511b | 3fe217d83e12773ac0ccfaae44d73d6f6c7c811d | |
refs/heads/master | <repo_name>chary12345/java-1.8<file_sep>/src/main/java/com/multithread/MyImpl.java
package com.multithread;
public class MyImpl {
public static void main(String[] args) {
char[] c= new char[]{103,111,111,100,0,110,105,103,104,116,160,98,114,111};
for (char t : c) {
System.out.print(t);
}
}
}
<file_sep>/src/main/java/com/task/sudocodes/ArrayCompare.java
package com.task.sudocodes;
import java.util.Arrays;
public class ArrayCompare {
public static void main(String[] args) {
int[] a = {1,2,3};
int[] b = {1,2,3};
if(Arrays.equals(a, b)){
System.out.println("two arrays are same");
}
else{
System.out.println("two arrays are not same");
}
ArrayConcat.arraymerge();
}
}
/**
* array concatanation in java(binding two arrays)
* @author ADMIN
*
*/
class ArrayConcat{
public static void arraymerge(){
int[] x= {1,2,3,4};
int[] y={5,6,7,8};
int l1 = x.length;
int l2 = y.length;
int[] result = new int[l1+l2];
System.arraycopy(x, 0, result, 0, l1);
System.arraycopy(y, 0, result, l1, l2);
System.out.println(Arrays.toString(result));
}
}
<file_sep>/src/main/java/com/practice/sudocodes/SumOfTwoNumbers.java
//To print sum of two digits of x+y;
package practice.sudocodes;
import java.util.Scanner;
public class SumOfTwoNumbers {
@SuppressWarnings("resource")
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("please enter a value of x=");
int x = sc.nextInt();
System.out.print("please enter a value of y=");
int y = sc.nextInt();
int z = x+y;
System.out.println("sum of x+y = "+ x+y);
}
}
<file_sep>/src/main/java/com/com/constructor/CustOwnSinglton.java
package com.constructor;
public class CustOwnSinglton {
private static CustOwnSinglton cs = null;
private static int id;
private static String name;
public int getId() {
return id;
}
public String getName() {
return name;
}
//write getInstance method for(facory method) create own single tone
public static CustOwnSinglton getCustOwnSinglton(){
if(cs==null)
return cs=new CustOwnSinglton();
else
return cs;
}
}
class CTest{
public static void main(String[] args) {
System.out.println(CustOwnSinglton.getCustOwnSinglton().hashCode());
System.out.println(CustOwnSinglton.class.hashCode());
}
}<file_sep>/src/main/java/com/java/TestDivision.java
package com.java;
import com.list.DivisionImpl;
import com.list.inter.IDivision;
public class TestDivision {
public static void main(String[] args) {
IDivision div = new DivisionImpl();
div.division(22, 11);
}
}
<file_sep>/src/main/java/com/java/TestAnonymus.java
package com.java;
import com.list.CaluculatorImpl;
import com.list.inter.IParent;
public class TestAnonymus {
public static void main(String[] args) {
IParent ip = new CaluculatorImpl(){
};
}
}
<file_sep>/src/main/java/com/practice/sudocodes/Swapping.java
//swapping 2 numbers
package com.practice.sudocodes;
import java.util.Scanner;
/**
*
* @author ADMIN
*swapping o inteegers
*with out using third variable
*/
public class Swapping {
@SuppressWarnings("resource")
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("enter value of x :");
int x = sc.nextInt();
System.out.println("enter value of y :");
int y = sc.nextInt();
System.out.println("--------------------------------------");
x=x+y;
y=x-y;
x=x-y;
System.out.println("--------------------------------------");
System.out.println("after swapping x= "+x);
System.out.println("after swapping y = "+y);
}
}
/*//swapping theory : assume two numbers x&y. add two nubers.do result substract second number,
//keep aside the value(1).these value substracted from addition of two numbers.
//see the out puts=swapped.value is 2,result is 1.*/<file_sep>/src/main/java/com/list/DivisionImpl.java
package com.list;
import com.list.inter.IDivision;
public class DivisionImpl implements IDivision {
@Override
public void division(int a, int b) {
System.out.println("The division Value is"+(a/b));
}
}
<file_sep>/src/main/java/com/list/inter/ISquare.java
package com.list.inter;
@FunctionalInterface
public interface ISquare {
public int squareNum(int n);
}
<file_sep>/src/main/java/com/task/sudocodes/ReverseNumbers.java
package task.sudocodes;
//reverse number using whike loop
import java.util.Scanner;
public class ReverseNumbers {
static double number;
static double reverseNumber;
@SuppressWarnings("resource")
public static void main(String[] args) {
//checkstt();
System.out.print("enter number to reverse :");
Scanner scanner = new Scanner(System.in);
number = scanner.nextDouble();
while(number != 0){//25
reverseNumber = reverseNumber*10.00;//0//50//
reverseNumber = reverseNumber+number%10.00;//5//50+0.25=52.5
number = number/10;//2.5//2.5/10=0.25//
}//int ignores decimals
System.out.println("reverse number is : "+reverseNumber);
}
private static void checkstt() {
final String s10 = "ravi";
String s11 = s10.concat("ravi");
System.out.println(s11);
Integer i=10;
Integer i1=10;
System.out.println(i==i1);
Integer i2=127;
Integer i3=127;
System.out.println(i2==i3);
String s1 = new String("Java");
s1.concat("developer");
String s6 =s1.concat("java");
System.out.println("s1 is : "+s1);
String s2 = new String("today");
final String s3= new String("today");
String s4 = s2+"exam";
String s5 = s3+"exam";
System.out.println(" double equals is "+s4==s5);
System.out.println("s4.equals s5 is :"+s4.equals(s5));
System.out.println("double equals "+s2==s3);
System.out.println(s1.intern());
System.out.println(s1==s1.intern());
System.out.println(s1.intern());
System.out.println(s2.intern()==s3.intern());
System.out.println( "intern method "+s6.intern());
}
}
<file_sep>/src/main/java/com/list/SquareImpl.java
package com.list;
import com.list.inter.ISquare;
public class SquareImpl implements ISquare{
@Override
public int squareNum(int n) {
return n*n;
}
}
<file_sep>/src/main/java/com/serializable/EvenArrayOddArray.java
package com.serializable;
import java.util.Arrays;
import java.util.stream.IntStream;
public class EvenArrayOddArray {
public static void main(String[] args) {
int[] a= {12,6,51};int[] b = {22,19,63,84,65};
int alen = a.length;
int blen = b.length;
int clen = alen+blen;
int[] c = new int[clen];
for (int i = 0; i < a.length; i++) {
c[i]=a[i];
}
for (int i = 0; i < b.length; i++) {
c[alen+i]=b[i];
}
for (int i = 0; i < c.length; i++) {
if(c[i]<=c[i]){
System.out.println(c[i]);
}
}
Arrays.sort(c);
String string = Arrays.toString(c);
System.out.println(Arrays.toString(c));
// System.out.println(string);
int[] i = {1,6,4,2,3,551,62,96,45};
EvenArrayOddArray eo = new EvenArrayOddArray();
System.out.println("max array is"+eo.maxArray(i));
int q = (int) EvenArrayOddArray.maxArray(i);
int p = EvenArrayOddArray.minArray(i);
int result = q-p;
System.out.println(result);
IntStream sorted = Arrays.stream(i).sorted();
System.out.println(sorted.toArray());
System.out.print(false);
}
private static int minArray(int[] min) {
int minValue = min[0];
for (int i = 1; i < min.length; i++) {
if(min[i]<minValue){
minValue = min[i];
}
}
return minValue;
}
private static int maxArray(int[] max) {
int maxValue = max[0];
for (int i = 1; i < max.length; i++) {
if(max[i]>maxValue){
maxValue = max[i];
}
}
return maxValue;
}
}
<file_sep>/src/main/java/com/task/sudocodes/ArraySum.java
//sum of arrays using with for loop
package task.sudocodes;
public class ArraySum {
public static void main(String[] args) {
int[] a= new int[]{1,2,3,4,5};
int result = 0;
for(int i =0; i<a.length;i++){
result = result+a[i];
}
System.out.println("sum of arrays is : "+result);
}
}
<file_sep>/src/main/java/com/supplier/Number50List.java
package com.supplier;
import java.util.LinkedList;
import java.util.function.Function;
import java.util.stream.Collectors;
public class Number50List {
public static void main(String[] args) {
LinkedList<Integer> list = new LinkedList<>();
//ArrayList<Integer> list = new ArrayList<>();
// HashSet<Integer> list = new HashSet<>();
// LinkedHashSet<Integer> list = new LinkedHashSet<>();
//TreeSet<Integer> list = new TreeSet<>();
list.add(22);
list.add(25);
list.add(36);
list.add(41);
list.add(42);
list.add(46);
list.add(49);
list.add(56);
list.add(57);
list.add(58);
list.add(69);
list.add(76);
Function<Integer, Integer> f = t -> {
if (t < 50)
return (t + 10);
else
return t;
};
System.out.println("the final output: ");
//Consumer<Integer> c = t->System.out.println(t);
/**
* even list of integers,add 10 to <50 integers,&print greternthan 50 numbers with non duplicates.
*/
list.stream().filter(i->i%2==0).map(f).filter(t->t>50).collect(Collectors.toSet()).forEach(t->System.out.println(t));
}
}
<file_sep>/src/main/java/com/task/sudocodes/ReverseRightAnleStar.java
package task.sudocodes;
/**
*
* @author ADMIN * * *
*/
public class ReverseRightAnleStar {
public static void main(String[] args) {
for(int i=0; i<4; i++){
for(int j=2*(4-i); j>=0; j--){
System.out.print(" ");
}
for(int j=0;j<=i;j++){
System.out.print(" *");
}
System.out.println();
}
}
}
<file_sep>/src/main/java/com/list/inter/IParent.java
package com.list.inter;
@FunctionalInterface
public interface IParent {
public void m1(int a, int b);
}
<file_sep>/src/main/java/com/multithread/B.java
package com.multithread;
class A{
int x;
public A(int y){
x=x+y;
}
public String toString(){
return "x";
}
}
public class B{
public static void main(String[] args) {
System.out.println(new A(10));
}
}
<file_sep>/src/main/java/com/predicate/PresicateForEmployeSalary.java
package com.predicate;
import java.awt.List;
import java.util.ArrayList;
import java.util.function.Predicate;
public class PresicateForEmployeSalary {
public static void main(String[] args) {
ArrayList<Employee> emplist = new ArrayList<>();
emplist.add(new Employee("raju", 100, 102000));
emplist.add(new Employee("mahendar", 103, 1180000));
emplist.add(new Employee("vijayender", 104, 1350000));
emplist.add(new Employee("devendar", 105, 990000));
emplist.add(new Employee("manikanta", 106, 1140000));
emplist.add(new Employee("dattatreya", 107, 1040000));
/**
* get the employee who having more than 104k
* in1.8 java
*/
Predicate<Employee> p = emp-> emp.getSalary()>10;
for (Employee emp : emplist) {
if(p.test(emp)){
System.out.println(emp);
}
}
/**
* performing predicate opperation to print only even number id employee
*/
Predicate<Employee> p1 = emp->emp.getId()%2==0;
System.out.println("even number employee list");
for (Employee empeven : emplist) {
if(p1.test(empeven))
System.out.println(empeven);
}
}
}
<file_sep>/src/main/java/com/practice/sudocodes/FibinocciSeries.java
/*//write a program to print fibinocci series
In fibonacci series, next number is the sum of previous two numbers .
for example 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55 etc. The first two numbers of fibonacci series are 0 and 1.*/
package com.practice.sudocodes;
public class FibinocciSeries {
public static void main(String[] args) {
/* int x=0;
int y = 1;
System.out.println("fibinoccseries of first 100 numbers are given below");
System.out.print(x+" "+y);
int z;
for(int i=2;i<=10;++i){
z=x+y;
System.out.print(" "+z);
x=y;
y=z;
}*/
int x = 0;
int y = 1;
int z;
for (int i=1;i<10;++i){
z=x+y;
System.out.println(" "+z);
x=y;
y=z;
}
}
}
<file_sep>/src/main/java/com/task/sudocodes/MapSream.java
package com.task.sudocodes;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.stream.Collectors;
public class MapSream {
public static void main(String[] args) {
HashMap<Integer, String> m1 = new HashMap<>();
m1.put(1, "vikaram");
m1.put(15, "vikaram");
m1.put(19, "vikaram");
m1.put(29, "vikaram");
m1.put(86, "vikaram");
m1.put(33, "vikaram");
ArrayList<Integer> al = new ArrayList<Integer>(m1.keySet());
HashSet<Integer> hs = new HashSet<>(m1.keySet());
hs.stream().sorted().collect(Collectors.toSet()).forEach(t->System.out.println(t));
//al.stream().sorted().collect(Collectors.toSet()).forEach(t->System.out.println(t));
//m1.keySet(). stream().forEach(t->System.out.println(t));
//m1.keySet().stream().map(e->e.compareTo(e)).collect(Collectors.toList()).forEach(t->System.out.println(t));
}
}<file_sep>/src/main/java/com/supplier/NumberPlus50.java
package com.supplier;
import java.util.Arrays;
import java.util.function.IntPredicate;
import java.util.function.IntUnaryOperator;
public class NumberPlus50 {
public static void main(String[] args) {
// std();
int[] values = new int[] { 22, 36, 42, 46, 49, 51, 67, 68, 71, 76, 84, 89, 92, 96 };
// IntStream stream1 = Arrays.stream(values);
// IntPredicate intP = t -> t % 2 == 0;
IntUnaryOperator f = t -> {
if (t < 50)
return (t + 10);
else
return t;
};
IntPredicate intP1 = t -> t > 50;
/*System.out.println("the final out put as");
for (int i : values) {
if (intP.test(i)) {
int j = f.apply(i);
if (intP1.test(j))
System.out.println(j);
}
}*/
Arrays.stream(values).filter(t->t%2==0).map(f).filter(intP1).map(t->t+10).filter(t->t<100).forEach(x -> System.out.println(x));
}
}
<file_sep>/src/main/java/com/java/TestCalLambda.java
package com.java;
import com.list.inter.IParent;
public class TestCalLambda {
public static void main(String[] args) {
IParent cal =( a, b)->{
System.out.println(a+b);
};
cal.m1(10, 20);
}
}
<file_sep>/src/main/java/com/predicate/Predicatest.java
package com.predicate;
public class Predicatest {
public static void main(String[] args) {
PredicateImpl p = new PredicateImpl();//predicate always returns the boolean value
System.out.println(p.test(12365));
System.out.println(p.test(125));
System.out.println(p.test(365));
System.out.println(p.test(12));
}
}
<file_sep>/src/main/java/com/predicate/CharacterCountInString.java
package com.predicate;
public class CharacterCountInString {
static final int MAX_CHAR = 200;
public static void main(String[] args) {
String s = "<NAME>";
int count[] = new int[MAX_CHAR];
int l = s.length();
//intialize count array Index
for (int i = 0; i < l; i++) {
count[s.charAt(i)]++;
/*for (int i : count) {
count[s.charAt(i)]++;*/
}
//create an array of given to string size
char ch[] = new char[s.length()];
System.out.println("given string is :"+s);
for (int i = 0; i < l; i++) {
// for (char i : ch) {
ch[i] = s.charAt(i);
int k = 0;
for(int j=0; j<=i; j++){
if(s.charAt(i) == ch[j])
k++;
}
if(k == 1)
System.out.println("Number of times repeated character "+s.charAt(i) +" is :"+count[s.charAt(i)]);
}
}
}
<file_sep>/src/main/java/com/practice/sudocodes/PasswordValidator.java
package com.practice.sudocodes;
/**
*
* @author ADMIN
*Must contain at least one digit
Must contain at least one of the following special characters @, #, $
Length should be between 6 to 8 characters.
*/
public class PasswordValidator {
public static int display(String password){
//copied from google
if(password.matches(".*[0-9]{1,}.*") && password.matches(".*[@#$]{1,}.*") && password.length()>=6 && password.length()<=8)
{
return 1;
}
else
{
return -1;
}
}
public static int dis(String pass){
if(pass.matches(".*[0-9]{1,}.*")&&pass.length()==6){
return 1;
}
else{
}
return -1;
}
}
<file_sep>/src/main/java/com/task/sudocodes/EvenAndOddNumbers.java
package task.sudocodes;
import java.util.Scanner;
import java.util.function.Predicate;
public class EvenAndOddNumbers {
static int x;
@SuppressWarnings("resource")
public static void main(String[] args) {
System.out.print("please enter value of x(integers) :");
Scanner scanner = new Scanner(System.in);
int x = scanner.nextInt();
if(x%2==0){
System.out.println
(x+" is a even number");
}
else{
System.out.println(x+" is a odd number");
}
}
}
<file_sep>/src/main/java/com/practice/sudocodes/WelcomeJava.java
package practice.sudocodes;
public class WelcomeJava {
public static void main(String[] args) {
System.out.println("Welcome to java.");
System.out.println("ready to write java programs.");
}
}
<file_sep>/src/main/java/com/task/sudocodes/ReverseString.java
package task.sudocodes;
//reverse string in these program using for loop
import java.util.Scanner;
public class ReverseString {
static String s;
@SuppressWarnings("resource")
public static void main(String[] args) {
System.out.print("enter a string reverse :");
Scanner scanner = new Scanner(System.in);
String s = scanner.nextLine();
String reverse = " ";
for(int i= s.length()-1 ; i>=0;i--){
reverse = reverse+s.charAt(i);
}
System.out.println("reverse of above string is : "+reverse);
}
}
/* System.out.print("enter a string :");
Scanner scanner = new Scanner(System.in);
String string = scanner.nextLine();
String rev= "";
System.out.print("reverse of string is :");
for(int i =string.length()-1; i>=0; i--){
rev = rev+string.charAt(i);
}
System.out.println(rev);*/<file_sep>/src/main/java/com/predicate/PredicateForEmpty.java
package com.predicate;
import java.util.function.Predicate;
public class PredicateForEmpty {
public static void main(String[] args) {
String name ="rakesh";
String id = "";
String sal = null;
Predicate<String> p = t->t.isEmpty() || t==null;
System.out.println(p.test(name));
System.out.println(p.test(id));
//System.out.println(p.test(sal));
System.out.println("mahesh");
}
}
<file_sep>/src/main/java/com/task/sudocodes/TablesMaths.java
package com.task.sudocodes;
import java.util.Scanner;
public class TablesMaths {
private static final Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
/* Integer N = scanner.nextInt();
// scanner.skip("(\r\n|[\n\r\u2028\u2029\u0085])?");
String x1 = scanner.next();
for(Integer c= 1; c<=10; c++){
Integer x=N*c;
String s = x.toString();
if(s.contains(x1)){
System.out.println();
}else {
System.out.println(N+" x "+c+" = "+x);}
}*/
//like();
reverse();
scanner.close();
}
private static void reverse() {
// TODO Auto-generated method stub
}
private static void like() {
// TODO Auto-generated method stub
String x1 = scanner.next();
Integer x = scanner.nextInt();
for(x=x;x>=0;--x){
String s1 = x.toString();
for(int i=s1.length();i>=0;i--){
int s2=s1.charAt(i);
}
if(s2.contains(x1))
System.out.print("");
else
System.out.println(x);
}
}
}
<file_sep>/src/main/java/com/task/sudocodes/DeadLockAvoid.java
package com.task.sudocodes;
public class DeadLockAvoid extends Thread{
@Override
public void run() {
m2();
m1();
}
public static void main(String[] args) {
Thread t = new Thread();
t.start();
}
public void m1() {
synchronized (Integer.class) {
System.out.println("aquired string class");
synchronized (String.class) {
System.out.println("aquired integer class");
}
}
}
public void m2() {
synchronized (Integer.class) {
System.out.println("aquired integer class");
synchronized (String.class) {
System.out.println("aquired string class");
}
}
}
}
<file_sep>/src/main/java/com/immutable/CustomArraylist.java
package com.immutable;
import java.util.ArrayList;
import java.util.Comparator;
public class CustomArraylist implements Comparator<CustomArraylist> {
public static void main(String[] args) {
ArrayList<Integer> l = new ArrayList<>();
l.add(12);
l.add(1265);
l.add(162);
l.add(120);
System.out.println();
//Collections.sort(l, );
System.out.println(l);
}
@Override
public int compare(CustomArraylist a, CustomArraylist b) {
// TODO Auto-generated method stub
if (a == b) {
return 0;
}
if (a != b) {
System.out.println();
return -1;
} else {
return 1;
}
}
}
<file_sep>/src/main/java/com/immutable/MyImmutable.java
package com.immutable;
public final class MyImmutable {
private int mobile;
private String name;
public int getMobile() {
return mobile;
}
public String getName() {
return name;
}
public MyImmutable(int mobile, String name) {
super();
this.mobile = mobile;
this.name = name;
}
public int modifydetails(int mobile){
if(this.mobile==mobile)
return mobile;
else
return new MyImmutable(mobile, name);
/*if(this.mobile==mobile)
return mobile;
else
return new MyImmutable(mobile, name);
*/
}
}
class test{
public static void main(String[] args) {
MyImmutable im = new MyImmutable(954545, "battu");
MyImmutable mm = im.modifydetails(954545);
System.out.println(mm.getMobile());
}
}<file_sep>/src/main/java/com/function/Studentgrades.java
package com.function;
import java.util.ArrayList;
import java.util.function.Function;
public class Studentgrades {
public static void main(String[] args) {
ArrayList<StudentPojo> list = new ArrayList<>();
list.add(new StudentPojo("ameer", 100, "vittalacharya", 960));
list.add(new StudentPojo("gareeb", 101, "vittalacharya", 460));
list.add(new StudentPojo("jareeb", 104, "vittalacharya", 860));
list.add(new StudentPojo("kareeb", 102, "vittalacharya", 679));
list.add(new StudentPojo("aayub", 106, "vittalacharya", 963));
list.add(new StudentPojo("masthan", 105, "vittalacharya", 954));
list.add(new StudentPojo("mujafar", 103, "vittalacharya", 500));
Function<StudentPojo, Character> grade = s->{
if(s.getMarks()>850)
return 'A';
else if(s.getMarks()>750)
return 'B';
else if(s.getMarks()>=500)
return 'c';
else
return 'F';
};
for (StudentPojo gradelist : list) {
System.out.println(gradelist+" grade-"+grade.apply(gradelist));
}
}
}
<file_sep>/src/main/java/com/practice/sudocodes/FactorialProgram.java
//Factorial Program in Java: Factorial of n is the product of all positive descending integers. Factorial of n is denoted by n!. For example:
package com.practice.sudocodes;
import java.util.Scanner;
public class FactorialProgram {
@SuppressWarnings("resource")
public static void main(String[] args) {
System.out.println("enter a number to find facorial");
Scanner sc = new Scanner(System.in);
int number = sc.nextInt();
int factorial = 1;
for (int i = 1; i <= number; i++) {
factorial=factorial*i;
}
System.out.println(number+" factorial is : "+factorial);
}
}
<file_sep>/src/main/java/com/serializable/Serialize.java
package com.serializable;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.ObjectOutputStream.PutField;
public class Serialize {
@SuppressWarnings("resource")
public static void main(String[] args) throws Exception{
/*Student s1 = new Student("Raju", 100, "Siei", "eeraptnam");
FileOutputStream fio = new FileOutputStream("F:\\New folder (3)\\Peru.txt");
System.out.println("file created");
ObjectOutputStream obj = new ObjectOutputStream(fio);
obj.writeObject(s1);
System.out.println("end");
obj.close(); */
FileInputStream fis = new FileInputStream("F:\\New folder (3)\\Peru.txt");
System.out.println("file loaded");
ObjectInputStream oi= new ObjectInputStream(fis);
Student s2 = (Student) oi.readObject();
System.out.println(s2);
System.out.println("file read succesefull");
}}<file_sep>/src/main/java/com/predicate/PredicateLambda.java
package com.predicate;
import java.util.function.Predicate;
public class PredicateLambda {
public static void main(String[] args) {
Predicate<Integer> p = i->i%2==0;
System.out.println(p.test(2561
+6469597));
System.out.println(p.test(256119665));
System.out.println(p.test(25666));
}
}
| 86204e04b6cd83a48166c66bb7238a6950781279 | [
"Java"
] | 37 | Java | chary12345/java-1.8 | aa997d36edcdd467bd85162721a2c00a5101f867 | de1edb495c68ffcf0236f99683c6c60b8d1725ba | |
refs/heads/main | <repo_name>Loan555/Partent-Locking-CustomView<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/views/LockCustomView.kt
package com.loan555.customview_canvas_animation.views
import android.content.Context
import android.graphics.*
import android.util.AttributeSet
import android.util.Log
import android.view.MotionEvent
import android.view.View
import androidx.core.content.ContextCompat
import androidx.lifecycle.LiveData
import androidx.lifecycle.MutableLiveData
import com.loan555.customview_canvas_animation.R
const val PARTITION_RATIO = 1 / 3f
class LockCustomView(context: Context?, attrs: AttributeSet?) : View(context, attrs) {
private var myPassword = ""
private val _password = MutableLiveData<String>().apply {
value = myPassword
}
val password: LiveData<String> = _password
private val countRow = 3
private val countColumn = 3
private var rectIndex = Pair(-1, -1)
private var touching = false
private var startPoint: Point? = null
private var endPoint: Point? = null
private val paint = Paint(Paint.ANTI_ALIAS_FLAG).apply {
style = Paint.Style.FILL
strokeWidth = 12f
color = Color.DKGRAY
}
private val highLightPaint = Paint().apply {
color = ContextCompat.getColor(context!!, R.color.hilight)
style = Paint.Style.FILL
isAntiAlias = true
}
private lateinit var squares: Array<Array<Rect>>
private lateinit var squaresData: Array<Array<String>>
private val listPoint: ArrayList<Point> = ArrayList()
override fun onDraw(canvas: Canvas?) {
Log.d("aaa", "onDraw")
super.onDraw(canvas)
initializeTicTacToeSquares()
if (touching) {
drawHighlightDot(canvas)
}
squaresData.forEachIndexed { i, strings ->
strings.forEachIndexed { j, s ->
drawDot(canvas, squares[i][j])
if (s != "")
drawTextInsideRectangle(canvas, squares[i][j], s)
}
}
if (startPoint != null && endPoint != null)
drawLine(canvas, startPoint!!, endPoint!!)
drawAllLine(canvas)
}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
Log.d("aaa", "onMeasure")
super.onMeasure(widthMeasureSpec, heightMeasureSpec)
squaresData = Array(countRow) { Array(countColumn) { "" } }
}
override fun onTouchEvent(event: MotionEvent?): Boolean {
val x = event?.x
val y = event?.y
return when (event?.action) {
MotionEvent.ACTION_DOWN -> {
if (x != null && y != null) {
listPoint.clear()
myPassword = ""
rectIndex = getRectIndexesFor(x, y)
if (rectIndex.first != -1 && rectIndex.second != -1) {
touching = true
addKey(rectIndex.first, rectIndex.second)
postInvalidate()
}
}
true
}
MotionEvent.ACTION_MOVE -> {
if (x != null && y != null) {
endPoint = Point(x.toInt(), y.toInt())
rectIndex = getRectIndexesFor(x, y)
if (rectIndex.first != -1 && rectIndex.second != -1) {
touching = true
addKey(rectIndex.first, rectIndex.second)
}
postInvalidate()
}
true
}
MotionEvent.ACTION_UP -> {
touching = false
if (myPassword.length < 4) {
squaresData = Array(countRow) { Array(countColumn) { "" } }
myPassword = ""
Log.e("aaa", "mat khau phai >= 4 ky tu")
}
listPoint.clear()
_password.value = myPassword
startPoint = null
endPoint = null
postInvalidate()
true
}
MotionEvent.ACTION_CANCEL -> {
true
}
else -> super.onTouchEvent(event)
}
}
private fun drawAllLine(canvas: Canvas?) {
if (listPoint.size >= 2) {
for (i in 0..listPoint.size - 2) {
drawLine(canvas, listPoint[i], listPoint[i + 1])
}
}
}
private fun addKey(i: Int, j: Int) {
val newKey = (i * 3 + j + 1).toString()
var count = false
myPassword.forEach {
if (it.toString() == newKey) {
count = true
return@forEach
}
}
if (!count) {
val rect = squares[rectIndex.first][rectIndex.second]
val point = Point(rect.centerX(), rect.centerY())
listPoint.add(point)
startPoint = point
squaresData[i][j] = newKey
myPassword += squaresData[i][j]
Log.d("aaa", "pass = $<PASSWORD>")
}
}
private fun drawHighlightDot(canvas: Canvas?) {
if (rectIndex.first != -1 && rectIndex.second != -1) {
val rect = squares[rectIndex.first][rectIndex.second]
canvas?.drawCircle(
rect.exactCenterX(),
rect.exactCenterY(),
(rect.right - rect.left).toFloat(),
highLightPaint
)
}
}
private fun getRectIndexesFor(x: Float, y: Float): Pair<Int, Int> {
squares.forEachIndexed { i, arrayOfRects ->
arrayOfRects.forEachIndexed { j, rect ->
if (rect.contains(x.toInt(), y.toInt()))
return Pair(i, j)
}
}
return Pair(-1, -1)// x, y do not lie in our view
}
private fun drawDot(canvas: Canvas?, rect: Rect) {
val radio = (rect.right - rect.left) / 4f
canvas?.drawCircle(
rect.left.toFloat() + (radio * 2),
rect.top.toFloat() + (2 * radio),
radio,
paint
)
}
private fun drawLine(canvas: Canvas?, startPoint: Point, endPoint: Point) {
canvas?.drawLine(
startPoint.x.toFloat(),
startPoint.y.toFloat(),
endPoint.x.toFloat(),
endPoint.y.toFloat(),
paint
)
}
private fun initializeTicTacToeSquares() {
squares = Array(countRow) { Array(countColumn) { Rect() } }
val xUnit = (width * PARTITION_RATIO).toInt() // one unit on x-axis
val yUnit = (height * PARTITION_RATIO).toInt() // one unit on y-axis
for (j in 0 until countRow) {
for (i in 0 until countColumn) {
val cx = (xUnit * (i + 0.5f)).toInt()
val cy = (yUnit * (j + 0.5f)).toInt()
val radio = (width * PARTITION_RATIO * 0.1).toInt()
squares[j][i] =
Rect(
cx - radio, cy - radio, cx + radio, cy + radio
)
}
}
}
private fun drawTextInsideRectangle(canvas: Canvas?, rect: Rect, str: String) {
val xOffset = paint.measureText(str) * 0.5f
val yOffset = paint.fontMetrics.ascent * -0.4f
val textX = (rect.exactCenterX()) - xOffset
val textY = (rect.exactCenterY()) + yOffset
canvas?.drawText(str, textX, textY, paint)
}
}<file_sep>/settings.gradle
rootProject.name = "CustomView-Canvas-Animation"
include ':app'
<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/AppPreferences.kt
package com.loan555.customview_canvas_animation
import android.content.Context
import android.content.SharedPreferences
import androidx.core.content.edit
object AppPreferences {
private const val NAME = "SpinKotlin"
private const val MODE = Context.MODE_PRIVATE
lateinit var preferences: SharedPreferences
private val password = Pair("<PASSWORD>", null)
fun init(context: Context) {
preferences = context.getSharedPreferences(NAME, MODE)
}
var myPassword: String?
get() = preferences.getString(password.first, password.second)
set(value) = preferences.edit {
this.putString(password.first, value)
}
}<file_sep>/README.md
"# Partent-Locking-CustomView"
<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/views/EmotionalFaceView.kt
package com.loan555.customview_canvas_animation.views
import android.content.Context
import android.graphics.*
import android.util.AttributeSet
import android.util.Log
import android.view.View
const val myTag = "aaa"
class EmotionalFaceView(context: Context?, attrs: AttributeSet?) : View(context, attrs) {
// Paint object for coloring and styling
private val paint = Paint(Paint.ANTI_ALIAS_FLAG)
// Some colors for the face background, eyes and mouth.
private var faceColor = Color.YELLOW
private var eyesColor = Color.BLACK
private var mouthColor = Color.WHITE
private var borderColor = Color.BLACK
// Face border width in pixels
private var borderWidth = 4.0f
// View size in pixels
var size = 320
private val mouthPath = Path()
override fun draw(canvas: Canvas?) {
Log.d(myTag,"draw")
super.draw(canvas)
drawFaceBackground(canvas)
drawEyes(canvas)
drawMouth(canvas)
}
override fun onMeasure(widthMeasureSpec: Int, heightMeasureSpec: Int) {
Log.d(myTag,"onMeasure")
super.onMeasure(widthMeasureSpec, heightMeasureSpec)
setMeasuredDimension(size, size)
// setMeasuredDimension(size,size)
}
private fun drawFaceBackground(canvas: Canvas?) {
// 1
paint.color = faceColor
paint.style = Paint.Style.FILL
// 2
val radius = size / 2f
// 3
canvas?.drawCircle(size / 2f, size / 2f, radius, paint)
// 4
paint.color = borderColor
paint.style = Paint.Style.STROKE
paint.strokeWidth = borderWidth
// 5
canvas?.drawCircle(size / 2f, size / 2f, radius - borderWidth / 2f, paint)
}
private fun drawEyes(canvas: Canvas?) {
// 1
paint.color = eyesColor
paint.style = Paint.Style.FILL
// 2
val leftEyeRect = RectF(size * 0.32f, size * 0.23f, size * 0.43f, size * 0.50f)
canvas?.drawOval(leftEyeRect, paint)
// 3
val rightEyeRect = RectF(size * 0.57f, size * 0.23f, size * 0.68f, size * 0.50f)
canvas?.drawOval(rightEyeRect, paint)
}
private fun drawMouth(canvas: Canvas?) {
// 1 move to (x0,y0)
mouthPath.moveTo(size * 0.22f, size * 0.7f)
// 2 vẽ một đường cong bắt đầu từ điểm (x0, y0) đi qua các điểm (x1, y1) và (x2, y2)
mouthPath.quadTo(size * 0.50f, size * 0.70f, size * 0.78f, size * 0.70f)
// 3 vẽ 1 đường cong từ điểm (x2, y2) tới điẻm (x0, y0) đi qua điểm (x3, y3)
mouthPath.quadTo(size * 0.50f, size * 0.90f, size * 0.22f, size * 0.70f)
// 4
paint.color = mouthColor
paint.style = Paint.Style.FILL
// 5
canvas?.drawPath(mouthPath, paint)
}
}<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/model/Dot.kt
package com.loan555.customview_canvas_animation.model
data class Dot(
val rowIndex: Int = 0,
val columnIndex: Int = 0,
val leftPoint: Int = 0,
val rightPoint: Int = 0,
val topPoint: Int = 0,
val bottomPoint: Int = 0,
val key: String? = null
)
<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/AppViewModel.kt
package com.loan555.customview_canvas_animation
import androidx.lifecycle.LiveData
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
class AppViewModel : ViewModel() {
private val _pass = MutableLiveData<String>().apply {
value = ""
}
private val _page = MutableLiveData<Int>().apply {
value = 0
}
val page: LiveData<Int> = _page
fun setPass(newPass: String) {
_pass.value = newPass
}
fun checkPass(commitPass: String): Boolean {
if (commitPass == _pass.value)
return true
return false
}
fun currentPage(number: Int) {
_page.value = number
}
}<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/ui/home/HomeFragment.kt
package com.loan555.customview_canvas_animation.ui.home
import android.animation.*
import android.graphics.drawable.AnimatedImageDrawable
import android.graphics.drawable.AnimatedVectorDrawable
import android.graphics.drawable.AnimationDrawable
import android.os.Build
import android.os.Bundle
import android.transition.Transition
import android.transition.TransitionManager
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.view.animation.Animation
import android.view.animation.AnimationSet
import android.view.animation.AnimationUtils
import android.widget.Toast
import androidx.annotation.RequiresApi
import androidx.fragment.app.Fragment
import androidx.lifecycle.ViewModelProvider
import androidx.lifecycle.ViewModelProviders
import com.loan555.customview_canvas_animation.AppViewModel
import com.loan555.customview_canvas_animation.R
import com.loan555.customview_canvas_animation.databinding.FragmentHomeBinding
import java.lang.Exception
class HomeFragment : Fragment() {
private lateinit var viewModel: AppViewModel
private var _binding: FragmentHomeBinding? = null
private lateinit var animationDrawable: AnimationDrawable
// This property is only valid between onCreateView and
// onDestroyView.
private val binding get() = _binding!!
private lateinit var animation: Animation
@RequiresApi(Build.VERSION_CODES.LOLLIPOP)
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
viewModel = activity?.let {
ViewModelProviders.of(it).get(AppViewModel::class.java)
} ?: throw Exception("Activity is null")
_binding = FragmentHomeBinding.inflate(inflater, container, false)
binding.fadeIn.setOnClickListener {
animation = AnimationUtils.loadAnimation(this.context, R.anim.fade_in)
binding.img.startAnimation(animation)
}
binding.fadeOut.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.fade_out))
}
binding.blink.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.blink))
}
binding.zoomIn.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.zoom_in))
}
binding.zoomOut.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.zoom_out))
}
binding.rotate.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.rotate))
}
binding.move.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.move))
}
binding.slideUp.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.slide_up))
}
binding.slideIn.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.slide_in))
}
binding.slideDown.setOnClickListener {
binding.img.startAnimation(
AnimationUtils.loadAnimation(
this.context,
R.anim.slide_down
)
)
}
binding.slideOut.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.slide_out))
}
binding.sequential.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.squential))
}
binding.together.setOnClickListener {
binding.img.startAnimation(AnimationUtils.loadAnimation(this.context, R.anim.together))
}
binding.valueAnimator.setOnClickListener {
ValueAnimator.ofFloat(0f, 100f).apply {
duration = 1000
repeatCount = Animation.INFINITE
start()
addUpdateListener {
binding.img.translationX = it.animatedValue as Float
}
}
}
binding.objectAnimation.setOnClickListener {
ObjectAnimator.ofFloat(binding.img, "translationX", -100f).apply {
duration = 1000
start()
}
}
binding.animatorSet.setOnClickListener {
val bouncer = AnimatorSet().apply {
play(ObjectAnimator.ofFloat(binding.img, "translationX", 100f).apply {
duration = 1000
}).before(ObjectAnimator.ofFloat(binding.img, "alpha", 1f, 0f).apply {
duration = 1000
})
}
AnimatorSet().apply {
play(bouncer).before(ObjectAnimator.ofFloat(binding.img, "alpha", 0f, 1f).apply {
duration = 1000
})
start()
}
}
binding.animationLayoutChange.setOnClickListener {
val animFadeOut = ObjectAnimator.ofFloat(binding.linearLayout, "scaleY", 1f, 0f).apply {
duration = 1000
}
val animSlideUp =
ObjectAnimator.ofFloat(binding.linearLayout, "scaleY", 0.0f, 1.0f).apply {
duration = 1000
}
AnimatorSet().apply {
play(animFadeOut).before(animSlideUp)
start()
addListener(object : AnimatorListenerAdapter() {
override fun onAnimationEnd(animation: Animator?) {
binding.linearLayout.visibility = View.GONE
}
})
}
}
binding.img.apply {
setBackgroundResource(R.drawable.rocket_image)
animationDrawable = background as AnimationDrawable
}
binding.img.setOnClickListener { animationDrawable.start() }
binding.vectorAnimation.apply {
setBackgroundResource(R.drawable.animatorvectordrawable)
val anim = background as AnimatedVectorDrawable
setOnClickListener { anim.start() }
}
binding.resetPass.setOnClickListener {
viewModel.currentPage(0)
}
return binding.root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/ui/login/LoginFragment.kt
package com.loan555.customview_canvas_animation.ui.login
import android.graphics.Color
import android.os.Bundle
import androidx.fragment.app.Fragment
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.Toast
import androidx.lifecycle.ViewModelProviders
import com.loan555.customview_canvas_animation.AppPreferences
import com.loan555.customview_canvas_animation.AppViewModel
import com.loan555.customview_canvas_animation.databinding.FragmentLoginBinding
import java.lang.Exception
class LoginFragment : Fragment() {
private lateinit var viewModel: AppViewModel
private var _binding: FragmentLoginBinding? = null
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
viewModel = activity?.let {
ViewModelProviders.of(it).get(AppViewModel::class.java)
} ?: throw Exception("Activity is null")
// Inflate the layout for this fragment
_binding = FragmentLoginBinding.inflate(inflater, container, false)
binding.lockCustomView.password.observe(viewLifecycleOwner, {
if (it != "") {
if (checkMyPass(it)) {
Toast.makeText(this.context, "Đăng nhập thành công", Toast.LENGTH_SHORT).show()
viewModel.currentPage(3)
}else{
binding.message.setTextColor(Color.RED)
binding.message.text = "Mật khẩu không đúng"
}
}
})
return binding.root
}
private fun checkMyPass(pass: String?): Boolean {
AppPreferences.init(this.requireContext())
if (pass == AppPreferences.myPassword) {
return true
}
return false
}
}<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/ui/notifications/NotificationsFragment.kt
package com.loan555.customview_canvas_animation.ui.notifications
import android.graphics.Color
import android.os.Bundle
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.Toast
import androidx.fragment.app.Fragment
import androidx.lifecycle.ViewModelProviders
import com.loan555.customview_canvas_animation.AppPreferences
import com.loan555.customview_canvas_animation.AppViewModel
import com.loan555.customview_canvas_animation.databinding.FragmentNotificationsBinding
import java.lang.Exception
class NotificationsFragment : Fragment() {
private lateinit var viewModel: AppViewModel
private var _binding: FragmentNotificationsBinding? = null
// This property is only valid between onCreateView and
// onDestroyView.
private val binding get() = _binding!!
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
viewModel = activity?.let {
ViewModelProviders.of(it).get(AppViewModel::class.java)
} ?: throw Exception("Activity is null")
_binding = FragmentNotificationsBinding.inflate(inflater, container, false)
binding.lockCustomView.password.observe(viewLifecycleOwner, {
if (it != "") {
if (viewModel.checkPass(it)) {
Toast.makeText(this.context, "Đặt mật khẩu thành công", Toast.LENGTH_SHORT)
.show()
AppPreferences.init(this.requireContext())
AppPreferences.myPassword = it
viewModel.currentPage(2)
viewModel.setPass("")
} else {
binding.message.setTextColor(Color.RED)
binding.message.text = "Vui lòng nhập đúng mật khẩu mới"
}
}
})
return binding.root
}
override fun onDestroyView() {
super.onDestroyView()
_binding = null
}
}<file_sep>/app/src/main/java/com/loan555/customview_canvas_animation/MainActivity.kt
package com.loan555.customview_canvas_animation
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.fragment.app.commit
import androidx.fragment.app.replace
import androidx.lifecycle.ViewModelProvider
import com.loan555.customview_canvas_animation.ui.dashboard.DashboardFragment
import com.loan555.customview_canvas_animation.ui.home.HomeFragment
import com.loan555.customview_canvas_animation.ui.login.LoginFragment
import com.loan555.customview_canvas_animation.ui.notifications.NotificationsFragment
class MainActivity : AppCompatActivity() {
private lateinit var viewModel: AppViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
viewModel = ViewModelProvider(this).get(AppViewModel::class.java)
AppPreferences.init(this)
val myPassword = AppPreferences.myPassword
if (myPassword != null) {
viewModel.currentPage(2)
}
viewModel.page.observe(this, {
when (it) {
0 -> {
supportFragmentManager.commit {
setCustomAnimations(
R.anim.slide_in,
R.anim.fade_out,
R.anim.slide_out,
R.anim.fade_in
)
setReorderingAllowed(true)
replace<DashboardFragment>(R.id.viewFragment)
addToBackStack("setPass")
}
}
1 -> {
supportFragmentManager.commit {
setCustomAnimations(
R.anim.slide_in,
R.anim.fade_out,
R.anim.slide_out,
R.anim.fade_in
)
setReorderingAllowed(true)
replace<NotificationsFragment>(R.id.viewFragment)
addToBackStack("commitPass")
}
}
2 -> {
supportFragmentManager.commit {
setCustomAnimations(
R.anim.slide_in,
R.anim.slide_out
)
setReorderingAllowed(true)
replace<LoginFragment>(R.id.viewFragment)
addToBackStack("animationDemo")
}
}
3 -> {
supportFragmentManager.commit {
setCustomAnimations(
R.anim.slide_in,
R.anim.slide_out
)
setReorderingAllowed(true)
replace<HomeFragment>(R.id.viewFragment)
addToBackStack("animationDemo")
}
}
}
})
setContentView(R.layout.activity_main)
}
override fun onBackPressed() {
when (viewModel.page.value) {
1 -> {
supportFragmentManager.popBackStack()
}
2 -> {
this.finish()
}
3 -> {
this.finish()
}
}
}
} | 638dd11caa74b2f1f79aae9ac1ba6073a07f5683 | [
"Markdown",
"Kotlin",
"Gradle"
] | 11 | Kotlin | Loan555/Partent-Locking-CustomView | 93d9f3b5359dc9cec96b6824376f2064035d149e | c4a89b81ee1bdf52f9a029c2ac4dbfb816633a7e | |
refs/heads/master | <file_sep>var noofsquares=6;
var colors=generatecolorrandom(noofsquares);
var squares=document.querySelectorAll(".square");
var pickedcolor=pickran();
var msgdisplay=document.querySelector("#rgb");
var threecode=document.querySelector("#color");
var head=document.querySelector("h1");
var ec=document.querySelector("#reset");
var hard=document.querySelector("#hard");
ec.addEventListener("click",function(){
ec.classList.add("ec2hd");
hard.classList.remove("ec2hd");
noofsquares=3;
colors=generatecolorrandom(noofsquares);
msgdisplay.textContent="Reset";
pickedcolor=pickran();
threecode.textContent=pickedcolor;
head.style.backgroundColor="steelblue";
for(var i=0;i<squares.length;i++){
if(colors[i]){
squares[i].style.backgroundColor=colors[i];
}
else{
squares[i].style.display="none";
}
}
})
hard.addEventListener("click",function(){
ec.classList.remove("ec2hd");
hard.classList.add("ec2hd");
noofsquares=6;
colors=generatecolorrandom(noofsquares);
msgdisplay.textContent="Reset";
head.style.backgroundColor="steelblue";
pickedcolor=pickran();
threecode.textContent=pickedcolor;
for(var i=0;i<squares.length;i++){
squares[i].style.backgroundColor=colors[i];
squares[i].style.display="block";
}
})
threecode.textContent=pickedcolor;
msgdisplay.textContent="Reset";
msgdisplay.addEventListener("click",function(){
colors=generatecolorrandom(noofsquares);
msgdisplay.textContent="Reset";
head.style.backgroundColor="steelblue";
pickedcolor=pickran();
threecode.textContent=pickedcolor;
for(var i=0;i<squares.length;i++){
squares[i].style.backgroundColor=colors[i];
}
})
for(var i=0;i<squares.length;i++){
squares[i].style.backgroundColor=colors[i];
squares[i].addEventListener("click",function(){
var clickedcolor=this.style.backgroundColor;
if(pickedcolor === clickedcolor){
msgdisplay.textContent="Correct/Reset";
changecolor(pickedcolor);
head.style.backgroundColor=pickedcolor;
}
else{
this.style.backgroundColor="#222222";
msgdisplay.textContent="InCorrect";
}
}
)
}
function changecolor(color){
for(var i=0;i<squares.length;i++){
squares[i].style.backgroundColor=color;
}
}
function pickran(){
var random=Math.floor(Math.random()*colors.length);
return colors[random];
}
function generatecolorrandom(num){
var arr=[];
for(var i=0;i<num;i++){
arr.push(sixran());
}
return arr;
}
function sixran(){
var r=Math.floor(Math.random()*256)
var g=Math.floor(Math.random()*256)
var b=Math.floor(Math.random()*256)
var d="rgb("+r+", "+g+", "+b+")";
return d;
}
| 22b21e6609c59bf650afcd9c66de2331cf1acc84 | [
"JavaScript"
] | 1 | JavaScript | Neethimani/Color-Guessor-Game | fcc3620476987c0509bf871d93201620e3346b8f | c7f2e23747b8036d378e22224f2b3465f1c97ec2 | |
refs/heads/master | <file_sep>import { HTTPHandler } from '@ifit/wool';
export class ExampleHandler extends HTTPHandler {
async run() {
return {
statusCode: 200,
body: { result: 'Hello world!' }
};
}
}
export default ExampleHandler.create();
| f55191e29ea896560050d0df0cf324b526fd2be6 | [
"JavaScript"
] | 1 | JavaScript | RKaneda9/test | 49243454447e5273da63b96360acc203f241335c | b6f465e7c7a78a2713025cd539c92fb522d121b2 | |
refs/heads/main | <repo_name>kimbcheh/anzsic-fairy<file_sep>/src/components/MainFooter.js
import { Footer, Text } from 'grommet'
function MainFooter() {
return (
<Footer background='brand' direction='column' pad='small'>
<Text as='small'>
DISCLAIMER: This is a just personal coding project and should not be relied
on for making any business or legal decisions
</Text>
<Text as='small'>MADE BY <NAME></Text>
</Footer>
)
}
export default MainFooter
<file_sep>/src/App.js
import './App.css'
import { Grommet } from 'grommet'
import Main from './components/Main'
const theme = {
global: {
font: {
family: 'Poppins',
},
},
}
function App() {
return (
<Grommet theme={theme}>
<Main />
</Grommet>
)
}
export default App
<file_sep>/src/components/MainHeader.js
import { Header, Heading } from 'grommet'
function MainHeader() {
return (
<Header background='brand' direction='column'>
<Heading size='small'>🧚 ANZSIC Fairy</Heading>
</Header>
)
}
export default MainHeader
<file_sep>/src/components/SearchForm.js
import { Box, Button, Form, FormField, TextInput } from 'grommet'
function SearchForm({ setSearchTerm }) {
return (
<Box
margin='medium'
direction='row-responsive'
justify='center'
align='center'
>
<Form
onSubmit={(e) => {
let trimmedSearch = e.target.search.value.trim()
setSearchTerm(trimmedSearch)
}}
>
<FormField
label="What's your primary business activity?"
name='search'
htmlFor='search'
required
>
<TextInput
id='search'
name='search'
placeholder='e.g. fishing'
></TextInput>
</FormField>
<Box direction='row' justify='between'>
<Button
type='reset'
label='Reset'
onClick={() => {
setSearchTerm('')
}}
/>
<Button type='submit' label='Find your code!' primary />
</Box>
</Form>
</Box>
)
}
export default SearchForm
<file_sep>/src/components/Main.js
import MainHeader from './MainHeader'
import SearchForm from './SearchForm'
import MainAccordion from './MainAccordion'
import MainFooter from './MainFooter'
import { useState } from 'react'
function Main() {
const [searchTerm, setSearchTerm] = useState('')
return (
<div>
<MainHeader />
<SearchForm setSearchTerm={setSearchTerm} />
<MainAccordion />
<MainFooter />
</div>
)
}
export default Main
<file_sep>/src/components/MainAccordion.js
import { Accordion, AccordionPanel, Anchor, Box, Text } from 'grommet'
import { CircleQuestion } from 'grommet-icons'
function MainAccordion() {
return (
<Box margin='medium' direction='column' justify='center' align='center'>
<Text size='xlarge'>
<CircleQuestion color='brand' /> Frequently Asked Questions
</Text>
<Accordion fill='true'>
<AccordionPanel label='Is ANZSIC Fairy an official tool?'>
<Box pad='small'>
<Text>
No! ANZSIC Fairy is a personal coding project by <NAME> using the
ANZSIC code search API. For official information about ANZSIC codes
please check out:
<ul>
<li>
<Anchor
href='https://www.abs.gov.au/ausstats/[email protected]/mf/1292.0'
label='Australian Bureau of Statistics website'
/>
</li>
<li>
<Anchor
href='https://www.ato.gov.au/calculators-and-tools/business-industry-code-tool/anzsiccoder.aspx'
label='Australian Taxation Office website'
/>
</li>
</ul>{' '}
</Text>
</Box>
</AccordionPanel>
<AccordionPanel label='What does ANZSIC stand for?'>
<Box pad='small'>
<Text>
ANZSIC stands for the 'Australia and New Zealand Standard Industrial
Classification' system.
</Text>
</Box>
</AccordionPanel>
<AccordionPanel label='What are ANZSIC codes used for?'>
<Box pad='small'>
<Text>
The ANZSIC system classifies entities based on their main business
activty and is used to collect and analyse data across industries. The
system has four levels with increasing detail at each level:
<ul>
<li>broad industry classification denoted by a letter</li>
<li>industry subdivision denoted by a two-digit code</li>
<li>industry group denoted by a three-digit code</li>
<li>industry class denoted by a four-digit code</li>
</ul>
</Text>
</Box>
</AccordionPanel>
<AccordionPanel label='How do I decide which ANZSIC code to use?'>
<Box pad='small'>
<Text>
Your primary ANZSIC code is pre-populated from your business' records on
the Australian Business Register (ABR). You should ensure this is the
four-digit industry class that best describes your main business
activity, especially if your business works in multiple industries. For
example, if 60% of your employees work in one section of the business,
the day-to-day duties of this section would be your main activity.
</Text>
</Box>
</AccordionPanel>
</Accordion>
</Box>
)
}
export default MainAccordion
| 2dce8c9bc0ad49662d879bea0a85d1535a2dc9a2 | [
"JavaScript"
] | 6 | JavaScript | kimbcheh/anzsic-fairy | f4623dded8191f943d22dd2a3c41ed4748b3a3ed | eb976516de9714f88ca040e498677c70b17409bc | |
refs/heads/master | <repo_name>annazhangg/text-mining<file_sep>/movie_rating/extractimdb.py
from imdbpie import Imdb
import pickle
def review_extracter(movie):
"""input movie name, outputs txt file with reviews on the front page of imdb"""
### extracts content from movie page ###
imdb = Imdb()
url = imdb.search_for_title(movie)[0]
reviews = imdb.get_title_user_reviews(url["imdb_id"])
### creates list of all reviews present on the front page###
imdb = Imdb()
url = imdb.search_for_title(movie)[0]
reviews = imdb.get_title_user_reviews(url["imdb_id"])
### creates txt file for all reviews present on the front page###
review_list = []
for i in range(len(reviews["reviews"])):
line = reviews["reviews"][i]["reviewText"]
review_list.append(line)
###pickles list###
with open(f"{movie}_imdb.pickle", "wb") as f:
pickle.dump(review_list, f)
# with open(f"{movie}.pickle", "rb") as input_file:
# copy = pickle.load(input_file)
# print(copy)
def main():
review_extracter("Mulan")
if __name__ == "__main__":
main()<file_sep>/movie_rating/review.py
import extractimdb
import extracttwitter
import analyze_nltk
import analyze_text
import os
import matplotlib.pyplot as plt
import scatterplot
#This is a master function for all of our analysis tools.
def analyze_movie(movie):
"""shows stats for movie reviews"""
source1 = "imdb"
source2 = "twitter"
# if imdb pickle does not exist, extracts pickle
if not os.path.isfile(f"{movie}_imdb.pickle"):
extractimdb.review_extracter(movie)
# if twitter pickle does not exist, extracts pickle
if not os.path.isfile(f"{movie}_twitter.pickle"):
extracttwitter.twitter_extracter(movie)
# process and analyze data
for source in [source1, source2]:
hist = analyze_text.process_file(movie, source)
t = analyze_text.most_common(hist, excluding_stopwords=True)
print()
print(f"The most common words in {source} reviews:")
for freq, word in t[0:20]:
print(f"{word:<10} {freq}")
print()
print(f"Histogram of top 10 words in {source} reviews")
analyze_text.print_most_common(hist, num=10)
print()
average, sentiment_list = analyze_nltk.sentiment_analysis(movie, source)
print(f"The average sentiment on {source} is {average}.")
print("Individual scores are:")
print(sentiment_list)
# create sentiment scatter plot
mulan1 = scatterplot.sentiment_scatter("Parasite", source1)
mulan2 = scatterplot.sentiment_scatter("Parasite", source2)
scatterplot.scatter(movie, source1, source2, mulan1, mulan2)
def main():
movie = "Parasite"
analyze_movie(movie)
if __name__ == "__main__":
main()
<file_sep>/movie_rating/extracttwitter.py
from twython import Twython
import pickle
def twitter_extracter(movie, tweet_number=50):
"""extracts twitter posts related to input: movie, and # of tweets. outputs: pickle of tweets"""
###enters API keys###
APP_KEY = "9iNbqBABwgvjucc9dE2pW7mTm"
APP_SECRET = "<KEY>"
twitter = Twython(APP_KEY, APP_SECRET)
###searches###
data = twitter.search(q=f"{movie} review", count=tweet_number)
###creates list of tweets###
twitter_list = []
for status in data["statuses"]:
twitter_list.append(status["text"])
###pickles tweets###
with open(f"{movie}_twitter.pickle", "wb") as f:
pickle.dump(twitter_list, f)
def main():
twitter_extracter("Mulan")
if __name__ == "__main__":
main()
<file_sep>/movie_rating/scatterplot.py
from nltk.sentiment.vader import SentimentIntensityAnalyzer
import pickle
import matplotlib.pyplot as plt
# python -m pip install -U pip
# python -m pip install -U matplotlib
def sentiment_scatter(movie, source):
"""senitment analysis, input str movie and source, output sentiment analysis"""
# opens pickle
with open(f"{movie}_{source}.pickle", "rb") as input_file:
copy = pickle.load(input_file)
# stores sentiment score in list
sentiment_pos = []
sentiment_neg = []
for line in copy:
score = SentimentIntensityAnalyzer().polarity_scores(line)
sentiment_pos.append(score["pos"])
sentiment_neg.append(score["neg"])
return sentiment_pos, sentiment_neg
def scatter(movie, source1, source2, source1_list, source2_list):
"""prints scatter plot when 2 data sources are inputted"""
source1_pos, source1_neg = source1_list
source2_pos, source2_neg = source2_list
one = plt.scatter(source1_pos, source1_neg, color="r", marker="x")
two = plt.scatter(source2_pos, source2_neg, color="b")
plt.xlabel("Positive Words")
plt.ylabel("Negative Words")
plt.title(f"Postive vs. Negative words in {movie} reviews.")
plt.legend((one, two), (source1, source2))
plt.show()
def main():
movie = "Parasite"
source1 = "twitter"
source2 = "imdb"
mulan1 = sentiment_scatter(movie, source1)
mulan2 = sentiment_scatter(movie, source2)
scatter(movie, source1, source2, mulan1, mulan2)
if __name__ == "__main__":
main()
<file_sep>/movie_rating/analyze_nltk.py
from nltk.sentiment.vader import SentimentIntensityAnalyzer
import pickle
def sentiment_analysis(movie, source):
"""senitment analysis, input str movie and source, output sentiment analysis"""
# opens pickle
with open(f"{movie}_{source}.pickle", "rb") as input_file:
copy = pickle.load(input_file)
# stores sentiment score in list
sentiment_list = []
for line in copy:
score = SentimentIntensityAnalyzer().polarity_scores(line)
sentiment_list.append(score["compound"])
# finds average of sentiment score
average = sum(sentiment_list) / len(sentiment_list)
# returns average and list
return average, sentiment_list
def main():
average, sentiment_list = sentiment_analysis("Mulan", "imdb")
print(f"The average sentiment is {average}.")
print("Individual scores are:")
print(sentiment_list)
if __name__ == "__main__":
main()<file_sep>/movie_rating/analyze_text.py
import string
import pickle
def process_file(movie, source):
"""Makes a histogram that contains the words from a file.
movie: string
source: string
returns: map from each word to the number of times it appears.
"""
hist = {}
# opens pickle
with open(f"{movie}_{source}.pickle", "rb") as input_file:
fp = pickle.load(input_file)
# removes extra chracters and stores into dictionary by frequency
strippables = string.punctuation + string.whitespace
for line in fp:
line = line.replace("-", " ")
line = line.replace('"', " ")
for word in line.split():
word = word.strip(strippables)
word = word.lower()
hist[word] = hist.get(word, 0) + 1
if "" in hist:
del hist[""]
return hist
def most_common(hist, excluding_stopwords=False):
"""Makes a list of word-freq pairs in descending order of frequency.
hist: map from word to frequency
returns: list of (frequency, word) pairs
"""
# imports stopword.txt and creates lists
common_words = []
stopwords = open("stopword.txt")
stopwords_list = []
# turns stopwords.txt into list
for line in stopwords:
lin = line.strip()
stopwords_list.append(lin)
# sorts dictionary by frequency and returns list of tupples
for word, freq in hist.items():
if not excluding_stopwords or not word in stopwords_list:
common_words.append((freq, word))
common_words.sort(reverse=True)
return common_words
def print_most_common(hist, num=10):
"""Prints the most commons words in a histgram and their frequencies.
hist: histogram (map from word to frequency)
num: number of words to print
"""
t = most_common(hist, excluding_stopwords=True)
for freq, word in t[0:num]:
print(f"{word:<10}", "*" * int((freq)))
def main():
movie = "Mulan"
hist = process_file(movie, "imdb")
t = most_common(hist, excluding_stopwords=True)
print("The most common words are:")
for freq, word in t[0:20]:
print(f"{word:<10} {freq}")
print_most_common(hist, num=10)
if __name__ == "__main__":
main()
| 06a4fd20de78f7ac674ee2f40c144392c9c4cf25 | [
"Python"
] | 6 | Python | annazhangg/text-mining | f06cb328719edcf7ef306ed982c22391c5e77af1 | 06cf40e781d3bdba1eff22a2d03faa7466936803 | |
refs/heads/main | <repo_name>wlgfour/eqnets<file_sep>/e3nn/install.sh
pip install torch==1.7.1+cu101 torchvision==0.8.2+cu101 torchaudio==0.7.2 -f https://download.pytorch.org/whl/torch_stable.html
pip install --no-index torch-scatter -f https://pytorch-geometric.com/whl/torch-1.7.0+cu101.html
pip install --no-index torch-sparse -f https://pytorch-geometric.com/whl/torch-1.7.0+cu101.html
pip install --no-index torch-cluster -f https://pytorch-geometric.com/whl/torch-1.7.0+cu101.html
pip install --no-index torch-spline-conv -f https://pytorch-geometric.com/whl/torch-1.7.0+cu101.html
pip install torch-geometric
pip install e3nn
<file_sep>/e3nn/net1.py
import os
from typing import List
import matplotlib as mpl
import numpy as np
from torch.utils import data
from e3nn import non_linearities
from e3nn.networks import GatedConvNetwork
from e3nn.point.data_helpers import DataNeighbors
from e3nn.point.message_passing import Convolution as MessageConv
from utils import get_data_loader, mask_by_len, N_FEATURES
import torch
from setproctitle import setproctitle
import argparse
from torch.utils.tensorboard import SummaryWriter
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
class MaskedAverageNet(torch.nn.Module):
def __init__(self, *args, **kwargs):
super().__init__()
self.network = GatedConvNetwork(*args, **kwargs)
def forward(self, lengths, *args, **kwargs):
output = self.network(*args, **kwargs)
output = mask_by_len(output, lengths)
return output.sum(1)[:, 0] / lengths
class GatedConvNeighbors(torch.nn.Module):
def __init__(self, *args, **kwargs):
super().__init__()
kwargs['convolution'] = MessageConv
self.network = GatedConvNetwork(*args, **kwargs)
def forward(self, nbrs):
""" nbrs is a neighbors list generated by e3nn.point.data_helpers.DataNeighbors
"""
output = self.network(nbrs.x, nbrs.edge_index, nbrs.edge_attr)
return output
def main(
name: str = 'GatedConvDefault',
data_path: str='',
save_dir: str='',
save_every: int=10000,
tblog: str = '',
tbinterval: int = 100,
gpu: int = -1,
lmax: int = 3,
rmax: float = 1000,
layers: int = 3,
lr: float = 1e-2,
batch_size: int = 1,
visualization_structs: List[str] = [],
):
""" Try to do the same as main1, but with the conbolution calculated only over a fixed-radius
neighborhood around a certain point.
"""
if tbinterval != 0:
ldir = os.path.join(tblog, name)
print(f'Logging in {ldir}...')
tbwriter = SummaryWriter(log_dir=ldir, comment=f'pid_{os.getpid()}')
else:
tbwriter = None
torch.set_default_dtype(torch.float64)
if gpu == -1:
device = 'cpu'
else:
device = f'cuda:{gpu}'
device = torch.device(device)
dataset, data_loader = get_data_loader(data_path, batch_size=batch_size, max_length=-1)
Rs_in = [(N_FEATURES, 0)]
Rs_hidden = [(16, 0), (8, 1), (4, 2)]
Rs_out = [(1, 0)]
net = GatedConvNeighbors(Rs_in, Rs_hidden, Rs_out, lmax=lmax, max_radius=rmax, layers=layers)
net = net.to(device)
optimizer = torch.optim.Adam(net.parameters(), lr=lr)
epoch = 0
global_step = 0
metrics = {
'Loss': [],
'Avg_Neighbors': [],
'Out/range': [],
'Out/var': []
}
while True:
epoch += 1
for batch in data_loader:
for j in range(batch_size):
global_step += 1
coords = batch['coords'][j]
feature = batch['features'][j]
# lengths = batch['length'][j]
nbrs = DataNeighbors(feature, coords, rmax)
# for tens in [nbrs]: # coords, feature, labels, lengths,
# tens.to(device)
nbrs = nbrs.to(device)
out = net(nbrs)
# if global_step == 1 and tbwriter != None:
# tbwriter.add_graph(net, [nbrs.x, nbrs.edge_index, nbrs.edge_attr])
# https://discuss.pytorch.org/t/why-do-we-need-to-set-the-gradients-manually-to-zero-in-pytorch/4903/20
labels = batch['drmsd'][j].to(device)
loss = (out.mean() - labels) ** 2
loss.backward()
if global_step % batch_size == 0:
optimizer.step()
optimizer.zero_grad()
# update metrics
metrics['Loss'].append(loss.detach().cpu())
metrics['Avg_Neighbors'].append(nbrs.edge_attr.shape[0] / nbrs.x.shape[0])
metrics['Out/range'].append((out.max() - out.min()).cpu())
metrics['Out/var'].append(out.var().cpu())
if global_step % save_every == 0 and save_dir != '' and save_every != 0:
torch.save(net.state_dict(), os.path.join(save_dir, sdir))
if tbwriter is not None:
pass
# tbwriter.add_hparams({'lr': lr, 'lmax': lmax, 'rmax': rmax, 'layers': layers, 'epochs': epoch, 'steps': i},
# {'Loss': 0., 'Avg_Neighbors': 0., 'Out/var': 0.},
# )
if global_step % tbinterval == 0:
if tbwriter is None:
print(f"epoch:step={epoch}:{global_step} loss={loss:.2f}")
for m in metrics:
val = sum(metrics[m]) / max(1, len(metrics[m]))
if tbwriter is not None:
tbwriter.add_scalar(m, val, global_step)
else:
print(f'\t{m}: {val:.2f}')
metrics = {m: [] for m in metrics}
# plot backbone colored by predictions
# net.eval()
# with torch.no_grad():
# for s in visualization_structs:
# if s not in data_loader:
# coords = dataset[s]
# nbrs = DataNeighbors(
# coords['features'],
# coords['coords'],
# rmax
# )
# nbrs.to(device)
# out = net(nbrs).detach().cpu().numpy()
# coords = coords['coords'].numpy()
# fig = plt.figure(figsize=(6, 6))
# ax = fig.add_subplot(1, 1, 1, projection='3d')
# _cmap = mpl.cm.get_cmap('cool')
# sm = mpl.cm.ScalarMappable(norm=mpl.colors.Normalize(vmin=0, vmax=750), cmap=_cmap)
# cmap = lambda c: _cmap(min(c, 750) / 750)
# # cmap = sm.cmap
# colors = [np.array(cmap(o)) for o in out.flatten()]
# ax.scatter(coords[:, 0], coords[:, 1], coords[:, 2], c=colors)
# for i in range(len(coords) - 1):
# seg = coords[i:i+2]
# ax.plot(seg[:, 0], seg[:, 1], seg[:, 2], c=(colors[i] + colors[i+1]) / 2)
# fig.colorbar(sm)
# tbwriter.add_figure(s, fig, global_step=global_step)
# net.train()
if __name__ == '__main__':
# command line interface
# Parse inputs
parser = argparse.ArgumentParser(
formatter_class=argparse.ArgumentDefaultsHelpFormatter)
parser.add_argument('--vs', dest='visualization_structs', type=str, nargs='+', default=[''],
help='List fo structures from the training set to include in the tensorboard plots.')
parser.add_argument('--data', type=str,
help=f'Direcotry to find the representations dataset outputted by protein_geometry/src/scripts/rgn_data.py')
parser.add_argument('--name', type=str, default='',
help='Override the name of the run. Affects the save file name and the name of the tensorboard run. '
'Will default to depend on the model specs.')
parser.add_argument('--load', type=None, default=None,
help='Not Implemented')
parser.add_argument('--save-dir', dest='save_dir', type=str, default=os.path.join('.', 'save'),
help='Where to save the model.')
parser.add_argument('--save-interval', dest='save_interval', type=int, default=10000,
help='How often to save the model. Setting to 0 will disable saving.')
parser.add_argument('--tb', type=str, default=os.path.join('~', 'eqnetworks', 'runs'),
help='Where to log tensorboard output. Will create a directory inside of the tb directory for this run.')
parser.add_argument('--tb-interval', dest='tb_interval', type=int, default=100,
help='How often to log to tensorboard. Setting to 0 will disable logging.')
parser.add_argument('--gpu', type=int, default=-1,
help='Which gpu to use. -1 means cpu.')
parser.add_argument('--lmax', type=int, default=3,
help='Maximum l to use for spherical hermonics.')
parser.add_argument('--rmax', type=float, default=1000,
help='Radius (Picometer) for convolution scope.')
parser.add_argument('--layers', type=int, default=3,
help='Number of gated equivariant convolutional layers.')
parser.add_argument('--reps', type=None, default=None,
help='Not Implemented')
parser.add_argument('--features', type=None, default=None,
help='Not Implemented')
parser.add_argument('--bs', type=int, default=1,
help='Batch size.')
parser.add_argument('--lr', type=float, default=1e-2,
help='Batch size.')
opts = parser.parse_args()
data_path = opts.data # '~/protein_geometry/data/representations/rgn'
save_dir = opts.save_dir
if opts.name == '':
name = f'lmax:{opts.lmax}-rmax:{opts.rmax}-layers:{opts.layers}-bs:{opts.bs}-lr:{opts.lr}'
else:
name = opts.name
# Considering parameters to a file that can be loaded.
kwargs = dict(
data_path=data_path,
save_every=opts.save_interval,
save_dir=save_dir,
tblog=opts.tb,
gpu=opts.gpu,
lmax=opts.lmax,
rmax=opts.rmax,
name=name,
layers=opts.layers,
tbinterval=opts.tb_interval,
visualization_structs=opts.visualization_structs,
batch_size=opts.bs,
lr=opts.lr,
)
setproctitle(name)
# save dir
if kwargs['save_every'] != 0:
i = 0
sdir = os.path.join(kwargs['save_dir'], f'{name}{i}')
while os.path.exists(sdir):
i += 1
sdir = os.path.join(kwargs['save_dir'], f'{name}{i}')
os.mkdir(sdir)
kwargs['save_dir'] = sdir
print(f'Starting {name} with pid {os.getpid()}')
main(**kwargs)
<file_sep>/e3nn/utils.py
from collections import defaultdict
from torch import Tensor
from torch.utils.data import Dataset, DataLoader
import torch
import numpy as np
import pandas as pd
import os
from typing import Any, Dict, List, Tuple, Union
DSSP_CODES = ['H', 'G', 'I', 'E', 'O', 'S', 'B', 'T', '-', 'L']
AAs = ['A', 'R', 'N', 'D', 'C', 'Q', 'E', 'G', 'H', 'I', 'L', 'K', 'M', 'F', 'P', 'S', 'T', 'W', 'Y', 'V', 'B', 'Z']
N_FEATURES = len(AAs) + len(DSSP_CODES)
class ProteinLoader(Dataset):
"""
ref: https://pytorch.org/tutorials/beginner/data_loading_tutorial.html
Loads a list of protein representations and their corresponding ground-truth drmsds.
Inheritance:
Dataset:
Args:
directory (str):
representation (str='Euclidean'):
>>>
"""
def __init__(self, dir: str, representation: str='Euclidean', max_length: int=-1):
"""
Args:
self (undefined):
directory (str):
representation (str='Euclidean'):
"""
self.dir = dir
self.labels = pd.read_csv(os.path.join(dir, 'labels.csv'))
self.rep = representation
self.max_length = max_length
def __len__(self):
return len(self.labels)
def __getitem__(self, i: Union[int, str]):
if torch.is_tensor(i):
i = i.tolist()
if isinstance(i, int):
name = self.labels.iloc[i, 0]
elif isinstance(i, str):
name = i
i = self.labels[self.labels == i].index[0]
else:
raise TypeError(f'Type ({type(i)}) not supported by ProteinLoader.')
dpath = os.path.join(self.dir, self.rep, f'{name}.npy')
fpath = os.path.join(self.dir, 'Features', f'{int(name[1:name.index("_")])}.csv')
coords = np.load(dpath)
if os.path.isfile(fpath):
with open(fpath, 'r') as f:
lines = f.readlines()
features = read_features(lines) # concatenated 1-hots for each position
else:
features = torch.from_numpy(np.ones_like(coords))[:, 0:1] # a single '1' at each position
if (self.max_length != -1) and (len(coords) > self.max_length):
coords = coords[:self.max_length]
drmsd = self.labels.iloc[i, 1]
return {'name': name, 'coords': torch.from_numpy(coords), 'drmsd': torch.tensor(drmsd), 'features': features}
def __contains__(self, key: Any):
if isinstance(key, str):
return (self.labels['protein.iteration'] == key).any()
else:
raise TypeError(f'Type ({type(i)}) not supported by ProteinLoader.')
def _collate(batch: Dict[str, Union[str, Tensor]]) -> List[Union[Tensor, List[str]]]:
ret = defaultdict(list)
for sample in batch:
ret['coords'].append(sample['coords'])
ret['features'].append(sample['features'])
ret['length'].append(torch.tensor(sample['coords'].shape[0]))
ret['drmsd'].append(sample['drmsd'])
ret['name'].append(sample['name'])
for k in ['coords', 'features']:
ret[k] = torch.nn.utils.rnn.pad_sequence(ret[k], batch_first=True)
for k in ['length', 'drmsd']:
ret[k] = torch.stack(ret[k])
return ret
def read_features(lines: List[str]) -> torch.Tensor:
""" Takes a list of lines read from a file containing features with the columns 'DSSP' and 'Sequence' and outputs
a concatenated list of one-hot vectors that is consistent no matter what parameters are passed.
"""
cols = {k: i for (i, k) in enumerate(lines[0].strip().split(','))}
features = np.zeros((len(lines) - 1, len(AAs) + len(DSSP_CODES)))
for i, line in enumerate(lines[1:]):
l = line.strip().split(',')
# index in AAs of the sequence of this residue
features[i, AAs.index(l[cols['Sequence']])] = 1.
features[i, len(AAs) + DSSP_CODES.index(l[cols['DSSP']])] = 1.
return torch.from_numpy(features)
def mask_by_len(inputs: Tensor, lens: Tensor) -> Tensor:
ml = torch.max(lens)
masks = torch.arange(ml)[None, :] < lens[:, None]
return inputs * masks[:, :, None]
def get_data_loader(dir: str, representation: str='Euclidean', batch_size: int=1, max_length: int=100) -> Tuple[Dataset, DataLoader]:
"""
Description of get_data_loader
Args:
dir (str):
representation (str='Euclidean'):
Returns:
DataLoader
"""
loader = ProteinLoader(dir=dir, representation=representation, max_length=max_length)
return loader, DataLoader(loader, batch_size=batch_size, shuffle=True, collate_fn=_collate, pin_memory=True)
if __name__ == '__main__':
d = '/home/wlg/development/HMS/protein_geometry/data/representations/150_1500'
dataset, data_loader = get_data_loader(d)
for batch in data_loader:
pass<file_sep>/e3nn/uninstall.sh
pip uninstall -y e3nn torch-grometric torch-scatter torch-sparse torch-cluster torch-spline-conv | a07b05f7776b030aa3a8eaf6c8590a47a75932ba | [
"Python",
"Shell"
] | 4 | Shell | wlgfour/eqnets | 08eeab24227fa572c434cf805d8bb6833f035282 | fafcb45222201bb4e7dac66ff94e786228ae379b | |
refs/heads/master | <repo_name>WillianLevandoski/Java-8-Examples<file_sep>/Java 8 Examples/src/StreamExample.java
import java.util.Arrays;
import java.util.List;
import java.util.regex.Pattern;
import java.util.stream.IntStream;
import java.util.stream.Stream;
public class StreamExample {
public static void main(String[] args) {
//Collection
List<Integer> list = Arrays.asList(1,2,3);
list.stream().forEach(System.out::println);
//Arrays
Integer[] intArray = new Integer[] {1,2,3};
Arrays.stream(intArray).forEach(System.out::println);
//Stream.of
Stream.of("Test", "print", "line").forEach(System.out::println);
//Range
System.out.println("Range");
IntStream.range(0,3).forEach(System.out::println);//0,1,2
//RangeClosed
System.out.println("RangeClosed");
IntStream.rangeClosed(0,3).forEach(System.out::println);//0,1,2,3
//Interate
Stream.iterate(5, n -> n*2).limit(3).forEach(System.out::println);
String s = "Pattern example";
Pattern pa = Pattern.compile(" ");
pa.splitAsStream(s).forEach(System.out::println);
}
}
| 1b25b5b754d27970244a8ba649b7edac7ef864da | [
"Java"
] | 1 | Java | WillianLevandoski/Java-8-Examples | df25dba0d267c1b078fd758120f3e2a07dd3a98a | 046a95fc95f1a8a34e7787a47b37edbe3bf75157 | |
refs/heads/master | <repo_name>diablo8226/user_logs<file_sep>/user_insights/migrations/0001_initial.py
# Generated by Django 2.0.9 on 2018-11-19 17:32
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
]
operations = [
migrations.CreateModel(
name='user',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('user_name', models.TextField(blank=True, max_length=1000)),
],
),
migrations.CreateModel(
name='user_app',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('app_name', models.TextField(blank=True, max_length=100)),
('app_id', models.TextField(blank=True, max_length=100)),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='user_insights.user')),
],
),
migrations.CreateModel(
name='user_log',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('call_timestamp', models.DateTimeField()),
('call_duration', models.IntegerField()),
('call_type', models.TextField(blank=True, max_length=100)),
('phone_number', models.TextField(blank=True, max_length=15)),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='user_insights.user')),
],
),
]
<file_sep>/README.md
# user_logs
#end point to upload call log csv
http://127.0.0.1:8000/upload_call_log_csv
#end point to upload app log csv
http://127.0.0.1:8000/upload_app_log_csv
#end point to view app log dasboard reponse
http://127.0.0.1:8000/user_app_analytics?user_name=user1
#end point to view call log dasboard reponse
http://127.0.0.1:8000/user_call_analytics?user_name=user1<file_sep>/user_insights/views.py
from django.http import HttpResponse
from django.http import *
from django.conf import settings
import json
from user_insights.models import user,user_log, user_app
from django.core.exceptions import ValidationError
from django.shortcuts import render, redirect, get_object_or_404
import datetime
from datetime import datetime
from collections import Counter
from django.http import JsonResponse
from collections import OrderedDict
import re
import requests
def upload_app_log_csv(request):
all_app_log = []
if request.POST and request.FILES:
if not request.POST.get('user_name'):
return JsonResponse({'Error':'user not present'},status=400)
get_user = user.objects.filter(user_name=request.POST.get('user_name'))
if not get_user:
get_user = user.objects.create(user_name=request.POST.get('user_name'))
else:
get_user = get_user[0]
for each_line in request.FILES['csv_file']:
each_record = each_line.strip().decode('utf-8')
if each_record:
record = each_record.split(",")
app_name = re.sub(r'"','', record[0])
app_id = re.sub(r'"','', record[1])
app_record = user_app(app_name=app_name, app_id=app_id, user=get_user)
all_app_log.append(app_record)
user_app.objects.bulk_create(all_app_log)
return JsonResponse({'success':'Uploaded file sucessfully'},status=200)
else:
return render(request, "user_insights/upload_app_log_csv.html", locals())
def upload_call_log_csv(request):
all_call_log = []
if request.POST and request.FILES:
if not request.POST.get('user_name'):
return JsonResponse({'Error':'user not present'},status=400)
get_user = user.objects.filter(user_name=request.POST.get('user_name'))
if not get_user:
get_user = user.objects.create(user_name=request.POST.get('user_name'))
else:
get_user = get_user[0]
first_line_count = 0
for each_line in request.FILES['csv_file']:
if first_line_count == 0:
first_line_count+=1
else:
each_record = each_line.strip().decode('utf-8')
if each_record:
record = re.split('\t|,',each_record)
call_timestamp = datetime.strptime(record[1], '%m/%d/%Y %H:%M')
call_record = user_log(call_timestamp= call_timestamp, call_duration = record[2], call_type =record[3], phone_number = record[4], user = get_user)
all_call_log.append(call_record)
user_log.objects.bulk_create(all_call_log)
return JsonResponse({'success':'Uploaded file sucessfully'},status=200)
else:
return render(request, "user_insights/upload_call_log_csv.html", locals())
def user_call_analytics(request):
response_payload = {}
if request.GET:
user_name = request.GET.get("user_name").strip()
phone_numbers = []
call_type = {}
call_pick_up = []
call_type_freq = []
total_call_duration = 0
user_call_logs = user_log.objects.filter(user__user_name__icontains=user_name)
for log in user_call_logs.iterator():
if "-" not in log.call_type:
phone_numbers.append(log.phone_number)
call_type_freq.append(log.call_type)
total_call_duration += int(log.call_duration)
if log.call_type in call_type.keys():
call_type[log.call_type]+= int(log.call_duration)
else:
call_type[log.call_type] = int(log.call_duration)
# this gives the logic of percentage distribution of incoming, outgoing and missed calls
call_type_freuency = Counter(call_type_freq)
all_calls = sum(call_type_freuency.values())
for k, v in call_type_freuency.items():
call_type_freuency[k] = float(call_type_freuency[k]/all_calls)*100
#converting to phone no format
friends_family = OrderedDict()
for key,value in Counter(phone_numbers).most_common(5):
phone_field = str(key)
phone_number = str(int(float(phone_field)))
if len(phone_number) > 10:
phone_number = phone_number[2:]
friends_family[phone_number]= value
response_payload = {
"friends_family_no_of_calls":friends_family,
"time_spend_on_call": total_call_duration,
"total_call_time_distibution": call_type,
"hit_miss_call_percent": call_type_freuency
}
return JsonResponse(response_payload)
else:
return render(request, "user_insights/user_call_analytics.html")
def user_app_analytics(request):
response_payload = {}
if request.GET:
user_name = request.GET.get("user_name").strip()
user_app_logs = user_app.objects.filter(user__user_name__icontains=user_name)[21:50]
base_url = "https://api.apptweak.com/android/applications/"
edge_url = "/information.json"
headers = {'X-Apptweak-Key': '<KEY>'}
fail = []
user_app_category = []
#logic to get app categories
for log in user_app_logs.iterator():
if len(log.app_category) == 0:
url = base_url + log.app_id + edge_url
try:
r = requests.get(url, headers=headers)
print(r.status_code)
if r.status_code == 200:
result = r.json()
if result:
log.app_category = result['content']['genres'][0]
log.save()
user_app_category.append(log.app_category)
else:
fail.append(log.app_name)
except:
fail.append(log.app_name)
else:
user_app_category.append(log.app_category)
#user type based on category freq
user_type = Counter(user_app_category).most_common(1)
print(Counter(user_app_category).most_common(5))
response_payload = {
"user_app_category":user_type,
"failed_to_get_info": fail
}
return JsonResponse(response_payload)
else:
return render(request, "user_insights/user_app_analytics.html")<file_sep>/user_insights/apps.py
from django.apps import AppConfig
class UserInsightsConfig(AppConfig):
name = 'user_insights'
<file_sep>/user_insights/models.py
from django.db import models
from django.core.validators import MaxValueValidator,MinValueValidator
from django.core.exceptions import ValidationError
from django.db.models.signals import pre_save
from phonenumber_field.modelfields import PhoneNumberField
class user(models.Model):
user_name = models.TextField(max_length=1000, blank=True)
def __str__(self):
return self.user_name
class user_log(models.Model):
call_timestamp = models.DateTimeField()
call_duration = models.IntegerField()
call_type = models.TextField(max_length=100, blank=True)
phone_number = models.TextField(max_length=15, blank=True)
# phone_number = PhoneNumberField(null=True, blank=True, unique=False)
user = models.ForeignKey(user, on_delete=models.CASCADE)#, related_name='tags')
def __str__(self):
return self.call_type
class user_app(models.Model):
app_name = models.TextField(max_length=100, blank=True)
app_id = models.TextField(max_length=100, blank=True)
app_category = models.TextField(max_length=100, blank=True)
user = models.ForeignKey(user, on_delete=models.CASCADE)
def __str__(self):
return self.app_name
# Created At Call Date Call Duration Call Type Phone Number
# def __str__(self):
# return self.message_type
# def clean(self):
# error_dict = {}
# for field in self._meta.get_fields():
# if field.name != "id":
# field_value = getattr(self, field.name)
# if not field_value:
# msg = field.name + " should not be blank"
# error_dict[field.name] = msg
# raise ValidationError(error_dict)
# def save(self, *args, **kwargs):
# self.full_clean()
# return super().save(*args, **kwargs) | 2bfe6c4dc9f61b9327c5d5f055dec794917bed3d | [
"Markdown",
"Python"
] | 5 | Python | diablo8226/user_logs | a2caee8e65abe356d27ba7ccd7e114b2720d62eb | acfcb174b5345f619ea07f9c808028f710b86d8a | |
refs/heads/master | <file_sep># Weatherfy
A **very** simple weather web application.
*Created by:*
<NAME> & <NAME>
As a project for Google CSSI: Online<file_sep>// Create elements to link to the classes in index.html
const notificationElement = document.querySelector(".notification");
const tempElement = document.querySelector(".temperature-value p");
const descElement = document.querySelector(".temperature-description p");
const locationElement = document.querySelector(".location p");
const KELVIN = 273;
// OpenSky Key
const key = "63739e5eab0f60ef4ab9478884e4077c";
// Store the weather data.
const weather = {
temperature : {
value : 43,
unit : "celsius"
},
description : "Sunny",
city : "Las Vegas",
country : "United States"
};
// To change the inner html.
tempElement.innerHTML =
`${weather.temperature.value}° <span>C</span>`;
descElement.innerHTML =
weather.description;
locationElement.innerHTML =
`${weather.city}, ${weather.country}`;
// When clicked, convert from C to F and vice versa.
tempElement.addEventListener("click", function(){
if(weather.temperature.value === undefined) return;
if(weather.temperature.unit === "celsius"){
let fahrenheit = celsiusToFahrenheit(weather.temperature.value);
fahrenheit = Math.floor(fahrenheit);
tempElement.innerHTML = `${fahrenheit}° <span>F</span>`;
weather.temperature.unit = "fahrenheit";
} else {
tempElement.innerHTML = `${weather.temperature.value}° <span>C</span>`;
weather.temperature.unit = "celsius";
}
})
// Convert Celsius to fahrenheit when called.
function celsiusToFahrenheit (temperature) {
return (temperature * 9/5) + 32;
}
// Get user location IF geolocation is enabled.
if("geolocation" in navigator){
navigator.geolocation.getCurrentPosition(setPosition, showError);
} else {
notificationElement.style.display = "block";
notificationElement.innerHTML = "<p>No location data could be found.</p>"
}
// Grab user location using coordinates.
function setPosition(position){
let latitude = position.coords.latitude;
let longitude = position.coords.longitude;
getWeather(latitude, longitude);
}
// If coordinates not found, show an error message.
function showError(error){
notificationElement.style.display = "block";
notificationElement.innerHTML = `<p> ${error.message} </p>`;
}
// Get geolocation data from the OpenSky Weather API.
function getWeather(latitude, longitude){
let api = `http://api.openweathermap.org/data/2.5/weather?lat=${latitude}&lon=${longitude}&appid=${key}`;
fetch(api).then(function(response){
let data = response.json();
return data;
})
// Then replace placeholder/old data with new data.
.then(function(data){
weather.temperature.value = Math.floor(data.main.temp - KELVIN);
weather.description = data.weather[0].description;
weather.city = data.name;
weather.country = data.sys.country;
})
// Then display the updated information.
.then(function(){
displayWeather();
});
}
// Display weather data to the interface.
function displayWeather(){
tempElement.innerHTML = `${weather.temperature.value}°<span>C</span>`;
descElement.innerHTML = weather.description;
locationElement.innerHTML = `${weather.city}, ${weather.country}`;
} | e2c586122290f4cb84002eaf0551b8e76c929095 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | therls/Weatherfy | 0b840a3c258278c331d53c3a4b5bd738bf446037 | 53806f5a811c3cb550debdb62065c41da29c60e9 | |
refs/heads/master | <repo_name>noahhusby/MachineFlow-iOS<file_sep>/Main.cs
using System;
using System.Drawing;
using CoreGraphics;
using Foundation;
using UIKit;
namespace MachineFlow_iOS
{
[Register("Main")]
public class Main : UIView
{
public Main()
{
Initialize();
}
public Main(RectangleF bounds) : base(bounds)
{
Initialize();
}
void Initialize()
{
BackgroundColor = UIColor.Red;
}
}
} | 0007026fbc8b098a1204069e5c0b4be90a4b55fb | [
"C#"
] | 1 | C# | noahhusby/MachineFlow-iOS | 27ec2169aa0411bad8a38db2b857a1d7e8c043e4 | 6e738ef598416ee4d7e6f7c1bdf531fffc01c3b6 | |
refs/heads/master | <file_sep>import os
import celery
import raven
from raven.contrib.celery import register_signal, register_logger_signal
from django.conf import settings # noqa
# set the default Django settings module for the 'celery' program.
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'falstartexample.settings')
class Celery(celery.Celery):
""" Configure celery with raven """
def on_configure(self):
if not getattr(settings, 'CELERY_RAVEN_DSN'):
# for no send raven reports from dev server
return
client = raven.Client(settings.CELERY_RAVEN_DSN)
# register a custom filter to filter out duplicate logs
register_logger_signal(client)
# hook into the Celery error handler
register_signal(client)
app = Celery('falstartexample')
# Using a string here means the worker will not have to
# pickle the object when using Windows.
app.config_from_object('django.conf:settings')
app.autodiscover_tasks(lambda: settings.INSTALLED_APPS)
<file_sep># falstart-example
Example of the [falstart](https://github.com/Samael500/falstart)-utility result to create a basic project in Django

```Shell
$ falstart falstart-example
> Django version ['1.9.5'] 1.10.2
> Debian version (for vagrant box) ['jessie64']
> Python version ['3.5.1'] 3.5.2
> Vagrant box IP-addr ['10.1.1.123'] 10.1.1.111
> Do you nead a POSTGRES? [Y/n]
> Do you nead a CELERY? [y/N] y
> Do you nead a REDIS? [y/N] y
> Do you nead a SENTRY? [y/N] y
> Database name ['falstartexample_db']
> Database user ['falstartexample_user']
> Database pass ['<PASSWORD>']
[falstart] > make dir: "/home/maks/temp/falstart-example"
[falstart] > render "Vagrantfile.j2" > "Vagrantfile"
[falstart] > render "Makefile.j2" > "Makefile"
[falstart] > run: "chmod +x Makefile"
[falstart] > make dir: "provision"
[falstart] > make dir: "provision"
[falstart] > render "provision_fabfile.j2" > "provision/fabric_provisioner.py"
[falstart] > render "requirements.j2" > "requirements.txt"
[falstart] > render "requirements.j2" > "requirements-remote.txt"
[falstart] > render "lintrc.j2" > ".lintrc"
[falstart] > render "coveragerc.j2" > ".coveragerc"
[falstart] > make dir: "falstartexample"
[falstart] > render "py_codes/settings_local.j2" > "falstartexample/settings_local.py.example"
[falstart] > make dir: "falstartexample"
[falstart] > render "py_codes/celery.j2" > "falstartexample/celery.py"
[falstart] > copy: "/home/maks/s50/falstart/falstart/templates/vagrant_templates" > "provision/templates"
[falstart] > run: "vagrant up"
Bringing machine 'falstartexample_vagrant' up with 'virtualbox' provider...
==> falstartexample_vagrant: Checking if box 'debian/jessie64' is up to date...
==> falstartexample_vagrant: A newer version of the box 'debian/jessie64' is available! You currently
==> falstartexample_vagrant: have version '8.4.0'. The latest is version '8.6.1'. Run
==> falstartexample_vagrant: `vagrant box update` to update.
==> falstartexample_vagrant: Clearing any previously set forwarded ports...
==> falstartexample_vagrant: Fixed port collision for 22 => 2222. Now on port 2200.
==> falstartexample_vagrant: Clearing any previously set network interfaces...
==> falstartexample_vagrant: Preparing network interfaces based on configuration...
falstartexample_vagrant: Adapter 1: nat
falstartexample_vagrant: Adapter 2: hostonly
==> falstartexample_vagrant: Forwarding ports...
falstartexample_vagrant: 22 (guest) => 2200 (host) (adapter 1)
==> falstartexample_vagrant: Running 'pre-boot' VM customizations...
==> falstartexample_vagrant: Booting VM...
==> falstartexample_vagrant: Waiting for machine to boot. This may take a few minutes...
falstartexample_vagrant: SSH address: 127.0.0.1:2200
falstartexample_vagrant: SSH username: vagrant
falstartexample_vagrant: SSH auth method: private key
==> falstartexample_vagrant: Machine booted and ready!
==> falstartexample_vagrant: Checking for guest additions in VM...
falstartexample_vagrant: No guest additions were detected on the base box for this VM! Guest
falstartexample_vagrant: additions are required for forwarded ports, shared folders, host only
falstartexample_vagrant: networking, and more. If SSH fails on this machine, please install
falstartexample_vagrant: the guest additions and repackage the box to continue.
falstartexample_vagrant:
falstartexample_vagrant: This is not an error message; everything may continue to work properly,
falstartexample_vagrant: in which case you may ignore this message.
==> falstartexample_vagrant: Setting hostname...
==> falstartexample_vagrant: Configuring and enabling network interfaces...
==> falstartexample_vagrant: Exporting NFS shared folders...
==> falstartexample_vagrant: Preparing to edit /etc/exports. Administrator privileges will be required...
[sudo] пароль для maks:
● nfs-server.service - NFS server and services
Loaded: loaded (/lib/systemd/system/nfs-server.service; enabled; vendor preset: enabled)
Active: active (exited) since Вс 2016-10-09 14:40:14 MSK; 4min 16s ago
Process: 1081 ExecStart=/usr/sbin/rpc.nfsd $RPCNFSDARGS (code=exited, status=0/SUCCESS)
Process: 1073 ExecStartPre=/usr/sbin/exportfs -r (code=exited, status=0/SUCCESS)
Main PID: 1081 (code=exited, status=0/SUCCESS)
CGroup: /system.slice/nfs-server.service
окт 09 14:40:13 zlm systemd[1]: Starting NFS server and services...
окт 09 14:40:14 zlm systemd[1]: Started NFS server and services.
==> falstartexample_vagrant: Mounting NFS shared folders...
==> falstartexample_vagrant: Running provisioner: fabric...
[127.0.0.1] Executing task 'common'
[127.0.0.1] put: <file obj> -> /etc/environment
[127.0.0.1] sudo: localedef ru_RU.UTF-8 -i ru_RU -fUTF-8
[127.0.0.1] sudo: touch /usr/locale_8913242974480500368
[127.0.0.1] sudo: apt-get -y update
[127.0.0.1] sudo: apt-get -y install libcurl4-gnutls-dev libexpat1-dev gettext libz-dev libssl-dev git redis-server
[127.0.0.1] sudo: touch /usr/apt_packages_-3330926058430280198
[127.0.0.1] sudo: apt-get -y install python-dev python-pip python-virtualenv build-essential libncurses5-dev libncursesw5-dev libreadline6-dev libgdbm-dev libsqlite3-dev libssl-dev libbz2-dev libexpat1-dev liblzma-dev zlib1g-dev libxml2-dev libxslt1-dev
[127.0.0.1] run: wget -O python.tgz https://www.python.org/ftp/python/3.5.2/Python-3.5.2.tgz
[127.0.0.1] run: tar -xf python.tgz
[127.0.0.1] run: mv Python-3.5.2 python
[127.0.0.1] run: ./configure --prefix=/usr/local/python-3.5.2
[127.0.0.1] run: make
[127.0.0.1] sudo: make altinstall
[127.0.0.1] sudo: rm /tmp/* -rf
[127.0.0.1] sudo: ln -sf /usr/local/python-3.5.2/bin/* /usr/local/bin/
[127.0.0.1] sudo: touch /usr/python_packages_-535002765752534493
[127.0.0.1] Executing task 'database'
[127.0.0.1] sudo: apt-get -y install postgresql postgresql-server-dev-all python-psycopg2
[127.0.0.1] run: sudo -u postgres psql -c "CREATE USER falstartexample_user;"
[127.0.0.1] run: sudo -u postgres psql -c "ALTER USER falstartexample_user WITH PASSWORD '<PASSWORD>';"
[127.0.0.1] run: sudo -u postgres psql -c "ALTER USER falstartexample_user CREATEDB;"
[127.0.0.1] run: sudo -u postgres psql -c "CREATE DATABASE falstartexample;"
[127.0.0.1] run: sudo -u postgres psql -c "GRANT ALL PRIVILEGES ON DATABASE falstartexample TO falstartexample_user;"
[127.0.0.1] sudo: touch /usr/database_-8337542331712744645
[127.0.0.1] Executing task 'nginx'
[127.0.0.1] sudo: apt-get -y install nginx
[127.0.0.1] sudo: touch /usr/nginx_install_1687306290436383039
[127.0.0.1] put: <file obj> -> /etc/nginx/sites-available/falstartexample
[127.0.0.1] sudo: ln -sf /etc/nginx/sites-available/falstartexample /etc/nginx/sites-enabled/falstartexample
[127.0.0.1] sudo: service nginx restart
[127.0.0.1] Executing task 'app'
[127.0.0.1] run: pyvenv-3.5 /home/vagrant/venv
[127.0.0.1] run: /home/vagrant/venv/bin/pip install -U pip wheel
[127.0.0.1] run: mkdir -p wheels
[127.0.0.1] run: /home/vagrant/venv/bin/pip wheel -w wheels/ -r requirements-remote.txt --pre
[127.0.0.1] run: make wheel_install
[127.0.0.1] run: /home/vagrant/venv/bin/django-admin startproject falstartexample .
[127.0.0.1] run: cd falstartexample && cp settings_local.py.example settings_local.py
[127.0.0.1] Executing task 'localserver'
[127.0.0.1] run: /home/vagrant/venv/bin/python manage.py migrate --noinput
[127.0.0.1] run: /home/vagrant/venv/bin/python manage.py collectstatic --noinput
[127.0.0.1] run: echo "from django.contrib.auth import get_user_model
User = get_user_model()
User.objects.create_superuser(**{'username': 'root', 'password': '<PASSWORD>', 'email': '<EMAIL>@e.co'})
" | /home/vagrant/venv/bin/python manage.py shell
[127.0.0.1] run: mkdir var -p
[127.0.0.1] run: make start
[127.0.0.1] run: make runcelery_multi
Done.
Disconnecting from 127.0.0.1:2200... done.
==> falstartexample_vagrant: Machine 'falstartexample_vagrant' has a post `vagrant up` message. This is a message
==> falstartexample_vagrant: from the creator of the Vagrantfile, and not from Vagrant itself:
==> falstartexample_vagrant:
==> falstartexample_vagrant: falstartexample dev server successfuly started.
==> falstartexample_vagrant: Connect to host with:
==> falstartexample_vagrant: http://10.1.1.111/
==> falstartexample_vagrant: or over ssh with `vagrant ssh`
==> falstartexample_vagrant:
==> falstartexample_vagrant: Admin user credentials:
==> falstartexample_vagrant: login: root
==> falstartexample_vagrant: password: <PASSWORD>
==> falstartexample_vagrant:
[falstart] > make dir: "provision"
[falstart] > render "provision_fabfile.j2" > "provision/fabric_provisioner.py"
[falstart] > render "gitignore.j2" > ".gitignore"
[falstart] > Update .gitignore
[falstart] > run: "git add . && git commit -m ":rocket: falstart init commit""
[master 0b93d53] :rocket: falstart init commit
39 files changed, 690 insertions(+), 4 deletions(-)
create mode 100644 .coveragerc
create mode 100644 .falstart.json
create mode 100644 .lintrc
create mode 100755 Makefile
create mode 100644 Vagrantfile
create mode 100644 falstartexample/__init__.py
create mode 100644 falstartexample/celery.py
create mode 100644 falstartexample/settings.py
create mode 100644 falstartexample/settings_local.py.example
create mode 100644 falstartexample/urls.py
create mode 100644 falstartexample/wsgi.py
create mode 100755 manage.py
create mode 100644 provision/fabric_provisioner.py
create mode 100644 provision/templates/environment.j2
create mode 100644 provision/templates/locale.gen.j2
create mode 100644 provision/templates/nginx-host.j2
create mode 100644 requirements-remote.txt
create mode 100644 requirements.txt
create mode 100644 wheels/Django-1.10.2-py2.py3-none-any.whl
create mode 100644 wheels/amqp-1.4.9-py2.py3-none-any.whl
create mode 100644 wheels/anyjson-0.3.3-py3-none-any.whl
create mode 100644 wheels/billiard-3.3.0.23-py3-none-any.whl
create mode 100644 wheels/celery-3.1.23-py2.py3-none-any.whl
create mode 100644 wheels/contextlib2-0.5.4-py2.py3-none-any.whl
create mode 100644 wheels/coverage-4.2-cp35-cp35m-linux_x86_64.whl
create mode 100644 wheels/coverage_badge-0.1.2-py3-none-any.whl
create mode 100644 wheels/django_rainbowtests-0.5.1-py3-none-any.whl
create mode 100644 wheels/gunicorn-19.4.5-py2.py3-none-any.whl
create mode 100644 wheels/kombu-3.0.37-py2.py3-none-any.whl
create mode 100644 wheels/mccabe-0.4.0-py2.py3-none-any.whl
create mode 100644 wheels/pep257-0.7.0-py2.py3-none-any.whl
create mode 100644 wheels/pep8-1.7.0-py2.py3-none-any.whl
create mode 100644 wheels/psycopg2-2.6.1-cp35-cp35m-linux_x86_64.whl
create mode 100644 wheels/pyflakes-1.0.0-py2.py3-none-any.whl
create mode 100644 wheels/pylama-7.0.7-py2.py3-none-any.whl
create mode 100644 wheels/pytz-2016.7-py2.py3-none-any.whl
create mode 100644 wheels/raven-5.26.0-py2.py3-none-any.whl
create mode 100644 wheels/redis-2.10.5-py2.py3-none-any.whl
```<file_sep>VENV_PATH := $(HOME)/venv/bin
PROJ_NAME := falstartexample
runserver:
$(VENV_PATH)/python manage.py runserver 0.0.0.0:8000
start:
mkdir -p var
$(VENV_PATH)/gunicorn --preload --pid var/gunicorn.pid \
-D -b 127.0.0.1:8000 $(PROJ_NAME).wsgi:application
stop:
kill `cat var/gunicorn.pid` || true
restart: stop start
pep8:
$(VENV_PATH)/pep8 --exclude=*migrations*,*settings_local.py* \
--max-line-length=119 --show-source $(PROJ_NAME)/
pyflakes:
$(VENV_PATH)/pylama --skip=*migrations* -l pyflakes $(PROJ_NAME)/
lint: pep8 pyflakes
test:
$(VENV_PATH)/python manage.py test -v 2 --noinput
cover_test:
$(VENV_PATH)/coverage run --source=$(PROJ_NAME) manage.py test -v 2 --noinput
cover_report:
$(VENV_PATH)/coverage report -m
$(VENV_PATH)/coverage html
$(VENV_PATH)/coverage-badge > htmlcov/coverage.svg
ci_test: cover_test cover_report lint
wheel_install:
$(VENV_PATH)/pip install --no-index -f wheels/ -r requirements.txt
runcelery:
$(VENV_PATH)/celery -A $(PROJ_NAME) worker -l info -B -s ./var/celerybeat-schedule
runcelery_multi:
$(VENV_PATH)/celery multi restart $(PROJ_NAME)_worker \
-A $(PROJ_NAME) -l info -B -s ./var/celerybeat-schedule \
--logfile="./var/celery_%n.log" \
--pidfile="./var/celery_%n.pid"
stopcelery_multi:
kill `cat var/celery_$(PROJ_NAME)_worker.pid` || true
restart_celery: stopcelery_multi runcelery_multi
<file_sep># vi: syntax=python
import os
import fabric
from inspect import getsource
from textwrap import dedent
from fabric.api import run, sudo, cd
fabric.state.env.colorize_errors = True
fabric.state.output['stdout'] = False
# task const variables
VARS = dict(
current_user=fabric.state.env.user,
# project settings
project_name='falstartexample',
user_data=dict(
username='root',
email='<EMAIL>',
password='<PASSWORD>'
),
# dirs configs
templates_dir='./provision/templates',
root_dir='/home/vagrant/proj',
venv_path='/home/vagrant/venv',
base_dir='/home/vagrant',
# nginx config
http_host='10.1.1.111',
http_port='80',
# database config
db_name='falstartexample',
db_password='<PASSWORD>',
db_user='falstartexample_user',
# locale conf
locale='ru_RU',
encoding='UTF-8',
)
def sentinel(sentinel_name=None):
def sentinel_wrapp(function):
hashed_func_name = '_'.join((function.__name__, str(hash(getsource(function)))))
def wrapped():
sentinel_path = '/usr/{}'.format(sentinel_name or hashed_func_name)
if fabric.contrib.files.exists(sentinel_path):
fabric.utils.warn('skip {}'.format(sentinel_name or hashed_func_name))
return
function()
sudo('touch {}'.format(sentinel_path))
return wrapped
return sentinel_wrapp
def common():
""" Common tasks """
locale()
apt_packages()
python_packages()
@sentinel()
def locale():
""" Set locale to enviroment """
fabric.contrib.files.upload_template(
'environment.j2', '/etc/environment',
context=VARS, use_jinja=True, backup=False, use_sudo=True, template_dir=VARS['templates_dir'])
sudo('localedef {locale}.{encoding} -i {locale} -f{encoding}'.format(**VARS))
# sudo('locale-gen')
@sentinel()
def apt_packages():
""" Install common packages """
sudo('apt-get -y update')
sudo('apt-get -y install libcurl4-gnutls-dev libexpat1-dev gettext libz-dev libssl-dev git redis-server')
@sentinel()
def python_packages():
""" Install python components """
sudo('apt-get -y install python-dev python-pip python-virtualenv build-essential '
'libncurses5-dev libncursesw5-dev libreadline6-dev libgdbm-dev libsqlite3-dev '
'libssl-dev libbz2-dev libexpat1-dev liblzma-dev zlib1g-dev libxml2-dev libxslt1-dev')
with cd('/tmp'):
# download python source code
run('wget -O python.tgz https://www.python.org/ftp/python/3.5.2/Python-3.5.2.tgz')
# extract python tarball
run('tar -xf python.tgz')
run('mv Python-3.5.2 python')
with cd('/tmp/python'):
# configuring python 3.4 Makefile
run('./configure --prefix=/usr/local/python-3.5.2')
# compiling the python 3.4 source code and install
run('make')
sudo('make altinstall')
sudo('rm /tmp/* -rf')
# make link to python
sudo('ln -sf /usr/local/python-3.5.2/bin/* /usr/local/bin/')
def nginx():
""" Install and configure nginx tasks """
nginx_install()
# create nginx config file for project
fabric.contrib.files.upload_template(
'nginx-host.j2', '/etc/nginx/sites-available/{project_name}'.format(**VARS),
context=VARS, use_jinja=True, backup=False, use_sudo=True, template_dir=VARS['templates_dir'])
# make s-link to enabled sites
sudo('ln -sf /etc/nginx/sites-available/{project_name} /etc/nginx/sites-enabled/{project_name}'.format(**VARS))
# restart nginx
sudo('service nginx restart')
@sentinel()
def nginx_install():
""" Install nginx """
# install nginx
sudo('apt-get -y install nginx')
@sentinel()
def database():
""" Install database tasks """
# install postgres apt
sudo('apt-get -y install postgresql postgresql-server-dev-all python-psycopg2')
# make postgers password
with fabric.context_managers.settings(warn_only=True):
commands = (
'CREATE USER {db_user};',
'ALTER USER {db_user} WITH PASSWORD \'{<PASSWORD>}\';',
'ALTER USER {db_user} CREATEDB;',
'CREATE DATABASE {db_name};',
'GRANT ALL PRIVILEGES ON DATABASE {db_name} TO {db_user};',
)
for command in commands:
run('sudo -u postgres psql -c "%s"' % command.format(**VARS))
def app():
""" Run application tasks """
with cd(VARS['root_dir']):
# Create venv and install requirements
run('pyvenv-3.5 {venv_path}'.format(**VARS))
# Install required python packages with pip from wheels archive
run('make wheel_install')
# run app tasks for devserver start
# Copy settings local
run('cd {project_name} && cp settings_local.py.example settings_local.py'.format(**VARS))
def localserver():
with cd(VARS['root_dir']):
# collect static files
for command in ('migrate --noinput', 'collectstatic --noinput', ): # 'compilemessages', ):
run('{venv_path}/bin/python manage.py {command}'.format(command=command, **VARS))
# make root dir available to read
# sudo('chmod 755 {base_dir}/static -R'.format(**VARS))
# Create user
create_user_py = dedent('''\
from django.contrib.auth import get_user_model
User = get_user_model()
User.objects.create_superuser(**{user_data})
''').format(**VARS)
run('echo "{create_user_py}" | {venv_path}/bin/python manage.py shell'.format(
create_user_py=create_user_py, **VARS))
run('mkdir var -p')
# Start celery and runserver
run('make start', pty=False)
run('make runcelery_multi', pty=False)
<file_sep>Django==1.10.2
django-rainbowtests==0.5.1
gunicorn==19.4.5
pep8==1.7.0
coverage==4.2
coverage-badge==0.1.2
pylama==7.0.7
psycopg2==2.6.1
Celery==3.1.23
redis==2.10.5
raven==5.26.0
| 6a261efdfd10c10652d824e6da39bcdaa4cb57a7 | [
"Markdown",
"Python",
"Makefile",
"Text"
] | 5 | Python | Samael500/falstart-example | 53745e17a8619c49b7cdd14129427236af04d187 | cd6ab7ab4008bfadc560337db05c9d1b0f90fb71 | |
refs/heads/master | <repo_name>E-Sangho/Baekjoon_Algorithm<file_sep>/9461.cpp
#include <iostream>
using namespace std;
int sequence[101];
int padovan(const int &n)
{
if(n <= 3) return 1;
else if(n <= 5) return 2;
else if(sequence[n] == 0){
sequence[n] = padovan(n-1) + padovan(n-5);
}
return sequence[n];
}
int main()
{
int T, N;
cin >> T;
for(int t = 0; t < T; ++t){
cin >> N;
cout << padovan(N) << '\n';
}
}<file_sep>/2292.cpp
#include <stdio.h>
int main(){
int N, count = 0;
scanf("%d", &N);
if(N == 1) count = 0;
else{
while(1 + count*(count + 1) * 3 < N){
++count;
}
}
printf("%d", count + 1);
}
<file_sep>/1874.cpp
#include <iostream>
#include <stack>
#include <vector>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, cnt = 0;
cin >> n;
stack<int> s;
vector<char> v;
int arr[n];
for(int i = 0; i < n; ++i)
{
cin >> arr[i];
}
for(int i = 1; i <= n; ++i)
{
s.push(i);
v.push_back('+');
while(!s.empty() && s.top() == arr[cnt])
{
s.pop();
v.push_back('-');
++cnt;
}
}
if(!s.empty()) cout << "NO";
else
{
int length = v.size();
for(int i = 0; i < length; ++i)
{
cout << v[i] << '\n';
}
}
return 0;
}<file_sep>/1010.cpp
#include <stdio.h>
using namespace std;
int dp[30][30];
int C(const int &n, const int &k)
{
if(n == k) return 1;
if(k == 0) return 1;
if(dp[n][k] != 0) return dp[n][k];
else
{
dp[n][k] = C(n-1, k-1) + C(n-1, k);
return dp[n][k];
}
}
int main()
{
int T, N, M;
scanf("%d", &T);
for(int t = 0; t < T; ++t)
{
scanf("%d %d", &N, &M);
printf("%d\n", C(M, N));
}
return 0;
}<file_sep>/2941.cpp
#include <stdio.h>
#include <string.h>
int main(){
char input[101];
int num = 0;
scanf("%s", input);
int length = strlen(input);
int i = 0;
while(i < length){
if(input[i] == 'c' && (input[i+1] == '=' || input[i+1] == '-')){
i += 2;
++num;
}
else if(input[i] == 'd' && input[i+1] == 'z' && input[i+2] == '='){
i += 3;
++num;
}
else if(input[i] == 'd' && input[i+1] == '-'){
i += 2;
++num;
}
else if((input[i] == 'l' || input[i] == 'n') && input[i+1] == 'j'){
i += 2;
++num;
}
else if((input[i] == 's' || input[i] == 'z') && input[i+1] == '='){
i += 2;
++num;
}
else{
++i;
++num;
}
}
printf("%d", num);
}<file_sep>/1655.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, temp, first, second;
cin >> N;
priority_queue<int> pq_left;
priority_queue<int, vector<int>, greater<int>> pq_right;
cin >> first >> second;
if(first < second){
pq_left.push(first);
pq_right.push(second);
}
else{
pq_left.push(second);
pq_right.push(first);
}
cout << first << '\n';
cout << pq_left.top() << '\n';
for(int n = 0; n < N - 2; ++n)
{
cin >> temp;
if(pq_left.size() == pq_right.size())
{
if(pq_right.top() < temp)
{
pq_right.push(temp);
pq_left.push(pq_right.top());
pq_right.pop();
}
else pq_left.push(temp);
}
else if(pq_left.size() > pq_right.size())
{
if(temp < pq_left.top())
{
pq_left.push(temp);
pq_right.push(pq_left.top());
pq_left.pop();
}
else pq_right.push(temp);
}
/*
else
{
if(pq_right.top() < temp)
{
pq_right.push(temp);
pq_left.push(pq_right.top());
pq_right.pop();
}
else pq_left.push(temp);
}
*/
cout << pq_left.top() << '\n';
}
return 0;
}
/*
1. pq_left.size() != pq_right.size();
1) pq_left.size() > pq_right.size();
(1) temp < pq_left.top()
pq_left.push(temp)
pq_right.push(pq_left.top())
pq_left.pop();
(2) pq_left.top() < temp < pq_right.top()
pq_rifht.push(temp);
(3) pq_right.top() < temp
pq_right.push(temp)
2) pq_right_size() > pq_left.size();
(1) temp < pq_left.top()
pq_left.push(temp);
(2) pq_left.top() < temp < pq_right.top()
pq_left.push(temp);
(3) pq_right.top() < temp
pq_right.push(temp);
pq_left.push(pq_rignt.top());
pq_right.pop();
2. pq_right.size() = pq_left.size()
1) temp < pq_left.top()
pq_left.push(temp)
2) pq_left.top() < temp < pq_right.top()
pq_left.push(temp)
3) pq_right.top() < temp
pq_right.push(temp)
pq_left.push(pq_right.top())
pq_right.pop()
*/ <file_sep>/11057.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
int mod = 10007, ans = 0;
int dp[1001][10] = {0};
for(int i = 1; i <= N; ++i)
{
for(int j = 0; j < 10; ++j)
{
if(i == 1) dp[i][j] = 1;
else
{
for(int k = 9; k >= j; --k)
{
dp[i][j] += dp[i-1][k] % mod;
}
}
}
}
for(int i = 0; i < 10; ++i)
{
ans += dp[N][i] % mod;
}
cout << ans % mod;
return 0;
}<file_sep>/2565.cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool compare(vector<int> &a, vector<int> &b)
{
return a[0] < b[0];
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, len = 0;
cin >> n;
vector<vector<int>> v(n, vector<int>(2));
int dp[n];
for(int i = 0; i < n; ++i)
{
cin >> v[i][0] >> v[i][1];
}
sort(v.begin(), v.end(), compare);
for(int i = 0; i < n; ++i)
{
dp[i] = 1;
for(int j = 0; j < i; ++j)
{
if(v[i][1] > v[j][1])
{
dp[i] = max(dp[i], dp[j] + 1);
}
}
len = max(len, dp[i]);
}
cout << n - len;
return 0;
}<file_sep>/4963.cpp
#include <iostream>
#include <stack>
#include <string.h>
using namespace std;
#define MAX_SIZE 50
int w, h;
int num_island = 0;
int arr[MAX_SIZE][MAX_SIZE];
bool visited[MAX_SIZE][MAX_SIZE];
int idx[8] = {-1, -1, -1, 0, 0, 1, 1, 1};
int idy[8] = {-1, 0, 1, -1, 1, -1, 0, 1};
bool in_map(int row, int column)
{
return (0 <= row && row < h && 0 <= column && column < w);
}
void DFS(int r, int c)
{
stack<pair<int, int>> s;
s.push({r, c});
visited[r][c] = true;
++num_island;
while(!s.empty())
{
int p_row = s.top().first;
int p_col = s.top().second;
s.pop();
for(int i = 0; i < 8; ++i)
{
int c_row = p_row + idx[i];
int c_col = p_col + idy[i];
if(in_map(c_row, c_col) &&
arr[c_row][c_col] == 1 &&
!visited[c_row][c_col])
{
s.push({c_row, c_col});
visited[c_row][c_col] = true;
}
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
while(1)
{
cin >> w >> h;
if(w == 0 && h == 0) break;
for(int row = 0; row < h; ++row)
{
for(int column = 0; column < w; ++column)
{
cin >> arr[row][column];
}
}
for(int row = 0; row < h; ++row)
{
for(int column = 0; column < w; ++column)
{
if(!visited[row][column] && arr[row][column] == 1)
{
DFS(row, column);
}
}
}
cout << num_island << endl;
memset(arr, 0, sizeof(arr));
memset(visited, false, sizeof(visited));
num_island = 0;
}
}<file_sep>/1931.cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool compare(pair<int,int> a, pair<int,int> b)
{
return a.second < b.second;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, ans = 1;
cin >> N;
vector<pair<int, int>> v(N);
for(int n = 0; n < N; ++n)
{
cin >> v[n].first >> v[n].second;
}
sort(v.begin(), v.end(), compare);
int s_time = v[0].first, e_time = v[0].second;
for(int n = 1; n < N; ++n)
{
if(e_time <= v[n].first)
{
s_time = v[n].first;
e_time = v[n].second;
++ans;
}
}
cout << ans;
return 0;
}<file_sep>/1789.cpp
#include <stdio.h>
using namespace std;
int main()
{
long long S, n = 0;
scanf("%d", &S);
while(!(n * (n + 1) <= 2 * S && 2 * S < (n + 1) * (n + 2)))
{
++n;
}
printf("%d", n);
return 0;
}<file_sep>/17225.cpp
#include <iostream>
#include <queue>
using namespace std;
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int A, B, N;
cin >> A >> B >> N;
int t, m;
char c;
priority_queue<int> pq_Sangmin, pq_Jisu;
for(int n = 0; n < N; ++n){
cin >> t >> c >> m;
if(c == 'B'){
for(int i = 1; i <= m; ++i){
if(!pq_Sangmin.empty() && t >= pq_Sangmin.top()){
t += A;
pq_Sangmin.push(t);
}
else if(pq_Sangmin.empty()){
t += A;
pq_Sangmin.push(t);
}
else{
t = pq_Sangmin.top() + A;
pq_Sangmin.push(t);
}
}
}
else if(c == 'R'){
for(int i = 1; i <= m; ++i){
if(!pq_Jisu.empty() && t >= pq_Jisu.top()){
t += B;
pq_Jisu.push(t);
}
else if(pq_Jisu.empty()){
t += B;
pq_Jisu.push(t);
}
else{
t = pq_Jisu.top() + B;
pq_Jisu.push(t);
}
}
}
}
int count = pq_Sangmin.size() + pq_Jisu.size();
priority_queue<int, vector<int>, greater<int>> Sangmin, Jisu;
while(!pq_Sangmin.empty() && !pq_Jisu.empty()){
if(pq_Sangmin.top() - A > pq_Jisu.top() - B){
Sangmin.push(count--);
pq_Sangmin.pop();
}
else{
Jisu.push(count--);
pq_Jisu.pop();
}
}
while(!pq_Sangmin.empty()){
Sangmin.push(count--);
pq_Sangmin.pop();
}
while(!pq_Jisu.empty()){
Jisu.push(count--);
pq_Jisu.pop();
}
int s = Sangmin.size(), j = Jisu.size();
cout << s << '\n';
for(int a = 0; a < s - 1; ++a){
cout << Sangmin.top() << " ";
Sangmin.pop();
}
cout << Sangmin.top() << '\n';
cout << j << '\n';
for(int b = 0; b < j - 1; ++b){
cout << Jisu.top() << " ";
Jisu.pop();
}
cout << Jisu.top();
}<file_sep>/1012.cpp
#include <iostream>
#include <queue>
using namespace std;
int idx[4] = {-1, 1, 0, 0};
int idy[4] = {0, 0, -1, 1};
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T, M, N, K;
cin >> T;
for(int t = 0; t < T; ++t)
{
cin >> M >> N >> K;
int arr[M][N];
bool visited[M][N];
for(int p = 0; p < M; ++p){
for(int q = 0; q < N; ++q)
{
arr[p][q] = 0;
visited[p][q] = false;
}
}
int x, y, count = 0;
for(int k = 0; k < K; ++k)
{
cin >> x >> y;
arr[x][y] = 1;
}
for(int a = 0; a < M; ++a){
for(int b = 0; b < N; ++b)
{
if(!visited[a][b] && arr[a][b] == 1)
{
queue<pair<int, int>> queue;
queue.push({a, b});
visited[a][b] = true;
++count;
while(!queue.empty())
{
pair<int, int> parent = queue.front();
queue.pop();
int p_row = parent.first;
int p_column = parent.second;
for(int i = 0; i < 4; ++i)
{
int c_row = p_row + idx[i];
int c_column = p_column + idy[i];
if(!visited[c_row][c_column] &&
0 <= c_row && c_row < M &&
0 <= c_column && c_column < N &&
arr[c_row][c_column] == 1)
{
queue.push({c_row, c_column});
visited[c_row][c_column] = true;
}
}
}
}
}
}
cout << count << '\n';
}
}<file_sep>/2164.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
int N;
cin >> N;
queue<int> deck;
for(int i = 1; i <= N; ++i)
{
deck.push(i);
}
while(deck.size() != 1)
{
deck.pop();
deck.push(deck.front());
deck.pop();
}
cout << deck.front();
return 0;
}<file_sep>/1149.cpp
#include <iostream>
using namespace std;
int color[1000][3];
int dp[1000][3];
int min(const int &a, const int &b){
return a > b ? b : a;
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, R, G, B;
cin >> N;
for(int n = 0; n < N; ++n){
cin >> color[n][0] >> color[n][1] >> color[n][2];
}
dp[0][0] = color[0][0];
dp[0][1] = color[0][1];
dp[0][2] = color[0][2];
for(int n = 1; n < N; ++n){
dp[n][0] = color[n][0] + min(dp[n-1][1], dp[n-1][2]);
dp[n][1] = color[n][1] + min(dp[n-1][0], dp[n-1][2]);
dp[n][2] = color[n][2] + min(dp[n-1][0], dp[n-1][1]);
}
cout << min(dp[N-1][0], min(dp[N-1][1], dp[N-1][2]));
}
<file_sep>/15577.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
double N, temp, first, second;
cin >> N;
priority_queue<double, vector<double>, greater<double>> pq;
for(int i = 0; i < N; ++i)
{
cin >> temp;
pq.push(temp);
}
while(N > 1)
{
first = pq.top();
pq.pop();
second = pq.top();
pq.pop();
pq.push((first + second)/2);
N--;
}
cout << fixed;
cout.precision(6);
cout << pq.top();
}<file_sep>/11051.cpp
#include <iostream>
int p = 10007;
int dp[1001][1001];
using namespace std;
int C(int N, int K) {
if(dp[N][K] != 0) return dp[N][K];
if(N == K || K == 0) {
dp[N][K] = 1;
return 1;
}
dp[N][K] = (C(N-1, K) + C(N-1, K-1)) % p;
return dp[N][K];
}
int main() {
int N, K;
cin >> N >> K;
cout << C(N, K) << endl;
}<file_sep>/2675.cpp
#include <stdio.h>
#include <string.h>
int main(){
int T, R;
char S[20];
scanf("%d", &T);
for(int i = 0; i < T; ++i){
scanf("%d %s", &R, S);
for(int j = 0; j <strlen(S); ++j){
for(int k = 0; k < R; ++k){
printf("%c", S[j]);
}
}
printf("\n");
}
}
<file_sep>/16395.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, k;
cin >> n >> k;
int C[n][k];
for(int i = 0; i < n; ++i)
{
for(int j = 0; j < k; ++j)
{
if(i == j || j == 0) C[i][j] = 1;
else
{
C[i][j] = C[i-1][j] + C[i-1][j-1];
}
}
}
cout << C[n-1][k-1];
return 0;
}<file_sep>/19638.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, H, T, count = 0;
cin >> N >> H >> T;
priority_queue<int> pq;
for(int i = 0; i < N; ++i)
{
int giant_Height;
cin >> giant_Height;
pq.push(giant_Height);
}
for(int i = 0; i < T; ++i)
{
int tallest = pq.top();
if(tallest < H)
{
break;
}
if(tallest > 1)
{
++count;
pq.pop();
pq.push(tallest / 2);
}
}
if(pq.top() < H)
{
cout << "YES\n" << count << "\n";
}
else
{
cout << "NO\n" << pq.top() << "\n";
}
}<file_sep>/2468.cpp
#include <iostream>
#include <string.h>
using namespace std;
int arr[100][100];
int N;
bool visited[100][100];
int idx[4] = {0, 0, -1, 1};
int idy[4] = {-1, 1, 0, 0};
void DFS(int i, int j, int h)
{
visited[i][j] = true;
for(int k = 0; k < 4; ++k){
int r = i + idx[k];
int c = j + idy[k];
if(!visited[r][c] &&
0 <= r && r < N &&
0 <= c && c < N &&
arr[r][c] > h)
DFS(r, c, h);
}
}
int main()
{
ios::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int max_height = 0, ans = 0;
cin >> N;
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j){
cin >> arr[i][j];
max_height = max(max_height, arr[i][j]);
}
}
for(int h = 0; h < max_height; ++h){
memset(visited, false, sizeof(visited));
int cnt = 0;
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j){
if(!visited[i][j] && arr[i][j] > h){
DFS(i, j, h);
++cnt;
}
}
}
ans = max(ans, cnt);
}
cout << ans;
}<file_sep>/1463.cpp
#include <iostream>
#include <algorithm>
using namespace std;
int goUp(int n){
if(n <= 1) return 0;
else{
return min(n % 3 + goUp(n/3) + 1, n % 2 + goUp(n/2) + 1);
}
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
cout << goUp(N);
return 0;
}<file_sep>/1427_1.cpp
#include <stdio.h>
#include <string.h>
void merge(int *arr, int *sorted, int start, int middle, int end){
int l = start, r = middle + 1, i = start;
while(l <= middle && r <= end){
if(arr[l] < arr[r]){
sorted[i] = arr[r];
++r;
}
else{
sorted[i] = arr[l];
++l;
}
++i;
}
if(l > middle){
while(r <= end){
sorted[i] = arr[r];
++r;
++i;
}
}
else{
while(l <= middle){
sorted[i] = arr[l];
++l;
++i;
}
}
for(int k = start; k <= end; ++k){
arr[k] = sorted[k];
}
}
void merge_sort(int *arr, int *sorted, int start, int end){
if(start < end){
int middle = (start + end) / 2;
merge_sort(arr, sorted, start, middle);
merge_sort(arr, sorted, middle + 1, end);
merge(arr, sorted, start, middle, end);
}
}
int main(){
char arr[10];
int ans[10];
int sorted[10] = {0};
scanf("%s", arr);
int len = strlen(arr);
for(int i = 0; i < len; ++i){
ans[i] = (int)arr[i] - 48;
}
merge_sort(ans, sorted, 0, len - 1);
for(int i = 0; i < len; ++i){
printf("%d",ans[i]);
}
}<file_sep>/4949.cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool check_balance(const string &str)
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
stack<char> s;
int length = str.length();
for(int i = 0; i < length; ++i)
{
if(str[i] == '(')
{
s.push('(');
}
else if(str[i] == '[')
{
s.push('[');
}
else if(str[i] == ')')
{
if(!s.empty() && s.top() == '(') s.pop();
else return false;
}
else if(str[i] == ']')
{
if(!s.empty() && s.top() =='[') s.pop();
else return false;
}
}
return s.empty();
}
int main()
{
while(1)
{
string str;
getline(cin, str);
if(str[0] == '.') break;
if(check_balance(str)) cout << "yes" << endl;
else cout << "no" << endl;
}
}<file_sep>/11074.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, K, ans = 0;
cin >> N >> K;
int A[N];
for(int n = 0; n < N; ++n)
{
cin >> A[n];
}
for(int i = N-1; i >= 0; --i)
{
if(A[i] <= K)
{
ans += K / A[i];
K = K - (K / A[i]) * A[i];
}
}
cout << ans;
return 0;
}<file_sep>/2231.cpp
#include <stdio.h>
int dividesum(int &input){
int arr[7] = {0}, temp = input, i = 0, result = input;
while(temp > 0){
arr[i] = temp % 10;
++i;
temp /= 10;
}
for(int i = 0; i < 7; ++i){
result += arr[i];
}
return result;
}
int main(){
int N;
scanf("%d", &N);
int length = 0, temp = N;
bool check = true;
while(temp > 0){
temp /= 10;
++length;
}
int start = N - length * 10;
for(int i = start; i < N; ++i){
if(dividesum(i) == N){
printf("%d", i);
check = false;
break;
}
}
if(check) printf("%d", 0);
}<file_sep>/1699.cpp
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
int dp[100001] = {0};
for(int i = 1; i <= N; ++i){
dp[i] = i;
for(int j = 1; j * j <= i; ++j)
{
dp[i] = min(dp[i], dp[i-j*j] + 1);
}
}
cout << dp[N];
return 0;
}<file_sep>/9012.cpp
#include <iostream>
#include <string>
#include <stack>
using namespace std;
bool check_VPS(const string input)
{
int length = input.length();
stack<char> s;
for(int i = 0; i < length; ++i)
{
if(input[i] == '(')
{
s.push('(');
}
else if(input[i] == ')')
{
if(s.empty()) return false;
else s.pop();
}
}
return s.empty();
}
int main()
{
ios_base:: sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T;
cin >> T;
while(T--)
{
string input;
cin >> input;
if(check_VPS(input)) cout << "YES" << endl;
else cout << "NO" << endl;
}
return 0;
}<file_sep>/10814.cpp
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
class Person{
private:
string name;
int age;
int order;
public:
Person(const string &name_input = "Noname", const int &age_input = 0, const int &order_input = 0)
: name(name_input)
, age(age_input)
, order(order_input)
{}
void setPersonInfo(int age_input, string name_input, int order_input)
{
name = name_input;
age = age_input;
order = order_input;
}
int getage(){
return age;
}
int getorder(){
return order;
}
string getname(){
return name;
}
};
bool compare(Person A, Person B){
if(A.getage() == B.getage()) return A.getorder() < B.getorder();
else return A.getage() < B.getage();
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, age;
string name;
cin >> N;
Person member[N];
for(int i = 0; i < N; ++i){
cin >> age >> name;
member[i].setPersonInfo(age, name, i);
}
sort(member, member + N , compare);
for(int i = 0; i < N; ++i){
cout << member[i].getage() << ' ' << member[i].getname() << '\n';
}
}<file_sep>/11650.cpp
#include <stdio.h>
#include <algorithm>
#include <vector>
class Point
{
public:
int x;
int y;
Point(const int &x_input, const int &y_input)
{
x = x_input;
y = y_input;
}
};
bool compare(Point &a, Point &b){
if(a.x == b.x) return a.y < b.y;
else return a.x < b.x;
}
int main(){
int N;
scanf("%d", &N);
std::vector<Point> p;
int x, y;
for(int i = 0; i < N; ++i){
scanf("%d %d", &x, &y);
p.push_back(Point(x,y));
}
sort(p.begin(), p.end(), compare);
for(int i = 0; i < N; ++i){
printf("%d %d\n", p[i].x, p[i].y);
}
}<file_sep>/1202.cpp
#include <iostream>
#include <queue>
#include <algorithm>
#include <set>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, K, m, v, c;
long long ans = 0;
cin >> N >> K;
priority_queue<pair<int, int>> jewerlly;
multiset<int> bag;
for(int n = 0; n < N; ++n)
{
cin >> m >> v;
jewerlly.push({v, m});
}
for(int k = 0; k < K; ++k)
{
cin >> c;
bag.insert(c);
}
while(!bag.empty() && !jewerlly.empty())
{
multiset<int>::iterator iter = bag.lower_bound(jewerlly.top().second);
if(iter != bag.end())
{
bag.erase(iter);
ans += jewerlly.top().first;
jewerlly.pop();
}
else jewerlly.pop();
}
cout << ans;
}<file_sep>/2798.cpp
#include <stdio.h>
int main(){
int N, M;
scanf("%d %d", &N, &M);
int arr[100], sum = 0, max = 0;
for(int i = 0; i < N; ++i){
scanf("%d", &arr[i]);
}
for(int i = 0; i < N; ++i){
for(int j = i + 1; j < N; ++j){
for(int k = j + 1; k < N; ++k){
sum = arr[i] + arr[j]+ arr[k];
if(sum <= M && max < sum){
max = sum;
}
}
}
}
printf("%d", max);
}<file_sep>/11651.cpp
#include <stdio.h>
#include <algorithm>
#include <vector>
class Point{
private:
int x;
int y;
public:
Point(const int &x_input, const int &y_input){
x = x_input;
y = y_input;
}
const int getx(){
int a = x;
return a;
}
const int gety(){
int b = y;
return b;
}
};
bool compare(Point &a, Point &b){
if(a.gety() == b.gety()) return a.getx() < b.getx();
else return a.gety() < b.gety();
}
int main(){
int N;
scanf("%d", &N);
int x = 0, y = 0;
std::vector<Point> p;
for(int i = 0; i < N; ++i){
scanf("%d %d", &x, &y);
p.push_back(Point(x,y));
}
sort(p.begin(), p.end(), compare);
for(int i = 0; i < N; ++i){
printf("%d %d\n", p[i].getx(), p[i].gety());
}
}<file_sep>/1427_3.cpp
#include <stdio.h>
#include <algorithm>
#include <string.h>
bool compare(char a, char b){
return a > b;
}
int main(){
char arr[11];
scanf("%s", arr);
std::sort(arr, arr + strlen(arr), compare);
printf("%s", arr);
}<file_sep>/15903.cpp
#include <iostream>
#include <queue>
using namespace std;
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
priority_queue<long long, vector<long long>, greater<long long>> pq;
long long n, m, temp;
long long ans = 0;
cin >> n >> m;
for(int i = 0; i < n; ++i){
cin >> temp;
pq.push(temp);
}
while(m--){
long long first = pq.top();
pq.pop();
long long second = pq.top();
pq.pop();
pq.push(first + second);
pq.push(first + second);
}
while(!pq.empty()){
ans += pq.top();
pq.pop();
}
cout << ans;
}<file_sep>/10809.cpp
#include <stdio.h>
#include <string.h>
int main(){
char input[101];
char alphabet[26];
scanf("%s", input);
int length = strlen(input);
for(char i = 'A'; i <= 'Z' ; ++i){
alphabet[i - 'A'] = -1;
for(int j = 0; j < length; ++j){
if('a' <= input[j]){
input[j] -= 32;
}
if(input[j] == i){
alphabet[i - 'A'] = j;
break;
}
}
}
for(int i = 0; i < 26; ++i){
printf("%d ", alphabet[i]);
}
}<file_sep>/2750.cpp
#include <stdio.h>
int main(){
int N;
scanf("%d", &N);
int arr[N];
for(int i =0; i < N; ++i){
scanf("%d", &arr[i]);
}
for(int i = 1; i < N; ++i){
int key = arr[i];
int j = i - 1;
while(j >= 0 && arr[j]> key){
arr[j+1] = arr[j];
j = j - 1;
}
arr[j+1] = key;
}
for(int i = 0; i < N; ++i){
printf("%d ", arr[i]);
}
}<file_sep>/10845.cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Queue
{
private:
vector<int> m_v;
public:
void push(const int &input)
{
m_v.push_back(input);
}
int pop()
{
if(empty()) return -1;
else
{
int temp;
temp = m_v.front();
m_v.erase(m_v.begin());
return temp;
}
}
int size()
{
return m_v.size();
}
int empty()
{
if(m_v.empty()) return 1;
else return 0;
}
int front()
{
if(m_v.empty()) return -1;
else return m_v.front();
}
int back()
{
if(m_v.empty()) return -1;
else return m_v.back();
}
void order(string s_in)
{
if(s_in == "push")
{
int temp;
cin >> temp;
push(temp);
}
else if(s_in == "pop")
{
cout << pop() << '\n';
}
else if(s_in == "size")
{
cout << size() << '\n';
}
else if(s_in == "empty")
{
cout << empty() << '\n';
}
else if(s_in == "front")
{
cout << front() << '\n';
}
else if(s_in == "back")
{
cout << back() << '\n';
}
}
};
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
string s_in;
cin >> N;
Queue q;
for(int i = 0; i < N; ++i)
{
cin >> s_in;
q.order(s_in);
}
return 0;
}<file_sep>/1966.cpp
#include <iostream>
#include <queue>
#include <vector>
#include <algorithm>
using namespace std;
bool compare(const int i, const int j)
{
return i > j;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
int T;
cin >> T;
for(int i = 0; i < T; ++i)
{
int N, M, count = 0;
cin >> N >> M;
queue<int> q;
queue<int> letter;
vector<int> v;
for(int j = 0; j < N; ++j)
{
int temp;
cin >> temp;
v.push_back(temp);
q.push(temp);
letter.push(j);
}
sort(v.begin(),v.end(), compare);
while(!q.empty())
{
if(v.front() == q.front())
{
++count;
if(letter.front() == M)
{
cout << count << '\n';
break;
}
v.erase(v.begin());
q.pop();
letter.pop();
}
else{
q.push(q.front());
q.pop();
letter.push(letter.front());
letter.pop();
}
}
}
return 0;
}<file_sep>/10828.cpp
#include <iostream>
#include <string>
using namespace std;
class Stack
{
private:
int *m_arr;
int m_top;
int m_size;
public:
Stack(const int& max_size)
{
m_arr = new int[max_size];
m_top = -1;
m_size = 0;
}
~Stack()
{
delete [] m_arr;
}
void push(const int &input)
{
m_arr[m_size] = input;
++m_size;
++m_top;
}
void pop()
{
if(empty()) cout << -1 << endl;
else
{
cout << m_arr[m_size - 1] << endl;
--m_size;
--m_top;
}
}
void top()
{
if(empty()) cout << -1 << endl;
else cout << m_arr[m_size - 1] << endl;
}
int empty()
{
if(m_top < 0) return 1;
else return 0;
}
void size()
{
cout << m_size << endl;
}
};
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
Stack stack(10000);
string str_in;
int push_in;
for(int i = 0; i < N; ++i)
{
cin >> str_in;
if(str_in == "push")
{
cin >> push_in;
stack.push(push_in);
}
else if(str_in == "top") stack.top();
else if(str_in == "size") stack.size();
else if(str_in == "empty")
{
if(stack.empty() == 1) cout << 1 << endl;
else cout << 0 << endl;
}
else if(str_in == "pop") stack.pop();
}
}<file_sep>/1946.cpp
#include <iostream>
#include <vector>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T;
cin >> T;
int ans[T];
for(int t = 0; t < T; ++t)
{
int N;
cin >> N;
vector<int> v(N+1);
int document, interview;
for(int n = 0; n < N; ++n)
{
cin >> document >> interview;
v[document] = interview;
}
int member = 1, current_ranking = v[1];
for(int i = 2; i <= N; ++i)
{
if(v[i] < current_ranking)
{
current_ranking = v[i];
++member;
}
}
cout << member << '\n';
}
return 0;
}<file_sep>/11720.cpp
#include <stdio.h>
int main(){
int N, sum = 0;
char arr[100];
scanf("%d %s", &N, arr);
for(int i = 0; i < N; ++i){
sum += (int)arr[i] - 48;
}
printf("%d", sum);
}<file_sep>/1157.cpp
#include <stdio.h>
#include <string.h>
int main(){
char a[1000001];
int alphabet[26] = {0};
scanf("%s", a);
int length = strlen(a);
for(int i = 0; i < length; ++i){// 알파벳 대문자로 바꾸기
if('a' <= a[i]) a[i] = a[i] - 32;
++alphabet[a[i] - 'A'];
}
int max = 0, max_num = 0, position = 0;
for(int i = 0; i < 26; ++i){
if(max < alphabet[i]) max = alphabet[i];
}
for(int i = 0; i < 26; ++i){
if(max == alphabet[i]){
++max_num;
position = i;
}
}
printf("%c",(max_num > 1)? '?' : 'A' + position);
}<file_sep>/1260.cpp
#include <iostream>
#include <vector>
#include <queue>
#include <algorithm>
using namespace std;
void BFS(int parent, vector<int> adj[], bool visited[])
{
queue<int> queue;
queue.push(parent);
while(!queue.empty())
{
parent = queue.front();
queue.pop();
if(!visited[parent])
{
visited[parent] = true;
cout << parent << " ";
}
for(int i = 0; i < adj[parent].size(); ++i)
{
int child = adj[parent][i];
if(!visited[child])
{
queue.push(child);
}
}
}
}
void DFS(int parent, vector<int> adj[], bool visited[])
{
visited[parent] = true;
cout << parent << " ";
for(int i = 0; i < adj[parent].size(); ++i)
{
int child = adj[parent][i];
if(!visited[child])
{
DFS(child, adj, visited);
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, M, V, v, w;
cin >> N >> M >> V;
vector<int> adj[1001];
bool visited[1001];
bool visited2[1001];
for(int m = 0; m < M; ++m)
{
cin >> v >> w;
adj[v].push_back(w);
adj[w].push_back(v);
}
for(int i = 1; i <= N; ++i)
{
sort(adj[i].begin(), adj[i].end());
}
DFS(V, adj, visited);
cout << '\n';
BFS(V, adj, visited2);
}<file_sep>/2293.cpp
#include <stdio.h>
using namespace std;
int main()
{
int n, k;
int input[100];
int dp[10001] = {0};
scanf("%d %d", &n, &k);
for(int i = 0; i < n; ++i) scanf("%d", &input[i]);
dp[0] = 1;
for(int i = 0; i < n; ++i)
{
for(int j = 1; j <= k; ++j)
{
if(input[i] <= j)
{
dp[j] += dp[j - input[i]];
}
}
}
printf("%d", dp[k]);
return 0;
}<file_sep>/1697.cpp
#include <iostream>
#include <queue>
using namespace std;
bool visited[100001] = {false,};
void BFS(int subin, int sister)
{
queue<pair<int, int>> graph;
graph.push({subin, 0});
visited[subin] = true;
if(graph.front().first == sister)
{
cout << graph.front().second;
return;
}
while(!graph.empty())
{
pair<int, int> x = graph.front();
graph.pop();
if(x.first-1 == sister || x.first+1 == sister || 2*x.first == sister)
{
cout << x.second + 1;
return;
}
if(0< x.first && x.first - 1<= 100000 && !visited[x.first-1])
{
graph.push({x.first-1, x.second + 1});
visited[x.first-1] = true;
}
if(x.first + 1 <= 100000 && !visited[x.first+1])
{
graph.push({x.first+1, x.second + 1});
visited[x.first+1] = true;
}
if(2*x.first <= 100000 && !visited[2*x.first])
{
graph.push({2*x.first, x.second + 1});
visited[2*x.first] = true;
}
}
}
int main()
{
ios::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, K;
cin >> N >> K;
BFS(N, K);
}<file_sep>/2667.cpp
#include <stdio.h>
#include <stack>
#include <vector>
#include <algorithm>
using namespace std;
int N, temp;
int arr[25][25];
bool visited[25][25];
vector<int> ans;
int idx[4] = {-1, 1, 0, 0};
int idy[4] = {0, 0, -1, 1};
bool inSquare(int row, int column)
{
if(0 <= row && row < N && 0 <= column && column < N)
{
return true;
}
else return false;
}
void dfs(int row, int column)
{
if(arr[row][column] == 1 && visited[row][column] == false)
{
int count = 0;
stack<pair<int, int>> stack;
stack.push({row, column});
while(!stack.empty())
{
row = stack.top().first;
column = stack.top().second;
stack.pop();
if(!visited[row][column])
{
visited[row][column] = true;
++count;
}
for(int i = 0; i < 4; ++i)
{
int child_row = row + idx[i];
int child_column = column + idy[i];
if(inSquare(child_row, child_column) && arr[child_row][child_column] == 1)
{
if(!visited[child_row][child_column])
{
stack.push({child_row, child_column});
}
}
}
}
ans.push_back(count);
count = 0;
}
}
int main()
{
scanf("%d", &N);
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j){
scanf("%1d", &arr[i][j]);
}
}
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j)
{
dfs(i, j);
}
}
sort(ans.begin(), ans.end());
printf("%d\n", ans.size());
for(int i = 0; i < ans.size(); ++i)
{
printf("%d\n", ans[i]);
}
}<file_sep>/6603.cpp
#include <iostream>
#define MAX_SIZE 13
using namespace std;
int lotto[MAX_SIZE];
int memory[6];
int K;
void dfs(int start, int depth) {
if(depth == 6) {
for(int i = 0; i < 6; ++i) {
cout << memory[i] << " ";
}
cout << endl;
return;
}
for(int i = start; i < K; ++i) {
memory[depth] = lotto[i];
dfs(i + 1, depth + 1);
}
}
int main()
{
while(1) {
cin >> K;
if(K == 0) break;
for(int k = 0; k < K; ++k) {
cin >> lotto[k];
}
dfs(0, 0);
cout << endl;
}
return 0;
}<file_sep>/1715.cpp
#include <iostream>
using namespace std;
class Heap
{
private:
int heap[100001];
int heap_count;
public:
Heap() {
heap_count = 0;
}
bool empty();
int top();
void pop();
void push(const int &a);
void heapify(int i);
};
bool Heap::empty()
{
return heap_count == 0;
}
int Heap::top()
{
return heap[0];
}
void Heap::pop()
{
heap[0] = heap[--heap_count];
heapify(0);
}
void Heap::push(const int &a)
{
heap[heap_count++] = a;
for(int i = heap_count - 1; i > 0;){
int parent = (i - 1) >> 1;
if(heap[parent] < heap[i]) break;
swap(heap[parent], heap[i]);
i = parent;
}
}
void Heap::heapify(int i)
{
int min = i;
int left = 2*i + 1;
int right = 2*(i + 1);
if(left < heap_count && heap[min] > heap[left]){
min = left;
}
if(right < heap_count && heap[min] > heap[right]){
min = right;
}
if(min != i){
swap(heap[min], heap[i]);
heapify(min);
}
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
Heap H;
int N, temp, ans = 0;
cin >> N;
for(int n = 0; n < N; ++n){
cin >> temp;
H.push(temp);
}
for(int n = 0; n < N - 1; ++n){
int first = H.top();
H.pop();
int second = H.top();
H.pop();
ans += first + second;
H.push(first + second);
}
cout << ans;
return 0;
}<file_sep>/5430.cpp
#include <iostream>
#include <deque>
#include <string>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T;
cin >> T;
for(int i = 0; i < T; ++i)
{
string p, input;
int n;
cin >> p >> n >> input;
int length = input.length();
deque<int> deq;
string temp;
for(int j = 0; j < length; ++j)
{
if('0' <= input[j] && input[j] <= '9')
{
temp += input[j];
}
else if(input[j] == ',' || input[j] == ']')
{
if(!temp.empty())
{
deq.push_back(stoi(temp));
temp.clear();
}
}
}
int start = 0, end = n;
bool reverse = false, error = false;
for(int j = 0; j < p.length(); ++j)
{
if(p[j] == 'R')
{
reverse = !reverse;
}
else
{
if(deq.empty())
{
error = true;
break;
}
else if(reverse)
{
deq.pop_back();
}
else
{
deq.pop_front();
}
}
}
if(error) cout << "error\n";
else
{
cout << '[';
if(!reverse)
{
while(!deq.empty())
{
cout << deq.front();
deq.pop_front();
if(!deq.empty())
cout << ',';
}
}
else
{
while(!deq.empty())
{
cout << deq.back();
deq.pop_back();
if(!deq.empty())
cout << ',';
}
}
cout << "]\n";
}
}
return 0;
}<file_sep>/15649.cpp
#include <iostream>
using namespace std;
int N, M;
bool visited[9]{0};
int arr[9]{0};
void backtrack(int cnt){
if(cnt == M){
for(int i = 0; i < M; ++i){
cout << arr[i] << ' ';
}
cout << '\n';
return;
}
for(int i = 1; i <= N; ++i){
if(!visited[i]){
visited[i] = true;
arr[cnt] = i;
backtrack(cnt + 1);
visited[i] = false;
}
}
return;
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
cin >> N >> M;
int cnt = 0;
backtrack(cnt);
}<file_sep>/14241.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, sum = 0, score = 0, first, second;
cin >> N;
cin >> first;
for(int n = 0; n < N - 1; ++n)
{
cin >> second;
score += first * second;
first += second;
}
cout << score << endl;
}
<file_sep>/1021.cpp
#include <iostream>
#include <deque>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, M, count = 0, index = 0;;
cin >> N >> M;
deque<int> deq;
for(int i = 1; i <= N; ++i)
{
deq.push_back(i);
}
while(M--)
{
int temp;
cin >> temp;
for(int i = 0; i < deq.size(); ++i)
{
if(deq[i] == temp)
{
index = i;
break;
}
}
if(deq.size() - index > index)
{
while(deq.front() != temp)
{
deq.push_back(deq.front());
deq.pop_front();
++count;
}
}
else
{
while(deq.front() != temp)
{
deq.push_front(deq.back());
deq.pop_back();
++count;
}
}
deq.pop_front();
}
cout << count;
}<file_sep>/1912.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, seq, ans, input;
cin >> n >> seq;
ans = seq;
for(int i = 1; i < n; ++i)
{
cin >> input;
seq = seq < 0 ? input : seq + input;
ans = ans > seq ? ans : seq;
}
cout << ans;
return 0;
}<file_sep>/[try]6549.cpp
#include <iostream>
#include <vector>
using namespace std;
int print(const vector<int> &h, const int &n)
{
int area = 0, height = 1000000001, width = 0;
for(int i = 0; i < n; ++i)
{
if(h[i] < height)
{
height = h[i];
++width;
if(area < h[i] * (width + 1))
{
area = height * width;
}
}
else
{
if(area <= height * (width + 1))
{
++width;
area = height * width;
}
else
{
}
}
}
return area;
}
int main()
{
int n = 0;
while(true)
{
cin >> n;
if(n == 0) break;
vector<int> h(n);
for(int i = 0; i < n; ++i)
{
cin >> h[i];
}
cout << '\n' << print(h,n);
}
return 0;
}<file_sep>/16953.cpp
#include <stdio.h>
using namespace std;
int main()
{
int A, B, ans = 0;;
scanf("%d %d", &A, &B);
while(B > A)
{
if(B % 2 == 0){
B /= 2;
++ans;
}
else if(B % 10 == 1){
B /= 10;
++ans;
}
else{
break;
}
}
A == B ? printf("%d", ans + 1) : printf("%d", -1);
return 0;
}<file_sep>/18870.cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
vector<int> v;
int temp = 0;
for(int i = 0; i < N; ++i){
cin >> temp;
v.push_back(temp);
}
vector<int> copy(v);
sort(v.begin(), v.end());
v.erase(unique(v.begin(), v.end()), v.end());
for(int i = 0; i < N; ++i){
int ans = lower_bound(v.begin(), v.end(), copy[i]) - v.begin();
cout << ans << ' ';
}
}<file_sep>/11399.cpp
#include <stdio.h>
#include <vector>
#include <algorithm>
using namespace std;
int main()
{
int N, ans = 0;
scanf("%d", &N);
vector<int> P(N);
for(int i = 0; i < N; ++i)
{
scanf("%d", &P[i]);
}
sort(P.begin(), P.end());
for(int i = 0; i < N; ++i)
{
for(int j = 0; j <= i; ++j)
{
ans += P[j];
}
}
printf("%d", ans);
return 0;
}<file_sep>/2869.cpp
#include <stdio.h>
int main(){
int A, B, V, day = 0;
scanf("%d %d %d", &A, &B, &V);
V -= A;
if(V % ( A - B ) == 0){
day = V / ( A - B ) + 1;
}
else{
day = V / ( A - B ) + 2;
}
printf("%d", day);
}
/* V - A + B
* ...
* V - n(A - B) - A < 0 : day = n + 1
* V - n(A - B) - A = 0 : day = n + 1
*/<file_sep>/[try]2261.cpp
#include <iostream>
#include <cmath>
#include <vector>
using namespace std;
int distance(pair<int, int> a, pair<int, int> b)
{
return sqrt(pow(a.first - b.first, 2) + pow(a.second - b.second, 2));
}
bool compare(const pair<int, int> a, const pair<int, int> b)
{
if(a.first < b.first) return a < b;
else if(a.first > b.first) return a > b;
else if(a.second < b.second) return a < b;
}
int main()
{
int n;
vector<pair<int, int>> v(n);
for(int i = 0; i < n; ++i)
{
cin >> v[i].first >> v[i].second;
}
sort(v.begin(), v.end(), compare);
return 0;
}<file_sep>/2225.cpp
#include <iostream>
using namespace std;
int dp[201][201];
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, K, mod = 1000000000;
cin >> N >> K;
for(int k = 1; k <= K; ++k)
{
dp[0][k] = 1;
}
for(int n = 1; n <= N; ++n)
{
for(int k = 0; k <= K; ++k)
{
if(k == 0) dp[n][k] = 0;
else if(k == 1) dp[n][k] = 1;
else
{
dp[n][k] = (dp[n][k-1] + dp[n-1][k]) % mod;
}
}
}
cout << dp[N][K];
return 0;
}
// dp[n][k] = dp[n][k-1] + dp[n-1][k-1] + dp[n-2][k-1] + dp[n-3][k-1] ... + dp[1][k-1] + dp[0][k-1];
// dp[n-1][k] = dp[n-1][k-1] + ... + dp[0][k-1];
// dp[k][n] = dp[k-1][n] + ... + d[k-1][0];<file_sep>/11866.cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
void josephus(const int &n, const int &k)
{
}
int main()
{
int N, K, count = 1;
cin >> N >> K;
queue<int> q;
vector<int> ans;
for(int i = 1; i <= N; ++i)
{
q.push(i);
}
while(!q.empty())
{
if(count == K)
{
ans.push_back(q.front());
q.pop();
count = 1;
}
else
{
q.push(q.front());
q.pop();
++count;
}
}
cout << '<';
for(int i = 0; i < N - 1; ++i)
{
cout << ans[i] << ", ";
}
cout << ans[N-1] << '>';
return 0;
}<file_sep>/2775.cpp
#include <iostream>
int main(){
using namespace std;
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T;
scanf("%d", &T);
int arr[15][15];
for(int i = 0; i < 15; ++i){
arr[0][i] = i + 1;
arr[i][0] = 1;
}
for(int i = 1; i < 15; ++i){
for(int j = 1; j < 15; ++j){
arr[i][j] = arr[i][j-1] + arr[i-1][j];
}
}
for(int i = 0; i < T; ++i){
int k, n;
scanf("%d %d", &k, &n);
printf("%d\n",arr[k][n-1]);
}
}
<file_sep>/11286.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, x;
priority_queue<int, vector<int>, greater<int>> pq_pos, pq_neg;
cin >> N;
while(N--){
cin >> x;
if(x > 0) pq_pos.push(x);
else if(x < 0) pq_neg.push(-x);
else{
if(pq_pos.empty() && pq_neg.empty()) cout << "0\n";
else if(pq_pos.empty()){
cout << -pq_neg.top() << '\n';
pq_neg.pop();
}
else if(pq_neg.empty()){
cout << pq_pos.top() << '\n';
pq_pos.pop();
}
else{
if(pq_pos.top() < pq_neg.top()){
cout << pq_pos.top() << '\n';
pq_pos.pop();
}
else{
cout << -pq_neg.top() << '\n';
pq_neg.pop();
}
}
}
}
return 0;
}<file_sep>/1541.cpp
#include <stdio.h>
using namespace std;
int main()
{
int sign = 1, number = 0, ans = 0;
char oper;
scanf("%d", &number);
while(scanf("%c", &oper) != EOF)
{
ans = ans + number * sign;
if(oper == '-') sign = -1;
scanf("%d", &number);
}
printf("%d", ans);
return 0;
}<file_sep>/18258.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
string s_in;
cin >> N;
queue<int> q;
for(int i = 0; i < N; ++i)
{
cin >> s_in;
if(s_in == "push")
{
int temp;
cin >> temp;
q.push(temp);
}
else if(s_in == "pop")
{
if(q.empty()) cout << -1 << '\n';
else
{
cout << q.front() << '\n';
q.pop();
}
}
else if(s_in == "size")
{
cout << q.size() << '\n';
}
else if(s_in == "empty")
{
cout << (int)q.empty() << '\n';
}
else if(s_in == "front")
{
if(q.empty()) cout << -1 << '\n';
else
{
cout << q.front() << '\n';
}
}
else if(s_in == "back")
{
if(q.empty()) cout << -1 << '\n';
else
{
cout << q.back() << '\n';
}
}
}
return 0;
}<file_sep>/7576.cpp
#include <iostream>
#include <queue>
using namespace std;
int N, M;
int arr[1000][1000];
bool visited[1000][1000];
int ans = 0, cnt = 0;
queue<pair<int, int>> q;
int idx[4] = {-1, 1, 0, 0};
int idy[4] = {0, 0, -1, 1};
void BFS()
{
while(!q.empty())
{
pair<int, int> parent = q.front();
int p_row = parent.first, p_column = parent.second;
q.pop();
for(int i = 0; i < 4; ++i)
{
int c_row = p_row + idx[i], c_column = p_column + idy[i];
if(!visited[c_row][c_column] &&
0 <= c_row && c_row < N &&
0 <= c_column && c_column < M &&
arr[c_row][c_column] == 0
)
{
q.push({c_row, c_column});
visited[c_row][c_column] = true;
arr[c_row][c_column] = arr[p_row][p_column] + 1;
ans = max(ans, arr[c_row][c_column] - 1);
++cnt;
}
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
cin >> M >> N;
for(int n = 0; n < N; ++n)
{
for(int m = 0; m < M; ++m)
{
cin >> arr[n][m];
}
}
for(int n = 0; n < N; ++n)
{
for(int m = 0; m < M; ++m)
{
if(arr[n][m] == 1)
{
q.push({n, m});
visited[n][m] = true;
++cnt;
}
if(arr[n][m] == -1)
{
++cnt;
}
}
}
BFS();
if(cnt != M*N) cout << -1 << endl;
else cout << ans;
}<file_sep>/[try]15881.cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
vector<int> getPi(string p)
{
int m = p.size(), j = 0;
vector<int> pi(m, 0);
for(int i = 1; i < m; ++i)
{
while(j > 0 && p[i] != p[j]) j = pi[i-1];
if(p[i] == p[j]) p[i] = ++j;
}
return pi;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, ans = 0, count = 0;
cin >> n;
string s;
cin >> s;
return 0;
}<file_sep>/14501.cpp
#include <stdio.h>
using namespace std;
int maximize(const int &a, const int &b)
{
return a > b ? a : b;
}
int main()
{
int N;
scanf("%d", &N);
int T[N+1], P[N+1], dp[N+1], max = 0; //dp[N]은 N일을 반드시 포함하면서 N일까지의 최댓값이다.
for(int i = 1; i <= N; ++i) scanf("%d %d", &T[i], &P[i]);
for(int i = 1; i <= N; ++i)
{
dp[i] = P[i];
if(i + T[i] > N + 1) dp[i] = 0;
else
{
for(int j = 1; j < i; ++j)
{
if(j + T[j] <= i) dp[i] = maximize(dp[i], dp[j] + P[i]);
}
}
max = maximize(max, dp[i]);
}
printf("%d", max);
return 0;
}<file_sep>/11053.cpp
#include <iostream>
using namespace std;
int A[1001];
int dp[1001];
int max(const int &a, const int &b)
{
return a > b ? a : b;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, max_len = 0;
cin >> N;
for(int i = 1; i <= N; ++i)
{
cin >> A[i];
}
for(int i = 1; i <= N; ++i)
{
for(int j = 1; j <= i; ++j)
{
if(A[i] > A[j] && dp[j] > dp[i])
{
dp[i] = dp[j];
}
}
++dp[i];
max_len = max(max_len, dp[i]);
}
cout << max_len;
return 0;
}<file_sep>/1417.cpp
#include <iostream>
#include <queue>
using namespace std;
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, dasom, ans = 0;
priority_queue<int> pq;
cin >> N;
cin >> dasom;
for(int n = 1; n < N; ++n){
int temp;
cin >> temp;
pq.push(temp);
}
while(!pq.empty() && dasom <= pq.top()){
++dasom;
++ans;
int temp = pq.top();
pq.pop();
pq.push(temp-1);
}
cout << ans;
}<file_sep>/[try]12015.cpp
#include <stdio.h>
using namespace std;
int max(const int &a, const int &b)
{
return a > b ? a : b;
}
int main()
{
int N;
scanf("%d", &N);
int A[N], dp[1000000] = {0}, ans = 0;
for(int n = 0; n < N; ++n) scanf("%d", &A[n]);
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < i; ++j)
{
if(A[j] < A[i]) dp[i] = max(dp[i], dp[j]);
}
++dp[i];
ans = max(ans, dp[i]);
}
printf("%d", ans);
return 0;
}<file_sep>/1780.cpp
#include <iostream>
using namespace std;
int N, m_one, zero, p_one;
int M[2187][2187];
void getinput()
{
cin >> N;
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
{
cin >> M[i][j];
}
}
}
void dc(int x, int y, int size)
{
int temp = M[x][y];
bool check = true;
for(int i = x; i < x + size; ++i)
{
for(int j = y; j < y + size; ++j)
{
if(temp != M[i][j])
{
check = false;
break;
}
}
}
if(check)
{
if(M[x][y] == -1)
{
++m_one;
}
if(M[x][y] == 0)
{
++zero;
}
if(M[x][y] == 1)
{
++p_one;
}
}
else
{
for(int i = 0; i < 3; ++i)
{
for(int j = 0; j < 3; ++j)
{
dc(x + i * size/3, y + j * size/3, size/3);
}
}
}
}
void solve()
{
getinput();
dc(0,0,N);
cout << m_one << '\n' << zero << '\n' << p_one;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(0);
cout.tie(0);
solve();
return 0;
}<file_sep>/1992.cpp
#include <iostream>
using namespace std;
int N = 0;
int M[64][64];
void getinput()
{
cin >> N;
char temp;
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j){
cin >> temp;
M[i][j] = int(temp) - 48;
}
}
}
void quadtree(int x, int y, int size)
{
int temp = M[x][y];
bool check = true;
for(int i = x; i < x + size; ++i){
for(int j = y; j < y + size; ++j){
if(temp != M[i][j]){
check = false;
break;
}
}
}
if(check)
{
cout << temp;
}
else
{
cout << '(';
int half = size / 2;
quadtree(x, y, half);
quadtree(x, y + half, half);
quadtree(x + half, y ,half);
quadtree(x + half, y + half , half);
cout << ')';
}
}
void solve()
{
getinput();
quadtree(0, 0, N);
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
solve();
return 0;
}<file_sep>/12865.cpp
#include <stdio.h>
#include <algorithm>
using namespace std;
int dp[100001];
int main()
{
int N, K, W, V;
scanf("%d %d", &N, &K);
for(int n = 0; n < N; ++n)
{
scanf("%d %d", &W, &V);
for(int j = K; j >= W; --j)
{
dp[j] = max(dp[j-W] + V, dp[j]);
}
}
printf("%d", dp[K]);
return 0;
}<file_sep>/1766.cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, M, a, b;
priority_queue<int, vector<int>, greater<int>> queue;
cin >> N >> M;
int in_degree[N+1];
vector<int> edge[N+1];
for(int i = 0; i <= N; ++i)
{
in_degree[i] = 0;
}
for(int m = 0; m < M; ++m)
{
cin >> a >> b;
edge[a].push_back(b);
in_degree[b]++;
}
for(int i = 1; i <= N; ++i)
{
if(!in_degree[i])
{
queue.push(i);
}
}
while(!queue.empty())
{
int vec = queue.top();
queue.pop();
cout << vec << " ";
for(int i : edge[vec])
{
in_degree[i]--;
if(!in_degree[i])
{
queue.push(i);
}
}
}
}
<file_sep>/15651.cpp
#include <iostream>
using namespace std;
int N, M;
int arr[7] = {0,};
void backTrack(int height) {
if(height == M) {
for(int i = 0; i < M; ++i) {
cout << arr[i] << " ";
}
cout << '\n';
return;
}
for(int i = 1; i <= N; ++i) {
arr[height] = i;
backTrack(height + 1);
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
cin >> N >> M;
backTrack(0);
return 0;
}
<file_sep>/1904.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
if(N <= 3) cout << N;
else{
int a = 1, b = 2, c;
for(int i = 3; i <= N; ++i){
c = (a + b) % 15746;
a = b;
b = c;
}
cout << c;
}
return 0;
}<file_sep>/11401.cpp
#include <iostream>
using namespace std;
int p = 1e9 + 7;
long long power(long long a, long long b)
{
if(b == 0) return 1;
long long ans = power(a, b / 2);
ans = (ans * ans) % p;
if(b % 2 != 0) ans = (ans * a) % p;
return ans;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, K;
cin >> N >> K;
long long A = 1, B = 1;
if(N - K < K) K = N - K;
for(int n = N-K+1; n <= N; ++n)
{
A *= n;
A %= p;
}
for(int k = 1; k <= K; ++k)
{
B *= k;
B %= p;
}
long long cal = A * power(B, p-2);
cal %= p;
cout << cal;
return 0;
}<file_sep>/1629.cpp
#include <iostream>
using namespace std;
long long power(int a, int b, int c)
{
if(b == 0) return 1;
long long ans = power(a, b / 2, c);
ans = (ans * ans) % c;
if(b % 2 == 0)
{
return ans;
}
else
{
ans = (ans * a) % c;
return ans;
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int A, B, C;
cin >> A >> B >> C;
cout << power(A,B,C);
}<file_sep>/13305.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, price, min = 1e9 + 1;
long long ans = 0;
cin >> N;
int length[N-1];
for(int i = 0; i < N-1; ++i) cin >> length[i];
for(int i = 0; i < N-1; ++i)
{
cin >> price;
min = min < price ? min : price;
ans += (long long)min * length[i];
}
cout << ans;
return 0;
}<file_sep>/15652.cpp
#include <iostream>
#include <vector>
using namespace std;
void backTrack(int height, int N, int M, int * arr) {
if(height == M) {
for(int i = 0; i < M; ++i) {
cout << arr[i] << " ";
}
cout << endl;
return;
}
for(int i = 1; i <= N; ++i) {
if(arr[0] == 0 || arr[height-1] <= i) {
arr[height] = i;
backTrack(height+1, N, M, arr);
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, M;
cin >> N >> M;
int arr[M];
fill(arr, arr + M, 0);
backTrack(0, N, M, arr);
return 0;
}<file_sep>/1449.cpp
#include <stdio.h>
#include <algorithm>
using namespace std;
int main()
{
int N, L;
scanf("%d %d", &N, &L);
int input[N], start = -2000, ans = 0;
for(int n = 0; n < N; ++n){
scanf("%d", &input[n]);
}
sort(input, input + N);
for(int n = 0; n < N; ++n){
if(input[n] - start > L - 1){
start = input[n];
++ans;
}
}
printf("%d", ans);
return 0;
}<file_sep>/9184.cpp
#include <iostream>
using namespace std;
const int maxn = 21;
int v[maxn][maxn][maxn];
int w(int a, int b, int c)
{
if(a <= 0 || b <= 0 || c <= 0) return 1;
else if(a > 20 || b > 20 || c > 20) return w(20, 20, 20);
if(!v[a][b][c]){
if(a < b && b < c){
v[a][b][c] = w(a, b, c-1) + w(a, b-1, c-1) - w(a, b-1, c);
}
else{
v[a][b][c] = w(a-1, b, c) + w(a-1, b-1, c) + w(a-1, b, c-1) - w(a-1, b-1, c-1);
}
}
return v[a][b][c];
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int a, b, c;
while(true)
{
cin >> a >> b >> c;
if(a == -1 && b == -1 && c == -1) break;
cout << "w(" << a << ", " << b << ", " << c << ") = " << w(a, b, c) << '\n';
}
}<file_sep>/1193.cpp
#include <stdio.h>
int main(){
int numerator = 1, denominator = 1, count = 0, X;
scanf("%d", &X);
while((count * (count + 1)) / 2 < X){
++count;
}
X -= (((count-1) * count) / 2 + 1);
if(count % 2 == 0){
numerator = 1;
denominator = count;
while(X != 0){
++numerator;
--denominator;
--X;
}
}
else{
numerator = count;
denominator = 1;
while(X != 0){
--numerator;
++denominator;
--X;
}
}
printf("%d/%d",numerator,denominator);
}<file_sep>/1181.cpp
#include <iostream>
#include <string>
#include <algorithm>
#include <vector>
using namespace std;
bool compare(string a, string b){
if(a.length() == b.length()) return a < b;
else return a.length() < b.length();
}
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
vector<string> arr;
string temp;
for(int i = 0; i < N; ++i){
cin >> temp;
arr.push_back(temp);
}
sort(arr.begin(), arr.end(), compare);
for(int i = 0; i < arr.size() - 1;){
if(arr[i] == arr[i+1]) arr.erase(arr.begin()+i);
else ++i;
}
for(int i = 0; i < arr.size(); ++i){
cout << arr[i] << '\n';
}
}<file_sep>/1316.cpp
#include <stdio.h>
#include <string.h>
int main(){
/* There are three cases when we check each alphabet.
* 1. First met but not a last letter.
* 2. First met and last letter.
* 3. Alread met
*
* The case.1 We just do next iteration, because it's not a
* last so we can act on case.2.
*
* The case.2 We 'mark' the letter was encountered.
*
* The case.3 Erase the 'mark' which we've done on case.2
*/
// get input N
// For each iteration, get input[101] and alphabet[26] = {0}
// If (alphabet[a[i] - 'a'] > 0), count = 0; break;
// else If input[i] != input[i+1], alphabet[array[i] - 'a'] = 1
// sum += count;
int N = 0, sum = 0;
scanf("%d", &N);
for(int n = 0; n < N; ++n){
char input[101];
scanf("%s", input);
int alphabet[26] = {0}, count = 1;
int length = strlen(input);
for(int i = 0; i < length; ++i){
if(alphabet[input[i] - 'a'] > 0){
count = 0;
break;
}
else if(input[i] != input[i+1]){
alphabet[input[i] - 'a'] = 1;
}
}
sum += count;
}
printf("%d", sum);
}<file_sep>/9251.cpp
#include <iostream>
#include <string>
using namespace std;
int dp[1001][1001];
int LCS(string s1, string s2)
{
int n = s1.length();
int m = s2.length();
for(int x = 0; x < n; ++x) dp[x][0] = 0;
for(int y = 0; y < m; ++y) dp[0][y] = 0;
for(int i = 0; i < n; ++i)
{
for(int j = 0; j < m; ++j)
{
if(s1[i] == s2[j]){
dp[i+1][j+1] = dp[i][j] + 1;
}
else if(dp[i][j+1] >= dp[i+1][j])
{
dp[i+1][j+1] = dp[i][j+1];
}
else dp[i+1][j+1] = dp[i+1][j];
}
}
return dp[n][m];
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
string s1, s2;
cin >> s1 >> s2;
cout << LCS(s1, s2);
return 0;
}<file_sep>/17298.cpp
#include <iostream>
#include <stack>
#include <vector>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, temp = 0, index = 0;
cin >> N;
stack<int> s;
vector<int> v, ans(N, -1);
for(int i = 0; i < N; ++i)
{
cin >> temp;
v.push_back(temp);
}
s.push(0);
for(int i = 1; i < N; ++i)
{
while(!s.empty() && v[s.top()] < v[i])
{
ans[s.top()] = v[i];
s.pop();
}
s.push(i);
}
for(int i = 0; i < N; ++i)
{
cout << ans[i] << ' ';
}
}
/*
* stack s = {s1, s2, ... , s.top} s.t. v[s1] >= v[s2] >= ... >= v[s.top]
* 1. v[i] <= v[s.top] --> s = {s1, s2, ... , s.top, s[i]} s.t. v[s1] >= v[s2] >= ... >= v[s.top] >= v[input]
* 2. v[i] > v[s.top] --> while(!s.empty() && v[s.top] < v[i]) ans[s.top] = v[i], s.pop()
*/ <file_sep>/7568.cpp
#include <stdio.h>
int main(){
int N;
scanf("%d", &N);
int arr[2 * N], rank[N];
for(int i = 0; i < N; ++i){
rank[i] = 0;
}
for(int i = 0; i < N; ++i){
scanf("%d %d", &arr[i], &arr[i + N]);
}
for(int i = 0; i < N; ++i){
for(int j = 0; j < N; ++j){
if(arr[i] < arr[j] && arr[i + N] < arr[j + N]){
++rank[i];
}
}
}
for(int i = 0; i < N; ++i){
printf("%d ", rank[i] + 1);
}
}<file_sep>/10250.cpp
#include <iostream>
int main(){
using namespace std;
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T;
cin >> T;
for(int i = 0; i < T; ++i){
int H = 0, W = 0, N = 0;
cin >> H >> W >> N;
int floor = 0, room = 0;
floor = (N + (H - 1)) % H + 1;
room = (N - 1) / H + 1;
int num = floor * 100 + room;
cout << num << "\n";
}
}
/*
* H01 H02 HW
* ...
* 301
* 201 202 2W
* 101 102 ... 1W
*
* n(mod H) : 0 ~ H - 1, n = 1 -> 1
* n(mod H) + 1 : 1 ~ H , n = 1 -> 2
* n + H - 1(mod H) + 1 : 0 ~ H - 1, n = 1 -> 1
*/ <file_sep>/1080.cpp
#include <stdio.h>
using namespace std;
int A[50][50], B[50][50];
void change(int i, int j)
{
for(int x = i; x < i + 3; ++x){
for(int y = j; y < j + 3; ++y)
{
if(A[x][y] == 1) A[x][y] = 0;
else if(A[x][y] == 0) A[x][y] = 1;
}
}
}
bool check_matrix(int i, int j)
{
for(int x = 0; x < i; ++x){
for(int y = 0; y < j; ++y)
{
if(A[x][y] != B[x][y]) return false;
}
}
return true;
}
int main(){
int N, M, ans = 0;
scanf("%d %d", &N, &M);
for(int i = 0; i < N; ++i){
for(int j = 0; j < M; ++j){
scanf("%1d", &A[i][j]);
}
}
for(int i = 0; i < N; ++i){
for(int j = 0; j < M; ++j){
scanf("%1d", &B[i][j]);
}
}
for(int i = 0; i < N - 2; ++i){
for(int j = 0; j < M - 2; ++j){
if(A[i][j] != B[i][j]){
change(i, j);
++ans;
}
}
}
if(!check_matrix(N, M)){
ans = -1;
}
printf("%d", ans);
return 0;
}<file_sep>/1543.cpp
#include <iostream>
#include <string>
using namespace std;
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
string document, search;
getline(cin, document);
getline(cin, search);
int s_len = search.length(), jump = -1, d_len = document.length(), ans = 0;
bool flag = true;
for(int i = 1; i < s_len; ++i){
if(search[0] == search[i]){
jump = i;
break;
}
}
for(int i = 0; i < d_len;){
for(int j = 0; j < s_len; ++j){
if(document[i+j] != search[j]){
if(j <= 1) ++i;
else if(jump == -1 || j < jump) i += j;
else i += jump;
flag = false;
break;
}
}
if(flag){
++ans;
i += s_len;
}
flag = true;
}
cout << ans;
return 0;
}<file_sep>/14325.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, a;
priority_queue<int> pq;
cin >> n;
while(n--){
cin >> a;
if(a == 0)
{
if(pq.empty())
{
cout << -1 << '\n';
}
else
{
cout << pq.top() << '\n';
pq.pop();
}
}
else
{
while(a--)
{
int temp;
cin >> temp;
pq.push(temp);
}
}
}
}<file_sep>/2178.cpp
#include <stdio.h>
#include <queue>
using namespace std;
int N, M;
int arr[100][100];
bool visited[100][100] = {false,};
int idx[4] = {-1, 1, 0, 0};
int idy[4] = {0, 0, -1, 1};
bool in_matrix(int row, int column)
{
return (0 <= row && row < N && 0 <= column && column < M);
}
void BFS(int row, int column)
{
queue<pair<int, int>> queue;
queue.push({row, column});
visited[row][column] = true;
while(!queue.empty())
{
pair<int, int> parent = queue.front();
queue.pop();
int p_row = parent.first;
int p_column = parent.second;
for(int i = 0; i < 4; ++i)
{
int c_row = p_row + idx[i];
int c_column = p_column + idy[i];
if(in_matrix(c_row, c_column) && arr[c_row][c_column] && !visited[c_row][c_column])
{
queue.push({c_row, c_column});
visited[c_row][c_column] = true;
arr[c_row][c_column] = arr[p_row][p_column] + 1;
}
}
}
}
int main()
{
scanf("%d %d", &N, &M);
for(int n = 0; n < N; ++n){
for(int m = 0; m < M; ++m){
scanf("%1d", &arr[n][m]);
}
}
BFS(0, 0);
printf("%d", arr[N-1][M-1]);
}<file_sep>/1932.cpp
#include <stdio.h>
#include <algorithm>
using namespace std;
int dp[500][500];
int main(){
int n;
scanf("%d", &n);
for(int i = 0; i < n; ++i){
for(int j = 0; j <= i; ++j){
scanf("%d", &dp[i][j]);
}
}
for(int i = 1; i < n; ++i){
dp[i][0] = dp[i-1][0] + dp[i][0];
dp[i][i] = dp[i-1][i-1] + dp[i][i];
for(int j = 1; j < i; ++j){
dp[i][j] = max(dp[i-1][j-1], dp[i-1][j]) + dp[i][j];
}
}
int temp = dp[n-1][0];
for(int i = 1; i < n; ++i){
temp = max(temp, dp[n-1][i]);
}
printf("%d",temp);
return 0;
}<file_sep>/10844.cpp
#include <iostream>
using namespace std;
long long dp[101][10]; //dp[x][y]는 길이가 x이고 앞자리가 y인 수의 갯수
int mod = 1000000000;
void stair(const int &n){
for(int i = 1; i < 9; ++i){
dp[n][i] = (dp[n-1][i-1] + dp[n-1][i+1]) % mod;
}
dp[n][0] = dp[n-1][1] % mod;
dp[n][9] = dp[n-1][8] % mod;
}
int main()
{
int N;
long long sum = 0;
cin >> N;
for(int i = 0; i < 10; ++i){
dp[1][i] = 1;
}
for(int n = 2; n <= N; ++n){
stair(n);
}
for(int i = 1; i <= 9; ++i){
sum += dp[N][i] % mod;
}
cout << sum % mod;
return 0;
}<file_sep>/2606.cpp
#include <iostream>
#include <vector>
using namespace std;
int cnt = 0;
vector<int> edge[101];
bool visited[101] ={false,};
void DFS(int parent)
{
visited[parent] = true;
for(auto child : edge[parent]){
if(!visited[child]){
DFS(child);
++cnt;
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int num_com, num_pair, a, b;
cin >> num_com >> num_pair;
for(int i = 0; i < num_pair; ++i){
cin >> a >> b;
edge[a].push_back(b);
edge[b].push_back(a);
}
DFS(1);
cout << cnt;
}<file_sep>/2579.cpp
#include <iostream>
#include <algorithm>
using namespace std;
int stair[301];
int dp[301];
int main(){
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n;
cin >> n;
for(int i = 0; i < n; ++i){
cin >> stair[i];
}
dp[0] = stair[0];
dp[1] = stair[1] + dp[0];
dp[2] = max(stair[2] + stair[0], stair[2] + stair[1]);
for(int i = 3; i < n; ++i){
dp[i] = stair[i] + max(stair[i-1] + dp[i-3], dp[i-2]);
}
cout << dp[n-1];
return 0;
}<file_sep>/13301.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, ans;
cin >> N;
int dp[N];
dp[0] = 1;
dp[1] = 1;
for(int i = 2; i < N; ++i)
{
dp[i] = dp[i-1] + dp[i-2];
}
if(N == 1) ans = 4;
else if(N == 2) ans = 6;
else
{
ans = 2 * (2 * dp[N-1] + dp[N-2]);
}
cout << ans;
return 0;
}<file_sep>/10830.cpp
#include <iostream>
#include <vector>
using namespace std;
vector<vector<long long>> matrix_multi(vector<vector<long long>> A, vector<vector<long long>> B)
{
long long length = A.size();
vector<vector<long long>> temp(length, vector<long long>(length));
for(long long i = 0; i < length; ++i)
{
for(long long j = 0; j < length; ++j)
{
long long term = 0;
for(long long k = 0; k < length; ++k)
{
term += A[i][k] * B[k][j];
}
temp[i][j] = (term % 1000);
}
}
return temp;
}
vector<vector<long long>> power(vector<vector<long long>> A, long long n, long long B)
{
vector<vector<long long>> I(n, vector<long long>(n));
for(long long i = 0; i < n; ++i)
{
I[i][i] = 1;
}
if(B == 0) return I;
vector<vector<long long>> temp = power(A, n, B / 2);
temp = matrix_multi(temp, temp);
if(B % 2 != 0)
{
temp = matrix_multi(temp, A);
}
return temp;
}
void print(vector<vector<long long>> A)
{
int n = A.size();
for(long long i = 0; i < n; ++i)
{
for(long long j = 0; j < n; ++j)
{
cout << A[i][j] << ' ';
}
cout << '\n';
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
long long N, B;
cin >> N >> B;
vector<vector<long long>> m(N, vector<long long>(N));
for(long long i = 0; i < N; ++i)
{
for(long long j = 0; j < N; ++j)
{
cin >> m[i][j];
}
}
print(power(m, N, B));
}<file_sep>/12018.cpp
#include <iostream>
#include <queue>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n, m, mileage = 0, ans = 0;
cin >> n >> m;
int P, L;
priority_queue<int, vector<int>, greater<int>> pq;
for(int i = 0; i < n; ++i){
cin >> P >> L;
int temp;
priority_queue<int, vector<int>, greater<int>> tempq;
for(int p = 0; p < P; ++p){
cin >> temp;
tempq.push(temp);
}
if(P >= L){
while(P > L){
tempq.pop();
--P;
}
pq.push(tempq.top());
}
else{
pq.push(1);
}
}
while(mileage + pq.top() <= m && ans < n){
mileage += pq.top();
++ans;
pq.pop();
}
cout << ans;
}<file_sep>/9625.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int K;
cin >> K;
pair<int, int> dp[K+1];
dp[0] = make_pair(1,0);
for(int i = 1; i <= K; ++i)
{
dp[i] = make_pair(dp[i-1].second, dp[i-1].first + dp[i-1].second);
}
cout << dp[K].first << " " << dp[K].second;
return 0;
}<file_sep>/9465.cpp
#include <iostream>
#include <algorithm>
using namespace std;
int sticker[100001][2];
int dp[100001][2];
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int T, n;
cin >> T;
while(T--){
cin >> n;
for(int j = 0; j < 2; ++j){
for(int i = 0; i < n; ++i){
cin >> sticker[i][j];
}
}
dp[0][0] = sticker[0][0];
dp[0][1] = sticker[0][1];
dp[1][0] = sticker[1][0] + dp[0][1];
dp[1][1] = sticker[1][1] + dp[0][0];
for(int i = 2; i < n; ++i){
dp[i][0] = max(dp[i-1][1], max(dp[i-2][0], dp[i-2][1])) + sticker[i][0];
dp[i][1] = max(dp[i-1][0], max(dp[i-2][0], dp[i-2][1])) + sticker[i][1];
}
cout << max(dp[n-1][0], dp[n-1][1]) << '\n';
}
return 0;
}<file_sep>/2630.cpp
#include <iostream>
#include <cmath>
using namespace std;
int N, blue, white;
int M[128][128];
void getinput()
{
cin >> N;
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
{
cin >> M[i][j];
}
}
}
void quadtree(int x, int y, int size)
{
int temp_blue = 0;
for(int i = x; i < x + size; ++i)
{
for(int j = y; j < y + size; ++j)
{
if(M[i][j] == 1) ++temp_blue;
}
}
if(temp_blue == 0)
{
++white;
return;
}
else if(temp_blue == size * size)
{
++blue;
return;
}
int half = size / 2;
quadtree(x, y, half);
quadtree(x, y + half, half);
quadtree(x + half, y, half);
quadtree(x + half, y + half, half);
}
void solve()
{
quadtree(0,0,N);
cout << white << '\n' << blue << '\n';
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
getinput();
solve();
return 0;
}<file_sep>/11054_2.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
int A[N], front_len[1000] = {0}, back_len[1000] = {0}, bitonic_len = 0;
for(int i = 0; i < N; ++i)
{
cin >> A[i];
}
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < i; ++j)
{
if(A[j] < A[i])
{
front_len[i] = max(front_len[i], front_len[j]);
}
if(A[N-1-j] < A[N-1-i])
{
back_len[N-1-i] = max(back_len[N-1-i], back_len[N-1-j]);
}
}
++front_len[i];
++back_len[N-1-i];
}
for(int i = 0; i < N; ++i)
{
bitonic_len = max(bitonic_len, front_len[i] + back_len[i]);
}
cout << bitonic_len - 1;
return 0;
}<file_sep>/1439.cpp
#include <iostream>
#include <string>
using namespace std;
int main(){
int cnt1 = 0, cnt2 = 0;
string S;
char c;
cin >> S;
bool flag = true;
int n = S.length();
for(int i = 0; i < n;){
c = S[i];
while(c == S[i] && i < n)
{
++i;
}
if(flag){
++cnt1;
flag = false;
}
else{
++cnt2;
flag = true;
}
}
cout << (cnt1 < cnt2 ? cnt1 : cnt2);
return 0;
}<file_sep>/1427_2.cpp
#include <stdio.h>
#include <algorithm>
bool compare(int a, int b){
return b < a;
}
int main(){
char arr[11];
int i;
for(i = 0; i < 10; ++i){
char c = getchar();
if(c == '\n'){
arr[i] = 0;
break;
}
arr[i] = c;
}
std::sort(arr, arr + i, compare);
printf("%s", arr);
}<file_sep>/2217.cpp
#include <iostream>
#include <algorithm>
using namespace std;
bool compare(const int a, const int b)
{
return a > b;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, ans = 0;
cin >> N;
int W[N];
for(int i = 0; i < N; ++i) cin >> W[i];
sort(W, W + N, compare);
for(int i = 0; i < N; ++i)
{
if(ans < W[i] * (i+1))
{
ans = W[i] * (i+1) ;
}
}
cout << ans;
return 0;
}<file_sep>/11052.cpp
#include <iostream>
#include <algorithm>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N;
cin >> N;
int p[N+1], dp[N+1];
for(int i = 1; i <= N; ++i)
{
cin >> p[i];
}
for(int i = 1; i <= N; ++i)
{
dp[i] = p[i];
for(int j = 1; j < i; ++j)
{
dp[i] = max(dp[i], dp[j] + dp[i-j]);
}
}
cout << dp[N];
return 0;
}<file_sep>/5622.cpp
#include <stdio.h>
#include <string.h>
int main(){
char input[15];
scanf("%s", input);
int sum = 0;
int length = strlen(input);
for(int i = 0; i < length; ++i){
if('A' <= input[i] && input[i] <= 'O') sum += (input[i] - 'A') / 3 + 3;
else if('P' <= input[i] && input[i] <= 'S') sum += 8;
else if('T' <= input[i] && input[i] <= 'V') sum += 9;
else if('W' <= input[i] && input[i] <= 'Z') sum += 10;
}
printf("%d", sum);
}<file_sep>/2075.cpp
#include <iostream>
#include <queue>
#include <algorithm>
using namespace std;
int main()
{
ios::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, temp;
cin >> N;
priority_queue<int, vector<int>, greater<int>> pq;
for(int n = 0; n < N; ++n){
for(int m = 0; m < N; ++m){
cin >> temp;
if(pq.size() == N && temp <= pq.top()) continue;
pq.push(temp);
if(pq.size() > N) pq.pop();
}
}
cout << pq.top();
}<file_sep>/11444.cpp
#include <iostream>
#include <vector>
using namespace std;
typedef vector<vector<long long>> matrix;
int p = 1000000007;
matrix I = {{1,0},{0,1}};
matrix m = {{1,1},{1,0}};
matrix operator *(matrix a, matrix b)
{
matrix temp(2, vector<long long>(2));
for(int i = 0; i < 2; ++i){
for(int j = 0; j < 2; ++j){
for(int k = 0; k < 2; ++k){
temp[i][j] += a[i][k] * b[k][j];
}
temp[i][j] %= p;
}
}
return temp;
}
matrix Fibonacci(const matrix a, long long n)
{
if(n == 1) return m;
matrix temp = Fibonacci(a, n / 2);
temp = temp * temp;
if(n % 2 != 0)
{
temp = temp * a;
}
return temp;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
long long N;
cin >> N;
cout << Fibonacci(m, N)[0][1];
}
/*
* Fn = Fn-1 + Fn-2
* Fn Fn-1 (1 1) Fn-1 Fn-2 ... (1 1)^n-1 F2 F1 = (1 1)^n
* Fn-1 Fn-2 (1 0) Fn-2 Fn-3 ... (1 0) F1 F0 (1 0)
*
*
*
*
*/<file_sep>/15650.cpp
#include <iostream>
#include <vector>
using namespace std;
void backTrack(int height, int N, int M, bool *visited, vector<int> answer) {
if(height == M) {
for(int i = 0; i < M; ++i) {
cout << answer[i] << " ";
}
cout << endl;
return;
}
for(int i = 1; i <= N; ++i) {
if(!visited[i] && (answer.empty() || answer.back() < i)) {
visited[i] = true;
answer.push_back(i);
backTrack(height+1, N, M, visited, answer);
visited[i] = false;
answer.pop_back();
}
}
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, M;
cin >> N >> M;
bool visited[9]{0};
vector<int> answer;
backTrack(0, N, M, visited, answer);
return 0;
}<file_sep>/2108.cpp
#include <stdio.h>
void merge(int *arr, int *sorted, int start, int middle, int end){
int l = start, r = middle + 1, i = start;
while(l <= middle && r <= end){
if(arr[l] > arr[r]){
sorted[i] = arr[r];
++r;
}
else{
sorted[i] = arr[l];
++l;
}
++i;
}
if(l > middle){
while(r <= end){
sorted[i] = arr[r];
++r;
++i;
}
}
else{
while(l <= middle){
sorted[i] = arr[l];
++l;
++i;
}
}
for(int k = start; k <= end; ++k){
arr[k] = sorted[k];
}
}
void merge_sort(int *arr, int *sorted, int start, int end){
if(start < end){
int middle = (start + end) / 2;
merge_sort(arr, sorted, start, middle);
merge_sort(arr, sorted, middle + 1, end);
merge(arr, sorted, start, middle, end);
}
}
int main(){
int N;
scanf("%d", &N);
int arr[N], sorted[500000] = {0}, min = 4000, max = -4000;
int count[8001] = {0}, max_count = 0, check = 0, mode = 0;
double mean = 0;
for(int i = 0; i < N; ++i){
scanf("%d", &arr[i]);
mean += arr[i];
if(arr[i] > max) max = arr[i];
if(arr[i] < min) min = arr[i];
++count[arr[i] + 4000];
if(count[arr[i] + 4000] > max_count) max_count = count[arr[i] + 4000];
}
for(int k = 0; k <= 8000; ++k){
if(count[k] == max_count){
++check;
}
}
for(int k = 0; k <= 8000; ++k){
if(check == 1 && count[k] == max_count){
mode = k - 4000;
break;
}
else{
if(count[k] == max_count){
check = 1;
}
}
}
merge_sort(arr, sorted, 0, N - 1);
// print arithmetic mean
mean /= N;
printf("%.0f\n", mean);
// print median
printf("%d\n", arr[N/2]);
// print mode
printf("%d\n", mode);
// print range
printf("%d\n", max - min);
}<file_sep>/2748.cpp
#include <iostream>
using namespace std;
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int n;
cin >> n;
long long Fn[n+1];
Fn[0] = 0;
Fn[1] = 1;
for(int i = 2; i <= n; ++i)
{
Fn[i] = Fn[i-1] + Fn[i-2];
}
cout << Fn[n];
return 0;
}<file_sep>/1339.cpp
#include <iostream>
#include <algorithm>
#include <string>
#include <cmath>
using namespace std;
bool rev(const int a, const int b){
return a > b;
}
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
int N, alphabet[26]={0,}, ans = 0;
cin >> N;
string temp;
for(int n = 0; n < N; ++n){
cin >> temp;
int len = temp.length();
for(int j = 0; j < len; ++j){
alphabet[temp[j]-'A'] += pow(10, len-1-j);
}
}
sort(alphabet, alphabet + 26, rev);
for(int i = 0; i < 10; ++i){
ans += alphabet[i] * (9-i);
}
cout << ans;
}<file_sep>/2751.cpp
#include <stdio.h>
// merge() is a function that combine splited L-array and R-array into the one array;
void merge(int *arr, int *sorted, int &start, int &middle, int &end){
int l = start;
int r = middle + 1;
int i = start;
while(l <= middle && r <= end){
if(arr[l] > arr[r]){
sorted[i] = arr[r];
++i;
++r;
}
else{
sorted[i] = arr[l];
++i;
++l;
}
}
if(l > middle){
while(r <= end){
sorted[i] = arr[r];
++i;
++r;
}
}
else{
while(l <= middle){
sorted[i] = arr[l];
++i;
++l;
}
}
for(int k = start; k <= end; ++k){
arr[k] = sorted[k];
}
}
// merge-sort() is a function that splite the array and combine them again to use merge();
void merge_sort(int *arr, int *sorted, int &begin, int &end){
if(begin < end){
int center = (end + begin) / 2;
int center2 = center + 1;
merge_sort(arr, sorted, begin, center);
merge_sort(arr, sorted, center2, end);
merge(arr, sorted, begin, center, end);
}
}
int main(){
int N;
int begin = 0;
scanf("%d", &N);
int arr[N];
int sorted[N];
for(int i = 0; i < N; ++i){
scanf("%d", &arr[i]);
}
int end = N - 1;
merge_sort(arr, sorted, begin, end);
for(int i = 0; i < N; ++i){
printf("%d\n", arr[i]);
}
}<file_sep>/17503.cpp
#include <iostream>
#include <queue>
using namespace std;
struct compare_list{
bool operator()(pair<int, int> a, pair<int, int> b)
{
if(a.second == b.second) return a.first < b.first;
else return a.second > b.second;
}
};
struct compare_chosen{
bool operator()(pair<int, int> a, pair<int, int> b)
{
if(a.first == b.first) return a.second < b.second;
else return a.first > b.first;
}
};
int main()
{
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
priority_queue<pair<int, int>, vector<pair<int, int>>, compare_list> list;
priority_queue<pair<int, int>, vector<pair<int, int>>, compare_chosen> chosen;
int N, M, K, v, c, level = 0, preference = 0;
cin >> N >> M >> K;
for(int i = 0; i < K; ++i)
{
cin >> v >> c;
list.push(pair<int, int>(v, c));
}
for(int i = 0; i < K; ++i)
{
chosen.push(list.top());
preference += list.top().first;
level = max(level, list.top().second);
list.pop();
if(chosen.size() > N)
{
preference -= chosen.top().first;
chosen.pop();
}
if(chosen.size() == N && preference >= M)
{
cout << level;
return 0;
}
}
if(preference < M)
{
cout << -1;
return 0;
}
}<file_sep>/1003.cpp
#include <stdio.h>
using namespace std;
int fibo[41];
int fibonacci(const int &n)
{
if(fibo[n] != 0) return fibo[n];
else if(n <= 0){
fibo[0] = 0;
return 0;
}
else if(n == 1)
{
fibo[1] = 1;
return 1;
}
else{
return fibo[n] = fibonacci(n-1) + fibonacci(n-2);
}
}
int main()
{
int T, N;
scanf("%d",&T);
for(int t = 0; t < T; ++t)
{
scanf("%d", &N);
if(N == 0) printf("%d %d\n", 1, 0);
else if(N == 1) printf("%d %d\n", 0, 1);
else{
printf("%d %d\n", fibonacci(N-1), fibonacci(N));
}
}
} <file_sep>/10872.cpp
#include <stdio.h>
int main(){
int N, answer = 1;
scanf("%d", &N);
for(int i = 1; i <= N; ++i){
answer *= i;
}
printf("%d", answer);
}<file_sep>/2980.cpp
#include <stdio.h>
int main(){
int input1 = 0, input2 = 0;
scanf("%d %d", &input1, &input2);
input1 = 100 * (input1 % 10) + 10 * ((input1 % 100) / 10) + input1 / 100;
input2 = 100 * (input2 % 10) + 10 * ((input2 % 100) / 10) + input2 / 100;
if(input1 > input2) printf("%d", input1);
else printf("%d", input2);
} | d7d3134b64c139e4c38181f840eecaf4ac41e8e7 | [
"C++"
] | 122 | C++ | E-Sangho/Baekjoon_Algorithm | f51a0cd7562f8d3a6168d2aeecdb3e3b46b8d981 | bb1770740d655063780b306f9ca209473761250f | |
refs/heads/master | <file_sep># Playing-around-with-Machine-Learning
In this repository some smaller projects will be uploaded. If you would like to make a contribution and have suggestions for improvement, you are welcome
to make a contribution.
The contents are:
1. Linear Regression
1. K-Means
1. Decision Tree (coming soon)
<file_sep>###########################################################################
###########Playing around with Linear Regression############
###########################################################################
#####Required packages#####
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import RepeatedKFold
from sklearn.model_selection import cross_val_score
#Read in data, explore data, prepare data
df = pd.read_csv(r"hotel_daten.csv")
df = pd.get_dummies(df, columns=["city"])
print(df.dtypes)
print(df.describe())
print(df.nunique())
print(df.corr())
#Evaluating some linear regression with Repeated K-Fold Cross-Validation
'''Model 1'''
X = df[["profit", "square meter"]].values
y = df[["price in millions"]].values
score = cross_val_score(LinearRegression(), X, y, cv=RepeatedKFold(n_repeats=200))
print(np.mean(score))
'''Model 2'''
X = df[["profit", "square meter", "city_Berlin", "city_Köln"]].values
y = df[["price in millions"]].values
score = cross_val_score(LinearRegression(), X, y, cv=RepeatedKFold(n_repeats=200))
print(np.mean(score))
'''Model 3'''
X = df[["square meter", "city_Berlin", "city_Köln"]].values
y = df[["price in millions"]].values
score = cross_val_score(LinearRegression(), X, y, cv=RepeatedKFold(n_repeats=200))
print(np.mean(score))
'''Model 4'''
X = df[["square meter"]].values
y = df[["price in millions"]].values
score = cross_val_score(LinearRegression(), X, y, cv=RepeatedKFold(n_repeats=200))
print(np.mean(score))
#Single parameter "square meter" seems to explain the price of asset very well on its own
single_model = LinearRegression().fit(X,y)
x_min = min(X)
x_max = max(X)
predicted = single_model.predict([x_min,x_max])
plt.scatter(X, y, color="b")
plt.plot([x_min,x_max], predicted, color="r")
plt.title(" Simple Regression ")
plt.ylabel("Price of asset in millions")
plt.xlabel("Total square meters of building")
plt.legend(loc="upper left", labels=["Regression line", "True values"])
plt.show()
| 003afe01340c30a1529a8b2759e397dcf0d90ee9 | [
"Markdown",
"Python"
] | 2 | Markdown | Andrija-Jelic/Playing-around-with-Machine-Learning | c603eb046b79136f5498f5c625a6e486702b7b02 | f649add8d17cf9404614d5178133c8e8a4592f7a | |
refs/heads/master | <repo_name>akitekt/k8s-network-blog<file_sep>/trunks-iptables.cs
root@ip-101-202-7-202:/home/pie# iptables -L -t nat | grep -B 1 -A 4 -E 'KUBE-SVC-3C2I2DNJ4VWZY5PF|KUBE-SEP-P6JNEFEXMECE2WS6|KUBE-SEP-DX25GZBAXCASAQMI|KUBE-SEP-HI43CU4ZL6YUQHDB'
KUBE-MARK-MASQ tcp -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ tcp dpt:30998
KUBE-SVC-3C2I2DNJ4VWZY5PF tcp -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ tcp dpt:30998
--
Chain KUBE-SEP-DX25GZBAXCASAQMI (1 references)
target prot opt source destination
KUBE-MARK-MASQ all -- ip-10-0-11-27.ec2.internal anywhere /* performanceks1/performanceks1-external-ingress-controller:https */
DNAT tcp -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ tcp to:10.0.11.27:80
--
Chain KUBE-SEP-HI43CU4ZL6YUQHDB (1 references)
target prot opt source destination
KUBE-MARK-MASQ all -- ip-10-0-35-21.ec2.internal anywhere /* performanceks1/performanceks1-external-ingress-controller:https */
DNAT tcp -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ tcp to:10.0.35.21:80
--
Chain KUBE-SEP-P6JNEFEXMECE2WS6 (1 references)
target prot opt source destination
KUBE-MARK-MASQ all -- ip-10-0-11-26.ec2.internal anywhere /* performanceks1/performanceks1-external-ingress-controller:https */
DNAT tcp -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ tcp to:10.0.11.26:80
--
KUBE-MARK-MASQ tcp -- !ip-10-0-0-0.ec2.internal/16 ip-10-1-60-159.ec2.internal /* performanceks1/performanceks1-external-ingress-controller:https cluster IP */ tcp dpt:https
KUBE-SVC-3C2I2DNJ4VWZY5PF tcp -- anywhere ip-10-1-60-159.ec2.internal /* performanceks1/performanceks1-external-ingress-controller:https cluster IP */ tcp dpt:https
--
Chain KUBE-SVC-3C2I2DNJ4VWZY5PF (2 references)
target prot opt source destination
KUBE-SEP-P6JNEFEXMECE2WS6 all -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ statistic mode random probability 0.33332999982
KUBE-SEP-DX25GZBAXCASAQMI all -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */ statistic mode random probability 0.50000000000
KUBE-SEP-HI43CU4ZL6YUQHDB all -- anywhere anywhere /* performanceks1/performanceks1-external-ingress-controller:https */
<file_sep>/trunks-ip-route.cs
10.0.183.0/24 dev kube-bridge proto kernel scope link src 10.0.183.1
10.0.184.0/24 via 172.16.58.3 dev eth0 proto 17
10.0.185.0/24 via 192.168.3.11 dev eth0 proto 17
10.0.186.0/24 via 192.168.127.12 dev eth0 proto 17
10.0.188.0/24 via 172.16.58.3 dev eth0 proto 17
10.0.195.0/24 dev tun-102050124 proto 17 src 172.16.31.10
10.0.196.0/24 dev tun-102051237 proto 17 src 172.16.31.10
10.0.230.0/24 dev tun-1020514194 proto 17 src 172.16.31.10
10.0.231.0/24 dev tun-1020514171 proto 17 src 172.16.31.10
<file_sep>/gogeta-ip-route.cs
default via 192.168.3.11 dev eth0
10.0.0.0/24 via 192.168.3.11 dev tunl0 proto bird onlink
10.0.1.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.2.0/24 via 192.168.3.11 dev eth0 proto bird
10.0.3.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.4.0/24 via 172.16.31.10 dev tunl0 proto bird onlink
10.0.5.0/24 via 172.16.31.10 dev eth0 proto bird
10.0.6.0/24 via 172.16.17.32 dev tunl0 proto bird onlink
blackhole 10.0.7.0/24 proto bird
10.0.7.2 dev cali77ec83574f4 scope link
10.0.7.3 dev cali09a12e605e2 scope link
10.0.7.4 dev calid9fe6681678 scope link
10.0.7.5 dev cali00eb81c9259 scope link
10.0.7.8 dev calia645991a7fb scope link
10.0.7.9 dev cali8e98e4eb396 scope link
10.0.7.11 dev cali15c7619fccc scope link
10.0.7.12 dev calid75abf4f5e0 scope link
192.168.127.12/23 dev eth0 proto kernel scope link src 172.16.31.10
172.17.0.0/16 dev docker0 proto kernel scope link src 172.17.0.1 linkdown
<file_sep>/vegata-ip-route.cs
10.0.100.0/24 via 192.168.127.12 dev tunl0 proto bird onlink
10.0.101.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.102.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.103.0/24 via 192.168.3.11 dev tunl0 proto bird onlink
blackhole 10.0.104.0/24 proto bird
10.0.104.2 dev calibeec4c34496 scope link
10.0.104.7 dev calia08627eee93 scope link
10.0.104.8 dev cali7f75471adc7 scope link
10.0.104.9 dev cali9066bf87c18 scope link
10.0.104.36 dev calibb5dd9f41de scope link
10.0.104.43 dev calicf6bd8e667d scope link
10.0.104.44 dev califfcbcf93207 scope link
10.0.104.50 dev caliaa698084a1f scope link
10.0.104.53 dev calib424fcbd49c scope link
10.0.105.0/24 via 192.168.127.12 dev tunl0 proto bird onlink
10.0.106.0/24 via 192.168.127.12 dev tunl0 proto bird onlink
10.0.107.0/24 via 172.16.58.346 dev tunl0 proto bird onlink
10.0.108.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.109.0/24 via 172.16.58.3 dev tunl0 proto bird onlink
10.0.110.0/24 via 172.16.31.10 dev tunl0 proto bird onlink
172.16.31.10/23 dev eth0 proto kernel scope link src 172.16.31.10
172.17.0.0/16 dev docker0 proto kernel scope link src 172.17.0.1 linkdown
<file_sep>/vegeta-crictl-inspectp.cs
root@ip-101-202-5-39:/home/pie# crictl pods
POD ID CREATED STATE NAME NAMESPACE ATTEMPT
843aae920308e 2 weeks ago Ready splunk-universal-forwarder-mhqxk kube-system 0
3c825484d2734 2 weeks ago Ready jaeger-agent-2x26m jaeger 0
6a4731b67a62c 2 weeks ago Ready kube2iam-6wkjc kube-system 0
15fd1a68b837d 2 weeks ago Ready calico-node-j8nf9 kube-system 0
254a9cc452cfd 2 weeks ago Ready kube-proxy-cshd9 kube-system 0
root@ip-101-202-5-39:/home/pie# crictl inspectp 3c825484d2734
{
"status": {
"id": "3c825484d2734e4fe1c54f2ecdc9d34bb54e4330ab48c9426fc90fdd02d2335d",
"metadata": {
"attempt": 0,
"name": "jaeger-agent-2x26m",
"namespace": "jaeger",
"uid": "6dc97082-095c-11e9-a5bc-0258965a256c"
},
"state": "SANDBOX_READY",
"createdAt": "2018-12-26T22:20:50.673959575Z",
"network": {
"ip": "10.0.104.2"
},
"linux": {
"namespaces": {
"options": {
"ipc": "POD",
"network": "POD",
"pid": "CONTAINER"
}
}
},
"labels": {
"app": "jaeger",
"component": "agent",
"controller-revision-hash": "957477944",
"io.kubernetes.pod.name": "jaeger-agent-2x26m",
"io.kubernetes.pod.namespace": "jaeger",
"io.kubernetes.pod.uid": "6dc97082-095c-11e9-a5bc-0258965a256c",
"jaeger-infra": "agent-instance",
"pod-template-generation": "1",
"release": "jaeger"
},
"annotations": {
"kubernetes.io/config.seen": "2018-12-26T22:20:18.48411321Z",
"kubernetes.io/config.source": "api"
}
}
} | 037df9476acb4dfa6c865a75f2737b33ce520325 | [
"C#"
] | 5 | C# | akitekt/k8s-network-blog | b6583966421d1e04dccdf4d5c1251361a47f23c8 | f6b2a3bf284b2885ae4dd0ebeab5c548a4dcc0bb | |
refs/heads/master | <repo_name>Nitei/wishList<file_sep>/src/app/agregar/agregar.page.ts
import { Component, OnInit } from '@angular/core';
import { ActivatedRoute } from '@angular/router';
import { ListaItem, Lista } from 'src/models';
import { DeseosService } from '../servicios/deseos.service';
import { AlertController } from '@ionic/angular';
@Component( {
selector: 'app-agregar',
templateUrl: './agregar.page.html',
styleUrls: [ './agregar.page.scss' ],
} )
export class AgregarPage implements OnInit {
titulo: string;
indexOfList: number;
lista: Lista;
nombreItem: string;
constructor (
private route: ActivatedRoute, private deseosService: DeseosService, private alertController: AlertController ) {
this.route.params.subscribe( ( data: any ) => {
this.titulo = data[ 'titulo' ];
if ( this.deseosService.newListInListas( this.titulo ) ) {
// If the list not exist we create an new list and push into the array of lists.
this.lista = new Lista( this.titulo );
this.deseosService.agregarLista( this.lista );
} else {
// If the list exist we find it in the array of list with the index passed in the url by param.
this.indexOfList = data[ 'lista' ];
this.lista = this.deseosService.listas[ this.indexOfList ];
}
} );
}
agregarItem() {
if ( this.nombreItem !== '' ) {
const nuevoItem = new ListaItem( this.nombreItem );
if ( this.indexOfList === undefined ) {
// If the list not exist we will find the lastone list in the array and we push the new item.
this.deseosService.listas[ this.deseosService.listas.length - 1 ].items.push( nuevoItem );
} else {
// if the list exist we find the list in the array by index and push the new item.
this.deseosService.listas[ this.indexOfList ].items.push( nuevoItem );
}
this.nombreItem = '';
this.deseosService.guardarStorage();
}
}
actualizaItem( item: ListaItem ) {
item.completado = !item.completado;
const pendientes = this.lista.items.filter( itemData => {
return !itemData.completado;
} ).length;
if ( pendientes === 0 ) {
this.lista.terminada = true;
this.lista.terminadaEn = new Date();
// console.log( this.lista );
} else {
this.lista.terminada = false;
this.lista.terminadaEn = null;
// console.log( this.lista );
}
this.deseosService.guardarStorage();
}
borrarItem( item: number ) {
this.lista.items.splice( item, 1 );
console.log( item );
}
async editarLista( listaItemsItem: Lista ) {
console.log( listaItemsItem );
const editarNombre = await this.alertController.create( {
header: 'Cambiar nombre',
subHeader: 'Nuevo nombre',
message: 'Nuevo nombre del item',
buttons: [ {
text: 'Cancelar',
}, {
text: 'Guardar',
handler: nuevoNombre => {
if ( nuevoNombre[ 0 ] !== '' ) {
console.log( nuevoNombre );
listaItemsItem[ 'desc' ] = nuevoNombre[ 0 ];
this.deseosService.guardarStorage();
}
}
} ],
inputs: [ {
placeholder: 'Nuevo nombre de la lista'
} ]
} );
await editarNombre.present();
}
ngOnInit() {
}
}
<file_sep>/src/app/pendientes/pendientes.page.ts
import { Component, OnInit } from '@angular/core';
import { DeseosService } from '../servicios/deseos.service';
import { Lista } from 'src/models';
import { AlertController } from '@ionic/angular';
import { Router } from '@angular/router';
@Component( {
selector: 'app-pendientes',
templateUrl: './pendientes.page.html',
styleUrls: [ './pendientes.page.scss' ],
} )
export class PendientesPage implements OnInit {
listas: Lista[];
constructor (
public deseosService: DeseosService,
private alertController: AlertController,
private route: Router
) {
this.listas = this.deseosService.listas;
}
listaSeleccionada( lista: Lista, idx: number ) {
this.route.navigate( [ '/tabs/agregar/', lista.titulo, idx ] );
}
async editarLista( lista: Lista ) {
const editarNombre = await this.alertController.create( {
header: 'Cambiar nombre',
subHeader: 'Nuevo nombre',
message: 'Nuevo nombre de la lista',
buttons: [ {
text: 'Cancelar',
}, {
text: 'Guardar',
handler: data => {
if ( data[ 0 ] !== '' ) {
console.log( data );
lista.titulo = data[ 0 ];
this.deseosService.guardarStorage();
}
}
} ],
inputs: [ {
placeholder: 'Nuevo nombre de la lista'
} ]
} );
await editarNombre.present();
}
async agregarLista() {
const alerta = await this.alertController.create( {
header: 'Nueva lista',
subHeader: 'Nombre de la nueva lista que se desea',
message: 'Nombre de la nueva lista',
buttons: [ {
text: 'Cancelar',
}, {
text: 'Agregar',
handler: lista => {
if ( this.deseosService.newListInListas( lista.titulo ) ) {
this.route.navigate( [ '/tabs/agregar/', lista.titulo ] );
}
}
} ],
inputs: [ {
name: 'titulo',
placeholder: 'Nombre de la lista'
} ]
} );
await alerta.present();
}
borrarLista( item: Lista ) {
this.deseosService.listas.splice( this.deseosService.listas.indexOf( item ), 1 );
this.deseosService.guardarStorage();
}
ngOnInit() {
console.log( this.deseosService.listas );
}
}
<file_sep>/src/app/shared/shared.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { HeaderComponent } from './header/header.component';
import { IonicModule } from '@ionic/angular';
import { FormsModule } from '@angular/forms';
import { FiltroCompletadoPipe } from './pipes/filtro-completado.pipe';
@NgModule( {
declarations: [
HeaderComponent,
FiltroCompletadoPipe
],
entryComponents: [
HeaderComponent
],
imports: [
IonicModule,
FormsModule,
CommonModule
],
exports: [ HeaderComponent, FiltroCompletadoPipe ]
} )
export class SharedModule { }
<file_sep>/src/models/lista.model.ts
import { ListaItem } from "./lista-item.model";
export class Lista {
constructor (
public titulo: string,
public id: number = new Date().getTime(),
public creadaEn: Date = new Date(),
public terminadaEn?: Date,
public terminada: boolean = false,
public items: ListaItem[] = []
) {
}
}
<file_sep>/src/app/servicios/deseos.service.ts
import { Injectable } from '@angular/core';
import { Lista } from 'src/models/index';
const enum booleans {
Yes = 1,
No = -1
}
@Injectable( {
providedIn: 'root'
} )
export class DeseosService {
listas: Lista[];
constructor () {
this.cargarStorage();
}
guardarStorage() {
localStorage.setItem( 'itemLista', JSON.stringify( this.listas ) );
}
cargarStorage() {
if ( localStorage.getItem( 'itemLista' ) ) {
this.listas = JSON.parse( localStorage.getItem( 'itemLista' ) );
}
}
agregarLista( lista: Lista ) {
this.listas.push( lista );
this.guardarStorage();
}
// true if is a new List into this.listas.
// false if are repeat into this.listas.
// false if the title is void.
newListInListas( titulo: string ) {
let res = true;
for ( const index of this.listas ) {
if ( index.titulo === titulo ) {
res = false;
console.log( `Existe en la lista: ${ titulo }` );
break;
}
}
if ( titulo === '' ) {
res = false;
console.error( 'El título no puede estar vacio' );
}
return res;
}
}
<file_sep>/src/app/terminados/terminados.page.ts
import { Component, OnInit } from '@angular/core';
import { Lista } from 'src/models';
import { DeseosService } from '../servicios/deseos.service';
import { Router } from '@angular/router';
@Component( {
selector: 'app-terminados',
templateUrl: './terminados.page.html',
styleUrls: [ './terminados.page.scss' ],
} )
export class TerminadosPage implements OnInit {
constructor ( public deseosService: DeseosService, private route: Router ) {
}
listaSeleccionada( lista: Lista, idx: number ) {
console.log( idx );
this.route.navigate( [ '/tabs/agregar/', lista.titulo, idx ] );
}
borrarLista( item: Lista ) {
this.deseosService.listas.splice( this.deseosService.listas.indexOf( item ), 1 );
this.deseosService.guardarStorage();
}
ngOnInit() {
}
}
<file_sep>/src/app/servicios/deseos.service.spec.ts
import { TestBed } from '@angular/core/testing';
import { DeseosService } from './deseos.service';
import { Lista } from 'src/models';
const service: DeseosService = TestBed.get( DeseosService );
describe( 'DeseosService', () => {
beforeEach( function () {
TestBed.configureTestingModule( {} );
service.listas = [ new Lista( 'Compras de invierno' ) ];
} );
it( 'newListInListas("Compras de invierno") or newListInListas( "" ) should return false', () => {
expect( service.newListInListas( 'Compras de invierno' ) ).toBeFalsy();
expect( service.newListInListas( '' ) ).toBeFalsy();
console.log( service.listas );
} );
it( 'newListInListas("Compras a lo loco") should return false', () => {
expect( service.newListInListas( 'Compras a lo loco' ) ).toBeTruthy();
console.log( service.listas );
} );
} );
<file_sep>/src/app/agregar/agregar.page.spec.ts
import { CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
import { async, ComponentFixture, TestBed } from '@angular/core/testing';
import { AgregarPage } from './agregar.page';
xdescribe( 'AgregarPage', () => {
let component: AgregarPage;
let fixture: ComponentFixture<AgregarPage>;
beforeEach( async( () => {
TestBed.configureTestingModule( {
declarations: [ AgregarPage ],
schemas: [ CUSTOM_ELEMENTS_SCHEMA ],
} )
.compileComponents();
} ) );
beforeEach( () => {
fixture = TestBed.createComponent( AgregarPage );
component = fixture.componentInstance;
fixture.detectChanges();
} );
it( 'should create', () => {
expect( component ).toBeTruthy();
} );
} );
| 41dd375d15662e140500d6a3014ae5bd7108f965 | [
"TypeScript"
] | 8 | TypeScript | Nitei/wishList | d90b502d61019a0fec23f5b6b53e12842ecc5dbf | d6fea4d677ff6a3e17d8e15ca1a742ec2e990858 | |
refs/heads/master | <file_sep>"""
distutils commands for riak-python-client
"""
__all__ = ['create_bucket_types']
from distutils import log
from distutils.core import Command
from distutils.errors import DistutilsOptionError
from subprocess import CalledProcessError, Popen, PIPE
try:
from subprocess import check_output
except ImportError:
def check_output(*popenargs, **kwargs):
"""Run command with arguments and return its output as a byte string.
If the exit code was non-zero it raises a CalledProcessError. The
CalledProcessError object will have the return code in the returncode
attribute and output in the output attribute.
The arguments are the same as for the Popen constructor. Example:
>>> check_output(["ls", "-l", "/dev/null"])
'crw-rw-rw- 1 root root 1, 3 Oct 18 2007 /dev/null\n'
The stdout argument is not allowed as it is used internally.
To capture standard error in the result, use stderr=STDOUT.
>>> import sys
>>> check_output(["/bin/sh", "-c",
... "ls -l non_existent_file ; exit 0"],
... stderr=sys.stdout)
'ls: non_existent_file: No such file or directory\n'
"""
if 'stdout' in kwargs:
raise ValueError('stdout argument not allowed, it will be '
'overridden.')
process = Popen(stdout=PIPE, *popenargs, **kwargs)
output, unused_err = process.communicate()
retcode = process.poll()
if retcode:
cmd = kwargs.get("args")
if cmd is None:
cmd = popenargs[0]
raise CalledProcessError(retcode, cmd, output=output)
return output
try:
import simplejson as json
except ImportError:
import json
class create_bucket_types(Command):
"""
Creates bucket-types appropriate for testing. By default this will create:
* `pytest-maps` with ``{"datatype":"map"}``
* `pytest-sets` with ``{"datatype":"set"}``
* `pytest-counters` with ``{"datatype":"counter"}``
* `pytest-consistent` with ``{"consistent":true}``
* `pytest` with ``{"allow_mult":false}``
"""
description = "create bucket-types used in integration tests"
user_options = [
('riak-admin=', None, 'path to the riak-admin script')
]
_props = {
'pytest-maps': {'datatype': 'map'},
'pytest-sets': {'datatype': 'set'},
'pytest-counters': {'datatype': 'counter'},
'pytest-consistent': {'consistent': True},
'pytest': {'allow_mult': False}
}
def initialize_options(self):
self.riak_admin = None
def finalize_options(self):
if self.riak_admin is None:
raise DistutilsOptionError("riak-admin option not set")
def run(self):
if self._check_available():
for name in self._props:
self._create_and_activate_type(name, self._props[name])
def check_output(self, *args, **kwargs):
if self.dry_run:
log.info(' '.join(args))
return bytearray()
else:
return check_output(*args, **kwargs)
def _check_available(self):
try:
self.check_btype_command("list")
return True
except CalledProcessError:
log.error("Bucket types are not supported on this Riak node!")
return False
def _create_and_activate_type(self, name, props):
# Check status of bucket-type
exists = False
active = False
try:
status = self.check_btype_command('status', name)
except CalledProcessError as e:
status = e.output
exists = ('not an existing bucket type' not in status)
active = ('is active' in status)
if exists or active:
log.info("Updating {!r} bucket-type with props {!r}".format(name,
props))
self.check_btype_command("update", name,
json.dumps({'props': props},
separators=(',', ':')))
else:
log.info("Creating {!r} bucket-type with props {!r}".format(name,
props))
self.check_btype_command("create", name,
json.dumps({'props': props},
separators=(',', ':')))
if not active:
log.info('Activating {!r} bucket-type'.format(name))
self.check_btype_command("activate", name)
def check_btype_command(self, *args):
cmd = self._btype_command(*args)
return self.check_output(cmd)
def run_btype_command(self, *args):
self.spawn(self._btype_command(*args))
def _btype_command(self, *args):
cmd = [self.riak_admin, "bucket-type"]
cmd.extend(args)
return cmd
<file_sep>import os
from riak.test_server import TestServer
USE_TEST_SERVER = int(os.environ.get('USE_TEST_SERVER', '0'))
if USE_TEST_SERVER:
HTTP_PORT = 9000
PB_PORT = 9002
test_server = TestServer()
test_server.cleanup()
test_server.prepare()
test_server.start()
try:
__import__('riak_pb')
HAVE_PROTO = True
except ImportError:
HAVE_PROTO = False
HOST = os.environ.get('RIAK_TEST_HOST', '127.0.0.1')
PB_HOST = os.environ.get('RIAK_TEST_PB_HOST', HOST)
PB_PORT = int(os.environ.get('RIAK_TEST_PB_PORT', '8087'))
HTTP_HOST = os.environ.get('RIAK_TEST_HTTP_HOST', HOST)
HTTP_PORT = int(os.environ.get('RIAK_TEST_HTTP_PORT', '8098'))
# these ports are used to simulate errors, there shouldn't
# be anything listening on either port.
DUMMY_HTTP_PORT = int(os.environ.get('DUMMY_HTTP_PORT', '1023'))
DUMMY_PB_PORT = int(os.environ.get('DUMMY_PB_PORT', '1022'))
SKIP_SEARCH = int(os.environ.get('SKIP_SEARCH', '1'))
RUN_YZ = int(os.environ.get('RUN_YZ', '0'))
SKIP_INDEXES = int(os.environ.get('SKIP_INDEXES', '1'))
SKIP_POOL = os.environ.get('SKIP_POOL')
SKIP_RESOLVE = os.environ.get('SKIP_RESOLVE', '0')
SKIP_BTYPES = os.environ.get('SKIP_BTYPES', '0')
<file_sep>"""
Copyright 2010 <NAME> <<EMAIL>>
Copyright 2010 <NAME> <<EMAIL>>
Copyright 2009 <NAME> <<EMAIL>>
This file is provided to you under the Apache License,
Version 2.0 (the "License"); you may not use this file
except in compliance with the License. You may obtain
a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
"""
import httplib
import socket
from riak.transports.pool import Pool
from riak.transports.http.transport import RiakHttpTransport
class NoNagleHTTPConnection(httplib.HTTPConnection):
"""
Setup a connection class which does not use Nagle - deal with
latency on PUT requests lower than MTU
"""
def connect(self):
"""
Set TCP_NODELAY on socket
"""
httplib.HTTPConnection.connect(self)
self.sock.setsockopt(socket.IPPROTO_TCP, socket.TCP_NODELAY, 1)
class RiakHttpPool(Pool):
"""
A pool of HTTP(S) transport connections.
"""
def __init__(self, client, **options):
self.client = client
self.options = options
if client.protocol == 'https':
self.connection_class = httplib.HTTPSConnection
else:
self.connection_class = NoNagleHTTPConnection
super(RiakHttpPool, self).__init__()
def create_resource(self):
node = self.client._choose_node()
return RiakHttpTransport(node=node,
client=self.client,
connection_class=self.connection_class,
**self.options)
def destroy_resource(self, transport):
transport.close()
CONN_CLOSED_ERRORS = (
httplib.NotConnected,
httplib.IncompleteRead,
httplib.ImproperConnectionState,
httplib.BadStatusLine
)
def is_retryable(err):
"""
Determines if the given exception is something that is
network/socket-related and should thus cause the HTTP connection
to close and the operation retried on another node.
:rtype: boolean
"""
for errtype in CONN_CLOSED_ERRORS:
if isinstance(err, errtype):
return True
return False
<file_sep>"""
Copyright 2012 <NAME>, Inc.
This file is provided to you under the Apache License,
Version 2.0 (the "License"); you may not use this file
except in compliance with the License. You may obtain
a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing,
software distributed under the License is distributed on an
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
KIND, either express or implied. See the License for the
specific language governing permissions and limitations
under the License.
"""
import socket
import struct
from riak import RiakError
from riak_pb.messages import (
MESSAGE_CLASSES,
MSG_CODE_ERROR_RESP
)
class RiakPbcConnection(object):
"""
Connection-related methods for RiakPbcTransport.
"""
def _encode_msg(self, msg_code, msg=None):
if msg is None:
return struct.pack("!iB", 1, msg_code)
msgstr = msg.SerializeToString()
slen = len(msgstr)
hdr = struct.pack("!iB", 1 + slen, msg_code)
return hdr + msgstr
def _request(self, msg_code, msg=None, expect=None):
self._send_msg(msg_code, msg)
return self._recv_msg(expect)
def _send_msg(self, msg_code, msg):
self._connect()
self._socket.send(self._encode_msg(msg_code, msg))
def _recv_msg(self, expect=None):
self._recv_pkt()
msg_code, = struct.unpack("B", self._inbuf[:1])
if msg_code is MSG_CODE_ERROR_RESP:
err = self._parse_msg(msg_code, self._inbuf[1:])
raise RiakError(err.errmsg)
elif msg_code in MESSAGE_CLASSES:
msg = self._parse_msg(msg_code, self._inbuf[1:])
else:
raise Exception("unknown msg code %s" % msg_code)
if expect and msg_code != expect:
raise RiakError("unexpected protocol buffer message code: %d, %r"
% (msg_code, msg))
return msg_code, msg
def _recv_pkt(self):
nmsglen = self._socket.recv(4)
if len(nmsglen) != 4:
raise RiakError(
"Socket returned short packet length %d - expected 4"
% len(nmsglen))
msglen, = struct.unpack('!i', nmsglen)
self._inbuf_len = msglen
self._inbuf = ''
while len(self._inbuf) < msglen:
want_len = min(8192, msglen - len(self._inbuf))
recv_buf = self._socket.recv(want_len)
if not recv_buf:
break
self._inbuf += recv_buf
if len(self._inbuf) != self._inbuf_len:
raise RiakError("Socket returned short packet %d - expected %d"
% (len(self._inbuf), self._inbuf_len))
def _connect(self):
if not self._socket:
if self._timeout:
self._socket = socket.create_connection(self._address,
self._timeout)
else:
self._socket = socket.create_connection(self._address)
def close(self):
"""
Closes the underlying socket of the PB connection.
"""
if self._socket:
self._socket.shutdown(socket.SHUT_RDWR)
def _parse_msg(self, code, packet):
try:
pbclass = MESSAGE_CLASSES[code]
except KeyError:
pbclass = None
if pbclass is None:
return None
pbo = pbclass()
pbo.ParseFromString(packet)
return pbo
# These are set in the RiakPbcTransport initializer
_address = None
_timeout = None
<file_sep>========================
Python Client for Riak
========================
Documentation
=============
`Documentation for the Riak Python Client Library
<http://basho.github.io/riak-python-client/index.html>`_ is available
here. The documentation source is found in `docs/ subdirectory
<https://github.com/basho/riak-python-client/tree/master/docs>`_ and
can be built with `Sphinx <http://sphinx.pocoo.org/>`_.
Documentation for Riak is available at http://docs.basho.com/riak/latest
Install
=======
The recommended version of Python for use with this client is Python
2.7.
You must have `Protocol Buffers`_ installed before you can install the
Riak Client. From the Riak Python Client root directory, execute::
python setup.py install
There is an additional dependency on the Python package `setuptools`.
Please install `setuptools` first, e.g. ``port install
py27-setuptools`` for OS X and MacPorts.
Testing
=======
To run the tests against a Riak server (with default TCP port
configuration) on localhost, execute::
python setup.py test
Connections to Riak in Tests
----------------------------
If your Riak server isn't running on localhost or you have built a
Riak devrel from source, use the environment variables
``RIAK_TEST_HOST``, ``RIAK_TEST_HTTP_PORT`` and
``RIAK_TEST_PB_PORT=8087`` to specify where to find the Riak server.
Some of the connection tests need port numbers that are NOT in use. If
ports 1023 and 1022 are in use on your test system, set the
environment variables ``DUMMY_HTTP_PORT`` and ``DUMMY_PB_PORT`` to
unused port numbers.
Testing Search
--------------
If you don't have `Riak Search
<http://docs.basho.com/riak/latest/dev/using/search/>`_ enabled, you
can set the ``SKIP_SEARCH`` environment variable to 1 skip those
tests.
If you don't have `Search 2.0 <https://github.com/basho/yokozuna>`_
enabled, you can set the ``RUN_YZ`` environment variable to 0 to skip
those tests.
Testing Bucket Types (Riak 2+)
------------------------------
To test bucket-types, you must run the ``create_bucket_types`` setup
command, which will create the bucket-types used in testing, or create
them manually yourself. It can be run like so (substituting ``$RIAK``
with the root of your Riak install)::
./setup.py create_bucket_types --riak-admin=$RIAK/bin/riak-admin
You may alternately add these lines to `setup.cfg`::
[create_bucket_types]
riak-admin=/Users/sean/dev/riak/rel/riak/bin/riak-admin
To skip the bucket-type tests, set the ``SKIP_BTYPES`` environment
variable to ``1``.
| c75ecf1ead21ef147e2c086f71e66cf3af18f3df | [
"Python",
"reStructuredText"
] | 5 | Python | linearregression/riak-python-client | 773e7bc5027ed7e01471475c8bd342881e98398c | dbc65c4255f693b9665c0fbed8e8545f25c82e80 | |
refs/heads/master | <repo_name>ianhi/jupyterlab-git<file_sep>/jupyterlab_git/tests/test_remote.py
import subprocess
from unittest.mock import Mock, patch
from jupyterlab_git.handlers import GitRemoteAddHandler
from .testutils import assert_http_error, ServerTest, maybe_future
class TestAddRemote(ServerTest):
@patch("jupyterlab_git.git.execute")
def test_git_add_remote_success_no_name(self, mock_execute):
# Given
path = "test_path"
url = "http://github.com/myid/myrepository.git"
mock_execute.return_value = maybe_future((0, "", ""))
# When
body = {
"top_repo_path": path,
"url": url,
}
response = self.tester.post(["remote", "add"], body=body)
# Then
command = ["git", "remote", "add", "origin", url]
mock_execute.assert_called_once_with(command, cwd=path)
assert response.status_code == 201
payload = response.json()
assert payload == {
"code": 0,
"command": " ".join(command),
}
@patch("jupyterlab_git.git.execute")
def test_git_add_remote_success(self, mock_execute):
# Given
path = "test_path"
url = "http://github.com/myid/myrepository.git"
name = "distant"
mock_execute.return_value = maybe_future((0, "", ""))
# When
body = {"top_repo_path": path, "url": url, "name": name}
response = self.tester.post(["remote", "add"], body=body)
# Then
command = ["git", "remote", "add", name, url]
mock_execute.assert_called_once_with(command, cwd=path)
assert response.status_code == 201
payload = response.json()
assert payload == {
"code": 0,
"command": " ".join(command),
}
@patch("jupyterlab_git.git.execute")
def test_git_add_remote_failure(self, mock_execute):
# Given
path = "test_path"
url = "http://github.com/myid/myrepository.git"
error_msg = "Fake failure"
error_code = 128
mock_execute.return_value = maybe_future((error_code, "", error_msg))
# When
body = {
"top_repo_path": path,
"url": url,
}
with assert_http_error(500):
self.tester.post(["remote", "add"], body=body)
# Then
mock_execute.assert_called_once_with(
["git", "remote", "add", "origin", url], cwd=path
)
<file_sep>/ROADMAP.md
## CI/CD:
- Integration Testing
- **What?** Test suite that actually exercises UI and API against an actual Git repo without mocking out Git calls.
- **Why?** Enables us to better test against an actual Git process. Stepping stone to x-OS testing such as Windows.
## Features
- Plaintext diffs
- **What?** Diff of non-notebook files such as .py files
- **Why?** Fully featured diff experience for most types of files
- Credentials
- **What?** Credential management during push/pull/clone without actually storing credentials
- **Why?** Better UX when a user doesn't have credentials for accessing a Git repo.
- Merge resolution
- **What?** UI to handle the conflicts for notebook and plain text files.
- **Why?** Various Git operations may result in conflicts.
## Maintenance
- Issue & Bug triage
- **What?** Triage issues and fix bugs in existing featutes
- **Why?** Operational maintenance of the extension
<file_sep>/jupyterlab_git/tests/test_settings.py
import os
from unittest.mock import Mock, patch
from packaging.version import parse
from jupyterlab_git import __version__
from jupyterlab_git.handlers import GitSettingsHandler
from .testutils import ServerTest, assert_http_error, maybe_future
class TestSettings(ServerTest):
@patch("jupyterlab_git.git.execute")
def test_git_get_settings_success(self, mock_execute):
# Given
git_version = "2.10.3"
jlab_version = "2.1.42-alpha.24"
mock_execute.return_value = maybe_future(
(0, "git version {}.os_platform.42".format(git_version), "")
)
# When
response = self.tester.get(["settings"], params={"version": jlab_version})
# Then
mock_execute.assert_called_once_with(["git", "--version"], cwd=os.curdir)
assert response.status_code == 200
payload = response.json()
assert payload == {
"frontendVersion": str(parse(jlab_version)),
"gitVersion": git_version,
"serverRoot": self.notebook.notebook_dir,
"serverVersion": str(parse(__version__)),
}
@patch("jupyterlab_git.git.execute")
def test_git_get_settings_no_git(self, mock_execute):
# Given
jlab_version = "2.1.42-alpha.24"
mock_execute.side_effect = FileNotFoundError(
"[Errno 2] No such file or directory: 'git'"
)
# When
response = self.tester.get(["settings"], params={"version": jlab_version})
# Then
mock_execute.assert_called_once_with(["git", "--version"], cwd=os.curdir)
assert response.status_code == 200
payload = response.json()
assert payload == {
"frontendVersion": str(parse(jlab_version)),
"gitVersion": None,
"serverRoot": self.notebook.notebook_dir,
"serverVersion": str(parse(__version__)),
}
@patch("jupyterlab_git.git.execute")
def test_git_get_settings_no_jlab(self, mock_execute):
# Given
git_version = "2.10.3"
mock_execute.return_value = maybe_future(
(0, "git version {}.os_platform.42".format(git_version), "")
)
# When
response = self.tester.get(["settings"])
# Then
mock_execute.assert_called_once_with(["git", "--version"], cwd=os.curdir)
assert response.status_code == 200
payload = response.json()
assert payload == {
"frontendVersion": None,
"gitVersion": git_version,
"serverRoot": self.notebook.notebook_dir,
"serverVersion": str(parse(__version__)),
}
<file_sep>/jupyterlab_git/__init__.py
"""Initialize the backend server extension
"""
# need this in order to show version in `jupyter serverextension list`
from ._version import __version__
from traitlets import List, Dict, Unicode
from traitlets.config import Configurable
from jupyterlab_git.handlers import setup_handlers
from jupyterlab_git.git import Git
class JupyterLabGit(Configurable):
"""
Config options for jupyterlab_git
Modeled after: https://github.com/jupyter/jupyter_server/blob/9dd2a9a114c045cfd8fd8748400c6a697041f7fa/jupyter_server/serverapp.py#L1040
"""
actions = Dict(
help="Actions to be taken after a git command. Each action takes a list of commands to execute (strings). Supported actions: post_init",
config=True,
trait=List(
trait=Unicode(), help='List of commands to run. E.g. ["touch baz.py"]'
)
# TODO Validate
)
def _jupyter_server_extension_paths():
"""Declare the Jupyter server extension paths."""
return [{"module": "jupyterlab_git"}]
def load_jupyter_server_extension(nbapp):
"""Load the Jupyter server extension."""
config = JupyterLabGit(config=nbapp.config)
git = Git(nbapp.web_app.settings["contents_manager"], config)
nbapp.web_app.settings["git"] = git
setup_handlers(nbapp.web_app)
<file_sep>/binder/postBuild
#!/usr/bin/env bash
pip install .
jupyter serverextension enable --sys-prefix --py nbgitpuller
jupyter lab build --dev-build=False --minimize=False --debug
| 392927be800d32746d8c790de98a6cbad7c9133b | [
"Markdown",
"Python",
"Shell"
] | 5 | Python | ianhi/jupyterlab-git | 6043cf33ce1e5d02a66399f099ea0e4ae0a15f9a | 649c689425ee5dbfc2038baf3f4a40a491a9dec6 | |
refs/heads/master | <file_sep>package com.android.tugaskotlin2
import android.content.Intent
import android.net.Uri
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import android.widget.ImageView
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
fb.setOnClickListener {
val fb = Intent(android.content.Intent.ACTION_VIEW)
fb.data= Uri.parse("https://id-id.facebook.com/")
startActivity(fb)
}
ig.setOnClickListener {
val ig = Intent(android.content.Intent.ACTION_VIEW)
ig.data= Uri.parse("https://www.instagram.com/armandoputra77")
startActivity(ig)
}
tw.setOnClickListener {
val tw = Intent(android.content.Intent.ACTION_VIEW)
tw.data= Uri.parse("https://twitter.com/login?lang=id")
startActivity(tw)
}
gh.setOnClickListener {
val gh = Intent(android.content.Intent.ACTION_VIEW)
gh.data= Uri.parse("https://github.com/armandoutamaputra")
startActivity(gh)
}
}
}
| ccf4696c1a7b1392a9eac079d58ec24861fac212 | [
"Kotlin"
] | 1 | Kotlin | ArmandoUtamaPutra/tugaskotlin2 | 397b7673140196cff8d9035f18119566e1a81bb1 | 0e75a006a7ba3e50155265dafeea51b3671945f5 | |
refs/heads/main | <repo_name>omer0909/blender-correct-uv-addon<file_sep>/__init__.py
bl_info = {
"name": "Correct Uv",
"author": "<NAME>",
"version": (1, 0),
"blender": (2, 90, 0),
"location": "View3D > Edit > UV > Correct Uv",
"description": "make a uv",
"warning": "",
"doc_url": "",
"category": "Edit",
}
import bpy
import bmesh
deafults={
"Angle_Limit":66.0,
"Method":'ANGLE_BASED',
"Fill_Holes":True,
"Correct_Aspect":True,
"Use_Subdivision_Surface":False,
"Margin":0.001,
}
def mainCorrectUv():
objects=[]
objects.append(bpy.context.active_object.name)
for object in bpy.context.selected_objects:
if not object==bpy.context.active_object:
objects.append(object.name)
active=bpy.context.active_object
edges=objects[:]
for index in range(len(objects)):
bpy.context.view_layer.objects.active=bpy.context.view_layer.objects[objects[index]]
me = bpy.context.edit_object.data
bm = bmesh.from_edit_mesh(me)
edges[index]=[]
number=0
for v in bm.edges:
edges[index].append(v.seam)
number+=1
bpy.ops.uv.smart_project(angle_limit=deafults["Angle_Limit"])
area=bpy.context.area.ui_type
bpy.context.area.ui_type = 'UV'
bpy.ops.uv.select_all(action='SELECT')
bpy.context.area.ui_type =area
bpy.ops.uv.seams_from_islands()
bpy.ops.uv.unwrap(method=deafults["Method"], fill_holes=deafults["Fill_Holes"], correct_aspect=deafults["Correct_Aspect"], use_subsurf_data=deafults["Use_Subdivision_Surface"], margin=deafults["Margin"])
bpy.ops.mesh.mark_seam(clear=True)
for index in range(len(objects)):
bpy.context.view_layer.objects.active=bpy.context.view_layer.objects[objects[index]]
me = bpy.context.edit_object.data
bm = bmesh.from_edit_mesh(me)
number=0
for v in bm.edges:
v.seam=edges[index][number]
number+=1
bmesh.update_edit_mesh(me, True)
even=[False]
class CorrectUv(bpy.types.Operator):
bl_label="Correct Uv"
bl_idname="wm.correct_uv"
Angle_Limit=bpy.props.FloatProperty(name="Angle Limit",default=66.0,min=1,max=89,precision=3)
Method :bpy.props.EnumProperty(
name="Method",
default='ANGLE_BASED',
items=[
('ANGLE_BASED',"Angle Based",""),
('CONFORMAL',"Conformal",""),
]
)
Fill_Holes=bpy.props.BoolProperty(name="Fill Holes",default=True)
Correct_Aspect=bpy.props.BoolProperty(name="Correct Aspect",default=True)
Use_Subdivision_Surface=bpy.props.BoolProperty(name="Use Subdivision Surface",default=False)
Margin=bpy.props.FloatProperty(name="Margin",default=0.001,min=0,max=1,precision=3)
def execute(self,context):
if even[0]:
even[0]=False
deafults["Angle_Limit"]=self.Angle_Limit
deafults["Method"]=self.Method
deafults["Fill_Holes"]=self.Fill_Holes
deafults["Correct_Aspect"]=self.Correct_Aspect
deafults["Use_Subdivision_Surface"]=self.Use_Subdivision_Surface
deafults["Margin"]=self.Margin
mainCorrectUv()
else:
even[0]=False
bpy.ops.wm.correct_uv("INVOKE_DEFAULT")
return {"FINISHED"}
def invoke(self,context,event):
self.Angle_Limit=deafults["Angle_Limit"]
self.Method=deafults["Method"]
self.Fill_Holes=deafults["Fill_Holes"]
self.Correct_Aspect=deafults["Correct_Aspect"]
self.Use_Subdivision_Surface=deafults["Use_Subdivision_Surface"]
self.Margin=deafults["Margin"]
even[0]=True
return context.window_manager.invoke_props_dialog(self)
def cancel(self, context):
even[0]=False
def menu_function(self, context):
layout = self.layout
layout.separator()
layout.operator("wm.correct_uv")
def register():
bpy.utils.register_class(CorrectUv)
bpy.types.VIEW3D_MT_uv_map.append(menu_function)
def unregister():
bpy.utils.unregister_class(CorrectUv)
bpy.types.VIEW3D_MT_uv_map.remove(menu_function)
if __name__ == "__main__":
register()
<file_sep>/README.md
# blender-correct-uv-addon
this is a Blender addon for uv unwrap<br/>
[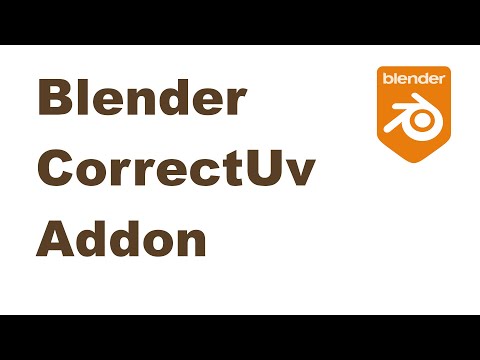](https://youtu.be/FWNjvWWPC8o)
| ad2deec4d1f99aff4127dff61502fd09f214e7cc | [
"Markdown",
"Python"
] | 2 | Python | omer0909/blender-correct-uv-addon | 3f3b047c6c192d9d4fc3ccf9d8e53d3e7091cc7a | 9168cae4b5e441497b80b3bfb58abd53e8703d76 | |
refs/heads/master | <file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
import datetime
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0011_auto_20150505_0008'),
]
operations = [
migrations.AddField(
model_name='logcontent',
name='state',
field=models.CharField(default=datetime.datetime(2015, 5, 7, 20, 11, 42, 875000), max_length=10),
preserve_default=False,
),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0006_auto_20150504_1403'),
]
operations = [
migrations.RenameField(
model_name='logcontent',
old_name='Value',
new_name='value',
),
migrations.AlterField(
model_name='log',
name='log_contents',
field=models.TextField(),
),
migrations.AlterField(
model_name='log',
name='pub_date',
field=models.DateTimeField(auto_now_add=True),
),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
]
operations = [
migrations.CreateModel(
name='Information',
fields=[
('id', models.AutoField(verbose_name='ID', serialize=False, auto_created=True, primary_key=True)),
('info_type', models.CharField(max_length=200)),
('priority', models.IntegerField(default=0)),
],
),
migrations.CreateModel(
name='Space',
fields=[
('id', models.AutoField(verbose_name='ID', serialize=False, auto_created=True, primary_key=True)),
('space_name', models.CharField(max_length=200)),
('pub_date', models.DateTimeField(verbose_name=b'date published')),
],
),
migrations.AddField(
model_name='information',
name='space',
field=models.ForeignKey(to='LogTool.Space'),
),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0014_auto_20150509_0102'),
]
operations = [
migrations.RemoveField(
model_name='state',
name='log',
),
migrations.AddField(
model_name='log',
name='state',
field=models.CharField(default=b'Todo', max_length=15, choices=[(b'TODO', b'Todo'), (b'IN_PROGRESS', b'In progress'), (b'DONE', b'Done')]),
),
migrations.AddField(
model_name='log',
name='text',
field=models.TextField(blank=True),
),
migrations.AlterField(
model_name='log',
name='pub_date',
field=models.DateTimeField(default=django.utils.timezone.now),
),
migrations.DeleteModel(
name='State',
),
]
<file_sep>from django.conf.urls import include, url
from django.contrib import admin
urlpatterns = [
# Examples:
# url(r'^$', 'DayLog.views.home', name='home'),
# url(r'^blog/', include('blog.urls')),
url(r'^admin/', include(admin.site.urls)),
url(r'^logtool/', include('LogTool.urls',
namespace='logtool')),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
import datetime
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0010_auto_20150504_2354'),
]
operations = [
migrations.RemoveField(
model_name='logcontent',
name='pub_date',
),
migrations.AddField(
model_name='log',
name='pub_date',
field=models.DateTimeField(default=datetime.datetime(2015, 5, 5, 0, 8, 3, 928000), verbose_name=b'Date published'),
preserve_default=False,
),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0007_auto_20150504_1408'),
]
operations = [
migrations.AlterField(
model_name='log',
name='log_contents',
field=models.TextField(max_length=500),
),
]
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0013_auto_20150508_1350'),
]
operations = [
migrations.CreateModel(
name='State',
fields=[
('id', models.AutoField(verbose_name='ID', serialize=False, auto_created=True, primary_key=True)),
('state', models.CharField(max_length=15, choices=[(b'TODO', b'To do'), (b'IN_PROGRESS', b'In progress'), (b'DONE', b'Done')])),
('log', models.ForeignKey(to='LogTool.Log')),
],
),
migrations.RemoveField(
model_name='logcontent',
name='state',
),
]
<file_sep>from datetime import datetime
from django.shortcuts import render, redirect, get_object_or_404
from django.utils import timezone
from .forms import LogForm
from .models import Log
def index(request):
logs = Log.objects.filter(start_date__lte=timezone.now()).order_by('start_date')
#for log in logs
#time_left = _days_left(end_date)
return render(request, 'LogTool/index.html', {'logs': logs})
def log_new(request):
if request.method == "POST":
form = LogForm(request.POST)
if form.is_valid():
log = form.save(commit=False)
log.save()
return redirect('LogTool.views.index')
else:
form = LogForm()
return render(request, 'LogTool/log_edit.html', {'form': form})
def log_detail(request, pk):
log = get_object_or_404(Log, pk=pk)
return render(request, 'LogTool/log_edit.html', {'log': log})
def _days_left(end_date):
end_date - datetime.now()
<file_sep>from django.conf.urls import url
from . import views
urlpatterns = [
url(r'^$', views.index, name='index'),
#url(r'^log/new_log/$', views.log_new, name='log_new'),
]<file_sep>from django.contrib import admin
from .models import Log, LogContent
class LogInline(admin.TabularInline):
model = LogContent
extra = 1
class LogAdmin(admin.ModelAdmin):
fieldsets = [
('Name it', {'fields': ['log_name']}),
('Time issued', {'fields': ['start_date'],
'classes': ['collapse']}),
]
inlines = [LogInline]
list_display = ['log_name', 'start_date']
list_filter = ['start_date']
search_fields = ['question_text']
admin.site.register(Log, LogAdmin)
admin.site.register(LogContent)
# Register your models here.
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0012_logcontent_state'),
]
operations = [
migrations.AlterField(
model_name='logcontent',
name='state',
field=models.CharField(default=1, max_length=2, choices=[(1, b'To do'), (2, b'In progress'), (3, b'Done')]),
),
]
<file_sep># DayLog
This project - web application is about storing all your daily activities , tasks and everything what matters for you.
Also it will include to do function when, where and what you need to do or accomplish tomorrow or any time in the future ... or past .
DayLog will be fast, simple and very useful tool for everyday use.
I have made a research of what people want and need and have an abstract concept of how this application will look like. I am planning to have first version in few weeks.
This application will be my first pancake built with Django but I hope it wont be the worst ..
Wish me luck :)
Functions:
* Date/time
* Description
* Importance / value
* State (done, predicted, ongoing, to do)
* Duration
Look:
* One page application
* Text field where to log
* Table
* Status bar
Objects:
Log(name , date_published, state)
functionality
add_log, edit_log(change description, change state), remove_log
UI
table of logs sorted by states(each state has its own color)
1. add button
2. Pop up window when pressed on a new or existing log.
On this window all information of log is displayed.
<file_sep>from django.db import models
from django.utils import timezone
#
STATE_CHOICES = (
('TODO', 'Todo'),
('IN_PROGRESS', 'In progress'),
('DONE', 'Done'),
)
class Log(models.Model):
log_name = models.CharField(max_length=30)
start_date = models.DateTimeField(default=timezone.now)
end_date = models.DateTimeField(default=timezone.now)
text = models.TextField(blank=True)
state = models.CharField(
default='Todo',
max_length=15,
choices=STATE_CHOICES,
)
def log_save(self):
self.start_date = timezone.now()
self.save()
def __str__(self):
return self.log_name
class LogContent(models.Model):
log = models.ForeignKey(Log)
log_contents = models.TextField()
value = models.IntegerField(default=0)
def __str__(self):
return self.log<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
import datetime
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0008_auto_20150504_1414'),
]
operations = [
migrations.RemoveField(
model_name='log',
name='log_contents',
),
migrations.RemoveField(
model_name='log',
name='pub_date',
),
migrations.RemoveField(
model_name='logcontent',
name='info_list',
),
migrations.AddField(
model_name='logcontent',
name='log_contents',
field=models.TextField(default=datetime.datetime(2015, 5, 4, 23, 35, 53, 25000)),
preserve_default=False,
),
migrations.AddField(
model_name='logcontent',
name='pub_date',
field=models.DateTimeField(default=datetime.datetime(2015, 5, 4, 23, 35, 59, 586000), auto_now_add=True),
preserve_default=False,
),
]
<file_sep>from django import forms
from .models import Log
class LogForm(forms.ModelForm):
class Meta:
model = Log
fields = ('log_name', 'text', 'state')
<file_sep># -*- coding: utf-8 -*-
from __future__ import unicode_literals
from django.db import models, migrations
class Migration(migrations.Migration):
dependencies = [
('LogTool', '0009_auto_20150504_2335'),
]
operations = [
migrations.AlterField(
model_name='logcontent',
name='pub_date',
field=models.DateTimeField(verbose_name=b'Date published'),
),
]
| 5127125147061a76fc7df8acf7e694abab945c07 | [
"Markdown",
"Python"
] | 17 | Python | Dambre/DayLog | 06c4de97b163dc7ee9c1a8bc0bb5694436d88d8c | 3362c1a724f17d2bac86d26e4a0c8c8ef019615b | |
refs/heads/master | <file_sep>var random = require("../tools/random")
module.exports = (values, apiKey, subdomains) => {
var temp, host, protocol, port
[temp, protocol, host, temp, port] = /^(https?:\/\/)([^:\/]+)(:(\d+))?/.exec(values.urls.primary)
// cast port to number
var portNum = port ? Number.parseInt(port) : null
var domainFromHost = (host) => {
var temp, domain
[temp, domain] = /^([^\.]+)/.exec(host)
return domain
}
var genHost = (prefix) => prefix ? `${prefix}.${host}` : host
var genUrl = (prefix,
port = portNum,
isWebStandardPort = true,
webStandardPort = values.tls.enable
? values.ports.web.https
: values.ports.web.http) => {
const tmp = `${protocol}${genHost(prefix)}`
// return early with not defined port
if (! port) return tmp
// cut protocols default patturn
switch (protocol) {
case "http:":
if (port === 80) return tmp
break
case "https:":
if (port === 443) return tmp
}
// prior port than webStandardPort, when webStandardPort enabled or port isn't equal to webStandardPort.
if (! webStandardPort || port !== webStandardPort) return `${tmp}:${port}`
// join webStandardPort...
return `${tmp}:${webStandardPort}`
}
/** base */
var response = {
mongo: {
uri: "mongodb://" + values.mongo.host + "/misskey-web"
},
redis: {
host: values.redis.host
},
port: {
http: values.ports.web.http
},
https: {
enable: values.tls.enable
},
apiPasskey: apiKey,
apiServerIp: "127.0.0.1",
apiServerPort: values.ports.api,
cookiePass: random(),
sessionKey: "hmsk",
sessionSecret: random(),
googleRecaptchaSecret: values.recaptcha.secret,
publicConfig: {
themeColor: values.themeColor,
domain: domainFromHost(host),
host: host,
url: genUrl(null),
apiHost: genHost("api"),
apiUrl: genUrl("api", values.ports.api, false),
googleRecaptchaSiteKey: values.recaptcha.site,
}
}
/** add subdomains to response */
Object.keys(subdomains["web"]).forEach(name => {
response.publicConfig[name + "Domain"] = subdomains["web"][name]
response.publicConfig[name + "Url"] = genUrl(subdomains["web"][name])
})
Object.keys(subdomains["web-host"]).forEach(name => {
response.publicConfig[name + "Host"] = genHost(subdomains["web-host"][name]),
response.publicConfig[name + "Url"] = genUrl(subdomains["web-host"][name])
})
/** mongo section */
if (values.mongo.auth) {
response.mongo.options = {
user: values.mongo.authUser,
password: <PASSWORD>
}
}
/** redis section */
if (values.redis.auth) {
response.redis.password = <PASSWORD>.<PASSWORD>
}
/** https section */
if (values.tls.enable) {
response.https.keyPath = values.tls.key
response.https.certPath = values.tls.cert
response.port.https = values.ports.web.https
}
return response
}
<file_sep>var crypto = require('crypto')
/**
* create radom string.
*
* http://qiita.com/sifue/items/06032a373a1168e5b6e0
*/
module.exports = () => crypto.randomBytes(15).toString('hex')<file_sep>misskey-gen
=====
generate configurations for below projects under misskey-delta.
- [misskey-api](https://github.com/misskey-delta/misskey-api)
- [misskey-web](https://github.com/misskey-delta/misskey-web)
- [misskey-file](https://github.com/misskey-delta/misskey-file)
Usage
-----
1. clone this repository.
2. run `npm start`. after you answered 10-20 questions, misskey-gen generates configurations to `store` directory.
3. move json files in `store` to your `$HOME/.misskey`.
4. add `store/hosts-snippet` to your hosts file. (_optional_)
### input
you can input settings by interacting, or environment variables. want list of environment variables, see below.
| name | required | type | sample |
| :-- | :-- | :-- | :-- |
| MISSKEY_GEN_URLS_PRIMARY | yes | url string | http://example.com:8081/ |
| MISSKEY_GEN_URLS_SECONDARY | yes | url string | http://example.com:8084/ |
| MISSKEY_GEN_TLS_ENABLE | yes | bool (0 or 1) | 0 |
| MISSKEY_GEN_TLS_KEY | if TLS enabled, yes | path string | /etc/letsencrypt/live/example.com/privkey.pem |
| MISSKEY_GEN_TLS_CERT | if TLS enabled, yes | path string | /etc/letsencrypt/live/example.com/fullchain.pem |
| MISSKEY_GEN_MONGO_HOST | yes | host string | localhost:27017 |
| MISSKEY_GEN_MONGO_AUTH | yes | bool (0 or 1) | 0 |
| MISSKEY_GEN_MONGO_USER | if MONGO auth enabled, yes | string | sample |
| MISSKEY_GEN_MONGO_PASSWORD | if MONGO auth enabled, yes | string | sample |
| MISSKEY_GEN_REDIS_HOST | yes | host string | localhost:6379 |
| MISSKEY_GEN_REDIS_AUTH | yes | bool (0 or 1) | 0 |
| MISSKEY_GEN_REDIS_PASSWORD | yes | string | sample |
| MISSKEY_GEN_PORTS_API | yes | number | 8080 |
| MISSKEY_GEN_PORTS_WEB_HTTP | yes | number | 8081 |
| MISSKEY_GEN_PORTS_WEB_HTTPS | if TLS enabled, yes | number | 8082 |
| MISSKEY_GEN_PORTS_FILE_INTERNAL | yes | number | 8083 |
| MISSKEY_GEN_PORTS_FILE_HTTP | yes | number | 8084 |
| MISSKEY_GEN_PORTS_FILE_HTTPS | if TLS enabled, yes | number | 8085 |
| MISSKEY_GEN_RECAPTCHA_SITE | yes | reCAPTHCHA Site key | <KEY> |
| MISSKEY_GEN_RECAPTCHA_SECRET | yes | reCAPTCHA Secret key | <KEY> |
| MISSKEY_GEN_THEME_COLOR | yes | color string | #666666 |
| MISSKEY_GEN_FILE_STORAGE_PATH | yes | path string | /home/misskey/storage |
Tips
-----
### domains
web uses subdomains under primary domain:
| subdomain name | description | implemented | need to open |
| :-- | :-- | :-- | :-- |
| auth | auth use applications | no | no |
| signup | user signup to misskey | yes | yes |
| signin | user signin point | yes | yes |
| signout | user signout point | yes | yes |
| resources | delivery css & js | yes | yes |
| about | about page | yes | yes |
| search | search posts | yes | yes |
| talk | direct messages | yes | yes |
| himasaku | api proxy | yes | yes |
| share | providing embedding share script | yes | no |
api uses subdomain under primary domain:
| subdomain name | description | used by program | need to open |
| :-- | :-- |:-- | :-- |
| api | internal api domain | yes | no, **must not**. |
file uses secondary domain.
License
-----
public license.
<file_sep>module.exports = (values, apiKey, fileKey) => {
var host, protocol, temp
[temp, protocol, host] = /^(https?:\/\/)(.*)$/.exec(values.urls.secondary)
// split magic (remove end slash)
host = host.split("/")[0]
var response = {
mongo: {
uri: "mongodb://" + values.mongo.host + "/misskey-api"
},
redis: {
host: values.redis.host,
port: 6379
},
fileServer: {
passkey: fileKey,
url: protocol + host,
ip: "127.0.0.1",
port: values.ports.file.internal
},
apiPasskey: apiKey,
port: {
http: values.ports.api
},
https: {
enable: false
}
}
/** mongo section */
if (values.mongo.auth) {
response.mongo.options = {
user: values.mongo.authUser,
password: <PASSWORD>
}
}
/** redis section */
if (values.redis.auth) {
response.redis.password = values.redis.authPassword
}
return response
}<file_sep>var question = require("./tools/question")
module.exports = async () => {
var isValidURL = (value) => {
const regExp = /^(http)|(https):.+\/?$/
return regExp.test(value)
}
var values = {
urls: {},
tls: {},
mongo: {},
redis: {},
ports: {
web: {},
file: {}
},
recaptcha: {}
}
/** url section */
values.urls.primary =
process.env.MISSKEY_GEN_URLS_PRIMARY ||
await question.input("what is primary URL for api & web servers? (ex. https://misskey.cf/)", isValidURL)
values.urls.secondary =
process.env.MISSKEY_GEN_URLS_SECONDARY ||
await question.input("what is secondary url for file server? (ex. https://uc-misskey.cf/)", isValidURL)
/** tls section */
values.tls.enable =
process.env.MISSKEY_GEN_TLS_ENABLE
? Boolean(parseInt(process.env.MISSKEY_GEN_TLS_ENABLE))
: await question.confirm("do you want to use Node.js providing TLS?")
if (values.tls.enable) {
values.tls.key =
process.env.MISSKEY_GEN_TLS_KEY ||
await question.input("where is TLS key file?")
values.tls.cert =
process.env.MISSKEY_GEN_TLS_CERT ||
await question.input("where is TLS cert file?")
}
/** mongoDB section */
values.mongo.host =
process.env.MISSKEY_GEN_MONGO_HOST ||
await question.input("what is MongoDB server hostname? (ex. localhost)")
values.mongo.auth =
process.env.MISSKEY_GEN_MONGO_AUTH
? Boolean(parseInt(process.env.MISSKEY_GEN_MONGO_AUTH))
: await question.confirm("do you want to use MongoDB authentication?")
if (values.mongo.auth) {
values.mongo.authUser =
process.env.MISSKEY_GEN_MONGO_USER ||
await question.confirm("what is MongoDB's username?")
values.mongo.authPassword =
process.env.MISSKEY_GEN_MONGO_PASSWORD ||
await question.input("what is MongoDB's password?")
}
/** redis section */
values.redis.host =
process.env.MISSKEY_GEN_REDIS_HOST ||
await question.input("what is Redis server hostname? (ex. localhost)")
values.redis.auth =
process.env.MISSKEY_GEN_REDIS_AUTH
? Boolean(parseInt(process.env.MISSKEY_GEN_REDIS_AUTH))
: await question.confirm("do you want to use Redis authentication?")
if (values.redis.auth) {
values.redis.authPassword =
process.env.MISSKEY_GEN_REDIS_PASSWORD ||
await question.input("what is Redis's password?")
}
/** port section */
values.ports.api =
process.env.MISSKEY_GEN_PORTS_API
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_API)
: await question.inputNumber("what is http port to run misskey-api?")
values.ports.web.http =
process.env.MISSKEY_GEN_PORTS_WEB_HTTP
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_WEB_HTTP)
: await question.inputNumber("what is http port to run misskey-web?")
if (values.tls.enable) {
values.ports.web.https =
process.env.MISSKEY_GEN_PORTS_WEB_HTTPS
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_WEB_HTTPS)
: await question.inputNumber("what is https port to run misskey-web?")
}
values.ports.file.internal =
process.env.MISSKEY_GEN_PORTS_FILE_INTERNAL
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_FILE_INTERNAL)
: await question.inputNumber("what is internal http port to run misskey-file?")
values.ports.file.http =
process.env.MISSKEY_GEN_PORTS_FILE_HTTP
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_FILE_HTTP)
: await question.inputNumber("what is http port to run misskey-file?")
if (values.tls.enable) {
values.ports.file.https =
process.env.MISSKEY_GEN_PORTS_FILE_HTTPS
? Number.parseInt(process.env.MISSKEY_GEN_PORTS_FILE_HTTPS)
: await question.inputNumber("what is https port to run misskey-file?")
}
/** recaptcha section */
values.recaptcha.site =
process.env.MISSKEY_GEN_RECAPTCHA_SITE ||
await question.input("please input recaptcha site key.")
values.recaptcha.secret =
process.env.MISSKEY_GEN_RECAPTCHA_SECRET ||
await question.input("please input recaptcha secret key.")
/** theme color */
values.themeColor =
process.env.MISSKEY_GEN_THEME_COLOR ||
await question.input("what color do you want use in misskey? (ex. #666666)")
/** file storage */
values.fileStorage =
process.env.MISSKEY_GEN_FILE_STORAGE_PATH ||
await question.input("please input path for misskey-file storage.")
return values
}<file_sep>/* require modules */
var readline = require('readline')
/**
* question prover: input
*
* @param query {string} question sentence
* @param callback {function} function to test inputted value, taking an argument of inputted value. (option)
* @param reInputNotifier {string} re-input error sentence (option)
* @returns {string} inputted value that passed callback.
*/
var question = async (query, callback, reInputNotifier = "not a valid input value") => {
/* interface.question promise wrapper */
var q = (query, noReturn = false, retryTimes) => new Promise((resolve, reject) => {
if (retryTimes) {
console.log(`${query} (retry ${retryTimes} times)`)
} else {
console.log(query)
}
process.stdout.write(" > ")
if (process.stdin.isTTY) process.stdin.setRawMode(true)
readline.emitKeypressEvents(process.stdin)
// handler for process.stdin event named 'keypress'
var union = ""
var stdinHandler = (ch, key) => {
var handler = (input) => {
process.stdin.removeListener("keypress", stdinHandler)
if (process.stdin.isTTY) process.stdin.setRawMode(false)
// clear previous line
readline.cursorTo(process.stdout, 0)
// noReturn time, remove input in this line
if (noReturn) readline.clearLine(process.stdout, 1)
readline.moveCursor(process.stdout, 0, -1)
readline.clearLine(process.stdout, 1)
resolve(input)
}
if (key.ctrl === true & key.name === "c") {
process.exit()
}
// magic with noReturn
else if (key.name === "return") {
if (! noReturn) process.stdout.write("\n")
handler(union)
}
// OH LEGACY!!!
else if (key.name === key.sequence.toLowerCase() || key.name === undefined || key.name === "space") {
union += key.sequence
process.stdout.write(key.sequence)
} else if (key.name === "backspace" && union.length > 0) {
readline.moveCursor(process.stdout, -1)
readline.clearLine(process.stdout, 1)
union = union.slice(0, union.length - 1)
}
}
process.stdin.on("keypress", stdinHandler)
})
var result = await q(`[?] ${query}`)
/* if callback exists, check with it! */
if (callback && typeof callback === "function") {
var fuck = 0
while (! callback(result) === true) {
result = await q(`[!] ${reInputNotifier}, please re-input...`, true, fuck)
fuck++
}
}
return result
}
exports.input = question
/**
* question prover: confirm
*
* @param query {string} question sentence
* @returns {boolean}
*/
exports.confirm = async (query) => {
var result = await question(`${query} (y/n)`, (answer) => /^y|n$/.test(answer), "not y/n")
return "y" === result
}
/**
* question provider: inputNumber
*
* @param query {string} question sentence
* @returns {number}
*/
exports.inputNumber = async (query) => {
var result = await question(`${query} (number)`, (answer) => /^\d+$/.test(answer), "not a valid input number")
return Number.parseInt(result)
}
| c92ff893934049de1487e8cda54cd916c7fdb7af | [
"JavaScript",
"Markdown"
] | 6 | JavaScript | tosuke/misskey-gen | a75fd3af4b3ef5e07e1d587b94253e22136c57ee | 55a582523022c7f85a5344c70a9c0d2954dfc777 | |
refs/heads/master | <file_sep># supergoose
Example App using SuperTest and Mockgoose for API & Mongoose model testing
[](https://travis-ci.com/JB-Tellez/supergoose)
<file_sep>import mongoose, {Schema} from 'mongoose';
const schema = new Schema({
name : String,
instrument: { type: String, default: 'singer'},
band: { type: Schema.Types.ObjectId, ref : 'Band'},
});
export default mongoose.model('Musician', schema);<file_sep>import mongoose, {Schema} from 'mongoose';
const schema = new Schema({
name : String,
});
export default mongoose.model('Band', schema);<file_sep>import mongoose from 'mongoose';
import {
Mockgoose,
} from 'mockgoose-fix';
// Give a longer timeout for when Travis runs slow
jest.setTimeout(60000);
const mockgoose = new Mockgoose(mongoose);
export default {
prepare: () => {
return mockgoose.prepareStorage().then(() => {
return mongoose.connect('mongodb://localhost/supergoose').catch(err => {
console.log('already connected, no sweat', err);
});
}).catch(console.error);
},
};
afterEach((done) => {
// console.log('after each');
mockgoose.helper.reset().then(done);
});<file_sep>import { Router } from 'express';
import Musician from './model';
import auth from '../auth/middleware';
const router = new Router();
router.get('/', (req, res, next) => {
Musician
.find()
.then(bands => res.send(bands))
.catch(err => next(err));
});
router.post('/', (req, res, next) => {
Musician
.create(req.body)
.then(band => res.send(band))
.catch(next);
});
router.put('/:id', (req, res, next) => {
Musician.findByIdAndUpdate(req.params.id, req.body)
.then(band => res.send(band))
.catch(next);
});
router.delete('/:id', (req, res, next) => {
Musician.findByIdAndRemove(req.params.id)
.then(results => res.send(results))
.catch(next);
});
router.get('/:id', (req, res, next) => {
Musician
.findById(req.params.id)
.then(band => res.send(band))
.catch(next);
});
router.get('/protected', auth, (req, res) => {
res.send('the keys to the Musician kingdom');
});
export default router;
<file_sep>import supertest from 'supertest';
import superagent from 'superagent';
const {
app,
} = require('../../src/app.js');
const mockRequest = supertest(app);
const API_STUB = '/api/v1';
describe('app module', () => {
it('supertest should exist', () => {
expect(supertest);
});
it('app should exist', () => {
expect(app);
});
it('should sign up and return token', async () => {
const token = await mockRequest
.post('/signup')
.send({
username: 'foo',
password: 'bar',
})
.then(response => response.text)
.catch(fail);
expect(token).toBeDefined();
});
it('should sign in', async () => {
await mockRequest
.post('/signup')
.send({
username: 'foo',
password: 'bar',
});
await mockRequest
.get('/signin')
.auth('foo', 'bar')
.then(response => {
expect(response.text).toBe('Hi');
});
});
it('should fail sign in with bad password', async () => {
await mockRequest
.post('/signup')
.send({
username: 'foo',
password: 'bar',
});
await mockRequest
.get('/signin')
.auth('foo', 'badpassword')
.then(response => {
expect(response.status).toBe(401);
});
});
it('should fail sign in with unknown name', async () => {
await mockRequest
.post('/signup')
.send({
username: 'foo',
password: 'bar',
});
const response = await mockRequest
.get('/signin')
.auth('nobody', 'bar');
expect(response.status).toBe(401);
});
it('should give access to protected route with token', async () => {
const token = await mockRequest
.post('/signup')
.send({
username: 'foo',
password: 'bar',
})
.then(response => response.text);
const response = await mockRequest
.get('/api/v1/bands/protected')
.set('Authorization', 'Bearer ' + token)
.expect(200)
.catch(fail);
expect(response.text).toBe('the keys to the band kingdom');
});
it('should protect route when no token', async () => {
const response = await mockRequest.get('/api/v1/bands/protected');
expect(response.status).toBe(401);
});
it('should protect route when bad token', async () => {
const response = await mockRequest
.get('/api/v1/bands/protected')
.set('Authorization', 'Bearer ' + 'badtoken');
expect(response.status).toBe(401);
});
it('should get [] for initial request to GET bands', async () => {
const response = await mockRequest.get(API_STUB + '/bands');
expect(JSON.parse(response.text)).toEqual([]);
});
it('should get populated list after creating', async () => {
let response = await mockRequest.post(API_STUB + '/bands')
.send({
name: 'The Who',
});
expect(response.body.name).toBe('The Who');
response = await mockRequest.get(API_STUB + '/bands');
expect(response.body.length).toBe(1);
});
it('should get error 404 if unfound id', async () => {
const response = await mockRequest.get(API_STUB + '/bands/unknown_id');
expect(response.status).toBe(404);
});
it('should update resource', async () => {
let response = await mockRequest.post(API_STUB + '/bands')
.send({
name: 'The Who',
});
const band = response.body;
response = await mockRequest
.put(API_STUB + '/bands/' + band._id)
.send({name : 'The Whom'})
.catch(fail);
expect(response.status).toBe(200);
expect(response.body.name).toBe('The Whom');
});
});<file_sep>import mongoose, {Schema} from 'mongoose';
const schema = new Schema({
name : String,
band: { type: Schema.Types.ObjectId, ref : 'Band'},
});
export default mongoose.model('Roadie', schema);<file_sep>import Roadie from '../../../src/roadies/model';
describe('Roadie model', () => {
it('should be defined', () => {
expect(Roadie).toBeDefined();
});
});<file_sep>import Musician from '../../../src/musicians/model';
import Band from '../../../src/bands/model';
import Roadie from '../../../src/roadies/model';
describe('band + roadie', () => {
it('should have a Roadie as Id', async () => {
const band = await Band.create({name:'<NAME>'});
const roadie = await Roadie.create({name: '<NAME>', band: band._id});
expect(roadie.band._id).toBe(band._id);
});
it('should have Roadie as populated object', async () => {
const band = await Band.create({name:'<NAME>'});
const roadie = await Roadie.create({name: '<NAME>', band: band._id});
const foundRoadie = await Roadie.findById(roadie._id).populate('band').exec();
expect(foundRoadie.band.name).toBe(band.name);
});
});
describe('band + musician', () => {
it('should do musician + band - async style', async () => {
const fooFighters = await Band.create({name:'<NAME>'});
const dave = await Musician.create({name: '<NAME>', band: fooFighters._id});
const popDave = await Musician.findById(dave._id).populate('band').exec();
expect(popDave.band.name).toBe('Foo Fighters');
});
it('should find the musicians in a band', async () => {
const beeGees = await Band.create({name: '<NAME>'});
await Musician.create({name : 'Barry', band: beeGees._id});
await Musician.create({name : 'Robin', band: beeGees._id});
await Musician.create({name : 'Maurice', band: beeGees._id});
const stooges = await Band.create({name: 'The Stooges'});
await Musician.create({name: '<NAME>', band: stooges._id});
const musicians = await Musician.find({band:beeGees._id}).populate('band').exec();
expect(musicians.length).toEqual(3);
expect(musicians[0].band.name).toBe('Bee Gees');
});
});
describe('Band model', () => {
/* NOTE: you probably won't do tests like these
* since they're really testing Mongoose internals
* where we want to test our code.
* But including them for instructional purposes */
it('should be defined', () => {
expect(Band).toBeDefined();
});
it('should create', async () => {
const band = await Band.create({name: 'Spinal Tap'});
expect(band.name).toBe('Spinal Tap');
});
it('should get one', async () => {
const rawBand = {
name: 'Spinal Tap',
};
const band = await Band.create(rawBand);
const foundBand = await Band.findById(band._id);
expect(foundBand.name).toEqual(band.name);
});
it('should track creations when getting all - async/await style', async () => {
let bands = await Band.find();
expect(bands.length).toBe(0);
await Band.create({
name: 'Fugazi',
});
bands = await Band.find();
expect(bands.length).toBe(1);
});
it('should track creations when getting all - promise style', () => {
return Band
.find()
.then(bands => expect(bands.length).toBe(0))
.then(() => Band.create({ name: 'Fugazi' }))
.then(() => Band.find())
.then(bands => expect(bands.length).toBe(1));
});
});
<file_sep>'use strict';
import mongoose from 'mongoose';
import { JsonWebTokenError } from 'jsonwebtoken';
// Custom Error Handler because we always want to return a JSON response
export default (err,req,res,next) => {
if(err instanceof mongoose.Error) {
res.statusCode = 404;
res.send('Database Error');
} else if (err instanceof JsonWebTokenError) {
res.statusCode = 401;
res.send(err);
} else {
res.statusCode = err.status || 500;
res.statusMessage = err.statusMessage || 'Server Error';
res.message = err.message;
res.send(err);
}
};<file_sep>
import helper from './test-helper';
helper.prepare();<file_sep>import express from 'express';
import bandsRouter from './bands/router';
import musiciansRouter from './musicians/router';
import roadiesRouter from './roadies/router';
import errorHandler from './middleware/error';
import notFound from './middleware/404';
import authRouter from './auth/router';
const app = express();
app.use(express.json());
app.use(authRouter);
app.use('/api/v1/bands', bandsRouter);
app.use('/api/v1/musicians', musiciansRouter);
app.use('/api/v1/roadies', roadiesRouter);
app.use(notFound);
app.use(errorHandler);
module.exports = {
start: port => {
app.listen(port, () => console.log('Listening on port', port));
},
stop: () => app.close(),
app,
}; | 01a1f62b4c49ed92728edecd19c87a1c57eefa3b | [
"Markdown",
"JavaScript"
] | 12 | Markdown | JB-Tellez/supergoose | 72a7a64543193f89a8fa7cd0293839f6417bf954 | 1fd744cd4834895d2c5fb752b263084ac20ebed7 | |
refs/heads/main | <file_sep>import { ModelInit, MutableModel, PersistentModelConstructor } from "@aws-amplify/datastore";
type PostMetaData = {
readOnlyFields: 'createdAt' | 'updatedAt';
}
type VoteMetaData = {
readOnlyFields: 'createdAt' | 'updatedAt';
}
type CommentMetaData = {
readOnlyFields: 'createdAt' | 'updatedAt';
}
export declare class Post {
readonly id: string;
readonly title: string;
readonly contents: string;
readonly image?: string;
readonly votes: Vote[];
readonly comments?: (Comment | null)[];
readonly createdAt?: string;
readonly updatedAt?: string;
constructor(init: ModelInit<Post, PostMetaData>);
static copyOf(source: Post, mutator: (draft: MutableModel<Post, PostMetaData>) => MutableModel<Post, PostMetaData> | void): Post;
}
export declare class Vote {
readonly id: string;
readonly vote: string;
readonly post?: Post;
readonly createdAt?: string;
readonly updatedAt?: string;
constructor(init: ModelInit<Vote, VoteMetaData>);
static copyOf(source: Vote, mutator: (draft: MutableModel<Vote, VoteMetaData>) => MutableModel<Vote, VoteMetaData> | void): Vote;
}
export declare class Comment {
readonly id: string;
readonly post?: Post;
readonly content: string;
readonly createdAt?: string;
readonly updatedAt?: string;
constructor(init: ModelInit<Comment, CommentMetaData>);
static copyOf(source: Comment, mutator: (draft: MutableModel<Comment, CommentMetaData>) => MutableModel<Comment, CommentMetaData> | void): Comment;
} | 9ca45d057f0435a8402e5661b9e1e66a0cd6394e | [
"TypeScript"
] | 1 | TypeScript | alexwendte/reddit-clone | 85b7e7dbdd8871caedd9240d4fa8f3dfd8b506e4 | cf5a435736df2a3611c2965977f4511b5a206a2d | |
refs/heads/master | <file_sep>var baseURL = '/api'; // 根路径
var latestNews =baseURL+'/api/4/news/latest' // 最新消息
var beforeNews = baseURL+'/api/4/news/before/' // 往日消息
var detail = baseURL+'/api/4/news/' // 新闻详情
var other = baseURL+'/api/4/story-extra/' // 新闻额外信息
var pinglun = baseURL+'/api/4/story/' // 评论
export default{
latestNews,
beforeNews,
detail,
other,
pinglun
}<file_sep>import filterDate from './filterDate' // 过滤首页标题日期
import filterTime from './filterTime' // 过滤评论日期
export default {
filterDate,
filterTime
} | f8f4f8afba3369b264bd1152a6297b5539a11ba4 | [
"JavaScript"
] | 2 | JavaScript | 18501375350/zhihu | a97be10355f23fc4416c8f34dd213e49af9733c5 | c0f8b925f3c31823f7424fc96cae7949cd2686c5 | |
refs/heads/master | <file_sep>platform :ios, '8.0'
target ‘CustomNavAndTabBar’ do
pod 'Masonry', '~> 1.1.0'
pod 'JSONModel', '~> 1.2.0'
pod 'SDWebImage', '~> 4.2.3'
end
#去掉警告方法
#pod 'AFNetworking', '~> 2.0', :inhibit_warnings => true
| 3b1ecb27a97c9ba5ce0614573eeddd47b1e865a6 | [
"Ruby"
] | 1 | Ruby | yataoli/iOSProject | 4ba614bea22b7a59c1732588505c22f347f90cfd | 5096d4bdbb481dca60930ca4dc0cba7fb2153989 | |
refs/heads/master | <file_sep>import React from "react";
import {connect} from "react-redux";
const MoviesPage = () => {
return <div>
</div>
};
let mapStateToProps = (state) => ({
films: state.filmsPage.films,
currentPage:state.filmsPage.currentPage
});
export default connect()(MoviesPage)<file_sep>import * as axios from 'axios'
export const API = {
getFilm(page, genreId) {
return axios.get(`https://api.themoviedb.org/3/discover/movie?page=${page}&api_key=<KEY>&with_genres=${genreId}`)
},
getGenres() {
return axios.get(`http://api.themoviedb.org/3/genre/movie/list?api_key=<KEY>`).then(genres => {
return genres.data.genres
})
},
getByTitle(title) {
return axios.get(`https://api.themoviedb.org/3/search/movie?api_key=<KEY>&query=${title}`)
},
getByAll(page, genreId, title) {
return axios.get(`https://api.themoviedb.org/3/search/movie?api_key=<KEY>&query=${title}&with_genres=${genreId}&page=${page}`)
}
};
export const MovieAPI = {
getMovie(movieId) {
return axios.get(`https://api.themoviedb.org/3/movie/${movieId}?api_key=<KEY>&language=en-US`)
}
};
<file_sep>import {applyMiddleware, combineReducers, createStore} from "redux";
import filmsReducer from "./filmsReducer";
import MovieReducer from "./MoviePageReducer";
import thunkMiddleware from "redux-thunk"
import {reducer as formReducer} from "redux-form"
let reducers = combineReducers({
filmsPage: filmsReducer,
moviePage: MovieReducer,
form:formReducer
});
let store = createStore(reducers, applyMiddleware(thunkMiddleware));
export default store<file_sep>import React from "react";
import StarRatings from "react-star-ratings";
import {NavLink} from "react-router-dom";
import style from './MoviesListCard.module.css'
const MoviesListCard = (props) => {
return <div className={style.movieCard}>
<NavLink to={`/film/${props.film.id}`} className={style.movieBlock}>
<div onClick={()=>props.setFilm(props.film)}>
<img src={`https://image.tmdb.org/t/p/w500${props.film.backdrop_path}`} alt="filmPhoto"/>
<h2>{props.film.title}</h2>
</div>
</NavLink>
<div>rating {props.film.vote_average}/10</div>
<StarRatings
rating={props.film.vote_average}
starDimension="20px"
starRatedColor="gold"
numberOfStars={10}
name='rating'
/>
<br/>
<div>{props.film.overview}</div>
</div>
};
export default MoviesListCard<file_sep>import React from 'react';
import './App.css';
import {Provider} from "react-redux";
import store from "./Redux/store";
import MoviesList from "./components/MoviesList";
import {BrowserRouter, Route} from "react-router-dom";
import Movie from "./components/Movie/Movie";
import Header from "./components/Header/Header";
function App() {
return (
<BrowserRouter>
<Provider store={store}>
<Header/>
<Route exact path={'/'} render={() => <MoviesList/>}/>
<Route path={'/film/:id'} render={() => <Movie/>}/>
</Provider>
</BrowserRouter>
);
}
export default App;
<file_sep>import React from "react";
import {compose} from "redux";
import {NavLink, withRouter} from "react-router-dom";
import {connect} from "react-redux";
import {getMoviePage} from "../../Redux/MoviePageReducer";
import StarRatings from "react-star-ratings";
import style from './Movie.module.css'
import cn from 'classnames'
import {changeTheme} from "../../Redux/filmsReducer";
class Movie extends React.Component {
componentDidMount() {
this.props.getMoviePage(this.props.match.params.id)
}
render() {
return <div className={cn({[style.darkTheme]: this.props.darkTheme}, style.moviePage)}>
<div className={style.movieImg}><img src={`https://image.tmdb.org/t/p/w500${this.props.movie.poster_path}`}
alt="poster"/></div>
<div className={style.movieDescription}>
<h2>{this.props.movie.title}</h2>
<StarRatings
rating={this.props.movie.vote_average}
starDimension="20px"
starRatedColor="gold"
numberOfStars={10}
name='rating'
/>
<div className={style.movieOverview}>{this.props.movie.overview}</div>
<div className={style.releaseDate}><span>Release date: </span>{this.props.movie.release_date}</div>
<div className={style.anotherInfo}>
<div><b>Companies:</b> {this.props.movie.production_companies.map(company => <div
key={company.id}>{company.name}, </div>)}</div>
<div><b>Countries:</b> {this.props.movie.production_countries.map(country => <div key={country.id}>
{country.name}, </div>)}</div>
<div><b>Genres: </b>{this.props.movie.genres.map(genre => <div
key={genre.id}>{genre.name},</div>)}</div>
<div><b>Run time: </b>{this.props.movie.runtime} min</div>
<div className={cn({[style.linkDark]: this.props.darkTheme}, style.links)}><a
href={this.props.movie.homepage}>Movie Home page</a></div>
<NavLink to={'/'} className={cn({[style.linkDark]: this.props.darkTheme}, style.links)}>
Go to Home Page
</NavLink>
</div>
</div>
</div>
}
}
let mapStateToProps = (state) => ({
movie: state.moviePage.movie,
darkTheme: state.filmsPage.darkTheme
});
export default compose(
connect(mapStateToProps, {getMoviePage, changeTheme}),
withRouter
)(Movie)<file_sep>import React from "react";
import style from "./Switcher.module.css";
const Switcher = (props) => {
return <div className={style.switch} onClick={() => props.changeTheme(props.darkTheme)}>
<input type="checkbox" checked={props.darkTheme}/>
<span className={style.slider}></span>
</div>
};
export default Switcher<file_sep>import React from "react";
import style from './GenreBadge.module.css'
import cn from 'classnames'
const GenreBadge = (props) => {
return <div className={style.genreList}>
{props.genres.map(genre => <div key={genre.id}
className={cn({[style.darkTheme]:props.darkTheme},{[style.activeGenre]: props.activeGenre === genre.id}, style.genreItem)}
onClick={() => props.getFilmsForGenre(genre.id)}>
{genre.name}
</div>)}
</div>
};
export default GenreBadge<file_sep>import React from "react";
import {Field, reduxForm} from "redux-form";
const InputForm = (props) => {
return <form onSubmit={props.handleSubmit}>
<Field placeholder={'Search film'} name={'filmQuery'} component={'input'}/>
<button>Search</button>
</form>
};
const InputReduxForm = reduxForm({form: 'search'})(InputForm);
export default InputReduxForm | 851e86f039a71eaa980cca48fe06a3201fb4c19c | [
"JavaScript"
] | 9 | JavaScript | Igor051/Movie | 09fe7a8e39afa8ed8ffb2803a54ba64c3794110b | 9d74b97476d261511ea8403b20044418c042ec17 | |
refs/heads/master | <file_sep>$PRIVATE_KEY = "62c7a478061f92f227a0425e56748f2"<file_sep>class Pets
include Mongoid::Document
field :name, type: String
public
def self.get_pets(shelter_id, count)
# petfinder = Petfinder::Client.new('f77a4c7581f1896f99d6e4764a7cffe6', "62c7a478061f92f227a0425e56748f2")
# petfinder.shelter('CA123').getPets
# test_url = "http://api.petfinder.com/shelter.getPets?key=f77a4c7581f1896f99d6e4764a7cffe6&id=KY
# 413&count=25&format=json"
response = HTTParty.get(URI.encode("http://api.petfinder.com/shelter.getPets?key=f77a4c7581f1896f99d6e4764a7cffe6&id=#{shelter_id}&count=#{count}&format=json"))
end
end
# puts response.body, response.code, response.message, response.headers.inspect
# # Or wrap things up in your own class
# class StackExchange
# include HTTParty
# base_uri 'api.stackexchange.com'
# def initialize(service, page)
# @options = { query: {site: service, page: page} }
# end
# def questions
# self.class.get("/2.2/questions", @options)
# end
# def users
# self.class.get("/2.2/users", @options)
# end
# end
# stack_exchange = StackExchange.new("stackoverflow", 1)
# puts stack_exchange.questions
# puts stack_exchange.users
# end
# end<file_sep>class PagesController < ApplicationController
def index
end
def create
end
def list
@pets = Pets.get_pets(params["shelter"],params["count"])["petfinder"]["pets"]["pet"]
create_all(@pets)
end
end | 77ab88cb821d3c9cd5e2c27f45c890952fa9def7 | [
"Ruby"
] | 3 | Ruby | dgoodman1224/pet | 5b1b67b2615c0aacafc442a0dbcbc81653f35c3b | a8b4ac81707d3ce83ebc7b8486f0e2f54a154dea | |
refs/heads/master | <repo_name>limingshang/tree<file_sep>/README.md
# tree
依据Golang实现各种树的包
二叉树即实现
平衡二叉树即实现
红黑树即实现
<file_sep>/tree.go
// +----------------------------------------------------------------------
// | 功能: 各种树调用
// +----------------------------------------------------------------------
// | Copyright (c) <EMAIL> All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( https://github.com/limingshang )
// +----------------------------------------------------------------------
// | Author: apple
// +----------------------------------------------------------------------
// | Date: 5:09 下午
// +----------------------------------------------------------------------
package main
import "tree/balance"
func main() {
balanceTree()
}
func balanceTree() {
node:= balance.CreateNode(10)
balance.InsertNode(&node, 50)
balance.InsertNode(&node, 60)
balance.InsertNode(&node, 30)
balance.InsertNode(&node, 70)
balance.InsertNode(&node, 20)
balance.InsertNode(&node, 40)
balance.InsertNode(&node, 120)
node.MiddleRange()
}
<file_sep>/balance/readme.md
### 首先得了解一些简单的基础知识<file_sep>/balance/balance.go
// +----------------------------------------------------------------------
// | 功能: 平衡二叉树
// +----------------------------------------------------------------------
// | Copyright (c) <EMAIL> All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( https://github.com/limingshang )
// +----------------------------------------------------------------------
// | Author: apple
// +----------------------------------------------------------------------
// | Date: 5:50 下午
// +----------------------------------------------------------------------
package balance
import (
"math"
"tree/BaseNode"
)
//type Node struct {
// Value int // 当前节点值
// Left *Node // 当前节点的左节点
// Right *Node // 当前节点的右节点
// height float64 // 当前节点的高度
//}
// 初始化创建根节点
func CreateNode(Value int) BaseNode.Node {
return BaseNode.Node{
Value: Value,
Left: &BaseNode.Node{},
Right: &BaseNode.Node{},
Height: 0,
}
}
// 右右型数据左旋转操作此操作做了一次左旋转之后,返回当前数据值,重新赋值给父节点
func rrTurnLeft(node BaseNode.Node) BaseNode.Node {
x := node.Right
node.Right = x.Left
x.Left = &node
return *x
}
// 左左型数据左旋转操作此操作做了一次右旋转之后,返回当前数据值,重新赋值给父节点
func llTrunRight(node BaseNode.Node) BaseNode.Node {
x := node.Left
node.Left = x.Right
x.Right = &node
x.Height = getHeight(*x)
return *x
}
// 获取当前节点的高度
func getHeight(node BaseNode.Node) float64 {
if node == (BaseNode.Node{}) {
return 0
}
leftHeight := getHeight(*node.Left)
rightHeight := getHeight(*node.Right)
if leftHeight > rightHeight {
return leftHeight + 1
} else {
return rightHeight + 1
}
}
// 平衡二叉树的插入操作
func InsertNode(node *BaseNode.Node, Value int) {
if *node == (BaseNode.Node{}) {
// 检测如果当前节点是空创建一个节点值返回
*node = CreateNode(Value)
} else if Value > node.Value {
InsertNode(node.Right, Value)
height := getHeight(*node.Right) - getHeight(*node.Left)
if math.Abs(height) >= 2 {
// 当前插入数据大于右侧节点的值为右右插入RR型,需要进行一次左旋转
if Value > node.Right.Value {
*node = rrTurnLeft(*node)
// // 右侧插入 RL 型先进行一次右旋转,在进行一次左旋转
} else if Value < node.Right.Value {
*node.Right = llTrunRight(*node.Right)
*node = rrTurnLeft(*node)
}
}
} else if Value < node.Value {
InsertNode(node.Left, Value)
height := getHeight(*node.Right) - getHeight(*node.Left)
if math.Abs(height) >= 2 {
// 当前插入数据大于右侧节点的值为右右插入RR型,需要进行一次左旋转
if Value < node.Left.Value {
*node = llTrunRight(*node)
// 当前插入数据小于右侧节点的值为右右插入RL型,需要现进行一次右旋转然后左旋转一次
} else if Value > node.Left.Value {
*node.Left = rrTurnLeft(*node)
*node = llTrunRight(*node)
}
}
// 否则是等于当前数据重复插入,不做保存
} else {
}
}
//
//func AfterRange(node BaseNode.Node) {
// if node != node {
// fmt.Println(node.Value)
// AfterRange(*node.Left)
// AfterRange(*node.Right)
// }
//}
//func MiddleRange(node BaseNode.Node) {
// if node != (BaseNode.Node{}) {
// MiddleRange(*node.Left)
// fmt.Println(node.Value)
// MiddleRange(*node.Right)
// }
//}
//func FloorRange(node BaseNode.Node) {
// if node != (BaseNode.Node{}) {
// FloorRange(*node.Left)
// FloorRange(*node.Right)
// fmt.Println(node.Value)
// }
//}
<file_sep>/preface/preface.go
// +----------------------------------------------------------------------
// | 功能: 普通二叉树实现
// +----------------------------------------------------------------------
// | Copyright (c) <EMAIL> All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( https://github.com/limingshang )
// +----------------------------------------------------------------------
// | Author: apple
// +----------------------------------------------------------------------
// | Date: 11:29 上午
// +----------------------------------------------------------------------
package preface
import "github.com/limingshang/tree/node"
//type Node struct {
// value int // 当前节点值
// left *Node // 当前节点的左节点
// right *Node // 当前节点的右节点
//}
func createNode(value int) node.Node {
return node.Node{
Value,
&node.Node{},
&node.Node{},
0,
&node.Node{},
}
}
// 此是插入操作,删除很简单,左节点的最右节点上移活着右节点最左节点上移到要删除的节点就可以了
func Insert(node *Node, value int) {
if *node == (Node{}) {
*node = createNode(value)
} else {
if value > node.value {
if *node.right == (Node{}) {
*node.right = createNode(value)
} else {
Insert(node.right, value)
}
} else if value < node.value {
if *node.left == (Node{}) {
*node.left = createNode(value)
} else {
//*node.left = createNode(value)
Insert(node.left, value)
}
}
}
}
<file_sep>/BaseNode/BaseNode.go
// +----------------------------------------------------------------------
// | 功能:
// +----------------------------------------------------------------------
// | Copyright (c) <EMAIL> All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( https://github.com/limingshang )
// +----------------------------------------------------------------------
// | Author: apple
// +----------------------------------------------------------------------
// | Date: 8:24 下午
// +----------------------------------------------------------------------
package BaseNode
import "fmt"
type Node struct {
Value int // 当前节点值
Left *Node // 当前节点的左节点
Right *Node // 当前节点的右节点
Height float64 // 当前节点的高度
Parent *Node // 当前节点的父节点
}
// 前序遍历
func (n Node) AfterRange() {
if n != (Node{}) {
fmt.Println(n.Value)
n.Left.AfterRange()
n.Right.AfterRange()
}
}
// 中序遍历
func (n Node) MiddleRange() {
if n != (Node{}) {
n.Left.MiddleRange()
fmt.Println(n.Value)
n.Right.MiddleRange()
}
}
// 后序遍历
func (n Node) FloorRange() {
if n != (Node{}) {
n.Left.FloorRange()
n.Right.FloorRange()
fmt.Println(n.Value)
}
}
// 定义接口
type Tree interface {
CreateNode(int) Node // 创建节点方法
Insert(*Node, int) Node // 插入节点方法
Delete(*Node, int) Node // 删除节点方法
Find(*Node, int) Node // 查找节点方法
}
| c35d245a8336adfb4107df651dc4af127c83dc0d | [
"Markdown",
"Go"
] | 6 | Markdown | limingshang/tree | ebaf537b189fb6075b3a7dab67e1dc436075ca18 | d0791f1435bd23d2cba054bcc6636281e8012991 | |
refs/heads/master | <repo_name>Shikhar10000/koalixcrm<file_sep>/koalixcrm/crm/product/unit.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class Unit(models.Model):
description = models.CharField(verbose_name=_("Description"),
max_length=100)
short_name = models.CharField(verbose_name=_("Displayed Name After Quantity In The Position"),
max_length=3)
is_a_fraction_of = models.ForeignKey('self',
blank=True,
null=True,
verbose_name=_("Is A Fraction Of"))
fraction_factor_to_next_higher_unit = models.DecimalField(verbose_name=_("Factor Between This And Next Higher Unit"),
max_digits=20,
decimal_places=10,
blank=True,
null=True)
def __str__(self):
return self.short_name
class Meta:
app_label = "crm"
verbose_name = _('Unit')
verbose_name_plural = _('Units')
class OptionUnit(admin.ModelAdmin):
list_display = ('id',
'description',
'short_name',
'is_a_fraction_of',
'fraction_factor_to_next_higher_unit')
fieldsets = (('', {'fields': ('description',
'short_name',
'is_a_fraction_of',
'fraction_factor_to_next_higher_unit')}),)
allow_add = True
<file_sep>/koalixcrm/crm/views/work_entry_form.py
# -*- coding: utf-8 -*-
import datetime
from django.forms import NumberInput
from koalixcrm.crm.reporting.task import Task
from koalixcrm.crm.reporting.project import Project
from django.contrib.admin.widgets import *
from koalixcrm.global_support_functions import limit_string_length
from koalixcrm.djangoUserExtension.models import UserExtension
from koalixcrm.crm.reporting.reporting_period import ReportingPeriod
from koalixcrm.crm.reporting.human_resource import HumanResource
class WorkEntry(forms.Form):
"""
Form which allows to fill out a full Work. Instead of only showing the task, it is possible
to select the task based on the input from the project
"""
project = forms.ModelChoiceField(queryset=Project.objects.filter(reportingperiod__status__is_done=False).distinct(),
required=True)
task = forms.ModelChoiceField(queryset=Task.objects.filter(status__is_done=False),
required=True)
date = forms.DateField(widget=AdminDateWidget,
required=True)
start_time = forms.TimeField(widget=AdminTimeWidget,
required=False)
stop_time = forms.TimeField(widget=AdminTimeWidget,
required=False)
worked_hours = forms.DecimalField(widget=NumberInput(attrs={'step': 0.1,
'min': 0,
'max': 24}),
required=False)
description = forms.CharField(widget=AdminTextareaWidget,
required=True)
work_id = forms.IntegerField(widget=forms.HiddenInput(),
required=False)
def __init__(self, *args, **kwargs):
self.from_date = kwargs.pop('from_date')
self.to_date = kwargs.pop('to_date')
self.original_from_date = self.from_date
self.original_to_date = self.to_date
super(WorkEntry, self).__init__(*args, **kwargs)
@staticmethod
def check_working_hours(cleaned_data):
"""This method checks that the working hour is correctly proved either using the start_stop pattern
or by providing the worked_hours in total.
Args:
cleaned_data (Dict): The cleaned_data must contain the values form the form validation.
The django built in form validation must already have been passed
Returns:
True when no ValidationError was raised
Raises:
may raise ValidationError exception"""
if ("start_time" in cleaned_data) & ("stop_time" in cleaned_data) & ("worked_hours" in cleaned_data):
start_stop_pattern_complete = bool(cleaned_data["start_time"]) & bool(cleaned_data["stop_time"])
start_stop_pattern_stop_missing = bool(cleaned_data["start_time"]) & (not bool(cleaned_data["stop_time"]))
start_stop_pattern_start_missing = (not bool(cleaned_data["start_time"])) & bool(cleaned_data["stop_time"])
worked_hours_pattern = bool(cleaned_data["worked_hours"])
else:
raise forms.ValidationError('Programming error', code='invalid')
if start_stop_pattern_complete & worked_hours_pattern:
raise forms.ValidationError('Please either set the start, stop time or worked hours (not both)',
code='invalid')
elif start_stop_pattern_start_missing or start_stop_pattern_stop_missing:
raise forms.ValidationError('Set start and stop time',
code='invalid')
elif not start_stop_pattern_complete and not worked_hours_pattern:
raise forms.ValidationError('Either fill out the start_time and stop_time or the worked_hours',
code='invalid')
return True
def clean(self):
cleaned_data = super(WorkEntry, self).clean()
if 'date' in cleaned_data:
date = cleaned_data['date']
if date < self.from_date:
raise forms.ValidationError('date is not within the selected range', code='invalid')
elif self.to_date < date:
raise forms.ValidationError('date is not within the selected range', code='invalid')
if not cleaned_data["project"].is_reporting_allowed():
raise forms.ValidationError('The project is either closed or there is not '
'reporting period available', code='invalid')
WorkEntry.check_working_hours(cleaned_data)
return cleaned_data
def update_work(self, request):
from koalixcrm.crm.reporting.work import Work
if self.has_changed():
if self.cleaned_data['work_id']:
work = Work.objects.get(id=self.cleaned_data['work_id'])
else:
work = Work()
if self.cleaned_data['DELETE']:
work.delete()
else:
work.task = self.cleaned_data['task']
work.reporting_period = ReportingPeriod.get_reporting_period(project=self.cleaned_data['task'].project,
search_date=self.cleaned_data['date'])
work.human_resource = HumanResource.objects.get(user=UserExtension.get_user_extension(request.user))
work.date = self.cleaned_data['date']
if bool(self.cleaned_data['start_time']) & bool(self.cleaned_data['stop_time']):
work.start_time = datetime.datetime.combine(self.cleaned_data['date'],
self.cleaned_data['start_time'])
work.stop_time = datetime.datetime.combine(self.cleaned_data['date'],
self.cleaned_data['stop_time'])
else:
work.worked_hours = self.cleaned_data['worked_hours']
work.description = self.cleaned_data['description']
work.short_description = limit_string_length(work.description, 100)
work.save()
<file_sep>/koalixcrm/crm/exceptions.py
# -*- coding: utf-8 -*-
class TemplateSetMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class TemplateMissingInTemplateSet(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class TemplateSetMissingInContract(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class TemplateFOPConfigFileMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class TemplateXSLTFileMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class NoSerializationPatternFound(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class OpenInterestAccountMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class IncompleteInvoice(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class InvoiceAlreadyRegistered(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class UserIsNoHumanResource(Exception):
def __init__(self, value):
self.value = value
self.view = "/koalixcrm/crm/reporting/user_is_not_human_resource"
def __str__(self):
return repr(self.value)
class ReportingPeriodDoneDeleteNotPossible(Exception):
def __init__(self, value=None):
self.value = value
self.view = "/koalixcrm/crm/reporting/reporting_period_done_delete_not_possible"
def __str__(self):
return repr(self.value)
class ReportingPeriodNotFound(Exception):
def __init__(self, value):
self.value = value
self.view = "/koalixcrm/crm/reporting/reporting_period_missing"
def __str__(self):
return repr(self.value)
<file_sep>/koalixcrm/crm/migrations/0020_auto_20180116_2056.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-16 20:56
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0019_auto_20180112_2020'),
]
operations = [
migrations.DeleteModel(
name='PurchaseOrder',
),
migrations.DeleteModel(
name='EmailAddressForPurchaseOrder',
),
migrations.DeleteModel(
name='PhoneAddressForPurchaseOrder',
),
migrations.DeleteModel(
name='PostalAddressForPurchaseOrder',
),
migrations.DeleteModel(
name='PurchaseOrderPosition',
),
]
<file_sep>/koalixcrm/crm/documents/quote.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from django.utils.html import format_html
from koalixcrm.crm.const.status import *
from koalixcrm.plugin import *
from koalixcrm.crm.documents.sales_document import SalesDocument, OptionSalesDocument
from koalixcrm.global_support_functions import limit_string_length
class Quote(SalesDocument):
valid_until = models.DateField(verbose_name=_("Valid until"))
status = models.CharField(max_length=1, choices=QUOTESTATUS, verbose_name=_('Status'))
def link_to_quote(self):
if self.id:
return format_html("<a href='/admin/crm/quote/%s' >%s</a>" % (str(self.id),
limit_string_length(str(self.description),
30)))
else:
return "Not present"
link_to_quote.short_description = _("Quote");
def create_from_reference(self, calling_model):
self.create_sales_document(calling_model)
self.status = 'I'
self.valid_until = date.today().__str__()
self.date_of_creation = date.today().__str__()
self.template_set = self.contract.get_template_set(self)
self.save()
self.attach_sales_document_positions(calling_model)
self.attach_text_paragraphs()
def __str__(self):
return _("Quote") + ": " + self.id.__str__() + " " + _("from Contract") + ": " + self.contract.id.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Quote')
verbose_name_plural = _('Quotes')
class OptionQuote(OptionSalesDocument):
list_display = OptionSalesDocument.list_display + ('valid_until',
'status',)
list_filter = OptionSalesDocument.list_filter + ('status',)
ordering = OptionSalesDocument.ordering
search_fields = OptionSalesDocument.search_fields
fieldsets = OptionSalesDocument.fieldsets + (
(_('Quote specific'), {
'fields': ('valid_until',
'status', )
}),
)
save_as = OptionSalesDocument.save_as
inlines = OptionSalesDocument.inlines
actions = ['create_purchase_confirmation',
'create_invoice',
'create_delivery_note',
'create_purchase_order',
'create_project',
'create_pdf']
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("quoteInlines"))
class InlineQuote(admin.TabularInline):
model = Quote
classes = ['collapse']
show_change_link = True
can_delete = True
extra = 1
readonly_fields = ('link_to_quote',
'contract',
'customer',
'valid_until',
'status',
'last_pricing_date',
'last_calculated_price',
'last_calculated_tax',)
fieldsets = (
(_('Quote'), {
'fields': ('link_to_quote',
'contract',
'customer',
'valid_until',
'status',
'last_pricing_date',
'last_calculated_price',
'last_calculated_tax',)
}),
)
allow_add = False
<file_sep>/koalixcrm/crm/product/price.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.product.currency import Currency
from koalixcrm.crm.product.unit import Unit
from koalixcrm.crm.contact.customer_group import CustomerGroup
from koalixcrm.crm.product.unit_transform import UnitTransform
from koalixcrm.crm.product.customer_group_transform import CustomerGroupTransform
from koalixcrm.crm.product.currency_transform import CurrencyTransform
class Price(models.Model):
unit = models.ForeignKey(Unit,
blank=False,
verbose_name=_("Unit"))
currency = models.ForeignKey(Currency,
verbose_name='Currency',
blank=False,
null=False)
customer_group = models.ForeignKey(CustomerGroup,
verbose_name=_("Customer Group"),
blank=True,
null=True)
price = models.DecimalField(max_digits=17,
decimal_places=2,
verbose_name=_("Price Per Unit"))
valid_from = models.DateField(verbose_name=_("Valid from"),
blank=True,
null=True)
valid_until = models.DateField(verbose_name=_("Valid until"),
blank=True,
null=True)
def __str__(self):
return str(self.id) + " " +str(self.price) + " " + str(self.currency.short_name)
def is_valid_from_criteria_fulfilled(self, date):
if self.valid_from is None:
return True
elif (self.valid_from - date).days <= 0:
return True
else:
return False
def is_valid_until_criteria_fulfilled(self, date):
if self.valid_until is None:
return True
elif (date - self.valid_until).days <= 0:
return True
else:
return False
def is_customer_group_criteria_fulfilled(self, customer_group):
if self.customer_group is None:
return True
elif self.customer_group == customer_group:
return True
else:
return False
def is_currency_criteria_fulfilled(self, currency):
if self.currency == currency:
return True
else:
return False
def is_unit_criteria_fulfilled(self, unit):
if self.unit == unit:
return True
else:
return False
def is_date_in_range(self, date):
if (self.valid_from is None) and (self.valid_until is None):
return True
elif self.valid_until is None:
if self.valid_from <= date:
return True
else:
return False
elif self.valid_from is None:
if date <= self.valid_until:
return True
else:
return False
elif (self.valid_from <= date) and (date <= self.valid_until):
return True
else:
return False
def get_currency_transform_factor(self, currency, product_type):
"""check currency conditions and factor"""
currency_factor = 0
if self.currency == currency:
currency_factor = 1
else:
currency_transform = CurrencyTransform.objects.get(from_currency=self.currency,
to_currency=currency,
product_type=product_type)
if currency_transform:
currency_factor = currency_transform.get_transform_factor()
return currency_factor
def get_unit_transform_factor(self, unit, product_type):
"""check unit conditions and factor"""
unit_factor = 0
if self.unit == unit:
unit_factor = 1
else:
unit_transform = UnitTransform.objects.get(from_unit=self.unit,
to_unit=unit,
product_type=product_type)
if unit_transform:
unit_factor = unit_transform.get_transform_factor()
return unit_factor
def get_customer_group_transform_factor(self, customer, product_type):
"""The function searches through all customer_groups in which the customer is member of
from these customer_groups, the function returns the customer_group with the perfect match
or it returns the factor with the lowest transform factor
Args:
koalixcrm.crm.contact.customer customer
koalixcrm.crm.product.product product
Returns:
Decimal factor
Raises:
No exceptions planned"""
customer_group_factor = 0
if self.customer_group is None:
customer_group_factor = 1
elif customer is not None:
customer_groups = customer.is_member_of.all()
if customer_groups is not None:
for customer_group in customer_groups:
if self.customer_group == customer_group:
customer_group_factor = 1
# Stop for loop when a perfect match is found
break
else:
customer_group_transform = CustomerGroupTransform.objects.get(
from_customer_group=self.customer_group,
to_customer_group=customer_group,
product_type=product_type)
if customer_group_transform:
transform_factor = customer_group_transform.get_transform_factor()
if customer_group_factor > transform_factor or customer_group_factor == 0:
customer_group_factor = transform_factor
return customer_group_factor
class Meta:
app_label = "crm"
verbose_name = _('Price')
verbose_name_plural = _('Prices')
<file_sep>/koalixcrm/crm/migrations/0028_auto_20180611_1835.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-11 18:35
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0027_auto_20180606_2034'),
]
operations = [
migrations.AlterField(
model_name='task',
name='planned_end_date',
field=models.DateField(blank=True, null=True, verbose_name='Planned End Date'),
),
migrations.AlterField(
model_name='task',
name='planned_start_date',
field=models.DateField(blank=True, null=True, verbose_name='Planned Start Date'),
),
migrations.AlterField(
model_name='task',
name='status',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.TaskStatus', verbose_name='Task Status'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_unit_transform.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import UnitTransform
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory, SmallUnitFactory
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
class StandardUnitTransformFactory(factory.django.DjangoModelFactory):
class Meta:
model = UnitTransform
django_get_or_create = ('from_unit',
'to_unit')
from_unit = factory.SubFactory(StandardUnitFactory)
to_unit = factory.SubFactory(SmallUnitFactory)
product_type = factory.SubFactory(StandardProductTypeFactory)
factor = 1.10
<file_sep>/koalixcrm/crm/migrations/0031_auto_20180612_1924.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-12 19:24
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0030_auto_20180611_1930'),
]
operations = [
migrations.AlterField(
model_name='task',
name='short_description',
field=models.CharField(blank=True, max_length=100, null=True, verbose_name='Short Description'),
),
]
<file_sep>/koalixcrm/crm/admin.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from koalixcrm.crm.documents.quote import Quote, OptionQuote
from koalixcrm.crm.documents.purchase_confirmation import PurchaseConfirmation, OptionPurchaseConfirmation
from koalixcrm.crm.documents.delivery_note import DeliveryNote, OptionDeliveryNote
from koalixcrm.crm.documents.invoice import Invoice, OptionInvoice
from koalixcrm.crm.documents.payment_reminder import PaymentReminder, OptionPaymentReminder
from koalixcrm.crm.documents.purchase_order import PurchaseOrder, OptionPurchaseOrder
from koalixcrm.crm.documents.contract import Contract, OptionContract
from koalixcrm.crm.product.tax import Tax, OptionTax
from koalixcrm.crm.product.unit import Unit, OptionUnit
from koalixcrm.crm.product.product_type import ProductType, ProductTypeAdminView
from koalixcrm.crm.product.currency import Currency, OptionCurrency
from koalixcrm.crm.contact.customer import Customer, OptionCustomer
from koalixcrm.crm.contact.supplier import Supplier, OptionSupplier
from koalixcrm.crm.contact.customer_group import CustomerGroup, OptionCustomerGroup
from koalixcrm.crm.contact.customer_billing_cycle import CustomerBillingCycle, OptionCustomerBillingCycle
from koalixcrm.crm.contact.person import Person
from koalixcrm.crm.contact.contact import OptionPerson, CallForContact, VisitForContact
from koalixcrm.crm.contact.call import OptionCall, OptionVisit
from koalixcrm.crm.reporting.task import Task, TaskAdminView
from koalixcrm.crm.reporting.task_link_type import TaskLinkType, OptionTaskLinkType
from koalixcrm.crm.reporting.task_status import TaskStatus, OptionTaskStatus
from koalixcrm.crm.reporting.estimation_status import EstimationStatus, EstimationStatusAdminView
from koalixcrm.crm.reporting.agreement_status import AgreementStatus, AgreementStatusAdminView
from koalixcrm.crm.reporting.agreement_type import AgreementType, AgreementTypeAdminView
from koalixcrm.crm.reporting.resource_type import ResourceType, ResourceTypeAdminView
from koalixcrm.crm.reporting.human_resource import HumanResource, HumanResourceAdminView
from koalixcrm.crm.reporting.resource_manager import ResourceManager, ResourceManagerAdminView
from koalixcrm.crm.reporting.project import Project, ProjectAdminView
from koalixcrm.crm.reporting.project_link_type import ProjectLinkType, OptionProjectLinkType
from koalixcrm.crm.reporting.project_status import ProjectStatus, OptionProjectStatus
from koalixcrm.crm.reporting.work import Work, WorkAdminView
from koalixcrm.crm.reporting.reporting_period import ReportingPeriod, ReportingPeriodAdmin
from koalixcrm.crm.reporting.reporting_period_status import ReportingPeriodStatus, OptionReportingPeriodStatus
admin.site.register(Customer, OptionCustomer)
admin.site.register(CustomerGroup, OptionCustomerGroup)
admin.site.register(CustomerBillingCycle, OptionCustomerBillingCycle)
admin.site.register(Supplier, OptionSupplier)
admin.site.register(Person, OptionPerson)
admin.site.register(CallForContact, OptionCall)
admin.site.register(VisitForContact, OptionVisit)
admin.site.register(Contract, OptionContract)
admin.site.register(Quote, OptionQuote)
admin.site.register(PurchaseConfirmation, OptionPurchaseConfirmation)
admin.site.register(DeliveryNote, OptionDeliveryNote)
admin.site.register(Invoice, OptionInvoice)
admin.site.register(PaymentReminder, OptionPaymentReminder)
admin.site.register(PurchaseOrder, OptionPurchaseOrder)
admin.site.register(Unit, OptionUnit)
admin.site.register(Currency, OptionCurrency)
admin.site.register(Tax, OptionTax)
admin.site.register(ProductType, ProductTypeAdminView)
admin.site.register(Task, TaskAdminView)
admin.site.register(TaskLinkType, OptionTaskLinkType)
admin.site.register(TaskStatus, OptionTaskStatus)
admin.site.register(EstimationStatus, EstimationStatusAdminView)
admin.site.register(AgreementStatus, AgreementStatusAdminView)
admin.site.register(AgreementType, AgreementTypeAdminView)
admin.site.register(Work, WorkAdminView)
admin.site.register(HumanResource, HumanResourceAdminView)
admin.site.register(ResourceType, ResourceTypeAdminView)
admin.site.register(ResourceManager, ResourceManagerAdminView)
admin.site.register(Project, ProjectAdminView)
admin.site.register(ProjectLinkType, OptionProjectLinkType)
admin.site.register(ProjectStatus, OptionProjectStatus)
admin.site.register(ReportingPeriod, ReportingPeriodAdmin)
admin.site.register(ReportingPeriodStatus, OptionReportingPeriodStatus)
<file_sep>/koalixcrm/crm/factories/factory_estimation.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import Estimation
from koalixcrm.crm.factories.factory_resource import StandardResourceFactory
from koalixcrm.crm.factories.factory_human_resource import StandardHumanResourceFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.crm.factories.factory_estimation_status import StartedEstimationStatusFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardEstimationToTaskFactory(factory.django.DjangoModelFactory):
class Meta:
model = Estimation
amount = "112.50"
task = factory.SubFactory(StandardTaskFactory)
resource = factory.SubFactory(StandardResourceFactory)
date_from = make_date_utc(datetime.datetime(2018, 5, 2, 00))
date_until = make_date_utc(datetime.datetime(2018, 6, 15, 00))
status = factory.SubFactory(StartedEstimationStatusFactory)
reporting_period = factory.SubFactory(StandardReportingPeriodFactory)
class StandardHumanResourceEstimationToTaskFactory(factory.django.DjangoModelFactory):
class Meta:
model = Estimation
amount = "112.50"
task = factory.SubFactory(StandardTaskFactory)
resource = factory.SubFactory(StandardHumanResourceFactory)
date_from = make_date_utc(datetime.datetime(2018, 5, 2, 00))
date_until = make_date_utc(datetime.datetime(2018, 6, 15, 00))
status = factory.SubFactory(StartedEstimationStatusFactory)
reporting_period = factory.SubFactory(StandardReportingPeriodFactory)
<file_sep>/koalixcrm/crm/views/pdfexport.py
# -*- coding: utf-8 -*-
from os import path
from wsgiref.util import FileWrapper
from subprocess import CalledProcessError
from django.http import Http404
from django.http import HttpResponse
from django.http import HttpResponseRedirect
from django.utils.translation import ugettext as _
from koalixcrm.crm.exceptions import *
from koalixcrm.djangoUserExtension.exceptions import *
from django.contrib import messages
class PDFExportView:
@staticmethod
def export_pdf(calling_model_admin, request, source, redirect_to, template_to_use, *args, **kwargs):
"""This method exports PDFs provided by different Models in the crm application
Args:
calling_model_admin (ModelAdmin): The calling ModelAdmin must be provided for error message response.
request: The request User is to know where to save the error message
source: The model from which a PDF should be exported
redirect_to (str): String that describes to where the method should redirect in case of an error
template_to_use (Template Set): For some documents that need to be created there exists more
than one template with this parameter the template set can be set during the export function
Returns:
HTTpResponse with a PDF when successful
HTTpResponseRedirect when not successful
Raises:
raises Http404 exception if anything goes wrong"""
try:
pdf = source.create_pdf(template_to_use, request.user, *args, **kwargs)
response = HttpResponse(FileWrapper(open(pdf, 'rb')), content_type='application/pdf')
response['Content-Length'] = path.getsize(pdf)
except (TemplateSetMissing,
TemplateSetMissingInContract,
UserExtensionMissing,
CalledProcessError,
UserExtensionEmailAddressMissing,
UserExtensionPhoneAddressMissing,
TemplateSetMissingForUserExtension) as e:
if isinstance(e, UserExtensionMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("User Extension Missing"),
level=messages.ERROR)
elif isinstance(e, UserExtensionEmailAddressMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("User Extension Email Missing"),
level=messages.ERROR)
elif isinstance(e, UserExtensionPhoneAddressMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("User Extension Phone Missing"),
level=messages.ERROR)
elif isinstance(e, TemplateSetMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("Template-set Missing"),
level=messages.ERROR)
elif isinstance(e, TemplateSetMissingInContract):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("Template-set Missing"),
level=messages.ERROR)
elif isinstance(e, TemplateFOPConfigFileMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("Fop Config File Missing in TemplateSet"),
level=messages.ERROR)
elif isinstance(e, TemplateXSLTFileMissing):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("XSLT File Missing in TemplateSet"),
level=messages.ERROR)
elif isinstance(e, TemplateSetMissingForUserExtension):
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, _("Work report template missing in the user extension"),
level=messages.ERROR)
elif type(e) == CalledProcessError:
response = HttpResponseRedirect(redirect_to)
calling_model_admin.message_user(request, e.output)
else:
raise Http404
return response
<file_sep>/koalixcrm/djangoUserExtension/factories/factory_user_extension.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.djangoUserExtension.models import UserExtension
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.djangoUserExtension.factories.factory_template_set import StandardTemplateSetFactory
class StandardUserExtensionFactory(factory.django.DjangoModelFactory):
class Meta:
model = UserExtension
django_get_or_create = ('user',)
user = factory.SubFactory(StaffUserFactory)
default_template_set = factory.SubFactory(StandardTemplateSetFactory)
default_currency = factory.SubFactory(StandardCurrencyFactory)
<file_sep>/koalixcrm/crm/factories/factory_unit.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Unit
class StandardUnitFactory(factory.django.DjangoModelFactory):
class Meta:
model = Unit
django_get_or_create = ('short_name',)
description = "Kilogram"
short_name = "kg"
is_a_fraction_of = None
fraction_factor_to_next_higher_unit = None
class SmallUnitFactory(factory.django.DjangoModelFactory):
class Meta:
model = Unit
django_get_or_create = ('short_name',)
description = "Gram"
short_name = "g"
is_a_fraction_of = factory.SubFactory(StandardUnitFactory)
fraction_factor_to_next_higher_unit = 1000
<file_sep>/koalixcrm/crm/factories/factory_human_resource.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import HumanResource
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
from koalixcrm.crm.factories.factory_resource_type import StandardResourceTypeFactory
from koalixcrm.crm.factories.factory_resource_manager import StandardResourceManagerFactory
class StandardHumanResourceFactory(factory.django.DjangoModelFactory):
class Meta:
model = HumanResource
user = factory.SubFactory(StandardUserExtensionFactory)
resource_type = factory.SubFactory(StandardResourceTypeFactory)
resource_manager = factory.SubFactory(StandardResourceManagerFactory)
<file_sep>/koalixcrm/crm/reporting/resource.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
class Resource(models.Model):
resource_manager = models.ForeignKey("ResourceManager",
verbose_name=_("Manager"),
blank=True,
null=True)
resource_type = models.ForeignKey("ResourceType",
verbose_name=_("Resource Type"),
blank=True,
null=True)
def __str__(self):
from koalixcrm.crm.reporting.human_resource import HumanResource
human_resource = HumanResource.objects.get(id=self.id)
if human_resource:
return human_resource.__str__()
else:
return "Resource"
<file_sep>/koalixcrm/accounting/accounting/accounting_period.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from django import forms
from koalixcrm.accounting.models import Account
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.accounting.exceptions import AccountingPeriodNotFound
from koalixcrm.accounting.exceptions import TemplateSetMissingInAccountingPeriod
from koalixcrm.accounting.models import InlineBookings
class AccountingPeriod(models.Model):
"""Accounting period represents the equivalent of the business logic element of a fiscal year
the accounting period is referred in the booking and is used as a supporting object to generate
balance sheets and profit/loss statements"""
title = models.CharField(max_length=200, verbose_name=_("Title")) # For example "Year 2009", "1st Quarter 2009"
begin = models.DateField(verbose_name=_("Begin"))
end = models.DateField(verbose_name=_("End"))
template_set_balance_sheet = models.ForeignKey("djangoUserExtension.DocumentTemplate",
verbose_name=_("Referred template for balance sheet"),
related_name='db_balancesheet_template_set',
null=True,
blank=True)
template_profit_loss_statement = models.ForeignKey("djangoUserExtension.DocumentTemplate",
verbose_name=_("Referred template for profit, loss statement"),
related_name='db_profit_loss_statement_template_set',
null=True,
blank=True)
def get_template_set(self, template_set):
if template_set == self.template_set_balance_sheet:
if self.template_set_balance_sheet:
return self.template_set_balance_sheet
else:
raise TemplateSetMissingInAccountingPeriod((_("Template Set for balance sheet " +
"is missing in Accounting Period" + str(self))))
elif template_set == self.template_profit_loss_statement:
if self.template_profit_loss_statement:
return self.template_profit_loss_statement
else:
raise TemplateSetMissingInAccountingPeriod((_("Template Set for profit loss statement" +
" is missing in Accounting Period" + str(self))))
def get_fop_config_file(self, template_set):
template_set = self.get_template_set(template_set)
return template_set.get_fop_config_file()
def get_xsl_file(self, template_set):
template_set = self.get_template_set(template_set)
return template_set.get_xsl_file()
def create_pdf(self, template_set, printed_by):
import koalixcrm.crm
return koalixcrm.crm.documents.pdfexport.PDFExport.create_pdf(self, template_set, printed_by)
def overall_earnings(self):
earnings = 0
accounts = Account.objects.all()
for account in list(accounts):
if account.account_type == "E":
earnings += account.sum_of_all_bookings_within_accounting_period(self)
return earnings
def overall_spendings(self):
spendings = 0
accounts = Account.objects.all()
for account in list(accounts):
if account.account_type == "S":
spendings += account.sum_of_all_bookings_within_accounting_period(self)
return spendings
def overall_assets(self):
assets = 0
accounts = Account.objects.all()
for account in list(accounts):
if account.account_type == "A":
assets += account.sum_of_all_bookings_through_now(self)
return assets
def overall_liabilities(self):
liabilities = 0
accounts = Account.objects.all()
for account in list(accounts):
if account.account_type == "L":
liabilities += account.sum_of_all_bookings_through_now(self)
return liabilities
def serialize_to_xml(self):
objects = [self, ]
main_xml = PDFExport.write_xml(objects)
accounts = Account.objects.all()
for account in accounts:
account_xml = account.serialize_to_xml(self)
main_xml = PDFExport.merge_xml(main_xml, account_xml)
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Overall_Earnings",
self.overall_earnings())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Overall_Spendings",
self.overall_spendings())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>']",
"Overall_Assets",
self.overall_assets())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@<EMAIL>='<EMAIL>']",
"Overall_Liabilities",
self.overall_liabilities())
return main_xml
@staticmethod
def get_current_valid_accounting_period():
"""Returns the accounting period that is currently valid. Valid is an accounting_period when the current date
lies between begin and end of the accounting_period
Args:
no arguments
Returns:
accounting_period (AccoutingPeriod)
Raises:
AccountingPeriodNotFound when there is no valid accounting Period"""
current_valid_accounting_period = None
for accounting_period in AccountingPeriod.objects.all():
if accounting_period.begin < date.today() and accounting_period.end > date.today():
return accounting_period
if not current_valid_accounting_period:
raise AccountingPeriodNotFound("The accounting period was not found")
def get_all_prior_accounting_periods(self):
"""Returns the accounting period that is currently valid. Valid is an accountingPeriod when the current date
lies between begin and end of the accountingPeriod
Args:
no arguments
Returns:
accounting_period (List of AccoutingPeriod)
Raises:
AccountingPeriodNotFound when there is no valid accounting Period"""
accounting_periods = []
for accounting_period in AccountingPeriod.objects.all():
if accounting_period.end < self.begin:
accounting_periods.append(accounting_period)
if accounting_periods == []:
raise AccountingPeriodNotFound("Accounting Period does not exist")
return accounting_periods
def __str__(self):
return self.title
# TODO: def createNewAccountingPeriod() Neues Geschäftsjahr erstellen
class Meta:
app_label = "accounting"
verbose_name = _('Accounting Period')
verbose_name_plural = _('Accounting Periods')
class AccountingPeriodForm(forms.ModelForm):
"""AccountingPeriodForm is used to overwrite the clean method of the
original form and to add an additional check to the model"""
class Meta:
model = AccountingPeriod
fields = '__all__'
def clean(self):
super(AccountingPeriodForm, self).clean()
errors = []
try:
if self.cleaned_data['begin'] > self.cleaned_data['end']:
errors.append(_('The begin date cannot be later than the end date.'))
except KeyError:
errors.append(_('The begin and the end date may not be empty'))
if errors:
raise forms.ValidationError(errors)
return self.cleaned_data
class OptionAccountingPeriod(admin.ModelAdmin):
list_display = ('title',
'begin',
'end',
'template_set_balance_sheet',
'template_profit_loss_statement')
list_display_links = ('title',
'begin',
'end',
'template_set_balance_sheet',
'template_profit_loss_statement')
fieldsets = (
(_('Basics'), {
'fields': ('title',
'begin',
'end',
'template_set_balance_sheet',
'template_profit_loss_statement')
}),
)
inlines = [InlineBookings, ]
save_as = True
form = AccountingPeriodForm
def save_formset(self, request, form, formset, change):
instances = formset.save(commit=False)
for instance in instances:
if change:
instance.last_modified_by = request.user
else:
instance.last_modified_by = request.user
instance.staff = request.user
instance.save()
def create_pdf_of_balance_sheet(self, request, queryset):
from koalixcrm.crm.views.pdfexport import PDFExportView
for obj in queryset:
response = PDFExportView.export_pdf(self,
request,
obj,
("/admin/accounting/"+obj.__class__.__name__.lower()+"/"),
obj.template_set_balance_sheet)
return response
create_pdf_of_balance_sheet.short_description = _("Create PDF of Balance Sheet")
def create_pdf_of_profit_loss_statement(self, request, queryset):
from koalixcrm.crm.views.pdfexport import PDFExportView
for obj in queryset:
response = PDFExportView.export_pdf(self,
request,
obj,
("/admin/accounting/"+obj.__class__.__name__.lower()+"/"),
obj.template_profit_loss_statement,)
return response
create_pdf_of_profit_loss_statement.short_description = _("Create PDF of Profit Loss Statement Sheet")
actions = ['create_pdf_of_balance_sheet',
'create_pdf_of_profit_loss_statement']
<file_sep>/koalixcrm/crm/migrations/0055_auto_20181022_1937.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-22 19:37
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0054_auto_20181020_1845'),
]
operations = [
migrations.AlterField(
model_name='currencytransform',
name='factor',
field=models.DecimalField(decimal_places=2, default=1, max_digits=17, verbose_name='Factor between From and To Currency'),
preserve_default=False,
),
migrations.AlterField(
model_name='customergrouptransform',
name='factor',
field=models.DecimalField(decimal_places=2, default=1, max_digits=17, verbose_name='Factor between From and To Customer Group'),
preserve_default=False,
),
migrations.AlterField(
model_name='unittransform',
name='factor',
field=models.DecimalField(decimal_places=2, default=1, max_digits=17, verbose_name='Factor between From and To Unit'),
preserve_default=False,
),
]
<file_sep>/koalixcrm/crm/documents/purchase_order.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.status import *
from koalixcrm.crm.documents.sales_document import SalesDocument, OptionSalesDocument
from koalixcrm.plugin import *
class PurchaseOrder(SalesDocument):
supplier = models.ForeignKey("Supplier", verbose_name=_("Supplier"), null=True)
status = models.CharField(max_length=1, choices=PURCHASEORDERSTATUS)
def create_from_reference(self, calling_model):
self.create_sales_document(calling_model)
self.status = 'O'
self.template_set = self.contract.get_template_set(self)
self.save()
self.attach_sales_document_positions(calling_model)
self.attach_text_paragraphs()
self.staff = calling_model.staff
def __str__(self):
return _("Purchase Order") + ": " + self.id.__str__() + " " + _("from Contract") + ": " + self.contract.id.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Purchase Order')
verbose_name_plural = _('Purchase Orders')
class OptionPurchaseOrder(OptionSalesDocument):
list_display = OptionSalesDocument.list_display + ('supplier', 'status',)
list_filter = OptionSalesDocument.list_filter + ('status',)
ordering = OptionSalesDocument.ordering
search_fields = OptionSalesDocument.search_fields
fieldsets = OptionSalesDocument.fieldsets + (
(_('Purchase Order specific'), {
'fields': ('supplier', 'status',)
}),
)
save_as = OptionSalesDocument.save_as
inlines = OptionSalesDocument.inlines
actions = ['create_purchase_confirmation', 'create_invoice', 'create_quote',
'create_delivery_note', 'create_pdf',
'register_invoice_in_accounting', 'register_payment_in_accounting',]
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("quoteInlines"))
<file_sep>/koalixcrm/crm/reporting/resource_manager.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from koalixcrm.djangoUserExtension.user_extension.user_extension import UserExtension
from django.utils.translation import ugettext as _
class ResourceManager(models.Model):
user = models.ForeignKey(UserExtension,
verbose_name=_("User"))
class ResourceManagerAdminView(admin.ModelAdmin):
list_display = ('id',
'user',)
fieldsets = (
(_('Basics'), {
'fields': ('user',)
}),
)<file_sep>/koalixcrm/crm/tests/test_calculations_document.py
import pytest
import datetime
from django.test import TestCase
from koalixcrm.crm.documents.calculations import Calculations
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_quote import StandardQuoteFactory
from koalixcrm.crm.factories.factory_sales_document_position import StandardSalesDocumentPositionFactory
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
from koalixcrm.crm.factories.factory_product_price import StandardPriceFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory, AdvancedCustomerGroupFactory
from koalixcrm.crm.factories.factory_tax import StandardTaxFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory, SmallUnitFactory
from koalixcrm.crm.factories.factory_customer_group_transform import StandardCustomerGroupTransformFactory
from koalixcrm.crm.factories.factory_unit_transform import StandardUnitTransformFactory
from koalixcrm.crm.factories.factory_currency_transform import StandardCurrencyTransformFactory
from koalixcrm.test_support_functions import make_date_utc
class DocumentCalculationsTest(TestCase):
def setUp(self):
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
start_date = (datetime_now - datetime.timedelta(days=30)).date()
end_date = (datetime_now + datetime.timedelta(days=30)).date()
self.tax = StandardTaxFactory.create(tax_rate=10)
self.test_currency_with_rounding = StandardCurrencyFactory.create(rounding=1)
self.test_currency_without_rounding = StandardCurrencyFactory.create(
rounding=None,
description='Euro',
short_name='EUR'
)
self.alternative_currency = self.test_currency_without_rounding
self.customer_group = StandardCustomerGroupFactory.create()
self.alternative_customer_group = AdvancedCustomerGroupFactory.create()
self.customer = StandardCustomerFactory.create()
self.customer.is_member_of.add(self.customer_group)
self.customer.save()
self.unit = StandardUnitFactory.create()
self.alternative_unit = SmallUnitFactory.create()
self.product_without_dates = StandardProductTypeFactory.create(
product_type_identifier="A",
tax=self.tax
)
self.product_without_date_from = StandardProductTypeFactory.create(
product_type_identifier="B",
tax=self.tax
)
self.product_without_date_until = StandardProductTypeFactory.create(
product_type_identifier="C",
tax=self.tax
)
self.product_without_customer_group = StandardProductTypeFactory.create(
product_type_identifier="D",
tax=self.tax
)
self.product_with_currency_rounding = StandardProductTypeFactory.create(
product_type_identifier="E",
tax=self.tax
)
self.product_without_currency_rounding = StandardProductTypeFactory.create(
product_type_identifier="F",
tax=self.tax
)
self.product_with_alternative_customer_group = StandardProductTypeFactory.create(
product_type_identifier="G",
tax=self.tax
)
self.product_with_alternative_unit = StandardProductTypeFactory.create(
product_type_identifier="H",
tax=self.tax
)
self.product_with_alternative_currency = StandardProductTypeFactory.create(
product_type_identifier="I",
tax=self.tax
)
self.price_without_customer_group = StandardPriceFactory.create(
product_type=self.product_without_customer_group,
customer_group=None,
price=100,
unit=self.unit,
currency=self.test_currency_with_rounding,
valid_from=start_date,
valid_until=end_date
)
self.price_without_dates = StandardPriceFactory.create(
product_type=self.product_without_dates,
customer_group=self.customer_group,
valid_from=None,
valid_until=None,
unit=self.unit,
currency=self.test_currency_with_rounding,
price=80
)
self.price_without_date_to = StandardPriceFactory.create(
product_type=self.product_without_date_until,
customer_group=self.customer_group,
valid_from=start_date,
valid_until=None,
unit=self.unit,
currency=self.test_currency_with_rounding,
price=130
)
self.price_without_date_from = StandardPriceFactory.create(
product_type=self.product_without_date_from,
customer_group=self.customer_group,
valid_from=None,
valid_until=end_date,
unit=self.unit,
currency=self.test_currency_with_rounding,
price=50
)
self.price_without_date_from = StandardPriceFactory.create(
product_type=self.product_without_currency_rounding,
currency=self.test_currency_without_rounding,
customer_group=self.customer_group,
price=25,
unit=self.unit,
valid_from=start_date,
valid_until=end_date
)
self.price_without_date_from = StandardPriceFactory.create(
product_type=self.product_with_currency_rounding,
currency=self.test_currency_with_rounding,
customer_group=self.customer_group,
price=33,
unit=self.unit,
valid_from=start_date,
valid_until=end_date
)
self.price_with_alternative_customer_group = StandardPriceFactory.create(
product_type=self.product_with_alternative_customer_group,
customer_group=self.alternative_customer_group,
valid_from=start_date,
valid_until=end_date,
unit=self.unit,
currency=self.test_currency_with_rounding,
price=80
)
self.customer_group_transform = StandardCustomerGroupTransformFactory.create(
from_customer_group=self.alternative_customer_group,
to_customer_group=self.customer_group,
product_type=self.product_with_alternative_customer_group,
factor=0.50
)
self.price_with_alternative_currency = StandardPriceFactory.create(
product_type=self.product_with_alternative_currency,
customer_group=self.customer_group,
valid_from=start_date,
valid_until=end_date,
unit=self.unit,
currency=self.alternative_currency,
price=80
)
self.currency_transform = StandardCurrencyTransformFactory.create(
from_currency=self.alternative_currency,
to_currency=self.test_currency_with_rounding,
product_type=self.product_with_alternative_currency,
factor=0.50
)
self.price_with_alternative_unit = StandardPriceFactory.create(
product_type=self.product_with_alternative_unit,
customer_group=self.customer_group,
valid_from=start_date,
valid_until=end_date,
unit=self.alternative_unit,
currency=self.test_currency_with_rounding,
price=80
)
self.currency_transform = StandardUnitTransformFactory.create(
from_unit=self.alternative_unit,
to_unit=self.unit,
product_type=self.product_with_alternative_unit,
factor=0.50
)
@pytest.mark.back_end_tests
def test_calculate_document_price_without_customer_group(self):
quote_1 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
product_type=self.product_without_customer_group,
overwrite_product_price=False,
unit=self.unit,
sales_document=quote_1
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_1,
pricing_date=date_now)
self.assertEqual(
quote_1.last_calculated_price.__str__(), "90.00")
self.assertEqual(
quote_1.last_calculated_tax.__str__(), "10.00")
@pytest.mark.back_end_tests
def test_calculate_document_price_without_date_from(self):
quote_2 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_without_date_from,
overwrite_product_price=False,
sales_document=quote_2
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_2,
pricing_date=date_now)
self.assertEqual(
quote_2.last_calculated_price.__str__(), "45.00")
self.assertEqual(
quote_2.last_calculated_tax.__str__(), "5.00")
@pytest.mark.back_end_tests
def test_calculate_document_price_without_date_until(self):
quote_3 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_without_date_until,
overwrite_product_price=False,
sales_document=quote_3
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_3,
pricing_date=date_now)
self.assertEqual(
quote_3.last_calculated_price.__str__(), "117.00")
self.assertEqual(
quote_3.last_calculated_tax.__str__(), "13.00")
@pytest.mark.back_end_tests
def test_calculate_document_price_without_dates(self):
quote_4 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_without_dates,
overwrite_product_price=False,
sales_document=quote_4
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_4,
pricing_date=date_now)
self.assertEqual(
quote_4.last_calculated_price.__str__(), "72.00")
self.assertEqual(
quote_4.last_calculated_tax.__str__(), "8.00")
@pytest.mark.back_end_tests
def test_calculate_document_price_with_currency_rounding(self):
quote_5 = StandardQuoteFactory.create(
customer=self.customer,
currency=self.test_currency_with_rounding
)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=7.5,
unit=self.unit,
product_type=self.product_with_currency_rounding,
overwrite_product_price=False,
sales_document=quote_5
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_5,
pricing_date=date_now)
self.assertEqual(
quote_5.last_calculated_price.__str__(), "27")
self.assertEqual(
quote_5.last_calculated_tax.__str__(), "3")
@pytest.mark.back_end_tests
def test_calculate_document_price_without_currency_rounding(self):
quote_6 = StandardQuoteFactory.create(
customer=self.customer,
currency=self.test_currency_without_rounding
)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=7.5,
unit=self.unit,
product_type=self.product_without_currency_rounding,
overwrite_product_price=False,
sales_document=quote_6
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_6,
pricing_date=date_now)
self.assertEqual(
quote_6.last_calculated_price.__str__(), "20.80")
self.assertEqual(
quote_6.last_calculated_tax.__str__(), "2.30")
@pytest.mark.back_end_tests
def test_calculate_document_price_with_document_discount(self):
quote_7 = StandardQuoteFactory.create(
customer=self.customer,
currency=self.test_currency_without_rounding,
discount=10
)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=7.5,
unit=self.unit,
product_type=self.product_without_currency_rounding,
overwrite_product_price=False,
sales_document=quote_7
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_7,
pricing_date=date_now)
self.assertEqual(
quote_7.last_calculated_price.__str__(), "18.70")
self.assertEqual(
quote_7.last_calculated_tax.__str__(), "2.05")
@pytest.mark.back_end_tests
def test_calculate_document_with_customer_group_transform(self):
quote_8 = StandardQuoteFactory.create(
customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_with_alternative_customer_group,
overwrite_product_price=False,
sales_document=quote_8
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_8,
pricing_date=date_now)
self.assertEqual(
quote_8.last_calculated_price.__str__(), "36.00")
self.assertEqual(
quote_8.last_calculated_tax.__str__(), "4.00")
@pytest.mark.back_end_tests
def test_calculate_document_with_currency_transform(self):
quote_9 = StandardQuoteFactory.create(
currency=self.test_currency_with_rounding,
customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_with_alternative_currency,
overwrite_product_price=False,
sales_document=quote_9
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_9,
pricing_date=date_now)
self.assertEqual(
quote_9.last_calculated_price.__str__(), "36")
self.assertEqual(
quote_9.last_calculated_tax.__str__(), "4")
@pytest.mark.back_end_tests
def test_calculate_document_with_unit_transform(self):
quote_10 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
unit=self.unit,
product_type=self.product_with_alternative_unit,
overwrite_product_price=False,
sales_document=quote_10
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_10,
pricing_date=date_now)
self.assertEqual(
quote_10.last_calculated_price.__str__(), "36.00")
self.assertEqual(
quote_10.last_calculated_tax.__str__(), "4.00")
<file_sep>/koalixcrm/crm/factories/factory_purchase_order.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import PurchaseOrder
from koalixcrm.crm.factories.factory_supplier import StandardSupplierFactory
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardPurchaseOrderFactory(StandardSalesDocumentFactory):
class Meta:
model = PurchaseOrder
supplier = factory.SubFactory(StandardSupplierFactory)
status = "C"
<file_sep>/koalixcrm/crm/factories/factory_task.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import Task
from koalixcrm.crm.factories.factory_project import StandardProjectFactory
from koalixcrm.crm.factories.factory_task_status import StartedTaskStatusFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardTaskFactory(factory.django.DjangoModelFactory):
class Meta:
model = Task
title = 'This is a test Task'
project = factory.SubFactory(StandardProjectFactory)
description = "This is a description"
status = factory.SubFactory(StartedTaskStatusFactory)
last_status_change = make_date_utc(datetime.datetime(2018, 6, 15, 00))
<file_sep>/koalixcrm/crm/tests/test_task_effective_duration.py
import datetime
import pytest
from django.test import TestCase
from koalixcrm.crm.factories.factory_customer_billing_cycle import StandardCustomerBillingCycleFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_work import StandardWorkFactory
from koalixcrm.crm.factories.factory_task_status import DoneTaskStatusFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.crm.factories.factory_human_resource import StandardHumanResourceFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.test_support_functions import make_date_utc
from koalixcrm.crm.factories.factory_estimation import StandardHumanResourceEstimationToTaskFactory
@pytest.fixture()
def freeze(monkeypatch):
""" Now() manager patches date return a fixed, settable, value
(freezes date)
"""
original = datetime.date
class FreezeMeta(type):
def __instancecheck__(self, instance):
if instance.isinstance(original) or instance.isinstance(Freeze):
return True
class Freeze(datetime.datetime):
__metaclass__ = FreezeMeta
@classmethod
def freeze(cls, val):
cls.frozen = val
@classmethod
def today(cls):
return cls.frozen
@classmethod
def delta(cls, timedelta=None, **kwargs):
""" Moves time fwd/bwd by the delta"""
from datetime import timedelta as td
if not timedelta:
timedelta = td(**kwargs)
cls.frozen += timedelta
monkeypatch.setattr(datetime, 'date', Freeze)
Freeze.freeze(original.today())
return Freeze
class TaskEffectiveDuration(TestCase):
@pytest.fixture(autouse=True)
def freeze_time(self, freeze):
self._freeze = freeze
def setUp(self):
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
start_date = (datetime_now - datetime.timedelta(days=30))
end_date_first_task = (datetime_now + datetime.timedelta(days=30))
end_date_second_task = (datetime_now + datetime.timedelta(days=60))
self.test_billing_cycle = StandardCustomerBillingCycleFactory.create()
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.human_resource = StandardHumanResourceFactory.create()
self.test_reporting_period = StandardReportingPeriodFactory.create()
self.test_1st_task = StandardTaskFactory.create(title="1st Test Task",
project=self.test_reporting_period.project)
self.estimation_1st_task = StandardHumanResourceEstimationToTaskFactory(task=self.test_1st_task,
date_from=start_date,
date_until=end_date_first_task)
self.test_2nd_task = StandardTaskFactory.create(title="2nd Test Task",
project=self.test_reporting_period.project)
self.estimation_2nd_task = StandardHumanResourceEstimationToTaskFactory(task=self.test_2nd_task,
date_from=start_date,
date_until=end_date_second_task)
@pytest.mark.back_end_tests
def test_effective_duration(self):
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
datetime_later_1 = make_date_utc(datetime.datetime(2024, 1, 2, 2, 00))
datetime_later_2 = make_date_utc(datetime.datetime(2024, 1, 2, 3, 30))
datetime_later_3 = make_date_utc(datetime.datetime(2024, 1, 4, 5, 45))
datetime_later_4 = make_date_utc(datetime.datetime(2024, 1, 4, 6, 15))
self.assertEqual(
(self.test_1st_task.planned_duration()).__str__(), "60")
self.assertEqual(
(self.test_1st_task.effective_duration()).__str__(), "0")
self.assertEqual(
(self.test_2nd_task.planned_duration()).__str__(), "90")
self.assertEqual(
(self.test_2nd_task.effective_duration()).__str__(), "0")
new_status = DoneTaskStatusFactory.create()
self._freeze.freeze(datetime.date(2024, 2, 2))
self.test_1st_task.status = new_status
self.test_1st_task.save()
self._freeze.freeze(datetime.date(2024, 2, 5))
self.test_2nd_task.status = new_status
self.test_2nd_task.save()
self.assertEqual(
(self.test_1st_task.planned_duration()).__str__(), "60")
self.assertEqual(
(self.test_1st_task.effective_duration()).__str__(), "62")
self.assertEqual(
(self.test_2nd_task.planned_duration()).__str__(), "90")
self.assertEqual(
(self.test_2nd_task.effective_duration()).__str__(), "65")
StandardWorkFactory.create(
human_resource=self.human_resource,
date=datetime_now,
start_time=datetime_now,
stop_time=datetime_later_1,
task=self.test_1st_task,
reporting_period=self.test_reporting_period
)
StandardWorkFactory.create(
human_resource=self.human_resource,
date=datetime_later_2,
start_time=datetime_later_1,
stop_time=datetime_later_2,
task=self.test_1st_task,
reporting_period=self.test_reporting_period
)
StandardWorkFactory.create(
human_resource=self.human_resource,
date=datetime_now,
start_time=datetime_now,
stop_time=datetime_later_3,
task=self.test_2nd_task,
reporting_period=self.test_reporting_period
)
StandardWorkFactory.create(
human_resource=self.human_resource,
date=datetime_later_4,
start_time=datetime_now,
stop_time=datetime_later_4,
task=self.test_2nd_task,
reporting_period=self.test_reporting_period
)
self.assertEqual(
(self.test_1st_task.planned_duration()).__str__(), "60")
self.assertEqual(
(self.test_1st_task.effective_duration()).__str__(), "1")
self.assertEqual(
(self.test_2nd_task.planned_duration()).__str__(), "90")
self.assertEqual(
(self.test_2nd_task.effective_duration()).__str__(), "3")
<file_sep>/koalixcrm/crm/documents/invoice.py
# -*- coding: utf-8 -*-
from datetime import *
from django import forms
from django.db import models
from django.contrib import admin
from django.http import HttpResponseRedirect
from django.utils.translation import ugettext as _
from django.utils.html import format_html
from django.contrib.admin import helpers
from django.shortcuts import render
from django.contrib import messages
from django.template.context_processors import csrf
from koalixcrm.crm.const.status import *
from koalixcrm.crm.exceptions import *
from koalixcrm import accounting
from koalixcrm.crm.documents.sales_document import SalesDocument, OptionSalesDocument
from koalixcrm.crm.documents.sales_document_position import SalesDocumentPosition
from koalixcrm.plugin import *
from koalixcrm.accounting.models import Account
from koalixcrm.global_support_functions import limit_string_length
class Invoice(SalesDocument):
payable_until = models.DateField(verbose_name=_("To pay until"))
payment_bank_reference = models.CharField(verbose_name=_("Payment Bank Reference"), max_length=100, blank=True,
null=True)
status = models.CharField(max_length=1, choices=INVOICESTATUS)
def link_to_invoice(self):
if self.id:
return format_html("<a href='/admin/crm/invoice/%s' >%s</a>" % (str(self.id),
limit_string_length(str(self.description),
30)))
else:
return "Not present"
link_to_invoice.short_description = _("Invoice")
def create_from_reference(self, calling_model):
self.create_sales_document(calling_model)
self.status = 'C'
self.payable_until = date.today() + \
timedelta(days=self.customer.default_customer_billing_cycle.time_to_payment_date)
self.date_of_creation = date.today().__str__()
self.template_set = self.contract.get_template_set(self)
self.save()
self.attach_sales_document_positions(calling_model)
self.attach_text_paragraphs()
def register_invoice_in_accounting(self, request):
dict_prices = dict()
dict_tax = dict()
current_valid_accounting_period = accounting.models.AccountingPeriod.get_current_valid_accounting_period()
activa_account = accounting.models.Account.objects.filter(isopeninterestaccount=True)
if not self.is_complete_with_price():
raise IncompleteInvoice(_("Complete invoice and run price recalculation. Price may not be Zero"))
if len(activa_account) == 0:
raise OpenInterestAccountMissing(_("Please specify one open interest account in the accounting"))
for position in list(SalesDocumentPosition.objects.filter(sales_document=self.id)):
profit_account = position.product.accounting_product_categorie.profitAccount
dict_prices[profit_account] = position.last_calculated_price
dict_tax[profit_account] = position.last_calculated_tax
for booking in accounting.models.Booking.objects.filter(accountingPeriod=current_valid_accounting_period):
if booking.bookingReference == self:
raise InvoiceAlreadyRegistered("The invoice is already registered")
for profit_account, amount in iter(dict_prices.items()):
booking = accounting.models.Booking()
booking.toAccount = activa_account[0]
booking.fromAccount = profit_account
booking.bookingReference = self
booking.accountingPeriod = current_valid_accounting_period
booking.bookingDate = date.today().__str__()
booking.staff = request.user
booking.amount = amount
booking.lastmodifiedby = request.user
booking.save()
def register_payment_in_accounting(self, request, amount, payment_account):
current_valid_accounting_period = accounting.models.AccountingPeriod.get_current_valid_accounting_period()
activa_account = accounting.models.Account.objects.filter(isopeninterestaccount=True)
booking = accounting.models.Booking()
booking.toAccount = payment_account
booking.fromAccount = activa_account[0]
booking.bookingDate = date.today().__str__()
booking.bookingReference = self
booking.accountingPeriod = current_valid_accounting_period
booking.amount = amount
booking.staff = request.user
booking.lastmodifiedby = request.user
booking.save()
def __str__(self):
return _("Invoice") + ": " + self.id.__str__() + " " + _("from Contract") + ": " + self.contract.id.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Invoice')
verbose_name_plural = _('Invoices')
class OptionInvoice(OptionSalesDocument):
list_display = OptionSalesDocument.list_display + ('payable_until', 'status',)
list_filter = OptionSalesDocument.list_filter + ('status',)
ordering = OptionSalesDocument.ordering
search_fields = OptionSalesDocument.search_fields
fieldsets = OptionSalesDocument.fieldsets + (
(_('Invoice specific'), {
'fields': ('payable_until', 'status', 'payment_bank_reference' )
}),
)
class PaymentForm(forms.Form):
payment_amount = forms.DecimalField()
_selected_action = forms.CharField(widget=forms.MultipleHiddenInput)
payment_account = forms.ModelChoiceField(Account.objects.filter(account_type="A"))
def register_invoice_in_accounting(self, request, queryset):
try:
for obj in queryset:
obj.register_invoice_in_accounting(request)
self.message_user(request, _("Successfully registered Invoice in the Accounting"))
return;
except OpenInterestAccountMissing as e:
self.message_user(request, "Did not register Invoice in Accounting: " + e.__str__(), level=messages.ERROR)
return;
except IncompleteInvoice as e:
self.message_user(request, "Did not register Invoice in Accounting: " + e.__str__(), level=messages.ERROR)
return;
register_invoice_in_accounting.short_description = _("Register Invoice in Accounting")
# def unregisterInvoiceInAccounting(self, request, queryset):
# for obj in queryset:
# obj.createPDF(deliveryorder=True)
# self.message_user(request, _("Successfully unregistered Invoice in the Accounting"))
# unregisterInvoiceInAccounting.short_description = _("Unregister Invoice in Accounting")
def register_payment_in_accounting(self, request, queryset):
form = None
if request.POST.get('post'):
if 'cancel' in request.POST:
self.message_user(request, _("Canceled registration of payment in the accounting"), level=messages.ERROR)
return
elif 'register' in request.POST:
form = self.PaymentForm(request.POST)
if form.is_valid():
payment_amount = form.cleaned_data['payment_amount']
payment_account = form.cleaned_data['payment_account']
for obj in queryset:
obj.register_payment_in_accounting(request, payment_amount, payment_account)
self.message_user(request, _("Successfully registered Payment in the Accounting"))
return HttpResponseRedirect(request.get_full_path())
else:
form = self.PaymentForm
c = {'action_checkbox_name': helpers.ACTION_CHECKBOX_NAME,
'queryset': queryset,
'form': form}
c.update(csrf(request))
return render(request, 'crm/admin/register_payment.html', c)
register_payment_in_accounting.short_description = _("Register Payment in Accounting")
save_as = OptionSalesDocument.save_as
inlines = OptionSalesDocument.inlines
actions = ['create_purchase_confirmation', 'create_quote',
'create_delivery_note', 'create_purchase_order', 'create_pdf', 'create_payment_reminder',
'register_invoice_in_accounting', 'register_payment_in_accounting',]
pluginProcessor = PluginProcessor()
actions.extend(pluginProcessor.getPluginAdditions("invoiceActions"))
inlines.extend(pluginProcessor.getPluginAdditions("invoiceInlines"))
class InlineInvoice(admin.TabularInline):
model = Invoice
classes = ['collapse']
show_change_link = True
can_delete = True
extra = 1
readonly_fields = ('link_to_invoice',
'contract',
'customer',
'payable_until',
'status',
'last_pricing_date',
'last_calculated_price',
'last_calculated_tax')
fieldsets = (
(_('Invoice'), {
'fields': ('link_to_invoice',
'contract',
'customer',
'payable_until',
'status',
'last_pricing_date',
'last_calculated_price',
'last_calculated_tax')
}),
)
allow_add = False
<file_sep>/koalixcrm/crm/product/product_type.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.product.product_price import ProductPrice
from koalixcrm.crm.product.product_price import ProductPriceInlineAdminView
from koalixcrm.crm.product.unit_transform import UnitTransformInlineAdminView
from koalixcrm.crm.product.customer_group_transform import CustomerGroupTransformInlineAdminView
from koalixcrm.crm.product.currency_transform import CurrencyTransformInlineAdminView
class ProductType(models.Model):
description = models.TextField(verbose_name=_("Description"),
null=True,
blank=True)
title = models.CharField(verbose_name=_("Title"),
max_length=200)
product_type_identifier = models.CharField(verbose_name=_("Product Number"),
max_length=200,
null=True,
blank=True)
default_unit = models.ForeignKey("Unit", verbose_name=_("Unit"))
tax = models.ForeignKey("Tax",
blank=False,
null=False)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"),
null=True,
blank=True)
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
accounting_product_category = models.ForeignKey('accounting.ProductCategory',
verbose_name=_("Accounting Product Category"),
null=True,
blank=True)
def get_price(self, date, unit, customer, currency):
"""The function searches for a valid price and returns the price of the product as a decimal value.
Args:
koalixcrm.crm.contact.customer customer
koalixcrm.crm.product.unit unit
koalixcrm.crm.product.currency currency
datetime.date date
Returns:
when a match is found: dict customer_group_factors name=customer_group, value=factor
when no match is found: customer_group_factors is None
Raises:
In case the algorithm does not find a valid product price, the function raises a
NoPriceFound Exception"""
prices = ProductPrice.objects.filter(product_type=self)
valid_prices = list()
for price in list(prices):
currency_factor = price.get_currency_transform_factor(currency, self.id)
unit_factor = price.get_unit_transform_factor(unit, self.id)
group_factor = price.get_customer_group_transform_factor(customer, self.id)
date_in_range = price.is_date_in_range(date)
if date_in_range \
and currency_factor != 0 \
and unit_factor != 0 \
and group_factor != 0:
transformed_price = price.price*group_factor*unit_factor*currency_factor
valid_prices.append(transformed_price)
if len(valid_prices) > 0:
lowest_price = valid_prices[0]
for price in valid_prices:
if price < lowest_price:
lowest_price = price
return lowest_price
else:
raise ProductType.NoPriceFound(customer, unit, date, currency, self)
def get_tax_rate(self):
return self.tax.get_tax_rate()
def __str__(self):
return str(self.product_type_identifier) + ' ' + self.title.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Product Type')
verbose_name_plural = _('Product Types')
class NoPriceFound(Exception):
def __init__(self, customer, unit, date, currency, product):
self.customer = customer
self.unit = unit
self.date = date
self.product = product
self.currency = currency
return
def __str__(self):
return _("There is no Price for this product type") + ": " + self.product.__str__() + _(
"that matches the date") + ": " + self.date.__str__() + " ," + _(
"customer") + ": " + self.customer.__str__() + " ," + _(
"currency") + ": " + self.currency.__str__() + _(" and unit") + ":" + self.unit.__str__()
class ProductTypeAdminView(admin.ModelAdmin):
list_display = (
'product_type_identifier',
'title',
'default_unit',
'tax',
'accounting_product_category')
list_display_links = ('product_type_identifier',)
fieldsets = (
(_('Basics'), {
'fields': (
'product_type_identifier',
'title',
'description',
'default_unit',
'tax',
'accounting_product_category')
}),
)
inlines = [ProductPriceInlineAdminView,
UnitTransformInlineAdminView,
CurrencyTransformInlineAdminView,
CustomerGroupTransformInlineAdminView]
<file_sep>/koalixcrm/accounting/views.py
# -*- coding: utf-8 -*-
from os import path
from wsgiref.util import FileWrapper
from django.http import Http404
from django.http import HttpResponse
from django.http import HttpResponseRedirect
from koalixcrm.crm.exceptions import *
from koalixcrm.djangoUserExtension.exceptions import *
from django.utils.translation import ugettext as _
def export_pdf(calling_model_admin, request, whereToCreateFrom, whatToCreate, redirectTo):
"""This method exports PDFs provided by different Models in the accounting application
Args:
calling_model_admin (ModelAdmin): The calling ModelAdmin must be provided for error message response.
request: The request User is required to get the Calling User TemplateSets and to know where to
save the error message whereToCreateFrom (Model): The model from which a PDF should be exported
whatToCreate (str): What document Type that has to be created
redirectTo (str): String that describes to where the method sould redirect in case of an error
Returns:
HTTpResponse with a PDF when successful
HTTpResponseRedirect when not successful
Raises:
Http404 exception if anything goes wrong"""
try:
pdf = whereToCreateFrom.createPDF(request.user, whatToCreate)
response = HttpResponse(FileWrapper(open(pdf, 'rb')), content_type='application/pdf')
response['Content-Length'] = path.getsize(pdf)
except (TemplateSetMissing, UserExtensionMissing) as e:
if e.isinstance(UserExtensionMissing):
response = HttpResponseRedirect(redirectTo)
calling_model_admin.message_user(request, _("User Extension Missing"))
elif e.isinstance(TemplateSetMissing):
response = HttpResponseRedirect(redirectTo)
calling_model_admin.message_user(request, _("Templateset Missing"))
else:
raise Http404
return response
def export_xml(callingModelAdmin, request, whereToCreateFrom, whatToCreate, redirectTo):
"""This method exports XMLs provided by different Models in the accounting application
Args:
callingModelAdmin (ModelAdmin): The calling ModelAdmin must be provided for error message response.
request: The request User is required to get the Calling User TemplateSets and to know where
to save the error message hereToCreateFrom (Model): The model from which a PDF should be exported
whatToCreate (str): What objects that have to be serialized
redirectTo (str): String that describes to where the method sould redirect in case of an error
Returns:
HTTpResponse with a PDF when successful
HTTpResponseRedirect when not successful
Raises:
raises Http404 exception if anything goes wrong"""
try:
xml = whereToCreateFrom.createXML(request.user, whatToCreate)
response = HttpResponse(FileWrapper(open(xml, 'rb')), mimetype='application/xml')
response['Content-Length'] = path.getsize(xml)
except (TemplateSetMissing, UserExtensionMissing) as e:
if e.isinstance(UserExtensionMissing):
response = HttpResponseRedirect(redirectTo)
callingModelAdmin.message_user(request, _("User Extension Missing"))
elif e.isinstance(TemplateSetMissing):
response = HttpResponseRedirect(redirectTo)
callingModelAdmin.message_user(request, _("Templateset Missing"))
else:
raise Http404
return response
<file_sep>/koalixcrm/djangoUserExtension/migrations/0004_auto_20171210_2126.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-12-10 21:26
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0003_auto_20171110_1732'),
]
operations = [
migrations.CreateModel(
name='DocumentTemplate',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(blank=True, max_length=100, null=True, verbose_name='Title')),
('xsl_file', filebrowser.fields.FileBrowseField(max_length=200, verbose_name='XSL File')),
('fop_config_file', filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='FOP Configuration File')),
('logo', filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='Logo for the PDF generation')),
],
options={
'verbose_name': 'Document template',
'verbose_name_plural': 'Document templates',
},
),
migrations.RemoveField(
model_name='templateset',
name='addresser',
),
migrations.RemoveField(
model_name='templateset',
name='balancesheetXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='bankingaccountref',
),
migrations.RemoveField(
model_name='templateset',
name='deilveryorderXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='footerTextpurchaseorders',
),
migrations.RemoveField(
model_name='templateset',
name='footerTextsalesorders',
),
migrations.RemoveField(
model_name='templateset',
name='fopConfigurationFile',
),
migrations.RemoveField(
model_name='templateset',
name='headerTextpurchaseorders',
),
migrations.RemoveField(
model_name='templateset',
name='headerTextsalesorders',
),
migrations.RemoveField(
model_name='templateset',
name='invoiceXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='logo',
),
migrations.RemoveField(
model_name='templateset',
name='organisationname',
),
migrations.RemoveField(
model_name='templateset',
name='pagefooterleft',
),
migrations.RemoveField(
model_name='templateset',
name='pagefootermiddle',
),
migrations.RemoveField(
model_name='templateset',
name='profitLossStatementXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='purchaseconfirmationXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='purchaseorderXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='quoteXSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='reminder1XSLFile',
),
migrations.RemoveField(
model_name='templateset',
name='reminder2XSLFile',
),
migrations.CreateModel(
name='BalanceSheetTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Balance sheet template',
'verbose_name_plural': 'Balance sheet templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='DeliveryNoteTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Delivery note template',
'verbose_name_plural': 'Delivery note templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='InvoiceTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Invoice template',
'verbose_name_plural': 'Invoice templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='PaymentReminderTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Payment reminder template',
'verbose_name_plural': 'Payment reminder templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='ProfitLossStatementTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Profit loss statement template',
'verbose_name_plural': 'Profit loss statement templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='PurchaseConfirmationTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Purchase confirmation template',
'verbose_name_plural': 'Purchase confirmation templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='PurchaseOrderTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Purchase order template',
'verbose_name_plural': 'Purchase order templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.CreateModel(
name='QuoteTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Quote template',
'verbose_name_plural': 'Quote templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.DeleteModel(
name='XSLFile',
),
migrations.AddField(
model_name='templateset',
name='balance_sheet_statement_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.BalanceSheetTemplate'),
),
migrations.AddField(
model_name='templateset',
name='delivery_note_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.DeliveryNoteTemplate'),
),
migrations.AddField(
model_name='templateset',
name='invoice_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.InvoiceTemplate'),
),
migrations.AddField(
model_name='templateset',
name='payment_reminder_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.PaymentReminderTemplate'),
),
migrations.AddField(
model_name='templateset',
name='profit_loss_statement_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.ProfitLossStatementTemplate'),
),
migrations.AddField(
model_name='templateset',
name='purchase_confirmation_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.PurchaseConfirmationTemplate'),
),
migrations.AddField(
model_name='templateset',
name='purchase_order_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.PurchaseOrderTemplate'),
),
migrations.AddField(
model_name='templateset',
name='quote_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.QuoteTemplate'),
),
]
<file_sep>/documentation/source/code_documentation/task.rst
.. highlight:: rst
Task
----
.. automodule:: koalixcrm.crm.reporting.task
:members:
<file_sep>/koalixcrm/djangoUserExtension/migrations/0001_initial.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.2 on 2017-07-05 17:02
from __future__ import unicode_literals
import django.db.models.deletion
import filebrowser.fields
from django.conf import settings
from django.db import migrations, models
class Migration(migrations.Migration):
initial = True
dependencies = [
('crm', '0001_initial'),
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
]
operations = [
migrations.CreateModel(
name='TemplateSet',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('organisationname', models.CharField(max_length=200, verbose_name='Name of the Organisation')),
('title', models.CharField(max_length=100, verbose_name='Title')),
('logo', filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True,
verbose_name='Logo for the PDF generation')),
('bankingaccountref',
models.CharField(blank=True, max_length=60, null=True, verbose_name='Reference to Banking Account')),
('addresser', models.CharField(blank=True, max_length=200, null=True, verbose_name='Addresser')),
('fopConfigurationFile', filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True,
verbose_name='FOP Configuration File')),
('footerTextsalesorders',
models.TextField(blank=True, null=True, verbose_name='Footer Text On Salesorders')),
('headerTextsalesorders',
models.TextField(blank=True, null=True, verbose_name='Header Text On Salesorders')),
('headerTextpurchaseorders',
models.TextField(blank=True, null=True, verbose_name='Header Text On Purchaseorders')),
('footerTextpurchaseorders',
models.TextField(blank=True, null=True, verbose_name='Footer Text On Purchaseorders')),
('pagefooterleft',
models.CharField(blank=True, max_length=40, null=True, verbose_name='Page Footer Left')),
('pagefootermiddle',
models.CharField(blank=True, max_length=40, null=True, verbose_name='Page Footer Middle')),
],
options={
'verbose_name': 'Templateset',
'verbose_name_plural': 'Templatesets',
},
),
migrations.CreateModel(
name='UserExtension',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('defaultCurrency', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Currency')),
('defaultTemplateSet',
models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.TemplateSet')),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL)),
],
options={
'verbose_name': 'User Extention',
'verbose_name_plural': 'User Extentions',
},
),
migrations.CreateModel(
name='UserExtensionEmailAddress',
fields=[
('emailaddress_ptr',
models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True,
primary_key=True, serialize=False, to='crm.EmailAddress')),
('purpose', models.CharField(
choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')],
max_length=1, verbose_name='Purpose')),
('userExtension', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
to='djangoUserExtension.UserExtension')),
],
options={
'verbose_name': 'Email Address for User Extention',
'verbose_name_plural': 'Email Address for User Extention',
},
bases=('crm.emailaddress',),
),
migrations.CreateModel(
name='UserExtensionPhoneAddress',
fields=[
('phoneaddress_ptr',
models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True,
primary_key=True, serialize=False, to='crm.PhoneAddress')),
('purpose', models.CharField(
choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')],
max_length=1, verbose_name='Purpose')),
('userExtension', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
to='djangoUserExtension.UserExtension')),
],
options={
'verbose_name': 'Phonenumber for User Extention',
'verbose_name_plural': 'Phonenumber for User Extention',
},
bases=('crm.phoneaddress',),
),
migrations.CreateModel(
name='UserExtensionPostalAddress',
fields=[
('postaladdress_ptr',
models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True,
primary_key=True, serialize=False, to='crm.PostalAddress')),
('purpose', models.CharField(
choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')],
max_length=1, verbose_name='Purpose')),
('userExtension', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
to='djangoUserExtension.UserExtension')),
],
options={
'verbose_name': 'Postal Address for User Extention',
'verbose_name_plural': 'Postal Address for User Extention',
},
bases=('crm.postaladdress',),
),
migrations.CreateModel(
name='XSLFile',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(blank=True, max_length=100, null=True, verbose_name='Title')),
('xslfile', filebrowser.fields.FileBrowseField(max_length=200, verbose_name='XSL File')),
],
options={
'verbose_name': 'XSL File',
'verbose_name_plural': 'XSL Files',
},
),
migrations.AddField(
model_name='templateset',
name='balancesheetXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
related_name='db_reltemplatebalancesheet', to='djangoUserExtension.XSLFile',
verbose_name='XSL File for Balancesheet'),
),
migrations.AddField(
model_name='templateset',
name='deilveryorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
related_name='db_reltemplatedeliveryorder', to='djangoUserExtension.XSLFile',
verbose_name='XSL File for Deilvery Order'),
),
migrations.AddField(
model_name='templateset',
name='invoiceXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplateinvoice',
to='djangoUserExtension.XSLFile', verbose_name='XSL File for Invoice'),
),
migrations.AddField(
model_name='templateset',
name='profitLossStatementXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
related_name='db_reltemplateprofitlossstatement', to='djangoUserExtension.XSLFile',
verbose_name='XSL File for Profit Loss Statement'),
),
migrations.AddField(
model_name='templateset',
name='purchaseconfirmationXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
related_name='db_reltemplatepurchaseconfirmation', to='djangoUserExtension.XSLFile',
verbose_name='XSL File for Purchase Confirmation'),
),
migrations.AddField(
model_name='templateset',
name='purchaseorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE,
related_name='db_reltemplatepurchaseorder', to='djangoUserExtension.XSLFile',
verbose_name='XSL File for Purchaseorder'),
),
migrations.AddField(
model_name='templateset',
name='quoteXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatequote',
to='djangoUserExtension.XSLFile', verbose_name='XSL File for Quote'),
),
]
<file_sep>/documentation/source/architecture.rst
.. highlight:: rst
Architecture
========
Standard installation with docker, postgres
-------------------------------------------
.. image:: /images/koalixcrm_architecture_django_webserver.png
Standard installation with docker, postgres and apache2
-------------------------------------------------------
.. image:: /images/koalixcrm_architecture_dedicated_webserver.png
<file_sep>/koalixcrm/djangoUserExtension/migrations/0007_auto_20180702_1628.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-07-02 16:28
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0006_auto_20180701_2128'),
]
operations = [
migrations.RenameField(
model_name='userextension',
old_name='defaultCurrency',
new_name='default_currency',
),
migrations.RenameField(
model_name='userextension',
old_name='defaultTemplateSet',
new_name='default_template_set',
),
]
<file_sep>/koalixcrm/djangoUserExtension/user_extension/text_paragraph.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.purpose import *
class TextParagraphInDocumentTemplate(models.Model):
document_template = models.ForeignKey("djangoUserExtension.DocumentTemplate")
purpose = models.CharField(verbose_name=_("Purpose"), max_length=2, choices=PURPOSESTEXTPARAGRAPHINDOCUMENTS)
text_paragraph = models.TextField(verbose_name=_("Text"), blank=False, null=False)
class Meta:
app_label = "crm"
verbose_name = _('TextParagraphInDocumentTemplate')
verbose_name_plural = _('TextParagraphInDocumentTemplates')
def __str__(self):
return str(self.id)
class InlineTextParagraph(admin.TabularInline):
model = TextParagraphInDocumentTemplate
extra = 1
classes = ('collapse-open',)
fieldsets = (
(_('Basics'), {
'fields': ('purpose', 'text_paragraph',)
}),
)
allow_add = True<file_sep>/koalixcrm/djangoUserExtension/migrations/0005_auto_20180612_1924.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-12 19:24
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
]
operations = [
migrations.CreateModel(
name='MonthlyProjectSummaryTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Monthly project summary template',
'verbose_name_plural': 'Monthly project summary templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.AddField(
model_name='templateset',
name='monthly_project_summary_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.MonthlyProjectSummaryTemplate'),
),
]
<file_sep>/koalixcrm/crm/tests/test_view_work_entry_form.py
# -*- coding: utf-8 -*-
import pytest
import datetime
from django.test import LiveServerTestCase
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from koalixcrm.test_support_functions import *
from koalixcrm.crm.factories.factory_user import AdminUserFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.crm.reporting.work import Work
from koalixcrm.crm.factories.factory_human_resource import StandardHumanResourceFactory
class TimeTrackingWorkEntry(LiveServerTestCase):
def setUp(self):
firefox_options = webdriver.firefox.options.Options()
firefox_options.set_headless(headless=True)
self.selenium = webdriver.Firefox(firefox_options=firefox_options)
self.test_user = AdminUserFactory.create()
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.test_user_extension = StandardUserExtensionFactory.create(user=self.test_user)
self.test_human_resource = StandardHumanResourceFactory.create(user=self.test_user_extension)
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.test_reporting_period = StandardReportingPeriodFactory.create()
self.test_1st_task = StandardTaskFactory.create(title="1st Test Task",
project=self.test_reporting_period.project,
)
def tearDown(self):
self.selenium.quit()
@pytest.mark.front_end_tests
def test_registration_of_work(self):
selenium = self.selenium
# login
selenium.get('%s%s' % (self.live_server_url, '/koalixcrm/crm/reporting/time_tracking/'))
# the browser will be redirected to the login page
timeout = 5
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_username'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
username = selenium.find_element_by_xpath('//*[@id="id_username"]')
password = selenium.find_element_by_xpath('//*[@id="id_password"]')
submit_button = selenium.find_element_by_xpath('/html/body/div/article/div/div/form/div/ul/li/input')
username.send_keys("admin")
password.send_keys("<PASSWORD>")
submit_button.send_keys(Keys.RETURN)
# after the login, the browser is redirected to the target url /koalixcrm/crm/reporting/time_tracking
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_form-0-project'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
# find the form element
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-project"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-task"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-date"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-start_time"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-stop_time"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-worked_hours"]')
assert_when_element_does_not_exist(self, '//*[@id="id_form-0-description"]')
assert_when_element_does_not_exist(self, 'save')
project = selenium.find_element_by_xpath('//*[@id="id_form-0-project"]')
task = selenium.find_element_by_xpath('//*[@id="id_form-0-task"]')
date = selenium.find_element_by_xpath('//*[@id="id_form-0-date"]')
start_time = selenium.find_element_by_xpath('//*[@id="id_form-0-start_time"]')
stop_time = selenium.find_element_by_xpath('//*[@id="id_form-0-stop_time"]')
description = selenium.find_element_by_xpath('//*[@id="id_form-0-description"]')
project.send_keys(self.test_reporting_period.project.id.__str__())
date.send_keys(datetime.date.today().__str__())
start_time.send_keys(datetime.time(11, 55).__str__())
stop_time.send_keys(datetime.time(12, 55).__str__())
description.send_keys("This is a test work entered through the front-end")
task.send_keys(self.test_1st_task.id.__str__())
save_button = selenium.find_element_by_name('save')
save_button.send_keys(Keys.RETURN)
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_form-1-project'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
assert_when_element_does_not_exist(self, '//*[@id="id_form-1-task"]')
work = Work.objects.get(description="This is a test work entered through the front-end")
self.assertEqual(type(work), Work)
<file_sep>/koalixcrm/crm/migrations/0002_auto_20170927_2042.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-09-27 20:42
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0001_initial'),
]
operations = [
migrations.AlterField(
model_name='salescontract',
name='lastCalculatedPrice',
field=models.DecimalField(blank=True, decimal_places=2, max_digits=17, null=True, verbose_name='Last Calculated Price With Tax'),
),
]
<file_sep>/koalixcrm/crm/contact/call.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from django.utils import timezone
from koalixcrm.crm.const.status import *
class Call(models.Model):
staff = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Staff"),
related_name="db_relcallstaff",
blank=True,
null=True)
description = models.TextField(verbose_name=_("Description"))
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
date_due = models.DateTimeField(verbose_name=_("Date due"),
default=datetime.now,
blank=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
blank=True,
null=True,
verbose_name=_("Last modified by"),
related_name="db_calllstmodified")
status = models.CharField(verbose_name=_("Status"),
max_length=1,
choices=CALLSTATUS,
default="P")
def __str__(self):
return _("Call") + " " + str(self.id)
class CallOverdueFilter(admin.SimpleListFilter):
title = _('Is call overdue')
parameter_name = 'date_due'
def lookups(self, request, model_admin):
return (
('overdue', _('Overdue')),
('planned', _('Planned')),
)
def queryset(self, request, queryset):
if self.value() == 'planned':
return queryset.filter(date_due__gt=timezone.now())
elif self.value() == 'overdue':
return queryset.filter(date_due__lt=timezone.now()).exclude(status__in=['F', 'S'])
else:
return queryset
class OptionCall(admin.ModelAdmin):
list_display = ('id',
'description',
'date_due',
'purpose',
'status',
'cperson',
'is_call_overdue',
'get_contact_name')
fieldsets = (('', {'fields': ('staff',
'description',
'date_due',
'purpose',
'company',
'cperson',
'status')}),)
list_filter = [CallOverdueFilter]
@staticmethod
def get_contact_name(obj):
return obj.company.name
get_contact_name.short_description = _("Company")
@staticmethod
def is_call_overdue(obj):
if obj.date_due < timezone.now() and obj.status not in ['F', 'S']:
overdue = True
else:
overdue = False
return overdue
is_call_overdue.short_description = _("Is call overdue")
class OptionVisit(admin.ModelAdmin):
list_display = ('id',
'description',
'cperson',
'date_due',
'purpose',
'get_contact_name',
'status',
'ref_call',)
fieldsets = (('', {'fields': ('staff',
'description',
'date_due',
'purpose',
'company',
'cperson',
'status')}),)
@staticmethod
def get_contact_name(obj):
return obj.company.name
get_contact_name.short_description = _("Company")
<file_sep>/koalixcrm/crm/rest/product_rest.py
from rest_framework import serializers
from koalixcrm.accounting.accounting.product_category import ProductCategory
from koalixcrm.accounting.rest.product_categorie_rest import ProductCategoryMinimalJSONSerializer
from koalixcrm.crm.product.product_type import ProductType
from koalixcrm.crm.product.tax import Tax
from koalixcrm.crm.product.unit import Unit
from koalixcrm.crm.rest.tax_rest import OptionTaxJSONSerializer
from koalixcrm.crm.rest.unit_rest import OptionUnitJSONSerializer
class ProductJSONSerializer(serializers.HyperlinkedModelSerializer):
productNumber = serializers.IntegerField(source='product_number', allow_null=False)
unit = OptionUnitJSONSerializer(source='default_unit', allow_null=False)
tax = OptionTaxJSONSerializer(allow_null=False)
productCategory = ProductCategoryMinimalJSONSerializer(source='accounting_product_categorie', allow_null=False)
class Meta:
model = ProductType
fields = ('id',
'productNumber',
'title',
'unit',
'tax',
'productCategory')
depth = 1
def create(self, validated_data):
product = ProductType()
product.product_number = validated_data['product_number']
product.title = validated_data['title']
# Deserialize default_unit
default_unit = validated_data.pop('default_unit')
if default_unit:
if default_unit.get('id', None):
product.default_unit = Unit.objects.get(id=default_unit.get('id', None))
else:
product.default_unit = None
# Deserialize tax
tax = validated_data.pop('tax')
if tax:
if tax.get('id', None):
product.tax = Tax.objects.get(id=tax.get('id', None))
else:
product.tax = None
# Deserialize product category
product_category = validated_data.pop('accounting_product_categorie')
if product_category:
if product_category.get('id', None):
product.accounting_product_category = ProductCategory.objects.get(id=product_category.get('id', None))
else:
product.accounting_product_category = None
product.save()
return product
def update(self, instance, validated_data):
instance.title = validated_data['title']
instance.product_number = validated_data['product_number']
# Deserialize default_unit
default_unit = validated_data.pop('default_unit')
if default_unit:
if default_unit.get('id', instance.default_unit):
instance.default_unit = Unit.objects.get(id=default_unit.get('id', None))
else:
instance.default_unit = instance.default_unit
else:
instance.default_unit = None
# Deserialize tax
tax = validated_data.pop('tax')
if tax:
if tax.get('id', instance.default_unit):
instance.tax = Tax.objects.get(id=tax.get('id', None))
else:
instance.tax = instance.tax
else:
instance.tax = None
# Deserialize product category
product_category = validated_data.pop('accounting_product_categorie')
if product_category:
if product_category.get('id', instance.accounting_product_categorie):
instance.accounting_product_categorie = ProductCategory.objects.get(
id=product_category.get('id', None))
else:
instance.accounting_product_categorie = instance.accounting_product_categorie
else:
instance.accounting_product_categorie = None
instance.save()
return instance
<file_sep>/koalixcrm/crm/reporting/resource_price.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.product.price import Price
from koalixcrm.crm.reporting.resource import Resource
class ResourcePrice(Price):
resource = models.ForeignKey(Resource,
verbose_name=_('Resource'),
blank=False,
null=False)
def __str__(self):
return str(self.price) + " " + str(self.currency.short_name)
class ResourcePriceInlineAdminView(admin.TabularInline):
model = ResourcePrice
extra = 1
classes = ['collapse']
fieldsets = (
('Basic', {
'fields': ('price',
'currency',
'unit',
'valid_from',
'valid_until',
'customer_group')
}),
)
allow_add = True
<file_sep>/koalixcrm/crm/reporting/work.py
# -*- coding: utf-8 -*-
from django.db import models
from django.forms import ValidationError
from django.contrib import admin
from django.utils.html import format_html
from django.utils.translation import ugettext as _
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.global_support_functions import *
from koalixcrm.crm.exceptions import ReportingPeriodDoneDeleteNotPossible
from django.contrib import messages
class Work(models.Model):
human_resource = models.ForeignKey("HumanResource")
date = models.DateField(verbose_name=_("Date"),
blank=False,
null=False)
start_time = models.DateTimeField(verbose_name=_("Start Time"),
blank=True,
null=True)
stop_time = models.DateTimeField(verbose_name=_("Stop Time"),
blank=True,
null=True)
worked_hours = models.DecimalField(verbose_name=_("Worked Hours"),
max_digits=5,
decimal_places=2,
blank=True,
null=True)
short_description = models.CharField(verbose_name=_("Short Description"),
max_length=300,
blank=False,
null=False)
description = models.TextField(verbose_name=_("Text"),
blank=True,
null=True)
task = models.ForeignKey("Task",
verbose_name=_('Task'),
blank=False,
null=False)
reporting_period = models.ForeignKey("ReportingPeriod",
verbose_name=_('Reporting Period'),
blank=False,
null=False)
def link_to_work(self):
if self.id:
return format_html("<a href='/admin/crm/work/%s' >%s</a>" % (str(self.id), str(self.id)))
else:
return "Not present"
link_to_work.short_description = _("Work")
def get_short_description(self):
if self.short_description:
return self.short_description
elif self.description:
return limit_string_length(self.description, 100)
else:
return _("Please add description")
get_short_description.short_description = _("Short description");
def serialize_to_xml(self):
objects = [self, ]
main_xml = PDFExport.write_xml(objects)
return main_xml
def effort_hours(self):
if self.effort_seconds() != 0:
return self.effort_seconds()/3600
else:
return 0
def effort_seconds(self):
if not self.start_stop_pattern_complete() and not bool(self.worked_hours):
return 0
elif (not bool(self.stop_time)) or (not bool(self.start_time)):
return float(self.worked_hours)*3600
else:
return (self.stop_time - self.start_time).total_seconds()
def effort_as_string(self):
return str(self.effort_hours()) + " h"
def start_stop_pattern_complete(self):
return bool(self.start_time) & bool(self.stop_time)
def start_stop_pattern_stop_missing(self):
return bool(self.start_time) & (not bool(self.stop_time))
def start_stop_pattern_start_missing(self):
return bool(self.stop_time) & (not bool(self.start_time))
def __str__(self):
return _("Work") + ": " + str(self.id) + " " + _("from Person") + ": " + str(self.human_resource.id)
def check_working_hours(self):
"""This method checks that the working hour is correctly provided either using the start_stop pattern
or by providing the worked_hours in total.
Args:
Returns:
True when no ValidationError was raised
Raises:
may raise ValidationError exception"""
if self.start_stop_pattern_complete() & bool(self.worked_hours):
raise ValidationError('Please either set the start, stop time or worked hours (not both)', code='invalid')
elif self.start_stop_pattern_start_missing() or self.start_stop_pattern_stop_missing():
raise ValidationError('Set start and stop time', code='invalid')
return True
def clean(self):
cleaned_data = super(Work, self).clean()
self.check_working_hours()
return cleaned_data
def delete(self, using=None, keep_parents=False):
if self.reporting_period.status.is_done:
raise ReportingPeriodDoneDeleteNotPossible("It was not possible to delete the work")
else:
super(Work, self).delete(using, keep_parents)
def save(self, *args, **kwargs):
if self.reporting_period.status.is_done:
raise ReportingPeriodDoneDeleteNotPossible("It was not possible to update the work")
else:
super(Work, self).save(*args, **kwargs)
class Meta:
app_label = "crm"
verbose_name = _('Work')
verbose_name_plural = _('Work')
class WorkAdminView(admin.ModelAdmin):
list_display = ('link_to_work',
'human_resource',
'task',
'get_short_description',
'date',
'reporting_period',
'effort_as_string')
list_filter = ('task', 'date')
ordering = ('-id',)
fieldsets = (
(_('Work'), {
'fields': ('human_resource',
'date',
'start_time',
'stop_time',
'worked_hours',
'short_description',
'description',
'task',
'reporting_period',)
}),
)
save_as = True
actions = ['delete_selected', ]
def delete_selected(self, request, queryset):
for obj in queryset:
if obj.reporting_period.status.is_done:
self.message_user(request, _("Delete is not allowed because the work"
" is used in a reporting period which is marked "
"'as done'"),
level=messages.ERROR)
else:
obj.delete()
delete_selected.short_description = _("Delete Selected Work")
class WorkInlineAdminView(admin.TabularInline):
model = Work
readonly_fields = ('link_to_work',
'get_short_description',
'human_resource',
'date',
'effort_as_string',)
fieldsets = (
(_('Work'), {
'fields': ('link_to_work',
'get_short_description',
'human_resource',
'date',
'effort_as_string',)
}),
)
extra = 0
def has_add_permission(self, request, obj=None):
return False
def has_delete_permission(self, request, obj=None):
return False
<file_sep>/koalixcrm/crm/const/status.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
INVOICESTATUS = (
('P', _('Payed')),
('C', _('Invoice created')),
('I', _('Invoice sent')),
('F', _('First reminder sent')),
('R', _('Second reminder sent')),
('U', _('Customer cant pay')),
('D', _('Deleted')),
)
QUOTESTATUS = (
('S', _('Success')),
('I', _('Quote created')),
('Q', _('Quote sent')),
('F', _('First reminder sent')),
('R', _('Second reminder sent')),
('D', _('Deleted')),
)
PURCHASEORDERSTATUS = (
('O', _('Ordered')),
('D', _('Delayed')),
('Y', _('Delivered')),
('I', _('Invoice registered')),
('P', _('Invoice payed')),
)
DELIVERYNOTESTATUS = (
('C', _('Created')),
('S', _('Sent')),
('R', _('Received')),
('R', _('Lost')),
)
CALLSTATUS = (
('P', _('Planned')),
('D', _('Delayed')),
('R', _('ToRecall')),
('F', _('Failed')),
('S', _('Success')),
)
<file_sep>/koalixcrm/crm/factories/factory_agreement_status.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import AgreementStatus
class AgreedAgreementStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = AgreementStatus
django_get_or_create = ('title',)
title = "Done"
description = "This agreement is agreed"
is_agreed = True
class PlannedAgreementStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = AgreementStatus
django_get_or_create = ('title',)
title = "Planned"
description = "This agreement is planned"
is_agreed = False
class StartedAgreementStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = AgreementStatus
django_get_or_create = ('title',)
title = "Started"
description = "This agreement is started"
is_agreed = False
<file_sep>/koalixcrm/crm/migrations/0014_auto_20180108_2048.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-08 20:48
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0013_auto_20180105_2159'),
]
operations = [
migrations.RemoveField(
model_name='invoice',
name='derived_from_quote',
),
migrations.AddField(
model_name='salescontract',
name='derived_from_sales_contract',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.SalesContract'),
),
]
<file_sep>/koalixcrm/crm/tests/test_version_increase.py
from django.test import TestCase
from koalixcrm.global_support_functions import get_string_between
from subprocess import Popen, PIPE
from koalixcrm.version import KOALIXCRM_VERSION
import pytest
class VersionIncreaseTest(TestCase):
@staticmethod
def get_all_koalixcrm_version_from_pip():
process_out = Popen(['pip install koalix-crm=='], shell=True, stderr=PIPE)
output, string_containing_all_version = process_out.communicate()
all_koalixcrm_version_csv = get_string_between(string_containing_all_version.__str__(), "koalix-crm== (", ")")
all_koalixcrm_version = all_koalixcrm_version_csv.split(", ")
return all_koalixcrm_version
def setUp(self):
self.available_versions = VersionIncreaseTest.get_all_koalixcrm_version_from_pip()
@pytest.mark.version_increase
def test_version_increase(self):
last_version = ""
for version in self.available_versions:
last_version = version
if version == KOALIXCRM_VERSION:
break
self.assertNotEqual(last_version, KOALIXCRM_VERSION)
<file_sep>/koalixcrm/crm/documents/sales_document_position.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.core.validators import MinValueValidator
from django.utils.translation import ugettext as _
class Position(models.Model):
position_number = models.PositiveIntegerField(verbose_name=_("Position Number"),
validators=[MinValueValidator(1)])
quantity = models.DecimalField(verbose_name=_("Quantity"),
decimal_places=3,
max_digits=10)
description = models.TextField(verbose_name=_("Description"),
blank=True, null=True)
discount = models.DecimalField(max_digits=5, decimal_places=2,
verbose_name=_("Discount"),
blank=True,
null=True)
product_type = models.ForeignKey("ProductType",
verbose_name=_("Product"),
blank=False,
null=True)
unit = models.ForeignKey("Unit", verbose_name=_("Unit"),
blank=True,
null=True)
sent_on = models.DateField(verbose_name=_("Shipment on"),
blank=True,
null=True)
overwrite_product_price = models.BooleanField(verbose_name=_('Overwrite Product Price'))
position_price_per_unit = models.DecimalField(verbose_name=_("Price Per Unit"),
max_digits=17,
decimal_places=2,
blank=True, null=True)
last_pricing_date = models.DateField(verbose_name=_("Last Pricing Date"),
blank=True,
null=True)
last_calculated_price = models.DecimalField(max_digits=17,
decimal_places=2,
verbose_name=_("Last Calculated Price"),
blank=True, null=True)
last_calculated_tax = models.DecimalField(max_digits=17,
decimal_places=2,
verbose_name=_("Last Calculated Tax"),
blank=True,
null=True)
def __str__(self):
return _("Position") + ": " + self.id.__str__()
class Meta:
app_label = "crm"
ordering = ["position_number"]
verbose_name = _('Position')
verbose_name_plural = _('Positions')
class SalesDocumentPosition(Position):
sales_document = models.ForeignKey("SalesDocument", verbose_name=_("Contract"))
class Meta:
app_label = "crm"
verbose_name = _('Position in Sales Document')
verbose_name_plural = _('Positions Sales Document')
@staticmethod
def add_positions(position_class, object_to_create_pdf):
from koalixcrm.crm.product.unit import Unit
from koalixcrm.crm.product.product_type import ProductType
objects = list(position_class.objects.filter(sales_document=object_to_create_pdf.id))
for position in list(position_class.objects.filter(sales_document=object_to_create_pdf.id)):
objects += list(Position.objects.filter(id=position.id))
objects += list(ProductType.objects.filter(id=position.product_type.id))
objects += list(Unit.objects.filter(id=position.unit.id))
return objects
def create_position(self, calling_model, attach_to_model):
"""Copies all the content of the calling model and attaches
links itself to the attach_to_model, this function is usually
used within the create invoice, quote, reminder,... functions"""
self.product_type = calling_model.product_type
self.position_number = calling_model.position_number
self.quantity = calling_model.quantity
self.description = calling_model.description
self.discount = calling_model.discount
self.unit = calling_model.unit
self.sent_on = calling_model.sent_on
self.overwrite_product_price = calling_model.overwrite_product_price
self.position_price_per_unit = calling_model.position_price_per_unit
self.last_pricing_date = calling_model.last_pricing_date
self.last_calculated_price = calling_model.last_calculated_price
self.last_calculated_tax = calling_model.last_calculated_tax
self.sales_document = attach_to_model
self.save()
def __str__(self):
return _("Sales Document Position") + ": " + str(self.id)
class SalesDocumentInlinePosition(admin.TabularInline):
model = SalesDocumentPosition
extra = 1
classes = ['expand']
fieldsets = (
('', {
'fields': (
'position_number',
'quantity',
'unit',
'product_type',
'description',
'discount',
'overwrite_product_price',
'position_price_per_unit',
'sent_on')
}),
)
allow_add = True
<file_sep>/koalixcrm/accounting/rest/product_categorie_rest.py
# -*- coding: utf-8 -*-
from koalixcrm.accounting.rest.account_rest import OptionAccountJSONSerializer
from rest_framework import serializers
from koalixcrm.accounting.accounting.product_category import ProductCategory
from koalixcrm.accounting.models import Account
class ProductCategoryMinimalJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
title = serializers.CharField(read_only=True)
class Meta:
model = Account
fields = ('id',
'title')
class ProductCategoryJSONSerializer(serializers.HyperlinkedModelSerializer):
profitAccount = OptionAccountJSONSerializer(source='profit_account')
lossAccount = OptionAccountJSONSerializer(source='loss_account')
class Meta:
model = ProductCategory
fields = ('id',
'title',
'profitAccount',
'lossAccount')
depth = 1
def create(self, validated_data):
product_category = ProductCategory()
product_category.title = validated_data['title']
# Deserialize profit account
profit_account = validated_data.pop('profit_account')
if profit_account:
if profit_account.get('id', None):
product_category.profit_account = Account.objects.get(id=profit_account.get('id', None))
else:
product_category.profit_account = None
# Deserialize loss account
loss_account = validated_data.pop('loss_account')
if loss_account:
if loss_account.get('id', None):
product_category.loss_account = Account.objects.get(id=loss_account.get('id', None))
else:
product_category.loss_account = None
product_category.save()
return product_category
def update(self, instance, validated_data):
instance.title = validated_data.get('title', instance.title)
# Deserialize profit account
profit_account = validated_data.pop('profit_account')
if profit_account:
if profit_account.get('id', instance.profit_account_id):
instance.profit_account = Account.objects.get(id=profit_account.get('id', None))
else:
instance.profit_account = instance.profit_account_id
else:
instance.profit_account = None
# Deserialize loss account
loss_account = validated_data.pop('loss_account')
if loss_account:
if loss_account.get('id', instance.loss_account_id):
instance.loss_account = Account.objects.get(id=loss_account.get('id', None))
else:
instance.loss_account = instance.loss_account_id
else:
instance.loss_account = None
instance.save()
return instance
<file_sep>/koalixcrm/crm/contact/person.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.postaladdressprefix import *
class Person(models.Model):
prefix = models.CharField(max_length=1,
choices=POSTALADDRESSPREFIX,
verbose_name=_("Prefix"),
blank=True,
null=True)
name = models.CharField(max_length=100,
verbose_name=_("Name"),
blank=True,
null=True)
pre_name = models.CharField(max_length=100,
verbose_name=_("Pre-name"),
blank=True,
null=True)
email = models.EmailField(max_length=200,
verbose_name=_("Email Address"))
phone = models.CharField(max_length=20,
verbose_name=_("Phone Number"))
role = models.CharField(max_length=100,
verbose_name=_("Role"),
blank=True,
null=True)
companies = models.ManyToManyField("Contact",
through='ContactPersonAssociation',
verbose_name=_('Works at'), blank=True)
def get_pre_name(self):
if self.pre_name:
return self.pre_name
else:
return "n/a"
def get_name(self):
if self.name:
return self.name
else:
return "n/a"
def get_email(self):
if self.email:
return self.email
else:
return "n/a"
def __str__(self):
return self.get_pre_name() + ' ' + self.get_name() + ' - ' + self.get_email()
class Meta:
app_label = "crm"
verbose_name = _('Person')
verbose_name_plural = _('People')
<file_sep>/koalixcrm/crm/rest/restinterface.py
# -*- coding: utf-8 -*-
from django.conf import settings
from django.contrib.auth.decorators import login_required
from django.utils.decorators import method_decorator
from rest_framework import viewsets
from rest_framework.renderers import JSONRenderer, BrowsableAPIRenderer
from rest_framework_xml.renderers import XMLRenderer
from koalixcrm.crm.documents.contract import Contract, ContractJSONSerializer
from koalixcrm.crm.product.currency import Currency
from koalixcrm.crm.product.product_type import ProductType
from koalixcrm.crm.product.tax import Tax
from koalixcrm.crm.product.unit import Unit
from koalixcrm.crm.reporting.project import Project, ProjectJSONSerializer
from koalixcrm.crm.reporting.task import Task, TaskSerializer
from koalixcrm.crm.reporting.task_status import TaskStatus
from koalixcrm.crm.rest.currency_rest import CurrencyJSONSerializer
from koalixcrm.crm.rest.product_rest import ProductJSONSerializer
from koalixcrm.crm.rest.tax_rest import TaxJSONSerializer
from koalixcrm.crm.rest.unit_rest import UnitJSONSerializer
from koalixcrm.crm.views.renderer import XSLFORenderer
from koalixcrm.global_support_functions import ConditionalMethodDecorator
class TaskAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows users to be viewed.
"""
queryset = Task.objects.all()
serializer_class = TaskSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
filter_fields = ('project',)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(TaskAsJSON, self).dispatch(*args, **kwargs)
class ContractAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows users to be viewed.
"""
queryset = Contract.objects.all()
serializer_class = ContractJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(ContractAsJSON, self).dispatch(*args, **kwargs)
class TaskStatusAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows users to be viewed.
"""
queryset = TaskStatus.objects.all()
serializer_class = TaskSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(TaskStatusAsJSON, self).dispatch(*args, **kwargs)
class CurrencyAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows currencies to be viewed.
"""
queryset = Currency.objects.all()
serializer_class = CurrencyJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(CurrencyAsJSON, self).dispatch(*args, **kwargs)
class TaxAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows taxes to be viewed.
"""
queryset = Tax.objects.all()
serializer_class = TaxJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(TaxAsJSON, self).dispatch(*args, **kwargs)
class UnitAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows units to be viewed.
"""
queryset = Unit.objects.all()
serializer_class = UnitJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(UnitAsJSON, self).dispatch(*args, **kwargs)
class ProductAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows products to be viewed.
"""
queryset = ProductType.objects.all()
serializer_class = ProductJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(ProductAsJSON, self).dispatch(*args, **kwargs)
class ProjectAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows users to be viewed.
"""
queryset = Project.objects.all()
serializer_class = ProjectJSONSerializer
renderer_classes = (BrowsableAPIRenderer, JSONRenderer, XMLRenderer, XSLFORenderer)
file_name = "this_is_the_ProjectList.xml"
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(ProjectAsJSON, self).dispatch(*args, **kwargs)
<file_sep>/koalixcrm/crm/factories/factory_currency_transform.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import CurrencyTransform
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory, SecondStandardCurrencyFactory
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
class StandardCurrencyTransformFactory(factory.django.DjangoModelFactory):
class Meta:
model = CurrencyTransform
django_get_or_create = ('from_currency',
'to_currency')
from_currency = factory.SubFactory(StandardCurrencyFactory)
to_currency = factory.SubFactory(SecondStandardCurrencyFactory)
product_type = factory.SubFactory(StandardProductTypeFactory)
factor = 0.90
<file_sep>/koalixcrm/crm/factories/factory_reporting_period.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ReportingPeriod
from koalixcrm.crm.factories.factory_project import StandardProjectFactory
from koalixcrm.crm.factories.factory_reporting_period_status import ReportingReportingPeriodStatusFactory
class StandardReportingPeriodFactory(factory.django.DjangoModelFactory):
class Meta:
model = ReportingPeriod
django_get_or_create = ('title',)
project = factory.SubFactory(StandardProjectFactory)
title = "This is a test project"
begin = '2018-06-15'
end = '2044-06-15'
status = factory.SubFactory(ReportingReportingPeriodStatusFactory)
<file_sep>/koalixcrm/crm/factories/factory_payment_reminder.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.models import PaymentReminder
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardPaymentReminderFactory(StandardSalesDocumentFactory):
class Meta:
model = PaymentReminder
payable_until = "2018-05-20"
payment_bank_reference = "This is a bank account reference"
iteration_number = "1"
status = "C"
<file_sep>/koalixcrm/crm/migrations/0053_auto_20181014_2305.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
def initiate_human_resource(apps, schema_editor):
Work = apps.get_model("crm", "Work")
HumanResource = apps.get_model("crm", "HumanResource")
db_alias = schema_editor.connection.alias
all_works = Work.objects.using(db_alias).all()
for work in all_works:
all_human_resources = HumanResource.objects.using(db_alias).all()
human_resource_found = False
for human_resource in all_human_resources:
if work.employee == human_resource.user:
human_resource_found = True
work.human_resource = human_resource
break
if not human_resource_found:
new_human_resource = HumanResource.objects.using(db_alias).create(user=work.employee)
new_human_resource.save()
work.human_resource = new_human_resource
work.save()
def reverse_func(apps, schema_editor):
return 1
class Migration(migrations.Migration):
dependencies = [
('crm', '0052_auto_20181014_2304'),
]
operations = [
migrations.AlterField(
model_name='customergrouptransform',
name='product_type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
migrations.AlterField(
model_name='unittransform',
name='product_type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
migrations.RemoveField(
model_name='customergrouptransform',
name='product_backup',
),
migrations.RemoveField(
model_name='position',
name='product_backup',
),
migrations.RemoveField(
model_name='price',
name='product_backup',
),
migrations.RemoveField(
model_name='unittransform',
name='product_backup',
),
migrations.AddField(
model_name='producttype',
name='accounting_product_category',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='accounting.ProductCategory', verbose_name='Accounting Product Category'),
preserve_default=False,
),
migrations.RemoveField(model_name='producttype',
name='accounting_product_categorie'),
migrations.AlterModelOptions(
name='producttype',
options={'verbose_name': 'Product Type', 'verbose_name_plural': 'Product Types'},
),
migrations.RemoveField(
model_name='producttype',
name='product_number',
),
migrations.AddField(
model_name='producttype',
name='product_type_identifier',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Product Number'),
),
migrations.AlterField(
model_name='position',
name='product_type',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product'),
),
migrations.AlterField(
model_name='productprice',
name='product_type',
field=models.ForeignKey(default=1, on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
migrations.AlterField(
model_name='producttype',
name='last_modified_by',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to=settings.AUTH_USER_MODEL, verbose_name='Last modified by'),
),
migrations.CreateModel(
name='Product',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('identifier', models.CharField(verbose_name="Product Number",
max_length=200,
null=True,
blank=True)),
('product_type', models.ForeignKey("ProductType", verbose_name='Product Type')),
],
options={
'verbose_name': 'Product',
'verbose_name_plural': 'Products',
},
),
migrations.CreateModel(
name='Agreement',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('date_from', models.DateField(verbose_name='Agreement From')),
('date_until', models.DateField(verbose_name='Agreement To')),
('amount', models.DecimalField(blank=True, decimal_places=2, max_digits=5, null=True, verbose_name='Amount')),
],
options={
'verbose_name': 'Agreement',
'verbose_name_plural': 'Agreements',
},
),
migrations.CreateModel(
name='AgreementStatus',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=250, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
('is_agreed', models.BooleanField(verbose_name='Status represents agreement exists')),
],
options={
'verbose_name': 'Agreement Status',
'verbose_name_plural': 'Agreement Status',
},
),
migrations.CreateModel(
name='AgreementType',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=300, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
],
options={
'verbose_name': 'Agreement Type',
'verbose_name_plural': 'Agreement Type',
},
),
migrations.CreateModel(
name='CurrencyTransform',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('factor', models.IntegerField(blank=True, null=True, verbose_name='Factor between From and To Currency')),
('from_currency', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltransformfromcurrency', to='crm.Currency', verbose_name='From Currency')),
],
options={
'verbose_name': 'Currency Transform',
'verbose_name_plural': 'Currency Transforms',
},
),
migrations.CreateModel(
name='Estimation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('date_from', models.DateField(verbose_name='Estimation From')),
('date_until', models.DateField(verbose_name='Estimation To')),
('amount', models.DecimalField(blank=True, decimal_places=2, max_digits=5, null=True, verbose_name='Amount')),
('reporting_period', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ReportingPeriod', verbose_name='Reporting Period based on which the estimation was done')),
],
options={
'verbose_name': 'Estimation of Resource Consumption',
'verbose_name_plural': 'Estimation of Resource Consumptions',
},
),
migrations.CreateModel(
name='EstimationStatus',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=250, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
('is_obsolete', models.BooleanField(verbose_name='Status represents estimation is obsolete')),
],
options={
'verbose_name': 'Estimation Status',
'verbose_name_plural': 'Estimation Status',
},
),
migrations.CreateModel(
name='Resource',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
],
),
migrations.CreateModel(
name='ResourceManager',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.UserExtension', verbose_name='User')),
],
),
migrations.CreateModel(
name='ResourcePrice',
fields=[
('price_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.Price')),
],
bases=('crm.price',),
),
migrations.CreateModel(
name='ResourceType',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=300, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
],
options={
'verbose_name': 'Resource Link Type',
'verbose_name_plural': 'Resource Link Type',
},
),
migrations.RemoveField(
model_name='employeeassignmenttotask',
name='employee',
),
migrations.RemoveField(
model_name='employeeassignmenttotask',
name='task',
),
migrations.AlterModelOptions(
name='customergrouptransform',
options={'verbose_name': 'Customer Group Price Transform', 'verbose_name_plural': 'Customer Group Price Transforms'},
),
migrations.AlterModelOptions(
name='product',
options={},
),
migrations.AlterModelOptions(
name='unittransform',
options={'verbose_name': 'Unit Transform', 'verbose_name_plural': 'Unit Transforms'},
),
migrations.RenameField(
model_name='genericprojectlink',
old_name='task',
new_name='project',
),
migrations.RemoveField(
model_name='task',
name='planned_end_date',
),
migrations.RemoveField(
model_name='task',
name='planned_start_date',
),
migrations.AddField(
model_name='project',
name='default_currency',
field=models.ForeignKey(default=1, on_delete=django.db.models.deletion.CASCADE, to='crm.Currency', verbose_name='Default Currency'),
preserve_default=False,
),
migrations.AlterField(
model_name='customergrouptransform',
name='from_customer_group',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltransfromfromcustomergroup', to='crm.CustomerGroup', verbose_name='From Customer Group'),
),
migrations.AlterField(
model_name='customergrouptransform',
name='to_customer_group',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltransfromtocustomergroup', to='crm.CustomerGroup', verbose_name='To Customer Group'),
),
migrations.AlterField(
model_name='salesdocument',
name='custom_date_field',
field=models.DateField(blank=True, null=True, verbose_name='Custom Date'),
),
migrations.CreateModel(
name='HumanResource',
fields=[
('resource_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.Resource')),
('user', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.UserExtension', verbose_name='User')),
],
bases=('crm.resource',),
),
migrations.DeleteModel(
name='EmployeeAssignmentToTask',
),
migrations.AddField(
model_name='resourceprice',
name='resource',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Resource', verbose_name='Resource'),
),
migrations.AddField(
model_name='resource',
name='resource_manager',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ResourceManager', verbose_name='Manager'),
),
migrations.AddField(
model_name='resource',
name='resource_type',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ResourceType', verbose_name='Resource Type'),
),
migrations.AddField(
model_name='estimation',
name='resource',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Resource'),
),
migrations.AddField(
model_name='estimation',
name='status',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.EstimationStatus', verbose_name='Status of the estimation'),
),
migrations.AddField(
model_name='estimation',
name='task',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Task', verbose_name='Task'),
),
migrations.AddField(
model_name='currencytransform',
name='to_currency',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltransformtocurrency', to='crm.Currency', verbose_name='To Currency'),
),
migrations.AddField(
model_name='agreement',
name='costs',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ResourcePrice'),
),
migrations.AddField(
model_name='agreement',
name='resource',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Resource'),
),
migrations.AddField(
model_name='agreement',
name='status',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.AgreementStatus'),
),
migrations.AddField(
model_name='agreement',
name='task',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Task', verbose_name='Task'),
),
migrations.AddField(
model_name='agreement',
name='type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.AgreementType'),
),
migrations.AddField(
model_name='agreement',
name='unit',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Unit'),
),
migrations.AddField(
model_name='work',
name='human_resource',
field=models.ForeignKey(null=True, to='crm.HumanResource', on_delete=django.db.models.deletion.SET_NULL),
preserve_default=False,
),
migrations.AddField(
model_name='currencytransform',
name='product_type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product'),
),
migrations.RunPython(initiate_human_resource, reverse_func),
migrations.RemoveField(
model_name='work',
name='employee',
),
migrations.AlterField(
model_name='product',
name='product_type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
migrations.AlterField(
model_name='work',
name='human_resource',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.HumanResource'),
),
]
<file_sep>/koalixcrm/accounting/rest/restinterface.py
# -*- coding: utf-8 -*-
from django.conf import settings
from django.contrib.auth.decorators import login_required
from django.utils.decorators import method_decorator
from rest_framework import viewsets
from koalixcrm.accounting.models import Account, AccountingPeriod, Booking, ProductCategory
from koalixcrm.accounting.rest.account_rest import AccountJSONSerializer
from koalixcrm.accounting.rest.accounting_period_rest import AccountingPeriodJSONSerializer
from koalixcrm.accounting.rest.booking_rest import BookingJSONSerializer
from koalixcrm.accounting.rest.product_categorie_rest import ProductCategoryJSONSerializer
from koalixcrm.global_support_functions import ConditionalMethodDecorator
class AccountAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows accounts to be created, viewed and modified.
"""
queryset = Account.objects.all()
serializer_class = AccountJSONSerializer
filter_fields = ('account_type',)
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(AccountAsJSON, self).dispatch(*args, **kwargs)
class AccountingPeriodAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows accounting periods to be created, viewed and modified.
"""
queryset = AccountingPeriod.objects.all()
serializer_class = AccountingPeriodJSONSerializer
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(AccountingPeriodAsJSON, self).dispatch(*args, **kwargs)
class BookingAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows bookings to be created, viewed and modified.
"""
queryset = Booking.objects.all()
serializer_class = BookingJSONSerializer
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(BookingAsJSON, self).dispatch(*args, **kwargs)
class ProductCategoryAsJSON(viewsets.ModelViewSet):
"""
API endpoint that allows product categories to be created, viewed and modified.
"""
queryset = ProductCategory.objects.all()
serializer_class = ProductCategoryJSONSerializer
@ConditionalMethodDecorator(method_decorator(login_required), settings.KOALIXCRM_REST_API_AUTH)
def dispatch(self, *args, **kwargs):
return super(ProductCategoryAsJSON, self).dispatch(*args, **kwargs)
<file_sep>/koalixcrm/crm/rest/unit_rest.py
from rest_framework import serializers
from koalixcrm.crm.product.unit import Unit
class OptionUnitJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
description = serializers.CharField(read_only=True)
shortName = serializers.CharField(source='short_name', read_only=True)
class Meta:
model = Unit
fields = ('id',
'description',
'shortName')
class UnitJSONSerializer(serializers.HyperlinkedModelSerializer):
shortName = serializers.CharField(source='short_name')
description = serializers.CharField()
isFractionOf = OptionUnitJSONSerializer(source='is_a_fraction_of',
allow_null=True)
fractionFactor = serializers.DecimalField(source='fraction_factor_to_next_higher_unit',
max_digits=20,
decimal_places=10,
required=False,
allow_null=True)
class Meta:
model = Unit
fields = ('id',
'description',
'shortName',
'isFractionOf',
'fractionFactor')
depth = 1
def create(self, validated_data):
unit = Unit()
unit.description = validated_data['description']
unit.short_name = validated_data['short_name']
if 'fraction_factor_to_next_higher_unit' in validated_data:
unit.fraction_factor_to_next_higher_unit = validated_data['fraction_factor_to_next_higher_unit']
parent_unit = validated_data.pop('is_a_fraction_of')
if parent_unit:
if parent_unit.get('id', None):
unit.is_a_fraction_of = Unit.objects.get(id=parent_unit.get('id', None))
else:
unit.is_a_fraction_of = None
unit.save()
return unit
def update(self, instance, validated_data):
instance.description = validated_data.get('description', instance.description)
instance.short_name = validated_data.get('short_name', instance.short_name)
parent_unit = validated_data.pop('is_a_fraction_of')
if parent_unit:
if parent_unit.get('id', instance.is_a_fraction_of_id):
instance.is_a_fraction_of = Unit.objects.get(id=parent_unit.get('id', None))
else:
instance.is_a_fraction_of = instance.is_a_fraction_of_id
else:
instance.is_a_fraction_of = None
instance.fraction_factor_to_next_higher_unit = validated_data.get('fraction_factor_to_next_higher_unit',
instance.fraction_factor_to_next_higher_unit)
instance.save()
return instance
<file_sep>/koalixcrm/djangoUserExtension/migrations/0002_auto_20171009_1949.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-10-09 19:49
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0001_initial'),
]
operations = [
migrations.AlterModelOptions(
name='templateset',
options={'verbose_name': 'Vorlagenset', 'verbose_name_plural': 'Vorlagensets'},
),
migrations.AlterModelOptions(
name='userextension',
options={'verbose_name': 'Benutzer Erweiterung', 'verbose_name_plural': 'Benutzer Erweiterungen'},
),
migrations.AlterModelOptions(
name='xslfile',
options={'verbose_name': 'XSL Datei', 'verbose_name_plural': 'XSL Dateien'},
),
migrations.AlterField(
model_name='templateset',
name='addresser',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Absender'),
),
migrations.AlterField(
model_name='templateset',
name='balancesheetXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatebalancesheet', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für die Billanz'),
),
migrations.AlterField(
model_name='templateset',
name='bankingaccountref',
field=models.CharField(blank=True, max_length=60, null=True, verbose_name='Bankverbindung'),
),
migrations.AlterField(
model_name='templateset',
name='deilveryorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatedeliveryorder', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für den Lieferschein'),
),
migrations.AlterField(
model_name='templateset',
name='footerTextpurchaseorders',
field=models.TextField(blank=True, null=True, verbose_name='Grussfromel für die Kaufaufträge'),
),
migrations.AlterField(
model_name='templateset',
name='footerTextsalesorders',
field=models.TextField(blank=True, null=True, verbose_name='Grussfromel für die Verkaufsaufträge'),
),
migrations.AlterField(
model_name='templateset',
name='fopConfigurationFile',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='FOP Configurations Datei'),
),
migrations.AlterField(
model_name='templateset',
name='headerTextpurchaseorders',
field=models.TextField(blank=True, null=True, verbose_name='Begrüssungsformel für die Kaufaufträge'),
),
migrations.AlterField(
model_name='templateset',
name='headerTextsalesorders',
field=models.TextField(blank=True, null=True, verbose_name='Begrüssungsfromel für die Verkaufsaufträge'),
),
migrations.AlterField(
model_name='templateset',
name='invoiceXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplateinvoice', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für die Rechnung'),
),
migrations.AlterField(
model_name='templateset',
name='logo',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name="Logo für die PDF's"),
),
migrations.AlterField(
model_name='templateset',
name='organisationname',
field=models.CharField(max_length=200, verbose_name='Name der Organisation'),
),
migrations.AlterField(
model_name='templateset',
name='pagefooterleft',
field=models.CharField(blank=True, max_length=40, null=True, verbose_name='Fussnote Links'),
),
migrations.AlterField(
model_name='templateset',
name='pagefootermiddle',
field=models.CharField(blank=True, max_length=40, null=True, verbose_name='Fussnote Mitte'),
),
migrations.AlterField(
model_name='templateset',
name='profitLossStatementXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplateprofitlossstatement', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für die Erfolgsrechung'),
),
migrations.AlterField(
model_name='templateset',
name='purchaseorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatepurchaseorder', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für die Kaufsbestätigung'),
),
migrations.AlterField(
model_name='templateset',
name='quoteXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatequote', to='djangoUserExtension.XSLFile', verbose_name='XSL Datei für die Offerte'),
),
migrations.AlterField(
model_name='templateset',
name='title',
field=models.CharField(max_length=100, verbose_name='Titel'),
),
migrations.AlterField(
model_name='userextensionemailaddress',
name='purpose',
field=models.CharField(choices=[('H', 'Privat'), ('O', 'Geschäftlich'), ('P', 'Mobil Privat'), ('B', 'Mobil Geschäftlich')], max_length=1, verbose_name='Verwendungszweck'),
),
migrations.AlterField(
model_name='userextensionphoneaddress',
name='purpose',
field=models.CharField(choices=[('H', 'Privat'), ('O', 'Geschäftlich'), ('P', 'Mobil Privat'), ('B', 'Mobil Geschäftlich')], max_length=1, verbose_name='Verwendungszweck'),
),
migrations.AlterField(
model_name='userextensionpostaladdress',
name='purpose',
field=models.CharField(choices=[('H', 'Privat'), ('O', 'Geschäftlich'), ('P', 'Mobil Privat'), ('B', 'Mobil Geschäftlich')], max_length=1, verbose_name='Verwendungszweck'),
),
migrations.AlterField(
model_name='xslfile',
name='title',
field=models.CharField(blank=True, max_length=100, null=True, verbose_name='Titel'),
),
migrations.AlterField(
model_name='xslfile',
name='xslfile',
field=filebrowser.fields.FileBrowseField(max_length=200, verbose_name='XSL Datei'),
),
]
<file_sep>/koalixcrm/accounting/models.py
# -*- coding: utf-8 -*-
from koalixcrm.accounting.accounting.account import Account
from koalixcrm.accounting.accounting.booking import Booking
from koalixcrm.accounting.accounting.booking import InlineBookings
from koalixcrm.accounting.accounting.accounting_period import AccountingPeriod
from koalixcrm.accounting.accounting.product_category import ProductCategory
<file_sep>/koalixcrm/crm/product/currency_transform.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class CurrencyTransform(models.Model):
from_currency = models.ForeignKey('Currency',
blank=False,
null=False,
verbose_name=_("From Currency"),
related_name="db_reltransformfromcurrency")
to_currency = models.ForeignKey('Currency',
blank=False,
null=False,
verbose_name=_("To Currency"),
related_name="db_reltransformtocurrency")
product_type = models.ForeignKey('ProductType',
blank=False,
null=False,
verbose_name=_("Product"))
factor = models.DecimalField(verbose_name=_("Factor between From and To Currency"),
blank=False,
null=False,
max_digits=17,
decimal_places=2,)
def get_transform_factor(self):
return self.factor
def __str__(self):
return "From " + self.from_currency.short_name + " to " + self.to_currency.short_name
class Meta:
app_label = "crm"
verbose_name = _('Currency Transform')
verbose_name_plural = _('Currency Transforms')
class CurrencyTransformInlineAdminView(admin.TabularInline):
model = CurrencyTransform
extra = 1
classes = ['collapse']
fieldsets = (
('', {
'fields': ('from_currency',
'to_currency',
'factor',)
}),
)
allow_add = True
<file_sep>/koalixcrm/crm/migrations/0011_auto_20180104_2152.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-04 21:52
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0010_auto_20180104_2138'),
]
operations = [
migrations.RenameField(
model_name='currency',
old_name='shortName',
new_name='short_name',
),
migrations.RenameField(
model_name='customergrouptransform',
old_name='fromCustomerGroup',
new_name='from_customer_group',
),
migrations.RenameField(
model_name='customergrouptransform',
old_name='toCustomerGroup',
new_name='to_customer_group',
),
migrations.RenameField(
model_name='price',
old_name='customerGroup',
new_name='customer_group',
),
migrations.RenameField(
model_name='price',
old_name='validfrom',
new_name='valid_from',
),
migrations.RenameField(
model_name='price',
old_name='validuntil',
new_name='valid_until',
),
migrations.RenameField(
model_name='tax',
old_name='accountActiva',
new_name='account_activa',
),
migrations.RenameField(
model_name='tax',
old_name='accountPassiva',
new_name='account_passiva',
),
migrations.RenameField(
model_name='tax',
old_name='taxrate',
new_name='tax_rate',
),
migrations.RenameField(
model_name='unit',
old_name='fractionFactorToNextHigherUnit',
new_name='fraction_factor_to_next_higher_unit',
),
migrations.RenameField(
model_name='unit',
old_name='isAFractionOf',
new_name='is_a_fraction_of',
),
migrations.RenameField(
model_name='unit',
old_name='shortName',
new_name='short_name',
),
migrations.RenameField(
model_name='unittransform',
old_name='fromUnit',
new_name='from_unit',
),
migrations.RenameField(
model_name='unittransform',
old_name='toUnit',
new_name='to_unit',
),
]
<file_sep>/koalixcrm/accounting/migrations/0005_auto_20171110_1732.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-11-10 17:32
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('accounting', '0004_auto_20171009_1949'),
]
operations = [
migrations.AlterModelOptions(
name='account',
options={'ordering': ['accountNumber'], 'verbose_name': 'Account', 'verbose_name_plural': 'Account'},
),
migrations.AlterModelOptions(
name='accountingperiod',
options={'verbose_name': 'Accounting Period', 'verbose_name_plural': 'Accounting Periods'},
),
migrations.AlterModelOptions(
name='booking',
options={'verbose_name': 'Booking', 'verbose_name_plural': 'Bookings'},
),
migrations.AlterModelOptions(
name='productcategorie',
options={'verbose_name': 'Product Categorie', 'verbose_name_plural': 'Product Categories'},
),
migrations.AlterField(
model_name='account',
name='accountNumber',
field=models.IntegerField(verbose_name='Account Number'),
),
migrations.AlterField(
model_name='account',
name='accountType',
field=models.CharField(choices=[('E', 'Earnings'), ('S', 'Spendings'), ('L', 'Liabilities'), ('A', 'Assets')], max_length=1, verbose_name='Account Type'),
),
migrations.AlterField(
model_name='account',
name='description',
field=models.TextField(blank=True, null=True, verbose_name='Description'),
),
migrations.AlterField(
model_name='account',
name='isACustomerPaymentAccount',
field=models.BooleanField(verbose_name='Is a Customer Payment Account'),
),
migrations.AlterField(
model_name='account',
name='isProductInventoryActiva',
field=models.BooleanField(verbose_name='Is a Product Inventory Account'),
),
migrations.AlterField(
model_name='account',
name='isopeninterestaccount',
field=models.BooleanField(verbose_name='Is The Open Interests Account'),
),
migrations.AlterField(
model_name='account',
name='isopenreliabilitiesaccount',
field=models.BooleanField(verbose_name='Is The Open Liabilities Account'),
),
migrations.AlterField(
model_name='account',
name='title',
field=models.CharField(max_length=50, verbose_name='Account Title'),
),
migrations.AlterField(
model_name='accountingperiod',
name='begin',
field=models.DateField(verbose_name='Begin'),
),
migrations.AlterField(
model_name='accountingperiod',
name='end',
field=models.DateField(verbose_name='End'),
),
migrations.AlterField(
model_name='accountingperiod',
name='title',
field=models.CharField(max_length=200, verbose_name='Title'),
),
migrations.AlterField(
model_name='booking',
name='accountingPeriod',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='accounting.AccountingPeriod', verbose_name='AccountingPeriod'),
),
migrations.AlterField(
model_name='booking',
name='amount',
field=models.DecimalField(decimal_places=2, max_digits=20, verbose_name='Amount'),
),
migrations.AlterField(
model_name='booking',
name='bookingDate',
field=models.DateTimeField(verbose_name='Booking at'),
),
migrations.AlterField(
model_name='booking',
name='bookingReference',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Invoice', verbose_name='Booking Reference'),
),
migrations.AlterField(
model_name='booking',
name='dateofcreation',
field=models.DateTimeField(auto_now=True, verbose_name='Created at'),
),
migrations.AlterField(
model_name='booking',
name='description',
field=models.CharField(blank=True, max_length=120, null=True, verbose_name='Description'),
),
migrations.AlterField(
model_name='booking',
name='fromAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_fromaccount', to='accounting.Account', verbose_name='From Account'),
),
migrations.AlterField(
model_name='booking',
name='lastmodification',
field=models.DateTimeField(auto_now_add=True, verbose_name='Last modified'),
),
migrations.AlterField(
model_name='booking',
name='lastmodifiedby',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_lstmodified', to=settings.AUTH_USER_MODEL, verbose_name='Last modified by'),
),
migrations.AlterField(
model_name='booking',
name='staff',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_refstaff', to=settings.AUTH_USER_MODEL, verbose_name='Reference Staff'),
),
migrations.AlterField(
model_name='booking',
name='toAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_toaccount', to='accounting.Account', verbose_name='To Account'),
),
migrations.AlterField(
model_name='productcategorie',
name='lossAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_loss_account', to='accounting.Account', verbose_name='Loss Account'),
),
migrations.AlterField(
model_name='productcategorie',
name='profitAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_profit_account', to='accounting.Account', verbose_name='Profit Account'),
),
migrations.AlterField(
model_name='productcategorie',
name='title',
field=models.CharField(max_length=50, verbose_name='Product Categorie Title'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_resource.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Resource
from koalixcrm.crm.factories.factory_resource_type import StandardResourceTypeFactory
from koalixcrm.crm.factories.factory_resource_manager import StandardResourceManagerFactory
class StandardResourceFactory(factory.django.DjangoModelFactory):
class Meta:
model = Resource
resource_type = factory.SubFactory(StandardResourceTypeFactory)
resource_manager = factory.SubFactory(StandardResourceManagerFactory)
<file_sep>/koalixcrm/accounting/tests/test_accountingModelTest.py
import datetime
from django.test import TestCase
from django.contrib.auth.models import User
from koalixcrm.accounting.models import Account
from koalixcrm.accounting.models import AccountingPeriod
from koalixcrm.accounting.models import Booking
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.test_support_functions import make_date_utc
class AccountingModelTest(TestCase):
def setUp(self):
user = User.objects.create(username='Username',
password="<PASSWORD>")
cash = Account.objects.create(account_number="1000",
title="Cash",
account_type="A",
description="Highest liquid asset",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=True)
bank_account = Account.objects.create(account_number="1300",
title="Bank Account",
account_type="A",
description="Moderate liquid asset",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=True)
bank_loan = Account.objects.create(account_number="2000",
title="Shortterm bankloans",
account_type="L",
description="Shortterm loan",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=False)
investment_capital = Account.objects.create(account_number="2900",
title="Investment capital",
account_type="L",
description="Very longterm loan",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=False)
spendings = Account.objects.create(account_number="3000",
title="Spendings",
account_type="S",
description="Purchase spendings",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=False)
earnings = Account.objects.create(account_number="4000",
title="Earnings",
account_type="E",
description="Sales account",
is_open_interest_account=False,
is_open_reliabilities_account=False,
is_product_inventory_activa=False,
is_a_customer_payment_account=False)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
from_date = datetime_now.date()
to_date = (datetime_now + datetime.timedelta(days=365)).date()
accounting_period_2024 = AccountingPeriod.objects.create(title="Fiscal year 2024",
begin=from_date,
end=to_date)
from_date = (datetime_now + datetime.timedelta(days=(365+1))).date()
to_date = (datetime_now + datetime.timedelta(days=(365*2))).date()
accounting_period_2025 = AccountingPeriod.objects.create(title="Fiscal year 2025",
begin=from_date,
end=to_date)
from_date = (datetime_now + datetime.timedelta(days=(365*2+1))).date()
to_date = (datetime_now + datetime.timedelta(days=(365*3))).date()
AccountingPeriod.objects.create(title="Fiscal year 2026",
begin=from_date,
end=to_date)
Booking.objects.create(from_account=cash,
to_account=spendings,
amount="1000",
description="This is the first booking",
booking_date=make_date_utc(datetime.datetime(2025, 1, 1, 0, 00)),
accounting_period=accounting_period_2025,
staff=user,
last_modified_by=user)
Booking.objects.create(from_account=earnings,
to_account=cash,
amount="500",
description="This is the first booking",
booking_date=make_date_utc(datetime.datetime(2025, 1, 1, 0, 00)),
accounting_period=accounting_period_2025,
staff=user,
last_modified_by=user)
Booking.objects.create(from_account=bank_loan,
to_account=cash,
amount="5000",
description="This is the first booking",
booking_date=make_date_utc(datetime.datetime(2025, 1, 1, 0, 00)),
accounting_period=accounting_period_2025,
staff=user,
last_modified_by=user)
Booking.objects.create(from_account=investment_capital,
to_account=bank_account,
amount="490000",
description="This is the first booking",
booking_date=make_date_utc(datetime.datetime(2025, 1, 1, 0, 00)),
accounting_period=accounting_period_2024,
staff=user,
last_modified_by=user)
def test_sumOfAllBookings(self):
cash_account = Account.objects.get(title="Cash")
spendings_account = Account.objects.get(title="Spendings")
earnings_account = Account.objects.get(title="Earnings")
self.assertEqual((cash_account.sum_of_all_bookings()).__str__(), "4500.00")
self.assertEqual((spendings_account.sum_of_all_bookings()).__str__(), "1000.00")
self.assertEqual((earnings_account.sum_of_all_bookings()).__str__(), "500.00")
def test_sumOfAllBookingsBeforeAccountPeriod(self):
cash_account = Account.objects.get(title="Cash")
spendings_account = Account.objects.get(title="Spendings")
earnings_account = Account.objects.get(title="Earnings")
accounting_period_2026 = AccountingPeriod.objects.get(title="Fiscal year 2026")
self.assertEqual((cash_account.sum_of_all_bookings_before_accounting_period(accounting_period_2026)).__str__(), "4500.00")
self.assertEqual((spendings_account.sum_of_all_bookings_before_accounting_period(accounting_period_2026)).__str__(), "1000.00")
self.assertEqual((earnings_account.sum_of_all_bookings_before_accounting_period(accounting_period_2026)).__str__(), "500.00")
def test_sumOfAllBookingsWithinAccountgPeriod(self):
cash_account = Account.objects.get(title="Cash")
spendings_account = Account.objects.get(title="Spendings")
earnings_account = Account.objects.get(title="Earnings")
accounting_period_2024 = AccountingPeriod.objects.get(title="Fiscal year 2024")
self.assertEqual((cash_account.sum_of_all_bookings_within_accounting_period(accounting_period_2024)).__str__(), "0")
self.assertEqual((spendings_account.sum_of_all_bookings_within_accounting_period(accounting_period_2024)).__str__(), "0")
self.assertEqual((earnings_account.sum_of_all_bookings_within_accounting_period(accounting_period_2024)).__str__(), "0")
def test_overall_liabilities(self):
accounting_period_2025 = AccountingPeriod.objects.get(title="Fiscal year 2025")
self.assertEqual(
(accounting_period_2025.overall_liabilities()).__str__(), "495000.00")
def test_overall_assets(self):
accounting_period_2025 = AccountingPeriod.objects.get(title="Fiscal year 2025")
self.assertEqual(
(accounting_period_2025.overall_assets()).__str__(), "494500.00")
def test_overall_earnings(self):
accounting_period_2025 = AccountingPeriod.objects.get(title="Fiscal year 2025")
self.assertEqual(
(accounting_period_2025.overall_earnings()).__str__(), "500.00")
def test_overall_spendings(self):
accounting_period_2025 = AccountingPeriod.objects.get(title="Fiscal year 2025")
self.assertEqual(
(accounting_period_2025.overall_spendings()).__str__(), "1000.00")
def test_serialize_to_xml(self):
accounting_period_2025 = AccountingPeriod.objects.get(title="Fiscal year 2025")
xml = accounting_period_2025.serialize_to_xml()
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>']/field[@name='title']", 'Earnings')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>']/field[@name='title']", 'Spendings')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>']/field[@name='title']", 'Investment capital')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>']/field[@name='title']", 'Shortterm bankloans')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>']/field[@name='title']",'Cash')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>='<EMAIL>']/field[@name='title']",'Bank Account')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>='<EMAIL>']/Overall_Spendings", '1000.00')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>='<EMAIL>']/Overall_Earnings", '500.00')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>='<EMAIL>']/Overall_Assets", '494500.00')
self.assertEqual(result, 1)
result=PDFExport.find_element_in_xml(xml, "object/[<EMAIL>='<EMAIL>']/Overall_Liabilities", '495000.00')
self.assertEqual(result, 1)
<file_sep>/koalixcrm/crm/documents/calculations.py
# -*- coding: utf-8 -*-
from decimal import *
from koalixcrm.crm.documents.sales_document_position import SalesDocumentPosition
class Calculations:
@staticmethod
def calculate_document_price(document, pricing_date):
"""Performs a price recalculation on contact documents.
The calculated price is stored in the last_calculated_price and last_calculated_tax.
The date when the price was calculated is stored in last_pricing_date
Args:
document: possible document classes must be derived from Contract
pricing_date: must be a python date object
Returns:
1 (Boolean) when passed
or raises exception
Raises:
Can trow Product.NoPriceFound when Product Price could not be found"""
price = 0
tax = 0
positions = SalesDocumentPosition.objects.filter(sales_document=document.id)
contact_for_price_calculation = document.customer
if positions.exists():
for position in positions:
price += Calculations.calculate_position_price(position,
pricing_date,
contact_for_price_calculation,
document.currency)
tax += Calculations.calculate_position_tax(position, document.currency)
if document.discount is not None:
discount = Decimal(document.discount)
total_price = price * (1 - discount / 100)
total_tax = tax * (1 - discount / 100)
getcontext().prec = 5
total_price = Decimal(total_price)
total_tax = Decimal(total_tax)
price = document.currency.round(total_price)
tax = document.currency.round(total_tax)
document.last_calculated_price = price
document.last_calculated_tax = tax
document.last_pricing_date = pricing_date
document.save()
return 1
@staticmethod
def calculate_position_price(position, pricing_date, contact, currency):
"""Performs a price calculation a position.
The calculated price is stored in the last_calculated_price
The date when the price was calculated is stored in last_pricing_date
Args:
position: possible document classes must be derived from Contract class
pricing_date: must be a python date object
contact: is used for the lookup of the correct price
currency: is used for the lookup of the correct price and for the definition of the rounding
Returns:
calculated price
or raises exception
Raises:
Can trow Product.NoPriceFound when Product Price could not be found"""
if not position.overwrite_product_price:
position.position_price_per_unit = position.product_type.get_price(pricing_date,
position.unit,
contact,
currency)
nominal_total = position.position_price_per_unit * position.quantity
if isinstance(position.discount, Decimal):
nominal_minus_discount = nominal_total * (1 - position.discount / 100)
else:
nominal_minus_discount = nominal_total
total_with_tax = nominal_minus_discount * ((100-position.product_type.get_tax_rate()) / 100)
getcontext().prec = 5
total_with_tax = Decimal(total_with_tax)
position.last_calculated_price = currency.round(total_with_tax)
position.last_pricing_date = pricing_date
position.save()
return position.last_calculated_price
@staticmethod
def calculate_position_tax(position, currency):
"""Performs a tax calculation a position.
The calculated tax is stored in the last_calculated_tax
Args:
position: possible document classes must be derived from Position class
currency: is used for the definition of the rounding
Returns:
calculated tax
or raises exception
Raises:
Can trow Product.NoPriceFound when Product Price could not be found"""
nominal_total = position.position_price_per_unit * position.quantity
if isinstance(position.discount, Decimal):
nominal_minus_discount = nominal_total * (1 - position.discount / 100)
else:
nominal_minus_discount = nominal_total
total_tax = nominal_minus_discount * position.product_type.get_tax_rate() / 100
getcontext().prec = 5
total_tax = Decimal(total_tax)
position.last_calculated_tax = currency.round(total_tax)
position.save()
return position.last_calculated_tax
<file_sep>/documentation/source/intro.rst
.. highlight:: rst
Intro
=====
This Intro should give you an overview of koalixcrm's basic functions and structure.
Basic Structure
---------------
koalixcrm tries to keep its structure near the real world objects it represents.
Lets say you want to register a customer in koalixcrm then you find a customer
in your koalixcrm. When you receive a question for a quote, you find the quote
in your koalixcrm. Same for many kind of business objects you know in your company.
Every such real world objects is represented in the admin start page
(called "dashboard") as row of the Applications table.
Every object has a name - of cause - an Add and a Change button.
When you want to create a customer you just press the "Add" button in the
Customers row and you will see a form (called "object_modify_view") to fill
out for your customer. By pressing the Change or name button (eg "Customers")
of the object you see a list of all objects (called "objects_list") you have
already saved.
dashboard
---------
The dashboard ("Site Administration") is the place you start after logging in.
In the dashboard you find a list of all object types (called objectlist)
in three groups (called applications): crm, accounting and djangoUserExtentions.
.. image:: /images/dashboard.png
Administrator only
^^^^^^^^^^^^^^^^^^
When you are administrator, you can also see a group called auth where you
can manage users and groups. During the installation you are asked to
create a first, administrator user. Every new user after that starts with no privileges.
To be able to log in to koalixcrm the new user must be moderator.
When you want to create an accounting-only user you must
select all accounting privileges in the user_modification_view.
.. image:: /images/userprivileges.png
objects_list
------------
From the dashboard you are able to select one object type by clicking
on the name of it. What you get is a quite detailed list
of objects of this object type.
To modify an object you simply press press on the id of the object to
get to a form-view of the object.
Adding A New Object To The List
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To add a new object to the list you simply have to press the "Add button"
on the top right of the page
.. image:: /images/addbutton.png
Apply An Action On An Object
^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Applying actions to objects is a basic point of using koalixcrm.
To be able to remove objects from your list you have to select all objects
you want to remove by clicking on the checkbox on the left side of the object list.
When you finish making your selection you will see a new selection field rising
from the bottom of your page. Here you can select what action you would
like to to apply to the objects. Serveral things can be done with these actions.
.. image:: /images/checkboxobjectlist.png
.. image:: /images/actionslist.png
Apply A Filter On The List Of Objects
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
On some list of objects you may apply filter functions.
This filter function can be found on the right side of the page.
Depending on the filter you apply on the list you get a smaller number of objects
.. image:: /images/filter.png
Do A Search Through All Objects
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
The more objects you registered in your system, the more difficult it becomes
to find the one you are looking for. On some object lists you will find a search box.
The keywords you enter in this searchbox will be searched in
the objects name, id, description and so on.
All objects that include your selected keywords will be displayed in the list.
.. image:: /images/searchbox.png
object_modify_view
------------------
Lets say you logged into the system as a moderator with enough
privileges to modify a customer. You first access the
customer objects list. You will find your exiting list of customers on the screen
.. image:: /images/customer_object_list.png
As soon as you press on the button "ADD CUSTOMER", the browser will load a new screen: The
"object modify view". In the modify view you will find several fields to fill out.
As soon as the fields have been filled out you can press the "SAVE" button at the
bottom of the page. The field entries are then transmit and validated by the
application.
The fields which have a bold title are "must"-fields - they have to be filled out before
saving the customer.
.. image:: /images/customer_modify_view_collapsed.png
In case you have entered something wrong or invalid, the application will
complain and ask you to correct the invalid data.
.. image:: /images/customer_modify_view_error.png
Some of the optional fields are hidden (collapsed) by default.
By pressing the "SHOW" button beside "POSTAL ADDRESS FOR CONTACT" you can expand these
fields.
.. image:: /images/customer_modify_view_expand.png<file_sep>/koalixcrm/crm/factories/factory_product.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Product
class StandardProductFactory(factory.django.DjangoModelFactory):
class Meta:
model = Product
django_get_or_create = ('product_number',)
<file_sep>/koalixcrm/crm/reporting/estimation.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.reporting.resource_price import ResourcePrice
class Estimation(models.Model):
task = models.ForeignKey("Task",
verbose_name=_('Task'),
blank=False,
null=False)
resource = models.ForeignKey("Resource")
date_from = models.DateField(verbose_name=_("Estimation From"),
blank=False,
null=False)
date_until = models.DateField(verbose_name=_("Estimation To"),
blank=False,
null=False)
amount = models.DecimalField(verbose_name=_("Amount"),
max_digits=5,
decimal_places=2,
blank=True,
null=True)
status = models.ForeignKey("EstimationStatus",
verbose_name=_('Status of the estimation'),
blank=False,
null=False)
reporting_period = models.ForeignKey("ReportingPeriod",
verbose_name=_('Reporting Period based on which the estimation was done'),
blank=False,
null=False)
def calculated_costs(self):
default_resource_price = ResourcePrice.objects.filter(id=self.resource.id)
if len(default_resource_price) == 0:
costs = 0
else:
for resource_price in default_resource_price:
costs = self.amount*resource_price.price
break
return costs
def __str__(self):
return _("Estimation of Resource Consumption") + ": " + str(self.id)
class Meta:
app_label = "crm"
verbose_name = _('Estimation of Resource Consumption')
verbose_name_plural = _('Estimation of Resource Consumptions')
class EstimationInlineAdminView(admin.TabularInline):
model = Estimation
fieldsets = (
(_('Work'), {
'fields': ('task',
'resource',
'amount',
'date_from',
'date_until',
'status',
'reporting_period')
}),
)
extra = 1
<file_sep>/koalixcrm/accounting/migrations/0008_auto_20181012_2056.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('accounting', '0007_auto_20180422_2105'),
]
operations = [
migrations.CreateModel(
name='ProductCategory',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=50, verbose_name='Product Category Title')),
],
options={
'verbose_name': 'Product Category',
'verbose_name_plural': 'Product Categories',
},
),
]
<file_sep>/koalixcrm/djangoUserExtension/const/purpose.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
PURPOSESADDRESSINUSEREXTENTION = (
('H', _('Private')),
('O', _('Business')),
('P', _('Mobile Private')),
('B', _('Mobile Business')),
)
<file_sep>/koalixcrm/crm/migrations/0021_purchaseorder.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-16 20:59
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0020_auto_20180116_2056'),
]
operations = [
migrations.CreateModel(
name='PurchaseOrder',
fields=[
('salesdocument_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.SalesDocument')),
('status', models.CharField(choices=[('O', 'Ordered'), ('D', 'Delayed'), ('Y', 'Delivered'), ('I', 'Invoice registered'), ('P', 'Invoice payed')], max_length=1)),
('supplier', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Supplier', verbose_name='Supplier')),
],
options={
'verbose_name': 'Payment Reminder',
'verbose_name_plural': 'Payment Reminders',
},
bases=('crm.salesdocument',),
),
]
<file_sep>/koalixcrm/crm/factories/factory_task_link_type.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import TaskLinkType
class RelatedToTaskLinkTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = TaskLinkType
django_get_or_create = ('title',)
title = "Is related to"
description = "This task is related with ...."
class RequiresLinkTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = TaskLinkType
django_get_or_create = ('title',)
title = "This task requires"
description = "This task requires the completion or the existence of ...."
<file_sep>/setup.py
from setuptools import setup, find_packages
from koalixcrm.version import KOALIXCRM_VERSION
setup(name='koalix-crm',
version=KOALIXCRM_VERSION,
description='koalixcrm is a tiny and easy to use Customer-Relationship-Management '
'Software (CRM) including tiny and easy to use Accounting Software',
url='http://github.com/scaphilo/koalixcrm',
author='<NAME>',
author_email='<EMAIL>',
license='BSD',
packages=find_packages(exclude=["projectsettings", "documentation"]),
install_requires=['Django==1.11.21',
'django-filebrowser==3.9.1',
'lxml>=3.8.0',
'olefile>=0.44',
'Pillow==4.2.1',
'psycopg2_binary>=2.7.5',
'pytz==2017.2',
'django-grappelli==2.10.1',
'djangorestframework>=3.9.1',
'markdown==2.6.11',
'django-filter==1.1.0',
'djangorestframework-xml==1.3.0'
],
zip_safe=False,
classifiers=['Development Status :: 4 - Beta',
'Programming Language :: Python :: 3.5',
],
python_requires='~=3.5',
include_package_data=True,
)
<file_sep>/koalixcrm/crm/migrations/0010_auto_20180104_2138.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-04 21:38
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0009_auto_20180104_2111'),
]
operations = [
migrations.RenameField(
model_name='position',
old_name='positionPricePerUnit',
new_name='position_price_per_unit',
),
]
<file_sep>/koalixcrm/crm/factories/factory_customer_group_transform.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.product.customer_group_transform import CustomerGroupTransform
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
from koalixcrm.crm.factories.factory_customer_group import AdvancedCustomerGroupFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
class StandardCustomerGroupTransformFactory(factory.django.DjangoModelFactory):
class Meta:
model = CustomerGroupTransform
from_customer_group = factory.SubFactory(AdvancedCustomerGroupFactory)
to_customer_group = factory.SubFactory(StandardCustomerGroupFactory)
product_type = factory.SubFactory(StandardProductTypeFactory)
factor = "10"
<file_sep>/requirements/heroku_requirements.txt
-r base_requirements.txt
-r test_requirements.txt
gunicorn==19.7.1
dj-database-url==0.4.2
<file_sep>/koalixcrm/crm/reporting/project.py
# -*- coding: utf-8 -*-
from decimal import *
from datetime import *
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from django.utils.html import format_html
from koalixcrm.crm.reporting.generic_project_link import GenericLinkInlineAdminView
from koalixcrm.crm.reporting.reporting_period import ReportingPeriodInlineAdminView, ReportingPeriod
from koalixcrm.crm.reporting.task import TaskInlineAdminView
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.crm.exceptions import TemplateSetMissingInContract
from koalixcrm.crm.models import Task
from rest_framework import serializers
class Project(models.Model):
project_manager = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True},
verbose_name=_("Staff"),
related_name="db_rel_project_staff",
blank=True,
null=True)
project_name = models.CharField(verbose_name=_("Project name"),
max_length=100,
null=True,
blank=True)
description = models.TextField(verbose_name=_("Description"),
null=True,
blank=True)
project_status = models.ForeignKey("ProjectStatus",
verbose_name=_('Project Status'),
blank=True,
null=True)
default_template_set = models.ForeignKey("djangoUserExtension.TemplateSet",
verbose_name=_("Default Template Set"),
null=True,
blank=True)
default_currency = models.ForeignKey("Currency",
verbose_name=_("Default Currency"),
null=False,
blank=False)
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"),
related_name="db_project_last_modified")
def link_to_project(self):
if self.id:
return format_html("<a href='/admin/crm/project/%s' >%s</a>" % (str(self.id), str(self.project_name)))
else:
return "Not present"
link_to_project.short_description = _("Project")
def create_pdf(self, template_set, printed_by):
self.last_print_date = datetime.now()
self.save()
return PDFExport.create_pdf(self, template_set, printed_by)
def get_template_set(self):
if self.default_template_set.monthly_project_summary_template:
return self.default_template_set.monthly_project_summary_template
else:
raise TemplateSetMissingInContract((_("Template Set missing in Project" + str(self))))
def get_fop_config_file(self, template_set):
template_set = self.get_template_set()
return template_set.get_fop_config_file()
def get_xsl_file(self, template_set):
template_set = self.get_template_set()
return template_set.get_xsl_file()
def get_reporting_period(self, search_date):
from koalixcrm.crm.reporting.reporting_period import ReportingPeriod
"""Returns the reporting period that is valid. Valid is a reporting period when the provided date
lies between begin and end of the reporting period
Args:
no arguments
Returns:
accounting_period (ReportPeriod)
Raises:
ReportPeriodNotFound when there is no valid reporting Period"""
return ReportingPeriod.get_reporting_period(self, search_date)
def serialize_to_xml(self, **kwargs):
reporting_period = kwargs.get('reporting_period', None)
from koalixcrm.djangoUserExtension.models import UserExtension
objects = [self, ]
objects += UserExtension.objects_to_serialize(self, self.project_manager)
main_xml = PDFExport.write_xml(objects)
for task in Task.objects.filter(project=self.id):
task_xml = task.serialize_to_xml(reporting_period=reporting_period)
main_xml = PDFExport.merge_xml(main_xml, task_xml)
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Effective_Effort_Overall",
self.effective_costs(reporting_period=None))
if reporting_period:
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>']",
"Effective_Effort_InPeriod",
self.effective_costs(reporting_period=reporting_period))
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Planned_Effort",
self.planned_costs())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Effective_Duration",
self.effective_duration())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Planned_Duration",
self.planned_duration())
return main_xml
def effective_accumulated_costs(self, reporting_period=None):
if reporting_period:
reporting_periods = ReportingPeriod.get_all_predecessors(target_reporting_period=reporting_period,
project=self)
else:
reporting_periods = ReportingPeriod.objects.filter(project=self.id)
effective_accumulated_costs = 0
for single_reporting_period in reporting_periods:
all_project_tasks = Task.objects.filter(project=self.id)
for task in all_project_tasks:
effective_accumulated_costs += float(task.effective_costs(reporting_period=single_reporting_period))
getcontext().prec = 5
effective_accumulated_costs = Decimal(effective_accumulated_costs)
self.default_currency.round(effective_accumulated_costs)
return effective_accumulated_costs
effective_accumulated_costs.short_description = _("Effective Accumulated costs")
effective_accumulated_costs.tags = True
def effective_costs(self, reporting_period):
effective_cost = 0
for task in Task.objects.filter(project=self.id):
effective_cost += task.effective_costs(reporting_period=reporting_period)
self.default_currency.round(effective_cost)
return effective_cost
def planned_costs(self, reporting_period=None):
"""The function return the planned overall costs
Args:
no arguments
Returns:
planned costs (String)
Raises:
No exceptions planned"""
planned_effort_accumulated = 0
all_project_tasks = Task.objects.filter(project=self.id)
if all_project_tasks:
for task in all_project_tasks:
planned_effort_accumulated += task.planned_costs(reporting_period)
getcontext().prec = 5
planned_effort_accumulated = Decimal(planned_effort_accumulated)
self.default_currency.round(planned_effort_accumulated)
return planned_effort_accumulated
planned_costs.short_description = _("Planned Costs")
planned_costs.tags = True
def effective_start(self):
"""The function return the effective start of a project as a date
Args:
no arguments
Returns:
effective_start (Date) or None when not yet started
Raises:
No exceptions planned"""
no_tasks_started = True
all_project_tasks = Task.objects.filter(project=self.id)
effective_project_start = None
if len(all_project_tasks) == 0:
effective_project_start = None
else:
for task in all_project_tasks:
if not effective_project_start:
if task.effective_start():
effective_project_start = task.effective_start()
no_tasks_started = False
effective_task_start = task.effective_start()
if effective_task_start:
if effective_task_start < effective_project_start:
effective_project_start = effective_task_start
if no_tasks_started:
effective_project_start = None
return effective_project_start
effective_start.short_description = _("Effective Start")
effective_start.tags = True
def effective_end(self):
"""The function return the effective end of a project as a date
Args:
no arguments
Returns:
effective_end (Date) or None when not yet ended
Raises:
No exceptions planned"""
all_tasks_done = True
all_project_tasks = Task.objects.filter(project=self.id)
effective_project_end = None
if len(all_project_tasks) == 0:
effective_project_end = None
else:
i = 0
for task in all_project_tasks:
if not effective_project_end:
if not task.effective_start():
all_tasks_done = False
break
else:
effective_project_end = task.effective_start()
effective_task_end = task.effective_end()
if not effective_task_end:
all_tasks_done = False
break
elif effective_task_end > effective_project_end:
effective_project_end = effective_task_end
i = i+1
if not all_tasks_done:
effective_project_end = None
return effective_project_end
effective_end.short_description = _("Effective End")
effective_end.tags = True
def effective_duration(self):
"""The function return the effective overall duration of a project as a string in days
The function is reading the effective_starts and effective_ends of the project and
subtract them from each other.
Args:
no arguments
Returns:
duration_in_days or description (String)
Raises:
No exceptions planned"""
effective_end = self.effective_end()
effective_start = self.effective_start()
if not effective_start:
duration_as_string = "Project has not yet started"
elif not effective_end:
duration_as_string = "Project has not yet ended"
else:
duration_as_date = self.effective_end()-self.effective_start()
duration_as_string = duration_as_date.days.__str__()
return duration_as_string
effective_duration.short_description = _("Effective Duration [dys]")
effective_duration.tags = True
def planned_start(self):
""" The function return planned overall start of a project as a date
the function finds all tasks within this project and finds the earliest start date.
when no task is attached the task has which are attached have no start_date set, the
function returns a None value
Args:
no arguments
Returns:
planned_end (datetime.Date) or None
Raises:
No exceptions planned"""
tasks = Task.objects.filter(project=self.id)
if tasks:
i = 0
project_start = None
for task in tasks:
if task.planned_start():
if i == 0:
project_start = task.planned_start()
elif task.planned_start() < project_start:
project_start = task.planned_start()
i += 1
return project_start
else:
return None
def planned_end(self):
"""T he function return planned overall end of a project as a date
the function finds all tasks within this project and finds the latest start end_date.
when no task is attached the task has which are attached have no end_date set, the
function returns a None value
Args:
no arguments
Returns:
planned_end (datetime.Date)
Raises:
No exceptions planned"""
tasks = Task.objects.filter(project=self.id)
if tasks:
i = 0
project_end = None
for task in tasks:
if task.planned_end():
if i == 0:
project_end = task.planned_end()
elif task.planned_end() > project_end:
project_end = task.planned_end()
i += 1
return project_end
else:
return None
def planned_duration(self):
"""The function return planned overall duration of a project as a string in days
Args:
no arguments
Returns:
duration_in_days (String)
Raises:
No exceptions planned"""
if (not self.planned_start()) or (not self.planned_end()):
duration_in_days = "n/a"
elif self.planned_start() > self.planned_end():
duration_in_days = "n/a"
else:
duration_in_days = (self.planned_end()-self.planned_start()).days.__str__()
return duration_in_days
planned_duration.short_description = _("Planned Duration [dys]")
planned_duration.tags = True
def get_project_name(self):
"""The function safely returns a project_name even if the values is empty, in such a case
the function returns a n/a"
Args:
no arguments
Returns:
project_name (String)
Raises:
No exceptions planned"""
if self.project_name:
return self.project_name
else:
return "n/a"
def is_reporting_allowed(self):
"""The function returns a boolean True when it is allowed to report on the project and
on one of the reporting periods does also allow reporting
Args:
no arguments
Returns:
reporting_allowed (Boolean)
Raises:
No exceptions planned"""
from koalixcrm.crm.reporting.reporting_period import ReportingPeriod
reporting_periods = ReportingPeriod.objects.filter(project=self.id, status__is_done=False)
if len(reporting_periods) != 0:
if not self.project_status.is_done:
return True
else:
return False
else:
return False
def __str__(self):
return str(self.id)+" "+self.get_project_name()
class Meta:
app_label = "crm"
verbose_name = _('Project')
verbose_name_plural = _('Projects')
class ProjectAdminView(admin.ModelAdmin):
list_display = ('id',
'project_status',
'project_name',
'project_manager',
'default_currency',
'planned_duration',
'planned_costs',
'effective_duration',
'effective_accumulated_costs'
)
list_display_links = ('id',)
ordering = ('-id',)
fieldsets = (
(_('Project'), {
'fields': ('project_status',
'project_manager',
'project_name',
'description',
'default_currency',
'default_template_set',)
}),
)
inlines = [TaskInlineAdminView,
GenericLinkInlineAdminView,
ReportingPeriodInlineAdminView]
actions = ['create_report_pdf', ]
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
def create_report_pdf(self, request, queryset):
from koalixcrm.crm.views.pdfexport import PDFExportView
for obj in queryset:
response = PDFExportView.export_pdf(self,
request,
obj,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"),
obj.default_template_set.monthly_project_summary_template)
return response
create_report_pdf.short_description = _("Create Report PDF")
class ProjectInlineAdminView(admin.TabularInline):
model = Project
readonly_fields = ('link_to_project',
'project_status',
'project_manager',
'description',
'default_template_set')
fieldsets = (
(_('Project'), {
'fields': ('link_to_project',
'project_status',
'project_manager',
'description',
'default_template_set')
}),
)
extra = 0
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
class ProjectJSONSerializer(serializers.ModelSerializer):
from koalixcrm.crm.reporting.task import TaskSerializer
tasks = TaskSerializer(many=True, read_only=True)
is_reporting_allowed = serializers.SerializerMethodField()
def get_is_reporting_allowed(self, obj):
if obj.is_reporting_allowed():
return "True"
else:
return "False"
class Meta:
model = Project
fields = ('id',
'project_manager',
'project_name',
'tasks',
'is_reporting_allowed')<file_sep>/koalixcrm/crm/factories/factory_currency.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Currency
class StandardCurrencyFactory(factory.django.DjangoModelFactory):
class Meta:
model = Currency
django_get_or_create = ('description',)
description = "Swiss Francs"
short_name = "CHF"
rounding = "0.05"
class SecondStandardCurrencyFactory(factory.django.DjangoModelFactory):
class Meta:
model = Currency
django_get_or_create = ('description',)
description = "Euro"
short_name = "EUR"
rounding = "0.01"
<file_sep>/koalixcrm/subscriptions/models.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.utils.translation import ugettext as _
from filebrowser.fields import FileBrowseField
from koalixcrm.subscriptions.const.events import *
import koalixcrm.crm.documents
class Subscription(models.Model):
contract = models.ForeignKey('crm.Contract', verbose_name=_('Subscription Type'))
subscription_type = models.ForeignKey('SubscriptionType', verbose_name=_('Subscription Type'), null=True)
def create_subscription_from_contract(self, contract):
self.contract = contract
self.save()
return self
def create_quote(self):
quote = koalixcrm.crm.documents.quote.Quote()
quote.contract = self.contract
quote.discount = 0
quote.staff = self.contract.staff
quote.customer = self.contract.defaultcustomer
quote.status = 'C'
quote.currency = self.contract.defaultcurrency
quote.valid_until = date.today().__str__()
quote.date_of_creation = date.today().__str__()
quote.save()
return quote
def create_invoice(self):
invoice = koalixcrm.crm.documents.invoice.Invoice()
invoice.contract = self.contract
invoice.discount = 0
invoice.staff = self.contract.staff
invoice.customer = self.contract.default_customer
invoice.status = 'C'
invoice.currency = self.contract.default_currency
invoice.payable_until = date.today() + timedelta(
days=self.contract.defaultcustomer.defaultCustomerBillingCycle.timeToPaymentDate)
invoice.date_of_creation = date.today().__str__()
invoice.save()
return invoice
class Meta:
app_label = "subscriptions"
verbose_name = _('Subscription')
verbose_name_plural = _('Subscriptions')
class SubscriptionEvent(models.Model):
subscriptions = models.ForeignKey('Subscription',
verbose_name=_('Subscription'))
event_date = models.DateField(verbose_name=_("Event Date"),
blank=True, null=True)
event = models.CharField(max_length=1, choices=SUBSCRITIONEVENTS,
verbose_name=_('Event'))
def __str__(self):
return self.event
class Meta:
app_label = "subscriptions"
verbose_name = _('Subscription Event')
verbose_name_plural = _('Subscription Events')
class SubscriptionType(models.Model):
product_type = models.ForeignKey('crm.ProductType',
verbose_name=_('Product Type'),
on_delete=models.deletion.SET_NULL,
null=True,
blank=True)
cancellation_period = models.IntegerField(verbose_name=_("Cancellation Period (months)"),
blank=True,
null=True)
automatic_contract_extension = models.IntegerField(verbose_name=_("Automatic Contract Extension (months)"),
blank=True,
null=True)
automatic_contract_extension_reminder = models.IntegerField(
verbose_name=_("Automatic Contract Extension Reminder (days)"),
blank=True,
null=True)
minimum_duration = models.IntegerField(verbose_name=_("Minimum Contract Duration"),
blank=True,
null=True)
payment_interval = models.IntegerField(verbose_name=_("Payment Interval (days)"),
blank=True,
null=True)
contract_document = FileBrowseField(verbose_name=_("Contract Documents"),
blank=True,
null=True,
max_length=200)
class Meta:
app_label = "subscriptions"
verbose_name = _('Subscription Type')
verbose_name_plural = _('Subscription Types')
<file_sep>/koalixcrm/crm/migrations/0019_auto_20180112_2020.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-12 20:20
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0018_auto_20180111_2031'),
]
operations = [
migrations.AlterModelOptions(
name='emailaddressforsalesdocument',
options={'verbose_name': 'Email Address For Sales Documents', 'verbose_name_plural': 'Email Address For Sales Documents'},
),
migrations.AlterModelOptions(
name='phoneaddressforsalesdocument',
options={'verbose_name': 'Phone Address For Sales Documents', 'verbose_name_plural': 'Phone Address For Sales Documents'},
),
migrations.AlterModelOptions(
name='postaladdressforsalesdocument',
options={'verbose_name': 'Postal Address For Sales Documents', 'verbose_name_plural': 'Postal Address For Sales Documents'},
),
migrations.RenameField(
model_name='customerbillingcycle',
old_name='timeToPaymentDate',
new_name='time_to_payment_date',
),
migrations.RemoveField(
model_name='deliverynote',
name='derived_from_invoice',
),
migrations.RemoveField(
model_name='deliverynote',
name='derived_from_quote',
),
migrations.RemoveField(
model_name='paymentreminder',
name='derived_from_invoice',
),
migrations.RemoveField(
model_name='purchaseconfirmation',
name='derived_from_quote',
),
migrations.AddField(
model_name='customerbillingcycle',
name='payment_reminder_time_to_payment',
field=models.IntegerField(default=10, verbose_name='Payment Reminder, Days To Payment Date '),
preserve_default=False,
),
]
<file_sep>/koalixcrm/subscriptions/migrations/0003_auto_20171110_1732.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-11-10 17:32
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('subscriptions', '0002_auto_20171009_1949'),
]
operations = [
migrations.AlterModelOptions(
name='subscription',
options={'verbose_name': 'Subscription', 'verbose_name_plural': 'Subscriptions'},
),
migrations.AlterModelOptions(
name='subscriptionevent',
options={'verbose_name': 'Subscription Event', 'verbose_name_plural': 'Subscription Events'},
),
migrations.AlterModelOptions(
name='subscriptiontype',
options={'verbose_name': 'Subscription Type', 'verbose_name_plural': 'Subscription Types'},
),
migrations.AlterField(
model_name='subscription',
name='contract',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contract', verbose_name='Subscription Type'),
),
migrations.AlterField(
model_name='subscription',
name='subscriptiontype',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE, to='subscriptions.SubscriptionType', verbose_name='Subscription Type'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='event',
field=models.CharField(choices=[('O', 'Offered'), ('C', 'Canceled'), ('S', 'Signed')], max_length=1, verbose_name='Event'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='eventdate',
field=models.DateField(blank=True, null=True, verbose_name='Event Date'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='subscriptions',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='subscriptions.Subscription', verbose_name='Subscription'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtension',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatic Contract Extension (months)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtensionReminder',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatic Contract Extensoin Reminder (days)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='cancelationPeriod',
field=models.IntegerField(blank=True, null=True, verbose_name='Cancelation Period (months)'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='contractDocument',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='Contract Documents'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='minimumDuration',
field=models.IntegerField(blank=True, null=True, verbose_name='Minimum Contract Duration'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='paymentIntervall',
field=models.IntegerField(blank=True, null=True, verbose_name='Payment Intervall (days)'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_generic_project_link.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import GenericProjectLink
from koalixcrm.crm.factories.factory_project import StandardProjectFactory
from koalixcrm.crm.factories.factory_task_link_type import RelatedToTaskLinkTypeFactory
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from django.contrib.contenttypes.models import ContentType
class StandardGenericTaskLinkFactory(factory.django.DjangoModelFactory):
class Meta:
exclude = ['generic_crm_object']
task = factory.SubFactory(StandardProjectFactory)
task_link_type = factory.SubFactory(RelatedToTaskLinkTypeFactory)
content_type = factory.LazyAttribute(
lambda o: ContentType.objects.get_for_model(o.generic_crm_object))
object_id = factory.SelfAttribute('generic_crm_object.id')
date_of_creation = "2018-05-01"
last_modified_by = factory.SubFactory(StaffUserFactory)
class LinkToTaskGenericTaskLinkFactory(StandardGenericTaskLinkFactory):
class Meta:
model = GenericProjectLink
generic_crm_object = factory.SubFactory(StandardTaskFactory)
<file_sep>/koalixcrm/crm/tests/test_task_constructor.py
import datetime
import pytest
from django.test import TestCase
from koalixcrm.crm.factories.factory_user import AdminUserFactory
from koalixcrm.crm.factories.factory_customer_billing_cycle import StandardCustomerBillingCycleFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
from koalixcrm.crm.reporting.task import Task
@pytest.fixture()
def freeze(monkeypatch):
""" Now() manager patches date return a fixed, settable, value
(freezes date)
"""
original = datetime.date
class FreezeMeta(type):
def __instancecheck__(self, instance):
if instance.isinstance(original) or instance.isinstance(Freeze):
return True
class Freeze(datetime.datetime):
__metaclass__ = FreezeMeta
@classmethod
def freeze(cls, val):
cls.frozen = val
@classmethod
def today(cls):
return cls.frozen
@classmethod
def delta(cls, timedelta=None, **kwargs):
""" Moves time fwd/bwd by the delta"""
from datetime import timedelta as td
if not timedelta:
timedelta = td(**kwargs)
cls.frozen += timedelta
monkeypatch.setattr(datetime, 'date', Freeze)
Freeze.freeze(original.today())
return Freeze
class TaskConstructorTest(TestCase):
@pytest.fixture(autouse=True)
def freeze_time(self, freeze):
self._freeze = freeze
def setUp(self):
self.test_billing_cycle = StandardCustomerBillingCycleFactory.create()
self.test_user = AdminUserFactory.create()
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.test_user_extension = StandardUserExtensionFactory.create(user=self.test_user)
self.test_reporting_period = StandardReportingPeriodFactory.create()
def test_task_constructor(self):
self._freeze.freeze(datetime.date(2024, 6, 2))
task_minimal_1 = Task.objects.create(
project=self.test_reporting_period.project,
)
task_minimal_1.save()
self.assertEquals(task_minimal_1.last_status_change, datetime.date(2024, 6, 2))
self._freeze.freeze(datetime.date(2018, 6, 15))
task_minimal_2 = Task.objects.create(
project=self.test_reporting_period.project,
)
task_minimal_2.save()
self.assertEquals(task_minimal_2.last_status_change, datetime.date(2018, 6, 15))
<file_sep>/koalixcrm/crm/factories/factory_product_type.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import ProductType
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_tax import StandardTaxFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardProductTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProductType
django_get_or_create = ('product_type_identifier',)
title = "This is a test Product"
description = "This is a test Product"
product_type_identifier = "123456"
default_unit = factory.SubFactory(StandardUnitFactory)
date_of_creation = make_date_utc(datetime.datetime(2018, 6, 15, 00))
last_modification = make_date_utc(datetime.datetime(2018, 6, 15, 00))
last_modified_by = factory.SubFactory(StaffUserFactory)
tax = factory.SubFactory(StandardTaxFactory)
<file_sep>/requirements/base_requirements.txt
Django==1.11.21
django-filebrowser==3.9.1
lxml==3.8.0
olefile==0.44
Pillow==6.2.0
psycopg2-binary==2.7.5
pytz==2017.2
django-grappelli==2.10.1
djangorestframework>=3.9.1
djangorestframework-xml==1.4.0
markdown==2.6.11
django-filter==1.1.0
<file_sep>/koalixcrm/crm/contact/customer_billing_cycle.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class CustomerBillingCycle(models.Model):
name = models.CharField(max_length=300,
verbose_name=_("Name"))
time_to_payment_date = models.IntegerField(verbose_name=_("Days To Payment Date"))
payment_reminder_time_to_payment = models.IntegerField(verbose_name=_("Payment Reminder, Days To Payment Date "))
class Meta:
app_label = "crm"
verbose_name = _('Customer Billing Cycle')
verbose_name_plural = _('Customer Billing Cycle')
def __str__(self):
return self.id.__str__() + ' ' + self.name
class OptionCustomerBillingCycle(admin.ModelAdmin):
list_display = ('id',
'name',
'time_to_payment_date',
'payment_reminder_time_to_payment')
fieldsets = (('', {'fields': ('name',
'time_to_payment_date',
'payment_reminder_time_to_payment',
)}),)
allow_add = True
<file_sep>/koalixcrm/crm/reporting/human_resource.py
# -*- coding: utf-8 -*-
import datetime
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.djangoUserExtension.user_extension.user_extension import UserExtension
from koalixcrm.crm.reporting.resource import Resource
from koalixcrm.crm.reporting.resource_price import ResourcePriceInlineAdminView
from koalixcrm.crm.reporting.work import Work
from koalixcrm.crm.documents.pdf_export import PDFExport
class HumanResource(Resource):
user = models.ForeignKey(UserExtension,
verbose_name=_("User"))
def __str__(self):
return self.user.__str__()
def serialize_to_xml(self, **kwargs):
date_from = kwargs.get('date_from', datetime.date.today()-datetime.timedelta(days=60))
date_to = kwargs.get('date_to', datetime.date.today())
date_first_of_the_month = date_from.replace(day=1)
date_to_month = date_to.month
date_end_of_the_month = date_from.replace(day=1).replace(month=date_to_month+1) - datetime.timedelta(days=1)
date = date_first_of_the_month
days = dict()
weeks = dict()
months = dict()
projects = self.resource_contribution_project(date_from, date_to)
objects = [self, self.user]
objects.extend(projects)
main_xml = PDFExport.write_xml(objects)
while date < date_from:
project_efforts = dict()
for project in projects:
project_efforts[project] = {'effort': "-",
'project': project.id.__str__()}
days[date] = {'effort': "-",
"day": str(date.day),
"week": str(date.isocalendar()[1]),
"week_day": str(date.isoweekday()),
"month": str(date.month),
"year": str(date.year),
"project_efforts": project_efforts}
date += datetime.timedelta(days=1)
while date <= date_to:
project_efforts_day = dict()
project_efforts_week = dict()
project_efforts_month = dict()
for project in projects:
project_efforts_day[project] = {'effort': 0,
'project': project.id.__str__()}
project_efforts_week[project] = {'effort': 0,
'project': project.id.__str__()}
project_efforts_month[project] = {'effort': 0,
'project': project.id.__str__()}
days[date] = {'effort': 0,
"day": str(date.day),
"week": str(date.isocalendar()[1]),
"week_day": str(date.isoweekday()),
"month": str(date.month),
"year": str(date.year),
"project_efforts": project_efforts_day}
month_key = str(date.month)+"/"+str(date.year)
week_key = str(date.isocalendar()[1])+"/"+str(date.year)
if not (week_key in weeks):
weeks[week_key] = {'effort': 0,
'week': str(date.isocalendar()[1]),
'year': str(date.year),
"project_efforts": project_efforts_week}
if not (month_key in months):
months[month_key] = {'effort': 0,
'month': str(date.month),
'year': str(date.year),
"project_efforts": project_efforts_month}
date += datetime.timedelta(days=1)
while date < date_end_of_the_month:
project_efforts = dict()
for project in projects:
project_efforts[project] = {'effort': "-",
'project': project.id.__str__()}
days[date] = {'effort': "-",
"day": str(date.day),
"week": str(date.isocalendar()[1]),
"week_day": str(date.isoweekday()),
"month": str(date.month),
"year": str(date.year),
"project_efforts": project_efforts}
date += datetime.timedelta(days=1)
main_xml = PDFExport.append_element_to_pattern(main_xml,
".",
"range_from",
date_from.__str__(),
attributes={"day": str(date_from.day),
"week": str(date_from.isocalendar()[1]),
"week_day": str(date_from.isoweekday()),
"month": str(date_from.month),
"year": str(date_from.year)})
main_xml = PDFExport.append_element_to_pattern(main_xml,
".",
"range_to",
date_to.__str__(),
attributes={"day": str(date_to.day),
"week": str(date_to.isocalendar()[1]),
"week_day": str(date_to.isoweekday()),
"month": str(date_to.month),
"year": str(date_to.year)})
works = Work.objects.filter(human_resource=self, date__range=(date_from, date_to))
for work in works:
days[work.date]['effort'] += work.effort_hours()
days[work.date]['project_efforts'][work.task.project]['effort'] += work.effort_hours()
month_key = str(work.date.month)+"/"+str(work.date.year)
week_key = str(work.date.isocalendar()[1])+"/"+str(work.date.year)
weeks[week_key]['effort'] += work.effort_hours()
weeks[week_key]['project_efforts'][work.task.project]['effort'] += work.effort_hours()
months[month_key]['effort'] += work.effort_hours()
months[month_key]['project_efforts'][work.task.project]['effort'] += work.effort_hours()
work_xml = work.serialize_to_xml()
main_xml = PDFExport.merge_xml(main_xml, work_xml)
for day_key in days.keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@<EMAIL>='django<EMAIL>']",
"Day_Work_Hours",
str(days[day_key]['effort']),
attributes={"day": days[day_key]['day'],
"week": days[day_key]['week'],
"week_day": days[day_key]['week_day'],
"month": days[day_key]['month'],
"year": days[day_key]['year']})
for project_key in days[day_key]['project_efforts'].keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@<EMAIL>='<EMAIL>']",
"Day_Project_Work_Hours",
str(days[day_key]['project_efforts'][project_key]['effort']),
attributes={"day": days[day_key]['day'],
"week": days[day_key]['week'],
"week_day": days[day_key]['week_day'],
"month": days[day_key]['month'],
"year": days[day_key]['year'],
"project": days[day_key]['project_efforts'][project_key]['project']})
for week_key in weeks.keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@model='<EMAIL>']",
"Week_Work_Hours",
str(weeks[week_key]['effort']),
attributes={"week": weeks[week_key]['week'],
"year": weeks[week_key]['year']})
for project_key in weeks[week_key]['project_efforts'].keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@model='django<EMAIL>']",
"Week_Project_Work_Hours",
str(weeks[week_key]['project_efforts'][project_key]['effort']),
attributes={"week": weeks[week_key]['week'],
"year": weeks[week_key]['year'],
"project": weeks[week_key]['project_efforts'][project_key]['project']})
for month_key in months.keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@model='django<EMAIL>']",
"Month_Work_Hours",
str(months[month_key]['effort']),
attributes={"month": months[month_key]['month'],
"year": months[month_key]['year']})
for project_key in months[month_key]['project_efforts'].keys():
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[@model='django<EMAIL>']",
"Month_Project_Work_Hours",
str(months[month_key]['project_efforts'][project_key]['effort']),
attributes={"month": months[month_key]['month'],
"year": months[month_key]['year'],
"project": months[month_key]['project_efforts'][project_key]['project']})
return main_xml
def create_pdf(self, template_set, printed_by, *args, **kwargs):
return PDFExport.create_pdf(self, template_set, printed_by, *args, **kwargs)
def get_template_set(self, template_set):
return self.user.get_template_set(template_set)
def get_fop_config_file(self, template_set):
return self.user.get_fop_config_file(template_set)
def get_xsl_file(self, template_set):
return self.user.get_xsl_file(template_set)
def resource_contribution_project(self, date_from, date_to):
works = Work.objects.filter(human_resource=self,
date__range=(date_from, date_to))
projects = []
for work in works:
if work.task.project not in projects:
projects.append(work.task.project)
return projects
class HumanResourceAdminView(admin.ModelAdmin):
list_display = ('id',
'user',
'resource_manager',
'resource_type')
list_display_links = ('id',
'user')
list_filter = ('user',)
ordering = ('id',)
search_fields = ('id',
'user')
fieldsets = (
(_('Basics'), {
'fields': ('user',
'resource_manager',
'resource_type')
}),
)
def create_work_report_pdf(self, request, queryset):
from koalixcrm.crm.views.create_work_report import create_work_report
return create_work_report(self, request, queryset)
create_work_report_pdf.short_description = _("Work Report PDF")
save_as = True
actions = [create_work_report_pdf]
inlines = [ResourcePriceInlineAdminView]
<file_sep>/koalixcrm/crm/views/user_is_not_human_resource.py
# -*- coding: utf-8 -*-
from django.http import HttpResponseRedirect, Http404
from django.shortcuts import render
from django.template.context_processors import csrf
from django.contrib.admin import helpers
from django.contrib.admin.widgets import *
from koalixcrm.djangoUserExtension.exceptions import TooManyUserExtensionsAvailable
class ReportingPeriodMissingForm(forms.Form):
NEXT_STEPS = (
('create_human_resource', 'Create Human Resource'),
('return_to_start', 'Return To Start'),
)
next_steps = forms.ChoiceField(required=True,
widget=forms.Select,
choices=NEXT_STEPS)
def user_is_not_human_resource(request):
try:
if request.POST.get('post'):
if 'confirm_selection' in request.POST:
reporting_period_missing_form = ReportingPeriodMissingForm(request.POST)
if reporting_period_missing_form.is_valid():
if reporting_period_missing_form.cleaned_data['next_steps'] == 'return_to_start':
return HttpResponseRedirect('/admin/')
else:
return HttpResponseRedirect('/admin/crm/humanresource/add/')
else:
reporting_period_missing_form = ReportingPeriodMissingForm(initial={'next_steps': 'create_user_extension'})
title = "User is not registered as Human Resource"
description = "The operation you have selected requires that the currently active user is a registered " \
"as a Human Resource. "
c = {'action_checkbox_name': helpers.ACTION_CHECKBOX_NAME,
'form': reporting_period_missing_form,
'description': description,
'title': title}
c.update(csrf(request))
return render(request, 'crm/admin/exception.html', c)
except TooManyUserExtensionsAvailable:
raise Http404
<file_sep>/koalixcrm/crm/contact/postal_address.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.country import *
from koalixcrm.crm.const.postaladdressprefix import *
class PostalAddress(models.Model):
prefix = models.CharField(max_length=1,
choices=POSTALADDRESSPREFIX,
verbose_name=_("Prefix"), blank=True,
null=True)
name = models.CharField(max_length=100,
verbose_name=_("Name"),
blank=True,
null=True)
pre_name = models.CharField(max_length=100,
verbose_name=_("Pre-name"),
blank=True,
null=True)
address_line_1 = models.CharField(max_length=200,
verbose_name=_("Address line 1"),
blank=True,
null=True)
address_line_2 = models.CharField(max_length=200,
verbose_name=_("Address line 2"),
blank=True,
null=True)
address_line_3 = models.CharField(max_length=200,
verbose_name=_("Address line 3"),
blank=True,
null=True)
address_line_4 = models.CharField(max_length=200,
verbose_name=_("Address line 4"),
blank=True,
null=True)
zip_code = models.IntegerField(verbose_name=_("Zip Code"),
blank=True,
null=True)
town = models.CharField(max_length=100,
verbose_name=_("City"),
blank=True,
null=True)
state = models.CharField(max_length=100,
verbose_name=_("State"),
blank=True,
null=True)
country = models.CharField(max_length=2,
choices=[(x[0], x[3]) for x in COUNTRIES],
verbose_name=_("Country"),
blank=True,
null=True)
class Meta:
app_label = "crm"
verbose_name = _('Postal Address')
verbose_name_plural = _('Postal Address')
<file_sep>/koalixcrm/crm/tests/test_create_task.py
# -*- coding: utf-8 -*-
import pytest
from django.test import TestCase
from koalixcrm.crm.factories.factory_sales_document_position import StandardSalesDocumentPositionFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_quote import StandardQuoteFactory
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
from koalixcrm.crm.factories.factory_product_price import StandardPriceFactory
from koalixcrm.crm.models import Task
from koalixcrm.crm.views.create_task import CreateTaskView
class DocumentCalculationsTest(TestCase):
def setUp(self):
self.test_currency = StandardCurrencyFactory.create()
self.test_quote = StandardQuoteFactory.create()
self.test_user = StaffUserFactory.create()
for i in range(10):
test_product = StandardProductTypeFactory.create(
description="This is a test product " + i.__str__(),
title="This is a test product " + i.__str__(),
product_type_identifier=12334235+i,
)
StandardPriceFactory.create(
product_type=test_product,
)
StandardSalesDocumentPositionFactory.create(
sales_document=self.test_quote,
position_number=i*10,
quantity=0.333*i,
description="This is a test position " + i.__str__(),
discount=i*5
)
@pytest.mark.back_end_tests
def test_create_task(self):
project = CreateTaskView.create_project_from_document(self.test_user, self.test_quote)
tasks = Task.objects.filter(project=project.id)
task_counter = 0
for task_current in tasks:
self.assertEqual(
task_current.title.__str__()[:24], "This is a test position ")
task_counter += 1
self.assertEqual(
task_counter.__str__(), "10")
<file_sep>/koalixcrm/crm/factories/factory_sales_document.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import SalesDocument
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_contract import StandardContractFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.djangoUserExtension.factories.factory_document_template import StandardQuoteTemplateFactory
class StandardSalesDocumentFactory(factory.django.DjangoModelFactory):
class Meta:
model = SalesDocument
contract = factory.SubFactory(StandardContractFactory)
external_reference = "This is an external Reference"
discount = "0"
description = "This is the description of a sales document"
last_pricing_date = "2018-05-01"
last_calculated_price = "220.00"
last_calculated_tax = "10.00"
customer = factory.SubFactory(StandardCustomerFactory)
staff = factory.SubFactory(StaffUserFactory)
currency = factory.SubFactory(StandardCurrencyFactory)
date_of_creation = "2018-05-01"
custom_date_field = "2018-05-20"
last_modification = "2018-05-25"
last_modified_by = factory.SubFactory(StaffUserFactory)
template_set = factory.SubFactory(StandardQuoteTemplateFactory)
derived_from_sales_document = None
last_print_date = "2018-05-26"
<file_sep>/koalixcrm/crm/factories/factory_project_status.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ProjectStatus
class DoneProjectStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProjectStatus
title = "Done"
description = "This project is done"
is_done = True
class StartedProjectStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProjectStatus
title = "Started"
description = "This project has started"
is_done = False
<file_sep>/koalixcrm/crm/factories/factory_quote.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.models import Quote
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardQuoteFactory(StandardSalesDocumentFactory):
class Meta:
model = Quote
valid_until = "2018-05-20"
status = "C"
<file_sep>/koalixcrm/crm/product/customer_group_transform.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class CustomerGroupTransform(models.Model):
from_customer_group = models.ForeignKey('CustomerGroup',
verbose_name=_("From Customer Group"),
related_name="db_reltransfromfromcustomergroup",
blank=False,
null=False)
to_customer_group = models.ForeignKey('CustomerGroup',
verbose_name=_("To Customer Group"),
related_name="db_reltransfromtocustomergroup",
blank=False,
null=False)
product_type = models.ForeignKey('ProductType',
verbose_name=_("Product Type"),
blank=False,
null=False)
factor = models.DecimalField(verbose_name=_("Factor between From and To Customer Group"),
blank=False,
null=False,
max_digits=17,
decimal_places=2,)
def transform(self, customer_group):
"""The transform function verifies whether the provided argument customer_group
is corresponding with the "from_customer_group" variable of the CustomerGroupTransform class
When this is ok, the function returns the "to_customer_group". When the provided customer_group
argument is not corresponding, the function returns a "None"
Args:
customer_group: CustomerGroup object
Returns:
CustomerGroup object or None
Raises:
No exceptions planned"""
if self.from_customer_group == customer_group:
return self.to_customer_group
else:
return None
def get_transform_factor(self):
return self.factor
def __str__(self):
return "From " + self.from_customer_group.name + " to " + self.to_customer_group.name
class Meta:
app_label = "crm"
verbose_name = _('Customer Group Price Transform')
verbose_name_plural = _('Customer Group Price Transforms')
class CustomerGroupTransformInlineAdminView(admin.TabularInline):
model = CustomerGroupTransform
extra = 1
classes = ['collapse']
fieldsets = (
('', {
'fields': ('from_customer_group',
'to_customer_group',
'factor',)
}),
)
allow_add = True
<file_sep>/koalixcrm/crm/factories/factory_user.py
# -*- coding: utf-8 -*-
import factory
from django.contrib.auth.models import User
class StaffUserFactory(factory.django.DjangoModelFactory):
class Meta:
model = User
django_get_or_create = ('username',)
username = 'staff_user'
email = '<EMAIL>'
password = factory.PostGenerationMethodCall('set_password', '<PASSWORD>')
is_superuser = False
is_staff = True
is_active = True
class AdminUserFactory(factory.django.DjangoModelFactory):
class Meta:
model = User
django_get_or_create = ('username',)
username = 'admin'
email = '<EMAIL>'
password = factory.PostGenerationMethodCall('set_password', '<PASSWORD>')
is_superuser = True
is_staff = True
is_active = True
class StandardUserFactory(factory.django.DjangoModelFactory):
class Meta:
model = User
django_get_or_create = ('username',)
username = 'standard'
email = '<EMAIL>'
password = factory.PostGenerationMethodCall('set_password', '<PASSWORD>')
is_superuser = False
is_staff = False
is_active = True
<file_sep>/koalixcrm/crm/tests/test_project_planned_costs.py
import datetime
import pytest
from django.test import TestCase
from koalixcrm.crm.factories.factory_user import AdminUserFactory
from koalixcrm.crm.factories.factory_customer_billing_cycle import StandardCustomerBillingCycleFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.crm.factories.factory_estimation import StandardEstimationToTaskFactory
from koalixcrm.crm.factories.factory_human_resource import StandardHumanResourceFactory
from koalixcrm.crm.factories.factory_resource_price import StandardResourcePriceFactory
from koalixcrm.crm.factories.factory_project import StandardProjectFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
from koalixcrm.crm.factories.factory_estimation import StandardHumanResourceEstimationToTaskFactory
from koalixcrm.test_support_functions import make_date_utc
class TaskPlannedEffort(TestCase):
def setUp(self):
self.test_billing_cycle = StandardCustomerBillingCycleFactory.create()
self.test_user = AdminUserFactory.create()
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_unit = StandardUnitFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.test_user_extension = StandardUserExtensionFactory.create(user=self.test_user)
self.test_project = StandardProjectFactory.create()
self.test_human_resource = StandardHumanResourceFactory.create()
@pytest.mark.back_end_tests
def test_project_planned_costs(self):
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
start_date = (datetime_now - datetime.timedelta(days=30)).date()
end_date_first_task = (datetime_now + datetime.timedelta(days=30)).date()
end_date_second_task = (datetime_now + datetime.timedelta(days=60)).date()
self.assertEqual(
(self.test_project.planned_costs()).__str__(), "0")
self.test_reporting_period = StandardReportingPeriodFactory.create(
project=self.test_project
)
self.assertEqual(
(self.test_project.planned_costs()).__str__(), "0")
self.test_1st_task = StandardTaskFactory.create(title="1st Test Task",
project=self.test_project)
self.test_2nd_task = StandardTaskFactory.create(title="2nd Test Task",
project=self.test_project)
self.assertEqual(
(self.test_project.planned_costs()).__str__(), "0")
self.estimation_1st_task = StandardHumanResourceEstimationToTaskFactory(task=self.test_1st_task,
date_from=start_date,
date_until=end_date_first_task,
amount=0)
self.estimation_2nd_task = StandardHumanResourceEstimationToTaskFactory(task=self.test_2nd_task,
date_from=start_date,
date_until=end_date_second_task,
amount=0)
self.resource_price = StandardResourcePriceFactory.create(
resource=self.test_human_resource,
unit=self.test_unit,
currency=self.test_currency,
customer_group=self.test_customer_group,
price="120",
)
self.assertEqual(
(self.test_project.planned_costs()).__str__(), "0")
StandardEstimationToTaskFactory.create(resource=self.test_human_resource,
amount="2.00",
task=self.test_1st_task,
reporting_period=self.test_reporting_period)
StandardEstimationToTaskFactory.create(resource=self.test_human_resource,
amount="1.50",
task=self.test_1st_task,
reporting_period=self.test_reporting_period)
StandardEstimationToTaskFactory.create(resource=self.test_human_resource,
amount="4.75",
task=self.test_2nd_task,
reporting_period=self.test_reporting_period)
StandardEstimationToTaskFactory.create(resource=self.test_human_resource,
amount="3.25",
task=self.test_2nd_task,
reporting_period=self.test_reporting_period)
self.assertEqual(
(self.test_project.planned_costs(reporting_period=self.test_reporting_period)).__str__(), "1380.0")
<file_sep>/koalixcrm/crm/reporting/urls.py
# coding: utf-8
# DJANGO IMPORTS
from django.conf.urls import url
from koalixcrm.crm.views.time_tracking import work_report
from koalixcrm.crm.views.user_extension_missing import user_extension_missing
from koalixcrm.crm.views.reporting_period_missing import reporting_period_missing
from koalixcrm.crm.views.user_is_not_human_resource import user_is_not_human_resource
urlpatterns = [
url(r'^time_tracking/$', work_report, name="monthly_report"),
url(r'^user_extension_missing/$', user_extension_missing, name="user_extension_missing"),
url(r'^reporting_period_missing/$', reporting_period_missing, name="reporting_period_missing"),
url(r'^user_is_not_human_resource/$', user_is_not_human_resource, name="user_is_not_human_resource"),
]<file_sep>/CONTRIBUTING.md
# Contributing
When contributing to this repository, please first discuss the change you wish to make via issue,
email, or any other method with the owners of this repository before making a change.
Please note we have a code of conduct, please follow it in all your interactions with the project.
## Pull Request Process
1. Ensure any install or build dependencies are removed before the end of the layer when doing a
build.
2. Update the README.md with details of changes to the interface, this includes new environment
variables, exposed ports, useful file locations and container parameters.
3. Increase the version numbers in all version.py files to the new version that this
Pull Request would represent. The versioning scheme (see next section)
4. Crate a Pull Request towards the "development" branch, ensure that your pull-request
has at least 80% test-coverage and the Pull Request does not show issues in Travis
5. Once the Pull Request is reviewed it will be merged by one of the core developers
## Versioning scheme
- 1.2.0.dev1 - Development release
- 1.2.0a1 - Alpha Release
- 1.2.0b1 - Beta Release
- 1.2.0rc1 - Release Candidate
- 1.2.0 - Final Release
- 1.2.0.post1 - Post Release
<file_sep>/koalixcrm/crm/migrations/0006_auto_20171210_1805.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-12-10 18:05
from __future__ import unicode_literals
import django.core.validators
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0003_auto_20171110_1732'),
('crm', '0005_auto_20171110_1732'),
]
operations = [
migrations.CreateModel(
name='DeliveryNote',
fields=[
('salescontract_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.SalesContract')),
('status', models.CharField(choices=[('C', 'Created'), ('S', 'Sent'), ('R', 'Received'), ('R', 'Lost')], max_length=1)),
('derived_from_invoice', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Invoice')),
],
options={
'verbose_name_plural': 'Delivery Notes',
'verbose_name': 'Delivery Note',
},
bases=('crm.salescontract',),
),
migrations.CreateModel(
name='PaymentReminder',
fields=[
('salescontract_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.SalesContract')),
('iteration_number', models.IntegerField(validators=[django.core.validators.MinValueValidator(1), django.core.validators.MaxValueValidator(3)], verbose_name='Iteration Number')),
('status', models.CharField(choices=[('P', 'Payed'), ('C', 'Invoice created'), ('I', 'Invoice sent'), ('F', 'First reminder sent'), ('R', 'Second reminder sent'), ('U', 'Customer cant pay'), ('D', 'Deleted')], max_length=1)),
('derived_from_invoice', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Invoice')),
],
options={
'verbose_name_plural': 'Payment Reminders',
'verbose_name': 'Payment Reminder',
},
bases=('crm.salescontract',),
),
migrations.CreateModel(
name='PurchaseConfirmation',
fields=[
('salescontract_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.SalesContract')),
('validuntil', models.DateField(verbose_name='Valid until')),
('status', models.CharField(choices=[('S', 'Success'), ('I', 'Quote created'), ('Q', 'Quote sent'), ('F', 'First reminder sent'), ('R', 'Second reminder sent'), ('D', 'Deleted')], max_length=1, verbose_name='Status')),
],
options={
'verbose_name_plural': 'Purchase Confirmations',
'verbose_name': 'Purchase Confirmation',
},
bases=('crm.salescontract',),
),
migrations.CreateModel(
name='TextParagraphInSalesContract',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('purpose', models.CharField(choices=[('BS', 'Before subject'), ('AS', 'After subject'), ('BT', 'Before total'), ('AT', 'After total'), ('BW', 'Before wishes'), ('AW', 'After wishes'), ('C1', 'Custom 1'), ('C2', 'Custom 2'), ('C3', 'Custom 3'), ('C4', 'Custom 4')], max_length=1, verbose_name='Purpose')),
('text_paragraph', models.TextField(verbose_name='Text')),
],
options={
'verbose_name_plural': 'TextParagraphsInSalesContract',
'verbose_name': 'TextParagraphInSalesContract',
},
),
migrations.AlterModelOptions(
name='supplier',
options={'verbose_name': 'Supplier', 'verbose_name_plural': 'Suppliers'},
),
migrations.RemoveField(
model_name='position',
name='shipmentID',
),
migrations.RemoveField(
model_name='position',
name='supplier',
),
migrations.AddField(
model_name='salescontract',
name='template_set',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.TemplateSet', verbose_name='Referred Template Set'),
),
migrations.AddField(
model_name='textparagraphinsalescontract',
name='SalesContract',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.SalesContract'),
),
]
<file_sep>/koalixcrm/crm/migrations/0033_auto_20180612_1936.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-12 19:36
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0032_auto_20180612_1925'),
]
operations = [
migrations.AlterModelOptions(
name='projectstatus',
options={'verbose_name': 'Project Status', 'verbose_name_plural': 'Project Status'},
),
migrations.AlterField(
model_name='task',
name='project',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Project', verbose_name='Project'),
),
]
<file_sep>/documentation/source/accounting.rst
.. highlight:: rst
Accounting
============
Accouting Periods
-------------------------
An Accounting Period is the timeframe over which you make your Profit and Loss calculation.
Its often called Fiscal Year, Business Year or Business Quarter. What ever you like.
Accounting Periods have nothing to do with the Accounts but with the Bookings.
When you open an existing Accounting Period you find a list of all
Bookings during the period below as an inline table on the page.
Feel free to add your bookings here. Be careful when you set up a new
Accounting Period - koalixcrm does not check if you create bookings that are outside the
possible Accounting Period range nor does it check if there are two accounting periods
for the same time period. It is up to the user to check that.
Account section
---------------
Is a Customer Payment Account
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
These are the accounts you are able to select when you choose "register payment"
in your invoice detail view as destinations for Customers to pay into.
Is the Open Liabilities Account
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
This is not implemented yet because the link between Accounting and CRM
is not yet finised for the purchase order side
(where you were buying not selling).
This is usually not used by companies because its to much effort to copy
all the invoices they receive from suppliers into your system.
Normally you will only book this manually and store the invoice
you got from your supplier physically in a filer in your office.
If you do so think about using a good description so that you find
the invoice in case of a tax audit (I have never had a tax audit in my
company but I think they are really interested in such things :-) ).
Is the Open Intrest Account
^^^^^^^^^^^^^^^^^^^^^^^^^^^
There should only be one Open Intrest Account in your Accounting
and it is used to book invoiced contract amounts. You will have to set
this open intrest account. If this is not set on at least one account
the link between Accounting and CRM will not work correctly.
Is a Product Inventory Account
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
You may choose this option but it has no effect yet
because Product inventory is not yet implemented.
Bookings
--------
A booking is a common part of all accounting. Link to wikipage.
Product Categories
------------------
Product Categories are needed for the interface between the CRM and Accounting.
You can introduce different product Categories for different kinds of companies.
In this example, we have an "IT support company". An IT Support company usually
provides Support and Hardware. Support can be for on-site support or in-house support.
Its possible that the support must be bought from an other company because
you were not able to do everything yourself. Then the Hardware, you normally
don't produce hardware on your own, you buy them from a supplier.
Now lets record that as the following products you may offer to your customers.
+-------------+---------------------+
| Product Nr. | Description |
+-------------+---------------------+
| 1 | in-house work |
| 2 | on-site work |
| 3 | computer hardware |
| 4 | voip hardware |
+-------------+---------------------+
To be able to do automatic accounting you will have to set the accounts where
you want to book your income and spending for each product.
+------------+------------------+------------------------------------+
|Product Nr. | Earnings Acc. | Spendings Acc. |
+------------+------------------+------------------------------------+
|1. | Income Support | Spending Support from third |
|2. | Income Support | Spending Support from third |
|3. | Income Hardware | Spendings Hardware |
|4. | Income Hardware | Spendings Hardware |
+------------+------------------+------------------------------------+
Of course, there are more than four products in your inventory - I hope -
and depending on your "love of details" you will have hundreds of them.
Instead of setting the Earnings and Spendings Accounts for every Product
individually koalixcrm has useful product categories where you only
have to set it once. After that you are able to link the product
categories when you add a product in your CRM. Its also possible to
leave this blank if you don't want to make the link between CRM and Accounting
<file_sep>/documentation/source/code_documentation.rst
Code Documentation
==================
.. toctree::
:maxdepth: 2
code_documentation/project
code_documentation/task
code_documentation/work
code_documentation/estimation
code_documentation/agreement
code_documentation/resource
<file_sep>/koalixcrm/crm/reporting/agreement.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.reporting.resource_price import ResourcePrice
class Agreement(models.Model):
task = models.ForeignKey("Task",
verbose_name=_('Task'),
blank=False,
null=False)
resource = models.ForeignKey("Resource")
unit = models.ForeignKey("Unit")
costs = models.ForeignKey(ResourcePrice)
type = models.ForeignKey("AgreementType")
status = models.ForeignKey("AgreementStatus")
date_from = models.DateField(verbose_name=_("Agreement From"),
blank=False,
null=False)
date_until = models.DateField(verbose_name=_("Agreement To"),
blank=False,
null=False)
amount = models.DecimalField(verbose_name=_("Amount"),
max_digits=5,
decimal_places=2,
blank=True,
null=True)
def calculated_costs(self):
return 0
def match_with_work(self, work):
"""This method checks whether the provided work can be covered by the agreement.
the method checks whether the reported work corresponds with the resource and whether the
reported work was within the time-frame of the agreement.
The method returns False when the Agreement is not yet in status agreed
Args:
work (koalixcrm.crm.reporting.work.Work)
Returns:
True when no ValidationError was raised
Raises:
should not raise exceptions"""
matches = False
if self.status.is_agreed:
if (work.date >= self.date_from) and (work.date <= self.date_until):
if self.resource.id == work.human_resource.id:
matches = True
return matches
def __str__(self):
return _("Agreement of Resource Consumption") + ": " + str(self.id)
class Meta:
app_label = "crm"
verbose_name = _('Agreement')
verbose_name_plural = _('Agreements')
class AgreementInlineAdminView(admin.TabularInline):
model = Agreement
fieldsets = (
(_('Work'), {
'fields': ('task',
'resource',
'amount',
'unit',
'costs',
'date_from',
'date_until',
'type',
'status')
}),
)
extra = 1
<file_sep>/requirements/development_requirements.txt
-r base_requirements.txt
<file_sep>/koalixcrm/crm/product/product_price.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.product.price import Price
class ProductPrice(Price):
product_type = models.ForeignKey("ProductType",
verbose_name=_("Product Type"))
def __str__(self):
return str(self.price) + " " + str(self.currency.short_name)
class Meta:
app_label = "crm"
verbose_name = _('Product Price')
verbose_name_plural = _('Product Prices')
class ProductPriceInlineAdminView(admin.TabularInline):
model = ProductPrice
extra = 1
classes = ['collapse']
fieldsets = (
('', {
'fields': ('price',
'currency',
'unit',
'valid_from',
'valid_until',
'customer_group')
}),
)
allow_add = True
<file_sep>/koalixcrm/crm/migrations/0007_auto_20171210_2126.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-12-10 21:26
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
('crm', '0006_auto_20171210_1805'),
]
operations = [
migrations.CreateModel(
name='TextParagraphInDocumentTemplate',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('purpose', models.CharField(choices=[('BS', 'Before subject'), ('AS', 'After subject'), ('BT', 'Before total'), ('AT', 'After total'), ('BW', 'Before wishes'), ('AW', 'After wishes'), ('C1', 'Custom 1'), ('C2', 'Custom 2'), ('C3', 'Custom 3'), ('C4', 'Custom 4')], max_length=2, verbose_name='Purpose')),
('text_paragraph', models.TextField(verbose_name='Text')),
('document_template', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'TextParagraphInDocumentTemplate',
'verbose_name_plural': 'TextParagraphInDocumentTemplates',
},
),
migrations.AlterField(
model_name='textparagraphinsalescontract',
name='purpose',
field=models.CharField(choices=[('BS', 'Before subject'), ('AS', 'After subject'), ('BT', 'Before total'), ('AT', 'After total'), ('BW', 'Before wishes'), ('AW', 'After wishes'), ('C1', 'Custom 1'), ('C2', 'Custom 2'), ('C3', 'Custom 3'), ('C4', 'Custom 4')], max_length=2, verbose_name='Purpose'),
),
]
<file_sep>/koalixcrm/crm/migrations/0013_auto_20180105_2159.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-05 21:59
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
('crm', '0012_auto_20180105_1840'),
]
operations = [
migrations.RemoveField(
model_name='purchaseconfirmation',
name='status',
),
migrations.RemoveField(
model_name='purchaseconfirmation',
name='valid_until',
),
migrations.AddField(
model_name='deliverynote',
name='derived_from_quote',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Quote'),
),
migrations.AddField(
model_name='deliverynote',
name='tracking_reference',
field=models.CharField(blank=True, max_length=100, verbose_name='Tracking Reference'),
),
migrations.AddField(
model_name='paymentreminder',
name='payable_until',
field=models.DateField(default=django.utils.timezone.now, verbose_name='To pay until'),
preserve_default=False,
),
migrations.AddField(
model_name='paymentreminder',
name='payment_bank_reference',
field=models.CharField(blank=True, max_length=100, null=True, verbose_name='Payment Bank Reference'),
),
migrations.AddField(
model_name='purchaseconfirmation',
name='derived_from_quote',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Quote'),
),
migrations.AddField(
model_name='purchaseorder',
name='template_set',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.DocumentTemplate', verbose_name='Referred Template'),
),
]
<file_sep>/koalixcrm/crm/views/renderer.py
# -*- coding: utf-8 -*-
"""
Provides XML rendering support.
"""
import os
from subprocess import check_output, STDOUT
from django.conf import settings
from django.core.exceptions import ImproperlyConfigured
from rest_framework.renderers import TemplateHTMLRenderer
from rest_framework_xml.renderers import XMLRenderer
class XSLFORenderer(TemplateHTMLRenderer):
"""
Renderer which serializes to PDF using XSLT and FO
"""
media_type_unused = 'application/pdf'
media_type = 'plain/text'
format = 'pdf'
charset = 'utf-8'
def get_template_names(self, response, view):
"""Override with xslt_names. This allows for you
to still render HTML if you want
"""
if hasattr(response, 'xslt_name'):
return [response.latex_name]
elif self.xslt_name:
return [self.xslt_name]
elif hasattr(view, 'get_xslt_names'):
return view.get_xslt_names()
elif hasattr(view, 'xslt_name'):
return [view.xslt_name]
raise ImproperlyConfigured(
u'Returned a template response with no `xslt_name` attribute '
u'set on either the view or response')
def perform_xsl_transformation(self, file_with_serialized_xml, xsl_file, fop_config_file, file_output_pdf):
check_output([settings.FOP_EXECUTABLE,
'-c', fop_config_file.path_full,
'-xml', os.path.join(settings.PDF_OUTPUT_ROOT, file_with_serialized_xml),
'-xsl', xsl_file.path_full,
'-pdf', file_output_pdf], stderr=STDOUT)
def render(self, data, accepted_media_type=None, renderer_context=None):
"""
First renders `data` into serialized XML, afterwards start fop and return PDF
"""
if data is None:
return ''
super(XSLFORenderer, self).render(data, accepted_media_type, renderer_context)
xml_renderer = XMLRenderer()
xml_string = xml_renderer.render(data, accepted_media_type, renderer_context)
xml_file = open(renderer_context['view'].file_name, "wb+")
xml_file.truncate()
xml_file.write(xml_string.encode('utf-8'))
xml_file.close()
# perform xsl transformation
self.perform_xsl_transformation(xml_file,
self.xsl_file,
self.fop_config_file,
self.file_output_pdf)
# Read file
with open(self.file_output_pdf, 'rb') as f:
rendered_output = f.read()
return rendered_output<file_sep>/koalixcrm/crm/migrations/0036_auto_20180626_2059.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-26 20:59
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0035_auto_20180619_1924'),
]
operations = [
migrations.CreateModel(
name='ReportingPeriod',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=200, verbose_name='Title')),
('begin', models.DateField(verbose_name='Begin')),
('end', models.DateField(verbose_name='End')),
('project', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Project', verbose_name='Project')),
],
options={
'verbose_name': 'Project',
'verbose_name_plural': 'Projects',
},
),
migrations.AddField(
model_name='work',
name='reporting_period',
field=models.ForeignKey(default=1, on_delete=django.db.models.deletion.CASCADE, to='crm.ReportingPeriod', verbose_name='Reporting Period'),
preserve_default=False,
),
]
<file_sep>/koalixcrm/subscriptions/const/events.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
SUBSCRITIONEVENTS = (
('O', _('Offered')),
('C', _('Canceled')),
('S', _('Signed')),
)
<file_sep>/koalixcrm/accounting/rest/account_rest.py
# -*- coding: utf-8 -*-
from rest_framework import serializers
from koalixcrm.accounting.accounting.account import Account
class OptionAccountJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
accountNumber = serializers.IntegerField(source='account_number', read_only=True)
title = serializers.CharField(read_only=True)
class Meta:
model = Account
fields = ('id',
'accountNumber',
'title')
class AccountJSONSerializer(serializers.HyperlinkedModelSerializer):
accountNumber = serializers.IntegerField(source='account_number', allow_null=False)
accountType = serializers.CharField(source='account_type', allow_null=False)
isOpenReliabilitiesAccount = serializers.BooleanField(source='is_open_reliabilities_account')
isOpenInterestAccount = serializers.BooleanField(source='is_open_interest_account')
isProductInventoryActiva = serializers.BooleanField(source='is_product_inventory_activa')
isCustomerPaymentAccount = serializers.BooleanField(source='is_a_customer_payment_account')
class Meta:
model = Account
fields = ('id',
'accountNumber',
'title',
'accountType',
'description',
'isOpenReliabilitiesAccount',
'isOpenInterestAccount',
'isProductInventoryActiva',
'isCustomerPaymentAccount')
depth = 1
<file_sep>/koalixcrm/crm/migrations/0052_auto_20181014_2304.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
def reverse_func(apps, schema_editor):
return 1
def restore_from_backup(apps, schema_editor):
Position = apps.get_model("crm", "Position")
ProductType = apps.get_model("crm", "ProductType")
CustomerGroupTransform = apps.get_model("crm", "CustomerGroupTransform")
Price = apps.get_model("crm", "Price")
ProductPrice = apps.get_model("crm", "ProductPrice")
UnitTransform = apps.get_model("crm", "UnitTransform")
db_alias = schema_editor.connection.alias
all_positions = Position.objects.using(db_alias).all()
for position in all_positions:
product_type = ProductType.objects.using(db_alias).get(id=position.product_backup)
position.product_type = product_type
position.save()
all_customer_group_transforms = CustomerGroupTransform.objects.using(db_alias).all()
for customer_group_transform in all_customer_group_transforms:
product_type = ProductType.objects.using(db_alias).get(id=customer_group_transform.product_backup)
customer_group_transform.product_type = product_type
customer_group_transform.save()
all_prices = Price.objects.using(db_alias).all()
for price in all_prices:
product_type = ProductType.objects.using(db_alias).get(id=price.product_backup)
new_product_price = ProductPrice.objects.using(db_alias).create(unit=price.unit,
currency=price.currency,
customer_group=price.customer_group,
price=price.price,
valid_from=price.valid_from,
valid_until=price.valid_until,
product_type=product_type)
new_product_price.save()
price.delete()
all_unit_transforms = UnitTransform.objects.using(db_alias).all()
for unit_transform in all_unit_transforms:
product_type = ProductType.objects.using(db_alias).get(id=unit_transform.product_backup)
unit_transform.product_type = product_type
unit_transform.save()
class Migration(migrations.Migration):
dependencies = [
('crm', '0051_auto_20181014_2302'),
]
operations = [
migrations.RunPython(restore_from_backup, reverse_func),
]
<file_sep>/koalixcrm/crm/migrations/0032_auto_20180612_1925.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-12 19:25
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('djangoUserExtension', '0005_auto_20180612_1924'),
('crm', '0031_auto_20180612_1924'),
]
operations = [
migrations.CreateModel(
name='Project',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('project_name', models.CharField(blank=True, max_length=100, null=True, verbose_name='Project name')),
('description', models.TextField(blank=True, null=True, verbose_name='Description')),
('date_of_creation', models.DateTimeField(auto_now_add=True, verbose_name='Created at')),
('last_modification', models.DateTimeField(auto_now=True, verbose_name='Last modified')),
('default_template_set', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.TemplateSet', verbose_name='Default Template Set')),
('last_modified_by', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_project_last_modified', to=settings.AUTH_USER_MODEL, verbose_name='Last modified by')),
('project_manager', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_rel_project_staff', to=settings.AUTH_USER_MODEL, verbose_name='Staff')),
],
options={
'verbose_name': 'Project',
'verbose_name_plural': 'Projects',
},
),
migrations.CreateModel(
name='ProjectLinkType',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=300, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
],
options={
'verbose_name': 'Project Link Type',
'verbose_name_plural': 'Project Link Type',
},
),
migrations.CreateModel(
name='ProjectStatus',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=250, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
('is_done', models.BooleanField(verbose_name='Status represents project is done')),
],
options={
'verbose_name': 'Task Status',
'verbose_name_plural': 'Task Status',
},
),
migrations.AddField(
model_name='project',
name='project_status',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ProjectStatus', verbose_name='Project Status'),
),
]
<file_sep>/koalixcrm/crm/management/commands/koalixcrm_install_defaulttemplates.py
# -*- coding: utf-8 -*-
from os import path
from koalixcrm import crm
from koalixcrm import djangoUserExtension
from django.contrib.auth.models import User
from django.core.management.base import BaseCommand
from filebrowser.base import FileObject
from django.utils.translation import ugettext as _
from django.conf import settings
DEFAULT_FILE = 'dashboard.py'
class Command(BaseCommand):
help = (
'This Command is going to install the default Templates, given by the koalixcrm base installation, in your django instance. Be sure you first run syncdb')
args = '[]'
label = 'application name'
@staticmethod
def store_default_template_xsl_file(language, file_name):
file_path = Command.path_of_default_template_file(language, file_name)
xsl_file = Command.store_xsl_file(file_path)
return xsl_file
@staticmethod
def path_of_default_template_file(language, file_name):
file_path = path.join(settings.STATIC_ROOT, "default_templates", language, file_name)
f = None;
try:
f = open(file_path,'r')
except (FileNotFoundError) as e:
print(_("File not found:") + file_path)
print(_("Run collectstatic command and fix potential errors"))
finally:
if f is not None:
f.close()
return file_path
@staticmethod
def store_xsl_file(xsl_file_path):
xsl_file = djangoUserExtension.models.XSLFile()
xsl_file.title = path.basename(xsl_file_path)
xsl_file.xslfile = FileObject(xsl_file_path)
xsl_file.save()
return xsl_file
def handle(self, *args, **options):
template_set = djangoUserExtension.models.TemplateSet()
template_set.title = 'default_template_set'
template_set.invoiceXSLFile = Command.store_default_template_xsl_file("en", "invoice.xsl")
template_set.quoteXSLFile = Command.store_default_template_xsl_file("en", "quote.xsl")
template_set.purchaseconfirmationXSLFile = Command.store_default_template_xsl_file("en", "purchaseconfirmation.xsl")
template_set.purchaseorderXSLFile = Command.store_default_template_xsl_file("en", "purchaseorder.xsl")
template_set.deilveryorderXSLFile = Command.store_default_template_xsl_file("en", "deliveryorder.xsl")
if 'koalixcrm.accounting' in settings.INSTALLED_APPS:
template_set.profitLossStatementXSLFile = Command.store_default_template_xsl_file("en", "profitlossstatement.xsl")
template_set.balancesheetXSLFile = Command.store_default_template_xsl_file("en", "balancesheet.xsl")
template_set.logo = FileObject(Command.path_of_default_template_file("generic", "logo.jpg"))
template_set.fopConfigurationFile = FileObject(Command.path_of_default_template_file("generic", "fontconfig.xml"))
template_set.bankingaccountref = "xx-xxxxxx-x"
template_set.addresser = _("<NAME>, Sample Company, 8976 Smallville")
template_set.headerTextsalesorders = _(
"According to your wishes the contract consists of the following positions:")
template_set.footerTextsalesorders = _("Thank you for your interest in our company \n Best regards")
template_set.headerTextpurchaseorders = _("We would like to order the following positions:")
template_set.footerTextpurchaseorders = _("Best regards")
template_set.pagefooterleft = _("Sample Company")
template_set.pagefootermiddle = _("Sample Address")
template_set.save()
currency = crm.models.Currency()
currency.description = "US Dollar"
currency.shortName = "USD"
currency.rounding = "0.10"
currency.save()
user_extension = djangoUserExtension.models.UserExtension()
user_extension.defaultTemplateSet = template_set
user_extension.defaultCurrency = currency
user_extension.user = User.objects.all()[0]
user_extension.save()
postaladdress = djangoUserExtension.models.UserExtensionPostalAddress()
postaladdress.purpose = 'H'
postaladdress.name = "John"
postaladdress.prename = "Smith"
postaladdress.addressline1 = "Ave 1"
postaladdress.zipcode = 899887
postaladdress.town = "Smallville"
postaladdress.userExtension = user_extension
postaladdress.save()
phoneaddress = djangoUserExtension.models.UserExtensionPhoneAddress()
phoneaddress.phone = "1293847"
phoneaddress.purpose = 'H'
phoneaddress.userExtension = user_extension
phoneaddress.save()
emailaddress = djangoUserExtension.models.UserExtensionEmailAddress()
emailaddress.email = "<EMAIL>"
emailaddress.purpose = 'H'
emailaddress.userExtension = user_extension
emailaddress.save()
<file_sep>/koalixcrm/accounting/rest/accounting_period_rest.py
# -*- coding: utf-8 -*-
from rest_framework import serializers
from koalixcrm.accounting.accounting.accounting_period import AccountingPeriod
from koalixcrm.accounting.models import Account
class OptionAccountingPeriodJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
title = serializers.CharField(read_only=True)
class Meta:
model = Account
fields = ('id',
'title')
class AccountingPeriodJSONSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = AccountingPeriod
fields = ('id',
'title',
'begin',
'end')
depth = 1
<file_sep>/koalixcrm/subscriptions/migrations/0005_auto_20181013_2228.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-13 22:28
from __future__ import unicode_literals
from django.db import migrations, models
from filebrowser.fields import FileBrowseField
import django.db.models.deletion
def fill_subscription_type_from_backup(apps, schema_editor):
Subscription = apps.get_model("subscriptions", "Subscription")
SubscriptionType = apps.get_model("subscriptions", "SubscriptionType")
SubscriptionTypeBackup = apps.get_model("subscriptions", "SubscriptionTypeBackup")
ProductType = apps.get_model("crm", "ProductType")
db_alias = schema_editor.connection.alias
all_subscription_type_backups = SubscriptionTypeBackup.objects.using(db_alias).all()
for subscription_type_backup in all_subscription_type_backups:
product_type = ProductType.objects.using(db_alias).create(
description=subscription_type_backup.description,
title=subscription_type_backup.title,
default_unit=subscription_type_backup.default_unit,
date_of_creation=subscription_type_backup.date_of_creation,
last_modification=subscription_type_backup.last_modification,
last_modified_by=subscription_type_backup.last_modified_by,
tax=subscription_type_backup.tax)
product_type.save()
subscription_type = SubscriptionType.objects.using(db_alias).create(
product_type=product_type,
contract_document=subscription_type_backup.contract_document,
payment_interval=subscription_type_backup.payment_interval,
minimum_duration=subscription_type_backup.minimum_duration,
automatic_contract_extension_reminder=subscription_type_backup.automatic_contract_extension_reminder,
automatic_contract_extension=subscription_type_backup.automatic_contract_extension,
cancellation_period=subscription_type_backup.cancellation_period)
subscription_type.save()
all_subscriptions = Subscription.objects.using(db_alias).filter(
subscription_type_backup=subscription_type_backup.old_id)
for subscription in all_subscriptions:
subscription.subscription_type=subscription_type
subscription.save()
def reverse_func(apps, schema_editor):
return 1
class Migration(migrations.Migration):
dependencies = [
('crm', '0053_auto_20181014_2305'),
('subscriptions', '0004_auto_20181013_2213'),
]
operations = [
migrations.CreateModel(
name='SubscriptionType',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('cancellation_period', models.IntegerField(verbose_name="Cancellation Period (months)",
blank=True,
null=True)),
('automatic_contract_extension', models.IntegerField(
verbose_name="Automatic Contract Extension (months)",
blank=True,
null=True)),
('automatic_contract_extension_reminder', models.IntegerField(
verbose_name="Automatic Contract Extension Reminder (days)",
blank=True,
null=True)),
('minimum_duration', models.IntegerField(verbose_name="Minimum Contract Duration",
blank=True,
null=True)),
('payment_interval', models.IntegerField(verbose_name="Payment Interval (days)",
blank=True,
null=True)),
('contract_document', FileBrowseField(verbose_name="Contract Documents",
blank=True,
null=True,
max_length=200)),
('product_type', models.ForeignKey('crm.ProductType',
verbose_name='Product Type',
on_delete=models.deletion.SET_NULL,
null=True,
blank=True)),
],
options={
'verbose_name': 'Subscription Type',
'verbose_name_plural': 'Subscription Types',
},
),
migrations.AddField(
model_name='subscription',
name='subscription_type',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.SET_NULL,
to='SubscriptionType',
verbose_name='Subscription Type'),
preserve_default=False,
),
migrations.RunPython(fill_subscription_type_from_backup, reverse_func),
migrations.AlterField(
model_name='subscription',
name='subscription_type',
field=models.ForeignKey(null=True,
on_delete=django.db.models.deletion.CASCADE,
to='subscriptions.SubscriptionType',
verbose_name='Subscription Type'),
),
migrations.DeleteModel('SubscriptionTypeBackup'),
migrations.RemoveField(
model_name='subscription',
name='subscription_type_backup',
),
]
<file_sep>/koalixcrm/crm/views/create_task.py
# -*- coding: utf-8 -*-
from django.http import Http404
from django.http import HttpResponseRedirect
from django.core.exceptions import ObjectDoesNotExist
from django.utils.translation import ugettext as _
from django.contrib.contenttypes.models import ContentType
from koalixcrm.crm.exceptions import *
from koalixcrm.djangoUserExtension.exceptions import *
from koalixcrm.crm.documents.sales_document import SalesDocument
from koalixcrm.crm.documents.sales_document_position import SalesDocumentPosition
from koalixcrm.crm.reporting.task import Task
from koalixcrm.crm.reporting.generic_task_link import GenericTaskLink
from koalixcrm.crm.reporting.project import Project
from koalixcrm.global_support_functions import *
from datetime import date
class CreateTaskView:
@staticmethod
def create_task_from_sales_document_position(sales_document_position,
user,
document,
project):
date_now = date.today()
content_type_sales_document_position = ContentType.objects.get_for_model(SalesDocumentPosition)
task_title = limit_string_length(sales_document_position.description, 30)
try:
existing_task = GenericTaskLink.objects.get(content_type=content_type_sales_document_position,
object_id=sales_document_position.id)
task_id = existing_task.task.id
task = Task.objects.filter(id=task_id).update(
title=task_title,
planned_start_date=date_now,
project=project,
description=sales_document_position.description,
last_status_change=date_now
)
except ObjectDoesNotExist:
task = Task.objects.create(
title=task_title,
project=project,
description=sales_document_position.description,
last_status_change=date_now
)
GenericTaskLink.objects.create(
task=task,
content_type=content_type_sales_document_position,
object_id=sales_document_position.id,
last_modified_by=user
)
GenericTaskLink.objects.create(
task=task,
content_type=ContentType.objects.get_for_model(SalesDocument),
object_id=document.id,
last_modified_by=user
)
return task
@staticmethod
def create_project_from_document(user, document):
sales_document_positions = SalesDocumentPosition.objects.filter(sales_document=document)
project_name = limit_string_length(document.contract.description, 30)
project = Project.objects.create(project_manager=user,
project_name=project_name,
description=document.contract.description,
default_template_set=document.contract.default_template_set,
date_of_creation=date.today(),
last_modification=date.today(),
last_modified_by=user,
default_currency=document.currency)
for sales_document_position in sales_document_positions:
CreateTaskView.create_task_from_sales_document_position(sales_document_position,
user,
document,
project)
return project
@staticmethod
def create_project(calling_model_admin, request, document, redirect_to):
"""This method creates tasks from the positions of a sales document
Args:
calling_model_admin (ModelAdmin): The calling ModelAdmin must be provided for error message response.
request: The request User is to know where to save the error message
document (SalesDocument): The model from which a tasks shall be created
redirect_to (str): String that describes to where the method should redirect in case of an error
Returns:
HTTpResponse with a PDF when successful
HTTpResponseRedirect when not successful
Raises:
raises Http404 exception if anything goes wrong"""
try:
project = CreateTaskView.create_project_from_document(request.user, document)
calling_model_admin.message_user(request, _("Successfully created Project and Tasks for this contract"))
response = HttpResponseRedirect('/admin/crm/'+
project.__class__.__name__.lower()+
'/'+
str(project.id))
except (TemplateSetMissing,
UserExtensionMissing,
UserExtensionEmailAddressMissing,
UserExtensionPhoneAddressMissing) as e:
if isinstance(e, UserExtensionMissing):
return render(request, 'crm/admin/exception.html')
elif isinstance(e, UserExtensionEmailAddressMissing):
return render(request, 'crm/admin/exception.html')
else:
raise Http404
return response
<file_sep>/koalixcrm/crm/migrations/0042_auto_20180724_1816.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-07-24 18:16
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0041_auto_20180724_1657'),
]
operations = [
migrations.AlterField(
model_name='unit',
name='fraction_factor_to_next_higher_unit',
field=models.DecimalField(blank=True, decimal_places=10, max_digits=20, null=True, verbose_name='Factor Between This And Next Higher Unit'),
),
]
<file_sep>/koalixcrm/djangoUserExtension/migrations/0006_auto_20180701_2128.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-07-01 21:28
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0005_auto_20180612_1924'),
]
operations = [
migrations.CreateModel(
name='WorkReportTemplate',
fields=[
('documenttemplate_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='djangoUserExtension.DocumentTemplate')),
],
options={
'verbose_name': 'Work report template',
'verbose_name_plural': 'Work report templates',
},
bases=('djangoUserExtension.documenttemplate',),
),
migrations.AlterModelOptions(
name='templateset',
options={'verbose_name': 'Template-set', 'verbose_name_plural': 'Template-sets'},
),
migrations.AlterModelOptions(
name='userextension',
options={'verbose_name': 'User Extension', 'verbose_name_plural': 'User Extension'},
),
migrations.AlterModelOptions(
name='userextensionemailaddress',
options={'verbose_name': 'Email Address for User Extension', 'verbose_name_plural': 'Email Address for User Extension'},
),
migrations.AlterModelOptions(
name='userextensionphoneaddress',
options={'verbose_name': 'Phone number for User Extension', 'verbose_name_plural': 'Phone number for User Extension'},
),
migrations.AlterModelOptions(
name='userextensionpostaladdress',
options={'verbose_name': 'Postal Address for User Extension', 'verbose_name_plural': 'Postal Address for User Extension'},
),
migrations.AddField(
model_name='templateset',
name='work_report_template',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.WorkReportTemplate'),
),
]
<file_sep>/koalixcrm/crm/contact/supplier.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.contact.contact import Contact
from koalixcrm.crm.contact.contact import ContactPostalAddress
from koalixcrm.crm.contact.contact import ContactPhoneAddress
from koalixcrm.crm.contact.contact import ContactEmailAddress
class Supplier(Contact):
offersShipmentToCustomers = models.BooleanField(verbose_name=_("Offers Shipment to Customer"))
class Meta:
app_label = "crm"
verbose_name = _('Supplier')
verbose_name_plural = _('Suppliers')
def __str__(self):
return str(self.id) + ' ' + self.name
class OptionSupplier(admin.ModelAdmin):
list_display = ('id', 'name', 'offersShipmentToCustomers')
fieldsets = (('', {'fields': ('name', 'offersShipmentToCustomers')}),)
inlines = [ContactPostalAddress, ContactPhoneAddress, ContactEmailAddress]
allow_add = True
def save_model(self, request, obj, form, change):
if (change == True):
obj.lastmodifiedby = request.user
else:
obj.lastmodifiedby = request.user
obj.staff = request.user
obj.save()<file_sep>/koalixcrm/crm/factories/factory_sales_document_position.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import SalesDocumentPosition
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
class StandardSalesDocumentPositionFactory(factory.django.DjangoModelFactory):
class Meta:
model = SalesDocumentPosition
position_number = 5
quantity = 5
description = "This is a test sales document position"
discount = 10
product_type = factory.SubFactory(StandardProductTypeFactory)
unit = factory.SubFactory(StandardUnitFactory)
overwrite_product_price = True
position_price_per_unit = 155
<file_sep>/koalixcrm/accounting/migrations/0009_auto_20181012_2056.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0053_auto_20181014_2305'),
('accounting', '0008_auto_20181012_2056'),
]
operations = [
migrations.DeleteModel(
name='ProductCategorie',
),
migrations.AddField(
model_name='productcategory',
name='loss_account',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_loss_account', to='accounting.Account', verbose_name='Loss Account'),
),
migrations.AddField(
model_name='productcategory',
name='profit_account',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_profit_account', to='accounting.Account', verbose_name='Profit Account'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_agreement.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import Agreement
from koalixcrm.crm.factories.factory_resource import StandardResourceFactory
from koalixcrm.crm.factories.factory_human_resource import StandardHumanResourceFactory
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
from koalixcrm.crm.factories.factory_resource_price import StandardResourcePriceFactory
from koalixcrm.crm.factories.factory_agreement_type import StandardAgreementTypeFactory
from koalixcrm.crm.factories.factory_agreement_status import AgreedAgreementStatusFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardAgreementToTaskFactory(factory.django.DjangoModelFactory):
class Meta:
model = Agreement
date_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
date_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
amount = "112.50"
task = factory.SubFactory(StandardTaskFactory)
resource = factory.SubFactory(StandardResourceFactory)
unit = factory.SubFactory(StandardUnitFactory)
status = factory.SubFactory(AgreedAgreementStatusFactory)
costs = factory.SubFactory(StandardResourcePriceFactory)
type = factory.SubFactory(StandardAgreementTypeFactory)
class StandardHumanResourceAgreementToTaskFactory(factory.django.DjangoModelFactory):
class Meta:
model = Agreement
date_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
date_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
amount = "112.50"
task = factory.SubFactory(StandardTaskFactory)
resource = factory.SubFactory(StandardHumanResourceFactory)
unit = factory.SubFactory(StandardUnitFactory)
status = factory.SubFactory(AgreedAgreementStatusFactory)
costs = factory.SubFactory(StandardResourcePriceFactory)
type = factory.SubFactory(StandardAgreementTypeFactory)
<file_sep>/koalixcrm/crm/migrations/0022_auto_20180117_2020.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-17 20:20
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0021_purchaseorder'),
]
operations = [
migrations.AlterModelOptions(
name='purchaseorder',
options={'verbose_name': 'Purchase Order', 'verbose_name_plural': 'Purchase Orders'},
),
migrations.AlterField(
model_name='purchaseorder',
name='supplier',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Supplier', verbose_name='Supplier'),
),
]
<file_sep>/documentation/source/tutorial.rst
.. highlight:: rst
Tutorial
========
This tutorial is going to teach you how to
1. Create a customer
2. Create a currency
3. Create a product
4. Create a contract
5. Create a quote
6. Create an invoice
7. Set up your accounting
8. Record an invoice in your accounting
9. Record a customer payment in the accounting
Before you start I recommend you first have a look into the :doc:`intro`.
This gives you an idea of the basic structure of koalixcrm.
When you understand the structure and functions of koalixcrm or any other
large Django application you are ready to start with the tutorial.
Further, have a look at the :doc:`installation` section
Create Your First Customer
--------------------------
Let say you want to Register the following Customer:
Private address::
<NAME>
Ave 1
90990 Smallville
Phone:
Mobile:
Email:
Business address::
<NAME>
Ave 2
120987 Largeville
Phone:
Mobile:
Email:
Logging In Adminpage
^^^^^^^^^^^^^^^^^^^^
Depending on where you installed your koalixcrm - in this tutorial I assume
you installed it on your localhost (the computer you are sitting in front of)
- visit http://localhost/admin in your browser.
.. image:: /images/adminlogin.png
fill out the username and password you set during :doc:`installation`
in the syncdb procedure.
Create a Currency
^^^^^^^^^^^^^^^^^
.. image:: /images/addcurrencyform.png
Select "Add Customer"
^^^^^^^^^^^^^^^^^^^^^
After login you will see the dashboard you already know from
the :doc:`intro`. In the Applications list select the "Customer" - add button
.. image:: /images/customeraddbutton.png
Fill out Fields
^^^^^^^^^^^^^^^
After adding the customer you will have to fill out all required values
to get a valid registered customer. You will have to fill out "postal address"
and "phone address". Further you will need to add a new "Default Billing Cycle"
This can be added by pressing the greeen "plus" sign beside the "Default billing cycle"
drop down box.
A new page will open up and give you the ability to add a new billing cycle.
Fill in the values as described in the image below:
.. image:: /images/addcustomerbillingcycleform.png
After you filled in all required values you will have to click on the save button.
This click will bring you back to the customer adding view ... and you will
see that the default payment method is now set. Next is the customer group, we
have to do exactly the same. Click on the green "plus" sign beside the groups
list and you will soon get a pop up where you can add your first default customer group.
Fill in the values as described in the image below
.. image:: /images/addcustomergroupform.png
After that you have to fill out all the addresses defined in the header of this tutorial.
Finish the customer registration by pressing the save button
.. image:: /images/addcustomerform.png
Look at your first Customer
^^^^^^^^^^^^^^^^^^^^^^^^^^^
Ok now we have registered your first Customer.
Of course to have a customer is not that interesting, we hope that a customer is going
to order something, or needs an invoice, a quote or something else.
This will be the next step of the tutorial. But first have a look at your bright shiny
customer by selecting customer's id the dashboard as described in the :doc:`intro`.
Create Your First Contract
--------------------------
I expect you are still looking at your first customer we just created.
The next step is to click the checkbox to the left of your customer on
the CRM customer list and look at the the actions list that appears on the
bottom of the page.
.. image:: /images/customeractions.png
Select "Create Contract" from this list. This will bring you to the "add contract" form.
The advantage of doing it this way - instead of adding a new contract through
the dashboard - is that you have some values, like the default customer, already
set. this will give you some additional seconds for your daily work.
I expect you are a little bit surprised ... where do I select that I want
to have a quote or an invoice? Well, in koalixcrm a contract is not an invoice
and a contract is not a quote. A contract is simply a place to store all kind
of documents that are related to the contract. This can of course be a invoice
or a quote but also purchase orders and so on.
Fill in the description field, then by clicking on the save button you have
finished the creation of a contract.
Create Your First Quote
-----------------------
Until now there are no products, no prices and no units registered. In order to
be able to offer a product to a customer we need some products first.... you
could do it the lazy way by adding the product while registering the
quote but in this case we are going to register the products, units and prices
before we create the quote.
Create Your First Product
^^^^^^^^^^^^^^^^^^^^^^^^^
To create your first product visit the dashboard by either following the
breadcrumps back to the dashboard
.. image:: /images/breadcrumps.png
or visit http://localhost/admin again. Press the Add button next to Units to
access the Unit adding form. Now fill out all the
required fields to register the unit "hours"
.. image:: /images/addunitformhour.png
Press save, add an other unit by again pressing the add button.
Now we create a unit "minute".
.. image:: /images/addunitformminute.png
Press save and go back to the dashboard
As we have registered some units, now we are able to create a product.
Press the Products add button to get to the products adding form. We start with
a common product called Manpower.
Fill all fields with the following values:
.. image:: /images/addproductform1.png
as you know every product has its price specially manpower - time is money.
That's why we have to add at least one price for this product by giving
the Prices fields the following values.
.. image:: /images/addproductform2.png
You will see an other part of this form called Unit Transfroms.
Unit Transforms are sometimes needed when, for example, you have
stacks of certain products but only one price per piece. Leave this
blank when you only have one unit for one product.
After adding this product you are ready to create your first Quote by going
to your dashboard. Open Contracts, select the check box beside the contract
you want to change and select "Create Quote" from the Actions list.
A form will open for you to fill out your fist quote.
.. image:: /images/addquoteform1.png
As you can see, there are lots of predefined values because we created
the quote with the action instead of via dashboard
and Quote Add. There are three major parts of a quote:
* First the general values like "valid until", "description" and so on
* Second are role positions of the quote
* Lastly are Addresses related to the Contract
By pressing the "+" sign you can add as many positions as you like.
Fill in the values as described below.
.. image:: /images/addquoteform2.png
Click on the save button to finish your first quote. Go back to the dashboard,
go to quotes and select the newly created quote's checkbox.
From the actions select "Create PDF of Quote" to generate a pdf of this new quote.
Create Your First Invoice
-------------------------
This is going to be a very short chapter because all you have to do is
repeat the steps above but instead of selecting
"Create Quote" in the Contract Actions list you select "Create Invoice";
alternatively by selecting your new Quote and use the action
"Create Invoice". The second way is much easier and faster because the
program just takes all values and positions from the
quote and transforms it into a invoice.
<file_sep>/graph.py
# libraries and data
<file_sep>/koalixcrm/version.py
# -*- coding: utf-8 -*-
KOALIXCRM_VERSION = "1.12.1"<file_sep>/koalixcrm/crm/migrations/0046_auto_20180805_2051.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-08-05 20:51
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0045_auto_20180805_2047'),
]
operations = [
migrations.RemoveField(
model_name='work',
name='worked_hours')
]
<file_sep>/koalixcrm/crm/product/unit_transform.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class UnitTransform(models.Model):
from_unit = models.ForeignKey('Unit',
verbose_name=_("From Unit"),
blank=False,
null=False,
related_name="db_reltransfromfromunit")
to_unit = models.ForeignKey('Unit',
verbose_name=_("To Unit"),
blank=False,
null=False,
related_name="db_reltransfromtounit")
product_type = models.ForeignKey('ProductType',
blank=False,
null=False,
verbose_name=_("Product Type"))
factor = models.DecimalField(verbose_name=_("Factor between From and To Unit"),
blank=False,
null=False,
max_digits=17,
decimal_places=2,)
def transform(self, unit):
if self.from_unit == unit:
return self.to_unit
else:
return None
def get_transform_factor(self):
return self.factor
def __str__(self):
return "From " + self.from_unit.short_name + " to " + self.to_unit.short_name
class Meta:
app_label = "crm"
verbose_name = _('Unit Transform')
verbose_name_plural = _('Unit Transforms')
class UnitTransformInlineAdminView(admin.TabularInline):
model = UnitTransform
extra = 1
classes = ['collapse']
fieldsets = (
('', {
'fields': ('from_unit',
'to_unit',
'factor',)
}),
)
allow_add = True
<file_sep>/koalixcrm/crm/tests/test_user_is_not_human_resource.py
import pytest
from django.test import LiveServerTestCase
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support.ui import Select
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from koalixcrm.test_support_functions import *
from koalixcrm.crm.factories.factory_user import AdminUserFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
class TimeTrackingAddRow(LiveServerTestCase):
def setUp(self):
firefox_options = webdriver.firefox.options.Options()
firefox_options.set_headless(headless=True)
self.selenium = webdriver.Firefox(firefox_options=firefox_options)
self.test_user = AdminUserFactory.create()
self.test_customer_group = StandardCustomerGroupFactory.create()
self.test_customer = StandardCustomerFactory.create(is_member_of=(self.test_customer_group,))
self.test_currency = StandardCurrencyFactory.create()
self.test_user_extension = StandardUserExtensionFactory.create(user=self.test_user)
def tearDown(self):
self.selenium.quit()
@pytest.mark.front_end_tests
def test_add_new_row(self):
selenium = self.selenium
# login
selenium.get('%s%s' % (self.live_server_url, '/koalixcrm/crm/reporting/time_tracking/'))
# the browser will be redirected to the login page
timeout = 5
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_username'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
username = selenium.find_element_by_xpath('//*[@id="id_username"]')
password = selenium.find_element_by_xpath('//*[@id="id_password"]')
submit_button = selenium.find_element_by_xpath('/html/body/div/article/div/div/form/div/ul/li/input')
username.send_keys("admin")
password.send_keys("<PASSWORD>")
submit_button.send_keys(Keys.RETURN)
# after the login, the browser is redirected to the target url /koalixcrm/crm/reporting/time_tracking
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_next_steps'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
# Because the user is not equipped with a corresponding human_resource, the user must receive an exception
# screen giving im the possibility to select what he wants to do next
# In this test_step it is checked whether the form contains the required fields. the test will then select
# to return to the start page instead of defining a new human resource
assert_when_element_does_not_exist(self, '//*[@id="id_next_steps"]')
assert_when_element_does_not_exist(self, 'confirm_selection')
submit_button = selenium.find_element_by_xpath('/html/body/div/article/div/form/table/tbody/tr[3]/td/input[2]')
selection = Select(selenium.find_element_by_xpath('//*[@id="id_next_steps"]'))
selection.select_by_value("return_to_start")
submit_button.send_keys(Keys.RETURN)
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'grp-content-title'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
assert_when_element_is_not_equal_to(self, 'grp-content-title', "<h1>Site administration</h1>")
# Because the user is still not equipped with a corresponding human_resource, the user must
# receive an exception screen giving im the possibility to select what he wants to do next
# In this test_step it is checked whether the form contains the required fields. the test will then select
# to return to the start page instead of defining a new human resource
selenium.get('%s%s' % (self.live_server_url, '/koalixcrm/crm/reporting/time_tracking/'))
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_next_steps'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
assert_when_element_does_not_exist(self, '//*[@id="id_next_steps"]')
assert_when_element_does_not_exist(self, 'confirm_selection')
submit_button = selenium.find_element_by_xpath('/html/body/div/article/div/form/table/tbody/tr[3]/td/input[2]')
selection = Select(selenium.find_element_by_xpath('//*[@id="id_next_steps"]'))
selection.select_by_value("create_human_resource")
submit_button.send_keys(Keys.RETURN)
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'grp-content-title'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
assert_when_element_is_not_equal_to(self, 'grp-content-title', "<h1>Add human resource</h1>")
# This test is passed here, other testcase are performed to ensure proper functionality of this view
# when the human resource is properly set"
<file_sep>/koalixcrm/crm/migrations/0026_auto_20180507_1957.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-05-07 19:57
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0025_auto_20180413_1937'),
]
operations = [
migrations.RenameField(
model_name='contact',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='contact',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='contact',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='customer',
old_name='defaultCustomerBillingCycle',
new_name='default_customer_billing_cycle',
),
migrations.RenameField(
model_name='customer',
old_name='ismemberof',
new_name='is_member_of',
),
]
<file_sep>/koalixcrm/crm/contact/customer.py
# -*- coding: utf-8 -*-
from django.http import HttpResponseRedirect
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.plugin import *
from koalixcrm.crm.contact.contact import Contact, ContactCall, ContactVisit,\
PeopleInlineAdmin, PostalAddressForContact, ContactPostalAddress, \
ContactPhoneAddress, ContactEmailAddress, CityFilter, StateFilter
from koalixcrm.crm.documents.contract import Contract
class Customer(Contact):
default_customer_billing_cycle = models.ForeignKey('CustomerBillingCycle',
verbose_name=_('Default Billing Cycle'))
is_member_of = models.ManyToManyField("CustomerGroup",
verbose_name=_('Is member of'),
blank=True)
is_lead = models.BooleanField(default=True)
def create_contract(self, request):
contract = Contract()
contract.create_from_reference(self, request.user)
return contract
def create_invoice(self, request):
contract = self.create_contract(request)
invoice = contract.create_invoice()
return invoice
def create_quote(self, request):
contract = self.create_contract(request)
quote = contract.create_quote()
return quote
def is_in_group(self, customer_group):
for customer_group_membership in self.is_member_of.all():
if customer_group_membership.id == customer_group.id:
return 1
return 0
class Meta:
app_label = "crm"
verbose_name = _('Customer')
verbose_name_plural = _('Customers')
def __str__(self):
return str(self.id) + ' ' + self.name
class IsLeadFilter(admin.SimpleListFilter):
title = _('Is lead')
parameter_name = 'is_lead'
def lookups(self, request, model_admin):
return (
('lead', _('Lead')),
('customer', _('Customer')),
)
def queryset(self, request, queryset):
if self.value() == 'lead':
return queryset.filter(is_lead=True)
elif self.value() == 'customer':
return queryset.filter(is_lead=False)
else:
return queryset
class OptionCustomer(admin.ModelAdmin):
list_display = ('id',
'name',
'default_customer_billing_cycle',
'get_state',
'get_town',
'date_of_creation',
'get_is_lead',)
list_filter = ('is_member_of', StateFilter, CityFilter, IsLeadFilter)
fieldsets = (('', {'fields': ('name',
'default_customer_billing_cycle',
'is_member_of',)}),)
allow_add = True
ordering = ('id',)
search_fields = ('id', 'name')
inlines = [ContactPostalAddress,
ContactPhoneAddress,
ContactEmailAddress,
PeopleInlineAdmin,
ContactCall,
ContactVisit]
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("customerInline"))
@staticmethod
def get_postal_address(obj):
return PostalAddressForContact.objects.filter(person=obj.id).first()
def get_state(self, obj):
address = self.get_postal_address(obj)
return address.state if address is not None else None
get_state.short_description = _("State")
def get_town(self, obj):
address = self.get_postal_address(obj)
return address.town if address is not None else None
get_town.short_description = _("City")
@staticmethod
def get_is_lead(obj):
return obj.is_lead
get_is_lead.short_description = _("Is Lead")
def create_contract(self, request, queryset):
for obj in queryset:
contract = obj.create_contract(request)
response = HttpResponseRedirect('/admin/crm/contract/' + str(contract.id))
return response
create_contract.short_description = _("Create Contract")
@staticmethod
def create_quote(self, request, queryset):
for obj in queryset:
quote = obj.create_quote(request)
response = HttpResponseRedirect('/admin/crm/quote/' + str(quote.id))
return response
create_quote.short_description = _("Create Quote")
@staticmethod
def create_invoice(self, request, queryset):
for obj in queryset:
invoice = obj.create_invoice(request)
response = HttpResponseRedirect('/admin/crm/invoice/' + str(invoice.id))
return response
create_invoice.short_description = _("Create Invoice")
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
actions = ['create_contract', 'create_invoice', 'create_quote']
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("customerActions"))<file_sep>/koalixcrm/crm/reporting/task.py
# -*- coding: utf-8 -*-
from decimal import *
from django.db import models
from django.utils.translation import ugettext as _
from django.contrib import admin
from django.utils.html import format_html
from koalixcrm.crm.reporting.agreement import Agreement
from koalixcrm.crm.reporting.agreement import AgreementInlineAdminView
from koalixcrm.crm.reporting.estimation import EstimationInlineAdminView
from koalixcrm.crm.reporting.generic_task_link import InlineGenericTaskLink
from koalixcrm.crm.reporting.work import WorkInlineAdminView, Work
from koalixcrm.crm.reporting.reporting_period import ReportingPeriod
from koalixcrm.crm.reporting.resource_price import ResourcePrice
from koalixcrm.crm.reporting.estimation import Estimation
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.crm.exceptions import ReportingPeriodNotFound
from rest_framework import serializers
from koalixcrm import global_support_functions
class Task(models.Model):
""" The Task model"""
title = models.CharField(verbose_name=_("Title"),
max_length=100,
blank=True,
null=True)
project = models.ForeignKey("Project",
verbose_name=_('Project'),
related_name='tasks',
blank=False,
null=False)
description = models.TextField(verbose_name=_("Description"),
blank=True,
null=True)
status = models.ForeignKey("TaskStatus", verbose_name=_('Status'),
blank=True,
null=True)
last_status_change = models.DateField(verbose_name=_("Last Status Change"),
blank=True,
null=False)
previous_status = None
def __init__(self, *args, **kwargs):
super(Task, self).__init__(*args, **kwargs)
self.previous_status = self.status
def save(self, *args, **kwargs):
if self.id is not None:
if self.status != self.previous_status:
self.last_status_change = global_support_functions.get_today_date()
elif self.last_status_change is None:
self.last_status_change = global_support_functions.get_today_date()
super(Task, self).save(*args, **kwargs)
def link_to_task(self):
if self.id:
return format_html("<a href='/admin/crm/task/%s' >%s</a>" % (str(self.id), str(self.title)))
else:
return "Not present"
link_to_task.short_description = _("Task")
def planned_duration(self):
if (not self.planned_start()) or (not self.planned_end()):
duration_in_days = "n/a"
elif self.planned_start() > self.planned_end():
duration_in_days = "n/a"
else:
duration_in_days = (self.planned_end()-self.planned_start()).days
return duration_in_days
planned_duration.short_description = _("Planned Duration [dys]")
planned_duration.tags = True
def planned_start(self):
""" The function return the planned start of a task as a date based on the estimations which are
attached to the task in case there was no estimation attached to this task, the function returns None
Args:
no arguments
Returns:
planned_start (Date) or None
Raises:
No exceptions planned"""
all_task_estimations = Estimation.objects.filter(task=self.id)
planned_task_start = None
if len(all_task_estimations) == 0:
planned_task_start = None
else:
for estimation in all_task_estimations:
if planned_task_start is None:
planned_task_start = estimation.date_from
elif estimation.date_from < planned_task_start:
planned_task_start = estimation.date_from
return planned_task_start
planned_start.short_description = _("Planned Start")
planned_start.tags = True
def planned_end(self):
""" The function return the planned end of a task as a date based on the estimations which are
attached to the task in case there was no estimation attached to this task, the function returns None
Args:
no arguments
Returns:
planned_end (Date) or None
Raises:
No exceptions planned"""
all_task_estimations = Estimation.objects.filter(task=self.id)
planned_task_end = None
if len(all_task_estimations) == 0:
planned_task_end = None
else:
for estimation in all_task_estimations:
if not planned_task_end:
planned_task_end = estimation.date_until
elif estimation.date_until < planned_task_end:
planned_task_end = estimation.date_until
return planned_task_end
planned_end.short_description = _("Planned End")
planned_end.tags = True
def planned_effort(self, reporting_period=None):
"""The function return the planned effort of resources which have been estimated for this task
at a specific reporting period. When no reporting_period is provided, the last reporting period
is selected
Args:
no arguments
Returns:
planned effort (Decimal) [hrs], 0 if when no estimation are present
Raises:
no exceptions expected"""
try:
if not reporting_period:
reporting_period_internal = ReportingPeriod.get_latest_reporting_period(
self.project)
else:
reporting_period_internal = reporting_period
estimations_to_this_task = Estimation.objects.filter(task=self.id,
reporting_period=reporting_period_internal)
effort = 0
for estimation_to_this_task in estimations_to_this_task:
effort += estimation_to_this_task.amount
except ReportingPeriodNotFound:
effort = 0
return effort
planned_effort.short_description = _("Planned Effort")
planned_effort.tags = True
def planned_costs(self, reporting_period=None):
"""The function returns the planned costs of resources which have been estimated for this task
at a specific reporting period plus the costs of the effective effort before the provided reporting_period
When no reporting_period is provided, the last reporting period
is selected.
Args:
no arguments
Returns:
planned costs (Decimal), 0 if when no estimation or no reporting period is present
Raises:
No exceptions planned"""
try:
if not reporting_period:
reporting_period_internal = ReportingPeriod.get_latest_reporting_period(self.project)
else:
reporting_period_internal = ReportingPeriod.objects.get(id=reporting_period.id)
predecessor_reporting_periods = ReportingPeriod.get_all_predecessors(reporting_period_internal,
self.project)
estimations_to_this_task = Estimation.objects.filter(task=self.id,
reporting_period=reporting_period_internal)
sum_costs = 0
if len(estimations_to_this_task) != 0:
for estimation_to_this_task in estimations_to_this_task:
sum_costs += estimation_to_this_task.calculated_costs()
if len(predecessor_reporting_periods) != 0:
for predecessor_reporting_period in predecessor_reporting_periods:
sum_costs += self.effective_costs(reporting_period=predecessor_reporting_period)
except ReportingPeriodNotFound:
sum_costs = 0
return sum_costs
planned_costs.short_description = _("Planned Costs")
planned_costs.tags = True
def effective_start(self):
"""The function return the effective start of a task as a date. The
function return the effective start of a task as a date based on the reported work
in case there was no work reported to this task, the planned start is used as a
fall-back.
Args:
no arguments
Returns:
effective_start (Date)
Raises:
No exceptions planned"""
all_task_works = Work.objects.filter(task=self.id)
effective_task_start = None
if len(all_task_works) == 0:
effective_task_start = self.planned_start()
else:
for work in all_task_works:
if not effective_task_start:
effective_task_start = work.date
elif work.date < effective_task_start:
effective_task_start = work.date
return effective_task_start
effective_start.short_description = _("Effective Start")
effective_start.tags = True
def task_end(self):
"""The function returns a boolean value True when the task is on
status done. In all other case (example in no status case) the function
returns False
Args:
no arguments
Returns:
task_ended (Boolean)
Raises:
No exceptions planned"""
if not self.status:
task_ended = False
elif self.status.is_done:
task_ended = self.status.is_done
else:
task_ended = False
return task_ended
def effective_end(self):
"""When the task has already ended, the
function return the effective end of a task as a date based on the reported work
in case there was no work reported to this task, the last status change is used as a
fall-back. When the task did not yet end, the function returns None
Args:
no arguments
Returns:
effective_end (Date) or None when not yet ended
Raises:
No exceptions planned"""
all_task_works = Work.objects.filter(task=self.id)
effective_task_end = None
if self.task_end():
if len(all_task_works) == 0:
effective_task_end = self.last_status_change
else:
for work in all_task_works:
if not effective_task_end:
effective_task_end = work.date
elif work.date > effective_task_end:
effective_task_end = work.date
else:
effective_task_end = None
return effective_task_end
effective_end.short_description = _("Effective End")
effective_end.tags = True
def effective_duration(self):
"""The function return the effective overall duration of a task as a string in days
The function is reading the effective_starts and effective_ends of the task and
subtract them from each other.
Args:
no arguments
Returns:
duration [dys]
Raises:
No exceptions planned"""
effective_end = self.effective_end()
effective_start = self.effective_start()
if effective_start is None:
duration_as_string = 0
elif effective_end is None:
duration_as_string = 0
else:
duration_as_date = effective_end-effective_start
duration_as_string = duration_as_date.days.__str__()
return duration_as_string
effective_duration.short_description = _("Effective Duration [dys]")
effective_duration.tags = True
def serialize_to_xml(self, reporting_period):
objects = [self, ]
main_xml = PDFExport.write_xml(objects)
if reporting_period:
works = Work.objects.filter(task=self.id,
reporting_period=reporting_period)
else:
works = Work.objects.filter(task=self.id)
for work in works:
work_xml = work.serialize_to_xml()
main_xml = PDFExport.merge_xml(main_xml, work_xml)
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='crm.<EMAIL>']",
"Effective_Effort_Overall",
self.effective_costs(reporting_period=None))
if reporting_period:
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Effective_Effort_InPeriod",
self.effective_costs(reporting_period=reporting_period))
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Planned_Effort",
self.planned_costs())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='crm.<EMAIL>']",
"Effective_Duration",
self.effective_duration())
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"Planned_Duration",
self.planned_duration())
return main_xml
def effective_effort_overall(self):
return self.effective_effort(reporting_period=None)
effective_effort_overall.short_description = _("Effective Effort [hrs]")
effective_effort_overall.tags = True
def effective_effort(self, reporting_period=None):
""" Effective effort returns the effective effort on a task
when reporting_period is None, the effective effort overall is calculated
when reporting_period is specified, the effective effort in this period is calculated"""
if reporting_period:
work_objects = Work.objects.filter(task=self.id,
reporting_period=reporting_period)
else:
work_objects = Work.objects.filter(task=self.id)
sum_effort = 0
for work_object in work_objects:
sum_effort += work_object.effort_seconds()
sum_effort_in_hours = sum_effort / 3600
return sum_effort_in_hours
def effective_costs(self, reporting_period=None):
"""Returns the effective costs on a task
when reporting_period is None, the effective costs overall is calculated
when reporting_period is specified, the effective effort in this period is calculated
the costs are calculated in the project currency
Args:
no arguments
Returns:
costs [Currency] (Decimal)
Raises:
no exception planned
"""
agreements = Agreement.objects.filter(task=self)
if reporting_period:
all_work_in_task = Work.objects.filter(task=self.id,
reporting_period=reporting_period)
else:
all_work_in_task = Work.objects.filter(task=self.id)
sum_costs = 0
work_with_agreement = list()
work_without_agreement = list()
work_calculated = list()
human_resource_list = dict()
for work_object in all_work_in_task:
if work_object.human_resource not in human_resource_list:
human_resource_list[work_object.human_resource] = dict()
for agreement in agreements:
agreement_matches = agreement.match_with_work(work_object)
if agreement_matches:
if work_object not in work_with_agreement:
work_with_agreement.append(work_object)
if agreement not in human_resource_list.get(work_object.human_resource):
human_resource_list.get(work_object.human_resource)[agreement] = list()
human_resource_list.get(work_object.human_resource).get(agreement).append(work_object)
if work_object not in work_with_agreement:
work_without_agreement.append(work_object)
for human_resource_dict in human_resource_list:
agreement_list = Agreement.objects.filter(task=self, resource=human_resource_dict).order_by('costs__price')
for agreement in agreement_list:
agreement_remaining_amount = agreement.amount
if human_resource_list[human_resource_dict].get(agreement):
for work in human_resource_list[human_resource_dict].get(agreement):
if work in work_with_agreement:
if (agreement_remaining_amount - work.worked_hours) > 0:
agreement_remaining_amount -= work.worked_hours
getcontext().prec = 5
sum_costs += Decimal(work.effort_hours())*agreement.costs.price
work_calculated.append(work)
for work in all_work_in_task:
if work not in work_calculated:
if work not in work_without_agreement:
work_without_agreement.append(work)
for work in work_without_agreement:
default_resource_prices = ResourcePrice.objects.filter(resource=work.human_resource.id).order_by('price')
if default_resource_prices:
default_resource_price = default_resource_prices[0]
getcontext().prec = 5
sum_costs += Decimal(work.effort_hours())*default_resource_price.price
else:
sum_costs = 0
break
sum_costs = self.project.default_currency.round(sum_costs)
return sum_costs
effective_costs.short_description = _("Effective costs")
effective_costs.tags = True
def is_reporting_allowed(self):
"""Returns True when the task is available for reporting,
Returns False when the task is not available for reporting,
The decision whether the task is available for reporting is purely depending
on the task_status. When the status is done or when the status is unknown,
the task is not longer available for reporting.
Args:
no arguments
Returns:
allowed (Boolean)
Raises:
when there is no valid reporting Period"""
if self.status:
if self.status.is_done:
allowed = False
else:
allowed = True
else:
allowed = False
return allowed
is_reporting_allowed.short_description = _("Reporting")
is_reporting_allowed.tags = True
def get_title(self):
if self.title:
return self.title
else:
return "n/a"
def __str__(self):
return str(self.id) + " " + self.get_title()
class Meta:
app_label = "crm"
verbose_name = _('Task')
verbose_name_plural = _('Tasks')
class TaskAdminView(admin.ModelAdmin):
list_display = ('link_to_task',
'planned_start',
'planned_end',
'project',
'status',
'last_status_change',
'planned_duration',
'planned_costs',
'effective_duration',
'effective_effort_overall',
'effective_costs')
list_display_links = ('link_to_task',)
list_filter = ('project',)
ordering = ('-id',)
fieldsets = (
(_('Work'), {
'fields': ('title',
'project',
'description',
'status')
}),
)
save_as = True
inlines = [AgreementInlineAdminView,
EstimationInlineAdminView,
InlineGenericTaskLink,
WorkInlineAdminView]
class TaskInlineAdminView(admin.TabularInline):
model = Task
readonly_fields = ('link_to_task',
'last_status_change',
'planned_start',
'planned_end',
'planned_duration',
'planned_costs',
'effective_duration',
'effective_effort_overall',
'effective_costs')
fieldsets = (
(_('Task'), {
'fields': ('link_to_task',
'title',
'planned_start',
'planned_end',
'status',
'last_status_change',
'planned_duration',
'planned_costs',
'effective_duration',
'effective_effort_overall',
'effective_costs')
}),
)
extra = 1
def has_add_permission(self, request):
return True
def has_delete_permission(self, request, obj=None):
return False
class TaskSerializer(serializers.ModelSerializer):
is_reporting_allowed = serializers.SerializerMethodField()
def get_is_reporting_allowed(self, obj):
if obj.is_reporting_allowed():
return "True"
else:
return "False"
class Meta:
model = Task
fields = ('id',
'title',
'planned_end',
'planned_start',
'project',
'description',
'status',
'is_reporting_allowed')
<file_sep>/koalixcrm/accounting/rest/booking_rest.py
# -*- coding: utf-8 -*-
from django.contrib.auth.models import User
from rest_framework import serializers
from koalixcrm.accounting.accounting.accounting_period import AccountingPeriod
from koalixcrm.accounting.accounting.booking import Booking
from koalixcrm.accounting.models import Account
from koalixcrm.accounting.rest.account_rest import OptionAccountJSONSerializer
from koalixcrm.accounting.rest.accounting_period_rest import OptionAccountingPeriodJSONSerializer
class UserJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
username = serializers.CharField(read_only=True)
class Meta:
model = User
fields = (
'id',
'username')
class BookingJSONSerializer(serializers.HyperlinkedModelSerializer):
fromAccount = OptionAccountJSONSerializer(source='from_account', allow_null=False)
toAccount = OptionAccountJSONSerializer(source='to_account', allow_null=False)
bookingDate = serializers.DateTimeField(source='booking_date', format="%Y-%m-%dT%H:%M", input_formats=None,
allow_null=False)
bookingReference = serializers.CharField(source='booking_reference', allow_null=True)
accountingPeriod = OptionAccountingPeriodJSONSerializer(source='accounting_period', allow_null=False)
staff = UserJSONSerializer(allow_null=False)
class Meta:
model = Booking
fields = ('id',
'fromAccount',
'toAccount',
'description',
'amount',
'bookingDate',
'staff',
'bookingReference',
'accountingPeriod')
depth = 1
def create(self, validated_data):
booking = Booking()
booking.description = validated_data['description']
booking.amount = validated_data['amount']
booking.booking_date = validated_data['booking_date']
booking.booking_reference = validated_data['booking_reference']
# Deserialize from staff
staff = validated_data.pop('staff')
if staff:
if staff.get('id', None):
user = User.objects.get(id=staff.get('id', None))
booking.staff = user
booking.last_modified_by = user
else:
booking.staff = None
# Deserialize from account
from_account = validated_data.pop('from_account')
if from_account:
if from_account.get('id', None):
booking.from_account = Account.objects.get(id=from_account.get('id', None))
else:
booking.from_account = None
# Deserialize to account
to_account = validated_data.pop('to_account')
if to_account:
if to_account.get('id', None):
booking.to_account = Account.objects.get(id=to_account.get('id', None))
else:
booking.to_account = None
# Deserialize to accounting period
accounting_period = validated_data.pop('accounting_period')
if accounting_period:
if accounting_period.get('id', None):
booking.accounting_period = AccountingPeriod.objects.get(id=accounting_period.get('id', None))
else:
booking.accounting_period = None
booking.save()
return booking
def update(self, instance, validated_data):
instance.description = validated_data['description']
instance.amount = validated_data['amount']
instance.booking_date = validated_data['booking_date']
instance.booking_reference = validated_data['booking_reference']
# Deserialize from staff
staff = validated_data.pop('staff')
if staff:
if staff.get('id', instance.staff):
instance.staff = User.objects.get(id=staff.get('id', None))
else:
instance.staff = instance.staff_id
else:
instance.staff = None
# Deserialize from account
from_account = validated_data.pop('from_account')
if from_account:
if from_account.get('id', instance.from_account):
instance.from_account = Account.objects.get(id=from_account.get('id', None))
else:
instance.from_account = instance.from_account_id
else:
instance.from_account = None
# Deserialize to account
to_account = validated_data.pop('to_account')
if to_account:
if to_account.get('id', instance.to_account):
user = User.objects.get(id=staff.get('id', None))
instance.staff = user
instance.last_modified_by = user
else:
instance.to_account = instance.to_account_id
else:
instance.to_account = None
# Deserialize to accounting period
accounting_period = validated_data.pop('accounting_period')
if accounting_period:
if accounting_period.get('id', instance.accounting_period):
instance.accounting_period = AccountingPeriod.objects.get(id=accounting_period.get('id', None))
else:
instance.accounting_period = instance.accounting_period_id
else:
instance.accounting_period = None
instance.save()
return instance
<file_sep>/koalixcrm/crm/factories/factory_resource_type.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ResourceType
class StandardResourceTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = ResourceType
title = 'This is a test Resource Type'
description = "This is a description of such a Resource Type"
<file_sep>/requirements.txt
-r requirements/heroku_requirements.txt<file_sep>/koalixcrm/subscriptions/admin.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from django.http import HttpResponseRedirect
from django.utils.translation import ugettext as _
from koalixcrm.subscriptions.models import *
class AdminSubscriptionEvent(admin.TabularInline):
model = SubscriptionEvent
extra = 1
classes = ('collapse-open',)
fieldsets = (
('Basics', {
'fields': (
'event_date',
'event',)
}),
)
allow_add = True
class InlineSubscription(admin.TabularInline):
model = Subscription
extra = 1
classes = ('collapse-open',)
readonly_fields = ('contract',
'subscription_type')
fieldsets = (
(_('Basics'), {
'fields': (
'contract',
'subscription_type')
}),
)
allow_add = False
class OptionSubscription(admin.ModelAdmin):
list_display = ('id',
'contract',
'subscription_type',)
ordering = ('id',
'contract',
'subscription_type')
search_fields = ('id',
'contract',)
fieldsets = (
(_('Basics'), {
'fields': (
'contract',
'subscription_type',)
}),
)
inlines = [AdminSubscriptionEvent]
@staticmethod
def create_invoice(queryset):
for obj in queryset:
invoice = obj.create_invoice()
response = HttpResponseRedirect('/admin/crm/invoice/' + str(invoice.id))
return response
create_invoice.short_description = _("Create Invoice")
@staticmethod
def create_quote(queryset):
for obj in queryset:
invoice = obj.create_invoice()
response = HttpResponseRedirect('/admin/crm/invoice/' + str(invoice.id))
return response
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
actions = ['create_subscription_pdf', 'create_invoice']
class OptionSubscriptionType(admin.ModelAdmin):
list_display = ('id',)
list_display_links = ('id',)
ordering = ('id', )
search_fields = ('id',)
fieldsets = (
(_('Basics'), {
'fields': (
'cancellation_period',
'automatic_contract_extension',
'automatic_contract_extension_reminder',
'minimum_duration',
'payment_interval',
'contract_document')
}),
)
def create_subscription(a, request, queryset):
for contract in queryset:
subscription = Subscription()
subscription.create_subscription_from_contract(crmmodels.Contract.objects.get(id=contract.id))
response = HttpResponseRedirect('/admin/subscriptions/' + str(subscription.id))
return response
create_subscription.short_description = _("Create Subscription")
class KoalixcrmPluginInterface(object):
contractInlines = [InlineSubscription]
contractActions = [create_subscription]
invoiceInlines = []
invoiceActions = []
quoteInlines = []
quoteActions = []
customerInlines = []
customerActions = []
admin.site.register(Subscription, OptionSubscription)
admin.site.register(SubscriptionType, OptionSubscriptionType)
<file_sep>/koalixcrm/crm/views/range_selection_form.py
# -*- coding: utf-8 -*-
from django.contrib.admin.widgets import *
class RangeSelectionForm(forms.Form):
"""
Form which allows to select the Range in which the work item can be registered
and which define which range of work items are loaded from the database and shown
to the user
"""
from_date = forms.DateField(widget=AdminDateWidget)
to_date = forms.DateField(widget=AdminDateWidget)
original_from_date = forms.DateField(widget=forms.HiddenInput(),
required=False)
original_to_date = forms.DateField(widget=forms.HiddenInput(),
required=False)
def evaluate_pre_check_from_date(self):
original_from_date = self.cleaned_data['original_from_date']
new_from_date = self.cleaned_data['from_date']
if original_from_date < new_from_date:
from_date = original_from_date
else:
from_date = new_from_date
return from_date
def evaluate_pre_check_to_date(self):
original_to_date = self.cleaned_data['original_to_date']
new_to_date = self.cleaned_data['to_date']
if original_to_date > new_to_date:
to_date = original_to_date
else:
to_date = new_to_date
return to_date
def update_from_input(self):
self.from_date = self.cleaned_data['from_date']
self.to_date = self.cleaned_data['to_date']
self.original_from_date = self.from_date
self.original_to_date = self.to_date
@staticmethod
def create_range_selection_form(from_date, to_date):
initial_form_data = {'from_date': from_date,
'to_date': to_date,
'original_from_date': from_date,
'original_to_date': to_date}
range_selection_form = RangeSelectionForm(initial=initial_form_data)
return range_selection_form
<file_sep>/koalixcrm/crm/factories/factory_work.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import Work
from koalixcrm.crm.factories.factory_task import StandardTaskFactory
from koalixcrm.crm.factories.factory_reporting_period import StandardReportingPeriodFactory
from koalixcrm.crm.factories.factory_human_resource import StandardUserExtensionFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardWorkFactory(factory.django.DjangoModelFactory):
class Meta:
model = Work
human_resource = factory.SubFactory(StandardUserExtensionFactory)
date = make_date_utc(datetime.datetime(2018, 5, 1, 0, 00))
start_time = None
stop_time = None
worked_hours = "1.50"
short_description = "The employee did some work"
description = "And here he describes some more about his work"
task = factory.SubFactory(StandardTaskFactory)
reporting_period = factory.SubFactory(StandardReportingPeriodFactory)
<file_sep>/koalixcrm/crm/factories/factory_contact.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Contact
from koalixcrm.crm.factories.factory_user import StaffUserFactory
class StandardContactFactory(factory.django.DjangoModelFactory):
class Meta:
model = Contact
django_get_or_create = ('name',)
name = "<NAME>"
date_of_creation = "2018-05-01"
last_modification = "2018-05-03"
last_modified_by = factory.SubFactory(StaffUserFactory)
<file_sep>/koalixcrm/accounting/migrations/0003_remove_account_originalamount.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.2 on 2017-07-07 12:40
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('accounting', '0002_auto_20170705_1702'),
]
operations = [
migrations.RemoveField(
model_name='account',
name='originalAmount',
),
]
<file_sep>/koalixcrm/crm/product/tax.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class Tax(models.Model):
tax_rate = models.DecimalField(max_digits=5,
decimal_places=2,
verbose_name=_("Taxrate in Percentage"))
name = models.CharField(verbose_name=_("Taxname"),
max_length=100)
account_activa = models.ForeignKey('accounting.Account',
verbose_name=_("Activa Account"),
related_name="db_relaccountactiva",
null=True,
blank=True)
account_passiva = models.ForeignKey('accounting.Account',
verbose_name=_("Passiva Account"),
related_name="db_relaccountpassiva",
null=True,
blank=True)
def get_tax_rate(self):
return self.tax_rate;
def __str__(self):
return self.name
class Meta:
app_label = "crm"
verbose_name = _('Tax')
verbose_name_plural = _('Taxes')
class OptionTax(admin.ModelAdmin):
list_display = ('id',
'tax_rate',
'name',
'account_activa',
'account_passiva')
fieldsets = (('', {'fields': ('tax_rate',
'name',
'account_activa',
'account_passiva')}),)
allow_add = True
<file_sep>/koalixcrm/accounting/migrations/0007_auto_20180422_2105.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-04-22 21:05
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
('accounting', '0006_auto_20180422_2048'),
]
operations = [
migrations.RemoveField(
model_name='accountingperiod',
name='template_set',
),
migrations.AddField(
model_name='accountingperiod',
name='template_profit_loss_statement',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_profit_loss_statement_template_set', to='djangoUserExtension.DocumentTemplate', verbose_name='Referred template for profit, loss statement'),
),
migrations.AddField(
model_name='accountingperiod',
name='template_set_balance_sheet',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_balancesheet_template_set', to='djangoUserExtension.DocumentTemplate', verbose_name='Referred template for balance sheet'),
),
]
<file_sep>/koalixcrm/crm/documents/sales_document.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.contrib import admin, messages
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.purpose import *
from koalixcrm.global_support_functions import xstr
from koalixcrm.crm.contact.phone_address import PhoneAddress
from koalixcrm.crm.contact.email_address import EmailAddress
from koalixcrm.crm.contact.postal_address import PostalAddress
from koalixcrm.crm.documents.sales_document_position import SalesDocumentPosition, SalesDocumentInlinePosition
from koalixcrm.djangoUserExtension.models import TextParagraphInDocumentTemplate, UserExtension
from koalixcrm.crm.product.product_type import ProductType
from koalixcrm.crm.exceptions import TemplateSetMissingInContract
import koalixcrm.crm.documents.calculations
from koalixcrm.crm.documents.pdf_export import PDFExport
class TextParagraphInSalesDocument(models.Model):
sales_document = models.ForeignKey("SalesDocument")
purpose = models.CharField(verbose_name=_("Purpose"), max_length=2, choices=PURPOSESTEXTPARAGRAPHINDOCUMENTS)
text_paragraph = models.TextField(verbose_name=_("Text"), blank=False, null=False)
def create_paragraph(self, default_paragraph, sales_document):
self.sales_document = sales_document
self.purpose = default_paragraph.purpose
self.text_paragraph = default_paragraph.text_paragraph
self.save()
return
class Meta:
app_label = "crm"
verbose_name = _('Text Paragraph In Sales Document')
verbose_name_plural = _('Text Paragraphs In Sales Documents')
def __str__(self):
return self.id.__str__()
class SalesDocument(models.Model):
contract = models.ForeignKey("Contract",
verbose_name=_('Contract'))
external_reference = models.CharField(verbose_name=_("External Reference"),
max_length=100,
blank=True)
discount = models.DecimalField(max_digits=5,
decimal_places=2,
verbose_name=_("Discount"),
blank=True,
null=True)
description = models.CharField(verbose_name=_("Description"),
max_length=100,
blank=True,
null=True)
last_pricing_date = models.DateField(verbose_name=_("Pricing Date"),
blank=True,
null=True)
last_calculated_price = models.DecimalField(max_digits=17,
decimal_places=2,
verbose_name=_("Price without Tax "),
blank=True,
null=True)
last_calculated_tax = models.DecimalField(max_digits=17,
decimal_places=2,
verbose_name=_("Tax"),
blank=True,
null=True)
customer = models.ForeignKey("Customer",
verbose_name=_("Customer"))
staff = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
blank=True,
verbose_name=_("Staff"),
related_name="db_relscstaff",
null=True)
currency = models.ForeignKey("Currency", verbose_name=_("Currency"),
blank=False, null=False)
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
custom_date_field = models.DateField(verbose_name=_("Custom Date"),
blank=True,
null=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"),
related_name="db_lstscmodified",
null=True,
blank="True")
template_set = models.ForeignKey("djangoUserExtension.DocumentTemplate",
verbose_name=_("Referred Template"),
null=True,
blank=True)
derived_from_sales_document = models.ForeignKey("SalesDocument",
blank=True,
null=True)
last_print_date = models.DateTimeField(verbose_name=_("Last printed"),
blank=True,
null=True)
class Meta:
app_label = "crm"
verbose_name = _('Sales Document')
verbose_name_plural = _('Sales Documents')
def serialize_to_xml(self):
from koalixcrm.crm.models import PostalAddressForContact
from koalixcrm.crm.models import Currency
from koalixcrm.crm.models import PurchaseOrder
from koalixcrm.crm.models import SalesDocument
from koalixcrm.crm.models import SalesDocumentPosition
from koalixcrm.crm.models import Contact
from django.contrib import auth
objects = [self, ]
position_class = SalesDocumentPosition
objects += list(SalesDocument.objects.filter(id=self.id))
if isinstance(self, PurchaseOrder):
objects += list(Contact.objects.filter(id=self.supplier.id))
objects += list(PostalAddressForContact.objects.filter(person=self.supplier.id))
for address in list(PostalAddressForContact.objects.filter(person=self.supplier.id)):
objects += list(PostalAddress.objects.filter(id=address.id))
else:
objects += list(Contact.objects.filter(id=self.customer.id))
objects += list(PostalAddressForContact.objects.filter(person=self.customer.id))
for address in list(PostalAddressForContact.objects.filter(person=self.customer.id)):
objects += list(PostalAddress.objects.filter(id=address.id))
objects += list(TextParagraphInSalesDocument.objects.filter(sales_document=self.id))
objects += list(Currency.objects.filter(id=self.currency.id))
objects += SalesDocumentPosition.add_positions(position_class, self)
objects += list(auth.models.User.objects.filter(id=self.staff.id))
objects += UserExtension.objects_to_serialize(self, self.staff)
main_xml = PDFExport.write_xml(objects)
return main_xml
def is_complete_with_price(self):
""" Checks whether the SalesContract is completed with a price, in case the
SalesContract was not completed or the price calculation was not performed,
the method returns false"""
if self.last_pricing_date and self.last_calculated_price:
return True
else:
return False
def create_sales_document(self, calling_model):
self.staff = calling_model.staff
if isinstance(calling_model, koalixcrm.crm.documents.contract.Contract):
self.contract = calling_model
self.customer = calling_model.default_customer
self.currency = calling_model.default_currency
self.description = calling_model.description
self.discount = 0
elif isinstance(calling_model, SalesDocument):
self.derived_from_sales_document = calling_model
self.contract = calling_model.contract
self.customer = calling_model.customer
self.currency = calling_model.currency
self.description = calling_model.description
self.discount = calling_model.discount
def attach_text_paragraphs(self):
default_paragraphs = TextParagraphInDocumentTemplate.objects.filter(document_template=self.template_set)
for default_paragraph in list(default_paragraphs):
invoice_paragraph = TextParagraphInSalesDocument()
invoice_paragraph.create_paragraph(default_paragraph, self)
def attach_sales_document_positions(self, calling_model):
if isinstance(calling_model, SalesDocument):
sales_document_positions = SalesDocumentPosition.objects.filter(sales_document=calling_model.id)
for sales_document_position in list(sales_document_positions):
new_position = SalesDocumentPosition()
new_position.create_position(sales_document_position, self)
def create_pdf(self, template_set, printed_by):
self.last_print_date = datetime.now()
self.save()
return koalixcrm.crm.documents.pdf_export.PDFExport.create_pdf(self, template_set, printed_by)
def get_template_set(self):
if self.template_set:
return self.template_set
else:
raise TemplateSetMissingInContract((_("Template Set missing in Sales Document" + str(self))))
def get_fop_config_file(self, template_set):
template_set = self.get_template_set()
return template_set.get_fop_config_file()
def get_xsl_file(self, template_set):
template_set = self.get_template_set()
return template_set.get_xsl_file()
def __str__(self):
return _("Sales Contract") + ": " + str(self.id) + " " + _("from Contract") + ": " + str(self.contract.id)
class PostalAddressForSalesDocument(PostalAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
sales_document = models.ForeignKey("SalesDocument")
class Meta:
app_label = "crm"
verbose_name = _('Postal Address For Sales Documents')
verbose_name_plural = _('Postal Address For Sales Documents')
def __str__(self):
return xstr(self.pre_name) + ' ' + xstr(self.name) + ' ' + xstr(self.address_line_1)
class EmailAddressForSalesDocument(EmailAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
sales_document = models.ForeignKey("SalesDocument")
class Meta:
app_label = "crm"
verbose_name = _('Email Address For Sales Documents')
verbose_name_plural = _('Email Address For Sales Documents')
def __str__(self):
return str(self.email)
class PhoneAddressForSalesDocument(PhoneAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
sales_document = models.ForeignKey("SalesDocument")
class Meta:
app_label = "crm"
verbose_name = _('Phone Address For Sales Documents')
verbose_name_plural = _('Phone Address For Sales Documents')
def __str__(self):
return str(self.phone)
class SalesDocumentTextParagraph(admin.StackedInline):
model = TextParagraphInSalesDocument
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('text_paragraph', 'purpose', )
}),
)
allow_add = True
class SalesDocumentPostalAddress(admin.StackedInline):
model = PostalAddressForSalesDocument
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('prefix',
'pre_name',
'name',
'address_line_1',
'address_line_2',
'address_line_3',
'address_line_4',
'zip_code',
'town',
'state',
'country',
'purpose')
}),
)
allow_add = True
class SalesDocumentPhoneAddress(admin.TabularInline):
model = PhoneAddressForSalesDocument
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('phone', 'purpose',)
}),
)
allow_add = True
class SalesDocumentEmailAddress(admin.TabularInline):
model = EmailAddressForSalesDocument
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('email', 'purpose',)
}),
)
allow_add = True
class OptionSalesDocument(admin.ModelAdmin):
list_display = ('id',
'description',
'contract',
'customer',
'currency',
'staff',
'last_modified_by',
'last_calculated_price',
'last_calculated_tax',
'last_pricing_date',
'last_modification',
'last_print_date')
list_display_links = ('id',)
list_filter = ('customer',
'contract',
'currency',
'staff',
'last_modification')
ordering = ('-id',)
search_fields = ('contract__id',
'customer__name',
'currency__description')
fieldsets = (
(_('Sales Contract'), {
'fields': ('contract',
'description',
'customer',
'currency',
'discount',
'staff',
'external_reference',
'template_set',
'custom_date_field')
}),
)
save_as = True
inlines = [SalesDocumentInlinePosition, SalesDocumentTextParagraph,
SalesDocumentPostalAddress, SalesDocumentPhoneAddress,
SalesDocumentEmailAddress]
def response_add(self, request, obj, post_url_continue=None):
new_obj = self.after_saving_model_and_related_inlines(request, obj)
new_obj.custom_date_field = date.today().__str__()
return super(OptionSalesDocument, self).response_add(request=request,
obj=new_obj,
post_url_continue=post_url_continue)
def response_change(self, request, new_object):
obj = self.after_saving_model_and_related_inlines(request, new_object)
return super(OptionSalesDocument, self).response_change(request, obj)
def after_saving_model_and_related_inlines(self, request, obj):
try:
koalixcrm.crm.documents.calculations.Calculations.calculate_document_price(obj, date.today())
self.message_user(request, "Successfully calculated Prices")
except ProductType.NoPriceFound as e:
self.message_user(request, "Unsuccessful in updating the Prices " + e.__str__(), level=messages.ERROR)
return obj
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
def create_quote(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.quote.Quote,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_quote.short_description = _("Create Quote")
def create_invoice(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.invoice.Invoice,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_invoice.short_description = _("Create Invoice")
def create_purchase_confirmation(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.purchaseconfirmation.PurchaseConfirmation,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_purchase_confirmation.short_description = _("Create Purchase Confirmation")
def create_delivery_note(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.deliverynote.DeliveryNote,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_delivery_note.short_description = _("Create Delivery note")
def create_payment_reminder(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.paymentreminder.PaymentReminder,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_payment_reminder.short_description = _("Create Payment Reminder")
def create_purchase_order(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.purchaseorder.PurchaseOrder,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_purchase_order.short_description = _("Create Purchase Order")
def create_pdf(self, request, queryset):
from koalixcrm.crm.views.pdfexport import PDFExportView
for obj in queryset:
response = PDFExportView.export_pdf(self,
request,
obj,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"),
obj.template_set)
return response
create_pdf.short_description = _("Create PDF")
def create_project(self, request, queryset):
from koalixcrm.crm.views.create_task import CreateTaskView
for obj in queryset:
response = CreateTaskView.create_project(self,
request,
obj,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_project.short_description = _("Create Project")
<file_sep>/koalixcrm/crm/documents/contract.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.plugin import *
from koalixcrm.crm.contact.phone_address import PhoneAddress
from koalixcrm.crm.contact.email_address import EmailAddress
from koalixcrm.crm.contact.postal_address import PostalAddress
from koalixcrm.crm.documents.invoice import Invoice
from koalixcrm.crm.documents.quote import Quote
from koalixcrm.crm.documents.purchase_order import PurchaseOrder
from koalixcrm.global_support_functions import xstr
from koalixcrm.crm.const.purpose import *
from koalixcrm.crm.documents.invoice import InlineInvoice
from koalixcrm.crm.documents.quote import InlineQuote
from koalixcrm.crm.reporting.generic_project_link import InlineGenericProjectLink
from koalixcrm.crm.exceptions import *
from koalixcrm.djangoUserExtension.models import UserExtension
import koalixcrm.crm.documents.calculations
import koalixcrm.crm.documents.pdf_export
from rest_framework import serializers
class PostalAddressForContract(PostalAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
contract = models.ForeignKey('Contract')
class Meta:
app_label = "crm"
verbose_name = _('Postal Address For Contracts')
verbose_name_plural = _('Postal Address For Contracts')
def __str__(self):
return xstr(self.prename) + ' ' + xstr(self.name) + ' ' + xstr(self.addressline1)
class PhoneAddressForContract(PhoneAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
contract = models.ForeignKey('Contract')
class Meta:
app_label = "crm"
verbose_name = _('Phone Address For Contracts')
verbose_name_plural = _('Phone Address For Contracts')
def __str__(self):
return str(self.phone)
class EmailAddressForContract(EmailAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINCONTRACT)
contract = models.ForeignKey('Contract')
class Meta:
app_label = "crm"
verbose_name = _('Email Address For Contracts')
verbose_name_plural = _('Email Address For Contracts')
def __str__(self):
return str(self.email)
class ContractPostalAddress(admin.StackedInline):
model = PostalAddressForContract
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('prefix',
'pre_name',
'name',
'address_line_1',
'address_line_2',
'address_line_3',
'address_line_4',
'zip_code',
'town',
'state',
'country',
'purpose'),
}),
)
allow_add = True
class ContractPhoneAddress(admin.TabularInline):
model = PhoneAddressForContract
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('phone', 'purpose',)
}),
)
allow_add = True
class ContractEmailAddress(admin.TabularInline):
model = EmailAddressForContract
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('email',
'purpose',)
}),
)
allow_add = True
class Contract(models.Model):
staff = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Staff"),
related_name="db_relcontractstaff",
blank=True,
null=True)
description = models.TextField(verbose_name=_("Description"))
default_customer = models.ForeignKey("Customer",
verbose_name=_("Default Customer"),
null=True,
blank=True)
default_supplier = models.ForeignKey("Supplier",
verbose_name=_("Default Supplier"),
null=True,
blank=True)
default_currency = models.ForeignKey("Currency",
verbose_name=_("Default Currency"),
blank=False,
null=False)
default_template_set = models.ForeignKey("djangoUserExtension.TemplateSet",
verbose_name=_("Default Template Set"), null=True, blank=True)
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"),
related_name="db_contractlstmodified")
class Meta:
app_label = "crm"
verbose_name = _('Contract')
verbose_name_plural = _('Contracts')
def get_template_set(self, calling_model):
if self.default_template_set:
required_template_set = str(type(calling_model).__name__)
return self.default_template_set.get_template_set(required_template_set)
else:
raise TemplateSetMissingInContract("The Contract has no Default Template Set selected")
def create_from_reference(self, calling_model, staff):
staff_user_extension = UserExtension.get_user_extension(staff.id)
self.default_customer = calling_model
self.default_currency = staff_user_extension.defaultCurrency
self.default_template_set = staff_user_extension.defaultTemplateSet
self.last_modified_by = staff
self.staff = staff
self.save()
return self
def create_invoice(self):
invoice = Invoice()
invoice.create_from_reference(self)
return invoice
def create_quote(self):
quote = Quote()
quote.create_from_reference(self)
return quote
def create_purchase_order(self):
purchase_order = PurchaseOrder()
purchase_order.create_from_reference(self)
return purchase_order
def __str__(self):
return _("Contract") + " " + str(self.id)
class OptionContract(admin.ModelAdmin):
list_display = ('id',
'description',
'default_customer',
'default_supplier',
'staff',
'default_currency',
'date_of_creation',
'last_modification',
'last_modified_by')
list_display_links = ('id',)
list_filter = ('default_customer',
'default_supplier',
'staff',
'default_currency')
ordering = ('id', )
search_fields = ('id',
'contract')
fieldsets = (
(_('Basics'), {
'fields': ('description',
'default_customer',
'staff',
'default_supplier',
'default_currency',
'default_template_set')
}),
)
inlines = [ContractPostalAddress,
ContractPhoneAddress,
ContractEmailAddress,
InlineQuote,
InlineInvoice,
InlineGenericProjectLink]
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("contractInlines"))
def create_quote(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.quote.Quote,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_quote.short_description = _("Create Quote")
def create_invoice(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.invoice.Invoice,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_invoice.short_description = _("Create Invoice")
def create_purchase_confirmation(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.purchaseconfirmation.PurchaseConfirmation,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_purchase_confirmation.short_description = _("Create Purchase Confirmation")
def create_delivery_note(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.deliverynote.DeliveryNote,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_delivery_note.short_description = _("Create Delivery note")
def create_payment_reminder(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.paymentreminder.PaymentReminder,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_payment_reminder.short_description = _("Create Payment Reminder")
def create_purchase_order(self, request, queryset):
from koalixcrm.crm.views.newdocument import CreateNewDocumentView
for obj in queryset:
response = CreateNewDocumentView.create_new_document(self, request, obj,
koalixcrm.crm.documents.purchaseorder.PurchaseOrder,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"))
return response
create_purchase_order.short_description = _("Create Purchase Order")
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
actions = ['create_quote', 'create_invoice', 'create_purchase_order']
pluginProcessor = PluginProcessor()
actions.extend(pluginProcessor.getPluginAdditions("contractActions"))
class ContractJSONSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = Contract
fields = ('id',
'description')
<file_sep>/koalixcrm/crm/factories/factory_purchase_confirmation.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.models import PurchaseConfirmation
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardPurchaseConfirmationFactory(StandardSalesDocumentFactory):
class Meta:
model = PurchaseConfirmation
<file_sep>/koalixcrm/accounting/factories/factory_product_category.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.accounting.models import ProductCategory
class StandardProductCategoryFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProductCategory
django_get_or_create = ('title',)
title = "Service"
profit_account = ""
loss_account = ""
<file_sep>/koalixcrm/djangoUserExtension/exceptions.py
# -*- coding: utf-8 -*-
from django import forms
class TemplateSetMissingForUserExtension(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class UserExtensionMissing(Exception):
def __init__(self, value):
self.value = value
self.view = "/koalixcrm/crm/reporting/user_extension_missing"
def __str__(self):
return repr(self.value)
class TooManyUserExtensionsAvailable(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class UserExtensionPhoneAddressMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)
class UserExtensionEmailAddressMissing(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return repr(self.value)<file_sep>/documentation/source/code_documentation/resource.rst
.. highlight:: rst
Resource
--------
.. automodule:: koalixcrm.crm.reporting.resource
:members:
<file_sep>/koalixcrm/subscriptions/migrations/0004_auto_20181013_2213.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-13 22:13
from __future__ import unicode_literals
from django.db import migrations, models
from filebrowser.fields import FileBrowseField
def fill_subscription_type_backup_table(apps, schema_editor):
Subscription = apps.get_model("subscriptions", "Subscription")
SubscriptionType = apps.get_model("subscriptions", "SubscriptionType")
SubscriptionTypeBackup = apps.get_model("subscriptions", "SubscriptionTypeBackup")
db_alias = schema_editor.connection.alias
all_subscription_types = SubscriptionType.objects.using(db_alias).all()
for subscription_type in all_subscription_types:
subscription_type_backup = SubscriptionTypeBackup.objects.using(db_alias).create(
cancellation_period=subscription_type.cancelationPeriod,
automatic_contract_extension=subscription_type.automaticContractExtension,
automatic_contract_extension_reminder=subscription_type.automaticContractExtensionReminder,
minimum_duration=subscription_type.minimumDuration,
payment_interval=subscription_type.paymentIntervall,
contract_document=subscription_type.contractDocument,
description=subscription_type.description,
title=subscription_type.title,
product_number=subscription_type.product_number,
default_unit=subscription_type.default_unit,
date_of_creation=subscription_type.date_of_creation,
last_modification=subscription_type.last_modification,
last_modified_by=subscription_type.last_modified_by,
tax=subscription_type.tax,
old_id=subscription_type.id)
subscription_type_backup.save()
subscriptions = Subscription.objects.using(db_alias).all()
if len(subscriptions) > 0:
for subscription in subscriptions:
if subscription.subscriptiontype is not None:
subscription.subscription_type_backup = subscription.subscriptiontype.id
subscription.save()
def reverse_func(apps, schema_editor):
return 1
class Migration(migrations.Migration):
dependencies = [
('crm', '0047_work_worked_hours'),
('subscriptions', '0003_auto_20171110_1732'),
]
operations = [
migrations.RenameField(
model_name='subscriptionevent',
old_name='eventdate',
new_name='event_date',
),
migrations.AddField(
model_name='Subscription',
name='subscription_type_backup',
field=models.IntegerField(blank=True, null=True, verbose_name='Subscription Type Backup'),
),
migrations.CreateModel(
name='SubscriptionTypeBackup',
fields=[
('cancellation_period', models.IntegerField(verbose_name="Cancellation Period (months)",
blank=True,
null=True)),
('automatic_contract_extension', models.IntegerField(
verbose_name="Automatic Contract Extension (months)",
blank=True,
null=True)),
('automatic_contract_extension_reminder', models.IntegerField(
verbose_name="Automatic Contract Extension Reminder (days)",
blank=True,
null=True)),
('minimum_duration', models.IntegerField(verbose_name="Minimum Contract Duration",
blank=True,
null=True)),
('payment_interval', models.IntegerField(verbose_name="Payment Interval (days)",
blank=True,
null=True)),
('contract_document', FileBrowseField(verbose_name="Contract Documents",
blank=True,
null=True,
max_length=200)),
('description', models.TextField(verbose_name="Description",
null=True,
blank=True)),
('title', models.CharField(verbose_name="Title",
max_length=200)),
('product_number', models.IntegerField(verbose_name="Product Number")),
('default_unit', models.ForeignKey("crm.Unit",
verbose_name="Unit")),
('date_of_creation', models.DateTimeField(verbose_name="Created at",
auto_now_add=True)),
('last_modification', models.DateTimeField(verbose_name="Last modified",
auto_now=True)),
('last_modified_by', models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name="Last modified by",
null=True,
blank="True")),
('tax', models.ForeignKey("crm.Tax",
blank=False)),
('old_id', models.IntegerField(blank=True,
null=True,
verbose_name='Subscription Type Backup')),
],
options={
'verbose_name': 'SubscriptionTypeBackup',
'verbose_name_plural': 'SubscriptionTypeBackups',
},
),
migrations.RunPython(fill_subscription_type_backup_table, reverse_func),
migrations.RemoveField(
model_name='subscription',
name='subscriptiontype',
),
migrations.DeleteModel('SubscriptionType'),
]
<file_sep>/koalixcrm/crm/views/newdocument.py
# -*- coding: utf-8 -*-
from django.http import Http404
from django.http import HttpResponseRedirect
from django.utils.translation import ugettext as _
from django.contrib import messages
from koalixcrm.crm.exceptions import *
class CreateNewDocumentView:
def create_new_document(calling_model_admin, request, calling_model, requested_document_type, redirect_to):
"""This method exports PDFs provided by different Models in the crm application
Args:
calling_model_admin (ModelAdmin): The calling ModelAdmin must be provided for error message response.
request: The request User is to know where to save the error message
calling_model (Contract or SalesDocument): The model from which a new document shall be created
requested_document_type (str): The document type name that shall be created
redirect_to (str): String that describes to where the method should redirect in case of an error
Returns:
HTTpResponse with a PDF when successful
HTTpResponseRedirect when not successful
Raises:
raises Http404 exception if anything goes wrong"""
from koalixcrm.crm.documents.contract import Contract
try:
new_document = requested_document_type()
new_document.create_from_reference(calling_model)
calling_model_admin.message_user(request, _(str(new_document) +
" created"))
response = HttpResponseRedirect('/admin/crm/'+
new_document.__class__.__name__.lower()+
'/'+
str(new_document.id))
except (TemplateSetMissingInContract, TemplateMissingInTemplateSet) as e:
if isinstance(calling_model, Contract):
contract = calling_model
else:
contract = calling_model.contract
if isinstance(e, TemplateSetMissingInContract):
response = HttpResponseRedirect('/admin/crm/contract/'+
str(contract.id))
calling_model_admin.message_user(request, _("Missing Templateset "),
level=messages.ERROR)
elif isinstance(e, TemplateMissingInTemplateSet):
response = HttpResponseRedirect('/admin/djangoUserExtension/templateset/' +
str(contract.default_template_set.id))
calling_model_admin.message_user(request,
(_("Missing template for ")+
new_document.__class__.__name__),
level=messages.ERROR)
else:
raise Http404
return response
<file_sep>/documentation/source/code_documentation/project.rst
.. highlight:: rst
Project
-------
.. automodule:: koalixcrm.crm.reporting.project
:members:
<file_sep>/koalixcrm/crm/views/time_tracking.py
# -*- coding: utf-8 -*-
import datetime
from django.http import HttpResponseRedirect, Http404
from django.contrib import messages
from django.shortcuts import render
from django.utils.translation import ugettext as _
from django.template.context_processors import csrf
from django.contrib.auth.decorators import login_required
from koalixcrm.djangoUserExtension.models import UserExtension
from koalixcrm.crm.exceptions import ReportingPeriodNotFound
from koalixcrm.crm.exceptions import UserIsNoHumanResource
from koalixcrm.djangoUserExtension.exceptions import UserExtensionMissing, TooManyUserExtensionsAvailable
from koalixcrm.crm.views.range_selection_form import RangeSelectionForm
from koalixcrm.crm.views.work_entry_formset import BaseWorkEntryFormset
from koalixcrm.crm.reporting.human_resource import HumanResource
@login_required
def work_report(request):
try:
human_resource = HumanResource.objects.filter(user=UserExtension.get_user_extension(request.user))
if len(human_resource) == 0:
error_message = "User "+request.user.__str__()+" is not registered as human resource"
raise UserIsNoHumanResource(error_message)
if request.POST.get('post'):
if 'cancel' in request.POST:
return HttpResponseRedirect('/admin/')
elif 'save' in request.POST:
range_selection_form = RangeSelectionForm(request.POST)
if range_selection_form.is_valid():
formset = BaseWorkEntryFormset.load_formset(range_selection_form,
request)
if not formset.is_valid():
c = {'range_selection_form': range_selection_form,
'formset': formset}
c.update(csrf(request))
return render(request, 'crm/admin/time_reporting.html', c)
else:
for form in formset:
form.update_work(request)
messages.success(request, _('you have successfully updated your work'))
formset = BaseWorkEntryFormset.create_updated_formset(range_selection_form,
human_resource)
range_selection_form.update_from_input()
c = {'range_selection_form': range_selection_form,
'formset': formset}
c.update(csrf(request))
return render(request, 'crm/admin/time_reporting.html', c)
return HttpResponseRedirect('/admin/')
else:
datetime_now = datetime.datetime.today()
to_date = (datetime_now + datetime.timedelta(days=30)).date()
from_date = datetime_now.date()
range_selection_form = RangeSelectionForm.create_range_selection_form(from_date, to_date)
formset = BaseWorkEntryFormset.create_new_formset(from_date,
to_date,
human_resource)
c = {'formset': formset,
'range_selection_form': range_selection_form}
c.update(csrf(request))
return render(request, 'crm/admin/time_reporting.html', c)
except (UserExtensionMissing,
ReportingPeriodNotFound,
TooManyUserExtensionsAvailable,
UserIsNoHumanResource) as e:
if isinstance(e, UserExtensionMissing):
return HttpResponseRedirect(e.view)
elif isinstance(e, ReportingPeriodNotFound):
return HttpResponseRedirect(e.view)
elif isinstance(e, TooManyUserExtensionsAvailable):
return HttpResponseRedirect(e.view)
elif isinstance(e, UserIsNoHumanResource):
return HttpResponseRedirect(e.view)
else:
raise Http404
<file_sep>/koalixcrm/crm/factories/factory_resource_price.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import ResourcePrice
from koalixcrm.crm.factories.factory_resource import StandardResourceFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardResourcePriceFactory(factory.django.DjangoModelFactory):
class Meta:
model = ResourcePrice
resource = factory.SubFactory(StandardResourceFactory)
unit = factory.SubFactory(StandardUnitFactory)
currency = factory.SubFactory(StandardCurrencyFactory)
customer_group = factory.SubFactory(StandardCustomerGroupFactory)
price = "100.50"
valid_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
valid_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
class HighResourcePriceFactory(factory.django.DjangoModelFactory):
class Meta:
model = ResourcePrice
resource = factory.SubFactory(StandardResourceFactory)
unit = factory.SubFactory(StandardUnitFactory)
currency = factory.SubFactory(StandardCurrencyFactory)
customer_group = factory.SubFactory(StandardCustomerGroupFactory)
price = "250.50"
valid_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
valid_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
<file_sep>/koalixcrm/crm/models.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.contact.contact import *
from koalixcrm.crm.contact.customer_group import *
from koalixcrm.crm.contact.customer import *
from koalixcrm.crm.contact.postal_address import *
from koalixcrm.crm.contact.customer_billing_cycle import *
from koalixcrm.crm.contact.email_address import *
from koalixcrm.crm.contact.phone_address import *
from koalixcrm.crm.contact.supplier import *
from koalixcrm.crm.documents.contract import Contract, PostalAddressForContract
from koalixcrm.crm.documents.contract import PhoneAddressForContract, EmailAddressForContract
from koalixcrm.crm.documents.sales_document_position import Position, SalesDocumentPosition
from koalixcrm.crm.documents.sales_document import SalesDocument, PostalAddressForSalesDocument
from koalixcrm.crm.documents.sales_document import EmailAddressForSalesDocument, PhoneAddressForSalesDocument
from koalixcrm.crm.documents.sales_document import TextParagraphInSalesDocument
from koalixcrm.crm.documents.invoice import Invoice
from koalixcrm.crm.documents.purchase_confirmation import PurchaseConfirmation
from koalixcrm.crm.documents.purchase_order import PurchaseOrder
from koalixcrm.crm.documents.quote import Quote
from koalixcrm.crm.documents.payment_reminder import PaymentReminder
from koalixcrm.crm.documents.delivery_note import DeliveryNote
from koalixcrm.crm.product.currency import *
from koalixcrm.crm.product.price import *
from koalixcrm.crm.product.product import *
from koalixcrm.crm.product.product_type import *
from koalixcrm.crm.product.product_price import *
from koalixcrm.crm.product.customer_group_transform import *
from koalixcrm.crm.product.unit_transform import *
from koalixcrm.crm.product.tax import *
from koalixcrm.crm.product.unit import *
from koalixcrm.crm.reporting.agreement import *
from koalixcrm.crm.reporting.agreement_status import *
from koalixcrm.crm.reporting.agreement_type import *
from koalixcrm.crm.reporting.agreement_status import *
from koalixcrm.crm.reporting.estimation import *
from koalixcrm.crm.reporting.estimation_status import *
from koalixcrm.crm.reporting.human_resource import *
from koalixcrm.crm.reporting.resource_manager import *
from koalixcrm.crm.reporting.resource_type import *
from koalixcrm.crm.reporting.resource_price import *
from koalixcrm.crm.reporting.generic_task_link import *
from koalixcrm.crm.reporting.task import *
from koalixcrm.crm.reporting.task_link_type import *
from koalixcrm.crm.reporting.task_status import *
from koalixcrm.crm.reporting.work import *
from koalixcrm.crm.reporting.project import *
from koalixcrm.crm.reporting.project_link_type import *
from koalixcrm.crm.reporting.project_status import *
from koalixcrm.crm.reporting.generic_project_link import *
from koalixcrm.crm.reporting.reporting_period import *
from koalixcrm.crm.reporting.reporting_period_status import *
<file_sep>/koalixcrm/crm/views/reporting_period_missing.py
# -*- coding: utf-8 -*-
from django.http import HttpResponseRedirect, Http404
from django.shortcuts import render
from django.template.context_processors import csrf
from django.contrib.admin import helpers
from django.contrib.admin.widgets import *
from koalixcrm.djangoUserExtension.exceptions import TooManyUserExtensionsAvailable
class ReportingPeriodMissingForm(forms.Form):
NEXT_STEPS = (
('add_reporting_period', 'Add Reporting Period'),
('return_to_start', 'Return To Start'),
)
next_steps = forms.ChoiceField(required=True,
widget=forms.Select,
choices=NEXT_STEPS)
def reporting_period_missing(request):
try:
if request.POST.get('post'):
if 'confirm_selection' in request.POST:
reporting_period_missing_form = ReportingPeriodMissingForm(request.POST)
if reporting_period_missing_form.is_valid():
if reporting_period_missing_form.cleaned_data['next_steps'] == 'return_to_start':
return HttpResponseRedirect('/admin/')
else:
return HttpResponseRedirect('/admin/crm/reportingperiod/add/')
else:
reporting_period_missing_form = ReportingPeriodMissingForm(initial={'next_steps': 'create_user_extension'})
title = "User Extension Missing"
description = "The operation you have selected requires an existing 'Reporting Period' element to exist" \
"for the project. For one of the required project this 'Reporting Period' did not exist" \
"Please choose one of the available options and proceed with the intended " \
"operation afterwards"
c = {'action_checkbox_name': helpers.ACTION_CHECKBOX_NAME,
'form': reporting_period_missing_form,
'description': description,
'title': title}
c.update(csrf(request))
return render(request, 'crm/admin/exception.html', c)
except TooManyUserExtensionsAvailable:
raise Http404
<file_sep>/koalixcrm/crm/factories/factory_agreement_type.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import AgreementType
class StandardAgreementTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = AgreementType
title = 'This is a test Agreement Type'
description = "This is a description of such a Agreement Type"
<file_sep>/koalixcrm/accounting/migrations/0001_initial.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.2 on 2017-07-05 17:02
from __future__ import unicode_literals
import django.db.models.deletion
from django.db import migrations, models
class Migration(migrations.Migration):
initial = True
dependencies = [
]
operations = [
migrations.CreateModel(
name='Account',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('accountNumber', models.IntegerField(verbose_name='Account Number')),
('title', models.CharField(max_length=50, verbose_name='Account Title')),
('accountType', models.CharField(
choices=[('E', 'Earnings'), ('S', 'Spendings'), ('L', 'Liabilities'), ('A', 'Assets')],
max_length=1, verbose_name='Account Type')),
('description', models.TextField(blank=True, null=True, verbose_name='Description')),
('originalAmount',
models.DecimalField(decimal_places=2, default=0.0, max_digits=20, verbose_name='Original Amount')),
('isopenreliabilitiesaccount', models.BooleanField(verbose_name='Is The Open Liabilities Account')),
('isopeninterestaccount', models.BooleanField(verbose_name='Is The Open Interests Account')),
('isProductInventoryActiva', models.BooleanField(verbose_name='Is a Product Inventory Account')),
('isACustomerPaymentAccount', models.BooleanField(verbose_name='Is a Customer Payment Account')),
],
options={
'ordering': ['accountNumber'],
'verbose_name': 'Account',
'verbose_name_plural': 'Account',
},
),
migrations.CreateModel(
name='AccountingPeriod',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=200, verbose_name='Title')),
('begin', models.DateField(verbose_name='Begin')),
('end', models.DateField(verbose_name='End')),
],
options={
'verbose_name': 'Accounting Period',
'verbose_name_plural': 'Accounting Periods',
},
),
migrations.CreateModel(
name='Booking',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('amount', models.DecimalField(decimal_places=2, max_digits=20, verbose_name='Amount')),
('description', models.CharField(blank=True, max_length=120, null=True, verbose_name='Description')),
('bookingDate', models.DateTimeField(verbose_name='Booking at')),
('dateofcreation', models.DateTimeField(auto_now=True, verbose_name='Created at')),
('lastmodification', models.DateTimeField(auto_now_add=True, verbose_name='Last modified')),
('accountingPeriod',
models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='accounting.AccountingPeriod',
verbose_name='AccountingPeriod')),
],
options={
'verbose_name': 'Booking',
'verbose_name_plural': 'Bookings',
},
),
migrations.CreateModel(
name='ProductCategorie',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=50, verbose_name='Product Categorie Title')),
('lossAccount',
models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_loss_account',
to='accounting.Account', verbose_name='Loss Account')),
('profitAccount',
models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_profit_account',
to='accounting.Account', verbose_name='Profit Account')),
],
options={
'verbose_name': 'Product Categorie',
'verbose_name_plural': 'Product Categories',
},
),
]
<file_sep>/koalixcrm/accounting/migrations/0004_auto_20171009_1949.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-10-09 19:49
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('accounting', '0003_remove_account_originalamount'),
]
operations = [
migrations.AlterModelOptions(
name='account',
options={'ordering': ['accountNumber'], 'verbose_name': 'Konto', 'verbose_name_plural': 'Konto'},
),
migrations.AlterModelOptions(
name='accountingperiod',
options={'verbose_name': 'Abrechnungszeitraum', 'verbose_name_plural': 'Abrechnungszeiträume'},
),
migrations.AlterModelOptions(
name='booking',
options={'verbose_name': 'Buchung', 'verbose_name_plural': 'Buchungen'},
),
migrations.AlterModelOptions(
name='productcategorie',
options={'verbose_name': 'Produktekategorie', 'verbose_name_plural': 'Produktekategorien'},
),
migrations.AlterField(
model_name='account',
name='accountNumber',
field=models.IntegerField(verbose_name='Kontonummer'),
),
migrations.AlterField(
model_name='account',
name='accountType',
field=models.CharField(choices=[('E', 'Ertrag (Einnahmen)'), ('S', 'Aufwand (Ausgaben)'), ('L', 'Passiva (Schulden)'), ('A', 'Aktiva (Vermögen)')], max_length=1, verbose_name='Kontotyp'),
),
migrations.AlterField(
model_name='account',
name='description',
field=models.TextField(blank=True, null=True, verbose_name='Beschreibung'),
),
migrations.AlterField(
model_name='account',
name='isACustomerPaymentAccount',
field=models.BooleanField(verbose_name='Konto für Kundeneinzahlungen'),
),
migrations.AlterField(
model_name='account',
name='isProductInventoryActiva',
field=models.BooleanField(verbose_name='Konto für Lager'),
),
migrations.AlterField(
model_name='account',
name='isopeninterestaccount',
field=models.BooleanField(verbose_name='Konto für offene Forderungen'),
),
migrations.AlterField(
model_name='account',
name='isopenreliabilitiesaccount',
field=models.BooleanField(verbose_name='Konto für offene Rechungen'),
),
migrations.AlterField(
model_name='account',
name='title',
field=models.CharField(max_length=50, verbose_name='Kontobezeichnung'),
),
migrations.AlterField(
model_name='accountingperiod',
name='begin',
field=models.DateField(verbose_name='Anfang'),
),
migrations.AlterField(
model_name='accountingperiod',
name='end',
field=models.DateField(verbose_name='Ende'),
),
migrations.AlterField(
model_name='accountingperiod',
name='title',
field=models.CharField(max_length=200, verbose_name='Titel'),
),
migrations.AlterField(
model_name='booking',
name='accountingPeriod',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='accounting.AccountingPeriod', verbose_name='Abrechnungszeitraum'),
),
migrations.AlterField(
model_name='booking',
name='amount',
field=models.DecimalField(decimal_places=2, max_digits=20, verbose_name='Betrag'),
),
migrations.AlterField(
model_name='booking',
name='bookingDate',
field=models.DateTimeField(verbose_name='Buchung am'),
),
migrations.AlterField(
model_name='booking',
name='bookingReference',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Invoice', verbose_name='Buchungsreferenz'),
),
migrations.AlterField(
model_name='booking',
name='dateofcreation',
field=models.DateTimeField(auto_now=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='booking',
name='description',
field=models.CharField(blank=True, max_length=120, null=True, verbose_name='Beschreibung'),
),
migrations.AlterField(
model_name='booking',
name='fromAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_fromaccount', to='accounting.Account', verbose_name='Vom Konto (Soll)'),
),
migrations.AlterField(
model_name='booking',
name='lastmodification',
field=models.DateTimeField(auto_now_add=True, verbose_name='Zuletzt geändert'),
),
migrations.AlterField(
model_name='booking',
name='lastmodifiedby',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_lstmodified', to=settings.AUTH_USER_MODEL, verbose_name='Zuletzt geändert durch'),
),
migrations.AlterField(
model_name='booking',
name='staff',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_refstaff', to=settings.AUTH_USER_MODEL, verbose_name='Zuständiger Mitarbeiter'),
),
migrations.AlterField(
model_name='booking',
name='toAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_toaccount', to='accounting.Account', verbose_name='Zu Konto (Haben)'),
),
migrations.AlterField(
model_name='productcategorie',
name='lossAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_loss_account', to='accounting.Account', verbose_name='Aufwand Konto'),
),
migrations.AlterField(
model_name='productcategorie',
name='profitAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_profit_account', to='accounting.Account', verbose_name='Ertrag Konto'),
),
migrations.AlterField(
model_name='productcategorie',
name='title',
field=models.CharField(max_length=50, verbose_name='Produktekategorie'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_tax.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Tax
class StandardTaxFactory(factory.django.DjangoModelFactory):
class Meta:
model = Tax
django_get_or_create = ('name',)
tax_rate = "7.7"
name = "Swiss MwSt 7.7%"
account_activa = None
account_passiva = None
<file_sep>/koalixcrm/crm/migrations/0049_auto_20181014_2258.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.db import migrations
def reverse_func(apps, schema_editor):
return 1
def backup_identifiers(apps, schema_editor):
Position = apps.get_model("crm", "Position")
CustomerGroupTransform = apps.get_model("crm", "CustomerGroupTransform")
Price = apps.get_model("crm", "Price")
UnitTransform = apps.get_model("crm", "UnitTransform")
db_alias = schema_editor.connection.alias
all_positions = Position.objects.using(db_alias).all()
for position in all_positions:
position.product_backup = position.product.id
position.save()
all_customer_group_transforms = CustomerGroupTransform.objects.using(db_alias).all()
for customer_group_transform in all_customer_group_transforms:
customer_group_transform.product_backup = customer_group_transform.product.id
customer_group_transform.save()
all_prices = Price.objects.using(db_alias).all()
for price in all_prices:
price.product_backup = price.product.id
price.save()
all_unit_transforms = UnitTransform.objects.using(db_alias).all()
for unit_transform in all_unit_transforms:
unit_transform.product_backup = unit_transform.product.id
unit_transform.save()
class Migration(migrations.Migration):
dependencies = [
('crm', '0048_auto_20181012_2056'),
]
operations = [
migrations.RunPython(backup_identifiers, reverse_func),
]
<file_sep>/projectsettings/dashboard.py
"""
This file was generated with the customdashboard management command and
contains the class for the main dashboard.
To activate your index dashboard add the following to your settings.py::
GRAPPELLI_INDEX_DASHBOARD = 'koalixcrm.dashboard.CustomIndexDashboard'
"""
from django.utils.translation import ugettext_lazy as _
from grappelli.dashboard import modules, Dashboard
from grappelli.dashboard.utils import get_admin_site_name
from koalixcrm.version import KOALIXCRM_VERSION
class CustomIndexDashboard(Dashboard):
"""
Custom index dashboard for www.
"""
def init_with_context(self, context):
self.children.append(modules.Group(
_('koalixcrm Version' + KOALIXCRM_VERSION),
column=1,
collapsible=True,
children=[
modules.ModelList(
_('Sales Documents and Contracts'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.documents.contract.Contract',
'koalixcrm.crm.documents.quote.Quote',
'koalixcrm.crm.documents.purchaseconfirmation.PurchaseConfirmation',
'koalixcrm.crm.documents.deliverynote.DeliveryNote',
'koalixcrm.crm.documents.invoice.Invoice',
'koalixcrm.crm.documents.paymentreminder.PaymentReminder',),
),
modules.ModelList(
_('Scheduler'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.contact.contact.CallForContact',
'koalixcrm.crm.contact.contact.VisitForContact',),
),
modules.ModelList(
_('Products'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.product.product.Product',),
),
modules.ModelList(
_('Contacts'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.contact.customer.Customer',
'koalixcrm.crm.contact.supplier.Supplier',
'koalixcrm.crm.contact.person.Person',),
),
modules.ModelList(
_('Accounting'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.accounting.*',),
),
modules.ModelList(
_('Projects'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.reporting.*',
'koalixcrm.crm.documents.purchaseorder.PurchaseOrder',),
),
modules.LinkList(
_('Report Work And Expenses'),
column=1,
children=[{'title': _('Time Tracking'),
'url': '/koalixcrm/crm/reporting/time_tracking/',
'external': False}]
)
]
))
# append a group for "Administration" & "Applications"
self.children.append(modules.Group(
_('Group: Administration & Applications'),
column=1,
collapsible=True,
children=[
modules.ModelList(
_('Administration'),
column=1,
collapsible=False,
models=('django.contrib.*',),
),
modules.ModelList(
_('koalixcrm Settings'),
column=1,
css_classes=('collapse closed',),
models=('koalixcrm.crm.contact.customerbillingcycle.CustomerBillingCycle',
'koalixcrm.crm.contact.customergroup.CustomerGroup',
'koalixcrm.crm.product.tax.Tax',
'koalixcrm.crm.product.unit.Unit',
'koalixcrm.crm.product.currency.Currency',
'koalixcrm.djangoUserExtension.*'),
),
]
))
# append another link list module for "support".
self.children.append(modules.LinkList(
_('Media Management'),
column=2,
children=[
{
'title': _('FileBrowser'),
'url': '/admin/filebrowser/browse/',
'external': False,
},
]
))
# append another link list module for "support".
self.children.append(modules.LinkList(
_('Support'),
column=2,
children=[
{
'title': _('koalixcrm on github'),
'url': 'https://github.com/scaphilo/koalixcrm/',
'external': True,
},
{
'title': _('Django Documentation'),
'url': 'http://docs.djangoproject.com/',
'external': True,
},
{
'title': _('Grappelli Documentation'),
'url': 'http://packages.python.org/django-grappelli/',
'external': True,
},
{
'title': _('Grappelli Google-Code'),
'url': 'http://code.google.com/p/django-grappelli/',
'external': True,
},
]
))
# append a feed module
self.children.append(modules.Feed(
_('Latest Django News'),
column=2,
feed_url='http://www.djangoproject.com/rss/weblog/',
limit=5
))
# append a recent actions module
self.children.append(modules.RecentActions(
_('Recent Actions'),
limit=5,
collapsible=False,
column=3,
))
<file_sep>/koalixcrm/crm/const/postaladdressprefix.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
POSTALADDRESSPREFIX = (
('F', _('Company')),
('W', _('Mrs')),
('H', _('Mr')),
('G', _('Ms')),
)
<file_sep>/koalixcrm/crm/migrations/0030_auto_20180611_1930.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-11 19:30
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
import django.utils.timezone
class Migration(migrations.Migration):
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('crm', '0029_auto_20180611_1903'),
]
operations = [
migrations.AddField(
model_name='generictasklink',
name='date_of_creation',
field=models.DateTimeField(auto_now_add=True, default=django.utils.timezone.now, verbose_name='Created at'),
preserve_default=False,
),
migrations.AddField(
model_name='generictasklink',
name='last_modified_by',
field=models.ForeignKey(default=2, on_delete=django.db.models.deletion.CASCADE, related_name='db_task_link_last_modified', to=settings.AUTH_USER_MODEL, verbose_name='Last modified by'),
preserve_default=False,
),
]
<file_sep>/koalixcrm/crm/factories/factory_reporting_period_status.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ReportingPeriodStatus
class InPreparationReportingPeriodStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = ReportingPeriodStatus
django_get_or_create = ('title',)
title = "In Preparation"
description = "In Preparation, reporting not yet enabled"
is_done = False
class ReportingReportingPeriodStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = ReportingPeriodStatus
django_get_or_create = ('title',)
title = "Reporting"
description = "Reporting is enabled"
is_done = False
class DoneReportingPeriodStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = ReportingPeriodStatus
django_get_or_create = ('title',)
title = "Done"
description = "This reporting period is done"
is_done = True
<file_sep>/koalixcrm/crm/migrations/0047_work_worked_hours.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-08-06 20:57
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0046_auto_20180805_2051'),
]
operations = [
migrations.AddField(
model_name='work',
name='worked_hours',
field=models.DecimalField(blank=True, decimal_places=2, max_digits=5, null=True, verbose_name='Worked Hours'),
),
]
<file_sep>/koalixcrm/crm/migrations/0018_auto_20180111_2031.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-11 20:31
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0017_auto_20180111_2022'),
]
operations = [
migrations.AlterModelOptions(
name='textparagraphinsalesdocument',
options={'verbose_name': 'Text Paragraph In Sales Document', 'verbose_name_plural': 'Text Paragraphs In Sales Documents'},
),
migrations.RenameField(
model_name='deliverynote',
old_name='salescontract_ptr',
new_name='salesdocument_ptr',
),
migrations.RenameField(
model_name='emailaddressforsalesdocument',
old_name='contract',
new_name='sales_document',
),
migrations.RenameField(
model_name='invoice',
old_name='salescontract_ptr',
new_name='salesdocument_ptr',
),
migrations.RenameField(
model_name='paymentreminder',
old_name='salescontract_ptr',
new_name='salesdocument_ptr',
),
migrations.RenameField(
model_name='phoneaddressforsalesdocument',
old_name='contract',
new_name='sales_document',
),
migrations.RenameField(
model_name='postaladdressforsalesdocument',
old_name='contract',
new_name='sales_document',
),
migrations.RenameField(
model_name='purchaseconfirmation',
old_name='salescontract_ptr',
new_name='salesdocument_ptr',
),
migrations.RenameField(
model_name='quote',
old_name='salescontract_ptr',
new_name='salesdocument_ptr',
),
migrations.RenameField(
model_name='salesdocumentposition',
old_name='contract',
new_name='sales_document',
),
migrations.RenameField(
model_name='textparagraphinsalesdocument',
old_name='sales_contract',
new_name='sales_document',
),
]
<file_sep>/koalixcrm/djangoUserExtension/user_extension/document_template.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from filebrowser.fields import FileBrowseField
from koalixcrm.djangoUserExtension.user_extension.text_paragraph import InlineTextParagraph
from koalixcrm.global_support_functions import xstr
from koalixcrm.crm.exceptions import *
class DocumentTemplate(models.Model):
title = models.CharField(verbose_name=_("Title"),
max_length=100,
blank=True,
null=True)
xsl_file = FileBrowseField(verbose_name=_("XSL File"),
max_length=200)
fop_config_file = FileBrowseField(verbose_name=_("FOP Configuration File"),
blank=True,
null=True,
max_length=200)
logo = FileBrowseField(verbose_name=_("Logo for the PDF generation"),
blank=True,
null=True,
max_length=200)
def get_fop_config_file(self):
if self.fop_config_file:
return self.fop_config_file
else:
raise TemplateFOPConfigFileMissing(_("Fop Config File missing in Document Template"+str(self)))
def get_xsl_file(self):
if self.xsl_file:
return self.xsl_file
else:
raise TemplateXSLTFileMissing(_("XSL Template missing in Document Template"+str(self)))
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Document template')
verbose_name_plural = _('Document templates')
def __str__(self):
return xstr(self.id) + ' ' + xstr(self.title.__str__())
class InvoiceTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Invoice template')
verbose_name_plural = _('Invoice templates')
class QuoteTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Quote template')
verbose_name_plural = _('Quote templates')
class DeliveryNoteTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Delivery note template')
verbose_name_plural = _('Delivery note templates')
class PaymentReminderTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Payment reminder template')
verbose_name_plural = _('Payment reminder templates')
class PurchaseOrderTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Purchase order template')
verbose_name_plural = _('Purchase order templates')
class PurchaseConfirmationTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Purchase confirmation template')
verbose_name_plural = _('Purchase confirmation templates')
class ProfitLossStatementTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Profit loss statement template')
verbose_name_plural = _('Profit loss statement templates')
class BalanceSheetTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Balance sheet template')
verbose_name_plural = _('Balance sheet templates')
class MonthlyProjectSummaryTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Monthly project summary template')
verbose_name_plural = _('Monthly project summary templates')
class WorkReportTemplate(DocumentTemplate):
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Work report template')
verbose_name_plural = _('Work report templates')
class OptionDocumentTemplate(admin.ModelAdmin):
list_display = ('id', 'title')
list_display_links = ('id', 'title')
ordering = ('id',)
search_fields = ('id', 'title')
fieldsets = (
(_('Basics'), {
'fields': ('title',
'xsl_file',
'fop_config_file',
'logo')
}),
)
inlines = [InlineTextParagraph]
<file_sep>/koalixcrm/djangoUserExtension/migrations/0003_auto_20171110_1732.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-11-10 17:32
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0002_auto_20171009_1949'),
]
operations = [
migrations.AlterModelOptions(
name='templateset',
options={'verbose_name': 'Templateset', 'verbose_name_plural': 'Templatesets'},
),
migrations.AlterModelOptions(
name='userextension',
options={'verbose_name': 'User Extention', 'verbose_name_plural': 'User Extentions'},
),
migrations.AlterModelOptions(
name='xslfile',
options={'verbose_name': 'XSL File', 'verbose_name_plural': 'XSL Files'},
),
migrations.AddField(
model_name='templateset',
name='reminder1XSLFile',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatepaymentreminder1', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Payment Reminder 1'),
),
migrations.AddField(
model_name='templateset',
name='reminder2XSLFile',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatepaymentreminder2', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Payment Reminder 2'),
),
migrations.AlterField(
model_name='templateset',
name='addresser',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Addresser'),
),
migrations.AlterField(
model_name='templateset',
name='balancesheetXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatebalancesheet', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Balancesheet'),
),
migrations.AlterField(
model_name='templateset',
name='bankingaccountref',
field=models.CharField(blank=True, max_length=60, null=True, verbose_name='Reference to Banking Account'),
),
migrations.AlterField(
model_name='templateset',
name='deilveryorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatedeliveryorder', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Deilvery Order'),
),
migrations.AlterField(
model_name='templateset',
name='footerTextpurchaseorders',
field=models.TextField(blank=True, null=True, verbose_name='Footer Text On Purchaseorders'),
),
migrations.AlterField(
model_name='templateset',
name='footerTextsalesorders',
field=models.TextField(blank=True, null=True, verbose_name='Footer Text On Salesorders'),
),
migrations.AlterField(
model_name='templateset',
name='fopConfigurationFile',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='FOP Configuration File'),
),
migrations.AlterField(
model_name='templateset',
name='headerTextpurchaseorders',
field=models.TextField(blank=True, null=True, verbose_name='Header Text On Purchaseorders'),
),
migrations.AlterField(
model_name='templateset',
name='headerTextsalesorders',
field=models.TextField(blank=True, null=True, verbose_name='Header Text On Salesorders'),
),
migrations.AlterField(
model_name='templateset',
name='invoiceXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplateinvoice', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Invoice'),
),
migrations.AlterField(
model_name='templateset',
name='logo',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='Logo for the PDF generation'),
),
migrations.AlterField(
model_name='templateset',
name='organisationname',
field=models.CharField(max_length=200, verbose_name='Name of the Organisation'),
),
migrations.AlterField(
model_name='templateset',
name='pagefooterleft',
field=models.CharField(blank=True, max_length=40, null=True, verbose_name='Page Footer Left'),
),
migrations.AlterField(
model_name='templateset',
name='pagefootermiddle',
field=models.CharField(blank=True, max_length=40, null=True, verbose_name='Page Footer Middle'),
),
migrations.AlterField(
model_name='templateset',
name='profitLossStatementXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplateprofitlossstatement', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Profit Loss Statement'),
),
migrations.AlterField(
model_name='templateset',
name='purchaseorderXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatepurchaseorder', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Purchaseorder'),
),
migrations.AlterField(
model_name='templateset',
name='quoteXSLFile',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_reltemplatequote', to='djangoUserExtension.XSLFile', verbose_name='XSL File for Quote'),
),
migrations.AlterField(
model_name='templateset',
name='title',
field=models.CharField(max_length=100, verbose_name='Title'),
),
migrations.AlterField(
model_name='userextensionemailaddress',
name='purpose',
field=models.CharField(choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')], max_length=1, verbose_name='Purpose'),
),
migrations.AlterField(
model_name='userextensionphoneaddress',
name='purpose',
field=models.CharField(choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')], max_length=1, verbose_name='Purpose'),
),
migrations.AlterField(
model_name='userextensionpostaladdress',
name='purpose',
field=models.CharField(choices=[('H', 'Private'), ('O', 'Business'), ('P', 'Mobile Private'), ('B', 'Mobile Business')], max_length=1, verbose_name='Purpose'),
),
migrations.AlterField(
model_name='xslfile',
name='title',
field=models.CharField(blank=True, max_length=100, null=True, verbose_name='Title'),
),
migrations.AlterField(
model_name='xslfile',
name='xslfile',
field=filebrowser.fields.FileBrowseField(max_length=200, verbose_name='XSL File'),
),
]
<file_sep>/koalixcrm/djangoUserExtension/factories/factory_document_template.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.djangoUserExtension.models import QuoteTemplate
from filebrowser.base import FileObject
class StandardQuoteTemplateFactory(factory.django.DjangoModelFactory):
class Meta:
model = QuoteTemplate
title = "This is a test Quote Template"
xsl_file = FileObject("~/path/to/xsl_file.xsl")
fop_config_file = FileObject("~/path/to/fop_config_file.xml")
logo = FileObject("~/path/to/logo_file.jpg")
<file_sep>/koalixcrm/crm/contact/phone_address.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
class PhoneAddress(models.Model):
phone = models.CharField(max_length=20,
verbose_name=_("Phone Number"))
class Meta:
app_label = "crm"
verbose_name = _('Phone Address')
verbose_name_plural = _('Phone Address')
<file_sep>/pytest.ini
[pytest]
DJANGO_SETTINGS_MODULE=projectsettings.settings.development_sqlite_settings
<file_sep>/koalixcrm/crm/migrations/0024_auto_20180122_2121.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-22 21:21
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0023_auto_20180119_2102'),
]
operations = [
migrations.AlterModelOptions(
name='position',
options={'ordering': ['position_number'], 'verbose_name': 'Position', 'verbose_name_plural': 'Positions'},
),
migrations.AddField(
model_name='salesdocument',
name='custom_date_field',
field=models.DateTimeField(blank=True, null=True, verbose_name='Custom Date/Time'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_postal_address.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import PostalAddress
class StandardPostalAddressFactory(factory.django.DjangoModelFactory):
class Meta:
model = PostalAddress
prefix = "M"
name = "Smith"
pre_name = "John"
address_line_1 = "Main-street 5"
address_line_2 = None
address_line_3 = None
address_line_4 = None
zip_code = 8000
town = "Zürich"
state = "ZH"
country = "CH"
<file_sep>/koalixcrm/djangoUserExtension/user_extension/user_extension.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.contact.postal_address import PostalAddress
from koalixcrm.crm.contact.phone_address import PhoneAddress
from koalixcrm.crm.contact.email_address import EmailAddress
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.djangoUserExtension.const.purpose import *
from koalixcrm.djangoUserExtension.exceptions import *
from koalixcrm.global_support_functions import xstr
class UserExtension(models.Model):
user = models.ForeignKey("auth.User",
blank=False,
null=False)
default_template_set = models.ForeignKey("TemplateSet")
default_currency = models.ForeignKey("crm.Currency")
@staticmethod
def objects_to_serialize(object_to_create_pdf, reference_user):
from koalixcrm.crm.contact.phone_address import PhoneAddress
from koalixcrm.crm.contact.email_address import EmailAddress
from django.contrib import auth
objects = list(auth.models.User.objects.filter(id=reference_user.id))
user_extension = UserExtension.objects.filter(user=reference_user.id)
if len(user_extension) == 0:
raise UserExtensionMissing(_("During "+str(object_to_create_pdf)+" PDF Export"))
phone_address = UserExtensionPhoneAddress.objects.filter(
userExtension=user_extension[0].id)
if len(phone_address) == 0:
raise UserExtensionPhoneAddressMissing(_("During "+str(object_to_create_pdf)+" PDF Export"))
email_address = UserExtensionEmailAddress.objects.filter(
userExtension=user_extension[0].id)
if len(email_address) == 0:
raise UserExtensionEmailAddressMissing(_("During "+str(object_to_create_pdf)+" PDF Export"))
objects += list(user_extension)
objects += list(EmailAddress.objects.filter(id=email_address[0].id))
objects += list(PhoneAddress.objects.filter(id=phone_address[0].id))
return objects
@staticmethod
def get_user_extension(django_user):
user_extensions = UserExtension.objects.filter(user=django_user)
if len(user_extensions) > 1:
raise TooManyUserExtensionsAvailable(_("More than one User Extension define for user ") + django_user.__str__())
elif len(user_extensions) == 0:
raise UserExtensionMissing(_("No User Extension define for user ") + django_user.__str__())
return user_extensions[0]
def get_template_set(self, template_set):
if template_set == self.default_template_set.work_report_template:
if self.default_template_set.work_report_template:
return self.default_template_set.work_report_template
else:
raise TemplateSetMissingForUserExtension((_("Template Set for work report " +
"is missing for User Extension" + str(self))))
def get_fop_config_file(self, template_set):
template_set = self.get_template_set(template_set)
return template_set.get_fop_config_file()
def get_xsl_file(self, template_set):
template_set = self.get_template_set(template_set)
return template_set.get_xsl_file()
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('User Extension')
verbose_name_plural = _('User Extension')
def __str__(self):
return xstr(self.id) + ' ' + xstr(self.user.__str__())
class UserExtensionPostalAddress(PostalAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINUSEREXTENTION)
userExtension = models.ForeignKey(UserExtension)
def __str__(self):
return xstr(self.name) + ' ' + xstr(self.pre_name)
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Postal Address for User Extension')
verbose_name_plural = _('Postal Address for User Extension')
class UserExtensionPhoneAddress(PhoneAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINUSEREXTENTION)
userExtension = models.ForeignKey(UserExtension)
def __str__(self):
return xstr(self.phone)
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Phone number for User Extension')
verbose_name_plural = _('Phone number for User Extension')
class UserExtensionEmailAddress(EmailAddress):
purpose = models.CharField(verbose_name=_("Purpose"), max_length=1, choices=PURPOSESADDRESSINUSEREXTENTION)
userExtension = models.ForeignKey(UserExtension)
def __str__(self):
return xstr(self.email)
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Email Address for User Extension')
verbose_name_plural = _('Email Address for User Extension')
class InlineUserExtensionPostalAddress(admin.StackedInline):
model = UserExtensionPostalAddress
extra = 1
classes = ('collapse-open',)
fieldsets = (
(_('Basics'), {
'fields': (
'prefix',
'pre_name',
'name',
'address_line_1',
'address_line_2',
'address_line_3',
'address_line_4',
'zip_code',
'town',
'state',
'country',
'purpose')
}),
)
allow_add = True
class InlineUserExtensionPhoneAddress(admin.StackedInline):
model = UserExtensionPhoneAddress
extra = 1
classes = ('collapse-open',)
fieldsets = (
(_('Basics'), {
'fields': ('phone',
'purpose',)
}),
)
allow_add = True
class InlineUserExtensionEmailAddress(admin.StackedInline):
model = UserExtensionEmailAddress
extra = 1
classes = ('collapse-open',)
fieldsets = (
(_('Basics'), {
'fields': ('email',
'purpose',)
}),
)
allow_add = True
class OptionUserExtension(admin.ModelAdmin):
list_display = ('id',
'user',
'default_template_set',
'default_currency')
list_display_links = ('id',
'user')
list_filter = ('user',
'default_template_set',)
ordering = ('id',)
search_fields = ('id',
'user')
fieldsets = (
(_('Basics'), {
'fields': ('user',
'default_template_set',
'default_currency')
}),
)
def create_work_report_pdf(self, request, queryset):
from koalixcrm.crm.views.create_work_report import create_work_report
return create_work_report(self, request, queryset)
create_work_report_pdf.short_description = _("Work Report PDF")
save_as = True
actions = [create_work_report_pdf]
inlines = [InlineUserExtensionPostalAddress,
InlineUserExtensionPhoneAddress,
InlineUserExtensionEmailAddress]
<file_sep>/README.md
# Welcome to the koalixcrm
## Why koalixcrm
<table><tr><th>Values:</th><th>Features:</th></tr>
<tr><td><ul>
<li><b>Free and open</b></li>
<li>REST interface to many entities </li>
<li>Open source </li>
<li>BSD license </li>
<li>Simple and beautiful user interface </li>
<li>High quality output documents </li>
<li>Small business <10 employees with access </li>
<li>Cloud hosted, Self-hosted, Not hosted (single-user, offline)</li></ul></td>
<td><ul>
<li> Manage Contacts, Leads, Persons</li>
<li> Manage Products and Prices</li>
<li> Manage Documents such as Invoices, Quotes, Purchase Orders, ...</li>
<li> Manage Projects, Tasks, Work (Traditional project management)</li>
<li> Manage Document Tempaltes</li>
<li>Double Entry Accounting</li>
<li> Create Project Reports</li>
<li> Adjust Access Rights </li></ul></td>
</tr></table>
## Quality badges on master
| Project build: | Codacy results: |Docker: | Social Networks: |
| --- | --- | --- | --- |
| [](https://travis-ci.org/scaphilo/koalixcrm) | [](https://www.codacy.com/app/simon.riedener/koalixcrm?utm_source=github.com&utm_medium=referral&utm_content=scaphilo/koalixcrm&utm_campaign=badger) [](https://www.codacy.com/app/simon.riedener/koalixcrm?utm_source=github.com&utm_medium=referral&utm_content=scaphilo/koalixcrm&utm_campaign=Badge_Coverage) | []() []()<br/> []() []() | [](https://gitter.im/koalix-crm/Lobby) |
## Demos
The demo-branch is automatically deployed and can be found here:
[Demo-Deployment](https://demo.koalixcrm.koalix.net/admin/).
To be able to log in use the following credentials
<ul>
<li><b>user: guest</b></li>
<li><b>password: <PASSWORD></b></li>
</ul>
## Documentation
You can find the documentation of koalixcrm here: [doc](http://readthedocs.org/docs/koalixcrm/en/master/)
## Installation
Some information about the installation of koalixcrm: [installation](https://github.com/scaphilo/koalixcrm/wiki/Installation)
## Development environment setup
Information about the development environment setup for this and similar projects can be found here: [Development environment setup](https://github.com/scaphilo/koalixcrm/wiki/Development-Environment-Setup)
## Release Process
Information about the release process: [Release Process](https://github.com/scaphilo/koalixcrm/wiki/Release-Process)
## Update from version 1.11 to 1.12
Some information about the update procedure from Version 1.11 to Version 1.12: [update](https://github.com/scaphilo/koalixcrm/wiki/Update)
<file_sep>/koalixcrm/accounting/const/accountTypeChoices.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
ACCOUNTTYPECHOICES = (
('E', _('Earnings')),
('S', _('Spendings')),
('L', _('Liabilities')),
('A', _('Assets')),
)
<file_sep>/koalixcrm/accounting/accounting/account.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from django import forms
from koalixcrm.accounting.const.accountTypeChoices import *
from koalixcrm.accounting.exceptions import AccountingPeriodNotFound
from koalixcrm.crm.documents.pdf_export import PDFExport
class Account(models.Model):
account_number = models.IntegerField(verbose_name=_("Account Number"))
title = models.CharField(verbose_name=_("Account Title"),
max_length=50)
account_type = models.CharField(verbose_name=_("Account Type"),
max_length=1,
choices=ACCOUNTTYPECHOICES)
description = models.TextField(verbose_name=_("Description"), null=True, blank=True)
is_open_reliabilities_account = models.BooleanField(verbose_name=_("Is The Open Liabilities Account"))
is_open_interest_account = models.BooleanField(verbose_name=_("Is The Open Interests Account"))
is_product_inventory_activa = models.BooleanField(verbose_name=_("Is a Product Inventory Account"))
is_a_customer_payment_account = models.BooleanField(verbose_name=_("Is a Customer Payment Account"))
def sum_of_all_bookings(self):
calculated_sum = self.all_bookings(from_account=False) - self.all_bookings(from_account=True)
if self.account_type == 'E' or self.account_type == 'L':
calculated_sum = 0 - calculated_sum
return calculated_sum
sum_of_all_bookings.short_description = _("Value")
def sum_of_all_bookings_within_accounting_period(self, accounting_period):
calculated_sum = self.all_bookings_within_accounting_period(from_account=False,
accounting_period=accounting_period) - \
self.all_bookings_within_accounting_period(from_account=True,
accounting_period=accounting_period)
if self.account_type == 'E' or self.account_type == 'L':
calculated_sum = -calculated_sum
return calculated_sum
def sum_of_all_bookings_before_accounting_period(self, current_accounting_period):
try:
accounting_periods = current_accounting_period.get_all_prior_accounting_periods()
except AccountingPeriodNotFound:
return 0
sum_of_all_bookings = 0
for accounting_period in accounting_periods:
sum_of_all_bookings += self.all_bookings_within_accounting_period(from_account=False,
accounting_period=accounting_period) - self.all_bookings_within_accounting_period(
from_account=True, accounting_period=accounting_period)
if self.account_type == 'E' or self.account_type == 'L':
sum_of_all_bookings = -sum_of_all_bookings
return sum_of_all_bookings
def sum_of_all_bookings_through_now(self, current_accounting_period):
within_accounting_period = self.sum_of_all_bookings_within_accounting_period(current_accounting_period)
before_accounting_period = self.sum_of_all_bookings_before_accounting_period(current_accounting_period)
current_value = within_accounting_period + before_accounting_period
return current_value
def all_bookings(self, from_account):
from koalixcrm.accounting.models import Booking
sum_all = 0
if from_account:
bookings = Booking.objects.filter(from_account=self.id)
else:
bookings = Booking.objects.filter(to_account=self.id)
for booking in list(bookings):
sum_all += booking.amount
return sum_all
def all_bookings_within_accounting_period(self, from_account, accounting_period):
from koalixcrm.accounting.models import Booking
sum = 0
if from_account:
bookings = Booking.objects.filter(from_account=self.id, accounting_period=accounting_period.id)
else:
bookings = Booking.objects.filter(to_account=self.id, accounting_period=accounting_period.id)
for booking in list(bookings):
sum += booking.amount
return sum
def serialize_to_xml(self, accounting_period):
objects = [self, ]
main_xml = PDFExport.write_xml(objects)
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>']",
"sum_of_all_bookings_within_accounting_period",
self.sum_of_all_bookings_within_accounting_period(accounting_period))
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>']",
"sum_of_all_bookings_through_now",
self.sum_of_all_bookings_through_now(accounting_period))
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>']",
"sum_of_all_bookings_before_accounting_period",
self.sum_of_all_bookings_before_accounting_period(accounting_period))
main_xml = PDFExport.append_element_to_pattern(main_xml,
"object/[<EMAIL>='<EMAIL>']",
"sum_of_all_bookings_through_now",
self.sum_of_all_bookings())
return main_xml
def __str__(self):
return self.account_number.__str__() + " " + self.title
class Meta:
app_label = "accounting"
verbose_name = _('Account')
verbose_name_plural = _('Account')
ordering = ['account_number']
class AccountForm(forms.ModelForm):
"""AccountForm is used to overwrite the clean method of the
original form and to add an additional checks to the model"""
class Meta:
model = Account
fields = '__all__'
def clean(self):
super(AccountForm, self).clean()
errors = []
if self.cleaned_data['is_open_reliabilities_account']:
open_reliabilities_account = Account.objects.filter(is_open_reliabilities_account=True)
if self.cleaned_data['account_type'] != "L":
errors.append(_('The open liabilities account must be a liabities account'))
elif open_reliabilities_account:
errors.append(_('There may only be one open liablities account in the system'))
if self.cleaned_data['is_open_interest_account']:
open_interest_account = Account.objects.filter(is_open_interest_account=True)
if self.cleaned_data['account_type'] != "A":
errors.append(_('The open interests account must be an asset account'))
elif open_interest_account:
errors.append(_('There may only be one open intrests account in the system'))
if self.cleaned_data['is_a_customer_payment_account']:
if self.cleaned_data['account_type'] != "A":
errors.append(_('A customer payment account must be an asset account'))
if self.cleaned_data['is_product_inventory_activa']:
if self.cleaned_data['account_type'] != "A":
errors.append(_('A product inventory account must be an asset account'))
if len(errors) > 0:
raise forms.ValidationError(errors)
return self.cleaned_data
class OptionAccount(admin.ModelAdmin):
list_display = ('account_number',
'account_type',
'title',
'sum_of_all_bookings')
list_display_links = ('account_number',
'account_type',
'title',
'sum_of_all_bookings')
fieldsets = ((_('Basic'),
{'fields': ('account_number',
'account_type',
'title',
'description',
'is_open_reliabilities_account',
'is_open_interest_account',
'is_product_inventory_activa',
'is_a_customer_payment_account')}),)
save_as = True
form = AccountForm
<file_sep>/koalixcrm/crm/migrations/0016_auto_20180111_2011.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-11 20:11
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0015_auto_20180111_2043'),
]
operations = [
migrations.RenameModel(
old_name='EmailAddressForSalesContract',
new_name='EmailAddressForSalesDocument'
),
migrations.RenameModel(
old_name='PhoneAddressForSalesContract',
new_name='PhoneAddressForSalesDocument',
),
migrations.RenameModel(
old_name='PostalAddressForSalesContract',
new_name='PostalAddressForSalesDocument',
),
migrations.RenameModel(
old_name='SalesContractPosition',
new_name='SalesDocumentPosition',
),
migrations.RenameModel(
old_name='TextParagraphInSalesContract',
new_name='TextParagraphInSalesDocument',
),
migrations.AddField(
model_name='salesdocument',
name='derived_from_sales_document',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.SalesDocument'),
),
]
<file_sep>/koalixcrm/crm/contact/customer_group.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class CustomerGroup(models.Model):
name = models.CharField(max_length=300)
def __str__(self):
return str(self.id) + ' ' + self.name
class Meta:
app_label = "crm"
verbose_name = _('Customer Group')
verbose_name_plural = _('Customer Groups')
class OptionCustomerGroup(admin.ModelAdmin):
list_display = ('id', 'name')
fieldsets = (('', {'fields': ('name',)}),)
allow_add = True<file_sep>/koalixcrm/crm/factories/factory_resource_manager.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ResourceManager
from koalixcrm.djangoUserExtension.factories.factory_user_extension import StandardUserExtensionFactory
class StandardResourceManagerFactory(factory.django.DjangoModelFactory):
class Meta:
model = ResourceManager
user = factory.SubFactory(StandardUserExtensionFactory)
<file_sep>/documentation/source/code_documentation/agreement.rst
.. highlight:: rst
Agreement
---------
.. automodule:: koalixcrm.crm.reporting.agreement
:members:
<file_sep>/koalixcrm/accounting/accounting/booking.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from django.db import models
from django.utils.translation import ugettext as _
class Booking(models.Model):
from_account = models.ForeignKey('Account', verbose_name=_("From Account"), related_name="db_booking_fromaccount")
to_account = models.ForeignKey('Account', verbose_name=_("To Account"), related_name="db_booking_toaccount")
amount = models.DecimalField(max_digits=20, decimal_places=2, verbose_name=_("Amount"))
description = models.CharField(verbose_name=_("Description"), max_length=120, null=True, blank=True)
booking_reference = models.ForeignKey('crm.Invoice', verbose_name=_("Booking Reference"), null=True, blank=True)
booking_date = models.DateTimeField(verbose_name=_("Booking at"))
accounting_period = models.ForeignKey('AccountingPeriod', verbose_name=_("AccountingPeriod"))
staff = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True}, blank=True,
verbose_name=_("Reference Staff"), related_name="db_booking_refstaff")
date_of_creation = models.DateTimeField(verbose_name=_("Created at"), auto_now=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"), auto_now_add=True)
last_modified_by = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True}, blank=True,
verbose_name=_("Last modified by"), related_name="db_booking_lstmodified")
def booking_date_only(self):
return self.booking_date.date()
booking_date_only.short_description = _("Date");
def __str__(self):
return self.from_account.__str__() + " " + self.to_account.__str__() + " " + self.amount.__str__()
class Meta:
app_label = "accounting"
verbose_name = _('Booking')
verbose_name_plural = _('Bookings')
class OptionBooking(admin.ModelAdmin):
list_display = ('from_account',
'to_account',
'amount',
'booking_date_only',
'staff')
fieldsets = ((_('Basic'), {'fields': ('from_account',
'to_account',
'amount',
'booking_date',
'staff',
'description',
'booking_reference',
'accounting_period')}),)
save_as = True
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
class InlineBookings(admin.TabularInline):
model = Booking
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('from_account',
'to_account',
'description',
'amount',
'booking_date',
'staff',
'booking_reference',)
}),
)
allow_add = False
<file_sep>/koalixcrm/crm/factories/factory_customer_billing_cycle.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import CustomerBillingCycle
class StandardCustomerBillingCycleFactory(factory.django.DjangoModelFactory):
class Meta:
model = CustomerBillingCycle
django_get_or_create = ('name',)
name = "This is a test billing cycle"
time_to_payment_date = 30
payment_reminder_time_to_payment = 20
<file_sep>/koalixcrm/crm/contact/contact.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.contact.phone_address import PhoneAddress
from koalixcrm.crm.contact.email_address import EmailAddress
from koalixcrm.crm.contact.postal_address import PostalAddress
from koalixcrm.crm.contact.call import Call
from koalixcrm.crm.contact.person import *
from koalixcrm.crm.const.purpose import *
from koalixcrm.global_support_functions import xstr
from koalixcrm.crm.inlinemixin import LimitedAdminInlineMixin
class Contact(models.Model):
name = models.CharField(max_length=300,
verbose_name=_("Name"))
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
last_modification = models.DateTimeField(verbose_name=_("Last modified"),
auto_now=True)
last_modified_by = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True},
blank=True,
verbose_name=_("Last modified by"),
editable=True)
class Meta:
app_label = "crm"
verbose_name = _('Contact')
verbose_name_plural = _('Contact')
def __str__(self):
return self.name
class PhoneAddressForContact(PhoneAddress):
purpose = models.CharField(verbose_name=_("Purpose"),
max_length=1,
choices=PURPOSESADDRESSINCUSTOMER)
person = models.ForeignKey(Contact)
class Meta:
app_label = "crm"
verbose_name = _('Phone Address For Contact')
verbose_name_plural = _('Phone Address For Contact')
def __str__(self):
return str(self.phone)
class EmailAddressForContact(EmailAddress):
purpose = models.CharField(verbose_name=_("Purpose"),
max_length=1,
choices=PURPOSESADDRESSINCUSTOMER)
person = models.ForeignKey(Contact)
class Meta:
app_label = "crm"
verbose_name = _('Email Address For Contact')
verbose_name_plural = _('Email Address For Contact')
def __str__(self):
return str(self.email)
class PostalAddressForContact(PostalAddress):
purpose = models.CharField(verbose_name=_("Purpose"),
max_length=1,
choices=PURPOSESADDRESSINCUSTOMER)
person = models.ForeignKey(Contact)
class Meta:
app_label = "crm"
verbose_name = _('Postal Address For Contact')
verbose_name_plural = _('Postal Address For Contact')
def __str__(self):
return xstr(self.pre_name) + ' ' + xstr(self.name) + ' ' + xstr(self.address_line_1)
class ContactPostalAddress(admin.StackedInline):
model = PostalAddressForContact
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('prefix',
'pre_name',
'name',
'address_line_1',
'address_line_2',
'address_line_3',
'address_line_4',
'zip_code',
'town',
'state',
'country',
'purpose')
}),
)
allow_add = True
class ContactPhoneAddress(admin.TabularInline):
model = PhoneAddressForContact
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('phone', 'purpose',)
}),
)
allow_add = True
class ContactEmailAddress(admin.TabularInline):
model = EmailAddressForContact
extra = 1
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': ('email', 'purpose',)
}),
)
allow_add = True
class ContactPersonAssociation(models.Model):
contact = models.ForeignKey(Contact, related_name='person_association', blank=True, null=True)
person = models.ForeignKey(Person, related_name='contact_association', blank=True, null=True)
class Meta:
app_label = "crm"
verbose_name = _('Contacts')
verbose_name_plural = _('Contacts')
def __str__(self):
return ''
class PeopleInlineAdmin(admin.TabularInline):
model = ContactPersonAssociation
extra = 0
show_change_link = True
class CompaniesInlineAdmin(admin.TabularInline):
model = ContactPersonAssociation
extra = 0
show_change_link = True
class OptionPerson(admin.ModelAdmin):
list_display = ('id',
'name',
'pre_name',
'email',
'role',
'get_companies',)
fieldsets = (('', {'fields': ('prefix',
'name',
'pre_name',
'role',
'email',
'phone',)}),)
allow_add = True
inlines = [CompaniesInlineAdmin]
def get_companies(self, obj):
items = []
for c in obj.companies.all():
items.append(c.name)
return ','.join(items)
get_companies.short_description = _("Works at")
class CallForContact(Call):
company = models.ForeignKey(Contact)
cperson = models.ForeignKey(Person, verbose_name=_("Person"),
blank=True,
null=True)
purpose = models.CharField(verbose_name=_("Purpose"),
max_length=1,
choices=PURPOSECALLINCUSTOMER)
class Meta:
app_label = "crm"
verbose_name = _('Call')
verbose_name_plural = _('Calls')
def __str__(self):
return xstr(self.description) + ' ' + xstr(self.date_due)
class VisitForContact(Call):
company = models.ForeignKey(Contact)
cperson = models.ForeignKey(Person,
verbose_name=_("Person"),
blank=True,
null=True)
purpose = models.CharField(verbose_name=_("Purpose"),
max_length=1,
choices=PURPOSEVISITINCUSTOMER)
ref_call = models.ForeignKey(CallForContact,
verbose_name=_("Reference Call"),
blank=True,
null=True)
class Meta:
app_label = "crm"
verbose_name = _('Visit')
verbose_name_plural = _('Visits')
def __str__(self):
return xstr(self.description) + ' ' + xstr(self.date_due)
class ContactCall(LimitedAdminInlineMixin, admin.StackedInline):
model = CallForContact
extra = 0
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': (
'description',
'date_due',
'purpose',
'status',
'cperson',)
}),
)
allow_add = True
def get_filters(self, request, obj):
return getattr(self, 'filters', ()) if obj is None else (('cperson', dict(companies=obj.id)),)
class ContactVisit(LimitedAdminInlineMixin, admin.StackedInline):
model = VisitForContact
extra = 0
classes = ['collapse']
fieldsets = (
('Basics', {
'fields': (
'description',
'date_due',
'purpose',
'status',
'cperson',
'ref_call',)
}),
)
allow_add = True
def get_filters(self, request, obj):
return getattr(self, 'filters', ()) if obj is None else (('cperson', dict(companies=obj.id)),('ref_call', dict(company=obj.id, status='S')))
class StateFilter(admin.SimpleListFilter):
title = _('State')
parameter_name = 'state'
def lookups(self, request, model_admin):
items = []
for a in PostalAddressForContact.objects.values('state').distinct():
if a['state']:
items.append((a['state'], _(a['state'])))
return (
items
)
def queryset(self, request, queryset):
if self.value():
matching_addresses = PostalAddressForContact.objects.filter(state=self.value())
ids = [a.person.id for a in matching_addresses]
return queryset.filter(pk__in=ids)
return queryset
class CityFilter(admin.SimpleListFilter):
title = _('City')
parameter_name = 'city'
def lookups(self, request, model_admin):
items = []
state = request.GET.get('state', None)
unique_list = PostalAddressForContact.objects.all().order_by('town')
adjusted_queryset = unique_list if state is None else unique_list.filter(state=state)
for a in adjusted_queryset.values('town').distinct():
if a['town']:
items.append((a['town'], _(a['town'])))
return (
items
)
def queryset(self, request, queryset):
if self.value():
matching_addresses = PostalAddressForContact.objects.filter(town=self.value())
ids = [(a.person.id) for a in matching_addresses]
return queryset.filter(pk__in=ids)
return queryset
<file_sep>/koalixcrm/accounting/migrations/0006_auto_20180422_2048.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-04-22 20:48
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
('accounting', '0005_auto_20171110_1732'),
]
operations = [
migrations.AlterModelOptions(
name='account',
options={'ordering': ['account_number'], 'verbose_name': 'Account', 'verbose_name_plural': 'Account'},
),
migrations.RenameField(
model_name='account',
old_name='accountNumber',
new_name='account_number',
),
migrations.RenameField(
model_name='account',
old_name='accountType',
new_name='account_type',
),
migrations.RenameField(
model_name='account',
old_name='isACustomerPaymentAccount',
new_name='is_a_customer_payment_account',
),
migrations.RenameField(
model_name='account',
old_name='isopeninterestaccount',
new_name='is_open_interest_account',
),
migrations.RenameField(
model_name='account',
old_name='isopenreliabilitiesaccount',
new_name='is_open_reliabilities_account',
),
migrations.RenameField(
model_name='account',
old_name='isProductInventoryActiva',
new_name='is_product_inventory_activa',
),
migrations.RenameField(
model_name='booking',
old_name='accountingPeriod',
new_name='accounting_period',
),
migrations.RenameField(
model_name='booking',
old_name='bookingDate',
new_name='booking_date',
),
migrations.RenameField(
model_name='booking',
old_name='bookingReference',
new_name='booking_reference',
),
migrations.RenameField(
model_name='booking',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='booking',
old_name='fromAccount',
new_name='from_account',
),
migrations.RenameField(
model_name='booking',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='booking',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='booking',
old_name='toAccount',
new_name='to_account',
),
migrations.RenameField(
model_name='productcategorie',
old_name='lossAccount',
new_name='loss_account',
),
migrations.RenameField(
model_name='productcategorie',
old_name='profitAccount',
new_name='profit_account',
),
migrations.AddField(
model_name='accountingperiod',
name='template_set',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.DocumentTemplate', verbose_name='Referred Template'),
),
]
<file_sep>/entrypoint.prod.sh
#!/bin/bash
# Install dependencies
pip install -r base_requirements.txt
# Install FOP 2.2
wget http://archive.apache.org/dist/xmlgraphics/fop/binaries/fop-2.2-bin.tar.gz
tar -xzf fop-2.2-bin.tar.gz -C ../usr/bin
rm -rf fop-2.2-bin.tar.gz
chmod 755 ../usr/bin/fop-2.2/fop
# Install Java 8
wget --no-check-certificate -c --header "Cookie: oraclelicense=accept-securebackup-cookie" http://download.oracle.com/otn-pub/java/jdk/8u162-b12/0da788060d494f5095bf8624735fa2f1/jdk-8u162-linux-x64.tar.gz
tar -xzf jdk-8u162-linux-x64.tar.gz -C ../usr/bin
rm -rf jdk-8u162-linux-x64.tar.gz
# Create /media/uploads/ directory which is required by django filebrowser
mkdir -p projectsettings/media/uploads
chmod -R 755 projectsettings/media
# Create /static/pdf for FOP PDF export
mkdir -p projectsettings/static/pdf
chmod -R 755 projectsettings/static/pdf
# Execute startup scripts
python manage.py collectstatic --noinput
python manage.py migrate
python manage.py runserver 0.0.0.0:8000<file_sep>/koalixcrm/crm/product/currency.py
# -*- coding: utf-8 -*-
from decimal import Decimal
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
class Currency(models.Model):
description = models.CharField(verbose_name=_("Description"),
max_length=100)
short_name = models.CharField(verbose_name=_("Displayed Name After Prices"),
max_length=3)
rounding = models.DecimalField(max_digits=5,
decimal_places=2,
verbose_name=_("Rounding"),
blank=True,
null=True)
def get_rounding(self):
"""Returns either the stored rounding value for a currency or a default rounding value of 0.05
Args: no arguments
Returns: Decimal value
Raises: should not return exceptions"""
if self.rounding is None:
return Decimal('0.05')
else:
return self.rounding
def round(self, value):
"""Rounds the input value to the rounding resolution which is defined in the variable "rounding"
Args: Decimal value value
Returns: Decimal value
Raises: should not return exceptions"""
rounded_value = int(value / self.get_rounding()) * self.get_rounding()
return rounded_value
def __str__(self):
return self.short_name
class Meta:
app_label = "crm"
verbose_name = _('Currency')
verbose_name_plural = _('Currency')
class OptionCurrency(admin.ModelAdmin):
list_display = ('id',
'description',
'short_name',
'rounding')
fieldsets = (('', {'fields': ('description',
'short_name',
'rounding')}),)
allow_add = True
<file_sep>/koalixcrm/crm/migrations/0038_auto_20180702_1628.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-07-02 16:28
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0037_auto_20180701_2128'),
]
operations = [
migrations.RemoveField(
model_name='postaladdress',
name='addressline1',
),
migrations.RemoveField(
model_name='postaladdress',
name='addressline2',
),
migrations.RemoveField(
model_name='postaladdress',
name='addressline3',
),
migrations.RemoveField(
model_name='postaladdress',
name='addressline4',
),
migrations.RemoveField(
model_name='postaladdress',
name='prename',
),
migrations.RemoveField(
model_name='postaladdress',
name='zipcode',
),
migrations.AddField(
model_name='postaladdress',
name='address_line_1',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Address line 1'),
),
migrations.AddField(
model_name='postaladdress',
name='address_line_2',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Address line 2'),
),
migrations.AddField(
model_name='postaladdress',
name='address_line_3',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Address line 3'),
),
migrations.AddField(
model_name='postaladdress',
name='address_line_4',
field=models.CharField(blank=True, max_length=200, null=True, verbose_name='Address line 4'),
),
migrations.AddField(
model_name='postaladdress',
name='pre_name',
field=models.CharField(blank=True, max_length=100, null=True, verbose_name='Pre-name'),
),
migrations.AddField(
model_name='postaladdress',
name='zip_code',
field=models.IntegerField(blank=True, null=True, verbose_name='Zip Code'),
),
]
<file_sep>/koalixcrm/crm/migrations/0017_auto_20180111_2022.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-11 20:22
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0016_auto_20180111_2011'),
]
operations = [
migrations.AlterModelOptions(
name='emailaddressforsalesdocument',
options={'verbose_name': 'Email Address For Contract', 'verbose_name_plural': 'Email Address For Contracts'},
),
migrations.AlterModelOptions(
name='phoneaddressforsalesdocument',
options={'verbose_name': 'Phone Address For Sales Contract', 'verbose_name_plural': 'Phone Address For Contracts'},
),
migrations.AlterModelOptions(
name='postaladdressforsalesdocument',
options={'verbose_name': 'Postal Address For Contract', 'verbose_name_plural': 'Postal Address For Contracts'},
),
migrations.AlterModelOptions(
name='salesdocument',
options={'verbose_name': 'Sales Document', 'verbose_name_plural': 'Sales Documents'},
),
migrations.AlterModelOptions(
name='salesdocumentposition',
options={'verbose_name': 'Position in Sales Document', 'verbose_name_plural': 'Positions Sales Document'},
),
migrations.AlterField(
model_name='position',
name='last_calculated_price',
field=models.DecimalField(blank=True, decimal_places=2, max_digits=17, null=True, verbose_name='Last Calculated Price'),
),
migrations.AlterField(
model_name='position',
name='last_calculated_tax',
field=models.DecimalField(blank=True, decimal_places=2, max_digits=17, null=True, verbose_name='Last Calculated Tax'),
),
]
<file_sep>/koalixcrm/crm/reporting/generic_task_link.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from django.contrib.contenttypes.fields import GenericForeignKey
from django.contrib.contenttypes.models import ContentType
from django.contrib import admin
class GenericTaskLink(models.Model):
task = models.ForeignKey("Task", verbose_name=_('Task'), blank=False, null=False)
task_link_type = models.ForeignKey("TaskLinkType", verbose_name=_('Task Link Type'), blank=True, null=True)
content_type = models.ForeignKey(ContentType, on_delete=models.CASCADE)
object_id = models.PositiveIntegerField()
generic_crm_object = GenericForeignKey('content_type', 'object_id')
date_of_creation = models.DateTimeField(verbose_name=_("Created at"), auto_now_add=True)
last_modified_by = models.ForeignKey('auth.User', limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"), related_name="db_task_link_last_modified")
def __str__(self):
return str(self.id) + " " + str(self.title)
class Meta:
app_label = "crm"
verbose_name = _('Task Link')
verbose_name_plural = _('Task Links')
class InlineGenericTaskLink(admin.TabularInline):
model = GenericTaskLink
readonly_fields = ('task_link_type', 'content_type', 'object_id', 'date_of_creation', 'last_modified_by')
extra = 0
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False<file_sep>/koalixcrm/crm/product/product.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
class Product(models.Model):
identifier = models.CharField(verbose_name=_("Product Number"),
max_length=200,
null=True,
blank=True)
product_type = models.ForeignKey("ProductType", verbose_name=_("Product Type"))
<file_sep>/koalixcrm/crm/reporting/reporting_period.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.crm.documents.pdf_export import PDFExport
from koalixcrm.crm.exceptions import ReportingPeriodNotFound
from koalixcrm.crm.reporting.work import WorkInlineAdminView
from rest_framework import serializers
from django.core.exceptions import ValidationError
from django.forms import ModelForm
class ReportingPeriod(models.Model):
"""The reporting period is referred in the work, in the expenses and purchase orders, it is used as a
supporting object to generate project reports"""
project = models.ForeignKey("Project",
verbose_name=_("Project"),
blank=False,
null=False)
title = models.CharField(max_length=200,
verbose_name=_("Title"),
blank=False,
null=False) # For example "March 2018", "1st Quarter 2019"
begin = models.DateField(verbose_name=_("Begin"),
blank=False,
null=False)
end = models.DateField(verbose_name=_("End"),
blank=False,
null=False)
status = models.ForeignKey("ReportingPeriodStatus",
verbose_name=_("Reporting Period Status"),
blank=True,
null=True)
@staticmethod
def get_reporting_period(project, search_date):
"""Returns the reporting period that is currently valid. Valid is a reporting period when the provided date
lies between begin and end of the reporting period
Args:
no arguments
Returns:
reporting_period (ReportPeriod)
Raises:
ReportPeriodNotFound when there is no valid reporting Period"""
current_valid_reporting_period = None
for reporting_period in ReportingPeriod.objects.filter(project=project):
if reporting_period.begin <= search_date <= reporting_period.end:
return reporting_period
if not current_valid_reporting_period:
raise ReportingPeriodNotFound("Reporting Period does not exist")
@staticmethod
def get_latest_reporting_period(project):
"""Returns the latest reporting period
Args:
no arguments
Returns:
reporting_period (ReportPeriod)
Raises:
ReportPeriodNotFound when there is no valid reporting Period"""
latest_reporting_period = None
reporting_periods = ReportingPeriod.objects.filter(project=project)
for reporting_period in reporting_periods:
if latest_reporting_period is None:
latest_reporting_period = reporting_period
else:
if latest_reporting_period.end <= reporting_period.begin:
latest_reporting_period = reporting_period
if not latest_reporting_period:
raise ReportingPeriodNotFound("Reporting Period does not exist")
return latest_reporting_period
@staticmethod
def get_predecessor(target_reporting_period, project):
"""Returns the reporting period which was valid right before the provided target_reporting_period
Args:
no arguments
Returns:
predecessor_reporting_period (ReportPeriod)
Raises:
ReportPeriodNotFound when there is no valid reporting Period"""
predecessor_reporting_period = None
reporting_periods = ReportingPeriod.objects.filter(project=project)
for reporting_period in reporting_periods:
if reporting_period.end <= target_reporting_period.begin and\
predecessor_reporting_period is None:
predecessor_reporting_period = reporting_period
elif reporting_period.end <= target_reporting_period.begin and\
reporting_period.end <= predecessor_reporting_period.begin:
predecessor_reporting_period = reporting_period
if predecessor_reporting_period is None:
raise ReportingPeriodNotFound("Reporting Period does not exist")
return predecessor_reporting_period
@staticmethod
def get_all_predecessors(target_reporting_period, project):
"""Returns all reporting periods which have been valid before the provided target_reporting_period
Args:
no arguments
Returns:
predecessor_reporting_periods[] (Array of ReportPeriod)
Raises:
ReportPeriodNotFound when there is no valid reporting Period"""
predecessor_reporting_periods = list()
reporting_periods = ReportingPeriod.objects.filter(project=project)
for reporting_period in reporting_periods:
if reporting_period.end <= target_reporting_period.begin:
predecessor_reporting_periods.append(reporting_period)
return predecessor_reporting_periods
def is_reporting_allowed(self):
"""Returns True when the reporting period is available for reporting,
Returns False when the reporting period is not available for reporting,
The decision whether the reporting period is available for reporting is purely depending
on the reporting_period_status. When the status is done, the reporting period is not longer
available for reporting. In all other cases the reporting is allowed.
Args:
no arguments
Returns:
allowed (Boolean)
Raises:
when there is no valid reporting Period"""
if self.status:
if self.status.is_done:
allowed = False
else:
allowed = True
else:
allowed = False
return allowed
def create_pdf(self, template_set, printed_by):
self.last_print_date = datetime.now()
self.save()
return PDFExport.create_pdf(self, template_set, printed_by)
def get_template_set(self):
return self.project.get_template_set()
def get_fop_config_file(self, template_set):
return self.project.get_fop_config_file(template_set=None)
def get_xsl_file(self, template_set):
return self.project.get_xsl_file(template_set=None)
def serialize_to_xml(self):
objects = [self, ]
main_xml = PDFExport.write_xml(objects)
project_xml = self.project.serialize_to_xml(reporting_period=self)
main_xml = PDFExport.merge_xml(main_xml, project_xml)
return main_xml
def __str__(self):
return str(self.id)+" "+self.title
class Meta:
app_label = "crm"
verbose_name = _('Reporting Period')
verbose_name_plural = _('Reporting Periods')
class ReportingPeriodAdminForm(ModelForm):
def clean(self):
"""Check that the begin of the new reporting period is not located within an existing
reporting period, Checks that the begin is date earlier than the end"""
cleaned_data = super().clean()
project = cleaned_data['project']
end = cleaned_data['end']
begin = cleaned_data['begin']
reporting_periods = ReportingPeriod.objects.filter(project=project)
for reporting_period in reporting_periods:
if reporting_period.pk != self.instance.pk:
if (begin < reporting_period.end) and (begin > reporting_period.begin):
raise ValidationError('The Reporting Period overlaps with an existing '
'Reporting Period within the same project')
if (end < reporting_period.end) and (end > reporting_period.begin):
raise ValidationError('The Reporting Period overlaps with an existing '
'Reporting Period within the same project')
if end < begin:
raise ValidationError('Begin date must be earlier than end date')
class ReportingPeriodAdmin(admin.ModelAdmin):
form = ReportingPeriodAdminForm
list_display = ('id',
'project',
'title',
'begin',
'end',
'status')
list_display_links = ('id',)
ordering = ('-id',)
fieldsets = (
(_('ReportingPeriod'), {
'fields': ('project',
'title',
'begin',
'end',
'status')
}),
)
inlines = [WorkInlineAdminView, ]
actions = ['create_report_pdf', ]
def save_model(self, request, obj, form, change):
if change:
obj.last_modified_by = request.user
else:
obj.last_modified_by = request.user
obj.staff = request.user
obj.save()
def create_report_pdf(self, request, queryset):
from koalixcrm.crm.views.pdfexport import PDFExportView
for obj in queryset:
response = PDFExportView.export_pdf(self,
request,
obj,
("/admin/crm/"+obj.__class__.__name__.lower()+"/"),
obj.project.default_template_set.monthly_project_summary_template)
return response
create_report_pdf.short_description = _("Create Report PDF")
class ReportingPeriodInlineAdminView(admin.TabularInline):
model = ReportingPeriod
fieldsets = (
(_('ReportingPeriod'), {
'fields': ('project',
'title',
'begin',
'end',
'status')
}),
)
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
class ProjectJSONSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = ReportingPeriod
fields = ('id',
'project',
'title',
'begin',
'end')<file_sep>/koalixcrm/crm/const/country.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
COUNTRIES = (
('AF', 'AFG', '004', _('Afghanistan')),
('AX', 'ALA', '248', _('Aland Islands')),
('AL', 'ALB', '008', _('Albania')),
('DZ', 'DZA', '012', _('Algeria')),
('AS', 'ASM', '016', _('American Samoa')),
('AD', 'AND', '020', _('Andorra')),
('AO', 'AGO', '024', _('Angola')),
('AI', 'AIA', '660', _('Anguilla')),
('AQ', 'ATA', '010', _('Antarctica')),
('AG', 'ATG', '028', _('Antigua and Barbuda')),
('AR', 'ARG', '032', _('Argentina')),
('AM', 'ARM', '051', _('Armenia')),
('AW', 'ABW', '533', _('Aruba')),
('AU', 'AUS', '036', _('Australia')),
('AT', 'AUT', '040', _('Austria')),
('AZ', 'AZE', '031', _('Azerbaijan')),
('BS', 'BHS', '044', _('the Bahamas')),
('BH', 'BHR', '048', _('Bahrain')),
('BD', 'BGD', '050', _('Bangladesh')),
('BB', 'BRB', '052', _('Barbados')),
('BY', 'BLR', '112', _('Belarus')),
('BE', 'BEL', '056', _('Belgium')),
('BZ', 'BLZ', '084', _('Belize')),
('BJ', 'BEN', '204', _('Benin')),
('BM', 'BMU', '060', _('Bermuda')),
('BT', 'BTN', '064', _('Bhutan')),
('BO', 'BOL', '068', _('Bolivia')),
('BA', 'BIH', '070', _('Bosnia and Herzegovina')),
('BW', 'BWA', '072', _('Botswana')),
('BV', 'BVT', '074', _('Bouvet Island')),
('BR', 'BRA', '076', _('Brazil')),
('IO', 'IOT', '086', _('British Indian Ocean Territory')),
('BN', 'BRN', '096', _('Brunei Darussalam')),
('BG', 'BGR', '100', _('Bulgaria')),
('BF', 'BFA', '854', _('Burkina Faso')),
('BI', 'BDI', '108', _('Burundi')),
('KH', 'KHM', '116', _('Cambodia')),
('CM', 'CMR', '120', _('Cameroon')),
('CA', 'CAN', '124', _('Canada')),
('CV', 'CPV', '132', _('Cape Verde')),
('KY', 'CYM', '136', _('Cayman Islands')),
('CF', 'CAF', '140', _('Central African Republic')),
('TD', 'TCD', '148', _('Chad')),
('CL', 'CHL', '152', _('Chile')),
('CN', 'CHN', '156', _('China')),
('CX', 'CXR', '162', _('Christmas Island')),
('CC', 'CCK', '166', _('Cocos (Keeling) Islands')),
('CO', 'COL', '170', _('Colombia')),
('KM', 'COM', '174', _('Comoros')),
('CG', 'COG', '178', _('Congo')),
('CD', 'COD', '180', _('Democratic Republic of the Congo')),
('CK', 'COK', '184', _('Cook Islands')),
('CR', 'CRI', '188', _('Costa Rica')),
('CI', 'CIV', '384', _('Cote d\'Ivoire')),
('HR', 'HRV', '191', _('Croatia')),
('CU', 'CUB', '192', _('Cuba')),
('CY', 'CYP', '196', _('Cyprus')),
('CZ', 'CZE', '203', _('Czech Republic')),
('DK', 'DNK', '208', _('Denmark')),
('DJ', 'DJI', '262', _('Djibouti')),
('DM', 'DMA', '212', _('Dominica')),
('DO', 'DOM', '214', _('Dominican Republic')),
('EC', 'ECU', '218', _('Ecuador')),
('EG', 'EGY', '818', _('Egypt')),
('SV', 'SLV', '222', _('El Salvador')),
('GQ', 'GNQ', '226', _('Equatorial Guinea')),
('ER', 'ERI', '232', _('Eritrea')),
('EE', 'EST', '233', _('Estonia')),
('ET', 'ETH', '231', _('Ethiopia')),
('FK', 'FLK', '238', _('Falkland Islands (Malvinas)')),
('FO', 'FRO', '234', _('Faroe Islands')),
('FJ', 'FJI', '242', _('Fiji')),
('FI', 'FIN', '246', _('Finland')),
('FR', 'FRA', '250', _('France')),
('GF', 'GUF', '254', _('French Guiana')),
('PF', 'PYF', '258', _('French Polynesia')),
('TF', 'ATF', '260', _('French Southern and Antarctic Lands')),
('GA', 'GAB', '266', _('Gabon')),
('GM', 'GMB', '270', _('Gambia')),
('GE', 'GEO', '268', _('Georgia')),
('DE', 'DEU', '276', _('Germany')),
('GH', 'GHA', '288', _('Ghana')),
('GI', 'GIB', '292', _('Gibraltar')),
('GR', 'GRC', '300', _('Greece')),
('GL', 'GRL', '304', _('Greenland')),
('GD', 'GRD', '308', _('Grenada')),
('GP', 'GLP', '312', _('Guadeloupe')),
('GU', 'GUM', '316', _('Guam')),
('GT', 'GTM', '320', _('Guatemala')),
('GG', 'GGY', '831', _('Guernsey')),
('GN', 'GIN', '324', _('Guinea')),
('GW', 'GNB', '624', _('Guinea-Bissau')),
('GY', 'GUY', '328', _('Guyana')),
('HT', 'HTI', '332', _('Haiti')),
('HM', 'HMD', '334', _('Heard Island and McDonald Islands')),
('VA', 'VAT', '336', _('Vatican City Holy See')),
('HN', 'HND', '340', _('Honduras')),
('HK', 'HKG', '344', _('Hong Kong')),
('HU', 'HUN', '348', _('Hungary')),
('IS', 'ISL', '352', _('Iceland')),
('IN', 'IND', '356', _('India')),
('ID', 'IDN', '360', _('Indonesia')),
('IR', 'IRN', '364', _('Iran')),
('IQ', 'IRQ', '368', _('Iraq')),
('IE', 'IRL', '372', _('Ireland')),
('IM', 'IMN', '833', _('Isle of Man')),
('IL', 'ISR', '376', _('Israel')),
('IT', 'ITA', '380', _('Italy')),
('JM', 'JAM', '388', _('Jamaica')),
('JP', 'JPN', '392', _('Japan')),
('JE', 'JEY', '832', _('Jersey')),
('JO', 'JOR', '400', _('Jordan')),
('KZ', 'KAZ', '398', _('Kazakhstan')),
('KE', 'KEN', '404', _('Kenya')),
('KI', 'KIR', '296', _('Kiribati')),
('KP', 'PRK', '408', _('North Korea')),
('KR', 'KOR', '410', _('South Korea')),
('KW', 'KWT', '414', _('Kuwait')),
('KG', 'KGZ', '417', _('Kyrgyzstan')),
('LA', 'LAO', '418', _('Laos Lao')),
('LV', 'LVA', '428', _('Latvia')),
('LB', 'LBN', '422', _('Lebanon')),
('LS', 'LSO', '426', _('Lesotho')),
('LR', 'LBR', '430', _('Liberia')),
('LY', 'LBY', '434', _('Libya Libyan Arab Jamahiriya')),
('LI', 'LIE', '438', _('Liechtenstein')),
('LT', 'LTU', '440', _('Lithuania')),
('LU', 'LUX', '442', _('Luxembourg')),
('MO', 'MAC', '446', _('Macau Macao')),
('MK', 'MKD', '807', _('Macedonia')),
('MG', 'MDG', '450', _('Madagascar')),
('MW', 'MWI', '454', _('Malawi')),
('MY', 'MYS', '458', _('Malaysia')),
('MV', 'MDV', '462', _('Maldives')),
('ML', 'MLI', '466', _('Mali')),
('MT', 'MLT', '470', _('Malta')),
('MH', 'MHL', '584', _('Marshall Islands')),
('MQ', 'MTQ', '474', _('Martinique')),
('MR', 'MRT', '478', _('Mauritania')),
('MU', 'MUS', '480', _('Mauritius')),
('YT', 'MYT', '175', _('Mayotte')),
('MX', 'MEX', '484', _('Mexico')),
('FM', 'FSM', '583', _('Micronesia')),
('MD', 'MDA', '498', _('Moldova')),
('MC', 'MCO', '492', _('Monaco')),
('MN', 'MNG', '496', _('Mongolia')),
('ME', 'MNE', '499', _('Montenegro')),
('MS', 'MSR', '500', _('Montserrat')),
('MA', 'MAR', '504', _('Morocco')),
('MZ', 'MOZ', '508', _('Mozambique')),
('MM', 'MMR', '104', _('Myanmar')),
('NA', 'NAM', '516', _('Namibia')),
('NR', 'NRU', '520', _('Nauru')),
('NP', 'NPL', '524', _('Nepal')),
('NL', 'NLD', '528', _('Netherlands')),
('AN', 'ANT', '530', _('Netherlands Antilles')),
('NC', 'NCL', '540', _('New Caledonia')),
('NZ', 'NZL', '554', _('New Zealand')),
('NI', 'NIC', '558', _('Nicaragua')),
('NE', 'NER', '562', _('Niger')),
('NG', 'NGA', '566', _('Nigeria')),
('NU', 'NIU', '570', _('Niue')),
('NF', 'NFK', '574', _('Norfolk Island Norfolk Island')),
('MP', 'MNP', '580', _('Northern Mariana Islands')),
('NO', 'NOR', '578', _('Norway')),
('OM', 'OMN', '512', _('Oman')),
('PK', 'PAK', '586', _('Pakistan')),
('PW', 'PLW', '585', _('Palau')),
('PS', 'PSE', '275', _('Palestinian Territory')),
('PA', 'PAN', '591', _('Panama')),
('PG', 'PNG', '598', _('Papua New Guinea')),
('PY', 'PRY', '600', _('Paraguay')),
('PE', 'PER', '604', _('Peru')),
('PH', 'PHL', '608', _('Philippines')),
('PN', 'PCN', '612', _('Pitcairn Islands')),
('PL', 'POL', '616', _('Poland')),
('PT', 'PRT', '620', _('Portugal')),
('PR', 'PRI', '630', _('Puerto Rico')),
('QA', 'QAT', '634', _('Qatar')),
('RE', 'REU', '638', _('Reunion')),
('RO', 'ROU', '642', _('Romania')),
('RU', 'RUS', '643', _('Russia')),
('RW', 'RWA', '646', _('Rwanda')),
('SH', 'SHN', '654', _('Saint Helena')),
('KN', 'KNA', '659', _('Saint Kitts and Nevis')),
('LC', 'LCA', '662', _('Saint Lucia')),
('PM', 'SPM', '666', _('Saint Pierre and Miquelon')),
('VC', 'VCT', '670', _('Saint Vincent and the Grenadines')),
('WS', 'WSM', '882', _('Samoa')),
('SM', 'SMR', '674', _('San Marino')),
('ST', 'STP', '678', _('Sao Tome and Principe')),
('SA', 'SAU', '682', _('Saudi Arabia')),
('SN', 'SEN', '686', _('Senegal')),
('RS', 'SRB', '688', _('Serbia')),
('SC', 'SYC', '690', _('Seychelles')),
('SL', 'SLE', '694', _('Sierra Leone')),
('SG', 'SGP', '702', _('Singapore')),
('SK', 'SVK', '703', _('Slovakia')),
('SI', 'SVN', '705', _('Slovenia')),
('SB', 'SLB', '090', _('Solomon Islands')),
('SO', 'SOM', '706', _('Somalia')),
('ZA', 'ZAF', '710', _('South Africa')),
('GS', 'SGS', '239', _('South Georgia and the South Sandwich Islands')),
('ES', 'ESP', '724', _('Spain')),
('LK', 'LKA', '144', _('Sri Lanka')),
('SD', 'SDN', '736', _('Sudan')),
('SR', 'SUR', '740', _('Suriname')),
('SJ', 'SJM', '744', _('Svalbard and Jan Mayen')),
('SZ', 'SWZ', '748', _('Swaziland')),
('SE', 'SWE', '752', _('Sweden')),
('CH', 'CHE', '756', _('Switzerland')),
('SY', 'SYR', '760', _('Syria')),
('TW', 'TWN', '158', _('Taiwan')),
('TJ', 'TJK', '762', _('Tajikistan')),
('TZ', 'TZA', '834', _('Tanzania')),
('TH', 'THA', '764', _('Thailand')),
('TL', 'TLS', '626', _('East Timor')),
('TG', 'TGO', '768', _('Togo')),
('TK', 'TKL', '772', _('Tokelau')),
('TO', 'TON', '776', _('Tonga')),
('TT', 'TTO', '780', _('Trinidad and Tobago')),
('TN', 'TUN', '788', _('Tunisia')),
('TR', 'TUR', '792', _('Turkey')),
('TM', 'TKM', '795', _('Turkmenistan')),
('TC', 'TCA', '796', _('Turks and Caicos Islands')),
('TV', 'TUV', '798', _('Tuvalu')),
('UG', 'UGA', '800', _('Uganda')),
('UA', 'UKR', '804', _('Ukraine')),
('AE', 'ARE', '784', _('United Arab Emirates')),
('GB', 'GBR', '826', _('United Kingdom')),
('US', 'USA', '840', _('United States')),
('UM', 'UMI', '581', _('United States Minor Outlying Islands')),
('UY', 'URY', '858', _('Uruguay')),
('UZ', 'UZB', '860', _('Uzbekistan')),
('VU', 'VUT', '548', _('Vanuatu')),
('VE', 'VEN', '862', _('Venezuela')),
('VN', 'VNM', '704', _('Vietnam Viet Nam')),
('VG', 'VGB', '092', _('British Virgin Islands')),
('VI', 'VIR', '850', _('United States Virgin Islands')),
('WF', 'WLF', '876', _('Wallis and Futuna')),
('EH', 'ESH', '732', _('Western Sahara')),
('YE', 'YEM', '887', _('Yemen')),
('ZM', 'ZMB', '894', _('Zambia')),
('ZW', 'ZWE', '716', _('Zimbabwe')),
)
<file_sep>/koalixcrm/crm/documents/payment_reminder.py
# -*- coding: utf-8 -*-
from datetime import *
from django.db import models
from django.utils.translation import ugettext as _
from koalixcrm.crm.const.status import *
from django.core.validators import MaxValueValidator, MinValueValidator
from koalixcrm.crm.documents.sales_document import SalesDocument, OptionSalesDocument
from koalixcrm.plugin import *
class PaymentReminder(SalesDocument):
payable_until = models.DateField(verbose_name=_("To pay until"))
payment_bank_reference = models.CharField(verbose_name=_("Payment Bank Reference"), max_length=100, blank=True,
null=True)
iteration_number = models.IntegerField(blank=False, null=False, verbose_name=_("Iteration Number"),
validators=[MinValueValidator(1), MaxValueValidator(3)])
status = models.CharField(max_length=1, choices=INVOICESTATUS)
def create_from_reference(self, calling_model):
self.create_sales_document(calling_model)
self.status = 'C'
self.iteration_number = 1
self.payable_until = date.today() + \
timedelta(days=self.customer.defaultCustomerBillingCycle.payment_reminder_time_to_payment)
self.template_set = self.contract.get_template_set(self)
self.save()
self.attach_sales_document_positions(calling_model)
self.attach_text_paragraphs()
self.staff = calling_model.staff
def __str__(self):
return _("Payment Reminder") + ": " + self.id.__str__() + " " + _("from Contract") + ": " + self.contract.id.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Payment Reminder')
verbose_name_plural = _('Payment Reminders')
class OptionPaymentReminder(OptionSalesDocument):
list_display = OptionSalesDocument.list_display + ('payable_until', 'status', 'iteration_number')
list_filter = OptionSalesDocument.list_filter + ('status',)
ordering = OptionSalesDocument.ordering
search_fields = OptionSalesDocument.search_fields
fieldsets = OptionSalesDocument.fieldsets + (
(_('Quote specific'), {
'fields': ( 'payable_until', 'status', 'payment_bank_reference', 'iteration_number' )
}),
)
save_as = OptionSalesDocument.save_as
inlines = OptionSalesDocument.inlines
actions = ['create_purchase_confirmation', 'create_invoice', 'create_quote',
'create_delivery_note', 'create_pdf',
'register_invoice_in_accounting', 'register_payment_in_accounting',]
pluginProcessor = PluginProcessor()
inlines.extend(pluginProcessor.getPluginAdditions("quoteInlines"))
<file_sep>/koalixcrm/crm/migrations/0045_auto_20180805_2047.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-08-05 20:47
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0044_reportingperiod_status'),
]
operations = [
migrations.AddField(
model_name='work',
name='worked_hours',
field=models.DateTimeField(blank=True, null=True, verbose_name='Stop Time'),
),
migrations.AlterField(
model_name='task',
name='planned_end_date',
field=models.DateField(blank=True, null=True, verbose_name='Planned End'),
),
migrations.AlterField(
model_name='task',
name='planned_start_date',
field=models.DateField(blank=True, null=True, verbose_name='Planned Start'),
),
migrations.AlterField(
model_name='task',
name='status',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.TaskStatus', verbose_name='Status'),
),
migrations.AlterField(
model_name='work',
name='start_time',
field=models.DateTimeField(blank=True, null=True, verbose_name='Start Time'),
),
migrations.AlterField(
model_name='work',
name='stop_time',
field=models.DateTimeField(blank=True, null=True, verbose_name='Stop Time'),
),
]
<file_sep>/koalixcrm/crm/migrations/0015_auto_20180111_2043.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-08 20:48
from __future__ import unicode_literals
from django.db import migrations
from django.db.migrations.operations.base import Operation
from django.db.models.fields.related import RECURSIVE_RELATIONSHIP_CONSTANT
class MigrateSalesContractToSalesDocument(Operation):
# If this is False, it means that this operation will be ignored by
# sqlmigrate; if true, it will be run and the SQL collected for its output.
reduces_to_sql = False
# If this is False, Django will refuse to reverse past this operation.
reversible = False
def __init__(self, arg1, arg2):
# Operations are usually instantiated with arguments in migration
# files. Store the values of them on self for later use.
pass
def _get_model_tuple(self, remote_model, app_label, model_name):
if remote_model == RECURSIVE_RELATIONSHIP_CONSTANT:
return app_label, model_name.lower()
elif '.' in remote_model:
return tuple(remote_model.lower().split('.'))
else:
return app_label, remote_model.lower()
def state_forwards(self, app_label, state):
# Add a new model.
renamed_model = state.models["crm", "salescontract"].clone()
renamed_model.name = "SalesDocument"
state.models["crm", "salesdocument"] = renamed_model
# Repoint all fields pointing to the old model to the new one.
old_model_tuple = "crm", "salescontract"
old_remote_model = '%s.%s' % ("crm", "salescontract")
new_remote_model = '%s.%s' % ("crm", "SalesDocument")
to_reload = []
for (model_app_label, model_name), model_state in state.models.items():
if model_name != "salescontract" and model_name != "salesdocument":
new_model_state = model_state
model_with_new_base = state.models[model_app_label, model_name]
for index, (name, field) in enumerate(model_state.fields):
changed_field = None
remote_field = field.remote_field
if remote_field:
remote_model_tuple = self._get_model_tuple(
remote_field.model, model_app_label, model_name
)
if remote_model_tuple == old_model_tuple:
changed_field = field.clone()
changed_field.remote_field.model = new_remote_model
through_model = getattr(remote_field, 'through', None)
if through_model:
through_model_tuple = self._get_model_tuple(
through_model, model_app_label, model_name
)
if through_model_tuple == old_model_tuple:
if changed_field is None:
changed_field = field.clone()
changed_field.remote_field.through = new_remote_model
if changed_field:
new_model_state = model_state.clone()
new_model_state.fields[index] = name, changed_field
model_changed = True
if old_remote_model in model_state.bases:
new_bases = []
for base in model_state.bases:
if old_remote_model == base:
new_bases.append(new_remote_model.lower())
new_model_state.bases = tuple(new_bases)
if model_changed:
state.remove_model(model_app_label, model_name)
state.models[model_app_label, model_name] = new_model_state
to_reload.append((model_app_label, model_name))
# Reload models related to old model before removing the old model.
state.remove_model("crm", "salescontract")
state.reload_model("crm", "salesdocument", delay=True)
state.reload_models(to_reload, delay=True)
# Remove the old model.
def database_forwards(self, app_label, schema_editor, from_state, to_state):
# The Operation should use schema_editor to apply any changes it
# wants to make to the database.
new_model = to_state.apps.get_model("crm", "SalesDocument")
if self.allow_migrate_model(schema_editor.connection.alias, new_model):
old_model = from_state.apps.get_model("crm", "SalesContract")
# Move the main table
schema_editor.alter_db_table(
new_model,
old_model._meta.db_table,
new_model._meta.db_table,
)
# Alter the fields pointing to us
for related_object in old_model._meta.related_objects:
if related_object.related_model == old_model:
model = new_model
related_key = ("crm", "salesdocument")
else:
model = related_object.related_model
related_key = (
related_object.related_model._meta.app_label,
related_object.related_model._meta.model_name,
)
to_field = to_state.apps.get_model(*related_key)._meta.get_field(related_object.field.name)
schema_editor.alter_field(
model,
related_object.field,
to_field,
)
# Rename M2M fields whose name is based on this model's name.
fields = zip(old_model._meta.local_many_to_many, new_model._meta.local_many_to_many)
for (old_field, new_field) in fields:
# Skip self-referential fields as these are renamed above.
if new_field.model == new_field.related_model or not new_field.remote_field.through._meta.auto_created:
continue
# Rename the M2M table that's based on this model's name.
old_m2m_model = old_field.remote_field.through
new_m2m_model = new_field.remote_field.through
schema_editor.alter_db_table(
new_m2m_model,
old_m2m_model._meta.db_table,
new_m2m_model._meta.db_table,
)
# Rename the column in the M2M table that's based on this
# model's name.
schema_editor.alter_field(
new_m2m_model,
old_m2m_model._meta.get_field(old_model._meta.model_name),
new_m2m_model._meta.get_field(new_model._meta.model_name),
)
def database_backwards(self, app_label, schema_editor, from_state, to_state):
# If reversible is True, this is called when the operation is reversed.
pass
def describe(self):
# This is used to describe what the operation does in console output.
return "Custom Operation"
class Migration(migrations.Migration):
dependencies = [
('crm', '0014_auto_20180108_2048'),
]
operations = [
migrations.RemoveField(
model_name='salescontract',
name='derived_from_sales_contract',
),
MigrateSalesContractToSalesDocument("crm", "salescontract")
]
<file_sep>/documentation/source/crm.rst
.. highlight:: rst
CRM
===
Introduction
------------
Products
--------
Contact
--------
Customer
^^^^^^^^
Documents
---------
Sales Contract
^^^^^^^^^^^^^^
Purchase Order
^^^^^^^^^^^^^^
Invoice
^^^^^^^
Quote
^^^^^
Reporting
---------
<file_sep>/koalixcrm/crm/migrations/0034_genericprojectlink.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-12 20:01
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('contenttypes', '0002_remove_content_type_name'),
('crm', '0033_auto_20180612_1936'),
]
operations = [
migrations.CreateModel(
name='GenericProjectLink',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('object_id', models.PositiveIntegerField()),
('date_of_creation', models.DateTimeField(auto_now_add=True, verbose_name='Created at')),
('content_type', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='contenttypes.ContentType')),
('last_modified_by', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_project_link_last_modified', to=settings.AUTH_USER_MODEL, verbose_name='Last modified by')),
('project_link_type', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ProjectLinkType', verbose_name='Project Link Type')),
('task', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Project', verbose_name='Project')),
],
options={
'verbose_name': 'Project Link',
'verbose_name_plural': 'Project Links',
},
),
]
<file_sep>/koalixcrm/crm/reporting/estimation_status.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from django.contrib import admin
from rest_framework import serializers
class EstimationStatus(models.Model):
title = models.CharField(verbose_name=_("Title"),
max_length=250,
blank=False,
null=False)
description = models.TextField(verbose_name=_("Text"),
blank=True,
null=True)
is_obsolete = models.BooleanField(verbose_name=_("Status represents estimation is obsolete"),)
class Meta:
app_label = "crm"
verbose_name = _('Estimation Status')
verbose_name_plural = _('Estimation Status')
def __str__(self):
return str(self.id) + " " + str(self.title)
class EstimationStatusAdminView(admin.ModelAdmin):
list_display = ('id',
'title',
'description',
'is_obsolete')
fieldsets = (
(_('Agreement Status'), {
'fields': ('title',
'description',
'is_obsolete')
}),
)
save_as = True
class EstimationStatusJSONSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = EstimationStatus
fields = ('id',
'title',
'description',)
<file_sep>/koalixcrm/crm/migrations/0054_auto_20181020_1845.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-20 18:45
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0053_auto_20181014_2305'),
]
operations = [
migrations.AlterField(
model_name='currency',
name='short_name',
field=models.CharField(max_length=3, verbose_name='Displayed Name After Prices'),
),
]
<file_sep>/koalixcrm/crm/tests/test_poject_admin.py
# -*- coding: utf-8 -*-
import pytest
from django.test import LiveServerTestCase
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
from koalixcrm.crm.factories.factory_user import AdminUserFactory
class TestProjectAdminView(LiveServerTestCase):
def setUp(self):
firefox_options = webdriver.firefox.options.Options()
firefox_options.set_headless(headless=True)
self.selenium = webdriver.Firefox(firefox_options=firefox_options)
self.test_user = AdminUserFactory.create()
def tearDown(self):
self.selenium.quit()
@pytest.mark.front_end_tests
def test_project_admin(self):
selenium = self.selenium
# login
selenium.get('%s%s' % (self.live_server_url, '/admin/crm/project/'))
# the browser will be redirected to the login page
timeout = 5
try:
element_present = expected_conditions.presence_of_element_located((By.ID, 'id_username'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
username = selenium.find_element_by_xpath('//*[@id="id_username"]')
password = selenium.find_element_by_xpath('//*[@id="id_password"]')
submit_button = selenium.find_element_by_xpath('/html/body/div/article/div/div/form/div/ul/li/input')
username.send_keys("admin")
password.send_keys("<PASSWORD>")
submit_button.send_keys(Keys.RETURN)
try:
element_present = expected_conditions.presence_of_element_located((By.ID,
'/html/body/div/article/header/ul/li/a'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
# find the form element
selenium.get('%s%s' % (self.live_server_url, '/admin/crm/project/add'))
try:
element_present = expected_conditions.presence_of_element_located((By.ID, '//*[@id="id_project_status"]'))
WebDriverWait(selenium, timeout).until(element_present)
except TimeoutException:
print("Timed out waiting for page to load")
<file_sep>/koalixcrm/crm/factories/factory_delivery_note.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.models import DeliveryNote
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardDeliveryNoteFactory(StandardSalesDocumentFactory):
class Meta:
model = DeliveryNote
tracking_reference = "This is a tracking reference"
status = "S"
<file_sep>/koalixcrm/crm/factories/factory_customer_group.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import CustomerGroup
class StandardCustomerGroupFactory(factory.django.DjangoModelFactory):
class Meta:
model = CustomerGroup
django_get_or_create = ('name',)
name = factory.Sequence(lambda n: "Customer Group #%s" % n)
class AdvancedCustomerGroupFactory(factory.django.DjangoModelFactory):
class Meta:
model = CustomerGroup
name = factory.Sequence(lambda n: "Customer Group #%s" % n)
<file_sep>/koalixcrm/crm/migrations/0027_auto_20180606_2034.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-06-06 20:34
from __future__ import unicode_literals
import datetime
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('crm', '0026_auto_20180507_1957'),
]
operations = [
migrations.CreateModel(
name='Call',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('description', models.TextField(verbose_name='Description')),
('date_of_creation', models.DateTimeField(auto_now_add=True, verbose_name='Created at')),
('date_due', models.DateTimeField(blank=True, default=datetime.datetime.now, verbose_name='Date due')),
('last_modification', models.DateTimeField(auto_now=True, verbose_name='Last modified')),
('status', models.CharField(choices=[('P', 'Planned'), ('D', 'Delayed'), ('R', 'ToRecall'), ('F', 'Failed'), ('S', 'Success')], default='P', max_length=1, verbose_name='Status')),
],
),
migrations.CreateModel(
name='ContactPersonAssociation',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('contact', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='person_association', to='crm.Contact')),
],
options={
'verbose_name': 'Contacts',
'verbose_name_plural': 'Contacts',
},
),
migrations.CreateModel(
name='Person',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('prefix', models.CharField(blank=True, choices=[('F', 'Company'), ('W', 'Mrs'), ('H', 'Mr'), ('G', 'Ms')], max_length=1, null=True, verbose_name='Prefix')),
('name', models.CharField(blank=True, max_length=100, null=True, verbose_name='Name')),
('prename', models.CharField(blank=True, max_length=100, null=True, verbose_name='Prename')),
('email', models.EmailField(max_length=200, verbose_name='Email Address')),
('phone', models.CharField(max_length=20, verbose_name='Phone Number')),
('role', models.CharField(blank=True, max_length=100, null=True, verbose_name='Role')),
('companies', models.ManyToManyField(blank=True, through='crm.ContactPersonAssociation', to='crm.Contact', verbose_name='Works at')),
],
options={
'verbose_name': 'Person',
'verbose_name_plural': 'People',
},
),
migrations.AddField(
model_name='customer',
name='is_lead',
field=models.BooleanField(default=True),
),
migrations.CreateModel(
name='CallForContact',
fields=[
('call_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.Call')),
('purpose', models.CharField(choices=[('F', 'First commercial call'), ('S', 'Planned commercial call'), ('A', 'Assistance call')], max_length=1, verbose_name='Purpose')),
('company', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contact')),
('cperson', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Person', verbose_name='Person')),
],
options={
'verbose_name': 'Call',
'verbose_name_plural': 'Calls',
},
bases=('crm.call',),
),
migrations.CreateModel(
name='VisitForContact',
fields=[
('call_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.Call')),
('purpose', models.CharField(choices=[('F', 'First commercial visit'), ('S', 'Installation')], max_length=1, verbose_name='Purpose')),
('company', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contact')),
('cperson', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.Person', verbose_name='Person')),
('ref_call', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.CallForContact', verbose_name='Reference Call')),
],
options={
'verbose_name': 'Visit',
'verbose_name_plural': 'Visits',
},
bases=('crm.call',),
),
migrations.AddField(
model_name='contactpersonassociation',
name='person',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='contact_association', to='crm.Person'),
),
migrations.AddField(
model_name='call',
name='last_modified_by',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_calllstmodified', to=settings.AUTH_USER_MODEL, verbose_name='Last modified by'),
),
migrations.AddField(
model_name='call',
name='staff',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, related_name='db_relcallstaff', to=settings.AUTH_USER_MODEL, verbose_name='Staff'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_estimation_status.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import EstimationStatus
class ObsoleteEstimationStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = EstimationStatus
django_get_or_create = ('title',)
title = "Done"
description = "This estimation is obsolete"
is_obsolete = True
class PlannedEstimationStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = EstimationStatus
django_get_or_create = ('title',)
title = "Planned"
description = "This estimation is planned"
is_obsolete = False
class StartedEstimationStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = EstimationStatus
django_get_or_create = ('title',)
title = "Started"
description = "This estimation is started"
is_obsolete = False
<file_sep>/koalixcrm/crm/migrations/0025_auto_20180413_1937.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-04-13 19:37
from __future__ import unicode_literals
import django.core.validators
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0004_auto_20171210_2126'),
('contenttypes', '0002_remove_content_type_name'),
('crm', '0024_auto_20180122_2121'),
]
operations = [
migrations.CreateModel(
name='EmployeeAssignmentToTask',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('planned_effort', models.DecimalField(decimal_places=2, max_digits=10, verbose_name='Effort')),
('employee', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.UserExtension')),
],
options={
'verbose_name': 'Employee Assignment',
'verbose_name_plural': 'Employee Assignments',
},
),
migrations.CreateModel(
name='GenericTaskLink',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('object_id', models.PositiveIntegerField()),
('content_type', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='contenttypes.ContentType')),
],
options={
'verbose_name': 'Task Link',
'verbose_name_plural': 'Task Links',
},
),
migrations.CreateModel(
name='Task',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('short_description', models.CharField(blank=True, max_length=100, null=True, verbose_name='Description')),
('planned_start_date', models.DateField(verbose_name='Planned Start Date')),
('planned_end_date', models.DateField(verbose_name='Planned End Date')),
('description', models.TextField(blank=True, null=True, verbose_name='Description')),
('last_status_change', models.DateField(blank=True, verbose_name='Last Status Change')),
('project', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contract', verbose_name='Contract')),
],
options={
'verbose_name': 'Task',
'verbose_name_plural': 'Tasks',
},
),
migrations.CreateModel(
name='TaskLinkType',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=300, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
],
options={
'verbose_name': 'Task Link Type',
'verbose_name_plural': 'Task Link Type',
},
),
migrations.CreateModel(
name='TaskStatus',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('title', models.CharField(max_length=250, verbose_name='Title')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
('is_done', models.BooleanField(verbose_name='Status represents task done')),
],
options={
'verbose_name': 'Task Status',
'verbose_name_plural': 'Task Status',
},
),
migrations.CreateModel(
name='Work',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('date', models.DateField(verbose_name='Date')),
('start_time', models.DateTimeField(verbose_name='Start Time')),
('stop_time', models.DateTimeField(verbose_name='Stop Time')),
('short_description', models.CharField(max_length=300, verbose_name='Short Description')),
('description', models.TextField(blank=True, null=True, verbose_name='Text')),
('employee', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.UserExtension')),
('task', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Task', verbose_name='Task')),
],
options={
'verbose_name': 'Work',
'verbose_name_plural': 'Work',
},
),
migrations.AlterField(
model_name='position',
name='position_number',
field=models.PositiveIntegerField(validators=[django.core.validators.MinValueValidator(1)], verbose_name='Position Number'),
),
migrations.AddField(
model_name='task',
name='status',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.TaskStatus', verbose_name='Task Status'),
),
migrations.AddField(
model_name='generictasklink',
name='task',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Task', verbose_name='Task'),
),
migrations.AddField(
model_name='generictasklink',
name='task_link_type',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.TaskLinkType', verbose_name='Task Link Type'),
),
migrations.AddField(
model_name='employeeassignmenttotask',
name='task',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Task', verbose_name='Task'),
),
]
<file_sep>/koalixcrm/crm/rest/tax_rest.py
from rest_framework import serializers
from koalixcrm.crm.product.tax import Tax
class OptionTaxJSONSerializer(serializers.HyperlinkedModelSerializer):
id = serializers.IntegerField(required=False)
description = serializers.CharField(source='name', read_only=True)
class Meta:
model = Tax
fields = ('id',
'description')
class TaxJSONSerializer(serializers.HyperlinkedModelSerializer):
rate = serializers.CharField(source='tax_rate')
description = serializers.CharField(source='name')
class Meta:
model = Tax
fields = ('id',
'rate',
'description')
<file_sep>/documentation/source/code_documentation/estimation.rst
.. highlight:: rst
Estimation
----------
.. automodule:: koalixcrm.crm.reporting.estimation
:members:
<file_sep>/koalixcrm/crm/factories/factory_customer.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Customer
from koalixcrm.crm.factories.factory_contact import StandardContactFactory
from koalixcrm.crm.factories.factory_customer_billing_cycle import StandardCustomerBillingCycleFactory
class StandardCustomerFactory(StandardContactFactory):
class Meta:
model = Customer
default_customer_billing_cycle = factory.SubFactory(StandardCustomerBillingCycleFactory)
is_lead = True
@factory.post_generation
def is_member_of(self, create, extracted):
if not create:
# Simple build, do nothing.
return
if extracted:
# A list of groups were passed in, use them
for group in extracted:
self.is_member_of.add(group)
<file_sep>/koalixcrm/djangoUserExtension/admin.py
# -*- coding: utf-8 -*-
from koalixcrm.djangoUserExtension.models import *
from koalixcrm.djangoUserExtension.user_extension.user_extension import UserExtension, OptionUserExtension
from koalixcrm.djangoUserExtension.user_extension.template_set import TemplateSet, OptionTemplateSet
from koalixcrm.djangoUserExtension.user_extension.document_template import InvoiceTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import QuoteTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import DeliveryNoteTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import PaymentReminderTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import PurchaseOrderTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import ProfitLossStatementTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import BalanceSheetTemplate
from koalixcrm.djangoUserExtension.user_extension.document_template import OptionDocumentTemplate
admin.site.register(UserExtension, OptionUserExtension)
admin.site.register(TemplateSet, OptionTemplateSet)
admin.site.register(InvoiceTemplate, OptionDocumentTemplate)
admin.site.register(QuoteTemplate, OptionDocumentTemplate)
admin.site.register(DeliveryNoteTemplate, OptionDocumentTemplate)
admin.site.register(PaymentReminderTemplate, OptionDocumentTemplate)
admin.site.register(PurchaseOrderTemplate, OptionDocumentTemplate)
admin.site.register(PurchaseConfirmationTemplate, OptionDocumentTemplate)
admin.site.register(ProfitLossStatementTemplate, OptionDocumentTemplate)
admin.site.register(BalanceSheetTemplate, OptionDocumentTemplate)
admin.site.register(MonthlyProjectSummaryTemplate, OptionDocumentTemplate)
admin.site.register(WorkReportTemplate, OptionDocumentTemplate)
<file_sep>/koalixcrm/crm/rest/currency_rest.py
from rest_framework import serializers
from koalixcrm.crm.product.currency import Currency
class CurrencyJSONSerializer(serializers.HyperlinkedModelSerializer):
shortName = serializers.CharField(source='short_name')
class Meta:
model = Currency
fields = ('id',
'description',
'shortName',
'rounding')
<file_sep>/koalixcrm/subscriptions/migrations/0001_initial.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.2 on 2017-07-05 17:02
from __future__ import unicode_literals
import django.db.models.deletion
import filebrowser.fields
from django.db import migrations, models
class Migration(migrations.Migration):
initial = True
dependencies = [
('crm', '0001_initial'),
]
operations = [
migrations.CreateModel(
name='Subscription',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('contract', models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contract',
verbose_name='Subscription Type')),
],
options={
'verbose_name': 'Subscription',
'verbose_name_plural': 'Subscriptions',
},
),
migrations.CreateModel(
name='SubscriptionEvent',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('eventdate', models.DateField(blank=True, null=True, verbose_name='Event Date')),
('event', models.CharField(choices=[('O', 'Offered'), ('C', 'Canceled'), ('S', 'Signed')], max_length=1,
verbose_name='Event')),
('subscriptions',
models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='subscriptions.Subscription',
verbose_name='Subscription')),
],
options={
'verbose_name': 'Subscription Event',
'verbose_name_plural': 'Subscription Events',
},
),
migrations.CreateModel(
name='SubscriptionType',
fields=[
('product_ptr',
models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True,
primary_key=True, serialize=False, to='crm.Product')),
('cancelationPeriod',
models.IntegerField(blank=True, null=True, verbose_name='Cancelation Period (months)')),
('automaticContractExtension',
models.IntegerField(blank=True, null=True, verbose_name='Automatic Contract Extension (months)')),
('automaticContractExtensionReminder', models.IntegerField(blank=True, null=True,
verbose_name='Automatic Contract Extensoin Reminder (days)')),
('minimumDuration',
models.IntegerField(blank=True, null=True, verbose_name='Minimum Contract Duration')),
('paymentIntervall',
models.IntegerField(blank=True, null=True, verbose_name='Payment Intervall (days)')),
('contractDocument', filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True,
verbose_name='Contract Documents')),
],
options={
'verbose_name': 'Subscription Type',
'verbose_name_plural': 'Subscription Types',
},
bases=('crm.product',),
),
migrations.AddField(
model_name='subscription',
name='subscriptiontype',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE,
to='subscriptions.SubscriptionType', verbose_name='Subscription Type'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_invoice.py
# -*- coding: utf-8 -*-
from koalixcrm.crm.models import Invoice
from koalixcrm.crm.factories.factory_sales_document import StandardSalesDocumentFactory
class StandardInvoiceFactory(StandardSalesDocumentFactory):
class Meta:
model = Invoice
payable_until = "2018-05-20"
payment_bank_reference = "This is a bank account reference"
status = "C"
<file_sep>/koalixcrm/accounting/migrations/0002_auto_20170705_1702.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.2 on 2017-07-05 17:02
from __future__ import unicode_literals
import django.db.models.deletion
from django.conf import settings
from django.db import migrations, models
class Migration(migrations.Migration):
initial = True
dependencies = [
('crm', '0001_initial'),
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('accounting', '0001_initial'),
]
operations = [
migrations.AddField(
model_name='booking',
name='bookingReference',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE,
to='crm.Invoice', verbose_name='Booking Reference'),
),
migrations.AddField(
model_name='booking',
name='fromAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_fromaccount',
to='accounting.Account', verbose_name='From Account'),
),
migrations.AddField(
model_name='booking',
name='lastmodifiedby',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE,
related_name='db_booking_lstmodified', to=settings.AUTH_USER_MODEL,
verbose_name='Last modified by'),
),
migrations.AddField(
model_name='booking',
name='staff',
field=models.ForeignKey(blank=True, on_delete=django.db.models.deletion.CASCADE,
related_name='db_booking_refstaff', to=settings.AUTH_USER_MODEL,
verbose_name='Reference Staff'),
),
migrations.AddField(
model_name='booking',
name='toAccount',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, related_name='db_booking_toaccount',
to='accounting.Account', verbose_name='To Account'),
),
]
<file_sep>/koalixcrm/crm/factories/factory_product_price.py
# -*- coding: utf-8 -*-
import factory
import datetime
from koalixcrm.crm.models import ProductPrice
from koalixcrm.crm.factories.factory_product import StandardProductFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory
from koalixcrm.test_support_functions import make_date_utc
class StandardPriceFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProductPrice
django_get_or_create = ('product_type',
'unit',
'currency',
'customer_group',
'price',
'valid_from',
'valid_until')
product_type = factory.SubFactory(StandardProductFactory)
unit = factory.SubFactory(StandardUnitFactory)
currency = factory.SubFactory(StandardCurrencyFactory)
customer_group = factory.SubFactory(StandardCustomerGroupFactory)
price = "100.50"
valid_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
valid_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
class HighPriceFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProductPrice
product_type = factory.SubFactory(StandardProductFactory)
unit = factory.SubFactory(StandardUnitFactory)
currency = factory.SubFactory(StandardCurrencyFactory)
customer_group = factory.SubFactory(StandardCustomerGroupFactory)
price = "250.50"
valid_from = make_date_utc(datetime.datetime(2018, 6, 15, 00))
valid_until = make_date_utc(datetime.datetime(2024, 6, 15, 00))
<file_sep>/koalixcrm/crm/migrations/0048_auto_20181012_2056.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('djangoUserExtension', '0007_auto_20180702_1628'),
('accounting', '0008_auto_20181012_2056'),
migrations.swappable_dependency(settings.AUTH_USER_MODEL),
('crm', '0047_work_worked_hours'),
('subscriptions', '0004_auto_20181013_2213')
]
operations = [
migrations.AddField(
model_name='customergrouptransform',
name='product_backup',
field=models.IntegerField(blank=True, null=True, verbose_name='Product Backup Identifier'),
),
migrations.AddField(
model_name='position',
name='product_backup',
field=models.IntegerField(blank=True, null=True, verbose_name='Product Backup Identifier'),
),
migrations.AddField(
model_name='unittransform',
name='product_backup',
field=models.IntegerField(blank=True, null=True, verbose_name='Product Backup Identifier'),
),
migrations.AddField(
model_name='price',
name='product_backup',
field=models.IntegerField(blank=True, null=True, verbose_name='Product Backup Identifier'),
),
]
<file_sep>/documentation/source/customisation.rst
.. highlight:: rst
Customising koalixcrm
=====================
There are several ways in which to customise koalixcrm, depending on your requirements.
In all cases contributions can be submitted back to koalixcrm by opening an
issue in `GitHub <https://github.com/scaphilo/koalixcrm>`_ - see our
repositories CONTRIBUTING.md file for more information.
Translators
-----------
The Application itself
^^^^^^^^^^^^^^^^^^^^^^
To translate the application itself the only thing you have to do is to install koalixcrm on a Linux or Windows Machine. Please excuse that I can't give you good advice in how to install
the software under Windows I simply do not have the nerves to play around with the Windows powershell or the cmd :-).
::
cd /var/www/koalixcrm/crm
django-admin makemessages -l yourlanguage
this creates a new directory in the folder called ``/var/www/koalixcrm/crm/locale/yourlanguage/LC_MESSAGES/``
in this directory you will find one file called django.po - edit this file in the following way:
Lets say you want to translate koalixcrm to French. Find all msgid's in django.po and look at the english text.
The msgstr following a msgid will be the place you write your translation.
Example::
msgid "phonenumber"
msgstr "phonenumber"
Adjust it to the following::
msgid "phonenumber"
msgstr "nombre de telefon"
After you finised translating the whole file test your translation by compiling it.
::
django-admin compilemessages
After this you are able to use your translation. Sometimes setting your language in ``/var/www/koalixcrm/settings.py`` is required.
Go on with your translation for accounting and for djangouserextention folders.
As soon as you finish this part you will be able to work with koalixcrm in your own language. But there is still something missing: the templatefiles for pdf creation have to be translated as well.
Custom template files for PDF creation
--------------------------------------
For translators or localisers
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To translate the templatefiles for PDF creation you will have to adjust the templatefiles in the ``/var/www/koalixcrm/templatefiles`` folder. There you will find a folder for every language code that is already
translated by the koalixcrm comunity or me. To add your own language type the following::
cp -R en yourlanguage
now open yourlanguage folder and adjust the content of every xml file.
Site specific customisation
^^^^^^^^^^^^^^^^^^^^^^^^^^^
As with customisations for translators, the focus for site specific
customisation is koalixcrm's install path/templatefiles/yourlanguage. Entries
in the yourlanguage folder can be edited as you wish, to produce documents with
your letterhead, contact details or other modifications.
Modders
-------
There is currently no API available ... I'm going to start with that as soon as possible but certainly after I finish
the rest of the user documentation.
<file_sep>/koalixcrm/djangoUserExtension/factories/factory_template_set.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.djangoUserExtension.models import TemplateSet
class StandardTemplateSetFactory(factory.django.DjangoModelFactory):
class Meta:
model = TemplateSet
title = "Just an empty Template Set"
<file_sep>/koalixcrm/accounting/admin.py
# -*- coding: utf-8 -*-
from django.contrib import admin
from koalixcrm.accounting.accounting.accounting_period import AccountingPeriod, OptionAccountingPeriod
from koalixcrm.accounting.accounting.booking import Booking, OptionBooking
from koalixcrm.accounting.accounting.account import Account, OptionAccount
from koalixcrm.accounting.accounting.product_category import ProductCategory, OptionProductCategory
admin.site.register(Account, OptionAccount)
admin.site.register(Booking, OptionBooking)
admin.site.register(ProductCategory, OptionProductCategory)
admin.site.register(AccountingPeriod, OptionAccountingPeriod)
<file_sep>/koalixcrm/global_support_functions.py
# -*- coding: utf-8 -*-
import datetime
def limit_string_length(input_string, maximum_length):
if len(input_string) > maximum_length:
output_string = input_string[:maximum_length]
else:
output_string = input_string
return output_string
def get_string_between(input_string, search_start, search_end):
pos_start = input_string.index(search_start)
pos_end = input_string.index(search_end)
output_string = input_string[pos_start:pos_end]
return output_string
def xstr(s):
if s is None:
return ""
else:
return str(s)
def get_today_date():
return datetime.date.today()
def get_today_datetime():
return datetime.datetime.today()
class ConditionalMethodDecorator(object):
def __init__(self, dec, condition):
self.decorator = dec
self.condition = condition
def __call__(self, func):
if not self.condition:
# Return the function unchanged, not decorated.
return func
return self.decorator(func)
<file_sep>/koalixcrm/subscriptions/migrations/0002_auto_20171009_1949.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-10-09 19:49
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
import filebrowser.fields
class Migration(migrations.Migration):
dependencies = [
('subscriptions', '0001_initial'),
]
operations = [
migrations.AlterModelOptions(
name='subscription',
options={'verbose_name': 'Abonnement', 'verbose_name_plural': 'Abonnemente'},
),
migrations.AlterModelOptions(
name='subscriptionevent',
options={'verbose_name': 'Abonnement Ereignis', 'verbose_name_plural': 'Abonnement Ereignisse'},
),
migrations.AlterModelOptions(
name='subscriptiontype',
options={'verbose_name': 'Abonnement Typ', 'verbose_name_plural': 'Abonnement Typen'},
),
migrations.AlterField(
model_name='subscription',
name='contract',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='crm.Contract', verbose_name='Abonnement Typ'),
),
migrations.AlterField(
model_name='subscription',
name='subscriptiontype',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE, to='subscriptions.SubscriptionType', verbose_name='Abonnement Typ'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='event',
field=models.CharField(choices=[('O', 'Offeriert'), ('C', 'Aufgelöst'), ('S', 'Unterschrieben')], max_length=1, verbose_name='Ereignis'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='eventdate',
field=models.DateField(blank=True, null=True, verbose_name='Ereignis Datum'),
),
migrations.AlterField(
model_name='subscriptionevent',
name='subscriptions',
field=models.ForeignKey(on_delete=django.db.models.deletion.CASCADE, to='subscriptions.Subscription', verbose_name='Abonnement'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtension',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatische Vertragsverlängerung in Monaten'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='automaticContractExtensionReminder',
field=models.IntegerField(blank=True, null=True, verbose_name='Automatische Vertragsverlängerung Erinnerung in Tagen'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='cancelationPeriod',
field=models.IntegerField(blank=True, null=True, verbose_name='Kündigungsfirst in Monaten'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='contractDocument',
field=filebrowser.fields.FileBrowseField(blank=True, max_length=200, null=True, verbose_name='Vertragsdokumente'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='minimumDuration',
field=models.IntegerField(blank=True, null=True, verbose_name='Minimale Vertragsdauer'),
),
migrations.AlterField(
model_name='subscriptiontype',
name='paymentIntervall',
field=models.IntegerField(blank=True, null=True, verbose_name='Zahlungsintervall'),
),
]
<file_sep>/koalixcrm/crm/migrations/0037_auto_20180701_2128.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-07-01 21:28
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0036_auto_20180626_2059'),
]
operations = [
migrations.AlterModelOptions(
name='reportingperiod',
options={'verbose_name': 'Reporting Period', 'verbose_name_plural': 'Reporting Periods'},
),
]
<file_sep>/koalixcrm/crm/documents/purchase_confirmation.py
# -*- coding: utf-8 -*-
from django.utils.translation import ugettext as _
from koalixcrm.crm.documents.sales_document import SalesDocument, OptionSalesDocument
class PurchaseConfirmation(SalesDocument):
def create_from_reference(self, calling_model):
self.create_sales_document(calling_model)
self.template_set = self.contract.get_template_set(self)
self.save()
self.attach_sales_document_positions(calling_model)
self.attach_text_paragraphs()
def __str__(self):
return _("Purchase Confirmation") + ": " + self.id.__str__() + " " + _("from Contract") + ": " + self.contract.id.__str__()
class Meta:
app_label = "crm"
verbose_name = _('Purchase Confirmation')
verbose_name_plural = _('Purchase Confirmations')
class OptionPurchaseConfirmation(OptionSalesDocument):
list_display = OptionSalesDocument.list_display
list_filter = OptionSalesDocument.list_filter
ordering = OptionSalesDocument.ordering
search_fields = OptionSalesDocument.search_fields
fieldsets = OptionSalesDocument.fieldsets
save_as = OptionSalesDocument.save_as
inlines = OptionSalesDocument.inlines
actions = ['create_invoice', 'create_quote',
'create_delivery_note', 'create_purchase_order', 'create_', 'create_pdf']
<file_sep>/koalixcrm/crm/const/purpose.py
# -*- coding: utf-8 -*
from django.utils.translation import ugettext as _
PURPOSESADDRESSINCONTRACT = (
('D', _('Delivery Address')),
('B', _('Billing Address')),
('C', _('Contact Address')),
)
PURPOSESADDRESSINCUSTOMER = (
('H', _('Private')),
('O', _('Business')),
('P', _('Mobile Private')),
('B', _('Mobile Business')),
)
PURPOSESTEXTPARAGRAPHINDOCUMENTS = (
('BS', _('Before subject')),
('AS', _('After subject')),
('BT', _('Before total')),
('AT', _('After total')),
('BW', _('Before wishes')),
('AW', _('After wishes')),
('C1', _('Custom 1')),
('C2', _('Custom 2')),
('C3', _('Custom 3')),
('C4', _('Custom 4')),
)
PURPOSECALLINCUSTOMER = (
('F', _('First commercial call')),
('S', _('Planned commercial call')),
('A', _('Assistance call')),
)
PURPOSEVISITINCUSTOMER = (
('F', _('First commercial visit')),
('S', _('Installation')),
)<file_sep>/koalixcrm/crm/tests/test_incomplete_sales_document_position.py
import pytest
import datetime
from django.test import TestCase
from koalixcrm.crm.documents.calculations import Calculations
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.crm.factories.factory_quote import StandardQuoteFactory
from koalixcrm.crm.factories.factory_sales_document_position import StandardSalesDocumentPositionFactory
from koalixcrm.crm.factories.factory_product_type import StandardProductTypeFactory
from koalixcrm.crm.factories.factory_product_price import StandardPriceFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_customer_group import StandardCustomerGroupFactory, AdvancedCustomerGroupFactory
from koalixcrm.crm.factories.factory_tax import StandardTaxFactory
from koalixcrm.crm.factories.factory_unit import StandardUnitFactory, SmallUnitFactory
from koalixcrm.test_support_functions import make_date_utc
class DocumentSalesDocumentPosition(TestCase):
def setUp(self):
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
start_date = (datetime_now - datetime.timedelta(days=30)).date()
end_date = (datetime_now + datetime.timedelta(days=30)).date()
self.tax = StandardTaxFactory.create(tax_rate=10)
self.test_currency_with_rounding = StandardCurrencyFactory.create(rounding=1)
self.test_currency_without_rounding = StandardCurrencyFactory.create(
rounding=None,
description='Euro',
short_name='EUR'
)
self.alternative_currency = self.test_currency_without_rounding
self.customer_group = StandardCustomerGroupFactory.create()
self.alternative_customer_group = AdvancedCustomerGroupFactory.create()
self.customer = StandardCustomerFactory.create()
self.customer.is_member_of.add(self.customer_group)
self.customer.save()
self.unit = StandardUnitFactory.create()
self.alternative_unit = SmallUnitFactory.create()
self.product_without_dates = StandardProductTypeFactory.create(
product_type_identifier="A",
tax=self.tax
)
self.price_without_customer_group = StandardPriceFactory.create(
product_type=self.product_without_dates,
customer_group=None,
price=100,
unit=self.unit,
currency=self.test_currency_with_rounding,
valid_from=start_date,
valid_until=end_date
)
@pytest.mark.back_end_tests
def test_calculate_document_price_overwritten(self):
quote_1 = StandardQuoteFactory.create(customer=self.customer)
StandardSalesDocumentPositionFactory.create(
quantity=1,
discount=0,
product_type=self.product_without_dates,
overwrite_product_price=True,
position_price_per_unit=90,
unit=self.unit,
sales_document=quote_1
)
datetime_now = make_date_utc(datetime.datetime(2024, 1, 1, 0, 00))
date_now = datetime_now.date()
Calculations.calculate_document_price(
document=quote_1,
pricing_date=date_now)
self.assertEqual(
quote_1.last_calculated_price.__str__(), "81.00")
self.assertEqual(
quote_1.last_calculated_tax.__str__(), "9.00")
<file_sep>/koalixcrm/djangoUserExtension/models.py
# -*- coding: utf-8 -*-
from koalixcrm.djangoUserExtension.user_extension.document_template import *
from koalixcrm.djangoUserExtension.user_extension.template_set import *
from koalixcrm.djangoUserExtension.user_extension.user_extension import *
from koalixcrm.djangoUserExtension.user_extension.text_paragraph import *
<file_sep>/koalixcrm/crm/factories/factory_project_link_type.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import ProjectLinkType
class RelatedToProjectLinkTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProjectLinkType
title = "Is related to"
description = "This project is related with ...."
class RequiresProjectLinkTypeFactory(factory.django.DjangoModelFactory):
class Meta:
model = ProjectLinkType
title = "This project requires"
description = "This project requires the completion or the existence of ...."
<file_sep>/koalixcrm/crm/reporting/agreement_status.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from django.contrib import admin
from rest_framework import serializers
class AgreementStatus(models.Model):
title = models.CharField(verbose_name=_("Title"),
max_length=250,
blank=False,
null=False)
description = models.TextField(verbose_name=_("Text"),
blank=True,
null=True)
is_agreed = models.BooleanField(verbose_name=_("Status represents agreement exists"),)
class Meta:
app_label = "crm"
verbose_name = _('Agreement Status')
verbose_name_plural = _('Agreement Status')
def __str__(self):
return str(self.id) + " " + str(self.title)
class AgreementStatusAdminView(admin.ModelAdmin):
list_display = ('id',
'title',
'description',
'is_agreed')
fieldsets = (
(_('Agreement Status'), {
'fields': ('title',
'description',
'is_agreed')
}),
)
save_as = True
class AgreementStatusJSONSerializer(serializers.HyperlinkedModelSerializer):
class Meta:
model = AgreementStatus
fields = ('id',
'title',
'description',)
<file_sep>/koalixcrm/crm/migrations/0050_auto_20181014_2300.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-10-12 20:56
from __future__ import unicode_literals
from django.conf import settings
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0049_auto_20181014_2258'),
]
operations = [
migrations.RemoveField(
model_name='customergrouptransform',
name='product',
),
migrations.RemoveField(
model_name='position',
name='product',
),
migrations.RemoveField(
model_name='unittransform',
name='product',
),
migrations.RemoveField(
model_name='Price',
name='product',
),
migrations.RenameModel(old_name='Product',
new_name='ProductType'),
migrations.CreateModel(
name='ProductPrice',
fields=[
('price_ptr', models.OneToOneField(auto_created=True, on_delete=django.db.models.deletion.CASCADE, parent_link=True, primary_key=True, serialize=False, to='crm.Price')),
('product_type', models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='crm.ProductType', verbose_name='Product'),)
],
options={
'verbose_name': 'Product Price',
'verbose_name_plural': 'Product Prices',
},
bases=('crm.price',),
),
migrations.AddField(
model_name='customergrouptransform',
name='product_type',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.SET_NULL, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
migrations.AddField(
model_name='position',
name='product_type',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.SET_NULL, to='crm.ProductType', verbose_name='Product'),
),
migrations.AddField(
model_name='unittransform',
name='product_type',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.SET_NULL, to='crm.ProductType', verbose_name='Product Type'),
preserve_default=False,
),
]
<file_sep>/koalixcrm/djangoUserExtension/user_extension/template_set.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.global_support_functions import xstr
from koalixcrm.crm.exceptions import *
class TemplateSet(models.Model):
title = models.CharField(verbose_name=_("Title"),
max_length=100)
invoice_template = models.ForeignKey("InvoiceTemplate",
blank=True,
null=True)
quote_template = models.ForeignKey("QuoteTemplate",
blank=True,
null=True)
delivery_note_template = models.ForeignKey("DeliveryNoteTemplate",
blank=True, null=True)
payment_reminder_template = models.ForeignKey("PaymentReminderTemplate",
blank=True,
null=True)
purchase_confirmation_template = models.ForeignKey("PurchaseConfirmationTemplate",
blank=True, null=True)
purchase_order_template = models.ForeignKey("PurchaseOrderTemplate",
blank=True,
null=True)
profit_loss_statement_template = models.ForeignKey("ProfitLossStatementTemplate",
blank=True, null=True)
balance_sheet_statement_template = models.ForeignKey("BalanceSheetTemplate",
blank=True,
null=True)
monthly_project_summary_template = models.ForeignKey("MonthlyProjectSummaryTemplate",
blank=True,
null=True)
work_report_template = models.ForeignKey("WorkReportTemplate",
blank=True,
null=True)
class Meta:
app_label = "djangoUserExtension"
verbose_name = _('Template-set')
verbose_name_plural = _('Template-sets')
def __str__(self):
return xstr(self.id) + ' ' + xstr(self.title)
def get_template_set(self, required_template_set):
mapping_class_to_templates = {"Invoice": self.invoice_template,
"Quote": self.quote_template,
"DeliveryNote": self.delivery_note_template,
"PaymentReminder": self.payment_reminder_template,
"PurchaseConfirmation": self.purchase_confirmation_template,
"PurchaseOrder": self.purchase_order_template,
"ProfitLossStatement": self.profit_loss_statement_template,
"BalanceSheet": self.balance_sheet_statement_template,
"MonthlyProjectSummaryTemplate": self.monthly_project_summary_template,
"WorkReport": self.work_report_template}
try:
if mapping_class_to_templates[required_template_set]:
return mapping_class_to_templates[required_template_set]
else:
raise TemplateMissingInTemplateSet("The TemplateSet does not contain a template for " +
required_template_set)
except KeyError:
raise IncorrectUseOfAPI("")
class OptionTemplateSet(admin.ModelAdmin):
list_display = ('id', 'title')
list_display_links = ('id', 'title')
ordering = ('id',)
search_fields = ('id', 'title')
fieldsets = (
(_('Basics'), {
'fields': ('title',
'invoice_template',
'quote_template',
'delivery_note_template',
'payment_reminder_template',
'purchase_confirmation_template',
'purchase_order_template',
'profit_loss_statement_template',
'balance_sheet_statement_template',
'monthly_project_summary_template',
'work_report_template')
}),
)
<file_sep>/documentation/source/installation.rst
.. highlight:: rst
koalixcrm Installation
======================
on Windows 10 Version 1703
---------------------------
Install Python 3.6.2
^^^^^^^^^^^^^^^^^^^^
Download from https://www.python.org/downloads/
Install python with the defaults
Install FOP 2.2
^^^^^^^^^^^^^^^
Download and install java from oracle website
Download fop2.2 from apache fop website and install
Install koalixcrm app
^^^^^^^^^^^^^^^^^^^^^
Run command
C:\Users\YourUser\AppData\Local\Programs\Python\Python36-32\Scripts\pip.exe install koalix-crm
Setup django project
^^^^^^^^^^^^^^^^^^^^
Run command
C:\Users\YourUser\AppData\Local\Programs\Python\Python36-32\Scripts\django-admin.exe startproject test_koalixcrm
mkdir test_koalixcrm\media
mkdir test_koalixcrm\media\uploads
on ubuntu 17.04
---------------
Install required programs on your ubuntu
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
sudo bash
apt-get install fop virtualenv python3.5
exit
Create a virtual python environment for the koalixcrm project
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
mkdir ~/test_koalixcrm_env
virtualenv --no-site-package --python=/usr/bin/python3.5 ~/test_koalixcrm_env
source /test_koalixcrm_env/bin/activate
pip install koalix-crm
Create a generic django project
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
cd ~
django-admin startproject test_koalixcrm
Common on all Operating Systems
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Import koalixcrm to your project
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Open the file called settings.py
Search in the file the variable definition "INSTALLED_APPS"
Add following lines to the at the end of INSTALLED_APPS:
'koalixcrm.crm',
'koalixcrm.accounting',
'koalixcrm.djangoUserExtension',
'koalixcrm.subscriptions',
'filebrowser'
Create a new variable defintion "KOALIXCRM_PLUGIN"
KOALIXCRM_PLUGINS = (
'koalixcrm.subscriptions',
)
At the very end of the seetings.py file add the following lines:
STATIC_URL = '/static/'
STATIC_ROOT = os.path.join(BASE_DIR, 'static/')
MEDIA_URL = "/media/"
MEDIA_ROOT = os.path.join(BASE_DIR, '')
PROJECT_ROOT = BASE_DIR
# Settings specific for koalixcrm
PDF_OUTPUT_ROOT = os.path.join(STATIC_ROOT, 'pdf/')
FOP_
# Settings specific for filebrowser
FILEBROWSER_DIRECTORY = 'uploads/'
Enable the customized additional view for filebrowser
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Open the file called urls.py
Completely rewrite the file with following content
from django.conf.urls.static import *
from django.contrib.staticfiles.urls import static
from django.contrib import admin
from filebrowser.sites import FileBrowserSite
from django.core.files.storage import DefaultStorage
site = FileBrowserSite(name="filebrowser", storage=DefaultStorage())
customsite = FileBrowserSite(name='custom_filebrowser', storage=DefaultStorage())
customsite.directory = "uploads/"
admin.autodiscover()
urlpatterns = [
url(r'^admin/filebrowser/', customsite.urls),
url(r'^admin/', admin.site.urls),
]
urlpatterns += static(settings.STATIC_URL, document_root=settings.STATIC_ROOT)
Afterwards start the django application
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
cd ~/test_koalixcrm
python manage.py makemigrations
python manage.py migrate
python manage.py createsuperuser
python manage.py runserver 127.0.0.1:8000
Log in to the admin website
^^^^^^^^^^^^^^^^^^^^^^^^^^^
What you want to do next is of cause the test the software. Visit your http://127.0.0.1:8000/admin, log in and start testing.
<file_sep>/koalixcrm/crm/migrations/0009_auto_20180104_2111.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-04 21:11
from __future__ import unicode_literals
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('crm', '0008_auto_20180104_2042'),
]
operations = [
migrations.RenameField(
model_name='contract',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='contract',
old_name='defaultcurrency',
new_name='default_currency',
),
migrations.RenameField(
model_name='contract',
old_name='defaultcustomer',
new_name='default_customer',
),
migrations.RenameField(
model_name='contract',
old_name='defaultSupplier',
new_name='default_supplier',
),
migrations.RenameField(
model_name='contract',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='contract',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='invoice',
old_name='derivatedFromQuote',
new_name='derived_from_quote',
),
migrations.RenameField(
model_name='invoice',
old_name='payableuntil',
new_name='payable_until',
),
migrations.RenameField(
model_name='invoice',
old_name='paymentBankReference',
new_name='payment_bank_reference',
),
migrations.RenameField(
model_name='position',
old_name='lastCalculatedPrice',
new_name='last_calculated_price',
),
migrations.RenameField(
model_name='position',
old_name='lastCalculatedTax',
new_name='last_calculated_tax',
),
migrations.RenameField(
model_name='position',
old_name='lastPricingDate',
new_name='last_pricing_date',
),
migrations.RenameField(
model_name='position',
old_name='overwriteProductPrice',
new_name='overwrite_product_price',
),
migrations.RenameField(
model_name='position',
old_name='positionNumber',
new_name='position_number',
),
migrations.RenameField(
model_name='position',
old_name='sentOn',
new_name='sent_on',
),
migrations.RenameField(
model_name='product',
old_name='accoutingProductCategorie',
new_name='accounting_product_categorie',
),
migrations.RenameField(
model_name='product',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='product',
old_name='defaultunit',
new_name='default_unit',
),
migrations.RenameField(
model_name='product',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='product',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='product',
old_name='productNumber',
new_name='product_number',
),
migrations.RenameField(
model_name='purchaseconfirmation',
old_name='validuntil',
new_name='valid_until',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='externalReference',
new_name='external_reference',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='lastCalculatedPrice',
new_name='last_calculated_price',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='lastCalculatedTax',
new_name='last_calculated_tax',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='purchaseorder',
old_name='lastPricingDate',
new_name='last_pricing_date',
),
migrations.RenameField(
model_name='quote',
old_name='validuntil',
new_name='valid_until',
),
migrations.RenameField(
model_name='salescontract',
old_name='dateofcreation',
new_name='date_of_creation',
),
migrations.RenameField(
model_name='salescontract',
old_name='externalReference',
new_name='external_reference',
),
migrations.RenameField(
model_name='salescontract',
old_name='lastCalculatedPrice',
new_name='last_calculated_price',
),
migrations.RenameField(
model_name='salescontract',
old_name='lastCalculatedTax',
new_name='last_calculated_tax',
),
migrations.RenameField(
model_name='salescontract',
old_name='lastmodification',
new_name='last_modification',
),
migrations.RenameField(
model_name='salescontract',
old_name='lastmodifiedby',
new_name='last_modified_by',
),
migrations.RenameField(
model_name='salescontract',
old_name='lastPricingDate',
new_name='last_pricing_date',
),
]
<file_sep>/koalixcrm/crm/factories/factory_contract.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Contract
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_customer import StandardCustomerFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
from koalixcrm.djangoUserExtension.factories.factory_template_set import StandardTemplateSetFactory
class StandardContractFactory(factory.django.DjangoModelFactory):
class Meta:
model = Contract
staff = factory.SubFactory(StaffUserFactory)
description = "This is the description to a test contract"
default_customer = factory.SubFactory(StandardCustomerFactory)
default_currency = factory.SubFactory(StandardCurrencyFactory)
default_template_set = factory.SubFactory(StandardTemplateSetFactory)
date_of_creation = "2018-05-01"
last_modification = "2018-05-03"
last_modified_by = factory.SubFactory(StaffUserFactory)
<file_sep>/koalixcrm/crm/inlinemixin.py
class LimitedAdminInlineMixin(object):
"""
InlineAdmin mixin limiting the selection of related items according to
criteria which can depend on the current parent object being edited.
A typical use case would be selecting a subset of related items from
other inlines, ie. images, to have some relation to other inlines.
Use as follows::
class MyInline(LimitedAdminInlineMixin, admin.TabularInline):
def get_filters(self, request, obj):
return (('<field_name>', dict(<filters>)),)
Here, <field_name> is the name of the FK field to be filtered and <filters>
is a dict of parameters as you would normally specify them in the filter()
method of QuerySets.
Modified from:
https://stackoverflow.com/a/5008984
https://gist.github.com/dokterbob/828117
"""
@staticmethod
def limit_inline_choices(formset, field, empty=False, **filters):
"""
This function fetches the queryset with available choices for a given
`field` and filters it based on the criteria specified in filters,
unless `empty=True`. In this case, no choices will be made available.
"""
assert field in formset.form.base_fields
qs = formset.form.base_fields[field].queryset
if empty:
formset.form.base_fields[field].queryset = qs.none()
else:
qs = qs.filter(**filters)
formset.form.base_fields[field].queryset = qs
def get_formset(self, request, obj=None, **kwargs):
"""
Make sure we can only select variations that relate to the current
item.
"""
formset = super(LimitedAdminInlineMixin, self).get_formset(request,
obj,
**kwargs)
inline_filters = self.get_filters(request, obj)
if inline_filters:
for (field, filters,) in inline_filters:
self.limit_inline_choices(formset, field, **filters)
return formset<file_sep>/koalixcrm/accounting/accounting/product_category.py
# -*- coding: utf-8 -*-
from django.db import models
from django.contrib import admin
from django.utils.translation import ugettext as _
from koalixcrm.accounting.models import Account
class ProductCategory(models.Model):
title = models.CharField(verbose_name=_("Product Category Title"),
max_length=50)
profit_account = models.ForeignKey(Account,
verbose_name=_("Profit Account"),
limit_choices_to={"account_type": "E"},
related_name="db_profit_account")
loss_account = models.ForeignKey(Account,
verbose_name=_("Loss Account"),
limit_choices_to={"account_type": "S"},
related_name="db_loss_account")
class Meta:
app_label = "accounting"
verbose_name = _('Product Category')
verbose_name_plural = _('Product Categories')
def __str__(self):
return self.title
class OptionProductCategory(admin.ModelAdmin):
list_display = ('title',
'profit_account',
'loss_account')
list_display_links = ('title',
'profit_account',
'loss_account')
fieldsets = (
(_('Basics'), {
'fields': ('title',
'profit_account',
'loss_account')
}),
)
save_as = True
<file_sep>/koalixcrm/crm/factories/factory_project.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import Project
from koalixcrm.crm.factories.factory_project_status import StartedProjectStatusFactory
from koalixcrm.djangoUserExtension.factories.factory_template_set import StandardTemplateSetFactory
from koalixcrm.crm.factories.factory_user import StaffUserFactory
from koalixcrm.crm.factories.factory_currency import StandardCurrencyFactory
class StandardProjectFactory(factory.django.DjangoModelFactory):
class Meta:
model = Project
django_get_or_create = ('project_name',)
project_manager = factory.SubFactory(StaffUserFactory)
project_name = "This is a Test Project"
description = "This is description of a test Project"
project_status = factory.SubFactory(StartedProjectStatusFactory)
default_template_set = factory.SubFactory(StandardTemplateSetFactory)
default_currency = factory.SubFactory(StandardCurrencyFactory)
date_of_creation = "2018-05-01"
last_modification = "2018-05-02"
last_modified_by = factory.SubFactory(StaffUserFactory)
<file_sep>/koalixcrm/crm/views/create_work_report.py
# -*- coding: utf-8 -*-
import datetime
from django.http import HttpResponseRedirect, Http404
from django.shortcuts import render
from django.contrib.admin.widgets import *
from django.contrib.admin import helpers
from django.template.context_processors import csrf
from koalixcrm.djangoUserExtension.exceptions import TooManyUserExtensionsAvailable
from koalixcrm.crm.views.pdfexport import PDFExportView
class CreateWorkReportForm(forms.Form):
range_from = forms.DateField(widget=AdminDateWidget)
range_to = forms.DateField(widget=AdminDateWidget)
def create_work_report(calling_model_admin, request, queryset):
try:
if request.POST.get('post'):
if 'create_pdf' in request.POST:
create_work_report_form = CreateWorkReportForm(request.POST)
if create_work_report_form.is_valid():
for obj in queryset:
return PDFExportView.export_pdf(
calling_model_admin=calling_model_admin,
request=request,
source=obj,
redirect_to="/admin/",
template_to_use=obj.user.default_template_set.work_report_template,
date_from=create_work_report_form.cleaned_data["range_from"],
date_to=create_work_report_form.cleaned_data["range_to"])
else:
datetime_now = datetime.datetime.today()
range_from_date = (datetime_now - datetime.timedelta(days=30)).date()
range_to_date = datetime_now.date()
create_work_report_form = CreateWorkReportForm(initial={'range_from': range_from_date,
'range_to': range_to_date})
c = {'action_checkbox_name': helpers.ACTION_CHECKBOX_NAME,
'queryset': queryset,
'form': create_work_report_form}
c.update(csrf(request))
return render(request, 'crm/admin/create_work_report.html', c)
except TooManyUserExtensionsAvailable:
raise Http404
<file_sep>/documentation/source/code_documentation/work.rst
.. highlight:: rst
Work
----
.. automodule:: koalixcrm.crm.reporting.work
:members:
<file_sep>/documentation/source/index.rst
Welcome to koalixcrm's documentation!
=====================================
Contents:
.. toctree::
:maxdepth: 2
installation
intro
tutorial
accounting
crm
djangoUserExtention
customisation
architecture
faq
code_documentation
Indices and tables
==================
* :ref:`genindex`
* :ref:`modindex`
* :ref:`search`
<file_sep>/koalixcrm/crm/migrations/0004_auto_20171009_2008.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2017-10-09 20:08
from __future__ import unicode_literals
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('crm', '0003_auto_20171009_1949'),
]
operations = [
migrations.AlterField(
model_name='contact',
name='dateofcreation',
field=models.DateTimeField(auto_now_add=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='contact',
name='lastmodification',
field=models.DateTimeField(auto_now=True, verbose_name='Zuletzt geändert'),
),
migrations.AlterField(
model_name='contract',
name='dateofcreation',
field=models.DateTimeField(auto_now_add=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='contract',
name='lastmodification',
field=models.DateTimeField(auto_now=True, verbose_name='Zuletzt geändert'),
),
migrations.AlterField(
model_name='product',
name='dateofcreation',
field=models.DateTimeField(auto_now_add=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='product',
name='lastmodification',
field=models.DateTimeField(auto_now=True, verbose_name='Zuletzt geändert'),
),
migrations.AlterField(
model_name='purchaseorder',
name='dateofcreation',
field=models.DateTimeField(auto_now_add=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='purchaseorder',
name='last_print_date',
field=models.DateTimeField(blank=True, null=True, verbose_name='Zuletzt gedruckt'),
),
migrations.AlterField(
model_name='purchaseorder',
name='lastmodification',
field=models.DateTimeField(auto_now=True, verbose_name='Zuletzt geändert'),
),
migrations.AlterField(
model_name='salescontract',
name='dateofcreation',
field=models.DateTimeField(auto_now_add=True, verbose_name='Erstelldatum'),
),
migrations.AlterField(
model_name='salescontract',
name='last_print_date',
field=models.DateTimeField(blank=True, null=True, verbose_name='Zuletzt gedruckt'),
),
migrations.AlterField(
model_name='salescontract',
name='lastmodification',
field=models.DateTimeField(auto_now=True, verbose_name='Zuletzt geändert'),
),
]
<file_sep>/requirements/test_requirements.txt
pytest==3.7.0
pytest-cov==2.5.1
pytest-django==3.1.2
codacy-coverage==1.3.6
selenium==3.14.0
factory_boy==2.11.1<file_sep>/koalixcrm/crm/reporting/generic_project_link.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
from django.contrib.contenttypes.fields import GenericForeignKey
from django.contrib.contenttypes.models import ContentType
from django.contrib import admin
from django.contrib.contenttypes.admin import GenericTabularInline
class GenericProjectLink(models.Model):
project = models.ForeignKey("Project",
verbose_name=_('Project'),
blank=False,
null=False)
project_link_type = models.ForeignKey("ProjectLinkType",
verbose_name=_('Project Link Type'),
blank=True,
null=True)
content_type = models.ForeignKey(ContentType, on_delete=models.CASCADE)
object_id = models.PositiveIntegerField()
generic_crm_object = GenericForeignKey('content_type',
'object_id')
date_of_creation = models.DateTimeField(verbose_name=_("Created at"),
auto_now_add=True)
last_modified_by = models.ForeignKey('auth.User',
limit_choices_to={'is_staff': True},
verbose_name=_("Last modified by"),
related_name="db_project_link_last_modified")
def __str__(self):
return _("Link to") + " " + str(self.project)
class Meta:
app_label = "crm"
verbose_name = _('Project Link')
verbose_name_plural = _('Project Links')
class GenericLinkInlineAdminView(admin.TabularInline):
model = GenericProjectLink
readonly_fields = ('project_link_type',
'content_type',
'object_id',
'date_of_creation',
'last_modified_by')
extra = 0
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
class InlineGenericProjectLink(GenericTabularInline):
model = GenericProjectLink
readonly_fields = ('project_link_type',
'content_type',
'object_id',
'date_of_creation',
'last_modified_by')
extra = 0
def has_add_permission(self, request):
return False
def has_delete_permission(self, request, obj=None):
return False
<file_sep>/koalixcrm/test_support_functions.py
# -*- coding: utf-8 -*-
import pytz
from selenium.common.exceptions import NoSuchElementException
def assert_when_element_does_not_exist(testcase, xpath):
try:
testcase.selenium.find_element_by_xpath(xpath)
except NoSuchElementException:
testcase.assertTrue(True, xpath+" does not exist")
def assert_when_element_exists(testcase, xpath):
try:
testcase.selenium.find_element_by_xpath(xpath)
except NoSuchElementException:
testcase.assertTrue(True, xpath+" does exist")
def assert_when_element_is_not_equal_to(testcase, xpath, string):
try:
element = testcase.selenium.find_element_by_xpath(xpath)
if element.text != string:
print("issue")
except NoSuchElementException:
return
def make_date_utc(input_date):
output_date = pytz.timezone("UTC").localize(input_date, is_dst=None)
return output_date
<file_sep>/koalixcrm/crm/factories/factory_task_status.py
# -*- coding: utf-8 -*-
import factory
from koalixcrm.crm.models import TaskStatus
class DoneTaskStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = TaskStatus
django_get_or_create = ('title',)
title = "Done"
description = "This task is done"
is_done = True
class PlannedTaskStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = TaskStatus
django_get_or_create = ('title',)
title = "Planned"
description = "This task is planned"
is_done = False
class StartedTaskStatusFactory(factory.django.DjangoModelFactory):
class Meta:
model = TaskStatus
django_get_or_create = ('title',)
title = "Started"
description = "This task is started"
is_done = False
<file_sep>/koalixcrm/crm/documents/pdf_export.py
# -*- coding: utf-8 -*-
import os
from subprocess import check_output
from subprocess import STDOUT
from django.conf import settings
from django.core import serializers
from lxml import etree
import koalixcrm.crm.documents.sales_document
import koalixcrm.djangoUserExtension.models
class PDFExport:
@staticmethod
def find_element_in_xml(xml_string, find_pattern, find_value):
parser = etree.XMLParser(encoding='utf-8', remove_blank_text=True)
root_element = etree.fromstring(xml_string.encode('utf-8'), parser=parser)
found_element = root_element.findall(find_pattern)
if found_element is None:
return 0
else:
for element in found_element:
if element.text == find_value:
return 1
return 0
@staticmethod
def append_element_to_pattern(xml_string, find_pattern, name_of_element, value_of_element, **kwargs):
attributes = kwargs.get('attributes', None)
parser = etree.XMLParser(encoding='utf-8', remove_blank_text=True)
root_element = etree.fromstring(xml_string.encode('utf-8'), parser=parser)
found_element = root_element.find(find_pattern)
new_element = etree.SubElement(found_element, name_of_element, attrib=attributes)
new_element.text = value_of_element.__str__()
return (etree.tostring(root_element,
encoding='UTF-8',
xml_declaration=True,
pretty_print=True)).decode('utf-8')
@staticmethod
def merge_xml(xml_string_1, xml_string_2):
parser = etree.XMLParser(encoding='utf-8', remove_blank_text=True)
root_element_1 = etree.fromstring(xml_string_1.encode('utf-8'), parser=parser)
root_element_2 = etree.fromstring(xml_string_2.encode('utf-8'), parser=parser)
for child in root_element_2:
root_element_1.append(child)
return (etree.tostring(root_element_1,
encoding='UTF-8',
xml_declaration=True,
pretty_print=True)).decode('utf-8')
@staticmethod
def write_xml(objects_to_serialize):
xml = serializers.serialize("xml", objects_to_serialize, indent=3)
return xml
@staticmethod
def write_xml_file(xml, file_path):
f = open(file_path, "wb+")
f.truncate()
f.write(xml.encode('utf-8'))
f.close()
@staticmethod
def perform_xsl_transformation(file_with_serialized_xml, xsl_file, fop_config_file, file_output_pdf):
check_output([settings.FOP_EXECUTABLE,
'-c', fop_config_file.path_full,
'-xml', os.path.join(settings.PDF_OUTPUT_ROOT, file_with_serialized_xml),
'-xsl', xsl_file.path_full,
'-pdf', file_output_pdf], stderr=STDOUT)
@staticmethod
def create_pdf(object_to_create_pdf, template_set, printed_by, *args, **kwargs):
# define the files which are involved in pdf creation process
fop_config_file = object_to_create_pdf.get_fop_config_file(template_set)
xsl_file = object_to_create_pdf.get_xsl_file(template_set)
file_with_serialized_xml = os.path.join(settings.PDF_OUTPUT_ROOT, (str(type(object_to_create_pdf).__name__) +
"_" + str(object_to_create_pdf.id) + ".xml"))
file_output_pdf = os.path.join(settings.PDF_OUTPUT_ROOT, (str(type(object_to_create_pdf).__name__) +
"_" + str(object_to_create_pdf.id) + ".pdf"))
# list the sub-objects which have to be serialized
xml_string = object_to_create_pdf.serialize_to_xml(*args, **kwargs)
objects_to_serialize = list(koalixcrm.djangoUserExtension.models.DocumentTemplate.objects.filter(id=template_set.id))
xml_string_temp = PDFExport.write_xml(objects_to_serialize)
xml_string = PDFExport.merge_xml(xml_string, xml_string_temp)
objects_to_serialize = koalixcrm.djangoUserExtension.models.UserExtension.objects_to_serialize(object_to_create_pdf, printed_by)
xml_string_temp = PDFExport.write_xml(objects_to_serialize)
xml_string = PDFExport.merge_xml(xml_string, xml_string_temp)
# extend the xml-string with required basic settings
xml_string = PDFExport.append_element_to_pattern(xml_string,
".",
"filebrowser_directory",
settings.MEDIA_ROOT)
# write xml-string to xml-file
PDFExport.write_xml_file(xml_string, file_with_serialized_xml)
# perform xsl transformation
PDFExport.perform_xsl_transformation(file_with_serialized_xml, xsl_file, fop_config_file, file_output_pdf)
return file_output_pdf
<file_sep>/koalixcrm/crm/contact/email_address.py
# -*- coding: utf-8 -*-
from django.db import models
from django.utils.translation import ugettext as _
class EmailAddress(models.Model):
email = models.EmailField(max_length=200,
verbose_name=_("Email Address"))
class Meta:
app_label = "crm"
verbose_name = _('Email Address')
verbose_name_plural = _('Email Address')
<file_sep>/koalixcrm/crm/migrations/0012_auto_20180105_1840.py
# -*- coding: utf-8 -*-
# Generated by Django 1.11.4 on 2018-01-05 18:40
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
dependencies = [
('crm', '0011_auto_20180104_2152'),
]
operations = [
migrations.AlterField(
model_name='salescontract',
name='template_set',
field=models.ForeignKey(blank=True, null=True, on_delete=django.db.models.deletion.CASCADE, to='djangoUserExtension.DocumentTemplate', verbose_name='Referred Template'),
),
]
<file_sep>/koalixcrm/crm/views/work_entry_formset.py
# -*- coding: utf-8 -*-
from django import forms
from koalixcrm.crm.views.work_entry_form import WorkEntry
class BaseWorkEntryFormset(forms.BaseFormSet):
def __init__(self, *args, **kwargs):
super(BaseWorkEntryFormset, self).__init__(*args, **kwargs)
@staticmethod
def generate_initial_data(start_date, stop_date, human_resource):
from koalixcrm.crm.reporting.work import Work
list_of_work = Work.objects.filter(human_resource=human_resource).filter(date__lte=stop_date).filter(date__gte=start_date).order_by("date")
initial = []
for work in list_of_work:
initial.append({'work_id': work.id,
'task': work.task,
'project': work.task.project,
'date': work.date,
'start_time': work.start_time,
'stop_time': work.stop_time,
'worked_hours': work.worked_hours,
'description': work.description})
return initial
@staticmethod
def load_formset(range_selection_form, request):
WorkEntryFormSet = forms.formset_factory(WorkEntry,
extra=1,
max_num=60,
can_delete=True,
formset=BaseWorkEntryFormset)
from_date = range_selection_form.evaluate_pre_check_from_date()
to_date = range_selection_form.evaluate_pre_check_to_date()
form_kwargs = BaseWorkEntryFormset.compose_form_kwargs(from_date, to_date)
pre_check_formset = WorkEntryFormSet(request.POST,
form_kwargs=form_kwargs)
return pre_check_formset
@staticmethod
def compose_form_kwargs(from_date, to_date):
form_kwargs = {'from_date': from_date, 'to_date': to_date}
return form_kwargs
@staticmethod
def create_updated_formset(range_selection_form, human_resource):
WorkEntryFormSet = forms.formset_factory(WorkEntry,
extra=1,
max_num=60,
can_delete=True,
formset=BaseWorkEntryFormset)
from_date = range_selection_form.cleaned_data['from_date']
to_date = range_selection_form.cleaned_data['to_date']
initial_formset_data = BaseWorkEntryFormset.generate_initial_data(from_date,
to_date,
human_resource)
form_kwargs = BaseWorkEntryFormset.compose_form_kwargs(from_date, to_date)
formset = WorkEntryFormSet(initial=initial_formset_data,
form_kwargs=form_kwargs)
return formset
@staticmethod
def create_new_formset(from_date, to_date, human_resource):
WorkEntryFormSet = forms.formset_factory(WorkEntry,
extra=1,
max_num=60,
can_delete=True,
formset=BaseWorkEntryFormset)
initial_formset_data = BaseWorkEntryFormset.generate_initial_data(from_date,
to_date,
human_resource)
form_kwargs = BaseWorkEntryFormset.compose_form_kwargs(from_date,
to_date)
formset = WorkEntryFormSet(initial=initial_formset_data,
form_kwargs=form_kwargs)
return formset
| 74a0372356b332098a29dde176d3ea0dc9d2ee9d | [
"reStructuredText",
"Markdown",
"INI",
"Python",
"Text",
"Shell"
] | 246 | Python | Shikhar10000/koalixcrm | 6d0230a0f192bb7126c42db59ecfc4369a765a7a | 6fd1d6d3e341af108d1eb090c9a00a7f815edd32 | |
refs/heads/master | <repo_name>AjdinGaco/3DGameFinalProject<file_sep>/README.md
# 3DGameFinalProject
Ajdin 3DGame final project
<file_sep>/Assets/Scripts/EnemyAi.cs
using System.Collections;
using System.Collections.Generic;
using System.Threading;
using UnityEditor;
using UnityEngine;
public class EnemyAi : MonoBehaviour
{
public GameObject enemyHead;
public GameObject enemyBullet;
public GameObject bulletspawnplace;
public GameObject targeter;
public string tagToTarget = "Player";
public float detectionRange = 20f;
public float dmgBullet = 10f;
public float speedBullet = 100f;
public float cooldownShoot = 1f;
public float aimSpeed = 10f;
private bool canShoot = true;
private bool TagWithinRange;
private GameObject tagTarget;
public AudioSource shootingEffect;
private void FixedUpdate()
{
CheckForNearbyTag();
}
void CheckForNearbyTag()
{
GameObject[] plist = GameObject.FindGameObjectsWithTag(tagToTarget);
TagWithinRange = false;
foreach (GameObject item in plist)
{
if (Vector3.Distance(this.transform.position, item.transform.position) < detectionRange)
{
TagWithinRange = true;
tagTarget = item;
}
}
}
void AimAtTag()
{
if (tagTarget != null)
{
targeter.transform.LookAt(tagTarget.transform.position);
float step = aimSpeed * Time.deltaTime;
enemyHead.transform.rotation = Quaternion.RotateTowards(enemyHead.transform.rotation, targeter.transform.rotation, step);
}
}
bool CanHitTag()
{
RaycastHit hit;
if (Physics.Raycast(enemyHead.transform.position, enemyHead.transform.forward, out hit, detectionRange * 1.2f))
{
if (hit.transform.tag == tagToTarget)
{
return true;
}
else
{
return false;
}
}
else
{
return false;
}
}
// Update is called once per frame
void Shoot()
{
GameObject Shot = (GameObject)Instantiate(enemyBullet, bulletspawnplace.transform.position, bulletspawnplace.transform.rotation);
//Here i will adjust the dmg the shot does based on the charge
Damage shotdamage = Shot.GetComponent<Damage>();
BulletLogic shotlogic = Shot.GetComponent<BulletLogic>();
shotdamage.damageAmount = dmgBullet;
shotlogic.m_Speed = speedBullet;
canShoot = false;
cooldowntimer = cooldownShoot;
shootingEffect.GetComponent<AudioSource>().Play();
}
private float cooldowntimer;
void ShootCooldown()
{
cooldowntimer -= Time.deltaTime;
if (cooldowntimer < 0)
{
canShoot = true;
}
}
void Update()
{
if (TagWithinRange)
{
AimAtTag();
}
if (CanHitTag() && canShoot)
{
Shoot();
}
if (!canShoot)
{
ShootCooldown();
}
}
}
<file_sep>/Assets/Scripts/BulletLogic.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BulletLogic : MonoBehaviour
{
public float m_Speed = 10f; // this is the projectile's speed
public float m_Lifespan = 3f; // this is the projectile's lifespan (in seconds)
public float accel = 1f;
public float StormtrooperVariable = 0;
private Rigidbody m_Rigidbody;
void Awake()
{
m_Rigidbody = GetComponent<Rigidbody>();
}
void Start()
{
Vector3 posoffset = new Vector3(Random.Range(-StormtrooperVariable, StormtrooperVariable), 0, Random.Range(-StormtrooperVariable, StormtrooperVariable));
m_Rigidbody.AddForce((m_Rigidbody.transform.forward + posoffset) * m_Speed);
if (gameObject.name.Contains("(Clone)"))
{
//If this is a clone then it must DIE!
Destroy(gameObject, m_Lifespan);
}
else
{
}
}
private void Update()
{
m_Rigidbody.AddForce(m_Rigidbody.transform.forward * accel * Time.deltaTime);
}
}
<file_sep>/Assets/Scripts/MenuItem.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class MenuItem : MonoBehaviour
{
public string LeveltoLoadOnPress;
// Start is called before the first frame update
void Start()
{
this.GetComponent<Renderer>().material.color = Color.black;
}
void OnMouseEnter()
{
this.GetComponent<Renderer>().material.color = Color.red;
}
void OnMouseExit()
{
this.GetComponent<Renderer>().material.color = Color.black;
}
private void OnMouseDown()
{
SceneManager.LoadScene(LeveltoLoadOnPress.ToString());
}
}
<file_sep>/Assets/Scripts/PlayerController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEditorInternal;
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public Transform playerHead;
public Rigidbody playerBody;
public float moveSpeed = 1000f;
public float maxspeed = 100f;
public float CameraSpeed = 3f;
public bool grouded = false;
//Jumping vars
private bool jumping = false;
private bool jumpReady = true;
private float jumpCooldown = 0.20f;
public float jumpforce = 100f;
public KeyCode ControlForward = KeyCode.Z, ControlBack = KeyCode.S, ControlRight = KeyCode.D, ControlLeft = KeyCode.Q, ControlJump = KeyCode.Space;
private bool noinput = false;
private int CX = 0, CY = 0;
void Start()
{
Cursor.lockState = CursorLockMode.Locked;
Cursor.visible = false;
}
// Update is called once per frame
void Update()
{
ReadController();
CameraLook();
Movement();
}
Vector2 rotation = new Vector2(0, 0);
private void CameraLook()
{
rotation.y += Input.GetAxis("Mouse X");
rotation.x += -Input.GetAxis("Mouse Y");
playerHead.transform.eulerAngles = (Vector2)rotation * CameraSpeed;
//WILL FIX LATER? GAVE UP DUE TO MY OWN LAZYNESS
playerBody.angularVelocity = new Vector3(0, 0, 0);
playerBody.transform.rotation = Quaternion.Euler(0, rotation.y * CameraSpeed, 0);
}
private void ReadController()
{
if (Input.GetKey(ControlForward) && !Input.GetKey(ControlBack))
CY = 1;
else if (!Input.GetKey(ControlForward) && Input.GetKey(ControlBack))
CY = -1;
else
CY = 0;
if (Input.GetKey(ControlLeft) && !Input.GetKey(ControlRight))
CX = -1;
else if (!Input.GetKey(ControlLeft) && Input.GetKey(ControlRight))
CX = 1;
else
CX = 0;
if (Input.GetKeyDown(ControlJump))
jumping = true;
else
jumping = false;
if (CX == 0 && CY == 0 && !jumping)
noinput = true;
else
noinput = false;
}
private void Movement()
{
//Better gravity
playerBody.AddForce(Vector3.down * Time.deltaTime * 10);
ContraMovement();
if (jumping && grouded)
Jump();
float multiplier = 1;
if (!grouded)
multiplier = 0.5f;
playerBody.AddForce(playerBody.transform.forward * CY * moveSpeed * Time.deltaTime * multiplier);
playerBody.AddForce(playerBody.transform.right * CX * moveSpeed * Time.deltaTime * multiplier);
}
public Vector3 GetVelocity()
{
return playerBody.velocity;
}
private void ContraMovement()
{
if (noinput && grouded)
{
playerBody.velocity = playerBody.velocity * 0.9f;
}
if (playerBody.velocity.y > 0 && CY < 0)
playerBody.AddForce(playerBody.transform.forward * Time.deltaTime * CY * 0.5f);
if (playerBody.velocity.y < 0 && CY > 0)
playerBody.AddForce(playerBody.transform.forward * Time.deltaTime * CY * 0.5f);
if (playerBody.velocity.x > 0 && CX < 0)
playerBody.AddForce(playerBody.transform.right * Time.deltaTime * CX * 0.5f);
if (playerBody.velocity.x < 0 && CX > 0)
playerBody.AddForce(playerBody.transform.right * Time.deltaTime * CX * 0.5f);
}
private void Jump()
{
if (jumpReady && grouded)
{
jumpReady = false;
playerBody.AddForce(Vector2.up * jumpforce * 2f);
// make it a bit smoother
playerBody.AddForce(Vector2.up * jumpforce);
Invoke(nameof(ResetJump), jumpCooldown);
}
}
private void ResetJump()
{
jumpReady = true;
}
public void AddForce(Vector3 AddedForce)
{
playerBody.AddForce(AddedForce);
}
private void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.tag == "Floor")
grouded = true;
}
private void OnCollisionExit(Collision collision)
{
if (collision.gameObject.tag == "Floor")
grouded = false;
}
}
<file_sep>/Assets/Scripts/GunControll.cs
using System.Collections;
using System.Collections.Generic;
using TMPro;
using UnityEditorInternal;
using UnityEngine;
public class GunControll : MonoBehaviour
{
//This gun has both the functions of an grapling and and chargeable gun :D
public GameObject playerCam;
public GameObject playerBody;
public GameObject playerGun; // For turning it and stuff
public float maxGrapleDis = 10f;
public string tagForGrapplable = "AbleToGrapTo";
public Transform gunPoint;
private SpringJoint grapjoint;
public LineRenderer grapRope;
private Vector3 grappleEndpoint;
//Gun Setttings
private float charge = 0f;
public float chargeSpeed = 1f;
public float chargeMax = 10f;
public float chargeStartScale = 1f;
public float chargeToScaleRation = 1f;
public float chargeToDamage = 1f;
public float chargedShotSpeed = 10;
public float chargeToKnockBackValue = 100f;
public GameObject projectile;
public GameObject graplingHitPrefab;
public AudioSource chargingSoundEffect;
public AudioSource shootingSoundEffect;
public bool canGrapple;
private bool fireGrapple = false, chargeShot = false;
public float GetChargePercentage()
{
return (charge / chargeMax);
}
void Update()
{
canGrapple = CheckIfCanGrapple();
MouseInput();
GunControl();
DrawRope();
}
void GunControl()
{
if (fireGrapple)
GraplingFire();
else
{
ResetLookGun();
GraplingStop();
}
if (chargeShot)
ChargeShot();
else if (charge > 0) // That means there is a shot ready to fire
{
ShootChargedShot();
}
}
private void ChargeShot()
{
if (charge == 0)
{
chargingSoundEffect.GetComponent<AudioSource>().Play();
}
if (charge < chargeMax)
{
charge += chargeSpeed * Time.deltaTime;
}
else
{
chargingSoundEffect.GetComponent<AudioSource>().Stop();
}
}
private void ShootChargedShot()
{
GameObject Shot = (GameObject)Instantiate(projectile, gunPoint.position, gunPoint.rotation);
//Here i will adjust the dmg the shot does based on the charge
Damage shotdamage = Shot.GetComponent<Damage>();
BulletLogic shotlogic = Shot.GetComponent<BulletLogic>();
shotdamage.damageAmount = charge * chargeToDamage;
Shot.transform.localScale = Shot.transform.localScale * (chargeStartScale + charge) * chargeToScaleRation;
shotlogic.m_Speed = chargedShotSpeed;
playerBody.GetComponent<PlayerController>().AddForce(-playerCam.transform.forward * charge * chargeToKnockBackValue); // KnockBaco
chargingSoundEffect.GetComponent<AudioSource>().Stop();
shootingSoundEffect.GetComponent<AudioSource>().Play();
charge = 0f;
}
public bool CheckIfCanGrapple() // This is mostly meant for the Hud
{
RaycastHit hit;
if (Physics.Raycast(playerCam.transform.position, playerCam.transform.forward, out hit, maxGrapleDis))
{
if (hit.transform.gameObject.tag == tagForGrapplable && !playerBody.GetComponent<SpringJoint>())
{
return true;
}
else
{
return false;
}
}
else
{
return false;
}
}
private RaycastHit lastHit;
void GraplingFire()
{
RaycastHit hit;
if (Physics.Raycast(playerCam.transform.position, playerCam.transform.forward, out hit, maxGrapleDis))
{
if (hit.transform.gameObject.tag == tagForGrapplable && !playerBody.GetComponent<SpringJoint>())
{
lastHit = hit;
grappleEndpoint = hit.point;
grapjoint = playerBody.gameObject.AddComponent<SpringJoint>();
grapjoint.autoConfigureConnectedAnchor = false;
grapjoint.connectedAnchor = hit.point;
float distance = Vector3.Distance(playerBody.transform.position, hit.point);
grapjoint.maxDistance = distance * 0.8f;
grapjoint.minDistance = 0;
grapjoint.spring = 1f;
grapjoint.damper = 5f;
grapjoint.massScale = 4.5f;
//Enable the rope thing to work
grapRope.positionCount = 2;
// Hit Effect
Instantiate(graplingHitPrefab, gunPoint.position, gunPoint.rotation);
}
else if (playerBody.GetComponent<SpringJoint>())
{
//Lenght Adjust so it feels a bit better
float distance = Vector3.Distance(playerBody.transform.position, lastHit.point);
if (distance < grapjoint.maxDistance)
{
grapjoint.maxDistance = distance * 0.9f;
}
grapjoint.minDistance = 0;
}
}
}
void GraplingStop()
{
if (playerBody.GetComponent<SpringJoint>())
{
grapRope.positionCount = 0;
Destroy(grapjoint);
}
}
void ResetLookGun()
{
playerGun.transform.localRotation = Quaternion.Euler(0, 0, 0);
}
private Vector3 grappledPos;
void DrawRope()
{
if (grapjoint && grapRope.positionCount > 0)
{
grappledPos = Vector3.Lerp(grappledPos, grappleEndpoint, Time.deltaTime * 8f);
grapRope.SetPosition(0, gunPoint.position);
grapRope.SetPosition(1, grappledPos);
}
}
void MouseInput()
{
if (Input.GetMouseButton(0))
fireGrapple = true;
else
fireGrapple = false;
if (Input.GetMouseButton(1))
chargeShot = true;
else
chargeShot = false;
}
}
<file_sep>/Assets/Scripts/ParticleControll.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ParticleControll : MonoBehaviour
{
// This is mostly for controlling the particles of the player, depending on varius things. This is only meant o make it looks a bit fancier :D
private ParticleSystem playerParticles;
private PlayerController playerControl;
private void Start()
{
playerParticles = this.GetComponent<ParticleSystem>();
playerControl = this.GetComponent<PlayerController>();
}
//= playerControl.GetVelocity().x + playerControl.GetVelocity().y;
void Update()
{
if (!playerControl.grouded)
{
playerParticles.enableEmission = true;
playerParticles.emissionRate = 5f + playerControl.GetVelocity().x + playerControl.GetVelocity().y;
}
else
{
playerParticles.enableEmission = false;
}
}
}
<file_sep>/Assets/Scripts/HudController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEditor;
using UnityEngine;
using UnityEngine.UI;
public class HudController : MonoBehaviour
{
public GameObject player;
public GameObject cursor;
public RectTransform chargeRect; // This is the Rect Tranform of the charge meter
public RectTransform hpRect; // This is the Rect Tranform of the HP meter
public Text livesText;
//Due to my inability to work with children i have taken the easy route to just manualy select them
private GunControll playerguncontroll;
// Start is called before the first frame update
void Start()
{
if (player == null)
{
GameObject[] plist = GameObject.FindGameObjectsWithTag("Player");
player = plist[0];
}
playerguncontroll = player.GetComponent<GunControll>();
}
// Update is called once per frame
void Update()
{
UpdateWeaponCharge();
UpdatePlayerHPandLives();
UpdateCursor();
}
void UpdateCursor()
{
// This changes the cursor color depending if the target can be grappled
if (playerguncontroll.CheckIfCanGrapple())
cursor.GetComponent<Image>().color = new Color32(0, 255, 0, 100);
else
cursor.GetComponent<Image>().color = new Color32(255, 255, 225, 50);
}
void UpdateWeaponCharge()
{
float chargepercentage = playerguncontroll.GetChargePercentage() * 100;
float num = chargeRect.transform.parent.gameObject.GetComponent<RectTransform>().sizeDelta.y / 2;
chargeRect.transform.localPosition = new Vector3(0, -num + (chargepercentage * (num / 100)), 0);
chargeRect.sizeDelta = new Vector2(20f, chargepercentage);
}
void UpdatePlayerHPandLives()
{
float chargepercentage = player.GetComponent<Health>().healthPoints / player.GetComponent<Health>().respawnHealthPoints * 100;
float num = hpRect.transform.parent.gameObject.GetComponent<RectTransform>().sizeDelta.y / 2;
hpRect.transform.localPosition = new Vector3(0, num - (chargepercentage * (num / 100)), 0);
hpRect.sizeDelta = new Vector2(20f, chargepercentage * 2);
livesText.text = player.GetComponent<Health>().numberOfLives.ToString();
}
}
<file_sep>/Assets/Scripts/Goal.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Goal : MonoBehaviour
{
public string LeveltoLoadOnPress;
private void Start()
{
if (LeveltoLoadOnPress == null)
{
LeveltoLoadOnPress = Application.loadedLevelName;
}
}
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.tag == "Player")
{
print("Level Completed");
SceneManager.LoadScene(LeveltoLoadOnPress.ToString());
}
}
}
| 5e545b29b6f752ba9bc8c759fc9c34919696725f | [
"Markdown",
"C#"
] | 9 | Markdown | AjdinGaco/3DGameFinalProject | 2fabd8178a5e6cf6d7bd1bac0fb505c09da3159c | 8ba59f8afac561d3bda051baf27b8e7efd16dc90 | |
refs/heads/main | <file_sep>from bs4 import BeautifulSoup
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKCYAN = '\033[96m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
class Sito:
def __init__(self,h1,h2,h3,h4,link,div,script):
self.h1 = h1
self.h2 = h2
self.h3 = h3
self.h4 = h4
self.link = link
self.div = div
self.script = script
def BootStrap(self):
bsurl = "maxcdn.bootstrapcdn.com"
for x in self.link:
if bsurl in str(x.get("href")):
return(bcolors.OKGREEN + "BootStrap"+ bcolors.ENDC)
return(bcolors.FAIL + "BootStrap"+ bcolors.ENDC)
def jQuery(self):
jqurl = "code.jquery.com"
for x in self.script:
if jqurl in str(x.get("src")):
return(bcolors.OKGREEN + "Jquery"+ bcolors.ENDC)
return(bcolors.FAIL + "Jquery"+ bcolors.ENDC)
def Dati(self):
print(f"""
--- RESULT ---
H1 : {len(self.h1)}
H2 : {len(self.h2)}
H3 : {len(self.h3)}
H4 : {len(self.h4)}
Link : {len(self.link)}
Div : {len(self.div)}
Script : {len(self.script)}
--- LIBRARY ---
{self.BootStrap()}
{self.jQuery()}
--- RESULT ---
""")
def Search(site):
h1 = site.find_all(name = "h1")
h2 = site.find_all(name = "h2")
h3 = site.find_all(name = "h3")
h4 = site.find_all(name = "h4")
link = site.find_all(name = "link")
div = site.find_all(name = "div")
script = site.find_all(name = "script")
return Sito(h1,h2,h3,h4,link,div,script)
<file_sep>from bs4 import BeautifulSoup
import search
import requests
# Request the URL of The Page
url = input(":: Inserire Indirizzo :> ")
# Open a Session and clone the page
response = requests.get(url)
# Generate a Soup Object of The Source Code
soup = BeautifulSoup(response.text,'html.parser')
sito = search.Search(soup)
sito.Dati()
# bsurl = "maxcdn.bootstrapcdn.com"
# link = soup.find_all(name = "link")
# bootstrap = False
# for x in link:
# if bsurl in str(x.get("href")):
# print("ciao")<file_sep># Web_Scraper
Python Web Scraper
<h1> Victory Web Scraper </h1>
<p> python project </p>
<h2> Features </h2>
<ul>
<li> How Many <strong>H1</strong> are Inside </li>
<li> How Many <strong>H2</strong> are Inside </li>
<li> How Many <strong>H3</strong> are Inside </li>
<li> How Many <strong>H4</strong> are Inside </li>
<li> How Many <strong>Link</strong> are Inside</li>
<li> How Many <strong>Div</strong> are Inside</li>
<li> How Many <strong>Script</strong> are Inside</li>
<li> If the Site use <strong>Bootstrap</strong></li>
<li> If the Site use <strong>Jquery</strong></li>
</ul>
| 6a2a9f756b8b39993f756232d738fa175a33bfb9 | [
"Markdown",
"Python"
] | 3 | Python | GrandeVx/Web_Scraper | 4f175347c1d611b4ca03346dbcb4b904a9fb121f | a38b8fc1ec1919095a06c9a606ada8fb1f479ef9 | |
refs/heads/master | <repo_name>kmillns/js-interview<file_sep>/questions/simple_click_handler.js
<a href="#">Show it.</a>
<div style="display: none;">
<span>Hidden stuff.</span>
</div>
// write a simple click handler to show the hidden div<file_sep>/solutions/scope_and_hoisting.js
// What gets logged to the console?
var foo = 'hello';
(function() {
var foo = foo || 'world';
console.log(foo);
})();
// Answer: 'world'
// This is a bog simple case of variable hoisting and scope precendence
// It's much clearer once you break it down into what the interpreter is doing
// Step one: variables are hoisted to the top of their scope.
var foo = 'hello';
(function() {
var foo;
foo = foo || 'world';
console.log(foo);
})();
// Step two, unassigned variables are undefined
var foo = 'hello';
(function() {
var foo;
foo = undefined || 'world';
console.log(foo);
})();
// Step three and undefined is falsey, therefore assign 'world' to foo
var foo = 'hello';
(function() {
var foo;
foo = false || 'world';
console.log(foo);
})();
// For bonus points:
// What's logged to the console here
var foo = 'hello';
(function() {
var foo = foo || 'world';
console.log(foo);
})();
console.log(foo);
// Answer:
// 'world'
// 'hello'
// Because the inner function scoped foo doesn't affect the outer variable
// just overwrites it locally.
<file_sep>/questions/functional_programming.js
// Write code such that the following alerts "Hello World"
say('Hello')('World');<file_sep>/solutions/preventDefault_return_false.js
<div>
<a href="/doStuff">foo</a>
</div>
// what's the difference between these three click handlers?
// This version will prevent the default action (following the link)
// but will also stop propogation (events bound up the DOM won't recieve it),
// and stop immediate propogation (subsequent events on this element won't
// receive it, either)
$('a').on('click', function (event) {
$(this).doStuff();
return false;
});
// Both of these two examples only prevent default, which allows for
// propogation normally.
// The difference is subtle, and relates to what happens in a failure case.
// In the first example, if doStuff() fails, you still click through to the
// underlying link action.
// In the second, if doStuff() fails, your link is dead, and does nothing.
// Thus, the second example (preventDefault *last* in the event handler) is
// the preferable option for progressive enhancement purposes.
$('a').on('click', function (event) {
$(this).doStuff();
event.preventDefault();
});
$('a').on('click', function (event) {
event.preventDefault();
$(this).doStuff();
});
<file_sep>/solutions/prototypes.js
// 3: given the following code, how would you override the value of the
// bar property for the variable foo without affecting the value of the
// bar property for the variable bim? how would you affect the value of
// the bar property for both foo and bim? how would you add a method to
// foo and bim to console.log the value of each object's bar property? how
// would you tell if the object's bar property had been overridden for the
// particular object?
var Thinger = function() {
return this;
};
Thinger.prototype = {
bar: 'baz'
};
var foo = new Thinger(),
bim = new Thinger();
// how would you override the value of the
// bar property for the variable foo without affecting the value of the
// bar property for the variable bim?
// What we're looking for here is if you understand the property lookup chain.
// Local properties are tried first and don't affect other objects' properties.
foo.bar = 'override';
bim.bar === 'baz'; // true
// how would you affect the value of
// the bar property for both foo and bim?
// Note: this only affects the value if it *hasn't* been set locally
// This time we're asking for the other side of things.
// Altering a prototype alters the objects that have been created with that
// prototype *even after they've been created*.
Thinger.prototype.bar = 'woo';
bim.bar === 'woo'; // true
foo.bar === 'woo'; // false (foo is using its local value)
foo.bar === 'override'; // true
// how would you add a method to
// foo and bim to console.log the value of each object's bar property?
// Here we're testing adding objects to the prototype after the objects
// were created.
// Also, we're testing if you understand the scope of 'this' inside prototype
// properties points to the instance of the object.
Thinger.prototype.logger = function () {
console.log(this.bar);
}
foo.logger(); // logs 'override'
bar.logger(); // logs 'woo'
// how
// would you tell if the object's bar property had been overridden for the
// particular object?
// Testing if you understand 'hasOwnProperty'
foo.hasOwnProperty('bar'); // true
bim.hasOwnProperty('bar'); // false
// BONUS: When would you use 'hasOwnProperty' in practice?
// Crockford-ian Answer:
// As a filter when enumerating over an object's properties with
// for ( in ) {} loops to defend against taking prototype properties
Array.prototype.badIdea = function () {
console.log('oh god why are you modifying globals?!');
};
var test = [1, 2, 3];
var i;
for (i in test) {
console.log(i);
}
// Oh no you broke it.
for (i in test) {
if (test.hasOwnProperty(i)) {
console.log(i);
}
}
// jQuery answer:
// Test if an object is a plain object based on the presence of a constructor
// or prototype
core_hasOwn = Object.prototype.hasOwnProperty
isPlainObject: function( obj ) {
// Must be an Object.
// Because of IE, we also have to check the presence of the constructor property.
// Make sure that DOM nodes and window objects don't pass through, as well
if ( !obj || jQuery.type(obj) !== "object" || obj.nodeType || jQuery.isWindow( obj ) ) {
return false;
}
try {
// Not own constructor property must be Object
if ( obj.constructor &&
!core_hasOwn.call(obj, "constructor") &&
!core_hasOwn.call(obj.constructor.prototype, "isPrototypeOf") ) {
return false;
}
} catch ( e ) {
// IE8,9 Will throw exceptions on certain host objects #9897
return false;
}
// Own properties are enumerated firstly, so to speed up,
// if last one is own, then all properties are own.
var key;
for ( key in obj ) {}
return key === undefined || core_hasOwn.call( obj, key );
}
<file_sep>/questions/module_pattern.js
// How would you update this function to make the last two lines true
var first = '1';
var second = '2';
var name = 'foo';
var foo = (function () {
})();
foo.one === '1foo';
foo.two === '2foo';<file_sep>/solutions/jQuery_refactoring.js
// 6: how could you improve the following code?
$(document).ready(function () {
$('.foo #bar').css('color', 'red');
$('.foo #bar').css('border', '1px solid blue');
$('.foo #bar').text('new text!');
$('.foo #bar').click(function() {
$(this).attr('title', 'new title');
$(this).width('100px');
});
$('.foo #bar').click();
});
// First thing I see is selector caching:
// 6: how could you improve the following code?
$(document).ready(function () {
var $fooBar = $('.foo #bar');
$fooBar.css('color', 'red');
$fooBar.css('border', '1px solid blue');
$fooBar.text('new text!');
$fooBar.click(function () {
$(this).attr('title', 'new title');
$(this).width('100px');
});
$fooBar.click();
});
// Don't forget to cache $(this)
$(document).ready(function () {
var $fooBar = $('.foo #bar');
$fooBar.css('color', 'red');
$fooBar.css('border', '1px solid blue');
$fooBar.text('new text!');
$fooBar.click(function () {
var $this = $(this);
$this.attr('title', 'new title');
$this.width('100px');
});
$fooBar.click();
});
// The $ prefix is a psuedo-Hungarian notation convention that says that the
// object is a jQuery selector result. Not necessary, but idiomatic
// and a standard for us.
// Second thing is that we can chain the calls
$(document).ready(function () {
var $fooBar = $('.foo #bar');
$fooBar
.css('color', 'red')
.css('border', '1px solid blue')
.text('new text!')
.click(function () {
var $this = $(this);
$this
.attr('title', 'new title')
.width('100px');
})
.click();
});
// and yes, the chaining eliminates the need for selector caching in this case.
// The selector caching is still a good idea, and readability is preferred over
// clever chaining.
// Finally, you can condense the multiple CSS calls:
$(document).ready(function () {
var $fooBar = $('.foo #bar');
$fooBar
.css({
color: 'red',
border: '1px solid blue'
})
.text('new text!')
.click(function () {
var $this = $(this);
$this
.attr('title', 'new title')
.width('100px');
})
.click();
});
// Bonus points:
// An id selector should very very rarely depend on being inside a class,
// since a given id should be extremely specific.
var $fooBar = $('.foo #bar');
// becomes
var $bar = $('#bar');
// The CSS manipulation in JS creates mainentance and reuse problems
$fooBar
.css({
color: 'red',
border: '1px solid blue'
})
// becomes
$fooBar
.addClass('funkyColor')
// with some CSS
.funkyColor {
color: red;
border: 1px solid blue;
}
// Triple testability bonus points:
// Extract the click handler into a named function:
$(document).ready(function () {
var titleIt = function () {
var $this = $(this);
$this
.attr('title', 'new title')
.width('100px');
};
var $fooBar = $('#bar');
$fooBar
.addClass('funkyColor')
.text('new text!')
.click(titleIt)
.click();
});
// Expose the whole thing outside the ready function as a testable object
window.funkifier = {
titler: function () {
var $this = $(this);
$this
.attr('title', 'new title')
.width('100px');
},
makeFunky: function (elementToFunkify) {
var $target = $(elementToFunkify);
$target
.addClass('funkyColor')
.text('new text!')
.click(funkifier.titler)
.click();
}
};
$(document).on('ready', function () {
window.funkifier.makeFunky('#bar');
});
// Now we can test 'titler' and 'makeFunky' individually, rather than just
// testing their side effects
// Alternatively you could create a Funkifier object to be constructed
// with the properties you want to expose.
window.Funkifier = function (elementToFunkify) {
var self = this;
self.titler = function () {
var $this = $(this);
$this
.attr('title', 'new title')
.width('100px');
};
self.makeFunky = function () {
var $target = $(elementToFunkify);
$target
.addClass('funkyColor')
.text('new text!')
.click(self.titler)
.click();
};
return {
titler: self.titler,
makeFunky: self.makeFunky
};
};
$(document).on('ready', function () {
var funkifier = new Funkifier('#bar');
funkifier.makeFunky();
});
<file_sep>/questions/ternary_and_bracket_property_access.js
// 1: how could you rewrite the following to make it shorter?
if (foo) {
bar.doSomething(el);
} else {
bar.doSomethingElse(el);
}<file_sep>/questions/scope_and_hoisting.js
// What gets logged to the console?
var foo = 'hello';
(function() {
var foo = foo || 'world';
console.log(foo);
})(); | b32d30e6c0f6cea754214a4b14c9d19f4b69ebdc | [
"JavaScript"
] | 9 | JavaScript | kmillns/js-interview | f3b5d5c2dd5690f9f587d1360e3e5ebc1c2df24a | 842ca4f46d71057de3aefe30409ab985ce9db306 | |
refs/heads/main | <repo_name>Mallaxy/NextJS<file_sep>/pages/api/echo/[id].ts
import {NextApiRequest, NextApiResponse} from 'next'
type Data = {
yourId: string | string[]
}
export default function getById(req: NextApiRequest, res: NextApiResponse<Data>) {
// res.statusCode = 200
// res.setHeader('Content-Type', 'application/json')
// res.end(req.query.id)
res.json({yourId: req.query.id})
}
<file_sep>/pages/api/echo.ts
import {NextApiRequest, NextApiResponse} from 'next'
type Data = {
message: string
}
export default function echo(req: NextApiRequest, res: NextApiResponse<Data>) {
res.statusCode = 200
res.setHeader('Content-Type', 'application/json')
res.end(JSON.stringify({
message: req.query.message ?? 'Base Message'
}))
}
| 80a577a4ebd0e2e13f518b3aeeed085ce54f8bdf | [
"TypeScript"
] | 2 | TypeScript | Mallaxy/NextJS | b92206f0759ba4ec45d3526d410ed971681bb273 | 5defe6a8c191f5c8709f2e1414e9657daa95e674 | |
refs/heads/master | <repo_name>pop0030/vue-webview-based-player<file_sep>/src/js/fbSDK.js
import axios from 'axios'
import auth from './auth'
const fields = {
"fields": "name,id,picture,email,cover,age_range,link,gender,locale,timezone,verified"
}
window.fbAsyncInit = ()=>{
fbAsyncInit()
}
function fbAsyncInit() {
console.log('[facebook]=>FB套件建立')
FB.init({
appId: '1925894757644354',
cookie: true, // enable cookies to allow the server to access
// the session
xfbml: true, // parse social plugins on this page
version: 'v2.8' // use graph api version 2.8
});
// Now that we've initialized the JavaScript SDK, we call
// FB.getLoginStatus(). This function gets the state of the
// person visiting this page and can return one of three states to
// the callback you provide. They can be:
//
// 1. Logged into your app ('connected')
// 2. Logged into Facebook, but not your app ('not_authorized')
// 3. Not logged into Facebook and can't tell if they are logged into
// your app or not.
//
// These three cases are handled in the callback function.
auth.stateCheck()
};
// This is called with the results from from FB.getLoginStatus().
function statusChangeCallback(response) {
console.log('[狀態檢查]=>檢查中...')
// The response object is returned with a status field that lets the
// app know the current login status of the person.
// Full docs on the response object can be found in the documentation
// for FB.getLoginStatus().
if (response.status === 'connected') {
console.log('[狀態檢查]=>已連結')
// Logged into your app and Facebook.
} else if (response.status === 'not_authorized') {
// The person is logged into Facebook, but not your app.
console.log('[狀態檢查]=>未授權')
} else {
// The person is not logged into Facebook, so we're not sure if
// they are logged into this app or not.
console.log('[狀態檢查]=>未登入')
}
}
function getData() {
return new Promise(function(resolve, reject) {
console.log('[資料連線]=>連線中...')
let str = new Date()
FB.getLoginStatus(function(response){
statusChangeCallback(response);
if (response.status === 'connected') {
FB.api('/me', 'GET', fields, function(res) {
console.log('[資料連線]=>取得資料')
let end = new Date()
console.log('[資料連線]=>時間:'+(end-str)+'ms')
resolve(res)
})
} else {
resolve('unconnected')
}
})
})
}
// This function is called when someone finishes with the Login
// Button. See the onlogin handler attached to it in the sample
// code below.
function checkLoginState() {
FB.getLoginStatus(function(response) {
statusChangeCallback(response);
});
}
// Here we run a very simple test of the Graph API after login is
// successful. See statusChangeCallback() for when this call is made.
function login() {
console.log('[登入]連線中...')
FB.login(async function(res){
console.log('[登入]連線完成')
statusChangeCallback(res)
auth.stateCheck()
}, {scope: 'public_profile,email'})
//checkLoginState()
}
function logout() {
return new Promise((resolve,reject)=>{
console.log('[登出]連線中...')
FB.api('/me/permissions', 'DELETE', function(res) {
console.log('[登出]連線完成')
statusChangeCallback(res)
resolve()
})
})
}
export default {
fbAsyncInit,
logout,
login,
getData
}<file_sep>/src/js/contentSDK.js
import axios from 'axios'
const contentSDK = {}
const nav = navigator.userAgent
let canDownload = true
const os = function() {
var os = ""
if (/(Android)/i.test(nav)) {
//console.log('android')
os = "android"
} else if (/(iPhone|iPad|iPod|iOS)/i.test(nav)) {
//console.log('ios')
os = "ios"
} else {
os = "pc"
}
return os
}
contentSDK.getDeviceId = function() {
if (os == 'android') {
//console.log("android")
window.JSInterface.getDeviceId()
} else if (os == 'ios') {
//console.log("ios")
location.href = 'app:$getDeviceId'
} else {
//console.log("pc")
}
}
contentSDK.genToken = async function(deviceId, userId, getJWTTokenURL) {
let jsonData = JSON.stringify({ "iss": "ubn.com", "company": "ubn", "awesome": true, "account": "u7481722", "deviceId": deviceId, "userId": userId })
let strTime = new Date()
let res = await axios({
url: getJWTTokenURL,
method: 'POST',
responseType: "json",
header: {
'Content-Type': 'application/json',
},
data: jsonData
})
let endTime = new Date()
let transTime = endTime - strTime
//console.log(transTime+ 'ms')
contentSDK.saveToken(res.returnToken)
return res.data
}
contentSDK.sendAllContentId = function(strIds) {
console.log(strIds)
if (os == 'android') {
//console.log("android")
window.JSInterface.checkBookDownload(strIds)
} else if (os == 'ios') {
//console.log("ios")
location.href = 'app:$checkBookDownload:$strIds=' + strIds
} else {
//console.log("pc")
}
}
contentSDK.saveToken = function(token) {
//console.log("os=" + os + "saveToken=" + token)
if (os == 'android') {
window.JSInterface.saveToken(token)
} else if (os == 'ios') {
location.href = 'app:$saveToken:$token=' + token
}
}
contentSDK.checkBookDownload = function(strIds) {
}
contentSDK.downloadBookFile = function(contentId, token, cdn_url, imagePath) {
//console.log('contentId==>' + contentId)
//console.log('token==>' + token)
//console.log('cdn_url==>' + cdn_url)
//console.log('imagePath==>' + imagePath)
if (canDownload) {
if (os == 'android') {
window.JSInterface.downloadBookFile(contentId, token, cdn_url, imagePath)
} else if (os == 'ios') {
location.href = 'app:$downloadBookFile:$contentId=' + contentId + '&token=' + token + '&cdn_url=' + encodeURI(cdn_url) + '&image_url=' + encodeURI(imagePath)
}
canDownload = false
} else {
alert('檔案尚在下載中,請稍後')
return
}
}
contentSDK.bindDownloadChanged = function(contentId, percent) {
//console.log("percent=" + percent)
if (percent == 100) {
canDownload = true
}
bindDownloadChanged(contentId, percent)
}
contentSDK.openEPUB = function(contentId) {
//console.log('open epub contentId=' + contentId)
if (os == 'android') {
window.JSInterface.openEPUB(contentId)
} else if (os == 'ios') {
location.href = 'app:$openEPUB:$contentId=' + contentId
}
}
contentSDK.deleteBookFile = function(contentId) {
if (os == 'android') {
window.JSInterface.deleteBookFile(contentId)
} else if (os == 'ios') {
location.href = 'app:$deleteBookFile:$contentId=' + contentId
}
}
contentSDK.deletedContentId = function(contentId) {
}
contentSDK.playMovie = function(contentPath) {
if (os == 'android') {
window.JSInterface.playMovie(contentPath)
} else if (os == 'ios') {
location.href = 'app:$playMovie:$contentPath=' + contentPath
}
}
contentSDK.errorCallback = function(errCode) {
//console.log('JS errorCallback:' + errCode)
canDownload = true
errorCallback(errCode)
}
contentSDK.openQRCode = function() {
//console.log('JS openQRCode.....')
if (os == 'android') {
window.JSInterface.openQRCode()
} else if (os == 'ios') {
}
}
export default contentSDK
<file_sep>/src/js/auth.js
import axios from 'axios'
import fb from './fbSDK'
import store from './store'
const stateCheck = async function(){
console.log('[檢查登入]開始檢查...')
let apiId = '<KEY>'
let apiUrl = 'https://script.google.com/macros/s/'+ apiId +'/exec'
let fbDataObj = await fb.getData()
if ( fbDataObj === 'unconnected' ) {
console.log('[檢查登入]FB未登入或其他問題')
} else {
console.log('[檢查登入]FB已授權連線')
let str = new Date()
let fbDataText = JSON.stringify(fbDataObj)
console.log('[檢查登入]確認會員資料...')
axios({
method: 'POST',
url: apiUrl,
params : {
action: 'loginFromFacbook'
},
data: fbDataText
}).then((res)=>{
let userData = res.data[fbDataObj.id]
console.log('[檢查登入]完成確認')
console.log('歡迎!'+userData.name)
let end = new Date()
console.log('[檢查登入]=>時間:'+(end - str)+'ms')
store.commit('updateUser', userData)
}).catch((err)=>{
console.log(err)
})
}
}
const login = function(){
fb.login()
}
const logout = async function(){
let logout = await fb.logout()
store.commit('clearUser')
}
export default {
stateCheck,
login,
logout
} | 63b0623824c3db1a5b49471af56e17be2c9962e1 | [
"JavaScript"
] | 3 | JavaScript | pop0030/vue-webview-based-player | ed39439b17619ca3b7c29e777fc3e1b481d27214 | 14218938d921b8acf44a57cc6bb24f38e97f2a26 | |
refs/heads/master | <file_sep>using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace Hemming
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
double[,] files;
double[,] error_files;
double[,] W;
public double[,] Load_img(string[] directory)
{
Bitmap img = new Bitmap(directory[0]);
/*using (Graphics g = Graphics.FromImage(img))
g.Clear(Color.White);*/
double[,] file = new double[directory.Length, img.Width * img.Height];
for(int k = 0; k < directory.Length; k++)
{
int n = 0;
for (int i = 0; i < img.Width; i++)
for (int j = 0; j < img.Height; j++)
{
if (img.GetPixel(i, j) == Color.FromArgb(0, 0, 0))
{
file[k, n] = 1;
}
else
{
file[k, n] = 0;
}
n++;
}
}
//pictureBox1.Image = img;
return file;
}
private void button1_Click(object sender, EventArgs e)
{
comboBox1.Items.Clear();
string[] dir_error_file = Directory.GetFiles(@"C:\NewralNetwork\Hemming\Error");
error_files = Load_img(dir_error_file);
string[] dir_file = Directory.GetFiles(@"C:\NewralNetwork\Hemming\Example");
files = Load_img(dir_file);
W = new double[files.GetLength(0), files.GetLength(1)];
set_combo(dir_error_file);
}
public void init_W()
{
for (int i = 0; i < W.GetLength(0); i++)
for (int j = 0; j < W.GetLength(1); j++)
W[i, j] = files[i, j];
}
private void button2_Click(object sender, EventArgs e)
{
init_W();
}
private double[] classif(double[] x_new)
{
double[] y = first_layer(x_new);
double[] y_new = second_layer(y);
int t = 0;
double len = length_hem(y, y_new);
while (t < 500 && length_hem(y, y_new) > 0)
{
y = y_new;
y_new = second_layer(y);
t++;
}
int g = t;
return y_new;
}
private double[] first_layer(double[] x_new)
{
double[] y = new double[files.GetLength(0)];
for (int i = 0; i < files.GetLength(0); i++)
{
double temp = 0;
for (int j = 0; j < files.GetLength(1); j++)
{
temp += W[i, j] * x_new[i];
}
y[i] = temp + files.GetLength(1) / 2.0;
}
return y;
}
private double[] second_layer(double[] y_old)
{
double[] y = new double[files.GetLength(0)];
double eps = 1.0 / files.GetLength(0);
for (int j = 0; j < y.Length; j++)
{
double temp = 0;
for (int i = 0; i < y.Length; i++)
{
if (i != j)
temp += y_old[i];
}
temp *= eps;
y[j] = f(y_old[j] - temp, 200);
}
return y;
}
double f(double x, int N = 100)
{
if (x < 0) return 0;
else if (x > 0 && x < N)
return x;
else return N;
}
private double length_hem(double[] y, double[] d)
{
double temp = 0;
for (int i = 0; i < y.Length; i++)
{
if (y[i] != d[i])
temp += 1;
}
return temp;
}
private void set_combo(string[] a)
{
for (int i = 0; i < a.Length; i++)
{
string s = a[i].Substring(a[i].LastIndexOf('\\') + 1);
comboBox1.Items.Add(s);
}
}
private double[] arr_from_matr(double[,] y, int t)
{
double[] temp = new double[y.GetLength(1)];
for (int i = 0; i < temp.Length; i++)
{
temp[i] = y[t, i];
}
return temp;
}
private void button3_Click(object sender, EventArgs e)
{
textBox1.Text = "";
double[] result = classif(arr_from_matr(error_files, comboBox1.SelectedIndex));
for (int i = 0; i < result.Length; i++)
{
if (result[i] == result.Max())
textBox1.Text += i.ToString() + " , ";
}
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
string dir = @"C:\NewralNetwork\Hemming\Error\" + (comboBox1.SelectedIndex + 1) + ".bmp";
Bitmap img = new Bitmap(pictureBox1.Width,pictureBox1.Height);
using (Graphics g = Graphics.FromImage(img))
g.Clear(Color.White);
pictureBox1.Image = img;
int step = 20; // порог
img = new Bitmap(dir);
int average = (int)(pictureBox1.BackColor.R + pictureBox1.BackColor.G + pictureBox1.BackColor.B) / 3;
double[,] result = new double[img.Width, img.Height];
for (int i = 0; i < img.Width; i++)
for (int j = 0; j < img.Height; j++)
{
Color pixel = img.GetPixel(i, j);
int average2 = (int)(pixel.R + pixel.G + pixel.B) / 3;
if (average2 >= average - step && average2 <= average + step)
{
img.SetPixel(i, j, Color.White);
result[i, j] = 0;
}
else
{
img.SetPixel(i, j, Color.Black);
result[i, j] = 1;
}
}
pictureBox1.Image = img;
}
}
}
| 39b5af633af7477a87a6db7de8fbd6ca9f427788 | [
"C#"
] | 1 | C# | Fantom426/Hemming_Network | 0c3bbca9b361e674c3b393acbfcd923e37c62702 | 27c9e0d82f227c376224e87f014cdc466fcac479 | |
refs/heads/master | <repo_name>sheilazpy/agile-training-java<file_sep>/src/test/java/com/chikli/training/cucumber/features/RunCompletedCukeTest.java
package com.chikli.training.cucumber.features;
import org.junit.Ignore;
import org.junit.runner.RunWith;
import cucumber.junit.Cucumber;
@Ignore
@RunWith(Cucumber.class)
@Cucumber.Options(tags = { "@ok" }, format = { "pretty", "html:target/cucumber-html-report" })
public class RunCompletedCukeTest {
} | 43bf8dfe00bc496d1de0721e6eb8ee10d0fd76f4 | [
"Java"
] | 1 | Java | sheilazpy/agile-training-java | 2a17131fa40ac6dd878c1bdbc5252e78f86b7a02 | 421e548758bd8cd423899688ac549d4fa36d7c42 | |
refs/heads/master | <repo_name>TeamNavdeep/LetsBrawl<file_sep>/2dFinal GIT/Assets/Scripts/Main/TestHealth.cs
using UnityEngine;
using System.Collections;
public class TestHealth : MonoBehaviour {
private float health;
public GameObject healthbar;
private GameObject healthbar2;
private bool isAlive;
// Use this for initialization
void Start () {
print(gamestart.healthPower);
health = gamestart.healthPower;
isAlive = true;
healthbar2 = (GameObject)Instantiate (healthbar, transform.position, Quaternion.identity);
}
// Update is called once per frame
void Update () {
if (health <= 0) {
isAlive =false;
Destroy(healthbar);
}
healthbar2.transform.localScale = new Vector3 (health/100, 0.05f, 1);
healthbar2.transform.position = new Vector3(this.transform.position.x, this.transform.position.y+ 2.0f, this.transform.position.z);
//For Testing
if (Input.GetKeyDown(KeyCode.F5))
{
health -= 20;
}
if (!Network.isServer && !Network.isClient){
Destroy(healthbar);
}
}
public bool getAliveStatus(){
return isAlive;
}
public void setAliveStatus(bool status)
{
isAlive = status;
}
void OnCollisionEnter(Collision col){
if (col.gameObject.tag == "Projectile") {
health -= 10.0f;
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/PlayerController.cs
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
public class PlayerController : MonoBehaviour {
public GameObject player;
//buttons
public GameObject leftBtn,rightBtn,jumpBtn, shootBtn, gunBtn;
//projectiles
public GameObject pistolprojectile;
private int slotNum;
//movespeed
private Vector3 movement = Vector3.forward * 0.1f;
private WeaponManager wepManage;
//shooting variables
private float timer = 0f;
private float limit;
private bool canshoot = false;
private float speed = 10f;
//jump and ground check
public static bool isGrounded;
public static bool doubleJump;
public bool checkWalking;
public bool checkJump;
public bool checkDoubleJump;
private bool isleft = false;
private float xoffset = 0.7f;
//multi touch support
public static Touch[] touches;
private int fingertouch= 0;
//animation
private Animator anim;
private NetworkView netView;
//Sound variables
public AudioClip hgSound;
public AudioClip arSound;
public AudioClip sgSound;
void shoot(){
if (isleft) {
xoffset = -0.7f;
} else {
xoffset = 0.7f;
}
Vector3 bulletspawnpos = new Vector3 (
player.transform.position.x + xoffset,
player.transform.position.y + 0.7f,
player.transform.position.z
);
GameObject bullet =
(GameObject) Network.Instantiate(pistolprojectile, bulletspawnpos, player.transform.rotation, 0);
}
void Start ()
{
anim = this.GetComponent<Animator> ();
netView = this.GetComponent<NetworkView> ();
leftBtn = GameObject.Find ("Left_Btn");
rightBtn = GameObject.Find ("Right_Btn");
jumpBtn = GameObject.Find ("Jump_Btn");
shootBtn = GameObject.Find ("Shoot_Btn");
gunBtn = GameObject.Find ("Gun_Btn");
wepManage = GetComponent<WeaponManager> ();
}
void Update ()
{
timer += Time.deltaTime;
switch (wepManage.GetSlotNum()) {
case 0:
limit = 1.0f - gamestart.fireRate;
break;
case 1:
limit = 1.5f - gamestart.fireRate;
break;
case 2:
limit = 0.3f - gamestart.fireRate;
break;
case 5:
limit = 1.0f - gamestart.fireRate;
break;
}
if (timer >= limit) {
canshoot = true;
}
// for single touch use this code
//Input.GetTouch (0).phase == TouchPhase.Began
//for touch and hold
//Input.GetTouch (0).phase != TouchPhase.Ended && Input.GetTouch (0).phase != TouchPhase.Canceled
// to check if ray cast is hitting an object via touch
// if ( Physics.Raycast(ray, out hit) && hit.transform.gameObject == GAMEOBJECT)
if (netView.isMine){
if (Input.touchCount > 0 )
{
foreach(Touch t in Input.touches)
{
Ray ray = Camera.main.ScreenPointToRay( t.position );
RaycastHit hit;
if(t.phase != TouchPhase.Ended && t.phase != TouchPhase.Canceled)
{
if ( Physics.Raycast(ray, out hit) && hit.transform.gameObject == leftBtn)
{
isleft = true;
//Debug.Log("CLICK LEFT");
anim.SetBool("Moving", true);
checkWalking = true;
player.transform.rotation = Quaternion.Euler(0.0f, 270.0f, 0.0f);
player.transform.Translate(movement);
}
else if ( Physics.Raycast(ray, out hit) && hit.transform.gameObject == rightBtn)
{
isleft = false;
//Debug.Log("CLICK RIGHT");
anim.SetBool("Moving", true);
checkWalking = true;
player.transform.rotation = Quaternion.Euler(0.0f, -270.0f, 0.0f);
player.transform.Translate(movement);
}
//For Testing - START -
if (Input.GetKeyDown(KeyCode.F8))
{
{
anim.SetTrigger("Fire");
Debug.Log("SHOOTING");
if (wepManage.GetSlotNum() != 5)
{
shoot();
switch (wepManage.GetSlotNum())
{
case 0:
audio.PlayOneShot(hgSound);
break;
case 1:
audio.PlayOneShot(arSound);
break;
case 2:
audio.PlayOneShot(sgSound);
break;
}
}
canshoot = false;
timer = 0.0f;
}
}
//For Testing - END -
if (Physics.Raycast(ray, out hit) && hit.transform.gameObject == shootBtn)
{
if(canshoot == true){
anim.SetTrigger("Fire");
Debug.Log("SHOOTING");
if(wepManage.GetSlotNum() != 5){
shoot ();
switch (wepManage.GetSlotNum())
{
case 0:
audio.PlayOneShot(hgSound);
break;
case 1:
audio.PlayOneShot(arSound);
break;
case 2:
audio.PlayOneShot(sgSound);
break;
}
}
canshoot = false;
timer = 0.0f;
}
}
}
else
{
anim.SetBool("Moving", false);
checkWalking = false;
}
if(t.phase == TouchPhase.Began)
{
if (Physics.Raycast(ray, out hit) && hit.transform.gameObject == gunBtn)
{
slotNum++;
if(slotNum > 2)
{
slotNum = 0;
}
wepManage.SwitchWeapon(slotNum);
switch(wepManage.GetSlotNum())
{
case 0:
wepManage.SwitchWeapon(0);
break;
case 1:
wepManage.SwitchWeapon(1);
break;
case 2:
wepManage.SwitchWeapon(2);
break;
}
}
if (Physics.Raycast(ray, out hit) && hit.transform.gameObject == jumpBtn && isGrounded)
{
anim.SetTrigger("Jump");
checkJump = true;
isGrounded = false;
//Debug.Log("CLICK JUMP");
player.rigidbody.AddForce(new Vector2(0,1000f));
}
else if(Physics.Raycast(ray, out hit) && hit.transform.gameObject == jumpBtn && !isGrounded && doubleJump)
{
anim.SetTrigger("Double Jump");
checkDoubleJump = true;
player.rigidbody.AddForce(new Vector2(0,750f));
doubleJump=false;
}
}
}
}
}
syncAnimation ();
}
[RPC]
void syncAnimation(){
if (checkJump){
anim.SetTrigger("Jump");
checkJump = false;
}
if (checkDoubleJump){
anim.SetTrigger("Double Jump");
checkDoubleJump = false;
}
if (checkWalking){
anim.SetBool("Moving", true);
}
else{
anim.SetBool("Moving", false);
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Testing/LivesTest.cs
using UnityEngine;
using System.Collections;
public class LivesTest : MonoBehaviour {
private BattleResultsManager brmScript;
// Use this for initialization
void Start () {
brmScript = GetComponent<BattleResultsManager>();
}
// Update is called once per frame
void Update () {
if (Input.GetKeyDown(KeyCode.F6))
{
brmScript.setPlayerLives(brmScript.getPlayerLives() - 1);
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/gamestate.cs
using UnityEngine;
using System.Collections;
public class gamestate : MonoBehaviour {
// Declare properties
private static gamestate instance;
private string activeLevel;
private float healthPower,defence,moveSpeed,jumpStats,demageState,attackingSpeed;
private string playerName;
private bool winLoss;
UserStats userProp = new UserStats();
// ---------------------------------------------------------------------------------------------------
// gamestate()
// ---------------------------------------------------------------------------------------------------
// Creates an instance of gamestate as a gameobject if an instance does not exist
// ---------------------------------------------------------------------------------------------------
public static gamestate Instance
{
get
{
if(instance == null)
{
instance = new GameObject("gamestate").AddComponent<gamestate>();
}
return instance;
}
}
// Sets the instance to null when the application quits
public void OnApplicationQuit()
{
instance = null;
}
// startState()
// ---------------------------------------------------------------------------------------------------
// Creates a new game state
// ---------------------------------------------------------------------------------------------------
public void startState()
{
print ("Creating a new game state");
// Set default properties:
activeLevel = "Level 1";
playerName = userProp.PlayerName;
healthPower = userProp.HealthPower;
defence = userProp.Defence;
moveSpeed = userProp.MoveSpeed;
jumpStats = userProp.JumpStats;
demageState = userProp.DemageStats;
attackingSpeed = userProp.AttackingSpeed;
Application.LoadLevel("network_test");
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/PlatformGenerator.cs
using UnityEngine;
using System.Collections;
public class PlatformGenerator : MonoBehaviour {
GameObject[] platformsobj;
public Transform platform;
private bool isServer = false, isClient = false;
//private Vector3 lfstplatform, mfstplatform, rfstplatform;
private Vector3 lsndplatform, msndplatform, rsndplatform;
private Vector3 ltrdplatform, mtrdplatform, rtrdplatform;
private Vector3 lfthplatform, mfthplatform, rfthplatform;
void GeneratePlatform(){
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
// Upper most platforms
//lfstplatform = new Vector3 (-4.35f, 8f, -13.5f);
//mfstplatform = new Vector3 (0, 8f, -12.5f);
//rfstplatform = new Vector3 (4.35f, 8f, -13.5f);
// 2nd Upper platforms
lsndplatform = new Vector3 (-7.35f, 8f, -12f);
msndplatform = new Vector3 (0, 8f, -12f);
rsndplatform = new Vector3 (7.35f, 8f, -12f);
// 3rd lower most platforms
ltrdplatform = new Vector3 (-7.35f, 5f, -12f);
mtrdplatform = new Vector3 (0, 5f, -12f);
rtrdplatform = new Vector3 (7.35f, 5f, -12f);
// 4th lowest most platforms
lfthplatform = new Vector3 (-7.35f, 0f, -12f);
mfthplatform = new Vector3 (0, 0f, -12f);
rfthplatform = new Vector3 (7.35f, 0f, -12f);
//int fstplatformrng = Random.Range (0,50);
int sndplatformrng = Random.Range (0,50);
int trdplatformrng = Random.Range (0,50);
int fthplatformrng = Random.Range (0,50);
int slantedplatformrng = Random.Range(0, 10);
//int platformpicker = 0;
int platformpicker2 = 0;
int platformpicker3 = 0;
int platformpicker4 = 0;
/*
// First Row
if (fstplatformrng > 20) {
Debug.Log ("Spawning 1st row platform");
platformpicker = Random.Range (1,4);
switch(platformpicker)
{
case 1:
if(CheckPlatforms(lfstplatform) == true){
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, lfstplatform, Quaternion.identity);
}else{
Network.Instantiate(platform, lfstplatform, Quaternion.Euler(0,0,-10));
}
}
break;
case 2:
if(CheckPlatforms(mfstplatform) == true){
Network.Instantiate(platform, mfstplatform, Quaternion.identity);
}
break;
case 3:
if(CheckPlatforms(rfstplatform) == true){
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, rfstplatform, Quaternion.identity);
}else{
Network.Instantiate(platform, rfstplatform, Quaternion.Euler(0,0,10));
}
}
break;
}
Debug.Log ("1st Row = " + platformpicker);
}
*/
// Second Row
if (sndplatformrng > 10) {
Debug.Log ("Spawning 2nd row platform");
platformpicker2 = Random.Range (1,4);
switch(platformpicker2)
{
case 1:
if(CheckPlatforms(lsndplatform)==true)
{
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, lsndplatform, Quaternion.identity, 0);
}else{
Network.Instantiate(platform, lsndplatform, Quaternion.Euler(0,0,-10), 0);
}
}else{
if(CheckPlatforms(msndplatform)==true){
platformpicker2 = 2;
Network.Instantiate(platform, msndplatform, Quaternion.identity, 0);
}
}
break;
case 2:
if(CheckPlatforms(msndplatform)==true)
{
Network.Instantiate(platform, msndplatform, Quaternion.identity, 0);
}else if(CheckPlatforms(rsndplatform)==true){
platformpicker2 = 3;
Network.Instantiate(platform, rsndplatform, Quaternion.identity, 0);
}else if(CheckPlatforms(lsndplatform)==true){
platformpicker2 = 1;
Network.Instantiate(platform, lsndplatform, Quaternion.identity, 0);
}
break;
case 3:
if(CheckPlatforms(msndplatform)==true)
{
//platformpicker = 3;
Network.Instantiate(platform, msndplatform, Quaternion.identity, 0);
}else if(CheckPlatforms(rsndplatform)==true){
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, rsndplatform, Quaternion.identity, 0);
}else{
Network.Instantiate(platform, rsndplatform, Quaternion.Euler(0,0,10), 0);
}
}
break;
}
Debug.Log ("2nd Row = " + platformpicker2);
}
// Third Row
if (trdplatformrng > 10) {
Debug.Log ("Spawning 3rd row platform");
platformpicker3 = Random.Range (1,4);
switch(platformpicker3)
{
case 1:
if(platformpicker2 != 1 && CheckPlatforms(ltrdplatform)==true)
{
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, ltrdplatform, Quaternion.identity, 0);
}else{
Network.Instantiate(platform, ltrdplatform, Quaternion.Euler(0,0,-10), 0);
}
}else{
if(CheckPlatforms(mtrdplatform)==true){
platformpicker3 = 2;
Network.Instantiate(platform, mtrdplatform, Quaternion.identity, 0);
}
}
break;
case 2:
if(platformpicker2 != 2 && CheckPlatforms(mtrdplatform)==true)
{
Network.Instantiate(platform, mtrdplatform, Quaternion.identity, 0);
}else if(platformpicker2 == 1){
if(CheckPlatforms(rtrdplatform)==true){
platformpicker3 = 3;
Network.Instantiate(platform, rtrdplatform, Quaternion.identity, 0);
}
}else{
if(CheckPlatforms(ltrdplatform)==true){
platformpicker3 = 1;
Network.Instantiate(platform, ltrdplatform, Quaternion.identity, 0);
}
}
break;
case 3:
if(platformpicker2 == 3 && CheckPlatforms(mtrdplatform)==true)
{
platformpicker3 = 3;
Network.Instantiate(platform, mtrdplatform, Quaternion.identity, 0);
}else{
if(CheckPlatforms(rtrdplatform)==true){
slantedplatformrng = Random.Range (0, 10);
if(slantedplatformrng >= 5){
Network.Instantiate(platform, rtrdplatform, Quaternion.identity, 0);
}else{
Network.Instantiate(platform, rtrdplatform, Quaternion.Euler(0,0,10), 0);
}
}
}
break;
}
Debug.Log ("3rd Row = " + platformpicker3);
}
// Fourth Row
if (fthplatformrng > 10) {
Debug.Log ("Spawning 4th row platform");
platformpicker4 = Random.Range (1,4);
switch(platformpicker4)
{
case 1:
if(platformpicker3 != 1 && CheckPlatforms(lfthplatform)==true)
{
Network.Instantiate(platform, lfthplatform, Quaternion.identity, 0);
}else{
if(CheckPlatforms(mfthplatform)==true){
platformpicker4 = 2;
Network.Instantiate(platform, mfthplatform, Quaternion.identity, 0);
}
}
break;
case 2:
if(platformpicker3 != 2 && CheckPlatforms(mfthplatform)==true)
{
Network.Instantiate(platform, mfthplatform, Quaternion.identity, 0);
}else if(platformpicker3 == 1 || platformpicker2 == 1){
if(CheckPlatforms(rfthplatform)==true){
platformpicker4 = 3;
Network.Instantiate(platform, rfthplatform, Quaternion.identity, 0);
}
}else{
if(CheckPlatforms(lfthplatform)==true){
platformpicker4 = 1;
Network.Instantiate(platform, lfthplatform, Quaternion.identity, 0);
}
}
break;
case 3:
if(platformpicker3 == 3 && CheckPlatforms(mfthplatform)==true)
{
platformpicker4 = 3;
Network.Instantiate(platform, mfthplatform, Quaternion.identity, 0);
}else{
if(CheckPlatforms(rfthplatform)==true){
Network.Instantiate(platform, rfthplatform, Quaternion.identity, 0);
}
}
break;
}
Debug.Log ("4th Row = " + platformpicker4);
}
}
void DestroyPlatforms(){
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
Debug.Log ("Removing Platforms");
foreach (GameObject item in platformsobj) {
Destroy (item);
}
}
bool CheckPlatforms(Vector3 chosenplatform){
bool isfree = true;
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
if(platformsobj.Length > 0){
for (int i=0; i < platformsobj.Length; i++){
if(platformsobj[i].transform.position.x == chosenplatform.x &&
platformsobj[i].transform.position.y == chosenplatform.y &&
platformsobj[i].transform.position.z == chosenplatform.z){
isfree = false;
Debug.Log ("Detected overlapping on " + chosenplatform.x + " " + chosenplatform.y + " " + chosenplatform.z);
Debug.Log ("There are " + platformsobj.Length + " left");
}
}
}
return isfree;
}
// Use this for initialization
void Start () {
// REMOVE ORIENTATION LINE UNDER THIS COMMENT UPON MERGING
Screen.orientation = ScreenOrientation.Landscape;
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
// Generate the platforms
//TotalPlatforms ();
}
void TotalPlatforms(){
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
while(GameObject.FindGameObjectsWithTag("Platform").Length <= 3){
Debug.Log ("Less than 4 platforms!");
GeneratePlatform();
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
}
platformsobj = GameObject.FindGameObjectsWithTag ("Platform");
Debug.Log ("Platforms total is " + platformsobj.Length);
foreach (GameObject item in platformsobj) {
Debug.Log ("Platform: X=" + item.transform.position.x + " Y=" + item.transform.position.y + " Z=" + item.transform.position.z);
}
}
// Update is called once per frame
void Update () {
if (Network.isServer){
if (Input.GetKeyDown (KeyCode.T) || !isServer) {
Debug.Log ("Creating Level!!");
TotalPlatforms();
isServer = true;
}
// Reset the levels debug key
if (Input.GetKeyDown (KeyCode.R)) {
Debug.Log ("Destroying level!!");
DestroyPlatforms();
}
}
else{
if (isServer){
Debug.Log ("Destroying level!!");
DestroyPlatforms();
isServer = false;
}
}
if (Network.isClient){
isClient = true;
}
else{
if (isClient){
Debug.Log ("Destroying level!!");
DestroyPlatforms();
isClient = false;
}
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Testing/DC_Test.cs
using UnityEngine;
using System.Collections;
public class DC_Test : MonoBehaviour {
private WeaponManager wepManage;
private Animator anim;
public GameObject guiText;
// Use this for initialization
void Start () {
anim = this.GetComponent<Animator> ();
wepManage = GetComponent<WeaponManager> ();
}
// Update is called once per frame
void Update () {
if(Input.GetKeyDown(KeyCode.Space))
{
wepManage.SwitchWeapon(0);
guiText.SetActive(true);
this.transform.rotation = Quaternion.Euler(0.0f, 180.0f, 0.0f);
anim.SetTrigger("Disconnected");
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/UserStats.cs
using System;
using UnityEngine;
using System.Collections;
public class UserStats
{
private float healthPower,defence,moveSpeed,jumpStats,demageState,attackingSpeed;
private int playerLives = 3;
private string playerName;
private bool winLoss;
public UserStats(){}
public UserStats(float hp,float def,float mSpeed,float jStat,float dStat,string pName,bool wLoss,float aSpeed, int lives)
{
healthPower = hp;
defence = def;
moveSpeed = mSpeed;
jumpStats = jStat;
demageState = dStat;
playerName = pName;
winLoss = wLoss;
attackingSpeed = aSpeed;
playerLives = lives;
}
public float HealthPower
{
get { return healthPower; }
set { healthPower = value; }
}
public float Defence {
get { return defence; }
set { defence = value; }
}
public float MoveSpeed{
get { return moveSpeed; }
set { moveSpeed = value; }
}
public float JumpStats {
get { return jumpStats; }
set { jumpStats = value; }
}
public float DemageStats {
get { return demageState; }
set { demageState = value; }
}
public string PlayerName
{
get { return playerName; }
set { playerName = value; }
}
public bool WinLoss {
get { return winLoss; }
set { winLoss = value; }
}
public float AttackingSpeed {
get { return attackingSpeed; }
set { attackingSpeed = value; }
}
public int PlayerLives
{
get { return playerLives; }
set { playerLives = value; }
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/GameOverScript.cs
using UnityEngine;
using System.Collections;
public class GameOverScript : MonoBehaviour {
//Global Objects/Variables
public GameObject gameover;
//multi touch support
public static Touch[] touches;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
}
void OnGUI(){
Rect hg1 = new Rect (0, 0, Screen.width / 2, Screen.height);
Rect hg2 = new Rect (Screen.width / 2, 0, Screen.width / 2, Screen.height);
Rect info = new Rect (hg1.width / 2 - ((hg1.width - 50) / 2), 100, hg1.width - 100, hg1.height - 400);
if (GUI.Button(new Rect(hg2.width / 2 - 100, hg2.height - 150, 200, 100), "Menu")){
Application.LoadLevel("MainMenu");
}
}
}<file_sep>/2dFinal GIT/Assets/Scripts/Main/Projectile.cs
using UnityEngine;
using System.Collections;
public class Projectile : MonoBehaviour {
private float speed = 15f;
private float timer = 0.0f;
private float timerlimit = 5.0f;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
rigidbody.velocity = transform.forward * speed;
if (!renderer.isVisible) {
Debug.Log ("Destroying bullet");
Destroy (this.gameObject);
}
}
void OnCollisionEnter(Collision col){
Destroy (this.gameObject);
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/WeaponManager.cs
using UnityEngine;
using System.Collections;
public class WeaponManager : MonoBehaviour {
private Animator anim;
private int slotNum;
public GameObject slot_HG;
public GameObject slot_SG;
public GameObject slot_AR;
public GameObject slot_ME01;
// Use this for initialization
void Start () {
anim = this.GetComponent<Animator> ();
slotNum = 0;
}
// Update is called once per frame
void Update ()
{
}
public void SwitchWeapon(int newSlot)
{
slotNum = newSlot;
slot_HG.SetActive (false);
slot_SG.SetActive (false);
slot_AR.SetActive (false);
slot_ME01.SetActive (false);
switch(slotNum)
{
case 0:
slot_HG.SetActive (true);
anim.SetFloat("CurStyle", slotNum);
Debug.Log("HG equipped!");
break;
case 1:
slot_SG.SetActive (true);
anim.SetFloat("CurStyle", slotNum);
Debug.Log("SG equipped!");
break;
case 2:
slot_AR.SetActive (true);
anim.SetFloat("CurStyle", slotNum);
Debug.Log("AR equipped!");
break;
case 5:
slot_ME01.SetActive (true);
anim.SetFloat("CurStyle", slotNum);
Debug.Log("ME equipped!");
break;
}
}
public int GetSlotNum()
{
return slotNum;
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/ShootBtnManager.cs
using UnityEngine;
using System.Collections;
public class ShootBtnManager : MonoBehaviour {
private int curWep;
private WeaponManager wepManage;
public Texture HG_TEX;
public Texture SG_TEX;
public Texture AR_TEX;
public Texture ME_TEX;
// Use this for initialization
void Start () {
// wepManage = GameObject.FindWithTag ("Player").GetComponent<WeaponManager> ();
}
// Update is called once per frame
void Update () {
// curWep = wepManage.GetSlotNum ();
switch(curWep)
{
case 0:
this.renderer.material.mainTexture = HG_TEX;
break;
case 1:
this.renderer.material.mainTexture = SG_TEX;
break;
case 2:
this.renderer.material.mainTexture = AR_TEX;
break;
case 5:
this.renderer.material.mainTexture = ME_TEX;
break;
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/BattleResultsManager.cs
using UnityEngine;
using System.Collections;
public class BattleResultsManager : MonoBehaviour {
public GameObject life01;
public GameObject life02;
public GameObject life03;
private int playerLives = 3;
private TestHealth thScript;
private bool livesLeft;
// Use this for initialization
void Start () {
livesLeft = true;
thScript = GetComponent<TestHealth>();
}
// Update is called once per frame
void Update () {
thScript.getAliveStatus();
switch (playerLives)
{
case 0:
life01.SetActive(false);
life02.SetActive(false);
life03.SetActive(false);
break;
case 1:
life01.SetActive(true);
life02.SetActive(false);
life03.SetActive(false);
break;
case 2:
life01.SetActive(true);
life02.SetActive(true);
life03.SetActive(false);
break;
case 3:
life01.SetActive(true);
life02.SetActive(true);
life03.SetActive(true);
break;
}
if (thScript.getAliveStatus() == false)
{
thScript.setAliveStatus(true);
playerLives--;
}
if (playerLives <= 0)
{
livesLeft = false;
Application.LoadLevel("Lose_Screen");
}
}
public bool getLivesLeft()
{
return livesLeft;
}
public void setLivesLeft(bool newStatus)
{
livesLeft = newStatus;
}
//For testing only
public void setPlayerLives(int newValue)
{
playerLives = newValue;
}
public int getPlayerLives()
{
return playerLives;
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Testing/WeaponChange_Test.cs
using UnityEngine;
using System.Collections;
public class WeaponChange_Test : MonoBehaviour {
private WeaponManager wepManage;
// Use this for initialization
void Start () {
wepManage = GetComponent<WeaponManager> ();
}
// Update is called once per frame
void Update () {
if(Input.GetKeyDown(KeyCode.F1))
{
wepManage.SwitchWeapon(0);
}
if(Input.GetKeyDown(KeyCode.F2))
{
wepManage.SwitchWeapon(1);
}
if(Input.GetKeyDown(KeyCode.F3))
{
wepManage.SwitchWeapon(2);
}
if(Input.GetKeyDown(KeyCode.F4))
{
wepManage.SwitchWeapon(5);
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/PlayerCustomization.cs
using System.Collections.Generic;
using UnityEngine;
using System.Collections;
public class PlayerCustomization
{
public static int totalAttack, totalDefence, totalSpeed;
public enum Attack
{
PowerGloves,BrawnBadge,XAttack
}
public enum Defence
{
ProtectionBadge,Jacket,Suit,Helmet
}
public enum Speed
{
AgilityBadge,Boots,Booster,Shoes
}
public int[] EquipAttack(Attack att)
{
switch (att)
{
case Attack.PowerGloves:
totalAttack += 85;
totalDefence -= 35;
break;
case Attack.BrawnBadge:
totalAttack += 45;
totalDefence -= 25;
break;
case Attack.XAttack:
totalAttack += 65;
totalDefence -= 45;
break;
}
return new int[] { totalAttack, totalDefence}; // returning 2 values final attack , final defence
}
public int[] EquipDefence(Defence def)
{
switch (def)
{
case Defence.Helmet:
totalDefence += 55;
totalSpeed -= 25;
break;
case Defence.Jacket:
totalDefence += 85;
totalSpeed -= 35;
break;
case Defence.ProtectionBadge:
totalDefence += 45;
totalSpeed -= 22;
break;
case Defence.Suit:
totalDefence += 65;
totalSpeed -= 30;
break;
}
return new int[] {totalDefence, totalSpeed}; // returning two values | final defence and final speed;
}
public int[] EquipSpeed(Speed spd)
{
switch (spd)
{
case Speed.AgilityBadge:
totalSpeed += 55;
totalAttack -= 20;
break;
case Speed.Booster:
totalSpeed += 70;
totalAttack -= 45;
break;
case Speed.Boots:
totalSpeed += 40;
totalAttack-= 35;
break;
case Speed.Shoes:
totalSpeed += 35;
totalAttack -= 35;
break;
}
return new int[] { totalSpeed, totalAttack }; // returning two values | final speed and final attack
}
// void Start ()
// {
//
// //Debug.Log("First I choose helmet from defence equipment - Final Defence: " + EquipDefence(Defence.Helmet)[0]);
//
// //Debug.Log("second I choose boots from Speed equipment - Final Speed: " + EquipSpeed(Speed.Boots)[0]);
//
// EquipAttack(Attack.XAttack);
// EquipDefence(Defence.Helmet);
// EquipSpeed(Speed.Booster);
//
// Debug.Log("Total Attack : " + totalAttack);
// Debug.Log("Total speed : " + totalSpeed);
// Debug.Log("Total defence: " + totalDefence);
//
// }
//
// void Update () {
//
// }
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/GroundCheck.cs
using UnityEngine;
using System.Collections;
public class GroundCheck : MonoBehaviour {
public static Animator anim;
// Use this for initialization
void Start () {
anim = this.GetComponent<Animator> ();
}
// Update is called once per frame
void Update () {
}
void OnCollisionEnter(Collision col)
{
if (col.gameObject.tag == "Platform" || col.gameObject.tag == "Ground")
{
anim.SetBool("Grounded", true);
PlayerController.isGrounded = true;
PlayerController.doubleJump = true;
Debug.Log("GROUNDED");
}
}
void OnCollisionStay(Collision col)
{
if (col.gameObject.tag == "Platform" || col.gameObject.tag == "Ground")
{
anim.SetBool("Grounded", true);
PlayerController.isGrounded = true;
PlayerController.doubleJump = true;
Debug.Log("GROUNDED");
}
}
void OnCollisionExit(Collision col)
{
if (col.gameObject.tag == "Platform" || col.gameObject.tag == "Ground")
{
anim.SetBool("Grounded", false);
PlayerController.isGrounded = false;
PlayerController.doubleJump = true;
Debug.Log("NOT GROUNDED");
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/NetworkScript.cs
using UnityEngine;
using System.Collections;
public class NetworkScript : MonoBehaviour {
//Global Objects/Variables
public GUISkin skinGUI;
public string gameName = "Let's Brawl ver 0.5";//Name of the Game
public string serverName = "Game Match";//Custom name of the server
public string hostName = "John";//Host Name
private string password = "";//The password that the player enters
private bool wantPass = false;//If the player hosting a game wants to add a password
private string errorMessage = "";
private bool refreshing = true, searching = false;
private HostData[] hData = new HostData[6];
private int minPortNum = 25001, maxPortNum = 25555;
public int portNum = 25001;
private bool taken = false;
private string waitMsg = "";
private int[] playerNum = new int[6];
public int game;
public GameObject player;
public GameObject startPos;
void startServer(){
if (serverName == null || serverName == "")
errorMessage = "Enter a name in the Server Name field.";
else if (wantPass && password == "")
errorMessage = "Enter a Password";
else{
if (wantPass)
Network.incomingPassword = <PASSWORD>;
portNum = Random.Range(minPortNum, maxPortNum);
Network.InitializeServer (3, portNum, !Network.HavePublicAddress());//Number of players, port number, makes sure the port isn't taken
MasterServer.RegisterHost (gameName, serverName, hostName);
Debug.Log("Starting Server");
}
}
void startServer(int port){
if (serverName == null || serverName == "")
errorMessage = "Enter a name in the Server Name field.";
else if (wantPass && password == "")
errorMessage = "Enter a Password";
else{
if (wantPass)
Network.incomingPassword = <PASSWORD>;
Network.InitializeServer (3, port, !Network.HavePublicAddress());//Number of players, port number, makes sure the port isn't taken
MasterServer.RegisterHost (gameName, serverName, hostName);
Debug.Log("Starting Server");
}
}
void refreshHostList(){
MasterServer.RequestHostList (gameName);
refreshing = true;
for (int i = 0; i < hData.Length; i++){
hData[i] = null;
}
}
void createPlayer(int playerNum){
if (Network.isServer){
Network.Instantiate (player, startPos.transform.position, player.transform.rotation, 0);
}
else if (Network.isClient)
Network.Instantiate (player, startPos.transform.position, player.transform.rotation, playerNum);
}
void Update () {
if (refreshing){
if (MasterServer.PollHostList().Length > 0){
refreshing = false;
Debug.Log(MasterServer.PollHostList ().Length);
hData = MasterServer.PollHostList ();
waitMsg = "";
}
else{
waitMsg = "<size=20>Finding Games...</size>";
//refreshing = false;
}
}
else{
if (hData.Length <= 0){
waitMsg = "<size=20>Can't Find Games</size>";
}
else{
hData = MasterServer.PollHostList ();
}
}
}
void OnServerInitialized(){
Debug.Log ("Server Initialized");
createPlayer (game);
}
void OnConnectedToServer(){
createPlayer (game);
}
void OnPlayerConnected(NetworkPlayer player){
Debug.Log ("Player:" + player.port);
}
void OnPlayerDisconnected(NetworkPlayer player) {
Debug.Log("Clean up after player " + player);
Network.RemoveRPCs(player);
Network.DestroyPlayerObjects(player);
}
void OnDisconnectedFromServer(){
GameObject[] players = GameObject.FindGameObjectsWithTag("Player");
GameObject[] hpBars = GameObject.FindGameObjectsWithTag("HP");
for (int i = 0; i < players.Length; i++){
Destroy(players[i]);
Destroy(hpBars[i]);
}
}
void OnMasterServerEvent(MasterServerEvent mse){
if (searching){
if(mse == MasterServerEvent.RegistrationSucceeded){
taken = false;
Debug.Log("Registered Server!");
searching = false;
}
else{
errorMessage = "Server already taken.";
taken = true;
Debug.Log("Registration Failed");
startServer();
}
}
}
void OnGUI(){
//Determined menu sizes
Rect hg1 = new Rect (0, 0, Screen.width / 2, Screen.height+150);
Rect hg2 = new Rect (Screen.width / 2, 0, Screen.width / 2, Screen.height+150);
Rect info = new Rect (hg1.width / 2 - ((hg1.width - 50) / 2), 100, hg1.width - 100, hg1.height - 100);
GUI.skin = skinGUI;
//If you're not in a game
if (!Network.isServer && !Network.isClient){
GUI.BeginGroup (hg1);//Gives the GUI a default size
GUI.Box (new Rect(0, 0, hg1.width, hg1.height), "<size=20>Host Game</size>");
//Hosting game menu========================================================================================================
GUI.BeginGroup (info);
GUI.Label (new Rect (20, 1, 200, 80), "<size=20>Server Name:</size>");
serverName = GUI.TextField (new Rect(150, 10, 200, 30), serverName, 15);
//Toggle whether you want a password or not
wantPass = GUI.Toggle (new Rect (20, 30, 150, 100), wantPass, "<size=20>Password</size>");
//If you want a password with your game
if (wantPass){
GUI.Label(new Rect(20, 60, 200, 40), "<size=20>Enter Password:</size>");
password = GUI.PasswordField(new Rect(180, 60, 140, 30), password, '#', 15);
}
GUI.Label (new Rect (20, info.height - 80, info.width - 40, 20), errorMessage);
//Rect btnSize1 = new Rect (info.width / 2 - 50, info.height - 50, 100, 50);
GUI.EndGroup ();
if (GUI.Button(new Rect(hg1.width / 2-90, hg1.height/ 2-10, 200, 100), "<size=20>Start Server</size>")){
searching = true;
startServer();
}
GUI.EndGroup ();
//Finding game menu========================================================================================================
GUI.BeginGroup (hg2);
GUI.Box (new Rect (0, 0, hg2.width, hg2.height), "<size=20>Join Game</size>");
if (GUI.Button(new Rect(20, 20, 120, 80), "<size=20>Refresh</size>")){
refreshHostList();
Debug.Log("Refresh");
}
GUI.Label (new Rect (hg2.width / 2 - 70, hg2.height / 2 - 120, 200, 80), waitMsg);
Rect gameBox;
if (!refreshing){
for (int i = 0; i < hData.Length; i++){
if (hData[i] != null){
gameBox = new Rect (hg2.width / 2 - ((hg2.width - 200) / 2), 60 + (60 * i), hg2.width - 50, 60);
GUI.BeginGroup(gameBox);
GUI.Box(gameBox, "" + (1 + 1));
GUI.Label(new Rect(gameBox.width / 2 - 100, gameBox.height / 2 - 10, 200, 20), hData[i].gameName);
GUI.Label(new Rect(20, 10, 200, 20), "Host: " + hData[i].comment);
GUI.Label(new Rect(20, gameBox.height - 30, 50, 20), hData[i].connectedPlayers + "/" + hData[i].playerLimit);
playerNum[i] = hData[i].connectedPlayers;
if (GUI.Button(new Rect(gameBox.width - 100, 0, 40, 40), "Join")){
if (hData[i].passwordProtected){
//This is not important right now
}
else{
game = playerNum[i];
Network.Connect(hData[i]);
}
}
GUI.EndGroup();
}
}
}
if (GUI.Button(new Rect(hg2.width / 2-90, hg2.height/ 2-10, 200, 100), "<size=20>Menu</size>")){
Application.LoadLevel("MainMenu");
}
GUI.EndGroup ();
//End of game menu========================================================================================================
}
else{
}
if (Network.isServer){
if (GUI.Button(new Rect(20, 20, 200, 100), "Close Server")){
Network.Disconnect();
}
}
if (Network.isClient){
if (GUI.Button(new Rect(20, 20, 200, 100), "Disconnect")){
Network.Disconnect();
}
}
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Testing/Gui_Test.cs
using UnityEngine;
using System.Collections;
public class Gui_Test : MonoBehaviour
{
// we dont need to attach this script to camera. As Navdeep said just show something on the screen. SO I just
// added this Gui_Test to camera for testing purpose and created 2 UserStats object and passed some data.
private UserStats player1,player2;
void Start ()
{
// since we will allow 4 player to connect therefore we can just create 4 user stats on any script
// and modify values using proerties.
//nPlayer = new Userstats(float hp,float def,float mSpeed,float jStat,float dStat,string pName,bool wLoss,float aSpeed)
player1 = new UserStats(45,20,23,12,32,"Red Guy",true,32, 3);
player2 = new UserStats(20, 10, 40, 00, 12, "Bowser", true, 22, 3);
}
void Update () {
}
void OnGUI()
{
/////////////////// 1st player ////////////
GUILayout.BeginArea(new Rect(10, 10, 180, Screen.width / 2));
GUILayout.BeginVertical("box");
GUILayout.Label("Player Name: "+player1.PlayerName);
GUILayout.Label("Health Power: " + player1.HealthPower);
GUILayout.Label("Defence: "+player1.Defence);
GUILayout.Label("Move Speed: "+player1.MoveSpeed);
GUILayout.Label("Jump: "+player1.JumpStats);
GUILayout.Label("Demage: "+player1.DemageStats);
GUILayout.Label("win/lose: "+player1.WinLoss);
GUILayout.Label("Atacking Speed: "+player1.AttackingSpeed);
GUILayout.EndVertical();
GUILayout.EndArea();
/////////////////// 2nd player ////////////
GUILayout.BeginArea(new Rect(200, 10, 180, Screen.width / 2));
GUILayout.BeginVertical("box");
GUILayout.Label("Player Name: " + player2.PlayerName);
GUILayout.Label("Health Power: " + player2.HealthPower);
GUILayout.Label("Defence: " + player2.Defence);
GUILayout.Label("Move Speed: " + player2.MoveSpeed);
GUILayout.Label("Jump: " + player2.JumpStats);
GUILayout.Label("Demage: " + player2.DemageStats);
GUILayout.Label("win/lose: " + player2.WinLoss);
GUILayout.Label("Atacking Speed: " + player2.AttackingSpeed);
GUILayout.EndVertical();
GUILayout.EndArea();
}
}
<file_sep>/2dFinal GIT/Assets/Scripts/Main/gamestart.cs
using UnityEngine.UI;
using UnityEngine;
using System.Collections;
public class gamestart : MonoBehaviour
{
// Our Startscreen GUI
//healthPower being distributed to TestHealthPower, firerate changes firerate of each gun check player Controller switch
//statement
public static float healthPower = 100.00f;
public static float fireRate;
GameObject customizeMenu,GUIButton;
Slider headGearSlider,chestGearSlider,glovesSlider,fireRateSlider;
UserStats userStats = new UserStats();
void Start(){
customizeMenu = GameObject.Find("CustomizeMenu");
GUIButton = GameObject.Find("GUI");
customizeMenu.SetActive(false);
//GUI.skin.label.fontSize = Screen.height / 1080 * 64;
}
public void BackButton(){
customizeMenu.SetActive(false);
GUIButton.SetActive(true);
}
void OnGUI ()
{
if(GUI.Button(new Rect (Screen.width/2 - 150, Screen.height/2 - 40, 290, 90), "<size=28>Play Game</size>"))
{
startGame();
}
if (GUI.Button (new Rect (Screen.width/2 - 150, Screen.height/2 + 60, 290, 90), "<size=28>Customize Character</size>"))
{
customizeMenu.SetActive(true);
headGearSlider = GameObject.Find("headGearSlider").GetComponent<Slider>();
headGearSlider.onValueChanged.AddListener(HeadGearListener);
chestGearSlider = GameObject.Find("chestGearSlider").GetComponent<Slider>();
chestGearSlider.onValueChanged.AddListener(ChestGearListener);
glovesSlider = GameObject.Find("glovesSlider").GetComponent<Slider>();
glovesSlider.onValueChanged.AddListener(GlovesGearListener);
fireRateSlider = GameObject.Find("fireRateSlider").GetComponent<Slider>();
fireRateSlider.onValueChanged.AddListener(FireRateListener);
GUIButton.SetActive(false);
}
}
public void HeadGearListener(float value)
{
if(headGearSlider.value >= 0.7){
chestGearSlider.value = 0.5f;
glovesSlider.value = 0.2f;
healthPower = 90;
}
if(headGearSlider.value <= 0.5){
chestGearSlider.value = 0.6f;
glovesSlider.value = 0.3f;
healthPower = 80;
}
}
public void ChestGearListener(float value)
{
if(chestGearSlider.value >= 0.7){
headGearSlider.value = 0.5f;
glovesSlider.value = 0.4f;
healthPower = 90;
}
}
public void GlovesGearListener(float value)
{
if(glovesSlider.value >= 0.7){
chestGearSlider.value = 0.4f;
headGearSlider.value = 0.3f;
healthPower = 85;
}
if(glovesSlider.value <= 0.3){
chestGearSlider.value = 0.6f;
headGearSlider.value = 0.8f;
healthPower = 80;
}
}
public void FireRateListener(float value)
{
fireRate = fireRateSlider.value;
}
private void startGame()
{
//Launch Louis Script
print("Starting game scene");
DontDestroyOnLoad(gamestate.Instance);
gamestate.Instance.startState();
}
private void Achievements()
{
print("Achievements scene");
DontDestroyOnLoad(gamestate.Instance);
gamestate.Instance.startState();
}
}
| 1bdce5485991fef43942b72edd7cc66719dc58bf | [
"C#"
] | 18 | C# | TeamNavdeep/LetsBrawl | 9e9b381e73b19ef1f135a936c5d87649fe3c8f80 | f9cad58f676c14598c8822d74e92e203877b1c32 | |
refs/heads/master | <file_sep>using System;
namespace Appie
{
public static class SignalRDelegate
{
public delegate void SRAction(SignalRClient sender);
public delegate void SRException(SignalRClient sender, Exception exception);
}
}
<file_sep>using Appie;
using Microsoft.AspNetCore.Http.Connections;
using Microsoft.AspNetCore.Http.Connections.Client;
using Microsoft.AspNetCore.SignalR.Client;
using System;
namespace SignalRApp
{
public class ChatHub : SignalRClient
{
#region Constructors
public ChatHub(string url) : base(url) { }
public ChatHub(string url, Action<HttpConnectionOptions> configureHttpConnection) : base(url, configureHttpConnection) { }
public ChatHub(string url, HttpTransportType transports) : base(url, transports) { }
public ChatHub(string url, HttpTransportType transports, Action<HttpConnectionOptions> configureHttpConnection) : base(url, transports, configureHttpConnection) { }
public ChatHub(Uri url) : base(url) { }
public ChatHub(Uri url, Action<HttpConnectionOptions> configureHttpConnection) : base(url, configureHttpConnection) { }
public ChatHub(Uri url, HttpTransportType transports) : base(url, transports) { }
public ChatHub(Uri url, HttpTransportType transports, Action<HttpConnectionOptions> configureHttpConnection) : base(url, transports, configureHttpConnection) { }
#endregion
public void OnReceiveMessage(Action<string> method)
{
Connection.On("ReceiveMessage", method);
}
public void SendMessage(string message)
{
Connection.SendCoreAsync("SendMessage", new object[] { message });
}
}
}
<file_sep>using Microsoft.AspNetCore.Http.Connections;
using Microsoft.AspNetCore.Http.Connections.Client;
using Microsoft.AspNetCore.SignalR.Client;
using NStandard;
using System;
using System.Net.Http;
using System.Threading;
using System.Threading.Tasks;
namespace Appie
{
public class SignalRClient
{
public TimeSpan ExceptionRetryDelay { get; set; } = TimeSpan.FromSeconds(10);
/// <summary>
/// Maximum number of retries. If set to 0, there is no limit. (The default value is 5.)
/// </summary>
public int MaxRetry { get; set; } = 5;
public int RetryCount { get; private set; } = 0;
private volatile bool Running;
private bool AutomaticRetry = false;
public HubConnection Connection { get; private set; }
public event SignalRDelegate.SRAction Connecting;
public event SignalRDelegate.SRAction Connected;
public event SignalRDelegate.SRException Reconnecting;
public event SignalRDelegate.SRException Reconnected;
public event SignalRDelegate.SRException Closed;
public event SignalRDelegate.SRException Exception;
public event SignalRDelegate.SRException Failed;
#region Constructors
public SignalRClient(string url)
{
Connection = new HubConnectionBuilder().WithUrl(url).Build();
InitConnection();
}
public SignalRClient(string url, Action<HttpConnectionOptions> configureHttpConnection)
{
Connection = new HubConnectionBuilder().WithUrl(url, configureHttpConnection).Build();
InitConnection();
}
public SignalRClient(string url, HttpTransportType transports)
{
Connection = new HubConnectionBuilder().WithUrl(url, transports).Build();
InitConnection();
}
public SignalRClient(string url, HttpTransportType transports, Action<HttpConnectionOptions> configureHttpConnection)
{
Connection = new HubConnectionBuilder().WithUrl(url, transports, configureHttpConnection).Build();
InitConnection();
}
public SignalRClient(Uri url)
{
Connection = new HubConnectionBuilder().WithUrl(url).Build();
InitConnection();
}
public SignalRClient(Uri url, Action<HttpConnectionOptions> configureHttpConnection)
{
Connection = new HubConnectionBuilder().WithUrl(url, configureHttpConnection).Build();
InitConnection();
}
public SignalRClient(Uri url, HttpTransportType transports)
{
Connection = new HubConnectionBuilder().WithUrl(url, transports).Build();
InitConnection();
}
public SignalRClient(Uri url, HttpTransportType transports, Action<HttpConnectionOptions> configureHttpConnection)
{
Connection = new HubConnectionBuilder().WithUrl(url, transports, configureHttpConnection).Build();
InitConnection();
}
#endregion
protected void HandleClosed(Exception exception)
{
Closed?.Invoke(this, exception);
RetryCount = 0;
Running = false;
}
protected void HandleFailed(Exception exception)
{
Failed?.Invoke(this, exception);
RetryCount = 0;
Running = false;
}
protected void InitConnection()
{
Connection.Closed += ex0 => Task.Run(() =>
{
if (ex0 == null || !AutomaticRetry)
{
HandleClosed(ex0);
return;
}
Exception?.Invoke(this, ex0);
Thread.Sleep(ExceptionRetryDelay);
try
{
Running = false;
StartAsync(AutomaticRetry, ex0).Wait();
Reconnected?.Invoke(this, ex0);
}
catch (Exception ex)
{
HandleClosed(ex);
}
});
}
public async Task StartAsync(bool automaticRetry, Exception exception = null)
{
if (Running) throw new InvalidOperationException("The SignalR client is running.");
Running = true;
AutomaticRetry = automaticRetry;
await Task.Run(() =>
{
while (true)
{
if (exception is null)
Connecting?.Invoke(this);
else Reconnecting?.Invoke(this, exception);
try
{
Connection.StartAsync().Wait();
Connected?.Invoke(this);
RetryCount = 0;
return;
}
catch (Exception ex)
{
RetryCount++;
Exception?.Invoke(this, ex);
if (!AutomaticRetry || RetryCount >= MaxRetry)
{
HandleFailed(ex);
throw;
}
Thread.Sleep(ExceptionRetryDelay);
continue;
}
}
});
}
public async Task StopAsync()
{
await Task.Run(() =>
{
if (!Running) throw new InvalidOperationException("The SignalR client is not running.");
AutomaticRetry = false;
Connection.StopAsync().Wait();
});
}
}
}
<file_sep>using NStandard;
using System;
namespace SignalRApp
{
class Program
{
static void Main(string[] args)
{
var chatHub = new ChatHub("http://localhost:60210/ChatHub")
{
ExceptionRetryDelay = TimeSpan.FromSeconds(1),
};
chatHub.OnReceiveMessage(message =>
{
Console.WriteLine(message);
});
chatHub.Connecting += sender => Console.WriteLine("Connecting...");
chatHub.Connected += sender => Console.WriteLine("Connected...");
chatHub.Reconnecting += (sender, exception) => Console.WriteLine($"Reconnecting... {chatHub.RetryCount + 1}");
chatHub.Reconnected += (sender, exception) => Console.WriteLine("Reconnected...");
chatHub.Closed += (sender, exception) => Console.WriteLine("Closed...");
chatHub.Exception += (sender, exception) => Console.WriteLine($"Exception...");
chatHub.Failed += (sender, exception) => Console.WriteLine("Failed...");
chatHub.StartAsync(true).CatchAsync(ex => Console.WriteLine(ex.Message));
while (true)
{
var line = Console.ReadLine();
if (line == "stop")
{
chatHub.StopAsync().CatchAsync(ex => Console.WriteLine(ex.Message));
}
else if (line == "start")
{
chatHub.StartAsync(true).CatchAsync(ex => Console.WriteLine(ex.Message));
}
else chatHub.SendMessage(line);
}
}
}
}
| a30e6bcd92478454e3fc52c929d30ff6e5919394 | [
"C#"
] | 4 | C# | zmjack/SignalRSharp | 87b8042041698e85f14a5d303e6bd60eca9354bf | 7a40fe02ad9cb9862d6184bfbe99e5fadccd8270 | |
refs/heads/master | <repo_name>xwwwwww/text-classification<file_sep>/README.md
### 1. 文件结构

```
-- bert/ bert模型实现
-- chinese_wwm_ext_pytorch是bert的预训练数据
-- main.py utils.py 是head+tail做法的Bert实现
-- myBert目录下是分段输入Bert的实现,如报告中所说,我因显存限制未能测试模型效果,因此报告中没有汇报
这一方法的性能,也没有提供模型参数,无需复现。
-- train.py model.py solve.py提供了RNN,MLP,CNN,RNN+Attention的实现
-- newdata目录下是数据,已处理完毕
-- model_save目录下是保存的模型数据,提交时会删除掉,需下载此目录,注意保持目录结构,不要新增嵌套。
清华云盘下载链接: https://cloud.tsinghua.edu.cn/d/f885293c5c444630a084/
-- word2vec目录下是词向量,提交时会删除掉,需下载至此目录,注意保持目录结构,不要新增嵌套。
清华云盘下载链接:https://cloud.tsinghua.edu.cn/d/491f0e4168fb433ba960/
```
### 2. 复现方式
-e开启evaluate mode, --cuda指定GPU
#### MLP
```
python train.py --model_type MLP --lr 0.0001 -b 128 --wd 0.001 --name mixed_vector --vector mixed --fix_length 1560 -e --cuda 3
```
#### RNN
```
python train.py --model_type RNN --lr 1e-3 -b 16 --wd 1e-5 --name 1 --vector sogou --min_freq 1 -e --cuda 3
```
#### RNN+Attention
##### biGRU+Attention
```
python train.py --model_type AttRNN --lr 1e-3 -b 32 --wd 1e-4 --name gru_1e-3_att --vector sogou --min_freq 1 --max_epoch 100 -e --cuda 3
```
##### biLSTM+Attention
```
python train.py --model_type AttRNN --lr 1e-4 -b 32 --wd 1e-4 --name lstm --vector sogou --min_freq 1 --cell LSTM --max_epoch 100 -e --cuda 3
```
#### BERT
```
cd bert/
python main.py --lr 1e-6 -b 6 --wd 0.0005 --max_epoch 20 --name fix_np --cuda 1 -e
```
<file_sep>/bert/myBert/utils_myBert.py
import torch
import torch.autograd as autograd
import torch.nn.functional as F
from torchtext import data
from torchtext.vocab import Vectors, Vocab
from collections import Counter
import numpy as np
import sys
from scipy.stats import pearsonr
from sklearn.metrics import f1_score
import numpy as np
CLS = '[CLS]'
UNK = '[UNK]'
SEP = '[SEP]'
PAD = '[PAD]'
def field_load_vocab(field, vocab_path, novector=False):
if not novector:
vector=Vectors(name=vocab_path)
counter=Counter()
with open(vocab_path,'r',encoding='utf-8') as file:
# file head
file.readline()
q=file.readline()
while q:
q=q.strip()
counter[q.split()[0]]=1
q=file.readline()
field.vocab=Vocab(counter,vectors=vector)
else:
counter=Counter()
with open(vocab_path,'r',encoding='utf-8') as file:
lines=file.readlines()
sum=1000000
for index,line in enumerate(lines):
line=line.strip()
# 构造Vocab时,词频大的词排在词表的前面
counter[line]=sum-index
field.vocab=Vocab(counter,specials=[])
def train_one_epoch(model, train_iter, criterion, optimizer, epoch, args):
model.train()
train_loss = 0
train_acc = 0
times = 0
print_loss = 0
print_acc = 0
for batch in train_iter:
text, ori_label = batch.text, batch.label
label = torch.argmax(ori_label,dim=1)
model.zero_grad()
output = model(text)
loss = criterion(output,label)
_, pred = torch.max(output.data, dim=1)
acc = (pred == label).sum().item() / label.shape[0]
train_loss += loss.item()
train_acc += acc
print_loss += loss.item()
print_acc += acc
times += 1
if times % args.print_frequency == 0:
print('Train Epoch: {}, Iteration:{}, loss: {:.6f}, acc: {:.6f}'.format(
epoch, times, print_loss / args.print_frequency, print_acc / args.print_frequency))
sys.stdout.flush()
print_loss = 0
print_acc = 0
loss.backward()
optimizer.step()
train_loss /= times
train_acc /= times
return train_loss, train_acc
def evaluate(model, eval_iter,criterion):
model.eval()
test_loss = 0
test_acc = 0
times = 0
f1 = 0
corr = 0
for batch in eval_iter:
text, ori_label = batch.text, batch.label
label = torch.argmax(ori_label,dim=1)
output = model(text)
loss = criterion(output,label)
_, pred = torch.max(output.data, dim=1)
acc = (pred == label).sum().item() / label.shape[0] # 平均值
temp_corr = 0
for i in range(output.shape[0]):
temp_corr += pearsonr(output[i].cpu().detach().numpy(), ori_label[i].cpu().detach().numpy())[0]
temp_corr /= output.shape[0]
corr += temp_corr
f1 += f1_score(label.cpu().detach().numpy(), pred.cpu().detach().numpy(), np.arange(8),average='micro')
test_loss += loss.item()
test_acc += acc
times += 1
test_loss /= times
test_acc /= times
corr /= times
f1 /= times
print('\nValidation set: Average loss: {:.4f}, Accuracy: {:.4f} ({:.2f}%, Correlation:{:4f}), F-score:{:4f})\n'.format(
test_loss, test_acc, 100. * test_acc, corr, f1))
return test_loss, test_acc,corr,f1
if __name__=='__main__':
test()
<file_sep>/model.py
import torch
import torch.nn as nn
import torch.nn.functional as F
class RNN(nn.Module):
def __init__(self, vocab, input_size, hidden_size, fc_size, num_layers, cell,dropout):
super(RNN, self).__init__()
self.embedding = nn.Embedding(len(vocab), input_size)
self.embedding.weight.requires_grad = False
self.dropout = nn.Dropout(dropout)
if cell == 'GRU':
self.rnn = nn.GRU(
input_size=input_size,
hidden_size=hidden_size,
num_layers=num_layers,
#dropout=dropout,
bias=True,
#batch_first = True,
bidirectional=True,
)
elif cell == 'LSTM':
self.rnn = nn.LSTM(
input_size =input_size,
hidden_size = hidden_size,
num_layers = num_layers,
#dropout = dropout,
bias=True,
bidirectional =True
)
self.fc1 = nn.Linear(
in_features=hidden_size * 2,
out_features=8,
bias=True
)
def forward(self, x):
x = self.embedding(x)
x = self.dropout(x)
output,h = self.rnn(x)
x = output[-1]
x = self.fc1(x)
return x
class AttentionRNN(nn.Module):
def __init__(self, vocab, input_size, hidden_size, fc_size, num_layers,cell, dropout):
super(AttentionRNN, self).__init__()
self.embedding = nn.Embedding(len(vocab), input_size)
self.embedding.weight.data.copy_(vocab.vectors)
self.embedding.weight.requires_grad = False
self.dropout = nn.Dropout(dropout)
self.w = nn.Parameter(torch.randn((input_size*2,1)))
if cell =='GRU':
self.rnn = nn.GRU(
input_size=input_size,
hidden_size=hidden_size,
num_layers=num_layers,
#dropout=dropout,
bias=True,
batch_first = True,
bidirectional=True,
)
elif cell =='LSTM':
self.rnn = nn.LSTM(
input_size =input_size,
hidden_size = hidden_size,
num_layers = num_layers,
#dropout = dropout,
bias=True,
bidirectional =True
)
self.fc = nn.Linear(
in_features=hidden_size*2,
out_features=8, #8种情感
bias=True
)
def forward(self, x):
x = self.embedding(x)
x = self.dropout(x)
output,h = self.rnn(x)
output = torch.transpose(output, 1,2)
m = torch.tanh(output)
a = F.softmax(torch.matmul(self.w.t(),m), dim=2) #w: []
r = torch.matmul(output, torch.transpose(a,1,2))
r = r.squeeze(2)
r = torch.tanh(r)
x = self.fc(r)
return x
class CNN(nn.Module):
def __init__(self, vocab, input_size,fix_length, dropout):
super(CNN, self).__init__()
self.fix_length = fix_length
self.embedding = nn.Embedding(len(vocab), input_size)
self.embedding.weight.data.copy_(vocab.vectors)
self.embedding.weight.requires_grad = True #默认是false 不参与训练
self.filter_sizes=[3,4,5]
self.features = 100#300
self.convs = nn.ModuleList([nn.Conv2d(1,self.features,(k, input_size) )for k in self.filter_sizes])
self.dropout = nn.Dropout(dropout)
self.fc = nn.Linear(self.features * len(self.filter_sizes), 8)
def conv_and_pool(self,x,conv):
x = conv(x)
x = F.relu(x).squeeze(3)
x = F.max_pool1d(x,x.size(2)).squeeze(2)
return x
def forward(self,x):
x = self.embedding(x)
x = x.unsqueeze(1)
x = torch.cat([self.conv_and_pool(x,conv) for conv in self.convs],1)
x = self.dropout(x)
x = self.fc(x)
return x
class MLP(nn.Module):
def __init__(self, vocab, input_size,fix_length, dropout):
super(MLP, self).__init__()
self.fix_length = fix_length
self.input_size = input_size
self.embedding = nn.Embedding(len(vocab), input_size)
self.embedding.weight.data.copy_(vocab.vectors)
self.embedding.weight.requires_grad = False #默认是false 不参与训练
self.fc1 = nn.Linear(fix_length*input_size,512)
self.fc2 = nn.Linear(512,32)
self.dropout = nn.Dropout(dropout)
self.fc3 = nn .Linear(32,8)
def forward(self,x):
x = self.embedding(x)
x = x.view(-1,self.fix_length*self.input_size)
x = self.fc1(x)
x = F.relu(x)
x = self.dropout(x)
x = self.fc2(x)
x = F.relu(x)
x = self.fc3(x)
return x
<file_sep>/bert/myBert/bert.py
import torch
import torch.nn as nn
import torch.nn.functional as F
class BERT(nn.Module):
def __init__(self, baseBert, batch_size,device):
super(BERT,self).__init__()
self.baseBert = baseBert #加载的预训练BERT
print(device)
self.device = device
self.batch_size =batch_size
self.fc = nn.Linear(192,8)
#self.fc = nn.Linear(768,8)
def forward(self, x):
text_len = x.shape[1]
output_list = []
step = 256-32 #
length = 256
start = -1*step
end = -1
flag = False
while not flag and (start < text_len): #128区间 64步长滑动
if (start+step) >= text_len:
start = text_len
else:
start = start+step
if (start+length) >= text_len:
end = text_len
else:
end = start+length
if end == text_len: #标记本次为最后一次滑动
flag = True
temp_text = x[:,start:end]
self.cls_column = torch.LongTensor([[101] for i in range(x.shape[0])]).to(self.device)
self.sep_column = torch.LongTensor([[102] for i in range(x.shape[0])]).to(self.device)
temp_text = torch.cat([self.cls_column, temp_text, self.sep_column], dim = 1)
#在一整个batch加入clk和sep的id
mask = temp_text>0
#print('temp_text.shape =',temp_text.shape)
output = self.baseBert(
temp_text,
attention_mask=mask,
)
output = output[1] #output[1]是clk的输出 维度是[batch_size, hidden_size]
output_list.append(output)
#print('list len = ', len(output_list))
kernel_size = len(output_list)*4
concat_output = torch.cat(output_list,1)
concat_output = concat_output.unsqueeze(1)
final_output = F.max_pool1d(concat_output, kernel_size=kernel_size) #max_pooling
final_output = final_output.squeeze(1)
x = self.fc(final_output)
return x
<file_sep>/train.py
import torch
import torch.nn as nn
import argparse
import time
import numpy as np
from torchtext import data
from torchtext.vocab import Vectors, GloVe
import jieba
import os
from model import RNN,CNN,MLP,AttentionRNN
from solve import train_one_epoch, evaluate
parser = argparse.ArgumentParser()
# learning
parser.add_argument('--dropout',default=0.5,type=float)
parser.add_argument('--fix_length',default='512',type=int)
parser.add_argument('--model_type',default='RNN',type=str,help="model used, model list = ['RNN','CNN','MLP','AttRNN']")
parser.add_argument('--max_epoch', default=20, type=int, metavar='N', help='number of total epochs to run')
parser.add_argument('-b', '--batch-size', default=64, type=int, metavar='N', help='mini-batch size(default: 1')
parser.add_argument('--lr', '--learning-rate', default=0.001, type=float, metavar='LR', help='initial learning rate',
dest='lr')
parser.add_argument('--momentum', default=0.9, type=float, metavar='M', help='momentum')
parser.add_argument('--wd', '--weight-decay', default=0.0005, type=float, metavar='W',
help='weight decay(default: 1e-4)', dest='weight_decay')
parser.add_argument('-print-frequency', type=int, default=10,
help='how many steps to wait before logging training status [default: 50]')
parser.add_argument('-e', '--evaluate', dest='evaluate', action='store_true', help='evaluate model on validation set')
parser.add_argument('--cuda',default=None)
parser.add_argument('--name', default='name',help = 'name of the experiment')
parser.add_argument('--vector', type=str, default='sogou')
parser.add_argument('--min_freq',type=int,default=1)
parser.add_argument('--cell',default='GRU')
# device
# parser.add_argument('--device', type=int, default=0, help='device to use for iterate data, -1 mean cpu [default: 0]')
parser.add_argument('--no-cuda', action='store_true', default=False, help='disable the gpu')
args = parser.parse_args()
#args.cuda = (not args.no_cuda) and torch.cuda.is_available()
def tokenizer(text):
return text.split(' ')
def main():
# load data
fix_length = args.fix_length
if args.model_type != 'RNN' : #batch first && fix_length
LABEL = data.Field(sequential=False,use_vocab=False,batch_first=True, dtype=torch.float32)
TEXT = data.Field(sequential=True,fix_length = fix_length, tokenize=tokenizer, batch_first=True)
else:
LABEL = data.Field(sequential=False, use_vocab=False,dtype=torch.float32)
TEXT = data.Field(sequential=True,tokenize=tokenizer)
fields = {'label': ('label', LABEL), 'text': ('text', TEXT)}
print("ready to load data")
train, val, test = data.TabularDataset.splits(path='newdata', train='train.json', validation='val.json',
test='test.json', format='json', fields=fields)
train_length=len(train.examples)
val_length=len(val.examples)
test_length=len(test.examples)
print('train_length:{}\nval_length:{}\ntest_length:{}'.format(train_length,val_length,test_length))
print("ready to build vocab")
# build vocab
print('vector = ', args.vector)
if args.vector == 'sogou':
cache = 'word2vec/sogou_vector_cache'
vector_path = 'word2vec/sgns.sogou.word'
elif args.vector == 'baidu':
cache = 'word2vec/baidu_vector_cache'
vector_path = 'word2vec/sgns.target.word-word.dynwin5.thr10.neg5.dim300.iter5'
elif args.vector == 'mixed':
cache= 'word2vec/mixed_vector_cache'
vector_path = 'word2vec/sgns.merge.word'
#cache = '.vector_cache'
if not os.path.exists(cache):
print('cache not found')
os.mkdir(cache)
else:
print('found cache')
TEXT.build_vocab(train, min_freq=args.min_freq,vectors=Vectors(name = vector_path,cache=cache))
#TEXT.build_vocab(train, val, test, min_freq=5,vectors=Vectors(name = '../sgns.target.word-word.dynwin5.thr10.neg5.dim300.iter5',cache=cache))
#TEXT.build_vocab(train, val, test, min_freq=1,vectors=Vectors(name = '../sgns.merge.word',cache=cache))
print("finish build vocab")
vocab = TEXT.vocab
print('vocab len = ', len(vocab))
# 迭代器 返回batch
device = torch.device('cuda:{}'.format(args.cuda) if args.cuda != None else 'cpu')
train_iter, val_iter, test_iter = data.BucketIterator.splits(
(train, val, test), batch_size=args.batch_size, device=device, shuffle=True,sort_key=lambda x: len(x.text), sort_within_batch=False)
# 迭代器 返回batch
# define model
dropout = args.dropout
model = 0
print('model = ', args.model_type)
if args.model_type == 'CNN':
model = CNN(
vocab = TEXT.vocab,
input_size = 300,
fix_length = fix_length,
dropout = dropout
)
elif args.model_type == 'MLP':
model = MLP(
vocab = TEXT.vocab,
input_size = 300,
fix_length = fix_length,
dropout = dropout
)
elif args.model_type == 'RNN':
model = RNN(
vocab=TEXT.vocab,
input_size=300,
hidden_size=300,
num_layers=1,
fc_size=64,
cell = args.cell,
dropout=dropout
)
elif args.model_type == 'AttRNN':
model = AttentionRNN(
vocab=TEXT.vocab,
input_size=300,
hidden_size=300,
num_layers=1,
fc_size=64,
cell = args.cell,
dropout=dropout
)
print(type(model))
#weight = torch.Tensor([0.177625, 0.052946,0.061913,0.420154, 0.15670,0.07686, 0.042271,0.011528])
if args.cuda != None:
print('to cuda! device = ', device)
model.to(device)
#weight = torch.Tensor([0.177625, 0.052946,0.061913,0.420154, 0.15670, 0.07686, 0.042271, 0.011528]).to(device)
print('device = ', device)
#model.to(device)
# define loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=args.lr, weight_decay=args.weight_decay)
# train & val & test
train_loss_list = []
train_acc_list = []
val_loss_list = []
val_acc_list = []
if not args.evaluate:
best_val_acc = 0.0
test_loss, test_acc,test_corr,test_f1= evaluate(model, test_iter, criterion)
for epoch in range(args.max_epoch):
train_loss, train_acc = train_one_epoch(model, train_iter, criterion, optimizer, epoch, args)
val_loss, val_acc,val_corr,val_f1= evaluate(model, val_iter, criterion)
train_loss_list.append(train_loss)
train_acc_list.append(train_acc)
val_loss_list.append(val_loss)
val_acc_list.append(val_acc)
if val_acc > best_val_acc:
best_val_acc = val_acc
print('ready to save data')
save_path = 'model_save/{}'.format(args.model_type)
if not os.path.exists(save_path):
os.mkdir(save_path)
print('mkdir ',save_path)
else:
print('{} has existed'.format(save_path))
torch.save(model.state_dict(), '{}/parameter_{}.pkl'.format(save_path, args.name))
print('Epoch {} result is: train_acc={}, train_loss={}, val_acc={}, val_loss={}'.format(epoch, train_acc,
train_loss, val_acc,
val_loss))
test_loss, test_acc,test_corr,testf1= evaluate(model, test_iter, criterion)
'''
np.save('{}_{}_train_loss.npy'.format(args.model_type, args.name), train_loss_list)
np.save('{}_{}_train_acc.npy'.format(args.model_type, args.name), train_acc_list)
np.save('{}_{}_val_loss.npy'.format(args.model_type, args.name), val_loss_list)
np.save('{}_{}_val_acc.npy'.format(args.model_type, args.name), val_acc_list)
'''
else:
print('evaluate mode!')
#model.load_state_dict(torch.load('net_parameters.pkl'))
if args.cuda == None:
model.load_state_dict(torch.load('model_save/{}/parameter_{}.pkl'.format(args.model_type, args.name), map_location=device))
else:
model.load_state_dict(torch.load('model_save/{}/parameter_{}.pkl'.format(args.model_type, args.name)))
test_loss, test_acc,test_corr, test_f1 = evaluate(model, test_iter, criterion)
if __name__ == '__main__':
main()
<file_sep>/solve.py
import torch
import torch.autograd as autograd
import torch.nn.functional as F
from scipy.stats import pearsonr
from sklearn.metrics import f1_score
import numpy as np
def train_one_epoch(model, train_iter, criterion, optimizer, epoch, args):
model.train()
train_loss = 0
train_acc = 0
times = 0
print_loss = 0
print_acc = 0
for batch in train_iter:
optimizer.zero_grad()
text, ori_label = batch.text, batch.label
label = torch.argmax(ori_label, dim = 1) #分类任务计算
output = model(text)
loss = criterion(output, label) #label用regression的形式和classification(one hot)的形式试一下吧
#乘一个权重
_, pred = torch.max(output.data, dim=1)
acc = (pred == label).sum().item() / label.shape[0]
train_loss += loss.item()
train_acc += acc
print_loss += loss.item()
print_acc += acc
times += 1
if times % args.print_frequency == 0:
print('Train Epoch: {}, Iteration:{}, loss: {:.6f}, acc: {:.6f}'.format(
epoch, times, print_loss / args.print_frequency, print_acc / args.print_frequency))
print_loss = 0
print_acc = 0
loss.backward()
optimizer.step()
train_loss /= times
train_acc /= times
return train_loss, train_acc
def evaluate(model, eval_iter, criterion):
model.eval()
test_loss = 0
test_acc = 0
times = 0
f1 = 0
corr = 0
for batch in eval_iter:
text, ori_label = batch.text, batch.label #归一化的label用于计算cor
label = torch.argmax(ori_label,dim=1)
output = model(text)
loss = criterion(output, label)
_, pred = torch.max(output.data, dim=1)
acc = (pred == label).sum().item() / label.shape[0] # 平均值
temp_corr = 0
for i in range(output.shape[0]):
temp_corr += pearsonr(output[i].cpu().detach().numpy(), ori_label[i].cpu().detach().numpy())[0]
temp_corr /= output.shape[0]
corr += temp_corr
f1 += f1_score(label.cpu().detach().numpy(), pred.cpu().detach().numpy(), np.arange(8),average='micro')
test_loss += loss.item()
test_acc += acc
times += 1
test_loss /= times
test_acc /= times
corr /= times
f1 /= times
print('\nValidation set: Average loss: {:.4f}, Accuracy: {:.4f} ({:.2f}%), Correlation:{:4f}, F-score:{:4f})\n'.format(
test_loss, test_acc, 100. * test_acc, corr, f1))
return test_loss, test_acc,corr,f1
<file_sep>/bert/myBert/myBert.py
import torch
import torch.nn as nn
import argparse
from torchtext import data
import os
import transformers
from utils_myBert import train_one_epoch, evaluate, field_load_vocab, CLS, UNK, PAD, SEP
from bert import BERT
parser = argparse.ArgumentParser()
# learning
parser.add_argument('--max_epoch', default=20, type=int, metavar='N', help='number of total epochs to run')
parser.add_argument('-b', '--batch-size', default=16, type=int, metavar='N', help='mini-batch size(default: 1')
parser.add_argument('--lr', '--learning-rate', default=0.0001, type=float, metavar='LR', help='initial learning rate',
dest='lr')
parser.add_argument('--momentum', default=0.9, type=float, metavar='M', help='momentum')
parser.add_argument('--wd', '--weight-decay', default=0.0005, type=float, metavar='W',
help='weight decay(default: 1e-4)', dest='weight_decay')
parser.add_argument('-print-frequency', type=int, default=10,
help='how many steps to wait before logging training status [default: 50]')
parser.add_argument('-e', '--evaluate', dest='evaluate', action='store_true', help='evaluate model on validation set')
parser.add_argument('--model', default='rnn_cache', help='model cache')
parser.add_argument('--name',default=1)
parser.add_argument('--no-cuda', action='store_true', default=False, help='disable the gpu')
args = parser.parse_args()
#从预训练词表加载tokenizer
tokenizer = transformers.BertTokenizer.from_pretrained('../chinese_wwm_ext_pytorch/vocab.txt')
def myTokenizer(text):
t = ''.join(text.split(' '))
t = tokenizer.tokenize(t)[:1024]#max
#t.insert(0, CLS) #加入clk和sep
#t.append(SEP)
return t
def main():
# load data
LABEL = data.Field(sequential=False, use_vocab=False, batch_first=True, dtype=torch.float32)
TEXT = data.Field(sequential=True, tokenize=myTokenizer, lower=False, batch_first=True, unk_token=UNK, pad_token=PAD)
fields = {'label': ('label', LABEL), 'text': ('text', TEXT)}
train, val, test = data.TabularDataset.splits(path='../../newdata', train='train.json', validation='val.json',
test='test.json',format='json', fields=fields)
train_length = len(train.examples)
val_length = len(val.examples)
test_length = len(test.examples)
print('train_length:{}\nval_length:{}\ntest_length:{}'.format(train_length, val_length, test_length))
#加载与训练数据的词表
vocab_path = '../chinese_wwm_ext_pytorch/vocab.txt'
with open(vocab_path, 'r', encoding='utf-8') as file:
original_vocab_size = len(file.readlines())
field_load_vocab(TEXT, vocab_path, novector=True)
vocab_size = len(TEXT.vocab.stoi)
print('original vocab size:{}'.format(original_vocab_size))
print('vocab size:{}'.format(vocab_size))
assert vocab_size == original_vocab_size
cls_id = TEXT.vocab.stoi[CLS]
sep_id = TEXT.vocab.stoi[SEP]
print('cls id = {}, sep_id = {}'.format(cls_id, sep_id))
device = torch.device('cuda:{}'.format(args.cuda) if args.cuda else 'cpu')
train_iter, val_iter, test_iter = data.BucketIterator.splits(
(train, val, test), batch_size=args.batch_size, device=device, shuffle=True,sort_key=lambda x: len(x.text), sort_within_batch=False)
# define model
config = transformers.BertConfig.from_pretrained('../chinese_wwm_ext_pytorch/bert_config.json',output_attentions=False,output_hidden_states=False)
baseBert = transformers.BertModel.from_pretrained(
'../chinese_wwm_ext_pytorch/pytorch_model.bin',
config=config
)
model = BERT(baseBert,args.batch_size, device)
if args.cuda:
model.to(device)
# define loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=args.lr, weight_decay=args.weight_decay)
# train & val & test
best_val_acc = 0.0
print('begin to train!')
val_loss, val_acc,val_corr,val_f1 = evaluate(model, val_iter , criterion)
for epoch in range(args.max_epoch):
train_loss, train_acc = train_one_epoch(model, train_iter, criterion,optimizer, epoch, args)
val_loss, val_acc,val_corr,val_f1= evaluate(model, val_iter,criterion )
'''
if val_acc > best_val_acc:
best_val_acc = val_acc
if not os.path.exists(sample_method + '/'):
os.makedirs(sample_method + '/')
torch.save(model.state_dict(), '{}/net_parameters.pkl'.format(sample_method))
'''
print('Epoch {} result is: train_acc={}, train_loss={}, val_acc={}, val_loss={}'.format(epoch, train_acc,
train_loss, val_acc,
val_loss))
test_loss, test_acc,test_corr, test_f1 = evaluate(model, test_iter, criterion)
print('\nTest set: Average loss: {:.4f}, Accuracy: {:.4f} ({:.2f}%)\n'.format(test_loss, test_acc, test_acc * 100))
if __name__ == '__main__':
main()
| d158f8c440708fbd07ef9d5ebf958f1f1c7d4d89 | [
"Markdown",
"Python"
] | 7 | Markdown | xwwwwww/text-classification | 8b887f69335eb9355f868ce7a5970e7dd78f4023 | b92d5bfb841a005f22b87b5d55b91bb56536f1ad | |
refs/heads/master | <file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class UpdateScore : MonoBehaviour {
public int score;
public Text text;
// Use this for initialization
void Start () {
score= 0;
score = ZombieController.zombieKillCount;
}
// Update is called once per frame
void Update () {
text.text = "Score: "+ score;
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class PauseScript : MonoBehaviour {
public Button exitButton;
public Text text;
public void PauseGame()
{
if(Time.timeScale == 1){
Time.timeScale = 0;
text.color = new Color (255,0,0,255);
GetComponent<PlayerController>().enabled = false;
GetComponent<ZombieController>().enabled = false;
GetComponent<BulletControl>().enabled = false;
}
else if(Time.timeScale == 0){
Time.timeScale = 1;
text.color = new Color(0,0,0,0);
GetComponent<PlayerController>().enabled = true;
GetComponent<ZombieController>().enabled = true;
GetComponent<BulletControl>().enabled = true;
}
}
public void leaveButtonPause(){
Time.timeScale = 1;
SceneManager.LoadScene("planetSelect");
}
}<file_sep>
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ZombieController : MonoBehaviour {
public Rigidbody2D sprite;
public Sprite zombieSprite;
public GameObject zombieTemplate;
//Variables to check if the zombie is on the bullet
public bool onBullet;
public LayerMask whatIsBullet;
public float bulletCheckRadius;
public List<GameObject> zombieList = new List<GameObject>();
public List<int>zombieEraseList = new List<int>();
public int zombieCount=0;
public static int zombieKillCount = 0;
public int zombieSpawnCount = 2;
public float zombieSpeed = 0.2f;
//Text that updates the Player's score
public Text text;
public int getScore(){
return zombieKillCount;
}
//FUnction to lay the framework of the zombie
public void spawnZombie(){
GameObject zombie = Instantiate(zombieTemplate);
zombie.transform.parent = GameObject.Find("Main Camera").transform;
zombie.transform.position = new Vector3(Random.Range(-207,212), Random.Range(-164,168), 1);
zombie.AddComponent<SpriteRenderer>();
zombie.AddComponent<Rigidbody2D>();
zombie.GetComponent<Rigidbody2D>().isKinematic = true;
zombie.AddComponent<BoxCollider2D>();
zombie.GetComponent<BoxCollider2D>().size = new Vector2(5,5);
zombie.layer = 8;
zombie.transform.localScale = new Vector3(sprite.transform.localScale.x,sprite.transform.localScale.y,1);
zombie.GetComponent<SpriteRenderer>().sprite = zombieSprite;
zombieList.Add(zombie);//Adds zombie to an array list instead of a public varibale so multiple zombies can be manipulated
zombieCount++;
}
//THis function checks all the active zombies
public void checkDeadZombies(){
//This variableness goes through all the zombies in the array list and makes them chase the player
for(int i = 0; i < zombieList.Count; i++){
onBullet = Physics2D.OverlapCircle(zombieList[i].transform.position, bulletCheckRadius, whatIsBullet); //checks collision between zombies and pullets
zombieList[i].transform.position = Vector3.MoveTowards(zombieList[i].transform.position, sprite.position, zombieSpeed);//chases player
//If there is a collision between bullets and zombies it deletes it from the array list
if(onBullet){
//zombieEraseList.Add(i);
Destroy(zombieList[i]);//Come from the foreach loop
zombieList.Remove(zombieList[i]);//Come from foreach loop
zombieCount--;
zombieKillCount++;
}
}
/*
foreach(int i in zombieEraseList){
Destroy(zombieList[i]);
zombieList.Remove(zombieList[i]);
}
zombieEraseList = new List<int>();
*/
}
public void updateSpawnSpeed(){
if((zombieKillCount % 8 == 0)){
zombieSpawnCount += 2;
zombieSpeed += 0.1f;
}
}
// Use this for initialization
void Start () {
zombieTemplate = new GameObject();
spawnZombie();
spawnZombie();
zombieKillCount = 0;
}
// Update is called once per frame
void Update () {
text.text = "Score: " + zombieKillCount;
checkDeadZombies();
if(zombieCount == 0){
//Adds difficulty curve
updateSpawnSpeed();
for(int i = 0; i < zombieSpawnCount; i++){
spawnZombie();
}
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class PlayerController : MonoBehaviour {
public Rigidbody2D sprite;
public Button up;
public Button down;
public Button left;
public Button right;
public bool onZombie;
public LayerMask whatIsZombie;
public float zombieCheckRadius;
//Boolean values to check what direction the main character is facing
public static bool isRight;
public static bool isLeft;
public static bool isFacingRight;
public static bool isFacingLeft;
public static bool isDown;
public static bool isUp;
public static bool upRight;
public static bool upLeft;
public static bool downRight;
public static bool downLeft;
public Sprite bulletSprite;
public void goLeft(){
//coditional to make sure the character is facing the opposite direction, if passed, it goes in the opposite direction and flips the sprite to match direction
if(isFacingRight == true && isFacingLeft == false){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingLeft = true;
isFacingRight = false;
}
isRight = false;
isLeft = true;
isUp = false;
isDown = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(-30, 0);
}
public void goRight(){
//coditional to make sure the character is facing the opposite direction, if passed, it goes in the opposite direction and flips the sprite to match direction
if(isFacingLeft == true && isFacingRight == false){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingRight = true;
isFacingLeft = false;
}
isRight = true;
isLeft = false;
isUp = false;
isDown = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(30, 0);
}
public void goUp(){
isUp = true;
isDown = false;
isLeft = false;
isRight = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(0, 30);
}
public void goDown(){
isUp = false;
isDown = true;
isLeft = false;
isRight = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(0, -30);
}
public void goUpRight(){
if(isFacingLeft == true && isFacingRight == false){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingRight = true;
isFacingLeft = false;
}
isRight = false;
isLeft = false;
isUp = false;
isDown = false;
upRight = true;
upLeft = false;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(21.2f, 21.2f);
}
public void goUpLeft(){
if(isFacingLeft == false && isFacingRight == true){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingRight = false;
isFacingLeft = true;
}
isRight = false;
isLeft = false;
isUp = false;
isDown = false;
upRight = false;
upLeft = true;
downRight = false;
downLeft = false;
sprite.velocity = new Vector2(-21.2f, 21.2f);
}
public void goDownLeft(){
if(isFacingLeft == false && isFacingRight == true){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingRight = false;
isFacingLeft = true;
}
isRight = false;
isLeft = false;
isUp = false;
isDown = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = true;
sprite.velocity = new Vector2(-21.2f, -21.2f);
}
public void goDownRight(){
if(isFacingLeft == true && isFacingRight == false){
sprite.GetComponent<Transform>().localScale = new Vector3( sprite.GetComponent<Transform>().localScale.x * -1, sprite.GetComponent<Transform>().localScale.y, sprite.GetComponent<Transform>().localScale.z );
isFacingRight = true;
isFacingLeft = false;
}
isRight = false;
isLeft = false;
isUp = false;
isDown = false;
upRight = false;
upLeft = false;
downRight = true;
downLeft = false;
sprite.velocity = new Vector2(21.2f, -21.2f);
}
// Use this for initialization
void Start () {
isRight = false;
isLeft = true;
isDown = false;
isUp = false;
isFacingLeft = true;
isFacingRight = false;
upRight = false;
upLeft = false;
downRight = false;
downLeft = false;
}
// Update is called once per frame
void Update () {
//Check if player is on the zombie by a certain radius (zombieCheckRadius), from a rigidbody (sprite) for certain layers (whatIsZombie)
onZombie = Physics2D.OverlapCircle(sprite.transform.position, zombieCheckRadius, whatIsZombie);
if(onZombie){
SceneManager.LoadScene ("Lose");
}
}
}
<file_sep>using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class BulletControl : MonoBehaviour {
public Rigidbody2D sprite;
public Sprite bulletSprite;
public bool pistolShotBullet;
public bool pistolBulletPending;
public GameObject bullet;
//This is the function that repsonds to the shoot button, it makes the boolean true to help succed to if statement in update
public void pistolShoot(){
pistolShotBullet = true;
}
/*public void shootBullet(){
GameObject bullet;
bullet = new GameObject();
bullet.AddComponent<SpriteRenderer>();
bullet.AddComponent<Rigidbody2D>();
bullet.AddComponent<BoxCollider2D>();
bullet.GetComponent<Rigidbody2D>().bodyType = RigidbodyType2D.Kinematic;
bullet.transform.localScale = new Vector3(6,6,1);
Vector2 tempColl = new Vector2(1,1);
bullet.GetComponent<BoxCollider2D>().size = tempColl;
Vector3 temp = new Vector3(sprite.position.x,sprite.position.y,0);
bullet.transform.position = temp;
bullet.GetComponent<SpriteRenderer>().sprite = bulletSprite;
if(PlayerController.isRight == true && PlayerController.isLeft == false){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(230, bullet.GetComponent<Rigidbody2D>().velocity.y);
}
else if(PlayerController.isRight == false && PlayerController.isLeft == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(-230, bullet.GetComponent<Rigidbody2D>().velocity.y);
}
}*/
void Start(){
pistolShotBullet = false;
pistolBulletPending = false;
bullet = new GameObject();
}
void Update(){
//This part of the if statement checks if a the pistolShot() method is active and if there is any pistol bullets in the game. REMEBER: Pistols can only be shot after all other pistol bullets are gone
if(pistolShotBullet == true && pistolBulletPending == false){
//This part of the code creates the bullet object
bullet = new GameObject();
pistolBulletPending = true;
bullet.AddComponent<SpriteRenderer>();
bullet.AddComponent<Rigidbody2D>();
bullet.AddComponent<BoxCollider2D>();
bullet.transform.parent = GameObject.Find("Main Camera").transform;
bullet.GetComponent<Rigidbody2D>().bodyType = RigidbodyType2D.Kinematic;
bullet.layer = 9;
bullet.transform.localScale = new Vector3(6,6,1);
Vector2 tempColl = new Vector2(6,5);
bullet.GetComponent<BoxCollider2D>().size = tempColl;
Vector3 temp = new Vector3(sprite.position.x,sprite.position.y,0);
bullet.transform.position = temp;
bullet.GetComponent<SpriteRenderer>().sprite = bulletSprite;
//This part determines which direction to shoot the bullet based on the sprite from PLayerController and what direction he is facing
if(PlayerController.isRight == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(500,0);
}
else if(PlayerController.isLeft == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(-500,0);
}
else if(PlayerController.isDown == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(0,-500);
}
else if(PlayerController.isUp == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(0,500);
}
else if(PlayerController.upRight == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(354,354);
}
else if(PlayerController.upLeft == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(-354,354);
}
else if(PlayerController.downLeft == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(-354,-354);
}
else if(PlayerController.downRight == true){
bullet.GetComponent<Rigidbody2D>().velocity = new Vector2(354,-354);
}
}
//
if(pistolBulletPending == true &&(bullet.GetComponent<Rigidbody2D>().position.x < 20 || bullet.GetComponent<Rigidbody2D>().position.x > 465)){
Destroy(bullet.GetComponent<Rigidbody2D>());
Destroy(bullet.GetComponent<SpriteRenderer>());
Destroy(bullet.GetComponent<BoxCollider2D>());
pistolBulletPending = false;
pistolShotBullet = false;
}
else if(pistolBulletPending == true &&(bullet.GetComponent<Rigidbody2D>().position.y < -4 || bullet.GetComponent<Rigidbody2D>().position.y > 356)){
Destroy(bullet.GetComponent<Rigidbody2D>());
Destroy(bullet.GetComponent<SpriteRenderer>());
Destroy(bullet.GetComponent<BoxCollider2D>());
pistolBulletPending = false;
pistolShotBullet = false;
}
}
} | 4ee9a3e397230d54bfd9095901e1777abc956c46 | [
"C#"
] | 5 | C# | JarifAftab/RetroZombieSurvivor | eb9183167a89ea533afd4f63925aefe502393b8d | 1ed91c3a8d62df6dd5b70c95089b6924b91d6b6e | |
refs/heads/master | <file_sep>require 'open-uri'
require 'pry'
require 'nokogiri'
class Scraper
def self.scrape_index_page(index_url)
student_info=[]
web = Nokogiri::HTML(open(index_url))
roster = web.css(".student-card")
roster.each do |info|
student_info << {:name=>info.css("h4").text,:location=>info.css("p").text,:profile_url=>info.xpath('a').attr('href').text.strip}
end
student_info
end
def self.scrape_profile_page(profile_url)
profile_info={}
web = Nokogiri::HTML(open(profile_url))
profile = web.css("div.social-icon-container a")
profile.each do |info|
if info.attr("href").include?("twitter")
profile_info[:twitter]=info.attribute('href').value
elsif info.attr("href").include?("linkedin")
profile_info[:linkedin]=info.attribute('href').value
elsif info.attr("href").include?("github")
profile_info[:github]=info.attribute('href').value
else
profile_info[:blog]=info.attribute('href').value
end
end
profile_info[:profile_quote]=web.css(".profile-quote").text
profile_info[:bio]=web.css(".description-holder p")[0].text
profile_info
end
end
| e10ed5476a2c1592ee3bca83c8d46eea3703469e | [
"Ruby"
] | 1 | Ruby | MarieHughes93/oo-student-scraper-online-web-ft-110419 | c4275ffd0de65eeba49f2fb1c80f3badc4eb3aff | 918cdc6a4ef19f4777792fdd074de577ea17e4bf | |
refs/heads/master | <repo_name>saqemlas/NewsApp<file_sep>/src/components/Header.js
import React from 'react'
import { NavLink } from 'react-router-dom'
import { Transition } from 'semantic-ui-react'
const Header = (props) => {
return (
<NavLink exact to="/about" onClick={props.onClick}>
<div className="ui centered grid" style={{margin: 10}}>
<h2 className="ui icon header">
<Transition animation={'shake'} duration={500} visible={props.visible}>
<i className="globe icon" />
</Transition>
<div className="content">
Global News
<div className="sub header">
For all your current information needs
</div>
</div>
</h2>
</div>
</NavLink>
)}
export default Header
<file_sep>/src/components/topheadlines/TopHeadlinesList.js
import React from 'react'
import HeadlineCard from './HeadlineCard'
const TopHeadlinesList = (props) => {
return (
<div className="ui centered equal width grid" style={{ margin: 10 }} >
{props.headlines.map((headline, index) => (
<HeadlineCard
key={index}
headline={headline}
selectHeadline={props.selectHeadline}
/>
))}
</div>
)}
export default TopHeadlinesList
<file_sep>/src/components/sources/SourceList.js
import React from 'react'
import SourceCard from './SourceCard'
const SourceList = (props) => {
return (
<div className="ui centered equal width grid" style={{ margin: 10 }}>
{props.sources.map((source, index) => (
<SourceCard
key={index}
source={source}
/>
))}
</div>
)}
export default SourceList
<file_sep>/src/components/InitialSearch.js
import React from 'react'
const InitialSearch = (props) => {
return (
<>
<div className="ui labeled input" style={{ width: '50%' }}>
<div className="ui label">
<i className="quote left icon" />
</div>
<input
className="prompt"
type="text"
name="initialSearch"
placeholder="Search..."
value={props.searchTerm}
onChange={props.updateSearchTerm}
style={{ fontWeight: 'bold' }}
/>
<div className="ui label">
<i className="quote right icon" />
</div>
<button
className="ui teal right labeled icon button"
onClick={() => props.fetchData()}>
<i className="sync alternate icon" />
Initial Search
</button>
</div>
</>
)}
export default InitialSearch
<file_sep>/README.md
<a href="https://newsapp.saqemlas.now.sh/"> Live Demo </a>
<a href="https://github.com/saqemlas/NewsApp-api"> Global News API </a>
# Global News
An application for people to read and share news articles.
Users are able to sign in, search news articles by sources, top headlines, and all news. Users are then able to share news articles via various social media mediums and save articles to their account.
User and article information stored in mySQL database, with email/password authentication to implement Sign Up and Log In for users. React Router navigation to allow users to bookmark components, and React Pose animation on components for added user experience.
<table>
<tr>
<th>
Frontend
</th>
<th>
Backend
</th>
</tr>
<tr>
<td>
React - version 16.8.6
</td>
<td>
Express - version 4.17.1
</td>
</tr>
<tr>
<td>
React Router - version 5.0.1
</td>
<td>
MySQL2 - version 1.6.5
</td>
</tr>
<tr>
<td>
React Pose - version 4.0.8
</td>
<td>
Sequelize - version 5.8.9
</td>
</tr>
<tr>
<td>
Semantic UI - version 0.87.1
</td>
<td>
JSONwebtokens - version 8.5.1
</td>
</tr>
</table>
<file_sep>/src/components/everything/EverythingList.js
import React from 'react'
import EverythingCard from './EverythingCard'
const EverythingList = (props) => {
return (
<div className="ui centered equal width grid" style={{ margin: 10 }} >
{props.everything.map((article, index) => (
<EverythingCard
key={index}
article={article}
selectArticle={props.selectArticle}
/>
))}
</div>
)}
export default EverythingList
<file_sep>/src/components/everything/EverythingCard.js
import React from 'react'
import { Link } from 'react-router-dom'
import posed from 'react-pose'
const Button = posed.div({
pressable: true,
init: { scale: 1 },
press: { scale: 0.8 }
})
const CardContainer = posed.div({
hoverable: true,
init: {
scale: 0.95,
},
hover: {
scale: 1.05,
},
})
const EverythingCard = (props) => {
const datetimeFormat = () => {
const data = props.article.publishedAt
const datetime = data.slice(0, -1).split('T')
const result = 'At : ' + datetime[1] + ', ' + datetime[0]
return result
}
return (
<CardContainer className="box">
<div className="ui card" style={{ margin: 10}}>
<div className="image">
<img
alt={props.article.title}
src={props.article.urlToImage || "https://www.albertadoctors.org/images/ama-master/feature/News.jpg"}
/>
</div>
<div className="content">
<div className="header">
{props.article.title}
</div>
<div className="meta">
{props.article.source.name || "Unknown"}
</div>
<div className="description" style={{ fontStyle: 'italic' }}>
{datetimeFormat()}
</div>
</div>
<div className="extra content">
<div className="ui centered grid">
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<Link
key={props.article.title}
to={{
pathname: `/everything/${props.article.title}`,
state: {
article: props.article
}}}
>
<button className="ui teal button">
Details
</button>
</Link>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<button className="ui secondary button">
<a href={props.article.url} style={{color: 'white'}}>
Visit Article
</a>
</button>
</Button>
</div>
</div>
</div>
</CardContainer >
)}
export default EverythingCard
<file_sep>/src/containers/TopHeadlines.js
import React, { Component } from 'react'
import SearchBar from '../components/SearchBar'
import TopHeadlinesList from '../components/topheadlines/TopHeadlinesList'
import InitialSearch from '../components/InitialSearch'
import NoResults from '../components/NoResults'
const apiKey = process.env.REACT_APP_NEWS_API_KEY
export default class TopHeadlines extends Component {
state = {
headlines: [],
initialSearch: "",
searchTerm: "",
}
fetchHeadlines = () => {
fetch('https://newsapi.org/v2/top-headlines?pageSize=100&q=' + this.state.initialSearch + '&apiKey=' + apiKey)
.then(response => response.json())
.then(data => {
this.setState({
headlines: data.articles })
})
}
updateInput = e => {
this.setState({
[e.target.name]: e.target.value })
}
filterHeadlines = () => {
const searchFilter = this.state.headlines.filter(headline =>
headline.title.toLowerCase().includes(this.state.searchTerm.toLowerCase()))
const filtered = searchFilter
return filtered
}
render(){
return (
<div style={{ marginTop: '1%'}}>
<div
className="ui centered grid"
style={{ marginTop: 10, marginBottom: 10 }}>
<InitialSearch
searchTerm={this.state.initialSearch}
updateSearchTerm={this.updateInput}
fetchData={this.fetchHeadlines}
/>
</div>
{ this.state.headlines.length === 0
?
< NoResults />
:
<>
<div
className="ui centered grid"
style={{ marginTop: 10, marginBottom: 10}}>
<SearchBar
searchTerm={this.state.searchTerm}
updateSearchTerm={this.updateInput}
/>
</div>
< TopHeadlinesList
headlines={this.filterHeadlines()}
/>
</>
}
</div>
)}
}
<file_sep>/src/components/SearchBar.js
import React from 'react'
import posed from 'react-pose'
const Input = posed.input({
focusable: true,
init: {
scale: 1
},
focus: {
scale: 1.1
}
})
const SearchBar = (props) => {
return (
<div className="ui search" style={{ width: '40%' }}>
<div className="ui fluid icon input">
<Input
className="prompt"
type="text"
name="searchTerm"
placeholder="Filter results..."
value={props.searchTerm}
onChange={props.updateSearchTerm}
/>
<i
className="search icon"
/>
</div>
</div>
)}
export default SearchBar
<file_sep>/src/App.js
import React, { Component } from 'react'
import { Route, Switch, Redirect, withRouter } from 'react-router-dom'
import ErrorHandler from './components/ErrorHandler'
import Toolbar from './components/Toolbar'
import MainNavigation from './components/MainNavigation'
import Header from './components/Header'
import LoginPage from './containers/Auth/Login'
import SignupPage from './containers/Auth/Signup'
import NavBar from './components/NavBar'
import About from './containers/About'
import Saved from './containers/Saved'
import Sources from './containers/Sources'
import Everything from './containers/Everything'
import TopHeadlines from './containers/TopHeadlines'
import HeadlineDetails from './components/topheadlines/HeadlineDetails'
import EverythingDetails from './components/everything/EverythingDetails'
import SavedDetails from './components/saved/SavedDetails'
class App extends Component {
state = {
visible: true,
isAuth: false,
token: null,
userId: null,
authLoading: false,
error: null
}
componentDidMount() {
const token = localStorage.getItem('token')
const expiryDate = localStorage.getItem('expiryDate')
if (!token || !expiryDate) {
return
}
if (new Date(expiryDate) <= new Date()) {
this.logoutHandler()
return
}
const userId = localStorage.getItem('userId')
const remainingMilliseconds =
new Date(expiryDate).getTime() - new Date().getTime()
this.setState({ isAuth: true, token: token, userId: userId })
this.setAutoLogout(remainingMilliseconds)
}
logoutHandler = () => {
this.setState({ isAuth: false, token: null });
localStorage.removeItem('token');
localStorage.removeItem('expiryDate');
localStorage.removeItem('userId');
};
loginHandler = (event, authData) => {
event.preventDefault();
this.setState({ authLoading: true });
fetch('https://newsappapi.saqemlas.now.sh/api/auth/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
email: authData.email,
password: <PASSWORD>
})
})
.then(res => {
if (res.status === 422) {
throw new Error('Validation failed.');
}
if (res.status !== 200 && res.status !== 201) {
console.log('Error!');
throw new Error('Could not authenticate you!');
}
return res.json();
})
.then(resData => {
this.setState({
isAuth: true,
token: resData.token,
authLoading: false,
userId: resData.userId
});
localStorage.setItem('token', resData.token);
localStorage.setItem('userId', resData.userId);
const remainingMilliseconds = 60 * 60 * 1000;
const expiryDate = new Date(
new Date().getTime() + remainingMilliseconds
);
localStorage.setItem('expiryDate', expiryDate.toISOString());
this.setAutoLogout(remainingMilliseconds);
})
.catch(err => {
console.log(err);
this.setState({
isAuth: false,
authLoading: false,
error: err
})
})
}
signupHandler = (event, authData) => {
event.preventDefault();
this.setState({ authLoading: true });
fetch('https://newsappapi.saqemlas.now.sh/api/auth/signup', {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
email: authData.signupForm.email.value,
password: <PASSWORD>,
name: authData.signupForm.name.value
})
})
.then(res => {
if (res.status === 422) {
throw new Error(
"Validation failed. Make sure the email address isn't used yet!"
);
}
if (res.status !== 200 && res.status !== 201) {
console.log('Error!');
throw new Error('Creating a user failed!');
}
return res.json();
})
.then(resData => {
console.log(resData);
this.setState({ isAuth: false, authLoading: false });
this.props.history.replace('/');
})
.catch(err => {
console.log(err);
this.setState({
isAuth: false,
authLoading: false,
error: err
});
});
};
setAutoLogout = milliseconds => {
setTimeout(() => {
this.logoutHandler()
}, milliseconds)
}
errorHandler = () => {
this.setState({ error: null })
}
onClick = () => {
this.setState({
visible: !this.state.visible })
}
render() {
let routes = (
<Switch>
<Route
path="/"
exact
render={props => (
<LoginPage
{...props}
onLogin={this.loginHandler}
loading={this.state.authLoading}
/>
)}
/>
<Route
path="/signup"
exact
render={props => (
<SignupPage
{...props}
onSignup={this.signupHandler}
loading={this.state.authLoading}
/>
)}
/>
<Redirect to="/" />
</Switch>
)
if (this.state.isAuth) {
routes = (
<>
<NavBar
onClick={this.onClick}
/>
<Switch>
<Route exact path="/about" component={About} />
<Route exact path="/saved" component={props => (
< Saved userId={this.state.userId} token={this.state.token} /> )} />
<Route exact path="/sources" component={Sources} />
<Route exact path="/topheadlines" component={TopHeadlines} />
<Route exact path="/everything" component={Everything} />
<Route exact path={"/topheadlines/:headline"} component={props => (
< HeadlineDetails userId={this.state.userId} token={this.state.token} /> )} />
<Route exact path={"/everything/:article"} component={props => (
< EverythingDetails userId={this.state.userId} token={this.state.token} /> )} />
<Route exact path={"/saved/:article"} component={props => (
< SavedDetails userId={this.state.userId} token={this.state.token} /> )} />
<Redirect to="/" />
</Switch>
</>
);
}
return (
<div>
<>
<Toolbar>
<MainNavigation
onLogout={this.logoutHandler}
isAuth={this.state.isAuth}
/>
</Toolbar>
<ErrorHandler
error={this.state.error}
onHandle={this.errorHandler}
/>
<Header
visible={this.state.visible}
onClick={this.onClick}
/>
</>
<div>
{routes}
</div>
</div>
)}
}
export default withRouter(App)
<file_sep>/src/components/NoResults.js
import React from 'react'
const NoResults = () => {
return (
<div className="ui centered grid" style={{marginTop: '2%'}}>
<h1>
Please Submit Search
</h1>
<span>
<i className="angle double up big icon" />
</span>
</div>
)}
export default NoResults
<file_sep>/src/components/saved/SavedDetails.js
import React, { Component } from 'react'
import { withRouter, Link } from 'react-router-dom'
import {
FacebookShareButton, FacebookIcon,
LinkedinShareButton, LinkedinIcon,
TwitterShareButton, TwitterIcon,
WhatsappShareButton, WhatsappIcon,
RedditShareButton, RedditIcon,
EmailShareButton, EmailIcon
} from 'react-share'
import posed from 'react-pose'
const Button = posed.div({
pressable: true,
init: { scale: 1 },
press: { scale: 0.9 }
})
class SavedDetails extends Component {
deleteHandler = postId => {
const userId = this.props.userId
fetch(`http://localhost:3000/api/feed/post/${postId}/${userId}`, {
method: 'DELETE',
headers: {
Authorization: 'Bearer ' + this.props.token
},
})
.then(res => {
if (res.status !== 200 && res.status !== 201) {
throw new Error('Deleting a post failed!')
}
return res.json()
})
.catch(err => {
console.log(err)
})
}
render() {
const { saved } = this.props.location.state
return (
<div className="ui centered grid" >
<div className="ui fluid card" style={{ margin: 10, width: '50%'}}>
<div className="image">
<img
alt={saved.title}
src={saved.urlToImage || "https://www.albertadoctors.org/images/ama-master/feature/News.jpg"}
/>
</div>
<div className="content">
<div className="header">
{saved.title}
</div>
<div className="meta">
<div className="left floated">
Author : {saved.author || "Unknown"}
</div>
<span>
<div className="right floated">
Source : {saved.source.name || "Unknown"}
</div>
</span>
</div>
<div className="description" style={{ fontStyle: 'italic'}}>
{saved.description}
</div>
<div className="description">
{saved.content}
</div>
</div>
<div className="extra content">
<div className="ui centered grid">
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<button
className="ui teal button"
onClick={() => this.props.history.goBack()}>
Go Back
</button>
</Button>
<Link to="/saved">
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<button
className="ui red button"
onClick={() => this.deleteHandler(saved.id)}>
Delete
</button>
</Button>
</Link>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<button className="ui secondary button">
<a href={saved.url} style={{color: 'white'}}>
Visit Article
</a>
</button>
</Button>
</div>
</div>
<div className="extra content">
Share!
<div className="ui centered grid" style={{ marginTop: '1%', marginBottom: '1%'}}>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<FacebookShareButton url={saved.url} quote={saved.title} >
<FacebookIcon size={35} round />
</FacebookShareButton>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<LinkedinShareButton url={saved.url} quote={saved.title}>
<LinkedinIcon size={35} round />
</LinkedinShareButton>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<TwitterShareButton url={saved.url} quote={saved.title}>
<TwitterIcon size={35} round />
</TwitterShareButton>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<RedditShareButton url={saved.url} quote={saved.title}>
<RedditIcon size={35} round />
</RedditShareButton>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<WhatsappShareButton url={saved.url} quote={saved.title}>
<WhatsappIcon size={35} round />
</WhatsappShareButton>
</Button>
<Button className="box" style={{marginTop: 5, marginBottom: 5}}>
<EmailShareButton url={saved.url} quote={saved.title}>
<EmailIcon size={35} round />
</EmailShareButton>
</Button>
</div>
</div>
</div>
</div>
)}
}
export default withRouter(SavedDetails)
<file_sep>/src/containers/Everything.js
import React, { Component } from 'react'
import SearchBar from '../components/SearchBar'
import InitialSearch from '../components/InitialSearch'
import EverythingList from '../components/everything/EverythingList'
import NoResults from '../components/NoResults'
const apiKey = process.env.REACT_APP_NEWS_API_KEY
export default class Everything extends Component {
state = {
everything: [],
initialSearch: "",
searchTerm: "",
}
fetchEverything = () => {
fetch('https://newsapi.org/v2/everything?q=' + this.state.initialSearch + '&apiKey=' + apiKey)
.then(response => response.json())
.then(data => {
this.setState({
everything: data.articles })
})
}
updateInput = e => {
this.setState({
[e.target.name]: e.target.value })
}
filterEverything = () => {
const filtered = this.state.everything.filter(article =>
article.title.toLowerCase().includes(this.state.searchTerm.toLowerCase()))
return filtered
}
render(){
return (
<div style={{ marginTop: '1%'}}>
<div
className="ui centered grid"
style={{ marginTop: 10, marginBottom: 10 }}>
<InitialSearch
searchTerm={this.state.initialSearch}
updateSearchTerm={this.updateInput}
fetchData={this.fetchEverything}
/>
</div>
{ this.state.everything.length === 0
?
< NoResults />
:
<>
<div
className="ui centered grid"
style={{ marginTop: 10, marginBottom: 10 }}>
<SearchBar
searchTerm={this.state.searchTerm}
updateSearchTerm={this.updateInput}
/>
</div>
<EverythingList
everything={this.filterEverything()}
/>
</>
}
</div>
)}
}
<file_sep>/src/components/CategoryFilter.js
import React from 'react'
import { Dropdown } from 'semantic-ui-react'
import categoryIcons from './CategoryIcons'
const categories = ["general", "business", "entertainment", "health", "science", "sports", "technology"]
const categoryOptions = categories
.map((category, index) => ({
key: index,
text: category,
value: category,
icon: categoryIcons(category)
}))
const CategoryFilter = (props) => {
return (
<Dropdown
fluid
selection
search
clearable
floating
name="category"
placeholder="Select Category"
options={categoryOptions}
value={props.category}
onChange={props.updateCategory}
style={{ width: '40%', marginLeft: '1%', marginRight: '1%' }}
/>
)
}
export default CategoryFilter
<file_sep>/src/containers/About.js
import React from 'react'
const About = () => {
return (
<div
className="ui center aligned header"
style={{ marginTop: '3%'}}>
<h3>
Global News uses the Google News API to search articles from over 30,000 news sources and blogs!
</h3>
<h3>
With Global News at your disposal, find the right article for your daily news needs.
</h3>
</div>
)}
export default About
<file_sep>/src/components/saved/SavedList.js
import React from 'react'
import SavedCard from './SavedCard'
const SavedList = (props) => {
return (
<div className="ui centered equal width grid" style={{ margin: 10 }} >
{props.saved.map((article, index) => (
<SavedCard
key={index}
article={article}
selectArticle={props.selectArticle}
/>
))}
</div>
)}
export default SavedList
| 44300cff1b36ecb51f37f3bac4b80ab1c55f560e | [
"JavaScript",
"Markdown"
] | 16 | JavaScript | saqemlas/NewsApp | 90517b268853d99cb89f559c5ef4eeea53953730 | ea0719cda43f48f873d18e945b1925da8fd785ac | |
refs/heads/master | <file_sep>const REFRESH_UI = "REFRESH_UI";
export {
REFRESH_UI
}<file_sep>import React, { Component } from 'react';
import { NavLink, BrowserRouter, Route, Redirect} from 'react-router-dom'
class Culture extends Component {
render() {
return (
<div>
Culture
</div>
);
}
}
class Contact extends Component {
render() {
return (
<div>
Contact
</div>
);
}
}
class History extends Component {
render() {
return (
<div>
History
</div>
);
}
}
class Recommend extends Component {
render() {
return (
<div>
Recommend123
</div>
);
}
}
class Music extends Component {
render() {
return (
<div>
Music
</div>
);
}
}
class Home extends Component {
render() {
return (
<div>
<NavLink exact to="/" activeStyle={{ color: "purple", fontSize: "30px" }}>Recommend</NavLink>
<NavLink to="/home/music" activeStyle={{ color: "purple", fontSize: "30px" }}>Music</NavLink>
<Route exact path="/" component={Recommend}></Route>
<Route path="/home/music" component={Music}></Route>
</div>
);
}
}
class About extends Component {
render() {
return (
<div>
<NavLink exact to="/about" activeStyle={{ color: "purple", fontSize: "30px" }}>Culture</NavLink>
<NavLink to="/about/history" activeStyle={{ color: "purple", fontSize: "30px" }}>History</NavLink>
<NavLink to="/about/contact" activeStyle={{ color: "purple", fontSize: "30px" }}>Contact</NavLink>
<Route path="/about" component={Culture}></Route>
<Route path="/about/history" component={History}></Route>
<Route path="/about/contact" component={Contact}></Route>
</div>
);
}
}
class Me extends Component {
constructor(props) {
super(props)
this.state = {
isLogin: false,
}
}
render() {
const { isLogin } = this.state
return isLogin ? (
<div>
Me
</div>
) : <Redirect to="/login" />
}
}
class Detail extends Component {
render() {
const location = this.props.location
console.log(location.state);
return (
<div>
{"name:" + location.state.name + " age:" + location.state.age}
</div>
)
}
}
class Login extends Component {
render() {
return (
<div>
Login
</div>
);
}
}
class App extends Component {
render() {
return (
<div>
<BrowserRouter>
{/* <Link to="/">Home</Link>
<Link to="/about">About</Link>
<Link to="/me">Me</Link> */}
{/* NavLink模糊匹配 */}
<NavLink exact to="/" activeStyle={{ color: "red", fontSize: "30px" }}>Home</NavLink>
{/* 传参方式1
<NavLink to="/detail?name=mjk" activeStyle={{ color: "red", fontSize: "30px" }}>Detail</NavLink>
*/}
{/* 传参方式2 */}
<NavLink to= {{
pathname : "/detail",
search: "?name=mjk",
state : {name : "mjk", age : 25}
}}
activeStyle={{ color: "red", fontSize: "30px" }}>Detail</NavLink>
<NavLink to="/about" activeStyle={{ color: "red", fontSize: "30px" }}>About</NavLink>
<NavLink to="/me" activeStyle={{ color: "red", fontSize: "30px" }}>Me</NavLink>
<Route path="/" component={Home}></Route>
<Route path="/detail" component={Detail}></Route>
<Route path="/about" component={About}></Route>
<Route path="/me" component={Me}></Route>
<Route path="/login" component={Login}></Route>
</BrowserRouter>
</div>
);
}
}
export default App;<file_sep>import {
REFRESH_UI
} from './constants'
const defaultState = {
items: ["a"]
}
export default function reducer(state = defaultState, action) {
switch(action.type) {
case REFRESH_UI: {
return {...state, items: action.items}
}
default: {
return state
}
}
}<file_sep>import { createStore } from 'redux'
import reducer from './reducer'
const store = createStore(reducer);
//patch logging
function patchLogging(store) {
const next = store.dispatch;
function dispatchAndLogging(action) {
console.log("logging before---dispatching action:", action);
next(action);
console.log("logging after---new state:", store.getState());
}
return dispatchAndLogging;
}
// patch thunk
function patchThunk(store) {
const next = store.dispatch;
function dispatchAndThunk(action) {
console.log("thunk before---dispatching action:", action);
if (typeof action === "function") {
action(store.dispatch, store.getState)
} else {
next(action);
}
console.log("thunk after---new state:", store.getState());
}
return dispatchAndThunk;
}
// 5.封装applyMiddleware
function applyMiddlewares(...middlewares) {
const newMiddlewares = middlewares.reverse()
newMiddlewares.forEach(middleware => {
store.dispatch = middleware(store);
})
}
applyMiddlewares(patchLogging, patchThunk);
export default store;<file_sep>import React, { PureComponent } from 'react'
import connect from './utils/connect'
import {
incrementAction,
} from './store/actionCreators'
class Header extends PureComponent {
render() {
return (
<div>
<h1>计数器:{this.props.counter}</h1>
<button onClick={(e) => this.props.increment(2)}>+2</button>
</div>
);
}
}
const mapStateToProps = (state) => ({
counter: state.counter
})
const mapDispachToProps = (dispatch) => ({
increment(num) {
dispatch(incrementAction(num))
}
})
export default connect(mapStateToProps, mapDispachToProps)(Header)<file_sep># ReactStudy
每个项目下执行下面的两个命令就OK
1. yarn install
2. yarn start
<file_sep>import {createStore, applyMiddleware, compose} from 'redux'
import thunkMiddleware from 'redux-thunk';
import reducer from './reducer'
//react devtools
const composeEnhancers = window.__REDUX_DEVTOOLS_EXTENSION_COMPOSE__({trace: true}) || compose;
const storeEnhancer = applyMiddleware(thunkMiddleware);
const store = createStore(reducer, composeEnhancers(storeEnhancer));
export default store;<file_sep>import React, { PureComponent } from 'react'
import connect from './utils/connect'
import {
decrementAction,
} from './store/actionCreators'
class Bottom extends PureComponent {
render() {
return (
<div>
<h1>计数器:{this.props.counter}</h1>
<button onClick={(e) => this.props.decrement(2)}>-2</button>
</div>
);
}
}
const mapStateToProps = (state) => ({
counter: state.counter
})
const mapDispachToProps = (dispatch) => ({
decrement(num) {
dispatch(decrementAction(num))
}
})
export default connect(mapStateToProps, mapDispachToProps)(Bottom)<file_sep>import React, { memo, useState, useCallback } from 'react';
const MJKButton = memo((props) => {
console.log("MJKButton重新渲染: " + props.title);
return (
<button onClick={props.increment}>{props.title} +1</button>
)
});
function App() {
console.log("App重新渲染");
const [count, setCount] = useState(0);
const [show, setShow] = useState(true);
const increment1 = () => {
console.log("执行increment1函数");
setCount(count + 1);
}
const increment2 = useCallback(() => {
console.log("执行increment2函数");
setCount(count + 1);
}, [count]);
return (
<div>
<h2>HookCallback: {count}</h2>
<MJKButton title="btn1" increment={increment1}/>
<MJKButton title="btn2" increment={increment2}/>
<button onClick={e => setShow(!show)}>show切换</button>
</div>
)
}
export default App;<file_sep>import React, { PureComponent } from 'react'
import { connect } from 'react-redux'
import {
incrementAction,
} from './store/counter/actionCreators'
class Counter extends PureComponent {
render() {
return (
<div>
<h1>计数器:{this.props.counter}</h1>
<button onClick={(e) => this.props.increment(2)}>+2</button>
</div>
);
}
}
const mapStateToProps = (state) => ({
counter: state.counterInfo.counter
})
const mapDispachToProps = (dispatch) => ({
increment(num) {
dispatch(incrementAction(num))
}
})
export default connect(mapStateToProps, mapDispachToProps)(Counter)<file_sep>import React, { Component } from 'react'
import { connect } from 'react-redux'
import {
requestDataAction
} from './store/home/actionCreators'
class Home extends Component {
render() {
return (
<div>
<ul>
{
this.props.items.map((item, index, arr) => {
return (
<li key={item}> {item}</li>
)
})
}
</ul>
<button onClick={(e) => this.props.requestData()}>请求数据</button>
</div>
)
}
}
const mapStateToProps = (state) => ({
items: state.homeInfo.items
})
//redux-thunk传的是函数
const mapDispatchToProps = (dispatch) => ({
requestData() {
dispatch(requestDataAction)
}
})
export default connect(mapStateToProps, mapDispatchToProps)(Home)<file_sep>import React, { Component } from 'react'
import axios from 'axios'
export default class App extends Component {
render() {
return (
<div>
Hello
</div>
)
}
componentDidMount() {
// axios.post("https://httpbin.org/post", {
// name: "lucy",
// age: 28
// }).then(response => {
// console.log(response);
// })(error => {
// console.log(error);
// });
axios({
url: "https://httpbin.org/post",
data: {
name: "mjk",
age: 40
},
method: "post"
}).then(res => {
console.log(res);
}).catch(err => {
console.error(err);
})
}
}
<file_sep>import React, { PureComponent } from 'react'
import store from './store/'
import {
incrementAction,
decrementAction
} from './store/actionCreators'
class Header extends PureComponent {
constructor(props) {
super(props)
this.state = {
counter: store.getState().counter
}
}
componentDidMount() {
this.unsubscribue = store.subscribe(() => {
this.setState({
counter: store.getState().counter
})
})
}
componentWillUnmount() {
this.unsubscribue()
}
render() {
return (
<div>
<h1>计数器:{this.state.counter}</h1>
<button onClick={(e) => store.dispatch(incrementAction(2))}>+2</button>
</div>
);
}
}
class Bottom extends PureComponent {
constructor(props) {
super(props)
this.state = {
counter: store.getState().counter
}
}
componentDidMount() {
this.unsubscribue = store.subscribe(() => {
this.setState({
counter: store.getState().counter
})
})
}
componentWillUnmount() {
this.unsubscribue()
}
render() {
return (
<div>
<h1>计数器:{this.state.counter}</h1>
<button onClick={(e) => store.dispatch(decrementAction(2))}>-2</button>
</div>
);
}
}
export default class App extends PureComponent {
render() {
console.log(store.getState().counter)
return (
<div>
<Header/>
<Bottom/>
</div>
)
}
}
| 63caf7898821e9d67ea72826882c9c85332bf0ec | [
"JavaScript",
"Markdown"
] | 13 | JavaScript | majiakun111/ReactStudy | 63ac60fdf3499d6dd1b7067ce8ba89a5c899a17d | 8780df229ab5658d7d4068db70036b50102c8a17 | |
refs/heads/master | <repo_name>ssang1105/my-book<file_sep>/src/main/java/com/supersw/mybook/client/book/kakao/resposne/Meta.java
package com.supersw.mybook.client.book.kakao.resposne;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Getter;
@Getter
public class Meta {
@JsonProperty("is_end")
private boolean isEnd;
@JsonProperty("pageable_count")
private int pagableCount;
@JsonProperty("total_count")
private int totalCount;
}<file_sep>/src/main/java/com/supersw/mybook/error/exceptions/NoAuthorityException.java
package com.supersw.mybook.error.exceptions;
public class NoAuthorityException extends RuntimeException {
public NoAuthorityException(String message) {
super(message);
}
}
<file_sep>/src/main/java/com/supersw/mybook/client/exceptions/ClientException.java
package com.supersw.mybook.client.exceptions;
public class ClientException extends RuntimeException {
private static final long serialVersionUID = 8256583033452927847L;
public ClientException(int statusCode, String request, String response) {
super("statusCode: " + statusCode + "\nrequest: " + request + "\nresponse: " + response);
}
public ClientException(String message, Throwable t) {
super(message, t);
}
}
<file_sep>/src/main/java/com/supersw/mybook/member/domain/MemberJoinRequest.java
package com.supersw.mybook.member.domain;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Getter;
@Getter
public class MemberJoinRequest {
private final String id;
private final String password;
@JsonCreator
public MemberJoinRequest(@JsonProperty("username") String id, @JsonProperty("password") String password) {
this.id = id;
this.password = <PASSWORD>;
}
}
<file_sep>/src/main/java/com/supersw/mybook/config/JacksonConfig.java
package com.supersw.mybook.config;
import com.fasterxml.jackson.annotation.JsonAutoDetect.Visibility;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.DeserializationConfig;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.datatype.jdk8.Jdk8Module;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import com.fasterxml.jackson.module.paramnames.ParameterNamesModule;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
return new ObjectMapper()
.registerModule(new ParameterNamesModule())
.registerModule(new Jdk8Module())
.registerModule(new JavaTimeModule())
.setVisibility(PropertyAccessor.FIELD, Visibility.ANY)
.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
}
}
<file_sep>/src/main/java/com/supersw/mybook/member/service/MemberService.java
package com.supersw.mybook.member.service;
import com.supersw.mybook.error.exceptions.AlreadyExistMemberException;
import com.supersw.mybook.error.exceptions.NotExistMemberException;
import com.supersw.mybook.member.domain.Member;
import com.supersw.mybook.member.repository.MemberRepository;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import org.springframework.stereotype.Service;
import org.springframework.util.Base64Utils;
@RequiredArgsConstructor
@Service
public class MemberService {
private final MemberRepository memberRepository;
public Member join(@NonNull String id, @NonNull String password) {
if (memberRepository.findByMemberId(id) != null) {
throw new AlreadyExistMemberException("이미 존재하는 회원 아이디입니다. 회원 아이디 : " + id);
}
Member member = Member.create(id, Base64Utils.encodeToString(password.getBytes()));
return memberRepository.save(member);
}
public Member login(@NonNull String id, @NonNull String password) {
Member member = memberRepository.findByMemberIdAndPassword(id, Base64Utils.encodeToString(password.getBytes()));
if (member == null) {
throw new NotExistMemberException("존재하지 않는 회원입니다. 회원 아이디 : " + id);
}
return member;
}
public Member find(@NonNull String id) {
return memberRepository.findByMemberId(id);
}
}<file_sep>/src/main/java/com/supersw/mybook/book/service/BookService.java
package com.supersw.mybook.book.service;
import com.supersw.mybook.book.domain.BookSearchResult;
import com.supersw.mybook.client.book.BookSearchClientResponse;
import com.supersw.mybook.client.book.kakao.KakaoBookClient;
import com.supersw.mybook.client.book.naver.NaverBookClient;
import com.supersw.mybook.client.book.request.BookSearchParam;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import org.springframework.stereotype.Service;
@RequiredArgsConstructor
@Service
@Slf4j
public class BookService {
private final KakaoBookClient kakaoBookClient;
private final NaverBookClient naverBookClient;
private final BookSearchHistoryService bookSearchHistoryService;
public BookSearchResult searchBooks(@NonNull String query, int page, int size, String memberId) {
BookSearchParam bookSearchParam = BookSearchParam.create(query, page, size);
bookSearchHistoryService.save(query, memberId);
BookSearchClientResponse bookSearchClientResponse = null;
try {
bookSearchClientResponse = kakaoBookClient.searchBooks(bookSearchParam);
} catch (Exception e) {
log.error(e.getMessage(), e);
log.info("### KAKAO 책 API 가 실패하여 NAVER 책 API 를 호출합니다.");
bookSearchClientResponse = naverBookClient.searchBooks(bookSearchParam);
}
return BookSearchResult.of(bookSearchClientResponse);
}
}
<file_sep>/src/main/java/com/supersw/mybook/client/book/kakao/KakaoBookClient.java
package com.supersw.mybook.client.book.kakao;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.supersw.mybook.client.ClientTemplate;
import com.supersw.mybook.client.book.BookClient;
import com.supersw.mybook.client.book.BookSearchClientResponse;
import com.supersw.mybook.client.book.kakao.resposne.KakaoResponse;
import com.supersw.mybook.client.book.kakao.resposne.KakaoBookSearchResponse;
import com.supersw.mybook.client.book.request.BookSearchParam;
import java.net.URI;
import lombok.Getter;
import lombok.NonNull;
import org.apache.http.client.fluent.Request;
import org.apache.http.client.utils.URIBuilder;
public class KakaoBookClient extends ClientTemplate<KakaoResponse> implements BookClient {
private final int connectTimeout;
private final int socketTimeout;
private final String appKey;
@Getter
private final ObjectMapper objectMapper;
private final URI baseUri;
private static final String searchBookUri = "/v3/search/book";
private static final TypeReference<KakaoBookSearchResponse> kakaoSearchBookResponseTypeReference = new TypeReference<KakaoBookSearchResponse>() {
};
public KakaoBookClient(@NonNull ObjectMapper objectMapper, @NonNull KakaoBookClientProperties properties) {
this.objectMapper = objectMapper;
this.connectTimeout = properties.getConnectTimeout();
this.socketTimeout = properties.getSocketTimeout();
this.appKey = properties.getAppKey();
this.baseUri = properties.getBaseUri();
}
@Override
public BookSearchClientResponse searchBooks(BookSearchParam param) {
String uri = new URIBuilder(baseUri).setPath(searchBookUri)
.addParameter("query", param.getQuery())
.addParameter("page", String.valueOf(param.getPage()))
.addParameter("size", String.valueOf(param.getSize()))
.toString();
Request request = Request.Get(uri)
.addHeader("Authorization", "KakaoAK " + appKey);
KakaoBookSearchResponse response = execute(request, kakaoSearchBookResponseTypeReference);
return BookSearchClientResponse.of(response);
}
@Override
protected void initRequestHeader(Request request) {
super.initRequestHeader(request);
request.connectTimeout(this.connectTimeout)
.socketTimeout(this.socketTimeout);
}
}
<file_sep>/src/main/resources/static/js/mybook.main.js
(function () {
$('#join-btn').click(function () {
var username = $("input[name=username]").val();
if (!username) {
alert("아이디를 입력해주세요.");
return;
}
var password = $("input[name=password]").val();
if (!username) {
alert("패스워드를 입력해주세요.");
return;
}
var data = {
username: username,
password: <PASSWORD>
};
$.ajax({
url: location.protocol + '//' + location.host + '/api/members',
method: 'POST',
contentType: 'application/json',
data: JSON.stringify(data),
success: function (response) {
alert("회원가입 성공!");
window.location.reload();
},
error: function (result) {
if (result.responseJSON.userMessage) {
alert(result.responseJSON.userMessage);
} else {
alert("서버 오류입니다.\n잠시 후 다시 시도해주세요.");
}
}
});
});
$('#login-btn').click(function () {
var username = $("input[name=username]").val();
if (!username) {
alert("아이디를 입력해주세요.");
return;
}
var password = $("input[name=password]").val();
if (!username) {
alert("패스워드를 입력해주세요.");
return;
}
var data = {
username: username,
password: <PASSWORD>
};
$.ajax({
url: location.protocol + '//' + location.host + '/api/members/login',
method: 'POST',
contentType: 'application/json',
data: JSON.stringify(data),
success: function (response) {
alert("로그인 성공!");
window.location.reload();
},
error: function (result) {
if (result.responseJSON.userMessage) {
alert(result.responseJSON.userMessage);
} else {
alert("서버 오류입니다.\n잠시 후 다시 시도해주세요.");
}
}
});
});
$('#search-btn').click(function () {
var query = $("input[name=bookName]").val();
if (!query) {
alert("검색할 책 제목을 입력해주세요.");
return;
}
searchBook(query, 1);
});
$('#search-hist-btn').click(function () {
var $searchHistList = $('.search-hist-list');
if ($searchHistList.is(":visible")) {
$searchHistList.hide();
$searchHistList.children().remove();
return;
} else {
$searchHistList.show();
}
var memberId = $("input[name=memberId]").val();
$.ajax({
url: location.protocol + '//' + location.host + '/api/book-search-histories?memberId=' + memberId,
method: 'GET',
success: function (response) {
if ($.isEmptyObject(response)) {
$('.search-hist-list').append('<strong">검색 내역이 없습니다.</strong>');
return;
}
$.each(response, function (i, hist) {
appendHistToList(hist);
});
},
error: function (result) {
if (result.responseJSON.userMessage) {
alert(result.responseJSON.userMessage);
} else {
alert("서버 오류입니다.\n잠시 후 다시 시도해주세요.");
}
}
});
});
$('#popular-keyword-btn').click(function () {
var popularKeywordList = $('.popular-keyword-list');
if (popularKeywordList.is(":visible")) {
popularKeywordList.hide();
popularKeywordList.children().remove();
return;
} else {
popularKeywordList.show();
}
$.ajax({
url: location.protocol + '//' + location.host + '/api/book-search-histories/summary',
method: 'GET',
success: function (response) {
if ($.isEmptyObject(response)) {
$('.search-hist-list').append('<strong">아직 검색한 내역이 없습니다.</strong>');
return;
}
$.each(response, function (i, summary) {
appendSummaryToList(summary);
});
},
error: function (result) {
if (result.responseJSON.userMessage) {
alert(result.responseJSON.userMessage);
} else {
alert("서버 오류입니다.\n잠시 후 다시 시도해주세요.");
}
}
});
});
$(document).on("click", '#next-page-btn', function () {
var nextPageNum = parseInt($("input[name=pageNum]").val()) + 1;
$("input[name=pageNum]").val(nextPageNum);
searchBook($("input[name=query]").val(), nextPageNum);
});
$(document).on("click", '#prev-page-btn', function () {
var prevPageNum = parseInt($("input[name=pageNum]").val()) - 1;
$("input[name=pageNum]").val(prevPageNum);
searchBook($("input[name=query]").val(), prevPageNum);
});
$(document).on("click", '.book-title', function () {
var $bookInfo = $(this).parent().find('.book-info');
if ($bookInfo.is(":visible")) {
$bookInfo.hide();
} else {
$bookInfo.show();
}
});
function searchBook(query, page) {
$.ajax({
url: location.protocol + '//' + location.host + '/api/books?query=' + query + '&page=' + page,
method: 'GET',
success: function (response) {
$('.book-list').children().remove();
if ($.isEmptyObject(response.books)) {
$('.book-list').append('<strong">검색 결과가 없습니다.</strong>');
return;
}
$.each(response.books, function (i, book) {
appendBookToList(book);
});
if (page === 0 && $('.book-list').has('#prev-page-btn').length > 0) {
$('#prev-page-btn').remove();
} else if (page > 1) {
$('.book-list').append('<strong id="prev-page-btn" style="color: red">이전 페이지</strong>\t');
}
if (response.page.isEnd) {
$('#next-page-btn').remove();
} else if ($('.book-list').has('#next-page-btn').length === 0) {
$('.book-list').append('<strong id="next-page-btn" style="color: darkblue">다음 페이지</strong>');
}
$('.book-list').append("<input type='hidden' name='pageNum' value='" + page + "'/>");
$('.book-list').append("<input type='hidden' name='query' value='" + query + "'/>");
},
error: function (result) {
if (result.responseJSON.userMessage) {
alert(result.responseJSON.userMessage);
} else {
alert("서버 오류입니다.\n잠시 후 다시 시도해주세요.");
}
}
});
}
function appendSummaryToList(summary) {
$('.popular-keyword-list').append(
'<li>\n'
+ ' <strong class="keyword-name">' + summary.keyword + '</strong>\n'
+ ' <strong class="hist-datetime">' + summary.searchCount + '</strong>\n'
+ ' </li>'
);
}
function appendHistToList(hist) {
$('.search-hist-list').append(
'<li>\n'
+ ' <strong class="hist-keyword">' + hist.keyword + '</strong>\n'
+ ' <strong class="hist-datetime">' + hist.registeredDateTime + '</strong>\n'
+ ' </li>'
);
}
function appendBookToList(book) {
$('.book-list').append(
'<li>\n'
+ ' <strong class="book-title">' + book.title + '</strong>\n'
+ ' <div class="book-info" style="display: none">\n'
+ ' <dl>'
+ ' <img src="' + book.thumbnail + '">\n'
+ ' <dd>' + book.contents + '</dd>\n'
+ ' </dl>\n'
+ ' <dl>\n'
+ ' <dt><span>저자</span></dt>\n'
+ ' <dd>' + book.authors + '</dd>\n'
+ ' <dt><span">ISBN</span></dt>\n'
+ ' <dd>' + book.isbn + '</dd>\n'
+ ' <dt><span>출판사</span></dt>\n'
+ ' <dd>' + book.publisher + '</dd>\n'
+ ' <dt><span>출판일</span></dt>\n'
+ ' <dd>' + book.datetime + '</dd>\n'
+ ' <dt><span>정가</span></dt>\n'
+ ' <dd>' + book.price + '</dd>\n'
+ ' <dt><span>판매가</span></dt>\n'
+ ' <dd>' + book.salePrice + '</dd>\n'
+ ' </dl>\n'
+ ' </div>\n'
+ ' </li>'
);
}
}
)();<file_sep>/src/main/java/com/supersw/mybook/client/book/BookClient.java
package com.supersw.mybook.client.book;
import com.supersw.mybook.client.book.request.BookSearchParam;
public interface BookClient {
BookSearchClientResponse searchBooks(BookSearchParam param);
}
<file_sep>/src/main/java/com/supersw/mybook/member/domain/MemberBookSearchRequest.java
package com.supersw.mybook.member.domain;
public class MemberBookSearchRequest {
}
<file_sep>/README.md
# my-book
## executable-jar 다운로드 URL
* https://github.com/ssang1105/my-book/raw/master/my-book-0.0.1-SNAPSHOT.jar
## 사용한 라이브러리
* spring-boot-starter-* : 스프링 의존성과 설정 자동화
* fluentHc : HttpClient 생성
* randomBeansVersion : 테스트환경에서 랜덤 객체 생성
* lombok : 어노테이션을 이용한 코드 추가
<file_sep>/src/main/java/com/supersw/mybook/client/book/naver/response/NaverBookSearchResponse.java
package com.supersw.mybook.client.book.naver.response;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.supersw.mybook.client.book.naver.response.NaverBookSearchResponse.NaverBookSearchResponseData;
import java.time.LocalDate;
import java.util.List;
import lombok.Getter;
public class NaverBookSearchResponse extends NaverResponse<NaverBookSearchResponseData.Item> {
@Getter
public static class NaverBookSearchResponseData {
@Getter
private List<Item> items;
@Getter
public static class Item {
private String title;
private String link;
private String image;
private String author;
private int price;
private int discount;
private String publisher;
private String isbn;
private String description;
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyyMMdd")
private LocalDate pubdate;
}
}
}
<file_sep>/src/main/java/com/supersw/mybook/client/book/request/BookSearchParam.java
package com.supersw.mybook.client.book.request;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
@Getter
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
public class BookSearchParam {
private final String query;
private final int page;
private final int size;
public static BookSearchParam create(String query, int page, int size) {
return new BookSearchParam(query, page, size);
}
}
<file_sep>/src/main/java/com/supersw/mybook/error/exceptions/AlreadyExistMemberException.java
package com.supersw.mybook.error.exceptions;
public class AlreadyExistMemberException extends RuntimeException {
private static final long serialVersionUID = 2622515567353191285L;
public AlreadyExistMemberException(String message) {
super(message);
}
}
<file_sep>/src/main/java/com/supersw/mybook/book/domain/BookSearchHistorySummary.java
package com.supersw.mybook.book.domain;
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public class BookSearchHistorySummary {
private final String keyword;
private final long searchCount;
}
| ea32a5cd1ad892f3855254d3961e310dd8985c91 | [
"JavaScript",
"Java",
"Markdown"
] | 16 | Java | ssang1105/my-book | 820a645dbf31da4b678e2b3a9322e6dbccc61ed7 | f97e898c5a605d2cd36adb08cca270fbbd40c1bb | |
refs/heads/master | <repo_name>angelorobsonmelo/WorkerRequestSample<file_sep>/settings.gradle
rootProject.name='WorkkerRequestSample'
include ':app'
<file_sep>/app/src/main/java/br/com/angelorobson/www/workkerrequestsample/MainActivity.kt
package br.com.angelorobson.www.workkerrequestsample
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import android.widget.Toast
import androidx.lifecycle.Observer
import androidx.work.OneTimeWorkRequestBuilder
import androidx.work.WorkInfo
import androidx.work.WorkManager
import androidx.work.workDataOf
import kotlinx.android.synthetic.main.activity_main.*
import java.util.concurrent.TimeUnit
class MainActivity : AppCompatActivity() {
companion object {
const val ID = "id"
}
var time = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
btn_start_worker.setOnClickListener {
executeWorker(time++)
}
}
private fun executeWorker(time: Int) {
val workManager = WorkManager.getInstance(this)
// workDataOf (part of KTX) converts a list of pairs to a [Data] object.
val imageData = workDataOf(ID to time)
val uploadWorkRequest = OneTimeWorkRequestBuilder<UploadWorker>()
.setInputData(imageData)
.setInitialDelay(10, TimeUnit.SECONDS)
.build()
workManager.enqueue(uploadWorkRequest)
val status = workManager.getWorkInfoByIdLiveData(uploadWorkRequest.id)
status.observe(this, Observer {
if (it.state == WorkInfo.State.SUCCEEDED) {
Log.d("Times", "time= ${it.outputData.getInt(ID, 0)}")
Toast.makeText(
this@MainActivity,
"time= ${it.outputData.getInt(ID, 0)}",
Toast.LENGTH_SHORT
).show()
}
})
}
}
<file_sep>/app/src/main/java/br/com/angelorobson/www/workkerrequestsample/UploadWorker.kt
package br.com.angelorobson.www.workkerrequestsample
import android.app.Notification
import android.app.NotificationChannel
import android.app.NotificationManager
import android.content.Context
import android.os.Build
import androidx.core.app.NotificationCompat
import androidx.work.Worker
import androidx.work.WorkerParameters
import androidx.work.workDataOf
class UploadWorker(private val appContext: Context, workerParams: WorkerParameters) :
Worker(appContext, workerParams) {
override fun doWork(): Result {
// Get the input
val idInput = inputData.getInt(MainActivity.ID, 0)
// Create the output of the work
val outputData = workDataOf(MainActivity.ID to idInput)
val notificationManager =
appContext.getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager
val notification =
getNotification(
idInput.toString(),
appContext,
notificationManager,
id.toString()
)
notificationManager.notify(idInput, notification)
// Return the output
return Result.success(outputData)
}
private fun getNotification(
content: String,
context: Context?,
manager: NotificationManager,
id: String
): Notification {
val builder = NotificationCompat.Builder(context?.applicationContext)
.setContentText(content)
.setTicker("Alerta")
.setAutoCancel(false)
.setSmallIcon(R.mipmap.ic_launcher)
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val chanel =
NotificationChannel(id, id, NotificationManager.IMPORTANCE_DEFAULT)
manager.createNotificationChannel(chanel)
builder.setChannelId(id)
}
return builder.build()
}
} | 0947b55f450bbca264d49bd92c0c46fc9a9a3678 | [
"Kotlin",
"Gradle"
] | 3 | Gradle | angelorobsonmelo/WorkerRequestSample | f1486fb3ccf4a40f44f86697b70d943df9cdb979 | 8c1be39e7849a9184e58b8f10152e7e13f51911e | |
refs/heads/main | <file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.vendas.model;
/**
*
* @author guilherme
*/
public class PagamentoAVista implements RegraDePagamento{
@Override
public double pagar(Venda venda) {
double total = 0;
if (venda.getTotalVendido() > 500) {
total = venda.getTotalVendido() - (0.15 * venda.getTotalVendido());
} else if (venda.getTotalVendido() > 300) {
total = venda.getTotalVendido() - (0.1 * venda.getTotalVendido());
}else{
total = venda.getTotalVendido();
}
return total;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.vendas.model;
/**
*
* @author wolleyws
*/
public class Venda {
private double totalVendido;
private RegraDePagamento pagamento;
//SETS E GETS
public double getTotalVendido() {
return totalVendido;
}
public void setTotalVendido(double totalVendido) {
this.totalVendido = totalVendido;
}
public void setPagamento(RegraDePagamento pagamento){
this.pagamento = pagamento;
}
public double calcularTotal() {
return pagamento.pagar(this);
}
}
| 335448e02bb2cf90be1260d328bdf4aa76f589ec | [
"Java"
] | 2 | Java | GuilhermeTerriaga/DesignPatternStrategy | 1c72cbbc72009dd65746115c7f3b132363c4f220 | 4af30b04568233da22ada65f8a76eb6d259d35b2 | |
refs/heads/master | <repo_name>Matshisela/Boston-Housing-with-R<file_sep>/README.md
# Boston-Housing-with-R
Application of various regression methods
<file_sep>/Bostong Housing in R.R
#The Boston Housing data in the mlbench R package with 506 data points
#is being analysed uing Linear regression, Ridge, Elastic and LASSO
#Loading the packages
library(glmnet)
library(mlbench)
library(dplyr)
library(KernelKnn)
library(DAAG)
library(GGally)
#Loading the Boston Housing Data using the mlbench package
data("BostonHousing")
bdata <- BostonHousing
View(bdata)
str(bdata)
#Visualizing the data
ggpairs(bdata) #It is clear the data is not a normal distribution & Multicolinear
#Spliting the dataset
set.seed(263)
trainIndex <- sample(1:nrow(bdata), 0.8*nrow(bdata))
train <- bdata[trainIndex,]
test <- bdata[-trainIndex,]
lambda_seq <- 10^seq(2, -2, by=-.1)
set.seed(263)
train3 = bdata2 %>%
sample_frac(0.8)
test3 = bdata2 %>%
setdiff(train3)
x_train3 = model.matrix(medv~., train3)[,-14]
x_test3 = model.matrix(medv~., test3)[,-14]
y_train3 = train3 %>%
select(medv) %>%
unlist() %>%
as.numeric()
y_test3 = test3 %>%
select(medv) %>%
unlist() %>%
as.numeric()
#Building the Models
lin1 <- lm(medv~., data = train) #Modeling using Linear model= 73.26%
linpred <- predict(lin1, test) #Predicting using Linear model
AIC(lin1) #2418.523
#Predicting using Ridge
cv_output1 <- cv.glmnet(x_train3, y_train3,
alpha = 0, lambda=lambda_seq)
plot(cv_output1) #Best Lambda
best_lam1 <- cv_output1$lambda.min #0.0126
ridge_best <- glmnet(x_train3, y_train3, alpha = 0, lambda = best_lam1)
ridge_pred <- predict(ridge_best, s=best_lam1, newx = x_test3)
coef(ridge_best)
AIC(ridge_best)
#Predicting using Elastic net
cv_output2 <- cv.glmnet(x_train3, y_train3,
alpha = 0.5, lambda=lambda_seq)
plot(cv_output2) #Best Lambda
best_lam2 <- cv_output2$lambda.min #0.02512
elastic_best <- glmnet(x_train3, y_train3, alpha = 0.5, lambda = best_lam2)
elastic_pred <- predict(elastic_best, s=best_lam2, newx = x_test3)
coef(elastic_best)
#Predicting using LAsso
cv_output <- cv.glmnet(x_train3, y_train3,
alpha = 1, lambda=lambda_seq)
plot(cv_output) #Best Lambda
best_lam <- cv_output$lambda.min #0.0126
lasso_best <- glmnet(x_train3, y_train3, alpha = 1, lambda = best_lam)
lasso_pred <- predict(lasso_best, s=best_lam, newx = x_test3)
coef(lasso_best)
#Prediction test
actpred1 <- data.frame(cbind(actuals=test$medv, predicted=linpred))
cor_ac <- cor(actpred1) #85.6%
actpred2 <- data.frame(cbind(y_test3, lasso_pred))
cor_ac2 <- cor(actpred2) #66.39%
actpred3 <- data.frame(cbind(y_test3, ridge_pred))
cor_ac3 <- cor(actpred3) #66.27%
actpred4 <- data.frame(cbind(y_test3, elastic_pred))
cor_ac4 <- cor(actpred4) #66.36%
#K-Fold Cross Validation
#Linear Regression
cvResults1 <- suppressWarnings(CVlm(data.frame(bdata), form.lm = medv~., m=5,
dots = FALSE, seed=263, legend.pos = "topleft",
printit = FALSE))
attr(cvResults1, 'ms') #835.61
#Ridge Regression
| 2a74fe721aff42a50dbb2a96071de80d1685f490 | [
"Markdown",
"R"
] | 2 | Markdown | Matshisela/Boston-Housing-with-R | 3365cd65d4c491ec85af56936509780fff1673f1 | 95b9d4d0aee0dd613fe704bd2ffa6ea4a0d92cd0 | |
refs/heads/master | <file_sep>package consumer
import (
"fmt"
"time"
)
// Waiter ожидающий
type Waiter struct {
*time.Ticker
}
// создает нового ожидающего
func newWaiter() *Waiter {
waiter := &Waiter{time.NewTicker(time.Millisecond * 250)}
go waiter.run()
return waiter
}
// запускает нового ожидающего
func (w *Waiter) run() {
commas := []string{
". ",
" . ",
" .",
}
i := 0
for {
<-w.C
fmt.Printf("\rgetting failure messages, please wait%s", commas[i])
if i == 2 {
i = 0
} else {
i++
}
}
}
<file_sep>package consumer
import (
"encoding/json"
"fmt"
"regexp"
"sync"
"github.com/streadway/amqp"
"github.com/Halfi/postmanq/common"
"github.com/Halfi/postmanq/logger"
)
var (
// обработчики результата отправки письма
resultHandlers = map[common.SendEventResult]func(*Consumer, *amqp.Channel, *common.MailMessage){
common.ErrorSendEventResult: (*Consumer).handleErrorSend,
common.DelaySendEventResult: (*Consumer).handleDelaySend,
common.OverlimitSendEventResult: (*Consumer).handleOverlimitSend,
}
)
// получатель сообщений из очереди
type Consumer struct {
id int
connector *amqpConnector
binding *Binding
}
// создает нового получателя
func NewConsumer(id int, connect *amqpConnector, binding *Binding) *Consumer {
app := new(Consumer)
app.id = id
app.connector = connect
app.binding = binding
return app
}
// запускает получение сообщений из очереди в заданное количество потоков
func (c *Consumer) run() {
for i := 0; i < c.binding.Handlers; i++ {
go c.consume(i)
}
}
// подключается к очереди для получения сообщений
func (c *Consumer) consume(id int) {
channel, err := c.connector.GetConnect().Channel()
if err != nil {
logger.All().Warn("consumer#%d, handler#%d can't get channel %s", c.id, id, c.binding.Queue)
return
}
// выбираем из очереди сообщения с запасом
// это нужно для того, чтобы после отправки письма новое уже было готово к отправке
// в тоже время нельзя выбираеть все сообщения из очереди разом, т.к. можно упереться в память
err = channel.Qos(c.binding.PrefetchCount, 0, false)
if err != nil {
logger.All().Warn("consumer#%d, handler#%d can't set qos %s", c.id, id, c.binding.Queue)
return
}
deliveries, err := channel.Consume(
c.binding.Queue, // name
"", // consumerTag,
false, // noAck
false, // exclusive
false, // noLocal
false, // noWait
nil, // arguments
)
if err == nil {
go c.consumeDeliveries(id, channel, deliveries)
} else {
logger.All().Warn("consumer#%d, handler#%d can't consume queue %s", c.id, id, c.binding.Queue)
}
}
// получает сообщения из очереди и отправляет их другим сервисам
func (c *Consumer) consumeDeliveries(id int, channel *amqp.Channel, deliveries <-chan amqp.Delivery) {
for delivery := range deliveries {
message := new(common.MailMessage)
err := json.Unmarshal(delivery.Body, message)
if err == nil {
// инициализируем параметры письма
message.Init()
logger.
By(message.HostnameFrom).
Info(
"consumer#%d-%d, handler#%d send mail#%d: envelope - %s, recipient - %s to mailer",
c.id,
message.Id,
id,
message.Id,
message.Envelope,
message.Recipient,
)
event := common.NewSendEvent(message)
logger.By(message.HostnameFrom).Debug("consumer#%d-%d send event", c.id, message.Id)
event.Iterator.Next().(common.SendingService).Event(event)
// ждем результата,
// во время ожидания поток блокируется
// если этого не сделать, тогда невозможно будет подтвердить получение сообщения из очереди
if handler, ok := resultHandlers[<-event.Result]; ok {
handler(c, channel, message)
}
message = nil
event = nil
} else {
failureBinding := c.binding.failureBindings[TechnicalFailureBindingType]
err := channel.Publish(
failureBinding.Exchange,
failureBinding.Routing,
false,
false,
amqp.Publishing{
ContentType: "text/plain",
Body: delivery.Body,
DeliveryMode: amqp.Transient,
},
)
logger.All().WarnWithErr(err, "consumer#%d can't unmarshal delivery body, body should be json, %s given", c.id, string(delivery.Body))
}
// всегда подтверждаем получение сообщения
// даже если во время отправки письма возникли ошибки,
// мы уже положили это письмо в другую очередь
_ = delivery.Ack(true)
}
}
// обрабатывает письма, которые не удалось отправить
func (c *Consumer) handleErrorSend(channel *amqp.Channel, message *common.MailMessage) {
// если есть ошибка при отправке, значит мы попали в серый список
// https://ru.wikipedia.org/wiki/%D0%A1%D0%B5%D1%80%D1%8B%D0%B9_%D1%81%D0%BF%D0%B8%D1%81%D0%BE%D0%BA
// или получили какую то ошибку от почтового сервиса, что он не может
// отправить письмо указанному адресату или выполнить какую то команду
var failureBinding *Binding
// если ошибка связана с невозможностью отправить письмо адресату
// перекладываем письмо в очередь для плохих писем
// и пусть отправители сами с ними разбираются
switch {
case message.Error.Code >= 500 && message.Error.Code < 600:
failureBinding = c.binding.failureBindings[errorSignsMap.BindingType(message)]
case message.Error.Code == 450 || message.Error.Code == 451:
failureBinding = delayedBindings[common.ThirtyMinutesDelayedBinding]
default:
failureBinding = c.binding.failureBindings[UnknownFailureBindingType]
}
jsonMessage, err := json.Marshal(message)
if err == nil {
// кладем в очередь
err = channel.Publish(
failureBinding.Exchange,
failureBinding.Routing,
false,
false,
amqp.Publishing{
ContentType: "text/plain",
Body: jsonMessage,
DeliveryMode: amqp.Transient,
},
)
if err == nil {
logger.
By(message.HostnameFrom).
Debug(
"consumer#%d-%d publish failure mail to queue %s, message: %s, code: %d",
c.id,
message.Id,
failureBinding.Queue,
message.Error.Message,
message.Error.Code,
)
} else {
logger.
By(message.HostnameFrom).
Debug(
"consumer#%d-%d can't publish failure mail to queue %s, message: %s, code: %d, publish error %v",
c.id,
message.Id,
failureBinding.Queue,
message.Error.Message,
message.Error.Code,
err,
)
logger.By(message.HostnameFrom).WarnErr(err)
}
} else {
logger.By(message.HostnameFrom).WarnErr(err)
}
}
// обрабатывает письма, которые нужно отправить позже
func (c *Consumer) handleDelaySend(channel *amqp.Channel, message *common.MailMessage) {
logger.
By(message.HostnameFrom).
Debug(
"consumer%d-%d find dlx queue",
c.id,
message.Id,
)
bindingType := common.UnknownDelayedBinding
if message.Error != nil {
logger.
By(message.HostnameFrom).
Debug(
"consumer%d-%d detect error, message: %s, code: %d",
c.id,
message.Id,
message.Error.Message,
message.Error.Code,
)
}
logger.
By(message.HostnameFrom).
Debug(
"consumer%d-%d detect old dlx queue type#%v",
c.id,
message.Id,
message.BindingType,
)
// если нам просто не удалось отправить письмо, берем следующую очередь из цепочки
if chainBinding, ok := bindingsChain[message.BindingType]; ok {
bindingType = chainBinding
}
c.publishDelayedMessage(channel, bindingType, message)
}
// обрабатывает письма, которые превысили лимит отправки
func (c *Consumer) handleOverlimitSend(channel *amqp.Channel, message *common.MailMessage) {
bindingType := common.UnknownDelayedBinding
logger.By(message.HostnameFrom).Debug("consumer#%d-%d detect overlimit, find dlx queue", c.id, message.Id)
for i := 0; i < limitBindingsLen; i++ {
if limitBindings[i] == message.BindingType {
bindingType = limitBindings[i]
break
}
}
c.publishDelayedMessage(channel, bindingType, message)
}
// кладет письмо обратно в одну из отложенных очередей
func (c *Consumer) publishDelayedMessage(channel *amqp.Channel, bindingType common.DelayedBindingType, message *common.MailMessage) {
// получаем очередь, проверяем, что она реально есть
// а что? а вдруг нет)
if delayedBinding, ok := c.binding.delayedBindings[bindingType]; ok {
message.BindingType = bindingType
jsonMessage, err := json.Marshal(message)
if err == nil {
// кладем в очередь
err = channel.Publish(
delayedBinding.Exchange,
delayedBinding.Routing,
false,
false,
amqp.Publishing{
ContentType: "text/plain",
Body: jsonMessage,
DeliveryMode: amqp.Transient,
},
)
if err == nil {
logger.By(message.HostnameFrom).Debug("consumer#%d-%d publish failure mail to queue %s", c.id, message.Id, delayedBinding.Queue)
} else {
logger.All().WarnWithErr(err, "consumer#%d-%d can't publish failure mail to queue %s", c.id, message.Id, delayedBinding.Queue)
}
} else {
logger.All().Warn("consumer#%d-%d can't marshal mail to json", c.id, message.Id)
}
} else {
logger.All().Warn("consumer#%d-%d unknow delayed type#%v", c.id, message.Id, bindingType)
}
}
// получает письма из всех очередей с ошибками
func (c *Consumer) consumeFailureMessages(group *sync.WaitGroup) {
channel, err := c.connector.GetConnect().Channel()
if err == nil {
for _, failureBinding := range c.binding.failureBindings {
for {
delivery, ok, _ := channel.Get(failureBinding.Queue, false)
if ok {
message := new(common.MailMessage)
err = json.Unmarshal(delivery.Body, message)
if err == nil {
sendEvent := common.NewSendEvent(message)
sendEvent.Iterator.Next().(common.ReportService).Event(sendEvent)
}
} else {
break
}
}
}
group.Done()
} else {
logger.All().WarnErr(err)
}
}
// получает сообщения из одной очереди и кладет их в другую
func (c *Consumer) consumeAndPublishMessages(event *common.ApplicationEvent, group *sync.WaitGroup) {
channel, err := c.connector.GetConnect().Channel()
if err == nil {
var envelopeRegex, recipientRegex *regexp.Regexp
srcBinding := c.findBindingByQueueName(event.GetStringArg("srcQueue"))
if srcBinding == nil {
fmt.Println("source queue should be defined")
common.App.SendEvents(common.NewApplicationEvent(common.FinishApplicationEventKind))
}
destBinding := c.findBindingByQueueName(event.GetStringArg("destQueue"))
if destBinding == nil {
fmt.Println("destination queue should be defined")
common.App.SendEvents(common.NewApplicationEvent(common.FinishApplicationEventKind))
}
if srcBinding == destBinding {
fmt.Println("source and destination queue should be different")
common.App.SendEvents(common.NewApplicationEvent(common.FinishApplicationEventKind))
}
if len(event.GetStringArg("envelope")) > 0 {
envelopeRegex, _ = regexp.Compile(event.GetStringArg("envelope"))
}
if len(event.GetStringArg("recipient")) > 0 {
recipientRegex, _ = regexp.Compile(event.GetStringArg("recipient"))
}
publishDeliveries := make([]amqp.Delivery, 0)
for {
delivery, ok, _ := channel.Get(srcBinding.Queue, false)
if ok {
message := new(common.MailMessage)
err = json.Unmarshal(delivery.Body, message)
if err == nil {
var necessaryPublish bool
if (event.GetIntArg("code") > common.InvalidInputInt && event.GetIntArg("code") == message.Error.Code) ||
(envelopeRegex != nil && envelopeRegex.MatchString(message.Envelope)) ||
(recipientRegex != nil && recipientRegex.MatchString(message.Recipient)) ||
(event.GetIntArg("code") == common.InvalidInputInt && envelopeRegex == nil && recipientRegex == nil) {
necessaryPublish = true
}
if necessaryPublish {
fmt.Printf(
"find mail#%d: envelope - %s, recipient - %s\n",
message.Id,
message.Envelope,
message.Recipient,
)
publishDeliveries = append(publishDeliveries, delivery)
}
}
} else {
break
}
}
for _, delivery := range publishDeliveries {
err = channel.Publish(
destBinding.Exchange,
destBinding.Routing,
false,
false,
amqp.Publishing{
ContentType: "text/plain",
Body: delivery.Body,
DeliveryMode: amqp.Transient,
},
)
if err == nil {
err := delivery.Ack(true)
if err != nil {
logger.All().WarnWithErr(err, "can't acknowledge message")
}
} else {
err := delivery.Nack(true, true)
if err != nil {
logger.All().WarnWithErr(err, "can't not acknowledge message")
}
}
}
group.Done()
} else {
logger.All().WarnErr(err)
}
}
// ищет связку по имени
func (c *Consumer) findBindingByQueueName(queueName string) *Binding {
var binding *Binding
if c.binding.Queue == queueName {
binding = c.binding
}
if binding == nil {
for _, failureBinding := range c.binding.failureBindings {
if failureBinding.Queue == queueName {
binding = failureBinding
break
}
}
}
if binding == nil {
for _, delayedBinding := range c.binding.delayedBindings {
if delayedBinding.Queue == queueName {
binding = delayedBinding
break
}
}
}
return binding
}
<file_sep>package common
import "sync"
// Queue потоко-безопасная очередь
type Queue struct {
// флаг, сигнализирующий, что очередь пуста
empty bool
// элементы очереди
items []interface{}
// семафор
mutex *sync.Mutex
}
// NewQueue создает новую очередь
func NewQueue() *Queue {
return &Queue{
empty: true,
items: make([]interface{}, 0),
mutex: new(sync.Mutex),
}
}
// Push добавляет элемент в конец очереди
func (q *Queue) Push(item interface{}) {
q.mutex.Lock()
if q.empty {
q.empty = false
}
q.items = append(q.items, item)
q.mutex.Unlock()
}
// Pop достает первый элемент из очереди
func (q *Queue) Pop() interface{} {
var item interface{}
q.mutex.Lock()
if !q.empty {
oldItems := q.items
oldItemsLen := len(oldItems)
if oldItemsLen > 0 {
item = oldItems[oldItemsLen-1]
q.items = oldItems[0 : oldItemsLen-1]
} else {
q.empty = true
}
}
q.mutex.Unlock()
return item
}
// Empty сигнализирует, что очередь пуста
func (q *Queue) Empty() bool {
var empty bool
q.mutex.Lock()
empty = q.empty
q.mutex.Unlock()
return empty
}
// Len возвращает длину очереди
func (q *Queue) Len() int {
var itemsLen int
q.mutex.Lock()
itemsLen = len(q.items)
q.mutex.Unlock()
return itemsLen
}
// статус очереди
type queueStatus int
const (
// лимитированная очередь
limitedQueueStatus queueStatus = iota
// безлимитная очередь
unlimitedQueueStatus
)
// LimitedQueue лимитированная очередь, в ней будут храниться клиенты к почтовым сервисам
type LimitedQueue struct {
*Queue
// статус, говорящий заблокирована очередь или нет
status queueStatus
// максимальное количество элементов, которое было в очереди
maxLen int
}
// NewLimitQueue создает новую лимитированную очередь
func NewLimitQueue() *LimitedQueue {
return &LimitedQueue{
Queue: NewQueue(),
status: unlimitedQueueStatus,
}
}
// HasLimit сигнализирует, что очередь имеет лимит
func (l *LimitedQueue) HasLimit() bool {
l.mutex.Lock()
hasLimit := l.status == limitedQueueStatus
l.mutex.Unlock()
return hasLimit
}
// HasLimitOn устанавливает лимит очереди
func (l *LimitedQueue) HasLimitOn() {
if l.MaxLen() > 0 && !l.HasLimit() {
l.setStatus(limitedQueueStatus)
}
}
// HasLimitOff снимает лимит очереди
func (l *LimitedQueue) HasLimitOff() {
l.setStatus(unlimitedQueueStatus)
}
// устанавливает статус очереди
func (l *LimitedQueue) setStatus(status queueStatus) {
l.mutex.Lock()
l.status = status
l.mutex.Unlock()
}
// MaxLen максимальная длина очереди до того момента, как был установлен лимит
func (l *LimitedQueue) MaxLen() int {
l.mutex.Lock()
maxLen := l.maxLen
l.mutex.Unlock()
return maxLen
}
// AddMaxLen увеличивает максимальную длину очереди
func (l *LimitedQueue) AddMaxLen() {
l.mutex.Lock()
l.maxLen++
l.mutex.Unlock()
}
<file_sep>package mailer
import (
"bytes"
"fmt"
"io"
"strconv"
"strings"
"github.com/emersion/go-msgauth/dkim"
"github.com/Halfi/postmanq/common"
"github.com/Halfi/postmanq/logger"
)
// Mailer отправитель письма
type Mailer struct {
// идентификатор для логов
id int
service *Service
}
// создает нового отправителя
func newMailer(id int, service *Service) {
mailer := &Mailer{id: id, service: service}
mailer.run()
}
// запускает отправителя
func (m *Mailer) run() {
for event := range m.service.events {
m.sendMail(event)
}
}
// подписывает dkim и отправляет письмо
func (m *Mailer) sendMail(event *common.SendEvent) {
message := event.Message
if common.EmailRegexp.MatchString(message.Envelope) && common.EmailRegexp.MatchString(message.Recipient) {
m.prepare(message)
m.send(event)
} else {
ReturnMail(event, fmt.Errorf("511 service#%d can't send mail#%d, envelope or ricipient is invalid", m.id, message.Id))
}
}
// подписывает dkim
func (m *Mailer) prepare(message *common.MailMessage) {
options := &dkim.SignOptions{
Domain: message.HostnameFrom,
Identifier: message.Envelope,
Selector: m.service.getDkimSelector(message.HostnameFrom),
Signer: m.service.getPrivateKey(message.HostnameFrom),
HeaderCanonicalization: dkim.CanonicalizationRelaxed,
BodyCanonicalization: dkim.CanonicalizationRelaxed,
HeaderKeys: []string{
"From",
"To",
"Subject",
},
}
var b bytes.Buffer
if err := dkim.Sign(&b, bytes.NewReader(message.Body), options); err != nil {
logger.By(message.HostnameFrom).WarnWithErr(err, "mailer#%d-%d can't sign mail", m.id, message.Id)
return
}
message.Body = b.Bytes()
logger.By(message.HostnameFrom).Debug("mailer#%d-%d success sign mail", m.id, message.Id)
}
// отправляет письмо
func (m *Mailer) send(event *common.SendEvent) {
message := event.Message
worker := event.Client.Worker
logger.By(event.Message.HostnameFrom).Info("mailer#%d-%d begin sending mail", m.id, message.Id)
logger.By(message.HostnameFrom).Debug("mailer#%d-%d receive smtp client#%d", m.id, message.Id, event.Client.Id)
success := false
toErr := event.Client.SetTimeout(common.App.Timeout().Mail)
if toErr != nil {
logger.By(message.HostnameFrom).ErrErr(toErr)
}
err := worker.Mail(message.Envelope)
if err == nil {
logger.By(message.HostnameFrom).Debug("mailer#%d-%d send command MAIL FROM: %s", m.id, message.Id, message.Envelope)
toErr := event.Client.SetTimeout(common.App.Timeout().Rcpt)
if toErr != nil {
logger.By(message.HostnameFrom).ErrErr(toErr)
}
err = worker.Rcpt(message.Recipient)
if err == nil {
logger.By(message.HostnameFrom).Debug("mailer#%d-%d send command RCPT TO: %s", m.id, message.Id, message.Recipient)
toErr := event.Client.SetTimeout(common.App.Timeout().Data)
if toErr != nil {
logger.By(message.HostnameFrom).ErrErr(toErr)
}
var wc io.WriteCloser
wc, err = worker.Data()
if err == nil {
logger.By(message.HostnameFrom).Debug("mailer#%d-%d send command DATA", m.id, message.Id)
_, err = wc.Write(message.Body)
if err == nil {
_ = wc.Close()
logger.By(message.HostnameFrom).Debug("%s", message.Body)
logger.By(message.HostnameFrom).Debug("mailer#%d-%d send command .", m.id, message.Id)
// стараемся слать письма через уже созданное соединение,
// поэтому после отправки письма не закрываем соединение
err = worker.Reset()
if err == nil {
logger.By(message.HostnameFrom).Debug("mailer#%d-%d send command RSET", m.id, message.Id)
logger.By(event.Message.HostnameFrom).Info("mailer#%d-%d success send mail#%d", m.id, message.Id, message.Id)
success = true
}
}
}
}
}
event.Client.Wait()
event.Queue.Push(event.Client)
if success {
// отпускаем поток получателя сообщений из очереди
event.Result <- common.SuccessSendEventResult
} else {
ReturnMail(event, err)
}
}
// возвращает письмо обратно в очередь после ошибки во время отправки
func ReturnMail(event *common.SendEvent, err error) {
// необходимо проверить сообщение на наличие кода ошибки
// обычно код идет первым
if err != nil {
errorMessage := err.Error()
parts := strings.Split(errorMessage, " ")
if len(parts) > 0 {
// пытаемся получить код
code, e := strconv.Atoi(strings.TrimSpace(parts[0]))
// и создать ошибку
// письмо с ошибкой вернется в другую очередь, отличную от письмо без ошибки
if e == nil {
event.Message.Error = &common.MailError{Message: errorMessage, Code: code}
}
} else {
logger.All().Err("can't get err code from error: %s", err)
}
}
// если в событии уже создан клиент
if event.Client != nil {
if event.Client.Worker != nil {
// сбрасываем цепочку команд к почтовому сервису
err := event.Client.Worker.Reset()
if err != nil {
logger.All().WarnErr(err)
}
}
}
// отпускаем поток получателя сообщений из очереди
if event.Message.Error == nil {
event.Result <- common.DelaySendEventResult
logger.All().Warn("message delayed")
} else {
if event.Message.Error.Code == 421 {
logger.All().Warn("message delayed with error %s", event.Message.Error.Message)
event.Result <- common.DelaySendEventResult
} else {
event.Result <- common.ErrorSendEventResult
}
}
}
<file_sep>package consumer
import (
"strings"
"github.com/Halfi/postmanq/common"
)
var (
// карта признаков ошибок, используется для распределения неотправленных сообщений по очередям для ошибок
errorSignsMap = ErrorSignsMap{
501: ErrorSigns{
ErrorSign{RecipientFailureBindingType, []string{
"bad address syntax",
}},
},
502: ErrorSigns{
ErrorSign{TechnicalFailureBindingType, []string{
"syntax error",
}},
},
503: ErrorSigns{
ErrorSign{TechnicalFailureBindingType, []string{
"sender not yet given",
"sender already",
"bad sequence",
"commands were rejected",
"rcpt first",
"rcpt command",
"mail first",
"mail command",
"mail before",
}},
ErrorSign{RecipientFailureBindingType, []string{
"account blocked",
"user unknown",
}},
},
504: ErrorSigns{
ErrorSign{RecipientFailureBindingType, []string{
"mailbox is disabled",
}},
},
511: ErrorSigns{
ErrorSign{RecipientFailureBindingType, []string{
"can't lookup",
}},
},
540: ErrorSigns{
ErrorSign{RecipientFailureBindingType, []string{
"recipient address rejected",
"account has been suspended",
"account deleted",
}},
},
550: ErrorSigns{
ErrorSign{TechnicalFailureBindingType, []string{
"sender verify failed",
"callout verification failed:",
"relay",
"verification failed",
"unnecessary spaces",
"host lookup failed",
"client host rejected",
"backresolv",
"can't resolve hostname",
"reverse",
"authentication required",
"bad commands",
"double-checking",
"system has detected that",
"more information",
"message has been blocked",
"unsolicited mail",
"blacklist",
"black list",
"not allowed to send",
"dns operator",
}},
ErrorSign{RecipientFailureBindingType, []string{
"unknown",
"no such",
"not exist",
"disabled",
"invalid mailbox",
"not found",
"mailbox unavailable",
"has been suspended",
"inactive",
"account unavailable",
"addresses failed",
"mailbox is frozen",
"address rejected",
"administrative prohibition",
"cannot deliver",
"unrouteable address",
"user banned",
"policy rejection",
"verify recipient",
"mailbox locked",
"blocked",
"no mailbox",
"bad destination mailbox",
"not stored this user",
"homo hominus",
}},
ErrorSign{ConnectionFailureBindingType, []string{
"spam",
"is full",
"over quota",
"quota exceeded",
"message rejected",
"was not accepted",
"content denied",
"timeout",
"support.google.com",
}},
},
552: ErrorSigns{
ErrorSign{ConnectionFailureBindingType, []string{
"receiving disabled",
"is full",
"over quot",
"to big",
}},
},
553: ErrorSigns{
ErrorSign{RecipientFailureBindingType, []string{
"list of allowed",
"ecipient has been denied",
}},
ErrorSign{TechnicalFailureBindingType, []string{
"relay",
}},
ErrorSign{ConnectionFailureBindingType, []string{
"does not accept mail from",
}},
},
554: ErrorSigns{
ErrorSign{TechnicalFailureBindingType, []string{
"relay access denied",
"unresolvable address",
"blocked using",
}},
ErrorSign{RecipientFailureBindingType, []string{
"recipient address rejected",
"user doesn't have",
"no such user",
"inactive user",
"user unknown",
"has been disabled",
"should log in",
"no mailbox here",
}},
ErrorSign{ConnectionFailureBindingType, []string{
"spam message rejected",
"suspicion of spam",
"synchronization error",
"refused",
}},
},
571: ErrorSigns{
ErrorSign{ConnectionFailureBindingType, []string{
"relay",
}},
},
578: ErrorSigns{
ErrorSign{ConnectionFailureBindingType, []string{
"address rejected with reverse-check",
}},
},
}
)
// карта признаков ошибок, в качестве ключа используется код ошибки, полученной от почтового сервиса
type ErrorSignsMap map[int]ErrorSigns
// отдает идентификатор очереди, в которую необходимо положить письмо с ошибкой
func (e ErrorSignsMap) BindingType(message *common.MailMessage) FailureBindingType {
if signs, ok := e[message.Error.Code]; ok {
return signs.BindingType(message)
} else {
return UnknownFailureBindingType
}
}
// признаки ошибок
type ErrorSigns []ErrorSign
// отдает идентификатор очереди, в которую необходимо положить письмо с ошибкой
func (e ErrorSigns) BindingType(message *common.MailMessage) FailureBindingType {
bindingType := UnknownFailureBindingType
for _, sign := range e {
if sign.resemble(message) {
bindingType = sign.bindingType
break
}
}
return bindingType
}
// признак ошибки
type ErrorSign struct {
// идентификатор очереди
bindingType FailureBindingType
// возможные части сообщения, по которым ошибка соотносится с очередью для ошибок
parts []string
}
// ищет возможные части сообщения в сообщении ошибки
func (e ErrorSign) resemble(message *common.MailMessage) bool {
hasPart := false
for _, part := range e.parts {
if strings.Contains(message.Error.Message, part) {
hasPart = true
break
}
}
return hasPart
}
<file_sep>module github.com/Halfi/postmanq
go 1.16
require (
github.com/alexliesenfeld/health v0.6.0
github.com/byorty/clitable v0.0.0-20150722055417-9f60651b8308
github.com/emersion/go-msgauth v0.6.5
github.com/kr/pretty v0.3.0 // indirect
github.com/prometheus/client_golang v1.11.0
github.com/rogpeppe/go-internal v1.8.0 // indirect
github.com/rs/zerolog v1.23.0
github.com/streadway/amqp v1.0.0
golang.org/x/crypto v0.0.0-20210813211128-0a44fdfbc16e // indirect
gopkg.in/check.v1 v1.0.0-20201130134442-10cb98267c6c // indirect
gopkg.in/yaml.v3 v3.0.0-20210107192922-496545a6307b
)
<file_sep>package connector
import (
"errors"
"fmt"
"time"
"github.com/Halfi/postmanq/common"
"github.com/Halfi/postmanq/logger"
"github.com/Halfi/postmanq/mailer"
)
// заготовщик, подготавливает событие соединения
type Preparer struct {
// Идентификатор для логов
id int
events chan *common.SendEvent
connectorEvents chan *ConnectionEvent
seekerEvents chan *ConnectionEvent
}
// создает и запускает нового заготовщика
func newPreparer(id int, events chan *common.SendEvent, connectorEvents, seekerEvents chan *ConnectionEvent) *Preparer {
return &Preparer{id: id, events: events, connectorEvents: connectorEvents, seekerEvents: seekerEvents}
}
// запускает прослушивание событий отправки писем
func (p *Preparer) run() {
for event := range p.events {
p.prepare(event)
}
}
// подготавливает и запускает событие создание соединения
func (p *Preparer) prepare(event *common.SendEvent) {
logger.By(event.Message.HostnameFrom).Info("preparer#%d-%d try create connection", p.id, event.Message.Id)
connectionEvent := &ConnectionEvent{
SendEvent: event,
servers: make(chan *MailServer, 1),
connectorId: p.id,
address: service.getAddress(event.Message.HostnameFrom, p.id),
}
goto connectToMailServer
connectToMailServer:
// отправляем событие сбора информации о сервере
p.seekerEvents <- connectionEvent
server := <-connectionEvent.servers
switch server.status {
case LookupMailServerStatus:
goto waitLookup
case SuccessMailServerStatus:
connectionEvent.server = server
p.connectorEvents <- connectionEvent
case ErrorMailServerStatus:
mailer.ReturnMail(
event,
errors.New(fmt.Sprintf("511 preparer#%d-%d can't lookup %s", p.id, event.Message.Id, event.Message.HostnameTo)),
)
}
waitLookup:
logger.By(event.Message.HostnameFrom).Debug("preparer#%d-%d wait ending look up mail server %s...", p.id, event.Message.Id, event.Message.HostnameTo)
time.Sleep(common.App.Timeout().Sleep)
goto connectToMailServer
}
<file_sep>package consumer
import (
"fmt"
"net/url"
"sync"
"github.com/streadway/amqp"
"gopkg.in/yaml.v3"
"github.com/Halfi/postmanq/common"
"github.com/Halfi/postmanq/logger"
)
type amqpConnector struct {
connect *amqp.Connection
rwm sync.RWMutex
}
func (ac *amqpConnector) SetConnect(connect *amqp.Connection) {
ac.rwm.Lock()
defer ac.rwm.Unlock()
ac.connect = connect
}
func (ac *amqpConnector) GetConnect() *amqp.Connection {
ac.rwm.RLock()
defer ac.rwm.RUnlock()
return ac.connect
}
// сервис получения сообщений
type Service struct {
// настройка получателей сообщений
Configs []*Config `yaml:"consumers"`
// подключения к очередям
connections map[string]*amqpConnector
// получатели сообщений из очереди
consumers map[string][]*Consumer
assistants map[string][]*Assistant
finish bool
}
// создает новый сервис получения сообщений
func Inst() common.SendingService {
return &Service{
connections: make(map[string]*amqpConnector),
consumers: make(map[string][]*Consumer),
assistants: make(map[string][]*Assistant),
}
}
// OnInit инициализирует сервис
func (s *Service) OnInit(event *common.ApplicationEvent) {
logger.All().Debug("init consumer service")
// получаем настройки
err := yaml.Unmarshal(event.Data, s)
if err != nil {
logger.All().ErrWithErr(err, "consumer service can't unmarshal config")
}
if len(s.Configs) == 0 {
logger.All().FailExit("consumer config is empty")
return
}
consumersCount := 0
assistantsCount := 0
for _, config := range s.Configs {
connect, err := amqp.Dial(config.URI)
if err != nil {
logger.All().ErrWithErr(err, "consumer service can't connect to %s", config.URI)
continue
}
connector := new(amqpConnector)
connector.SetConnect(connect)
channel, err := connector.GetConnect().Channel()
if err != nil {
logger.All().ErrWithErr(err, "consumer service can't get channel to %s", config.URI)
continue
}
consumers := make([]*Consumer, len(config.Bindings))
for i, binding := range config.Bindings {
binding.init()
// объявляем очередь
binding.declare(channel)
binding.delayedBindings = make(map[common.DelayedBindingType]*Binding)
// объявляем отложенные очереди
for delayedBindingType, delayedBinding := range delayedBindings {
delayedBinding.declareDelayed(binding, channel)
binding.delayedBindings[delayedBindingType] = delayedBinding
}
binding.failureBindings = make(map[FailureBindingType]*Binding)
for failureBindingType, tplName := range failureBindingTypeTplNames {
failureBinding := new(Binding)
failureBinding.Exchange = fmt.Sprintf(tplName, binding.Exchange)
failureBinding.Queue = fmt.Sprintf(tplName, binding.Queue)
failureBinding.Type = binding.Type
failureBinding.declare(channel)
binding.failureBindings[failureBindingType] = failureBinding
}
consumersCount++
consumers[i] = NewConsumer(consumersCount, connector, binding)
}
assistants := make([]*Assistant, len(config.Assistants))
for i, assistantBinding := range config.Assistants {
assistantBinding.Binding.init()
// объявляем очередь
assistantBinding.Binding.declare(channel)
destBindings := make(map[string]*Binding)
for domain, exchange := range assistantBinding.Dest {
for _, consumer := range consumers {
if consumer.binding.Exchange == exchange {
destBindings[domain] = consumer.binding
break
}
}
}
assistantsCount++
assistants[i] = &Assistant{
id: assistantsCount,
connector: connector,
srcBinding: assistantBinding,
destBindings: destBindings,
}
}
s.connections[config.URI] = connector
s.consumers[config.URI] = consumers
s.assistants[config.URI] = assistants
// слушаем закрытие соединения
s.reconnect(connector, config)
}
}
// объявляет слушателя закрытия соединения
func (s *Service) reconnect(connector *amqpConnector, config *Config) {
closeErrors := connector.GetConnect().NotifyClose(make(chan *amqp.Error))
go s.notifyCloseError(config, closeErrors)
}
// слушает закрытие соединения
func (s *Service) notifyCloseError(config *Config, closeErrors chan *amqp.Error) {
for closeError := range closeErrors {
if s.finish {
return
}
logger.All().Warn("consumer service close connection %s with error - %v, restart...", config.URI, closeError)
connect, err := amqp.Dial(config.URI)
if err == nil {
connector, ok := s.connections[config.URI]
if !ok {
s.connections[config.URI] = new(amqpConnector)
}
connector.SetConnect(connect)
s.reconnect(connector, config)
logger.All().Debug("consumer service reconnect to amqp server %s", config.URI)
} else {
logger.All().WarnWithErr(err, "consumer service can't reconnect to amqp server %s", config.URI)
}
}
}
// запускает сервис
func (s *Service) OnRun() {
logger.All().Debug("run consumers...")
for _, consumers := range s.consumers {
s.runConsumers(consumers)
}
for _, assistants := range s.assistants {
s.runAssistants(assistants)
}
}
// запускает получателей
func (s *Service) runConsumers(consumers []*Consumer) {
for _, consumer := range consumers {
go consumer.run()
}
}
func (s *Service) runAssistants(assistants []*Assistant) {
for _, assistant := range assistants {
go assistant.run()
}
}
// останавливает получателей
func (s *Service) OnFinish() {
logger.All().Debug("stop consumers...")
s.finish = true
for _, connector := range s.connections {
if connector != nil && connector.GetConnect() != nil {
err := connector.GetConnect().Close()
if err != nil {
logger.All().WarnErr(err)
}
}
}
s.connections = make(map[string]*amqpConnector)
s.consumers = make(map[string][]*Consumer)
s.assistants = make(map[string][]*Assistant)
}
// Event send event
func (s *Service) Event(_ *common.SendEvent) bool {
return true
}
// запускает получение сообщений с ошибками и пересылает их другому сервису
func (s *Service) OnShowReport() {
waiter := newWaiter()
group := new(sync.WaitGroup)
var delta int
for _, apps := range s.consumers {
for _, app := range apps {
delta += app.binding.Handlers
}
}
group.Add(delta)
for _, apps := range s.consumers {
go func(apps []*Consumer) {
for _, app := range apps {
for i := 0; i < app.binding.Handlers; i++ {
go app.consumeFailureMessages(group)
}
}
}(apps)
}
group.Wait()
waiter.Stop()
sendEvent := common.NewSendEvent(nil)
sendEvent.Iterator.Next().(common.ReportService).Event(sendEvent)
}
// перекладывает сообщения из очереди в очередь
func (s *Service) OnPublish(event *common.ApplicationEvent) {
group := new(sync.WaitGroup)
delta := 0
for uri, apps := range s.consumers {
var necessaryPublish bool
if len(event.GetStringArg("host")) > 0 {
parsedUri, err := url.Parse(uri)
if err == nil && parsedUri.Host == event.GetStringArg("host") {
necessaryPublish = true
} else {
necessaryPublish = false
}
} else {
necessaryPublish = true
}
if necessaryPublish {
for _, app := range apps {
delta += app.binding.Handlers
for i := 0; i < app.binding.Handlers; i++ {
go app.consumeAndPublishMessages(event, group)
}
}
}
}
group.Add(delta)
group.Wait()
fmt.Println("done")
common.App.SendEvents(common.NewApplicationEvent(common.FinishApplicationEventKind))
}
// получатель сообщений из очереди
type Config struct {
URI string `yaml:"uri"`
Assistants []*AssistantBinding `yaml:"assistants"`
Bindings []*Binding `yaml:"bindings"`
}
| 17c4960284a4ff5d1475b1545015b750e4904874 | [
"Go Module",
"Go"
] | 8 | Go | plyo/postmanq | 766d0feaf3f54a3aea9a863bb8a2b82b6367fb91 | 528c9e5a097e2da211de88098c1ca1f864af0b5b | |
refs/heads/master | <repo_name>emrissol18/galleryspringapi<file_sep>/src/main/java/com/gallery/util/ResponseModel.java
package com.gallery.util;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.springframework.http.HttpStatus;
import java.util.HashMap;
import java.util.Map;
//import lombok.ToString;
@ApiModel(value = "Response Model")
@Getter
@Setter
public class ResponseModel {
public static final String ATTR_USERS = "users";
public static final String ATTR_USER = "user";
public static final String ATTR_SUBSCRIPTIONS = "subscriptions";
public static final String ATTR_SUBSCRIBED = "subscribed";
public static final String ATTR_ALBUMS = "albums";
public static final String ATTR_ALBUM = "album";
public static final String ATTR_IMAGES = "images";
public static final String ATTR_PAGINATION = "pagination";
public static final String ATTR_FORM_ERRORS = "formErrors";
public static final String ATTR_UPDATES = "updates";
public static final String ATTR_UPDATES_COUNT = "updatesCount";
public static final String ATTR_THEME = "theme";
@ApiModelProperty(name = "attributes to hold data")
@ToString.Exclude
private Map<String, Object> attributes = new HashMap<>(4, 1);
@ApiModelProperty(name = "status code", example = "200", allowEmptyValue = true)
@Setter(AccessLevel.NONE)
private int statusCode;
@ApiModelProperty(name = "status message")
@Setter(AccessLevel.NONE)
private String statusMessage;
public ResponseModel() {
defaultStatus();
}
public void setStatus(HttpStatus httpStatus) {
statusCode = httpStatus.value();
statusMessage = httpStatus.getReasonPhrase();
}
public ResponseModel(HttpStatus status) {
setStatus(status);
}
public ResponseModel(String key, Object object) {
defaultStatus();
addAttribute(key, object);
}
/*public ResponseModel(String key, Iterable<Object> objects) {
defaultStatus();
addAttribute(key, objects);
}*/
public ResponseModel(Map<String, Object> attributes) {
defaultStatus();
this.attributes.putAll(attributes);
}
public void addAttribute(String key, Object object) {
attributes.put(key, object);
}
public void addAttribute(String key, Iterable<Object> object) {
attributes.put(key, object);
}
private void defaultStatus() {
setStatus(HttpStatus.OK);
}
}
<file_sep>/src/main/java/com/gallery/web/AlbumController.java
package com.gallery.web;
import com.gallery.model.dto.PaginationData;
import com.gallery.model.dto.album.AlbumDto;
import com.gallery.model.dto.album.AlbumDtoForm;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.user.User;
import com.gallery.model.entity.user.UserData;
import com.gallery.service.AlbumService;
import com.gallery.service.ImageService;
import com.gallery.service.UserDataService;
import com.gallery.service.UserService;
import com.gallery.service.delayedtask.DelayedEntityRemover;
import com.gallery.util.FunctionUtil;
import com.gallery.util.MailSenderService;
import com.gallery.util.ResponseModel;
import com.gallery.util.factory.MultipartFileImageFactoryImpl;
import com.gallery.util.image.MultipartFileImage;
import com.gallery.validation.ImageExtensionValidator;
import io.swagger.annotations.*;
import org.hibernate.HibernateException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.web.bind.annotation.*;
import springfox.documentation.annotations.ApiIgnore;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.Tuple;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
//@Slf4j
@RestController
@RequestMapping("/album")
public class AlbumController {
private static Logger logger = LoggerFactory.getLogger(AlbumController.class);
@Value("${pagination.page-items-size}")
private int pageItemsSize;
@PersistenceContext
private EntityManager entityManager;
private UserService userService;
private AlbumService albumService;
private ImageService imageService;
private MultipartFileImageFactoryImpl multipartFileImageFactory;
private ImageExtensionValidator extensionValidator;
private MailSenderService mailSender;
private DelayedEntityRemover<Album> albumDelayedRemover;
private UserDataService userDataService;
@Autowired
public AlbumController(UserService userService,
AlbumService albumService,
ImageService imageService,
MultipartFileImageFactoryImpl multipartFileImageFactory,
ImageExtensionValidator extensionValidator,
MailSenderService mailSender,
@Qualifier("albumRemover") DelayedEntityRemover albumDelayedRemover,
UserDataService userDataService) {
this.userService = userService;
this.albumService = albumService;
this.imageService = imageService;
this.multipartFileImageFactory = multipartFileImageFactory;
this.extensionValidator = extensionValidator;
this.mailSender = mailSender;
// this.entityServiceMulti = entityServiceMulti;
// this.entityServiceSingle = entityServiceSingle;
this.albumDelayedRemover = albumDelayedRemover;
this.userDataService = userDataService;
}
/**
* Fetch all albums and images
*/
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get albums")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "page"),
@ApiImplicitParam(name = "offset"),
@ApiImplicitParam(name = "limit"),
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "get albums", responseContainer = "List")
})
@GetMapping("/all")
public ResponseModel albumsAll(
@RequestParam(value = "page", defaultValue = "0", required = false) Integer page,
@RequestParam(value = "offset", defaultValue = "0", required = false) Integer offset,
@RequestParam(value = "limit", required = false) Integer limit) {
logger.info("get all albums");
long itemsTotal = albumService.countNoDeleted();
offset = FunctionUtil.resolveOffset(offset, page, pageItemsSize);
limit = FunctionUtil.resolveLimit(limit, pageItemsSize);
Collection<AlbumDto> albumDtos = albumService.findAllAndConstructAlbumDto(offset, limit);
PaginationData paginationData = new PaginationData(offset, page, itemsTotal, albumDtos.size(), pageItemsSize);
ResponseModel responseModel = new ResponseModel();
responseModel.addAttribute(ResponseModel.ATTR_ALBUMS, albumDtos);
responseModel.addAttribute(ResponseModel.ATTR_PAGINATION, paginationData);
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get albums by username")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "page"),
@ApiImplicitParam(name = "offset"),
@ApiImplicitParam(name = "limit"),
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "get albums", responseContainer = "List")
})
@GetMapping("/{username}/all")
public ResponseModel albumsByUser(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiIgnore @PathVariable String username,
@ApiParam("page") @RequestParam(value = "page", defaultValue = "0", required = false) Integer page,
@ApiParam("offset") @RequestParam(value = "offset", defaultValue = "0", required = false) Integer offset,
@ApiParam("limit") @RequestParam(value = "limit", required = false) Integer limit) {
logger.info("get albums by User");
ResponseModel responseModel = new ResponseModel();
User user = null;
long itemsTotal = 0;
boolean subscribed = false;
if (principal != null) {
Optional<Tuple> tupleOptional =
Optional.ofNullable(userService.findUserTupleWithAlbumsCountAndIsSubscribed(username, principal.getId()));
if(tupleOptional.isPresent()) {
try {
user = (User) tupleOptional.get().get(0);
itemsTotal = (Long) tupleOptional.get().get(1);
subscribed = (Boolean) tupleOptional.get().get(2);
}
catch (IllegalArgumentException | ClassCastException e) {
e.printStackTrace();
responseModel.setStatus(HttpStatus.INTERNAL_SERVER_ERROR);
return responseModel;
}
}
}
else {
user = userService.findByUsernameWithAlbums(username);
}
offset = FunctionUtil.resolveOffset(offset, page, pageItemsSize);
limit = FunctionUtil.resolveLimit(limit, pageItemsSize);
Collection<AlbumDto> albumDtos = albumService.findAllAndConstructAlbumDto(
FunctionUtil.getWhereClauseEntry("user", "id", user.getId().toString()),
offset, limit);
PaginationData paginationData = new PaginationData(offset, page, itemsTotal, albumDtos.size(), pageItemsSize);
responseModel.addAttribute(ResponseModel.ATTR_ALBUMS, albumDtos);
responseModel.addAttribute(ResponseModel.ATTR_SUBSCRIBED, subscribed);
responseModel.addAttribute(ResponseModel.ATTR_PAGINATION, paginationData);
return responseModel;
}
@ResponseStatus(HttpStatus.CREATED)
@ApiOperation(value = "create album", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "formData[cover, title, description]"),
})
@ApiResponses(value = {
@ApiResponse(code = 201, message = "album created"),
@ApiResponse(code = 500, message = "internal server error (exception)")
})
@PostMapping("/create")
public ResponseModel albumCreate(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiParam("formData") @ModelAttribute AlbumDtoForm albumDto) {
ResponseModel responseModel = new ResponseModel(HttpStatus.CREATED);
Album album = new Album(albumDto.getTitle(), albumDto.getDescription());
album.setUser(principal);
try {
albumService.save(album);
if ( ( ! albumDto.getCover().isEmpty() ) && this.extensionValidator.validate(albumDto.getCover()) ) {
MultipartFileImage multipartFileImage = multipartFileImageFactory.create(
FunctionUtil.buildAlbumPath(principal.getUsername(), album.getId()));
try {
album.setCover(multipartFileImage.saveImageAsSmall(albumDto.getCover()));
}
catch (IOException e) {
e.printStackTrace();
}
albumService.save(album);
}
}
catch (HibernateException e) {
e.printStackTrace();
multipartFileImageFactory.create(album.getCover()).deleteDirectory();
responseModel.setStatus(HttpStatus.INTERNAL_SERVER_ERROR);
}
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "edit album")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "formData [id, cover, title, description]", required = true),
@ApiImplicitParam(name = "coverRemoveRequest - boolean value indicates to remove album cover")
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "album edited successfully"),
@ApiResponse(code = 404, message = "album not found by formData.id")
})
@PutMapping("/edit")
public ResponseModel albumEdit(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiParam("formData") @ModelAttribute(name = "album") AlbumDtoForm albumDto,
@ApiParam("coverRemoveRequest") @RequestParam(required = false, defaultValue = "0") boolean coverRemoveRequest) {
logger.info("edit albumd");
logger.info(albumDto.toString());
ResponseModel responseModel = new ResponseModel();
String username = principal.getUsername();
Album album = albumService.findByIdAndUserUsernameWithUser(albumDto.getId(), username);
if ( album == null ) {
responseModel.setStatus(HttpStatus.NOT_FOUND);
return responseModel;
}
MultipartFileImage albumMultipartFileImageNew = multipartFileImageFactory.create(FunctionUtil.buildAlbumPath(username, album.getId()));
if (coverRemoveRequest) {
multipartFileImageFactory.create(album.getCover()).deleteImage();
album.setCover(null);
}
else if ( albumDto.getCover() != null && ! albumDto.getCover().isEmpty()) {
try {
//delete old images
multipartFileImageFactory.create(album.getCover()).deleteImage();
//save new
String coverPath = albumMultipartFileImageNew.saveImageAsSmall(albumDto.getCover());
album.setCover(coverPath);
}
catch (IOException e) {
e.printStackTrace();
}
}
album.setTitle(albumDto.getTitle());
album.setDescription(albumDto.getDescription());
if (albumService.save(album) == null) {
// delete images for unsaved album
albumMultipartFileImageNew.deleteImages();
}
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "delete album")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "album deleted successfully"),
@ApiResponse(code = 404, message = "album not found by id")
})
@DeleteMapping("/{id}/delete")
public ResponseModel deleteAlbum(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiIgnore @PathVariable("id") Long albumId,
@ApiIgnore HttpServletResponse response) {
ResponseModel responseModel = new ResponseModel();
Optional<Album> albumOptional = Optional.ofNullable(albumService.findByIdAndUserUsername(albumId, principal.getUsername()));
albumOptional.ifPresentOrElse(
(album) -> {
albumDelayedRemover.addEntity(album);
},
() -> {
responseModel.setStatus(HttpStatus.NOT_FOUND);
}
);
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "cancel album deletion (undo)")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "album deletion canceled")
})
@PostMapping("/delete/cancel/{id}")
public void cancelDelete(@PathVariable Long id) {
Optional<Album> optionalAlbum = albumService.findById(id);
if (optionalAlbum.isPresent()) {
// remove from list to be deleted
albumDelayedRemover.removeEntity(optionalAlbum.get());
}
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get most new albums", responseContainer = "List")
@GetMapping("/new")
public ResponseModel getMostNew(){
Collection<AlbumDto> albumDtos = albumService.findNew();
/*System.out.println("New:");
albumDtos.forEach(System.out::println);*/
return new ResponseModel("albums", albumDtos);
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get popular albums", responseContainer = "List")
@GetMapping("/pop")
public ResponseModel getMostPopular(){
Collection<AlbumDto> albumDtos = albumService.findPopular();
/*System.out.println("Popular:");
albumDtos.forEach(System.out::println);*/
return new ResponseModel("albums", albumDtos);
}
/**
* Fetch all albums of current user subscriptions with images.<br/>
* period values filter by: t (today) default; lw (last week); lm (last month)
*/
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get subscriptions albums")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "page"),
@ApiImplicitParam(name = "offset"),
@ApiImplicitParam(name = "limit"),
@ApiImplicitParam(name = "f - filter by date")
})
@GetMapping("/sub-all")
public ResponseModel albumsSubAll(
@ApiIgnore @AuthenticationPrincipal User principal,
@ApiParam("page") @RequestParam(value = "page", defaultValue = "0") Integer page,
@ApiParam("offset") @RequestParam(value = "offset", defaultValue = "0") Integer offset,
@ApiParam("limit") @RequestParam(value = "limit", required = false) Integer limit,
@ApiParam("f") @RequestParam(value = "f", defaultValue = "lw") String dateFilter
) {
Collection<User> subsFound = userService.findSubscriptionsOfUser(1L);
UserData principalUserData = userDataService.findById(principal.getId()).get();
try {
principalUserData.setLastUpdateCheck(new Date());
userDataService.save(principalUserData);
}
catch (NullPointerException e) {
e.printStackTrace();
}
if (subsFound.size() == 0) {
return new ResponseModel();
}
List<Long> ids = subsFound.stream().map(User::getId).collect(Collectors.toList());
// long itemsTotal = albumService.countAllSubAlbums(ids);
long itemsTotal = albumService.countNewUpdates(ids, principalUserData.getLastUpdateCheckSortStart());
offset = FunctionUtil.resolveOffset(offset, page, pageItemsSize);
limit = FunctionUtil.resolveLimit(limit, pageItemsSize);
Map<String, List<AlbumDto>> usernameWithAlbums = albumService.findAllSubs(ids, offset, limit, principalUserData.getLastUpdateCheckSortStart(), dateFilter);
ResponseModel responseModel = new ResponseModel(ResponseModel.ATTR_UPDATES, usernameWithAlbums);
responseModel.addAttribute(ResponseModel.ATTR_PAGINATION,
new PaginationData(offset, page, itemsTotal, usernameWithAlbums.values().size(), pageItemsSize));
return responseModel;
}
}
<file_sep>/src/main/java/com/gallery/model/dto/user/UserDto.java
package com.gallery.model.dto.user;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.user.User;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Set;
//@ApiModel(value = "DTO for User entity model")
@JsonInclude(value = JsonInclude.Include.NON_NULL)
@Getter
@Setter
//@ToString(exclude = {"albums"})
@NoArgsConstructor
public class UserDto {
private Long id=0L;
private String username="";
private String email="";
private String password="";
@JsonProperty("password_confirm")
private String passwordConfirm="";
private Date createdAt;
private boolean enabled;
private Collection<Album> albums;
private Set<User> subscribers;
public UserDto(String username, String password, String email) {
this.username = username;
this.password = <PASSWORD>;
this.email = email;
}
public UserDto(User user) {
if (user == null) {
return;
}
this.id = user.getId();
this.username = user.getUsername();
this.email = user.getEmail();
this.createdAt = user.getCreatedAt();
this.enabled = user.isEnabled();
}
public UserDto(User user, List<Album> albums) {
this(user);
this.albums = albums;
}
public UserDto(User user, Set<User> subscribers) {
this(user);
this.subscribers = subscribers;
}
public UserDto(User user, List<Album> albums, Set<User> subscribers) {
this(user);
this.albums = albums;
this.subscribers = subscribers;
}
public User toUser() {
return new User(this.username, this.password, this.email);
}
public UserDto toDto(User user) {
return new UserDto(user);
}
@Override
public String toString() {
return "UserDto{" +
"id=" + id +
", username='" + username + '\'' +
", email='" + email + '\'' +
", password='" + <PASSWORD> + '\'' +
", passwordConfirm='" + <PASSWORD> + '\'' +
", createdAt=" + createdAt +
'}';
}
}
<file_sep>/build.gradle
/*
* This file was generated by the Gradle 'init' task.
*
* This is a general purpose Gradle build.
* Learn how to create Gradle builds at https://guides.gradle.org/creating-new-gradle-builds
*/
plugins {
id 'org.springframework.boot' version '2.3.3.RELEASE'
id 'io.spring.dependency-management' version '1.0.10.RELEASE'
id 'java'
}
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-security'
//implementation 'org.springframework.boot:spring-boot-starter-mail:2.2.6'
implementation 'org.springframework.boot:spring-boot-starter-mail:2.2.6.RELEASE'
compileOnly 'org.projectlombok:lombok'
annotationProcessor 'org.projectlombok:lombok'
runtimeOnly 'com.h2database:h2'
runtimeOnly 'mysql:mysql-connector-java'
compileOnly 'org.springframework.boot:spring-boot-devtools'
//compile 'com.auth0:java-jwt'
//compile 'com.auth0:java-jwt:3.3.0'
compile 'io.jsonwebtoken:jjwt:0.9.1'
//implementation 'org.thymeleaf:thymeleaf:3.0.11.RELEASE'
implementation 'org.thymeleaf:thymeleaf-spring5:3.0.11.RELEASE'
//implementation 'org.springframework.boot:spring-boot-starter-amqp:2.3.12.RELEASE'
implementation 'org.springframework.boot:spring-boot-starter-activemq:2.0.5.RELEASE'
// implementation 'com.blazebit:blaze-persistence-core-api:1.6.0'
// implementation 'com.blazebit:blaze-persistence-entity-view-api:1.6.0'
// runtimeOnly 'com.blazebit:blaze-persistence-entity-view-impl:1.6.0'
// compileOnly 'org.hibernate:hibernate-jpamodelgen:5.4.32.Final'
// annotationProcessor('org.hibernate:hibernate-jpamodelgen:5.4.32.Final')
testImplementation('org.springframework.boot:spring-boot-starter-test') {
// exclude group: 'org.junit.vintage', module: 'junit-vintage-engine'
}
//testImplementation 'org.springframework.security:spring-security-test:5.3.9.RELEASE'
//testImplementation 'org.testng:testng:7.4.0'
implementation 'io.springfox:springfox-swagger2:2.9.2'
implementation 'io.springfox:springfox-swagger-ui:2.9.2'
}
test {
useJUnitPlatform()
}<file_sep>/src/test/resources/hibernate.properties
#IN MEMORY
#jdbc.driverClassName=org.h2.Driver
#jdbc.url=jdbc:h2:mem:gallery;DB_CLOSE_DELAY=-1
#hibernate.dialect=org.hibernate.dialect.H2Dialect
#hibernate.hbm2ddl.auto=create<file_sep>/src/test/java/com/gallery/service/UserServiceTest.java
package com.gallery.service;
import com.gallery.model.entity.user.User;
import com.gallery.service.common.ServiceTest;
import org.junit.Assert;
import org.junit.jupiter.api.Order;
import org.junit.jupiter.api.Test;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.annotation.Rollback;
import java.util.Collection;
import java.util.Set;
public class UserServiceTest implements ServiceTest {
private Logger logger = LoggerFactory.getLogger(UserServiceTest.class);
@Autowired
private UserService userService;
@Test
public void findAll() {
Collection<User> users = (Collection<User>) userService.findAll();
Assert.assertEquals(users.size(), 4);
}
@Test
public void save() {
long countBefore = count(userService);
User user = new User("new User", "new-user-password", "<EMAIL>");
User savedUser = userService.save(user);
long countAfter = count(userService);
Assert.assertNotNull(savedUser);
Assert.assertEquals(countBefore + 1, countAfter);
}
@Test
public void delete() {
long countBefore = count(userService);
User user1 = userService.findByUsername("Solomon");
Assert.assertNotNull(user1);
userService.delete(user1);
long countAfter = count(userService);
Assert.assertEquals(countBefore - 1, countAfter);
}
@Test
@Order(Order.DEFAULT + 1)
public void findByUsername() {
String username = "Solomon";
User user = userService.findByUsername(username);
Assert.assertNotNull(user);
Assert.assertEquals(username, user.getUsername());
}
@Test
@Order(Order.DEFAULT + 2)
// @Commit
@Rollback(false)
public void subscribeUser() {
// Elaine subscribes to Dominick
User elaine_Sexton = userService.findByUsername("Elaine");
User dominick_Garza = userService.findByUsername("Dominick");
Assert.assertNotNull(elaine_Sexton);
Assert.assertNotNull(dominick_Garza);
int elaine_SextonSubscriptionsBefore = userService.findSubscriptionsOfUser(elaine_Sexton.getId()).size();
Assert.assertFalse(
dominick_Garza.getSubscribers().contains(elaine_Sexton)
);
dominick_Garza.getSubscribers().add(elaine_Sexton);
userService.save(dominick_Garza);
Set<User> dominick_GarzaSubscribers = userService.findSubscribersByUserId(dominick_Garza.getId());
Assert.assertTrue(
dominick_GarzaSubscribers.contains(elaine_Sexton)
);
int elaine_SextonSubscriptionsAfter = userService.findSubscriptionsOfUser(elaine_Sexton.getId()).size();
Assert.assertEquals(elaine_SextonSubscriptionsBefore + 1, elaine_SextonSubscriptionsAfter);
}
@Test
@Order(Order.DEFAULT + 3)
// @Commit
@Rollback(false)
public void unsubscribeUser() {
// Elaine unsubscribes from <NAME>
User elaine_Sexton = userService.findByUsername("elaine");
User dominick_Garza = userService.findByUsername("dominick");
Assert.assertNotNull(elaine_Sexton);
Assert.assertNotNull(dominick_Garza);
Set<User> dominick_GarzaSubs = userService.findSubscribersByUserId(dominick_Garza.getId());
Assert.assertTrue(
dominick_GarzaSubs.contains(elaine_Sexton)
);
dominick_GarzaSubs.remove(elaine_Sexton);
dominick_Garza.setSubscribers(dominick_GarzaSubs);
userService.save(dominick_Garza);
dominick_GarzaSubs = userService.findSubscribersByUserId(dominick_Garza.getId());
Assert.assertFalse(
dominick_GarzaSubs.contains(elaine_Sexton)
);
}
}
<file_sep>/src/main/java/com/gallery/config/ResponseModelErrorHandler.java
package com.gallery.config;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.gallery.util.ResponseModel;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ExceptionHandler;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
//@RestControllerAdvice
public class ResponseModelErrorHandler {
private int errorStatus = HttpStatus.INTERNAL_SERVER_ERROR.value();
@Autowired
private ObjectMapper objectMapper;
@ExceptionHandler(value = Exception.class)
public void handle(HttpServletRequest request, HttpServletResponse response) {
if(response.getStatus() == errorStatus) {
try ( PrintWriter printWriter = response.getWriter() ) {
printWriter.write(objectMapper.writeValueAsString(new ResponseModel(HttpStatus.INTERNAL_SERVER_ERROR)));
}
catch (IOException e) {
e.printStackTrace();
}
}
}
}
<file_sep>/src/main/java/com/gallery/model/entity/user/Role.java
package com.gallery.model.entity.user;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import javax.persistence.*;
//@ApiModel
@Data
@EqualsAndHashCode(callSuper = false)
@NoArgsConstructor
@Entity(name = "Role")
@Table(name = "role")
public class Role {
public static String ROLE_USER = "USER";
public static String ROLE_ADMIN = "ADMIN";
public static String ROLE_USER_VALUE = "ROLE_USER";
public static String ROLE_ADMIN_VALUE = "ROLE_ADMIN";
// @ApiModelProperty(name = "id", dataType = "Long", required = true)
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
// @ApiModelProperty(name = "name", dataType = "String", required = true, position = 1)
@Column(name = "name", nullable = false)
private String name;
public Role(String name) {
this.name = name;
}
}
<file_sep>/src/main/java/com/gallery/service/entity/SingleEntityService.java
package com.gallery.service.entity;
public interface SingleEntityService extends Fetchable{
<T> T findOneByAttrWithOptions(Class<T> entityClass, String attrName, String attrVal, String... fetchOptions);
/*default <T> void applyOptionsForCriteriaBuilder(CriteriaBuilder cb, CriteriaQuery<T> criteriaQuery, Root<T> root, EntityServiceOptions options) {
if (options.hasOrderAsc()) {
criteriaQuery.orderBy(cb.asc(root.get(options.getOrderAscValue())));
} else if (options.hasOrderDesc()) {
criteriaQuery.orderBy(cb.desc(root.get(options.getOrderDescValue())));
}
}
default <T> void applyOptionsForTypedQuery(TypedQuery<T> typedQuery, EntityServiceOptions options) {
if (options.hasOffset()) {
try {
typedQuery.setFirstResult(options.getOffsetValue());
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
if (options.hasLimit()) {
try {
typedQuery.setMaxResults(options.getLimitValue());
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
}*/
}
<file_sep>/src/main/java/com/gallery/service/delayedtask/AlbumDelayedRemover.java
package com.gallery.service.delayedtask;
import com.gallery.model.entity.album.Album;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.data.repository.CrudRepository;
import org.springframework.stereotype.Service;
import java.util.LinkedList;
import java.util.Queue;
@Service("albumRemover")//albumDelayedRemover
public class AlbumDelayedRemover extends AbstractDelayedEntityRemover<Album> {
private static Logger logger = LoggerFactory.getLogger(AlbumDelayedRemover.class);
private volatile Queue<TaskItem<Album>> items = new LinkedList<>();
@Value("${undo.album.lifetime.millis}")
protected Long lifeTime;
@Autowired
public AlbumDelayedRemover(@Qualifier("albumRepository") CrudRepository<Album, Long> repository) {
super(repository);
}
@Override
public void addEntity(Album entity) {
entity.setDeleted(true);
repository.save(entity);
TaskItem<Album> taskItem = new TaskItem<>(lifeTime, entity);
getItems().add(taskItem);
System.out.println("entity added: " + entity.toString());
if ( ! running) {
running = true;
run();
}
}
@Override
public Queue<TaskItem<Album>> getItems() {
return items;
}
@Override
public boolean removeEntity(Album entity) {
boolean isRemoved = getItems().removeIf(taskItem -> taskItem.getKeyId().equals(entity.getId()));
if(isRemoved){
entity.setDeleted(false);
repository.save(entity);
}
return isRemoved;
}
}
<file_sep>/src/main/java/com/gallery/App.java
package com.gallery;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.jms.activemq.ActiveMQAutoConfiguration;
import org.springframework.context.MessageSource;
import org.springframework.context.annotation.Bean;
import org.springframework.context.support.ResourceBundleMessageSource;
//@SpringBootApplication//(exclude = {DataSourceAutoConfiguration.class})
//@EnableJpaRepositories(basePackages = {"emrissol.repository"})
//@EnableAutoConfiguration
@SpringBootApplication(exclude = ActiveMQAutoConfiguration.class)
//@EnableJms
public class App {
// @Qualifier("entityManager")
// @PersistenceContext
// EntityManager entityManager;
// @PersistenceContext
// private EntityManager entityManager;
// @Autowired
// MailSenderUtil mailSenderUtil;
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
@Bean("validationMessageSource")
public MessageSource resourceBundleMessageSourceBean() {
ResourceBundleMessageSource messageSource = new ResourceBundleMessageSource();
messageSource.setBasename("validation/validation");
messageSource.setUseCodeAsDefaultMessage(true);
return messageSource;
}
}<file_sep>/src/main/java/com/gallery/jms/MessageingService.java
package com.gallery.jms;
public interface MessageingService<T> {
void send(T object);
}
<file_sep>/src/main/java/com/gallery/model/dto/album/AlbumDtoForm.java
package com.gallery.model.dto.album;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.ToString;
import org.springframework.web.multipart.MultipartFile;
@Getter
@Setter
@ToString
@NoArgsConstructor
public class AlbumDtoForm {
private Long id;
private String title;
private String description;
private MultipartFile cover;
}
<file_sep>/src/main/java/com/gallery/util/MailSenderService.java
package com.gallery.util;
import lombok.AccessLevel;
import lombok.Getter;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.MailException;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Component;
import org.thymeleaf.context.Context;
import org.thymeleaf.spring5.SpringTemplateEngine;
import javax.mail.MessagingException;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.io.File;
@Component(value = "commonMailSenderService")
public class MailSenderService {
@Getter(AccessLevel.PROTECTED)
private JavaMailSender mailSender;
private SpringTemplateEngine templateEngine;
@Value("${client.host.url}")
private String clientHostUrl;
@Autowired
public MailSenderService(JavaMailSender mailSender, SpringTemplateEngine springTemplateEngine) {
this.mailSender = mailSender;
this.templateEngine = springTemplateEngine;
}
public void sendMimeEmail(String text) {
MimeMessage message = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setTo(new InternetAddress("<EMAIL>"));
helper.setText("mime message");
FileSystemResource fileSystemResource = new FileSystemResource(new File(""));
helper.addAttachment("id12", fileSystemResource);
} catch (MessagingException e) {
e.printStackTrace();
}
}
public void sendTextEmail(String text) {
SimpleMailMessage simpleMailMessage = new SimpleMailMessage();
simpleMailMessage.setTo("<EMAIL>");
simpleMailMessage.setSubject("My Spring App");
simpleMailMessage.setText(text);
try {
mailSender.send(simpleMailMessage);
} catch (MailException e) {
e.printStackTrace();
}
}
public void sendChangeRequestEmail(String emailTo, String uri, String token, String changeSubject) {
String tokenLink = clientHostUrl + uri + token;
String tokenHtmlLink = new String("<a href='" + tokenLink + "'>link</a>");
String body = new String("You have been requested for change your " + changeSubject.toLowerCase() + ". Click the " + tokenHtmlLink + " to proceed.<br>Ignore this email if you did not send such a request!");
String footer = new String("<empty footer>");
sendEmail("email",
emailTo,
changeSubject + " change request",
changeSubject + " change request",
body,
footer);
}
public void sendPasswordChangeEmail(String emailTo, String token) {
sendChangeRequestEmail(emailTo, "/change-password-request/", token, "Password");
}
public void sendEmailChangeEmail(String emailTo, String token) {
sendChangeRequestEmail(emailTo, "/change-email-request/", token, "Email");
}
public void sendConfirmationEmail(String emailTo, String authToken) {
String header = new String(AppConfig.APP_NAME + " | Registration confirmation");
String tokenLink = clientHostUrl + "/register/" + authToken;
String tokenHtmlLink = new String("<a href='" + tokenLink + "'>link</a>");
String body = new String("Please follow this " + tokenHtmlLink + " to confirm your registration.");
sendEmail("email", emailTo, header, header, body, "");
}
public void sendEmail(String template, String emailTo, String subject, String header, String body, String footer) {
final MimeMessage mimeMessage = mailSender.createMimeMessage();
try {
final MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true, "UTF-8");
mimeMessageHelper.setTo(emailTo);
mimeMessageHelper.setSubject(subject);
Context context = new Context();
context.setVariable("header", header);
context.setVariable("body", body);
context.setVariable("footer", footer);
final String emailContent = templateEngine.process(template, context);
mimeMessageHelper.setText(emailContent, true);
mailSender.send(mimeMessageHelper.getMimeMessage());
} catch (MessagingException e) {
e.printStackTrace();
}
}
// public void sendHtmlEmail(){
// final MimeMessage mimeMessage = this.mailSender.createMimeMessage();
// Optional<MimeMessageHelper> optionalMimeMessageHelper = Optional.empty();
// Optional<String> optionalS = Optional.empty();
// try {
// final MimeMessageHelper mimeMessageHelper = new MimeMessageHelper(mimeMessage, true, "UTF-8");
// mimeMessageHelper.setTo("<EMAIL>");
// mimeMessageHelper.setSubject("My Spring App");
//
// Context context = new Context();
// String logoUrl = "media/album/regular/42/1607522691349DsL9sK.jpg";
// context.setVariable("logo", logoUrl);
//// context.setVariable("logo", "logo.jpg");
// context.setVariable("header", "HEADER CONTENT");
// context.setVariable("body", "BODY CONTENT");
// context.setVariable("footer", "FOOTER CONTENT");
// final String emailContent = this.templateEngine.process("email", context);
// System.out.println(emailContent);
//// mimeMessageHelper.setText(emailContent, true);
//// mimeMessageHelper.addInline("logo.jpg", new FileSystemResource("src/main/webapp/media/album/regular/42/1607522691349DsL9sK.jpg"));
//// optionalMimeMessageHelper = Optional.of(mimeMessageHelper);
// } catch (MessagingException e) {
// e.printStackTrace();
// }
// optionalMimeMessageHelper.ifPresentOrElse(
// (helper) -> {
// System.out.println("sending html email");
// this.mailSender.send(helper.getMimeMessage());
// },
// () -> System.out.println("MimeMessageHelper is null")
// );
// }
// @Bean
// public ResourceBundleMessageSource emailMessageSource(){
// final ResourceBundleMessageSource resourceBundleMessageSource = new ResourceBundleMessageSource();
// resourceBundleMessageSource.setBasename("mail/MailMessages");
// return resourceBundleMessageSource;
// }
}
<file_sep>/src/test/java/com/gallery/web/client/UnknownControllerTest.java
package com.gallery.web.client;
import com.gallery.FunctionUtilTest;
import com.gallery.model.entity.user.User;
import com.gallery.service.UserService;
import com.gallery.util.ResponseModel;
import com.gallery.web.client.common.ControllerClientTest;
import org.junit.Assert;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.Timeout;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.test.web.client.ExpectedCount;
import org.springframework.test.web.client.MockRestServiceServer;
import org.springframework.web.client.RestTemplate;
import java.util.List;
//@ContextConfiguration(classes = {App.class})
//@WebAppConfiguration
//public class UserControllerTest extends AbstractTestNGSpringContextTests implements AppTest {
public class UnknownControllerTest extends ControllerClientTest {
@Value("${controller.uri.user.all}")
protected String userFindAllUri;
// private MockMvc mockMvc;
// @Autowired
// private WebApplicationContext webContext;
// private WebApplicationContext webContext;
// @Autowired private MockRestServiceServer server;
// @BeforeAll
// void setup(){
// mockMvc = MockMvcBuilders.webAppContextSetup(webContext).build();
// }
/*@BeforeAll
private void setup() {
mockMvc = MockMvcBuilders
.webAppContextSetup(webContext)
.apply(SecurityMockMvcConfigurers.springSecurity()) // enable security for the mock set up
.build();
}*/
/*@Test
public void secur(WebApplicationContext webContext) throws Exception {
*//*mockMvc = MockMvcBuilders
.standaloneSetup(webContext)
// .apply(SecurityMockMvcConfigurers.springSecurity()) // enable security for the mock set up
.build();*//*
MockHttpServletResponse response = mockMvc.perform(
MockMvcRequestBuilders.get("/api/user/secur"))
.andExpect(MockMvcResultMatchers.content().contentType(MediaType.APPLICATION_JSON))
.andExpect(MockMvcResultMatchers.status().isOk())
.andReturn().getResponse();
System.out.println(response.getContentAsString());
}*/
@Autowired
private UserService userService;
@Test
@Timeout(5)
public void findAllUsers() {
List<User> usersFound = (List<User>) userService.findAll();
ResponseModel responseModelExpected = new ResponseModel("users", usersFound);
String expectedJsonContent = asJson(responseModelExpected);
RestTemplate restTemplate = new RestTemplate();
server = MockRestServiceServer.bindTo(restTemplate).build();
server.expect(ExpectedCount.once(), requestTo(userFindAllUri))
.andExpect(method(HttpMethod.GET))
// .andExpect(content().contentType(MediaType.APPLICATION_JSON))
// .andRespond(withSuccess(expectedJson, MediaType.APPLICATION_JSON))
.andRespond(
withStatus(HttpStatus.OK)
.contentType(MediaType.APPLICATION_JSON)
.body(expectedJsonContent));
ResponseModel responseModelResponse = restTemplate.getForObject(userFindAllUri, ResponseModel.class);
server.verify();
int usersSize = FunctionUtilTest.getResponseModelCollectionAttrSize(responseModelResponse, ResponseModel.ATTR_USERS);
Assert.assertEquals(HttpStatus.OK.value(), responseModelResponse.getStatusCode());
Assert.assertEquals(4, usersSize);
}
}
<file_sep>/src/main/java/com/gallery/service/entity/MultiEntityServiceImpl.java
package com.gallery.service.entity;
import org.hibernate.loader.MultipleBagFetchException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.stereotype.Service;
import javax.persistence.EntityManager;
import javax.persistence.NoResultException;
import javax.persistence.TypedQuery;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
import java.util.ArrayList;
import java.util.Collection;
//@Service
public class MultiEntityServiceImpl implements MultiEntityService {
protected ApplicationContext applicationContext;
protected EntityManager entityManager;
@Autowired
public MultiEntityServiceImpl(ApplicationContext applicationContext) {
this.applicationContext = applicationContext;
this.entityManager = this.applicationContext.getBean(EntityManager.class);
}
/**
* Find all entities with multiple join fetch options.
*
* @param entityClass entity class (e.g. User.class)
// * @param options query options
* @param fetchOptions entity associations
* @return List<T> of entitites or empty
*/
@Override
public <T> Collection<T> findAllByClassWithOptions(Class<T> entityClass, String... fetchOptions) {
CriteriaBuilder cb = this.entityManager.getCriteriaBuilder();
CriteriaQuery<T> cq = cb.createQuery(entityClass);
cq.distinct(true);
Root<T> root = cq.from(entityClass);
fetch(root, fetchOptions);
TypedQuery<T> tq = this.entityManager.createQuery(cq.select(root));
Collection<T> resultList = new ArrayList<>();
try {
resultList = tq.getResultList();
} catch (NoResultException | MultipleBagFetchException ignored) {
}
return resultList;
}
}
<file_sep>/src/main/java/com/gallery/model/entity/album/Image.java
package com.gallery.model.entity.album;
import com.gallery.model.entity.AbstractEntity;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.ToString;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Table;
//@ApiModel(value = "Image entity model", parent = AbstractEntity.class)
@Getter
@Setter
@NoArgsConstructor
@ToString(callSuper = true)
@Entity(name = "Image")
@Table(name = "image")
public class Image extends AbstractEntity {
// @ApiModelProperty(name = "resource file path", dataType = "String", required = true, position = 2)
@Column(name = "src")
private String src;
// @ApiModelProperty(name = "image view count", dataType = "String", allowEmptyValue = true, position = 3)
@Column(name = "view_count", nullable = false)
private Integer viewCount = 0;
/* @JsonIgnore
@ManyToOne(fetch = FetchType.LAZY)
private Album album;
*/
public Image(String src) {
this.src = src;
}
}
<file_sep>/src/main/resources/validation/validation.properties
field.string.length.max=24
field.string.length.min=3
field.password.length.min=6
field.password.length.max=28
field.required={0} field is required
field.short={0} field is too short
field.long={0} field is too long
field.email.format=wrong email format
user.field.duplicate=user with such {0} already exists
field.password.mismatch=passwords do not match
field.required={0} field is required
field.short={0} field is too short
field.long={0} field is too long
field.email.format=wrong email format
user.field.duplicate=user with such {0} already exists
field.password.mismatch=passwords do not match
extension.format=wrong extension format<file_sep>/src/main/java/com/gallery/validation/UserValidator.java
package com.gallery.validation;
import com.gallery.model.dto.user.UserDto;
import com.gallery.service.UserService;
import com.gallery.validation.field.EmailFieldValidator;
import com.gallery.validation.field.PasswordFieldValidator;
import com.gallery.validation.field.UsernameFieldValidator;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.context.MessageSource;
import org.springframework.stereotype.Component;
import org.springframework.validation.Errors;
import org.springframework.validation.Validator;
//spring classpath: /src/main/resources
//@PropertySource("classpath:validation.properties")
@Component
public class UserValidator implements Validator {
private MessageSource messageSource;
private UserService userService;
private UsernameFieldValidator usernameFieldValidator;
private PasswordFieldValidator passwordFieldValidator;
private EmailFieldValidator emailFieldValidator;
public UserValidator(
@Qualifier("validationMessageSource") MessageSource messageSource,
UserService userService,
UsernameFieldValidator usernameFieldValidator,
PasswordFieldValidator passwordFieldValidator,
EmailFieldValidator emailFieldValidator) {
this.messageSource = messageSource;
this.userService = userService;
usernameFieldValidator.setFieldName("username");
this.usernameFieldValidator = usernameFieldValidator;
passwordFieldValidator.setFieldName("password");
this.passwordFieldValidator = passwordFieldValidator;
emailFieldValidator.setFieldName("email");
this.emailFieldValidator = emailFieldValidator;
}
@Override
public boolean supports(Class<?> aClass) {
return UserDto.class.equals(aClass);
}
@Override
public void validate(Object o, Errors errors) {
UserDto user = (UserDto) o;
this.usernameFieldValidator.validate(user.getUsername(), errors);
this.emailFieldValidator.validate(user.getEmail(), errors);
this.passwordFieldValidator.validate(user.getPassword(), errors);
if (!user.getPasswordConfirm().equals(user.getPassword())) {
errors.rejectValue("passwordConfirm", this.usernameFieldValidator.getMessageSource().getMessage("field.password.mismatch", null, null));
}
if( ! errors.hasErrors()){
if (userService.findByUsername(user.getUsername()) != null) {
errors.rejectValue("username", this.messageSource.getMessage("user.field.duplicate", new String[]{"username"}, null));
}
else if (userService.findByEmail(user.getEmail()) != null) {
errors.rejectValue("email", this.messageSource.getMessage("user.field.duplicate", new String[]{"email"}, null));
}
}
}
}<file_sep>/src/main/java/com/gallery/util/factory/Factory.java
package com.gallery.util.factory;
public interface Factory<T> {
T create(String endPath);
}
<file_sep>/src/main/java/com/gallery/service/entity/option/EntityServiceOptions.java
package com.gallery.service.entity.option;
import lombok.Getter;
import java.util.HashMap;
import java.util.Map;
public class EntityServiceOptions {
public static String KEY_OFFSET = "offset";
public static String KEY_LIMIT = "limit";
public static String KEY_ORDER_ASC = "orderAsc";
public static String KEY_ORDER_DESC = "orderDesc";
@Getter
protected Map<String, String> options = new HashMap<>();
public EntityServiceOptions() {
}
protected EntityServiceOptions(Map<String, String> options) {
this.options = options;
}
public boolean hasValueForKey(String key) {
return options.containsKey(key);
}
public String getValue(String key) {
return options.get(key);
}
public boolean hasLimit() {
return hasValueForKey(KEY_LIMIT);
}
public int getLimitValue() throws NumberFormatException {
return Integer.parseInt(getValue(KEY_LIMIT));
}
public boolean hasOffset() {
return hasValueForKey(KEY_OFFSET);
}
public int getOffsetValue() throws NumberFormatException {
return Integer.parseInt(getValue(KEY_OFFSET));
}
public boolean hasOrderAsc() {
return hasValueForKey(KEY_ORDER_ASC);
}
public String getOrderAscValue() {
return getValue(KEY_ORDER_ASC);
}
public boolean hasOrderDesc() {
return hasValueForKey(KEY_ORDER_DESC);
}
public String getOrderDescValue() {
return getValue(KEY_ORDER_DESC);
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map<String, String> options = new HashMap<>();
public Builder withLimit(int limit) {
options.put(KEY_LIMIT, String.valueOf(limit));
return this;
}
public Builder withOffset(int offset) {
options.put(KEY_OFFSET, String.valueOf(offset));
return this;
}
public Builder withOrderAsc(String fieldName) {
options.put(KEY_ORDER_ASC, fieldName);
return this;
}
public Builder withOrderDesc(String fieldName) {
options.put(KEY_ORDER_DESC, fieldName);
return this;
}
public EntityServiceOptions build() {
return new EntityServiceOptions(options);
}
}
}
<file_sep>/src/main/java/com/gallery/web/UserController.java
package com.gallery.web;
import com.gallery.model.dto.user.UserDto;
import com.gallery.model.entity.user.User;
import com.gallery.model.entity.user.UserData;
import com.gallery.service.UserDataService;
import com.gallery.service.UserService;
import com.gallery.util.ResponseModel;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiResponse;
import io.swagger.annotations.ApiResponses;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.web.bind.annotation.*;
import springfox.documentation.annotations.ApiIgnore;
import javax.servlet.http.HttpServletRequest;
import java.util.List;
@RestController
@RequestMapping("/user")
public class UserController {
private UserService userService;
private UserDataService userDataService;
@Autowired
public UserController(UserService userService, UserDataService userDataService) {
this.userService = userService;
this.userDataService = userDataService;
}
// @Autowired
// EntityService entityService;
@PostMapping("/get")
public ResponseModel getUser(@AuthenticationPrincipal User user,
@RequestParam(name = "options", required = false) List<String> options) {
// if(user != null && options.size() > 0){
// User user1 = entityService.findOneByAttrWithOptions( User.class, User_.id, user.getId().toString(), (String[]) options.toArray() );
// }
UserDto userDto = null;
if (user != null) {
userDto = new UserDto(user);
}
ResponseModel responseModel = new ResponseModel("user", userDto);
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "save current logged in user's theme pref setting")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "theme preference setting saved")
})
@PostMapping("/theme")
public void setTheme(@ApiIgnore @AuthenticationPrincipal User principal, @ApiIgnore HttpServletRequest request) {
UserData principalUserData = userDataService.findById(principal.getId()).get();
String theme = request.getParameter("theme");
try {
principalUserData.setTheme(Integer.parseInt(theme));
}
catch (NullPointerException | NumberFormatException e) {
e.printStackTrace();
}
}
}
<file_sep>/src/main/java/com/gallery/util/FunctionUtil.java
package com.gallery.util;
import com.gallery.util.image.ImageSaver;
import io.jsonwebtoken.JwtBuilder;
import io.jsonwebtoken.Jwts;
import net.bytebuddy.utility.RandomString;
import org.springframework.util.StringUtils;
import org.springframework.validation.BindingResult;
import org.springframework.validation.FieldError;
import javax.persistence.Query;
import java.util.*;
import java.util.regex.Pattern;
public class FunctionUtil {
public static final Pattern CLEAN_STRING_PATTERN = Pattern.compile("[{}!<>.,;%&@#/\\]\\[\\\\$^*()?|_\\s]*");
// public static final Pattern BACKSLASHES_PATTERN = Pattern.compile("\\\\");
public static String buildAlbumPath(String albumUserUsername, Long id) {
return ImageSaver.ROOT_CATALOG + "/album/" + cleanString(albumUserUsername.toLowerCase()) + "/" + id;
}
public static String buildImagePath(String albumUserUsername, Long id) {
return buildAlbumPath(albumUserUsername.toLowerCase(), id) + "/images";
}
public static String cleanString(String string) {
return CLEAN_STRING_PATTERN.matcher(string).replaceAll("");
}
public static String getFileExtension(String fileName, boolean dot) {
// int lastIndexOf = fileName.lastIndexOf(".");
// if (lastIndexOf > 0) {
// return fileName.substring(dot ? lastIndexOf : (lastIndexOf + 1));
// }
// return "";
String ext = StringUtils.getFilenameExtension(fileName);
return dot ? ("." + ext) : ext;
}
public static Map<String, String> mapBindingResultFieldErrors(BindingResult errors) {
Map<String, String> errorsMap = new HashMap<>();
if (errors.hasErrors()) {
for (FieldError fieldError : errors.getFieldErrors()) {
if ( ! errorsMap.containsKey(fieldError.getField())) {//put only 1st error for each field
errorsMap.put(fieldError.getField(), fieldError.getCode());
}
}
}
return errorsMap;
}
public static Integer resolveOffset(Integer offset, Integer page, int itemsSizePerPage){
if(page != null && page > 0){
offset = page * itemsSizePerPage;
}
return offset;
}
public static Integer resolveLimit(Integer limit, int itemsSizePerPage){
if(limit == null || limit == 0){
limit = itemsSizePerPage;
}
return limit;
}
public static void applyOffsetAndLimit(Query typedQuery, Integer offset, Integer limit){
if (offset != null && offset > 0) {
typedQuery.setFirstResult(offset);
}
if (limit != null && limit > 1) {
typedQuery.setMaxResults(limit);
}
}
public static AbstractMap.SimpleEntry< String, AbstractMap.SimpleEntry<String, String> > getWhereClauseEntry(
String rootKey,
String attrKey, String attrVal) {
return new AbstractMap.SimpleEntry<>(
rootKey,
new AbstractMap.SimpleEntry(attrKey, attrVal)
);
}
// public static String removeBackslashes(String string) {
// return BACKSLASHES_PATTERN.matcher(string).replaceAll("\\/");
// }
}
<file_sep>/gallery.sql
-- phpMyAdmin SQL Dump
-- version 4.9.0.1
-- https://www.phpmyadmin.net/
--
-- Host: localhost
-- Generation Time: Jul 22, 2021 at 05:25 PM
-- Server version: 8.0.19
-- PHP Version: 7.1.19
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO";
SET AUTOCOMMIT = 0;
START TRANSACTION;
SET time_zone = "+00:00";
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8mb4 */;
--
-- Database: `gallery`
--
-- --------------------------------------------------------
--
-- Table structure for table `album`
--
CREATE TABLE `album` (
`id` bigint NOT NULL,
`created_at` date DEFAULT NULL,
`cover` varchar(255) DEFAULT NULL,
`deleted` tinyint(1) DEFAULT '0',
`description` text,
`title` varchar(255) NOT NULL,
`user_id` bigint DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `album`
--
INSERT INTO `album` (`id`, `created_at`, `cover`, `deleted`, `description`, `title`, `user_id`) VALUES
(1, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #1', 1),
(2, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #2', 1),
(3, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #3', 1),
(4, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #4', 1),
(5, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #5', 1),
(6, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #6', 1),
(7, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #7', 1),
(8, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #8', 1),
(9, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #9', 1),
(10, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #10', 1),
(11, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #11', 1),
(12, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #12', 1),
(13, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #13', 1),
(14, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #14', 1),
(15, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #15', 1),
(16, '2021-06-16', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #16', 1),
(17, '2021-07-13', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #39', 2),
(18, '2021-07-15', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #40', 2),
(19, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #41', 2),
(20, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #42', 2),
(21, '2021-07-06', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #43', 2),
(22, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #44', 2),
(23, '2021-07-07', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #45', 2),
(24, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #46', 2),
(25, '2021-06-01', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #47', 2),
(26, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #48', 2),
(27, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #49', 2),
(28, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #50', 2),
(29, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #51', 2),
(30, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #52', 2),
(31, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #53', 2),
(32, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #54', 2),
(33, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #55', 2),
(34, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #56', 2),
(35, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #17', 4),
(36, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #18', 4),
(37, '2021-07-18', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #19', 4),
(38, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #20', 4),
(39, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #21', 4),
(40, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #22', 4),
(41, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #23', 4),
(42, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #24', 4),
(43, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #25', 4),
(44, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #26', 4),
(45, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #27', 4),
(46, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #28', 4),
(47, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #29', 4),
(48, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #30', 4),
(49, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #31', 4),
(50, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #32', 4),
(51, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #33', 4),
(52, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #34', 4),
(53, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, NULL, 'album #35', 4),
(54, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #36', 4),
(55, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, NULL, 'album #37', 4),
(56, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, NULL, 'album #38', 4);
-- --------------------------------------------------------
--
-- Table structure for table `image`
--
CREATE TABLE `image` (
`id` bigint NOT NULL,
`created_at` date DEFAULT NULL,
`src` varchar(255) DEFAULT NULL,
`view_count` int NOT NULL,
`album_id` bigint NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `image`
--
INSERT INTO `image` (`id`, `created_at`, `src`, `view_count`, `album_id`) VALUES
(1, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 1),
(2, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 1),
(3, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 1),
(4, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 1),
(5, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 1),
(6, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 1),
(7, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 1),
(8, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 1),
(9, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 1),
(10, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 2),
(11, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 2),
(12, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 2),
(13, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 2),
(14, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 2),
(15, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 2),
(16, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 2),
(17, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 2),
(18, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 3),
(19, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 3),
(20, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 3),
(21, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 3),
(22, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 3),
(23, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 3),
(24, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 3),
(25, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(26, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 4),
(27, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(28, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(29, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 4),
(30, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(31, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(32, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(33, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(34, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 4),
(35, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(36, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(37, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(38, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(39, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 4),
(40, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(41, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 4),
(42, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 4),
(43, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 5),
(44, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(45, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 5),
(46, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 5),
(47, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 5),
(48, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(49, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(50, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(51, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 5),
(52, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 5),
(53, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(54, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(55, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(56, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 5),
(57, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 5),
(58, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 5),
(59, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 5),
(60, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 6),
(61, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 6),
(62, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 6),
(63, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 6),
(64, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 6),
(65, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 6),
(66, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 6),
(67, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 6),
(68, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 6),
(69, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 6),
(70, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 6),
(71, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 7),
(72, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 7),
(73, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 7),
(74, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 8),
(75, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 8),
(76, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 8),
(77, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 9),
(78, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(79, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 9),
(80, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(81, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 9),
(82, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(83, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(84, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(85, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 9),
(86, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 9),
(87, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 9),
(88, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 9),
(89, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 9),
(90, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(91, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(92, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(93, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 10),
(94, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(95, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(96, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(97, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(98, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 10),
(99, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(100, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(101, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(102, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 10),
(103, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 10),
(104, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(105, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 10),
(106, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 11),
(107, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 11),
(108, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 11),
(109, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 11),
(110, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 11),
(111, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(112, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(113, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(114, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(115, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 12),
(116, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(117, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(118, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 12),
(119, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(120, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 12),
(121, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 13),
(122, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 13),
(123, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 13),
(124, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 13),
(125, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 13),
(126, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 13),
(127, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 13),
(128, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 13),
(129, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 13),
(130, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(131, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(132, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 14),
(133, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(134, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 14),
(135, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(136, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 14),
(137, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 14),
(138, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(139, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(140, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 14),
(141, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(142, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(143, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(144, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(145, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(146, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(147, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 14),
(148, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 14),
(149, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 15),
(150, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 15),
(151, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 15),
(152, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 15),
(153, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 15),
(154, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(155, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(156, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(157, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(158, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(159, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(160, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(161, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 16),
(162, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(163, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 16),
(164, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(165, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(166, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(167, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 16),
(168, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(169, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(170, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(171, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 16),
(172, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 16),
(173, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 16),
(174, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 17),
(175, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 17),
(176, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 17),
(177, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 17),
(178, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 17),
(179, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 17),
(180, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 17),
(181, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 17),
(182, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 17),
(183, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 17),
(184, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 17),
(185, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 17),
(186, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 18),
(187, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 18),
(188, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 18),
(189, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 18),
(190, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 18),
(191, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 18),
(192, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 19),
(193, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 19),
(194, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 19),
(195, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 19),
(196, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 19),
(197, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 19),
(198, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 19),
(199, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 19),
(200, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(201, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(202, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(203, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(204, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(205, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(206, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(207, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(208, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(209, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(210, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(211, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(212, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(213, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(214, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(215, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(216, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 20),
(217, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(218, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(219, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 20),
(220, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 20),
(221, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 21),
(222, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 21),
(223, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(224, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(225, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(226, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 21),
(227, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 21),
(228, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(229, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 21),
(230, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 21),
(231, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(232, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 21),
(233, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 21),
(234, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(235, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 21),
(236, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 21),
(237, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(238, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(239, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 22),
(240, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 22),
(241, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 22),
(242, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(243, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 22),
(244, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(245, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 22),
(246, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 22),
(247, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(248, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 22),
(249, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 22),
(250, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 22),
(251, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 22),
(252, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 23),
(253, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 23),
(254, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 23),
(255, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 23),
(256, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 23),
(257, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(258, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(259, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 23),
(260, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 23),
(261, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(262, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(263, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 23),
(264, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(265, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 23),
(266, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 24),
(267, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 25),
(268, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 25),
(269, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 25),
(270, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 25),
(271, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 25),
(272, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 25),
(273, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 25),
(274, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 25),
(275, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 26),
(276, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 26),
(277, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 26),
(278, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 26),
(279, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 26),
(280, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 26),
(281, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 27),
(282, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 27),
(283, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(284, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(285, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(286, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(287, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(288, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(289, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(290, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(291, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(292, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(293, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(294, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(295, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(296, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(297, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(298, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(299, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(300, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 28),
(301, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 28),
(302, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 28),
(303, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 29),
(304, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 29),
(305, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 30),
(306, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 30),
(307, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 30),
(308, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(309, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 31),
(310, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 31),
(311, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(312, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(313, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 31),
(314, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(315, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 31),
(316, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(317, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 31),
(318, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 31),
(319, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 31),
(320, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(321, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(322, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(323, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 31),
(324, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(325, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 31),
(326, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 31),
(327, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 31),
(328, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(329, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(330, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(331, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(332, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(333, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(334, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(335, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(336, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(337, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(338, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(339, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(340, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(341, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(342, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(343, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(344, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 32),
(345, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(346, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(347, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 32),
(348, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 32),
(349, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(350, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 33),
(351, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(352, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(353, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(354, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(355, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 33),
(356, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(357, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 33),
(358, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(359, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(360, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(361, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 33),
(362, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(363, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 33),
(364, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 33),
(365, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 34),
(366, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(367, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(368, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 34),
(369, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(370, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(371, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(372, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(373, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(374, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 34),
(375, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(376, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(377, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(378, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 34),
(379, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 34),
(380, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 35),
(381, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 35),
(382, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 35),
(383, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 35),
(384, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 35),
(385, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 35),
(386, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 35),
(387, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 35),
(388, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 35),
(389, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 35),
(390, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 35),
(391, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 35),
(392, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 37),
(393, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 37),
(394, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 37),
(395, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 37),
(396, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 37),
(397, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 37),
(398, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 37),
(399, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(400, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(401, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(402, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(403, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(404, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(405, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 38),
(406, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(407, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(408, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(409, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(410, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 38),
(411, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(412, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(413, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(414, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(415, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(416, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(417, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(418, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 38),
(419, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 38),
(420, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(421, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(422, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(423, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 39),
(424, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 39),
(425, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 39),
(426, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 39),
(427, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(428, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 39),
(429, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 39),
(430, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(431, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 39),
(432, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(433, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(434, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(435, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(436, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(437, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(438, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(439, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 41),
(440, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(441, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 41),
(442, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 41),
(443, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(444, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(445, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(446, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(447, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 41),
(448, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(449, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 41),
(450, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 41),
(451, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 41),
(452, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 42),
(453, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 42),
(454, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 42),
(455, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 42),
(456, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 44),
(457, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 44),
(458, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 44),
(459, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 44),
(460, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 44),
(461, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 44),
(462, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 44),
(463, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 44),
(464, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 44),
(465, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 45),
(466, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 45),
(467, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 45),
(468, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 46),
(469, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(470, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 46),
(471, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 46),
(472, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(473, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(474, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 46),
(475, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 46),
(476, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(477, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(478, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 46),
(479, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 47),
(480, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 47),
(481, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 48),
(482, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 48),
(483, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 48),
(484, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 48),
(485, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 48),
(486, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 48),
(487, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 49),
(488, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(489, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 49),
(490, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(491, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 49),
(492, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 49),
(493, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(494, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(495, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(496, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 49),
(497, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 49),
(498, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 49),
(499, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 49),
(500, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(501, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(502, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(503, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(504, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(505, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(506, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 50),
(507, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(508, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(509, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(510, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(511, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 50),
(512, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(513, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(514, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 50),
(515, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(516, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 50),
(517, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 50),
(518, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(519, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 50),
(520, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 50),
(521, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 51),
(522, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 51),
(523, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 51),
(524, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 51),
(525, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 51),
(526, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 51),
(527, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 51),
(528, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 51),
(529, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 51),
(530, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 51),
(531, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 51),
(532, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 52),
(533, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 52),
(534, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 52),
(535, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 52),
(536, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 52),
(537, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 52),
(538, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 52),
(539, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 52),
(540, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(541, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 53),
(542, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(543, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 53),
(544, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(545, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(546, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(547, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 53),
(548, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(549, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 53),
(550, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(551, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 53),
(552, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(553, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 53),
(554, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 53),
(555, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 53),
(556, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 54),
(557, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 54),
(558, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 54),
(559, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 54),
(560, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 54),
(561, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 54),
(562, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 54),
(563, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 54),
(564, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 54),
(565, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 54),
(566, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 54),
(567, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 54),
(568, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(569, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 55),
(570, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(571, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 55),
(572, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(573, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(574, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 55),
(575, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 55),
(576, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 55),
(577, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(578, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 55),
(579, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 55),
(580, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 55),
(581, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 56),
(582, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 56),
(583, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 56),
(584, '2021-06-25', '/media/album/common/1619788949530zb3vrUgbtyBb.jpg', 0, 56),
(585, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 56),
(586, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 56),
(587, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 56),
(588, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 56),
(589, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 56),
(590, '2021-06-25', '/media/album/common/1619789224979BYakIw8GSYR4.jpg', 0, 56),
(591, '2021-06-25', '/media/album/common/1619789224952qv4J5dLfI5rI.jpg', 0, 56);
-- --------------------------------------------------------
--
-- Table structure for table `role`
--
CREATE TABLE `role` (
`id` bigint NOT NULL,
`name` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `role`
--
INSERT INTO `role` (`id`, `name`) VALUES
(1, 'ROLE_USER'),
(2, 'ROLE_ADMIN');
-- --------------------------------------------------------
--
-- Table structure for table `user`
--
CREATE TABLE `user` (
`id` bigint NOT NULL,
`created_at` date DEFAULT NULL,
`account_expired` tinyint(1) NOT NULL DEFAULT '0',
`auth_token` varchar(510) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci DEFAULT NULL,
`credentials_expired` tinyint(1) NOT NULL DEFAULT '0',
`email` varchar(255) NOT NULL,
`email_token` varchar(510) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci DEFAULT NULL,
`enabled` tinyint(1) NOT NULL DEFAULT '0',
`locked` tinyint(1) NOT NULL DEFAULT '0',
`password` varchar(255) NOT NULL,
`password_token` varchar(510) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci DEFAULT NULL,
`username` varchar(255) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `user`
--
INSERT INTO `user` (`id`, `created_at`, `account_expired`, `auth_token`, `credentials_expired`, `email`, `email_token`, `enabled`, `locked`, `password`, `password_token`, `username`) VALUES
(1, '2021-06-17', 0, NULL, 0, '<EMAIL>', NULL, 1, 0, '$2a$10$NRxYSRSPsHrqsqO8MrbmJ.pEMG6g.F8WvUBN2ZVPvWFhGvnfWjUxm', NULL, 'Raina'),
(2, '2021-06-17', 0, NULL, 0, '<EMAIL>', NULL, 1, 0, '$2a$10$7np8eohUED/R8ed7ijESfe2MTvopJSkebBl5u3CMLK5pnXle.4coy', NULL, 'Solomon'),
(3, '2021-06-17', 0, NULL, 0, '<EMAIL>', NULL, 1, 0, '$2a$10$u/jliGTMdmSDZgL0Xr83J.MWPxDfGhKT3oDf478OTyR3k688MqZlG', NULL, 'Elaine'),
(4, '2021-06-17', 0, NULL, 0, '<EMAIL>', NULL, 1, 0, '$2a$10$S5B1WzC8H206cbROUdubYO/Dg9D0n5ylpkXNTIgFXlSWrA77m6yWS', NULL, 'Dominick');
-- --------------------------------------------------------
--
-- Table structure for table `user_data`
--
CREATE TABLE `user_data` (
`last_login` date DEFAULT NULL,
`last_update_check` date DEFAULT NULL,
`user_id` bigint NOT NULL,
`theme` tinyint DEFAULT '1',
`last_update_check_sort` date DEFAULT NULL COMMENT 'date to be used when user checked updates and user uses sort to group updates (today, last week, ...)'
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `user_data`
--
INSERT INTO `user_data` (`last_login`, `last_update_check`, `user_id`, `theme`, `last_update_check_sort`) VALUES
(NULL, '2021-07-22', 1, 2, '2021-07-05'),
(NULL, NULL, 2, 1, NULL),
(NULL, NULL, 3, 1, NULL),
(NULL, NULL, 4, 1, NULL);
-- --------------------------------------------------------
--
-- Table structure for table `user_roles`
--
CREATE TABLE `user_roles` (
`user_id` bigint NOT NULL,
`roles_id` bigint NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `user_roles`
--
INSERT INTO `user_roles` (`user_id`, `roles_id`) VALUES
(1, 1),
(2, 1),
(3, 1),
(4, 1);
-- --------------------------------------------------------
--
-- Table structure for table `user_subscribers`
--
CREATE TABLE `user_subscribers` (
`user_id` bigint NOT NULL,
`subscribers_id` bigint NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
--
-- Dumping data for table `user_subscribers`
--
INSERT INTO `user_subscribers` (`user_id`, `subscribers_id`) VALUES
(2, 1),
(4, 1),
(2, 4);
--
-- Indexes for dumped tables
--
--
-- Indexes for table `album`
--
ALTER TABLE `album`
ADD PRIMARY KEY (`id`),
ADD KEY `FKmi5m81x9aswan1ci0wnw04dq1` (`user_id`);
--
-- Indexes for table `image`
--
ALTER TABLE `image`
ADD PRIMARY KEY (`id`),
ADD KEY `FKklgd5pxhpuh3nwik115myord` (`album_id`);
--
-- Indexes for table `role`
--
ALTER TABLE `role`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `user`
--
ALTER TABLE `user`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `user_data`
--
ALTER TABLE `user_data`
ADD PRIMARY KEY (`user_id`);
--
-- Indexes for table `user_roles`
--
ALTER TABLE `user_roles`
ADD PRIMARY KEY (`user_id`,`roles_id`),
ADD KEY `FKj9553ass9uctjrmh0gkqsmv0d` (`roles_id`);
--
-- Indexes for table `user_subscribers`
--
ALTER TABLE `user_subscribers`
ADD PRIMARY KEY (`user_id`,`subscribers_id`),
ADD KEY `FKsic6kr4t25xyvr8hobrpyehip` (`subscribers_id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `album`
--
ALTER TABLE `album`
MODIFY `id` bigint NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=62;
--
-- AUTO_INCREMENT for table `image`
--
ALTER TABLE `image`
MODIFY `id` bigint NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=616;
--
-- AUTO_INCREMENT for table `role`
--
ALTER TABLE `role`
MODIFY `id` bigint NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=6;
--
-- AUTO_INCREMENT for table `user`
--
ALTER TABLE `user`
MODIFY `id` bigint NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=19;
--
-- Constraints for dumped tables
--
--
-- Constraints for table `album`
--
ALTER TABLE `album`
ADD CONSTRAINT `FKmi5m81x9aswan1ci0wnw04dq1` FOREIGN KEY (`user_id`) REFERENCES `user` (`id`);
--
-- Constraints for table `image`
--
ALTER TABLE `image`
ADD CONSTRAINT `FKklgd5pxhpuh3nwik115myord` FOREIGN KEY (`album_id`) REFERENCES `album` (`id`);
--
-- Constraints for table `user_data`
--
ALTER TABLE `user_data`
ADD CONSTRAINT `FKelmpammgqm7p72tyao7p2vb00` FOREIGN KEY (`user_id`) REFERENCES `user` (`id`);
--
-- Constraints for table `user_roles`
--
ALTER TABLE `user_roles`
ADD CONSTRAINT `FK55itppkw3i07do3h7qoclqd4k` FOREIGN KEY (`user_id`) REFERENCES `user` (`id`),
ADD CONSTRAINT `FKj9553ass9uctjrmh0gkqsmv0d` FOREIGN KEY (`roles_id`) REFERENCES `role` (`id`);
--
-- Constraints for table `user_subscribers`
--
ALTER TABLE `user_subscribers`
ADD CONSTRAINT `FKbygtkeaaeadsse09tt411m1eh` FOREIGN KEY (`user_id`) REFERENCES `user` (`id`),
ADD CONSTRAINT `FKsic6kr4t25xyvr8hobrpyehip` FOREIGN KEY (`subscribers_id`) REFERENCES `user` (`id`);
COMMIT;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
<file_sep>/src/main/java/com/gallery/model/entity/AbstractEntity.java
package com.gallery.model.entity;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import org.hibernate.annotations.CreationTimestamp;
import javax.persistence.*;
import java.util.Date;
import java.util.Objects;
//@ApiModel(subTypes = {User.class, Album.class, Image.class})
@Getter
@ToString
@MappedSuperclass
public abstract class AbstractEntity {
// @ApiModelProperty(name = "id", dataType = "Long", example = "1", required = true)
@Setter
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
protected Long id;
// @ApiModelProperty(name = "creation datetime", dataType = "Date", allowEmptyValue = true, position = 1)
@Setter
@CreationTimestamp
@Temporal(value = TemporalType.DATE)
@Column(name = "created_at", updatable = false)
protected Date createdAt;
@PrePersist
private void prePersist() {
createdAt = new Date();
}
@Override
public boolean equals(Object o) {
// if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AbstractEntity abstractEntity = (AbstractEntity) o;
if (abstractEntity.getId() == null && this.getId() == null) {
return this == abstractEntity;
}
return Objects.equals(this.getId(), abstractEntity.getId());
}
@Override
public int hashCode() {
return Objects.hash(this.getId());
}
}
<file_sep>/src/main/java/com/gallery/util/TokenGenerator.java
package com.gallery.util;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.gallery.model.dto.user.UserDto;
import io.jsonwebtoken.*;
import net.bytebuddy.utility.RandomString;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.util.Calendar;
import java.util.Date;
import java.util.HashMap;
@Component
public class TokenGenerator {
@Value("${jwt.key.secret}")
private String secret;
@Value("${request.change.email.token.seconds}")
private Long emailChangeRequestExpiration;
@Value("${request.change.password.token.seconds}")
private Long passwordChangeRequestExpiration;
public boolean isTokenValid(String token) {
if (token == null || token.isEmpty()) {
return false;
}
Date tokenDate;
try {
tokenDate = new Date(extractTokenTime(token));
}
catch (NumberFormatException e) {
e.printStackTrace();
tokenDate = new Date(0);
}
Date nowDate = Calendar.getInstance().getTime();
System.out.println("nowDate = " + nowDate);
System.out.println("tokenDate = " + tokenDate);
return nowDate.before(tokenDate);
}
/**
* @param token email or password change request token
* @return object array with length of 2:<br/>[0] - UserDto object,<br/>[1] - expiration date.
* @throws SignatureException
*/
public Object[] getChangeRequestTokenData(String token) throws SignatureException, ExpiredJwtException, JsonProcessingException {
Claims claims = Jwts.parser().setSigningKey(secret).parseClaimsJws(token).getBody();
String subject = claims.getSubject();
Date expiration = claims.getExpiration();
Object[] data = new Object[2];
ObjectMapper objectMapper = new ObjectMapper();
data[0] = objectMapper.readValue(subject, UserDto.class);
data[1] = expiration;
return data;
}
public long extractTokenTime(String token) {
int startIndex = token.lastIndexOf('_');
String time = token.substring(startIndex + 1);
Long timeMillis = Long.parseLong(time);
return timeMillis;
}
public String generateEmailToken(UserDto userDto) throws JsonProcessingException {
return generateRequestToken(userDto, emailChangeRequestExpiration);
}
public String generatePasswordToken(UserDto userDto) throws JsonProcessingException {
return generateRequestToken(userDto, passwordChangeRequestExpiration);
}
public String generateRequestToken(UserDto userDto, long expirationSec) throws JsonProcessingException {
Date dateNow = new Date();
String subject = new ObjectMapper().writeValueAsString(userDto);
JwtBuilder jwtBuilder = Jwts.builder()
.setClaims(new HashMap<>())
.setSubject(subject)
.setIssuedAt(dateNow)
.setExpiration(new Date(dateNow.getTime() + (expirationSec * 1000)))
.signWith(SignatureAlgorithm.HS256, secret);
return jwtBuilder.compact();
}
public String generateSimpleToken(int length) {
if (length <= 5) {
throw new IllegalArgumentException("parameter \"length\" must be positive and greater than 5!");
}
// String token = new RandomString(length).nextString() + "_" + (System.currentTimeMillis() + AppConfig.TOKEN_LIFETIME_MILLIS);;
return new RandomString(length).nextString() + "_" + (System.currentTimeMillis() + (passwordChangeRequestExpiration * 1000));
// try {
// MessageDigest messageDigest = MessageDigest.getInstance("SHA-256");
// messageDigest.update(token.getBytes());
// token = new String(messageDigest.digest());
// }catch (NoSuchAlgorithmException e){
// System.out.println("NoSuchAlgorithmException");
// }
// return token;
}
}
<file_sep>/src/test/java/com/gallery/service/RoleRepositoryTest.java
package com.gallery.service;
import com.gallery.model.entity.user.Role;
import com.gallery.model.entity.user.User;
import com.gallery.repository.RoleRepository;
import com.gallery.service.common.ServiceTest;
import org.junit.Assert;
import org.junit.jupiter.api.Test;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
public class RoleRepositoryTest implements ServiceTest {
private static Logger logger = LoggerFactory.getLogger(RoleRepositoryTest.class);
@Autowired
private RoleRepository roleRepository;
@Autowired
private UserService userService;
@Test
public void saveAdminRole() {
long rolesCountBefore = roleRepository.count();
Role adminRole = new Role(Role.ROLE_ADMIN_VALUE);
Role savedAdminRole = roleRepository.save(adminRole);
long rolesCountAfter = roleRepository.count();
Assert.assertNotNull(savedAdminRole);
Assert.assertEquals("Assert Roles Count.", (rolesCountBefore + 1), rolesCountAfter);
}
@Test
public void deleteUserRole() {
long rolesCountBefore = roleRepository.count();
List<User> userList = (List<User>) userService.findAll();
for (User u : userList){
u.getRoles().clear();
}
userService.getUserRepository().saveAll(userList);
Role role = new Role(Role.ROLE_USER_VALUE);
role.setId(1L);
roleRepository.deleteById(role.getId());
long rolesCountAfter = roleRepository.count();
Assert.assertEquals("Assert Roles Count.", (rolesCountBefore - 1), rolesCountAfter);
}
// @Before public void onSetup() {}
// @After public void onTearDown() {}
}
<file_sep>/src/main/java/com/gallery/model/ApplicationProperties.java
package com.gallery.model;
import org.springframework.core.env.Environment;
import java.util.HashMap;
import java.util.Map;
//@Component(value = "applicationProperties")
//@PropertySource(value = {"classpath:application.properties"})
public class ApplicationProperties {
private Environment environment;
// @Autowired
public ApplicationProperties(Environment environment) {
this.environment = environment;
}
public String getProperty(String name) {
return this.environment.getProperty(name);
}
public Map<String, String> getH2ConfigProperties() {
Map<String, String> properties = new HashMap<>();
properties.put("connection.dialect", environment.getProperty("spring.jpa.database-platform"));
properties.put("hibernate.connection.url", environment.getProperty("spring.datasource.url"));
properties.put("hibernate.connection.username", environment.getProperty("spring.datasource.username"));
properties.put("hibernate.connection.password", environment.getProperty("spring.datasource.password"));
properties.put("hibernate.current_session_context_class", environment.getProperty("hibernate.current_session_context_class"));
return properties;
}
}
<file_sep>/src/test/java/com/gallery/service/common/ServiceTest.java
package com.gallery.service.common;
import com.gallery.AppTest;
import com.gallery.service.EntityService;
import org.springframework.test.annotation.Rollback;
import org.springframework.transaction.annotation.Transactional;
//@ActiveProfiles("test")
//@SpringBootTest(classes = {App.class, TestHibernateConfiguration.class})
//@SpringBootTest(classes = {App.class})
@Transactional
@Rollback
public interface ServiceTest extends AppTest {
default Long count(EntityService entityService){
return entityService.count();
}
}
<file_sep>/src/main/resources/hidden_hibernate.properties
#connection.driver_class=com.mysql.jdbc.Driver
#hibernate.connection.driver_class=com.mysql.jdbc.Driver
#hibernate.dialect=org.hibernate.dialect.MySQLDialect
#hibernate.connection.username=root
#hibernate.connection.password=<PASSWORD>
#hibernate.connection.url=jdbc:mysql://localhost/com.gallery?serverTimezone=UTC
#hibernate.hbm2ddl=update
#hibernate.show_sql=true
#hibernate.format_sql=true<file_sep>/src/main/java/com/gallery/web/PageController.java
package com.gallery.web;
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.security.core.context.SecurityContext;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.User;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class PageController {
@GetMapping({"/", "/index"})
public String index(@AuthenticationPrincipal User user) {
String token = null;
return "page/index";
}
@GetMapping("/welcome")
public String welcome(@AuthenticationPrincipal User user) {
SecurityContext securityContextHolder = SecurityContextHolder.getContext();
System.out.println("PRINCIPAL>>>>> " + user);
return "page/welcome";
}
}
<file_sep>/src/main/java/com/gallery/service/ImageService.java
package com.gallery.service;
import com.gallery.model.entity.album.Image;
import com.gallery.repository.ImageRepository;
import com.gallery.util.FunctionUtil;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import javax.persistence.TypedQuery;
import javax.persistence.criteria.CriteriaBuilder;
import javax.persistence.criteria.CriteriaQuery;
import javax.persistence.criteria.Root;
import java.util.Collection;
import java.util.Optional;
@Service
public class ImageService implements EntityService {
@PersistenceContext
private EntityManager entityManager;
private ImageRepository imageRepository;
@Autowired
public ImageService(ImageRepository imageRepository) {
this.imageRepository = imageRepository;
}
public Image save(Image image) {
return imageRepository.save(image);
}
public Iterable<Image> findAll() {
return imageRepository.findAll();
}
public Optional<Image> findById(Long id){
return imageRepository.findById(id);
}
public Collection<Image> findAllByAlbumId(Long albumId) {
return imageRepository.findAllByAlbum(albumId);
}
@Override
public long count() {
return imageRepository.count();
}
public void saveAll(Iterable<Image> entites) {
imageRepository.saveAll(entites);
}
public void delete(Long id) {
imageRepository.deleteById(id);
}
public void delete(Image entity) {
imageRepository.delete(entity);
}
public Collection<Image> findAll(Long albumId, Integer offset, Integer limit) {
CriteriaBuilder cb = entityManager.getCriteriaBuilder();
CriteriaQuery<Image> imageCriteriaQuery = cb.createQuery(Image.class);
Root<Image> imageRoot = imageCriteriaQuery.from(Image.class);
//SELECT case with JUNCTION TABLE (works)
/* Root<Album> albumRoot = imageCriteriaQuery.from(Album.class);
Join<Album, Image> albumImageJoin = albumRoot.join(Album_.images, JoinType.LEFT);
imageCriteriaQuery.select(albumImageJoin)
.where( cb.equal(albumRoot.get(Album_.id), albumId));*/
//SELECT case with join column
// i.e. adding @JoinColumn for Collection<Image> images in album entity class
// (does not work - return duplicates for each album)
/* Root<Album> albumRoot = imageCriteriaQuery.from(Album.class);
Join<Album, Image> albumImageJoin = albumRoot.join(Album_.IMAGES, JoinType.LEFT);
imageCriteriaQuery.select(albumImageJoin).where(cb.equal(albumRoot.get(Album_.ID), albumId));*/
// TypedQuery<Image> typedQuery = entityManager.createQuery(imageCriteriaQuery);
//SELECT case with query
TypedQuery<Image> query = entityManager
.createQuery("SELECT img FROM Album a inner join a.images img WHERE a.id=:id ORDER BY img.createdAt DESC", Image.class)
.setParameter("id", albumId);
FunctionUtil.applyOffsetAndLimit(query, offset, limit);
System.out.println(query.getResultList().getClass().getName());
return query.getResultList();
}
}
<file_sep>/src/test/java/com/gallery/AppTest.java
package com.gallery;
import org.junit.runner.RunWith;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.ActiveProfiles;
import org.springframework.test.context.junit4.SpringRunner;
/**
* Entry point for test classes.
* */
//@RunWith(SpringRunner.class)
//@DataJpaTest
//@WebAppConfiguration
//@AutoConfigureTestEntityManager
//@EnableJpaRepositories(basePackages = {"com.gallery.repository"})
//@ComponentScan(basePackages = {"com.gallery.model.entity", "com.gallery.respository", "com.gallery.service"})
//@ComponentScan(basePackages = {"com.gallery"})
//@SpringBootTest(classes = {App.class, TestHibernateConfiguration.class})
//@ActiveProfiles("test")
//@PropertySource("classpath:application-test.properties")
//@ContextConfiguration(classes = {BCrypConfig.class})
//@ContextConfiguration(classes = {App.class})
//@TestPropertySource("classpath:application-test.properties")
//@Transactional
@RunWith(SpringRunner.class)
@ActiveProfiles("test")
@SpringBootTest(classes = {App.class}, webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public interface AppTest {
}
<file_sep>/src/main/java/com/gallery/service/delayedtask/AbstractDelayedEntityRemover.java
package com.gallery.service.delayedtask;
import com.gallery.model.entity.AbstractEntity;
import org.springframework.data.repository.CrudRepository;
import java.util.Queue;
public abstract class AbstractDelayedEntityRemover<T extends AbstractEntity> implements DelayedEntityRemover<T> {
protected CrudRepository<T, Long> repository;
protected boolean running = false;
public AbstractDelayedEntityRemover(CrudRepository<T, Long> repository) {
this.repository = repository;
}
public void run() {
new Thread( () -> {
Queue<TaskItem<T>> items = getItems();
while ( ! items.isEmpty()) {
try {
Thread.sleep(TaskItem.SLEEP_TIME_MILLIS);
while ( ! items.isEmpty() && (items.peek().getLifeTimeMillis() <= System.currentTimeMillis()) ) {
TaskItem<T> item = items.peek();
removeEntity(item.getValue());
}
}
catch (InterruptedException e) {
e.printStackTrace();
}
}
running = false;
}).start();
}
}
<file_sep>/src/test/java/com/gallery/Persister.java
package com.gallery;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.album.Image;
import com.gallery.model.entity.user.Role;
import com.gallery.model.entity.user.User;
import com.gallery.repository.RoleRepository;
import com.gallery.service.AlbumService;
import com.gallery.service.UserService;
import org.junit.Assert;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import javax.management.relation.RoleNotFoundException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Random;
public class Persister implements AppTest {
@Autowired
private RoleRepository roleRepository;
@Autowired
private UserService userService;
@Autowired
private AlbumService albumService;
public static List<User> USERS = new ArrayList<>();
static {
USERS.addAll(
List.of(
// users to be persisted into test database
new User("Raina", "raina_mercer", "<EMAIL>"),
new User("Solomon", "solomon_rosales", "<EMAIL>"),
new User("Elaine", "elaine_sexton", "<EMAIL>"),
new User("Dominick", "dominick_garza", "<EMAIL>")
)
);
}
@Test
public void persistAlbums() {
User user1 = userService.findByUsernameWithAlbums("Raina"); // has 16 albums | subscribed on Solomon and Dominick
User user2 = userService.findByUsernameWithAlbums("Solomon"); // has 18 albums
User user4 = userService.findByUsernameWithAlbums("Dominick"); // has 22 albums | subscribed on Solomon
if( ! user1.getAlbums().isEmpty()) {
return;
}
Random random = new Random();
int rand = 0;
for (int i = 1; i <= 56; i++) {
Album album = new Album("album #" + i);
rand = random.nextInt(22);
if (i <= 16) {
for (int j = 0; j < rand; j++) {
album.getImages().add(new Image("image" + i));
}
user1.addAlbum(album);
}
else if (i >= 39) {
for (int j = 0; j < rand; j++) {
album.getImages().add(new Image("image" + i));
}
user2.addAlbum(album);
}
else {
for (int j = 0; j < rand; j++) {
album.getImages().add(new Image("image" + i));
}
user4.addAlbum(album);
}
}
userService.save(user1);
userService.save(user2);
userService.save(user4);
}
@Test
public void persistUsers() {
Collection<User> users = (Collection<User>) userService.findAll();
if(users.size() == 0){
Exception exception = null;
try {
for (User u : USERS) {
userService.saveNew(u);
}
}
catch (RoleNotFoundException e){
System.err.println("Consider insert USER_ROLE entity first.");
exception = e;
exception.printStackTrace();;
}
finally {
Assert.assertNull(exception);
}
}
}
public void persistRoles(){
roleRepository.save(new Role(Role.ROLE_USER_VALUE));
roleRepository.save(new Role(Role.ROLE_ADMIN_VALUE));
}
/*
user_id > albumd_id > images_count
|1|1|9
|1|2|8
|1|3|7
|1|4|18
|1|5|17
|1|6|11
|1|7|3
|1|8|3
|1|9|13
|1|10|16
|1|11|5
|1|12|10
|1|13|9
|1|14|19
|1|15|5
|1|16|20
|2|17|12
|2|18|6
|2|19|8
|2|20|21
|2|21|16
|2|22|15
|2|23|14
|2|24|1
|2|25|8
|2|26|6
|2|27|2
|2|28|20
|2|29|2
|2|30|3
|2|31|20
|2|32|21
|2|33|16
|2|34|15
|4|35|12
|4|37|7
|4|38|21
|4|39|12
|4|41|20
|4|42|4
|4|44|9
|4|45|3
|4|46|11
|4|47|2
|4|48|6
|4|49|13
|4|50|21
|4|51|11
|4|52|8
|4|53|16
|4|54|12
|4|55|13
|4|56|11
*/
}
<file_sep>/src/main/java/com/gallery/model/entity/album/Album_.java
package com.gallery.model.entity.album;
import com.gallery.model.entity.AbstractEntity_;
import com.gallery.model.entity.user.User;
import javax.annotation.Generated;
import javax.persistence.metamodel.ListAttribute;
import javax.persistence.metamodel.SingularAttribute;
import javax.persistence.metamodel.StaticMetamodel;
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(Album.class)
public class Album_ extends AbstractEntity_ {
public static volatile SingularAttribute<Album, String> cover;
public static volatile ListAttribute<Album, Image> images;
public static volatile SingularAttribute<Album, Boolean> deleted;
public static volatile SingularAttribute<Album, String> description;
public static volatile SingularAttribute<Album, String> title;
public static volatile SingularAttribute<Album, User> user;
public static final String COVER = "cover";
public static final String IMAGES = "images";
public static final String DELETED = "deleted";
public static final String DESCRIPTION = "description";
public static final String TITLE = "title";
public static final String USER = "user";
}
<file_sep>/src/main/java/com/gallery/config/JmsConfiguration.java
package com.gallery.config;
import org.apache.activemq.ActiveMQConnectionFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jms.core.JmsTemplate;
import javax.jms.ConnectionFactory;
@Configuration
public class JmsConfiguration {
@Value("${spring.activemq.broker-url}")
private String borkerUrl;
@Value("${spring.activemq.user}")
private String username;
@Value("${spring.activemq.password}")
private String password;
@Bean
public ConnectionFactory connectionFactory() {
return new ActiveMQConnectionFactory(username, password, borkerUrl);
}
@Bean
public JmsTemplate jmsTemplate() {
JmsTemplate template = new JmsTemplate();
template.setConnectionFactory(connectionFactory());
return template;
}
/*@Bean
public MessageConverter messageConverter(){
return new Jackson2JsonMessageConverter();
}*/
/* @Bean
public JmsListenerContainerFactory<?> myFactory(ConnectionFactory connectionFactory,
DefaultJmsListenerContainerFactoryConfigurer configurer) {
DefaultJmsListenerContainerFactory factory = new DefaultJmsListenerContainerFactory();
// This provides all boot's default to this factory, including the message converter
configurer.configure(factory, connectionFactory);
// You could still override some of Boot's default if necessary.
return factory;
}
@Bean // Serialize message content to json using TextMessage
public MessageConverter jacksonJmsMessageConverter() {
MappingJackson2MessageConverter converter = new MappingJackson2MessageConverter();
converter.setTargetType(MessageType.TEXT);
converter.setTypeIdPropertyName("_type");
return converter;
}*/
}
<file_sep>/src/main/java/com/gallery/jwt/JwtEntryPoint.java
package com.gallery.jwt;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.web.AuthenticationEntryPoint;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
//@Component
public class JwtEntryPoint implements AuthenticationEntryPoint {
@Override
public void commence(HttpServletRequest request, HttpServletResponse response, AuthenticationException authException) throws IOException {
response.sendError(HttpServletResponse.SC_UNAUTHORIZED, "Jwt token not provided.");
}
}
<file_sep>/src/main/java/com/gallery/service/entity/Fetchable.java
package com.gallery.service.entity;
import javax.persistence.criteria.JoinType;
import javax.persistence.criteria.Root;
public interface Fetchable {
default <T> void fetch(Root<T> root, String[] fetchOptions) {
for (String option : fetchOptions) {
try {
root.fetch(option, JoinType.LEFT);
} catch (IllegalArgumentException ignored) {
System.err.println("Illegal argument option: " + option);
}
}
}
}
<file_sep>/src/main/java/com/gallery/util/image/ImageSaver.java
package com.gallery.util.image;
import lombok.AllArgsConstructor;
import lombok.Getter;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.multipart.MultipartFile;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
@AllArgsConstructor
@Getter
public class ImageSaver {
public static String ROOT_CATALOG = "/media";
public static final String SMALL_IMAGE_PREFIX = "sm";
public static final int SMALL_IMAGE_MIN_WIDTH = 200;
public static final int SMALL_IMAGE_MAX_WIDTH = 280;
public static final int SMALL_IMAGE_MIN_HEIGHT = 200;
public static final int SMALL_IMAGE_MAX_HEIGHT = 360;
public String saveSourceImage(MultipartFile multipartFile, String destImagePath) throws IOException {
if (this.save(multipartFile, destImagePath)) {
return destImagePath;
}
return null;
}
public String saveSmallImage(MultipartFile multipartFile, String smallImageDestPath, String extensionNoDot) throws IOException {
return saveSmallImage(ImageIO.read(multipartFile.getInputStream()), smallImageDestPath, extensionNoDot);
}
public String saveSmallImage(File sourceImageFile, String smallImageDestPath, String extensionNoDot) throws IOException {
return saveSmallImage(ImageIO.read(sourceImageFile), smallImageDestPath, extensionNoDot);
}
private boolean save(MultipartFile multipartFile, String path) throws IOException {
int res = FileCopyUtils.copy(multipartFile.getInputStream(), Files.newOutputStream(Path.of(path)));
return res > 0;
}
public String saveSmallImage(BufferedImage originalImage, String destinationPath, String extensionNoDot) throws IOException{
float sizeCoefficient = this.getSizeCoefficient(originalImage.getWidth(), originalImage.getHeight());
int width = (int) (originalImage.getWidth() * sizeCoefficient);
int height = (int) (originalImage.getHeight() * sizeCoefficient);
int type = originalImage.getType() == 0 ? BufferedImage.TYPE_INT_ARGB : originalImage.getType();
BufferedImage miniImage = new BufferedImage(width, height, type);
Graphics2D graphics2D = miniImage.createGraphics();
graphics2D.drawImage(originalImage, 0, 0, width, height, null);
graphics2D.dispose();
if (ImageIO.write(miniImage, extensionNoDot, new File(destinationPath))) {
return destinationPath;
}
return null;
}
private float getSizeCoefficient(int width, int height) {
if (width <= SMALL_IMAGE_MIN_WIDTH && height <= SMALL_IMAGE_MIN_HEIGHT) {
return 1.0f;
}
else {
float coefficient = 0.98f;
float decrementStep = .02f;
float res = width * coefficient;
while (res > SMALL_IMAGE_MAX_WIDTH) {
res = width * coefficient;
coefficient = coefficient - decrementStep;
}
return coefficient;
}
}
}
<file_sep>/src/main/java/com/gallery/jms/AlbumMessagingService.java
package com.gallery.jms;
//import org.springframework.amqp.rabbit.core.RabbitTemplate;
//import org.springframework.amqp.support.converter.MessageConverter;
//import org.springframework.stereotype.Service;
//@Service
public class AlbumMessagingService
// implements MessageingService<Album>
{
/*@Value("${amqp.routing-key.album}")
private String routingKey;
private RabbitTemplate rabbitTemplate;
private MessageConverter messageConverter;
@Autowired
public AlbumMessagingService(RabbitTemplate rabbitTemplate, MessageConverter messageConverter) {
this.rabbitTemplate = rabbitTemplate;
this.messageConverter = messageConverter;
}
@Override
public void send(Album album) {
// MessageProperties mp = new MessageProperties();
// Message message = messageConverter.toMessage(album, mp);
// rabbitTemplate.send(message);
rabbitTemplate.convertAndSend(routingKey, album);
}*/
}
<file_sep>/src/main/java/com/gallery/web/ImageController.java
package com.gallery.web;
import com.gallery.model.dto.PaginationData;
import com.gallery.model.dto.album.AlbumDto;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.album.Album_;
import com.gallery.model.entity.album.Image;
import com.gallery.model.entity.user.User;
import com.gallery.service.AlbumService;
import com.gallery.service.ImageService;
import com.gallery.service.entity.SingleEntityServiceImpl;
import com.gallery.util.FunctionUtil;
import com.gallery.util.ResponseModel;
import com.gallery.util.factory.MultipartFileImageFactoryImpl;
import com.gallery.util.image.MultipartFileImage;
import io.swagger.annotations.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.http.HttpStatus;
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import springfox.documentation.annotations.ApiIgnore;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Optional;
import java.util.stream.Stream;
@RestController
@RequestMapping("/image")
public class ImageController {
private static Logger logger = LoggerFactory.getLogger(ImageController.class);
@Value("${pagination.page-items-size}")
private int pageItemsSize;
private AlbumService albumService;
private ImageService imageService;
private MultipartFileImageFactoryImpl multipartFileImageFactory;
private SingleEntityServiceImpl entityService;
@Autowired
public ImageController(AlbumService albumService, ImageService imageService, MultipartFileImageFactoryImpl multipartFileImageFactory, SingleEntityServiceImpl entityService) {
this.albumService = albumService;
this.imageService = imageService;
this.entityService = entityService;
this.multipartFileImageFactory = multipartFileImageFactory;
}
/**
* Get images by album id.
* */
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "get images by ablum")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "page"),
@ApiImplicitParam(name = "offset"),
@ApiImplicitParam(name = "limit"),
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "get images"),
@ApiResponse(code = 404, message = "album not found")
})
@GetMapping("/{albumId}/all")
public ResponseModel albumImages(@PathVariable Long albumId,
@ApiParam("page") @RequestParam(value = "page", defaultValue = "0", required = false) Integer page,
@ApiParam("offset") @RequestParam(value = "offset", defaultValue = "0", required = false) Integer offset,
@ApiParam("limit") @RequestParam(value = "limit", required = false) Integer limit) {
logger.info("get images by album");
ResponseModel responseModel = new ResponseModel();
offset = FunctionUtil.resolveOffset(offset, page, pageItemsSize);
limit = FunctionUtil.resolveLimit(limit, pageItemsSize);
AlbumDto album = albumService.findOneAndConstructAlbumDto(FunctionUtil.getWhereClauseEntry("album", "id", albumId.toString()));
if (album != null) {
Collection<Image> images = imageService.findAll(album.getId(), offset, limit);
PaginationData paginationData = new PaginationData(offset, page, album.getImagesCount(), images.size(), pageItemsSize);
responseModel.addAttribute(ResponseModel.ATTR_ALBUM, album);
responseModel.addAttribute(ResponseModel.ATTR_IMAGES, images);
responseModel.addAttribute(ResponseModel.ATTR_PAGINATION, paginationData);
}
else {
responseModel.setStatus(HttpStatus.NOT_FOUND);
}
return responseModel;
}
@ResponseStatus(HttpStatus.CREATED)
@ApiOperation(value = "upload images to album")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "images array"),
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "images uploaded successfully"),
@ApiResponse(code = 500, message = "internal server error (exception)")
})
//upload images
@PostMapping("/{albumId}/upload")
public ResponseModel albumImagesAdd(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiIgnore @PathVariable Long albumId,
@ApiParam("images array (multiple)") @RequestParam("images") MultipartFile[] images) {
logger.info("UPLOAD IMAGES");
ResponseModel responseModel = new ResponseModel(HttpStatus.CREATED);
Album album = entityService.findOneByAttrWithOptions(Album.class, Album_.ID, String.valueOf(albumId), Album_.USER, Album_.IMAGES);
if (album == null || !(album.isHost(principal))) {
return new ResponseModel(HttpStatus.NOT_FOUND);
}
List<Image> imagesList = new ArrayList<>();
try {
for (MultipartFile image : images) {
String endPath = FunctionUtil.buildImagePath(album.getUser().getUsername(), album.getId());
MultipartFileImage multipartFileImage = multipartFileImageFactory.create(endPath);
String savedImagePath = multipartFileImage.saveImages(image);
Image imageEntity = new Image(savedImagePath);
logger.info(imageEntity.toString());
imagesList.add(imageEntity);
}
}
catch (IOException e) {
responseModel.setStatus(HttpStatus.INTERNAL_SERVER_ERROR);
e.printStackTrace();
}
int length = album.getImages().size();
album.getImages().addAll(imagesList);
albumService.save(album);
return responseModel;
}
@ResponseStatus(HttpStatus.OK)
@ApiOperation(value = "delete image")
@ApiResponses(value = {
@ApiResponse(code = 200, message = "get images"),
@ApiResponse(code = 500, message = "internal server error (exception)")
})
// @DeleteMapping("/{id:\\d+}/delete")
// public void deleteImage(@PathVariable("id") Long imageId) {
@DeleteMapping("/{albumId}/delete/{imageId}")
public ResponseModel deleteImage(@ApiIgnore @AuthenticationPrincipal User principal,
@ApiIgnore @PathVariable Long albumId,
@ApiIgnore @PathVariable Long imageId) {
logger.info("DELETE IMAGE");
ResponseModel responseModel = new ResponseModel();
Album album = entityService.findOneByAttrWithOptions(Album.class, Album_.ID, String.valueOf(albumId), Album_.USER, Album_.IMAGES);
if (album == null || !(album.isHost(principal))) {
return new ResponseModel(HttpStatus.NOT_FOUND);
}
Stream<Image> imagesStream = (album.getImages().size() > 100)
? album.getImages().parallelStream()
: album.getImages().stream();
//find image
Optional<Image> optionalImageToDel = imagesStream.filter((img) -> img.getId().equals(imageId)).findFirst();
optionalImageToDel.ifPresentOrElse(
(image) -> {
//remove image from list and save album
album.getImages().remove(image);
albumService.save(album);
//delete image file
multipartFileImageFactory.create(image.getSrc()).deleteImages();
responseModel.addAttribute(ResponseModel.ATTR_IMAGES, album.getImages());
},
//no image found, send 500
() -> responseModel.setStatus(HttpStatus.NOT_FOUND)
);
return responseModel;
}
@ApiOperation(value = "increment image's \"view_count\" attribute")
@ApiImplicitParams(value = {
@ApiImplicitParam(name = "page"),
@ApiImplicitParam(name = "offset"),
@ApiImplicitParam(name = "limit"),
})
@ApiResponses(value = {
@ApiResponse(code = 200, message = "view_count incremented")
})
@PostMapping("/{id}/increment-vc")
public void count(@PathVariable Long id) {
Optional<Image> image = imageService.findById(id);
if (image.isPresent()) {
Integer viewCount = image.get().getViewCount();
image.get().setViewCount(++viewCount);
imageService.save(image.get());
}
}
}
<file_sep>/src/main/java/com/gallery/model/dto/PaginationData.java
package com.gallery.model.dto;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
//@ApiModel(value = "Pagination DTO", description = "DTO to hold data about page, offset and limit GET parameters")
@Setter
@Getter
@ToString
public class PaginationData {
private int offset;
private int page;
//all items total found
private long itemsTotal;
//items total for one page
private int pageItemsTotal;
@Setter(AccessLevel.NONE)
private int pageTotal;
public PaginationData(int offset, int page, long itemsTotal, int pageItemsTotal, int itemsSizePerPage) {
this.offset = offset;
//to prev page if no items found AND page is not first
if(pageItemsTotal == 0 && page > 0){
page--;
}
this.page = page;
this.itemsTotal = itemsTotal;
this.pageItemsTotal = pageItemsTotal;
int pageTotalVal = 1;
if( itemsTotal > itemsSizePerPage){
try {
pageTotalVal = (int) Math.floor((itemsTotal / (double) itemsSizePerPage)) + 1;
if(itemsTotal % itemsSizePerPage == 0){
pageTotalVal--;
}
}
catch (ArithmeticException e){
e.printStackTrace();
}
}
this.pageTotal = pageTotalVal;
}
}<file_sep>/src/main/java/com/gallery/model/entity/user/User_.java
package com.gallery.model.entity.user;
import com.gallery.model.entity.AbstractEntity_;
import com.gallery.model.entity.album.Album;
import javax.annotation.Generated;
import javax.persistence.metamodel.ListAttribute;
import javax.persistence.metamodel.SetAttribute;
import javax.persistence.metamodel.SingularAttribute;
import javax.persistence.metamodel.StaticMetamodel;
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(User.class)
public class User_ extends AbstractEntity_ {
public static volatile SingularAttribute<User, String> emailToken;
public static volatile ListAttribute<User, Album> albums;
public static volatile SingularAttribute<User, String> avaPath;
public static volatile SetAttribute<User, User> subscribers;
public static volatile SingularAttribute<User, String> authToken;
public static volatile SetAttribute<User, Role> roles;
public static volatile SingularAttribute<User, Boolean> enabled;
public static volatile SingularAttribute<User, String> password;
public static volatile SingularAttribute<User, String> passwordToken;
public static volatile SingularAttribute<User, Boolean> accountExpired;
public static volatile SingularAttribute<User, Boolean> locked;
public static volatile SingularAttribute<User, Boolean> credentialsExpired;
public static volatile SingularAttribute<User, String> email;
public static volatile SingularAttribute<User, String> username;
public static final String EMAIL_TOKEN = "emailToken";
public static final String ALBUMS = "albums";
public static final String AVA_PATH = "avaPath";
public static final String SUBSCRIBERS = "subscribers";
public static final String AUTH_TOKEN = "authToken";
public static final String ROLES = "roles";
public static final String ENABLED = "enabled";
public static final String PASSWORD = "<PASSWORD>";
public static final String PASSWORD_TOKEN = "<PASSWORD>";
public static final String ACCOUNT_EXPIRED = "accountExpired";
public static final String LOCKED = "locked";
public static final String CREDENTIALS_EXPIRED = "credentialsExpired";
public static final String EMAIL = "email";
public static final String USERNAME = "username";
}<file_sep>/src/main/java/com/gallery/service/delayedtask/DelayedEntityRemover.java
package com.gallery.service.delayedtask;
import com.gallery.model.entity.AbstractEntity;
import java.util.Queue;
/**
* Remove entity with delay. (see {@link TaskItem})
* @param <T> type of entity
*/
public interface DelayedEntityRemover<T extends AbstractEntity> {
Queue<TaskItem<T>> getItems();
void addEntity(T entity);
//remove from list
boolean removeEntity(T entity);
//delete
//void deleteEntity(T item);
}
<file_sep>/src/test/java/com/gallery/service/ImageServiceTest.java
package com.gallery.service;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.album.Image;
import com.gallery.service.common.ServiceTest;
import org.junit.Assert;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
import java.util.Optional;
public class ImageServiceTest implements ServiceTest {
@Autowired
private ImageService imageService;
@Autowired
private AlbumService albumService;
@Test
public void save(){
long albumId = 24;
Optional<Album> optionalAlbum = albumService.findByIdWithImages(albumId);
Assert.assertTrue(optionalAlbum.isPresent());
int countBefore = optionalAlbum.get().getImages().size();
Image image = new Image("image#111");
optionalAlbum.get().getImages().add(image);
albumService.save(optionalAlbum.get());
optionalAlbum = albumService.findByIdWithImages(albumId);
long countAfter = optionalAlbum.get().getImages().size();
Assert.assertEquals(countBefore + 1, countAfter);
}
@Test
public void delete() {
long albumId = 24;
Optional<Album> optionalAlbum = albumService.findByIdWithImages(albumId);
Assert.assertTrue(optionalAlbum.isPresent());
int countBefore = optionalAlbum.get().getImages().size();
Assert.assertTrue(countBefore > 0);
Image image = optionalAlbum.get().getImages().get(0);
optionalAlbum.get().getImages().remove(image);
albumService.save(optionalAlbum.get());
optionalAlbum = albumService.findByIdWithImages(albumId);
long countAfter = optionalAlbum.get().getImages().size();
Assert.assertEquals(countBefore - 1, countAfter);
}
}
<file_sep>/src/test/java/com/gallery/web/client/AlbumControllerTest.java
package com.gallery.web.client;
import com.gallery.FunctionUtilTest;
import com.gallery.model.entity.album.Album;
import com.gallery.model.entity.user.User;
import com.gallery.service.AlbumService;
import com.gallery.service.UserService;
import com.gallery.util.ResponseModel;
import com.gallery.web.client.common.ControllerClientTest;
import org.junit.Assert;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.test.web.client.ExpectedCount;
import org.springframework.test.web.client.MockRestServiceServer;
import org.springframework.web.client.RestTemplate;
import org.springframework.web.util.UriComponents;
import org.springframework.web.util.UriComponentsBuilder;
import java.net.URI;
import java.util.List;
public class AlbumControllerTest extends ControllerClientTest {
@Autowired
private AlbumService albumService;
@Autowired
private UserService userService;
@Test
public void findAll() throws Exception {
List<Album> albumsFound = (List<Album>) albumService.findAll();
int page = 4;
int foundSize = albumsFound.size();
ResponseModel responseModelExpected = new ResponseModel("albums", albumsFound);
// String expectedJsonContent = asJson(responseModelExpected);
RestTemplate restTemplate = new RestTemplate();
server = MockRestServiceServer.bindTo(restTemplate).build();
UriComponents uriComponents = UriComponentsBuilder.fromUri(URI.create(albumAllUri))
.queryParam("offset", page)
.queryParam("limit", 10000)
.build();
server.expect(ExpectedCount.once(), requestTo(uriComponents.toUriString()))
.andExpect(method(HttpMethod.GET))
.andRespond(withStatusOkAndJsonBody(responseModelExpected));
ResponseModel responseModelResponse = restTemplate.getForObject(uriComponents.toUriString(), ResponseModel.class);
server.verify();
int albumsSize = FunctionUtilTest.getResponseModelCollectionAttrSize(responseModelResponse, ResponseModel.ATTR_ALBUMS);
Assert.assertEquals(HttpStatus.OK.value(), responseModelResponse.getStatusCode());
Assert.assertEquals(foundSize, albumsSize);
}
@Test
public void findAllByUser() throws Exception {
String username = "Dominick";
User user = userService.findByUsernameWithAlbums(username);
Assert.assertNotNull(user);
int albumsFoundSize = user.getAlbums().size();
RestTemplate restTemplate = new RestTemplate();
server = MockRestServiceServer.bindTo(restTemplate).build();
String url = albumByUserUriWithUsername.replace("{username}", username);
server.expect(expectOnce(), requestTo(url))
.andExpect(method(HttpMethod.GET))
.andRespond(withStatusOkAndJsonBody(new ResponseModel("albums", user.getAlbums())));
ResponseModel responseModel = restTemplate.getForObject(url, ResponseModel.class);
int size = FunctionUtilTest.getResponseModelCollectionAttrSize(responseModel, ResponseModel.ATTR_ALBUMS);
Assert.assertEquals(albumsFoundSize, size);
}
}
<file_sep>/src/main/java/com/gallery/model/entity/AbstractEntity_.java
package com.gallery.model.entity;
import java.util.Date;
import javax.annotation.Generated;
import javax.persistence.metamodel.SingularAttribute;
import javax.persistence.metamodel.StaticMetamodel;
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(AbstractEntity.class)
public class AbstractEntity_ {
public static volatile SingularAttribute<AbstractEntity, Date> createdAt;
public static volatile SingularAttribute<AbstractEntity, Long> id;
public static final String CREATED_AT = "createdAt";
public static final String ID = "id";
}
| c3806ef283971db6ca01a871fd8fa4aed91522c8 | [
"Java",
"SQL",
"INI",
"Gradle"
] | 48 | Java | emrissol18/galleryspringapi | 155478b89df9ad9bfe5af7f43399c636f314bab3 | 3c20d0d59c87ac24785a75aec228309430ac053e | |
refs/heads/master | <file_sep>package assignment5;
public class Overloading {
static void print(){
System.out.println("Overload");
}
static void print(int a){
System.out.println("Overload"+a);
}
static void print(float a){
System.out.println("Overload"+a);
}
public static void main(String args[]){
int a=1;
float b=1F;
Overloading.print();
Overloading.print(b);
Overloading.print(a);
}
}
| d6958a7fe5541440f7110a1579f193324a9be00d | [
"Java"
] | 1 | Java | dayanidhisingla71/assignment-6th | 6579a21e66f3914d9d2e2be6834fbeee231866a8 | cb64b276a2ad1b621e66b95e02cefeac083a796b | |
refs/heads/master | <repo_name>Sapuraizu/MufiBox<file_sep>/index.php
<!--
Crée par Sapuraizu par pur ennuie,
vous pouvez réutiliser ce "projet" à titre personnel (Je vois pas ce que vous pourrez en faire autrement de toute manière.),
-->
<!DOCTYPE html>
<html>
<head>
<title>MufiBox</title>
<meta charset="UTF-8"/>
<!-- CSS -->
<link rel="stylesheet" href="css/bootstrap/bootstrap.min.css"/>
<link rel="stylesheet" href="css/bootstrap/bootstrap-theme.min.css"/>
<link rel="stylesheet" href="css/fontawesome/fontawesome.min.css"/>
<link rel="stylesheet" href="css/animate/animate.css"/>
<link rel="stylesheet" href="css/style.css"/>
</head>
<body>
<div class="container">
<div class="logo-text"><h1>MufiBox <small id="state">Non connecté</small></h1></div>
<div class="form-group">
<textarea id="snd_msg" class="form-control" type="text" placeholder="Saisissez votre message et appuyez sur entrer pour valider"></textarea>
<small class="input-msg">Vous pouvez également <a href="javascript:void(0)" data-type="snd_cmd" data-command="/plant">poser une bombe</a>, défier quelqu'un au /shifumute ou <a href="javascript:void(0)" data-type="snd_cmd" data-command="/help">avoir plus d'informations</a>.</small>
<small class="pull-right" data-type="toggleSmileys"><a href="javascript:void(0)" data-default-text="(Afficher les smileys)" data-toggle-text="(Masquer les smileys)">(Masquer les smileys)</a></small>
</div>
<div class="form-group well" id="smileys"></div>
<div class="shoutbox-historic">
<table id="historic" class="table table-hover table-condensed">
<thead>
<tr>
<!--<th>#</th>-->
<th>Username</th>
<th colspan="2">Message</th>
<th>Date d'envois</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
</div>
</div>
<!-- JavaScript -->
<script type="text/javascript" src="js/jquery-3.1.0.min.js"></script>
<script type="text/javascript" src="js/bootstrap/bootstrap.min.js"></script>
<script type="text/javascript" src="js/javascript.js"></script>
<script type="text/javascript" src="js/moments.js"></script>
<!-- Socket IO -->
<?php
$uid = (isset($_GET['uid']) && !empty($_GET['uid'])) ? $_GET['uid'] : "";
$token = (isset($_GET['token']) && !empty($_GET['token'])) ? $_GET['token'] : "";
echo '<script>var uid = "'. $uid .'", token = "'. $token .'";</script>';
?>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/socket.io/1.4.8/socket.io.min.js"></script>
<script>
connectToMufiShout(uid, token, "http://shoutbox.mufibot.net:8080/");
function connectToMufiShout(uid, token, url){
var shoutbox = io.connect(url),
isWritingMultipleLines = false,
windowIsActive = true,
unReadMessages = 0;
shoutbox.on('connect', function(){
console.log('%cConnecté au serveur MufiBot.', 'color: #16a085');
})
shoutbox.on('disconnect', function(){
console.log('%cDéconnecté du serveur MufiBot.', 'color: #e74c3c');
})
shoutbox.on('authentication required', function(){
console.log('%cConnexion requise.', 'color: #e67e22');
$('#state').html('<span class="text-orange">Authentification...</span>');
shoutbox.emit('authenticate', {user_id: uid, token: token});
})
shoutbox.on('authentication success', function(data){
console.log('%cConnexion effecutée.', 'color: #16a085');
$('#state').html('<span class="text-green">Connecté.</span>');
shoutbox.emit('get messages', {count: 100});
})
shoutbox.on('authentication failure', function(data){
$('#state').html('<span class="text-red">Vous n\'êtes pas connecté.</span>').add;
console.log('%cEchec de la connexion.', 'color: #e74c3c');
})
shoutbox.on('not authenticated', function(data){
console.log('%cVous n\'êtes pas connecté.', 'color: #e74c3c');
})
shoutbox.on('messages list', function(data){
console.log('%cHistorique des messages récupéré.', 'color: #16a085');
//console.log(data);
$.each(data, function(index, value){
showMessage(value);
})
})
shoutbox.on('new message', function(data){
//console.log(data);
showMessage(data);
})
shoutbox.on('delete message', function(data){
$('tr[data-mid="'+ data.id +'"] td[data-id="content"]').prepend('<span class="text-red">(Supprimé)</span> ');
})
shoutbox.on('popup', function(data){
alert(data.message);
})
function showMessage(data){
var result = "",
prefix = (data.deleted) ? "<span class=\"text-red\">(Supprimé)</span> " : "" || (data.edited) ? "<span class=\"text-red\">(Édité)</span> " : "",
rowColor = "";
if(!windowIsActive)
document.title = "("+ ++unReadMessages +") - Mufibox";
if(unReadMessages == 1)
rowColor = "bg-gray";
result += '<tr class="animated fadeIn '+ rowColor +'" data-mid="'+ data.id +'">';
//result += '<td>'+ data.id +'</td>';
result += '<td><span data-type="talk-to" data-uid="'+ data.user_id +'">@</span>'+ (data.username_link || '<a target="_blank" href="http://forum.mufibot.net/user-8385.html">[BOT] Stéphanie</a>') +'</td>';
result += '<td data-id="content">'+ prefix + data.message +'</td>';
if(data.user_id == uid)
result += '<td class="text-right"><i data-type="msg_delete" class="action-icon fa fa-trash"></i> <i data-type="msg_edit" class="action-icon fa fa-pencil"></i></td>';
else
result += '<td></td>';
result += '<td class="text-right">'+ moment.unix(data.timestamp).format('HH:mm:ss') +'</td>';
result += '</tr>';
$('#historic tbody').prepend(result);
}
/*
KeyCode:
Shift: 16
Enter: 13
*/
$(document).on('keydown', '#snd_msg', function(e){
if(e.keyCode == 16) isWritingMultipleLines = true;
})
$(document).on('keyup', '#snd_msg', function(e){
if(!isWritingMultipleLines && e.keyCode == 13){
var _this = $(this),
message = _this.val().trim();
_this.val('');
shoutbox.emit('new message', {message: message});
} else if(e.keyCode == 16) {
isWritingMultipleLines = false;
}
})
$(document).on('click', '[data-type="snd_cmd"]', function(){
var command = $(this).attr('data-command');
shoutbox.emit('new message', {message: command});
})
$(document).on('click', '[data-type="talk-to"]', function(){
var _this = $(this),
uid = _this.attr('data-uid'),
username = _this.next().text().trim(),
userprofile = _this.next().attr('href'),
talkTo = '[url='+ userprofile +']@'+username+'[/url] ';
$('#snd_msg').val(talkTo).focus();
})
$(document).on('click', '[data-type="msg_delete"]', function(){
var _this = $(this),
mid = parseInt(_this.closest('tr').attr('data-mid'));
shoutbox.emit('delete message', {id: mid});
})
$(window).on('focus', function(){
windowIsActive = true;
unReadMessages = 0;
document.title = "Mufibox";
})
$(window).on('blur', function(){
windowIsActive = false;
})
}
</script>
</body>
</html><file_sep>/README.md
<p align="center"><img src="http://image.prntscr.com/image/ffb89563426c4dbebe869c12985e5378.png" alt="Sapuraizu"/></p>
# MufiBox
MufiBox est un outil permettant d'accéder à la shoutbox [MufiBot](http://forum.mufibot.net) sans avoir à ce rendre sur le forum, de plus, elle occupe une grande partie de l'écran et est facilement utilisable.
Quelques features de base sont actuellement disponibles, d'autres feront sûrement leur apparition avec le temps, à part une ou deux features, toutes les autres sont également disponibles sur le forum officiel.
Version d'essaie disponible : [Cliquez ici](http://sapuraizu.esy.es/mufibox/)
#Features actuelles
* Connexion à un compte (uid & token)
* Récupération de l'historique des messages
* Ne supprime pas les anciens messages
* Affichage des messages supprimés
* Envois de messages + commandes
* Envois de messages sur plusieurs lignes
* Affichage de l'heure où le message a été envoyé
* Suppression de ses propres messages
* Ajout des smileys
#Capture d'écran

#Comment l'utiliser ?
Pour commencer, il vous faudra télécharger ce projet puis le déposer en local dans le dossier www/ de [wamp](http://www.wampserver.com/) (Si c'est ce que vous utilisez).
Une fois ça fais, vous devriez pouvoir y acceder depuis [http://localhost/MufiBox/](http://localhost/MufiBox/), mais vous aurez sûrement un message rouge "Vous n'êtes pas connecté." à la place de "Connecté." sur le screen ci-dessus, cependant, vous pourrez toujours voir les nouveaux shouts ! (Mais pas l'historique, il est disponible uniquement une fois connecté.)
Pour remedier à ça, rendez vous sur le [forum MufiBot](http://forum.mufibot.net/), connectez-vous, et utilisez une de ces 2 méthodes.
##Méthode 1:
Faîtes CTRL + U puis cherchez "socketshoutbox(".

Voilà, vous avez votre UID (ici 8748) et votre TOKEN (ici <PASSWORD>) qui vous serviront à vous connecter via MufiBox !
Rendez-vous maintenant sur la page MufiBox, et ajoutez ?uid=VOTRE_UID&token=VOTRE_TOKEN dans le lien ! [http://localhost/MufiBox/?uid=VOTRE_UID&token=VOTRE_TOKEN](http://localhost/MufiBox/?uid=VOTRE_UID&token=VOTRE_TOKEN).
##Méthode 2:
Ouvrez la console à l'aide de F12, saisissez le code ci-dessous dedans.
```javascript
//Récupération des informations
var scriptContent = $('script[src="http://shoutbox.mufibot.net:8080/socket.io/socket.io.js"]').next().next().next().html(),
step1 = scriptContent.substring(scriptContent.indexOf("socketshoutbox("), scriptContent.indexOf(":8080\")")),
step2 = step1.replace(/\"/g, '').split('(')[1].split(','),
user_uid = step2[0].trim(),
user_token = step2[1].trim(),
getLink = "?uid="+ user_uid +"&token="+ user_token;
console.clear();
console.log("Votre UID : " + user_uid);
console.log("Votre TOKEN : " + user_token);
console.log("Votre LIEN : " + getLink);
```
(Si vous avez un problème du genre ".next is not a function", raffraîchissez votre page et recommencez.)

Il ne vous reste qu'à récupérer le résultat ?uid=UID&token=TOKEN puis le mettre au bout de votre lien localhost (cf. Méthode 1)
/!\ Attention, le Token diffère à chaque nouvelle connexion sur le forum MufiBot, vous devrez recommencer la manip jusqu'à votre prochaine connexion. /!\
<file_sep>/js/javascript.js
$(document).ready(function(){
$.getJSON("js/smileys.json", function(data){
$.each(data, function(index, value){
var _this = $(value),
img = '<img src="'+ _this[0].src +'" alt="'+ _this[0].alt +'" width="20px" height="20px" data-toggle="tooltip" title="'+ _this[0].alt +'"/>';
$('#smileys').prepend(img);
})
$('[data-toggle="tooltip"]').tooltip();
})
})
$(document).on('click', '#smileys img', function(){
$('#snd_msg').val($('#snd_msg').val() + ' ' + this.alt + ' ').focus();
})
$('[data-type="toggleSmileys"] a').on('click', function(){
var _this = $(this);
_this.text((_this.text() == _this.attr('data-default-text')) ? _this.attr('data-toggle-text') : _this.attr('data-default-text'));
$('#smileys').fadeToggle();
}) | 7e6244b43b56e4c04c4f506ee0ac421b1e3b9f15 | [
"Markdown",
"JavaScript",
"PHP"
] | 3 | PHP | Sapuraizu/MufiBox | 5673616215e2e474f2a11db4b40706d9cac99754 | 00ae983ade9a21cb5c12bd6b9d818a001282554f | |
refs/heads/master | <file_sep># Crossword-Solver
<p align="center">
<img src="https://github.com/pncnmnp/Crossword-Solver/blob/master/screenshots/newspaper.jpg">
</p>
This solver guesses the probable solutions based on the clues provided and uses a SMT solver to predict the result
## How does it work ?
[I have written a blogpost explaining the working of this crossword-solver.](https://pncnmnp.github.io/blogs/crossword-1.html)
## Structure
The project has 4 main files:
* `words.py`: Uses Moby's thesaurus, gensim's glove-wiki-gigaword-100 and nltk's Wordnet to guess the solutions
* `words_offline.py`: Uses all-clues.bz2 to guess the solutions
* `solve.py`: Solves the crossword based on the solution stored in `clues.json` using a SMT solver (Z3)
* `schema.py`: This is where a user needs to enter the layout of the crossword
## How to run
To run `words_offline.py`, I highly recommend using [pypy](https://pypy.org/).
There is a considerable difference in performance between PyPy and native python's implementation (`./corpus/all-clues.bz2` is a large file when uncompressed).
I have observed certain libraries like `nltk` work flawlessly with PyPy whereas `z3` has some issues.
Native python (default) works reasonably well with `words.py` and `solve.py`.
**Important**: Decompress the `./corpus/all-clues.bz2` file before running `words_offline.py`
## Working
**Here is the format entered in `schema.py`**
<pre>
CROSSWORD_GRID = {
"__ of bad news": {"start":(0, 1), "direction":"D", "length": 6},
"Posture problem": {"start":(0, 3), "direction":"D", "length": 5},
"Loads": {"start":(0, 4), "direction":"D", "length": 6},
"Laundry appliance": {"start":(0, 5), "direction":"D", "length": 5},
"Lectured": {"start":(1, 0), "direction":"D", "length": 5},
"One who weeps": {"start":(1, 2), "direction":"D", "length": 5},
"Grassy clump": {"start":(0, 3), "direction":"A", "length": 3},
"Pie chart portion": {"start":(1, 0), "direction":"A", "length": 6},
"\"Scary Movie,\" e.g.": {"start":(2, 0), "direction":"A", "length": 6},
"Maryland's state bird": {"start":(3, 0), "direction":"A", "length": 6},
"Something worth saving": {"start":(4, 0), "direction":"A", "length": 6},
"\"To __ is human\"": {"start":(5, 0), "direction":"A", "length": 3}
}
</pre>
**Output (using `words_offline.py`)**

### I am getting error running `solve.py`
If the error being faced is: `z3.z3types.Z3Exception: model is not available`, it is because a solution with the clues in `clues.json` does not exist!
This is usually seen when trying fetching solutions for clues from `words.py`. I recommend running with `words_offline.py` in such cases.
## Attribution
* For corpus attribution, see [`./corpus/README`](https://github.com/pncnmnp/Crossword-Solver/blob/master/corpus/README.txt)
* [Wikipedia's MediaWiki API](https://www.mediawiki.org/wiki/API:Main_page): [Donate to Wikipedia](https://wikimediafoundation.org/support/)
* https://nlp.stanford.edu/projects/glove/
* The crossword image is by <a href="https://pixabay.com/users/stevepb-282134/?utm_source=link-attribution&utm_medium=referral&utm_campaign=image&utm_content=412452"><NAME></a> from <a href="https://pixabay.com/?utm_source=link-attribution&utm_medium=referral&utm_campaign=image&utm_content=412452">Pixabay</a>
<file_sep># Pattern is (initial_X, initial_Y), direction(D or A), length
# THE WORDS INSERTED SHOULD HAVE THEIR STARTING LETTER CAPITALIZED (For clue names)
# "": {"start":(), "direction":"", "length": },
CROSSWORD_GRID = {
"__ of bad news": {"start":(0, 1), "direction":"D", "length": 6},
"Posture problem": {"start":(0, 3), "direction":"D", "length": 5},
"Loads": {"start":(0, 4), "direction":"D", "length": 6},
"Laundry appliance": {"start":(0, 5), "direction":"D", "length": 5},
"Lectured": {"start":(1, 0), "direction":"D", "length": 5},
"One who weeps": {"start":(1, 2), "direction":"D", "length": 5},
"Grassy clump": {"start":(0, 3), "direction":"A", "length": 3},
"Pie chart portion": {"start":(1, 0), "direction":"A", "length": 6},
"\"Scary Movie,\" e.g.": {"start":(2, 0), "direction":"A", "length": 6},
"Maryland's state bird": {"start":(3, 0), "direction":"A", "length": 6},
"Something worth saving": {"start":(4, 0), "direction":"A", "length": 6},
"\"To __ is human\"": {"start":(5, 0), "direction":"A", "length": 3}
}
<file_sep>MOBY_PATH = "./corpus/moby_words.txt"
ALL_WORD_VECTOR_PATH = "./corpus/all_word_vectors.pickle"
CLUES_PATH = "./clues.json"
ALL_CLUES = "./corpus/all-clues"<file_sep>from nltk.corpus import wordnet as wn
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
from ast import literal_eval
import gensim.downloader as api
from file_path import *
from schema import CROSSWORD_GRID
import pickle
import string
import requests
import inflect # Library used to check whether a sentence is singular or plural
import json
"""
TODO: >> Plural detection and conversion [done]
>> Variable cutoff value
"""
class Words():
def __init__(self):
self.word_vectors = self.retrieve_all_word_vectors()
if self.word_vectors == None:
self.store_all_word_vectors()
self.word_vectors = self.retrieve_all_word_vectors()
def fetch_all_word_vectors(self):
return api.load("glove-wiki-gigaword-100")
def store_all_word_vectors(self):
all_word_vectors = self.fetch_all_word_vectors()
with open(ALL_WORD_VECTOR_PATH, "wb") as fp:
pickle.dump(all_word_vectors, fp)
def retrieve_all_word_vectors(self):
try:
with open(ALL_WORD_VECTOR_PATH, "rb") as fp:
all_word_vectors = pickle.load(fp)
return all_word_vectors
except:
return None
def wikipedia_solution(self, wikipedia_clues, clues):
WIKIPEDIA_API = "https://en.wikipedia.org/w/api.php?action=query&utf8=&format=json&list=search&srlimit=50&srsearch="
stop = stopwords.words('english') + list(string.punctuation)
clue_mapping = dict()
print(">>> STARTING WIKI FETCH.....")
for sentence in wikipedia_clues:
req = requests.get(WIKIPEDIA_API + sentence)
wiki_json = literal_eval(req.text)
# The following code finds all titles from the JSON response,
# The title is stripped of stop_words and punctuations
# The title is split by space
# Duplicates are removed and words are converted to a 1-D list
solutions = list(set([word for word in [[word for word in word_tokenize(info["title"].lower()) if word not in stop] for info in wiki_json["query"]["search"]] for word in word]))
for soln in solutions:
if len(soln) != clues[sentence]:
continue
try:
clue_mapping[sentence] += [soln]
except:
clue_mapping[sentence] = [soln]
return clue_mapping
def reduce_search_bound(self, all_words_wordnet, sentence_clues, clues):
"""Reduces the search space by finding most similar words from self.word_vectors
using the method 'most_similar'.
Common words found between self.word_vectors.most_similar() and all_words_wordnet
are returned.
Param: all_words_wordnet : has to be wn.words()
where wn is wordnet from nltk.corpus
"""
words = list(all_words_wordnet)
possible_words = list()
stop = stopwords.words('english') + list(string.punctuation)
topn = 4000
for sentence in sentence_clues:
pos = [word for word in word_tokenize(sentence.lower()) if word not in stop]
try:
search_words = [word[0] for word in self.word_vectors.most_similar(positive=pos, topn=topn) if len(word[0])==clues[sentence]]
except:
pass
possible_words += list(set(words).intersection(set(search_words)))
return sorted(list(set(possible_words)))
def sentence_solution(self, sentence_clues, clues):
all_words_wordnet = self.reduce_search_bound(wn.words(), sentence_clues, clues)
word_vectors = self.word_vectors
stop = stopwords.words('english') + list(string.punctuation)
clues_tokenized = dict()
clue_mapping = dict()
clue_plural = dict()
infl = inflect.engine()
# Tokenize all the clues, removing stopwords and punctuations
for clue in sentence_clues:
tokenized = [word for word in word_tokenize(clue.lower()) if word not in stop]
clues_tokenized[clue] = tokenized
# check if the clue is singular or plural
if infl.singular_noun(clue) == False:
clue_plural[clue] = "singular"
else:
clue_plural[clue] = "plural"
print(">>> STARTING SENTENCE LOCAL FETCH.....")
for word_wordnet in all_words_wordnet:
iter_val = len(wn.synsets(word_wordnet))
for syn_no in range(iter_val):
synset_tokenized = [word for word in word_tokenize(wn.synsets(word_wordnet)[syn_no].definition().lower()) if word not in stop]
for clue in sentence_clues:
# To prevent any modifications on original variable
# Can be caused if the clue is in plural form, and word_wordnet gets converted to plural form
# This happens if length of the plural form of word matches with clue's required length
# Example: "<NAME>" (PLURAL) (length - 7) -> filly -> fillies
temp_word_wordnet = word_wordnet
if len(temp_word_wordnet) != clues[clue]:
# Check if the plural form of the word is of same length as required by the crossword
if clue_plural[clue] == "plural" and len(infl.plural(temp_word_wordnet)) == clues[clue]:
temp_word_wordnet = infl.plural(temp_word_wordnet)
else:
continue
try:
similarity = word_vectors.n_similarity(clues_tokenized[clue], synset_tokenized)
except KeyError as e:
try:
# This error is caused by word_vectors.n_similarity()
# The keyerror is printed as "word 'XXX' not in vocabulary"
# We are giving the prediction another chance by removing the KeyError word
try_removing_a_word = e.args[0].replace("word ", "").replace("not in vocabulary", "").replace("'", "").strip()
synset_tokenized.remove(try_removing_a_word)
similarity = word_vectors.n_similarity(clues_tokenized[clue], synset_tokenized)
except:
continue
except:
continue
if similarity > 0.65:
try:
clue_mapping[clue] += [(temp_word_wordnet, similarity)]
except:
clue_mapping[clue] = [(temp_word_wordnet, similarity)]
clue_mapping[clue] = sorted(set(clue_mapping[clue]), key=lambda x: x[1], reverse=True)
return clue_mapping
def one_word_solution(self, one_word_clues, clues):
fp = open(MOBY_PATH)
moby_lines = fp.readlines()
clue_mapping = dict()
# Copy the contents of the 'clues' dict in a temp variable
# To prevent any modification changes appearing globally
temp_clues = dict()
for word in list(clues.keys()):
temp_clues[word] = clues[word]
print(">>> STARTING ONE-WORD LOCAL FETCH (V.1).....")
# splits and re-indexes clues such as 'extra-large' as 'large'
# this is done to maintain consistency with 'one_word_clues'
for word in list(temp_clues.keys()):
temp_clues[word.replace("-", " ").split()[-1].lower()] = temp_clues.pop(word)
for line in moby_lines:
guess_words = line.replace("\n", "").split(",")
# removing spaces between the same word: at first -> atfirst
guess_words = list(map(lambda word: word.replace(" ", ""), guess_words))
common = list(set(guess_words).intersection(set(one_word_clues)))
if len(common) == 0:
continue
else:
for word in common:
guess_words = [guess for guess in guess_words if len(guess)==temp_clues[word]]
try:
clue_mapping[word] += guess_words
except:
clue_mapping[word] = guess_words
clue_mapping[word] = sorted(list(set(clue_mapping[word])))
return clue_mapping
def one_word_solution_alternate(self, one_word_clues, clues):
clue_mapping = dict()
stop = stopwords.words('english') + list(string.punctuation)
# For common words, optimal is around 100, for proper nouns optimal is 1000-2000
topn = 100
# Copy the contents of the 'clues' dict in a temp variable
# To prevent any modification changes appearing globally
temp_clues = dict()
for word in list(clues.keys()):
temp_clues[word] = clues[word]
print(">>> STARTING ONE-WORD LOCAL FETCH (V.2).....")
# splits and re-indexes clues such as 'extra-large' as 'large'
# this is done to maintain consistency with 'one_word_clues'
for word in list(temp_clues.keys()):
temp_clues[word.replace("-", " ").split()[-1].lower()] = temp_clues.pop(word)
for clue in one_word_clues:
pos = [word for word in word_tokenize(clue.lower()) if word not in stop]
try:
clue_mapping[clue] = [word[0] for word in self.word_vectors.most_similar(positive=pos, topn=topn) if len(word[0]) == temp_clues[clue]]
except:
pass
return clue_mapping
def store_words(self, one_word_solved, one_word_clues, sentence_solved, wikipedia_solved):
clues = dict()
for key in list(one_word_solved.keys()):
actual_key = [val[1] for val in one_word_clues if val[0]==key][0]
try:
clues[actual_key] += one_word_solved[key]
except:
clues[actual_key] = list()
clues[actual_key] += one_word_solved[key]
for key in list(sentence_solved.keys()):
try:
clues[key] += [word[0] for word in sentence_solved[key]]
except:
clues[key] = list()
clues[key] += [word[0] for word in sentence_solved[key]]
for key in list(wikipedia_solved.keys()):
try:
clues[key] += wikipedia_solved[key]
except:
clues[key] = list()
clues[key] += wikipedia_solved[key]
print(">>> STORED CLUES.....")
with open(CLUES_PATH, "w") as fp:
json.dump(str(clues), fp)
def fetch_words(self, clues):
""" 1. The tense of the clues have to be guessed
2. We segregate the "one word" clues with "sentence" clues
3. Possible solutions of the "one word" clues (in the tenses required)
are searched in Moby's Thesaurus
4. "Sentence" clues are searched in Wordnet (NLTK's version)
5. If a "sentence" clue has less confidence level than expected,
Wikipedia's MediaWiki API is used.
6. All the possible solutions are searched with the criteria:
Word length is same as required ||in the correct tense||
Param: clues - dict
{clue_1: word_len_1, clue_2: word_len_2}
"""
all_clues = list(clues.keys())
one_word_clues = [(clue.lower(),clue) for clue in all_clues if len(clue.split(" ")) == 1]
# converting words such as extra-large into large
one_word_clues += [(clue.split("-")[-1].lower(),clue) for clue in all_clues
if ("-" in clue) and (len(clue.split("-"))) == 2]
one_word_solved = self.one_word_solution_alternate([clue[0] for clue in one_word_clues], clues)
sentence_clues = list(set(all_clues).difference(set(one_word_clues)))
sentence_solved = self.sentence_solution(sentence_clues, clues)
wikipedia_clues = list()
# Print top N results
N = 40
for clue in sentence_solved:
sentence_solved[clue] = sentence_solved[clue][:N]
wikipedia_solved = self.wikipedia_solution(sentence_clues, clues)
self.store_words(one_word_solved, one_word_clues, sentence_solved, wikipedia_solved)
if __name__ == '__main__':
grid = CROSSWORD_GRID
clues = dict()
for clue in CROSSWORD_GRID:
clues[clue] = CROSSWORD_GRID[clue]["length"]
Words().fetch_words(clues)
<file_sep>from nltk.corpus import stopwords
from collections import Counter
from schema import CROSSWORD_GRID
from file_path import *
import string
import math
import re
import json
class Words_Offline():
def __init__(self):
pass
def all_solution(self, clues):
stop = stopwords.words('english') + [""]
with open(ALL_CLUES, encoding="latin-1") as fp:
dict_guesses = fp.readlines()
clue_mapping = dict()
all_lengths = []
for clue in clues:
clue_mapping[clue] = list()
if clues[clue] not in all_lengths:
all_lengths.append(clues[clue])
clue_statements = list(clues.keys())
clue_vecs = dict()
for clue in clue_statements:
clue_vecs[clue] = [word for word in [word.strip(string.punctuation) for word in clue.lower().split()] if word not in stop]
print(">>> STARTING ALL CLUES FETCH (V.1).....")
for guess in dict_guesses:
if len(guess.split()[0]) not in all_lengths:
continue
guess_statement = " ".join(guess.split()[4:])
guess_vec = Counter([word for word in [word.strip(string.punctuation) for word in guess_statement.lower().split()] if word not in stop])
for clue in clue_statements:
if len(guess.split()[0]) == clues[clue]:
clue_vec = Counter(clue_vecs[clue])
# https://stackoverflow.com/questions/15173225/calculate-cosine-similarity-given-2-sentence-strings
intersection = set(guess_vec.keys()) & set(clue_vec.keys())
numerator = sum([guess_vec[x] * clue_vec[x] for x in intersection])
sum1 = sum([guess_vec[x]**2 for x in guess_vec.keys()])
sum2 = sum([clue_vec[x]**2 for x in clue_vec.keys()])
denominator = math.sqrt(sum1) * math.sqrt(sum2)
if not denominator:
sim = 0.0
else:
sim = float(numerator) / denominator
if sim > 0.65:
clue_mapping[clue] += [guess.split()[0].lower()]
for clue in clues:
clue_mapping[clue] = list(set(clue_mapping[clue]))
return clue_mapping
def fetch_words(self, clues):
all_solved = self.all_solution(clues)
print(">>> STORED CLUES.....")
with open(CLUES_PATH, "w") as fp:
json.dump(str(all_solved), fp)
if __name__ == '__main__':
grid = CROSSWORD_GRID
clues = dict()
for clue in CROSSWORD_GRID:
clues[clue] = CROSSWORD_GRID[clue]["length"]
Words_Offline().fetch_words(clues)
<file_sep>from ast import literal_eval
from tabulate import tabulate
from file_path import *
import json
import z3
from schema import CROSSWORD_GRID
"""
NOTE: No length-verification is required in Solve(),
as all clues retrieved from CLUES_PATH are of required length
"""
# Pattern is (initial_X, initial_Y), direction(D or A), length
# "": {"start":(), "direction":"", "length": },
GRID = CROSSWORD_GRID
class Solve():
def __init__(self):
pass
def fetch_clues(self):
"""Fetches the clues present in "clues.json"
"""
clues = dict()
with open(CLUES_PATH) as fp:
clues = literal_eval(json.load(fp))
return clues
def get_matrix(self):
clues = self.fetch_clues()
start_positions = dict()
# finding the square matrix size
max_val = 0
for clue in GRID:
start_positions[GRID[clue]["start"]] = clue
if GRID[clue]["start"][0] > max_val:
max_val = GRID[clue]["start"][0]
elif GRID[clue]["start"][1] > max_val:
max_val = GRID[clue]["start"][1]
# As the GRID starts from 0, we have to add 1 to get actual max_val
max_val += 1
matrix = [[None for index in range(max_val)] for index in range(max_val)]
# The following code encodes the matrix declared with z3.Int(VALUE)
# If at a position value is: (z3.Int(VALUE)_1, z3.Int(VALUE)_2), it signifies intersection is taking place there
# NOTE: _1 -> signifies the COLUMN index AND _2 -> signifies the ROW index
for x_index in range(max_val):
for y_index in range(max_val):
if (x_index, y_index) in list(start_positions.keys()):
pos_info = GRID[start_positions[(x_index, y_index)]]
for i in range(pos_info["length"]):
if pos_info["direction"] == "A":
if isinstance(matrix[x_index][y_index + i], z3.z3.ArithRef):
matrix[x_index][y_index + i] = (z3.Int("alpha_" + str(x_index) + "_" + str(y_index + i) + "_1"), z3.Int("alpha_" + str(x_index) + "_" + str(y_index + i) + "_2"))
else:
matrix[x_index][y_index + i] = z3.Int("alpha_" + str(x_index) + "_" + str(y_index + i))
elif pos_info["direction"] == "D":
if isinstance(matrix[x_index + i][y_index], z3.z3.ArithRef):
matrix[x_index + i][y_index] = (z3.Int("alpha_" + str(x_index + i) + "_" + str(y_index) + "_1"), z3.Int("alpha_" + str(x_index + i) + "_" + str(y_index) + "_2"))
else:
matrix[x_index + i][y_index] = z3.Int("alpha_" + str(x_index + i) + "_" + str(y_index))
return matrix, start_positions, max_val
def convert_clues_code(self):
"""converts all the clues (in string format) to a list of their ascii characters value
example: "mumbai" -> [109, 117, 109, 98, 97, 105]
"""
clues = self.fetch_clues()
clues_ord = dict()
for clue in clues:
for guess in clues[clue]:
try:
clues_ord[clue].append([ord(ch) for ch in guess.lower()])
except:
clues_ord[clue] = [[ord(ch) for ch in guess.lower()]]
return clues_ord
def make_guess_constraint(self):
clues = self.convert_clues_code()
matrix, start_positions, max_val = self.get_matrix()
clue_constraint = list()
for x_index in range(max_val):
for y_index in range(max_val):
if (x_index, y_index) in list(start_positions.keys()):
pos_info = GRID[start_positions[(x_index, y_index)]]
clue = start_positions[(x_index, y_index)]
guesses_ord = clues[clue]
all_guesses_constraint = list()
for guess_ord in guesses_ord:
guess_constraint = list()
x_i = x_index
y_i = y_index
for ch in guess_ord:
if isinstance(matrix[x_i][y_i], tuple):
# NOTE: _1 -> signifies the COLUMN index AND _2 -> signifies the ROW index
if pos_info["direction"] == "D":
matrix_val = matrix[x_i][y_i][0]
elif pos_info["direction"] == "A":
matrix_val = matrix[x_i][y_i][1]
else:
matrix_val = matrix[x_i][y_i]
guess_constraint.append(z3.And(matrix_val == ch))
if pos_info["direction"] == "D":
x_i += 1
elif pos_info["direction"] == "A":
y_i += 1
all_guesses_constraint.append(z3.And(guess_constraint))
clue_constraint.append(z3.Or(all_guesses_constraint))
clues_constraint = z3.And(clue_constraint)
return clues_constraint
def common_position_constraint(self):
clues = self.convert_clues_code()
matrix, start_positions, max_val = self.get_matrix()
equality_constraint = list()
for x_index in range(max_val):
for y_index in range(max_val):
if isinstance(matrix[x_index][y_index], tuple):
first = matrix[x_index][y_index][0]
second = matrix[x_index][y_index][1]
equality_constraint.append(z3.And(first == second))
common_position_constraint = z3.And(equality_constraint)
return common_position_constraint
def apply_constraints(self):
solver = z3.Solver()
solver.add(self.make_guess_constraint())
solver.add(self.common_position_constraint())
solver.check()
return solver.model()
def solution(self):
solved = self.apply_constraints()
solved_keys = [index.name() for index in solved]
matrix, start_positions, max_val = self.get_matrix()
for x_index in range(max_val):
for y_index in range(max_val):
if isinstance(matrix[x_index][y_index], tuple):
matrix_val = matrix[x_index][y_index][0]
elif matrix[x_index][y_index] != None:
matrix_val = matrix[x_index][y_index]
else:
continue
no = solved[matrix_val]
ch = chr(no.as_long())
matrix[x_index][y_index] = ch
return matrix
if __name__ == '__main__':
solve = Solve()
print(tabulate(solve.solution()))
<file_sep>gensim==3.8.1
tabulate==0.8.6
nltk==3.4.5
z3_solver==4.8.7.0
inflect==3.0.2
requests>=2.20.0
z3==0.2.0
| 37f2fa53180be91aaf878383e67b9877f94e5d70 | [
"Markdown",
"Python",
"Text"
] | 7 | Markdown | pncnmnp/Crossword-Solver | f36e93df2a38b7d4275ba569237e01a630a1eec9 | 012292eac00cfa75ae91ba0f238f9582320436fe | |
refs/heads/master | <repo_name>legaldev/USACO_Practice<file_sep>/section2/frac1.cpp
/*
ID: luglian1
PROG: frac1
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cstring>
using namespace std;
#define MAXSIZE 160
#define PV(v) cout << #v << " = " << v << endl;
struct Fraction
{
int numerator;
int denominator;
float value;
Fraction(int inumerator=0, int idenominator=1):
numerator(inumerator), denominator(idenominator)
{
value = (float)numerator / (float)idenominator;
}
};
int gcd(int a, int b)
{
if(a < b)
{
int temp = a;
a = b;
b = temp;
}
while(b != 0)
{
int reminder = a % b;
a = b;
b = reminder;
}
return a;
}
int main(){
ifstream file_in("frac1.in");
ofstream file_out("frac1.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n = 0;
file_in >> n;
int pre_size = 0;
int n_size = 0;
int total_size = 0;
Fraction pre[MAXSIZE * MAXSIZE];
Fraction cur[MAXSIZE * MAXSIZE];
Fraction ncur[MAXSIZE];
// initialize
total_size = 2;
cur[0] = Fraction(0, 1);
cur[1] = Fraction(1, 1);
PV(sizeof(Fraction));
for(int i=2; i<=n; i++)
{
// create n
n_size = 1;
ncur[0] = Fraction(1, i);
for(int p=2; p<i; p++)
{
if(gcd(i, p) > 1)
continue;
ncur[n_size] = Fraction(p, i);
++n_size;
}
// cout << "n_size = " << n_size << endl;
// for(int j=0; j<n_size; j++)
// {
// cout << ncur[j].numerator << "/" << ncur[j].denominator << endl;
// }
// copy cur to pre
memcpy(pre, cur, sizeof(Fraction) * total_size);
// for (int j = 0; j < total_size; ++j)
// {
// cout << pre[j].numerator << "/" << pre[j].denominator << endl;
// }
pre_size = total_size;
total_size += n_size;
// merge n to next
int pre_pos=0;
int n_pos=0;
int cur_pos=0;
while(n_pos < n_size)
{
if(pre[pre_pos].value < ncur[n_pos].value)
{
cur[cur_pos] = pre[pre_pos];
++pre_pos;
}
else
{
cur[cur_pos] = ncur[n_pos];
++n_pos;
}
++cur_pos;
}
cur[cur_pos] = pre[pre_pos];
++cur_pos;
if(total_size != cur_pos)
{
cout << "error: total size is not correct." << endl;
cout << "total_size = " << total_size << endl;
cout << "cur_pos = " << cur_pos << endl;
}
}
for(int i=0; i<total_size; i++)
{
// cout << cur[i].numerator << "/" << cur[i].denominator << endl;
file_out << cur[i].numerator << "/" << cur[i].denominator << endl;
}
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/butter.cpp
/*
ID: luglian1
PROG: butter
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
#include <map>
#include <cassert>
#include <set>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << (v)[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "butter"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define INF 0x7fffffff
#define MAXP 801
using namespace std;
int N, P, C;
unsigned int dist[801][801] = {0};
int cow[500] = {0};
int mind = INF;
map<int, vector<int> > edges;
bool load();
void save(ostream& out);
bool save();
void solve();
void solve2();
void solve3();
int main(){
load();
//solve();
//solve2();
solve3();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N >> P >> C;
for(int i=0; i<N; ++i)
{
file_in >> cow[i];
}
for(int i=0; i<=P; ++i)
{
for(int j=0; j<=P; ++j)
dist[i][j] = INF;
dist[i][i] = 0;
}
int a, b, d;
for(int i=0; i<C; ++i)
{
file_in >> a >> b >> d;
dist[a][b] = d;
dist[b][a] = d;
if(edges.count(a) == 0)
edges.insert(pair<int, vector<int> >(a, vector<int>()));
if(edges.count(b) == 0)
edges.insert(pair<int, vector<int> >(b, vector<int>()));
edges[a].push_back(b);
edges[b].push_back(a);
}
return true;
}
void save(ostream& out)
{
out << mind << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
// for(int i=1; i<=P; ++i)
// {
// PVL(dist[i], P+1)
// }
// cout << endl;
for(int k=1; k<=P; ++k)
for(int i=1; i<=P; ++i)
for(int j=1; j<=P; ++j)
{
if(dist[i][k] + dist[k][j] < dist[i][j])
dist[i][j] = dist[i][k] + dist[k][j];
}
// for(int i=1; i<=P; ++i)
// {
// PVL(dist[i], P+1)
// }
mind = INF;
int minid = 0;
for(int i=1; i<=P; ++i)
{
int newd = 0;
bool fail = false;
for(int j=0; j<N; ++j)
{
if(dist[i][cow[j]] == INF)
{
fail = true;
continue;
}
newd += dist[i][cow[j]];
}
if(fail)
continue;
if(newd < mind)
{
mind = newd;
}
}
PV(mind)
}
void dijkstra(unsigned int* q, int src)
{
int s[MAXP] = {0};
s[src] = 1;
for(int i=1; i<= P; ++i)
{
int minid = -1;
int minq = INF;
for(int j=1; j <= P; ++j)
{
if(s[j] == 0 && q[j] < minq)
{
minid = j;
minq = q[j];
}
}
//PV(minid)
if(minid < 0)
break;
s[minid] = 1;
for(int j=1; j<=P; ++j)
{
if(s[j] == 0 && dist[minid][j] < INF)
{
int newq = minq + dist[minid][j];
if(newq < q[j])
q[j] = newq;
}
}
}
// PVL(q, P+1)
return;
}
void solve2()
{
// for(int i=1; i<=P; ++i)
// {
// PVL(dist[i], P+1)
// }
// cout << endl;
//for(int i=0; i<N; ++i)
dijkstra(dist[cow[0]], cow[0]);
PVL(dist[cow[0]], P+1)
// for(int i=1; i<=P; ++i)
// {
// PVL(dist[i], P+1)
// }
mind = INF;
int minid = 0;
for(int i=1; i<=P; ++i)
{
int newd = 0;
bool fail = false;
for(int j=0; j<N; ++j)
{
if(dist[cow[j]][i] == INF)
{
fail = true;
continue;
}
newd += dist[cow[j]][i];
}
if(fail)
continue;
if(newd < mind)
{
mind = newd;
}
}
PV(mind)
}
int cur_src;
struct compare
{
bool operator()(const int& n1, const int& n2)
{
return dist[cur_src][n1] > dist[cur_src][n2];
};
};
int vn[800];
int mark[801];
int vton[801];
compare c;
set<int> ss;
inline void my_swap(int i, int j)
{
swap(vn[i], vn[j]);
swap(vton[vn[i]], vton[vn[j]]);
}
void update_heap(int i, int n)
{
if(n <= 0)
return;
int p=i;
if(i > 0)
{
do
{
p = (i - 1) / 2;
if(p >=0 && c(vn[p], vn[i]))
{
my_swap(p, i);
i = p;
}
else
{
break;
}
}while(i > 0);
}
else
{
p = i;
do
{
int ch1 = 2 * p + 1;
int ch2 = 2 * p + 2;
int min = p;
if(ch2 < n)
{
if(c(vn[p], vn[ch1]) && c(vn[ch2], vn[ch1]))
min = ch1;
else if(c(vn[p], vn[ch2]))
min = ch2;
}
else if(ch1 < n)
{
if(c(vn[p], vn[ch1]))
min = ch1;
}
else
{
break;
}
if(min == p || min >= n)
{
break;
}
assert(dist[cur_src][vn[min]] < dist[cur_src][vn[p]]);
my_swap(p, min);
p = min;
}while(p < n);
}
}
void my_pop_heap(int n)
{
my_swap(0, n-1);
update_heap(0, n-1);
}
void dijkstra_fast(int src)
{
cur_src = src;
for(int i=0; i<P; ++i)
{
vn[i] = i+1;
}
make_heap(vn, vn+P, c);
for(int i=0; i<P; ++i)
{
vton[vn[i]] = i;
}
for(int i=P; i>0; --i)
{
int n = vn[0];
my_pop_heap(i);
// pop_heap(vn, vn+i, c);
if(edges.count(n) > 0)
{
const vector<int>& e = edges[n];
for(vector<int>::const_iterator it=e.begin(); it!=e.end(); ++it)
{
if(vton[*it] <= i-1 && dist[src][n] + dist[n][*it] < dist[src][*it])
{
dist[src][*it] = dist[src][n] + dist[n][*it];
// make_heap(vn, vn+i-1, compare());
assert(vn[vton[*it]] == *it);
update_heap(vton[*it], i-1);
assert(vn[vton[*it]] == *it);
}
}
}
}
// PV(ss.size())
}
void solve3()
{
// int myints[] = {10,20,30,5,15};
// make_heap (myints, myints+5, greater<int>());
// for (unsigned i=0; i<5; i++) cout << " " << myints[i];
// cout << endl;
// for(int i=5; i>0; --i)
// {
// cout << endl;
// pop_heap(myints, myints+i, greater<int>());
// for (unsigned j=0; j<i-1; j++) cout << " " << myints[j];
// cout << endl;
// }
// for(int i=1; i<=P; ++i)
// {
// PVL(dist[i], P+1)
// }
// cout << endl;
for(int i=0; i<N; ++i)
dijkstra_fast(cow[i]);
// PVL((dist[cow[14]] + 1), P)
// for(int i=0; i<N; ++i)
// {
// PVL((dist[cow[i]] + 1), P)
// }
mind = INF;
int minid = 0;
for(int i=1; i<=P; ++i)
{
int newd = 0;
bool fail = false;
for(int j=0; j<N; ++j)
{
if(dist[cow[j]][i] == INF)
{
fail = true;
continue;
}
newd += dist[cow[j]][i];
}
if(fail)
continue;
if(newd < mind)
{
mind = newd;
}
}
PV(mind)
}
// We approach this problem directly, by calculating the distance from each cow to each pasture. Once this is done, we will simply have to sum the distances for each cow to get the total cost of putting the sugar cube at a given pasture. The key to a fast distance calculation is that our graph is quite sparse. Thus, we use Dijkstra with a heap to calculate the distance from a given cow to all pastures. This requires on the order of N*C*log(P), or about 7,000,000, operations.
// #include <stdio.h>
// #include <string.h>
// const int BIG = 1000000000;
// const int MAXV = 800;
// const int MAXC = 500;
// const int MAXE = 1450;
// int cows;
// int v,e;
// int cow_pos[MAXC];
// int degree[MAXV];
// int con[MAXV][MAXV];
// int cost[MAXV][MAXV];
// int dist[MAXC][MAXV];
// int heapsize;
// int heap_id[MAXV];
// int heap_val[MAXV];
// int heap_lookup[MAXV];
// bool validheap(void){
// for(int i = 0; i < heapsize; ++i){
// if(!(0 <= heap_id[i] && heap_id[i] < v)){
// return(false);
// }
// if(heap_lookup[heap_id[i]] != i){
// return(false);
// }
// }
// return(true);
// }
// void heap_swap(int i, int j){
// int s;
// s = heap_val[i];
// heap_val[i] = heap_val[j];
// heap_val[j] = s;
// heap_lookup[heap_id[i]] = j;
// heap_lookup[heap_id[j]] = i;
// s = heap_id[i];
// heap_id[i] = heap_id[j];
// heap_id[j] = s;
// }
// void heap_up(int i){
// if(i > 0 && heap_val[(i-1) / 2] > heap_val[i]){
// heap_swap(i, (i-1)/2);
// heap_up((i-1)/2);
// }
// }
// void heap_down(int i){
// int a = 2*i+1;
// int b = 2*i+2;
// if(b < heapsize){
// if(heap_val[b] < heap_val[a] && heap_val[b] < heap_val[i]){
// heap_swap(i, b);
// heap_down(b);
// return;
// }
// }
// if(a < heapsize && heap_val[a] < heap_val[i]){
// heap_swap(i, a);
// heap_down(a);
// }
// }
// int main(){
// FILE *filein = fopen("butter.in", "r");
// fscanf(filein, "%d %d %d", &cows, &v, &e);
// for(int i = 0; i < cows; ++i){
// fscanf(filein, "%d", &cow_pos[i]);
// --cow_pos[i];
// }
// for(int i = 0; i < v; ++i){
// degree[i] = 0;
// }
// for(int i = 0; i < e; ++i){
// int a,b,c;
// fscanf(filein, "%d %d %d", &a, &b, &c);
// --a;
// --b;
// con[a][degree[a]] = b;
// cost[a][degree[a]] = c;
// ++degree[a];
// con[b][degree[b]] = a;
// cost[b][degree[b]] = c;
// ++degree[b];
// }
// fclose(filein);
// for(int i = 0; i < cows; ++i){
// heapsize = v;
// for(int j = 0; j < v; ++j){
// heap_id[j] = j;
// heap_val[j] = BIG;
// heap_lookup[j] = j;
// }
// heap_val[cow_pos[i]] = 0;
// heap_up(cow_pos[i]);
// bool fixed[MAXV];
// memset(fixed, false, v);
// for(int j = 0; j < v; ++j){
// int p = heap_id[0];
// dist[i][p] = heap_val[0];
// fixed[p] = true;
// heap_swap(0, heapsize-1);
// --heapsize;
// heap_down(0);
// for(int k = 0; k < degree[p]; ++k){
// int q = con[p][k];
// if(!fixed[q]){
// if(heap_val[heap_lookup[q]] > dist[i][p] + cost[p][k]){
// heap_val[heap_lookup[q]] = dist[i][p] + cost[p][k];
// heap_up(heap_lookup[q]);
// }
// }
// }
// }
// }
// int best = BIG;
// for(int i = 0; i < v; ++i){
// int total = 0;
// for(int j = 0; j < cows; ++j){
// total += dist[j][i];
// }
// best <?= total;
// }
// FILE *fileout = fopen("butter.out", "w");
// fprintf(fileout, "%d\n", best);
// fclose(fileout);
// return(0);
// }
<file_sep>/section3/agrinet.cpp
/*
ID: luglian1
PROG: agrinet
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "agrinet"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAXN 100
#define INF 99999999
using namespace std;
int n;
int edge[MAXN][MAXN] = {0};
int dis[MAXN];
int tree[MAXN] = {0};
int res = INF;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> n;
for(int i=0; i<n; ++i)
for(int j=0; j<n; ++j)
file_in >> edge[i][j];
for(int i=0; i<n; ++i)
{
PVL(edge[i], n)
}
return true;
}
void save(ostream& out)
{
out << res << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
int solve_one(int src)
{
for(int i=0; i<n; ++i)
{
tree[i] = 0;
if(edge[src][i])
dis[i] = edge[src][i];
else
dis[i] = INF;
}
dis[src] = 0;
tree[src] = 1;
for(int i=0; i<n-1; ++i)
{
int mind = INF;
int minid = -1;
for(int j=0; j<n; ++j)
{
if(tree[j] == 0 && dis[j] < mind)
{
mind = dis[j];
minid = j;
}
}
tree[minid] = 1;
for(int j=0; j<n; ++j)
{
if(tree[j] == 0)
dis[j] = min(dis[j], edge[minid][j]);
}
}
PVL(dis, n)
int totald = 0;
for(int i=0; i<n; ++i)
totald += dis[i];
PV(totald)
return totald;
}
void solve()
{
for(int i=0; i<1; ++i)
{
int d = solve_one(i);
res = min(d, res);
}
}
// This problem requires finding the minimum spanning tree of the given graph. We use an algorithm that, at each step, looks to add to the spanning tree the closest node not already in the tree.
// Since the tree sizes are small enough, we don't need any complicated data structures: we just consider every node each time.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXFARM 100
// int nfarm;
// int dist[MAXFARM][MAXFARM];
// int isconn[MAXFARM];
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, j, nfarm, nnode, mindist, minnode, total;
// fin = fopen("agrinet.in", "r");
// fout = fopen("agrinet.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d", &nfarm);
// for(i=0; i<nfarm; i++)
// for(j=0; j<nfarm; j++)
// fscanf(fin, "%d", &dist[i][j]);
// total = 0;
// isconn[0] = 1;
// nnode = 1;
// for(isconn[0]=1, nnode=1; nnode < nfarm; nnode++) {
// mindist = 0;
// for(i=0; i<nfarm; i++)
// for(j=0; j<nfarm; j++) {
// if(dist[i][j] && isconn[i] && !isconn[j]) {
// if(mindist == 0 || dist[i][j] < mindist) {
// mindist = dist[i][j];
// minnode = j;
// }
// }
// }
// assert(mindist != 0);
// isconn[minnode] = 1;
// total += mindist;
// }
// fprintf(fout, "%d\n", total);
// exit(0);
// }
// Here is additional analysis from <NAME>:
// The solution given is O(N3); however, we can obtain O(N2) if we modify it by storing the distance from each node outside of the tree to the tree in an array, instead of recalculating it each time. Thus, instead of checking the distance from every node in the tree to every node outside of the tree each time that we add a node to the tree, we simply check the value in the array for each node outside of the tree.
// #include <fstream.h>
// #include <assert.h>
// const int BIG = 20000000;
// int n;
// int dist[1000][1000];
// int distToTree[1000];
// bool inTree[1000];
// main ()
// {
// ifstream filein ("agrinet.in");
// filein >> n;
// for (int i = 0; i < n; ++i) {
// for (int j = 0; j < n; ++j) {
// filein >> dist[i][j];
// }
// distToTree[i] = BIG;
// inTree[i] = false;
// }
// filein.close ();
// int cost = 0;
// distToTree[0] = 0;
// for (int i = 0; i < n; ++i) {
// int best = -1;
// for (int j = 0; j < n; ++j) {
// if (!inTree[j]) {
// if (best == -1 || distToTree[best] > distToTree[j]) {
// best = j;
// }
// }
// }
// assert (best != -1);
// assert (!inTree[best]);
// assert (distToTree[best] < BIG);
// inTree[best] = true;
// cost += distToTree[best];
// distToTree[best] = 0;
// for (int j = 0; j < n; ++j) {
// if (distToTree[j] > dist[best][j]) {
// distToTree[j] = dist[best][j];
// assert (!inTree[j]);
// }
// }
// }
// ofstream fileout ("agrinet.out");
// fileout << cost << endl;
// fileout.close ();
// exit (0);
// }<file_sep>/section 1/crypt1.cpp
/*
ID: luglian1
PROG: crypt1
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
bool checknum(int num, const vector<int>& nums){
while(num > 0){
int final = num % 10;
int i;
for(i=0; i!=nums.size(); ++i){
if(final == nums[i]){
break;
}
}
if(i == nums.size())
return false;
num /= 10;
}
return true;
}
int main(){
ifstream file_in("crypt1.in");
ofstream file_out("crypt1.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
file_in >> n;
vector<int> nums(n);
for(int i=0; i!=n; ++i){
file_in >> nums[i];
}
sort(nums.begin(), nums.end());
for(int i=0; i!=nums.size(); ++i)
cout << nums[i] << endl;
// complete search
int count=0;
for(int i=0; i!=n; ++i){
for(int j=0; j!=n; ++j){
for(int k=0; k!=n; ++k){
int num1 = nums[i]*100 + nums[j]*10 + nums[k];
for(int s=0; s!=n; ++s){
int pro2 = (num1 * nums[s]);
if(pro2 > 999 || !checknum(pro2, nums))
continue;
for(int t=0; t!=n; ++t){
int pro1 = (num1 * nums[t]);
if(pro1 > 999 || !checknum(pro1, nums))
continue;
int sum = (pro2 * 10 + pro1);
if(sum > 9999 || !checknum(sum, nums))
continue;
++count;
//cout << sum << endl;
}
}
}
}
}
cout << count << endl;
file_out << count << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/milk2.cpp
/*
ID: luglian1
PROG: milk2
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <list>
using namespace std;
typedef pair<int, int> timeInv;
// 0 no overlap, -1 t1 left overlap t2, 1 t1 right overlap t2
bool checkOverlap(const timeInv& t1, const timeInv& t2){
if(t1.second < t2.first || t1.first > t2.second)
return false;
return true;
}
// merge t1 into t2
void mergeTimeInv(timeInv& t1, timeInv& t2){
t2.first = t2.first < t1.first ? t2.first : t1.first;
t2.second = t2.second > t1.second ? t2.second : t1.second;
}
int main(){
ifstream file_in("milk2.in");
ofstream file_out("milk2.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
list<timeInv > milk_interval;
int n;
file_in >> n;
timeInv onetime;
for(int i=0; i!=n; ++i){
file_in >> onetime.first >> onetime.second;
list<timeInv>::iterator it;
bool overlap = false;
for( it=milk_interval.begin(); it != milk_interval.end(); ++it){
if( checkOverlap(onetime, *it) ){
overlap = true;
list<timeInv>::iterator itmp = it;
mergeTimeInv(onetime, *it);
for(int j=-1; j < 2; j+=2){
int newmeger = true;
while(newmeger){
newmeger = false;
itmp = it;
if(j<0)
--itmp;
else
++itmp;
if(itmp != milk_interval.end())
if( checkOverlap(*itmp, *it) ){
mergeTimeInv(*itmp, *it);
milk_interval.erase(itmp);
newmeger = true;
}
}
}
}
else{ // no overlap, insert
if(onetime.second < it->first){
break;
}
}
} // for
if(!overlap ){
milk_interval.insert(it, onetime);
}
}
cout << n << " " << milk_interval.size() << endl;
int milk=0, nomilk=0;
for(list<timeInv>::iterator it=milk_interval.begin(); it != milk_interval.end(); ++it){
cout << it->first << " " << it->second << endl;
if(milk < it->second - it->first)
milk = it->second - it->first;
list<timeInv>::iterator next = it;
++next;
if(next != milk_interval.end())
if(nomilk < next->first - it->second)
nomilk = next->first - it->second;
}
file_out << milk <<" " << nomilk << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section2/ttwo.cpp
/*
ID: luglian1
PROG: ttwo
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "ttwo"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int farm[10][10] = {0};
int fp[2] = {0};
int fd = 0;
int cp[2] = {0};
int cd = 0;
int dirs[4][2] = {{-1, 0}, {0, 1}, {1, 0}, {0, -1}};
int res = 0;
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
for(int i=0; i<10; ++i)
{
string line;
file_in >> line;
for(int j=0; j<10; ++j)
{
char c = line[j];
switch(c)
{
case '*':
farm[i][j] = 1;
break;
case 'F':
fp[0] = i;
fp[1] = j;
break;
case 'C':
cp[0] = i;
cp[1] = j;
break;
default:
break;
}
}
}
return true;
}
void save(ostream& out)
{
out << res << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void tick(int* pos, int* dir)
{
int nx = pos[0] + dirs[*dir][0];
int ny = pos[1] + dirs[*dir][1];
if(nx < 0 || nx > 9 || ny < 0 || ny > 9 || farm[nx][ny])
{
*dir = (*dir + 1) % 4;
}
else
{
pos[0] = nx;
pos[1] = ny;
}
}
void solve()
{
// find 1,000,000 times
for(int t=0; t< 1000000; ++t)
{
tick(fp, &fd);
tick(cp, &cd);
if(fp[0] == cp[0] && fp[1] == cp[1])
{
res = t + 1;
break;
}
}
}
// analysis
// We can simply simulate the movement of <NAME> and the two cows, but we need to find a way to detect loops. There are only 100 places where the cows can be, and 4 directions they can face. The same goes for <NAME>. This multiplies out to 400*400 = 160,000 different (place, direction) configurations for <NAME> and the cows. If we ever happen upon a configuration we have been in before, we are in an infinite loop, and John and the cows will never meet.
// In fact, we don't even need to keep track of which configurations we've seen. If they're going to meet, they're going to meet in fewer than 160,000 steps. Otherwise, they have to repeat some configuration, in which case they'll never meet.
// We take care of the simulation by keeping track of the position and direction of each. Direction is a number from 0 to 3, 0 = north, 1 = east, 2 = south, 3 = west. So turning clockwise is incrementing one. We calculate how to move given the direction by looking up offsets in the deltax and deltay arrays.
// /*
// PROG: ttwo
// ID: rsc001
// */
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// char grid[10][10];
// /* delta x, delta y position for moving north, east, south, west */
// int deltax[] = { 0, 1, 0, -1 };
// int deltay[] = { -1, 0, 1, 0 };
// void
// move(int *x, int *y, int *dir)
// {
// int nx, ny;
// nx = *x+deltax[*dir];
// ny = *y+deltay[*dir];
// if(nx < 0 || nx >= 10 || ny < 0 || ny >= 10 || grid[ny][nx] == '*')
// *dir = (*dir + 1) % 4;
// else {
// *x = nx;
// *y = ny;
// }
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// char buf[100];
// int i, x, y;
// int cowx, cowy, johnx, johny, cowdir, johndir;
// fin = fopen("ttwo.in", "r");
// fout = fopen("ttwo.out", "w");
// assert(fin != NULL && fout != NULL);
// cowx = cowy = johnx = johny = -1;
// for(y=0; y<10; y++) {
// fgets(buf, sizeof buf, fin);
// for(x=0; x<10; x++) {
// grid[y][x] = buf[x];
// if(buf[x] == 'C') {
// cowx = x;
// cowy = y;
// grid[y][x] = '.';
// }
// if(buf[x] == 'F') {
// johnx = x;
// johny = y;
// grid[y][x] = '.';
// }
// }
// }
// assert(cowx >= 0 && cowy >= 0 && johnx >= 0 && johny >= 0);
// cowdir = johndir = 0; /* north */
// for(i=0; i<160000 && (cowx != johnx || cowy != johny); i++) {
// move(&cowx, &cowy, &cowdir);
// move(&johnx, &johny, &johndir);
// }
// if(cowx == johnx && cowy == johny)
// fprintf(fout, "%d\n", i);
// else
// fprintf(fout, "0\n");
// exit(0);
// }<file_sep>/section 1/skidesign.cpp
/*
ID: luglian1
PROG: skidesign
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "skidesign"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
void anothersolve();
int N = 0;
vector<int> hs;
vector<int> curmove;
int sum = 0;
int main(){
load();
solve();
//anothersolve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
int n;
for(int i=0; i<N; i++)
{
file_in >> n;
hs.push_back(n);
curmove.push_back(0);
}
return true;
}
void save(ostream& out)
{
out << sum << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
int findnextlow(int curlow)
{
for(int i=0; i<N; i++)
{
if (hs[i] > curlow)
return hs[i];
}
}
int findnexthigh(int curhigh)
{
for(int i=N-1; i>=0; i--)
{
if (hs[i] < curhigh)
return hs[i];
}
}
int findcountlow(int low)
{
for(int i=0; i<N; i++)
{
if (hs[i] > low)
return i+1;
}
}
int findcounthigh(int high)
{
for(int i=N-1; i>=0; i--)
{
if (hs[i] < high)
return i;
}
}
template <typename T>
T dosum(std::vector<T>& list, int begin, int end)
{
T sum = 0;
for (int i=begin; i<end; i++)
{
sum += list[i];
}
return sum;
}
void updatecurmove(int low, int high)
{
for(int i=0; i<N; i++)
{
if(hs[i] < low)
curmove[i] = low - hs[i];
else if(hs[i] > high)
curmove[i] = hs[i] - high;
else
curmove[i] = 0;
}
}
void solve()
{
sort(hs.begin(), hs.end());
//PVL(hs, N)
int maxdiff = hs[N-1] - hs[0];
if (maxdiff <= 17)
return;
int curdiff = maxdiff;
int low = hs[0], high = hs[N-1];
PVL(curmove, N)
while (high - low > 17)
{
int nextlow = findnextlow(low);
int nexthigh = findnexthigh(high);
PV(nexthigh)
PV(nextlow)
int n = min(nextlow-low, min(high-nexthigh, curdiff-17));
PV(n)
int countlow = findcountlow(low);
int counthigh = findcounthigh(high);
int sumlow = dosum(curmove, 0, countlow);
int sumhigh = dosum(curmove, N-1-counthigh, N);
PV(sumhigh)
PV(sumlow)
int nexthighmove = max((n + sumlow - sumhigh) / 2, 0);
int realhighmove = min(n, min(nexthighmove, high-nexthigh));
int nextlowmove = max((n + sumhigh - sumlow) / 2, 0);
int reallowmove = min(n, min(nextlowmove, nextlow-low));
PV(nexthighmove)
PV(nextlowmove)
if (realhighmove == 0 && reallowmove == 0)
{
realhighmove = 1;
reallowmove = 1;
}
high -= realhighmove;
low += reallowmove;
PV(high)
PV(low)
updatecurmove(low, high);
cout << "==============" << endl;
}
PV(high)
PV(low)
PVL(curmove, N)
int countlow = findcountlow(low);
int counthigh = findcounthigh(high);
for(int i=0; i<N; i++)
{
sum += curmove[i] * curmove[i];
}
}
void anothersolve()
{
int minsum = 0x7fffffff;
int minlow=0, minhigh=100;
for (int low=0; low<=83; low++)
{
int high = low + 17;
//for (int high=low + 17; high <= 100; high++)
{
int onesum = 0;
for(int i=0; i<N; i++)
{
if(hs[i] < low)
onesum += (low - hs[i]) * (low - hs[i]);
else if(hs[i] > high)
onesum += (hs[i] - high) * (hs[i] - high);
}
if (onesum < minsum)
{
minsum = onesum;
minlow = low;
minhigh = high;
}
}
}
PV(minsum)
PV(minlow)
PV(minhigh)
sum = minsum;
}<file_sep>/section3/ratios.cpp
/*
ID: luglian1
PROG: ratios
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "ratios"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define INF 0x7fffffff
using namespace std;
int goal[3] = {0};
int other[3][3] = {0};
int mix[101][3][3] = {0};
int res[5] = {INF, INF, INF, INF, INF};
bool found = false;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> goal[0] >> goal[1] >> goal[2];
for(int i=0; i<3; ++i)
{
for(int j=0; j<3; ++j)
file_in >> other[i][j];
}
return true;
}
void save(ostream& out)
{
if(found)
{
for(int i=0; i<3; ++i)
out << res[i] << " ";
out << res[3] << endl;
}
else{
out << "NONE" << endl;
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
for(int i=0; i<=100; ++i)
{
for(int j=0; j<3; ++j)
{
for(int k=0; k<3; ++k)
{
mix[i][j][k] = other[j][k] * i;
}
}
}
// for(int j=0; j<3; ++j)
// {PVL(mix[4][j], 3)}
int tmp[3];
for(int i=0; i<=100; ++i)
{
for(int j=0; j<=100; ++j)
{
for(int k=0; k<=100; ++k)
{
if(i==0 && j==0 && k==0)
continue;
if(i+j+k > res[4])
continue;
for(int a=0; a<3; ++a)
{
tmp[a] = 0;
tmp[a] += mix[i][0][a] + mix[j][1][a] + mix[k][2][a];
}
int ratio = tmp[0] / goal[0];
if((tmp[0] == goal[0] *ratio) && (tmp[1] == goal[1] * ratio)&& (tmp[2] == goal[2] *ratio))
{
// cout << i <<", " << j <<", "<< k << endl;
// PVL(tmp, 3)
res[0] = i;
res[1] = j;
res[2] = k;
res[3] = tmp[0] / goal[0];
res[4] = i+j+k;
found = true;
}
}
}
}
}
// As there are only 1013 = 1,030,301 ways to do this, try them all and pick the best.
// #include <stdio.h>
// /* the goal ratio */
// int goal[3];
// /* the ratio of the feeds */
// int ratios[3][3];
// /* the best solution found so far */
// int min;
// int mloc[4]; /* amounts of ratio 1, 2, and 3, and the amount of ratio 4 prod */
// /* the amount of each type of component in the feed */
// int sum[3];
// int main(int argc, char **argv)
// {
// FILE *fout, *fin;
// int lv, lv2, lv3; /* loop variables */
// int t, s; /* temporary variables */
// int gsum; /* the sum of the amounts of components in the goal mixture */
// if ((fin = fopen("ratios.in", "r")) == NULL)
// {
// perror ("fopen fin");
// exit(1);
// }
// if ((fout = fopen("ratios.out", "w")) == NULL)
// {
// perror ("fopen fout");
// exit(1);
// }
// /* read in data */
// fscanf (fin, "%d %d %d", &goal[0], &goal[1], &goal[2]);
// for (lv = 0; lv < 3; lv++)
// fscanf (fin, "%d %d %d", ratios[lv]+0, ratios[lv]+1, ratios[lv]+2);
// gsum = goal[0] + goal[1] + goal[2];
// min = 301; /* worst than possible = infinity */
// /* boundary case (this ensures gsum != 0) */
// if (gsum == 0)
// {
// fprintf (fout, "0 0 0 0\n");
// return 0;
// }
// for (lv = 0; lv < 100; lv++)
// for (lv2 = 0; lv2 < 100; lv2++)
// { /* for each amout of the first two types of mixtures */
// sum[0] = lv*ratios[0][0] + lv2*ratios[1][0];
// sum[1] = lv*ratios[0][1] + lv2*ratios[1][1];
// sum[2] = lv*ratios[0][2] + lv2*ratios[1][2];
// if (lv + lv2 > min) break;
// for (lv3 = 0; lv3 < 100; lv3++)
// {
// s = lv + lv2 + lv3;
// if (s >= min) break; /* worse than we've found already */
// /* calculate a guess at the multiples of the goal we've obtained */
// /* use gsum so we don't have to worry about divide by zero */
// t = (sum[0] + sum[1] + sum[2]) / gsum;
// if (t != 0 && sum[0] == t*goal[0] &&
// sum[1] == t*goal[1] && sum[2] == t*goal[2])
// { we've found a better solution!
// /* update min */
// min = s;
// /* store the solution */
// mloc[0] = lv;
// mloc[1] = lv2;
// mloc[2] = lv3;
// mloc[3] = t;
// }
// /* add another 'bucket' of feed #2 */
// sum[0] += ratios[2][0];
// sum[1] += ratios[2][1];
// sum[2] += ratios[2][2];
// }
// }
// if (min == 301) fprintf (fout, "NONE\n"); /* no solution found */
// else fprintf (fout, "%d %d %d %d\n", mloc[0], mloc[1], mloc[2], mloc[3]);
// return 0;
// }
// <NAME> Solution
// When you combine multiples of mixtures, you can look at it as a multiplication of a matrix by a vector. For example, 8*(1:2:3) + 1*(3:7:1) + 5*(2:1:2) = (21:28:35) = 7*(3:4:5) can be seen as
// [ 1 3 2 ] [ 8 ]
// [ 2 7 1 ] * [ 1 ] = 7 [3 4 5]
// [ 3 1 2 ] [ 5 ]
// The matrix and the goal ratio vector (3:4:5 in this case) are given; what we have to find is the multiple vector (8:1:5 in this case) and the proportionality costant (7 here). This is like solving a system of linear equations. We can write it as
// AX = kB.
// Now, if we use Cramer's Rule, and let D = determinant of A, then
// X_1 = k D_1 / D
// X_2 = k D_2 / D
// X_3 = k D_3 / D,
// where D_1 is the determinant of the matrix A with the 1st column is replaced by B, D_2 is the determinant of the matrix A with the 2nd column is replaced by B, D_3 is the determinant of the matrix A with the 3rd column is replaced by B. (see a Linear Algebra textbook on why this works.) ,P> We are looking for integral solutions. If D = 0, no solutions. Otherwise, let k = D, and then X_1 = D_1, etc. If these values (X_1,X_2,X_3, _and_ k) all have a greatest common factor above 1, divide them all by that factor, as we are looking for the smallest possible solutions.
// Now, if some of the numbers is greater than 100, we have not found a feasible solution, so we output `NONE'. Otherwise, the triple (X_1,X_2,X_3) is output, as well as the value k. <file_sep>/mytest/test.cpp
/*
ID: luglian1
PROG: beads
LANG: C++
*/
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
using namespace std;
struct MeshData
{
int nVertices;
int nIndices;
int vertexSize; /// in byte
char* pVertexBuffer;
int* pIndexBuffer;
};
int main(){
string s("010");
int test;
//cout << test << endl;
stringstream ss;
ss << 1 << " ";
//ss >> test;
int * ip = new int[0];
cout << (int)ip <<endl;
*ip = 1;
cout << *ip <<endl;
float a=0.f;
a++;
cout << ++a <<endl;
char ca = -1;
unsigned char uca = ca;
cout << (int)uca << endl;
cout << (unsigned char)1 - (unsigned char)3 << endl;
cout << -1 % 50 << endl;
return 0;
}
<file_sep>/section 1/pprime.cpp
/*
ID: luglian1
PROG: pprime
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include <vector>
#include <cmath>
using namespace std;
class PrimeGenerator{
int cur;
int begin;
int over;
int index;
public:
static vector<int> prime;
PrimeGenerator(int a, int b): begin(a), over(b), cur(3), index(1){
prime.push_back(2);
prime.push_back(3);
int sq = sqrt((double)over) + 1;
cur += 2;
while( cur <= sq ){
if( checkPrime(cur) ){
prime.push_back(cur);
}
cur += 2;
}
cur = begin%2==0 ? begin -1 : begin-2;
}
int next(){
if(prime[prime.size()-1]>=cur && index<=prime.size()-1){
for(; prime[index]<cur && index<=prime.size()-1; ++index);
if(index<=prime.size()-1){
cur = prime[index];
++index;
return cur;
}
}
cur += 2;
while( cur <= over ){
if( checkPrime(cur) ){
//prime.push_back(cur);
if(cur >= begin)
return cur;
}
cur += 2;
}
return end();
}
int end(){
return 0;
}
static bool checkPrime(int n){
int sq = sqrt((double)n)+1;
for(int i=0; i!=prime.size(); ++i){
if(prime[i] > sq)
break;
if( n % prime[i] == 0)
return false;
}
return true;
}
};
vector<int> PrimeGenerator::prime;
class PalinGenerator{
int min;
int max;
int test;
string snum;
stringstream ss;
public:
PalinGenerator(int a, int b): min(a), max(b){}
void findPP(int pos, int len, ostream& file_out){
if(len <= 0){
ss.clear();
ss.str(snum);
//ss << snum;
ss >> test;
//cout << snum << " " << test <<endl;
if(test>=min && test <= max && PrimeGenerator::checkPrime(test))
file_out << test << endl;
return;
}
int i = pos == 0 ? 1 : 0;
for(; i<=9; ++i){
snum[pos] = snum[snum.size()-1-pos] = '0'+i;
findPP(pos+1, len-2, file_out);
}
}
void work(ostream& file_out){
for(int len=1; len<=8; ++len){
snum.resize(len);
findPP(0, len, file_out);
}
}
static bool checkPalin(int i){
string s;
while( i>0 ){
s += i%10;
i /= 10;
}
for(int k=0; k<s.size()/2; ++k){
if(s[k] != s[s.size()-k-1])
return false;
}
return true;
}
};
int a, b;
void read(istream& file_in){
file_in >> a >> b;
}
void palinwork(ostream& file_out){
//cout << a << " " << b << endl;
PrimeGenerator ppg(5, 100000000);
//cout << ppg.prime.size() << endl;
PalinGenerator pg(a, b);
pg.work(file_out);
}
int main(){
ifstream file_in("pprime.in");
ofstream file_out("pprime.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
read(file_in);
palinwork(file_out);
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section2/castle.cpp
/*
ID: luglian1
PROG: castle
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cstring>
using namespace std;
#define WEST 0x01
#define NORTH 0x02
#define EAST 0x04
#define SOUTH 0x08
typedef unsigned char byte;
const int max_size = 50;
struct Castle
{
int num_rooms;
int rooms_size[max_size*max_size];
int largest_size;
Castle(): num_rooms(0), largest_size(0) {}
};
byte wall[max_size][max_size];
int room_component[max_size][max_size];
int m, n;
Castle castle_flood_fill();
int castle_flood_fill(int i, int j, int roomId);
int main()
{
ifstream file_in("castle.in");
ofstream file_out("castle.out");
if(!file_in || !file_out)
{
cerr << "Error: Failed to open file" << endl;
return 0;
}
file_in >> n >> m;
for(int i=0; i<m; i++)
{
for(int j=0; j<n; j++)
{
int temp;
file_in >> temp;
wall[i][j] = (byte)temp;
}
}
Castle info = castle_flood_fill();
file_out << info.num_rooms << endl << info.largest_size << endl;
// for(int i=0; i<m; i++)
// {
// for(int j=0; j<n; j++)
// {
// cout << room_component[i][j] << " ";
// }
// cout<< endl;
// }
// find the wall to remove
int moduleRowId = -1;
int moduleColId = -1;
byte wall_to_remove = 0;
int merge_max_size = -1;
byte wall_to_check[2] = {NORTH, EAST};
byte wall_to_check_neighbor[2] = {SOUTH, WEST};
printf("%x\n", ~wall_to_check[0]);
for(int j=0; j < n; j++)
{
for(int i=m-1; i>=0; i--)
{
// north
if(i > 0 && room_component[i][j] != room_component[i-1][j])
{
int size =
info.rooms_size[room_component[i][j]] +
info.rooms_size[room_component[i-1][j]];
if(size > merge_max_size)
{
merge_max_size = size;
moduleRowId = i;
moduleColId = j;
wall_to_remove = NORTH;
}
}
// east
if(j < n-1 && room_component[i][j] != room_component[i][j+1])
{
int size =
info.rooms_size[room_component[i][j]] +
info.rooms_size[room_component[i][j+1]];
if(size > merge_max_size)
{
merge_max_size = size;
moduleRowId = i;
moduleColId = j;
wall_to_remove = EAST;
}
}
continue;
byte wall_backup = wall[i][j];
for(int wallId=0;
wallId < sizeof(wall_to_check)/sizeof(wall_to_check[0]);
wallId++)
{
if(j == n-1 && wall_to_check[wallId] == EAST)
continue;
if(i ==0 && wall_to_check[wallId] == NORTH)
continue;
if(wall[i][j] & wall_to_check[wallId])
{
byte* pNeighbor =
wall_to_check[wallId] == EAST ?
&wall[i][j+1] : &wall[i-1][j];
byte neighbor_wall = *pNeighbor;
*pNeighbor &= ~wall_to_check_neighbor[wallId];
wall[i][j] = wall[i][j] & (~wall_to_check[wallId]);
Castle remove_info = castle_flood_fill();
cout << i << " " << j << " " << (wall_to_check[wallId] == EAST ? "E" : "N") << " ";
cout << remove_info.num_rooms << " " << remove_info.largest_size << endl;;
if(remove_info.largest_size > merge_max_size)
{
merge_max_size = remove_info.largest_size;
moduleRowId = i;
moduleColId = j;
wall_to_remove = wall_to_check[wallId];
}
wall[i][j] = wall_backup;
*pNeighbor = neighbor_wall;
}
}
}
}
file_out << merge_max_size << endl;
file_out << moduleRowId+1 << " " << moduleColId+1 << " "
<< (wall_to_remove == EAST ? "E" : "N") << endl;
cout << m << " " << n << endl;
cout << info.num_rooms << endl << info.largest_size << endl;
cout << merge_max_size << endl;
cout << moduleRowId << " " << moduleColId << " " << (int)wall_to_remove << endl;
file_in.close();
file_out.close();
return 0;
}
Castle castle_flood_fill()
{
// initial all room component
memset(room_component, -1, max_size * max_size * sizeof(int));
// flood fill find component
Castle info;
for(int i=0; i<m; i++)
{
for(int j=0; j<n; j++)
{
if(room_component[i][j] == -1)
{
int size = castle_flood_fill(i, j, info.num_rooms);
info.rooms_size[info.num_rooms] = size;
++info.num_rooms;
info.largest_size = max(size, info.largest_size);
}
}
}
return info;
}
int castle_flood_fill(int i, int j, int roomId)
{
if(room_component[i][j] != -1)
{
return 0;
}
int size = 1;
room_component[i][j] = roomId;
if((wall[i][j] & WEST) == 0)
size += castle_flood_fill(i, j-1, roomId);
if((wall[i][j] & NORTH) == 0)
size += castle_flood_fill(i-1, j, roomId);
if((wall[i][j] & EAST) == 0)
size += castle_flood_fill(i, j+1, roomId);
if((wall[i][j] & SOUTH) == 0)
size += castle_flood_fill(i+1, j, roomId);
return size;
}<file_sep>/section 1/barn1.cpp
/*
ID: luglian1
PROG: barn1
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <list>
using namespace std;
struct board{
int start;
int end;
board(int s, int e): start(s), end(e) {}
};
bool compareboard(const board& b1, const board& b2){
return b1.start < b2.start;
}
typedef list<board>::iterator BoardIt;
list<board>::iterator findMinGap(list<board>& boardlist){
list<board>::iterator minit;
int mingap = 200;
list<board>::iterator it = boardlist.begin();
for(int i=0; i!=boardlist.size()-1; ++i){
int prend = it->end;
int curstart = (++it)->start;
if(curstart - prend < mingap ){
mingap = curstart - prend;
minit = it;
}
}
return minit;
}
void margeBoard(BoardIt& minit, list<board>& boardlist){
BoardIt tmpit = minit;
--tmpit;
if(tmpit != boardlist.end()){
tmpit->end = minit->end;
boardlist.erase(minit);
}
}
int main(){
ifstream file_in("barn1.in");
ofstream file_out("barn1.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int m, s, c;
file_in >> m >> s >> c;
list<board> boardlist;
for(int i=0; i!=c; ++i){
int t;
file_in >> t;
boardlist.push_back(board(t, t));
}
boardlist.sort(compareboard);
while(boardlist.size() > m){
BoardIt minit = findMinGap(boardlist);
margeBoard(minit, boardlist);
}
cout << boardlist.size() << endl;
int size=0;
for(BoardIt it = boardlist.begin(); it!=boardlist.end(); ++it){
cout << it->start << " " << it->end << endl;
size += it->end-it->start+1;
}
cout << size << endl;
file_out << size << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/fence.cpp
/*
ID: luglian1
PROG: fence
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <map>
#include <set>
#include <vector>
#include <sstream>
#include <algorithm>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "fence"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAXN 500
using namespace std;
struct compare
{
string itos(int i) const
{
string s;
s += char(i % 10 + '0');
i = i / 10;
while(i > 0)
{
s += char(i % 10 + '0');
i = i / 10;
};
return s;
};
bool operator()(const int& i, const int& j) const
{
return i<j;
string a = itos(i);
string b= itos(j);
return lexicographical_compare(a.begin(), a.end(), b.begin(), b.end());
};
};
int F;
set<int, compare> node;
map<int, int, compare> edges[MAXN+1];
vector<int> steps;
int degree[MAXN+1] = {0};
int adjacent[MAXN+1][MAXN+1] = {0};
bool load();
void save(ostream& out);
bool save();
void solve();
void solve2();
int main(){
load();
// solve();
solve2();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> F;
for(int i=0; i<F; ++i)
{
int n1, n2;
file_in >> n1 >> n2;
node.insert(n1);
node.insert(n2);
if(edges[n1].count(n2))
{
++edges[n1][n2];
}
else
{
edges[n1].insert(pair<int, int>(n2, 1));
}
if(edges[n2].count(n1))
{
++edges[n2][n1];
}
else
{
edges[n2].insert(pair<int, int>(n1, 1));
}
degree[n1]++;
degree[n2]++;
adjacent[n1][n2]++;
adjacent[n2][n1]++;
}
return true;
}
void save(ostream& out)
{
for(vector<int>::reverse_iterator it=steps.rbegin(); it!=steps.rend(); ++it)
{
out << *it << endl;
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void dfs(int i)
{
// PV(i)
if(edges[i].size() == 0)
{
steps.push_back(i);
// cout << "save " << i << endl;
return;
}
else
{
while(edges[i].size() > 0)
{
int en = edges[i].begin()->first;
--(edges[i].begin()->second);
if(edges[i].begin()->second == 0)
{
edges[i].erase(edges[i].begin());
}
map<int, int, compare>::iterator it = edges[en].find(i);
--(it->second);
if(it->second == 0)
edges[en].erase(it);
dfs(en);
}
steps.push_back(i);
// cout << "save " << i << endl;
}
}
void solve()
{
// find odd node
int start = 0;
for(set<int, compare>::const_iterator it=node.begin(); it!=node.end(); ++it)
{
if(edges[*it].size() % 2 != 0)
{
start = *it;
break;
}
}
PV(start)
PV(*--node.end());
string a = "01";
string b = "4";
PV(lexicographical_compare(a.begin(), a.end(), b.begin(), b.end()));
PV(compare().itos(123))
PV(compare()(1, 2))
PV(*(node.begin()))
if(!start)
start = *(node.begin());
dfs(start);
}
void dfs2(int i)
{
if(degree[i] == 0)
{
steps.push_back(i);
return;
}
else
{
for(int j=1; j<=MAXN; ++j)
{
if(adjacent[i][j] > 0)
{
degree[i]--;
degree[j]--;
adjacent[i][j]--;
adjacent[j][i]--;
dfs2(j);
}
}
steps.push_back(i);
}
}
void solve2()
{
int start = 0;
for(int i=1; i<=MAXN; ++i)
{
if(start == 0 && degree[i] > 0)
start = i;
if(degree[i] % 2 != 0)
{
start = i;
break;
}
}
dfs2(start);
}
// Assuming you pick the lowest index vertex connected to each node, the Eulerian Path algorithm actually determines the path requested, although in the reverse direction. You must start the path determination at the lowest legal vertex for this to work.
// /* Prob #5: Riding the Fences */
// #include <stdio.h>
// #include <string.h>
// #define MAXI 500
// #define MAXF 1200
// char conn[MAXI][MAXI];
// int deg[MAXI];
// int nconn;
// int touched[MAXI];
// int path[MAXF];
// int plen;
// /* Sanity check routine */
// void fill(int loc)
// {
// int lv;
// touched[loc] = 1;
// for (lv = 0; lv < nconn; lv++)
// if (conn[loc][lv] && !touched[lv])
// fill(lv);
// }
// /* Sanity check routine */
// int is_connected(int st)
// {
// int lv;
// memset(touched, 0, sizeof(touched));
// fill(st);
// for (lv = 0; lv < nconn; lv++)
// if (deg[lv] && !touched[lv])
// return 0;
// return 1;
// }
// /* this is exactly the Eulerian Path algorithm */
// void find_path(int loc)
// {
// int lv;
// for (lv = 0; lv < nconn; lv++)
// if (conn[loc][lv])
// {
// /* delete edge */
// conn[loc][lv]--;
// conn[lv][loc]--;
// deg[lv]--;
// deg[loc]--;
// /* find path from new location */
// find_path(lv);
// }
// /* add this node to the `end' of the path */
// path[plen++] = loc;
// }
// int main(int argc, char **argv)
// {
// FILE *fin, *fout;
// int nfen;
// int lv;
// int x, y;
// if (argc == 1)
// {
// if ((fin = fopen("fence.in", "r")) == NULL)
// {
// perror ("fopen fin");
// exit(1);
// }
// if ((fout = fopen("fence.out", "w")) == NULL)
// {
// perror ("fopen fout");
// exit(1);
// }
// } else {
// if ((fin = fopen(argv[1], "r")) == NULL)
// {
// perror ("fopen fin filename");
// exit(1);
// }
// fout = stdout;
// }
// fscanf (fin, "%d", &nfen);
// for (lv = 0; lv < nfen; lv++)
// {
// fscanf (fin, "%d %d", &x, &y);
// x--; y--;
// conn[x][y]++;
// conn[y][x]++;
// deg[x]++;
// deg[y]++;
// if (x >= nconn) nconn = x+1;
// if (y >= nconn) nconn = y+1;
// }
// /* find first node of odd degree */
// for (lv = 0; lv < nconn; lv++)
// if (deg[lv] % 2 == 1) break;
// /* if no odd-degree node, find first node with non-zero degree */
// if (lv >= nconn)
// for (lv = 0; lv < nconn; lv++)
// if (deg[lv]) break;
// #ifdef CHECKSANE
// if (!is_connected(lv)) /* input sanity check */
// {
// fprintf (stderr, "Not connected?!?\n");
// return 0;
// }
// #endif
// /* find the eulerian path */
// find_path(lv);
// /* the path is discovered in reverse order */
// for (lv = plen-1; lv >= 0; lv--)
// fprintf (fout, "%i\n", path[lv]+1);
// return 0;
// }<file_sep>/README.md
USACO_practise
=================
This is my practice program files on USACO TRAINING<file_sep>/section2/holstein.cpp
/*
ID: luglian1
PROG: holstein
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cstring>
using namespace std;
#define MAX_V 25
#define MAX_G 15
int v_num;
int varray[MAX_V];
int g_num;
int garrry[MAX_G][MAX_V];
int best_scoop_count;
bool best_scoops[MAX_G];
bool scoops[MAX_G];
int v_temp[MAX_V];
bool load()
{
ifstream file_in("holstein.in");
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> v_num;
for (int i = 0; i < v_num; ++i)
{
file_in >> varray[i];
}
file_in >> g_num;
for (int i = 0; i < g_num; ++i)
{
for (int j = 0; j < v_num; ++j)
{
file_in >> garrry[i][j];
}
}
return true;
}
void save(ostream& out)
{
out << best_scoop_count;
for (int i = 0; i < g_num; ++i)
{
if(best_scoops[i])
out << " " << i+1;
}
out << endl;
}
bool save()
{
ofstream file_out("holstein.out");
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void dfs_solve(int type_id, int scoops_count)
{
// check over
if(scoops_count >= best_scoop_count)
return;
bool find = true;
for (int i = 0; i < v_num; ++i)
{
if(v_temp[i] > 0)
{
find = false;
break;
}
}
if(find)
{
if(scoops_count < best_scoop_count)
{
memmove(best_scoops, scoops, g_num * sizeof(scoops[0]));
best_scoop_count = scoops_count;
}
}
else if(type_id < g_num)
{
// feed[type_id] is on
scoops[type_id] = true;
for (int i = 0; i < v_num; ++i)
{
v_temp[i] -= garrry[type_id][i];
}
dfs_solve(type_id+1, scoops_count+1);
// feed[type_id] is off
scoops[type_id] = false;
for (int i = 0; i < v_num; ++i)
{
v_temp[i] += garrry[type_id][i];
}
dfs_solve(type_id+1, scoops_count);
}
}
void solve()
{
best_scoop_count = g_num+2;
for (int i = 0; i < MAX_G; ++i)
{
best_scoops[i] = false;
}
memmove(v_temp, varray, v_num * sizeof(varray[0]));
dfs_solve(0, 0);
}
int main(){
load();
solve();
save();
return 0;
}
<file_sep>/section 1/calfflac.cpp
/*
ID: luglian1
PROG: calfflac
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <algorithm>
#include <vector>
using namespace std;
int main(){
ifstream file_in("calfflac.in");
ofstream file_out("calfflac.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
//return 0;
}
string all;
string line;
while(getline(file_in, line)){
all += "\n" + line;
}
all += "\n";
// get the char in [a-z][A-Z]
string origin;
vector<int> pos; // remember the char position
for(int i=0; i!=all.size(); ++i){
char c = all.at(i);
if( c >= 'A' && c <= 'Z'){
origin += 'a' + c - 'A';
pos.push_back(i);
}
else if( c >= 'a' && c<= 'z'){
origin += c;
pos.push_back(i);
}
}
cout << origin << endl;
// // reverse it
// string reverse(origin);
// reverse_copy(origin.begin(), origin.end(), reverse.begin());
// cout << reverse << endl;
// check the pos where origin[pos-1] == origin[pos+1]
int maxlength=0;
int tmplength;
int maxspos=0;
int endpos = -1;
int startpos=0;
for(int i=0; i!=origin.size()-1; ++i){
if(origin[i-1] == origin[i+1]){
startpos = endpos = i;
while(startpos >=-1 && endpos<=origin.size() &&
origin[startpos] == origin[endpos]){
--startpos;
++endpos;
}
tmplength = endpos - startpos -1;
if(tmplength > maxlength){
maxlength = tmplength;
maxspos = startpos+1;
}
}
if(origin[i] == origin[i+1]){
startpos = i;
endpos = i+1;
while(startpos >=-1 && endpos<=origin.size() &&
origin[startpos] == origin[endpos]){
--startpos;
++endpos;
}
tmplength = endpos - startpos - 1;
if(tmplength > maxlength){
maxlength = tmplength;
maxspos = startpos+1;
}
}
}
// // get the max equal in origin and reverse
// while(startpos < origin.size()){
// while(origin[startpos] != reverse[startpos] && startpos < origin.size())
// ++startpos;
// for(endpos = startpos;
// endpos < origin.size() && origin[endpos] == reverse[endpos];
// ++endpos);
// if(endpos - startpos > maxlength){
// maxlength = endpos - startpos;
// maxspos = startpos;
// }
// startpos = endpos;
// }
cout << startpos << endl;
cout << maxlength << endl;
// get the original string
int reallength = pos[maxspos+maxlength-1] - pos[maxspos]+1;
cout << all.substr(pos[maxspos], reallength) << endl;
file_out << maxlength << endl << all.substr(pos[maxspos], reallength) << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/sprime.cpp
/*
ID: luglian1
PROG: sprime
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cmath>
#include <vector>
using namespace std;
class PrimeRib{
vector<int> prime;
int N;
public:
PrimeRib(int n): N(n){
prime.push_back(2);
prime.push_back(3);
int sq = sqrt(pow(10, n)) + 1;
int cur = 5;
while( cur <= sq ){
if( checkPrime(cur) ){
prime.push_back(cur);
}
cur += 2;
}
}
bool checkPrime(int n){
if(n == 1) return false;
int sq = sqrt((double)n)+1;
for(int i=0; i!=prime.size(); ++i){
if(n == prime[i])
return true;
if(prime[i] > sq)
break;
if( n % prime[i] == 0)
return false;
}
return true;
}
bool checkPrimeRib(int n){
while(n > 0){
if(!checkPrime(n))
return false;
n /= 10;
}
return true;
}
void workByadd2(ostream& file_out){
int i=pow(10, N-1)+1;
int end = pow(10, N);
for(; i < end; i+=2){
if(checkPrimeRib(i))
file_out << i << endl;
}
}
void workBybfs(int len, int n, ostream& file_out){
if(n == 0 || checkPrime(n)){
if(len <= 0){
file_out << n << endl;
return;
}
for(int i = 1; i<=9; i+=1){
workBybfs(len-1, n*10+i, file_out);
}
}
}
void work(ostream& file_out){
//workByadd2(file_out);
workBybfs(N, 0, file_out);
//cout << checkPrime(2) << endl;
}
};
int main(){
ifstream file_in("sprime.in");
ofstream file_out("sprime.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int N;
file_in >> N;
PrimeRib pr(N);
pr.work(file_out);
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/template.cpp
/*
ID: luglian1
PROG: beads
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int main(){
ifstream file_in("beads.in");
ofstream file_out("beads.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/msquare.cpp
/*
ID: luglian1
PROG: msquare
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <map>
#include <deque>
#include <cassert>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "msquare"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
struct node
{
int move;
string parent;
int change;
node(int m=0, const string& p="", int c=0):move(m), parent(p), change(c) {};
};
const string start("12345678");
map<string, node> m;
string target;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
string s;
for(int i=0; i<8; ++i)
{
file_in >> s;
target += s;
}
PV(target)
return true;
}
void save(ostream& out)
{
if(m.count(target)==0)
return;
string s("ABC");
string outs;
string cur = target;
out << m[cur].move << endl;
while(cur != "12345678")
{
outs.push_back(s[m[cur].change]);
cur = m[cur].parent;
}
for(int i=outs.length()-1; i>=0; --i)
out << outs[i];
out << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
string movea(const string& s)
{
string news(s);
for(int i=0; i<4; ++i)
{
swap(news[i], news[7-i]);
}
return news;
}
string moveb(const string& s)
{
string news(8, '0');
news[0] = s[3]; news[1] = s[0]; news[2] = s[1]; news[3] = s[2];
news[4] = s[5]; news[5] = s[6]; news[6] = s[7]; news[7] = s[4];
return news;
}
string movec(const string& s)
{
string news(s);
news[1] = s[6]; news[2] = s[1]; news[5] = s[2]; news[6] = s[5];
return news;
}
string (*funcs[3])(const string& s) = {movea, moveb, movec};
void dfs(int move, const string& parent)
{
if(move > 19)
return;
for(int i=0; i<3; ++i)
{
string s = (*(funcs[i]))(parent);
if(m.count(s) == 0)
{
m.insert(pair<string, node>(s, node(move+1, parent, i)));
dfs(move+1, s);
}
else if(m[s].move > move + 1)
{
m[s].move = move+1;
m[s].parent = parent;
m[s].change = i;
dfs(move+1, s);
}
}
}
void bfs()
{
deque<string> ds;
ds.push_back(start);
while(ds.size() > 0)
{
string cur = ds[0];
ds.pop_front();
const node& n = m[cur];
for(int i=0; i<3; ++i)
{
string s = (*(funcs[i]))(cur);
if(m.count(s) == 0)
{
ds.push_back(s);
m.insert(pair<string, node>(s, node(n.move+1, cur, i)));
}
if(s == target)
return;
}
}
}
int encode(int *board)
{
static int mult[8] =
{1, 8, 8*7, 8*7*6, 8*7*6*5,
8*7*6*5*4, 8*7*6*5*4*3, 8*7*6*5*4*3*2};
/* used to calculate the position of a number within the
remaining ones */
int look[8] = {0, 1, 2, 3, 4, 5, 6, 7};
int rlook[8] = {0, 1, 2, 3, 4, 5, 6, 7};
/* rlook[look[p]] = p and look[rlook[p]] = p */
int lv, rv;
int t;
rv = 0;
for (lv = 0; lv < 8; lv++)
{
t = look[board[lv]]; /* the rank of the board position */
// PV(t)
assert(t < 8-lv); /* sanity check */
rv += t * mult[lv];
assert(look[rlook[7-lv]] == 7-lv); /* sanity check */
/* delete t */
look[rlook[7-lv]] = t;
rlook[t] = rlook[7-lv];
// PVL(look, 8)
// PVL(rlook, 8)
}
return rv;
}
void stob(const string& s, int* board)
{
for(int i=0; i<8; ++i)
{
board[i] = (s[i] - '0') % 8;
}
}
void solve()
{
node root(0);
m.insert(pair<string, node>(start, root));
int board[8] = {1,0,6,5,4,3,2,7};
PV(encode(board))
bfs();
map<int, string> mi;
for(map<string, node>::iterator it=m.begin(); it!=m.end(); ++it)
{
stob(it->first, board);
mi.insert(pair<int, string>(encode(board), it->first));
}
PV(mi[0])
PV(mi[1])
PV(mi[2])
//dfs(0, "12345678");
//PV(m.size())
// PV(m.count(target))
//while(m.size() < 40320)
}
// This is a shortest path problem, where the nodes of the graph are board arrangements, and edges are transformations. There are 8! = 40,320 possible board arrangements, so the problem can be solved using breadth-first search (since all edges are of unit length.
// Number the boards in increasing order lexicographically, so that indexing is simpler.
// In order to simplify the calculations, walk the path backward (start at the ending arrangement given, find the minimum path to the initial configuration following reverse transformations). Then, walk backwards in the resulting tree to determine the path.
// #include <stdio.h>
// #include <assert.h>
// /* the distance from the initial configuration (+1) */
// /* dist == 0 => no know path */
// int dist[40320];
// /* calculate the index of a board */
// int encode(int *board)
// {
// static int mult[8] =
// {1, 8, 8*7, 8*7*6, 8*7*6*5,
// 8*7*6*5*4, 8*7*6*5*4*3, 8*7*6*5*4*3*2};
// /* used to calculate the position of a number within the
// remaining ones */
// int look[8] = {0, 1, 2, 3, 4, 5, 6, 7};
// int rlook[8] = {0, 1, 2, 3, 4, 5, 6, 7};
// /* rlook[look[p]] = p and look[rlook[p]] = p */
// int lv, rv;
// int t;
// rv = 0;
// for (lv = 0; lv < 8; lv++)
// {
// t = look[board[lv]]; /* the rank of the board position */
// assert(t < 8-lv); /* sanity check */
// rv += t * mult[lv];
// assert(look[rlook[7-lv]] == 7-lv); /* sanity check */
// /* delete t */
// look[rlook[7-lv]] = t;
// rlook[t] = rlook[7-lv];
// }
// return rv;
// }
// /* the set of transformations, in order */
// static int tforms[3][8] = { {8, 7, 6, 5, 4, 3, 2, 1},
// {4, 1, 2, 3, 6, 7, 8, 5}, {1, 7, 2, 4, 5, 3, 6, 8} };
// void do_trans(int *inboard, int *outboard, int t)
// { /* calculate the board (into outboard) that results from doing
// the t'th transformation to inboard */
// int lv;
// int *tform = tforms[t];
// assert(t >= 0 && t < 3);
// for (lv = 0; lv < 8; lv++)
// outboard[lv] = inboard[tform[lv]-1];
// }
// void do_rtrans(int *inboard, int *outboard, int t)
// { /* calculate the board (into outboard) that which would result
// in inboard if the t'th transformation was applied to it */
// int lv;
// int *tform = tforms[t];
// assert(t >= 0 && t < 3);
// for (lv = 0; lv < 8; lv++)
// outboard[tform[lv]-1] = inboard[lv];
// }
// queue for breadth-first search
// int queue[40325][8];
// int qhead, qtail;
// /* calculate the distance from each board to the ending board */
// void do_dist(int *board)
// {
// int lv;
// int t1;
// int d, t;
// qhead = 0;
// qtail = 1;
// /* the ending board is 0 steps away from itself */
// for (lv = 0; lv < 8; lv++) queue[0][lv] = board[lv];
// dist[encode(queue[0])] = 1; /* 0 steps (+ 1 offset for dist array) */
// while (qhead < qtail)
// {
// t1 = encode(queue[qhead]);
// d = dist[t1];
// /* for each transformation */
// for (lv = 0; lv < 3; lv++)
// {
// /* apply the reverse transformation */
// do_rtrans(queue[qhead], queue[qtail], lv);
// t = encode(queue[qtail]);
// if (dist[t] == 0)
// { /* found a new board position! add it to queue */
// qtail++;
// dist[t] = d+1;
// }
// }
// qhead++;
// }
// }
// /* find the path from the initial configuration to the ending board */
// void walk(FILE *fout)
// {
// int newboard[8];
// int cboard[8];
// int lv, lv2;
// int t, d;
// for (lv = 0; lv < 8; lv++) cboard[lv] = lv;
// d = dist[encode(cboard)];
// /* start at the ending board */
// while (d > 1)
// {
// for (lv = 0; lv < 3; lv++)
// {
// do_trans(cboard, newboard, lv);
// t = encode(newboard);
// if (dist[t] == d-1) /* we found the previous board! */
// {
// /* output transformatino */
// fprintf (fout, "%c", lv+'A');
// /* find the rest of the path */
// for (lv2 = 0; lv2 < 8; lv2++) cboard[lv2] = newboard[lv2];
// break;
// }
// }
// assert(lv < 3);
// d--;
// }
// fprintf (fout, "\n");
// }
// int main(int argc, char **argv)
// {
// FILE *fout, *fin;
// int board[8];
// int lv;
// if ((fin = fopen("msquare.in", "r")) == NULL)
// {
// perror ("fopen fin");
// exit(1);
// }
// if ((fout = fopen("msquare.out", "w")) == NULL)
// {
// perror ("fopen fout");
// exit(1);
// }
// for (lv = 0; lv < 8; lv++)
// {
// fscanf (fin, "%d", &board[lv]);
// board[lv]--; /* use 0-based instead of 1-based */
// }
// /* calculate the distance from each board to the ending board */
// do_dist(board);
// for (lv = 0; lv < 8; lv++) board[lv] = lv;
// /* output the distance from and the path from the initial configuration */
// fprintf (fout, "%d\n", dist[encode(board)]-1);
// walk(fout);
// return 0;
// }<file_sep>/mytest/sequences.cpp
// print string sequence
#include <iostream>
#include <string>
#include <fstream>
#include <ctime>
using namespace std;
ofstream file_out("sequences.out");
ostream& out = cout;//file_out;//
int num_string = 0;
void print(char* s, int cur_pos, int n)
{
if(cur_pos == n)
{
s[cur_pos] = '\0';
// if(num_string != 0)
// out << ",";
// num_string++;
// out << "(";
// for(int i=0; i<n-1; i++)
// {
// out << s[i] << ",";
// }
// out << s[n-1] << ")";
return;
}
for(s[cur_pos] = s[cur_pos-1] + 1; s[cur_pos] <= 'z'; s[cur_pos]++)
{
// lop
if(n - cur_pos - 1 > 'z' - s[cur_pos])
return;
print(s, cur_pos+1, n);
}
}
void calc(int len)
{
// 初始化字符串(性质1)
char digs[27] = "abcdefghijklmnopqrstuvwxyz";
digs[len] = 0;
bool flag = true;
while (flag)
{
//printf("%s,", digs);
flag = false;
for (int i = len-1; i >= 0; i --)
{
// 字符串从低位往高位增,满足字典序(性质3)
digs[i] ++;
//满足该条件的,才能使字符依次递增
if (digs[i] <= 'z'-(len-1-i))
{
// 字符是依次递增的(性质2)
for (int j = i+1; j < len; j ++)
digs[j] = digs[j-1] + 1;
flag = true;
break;
}
}
}
printf("1\n");
}
int main()
{
clock_t begin = clock();
char s[1024];
for(int n=1; n<=26; n++)
{
num_string = 0;
for(char c = 'a'; c<='z'; c++)
{
s[0] = c;
print(s, 1, n);
}
printf("1\n");
//out << endl;
}
// for(int i=0; i<=26; i++)
// {
// calc(i);
// }
clock_t end = clock();
double time = (double)(end - begin) / CLOCKS_PER_SEC;
}<file_sep>/section2/subset.cpp
/*
ID: luglian1
PROG: subset
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
bool load();
void save(ostream& out);
bool save();
void buildTable();
void solve();
int M;
unsigned int num;
unsigned int table[40][801] = {0}; // table for num of set of sum n in m, table[m][n]
int main(){
load();
buildTable();
solve();
save();
return 0;
}
void buildTable()
{
table[1][0] = 1;
table[1][1] = 1;
for(int m=2; m <= 39; ++m)
{
int max = (m+1) * m / 2;
for(int n=0; n<=max; ++n)
{
unsigned int num = 0;
if(n - m >= 0)
num += table[m-1][n-m];
num += table[m-1][n];
table[m][n] = num;
}
}
// output table
// ofstream file_out("subset_table.txt");
// for(int m=1; m<40; ++m)
// {
// int max = (m+1) * m / 2;
// for(int n=1; n<=max; ++n)
// {
// file_out << table[m][n] << " ";
// }
// file_out << endl;
// }
// file_out.close();
}
void solve()
{
int sum = (M+1) * M / 2;
cout << sum << endl;
if(sum % 2 == 1)
{
num = 0;
return;
}
sum /= 2;
cout << sum << endl;
cout << table[M][sum] << endl;
num = table[M][sum] / 2;
}
bool load()
{
ifstream file_in("subset.in");
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> M;
return true;
}
void save(ostream& out)
{
out << num << endl;
}
bool save()
{
ofstream file_out("subset.out");
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
<file_sep>/section2/runround.cpp
/*
ID: luglian1
PROG: runround
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <string>
#include <sstream>
#include <cstdlib>
#define PV(v) std::cout << #v << " = " << v << std::endl;
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int N;
int main(){
load();
solve();
save();
return 0;
}
bool checkRunAround(int n)
{
stringstream ss;
for(int i=0; i<9; ++i)
{
ss << n;
}
string s;
ss >> s;
if(s.find('0') != s.npos) return false;
int count[10] = {0};
int length = s.length() / 9;
int curpos = 0;
for(int i=0; i<length+1; ++i)
{
//PV(curpos);
int curi = s[curpos]-'0';
if(i == length)
{
if(curpos != 0)
return false;
}
else
{
//PV(curi);
if(count[curi] != 0)
return false;
count[curi] = 1;
}
curpos = s.find_first_of(s[curpos + curi]);
}
int sum = 0;
for(int i=0; i<10; ++i)
{
sum += count[i];
}
if(sum != length)
return false;
return true;
}
int findLeastLarge(int a, int* count)
{
for(int i = a+1; i < 10; ++i)
{
//PV(count[i]);
if(count[i] == 0)
return i;
}
return 0;
}
int generateNext(int n)
{
stringstream ss;
ss << n;
string s = ss.str();
int count[10] = {0};
int replace_pos=0;
// find repeat and count each unique num
for(; replace_pos < s.length(); ++replace_pos)
{
int i = s[replace_pos] - '0';
if(i == 0)
break;
if(count[i] == 1)
break;
++count[i];
}
//PV(replace_pos);
// all nums are unique
if(replace_pos == s.length())
--replace_pos;
for(int i=replace_pos; i>=-1; --i)
{
replace_pos = i;
if(i == -1)
break;
int curi = s[i] - '0';
if(findLeastLarge(curi, count) != 0)
{
break;
}
count[curi] = 0;
//PV(curi);
}
if(replace_pos == -1)
{
s = "0" + s;
replace_pos = 0;
}
//PV(s)
//PV(s.length())
//PV(replace_pos);
int curi = s[replace_pos] - '0';
int replacei = findLeastLarge(curi, count);
//PV(curi);
//PV(replacei);
s[replace_pos] = (char)(replacei + '0');
count[curi] = 0;
count[replacei] = 1;
int curpos = replace_pos + 1;
for(int i = 1; i< 10; ++i)
{
if(count[i] == 0)
{
s[curpos] = (char)(i + '0');
++curpos;
if(curpos >= s.length())
break;
}
}
//PV(s)
ss.str("");
ss << s;
ss >> n;
return n;
}
void solve()
{
N = generateNext(N);
//PV(N);
while(!checkRunAround(N))
{
N = generateNext(N);
//PV(N);
}
//cout << __FUNCTION__ << endl;
}
bool load()
{
ifstream file_in("runround.in");
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
return true;
}
void save(ostream& out)
{
out << N << endl;
}
bool save()
{
ofstream file_out("runround.out");
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
//save(cout);
return true;
}
<file_sep>/section2/preface.cpp
/*
ID: luglian1
PROG: preface
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cmath>
using namespace std;
struct IV
{
int i_num = 0;
int v_num = 0;
int num9s = 0;
};
bool load();
void save(ostream& out);
bool save();
void solve();
void initial();
IV calculateIV(int n, int pos);
void print(int id);
void print(IV);
int N;
int I_table[10] = {0};
int I_allIn10;
int V_table[10] = {0};
int V_allin10;
IV iv_num[4];
int main(){
load();
solve();
save();
return 0;
}
// void print(int id)
// {
// cout << id;
// for(int i=0; i<4; ++i)
// {
// cout << (int)iv_num[i].i_name;
// cout << (int)iv_num[i].v_name;
// }
// cout << endl;
// }
ostream& operator<<(ostream& out, IV iv)
{
out << iv.i_num << " " << iv.v_num << " " << iv.num9s;
return out;
}
void solve()
{
initial();
int i=0;
int num9s=0;
int length = 0;
int n = N;
while(n>0)
{
++length;
n = n / 10;
}
for(int i=0; i<length; ++i)
{
iv_num[i] = calculateIV(N, i);
cout << i << ": " << iv_num[i] << endl;
if(i > 0)
iv_num[i].i_num += iv_num[i-1].num9s;
cout << i << ": " << iv_num[i] << endl;
}
}
IV calculateIV(int n, int pos)
{
int apow = pow(10, pos);
int cutNum = (n / apow) % 10;
int numof10 = n / apow / 10;
int rest = n % apow + 1;
cout << pos << ": " << numof10 << " " << cutNum << " " << rest << endl;
IV iv;
if(numof10 > 0)
{
iv.i_num += I_allIn10 * numof10;
iv.v_num += V_allin10 * numof10;
}
for(int i=0; i<cutNum; ++i)
{
iv.i_num += I_table[i];
iv.v_num += V_table[i];
}
iv.num9s = numof10;
iv.i_num *= apow;
iv.v_num *= apow;
iv.num9s *= apow;
cout << iv.i_num << endl;
iv.i_num += I_table[cutNum] * rest;
iv.v_num += V_table[cutNum] * rest;
cout << iv.i_num << endl;
if(cutNum == 9)
iv.num9s += rest;
return iv;
}
void initial()
{
for(int i=0; i<4; ++i)
I_table[i] = i;
I_table[4] = 1;
for(int i=5; i<10; ++i)
I_table[i] = I_table[i-5];
I_allIn10 = 14;
for(int i=4; i<=8; ++i)
V_table[i] = 1;
V_allin10 = 5;
}
bool load()
{
ifstream file_in("preface.in");
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
return true;
}
void save(ostream& out)
{
char names[] = "IVXLCDMM";
for(int i=0; i<4; ++i)
{
if(iv_num[i].i_num > 0)
out << names[i*2] << " " << iv_num[i].i_num << endl;
if(iv_num[i].v_num > 0)
out << names[i*2+1] << " " << iv_num[i].v_num << endl;
}
}
bool save()
{
ofstream file_out("preface.out");
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
<file_sep>/section 1/clocks.cpp
/*
ID: luglian1
PROG: clocks
LANG: C++
*/
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
#include <vector>
#include <deque>
#include <algorithm>
using namespace std;
template <typename T>
void printVector(const vector<T>& order){
for(int i=0; i!=order.size(); ++i)
cout << order[i] << " ";
cout << endl;
}
int move[9][9] = {
{3,3,0,3,3,0,0,0,0},
{3,3,3,0,0,0,0,0,0},
{0,3,3,0,3,3,0,0,0},
{3,0,0,3,0,0,3,0,0},
{0,3,0,3,3,3,0,3,0},
{0,0,3,0,0,3,0,0,3},
{0,0,0,3,3,0,3,3,0},
{0,0,0,0,0,0,3,3,3},
{0,0,0,0,3,3,0,3,3},
};
bool checkClocks(const vector<int>& clocks){
if(clocks.size() != 9){
cerr << "Error: clocks size is not accepted." << endl;
return false;
}
for(int i=0; i<clocks.size(); ++i){
if(clocks[i]%12 != 0)
return false;
}
return true;
}
void clockChange(vector<int>& clocks, int moveid){
for(int i=0; i!=9; ++i)
clocks[i] = clocks[i] + move[moveid][i];
}
void clockUnChange(vector<int>& clocks, int moveid){
for(int i=0; i!=9; ++i)
clocks[i] = clocks[i] + 12 - move[moveid][i];
}
vector<vector<int> > pathset;
bool DFSIDsearchPath(vector<int>& clocks, vector<int>& path, int depth){
if(depth == 0){
if(checkClocks(clocks)){
pathset.push_back(path);
return true;
}
else
return false;
}
for(int i=0; i!=9; ++i){
path.push_back(i);
clockChange(clocks, i);
if(DFSIDsearchPath(clocks, path, depth-1))
return true;
clockUnChange(clocks, i);
path.pop_back();
}
return false;
}
void DFSIDsearch(vector<int>& clocks){
int depth = 1;
vector<int> path;
while(true){
DFSIDsearchPath(clocks, path, depth);
if(pathset.size() != 0)
break;
++depth;
cout << "depth: " << depth << endl;
}
}
deque<vector<int> > pathqueue;
void BFSprocess(vector<int>& onepath){
for(int i=0; i!=9; ++i){
onepath.push_back(i);
pathqueue.push_back(onepath);
onepath.pop_back();
}
}
void BFSsearch(vector<int>& clocks){
vector<int> onepath;
pathqueue.push_back(onepath);
while(pathset.size() == 0){
onepath.swap(pathqueue.front());
pathqueue.pop_front();
vector<int> tmpc(clocks);
for(int i=0; i!=onepath.size(); ++i)
clockChange(tmpc, i);
if(checkClocks(tmpc))
break;
BFSprocess(onepath);
}
}
string pathToString(const vector<int>& path){
stringstream ss;
for(int i=8; i>=0; --i){
int t = path[i];
while(t>0){
ss << 8-i+1;
--t;
}
}
string result;
ss >> result;
return result;
}
bool compareStringPath(const string& p1, const string& p2){
if(p1.size() != p2.size())
return p1.size() < p2.size();
else
return p1 < p2;
}
bool checkClocks(const vector<int>& clocks, const vector<int>& path){
if(path.size() != 9 || clocks.size() != 9){
return false;
}
vector<int> tmpclocks(clocks);
//printVector(path);
for(int i=8; i>=0; --i){
int t = path[i];
while(t>0){
clockChange(tmpclocks, 8-i);
--t;
}
}
if(checkClocks(tmpclocks))
return true;
return false;
}
vector<int> origin_clocks;
vector<string> pathSetIns;
void generatePath(vector<int>& path){ // path show the times of each move, path[0]->move[8], path[8]->move[0]
if(path.size() >= 9){
if(checkClocks(origin_clocks, path)){
pathSetIns.push_back(pathToString(path));
//cout << pathSetIns.back() << endl;
}
}
else{
for(int i=0; i!=4; ++i){
path.push_back(i);
generatePath(path);
path.pop_back();
}
}
}
void OptimizedSearch(){
vector<int> path;
generatePath(path);
//cout << "over" << endl;
}
int main(){
ifstream file_in("clocks.in");
ofstream file_out("clocks.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
vector<int> clocks(9);
for(int i=0; i!=9; ++i){
file_in >> clocks[i];
clocks[i] = clocks[i]%12;
}
// the complexity of DFS and BFS is too high!!
//DFSIDsearch(clocks);
//BFSsearch(clocks);
// in fact it only needs 4^9 complexity
origin_clocks.insert(origin_clocks.begin(), clocks.begin(), clocks.end());
OptimizedSearch();
//cout << "over" << endl;
//cout << pathSetIns.size() << endl;
// for(int i=0; i!=pathset.size(); ++i){
// pathSetIns[i] = pathToString(pathset[i]);
// //printVector(pathset[i]);
// }
sort(pathSetIns.begin(), pathSetIns.end(), compareStringPath);
//printVector(pathSetIns);
for(int i=0; i!=pathSetIns[0].size()-1; ++i)
file_out << pathSetIns[0][i] << " ";
file_out << pathSetIns[0][pathSetIns[0].size()-1] << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/stamps.cpp
/*
ID: luglian1
PROG: stamps
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <algorithm>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "stamps"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAXS 50
#define MAX_LEN 12000000
using namespace std;
int k, n;
int cent[MAXS] = {0};
int m=0;
unsigned char mink[MAX_LEN] = {0};
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> k >> n;
for(int i=0; i<n; ++i)
file_in >> cent[i];
return true;
}
void save(ostream& out)
{
out << m << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
sort(cent, cent+n);
PVL(cent, n)
if(cent[0] != 1)
return;
for(int i=0; i<n; ++i)
{
mink[cent[i]] = 1;
}
for(m=2; m<MAX_LEN; ++m)
{
if(mink[m])
continue;
int mm = k+1;
for(int j=0; j<n; ++j)
{
if(m-cent[j] > 0)
{
mm = min(mm, mink[m-cent[j]] + 1);
}
}
if(mm <= k)
mink[m] = mm;
else
break;
}
m--;
}
// This problem is simply begging for a DP solution. We keep an array "minstamps" such that minstamps[i] is the minimum number of stamps needed to make i cents. For each stamp type, we try adding one stamp of that type to each number of cents that we have already made. After we have found the minimum number of stamps needed to make any given number of cents, we find the smallest number of cents that we cannot make with the given number of stamps, and then we output one less then that.
// #include <fstream.h>
// const int BIG = 10000;
// short minstamps[10000 * (200 + 3) + 3];
// int stamps;
// int value[200];
// int number;
// int
// main ()
// {
// ifstream filein ("stamps.in");
// filein >> number >> stamps;
// int biggest = -1;
// for (int i = 0; i < stamps; ++i) {
// filein >> value[i];
// if (biggest < value[i]) {
// biggest = value[i];
// }
// }
// filein.close ();
// for (int i = 0; i <= biggest * number + 3; ++i) {
// minstamps[i] = BIG;
// }
// minstamps[0] = 0;
// for (int i = 0; i < stamps; ++i) {
// for (int j = 0; j <= biggest * number; ++j) {
// if (minstamps[j] < number) {
// if (minstamps[j + value[i]] > 1 + minstamps[j]) {
// minstamps[j + value[i]] = 1 + minstamps[j];
// }
// }
// }
// }
// int string = 0;
// while (minstamps[string + 1] <= number) {
// ++string;
// }
// ofstream fileout ("stamps.out");
// fileout << string << endl;
// fileout.close ();
// return (0);
// }
<file_sep>/section2/money.cpp
/*
ID: luglian1
PROG: money
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "money"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int V;
int N;
int coin[26] = {0};
long long tb[26][10001] = {0};
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> V >> N;
for(int i=1; i<=V; ++i)
{
file_in >> coin[i];
}
// PV(V)
// PV(N)
// PVL(coin, V+1)
return true;
}
void save(ostream& out)
{
out << tb[V][N] << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
//init
for(int i=0; i<=N; ++i)
{
tb[1][i] = i % coin[1] == 0 ? 1 : 0;
}
for(int i=0; i<=V; ++i)
{
tb[i][0] = 1;
}
if(V == 1)
return;
for(int i=2; i<=V; ++i)
{
for(int j=coin[i]; j<=N; ++j)
{
tb[i][j] = tb[i-1][j];
if(j-coin[i] >=0)
tb[i][j] += tb[i][j-coin[i]];
// for(int k=1; k<=j/coin[i]; ++k)
// {
// tb[i][j] += tb[i-1][j-coin[i]*k];
// }
// if(j % coin[i] == 0)
// tb[i][j] += 1;
}
}
// PVL(tb[1], N+1)
// PVL(tb[2], N+1)
// PVL(tb[3], N+1)
}
// 范例
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXTOTAL 10000
// long long nway[MAXTOTAL+1];
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, j, n, v, c;
// fin = fopen("money.in", "r");
// fout = fopen("money.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d %d", &v, &n);
// nway[0] = 1;
// for(i=0; i<v; i++) {
// fscanf(fin, "%d", &c);
// for(j=c; j<=n; j++)
// nway[j] += nway[j-c];
// }
// fprintf(fout, "%lld\n", nway[n]);
// }<file_sep>/section2/sort3.cpp
/*
ID: luglian1
PROG: sort3
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
#define min(a, b) (a)<(b)?(a):(b)
int sequences[1000];
// not correct
int Sequence()
{
ifstream file_in("sort3.in");
ofstream file_out("sort3.out");
if(!file_in || !file_out)
{
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
file_in >> n;
int max=0;
bool appear[] = {false, false, false, false};
int count = 0;
// read all the data first
for(int i=0; i<n; i++)
{
file_in >> sequences[i];
}
// deal with 1 first
int insert_pos = 0;
int cur_pos = n-1;
while(true)
{
for(;sequences[insert_pos] == 1 && insert_pos<n; insert_pos++);
for(;sequences[cur_pos] != 1 && cur_pos>=0; cur_pos--);
if(cur_pos > insert_pos)
{
int temp = sequences[insert_pos];
sequences[insert_pos] = sequences[cur_pos];
sequences[cur_pos] = temp;
count ++;
}
else
break;
}
for(int i=0; i<n; i++)
{
cout << sequences[i] << " ";
}
cout << endl;
// deal with 2
//insert_pos = 0;
cur_pos = n-1;
while(true)
{
for(;sequences[insert_pos] == 2 && insert_pos<n; insert_pos++);
for(;sequences[cur_pos] != 2 && cur_pos>=0; cur_pos--);
if(cur_pos > insert_pos)
{
int temp = sequences[insert_pos];
sequences[insert_pos] = sequences[cur_pos];
sequences[cur_pos] = temp;
count ++;
}
else
break;
}
//cout << count << endl;
for(int i=0; i<n; i++)
{
cout << sequences[i] << " ";
}
file_out << count << endl;
file_in.close();
file_out.close();
return count;
}
void printCount(int (*pos_count)[4])
{
//return;
for(int i=1; i<4; i++)
{
for(int j=1; j<4; j++)
cout << pos_count[i][j] << " ";
cout << endl;
}
cout << endl;
}
//先交换那些一次交换就可以回到正确位置的数字
int Greed()
{
ifstream file_in("sort3.in");
ofstream file_out("sort3.out");
if(!file_in || !file_out)
{
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
file_in >> n;
int change_count = 0;
int count[4]={0};
// read all the data first
for(int i=0; i<n; i++)
{
file_in >> sequences[i];
count[sequences[i]]++;
}
// 先找出1,2,3的个数,贪婪地先把一次交换可以是两个数字回到正确的位置上
for(int i=1; i<4; i++)
cout << count[i] << " ";
cout << endl;
//统计1位置上2,3的个数,2位置上1,3个数,3位置上1,2个数
int pos_count[4][4] = {0};
for(int i=1, pos=0; i<4; i++)
{
for(int j=pos; j<pos+count[i]; j++)
{
pos_count[i][sequences[j]]++;
}
pos += count[i];
}
printCount(pos_count);
// 1, 3交换
int change_pos[3][2] =
{
{1, 3},
{1, 2},
{2, 3}
};
for(int i=0; i<3; i++)
{
int pos1 = change_pos[i][0];
int pos2 = change_pos[i][1];
int min_count = min(pos_count[pos1][pos2], pos_count[pos2][pos1]);
pos_count[pos1][pos2] -= min_count;
pos_count[pos2][pos1] -= min_count;
pos_count[pos1][pos1] += min_count;
pos_count[pos2][pos2] += min_count;
printCount(pos_count);
change_count += min_count;
}
for(int i=2; i<4; i++)
{
change_count += pos_count[1][i]*2;
}
cout << change_count << endl;
file_out << change_count << endl;
return change_count;
}
int main()
{
Greed();
//Sequence();
return 0;
}
<file_sep>/section 1/beads.cpp
/*
ID: luglian1
PROG: beads
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int main(){
ifstream file_in("beads.in");
ofstream file_out("beads.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
string beads;
file_in >> n >> beads;
beads += beads;
int maxn=0;
bool one_color = true;
for(int i=0; i!=beads.size()-1; ++i){
if( beads.at(i) != beads.at(i+1) ){ // try break
one_color = false;
int newn=0;
char onec = beads.at(i), otherc = beads.at(i+1);
if(onec == 'r') otherc = 'b';
if(onec == 'b') otherc = 'r';
if(otherc == 'r') onec = 'b';
if(otherc == 'b') onec = 'r';
for(int j=i; j>-1; --j){
if(beads.at(j) == onec || beads.at(j) == 'w')
++ newn;
else
break;
}
for(int j=i+1; j < beads.size(); ++j){
if(beads.at(j) == otherc || beads.at(j) == 'w')
++ newn;
else
break;
}
maxn = maxn > newn ? maxn : newn;
}
}
if(one_color || maxn > beads.size()/2)
maxn = beads.size() / 2;
file_out << maxn << endl;
cout << maxn << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/transform.cpp
/*
ID: luglian1
PROG: transform
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
class Square;
class Square{
int n;
char data[10][10];
public:
friend istream& operator>>(istream& ins, Square& s);
friend ostream& operator<<(ostream& outs, Square& s);
int size(){ return n; }
Square(int size): n(size) { };
Square(const Square& s): n(s.n){
for(int i=0; i!=n; ++i){
for(int j=0; j!=n; ++j){
data[i][j] = s.data[i][j];
}
}
}
void rotate90(){
// rotate in place
for(int i = 0; i!= (n+1)/2; ++i){
for(int j=0; j!=n/2; ++j){
int tmp = data[i][j];
data[i][j] = data[n-1-j][i];
data[n-1-j][i] = data[n-1-i][n-1-j];
data[n-1-i][n-1-j] = data[j][n-1-i];
data[j][n-1-i] = tmp;
}
}
}
void reflect(){
// reflect in place
for(int i=0; i!=n; ++i){
for(int j=0; j!=n/2; ++j){
int tmp = data[i][j];
data[i][j] = data[i][n-1-j];
data[i][n-1-j] = tmp;
}
}
}
Square& operator=(const Square& rhs){
n = rhs.n;
for(int i=0; i!=n; ++i){
for(int j=0; j!=n; ++j){
data[i][j] = rhs.data[i][j];
}
}
}
bool operator==(const Square& rhs) const{
if(n == rhs.n){
for(int i=0; i!=n; ++i){
for(int j=0; j!=n; ++j){
if(data[i][j] != rhs.data[i][j])
return false;
}
}
}
else
return false;
return true;
}
};
istream& operator>>(istream& ins, Square& s){
for(int i=0; i!=s.n; ++i){
string line;
ins >> line;
for(int j=0; j!=s.n; ++j){
s.data[i][j] = line.at(j);
}
}
return ins;
}
ostream& operator<<(ostream& outs, Square& s){
for(int i=0; i!=s.n; ++i){
for(int j=0; j!=s.n; ++j){
outs << s.data[i][j];
}
outs << endl;
}
return outs;
}
int main(){
ifstream file_in("transform.in");
ofstream file_out("transform.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
file_in >> n;
Square origin(n);
Square target(n);
file_in >> origin >> target;
cout << origin << target;
// // test rotate
// cout << origin << endl;
// origin.rotate90();
// cout << origin << endl;
// // test reflect
// origin.reflect();
// cout << origin << endl;
bool fit = false;
int state=0;
int return_s[] = {1, 2, 3, 4, 5, 5, 5, 6, 7};
for(int i=0; i!=2; ++i){
if(fit) break;
for(int j=0; j!=3; ++j){
origin.rotate90();
if( origin == target ){
fit = true;
break;
}
++state;
}
origin.rotate90();
origin.reflect();
if(origin == target){
fit = true;
break;
}
if(fit) break;
++state;
}
file_out << return_s[state] << endl;
cout << return_s[state] << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/checker.cpp
/*
ID: luglian1
PROG: checker
LANG: C++
*/
#include <iostream>
#include <string>
#include <sstream>
#include <fstream>
#include <vector>
using namespace std;
bool diagonal[200] = {false};
bool rediagonal[200] = {false};
bool col[100] = {false};
int n;
int count = 0;
int solution[100] = {0};
vector<string> prints;
void dfs(int row){
if(row == n){
++ count;
if(count<4){
stringstream ss;
for(int i=0; i!=n-1; ++i)
ss << solution[i]+1 << " ";
ss << solution[n-1]+1;
prints.push_back(ss.str());
}
return;
}
for(int i=0; i!=n; ++i){
if(!col[i] && !diagonal[row-i+n] && !rediagonal[n-row-i+n] ){
col[i] = true;
diagonal[row-i+n] = true;
rediagonal[n-row-i+n] = true;
solution[row] = i;
dfs(row+1);
col[i] = false;
diagonal[row-i+n] = false;
rediagonal[n-row-i+n] = false;
}
}
}
void work(){
dfs(0);
}
void print(ostream& file_out){
for(int i=0; i!=prints.size(); ++i)
file_out << prints[i] << endl;
file_out << count << endl;
}
int main(){
ifstream file_in("checker.in");
ofstream file_out("checker.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
file_in >> n;
work();
print(file_out);
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/dualpal.cpp
/*
ID: luglian1
PROG: dualpal
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
using namespace std;
bool checkPalindromic(const string& s){
for(int i=0; i!=s.size()/2; ++i){
if(s.at(i) != s.at(s.size()-i-1) )
return false;
}
return true;
}
string digits = "0123456789ABCDEFGHIJKLMN";
string buildString(int i, int base){
string res;
int quo = i;
while(quo > 0){
res = digits.at(quo % base)+res;
quo = quo / base;
}
return res;
}
int main(){
ifstream file_in("dualpal.in");
ofstream file_out("dualpal.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n, s;
file_in >> n >> s;
int num=s+1;
vector<int> nv;
while(nv.size() != n){
int count=0;
for(int base=2; base!=11; ++base){
if(checkPalindromic(buildString(num, base)))
++count;
if(count >= 2){
nv.push_back(num);
break;
}
}
++num;
}
for(int i=0; i!=nv.size(); ++i)
file_out << nv[i] << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/ride.cpp
/*
ID: luglian1
PROG: ride
LANG: C++
*/
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main(){
ifstream in("ride.in");
ofstream out("ride.out");
string comet, group;
in >> comet >> group;
int c=1, g=1;
for(int i=0; i!=comet.size(); ++i){
c = (c * (comet.at(i)-'A'+1)) % 47;
}
for(int i=0; i!=group.size(); ++i){
g = (g * (group.at(i)-'A'+1)) % 47;
}
if( c== g )
out << "GO" << endl;
else
out << "STAY" << endl;
in.close();
out.close();
return 0;
}<file_sep>/mytest/boost/array_test.cpp
#include <boost/array.hpp>
#include <iostream>
using namespace std;
int main()
{
boost::array<int, 2> a;
boost::array<int, 2> b = {1, 2};
cout << 1 << endl;
cout << a[0] << a[1] << endl;
return 0;
}<file_sep>/section 1/milk3.cpp
/*
ID: luglian1
PROG: milk3
LANG: C++
*/
#include <iostream>
#include <string>
#include <set>
#include <vector>
#include <fstream>
using namespace std;
set<int> cset;
enum buckets {A=0, B, C};
int Capacity[3] = {0};
int cleft[21] = {0};
// failed, over time
int tf[21][21] = {0};
void searcha(int* cur, int depth){
// if(depth <= 0)
// return;
if(tf[cur[0]][cur[1]] == 1)
return;
tf[cur[0]][cur[1]] = 1;
if(cur[0] == 0){
cleft[cur[2]] = 1;
}
for(int i=0; i!=3; ++i){
if(cur[i] !=0){
for(int j=0; j!=3; ++j){
if(j == i) continue;
int change = min(cur[i], Capacity[j]-cur[j]);
cur[j] += change;
cur[i] -= change;
searcha(cur, depth-1);
cur[i] += change;
cur[j] -= change;
}
}
}
}
// void searcha3(int i, int k){
// if()
// }
int gcd(int a, int b){
if(b > a)
swap(a, b);
int rem = a%b;
while(rem!=0){
a = b;
b = rem;
rem = a % b;
}
return b;
}
void pours(int* cur, int from, int to){
if(from == to)
return;
int change = min(cur[from], Capacity[to]-cur[to]);
cur[to] += change;
cur[from] -= change;
}
//
void searcha2(int* cur, int depth){
int tmpcur[3];
copy(cur, cur+3, tmpcur);
for(int i = depth*3+2; i>=1; --i){
if(tmpcur[0] == 0)
cleft[tmpcur[2]] = 1;
pours(tmpcur, i%3, (i-1)%3);
}
copy(cur, cur+3, tmpcur);
for(int i = 2; i<=depth*3+1; ++i){
if(tmpcur[0] == 0)
cleft[tmpcur[2]] = 1;
pours(tmpcur, i%3, (i+1)%3);
}
}
int main(){
ifstream file_in("milk3.in");
ofstream file_out("milk3.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
for(int i=0; i!=3; ++i)
file_in >> Capacity[i];
int cur[3] = {0};
cur[2] = Capacity[2];
searcha(cur, 13);
//searcha2(cur, 12);
// if(Capacity[0] <= Capacity[1]/2 ){
// for(int i=Capacity[2]-Capacity[1]; i<=Capacity[2]; ++i)
// cleft[i] = 1;
// }
vector<int> cvec;
for(int i=0; i!=21; ++i){
if(cleft[i] == 1){
cvec.push_back(i);
cout << i << endl;
}
}
cout << cvec.size() << endl;
for(int i=0; i!=cvec.size()-1; ++i)
file_out << cvec[i] << " ";
file_out << cvec.back() << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section2/nocows.cpp
/*
ID: luglian1
PROG: nocows
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cstring>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "nocows"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
int N = 0; // [3, 199]
int K = 0; // [2, 99]
int count = 0;
int tab[100][200]; // [k, n] count
int nc[100][200]; // [n, <=k] count
bool load();
void save(ostream& out);
bool save();
void solve();
int cc();
int main(){
load();
solve();
//cc();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N >> K;
for(int i=0; i<100; i++)
for(int j=0; j<200; j++)
{
tab[i][j] = 0;
nc[i][j] = 0;
}
return true;
}
void save(ostream& out)
{
out << tab[K][N] << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
tab[1][1] = 1;
//nc[2][1] = 1;
for(int k=2; k<100; k++)
{
int minn = k * 2 - 1;
int maxn = 200;
if(k < 8)
maxn = 1 << k;
int i = k-1;
//PV(k)
for(int m = 1; m < 200; m++)
{
for(int ni = i-1; ni>=0; ni--)
nc[i][m] += tab[ni][m];
nc[i][m] = nc[i][m] % 9901;
}
for(int n = minn; n < maxn; n += 2)
{
//PV(n)
int sum = 0;
for(int m = n-2; m >= 1; m--)
{
//PV(m)
int rightm = n-1-m;
int add = 0;
add += tab[i][m] * nc[i][rightm] % 9901;
add += tab[i][rightm] * nc[i][m] % 9901;
add += tab[i][m] * tab[i][rightm] % 9901;
sum += add % 9901;
}
tab[k][n] = sum % 9901;
//cout << k << ", " << n << ", " << tab[k][n] << endl;
}
}
for(int k=1; k<100; k++)
{
for(int n=0; n<200; n++)
cout << "[" << n << "]" << tab[k][n] << ", ";
cout << endl;
}
cout << endl;
for(int k=1; k<100; k++)
{
for(int n=0; n<200; n++)
cout << "[" << n << "]" << nc[k][n] << ", ";
cout << endl;
}
}
int cc()
{
ifstream fin("nocows.in");
ofstream fout("nocows.out");
int n,k,dp[200][200];
fin>>n>>k;
memset(dp,0,sizeof(dp));
dp[1][1]=1;
for (int i=3;i<=n;i+=2)
for (int j=1;j<=i-2;j+=2)
for (int k1=1;k1<=(j+1)/2;k1++)
for (int k2=1;k2<=(i-j)/2;k2++)
{
dp[i][max(k1,k2)+1]+=dp[j][k1]*dp[i-j-1][k2];
dp[i][max(k1,k2)+1]%=9901;
}
for(int k=1; k<100; k++)
{
for(int n=0; n<200; n++)
cout << "[" << n << "]" << dp[n][k] << ", ";
cout << endl;
}
fout<<dp[n][k]<<endl;
cout<<dp[n][k]<<endl;
return 0;
}<file_sep>/section2/concom.cpp
/*
ID: luglian1
PROG: concom
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "concom"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int N = 0;
int x[101][101] = {0};
int c[101][101] = {0};
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
for(int i=0; i<N; ++i)
{
int a, b, c;
file_in >> a >> b >> c;
x[a][b] = c;
}
return true;
}
void save(ostream& out)
{
for(int i=1; i<=100; ++i)
for(int j=1; j<=100; ++j)
{
if(i == j)
continue;
if(c[i][j])
{
out << i << " " << j << endl;
}
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
for(int t=0; t<100; ++t)
{
for(int i=1; i<=100; ++i)
for(int j=1; j<=100; ++j)
{
if(c[i][j])
continue;
int xall = x[i][j];
for(int k=1; k<=100; ++k)
{
if(c[i][k])
xall += x[k][j];
}
if(xall >= 50)
{
c[i][j] = 1;
}
}
}
}
// example
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define NCOM 101
// int owns[NCOM][NCOM]; /* [i,j]: how much of j do i and its
// controlled companies own? */
// int controls[NCOM][NCOM]; /* [i, j]: does i control j? */
// /* update info: now i controls j */
// void
// addcontroller(int i, int j)
// {
// int k;
// if(controls[i][j]) /* already knew that */
// return;
// controls[i][j] = 1;
// /* now that i controls j, add to i's holdings everything from j */
// for(k=0; k<NCOM; k++)
// owns[i][k] += owns[j][k];
// /* record the fact that controllers of i now control j */
// for(k=0; k<NCOM; k++)
// if(controls[k][i])
// addcontroller(k, j);
// /* if i now controls more companies, record that fact */
// for(k=0; k<NCOM; k++)
// if(owns[i][k] > 50)
// addcontroller(i, k);
// }
// /* update info: i owns p% of j */
// void
// addowner(int i, int j, int p)
// {
// int k;
// /* add p% of j to each controller of i */
// for(k=0; k<NCOM; k++)
// if(controls[k][i])
// owns[k][j] += p;
// /* look for new controllers of j */
// for(k=0; k<NCOM; k++)
// if(owns[k][j] > 50)
// addcontroller(k, j);
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, j, n, a, b, p;
// fin = fopen("concom.in", "r");
// fout = fopen("concom.out", "w");
// assert(fin != NULL && fout != NULL);
// for(i=0; i<NCOM; i++)
// controls[i][i] = 1;
// fscanf(fin, "%d", &n);
// for(i=0; i<n; i++) {
// fscanf(fin, "%d %d %d", &a, &b, &p);
// addowner(a, b, p);
// }
// for(i=0; i<NCOM; i++)
// for(j=0; j<NCOM; j++)
// if(i != j && controls[i][j])
// fprintf(fout, "%d %d\n", i, j);
// exit(0);
// }<file_sep>/section2/comehome.cpp
/*
ID: luglian1
PROG: comehome
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "comehome"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAX_DIS 10000000
#define LEN 58
using namespace std;
int n=0;
int g[LEN][LEN] = {0};
int path[LEN] = {0};
int res = 0;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> n;
for(int i=0; i<n; ++i)
{
char a, b;
int dis;
file_in >> a >> b >> dis;
a = a - 'A';
b = b - 'A';
if(g[a][b] == 0 || dis < g[a][b])
{
g[a][b] = g[b][a] = dis;
// cout << int(a) << ", " << int(b) << ", " << dis << endl;;
}
}
// PVL(g[0], LEN)
// PV(g[0][57])
return true;
}
void save(ostream& out)
{
out << char('A' + res) << " " << g[25][res] << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
for(int i=0; i<LEN; ++i)
{
path[i] = MAX_DIS;
for(int j=0; j<LEN; ++j)
if(g[i][j] == 0)
g[i][j] = MAX_DIS;
g[i][i] = 0;
}
int src = 25;
path[src] = 0;
for(int k=0; k<LEN; ++k)
for(int i=0; i<LEN; ++i)
{
for(int j=0; j<LEN; ++j)
{
int newd = g[i][k] + g[k][j];
if(newd < g[i][j])
g[i][j] = newd;
}
}
// PVL(g[src], LEN)
int mind = MAX_DIS;
for(int i=0; i<src; ++i)
{
if(i == src)
continue;
if(g[src][i] < mind)
{
mind = g[src][i];
res = i;
}
}
// PV(res)
}
// We use the Floyd-Warshall all pairs shortest path algorithm to calculate the minimum distance between the barn and all other points in the pasture. Then we scan through all the cow-containing pastures looking for the minimum distance.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #include <ctype.h>
// #define INF 60000 /* bigger than longest possible path */
// int dist[52][52];
// int
// char2num(char c)
// {
// assert(isalpha(c));
// if(isupper(c))
// return c-'A';
// else
// return c-'a'+26;
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, j, k, npath, d;
// char a, b;
// int m;
// fin = fopen("comehome.in", "r");
// fout = fopen("comehome.out", "w");
// assert(fin != NULL && fout != NULL);
// for(i=0; i<52; i++)
// for(j=0; j<52; j++)
// dist[i][j] = INF;
// for(i=0; i<26; i++)
// dist[i][i] = 0;
// fscanf(fin, "%d\n", &npath);
// for(i=0; i<npath; i++) {
// fscanf(fin, "%c %c %d\n", &a, &b, &d);
// a = char2num(a);
// b = char2num(b);
// if(dist[a][b] > d)
// dist[a][b] = dist[b][a] = d;
// }
// /* floyd warshall all pair shortest path */
// for(k=0; k<52; k++)
// for(i=0; i<52; i++)
// for(j=0; j<52; j++)
// if(dist[i][k]+dist[k][j] < dist[i][j])
// dist[i][j] = dist[i][k]+dist[k][j];
// /* find closest cow */
// m = INF;
// a = '#';
// for(i='A'; i<='Y'; i++) {
// d = dist[char2num(i)][char2num('Z')];
// if(d < m) {
// m = d;
// a = i;
// }
// }
// fprintf(fout, "%c %d\n", a, m);
// exit(0);
// }
// Analysis of and code for Bessie Come Home by <NAME> of The Netherlands
// When looking at the problem the first thing you can conclude is that for the solution you will need to know all the distances from the pastures to the barn. After calculating them you only have to check all these distances and pick out the nearest pasture with a cow in it, and that's all.
// Because the amount of vertices (=pastures+barn) is small, running Floyd/Warshall algorithm will solve the problem easily in time. If you think programming Floyd/Warshall is easier than Dijkstra, just do it. But you can also solve the problem running Dijkstra once, which of course speeds up your program quite a bit. Just initialise the barn as starting point, and the algorithm will find the distances from the barn to all the pastures which is the same as the distances from all the pastures to the barn because the graph is undirected. Using dijkstra for the solution would make far more complex data solvable within time. Here below you can see my implementation of this solution in Pascal. It might look big, but this way of partitioning your program keeps it easy to debug.
// Var Dist:Array [1..58] of LongInt; {Array with distances to barn}
// Vis :Array [1..58] of Boolean; {Array keeping track which
// pastures visited}
// Conn:Array [1..58,1..58] of Word; {Matrix with length of edges, 0 = no edge}
// Procedure Load;
// Var TF :Text;
// X,D,E:Word;
// P1,P2:Char;
// Begin
// Assign(TF,'comehome.in');
// Reset(TF);
// Readln(TF,E); {Read number of edges}
// For X:=1 to E do
// Begin
// Read(TF,P1); {Read both pastures and edge
// length}
// Read(TF,P2);
// Read(TF,P2); {Add edge in matrix if no edge between P1 and P2 yet or}
// Readln(TF,D); {this edge is shorter than the shortest till now}
// If (Conn[Ord(P1)-Ord('A')+1,Ord(P2)-Ord('A')+1]=0) or
// (Conn[Ord(P1)-Ord('A')+1,Ord(P2)-Ord('A')+1]>D) then
// Begin
// Conn[Ord(P1)-Ord('A')+1,Ord(P2)-Ord('A')+1]:=D;
// Conn[Ord(P2)-Ord('A')+1,Ord(P1)-Ord('A')+1]:=D;
// End;
// End;
// Close(TF);
// For X:=1 to 58 do
// Dist[X]:=2147483647; {Set all distances to infinity}
// Dist[Ord('Z')-Ord('A')+1]:=0; {Set distance from barn to barn to 0}
// End;
// Procedure Solve;
// Var X,P,D:LongInt; {P = pasture and D = distance}
// Begin
// Repeat
// P:=0;
// D:=2147483647;
// For X:=1 to 58 do {Find nearest pasture not
// visited yet}
// If Not Vis[X] and (Dist[X]<D) then
// Begin
// P:=X;
// D:=Dist[X];
// End;
// If (P<>0) then
// Begin
// Vis[P]:=True; {If there is one mark it
// visited}
// For X:=1 to 58 do {And update all distances}
// If (Conn[P,X]<>0) and (Dist[X]>Dist[P]+Conn[P,X]) then
// Dist[X]:=Dist[P]+Conn[P,X];
// End;
// Until (P=0); {Until no reachable and unvisited pastures
// left}
// End;
// Procedure Save;
// Var TF :Text;
// X,BD:LongInt; {BD = best distance}
// BP :Char; {BP = best pasture}
// Begin
// BD:=2147483647;
// For X:=1 to 25 do {Find neares pasture}
// If (Dist[X]<BD) then
// Begin
// BD:=Dist[X];
// BP:=Chr(Ord('A')+X-1);
// End;
// Assign(TF,'comehome.out');
// Rewrite(TF);
// Writeln(TF,BP,' ',BD); {Write outcome to disk}
// Close(TF);
// End;
// Begin
// Load;
// Solve;
// Save;
// End.<file_sep>/section3/camelot.cpp
/*
ID: luglian1
PROG: camelot
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <queue>
#include <set>
#include <cassert>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << (v)[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "camelot"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAX_ROW 30
#define MAX_COL 26
#define MAX_SIZE MAX_ROW * MAX_COL
#define INF 0x7ffffffe
typedef unsigned int uint;
using namespace std;
int row = 0, col = 0;
int maxs = 0;
uint res = 0;
uint grid[MAX_SIZE][MAX_SIZE] = {0};
int king = 0;
vector<int> knight;
bool load();
void save(ostream& out);
bool save();
void solve();
void solve2();
int main(){
load();
solve2();
save();
return 0;
}
int getPos(int r, const string& c)
{
return (r-1) * col + c[0] - 'A';
}
void getrc(int pos, int& r, int& c)
{
r = pos / col;
c = pos - r * col;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> row >> col;
maxs = row * col;
int r;
string c;
file_in >> c >> r;
king = getPos(r, c);
set<int> sk;
while(file_in.eof() == 0)
{
file_in >> c;
file_in >> r;
//knight.push_back(getPos(r, c));
sk.insert(getPos(r, c));
}
// for(int i=0; i<maxs; ++i)
// {
// if(sk.count(i) == 0)
// {
// PV(i)
// sk.insert(i);
// }
// }
for(set<int>::iterator it=sk.begin(); it!=sk.end(); ++it)
knight.push_back(*it);
PV(row)
PV(col)
PV(maxs)
PV(king)
PV(knight.size())
PVL(knight, knight.size())
return true;
}
void save(ostream& out)
{
out << res << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
int near[8][2] = {{-1, -2}, {-2, -1}, {-2, 1}, {-1, 2}, {1, -2}, {2, -1}, {1, 2}, {2, 1}};
int neardiff[8] = {0};
int vn[MAX_SIZE];
int mark[MAX_SIZE];
int vton[MAX_SIZE];
inline bool compare(int src, int i, int j)
{
return grid[src][i] > grid[src][j];
}
vector<int> find_near(int c)
{
vector<int> res;
for(int i=0; i<8; ++i)
{
int n = c + neardiff[i];
if(n < 0 || n >= maxs)
continue;
res.push_back(n);
}
return res;
}
inline void my_swap(int i, int j)
{
swap(vn[i], vn[j]);
swap(vton[vn[i]], vton[vn[j]]);
}
void goup_heap(int src, int i)
{
int p=i;
do
{
p = (i - 1) / 2;
if(p >=0 && compare(src, vn[p], vn[i]))
{
my_swap(p, i);
i = p;
}
else
{
break;
}
}while(i > 0);
}
void godown_heap(int src, int i, int n)
{
int p = i;
do
{
int ch1 = 2 * p + 1;
int ch2 = 2 * p + 2;
int min = p;
if(ch2 < n)
{
if(compare(src, vn[p], vn[ch1]) && compare(src, vn[ch2], vn[ch1]))
min = ch1;
else if(compare(src, vn[p], vn[ch2]))
min = ch2;
}
else if(ch1 < n)
{
if(compare(src, vn[p], vn[ch1]))
min = ch1;
}
else
{
break;
}
if(min == p || min >= n)
{
break;
}
//assert(dist[cur_src][vn[min]] < dist[cur_src][vn[p]]);
my_swap(p, min);
p = min;
}while(p < n);
}
void my_pop_heap(int src, int n)
{
my_swap(0, n-1);
godown_heap(src, 0, n-1);
}
void dijkstra(int src)
{
for(int i=0; i<maxs; ++i)
{
vn[i] = i;
}
for(int i=0; i<maxs; ++i)
{
vton[vn[i]] = i;
}
vector<int> ns = find_near(src);
grid[src][src] = 0;
goup_heap(src, src);
for(vector<int>::iterator it=ns.begin(); it!=ns.end(); ++it)
{
grid[src][*it] = 1;
goup_heap(src, *it);
}
for(int i=maxs; i>0; --i)
{
int n = vn[0];
my_pop_heap(src, i);
for(int j=0; j<8; ++j)
{
int next = n + neardiff[j];
if(next < 0 || next >= maxs)
continue;
if(vton[next] <= i-1 && grid[src][n] + 1 < grid[src][next])
{
grid[src][next] = grid[src][n] + 1;
goup_heap(src, vton[next]);
}
}
}
}
void solve()
{
for(int i=0; i<maxs; ++i)
for(int j=0; j<maxs; ++j)
grid[i][j] = INF;
for(int i=0; i<8; ++i)
{
neardiff[i] = near[i][0] * row + near[i][1];
}
PVL(neardiff, 8)
for(int i=0; i<knight.size(); ++i)
{
dijkstra(knight[i]);
}
for(int i=0; i<knight.size(); ++i)
{
PVL(grid[knight[i]], maxs)
}
}
void bfs(int src)
{
queue<int> node;
node.push(src);
grid[src][src] = 0;
while(!node.empty())
{
int cur = node.front();
int r, c;
getrc(cur, r, c);
node.pop();
int move = grid[src][cur];
for(int j=0; j<8; ++j)
{
// int nr = r + near[j][0];
// int nc = c + near[j][1];
// if (nr < 0 || nr >= row || nc < 0 || nc >=col)
// continue;
// int next = nr * col + nc;
// if(grid[src][next] < INF)
// continue;
int next = cur + neardiff[j];
if(next < 0 || next >= maxs || grid[src][next] < INF ||
r + near[j][0] < 0 || r + near[j][0] >= row ||
c + near[j][1] < 0 || c + near[j][1] >=col)
continue;
grid[src][next] = move + 1;
node.push(next);
// PV(next)
// PV(grid[src][next])
}
}
}
int near_knight[MAX_SIZE];
uint king_grid[MAX_SIZE][MAX_SIZE] = {0};
uint res_grid[MAX_SIZE] = {0};
int king_dist(int src, int dst)
{
int sr, sc;
getrc(src, sr, sc);
int dr, dc;
getrc(dst, dr, dc);
int r = abs(dr - sr);
int c = abs(dc - sc);
return r + c - min(r, c);
}
void king_path()
{
for(int i=0; i<maxs; ++i)
for(int j=0; j<maxs; ++j)
king_grid[i][j] = king_dist(i, j);
}
void solve2()
{
for(int i=0; i<maxs; ++i)
for(int j=0; j<maxs; ++j)
{
grid[i][j] = INF;
king_grid[i][j] = INF;
}
for(int i=0; i<8; ++i)
{
neardiff[i] = near[i][0] * col + near[i][1];
}
// PVL(neardiff, 8)
for(int i=0; i<maxs; ++i)
{
bfs(i);
}
// PVL(near_knight, maxs)
// for(int i=0; i<knight.size(); ++i)
// {
// PVL(grid[knight[i]], maxs)
// }
king_path();
PVL(king_grid[0], maxs)
if(knight.empty())
return;
for(int i=0; i<maxs; ++i)
{
res_grid[i] = 0;//king_grid[king][i];
for(int j=0; j<knight.size(); ++j)
{
res_grid[i] += grid[knight[j]][i];
}
// PV(res_grid[i])
}
PVL(res_grid, maxs)
vector<int> search_pos;
int kr, kc;
getrc(king, kr, kc);
int range = 3;
for(int i=-range; i<=range; ++i)
{
for(int j=-range; j<=range; ++j)
{
int r = kr + i;
int c = kc + j;
if(r < 0 || r >= row || c < 0 || c >=col)
continue;
search_pos.push_back(r * col + c);
}
}
PVL(search_pos, search_pos.size())
res = INF;
uint minp = 0;
for(int i=0; i<maxs; ++i)
{
int mind = INF;
for(vector<int>::iterator itj=search_pos.begin(); itj!=search_pos.end(); ++itj)
//for(int j=0; j<maxs; ++j)
{
int j = *itj;
for(int k=0; k<knight.size(); ++k)
{
int kn = knight[k];
long long d = king_grid[king][j] + grid[kn][j] + grid[j][i] - grid[kn][i];
if(d < mind)
mind = d;
}
}
// // if(mind < 0)
res_grid[i] = res_grid[i] + mind;
if(res_grid[i] < res)
{
res = res_grid[i];
minp = i;
}
}
int r, c;
getrc(minp, r, c);
PV(minp)
PV(r)
PV(c)
// bfs(knight[0]);
// PVL(grid[knight[0]], maxs)
}
// This is a modification of the shortest path algorithm. If there was no king, then the shortest path algorithm can determine the distance that each knight must travel to get to each square. Thus, the cost of gathering in a particular square is simply the sum of the distance that each knight must travel, which is fairly simple to calculate.
// In order to consider the king, consider a knight which 'picks-up' the king in some square and then travels to the gathering spot. This costs some number of extra moves than just traveling to the gathering spot. In particular, the king must move to the pick-up square, and the knight must travel to this square and then to the final gathering point. Consider the number of extra moves to be the `cost' for that knight to pick-up the king. It is simple to alter the shortest path algorithm to consider picking-up the king by augmenting the state with a boolean flag stating whether the knight has the king or not.
// In this case, the cost for gathering at a particular location is the sum of the distance that each knight must travel to get to that square plus the minimum cost for a knight picking up the king on the way.
// Thus, for each square, we keep two numbers, the sum of the distance that all the knights that we have seen thus far would have to travel to get to this square and the minimum cost for one of those knights picking up the king on the way (note that one way to 'pick-up' the king is to have the king travel all by itself to the gathering spot). Then, when we get a new knight, we run the shortest path algorithm and add the cost of getting that knight (without picking up the king) to each square to the cost of gathering at that location. Additionally, for each square, we check if the new knight can pick-up the king in fewer moves than any previous knight, and update that value if it can.
// After all the knights have been processed, we determine the minimum over all squares of the cost to get to that square plus the additional cost for a knight to pick-up the king on its way to that square.
// #include <stdio.h>
// #include <string.h>
// #include <stdlib.h>
// /* "infinity"... > maximum distance possible (for one knight) */
// #define MAXN 10400
// /* maximum number of rows */
// #define MAXR 40
// /* maximum number of columns */
// #define MAXC 26
// /* cost of collecting all knights here */
// int cost[MAXC][MAXR];
// /* cost of getting a knight to collect the king */
// int kingcost[MAXC][MAXR];
// /* distance the king must travel to get to this position */
// int kdist[MAXC][MAXR];
// /* distance to get for current knight to get to this square */
// /* third index: 0 => without king, 1 => with king */
// int dist[MAXC][MAXR][2];
// /* number of rows and columns */
// int nrow, ncol;
// int do_step(int x, int y, int kflag) {
// int f = 0; /* maximum distance added */
// int d = dist[x][y][kflag]; /* distance of current move */
// /* go through all possible moves that a knight can make */
// if (y > 0) {
// if (x > 1)
// if (dist[x-2][y-1][kflag] > d+1) {
// dist[x-2][y-1][kflag] = d+1;
// f = 1;
// }
// if (x < ncol-2) {
// if (dist[x+2][y-1][kflag] > d+1) {
// dist[x+2][y-1][kflag] = d+1;
// f = 1;
// }
// }
// if (y > 1) {
// if (x > 0)
// if (dist[x-1][y-2][kflag] > d+1) {
// dist[x-1][y-2][kflag] = d+1;
// f = 1;
// }
// if (x < ncol-1)
// if (dist[x+1][y-2][kflag] > d+1) {
// dist[x+1][y-2][kflag] = d+1;
// f = 1;
// }
// }
// }
// if (y < nrow-1) {
// if (x > 1)
// if (dist[x-2][y+1][kflag] > d+1) {
// dist[x-2][y+1][kflag] = d+1;
// f = 1;
// }
// if (x < ncol-2) {
// if (dist[x+2][y+1][kflag] > d+1) {
// dist[x+2][y+1][kflag] = d+1;
// f = 1;
// }
// }
// if (y < nrow-2) {
// if (x > 0)
// if (dist[x-1][y+2][kflag] > d+1) {
// dist[x-1][y+2][kflag] = d+1;
// f = 1;
// }
// if (x < ncol-1)
// if (dist[x+1][y+2][kflag] > d+1) {
// dist[x+1][y+2][kflag] = d+1;
// f = 1;
// }
// }
// }
// /* also check the 'pick up king here' move */
// if (kflag == 0 && dist[x][y][1] > d + kdist[x][y]) {
// dist[x][y][1] = d + kdist[x][y];
// if (kdist[x][y] > f) f = kdist[x][y];
// }
// return f; /* 1 if simple knight move made, 0 if no new move found */
// }
// void calc_dist(int col, int row) {
// int lv, lv2; /* loop variables */
// int d; /* current distance being checked */
// int max; /* maximum finite distance found so far */
// int f; /* temporary variable (returned value from do_step */
// /* initiate all positions to be infinite distance away */
// for (lv = 0; lv < ncol; lv++)
// for (lv2 = 0; lv2 < nrow; lv2++)
// dist[lv][lv2][0] = dist[lv][lv2][1] = MAXN;
// /* starting location is zero w/o king, kdist[col][row] with king */
// dist[col][row][0] = 0;
// max = dist[col][row][1] = kdist[col][row];
// for (d = 0; d <= max; d++) { /* for each distance away */
// for (lv = 0; lv < ncol; lv++)
// for (lv2 = 0; lv2 < nrow; lv2++) {
// /* for each position that distance away */
// if (dist[lv][lv2][0] == d) {
// /* update with moves through this square */
// f = do_step(lv, lv2, 0);
// if (d + f > max) /* update max if necessary */
// max = d + f;
// }
// if (dist[lv][lv2][1] == d) {
// /* same as above, except this time knight has king */
// f = do_step(lv, lv2, 1);
// if (d + f > max) max = d + f;
// }
// }
// }
// }
// int main(int argc, char **argv) {
// FILE *fout, *fin;
// char t[10];
// int pr, pc;
// int lv, lv2;
// int i, j;
// if ((fin = fopen("camelot.in", "r")) == NULL) {
// perror ("fopen fin");
// exit(1);
// }
// if ((fout = fopen("camelot.out", "w")) == NULL) {
// perror ("fopen fout");
// exit(1);
// }
// fscanf (fin, "%d %d", &nrow, &ncol);
// fscanf (fin, "%s %d", t, &pr);
// pc = t[0] - 'A';
// pr--;
// /* Calculate cost of moving king from starting position to
// * each board position. This is just the taxi-cab distance */
// for (lv = 0; lv < ncol; lv++)
// for (lv2 = 0; lv2 < nrow; lv2++) {
// i = abs(pc-lv);
// j = abs(pr-lv2);
// if (i < j) i = j;
// kingcost[lv][lv2] = kdist[lv][lv2] = i;
// }
// while (fscanf (fin, "%s %d", t, &pr) == 2) { /* for all knights */
// pc = t[0] - 'A';
// pr--;
// /* calculate distances */
// calc_dist(pc, pr);
// for (lv = 0; lv < ncol; lv++)
// for (lv2 = 0; lv2 < nrow; lv2++) {
// /* to collect here, we must also move knight here */
// cost[lv][lv2] += dist[lv][lv2][0];
// /* check to see if it's cheaper for the new knight to
// pick the king up instead of whoever is doing it now */
// if (dist[lv][lv2][1] - dist[lv][lv2][0] < kingcost[lv][lv2]) {
// kingcost[lv][lv2] = dist[lv][lv2][1] - dist[lv][lv2][0];
// }
// }
// }
// /* find best square to collect in */
// pc = cost[0][0] + kingcost[0][0];
// for (lv = 0; lv < ncol; lv++)
// for (lv2 = 0; lv2 < nrow; lv2++)
// if (cost[lv][lv2] + kingcost[lv][lv2] < pc) /* better square? */
// pc = cost[lv][lv2] + kingcost[lv][lv2];
// fprintf (fout, "%i\n", pc);
// return 0;
// }<file_sep>/section 1/ariprog.cpp
/*
ID: luglian1
PROG: ariprog
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <set>
#include <map>
#include <algorithm>
using namespace std;
template <typename T>
void printVector(const vector<T>& order){
for(int i=0; i!=order.size(); ++i)
cout << order[i] << " ";
cout << endl;
}
ostream& operator<<(ostream& out, const set<int>& s){
out << "{";
set<int>::const_iterator it;
for(it = s.begin(); it!=s.end(); ++it)
out << *it << ", ";
out << "}";
return out;
}
struct Progression{
int start;
int dict;
Progression(): start(0), dict(0){}
Progression(int s, int d): start(s), dict(d) {}
Progression& operator=(const Progression& rhs){
start = rhs.start;
dict = rhs.dict;
return *this;
}
};
bool operator<(const Progression& s1, const Progression& s2){
return s1.start == s2.start ? s1.dict < s2.dict : s1.start < s2.start;
}
class CompareProgression{
public:
bool operator()(const Progression& lhs, const Progression& rhs) const{
return lhs.start == rhs.start ?
lhs.dict < rhs.dict :
lhs.start < rhs.start;
}
};
struct Sequence{
int start;
int dict;
int length;
// bool operator<(const Sequence& s2) const {
// return start == s2.start ? dict < s2.dict : start < s2.start;
// }
};
bool operator<(const Sequence& s1, const Sequence& s2){
return s1.start == s2.start ? s1.dict < s2.dict : s1.start < s2.start;
}
bool compare(const Progression& lhs, const Progression& rhs){
return lhs.dict == rhs.dict ? lhs.start < rhs.start : lhs.dict < rhs.dict;
}
ostream& operator<<(ostream& out, const Progression& pg){
out << pg.start << " " << pg.dict;
}
int main(){
ifstream file_in("ariprog.in");
ofstream file_out("ariprog.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n, m;
file_in >> n >> m;
int exist[125000] = {0};
set<int> bisquares;
cout << "here"<< endl;
for (int i = 0; i <= m; ++i) {
for(int j=i; j <= m; ++j ){
bisquares.insert(i*i + j*j);
exist[i*i + j*j-1] = 1;
}
}
cout << "over" << endl;
int max = 2*m*m;
cout << bisquares.size() << endl;
vector<int> vbisquare;
vbisquare.insert(vbisquare.begin() ,bisquares.begin(), bisquares.end());
cout << vbisquare.size() << endl;
cout << "copy" << endl;
// 3. optimize 1
cout << "begin" << endl;
vector<Progression> result;
for(int i=0; i!=vbisquare.size(); ++i){
Progression pg;
for(int j=i+1; j<vbisquare.size(); ++j){
pg.start = vbisquare[i];
pg.dict = vbisquare[j] - vbisquare[i];
int count = 2;
int cur = vbisquare[j];
while(count < n){
cur += pg.dict;
if(cur > max)
break;
if(exist[cur-1] == 0)
break;
++count;
}
if(count == n)
result.push_back(pg);
}
}
cout << result.size() << endl;
sort(result.begin(), result.end(), compare);
//printVector(result);
if(result.size() == 0) file_out << "NONE" << endl;
for(int i=0; i!=result.size(); ++i){
file_out << result[i].start << " "<< result[i].dict << endl;
}
file_in.close();
file_out.close();
return 0;
// 1. try the foolish method, over time!
// int n, m;
// file_in >> n >> m;
// set<int> bisquares;
// for (int i = 0; i <= m; ++i) {
// for(int j=i; j <= m; ++j ){
// bisquares.insert(i*i + j*j);
// }
// }
// cout << bisquares << endl;
// cout << bisquares.size() << endl;
// vector<int> vbisquare(bisquares.begin(), bisquares.end());
// vector<Progression> result;
// for(int i=0; i!=vbisquare.size(); ++i){
// Progression pg;
// for(int j=i+1; j<vbisquare.size(); ++j){
// pg.start = vbisquare[i];
// pg.dict = vbisquare[j] - vbisquare[i];
// int count = 2;
// int cur = vbisquare[j];
// while(count < n){
// cur += pg.dict;
// if(bisquares.count(cur) == 0)
// break;
// ++count;
// }
// if(count == n)
// result.push_back(pg);
// }
// }
// // 2. another method, over memory
// map<Progression, int> seqmap;
// for(int i=vbisquare.size()-2; i>=0; --i){
// for(int j=i+1; j<vbisquare.size(); ++j){
// Progression pg(vbisquare[j], vbisquare[j] - vbisquare[i]);
// map<Progression, int>::iterator it = seqmap.find(pg);
// if( it == seqmap.end()){
// pg.start = vbisquare[i];
// seqmap.insert(make_pair(pg, 2));
// }
// else{
// cout << "find" << endl;
// pg = it->first;
// pg.start = vbisquare[i];
// int length = it->second+1;
// seqmap.erase(it);
// seqmap.insert(make_pair(pg, length));
// }
// }
// }
// for(map<Progression, int>::iterator it = seqmap.begin(); it!=seqmap.end(); ++it){
// cout << it->first << " " << it->second << endl;
// }
}
<file_sep>/section3/contact.cpp
/*
ID: luglian1
PROG: contact
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <map>
#include <set>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "contact"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
struct scmp
{
bool operator()(const string& lhs, const string& rhs) const
{
if(lhs.length() != rhs.length())
return lhs.length() < rhs.length();
return lhs<rhs;
};
};
class node
{
public:
int count;
node* ch[2];
node(): count(0)
{
ch[0] = NULL;
ch[1] = NULL;
};
void addStr(const char* s, int len)
{
if(len <= 0)
{
count++;
return;
}
int cid = s[0] - '0';
if(!ch[cid])
ch[cid] = new node;
if(len > 1)
ch[cid]->addStr(s+1, len-1);
else
ch[cid]->addStr(s, len-1);
}
void dfsCount(map<int, set<string, scmp> >& count_map, const string& s)
{
if(count > 0)
{
if(!count_map.count(count))
count_map.insert(pair<int, set<string, scmp> >(count, set<string, scmp>()));
count_map[count].insert(s);
}
for(int i=0; i<2; ++i)
{
if(ch[i])
ch[i]->dfsCount(count_map, s+char('0'+i));
}
}
~node()
{
for(int i=0; i<2; ++i)
if(ch[i])
delete ch[i];
}
};
int a, b, n;
string s;
map<int, set<string, scmp> > count_map;
ostream& operator<<(ostream& out, const set<string, scmp>& s)
{
for(set<string, scmp>::const_iterator it=s.begin(); it!=s.end(); ++it)
out << *it << ", ";
return out;
}
ostream& operator<<(ostream& out, const map<int, set<string, scmp> >& m)
{
for(map<int, set<string, scmp> >::const_iterator it=m.begin(); it!=m.end(); ++it)
out << (*it).first << ": " << (*it).second << endl;
return out;
}
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> a >> b >> n;
string line;
getline(file_in, line);
while(getline(file_in, line))
{
s += line;
}
PV(s)
return true;
}
void save(ostream& out)
{
map<int, set<string, scmp> >::reverse_iterator it=count_map.rbegin();
for(int i=0; i<n && it!=count_map.rend(); ++i, ++it)
{
out << (*it).first << endl;
set<string, scmp>::iterator it2 = (*it).second.begin();
int c=0;
out << *it2;
for(it2++;it2 != (*it).second.end(); ++it2)
{
c++;
if(c % 6 == 0)
out << endl;
else
out << " ";
out << *it2;
}
out << endl;
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
const char* cs = s.c_str();
node root;
for(int i=0; i<s.size(); ++i)
{
for(int j=a; j<=b; ++j)
{
if(i + 1 >= j)
{
root.addStr(cs+i+1-j, j);
}
}
}
root.dfsCount(count_map, "");
PV(count_map)
}
// For this problem, we keep track of every bit sequence we see. We could use the bit sequence itself as an index into a table of frequencies, but that would not distinguish between the 2-bit sequence "10" and the 4-bit sequence "0010". To solve this, we always add a 1 to the beginning of the number, so "10" becomes "110" and "0010" becomes "10010".
// After reading the entire bit string, we sort the frequency table and walk through it to print out the top sequences.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXBITS 12
// #define MAXSEQ (1<<(MAXBITS+1))
// typedef struct Seq Seq;
// struct Seq {
// unsigned bits;
// int count;
// };
// Seq seq[MAXSEQ];
// /* increment the count for the n-bit sequence "bits" */
// void
// addseq(unsigned bits, int n)
// {
// bits &= (1<<n)-1;
// bits |= 1<<n;
// assert(seq[bits].bits == bits);
// seq[bits].count++;
// }
// /* print the bit sequence, decoding the 1<<n stuff */
// /* recurse to print the bits most significant bit first */
// void
// printbits(FILE *fout, unsigned bits)
// {
// assert(bits >= 1);
// if(bits == 1) /* zero-bit sequence */
// return;
// printbits(fout, bits>>1);
// fprintf(fout, "%d", bits&1);
// }
// int
// seqcmp(const void *va, const void *vb)
// {
// Seq *a, *b;
// a = (Seq*)va;
// b = (Seq*)vb;
// /* big counts first */
// if(a->count < b->count)
// return 1;
// if(a->count > b->count)
// return -1;
// /* same count: small numbers first */
// if(a->bits < b->bits)
// return -1;
// if(a->bits > b->bits)
// return 1;
// return 0;
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, a, b, n, nbit, c, j, k;
// unsigned bit;
// char *sep;
// fin = fopen("contact.in", "r");
// fout = fopen("contact.out", "w");
// assert(fin != NULL && fout != NULL);
// nbit = 0;
// bit = 0;
// for(i=0; i<=MAXBITS; i++)
// for(j=0; j<(1<<i); j++)
// seq[(1<<i) | j].bits = (1<<i) | j;
// fscanf(fin, "%d %d %d", &a, &b, &n);
// while((c = getc(fin)) != EOF) {
// if(c != '0' && c != '1')
// continue;
// bit <<= 1;
// if(c == '1')
// bit |= 1;
// if(nbit < b)
// nbit++;
// for(i=a; i<=nbit; i++)
// addseq(bit, i);
// }
// qsort(seq, MAXSEQ, sizeof(Seq), seqcmp);
// /* print top n frequencies for number of bits between a and b */
// j = 0;
// for(i=0; i<n && j < MAXSEQ; i++) {
// if(seq[j].count == 0)
// break;
// c = seq[j].count;
// fprintf(fout, "%d\n", c);
// /* print all entries with frequency c */
// sep = "";
// for(k=0; seq[j].count == c; j++, k++) {
// fprintf(fout, sep);
// printbits(fout, seq[j].bits);
// if(k%6 == 5)
// sep = "\n";
// else
// sep = " ";
// }
// fprintf(fout, "\n");
// }
// exit(0);
// }
<file_sep>/mytest/test_swap.cpp
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
using namespace std;
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
int main()
{
vector<int> a{1,2,3,4,5,6,7,8};
a.push_back(9);
vector<int>::iterator ait = a.begin();
for(int i=0; i<7; ++i)
a.pop_back();
PV(a.size())
PV(a.capacity())
vector<int> b{10};
vector<int>::iterator bit = b.begin();
b.swap(a);
PV(b.size())
PV(b.capacity())
PV(*(++ait))
PV(*(++bit))
}
<file_sep>/section2/fracdec.cpp
/*
ID: luglian1
PROG: fracdec
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <cstring>
#include <map>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "fracdec"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
int a, b;
int integer = 0;
vector<int> decimal;
int remainder = 0;
map<int, int> remmap;
int resi = 0;
int resl = 0;
int divisible = 0;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> a >> b;
return true;
}
void save(ostream& out)
{
if(divisible)
{
out << integer << ".";
for(int i=0; i<decimal.size(); ++i)
out << decimal[i];
out << endl;
}
else
{
int line=0;
vector<int> outin;
do
{
outin.push_back(integer % 10);
integer = integer / 10;
}while(integer > 0);
for(int i=outin.size()-1; i>=0; --i)
out << outin[i];
out << ".";
line = outin.size() + 1;
for(int i=0; i<resi; ++i)
{
out << decimal[i];
line++;
if(line == 76)
{
line = 0;
out << endl;
}
}
out << "(";
line++;
if(line == 76)
{
line = 0;
out << endl;
}
for(int i=0; i<resl; ++i)
{
out << decimal[resi+i];
line++;
if(line == 76)
{
line = 0;
out << endl;
}
}
out << ")" << endl;
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
int check_loop(const vector<int>& num)
{
for(int i=0; i<num.size(); ++i)
{
for(int j=i+1; j<(num.size()-i)/3; ++j)
{
if(num[i] != num[j])
continue;
if(memcmp(num.data()+i, num.data()+j, (j-i)*sizeof(int)) == 0 &&
memcmp(num.data()+j, num.data()+j+j-i, (j-i)*sizeof(int)) == 0)
{
if (j-i == 1)
{
if(num.size()-j < 10)
return 0;
for(int k=j; k<num.size(); ++k)
if(num[k] != num[j])
return 0;
resi = i;
resl = j - i;
return 1;
}
else
{
resi = i;
resl = j - i;
return 1;
}
}
}
}
return 0;
}
void solve()
{
integer = a / b;
remainder = a % b;
for(int i=0; i<100000; ++i)
{
if(remmap.count (remainder))
{
resi = remmap[remainder];
resl = i - resi;
break;
}
if(remainder == 0)
{
divisible = 1;
break;
}
remmap.insert(pair<int,int>(remainder, i));
remainder *= 10;
decimal.push_back(remainder / b);
remainder = remainder % b;
// if(check_loop(decimal))
// break;
}
if(decimal.empty())
decimal.push_back(0);
// PV(remmap.size())
// PVL(decimal, decimal.size())
}
// Remember long division? We know that the decimal expansion is repeating when, after the decimal point, we see a remainder we've seen before. The repeating part will be all the digits we've calculated since the last time we saw that remainder.
// We read in the input and print the integer part. Then we do long division on the fractional part until we see a remainder more than once or the remainder becomes zero. If we see a remainder more than once, we're repeating, in which case we print the non-repeated and repeated part appropriately. If the remainder becomes zero, we finished, in which case we print the decimal expansion. When no digits of the decimal expansion have been generated, the correct answer seems to be to print a zero.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXDIGIT 100100
// char dec[MAXDIGIT];
// int lastrem[MAXDIGIT];
// char buf[MAXDIGIT];
// void
// main(void)
// {
// FILE *fin, *fout;
// int n, d, k, i, rem, len;
// fin = fopen("fracdec.in", "r");
// fout = fopen("fracdec.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d %d", &n, &d);
// sprintf(buf, "%d.", n/d);
// /* long division keeping track of if we've seem a remainder before */
// for(i=0; i<MAXDIGIT; i++)
// lastrem[i] = -1;
// rem = n % d;
// for(i=0;; i++) {
// if(rem == 0) {
// if(i == 0)
// sprintf(buf+strlen(buf), "0");
// else
// sprintf(buf+strlen(buf), "%s", dec);
// break;
// }
// if(lastrem[rem] != -1) {
// k = lastrem[rem];
// sprintf(buf+strlen(buf), "%.*s(%s)", k, dec, dec+k);
// break;
// }
// lastrem[rem] = i;
// n = rem * 10;
// dec[i] = n/d + '0';
// rem = n%d;
// }
// /* print buf 76 chars per line */
// len = strlen(buf);
// for(i=0; i<len; i+=76) {
// fprintf(fout, "%.76s\n", buf+i);
// }
// exit(0);
// }
// Here's a another, more elegant solution from <NAME>.
// Compute the number of digits before the repeat starts, and then you don't even have to store the digits or remainders, making the program use much less memory and go faster. We know that powers of 2 and 5 are the only numbers which do not result in a repeat, so to find the number of digits before the repeat, we just find the maximum of the differences between the powers of 2 and 5 in the denominator and numerator (see code snippet). Then we just use the first remainder, and output each digit as we calculate it:
// #include <iostream.h>
// #include <fstream.h>
// #include <math.h>
// ofstream out("fracdec.out");
// int colcount=0;
// int numBeforeRepeat(int n, int d) {
// int c2=0, c5=0;
// if (n == 0) return 1;
// while (d%2==0) { d/=2; c2++; }
// while (d%5==0) { d/=5; c5++; }
// while (n%2==0) { n/=2; c2--; } /* can go negative */
// while (n%5==0) { n/=5; c5--; } /* can go negative */
// if (c2>c5)
// if (c2>0) return c2;
// else return 0;
// else
// if (c5>0) return c5;
// else return 0;
// }
// void print (char c) {
// if (colcount==76) {
// out<<endl;
// colcount=0;
// }
// out<<c;
// colcount++;
// }
// void print (int n) {
// if (n>=10) print (n/10);
// print ((char)('0'+(n%10)));
// }
// int main() {
// int n, d;
// ifstream in("fracdec.in");
// in>>n>>d;
// in.close();
// print (n/d);
// print ('.');
// n=n%d;
// int m=numBeforeRepeat(n,d);
// for(int i=0;i<m;i++) {
// n*=10;
// print (n/d);
// n%=d;
// }
// int r=n;
// if(r!=0) {
// print ('(');
// do {
// n*=10;
// print (n/d);
// n%=d;
// } while (n!=r);
// print (')');
// }
// out<<endl;
// out.close();
// exit (0);
// }
<file_sep>/section 1/packrec.cpp
/*
ID: luglian1
PROG: packrec
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <set>
using namespace std;
vector<vector<int> > orderset;
template <typename T>
void printVector(const vector<T>& order){
for(int i=0; i!=order.size(); ++i)
cout << order[i] << " ";
cout << endl;
}
void buildOrder(int n, vector<int>& earlyorder, vector<bool>& mark){
if(earlyorder.size() == n){
orderset.push_back(earlyorder);
return;
}
for(int i=0; i!=n; ++i){
if(!mark[i]){
// printVector(earlyorder);
// printVector(mark);
earlyorder.push_back(i);
mark[i] = true;
buildOrder(n, earlyorder, mark);
earlyorder.pop_back();
mark[i] = false;
}
}
}
vector<vector<bool> > rotateset;
void buildRotate(int n, vector<bool>& earlyrotate){
if(earlyrotate.size() == 4){
rotateset.push_back(earlyrotate);
return;
}
earlyrotate.push_back(false);
buildRotate(n, earlyrotate);
earlyrotate.pop_back();
earlyrotate.push_back(true);
buildRotate(n, earlyrotate);
earlyrotate.pop_back();
}
class Rectangle{
public:
int width;
int height;
int area;
Rectangle(int w, int h): width(w), height(h), area(w*h) {}
Rectangle rotate(bool r=true){
if(r)
return Rectangle(height, width);
else
return Rectangle(width, height);
}
};
bool operator<(const Rectangle& lhs, const Rectangle& rhs){
return lhs.width < rhs.width;
}
ostream& operator<<(ostream& out, const Rectangle& r){
out << r.width << " " << r.height << " " << r.area;
}
void calMinRect(const Rectangle& r1, vector<Rectangle>& minrect){
if(minrect.size() == 0){
minrect.push_back(r1);
}
else if(r1.area < minrect[0].area){
minrect.clear();
minrect.push_back(r1);
}
else if(r1.area == minrect[0].area){
minrect.push_back(r1);
}
}
void calLayoutArea(const vector<Rectangle>& in, vector<Rectangle>& minrect){
if(in.size() != 4){
cerr << "Error: size of in is not equal 4" << endl;
return;
}
int h=0,w=0;
// first layout
h = max(max(max(in[0].height, in[1].height), in[2].height), in[3].height);
for(int i=0; i!=4; ++i)
w += in[i].width;
calMinRect(Rectangle(w, h), minrect);
// second
h = max(max(in[0].height, in[1].height), in[2].height) + in[3].height;
for(int i=0, w=0; i!=3; ++i)
w += in[i].width;
w = max(w, in[3].width);
calMinRect(Rectangle(w, h), minrect);
// third
h = max(in[3].height, max(in[0].height, in[1].height) + in[2].height);
w = max(in[0].width + in[1].width, in[2].width) + in[3].width;
calMinRect(Rectangle(w, h), minrect);
// fourth
h = max(in[0].height, max(in[1].height + in[2].height, in[3].height));
w = in[0].width + max(in[1].width, in[2].width) + in[3].width;
calMinRect(Rectangle(w, h), minrect);
// fifth
h = max(max(in[0].height+in[1].height, in[2].height), in[3].height);
w = max(in[0].width, in[1].width) + in[2].width + in[3].width;
calMinRect(Rectangle(w, h), minrect);
// sixth
h = max(in[0].height + in[1].height, in[2].height+in[3].height);
w = max(in[0].width, in[1].width) + max(in[2].width, in[3].width);
calMinRect(Rectangle(w, h), minrect);
// seventh
h = max(in[0].height, in[1].height + in[2].height + in[3].height);
w = in[0].width + max(in[1].width, max(in[2].width, in[3].width));
calMinRect(Rectangle(w, h), minrect);
// eighth
if(in[1].height >= in[3].height && in[2].width <= in[3].width){
h = max(in[0].height + in[1].height, in[2].height+in[3].height);
w = max(in[0].width + in[2].width, in[1].width + in[3].width);
}
// else{
// h = max(in[0].height + in[1].height, max(in[0].height+in[3].height,
// max(in[1].height+in[2].height, in[2].height+in[3].height)));
// w = max(in[0].width + in[2].width,
// max(in[0].width+in[3].width, in[1].width+in[3].width));
// }
// if(h*w==80){
// for(int i=0; i!=in.size(); ++i){
// cout << in[i].width << " " << in[i].height << endl;
// }
// cout << endl;
// }
calMinRect(Rectangle(w, h), minrect);
}
void cutResult(vector<Rectangle>& minrect){
set<Rectangle> minset;
vector<Rectangle> tmprect(minrect);
for(int i=0; i!=tmprect.size(); ++i){
if(tmprect[i].height < tmprect[i].width){
swap(tmprect[i].width, tmprect[i].height);
}
}
minset.insert(tmprect.begin(), tmprect.end());
minrect.clear();
minrect.insert(minrect.begin(), minset.begin(), minset.end());
}
int main(){
ifstream file_in("packrec.in");
ofstream file_out("packrec.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file." << endl;
return 0;
}
vector<Rectangle> rectset;
int w, h;
for(int i=0; i!=4; ++i){
file_in >> w >> h;
rectset.push_back(Rectangle(w, h));
//cout << rectset[i].width << " " << rectset[i].height << endl;
}
cout << rectset.size() << endl;
//printVector(rectset);
// build order set
vector<int> tmporder;
vector<bool> mark(4, false);
buildOrder(4, tmporder, mark);
// for(int i=0; i!=orderset.size(); ++i)
// printVector(orderset[i]);
// build rotate set
vector<bool> tmprotate;
buildRotate(4, tmprotate);
cout << rotateset.size() << endl;
// for(int i=0; i!=rotateset.size(); ++i)
// printVector(rotateset[i]);
// find all the min rect
vector<Rectangle> minrect;
for(int i=0; i!=orderset.size(); ++i){
for(int j=0; j!=rotateset.size(); ++j){
vector<Rectangle> testset;
for(int k=0; k!=4; ++k)
testset.push_back(rectset[orderset[i][k]].rotate(rotateset[j][k]));
calLayoutArea(testset, minrect);
}
}
cout << minrect.size() << endl;
cout << "before" << endl;
for(int i=0; i!=minrect.size(); ++i){
cout << minrect[i].width << " " << minrect[i].height << endl;
}
cout << "here" << endl;
cutResult(minrect);
cout << minrect.size() << endl;
// for(int i=0; i!=minrect.size(); ++i){
// cout << minrect[i] << endl;
// }
file_out << minrect[0].area << endl;
for (int i = 0; i < minrect.size(); ++i)
{
file_out << minrect[i].width << " " << minrect[i].height << endl;
}
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section 1/namenum.cpp
/*
ID: luglian1
PROG: namenum
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <queue>
using namespace std;
class TrieNode{
public:
TrieNode* children[26];
bool isLeaf;
TrieNode():isLeaf(false){
for(int i=0; i!=26; ++i)
children[i] = NULL;
}
};
int main(){
ifstream file_in("namenum.in");
ofstream file_out("namenum.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
string s;
file_in >> s;
char code[8][3] = {{'A','B','C'}, {'D','E','F'},{'G','H','I'},{'J','K','L'}, {'M','N','O'},
{'P','R','S'},{'T','U','V'},{'W','X','Y'}};
vector<int> num;
for(int i=0; i!=s.size(); ++i)
num.push_back(s.at(i)-'2');
// load name and build trie tree
ifstream dict_in("dict.txt");
if(!dict_in){
cerr << "Error: no file dict.txt" << endl;
return 0;
}
TrieNode root;
vector<TrieNode*> alloctn;
while(!dict_in.eof()){
dict_in >> s;
TrieNode* tp = &root;
for(int i=0; i!=s.size(); ++i){
if(tp->children[s.at(i)-'A'] == NULL){
tp->children[s.at(i)-'A'] = new TrieNode();
alloctn.push_back(tp->children[s.at(i)-'A']);
}
tp = tp->children[s.at(i)-'A'];
}
tp->isLeaf = true;
}
cout << alloctn.size() << endl;
for(int i=0; i!=num.size(); ++i){
cout << num[i] << endl;
}
// search it
queue<TrieNode*> tnq; // queue to be search
queue<string> tns; // word to match
tns.push("");
tnq.push(&root);
for(int pos=0; pos!=num.size(); ++pos){
int size = tnq.size();
if(size == 0)
break;
for(int i=0; i!=size; ++i){
TrieNode* tp = tnq.front();
tnq.pop();
string neww = tns.front();
tns.pop();
cout << neww << " ";
for(int j=0; j!=3; ++j){
if(tp->children[code[num[pos]][j]-'A'] != NULL){
cout << "find " << pos << endl;
tnq.push(tp->children[code[num[pos]][j]-'A']);
tns.push(neww + code[num[pos]][j]);
}
}
}
}
if(tns.size() != 0){
while(!tns.empty()){
if(tnq.front()->isLeaf)
file_out << tns.front() << endl;
tnq.pop();
tns.pop();
}
}
else
file_out << "NONE" << endl;
for(int i=0; i!=alloctn.size(); ++i){
delete alloctn[i];
}
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/inflate.cpp
/*
ID: luglian1
PROG: inflate
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "inflate"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAX_LEN 10000
using namespace std;
int m;
int n;
int cp[MAX_LEN] = {0};
int cm[MAX_LEN] = {0};
int a[MAX_LEN+1] = {0};
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> m >> n;
for(int i=0; i<n; ++i)
{
file_in >> cp[i] >> cm[i];
}
return true;
}
void save(ostream& out)
{
out << a[m] << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
for(int i=1; i<=m; ++i)
{
int maxa = 0;
for(int j=0; j<n; ++j)
{
if(i >= cm[j])
maxa = max(a[i - cm[j]] + cp[j], maxa);
}
a[i] = max(a[i-1], maxa);
}
PVL(a, m);
}
// We use dynamic programming to calculate the best way to use t minutes for all t from 0 to tmax.
// When we find out about a new category of problem with points p and length t, we update the best for j minutes by taking the better of what was there already and what we can do by using a p point problem with the best for time j - t.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXCAT 10000
// #define MAXTIME 10000
// int best[MAXTIME+1];
// void
// main(void)
// {
// FILE *fin, *fout;
// int tmax, ncat;
// int i, j, m, p, t;
// fin = fopen("inflate.in", "r");
// fout = fopen("inflate.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d %d", &tmax, &ncat);
// for(i=0; i<ncat; i++) {
// fscanf(fin, "%d %d", &p, &t);
// for(j=0; j+t <= tmax; j++)
// if(best[j]+p > best[j+t])
// best[j+t] = best[j]+p;
// }
// m = 0;
// for(i=0; i<=tmax; i++)
// if(m < best[i])
// m = best[i];
// fprintf(fout, "%d\n", m);
// exit(0);
// }
// <NAME> writes: After the main `for' loop that does the actual DP work, we don't need to look at the entire array of best point totals to find the highest one. The array is always nondecreasing, so we simply output the last element of the array.
// #include <fstream.h>
// ifstream fin("inflate.in");
// ofstream fout("inflate.out");
// const short maxm = 10010;
// long best[maxm], m, n;
// void
// main()
// {
// short i, j, len, pts;
// fin >> m >> n;
// for (j = 0; j <= m; j++)
// best[j] = 0;
// for (i = 0; i < n; i++) {
// fin >> pts >> len;
// for (j = len; j <= m; j++)
// if (best[j-len] + pts > best[j])
// best[j] = best[j-len] + pts;
// }
// fout << best[m] << endl; // This is always the highest total.
// }<file_sep>/section2/hamming.cpp
/*
ID: luglian1
PROG: hamming
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cstring>
#include <set>
#include <vector>
#include <cassert>
using namespace std;
bool load();
void save(ostream& out);
bool save();
#define MAX_B 256
int N, B, D;
int hamming_distance[MAX_B][MAX_B];
int search_path[MAX_B+1];
int depth[MAX_B];
int max_value;
bool isFound;
set<int> result;
void calculateDistance()
{
max_value = 1 << B;
// calculcate hammming distance
for (int i = 0; i < max_value; ++i)
{
for (int j = i; j < max_value; ++j)
{
int xorvalue = i ^ j;
hamming_distance[i][j] = 0;
while(xorvalue)
{
xorvalue = xorvalue & (xorvalue-1);
++ hamming_distance[i][j];
}
}
}
for (int i = 0; i < max_value; ++i)
{
for (int j = 0; j < i; ++j)
{
hamming_distance[i][j] = hamming_distance[j][i];
}
}
// cout
// for (int i = 0; i < max_value; ++i)
// {
// for (int j = 0; j < max_value; ++j)
// {
// cout << hamming_distance[i][j] << " ";
// }
// cout << endl;
// }
}
void dfs_solve(int cur_depth, int cur_id) // 接着要处理的数字和该数字所在的深度
{
// check
cout << "cur depth: " << cur_depth << endl;
search_path[cur_depth] = cur_id;
depth[cur_id] = cur_depth;
if(cur_depth > max_value)
return;
if(cur_depth == N) // check finish
{
result.clear();
for (int i = 1; i <= N; ++i)
{
result.insert(search_path[i]);
}
assert(result.size() == N);
isFound = true;
return;
}
else
{
for (int i = cur_id+1; i < max_value; ++i)
{
int j=1;
for (; j <= cur_depth; ++j)
{
if(hamming_distance[search_path[j]][i] < D)
break;
}
if(j> cur_depth)
dfs_solve(cur_depth+1, i);
if(isFound)
return;
}
depth[cur_id] = 0;
}
}
void solve()
{
calculateDistance();
memset(depth, 0, MAX_B * sizeof(int));
isFound = false;
dfs_solve(1, 0);
// for (int i = 0; i < max_value; ++i)
// {
// dfs_solve(1, i);
// if(isFound)
// return;
// }
}
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in("hamming.in");
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N >> B >> D;
cout << N << " " << B << " " << D << endl;
return true;
}
void save(ostream& out)
{
vector<int> resultv(result.begin(), result.end());
for (int i = 0; i < N; ++i)
{
out << resultv[i];
if(i % 10 == 9)
out << endl;
else if(i != N-1)
out << " ";
else
out << endl;
}
}
bool save()
{
ofstream file_out("hamming.out");
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
<file_sep>/section 1/palsquare.cpp
/*
ID: luglian1
PROG: palsquare
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
bool checkPalindromic(const string& s){
for(int i=0; i!=s.size()/2; ++i){
if(s.at(i) != s.at(s.size()-i-1) )
return false;
}
return true;
}
string digits = "0123456789ABCDEFGHIJKLMN";
string buildString(int i, int base){
string res;
int quo = i;
while(quo > 0){
res = digits.at(quo % base)+res;
quo = quo / base;
}
return res;
}
int main(){
ifstream file_in("palsquare.in");
ofstream file_out("palsquare.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n;
file_in >> n;
for(int i=1; i!=300; ++i){
int square = i * i;
string s = buildString(square, n);
if(checkPalindromic(s))
file_out << buildString(i, n) << " " << s << endl;
}
cout << buildString(300, 16) << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/humble.cpp
/*
ID: luglian1
PROG: humble
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <cmath>
#include <algorithm>
#include <vector>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "humble"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAXP 100
#define INF 0x7fffffff
using namespace std;
int n;
int m;
int p[MAXP]={0};
vector<int> num;
int next_num[MAXP];
int res;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> n >> m;
for(int i=0; i<n; ++i)
file_in >> p[i];
return true;
}
void save(ostream& out)
{
out << res << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
sort(p, p+n);
// PVL(p, n)
num.push_back(1);
for(int i=0; i<m; ++i)
{
int min_next = INF;
int min_p = 0;
for(int j=0; j<n; ++j)
{
int next = p[j] * num[next_num[j]];
while(next <= num[num.size()-1])
{
next_num[j]++;
next = p[j] * num[next_num[j]];
}
if(next < min_next)
{
min_next = next;
min_p = j;
}
}
next_num[min_p]++;
num.push_back(min_next);
}
res = num[num.size()-1];
// PVL(num, num.size())
}
// We compute the first n humble numbers in the "hum" array. For simplicity of implementation, we treat 1 as a humble number, and adjust accordingly.
// Once we have the first k humble numbers and want to compute the k+1st, we do the following:
// for each prime p
// find the minimum humble number h
// such that h * p is bigger than the last humble number.
// take the smallest h * p found: that's the next humble number.
// To speed up the search, we keep an index "pindex" of what h is for each prime, and start there rather than at the beginning of the list.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #include <ctype.h>
// #define MAXPRIME 100
// #define MAXN 100000
// long hum[MAXN+1];
// int nhum;
// int prime[MAXPRIME];
// int pindex[MAXPRIME];
// int nprime;
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, minp;
// long min;
// int n;
// fin = fopen("humble.in", "r");
// fout = fopen("humble.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d %d", &nprime, &n);
// for(i=0; i<nprime; i++)
// fscanf(fin, "%d", &prime[i]);
// hum[nhum++] = 1;
// for(i=0; i<nprime; i++)
// pindex[i] = 0;
// while(nhum < n+1) {
// min = 0x7FFFFFFF;
// minp = -1;
// for(i=0; i<nprime; i++) {
// while((double)prime[i] * hum[pindex[i]] <= hum[nhum-1])
// pindex[i]++;
// /* double to avoid overflow problems */
// if((double)prime[i] * hum[pindex[i]] < min) {
// min = prime[i] * hum[pindex[i]];
// minp = i;
// }
// }
// hum[nhum++] = min;
// pindex[minp]++;
// }
// fprintf(fout, "%d\n", hum[n]);
// exit(0);
// }
<file_sep>/section3/spin.cpp
/*
ID: luglian1
PROG: spin
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <set>
#include <cstring>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "spin"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
struct wedge
{
int begin;
int range;
wedge(int b, int r): begin(b), range(r) {};
};
class wheel
{
public:
vector<wedge> wedges;
int speed;
wheel(int s=0): speed(s) {};
void add_wedge(int begin, int range)
{
wedges.push_back(wedge(begin, range));
}
set<int> get_minute(int m)
{
set<int> s;
for(vector<wedge>::iterator w=wedges.begin(); w!=wedges.end(); w++)
{
int b = (*w).begin + m * speed;
for(int i=0; i<=(*w).range; ++i)
{
s.insert((b+i) % 360);
};
}
return s;
}
bool get_minute(int m, int n, int* count, bool& found)
{
bool match = false;
for(vector<wedge>::iterator w=wedges.begin(); w!=wedges.end(); w++)
{
int b = (*w).begin + m * speed;
for(int i=0; i<=(*w).range; ++i)
{
int id = (b+i) % 360;
count[id]++;
if(count[id] == 5)
{
found = true;
return true;
}
if(count[id] == n+1)
{
match = true;
}
};
}
return match;
}
};
ostream& operator<<(ostream& out, const set<int>& s)
{
for(set<int>::const_iterator it=s.begin(); it!=s.end(); ++it)
out << *it << ", ";
return out;
}
vector<wheel> whs(5);
int count[360] = {0};
int resout = -1;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
for(int i=0; i<5; ++i)
{
int speed, n;
file_in >> speed >> n;
wheel& w = whs[i];
w.speed = speed;
for(int j=0; j<n; ++j)
{
int begin, range;
file_in >> begin >> range;
w.add_wedge(begin, range);
}
PV(whs[i].speed)
PV(whs[i].wedges[0].begin)
PV(whs[i].wedges[0].range)
}
return true;
}
void save(ostream& out)
{
if(resout >= 0)
out << resout << endl;
else
out << "none" << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
bool found = false;
for(int i=0 ;i<=360; ++i)
{
memset(count, 0, 360 * sizeof(int));
for(int j=0; j<5; ++j)
{
bool match = whs[j].get_minute(i, j, count, found);
if(j==0)
continue;
if(!match)
break;
if(found)
{
resout = i;
break;
}
}
if(found)
break;
}
}
// The key observation for this problem is that after 360 seconds, the wheels have returned to their original locations, so if the wheels don't line up in 360 seconds, they will never line up.
// To determine if there is a location through which a light can be shine, mark, for each wheel, which angles between 0 and 359 a light can be shone through. If any location gets marked for all the wheels, then a light can be shone through the entire system. Otherwise, no light can be shone through all the wheels.
// #include <stdio.h>
// #include <assert.h>
// #include <string.h>
// int speed[5]; /* speed of each wheel */
// int wedgest[5][5]; /* start of each wedge (-1 == no wedge) */
// int wedglen[5][5]; /* length of each wedge */
// int pos[5]; /* angular position of each wheel */
// int t; /* time (in seconds) since start */
// /* (light[deg] >> wid) & 0x1 is true if and only if there
// is a wedge in wheel wid that a light can shine through at
// angle deg */
// int light[360];
// /* mark all the degrees we can see through wheel w */
// void mark_light(int w)
// {
// int lv, lv2; /* loop variables */
// int wpos; /* wedge position */
// for (lv = 0; lv < 5; lv++)
// {
// if (wedglen[w][lv] < 0) /* no more wedges for this wheel */
// break;
// /* start of wedge */
// wpos = (pos[w] + wedgest[w][lv]) % 360;
// for (lv2 = 0; lv2 <= wedglen[w][lv]; lv2++)
// { /* throughout extent of wedge */
// light[wpos] |= (1 << w); /* mark as hole in wheel */
// wpos = (wpos + 1) % 360; /* go to the next degree */
// }
// }
// }
// int main(int argc, char **argv)
// {
// FILE *fp;
// FILE *fout;
// int w, f;
// int lv, lv2;
// fp = fopen("spin.in", "r");
// fout = fopen("spin.out", "w");
// assert(fp);
// assert(fout);
// /* read in the data */
// for (lv = 0; lv < 5; lv++)
// {
// fscanf (fp, "%d %d", &speed[lv], &w);
// for (lv2 = 0; lv2 < w; lv2++)
// fscanf (fp, "%d %d", &wedgest[lv][lv2], &wedglen[lv][lv2]);
// /* mark the rest of the wedges as not existing for this wheel */
// for (; lv2 < 5; lv2++)
// wedglen[lv][lv2] = -1;
// }
// f = 0;
// while (t < 360) /* for each time step */
// {
// memset(light, 0, sizeof(light));
// /* mark the degrees we can see through each wheel */
// for (lv = 0; lv < 5; lv++)
// mark_light(lv);
// for (lv = 0; lv < 360; lv++)
// if (light[lv] == 31) /* we can shine a light through all five wheels */
// f = 1;
// if (f) break; /* we found a match! */
// /* make a time step */
// t++;
// for (lv = 0; lv < 5; lv++)
// pos[lv] = (pos[lv] + speed[lv]) % 360;
// }
// /* after 360 time steps, all the wheels have returned to their
// original location */
// if (t >= 360) fprintf (fout, "none\n");
// else fprintf (fout, "%i\n", t);
// return 0;
// }
<file_sep>/mytest/test_time.cpp
#include <iostream>
#include <time.h>
using namespace std;
int main()
{
time_t t;
cout << time(&t) << endl;
cout << daylight << endl;
cout << timezone << endl;
}<file_sep>/section 1/combo.cpp
/*
ID: luglian1
PROG: combo
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "combo"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define abs(a) (a) >= 0 ? (a) : -(a)
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int N;
int f[3]; // farmer
int m[3]; // master
int count = 0;
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
for(int i=0; i<3; i++)
file_in >> f[i];
for (int i=0; i<3; i++)
file_in >> m[i];
return true;
}
void save(ostream& out)
{
out << count << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
int dis(int a, int b)
{
int ab = abs(a-b);
if(ab < 5)
return 5-ab;
else if(ab > N - 5)
return 5-(N-ab);
return 0;
}
void solve()
{
if(N <= 5)
{
count = N*N*N;
return;
}
int minus = 1;
for(int i=0; i<3; i++)
{
int d = dis(f[i], m[i]);
minus *= d;
}
PV(minus)
count = 250 - minus;
}<file_sep>/section 1/wormhole.cpp
/*
ID: luglian1
PROG: wormhole
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
{std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;}
#define PROB_NAME "wormhole"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int N;
int pos[13][2];
int count=0;
int p[13] = {0};
int y[13][13] {};
int main(){
load();
solve();
save();
return 0;
}
int findy(int py)
{
int i=0;
for(; i<N; i++)
{
if(y[i][0] == py || y[i][0] == -1)
break;
}
return i;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
for(int i=1; i<=N; i++)
{
file_in >> pos[i][0] >> pos[i][1];
//PVL(pos[i], 2);
}
//PV(N)
// 构造一个y相同,以x排序的数组,插入排序
for(int i=0; i<13; i++)
for(int j=0; j<13; j++)
y[i][j]=-1;
for(int i=1; i<=N; i++)
{
int posx = pos[i][0], posy = pos[i][1];
int py = findy(posy);
//PV(py)
if(y[py][0] == -1)
{
y[py][0] = posy;
y[py][1] = i;
continue;
}
int j=12;
for(; j>=1 && y[py][j]<=0; j--);
for(; j>=1 && pos[y[py][j]][0] > posx; j--)
{
y[py][j+1] = y[py][j];
}
y[py][j+1] = i;
}
// for(int i=0; i<13; i++)
// {
// PVL(y[i], 13);
// }
return true;
}
void save(ostream& out)
{
out << count << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void check()
{
int pair[13][2] {0};
for(int i=1; i<=N; i++)
{
if(pair[p[i]][0] == 0)
pair[p[i]][0] = i;
else
pair[p[i]][1] = i;
}
// for(int i=0; i<13; i++)
// {
// PVL(pair[i], 2);
// }
int r[13] {};
//暴力遍历来找回路
for(int i=1; i<=N; i++)
{
for(int i=0; i<13; i++)
r[i] = 0;
int cur = i; //进入i
bool cir = false;
for(int j=1; j<=N; j++)
{
r[cur] = 1;
int next = 0;
if(pair[p[cur]][0] == cur)
next = pair[p[cur]][1];
else
next = pair[p[cur]][0];
//r[next] = 1;
//cout << cur << ", " << next << endl;
int posx = pos[next][0], posy = pos[next][1];
int py = findy(posy);
int sp = 0;
for(; y[py][sp] != next; sp++);
if(y[py][sp+1] > 0)
next = y[py][sp+1];
else
break;
if(r[next] == 1)
{
cir = true;
break;
}
cur = next;
}
if(cir)
{
count++;
// PV(i)
// PVL(p, 13)
break;
}
}
//PVL(r, 13)
}
// 选取第n对虫洞
void pick(int n)
{
if(n > N / 2)
{
check();
return;
}
int cur = 1;
for(; p[cur] != 0; cur++);
p[cur] = n;
for(int i=cur+1; i<=N; i++)
{
if(p[i] != 0)
continue;
p[i] = n;
pick(n+1);
p[i] = 0;
}
p[cur] = 0;
}
void solve()
{
pick(1);
}<file_sep>/section2/runround2.cpp
/*
ID: luglian1
PROG: runround
LANG: C++
*/
#include <fstream>
#include <string>
#include <vector>
#include <algorithm>
#define INPUT_FILE "runround.in"
#define OUTPUT_FILE "runround.out"
using namespace std;
void NextNumber(std::vector<int>& number, int Digits) {
number[Digits - 1]++;
for (int i = Digits - 1; i >= 0; i--) {
if (number[i] == 10) {
number[i] = 1;
if (i == 0) {
number.insert (number.begin(),1);
return;
} else
number[i - 1]++;
}
}
return;
}
bool CheckElement(std::vector<int>::iterator first,
std::vector<int>::iterator last, int val) {
while (first < last) {
if (*first == val)
return true;
++first;
}
return false;
}
void NextUniqueNumber(std::vector<int>& number) {
std::vector<int> old = number;
for (int i = 1; i < number.size(); ++i) {
if (number[i] == 0) number[i]++;
while (CheckElement (number.begin(),number.begin() + i,number[i])) {
number[i]++;
if (number[i] == 10) {
number[i] = 1;
NextNumber (number,i);
i = 1;
continue;
}
}
}
return;
}
bool IsRoundNumber(std::vector<int>& number) {
std::vector<bool> used(10,false);
for (int i = 0, pos = 0, val = number[0]; i < number.size(); i++) {
pos = (pos + val) % number.size();
val = number[pos];
if (used[pos] == true) return false;
used[pos] = true;
}
return true;
}
unsigned int NextRoundNumber(unsigned int number) {
std::vector<int> digits;
for (int i = 0, tens = 1; i <= 10; ++i, tens *= 10) {
int partial = number / tens;
if (partial == 0) break;
partial %= 10;
digits.push_back(partial);
}
std::reverse (digits.begin(),digits.end());
NextNumber (digits,digits.size());
NextUniqueNumber (digits);
while (!IsRoundNumber(digits)) {
NextNumber (digits,digits.size());
NextUniqueNumber (digits);
}
number = 0;
for (int i = 0; i < digits.size(); i++)
number = 10 * number + digits[i];
return number;
}
int main(int argc, char *argv[]) {
ifstream FileInput (INPUT_FILE);
ofstream FileOutput (OUTPUT_FILE);
unsigned int Number;
FileInput >> Number;
FileOutput << NextRoundNumber(Number) << "\n";
return 0;
}<file_sep>/section 1/friday.cpp
/*
ID: luglian1
PROG: friday
LANG: C++
*/
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
using namespace std;
bool cheakLeap(int year){
return ( (year % 4 == 0 && year % 100 != 0) || year % 400 == 0);
}
int main(){
int noleap_days[] = {31, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30};
int leap_days[] = {31, 31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30};
ifstream in("friday.in");
ofstream out("friday.out");
int n;
in >> n;
int count[7] = {0,0,0,0,0,0,0};
int start = (0-31)%7+7;
for(int i=1900; i < 1900 + n; ++i){
int* pdays;
if( cheakLeap(i) )
pdays = leap_days;
else
pdays = noleap_days;
for(int j=0; j != 12; ++j){
start = (start + pdays[j]) % 7;
++count[start];
}
}
for(int i=0; i!=6; ++i){
out << count[i] << " ";
}
out << count[6] << endl;
in.close();
out.close();
return 0;
}
<file_sep>/section3/kimbits.cpp
/*
ID: luglian1
PROG: kimbits
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <bitset>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PVB(v, n) std::cout << #v << " = " << bitset<n>(v) << std::endl;
#define PROB_NAME "kimbits"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
unsigned int N, L, I;
unsigned int maxk[33] = {0};
unsigned int one[33] = {0};
unsigned int res;
bitset<32> resb;
unsigned int count[33][33] = {0};
bool load();
void save(ostream& out);
bool save();
void solve();
void solve2();
int main(){
load();
//solve();
solve2();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N >> L >> I;
return true;
}
void save(ostream& out)
{
// bitset<32> b(res);
// for(int i=N-1; i>=0; --i)
// out << b[i];
// out << endl;
for(int i=N; i>=1; --i)
out << resb[i];
out << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
// n bits with most k 1s
unsigned int findnext(unsigned int cur, int n, int k)
{
if(n == 0)
{
return cur | one[0];
}
int realk = min(k, n);
int mini = max(0, n-k);
int seqk = 0;
int i=n-1;
// find continuous 1s
for(; i>=mini; --i)
{
if(!(cur & one[i]))
break;
seqk ++;
}
// find continuous 0s
if(seqk < realk)
{
for(; i >= 0; --i)
{
if(cur & one[i])
break;
}
return findnext(cur, i+1, k-seqk);
}
else
{
return cur & (~maxk[n-1]) | one[n];
}
}
void solve()
{
for(int i=0; i<32; ++i)
{
one[i] = 1 << i;
if(i > 0)
maxk[i] = maxk[i-1];
maxk[i] |= one[i];
}
// PVL(maxk, 33)
res = 0;
for(int i=0; i<I-1; ++i)
{
res = findnext(res, N, L);
// PVB(res, 32)
}
// PVB(res, 32)
}
void solve2()
{
for(int i=0; i<33; ++i)
{
count[i][0] = 1;
count[0][i] = 1;
}
for(int i=1; i<33; ++i)
{
for(int j=1; j<=i; ++j)
{
count[i][j] = count[i-1][j] + count[i-1][j-1];
}
for(int j=i+1; j<33; ++j)
count[i][j] = count[i][i];
}
for(int i=0; i<33; ++i)
{
PVL(count[i], 33)
}
unsigned int curi = I;
unsigned curl = L;
for(int i=N; i>0; --i)
{
// PV(i)
// PV(curi)
// PV(curl)
if(curi > count[i-1][curl])
{
resb[i] = true;
curi -= count[i-1][curl];
curl --;
if(curl == 0)
break;
}
}
PV(resb)
}
// Suppose we knew how to calculate the size of the set of binary numbers for a given nbits and nones. That is, suppose we have a function sizeofset(n, m) that returns the number of n-bit binary numbers that have at most m ones in them.
// Then we can solve the problem as follows. We're looking for the ith element in the set of size n with m bits. This set has two parts: the numbers the start with zero, and the numbers that start with one. There are sizeofset(n-1, m) numbers that start with zero and have at most m one bits, and there are sizeofset(n-1, m-1) numbers that start with one and have at most m one bits.
// So if the index is less than sizeofset(n-1, m), the number in question occurs in the part of the set that is numbers that start with zero. Otherwise, it starts with a one.
// This lends itself to a nice recursive solution, implemented by "printbits".
// The only difficult part left is calculating "sizeofset". We can do this by dynamic programming using the property described above:
// sizeofset(n, m) = sizeofset(n-1, m) + sizeofset(n-1, m-1)
// and sizeofset(0, m) = 1 for all m. We use double's throughout for bits, but that's overkill given the rewritten problem that requires only 31 bits intead of 32.
// /*
// PROG: kimbits
// ID: rsc001
// */
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// FILE *fout;
// /* calculate binomial coefficient (n choose k) */
// double sizeofset[33][33];
// void
// initsizeofset(void)
// {
// int i, j;
// for(j=0; j<=32; j++)
// sizeofset[0][j] = 1;
// for(i=1; i<=32; i++)
// for(j=0; j<=32; j++)
// if(j == 0)
// sizeofset[i][j] = 1;
// else
// sizeofset[i][j] = sizeofset[i-1][j-1] + sizeofset[i-1][j];
// }
// void
// printbits(int nbits, int nones, double index)
// {
// double s;
// if(nbits == 0)
// return;
// s = sizeofset[nbits-1][nones];
// if(s <= index) {
// fprintf(fout, "1");
// printbits(nbits-1, nones-1, index-s);
// } else {
// fprintf(fout, "0");
// printbits(nbits-1, nones, index);
// }
// }
// void
// main(void)
// {
// FILE *fin;
// int nbits, nones;
// double index;
// fin = fopen("kimbits.in", "r");
// fout = fopen("kimbits.out", "w");
// assert(fin != NULL && fout != NULL);
// initsizeofset();
// fscanf(fin, "%d %d %lf", &nbits, &nones, &index);
// printbits(nbits, nones, index-1);
// fprintf(fout, "\n");
// exit(0);
// }
<file_sep>/section 1/numtri.cpp
/*
ID: luglian1
PROG: numtri
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
int mat[1000][1000]={0};
int r=0;
void read(ifstream& file_in){
file_in >> r;
for(int i=0; i!=r; ++i){
for(int j=0; j<=i; ++j){
file_in >> mat[i][j];
}
}
}
void work(){
r=r-2;
while(r>=0){
for(int i=0; i<=r; ++i){
mat[r][i] += max(mat[r+1][i], mat[r+1][i+1]);
}
--r;
}
}
int main(){
ifstream file_in("numtri.in");
ofstream file_out("numtri.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
read(file_in);
//cout << r << endl;
work();
file_out << mat[0][0] << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section3/shopping.cpp
/*
ID: luglian1
PROG: shopping
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
#include <map>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << (v)[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "shopping"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
struct coffer
{
int code;
int num;
int price;
coffer(int c=0, int n=0, int p=0): code(c), num(n), price(p){};
};
struct offer
{
vector<coffer> cs;
int reduce;
};
bool cmp_coffer(const coffer& c1, const coffer& c2)
{
return c1.code < c2.code;
}
ostream& operator<<(ostream& out, const coffer& c)
{
out << "(" << c.code << ", " << c.num << ", " << c.price <<")";
return out;
}
int s;
int n;
vector<offer> offers;
vector<coffer> purchase;
int dp[6][6][6][6][6] = {0};
int res=0;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> s;
for(int i=0; i<s; ++i)
{
int n;
file_in >> n;
offer o;
for(int j=0; j<n; ++j)
{
int code, num;
file_in >> code >> num;
o.cs.push_back(coffer(code, num));
}
file_in >> o.reduce;
offers.push_back(o);
}
int p;
file_in >> p;
for(int i=0; i<p; ++i)
{
int code, num, price;
file_in >> code >> num >> price;
purchase.push_back(coffer(code, num, price));
}
PVL(purchase, purchase.size())
sort(purchase.begin(), purchase.end(), cmp_coffer);
map<int, int> code_map;
map<int, int> price_map;
for(int i=0; i<p; ++i)
{
code_map.insert(pair<int, int>(purchase[i].code, i));
PV(purchase[i].code)
PV(code_map[purchase[i].code])
purchase[i].code = i;
}
PV(p)
for(vector<offer>::iterator it=offers.begin(); it!=offers.end(); ++it)
{
int cost = 0;
bool can_buy=true;
for(vector<coffer>::iterator cit=it->cs.begin(); cit!=it->cs.end(); ++cit)
{
if(code_map.count(cit->code) == 0)
{
can_buy = false;
break;
}
cit->code = code_map[cit->code];
cost += purchase[cit->code].price * cit->num;
}
if(can_buy)
it->reduce = cost - it->reduce;
else
it->reduce = 0;
}
for(vector<offer>::iterator it=offers.begin(); it!=offers.end(); ++it)
{
PVL(it->cs, it->cs.size())
PV(it->reduce)
}
PVL(purchase, purchase.size())
return true;
}
void save(ostream& out)
{
out << res << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
int p[5] = {0};
for(int i=0; i<purchase.size(); ++i)
{
p[i] = 5;
}
PVL(p, 5)
for(int i=0; i<=p[4]; ++i)
{
for(int j=0; j<=p[3]; ++j)
{
for(int k=0; k<=p[2]; ++k)
{
for(int m=0; m<=p[1]; ++m)
{
for(int n=0; n<=p[0]; ++n)
{
int ids[5] = {n, m, k, j, i};
// PVL(ids, 5)
int maxr = 0;
for(vector<offer>::iterator it=offers.begin(); it!=offers.end(); ++it)
{
bool can_buy = true;
for(vector<coffer>::iterator it2=it->cs.begin(); it2!=it->cs.end(); ++it2)
{
if(ids[it2->code] < it2->num)
{
can_buy = false;
break;
}
}
if(!can_buy)
continue;
int nids[5] = {n, m, k, j, i};
for(vector<coffer>::iterator it2=it->cs.begin(); it2!=it->cs.end(); ++it2)
{
nids[it2->code] -= it2->num;
}
int r = it->reduce + dp[nids[0]][nids[1]][nids[2]][nids[3]][nids[4]];
maxr = max(r, maxr);
}
dp[n][m][k][j][i] = maxr;
}
}
}
}
}
// PVL((int*)dp, 3125)
int q[5] = {0};
int cost=0;
for(int i=0; i<purchase.size(); ++i)
{
q[purchase[i].code] = purchase[i].num;
cost += (purchase[i].num * purchase[i].price);
}
PV(cost)
PVL(q, 5)
PV(dp[q[0]][q[1]][q[2]][q[3]][q[4]])
res = cost - dp[q[0]][q[1]][q[2]][q[3]][q[4]];
}
// Shopping Offers
// <NAME>
// This is a shortest path problem. The goal is to find the shortest path from an empty basket to a basket containing the requested objects. Thus, Dijkstra's algorithm can be used.
// The nodes in the graph correspond to baskets and the edges correspond to offers (purchasing a single item can be considered a degenerate offer). The length of an edge is the cost of the offer. For each item type, there can be between 0 and 5 inclusive objects of that type in the basket, for a total of 65 = 7,776 possible baskets.
// #include <stdio.h>
// #include <string.h>
// /* maximum number of offers */
// /* 100 offers + 5 degenerate offers */
// #define MAXO 105
// typedef struct OFFER_T
// {
// int nitem; /* number of items in the offer */
// int itemid[5]; /* item's id */
// int itemamt[5]; /* item's amount */
// int cost; /* the cost of this offer */
// } offer_t;
// offer_t offers[MAXO];
// int noffer;
// /* the cost of each basket type */
// int cost[7776];
// /* the item statistics */
// int itemid[5]; /* the id */
// int itemcst[5]; /* the cost of buying just 1 */
// int nitem;
// /* heap used by Dijkstra's algorithm */
// int heap[7776];
// int hsize;
// int hloc[7776]; /* location of baskets within the heap */
// /* debugging routine */
// void check_heap(void)
// { /* ensure heap order is maintained */
// int lv;
// return;
// for (lv = 1; lv < hsize; lv++)
// {
// if (cost[heap[lv]] < cost[heap[(lv-1)/2]])
// {
// fprintf (stderr, "HEAP ERROR!\n");
// return;
// }
// }
// }
// /* delete the minimum element in the heap */
// void delete_min(void)
// {
// int loc, val;
// int p, t;
// /* take last item from the heap */
// loc = heap[--hsize];
// val = cost[loc];
// /* p is the current position of item (loc,val) in the heap */
// /* the item isn't actually there, but that's where we're
// considering putting it */
// p = 0;
// while (2*p+1 < hsize)
// { /* while one child is less than the last item,
// move the lesser child up */
// t = 2*p+1;
// /* pick lesser child */
// if (t+1 < hsize && cost[heap[t+1]] < cost[heap[t]]) t++;
// if (cost[heap[t]] < val)
// { /* if child is less than last item, move it up */
// heap[p] = heap[t];
// hloc[heap[p]] = p;
// p = t;
// } else break;
// }
// /* put the last item back into the heap */
// heap[p] = loc;
// hloc[loc] = p;
// check_heap();
// }
// /* we decreased the value corresponding to basket loc */
// /* alter heap to maintain heap order */
// void update(int loc)
// {
// int val;
// int p, t;
// val = cost[loc];
// p = hloc[loc];
// while (p > 0) /* while it's not at the root */
// {
// t = (p-1)/2; /* t = parent of node */
// if (cost[heap[t]] > val)
// { /* parent is higher cost than us, swap */
// heap[p] = heap[t];
// hloc[heap[p]] = p;
// p = t;
// } else break;
// }
// /* put basket back into heap */
// heap[p] = loc;
// hloc[loc] = p;
// check_heap();
// }
// /* add this element into the heap */
// void add_heap(int loc)
// {
// if (hloc[loc] == -1)
// { /* if it's not in the heap */
// /* add it to the end (same as provisionally setting it's value
// to infinity) */
// heap[hsize++] = loc;
// hloc[loc] = hsize-1;
// }
// /* set to correct value */
// update(loc);
// }
// /* given an id, calculate the index of it */
// int find_item(int id)
// {
// if (itemid[0] == id) return 0;
// if (itemid[1] == id) return 1;
// if (itemid[2] == id) return 2;
// if (itemid[3] == id) return 3;
// if (itemid[4] == id) return 4;
// return -1;
// }
// /* encoding constants 6^0, 6^1, 6^2, ..., 6^5 */
// const int mask[5] = {1, 6, 36, 216, 1296};
// void find_cost(void)
// {
// int p;
// int cst;
// int lv, lv2;
// int amt;
// offer_t *o;
// int i;
// int t;
// /* initialize costs to be infinity */
// for (lv = 0; lv < 7776; lv++) cost[lv] = 999*25+1;
// /* offer not in heap yet */
// for (lv = 0; lv < 7776; lv++) hloc[lv] = -1;
// /* add empty baset */
// cost[0] = 0;
// add_heap(0);
// while (hsize)
// {
// /* take minimum basket not checked yet */
// p = heap[0];
// cst = cost[p];
// /* delete it from the heap */
// delete_min();
// /* try adding each offer to it */
// for (lv = 0; lv < noffer; lv++)
// {
// o = &offers[lv];
// t = p; /* the index of the new heap */
// for (lv2 = 0; lv2 < o->nitem; lv2++)
// {
// i = o->itemid[lv2];
// /* amt = amt of item lv2 already in basket */
// amt = (t / mask[i]) % 6;
// if (amt + o->itemamt[lv2] <= 5)
// t += mask[i] * o->itemamt[lv2];
// else
// { /* if we get more than 5 items in the basket,
// this is an illegal move */
// t = 0; /* ensures we will ignore it, since cost[0] = 0 */
// break;
// }
// }
// if (cost[t] > cst + o->cost)
// { /* we found a better way to get this basket */
// /* update the cost */
// cost[t] = cst + o->cost;
// add_heap(t); /* add, if necessary, and reheap */
// }
// }
// }
// }
// int main(int argc, char **argv)
// {
// FILE *fout, *fin;
// int lv, lv2; /* loop variable */
// int amt[5]; /* goal amounts of each type */
// int a; /* temporary variable */
// if ((fin = fopen("shopping.in", "r")) == NULL)
// {
// perror ("fopen fin");
// exit(1);
// }
// if ((fout = fopen("shopping.out", "w")) == NULL)
// {
// perror ("fopen fout");
// exit(1);
// }
// fscanf (fin, "%d", &noffer);
// /* read offers */
// for (lv = 0; lv < noffer; lv++)
// {
// fscanf (fin, "%d", &offers[lv].nitem);
// for (lv2 = 0; lv2 < offers[lv].nitem; lv2++)
// fscanf (fin, "%d %d", &offers[lv].itemid[lv2], &offers[lv].itemamt[lv2]);
// fscanf (fin, "%d", &offers[lv].cost);
// }
// /* read item's information */
// fscanf (fin, "%d", &nitem);
// for (lv = 0; lv < nitem; lv++)
// fscanf (fin, "%d %d %d", &itemid[lv], &amt[lv], &cost[lv]);
// /* fill in rest of items will illegal data, if necessary */
// for (lv = nitem; lv < 5; lv++)
// {
// itemid[lv] = -1;
// amt[lv] = 0;
// cost[lv] = 0;
// }
// /* go through offers */
// /* make sure itemid's are of item's in goal basket */
// /* translate itemid's into indexes */
// for (lv = 0; lv < noffer; lv++)
// {
// for (lv2 = 0; lv2 < offers[lv].nitem; lv2++)
// {
// a = find_item(offers[lv].itemid[lv2]);
// if (a == -1)
// { /* offer contains an item which isn't in goal basket */
// /* delete offer */
// /* copy last offer over this one */
// memcpy (&offers[lv], &offers[noffer-1], sizeof(offer_t));
// noffer--;
// /* make sure we check this one again */
// lv--;
// break;
// }
// else
// offers[lv].itemid[lv2] = a; /* translate id to index */
// }
// }
// /* add in the degenerate offers of buying single items 8/
// for (lv = 0; lv < nitem; lv++)
// {
// offers[noffer].nitem = 1;
// offers[noffer].cost = cost[lv];
// offers[noffer].itemamt[0] = 1;
// offers[noffer].itemid[0] = lv;
// noffer++;
// }
// /* find the cost for all baskets */
// find_cost();
// /* calculate index of goal basket */
// a = 0;
// for (lv = 0; lv < 5; lv++)
// a += amt[lv] * mask[lv];
// /* output answer */
// fprintf (fout, "%i\n", cost[a]);
// return 0;
// }
// <NAME>'s Comments
// This problem can be solved using dynamic programming. This way is easier to code, and for the test cases it runs for much less than 0.1 seconds.
// We keep a five dimensional array sol (it's not that much, because its size is only 5*5*5*5*5*sizeof(special_offer).) Each configuration of the dimensions sol[a][b][c][d][e] corresponds to having a products from the first kind, b products from the second kind, c from the third, etc.
// Basically, the DP forumla is the following : sol[a][b][c][d][e]= min (a*price[1]+b*price[2]+c*price[3]+d*price[4]+e*price[5], so[k].price+ sol[a-so[k].prod[1].items] [b-so[k].prod[2].items] [c-so[k].prod[3].items] [d-so[k].prod[4].items] [e-so[k].prod[5].items] ) where k changes from 1 to the number of special offers. Or, in other words, for each field of the array we check which is better :
// Not to use any special offer
// To use some special offer
// It's very similliar to the knapsack problem. The complexity of this algorithm is O(5*5*5*5*5*100)=O(312,500), which is quite acceptable.
// #include <fstream>
// #include <cstring>
// using namespace std;
// ifstream fin ("shopping.in");
// ofstream fout("shopping.out");
// struct special_offer {
// int n;
// int price; // the price of that special offer
// struct product { // for each product we have to keep :
// int id; // the id of the product
// int items; // how many items it includes
// } prod[6];
// } so[100]; // here the special offers are kept
// int code[1000], /* Each code is 'hashed' from its real value
// to a smaller index. Example :
// If in the input we have code 111, 934, 55,
// 1, 66 we code them as 1,2,3,4,5. That is
// kept in code[1000];
// */
// price[6], // the price of each product
// many[6]; // how many of each product are to be bought
// int s, // the number of special offers
// b; // the number of different kinds of products to be bought
// int sol[6][6][6][6][6]; // here we keep the price of each configuration
// void init() { // reads the input
// fin>>s;
// for (int i=1;i<=s;i++) {
// fin>>so[i].n;
// for (int j=1;j<=so[i].n;j++)
// fin>>so[i].prod[j].id>>so[i].prod[j].items;
// fin>>so[i].price;
// }
// fin>>b;
// for (int i=1;i<=b;i++) {
// int tmp;
// fin>>tmp;
// code[tmp]=i; // here we convert the code to an id from 1..5
// fin>>many[i];
// fin>>price[i];
// }
// }
// void solve() { // the procedure that solves the problem
// for (int a=0;a<=many[1];a++)
// for (int b=0;b<=many[2];b++)
// for (int c=0;c<=many[3];c++)
// for (int d=0;d<=many[4];d++)
// for (int e=0;e<=many[5];e++)
// if ((a!=0)||(b!=0)||(c!=0)||(d!=0)||(e!=0)) {
// int min=a*price[1]+b*price[2]+c*price[3]+d*price[4]+e*price[5];
// /* in min we keep the lowest price at which we can buy a items
// from the 1st type, +b from the 2nd+c of the 3rd... e from the
// 5th */
// for (int k=1;k<=s;k++) { // for each special offer
// int can=1,hm[6];
// memset(&hm,0,sizeof(hm));
// for (int l=1;l<=so[k].n;l++)
// hm[code[so[k].prod[l].id]]=so[k].prod[l].items;
// if ((hm[1]>a)||(hm[2]>b)||(hm[3]>c)||(hm[4]>d)||(hm[5]>e))
// can=0;// we check if it is possible to use that offer
// if (can) { // if possible-> check if it is better
// // than the current min
// int pr=so[k].price+sol[a-hm[1]][b-hm[2]][c-hm[3]]
// [d-hm[4]][e-hm[5]];
// /* Those which are not included in the special offer */
// if (pr<min) min=pr;
// }
// }
// sol[a][b][c][d][e]=min;
// }
// }
// int main() {
// memset(&so,0,sizeof(so));
// init();
// solve();
// fout<<sol[many[1]][many[2]][many[3]][many[4]][many[5]]<<endl;
// return 0;
// }
// USACO Gateway | Comment or Question<file_sep>/section 1/milk.cpp
/*
ID: luglian1
PROG: milk
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
class farmer{
public:
int price;
int amount;
};
class FarmerCompare{
public:
bool operator()(const farmer& lhs, const farmer& rhs){
return lhs.price < rhs.price;
}
};
int main(){
ifstream file_in("milk.in");
ofstream file_out("milk.out");
if(!file_in || !file_out){
cerr << "Error: Failed to open file" << endl;
return 0;
}
int n, m;
file_in >> n >> m;
vector<farmer> farmers(m);
for(int i=0; i!=m; ++i){
file_in >> farmers[i].price >> farmers[i].amount;
}
sort(farmers.begin(), farmers.end(), FarmerCompare());
for(int i=0; i!=m; ++i){
cout << farmers[i].price << " " << farmers[i].amount << endl;
}
int price = 0;
int i=0;
while(n > 0){
if(n > farmers[i].amount){
price += farmers[i].amount * farmers[i].price;
n -= farmers[i].amount;
}
else{
price += n * farmers[i].price;
n = 0;
}
++i;
}
file_out << price << endl;
file_in.close();
file_out.close();
return 0;
}
<file_sep>/section2/zerosum.cpp
/*
ID: luglian1
PROG: zerosum
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <sstream>
#include <algorithm>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "zerosum"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
//int N = 5;
//int cal[20] = {1, 12, 2, 12, 3, 10, 4, 11, 5, 0, 0, 0}; // 10 = '+', 11 = '-', 12 = ' '
int N = 0;
int cal[20] = {0}; // 10 = '+', 11 = '-', 12 = ' '
vector<string> outstr;
bool load();
void save(ostream& out);
bool save();
void solve();
int parseResult();
void dfs(int);
string makestr();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
for(int i=1; i <= N; ++i)
{
cal[(i-1) * 2] = i;
}
return true;
}
string makestr()
{
stringstream ss;
for(int i=0; i < 2 * N - 1; ++i)
{
switch(cal[i])
{
case 10:
ss << '+';
break;
case 11:
ss << '-';
break;
case 12:
ss << ' ';
break;
default:
ss << cal[i];
}
}
return ss.str();
}
void save(ostream& out)
{
for(vector<string>::iterator begin=outstr.begin(); begin != outstr.end(); begin++)
{
out << *begin << endl;
}
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
sort(outstr.begin(), outstr.end());
save(file_out);
save(cout);
return true;
}
void solve()
{
dfs(1);
}
void dfs(int curpos)
{
if(curpos >= 2 * N - 1)
{
if(parseResult() == 0)
{
outstr.push_back(makestr());
}
}
else
{
for(int i=10; i<=12; ++i)
{
cal[curpos] = i;
dfs(curpos + 2);
}
}
}
int parseResult()
{
int re = 0;
int op = 0;
int lastop = 0;
int right = 0;
int recal[20] = {0};
int curpos = 0;
// first merge space and num
for(int i=0; i < 2 * N; ++i)
{
if (i % 2 == 0) // num
{
right = right * 10 + cal[i];
}
else
{
op = cal[i];
if(op != 12)
{
recal[curpos++] = right;
right = 0;
recal[curpos++] = op;
}
}
}
//PVL(recal, 20)
// secode calc result
re = recal[0];
right = 0;
op = 0;
for(int i=1; i < curpos; ++i)
{
if (i % 2 == 0) // num
{
right = recal[i];
if(op == 10) // +
{
re = re + right;
}
else if(op == 11) // -
{
re = re - right;
}
}
else
{
op = recal[i];
}
}
//PV(re)
return re;
}<file_sep>/section2/lamps.cpp
/*
ID: luglian1
PROG: lamps
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <set>
#include <string>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define INPUT_FILE "lamps.in"
#define OUTPUT_FILE "lamps.out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int N;
int C;
bool stats[101] = {true};
vector<int> onIds;
vector<int> offIds;
set<string> results;
int main(){
load();
solve();
save();
return 0;
}
void changeLamps(int begin, int step)
{
for(int i=begin; i<=N; i+=step)
{
stats[i] = !stats[i];
}
}
bool checkValid(int remainC)
{
for(int i=0; i<onIds.size(); ++i)
{
if(!stats[onIds[i]])
return false;
}
for(int i=0; i<offIds.size(); ++i)
{
if(stats[offIds[i]])
return false;
}
if(remainC % 2 == 1)
return false;
return true;
}
string statsToString()
{
char s[N+1];
for(int i=1; i<=N; ++i)
{
s[i-1] = stats[i] ? '1' : '0';
}
s[N] = '\0';
return string(s);
}
void dfs(int buttonId, int remainC)
{
if(checkValid(remainC))
{
results.insert(statsToString());
}
if(remainC <= 0 || buttonId > 4)
return;
int begin = 1;
int step = 1;
switch(buttonId)
{
case 1:
begin = 1;
step = 1;
break;
case 2:
begin = 1;
step = 2;
break;
case 3:
begin = 2;
step = 2;
break;
case 4:
begin = 1;
step = 3;
break;
default:
break;
}
if(buttonId < 4)
dfs(buttonId+1, remainC);
changeLamps(begin, step);
//PV(buttonId);
//PV(statsToString());
dfs(buttonId+1, remainC-1);
changeLamps(begin, step);
}
void solve()
{
for(int i=1; i<= N; ++i)
{
stats[i] = true;
}
dfs(1, C);
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> N;
file_in >> C;
int end;
file_in >> end;
while(end != -1)
{
onIds.push_back(end);
file_in >> end;
}
file_in >> end;
while(end != -1)
{
offIds.push_back(end);
file_in >> end;
}
return true;
}
void save(ostream& out)
{
for(set<string>::iterator it = results.begin(); it != results.end(); ++it)
{
out << *it << endl;
}
if(results.size() == 0)
out << "IMPOSSIBLE" << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
<file_sep>/section3/fact4.cpp
/*
ID: luglian1
PROG: fact4
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "fact4"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
int n;
int res = 1;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> n;
return true;
}
void save(ostream& out)
{
out << res % 10 << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void solve()
{
res = 1;
for(int i=1; i<=n; ++i)
{
res *= i;
while(res % 10 == 0)
res = res / 10;
if(res > 100000)
res = res % 100000;
PV(res)
}
}
// The insight for this problem is that 0's at the end of a number come from it being divisible by 10, or equivalently, by 2 and 5. Furthermore, there are always more 2s than 5s in a factorial.
// To get the last digit of the factorial, we can run a loop to calculate it modulo 10, as long as we don't include any 2s or 5s in the product. Of course, we want to exclude the same number of 2s and 5s, so that all we're really doing is ignoring 10s. So after the loop, we need to multiply in any extra 2s that didn't have 5s to cancel them out.
// PROG: fact4
// ID: rsc001
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// void
// main(void)
// {
// FILE *fin, *fout;
// int n2, n5, i, j, n, digit;
// fin = fopen("fact4.in", "r");
// fout = fopen("fact4.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d", &n);
// digit = 1;
// n2 = n5 = 0;
// for(i=2; i<=n; i++) {
// j = i;
// while(j%2 == 0) {
// n2++;
// j /= 2;
// }
// while(j%5 == 0) {
// n5++;
// j /= 5;
// }
// digit = (digit * j) % 10;
// }
// for(i=0; i<n2-n5; i++)
// digit = (digit * 2) % 10;
// fprintf(fout, "%d\n", digit);
// exit(0);
// }<file_sep>/section2/maze1.cpp
/*
ID: luglian1
PROG: maze1
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "maze1"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
int w = 0, h = 0, all = 0;
vector<vector<int> > e;
vector<int> ex;
int res[3800] = {0};
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> w >> h;
all = w * h;
e.resize(all, vector<int>());
// PV(e.size())
string line;
getline(file_in, line);
for(int x=0; x < 2 * h + 1; ++x)
{
string line;
getline(file_in, line);
// cout << line << endl;
if(line.length() < 2 * w + 1)
{
line += string(2 * w + 1 - line.length(), ' ');
}
for(int y=0; y < 2 * w + 1; ++y)
{
char c = line[y];
if(c != ' ')
continue;
if(x % 2 == 0)
{
if(x == 0)
{
int nid = (y - 1) / 2;
ex.push_back(nid);
}
else if(x == 2 * h)
{
int nid = w * (h-1) + (y - 1) / 2;
ex.push_back(nid);
}
else
{
int nid1 = (x - 2) / 2 * w + (y - 1) / 2;
int nid2 = x / 2 * w + (y - 1) / 2;
e[nid1].push_back(nid2);
e[nid2].push_back(nid1);
}
}
else
{
if(y == 0)
{
int nid = (x - 1) / 2 * w;
ex.push_back(nid);
}
else if(y == 2 * w)
{
int nid = (x - 1) / 2 * w + w -1;
ex.push_back(nid);
}
else if(y % 2 == 0)
{
int nid1 = (x - 1) / 2 * w + (y - 2) / 2;
int nid2 = nid1 + 1;
e[nid1].push_back(nid2);
e[nid2].push_back(nid1);
}
}
}
} // for
// for(int i=0; i < w * h; ++ i)
// {
// PVL(e[i], e[i].size())
// }
// PV(ex.size())
// PVL(ex, 2)
return true;
}
void save(ostream& out)
{
//out << res + 1 << endl;
// PVL(res, all)
//PV(res[0])
int max = 0;
for(int i=0; i< all; ++i)
{
if(res[i] > max)
max = res[i];
}
out << max + 1 << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void dijkstra(int src)
{
int q[3800] = {0};
int s[3800] = {0};
// init q
for(int i=0; i< all; ++i)
{
q[i] = 3800;
}
for(vector<int>::iterator i=e[src].begin(); i != e[src].end(); ++i)
q[*i] = 1;
q[src] = 0;
s[src] = 1;
for(int i=0; i< all; ++i)
{
int minid = -1;
int minq = 3800;
for(int j=0; j < all; ++j)
{
if(s[j] == 0 && q[j] < minq)
{
minid = j;
minq = q[j];
}
}
//PV(minid)
if(minid < 0)
break;
s[minid] = 1;
for(vector<int>::iterator j=e[minid].begin(); j != e[minid].end(); ++j)
{
if(s[*j] == 0)
{
int newq = minq + 1;
if(newq < q[*j])
q[*j] = newq;
}
}
}
// PVL(q, all)
for(int i=0; i<all; ++i)
{
if(q[i] < res[i])
res[i] = q[i];
}
return;
}
void solve()
{
for(int i=0; i < all; ++i)
res[i] = 3800;
for(vector<int>::iterator src=ex.begin(); src != ex.end(); src++)
{
dijkstra(*src);
}
}
// We can solve this with a standard flood fill, using a queue to implement breadth first search. It is convenient to leave the maze in its ASCII format and just look at it as a bunch of characters, with non-space characters being walls.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #define MAXWID 38
// #define MAXHT 100
// typedef struct Point Point;
// struct Point {
// int r, c;
// };
// int wid, ht;
// char maze[MAXHT*2+1][MAXWID*2+1+2]; /* extra +2 for "\n\0" */
// int dist[MAXHT*2+1][MAXWID*2+1];
// Point
// Pt(int r, int c)
// {
// Point p;
// p.r = r;
// p.c = c;
// return p;
// }
// typedef struct Queue Queue;
// struct Queue {
// Point p;
// int d;
// };
// Queue floodq[MAXHT*MAXWID];
// int bq, eq;
// /* if no wall between point p and point np, add np to queue with distance d+1 */
// void
// addqueue(int d, Point p, Point np)
// {
// if(maze[(p.r+np.r)/2][(p.c+np.c)/2] == ' ' && maze[np.r][np.c] == ' ') {
// maze[np.r][np.c] = '*';
// floodq[eq].p = np;
// floodq[eq].d = d+1;
// eq++;
// }
// }
// /* if there is an exit at point exitp, plug it and record a start point
// * at startp */
// void
// lookexit(Point exitp, Point startp)
// {
// if(maze[exitp.r][exitp.c] == ' ') {
// addqueue(0, startp, startp);
// maze[exitp.r][exitp.c] = '#';
// }
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// Point p;
// int i, r, c, m, d;
// fin = fopen("maze1.in", "r");
// fout = fopen("maze1.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d %d\n", &wid, &ht);
// wid = 2*wid+1;
// ht = 2*ht+1;
// for(i=0; i<ht; i++)
// fgets(maze[i], sizeof(maze[i]), fin);
// /* find exits */
// for(i=1; i<wid; i+=2) {
// lookexit(Pt(0, i), Pt(1, i));
// lookexit(Pt(ht-1, i), Pt(ht-2, i));
// }
// for(i=1; i<ht; i+=2) {
// lookexit(Pt(i, 0), Pt(i, 1));
// lookexit(Pt(i, wid-1), Pt(i, wid-2));
// }
// /* must have found at least one square with an exit */
// /* since two exits might open onto the same square, perhaps eq == 1 */
// assert(eq == 1 || eq == 2);
// for(bq = 0; bq < eq; bq++) {
// p = floodq[bq].p;
// d = floodq[bq].d;
// dist[p.r][p.c] = d;
// addqueue(d, p, Pt(p.r-2, p.c));
// addqueue(d, p, Pt(p.r+2, p.c));
// addqueue(d, p, Pt(p.r, p.c-2));
// addqueue(d, p, Pt(p.r, p.c+2));
// }
// /* find maximum distance */
// m = 0;
// for(r=0; r<ht; r++)
// for(c=0; c<wid; c++)
// if(dist[r][c] > m)
// m = dist[r][c];
// fprintf(fout, "%d\n", m);
// exit(0);
// }
<file_sep>/section2/cowtour.cpp
/*
ID: luglian1
PROG: cowtour
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#include <vector>
#include <cmath>
#include <iomanip>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << v[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "cowtour"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
#define MAX_DIS 1000000
using namespace std;
struct Point
{
int x;
int y;
};
ostream& operator<<(ostream& out, const Point& p)
{
out << "(" << p.x << ", " << p.y << ")";
}
// union find
class UF
{
public:
UF(int n)
{
id.resize(n, 0);
uni.resize(n, vector<int>());
for(int i=0; i<n; ++i)
{
id[i] = i;
uni[i].push_back(i);
};
c = n;
}
int find(int i) const
{
return id[i];
}
void merge(int i, int j)
{
int fi = id[i];
int fj = id[j];
if(fi == fj)
return;
for(int k = 0; k < id.size(); ++k)
{
if(id[k] == fj)
{
id[k] = fi;
uni[fi].push_back(k);
}
}
uni[fj].clear();
c--;
}
bool connected(int i, int j) const
{
return id[i] == id[j];
}
int count() const
{
return c;
}
const vector<int>& findUnion(int uid) const
{
return uni[uid];
}
public:
vector<int> id;
int c;
vector<vector<int> > uni;
};
int n = 0;
string g[150];
double path[150][150] = {0};
double dis[150][150] = {0};
Point pos[150];
double mindis = MAX_DIS;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
solve();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
file_in >> n;
for(int i=0; i < n; ++i)
{
file_in >> pos[i].x >> pos[i].y;
}
for(int i=0; i< n; ++i)
{
file_in >> g[i];
}
// PVL(g, n);
return true;
}
void save(ostream& out)
{
out << setiosflags(ios::fixed) << setprecision(6) << mindis << endl;
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
void dijkstra(int src, const UF& uf)
{
double* q = path[src];
int s[150] = {0};
int per[150] = {0};
// init q
for(int i=0; i< n; ++i)
{
q[i] = MAX_DIS;
}
for(int i=0; i < n; ++i)
if(g[src][i] == '1')
{
q[i] = dis[src][i];
per[i] = src;
}
q[src] = 0;
s[src] = 1;
const vector<int>& u = uf.findUnion(uf.find(src));
double pp = 0;
for(int i=0; i < u.size(); ++i)
{
int minid = -1;
double minq = MAX_DIS;
for(vector<int>::const_iterator j=u.begin(); j != u.end(); ++j)
{
if(s[*j] == 0 && q[*j] < minq)
{
minid = *j;
minq = q[*j];
}
}
//PV(minid)
// if(src == 15)
// {
// PV(minid)
// }
// if(minq == 39793)
// {
// PV(src)
// PV(minid)
// }
if(minid < 0)
break;
s[minid] = 1;
for(vector<int>::const_iterator j=u.begin(); j != u.end(); ++j)
{
if(s[*j] == 0 && g[minid][*j] == '1')
{
double newq = minq + dis[minid][*j];
if(newq < q[*j])
{
q[*j] = newq;
per[*j] = minid;
}
}
}
}
// if(src == 15)
// {
// int dst = 20;
// double dd = 0;
// while(per[dst] != 15)
// {
// dd += dis[dst][per[dst]];
// dst = per[dst];
// }
// PV(dd)
// PV(dis[dst][15])
// dd += dis[dst][15];
// PV(dd)
// }
return;
}
double addConnect(const UF& uf, int a, int b)
{
double maxdis = 0;
double dab = dis[a][b];
const vector<int>& ua = uf.findUnion(uf.find(a));
const vector<int>& ub = uf.findUnion(uf.find(b));
for(vector<int>::const_iterator i=ua.begin(); i!=ua.end(); ++i)
{
for(vector<int>::const_iterator j=ub.begin(); j!=ub.end(); ++j)
{
double d = path[*i][a] + dab + path[*j][b];
if(d > maxdis)
maxdis = d;
if(d >= MAX_DIS)
{
PV(uf.find(a))
PV(uf.find(b))
PVL(ua, ua.size())
PVL(ub, ub.size())
PV(a)
PV(b)
PV(*i)
PV(*j)
PV(dab)
PV(path[*i][a])
PV(path[*j][b])
PV(d)
exit(0);
}
}
}
// for(int i=0; i<n; ++i)
// {
// if(!uf.connected(i, a))
// continue;
// for(int j=0; j<n; ++j)
// {
// if(!uf.connected(j, b))
// continue;
// // recalc the dis
// double d = dis(i, a) + dab + dis(j, b);
// if(d > maxdis)
// maxdis = d;
// }
// }
return maxdis;
}
void solve()
{
UF uf(n);
for(int i=0; i < n; ++i)
for(int j=i+1; j < n; ++j)
{
if(g[i][j] == '1')
uf.merge(i, j);
double x = pos[i].x - pos[j].x;
double y = pos[i].y - pos[j].y;
dis[i][j] = dis[j][i] = sqrt((x * x) + (y * y));
}
// PV(uf.count())
for(int i=0; i < n; ++i)
dijkstra(i, uf);
vector<int> uid;
// PVL(uf.id, n)
for(int i=0; i<n; ++i)
{
//PVL(path[i], n);
if(uf.uni[i].size()>0)
{
uid.push_back(i);
//PVL(uf.uni[i], uf.uni[i].size());
// PV(uf.uni[i][0])
// PV(pos[uf.uni[i][0]])
// PV(uf.uni[i].size())
}
}
double maxdis = 0;
for(int i=0; i< n; ++i)
for(int j=i+1; j<n; ++j)
if(path[i][j] != MAX_DIS && path[i][j] > maxdis)
maxdis = path[i][j];
// PV(maxdis)
// PV(uid.size())
for(int i=0; i<uid.size(); ++i)
{
const vector<int>& ui = uf.findUnion(uid[i]);
for(int j=i+1; j<uid.size(); ++j)
{
const vector<int>& uj = uf.findUnion(uid[j]);
for(vector<int>::const_iterator ci=ui.begin(); ci!=ui.end(); ++ci)
{
for(vector<int>::const_iterator cj=uj.begin(); cj!=uj.end(); ++cj)
{
// PV(*ci)
// PV(*cj)
double res = addConnect(uf, *ci, *cj);
// PV(res)
res = max(maxdis, res);
if(res < mindis)
mindis = res;
}
}
}
}
}
// We do a fair bit of precomputation before choosing the path to add.
// First, we calculate the minimum distance between all connected points. Then, we use a recursive depth first search to identify the different fields. Then we fill in diam[i], which is defined to be the distance to the farthest pasture that pasture i is connected to. Fielddiam[j] is the diameter of field j, which is the maximum of diam[i] for all pastures i in the field j.
// Once we have all this, selecting a path is simple. If we add a path joining pastures i and j which are in different fields, the diameter of the new field is the maximum of:
// dist to point farthest from i + dist from i to j + dist to point farthest from j.
// old diameter of the field containing pasture i.
// old diameter of the field containing pasture j.
// #include <stdio.h>
// #include <stdlib.h>
// #include <string.h>
// #include <assert.h>
// #include <math.h>
// #define INF (1e40)
// typedef struct Point Point;
// struct Point {
// int x, y;
// };
// #define MAXPASTURE 150
// int n;
// double dist[MAXPASTURE][MAXPASTURE];
// double diam[MAXPASTURE];
// double fielddiam[MAXPASTURE];
// Point pt[MAXPASTURE];
// int field[MAXPASTURE];
// int nfield;
// double
// ptdist(Point a, Point b)
// {
// return sqrt((double)(b.x-a.x)*(b.x-a.x)+(double)(b.y-a.y)*(b.y-a.y));
// }
// /* mark the field containing pasture i with number m */
// void
// mark(int i, int m)
// {
// int j;
// if(field[i] != -1) {
// assert(field[i] == m);
// return;
// }
// field[i] = m;
// for(j=0; j<n; j++)
// if(dist[i][j] < INF/2)
// mark(j, m);
// }
// void
// main(void)
// {
// FILE *fin, *fout;
// int i, j, k, c;
// double newdiam, d;
// fin = fopen("cowtour.in", "r");
// fout = fopen("cowtour.out", "w");
// assert(fin != NULL && fout != NULL);
// fscanf(fin, "%d\n", &n);
// for(i=0; i<n; i++)
// fscanf(fin, "%d %d\n", &pt[i].x, &pt[i].y);
// for(i=0; i<n; i++) {
// for(j=0; j<n; j++) {
// c = getc(fin);
// if(i == j)
// dist[i][j] = 0;
// else if(c == '0')
// dist[i][j] = INF; /* a lot */
// else
// dist[i][j] = ptdist(pt[i], pt[j]);
// }
// assert(getc(fin) == '\n');
// }
// /* Floyd-Warshall all pairs shortest paths */
// for(k=0; k<n; k++)
// for(i=0; i<n; i++)
// for(j=0; j<n; j++)
// if(dist[i][k]+dist[k][j] < dist[i][j])
// dist[i][j] = dist[i][k]+dist[k][j];
// /* mark fields */
// for(i=0; i<n; i++)
// field[i] = -1;
// for(i=0; i<n; i++)
// if(field[i] == -1)
// mark(i, nfield++);
// /* find worst diameters involving pasture i, and for whole field */
// for(i=0; i<n; i++) {
// for(j=0; j<n; j++)
// if(diam[i] < dist[i][j] && dist[i][j] < INF/2)
// diam[i] = dist[i][j];
// if(diam[i] > fielddiam[field[i]])
// fielddiam[field[i]] = diam[i];
// }
// /* consider a new path between i and j */
// newdiam = INF;
// for(i=0; i<n; i++)
// for(j=0; j<n; j++) {
// if(field[i] == field[j])
// continue;
// d = diam[i]+diam[j]+ptdist(pt[i], pt[j]);
// if(d < fielddiam[field[i]])
// d = fielddiam[field[i]];
// if(d < fielddiam[field[j]])
// d = fielddiam[field[j]];
// if(d < newdiam)
// newdiam = d;
// }
// fprintf(fout, "%.6lf\n", newdiam);
// exit(0);
// }
<file_sep>/section 1/gift1.cpp
/*
ID: luglian1
PROG: gift1
LANG: C++
*/
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <map>
using namespace std;
struct person{
string name;
int initial_m;
int final_m;
//int gifts;
vector<string> friends;
};
void readPerson(person& p, ifstream& in){
in >> p.initial_m;
int fn;
in >> fn;
p.friends.resize(fn);
for(int i=0; i!=fn; ++i){
in >> p.friends[i];
}
p.final_m = 0;
}
int main(){
ifstream in("gift1.in");
ofstream out("gift1.out");
int n;
in >> n;
cout << n << endl;
//return 0;
map<string, int> person_id;
vector<person> person_list(n);
cout << person_list.size() << endl;
person ptmp;
for(int i=0; i!=n; ++i){
in >> person_list[i].name;
person_id.insert(pair<string, int>(person_list[i].name, i));
cout << person_id.size() << " " << person_list[i].name << endl;
}
string name;
for(int i=0; i!=n; ++i){
in >> name;
cout << person_id.find(name)->second << endl;
readPerson(person_list[person_id.find(name)->second], in);
}
cout << "i am here" << endl;
// give gifts
for(int i=0; i!=n; ++i){
if(person_list[i].friends.size() == 0)
continue;
int quo = person_list[i].initial_m / person_list[i].friends.size();
int rem = person_list[i].initial_m % person_list[i].friends.size();
person_list[i].final_m += rem;
cout << "i am here" << endl;
for(int j=0; j < person_list[i].friends.size(); ++j){
person_list[person_id.find(person_list[i].friends[j])->second].final_m += quo;
}
cout << "finish" << endl;
}
for(int i=0; i!=n; ++i){
out << person_list[i].name << " "
<< person_list[i].final_m - person_list[i].initial_m << endl;
}
in.close();
out.close();
return 0;
}
<file_sep>/template.cpp
/*
ID: luglian1
PROG: beads
LANG: C++
*/
#include <iostream>
#include <string>
#include <fstream>
#define PV(v) std::cout << #v << " = " << v << std::endl;
#define PVL(v, n) \
std::cout << #v << " = ";\
for(int _i=0; _i<n; ++_i) \
{std::cout << (v)[_i] << ", ";}\
std::cout << std::endl;
#define PROB_NAME "combo"
#define INPUT_FILE PROB_NAME".in"
#define OUTPUT_FILE PROB_NAME".out"
using namespace std;
bool load();
void save(ostream& out);
bool save();
void solve();
int main(){
load();
save();
return 0;
}
bool load()
{
ifstream file_in(INPUT_FILE);
if(!file_in)
{
cerr << "Error: Failed to load file." << endl;
return false;
}
return true;
}
void save(ostream& out)
{
}
bool save()
{
ofstream file_out(OUTPUT_FILE);
if(!file_out){
cerr << "Error: Failed to open file." << endl;
return false;
}
save(file_out);
save(cout);
return true;
}
| 53a41ea64f4c08230c30cf00db7be2c296af42f1 | [
"Markdown",
"C++"
] | 65 | C++ | legaldev/USACO_Practice | 766d792cd556ac544321a52ec72040f5b4fa3f82 | 35690e70134342d56652371684cfeb725763f77c | |
refs/heads/master | <repo_name>Sanchez056/Proyecto-Final-Aplicada-1-Sistema-De-VentasSm<file_sep>/Entidades/Proveedores.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
namespace Entidades
{
public class Proveedores
{
[Key]
public int ProveedorId { get; set; }
public string Nombre { get; set; }
public string Ciudad { get; set; }
public string Direccion { get; set; }
public string Telefono { get; set; }
public string Fax { get; set; }
public string Correo { get; set; }
public DateTime Fecha { get; set; }
public Proveedores( string nombre)
{
this.Nombre = nombre;
}
public Proveedores()
{
}
}
}
<file_sep>/Proyecto Final/Registros/Ejercicio de Estructura/7.2.cpp
#include <iostream>
#include <string.h>
using namespace std;
int polindromo(char *cad)
{
int tam = strlen(cad);
char aux[tam];
for(int x = tam-1,y = 0; x >= 0; x--, y++)
aux[y] = cad[x];
if(strcmp(cad,aux) == 0)
return 1;
else
return 0;
}
void esPolindromo(char *cad)
{
if(polindromo(cad))
cout << "es polindromo";
else
{
cout << "introduzca una cadena: ";
cin >> cad;
esPolindromo(cad);
}
}
int main()
{
char cad[5] = {'e','l','a',};
esPolindromo(cad);
return 0;
}
<file_sep>/Proyecto Final/MenuPrincipal.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using BLL;
using Entidades;
namespace ProyectoFinal_Aplicada1
{
public partial class MenuPrincipal : Form
{
public MenuPrincipal()
{
InitializeComponent();
}
private void RegistroarticuloToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosArticuloss ResUs = new Registros.RegistrosArticuloss();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void toolStripMenuItem1_Click(object sender, EventArgs e)
{
Registros.RegistrosVentass ResUs = new Registros.RegistrosVentass();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void RegistrosclientesToolStripMenuItem1_Click(object sender, EventArgs e)
{
Registros.RegistrosClientes ResUs = new Registros.RegistrosClientes();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void RegistrosusuariosToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosUsuarios ResUs = new Registros.RegistrosUsuarios();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void tiposUsuariosToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosTiposUsuarios ResUs = new Registros.RegistrosTiposUsuarios();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void RegistrosproveedorToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosProvvedores ResUs = new Registros.RegistrosProvvedores();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void registrosEmpleadoToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosEmpleados ResUs = new Registros.RegistrosEmpleados();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void categoriasToolStripMenuItem_Click(object sender, EventArgs e)
{
Registros.RegistrosCategorias ResUs = new Registros.RegistrosCategorias();
ResUs.MdiParent = this;
ResUs.Show();
ResUs.Location = new Point(40, 40);
}
private void ConsultausuariosToolStripMenuItem1_Click(object sender, EventArgs e)
{
Consultas.ConsultaUsuarios ConUs = new Consultas.ConsultaUsuarios();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void tipoDeUsuariosToolStripMenuItem_Click(object sender, EventArgs e)
{
Consultas.ConsultaTipoUsuarios ConUs = new Consultas.ConsultaTipoUsuarios();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void ConsultaclientesToolStripMenuItem2_Click(object sender, EventArgs e)
{
Consultas.ConsultaClientes ConUs = new Consultas.ConsultaClientes();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void ConsultaproveedoresToolStripMenuItem_Click(object sender, EventArgs e)
{
Consultas.ConsultaProvvedor ConUs = new Consultas.ConsultaProvvedor();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void ConsultaempleadosToolStripMenuItem_Click(object sender, EventArgs e)
{
Consultas.ConsultaEmpleados ConUs = new Consultas.ConsultaEmpleados();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void ConsultaarticulosToolStripMenuItem_Click(object sender, EventArgs e)
{
Consultas.ConsultasArticulos ConUs = new Consultas.ConsultasArticulos();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void ventasToolStripMenuItem1_Click(object sender, EventArgs e)
{
Consultas.ConsultaVentas ConUs = new Consultas.ConsultaVentas();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
private void categoriaDeArticulosToolStripMenuItem_Click(object sender, EventArgs e)
{
Consultas.ConsultaCategorias ConUs = new Consultas.ConsultaCategorias();
ConUs.MdiParent = this;
ConUs.Show();
ConUs.Location = new Point(40, 40);
}
}
}
<file_sep>/Entidades/TipoPagos.cs
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
namespace Entidades
{
public class TipoPagos
{
[Key]
public int TipoId { get; set; }
public string Descripcion { get; set; }
}
}
<file_sep>/Proyecto Final/Registros/Ejercicio de Estructura/7.4.cpp
#include <iostream>
#include <string.h>
#include <stdlib.h>
using namespace std;
int numeroVocales(char *cad, int x)
{
if(cad[x] == 'a' || cad[x] == 'e' || cad[x] == 'i' || cad[x] == 'o' || cad[x] == 'u' || cad[x] == 'A' || cad[x] == 'E' || cad[x] == 'I' || cad[x] == 'O' || cad[x] == 'U')
return 1 + numeroVocales(cad, x+1);
else
if(cad[x] != '\0')
return 0 + numeroVocales(cad, x+1);
}
int main()
{
char array[10] = {'h', 'o', 'l', 'a', 'm', 'i','j','o','s'};
cout << numeroVocales(array,0);
return 0;
}
<file_sep>/Proyecto Final/VentanasReportes/FacturaReporte.cs
using Entidades;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ProyectoFinal_Aplicada1.VentanasReportes
{
public partial class FacturaReporte : Form
{
public int VentaId { get; set; }
public FacturaReporte()
{
InitializeComponent();
}
private void FacturaReporte_Load(object sender, EventArgs e)
{
Ventas vent = BLL.VentasBLL.Buscar(VentaId);
VentasBindingSource.Add(vent);
foreach (var articul in BLL.ArticulosBLL.Articulo(VentaId))
{
ArticulosBindingSource.Add(articul);
}
this.reportViewer1.RefreshReport();
}
private void reportViewer1_Load(object sender, EventArgs e)
{
}
private void VentasBindingSource_CurrentChanged(object sender, EventArgs e)
{
}
private void ArticulosBindingSource_CurrentChanged(object sender, EventArgs e)
{
}
}
}
<file_sep>/Proyecto Final/Consultas/ConsultasArticulos.cs
using BLL;
using Entidades;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ProyectoFinal_Aplicada1.Consultas
{
public partial class ConsultasArticulos : Form
{
public ConsultasArticulos()
{
InitializeComponent();
CargarFiltrar();
}
public List<Articulos> lista = new List<Articulos>();
private void Buscarbutton_Click(object sender, EventArgs e)
{
if (validar() == true)
BuscarSelecionComBox();
}
private void CargarFiltrar()
{
FiltrarcomboBox.Items.Insert(0, "ArticuloId");
FiltrarcomboBox.Items.Insert(1, "NombreArticulo");
FiltrarcomboBox.Items.Insert(2, "MarcaArticulo");
FiltrarcomboBox.Items.Insert(3, "FechaIngreso");
FiltrarcomboBox.DataSource = FiltrarcomboBox.Items;
FiltrarcomboBox.DisplayMember = "Id";
ConsultaArticulosdataGridView.DataSource = ArticulosBLL.GetLista();
}
private void BuscarSelecionComBox()
{
if (FiltrarcomboBox.SelectedIndex == 0)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ArticulosBLL.GetLista(Utilidades.ToInt(FiltrotextBox.Text));
}
else
{
lista = ArticulosBLL.GetLista();
}
ConsultaArticulosdataGridView.DataSource = lista;
}
if (FiltrarcomboBox.SelectedIndex == 1)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ArticulosBLL.GetListaNombreArticulo(FiltrotextBox.Text);
}
else
{
lista = ArticulosBLL.GetLista();
}
ConsultaArticulosdataGridView.DataSource = lista;
}
if (FiltrarcomboBox.SelectedIndex == 2)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ArticulosBLL.GetListaMarcaArticulo(FiltrotextBox.Text);
}
else
{
lista = ArticulosBLL.GetLista();
}
ConsultaArticulosdataGridView.DataSource = lista;
}
if (FiltrarcomboBox.SelectedIndex == 4)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
//lista = ArticuloBLL.GetListaFecha(DesdeDateTimePicke.Value, HastadateTimePicker.Value);
}
else
{
lista = ArticulosBLL.GetLista();
}
ConsultaArticulosdataGridView.DataSource = lista;
}
}
private bool validar()
{
if (FiltrarcomboBox.SelectedIndex == 0 && ArticulosBLL.GetLista(Utilidades.ToInt(FiltrotextBox.Text)).Count == 0)
{
MessageBox.Show("No hay registros que coincidan con este campo de filtro..." + "\n" + "\n" + "Intente con otro campo");
return false;
}
if (string.IsNullOrEmpty(FiltrotextBox.Text))
{
BuscarerrorProvider.SetError(FiltrotextBox, "Ingresar el campo que desea filtar");
return false;
}
if (FiltrarcomboBox.SelectedIndex == 1 && ArticulosBLL.GetListaNombreArticulo(FiltrotextBox.Text).Count == 0)
{
MessageBox.Show("No hay registros que coincidan con este campo de filtro..." + "\n" + "\n" + "Intente con otro campo");
return false;
}
if (FiltrarcomboBox.SelectedIndex == 2 && ArticulosBLL.GetListaMarcaArticulo(FiltrotextBox.Text).Count == 0)
{
MessageBox.Show("No hay registros que coincidan con este campo de filtro..." + "\n" + "\n" + "Intente con otro campo");
return false;
}
if (FiltrarcomboBox.SelectedIndex == 4)
{
if (DesdeDateTimePicke.Value == HastadateTimePicker.Value)
{
MessageBox.Show("Favor definir un intervalo diferente entre las dos fechas");
return false;
}
else
{
return true;
}
}
BuscarerrorProvider.Clear();
return true;
}
private void Imprimirbutton_Click(object sender, EventArgs e)
{
MyViewerArticulos viewer = new MyViewerArticulos();
viewer.ArticulosreportViewer.Reset();
viewer.ArticulosreportViewer.ProcessingMode = Microsoft.Reporting.WinForms.ProcessingMode.Local;
viewer.ArticulosreportViewer.LocalReport.ReportPath = @"C:\Users\adolfo\Documents\Proyecto Final\Proyecto-Final-Aplicada-1-Sistema-De-VentasSm\Proyecto Final\Reportes\ListadoArticulos.rdlc";
viewer.ArticulosreportViewer.LocalReport.DataSources.Clear();
viewer.ArticulosreportViewer.LocalReport.DataSources.Add(
new Microsoft.Reporting.WinForms.ReportDataSource("DataSetArticulos",
ArticulosBLL.GetLista()));
viewer.ArticulosreportViewer.LocalReport.Refresh();
viewer.Show();
}
}
}
<file_sep>/BLL/ProveedoresBLL.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Entidades;
using DAL;
using System.Windows.Forms;
namespace BLL
{
public class ProveedoresBLL
{
public static bool Insertar(Proveedores proveedor)
{
bool resultado = false;
using (var conexion = new SistemaVentasDb())
{
try
{
conexion.Proveedore.Add(proveedor);
conexion.SaveChanges();
resultado = true;
}
catch (Exception)
{
throw;
}
return resultado;
}
}
public static List<Proveedores> GetLista()
{
List<Proveedores> lista = new List<Proveedores>();
using (var conexion = new SistemaVentasDb())
{
try
{
lista = conexion.Proveedore.ToList();
}
catch (Exception e)
{
MessageBox.Show(e.ToString());
}
return lista;
}
}
public static bool Modificar(int id, Proveedores prov)
{
bool retorno = false;
try
{
using (var db = new SistemaVentasDb())
{
Proveedores p = db.Proveedore.Find(id);
p.Nombre = prov.Nombre;
p.Telefono = prov.Telefono;
p.Ciudad = prov.Ciudad;
p.Correo = prov.Correo;
p.Direccion = prov.Direccion;
p.Fax = prov.Fax;
db.SaveChanges();
}
retorno = true;
}
catch (Exception)
{
throw;
}
return retorno;
}
public static Proveedores Buscar(int id)
{
var db = new SistemaVentasDb();
return db.Proveedore.Find(id);
}
public static bool Eliminar(int id)
{
try
{
using (var db = new SistemaVentasDb())
{
Proveedores p = new Proveedores();
p = db.Proveedore.Find(id);
db.Proveedore.Remove(p);
db.SaveChanges();
return false;
}
}
catch (Exception)
{
return true;
throw;
}
}
public static List<Proveedores> GetLista(int proveedorId)
{
List<Proveedores> lista = new List<Proveedores>();
var db = new SistemaVentasDb();
lista = db.Proveedore.Where(p => p.ProveedorId == proveedorId).ToList();
return lista;
}
public static List<Proveedores> GetListaNombreProveedor(string aux)
{
List<Proveedores> lista = new List<Proveedores>();
var db = new SistemaVentasDb();
lista = db.Proveedore.Where(p => p.Nombre == aux).ToList();
return lista;
}
public static List<Proveedores> GetListaFecha(DateTime aux)
{
List<Proveedores> lista = new List<Proveedores>();
var db = new SistemaVentasDb();
lista = db.Proveedore.Where(p => p.Fecha == aux).ToList();
return lista;
}
public static List<Proveedores> GetListaFecha(DateTime Desde, DateTime Hasta)
{
List<Proveedores> lista = new List<Proveedores>();
var db = new SistemaVentasDb();
lista = db.Proveedore.Where(p => p.Fecha >= Desde && p.Fecha <= Hasta).ToList();
return lista;
}
}
}
<file_sep>/Proyecto Final/Registros/RegistrosCategorias.cs
using Entidades;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ProyectoFinal_Aplicada1.Registros
{
public partial class RegistrosCategorias : Form
{
public RegistrosCategorias()
{
InitializeComponent();
}
private void Buscarbutton_Click(object sender, EventArgs e)
{
if (validarId("Favor ingresar el id de la Categoria que desea buscar") && ValidarBuscar())
LLenar(BLL.CategoriasBLL.Buscar(Utilidades.ToInt(CategoriaIdtextBox.Text)));
}
private void LLenar(Categorias categoria)
{
CategoriaIdtextBox.Text = categoria.CategoriaId.ToString();
DescripciontextBox.Text = categoria.Descripcion;
}
private bool ValidarExiste(string aux)
{
if (BLL.CategoriasBLL.GetListaDescripcion(aux).Count() > 0)
{
MessageBox.Show("Este Categoria ya existe, favor intentar con otra Descripcion de Categoria o Modificar...");
return false;
}
return true;
}
private bool ValidarBuscar()
{
if (BLL.CategoriasBLL.Buscar(Utilidades.ToInt(CategoriaIdtextBox.Text)) == null)
{
MessageBox.Show("Este registro no existe");
return false;
}
return true;
}
private bool validarId(string message)
{
if (string.IsNullOrEmpty(CategoriaIdtextBox.Text))
{
MessageBox.Show(message);
return false;
}
else
{
return true;
}
}
private void Nuevobutton_Click(object sender, EventArgs e)
{
Limpiar();
}
public void Limpiar()
{
CategoriaIdtextBox.Clear();
DescripciontextBox.Clear();
limpiarErroresProvider();
}
private void Guardarbutton_Click(object sender, EventArgs e)
{
Categorias categoria = new Categorias();
BuscarerrorProvider.Clear();
LlenarClase(categoria);
if (ValidarTextbox() && ValidarExiste(DescripciontextBox.Text))
{
BLL.CategoriasBLL.Insertar(categoria);
Limpiar();
limpiarErroresProvider();
MessageBox.Show("Guardado con exito");
}
}
private void LlenarClase(Categorias c)
{
c.Descripcion = DescripciontextBox.Text;
}
private bool ValidarTextbox()
{
if (string.IsNullOrEmpty(DescripciontextBox.Text)
)
{
DescripcionerrorProvider.SetError(DescripciontextBox, "Favor la descripcion de la Categoria de los articulo");
MessageBox.Show("Favor llenar todos los campos obligatorios");
return false;
}
return true;
}
private void button1_Click(object sender, EventArgs e)
{
if (validarId("Favor digitar el id de la Categoria que desea eliminar") && ValidarBuscar())
{
BLL.CategoriasBLL.Eliminar(Utilidades.ToInt(CategoriaIdtextBox.Text));
Limpiar();
MessageBox.Show("ELiminado con exito");
}
}
private void limpiarErroresProvider()
{
BuscarerrorProvider.Clear();
DescripcionerrorProvider.Clear();
}
private void Editarbutton_Click(object sender, EventArgs e)
{
Categorias categoria = new Categorias();
if (validarId("Favor Buscar el Id para que desea actualizar") && ValidarTextbox())
{
LlenarClase(categoria);
if (ValidarExiste(DescripciontextBox.Text))
{
BLL.CategoriasBLL.Modificar(Utilidades.ToInt(CategoriaIdtextBox.Text), categoria);
Limpiar();
limpiarErroresProvider();
MessageBox.Show("Actualizado con exito");
}
}
}
}
}
<file_sep>/Entidades/Ventas.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
namespace Entidades
{
public class Ventas
{
[Key]
public int VentaId { get; set; }
public string Empleado { get; set; }
public string Cliente { get; set; }
public int Cantidad { get; set; }
public double SubTotal { get; set; }
public double Itebis{ get; set; }
public double TotalItebis { get; set; }
public double Total { get; set; }
public double Abono { get; set; }
public double Deuda { get; set; }
public DateTime Fecha { get; set; }
[Browsable(false)]
public virtual List<Articulos> Articulos{ get; set; }
public Ventas(int VentaId)
{
this.VentaId = VentaId;
this.Articulos = new List<Articulos>();
}
public Ventas()
{
this.Articulos = new List<Articulos>();
}
}
}
<file_sep>/BLL/UsuariosBLL.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Entidades;
using DAL;
namespace BLL
{
public class UsuariosBLL
{
public static bool Insertar(Usuarios usuario)
{
bool retorno = false;
try
{
var db = new SistemaVentasDb();
db.Usuarios.Add(usuario);
db.SaveChanges();
retorno = true;
}
catch (Exception)
{
throw;
}
return retorno;
}
public static bool Eliminar(int id)
{
bool obtener = false;
using (var db = new SistemaVentasDb())
{
try
{
Usuarios us = db.Usuarios.Find(id);
db.Usuarios.Remove(us);
db.SaveChanges();
obtener = true;
}
catch (Exception)
{
throw;
}
return obtener;
}
}
public static Usuarios Buscar(int id)
{
var us = new Usuarios();
var conn = new SistemaVentasDb();
us = conn.Usuarios.Find(id);
return us;
}
public static List<Usuarios> GetLista()
{
List<Usuarios> lista = new List<Usuarios>();
var db = new SistemaVentasDb();
lista = db.Usuarios.ToList();
return lista;
}
public static List<Usuarios> GetLista(int usuarioId)
{
List<Usuarios> lista = new List<Usuarios>();
var db = new SistemaVentasDb();
lista = db.Usuarios.Where(p => p.UsuarioId == usuarioId).ToList();
return lista;
}
public static List<Usuarios> GetListaNombreUsuario(string aux)
{
List<Usuarios> lista = new List<Usuarios>();
var db = new SistemaVentasDb();
lista = db.Usuarios.Where(p => p.Nombre == aux).ToList();
return lista;
}
public static bool Modificar(int id, Usuarios us)
{
bool retorno = false;
try
{
using (var db = new SistemaVentasDb())
{
Usuarios usa = db.Usuarios.Find(id);
usa.Nombre = us.Nombre;
usa.Contrasena = us.Contrasena;
usa.Tipo = us.Tipo;
db.SaveChanges();
}
retorno = true;
}
catch (Exception)
{
throw;
}
return retorno;
}
public static List<Usuarios> getContraseña(string aux)
{
List<Usuarios> lista = new List<Usuarios>();
var db = new SistemaVentasDb();
lista = db.Usuarios.Where(p => p.Contrasena == aux).ToList();
return lista;
}
}
}
<file_sep>/Proyecto Final/Registros/Ejercicio de Estructura/7.8.cpp
#include <iostream>
using namespace std;
long funcionRecursiva(int n)
{
if(n == 0 || n == 1)
return 1;
else
if(n % 2 == 0)
return 2 + funcionRecursiva(n-1);
else
return 3 + funcionRecursiva(n-2);
}
int main()
{
cout << funcionRecursiva(10);
return 0;
}
<file_sep>/Proyecto Final/Consultas/ConsultaProvvedor.cs
using BLL;
using Entidades;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ProyectoFinal_Aplicada1.Consultas
{
public partial class ConsultaProvvedor : Form
{
public ConsultaProvvedor()
{
InitializeComponent();
CargarFiltrar();
}
public List<Proveedores> lista = new List<Proveedores>();
private void Buscarbutton_Click(object sender, EventArgs e)
{
if (validar() == true)
BuscarSelecionComBox();
}
private void CargarFiltrar()
{
FiltrarcomboBox.Items.Insert(0, "ProveedorId");
FiltrarcomboBox.Items.Insert(1, "NombreProveedor");
FiltrarcomboBox.Items.Insert(2, "FechaIngreso");
FiltrarcomboBox.DataSource = FiltrarcomboBox.Items;
FiltrarcomboBox.DisplayMember = "Id";
ConsultaProveedoressdataGridView.DataSource = ProveedoresBLL.GetLista();
}
private void BuscarSelecionComBox()
{
if (FiltrarcomboBox.SelectedIndex == 0)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ProveedoresBLL.GetLista(Utilidades.ToInt(FiltrotextBox.Text));
}
else
{
lista = ProveedoresBLL.GetLista();
}
ConsultaProveedoressdataGridView.DataSource = lista;
}
if (FiltrarcomboBox.SelectedIndex == 1)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ProveedoresBLL.GetListaNombreProveedor(FiltrotextBox.Text);
}
else
{
lista = ProveedoresBLL.GetLista();
}
ConsultaProveedoressdataGridView.DataSource = lista;
}
if (FiltrarcomboBox.SelectedIndex == 2)
{
if (!String.IsNullOrEmpty(FiltrotextBox.Text))
{
lista = ProveedoresBLL.GetListaFecha(DesdeDateTimePicke.Value, HastadateTimePicker.Value);
}
else
{
lista = ProveedoresBLL.GetLista();
}
ConsultaProveedoressdataGridView.DataSource = lista;
}
}
private bool validar()
{
if (FiltrarcomboBox.SelectedIndex == 2)
{
if (DesdeDateTimePicke.Value == HastadateTimePicker.Value)
{
MessageBox.Show("Favor definir un intervalo diferente entre las dos fechas");
return false;
}
else
{
return true;
}
}
if (string.IsNullOrEmpty(FiltrotextBox.Text))
{
BuscarerrorProvider.SetError(FiltrotextBox, "Ingresar el campo que desea filtar");
return false;
}
if (FiltrarcomboBox.SelectedIndex == 1 && ProveedoresBLL.GetListaNombreProveedor(FiltrotextBox.Text).Count == 0)
{
MessageBox.Show("No hay registros que coincidan con este campo de filtro..." + "\n" + "\n" + "Intente con otro campo");
return false;
}
if (FiltrarcomboBox.SelectedIndex == 0 && ProveedoresBLL.GetLista(Utilidades.ToInt(FiltrotextBox.Text)).Count == 0)
{
MessageBox.Show("No hay registros que coincidan con este campo de filtro..." + "\n" + "\n" + "Intente con otro campo");
return false;
}
BuscarerrorProvider.Clear();
return true;
}
private void Imprimirbutton_Click(object sender, EventArgs e)
{
MyViewerProveedores viewer = new MyViewerProveedores();
viewer.ProveedorreportViewer.Reset();
viewer.ProveedorreportViewer.ProcessingMode = Microsoft.Reporting.WinForms.ProcessingMode.Local;
viewer.ProveedorreportViewer.LocalReport.ReportPath = @"C:\Users\adolfo\Documents\Proyecto Final\Proyecto-Final-Aplicada-1-Sistema-De-VentasSm\Proyecto Final\Reportes\ListadoProveedores.rdlc";
viewer.ProveedorreportViewer.LocalReport.DataSources.Clear();
viewer.ProveedorreportViewer.LocalReport.DataSources.Add(
new Microsoft.Reporting.WinForms.ReportDataSource("DataSetProveedores",
ProveedoresBLL.GetLista()));
viewer.ProveedorreportViewer.LocalReport.Refresh();
viewer.Show();
}
}
}
<file_sep>/Proyecto Final/Registros/RegistrosArticuloss.cs
using BLL;
using DAL;
using Entidades;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ProyectoFinal_Aplicada1.Registros
{
public partial class RegistrosArticuloss : Form
{
private List<Proveedores> prove;
public RegistrosArticuloss()
{
InitializeComponent();
CargarConboBoxCategorias();
CargarConboBoxProveedores();
prove = new List<Proveedores>();
}
private void Limpiar()
{
ArticuloIdtextBox.Clear();
NombretextBox.Clear();
PrecioVentatextBox.Clear();
CantidadtextBox.Clear();
MarcatextBox.Clear();
DespcripciontextBox.Clear();
limpiarErroresProvider();
}
private Articulos LLenarClase()
{
Articulos Prod = new Articulos();
Prod.ArticuloId = Utilidades.ToInt(ArticuloIdtextBox.Text);
Prod.Cantidad = Utilidades.ToInt(CantidadtextBox.Text);
Prod.Precio = Utilidades.ToInt(PrecioVentatextBox.Text);
Prod.Nombre = NombretextBox.Text;
Prod.Marca = MarcatextBox.Text;
Prod.Descripcion = DespcripciontextBox.Text;
CargarConboBoxProveedores();
CargarConboBoxCategorias();
return Prod;
}
public List<Proveedores> lista = new List<Proveedores>();
private void CargarConboBoxProveedores()
{
var db = new SistemaVentasDb();
lista = db.Proveedore.ToList();
if (lista.Count > 0)
{
NombreProveedorcomboBox1.DataSource = lista;
NombreProveedorcomboBox1.ValueMember = "ProveedorId";
NombreProveedorcomboBox1.DisplayMember = "Nombre";
}
}
public List<Categorias> list = new List<Categorias>();
private void CargarConboBoxCategorias()
{
var db = new SistemaVentasDb();
list = db.Categorias.ToList();
if (list.Count > 0)
{
CategoriacomboBox.DataSource = list;
CategoriacomboBox.ValueMember = "CategoriaId";
CategoriacomboBox.DisplayMember = "Descripcion";
}
}
private void button4_Click(object sender, EventArgs e)
{
Limpiar();
}
private void button3_Click(object sender, EventArgs e)
{
Articulos articulo = new Articulos();
articulo = LLenarClase();
if (ValidarTextbox() && ValidarExiste(NombretextBox.Text))
{
if (articulo != null)
{
limpiarErroresProvider();
if (BLL.ArticulosBLL.Insertar(articulo))
{
MessageBox.Show("Gurardado con exito");
}
}
}
}
private void Buscarbutton_Click_1(object sender, EventArgs e)
{
var articulo = ArticulosBLL.Buscar(Utilidades.ToInt(ArticuloIdtextBox.Text));
if (articulo != null)
{
NombretextBox.Text = articulo.Nombre;
MarcatextBox.Text = articulo.Marca;
DespcripciontextBox.Text = articulo.Descripcion;
CantidadtextBox.Text = articulo.Cantidad.ToString();
PrecioVentatextBox.Text = articulo.Precio.ToString();
CargarConboBoxCategorias();
CargarConboBoxProveedores();
}
else
{
MessageBox.Show("Este Id no esta Registrado ");
}
}
private void Editarbutton_Click(object sender, EventArgs e)
{
Articulos articulo = new Articulos();
if (validarId("Favor Buscar el Id para que desea actualizar") && ValidarTextbox())
{
articulo= LLenarClase();
if (ValidarExiste(NombretextBox.Text))
{
ArticulosBLL.Modificar(Utilidades.ToInt(ArticuloIdtextBox.Text), articulo);
Limpiar();
limpiarErroresProvider();
MessageBox.Show("Actualizado con exito");
}
}
}
private void Eliminarbutton_Click_1(object sender, EventArgs e)
{
ArticulosBLL.Eliminar(ArticulosBLL.Buscar(Convert.ToInt32(ArticuloIdtextBox.Text)));
Limpiar();
MessageBox.Show("Vendedor ha sido eliminado");
}
//-----------------------------Validad-------------------------------------------------------
private bool ValidarTextbox()
{
if (string.IsNullOrEmpty(NombretextBox.Text) &&
string.IsNullOrEmpty(DespcripciontextBox.Text) &&
string.IsNullOrEmpty(MarcatextBox.Text) &&
string.IsNullOrEmpty(NombreProveedorcomboBox1.Text) &&
string.IsNullOrEmpty(CategoriacomboBox.Text) &&
string.IsNullOrEmpty(CantidadtextBox.Text) &&
string.IsNullOrEmpty(PrecioVentatextBox.Text)
)
{
NombreerrorProvider.SetError(NombretextBox, "Favor Ingresar el nombre del articulos");
DescripcionerrorProvider.SetError(DespcripciontextBox, "Favor Ingresar la descripcion del articulo");
MarcaerrorProvider.SetError(MarcatextBox, "Favor Ingresar la marca del articulo");
NombreProveedorerrorProvider.SetError(NombreProveedorcomboBox1, "Favor elegir el proveedor a que correponde el articulo");
CategoriaerrorProvider.SetError(CategoriacomboBox, "Favor elegir la categoria a que correponde el articulo");
CantidaderrorProvider.SetError(CantidadtextBox, "Favor Ingresar la cantidad del articulo");
PrecioVentaerrorProvider.SetError(PrecioVentatextBox, "Favor Ingresar el precio de venta del articulo");
MessageBox.Show("Favor llenar todos los campos obligatorios");
}
if (string.IsNullOrEmpty(NombretextBox.Text))
{
NombreerrorProvider.Clear();
NombreerrorProvider.SetError(NombretextBox, "Favor Ingresar el nombre del articulos");
return false;
}
if (string.IsNullOrEmpty(DespcripciontextBox.Text))
{
DescripcionerrorProvider.Clear();
DescripcionerrorProvider.SetError(DespcripciontextBox, "Favor Ingresar la descripcion del articulo");
return false;
}
if (string.IsNullOrEmpty(MarcatextBox.Text))
{
MarcaerrorProvider.Clear();
MarcaerrorProvider.SetError(MarcatextBox, "Favor Ingresar la marca del articulo");
return false;
}
if (string.IsNullOrEmpty(NombreProveedorcomboBox1.Text))
{
NombreProveedorerrorProvider.Clear();
NombreProveedorerrorProvider.SetError(NombreProveedorcomboBox1, "Favor elegir el proveedor a que correponde el articulo");
return false;
}
if (string.IsNullOrEmpty(CategoriacomboBox.Text))
{
CategoriaerrorProvider.Clear();
CategoriaerrorProvider.SetError(CategoriacomboBox, "Favor elegir la categoria a que correponde el articulo");
return false;
}
if (string.IsNullOrEmpty(CantidadtextBox.Text))
{
CantidaderrorProvider.Clear();
CantidaderrorProvider.SetError(CantidadtextBox, "Favor Ingresar la cantidad del articulo");
return false;
}
if (string.IsNullOrEmpty(PrecioVentatextBox.Text))
{
PrecioVentaerrorProvider.Clear();
PrecioVentaerrorProvider.SetError(PrecioVentatextBox, "Favor Ingresar el precio de venta del articulo");
return false;
}
return true;
}
private bool ValidarBuscar()
{
if (ArticulosBLL.Buscar(Utilidades.ToInt(ArticuloIdtextBox.Text)) == null)
{
MessageBox.Show("Este registro no existe");
return false;
}
return true;
}
private bool validarId(string message)
{
if (string.IsNullOrEmpty(ArticuloIdtextBox.Text))
{
MessageBox.Show(message);
return false;
}
else
{
return true;
}
}
private bool ValidarExiste(string aux)
{
if (ArticulosBLL.GetListaNombreArticulo(aux).Count() > 0)
{
MessageBox.Show("Este nombre ya existe, favor intentar con otra nombre o modificar ...");
return false;
}
return true;
}
//----------------------------- Limipiar Erro------------------------------------------------
private void limpiarErroresProvider()
{
BuscarerrorProvider.Clear();
NombreerrorProvider.Clear();
DescripcionerrorProvider.Clear();
MarcaerrorProvider.Clear();
CantidaderrorProvider.Clear();
PrecioCompraerrorProvider.Clear();
PrecioVentaerrorProvider.Clear();
}
private void NombreProveedorcomboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
}
}
}
<file_sep>/Entidades/Articulos.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Text;
namespace Entidades
{
public class Articulos
{
[Key]
public int ArticuloId{ get; set; }
public string Nombre { get; set; }
[Browsable(false)]
public string Descripcion { get; set; }
public Double Precio { get; set; }
[Browsable(false)]
public string Marca { get; set; }
public int Cantidad { get; set; }
public Double Importe { get; set; }
public virtual List<Ventas> Ventas { get; set; }
public Articulos()
{
this.Ventas = new List<Ventas>();
}
public Articulos(int articuloId, string nombre, string descripcion, int precio, int unidad,string marca)
{
this.ArticuloId = articuloId;
this.Nombre = nombre;
this.Descripcion = descripcion;
this.Precio = precio;
this.Cantidad= Cantidad;
this.Marca = marca;
this.Ventas = new List<Ventas>();
}
public Articulos(int articuloId, string nombre, Double precio, int cantidad)
{
this.ArticuloId = articuloId;
this.Nombre = nombre;
this.Precio = precio;
this.Cantidad = cantidad;
this.Importe = cantidad * precio;
this.Ventas = new List<Ventas>();
}
}
}
<file_sep>/Proyecto Final/Registros/Ejercicio de Estructura/7.6.cpp
#include <iostream>
using namespace std;
int hanoi(int Numero)
{
if(Numero == 1)
return 1;
else
return 2 * hanoi(Numero-1) + 1;
}
int main()
{
int Disco ;
cout<< "\n Torre de Hanoi \n\n";
cout<< "Numero de discos: ";
cin>> Disco;
cout<<"\nLos Movimientos Obligatorio Son: ";
cout<< hanoi(Disco);
return 0;
}
<file_sep>/README.md
# Proyecto-Final-Aplicada-1-Sistema-De-VentasSm
sss
<file_sep>/BLL/TipoPagosBLL.cs
using DAL;
using Entidades;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace BLL
{
public class TipoPagosBLL
{
public static bool Insertar(TipoPagos c)
{
bool retorna = false;
try
{
using (var db = new SistemaVentasDb())
{
db.CodicionPagos.Add(c);
db.SaveChanges();
retorna = true;
}
}
catch (Exception)
{
throw;
}
return retorna;
}
public static bool Modificar(int id, TipoPagos cps)
{
bool retorno = false;
try
{
using (var db = new SistemaVentasDb())
{
TipoPagos cp = db.CodicionPagos.Find(id);
cp.Descripcion = cps.Descripcion;
db.SaveChanges();
}
retorno = true;
}
catch (Exception)
{
throw;
}
return retorno;
}
public static TipoPagos Buscar(int id)
{
var db = new SistemaVentasDb();
return db.CodicionPagos.Find(id);
}
public static bool Eliminar(int id)
{
try
{
using (var db = new SistemaVentasDb())
{
TipoPagos cps = new TipoPagos();
cps = db.CodicionPagos.Find(id);
db.CodicionPagos.Remove(cps);
db.SaveChanges();
db.Dispose();
return false;
}
}
catch (Exception)
{
return true;
throw;
}
}
public static List<TipoPagos> GetListaDescripcion(string aux)
{
List<TipoPagos> lista = new List<TipoPagos>();
var db = new SistemaVentasDb();
lista = db.CodicionPagos.Where(p => p.Descripcion == aux).ToList();
return lista;
}
public static List<TipoPagos> GetLista(int id)
{
List<TipoPagos> lista = new List<TipoPagos>();
var db = new SistemaVentasDb();
lista = db.CodicionPagos.Where(p => p.TipoId == id).ToList();
return lista;
}
public static List<TipoPagos> GetLista()
{
List<TipoPagos> lista = new List<TipoPagos>();
var db = new SistemaVentasDb();
lista = db.CodicionPagos.ToList();
return lista;
}
}
}
<file_sep>/DAL/SistemaVentasDb.cs
using Entidades;
using SistemaDeVentas.Entidades;
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Text;
namespace DAL
{
public class SistemaVentasDb : DbContext
{
public SistemaVentasDb() : base("name=ConStr")
{
}
public virtual DbSet<Usuarios> Usuarios { get; set; }
public virtual DbSet<Articulos> Articulos { get; set; }
public virtual DbSet<Categorias> Categorias { get; set; }
public virtual DbSet<Clientes> Clientes{ get; set; }
public virtual DbSet<Empleados> Empleados { get; set; }
public virtual DbSet<TipoUsuarios> TipoUsuarios { get; set; }
public virtual DbSet<TipoPagos> CodicionPagos { get; set; }
public virtual DbSet<Proveedores> Proveedore { get; set; }
public virtual DbSet<Ventas> Ventas{ get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Entity<Ventas>()
.HasMany<Articulos>(v => v.Articulos)
.WithMany(a=> a.Ventas)
.Map(Va=>
{
Va.MapLeftKey("VentaId");
Va.MapRightKey("ArticuloId");
Va.ToTable("DetalleVentas");
});
}
}
}
| 7b874555302e132fe634f4b8ad61faf462bf14a5 | [
"Markdown",
"C#",
"C++"
] | 19 | C# | Sanchez056/Proyecto-Final-Aplicada-1-Sistema-De-VentasSm | e2e4c115a0c3d800dc66f3ea79c345c397667d79 | e19fd78d089e1ec721ba4c25795eb0550b155ddc | |
refs/heads/master | <repo_name>luna825/augblog-umi<file_sep>/src/utils/Authorized.js
import RenderAuthorized from '@/components/Authorized';
// 默认为用户guset
let Authorized = RenderAuthorized('guest'); // eslint-disable-line
// Reload the rights component
const reloadAuthorized = role => {
Authorized = RenderAuthorized(role);
};
export { reloadAuthorized };
export default Authorized;
<file_sep>/src/pages/Article/Edit.js
import React, { PureComponent } from 'react';
import { connect } from 'dva';
import RichEditor from '@/components/RichEditor/RichEditor';
import Pageloading from '@/components/PageLoading';
import { message } from 'antd';
import router from 'umi/router';
import { update } from '@/services/api';
@connect(({ article, loading }) => ({
article,
loading: loading.models.article,
}))
class Edit extends PureComponent {
onSubmit = async article => {
const { article: oldArticle, dispatch } = this.props;
const hide = message.loading('正在提交数据...');
try {
const response = await update(oldArticle.links.self, article);
if (response.status === 200) {
hide();
message.success('文章编辑成功');
dispatch({
type: 'article/save',
payload: response.data,
});
router.push(`/posts/${response.data.id}`);
}
} catch (e) {
hide();
message.error('文章编辑失败');
}
};
render() {
const { article, loading } = this.props;
return (
<>{loading ? <Pageloading /> : <RichEditor article={article} onSubmit={this.onSubmit} />}</>
);
}
}
export default Edit;
<file_sep>/src/utils/auth.js
import request from '@/utils/clientRequest';
export async function login(params) {
return request('/token', {
method: 'POST',
data: params,
});
}
export async function logout() {
return false;
}
export async function fetchCurrentUser() {
return request('/user');
}
<file_sep>/src/components/PageLoading/loading.js
import React from 'react';
import './index.css';
// loading components from code split
// https://umijs.org/plugin/umi-plugin-react.html#dynamicimport
export default () => (
<div className="lds-ring">
<div />
<div />
<div />
<div />
</div>
);
<file_sep>/src/models/auth.js
import { routerRedux } from 'dva/router';
import { getPageQuery } from '@/utils/utils';
import { login } from '@/services/auth';
import { reloadAuthorized } from '@/utils/Authorized';
export default {
namespace: 'auth',
state: {
isLogin: false,
},
effects: {
*login({ payload }, { call, put }) {
try {
const response = yield call(login, payload);
yield localStorage.setItem('aug-blog-user-token', response.data.token);
yield put({
type: 'user/fetchCurrent',
});
yield put({
type: 'changeLoginStatus',
isLogin: true,
});
if (response.status === 201) {
const urlParams = new URL(window.location.href);
const params = getPageQuery();
let { redirect } = params;
if (redirect) {
const redirectUrlParams = new URL(redirect);
if (redirectUrlParams.origin === urlParams.origin) {
redirect = redirect.substr(urlParams.origin.length);
if (redirect.match(/^\/.*#/)) {
redirect = redirect.substr(redirect.indexOf('#') + 1);
}
} else {
window.location.href = redirect;
return;
}
}
yield put(routerRedux.replace(redirect || '/'));
}
} catch (e) {
yield put({
type: 'changeLoginStatus',
isLogin: false,
error: e.data,
});
}
},
*logout(_, { put }) {
yield put({
type: 'changeLoginStatus',
isLogin: false,
});
yield put({
type: 'user/saveCurrentUser',
});
reloadAuthorized('guest');
localStorage.removeItem('aug-blog-user-token');
yield put(
routerRedux.push({
pathname: '/home',
})
);
},
},
reducers: {
changeLoginStatus(state, action) {
return {
...state,
...action,
};
},
},
};
<file_sep>/src/components/RichEditor/RichEditor.js
import 'braft-editor/dist/index.css';
import React from 'react';
import BraftEditor from 'braft-editor';
import HeaderId from 'braft-extensions/dist/header-id';
import { Form, Icon, message } from 'antd';
import styles from './RichText.less';
BraftEditor.use(HeaderId());
const FormItem = Form.Item;
const controls = [
'undo',
'redo',
'remove-styles',
'separator',
'headings',
'font-size',
'separator',
'bold',
'italic',
'underline',
'strike-through',
'separator',
'superscript',
'subscript',
'separator',
'text-indent',
'text-align',
'separator',
'list-ul',
'list-ol',
'blockquote',
'code',
'separator',
'link',
'separator',
'hr',
'separator',
'separator',
];
class Editor extends React.Component {
static defaultProps = {
article: {},
onSubmit: () => {},
};
handleSubmit = async event => {
event.preventDefault();
const { form, onSubmit } = this.props;
form.validateFields((error, values) => {
if (error) {
message.error(`博客内容或标题不能为空!`);
return;
}
const submitData = {
title: values.title,
body: values.content.toRAW(), // or values.content.toHTML()
bodyHtml: values.content.toHTML(),
};
onSubmit(submitData);
});
};
renderTitle = (getFieldDecorator, title = '') => (
<FormItem className={styles.editorTitle}>
{getFieldDecorator('title', {
validateTrigger: 'onBlur',
initialValue: title,
rules: [
{
required: true,
validator: (_, value, callback) => {
if (value === '') {
callback(false);
} else {
callback();
}
},
},
],
})(<input placeholder="无标题" />)}
</FormItem>
);
render() {
const { form, article } = this.props;
const { getFieldDecorator } = form;
const extendControls = [
'separator',
{
key: 'update-and-post', // 控件唯一标识,必传
type: 'button',
title: '更新发布', // 指定鼠标悬停提示文案
className: 'post-button', // 指定按钮的样式名
html: null, // 指定在按钮中渲染的html字符串
text: (
<span>
<Icon type="sync" />
{' 更新发布'}
</span>
), // 指定按钮文字,此处可传入jsx,若已指定html,则text不会显示
onClick: event => this.handleSubmit(event),
},
];
return (
<div className={styles.main}>
<Form>
<FormItem>
{getFieldDecorator('content', {
validateTrigger: 'onBlur',
initialValue: BraftEditor.createEditorState(article.bodyHtml),
rules: [
{
required: true,
validator: (_, value, callback) => {
if (value.isEmpty()) {
callback(false);
} else {
callback();
}
},
},
],
})(
<BraftEditor
controlBarClassName={styles.editorControl}
className={styles.editor}
contentClassName={styles.editorContent}
controls={controls}
placeholder="请输入正文内容"
componentBelowControlBar={this.renderTitle(getFieldDecorator, article.title)}
extendControls={extendControls}
/>
)}
</FormItem>
</Form>
</div>
);
}
}
export default Form.create()(Editor);
<file_sep>/src/models/user.js
import { fetchCurrentUser } from '@/services/auth';
import { reloadAuthorized } from '@/utils/Authorized';
export default {
namespace: 'user',
state: {
currentUser: {},
},
effects: {
*fetchCurrent(_, { call, put }) {
if (!localStorage.getItem('aug-blog-user-token')) {
yield put({
type: 'savaCurrentUser',
});
return;
}
try {
const { data } = yield call(fetchCurrentUser);
reloadAuthorized(data.role);
yield put({
type: 'saveCurrentUser',
payload: data,
});
} catch (e) {
localStorage.removeItem('aug-blog-user-token');
yield put({
type: 'savaCurrentUser',
});
}
},
},
reducers: {
saveCurrentUser(state, action) {
return {
...state,
currentUser: action.payload || {},
};
},
},
};
<file_sep>/src/pages/Home/PostList.js
import React, { memo } from 'react';
import Link from 'umi/link';
import { Card, List, Spin } from 'antd';
import InfiniteScroll from 'react-infinite-scroller';
import ArticleListContent from '@/components/ArticleListContent';
import styles from './postList.less';
const { Item } = List;
export default memo(({ articles, loading, handleInfiniteOnLoad }) => (
<Card bordered={false} bodyStyle={{ padding: '8px 32px 32px 32px' }}>
<InfiniteScroll
initialLoad={false}
pageStart={0}
loadMore={() => handleInfiniteOnLoad(articles.links.next)}
hasMore={!loading && !!articles.links.next}
>
<List
size="large"
rowKey="id"
itemLayout="vertical"
loading={loading}
dataSource={articles.items}
renderItem={item => (
<Item key={item.id} extra={item.images ? <div className={styles.listItemExtra} /> : null}>
<Item.Meta title={<Link to={`/posts/${item.id}`}>{item.title}</Link>} />
<ArticleListContent data={item} />
</Item>
)}
>
{loading && articles.links.next && (
<div className={styles.loadingContainer}>
<Spin />
</div>
)}
</List>
</InfiniteScroll>
</Card>
));
<file_sep>/src/pages/Account/model.js
import { message } from 'antd';
import { fetch, remove } from '@/services/api';
import removeArr from 'lodash/remove';
export default {
namespace: 'account',
state: {
articles: {
next: null,
items: [],
},
},
effects: {
*fetchArticles({ url, add }, { put, call }) {
try {
const { data } = yield call(fetch, url);
yield put({
type: 'saveArticles',
payload: data,
add,
});
} catch (e) {
// 出现错误,传递空数据
yield put({
type: 'save',
payload: { items: [] },
add: true,
});
}
},
*removeArticle({ url, id }, { put, call }) {
const hide = message.loading('正在删除...');
try {
yield call(remove, url);
yield put({
type: 'deleteArticle',
payload: id,
});
hide();
} catch (e) {
hide();
message.error('删除失败, 请稍后再试...');
}
},
},
reducers: {
saveArticles(state, { payload, add }) {
const { articles } = state;
return {
...state,
articles: {
...articles,
...payload,
items: add ? state.items.concat(payload.items) : payload.items,
},
};
},
deleteArticle(state, { payload }) {
const { articles } = state;
removeArr(articles.items, article => article.id === payload);
return {
...state,
articles: {
...articles,
},
};
},
},
};
<file_sep>/src/utils/clientRequest.js
import axios from 'axios';
import router from 'umi/router';
import { Message } from 'antd';
// const codeMessage = {
// 200: '服务器成功返回请求的数据。',
// 201: '新建或修改数据成功。',
// 202: '一个请求已经进入后台排队(异步任务)。',
// 204: '删除数据成功。',
// 400: '发出的请求有错误,服务器没有进行新建或修改数据的操作。',
// 401: '用户没有权限(令牌、用户名、密码错误)。',
// 403: '用户得到授权,但是访问是被禁止的。',
// 404: '发出的请求针对的是不存在的记录,服务器没有进行操作。',
// 406: '请求的格式不可得。',
// 410: '请求的资源被永久删除,且不会再得到的。',
// 422: '当创建一个对象时,发生一个验证错误。',
// 500: '服务器发生错误,请检查服务器。',
// 502: '网关错误。',
// 503: '服务不可用,服务器暂时过载或维护。',
// 504: '网关超时。',
// };
axios.defaults.timeout = 3000;
axios.defaults.withCredentials = true;
axios.interceptors.request.use(
config => {
const newConfig = { ...config };
// 从本地存储中取得 jwt 的验证 token
const token = localStorage.getItem('aug-blog-user-token');
if (token) {
newConfig.headers.Authorization = `Bearer ${token}`;
}
return newConfig;
},
err => Promise.reject(err)
);
axios.interceptors.response.use(
response => {
const { data, status } = response;
if (status >= 200 && status < 300) {
if (data.error_code === 1) {
Message.success('数据删除成功');
}
return response;
}
return Promise.reject(response);
},
error => Promise.reject(error)
);
export default function request(url, opt) {
return axios(url, opt).catch(error => {
// 请求配置发生的错误
if (!error.response) {
// eslint-disable-next-line no-console
return console.log('Error', error.message);
}
// 响应时的状态码处理
const { status } = error.response;
if (status === 401) {
// @HACK
/* eslint-disable no-underscore-dangle */
window.g_app._store.dispatch({
type: 'login/logout',
});
}
// environment should not be used
if (status === 403) {
router.push('/exception/403');
}
if (status <= 504 && status >= 500) {
router.push('/exception/500');
}
if (status >= 404 && status < 422) {
router.push('/exception/404');
}
return Promise.reject(error.response);
});
}
<file_sep>/src/pages/Authorized.js
import React from 'react';
import RenderAuthorized from '@/components/Authorized';
import Redirect from 'umi/redirect';
const Authorized = RenderAuthorized();
export default ({ children }) => (
<Authorized authority={children.props.route.authority} noMatch={<Redirect to="/login" />}>
{children}
</Authorized>
);
<file_sep>/src/pages/Article/ArticleWrapper.js
/* eslint-disable react/no-danger */
import 'github-markdown-css';
import React, { PureComponent } from 'react';
import { connect } from 'dva';
@connect()
class ArticleWrapper extends PureComponent {
componentDidMount() {
const { dispatch, match } = this.props;
dispatch({
type: 'article/fetch',
payload: `/api/v1/posts/${match.params.id}`,
});
}
render() {
const { children } = this.props;
return <>{children}</>;
}
}
export default ArticleWrapper;
<file_sep>/src/pages/Login/Login.js
import React, { Component } from 'react';
import { Checkbox, Alert } from 'antd';
import { formatMessage, FormattedMessage } from 'umi/locale';
import { connect } from 'dva';
import Login from '@/components/Login';
import styles from './login.less';
const { UserName, Password, Submit } = Login;
@connect(({ auth, loading }) => ({
auth,
submitting: loading.effects['auth/login'],
}))
class LoginPage extends Component {
state = {
autoLogin: true,
type: 100,
};
changeAutoLogin = e => {
this.setState({
autoLogin: e.target.checked,
});
};
handleSubmit = (err, values) => {
const { type } = this.state;
if (!err) {
const { dispatch } = this.props;
dispatch({
type: 'auth/login',
payload: {
...values,
type,
},
});
}
};
renderMessage = content => (
<Alert style={{ marginBottom: 24 }} message={content} type="error" showIcon />
);
render() {
const { autoLogin } = this.state;
const { submitting, auth = {} } = this.props;
return (
<div className={styles.main}>
{auth.error &&
!auth.isLogin &&
!submitting &&
this.renderMessage(formatMessage({ id: 'app.login.message-invalid-credentials' }))}
<Login
onSubmit={this.handleSubmit}
ref={form => {
this.loginForm = form;
}}
>
<UserName name="account" />
<Password name="<PASSWORD>" />
<div>
<Checkbox checked={autoLogin} onChange={this.changeAutoLogin}>
<FormattedMessage id="app.login.remember-me" />
</Checkbox>
<a style={{ float: 'right' }} href="">
<FormattedMessage id="app.login.forgot-password" />
</a>
</div>
<Submit loading={submitting}>
<FormattedMessage id="app.login.login" />
</Submit>
</Login>
</div>
);
}
}
export default LoginPage;
<file_sep>/src/components/ArticleListContent/index.js
import React from 'react';
import moment from 'moment';
import { Avatar } from 'antd';
import styles from './index.less';
const formatBody = body => {
try {
const bodyObj = JSON.parse(body);
const text = bodyObj.blocks.map(block => block.text).join(' ');
return text;
} catch (e) {
return body;
}
};
const ArticleListContent = ({ data: { body, timestamp, author } }) => (
<div className={styles.listContent}>
<p className={styles.description}>{`${formatBody(body).slice(0, 150)}...`}</p>
<div className={styles.extra}>
<Avatar src={author.avatarUrl} />
<a>{author.nickname}</a>
<em>{moment(timestamp).format('YYYY-MM-DD HH:mm')}</em>
</div>
</div>
);
export default ArticleListContent;
<file_sep>/src/pages/Home/Home.js
import React, { PureComponent } from 'react';
import { Layout, Divider, Icon, Card } from 'antd';
import { connect } from 'dva';
import GridContent from '@/components/PageHeaderWrapper/GridContent';
import PostList from './PostList';
import styles from './index.less';
const { Content, Sider } = Layout;
class Home extends PureComponent {
componentDidMount() {
const { dispatch } = this.props;
dispatch({
type: 'articles/fetch',
url: '/api/v1/posts',
});
}
onLoadMore = next => {
const { dispatch } = this.props;
dispatch({
type: 'articles/fetch',
url: next,
add: true,
});
};
render() {
const { articles, loading } = this.props;
return (
<GridContent>
<Content>
<PostList articles={articles} loading={loading} handleInfiniteOnLoad={this.onLoadMore} />
</Content>
<Sider
width={300}
className={styles.sider}
breakpoint="lg"
collapsedWidth={0}
trigger={null}
>
<Card bordered={false}>
<div className={styles.avatarHolder}>
<img alt="" src="https://avatars0.githubusercontent.com/u/10859713?s=460&v=4" />
<div className={styles.name}>August</div>
</div>
<div className={styles.detail}>
<p>欢迎来到我的博客!</p>
<p>我是一名计算机爱好者,目前居住于重庆</p>
<p>谢谢你的访问。</p>
</div>
<Divider dashed />
<div className={styles.link}>
<div>
{' '}
<Icon type="mail" /> <EMAIL>{' '}
</div>
<div>
{' '}
<Icon type="github" />{' '}
<a rel="noopener noreferrer" target="_blank" href="https://github.com/luna825">
Github{' '}
</a>
</div>
</div>
</Card>
</Sider>
</GridContent>
);
}
}
export default connect(({ articles, loading }) => ({
articles,
loading: loading.models.articles,
}))(Home);
<file_sep>/Dockerfile
FROM circleci/node:latest-browsers
WORKDIR /usr/src/app/
USER root
COPY package.json ./
RUN yarn
COPY ./ ./
RUN npm run build
CMD ["ls"]<file_sep>/src/pages/Home/models/articles.js
import { fetch } from '@/services/api';
export default {
namespace: 'articles',
state: {
links: {
next: null,
},
items: [],
},
effects: {
*fetch({ url, add }, { put, call }) {
try {
const { data } = yield call(fetch, url);
yield put({
type: 'save',
payload: data,
add,
});
} catch (e) {
// 出现错误,传递空数据
yield put({
type: 'save',
payload: { items: [] },
add: true,
});
}
},
},
reducers: {
save(state, { payload, add }) {
return {
...state,
...payload,
items: add ? state.items.concat(payload.items) : payload.items,
};
},
},
};
<file_sep>/src/pages/Article/Article.js
import React, { PureComponent } from 'react';
import { connect } from 'dva';
import { Card, Avatar, Row, Col, Skeleton } from 'antd';
import moment from 'moment';
import styles from './index.less';
@connect(({ article, loading }) => ({
article,
loading: loading.models.article,
}))
class Article extends PureComponent {
render() {
const { article, loading } = this.props;
return (
<Card style={{ maxWidth: '100rem', margin: 'auto' }}>
<Row className={styles.blog}>
<Col xs={24} md={18}>
<Skeleton loading={loading} active avatar paragraph={{ rows: 22 }}>
{Object.keys(article).length && article.bodyHtml && (
<>
<div className={styles.header}>
<Avatar size="large" src={article.author.avatarUrl} />
<div className={styles.avatarInfoBox}>
<a className={styles.username}>{article.author.nickname}</a>
<div className={styles.time}>
{moment(article.timestamp).format('YYYY年MM月DD日 HH:mm')}
</div>
</div>
</div>
<h1 className={styles.title}>{article.title}</h1>
<div
className="markdown-body"
dangerouslySetInnerHTML={{ __html: article.bodyHtml }}
/>
</>
)}
</Skeleton>
</Col>
</Row>
</Card>
);
}
}
export default Article;
| 7a196b6e02571e72ae10d43001dbed7d7430f5a2 | [
"JavaScript",
"Dockerfile"
] | 18 | JavaScript | luna825/augblog-umi | e0f5fe96930e2a09eb3ca600db46e0cfd86b6b0a | 6c97c0de09a3fd383b68b5514918c94ee7912c31 | |
refs/heads/master | <file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package telas;
import javax.swing.JOptionPane;
import br.com.biblisis.controller.*;
import java.sql.SQLException;
/**
*
* @author romeu
*/
public class TelaLogin extends javax.swing.JFrame {
/**
* Creates new form TelaLogin
*/
private String tipoDoUsuario;
private String login;
public TelaLogin() {
initComponents();
this.tipoDoUsuario = "Aluno/Professor";
}
public void setTipoDoUsuario(String tipoDoUsuario){
this.tipoDoUsuario = tipoDoUsuario;
}
private void verificaLoginAdministradorFuncionario(){
if(this.tipoDoUsuario.equals("Administrador") || this.tipoDoUsuario.equals("Funcionário") ){
btnNovoCadastro.setVisible(false);
}
}
private void chamaMenu(){
if (this.tipoDoUsuario.equals("Administrador")){
TelaAdministrador telaAdministrador = new TelaAdministrador();
telaAdministrador.setLogin(this.login);
telaAdministrador.setVisible(true);
telaAdministrador.setExtendedState(MAXIMIZED_BOTH);
dispose();
}else if(this.tipoDoUsuario.equals("Aluno/Professor")){
TelaAlunoProfessor telaAlunoProfessor = new TelaAlunoProfessor();
telaAlunoProfessor.setLogin(this.login);
telaAlunoProfessor.setVisible(true);
telaAlunoProfessor.setExtendedState(MAXIMIZED_BOTH);
dispose();
}else{
TelaFuncionario telaFuncionario = new TelaFuncionario();
telaFuncionario.setLogin(this.login);
telaFuncionario.setVisible(true);
telaFuncionario.setExtendedState(MAXIMIZED_BOTH);
dispose();
;
}
}
private void chamaTelaDeNovoCadastro (String tipo){
TelaCadastroUsuario novaTelaDeCadastro = new TelaCadastroUsuario ();
novaTelaDeCadastro.setTipoDoUsuario(tipo);
novaTelaDeCadastro.setVisible(true);
}
private boolean validaLogin (String loginId, String senha){
boolean resposta = false;
if (this.tipoDoUsuario.equals("Funcionário")){
try {
resposta = CFuncionario.login(loginId, senha, 0);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
} else if (this.tipoDoUsuario.equals("Administrador")){
try {
resposta = CFuncionario.login(loginId, senha, 1);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}else{
try {
resposta = CAlunoProfessor.login(loginId, senha);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}
return resposta;
}
private boolean validaCaseLogin (String loginId, String senha){
boolean resposta = false;
String [] usuario = null;
if (this.tipoDoUsuario.equals("Funcionário")){
try {
resposta = CFuncionario.login(loginId, senha, 0);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
try {
usuario = CFuncionario.search(loginId);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
if ( usuario == null || !usuario[0].equals(loginId)){
resposta = false;
}
} else if (this.tipoDoUsuario.equals("Administrador")){
try {
resposta = CFuncionario.login(loginId, senha, 1);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
try {
usuario = CFuncionario.search(loginId);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
if ( usuario == null || !usuario[0].equals(loginId)){
resposta = false;
}
}else{
try {
resposta = CAlunoProfessor.login(loginId, senha);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
try {
usuario = CAlunoProfessor.search(loginId);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
if ( usuario == null || !usuario[0].equals(loginId)){
resposta = false;
}
}
return resposta;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jLabel2 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
btnEntrar = new javax.swing.JButton();
btnNovoCadastro = new javax.swing.JButton();
campoPassSenha = new javax.swing.JPasswordField();
btnRetornar = new javax.swing.JButton();
jLabel4 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
lblAviso = new javax.swing.JLabel();
lblTipo = new javax.swing.JLabel();
campoTextoId = new javax.swing.JFormattedTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Autenticação");
setBounds(new java.awt.Rectangle(0, 0, 0, 0));
setFocusCycleRoot(false);
setFocusTraversalPolicyProvider(true);
setUndecorated(true);
setResizable(false);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowOpened(java.awt.event.WindowEvent evt) {
formWindowOpened(evt);
}
});
addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
formKeyPressed(evt);
}
public void keyTyped(java.awt.event.KeyEvent evt) {
formKeyTyped(evt);
}
});
jLabel1.setFont(new java.awt.Font("Tahoma", 0, 20)); // NOI18N
jLabel1.setText("LOGIN");
jLabel1.setBorder(javax.swing.BorderFactory.createEtchedBorder());
jLabel2.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel2.setText("ID");
jLabel3.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel3.setText("SENHA");
btnEntrar.setText("Entrar");
btnEntrar.setToolTipText("prosseguir com login");
btnEntrar.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnEntrarActionPerformed(evt);
}
});
btnNovoCadastro.setText("Novo Cadastro");
btnNovoCadastro.setToolTipText("ainda não é cadastrado?");
btnNovoCadastro.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnNovoCadastroActionPerformed(evt);
}
});
campoPassSenha.setToolTipText("digite sua senha");
campoPassSenha.setPreferredSize(new java.awt.Dimension(40, 23));
campoPassSenha.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
campoPassSenhaKeyPressed(evt);
}
});
btnRetornar.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icones/icons8-left-32.png"))); // NOI18N
btnRetornar.setToolTipText("retornar ao menu principal");
btnRetornar.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnRetornarActionPerformed(evt);
}
});
jLabel5.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel5.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icones/icons8-reading-filled-100.png"))); // NOI18N
lblAviso.setFont(new java.awt.Font("Tahoma", 1, 11)); // NOI18N
lblAviso.setForeground(new java.awt.Color(255, 0, 0));
lblAviso.setText("Senha incorreta ou usuário inexistente!");
lblAviso.setBorder(javax.swing.BorderFactory.createEtchedBorder());
lblTipo.setFont(new java.awt.Font("Tahoma", 1, 11)); // NOI18N
lblTipo.setText("Tipo do Usuário");
lblTipo.setBorder(javax.swing.BorderFactory.createEtchedBorder());
campoTextoId.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
campoTextoIdActionPerformed(evt);
}
});
campoTextoId.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
campoTextoIdKeyPressed(evt);
}
public void keyReleased(java.awt.event.KeyEvent evt) {
campoTextoIdKeyReleased(evt);
}
public void keyTyped(java.awt.event.KeyEvent evt) {
campoTextoIdKeyTyped(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(20, 20, 20)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel2)
.addComponent(jLabel3))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(lblAviso)
.addGap(0, 0, Short.MAX_VALUE))
.addComponent(campoPassSenha, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(campoTextoId, javax.swing.GroupLayout.Alignment.TRAILING))
.addGap(18, 18, 18)
.addComponent(jLabel4))
.addGroup(layout.createSequentialGroup()
.addComponent(btnEntrar, javax.swing.GroupLayout.PREFERRED_SIZE, 105, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(btnNovoCadastro)
.addGap(0, 0, Short.MAX_VALUE)))
.addContainerGap())
.addGroup(layout.createSequentialGroup()
.addComponent(btnRetornar)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(lblTipo))
.addGroup(layout.createSequentialGroup()
.addGap(127, 127, 127)
.addComponent(jLabel1)
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addGap(105, 105, 105)
.addComponent(jLabel5)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(0, 300, Short.MAX_VALUE)
.addComponent(jLabel4))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jLabel5)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(campoTextoId, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(campoPassSenha, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(lblAviso)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnEntrar)
.addComponent(btnNovoCadastro))))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(btnRetornar, javax.swing.GroupLayout.PREFERRED_SIZE, 29, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(lblTipo)))
);
pack();
setLocationRelativeTo(null);
}// </editor-fold>//GEN-END:initComponents
private void btnNovoCadastroActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnNovoCadastroActionPerformed
Object[] itens = { "Aluno", "Professor"};
Object tipoEscolhido = JOptionPane.showInputDialog(null, "Qual o tipo do usuário?", "Novo usuário",
JOptionPane.INFORMATION_MESSAGE, null,
itens, itens [0]); //
if (tipoEscolhido != null){
chamaTelaDeNovoCadastro (tipoEscolhido.toString());
}
}//GEN-LAST:event_btnNovoCadastroActionPerformed
private void btnEntrarActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnEntrarActionPerformed
boolean respostaDaValidacao;
respostaDaValidacao = validaCaseLogin(campoTextoId.getText(),campoPassSenha.getText());
if (respostaDaValidacao){
dispose ();
this.login = campoTextoId.getText();
chamaMenu();
}
lblAviso.setVisible(true);
}//GEN-LAST:event_btnEntrarActionPerformed
private void formWindowOpened(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowOpened
verificaLoginAdministradorFuncionario();
lblAviso.setVisible(false);
lblTipo.setText(tipoDoUsuario);
}//GEN-LAST:event_formWindowOpened
private void btnRetornarActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnRetornarActionPerformed
this.dispose();
TelaPrincipal novaTelaPrincipal = new TelaPrincipal();
novaTelaPrincipal.setVisible(true);
}//GEN-LAST:event_btnRetornarActionPerformed
private void campoTextoIdActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_campoTextoIdActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_campoTextoIdActionPerformed
private void campoTextoIdKeyTyped(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_campoTextoIdKeyTyped
}//GEN-LAST:event_campoTextoIdKeyTyped
private void formKeyTyped(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_formKeyTyped
campoTextoId.setText(campoTextoId.getText().toUpperCase());
}//GEN-LAST:event_formKeyTyped
private void formKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_formKeyPressed
campoTextoId.setText(campoTextoId.getText().toUpperCase());
}//GEN-LAST:event_formKeyPressed
private void campoTextoIdKeyReleased(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_campoTextoIdKeyReleased
//campoTextoId.setText(campoTextoId.getText().toUpperCase());
}//GEN-LAST:event_campoTextoIdKeyReleased
private void campoPassSenhaKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_campoPassSenhaKeyPressed
if(evt.getKeyCode() == evt.VK_ENTER){
boolean respostaDaValidacao;
respostaDaValidacao = validaCaseLogin(campoTextoId.getText(),campoPassSenha.getText());
if (respostaDaValidacao){
this.login = campoTextoId.getText();
chamaMenu();
}
lblAviso.setVisible(true);
}
}//GEN-LAST:event_campoPassSenhaKeyPressed
private void campoTextoIdKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_campoTextoIdKeyPressed
if(evt.getKeyCode() == evt.VK_ENTER){
boolean respostaDaValidacao;
respostaDaValidacao = validaCaseLogin(campoTextoId.getText(),campoPassSenha.getText());
if (respostaDaValidacao){
this.login = campoTextoId.getText();
chamaMenu();
}
lblAviso.setVisible(true);
}
}//GEN-LAST:event_campoTextoIdKeyPressed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(TelaLogin.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(TelaLogin.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(TelaLogin.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(TelaLogin.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new TelaLogin().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton btnEntrar;
private javax.swing.JButton btnNovoCadastro;
private javax.swing.JButton btnRetornar;
private javax.swing.JPasswordField campoPassSenha;
private javax.swing.JFormattedTextField campoTextoId;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel lblAviso;
private javax.swing.JLabel lblTipo;
// End of variables declaration//GEN-END:variables
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package telas;
import javax.swing.JOptionPane;
/**
*
* @author romeu
*/
public class TelaAlunoProfessor extends javax.swing.JFrame {
/**
* Creates new form TelaAlunoProfessor
*/
private String login = "Usuário";
public TelaAlunoProfessor() {
initComponents();
}
public void setLogin(String login) {
this.login = login;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jMenuBar1 = new javax.swing.JMenuBar();
menuUsuario = new javax.swing.JMenu();
jMenuItem1 = new javax.swing.JMenuItem();
menuItemVisualizarHistorico = new javax.swing.JMenuItem();
jMenu2 = new javax.swing.JMenu();
jMenuItem3 = new javax.swing.JMenuItem();
jMenuItem4 = new javax.swing.JMenuItem();
jMenuItem5 = new javax.swing.JMenuItem();
menuAcervo = new javax.swing.JMenu();
menuItemConsultarAcervo = new javax.swing.JMenuItem();
jMenu5 = new javax.swing.JMenu();
menuItemEncerrarSessao = new javax.swing.JMenuItem();
setDefaultCloseOperation(javax.swing.WindowConstants.DO_NOTHING_ON_CLOSE);
setTitle("Menu do Usuário");
setUndecorated(true);
setResizable(false);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowOpened(java.awt.event.WindowEvent evt) {
formWindowOpened(evt);
}
});
jLabel1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icones/backgroundPadrao.jpg"))); // NOI18N
menuUsuario.setText("Usuário");
jMenuItem1.setText("Editar dados cadastrais");
jMenuItem1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem1ActionPerformed(evt);
}
});
menuUsuario.add(jMenuItem1);
menuItemVisualizarHistorico.setText("Visualizar histórico");
menuItemVisualizarHistorico.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
menuItemVisualizarHistoricoActionPerformed(evt);
}
});
menuUsuario.add(menuItemVisualizarHistorico);
jMenuBar1.add(menuUsuario);
jMenu2.setText("Reserva");
jMenuItem3.setText("Fazer reserva");
jMenuItem3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem3ActionPerformed(evt);
}
});
jMenu2.add(jMenuItem3);
jMenuItem4.setText("Consultar reserva");
jMenuItem4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem4ActionPerformed(evt);
}
});
jMenu2.add(jMenuItem4);
jMenuItem5.setText("Cancelar reserva");
jMenu2.add(jMenuItem5);
jMenuBar1.add(jMenu2);
menuAcervo.setText("Acervo");
menuItemConsultarAcervo.setText("Consultar acervo");
menuItemConsultarAcervo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
menuItemConsultarAcervoActionPerformed(evt);
}
});
menuAcervo.add(menuItemConsultarAcervo);
jMenuBar1.add(menuAcervo);
jMenu5.setText("Logout");
menuItemEncerrarSessao.setText("Encerrar sessão");
menuItemEncerrarSessao.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
menuItemEncerrarSessaoActionPerformed(evt);
}
});
jMenu5.add(menuItemEncerrarSessao);
jMenuBar1.add(jMenu5);
setJMenuBar(jMenuBar1);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jLabel1))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 1189, Short.MAX_VALUE)
.addContainerGap())
);
pack();
setLocationRelativeTo(null);
}// </editor-fold>//GEN-END:initComponents
private void jMenuItem4ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem4ActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_jMenuItem4ActionPerformed
private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem1ActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_jMenuItem1ActionPerformed
private void jMenuItem3ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem3ActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_jMenuItem3ActionPerformed
private void menuItemConsultarAcervoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_menuItemConsultarAcervoActionPerformed
TelaConsultaAcervo novaTelaDeConsulta = new TelaConsultaAcervo();
novaTelaDeConsulta.setVisible(true);
}//GEN-LAST:event_menuItemConsultarAcervoActionPerformed
private void menuItemEncerrarSessaoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_menuItemEncerrarSessaoActionPerformed
int resposta = JOptionPane.showConfirmDialog(null, "Deseja encerrar sua sessão?");
if (resposta == 0){
TelaPrincipal novaTela = new TelaPrincipal ();
novaTela.setVisible(true);
this.dispose();
}
}//GEN-LAST:event_menuItemEncerrarSessaoActionPerformed
private void formWindowOpened(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowOpened
menuUsuario.setText("Olá, " + login+"!");
}//GEN-LAST:event_formWindowOpened
private void menuItemVisualizarHistoricoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_menuItemVisualizarHistoricoActionPerformed
TelaConsultaEmprestimo novaTelaDeConsulta = new TelaConsultaEmprestimo();
novaTelaDeConsulta.setVisible(true);
novaTelaDeConsulta.setLoginAlunoProfessor(login);
}//GEN-LAST:event_menuItemVisualizarHistoricoActionPerformed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(TelaAlunoProfessor.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(TelaAlunoProfessor.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(TelaAlunoProfessor.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(TelaAlunoProfessor.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new TelaAlunoProfessor().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel jLabel1;
private javax.swing.JMenu jMenu2;
private javax.swing.JMenu jMenu5;
private javax.swing.JMenuBar jMenuBar1;
private javax.swing.JMenuItem jMenuItem1;
private javax.swing.JMenuItem jMenuItem3;
private javax.swing.JMenuItem jMenuItem4;
private javax.swing.JMenuItem jMenuItem5;
private javax.swing.JMenu menuAcervo;
private javax.swing.JMenuItem menuItemConsultarAcervo;
private javax.swing.JMenuItem menuItemEncerrarSessao;
private javax.swing.JMenuItem menuItemVisualizarHistorico;
private javax.swing.JMenu menuUsuario;
// End of variables declaration//GEN-END:variables
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.model.bean;
/**
*
* @author romeu
*/
public class Editora {
private int codEdit;
private String nomeEdit;
public Editora(int codEdit, String nomeEdit){
this.codEdit = codEdit;
this.nomeEdit = nomeEdit;
}
public Editora(String nomeEdit) {
this.nomeEdit = nomeEdit;
}
public Editora(int codEdit) {
this.codEdit = codEdit;
this.nomeEdit = null;
}
public void setNomeEdit(String nomeEdit) {
this.nomeEdit = nomeEdit;
}
public int getCodEdit() {
return codEdit;
}
public String getNomeEdit() {
return nomeEdit;
}
@Override
public String toString() {
return "Editora{" + "codEdit=" + codEdit + ", nomeEdit=" + nomeEdit + '}';
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Editora)) {
return false;
}
Editora other = (Editora) obj;
return other.codEdit == this.codEdit;
}
}<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.model.bean;
import java.util.Calendar;
import java.util.Date;
public class Funcionario extends Usuario {
private int codCargo;
public Funcionario(String login, String senha, String nome, int codCargo) {
super(login, senha, nome);
this.codCargo = codCargo;
}
public Funcionario(String login) {
super(login);
}
public int getCargo() {
return this.codCargo;
}
public void setCargo(int codCargo) {
this.codCargo = codCargo;
}
@Override
public String toString() {
return "Funcionario{" + " funcionario = " + super.getNome() + " login = " + super.getLogin() + " senha = " + super.getSenha() + " codigo = " + this.codCargo + '}';
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Funcionario)) {
return false;
}
Funcionario other = (Funcionario) obj;
return other.getLogin().equals(this.getLogin());
}
/*
public Emprestimo fazEmprestimo (Usuario usuario, Exemplar exemplar, Obra obra){
Data dtEmprestimo, dtDevolucao;
dtEmprestimo = dtDevolucao = new Data("yyyy-MM-dd HH:mm:ss");
dtDevolucao.adicionarAnos(7);
return (new Emprestimo(this, usuario, exemplar, obra, dtEmprestimo, dtDevolucao));
}*/
/*
public Emprestimo fazEmprestimo (Usuario usuario, Exemplar exemplar){
Date dataAtual = new Date ();
Date dataLimite = geraDataLimite();
Emprestimo novoEmprestimo = new Emprestimo (this, usuario, exemplar, dataAtual, dataLimite );
return novoEmprestimo;
}
private Date geraDataLimite (){
Date dataLimite;
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date());
calendar.add(Calendar.DATE, +3);
dataLimite = calendar.getTime();
return dataLimite;
}
public boolean verificaAtrasoDeEmprestimo (Emprestimo emprestimo){
return emprestimo.isAtraso();
}
public Devolucao devolveEmprestimo (Emprestimo emprestimo, int codigoDaDevolucao){//EXPLICAR
Date dataAtual = new Date ();
Devolucao novaDevolucao = new Devolucao (codigoDaDevolucao, this, emprestimo.getExemplar(),dataAtual);
return novaDevolucao;
}
*/
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
/*Trocar o tipo DATE por Data*/
package br.com.biblisis.model.bean;
import java.util.Date;
public class Emprestimo {
private Funcionario funcionario;
private Usuario usuario;
private Exemplar exemplar;
private Obra obra;
private Data dataEmprestimo;
private Data dataDevolucao;
public Emprestimo(Funcionario funcionario, Usuario usuario, Exemplar exemplar, Obra obra, Data dataEmprestimo, Data dataDevolucao) {
this.funcionario = funcionario;
this.usuario = usuario;
this.exemplar = exemplar;
this.obra = obra;
this.dataEmprestimo = dataEmprestimo;
this.dataDevolucao = dataDevolucao;
}
public Funcionario getFuncionario() {
return funcionario;
}
public Usuario getUsuario() {
return usuario;
}
public Exemplar getExemplar() {
return exemplar;
}
public Data getDataEmprestimo() {
return dataEmprestimo;
}
public Data getDataDevolucao() {
return dataDevolucao;
}
public void setFuncionario(Funcionario funcionario) {
this.funcionario = funcionario;
}
public void setUsuario(Usuario usuario) {
this.usuario = usuario;
}
public void setExemplar(Exemplar exemplar) {
this.exemplar = exemplar;
}
public void setDataEmprestimo(Data dataEmprestimo) {
this.dataEmprestimo = dataEmprestimo;
}
public void setDataDevolucao(Data dataDevolucao) {
this.dataDevolucao = dataDevolucao;
}
public Obra getObra() {
return obra;
}
public void setObra(Obra obra) {
this.obra = obra;
}
public boolean isAtraso (){
return !dataDevolucao.depoisDaDataAtual();
}
public void calculaDataDevolucao(int dias) {
this.dataDevolucao.adicionarDias(dias);
}
@Override
public String toString() {
return "Emprestimo{" + "funcionario=" + funcionario.getLogin() + ", usuario=" + usuario.getLogin() + ", exemplar=" + exemplar.getCodExemplar() + ", obra=" + obra.getTitulo() + ", dataEmprestimo=" + dataEmprestimo + ", dataDevolucao=" + dataDevolucao + '}';
}
}
<file_sep>create database dbbiblisis;
use dbbiblisis;
show tables;
create table tbeditora(
codEdit int primary key auto_increment,
nomeEdit varchar(60) not null
);
create table tbobra(
codObra int primary key auto_increment,
tituloObra varchar(100) not null,
categoriaObra varchar(60) not null,
autorObra varchar(60),
dataPublicao date,
codEdit int not null,
foreign key (codEdit) references tbeditora(codEdit)
);
create table tblivro(
isbn varchar(20) not null,
codObra int not null,
foreign key(codObra) references tbobra(codObra),
primary key(isbn, codObra)
);
create table tbperiodico(
issn varchar(20) not null,
codObra int not null,
foreign key(codObra) references tbobra(codObra),
primary key(issn, codObra)
);
create table tbexemplar(
codEx int auto_increment,
codObra int not null,
isEmprestado bool,
foreign key(codObra) references tbobra(codObra),
primary key(codEx, codObra)
);
create table tbcargo(
codCargo int primary key,
nomeCargo varchar(80) not null
);
create table tbfuncionario(
loginFun varchar(15) not null unique primary key,
senhaFun varchar(15) not null,
nomeFun varchar(60) not null,
codCargo int not null references tbcargo(codCargo) /*Ele não adicionou o relacionamento a cargo*/
);
create table tbusuario(
loginUser varchar(15) not null unique primary key,
senhaUser varchar(15) not null,
nomeUser varchar(60) not null,
statusUser bool
);
create table tbreserva(
loginUser varchar(15) not null,
codObra int not null,
codEx int not null,
dataReserva timestamp default current_timestamp, /*dataTime*/
dataRetirada dateTime, /*YYYY-MM-DD HH:MM:SS*/
foreign key(loginUser) references tbusuario(loginUser),
foreign key(codObra) references tbobra(codObra),
foreign key(codEx) references tbexemplar(codEx),
primary key(loginUser, codObra, codEx)
);
create table tbemprestimo(
loginFun varchar(15) not null,
loginUser varchar(15) not null,
codEx int not null,
codObra int not null,
dataEmprestimo timestamp default current_timestamp,
dataDevolucao dateTime not null,
foreign key(loginFun) references tbfuncionario(LoginFun),
foreign key(loginUser) references tbusuario(loginUser),
foreign key(codEx) references tbexemplar(codEx),
foreign key(codObra) references tbobra(codObra),
primary key(loginFun, loginUser, codEx, codObra)
);
create table tbdevolucao (
codDevolucao int auto_increment primary key,
loginFun varchar(15) not null,
codEx int not null,
codObra int not null,
dataDevolucao dateTime not null,
foreign key(loginFun) references tbfuncionario(loginFun),
foreign key(codEx) references tbexemplar(codEx),
foreign key(codObra) references tbobra(codObra)
);
create table tbrenovacao (
codRenovacao int auto_increment primary key,
loginFun varchar(15) not null,
codEx int not null,
codObra int not null,
dataRenovacao dateTime not null,
foreign key(loginFun) references tbfuncionario(loginFun),
foreign key(codEx) references tbexemplar(codEx),
foreign key(codObra) references tbobra(codObra)
);
/*Inserindo Cargos, por hora somente 2*/
insert into tbcargo values(1, 'ATENDENTE'), (2, 'ADM');
/*Inserindo Funcionarios*/
insert into tbfuncionario values ('admin', 'admin', 'jock', 1);
insert into tbfuncionario values ('carllosveiga', '12456', 'carlos', 2), ('pedriHG', '9999', 'Pedro', 2);
insert into tbfuncionario values ('root', 'admin', 'administrador', 1);
/*Inserindo Usuarios*/
insert into tbusuario values ('jockfss', '343536', 'Jock', false);
insert into tbusuario values ('luquete', '8745', 'lucas', false);
insert into tbusuario values ('joao978', '4861556', '<NAME>', false);
insert into tbusuario values ('pedrinho@10', 'ped789', 'Pedro', false);
insert into tbusuario values ('ivavia#14', 'iva123', 'Ivan', false);
insert into tbusuario values ('ters@15', '89712', 'Terezina', false);
/*Inserindo as Principais Editoras*/
insert into tbeditora values (null, 'Veja'), (null, 'Escute'), (null, 'Ande');
insert into tbeditora values (null, 'PC&CIA');
/*Inserindo Obra*/
insert into tbobra values (null, 'As Aventuras de Pedro', 'Aventura', 'Jorge', '2018-03-02', 1);
insert into tbobra values (null, 'Algebra Linear - XXXX', 'Matemáica', 'Carlos', '2018-03-02', 2);
insert into tbobra values (null, 'Zip - ED de compactacao', 'Informatica', '<NAME>', '2018-02-01', 3);
insert into tbobra values (null, 'Raspeberry Pi', 'Informatica', 'PC&Cia', '2018-02-01', 4);
/*Inserindo Livros*/
insert into tblivro values ('ISBN388053101-3', 1);
insert into tblivro values ('ISBN012345689-0', 2);
insert into tblivro values ('ISBN095530100-9', 4);
/*Inserindo um Periodo*/
insert into tbperiodico values ('ISSN1511-3707', 5);
/*Inserindo Exemplares*/
-- exemplares das As Aventuras de Pedro
insert into tbexemplar values (1, 1, 0);
insert into tbexemplar values (2, 1, 0);
insert into tbexemplar values (3, 1, 0);
insert into tbexemplar values (4, 1, 0);
insert into tbexemplar values (5, 1, 0);
-- exemplares de Algebra Linear
insert into tbexemplar values (1, 2, 0);
insert into tbexemplar values (2, 2, 0);
insert into tbexemplar values (3, 2, 0);
insert into tbexemplar values (4, 2, 0);
-- exemplares de Zip - ED de compactacao
insert into tbexemplar values (1, 4, 0);
insert into tbexemplar values (2, 4, 0);
insert into tbexemplar values (3, 4, 0);
insert into tbexemplar values (4, 4, 0);
-- exemplares de Raspeberry - PI*/
insert into tbexemplar values (1, 5, 0);
insert into tbexemplar values (2, 5, 0);
insert into tbexemplar values (3, 5, 0);
insert into tbexemplar values (4, 5, 0);
/*************** Funções do Programa ***************************/
/******************* Reserva ***********************************/
/*Usuario solicita Reserva CREATE */
insert into tbreserva values ('jockfss', 1, 1, null, null);
insert into tbreserva values ('joao978', 1, 2, null, null);
insert into tbreserva values ('ivavia#14', 1, 3, null, null);
insert into tbreserva values ('ivavia#14', 1, 4, null, null);
/*Usuario Cancela uma Reseva - Delete*/
-- delete from tbreserva where loginUser = 'jockfss' and codObra = 1 and codEx = 1;
/*Consulta Reserva - Search*/
select * from tbreserva where loginUser = 'jockfss' and codObra = 1 and codEx = 1;
/*Atualiza uma Reserva - UPDATE (Quem Faz Isso e o Funcionario)*/
update tbreserva set dataRetirada = '2018-02-2 14:39:15' where loginUser = 'jockfss' and codObra = 1 and codEx = 1;
/*Visualisa Hitorico de Reserva*/
select * from tbreserva where loginUser = 'ivavia#14';
/*Reserva em Aberto?*/
select * from tbreserva where dataRetirada is null;
/*******Realizar um emprestimo************/
update tbexemplar set isEmprestado = true where codObra = 1 and codEx = 1;
update tbreserva set dataRetirada = '2018-02-2 14:39:15' where loginUser = 'jockfss' and codObra = 1 and codEx = 1;
insert into tbemprestimo values ('carllosveiga', 'jockfss', 1, 1, null, '2018-02-27 14:39:15'); /*Gera uma data de 7 dias pra devolucao*/
update tbexemplar set isEmprestado = true where codObra = 1 and codEx = 4;
update tbreserva set dataRetirada = '2018-02-27 14:39:15' where loginUser = 'ivavia#14' and codObra = 1 and codEx = 4;
insert into tbemprestimo values ('carllosveiga', 'ivavia#14', 1, 4, null, '2018-02-27 14:39:15'); /*Gera uma data de 7 dias pra devolucao*/
/*Visualisa Hitorico de Emprestimo*/
select * from tbemprestimo where loginUser = 'ivavia#14';
/*Consulta Acervo de Obras*/
select * from tbobra;
/*Consultar Situacao do Aluno/Professor*/
select * from tbusuario where loginUser = 'jockfss';
/***************/
select * from tbemprestimo;
select * from tbusuario;
select * from tbreserva;
select * from tbexemplar order by codObra, codEx;
select * from tbexemplar order by codObra, codEx;
select * from tbusuario;
/***************/
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package telas;
import br.com.biblisis.controller.CAlunoProfessor;
import br.com.biblisis.controller.CEmprestimo;
import br.com.biblisis.controller.CExemplar;
import br.com.biblisis.controller.CObra;
import java.awt.Color;
import java.awt.event.KeyEvent;
import java.text.SimpleDateFormat;
import java.sql.SQLException;
import java.text.DateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.Iterator;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.DefaultListModel;
import javax.swing.JOptionPane;
/**
*
* @author romeu
*/
public class TelaNovoEmprestimo extends javax.swing.JFrame {
/**
* Creates new form TelaNovoEmprestimo
*/
ArrayList <String[]> acervo;
ArrayList <String[]> acervoDeExemplares;
ArrayList <String[]> emprestimos;
private DefaultListModel modeloLista = new DefaultListModel();
private String loginFuncionario;
private String loginUsuario;
private int codigoDoExemplar;
private int codigoDaObra;
private int diasParaADevolucao;
public TelaNovoEmprestimo() {
initComponents();
this.acervo = new ArrayList<String[]>();
this.acervoDeExemplares = new ArrayList<String[]>();
this.emprestimos = new ArrayList<String[]>();
}
private void constroiListaDeEmprestimos() throws ParseException{
if (!this.emprestimos.isEmpty()){
this.emprestimos.clear();
}
try {
this.emprestimos = (ArrayList<String[]>) CEmprestimo.readAll();
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}
public void setLoginFuncionario(String loginFuncionario) {
this.loginFuncionario = loginFuncionario;
}
private void adicionaExemplaroNaLista (String codigoDoExemplar){
listaExemplares.setModel(modeloLista);
modeloLista.addElement(codigoDoExemplar);
}
private String[] buscaAlunoProfessor(String login){
String[] resposta = null;
try {
resposta = CAlunoProfessor.search(login);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
return resposta;
}
private void constroiAcervo(){
try {
this.acervo = (ArrayList<String[]>) CObra.read();
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}
private void constroiAcervoDeExemplares(){
try {
this.acervoDeExemplares = (ArrayList<String[]>) CExemplar.read();
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}
private String [] buscaExemplar(int codigoDoExemplar, int codigoDaObra){
String [] resposta = null;
try {
resposta = CExemplar.search(codigoDoExemplar, codigoDaObra);
}catch (SQLException e) {
System.out.println("Erro!" + e);
return resposta;
}
return resposta;
}
private String [] buscaObra (int codigoDaObra){
Iterator it = this.acervo.iterator();
String [] obra;
while (it.hasNext()){
obra = (String [])it.next();
if (Integer.parseInt(obra[0]) == codigoDaObra ){
return obra;
}
}
return null;
}
private ArrayList<String> buscaExemplares(int codigoDaObra){
Iterator it = this.acervoDeExemplares.iterator();
String [] exemplar;
String [] obra;
ArrayList<String> exemplaresDesejados = new ArrayList();
while (it.hasNext()){
exemplar = (String [])it.next();
obra = exemplar[1].split(",");
obra = obra[0].split("=");
if (codigoDaObra == Integer.parseInt(obra[1]) ){
exemplaresDesejados.add(exemplar[0]);
}
}
return exemplaresDesejados;
}
private boolean disponibilidadeDoExemplar (int codigoDoExemplar, int codigoDaObra){
String [] exemplarProcurado = buscaExemplar(codigoDoExemplar, codigoDaObra);
//System.out.println(exemplarProcurado[2]);
if (Boolean.parseBoolean(exemplarProcurado[2]) == true){
return false;
}else{
return true;
}
}
private void registraNovoEmprestimo (String loginFunc, String loginUsu, int codEx, int codOb, int dias){
try {
CEmprestimo.create(loginFunc, loginUsu, codEx, codOb, dias);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
try {
CExemplar.update(codEx, codOb, true);
}catch (SQLException e) {
System.out.println("Erro!" + e);
}
}
private Date geraDataLimite (int diasEmprestados , Date dataAtual){
Date dataLimite;
Calendar calendar = Calendar.getInstance();
calendar.setTime(dataAtual);
calendar.add(Calendar.DATE, + diasEmprestados);
dataLimite = calendar.getTime();
return dataLimite;
}
/*
private int buscaCodigoDoEmprestimo (String [] emprestimoProcurado){
int indiceProcurado;
int codigoDoEmprestimo;
if (this.emprestimos.contains(emprestimoProcurado)){
indiceProcurado = this.emprestimos.indexOf(emprestimoProcurado);
}else{
return -1;
}
codigoDoEmprestimo = this.emprestimos.size() - indiceProcurado;
return codigoDoEmprestimo;
}
private int buscaCodigoDoEmprestimo (String logFunc, String logUsu, String dataEmp ){
Iterator it = this.emprestimos.iterator();
String [] emprestimoDaLista;
while (it.hasNext()){
emprestimoDaLista = (String[]) it.next();
String dataDoEmprestimoDaLista = emprestimoDaLista[4];
String [] dataDividida = dataDoEmprestimoDaLista.split(" ");
String hora = dataDividida[1];
dataDividida = dataDividida[0].split("-");
String dataFormatada = dataDividida[2]+"/"+dataDividida[1] + "/" + dataDividida[0] +" "+ hora;
System.out.println(this.emprestimos.size() - this.emprestimos.indexOf(emprestimoDaLista));
System.out.println(emprestimoDaLista[0] + " - " + logFunc + emprestimoDaLista[1] + " - " + logUsu + dataFormatada + " - " + dataEmp );
System.out.println(emprestimoDaLista[0].equals(logFunc) + " " + emprestimoDaLista[1].equals(logUsu) + " " + dataEmp.equals(dataFormatada) );
if ( (emprestimoDaLista[0].equals(logFunc)) && (emprestimoDaLista[1].equals(logUsu)) && (dataEmp.equals(dataFormatada)) ){
return this.emprestimos.size() - this.emprestimos.indexOf(emprestimoDaLista);
}
}
return -1;
*/
private String geraCodigoDeEmprestimo (String data){
String [] dataDividida = data.split(" ");
String hora = dataDividida[1];
dataDividida = dataDividida[0].split("/");
String [] horaDividida = hora.split(":");
String codigoDoEmprestimo = "E" + dataDividida[0] + dataDividida[1] + dataDividida[2] + "-" + horaDividida[0] + horaDividida[1] + horaDividida[2];
return codigoDoEmprestimo;
}
private String [] buscaReferenciaDoEmprestimo (String logFunc, String logUsu, int codEx, int codObra, String dataEmp ){ //OK
Iterator it = this.emprestimos.iterator();
String [] emprestimoDaLista;
while (it.hasNext()){
emprestimoDaLista = (String[]) it.next();
System.out.println(dataEmp);
System.out.println(emprestimoDaLista[4]);
System.out.println(dataEmp.equals(emprestimoDaLista[4]));
if ( (emprestimoDaLista[0].equals(logFunc)) && (emprestimoDaLista[1].equals(logUsu)) && (emprestimoDaLista[2].equals(Integer.toString(codEx))) && (emprestimoDaLista[3].equals(Integer.toString(codObra))) && (dataEmp.equals(emprestimoDaLista[4])) ){
return emprestimoDaLista;
}
}
return null;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
jLabel1 = new javax.swing.JLabel();
jSeparator1 = new javax.swing.JSeparator();
txtLoginId = new javax.swing.JTextField();
jLabel3 = new javax.swing.JLabel();
btnVerificarSituacao = new javax.swing.JButton();
lblSituacaoDoUsuario = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
lblNome = new javax.swing.JLabel();
lblSituacaoDaObra = new javax.swing.JLabel();
btnVerificarDisponibilidade = new javax.swing.JButton();
jLabel7 = new javax.swing.JLabel();
txtCodigoDaObra = new javax.swing.JTextField();
lblTitulo = new javax.swing.JLabel();
jLabel9 = new javax.swing.JLabel();
jLabel10 = new javax.swing.JLabel();
jScrollPane2 = new javax.swing.JScrollPane();
listaExemplares = new javax.swing.JList<>();
btnConfirmarEmprestimo = new javax.swing.JButton();
lblCodigoDoExemplar = new javax.swing.JLabel();
jLabel11 = new javax.swing.JLabel();
btnFechar3 = new javax.swing.JButton();
lblConsultarAcervo = new javax.swing.JButton();
btnColarCodigoCopiado = new javax.swing.JButton();
lblDisponibilidadeDoExemplar = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
txtDiasParaDevolucao = new javax.swing.JLabel();
txtDiasParaADevolucao = new javax.swing.JTextField();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle("Novo Empréstimo");
setUndecorated(true);
setResizable(false);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowOpened(java.awt.event.WindowEvent evt) {
formWindowOpened(evt);
}
});
jLabel1.setFont(new java.awt.Font("Tahoma", 0, 20)); // NOI18N
jLabel1.setText("NOVO EMPRÉSTIMO");
jLabel1.setBorder(javax.swing.BorderFactory.createEtchedBorder());
txtLoginId.setToolTipText("seu ID pode conter letras ou números");
txtLoginId.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtLoginIdKeyPressed(evt);
}
public void keyReleased(java.awt.event.KeyEvent evt) {
txtLoginIdKeyReleased(evt);
}
});
jLabel3.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel3.setText("LOGIN/ID");
btnVerificarSituacao.setText("Verificar Situação");
btnVerificarSituacao.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnVerificarSituacaoActionPerformed(evt);
}
});
lblSituacaoDoUsuario.setText("Situação");
lblSituacaoDoUsuario.setBorder(javax.swing.BorderFactory.createEtchedBorder());
jLabel4.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel4.setText("NOME");
lblNome.setText("Digite o ID do usuário");
lblNome.setBorder(javax.swing.BorderFactory.createEtchedBorder());
lblSituacaoDaObra.setText("Situação");
lblSituacaoDaObra.setBorder(javax.swing.BorderFactory.createEtchedBorder());
btnVerificarDisponibilidade.setText("Verificar Disponibildade");
btnVerificarDisponibilidade.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnVerificarDisponibilidadeActionPerformed(evt);
}
});
jLabel7.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel7.setText("CÓDIGO DA OBRA");
txtCodigoDaObra.setToolTipText("seu ID pode conter letras ou números");
txtCodigoDaObra.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtCodigoDaObraKeyPressed(evt);
}
});
lblTitulo.setText("Digite o código da obra");
lblTitulo.setBorder(javax.swing.BorderFactory.createEtchedBorder());
jLabel9.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel9.setText("TÍTULO");
jLabel10.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel10.setText("EXEMPLARES DISPONÍVEIS");
listaExemplares.setModel(new javax.swing.AbstractListModel<String>() {
String[] strings = { "Exemplares" };
public int getSize() { return strings.length; }
public String getElementAt(int i) { return strings[i]; }
});
listaExemplares.setToolTipText("clique sobre o exemplar desejado para mais detalhes");
listaExemplares.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
listaExemplaresMouseClicked(evt);
}
});
listaExemplares.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
listaExemplaresKeyPressed(evt);
}
});
listaExemplares.addListSelectionListener(new javax.swing.event.ListSelectionListener() {
public void valueChanged(javax.swing.event.ListSelectionEvent evt) {
listaExemplaresValueChanged(evt);
}
});
jScrollPane2.setViewportView(listaExemplares);
btnConfirmarEmprestimo.setText("Confirmar Empréstimo");
btnConfirmarEmprestimo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnConfirmarEmprestimoActionPerformed(evt);
}
});
lblCodigoDoExemplar.setText("Digite o código da obra");
lblCodigoDoExemplar.setBorder(javax.swing.BorderFactory.createEtchedBorder());
jLabel11.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel11.setText("EXEMPLAR SELECIONADO");
btnFechar3.setIcon(new javax.swing.ImageIcon(getClass().getResource("/icones/icons8-close-window-24.png"))); // NOI18N
btnFechar3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnFechar3ActionPerformed(evt);
}
});
lblConsultarAcervo.setText("Consultar Acervo");
lblConsultarAcervo.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
lblConsultarAcervoActionPerformed(evt);
}
});
btnColarCodigoCopiado.setText("Colar Código Copiado");
btnColarCodigoCopiado.setToolTipText("acesse o acervo e copie um código para colar");
btnColarCodigoCopiado.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
btnColarCodigoCopiadoActionPerformed(evt);
}
});
lblDisponibilidadeDoExemplar.setText("Digite o código da obra");
lblDisponibilidadeDoExemplar.setBorder(javax.swing.BorderFactory.createEtchedBorder());
jLabel12.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel12.setText("DISPONIBILIDADE DO EXEMPLAR");
txtDiasParaDevolucao.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
txtDiasParaDevolucao.setText("DIAS PARA A DEVOLUÇÃO");
txtDiasParaADevolucao.addFocusListener(new java.awt.event.FocusAdapter() {
public void focusLost(java.awt.event.FocusEvent evt) {
txtDiasParaADevolucaoFocusLost(evt);
}
});
txtDiasParaADevolucao.addKeyListener(new java.awt.event.KeyAdapter() {
public void keyPressed(java.awt.event.KeyEvent evt) {
txtDiasParaADevolucaoKeyPressed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator1, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel3)
.addComponent(jLabel4))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(lblNome, javax.swing.GroupLayout.PREFERRED_SIZE, 213, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createSequentialGroup()
.addComponent(txtLoginId)
.addGap(18, 18, 18)
.addComponent(btnVerificarSituacao)
.addGap(18, 18, 18)
.addComponent(lblSituacaoDoUsuario, javax.swing.GroupLayout.PREFERRED_SIZE, 150, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(34, 34, 34))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(13, 13, 13)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 173, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(lblCodigoDoExemplar, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel11)
.addGap(165, 165, 165)))
.addGap(0, 200, Short.MAX_VALUE))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel12, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(lblDisponibilidadeDoExemplar, javax.swing.GroupLayout.PREFERRED_SIZE, 142, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(txtDiasParaDevolucao)
.addComponent(txtDiasParaADevolucao, javax.swing.GroupLayout.PREFERRED_SIZE, 31, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(89, 89, 89))))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(btnConfirmarEmprestimo)
.addComponent(jLabel10, javax.swing.GroupLayout.PREFERRED_SIZE, 329, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(81, 81, 81))))
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel9)
.addGap(18, 18, 18)
.addComponent(lblTitulo, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(lblConsultarAcervo, javax.swing.GroupLayout.PREFERRED_SIZE, 121, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(txtCodigoDaObra, javax.swing.GroupLayout.PREFERRED_SIZE, 90, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(btnVerificarDisponibilidade)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(lblSituacaoDaObra, javax.swing.GroupLayout.PREFERRED_SIZE, 147, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(35, 35, 35)))
.addComponent(btnColarCodigoCopiado)
.addGap(18, 18, 18))))
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup()
.addGap(0, 0, Short.MAX_VALUE)
.addComponent(jLabel1)
.addGap(216, 216, 216)
.addComponent(btnFechar3, javax.swing.GroupLayout.PREFERRED_SIZE, 21, javax.swing.GroupLayout.PREFERRED_SIZE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel1))
.addComponent(btnFechar3, javax.swing.GroupLayout.PREFERRED_SIZE, 19, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(txtLoginId, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(btnVerificarSituacao)
.addComponent(lblSituacaoDoUsuario)))
.addGap(13, 13, 13)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(lblNome))
.addGap(18, 18, 18)
.addComponent(jSeparator1, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel7)
.addComponent(txtCodigoDaObra, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(btnVerificarDisponibilidade)
.addComponent(lblSituacaoDaObra))
.addGap(18, 18, 18)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel9)
.addComponent(lblTitulo)
.addComponent(btnColarCodigoCopiado)
.addComponent(lblConsultarAcervo))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 83, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(btnConfirmarEmprestimo))
.addGroup(layout.createSequentialGroup()
.addComponent(jLabel11)
.addGap(11, 11, 11)
.addComponent(lblCodigoDoExemplar)
.addGap(14, 14, 14)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel12)
.addComponent(txtDiasParaDevolucao))
.addGap(5, 5, 5)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lblDisponibilidadeDoExemplar)
.addComponent(txtDiasParaADevolucao, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addContainerGap(37, Short.MAX_VALUE))
);
pack();
setLocationRelativeTo(null);
}// </editor-fold>//GEN-END:initComponents
private void formWindowOpened(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowOpened
constroiAcervo();
constroiAcervoDeExemplares();
try {
constroiListaDeEmprestimos();
} catch (ParseException ex) {
Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(Level.SEVERE, null, ex);
}
}//GEN-LAST:event_formWindowOpened
private void btnConfirmarEmprestimoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnConfirmarEmprestimoActionPerformed
try {
constroiListaDeEmprestimos ();
} catch (ParseException ex) {
Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(Level.SEVERE, null, ex);
}
if (!txtLoginId.getText().equals("") && lblSituacaoDoUsuario.getText().equals("Liberado")){
if (!txtCodigoDaObra.getText().equals("") && lblSituacaoDaObra.getText().equals("Disponível")){
if (lblDisponibilidadeDoExemplar.getText().equals("Disponível")){
if(!txtDiasParaADevolucao.getText().equals("") && !txtDiasParaADevolucao.getText().matches("[A-Za-z]{1,}")){
int resposta = JOptionPane.showConfirmDialog(null, "Deseja realizar o empréstimo?");
if (resposta == 0){
this.diasParaADevolucao = Integer.parseInt(txtDiasParaADevolucao.getText());
registraNovoEmprestimo(this.loginFuncionario, this.loginUsuario, this.codigoDoExemplar, this.codigoDaObra, this.diasParaADevolucao);
Date dataDoEmprestimo = new Date ();
Date dataLimite = geraDataLimite(this.diasParaADevolucao, dataDoEmprestimo);
SimpleDateFormat formato = new SimpleDateFormat ("dd/MM/yyyy");
try {//recarrega do banco para ArrayList de emprestimos
constroiListaDeEmprestimos();
} catch (ParseException ex) {
Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(Level.SEVERE, null, ex);
}
SimpleDateFormat formatoParaGerarCodigo = new SimpleDateFormat ("dd/MM/yyyy HH:mm:ss");
String codigoDoNovoEmprestimo = geraCodigoDeEmprestimo(formatoParaGerarCodigo.format(dataDoEmprestimo) );
System.out.println(codigoDoNovoEmprestimo);
JOptionPane.showMessageDialog(null,"Código do Empréstimo: " + codigoDoNovoEmprestimo + " Data de devolução: " + formato.format(dataLimite));
}
}else{
JOptionPane.showMessageDialog(null, "Digite os dias para a devolução!");
}
}else{
JOptionPane.showMessageDialog(null, "Exemplar indisponível!");
}
}else{
JOptionPane.showMessageDialog(null, "Obra indisponível ou código não digitado!");
}
}else{
JOptionPane.showMessageDialog(null, "Usuário bloqueado ou nome não digitado!");
}
}//GEN-LAST:event_btnConfirmarEmprestimoActionPerformed
private void listaExemplaresMouseClicked(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_listaExemplaresMouseClicked
if (!modeloLista.isEmpty()){
String codigoDoExemplar = listaExemplares.getSelectedValue();
lblCodigoDoExemplar.setText(codigoDoExemplar);
boolean isExemplarDisponivel = disponibilidadeDoExemplar(Integer.parseInt(codigoDoExemplar), this.codigoDaObra);
if (isExemplarDisponivel){
lblDisponibilidadeDoExemplar.setText("Disponível");
lblDisponibilidadeDoExemplar.setForeground(Color.blue);
this.codigoDoExemplar = Integer.parseInt(codigoDoExemplar);//ATRIBUTO
}else{
lblDisponibilidadeDoExemplar.setText("Não disponível");
lblDisponibilidadeDoExemplar.setForeground(Color.red);
}
}
}//GEN-LAST:event_listaExemplaresMouseClicked
private void btnFechar3ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnFechar3ActionPerformed
dispose();
}//GEN-LAST:event_btnFechar3ActionPerformed
private void btnVerificarSituacaoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnVerificarSituacaoActionPerformed
String loginDoUsuario = txtLoginId.getText();
String [] alunoProfessor;
if (!loginDoUsuario.equals("") ){
alunoProfessor = buscaAlunoProfessor(loginDoUsuario);
if (alunoProfessor != null){
lblNome.setText(alunoProfessor[1]);
lblNome.setForeground(Color.black);
if (Boolean.parseBoolean(alunoProfessor[2])){
lblSituacaoDoUsuario.setText("Bloqueado");
lblSituacaoDoUsuario.setForeground(Color.red);
}else{
lblSituacaoDoUsuario.setText("Liberado");
lblSituacaoDoUsuario.setForeground(Color.blue);
this.loginUsuario = txtLoginId.getText(); //ATRIBUTO
}
}else{
lblNome.setText("Usuário não encontrado!");
}
}else{
lblNome.setText("Digite um ID!");
lblNome.setForeground(Color.red);
}
}//GEN-LAST:event_btnVerificarSituacaoActionPerformed
private void lblConsultarAcervoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_lblConsultarAcervoActionPerformed
TelaConsultaAcervo novaTela = new TelaConsultaAcervo ();
novaTela.setVisible(true);
}//GEN-LAST:event_lblConsultarAcervoActionPerformed
private void btnColarCodigoCopiadoActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnColarCodigoCopiadoActionPerformed
TextTransfer clipboard = new TextTransfer();
txtCodigoDaObra.setText(clipboard.getClipboardContents());
}//GEN-LAST:event_btnColarCodigoCopiadoActionPerformed
private void btnVerificarDisponibilidadeActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_btnVerificarDisponibilidadeActionPerformed
if (!txtCodigoDaObra.getText().matches("[A-Za-z]{1,}")){
if (txtCodigoDaObra.getText().equals("")){
lblSituacaoDaObra.setText("Digite um código!");
lblSituacaoDaObra.setForeground(Color.red);
}
if (!txtCodigoDaObra.getText().equals("")){
modeloLista.clear();
String codigoDigitado = txtCodigoDaObra.getText();
String [] obraDesejada = null;
if (!codigoDigitado.equals("")){
obraDesejada = buscaObra(Integer.parseInt(codigoDigitado));
if (obraDesejada != null){
lblTitulo.setText(obraDesejada[1]);
lblTitulo.setForeground(Color.black);
}else{
lblTitulo.setText("Obra não encontrada!");
lblTitulo.setForeground(Color.red);
}
}else{
lblTitulo.setText("Digite um código para prosseguir!");
lblTitulo.setForeground(Color.red);
}
ArrayList<String > exemplaresEncontrados;
boolean isObraDisponivel = false;
if (obraDesejada != null){
exemplaresEncontrados = buscaExemplares(Integer.parseInt(codigoDigitado));
if (!exemplaresEncontrados.isEmpty()){
Iterator it = exemplaresEncontrados.iterator();
String exemplar;
while (it.hasNext()){
exemplar = (String)it.next();
if (isObraDisponivel == false){
isObraDisponivel = disponibilidadeDoExemplar(Integer.parseInt(exemplar), Integer.parseInt(codigoDigitado));//ARRUMAR ERRO!!!!!!!
}
listaExemplares.setModel(modeloLista);
modeloLista.addElement(exemplar);
}
}else{
lblCodigoDoExemplar.setText("Sem exemplares para a obra! Contate o administrador.");
}
}
if (isObraDisponivel){
lblSituacaoDaObra.setText("Disponível");
lblSituacaoDaObra.setForeground(Color.blue);
this.codigoDaObra = Integer.parseInt(codigoDigitado);//ATRIBUTO
}else{
this.codigoDaObra = Integer.parseInt(codigoDigitado);
lblSituacaoDaObra.setText("Não disponível");
lblSituacaoDaObra.setForeground(Color.red);
}
}
}
}//GEN-LAST:event_btnVerificarDisponibilidadeActionPerformed
private void listaExemplaresValueChanged(javax.swing.event.ListSelectionEvent evt) {//GEN-FIRST:event_listaExemplaresValueChanged
}//GEN-LAST:event_listaExemplaresValueChanged
private void txtLoginIdKeyReleased(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtLoginIdKeyReleased
//txtLoginId.setText(txtLoginId.getText().toUpperCase());
}//GEN-LAST:event_txtLoginIdKeyReleased
private void txtDiasParaADevolucaoFocusLost(java.awt.event.FocusEvent evt) {//GEN-FIRST:event_txtDiasParaADevolucaoFocusLost
if(!txtDiasParaADevolucao.getText().equals("") && !txtDiasParaADevolucao.getText().matches("[A-Za-z]{1,}")){
int diasDigitados = Integer.parseInt(txtDiasParaADevolucao.getText());
if(diasDigitados > 10){
JOptionPane.showMessageDialog(null, "Não é permitido um prazo maior que 10 dias!");
txtDiasParaADevolucao.setText("10");
}
}
}//GEN-LAST:event_txtDiasParaADevolucaoFocusLost
private void listaExemplaresKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_listaExemplaresKeyPressed
if (!modeloLista.isEmpty()){
String codigoDoExemplar = listaExemplares.getSelectedValue();
lblCodigoDoExemplar.setText(codigoDoExemplar);
boolean isExemplarDisponivel = disponibilidadeDoExemplar(Integer.parseInt(codigoDoExemplar), this.codigoDaObra);
if (isExemplarDisponivel){
lblDisponibilidadeDoExemplar.setText("Disponível");
lblDisponibilidadeDoExemplar.setForeground(Color.blue);
this.codigoDoExemplar = Integer.parseInt(codigoDoExemplar);//ATRIBUTO
}else{
lblDisponibilidadeDoExemplar.setText("Não disponível");
lblDisponibilidadeDoExemplar.setForeground(Color.red);
}
}
}//GEN-LAST:event_listaExemplaresKeyPressed
private void txtLoginIdKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtLoginIdKeyPressed
if(evt.getKeyCode() == KeyEvent.VK_ENTER){
String loginDoUsuario = txtLoginId.getText();
String [] alunoProfessor;
if (!loginDoUsuario.equals("") ){
alunoProfessor = buscaAlunoProfessor(loginDoUsuario);
if (alunoProfessor != null){
lblNome.setText(alunoProfessor[1]);
lblNome.setForeground(Color.black);
if (Boolean.parseBoolean(alunoProfessor[2])){
lblSituacaoDoUsuario.setText("Bloqueado");
lblSituacaoDoUsuario.setForeground(Color.red);
}else{
lblSituacaoDoUsuario.setText("Liberado");
lblSituacaoDoUsuario.setForeground(Color.blue);
this.loginUsuario = txtLoginId.getText(); //ATRIBUTO
}
}else{
lblNome.setText("Usuário não encontrado!");
}
}else{
lblNome.setText("Digite um ID!");
lblNome.setForeground(Color.red);
}
}
}//GEN-LAST:event_txtLoginIdKeyPressed
private void txtCodigoDaObraKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtCodigoDaObraKeyPressed
//tirar selecao
if(evt.getKeyCode() == KeyEvent.VK_ENTER){
if (!txtCodigoDaObra.getText().matches("[A-Za-z]{1,}")){
if (txtCodigoDaObra.getText().equals("")){
lblSituacaoDaObra.setText("Digite um código!");
lblSituacaoDaObra.setForeground(Color.red);
}
if (!txtCodigoDaObra.getText().equals("")){
modeloLista.clear();
String codigoDigitado = txtCodigoDaObra.getText();
String [] obraDesejada = null;
if (!codigoDigitado.equals("")){
obraDesejada = buscaObra(Integer.parseInt(codigoDigitado));
if (obraDesejada != null){
lblTitulo.setText(obraDesejada[1]);
lblTitulo.setForeground(Color.black);
}else{
lblTitulo.setText("Obra não encontrada!");
lblTitulo.setForeground(Color.red);
}
}else{
lblTitulo.setText("Digite um código para prosseguir!");
lblTitulo.setForeground(Color.red);
}
ArrayList<String > exemplaresEncontrados;
boolean isObraDisponivel = false;
if (obraDesejada != null){
exemplaresEncontrados = buscaExemplares(Integer.parseInt(codigoDigitado));
if (!exemplaresEncontrados.isEmpty()){
Iterator it = exemplaresEncontrados.iterator();
String exemplar;
while (it.hasNext()){
exemplar = (String)it.next();
if (isObraDisponivel == false){
isObraDisponivel = disponibilidadeDoExemplar(Integer.parseInt(exemplar), Integer.parseInt(codigoDigitado));//ARRUMAR ERRO!!!!!!!
}
listaExemplares.setModel(modeloLista);
modeloLista.addElement(exemplar);
}
}else{
lblCodigoDoExemplar.setText("Sem exemplares para a obra! Contate o administrador.");
}
}
if (isObraDisponivel){
lblSituacaoDaObra.setText("Disponível");
lblSituacaoDaObra.setForeground(Color.blue);
this.codigoDaObra = Integer.parseInt(codigoDigitado);//ATRIBUTO
}else{
this.codigoDaObra = Integer.parseInt(codigoDigitado);
lblSituacaoDaObra.setText("Não disponível");
lblSituacaoDaObra.setForeground(Color.red);
}
}
}
}
}//GEN-LAST:event_txtCodigoDaObraKeyPressed
private void txtDiasParaADevolucaoKeyPressed(java.awt.event.KeyEvent evt) {//GEN-FIRST:event_txtDiasParaADevolucaoKeyPressed
if(evt.getKeyCode() == KeyEvent.VK_ENTER){
try {
constroiListaDeEmprestimos ();
} catch (ParseException ex) {
Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(Level.SEVERE, null, ex);
}
if (!txtLoginId.getText().equals("") && lblSituacaoDoUsuario.getText().equals("Liberado")){
if (!txtCodigoDaObra.getText().equals("") && lblSituacaoDaObra.getText().equals("Disponível")){
if (lblDisponibilidadeDoExemplar.getText().equals("Disponível")){
if(!txtDiasParaADevolucao.getText().equals("") && !txtDiasParaADevolucao.getText().matches("[A-Za-z]{1,}")){
int resposta = JOptionPane.showConfirmDialog(null, "Deseja realizar o empréstimo?");
if (resposta == 0){
this.diasParaADevolucao = Integer.parseInt(txtDiasParaADevolucao.getText());
registraNovoEmprestimo(this.loginFuncionario, this.loginUsuario, this.codigoDoExemplar, this.codigoDaObra, this.diasParaADevolucao);
Date dataDoEmprestimo = new Date ();
Date dataLimite = geraDataLimite(this.diasParaADevolucao, dataDoEmprestimo);
SimpleDateFormat formato = new SimpleDateFormat ("dd/MM/yyyy");
try {//recarrega do banco para ArrayList de emprestimos
constroiListaDeEmprestimos();
} catch (ParseException ex) {
Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(Level.SEVERE, null, ex);
}
SimpleDateFormat formatoParaGerarCodigo = new SimpleDateFormat ("dd/MM/yyyy HH:mm:ss");
String codigoDoNovoEmprestimo = geraCodigoDeEmprestimo(formatoParaGerarCodigo.format(dataDoEmprestimo) );
System.out.println(codigoDoNovoEmprestimo);
JOptionPane.showMessageDialog(null,"Código do Empréstimo: " + codigoDoNovoEmprestimo + " Data de devolução: " + formato.format(dataLimite));
}
}else{
JOptionPane.showMessageDialog(null, "Digite os dias para a devolução!");
}
}else{
JOptionPane.showMessageDialog(null, "Exemplar indisponível!");
}
}else{
JOptionPane.showMessageDialog(null, "Obra indisponível ou código não digitado!");
}
}else{
JOptionPane.showMessageDialog(null, "Usuário bloqueado ou nome não digitado!");
}
}
}//GEN-LAST:event_txtDiasParaADevolucaoKeyPressed
/**
* @param args the command line arguments
*/
public static void main(String args[]) {
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(TelaNovoEmprestimo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new TelaNovoEmprestimo().setVisible(true);
}
});
}
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton btnColarCodigoCopiado;
private javax.swing.JButton btnConfirmarEmprestimo;
private javax.swing.JButton btnFechar3;
private javax.swing.JButton btnVerificarDisponibilidade;
private javax.swing.JButton btnVerificarSituacao;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel10;
private javax.swing.JLabel jLabel11;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel9;
private javax.swing.JScrollPane jScrollPane2;
private javax.swing.JSeparator jSeparator1;
private javax.swing.JLabel lblCodigoDoExemplar;
private javax.swing.JButton lblConsultarAcervo;
private javax.swing.JLabel lblDisponibilidadeDoExemplar;
private javax.swing.JLabel lblNome;
private javax.swing.JLabel lblSituacaoDaObra;
private javax.swing.JLabel lblSituacaoDoUsuario;
private javax.swing.JLabel lblTitulo;
private javax.swing.JList<String> listaExemplares;
private javax.swing.JTextField txtCodigoDaObra;
private javax.swing.JTextField txtDiasParaADevolucao;
private javax.swing.JLabel txtDiasParaDevolucao;
private javax.swing.JTextField txtLoginId;
// End of variables declaration//GEN-END:variables
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.controller;
import br.com.biblisis.model.bean.Data;
import br.com.biblisis.model.bean.Editora;
import br.com.biblisis.model.bean.Obra;
import br.com.biblisis.model.dao.DAOEditora;
import br.com.biblisis.model.dao.DAOObra;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author Jock
*/
public abstract class CObra {
private static Obra obra;
private static DAOObra daoObra;
private static DAOEditora daoEditora;
/**
* Rotina apenas o Administrador realiza - codCargo = 1
* Porem o Funcionario pode realizar um seu cadastro (Caso não exista!)
* Ao executar o cadastro faça sempre uma busca na base antes
*/
public static void create(int codObra, String titulo, String categoria, String autor, String dataDePublicacao, int codEdit) throws SQLException { //data no formato --> yyyy/MM/DD <--- ler cada campo como string e juntar '+'
daoObra = new DAOObra();
obra = new Obra(codObra, titulo, categoria, autor, new Data(dataDePublicacao), (new DAOEditora()).search(new Editora(codEdit)));
daoObra.create(obra);
}
/*Rotina apenas o Administrador realiza - codCargo = 1*/
public static List<String[]> read() throws SQLException {
daoObra = new DAOObra();
ArrayList<String[]> obras = new ArrayList<String[]>();
for (Obra obraEncontrada : daoObra.read()) {
String[] busca = new String[6];
busca[0] = String.valueOf(obraEncontrada.getCodigoObra());
busca[1] = obraEncontrada.getTitulo();
busca[2] = obraEncontrada.getCategoria();
busca[3] = obraEncontrada.getAutor();
busca[4] = obraEncontrada.getDataPublicacao().toString();
busca[5] = String.valueOf(obraEncontrada.getEditora().getCodEdit());
obras.add(busca);
}
return obras;
}
public static void update(int codObra, String titulo, String categoria, String autor, String dataDePublicao, int codEdit) throws SQLException {
daoObra = new DAOObra();
obra = new Obra(codObra, titulo, categoria, autor, new Data(dataDePublicao), (new DAOEditora()).search(new Editora(codEdit)));
daoObra.update(obra);
}
/** Rotina apenas o Administrador realiza - codCargo = 1
* Porem um funcionario pode excluir sua conta
*/
public static void delete(int codEdit) throws SQLException {
daoObra = new DAOObra();
obra = new Obra(codEdit);
daoObra.delete(obra);
}
public static String[] search(int codObra) throws SQLException {
daoObra = new DAOObra();
obra = daoObra.search(new Obra(codObra));
String[] retorno = new String[6];
if (obra != null){
retorno[0] = String.valueOf(obra.getCodigoObra());
retorno[1] = obra.getTitulo();
retorno[2] = obra.getCategoria();
retorno[3] = obra.getAutor();
retorno[4] = obra.getDataPublicacao().toString();
retorno[5] = String.valueOf(obra.getEditora().getCodEdit());
}else{
retorno = null;
}
return retorno;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.model.bean;
public class AlunoProfessor extends Usuario {
private boolean status;
public AlunoProfessor(String login, String senha, String nome, boolean status) {
super(login, senha, nome);
this.status = status;
}
public AlunoProfessor(String login) {
super(login);
}
public void setStaus(boolean status) {
this.status = status;
}
public boolean getStatus() {
return this.status;
}
public String toString() {
return super.toString() + " - " + status;
}
/*
public Emprestimo fazEmprestimo (Usuario usuario, Exemplar exemplar){
Date dataAtual = new Date ();
Date dataLimite = geraDataLimite();
Emprestimo novoEmprestimo = new Emprestimo (this, usuario, exemplar, exemplar.getObra(), dataAtual, dataLimite );
return novoEmprestimo;
}
private Date geraDataLimite (){
Date dataLimite;
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date());
calendar.add(Calendar.DATE, +3);
dataLimite = calendar.getTime();
return dataLimite;
}
public boolean verificaAtrasoDeEmprestimo (Emprestimo emprestimo){
return emprestimo.isAtraso();
}
*/
}
<file_sep>1 - Instale mysql e xampp
2 - Copie e cole o script no mysql e execute o raio sem barrinha.
(Crie o BD e todas as tabelas - dbbiblisis)
OBS: Software desenvolvido no NETBEANS <file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.model.bean;
public class Livro extends Obra {
private String isbn;
public Livro (int codObra, String titulo, String categoria, String autor, Data dataPublicacao, Editora editora, String isbn){
super(codObra, titulo, categoria, autor, dataPublicacao, editora);
this.isbn = isbn;
}
public String getIsbn() {
return this.isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
@Override
public String toString() {
return "Livro{" + "codObra=" + super.getCodigoObra() + ", titulo=" + super.getTitulo() + ", categoria=" + super.getCategoria() + ", autor=" + super.getAutor() + ", dataPublicacao=" + super.getDataPublicacao() + ", ISBN=" + this.isbn + '}';
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.controller;
import br.com.biblisis.model.bean.AlunoProfessor;
import br.com.biblisis.model.bean.Data;
import br.com.biblisis.model.bean.Emprestimo;
import br.com.biblisis.model.bean.Exemplar;
import br.com.biblisis.model.bean.Funcionario;
import br.com.biblisis.model.bean.Obra;
import br.com.biblisis.model.dao.DAOAlunoProfessor;
import br.com.biblisis.model.dao.DAOEmprestimo;
import br.com.biblisis.model.dao.DAOExemplar;
import br.com.biblisis.model.dao.DAOFuncionario;
import br.com.biblisis.model.dao.DAOObra;
import java.sql.SQLException;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author Jock
*/
public abstract class CEmprestimo {
private static Emprestimo emprestimo;
private static Exemplar exemplar;
private static Obra obra;
private static AlunoProfessor alunoProfessor;
private static Funcionario funcionario;
private static Data dtDevolucao, dtEmprestimo;
private static DAOEmprestimo daoEmprestimo;
private static DAOExemplar daoExemplar;
private static DAOObra daoObra;
private static DAOAlunoProfessor daoAlunoProfessor;
private static DAOFuncionario daoFuncionario;
private static Emprestimo cud(String loginFun, String loginUser, int codExemplar, int codObra) throws SQLException {
//Instancias de daos
daoExemplar = new DAOExemplar();
daoObra = new DAOObra();
daoAlunoProfessor = new DAOAlunoProfessor();
daoFuncionario = new DAOFuncionario();
//instancias do model
exemplar = daoExemplar.search(new Exemplar(codExemplar, new Obra(codObra))); // cuida com null pointerException
obra = daoObra.search(new Obra(codObra));
alunoProfessor = daoAlunoProfessor.search(new AlunoProfessor(loginUser));
funcionario = daoFuncionario.search(new Funcionario(loginFun));
dtDevolucao = dtEmprestimo = new Data("yyyy-MM-dd HH:mm:ss");
return (new Emprestimo(funcionario, alunoProfessor, exemplar, obra, dtEmprestimo, dtDevolucao) );
}
public static Data create(String loginFun, String loginUser, int codExemplar, int codObra, int diasParaDevolucao) throws SQLException {
try {
daoEmprestimo = new DAOEmprestimo();
emprestimo = cud(loginFun, loginUser, codExemplar, codObra);
emprestimo.getDataDevolucao().adicionarDias(diasParaDevolucao);
daoEmprestimo.create(emprestimo);
} catch (NullPointerException e) {
throw new IllegalArgumentException("ERRO ::: login(funcionario ou usuario), codExmplar ou codObra incorretos.\n" + e);
}
return emprestimo.getDataEmprestimo();
}
public static void update(String loginFun, String loginUser, int codExemplar, int codObra, int diasParaDevolucao) throws SQLException {
try {
daoEmprestimo = new DAOEmprestimo();
emprestimo = cud(loginFun, loginUser, codExemplar, codObra);
emprestimo.getDataDevolucao().adicionarDias(diasParaDevolucao);
daoEmprestimo.update(emprestimo);
} catch (NullPointerException e) {
throw new IllegalArgumentException("ERRO ::: login(funcionario ou usuario), codExmplar ou codObra incorretos.\n" + e);
}
}
/*Rotina apenas o Administrador realiza - codCargo = 1*/
public static void delete(String loginFun, String loginUser, int codExemplar, int codObra) throws SQLException {
try {
daoEmprestimo = new DAOEmprestimo();
emprestimo = cud(loginFun, loginUser, codExemplar, codObra);
daoEmprestimo.delete(emprestimo);
} catch (NullPointerException e) {
throw new IllegalArgumentException("ERRO ::: login(funcionario ou usuario), codExmplar ou codObra incorretos.\n" + e);
}
}
/*Rotina apenas o Administrador realiza - codCargo = 1*/
private static List<String[]> read(List<Emprestimo> read) throws SQLException, ParseException {
daoEmprestimo = new DAOEmprestimo();
ArrayList<String[]> emprestimos = new ArrayList<>();
for (Emprestimo emprestimo : read) {
String[] busca = new String[6];
busca[0] = emprestimo.getFuncionario().getLogin();
busca[1] = emprestimo.getUsuario().getLogin();
busca[2] = String.valueOf(emprestimo.getExemplar().getCodExemplar());
busca[3] = String.valueOf(emprestimo.getObra().getCodigoObra());
busca[4] = emprestimo.getDataEmprestimo().toString();
busca[5] = emprestimo.getDataDevolucao().toString();
emprestimos.add(busca);
}
return emprestimos;
}
public static List<String[]> readAll() throws SQLException, ParseException {
daoEmprestimo = new DAOEmprestimo();
ArrayList<String[]> emprestimos = new ArrayList<>();
for (Emprestimo emprestimo : daoEmprestimo.readAll()) {
String[] busca = new String[6];
busca[0] = emprestimo.getFuncionario().getLogin();
busca[1] = emprestimo.getUsuario().getLogin();
busca[2] = String.valueOf(emprestimo.getExemplar().getCodExemplar());
busca[3] = String.valueOf(emprestimo.getObra().getCodigoObra());
busca[4] = emprestimo.getDataEmprestimo().toString();
busca[5] = emprestimo.getDataDevolucao().toString();
emprestimos.add(busca);
}
return emprestimos;
}
public static List<String[]> allRead() throws SQLException, ParseException {
return read( (new DAOEmprestimo() ).allRead() );
}
public static List<String[]> userRead(String loginUser) throws SQLException, ParseException {
return read( (new DAOEmprestimo().userRead(loginUser)) );
}
public static List<String[]> funcionarioRead(String loginFun) throws SQLException, ParseException {
return read( (new DAOEmprestimo()).funcionarioRead(loginFun) );
}
/**
* Rotina apenas o Administrador realiza - codCargo = 1
* Porem o Funcionario pode realizar um seu cadastro (Caso não exista!)
* Ao executar o cadastro faça sempre uma busca na base antes
*/
/*public static void create(String loginFun, String loginUser, int codExemplar, int codObra, int diasParaDevolucao) {
//Instancias de daos
daoEmprestimo = new DAOEmprestimo();
daoExemplar = new DAOExemplar();
daoObra = new DAOObra();
daoAlunoProfessor = new DAOAlunoProfessor();
daoFuncionario = new DAOFuncionario();
try {
//instancias do model
exemplar = daoExemplar.search(new Exemplar(codExemplar, new Obra(codObra))); // cuida com null pointerException
obra = daoObra.search(new Obra(codObra));
alunoProfessor = daoAlunoProfessor.search(new AlunoProfessor(loginUser));
funcionario = daoFuncionario.search(new Funcionario(loginFun));
dtDevolucao = dtEmprestimo = new Data("yyyy-MM-dd HH:mm:ss");
//Altera o status do exemplar e atualiza no banco
//exemplar.setIsEmprestado(true); //quem deve fazer isso é o controler de fun (faz emprestimo)
//daoExemplar.update(exemplar); //quem deve fazer isso é o controler de fun (faz emprestimo)
//Altera a data de devolucao e guarda o emprestimo no banco
dtDevolucao.adicionarDias(diasParaDevolucao);
daoEmprestimo.create(new Emprestimo(funcionario, alunoProfessor, exemplar, obra, dtEmprestimo, dtDevolucao));
} catch (NullPointerException | SQLException e) {
System.err.println("ERRO, exemplar ou obra ou alunoProfessor ou funionario inexistente ou ERRO ao salvar no Banco de Dados ::: \n" + e);
}
}*/
/*public static void update(String loginFun, String loginUser, int codExemplar, int codObra, int diasParaDevolucao) throws SQLException {
try {
//Instancias de daos
daoEmprestimo = new DAOEmprestimo();
daoExemplar = new DAOExemplar();
daoObra = new DAOObra();
daoAlunoProfessor = new DAOAlunoProfessor();
daoFuncionario = new DAOFuncionario();
//instancias do model
exemplar = daoExemplar.search(new Exemplar(codExemplar, new Obra(codObra))); // cuida com null pointerException
obra = daoObra.search(new Obra(codObra));
alunoProfessor = daoAlunoProfessor.search(new AlunoProfessor(loginUser));
funcionario = daoFuncionario.search(new Funcionario(loginFun));
dtDevolucao = new Data("yyyy-MM-dd HH:mm:ss");
//Altera o status do exemplar e atualiza no banco
//exemplar.setIsEmprestado(true); //quem deve fazer isso é o controler de fun (faz emprestimo)
//daoExemplar.update(exemplar); //quem deve fazer isso é o controler de fun (faz emprestimo)
//Altera a data de devolucao e guarda o emprestimo no banco
dtDevolucao.adicionarDias(diasParaDevolucao);
daoEmprestimo.update(new Emprestimo(funcionario, alunoProfessor, exemplar, obra, dtEmprestimo, dtDevolucao));
} catch (NullPointerException | SQLException e) {
System.err.println("ERRO, exemplar ou obra ou alunoProfessor ou funionario inexistente ou ERRO ao salvar no Banco de Dados ::: \n" + e);
}
}*/
/** Rotina apenas o Administrador realiza - codCargo = 1
* Porem um funcionario pode excluir sua conta
*/
/*public static void delete(String loginFun, String loginUser, int codExemplar, int codObra) throws SQLException {
try {
//Instancias de daos
daoEmprestimo = new DAOEmprestimo();
daoExemplar = new DAOExemplar();
daoObra = new DAOObra();
daoAlunoProfessor = new DAOAlunoProfessor();
daoFuncionario = new DAOFuncionario();
//instancias do model
exemplar = daoExemplar.search(new Exemplar(codExemplar, new Obra(codObra))); // cuida com null pointerException
obra = daoObra.search(new Obra(codObra));
alunoProfessor = daoAlunoProfessor.search(new AlunoProfessor(loginUser));
funcionario = daoFuncionario.search(new Funcionario(loginFun));
//Altera o status do exemplar e atualiza no banco
//exemplar.setIsEmprestado(true); //quem deve fazer isso é o controler de fun (faz emprestimo)
//daoExemplar.update(exemplar); //quem deve fazer isso é o controler de fun (faz emprestimo)
//Altera a data de devolucao e guarda o emprestimo no banco
daoEmprestimo.delete(new Emprestimo(funcionario, alunoProfessor, exemplar, obra, null, null));
} catch (NullPointerException | SQLException e) {
System.err.println("ERRO, exemplar ou obra ou alunoProfessor ou funionario inexistente ou ERRO ao salvar no Banco de Dados ::: \n" + e);
}
}*/
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.controller;
import br.com.biblisis.model.bean.Editora;
import br.com.biblisis.model.dao.DAOEditora;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author Jock
*/
public abstract class CEditora {
private static Editora editora;
private static DAOEditora daoEditora;
/**
* Rotina apenas o Administrador realiza - codCargo = 1
* Porem o Funcionario pode realizar um seu cadastro (Caso não exista!)
* Ao executar o cadastro faça sempre uma busca na base antes
*/
public static void create(String nomeEdit) throws SQLException {
daoEditora = new DAOEditora();
editora = new Editora(nomeEdit);
daoEditora.create(editora);
}
/*Rotina apenas o Administrador realiza - codCargo = 1*/
public static List<String[]> read() throws SQLException {
daoEditora = new DAOEditora();
ArrayList<String[]> editoras = new ArrayList<>();
for (Editora editora : daoEditora.read()) {
String[] busca = new String[2];
busca[0] = String.valueOf(editora.getCodEdit());
busca[1] = editora.getNomeEdit();
editoras.add(busca);
}
return editoras;
}
public static void update(int codEdit, String nomeEdit) throws SQLException {
daoEditora = new DAOEditora();
editora = new Editora(codEdit, nomeEdit);
daoEditora.update(editora);
}
/** Rotina apenas o Administrador realiza - codCargo = 1
* Porem um funcionario pode excluir sua conta
*/
public static void delete(int codEdit) throws SQLException {
daoEditora = new DAOEditora();
editora = new Editora(codEdit);
daoEditora.delete(editora);
}
public static String[] search(int codEdit) throws SQLException {
daoEditora = new DAOEditora();
editora = daoEditora.search(new Editora(codEdit));
String[] retorno = new String[2];
if (editora!= null){
retorno[0] = String.valueOf(editora.getCodEdit());
retorno[1] = editora.getNomeEdit();
}else{
retorno = null;
}
return retorno;
}
}
<file_sep>/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package br.com.biblisis.model.dao;
import br.com.biblisis.model.bean.*;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.List;
/**
*
* @author Jock
*/
public class DAOReserva {
public void create(Reserva reserva) throws SQLException {
Connection con = ConnectionFactory.getConnection();
PreparedStatement stmt = null;
try {
stmt = con.prepareStatement("INSERT INTO tbreserva VALUES (?, ?, ?, ?, ?)");
} catch (SQLException e) {
System.err.println("ERRO ::: " + e);
} finally {
ConnectionFactory.closeConnection(con, stmt);
}
}
public List<Reserva> read() throws SQLException {
Connection con = ConnectionFactory.getConnection();
PreparedStatement stmt = null;
try {
stmt = con.prepareStatement("");
} catch (SQLException e) {
System.err.println("ERRO ::: " + e);
} finally {
ConnectionFactory.closeConnection(con, stmt);
}
return null;
}
public void update(Reserva reserva) throws SQLException {
Connection con = ConnectionFactory.getConnection();
PreparedStatement stmt = null;
try {
stmt = con.prepareStatement("");
} catch (SQLException e) {
System.err.println("ERRO ::: " + e);
} finally {
ConnectionFactory.closeConnection(con, stmt);
}
}
public void delete(Reserva reserva) throws SQLException {
Connection con = ConnectionFactory.getConnection();
PreparedStatement stmt = null;
try {
stmt = con.prepareStatement("");
} catch (SQLException e) {
System.err.println("ERRO ::: " + e);
} finally {
ConnectionFactory.closeConnection(con, stmt);
}
}
public Reserva search(Reserva reserva) throws SQLException {
Connection con = ConnectionFactory.getConnection();
PreparedStatement stmt = null;
ResultSet rs = null;
try {
stmt = con.prepareStatement("");
} catch (SQLException e) {
System.err.println("ERRO ::: " + e);
} finally {
ConnectionFactory.closeConnection(con, stmt);
}
return null;
}
}
| 296dee331f93afeb67a9f1d171dddabf87752137 | [
"Java",
"Text",
"SQL"
] | 14 | Java | daburajr/projeto_biblisis_JDBC | cf8d181129b6aa1d6c3dd8e7bdb01d9bd5921096 | 8b2696ba35da79c03fba1bd251aae515fb26ac91 | |
refs/heads/master | <repo_name>AceroJuan/React-App-Gif-Finder<file_sep>/src/Components/GifExpertApp/GifExpertApp.js
import React, { useState } from "react";
import AddCategory from "../AddCategory/AddCategory";
import GifGrid from "../GifGrid/GifGrid";
import "animate.css";
const GifExpertApp = ({ defaultCategories = [] }) => {
const [categories, setCategories] = useState(defaultCategories);
return (
<>
<h1 className="animate__animated animate__fadeIn">Hola</h1>
<AddCategory setCategories={setCategories} />
<hr />
<ul>
{categories.map((category) => (
<GifGrid key={category} category={category} />
))}
</ul>
</>
);
};
export default GifExpertApp;
<file_sep>/src/tests/helpers/getGifs.test.js
import getGifs from "../../utils/helpers/getGifs";
import "@testing-library/jest-dom";
describe("pruebas en getGifs.js", () => {
test("debe traer 15 elementos", async () => {
const gifs = await getGifs("goku");
expect(gifs.length).toBe(15);
});
test("debe verificar un string vacio", async () => {
const gifs = await getGifs("");
expect(gifs.length).toBe(0);
});
});
| 93c6b50ca5c70f002721a361be9d8a85e5525c64 | [
"JavaScript"
] | 2 | JavaScript | AceroJuan/React-App-Gif-Finder | daac7b9c1ae844fe6240715bbb0e133bd4ed2a54 | 0760c059b08e1ead7b8d57da223fe5824210ef4b | |
refs/heads/master | <repo_name>kekatoz/zxcv<file_sep>/zxcv12/testt/urls.py
from django.urls import path
from .views import get, fine
urlpatterns = [
path('', get),
path('fine/', fine)
]
<file_sep>/zxcv12/testt/forms.py
from django import forms
class Testform(forms.Form):
testfield= forms.CharField(label='testfield', max_length=255)
<file_sep>/zxcv12/testt/migrations/0002_auto_20210203_1727.py
# Generated by Django 3.0 on 2021-02-03 14:27
import django.contrib.postgres.fields.jsonb
from django.db import migrations, models
class Migration(migrations.Migration):
dependencies = [
('testt', '0001_initial'),
]
operations = [
migrations.CreateModel(
name='InputModel',
fields=[
('id', models.AutoField(auto_created=True, primary_key=True, serialize=False, verbose_name='ID')),
('field', models.CharField(max_length=255)),
('data', django.contrib.postgres.fields.jsonb.JSONField()),
],
),
migrations.DeleteModel(
name='TestModel',
),
]
<file_sep>/zxcv12/testt/views.py
from django.shortcuts import render, redirect
from .forms import Testform
from .models import Testmodel
import json
def get(request):
if request.method == 'POST':
form = Testform(request.POST)
if form.is_valid():
name_input = form.cleaned_data['testfield']
data = json.dumps(name_input, ensure_ascii=False)
Testmodel.objects.create(data=data, field='testfield')
for count in range(1, len(request.POST) - 1):
query = 'field' + str(count)
input_value = request.POST[query]
if input_value:
data = json.dumps(input_value, ensure_ascii=False)
field = json.dumps(query)
Testmodel.objects.create(data=data, field=field)
count += 1
return redirect('/fine/')
else:
form = Testform()
return render(request, 'base.html', {'form': form})
def fine(request):
# Take data from the db
query = Testmodel.objects.all().values('id', 'data', 'field')
json_list = list(query)
return render(request, 'fine.html', {'json_list': json_list})
<file_sep>/zxcv12/testt/models.py
from django.db import models
from django.contrib.postgres.fields import JSONField
# Create your models here.
class Testmodel(models.Model):
field = models.TextField(max_length=255)
data = JSONField()
def __str__(self):
return self.field, self.data
<file_sep>/zxcv12/testt/migrations/0005_auto_20210205_1031.py
# Generated by Django 3.0 on 2021-02-05 10:31
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('testt', '0004_auto_20210203_1901'),
]
operations = [
migrations.RenameModel(
old_name='InputModel',
new_name='Testmodel',
),
]
| 44f894d52973d38ecc2ccdcef02804c79f4c546c | [
"Python"
] | 6 | Python | kekatoz/zxcv | d39e13d086935e9af4a08c190b09252f8e6d5ad4 | 9d187801af0dc0134a6ad60b3bc65c9c427672b0 | |
refs/heads/master | <repo_name>chatard/Coursera-Data-Cleaning-Project<file_sep>/README.md
---
title: "Cleaning Data Course Project: Tidy dataset."
author: "Jacques.Chatard"
date: "25 février 2018"
output: html_document
---
this work is part of the assignment concerning the chapter "Getting and Cleaning Data"
of the Johns Hopkins University Data Science course on coursera.org.
<ins>The original document that contains the base files can be found on:</ins>
[UCI.EDU](http://archive.ics.uci.edu/ml/datasets/Human+Activity+Recognition+Using+Smartphones)
* Original data comes from : UCI Machine Learning Repository.
* Link (below) for original zipped data files is:
[zipped data files](https://d396qusza40orc.cloudfront.net/getdata%2Fprojectfiles%2FUCI%20HAR%20Dataset.zip)
I have downloaded this zip file in my working directory on february 18th 2018.
in my working directory unzipped files stand in a new directory named : "UCI HAR Dataset".
## The purpose of this assignment is:
.1 Merges the training and the test sets to create one data set.
.2 Extracts only the measurements on the mean and standard deviation for each measurement.
.3 Uses descriptive activity names to name the activities in the data set
.4 Appropriately labels the data set with descriptive variable names.
.5 From the data set in step 4, creates a second, independent tidy data set with the average of each variable for each activity and each subject.
## File structure in this document:
[README.md](https://github.com/chatard/Coursera-Data-Cleaning-Project/blob/master/README.md)
[run_analysis.R](https://github.com/chatard/Coursera-Data-Cleaning-Project/blob/master/run_analysis.R)
[CODEBOOK](https://github.com/chatard/Coursera-Data-Cleaning-Project/blob/master/CODEBOOK.md)
[New tidy dataset](https://github.com/chatard/Coursera-Data-Cleaning-Project/blob/master/tidydatasetG.txt)
<file_sep>/CODEBOOK.md
---
title: "CODEBOOK"
author: "john"
date: "27 février 2018"
output: html_document
---
## Codebook elements provided in the original files:
* ./UCI_HAR_Dataset/features_info.txt
this file lists and gives information on the main measured variables.
* ./UCI_HAR_Dataset/features.txt
this file provides the names of each one of 561 measured or estimated variables.
* ./UCI_HAR_Dataset/activity_labels.txt
this file provides the names of the different categories of activities for which measurements have been made.
* ./UCI_HAR_Dataset/train/y_train.txt and ./UCI_HAR_Dataset/test/y_test.txt
these 2 files provide an identification code in range [1, 30] to identify each trial participant for each observation.
## About variables related units of measure:
* <ins>For acceleration type variables along an axis:</ins> units of gravity expressed in g.
* <ins>For other variables (gyroscope angular speed):</ins> unit = gradians / second.
## Modified or added variables during the process for my "tidy data set":
### modified activity labels (line 32 of run_analysis.R) :
WALKING, WALKING_UPSTAIRS, WALKING_DOWNSTAIRS, SITTING, STANDING, LAYING.
respectively substituted by:
walking, walkingupstairs, walkingdownstairs, sitting, laying.
### added : "subjid"
* subjid is a subject identifier : line 16 in file run_analysis.R
### added : "activitycode"
* activitycode is a container for activity labels : line 27 in file run_analysis.R
<file_sep>/run_analysis.R
#run_analysis.R
# loading the libraries that I will use:
library(data.table)
library(dplyr)
# 1) Merges the training and the test sets to create one data set.
trainsubjects<-read.table("./UCI HAR Dataset/train/subject_train.txt")
testsubjects<-read.table("./UCI HAR Dataset/test/subject_test.txt")
# allsubjects will be the merged table of subjects in training sample and in test sample:
# using rbind() to create the merged table "allsubjects":
# this new object is a data table with 1 variable that contains the identifiers of the subjects
# who participated in the experiment:
# I chose to give a more meaningful name to this variable, namely: subjid.
# for more details about the choice of variable names: please see in my "codebook" file.
allsubjects<-data.table(rbind(trainsubjects,testsubjects))
allsubjects <- rename(allsubjects, subjid=V1)
# ..............................................................................
# 2) in the same way, "allactivities" will be a merged table of activities
# in training sample and in test sample :
# here, the variable "activitycode" designates the different phases of physical
# activities that gave rise to measurements.
# this variable : "activitycode" may have six different values according to the
# description of the original file "activity_labels.txt" ....
trainactivities<-data.table(read.table("./UCI HAR Dataset/train/y_train.txt"))
testactivities <- data.table(read.table("./UCI HAR Dataset/test/y_test.txt"))
allactivities<-data.table(rbind(trainactivities,testactivities))
allactivities<-rename(allactivities, activitycode=V1)
# naming activities levels in the data set:
# for more details about the choice of variable names: please see in my "codebook" file.
allactivities$activitycode<-factor(allactivities$activitycode,
levels=c(1:6),
labels=c("walking","walkingupstairs", "walkingdownstairs", "sitting", "standing", "laying"))
# ------------------------------------------------------------------- #
features<-read.table(file = "./UCI HAR Dataset/features.txt")
trainset <- data.table(read.table("./UCI HAR Dataset/train/X_train.txt"))
testset<- data.table(read.table(file = "./UCI HAR Dataset/test/X_test.txt"))
allset <- data.table(rbind(trainset,testset))
#featuressubset<-grep("mean()|std()", features[,2])
#featuressubset<-grep("mean()|std()|Mean", features[,2])
featuressubset<-grep("mean[^Freq]()|std()", features[,2])
meanandstdsubset<-allset[,..featuressubset]
subfea<-features[featuressubset,]
namesvec <- as.character(subfea$V2)
meanandstdsubset <- setnames(meanandstdsubset, namesvec)
#Creating a grouped tidydataset : step4:
# data set is already labeled with descriptive variable names...
# ... because labels added during each step.
tidydataset<-cbind(allsubjects, allactivities, meanandstdsubset)
#From the data set in step 4, creates a second, independent tidy data set
# with the average of each variable for each activity and each subject.
#tidyarr<-arrange(tidydataset, activitycode, subjid)
#newtidydata<-melt(tidydataset, id.vars = c("subjid", "activitycode"))
#aqw <-dcast(newtidydata, formula = subjid +activitycode ~variable)
# ds<-group_by(tidydataset, activitycode,subjid)
# ds.avg<-summarise_all(ds,funs(mean))
tidydatasetG<-group_by(tidydataset, activitycode,subjid)
tidydatasetG<-summarise_all(tidydatasetG, funs(mean))
write.table(tidydatasetG, "tidydatasetG.txt", row.names = FALSE)
| 1274ca757c316d5c3256fa473169fa7cdd929ea4 | [
"Markdown",
"R"
] | 3 | Markdown | chatard/Coursera-Data-Cleaning-Project | 04c38aadfaa17a6a6a48437150aff7c2eb0de64d | 1ae3098b47304ff9807dba52a692a68b2ed955ea | |
refs/heads/master | <repo_name>AnkeTarou/Anke<file_sep>/webserver/routes/follow.js
const dbo = require('../lib/mongo');
exports.get = function(req,res){
res.render("follow",{result:"ssss"});
}
<file_sep>/webserver/views/js/lib.js
var key = {
index:0,
topId:"1",
bottomId:"-1",
type:"search"
};
var conFlg = false;
var newResultBox = [];
function connect(uri,data,callback,error){
const req = new XMLHttpRequest();
//レスポンスが返ってきたときの処理
req.addEventListener("load",function(e) {
console.log("connect");
console.log("uri",uri);
console.log("送信データ",data);
console.log("受信データ",req.response);
callback(req.response);
})
req.addEventListener("error",function(e){
console.error(uri+"でエラーが起きています。");
console.error("入力値:",data);
if(error){
error()
}
})
req.responseType = "json"
req.open('POST', uri);
req.setRequestHeader("Content-type", "application/JSON");
req.send(JSON.stringify(data));
}
function updateResult(response){
const res = response.result;
const result = document.getElementById("result");
for(let index in res){
const card = createCard(res[index]);
result.appendChild(card);
createBody(res,res[index]);
}
setActionVote();
key.index += res.length;
key.bottomId = res[res.length-1]._id;
document.getElementById("loader").setAttribute('style','display:none;');
conFlg = response.conFlg;
console.log("done");
}
function addNewResult(){
const result = document.getElementById("result");
for(let index in newResultBox){
const child = document.getElementById(key.topId + "-card");
const card = createCard(newResultBox[index]);
result.insertBefore(card,child);
key.topId = newResultBox[index]._id;
createBody(newResultBox,newResultBox[index]);
}
setActionVote();
newResultBox.length = 0;
this.setAttribute("style","display: none;");
document.getElementById("noResulttext").setAttribute("style","display: none;");
}
function createCard(data){
const card = document.createElement("div");
card.setAttribute("id",data._id + "-card");
card.setAttribute("class","card");
card.setAttribute("style","margin-top:10px;");
card.setAttribute("key",data._id);
const header = document.createElement("div");
header.setAttribute("class","card-header");
header.setAttribute("role","tab");
header.setAttribute("style","background-color:white");
const img = document.createElement("img");
img.setAttribute("class","rounded-circle");
img.setAttribute("height","46");
img.setAttribute("src","/image/" + data.inventory.img);
img.setAttribute("align","top");
img.setAttribute("onerror","this.src='/image/default.jpg'")
header.appendChild(img);
const label1 = document.createElement("label");
label1.setAttribute("class","ml-3");
label1.innerHTML = data.inventory.nickname;
header.appendChild(label1);
const label2 = document.createElement("label");
label2.setAttribute("class","text-center text-muted");
label2.innerHTML = "@" + data.senderId;
header.appendChild(label2);
const a = document.createElement("a");
a.setAttribute("class","text-body ml-5");
a.setAttribute("data-toggle","collapse");
a.setAttribute("href","#" + data._id);
a.setAttribute("role","button");
a.setAttribute("aria-expanded","false");
a.setAttribute("aria-controls","collapseOne");
a.innerHTML = data.query;
header.appendChild(a);
const form = document.createElement("div");
form.setAttribute("class","form-check-inline");
header.appendChild(form);
const i = document.createElement("i");
i.setAttribute("class","far fa-chart-bar");
i.innerHTML = data.total;
form.appendChild(i);
const label3 = document.createElement("label");
label3.setAttribute("for",data._id + "-favorite");
const inp = document.createElement("input");
inp.setAttribute("id",data._id + "-favorite");
inp.setAttribute("type","checkbox");
inp.setAttribute("style","display:none;");
inp.setAttribute("onclick","favorite(this)");
if(data.myfavorite){
inp.setAttribute("checked","true");
}
label3.appendChild(inp);
const i2 = document.createElement("i");
i2.setAttribute("id",data._id + "-star");
if(data.myfavorite){
i2.setAttribute("class","fas fa-star");
i2.setAttribute("style","color:yellow");
}else {
i2.setAttribute("class","far fa-star");
i2.setAttribute("style","color:#000000");
}
i2.textContent = " " + data.favorite;
label3.appendChild(i2);
form.appendChild(label3);
const body = document.createElement("div");
body.setAttribute("id",data._id);
body.setAttribute("class","card-body collapse");
body.setAttribute("role","tabpanel");
body.setAttribute("aria-labelledby","headingOne");
body.setAttribute("data-parent","#accordion");
card.appendChild(header);
card.appendChild(body);
return card;
}
function createBody(array,data){
const contents = document.getElementById(data._id);
if(data.result){
const canvas = document.createElement("canvas");
contents.appendChild(canvas);
graph(canvas,data)
}else{
contents.innerHTML =
`<table class="table">
<tbody>`
+
function(){
let answers = "";
for(let j in data.answers){
answers +=
`<tr>
<td>
<div class="custom-control custom-` + data.type + `">
<input id=` + data._id + "-" + j + ` name=` + data._id + "-answer" + ` type=` + data.type + ` class="custom-control-input">
<label class="custom-control-label" for=` + data._id + "-" + j +`>` + data.answers[j].answer + `</label>
</div>
</td>
</tr>`
}
return answers;
}()
+
`</tbody>
</table>
<button type="button" class="btn btn-primary" name="vote">投票</button>
<div class="border-top"></div>`;
}
}
function setActionVote(){
const btns = document.getElementsByName("vote");
for(let btn of btns){
btn.addEventListener("click",function(e){
const card = e.target.parentElement;
const answers = document.getElementsByName(card.id + "-answer");
const obj = {
id:card.id,
index:[]
}
for(let answer of answers){
if(answer.checked){
obj.index.push(1);
}else{
obj.index.push(0);
}
}
connect("/vote/",obj,function(newResultBox){
card.innerHTML = "";
const canvas = document.createElement("canvas");
card.appendChild(canvas);
graph(canvas,newResultBox);
})
})
}
}
function graph(canvas,array){
canvas.parentNode.style.height= array.answers.length*60+"px";
const chartData = function(data){
const obj = {
labels:[],
datasets:[
{
label:"なし",
data:[],
backgroundColor: function(context) {
console.log(this)
var index = context.dataIndex;
var value = context.dataset.data[index];
return value < 0 ? 'red' : // 赤で負値を描画する
index % 2 ? 'blue' : // 負値でなければ、青と緑の交互
'green';
}
}
]
};
for(let d of data.answers){
obj.labels.push(d.answer);
obj.datasets[0].data.push(d.total);
}
return obj;
}(array)
new Chart(canvas, {
// 作成するグラフの種類
type: 'horizontalBar',
// ラベルとデータセットを設定
data: chartData,
//オプション設定
options: {
legend: {
display: false, //詳細ラベルの表示
},
scales: { //軸設定
yAxes: [{ //表示設定
stacked: false,
//y軸の数字
ticks: {
min: 0, //最小値
fontSize: 18, //フォントサイズ
stepSize: 10, //軸間隔
fontColor: "#00f"
},
//y軸グリッド
gridLines:{ //グリッド設定
display:true,
color:"rgba(0,0,0,0.8)"
}
}],
xAxes: [{ //x軸設定
stacked: false,
categoryPercentage: 0.4, //棒グラフ幅
scaleLabel: { //軸ラベル設定
display: true, //表示設定
fontSize: 18 //フォントサイズ
},
//x軸の数字
ticks: {
fontSize: 16, //フォントサイズ
fontColor:"#fff",
tickMarkLength:1
},
//x軸グリッド
gridLines:{ //グリッド設定
display:true,
color:"rgba(0,0,0,0.3)",
}
}],
},
animation:{
duration:1500, //アニメーションにかける時間
easing:"easeInQuad"
},
tooltips:{
mode:'label' //マウスオーバー時に表示されるtooltip
}
}
});
}
function favorite(btn){
const req = new XMLHttpRequest();
const data = {
targetId:btn.parentElement.parentElement.parentElement.parentElement.getAttribute("key"),
favorite:btn.checked ? 1 : 0
}
//レスポンスが返ってきたときの処理
req.addEventListener("load",function(e) {
console.log(req.response)
if(req.response.status == "success"){
document.getElementById(data.targetId + "-favorite").parentElement.lastElementChild.textContent = req.response.favorite;
if(data.favorite === 1){
document.getElementById(data.targetId + "-favorite").parentElement.lastElementChild.setAttribute("style","color:red;");
}else {
document.getElementById(data.targetId + "-favorite").parentElement.lastElementChild.setAttribute("style","color:#000000;");
}
}
})
req.responseType = "json"
req.open('POST', 'favorite');
req.setRequestHeader("Content-type", "application/JSON");
req.send(JSON.stringify(data));
}
<file_sep>/webserver/app.js
const express = require('express');
const cookieParser = require('cookie-parser')
const app = express();
const bodyParser = require('body-parser');
const indexRouter = require('./routes/index');
// 外部から参照できるファイルを指定
app.use(express.static('views'));
// clientから送られてくるデータを扱いやすい形式に変換
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ type: 'application/json' }));
// cookieの設定
app.use(cookieParser());
// アクセスしてきたときに最初に実行される処理
app.use(function(req,res,next){
console.log("url\n"+req.url);
console.log("セッション\n"+JSON.stringify(req.session));
console.log("cookie\n"+JSON.stringify(req.cookies));
console.log("受信データ\n");
console.log("POST\n",JSON.stringify(req.body));
console.log("GET\n",JSON.stringify(req.query));
next();
});
app.set('view engine', 'ejs');
app.use(indexRouter);
app.listen(80);
<file_sep>/webserver/routes/index.js
var express = require('express');
var router = express.Router();
const dbo = require('../lib/mongo');
const post = require('./post');
const search = require('./search');
const vote = require('./vote');
const favorite = require('./favorite');
const home = require('./home');
const login = require('./login');
const follow = require('./follow');
const insertuser = require('./insertuser');
router.get('/',function(req,res,next){
console.log("get",req.cookies._id && req.cookies.sessionkey);
if(req.cookies._id && req.cookies.sessionkey){
next();
}else{
if(req.url == '/login' || req.url == '/search'){
next();
}else {
res.redirect('/login');
}
}
});
router.post('/',function(req,res,next){
console.log("post",req.cookies._id && req.cookies.sessionkey);
if(req.cookies._id && req.cookies.sessionkey){
next();
}else{
if(req.url == '/login' || req.url == '/search' || req.url == '/home'){
next();
}else {
res.json('err');
}
}
})
router.get('/',home.get);
router.post('/home',home.post);
router.get('/search',search.get);
router.post('/search',search.post);
router.post('/post',post.post);
router.post('/vote',vote.post);
router.post('/favorite',favorite.post);
router.get('/follow',follow.get);
router.post('/insertuser',insertuser.post);
router.get('/usercreate',function(req,res){
res.render('usercreate');
});
router.get('/login',function(req,res){
if(req.cookies._id && req.cookies.sessionkey){
res.redirect('/');
}else {
res.render('login');
}
});
router.post('/login',login.post);
module.exports = router;
<file_sep>/webserver/routes/home.js
const dbo = require('../lib/mongo');
exports.get = function(req,res){
let user = req.cookies;
const key = [
{$lookup:{
from:"question",
let:{userId:"$_id"},
pipeline:[{$match:{$expr:{$eq:["$$userId","$senderId"]}}}],
as:"inventory"
}},
{$match:{_id:user._id}},
{$project:{
followcount:{$size:"$follow"},
followercount:{$size:"$follower"},
favoritecount:{$size:"$favorite"},
nickname:1,
img:1,
postcount:{$size:"$inventory"}
}}
]
dbo.aggregate("user",key)
.then(function(result){
console.log(result);
res.render("home",result[0]);
})
.catch(function(err){
console.log(err);
res.render("home");
})
}
exports.post = function(req,res){
let user = req.cookies;
if(!user){
user = {
_id:"",
sessionkey:""
};
}
const userKey = [
{$match:{_id:user._id}},
{$project:{
follow:1
}}
]
dbo.userCheck(user)
.then(function(usercheck){
if(usercheck){
return true;
}else{
return false;
}
})
.then(function(usercheck) {
if(usercheck){
// ユーザー認証成功
//検索して結果を返す
const index = indexCheck(req.body.index,req.body.type);
const questionKey = [
{$lookup:{
from:"user",
let:{senderId:"$senderId",follow:"$follow"},
pipeline:[{$match:{$expr:{$eq:["$$senderId","$_id"]}}}],
as:"inventory"
}},
{$match:{$or:[{"senderId":{$in:["testuser"]}}]}},
{$unwind:"$answers"},
{$unwind:"$inventory"},
{$group:{
_id:"$_id",
senderId:{$first:"$senderId"},
query:{$first:"$query"},
type:{$first:"$type"},
answers:{$push:{
answer:"$answers.answer",
total:{$size:"$answers.voter"}
}},
answer:{$push:{
answer:"$answers.answer"
}},
voters:{$first:"$voters"},
comment:{$first:{$size:"$comment"}},
favorite:{$first:"$favorite"},
date:{$first:"$date"},
inventory:{$first:"$inventory"}
}},
{$project:{
_id:1,
senderId:1,
query:1,
type:1,
total:{$size:"$voters"},
comment:1,
favorite:{$size:"$favorite"},
date:1,
"inventory.nickname":1,
"inventory.img":1,
"inventory.follow":1,
answers:{
$cond:{
if:{$in:[user._id,"$voters"]},
then:"$answers",
else:"$answer"
}
},
result:{
$cond:{
if:{$in:[user._id,"$voters"]},
then:true,
else:false
}
},
myfavorite:{
$cond:{
if:{$in:[user._id,"$favorite"]},
then:true,
else:false
}
}
}},
{$sort:{date:-1}},
{$skip:index},
{$limit:15}
];
dbo.aggregate("question",questionKey)
.then(function(result1){
/**** 検索結果を整形する ****/
let conFlg = (result1.length == 15); //次の該当項目が存在するかどうか
let size = result1.length;
for(let i = 0; i < size; i++){
// どのリクエストからか判別
if(req.body.type == "new"){
if(result1[i]._id == req.body.topId){
result1.splice(i, size-i);
break;
}
}else{
if(result1[i]._id == req.body.bottomId){
result1.splice(0, i+1);
i = 0;
size = result1.length;
}
}
}
// レスポンスオブジェクトの生成
const response = {
result:result1,
conFlg:conFlg
}
res.json(response);
})
.catch(function(err){
console.log(err);
res.json(null)
})
}else{
// ユーザー認証失敗ならnullを返す
res.json(null);
}
})
.catch(function(error) {
console.log(error);
res.json(null);
});
}
/**
*indexの形式をチェック
*引数param
*@ <int> req.body.index
*@ <String> req.body.type
*return <int> 0 or index
**/
function indexCheck(num,type){
let index;
if(Number.isNaN(index = parseInt(num)) || type == "new"){
return 0;
}
return index;
}
<file_sep>/webserver/ip.js
module.exports = {
ip:"192.168.5.5"
//ip:"127.0.0.1"
}
<file_sep>/webserver/routes/search.js
const dbo = require('../lib/mongo');
// 検索処理 1単語のみの検索に対応
exports.get = function(req,res){
let obj = {
sort:sortCheck(req.query.sort),
order:orderCheck(req.query.order),
text:textCheck(req.query.text),
page:"search",
index:0
}
res.render("search",obj);
}
exports.post = function(req,res){
// 検索キーを生成
let keyObj = createKeyObj(
sortCheck(req.body.sort),
orderCheck(req.body.order),
textCheck(req.body.text),
indexCheck(req.body.index,req.body.type),
pageCheck(req.body.page),
req.cookies
);
console.log(req.cookies);
// 未ログインなら検索結果だけを返す
if(!(req.cookies._id && req.cookies.sessionkey)){
dbo.aggregate("question",keyObj)
.then(function(result){
/**** 検索結果を整形する ****/
let conFlg = (result.length == 15); //次の該当項目が存在するかどうか
let size = result.length;
for(let i = 0; i < size; i++){
// どのリクエストからか判別
if(req.body.type == "new"){
if(result[i]._id == req.body.topId){
result.splice(i, size-i);
break;
}
}else{
if(result[i]._id == req.body.bottomId){
result.splice(0, i+1);
i = 0;
size = result.length;
}
}
}
// レスポンスオブジェクトの生成
const response = {
result:result,
conFlg:conFlg
}
res.json(response);
});
}
// ログイン状態かチェック
if(req.cookies._id && req.cookies.sessionkey){
dbo.userCheck(req.cookies)
.then(function(usercheck){
if(usercheck){
return true;
}else{
return false;
}
})
.then(function(usercheck) {
if(usercheck){
// ユーザー認証成功
//検索して結果を返す
dbo.aggregate("question",keyObj)
.then(function(result){
/**** 検索結果を整形する ****/
let conFlg = (result.length == 15); //次の該当項目が存在するかどうか
let size = result.length;
for(let i = 0; i < size; i++){
// どのリクエストからか判別
if(req.body.type == "new"){
if(result[i]._id == req.body.topId){
result.splice(i, size-i);
break;
}
}else{
if(result[i]._id == req.body.bottomId){
result.splice(0, i+1);
i = 0;
size = result.length;
}
}
}
// レスポンスオブジェクトの生成
const response = {
result:result,
conFlg:conFlg
}
res.json(response);
})
.catch(function(error) {
console.log("question aggregateエラー",error);
});
}else{
// ユーザー認証失敗ならnullを返す
console.log("user認証失敗",user);
res.json(null);
}
})
.catch(function(error) {
console.log("userCheckエラー",error);
});
}
};
/**
*textの形式をチェック
*引数param
*@ <String> req.body.text
*return <String> text
**/
function textCheck(text){
if(text == undefined){
text = "";
}
return text.toString();
}
/**
*sortの形式をチェック
*引数param
*@ <String> req.body.sort
*return <String> total or sort
**/
function sortCheck(sort) {
let check = (sort == "total") || (sort == "good") || (sort == "date");
if(!check){
return "date";
}else{
return sort;
}
}
/**
*orderの形式をチェック
*引数param
*@ <int> req.body.order
*return <int> -1 or order
**/
function orderCheck(order) {
let check = (order == 1) || (order == -1);
if(!check){
return -1;
}else{
return parseInt(order);
}
}
/**
*indexの形式をチェック
*引数param
*@ <int> req.body.index
*@ <String> req.body.type
*return <int> 0 or index
**/
function indexCheck(num,type){
let index;
if(Number.isNaN(index = parseInt(num)) || type == "new"){
return 0;
}
return index;
}
/**
*pageの形式をチェック
*引数param
*@ <String> req.body.page
*return <String> "search" or page
**/
function pageCheck(page){
if(page == "user" || page == "home"){
return page.toString();
}else{
return "search";
}
}
/**
*検索キーを生成して返す
*引数param
*@ <String> req.body.sort
*@ <int> req.body.order
*@ <String> req.body.text
*@ <int> req.body.index
*@ <object> req.cookies.user
*return <object> keyObj
**/
function createKeyObj(sort,order,text,index,page,user) {
if(!user){
user = {_id:""};
}
const key = {$regex:".*"+text+".*"};
let keyObj = [];
keyObj[0] = {$lookup:{
from:"user",
let:{senderId:"$senderId"},
pipeline:[{$match:{$expr:{$eq:["$$senderId","$_id"]}}}],
as:"inventory"
}};
if(page == "user"){
keyObj[1] = {$match:{"senderId":user._id}};
}else{
keyObj[1] = {$match:{$or:[{query:key},{"answers.answer":key},{"senderId":key}]}};
}
keyObj[2] = {$unwind:"$answers"};
keyObj[3] = {$unwind:"$inventory"};
keyObj[4] = {$group:{
_id:"$_id",
senderId:{$first:"$senderId"},
query:{$first:"$query"},
type:{$first:"$type"},
answers:{$push:{
answer:"$answers.answer",
total:{$size:"$answers.voter"}
}},
answer:{$push:{
answer:"$answers.answer"
}},
voters:{$first:"$voters"},
comment:{$first:{$size:"$comment"}},
favorite:{$first:"$favorite"},
date:{$first:"$date"},
inventory:{$first:"$inventory"}
}};
keyObj[5] = {$project:{
_id:1,
senderId:1,
query:1,
type:1,
total:{$size:"$voters"},
comment:1,
favorite:{$size:"$favorite"},
date:1,
"inventory.nickname":1,
"inventory.img":1,
answers:{
$cond:{
if:{$in:[user._id,"$voters"]},
then:"$answers",
else:"$answer"
}
},
result:{
$cond:{
if:{$in:[user._id,"$voters"]},
then:true,
else:false
}
},
myfavorite:{
$cond:{
if:{$in:[user._id,"$favorite"]},
then:true,
else:false
}
}
}};
if(sort == "date"){
keyObj[6] = {$sort:{date:order}};
}else if(sort == "favorite"){
keyObj[6] = {$sort:{favorite:order}};
}else if(sort == "total"){
keyObj[6] = {$sort:{total:order}};
}
keyObj[7] = {$skip:index};
keyObj[8] = {$limit:15};
return keyObj;
}
<file_sep>/webserver/lib/mongo.js
const Mongo = require('mongodb')
const MongoClient = Mongo.MongoClient;
const ip = require("../ip.js");
const url = 'mongodb://'+ ip.ip +':27017';
//callback関数に検索結果を適用する。
exports.aggregate = function(collectionName,key){
return new Promise(function(resolve,reject){
MongoClient.connect(url,{ useNewUrlParser:true },function(error, database) {
if (error) reject(error);
const dbo = database.db("Data");
dbo.collection(collectionName).aggregate(key).toArray(function(err, result) {
if (err) reject(err);
database.close();
resolve(result)
});
});
})
.catch(function(err){
console.log(err);
})
};
//ObjectをDBに挿入する
exports.insert = function(collection,key){
return new Promise(function(resolve,reject){
MongoClient.connect(url,{ useNewUrlParser:true },function(error, database) {
if (error) reject(error);
const dbo = database.db("Data");
dbo.collection(collection).insertOne(key, function(err, result) {
if (err) reject(err);
database.close();
resolve(result);
});
});
})
.catch(function(err){
console.log(err);
})
}
// userの認証
exports.userCheck = function(checkuser){
if(!(checkuser._id && checkuser.sessionkey)){
checkuser._id = "";
checkuser.sessionkey = "";
}
return new Promise(function(resolve,reject){
MongoClient.connect(url,{ useNewUrlParser:true },function(error, database) {
if(error) reject(error);
const dbo = database.db("Data");
const key = [{$match:{_id:checkuser._id,sessionkey:checkuser.sessionkey}}];
dbo.collection("user").aggregate(key).toArray(function(err, result) {
if (err) reject(err);
database.close();
if(result[0]){
resolve(result[0])
}else {
resolve(null);
}
});
});
})
.catch(function(err){
console.log(err);
})
}
exports.ObjectID = Mongo.ObjectID;
exports.favorite = function (userId,targetId,favorite){
let database = null;
let Data = null;
let targetObjId = Mongo.ObjectID(targetId);
return new Promise((resolve,reject)=>{
open().then((db)=>{
database = db;
return db.db("Data");
})
.then((data)=>{
Data = data;
if(favorite === 1){
return Promise.all([
Data.collection("question").updateOne({_id:targetObjId},{$addToSet:{"favorite":userId}}),
Data.collection("user").updateOne({_id:userId},{$addToSet:{"favorite":targetId}})
])
}else if(favorite === 0){
return Promise.all([
Data.collection("question").updateOne({_id:targetObjId},{$pull:{"favorite":userId}}),
Data.collection("user").updateOne({_id:userId},{$pull:{"favorite":targetId}})
])
}
})
.then(()=>{
const matchKey = [
{$match:{_id:targetObjId}},
{$project:{favorite:{$size:"$favorite"}}}
];
Data.collection("question").aggregate(matchKey).toArray((err,result)=>{
if(err) reject(err);
database.close()
resolve({status:"success",favorite:result[0].favorite})
})
})
.catch((err)=>{
if(database) database.close();
reject(err);
})
});
}
//投票
exports.vote = function (user,id,index){
let database = null;
let userCollection = null;
const key = {_id:Mongo.ObjectID(id)};
return new Promise((resolve,reject) => {
open().then((db)=>{
database = db;
return db.db("Data").collection('question')
})
.then((users)=>{
userCollection = users;
let prom = Promise.resolve();
for(let i = 0; i<index.length; i++){
if(index[i] == "1"){
let update = {$addToSet:{[`answers.${i}.voter`]: user._id}};
prom = prom.then(()=>{
return userCollection.updateOne(key,update);
})
}
}
return prom
})
.then(()=>{
return userCollection.update(key,{$addToSet:{voters:user._id}})
})
.then(()=>{
const matchKey = [
{$match:key},
{$unwind:"$answers"},
{$group:{
_id:"$_id",
answers:{$push:{
answer:"$answers.answer",
"total":{$size:"$answers.voter"}
}},
total:{$first:{$size:"$voters"}},
comment:{$first:{$size:"$comment"}},
favorite:{$first:{$size:"$favorite"}},
}}
]
userCollection.aggregate(matchKey).toArray((err,result)=>{
if(err) reject(err);
database.close()
resolve(result[0]);
});
})
.catch((err)=>{
if(database) database.close();
reject(err);
})
})
}
function open(){
return new Promise((resolve, reject)=>{
MongoClient.connect(url,{ useNewUrlParser: true },(err, db) => {
if (err) {
reject(err);
} else {
resolve(db);
}
});
});
}
// sessionkey挿入
exports.session = function(id,sessionkey){
return new Promise(function(resolve,reject){
MongoClient.connect(url,{ useNewUrlParser:true },function(error, database) {
if (error) throw error;
const dbo = database.db("Data");
dbo.collection("user").update({_id:id},{$set:{'sessionkey':sessionkey}},
function(err, res) {
if (err) throw err;
resolve();
});
});
})
.catch(function(err){
console.log(err);
})
}
| 7a19d14ec9feff73c6f4522515f6268418cac5d2 | [
"JavaScript"
] | 8 | JavaScript | AnkeTarou/Anke | 1e969a20628471f403d81be858d7601bf46cf55d | 15f3bfbaa95ad29e330d0525ef9ce5dc7daf80d1 | |
refs/heads/master | <file_sep>import Bricks from 'bricks.js'
const instance = Bricks({
container: '.categories-container',
packed: 'packed',
sizes: [
{ columns: 1, gutter: 20 },
{ mq: '940px', columns: 2, gutter: 20 }
]
})
setTimeout(() => {
instance.resize(true)
setTimeout(() => {
instance.pack()
}, 1)
}, 1)
<file_sep>require('dotenv').config()
const fs = require('fs')
const indentString = require('indent-string')
const R = require('ramda')
const inquirer = require('inquirer')
const YAML = require('yamljs')
const validUrl = require('valid-url')
const Twitter = require('twitter')
const client = new Twitter({
consumer_key: process.env.TWITTER_CONSUMER_KEY,
consumer_secret: process.env.TWITTER_CONSUMER_SECRET,
access_token_key: process.env.TWITTER_ACCESS_TOKEN_KEY,
access_token_secret: process.env.TWITTER_ACCESS_TOKEN_SECRET
})
const postTweet = status => {
client.post('statuses/update', {status}, function (error, tweet, response) {
if (error) {
console.log(error)
throw error
}
const tweetLink = `https://twitter.com/${tweet.user.screen_name}/status/${tweet.id_str}`
console.log('tweeted:', tweetLink)
})
}
const categories = YAML.load('./data/categories.yml')
const links = YAML.load('./data/links.yml')
const categoryIds = Object.keys(categories)
const categoryChoices = R.values(categories).map((o, i) => ({
// inquirer needs 'value' key
value: categoryIds[i], name: o.name
}))
const validateLink = link => {
if (!validUrl.isUri(link)) {
return 'valid URL please'
}
if (R.find(v => v.link === link, links)) {
return 'this link is already there'
}
return true
}
inquirer.prompt([
{
type: 'input',
name: 'link',
message: 'link',
validate: validateLink
},
{
type: 'input',
name: 'title',
message: 'title',
validate: str => !!str || 'provide a title'
},
{
type: 'input',
name: 'text',
message: 'text',
validate: str => !!str || 'provide a text'
},
{
type: 'list',
name: 'category',
message: 'category',
choices: categoryChoices
},
{
type: 'confirm',
name: 'tweet',
message: 'tweet it'
},
{
type: 'confirm',
name: 'confirm',
message: 'looks legit?'
}
]).then(function (answers) {
if (!answers.confirm) {
console.log('¯\\_(ツ)_/¯')
return
}
const link = R.omit(['confirm', 'tweet'], answers)
const yamlString = `${indentString('-\n', 2)}${indentString(YAML.stringify(link, 4, 2), 4)}`
fs.appendFile('./data/links.yml', yamlString, function (err) {
if (err) throw err
console.log('file saved 👏')
})
if (answers.tweet) {
const tweet = `Here's a nice website: ${link.title} ${link.link}`
postTweet(tweet)
}
})
<file_sep># personal links collection

[links.adamboro.com](https://links.adamboro.com)
<file_sep>###
# Page options, layouts, aliases and proxies
###
# Per-page layout changes:
#
# With no layout
page '/*.xml', layout: false
page '/*.json', layout: false
page '/*.txt', layout: false
# General configuration
# Reload the browser automatically whenever files change
configure :development do
# activate :livereload, apply_css_live: false, apply_js_live: false
end
# tell Middleman to create a folder for each .html file and place the built template file as the index of that folder
activate :directory_indexes
activate :autoprefixer
activate :external_pipeline,
name: :webpack,
command: build? ? './node_modules/webpack/bin/webpack.js -p' : './node_modules/webpack/bin/webpack.js --watch -d',
source: ".tmp/dist",
latency: 1
ignore 'javascripts/*'
###
# Helpers
###
# Build-specific configuration
configure :build do
# Use relative URLs
activate :relative_assets
activate :asset_hash
# Minify CSS on build
activate :minify_css
end
| 3371ca9c3de4d4554638c9045b6cf08f0ab09100 | [
"JavaScript",
"Ruby",
"Markdown"
] | 4 | JavaScript | adekbadek/links | 7dead01f4f79f156d89ad1496096ad3755e755d1 | 55a11572e2f66830357a963530fa12871bb96182 | |
refs/heads/master | <file_sep>import pexpect
import os
class Player:
def __init__(self, playIndex=None):
"""
When the Player is instantiated, spawn pianobar in the terminal.
If music defaults to playing, pause it and go to the station select screen.
"""
print 'Launching Pandora player...'
# First things first, clear that pesky state config file.
# Otherwise, pianobar will default to playing a station,
# which just throws everything off.
if os.path.isfile(os.path.expanduser("~" + "/.config/pianobar/state")):
os.system("rm ~/.config/pianobar/state")
# Spawn the Pianobar
self.child = pexpect.spawn('pianobar')
# Now that we're waiting for a station, get the station dict.
self.updateStations()
# Initialize a few variables up in this bitch.
self.waitingForStation = True
self.currentStationIndex = -1 # By convention, this means no station yet.
self.playing = False
self.track = None
self.artist = None
self.album = None
def playByIndex(self, index):
"""
Takes in the index of a station to be played, and plays that station.
"""
# Make sure the index supplied is valid.
if not self.validateIndex(index):
print "ERROR: Please supply a valid station number!"
return
# Make sure we're waiting for station.
if not self.waitingForStation:
self.child.send('s') # Change station
self.waitingForStation = True
else:
pass
self.child.sendline(str(index))
self.playing = True
self.waitingForStation = False
self.currentStationIndex = int(index)
self.currentStationName = self.indexDict[str(self.currentStationIndex)]
print "Now playing station: " + self.indexDict[str(index)]
def thumbsUp(self):
"""
Thumbs up the current track.
"""
self.updateTrack()
print "Liked track " + self.track
if self.waitingForStation:
self.child.send('\n')
self.waitingForStation = False
self.child.send('+')
def thumbsDown(self):
"""
Thumbs down the current track.
"""
self.updateTrack()
print "Disliked track " + self.track
if self.waitingForStation:
self.child.send('\n')
self.waitingForStation = False
self.child.send('-')
def next(self):
"""
Skips to the next track.
"""
print 'Skipping track...'
if self.waitingForStation:
self.child.send('\n')
self.waitingForStation = False
self.child.send('n')
def updateTrack(self):
"""
Figures out the name of the track currently playing.
"""
# Flip through all the tracks that have happened since last time.
newTrackFlag = False
while True:
i = self.child.expect(['\|>', pexpect.TIMEOUT], timeout=1)
if i == 0: # Found another track
newTrackFlag = True
elif i == 1: # That was the last one!
break
# If this track is different from the old one,
if newTrackFlag:
# Get ["'Track', 'Name'", 'by', '"Artist', 'Name"', 'on', '"Album', 'Name"', '<3'?, '\xlb[2K']
self.child.expect('#')
wordList = self.child.before.split()
hitByOn = 0
trackList = []
artistList = []
albumList = []
# parse that shit
for i in range(len(wordList)):
# if we're at the track
if hitByOn == 0:
# if we're changing to the artist (happens at "by")
if i != 0 and (wordList[i-1][-1] == '"' and wordList[i] == 'by' and wordList[i+1][0] == '"'):
hitByOn = 1
continue
else:
trackList.append(wordList[i])
# if we're at the artist
elif hitByOn == 1:
# if we're changing to the album (happens at "on")
if wordList[i-1][-1] == '"' and wordList[i] == 'on' and wordList[i+1][0] == '"':
hitByOn = 2
continue
else:
artistList.append(wordList[i])
# if we're at the album
elif hitByOn == 2:
# if we're done
if wordList[i] == '<3' or i == len(wordList)-1:
break
else:
albumList.append(wordList[i])
# Put that thing back where it came from, or so help me
# (So help me! So help me!)
self.track = " ".join(trackList)
self.artist = " ".join(artistList)
self.album = " ".join(albumList)
def quit(self):
"""
Exits gracefully from the Pianobar.
"""
if self.waitingForStation:
self.child.send('\n')
self.child.expect('#')
self.waitingForStation = False
self.currentStationIndex = 0
print "Exiting from Pandora player."
self.child.sendline('q')
def exit(self):
self.quit()
def validateIndex(self, index):
"""
Returns True if index is a viable station index,
and False if not.
"""
return str(index) in self.indexDict.keys()
def updateStations(self):
"""
To be called whenever we reach the Station Select screen.
Creates self.stationDict, which contains all stations:
{stationTitle: stationIndex}
and self.indexDict, which does it backward:
{stationIndex: stationTitle}
"""
self.child.expect('station:')
lines = self.child.before.split('\r\n')
stationList = [line for line in lines if "q" in line or "Q" in line]
stationDict = {}
indexDict = {}
for i in stationList:
name = " ".join(i.split()[3:])
index = i.split()[1][:-1]
stationDict[name] = index
indexDict[index] = name
self.stationDict = stationDict
self.indexDict = indexDict
def __str__(self):
self.updateTrack()
return 'Currently playing ' + self.track + " by " + self.artist + " on " + self.album
# Cool shit to show you that shit works!
if __name == "__main__":
import time
import tts
player = Player()
player.playByIndex(7)
print player
time.sleep(3)
player.playByIndex(3)
time.sleep(5)
player.next()
player.next()
print player
tts.say(player.__str__())
time.sleep(5)
player.quit()<file_sep>SmartRoom
=========
This repo will contain the source code to run on the Raspberry Pi which will control Smartroom in Brooks and Greg's room, 2013-2014.
Note: It might not wind up being called Smartroom. It's a working title.
Dependencies
------------
Pianobar: A Pandora app that runs in the terminal.
sudo apt-get install pianobar
cp /usr/share/doc/pianobar/contrib/config-example ~/.config/pianobar
vim ~/.config/pianobar
Edit the `tls\_fingerprint` to be:
```
2<PASSWORD>DAFA1<PASSWORD>
```
Notes
-----
I did not make the PyTTS speech synthesizer; <NAME> did. Thanks for being a pal, <NAME>!
<file_sep>#!/usr/bin/env python2
# -*- coding: utf-8 -*-
import httplib
import json
import sys
def speech_to_text(audio):
url = "www.google.com"
path = "/speech-api/v1/recognize?xjerr=1&client=chromium&lang=en"
headers = { "Content-type": "audio/x-flac; rate=16000" };
params = {"xjerr": "1", "client": "chromium"}
conn = httplib.HTTPSConnection(url)
conn.request("POST", path, audio, headers)
response = conn.getresponse()
data = response.read()
jsdata = json.loads(data)
return jsdata["hypotheses"][0]["utterance"]
if __name__ == "__main__":
if len(sys.argv) != 2 or "--help" in sys.argv:
print "Usage: stt.py <flac-audio-file>"
sys.exit(-1)
else:
with open(sys.argv[1], "r") as f:
speech = f.read()
text = speech_to_text(speech)
print text<file_sep>import os
from pytts import pytts
def say(string):
pytts().say(string)
def sayNB(string):
pytts().sayNB(string)
if __name__ == "__main__":
say("Look, it works! Even with 'apostrophes' and \"quotes\".")
<file_sep>#!/usr/bin/python
# -*- coding: utf-8 -*-
import sys
import gst
import gobject
from urllib2 import Request, urlopen
class pytts():
def on_finish(self, bus, message): #Callback triggered at the end of the stream
self.player.set_state(gst.STATE_NULL)
self.mainloop.quit()
def __init__(self):
self.player = None
self.mainloop = gobject.MainLoop() #Instantiate the mainloop even if not used
def say(self, text, lang="en",volume=5.0):
music_stream_uri = 'http://translate.google.com/translate_tts?'+'q='+text+'&tl='+lang+"&ie=UTF-8"
self.player = gst.element_factory_make("playbin", "player") #Create the player
self.player.set_property('uri', music_stream_uri) #Provide the source which is a stream
self.player.set_property('volume',volume) #Val from 0.0 to 10 (float)
self.player.set_state(gst.STATE_PLAYING) #Play
bus = self.player.get_bus() #Get the bus (to send catch connect signals..)
bus.add_signal_watch_full(1)
bus.connect("message::eos", self.on_finish) #Connect end of stream to the callback
self.mainloop.run() #Wait an event
def sayNB(self, text, lang="en",volume=5.0): #NB for Non-Blocking
music_stream_uri = 'http://translate.google.com/translate_tts?tl='+lang+'&q=' + text +"&ie=UTF-8"
self.player = gst.element_factory_make("playbin", "player") #Create the player
self.player.set_property('uri', music_stream_uri) #Provide the source which is a stream
self.player.set_property('volume',volume) #Val from 0.0 to 10 (float)
self.player.set_state(gst.STATE_PLAYING) #Play
def download(self, text, lang="en", filename="translate_tts.mp3"):
req = Request(url='http://translate.google.com/translate_tts')
req.add_header('User-Agent', 'My agent !') #Needed otherwise return 403 Forbidden
req.add_data("tl="+lang+"&q="+text+"&ie=UTF-8")
fin = urlopen(req)
mp3 = fin.read()
fout = file(filename, "wb")
fout.write(mp3)
fout.close()
def setVolume(self, val):
self.player.set_property('volume',val) #Val from 0 to 1 (float)
if __name__=="__main__":
input_string = sys.argv
if len(input_string) < 3:
print("Usage: ./tts.py language_code Your text separated with spaces.")
sys.exit(1)
input_string.pop(0) #remove the program name from the argv list
lang = input_string[0] #Take the language which should be now the first args
input_string.pop(0) #Pop it
tts_string = '+'.join(input_string) #convert to url all the rest (with + replacing spaces) Nice but not needed
pytts().say(tts_string, lang)
sys.exit(0) | 2506c0488739cf223b47a491306400cee80c3a1c | [
"Markdown",
"Python"
] | 5 | Python | Gredelston/SmartRoom | c3c3a6eaf437625d9af43b374ec127274e7b37e5 | 65ae4600c77dc4fe70a88dbf9e9fedb518117cfb | |
refs/heads/master | <repo_name>swissfiscal/iat<file_sep>/src/com/salomon/tools/Json2XML.java
package com.salomon.tools;
import java.io.File;
import java.io.FileWriter;
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
import org.dom4j.Document;
import org.dom4j.DocumentHelper;
import org.dom4j.Element;
import org.dom4j.io.OutputFormat;
import org.dom4j.io.XMLWriter;
public class Json2XML {
public static void JsonSchema2XML(JSONObject jsonStr, Element root) {
for (Object key : jsonStr.keySet()) {
Object value = jsonStr.get(key);
String name = key.toString();
String type = "";
Class valueClass = value.getClass();
if (valueClass.equals(String.class)) {
Element stringNode = root.addElement("String");
stringNode.addAttribute("Name", name);
} else if (valueClass.equals(Long.class)
|| valueClass.equals(java.lang.Integer.class)) {
Element stringNode = root.addElement("Long");
stringNode.addAttribute("Name", name);
} else if (valueClass.equals(Boolean.class)) {
Element stringNode = root.addElement("Boolean");
stringNode.addAttribute("Name", name);
} else if (valueClass.equals(JSONObject.class)) {
Element stringNode = root.addElement("JsonObject");
stringNode.addAttribute("Name", name);
JsonSchema2XML(jsonStr.getJSONObject(name), stringNode);
} else if (valueClass.equals(JSONArray.class)) {
JSONArray arrayObj = (JSONArray) value;
if (arrayObj.size() == 0) {
return;
}
if ((arrayObj.get(0).getClass() != JSONObject.class)
&& (arrayObj.get(0).getClass() != JSONArray.class)) {
Element stringNode = root.addElement("Array");
stringNode.addAttribute("Name", name);
} else {
Element stringNode = root.addElement("JsonArray");
stringNode.addAttribute("Name", name);
for (Object jsonO : arrayObj.toArray()) {
if (jsonO.getClass() == JSONObject.class) {
JsonSchema2XML(JSONObject.fromObject(jsonO),
stringNode);
}
}
}
}
}
}
public static boolean Doc2XmlFile(Document document, String filename) {
boolean flag = true;
try {
/* 将document中的内容写入文件中 */
// 默认为UTF-8格式,指定为"GB2312"
OutputFormat format = OutputFormat.createPrettyPrint();
format.setEncoding("GB2312");
XMLWriter writer = new XMLWriter(
new FileWriter(new File(filename)), format);
writer.write(document);
writer.close();
} catch (Exception ex) {
flag = false;
ex.printStackTrace();
}
return flag;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
String jsonStr = "{\"address\":[\"北京\"],\"city\":\"昌平\",\"createTime\":1381823621000,\"cxo\":\"\",\"description\":\"牛逼公司\",\"detail\":\"详情1\",\"id\":1,\"industry\":[{\"levelOneIndustry\":{\"id\":\"01\",\"name\":\"IT/互联网/通信/电子\",\"parentId\":\"-1\"},\"levelTwoIndustry\":{\"id\":\"011\",\"name\":\"互联网\",\"parentId\":\"01\"}}],\"level\":13,\"logoUrl\":\"/4/headpic/131104/19/jL6q1An.jpg\",\"managerId\":0,\"middleLogo\":\"http://hdn.mtimg.net//4/headpic/131104/19/jL6q1An_m.jpg\",\"name\":\"新浪微博\",\"orgRegisterTime\":1358154600000,\"origLogo\":\"http://hdn.mtimg.net//4/headpic/131104/19/jL6q1An_o.jpg\",\"province\":\"北京\",\"resourceId\":1,\"telephone\":[\"112233\"],\"type\":0,\"updateTime\":1383565988000,\"website\":[\"http://www.renren.com\"]}";
JSONObject jsonObj = JSONObject.fromObject(jsonStr);
// System.out.println(" type : " + jsonObj.get("arr").getClass());
Document document = DocumentHelper.createDocument();
Element root = document.addElement("Result");
JsonSchema2XML(jsonObj, root);
System.out.println(document.toString());
Doc2XmlFile(document, "a.xml");
}
}
<file_sep>/README.md
iat
===
Http和Https restful接口的全套自动化接口测试框架(包括一个代码生成器)。
didi.mao
xiaolong.xu
| 87d8de629039252576e0d86dee4e653a5ae1c169 | [
"Markdown",
"Java"
] | 2 | Java | swissfiscal/iat | dc1053e91ba3b789a94d71e3a41502fea767974b | 2e1ecc4337a9060ac3b95cafca1bdbb22033ec63 | |
refs/heads/master | <file_sep><?php
include "AESEncryptDecrypt.php";
// Como usar las funciones para encriptar y desencriptar.
$aes = new AESEncryptDecrypt();
$data = "q";
$en = $aes->encrypt($data);
$des = $aes->decrypt($en);
echo 'Dato encriptado: '. $en . '<br>';
echo 'Dato desencriptado: '. $des . '<br>';
?><file_sep><?php
class AESEncryptDecrypt {
public $password = '<PASSWORD>';
public $method = 'aes-256-cbc';
public $strong = false;
public $iv_length;
public $iv;
/**
* Función que encrypta
*/
public function encrypt($data) {
$this->iv_length = openssl_cipher_iv_length($this->method);
$this->iv = openssl_random_pseudo_bytes($this->iv_length, );
return base64_encode(openssl_encrypt($data, $this->method, $this->password, true, $this->iv));
}
/**
* Función que desencrypta
*/
public function decrypt($data){
$this->iv_length = openssl_cipher_iv_length($this->method);
$this->iv = openssl_random_pseudo_bytes($this->iv_length, $this->strong);
return openssl_decrypt(base64_decode($data), $this->method, $this->password, true, $this->iv);
}
}
?><file_sep><?php
class AESEncrypt {
public $password;
public $method;
public $strong;
public $iv_length;
public $iv;
public __construc(){
this->$password = "<PASSWORD>";
this->$method = 'aes-256-cbc';
this->$strong = false;
this->$iv_length = openssl_cipher_iv_length(this->$method);
this->$iv = openssl_random_pseudo_bytes(this->$iv_length, this->$strong);
}
function encrypt($data) { return base64_encode(openssl_encrypt($data, $method, $password, true, $iv)); }
function decrypt($data){ return openssl_decrypt(base64_decode($enc_data), $method, $password, true, $iv); }
}
?> | a81376330f0a05eb316d405ca9ab741ca209aad3 | [
"PHP"
] | 3 | PHP | CHimaLeo/Demo_AES | a7737554e7cb0ac01953c4f869debccb3f217422 | da1b3507e778bc2dc9437709c4d1f372bbae5c1e | |
refs/heads/master | <file_sep>#include <iostream>
#include "TTree.h"
#include "TClonesArray.h"
#include "VHTauTau/TreeMaker/plugins/GenJetBlock.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
#include "DataFormats/JetReco/interface/GenJetCollection.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
GenJetBlock::GenJetBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("genJetSrc"))
{
}
void GenJetBlock::beginJob() {
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneGenJet = new TClonesArray("vhtm::GenJet");
tree->Branch("GenJet", &cloneGenJet, 32000, 2);
tree->Branch("nGenJet", &fnGenJet, "fnGenJet/I");
}
void GenJetBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneGenJet->Clear();
fnGenJet = 0;
if (!iEvent.isRealData()) {
edm::Handle<reco::GenJetCollection> genJets;
iEvent.getByLabel(_inputTag, genJets);
if (genJets.isValid()) {
edm::LogInfo("GenJetBlock") << "Total # GenJets: " << genJets->size();
for (reco::GenJetCollection::const_iterator it = genJets->begin();
it != genJets->end(); ++it) {
if (fnGenJet == kMaxGenJet) {
edm::LogInfo("GenJetBlock") << "Too many Gen Jets, fnGenJet = " << fnGenJet;
break;
}
genJetB = new ((*cloneGenJet)[fnGenJet++]) vhtm::GenJet();
// fill in all the vectors
genJetB->eta = it->eta();
genJetB->phi = it->phi();
genJetB->p = it->p();
genJetB->pt = it->pt();
double energy = it->energy();
genJetB->energy = energy;
genJetB->emf = (energy>0) ? it->emEnergy()/energy : 0;
genJetB->hadf = (energy>0) ? it->hadEnergy()/energy : 0;
}
}
else {
edm::LogError("GenJetBlock") << "Error >> Failed to get GenJetCollection for label: "
<< _inputTag;
}
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(GenJetBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
triggerBlock = cms.EDAnalyzer("TriggerBlock",
verbosity = cms.int32(1),
l1InputTag = cms.InputTag('gtDigis'),
hltInputTag = cms.InputTag('TriggerResults','','HLT'),
hltPathsOfInterest = cms.vstring ("HLT_DoubleMu",
"HLT_Mu",
"HLT_IsoMu",
"HLT_TripleMu",
"IsoPFTau",
"TrkIsoT",
"HLT_Ele")
)
<file_sep>#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
namespace {
struct dictionary {
vhtm::Event rv1;
vhtm::GenEvent rv2;
vhtm::Electron rv3;
vhtm::GenParticle rv4;
vhtm::GenJet rv5;
vhtm::GenMET rv6;
vhtm::MET rv7;
vhtm::Tau rv8;
vhtm::Muon rva;
vhtm::Jet rvb;
vhtm::Vertex rvd;
vhtm::Track rvf;
vhtm::TriggerObject rvh;
};
}
<file_sep>#ifndef __VHTauTau_TreeMaker_MuonBlock_h
#define __VHTauTau_TreeMaker_MuonBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class Muon;
class MuonBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob(){}
public:
explicit MuonBlock(const edm::ParameterSet& iConfig);
virtual ~MuonBlock();
enum {
kMaxMuon = 100
};
private:
TClonesArray* cloneMuon;
int fnMuon;
int _verbosity;
edm::InputTag _muonInputTag;
edm::InputTag _vtxInputTag;
edm::InputTag _beamSpotInputTag;
bool _beamSpotCorr;
std::string _muonID;
vhtm::Muon* muonB;
};
#endif
<file_sep>void plotres2(){
hMCMatchBa->SetLineColor(1);
//h1PtReco->SetLineColor(4);
//h1PtReco->SetXTitle("GeV/c");
hMCMatchBa->SetYTitle("Events");
hMCMatchBa->GetYaxis()->SetTitleOffset(1.3);
hMCMatchBa->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchBa->GetXaxis()->SetTitleOffset(1.1);
hMCMatchBa->Draw(); //draw hist_2 first as it has a larger range
hMCMatchBa->Draw("c hist same"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_2.eps");
c1->SaveAs("plot_res_2.png");
//h1PtReco->Draw("same");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms");
leg_hist->AddEntry(hMCMatchEC,"Gen Pt","l");
leg_hist->AddEntry(h1PtReco,"Reco Pt","l");
leg_hist->Draw();*/
}
<file_sep>./copyfile.sh > copy.sh
sed -e "s|Pythia6_Tauola_GluGluH_tautau_mumu_14TeV_RECOSIM_2019_private_standardpat//cms/data/store/user/amohapat/||" copy.sh >> copy_1.sh ; mv copy_1.sh copy.sh
chmod +x copy.sh
source copy.sh
<file_sep>#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
#define NEL(x) (sizeof((x))/sizeof((x)[0]))
vhtm::Event::Event() :
run(0),
event(0),
lumis(0),
bunch(0),
orbit(0),
time(-1),
isdata(false),
isPhysDeclared(false),
isBPTX0(false),
isBSCMinBias(false),
isBSCBeamHalo(false),
isPrimaryVertex(false),
isBeamScraping(false)
{
nPU.clear();
bunchCrossing.clear();
trueNInt.clear();
}
vhtm::GenEvent::GenEvent() :
processID(0),
ptHat(-999)
{
pdfWeights.clear();
}
vhtm::Electron::Electron() :
eta(-999),
phi(-999),
pt(-999),
ecalDriven(false),
hasGsfTrack(false),
trackPt(-999),
trackPtError(-999),
energy(-999),
caloEnergy(-999),
caloEnergyError(-999),
charge(-999),
pixHits(-1),
trkHits(-1),
nValidHits(-1),
trkD0(-999),
trkD0Error(-999),
hoe(-999),
hoeDepth1(-999),
eop(-999),
sigmaEtaEta(-999),
sigmaIEtaIEta(-999),
deltaPhiTrkSC(-999),
deltaEtaTrkSC(-999),
classif(-1),
e1x5overe5x5(-999),
e2x5overe5x5(-99),
isoEcal03(-999),
isoHcal03(-999),
isoTrk03(-999),
isoEcal04(-999),
isoHcal04(-999),
isoTrk04(-999),
isoRel03(-999),
isoRel04(-999),
vx(-999),
vy(-999),
vz(-999),
scEn(-999),
scEta(-999),
scPhi(-999),
scET(-999),
scRawEnergy(-999),
vtxDist3D(-999),
vtxIndex(-1),
vtxDistZ(-999),
relIso(-999),
pfRelIso(-999),
chargedHadronIso(-999),
neutralHadronIso(-999),
photonIso(-999),
missingHits(-1),
dB(-999),
edB(-999),
dB3d(-999),
edB3d(-999),
scE1E9(-999),
scS4S1(-999),
sckOutOfTime(-999),
scEcalIso(-999),
scHEEPEcalIso(-999),
scHEEPTrkIso(-999),
nBrems(-1),
fbrem(-999),
dist_vec(-999),
dCotTheta(-99),
hasMatchedConv(false),
mva(-999),
mvaPOGTrig(-999),
mvaPOGNonTrig(-999),
mvaPreselection(false),
isTriggerElectron(false),
isoMVA(-999),
pfRelIso03v1(-999),
pfRelIso03v2(-999),
pfRelIsoDB03v1(-999),
pfRelIsoDB03v2(-999),
pfRelIsoDB03v3(-999),
pfRelIso04v1(-999),
pfRelIso04v2(-999),
pfRelIsoDB04v1(-999),
pfRelIsoDB04v2(-999),
pfRelIsoDB04v3(-999),
pfRelIso03(-999),
pfRelIso04(-999),
pfRelIsoDB03(-999),
pfRelIsoDB04(-999),
selbit(0),
fidFlag(0)
{}
vhtm::GenParticle::GenParticle() :
eta(-999),
phi(-999),
p(-999),
px(-999),
py(-999),
pz(-999),
pt(-999),
energy(-999),
pdgId(-999),
vx(-999),
vy(-999),
vz(-999),
status(-999),
charge(-999),
numDaught(-1),
numMother(-1),
motherIndex(-1)
{
motherIndices.clear();
daughtIndices.clear();
}
vhtm::GenJet::GenJet() :
eta(-999),
phi(-999),
p(-999),
pt(-999),
energy(-999),
emf(-999),
hadf(-999)
{}
vhtm::MET::MET() :
metx(-999),
mety(-999),
met(-999),
metphi(-999),
sumet(-999),
metuncorr(-999),
metphiuncorr(-999),
sumetuncorr(-999)
{}
vhtm::GenMET::GenMET() :
met(-999),
metphi(-999),
sumet(-999)
{}
vhtm::Tau::Tau():
eta(-999),
phi(-999),
pt(-999),
energy(-999),
charge(-999),
mass(-999),
px(-999),
py(-999),
pz(-999),
leadTrkPt(-999),
leadTrkPtError(-999),
leadTrkEta(-999),
leadTrkPhi(-999),
leadTrkCharge(-999),
leadTrkD0(-999),
leadTrkD0Error(-999),
leadTrkDz(-999),
leadTrkDzError(-999),
vtxIndex(-1),
vtxDxy(-999),
vtxDz(-999),
leadChargedParticlePt(-999),
leadNeutralParticlePt(-999),
leadParticlePt(-999),
numChargedHadronsSignalCone(-1),
numNeutralHadronsSignalCone(-1),
numPhotonsSignalCone(-1),
numParticlesSignalCone(-1),
numChargedHadronsIsoCone(-1),
numNeutralHadronsIsoCone(-1),
numPhotonsIsoCone(-1),
numParticlesIsoCone(-1),
ptSumPFChargedHadronsIsoCone(-999),
ptSumPFNeutralHadronsIsoCone(-999),
ptSumPhotonsIsoCone(-999),
decayModeFinding(-1),
decayModeFindingNewDMs(-1),
decayModeFindingOldDMs(-1),
chargedIsoPtSum(-1),
neutralIsoPtSum(-1),
puCorrPtSum(-1),
CombinedIsolationDeltaBetaCorrRaw3Hits(-1),
CombinedIsolationDeltaBetaCorrRaw(-1),
byIsolationMVA3newDMwLTraw(-1),
byIsolationMVA3newDMwoLTraw(-1),
byIsolationMVA3oldDMwLTraw(-1),
byIsolationMVA3oldDMwoLTraw(-1),
byLooseCombinedIsolationDeltaBetaCorr(-1),
byLooseCombinedIsolationDeltaBetaCorr3Hits(-1),
byMediumCombinedIsolationDeltaBetaCorr(-1),
byMediumCombinedIsolationDeltaBetaCorr3Hits(-1),
byTightCombinedIsolationDeltaBetaCorr(-1),
byTightCombinedIsolationDeltaBetaCorr3Hits(-1),
byLooseIsolationMVA3newDMwLT(-1),
byLooseIsolationMVA3newDMwoLT(-1),
byLooseIsolationMva3oldDMwLT(-1),
byLooseIsolationMVA3oldDMwoLT(-1),
byMediumIsolationMVA3newDMwLT(-1),
byMediumIsolationMVA3newDMwoLT(-1),
byMediumIsolationMva3oldDMwLT(-1),
byMediumIsolationMVA3oldDMwoLT(-1),
byTightIsolationMVA3newDMwLT(-1),
byTightIsolationMVA3newDMwoLT(-1),
byTightIsolationMva3oldDMwLT(-1),
byTightIsolationMVA3oldDMwoLT(-1),
// discriminators against electrons/muons
againstMuonLoose(-1),
againstMuonLoose2(-1),
againstMuonLoose3(-1),
againstMuonLooseMVA(-1),
againstMuonTight(-1),
againstMuonTight2(-1),
againstMuonTight3(-1),
againstMuonTightMVA(-1),
againstElectronLoose(-1),
againstElectronMedium(-1),
againstElectronTight(-1),
pfElectronMVA(-1),
// ElectronIDMVA, electron faking tau
againstElectronMVALooseMVA5(-1),
againstElectronMVAMediumMVA5(-1),
againstElectronMVATightMVA5(-1),
byVLooseCombinedIsolationDeltaBetaCorr(-1),
// MVA based isolation
byLooseIsolationMVA(-1),
byMediumIsolationMVA(-1),
byTightIsolationMVA(-1),
jetPt(-999),
jetEta(-999),
jetPhi(-999),
emFraction(-999),
maximumHCALPFClusterEt(-999),
ecalStripSumEOverPLead(-999),
bremsRecoveryEOverPLead(-999),
hcalTotOverPLead(-999),
hcalMaxOverPLead(-999),
hcal3x3OverPLead(-999),
etaetaMoment(-999),
phiphiMoment(-999),
etaphiMoment(-999),
vx(-999), vy(-999), vz(-999),
zvertex(-999), ltsipt(-999),
selbit(0)
{
for (int i = 0; i < kMaxPFChargedCand; ++i) {
sigChHadCandPt[i] = sigChHadCandEta[i] = sigChHadCandPhi[i] =
isoChHadCandPt[i] = isoChHadCandEta[i] = isoChHadCandPhi[i] = -10;
}
for (int i = 0; i < kMaxPFNeutralCand; ++i) {
sigNeHadCandPt[i] = sigNeHadCandEta[i] = sigNeHadCandPhi[i] =
isoNeHadCandPt[i] = isoNeHadCandEta[i] = isoNeHadCandPhi[i] =
sigGammaCandPt[i] = sigGammaCandEta[i] = sigGammaCandPhi[i] =
isoGammaCandPt[i] = isoGammaCandEta[i] = isoGammaCandPhi[i] = -10;
}
}
vhtm::Muon::Muon() :
isTrackerMuon(false),
isPFMuon(false),
ptgen(-999),
eta(-999),
phi(-999),
pt(-999),
ptError(-999),
px(-999),
py(-999),
pz(-999),
energy(-999),
charge(-999),
trkD0(-999),
trkD0Error(-999),
trkDz(-999),
trkDzError(-999),
globalChi2(-999),
trkIso(-999),
ecalIso(-999),
hcalIso(-999),
hoIso(-999),
relIso(-999),
passID(false),
vtxDist3D(-999),
vtxIndex(-1),
vtxDistZ(-999),
pixHits(-1),
trkHits(-1),
muoHits(-1),
matches(-1),
trackerLayersWithMeasurement(-1),
pfRelIso(-999),
vx(-999),
vy(-999),
vz(-999),
dB(-999),
edB(-999),
dB3d(-999),
edB3d(-999),
isGlobalMuonPromptTight(false),
isAllArbitrated(false),
nChambers(-1),
nMatches(-1),
nMatchedStations(-1),
stationMask(0),
stationGapMaskDistance(0),
stationGapMaskPull(0),
muonID(-1),
idMVA(-999),
isoRingsMVA(-999),
isoRingsRadMVA(-999),
idIsoCombMVA(-999),
pfRelIso03v1(-999),
pfRelIso03v2(-999),
pfRelIsoDB03v1(-999),
pfRelIsoDB03v2(-999),
pfRelIso04v1(-999),
pfRelIso04v2(-999),
pfRelIsoDB04v1(-999),
pfRelIsoDB04v2(-999),
selbit(0)
{}
vhtm::Jet::Jet() :
eta(-999),
phi(-999),
pt(-999),
pt_raw(-999),
energy(-999),
energy_raw(-999),
jecUnc(-999),
resJEC(-999),
partonFlavour(-1),
puIdMVA(-999),
puIdFlag(-1),
puIdBits(-1),
chargedEmEnergyFraction(-999),
chargedHadronEnergyFraction(-999),
chargedMuEnergyFraction(-999),
electronEnergyFraction(-999),
muonEnergyFraction(-999),
neutralEmEnergyFraction(-999),
neutralHadronEnergyFraction(-999),
photonEnergyFraction(-999),
chargedHadronMultiplicity(-1),
chargedMultiplicity(-1),
electronMultiplicity(-1),
muonMultiplicity(-1),
neutralHadronMultiplicity(-1),
neutralMultiplicity(-1),
photonMultiplicity(-1),
nConstituents(-1),
trackCountingHighEffBTag(-999),
trackCountingHighPurBTag(-999),
simpleSecondaryVertexHighEffBTag(-999),
simpleSecondaryVertexHighPurBTag(-999),
jetProbabilityBTag(-999),
jetBProbabilityBTag(-999),
combinedSecondaryVertexBTag(-999),
combinedSecondaryVertexMVABTag(-999),
combinedInclusiveSecondaryVertexBTag(-999),
combinedMVABTag(-999),
passLooseID(-1),
passTightID(-1),
selbit(0) {}
vhtm::Vertex::Vertex() :
x(-999),
y(-999),
z(-999),
xErr(-999),
yErr(-999),
zErr(-999),
rho(-999),
chi2(-999),
ndf(-1),
ntracks(-1),
ntracksw05(-1),
isfake(true),
isvalid(false),
sumPt(-999),
selbit(0) {}
vhtm::Track::Track()
: eta(-999),
etaError(-999),
theta(-999),
thetaError(-999),
phi(-999),
phiError(-999),
p(-999),
pt(-999),
ptError(-999),
qoverp(-999),
qoverpError(-999),
charge(-999),
nValidHits(-1),
nLostHits(-1),
validFraction(-999),
nValidTrackerHits(-1),
nValidPixelHits(-1),
nValidStripHits(-1),
trackerLayersWithMeasurement(-1),
pixelLayersWithMeasurement(-1),
stripLayersWithMeasurement(-1),
dxy(-999),
dxyError(-999),
dz(-999),
dzError(-999),
chi2(-999),
ndof(-1),
vx(-999), vy(-999), vz(-999),
selbit(0) {}
vhtm::TriggerObject::TriggerObject() :
energy(-999),
pt(-999),
eta(-999),
phi(-999)
{
pathList.clear();
}
<file_sep>import FWCore.ParameterSet.Config as cms
process = cms.Process("PAT")
## MessageLogger
process.load("FWCore.MessageLogger.MessageLogger_cfi")
## Options and Output Report
process.options = cms.untracked.PSet( wantSummary = cms.untracked.bool(True) )
## Source
process.source = cms.Source("PoolSource",
fileNames = cms.untracked.vstring()
)
## Maximal Number of Events
process.maxEvents = cms.untracked.PSet( input = cms.untracked.int32(-1) )
## Geometry and Detector Conditions (needed for a few patTuple production steps)
process.load('Configuration.Geometry.GeometryExtended2019Reco_cff')
process.load('Configuration.Geometry.GeometryExtended2019_cff')
process.load('Configuration.StandardSequences.MagneticField_38T_cff')
#process.load("RecoLocalCalo.EcalRecAlgos.EcalSeverityLevelESProducer_cfi")
process.load('Configuration.StandardSequences.Services_cff')
process.load('Configuration.StandardSequences.FrontierConditions_GlobalTag_cff')
#process.GlobalTag.globaltag = 'STAR17_61_V5A::All'
#process.GlobalTag.globaltag = 'DES17_61_V4::All'
process.GlobalTag.globaltag = 'DES19_62_V8::All'
process.load("Configuration.StandardSequences.MagneticField_cff")
## Output Module Configuration (expects a path 'p')
from PhysicsTools.PatAlgos.patEventContent_cff import patEventContentNoCleaning
process.out = cms.OutputModule("PoolOutputModule",
fileName = cms.untracked.string('patTuple.root'),
## save only events passing the full path
#SelectEvents = cms.untracked.PSet( SelectEvents = cms.vstring('p') ),
## save PAT output; you need a '*' to unpack the list of commands
## 'patEventContent'
outputCommands = cms.untracked.vstring('drop *', *patEventContentNoCleaning )
)
process.outpath = cms.EndPath(process.out)
#from PhysicsTools.PatAlgos.mcMatchLayer0 import *
#process.load("PhysicsTools.PatAlgos.patSequences_cff")
#process.load('PhysicsTools.PatAlgos.mcMatchLayer0.muonMatch_cfi')
process.load('PhysicsTools.PatAlgos.producersLayer1.muonProducer_cff')
#process.muMatch1 = process.muonMatch.clone(mcStatus = [1])
# verbose flags for the PF2PAT modules
process.options.allowUnscheduled = cms.untracked.bool(True)
#process.Tracer = cms.Service("Tracer")
# Configure PAT to use PF2PAT instead of AOD sources
# this function will modify the PAT sequences.
from PhysicsTools.PatAlgos.tools.pfTools import *
postfix = "PFlow"
jetAlgo="AK5"
usePF2PAT(process,runPF2PAT=True, jetAlgo=jetAlgo, runOnMC=True, postfix=postfix)
# to turn on type-1 MET corrections, use the following call
#usePF2PAT(process,runPF2PAT=True, jetAlgo=jetAlgo, runOnMC=True, postfix=postfix, typeIMetCorrections=True)
# to switch default tau (HPS) to old default tau (shrinking cone) uncomment
# the following:
# note: in current default taus are not preselected i.e. you have to apply
# selection yourself at analysis level!
#adaptPFTaus(process,"shrinkingConePFTau",postfix=postfix)
# Add PF2PAT output to the created file
from PhysicsTools.PatAlgos.patEventContent_cff import patEventContentNoCleaning
#process.load("CommonTools.ParticleFlow.PF2PAT_EventContent_cff")
#process.out.outputCommands = cms.untracked.vstring('drop *')
process.out.outputCommands = cms.untracked.vstring('drop *',
'keep recoPFCandidates_particleFlow_*_*',
'keep *_selectedPatJets*_*_*',
'drop *_selectedPatJets*_caloTowers_*',
'keep *_selectedPatElectrons*_*_*',
'keep *_selectedPatMuons*_*_*',
'keep *_selectedPatTaus*_*_*',
'keep *_patMETs*_*_*',
'keep *_selectedPatPhotons*_*_*',
'keep *_selectedPatTaus*_*_*',
'keep *_generalTracks_*_*',
'keep *_genParticles_*_*',
'keep *_offlineBeamSpot_*_*',
'keep *_offlinePrimaryVertices_*_*',
'keep *_gtDigis_*_*'
)
# top projections in PF2PAT:
#getattr(process,"pfNoPileUp"+postfix).enable = True
#getattr(process,"pfNoMuon"+postfix).enable = True
#getattr(process,"pfNoElectron"+postfix).enable = True
#getattr(process,"pfNoTau"+postfix).enable = False
#getattr(process,"pfNoJet"+postfix).enable = True
# to use tau-cleaned jet collection uncomment the following:
#getattr(process,"pfNoTau"+postfix).enable = True
# verbose flags for the PF2PAT modules
#getattr(process,"pfNoMuon"+postfix).verbose = False
# enable delta beta correction for muon selection in PF2PAT?
#getattr(process,"pfIsolatedMuons"+postfix).doDeltaBetaCorrection = cms.bool(False)
#MCTruthMatching
#getattr(process,muMatch1).enable = True
process.p=cms.Path(
process.muonMatch +
getattr(process,"pfNoPileUp"+postfix)+
getattr(process,"pfNoMuon"+postfix)+
getattr(process,"pfNoElectron"+postfix)+
#getattr(process,"pfNoTau"+postfix).enable =
getattr(process,"pfNoJet"+postfix)
#process.analyzePatMCMatching
)
#process.patDefaultSequence.replace(process.patDefaultSequence,
# getattr(process,"pfNoPileUp"+postfix)+
# getattr(process,"pfNoMuon"+postfix)+
# getattr(process,"pfNoElectron"+postfix)+
#getattr(process,"pfNoTau"+postfix).enable =
# getattr(process,"pfNoJet"+postfix)
# process.muonMatch
# )
#process.p=cms.Path(process.patDefaultSequence)
## ------------------------------------------------------
# In addition you usually want to change the following
# parameters:
## ------------------------------------------------------
#
# process.GlobalTag.globaltag = ... ## (according to https://twiki.cern.ch/twiki/bin/view/CMS/SWGuideFrontierConditions)
# ##
#from PhysicsTools.PatAlgos.patInputFiles_cff import filesRelValProdTTbarAODSIM
#process.source.fileNames = filesRelValProdTTbarAODSIM
process.source = cms.Source("PoolSource",
fileNames = cms.untracked.vstring(
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_100_1_uUb.root'
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_101_1_2qJ.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_102_2_Rqu.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_103_1_d85.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_104_1_yTR.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_105_1_BFe.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_106_1_z6e.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_107_1_bhH.root',
# 'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluGluH_tautau_14TeV_620slhc5_v5_gaussBeamSpot_108_3_AIL.root'
)
)
# ##
#process.maxEvents.input = 10
# ##
# process.out.outputCommands = [ ... ] ## (e.g. taken from PhysicsTools/PatAlgos/python/patEventContent_cff.py)
# ##
process.out.fileName = 'patTuple_PF2PAT.root'
# ##
# process.options.wantSummary = False ## (to suppress the long output at the end of the job)
<file_sep>#ifndef __VHTauTau_TreeMaker_GenEventBlock_h
#define __VHTauTau_TreeMaker_GenEventBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class GenEvent;
class GenEventBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(edm::Event const& iEvent, edm::EventSetup const& iSetup);
virtual void endJob() {}
public:
explicit GenEventBlock(const edm::ParameterSet& iConfig);
virtual ~GenEventBlock();
private:
TClonesArray* cloneGenEvent;
int _verbosity;
const edm::InputTag _genEvtInfoInputTag;
const bool _storePDFWeights;
const edm::InputTag _pdfWeightsInputTag;
std::vector<double> *_pdfWeights;
vhtm::GenEvent* genEventB;
};
#endif
<file_sep>#include "TPaveStats.h"
void plot_dimuoneta(){
//TCanvas *c1 = new TCanvas("c1","c1",59,80,1288,535);
//c1->Divide(3,1);
//c1->cd(1);
h1DiMuonPtReco->SetLineColor(1);
h1SumMuonPtReco->SetLineColor(4);
h1DiMuonPtReco->SetXTitle("Reco. Muon p_{T} in GeV/c");
h1SumMuonPtReco->SetXTitle("Reco. Muon p_{T} in GeV/c");
h1DiMuonPtReco->SetYTitle("Number of Events/GeV");
h1SumMuonPtReco->SetYTitle("Number of Events/GeV");
//h1SumMuonPtReco->GetXaxis()->SetTitleOffset(1.4);
//h1DiMuonPtReco->GetXaxis()->SetTitleOffset(1.4);
h1SumMuonPtReco->Draw();
gPad->Update();
h1DiMuonPtReco->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) h1DiMuonPtReco->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) h1SumMuonPtReco->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
h1DiMuonPtReco->Draw();
h1SumMuonPtReco->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4770115,0.6038136,0.7658046,0.7923729);
leg_hist->SetHeader("p_{T} histograms");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(h1DiMuonPtReco,"Di muon p_{T}","l");
leg_hist->AddEntry(h1SumMuonPtReco,"Sum of the muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("muonpt.png");
}
<file_sep>import FWCore.ParameterSet.Config as cms
genParticleBlock = cms.EDAnalyzer("GenParticleBlock",
verbosity = cms.int32(0),
genParticleSrc = cms.InputTag('genParticles')
)
<file_sep>import FWCore.ParameterSet.Config as cms
process = cms.Process("HTauTauTree")
#------------------------
# Message Logger Settings
#------------------------
process.load("FWCore.MessageLogger.MessageLogger_cfi")
process.MessageLogger.cerr.FwkReport.reportEvery = 1
#--------------------------------------
# Event Source & # of Events to process
#---------------------------------------
process.source = cms.Source("PoolSource",
fileNames = cms.untracked.vstring()
)
process.maxEvents = cms.untracked.PSet(
input = cms.untracked.int32(-1)
)
process.options = cms.untracked.PSet( wantSummary = cms.untracked.bool(False) )
#-----------------------------
# Geometry
#-----------------------------
process.load("Configuration.StandardSequences.Geometry_cff")
#-----------------------------
# Magnetic Field
#-----------------------------
process.load('Configuration.StandardSequences.MagneticField_AutoFromDBCurrent_cff')
#-------------
# Global Tag
#-------------
process.load('Configuration.StandardSequences.FrontierConditions_GlobalTag_cff')
process.GlobalTag.globaltag = 'START53_V11::All'
#-------------
# Output ROOT file
#-------------
process.TFileService = cms.Service("TFileService",
fileName = cms.string('test_higgs.root')
)
#--------------------------------------------------
# VHTauTau Tree Specific
#--------------------------------------------------
process.load("VHTauTau.TreeMaker.TreeCreator_cfi")
process.load("VHTauTau.TreeMaker.TreeWriter_cfi")
process.load("VHTauTau.TreeMaker.TreeContentConfig_cff")
#-------------------------------------------------------
# PAT
#------------------------------------------------------
process.load("RecoTauTag.Configuration.RecoPFTauTag_cff")
process.load("PhysicsTools.PatAlgos.patSequences_cff")
#import PhysicsTools.PatAlgos.tools.tauTools as tauTools
#tauTools.switchToPFTauHPS(process) # For HPS Taus
## --
## Switch on PAT trigger
## --
import PhysicsTools.PatAlgos.tools.trigTools as trigTools
trigTools.switchOnTrigger( process, outputModule='' ) # This is optional and can be omitted.
process.p = cms.Path(
process.treeCreator +
process.treeContentSequence +
process.treeWriter
)
# List File names here
#---------------------------------------
process.PoolSource.fileNames = [
'file:./patTuple_higgs.root'
]
<file_sep>void plot_accept_pt_3d_graph(){
//h2->SetLineColor(1);
TTree *MyTree = new TTree("MyTree", "MyTree");
MyTree->ReadFile("acceptance2.file", "lead:sublead:weight");
//MyTree->SetEstimate(MyTree->GetEntries());
MyTree->Draw("sublead:lead:weight>>htemp", "", "COLZ");
//htemp->Rebin(0.5);
//cout<<htemp->GetAize
htemp->SetXTitle("Reco leading muon p_{T} in GeV/c");
htemp->SetYTitle("Reco subleading muon p_{T} in GeV/c");
htemp->SetTitle("2-D acceptance plot");
htemp->SetStats(0);
htemp->Draw("COLZ");
c1->SaveAs("acceptance_2d_100.png");
//gROOT->ProcessLine(".q");
}
<file_sep>void ratio(){
TFile* f1= new TFile("histos_signal.root");
TH1F *h1=(TH1F*)f1->Get("h1DiMuonEtaReco");
TFile* f2=new TFile("/home/amohapatra/work/CMSSW_6_2_0_SLHC12/src/bkg_dymu_pu_50/histos_bkg.root");
TH1F *h2=(TH1F*)f2->Get("h1DiMuonEtaReco");
//h2->Draw("COLZ");
//TH2F *h3 = new TH2F("h3","S/B acceptance plot ",120,0,30,102,0,30);
h1->SetLineColor(1);
h2->SetLineColor(4);
h1->SetStats(0);
h2->SetStats(0);
h1->SetTitle("Dimuon Eta");
h1->SetXTitle("Dimuon Eta for reco muons");
//h1->GetXaxis()->SetTitleOffset(1.44);
h1->SetYTitle("Events");
//h1->GetYaxis()->SetTitleOffset(1.44);
//h1->SetZTitle("Ratio");
h1->Draw();
h2->Draw("SAME");
leg_hist = new TLegend(0.4770115,0.6038136,0.7658046,0.7923729);
leg_hist->SetFillColor(0);
leg_hist->AddEntry(h1,"Signal","l");
leg_hist->AddEntry(h2,"Background","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("dimuon_eta.png");
//h2->Draw("SAME");
}
<file_sep>import FWCore.ParameterSet.Config as cms
metBlock = cms.EDAnalyzer("METBlock",
verbosity = cms.int32(0),
metSrc = cms.InputTag('patMETsPFlow'),
#metSrc = cms.InputTag('patMETs')
#metSrc = cms.InputTag('patPFMETsTypeIcorrected'),
#corrmetSrc = cms.InputTag('patPFMETsTypeIcorrected'),
#mvametSrc = cms.InputTag('patPFMETsTypeIcorrected')
)
<file_sep>void plot_int_3(){
hLeadMuon3->ComputeIntegral();
Double_t *integral = (hLeadMuon3->GetIntegral());
hLeadMuon3->SetContent(integral);
//TH1F *hLeadMuon3=1-(*hLeadMuon3);
//TH1F *hLeadMuon3i = new TH1F("hLeadMuon3i","histogram with 1 as entries",100,0,60);
Int_t binsize=hLeadMuon3->GetSize();
for (int i=1;i<binsize-1;i++){
Double_t entry=hLeadMuon3->GetBinContent(i);
hLeadMuon3->SetBinContent(i,1-entry);
}
hSubLeadMuon3->ComputeIntegral();
Double_t *integral = (hSubLeadMuon3->GetIntegral());
hSubLeadMuon3->SetContent(integral);
binsize=hSubLeadMuon3->GetSize();
//hSubLeadMuon3=1-(*hSubLeadMuon3);
for (int i=1;i<binsize-1;i++){
entry=hSubLeadMuon3->GetBinContent(i);
hSubLeadMuon3->SetBinContent(i,1-entry);
}
hLeadMuon3->SetLineColor(1);
hSubLeadMuon3->SetLineColor(4);
hLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon3->SetYTitle("Kinematic Acceptance");
hSubLeadMuon3->SetYTitle("Kinematic Acceptance");
hSubLeadMuon3->Draw();
hLeadMuon3->Draw("same"); //draw hist_2 first as it has a larger range
leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 3");
leg_hist->AddEntry(hLeadMuon3,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon3,"subleading muon pt","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
//c1->SaveAs("case1.png");
}
<file_sep>void plot_imp_par_Ba(){
hImPEC->SetLineColor(1);
//h1PtReco->SetLineColor(4);
//h1PtReco->SetXTitle("GeV/c");
hImPBa->SetYTitle("Events");
hImPBa->GetYaxis()->SetTitleOffset(1.35);
hImPBa->SetXTitle("d_{xy} in cm");
hImPBa->Draw(); //draw hist_2 first as it has a larger range
hImPBa->Draw("c hist same"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_imp_2.eps");
c1->SaveAs("plot_imp_2.png");
//h1PtReco->Draw("same");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms");
leg_hist->AddEntry(hImPEC,"Gen Pt","l");
leg_hist->AddEntry(h1PtReco,"Reco Pt","l");
leg_hist->Draw();*/
}
<file_sep>void plot_int_2d_1(){
TH3F *h2 = new TH3F("h2","acceptance plot",100,0,60,100,0,60,100,0,1);
Double_t weight=0.;
hLeadMuon1->ComputeIntegral();
Double_t *integral = (hLeadMuon1->GetIntegral());
hLeadMuon1->SetContent(integral);
Int_t binsize=hLeadMuon1->GetSize();
for (int i=1;i<binsize-1;i++){
Double_t entry=hLeadMuon1->GetBinContent(i);
hLeadMuon1->SetBinContent(i,1-entry);
}
hSubLeadMuon1->ComputeIntegral();
Double_t *integral = (hSubLeadMuon1->GetIntegral());
hSubLeadMuon1->SetContent(integral);
binsize=hSubLeadMuon1->GetSize();
for (int i=1;i<binsize-1;i++){
entry=hSubLeadMuon1->GetBinContent(i);
hSubLeadMuon1->SetBinContent(i,1-entry);
}
for (int i=1;i<leadmuonpt.size();i++){
for (int j=1;j<subleadmuonpt.size();j++){
weight=0;
int k=hleadMuon1->GetXaxis()->FindBin(leadmuonpt[i]);
int m=hSubLeadMuon1->GetXaxis()->FindBin(subleadmuonpt[j]);
if (hLeadMuon1->GetBinContent(k) < hSubLeadMuon1->GetBinContent(m))
weight=hLeadMuon1->GetBinContent(k);
else if (hLeadMuon1->GetBinContent(k) > hSubLeadMuon1->GetBinContent(m))
weight=hSubLeadMuon1->GetBinContent(m);
else
weight=hSubLeadMuon1->GetBinContent(m);
h2->Fill(leadmuonpt[i],subleadmuonpt[j],weight);
}
}
}
<file_sep>import FWCore.ParameterSet.Config as cms
treeWriter = cms.EDAnalyzer("TreeMakerModule",
verbosity = cms.int32(0),
createTree = cms.bool(False)
)
<file_sep>void plot2(){
hMC
h1PtGen->SetLineColor(1);
h1PtReco->SetLineColor(4);
h1PtReco->SetXTitle("GeV/c");
h1PtGen->SetXTitle("GeV/c");
h1PtGen->Draw(); //draw hist_2 first as it has a larger range
h1PtReco->Draw("same");
leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms");
leg_hist->AddEntry(h1PtGen,"Gen Pt","l");
leg_hist->AddEntry(h1PtReco,"Reco Pt","l");
leg_hist->Draw();
}
<file_sep>#ifndef __VHTauTau_TreeMaker_TrackBlock_h
#define __VHTauTau_TreeMaker_TrackBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class Track;
class TrackBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob(){}
public:
explicit TrackBlock(const edm::ParameterSet& iConfig);
virtual ~TrackBlock() {}
enum {
kMaxTrack = 200
};
private:
TClonesArray* cloneTrack;
int fnTrack;
int _verbosity;
edm::InputTag _inputTag;
edm::InputTag _beamSpot;
vhtm::Track* trackB;
};
#endif
<file_sep>#ifndef __VHTauTau_TreeMaker_PhysicsObjects_h
#define __VHTauTau_TreeMaker_PhysicsObjects_h
#include <vector>
#include <map>
#include <string>
#include "TObject.h"
namespace vhtm {
class Event: public TObject {
public:
Event();
~Event() {}
unsigned int run;
unsigned int event;
unsigned int lumis;
unsigned int bunch;
unsigned int orbit;
double time;
bool isdata;
bool isPhysDeclared;
bool isBPTX0;
bool isBSCMinBias;
bool isBSCBeamHalo;
bool isPrimaryVertex;
bool isBeamScraping;
bool passHBHENoiseFilter;
std::vector<int> nPU;
std::vector<int> bunchCrossing;
std::vector<int> trueNInt;
ClassDef(Event,1)
};
class GenEvent: public TObject {
public:
GenEvent();
~GenEvent() {}
unsigned int processID;
double ptHat;
std::vector<double> pdfWeights;
ClassDef(GenEvent,1)
};
class Electron: public TObject {
public:
Electron();
~Electron() {}
double eta;
double phi;
double pt;
bool ecalDriven;
bool hasGsfTrack;
double trackPt;
double trackPtError;
double energy;
double caloEnergy;
double caloEnergyError;
int charge;
int pixHits;
int trkHits;
int nValidHits;
float trkD0;
float trkD0Error;
// ID variables
double hoe;
double hoeDepth1;
double eop;
double sigmaEtaEta;
double sigmaIEtaIEta;
double deltaPhiTrkSC;
double deltaEtaTrkSC;
int classif;
double e1x5overe5x5;
double e2x5overe5x5;
// Iso variables
double isoEcal03;
double isoHcal03;
double isoTrk03;
double isoEcal04;
double isoHcal04;
double isoTrk04;
double isoRel03;
double isoRel04;
// Vertex
double vx;
double vy;
double vz;
// SC associated with electron
double scEn;
double scEta;
double scPhi;
double scET;
double scRawEnergy;
// Vertex association variables
double vtxDist3D;
int vtxIndex;
double vtxDistZ;
double relIso;
double pfRelIso;
// PFlow isolation variable
float chargedHadronIso;
float neutralHadronIso;
float photonIso;
int missingHits;
double dB;
double edB;
double dB3d;
double edB3d;
double scE1E9;
double scS4S1;
double sckOutOfTime;
double scEcalIso;
double scHEEPEcalIso;
double scHEEPTrkIso;
int nBrems;
float fbrem;
float dist_vec;
float dCotTheta;
float hasMatchedConv;
float mva;
float mvaPOGTrig;
float mvaPOGNonTrig;
bool mvaPreselection;
bool isTriggerElectron;
float isoMVA;
float pfRelIso03v1;
float pfRelIso03v2;
float pfRelIsoDB03v1;
float pfRelIsoDB03v2;
float pfRelIsoDB03v3;
float pfRelIso04v1;
float pfRelIso04v2;
float pfRelIsoDB04v1;
float pfRelIsoDB04v2;
float pfRelIsoDB04v3;
float pfRelIso03;
float pfRelIso04;
float pfRelIsoDB03;
float pfRelIsoDB04;
int selbit;
int fidFlag;
ClassDef(Electron, 1)
};
class GenParticle: public TObject {
public:
GenParticle();
~GenParticle() {}
double eta;
double phi;
double p;
double px;
double py;
double pz;
double pt;
double energy;
int pdgId;
double vx;
double vy;
double vz;
int status;
double charge;
int numDaught;
int numMother;
int motherIndex;
std::vector<int> motherIndices;
std::vector<int> daughtIndices;
ClassDef(GenParticle,1)
};
class GenJet: public TObject {
public:
GenJet();
~GenJet() {}
double eta;
double phi;
double p;
double pt;
double energy;
double emf;
double hadf;
ClassDef(GenJet,1)
};
class MET: public TObject {
public:
MET();
~MET() {}
double metx;
double mety;
double met;
double metphi;
double sumet;
double metuncorr;
double metphiuncorr;
double sumetuncorr;
ClassDef(MET,2)
};
class Tau: public TObject {
public:
Tau();
~Tau() {}
enum {
kMaxPFChargedCand = 40,
kMaxPFNeutralCand = 20
};
double eta;
double phi;
double pt;
double energy;
int charge;
double mass;
double px;
double py;
double pz;
double leadTrkPt;
double leadTrkPtError;
double leadTrkEta;
double leadTrkPhi;
double leadTrkCharge;
double leadTrkD0;
double leadTrkD0Error;
double leadTrkDz;
double leadTrkDzError;
int vtxIndex;
double vtxDxy;
double vtxDz;
// Leading particle pT
double leadChargedParticlePt;
double leadNeutralParticlePt;
double leadParticlePt;
// Number of charged/neutral candidates and photons in different cones
int numChargedHadronsSignalCone;
int numNeutralHadronsSignalCone;
int numPhotonsSignalCone;
int numParticlesSignalCone;
int numChargedHadronsIsoCone;
int numNeutralHadronsIsoCone;
int numPhotonsIsoCone;
int numParticlesIsoCone;
double ptSumPFChargedHadronsIsoCone;
double ptSumPFNeutralHadronsIsoCone;
double ptSumPhotonsIsoCone;
double sigChHadCandPt[kMaxPFChargedCand];
double sigChHadCandEta[kMaxPFChargedCand];
double sigChHadCandPhi[kMaxPFChargedCand];
double sigNeHadCandPt[kMaxPFNeutralCand];
double sigNeHadCandEta[kMaxPFNeutralCand];
double sigNeHadCandPhi[kMaxPFNeutralCand];
double sigGammaCandPt[kMaxPFNeutralCand];
double sigGammaCandEta[kMaxPFNeutralCand];
double sigGammaCandPhi[kMaxPFNeutralCand];
double isoChHadCandPt[kMaxPFChargedCand];
double isoChHadCandEta[kMaxPFChargedCand];
double isoChHadCandPhi[kMaxPFChargedCand];
double isoNeHadCandPt[kMaxPFNeutralCand];
double isoNeHadCandEta[kMaxPFNeutralCand];
double isoNeHadCandPhi[kMaxPFNeutralCand];
double isoGammaCandPt[kMaxPFNeutralCand];
double isoGammaCandEta[kMaxPFNeutralCand];
double isoGammaCandPhi[kMaxPFNeutralCand];
/////////////////
float decayModeFinding;
float decayModeFindingNewDMs;
float decayModeFindingOldDMs;
float chargedIsoPtSum ;
float neutralIsoPtSum;
float puCorrPtSum;
float CombinedIsolationDeltaBetaCorrRaw3Hits;
float CombinedIsolationDeltaBetaCorrRaw;
float byIsolationMVA3newDMwLTraw;
float byIsolationMVA3newDMwoLTraw;
float byIsolationMVA3oldDMwLTraw;
float byIsolationMVA3oldDMwoLTraw;
float byLooseCombinedIsolationDeltaBetaCorr;
float byLooseCombinedIsolationDeltaBetaCorr3Hits;
float byMediumCombinedIsolationDeltaBetaCorr;
float byMediumCombinedIsolationDeltaBetaCorr3Hits;
float byTightCombinedIsolationDeltaBetaCorr;
float byTightCombinedIsolationDeltaBetaCorr3Hits;
float byLooseIsolationMVA3newDMwLT;
float byLooseIsolationMVA3newDMwoLT;
float byLooseIsolationMva3oldDMwLT;
float byLooseIsolationMVA3oldDMwoLT;
float byMediumIsolationMVA3newDMwLT;
float byMediumIsolationMVA3newDMwoLT;
float byMediumIsolationMva3oldDMwLT;
float byMediumIsolationMVA3oldDMwoLT;
float byTightIsolationMVA3newDMwLT;
float byTightIsolationMVA3newDMwoLT;
float byTightIsolationMva3oldDMwLT;
float byTightIsolationMVA3oldDMwoLT;
// discriminators against electrons/muons
float againstMuonLoose;
float againstMuonLoose2;
float againstMuonLoose3;
float againstMuonLooseMVA;
float againstMuonTight;
float againstMuonTight2;
float againstMuonTight3;
float againstMuonTightMVA;
float againstElectronLoose;
float againstElectronMedium;
float againstElectronTight;
float pfElectronMVA;
// ElectronIDMVA, electron faking tau
float againstElectronMVALooseMVA5;
float againstElectronMVAMediumMVA5;
float againstElectronMVATightMVA5;
float byVLooseCombinedIsolationDeltaBetaCorr;
// MVA based isolation
float byLooseIsolationMVA;
float byMediumIsolationMVA;
float byTightIsolationMVA;
////////////
// kinematic variables for PFJet associated to PFTau
double jetPt;
double jetEta;
double jetPhi;
float emFraction;
float maximumHCALPFClusterEt;
float ecalStripSumEOverPLead;
float bremsRecoveryEOverPLead;
float hcalTotOverPLead;
float hcalMaxOverPLead;
float hcal3x3OverPLead;
float etaetaMoment;
float phiphiMoment;
float etaphiMoment;
double vx;
double vy;
double vz;
double zvertex;
double ltsipt;
int selbit;
ClassDef(Tau, 2)
};
class Muon: public TObject {
public:
Muon();
~Muon() {}
bool isTrackerMuon;
bool isPFMuon;
double ptgen;
/* double DR_status1Match;
double DR_status3Match;
double DR_defaultMatch;*/
double eta;
double phi;
double pt;
double ptError;
double px;
double py;
double pz;
double energy;
int charge;
double trkD0;
double trkD0Error;
double trkDz;
double trkDzError;
double globalChi2;
double trkIso;
double ecalIso;
double hcalIso;
double hoIso;
double relIso;
int passID;
double vtxDist3D;
int vtxIndex;
double vtxDistZ;
int pixHits;
int trkHits;
int muoHits;
int matches;
int trackerLayersWithMeasurement;
double pfRelIso;
double vx;
double vy;
double vz;
double dB; // PV2D
double edB;
double dB3d; // PV3D
double edB3d;
// UW Recommendation
bool isGlobalMuonPromptTight;
bool isAllArbitrated;
int nChambers;
int nMatches;
int nMatchedStations;
unsigned int stationMask;
unsigned int stationGapMaskDistance;
unsigned int stationGapMaskPull;
int muonID;
float idMVA;
float isoRingsMVA;
float isoRingsRadMVA;
float idIsoCombMVA;
float pfRelIso03v1;
float pfRelIso03v2;
float pfRelIsoDB03v1;
float pfRelIsoDB03v2;
float pfRelIso04v1;
float pfRelIso04v2;
float pfRelIsoDB04v1;
float pfRelIsoDB04v2;
int selbit;
ClassDef(Muon, 4)
};
class Jet: public TObject {
public:
Jet();
~Jet() {}
double eta;
double phi;
double pt;
double pt_raw;
double energy;
double energy_raw;
double jecUnc;
double resJEC;
int partonFlavour;
float puIdMVA;
int puIdFlag;
int puIdBits;
double chargedEmEnergyFraction;
double chargedHadronEnergyFraction;
double chargedMuEnergyFraction;
double electronEnergyFraction;
double muonEnergyFraction;
double neutralEmEnergyFraction;
double neutralHadronEnergyFraction;
double photonEnergyFraction;
int chargedHadronMultiplicity;
int chargedMultiplicity;
int electronMultiplicity;
int muonMultiplicity;
int neutralHadronMultiplicity;
int neutralMultiplicity;
int photonMultiplicity;
int nConstituents;
double trackCountingHighEffBTag;
double trackCountingHighPurBTag;
double simpleSecondaryVertexHighEffBTag;
double simpleSecondaryVertexHighPurBTag;
double jetProbabilityBTag;
double jetBProbabilityBTag;
double combinedSecondaryVertexBTag;
double combinedSecondaryVertexMVABTag;
double combinedInclusiveSecondaryVertexBTag;
double combinedMVABTag;
int passLooseID;
int passTightID;
int selbit;
ClassDef(Jet, 1)
};
class Vertex: public TObject {
public:
Vertex();
~Vertex() {}
double x;
double y;
double z;
double xErr;
double yErr;
double zErr;
double rho;
double chi2;
double ndf;
int ntracks;
int ntracksw05;
bool isfake;
bool isvalid;
double sumPt; // vector sum
int selbit;
ClassDef(Vertex, 1)
};
class GenMET: public TObject {
public:
GenMET();
~GenMET() {}
double met;
double metphi;
double sumet;
ClassDef(GenMET, 1)
};
class Track: public TObject {
public:
Track();
~Track() {}
double eta;
double etaError;
double theta;
double thetaError;
double phi;
double phiError;
double p;
double pt;
double ptError;
double qoverp;
double qoverpError;
float charge;
int nValidHits;
int nLostHits;
double validFraction;
int nValidTrackerHits;
int nValidPixelHits;
int nValidStripHits;
int trackerLayersWithMeasurement;
int pixelLayersWithMeasurement;
int stripLayersWithMeasurement;
double dxy;
double dxyError;
double dz;
double dzError;
double chi2;
int ndof;
double vx;
double vy;
double vz;
int selbit;
ClassDef(Track, 1)
};
class TriggerObject: public TObject {
public:
TriggerObject();
~TriggerObject() {}
double energy;
double pt;
double eta;
double phi;
std::map< std::string, unsigned int > pathList;
ClassDef(TriggerObject, 1)
};
}
#endif
<file_sep>void plot_int_2(){
hLeadMuon2->ComputeIntegral();
Double_t *integral = (hLeadMuon2->GetIntegral());
hLeadMuon2->SetContent(integral);
//TH1F *hLeadMuon2=1-(*hLeadMuon2);
//TH1F *hLeadMuon2i = new TH1F("hLeadMuon2i","histogram with 1 as entries",100,0,60);
Int_t binsize=hLeadMuon2->GetSize();
for (int i=1;i<binsize-1;i++){
Double_t entry=hLeadMuon2->GetBinContent(i);
hLeadMuon2->SetBinContent(i,1-entry);
}
hSubLeadMuon2->ComputeIntegral();
Double_t *integral = (hSubLeadMuon2->GetIntegral());
hSubLeadMuon2->SetContent(integral);
binsize=hSubLeadMuon2->GetSize();
//hSubLeadMuon2=1-(*hSubLeadMuon2);
for (int i=1;i<binsize-1;i++){
entry=hSubLeadMuon2->GetBinContent(i);
hSubLeadMuon2->SetBinContent(i,1-entry);
}
hLeadMuon2->SetLineColor(1);
hSubLeadMuon2->SetLineColor(4);
hLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon2->SetYTitle("Kinematic Acceptance");
hSubLeadMuon2->SetYTitle("Kinematic Acceptance");
hSubLeadMuon2->Draw();
hLeadMuon2->Draw("same"); //draw hist_2 first as it has a larger range
leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 2");
leg_hist->AddEntry(hLeadMuon2,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon2,"subleading muon pt","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
//c1->SaveAs("case1.png");
}
<file_sep>void plot_accept_pt_3d(){
//hAcceptAll->SetLineColor(1);
hAcceptAll->GetXaxis()->SetRangeUser(5, 30);
hAcceptAll->GetYaxis()->SetRangeUser(5, 30);
hAcceptAll->SetXTitle("Reco. leading muon p_{T} in GeV/c");
hAcceptAll->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//hAcceptAll->SetZTitle("Kinematic acceptance");
hAcceptAll->SetStats(0);
hAcceptAll->Draw("cont3 colz");
//HLT_PATH Mu17_Mu8
//gStyle->SetPalette(0,100);
Int_t a=hAcceptAll->GetXaxis()->FindBin(17);
Int_t b = hAcceptAll->GetYaxis()->FindBin(8);
Double_t c = hAcceptAll->GetBinContent(a,b);
//Double_t a = hAcceptAll->GetBinContent(hAcceptAll->GetXaxis()->FindBin(17),hAcceptAll->GetYaxis()->FindBin(8)));
//HLT_PATH Mu13_Mu8
//Double_t f=hAcceptAll->GetBinContent(
//TPaletteAxis *pal = hAcceptAll->GetListOfFunctions()->FindObject("palette");
//pal->GetAxis()->SetName("A_kin");
cout<<"17-18 "<<c<<endl;
Int_t d = hAcceptAll->GetXaxis()->FindBin(13);
Int_t e = hAcceptAll->GetYaxis()->FindBin(8);
Double_t f = hAcceptAll->GetBinContent(d,e);
cout<<"13-18 "<<f<<endl;
//Offline Cuts
Int_t g=hAcceptAll->GetXaxis()->FindBin(20);
Int_t h=hAcceptAll->GetYaxis()->FindBin(10);
Double_t z=hAcceptAll->GetBinContent(g,h);
//Double_t i=GetBinContenthAcceptAll->GetXaxis()->FindBin(20),hAcceptAll->GetYaxis()->FindBin(10));
cout<<"20-10 "<<z<<endl;
//Double_t m=hAcceptAll->GetBinContent(k,l);
//draw hist_2 first as it has a larger range
TLatex latex;
//latex.DrawLatex(17,8,TString::Format("#bullet %f ",a));
//latex.DrawLatex(17,8,TString::Format("#bullet %2f %",c*100));
latex.DrawLatex(17,8,"#bullet 62%");
latex.DrawLatex(13,8,"#diamond 71%");
latex.DrawLatex(20,10,"#color[2]{#bullet} 42%");
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(13,8,TString::Format("#diamond %f ",f));
//latex.DrawLatex(20,10,TString::Format("#circ %f ",i));
latex.DrawLatex(3,22,"#bullet");
latex.DrawLatex(2.83,25,"#diamond");
latex.DrawLatex(4,28,"#scale[0.8]{HLT Paths(Run-1)}");
latex.DrawLatex(4,22,"#scale[0.8]{Mu17_Mu8 (Luminosity 19.1 fb^{-1})}");
latex.DrawLatex(4,25,"#scale[0.8]{Mu13_Mu8 (Luminosity 3.7 fb^{-1})}");
latex.DrawLatex(4,19,"#scale[0.8]{Offline selection}");
latex.DrawLatex(3,16,"#color[2]{#bullet}");
latex.DrawLatex(4,16,"#scale[0.8]{Mu20_Mu10}");
c1->SaveAs("acceptance_2d_all_cont3.png");
c1->SaveAs("acceptance_2d_all_cont3.eps");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 1 (both muons in endcap)");
leg_hist->AddEntry(hAcceptAll,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon pt","l");
leg_hist->Draw();
*/
}
<file_sep>#ifndef __VHTauTau_TreeMaker_METBlock_h
#define __VHTauTau_TreeMaker_METBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class MET;
class METBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit METBlock(const edm::ParameterSet& iConfig);
virtual ~METBlock() {}
enum {
kMaxMET = 100
};
//void fillMET(const edm::Event& iEvent,
// const edm::EventSetup& iSetup,
// TClonesArray* cloneMET,
// int fnMET,
// edm::InputTag iTag,
// vhtm::MET* metB);
private:
TClonesArray* clonePFMET;
int fnPFMET;
// TClonesArray* cloneCorrMET;
//int fnCorrMET;
//TClonesArray* cloneMVAMET;
//int fnMVAMET;
int _verbosity;
edm::InputTag _pfInputTag;
//edm::InputTag _corrinputTag;
//edm::InputTag _mvainputTag;
vhtm::MET* metB;
//vhtm::MET* corrmetB;
//vhtm::MET* mvametB;
};
#endif
<file_sep>#include <cassert>
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "TTree.h"
#include "VHTauTau/TreeMaker/plugins/TreeMakerModule.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
TreeMakerModule::TreeMakerModule(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_createTree(iConfig.getParameter<bool>("createTree"))
{
}
void TreeMakerModule::beginJob()
{
if (!_createTree) return;
edm::Service<TFileService> fs;
fs->file().cd("/");
TTree* tree = fs->make<TTree>("vhtree", "VH Analysis Tree");
assert(tree);
fs->file().ls();
}
//
// -- Analyze
//
void TreeMakerModule::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Get TTree pointer
if (_createTree) return;
TTree* tree = vhtm::Utility::getTree("vhtree");
tree->Fill();
}
void TreeMakerModule::endJob() {
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(TreeMakerModule);
<file_sep>void plot_int_1(){
hLeadMuon1->ComputeIntegral();
Double_t *integral = (hLeadMuon1->GetIntegral());
hLeadMuon1->SetContent(integral);
//TH1F *hLeadMuon1=1-(*hLeadMuon1);
//TH1F *hLeadMuon1i = new TH1F("hLeadMuon1i","histogram with 1 as entries",100,0,60);
Int_t binsize=hLeadMuon1->GetSize();
for (int i=1;i<binsize-1;i++){
// std::cout<<i<<"\t"<<(*integral)<<endl;
Double_t entry=hLeadMuon1->GetBinContent(i);
hLeadMuon1->SetBinContent(i,1-entry);
}
hSubLeadMuon1->ComputeIntegral();
Double_t *integral = (hSubLeadMuon1->GetIntegral());
hSubLeadMuon1->SetContent(integral);
binsize=hSubLeadMuon1->GetSize();
//hSubLeadMuon1=1-(*hSubLeadMuon1);
for (int i=1;i<binsize-1;i++){
entry=hSubLeadMuon1->GetBinContent(i);
hSubLeadMuon1->SetBinContent(i,1-entry);
}
hLeadMuon1->SetLineColor(1);
hSubLeadMuon1->SetLineColor(4);
hLeadMuon1->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon1->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon1->SetYTitle("kinematic acceptance");
hSubLeadMuon1->SetYTitle("kinematic acceptance");
hSubLeadMuon1->Draw();
hLeadMuon1->Draw("same"); //draw hist_2 first as it has a larger range
leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 1");
leg_hist->AddEntry(hLeadMuon1,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
//c1->SaveAs("case1.png");
}
<file_sep>#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/L1GlobalTrigger/interface/L1GlobalTriggerReadoutRecord.h"
#include "DataFormats/L1GlobalTrigger/interface/L1GtFdlWord.h"
#include "DataFormats/VertexReco/interface/Vertex.h"
#include "DataFormats/VertexReco/interface/VertexFwd.h"
#include "DataFormats/TrackReco/interface/Track.h"
#include "DataFormats/TrackReco/interface/TrackFwd.h"
#include "SimDataFormats/PileupSummaryInfo/interface/PileupSummaryInfo.h"
#include "TTree.h"
#include "TClonesArray.h"
#include "VHTauTau/TreeMaker/plugins/EventBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
EventBlock::EventBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_l1InputTag(iConfig.getParameter<edm::InputTag>("l1InputTag")),
_vtxInputTag(iConfig.getParameter<edm::InputTag>("vertexInputTag")),
_trkInputTag(iConfig.getParameter<edm::InputTag>("trkInputTag")),
_vtxMinNDOF(iConfig.getParameter<unsigned int>("vertexMinimumNDOF")),
_vtxMaxAbsZ(iConfig.getParameter<double>("vertexMaxAbsZ")),
_vtxMaxd0(iConfig.getParameter<double>("vertexMaxd0")),
_numTracks(iConfig.getParameter<unsigned int>("numTracks")),
_hpTrackThreshold(iConfig.getParameter<double>("hpTrackThreshold"))
{
}
EventBlock::~EventBlock() {
delete _nPU;
delete _bunchCrossing;
delete _trueNInt;
}
void EventBlock::beginJob() {
_nPU = new std::vector<int>();
_bunchCrossing = new std::vector<int>();
_trueNInt = new std::vector<int>();
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneEvent = new TClonesArray("vhtm::Event");
tree->Branch("Event", &cloneEvent, 32000, 2);
tree->Branch("nPU", "std::vector<int>", &_nPU);
tree->Branch("bunchCrossing", "std::vector<int>", &_bunchCrossing);
tree->Branch("trueNInt", "std::vector<int>", &_trueNInt);
}
void EventBlock::analyze(edm::Event const& iEvent, edm::EventSetup const& iSetup) {
// Reset the TClonesArray
cloneEvent->Clear();
// Clear the two independent vectors
_nPU->clear();
_bunchCrossing->clear();
_trueNInt->clear();
// Create Event Object
eventB = new ( (*cloneEvent)[0] ) vhtm::Event();
eventB->run = iEvent.id().run();
eventB->event = iEvent.id().event();
eventB->lumis = iEvent.id().luminosityBlock();
eventB->bunch = iEvent.bunchCrossing();
eventB->orbit = iEvent.orbitNumber();
double sec = iEvent.time().value() >> 32 ;
double usec = 0xFFFFFFFF & iEvent.time().value();
double conv = 1e6;
eventB->time = sec+usec/conv;
eventB->isdata = iEvent.isRealData();
edm::Handle<L1GlobalTriggerReadoutRecord> l1GtReadoutRecord;
iEvent.getByLabel(_l1InputTag, l1GtReadoutRecord);
// Technical Trigger Part
if (l1GtReadoutRecord.isValid()) {
edm::LogInfo("EventBlock") << "Successfully obtained L1GlobalTriggerReadoutRecord for label: "
<< _l1InputTag;
L1GtFdlWord fdlWord = l1GtReadoutRecord->gtFdlWord();
if (fdlWord.physicsDeclared() == 1)
eventB->isPhysDeclared = true;
// BPTX0
if (l1GtReadoutRecord->technicalTriggerWord()[0])
eventB->isBPTX0 = true;
// MinBias
if (l1GtReadoutRecord->technicalTriggerWord()[40] || l1GtReadoutRecord->technicalTriggerWord()[41])
eventB->isBSCMinBias = true;
// BeamHalo
if ( (l1GtReadoutRecord->technicalTriggerWord()[36] || l1GtReadoutRecord->technicalTriggerWord()[37] ||
l1GtReadoutRecord->technicalTriggerWord()[38] || l1GtReadoutRecord->technicalTriggerWord()[39]) ||
((l1GtReadoutRecord->technicalTriggerWord()[42] && !l1GtReadoutRecord->technicalTriggerWord()[43]) ||
(l1GtReadoutRecord->technicalTriggerWord()[43] && !l1GtReadoutRecord->technicalTriggerWord()[42])) )
eventB->isBSCBeamHalo = true;
}
else {
edm::LogError("EventBlock") << "Error >> Failed to get L1GlobalTriggerReadoutRecord for label:"
<< _l1InputTag;
}
// Good Primary Vertex Part
edm::Handle<reco::VertexCollection> primaryVertices;
iEvent.getByLabel(_vtxInputTag, primaryVertices);
if (primaryVertices.isValid()) {
edm::LogInfo("EventBlock") << "Total # Primary Vertices: " << primaryVertices->size();
for (reco::VertexCollection::const_iterator it = primaryVertices->begin();
it != primaryVertices->end(); ++it) {
if (!(it->isFake()) && it->ndof() > _vtxMinNDOF &&
fabs(it->z()) <= _vtxMaxAbsZ && fabs(it->position().rho()) <= _vtxMaxd0
)
{
eventB->isPrimaryVertex = true;
break;
}
}
}
else {
edm::LogError("EventBlock") << "Error >> Failed to get VertexCollection for label:"
<< _vtxInputTag;
}
// Scraping Events Part
edm::Handle<reco::TrackCollection> tracks;
iEvent.getByLabel(_trkInputTag, tracks);
if (tracks.isValid()) {
edm::LogInfo("EventBlock") << "Total # Tracks: " << tracks->size();
int numhighpurity = 0;
double fraction = 1.;
reco::TrackBase::TrackQuality trackQuality = reco::TrackBase::qualityByName("highPurity");
if (tracks->size() > _numTracks) {
for (reco::TrackCollection::const_iterator it = tracks->begin();
it != tracks->end(); ++it) {
if (it->quality(trackQuality)) numhighpurity++;
}
fraction = (double)numhighpurity/(double)tracks->size();
if (fraction < _hpTrackThreshold)
eventB->isBeamScraping = true;
}
}
else {
edm::LogError("EventBlock") << "Error >> Failed to get TrackCollection for label:"
<< _trkInputTag;
}
// Access PU information
/*if (!iEvent.isRealData()) {
edm::Handle<std::vector<PileupSummaryInfo> > PupInfo;
iEvent.getByLabel("addPileupInfo", PupInfo);
std::vector<PileupSummaryInfo>::const_iterator PVI;
for (PVI = PupInfo->begin(); PVI != PupInfo->end(); ++PVI) {
eventB->bunchCrossing.push_back(PVI->getBunchCrossing());
_bunchCrossing->push_back(PVI->getBunchCrossing());
eventB->nPU.push_back(PVI->getPU_NumInteractions());
_nPU->push_back(PVI->getPU_NumInteractions());
eventB->trueNInt.push_back(PVI->getTrueNumInteractions());
_trueNInt->push_back(PVI->getTrueNumInteractions());
}
// More info about PU is here:
// https://twiki.cern.ch/twiki/bin/viewauth/CMS/PileupInformation#Accessing_PileupSummaryInfo_in_r
}*/
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(EventBlock);
<file_sep>#include <iostream>
#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/Common/interface/Handle.h"
#include "DataFormats/Common/interface/Ref.h"
#include "DataFormats/PatCandidates/interface/Tau.h"
#include "DataFormats/ParticleFlowCandidate/interface/PFCandidate.h"
#include "DataFormats/ParticleFlowCandidate/interface/PFCandidateFwd.h"
#include "DataFormats/BeamSpot/interface/BeamSpot.h"
#include "DataFormats/VertexReco/interface/Vertex.h"
#include "DataFormats/VertexReco/interface/VertexFwd.h"
#include "Utilities/General/interface/FileInPath.h"
#include "VHTauTau/TreeMaker/plugins/TauBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
const reco::GenParticle* getGenTau(const pat::Tau& pt)
{
std::vector<reco::GenParticleRef> associatedGenParticles = pt.genParticleRefs();
for ( std::vector<reco::GenParticleRef>::const_iterator im = associatedGenParticles.begin();
im != associatedGenParticles.end(); ++im ) {
if ( im->isAvailable() ) {
const reco::GenParticleRef& genParticle = (*im);
if ( genParticle->pdgId() == -15 || genParticle->pdgId() == +15 ) return genParticle.get();
}
}
return 0;
}
namespace tb
{
template<typename T>
bool isValidRef(const edm::Ref<T>& ref)
// bool isValidRef(const PFCandidatePtr ref))
{
return ( (ref.isAvailable() || ref.isTransient()) && ref.isNonnull() );
}
}
TauBlock::TauBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("patTauSrc")),
_vtxInputTag(iConfig.getParameter<edm::InputTag>("vertexSrc"))
{
}
TauBlock::~TauBlock() { }
void TauBlock::beginJob()
{
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneTau = new TClonesArray("vhtm::Tau");
tree->Branch("Tau", &cloneTau, 32000, 2);
tree->Branch("nTau", &fnTau, "fnTau/I");
}
void TauBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneTau->Clear();
fnTau = 0;
edm::Handle<reco::VertexCollection> primaryVertices;
iEvent.getByLabel(_vtxInputTag, primaryVertices);
edm::Handle<std::vector<pat::Tau> > taus;
iEvent.getByLabel(_inputTag, taus);
if (taus.isValid()) {
edm::LogInfo("TauBlock") << "Total # PAT Taus: " << taus->size();
for (std::vector<pat::Tau>::const_iterator it = taus->begin();
it != taus->end(); ++it) {
if (fnTau == kMaxTau) {
edm::LogInfo("TauBlock") << "Too many PAT Taus, fnTau = " << fnTau;
break;
}
tauB = new ((*cloneTau)[fnTau++]) vhtm::Tau();
const reco::GenParticle* genTau = getGenTau(*it);
if (!genTau) continue;
// Store Tau variables
tauB->eta = it->eta();
tauB->phi = it->phi();
tauB->pt = it->pt();
tauB->energy = it->energy();
tauB->charge = it->charge();
tauB->px = it->px();
tauB->py = it->py();
tauB->pz = it->pz();
// if (tb::isValidRef(it->leadPFChargedHadrCand()) /*&& tb::isValidRef(it->leadPFChargedHadrCand()->trackRef())*/) {
/*
reco::TrackRef trk = it->leadPFChargedHadrCand()->trackRef();
tauB->leadTrkPt = trk->pt();
tauB->leadTrkPtError = trk->ptError();
tauB->leadTrkEta = trk->eta();
tauB->leadTrkPhi = trk->phi();
tauB->leadTrkCharge = trk->charge();
tauB->leadTrkD0 = trk->d0();
tauB->leadTrkD0Error = trk->d0Error();
tauB->leadTrkDz = trk->dz();
tauB->leadTrkDzError = trk->dzError();
if (0) std::cout << trk->pt() << " "
<< trk->eta() << " "
<< trk->phi() << " "
<< trk->charge() << " "
<< trk->d0() << " "
<< trk->dz()
<< std::endl;
// IP of leadPFChargedHadrCand wrt PV
// Vertex association
double minVtxDist3D = 9999.;
int indexVtx = -1;
double vertexDz = 9999.;
double vertexDxy = 9999.;
if (primaryVertices.isValid()) {
edm::LogInfo("TauBlock") << "Total # Primary Vertices: " << primaryVertices->size();
for (reco::VertexCollection::const_iterator vit = primaryVertices->begin();
vit != primaryVertices->end(); ++vit) {
double dxy = trk->dxy(vit->position());
double dz = trk->dz(vit->position());
double dist3D = std::sqrt(pow(dxy,2) + pow(dz,2));
if (dist3D < minVtxDist3D) {
minVtxDist3D = dist3D;
indexVtx = int(std::distance(primaryVertices->begin(), vit));
vertexDxy = dxy;
vertexDz = dz;
}
}
}
else {
edm::LogError("TauBlock") << "Error >> Failed to get VertexCollection for label: "
<< _vtxInputTag;
}
tauB->vtxIndex = indexVtx;
tauB->vtxDxy = vertexDxy;
tauB->vtxDz = vertexDz;
if (0) std::cout << indexVtx << " " << vertexDxy << " " << vertexDz << std::endl;
*/
// } //if is valid ref
// Leading particle pT
tauB->leadChargedParticlePt = it->leadPFChargedHadrCand().isNonnull()
? it->leadPFChargedHadrCand()->pt(): 0.;
tauB->leadNeutralParticlePt = it->leadPFNeutralCand().isNonnull()
? it->leadPFNeutralCand()->et(): 0.;
tauB->leadParticlePt = it->leadPFCand().isNonnull()
? it->leadPFCand()->et(): 0.;
// Number of charged/neutral candidates and photons in different cones
tauB->numChargedHadronsSignalCone = it->signalPFChargedHadrCands().size();
tauB->numNeutralHadronsSignalCone = it->signalPFNeutrHadrCands().size();
tauB->numPhotonsSignalCone = it->signalPFGammaCands().size();
tauB->numParticlesSignalCone = it->signalPFCands().size();
tauB->numChargedHadronsIsoCone = it->isolationPFChargedHadrCands().size();
tauB->numNeutralHadronsIsoCone = it->isolationPFNeutrHadrCands().size();
tauB->numPhotonsIsoCone = it->isolationPFGammaCands().size();
tauB->numParticlesIsoCone = it->isolationPFCands().size();
tauB->ptSumPFChargedHadronsIsoCone = it->isolationPFChargedHadrCandsPtSum();
tauB->ptSumPhotonsIsoCone = it->isolationPFGammaCandsEtSum();
// Signal Constituents
// Charged hadrons
// int indx = 0;
/*for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->signalPFChargedHadrCands().begin(); iCand != it->signalPFChargedHadrCands().end(); ++iCand,indx++) {
// for (reco::PFCandidateRefVector::const_iterator iCand = it->signalPFChargedHadrCands().begin();
// iCand != it->signalPFChargedHadrCands().end(); ++iCand,indx++) {
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFChargedCand) {
tauB->sigChHadCandPt[indx] = cand.pt();
tauB->sigChHadCandEta[indx] = cand.eta();
tauB->sigChHadCandPhi[indx] = cand.phi();
}
}
// Neutral hadrons
indx = 0; // reset
for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->signalPFNeutrHadrCands().begin(); iCand != it->signalPFNeutrHadrCands().end(); ++iCand,indx++) {
//for (reco::PFCandidateRefVector::const_iterator iCand = it->signalPFNeutrHadrCands().begin();
//iCand != it->signalPFNeutrHadrCands().end(); ++iCand,indx++) {
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFNeutralCand) {
tauB->sigNeHadCandPt[indx] = cand.pt();
tauB->sigNeHadCandEta[indx] = cand.eta();
tauB->sigNeHadCandPhi[indx] = cand.phi();
}
}
// Photons
indx = 0; // reset
// for (reco::PFCandidateRefVector::const_iterator iCand = it->signalPFGammaCands().begin();
//iCand != it->signalPFGammaCands().end(); ++iCand,indx++)
for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->signalPFGammaCands().begin();
iCand != it->signalPFGammaCands().end(); ++iCand,indx++){
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFNeutralCand) {
tauB->sigGammaCandPt[indx] = cand.pt();
tauB->sigGammaCandEta[indx] = cand.eta();
tauB->sigGammaCandPhi[indx] = cand.phi();
}
}
// Isolation Constituents
// Charged hadrons
indx = 0; // reset
// for (reco::PFCandidateRefVector::const_iterator iCand = it->isolationPFChargedHadrCands().begin();
// iCand != it->isolationPFChargedHadrCands().end(); ++iCand,indx++) {
for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->isolationPFChargedHadrCands().begin();
iCand != it->isolationPFChargedHadrCands().end(); ++iCand,indx++) {
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFChargedCand) {
tauB->isoChHadCandPt[indx] = cand.pt();
tauB->isoChHadCandEta[indx] = cand.eta();
tauB->isoChHadCandPhi[indx] = cand.phi();
}
}
// Neutral hadrons
double ptSum = 0;
indx = 0; // reset
// for (reco::PFCandidateRefVector::const_iterator iCand = it->isolationPFNeutrHadrCands().begin();
// iCand != it->isolationPFNeutrHadrCands().end(); ++iCand,indx++) {
for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->isolationPFNeutrHadrCands().begin();
iCand != it->isolationPFNeutrHadrCands().end(); ++iCand,indx++) {
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFNeutralCand) {
tauB->isoNeHadCandPt[indx] = cand.pt();
tauB->isoNeHadCandEta[indx] = cand.eta();
tauB->isoNeHadCandPhi[indx] = cand.phi();
}
ptSum += std::abs(cand.pt());
}
tauB->ptSumPFNeutralHadronsIsoCone = ptSum;
// Photons
indx = 0; // reset
//for (reco::PFCandidateRefVector::const_iterator iCand = it->isolationPFGammaCands().begin();
// iCand != it->isolationPFGammaCands().end(); ++iCand,indx++) {
for (std::vector<edm::Ptr<reco::PFCandidate> >::const_iterator iCand = it->isolationPFGammaCands().begin();
iCand != it->isolationPFGammaCands().end(); ++iCand,indx++) {
const reco::PFCandidate& cand = (**iCand);
if (indx < vhtm::Tau::kMaxPFNeutralCand) {
tauB->isoGammaCandPt[indx] = cand.pt();
tauB->isoGammaCandEta[indx] = cand.eta();
tauB->isoGammaCandPhi[indx] = cand.phi();
}
}
*/
// tau id. discriminators
/*
tauB->decayModeFinding = it->tauID("decayModeFinding");
//tauB->decayModeFindingNewDMs = it->tauID("decayModeFindingNewDMs");
//tauB->decayModeFindingOldDMs = it->tauID("decayModeFindingOldDMs");
tauB->chargedIsoPtSum =it->tauID("chargedIsoPtSum");
tauB->neutralIsoPtSum =it->tauID("neutralIsoPtSum");
tauB->puCorrPtSum =it->tauID("puCorrPtSum");
tauB->CombinedIsolationDeltaBetaCorrRaw3Hits = it->tauID("byCombinedIsolationDeltaBetaCorrRaw3Hits");
tauB->CombinedIsolationDeltaBetaCorrRaw = it->tauID("byCombinedIsolationDeltaBetaCorrRaw");
tauB->byIsolationMVA3newDMwLTraw = it->tauID("byIsolationMVA3newDMwLTraw");
tauB->byIsolationMVA3newDMwoLTraw= it->tauID("byIsolationMVA3newDMwoLTraw");
tauB->byIsolationMVA3oldDMwLTraw= it->tauID("byIsolationMVA3oldDMwLTraw");
tauB-> byIsolationMVA3oldDMwoLTraw = it->tauID("byIsolationMVA3oldDMwoLTraw");
tauB->byLooseCombinedIsolationDeltaBetaCorr=it->tauID("byLooseCombinedIsolationDeltaBetaCorr");
tauB->byLooseCombinedIsolationDeltaBetaCorr3Hits=it->tauID("byLooseCombinedIsolationDeltaBetaCorr3Hits");
tauB->byMediumCombinedIsolationDeltaBetaCorr=it->tauID("byMediumCombinedIsolationDeltaBetaCorr");
tauB->byMediumCombinedIsolationDeltaBetaCorr3Hits=it->tauID("byMediumCombinedIsolationDeltaBetaCorr3Hits");
tauB->byTightCombinedIsolationDeltaBetaCorr=it->tauID("byTightCombinedIsolationDeltaBetaCorr");
tauB->byTightCombinedIsolationDeltaBetaCorr3Hits=it->tauID("byTightCombinedIsolationDeltaBetaCorr3Hits");
tauB->byLooseIsolationMVA3newDMwLT=it->tauID("byLooseIsolationMVA3newDMwLT");
tauB->byLooseIsolationMVA3newDMwoLT=it->tauID("byLooseIsolationMVA3newDMwoLT");
tauB->byLooseIsolationMva3oldDMwLT=it->tauID("byLooseIsolationMVA3oldDMwLT");
tauB->byLooseIsolationMVA3oldDMwoLT=it->tauID("byLooseIsolationMVA3oldDMwoLT");
tauB->byMediumIsolationMVA3newDMwLT=it->tauID("byMediumIsolationMVA3newDMwLT");
tauB->byMediumIsolationMVA3newDMwoLT=it->tauID("byMediumIsolationMVA3newDMwoLT");
tauB->byMediumIsolationMva3oldDMwLT=it->tauID("byMediumIsolationMVA3oldDMwLT");
tauB->byMediumIsolationMVA3oldDMwoLT=it->tauID("byMediumIsolationMVA3oldDMwoLT");
tauB->byTightIsolationMVA3newDMwLT=it->tauID("byTightIsolationMVA3newDMwLT");
tauB->byTightIsolationMVA3newDMwoLT=it->tauID("byTightIsolationMVA3newDMwoLT");
tauB->byTightIsolationMva3oldDMwLT=it->tauID("byTightIsolationMVA3oldDMwLT");
tauB->byTightIsolationMVA3oldDMwoLT=it->tauID("byTightIsolationMVA3oldDMwoLT");
*/
// discriminators against electrons/muons
tauB->againstMuonLoose = it->tauID("againstMuonLoose");
tauB->againstMuonLoose2 = it->tauID("againstMuonLoose2");
tauB->againstMuonLoose3 = it->tauID("againstMuonLoose3");
//tauB->againstMuonLooseMVA = it->tauID("againstMuonLooseMVA");
tauB->againstMuonTight = it->tauID("againstMuonTight");
tauB->againstMuonTight2 = it->tauID("againstMuonTight2");
tauB->againstMuonTight3 = it->tauID("againstMuonTight3");
//tauB->againstMuonTightMVA = it->tauID("againstMuonTightMVA");
tauB->againstElectronLoose = it->tauID("againstElectronLoose");
tauB->againstElectronMedium = it->tauID("againstElectronMedium");
tauB->againstElectronTight = it->tauID("againstElectronTight");
tauB->pfElectronMVA = it->leadPFCand().isNonnull()
? it->leadPFCand()->mva_e_pi() : 1.;
// ElectronIDMVA, electron faking tau
// tauB->againstElectronMVALooseMVA5 = it->tauID("againstElectronLooseMVA5");
//tauB->againstElectronMVAMediumMVA5 = it->tauID("againstElectronLooseMVA5");
//tauB->againstElectronMVATightMVA5 = it->tauID("againstElectronLooseMVA5");
//tauB->byVLooseCombinedIsolationDeltaBetaCorr = it->tauID("byVLooseCombinedIsolationDeltaBetaCorr");
// MVA based isolation
/* tauB->byLooseIsolationMVA = it->tauID("byLooseIsolationMVA");
tauB->byMediumIsolationMVA = it->tauID("byMediumIsolationMVA");
tauB->byTightIsolationMVA = it->tauID("byTightIsolationMVA");
*/
// kinematic variables for PFJet associated to PFTau
// if (tb::isValidRef(it->pfJetRef())) {
/* const reco::PFJetRef &jtr = it->pfJetRef();
tauB->jetPt = jtr->pt();
tauB->jetEta = jtr->eta();
tauB->jetPhi = jtr->phi();
if (0) std::cout << jtr->pt() << " " << jtr->eta() << " " << jtr->phi() << std::endl;
//}
*/
// NEW quantities
tauB->emFraction = it->emFraction();
tauB->maximumHCALPFClusterEt = it->maximumHCALPFClusterEt();
tauB->ecalStripSumEOverPLead = it->ecalStripSumEOverPLead();
tauB->bremsRecoveryEOverPLead = it->bremsRecoveryEOverPLead();
tauB->hcalTotOverPLead = it->hcalTotOverPLead();
tauB->hcalMaxOverPLead = it->hcalMaxOverPLead();
tauB->hcal3x3OverPLead = it->hcal3x3OverPLead();
tauB->etaetaMoment = it->etaetaMoment();
tauB->phiphiMoment = it->phiphiMoment();
tauB->phiphiMoment = it->etaphiMoment();
// Vertex information
const reco::Candidate::Point& vertex = it->vertex();
tauB->vx = vertex.x();
tauB->vy = vertex.y();
tauB->vz = vertex.z();
tauB->zvertex = it->vz(); // distance from the primary vertex
tauB->mass = it->p4().M();
tauB->ltsipt = TMath::Abs(it->leadPFChargedHadrCandsignedSipt());
}
}
else {
edm::LogError("TauBlock") << "Error! Failed to get pat::Tau collection for label: "
<< _inputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(TauBlock);
<file_sep>void plot_accept_pt_3d_2(){
//hAccept1->SetLineColor(1);
hAccept2->SetXTitle("Reco. leading muon p_{T} in GeV/c");
hAccept2->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//hAccept2->SetZTitle("Kinematic acceptance");
hAccept2->SetStats(0);
hAccept2->Draw("cont3 colz");
//HLT_PATH Mu17_Mu8
Int_t a=hAccept2->GetXaxis()->FindBin(17);
Int_t b = hAccept2->GetYaxis()->FindBin(8);
Double_t c = hAccept2->GetBinContent(a,b);
cout<<"17-18 "<<c<<endl;
//Double_t a = hAccept2->GetBinContent(hAccept2->GetXaxis()->FindBin(17),hAccept2->GetYaxis()->FindBin(8)));
//HLT_PATH Mu13_Mu8
//Double_t f=hAccept2->GetBinContent(
Int_t d = hAccept2->GetXaxis()->FindBin(13);
Int_t e = hAccept2->GetYaxis()->FindBin(8);
Double_t f = hAccept2->GetBinContent(d,e);
cout<<"13-18 "<<f<<endl;
//Offline Cuts
Int_t g=hAccept2->GetXaxis()->FindBin(20);
Int_t h=hAccept2->GetYaxis()->FindBin(10);
Double_t z=hAccept2->GetBinContent(g,h);
cout<<"20-10 "<<z<<endl;
//Double_t i=GetBinContenthAccept2->GetXaxis()->FindBin(20),hAccept2->GetYaxis()->FindBin(10));
//Double_t m=hAccept2->GetBinContent(k,l);
//draw hist_2 first as it has a larger range
TLatex latex;
//latex.DrawLatex(17,8,TString::Format("#bullet %f ",a));
//latex.DrawLatex(17,8,TString::Format("#bullet %2f %",c*100));
latex.DrawLatex(17,8,"#bullet 63%");
latex.DrawLatex(13,8,"#diamond 72%");
latex.DrawLatex(20,10,"#color[2]{#bullet} 42%");
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(13,8,TString::Format("#diamond %f ",f));
//latex.DrawLatex(20,10,TString::Format("#circ %f ",i));
latex.DrawLatex(3,22,"#bullet");
latex.DrawLatex(2.83,25,"#diamond");
latex.DrawLatex(4,28,"#scale[0.8]{HLT Paths(Run-1)}");
latex.DrawLatex(4,22,"#scale[0.8]{Mu17_Mu8 (Luminosity 19.1 fb^{-1})}");
latex.DrawLatex(4,25,"#scale[0.8]{Mu13_Mu8 (Luminosity 3.7 fb^{-1})}");
latex.DrawLatex(4,19,"#scale[0.8]{Offline selection}");
latex.DrawLatex(3,16,"#color[2]{#bullet}");
latex.DrawLatex(4,16,"#scale[0.8]{Mu20_Mu10}");
c1->SaveAs("acceptance_2d_2.png");
c1->SaveAs("acceptance_2d_2.eps");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 1 (both muons in endcap)");
leg_hist->AddEntry(hAccept2,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon pt","l");
leg_hist->Draw();
*/
}
<file_sep>#include <iostream>
#include <algorithm>
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "TTree.h"
#include "TClonesArray.h"
#include "TPRegexp.h"
#include "VHTauTau/TreeMaker/plugins/TriggerBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
#include "DataFormats/L1GlobalTrigger/interface/L1GlobalTriggerReadoutRecord.h"
#include "DataFormats/Common/interface/TriggerResults.h"
static const unsigned int NmaxL1AlgoBit = 128;
static const unsigned int NmaxL1TechBit = 64;
// Constructor
TriggerBlock::TriggerBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_l1InputTag(iConfig.getParameter<edm::InputTag>("l1InputTag")),
_hltInputTag(iConfig.getParameter<edm::InputTag>("hltInputTag")),
_hltPathsOfInterest(iConfig.getParameter<std::vector<std::string> > ("hltPathsOfInterest"))
{
}
TriggerBlock::~TriggerBlock() {
delete _l1physbits;
delete _l1techbits;
delete _hltpaths;
delete _hltresults;
delete _hltprescales;
}
void TriggerBlock::beginJob()
{
_l1physbits = new std::vector<int>();
_l1techbits = new std::vector<int>();
_hltpaths = new std::vector<std::string>();
_hltresults = new std::vector<int>();
_hltprescales = new std::vector<int>();
std::string tree_name = "vhtree";
TTree* tree = vhtm::Utility::getTree(tree_name);
tree->Branch("l1physbits", "vector<int>", &_l1physbits);
tree->Branch("l1techbits", "vector<int>", &_l1techbits);
tree->Branch("hltpaths", "vector<string>", &_hltpaths);
tree->Branch("hltresults", "vector<int>", &_hltresults);
tree->Branch("hltprescales", "vector<int>", &_hltprescales);
}
void TriggerBlock::beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {
bool changed = true;
if (hltConfig.init(iRun, iSetup, _hltInputTag.process(), changed)) {
// if init returns TRUE, initialisation has succeeded!
edm::LogInfo("TriggerBlock") << "HLT config with process name "
<< _hltInputTag.process() << " successfully extracted";
}
else {
// if init returns FALSE, initialisation has NOT succeeded, which indicates a problem
// with the file and/or code and needs to be investigated!
edm::LogError("TriggerBlock") << "Error! HLT config extraction with process name "
<< _hltInputTag.process() << " failed";
// In this case, all access methods will return empty values!
}
}
void TriggerBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
//cloneTrigger->Clear();
_l1physbits->clear();
_l1techbits->clear();
_hltpaths->clear();
_hltresults->clear();
_hltprescales->clear();
edm::Handle<L1GlobalTriggerReadoutRecord> l1GtReadoutRecord;
iEvent.getByLabel(_l1InputTag, l1GtReadoutRecord);
if (l1GtReadoutRecord.isValid()) {
edm::LogInfo("TriggerBlock") << "Successfully obtained L1GlobalTriggerReadoutRecord for label: "
<< _l1InputTag;
for (unsigned int i = 0; i < NmaxL1AlgoBit; ++i) {
_l1physbits->push_back(l1GtReadoutRecord->decisionWord()[i] ? 1 : 0);
}
for (unsigned int i = 0; i < NmaxL1TechBit; ++i) {
_l1techbits->push_back(l1GtReadoutRecord->technicalTriggerWord()[i] ? 1 : 0 );
}
}
else {
edm::LogError("TriggerBlock") << "Error >> Failed to get L1GlobalTriggerReadoutRecord for label: "
<< _l1InputTag;
}
edm::Handle<edm::TriggerResults> triggerResults;
iEvent.getByLabel(_hltInputTag, triggerResults);
if (triggerResults.isValid()) {
edm::LogInfo("TriggerBlock") << "Successfully obtained " << _hltInputTag;
const std::vector<std::string>& pathList = hltConfig.triggerNames();
for (std::vector<std::string>::const_iterator it = pathList.begin();
it != pathList.end(); ++it) {
if (_hltPathsOfInterest.size()) {
int nmatch = 0;
for (std::vector<std::string>::const_iterator kt = _hltPathsOfInterest.begin();
kt != _hltPathsOfInterest.end(); ++kt) {
nmatch += TPRegexp(*kt).Match(*it);
}
if (!nmatch) continue;
}
_hltpaths->push_back(*it);
int fired = 0;
unsigned int index = hltConfig.triggerIndex(*it);
if (index < triggerResults->size()) {
if (triggerResults->accept(index)) fired = 1;
}
else {
edm::LogInfo("TriggerBlock") << "Requested HLT path \"" << (*it) << "\" does not exist";
}
_hltresults->push_back(fired);
int prescale = -1;
if (hltConfig.prescaleSet(iEvent, iSetup) < 0) {
edm::LogError("TriggerBlock") << "Error >> The prescale set index number could not be obtained";
}
else {
prescale = hltConfig.prescaleValue(iEvent, iSetup, *it);
}
_hltprescales->push_back(prescale);
if (_verbosity)
std::cout << ">>> Path: " << (*it)
<< ", prescale: " << prescale
<< ", fired: " << fired
<< std::endl;
}
} else {
edm::LogError("TriggerBlock") << "Error >> Failed to get TriggerResults for label: "
<< _hltInputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(TriggerBlock);
<file_sep>void plot_corr(){
//h2->SetLineColor(1);
TCanvas *c1 = new TCanvas("c1","c1",700,400);
c1->Divide(2,2);
c1->cd(1);
h1->SetXTitle("Reco. leading muon p_{T} in GeV/c");
h1->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//h2->SetZTitle("Kinematic acceptance");
//h2->GetZaxis()->SetRangeUser(0,1);
//h2->SetStats(0);
h1->Draw("COLZ"); //draw hist_2 first as it has a larger range
c1->cd(2);
h11->SetXTitle("Reco. leading muon p_{T} in GeV/c");
h11->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
h11->Draw("COLZ"); //draw hist_2 first as it has a larger range
c1->cd(3);
h12->SetXTitle("Reco. leading muon p_{T} in GeV/c");
h12->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
h12->Draw("COLZ"); //draw hist_2 first as it has a larger range
c1->cd(4);
h13->SetXTitle("Reco. leading muon p_{T} in GeV/c");
h13->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
h13->Draw("COLZ");
//draw hist_2 first as it has a larger range
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 1 (both muons in endcap)");
leg_hist->AddEntry(h2,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon pt","l");
leg_hist->Draw();
*/
}
<file_sep>#!/bin/sh
source ~/.bashrc
source /cmssoft/cms/cmsset_default.sh
export SCRAM_ARCH=slc6_amd64_gcc472
cd /home/amohapatra/samples_gem_studies/Backgrounds/CMSSW_6_2_0_SLHC23_patch1/src/
cmsenv
sed -e 's|file.txt|'$1'|' -e 's/dytaumu_14TeV_pu0.root/dytaumu_14TeV_pu0_'$1'.root/' test_skimmer_condor.py >& test_skimmer_condor_$1.py
cmsRun test_skimmer_condor_$1.py
sed -e "s|local|$1|g" condor_makeclass_local.C >& condor_makeclass_$1.C
root -b condor_makeclass_$1.C
sed -e "s|local|$1|g" condor_vhtree_local.C >& condor_vhtree_$1.C
root -b condor_vhtree_$1.C
<file_sep>#define GemAnalyze_cxx
#include "GemAnalyze.h"
#include <TH2.h>
#include <TStyle.h>
#include <TCanvas.h>
void GemAnalyze::Loop()
{
// In a ROOT session, you can do:
// Root > .L GemAnalyze.C
// Root > GemAnalyze t
// Root > t.GetEntry(12); // Fill t data members with entry number 12
// Root > t.Show(); // Show values of entry 12
// Root > t.Show(16); // Read and show values of entry 16
// Root > t.Loop(); // Loop on all entries
//
// This is the loop skeleton where:
// jentry is the global entry number in the chain
// ientry is the entry number in the current Tree
// Note that the argument to GetEntry must be:
// jentry for TChain::GetEntry
// ientry for TTree::GetEntry and TBranch::GetEntry
//
// To read only selected branches, Insert statements like:
// METHOD1:
// fChain->SetBranchStatus("*",0); // disable all branches
// fChain->SetBranchStatus("branchname",1); // activate branchname
// METHOD2: replace line
// fChain->GetEntry(jentry); //read all branches
//by b_branchname->GetEntry(ientry); //read only this branch
if (fChain == 0) return;
double delRMin=1000.;
double delR=0.;
//double GenPtMatch=0.;
double delphi=0.;
double deleta=0.;
double RecoMuonPtMatch=0.;
double MuonInvariantMass=0.;
TH1F *h1PtReco = new TH1F("h1PtReco","Reco Muon Pt",100,0,200);
TH1F *h1PtGen = new TH1F("h1PtGen","Gen Muon Pt",100,0,200);
TH1F *hMCMatch = new TH1F("hMCMatch","Muon Pt resolution",100,-3,3);
TH1F *hInvMuon = new TH1F("hInvMuon","Invariant Mass plot",100,0,3);
Long64_t nentries = fChain->GetEntriesFast();
cout<<"Number of Entries is: "<<nentries<<endl;
Long64_t nbytes = 0, nb = 0;
for (Long64_t jentry=0; jentry<nentries;jentry++) {
Long64_t ientry = LoadTree(jentry);
if (ientry < 0) break;
nb = fChain->GetEntry(jentry); nbytes += nb;
// cout<<nMuon<<endl;
/* for (int i=0 ; i<nMuon; i++){
h1PtReco->Fill(Muon_pt[i]);
}
//cout<<GenParticle_pdgId<<endl;
for (int i=0; i<nGenParticle; i++){
if (GenParticle_pdgId[i] == 13){
h1PtGen->Fill(GenParticle_pt[i]);
}
}*/
cout<<nGenParticle<<endl;
//MC Truth Matching
for (int i=0;i<nGenParticle; i++){
if (GenParticle_pdgId[i] == 13 && ((fabs(GenParticle_eta[i]) > 1.6) && (fabs(GenParticle_eta[i]) < 2.2))){
delRMin=1000;
delR=0.;
RecoMuonPtMatch=0.;
for (int j=0; j<nMuon; j++){
if ((fabs(Muon_eta[j]) > 1.6) && (fabs(Muon_eta[j]) < 2.2)){
delphi=Muon_phi[j]-GenParticle_phi[i];
deleta=Muon_eta[j]-GenParticle_eta[i];
delR=sqrt((delphi*delphi)+(deleta*deleta));
if (delR<=delRMin){
delRMin=delR;
RecoMuonPtMatch=Muon_pt[j];
//cout<<GenPtMatch<<endl;
}
}
}
hMCMatch->Fill(RecoMuonPtMatch-GenParticle_pt[i]);
h1PtGen->Fill(GenParticle_pt[i]);
h1PtReco->Fill(RecoMuonPtMatch);
}
}
/*for (int i=0; i<nGenParticle; i++){
if (GenParticle_pdgId[i]==15) cout<<nGenParticle<<endl;
}*/
/*
for (int i=0; i<nGenParticle; i++){
for (int j=0; j<nGenParticle; j++){
if (i != j){
if (GenParticle_pdgId[i] == 13 && GenParticle_pdgId[j]==13){
//cout<<"hi"<<endl;
MuonInvariantMass=sqrt(fabs(((GenParticle_energy[i]+GenParticle_energy[j])*(GenParticle_energy[i]+GenParticle_energy[j]))-((GenParticle_p[i]+GenParticle_p[j])*(GenParticle_p[i]+GenParticle_p[j]))));
if (MuonInvariantMass != 0) {hInvMuon->Fill(MuonInvariantMass);}
}
}
}
}*/
}
}
<file_sep>#define vhtree_cxx
#include "vhtree.h"
#include <TH2.h>
#include <TStyle.h>
#include <TCanvas.h>
#include <vector>
#include <fstream>
#include <TMath.h>
void vhtree::Loop()
{
// In a ROOT session, you can do:
// Root > .L vhtree.C
// Root > vhtree t
// Root > t.GetEntry(12); // Fill t data members with entry number 12
// Root > t.Show(); // Show values of entry 12
// Root > t.Show(16); // Read and show values of entry 16
// Root > t.Loop(); // Loop on all entries
//
// This is the loop skeleton where:
// jentry is the global entry number in the chain
// ientry is the entry number in the current Tree
// Note that the argument to GetEntry must be:
// jentry for TChain::GetEntry
// ientry for TTree::GetEntry and TBranch::GetEntry
//
// To read only selected branches, Insert statements like:
// METHOD1:
// fChain->SetBranchStatus("*",0); // disable all branches
// fChain->SetBranchStatus("branchname",1); // activate branchname
// METHOD2: replace line
// fChain->GetEntry(jentry); //read all branches
//by b_branchname->GetEntry(ientry); //read only this branch
if (fChain == 0) return;
double delRMin=0.03;
double delR=0.;
double GenMuonPt=0.;
double delphi=0.;
double deleta=0.;
Double_t RecoMuonPt=0.;
double RecoMuonEta=0.;
double inv_mass=0.;
double ImpParam=0.;
double MuonDZ=0.;
double MuonChi2=0.;
double SumMuonChi2=0.;
double e1=0.;
double e2=0.;
double p1x=0.;
double p2x=0.;
double p1y=0.;
double p2y=0.;
double p1z=0.;
double p2z=0.;
double phi_1=0.;
double phi_tau_1=0.;
double phi_2=0.;
double phi_tau_2=0.;
double theta_1=0;
double theta_2=0;
double metx=0.;
double mety=0.;
double p_1=0.;
double p_2=0.;
double x_1=0;
double x_2=0;
double pvis_1=0;
double pvis_2=0;
double inv_mass_vis=0;
Double_t total_events=0;
Double_t events=0;
Double_t mag=0;
Double_t dimuoneta=0;
Double_t dimuonratiopt=0;
Int_t counter=0;
Double_t GenMuonCharge=0;
Double_t RecoMuonCharge=0;
Double_t numerator=0;
Double_t denominator=0;
Int_t counter_ba=0;
Int_t counter_ec=0;
Int_t counter_out=0;
Double_t cost=0.;
double dimuonpt=0;
double summuonpt=0;
double count=0;
double dcasig[2]={0};
double phi_pmuon_met = 0;
ofstream myfile("acceptance.file",ios::app);
ofstream myfile2("acceptance2.file",ios::app);
//TH1F::SetDefaultSumw2();
TH1F *h1PtReco = new TH1F("h1PtReco","Reco Muon Pt",300,0,300);
TH1F *h1PtGen = new TH1F("h1PtGen","Gen Muon Pt",300,0,300);
TH1F *h1DiMuonPtReco = new TH1F("h1DiMuonPtReco","Reco DiMuon Pt",300,0,300);
TH1F *h1SumMuonPtReco = new TH1F("h1SumMuonPtReco","Reco Sum Muon Pt",300,0,300);
TH1F *h1DiMuonSumPtRatio = new TH1F("h1DiMuonSumPtRatio","Ratio of dimuon pT to the muon sum pT",200,0,2);
TH1F *h1MuonImpParSig = new TH1F("h1MuonImpParSig","DCA Significance of muons",100,-5,5);
TH1F *h1PhiPMuonMet = new TH1F("h1PhiPMuonMet","Azimuthal Angle between the positive muon and MET ",10,0,3);
TH1F *h1DecayAngleDiMuonRest = new TH1F("h1DecayAngleDiMuonRest","Decay Angle of the positive muon in the dimuon rest frame",10,-1,1);
TH2F *hSumMuonDiMuon=new TH2F("hSumMuonDiMuon","Corr",300,0,300,300,0,300);
TH1F *h1PtLeading = new TH1F("h1PtLeading","Leading Muon Pt",100,0,100);
TH1F *h1PtSubLeading = new TH1F("h1PtSubLeading","SubLeading Muon Pt",100,0,100);
TH1F *h1PtLeadingi = new TH1F("h1PtLeadingi","Integrated Leading Muon Pt",100,0,100);
TH1F *h1PtSubLeadingi = new TH1F("h1PtSubLeadingi","Integrated SubLeading Muon Pt",100,0,100);
TH1F *h1EtaReco = new TH1F("h1EtaReco","Reco Muon Eta",100,-7,+7);
//TH1F *h1EtaGen = new TH1F("h1EtaGen","Gen Muon Eta",100,-5,+5);
TH1F *h1DiMuonEtaReco = new TH1F("h1DiMuonEtaReco","Dimuon Eta (with GEMs)",100,-5,5);
TH1F *hPhiReco = new TH1F("hPhiReco","Difference in Phi between the two muons in multiples of #pi",100,-2,2);
TH1F *hMCMatchEC = new TH1F("hMCMatchEC","Muon pT resolution in 1.5<|#eta|<2.2",200,-0.1,0.1);
TH1F *hMCMatchBa = new TH1F("hMCMatchBa","Muon pT resolution in |#eta|<1.5",200,-0.1,0.1);
TH1F *hLeadMuon1 = new TH1F("hLeadMuon1","Leading Muon and Subleading Muon in 1.5<|#eta|<2.2",100,0,100);
TH1F *hLeadMuon2 = new TH1F("hLeadMuon2","Leading Muon in 1.5<|#eta|<2.2, subleading muon in |#eta|<1.5 ",100,0,100);
TH1F *hLeadMuon3 = new TH1F("hLeadMuon3","Leading Muon in |#eta|<1.5, subleading muon in 1.5<|#eta|<2.2",100,0,100);
//TH1F *hLeadMuonAll = new TH1F("hLeadMuonAll","Overall Leading muon distribution",100,0,100);
TH1F *hSubLeadMuon1 = new TH1F("hSubLeadMuon1","Leading Muon and Subleading Muon in 1.5<|#eta|<2.2",100,0,100);
TH1F *hSubLeadMuon2 = new TH1F("hSubLeadMuon2","Leading Muon in 1.5<|#eta|<2.2, subleading muon in |#eta|<1.5",100,0,100);
TH1F *hSubLeadMuon3 = new TH1F("hSubLeadMuon3","Leading Muon in |#eta|<1.5, subleading muon in 1.5<|#eta|<2.2",100,0,100);
//TH1F *hSubLeadMuonAll = new TH1F("hSubLeadMuonAll","Overall Subleading muon distribution",100,0,100);
TH2F *h1 = new TH2F("h1","Correlation plot between Subleading and leading muon Pt(Overall)",30,0,30,30,0,30);
TH2F *h11 = new TH2F("h11","Correlation plot between Subleading and leading muon Pt(EC(Lead), EC(sublead))",30,0,30,30,0,30);
TH2F *h12 = new TH2F("h12","Correlation plot between Subleading and leading muon Pt(EC(Lead),Barrel(Sublead))",30,0,30,30,0,30);
TH2F *h13 = new TH2F("h13","Correlation plot between Subleading and leading muon Pt(EC(sublead),Barrel(Lead))",30,0,30,30,0,30);
//TH3F *h2 = new TH3F("h2","acceptance plot",300,0,300,100,0,150,100,0,1);
TH2F *hAcceptAll = new TH2F("hAcceptAll","Overall acceptance plot",120,0,30,120,0,30);
TH2F *hAccept1 = new TH2F("hAccept1","Leading Muon and Subleading Muon in 1.5<|#eta|<2.2 ",120,0,30,120,0,30);
TH2F *hAccept2 = new TH2F("hAccept2","Leading Muon in 1.5<|#eta|<2.2, subleading muon in |#eta|<1.5",120,0,30,120,0,30);
TH2F *hAccept3 = new TH2F("hAccept3","Leading Muon in |#eta|<1.5, subleading muon in 1.5<|#eta|<2.2 ",120,0,30,120,0,30);
//TProfile2D *h2 = new TProfile2D("h2","acceptance plot",100,0,300,100,0,300,0,1);
//TGraph2D *t2 = new TGraph2D();
TH1F *hInvTau = new TH1F("hInvTau","Invariant Mass plot for Taus",100,0,300);
TH1F *hInvMuon = new TH1F("hInvMuon","Invariant Mass plot for Muons(visible)",100,0,300);
TH1F *hImPEC = new TH1F("hImPEC","Impact parameter plot in 1.5<|#eta|<2.2",100,-0.06,0.06);
TH1F *hImPBa = new TH1F("hImPBa","Impact parameter plot in |#eta|<1.5",100,-0.06,0.06);
TH1F *hDZEC = new TH1F("hDZEC","Muon DZ plot End cap",100,-10,10);
TH1F *hDZBa = new TH1F("hDZBa","Muon DZ plot barrel",100,-10,10);
TH1F *hChiEC = new TH1F("hChiEC","Muon Chi plot End cap",100,0,16);
TH1F *hChiBa = new TH1F("hChiBa","Muon Chi plot barrel",100,0,16);
TH1F *hImPECEr = new TH1F("hImPECEr","Error in Impact parameter plot End cap",100,0,16);
TH1F *hImPBaEr = new TH1F("hImPBaEr","Error in Impact parameter plot barrel",100,0,16);
//Muon eta plots for pT threshold !
TH1F *hEtaPt10 = new TH1F("hEtaPt10","Reco Muon Eta 10",30,0,3);
TH1F *hEtaPt15 = new TH1F("hEtaPt15","Reco Muon Eta 15",30,0,3);
TH1F *hEtaPt20 = new TH1F("hEtaPt20","Reco Muon Eta 20",30,0,3);
TH1F *hEtaPt25 = new TH1F("hEtaPt25","Reco Muon Eta 25",30,0,3);
TH1F *hEtaPt30 = new TH1F("hEtaPt30","Reco Muon Eta 30",30,0,3);
TH1F *hEtaPt35 = new TH1F("hEtaPt35","Reco Muon Eta 35",30,0,3);
//2-D histograms
TH2F *hEtaResEC = new TH2F("hEtaResEC","Eta vs MuonPt resolution in Endcap",100,-3,3,100,-5,5);
TH2F *hEtaResBa = new TH2F("hEtaResBa","Eta vs MuonPt resolution in barrel",100,-3,3,100,-5,5);
TH2F *hEtaResAll = new TH2F("hEtaResAll","Eta vs MuonPt resolution Overall",100,-3,3,100,-5,5);
TH2F *hEtaPtEC = new TH2F("hEtaPtEC","Eta vs MuonPt in Endcap",100,-3,3,100,0,200);
TH2F *hEtaPtBa = new TH2F("hEtaPtBa","Eta vs MuonPt in barrel",100,-3,3,100,0,200);
TH2F *hEtaPtAll = new TH2F("hEtaPtAll","Reco Muon |#eta| vs p_{T}",100,0,3,100,0,200);
//std::vector<double> leadmuonpt;
//std::vector<double> subleadmuonpt;
Double_t pt_cut=30.;
Double_t bin_width=0.25;
const int bin_size_sl=pt_cut/bin_width;
const int bin_size_l=pt_cut/bin_width;
Double_t leadmuonpt[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt[bin_size_sl]={0};
Double_t leadmuonpt1[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt1[bin_size_sl]={0};
Double_t leadmuonpt2[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt2[bin_size_sl]={0};
Double_t leadmuonpt3[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt3[bin_size_sl]={0};
//TFile f("~/histos_mva_dytt.root","recreate");
TFile *f = new TFile("~/histos_mva_dytt.root","recreate");
//cout<<"New file created"<<endl;
TTree *tree = new TTree("gluHsignal","variables");
//cout<<"New tree created"<<endl;
tree->Branch("dimuoneta",&dimuoneta,"dimuoneta/D");
//tree->Branch("h1DiMuonEtaReco","TH1F",&h1DiMuonEtaReco,32000,0);
tree->Branch("dimuonratiopt",&dimuonratiopt,"dimuonratiopt/D");
//tree->Branch("coll_approx",&dimuonratiopt,"coll_approx/D");
tree->Branch("dcasig",&dcasig,"dcasig[2]/D");
tree->Branch("phi_pmuon_met",&phi_pmuon_met,"phi_pmuon_met/D");
tree->Branch("cost",&dcasig,"cost/D");
Long64_t nentries = fChain->GetEntriesFast();
cout<<"Number of events "<<nentries<<endl;
int dimuon=0;
Long64_t nbytes = 0, nb = 0;
//for (Long64_t jentry=0;jentry<nentries;jentry++) {
for (Long64_t jentry=0;jentry<nentries;jentry++) {
Long64_t ientry = LoadTree(jentry);
if (ientry < 0) break;
nb = fChain->GetEntry(jentry); nbytes += nb;
if (nMuon<2) continue;
if ((fabs(Muon_eta[0]) >= 2.1))continue;
if ((fabs(Muon_eta[1]) >= 2.1))continue;
if ((fabs(Muon_pt[0]) <= 20))continue;
if ((fabs(Muon_pt[1]) <= 10))continue;
if ((Muon_nMatchedStations[0])<1)continue;
if ((Muon_nMatchedStations[1])<1)continue;
if ((Muon_trackerLayersWithMeasurement[0])<=5)continue;
if ((Muon_trackerLayersWithMeasurement[1])<=5)continue;
if ((Muon_pixHits[0])<1)continue;
if ((Muon_pixHits[1])<1)continue;
if ((Muon_globalChi2[0])>=10)continue;
if ((Muon_globalChi2[1])>=10)continue;
if (fabs(Muon_trkD0[0])>=0.04)continue;
if (fabs(Muon_trkD0[1])>=0.04)continue;
//if (fabs(Muon_trkDz[0])>=1)continue;
//if (fabs(Muon_trkDz[1])>=1)continue;
if (fabs(Muon_vtxDistZ[0])>=0.1)continue;
if (fabs(Muon_vtxDistZ[1])>=0.1)continue;
if ((Muon_ptError[0]/Muon_pt[0])*100>=10) continue;
if ((Muon_ptError[1]/Muon_pt[1])*100>=10) continue;
if (fabs((Muon_phi[0]-Muon_phi[1])) <= 2) continue;
//if ((fabs(Muon_pt[0]) < 5))continue;
//if ((fabs(Muon_pt[1]) < 5))continue;
//if (abs(Muon_phi[0]-Muon_phi[1] < 2.0))continue;
e1=Muon_energy[0];
e2=Muon_energy[1];
p1x=Muon_px[0];
p2x=Muon_px[1];
p1y=Muon_py[0];
p2y=Muon_py[1];
p1z=Muon_pz[0];
p2z=Muon_pz[1];
inv_mass_vis=sqrt((e1+e2)*(e1+e2)-((p1x+p2x)*(p1x+p2x)+(p1y+p2y)*(p1y+p2y)+(p1z+p2z)*(p1z+p2z)));
if (inv_mass_vis<=35) continue;
/*count=0;
for (int i=0;i<nJet;i++){
if (Jet_pt[i]<30) ++count;
}
if (count<1) continue;
*/
++dimuon;
phi_1=Muon_phi[0];
phi_2=Muon_phi[1];
phi_tau_1=Tau_phi[0];
phi_tau_2=Tau_phi[1];
//cout<<phi_tau_1<<endl;
hInvTau->Fill(phi_tau_1-phi_tau_2);
//phi_tau_2=Tau_phi[1];
theta_1=2*atan(exp(-1*Muon_eta[0]));
theta_2=2*atan(exp(-1*Muon_eta[1]));
metx=MET_metx[0];
mety=MET_mety[0];
//cout<<metx<<" "<<mety<<endl;
p_1=(metx*sin(phi_2)-mety*cos(phi_2))/(sin(theta_1)*(cos(phi_1)-sin(phi_1)));
p_2=(metx*sin(phi_1)-mety*cos(phi_1))/(sin(theta_2)*(cos(phi_2)-sin(phi_2)));
pvis_1=sqrt(Muon_px[0]*Muon_px[0]+Muon_py[0]*Muon_py[0]+Muon_pz[0]*Muon_pz[0]);
pvis_2=sqrt(Muon_px[1]*Muon_px[1]+Muon_py[1]*Muon_py[1]+Muon_pz[1]*Muon_pz[1]);
//cout<<p_1<<endl;
x_1=pvis_1/(pvis_1+p_1);
x_2=pvis_2/(pvis_2+p_2);
/*e1=Muon_energy[0];
e2=Muon_energy[1];
p1x=Muon_px[0];
p2x=Muon_px[1];
p1y=Muon_py[0];
p2y=Muon_py[1];
p1z=Muon_pz[0];
p2z=Muon_pz[1];
inv_mass_vis=sqrt((e1+e2)*(e1+e2)-((p1x+p2x)*(p1x+p2x)+(p1y+p2y)*(p1y+p2y)+(p1z+p2z)*(p1z+p2z)));*/
inv_mass=inv_mass_vis/sqrt(fabs(x_1*x_2));
hPhiReco->Fill(((Muon_phi[0]-Muon_phi[1])/(22.0/7.0)));
//if ((fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) >= 1.1 || (fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) <= 0.9) cout<<(Muon_phi[0]-Muon_phi[1])/(22.0/7.0)<<" Pi"<<endl;
if (inv_mass>0) {
/*if ((fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) >= 1.3 || (fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) <= 0.7)hInvTau->Fill(inv_mass);*/
hInvMuon->Fill(inv_mass_vis);
}
if (inv_mass<0) {
/*if ((fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) >= 1.3 || (fabs((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))) <= 0.7)hInvTau->Fill(inv_mass);*/
cout<<"less than zero"<<endl;
}
h1DiMuonPtReco->Fill(sqrt(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1]))));
h1SumMuonPtReco->Fill(Muon_pt[0]+Muon_pt[1]);
if ((Muon_pt[0]+Muon_pt[1])!=0) dimuonratiopt=(sqrt(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1])))/(Muon_pt[0]+Muon_pt[1]));
//dimuonratiopt=(dimuonpt/SumMuonPt);
h1DiMuonSumPtRatio->Fill(dimuonratiopt);
summuonpt=Muon_pt[0]+Muon_pt[1];
dimuonpt=sqrt(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1])));
hSumMuonDiMuon->Fill(summuonpt,dimuonpt);
//cout<<(Muon_pt[0]+Muon_pt[1])<<endl;
//cout<<dimuonratiopt<<endl;
//cout<<"DiMuon pT"<<sqrt(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1])))<<"Sum of pT "<<Muon_pt[0]+Muon_pt[1]<<endl;
mag=sqrt(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1]))+((Muon_pz[0]+Muon_pz[1])*(Muon_pz[0]+Muon_pz[1])));
/*if ((mag-Muon_pz[0]+Muon_pz[1])!=0)*/dimuoneta=0.5*log((mag+Muon_pz[0]+Muon_pz[1])/(mag-(Muon_pz[0]+Muon_pz[1])));
h1DiMuonEtaReco->Fill(dimuoneta);
//h1MuonImpParSig->Fill(log10(Muon_trkD0[0]/Muon_trkD0Error[0]));
//h1MuonImpParSig->Fill(log10(Muon_trkD0[1]/Muon_trkD0Error[1]));
dcasig[0]=log10(Muon_trkD0[0]/Muon_trkD0Error[0]);
dcasig[1]=log10(Muon_trkD0[1]/Muon_trkD0Error[1]);
if (Muon_charge[0]>0)h1PhiPMuonMet->Fill(Muon_phi[0]-MET_metphi[0]);
if (Muon_charge[1]>0)h1PhiPMuonMet->Fill(Muon_phi[1]-MET_metphi[1]);
phi_pmuon_met=Muon_phi[0]-MET_metphi[0];
//if (((Muon_phi[0]-Muon_phi[1])/(22.0/7.0))==1) cout<<"meow"<<endl;
//Routine to calculate dimuon rest frame
// --- Get the muons parameters in the CM frame
//
TLorentzVector pMu1CM(p1x,p1y,p1z,e1);
TLorentzVector pMu2CM(p2x,p2y,p2z,e2);
//
// --- Obtain the dimuon parameters in the CM frame
//
TLorentzVector pDimuCM=pMu1CM+pMu2CM;
//
// --- Translate the dimuon parameters in the dimuon rest frame
//
TVector3 beta=(-1./pDimuCM.E())*pDimuCM.Vect();
TLorentzVector pMu1Dimu=pMu1CM;
TLorentzVector pMu2Dimu=pMu2CM;
pMu1Dimu.Boost(beta);
pMu2Dimu.Boost(beta);
//
// --- Determine the z axis for the calculation of the polarization angle
// (i.e. the direction of the dimuon in the CM system)
//
TVector3 zaxis;
zaxis=(pDimuCM.Vect()).Unit();
if (Muon_charge[0]>0)cost = zaxis.Dot((pMu1Dimu.Vect()).Unit());
if (Muon_charge[1]>0)cost = zaxis.Dot((pMu2Dimu.Vect()).Unit());
//h1DecayAngleDiMuonRest->Fill(cost);
//cout<<cost<<endl;
//cout<<dimuoneta<<endl;
//if ((((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1]))+((Muon_pz[0]+Muon_pz[1])*(Muon_pz[0]+Muon_pz[1])))<0.)
//cout<<(((Muon_px[0]+Muon_px[1])*(Muon_px[0]+Muon_px[1]))+((Muon_py[0]+Muon_py[1])*(Muon_py[0]+Muon_py[1]))+((Muon_pz[0]+Muon_pz[1])*(Muon_pz[0]+Muon_pz[1])))<<endl;
//tree->Fill();
//if (((mag+Muon_pz[0]+Muon_pz[1])/(mag-Muon_pz[0]+Muon_pz[1]))<0)cout<<"neg"<<endl;
/*
if (Muon_pt[0]>=Muon_pt[1]){
h1PtLeading->Fill(Muon_pt[0]);
h1PtSubLeading->Fill(Muon_pt[1]);
}
else if (Muon_pt[0]<Muon_pt[1]){
h1->Fill(Muon_pt[1],Muon_pt[0]);
//cout<<Muon_pt[1]<<" "<<Muon_pt[0]<<endl;
}
//Different cases
if (Muon_pt[0]>Muon_pt[1]){
if (((fabs(Muon_eta[0]) > 1.5) && (fabs(Muon_eta[0]) < 2.2)) && ((fabs(Muon_eta[1]) > 1.5) && (fabs(Muon_eta[1]) < 2.2))){
hLeadMuon1->Fill(Muon_pt[0]);
hSubLeadMuon1->Fill(Muon_pt[1]);
h1->Fill(Muon_pt[0],Muon_pt[1]);
h11->Fill(Muon_pt[0],Muon_pt[1]);
if (Muon_pt[0]<pt_cut){
for (int i=0;i<bin_size_l;i++){
if (Muon_pt[0]>=i*bin_width && Muon_pt[0]<(i+1)*bin_width){
leadmuonpt[i]+=1;
leadmuonpt1[i]+=1;
}
}
}
if (Muon_pt[1]<pt_cut){
for (int i=0;i<bin_size_sl;i++){
if (Muon_pt[1]>i*bin_width && Muon_pt[1]<=(i+1)*bin_width){
subleadmuonpt[i]+=1;
subleadmuonpt1[i]+=1;
}
}
}
}
if (((fabs(Muon_eta[0]) > 1.5) && (fabs(Muon_eta[0]) < 2.2)) && (fabs(Muon_eta[1]) < 1.5)){
h1->Fill(Muon_pt[0],Muon_pt[1]);
h12->Fill(Muon_pt[0],Muon_pt[1]);
hLeadMuon2->Fill(Muon_pt[0]);
hSubLeadMuon2->Fill(Muon_pt[1]);
if (Muon_pt[0]<pt_cut){
for (int i=0;i<bin_size_l;i++){
if (Muon_pt[0]>=i*bin_width && Muon_pt[0]<(i+1)*bin_width){
leadmuonpt[i]+=1;
leadmuonpt2[i]+=1;
}
}
}
if (Muon_pt[1]<pt_cut){
for (int i=0;i<bin_size_sl;i++){
if (Muon_pt[1]>i*bin_width && Muon_pt[1]<=(i+1)*bin_width){
subleadmuonpt[i]+=1;
subleadmuonpt2[i]+=1;
}
}
}
}
if ((fabs(Muon_eta[0]) < 1.5) && ((fabs(Muon_eta[1]) > 1.5) && (fabs(Muon_eta[1]) < 2.2))){
h1->Fill(Muon_pt[0],Muon_pt[1]);
h13->Fill(Muon_pt[0],Muon_pt[1]);
hLeadMuon3->Fill(Muon_pt[0]);
hSubLeadMuon3->Fill(Muon_pt[1]);
if (Muon_pt[0]<pt_cut){
for (int i=0;i<bin_size_l;i++){
if (Muon_pt[0]>=i*bin_width && Muon_pt[0]<(i+1)*bin_width){
leadmuonpt[i]+=1;
leadmuonpt3[i]+=1;
}
}
}
if (Muon_pt[1]<pt_cut){
for (int i=0;i<bin_size_sl;i++){
if (Muon_pt[1]>i*bin_width && Muon_pt[1]<=(i+1)*bin_width){
subleadmuonpt[i]+=1;
subleadmuonpt3[i]+=1;
}
}
}
}
}
else{
if (((fabs(Muon_eta[1]) > 1.5) && (fabs(Muon_eta[1]) < 2.2)) && ((fabs(Muon_eta[0]) > 1.5) && (fabs(Muon_eta[0]) < 2.2))){
hLeadMuon1->Fill(Muon_pt[1]);
hSubLeadMuon1->Fill(Muon_pt[0]);
}
if (((fabs(Muon_eta[1]) > 1.5) && (fabs(Muon_eta[1]) < 2.2)) && (fabs(Muon_eta[0]) < 1.5)){
hLeadMuon2->Fill(Muon_pt[1]);
hSubLeadMuon2->Fill(Muon_pt[0]);
}
if ((fabs(Muon_eta[1]) < 1.5) && ((fabs(Muon_eta[0]) > 1.5) && (fabs(Muon_eta[0]) < 2.2))){
hLeadMuon3->Fill(Muon_pt[1]);
hSubLeadMuon3->Fill(Muon_pt[0]);
}
}
/*for (int j=0;j<nGenParticle;j++){
delRMin=0.03;
for (int i=0;i<nMuon; i++){
//counter=0;
RecoMuonEta=Muon_eta[i];
delphi=Muon_phi[i]-GenParticle_phi[j];
deleta=Muon_eta[i]-GenParticle_eta[j];
delR=sqrt(delphi*delphi+deleta*deleta);
if (delR < delRMin){
delRMin=delR;
if ((fabs(RecoMuonEta) > 1.5) && (fabs(RecoMuonEta) < 2.2)){
//cout<<"haha"<<endl;
denominator++;
//cout<<"Reco Muon Charge "<<Muon_charge[i]<<"Gen Muon Charge "<<GenParticle_charge[j]<<endl;
GenMuonCharge=GenParticle_charge[j];
RecoMuonCharge=Muon_charge[i];
if (GenMuonCharge-RecoMuonCharge != 0) cout<<"MISMATCH"<<endl;//numerator++;
}
}
//cout<<delR<<endl;
}
}
//if (denominator!=0)cout<<"MIS ID "<<numerator/denominator<<endl;
for (int i=0;i<nMuon; i++){
RecoMuonEta=Muon_eta[i];
if (fabs(RecoMuonEta)>2.4) cout<<"one"<<endl;
GenMuonPt=Muon_ptgen[i];
ImpParam=Muon_trkD0[i];
MuonDZ=Muon_trkDz[i];
MuonChi2=Muon_globalChi2[i];
RecoMuonPt=Muon_pt[i];
if ((fabs(RecoMuonEta) > 1.5) && (fabs(RecoMuonEta) < 2.2)){
hMCMatchEC->Fill((GenMuonPt-RecoMuonPt)/GenMuonPt);
hImPEC->Fill(ImpParam);
hDZEC->Fill(MuonDZ);
hChiEC->Fill(MuonChi2);
hEtaPtEC->Fill(RecoMuonEta,RecoMuonPt);
//if(Muon_charge[i]-Muon_chargegen[i]!=0) cout<<"MISMATCH"<<endl;
//hEtaResEC->Fill(RecoMuonPt-GenMuonPt,RecoMuonEta);
}
if ((fabs(Muon_eta[i]) < 1.5)){
hMCMatchBa->Fill((GenMuonPt-RecoMuonPt)/GenMuonPt);
hImPBa->Fill(ImpParam);
hDZBa->Fill(MuonDZ);
hChiBa->Fill(MuonChi2);
hEtaPtBa->Fill(RecoMuonEta,RecoMuonPt);
}
h1PtGen->Fill(GenMuonPt);
h1PtReco->Fill(RecoMuonPt);
h1EtaReco->Fill(RecoMuonEta);
hEtaPtAll->Fill(fabs(RecoMuonEta),RecoMuonPt);
if (RecoMuonPt>10.){
hEtaPt10->Fill(fabs(RecoMuonEta));
//if(fabs(RecoMuonEta) < 1.5)counter_barrel++;
}
if (RecoMuonPt>15.){
hEtaPt15->Fill(fabs(RecoMuonEta));
}
if (RecoMuonPt>20.){hEtaPt20->Fill(fabs(RecoMuonEta));}
if (RecoMuonPt>25.){hEtaPt25->Fill(fabs(RecoMuonEta));}
if (RecoMuonPt>30.){hEtaPt30->Fill(fabs(RecoMuonEta));}
if (RecoMuonPt>35.){hEtaPt35->Fill(fabs(RecoMuonEta));}
if(fabs(RecoMuonEta) < 1.5)counter_ba++;
if((fabs(RecoMuonEta) > 1.5) && (fabs(RecoMuonEta) < 2.2))counter_ec++;
if((fabs(RecoMuonEta) > 2.2) && (fabs(RecoMuonEta) < 2.4))counter_out++;
//if ()
}*/
tree->Fill();
//f->cd();
//f1->Write();
//f->Close();
}
//
//h1DiMuonSumPtRatio->Write();
//h1MuonImpParSig->Write();
//h1DiMuonEtaReco->Write();
//h1PhiPMuonMet->Write();
//hSumMuonDiMuon->Write();
//f->Close();
cout<<"Number of dimuon events "<<dimuon<<" BR of di tau to dimuon "<<((float)dimuon/(float)nentries)*100<<endl;
cout<<"Number of muons in barrel "<<(float)counter_ba/(float)(dimuon*2)<<" Number of muons in endcap "<<(float)counter_ec/(float)(dimuon*2)<<" Number of muons outside "<<(float)counter_out/(float)(dimuon*2)<<endl;
f->cd();
tree->Write();
f->Close();
//Overall
/*
total_events=0;
events=0;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
if(leadmuonpt[i]!=0 && subleadmuonpt[j]!=0)total_events+=leadmuonpt[i]*subleadmuonpt[j];
}
}
cout<<total_events<<endl;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
events=0;
for (int k=i;k<bin_size_l;k++){
for (int l=j;l<k;l++){
if(leadmuonpt[k]!=0 && subleadmuonpt[l]!=0)events+=leadmuonpt[k]*subleadmuonpt[l];
}
}
hAcceptAll->Fill(i*bin_width,j*bin_width,((Double_t)events/(Double_t)total_events)*100);
}
}
//Case-1
total_events=0;
events=0;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
if(leadmuonpt1[i]!=0 && subleadmuonpt1[j]!=0)total_events+=leadmuonpt1[i]*subleadmuonpt1[j];
}
}
cout<<total_events<<endl;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
events=0;
for (int k=i;k<bin_size_l;k++){
for (int l=j;l<k;l++){
if(leadmuonpt1[k]!=0 && subleadmuonpt1[l]!=0)events+=leadmuonpt1[k]*subleadmuonpt1[l];
}
}
hAccept1->Fill(i*bin_width,j*bin_width,((Double_t)events/(Double_t)total_events)*100);
}
}
//Case-2
total_events=0;
events=0;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
if(leadmuonpt2[i]!=0 && subleadmuonpt2[j]!=0)total_events+=leadmuonpt2[i]*subleadmuonpt2[j];
}
}
cout<<total_events<<endl;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
events=0;
for (int k=i;k<bin_size_l;k++){
for (int l=j;l<k;l++){
if(leadmuonpt2[k]!=0 && subleadmuonpt2[l]!=0)events+=leadmuonpt2[k]*subleadmuonpt2[l];
}
}
hAccept2->Fill(i*bin_width,j*bin_width,((Double_t)events/(Double_t)total_events)*100);
}
}
//Case-3
total_events=0;
events=0;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
if(leadmuonpt3[i]!=0 && subleadmuonpt3[j]!=0)total_events+=leadmuonpt3[i]*subleadmuonpt3[j];
}
}
cout<<total_events<<endl;
for (int i=0;i<bin_size_l;i++){
for (int j=0;j<i;j++){
events=0;
for (int k=i;k<bin_size_l;k++){
for (int l=j;l<k;l++){
if(leadmuonpt3[k]!=0 && subleadmuonpt3[l]!=0)events+=leadmuonpt3[k]*subleadmuonpt3[l];
}
}
//myfile2<<i<<" "<<j<<" "<<" "<<events<<" "<<total_events<<" "<<(Double_t)events/(Double_t)total_events<<endl;
//myfile2<<i*bin_width<<" "<<j*bin_width<<" "<<(Double_t)events/(Double_t)total_events<<endl;
hAccept3->Fill(i*bin_width,j*bin_width,((Double_t)events/(Double_t)total_events)*100);
//hAccept1->Fill(i*bin_width,j*bin_width);
}
}
*/
}
//hAcceptAll->Write();
/*
//Time to beautify most of the plots
hLeadMuon1->SetLineColor(1);
hSubLeadMuon1->SetLineColor(4);
hLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hSubLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hLeadMuon1->SetYTitle("Number of Events/GeV");
hSubLeadMuon1->SetYTitle("Number of Events/GeV");
hSubLeadMuon1->Draw();
gPad->Update();
hLeadMuon1->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon1->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon1->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon1->Draw();
hLeadMuon1->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 1");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon1,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case1.pdf");
c1->Clear();
hLeadMuon2->SetLineColor(1);
hSubLeadMuon2->SetLineColor(4);
hLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon2->SetYTitle("Number of Events/GeV");
hSubLeadMuon2->SetYTitle("Number of Events/GeV");
hSubLeadMuon2->Draw();
gPad->Update();
hLeadMuon2->Draw();
gPad->Update();
//draw hist_2 first as it has a larger range
TPaveStats *tps1 = (TPaveStats*) hLeadMuon2->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon2->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon2->Draw();
hLeadMuon2->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 2");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon2,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon2,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case2.pdf");
hLeadMuon3->SetLineColor(1);
hSubLeadMuon3->SetLineColor(4);
hLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->Draw();
gPad->Update();
hLeadMuon3->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon3->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon3->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon3->Draw();
hLeadMuon3->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 3");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon3,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon3,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case3.pdf");
c1->Clear();
//pT resolution plots
hMCMatchEC->SetLineColor(1);
hMCMatchEC->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchEC->Draw(); //draw hist_2 first as it has a larger range
hMCMatchEC->Draw("esame"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_1.pdf");
c1->Clear();
hMCMatchBa->SetLineColor(1);
hMCMatchBa->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchBa->Draw(); //draw hist_2 first as it has a larger range
hMCMatchBa->Draw("esame"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_2.pdf");
c1->Clear();
//Acceptance plots
//Overall Acceptance
hAcceptAll->SetXTitle("Reco. leading muon p_{T} in GeV/c");
hAcceptAll->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//hAcceptAll->SetZTitle("Kinematic acceptance");
hAcceptAll->SetStats(0);
hAcceptAll->Draw("cont3 colz");
//HLT_PATH Mu17_Mu8
Int_t a=hAcceptAll->GetXaxis()->FindBin(17);
Int_t b = hAcceptAll->GetYaxis()->FindBin(8);
Double_t c = hAcceptAll->GetBinContent(a,b);
//Double_t a = hAcceptAll->GetBinContent(hAcceptAll->GetXaxis()->FindBin(17),hAcceptAll->GetYaxis()->FindBin(8)));
//HLT_PATH Mu13_Mu8
//Double_t f=hAcceptAll->GetBinContent(
Int_t d = hAcceptAll->GetXaxis()->FindBin(13);
Int_t e = hAcceptAll->GetYaxis()->FindBin(8);
Double_t f = hAcceptAll->GetBinContent(d,e);
//Offline Cuts
Int_t g=hAcceptAll->GetXaxis()->FindBin(20);
Int_t h=hAcceptAll->GetYaxis()->FindBin(10);
Double_t z=hAcceptAll->GetBinContent(g,h);
//Double_t i=GetBinContenthAcceptAll->GetXaxis()->FindBin(20),hAcceptAll->GetYaxis()->FindBin(10));
cout<<c<<" "<<f<<" "<<z<<endl;
//Double_t m=hAcceptAll->GetBinContent(k,l);
//draw hist_2 first as it has a larger range
TLatex latex;
//latex.DrawLatex(17,8,TString::Format("#bullet %f ",a));
//latex.DrawLatex(17,8,TString::Format("#bullet %2f %",c*100));
latex.DrawLatex(17,8,"#bullet 64%");
latex.DrawLatex(13,8,"#diamond 72%");
latex.DrawLatex(20,10,"#color[2]{#bullet} 43%");
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(13,8,TString::Format("#diamond %f ",f));
//latex.DrawLatex(20,10,TString::Format("#circ %f ",i));
latex.DrawLatex(3,22,"#bullet");
latex.DrawLatex(2.83,25,"#diamond");
latex.DrawLatex(4,28,"#scale[0.8]{HLT Paths(Run-1)}");
latex.DrawLatex(4,22,"#scale[0.8]{Mu17_Mu8 (Luminosity 19.1 fb^{-1})}");
latex.DrawLatex(4,25,"#scale[0.8]{Mu13_Mu8 (Luminosity 3.7 fb^{-1})}");
latex.DrawLatex(4,19,"#scale[0.8]{Offline selection}");
latex.DrawLatex(3,16,"#color[2]{#bullet}");
latex.DrawLatex(4,16,"#scale[0.8]{Mu20_Mu10}");
c1->SaveAs("acceptance_2d_all_cont3.pdf");
//Case-1
*/
<file_sep>#include "TPaveStats.h"
void plot1(){
//TCanvas *c1 = new TCanvas("c1","c1",59,80,1288,535);
//c1->Divide(3,1);
//c1->cd(1);
hLeadMuon1->SetLineColor(1);
hSubLeadMuon1->SetLineColor(4);
hLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hSubLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hLeadMuon1->SetYTitle("Number of Events/GeV");
hSubLeadMuon1->SetYTitle("Number of Events/GeV");
//hSubLeadMuon1->GetXaxis()->SetTitleOffset(1.4);
//hLeadMuon1->GetXaxis()->SetTitleOffset(1.4);
hSubLeadMuon1->Draw();
gPad->Update();
hLeadMuon1->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon1->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon1->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon1->Draw();
hLeadMuon1->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4770115,0.6038136,0.7658046,0.7923729);
leg_hist->SetHeader("P_{T} histograms for case 1");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon1,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case1.png");
}
<file_sep>#include "TPaveStats.h"
void plot_dimuoneta(){
//TCanvas *c1 = new TCanvas("c1","c1",59,80,1288,535);
//c1->Divide(3,1);
//c1->cd(1);
h1DiMuonEtaReco->SetLineColor(1);
h1DiMuonEtaReco->SetXTitle("Reco. Muon #eta");
h1DiMuonEtaReco->SetYTitle("Number of Events/GeV");
//h1SumMuonPtReco->GetXaxis()->SetTitleOffset(1.4);
//h1DiMuonPtReco->GetXaxis()->SetTitleOffset(1.4);
//h1SumMuonPtReco->Draw();
//gPad->Update();
/* TPaveStats *tps1 = (TPaveStats*) h1DiMuonEtaReco->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
*/
h1DiMuonEtaReco->Draw();
//tps1->Draw("same");
c1->SaveAs("muoneta.png");
}
<file_sep>import FWCore.ParameterSet.Config as cms
from VHTauTau.TreeMaker.EventBlock_cfi import eventBlock
from VHTauTau.TreeMaker.VertexBlock_cfi import vertexBlock
from VHTauTau.TreeMaker.ElectronBlock_cfi import electronBlock
from VHTauTau.TreeMaker.MuonBlock_cfi import muonBlock
from VHTauTau.TreeMaker.TauBlock_cfi import tauBlock
from VHTauTau.TreeMaker.JetBlock_cfi import jetBlock
from VHTauTau.TreeMaker.METBlock_cfi import metBlock
from VHTauTau.TreeMaker.TriggerBlock_cfi import triggerBlock
from VHTauTau.TreeMaker.TriggerObjectBlock_cfi import triggerObjectBlock
treeContentSequence = cms.Sequence(
eventBlock
+ vertexBlock
+ electronBlock
+ muonBlock
+ tauBlock
+ jetBlock
+ metBlock
+ triggerBlock
+ triggerObjectBlock
)
<file_sep>#ifndef __VHTauTau_TreeMaker_GenMETBlock_h
#define __VHTauTau_TreeMaker_GenMETBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class GenMET;
class GenMETBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit GenMETBlock(const edm::ParameterSet& iConfig);
virtual ~GenMETBlock() {}
enum {
kMaxGenMET = 100
};
private:
TClonesArray* cloneGenMET;
int fnGenMET;
int _verbosity;
edm::InputTag _inputTag;
vhtm::GenMET* genMetB;
};
#endif
<file_sep>#ifndef __VHTauTau_TreeMaker_EventBlock_h
#define __VHTauTau_TreeMaker_EventBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class Event;
class EventBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(edm::Event const& iEvent, edm::EventSetup const& iSetup);
virtual void endJob(){}
public:
explicit EventBlock(const edm::ParameterSet& iConfig);
virtual ~EventBlock();
private:
TClonesArray* cloneEvent;
int _verbosity;
const edm::InputTag _l1InputTag;
const edm::InputTag _vtxInputTag;
const edm::InputTag _trkInputTag;
const unsigned int _vtxMinNDOF;
const double _vtxMaxAbsZ, _vtxMaxd0;
const unsigned int _numTracks;
const double _hpTrackThreshold;
std::vector<int> *_nPU;
std::vector<int> *_bunchCrossing;
std::vector<int> *_trueNInt;
vhtm::Event* eventB;
};
#endif
<file_sep>void plot_imp_par_EC(){
hImPEC->SetLineColor(1);
//h1PtReco->SetLineColor(4);
//h1PtReco->SetXTitle("GeV/c");
hImPEC->SetXTitle("d_{xy} in cm");
hImPEC->GetYaxis()->SetTitleOffset(1.3);
hImPEC->SetYTitle("Events");
hImPEC->Draw(); //draw hist_2 first as it has a larger range
hImPEC->Draw("c hist same"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_imp_1.eps");
c1->SaveAs("plot_imp_1.png");
//h1PtReco->Draw("same");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms");
leg_hist->AddEntry(hImPEC,"Gen Pt","l");
leg_hist->AddEntry(h1PtReco,"Reco Pt","l");
leg_hist->Draw();*/
}
<file_sep>#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/VertexReco/interface/Vertex.h"
#include "DataFormats/VertexReco/interface/VertexFwd.h"
#include "VHTauTau/TreeMaker/plugins/VertexBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
VertexBlock::VertexBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("vertexSrc"))
{
}
void VertexBlock::beginJob() {
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneVertex = new TClonesArray("vhtm::Vertex");
tree->Branch("Vertex", &cloneVertex, 32000, 2);
tree->Branch("nVertex", &fnVertex, "fnVertex/I");
}
void VertexBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneVertex->Clear();
fnVertex = 0;
edm::Handle<reco::VertexCollection> primaryVertices;
iEvent.getByLabel(_inputTag, primaryVertices);
if (primaryVertices.isValid()) {
edm::LogInfo("VertexBlock") << "Total # Primary Vertices: " << primaryVertices->size();
for (reco::VertexCollection::const_iterator it = primaryVertices->begin();
it != primaryVertices->end(); ++it) {
if (fnVertex == kMaxVertex) {
edm::LogInfo("VertexBlock") << "Too many Vertex, fnVertex = " << fnVertex;
break;
}
vertexB = new ((*cloneVertex)[fnVertex++]) vhtm::Vertex();
vertexB->x = it->x();
vertexB->y = it->y();
vertexB->z = it->z();
vertexB->xErr = it->xError();
vertexB->yErr = it->yError();
vertexB->zErr = it->zError();
vertexB->rho = it->position().rho();
vertexB->chi2 = it->chi2();
vertexB->ndf = it->ndof();
vertexB->ntracks = int(it->tracksSize());
vertexB->ntracksw05 = it->nTracks(0.5); // number of tracks in the vertex with weight above 0.5
vertexB->isfake = it->isFake();
vertexB->isvalid = it->isValid();
vertexB->sumPt = it->p4().pt();
}
}
else {
edm::LogError("VertexBlock") << "Error >> Failed to get VertexCollection for label: "
<< _inputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(VertexBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
treeCreator = cms.EDAnalyzer("TreeMakerModule",
verbosity = cms.int32(0),
createTree = cms.bool(True)
)
<file_sep>rm -f submit_skimmer-*.jdl
rm -f test_skimmer_condor_file*.py
cp file test/
rm -f file*
cp test/file .
cp condor_vhtree_local.C condor_makeclass_local.C test/
rm condor_vhtree*.C condor_makeclass_*.C
cp test/condor_vhtree_local.C test/condor_makeclass_local.C .
rm -f vhtree_file*
rm -f *~<file_sep>import FWCore.ParameterSet.Config as cms
muonBlock = cms.EDAnalyzer("MuonBlock",
verbosity = cms.int32(0),
#muonSrc = cms.InputTag('muonVariables'),
muonSrc = cms.InputTag('selectedPatMuonsPFlow'),
#muonSrc = cms.InputTag('selectedPatMuons'),
vertexSrc = cms.InputTag('offlinePrimaryVertices'),
offlineBeamSpot = cms.InputTag('offlineBeamSpot'),
beamSpotCorr = cms.bool(True),
muonID = cms.string('GlobalMuonPromptTight')
)
<file_sep>void plotres1(){
hMCMatchEC->SetLineColor(1);
//h1PtReco->SetLineColor(4);
//h1PtReco->SetXTitle("GeV/c");
hMCMatchEC->GetYaxis()->SetTitleOffset(1.3);
hMCMatchEC->SetYTitle("Events");
hMCMatchEC->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchEC->GetXaxis()->SetTitleOffset(1.1);
hMCMatchEC->Draw(); //draw hist_2 first as it has a larger range
hMCMatchEC->Draw("c hist same"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_1.eps");
c1->SaveAs("plot_res_1.png");
//h1PtReco->Draw("same");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms");
leg_hist->AddEntry(hMCMatchEC,"Gen Pt","l");
leg_hist->AddEntry(h1PtReco,"Reco Pt","l");
leg_hist->Draw();*/
}
<file_sep>void plot_eta_pt(){
hEtaPt10->SetLineColor(8);
hEtaPt15->SetLineColor(9);
hEtaPt20->SetLineColor(6);
hEtaPt25->SetLineColor(2);
hEtaPt30->SetLineColor(5);
hEtaPt35->SetLineColor(30);
hEtaPt10->SetStats(0);
hEtaPt10->SetTitle("|#eta| as a function of p_{T} threshold");
hEtaPt10->SetXTitle("|#eta_{#mu}^{Reco}|");
hEtaPt10->GetYaxis()->SetTitleOffset(1.23);
hEtaPt10->SetYTitle("Events/0.1");
hEtaPt10->Draw("hist e");
hEtaPt15->Draw("hist e SAME");
hEtaPt20->Draw("hist e SAME");
hEtaPt25->Draw("hist e SAME");
hEtaPt30->Draw("hist e SAME");
hEtaPt35->Draw("hist e SAME");
TPaveText *pt = new TPaveText(0.9856374,1222.805,1.427289,1392.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
TText *text = pt->AddText("0 < |#eta| < 1.5");
text = pt->AddText("77 %");
pt->Draw();
pt = new TPaveText(1.627644,1222.805,2.076191,1392.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
text = pt->AddText("1.5 < |#eta| < 2.2");
text = pt->AddText("20 %");
pt->Draw();
pt = new TPaveText(2.278276,822.805,2.7307,992.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
text = pt->AddText("2.2 < |#eta| < 2.4");
text = pt->AddText("3 %");
pt->Draw();
leg_hist = new TLegend(0.7586207,0.6772152,0.9382184,0.8649789);
//leg_hist->SetHeader("Pt histograms");
leg_hist->SetBorderSize(0);
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hEtaPt10,"p_{T} > 10 GeV","f");
leg_hist->AddEntry(hEtaPt15,"p_{T} > 15 GeV","f");
leg_hist->AddEntry(hEtaPt20,"p_{T} > 20 GeV","f");
leg_hist->AddEntry(hEtaPt25,"p_{T} > 25 GeV","f");
leg_hist->AddEntry(hEtaPt30,"p_{T} > 30 GeV","f");
leg_hist->AddEntry(hEtaPt35,"p_{T} > 35 GeV","f");
leg_hist->Draw();
TLine *line1=new TLine(1.5,0,1.5,1500);
line1->SetLineStyle(2);
line1->SetLineColor(9);
line1->SetLineWidth(3);
line1->Draw("SAME");
TLine *line2=new TLine(2.2,0,2.2,1500);
line2->SetLineStyle(2);
line2->SetLineColor(2);
line2->SetLineWidth(3);
line2->Draw("SAME");
TLine *line3=new TLine(2.4,0,2.4,1500);
line3->SetLineStyle(2);
line3->SetLineColor(8);
line3->SetLineWidth(3);
line3->Draw("SAME");
c1->SaveAs("plot_eta_pt.png");
}
<file_sep>#include <iostream>
#include <algorithm>
#include "TFile.h"
#include "TTree.h"
#include "TROOT.h"
#include "TClonesArray.h"
#include "TVector3.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/PatCandidates/interface/Electron.h"
#include "FWCore/Framework/interface/ESHandle.h"
#include "DataFormats/GsfTrackReco/interface/GsfTrack.h"
#include "DataFormats/TrackReco/interface/HitPattern.h"
#include "DataFormats/TrackReco/interface/Track.h"
#include "DataFormats/TrackReco/interface/TrackFwd.h"
#include "DataFormats/VertexReco/interface/Vertex.h"
#include "DataFormats/VertexReco/interface/VertexFwd.h"
#include "DataFormats/BeamSpot/interface/BeamSpot.h"
#include "VHTauTau/TreeMaker/plugins/ElectronBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
// Constructor
ElectronBlock::ElectronBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_bsInputTag(iConfig.getParameter<edm::InputTag>("offlineBeamSpot")),
_trkInputTag(iConfig.getParameter<edm::InputTag>("trackSrc")),
_vtxInputTag(iConfig.getParameter<edm::InputTag>("vertexSrc")),
_electronInputTag(iConfig.getParameter<edm::InputTag>("electronSrc"))
{
}
ElectronBlock::~ElectronBlock() {
}
void ElectronBlock::beginJob()
{
// Get TTree pointer
std::string tree_name = "vhtree";
TTree* tree = vhtm::Utility::getTree(tree_name);
cloneElectron = new TClonesArray("vhtm::Electron");
tree->Branch("Electron", &cloneElectron, 32000, 2);
tree->Branch("nElectron", &fnElectron, "fnElectron/I");
}
void ElectronBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneElectron->Clear();
fnElectron = 0;
edm::Handle<reco::BeamSpot> beamSpot;
iEvent.getByLabel(_bsInputTag, beamSpot);
edm::Handle<reco::VertexCollection> primaryVertices;
iEvent.getByLabel(_vtxInputTag, primaryVertices);
edm::Handle<std::vector<pat::Electron> > electrons;
iEvent.getByLabel(_electronInputTag, electrons);
if (electrons.isValid()) {
edm::LogInfo("ElectronBlock") << "Total # PAT Electrons: " << electrons->size();
for (std::vector<pat::Electron>::const_iterator it = electrons->begin();
it != electrons->end(); ++it) {
if (fnElectron == kMaxElectron) {
edm::LogInfo("ElectronBlock") << "Too many PAT Electrons, fnElectron = "
<< fnElectron;
break;
}
bool hasGsfTrack = it->gsfTrack().isNonnull() ? true : false;
reco::GsfTrackRef tk = it->gsfTrack();
electronB = new ((*cloneElectron)[fnElectron++]) vhtm::Electron();
electronB->ecalDriven = it->ecalDrivenSeed();
electronB->eta = it->eta();
electronB->phi = it->phi();
electronB->pt = it->pt();
electronB->hasGsfTrack = hasGsfTrack;
electronB->energy = it->energy();
electronB->caloEnergy = it->ecalEnergy();
electronB->caloEnergyError = it->ecalEnergyError();
electronB->charge = it->charge();
if (hasGsfTrack) {
electronB->trackPt = tk->pt();
electronB->trackPtError = tk->ptError();
// Hit pattern
const reco::HitPattern& hitp = tk->hitPattern();
electronB->pixHits = hitp.numberOfValidPixelHits();
electronB->trkHits = hitp.numberOfValidTrackerHits();
electronB->nValidHits = tk->numberOfValidHits();
//electronB->missingHits = tk->trackerExpectedHitsInner().numberOfHits();
electronB->trkD0 = tk->d0();
electronB->trkD0Error = tk->d0Error();
}
// ID variables
electronB->hoe = it->hcalOverEcal();
electronB->hoeDepth1 = it->hcalDepth1OverEcal();
electronB->eop = it->eSuperClusterOverP();
electronB->sigmaEtaEta = it->sigmaEtaEta();
electronB->sigmaIEtaIEta = it->sigmaIetaIeta();
electronB->deltaPhiTrkSC = it->deltaPhiSuperClusterTrackAtVtx();
electronB->deltaEtaTrkSC = it->deltaEtaSuperClusterTrackAtVtx();
electronB->classif = it->classification();
electronB->e1x5overe5x5 = (it->e5x5() > 0) ? (it->e1x5()/it->e5x5()) : 0;
electronB->e2x5overe5x5 = (it->e5x5() > 0) ? (it->e2x5Max()/it->e5x5()) : 0;
// Iso variables
electronB->isoEcal03 = it->dr03EcalRecHitSumEt();
electronB->isoHcal03 = it->dr03HcalTowerSumEt();
electronB->isoTrk03 = it->dr03TkSumPt();
electronB->isoEcal04 = it->dr04EcalRecHitSumEt(); // ecalIso
electronB->isoHcal04 = it->dr04HcalTowerSumEt(); // hcalIso
electronB->isoTrk04 = it->dr04TkSumPt(); // trackIso
electronB->isoRel03 = (it->dr03EcalRecHitSumEt()
+ it->dr03HcalTowerSumEt()
+ it->dr03TkSumPt())/it->pt();
electronB->isoRel04 = (it->dr04EcalRecHitSumEt()
+ it->dr04HcalTowerSumEt()
+ it->dr04TkSumPt())/it->pt();
// SC associated with electron
electronB->scEn = it->superCluster()->energy();
electronB->scEta = it->superCluster()->eta();
electronB->scPhi = it->superCluster()->phi();
electronB->scET = it->superCluster()->energy()/cosh(it->superCluster()->eta());
electronB->scRawEnergy = it->superCluster()->rawEnergy();
electronB->dist_vec = it->userFloat("dist");
electronB->dCotTheta = it->userFloat("dcot");
electronB->hasMatchedConv = (it->userInt("antiConv") ? true : false);
// Vertex association
double minVtxDist3D = 9999.;
int indexVtx = -1;
double vertexDistZ = 9999.;
if (hasGsfTrack) {
if (primaryVertices.isValid()) {
edm::LogInfo("ElectronBlock") << "Total # Primary Vertices: " << primaryVertices->size();
for (reco::VertexCollection::const_iterator vit = primaryVertices->begin();
vit != primaryVertices->end(); ++vit) {
double dxy = tk->dxy(vit->position());
double dz = tk->dz(vit->position());
double dist3D = std::sqrt(pow(dxy, 2) + pow(dz, 2));
if (dist3D < minVtxDist3D) {
minVtxDist3D = dist3D;
indexVtx = int(std::distance(primaryVertices->begin(), vit));
vertexDistZ = dz;
}
}
}
else {
edm::LogError("ElectronBlock") << "Error >> Failed to get VertexCollection for label: "
<< _vtxInputTag;
}
}
// Vertex association variables
electronB->vtxDist3D = minVtxDist3D;
electronB->vtxIndex = indexVtx;
electronB->vtxDistZ = vertexDistZ;
electronB->relIso = (it->trackIso() + it->ecalIso() + it->hcalIso())/it->pt();
// PF based isolation
electronB->pfRelIso = it->userFloat("PFRelIsoDB04");
// PFlow isolation information
electronB->chargedHadronIso = it->chargedHadronIso();
electronB->neutralHadronIso = it->neutralHadronIso();
electronB->photonIso = it->photonIso();
// IP information
electronB->dB = it->dB(pat::Electron::PV2D);
electronB->edB = it->edB(pat::Electron::PV2D);
electronB->dB3d = it->dB(pat::Electron::PV3D);
electronB->edB3d = it->edB(pat::Electron::PV3D);
// Bremstrahlung information
electronB->nBrems = it->numberOfBrems();
electronB->fbrem = it->fbrem();
// MVA
electronB->mva = it->userFloat("mva");
electronB->mvaPOGTrig = it->userFloat("mvaPOGTrig");
electronB->mvaPOGNonTrig = it->userFloat("mvaPOGNonTrig");
electronB->mvaPreselection = (it->userInt("mvaPreselection") ? true : false);
electronB->isTriggerElectron = (it->userInt("isTriggerElectron") ? true : false);
// MVA Iso
electronB->isoMVA = it->userFloat("eleIsoMVA");
// Fiducial flag
int fidFlag = 0;
if (it->isEB()) fidFlag |= (1 << 0);
if (it->isEE()) fidFlag |= (1 << 1);
if (it->isEBEtaGap()) fidFlag |= (1 << 2);
if (it->isEBPhiGap()) fidFlag |= (1 << 3);
if (it->isEERingGap()) fidFlag |= (1 << 4);
if (it->isEEDeeGap()) fidFlag |= (1 << 5);
if (it->isEBEEGap()) fidFlag |= (1 << 6);
electronB->fidFlag = fidFlag;
// Vertex information
const reco::Candidate::Point& vertex = it->vertex();
electronB->vx = vertex.x();
electronB->vy = vertex.y();
electronB->vz = vertex.z();
electronB->pfRelIso03v1 = it->userFloat("PFRelIso03v1");
electronB->pfRelIso03v2 = it->userFloat("PFRelIso03v2");
electronB->pfRelIsoDB03v1 = it->userFloat("PFRelIsoDB03v1");
electronB->pfRelIsoDB03v2 = it->userFloat("PFRelIsoDB03v2");
electronB->pfRelIsoDB03v3 = it->userFloat("PFRelIsoDB03v3");
electronB->pfRelIso04v1 = it->userFloat("PFRelIso04v1");
electronB->pfRelIso04v2 = it->userFloat("PFRelIso04v2");
electronB->pfRelIsoDB04v1 = it->userFloat("PFRelIsoDB04v1");
electronB->pfRelIsoDB04v2 = it->userFloat("PFRelIsoDB04v2");
electronB->pfRelIsoDB04v3 = it->userFloat("PFRelIsoDB04v3");
// 2012
electronB->pfRelIso03 = it->userFloat("PFRelIso03");
electronB->pfRelIso04 = it->userFloat("PFRelIso04");
electronB->pfRelIsoDB03 = it->userFloat("PFRelIsoDB03");
electronB->pfRelIsoDB04 = it->userFloat("PFRelIsoDB04");
}
}
else {
edm::LogError("ElectronBlock") << "Error >> Failed to get pat::Electron Collection for label: "
<< _electronInputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(ElectronBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
triggerObjectBlock = cms.EDAnalyzer("TriggerObjectBlock",
verbosity = cms.int32(0),
hltInputTag = cms.InputTag('TriggerResults','','HLT'),
triggerEventTag = cms.InputTag('patTriggerEvent'),
hltPathsOfInterest = cms.vstring ("HLT_DoubleMu",
"HLT_Mu13_Mu8_v",
"HLT_Mu17_Mu8_v",
"HLT_Mu1",
"HLT_Mu2",
"HLT_Mu3",
"HLT_Mu4",
"HLT_IsoMu1",
"HLT_IsoMu2",
"HLT_IsoMu3",
"HLT_IsoMu4",
"HLT_Mu17_Ele8_Calo",
"HLT_Mu8_Ele17_",
"HLT_Ele1",
"HLT_Ele2",
"HLT_Ele3",
"HLT_Ele4",
"IsoPFTau"),
May10ReRecoData = cms.bool(False)
)
<file_sep>void plot_dimuon_ratio(){
h1DiMuonEtaReco->SetLineColor(1);
h1DiMuonEtaReco->SetStats(0);
//h1DiMuonEtaReco->SetTitle("#eta as a function of p_{T} threshold");
h1DiMuonEtaReco->SetXTitle("Ratio of dimuon pT to scalar sum");
h1DiMuonEtaReco->SetYTitle("Events");
h1DiMuonEtaReco->Draw();
/*leg_hist = new TLegend(0.6724138,0.684322,0.8520115,0.8728814);
//leg_hist->SetHeader("Pt histograms");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hEtaPt10,"p_{T} > 10 GeV/c","l");
leg_hist->AddEntry(hEtaPt15,"p_{T} > 15 GeV/c","l");
leg_hist->AddEntry(hEtaPt20,"p_{T} > 20 GeV/c","l");
leg_hist->AddEntry(hEtaPt25,"p_{T} > 25 GeV/c","l");
leg_hist->AddEntry(hEtaPt30,"p_{T} > 30 GeV/c","l");
leg_hist->AddEntry(hEtaPt35,"p_{T} > 35 GeV/c","l");
leg_hist->Draw();*/
c1->SaveAs("plot_pt_ratio.png");
}
<file_sep>#!/bin/bash
echo -n "Enter the name of the file which you got after running the trick.sh file (exact path name)"
read text
echo "You entered: $text"
lines=$(cat $text | wc -l)
echo "Number of input files: $lines"
echo "How do you want to divide them? (if they have 200 lines/files, enter 50 to have 4 files with 50 files in them)"
read number
awk '{filename = "file" int((NR-1)/'$number'); print >> filename}' $text
loop_index=$((lines/number))
echo $loop_index
for((i=0;i<=loop_index;i++))
do
sed -e 's/file/file'$i'/' submit_skimmer >> submit_skimmer-$i.jdl
condor_submit submit_skimmer-$i.jdl
done
<file_sep>void plot_eta_pt_2d(){
hEtaPtAll->SetXTitle("|#eta_{#mu}^{Reco}|");
hEtaPtAll->SetYTitle("Reco Muon p_{T} in GeV/c");
hEtaPtAll->SetStats(0);
hEtaPtAll->Draw("COLZ");
c1->SaveAs("plot_eta_pt_2d.png");
}
<file_sep>#include <iostream>
#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "DataFormats/PatCandidates/interface/Muon.h"
#include "DataFormats/TrackReco/interface/HitPattern.h"
#include "DataFormats/BeamSpot/interface/BeamSpot.h"
#include "DataFormats/VertexReco/interface/Vertex.h"
#include "DataFormats/VertexReco/interface/VertexFwd.h"
#include "Math/GenVector/VectorUtil.h"
#include "DataFormats/GeometryVector/interface/GlobalPoint.h"
#include "DataFormats/GeometryVector/interface/VectorUtil.h"
#include "VHTauTau/TreeMaker/plugins/MuonBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
const reco::GenParticle* getGenMuon(const pat::Muon& pm)
{
std::vector<reco::GenParticleRef> associatedGenParticles = pm.genParticleRefs();
for ( std::vector<reco::GenParticleRef>::const_iterator im = associatedGenParticles.begin();
im != associatedGenParticles.end(); ++im ) {
if ( im->isAvailable() ) {
const reco::GenParticleRef& genParticle = (*im);
if ( genParticle->pdgId() == -13 || genParticle->pdgId() == +13 ) return genParticle.get();
}
}
return 0;
}
const reco::GenParticle* findMotherWithPdgId(const reco::GenParticle* genParticle, unsigned absPdgId)
{
// If this doesn't have any valid mother, return null
const reco::GenParticle* retVal = 0;
if ( genParticle ) {
// Get list of mothers. Not sure why this would be more than one.
size_t nGenMothers = genParticle->numberOfMothers();
for ( size_t iGenMother = 0; iGenMother < nGenMothers; ++iGenMother ) {
const reco::GenParticle* genMother = dynamic_cast<const reco::GenParticle*>(genParticle->mother(iGenMother));
if ( genMother ) {
unsigned genMotherPdgId = TMath::Abs(genMother->pdgId());
if ( genMotherPdgId == absPdgId ) {
retVal = genMother;
} else {
const reco::GenParticle* genGrandMother = findMotherWithPdgId(genMother, absPdgId);
if ( genGrandMother != 0 ) retVal = genGrandMother;
}
}
if ( retVal != 0 ) break;
}
}
return retVal;
}
MuonBlock::MuonBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_muonInputTag(iConfig.getParameter<edm::InputTag>("muonSrc")),
_vtxInputTag(iConfig.getParameter<edm::InputTag>("vertexSrc")),
_beamSpotInputTag(iConfig.getParameter<edm::InputTag>("offlineBeamSpot")),
_beamSpotCorr(iConfig.getParameter<bool>("beamSpotCorr")),
_muonID(iConfig.getParameter<std::string>("muonID"))
{
}
MuonBlock::~MuonBlock() {
}
void MuonBlock::beginJob()
{
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneMuon = new TClonesArray("vhtm::Muon");
tree->Branch("Muon", &cloneMuon, 32000, 2);
tree->Branch("nMuon", &fnMuon, "fnMuon/I");
}
void MuonBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneMuon->Clear();
fnMuon = 0;
edm::Handle<std::vector<pat::Muon> > muons;
iEvent.getByLabel(_muonInputTag, muons);
edm::Handle<reco::VertexCollection> primaryVertices;
iEvent.getByLabel(_vtxInputTag, primaryVertices);
edm::Handle<reco::BeamSpot> beamSpot;
iEvent.getByLabel(_beamSpotInputTag, beamSpot);
if (muons.isValid()) {
edm::LogInfo("MuonBlock") << "Total # Muons: " << muons->size();
for (std::vector<pat::Muon>::const_iterator it = muons->begin();
it != muons->end(); ++it) {
// consider only global muons
//if (muons->size() == 2){
//std::cout<<muons->size()<<std::endl;
if (!it->isGlobalMuon()) continue;
//remove those patmuons which were not matched with gen particles
//const reco::GenParticle* genMuon = getGenMuon(*it);
//if (!genMuon) continue;
// Choose only those pat muons which have taus as mother particles
const reco::GenParticle* genTau = findMotherWithPdgId(it->genLepton(), 15);
if(!genTau) continue;
if (fnMuon == kMaxMuon) {
edm::LogInfo("MuonBlock") << "Too many PAT Muons, fnMuon = " << fnMuon;
break;
}
reco::TrackRef tk = it->innerTrack(); // tracker segment only
reco::TrackRef gtk = it->globalTrack();
muonB = new ((*cloneMuon)[fnMuon++]) vhtm::Muon();
muonB->isTrackerMuon = (it->isTrackerMuon()) ? true : false;
muonB->isPFMuon = it->userInt("isPFMuon") ? true : false;
//kinematic variables for the gen lepton
muonB->ptgen = it->genLepton()->pt();
muonB->eta = it->eta();
muonB->phi = it->phi();
muonB->pt = it->pt();
muonB->ptError = tk->ptError();
muonB->px = it->px();
muonB->py = it->py();
muonB->pz = it->pz();
muonB->energy = it->energy();
muonB->charge = it->charge();
double trkd0 = tk->d0();
double trkdz = tk->dz();
if (_beamSpotCorr) {
if (beamSpot.isValid()) {
trkd0 = -(tk->dxy(beamSpot->position()));
trkdz = tk->dz(beamSpot->position());
}
else
edm::LogError("MuonsBlock") << "Error >> Failed to get BeamSpot for label: "
<< _beamSpotInputTag;
}
muonB->trkD0 = trkd0;
muonB->trkD0Error = tk->d0Error();
muonB->trkDz = trkdz;
muonB->trkDzError = tk->dzError();
muonB->globalChi2 = it->normChi2();
muonB->passID = (it->muonID(_muonID)) ? true : false;
// Vertex association
double minVtxDist3D = 9999.;
int indexVtx = -1;
double vertexDistZ = 9999.;
if (primaryVertices.isValid()) {
edm::LogInfo("MuonBlock") << "Total # Primary Vertices: " << primaryVertices->size();
for (reco::VertexCollection::const_iterator vit = primaryVertices->begin();
vit != primaryVertices->end(); ++vit) {
double dxy = tk->dxy(vit->position());
double dz = tk->dz(vit->position());
double dist3D = std::sqrt(pow(dxy,2) + pow(dz,2));
if (dist3D < minVtxDist3D) {
minVtxDist3D = dist3D;
indexVtx = int(std::distance(primaryVertices->begin(), vit));
vertexDistZ = dz;
}
}
}
else {
edm::LogError("MuonBlock") << "Error >> Failed to get VertexCollection for label: "
<< _vtxInputTag;
}
muonB->vtxDist3D = minVtxDist3D;
muonB->vtxIndex = indexVtx;
muonB->vtxDistZ = vertexDistZ;
// Hit pattern
const reco::HitPattern& hitp = gtk->hitPattern(); // innerTrack will not provide Muon Hits
muonB->pixHits = hitp.numberOfValidPixelHits();
muonB->trkHits = hitp.numberOfValidTrackerHits();
muonB->muoHits = hitp.numberOfValidMuonHits();
muonB->matches = it->numberOfMatches();
muonB->trackerLayersWithMeasurement = hitp.trackerLayersWithMeasurement();
// Isolation
muonB->trkIso = it->trackIso();
muonB->ecalIso = it->ecalIso();
muonB->hcalIso = it->hcalIso();
muonB->hoIso = it->isolationR03().hoEt;
double reliso = (it->trackIso() + it->ecalIso() + it->hcalIso())/it->pt();
muonB->relIso = reliso;
muonB->pfRelIso = it->userFloat("PFRelIsoDB04v2");
// IP information
muonB->dB = it->dB(pat::Muon::PV2D);
muonB->edB = it->edB(pat::Muon::PV2D);
// muonB->dB_BS = it->dB(pat::Muon::BS2D);
if (primaryVertices.isValid()) {
std::cout<<" db "<<it->dB(pat::Muon::PV2D)<<" db with bs "<<it->dB(pat::Muon::BS2D)<<std::endl;
// std::cout<<" dxy "<<it->globalTrack()->dxy((primaryVertices->begin())->position())<<std::endl;
}
muonB->dB3d = it->dB(pat::Muon::PV3D);
muonB->edB3d = it->edB(pat::Muon::PV3D);
// UW recommendation
muonB->isGlobalMuonPromptTight = muon::isGoodMuon(*it, muon::GlobalMuonPromptTight);
muonB->isAllArbitrated = muon::isGoodMuon(*it, muon::AllArbitrated);
muonB->nChambers = it->numberOfChambers();
muonB->nMatches = it->numberOfMatches();
muonB->nMatchedStations = it->numberOfMatchedStations();
muonB->stationMask = it->stationMask();
muonB->stationGapMaskDistance = it->stationGapMaskDistance();
muonB->stationGapMaskPull = it->stationGapMaskPull();
muonB->muonID = it->userInt("muonID");
// Vertex information
const reco::Candidate::Point& vertex = it->vertex();
muonB->vx = vertex.x();
muonB->vy = vertex.y();
muonB->vz = vertex.z();
muonB->idMVA = it->userFloat("muonIdMVA");
muonB->isoRingsMVA = it->userFloat("muonIsoRingsMVA");
muonB->isoRingsRadMVA = it->userFloat("muonIsoRingsRadMVA");
muonB->idIsoCombMVA = it->userFloat("muonIdIsoCombMVA");
muonB->pfRelIso03v1 = it->userFloat("PFRelIso03v1");
muonB->pfRelIso03v2 = it->userFloat("PFRelIso03v2");
muonB->pfRelIsoDB03v1 = it->userFloat("PFRelIsoDB03v1");
muonB->pfRelIsoDB03v2 = it->userFloat("PFRelIsoDB03v2");
muonB->pfRelIso04v1 = it->userFloat("PFRelIso04v1");
muonB->pfRelIso04v2 = it->userFloat("PFRelIso04v2");
muonB->pfRelIsoDB04v1 = it->userFloat("PFRelIsoDB04v1");
muonB->pfRelIsoDB04v2 = it->userFloat("PFRelIsoDB04v2");
}
}
else {
edm::LogError("MuonBlock") << "Error >> Failed to get pat::Muon collection for label: "
<< _muonInputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(MuonBlock);
<file_sep>#include <iostream>
#include <algorithm>
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "PhysicsTools/PatUtils/interface/TriggerHelper.h"
#include "DataFormats/PatCandidates/interface/TriggerEvent.h"
#include "VHTauTau/TreeMaker/plugins/TriggerObjectBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
#include "DataFormats/PatCandidates/interface/TriggerFilter.h"
#include "TMath.h"
#include "TTree.h"
#include "TClonesArray.h"
#include "TPRegexp.h"
// Constructor
TriggerObjectBlock::TriggerObjectBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_hltInputTag(iConfig.getParameter<edm::InputTag>("hltInputTag")),
_triggerEventTag(iConfig.getParameter<edm::InputTag>("triggerEventTag")),
_hltPathsOfInterest(iConfig.getParameter<std::vector<std::string> > ("hltPathsOfInterest")),
_may10ReRecoData(iConfig.getParameter<bool>("May10ReRecoData"))
{
std::cout << "==> TriggerObjectBlock::TriggerObjectBlock()" << std::endl;
}
TriggerObjectBlock::~TriggerObjectBlock() {
}
void TriggerObjectBlock::beginJob()
{
std::string tree_name = "vhtree";
TTree* tree = vhtm::Utility::getTree(tree_name);
cloneTriggerObject = new TClonesArray("vhtm::TriggerObject");
tree->Branch("TriggerObject", &cloneTriggerObject, 32000, 2);
tree->Branch("nTriggerObject", &fnTriggerObject, "fnTriggerObject/I");
_firingFlag = (_may10ReRecoData) ? false : true;
}
void TriggerObjectBlock::beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {
bool changed = true;
if (hltConfig.init(iRun, iSetup, _hltInputTag.process(), changed)) {
// if init returns TRUE, initialisation has succeeded!
edm::LogInfo("TriggerObjectBlock") << "HLT config with process name "
<< _hltInputTag.process()
<< " successfully extracted";
}
else {
// if init returns FALSE, initialisation has NOT succeeded, which indicates a problem
// with the file and/or code and needs to be investigated!
edm::LogError("TriggerObjectBlock") << "Error! HLT config extraction with process name "
<< _hltInputTag.process() << " failed";
// In this case, all access methods will return empty values!
}
}
void TriggerObjectBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneTriggerObject->Clear();
fnTriggerObject = 0;
if (_verbosity) {
std::cout << setiosflags(std::ios::fixed);
std::cout << "Indx Eta Phi Pt Energy =Trigger path list=" << std::endl;
}
// trigger event
edm::Handle<pat::TriggerEvent> triggerEvent;
iEvent.getByLabel(_triggerEventTag, triggerEvent);
// get the trigger objects corresponding to the used matching (HLT muons) and
// loop over selected trigger objects
pat::TriggerObjectRefVector myObjects(triggerEvent->objectRefs());
for (pat::TriggerObjectRefVector::const_iterator it = myObjects.begin();
it != myObjects.end();
++it) {
pat::TriggerPathRefVector myPaths = triggerEvent->objectPaths((*it));
std::map <std::string, unsigned int> pathInfoMap;
for (pat::TriggerPathRefVector::const_iterator ipath = myPaths.begin();
ipath != myPaths.end();
++ipath) {
std::string name = (**ipath).name();
for (std::vector<std::string>::const_iterator kt = _hltPathsOfInterest.begin();
kt != _hltPathsOfInterest.end();
++kt) {
std::string path_int = (*kt);
if (name.find(path_int) == std::string::npos) continue;
bool matched = true;
// Get the filters and access the L3 filter (needed for May10ReReco data)
if (_may10ReRecoData) {
matched = false;
pat::TriggerFilterRefVector filters( triggerEvent->pathFilters( name, _firingFlag) );
if ( filters.empty() ) continue;
pat::TriggerFilterRef lastFilter( filters.at( filters.size() - 1 ) );
if ( triggerEvent->objectInFilter( (*it), lastFilter->label() ) ) matched = true;
}
if (matched) {
unsigned int val = 0;
if (triggerEvent->path(name)->wasRun() && triggerEvent->path(name)->wasAccept()) val = 1;
pathInfoMap.insert(std::pair<std::string, unsigned int> (name, val));
}
}
}
if (pathInfoMap.size() > 0) {
if (fnTriggerObject == kMaxTriggerObject) {
edm::LogInfo("TriggerObjectBlock") << "Too many Trigger Muons (HLT), fnTriggerObject = "
<< fnTriggerObject;
break;
}
_triggerObject = new ((*cloneTriggerObject)[fnTriggerObject++]) vhtm::TriggerObject();
_triggerObject->eta = (**it).eta();
_triggerObject->phi = (**it).phi();
_triggerObject->pt = (**it).pt();
_triggerObject->energy = (**it).energy();
for (std::map <std::string, unsigned int>::iterator imap = pathInfoMap.begin();
imap != pathInfoMap.end();
++imap) {
_triggerObject->pathList.insert(std::pair<std::string, unsigned int> (imap->first, imap->second));
}
if (_verbosity) {
std::cout << std::setprecision(2);
std::cout << std::setw(4) << fnTriggerObject
<< std::setw(8) << _triggerObject->eta
<< std::setw(8) << _triggerObject->phi
<< std::setw(8) << _triggerObject->pt
<< std::setw(8) << _triggerObject->energy
<< std::endl;
for (std::map<std::string, unsigned int>::const_iterator jt = _triggerObject->pathList.begin();
jt != _triggerObject->pathList.end();
++jt)
{
std::cout << "\t\t\t\t\t" << jt->first << " " << jt->second << std::endl;
}
}
}
}
if (_verbosity) std::cout << " # of Trigger Objects " << fnTriggerObject << std::endl;
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(TriggerObjectBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
jetBlock = cms.EDAnalyzer("JetBlock",
verbosity = cms.int32(0),
jetSrc = cms.InputTag('patJets'),
jecUncertainty = cms.string('CondFormats/JetMETObjects/data/Spring10_Uncertainty_AK5PF.txt'),
applyResidualJEC = cms.bool(False),
residualJEC = cms.string('CondFormats/JetMETObjects/data/Spring10_L2L3Residual_AK5PF.txt')
)
<file_sep>#include <iostream>
#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "DataFormats/PatCandidates/interface/MET.h"
#include "VHTauTau/TreeMaker/plugins/METBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
METBlock::METBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_pfInputTag(iConfig.getParameter<edm::InputTag>("metSrc"))
// _corrinputTag(iConfig.getParameter<edm::InputTag>("corrmetSrc")),
//_mvainputTag(iConfig.getParameter<edm::InputTag>("mvametSrc"))
{
}
//METBlock::~METBlock(){
//}
void METBlock::beginJob()
{
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
clonePFMET = new TClonesArray("vhtm::MET");
tree->Branch("MET", &clonePFMET, 32000, 2);
tree->Branch("nMET", &fnPFMET, "fnPFMET/I");
/*cloneCorrMET = new TClonesArray("vhtm::MET");
tree->Branch("corrMET", &cloneCorrMET, 32000, 2);
tree->Branch("corrnMET", &fnCorrMET, "fnCorrMET/I");
cloneMVAMET = new TClonesArray("vhtm::MET");
tree->Branch("mvaMET", &cloneMVAMET, 32000, 2);
tree->Branch("mvanMET", &fnMVAMET, "fnMVAMET/I");*/
}
void METBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
//fillMET(iEvent, iSetup, clonePFMET, fnPFMET, _pfinputTag, pfmetB);
//fillMET(iEvent, iSetup, cloneCorrMET, fnCorrMET, _corrinputTag, corrmetB);
//fillMET(iEvent, iSetup, cloneMVAMET, fnMVAMET, _mvainputTag, mvametB);
// Reset the TClonesArray and the nObj variables
clonePFMET->Clear();
fnPFMET = 0;
edm::Handle<std::vector<pat::MET> > mets;
iEvent.getByLabel(_pfInputTag, mets);
if (mets.isValid()) {
edm::LogInfo("METBlock") << "Total # PAT METs: " << mets->size();
for (std::vector<pat::MET>::const_iterator it = mets->begin();
it != mets->end(); ++it) {
if (fnPFMET == kMaxMET) {
edm::LogInfo("METBlock") << "Too many PAT MET, nMET = " << fnPFMET
<< ", label: " << _pfInputTag;
break;
}
metB = new ((*clonePFMET)[fnPFMET++]) vhtm::MET();
// fill in all the vectors
metB->met = it->pt();
metB->metphi = it->phi();
metB->metx = it->px();
metB->mety = it->py();
metB->sumet = it->sumEt();
metB->metuncorr = it->uncorrectedPt(pat::MET::uncorrALL);
metB->metphiuncorr = it->uncorrectedPhi(pat::MET::uncorrALL);
metB->sumetuncorr = it->sumEt() - it->corSumEt(pat::MET::uncorrALL);
}
}
else {
edm::LogError("METBlock") << "Error >> Failed to get pat::MET collection for label: "
<< _pfInputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(METBlock);
<file_sep>void plot_accept_pt_3d_1(){
//hAccept1->SetLineColor(1);
hAccept1->SetXTitle("Reco. leading muon p_{T} in GeV/c");
hAccept1->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//hAccept1->SetZTitle("Kinematic acceptance");
hAccept1->SetStats(0);
hAccept1->Draw("cont3 colz");
//HLT_PATH Mu17_Mu8
Int_t a=hAccept1->GetXaxis()->FindBin(17);
Int_t b = hAccept1->GetYaxis()->FindBin(8);
Double_t c = hAccept1->GetBinContent(a,b);
cout<<"17-18 "<<c<<endl;
//Double_t a = hAccept1->GetBinContent(hAccept1->GetXaxis()->FindBin(17),hAccept1->GetYaxis()->FindBin(8)));
//HLT_PATH Mu13_Mu8
//Double_t f=hAccept1->GetBinContent(
Int_t d = hAccept1->GetXaxis()->FindBin(13);
Int_t e = hAccept1->GetYaxis()->FindBin(8);
Double_t f = hAccept1->GetBinContent(d,e);
cout<<"13-18 "<<f<<endl;
//Offline Cuts
Int_t g=hAccept1->GetXaxis()->FindBin(20);
Int_t h=hAccept1->GetYaxis()->FindBin(10);
Double_t z=hAccept1->GetBinContent(g,h);
cout<<"20-10 "<<z<<endl;
//Double_t i=GetBinContenthAccept1->GetXaxis()->FindBin(20),hAccept1->GetYaxis()->FindBin(10));
//Double_t m=hAccept1->GetBinContent(k,l);
//draw hist_2 first as it has a larger range
TLatex latex;
//latex.DrawLatex(17,8,TString::Format("#bullet %f ",a));
//latex.DrawLatex(17,8,TString::Format("#bullet %2f %",c*100));
latex.DrawLatex(17,8,"#bullet 64%");
latex.DrawLatex(13,8,"#diamond 72%");
latex.DrawLatex(20,10,"#color[2]{#bullet} 45%");
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(13,8,TString::Format("#diamond %f ",f));
//latex.DrawLatex(20,10,TString::Format("#circ %f ",i));
latex.DrawLatex(3,22,"#bullet");
latex.DrawLatex(2.83,25,"#diamond");
latex.DrawLatex(4,28,"#scale[0.8]{HLT Paths(Run-1)}");
latex.DrawLatex(4,22,"#scale[0.8]{Mu17_Mu8 (Luminosity 19.1 fb^{-1})}");
latex.DrawLatex(4,25,"#scale[0.8]{Mu13_Mu8 (Luminosity 3.7 fb^{-1})}");
latex.DrawLatex(4,19,"#scale[0.8]{Offline selection}");
latex.DrawLatex(3,16,"#color[2]{#bullet}");
latex.DrawLatex(4,16,"#scale[0.8]{Mu20_Mu10}");
c1->SaveAs("acceptance_2d_1.png");
c1->SaveAs("acceptance_2d_1.eps");
/*leg_hist = new TLegend(0.5,0.6,0.79,0.79);
leg_hist->SetHeader("Pt histograms for case 1 (both muons in endcap)");
leg_hist->AddEntry(hAccept1,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon pt","l");
leg_hist->Draw();
*/
}
<file_sep>import FWCore.ParameterSet.Config as cms
from VHTauTau.TreeMaker.EventBlock_cfi import eventBlock
from VHTauTau.TreeMaker.VertexBlock_cfi import vertexBlock
from VHTauTau.TreeMaker.JetBlock_cfi import jetBlock
from VHTauTau.TreeMaker.ElectronBlock_cfi import electronBlock
from VHTauTau.TreeMaker.METBlock_cfi import metBlock
from VHTauTau.TreeMaker.MuonBlock_cfi import muonBlock
from VHTauTau.TreeMaker.TauBlock_cfi import tauBlock
from VHTauTau.TreeMaker.GenParticleBlock_cfi import genParticleBlock
from VHTauTau.TreeMaker.TriggerBlock_cfi import triggerBlock
from VHTauTau.TreeMaker.TriggerObjectBlock_cfi import triggerObjectBlock
from VHTauTau.TreeMaker.TrackBlock_cfi import trackBlock
#from VHTauTau.TreeMaker.PatMCMatching_cfi import PatMCMatching
treeContentSequence = cms.Sequence(
eventBlock +
vertexBlock +
#+ electronBlock
trackBlock +
#genParticleBlock +
#+ jetBlock
metBlock +
muonBlock
#+ PatMCMatching
#tauBlock
#+ triggerBlock
)
<file_sep>void plot_int(){
h1->ComputeIntegral();
Double_t *integral = (h1->GetIntegral());
h1->SetContent(integral);
//TH1F *h1=1-(*h1);
//TH1F *h1i = new TH1F("h1i","histogram with 1 as entries",100,0,60);
//Int_t binsize=h1->GetSize();
//for (int i=1;i<binsize-1;i++){
// std::cout<<i<<"\t"<<(*integral)<<endl;
//Double_t entry=h1->GetBinContent(i);
//h1->SetBinContent(i,1-entry);
//}
//c1->SaveAs("case1.png");
}
<file_sep>{
//=========Macro generated from canvas: c1/c1
//========= (Tue Jan 13 17:15:16 2015) by ROOT version5.34/18
TCanvas *c1 = new TCanvas("c1", "c1",65,52,700,500);
c1->Range(-0.3770197,-211.4001,3.371634,1753.377);
c1->SetFillColor(0);
c1->SetBorderMode(0);
c1->SetBorderSize(2);
c1->SetTickx(1);
c1->SetTicky(1);
c1->SetFrameBorderMode(0);
c1->SetFrameBorderMode(0);
TH1F *hEtaPt10 = new TH1F("hEtaPt10","|#eta| as a function of p_{T} threshold",30,0,3);
hEtaPt10->SetBinContent(1,1458);
hEtaPt10->SetBinContent(2,1343);
hEtaPt10->SetBinContent(3,1234);
hEtaPt10->SetBinContent(4,1327);
hEtaPt10->SetBinContent(5,1353);
hEtaPt10->SetBinContent(6,1382);
hEtaPt10->SetBinContent(7,1280);
hEtaPt10->SetBinContent(8,1191);
hEtaPt10->SetBinContent(9,1186);
hEtaPt10->SetBinContent(10,1125);
hEtaPt10->SetBinContent(11,1061);
hEtaPt10->SetBinContent(12,1056);
hEtaPt10->SetBinContent(13,1051);
hEtaPt10->SetBinContent(14,914);
hEtaPt10->SetBinContent(15,848);
hEtaPt10->SetBinContent(16,811);
hEtaPt10->SetBinContent(17,764);
hEtaPt10->SetBinContent(18,683);
hEtaPt10->SetBinContent(19,641);
hEtaPt10->SetBinContent(20,581);
hEtaPt10->SetBinContent(21,517);
hEtaPt10->SetBinContent(22,466);
hEtaPt10->SetBinContent(23,359);
hEtaPt10->SetBinContent(24,343);
hEtaPt10->SetBinError(1,38.18377);
hEtaPt10->SetBinError(2,36.64696);
hEtaPt10->SetBinError(3,35.12834);
hEtaPt10->SetBinError(4,36.42801);
hEtaPt10->SetBinError(5,36.78315);
hEtaPt10->SetBinError(6,37.17526);
hEtaPt10->SetBinError(7,35.77709);
hEtaPt10->SetBinError(8,34.51087);
hEtaPt10->SetBinError(9,34.43835);
hEtaPt10->SetBinError(10,33.54102);
hEtaPt10->SetBinError(11,32.57299);
hEtaPt10->SetBinError(12,32.49615);
hEtaPt10->SetBinError(13,32.41913);
hEtaPt10->SetBinError(14,30.23243);
hEtaPt10->SetBinError(15,29.12044);
hEtaPt10->SetBinError(16,28.47806);
hEtaPt10->SetBinError(17,27.64055);
hEtaPt10->SetBinError(18,26.13427);
hEtaPt10->SetBinError(19,25.31798);
hEtaPt10->SetBinError(20,24.10394);
hEtaPt10->SetBinError(21,22.73763);
hEtaPt10->SetBinError(22,21.58703);
hEtaPt10->SetBinError(23,18.9473);
hEtaPt10->SetBinError(24,18.52026);
hEtaPt10->SetEntries(22974);
hEtaPt10->SetStats(0);
hEtaPt10->SetLineColor(8);
hEtaPt10->GetXaxis()->SetTitle("|#eta_{#mu}^{Reco}|");
hEtaPt10->GetYaxis()->SetTitle("Events/0.1");
hEtaPt10->GetYaxis()->SetTitleOffset(1.23);
hEtaPt10->Draw("hist e");
TH1F *hEtaPt15 = new TH1F("hEtaPt15","Reco Muon Eta 15",30,0,3);
hEtaPt15->SetBinContent(1,1224);
hEtaPt15->SetBinContent(2,1111);
hEtaPt15->SetBinContent(3,1031);
hEtaPt15->SetBinContent(4,1073);
hEtaPt15->SetBinContent(5,1114);
hEtaPt15->SetBinContent(6,1139);
hEtaPt15->SetBinContent(7,1075);
hEtaPt15->SetBinContent(8,979);
hEtaPt15->SetBinContent(9,980);
hEtaPt15->SetBinContent(10,928);
hEtaPt15->SetBinContent(11,868);
hEtaPt15->SetBinContent(12,853);
hEtaPt15->SetBinContent(13,896);
hEtaPt15->SetBinContent(14,751);
hEtaPt15->SetBinContent(15,693);
hEtaPt15->SetBinContent(16,632);
hEtaPt15->SetBinContent(17,632);
hEtaPt15->SetBinContent(18,538);
hEtaPt15->SetBinContent(19,513);
hEtaPt15->SetBinContent(20,471);
hEtaPt15->SetBinContent(21,429);
hEtaPt15->SetBinContent(22,386);
hEtaPt15->SetBinContent(23,266);
hEtaPt15->SetBinContent(24,262);
hEtaPt15->SetBinError(1,34.98571);
hEtaPt15->SetBinError(2,33.33167);
hEtaPt15->SetBinError(3,32.10919);
hEtaPt15->SetBinError(4,32.75668);
hEtaPt15->SetBinError(5,33.37664);
hEtaPt15->SetBinError(6,33.74907);
hEtaPt15->SetBinError(7,32.78719);
hEtaPt15->SetBinError(8,31.28898);
hEtaPt15->SetBinError(9,31.30495);
hEtaPt15->SetBinError(10,30.46309);
hEtaPt15->SetBinError(11,29.46184);
hEtaPt15->SetBinError(12,29.20616);
hEtaPt15->SetBinError(13,29.93326);
hEtaPt15->SetBinError(14,27.40438);
hEtaPt15->SetBinError(15,26.32489);
hEtaPt15->SetBinError(16,25.13961);
hEtaPt15->SetBinError(17,25.13961);
hEtaPt15->SetBinError(18,23.19483);
hEtaPt15->SetBinError(19,22.6495);
hEtaPt15->SetBinError(20,21.70253);
hEtaPt15->SetBinError(21,20.71232);
hEtaPt15->SetBinError(22,19.64688);
hEtaPt15->SetBinError(23,16.30951);
hEtaPt15->SetBinError(24,16.18641);
hEtaPt15->SetEntries(18844);
hEtaPt15->SetLineColor(9);
hEtaPt15->Draw("hist e SAME");
TH1F *hEtaPt20 = new TH1F("hEtaPt20","Reco Muon Eta 20",30,0,3);
hEtaPt20->SetBinContent(1,983);
hEtaPt20->SetBinContent(2,899);
hEtaPt20->SetBinContent(3,861);
hEtaPt20->SetBinContent(4,850);
hEtaPt20->SetBinContent(5,878);
hEtaPt20->SetBinContent(6,915);
hEtaPt20->SetBinContent(7,856);
hEtaPt20->SetBinContent(8,805);
hEtaPt20->SetBinContent(9,783);
hEtaPt20->SetBinContent(10,749);
hEtaPt20->SetBinContent(11,691);
hEtaPt20->SetBinContent(12,666);
hEtaPt20->SetBinContent(13,700);
hEtaPt20->SetBinContent(14,580);
hEtaPt20->SetBinContent(15,555);
hEtaPt20->SetBinContent(16,491);
hEtaPt20->SetBinContent(17,499);
hEtaPt20->SetBinContent(18,443);
hEtaPt20->SetBinContent(19,393);
hEtaPt20->SetBinContent(20,374);
hEtaPt20->SetBinContent(21,325);
hEtaPt20->SetBinContent(22,309);
hEtaPt20->SetBinContent(23,201);
hEtaPt20->SetBinContent(24,187);
hEtaPt20->SetBinError(1,31.35283);
hEtaPt20->SetBinError(2,29.98333);
hEtaPt20->SetBinError(3,29.3428);
hEtaPt20->SetBinError(4,29.15476);
hEtaPt20->SetBinError(5,29.63106);
hEtaPt20->SetBinError(6,30.24897);
hEtaPt20->SetBinError(7,29.25748);
hEtaPt20->SetBinError(8,28.37252);
hEtaPt20->SetBinError(9,27.98214);
hEtaPt20->SetBinError(10,27.36786);
hEtaPt20->SetBinError(11,26.28688);
hEtaPt20->SetBinError(12,25.80698);
hEtaPt20->SetBinError(13,26.45751);
hEtaPt20->SetBinError(14,24.08319);
hEtaPt20->SetBinError(15,23.55844);
hEtaPt20->SetBinError(16,22.15852);
hEtaPt20->SetBinError(17,22.33831);
hEtaPt20->SetBinError(18,21.04757);
hEtaPt20->SetBinError(19,19.82423);
hEtaPt20->SetBinError(20,19.33908);
hEtaPt20->SetBinError(21,18.02776);
hEtaPt20->SetBinError(22,17.5784);
hEtaPt20->SetBinError(23,14.17745);
hEtaPt20->SetBinError(24,13.67479);
hEtaPt20->SetEntries(14993);
hEtaPt20->SetLineColor(6);
hEtaPt20->Draw("hist e SAME");
TH1F *hEtaPt25 = new TH1F("hEtaPt25","Reco Muon Eta 25",30,0,3);
hEtaPt25->SetBinContent(1,770);
hEtaPt25->SetBinContent(2,684);
hEtaPt25->SetBinContent(3,673);
hEtaPt25->SetBinContent(4,666);
hEtaPt25->SetBinContent(5,674);
hEtaPt25->SetBinContent(6,709);
hEtaPt25->SetBinContent(7,649);
hEtaPt25->SetBinContent(8,640);
hEtaPt25->SetBinContent(9,610);
hEtaPt25->SetBinContent(10,597);
hEtaPt25->SetBinContent(11,544);
hEtaPt25->SetBinContent(12,503);
hEtaPt25->SetBinContent(13,551);
hEtaPt25->SetBinContent(14,441);
hEtaPt25->SetBinContent(15,434);
hEtaPt25->SetBinContent(16,379);
hEtaPt25->SetBinContent(17,380);
hEtaPt25->SetBinContent(18,328);
hEtaPt25->SetBinContent(19,308);
hEtaPt25->SetBinContent(20,281);
hEtaPt25->SetBinContent(21,248);
hEtaPt25->SetBinContent(22,242);
hEtaPt25->SetBinContent(23,146);
hEtaPt25->SetBinContent(24,130);
hEtaPt25->SetBinError(1,27.74887);
hEtaPt25->SetBinError(2,26.15339);
hEtaPt25->SetBinError(3,25.94224);
hEtaPt25->SetBinError(4,25.80698);
hEtaPt25->SetBinError(5,25.96151);
hEtaPt25->SetBinError(6,26.62705);
hEtaPt25->SetBinError(7,25.47548);
hEtaPt25->SetBinError(8,25.29822);
hEtaPt25->SetBinError(9,24.69818);
hEtaPt25->SetBinError(10,24.43358);
hEtaPt25->SetBinError(11,23.32381);
hEtaPt25->SetBinError(12,22.42766);
hEtaPt25->SetBinError(13,23.47339);
hEtaPt25->SetBinError(14,21);
hEtaPt25->SetBinError(15,20.83267);
hEtaPt25->SetBinError(16,19.46792);
hEtaPt25->SetBinError(17,19.49359);
hEtaPt25->SetBinError(18,18.11077);
hEtaPt25->SetBinError(19,17.54993);
hEtaPt25->SetBinError(20,16.76305);
hEtaPt25->SetBinError(21,15.74802);
hEtaPt25->SetBinError(22,15.55635);
hEtaPt25->SetBinError(23,12.08305);
hEtaPt25->SetBinError(24,11.40175);
hEtaPt25->SetEntries(11587);
hEtaPt25->SetLineColor(2);
hEtaPt25->Draw("hist e SAME");
TH1F *hEtaPt30 = new TH1F("hEtaPt30","Reco Muon Eta 30",30,0,3);
hEtaPt30->SetBinContent(1,606);
hEtaPt30->SetBinContent(2,514);
hEtaPt30->SetBinContent(3,514);
hEtaPt30->SetBinContent(4,487);
hEtaPt30->SetBinContent(5,517);
hEtaPt30->SetBinContent(6,539);
hEtaPt30->SetBinContent(7,474);
hEtaPt30->SetBinContent(8,496);
hEtaPt30->SetBinContent(9,463);
hEtaPt30->SetBinContent(10,459);
hEtaPt30->SetBinContent(11,414);
hEtaPt30->SetBinContent(12,382);
hEtaPt30->SetBinContent(13,423);
hEtaPt30->SetBinContent(14,341);
hEtaPt30->SetBinContent(15,344);
hEtaPt30->SetBinContent(16,293);
hEtaPt30->SetBinContent(17,274);
hEtaPt30->SetBinContent(18,239);
hEtaPt30->SetBinContent(19,233);
hEtaPt30->SetBinContent(20,226);
hEtaPt30->SetBinContent(21,176);
hEtaPt30->SetBinContent(22,167);
hEtaPt30->SetBinContent(23,103);
hEtaPt30->SetBinContent(24,92);
hEtaPt30->SetBinError(1,24.61707);
hEtaPt30->SetBinError(2,22.67157);
hEtaPt30->SetBinError(3,22.67157);
hEtaPt30->SetBinError(4,22.06808);
hEtaPt30->SetBinError(5,22.73763);
hEtaPt30->SetBinError(6,23.21637);
hEtaPt30->SetBinError(7,21.77154);
hEtaPt30->SetBinError(8,22.27106);
hEtaPt30->SetBinError(9,21.51743);
hEtaPt30->SetBinError(10,21.42429);
hEtaPt30->SetBinError(11,20.34699);
hEtaPt30->SetBinError(12,19.54482);
hEtaPt30->SetBinError(13,20.56696);
hEtaPt30->SetBinError(14,18.46619);
hEtaPt30->SetBinError(15,18.54724);
hEtaPt30->SetBinError(16,17.11724);
hEtaPt30->SetBinError(17,16.55295);
hEtaPt30->SetBinError(18,15.45962);
hEtaPt30->SetBinError(19,15.26434);
hEtaPt30->SetBinError(20,15.0333);
hEtaPt30->SetBinError(21,13.2665);
hEtaPt30->SetBinError(22,12.92285);
hEtaPt30->SetBinError(23,10.14889);
hEtaPt30->SetBinError(24,9.591663);
hEtaPt30->SetEntries(8776);
hEtaPt30->SetLineColor(5);
hEtaPt30->Draw("hist e SAME");
TH1F *hEtaPt35 = new TH1F("hEtaPt35","Reco Muon Eta 35",30,0,3);
hEtaPt35->SetBinContent(1,461);
hEtaPt35->SetBinContent(2,388);
hEtaPt35->SetBinContent(3,369);
hEtaPt35->SetBinContent(4,365);
hEtaPt35->SetBinContent(5,397);
hEtaPt35->SetBinContent(6,407);
hEtaPt35->SetBinContent(7,350);
hEtaPt35->SetBinContent(8,360);
hEtaPt35->SetBinContent(9,345);
hEtaPt35->SetBinContent(10,349);
hEtaPt35->SetBinContent(11,316);
hEtaPt35->SetBinContent(12,290);
hEtaPt35->SetBinContent(13,318);
hEtaPt35->SetBinContent(14,252);
hEtaPt35->SetBinContent(15,251);
hEtaPt35->SetBinContent(16,218);
hEtaPt35->SetBinContent(17,191);
hEtaPt35->SetBinContent(18,178);
hEtaPt35->SetBinContent(19,174);
hEtaPt35->SetBinContent(20,155);
hEtaPt35->SetBinContent(21,128);
hEtaPt35->SetBinContent(22,111);
hEtaPt35->SetBinContent(23,71);
hEtaPt35->SetBinContent(24,63);
hEtaPt35->SetBinError(1,21.47091);
hEtaPt35->SetBinError(2,19.69772);
hEtaPt35->SetBinError(3,19.20937);
hEtaPt35->SetBinError(4,19.10497);
hEtaPt35->SetBinError(5,19.92486);
hEtaPt35->SetBinError(6,20.17424);
hEtaPt35->SetBinError(7,18.70829);
hEtaPt35->SetBinError(8,18.97367);
hEtaPt35->SetBinError(9,18.57418);
hEtaPt35->SetBinError(10,18.68154);
hEtaPt35->SetBinError(11,17.77639);
hEtaPt35->SetBinError(12,17.02939);
hEtaPt35->SetBinError(13,17.83255);
hEtaPt35->SetBinError(14,15.87451);
hEtaPt35->SetBinError(15,15.84298);
hEtaPt35->SetBinError(16,14.76482);
hEtaPt35->SetBinError(17,13.82027);
hEtaPt35->SetBinError(18,13.34166);
hEtaPt35->SetBinError(19,13.19091);
hEtaPt35->SetBinError(20,12.4499);
hEtaPt35->SetBinError(21,11.31371);
hEtaPt35->SetBinError(22,10.53565);
hEtaPt35->SetBinError(23,8.42615);
hEtaPt35->SetBinError(24,7.937254);
hEtaPt35->SetEntries(6507);
hEtaPt35->SetLineColor(30);
hEtaPt35->Draw("hist e SAME");
TPaveText *pt = new TPaveText(0.9856374,1222.805,1.427289,1392.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
TText *text = pt->AddText("0 < |#eta| < 1.5");
text = pt->AddText("78 %");
pt->Draw();
pt = new TPaveText(1.627644,1222.805,2.076191,1392.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
text = pt->AddText("1.5 < |#eta| < 2.2");
text = pt->AddText("15 %");
pt->Draw();
pt = new TPaveText(2.278276,1222.805,2.7307,1392.754,"br");
pt->SetBorderSize(2);
pt->SetFillColor(0);
text = pt->AddText("2.2 < |#eta| < 2.4");
text = pt->AddText("7 %");
pt->Draw();
TLegend *leg = new TLegend(0.7586207,0.6772152,0.9382184,0.8649789,NULL,"brNDC");
leg->SetBorderSize(0);
leg->SetTextFont(62);
leg->SetLineColor(1);
leg->SetLineStyle(1);
leg->SetLineWidth(1);
leg->SetFillColor(0);
leg->SetFillStyle(1001);
TLegendEntry *entry=leg->AddEntry("hEtaPt10","p_{T} > 10 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(8);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
entry=leg->AddEntry("hEtaPt15","p_{T} > 15 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(9);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
entry=leg->AddEntry("hEtaPt20","p_{T} > 20 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(6);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
entry=leg->AddEntry("hEtaPt25","p_{T} > 25 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(2);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
entry=leg->AddEntry("hEtaPt30","p_{T} > 30 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(5);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
entry=leg->AddEntry("hEtaPt35","p_{T} > 35 GeV","f");
entry->SetFillStyle(1001);
entry->SetLineColor(30);
entry->SetLineStyle(1);
entry->SetLineWidth(1);
entry->SetMarkerColor(1);
entry->SetMarkerStyle(21);
entry->SetMarkerSize(1);
entry->SetTextFont(62);
leg->Draw();
TLine *line = new TLine(1.5,0,1.5,7000);
line->SetLineColor(9);
line->SetLineStyle(2);
line->SetLineWidth(3);
line->Draw();
line = new TLine(2.2,0,2.2,7000);
line->SetLineColor(2);
line->SetLineStyle(2);
line->SetLineWidth(3);
line->Draw();
line = new TLine(2.4,0,2.4,7000);
line->SetLineColor(8);
line->SetLineStyle(2);
line->SetLineWidth(3);
line->Draw();
pt = new TPaveText(0.01,0.9241139,0.496954,0.995,"blNDC");
pt->SetName("title");
pt->SetBorderSize(1);
pt->SetFillColor(0);
text = pt->AddText("|#eta| as a function of p_{T} threshold");
pt->Draw();
c1->Modified();
c1->cd();
c1->SetSelected(c1);
}
<file_sep>void plot3(){
hLeadMuon3->SetLineColor(1);
hSubLeadMuon3->SetLineColor(4);
hLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->Draw();
gPad->Update();
hLeadMuon3->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon3->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon3->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon3->Draw();
hLeadMuon3->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4770115,0.6038136,0.7658046,0.7923729);
leg_hist->SetHeader("Pt histograms for case 3");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon3,"leading muon Pt","l");
leg_hist->AddEntry(hSubLeadMuon3,"subleading muon pt","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case3.png");
}
<file_sep>#ifndef __VHTauTau_TreeMaker_TriggerObjectBlock_h
#define __VHTauTau_TreeMaker_TriggerObjectBlock_h
#include <string>
#include <vector>
#include <map>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "DataFormats/Common/interface/Ref.h"
#include "FWCore/Utilities/interface/InputTag.h"
#include "DataFormats/Common/interface/Handle.h"
#include "DataFormats/Provenance/interface/EventID.h"
#include "FWCore/ParameterSet/interface/ProcessDesc.h"
#include "HLTrigger/HLTcore/interface/HLTConfigProvider.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class TriggerObject;
class TriggerObjectBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup);
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit TriggerObjectBlock(const edm::ParameterSet& iConfig);
virtual ~TriggerObjectBlock();
enum {
kMaxTriggerObject = 100
};
private:
TClonesArray* cloneTriggerObject;
int fnTriggerObject;
int _verbosity;
const edm::InputTag _hltInputTag;
edm::InputTag _triggerEventTag;
const std::string _tagPathLabel;
const std::string _probePathLabel;
const std::vector<std::string> _hltPathsOfInterest;
bool _may10ReRecoData;
bool _firingFlag;
vhtm::TriggerObject* _triggerObject;
HLTConfigProvider hltConfig;
};
#endif
<file_sep>import FWCore.ParameterSet.Config as cms
trackBlock = cms.EDAnalyzer("TrackBlock",
verbosity = cms.int32(0),
trackSrc = cms.InputTag('generalTracks'),
offlineBeamSpot = cms.InputTag('offlineBeamSpot')
)
<file_sep>void condor_makeclass_local(){
gROOT->ProcessLine("TFile f(\"/home/amohapatra/ankit_storage/gem_physics/DYTauMu_PU0/dytaumu_14TeV_pu0_local.root\")");
gROOT->ProcessLine("treeCreator->cd()");
gROOT->ProcessLine("vhtree->MakeClass(\"vhtree_local\")");
gROOT->ProcessLine(".! cp vhtree.C vhtree_local.C");
gROOT->ProcessLine(".! sed -i 's|histos_mva_dytt.root|histos_mva_dytt_local.root|g' vhtree_local.C");
gROOT->ProcessLine(".! sed -i 's|vhtree|vhtree_local|g' vhtree_local.C");
gROOT->ProcessLine(".q");
}
<file_sep>void ankit(){
// gROOT->ProcessLine(".L vhtree_better_script.C");
gROOT->ProcessLine(".L vhtree.C");
gROOT->ProcessLine("vhtree gem");
gROOT->ProcessLine("gem.Loop()");
}
<file_sep>#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/HepMCCandidate/interface/GenParticle.h"
#include "VHTauTau/TreeMaker/plugins/GenParticleBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
GenParticleBlock::GenParticleBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("genParticleSrc"))
{
}
GenParticleBlock::~GenParticleBlock() {
}
void GenParticleBlock::beginJob() {
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneGenParticle = new TClonesArray("vhtm::GenParticle");
tree->Branch("GenParticle", &cloneGenParticle, 32000, 2);
tree->Branch("nGenParticle", &fnGenParticle, "fnGenParticle/I");
}
void GenParticleBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneGenParticle->Clear();
fnGenParticle = 0;
if (!iEvent.isRealData()) {
edm::Handle<reco::GenParticleCollection> genParticles;
iEvent.getByLabel(_inputTag, genParticles);
if (genParticles.isValid()) {
edm::LogInfo("GenParticleBlock") << "Total # GenParticles: " << genParticles->size();
for (reco::GenParticleCollection::const_iterator it = genParticles->begin();
it != genParticles->end(); ++it ) {
if (fnGenParticle == kMaxGenParticle) {
edm::LogInfo("GenParticleBlock") << "Too many GenParticles, fnGenParticle = "
<< fnGenParticle;
break;
}
// Doat store low energy gluons
int pdgid = it->pdgId();
double pt = it->pt();
genParticleB = new ((*cloneGenParticle)[fnGenParticle++]) vhtm::GenParticle();
// fill in all the vectors
genParticleB->eta = it->eta();
genParticleB->phi = it->phi();
genParticleB->p = it->p();
genParticleB->px = it->px();
genParticleB->py = it->py();
genParticleB->pz = it->pz();
genParticleB->pt = pt;
genParticleB->energy = it->energy();
genParticleB->pdgId = pdgid;
genParticleB->vx = it->vx();
genParticleB->vy = it->vy();
genParticleB->vz = it->vz();
genParticleB->status = it->status();
genParticleB->charge = it->charge();
genParticleB->numDaught = it->numberOfDaughters();
genParticleB->numMother = it->numberOfMothers();
int idx = -1;
for (reco::GenParticleCollection::const_iterator mit = genParticles->begin();
mit != genParticles->end(); ++mit ) {
if ( it->mother() == &(*mit) ) {
idx = std::distance(genParticles->begin(),mit);
break;
}
}
genParticleB->motherIndex = idx;
if (_verbosity > 0) std::cout << "pdgId=" << it->pdgId() << std::endl;
for (size_t j = 0; j < it->numberOfMothers(); ++j) {
const reco::Candidate* m = it->mother(j);
for (reco::GenParticleCollection::const_iterator mit = genParticles->begin();
mit != genParticles->end(); ++mit) {
if (m == &(*mit) ) { // -- keep all the entries && it->pdgId() != mit->pdgId() ) {
int idx = std::distance(genParticles->begin(), mit);
if (_verbosity > 0)
std::cout << "mother index/pdgId: " << idx << "/" << mit->pdgId() << std::endl;
genParticleB->motherIndices.push_back(idx);
break;
}
}
}
for (size_t j = 0; j < it->numberOfDaughters(); ++j) {
const reco::Candidate* d = it->daughter(j);
for (reco::GenParticleCollection::const_iterator mit = genParticles->begin();
mit != genParticles->end(); ++mit) {
if (d == &(*mit) ) { // -- keep all the entries && it->pdgId() != mit->pdgId() ) {
int idx = std::distance(genParticles->begin(), mit);
if (_verbosity > 0)
std::cout << "daughter index/pdgId: " << idx << "/" << mit->pdgId() << std::endl;
genParticleB->daughtIndices.push_back(idx);
break;
}
}
}
if (_verbosity > 0) std::cout << "# of mother/daughter: "
<< genParticleB->motherIndices.size() << "/"
<< genParticleB->daughtIndices.size() << std::endl;
}
}
else {
edm::LogError("GenParticleBlock") << "Error >> Failed to get GenParticleCollection for label: "
<< _inputTag;
}
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(GenParticleBlock);
<file_sep>#define vhtree_cxx
#include "vhtree.h"
#include <TH2.h>
#include <TStyle.h>
#include <TCanvas.h>
#include <vector>
#include <fstream>
void vhtree::Loop()
{
// In a ROOT session, you can do:
// Root > .L vhtree.C
// Root > vhtree t
// Root > t.GetEntry(12); // Fill t data members with entry number 12
// Root > t.Show(); // Show values of entry 12
// Root > t.Show(16); // Read and show values of entry 16
// Root > t.Loop(); // Loop on all entries
//
// This is the loop skeleton where:
// jentry is the global entry number in the chain
// ientry is the entry number in the current Tree
// Note that the argument to GetEntry must be:
// jentry for TChain::GetEntry
// ientry for TTree::GetEntry and TBranch::GetEntry
//
// To read only selected branches, Insert statements like:
// METHOD1:
// fChain->SetBranchStatus("*",0); // disable all branches
// fChain->SetBranchStatus("branchname",1); // activate branchname
// METHOD2: replace line
// fChain->GetEntry(jentry); //read all branches
//by b_branchname->GetEntry(ientry); //read only this branch
if (fChain == 0) return;
double delRMin=0.03;
double delR=0.;
double GenMuonPt=0.;
double delphi=0.;
double deleta=0.;
double RecoMuonPt=0.;
double RecoMuonEta=0.;
double inv_mass=0.;
double ImpParam=0.;
double MuonDZ=0.;
double MuonChi2=0.;
double SumMuonChi2=0.;
double e1=0.;
double e2=0.;
double p1x=0.;
double p2x=0.;
double p1y=0.;
double p2y=0.;
double p1z=0.;
double p2z=0.;
double phi_1=0.;
double phi_2=0;
double theta_1=0;
double theta_2=0;
double metx=0.;
double mety=0.;
double p_1=0.;
double p_2=0.;
double x_1=0;
double x_2=0;
double pvis_1=0;
double pvis_2=0;
double inv_mass_vis=0;
Double_t total_events=0;
Double_t events=0;
Double_t mag=0;
Double_t dimuoneta=0;
Double_t dimuonratiopt=0;
ofstream myfile("acceptance.file",ios::app);
ofstream myfile2("acceptance2.file",ios::app);
TH1F *h1PtReco = new TH1F("h1PtReco","Reco Muon Pt",100,0,300);
TH1F *h1PtGen = new TH1F("h1PtGen","Gen Muon Pt",100,0,300);
TH1F *h1DiMuonPtReco = new TH1F("h1DiMuonPtReco","Reco DiMuon Pt",100,0,300);
TH1F *h1SumMuonPtReco = new TH1F("h1SumMuonPtReco","Reco Sum Muon Pt",100,0,300);
TH1F *h1PtLeading = new TH1F("h1PtLeading","Leading Muon Pt",100,0,100);
TH1F *h1PtSubLeading = new TH1F("h1PtSubLeading","SubLeading Muon Pt",100,0,100);
TH1F *h1PtLeadingi = new TH1F("h1PtLeadingi","Integrated Leading Muon Pt",100,0,100);
TH1F *h1PtSubLeadingi = new TH1F("h1PtSubLeadingi","Integrated SubLeading Muon Pt",100,0,100);
TH1F *h1EtaReco = new TH1F("h1EtaReco","Reco Muon Eta",100,-5,+5);
//TH1F *h1EtaGen = new TH1F("h1EtaGen","Gen Muon Eta",100,-5,+5);
TH1F *h1DiMuonEtaReco = new TH1F("h1DiMuonEtaReco","Reco DiMuon Eta",100,-10,+10);
TH1F *hPhiReco = new TH1F("hPhiReco","Difference in Phi between the two muons in multiples of #pi",100,-2,2);
TH1F *hMCMatchEC = new TH1F("hMCMatchEC","Muon Pt resolution in End cap region",200,-0.5,0.5);
TH1F *hMCMatchBa = new TH1F("hMCMatchBa","Muon Pt resolution in barrel region",200,-0.5,0.5);
TH1F *hLeadMuon1 = new TH1F("hLeadMuon1","Leading Muon and Subleading Muon in EndCap",100,0,100);
TH1F *hLeadMuon2 = new TH1F("hLeadMuon2","Leading Muon in Endcap, subleading muon in barrel",100,0,100);
TH1F *hLeadMuon3 = new TH1F("hLeadMuon3","Leading Muon in Barrel, subleading muon in endcap",100,0,100);
//TH1F *hLeadMuonAll = new TH1F("hLeadMuonAll","Overall Leading muon distribution",100,0,100);
TH1F *hSubLeadMuon1 = new TH1F("hSubLeadMuon1","Leading Muon and Subleading Muon in EndCap",100,0,100);
TH1F *hSubLeadMuon2 = new TH1F("hSubLeadMuon2","Leading Muon in Endcap, subleading muon in barrel",100,0,100);
TH1F *hSubLeadMuon3 = new TH1F("hSubLeadMuon3","Leading Muon in Barrel, subleading muon in endcap",100,0,100);
//TH1F *hSubLeadMuonAll = new TH1F("hSubLeadMuonAll","Overall Subleading muon distribution",100,0,100);
TH2F *h1 = new TH2F("h1","Correlation plot between Subleading and leading muon Pt(Overall)",30,0,30,30,0,30);
TH2F *h11 = new TH2F("h11","Correlation plot between Subleading and leading muon Pt(EC(Lead), EC(sublead))",30,0,30,30,0,30);
TH2F *h12 = new TH2F("h12","Correlation plot between Subleading and leading muon Pt(EC(Lead),Barrel(Sublead))",30,0,30,30,0,30);
TH2F *h13 = new TH2F("h13","Correlation plot between Subleading and leading muon Pt(EC(sublead),Barrel(Lead))",30,0,30,30,0,30);
//TH3F *h2 = new TH3F("h2","acceptance plot",300,0,300,100,0,150,100,0,1);
TH2F *hAcceptAll = new TH2F("hAcceptAll","Overall acceptance plot",120,0,30,120,0,30);
TH2F *hAccept1 = new TH2F("hAccept1","Leading Muon and Subleading Muon in EndCap",120,0,30,120,0,30);
TH2F *hAccept2 = new TH2F("hAccept2","Leading Muon in Endcap, subleading muon in barrel",120,0,30,120,0,30);
TH2F *hAccept3 = new TH2F("hAccept3","Leading Muon in Barrel, subleading muon in endcap",120,0,30,120,0,30);
//TProfile2D *h2 = new TProfile2D("h2","acceptance plot",100,0,300,100,0,300,0,1);
//TGraph2D *t2 = new TGraph2D();
TH1F *hInvTau = new TH1F("hInvTau","Invariant Mass plot for Taus",100,0,300);
TH1F *hInvMuon = new TH1F("hInvMuon","Invariant Mass plot for Muons(visible)",100,0,300);
TH1F *hImPEC = new TH1F("hImPEC","Impact parameter plot End cap",100,-0.3,0.3);
TH1F *hImPBa = new TH1F("hImPBa","Impact parameter plot barrel",100,-0.3,0.3);
TH1F *hDZEC = new TH1F("hDZEC","Muon DZ plot End cap",100,-10,10);
TH1F *hDZBa = new TH1F("hDZBa","Muon DZ plot barrel",100,-10,10);
TH1F *hChiEC = new TH1F("hChiEC","Muon Chi plot End cap",100,0,16);
TH1F *hChiBa = new TH1F("hChiBa","Muon Chi plot barrel",100,0,16);
TH1F *hImPECEr = new TH1F("hImPECEr","Error in Impact parameter plot End cap",100,0,16);
TH1F *hImPBaEr = new TH1F("hImPBaEr","Error in Impact parameter plot barrel",100,0,16);
//Muon eta plots for pT threshold !
TH1F *hEtaPt10 = new TH1F("hEtaPt10","Reco Muon Eta 10",100,-3,3);
TH1F *hEtaPt15 = new TH1F("hEtaPt15","Reco Muon Eta 15",100,-3,3);
TH1F *hEtaPt20 = new TH1F("hEtaPt20","Reco Muon Eta 20",100,-3,3);
TH1F *hEtaPt25 = new TH1F("hEtaPt25","Reco Muon Eta 25",100,-3,3);
TH1F *hEtaPt30 = new TH1F("hEtaPt30","Reco Muon Eta 30",100,-3,3);
TH1F *hEtaPt35 = new TH1F("hEtaPt35","Reco Muon Eta 35",100,-3,3);
//2-D histograms
TH2F *hEtaResEC = new TH2F("hEtaResEC","Eta vs MuonPt resolution in Endcap",100,-3,3,100,-5,5);
TH2F *hEtaResBa = new TH2F("hEtaResBa","Eta vs MuonPt resolution in barrel",100,-3,3,100,-5,5);
TH2F *hEtaResAll = new TH2F("hEtaResAll","Eta vs MuonPt resolution Overall",100,-3,3,100,-5,5);
TH2F *hEtaPtEC = new TH2F("hEtaPtEC","Eta vs MuonPt in Endcap",100,-3,3,100,0,200);
TH2F *hEtaPtBa = new TH2F("hEtaPtBa","Eta vs MuonPt in barrel",100,-3,3,100,0,200);
TH2F *hEtaPtAll = new TH2F("hEtaPtAll","Eta vs MuonPt Overall",100,-3,3,100,0,200);
//std::vector<double> leadmuonpt;
//std::vector<double> subleadmuonpt;
Double_t pt_cut=30.;
Double_t bin_width=0.25;
const int bin_size_sl=pt_cut/bin_width;
const int bin_size_l=pt_cut/bin_width;
Double_t leadmuonpt[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt[bin_size_sl]={0};
Double_t leadmuonpt1[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt1[bin_size_sl]={0};
Double_t leadmuonpt2[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt2[bin_size_sl]={0};
Double_t leadmuonpt3[bin_size_l]={0}; //100 is the number of bins
Double_t subleadmuonpt3[bin_size_sl]={0};
//TFile *f1 = new TFile("histos_signal.root","RECREATE");
//f1->cd();
//TTree *tree = new TTree("gemtree","variables");
//tree->Branch("dimuoneta",&dimuoneta,"dimuoneta/D");
//tree->Branch("dimuonratiopt",&dimuonratiopt,"dimuonratiopt/D");
Long64_t nentries = fChain->GetEntriesFast();
cout<<"Number of events "<<nentries<<endl;
//int dimuon=0;
Long64_t nbytes = 0, nb = 0;
//for (Long64_t jentry=0;jentry<nentries;jentry++) {
for (Long64_t jentry=0;jentry<nentries;jentry++) {
Long64_t ientry = LoadTree(jentry);
if (ientry < 0) break;
nb = fChain->GetEntry(jentry); nbytes += nb;
//if (nMuon!=2) continue;
//if (abs(Muon_phi[0]-Muon_phi[1] < 2.0))continue;
//++dimuon;
for (int i=0;i<nMuon; i++){
if (Muon_pt[i]<0.3) cout<<"found it"<<endl;
RecoMuonPt=Muon_pt[i];
RecoMuonEta=Muon_eta[i];
GenMuonPt=Muon_ptgen[i];
ImpParam=Muon_trkD0[i];
MuonDZ=Muon_trkDz[i];
MuonChi2=Muon_globalChi2[i];
h1PtGen->Fill(GenMuonPt);
h1PtReco->Fill(RecoMuonPt);
h1EtaReco->Fill(RecoMuonEta);
hEtaPtAll->Fill(RecoMuonEta,RecoMuonPt);
}
//f1->cd();
//tree->Write();
//f1->Close();
}
}
//hAcceptAll->Write();
/*
//Time to beautify most of the plots
hLeadMuon1->SetLineColor(1);
hSubLeadMuon1->SetLineColor(4);
hLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hSubLeadMuon1->SetXTitle("Reco. Muon p_{T} in GeV/c");
hLeadMuon1->SetYTitle("Number of Events/GeV");
hSubLeadMuon1->SetYTitle("Number of Events/GeV");
hSubLeadMuon1->Draw();
gPad->Update();
hLeadMuon1->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon1->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon1->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon1->Draw();
hLeadMuon1->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 1");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon1,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon1,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case1.pdf");
c1->Clear();
hLeadMuon2->SetLineColor(1);
hSubLeadMuon2->SetLineColor(4);
hLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon2->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon2->SetYTitle("Number of Events/GeV");
hSubLeadMuon2->SetYTitle("Number of Events/GeV");
hSubLeadMuon2->Draw();
gPad->Update();
hLeadMuon2->Draw();
gPad->Update();
//draw hist_2 first as it has a larger range
TPaveStats *tps1 = (TPaveStats*) hLeadMuon2->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon2->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon2->Draw();
hLeadMuon2->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 2");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon2,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon2,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case2.pdf");
hLeadMuon3->SetLineColor(1);
hSubLeadMuon3->SetLineColor(4);
hLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hSubLeadMuon3->SetXTitle("Reco Muon p_{T} in GeV/c");
hLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->SetYTitle("Number of Events/GeV");
hSubLeadMuon3->Draw();
gPad->Update();
hLeadMuon3->Draw(); //draw hist_2 first as it has a larger range
gPad->Update();
TPaveStats *tps1 = (TPaveStats*) hLeadMuon3->FindObject("stats");
tps1->SetName("Hist1 Stats");
double X1 = tps1->GetX1NDC();
double Y1 = tps1->GetY1NDC();
double X2 = tps1->GetX2NDC();
double Y2 = tps1->GetY2NDC();
TPaveStats *tps2 = (TPaveStats*) hSubLeadMuon3->FindObject("stats");
tps2->SetTextColor(kRed);
tps2->SetLineColor(kRed);
tps2->SetX1NDC(X1);
tps2->SetX2NDC(X2);
tps2->SetY1NDC(Y1-(Y2-Y1));
tps2->SetY2NDC(Y1);
hSubLeadMuon3->Draw();
hLeadMuon3->Draw("same"); //draw hist_2 first as it has a larger range
tps2->Draw("same");
tps1->Draw("same");
leg_hist = new TLegend(0.4712644,0.5886076,0.7600575,0.778481,NULL,"brNDC");
leg_hist->SetHeader("p_{T} histograms for case 3");
leg_hist->SetFillColor(0);
leg_hist->AddEntry(hLeadMuon3,"leading muon p_{T}","l");
leg_hist->AddEntry(hSubLeadMuon3,"subleading muon p_{T}","l");
leg_hist->SetTextSize(0.03);
leg_hist->Draw();
c1->SaveAs("case3.pdf");
c1->Clear();
//pT resolution plots
hMCMatchEC->SetLineColor(1);
hMCMatchEC->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchEC->Draw(); //draw hist_2 first as it has a larger range
hMCMatchEC->Draw("esame"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_1.pdf");
c1->Clear();
hMCMatchBa->SetLineColor(1);
hMCMatchBa->SetXTitle("p_{T}^{gen}-p_{T}^{reco}/p_{T}^{gen}");
hMCMatchBa->Draw(); //draw hist_2 first as it has a larger range
hMCMatchBa->Draw("esame"); //draw hist_2 first as it has a larger range
c1->SaveAs("plot_res_2.pdf");
c1->Clear();
//Acceptance plots
//Overall Acceptance
hAcceptAll->SetXTitle("Reco. leading muon p_{T} in GeV/c");
hAcceptAll->SetYTitle("Reco. subleading muon p_{T} in GeV/c");
//hAcceptAll->SetZTitle("Kinematic acceptance");
hAcceptAll->SetStats(0);
hAcceptAll->Draw("cont3 colz");
//HLT_PATH Mu17_Mu8
Int_t a=hAcceptAll->GetXaxis()->FindBin(17);
Int_t b = hAcceptAll->GetYaxis()->FindBin(8);
Double_t c = hAcceptAll->GetBinContent(a,b);
//Double_t a = hAcceptAll->GetBinContent(hAcceptAll->GetXaxis()->FindBin(17),hAcceptAll->GetYaxis()->FindBin(8)));
//HLT_PATH Mu13_Mu8
//Double_t f=hAcceptAll->GetBinContent(
Int_t d = hAcceptAll->GetXaxis()->FindBin(13);
Int_t e = hAcceptAll->GetYaxis()->FindBin(8);
Double_t f = hAcceptAll->GetBinContent(d,e);
//Offline Cuts
Int_t g=hAcceptAll->GetXaxis()->FindBin(20);
Int_t h=hAcceptAll->GetYaxis()->FindBin(10);
Double_t z=hAcceptAll->GetBinContent(g,h);
//Double_t i=GetBinContenthAcceptAll->GetXaxis()->FindBin(20),hAcceptAll->GetYaxis()->FindBin(10));
cout<<c<<" "<<f<<" "<<z<<endl;
//Double_t m=hAcceptAll->GetBinContent(k,l);
//draw hist_2 first as it has a larger range
TLatex latex;
//latex.DrawLatex(17,8,TString::Format("#bullet %f ",a));
//latex.DrawLatex(17,8,TString::Format("#bullet %2f %",c*100));
latex.DrawLatex(17,8,"#bullet 64%");
latex.DrawLatex(13,8,"#diamond 72%");
latex.DrawLatex(20,10,"#color[2]{#bullet} 43%");
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(17,8,TString::Format("%f",c));
//latex.DrawLatex(13,8,TString::Format("#diamond %f ",f));
//latex.DrawLatex(20,10,TString::Format("#circ %f ",i));
latex.DrawLatex(3,22,"#bullet");
latex.DrawLatex(2.83,25,"#diamond");
latex.DrawLatex(4,28,"#scale[0.8]{HLT Paths(Run-1)}");
latex.DrawLatex(4,22,"#scale[0.8]{Mu17_Mu8 (Luminosity 19.1 fb^{-1})}");
latex.DrawLatex(4,25,"#scale[0.8]{Mu13_Mu8 (Luminosity 3.7 fb^{-1})}");
latex.DrawLatex(4,19,"#scale[0.8]{Offline selection}");
latex.DrawLatex(3,16,"#color[2]{#bullet}");
latex.DrawLatex(4,16,"#scale[0.8]{Mu20_Mu10}");
c1->SaveAs("acceptance_2d_all_cont3.pdf");
//Case-1
*/
<file_sep>{
// Set up FW Lite for automatic loading of CMS libraries
// and data formats. As you may have other user-defined setup
// in your rootlogon.C, the CMS setup is executed only if the CMS
// environment is set up.
//
TString cmsswbase = getenv("CMSSW_BASE");
if (cmsswbase.Length() > 0) {
//
// The CMSSW environment is defined (this is true even for FW Lite)
// so set up the rest.
//
cout << "Loading FW Lite setup." << endl;
gSystem->Load("libFWCoreFWLite.so");
AutoLibraryLoader::enable();
gSystem->Load("libDataFormatsFWLite.so");
gSystem->Load("libDataFormatsPatCandidates.so");
//TStyle *gStyle= new TStyle("BABAR","BaBar approved plots style");
gStyle->SetFrameBorderMode(0);
gStyle->SetCanvasBorderMode(0);
gStyle->SetPadBorderMode(0);
gStyle->SetPadColor(0);
gStyle->SetCanvasColor(0);
gStyle->SetStatColor(0);
// set the paper & margin sizes
gStyle->SetPaperSize(20,26);
gStyle->SetPadTopMargin(0.05);
gStyle->SetPadRightMargin(0.05);
gStyle->SetPadBottomMargin(0.16);
gStyle->SetPadLeftMargin(0.12);
// use large Times-Roman fonts
gStyle->SetTextFont(132);
gStyle->SetTextSize(0.08);
gStyle->SetLabelFont(132,"x");
gStyle->SetLabelFont(132,"y");
gStyle->SetLabelFont(132,"z");
gStyle->SetLabelSize(0.05,"x");
gStyle->SetTitleSize(0.06,"x");
gStyle->SetLabelSize(0.05,"y");
gStyle->SetTitleSize(0.06,"y");
gStyle->SetLabelSize(0.05,"z");
gStyle->SetTitleSize(0.06,"z");
// use bold lines and markers
gStyle->SetMarkerStyle(20);
gStyle->SetHistLineWidth(1.85);
gStyle->SetLineStyleString(2,"[12 12]"); // postscript dashes
// get rid of X error bars and y error bar caps
gStyle->SetErrorX(0.001);
// do not display any of the standard histogram decorations
gStyle->SetOptTitle(0);
gStyle->SetOptStat(0);
gStyle->SetOptFit(0);
// put tick marks on top and RHS of plots
gROOT->SetStyle("Plain");
gStyle->SetOptStat(1111111);
gStyle->SetPadTickX(1);
gStyle->SetPadTickY(1);
}
}
<file_sep>import FWCore.ParameterSet.Config as cms
## ---
## PAT trigger matching
## --
matchHLTMuons = cms.EDProducer(
# matching in DeltaR, sorting by best DeltaR
"PATTriggerMatcherDRLessByR"
# matcher input collections
, src = cms.InputTag( 'selectedPatMuons' )
, matched = cms.InputTag( 'patTrigger' )
# selections of trigger objects
, matchedCuts = cms.string( 'type( "TriggerMuon" ) && ( path( "HLT_IsoMu24_v*" ) || path( "HLT_IsoMu24" ) )' )
# selection of matches
, maxDPtRel = cms.double( 0.5 ) # no effect here
, maxDeltaR = cms.double( 0.5 )
, maxDeltaEta = cms.double( 0.2 ) # no effect here
# definition of matcher output
, resolveAmbiguities = cms.bool( True )
, resolveByMatchQuality = cms.bool( True )
)
<file_sep>#Automatically created by SCRAM
import os
__path__.append(os.path.dirname(os.path.abspath(__file__).rsplit('/VHTauTau/TreeMaker/',1)[0])+'/cfipython/slc6_amd64_gcc481/VHTauTau/TreeMaker')
<file_sep>#include <iostream>
#include <algorithm>
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "DataFormats/Common/interface/ValueMap.h"
#include "DataFormats/PatCandidates/interface/Jet.h"
#include "CondFormats/JetMETObjects/interface/FactorizedJetCorrector.h"
#include "CondFormats/JetMETObjects/interface/JetCorrectorParameters.h"
#include "CondFormats/JetMETObjects/interface/JetCorrectionUncertainty.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "PhysicsTools/SelectorUtils/interface/PFJetIDSelectionFunctor.h"
#include "TTree.h"
#include "TClonesArray.h"
#include "VHTauTau/TreeMaker/plugins/JetBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
PFJetIDSelectionFunctor pfjetIDLoose(PFJetIDSelectionFunctor::FIRSTDATA, PFJetIDSelectionFunctor::LOOSE);
PFJetIDSelectionFunctor pfjetIDTight(PFJetIDSelectionFunctor::FIRSTDATA, PFJetIDSelectionFunctor::TIGHT);
pat::strbitset retpf = pfjetIDLoose.getBitTemplate();
// Constructor
JetBlock::JetBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("jetSrc")),
_jecUncPath(iConfig.getParameter<std::string>("jecUncertainty")),
_applyResJEC (iConfig.getParameter<bool> ("applyResidualJEC")),
_resJEC (iConfig.getParameter<std::string> ("residualJEC"))
{}
void JetBlock::beginJob()
{
std::string tree_name = "vhtree";
TTree* tree = vhtm::Utility::getTree(tree_name);
cloneJet = new TClonesArray("vhtm::Jet");
tree->Branch("Jet", &cloneJet, 32000, 2);
tree->Branch("nJet", &fnJet, "fnJet/I");
}
void JetBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneJet->Clear();
fnJet = 0;
JetCorrectionUncertainty *jecUnc = 0;
JetCorrectorParameters *ResJetCorPar = 0;
FactorizedJetCorrector *JEC = 0;
bool applyResJECLocal = _applyResJEC;
if (_applyResJEC) {
try {
edm::FileInPath fipUnc(_jecUncPath);;
jecUnc = new JetCorrectionUncertainty(fipUnc.fullPath());
edm::FileInPath fipRes(_resJEC);
ResJetCorPar = new JetCorrectorParameters(fipRes.fullPath());
std::vector<JetCorrectorParameters> vParam;
vParam.push_back(*ResJetCorPar);
JEC = new FactorizedJetCorrector(vParam);
}
catch (std::exception& ex) {
edm::LogInfo("JetBlock") << "The following exception occurred:"
<< std::endl
<< ex.what();
applyResJECLocal = false;
}
}
edm::Handle<edm::View<pat::Jet> > jets;
iEvent.getByLabel(_inputTag, jets);
if (jets.isValid()) {
unsigned int njets = jets->size();
edm::LogInfo("JetBlock") << "Total # PAT Jets: " << njets;
for (size_t i = 0; i < njets; ++i) {
if (fnJet == kMaxJet) {
edm::LogInfo("JetBlock") << "Too many PAT Jets, fnJet = " << fnJet;
break;
}
const pat::Jet& jet = jets->at(i);
retpf.set(false);
int passjetLoose = (pfjetIDLoose(jet, retpf)) ? 1 : 0;
retpf.set(false);
int passjetTight = (pfjetIDTight(jet, retpf)) ? 1 : 0;
double corr = 1.;
if (applyResJECLocal && iEvent.isRealData() ) {
JEC->setJetEta(jet.eta());
JEC->setJetPt(jet.pt()); // here you put the L2L3 Corrected jet pt
corr = JEC->getCorrection();
}
if (jecUnc) {
jecUnc->setJetEta(jet.eta());
jecUnc->setJetPt(jet.pt()*corr); // the uncertainty is a function of the corrected pt
}
jetB = new ((*cloneJet)[fnJet++]) vhtm::Jet();
// fill in all the vectors
jetB->eta = jet.eta();
jetB->phi = jet.phi();
jetB->pt = jet.pt()*corr;
jetB->pt_raw = jet.correctedJet("Uncorrected").pt();
jetB->energy = jet.energy()*corr;
jetB->energy_raw = jet.correctedJet("Uncorrected").energy();
jetB->jecUnc = (jecUnc) ? jecUnc->getUncertainty(true) : -1;
jetB->resJEC = corr;
jetB->partonFlavour = jet.partonFlavour();
// Jet identification in high pile-up environment
float mvaValue = jet.userFloat("pileupJetIdProducer:fullDiscriminant");
jetB->puIdMVA = mvaValue;
jetB->puIdBits = jet.userInt("pileupJetIdProducer:fullId"); // Bits: 0:Tight,1:Medium,2:Loose
jetB->chargedEmEnergyFraction = jet.chargedEmEnergyFraction();
jetB->chargedHadronEnergyFraction = jet.chargedHadronEnergyFraction();
jetB->chargedMuEnergyFraction = jet.chargedMuEnergyFraction();
jetB->electronEnergyFraction = jet.electronEnergy()/jet.energy();
jetB->muonEnergyFraction = jet.muonEnergyFraction();
jetB->neutralEmEnergyFraction = jet.neutralEmEnergyFraction();
jetB->neutralHadronEnergyFraction = jet.neutralHadronEnergyFraction();
jetB->photonEnergyFraction = jet.photonEnergyFraction();
jetB->chargedHadronMultiplicity = jet.chargedHadronMultiplicity();
jetB->chargedMultiplicity = jet.chargedMultiplicity();
jetB->electronMultiplicity = jet.electronMultiplicity();
jetB->muonMultiplicity = jet.muonMultiplicity();
jetB->neutralHadronMultiplicity = jet.neutralHadronMultiplicity();
jetB->neutralMultiplicity = jet.neutralMultiplicity();
jetB->photonMultiplicity = jet.photonMultiplicity();
jetB->nConstituents = jet.numberOfDaughters();
jetB->trackCountingHighEffBTag = jet.bDiscriminator("trackCountingHighEffBJetTags");
jetB->trackCountingHighPurBTag = jet.bDiscriminator("trackCountingHighPurBJetTags");
jetB->simpleSecondaryVertexHighEffBTag = jet.bDiscriminator("simpleSecondaryVertexHighEffBJetTags");
jetB->simpleSecondaryVertexHighPurBTag = jet.bDiscriminator("simpleSecondaryVertexHighPurBJetTags");
jetB->jetProbabilityBTag = jet.bDiscriminator("jetProbabilityBJetTags");
jetB->jetBProbabilityBTag = jet.bDiscriminator("jetBProbabilityBJetTags");
jetB->combinedSecondaryVertexBTag = jet.bDiscriminator("combinedSecondaryVertexBJetTags");
jetB->combinedSecondaryVertexMVABTag = jet.bDiscriminator("combinedSecondaryVertexMVABJetTags");
jetB->combinedInclusiveSecondaryVertexBTag = jet.bDiscriminator("combinedInclusiveSecondaryVertexBJetTags");
jetB->combinedMVABTag = jet.bDiscriminator("combinedMVABJetTags");
jetB->passLooseID = passjetLoose;
jetB->passTightID = passjetTight;
if (_verbosity > 0)
std::cout << "JetBlock: trackCountingHighEffBJetTag = " << jetB->trackCountingHighEffBTag
<< ", trackCountingHighPurBJetTag = " << jetB->trackCountingHighPurBTag
<< ", jetProbabilityBTag = " << jetB->jetProbabilityBTag
<< ", jetBProbabilityBTag = " << jetB->jetBProbabilityBTag
<< std::endl;
}
}
else {
edm::LogError("JetBlock") << "Error >> Failed to get pat::Jet collection for label: "
<< _inputTag;
}
if (jecUnc) delete jecUnc;
if (ResJetCorPar) delete ResJetCorPar;
if (JEC) delete JEC;
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(JetBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
vertexBlock = cms.EDAnalyzer("VertexBlock",
verbosity = cms.int32(1),
vertexSrc = cms.InputTag('offlinePrimaryVertices')
)
<file_sep>#ifndef __VHTauTau_TreeMaker_TriggerBlock_h
#define __VHTauTau_TreeMaker_TriggerBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "DataFormats/Common/interface/Ref.h"
#include "FWCore/Utilities/interface/InputTag.h"
#include "DataFormats/Common/interface/Handle.h"
#include "DataFormats/Provenance/interface/EventID.h"
#include "FWCore/ParameterSet/interface/ProcessDesc.h"
#include "HLTrigger/HLTcore/interface/HLTConfigProvider.h"
class TClonesArray;
//class Trigger;
class TriggerBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup);
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit TriggerBlock(const edm::ParameterSet& iConfig);
virtual ~TriggerBlock();
private:
//TClonesArray* cloneTrigger;
int _verbosity;
edm::InputTag _inputTag;
const edm::InputTag _l1InputTag;
const edm::InputTag _hltInputTag;
const std::vector<std::string> _hltPathsOfInterest;
HLTConfigProvider hltConfig;
std::vector<int> *_l1physbits;
std::vector<int> *_l1techbits;
std::vector<std::string> *_hltpaths;
std::vector<int> *_hltresults;
std::vector<int> *_hltprescales;
};
#endif
<file_sep>#ifndef __VHTauTau_TreeMaker_GenJetBlock_h
#define __VHTauTau_TreeMaker_GenJetBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class GenJet;
class GenJetBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit GenJetBlock(const edm::ParameterSet& iConfig);
virtual ~GenJetBlock() {}
enum {
kMaxGenJet = 100
};
private:
TClonesArray* cloneGenJet;
int fnGenJet;
int _verbosity;
edm::InputTag _inputTag;
vhtm::GenJet* genJetB;
};
#endif
<file_sep>#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "DataFormats/Common/interface/View.h"
#include "DataFormats/TrackReco/interface/Track.h"
#include "DataFormats/TrackReco/interface/TrackFwd.h"
#include "DataFormats/TrackReco/interface/HitPattern.h"
#include "DataFormats/BeamSpot/interface/BeamSpot.h"
#include "Math/GenVector/VectorUtil.h"
#include "VHTauTau/TreeMaker/plugins/TrackBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
TrackBlock::TrackBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("trackSrc")),
_beamSpot(iConfig.getParameter<edm::InputTag>("offlineBeamSpot"))
{}
void TrackBlock::beginJob()
{
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneTrack = new TClonesArray("vhtm::Track");
tree->Branch("Track", &cloneTrack);
tree->Branch("nTrack", &fnTrack, "fnTrack/I");
}
void TrackBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneTrack->Clear();
fnTrack = 0;
// read the beam spot
edm::Handle<reco::BeamSpot> beamSpot;
iEvent.getByLabel(_beamSpot, beamSpot);
edm::Handle<reco::TrackCollection> tracks;
iEvent.getByLabel(_inputTag, tracks);
if (tracks.isValid()) {
edm::LogInfo("TrackBlock") << "Total # of Tracks: " << tracks->size();
reco::Track::TrackQuality quality = reco::Track::qualityByName("loose");
for (reco::TrackCollection::const_iterator it = tracks->begin();
it != tracks->end(); ++it) {
const reco::Track &track = *it;
if (!track.quality(quality)) continue;
if (fnTrack == kMaxTrack) {
edm::LogInfo("TrackBlock") << "Too many Tracks in the event, fnTrack = " << fnTrack;
break;
}
trackB = new ((*cloneTrack)[fnTrack++]) vhtm::Track();
trackB->eta = track.eta();
trackB->etaError = track.etaError();
trackB->theta = track.theta();
trackB->thetaError = track.thetaError();
trackB->phi = track.phi();
trackB->phiError = track.phiError();
trackB->p = track.p();
trackB->pt = track.pt();
trackB->ptError = track.ptError();
trackB->qoverp = track.qoverp();
trackB->qoverpError = track.qoverpError();
trackB->charge = track.charge();
trackB->nValidHits = track.numberOfValidHits();
trackB->nLostHits = track.numberOfLostHits();
trackB->validFraction = track.validFraction();
const reco::HitPattern& hitp = track.hitPattern();
trackB->nValidTrackerHits = hitp.numberOfValidTrackerHits();
trackB->nValidPixelHits = hitp.numberOfValidPixelHits();
trackB->nValidStripHits = hitp.numberOfValidStripHits();
trackB->trackerLayersWithMeasurement = hitp.trackerLayersWithMeasurement();
trackB->pixelLayersWithMeasurement = hitp.pixelLayersWithMeasurement();
trackB->stripLayersWithMeasurement = hitp.stripLayersWithMeasurement();
trackB->dxy = track.dxy(beamSpot->position());
trackB->dxyError = track.dxyError();
trackB->dz = track.dz(beamSpot->position());
trackB->dzError = track.dzError();
// trackB->d0 = track.d0();
trackB->chi2 = track.chi2();
trackB->ndof = track.ndof();
trackB->vx = track.vx();
trackB->vy = track.vy();
trackB->vz = track.vz();
}
}
else {
edm::LogError("TrackBlock") << "Error! Failed to get reco::Track collection, "
<< _inputTag;
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(TrackBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
electronBlock = cms.EDAnalyzer("ElectronBlock",
verbosity = cms.int32(0),
offlineBeamSpot = cms.InputTag('offlineBeamSpot'),
trackSrc = cms.InputTag('generalTracks'),
vertexSrc = cms.InputTag('offlinePrimaryVertices'),
electronSrc = cms.InputTag('electronVariables')
)
<file_sep>#include <algorithm>
#include <iostream>
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "SimDataFormats/GeneratorProducts/interface/GenEventInfoProduct.h"
#include "TTree.h"
#include "TClonesArray.h"
#include "VHTauTau/TreeMaker/plugins/GenEventBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
GenEventBlock::GenEventBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getUntrackedParameter<int>("verbosity", 0)),
_genEvtInfoInputTag(iConfig.getParameter<edm::InputTag>("GenEventInfoInputTag")),
_storePDFWeights(iConfig.getParameter<bool>("StorePDFWeights")),
_pdfWeightsInputTag(iConfig.getParameter<edm::InputTag>("PDFWeightsInputTag"))
{}
GenEventBlock::~GenEventBlock() {
delete _pdfWeights;
}
void GenEventBlock::beginJob()
{
_pdfWeights = new std::vector<double>();
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneGenEvent = new TClonesArray("vhtm::GenEvent");
tree->Branch("GenEvent", &cloneGenEvent, 32000, 2);
tree->Branch("pdfWeights", "vector<double>", &_pdfWeights);
}
void GenEventBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray
cloneGenEvent->Clear();
// Clear the two independent vectors
_pdfWeights->clear();
if (!iEvent.isRealData()) {
// Create Event Object
genEventB = new ( (*cloneGenEvent)[0] ) vhtm::GenEvent();
// GenEventInfo Part
edm::Handle<GenEventInfoProduct> genEvtInfoProduct;
iEvent.getByLabel(_genEvtInfoInputTag, genEvtInfoProduct);
if (genEvtInfoProduct.isValid()) {
edm::LogInfo("GenEventBlock") << "Success >> obtained GenEventInfoProduct for label:"
<< _genEvtInfoInputTag;
genEventB->processID = genEvtInfoProduct->signalProcessID();
genEventB->ptHat = genEvtInfoProduct->hasBinningValues()
? genEvtInfoProduct->binningValues()[0] : 0.;
}
else {
edm::LogError("GenEventBlock") << "Error >> Failed to get GenEventInfoProduct for label: "
<< _genEvtInfoInputTag;
}
// PDF Weights Part
if (_storePDFWeights) {
edm::Handle<std::vector<double> > pdfWeightsHandle;
iEvent.getByLabel(_pdfWeightsInputTag, pdfWeightsHandle);
if (pdfWeightsHandle.isValid()) {
edm::LogInfo("GenEventBlock") << "Success >> obtained PDF handle for label: "
<< _pdfWeightsInputTag;
copy(pdfWeightsHandle->begin(), pdfWeightsHandle->end(), genEventB->pdfWeights.begin());
copy(pdfWeightsHandle->begin(), pdfWeightsHandle->end(), _pdfWeights->begin());
}
else {
edm::LogError("GenEventBlock") << "Error >> Failed to get PDF handle for label: "
<< _pdfWeightsInputTag;
}
}
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(GenEventBlock);
<file_sep>#include "TTree.h"
#include "TClonesArray.h"
#include "FWCore/MessageLogger/interface/MessageLogger.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "DataFormats/METReco/interface/GenMET.h"
#include "DataFormats/METReco/interface/GenMETFwd.h"
#include "VHTauTau/TreeMaker/plugins/GenMETBlock.h"
#include "VHTauTau/TreeMaker/interface/Utility.h"
GenMETBlock::GenMETBlock(const edm::ParameterSet& iConfig) :
_verbosity(iConfig.getParameter<int>("verbosity")),
_inputTag(iConfig.getParameter<edm::InputTag>("genMETSrc"))
{
}
void GenMETBlock::beginJob()
{
// Get TTree pointer
TTree* tree = vhtm::Utility::getTree("vhtree");
cloneGenMET = new TClonesArray("vhtm::GenMET");
tree->Branch("GenMET", &cloneGenMET, 32000, 2);
tree->Branch("nGenMET", &fnGenMET, "fnGenMET/I");
}
void GenMETBlock::analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup) {
// Reset the TClonesArray and the nObj variables
cloneGenMET->Clear();
fnGenMET = 0;
if (!iEvent.isRealData()) {
edm::Handle<reco::GenMETCollection> mets;
iEvent.getByLabel(_inputTag, mets);
if (mets.isValid()) {
edm::LogInfo("GenMETBlock") << "Total # GenMETs: " << mets->size();
for (reco::GenMETCollection::const_iterator it = mets->begin();
it != mets->end(); ++it ) {
if (fnGenMET == kMaxGenMET) {
edm::LogInfo("GenMETBlock") << "Too many GenMET, fnGenMET = " << fnGenMET;
break;
}
genMetB = new ((*cloneGenMET)[fnGenMET++]) vhtm::GenMET();
// fill in all the vectors
genMetB->met = it->pt();
genMetB->metphi = it->phi();
genMetB->sumet = it->sumEt();
}
}
else {
edm::LogError("GenMETBlock") << "Error >> Failed to get GenMETCollection for label: "
<< _inputTag;
}
}
}
#include "FWCore/Framework/interface/MakerMacros.h"
DEFINE_FWK_MODULE(GenMETBlock);
<file_sep>import FWCore.ParameterSet.Config as cms
tauBlock = cms.EDAnalyzer("TauBlock",
verbosity = cms.int32(0),
#patTauSrc = cms.InputTag('tauVariables'),
patTauSrc = cms.InputTag('selectedPatTausPFlow'),
vertexSrc = cms.InputTag('offlinePrimaryVertices')
)
<file_sep>import FWCore.ParameterSet.Config as cms
genJetBlock = cms.EDAnalyzer("GenJetBlock",
verbosity = cms.int32(0),
genJetSrc = cms.InputTag('ak5GenJets')
)
<file_sep>import FWCore.ParameterSet.Config as cms
#channel = "BLABLA"
process = cms.Process("HTauTauTree")
## MessageLogger
process.load("FWCore.MessageLogger.MessageLogger_cfi")
process.MessageLogger.cerr.FwkReport.reportEvery = 1
#from CalibCalorimetry.EcalTrivialCondModules.EcalTrivialCondRetriever_cfi import *
#process.myCond = EcalTrivialConditionRetriever.clone()
#process.es_prefer_gt = cms.ESPrefer("PoolDBESSource","GlobalTag")
## Options and Output Report
process.options = cms.untracked.PSet( wantSummary = cms.untracked.bool(False) )
process.options.allowUnscheduled = cms.untracked.bool( True )
## Geometry and Detector Conditions (needed for a few patTuple production steps)
#process.load('Configuration.Geometry.GeometryExtended2017Reco_cff')
#process.load('Configuration.Geometry.GeometryExtended2017_cff')
process.load('Configuration.Geometry.GeometryExtended2019Reco_cff')
process.load('Configuration.Geometry.GeometryExtended2019_cff')
process.load('Configuration.StandardSequences.MagneticField_38T_cff')
#process.load("RecoLocalCalo.EcalRecAlgos.EcalSeverityLevelESProducer_cfi")
process.load('Configuration.StandardSequences.Services_cff')
process.load('Configuration.StandardSequences.FrontierConditions_GlobalTag_cff')
#process.GlobalTag.globaltag = 'STAR17_61_V5A::All'
#process.GlobalTag.globaltag = 'DES17_61_V4::All'
#for Archana di
process.GlobalTag.globaltag = 'DES19_62_V8::All'
process.load("Configuration.StandardSequences.MagneticField_cff")
process.source = cms.Source("PoolSource",
fileNames = cms.untracked.vstring(
#'root://xrootd.unl.edu//store/mc/Fall13dr/DYJetsToLL_M-50_13TeV-pythia6/AODSIM/tsg_PU40bx50_POSTLS162_V1-v1/00000/0659AA61-2175-E311-8B63-00266CFFA2B8.root'
#'root://xrootd.unl.edu//store/mc/Summer13/VBF_HToTauTau_M-125_14TeV-powheg-pythia6/GEN-SIM-RECO/UpgradePhase1Age0DES_DR61SLHCx_NoPileUp_DES17_61_V5-v1/10000/00A38233-7E02-E311-A483-002618943964.root'
#'root://xrootd.unl.edu//store/mc/Summer13/DYToTauTau_M-20_TuneZ2star_14TeV-pythia6-tauola/GEN-SIM-RECO/UpgradePhase1Age5H_DR61SLHCx_PU140Bx25_STAR17_61_V5A-v1/10000/0005C614-5DF2-E211-9BA8-0026189438ED.root'
#'file:/afs/cern.ch/work/a/archie/public/minbias/MinBias_TuneZ2star_14TeV_pythia6_CMSSW620_slhc5_996_1_Qy8.root',
#'file:/afs/cern.ch/work/a/archie/public/minbias/MinBias_TuneZ2star_14TeV_pythia6_CMSSW620_slhc5_997_1_1yO.root',
#'file:/afs/cern.ch/work/a/archie/public/minbias/MinBias_TuneZ2star_14TeV_pythia6_CMSSW620_slhc5_998_1_Tqc.root'
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_991_1_3qc.root',
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_996_1_kGt.root',
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_992_1_Ley.root',
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_997_1_cg0.root',
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_993_1_BdQ.root',
##'file:/afs/cern.ch/work/a/aabdelal/public/beamspotGauss/MinBias/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_998_1_QuQ.root',
'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1000_1_ESt.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1001_1_V3z.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1002_1_8rt.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1003_1_Jyg.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1004_1_cXq.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1005_1_YZ0.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1006_3_J9v.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1007_1_QBm.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1008_1_KS4.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1009_1_puE.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_100_1_En4.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1010_1_IFd.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1011_3_hFJ.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1012_1_dwz.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1013_1_xV0.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1014_1_Icy.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1015_1_tvI.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1016_1_SkU.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1017_1_jC3.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1018_1_NFq.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1019_1_YgP.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_101_1_L9f.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1020_1_Nz2.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1021_1_pds.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1022_1_Yg6.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1023_4_cu4.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1024_2_RF2.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1025_1_f4D.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1026_1_5NT.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1027_1_T4x.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1028_1_hqF.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1029_1_Vix.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1030_1_7Aj.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1031_1_flV.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1032_1_h62.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1033_1_Kvc.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1034_1_QOT.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1035_1_mWE.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1036_1_qYF.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1038_1_8CV.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1039_1_hTe.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_103_1_7NH.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1040_1_cAT.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1041_1_4N5.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1042_1_2La.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1043_1_5F0.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1044_1_hlt.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1045_1_2Pm.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1046_1_GHO.root',
#'root://xrootd.unl.edu//store/user/aabdelal/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/aabdelal_MinBias_TuneZ2star_14TeV_pythia6_14TeV_GaussBS_RECO_aod_CMSSW_6_2_0_SLHC5/4b73dbaf4bf75a21ad171c02dcd12bab/MinBias_TuneZ2star_14TeV_GaussBS_RECO_620slhc5_1047_1_ZpV.root'
)
)
process.maxEvents = cms.untracked.PSet( input = cms.untracked.int32(1) )
## Output Module Configuration (expects a path 'p')
#process.load("HighMassAnalysis.Configuration.patTupleEventContentForHiMassTau_cff")
###from HighMassAnalysis.Configuration.patTupleEventContentForHiMassTau_cff import *
###process.out = cms.OutputModule("PoolOutputModule",
### fileName = cms.untracked.string('skimPat.root'),
### # save only events passing the full path
### SelectEvents = cms.untracked.PSet( SelectEvents = cms.vstring('p') ),
### # outputCommands = cms.untracked.vstring('drop *', *patTupleEventContent ),
### fastCloning = cms.untracked.bool(False)
### )
#from PhysicsTools.PatAlgos.patEventContent_cff import patEventContentNoCleaning
#process.out = cms.OutputModule("PoolOutputModule",
#fileName = cms.untracked.string('patTuple.root'),
## save only events passing the full path
#SelectEvents = cms.untracked.PSet( SelectEvents = cms.vstring('p') ),
## save PAT output; you need a '*' to unpack the list of commands
## 'patEventContent'
#outputCommands = cms.untracked.vstring('drop *', *patEventContentNoCleaning )
#)
process.TFileService = cms.Service("TFileService",
fileName = cms.string('test_53x.root')
)
#process.scrapingVeto = cms.EDFilter("FilterOutScraping",
# applyfilter = cms.untracked.bool(True),
# debugOn = cms.untracked.bool(False),
# numtrack = cms.untracked.uint32(10),
# thresh = cms.untracked.double(0.2)
# )
#process.selectedVertices = cms.EDFilter("VertexSelector",
# src = cms.InputTag('offlinePrimaryVertices'),
# cut = cms.string('!isFake && isValid && ndof >= 4.0 && position.Rho < 2.0 && abs(z) < 24'),
# cut = cms.string('!isFake && isValid'),
# filter = cms.bool(True)
#)
#process.demo = cms.EDAnalyzer('TreeMaker',
# fillHZZInfo_ = cms.bool(True),
# patMuons = cms.InputTag("selectedPatMuons"),
# PFMET = cms.InputTag("pfMet"),
# Vertex=cms.InputTag("selectedVertices"),
# PileUpTag = cms.InputTag("addPileupInfo"),
# fastjetTag = cms.InputTag("kt6PFJets", "rho"),
# rhoConeSize = cms.double(0.5),
# rhoUEOffsetCorrection = cms.double(1.0),
# outputCommands = cms.untracked.vstring('drop *',
# 'keep *_*_*_ANA',
# 'keep *_gsfElectron*_*_*',
# 'keep *_muons_*_*',
# 'keep *_selectedPatMuons_*_*',
# 'keep *_zmm_*_*',
# 'keep *_pfMet_*_*',
# 'keep *_tcMet_*_*',
# 'keep *_ak5PFJets_*_*',
# 'keep *_TriggerResults_*_*',
# 'keep *_gtDigis_*_*',
# 'keep *_trackCountingHighEffBJetTags_*_*',
# 'keep *_ak5CaloJets_*_*',
# 'keep *_kt6PFJets_*_*',
# 'keep *_kt4PFJets_*_*',
# 'keep *_GlobalMuonPromptTight_*_*',
# 'keep *_offlinePrimaryVerticesWithBS_*_*',
# 'keep PileupSummaryInfos_*_*_*',
# 'keep *_offlinePrimaryVertices_*_*',
# 'keep *_selectedVertices_*_*',
# 'keep *_kt6PFJets_*_*',
# 'keep *_TriggerResults_*_*',
# 'keep triggerTriggerEvent_*_*_*',
# 'keep *_ak5CaloJets_*_*',
# 'keep recoPFClusters_*_*_*',
# 'keep *_selectedPatJets__*',
# 'keep *_selectedPatJetsFast__*',
# 'keep *_kt4PFJets_rho_PAT',
# 'keep *_kt4PFJetsIso_rho_PAT',
#
# )
#
#)
## Output Module Configuration (expects a path 'p')
#process.TFileService = cms.Service("TFileService",
# fileName = cms.string("analysis.root")
# )
#process.outpath = cms.EndPath(process.out)
# trigger + Skim sequence
###process.load("HighMassAnalysis.Skimming.triggerReq_cfi")
###process.load("HighMassAnalysis.Skimming.genLevelSequence_cff")
###if(channel == "BLABLA"):
### process.load("HighMassAnalysis.Skimming.TauSkimSequence_cff")
### process.theSkim = cms.Sequence( )
### process.hltFilter = cms.Sequence( )
### process.genLevelSelection = cms.Sequence( )
# Standard pat sequences
process.load('RecoJets.Configuration.RecoPFJets_cff')
process.load("PhysicsTools.PatAlgos.patSequences_cff")
# --------------------Modifications for taus--------------------
### process.load("PhysicsTools.JetMCAlgos.TauGenJets_cfi")
### process.load("RecoTauTag.Configuration.RecoPFTauTag_cff")
### from PhysicsTools.PatAlgos.tools.tauTools import *
### switchToPFTauHPS(process)
#from RecoJets.JetProducers.kt4PFJets_cfi import kt4PFJets
#process.kt6PFJetsForIsolation = kt4PFJets.clone( rParam = 0.6, doRhoFastjet = True )
#process.kt6PFJetsForIsolation.Rho_EtaMax = cms.double(2.5)
# --------------------Modifications for jets--------------------
#from PhysicsTools.PatAlgos.tools.jetTools import *
## uncomment the following lines to add ak5PFJets to your PAT output
#switchJetCollection(
# process,
# jetSource = cms.InputTag('ak5PFJets'),
# jetCorrections = ('AK5PF', cms.vstring(['L1FastJet', 'L2Relative', 'L3Absolute']), 'Type-2'),
# btagDiscriminators = [
# 'combinedSecondaryVertexBJetTags',
# 'combinedSecondaryVertexMVABJetTags',
# 'jetBProbabilityBJetTags',
# 'jetProbabilityBJetTags',
# 'simpleSecondaryVertexHighEffBJetTags',
# 'simpleSecondaryVertexHighPurBJetTags',
# ],
# )
#process.selectedPatJets.cut = cms.string("pt > 0 && abs(eta) < 5")
# --------------------Modifications for MET--------------------
### process.load("JetMETCorrections.Type1MET.pfMETCorrections_cff")
### process.load("JetMETCorrections.Type1MET.pfMETCorrectionType0_cfi")
### process.pfType1CorrectedMet.applyType0Corrections = cms.bool(False)
### process.pfType1CorrectedMet.srcType1Corrections = cms.VInputTag(
### cms.InputTag('pfMETcorrType0'),
### cms.InputTag('pfJetMETcorr', 'type1')
### )
# Let it run
process.load("VHTauTau.TreeMaker.TreeCreator_cfi")
process.load("VHTauTau.TreeMaker.TreeWriter_cfi")
process.load("VHTauTau.TreeMaker.TreeContentConfig_cff")
#-------------------------------------------------------
# PAT
#------------------------------------------------------
process.load("RecoTauTag.Configuration.RecoPFTauTag_cff")
process.load("PhysicsTools.PatAlgos.patSequences_cff")
import PhysicsTools.PatAlgos.tools.tauTools as tauTools
tauTools.switchToPFTauHPS(process) # For HPS Taus
process.p = cms.Path(
# process.type0PFMEtCorrection +
# process.producePFMETCorrections +
# process.tauGenJets +
# process.recoTauClassicHPSSequence +
process.patDefaultSequence
# process.treeCreator +
# process.treeContentSequence +
# process.treeWriter
# process.kt6PFJetsForIsolation +
# process.demo
)
<file_sep>import FWCore.ParameterSet.Config as cms
process = cms.Process("HTauTauTree")
#------------------------
# Message Logger Settings
#------------------------
process.load("FWCore.MessageLogger.MessageLogger_cfi")
process.MessageLogger.cerr.FwkReport.reportEvery = 1
#--------------------------------------
# Event Source & # of Events to process
#---------------------------------------
process.source = cms.Source("PoolSource",
fileNames = cms.untracked.vstring()
)
process.maxEvents = cms.untracked.PSet(
input = cms.untracked.int32(1)
)
process.options = cms.untracked.PSet( wantSummary = cms.untracked.bool(False) )
#-----------------------------
# Geometry
#-----------------------------
process.load("Configuration.StandardSequences.Geometry_cff")
#-----------------------------
# Magnetic Field
#-----------------------------
process.load('Configuration.StandardSequences.MagneticField_AutoFromDBCurrent_cff')
#-------------
# Global Tag
#-------------
process.load('Configuration.StandardSequences.FrontierConditions_GlobalTag_cff')
process.GlobalTag.globaltag = 'START53_V11::All'
#-------------
# Output ROOT file
#-------------
process.load("SimGeneral.HepPDTESSource.pythiapdt_cfi")
process.printTree = cms.EDAnalyzer("ParticleListDrawer",
maxEventsToPrint = cms.untracked.int32(1),
printVertex = cms.untracked.bool(False),
src = cms.InputTag("genParticles")
)
process.p = cms.Path(process.printTree)
process.PoolSource.fileNames = [
#'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/htotautomu_test.root',
#'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu_2/htotautomu_test_1_1_Hii.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/VHTauTau/TreeMaker/test/00E903E2-9FE9-E111-8B1E-003048FF86CA.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu_1/htotautomu_test_1_1_uIk.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu_1/htotautomu_test_3_1_wvk.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_1_1_zfD.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_2_1_1KO.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_6_1_Iwi.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_5_1_bow.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_12_1_DKN.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_13_1_lxR.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_7_1_r99.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_14_1_Y2O.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_4_1_7d9.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_10_1_72r.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_11_1_dgO.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_9_1_06x.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_15_1_36z.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_19_1_5Lg.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_22_1_Mi5.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_20_1_Fzy.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_17_1_O0L.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_16_1_fYJ.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_8_1_OgL.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_21_1_8jw.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_23_1_IkX.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_29_1_To5.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_28_1_zWe.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_30_1_WXO.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_18_1_ilY.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_25_1_UfF.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_24_1_j6W.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_26_1_csG.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_27_1_9DE.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_31_1_VR9.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_40_1_Eeb.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_38_1_ZYz.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_39_1_dq7.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_32_1_e7e.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_35_1_KDG.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_33_1_PJV.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_36_1_Nxk.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_37_1_Nq9.root',
'file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/htotautomu_test_34_1_6uG.root',
]
<file_sep>#ifndef __VHTauTau_TreeMaker_JetBlock_h
#define __VHTauTau_TreeMaker_JetBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "DataFormats/Common/interface/Ref.h"
#include "FWCore/Utilities/interface/InputTag.h"
#include "DataFormats/Common/interface/Handle.h"
#include "DataFormats/Provenance/interface/EventID.h"
#include "FWCore/ParameterSet/interface/ProcessDesc.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class Jet;
class JetBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit JetBlock(const edm::ParameterSet& iConfig);
virtual ~JetBlock() {}
enum {
kMaxJet = 100
};
private:
TClonesArray* cloneJet;
int fnJet;
int _verbosity;
edm::InputTag _inputTag;
std::string _jecUncPath;
bool _applyResJEC;
std::string _resJEC;
vhtm::Jet* jetB;
};
#endif
<file_sep>ls -ltr $1/| grep root | awk '{print ""$NF""}' > file
sed 's/^/file:/' file > newfile ; mv newfile file
sed -e "s|file:|file:$1|g" file > file1; mv file1 file
#sed -e "s/\(.*\)/'\1'/" file > file1; mv file1 file
#sed 's/$/,/' file > file1; mv file1 file
#xargs <file1 | sed -e "s|file:|file:/home/amohapatra/work/test/CMSSW_6_2_0_SLHC5/src/GluHTauMuMu/|g" > file<file_sep>#ifndef __VHTauTau_TreeMaker_TauBlock_h
#define __VHTauTau_TreeMaker_TauBlock_h
#include <string>
#include <vector>
#include "FWCore/Framework/interface/Frameworkfwd.h"
#include "FWCore/Framework/interface/EDAnalyzer.h"
#include "FWCore/Framework/interface/Event.h"
#include "FWCore/Framework/interface/MakerMacros.h"
#include "FWCore/ParameterSet/interface/ParameterSet.h"
#include "FWCore/ServiceRegistry/interface/Service.h"
#include "CommonTools/UtilAlgos/interface/TFileService.h"
#include "VHTauTau/TreeMaker/interface/PhysicsObjects.h"
class TClonesArray;
class Tau;
class TauBlock : public edm::EDAnalyzer
{
private:
virtual void beginJob();
virtual void beginRun(edm::Run const& iRun, edm::EventSetup const& iSetup) {}
virtual void analyze(const edm::Event& iEvent, const edm::EventSetup& iSetup);
virtual void endJob() {}
public:
explicit TauBlock(const edm::ParameterSet& iConfig);
virtual ~TauBlock();
static const reco::PFJetRef& getJetRef(const reco::PFTau& tau);
enum {
kMaxTau = 100
};
private:
TClonesArray* cloneTau;
int fnTau;
int _verbosity;
edm::InputTag _inputTag;
edm::InputTag _vtxInputTag;
vhtm::Tau* tauB;
};
#endif
| a86113a2e4403dd54b99de1d2d7b6a5e54095fa4 | [
"C",
"Python",
"C++",
"Shell"
] | 85 | C++ | tmoulik/HiggsTauTau | abcb19df959c3637a2ec929daa11c844142143ca | 873cdcdd0880067e7e0bfeaa75b2c874f9e26420 | |
refs/heads/master | <file_sep>
import { Injectable } from '@angular/core';
import { Router, CanActivate } from '@angular/router';
import { UserAuthService } from '../services/user-auth.service';
import { ROUTE } from '../app.constants';
@Injectable()
export class AuthGuard implements CanActivate {
constructor(
private router: Router,
private userAuthService: UserAuthService) { }
canActivate() {
if (!this.userAuthService.isTokenExpired()) {
return true;
}
this.router.navigate(['/' + ROUTE.SESSION_TIMEOUT]);
return false;
}
}<file_sep>/**
*
*/
const TOKEN_KEY: string = "tcc_auth_token";
const RESP_TOKEN_KEY: string = "token";
export class StorageHelper {
static get(key): string {
return localStorage.getItem(key);
}
static set(key, value): void {
localStorage.setItem(key, value);
}
static getToken(): string {
return this.get(TOKEN_KEY);
}
static setToken(value): void {
this.set(TOKEN_KEY, value);
}
static saveTokenFromResponse(resp): void {
let token = resp.headers.get(RESP_TOKEN_KEY);
if(token != undefined && token != null) {
this.setToken(token);
}
}
}<file_sep>import { Injectable } from '@angular/core';
import { HttpRequest, HttpHandler, HttpEvent, HttpInterceptor } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import { StorageHelper } from './storage-helper';
import { NGXLogger } from 'ngx-logger';
@Injectable()
export class AuthInterceptor implements HttpInterceptor {
constructor(private storage: StorageHelper, private logger: NGXLogger) { }
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
this.logger.info("AuthInterceptor:", request.url);
// Send token except for login & get user role:
if (request.url.indexOf('/auth') == -1) {
// add authorization header with jwt token if available
let token = StorageHelper.getToken();
if (token) {
request = request.clone({
setHeaders: {
Authorization: token
}
});
}
}
return next.handle(request);
}
}<file_sep>//Install express server
const express = require('express');
const path = require('path');
var bodyParser = require('body-parser')
const app = express();
// parse application/x-www-form-urlencoded
app.use(bodyParser.urlencoded({ extended: false }))
// parse application/json
app.use(bodyParser.json());
// Add headers
app.use(function (req, res, next) {
// Website you wish to allow to connect
res.setHeader('Access-Control-Allow-Origin', '*');
// Request methods you wish to allow
res.setHeader('Access-Control-Allow-Methods', 'GET, POST, OPTIONS, PUT, PATCH, DELETE');
// Request headers you wish to allow
res.setHeader('Access-Control-Allow-Headers', 'X-Requested-With,content-type, token_type, access_token, refresh_token, expiry_date');
// Set to true if you need the website to include cookies in the requests sent
// to the API (e.g. in case you use sessions)
res.setHeader('Access-Control-Allow-Credentials', true);
// Pass to next layer of middleware
next();
});
// Flatfile Database:
const low = require('lowdb')
const FileSync = require('lowdb/adapters/FileSync')
const adapter = new FileSync('db.json')
const db = low(adapter)
db.defaults({ users: [], count: 0 }).write()
/**** BACKEND SERVER ****/
app.get('/server/users', function(req, res) {
res.send({ status: 1, result: db.get('users').value() });
});
app.post('/server/users', function(req, res) {
db.get('users').push(req.body).write();
res.send({ status: 1 });
});
/**** FRONTEND ANGULAR APP ****/
// Serve only the static files form the dist directory
app.use(express.static(__dirname + '/dist/ng-bootstrap-starter'));
app.get('/*', function(req,res) {
res.sendFile(path.join(__dirname+'/dist/ng-bootstrap-starter/index.html'));
});
// Start the app by listening on the default Heroku port
var port = process.env.PORT || 8080;
app.listen(port, () => console.log(`Listening on port ${port}`));
<file_sep>import { Injectable } from '@angular/core';
import { HttpRequest, HttpResponse, HttpHandler, HttpEvent, HttpInterceptor, HTTP_INTERCEPTORS, HttpHeaders } from '@angular/common/http';
import { NGXLogger } from 'ngx-logger';
import { Observable } from 'rxjs';
import 'rxjs/add/observable/of';
import 'rxjs/add/observable/throw';
import 'rxjs/add/operator/delay';
import 'rxjs/add/operator/mergeMap';
import 'rxjs/add/operator/materialize';
import 'rxjs/add/operator/dematerialize';
import { URL, USER_ROLE } from '../app.constants';
@Injectable()
export class FakeBackendInterceptor implements HttpInterceptor {
dummyTokenHeader = new HttpHeaders({'token' : 'fake-token <PASSWORD>'});
constructor(private logger: NGXLogger) { }
intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
this.logger.info("FakeBackend:", request.url);
// wrap in delayed observable to simulate server api call
return Observable.of(null).mergeMap(() => {
if (request.url.indexOf(URL.GET_USER) != -1) {
let data = {
id: 1234,
username: 'manishg',
firstName: '<NAME>',
lastName: 'Ganta'
};
return Observable.of(new HttpResponse({ status: 200, body: data, headers: this.dummyTokenHeader }));
}
else if (request.url.indexOf(URL.REGISTER) != -1) {
let resp = {
status: 'success',
message: 'user created',
user_id: 1234
}
return Observable.of(new HttpResponse({ status: 200, body: resp }));
}
// authenticate
else if (request.url.indexOf(URL.LOGIN) != -1 && request.method === 'POST') {
// find if any user matches login credentials
let userPasswordValid = false;
if(request.body.user == 'demouser' && request.body.password == '<PASSWORD>') {
userPasswordValid = true;
}
if (userPasswordValid) {
// if login details are valid return 200 OK with user details and fake jwt token
let data = {
id: 1234,
username: 'demouser',
firstName: 'Demo',
lastName: 'User'
};
return Observable.of(new HttpResponse({ status: 200, body: data, headers: this.dummyTokenHeader }));
} else {
// else return 400 bad request
return Observable.throw('Username or password is incorrect');
}
}
// pass through any requests not handled above
return next.handle(request);
})
// call materialize and dematerialize to ensure delay even if an error is thrown (https://github.com/Reactive-Extensions/RxJS/issues/648)
.materialize()
.delay(500)
.dematerialize();
}
}
export let FakeBackendProvider = {
// use fake backend in place of Http service for backend-less development
provide: HTTP_INTERCEPTORS,
useClass: FakeBackendInterceptor,
multi: true
};<file_sep>
export const APP_TITLE = "Angular6 + Bootstrap";
// User Roles:
export const USER_ROLE = {
ADMIN: "admin",
USER: "user"
}
// URL constants:
export const URL = {
LOGIN : '/server/login',
REGISTER : '/server/create_user',
GET_USER : '/server/get_user',
UPDATE_USER : '/server/update_user'
};
// Routes:
export const ROUTE = {
SESSION_TIMEOUT: 'session-timeout',
HOME: 'home',
FEATURES: 'features',
CONTACT: 'contact',
REGISTER: 'register',
MY_PROFILE: 'my-profile'
}
<file_sep>// show-errors.component.ts
import { Component, Input } from '@angular/core';
import { AbstractControlDirective, AbstractControl, NgForm, NgModel } from '@angular/forms';
@Component({
selector: 'show-errors',
template: `
<ul *ngIf="shouldShowErrors()" style="list-style:none;padding:0;">
<li style="color: red" *ngFor="let error of listOfErrors()">{{error}}</li>
</ul>
`,
})
export class ShowErrorsComponent {
private static readonly errorMessages = {
'required': () => 'Entry required',
'minlength': (params) => 'Minimum characters are ' + params.requiredLength,
'maxlength': (params) => 'Maximum characters are ' + params.requiredLength,
'pattern': (params) => 'Invalid entry', // 'The required pattern is: ' + params.requiredPattern,
'years': (params) => params.message,
'countryCity': (params) => params.message,
'uniqueName': (params) => params.message,
'telephoneNumbers': (params) => params.message,
'telephoneNumber': (params) => params.message
};
@Input() private f: NgForm;
@Input() private control: NgModel;
@Input() private sameValue: NgModel;
@Input() private notSameValue: NgModel;
@Input() private other: NgModel;
@Input() private invalidVal: string;
@Input() private alreadyTaken:boolean
shouldShowErrors(): boolean {
let ret = this.control &&
this.control.errors &&
(this.control.dirty || this.control.touched || this.f.submitted) ||
(this.control.touched && this.sameValue != undefined && this.sameValue.value != this.control.value) ||
(this.control.touched && this.notSameValue != undefined && this.notSameValue.value == this.control.value) ||
(this.invalidVal != undefined && this.control.value == this.invalidVal)||this.alreadyTaken;
if (this.other != undefined) {
// ret - true means, there is an error, now check other field
ret = ret && (this.other.value == undefined || this.other.value == '');
}
return ret;
}
listOfErrors(): string[] {
if (this.other != undefined && (this.other.value == undefined || this.other.value == '')) {
// return ["Either " + this.other.name + " or " + this.control.name + " is required"];
return ["Entry Required"];
}
if (this.invalidVal != undefined && this.control.value == this.invalidVal) {
return ["Invalid value"];
}
if (this.sameValue != undefined) {
if (this.sameValue.valid && this.sameValue.value != this.control.value) {
let name = this.sameValue.name;
if(name == "newpassword") {
name = "New Password";
}
return [ name + " must match" ];
}
}
if (this.notSameValue != undefined) {
if (this.notSameValue.valid && this.notSameValue.value == this.control.value) {
return ["must not be same as " + this.notSameValue.name];
}
}
if(this.alreadyTaken){
return [this.control.name +" already in use"];
}
if(this.control.errors != undefined && this.control.errors != null) {
return Object.keys(this.control.errors)
.map(field => this.getMessage(field, this.control.errors[field]));
}
return [];
}
private getMessage(type: string, params: any) {
return ShowErrorsComponent.errorMessages[type](params);
}
}<file_sep>import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { HttpClientModule } from '@angular/common/http';
import { LoggerModule, NgxLoggerLevel } from 'ngx-logger';
import { AngularFontAwesomeModule } from 'angular-font-awesome';
import { LoadingBarRouterModule } from '@ngx-loading-bar/router';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { SocialLoginModule, AuthServiceConfig } from "angularx-social-login";
import { GoogleLoginProvider, FacebookLoginProvider, LinkedInLoginProvider} from "angularx-social-login";
import { AppComponent } from './app.component';
import { AppRouting } from './app.routing';
import { SessionTimeoutComponent } from './components/session-timeout.component';
import { HeaderComponent } from './components/partials/header/header.component';
import { FooterComponent } from './components/partials/footer/footer.component';
import { LoadingComponent } from './components/partials/loading/loading.component';
import { MyProfileComponent } from './components/my-profile/my-profile.component';
import { Utils } from './services/utils';
import { UserAuthService } from './services/user-auth.service';
import { FakeBackendProvider } from './helpers/fake-bakend';
import { environment } from '../environments/environment';
import { AuthGuard } from './helpers/auth.gaurd';
import { HomeComponent } from './components/home/home.component';
import { FeaturesComponent } from './components/features/features.component';
import { ContactComponent } from './components/contact/contact.component';
import { RegisterComponent } from './components/register/register.component';
import { ShowErrorsComponent } from './components/partials/show-errors.component';
export function getAuthServiceConfigs() {
let config = new AuthServiceConfig(
[
{
id: GoogleLoginProvider.PROVIDER_ID,
provider: new GoogleLoginProvider("1004357134903-s4mrfph41nhptm713rtun4h0v1t1svug.apps.googleusercontent.com")
},
{
id: FacebookLoginProvider.PROVIDER_ID,
provider: new FacebookLoginProvider("471510306668438")
},
// {
// id: LinkedInLoginProvider.PROVIDER_ID,
// provider: new LinkedInLoginProvider("81n843y6hvssco", true, 'en_US')
// },
]
);
return config;
}
@NgModule({
declarations: [
AppComponent,
SessionTimeoutComponent,
HeaderComponent,
FooterComponent,
LoadingComponent,
MyProfileComponent,
HomeComponent,
FeaturesComponent,
ContactComponent,
RegisterComponent,
ShowErrorsComponent
],
imports: [
BrowserModule,
BrowserAnimationsModule,
HttpClientModule,
FormsModule,
AppRouting,
AngularFontAwesomeModule,
SocialLoginModule,
LoggerModule.forRoot({
level: environment.production ? NgxLoggerLevel.LOG : NgxLoggerLevel.DEBUG,
serverLogLevel: NgxLoggerLevel.OFF
}),
LoadingBarRouterModule
],
providers: [
Utils,
AuthGuard,
UserAuthService,
{
provide: AuthServiceConfig,
useFactory: getAuthServiceConfigs
},
FakeBackendProvider
],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>import { Component, OnInit } from '@angular/core';
import { fadeAnimation } from './animations';
@Component({
selector: 'app-root',
template: `
<ngx-loading-bar [includeBar]='true' [includeSpinner]='false'></ngx-loading-bar>
<div [@fadeAnimation]="o.isActivated ? o.activatedRoute : ''">
<router-outlet #o="outlet"></router-outlet>
</div>`,
animations: [fadeAnimation]
})
export class AppComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
<file_sep>
import { ErrorHandler, Injectable, Injector } from '@angular/core';
import { Router } from '@angular/router';
import { NGXLogger } from 'ngx-logger';
import { ROUTE } from '../app.constants';
@Injectable()
export class AuthErrorHandler implements ErrorHandler {
constructor(private injector: Injector, private logger: NGXLogger) { }
handleError(error) {
if (error != undefined || error != null){
if (error.status === 401 || error.status === 403) {
this.logger.error("Auth error", error);
const router = this.injector.get(Router);
router.navigate(['/' + ROUTE.SESSION_TIMEOUT]);
} else if (error.status === 502) {
this.logger.error("Proxy error", error);
}
}
throw error;
}
}<file_sep>import { Component, OnInit, AfterViewInit } from '@angular/core';
import { AuthService } from "angularx-social-login";
import { FacebookLoginProvider, GoogleLoginProvider, LinkedInLoginProvider } from "angularx-social-login";
@Component({
selector: 'app-register',
templateUrl: './register.component.html',
styleUrls: ['./register.component.scss']
})
export class RegisterComponent implements OnInit, AfterViewInit {
menu: HTMLElement;
panelMenu: NodeListOf<HTMLElement>;
panelBoxes: NodeListOf<HTMLElement>;
signUp: HTMLElement;
signIn: HTMLElement;
emailLogin: boolean = false;
user: any = {
name: '',
email: '',
password: ''
}
constructor(private socialAuthService: AuthService) { }
ngOnInit() {
}
ngAfterViewInit() {
this.menu = document.getElementById('menu');
this.panelMenu = this.menu.querySelectorAll('li');
this.panelBoxes = document.querySelectorAll('.panel__box');
this.signUp = document.getElementById('signUp');
this.signIn = document.getElementById('signIn');
}
removeSelection() {
for (var i = 0, len = this.panelBoxes.length; i < len; i++) {
this.panelBoxes[i].classList.remove('active');
this.panelBoxes[i].classList.remove('inactive');
}
this.signUp.classList.remove("active");
this.signIn.classList.remove("active");
}
public socialSignIn(socialPlatform : string) {
let socialPlatformProvider;
if(socialPlatform == "facebook"){
socialPlatformProvider = FacebookLoginProvider.PROVIDER_ID;
}else if(socialPlatform == "google"){
socialPlatformProvider = GoogleLoginProvider.PROVIDER_ID;
} else if (socialPlatform == "linkedin") {
socialPlatformProvider = LinkedInLoginProvider.PROVIDER_ID;
}
this.socialAuthService.signIn(socialPlatformProvider).then(
(userData) => {
console.log(socialPlatform+" sign in data : " , userData);
// Now sign-in with userData
// ...
}
);
}
signOut(): void {
this.socialAuthService.signOut();
}
onClickSignIn(e) {
e.preventDefault();
this.removeSelection();
this.panelBoxes[0].classList.add('active');
this.panelBoxes[1].classList.add('inactive');
this.menu.classList.remove('second-box');
this.signIn.classList.add("active");
}
onClickSignUp(e) {
e.preventDefault();
this.removeSelection();
this.panelBoxes[1].classList.add('active');
this.panelBoxes[0].classList.add('inactive');
this.menu.classList.add('second-box');
this.signUp.classList.add("active");
}
signInSubmit() {
}
}
<file_sep>import { NGXLogger } from "ngx-logger";
import { HttpClient } from "@angular/common/http";
import { Router } from "@angular/router";
import { StorageHelper } from "../helpers/storage-helper";
import { Injectable } from "@angular/core";
import { ROUTE } from "../app.constants";
@Injectable()
export class Utils {
constructor(
private logger: NGXLogger,
private http: HttpClient,
private router: Router) { }
invokeHttp(url, type = "GET", payload = {}) {
let httpRsp;
if(type == "POST") {
httpRsp = this.http.post<any>(url, payload, { observe: 'response' });
} else {
httpRsp = this.http.get<any>(url, { observe: 'response' });
}
return httpRsp.map(resp => {
if (resp.status == 403) {
this.router.navigate(['/' + ROUTE.SESSION_TIMEOUT]);
return
}
StorageHelper.saveTokenFromResponse(resp);
let data: any = resp.body;
return data;
});
}
httpGet(url) {
return this.invokeHttp(url);
}
httpPost(url, postData) {
return this.invokeHttp(url, "POST", postData);
}
getParamsFromUrl(inputURL: string) {
var query = inputURL;
var result = {};
query.split("&").forEach(function (part) {
var item = part.split("=");
result[item[0]] = decodeURIComponent(item[1]);
});
return result;
}
}<file_sep>import { Injectable } from "@angular/core";
import * as jwt_decode from "jwt-decode";
import { StorageHelper } from '../helpers/storage-helper';
import { NGXLogger } from 'ngx-logger';
import { URL } from '../app.constants';
import { Utils } from './utils';
import { environment } from "../../environments/environment";
@Injectable()
export class UserAuthService {
constructor(private utils: Utils, private logger: NGXLogger) { }
getToken(): string {
return StorageHelper.getToken();
}
setToken(token: string): void {
StorageHelper.setToken(token);
}
getTokenExpirationDate(token: string): Date {
if (!environment.production && token.split(" ")[0] == 'fake-token') {
let date = new Date();
return new Date(date.getTime() + 10 * 60000);
}
// First split, becasue from the server we are getting "Bearer <>"
token = token.split(" ")[1];
const decoded = jwt_decode(token);
if (decoded['exp'] === undefined) {
return null;
}
this.logger.info("Token expires at: ", decoded['exp']);
const date = new Date(0);
date.setUTCSeconds(decoded['exp']);
return date;
}
isTokenExpired(token?: string): boolean {
if (!token) token = this.getToken();
if (!token || token == undefined || token == '' || token == null) return true;
const date = this.getTokenExpirationDate(token);
if (date === undefined) return false;
return !(date.valueOf() > new Date().valueOf());
}
}<file_sep>import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-session-timeout',
template: `<div class="text-center" style="padding-top: 200px; height: 100%;">
<h1>Your session has timedout! Please login again..</h1>
</div>`,
})
export class SessionTimeoutComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
<file_sep>import { RouterModule, Routes } from '@angular/router';
import { SessionTimeoutComponent } from './components/session-timeout.component';
import { AuthGuard } from './helpers/auth.gaurd';
import { ROUTE } from './app.constants';
import { MyProfileComponent } from './components/my-profile/my-profile.component';
import { HomeComponent } from './components/home/home.component';
import { FeaturesComponent } from './components/features/features.component';
import { ContactComponent } from './components/contact/contact.component';
import { RegisterComponent } from './components/register/register.component';
const routes: Routes = [
{ path: ROUTE.SESSION_TIMEOUT, component: SessionTimeoutComponent },
{ path: ROUTE.REGISTER, component: RegisterComponent },
{ path: ROUTE.HOME, component: HomeComponent },
{ path: ROUTE.FEATURES, component: FeaturesComponent },
{ path: ROUTE.CONTACT, component: ContactComponent },
// Private routes
{ path: ROUTE.MY_PROFILE, component: MyProfileComponent, canActivate: [AuthGuard] },
// Default Route:
{ path: '**', component: RegisterComponent },
];
export const AppRouting = RouterModule.forRoot(routes ,{useHash:true}); | f234694080c1b254b26564e86c6c52603e3706b2 | [
"JavaScript",
"TypeScript"
] | 15 | TypeScript | chbhargava/ng-bootstrap-starter | b8a1fd18f8b947e349f58f5b72a544acf119294e | edb4d6e8675b7bdfa78ea501f3fa4bdc0c6b8e27 | |
refs/heads/master | <file_sep>google-cloud-bigquery
pandas
google-cloud-pubsub
google-cloud-storage<file_sep># Data Pipeline
## Setup
There are multiple methods that can be used to setup the environment, however, the first option below is preferred due to providing an interactive experience and simple UI.
### Cloud - Automatic (Preferred)
Click [this link](https://console.cloud.google.com/cloudshell/open?cloudshell_image=gcr.io/graphite-cloud-shell-images/terraform:latest&cloudshell_git_repo=https://github.com/diggerdric/data_pipeline.git&cloudshell_git_branch=master&cloudshell_working_dir=./&open_in_editor=./main.tf&cloudshell_tutorial=./README.md) to open a Cloud Shell in GCP with this repo.
### Cloud - Manual
In the Cloud Console in GCP, enter the following code and then click "Open in Editor" when prompted:
```bash
cloudshell_open --repo_url "https://github.com/diggerdric/data_pipeline" --dir "./" --page "editor" --tutorial "./README.md" --open_in_editor "./main.tf" --git_branch "master"
```
### Local
Install Terraform and then clone this repo locally.
## Deployment
### Configuring GCP
In addition to a GCP account, you'll need to create a **GCP Project** to follow this guide.
Create or select the project you'd like to use throughout this guide below.
<walkthrough-project-billing-setup></walkthrough-project-billing-setup>
You can set the project id to use for the rest of this guide with the following command:
```bash
export GOOGLE_CLOUD_PROJECT={{project-id}}
```
### Configuring Terraform
Run the following steps to deploy the code:
```bash
terraform init
```
```bash
terraform apply -var 'project={{project-id}}'
```
To confirm the confgurations set in the main.tf file, a prompt appears asking to "*Enter a value*", simply type `yes`.
### Duration
The deployment process should take around five minutes to complete. Maybe a good time for a coffee?
## Ingest Pipeline
### Ingest Data
Run the following code to setup the project ID so it doesn't have to be re-entered every time. Replace `<PROJECT-ID>` with the ID of the project you just deployed to:
```bash
gcloud config set project {{project-id}}
```
Run the following code to push data to the Pub Sub queue:
```
gcloud pubsub topics publish ingest-topic --message '{
"mobileNum": "1234567890",
"url": "www.google.com",
"SessionStartTime": "2020-02-26 00:00:00",
"SessionEndTime": "2020-02-27 00:00:00",
"bytesIn": "1024",
"bytesOut": "1024"
}'
```
### Verify Data
Run the following code to check the data has been stored in the data lake (BigQuery):
```
bq query --use_legacy_sql=false 'SELECT * FROM `{{project-id}}.data_lake.subscriber_browsing` LIMIT 10'
```
## Reporting Pipeline
### Trigger Pipeline
Rather than wait until midnight, run this command to trigger the scheduler immediately:
```bash
gcloud scheduler jobs run scheduled-report
```
### View Reports
Find the bucket that the reports are saved to. It should be of the format: `gs://daily_reports_{{project-id}}`
```bash
gsutil ls gs://
```
Show all files in the bucket. There should be two reports: "report001...csv" and "report002...csv".
```bash
gsutil ls -r gs://daily_reports_{{project-id}}
```
Check the contents of the first report file.
```bash
gsutil cat gs://daily_reports_{{project-id}}/report001_<TIMESTAMP>.csv
```
Check the contents of the second report file.
```bash
gsutil cat gs://daily_reports_{{project-id}}/report002_<TIMESTAMP>.csv
```
## The End
Thank You! Have a great day!
<file_sep>from google.cloud import bigquery
from google.cloud import storage
import datetime
import os
def run_func(event, context):
"""Triggered from a message on a Cloud Pub/Sub topic.
Args:
event (dict): Event payload.
context (google.cloud.functions.Context): Metadata for the event.
"""
timestamp = datetime.datetime.today().strftime('%Y-%m-%dT%H-%M-%S')
# report 1
query = """select
mobileNum, url, sum(bytesIn) as totalBytesIn, sum(bytesOut) as totalBytesOut
from `{0}.data_lake.subscriber_browsing`
where url is not null
group by 1, 2""".format(os.environ.get('GCP_PROJECT'))
outputBucket = os.environ.get('BUCKETNAME')
outputFileName = 'report001_{0}.csv'.format(timestamp)
export_to_gcs(query, outputBucket, outputFileName)
# report 2
query = """select
mobileNum, url, sum(timestamp_diff(cast(SessionEndTime as timestamp), cast(SessionStartTime as timestamp), second)) as totalSessionTimeSeconds
from `{0}.data_lake.subscriber_browsing`
where url is not null
group by 1, 2""".format(os.environ.get('GCP_PROJECT'))
outputBucket = os.environ.get('BUCKETNAME')
outputFileName = 'report002_{0}.csv'.format(timestamp)
export_to_gcs(query, outputBucket, outputFileName)
def export_to_gcs(query, bucketName, fileName):
bq_client = bigquery.Client()
query_job = bq_client.query(query)
# Wait for query to finish and save to df
rows_df = query_job.result().to_dataframe()
storage_client = storage.Client()
bucket = storage_client.get_bucket(bucketName)
blob = bucket.blob(fileName)
blob.upload_from_string(rows_df.to_csv(sep=',',index=False, encoding='utf-8')
,content_type='application/octet-stream') | 7e5f94c5a0c475916be9126697d24fa2d171834f | [
"Markdown",
"Python",
"Text"
] | 3 | Text | diggerdric/data_pipeline | c5d81052c627a7409e701118e2bf5aa934f1d8bd | 7b3c103e84234e60e97f7ef6911a5d97ea97a20b | |
refs/heads/master | <repo_name>Vertalo/ec2-scripts<file_sep>/ec2_ephemeralswap
#!/bin/sh
# PROVIDE: ec2_ephemeralswap
# REQUIRE: NETWORKING
# BEFORE: savecore
# Define ec2_ephemeralswap_enable=YES in /etc/rc.conf to enable slicing
# of the ephemeral disks to create swap space when the system next boots.
#
# Define ec2_ephemeralswap_size=N in /etc/rc.conf to use N MB of swap space
# instead of auto-sizing based on the amount of RAM.
#
: ${ec2_ephemeralswap_enable=NO}
: ${ec2_ephemeralswap_size=AUTO}
. /etc/rc.subr
name="ec2_ephemeralswap"
rcvar=ec2_ephemeralswap_enable
start_cmd="ec2_ephemeralswap_run"
stop_cmd=":"
ec2_ephemeralswap_run()
{
local RAM RAMMB SWAPMB SWZONESTRUCTS SWZONEMAXMB
local EC2DISKS EC2DISK BLKDEV SWAPDISKS NSWAPDISKS
local SWAPPERDISK SWAPDISK
# Compute "ideal" swap size:
# 2*RAM if RAM <= 4 GB
# 8 GB if 4 GB <= RAM <= 8 GB
# RAM if 8 GB <= RAM
RAM=`sysctl -n hw.physmem`
RAMMB=`expr $RAM / 1048576`
if [ $RAMMB -lt 4096 ]; then
SWAPMB=`expr $RAMMB \* 2`
elif [ $RAMMB -lt 8192 ]; then
SWAPMB=8192
else
SWAPMB=$RAMMB
fi
# If a swap size was specified, use that instead of our "ideal" value.
case ${ec2_ephemeralswap_size} in
[Aa][Uu][Tt][Oo])
;;
*)
SWAPMB=${ec2_ephemeralswap_size}
;;
esac
# Reduce this size if the kernel hasn't reserved enough space to
# keep track of that much swap.
SWZONESTRUCTS=`vmstat -z | tr -d , | awk '/^SWAPMETA/ { print $3 }'`
SWZONEMAXMB=`expr $SWZONESTRUCTS / 16`
if [ $SWZONEMAXMB -lt $SWAPMB ]; then
echo -n "Reducing ephemeral swap target from $SWAPMB MB to"
echo -n " $SWZONEMAXMB MB since kernel SWAPMETA zone is"
echo " too small."
SWAPMB=$SWZONEMAXMB
fi
# Fetch a list of EC2 disks
EC2DISKS=`fetch -qo - http://169.254.169.254/latest/meta-data/block-device-mapping/ | grep -E '^ephemeral[0-9]+$'`
debug "EC2 ephemeral disks are $EC2DISKS"
# Figure out where they're mapped to
SWAPDISKS=""
NSWAPDISKS=0
for EC2DISK in $EC2DISKS; do
BLKDEV=`fetch -qo - http://169.254.169.254/latest/meta-data/block-device-mapping/$EC2DISK | sed 's|/dev/||'`
case "$BLKDEV" in
sd[a-j])
SWAPDISK="/dev/xbd`echo $BLKDEV | cut -c 3 | tr 'a-j' '0-9'`"
;;
xvd[a-z])
SWAPDISK="/dev/xbd`echo $BLKDEV | cut -c 4 | tr 'a-j' '0-9'`"
;;
*)
echo "Can't translate $EC2DISK to a FreeBSD device name"
continue
;;
esac
if [ -c $SWAPDISK ]; then
SWAPDISKS="$SWAPDISKS $SWAPDISK"
NSWAPDISKS=$(($NSWAPDISKS + 1))
else
debug "Ephemeral swap disk $SWAPDISK doesn't exist"
fi
done
# If we have no ephemeral disks, give up.
if [ $NSWAPDISKS -eq 0 ]; then
echo -n "No ephemeral disks are available,"
echo " so no swap space is being created."
return 0;
fi
# How much swap space do we want per disk?
SWAPPERDISK=`expr $SWAPMB / $NSWAPDISKS`
if [ $SWAPPERDISK -gt 32768 ]; then
# FreeBSD swap_pager code can't use more than 32 GB per device
SWAPPERDISK=32768
fi
debug "Want $SWAPPERDISK MB swap on each of $NSWAPDISKS disks: $SWAPDISKS"
# Slice and partition disks
for SWAPDISK in $SWAPDISKS; do
if [ `ls $SWAPDISK[a-z.]* 2>/dev/null | wc -l` -gt 1 ]; then
debug "Ephemeral swap disk $SWAPDISK already in use"
continue;
fi
# Figure out how large the disk is. Subtract off 16 MB for
# losses to "cylinder" alignment etc.
DISKSZ=`diskinfo $SWAPDISK | awk '{ print $3 }'`
DISKSZM=`expr $DISKSZ / 1048576 - 16`
# Use the size we want or the size we've got, whichever is less
if [ $DISKSZM -lt $SWAPPERDISK ]; then
SWAPSZ=$DISKSZM;
else
SWAPSZ=$SWAPPERDISK;
fi
# Try to create a $SWAPSZ MB slice on this disk.
echo -n "Attempting to create a $SWAPSZ MB swap area on $SWAPDISK..."
( echo "p 1 0xa5 64 ${SWAPSZ}M"; echo "p 2 0xa5 * *" ) |
fdisk -i -f /dev/stdin $SWAPDISK >/dev/null 2>/dev/null
# Attempt to create a swap partition on the new slice.
( echo "b: * 0 swap"; echo "c: * 0 unused" ) |
bsdlabel -R ${SWAPDISK}s1 /dev/stdin
# Did we succeed?
if [ -c ${SWAPDISK}s1b ]; then
echo " done."
else
echo " failed."
fi
done
# Turn on swap
for SWAPDISK in $SWAPDISKS; do
# If there isn't an s1b partition, this isn't one of ours.
if ! [ -c "${SWAPDISK}s1b" ]; then
continue;
fi
# Make sure that s1b is a swap partition.
if ! bsdlabel ${SWAPDISK}s1 | grep b: | grep -q swap; then
continue;
fi
# Enable swap on this disk
echo "Enabling swapping to ${SWAPDISK}s1b"
swapon ${SWAPDISK}s1b
done
# Turn on dumping, if ${dumpdev} is AUTO (the dumpon rc.d script runs
# early in the boot process, but we can't run until the network has
# been brought up -- so we duplicate here some of the work which that
# script does.
case ${dumpdev} in
[Aa][Uu][Tt][Oo])
for SWAPDISK in $SWAPDISKS; do
# Skip if this is not a disk we're swapping to.
if ! swapinfo | grep -Eq "^${SWAPDISK}s1b"; then
continue;
fi
# If we don't have a dump disk yet, use this one.
if ! [ -e /dev/dumpdev ]; then
echo "Enabling crash dumps to ${SWAPDISK}s1b"
dumpon ${SWAPDISK}s1b
ln -sf ${SWAPDISK}s1b /dev/dumpdev
fi
done
;;
esac
}
load_rc_config $name
run_rc_command "$1"
| 1f6c73f22d01f084c094a82ec50dfd1411cadda9 | [
"Shell"
] | 1 | Shell | Vertalo/ec2-scripts | 7fe5bdc0d5781faa07166069a396738fefd24547 | 16092a94635bea80303ad17e7e21fbee7c4de5f8 | |
refs/heads/master | <repo_name>zeroichi/sdc<file_sep>/catalog-be/src/main/resources/scripts/sdcBePy/common/sdcBeProxy.py
import json
from io import BytesIO
import pycurl
from sdcBePy.common.helpers import check_arguments_not_none
def get_url(ip, port, protocol):
return "%s://%s:%s" % (protocol, ip, port)
class SdcBeProxy:
BODY_SEPARATOR = "\r\n\r\n"
CHARTSET = 'UTF-8'
def __init__(self, be_ip, be_port, header, scheme, user_id="jh0003",
debug=False, connector=None):
if not check_arguments_not_none(be_ip, be_port, scheme, user_id):
raise AttributeError("The be_host, be_port, scheme or admin_user are missing")
url = get_url(be_ip, be_port, scheme)
self.con = connector if connector \
else CurlConnector(url, user_id, header, scheme=scheme, debug=debug)
def check_backend(self):
return self.con.get('/sdc2/rest/v1/user/jh0003')
def check_user(self, user_name):
return self.con.get("/sdc2/rest/v1/user" + user_name)
def create_user(self, first_name, last_name, user_id, email, role):
return self.con.post('/sdc2/rest/v1/user', json.dumps({
'firstName': first_name,
'lastName': last_name,
'userId': user_id,
'email': email,
'role': role
}))
def check_consumer(self, consumer_name):
return self.con.get("/sdc2/rest/v1/consumers" + consumer_name)
def create_consumer(self, consumer_name, slat, password):
return self.con.post("/sdc2/rest/v1/consumers", json.dumps({
'consumerName': consumer_name,
'consumerSalt': slat,
'consumerPassword': <PASSWORD>
}))
def get_normatives(self):
return self.con.get("/sdc2/rest/v1/screen", with_buffer=True)
def post_file(self, path, multi_part_form_data):
return self.con.post_file(path, multi_part_form_data)
def get_response_from_buffer(self):
value = self.con.buffer.getvalue()
self.con.buffer.truncate(0)
self.con.buffer.seek(0)
response = value.decode(self.CHARTSET).split(self.BODY_SEPARATOR)
return response[1] if len(response) == 2 else response[0]
class CurlConnector:
CONTENT_TYPE_HEADER = "Content-Type: application/json"
ACCEPT_HEADER = "Accept: application/json; charset=UTF-8"
def __init__(self, url, user_id_header, header, buffer=None, scheme="http", debug=False):
self.c = pycurl.Curl()
self.c.setopt(pycurl.HEADER, True)
self.user_header = "USER_ID: " + user_id_header
if not debug:
# disable printing not necessary logs in the terminal
self.c.setopt(pycurl.WRITEFUNCTION, lambda x: None)
else:
self.c.setopt(pycurl.VERBOSE, 1)
if not buffer:
self.buffer = BytesIO()
if header is None:
self.basicauth_header = ""
else:
self.basicauth_header = "Authorization: Basic " + header
self.url = url
self._check_schema(scheme)
def get(self, path, buffer=None, with_buffer=False):
try:
self.c.setopt(pycurl.URL, self.url + path)
self.c.setopt(pycurl.HTTPHEADER, [self.user_header,
CurlConnector.CONTENT_TYPE_HEADER,
CurlConnector.ACCEPT_HEADER,
self.basicauth_header])
if with_buffer:
write = self.buffer.write if not buffer else buffer.write
self.c.setopt(pycurl.WRITEFUNCTION, write)
self.c.perform()
return self.c.getinfo(pycurl.RESPONSE_CODE)
except pycurl.error:
return 111
def post(self, path, data):
try:
self.c.setopt(pycurl.URL, self.url + path)
self.c.setopt(pycurl.POST, 1)
self.c.setopt(pycurl.HTTPHEADER, [self.user_header,
CurlConnector.CONTENT_TYPE_HEADER,
CurlConnector.ACCEPT_HEADER,
self.basicauth_header])
self.c.setopt(pycurl.POSTFIELDS, data)
self.c.perform()
self.c.setopt(pycurl.POST, 0)
return self.c.getinfo(pycurl.RESPONSE_CODE)
except pycurl.error:
return 111
def post_file(self, path, post_body, buffer=None):
try:
self.c.setopt(pycurl.URL, self.url + path)
self.c.setopt(pycurl.POST, 1)
self.c.setopt(pycurl.HTTPHEADER, [self.user_header,
self.basicauth_header])
self.c.setopt(pycurl.HTTPPOST, post_body)
write = self.buffer.write if not buffer else buffer.write
self.c.setopt(pycurl.WRITEFUNCTION, write)
self.c.perform()
self.c.setopt(pycurl.POST, 0)
return self.c.getinfo(pycurl.RESPONSE_CODE)
except pycurl.error:
return 111
def _check_schema(self, scheme):
if scheme == 'https':
self.c.setopt(pycurl.SSL_VERIFYPEER, 0)
self.c.setopt(pycurl.SSL_VERIFYHOST, 0)
def __del__(self):
self.c.close()
<file_sep>/catalog-be/src/test/java/org/openecomp/sdc/be/components/health/HealthCheckBusinessLogicHealthTest.java
/*-
* ============LICENSE_START=======================================================
* SDC
* ================================================================================
* Copyright (C) 2020 AT&T Intellectual Property. All rights reserved.
* ================================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ============LICENSE_END=========================================================
*/
package org.openecomp.sdc.be.components.health;
import mockit.Deencapsulation;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.junit.MockitoJUnitRunner;
import org.onap.portalsdk.core.onboarding.exception.CipherUtilException;
import org.openecomp.sdc.be.catalog.impl.DmaapProducerHealth;
import org.openecomp.sdc.be.components.BeConfDependentTest;
import org.openecomp.sdc.be.components.distribution.engine.DistributionEngineClusterHealth;
import org.openecomp.sdc.be.components.distribution.engine.DmaapHealth;
import org.openecomp.sdc.be.switchover.detector.SwitchoverDetector;
import org.openecomp.sdc.common.api.HealthCheckInfo;
import org.openecomp.sdc.common.http.client.api.HttpExecuteException;
import org.springframework.test.util.ReflectionTestUtils;
import java.util.LinkedList;
import java.util.List;
import static org.mockito.ArgumentMatchers.eq;
import static org.mockito.Mockito.doReturn;
import static org.mockito.Mockito.doThrow;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.spy;
import static org.mockito.Mockito.when;
import static org.openecomp.sdc.common.api.Constants.HC_COMPONENT_ON_BOARDING;
@RunWith(MockitoJUnitRunner.class)
public class HealthCheckBusinessLogicHealthTest extends BeConfDependentTest {
private DmaapProducerHealth dmaapProducerHealth = mock(DmaapProducerHealth.class);
private HealthCheckInfo dmaapProducerHealthCheckInfo = mock(HealthCheckInfo.class);
private SwitchoverDetector switchoverDetector;
private HealthCheckBusinessLogic createTestSubject() {
HealthCheckBusinessLogic healthCheckBusinessLogic = new HealthCheckBusinessLogic();
DmaapHealth dmaapHealth = new DmaapHealth();
ReflectionTestUtils.setField(healthCheckBusinessLogic, "dmaapHealth", dmaapHealth);
PortalHealthCheckBuilder portalHealthCheckBuilder = new PortalHealthCheckBuilder();
ReflectionTestUtils.setField(healthCheckBusinessLogic, "portalHealthCheck", portalHealthCheckBuilder);
DistributionEngineClusterHealth distributionEngineClusterHealth = new DistributionEngineClusterHealth();
ReflectionTestUtils.setField(healthCheckBusinessLogic, "distributionEngineClusterHealth",
distributionEngineClusterHealth);
SwitchoverDetector switchoverDetector = new SwitchoverDetector();
ReflectionTestUtils.setField(healthCheckBusinessLogic, "switchoverDetector", switchoverDetector);
List<HealthCheckInfo> prevBeHealthCheckInfos = new LinkedList<>();
ReflectionTestUtils.setField(healthCheckBusinessLogic, "prevBeHealthCheckInfos", prevBeHealthCheckInfos);
ReflectionTestUtils.setField(healthCheckBusinessLogic, "dmaapProducerHealth", dmaapProducerHealth);
return healthCheckBusinessLogic;
}
@Before
public void beforeTest() {
when(dmaapProducerHealth.getHealthCheckInfo())
.thenReturn(dmaapProducerHealthCheckInfo);
}
@Test
public void testInit() throws Exception {
HealthCheckBusinessLogic testSubject = createTestSubject();
testSubject.init();
}
@Test
public void testIsDistributionEngineUp() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
testSubject.isDistributionEngineUp();
}
@Test
public void testGetBeHealthCheckInfosStatus() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
testSubject.getBeHealthCheckInfosStatus();
}
@Test
public void testGetBeHealthCheckInfos() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "getBeHealthCheckInfos");
}
@Test
public void testGetDmaapHealthCheck() throws Exception {
HealthCheckBusinessLogic testSubject;
List<HealthCheckInfo> healthCheckInfos = new LinkedList<>();
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "getDmaapHealthCheck");
}
@Test
public void testGetJanusGraphHealthCheck() throws Exception {
HealthCheckBusinessLogic testSubject;
List<HealthCheckInfo> healthCheckInfos = new LinkedList<>();
// default test
testSubject = createTestSubject();
// testSubject.getJanusGraphHealthCheck(healthCheckInfos);
healthCheckInfos.add(testSubject.getJanusGraphHealthCheck());
}
@Test
public void testGetCassandraHealthCheck() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "getCassandraHealthCheck");
}
@Test
public void testGetDistributionEngineCheck() throws Exception {
// HealthCheckBusinessLogic testSubject;
DistributionEngineClusterHealth testSubject = new DistributionEngineClusterHealth();
// default test
Deencapsulation.invoke(testSubject, "getHealthCheckInfo");
}
@Test
public void testGetAmdocsHealthCheck() throws Exception {
HealthCheckBusinessLogic testSubject;
List<HealthCheckInfo> healthCheckInfos = new LinkedList<>();
// default test
testSubject = createTestSubject();
String url = testSubject.buildOnBoardingHealthCheckUrl();
Deencapsulation.invoke(testSubject, "getHostedComponentsBeHealthCheck", HC_COMPONENT_ON_BOARDING, url);
}
@Test
public void testGetPortalHealthCheckSuccess() throws Exception {
PortalHealthCheckBuilder testSubject = spy(PortalHealthCheckBuilder.class);
String healthCheckURL = testSubject.buildPortalHealthCheckUrl();
int timeout = 3000;
doReturn(200).when(testSubject).getStatusCode(eq(healthCheckURL), eq(timeout));
testSubject.init();
testSubject.runTask();
HealthCheckInfo hci = testSubject.getHealthCheckInfo();
Assert.assertEquals("PORTAL", hci.getHealthCheckComponent());
Assert.assertEquals(HealthCheckInfo.HealthCheckStatus.UP, hci.getHealthCheckStatus());
Assert.assertEquals("OK", hci.getDescription());
}
@Test
public void testGetPortalHealthCheckFailureMissingConfig() throws Exception{
PortalHealthCheckBuilder testSubject = new PortalHealthCheckBuilder();
testSubject.init(null);
HealthCheckInfo hci = testSubject.getHealthCheckInfo();
Assert.assertEquals("PORTAL", hci.getHealthCheckComponent());
Assert.assertEquals(HealthCheckInfo.HealthCheckStatus.DOWN, hci.getHealthCheckStatus());
Assert.assertEquals("PORTAL health check configuration is missing", hci.getDescription());
}
@Test
public void testGetPortalHealthCheckFailureErrorResponse() throws HttpExecuteException, CipherUtilException {
PortalHealthCheckBuilder testSubject = spy(PortalHealthCheckBuilder.class);
String healthCheckURL = testSubject.buildPortalHealthCheckUrl();
int timeout = 3000;
// when(testSubject.getStatusCode(healthCheckURL,timeout)).thenReturn(404);
doReturn(404).when(testSubject).getStatusCode(eq(healthCheckURL), eq(timeout));
testSubject.init(testSubject.getConfiguration());
testSubject.runTask();
HealthCheckInfo hci = testSubject.getHealthCheckInfo();
Assert.assertEquals("PORTAL", hci.getHealthCheckComponent());
Assert.assertEquals(HealthCheckInfo.HealthCheckStatus.DOWN, hci.getHealthCheckStatus());
Assert.assertEquals("PORTAL responded with 404 status code", hci.getDescription());
}
@Test
public void testGetPortalHealthCheckFailureNoResponse() throws HttpExecuteException, CipherUtilException {
PortalHealthCheckBuilder testSubject = spy(PortalHealthCheckBuilder.class);
String healthCheckURL = testSubject.buildPortalHealthCheckUrl();
int timeout = 3000;
// when(testSubject.getStatusCode(healthCheckURL, timeout)).thenThrow(HttpExecuteException.class);
doThrow(HttpExecuteException.class).when(testSubject).getStatusCode(eq(healthCheckURL), eq(timeout));
testSubject.init(testSubject.getConfiguration());
testSubject.runTask();
HealthCheckInfo hci = testSubject.getHealthCheckInfo();
Assert.assertEquals("PORTAL", hci.getHealthCheckComponent());
Assert.assertEquals(HealthCheckInfo.HealthCheckStatus.DOWN, hci.getHealthCheckStatus());
Assert.assertEquals("PORTAL is not available", hci.getDescription());
}
@Test
public void testGetHostedComponentsBeHealthCheck() throws Exception {
HealthCheckBusinessLogic testSubject;
String componentName = "mock";
String healthCheckUrl = "mock";
// test 1
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "getHostedComponentsBeHealthCheck", componentName, healthCheckUrl);
// test 2
testSubject = createTestSubject();
healthCheckUrl = "";
Deencapsulation.invoke(testSubject, "getHostedComponentsBeHealthCheck", componentName, healthCheckUrl);
}
@Test
public void testDestroy() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "destroy");
}
@Test
public void testLogAlarm() throws Exception {
HealthCheckBusinessLogic testSubject;
String componentChangedMsg = "mock";
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "logAlarm", componentChangedMsg);
}
@Test
public void testGetSiteMode() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
testSubject.getSiteMode();
}
@Test
public void testAnyStatusChanged() throws Exception {
HealthCheckBusinessLogic testSubject;
List<HealthCheckInfo> beHealthCheckInfos = null;
List<HealthCheckInfo> prevBeHealthCheckInfos = null;
boolean result;
// test 1
testSubject = createTestSubject();
beHealthCheckInfos = null;
prevBeHealthCheckInfos = null;
result = testSubject.anyStatusChanged(beHealthCheckInfos, prevBeHealthCheckInfos);
Assert.assertEquals(false, result);
// test 2
testSubject = createTestSubject();
prevBeHealthCheckInfos = null;
beHealthCheckInfos = null;
result = testSubject.anyStatusChanged(beHealthCheckInfos, prevBeHealthCheckInfos);
Assert.assertEquals(false, result);
// test 3
testSubject = createTestSubject();
beHealthCheckInfos = null;
prevBeHealthCheckInfos = null;
result = testSubject.anyStatusChanged(beHealthCheckInfos, prevBeHealthCheckInfos);
Assert.assertEquals(false, result);
// test 4
testSubject = createTestSubject();
prevBeHealthCheckInfos = null;
beHealthCheckInfos = null;
result = testSubject.anyStatusChanged(beHealthCheckInfos, prevBeHealthCheckInfos);
Assert.assertEquals(false, result);
}
@Test
public void testBuildOnBoardingHealthCheckUrl() throws Exception {
HealthCheckBusinessLogic testSubject;
// default test
testSubject = createTestSubject();
Deencapsulation.invoke(testSubject, "buildOnBoardingHealthCheckUrl");
}
}
<file_sep>/catalog-be/src/test/java/org/openecomp/sdc/be/servlets/ModelServletTest.java
/*
* ============LICENSE_START=======================================================
* Copyright (C) 2021 Nordix Foundation
* ================================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* ============LICENSE_END=========================================================
*/
package org.openecomp.sdc.be.servlets;
import static org.assertj.core.api.Assertions.assertThat;
import static org.junit.jupiter.api.Assertions.assertThrows;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.when;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import javax.validation.ConstraintViolationException;
import javax.ws.rs.core.Response;
import org.apache.commons.lang3.StringUtils;
import org.eclipse.jetty.http.HttpStatus;
import org.glassfish.hk2.utilities.binding.AbstractBinder;
import org.glassfish.jersey.server.ResourceConfig;
import org.glassfish.jersey.test.JerseyTest;
import org.glassfish.jersey.test.TestProperties;
import org.junit.jupiter.api.AfterEach;
import org.junit.jupiter.api.BeforeAll;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.TestInstance;
import org.junit.jupiter.api.TestInstance.Lifecycle;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.openecomp.sdc.be.components.impl.ComponentInstanceBusinessLogic;
import org.openecomp.sdc.be.components.impl.ModelBusinessLogic;
import org.openecomp.sdc.be.components.impl.ResourceImportManager;
import org.openecomp.sdc.be.components.validation.UserValidations;
import org.openecomp.sdc.be.config.ConfigurationManager;
import org.openecomp.sdc.be.config.SpringConfig;
import org.openecomp.sdc.be.dao.api.ActionStatus;
import org.openecomp.sdc.be.exception.BusinessException;
import org.openecomp.sdc.be.impl.ComponentsUtils;
import org.openecomp.sdc.be.impl.ServletUtils;
import org.openecomp.sdc.be.impl.WebAppContextWrapper;
import org.openecomp.sdc.be.model.Model;
import org.openecomp.sdc.be.ui.model.ModelCreateRequest;
import org.openecomp.sdc.be.user.UserBusinessLogic;
import org.openecomp.sdc.common.api.ConfigurationSource;
import org.openecomp.sdc.common.api.Constants;
import org.openecomp.sdc.common.impl.ExternalConfiguration;
import org.openecomp.sdc.common.impl.FSConfigurationSource;
import org.openecomp.sdc.exception.ResponseFormat;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import org.springframework.web.context.WebApplicationContext;
@TestInstance(Lifecycle.PER_CLASS)
class ModelServletTest extends JerseyTest {
private static final String USER_ID = "cs0008";
@Mock
private HttpServletRequest request;
@Mock
private HttpSession session;
@Mock
private ServletContext servletContext;
@Mock
private WebAppContextWrapper webAppContextWrapper;
@Mock
private WebApplicationContext webApplicationContext;
@Mock
private UserBusinessLogic userBusinessLogic;
@Mock
private ComponentInstanceBusinessLogic componentInstanceBusinessLogic;
@Mock
private ComponentsUtils componentsUtils;
@Mock
private ServletUtils servletUtils;
@Mock
private ResourceImportManager resourceImportManager;
@Mock
private ModelBusinessLogic modelBusinessLogic;
@InjectMocks
private ModelServlet modelServlet;
@Mock
private ResponseFormat responseFormat;
@Mock
private UserValidations userValidations;
private Model model;
private Response response;
private ModelCreateRequest modelCreateRequest;
@BeforeAll
public void initClass() {
MockitoAnnotations.openMocks(this);
when(request.getSession()).thenReturn(session);
when(session.getServletContext()).thenReturn(servletContext);
when(servletContext.getAttribute(Constants.WEB_APPLICATION_CONTEXT_WRAPPER_ATTR))
.thenReturn(webAppContextWrapper);
when(webAppContextWrapper.getWebAppContext(servletContext)).thenReturn(webApplicationContext);
when(webApplicationContext.getBean(ModelBusinessLogic.class)).thenReturn(modelBusinessLogic);
when(request.getHeader(Constants.USER_ID_HEADER)).thenReturn(USER_ID);
when(webApplicationContext.getBean(ServletUtils.class)).thenReturn(servletUtils);
when(servletUtils.getComponentsUtils()).thenReturn(componentsUtils);
final String appConfigDir = "src/test/resources/config/catalog-be";
final ConfigurationSource configurationSource = new FSConfigurationSource(ExternalConfiguration.getChangeListener(), appConfigDir);
final ConfigurationManager configurationManager = new ConfigurationManager(configurationSource);
final org.openecomp.sdc.be.config.Configuration configuration = new org.openecomp.sdc.be.config.Configuration();
configuration.setJanusGraphInMemoryGraph(true);
configurationManager.setConfiguration(configuration);
ExternalConfiguration.setAppName("catalog-be");
}
@BeforeEach
void resetMock() throws Exception {
super.setUp();
initTestData();
}
@AfterEach
void after() throws Exception {
super.tearDown();
}
private void initTestData() {
final String modelName = "MY-INTEGRATION-TEST-MODEL";
model = new Model(modelName);
modelCreateRequest = new ModelCreateRequest();
modelCreateRequest.setName(modelName);
}
@Override
protected ResourceConfig configure() {
forceSet(TestProperties.CONTAINER_PORT, "0");
final ApplicationContext context = new AnnotationConfigApplicationContext(SpringConfig.class);
return new ResourceConfig(ModelServlet.class)
.register(new AbstractBinder() {
@Override
protected void configure() {
bind(request).to(HttpServletRequest.class);
bind(userBusinessLogic).to(UserBusinessLogic.class);
bind(componentInstanceBusinessLogic).to(ComponentInstanceBusinessLogic.class);
bind(componentsUtils).to(ComponentsUtils.class);
bind(servletUtils).to(ServletUtils.class);
bind(resourceImportManager).to(ResourceImportManager.class);
bind(modelBusinessLogic).to(ModelBusinessLogic.class);
}
})
.property("contextConfig", context);
}
@Test
void createModelSuccessTest() {
when(responseFormat.getStatus()).thenReturn(HttpStatus.OK_200);
when(componentsUtils.getResponseFormat(ActionStatus.CREATED)).thenReturn(responseFormat);
when(modelBusinessLogic.createModel(any(Model.class))).thenReturn(model);
response = modelServlet.createModel(modelCreateRequest, USER_ID);
assertThat(response.getStatus()).isEqualTo(HttpStatus.OK_200);
}
@Test
void createModelFailTest() {
when(responseFormat.getStatus()).thenReturn(HttpStatus.INTERNAL_SERVER_ERROR_500);
when(componentsUtils.getResponseFormat(ActionStatus.GENERAL_ERROR)).thenReturn(responseFormat);
when(modelBusinessLogic.createModel(any(Model.class))).thenReturn(model);
response = modelServlet.createModel(modelCreateRequest, USER_ID);
assertThat(response.getStatus()).isEqualTo(HttpStatus.INTERNAL_SERVER_ERROR_500);
}
@Test
void createModelFailWithModelNameEmptyTest() {
when(responseFormat.getStatus()).thenReturn(HttpStatus.INTERNAL_SERVER_ERROR_500);
when(componentsUtils.getResponseFormat(ActionStatus.GENERAL_ERROR)).thenReturn(responseFormat);
modelCreateRequest.setName(StringUtils.EMPTY);
final Exception exception = assertThrows(ConstraintViolationException.class, () -> modelServlet.createModel(modelCreateRequest, USER_ID));
assertThat(exception.getMessage()).isEqualTo("Model name cannot be empty");
}
@Test
void createModelFailWithModelNameNullTest() {
when(responseFormat.getStatus()).thenReturn(HttpStatus.INTERNAL_SERVER_ERROR_500);
when(componentsUtils.getResponseFormat(ActionStatus.GENERAL_ERROR)).thenReturn(responseFormat);
modelCreateRequest.setName(null);
final Exception exception = assertThrows(ConstraintViolationException.class, () -> modelServlet.createModel(modelCreateRequest, USER_ID));
assertThat(exception.getMessage()).isEqualTo("Model name cannot be null");
}
@Test
void createModelThrowsBusinessExceptionTest() {
when(modelBusinessLogic.createModel(model)).thenThrow(new BusinessException() {});
assertThrows(BusinessException.class, () -> modelServlet.createModel(modelCreateRequest, USER_ID));
}
}<file_sep>/catalog-model/src/main/java/org/openecomp/sdc/be/model/operations/impl/ModelOperation.java
/*
* ============LICENSE_START=======================================================
* Copyright (C) 2021 Nordix Foundation
* ================================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* ============LICENSE_END=========================================================
*/
package org.openecomp.sdc.be.model.operations.impl;
import fj.data.Either;
import java.util.Objects;
import org.openecomp.sdc.be.dao.api.ActionStatus;
import org.openecomp.sdc.be.dao.janusgraph.JanusGraphGenericDao;
import org.openecomp.sdc.be.dao.janusgraph.JanusGraphOperationStatus;
import org.openecomp.sdc.be.model.Model;
import org.openecomp.sdc.be.model.jsonjanusgraph.operations.exception.OperationException;
import org.openecomp.sdc.be.resources.data.ModelData;
import org.openecomp.sdc.common.log.enums.EcompLoggerErrorCode;
import org.openecomp.sdc.common.log.wrappers.Logger;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component("model-operation")
public class ModelOperation extends AbstractOperation {
private static final Logger log = Logger.getLogger(ModelOperation.class);
private final JanusGraphGenericDao genericDao;
@Autowired
public ModelOperation(final JanusGraphGenericDao janusGraphGenericDao) {
this.genericDao = janusGraphGenericDao;
}
public Model createModel(final Model model, final boolean inTransaction) {
Model result = null;
final ModelData modelData = new ModelData(model.getName(), UniqueIdBuilder.buildModelUid(model.getName()));
try {
final Either<ModelData, JanusGraphOperationStatus> createNode = genericDao.createNode(modelData, ModelData.class);
if (createNode.isRight()) {
final JanusGraphOperationStatus janusGraphOperationStatus = createNode.right().value();
log.error(EcompLoggerErrorCode.DATA_ERROR, ModelOperation.class.getName(), "Problem while creating model, reason {}",
janusGraphOperationStatus);
if (janusGraphOperationStatus == JanusGraphOperationStatus.JANUSGRAPH_SCHEMA_VIOLATION) {
throw new OperationException(ActionStatus.MODEL_ALREADY_EXISTS, model.getName());
}
throw new OperationException(ActionStatus.GENERAL_ERROR,
String.format("Failed to create model %s on JanusGraph with %s error", model, janusGraphOperationStatus));
}
result = new Model(createNode.left().value().getName());
return result;
} finally {
if (!inTransaction) {
if (Objects.nonNull(result)) {
genericDao.commit();
} else {
genericDao.rollback();
}
}
}
}
}
<file_sep>/openecomp-be/backend/openecomp-sdc-vendor-software-product-manager/src/test/java/org/openecomp/sdc/vendorsoftwareproduct/impl/orchestration/csar/validation/CsarSecurityValidatorTest.java
/*
* ============LICENSE_START=======================================================
* Copyright (C) 2019 Nordix Foundation
* ================================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
* ============LICENSE_END=========================================================
*/
package org.openecomp.sdc.vendorsoftwareproduct.impl.orchestration.csar.validation;
import static org.hamcrest.core.Is.is;
import static org.junit.Assert.assertThat;
import static org.junit.Assert.fail;
import static org.mockito.ArgumentMatchers.any;
import static org.mockito.Mockito.when;
import static org.mockito.MockitoAnnotations.initMocks;
import java.io.IOException;
import java.net.URISyntaxException;
import java.nio.file.Files;
import java.nio.file.Paths;
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.openecomp.sdc.vendorsoftwareproduct.impl.onboarding.OnboardingPackageProcessor;
import org.openecomp.sdc.vendorsoftwareproduct.impl.onboarding.validation.CnfPackageValidator;
import org.openecomp.sdc.vendorsoftwareproduct.security.SecurityManager;
import org.openecomp.sdc.vendorsoftwareproduct.security.SecurityManagerException;
import org.openecomp.sdc.vendorsoftwareproduct.types.OnboardPackageInfo;
import org.openecomp.sdc.vendorsoftwareproduct.types.OnboardSignedPackage;
public class CsarSecurityValidatorTest {
private static final String BASE_DIR = "/vspmanager.csar/";
private CsarSecurityValidator csarSecurityValidator;
@Mock
SecurityManager securityManager;
@Before
public void setUp() {
initMocks(this);
csarSecurityValidator = new CsarSecurityValidator(securityManager);
}
@Test
public void isSignatureValidTestCorrectStructureAndValidSignatureExists() throws SecurityManagerException {
final byte[] packageBytes = getFileBytesOrFail("signing/signed-package.zip");
final OnboardSignedPackage onboardSignedPackage = loadSignedPackage("signed-package.zip",
packageBytes, null);
when(securityManager.verifySignedData(any(), any(), any())).thenReturn(true);
final boolean isSignatureValid = csarSecurityValidator.verifyPackageSignature(onboardSignedPackage);
assertThat("Signature should be valid", isSignatureValid, is(true));
}
@Test(expected = SecurityManagerException.class)
public void isSignatureValidTestCorrectStructureAndNotValidSignatureExists() throws SecurityManagerException {
final byte[] packageBytes = getFileBytesOrFail("signing/signed-package-tampered-data.zip");
final OnboardSignedPackage onboardSignedPackage = loadSignedPackage("signed-package-tampered-data.zip",
packageBytes, null);
//no mocked securityManager
csarSecurityValidator = new CsarSecurityValidator();
csarSecurityValidator.verifyPackageSignature(onboardSignedPackage);
}
private byte[] getFileBytesOrFail(final String path) {
try {
return getFileBytes(path);
} catch (final URISyntaxException | IOException e) {
fail("Could not load file " + path);
return null;
}
}
private byte[] getFileBytes(final String path) throws URISyntaxException, IOException {
return Files.readAllBytes(Paths.get(
CsarSecurityValidatorTest.class.getResource(BASE_DIR + path).toURI()));
}
private OnboardSignedPackage loadSignedPackage(final String packageName, final byte[] packageBytes,
CnfPackageValidator cnfPackageValidator) {
final OnboardingPackageProcessor onboardingPackageProcessor =
new OnboardingPackageProcessor(packageName, packageBytes, cnfPackageValidator);
final OnboardPackageInfo onboardPackageInfo = onboardingPackageProcessor.getOnboardPackageInfo().orElse(null);
if (onboardPackageInfo == null) {
fail("Unexpected error. Could not load original package");
}
return (OnboardSignedPackage) onboardPackageInfo.getOriginalOnboardPackage();
}
}
<file_sep>/integration-tests/src/test/java/org/onap/sdc/frontend/ci/tests/pages/VspValidationPage.java
/**
* Copyright (c) 2019 Vodafone Group
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.onap.sdc.frontend.ci.tests.pages;
import com.aventstack.extentreports.Status;
import org.apache.commons.collections.CollectionUtils;
import org.onap.sdc.frontend.ci.tests.datatypes.DataTestIdEnum;
import org.onap.sdc.frontend.ci.tests.execute.setup.SetupCDTest;
import org.onap.sdc.frontend.ci.tests.utilities.GeneralUIUtils;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.testng.Assert;
import java.io.File;
import java.util.List;
public class VspValidationPage extends GeneralPageElements {
private VspValidationPage() {
super();
}
public static void navigateToVspValidationPageUsingNavbar() throws Exception {
clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_NAVBAR);
}
public static void navigateToVspValidationPageUsingBreadcrumbs() throws Exception {
clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_BREADCRUMBS);
}
public static void clickOnNextButton() throws Exception {
clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_PROCEED_TO_INPUTS_BUTTON);
}
public static void clickOnBackButton() throws Exception {
clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_PROCEED_TO_SETUP_BUTTON);
}
public static void clickOnSubmitButton() throws Exception {
clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_PROCEED_TO_RESULTS_BUTTON);
}
public static void loadVSPFile(String path, String filename) {
List<WebElement> checkboxes =
GeneralUIUtils.findElementsByXpath("//div[@class='validation-input-wrapper']//input");
boolean hasValue = CollectionUtils.isNotEmpty(checkboxes);
if (hasValue) {
WebElement browseWebElement = checkboxes.get(0);
browseWebElement.sendKeys(path + File.separator + filename);
GeneralUIUtils.ultimateWait();
} else {
Assert.fail("Did not find File input field in the page for loading VSP test file");
}
}
public static boolean checkNextButtonDisabled() throws Exception {
return GeneralUIUtils.isElementDisabled(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_PROCEED_TO_INPUTS_BUTTON.getValue());
}
public static void clickCertificationQueryAll() throws Exception {
List<WebElement> checkboxes = GeneralUIUtils.findElementsByXpath("//div[@data-test-id='vsp-validation-certifications-query-checkbox-tree']//label//span[@class='rct-checkbox']");
if (!checkboxes.isEmpty()) {
checkboxes.get(0).click();
} else {
Assert.fail("Did not find certification test checkbox in the page");
}
}
public static void clickComplianceChecksAll() throws Exception {
List<WebElement> vnfComplianceCheckboxes = GeneralUIUtils.findElementsByXpath("//div[@data-test-id='vsp-validation-compliance-checks-checkbox-tree']//span[@class='rct-text' and .//label//text()='vnf-compliance']//button");
if (!vnfComplianceCheckboxes.isEmpty()) {
vnfComplianceCheckboxes.get(vnfComplianceCheckboxes.size() - 1).click();
} else {
Assert.fail("Did not find vnf-compliance test checkbox in the page");
}
List<WebElement> checkboxes = GeneralUIUtils.findElementsByXpath("//div[@data-test-id='vsp-validation-compliance-checks-checkbox-tree']//label//span[@class='rct-title' and text()='csar-validate']");
if (!checkboxes.isEmpty()) {
checkboxes.get(checkboxes.size() - 1).click();
} else {
Assert.fail("Did not find csar-validate test Checkbox in the page");
}
}
public static boolean checkCertificationQueryExists() throws Exception {
WebElement parentDiv = GeneralUIUtils.getWebElementByTestID(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_CERTIFICATION_CHECKBOX_TREE.getValue());
List<WebElement> checkboxTreeDivs = getChildElements(parentDiv);
List<WebElement> orderedList = getChildElements(checkboxTreeDivs.get(0));
return (!orderedList.isEmpty());
}
public static boolean checkComplianceCheckExists() throws Exception {
WebElement parentDiv = GeneralUIUtils.getWebElementByTestID(DataTestIdEnum.VspValidationPage.VSP_VALIDATION_PAGE_COMPLIANCE_CHECKBOX_TREE.getValue());
List<WebElement> checkboxTreeDivs = getChildElements(parentDiv);
List<WebElement> orderedList = getChildElements(checkboxTreeDivs.get(0));
return (!orderedList.isEmpty());
}
public static boolean checkSelectedComplianceCheckExists() throws Exception {
WebElement selectedTests = GeneralUIUtils.findElementsByXpath("//div[contains(text(),'Selected Compliance Tests')]/..//select[@class='validation-setup-selected-tests']").get(0);
List<WebElement> options = getChildElements(selectedTests);
return (!options.isEmpty());
}
public static boolean checkSelectedCertificationQueryExists() throws Exception {
WebElement selectedTests = GeneralUIUtils.findElementsByXpath("//div[contains(text(),'Selected Certifications Query')]/..//select[@class='validation-setup-selected-tests']").get(0);
List<WebElement> options = getChildElements(selectedTests);
return (!options.isEmpty());
}
public static void clickOnElementUsingTestId(DataTestIdEnum.VspValidationPage elementTestId) throws Exception {
SetupCDTest.getExtendTest().log(Status.INFO, String.format("Clicking on %s", elementTestId.name()));
GeneralUIUtils.getWebElementByTestID(elementTestId.getValue()).click();
GeneralUIUtils.ultimateWait();
}
private static List<WebElement> getChildElements(WebElement webElement) throws Exception {
return webElement.findElements(By.xpath(".//*"));
}
}
<file_sep>/catalog-model/src/test/java/org/openecomp/sdc/be/model/operations/impl/PropertyOperationTest.java
/*-
* ============LICENSE_START=======================================================
* SDC
* ================================================================================
* Copyright (C) 2017 AT&T Intellectual Property. All rights reserved.
* ================================================================================
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* ============LICENSE_END=========================================================
*/
package org.openecomp.sdc.be.model.operations.impl;
import org.janusgraph.core.JanusGraphVertex;
import fj.data.Either;
import org.apache.commons.lang3.tuple.ImmutablePair;
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.openecomp.sdc.be.dao.impl.HealingPipelineDao;
import org.openecomp.sdc.be.dao.janusgraph.HealingJanusGraphGenericDao;
import org.openecomp.sdc.be.dao.janusgraph.JanusGraphClient;
import org.openecomp.sdc.be.dao.janusgraph.JanusGraphGenericDao;
import org.openecomp.sdc.be.dao.janusgraph.JanusGraphOperationStatus;
import org.openecomp.sdc.be.datatypes.elements.PropertyDataDefinition;
import org.openecomp.sdc.be.datatypes.elements.PropertyRule;
import org.openecomp.sdc.be.datatypes.enums.NodeTypeEnum;
import org.openecomp.sdc.be.model.*;
import org.openecomp.sdc.be.model.operations.api.StorageOperationStatus;
import org.openecomp.sdc.be.model.tosca.ToscaPropertyType;
import org.openecomp.sdc.be.model.tosca.ToscaType;
import org.openecomp.sdc.be.model.tosca.constraints.GreaterThanConstraint;
import org.openecomp.sdc.be.model.tosca.constraints.InRangeConstraint;
import org.openecomp.sdc.be.model.tosca.constraints.LessOrEqualConstraint;
import org.openecomp.sdc.be.resources.data.DataTypeData;
import org.openecomp.sdc.be.resources.data.PropertyData;
import org.openecomp.sdc.be.resources.data.PropertyValueData;
import java.util.*;
import static org.junit.Assert.*;
import static org.mockito.Mockito.mock;
public class PropertyOperationTest extends ModelTestBase {
HealingJanusGraphGenericDao janusGraphGenericDao = mock(HealingJanusGraphGenericDao.class);
PropertyOperation propertyOperation = new PropertyOperation(janusGraphGenericDao, null);
@Before
public void setup() {
propertyOperation.setJanusGraphGenericDao(janusGraphGenericDao);
}
private PropertyDefinition buildPropertyDefinition() {
PropertyDefinition property = new PropertyDefinition();
property.setDefaultValue("10");
property.setDescription("Size of the local disk, in Gigabytes (GB), available to applications running on the Compute node.");
property.setType(ToscaType.INTEGER.name().toLowerCase());
return property;
}
@Test
public void convertConstraintsTest() {
List<PropertyConstraint> constraints = buildConstraints();
List<String> convertedStringConstraints = propertyOperation.convertConstraintsToString(constraints);
assertEquals("constraints size", constraints.size(), convertedStringConstraints.size());
List<PropertyConstraint> convertedConstraints = propertyOperation.convertConstraints(convertedStringConstraints);
assertEquals("check size of constraints", constraints.size(), convertedConstraints.size());
Set<String> constraintsClasses = new HashSet<>();
for (PropertyConstraint propertyConstraint : constraints) {
constraintsClasses.add(propertyConstraint.getClass().getName());
}
for (PropertyConstraint propertyConstraint : convertedConstraints) {
assertTrue("check all classes generated", constraintsClasses.contains(propertyConstraint.getClass().getName()));
}
}
@Test
public void testIsPropertyDefaultValueValid_NoDefault() {
PropertyDefinition property = new PropertyDefinition();
property.setName("myProperty");
property.setType(ToscaPropertyType.BOOLEAN.getType());
assertTrue(propertyOperation.isPropertyDefaultValueValid(property, null));
}
@Test
public void testIsPropertyDefaultValueValid_ValidDefault() {
PropertyDefinition property = new PropertyDefinition();
property.setName("myProperty");
property.setType(ToscaPropertyType.INTEGER.getType());
property.setDefaultValue("50");
assertTrue(propertyOperation.isPropertyDefaultValueValid(property, null));
}
@Test
public void testIsPropertyDefaultValueValid_InvalidDefault() {
PropertyDefinition property = new PropertyDefinition();
property.setName("myProperty");
property.setType(ToscaPropertyType.BOOLEAN.getType());
property.setDefaultValue("50");
assertFalse(propertyOperation.isPropertyDefaultValueValid(property, null));
}
private List<PropertyConstraint> buildConstraints() {
List<PropertyConstraint> constraints = new ArrayList<>();
GreaterThanConstraint propertyConstraint1 = new GreaterThanConstraint("0");
LessOrEqualConstraint propertyConstraint2 = new LessOrEqualConstraint("10");
List<String> range = new ArrayList<>();
range.add("0");
range.add("100");
InRangeConstraint propertyConstraint3 = new InRangeConstraint(range);
constraints.add(propertyConstraint1);
constraints.add(propertyConstraint2);
constraints.add(propertyConstraint3);
return constraints;
}
@Test
public void findPropertyValueBestMatch1() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
instanceIdToValue.put("node2", instanceProperty2);
ComponentInstanceProperty instanceProperty3 = new ComponentInstanceProperty();
instanceProperty3.setValue("v1node3");
instanceIdToValue.put("node3", instanceProperty3);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", "v1node1", instanceProperty.getValue());
assertEquals("check default value", "v1node2", instanceProperty.getDefaultValue());
}
@Test
public void findPropertyValueBestMatch2() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
instanceProperty2.setValueUniqueUid("aaaa");
instanceIdToValue.put("node2", instanceProperty2);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", "v1node2", instanceProperty.getValue());
assertEquals("check default value", "vv1", instanceProperty.getDefaultValue());
assertNull("check value unique id is null", instanceProperty.getValueUniqueUid());
}
@Test
public void findPropertyValueBestMatch3() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
instanceProperty1.setValueUniqueUid("aaaa");
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty3 = new ComponentInstanceProperty();
instanceProperty3.setValue("v1node3");
instanceIdToValue.put("node3", instanceProperty3);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", "v1node1", instanceProperty.getValue());
assertEquals("check default value", "v1node3", instanceProperty.getDefaultValue());
assertEquals("check valid unique id", instanceProperty1.getValueUniqueUid(), instanceProperty.getValueUniqueUid());
}
@Test
public void findPropertyValueBestMatch1Rules() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
List<PropertyRule> rules = new ArrayList<>();
PropertyRule propertyRule = new PropertyRule();
String[] ruleArr = { "node1", ".+", "node3" };
List<String> rule1 = new ArrayList<>(Arrays.asList(ruleArr));
propertyRule.setRule(rule1);
propertyRule.setValue("88");
rules.add(propertyRule);
instanceProperty1.setRules(rules);
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
instanceIdToValue.put("node2", instanceProperty2);
ComponentInstanceProperty instanceProperty3 = new ComponentInstanceProperty();
instanceProperty3.setValue("v1node3");
instanceIdToValue.put("node3", instanceProperty3);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", propertyRule.getValue(), instanceProperty.getValue());
assertEquals("check default value", "v1node2", instanceProperty.getDefaultValue());
}
@Test
public void findPropertyValueBestMatch2Rules() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
List<PropertyRule> rules = new ArrayList<>();
PropertyRule propertyRule1 = new PropertyRule();
String[] ruleArr1 = { "node1", "node2", ".+" };
List<String> rule1 = new ArrayList<>(Arrays.asList(ruleArr1));
propertyRule1.setRule(rule1);
propertyRule1.setValue("88");
PropertyRule propertyRule2 = new PropertyRule();
String[] ruleArr2 = { "node1", "node2", "node3" };
List<String> rule2 = new ArrayList<>(Arrays.asList(ruleArr2));
propertyRule2.setRule(rule2);
propertyRule2.setValue("99");
rules.add(propertyRule2);
rules.add(propertyRule1);
instanceProperty1.setRules(rules);
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
instanceIdToValue.put("node2", instanceProperty2);
ComponentInstanceProperty instanceProperty3 = new ComponentInstanceProperty();
instanceProperty3.setValue("v1node3");
instanceIdToValue.put("node3", instanceProperty3);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", propertyRule2.getValue(), instanceProperty.getValue());
assertEquals("check default value", "v1node2", instanceProperty.getDefaultValue());
}
@Test
public void findPropertyValueBestMatch1RuleLowLevel() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
List<PropertyRule> rules = new ArrayList<>();
PropertyRule propertyRule1 = new PropertyRule();
String[] ruleArr1 = { "node1", "node2", ".+" };
List<String> rule1 = new ArrayList<>(Arrays.asList(ruleArr1));
propertyRule1.setRule(rule1);
propertyRule1.setValue("88");
PropertyRule propertyRule2 = new PropertyRule();
String[] ruleArr2 = { "node1", "node2", "node3" };
List<String> rule2 = new ArrayList<>(Arrays.asList(ruleArr2));
propertyRule2.setRule(rule2);
propertyRule2.setValue("99");
rules.add(propertyRule2);
rules.add(propertyRule1);
instanceProperty1.setRules(rules);
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
List<PropertyRule> rules3 = new ArrayList<>();
PropertyRule propertyRule3 = new PropertyRule();
String[] ruleArr3 = { "node2", "node3" };
List<String> rule3 = new ArrayList<>(Arrays.asList(ruleArr3));
propertyRule3.setRule(rule3);
propertyRule3.setValue("77");
rules3.add(propertyRule3);
instanceProperty2.setRules(rules3);
instanceIdToValue.put("node2", instanceProperty2);
ComponentInstanceProperty instanceProperty3 = new ComponentInstanceProperty();
instanceProperty3.setValue("v1node3");
instanceIdToValue.put("node3", instanceProperty3);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", propertyRule2.getValue(), instanceProperty.getValue());
assertEquals("check default value", propertyRule3.getValue(), instanceProperty.getDefaultValue());
}
@Test
public void findPropertyValueBestMatchDefaultValueNotChanged() {
String propertyUniqueId = "x1";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
instanceProperty.setValue("v1");
instanceProperty.setDefaultValue("vv1");
List<String> path = new ArrayList<>();
path.add("node1");
path.add("node2");
path.add("node3");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
ComponentInstanceProperty instanceProperty1 = new ComponentInstanceProperty();
instanceProperty1.setValue("v1node1");
List<PropertyRule> rules = new ArrayList<>();
PropertyRule propertyRule1 = new PropertyRule();
String[] ruleArr1 = { "node1", "node2", ".+" };
List<String> rule1 = new ArrayList<>(Arrays.asList(ruleArr1));
propertyRule1.setRule(rule1);
propertyRule1.setValue("88");
PropertyRule propertyRule2 = new PropertyRule();
String[] ruleArr2 = { "node1", "node2", "node3" };
List<String> rule2 = new ArrayList<>(Arrays.asList(ruleArr2));
propertyRule2.setRule(rule2);
propertyRule2.setValue("99");
rules.add(propertyRule2);
rules.add(propertyRule1);
instanceProperty1.setRules(rules);
instanceIdToValue.put("node1", instanceProperty1);
ComponentInstanceProperty instanceProperty2 = new ComponentInstanceProperty();
instanceProperty2.setValue("v1node2");
List<PropertyRule> rules3 = new ArrayList<>();
PropertyRule propertyRule3 = new PropertyRule();
String[] ruleArr3 = { "node2", "node333" };
List<String> rule3 = new ArrayList<>(Arrays.asList(ruleArr3));
propertyRule3.setRule(rule3);
propertyRule3.setValue("77");
rules3.add(propertyRule3);
instanceProperty2.setRules(rules3);
instanceIdToValue.put("node2", instanceProperty2);
propertyOperation.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
assertEquals("check value", propertyRule2.getValue(), instanceProperty.getValue());
assertEquals("check default value", "vv1", instanceProperty.getDefaultValue());
}
private PropertyOperation createTestSubject() {
return new PropertyOperation(new HealingJanusGraphGenericDao(new JanusGraphClient()), null);
}
@Test
public void testConvertPropertyDataToPropertyDefinition() throws Exception {
PropertyOperation testSubject;
PropertyData propertyDataResult = new PropertyData();
String propertyName = "";
String resourceId = "";
PropertyDefinition result;
// default test
testSubject = createTestSubject();
result = testSubject.convertPropertyDataToPropertyDefinition(propertyDataResult, propertyName, resourceId);
}
@Test
public void testAddProperty() throws Exception {
PropertyOperation testSubject;
String propertyName = "";
PropertyDefinition propertyDefinition = new PropertyDefinition();
String resourceId = "";
Either<PropertyData, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.addProperty(propertyName, propertyDefinition, resourceId);
}
@Test
public void testValidateAndUpdateProperty() throws Exception {
PropertyOperation testSubject;
IComplexDefaultValue propertyDefinition = new PropertyDefinition();
Map<String, DataTypeDefinition> dataTypes = new HashMap<>();
dataTypes.put("", new DataTypeDefinition());
StorageOperationStatus result;
// default test
testSubject = createTestSubject();
result = testSubject.validateAndUpdateProperty(propertyDefinition, dataTypes);
}
@Test
public void testAddPropertyToGraph() throws Exception {
PropertyOperation testSubject;
String propertyName = "";
PropertyDefinition propertyDefinition = new PropertyDefinition();
String resourceId = "";
Either<PropertyData, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.addPropertyToGraph(propertyName, propertyDefinition, resourceId);
}
@Test
public void testAddPropertyToGraphByVertex() throws Exception {
PropertyOperation testSubject;
JanusGraphVertex metadataVertex = null;
String propertyName = "";
PropertyDefinition propertyDefinition = new PropertyDefinition();
String resourceId = "";
JanusGraphOperationStatus result;
// default test
testSubject = createTestSubject();
result = testSubject.addPropertyToGraphByVertex(metadataVertex, propertyName, propertyDefinition, resourceId);
}
@Test
public void testGetJanusGraphGenericDao() throws Exception {
PropertyOperation testSubject;
JanusGraphGenericDao result;
// default test
testSubject = createTestSubject();
result = testSubject.getJanusGraphGenericDao();
}
@Test
public void testDeletePropertyFromGraph() throws Exception {
PropertyOperation testSubject;
String propertyId = "";
Either<PropertyData, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.deletePropertyFromGraph(propertyId);
}
@Test
public void testUpdateProperty() throws Exception {
PropertyOperation testSubject;
String propertyId = "";
PropertyDefinition newPropertyDefinition = new PropertyDefinition();
Map<String, DataTypeDefinition> dataTypes = new HashMap<>();
Either<PropertyData, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.updateProperty(propertyId, newPropertyDefinition, dataTypes);
}
@Test
public void testUpdatePropertyFromGraph() throws Exception {
PropertyOperation testSubject;
String propertyId = "";
PropertyDefinition propertyDefinition = null;
Either<PropertyData, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.updatePropertyFromGraph(propertyId, propertyDefinition);
}
@Test
public void testSetJanusGraphGenericDao() {
PropertyOperation testSubject;
HealingJanusGraphGenericDao janusGraphGenericDao = null;
// default test
testSubject = createTestSubject();
testSubject.setJanusGraphGenericDao(janusGraphGenericDao);
}
@Test
public void testAddPropertyToNodeType() {
PropertyOperation testSubject;
String propertyName = "";
PropertyDefinition propertyDefinition = new PropertyDefinition();
NodeTypeEnum nodeType = NodeTypeEnum.Attribute;
String uniqueId = "";
Either<PropertyData, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.addPropertyToNodeType(propertyName, propertyDefinition, nodeType, uniqueId);
}
@Test
public void testFindPropertiesOfNode() throws Exception {
PropertyOperation testSubject;
NodeTypeEnum nodeType = null;
String uniqueId = "";
Either<Map<String, PropertyDefinition>, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.findPropertiesOfNode(nodeType, uniqueId);
}
@Test
public void testDeletePropertiesAssociatedToNode() throws Exception {
PropertyOperation testSubject;
NodeTypeEnum nodeType = null;
String uniqueId = "";
Either<Map<String, PropertyDefinition>, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.deletePropertiesAssociatedToNode(nodeType, uniqueId);
}
@Test
public void testDeleteAllPropertiesAssociatedToNode() throws Exception {
PropertyOperation testSubject;
NodeTypeEnum nodeType = null;
String uniqueId = "";
Either<Map<String, PropertyDefinition>, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.deleteAllPropertiesAssociatedToNode(nodeType, uniqueId);
}
@Test
public void testIsPropertyExist() throws Exception {
PropertyOperation testSubject;
List<PropertyDefinition> properties = null;
String resourceUid = "";
String propertyName = "";
String propertyType = "";
boolean result;
// default test
testSubject = createTestSubject();
result = testSubject.isPropertyExist(properties, resourceUid, propertyName, propertyType);
}
@Test
public void testValidateAndUpdateRules() throws Exception {
PropertyOperation testSubject;
String propertyType = "";
List<PropertyRule> rules = null;
String innerType = "";
Map<String, DataTypeDefinition> dataTypes = null;
boolean isValidate = false;
ImmutablePair<String, Boolean> result;
// test 1
testSubject = createTestSubject();
rules = null;
result = testSubject.validateAndUpdateRules(propertyType, rules, innerType, dataTypes, isValidate);
}
@Test
public void testAddRulesToNewPropertyValue() throws Exception {
PropertyOperation testSubject;
PropertyValueData propertyValueData = new PropertyValueData();
ComponentInstanceProperty resourceInstanceProperty = new ComponentInstanceProperty();
String resourceInstanceId = "";
// default test
testSubject = createTestSubject();
testSubject.addRulesToNewPropertyValue(propertyValueData, resourceInstanceProperty, resourceInstanceId);
}
@Test
public void testFindPropertyValue() throws Exception {
PropertyOperation testSubject;
String resourceInstanceId = "";
String propertyId = "";
ImmutablePair<JanusGraphOperationStatus, String> result;
// default test
testSubject = createTestSubject();
result = testSubject.findPropertyValue(resourceInstanceId, propertyId);
}
@Test
public void testUpdateRulesInPropertyValue() throws Exception {
PropertyOperation testSubject;
PropertyValueData propertyValueData = new PropertyValueData();
ComponentInstanceProperty resourceInstanceProperty = new ComponentInstanceProperty();
String resourceInstanceId = "";
// default test
testSubject = createTestSubject();
testSubject.updateRulesInPropertyValue(propertyValueData, resourceInstanceProperty, resourceInstanceId);
}
@Test
public void testGetAllPropertiesOfResourceInstanceOnlyPropertyDefId() throws Exception {
PropertyOperation testSubject;
String resourceInstanceUid = "";
Either<List<ComponentInstanceProperty>, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getAllPropertiesOfResourceInstanceOnlyPropertyDefId(resourceInstanceUid);
}
@Test
public void testRemovePropertyOfResourceInstance() throws Exception {
PropertyOperation testSubject;
String propertyValueUid = "";
String resourceInstanceId = "";
Either<PropertyValueData, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.removePropertyOfResourceInstance(propertyValueUid, resourceInstanceId);
}
@Test
public void testRemovePropertyValueFromResourceInstance() throws Exception {
PropertyOperation testSubject;
String propertyValueUid = "";
String resourceInstanceId = "";
boolean inTransaction = false;
Either<ComponentInstanceProperty, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.removePropertyValueFromResourceInstance(propertyValueUid, resourceInstanceId,
inTransaction);
}
@Test
public void testBuildResourceInstanceProperty() throws Exception {
PropertyOperation testSubject;
PropertyValueData propertyValueData = new PropertyValueData();
ComponentInstanceProperty resourceInstanceProperty = new ComponentInstanceProperty();
ComponentInstanceProperty result;
// default test
testSubject = createTestSubject();
result = testSubject.buildResourceInstanceProperty(propertyValueData, resourceInstanceProperty);
}
@Test
public void testIsPropertyDefaultValueValid() throws Exception {
PropertyOperation testSubject;
IComplexDefaultValue propertyDefinition = null;
Map<String, DataTypeDefinition> dataTypes = null;
boolean result;
// test 1
testSubject = createTestSubject();
propertyDefinition = null;
result = testSubject.isPropertyDefaultValueValid(propertyDefinition, dataTypes);
Assert.assertEquals(false, result);
}
@Test
public void testIsPropertyTypeValid() throws Exception {
PropertyOperation testSubject;
IComplexDefaultValue property = null;
boolean result;
// test 1
testSubject = createTestSubject();
property = null;
result = testSubject.isPropertyTypeValid(property);
Assert.assertEquals(false, result);
}
@Test
public void testIsPropertyInnerTypeValid() throws Exception {
PropertyOperation testSubject;
IComplexDefaultValue property = null;
Map<String, DataTypeDefinition> dataTypes = null;
ImmutablePair<String, Boolean> result;
// test 1
testSubject = createTestSubject();
property = null;
result = testSubject.isPropertyInnerTypeValid(property, dataTypes);
}
@Test
public void testGetAllPropertiesOfResourceInstanceOnlyPropertyDefId_1() throws Exception {
PropertyOperation testSubject;
String resourceInstanceUid = "";
NodeTypeEnum instanceNodeType = null;
Either<List<ComponentInstanceProperty>, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getAllPropertiesOfResourceInstanceOnlyPropertyDefId(resourceInstanceUid, instanceNodeType);
}
@Test
public void testFindDefaultValueFromSecondPosition() throws Exception {
PropertyOperation testSubject;
List<String> pathOfComponentInstances = null;
String propertyUniqueId = "";
String defaultValue = "";
Either<String, JanusGraphOperationStatus> result;
// test 1
testSubject = createTestSubject();
pathOfComponentInstances = null;
result = testSubject.findDefaultValueFromSecondPosition(pathOfComponentInstances, propertyUniqueId,
defaultValue);
}
@Test
public void testUpdatePropertyByBestMatch() throws Exception {
PropertyOperation testSubject;
String propertyUniqueId = "";
ComponentInstanceProperty instanceProperty = new ComponentInstanceProperty();
List<String> path = new ArrayList<>();
path.add("path");
instanceProperty.setPath(path);
Map<String, ComponentInstanceProperty> instanceIdToValue = new HashMap<>();
instanceIdToValue.put("123", instanceProperty);
// default test
testSubject = createTestSubject();
testSubject.updatePropertyByBestMatch(propertyUniqueId, instanceProperty, instanceIdToValue);
}
@Test
public void testGetDataTypeByUid() throws Exception {
PropertyOperation testSubject;
String uniqueId = "";
Either<DataTypeDefinition, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getDataTypeByUid(uniqueId);
}
@Test
public void testAddDataType() throws Exception {
PropertyOperation testSubject;
DataTypeDefinition dataTypeDefinition = new DataTypeDefinition();
Either<DataTypeDefinition, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.addDataType(dataTypeDefinition);
}
@Test
public void testGetDataTypeByName() throws Exception {
PropertyOperation testSubject;
String name = "";
boolean inTransaction = false;
Either<DataTypeDefinition, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getDataTypeByName(name, inTransaction);
}
@Test
public void testGetDataTypeByName_1() throws Exception {
PropertyOperation testSubject;
String name = "";
Either<DataTypeDefinition, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getDataTypeByName(name);
}
@Test
public void testGetDataTypeByNameWithoutDerived() throws Exception {
PropertyOperation testSubject;
String name = "";
Either<DataTypeDefinition, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getDataTypeByNameWithoutDerived(name);
}
@Test
public void testGetDataTypeByUidWithoutDerivedDataTypes() throws Exception {
PropertyOperation testSubject;
String uniqueId = "";
Either<DataTypeDefinition, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getDataTypeByUidWithoutDerivedDataTypes(uniqueId);
}
@Test
public void testIsDefinedInDataTypes() throws Exception {
PropertyOperation testSubject;
String propertyType = "";
Either<Boolean, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.isDefinedInDataTypes(propertyType);
}
@Test
public void testGetAllDataTypes() throws Exception {
PropertyOperation testSubject;
Either<Map<String, DataTypeDefinition>, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getAllDataTypes();
}
@Test
public void testCheckInnerType() throws Exception {
PropertyOperation testSubject;
PropertyDataDefinition propDataDef = new PropertyDataDefinition();
Either<String, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.checkInnerType(propDataDef);
}
@Test
public void testGetAllDataTypeNodes() throws Exception {
PropertyOperation testSubject;
Either<List<DataTypeData>, JanusGraphOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.getAllDataTypeNodes();
}
@Test
public void testValidateAndUpdatePropertyValue() throws Exception {
PropertyOperation testSubject;
String propertyType = "";
String value = "";
boolean isValidate = false;
String innerType = "";
Map<String, DataTypeDefinition> dataTypes = null;
Either<Object, Boolean> result;
// default test
testSubject = createTestSubject();
result = testSubject.validateAndUpdatePropertyValue(propertyType, value, isValidate, innerType, dataTypes);
}
@Test
public void testValidateAndUpdatePropertyValue_1() throws Exception {
PropertyOperation testSubject;
String propertyType = "";
String value = "";
String innerType = "";
Map<String, DataTypeDefinition> dataTypes = new HashMap<>();
dataTypes.put("", new DataTypeDefinition());
Either<Object, Boolean> result;
// default test
testSubject = createTestSubject();
result = testSubject.validateAndUpdatePropertyValue(propertyType, value, innerType, dataTypes);
}
@Test
public void testAddPropertiesToElementType() throws Exception {
PropertyOperation testSubject;
String uniqueId = "";
NodeTypeEnum elementType = null;
List<PropertyDefinition> properties = null;
Either<Map<String, PropertyData>, JanusGraphOperationStatus> result;
// test 1
testSubject = createTestSubject();
properties = null;
result = testSubject.addPropertiesToElementType(uniqueId, elementType, properties);
}
@Test
public void testUpdateDataType() throws Exception {
PropertyOperation testSubject;
DataTypeDefinition newDataTypeDefinition = new DataTypeDefinition();
DataTypeDefinition oldDataTypeDefinition = new DataTypeDefinition();
Either<DataTypeDefinition, StorageOperationStatus> result;
// default test
testSubject = createTestSubject();
result = testSubject.updateDataType(newDataTypeDefinition, oldDataTypeDefinition);
}
}
| 7f2f26ac9465e77fc6b6b45ff88a2289b6c3996b | [
"Java",
"Python"
] | 7 | Python | zeroichi/sdc | b3d55d3dd8508919ae48d5f60425aa13e5731ac2 | ecda58e5b075248c3f550f5ae0dcce2750bdbda3 | |
refs/heads/master | <repo_name>furaga/SimplePMDViewer<file_sep>/SimplePMDViewer/SimplePMDViewer/MMDModel/ModelIK.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
using WORD = System.UInt16;
using DWORD = System.UInt32;
namespace SimplePMDViewer.MMDModel
{
class ModelIK
{
public WORD IKBoneIndex { get; set; }
public WORD IKTargetBoneIndex { get; set; }
public WORD Iterations {get; set;}
public float AngleLimit { get; set; }
public WORD[] IKChildBoneIndex { get; set; }
public ModelIK(BinaryReader reader)
{
Read(reader);
}
public void Read(BinaryReader reader)
{
IKBoneIndex = BitConverter.ToUInt16(reader.ReadBytes(2), 0);
IKTargetBoneIndex = BitConverter.ToUInt16(reader.ReadBytes(2), 0);
byte chainLength = reader.ReadByte();
Iterations = BitConverter.ToUInt16(reader.ReadBytes(2), 0);
AngleLimit = BitConverter.ToSingle(reader.ReadBytes(4), 0);
IKChildBoneIndex = new WORD[chainLength];
for (int i = 0; i < chainLength; i++)
{
IKChildBoneIndex[i] = BitConverter.ToUInt16(reader.ReadBytes(2), 0);
}
}
public void Write(StreamWriter writer)
{
writer.Write(IKBoneIndex + ",");
writer.Write(IKTargetBoneIndex + ",");
writer.Write(Iterations + ",");
writer.Write(AngleLimit + ",");
foreach (var e in IKChildBoneIndex) writer.Write(e + ",");
writer.WriteLine();
}
}
}
<file_sep>/SimplePMDViewer/SimplePMDViewer/MMDVertex.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework;
using System.Runtime.InteropServices;
namespace SimplePMDViewer
{
[StructLayout(LayoutKind.Sequential)]
struct VertexPositionNormal : IVertexType
{
public Vector3 Position;
public Vector3 Normal;
// メンバPosition, Normalがそれぞれどのようにメモリにマッピングされ、どのような用途で使われるかを指定
public readonly static VertexDeclaration vertexDeclaration =
new VertexDeclaration(
new VertexElement(0, VertexElementFormat.Vector3, VertexElementUsage.Position, 0),
new VertexElement(3 * sizeof(float), VertexElementFormat.Vector3, VertexElementUsage.Normal, 0)
);
public VertexDeclaration VertexDeclaration { get { return vertexDeclaration; } }
}
// struct VertexPositionNormalTexture はXNAのライブラリとしてすでに定義されている
}
<file_sep>/SimplePMDViewer/SimplePMDViewer/Game1.cs
using System;
using System.Collections.Generic;
using System.Linq;
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Audio;
using Microsoft.Xna.Framework.Content;
using Microsoft.Xna.Framework.GamerServices;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
using Microsoft.Xna.Framework.Media;
using System.IO;
using System.Drawing;
using System.Drawing.Imaging;
namespace SimplePMDViewer
{
public class Game1 : Microsoft.Xna.Framework.Game
{
GraphicsDeviceManager graphics;
SpriteBatch spriteBatch;
MMDModel.MMDModel model;
public Game1()
{
graphics = new GraphicsDeviceManager(this);
Content.RootDirectory = "Content";
graphics.PreferredBackBufferWidth = 1040;
graphics.PreferredBackBufferHeight = 1040;
}
protected override void Initialize()
{
base.Initialize();
}
byte[] buf;
Texture2D texture;
protected override void LoadContent()
{
spriteBatch = new SpriteBatch(GraphicsDevice);
model = new MMDModel.MMDModel(@"./Content/Lat式ミクVer2.3/Lat式ミクVer2.3_Sailor夏服.pmd", 1);
/*
var filename = @"Content\BLAZER.bmp";
int stride = 4; // iピクセル辺りの色情報を表すデータの数(RGBAなので4つ)
Bitmap image = (Bitmap)Image.FromFile(filename, true);
byte[] pixel_data = new byte[image.Width * image.Height * stride];
// 画像をロックする領域
System.Drawing.Rectangle lock_rect = new System.Drawing.Rectangle { X = 0, Y = 0, Width = image.Width, Height = image.Height };
// ロックする
// Rectangle rect ロック領域
// ImageLockMode flags ロック方法 今回は読み取るだけなのでReadOnlyを指定する
// PixelFormat format 画像のデータ形式 RGBAデータがほしいのでPixelFormat.Format32bppPArgbを指定する
BitmapData bitmap_data = image.LockBits(
lock_rect,
ImageLockMode.ReadOnly,
PixelFormat.Format32bppPArgb);
buf = new byte[4 * image.Height * image.Width];
// 色情報取得
for (int y = 0; y < image.Height; y++)
{
for (int x = 0; x < image.Width; x++)
{
int pixel_target = x * stride + bitmap_data.Stride * y;
int array_index = (y * image.Width + x) * stride;
// ロックしたポインタから色情報を取得(BGRAの順番で格納されてる)
for (int i = 0; i < stride; i++)
buf[array_index + i] =
System.Runtime.InteropServices.Marshal.ReadByte(
bitmap_data.Scan0,
pixel_target + stride - ((1 + i) % stride) - 1);
}
}
// ロックしたらアンロックを忘れずに!
image.UnlockBits(bitmap_data);
texture = new Texture2D(
GraphicsDevice,
image.Width,
image.Height,
false,
SurfaceFormat.Color);
// 色データ配列を設定
texture.SetData<byte>(buf);
*/
}
protected override void Update(GameTime gameTime)
{
if (Keyboard.GetState().IsKeyDown(Keys.Escape)) this.Exit();
base.Update(gameTime);
}
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Microsoft.Xna.Framework.Color.Black);
base.Draw(gameTime);
}
}
}
| 0c19014f76aeeb3c003fe00a1d685d0cef52bb22 | [
"C#"
] | 3 | C# | furaga/SimplePMDViewer | 917c64c7f58e0d206eaa37827bef6ce677e1aca5 | 46df4d9dc31f8185ab4fe1cb557b74cfc2e8bf3a | |
refs/heads/master | <file_sep>using System;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Runtime.Serialization;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Media;
using Microsoft.Phone.Controls;
using Microsoft.Phone.Shell;
using System.Collections.Generic;
#if WP8
using System.Threading.Tasks;
using Windows.ApplicationModel;
using Windows.Storage;
using Windows.System;
//Use alias in case Cordova File Plugin is enabled. Then the File class will be declared in both and error will occur.
using IOFile = System.IO.File;
#else
using Microsoft.Phone.Tasks;
#endif
namespace WPCordovaClassLib.Cordova.Commands
{
class HttpRequest : BaseCommand
{
public void echo(string options)
{
string argsString = JSON.JsonHelper.Deserialize<string[]>(options)[0];
string[] args = JSON.JsonHelper.Deserialize<string[]>(argsString);
string url = args[0];
string cookie = args[1];
KeyValuePair<string, string>[] headers = JSON.JsonHelper.Deserialize<KeyValuePair<string, string>[]>(args[2]);
string response = "{\"result\" : " + options + "}";
DispatchCommandResult(new PluginResult(PluginResult.Status.OK, response));
}
public void request(string options)
{
string argsString = JSON.JsonHelper.Deserialize<string[]>(options)[0];
string callbackId = JSON.JsonHelper.Deserialize<string[]>(options)[1];
string[] args = JSON.JsonHelper.Deserialize<string[]>(argsString);
string url = args[0];
string cookie = args[1];
KeyValuePair<string, string>[] headers = JSON.JsonHelper.Deserialize<KeyValuePair<string, string>[]>(args[2]);
Uri uri = new Uri(url);
HttpWebRequest request = (HttpWebRequest)HttpWebRequest.Create(url);
request.Method = "GET";
request.CookieContainer = new CookieContainer();
request.CookieContainer.SetCookies(uri, cookie); // 넘겨줄 쿠키가 있을때 CookiContainer 에 저장
WebHeaderCollection webHeaderCollection = request.Headers;
foreach (KeyValuePair<string, string> pair in headers)
{
webHeaderCollection[pair.Key] = pair.Value;
}
request.BeginGetResponse(responseCallback, new object[] {request, callbackId});
}
void responseCallback(IAsyncResult result)
{
object[] responseCallbackParams = (object[])result.AsyncState;
HttpWebRequest request = (HttpWebRequest)responseCallbackParams[0];
string callbackId = (string)responseCallbackParams[1];
if (request != null)
{
try
{
WebResponse response = request.EndGetResponse(result);
using (var reader = new StreamReader(response.GetResponseStream()))
{
string jsonString = reader.ReadToEnd();
PluginResult pluginResult = new PluginResult(PluginResult.Status.OK, jsonString);
pluginResult.KeepCallback = true;
DispatchCommandResult(pluginResult, callbackId);
}
}
catch (WebException e)
{
DispatchCommandResult(new PluginResult(PluginResult.Status.ERROR));
}
}
}
}
}
<file_sep>
function HttpRequest() {
}
HttpRequest.prototype.echo = function (params, callback) {
var options = [];
options.push(params.url);
options.push(params.cookie);
var temp = [];
var headers = params.headers;
for (var prop in headers) {
temp.push({Key:prop, Value: headers[prop]});
}
options.push(JSON.stringify(temp));
cordova.exec(callback, function (err) {
callback('Nothing to echo.');
}, "HttpRequest", "echo", [JSON.stringify(options)]);
};
HttpRequest.prototype.request = function (params, callback) {
var options = [];
options.push(params.url);
options.push(params.cookie);
var temp = [];
var headers = params.headers;
for (var prop in headers) {
temp.push({ Key: prop, Value: headers[prop] });
}
options.push(JSON.stringify(temp));
cordova.exec(callback, function (err) {
callback();
}, "HttpRequest", "request", [JSON.stringify(options)]);
};
module.exports.httpRequest = new HttpRequest();
| 8d0ba2901731397eb106ae2ce25cecc277a3c4d4 | [
"JavaScript",
"C#"
] | 2 | C# | transformersprimeabcxyz/cordova-http-request | 8bda1f22f11cd028ad4f921eb2ba7ba6fee8e5d6 | 735d294345983ecf4a1a2c0577004bb0b305ee64 | |
refs/heads/master | <file_sep>package com.qunb.geosearch.geoObject;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jclouds.aws.s3.blobstore.*;
import org.jclouds.blobstore.BlobMap;
import org.jclouds.blobstore.domain.Blob;
import org.jclouds.blobstore.domain.StorageMetadata;
import org.jclouds.blobstore.domain.StorageType;
import org.jclouds.blobstore.options.ListContainerOptions;
import org.json.simple.JSONObject;
import org.json.simple.JSONValue;
import com.google.appengine.api.datastore.DatastoreService;
import com.google.appengine.api.datastore.DatastoreServiceFactory;
import com.google.appengine.api.datastore.Entity;
import com.google.appengine.api.datastore.PreparedQuery;
import com.google.appengine.api.datastore.Query;
import com.google.appengine.api.datastore.Query.FilterOperator;
import com.qunb.fuzzymatch.LetterSimilarity;
import com.qunb.fuzzymatch.LetterSimilarity.CouplingLevel;
public class QunbTextSearch {
private volatile DatastoreService datastore;
private volatile String input;
private volatile String type;
private volatile String country;
private volatile String lang;
private volatile List<JSONObject> result;
public QunbTextSearch(String input,String type,String country,String lang) throws Exception{
if(input.contains(",")){
this.input =input.substring(0, input.indexOf(","));
}
else{
this.input=input;
}
if(lang!=null&&!lang.isEmpty()){
this.lang=lang;
}
else{
this.lang="en";
}
this.type = type;
this.country = country;
this.datastore= DatastoreServiceFactory.getDatastoreService();
this.result = this.constructResult();
}
public List<JSONObject> constructResult() throws Exception{
List<JSONObject> mydata = new ArrayList<JSONObject>();
mydata = this.getData();
if(mydata.isEmpty()){
return null;
}
return mydata;
}
@SuppressWarnings("deprecation")
public synchronized List<JSONObject> getData() throws IOException{
List<JSONObject> output = new ArrayList<JSONObject>();
List<Entity> entities = new ArrayList<Entity>();
if (this.input.length() == 2) {
entities = GeoDataStore.searchCountryCode(this.input);
}
if (entities.size() == 0) {
entities = GeoDataStore.searchName(this.input, this.country, this.type);
}
for(Entity geo:entities){
JSONObject mygeo = new JSONObject();
mygeo = GeoNameOperation.EntitytoJson(geo);
output.add(mygeo);
}
if(output.size()==0){
System.out.println("---Result Not Found at Qunb---");
}
return output;
}
public List<Map<String,Object>> getResult(){
List<Map<String,Object>> list = new ArrayList<Map<String,Object>>();
if(this.result!=null){
for(int i = 0;i<this.result.size();i++){
Map<String,Object> tmpmap = new HashMap<String,Object>();
tmpmap = GeoNameOperation.JsonToMap(this.result.get(i));
list.add(tmpmap);
}
}
return list;
}
public static List<Map<String,Object>> classResult(List<Map<String,Object>> mylist){
for(int i =0;i<mylist.size()-1;i++){
Map<String,Object> tmpmap_j = null;
Map<String,Object> tmpmap_j_1 = null;
for(int j=mylist.size()-1;j>i;j--){
if(Integer.valueOf(mylist.get(j).get("qunb:population").toString())!=0 &&Integer.valueOf(mylist.get(j-1).get("qunb:population").toString())!=0){
if(Integer.valueOf(mylist.get(j).get("qunb:population").toString())>Integer.valueOf(mylist.get(j-1).get("qunb:population").toString())){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
else if(Integer.valueOf(mylist.get(j).get("qunb:population").toString())==Integer.valueOf(mylist.get(j-1).get("qunb:population").toString())){
if(getfclordre(mylist.get(j))>getfclordre(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
else{
if(getfclordre(mylist.get(j))==0&&getfclordre(mylist.get(j-1))==0){
if(getfcodeorder_A(mylist.get(j))>getfcodeorder_A(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
}
else if(getfclordre(mylist.get(j))==1&&getfclordre(mylist.get(j-1))==1){
if(getfcodeorder_P(mylist.get(j))>getfcodeorder_P(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
}
}
}
}
else{
if(getfclordre(mylist.get(j))>getfclordre(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
else{
if(getfclordre(mylist.get(j))==0&&getfclordre(mylist.get(j-1))==0){
if(getfcodeorder_A(mylist.get(j))>getfcodeorder_A(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
}
else if(getfclordre(mylist.get(j))==1&&getfclordre(mylist.get(j-1))==1){
if(getfcodeorder_P(mylist.get(j))>getfcodeorder_P(mylist.get(j-1))){
tmpmap_j=mylist.get(j);
tmpmap_j_1=mylist.get(j-1);
mylist.set(j, tmpmap_j_1);
mylist.set(j-1,tmpmap_j);
}
}
}
}
}
}
return mylist;
}
public static int getfclordre(Map<String,Object> map){
if(map.get("qunb:fclcode").equals("A")){
return 2;
}
else if(map.get("qunb:fclcode").equals("P")){
return 1;
}
else {
return 0;
}
}
public static int getfcodeorder_P(Map<String,Object> map){
if(map.get("qunb:fcode").equals("PPLC")){
return 5;
}
else if(map.get("qunb:fcode").equals("PPLA")){
return 4;
}
else if(map.get("qunb:fcode").equals("PPLA2")){
return 3;
}
else if(map.get("qunb:fcode").equals("PPLA3")){
return 2;
}
else if(map.get("qunb:fcode").equals("PPLA4")){
return 1;
}
else {
return 0;
}
}
public static int getfcodeorder_A(Map<String,Object> map){
if(map.get("qunb:fcode").equals("PCL")){
return 8;
}
else if(map.get("qunb:fcode").equals("PCLD")){
return 7;
}
else if(map.get("qunb:fcode").equals("PCLF")){
return 6;
}
else if(map.get("qunb:fcode").equals("ADMD")){
return 5;
}
else if(map.get("qunb:fcode").equals("ADM1")){
return 4;
}
else if(map.get("qunb:fcode").equals("ADM2")){
return 3;
}
else if(map.get("qunb:fcode").equals("ADM3")){
return 2;
}
else if(map.get("qunb:fcode").equals("ADM4")){
return 1;
}
else {
return 0;
}
}
}
<file_sep>package com.qunb.geosearch.geoObject;
import javax.jdo.annotations.IdGeneratorStrategy;
import javax.jdo.annotations.PersistenceCapable;
import javax.jdo.annotations.Persistent;
import javax.jdo.annotations.PrimaryKey;
import org.json.JSONException;
import org.json.simple.JSONObject;
import com.google.appengine.api.datastore.Entity;
import com.google.appengine.api.datastore.Key;
import com.google.appengine.api.datastore.KeyFactory;
public class GeoName {
private String name;
private double lat;
private double lng;
private String geonameId;
private String countryCode;
private String countryName;
private String fcl;
private String fcode;
private String alternateNames;
private String population;
private String fclName;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setLat(String lat) {
this.lat = Double.valueOf(lat);
}
public double getLat() {
return this.lat;
}
public void setLng(String lng) {
this.lng = Double.valueOf(lng);
}
public double getLng() {
return this.lng;
}
public void setGeonameId(String geonameId) {
this.geonameId = geonameId;
}
public String getGeonameId() {
return this.geonameId;
}
public void setCountryName(String countryName) {
this.countryName = countryName;
}
public String getCountryName() {
return countryName;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
public String getCountryCode() {
return countryCode;
}
public void setFcode(String fcode) {
this.fcode = fcode;
}
public String getFcode() {
return fcode;
}
public void setFcl(String fcl) {
this.fcl = fcl;
}
public String getFcl() {
return fcl;
}
public void setPopulation(String ppl) {
this.population = ppl;
}
public String getPopulation() {
return this.population;
}
public String getFclName(){
return this.fclName;
}
public void setFclName(String fclname){
this.fclName = fclname;
}
public Entity toEntity(){
Key geo_key = KeyFactory.createKey("GeonameId", this.getGeonameId().toString());
Entity geo = new Entity("GeoName",geo_key);
geo.setProperty("geonameId", this.getGeonameId());
geo.setProperty("name", this.getName());
geo.setProperty("countryName", this.getCountryName());
geo.setProperty("countryCode", this.getCountryCode());
geo.setProperty("Lat", this.getLat());
geo.setProperty("Lng", this.getLng());
geo.setProperty("Fcl", this.getFcl());
geo.setProperty("Fcode", this.getFcode());
geo.setProperty("Population", this.getPopulation());
return geo;
}
public org.json.JSONObject toJson() throws JSONException{
org.json.JSONObject tmp = new org.json.JSONObject();
tmp.put("qunb:geoId", this.getGeonameId());
tmp.put("qunb:geoName",this.getName());
tmp.put("qunb:geoLat",this.getLat());
tmp.put("qunb:geoLng",this.getLng());
tmp.put("qunb:geoFcode",this.getFcode());
return tmp;
}
public String getAlternateNames() {
return alternateNames;
}
public void setAlternateNames(String alternateNames) {
this.alternateNames = alternateNames;
}
}
<file_sep>package com.qunb.rest.dimension;
import org.restlet.Application;
import org.restlet.Restlet;
import org.restlet.routing.Router;
import com.qunb.rest.unit.QunbUnitResource;
public class QunbDimensionWSApplication extends Application {
@Override
public Restlet createRoot() {
Router router = new Router(getContext());
router.attachDefault(QunbDimensionResource.class);
return router;
}
}
<file_sep>package com.qunb.geosearch.LatLngSearch;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import javax.ws.rs.Path;
import org.geonames.WebService;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import org.restlet.resource.Get;
import org.restlet.resource.ServerResource;
import com.qunb.geosearch.TextSearch.QunbGeoTextSearchResource;
import com.qunb.geosearch.geoObject.GeoName;
import com.qunb.geosearch.geoObject.GeoNameOperation;
import com.qunb.geosearch.geoObject.GeoNamesLatLngSearch;
import com.qunb.geosearch.geoObject.QunbLatLogSearch;
@Path("/geosearch/latlngsearch/search")
public class QunbGeoLatLngSearchResource extends ServerResource {
@Get
public JSONArray searchGeoID() throws Exception{
WebService svc = new WebService();
svc.setUserName("leipang");
double lat = Double.parseDouble(getQuery().getValues("lat"));
double lng = Double.parseDouble(getQuery().getValues("lng"));
String level = getQuery().getValues("level");
String limit = getQuery().getValues("limit");
List<JSONObject> result = new ArrayList<JSONObject>();
result = getResultList(lat,lng,level,limit);
JSONArray jsonresult = getSearchResult(result);
return jsonresult;
}
@SuppressWarnings("null")
public List<JSONObject> getResultList(double lat,double lng,String level,String limit) throws Exception{
List<JSONObject> list = new ArrayList<JSONObject>();
QunbLatLogSearch mysearch = new QunbLatLogSearch(lat,lng);
if(mysearch.getResult().isEmpty()){
GeoNamesLatLngSearch mysearch2 = new GeoNamesLatLngSearch(lat,lng,level);
mysearch2.storeData();
GeoName geo_search = mysearch2.getResult();
if(geo_search!=null){
JSONObject tmp = new JSONObject();
tmp = geo_search.toJson();
list.add(tmp);
}
else{
JSONObject tmp = new JSONObject();
tmp.put("error 400","The level is not a fcode!");
list.add(tmp);
}
}
else{
List<Map<String,Object>> qunb_search=mysearch.getResult();
List<Map<String,Object>> qunb_search_class=QunbLatLogSearch.classResult(qunb_search);
GeoNameOperation.toListJson(qunb_search_class, limit);
}
return list;
}
@SuppressWarnings("unchecked")
public JSONArray getSearchResult(List<JSONObject> mylist){
System.out.println(mylist.size());
JSONArray output = new JSONArray();
for(int i = 0;i<mylist.size();i++){
output.put(mylist.get(i));
}
return output;
}
}
<file_sep>package com.qunb.geosearch.TextSearch;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.geonames.WebService;
import org.json.*;
import org.restlet.resource.Get;
import org.restlet.resource.ServerResource;
import com.qunb.geosearch.geoObject.GeoNameOperation;
import com.qunb.geosearch.geoObject.GeoNamesTextSearch;
import com.qunb.geosearch.geoObject.QunbTextSearch;
import javax.ws.rs.Path;
@Path("/geosearch/textsearch/search")
public class QunbGeoTextSearchResource extends ServerResource{
@SuppressWarnings("static-access")
@Get
public JSONArray searchGeoID() throws Exception{
WebService svc = new WebService();
svc.setUserName("leipang");
String input = getQuery().getValues("q");
String type = getQuery().getValues("type");
String country = getQuery().getValues("country");
String lang = getQuery().getValues("lang");
String limit = getQuery().getValues("limit");
List<JSONObject> result = new ArrayList<JSONObject>();
result = getResultList(input,type,country,limit,lang);
JSONArray jsonresult = getSearchResult(result);
return jsonresult;
}
@SuppressWarnings("static-access")
public List<JSONObject> getResultList(String input,String type,String country,String limit,String lang) throws Exception{
List<JSONObject> list = new ArrayList<JSONObject>();
QunbTextSearch mysearch = new QunbTextSearch(input,type,country,lang);
if(mysearch.getResult().isEmpty()){
GeoNamesTextSearch mysearch2 = new GeoNamesTextSearch(input,limit,type,country,lang);
mysearch2.storeData();
List<Map<String, Object>> mylist_result = mysearch2.getReturnResult();
List<Map<String, Object>> mylist_result_class = QunbTextSearch.classResult(mylist_result);
list = GeoNameOperation.toListJson(mylist_result_class,limit);
}
else{
List<Map<String, Object>> mylist_result = mysearch.getResult();
List<Map<String, Object>> mylist_result_class = QunbTextSearch.classResult(mylist_result);
list = GeoNameOperation.toListJson(mylist_result_class,limit);
}
return list;
}
@SuppressWarnings("unchecked")
public JSONArray getSearchResult(List<JSONObject> mylist){
System.out.println(mylist.size());
JSONArray output = new JSONArray();
for(int i = 0;i<mylist.size();i++){
output.put(mylist.get(i));
}
return output;
}
}
| f4c2b81eba4e31a3ecc4e158281f56253a5a0619 | [
"Java"
] | 5 | Java | leipang/Qunbwebservice | dbd7a1600dffdfc32e1688d546b9da1f092c216a | 078e7f04773ac2a42b391bea1db0c2ab306c4b57 | |
refs/heads/master | <repo_name>artsiom-tsaryonau/pmc<file_sep>/build.gradle
apply plugin: 'eclipse'
apply plugin: 'java'
allprojects {
group = 'com.epam.app.pmc'
}
subprojects {
apply plugin: 'java'
apply plugin: 'eclipse'
repositories {
mavenCentral()
}
dependencies {
testCompile 'junit:junit:4.11'
}
}
buildscript {
repositories {
mavenCentral()
jcenter()
}
dependencies {
classpath 'net.saliman:gradle-liquibase-plugin:1.0.0'
classpath 'net.saliman:groovy-liquibase-dsl:1.0.0'
classpath 'org.postgresql:postgresql:9.3-1102-jdbc41'
classpath 'org.gradle.api.plugins:gradle-tomcat-plugin:0.9.9'
}
}
<file_sep>/pmc-web/src/main/resources/oauth_consumer.properties
graph.facebook.com.consumer_key = 774300602605348
graph.facebook.com.consumer_secret = 1001fe29d51104ea7b1a657a83278c9d<file_sep>/pmc-db/build.gradle
apply plugin: 'liquibase'
liquibase {
activities {
main {
changeLogFile 'src/main/liquibase/com/epam/app/pmc/db/changelog-1.groovy'
url 'jdbc:postgresql://localhost:5432/pmc'
username 'postgres'
password '<PASSWORD>'
}
}
runList = 'main'
}<file_sep>/README.md
pmc
===
project management system
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/usercomponent/experimental/elements/table/UserTaskTable.java
package com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements.table;
import com.epam.app.pmc.vaadin.component.table.ITable;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.data.util.IndexedContainer;
import com.vaadin.ui.Table;
import java.util.List;
public class UserTaskTable extends Table implements ITable<Object> {
@Override
public void attach() {
setVisibleColumns(StringValues.USER_TASKS_TABLE);
setSelectable(true);
setImmediate(true);
super.attach();
}
@Override
public <T> IndexedContainer loadData(List<T> data) {
// TODO Auto-generated method stub
return null;
}
@Override
public <T> IndexedContainer loadFilteredData(List<T> data) {
// TODO Auto-generated method stub
return null;
}
}
<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/api/AssignedDeveloperExtendedRepository.java
package com.epam.app.pmc.repository.api;
import com.epam.app.pmc.model.AssignedDeveloper;
import com.epam.app.pmc.model.AssignedDeveloperExtended;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Bring additional methods to Assigned developer repository.
*
*
* @author Artsiom_Tsaryonau
*/
@Repository("assignedDeveloperExtendedRepository")
public class AssignedDeveloperExtendedRepository {
protected static final String GET_DEVELOPERS_BY_PROJECT_ID = "AssignedDeveloper.getDevelopersByProjectId";
protected static final String GET_PROJECT_BY_DEVELOPER_ID = "AssignedDeveloper.getProjectByDeveloperId";
protected static final String GET_DEVELOPERS_BY_PROJECT_JOIN_INFORMATION = "AssignedDeveloper.getDevelopersByProjectJoinInformation";
protected static final String GET_ASSIGNED_DEVELOPER_BY_PROJECT_AND_DEVELOPER_ID = "AssignedDeveloper.getDeveloperByTwoIds";
protected static final String RESIGN_DEVELOPER_FROM_PROJECT = "AssignedDeveloper.resignDeveloper";
@Autowired
protected SqlSessionTemplate sqlSessionTemplate;
public List<AssignedDeveloper> getDevelopersByProject(String projectId) {
return sqlSessionTemplate.selectList(GET_DEVELOPERS_BY_PROJECT_ID, projectId);
}
public List<AssignedDeveloper> getProjectByDeveloper(String developerId) {
return sqlSessionTemplate.selectList(GET_PROJECT_BY_DEVELOPER_ID, developerId);
}
public List<AssignedDeveloperExtended> getDeveloperForProject(String projectId) {
return sqlSessionTemplate.selectList(GET_DEVELOPERS_BY_PROJECT_JOIN_INFORMATION, projectId);
}
public AssignedDeveloper getAssignedDeveloper(String developerId, String projectId) {
Map<String, String> map = new HashMap<>();
map.put("developerId", developerId);
map.put("projectId", projectId);
return sqlSessionTemplate.selectOne(GET_ASSIGNED_DEVELOPER_BY_PROJECT_AND_DEVELOPER_ID, map);
}
public int resignDeveloperFromProject(String developerId, String projectId) {
Map<String, String> map = new HashMap<>();
map.put("developerId", developerId);
map.put("projectId", projectId);
return sqlSessionTemplate.delete(RESIGN_DEVELOPER_FROM_PROJECT, map);
}
}
<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/api/IRepository.java
package com.epam.app.pmc.repository.api;
import java.util.List;
/**
* Interface for operating with different database objects.
*
* Date: Nov 5, 2014
*
* @param <T> object type to operate with
*
* @author Artsiom_Tsaryonau
*/
public interface IRepository<T> {
/**
* Stores object into data.
*
* @param object object to store
*/
void store(T object);
/**
* Returns object with certain id.
*
* @param id object's id
* @return object with id
*/
T get(String id);
/**
* Returns all objects in database.
*
* @return list of objects
*/
List<T> getAllItems();
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/util/StringValues.java
package com.epam.app.pmc.vaadin.component.util;
public final class StringValues {
public static final String ALL_PROJECTS_TABLE_CAPTION = "Projects";
public static final String[] ALL_PROJECTS_HEADERS = { "ID", "Project Name", "Project Owner" };
public static final String ALL_DEVELOPERS_TABLE_CAPTION = "Developers";
public static final String[] ALL_DEVELOPERS_HEADERS = { "ID", "Private information" };
public static final String ALL_TASKS_TABLE_CAPTION = "Tasks";
public static final String[] ALL_TASKS_TABLE_HEADERS = { "ID", "Description", "Developer", "Project", "Status", "Weight" };
public static final String[] ALL_MAIN_PAGE_TABS = { "Projects", "Developers", "Tasks" };
public static final String NEW_PROJECT_MODAL_WINDOW_CAPTION = "New Project";
public static final String PROJECT_DETAILS_WINDOW_CAPTION = "Project Details";
public static final String DEVELOPER_DETATILS_WINDOW_CAPTION = "Developer Details";
public static final String NEW_DEVELOPER_MODAL_WINDOW_CAPTION = "New Developer";
public static final String NEW_TASK_MODAL_WINDOW_CAPTION = "New Task";
public static final String TASK_DETAILS_WINDOW_CAPTION = "Task Details";
public static final String ALL_DEVELOPERS_FOR_PROJECT_TABLE_CAPTION = "Developers";
public static final String[] ALL_DEVELOPERS_FOR_PROJECT_HEADERS = { "ID", "Project ID", "Private information" };
public static final String ASSIGN_TASK_WINDOW_CAPTION = "Assign task";
public static final String[] PROJECT_TASK_HEADERS = { "ID", "Description", "Status", "Weight", "Developer" };
public static final String[] PROJECT_DEVELOPER_HEADERS = { "ID", "Private information" };
public static final String ASSIGN_DEVELOPER_WINDOW_CAPTION = "Assign developer";
public static final String[] PROCEDURAL_TAB_HEADERS = { "Details", "Search" };
public static final String LOGIN_FORM_CAPTION = "Authentication";
public static final String[] USER_TASKS_TABLE = { "ID", "Description", "Begin date", "End date", "Status" };
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/experimental/event/LoginFacebookEvent.java
package com.epam.app.pmc.vaadin.component.experimental.event;
public class LoginFacebookEvent {
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/experimental/view/MainView.java
package com.epam.app.pmc.vaadin.component.experimental.view;
import com.epam.app.pmc.vaadin.component.tab.MainTab;
import com.google.common.eventbus.EventBus;
import com.vaadin.navigator.Navigator;
import com.vaadin.navigator.ViewChangeListener.ViewChangeEvent;
import com.vaadin.ui.VerticalLayout;
public class MainView extends VerticalLayout implements IView {
private EventBus eventBus; //eventBus.post(new LogoutEvent())
@Override
public void setEventBus(EventBus eventBus) {
this.eventBus = eventBus;
addComponent(new MainTab()); //BAD SOLUTION
}
@Override
public void enter(ViewChangeEvent event) {
}
@Override
public void setNavigator(Navigator navigator) {
throw new UnsupportedOperationException("Not implemented");
}
}
<file_sep>/pmc-service/src/main/java/com/epam/app/pmc/service/project/ProjectService.java
package com.epam.app.pmc.service.project;
import com.epam.app.pmc.model.Project;
import com.epam.app.pmc.repository.ProjectRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
/**
* Service to operate with projects.
*
* Date: Nov 20, 2014
*
* @author Artsiom_Tsaryonau
*/
@Service("projectService")
public class ProjectService {
@Autowired
private ProjectRepository projectRepository;
public void insertProject(Project project) {
projectRepository.store(project);
}
public List<Project> findAllProjects() {
return projectRepository.getAllItems();
}
public Project getProject(String id) {
return projectRepository.get(id);
}
public Project getProjectByName(String name) {
return projectRepository.getProjectByName(name);
}
public List<Project> filterProrjects(String projectId, String projectName, String projectOwner) {
return projectRepository.getFilteredProjects(projectId, projectName, projectOwner);
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/modal/NewDeveloperModalWindow.java
package com.epam.app.pmc.vaadin.component.modal;
import com.epam.app.pmc.model.Developer;
import com.epam.app.pmc.vaadin.component.experimental.NavigatorUi;
import com.epam.app.pmc.vaadin.component.tab.AllDevelopersTabContent;
import com.epam.app.pmc.vaadin.component.util.DomainUtils;
import com.epam.app.pmc.vaadin.component.util.Generator;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.FormLayout;
import com.vaadin.ui.HorizontalLayout;
import com.vaadin.ui.TextArea;
import com.vaadin.ui.Window;
public class NewDeveloperModalWindow extends Window {
private TextArea privateInformation;
private Button createDeveloper;
private Button cancel;
private HorizontalLayout buttonLayout;
private AllDevelopersTabContent parentTab;
public void setParentTab(AllDevelopersTabContent parentTab) {
this.parentTab = parentTab;
}
@Override
public void attach() {
setCaption(StringValues.NEW_DEVELOPER_MODAL_WINDOW_CAPTION);
setSizeUndefined();
setResizable(false);
setModal(true);
setContent(createLayout());
super.attach();
}
private FormLayout createLayout() {
FormLayout mainLayout = null;
privateInformation = new TextArea("Private Information");
final NavigatorUi ui = (NavigatorUi) NavigatorUi.getCurrent();
createDeveloper = new Button("Create");
createDeveloper.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
Developer developer = DomainUtils.createDeveloper(Generator.gen(), privateInformation.getValue());
ui.getDeveloperService().insertDeveloper(developer);
parentTab.updateAllDevelopersTable(developer);
NewDeveloperModalWindow.this.close();
}
});
cancel = new Button("Cancel");
cancel.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
NewDeveloperModalWindow.this.close();
}
});
addCloseListener(new CloseListener(){
@Override
public void windowClose(CloseEvent e) {
ui.removeWindow(NewDeveloperModalWindow.this);
}
});
buttonLayout = new HorizontalLayout(createDeveloper, cancel);
mainLayout = new FormLayout(privateInformation, buttonLayout);
mainLayout.setMargin(true);
return mainLayout;
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/usercomponent/experimental/elements/modal/ProjectTasksModalWindow.java
package com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements.modal;
import com.vaadin.ui.Window;
public class ProjectTasksModalWindow extends Window {
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/modal/NewTaskForProjectModalWindow.java
package com.epam.app.pmc.vaadin.component.modal;
import com.epam.app.pmc.model.Project;
import com.epam.app.pmc.model.Task;
import com.epam.app.pmc.vaadin.component.experimental.NavigatorUi;
import com.epam.app.pmc.vaadin.component.util.DomainUtils;
import com.epam.app.pmc.vaadin.component.util.Generator;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.ComboBox;
import com.vaadin.ui.FormLayout;
import com.vaadin.ui.HorizontalLayout;
import com.vaadin.ui.TextArea;
import com.vaadin.ui.TextField;
/**
* Special extension of {@link NewTaskModalWindow}.
*
*
* @author Artsiom_Tsaryonau
*/
public class NewTaskForProjectModalWindow extends NewTaskModalWindow {
private Project project;
private TextField projectName;
private ProjectDetailModalWindow parentWindow;
public void setParentModalWindow(ProjectDetailModalWindow parentWindow) {
this.parentWindow = parentWindow;
}
@Override
protected FormLayout createLayout() {
FormLayout mainLayout = null;
projectName = new TextField("Project Name ", project.getProjectName());
projectName.setReadOnly(true);
developerId = new ComboBox("Developer ID");
description = new TextArea("Description");
weight = new TextField("Weight");
status = new TextField("Status");
final NavigatorUi ui = (NavigatorUi) NavigatorUi.getCurrent();
developerId.addItems(filterDevelopers(ui.getDeveloperService().findAllDevelopers()));
createTask = new Button("Create");
createTask.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
Task task = DomainUtils.createTask(Generator.gen(), description.getValue(),
developerId.getValue().toString(), status.getValue(),
ui.getProjectService().getProjectByName(projectName.getValue().toString()).getProjectId(),
weight.getValue());
ui.getTaskService().insertTask(task);
parentWindow.updateTasks(task);
NewTaskForProjectModalWindow.this.close();
}
});
cancel = new Button("Cancel");
cancel.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
NewTaskForProjectModalWindow.this.close();
}
});
addCloseListener(new CloseListener(){
@Override
public void windowClose(CloseEvent e) {
ui.removeWindow(NewTaskForProjectModalWindow.this);
}
});
buttonLayout = new HorizontalLayout(createTask, cancel);
mainLayout = new FormLayout(projectName, developerId, description, weight, status, buttonLayout);
mainLayout.setMargin(true);
return mainLayout;
}
public void setProject(Project project) {
this.project = project;
}
}
<file_sep>/pmc-domain/src/test/java/com/epam/app/pmc/model/TaskTest.java
package com.epam.app.pmc.model;
import static org.junit.Assert.assertEquals;
import org.junit.Before;
import org.junit.Test;
/**
* The unit test verifies {@link Task}.
*
* Date: Nov 5, 2014
*
* @author Artsiom_Tsaryonau
*/
public class TaskTest {
private static final String TASK_ID = "Task1234";
private static final String DESCRIPTION = "IMPLEMENT ME";
private static final String WEIGHT = "50";
private static final String STATUS = "IN PROGRESS";
private static final String TASK_PROJECT = "PROJECT_1";
private static final String TASK_DEVELOPER = "DEVELOPER";
private Task task;
@Before
public void setUp() {
task = new Task();
task.setTaskId(TASK_ID);
task.setDescription(DESCRIPTION);
task.setWeight(WEIGHT);
task.setStatus(STATUS);
task.setTaskProject(TASK_PROJECT);
task.setTaskDeveloper(TASK_DEVELOPER);
}
@Test
public void testPojo() {
assertEquals(TASK_ID, task.getTaskId());
assertEquals(DESCRIPTION, task.getDescription());
assertEquals(WEIGHT, task.getWeight());
assertEquals(STATUS, task.getStatus());
assertEquals(TASK_PROJECT, task.getTaskProject());
assertEquals(TASK_DEVELOPER, task.getTaskDeveloper());
}
}
<file_sep>/pmc-domain/src/test/java/com/epam/app/pmc/model/DeveloperTest.java
package com.epam.app.pmc.model;
import static org.junit.Assert.assertEquals;
import org.junit.Before;
import org.junit.Test;
/**
* The unit test verifies {@link Developer}.
*
* Date: Nov 5, 2014
*
* @author Artsiom_Tsaryonau
*/
public class DeveloperTest {
private static final String DEVELOPER_ID = "12345";
private static final String PRIVATE_INFORMATION = "FIO";
private Developer developer;
@Before
public void setUp() {
developer = new Developer();
developer.setDeveloperId(DEVELOPER_ID);
developer.setPrivateInformation(PRIVATE_INFORMATION);
}
@Test
public void testPojo() {
assertEquals(DEVELOPER_ID, developer.getDeveloperId());
assertEquals(PRIVATE_INFORMATION, developer.getPrivateInformation());
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/usercomponent/experimental/UserPage.java
package com.epam.app.pmc.vaadin.component.usercomponent.experimental;
import com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements.UserInformation;
import com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements.table.UserTaskTable;
import com.vaadin.event.Action;
import com.vaadin.event.Action.Handler;
import com.vaadin.ui.HorizontalLayout;
public class UserPage extends HorizontalLayout {
private UserInformation userInformation;
private UserTaskTable taskTable;
private static Action[] userTasksActions = { new Action("+Add...") };
public UserPage() {
userInformation = new UserInformation();
taskTable = new UserTaskTable();
taskTable.addActionHandler(new Handler() {
@Override
public Action[] getActions(Object target, Object sender) {
return userTasksActions;
}
@Override
public void handleAction(Action action, Object sender, Object target) {
switch(action.getCaption()) {
case "+Add...": {
break;
}
}
}
});
}
@Override
public void attach() {
addComponent(userInformation);
addComponent(taskTable);
super.attach();
}
}
<file_sep>/pmc-web/build.gradle
apply plugin: 'war'
apply plugin: 'tomcat'
repositories {
maven {
url 'http://maven.vaadin.com/vaadin-addons'
}
}
configurations {
provided
}
sourceSets {
main { compileClasspath += configurations.provided }
}
repositories {
maven {
url 'https://raw.github.com/fernandezpablo85/scribe-java/mvn-repo/'
}
}
dependencies {
compile project(':pmc-service')
compile 'jstl:jstl:1.2'
compile 'javax.servlet:jstl:1.2'
compile 'org.springframework:spring-core:4.1.1.RELEASE'
compile 'org.springframework:spring-context:4.1.1.RELEASE'
compile 'org.springframework:spring-webmvc:4.1.1.RELEASE'
compile 'org.springframework.security:spring-security-web:3.2.5.RELEASE'
compile 'org.springframework.security:spring-security-config:3.2.5.RELEASE'
// compile 'org.springframework.social:spring-social:1.1.0.RELEASE'
compile 'org.springframework.social:spring-social-facebook:1.1.1.RELEASE'
compile 'org.springframework.social:spring-social-core:1.1.0.RELEASE'
compile 'org.springframework.social:spring-social-web:1.1.0.RELEASE'
compile 'org.springframework.social:spring-social-config:1.1.0.RELEASE'
compile 'org.springframework.social:spring-social-security:1.1.0.RELEASE'
provided 'javax.servlet:servlet-api:2.5'
compile 'com.vaadin:vaadin-client:7.3.5'
compile 'com.vaadin:vaadin-server:7.3.5'
compile 'com.vaadin:vaadin-client-compiled:7.3.5'
compile 'com.vaadin:vaadin-themes:7.3.5'
compile('ru.xpoft.vaadin:spring-vaadin-integration:3.1') {
exclude group: 'org.slf4j'
}
compile 'com.google.guava:guava:18.0'
compile 'org.scribe:scribe:1.3.5'
compile ('org.brickred:socialauth-spring:2.3+') {
exclude group: 'javax.servlet'
}
compile 'org.apache.httpcomponents:httpclient:4.3.6'
compile 'org.apache.httpcomponents:httpcore:4.4'
def tomcatVersion = '7.0.56'
tomcat "org.apache.tomcat.embed:tomcat-embed-core:${tomcatVersion}"
tomcat "org.apache.tomcat.embed:tomcat-embed-logging-juli:${tomcatVersion}"
tomcat("org.apache.tomcat.embed:tomcat-embed-jasper:${tomcatVersion}") {
exclude group: 'org.eclipse.jdt.core.compiler', module: 'ecj'
}
runtime 'org.postgresql:postgresql:9.3-1102-jdbc41'
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/usercomponent/experimental/elements/modal/SetTaskWindow.java
package com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements.modal;
import com.vaadin.ui.Window;
public class SetTaskWindow extends Window {
}
<file_sep>/pmc-service/src/main/java/com/epam/app/pmc/service/project/AssignedDeveloperService.java
package com.epam.app.pmc.service.project;
import com.epam.app.pmc.model.AssignedDeveloper;
import com.epam.app.pmc.model.AssignedDeveloperExtended;
import com.epam.app.pmc.repository.AssignedDeveloperRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
/**
* Service to operate with assigned developers.
*
* Date: Nov 27, 2014
*
* @author Artsiom_Tsaryonau
*/
@Service("assignedDeveloperService")
public class AssignedDeveloperService {
@Autowired
private AssignedDeveloperRepository assignedDeveloperRepository;
public void insertAssignedDeveloper(AssignedDeveloper assignedDeveloper) {
assignedDeveloperRepository.store(assignedDeveloper);
}
public List<AssignedDeveloper> findAllAssignedDevelopers() {
return assignedDeveloperRepository.getAllItems();
}
public AssignedDeveloper getAssignedDeveloper(String id) {
return assignedDeveloperRepository.get(id);
}
public List<AssignedDeveloper> findProjectDeveloper(String projectId) {
return assignedDeveloperRepository.getDevelopersByProject(projectId);
}
public List<AssignedDeveloper> findDeveloperProjects(String developerId) {
return assignedDeveloperRepository.getProjectByDeveloper(developerId);
}
public List<AssignedDeveloperExtended> findProjectDevelopersExtended(String projectId) {
return assignedDeveloperRepository.getDeveloperForProject(projectId);
}
public int resignDeveloper(String projectId, String developerId) {
return assignedDeveloperRepository.resignDeveloperFromProject(developerId, projectId);
}
}
<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/api/DeveloperExtendedRepository.java
package com.epam.app.pmc.repository.api;
import static com.epam.app.pmc.repository.util.RepositoryUtils.nullifyEmpty;
import static com.epam.app.pmc.repository.util.RepositoryUtils.wrapLikeParameters;
import com.epam.app.pmc.model.Developer;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Repository("developerExtendedRepository")
public class DeveloperExtendedRepository {
protected static final String GET_NOT_ASSIGNED_TO_PROJECT = "Developer.getNotAssignedDevelopers";
protected static final String GET_FILTERED_DEVELOPERS = "Developer.getFilteredDevelopers";
@Autowired
protected SqlSessionTemplate sqlSessionTemplate;
public List<Developer> getNotAssignedDevelopers(String id) {
return sqlSessionTemplate.selectList(GET_NOT_ASSIGNED_TO_PROJECT, id);
}
public List<Developer> getFilteredDevelopers(String filterId, String filterPrivateInformation) {
Map<String, String> map = new HashMap<>();
map.put("filterId", filterId);
map.put("filterPrivateInformation", filterPrivateInformation);
return sqlSessionTemplate.selectList(GET_FILTERED_DEVELOPERS, wrapLikeParameters(nullifyEmpty(map)));
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/tab/AllDevelopersTabContent.java
package com.epam.app.pmc.vaadin.component.tab;
import com.epam.app.pmc.model.Developer;
import com.epam.app.pmc.vaadin.component.experimental.NavigatorUi;
import com.epam.app.pmc.vaadin.component.functional.DevelopersSearchForm;
import com.epam.app.pmc.vaadin.component.modal.DeveloperDetailModalWindow;
import com.epam.app.pmc.vaadin.component.modal.NewDeveloperModalWindow;
import com.epam.app.pmc.vaadin.component.table.AllDevelopersTable;
import com.vaadin.data.Item;
import com.vaadin.event.Action;
import com.vaadin.event.Action.Handler;
import com.vaadin.event.ItemClickEvent;
import com.vaadin.event.ItemClickEvent.ItemClickListener;
import com.vaadin.ui.HorizontalLayout;
import java.util.List;
public class AllDevelopersTabContent extends HorizontalLayout {
private AllDevelopersTable allDevelopersTable;
private NewDeveloperModalWindow newDeveloperModalWindow;
private DeveloperDetailModalWindow developerDetailModalWindow;
private DevelopersSearchForm developersSearchForm;
private static Action[] newAction = { new Action("+New...") };
public AllDevelopersTabContent() {
allDevelopersTable = new AllDevelopersTable();
developerDetailModalWindow = new DeveloperDetailModalWindow();
newDeveloperModalWindow = new NewDeveloperModalWindow();
newDeveloperModalWindow.setParentTab(this);
developersSearchForm = new DevelopersSearchForm();
developersSearchForm.setParentTabContent(this);
final NavigatorUi ui = (NavigatorUi) NavigatorUi.getCurrent();
allDevelopersTable.addActionHandler(new Handler() {
@Override
public Action[] getActions(Object target, Object sender) {
return newAction;
}
@Override
public void handleAction(Action action, Object sender, Object target) {
switch (action.getCaption()) {
case "+New...": {
ui.addWindow(newDeveloperModalWindow);
break;
}
}
}
});
allDevelopersTable.addItemClickListener(new ItemClickListener() {
@Override
public void itemClick(ItemClickEvent event) {
if (event.isDoubleClick()) {
Object currentItemId = event.getItemId();
developerDetailModalWindow.setDeveloperDetailsId(allDevelopersTable
.getItem(currentItemId).getItemProperty("ID").getValue().toString());
ui.addWindow(developerDetailModalWindow);
}
}
});
allDevelopersTable.setData(ui.getDeveloperService().findAllDevelopers()); // null pointer there
}
public void updateAllDevelopersTable(Developer newDeveloper) {
NavigatorUi currentUi = (NavigatorUi) NavigatorUi.getCurrent();
List<Developer> allDevelopers = currentUi.getDeveloperService().findAllDevelopers();
for (Developer d : allDevelopers) {
if (newDeveloper.getDeveloperId().equals(d.getDeveloperId())) {
Object itemId = allDevelopersTable.addItem();
Item item = allDevelopersTable.getItem(itemId);
item.getItemProperty("ID").setValue(d.getDeveloperId());
item.getItemProperty("Private information").setValue(d.getPrivateInformation());
}
}
}
public void filterTableItem(String filterId, String filterPrivateInformation) {
NavigatorUi currentUi = (NavigatorUi) NavigatorUi.getCurrent();
allDevelopersTable.removeAllItems();
List<Developer> list = currentUi.getDeveloperService().filterDevelopers(filterId, filterPrivateInformation);
for(Developer d: list) {
Object itemId = allDevelopersTable.addItem();
Item item = allDevelopersTable.getItem(itemId);
item.getItemProperty("ID").setValue(d.getDeveloperId());
item.getItemProperty("Private information").setValue(d.getPrivateInformation());
}
}
@Override
public void attach() {
addComponent(allDevelopersTable);
addComponent(developersSearchForm);
super.attach();
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/functional/ProjectSearchForm.java
package com.epam.app.pmc.vaadin.component.functional;
import com.epam.app.pmc.vaadin.component.functional.api.SearchForm;
import com.epam.app.pmc.vaadin.component.tab.AllProjectsTabContent;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.Button.ClickListener;
public class ProjectSearchForm extends SearchForm {
private AllProjectsTabContent parentTab;
public ProjectSearchForm() {
labels = StringValues.ALL_PROJECTS_HEADERS;
initComponents();
filterTable.addClickListener(new ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
parentTab.filterTableItem(fields[0].getValue(), fields[1].getValue(), fields[2].getValue());
}
});
}
public void setParentTabContent(AllProjectsTabContent parentTab) {
this.parentTab = parentTab;
}
}
<file_sep>/pmc-domain/src/main/java/com/epam/app/pmc/model/Task.java
package com.epam.app.pmc.model;
/**
*
*
* Date: Nov 5, 2014
*
* @author Artsiom_Tsaryonau
*/
public class Task {
private String taskId;
private String description;
private String weight;
private String status;
private String taskProject;
private String taskDeveloper;
public String getTaskId() {
return taskId;
}
public void setTaskId(String taskId) {
this.taskId = taskId;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getWeight() {
return weight;
}
public void setWeight(String weight) {
this.weight = weight;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getTaskProject() {
return taskProject;
}
public void setTaskProject(String taskProject) {
this.taskProject = taskProject;
}
public String getTaskDeveloper() {
return taskDeveloper;
}
public void setTaskDeveloper(String taskDeveloper) {
this.taskDeveloper = taskDeveloper;
}
}
<file_sep>/settings.gradle
rootProject.name = 'pmc'
include 'pmc-db'
include 'pmc-domain'
include 'pmc-repository'
include 'pmc-service'
include 'pmc-web'<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/AssignedDeveloperRepository.java
package com.epam.app.pmc.repository;
import com.epam.app.pmc.model.AssignedDeveloper;
import com.epam.app.pmc.repository.api.AssignedDeveloperExtendedRepository;
import com.epam.app.pmc.repository.api.IRepository;
import org.springframework.stereotype.Repository;
import java.util.List;
/**
* Implementation of {@link IRepository} for {@link AssignedDeveloper}.
*
* Date: Dec 2, 2014
*
* @author Artsiom_Tsaryonau
*/
@Repository("assignedDeveloperRepository")
public class AssignedDeveloperRepository extends AssignedDeveloperExtendedRepository implements
IRepository<AssignedDeveloper> {
private static final String STORE_ASSIGNED_DEVELOPER = "AssignedDeveloper.insertAssignedDeveloper";
private static final String GET_ASSIGNED_DEVELOPER_LIST = "AssignedDeveloper.getAssignedDeveloperList";
@Override
public void store(AssignedDeveloper object) {
AssignedDeveloper exists = this.getAssignedDeveloper(object.getDeveloperId(), object.getProjectId());
if (null != exists) {
if (exists.getProjectId().equals(object.getProjectId()) && exists.getDeveloperId().equals(object.getDeveloperId()))
return;
}
sqlSessionTemplate.insert(STORE_ASSIGNED_DEVELOPER, object);
}
@Override
public AssignedDeveloper get(String id) {
throw new UnsupportedOperationException("Cannot map ID in table with two primary IDs");
}
@Override
public List<AssignedDeveloper> getAllItems() {
return sqlSessionTemplate.selectList(GET_ASSIGNED_DEVELOPER_LIST);
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/experimental/view/UserView.java
package com.epam.app.pmc.vaadin.component.experimental.view;
import com.epam.app.pmc.vaadin.component.usercomponent.experimental.UserPage;
import com.google.common.eventbus.EventBus;
import com.vaadin.navigator.Navigator;
import com.vaadin.navigator.ViewChangeListener.ViewChangeEvent;
import com.vaadin.ui.VerticalLayout;
public class UserView extends VerticalLayout implements IView {
private EventBus eventBus;
@Override
public void setEventBus(EventBus eventBus) {
this.eventBus = eventBus;
addComponent(new UserPage());
}
@Override
public void enter(ViewChangeEvent event) {
}
@Override
public void setNavigator(Navigator navigator) {
throw new UnsupportedOperationException("Not implemented");
}
}
<file_sep>/pmc-service/README.md
Performs operation with application's objects<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/modal/NewTaskModalWindow.java
package com.epam.app.pmc.vaadin.component.modal;
import com.epam.app.pmc.model.Developer;
import com.epam.app.pmc.model.Project;
import com.epam.app.pmc.model.Task;
import com.epam.app.pmc.vaadin.component.experimental.NavigatorUi;
import com.epam.app.pmc.vaadin.component.tab.AllTasksTabContent;
import com.epam.app.pmc.vaadin.component.util.DomainUtils;
import com.epam.app.pmc.vaadin.component.util.Generator;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.ComboBox;
import com.vaadin.ui.FormLayout;
import com.vaadin.ui.HorizontalLayout;
import com.vaadin.ui.TextArea;
import com.vaadin.ui.TextField;
import com.vaadin.ui.Window;
import java.util.ArrayList;
import java.util.List;
public class NewTaskModalWindow extends Window {
private ComboBox projectName;
protected ComboBox developerId;
protected TextArea description;
protected TextField weight;
protected TextField status;
protected Button createTask;
protected Button cancel;
protected HorizontalLayout buttonLayout;
private AllTasksTabContent parentTab;
public void setParentTab(AllTasksTabContent parentTab) {
this.parentTab = parentTab;
}
@Override
public void attach() {
setCaption(StringValues.NEW_TASK_MODAL_WINDOW_CAPTION);
setSizeUndefined();
setResizable(false);
setModal(true);
setContent(createLayout());
super.attach();
}
protected FormLayout createLayout() {
FormLayout mainLayout = null;
projectName = new ComboBox("Project Name");
developerId = new ComboBox("Developer ID");
description = new TextArea("Description");
weight = new TextField("Weight");
status = new TextField("Status");
final NavigatorUi ui = (NavigatorUi) NavigatorUi.getCurrent();
projectName.addItems(filterProjects(ui.getProjectService().findAllProjects()));
developerId.addItems(filterDevelopers(ui.getDeveloperService().findAllDevelopers()));
createButtonCreate(ui);
createButtonCancel();
addCloseListener(new CloseListener(){
@Override
public void windowClose(CloseEvent e) {
ui.removeWindow(NewTaskModalWindow.this);
}
});
buttonLayout = new HorizontalLayout(createTask, cancel);
mainLayout = new FormLayout(projectName, developerId, description, weight, status, buttonLayout);
mainLayout.setMargin(true);
return mainLayout;
}
protected void createButtonCreate(final NavigatorUi ui) {
createTask = new Button("Create");
createTask.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
Task task = DomainUtils.createTask(Generator.gen(), description.getValue(),
developerId.getValue().toString(), status.getValue(),
ui.getProjectService().getProjectByName(projectName.getValue().toString()).getProjectId(),
weight.getValue());
ui.getTaskService().insertTask(task);
parentTab.updateAllTasksTable(task);
NewTaskModalWindow.this.close();
}
});
}
protected void createButtonCancel() {
cancel = new Button("Cancel");
cancel.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
NewTaskModalWindow.this.close();
}
});
}
private List<String> filterProjects(List<Project> list) {
List<String> names = new ArrayList<>();
for(Project item: list) {
names.add(item.getProjectName());
}
return names;
}
protected List<String> filterDevelopers(List<Developer> list) {
List<String> ids = new ArrayList<>();
for(Developer item: list) {
ids.add(item.getDeveloperId());
}
return ids;
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/usercomponent/experimental/elements/UserInformation.java
package com.epam.app.pmc.vaadin.component.usercomponent.experimental.elements;
import com.vaadin.ui.FormLayout;
import com.vaadin.ui.TextField;
public class UserInformation extends FormLayout {
private TextField idTextField;
private TextField privateInformationTextField;
public UserInformation() {
createLayout();
}
public void createLayout() {
idTextField = new TextField("ID");
privateInformationTextField = new TextField("Private information");
addComponents(idTextField, privateInformationTextField);
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/experimental/event/LogoutEvent.java
package com.epam.app.pmc.vaadin.component.experimental.event;
public class LogoutEvent {
}
<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/api/ProjectExtendedRepository.java
package com.epam.app.pmc.repository.api;
import static com.epam.app.pmc.repository.util.RepositoryUtils.nullifyEmpty;
import static com.epam.app.pmc.repository.util.RepositoryUtils.wrapLikeParameters;
import com.epam.app.pmc.model.Project;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Component("projectExtendedRepository")
public class ProjectExtendedRepository {
protected static final String GET_PROJECT_BY_NAME = "Project.getProjectByName";
protected static final String GET_FILTERED_PROJECTS = "Project.getFilteredProjects";
@Autowired
protected SqlSessionTemplate sqlSessionTemplate;
public Project getProjectByName(String name) {
return sqlSessionTemplate.selectOne(GET_PROJECT_BY_NAME, name);
}
public List<Project> getFilteredProjects(String projectId, String projectName, String projectOwner) {
Map<String, String> map = new HashMap<>();
map.put("projectId", projectId);
map.put("projectName", projectName);
map.put("projectOwner", projectOwner);
return sqlSessionTemplate.selectList(GET_FILTERED_PROJECTS, wrapLikeParameters(nullifyEmpty(map)));
}
}
<file_sep>/pmc-repository/build.gradle
dependencies {
compile project(':pmc-domain')
compile 'org.mybatis:mybatis:3.2.8'
compile 'org.mybatis:mybatis-spring:1.2.2'
compile 'org.springframework:spring-core:4.1.1.RELEASE'
compile 'org.springframework:spring-context:4.1.1.RELEASE'
compile 'org.springframework:spring-jdbc:4.1.1.RELEASE'
compile 'org.apache.commons:commons-lang3:3.0'
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/tab/MainTab.java
package com.epam.app.pmc.vaadin.component.tab;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.ui.TabSheet;
public class MainTab extends TabSheet {
private AllProjectsTabContent allProjectsTabContent;
private AllDevelopersTabContent allDevelopersTabContent;
private AllTasksTabContent allTasksTabContent;
public MainTab() {
allProjectsTabContent = new AllProjectsTabContent();
addTab(allProjectsTabContent, StringValues.ALL_MAIN_PAGE_TABS[0]);
allDevelopersTabContent = new AllDevelopersTabContent();
addTab(allDevelopersTabContent, StringValues.ALL_MAIN_PAGE_TABS[1]);
allTasksTabContent = new AllTasksTabContent();
addTab(allTasksTabContent, StringValues.ALL_MAIN_PAGE_TABS[2]);
}
// @Override
// public void attach() {
//
// allProjectsTabContent = new AllProjectsTabContent();
// addTab(allProjectsTabContent, StringValues.ALL_MAIN_PAGE_TABS[0]);
//
// allDevelopersTabContent = new AllDevelopersTabContent();
// addTab(allDevelopersTabContent, StringValues.ALL_MAIN_PAGE_TABS[1]);
//
// allTasksTabContent = new AllTasksTabContent();
// addTab(allTasksTabContent, StringValues.ALL_MAIN_PAGE_TABS[2]);
//
// super.attach();
// }
}
<file_sep>/pmc-web/src/main/resources/oauth.properties
oauth.callback = http://localhost:8080/pmc-web
oauth.application = facebook<file_sep>/pmc-repository/src/main/java/com/epam/app/pmc/repository/util/RepositoryUtils.java
package com.epam.app.pmc.repository.util;
import org.apache.commons.lang3.StringUtils;
import java.util.Map;
import java.util.Map.Entry;
public class RepositoryUtils {
public static String wrapLikeParameter(String parameter) {
return "%" + parameter + "%";
}
public static Map<String, String> nullifyEmpty(Map<String, String> parameters) {
for(Entry<String, String> entry: parameters.entrySet()) {
if (StringUtils.isBlank(entry.getValue())) {
parameters.put(entry.getKey(), null);
}
}
return parameters;
}
public static Map<String, String> wrapLikeParameters(Map<String, String> parameters) {
for(Entry<String, String> entry: parameters.entrySet()) {
if (StringUtils.isNotBlank(entry.getValue())) {
entry.setValue(wrapLikeParameter(entry.getValue()));
}
}
return parameters;
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/PmcServlet.java
package com.epam.app.pmc.vaadin;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import ru.xpoft.vaadin.SpringVaadinServlet;
public class PmcServlet extends SpringVaadinServlet {
@Override
protected void service(HttpServletRequest request, HttpServletResponse response) throws ServletException,
IOException {
// SecurityContextHolder.setContext(SecurityContextHolder.createEmptyContext());
RequestHolder.setRequest(request);
// SecurityContextHolder.clearContext();
super.service(request, response);
}
}
<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/experimental/view/DefaultView.java
package com.epam.app.pmc.vaadin.component.experimental.view;
import com.google.common.eventbus.EventBus;
import com.vaadin.navigator.Navigator;
import com.vaadin.navigator.ViewChangeListener.ViewChangeEvent;
import com.vaadin.ui.VerticalLayout;
public class DefaultView extends VerticalLayout implements IView {
private Navigator navigator;
@Override
public void setNavigator(Navigator navigator) {
this.navigator = navigator;
}
@Override
public void enter(ViewChangeEvent event) {
navigator.navigateTo("loginView");
}
@Override
public void setEventBus(EventBus eventBus) {
throw new UnsupportedOperationException("Not implemented for DefaulView");
}
}
<file_sep>/pmc-service/build.gradle
dependencies {
compile project(':pmc-repository')
compile 'org.springframework:spring-core:4.1.1.RELEASE'
compile 'org.springframework:spring-context:4.1.1.RELEASE'
}
<file_sep>/pmc-web/README.md
Responsible for operations with services.<file_sep>/pmc-web/src/main/java/com/epam/app/pmc/vaadin/component/modal/NewProjectModalWindow.java
package com.epam.app.pmc.vaadin.component.modal;
import com.epam.app.pmc.model.Project;
import com.epam.app.pmc.vaadin.component.experimental.NavigatorUi;
import com.epam.app.pmc.vaadin.component.tab.AllProjectsTabContent;
import com.epam.app.pmc.vaadin.component.util.DomainUtils;
import com.epam.app.pmc.vaadin.component.util.Generator;
import com.epam.app.pmc.vaadin.component.util.StringValues;
import com.vaadin.ui.Button;
import com.vaadin.ui.Button.ClickEvent;
import com.vaadin.ui.FormLayout;
import com.vaadin.ui.HorizontalLayout;
import com.vaadin.ui.TextField;
import com.vaadin.ui.Window;
public class NewProjectModalWindow extends Window {
private TextField projectName;
private TextField projectOwner;
private Button createProject;
private Button cancel;
private HorizontalLayout buttonLayout;
private AllProjectsTabContent parentTab;
public void setParentTab(AllProjectsTabContent parentTab) {
this.parentTab = parentTab;
}
@Override
public void attach() {
setCaption(StringValues.NEW_PROJECT_MODAL_WINDOW_CAPTION);
setSizeUndefined();
setResizable(false);
setModal(true);
setContent(createLayout());
super.attach();
}
private FormLayout createLayout() {
FormLayout mainLayout = null;
projectName = new TextField("Project Name");
projectOwner = new TextField("Project owner");
final NavigatorUi ui = (NavigatorUi) NavigatorUi.getCurrent();
createProject = new Button("Create");
createProject.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
Project project = DomainUtils.createProject(projectName.getValue(), Generator.gen(), projectOwner.getValue());
ui.getProjectService().insertProject(project);
parentTab.updateAllProjectsTable(project);
NewProjectModalWindow.this.close();
}
});
cancel = new Button("Cancel");
cancel.addClickListener(new Button.ClickListener() {
@Override
public void buttonClick(ClickEvent event) {
NewProjectModalWindow.this.close();
}
});
addCloseListener(new CloseListener(){
@Override
public void windowClose(CloseEvent e) {
ui.removeWindow(NewProjectModalWindow.this);
}
});
buttonLayout = new HorizontalLayout(createProject, cancel);
mainLayout = new FormLayout(projectName, projectOwner, buttonLayout);
mainLayout.setMargin(true);
return mainLayout;
}
}
| 6371d57622526459301efd4fe3d45e015250a485 | [
"Markdown",
"Java",
"INI",
"Gradle"
] | 41 | Gradle | artsiom-tsaryonau/pmc | 1ae49ac3ab767b2f265fb135ccb6770f037673e8 | 154f528300ed16452c6bbb00ea808c6e7642d4f7 | |
refs/heads/master | <file_sep>package com.game.gameobject;
import com.game.state.GameState;
public abstract class Bullet extends SpecificObject {
public Bullet(float posX, float posY, float width, float height, float mass, int healthPoint, int manaPoint,
GameState gameState) {
super(posX, posY, width, height, mass, healthPoint, manaPoint, gameState);
// TODO Auto-generated constructor stub
}
}
<file_sep>package com.game.gameobject;
import java.awt.Graphics;
import java.awt.Rectangle;
import com.game.gameinteface.Actable;
import com.game.state.GameState;
public abstract class HumanoidObject extends SpecificObject implements Actable{
private boolean isJumping;
private boolean isKneeling;
private boolean isLanding;
public HumanoidObject(float posX, float posY, float width, float height, float mass, int healthPoint, int manaPoint,
GameState gameState) {
super(posX, posY, width, height, mass, healthPoint, manaPoint, gameState);
setState(ALIVE);
}
@Override
public void Update() {
super.Update();
}
public boolean isJumping() {
return isJumping;
}
public void setJumping(boolean isJumping) {
this.isJumping = isJumping;
}
public boolean isKneeling() {
return isKneeling;
}
public void setKneeling(boolean isKneeling) {
this.isKneeling = isKneeling;
}
public boolean isLanding() {
return isLanding;
}
public void setLanding(boolean isLanding) {
this.isLanding = isLanding;
}
}
<file_sep>package com.game.gameinteface;
import java.awt.Graphics;
public interface ButtonState {
public static final int NONE = 0;
public static final int PRESS = 1;
public static final int HOVER = 2;
public abstract boolean isInButton();
public abstract void draw(Graphics g);
}
<file_sep>package com.game.userinterface;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JPanel;
import com.game.state.MenuState;
import com.game.state.State;
// Status: unfinished
// Con ham paint va ham run chua hoan thien
public class GamePanel extends JPanel implements KeyListener, Runnable {
State gameState;
KeyEventManager keyEventManager;
private Thread thread;
private boolean isRunning;
public GamePanel() {
gameState = new MenuState(this);
keyEventManager = new KeyEventManager(gameState);
}
@Override
public void paint(Graphics g) {
g.setColor(Color.GRAY);
g.fillRect(0, 0, GameFrame.SCREEN_WIDTH, GameFrame.SCREEN_HEIGHT);
}
public void startGame() {
if(thread == null) {
thread = new Thread(this);
isRunning = true;
thread.start();
}
}
public void setState(State state) {
this.gameState = state;
keyEventManager.setState(state);
}
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyPressed(KeyEvent e) {
// TODO Auto-generated method stub
System.out.println("You pressed");
keyEventManager.processKeyPressed(e.getKeyCode());
}
@Override
public void keyReleased(KeyEvent e) {
// TODO Auto-generated method stub
System.out.println("You released");
keyEventManager.processKeyReleased(e.getKeyCode());
}
@Override
public void run() {
// TODO Auto-generated method stub
long FPS = 80;
long period = 1000000000 / FPS;
long previousTime = System.nanoTime();
long sleepTime;
long currentTime;
while(isRunning) {
//Update
//Render
repaint();
currentTime = System.nanoTime();
sleepTime = period - (currentTime - previousTime);
try {
if(sleepTime > 0)
thread.sleep(sleepTime/1000000);
else thread.sleep(period/2000000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
<file_sep>package com.game.gameobject;
import java.awt.Rectangle;
import com.game.effect.Animation;
import com.game.gameinteface.Profile;
import com.game.gameinteface.Vulnerable;
import com.game.state.GameState;
// Status: Con PT isOutOfCameraView va Update
public abstract class SpecificObject extends GameObject implements Profile, Vulnerable {
private int state = ALIVE;
private int teamType;
private int direction;
private float width;
private float height;
private float speedX;
private float speedY;
private float mass;
private int healthPoint;;
private int manaPoint;
private int damage;
private long beginTimeToNoBeHurt;
private long timeForNoBeHurt;
protected Animation beHurtForward, beHurtBackward;;
public SpecificObject(float posX, float posY, float width, float height, float mass, int healthPoint, int manaPoint, GameState gameState) {
super(posX, posY, gameState);
setWidth(width);
setHeight(height);
setMass(mass);
setHealthPoint(healthPoint);
setManaPoint(manaPoint);
direction = RIGHT_DIR;
}
public boolean isOutOfCameraView() {
// Can Camera
return false;
}
public void beHurt (int damageRecieved) {
setHealthPoint(getHealthPoint() - damageRecieved);
state = BEHURT;
}
public Rectangle getBoundForCollisionWithMap() {
Rectangle rect = new Rectangle();
rect.x = (int) (getPosX() - getWidth()/2);
rect.y = (int) (getPosY() - getHeight()/2);
rect.width = (int) getWidth();
rect.height = (int) getHeight();
return rect;
}
@Override
public void Update() {
switch(state) {
case ALIVE:
// Cho SpecificObjectManager
break;
case BEHURT:
if(beHurtBackward == null) {
state = CANTBEHURT;
beginTimeToNoBeHurt = System.nanoTime();
if(getHealthPoint() == 0) {
state = FEY;
}
} else {
beHurtForward.Update(System.nanoTime());
if(beHurtForward.isLastFrame()) {
beHurtForward.reset();
state = CANTBEHURT;
if(getHealthPoint() == 0) {
state = FEY;
beginTimeToNoBeHurt = System.nanoTime();
}
}
}
break;
case FEY:
state = DEATH;
break;
case CANTBEHURT:
if(System.nanoTime() - beginTimeToNoBeHurt > timeForNoBeHurt) {
state = ALIVE;
}
break;
case DEATH:
break;
}
}
public int getState() {
return state;
}
public void setState(int state) {
this.state = state;
}
public int getTeamType() {
return teamType;
}
public void setTeamType(int teamType) {
this.teamType = teamType;
}
public int getDirection() {
return direction;
}
public void setDirection(int direction) {
this.direction = direction;
}
public float getWidth() {
return width;
}
public void setWidth(float width) {
this.width = width;
}
public float getHeight() {
return height;
}
public void setHeight(float height) {
this.height = height;
}
public float getSpeedX() {
return speedX;
}
public void setSpeedX(float speedX) {
this.speedX = speedX;
}
public float getSpeedY() {
return speedY;
}
public void setSpeedY(float speedY) {
this.speedY = speedY;
}
public float getMass() {
return mass;
}
public void setMass(float mass) {
this.mass = mass;
}
public int getHealthPoint() {
return healthPoint;
}
public void setHealthPoint(int healthPoint) {
if(healthPoint >= 0)
this.healthPoint = healthPoint;
else this.healthPoint = 0;
}
public int getManaPoint() {
return manaPoint;
}
public void setManaPoint(int manaPoint) {
if(manaPoint >= 0)
this.manaPoint = manaPoint;
else this.manaPoint = 0;
}
public int getDamage() {
return damage;
}
public void setDamage(int damage) {
this.damage = damage;
}
public long getBeginTimeToBeHurt() {
return beginTimeToNoBeHurt;
}
public void setBeginTimeToBeHurt(long beginTimeToBeHurt) {
this.beginTimeToNoBeHurt = beginTimeToBeHurt;
}
public long getTimeForNoBeHurt() {
return timeForNoBeHurt;
}
public void setTimeForNoBeHurt(long timeForNoBeHurt) {
this.timeForNoBeHurt = timeForNoBeHurt;
}
}
<file_sep>package com.game.state;
import com.game.userinterface.GamePanel;
public class MenuState extends State{
public MenuState(GamePanel gamePanel) {
super(gamePanel);
}
public void processPressButton(int keyEvent) {
}
public void processReleaseButton(int keyEvent) {
}
@Override
public void Update() {
// TODO Auto-generated method stub
}
@Override
public void Render() {
// TODO Auto-generated method stub
}
}
<file_sep>package com.game.state;
import com.game.userinterface.GamePanel;
public abstract class State {
public State(GamePanel gamePanel) {
// TODO Auto-generated constructor stub
}
public abstract void Update();
public abstract void Render();
public abstract void processPressButton(int keyEvent);
public abstract void processReleaseButton(int keyEvent);
}
<file_sep>package com.game.userinterface;
import java.io.IOException;
import javax.swing.JFrame;
import com.game.effect.DataLoader;
// Status: Completed
public class GameFrame extends JFrame {
public static final int SCREEN_WIDTH = 1000;
public static final int SCREEN_HEIGHT = 750;
GamePanel gamePanel;
public GameFrame() {
gamePanel = new GamePanel();
setSize(SCREEN_WIDTH, SCREEN_HEIGHT);
setLocationRelativeTo(null);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
try {
DataLoader.getInstance().LoadData();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
add(gamePanel);
addKeyListener(gamePanel);
}
public void startGame() {
gamePanel.startGame();
}
public static void main(String[] args) {
GameFrame gameFrame = new GameFrame();
gameFrame.startGame();
}
}
<file_sep>package com.game.objectmanager;
public class SpecificObjectManager {
}
<file_sep>package com.game.effect;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
// Status: Completed
public class FrameImage {
private String name;
private BufferedImage image;
public FrameImage() {
// TODO Auto-generated constructor stub
this.image = null;
this.name = null;
}
public FrameImage(String name, BufferedImage image) {
// TODO Auto-generated constructor stub
this.name = name;
this.image = image;
}
public FrameImage(FrameImage frameImage) {
// TODO Auto-generated constructor stub
image = new BufferedImage(frameImage.getImage().getWidth(), frameImage.getImage().getHeight(), frameImage.getImage().getType());
Graphics g = image.getGraphics();
g.drawImage(frameImage.getImage(), 0, 0, null);
this.name = frameImage.name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BufferedImage getImage() {
return image;
}
public void setImage(BufferedImage image) {
this.image = image;
}
}
<file_sep>package com.game.gameobject;
import java.awt.Graphics;
public class BackgroundMap extends GameObject{
@Override
public void Update() {
// TODO Auto-generated method stub
}
@Override
public void draw(Graphics g) {
// TODO Auto-generated method stub
}
}
| a354571a414b7ad508a5e932a552124712853fbc | [
"Java"
] | 11 | Java | phamducdat/Test2 | 1c85c3d4c68439c544e65dbae7b971f5f0fa9b69 | 7ac82f89ec754da6ca56d443059532a66096d03d | |
refs/heads/master | <file_sep>const restify = require('restify'),
jwt = require('express-jwt'),
jwks = require('jwks-rsa'),
dotenv = require('dotenv'),
corsMiddleware = require('restify-cors-middleware'),
server = restify.createServer({
name: 'rage-meter',
version: '1.0.0',
}),
auth = require('./middleware/Auth'),
cors = corsMiddleware({
origins: [
'http://localhost:4200',
],
}),
Rage = require('./model/Rage'),
RageConfig = require('./model/RageConfig'),
Person = require('./model/Person');
dotenv.config();
const jwtCheck = jwt({
secret: jwks.expressJwtSecret({
cache: true,
rateLimit: true,
jwksRequestsPerMinute: 5,
jwksUri: process.env.AUTH0_JWKS_URI,
}),
audience: process.env.AUTH0_API_AUDIENCE,
issuer: '',
algorithms: ['RS256'],
});
server.use(restify.plugins.bodyParser());
server.pre(cors.preflight);
server.use(cors.actual);
server.use(auth);
const rageConfig = new RageConfig({
maxRage: 10,
minRage: 0,
baseRoute: '/rage',
jwtConfig: jwtCheck,
});
let arrayPerson = [
{name: 'person1'},
{name: 'person2'},
{name: 'person3'},
{name: 'person4'},
{name: 'person5'},
{name: 'person6'},
{name: 'person7'},
];
arrayPerson = arrayPerson.map((p) => {
return new Person(p.name, 2);
});
const rageMeter = new Rage(arrayPerson, 0, 10, '/rage');
const routes = require('./routes/Routes');
routes(server, rageMeter, rageConfig);
server.listen(8005);
console.log('RAGE API started on port 8005');
module.exports = server;
<file_sep># Rage Meter API
[](https://circleci.com/gh/L4ngu0r/rage-meter/tree/master) [](https://greenkeeper.io/)
### Description
Used to troll in our team, decide to make a little API to integrate in our dev tools!
Today only in mem and with mocks.
### Features
Someone is on rage ? Vote for him to increase his level !!
Two votes are necessary to make his rage level up, also a cooldown
of 5 minutes between each vote.
### Services
[API](doc/api.html) : generated with raml2html
[RAML](doc/api.raml)
```
npm install
node index.js
```
##### Generate documentation
```
npm run gen-doc
```
### TODO
- reset on daily basis
- widget
- use database
- admin section security
- configuration (rage limits, cooldown, number of votes etc)
### License
MIT<file_sep>/* eslint linebreak-style: ["error", "windows"]*/
'use strict'
const http = require('http')
const ecstatic = require('ecstatic')({
root: `${__dirname}`,
showDir: false,
autoIndex: true,
defaultExt: 'html',
contentType: 'text/html',
cors: true,
});
http.createServer(ecstatic).listen(8080);
<file_sep>const crypto = require('crypto');
let mapIps = {};
/**
*
* @param {object} req
* @param {object} res
* @param {object} next
*/
module.exports = function Auth(req, res, next) {
// regex limited to 255
let idsReg = /\/rage\/([01]?[0-9]?[0-9]|2[0-4][0-9]|25[0-5])/,
reqPath = req.url,
reqMethod = req.method,
reqIp = req.headers['x-forwarded-for'] || req.connection.remoteAddress,
matches;
// use of regex to identify if we try to get a person
if ((matches = idsReg.exec(reqPath)) !== null) {
if (matches.index === idsReg.lastIndex) {
idsReg.lastIndex++;
}
}
let ip = null;
if (reqIp.length < 16) {
// fallback for ipv6 localhost (::1)
ip = req.headers.host.split(':')[0];
} else {
let ipSplit = reqIp.split(':');
ip = ipSplit[ipSplit.length - 1];
}
if (reqMethod !== 'POST') {
next();
} else {
// check if ip already requested something
let isPresent = Object.keys(mapIps).find((key) => {
return key === ip;
});
let now = new Date().getTime();
let accessAdmin = reqPath.indexOf('adm') !== -1;
if (accessAdmin) {
let ipTrying = isPresent || ip;
switch (ipTrying) {
case 'localhost':
case '::1':
case '127.0.0.1':
next();
break;
default:
res.status(401);
res.json({'Error': 'Unauthorized request!'});
}
} else if (!isPresent) {
// first time call
mapIps[ip] = {
hash: crypto.randomBytes(20).toString('hex'),
timestamp: now,
};
next();
} else if (mapIps[ip].timestamp + 5 * 60 * 1000 > now && matches && reqMethod === 'POST') {
// we block vote on POST method with regex matches id and for 5 minutes
// showing the remaining time in the response
let remainingTime = ((mapIps[ip].timestamp + 5 * 60 * 1000) - now) / 60;
res.status(401);
res.json({
'Warning': 'Too early sorry :)',
'Time remaining': Math.floor(remainingTime) + 's',
});
} else {
// can get data otherwise, and update timestamp
mapIps[isPresent].timestamp = now;
next();
}
}
};
<file_sep>/**
*
* @param {object} server
* @param {module.Rage} rage
* @param {module.RageConfig} rageConfig
* @param {Router} routerInstance
*/
module.exports = function Routes(server, rage, rageConfig) {
server.get(rage.baseRoute, rageConfig.jwtConfig, (req, res) => {
res.header('Content-Type', 'application/json');
res.json(rage.persons);
});
server.get(`${rage.baseRoute}/:id`, rageConfig.jwtConfig, (req, res) => {
let person = rage.findPersonById(req.params.id);
if (person) {
res.json(person);
} else {
res.status(404);
res.json({});
}
});
server.post(`${rage.baseRoute}/:id`, rageConfig.jwtConfig, (req, res) => {
let person = rage.findPersonById(req.params.id);
if (person) {
person.nbVote++;
switch (person.nbVote) {
case 1:
res.json({'Vote': 'added'});
break;
case 2:
person.nbVote = 0;
person.rageLevel++;
res.json({'Vote': 'added'});
break;
default:
res.json({'Error': 'too much vote'});
}
} else {
res.status(400);
res.json({'Error': 'id ' + req.params.id + ' not matching anyone'});
}
});
};
<file_sep>/**
*
* @type {module.RageConfig}
*/
module.exports = class RageConfig {
/**
*
* @param {object} options
*/
constructor(options) {
if (!options) {
throw new Error('No options found !');
}
this.maxRage = options.maxRage ? options.maxRage : 10;
this.minRage = options.minRage ? options.minRage : 0;
this.baseRoute = options.baseRoute ? options.baseRoute : '/';
this.minutesToWait = options.minutesToWait ? options.minutesToWait : 5;
this.jwtConfig = options.jwtConfig ? options.jwtConfig : null;
}
};
<file_sep>const chai = require('chai'),
chaiHttp = require('chai-http'),
server = require('../index'),
should = chai.should(),
dotenv = require('dotenv');
dotenv.config();
chai.use(chaiHttp);
describe('Persons', () => {
let token = {};
let persons = [];
before((done) => {
chai.request('https://languor.eu.auth0.com/oauth/token')
.post('/')
.send({
'client_id': process.env.AUTH0_API_ID,
'client_secret': process.env.AUTH0_API_SECRET,
'audience': process.env.AUTH0_API_AUDIENCE,
'grant_type': 'client_credentials',
})
.end((err, res) => {
res.should.have.status(200);
token = res.body.access_token;
done();
});
});
describe('/GET person', () => {
it('it should GET all the persons', (done) => {
chai.request(server)
.get('/rage')
.set('Authorization', `Bearer ${token}`)
.end((err, res) => {
if (err) {
throw err;
}
persons = res.body;
res.should.have.status(200);
res.body.should.be.a('array');
res.body.length.should.be.eql(7);
done();
});
});
});
describe('/GET/:id person', () => {
it('it should GET a person by the given id', (done) => {
const person = persons[0];
chai.request(server)
.get(`/rage/${person.id}`)
.set('Authorization', `Bearer ${token}`)
.end((err, res) => {
if (err) {
throw err;
}
res.should.have.status(200);
res.body.should.be.a('object');
res.body.should.have.property('name');
res.body.should.have.property('id');
res.body.should.have.property('id').eql(person.id);
done();
});
});
});
describe('/POST/:id', () => {
it('should POST a vote for a person', (done) => {
chai.request(server)
.post(`/rage/${persons[1].id}`)
.set('Authorization', `Bearer ${token}`)
.end((err, res) => {
if (err) {
throw err;
}
res.should.have.status(200);
res.body.should.be.a('object');
res.body.should.have.property('Vote');
res.body.should.have.property('Vote').eql('added');
done();
});
});
it('should not add a vote for a person before 5 min', (done) => {
chai.request(server)
.post(`/rage/${persons[1].id}`)
.set('Authorization', `Bearer ${token}`)
.end((err, res) => {
res.should.have.status(401);
res.body.should.be.a('object');
res.body.should.have.property('Warning');
res.body.should.have.property('Warning').eql('Too early sorry :)');
res.body.should.have.property('Time remaining');
done();
});
});
});
});
| d3e8b3624e6e27f5eaf2bf49e21b551795a6b5b9 | [
"JavaScript",
"Markdown"
] | 7 | JavaScript | L4ngu0r/rage-meter | 78e7928321b24abcd2fe8a4924d1e1ee3fc47d2b | e49a3d6c053e11ce44a6befe8947eb427095e698 | |
refs/heads/master | <file_sep>import React, { Component } from 'react'
import './App.css';
const calculate = array => {
const operators = {
"+": (a, b) => a + b,
"-": (a, b) => a - b,
"*": (a, b) => a * b,
"/": (a, b) => a / b,
}
let result;
let currentOp;
array.forEach(numOrOp => {
if (!result) {
result = numOrOp;
}
if (currentOp && typeof numOrOp === "number") {
result = operators[currentOp](result, numOrOp);
}
if (typeof numOrOp === "string") {
currentOp = numOrOp;
}
});
return result;
};
const operatorCheck = check => {
const operators = ["+", "-", "*", "/"];
return operators.includes(check);
};
const decimalCheck = array => {
let result = false;
for (let i = 0; i < array.length; i++) {
if (typeof array[i] === "string") {
if (array[i].indexOf('.') > -1) {
result = true;
break
}
}
}
return result;
};
const NumPad = props => {
const { func } = props;
const numbers = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"];
return numbers.map((num, i) => {
return (
<button
id={num}
className={`numbers-${i} ${num} pad-btn`}
style={{ gridArea: num }}
key={num}
onClick={() => func(`${i}`)}>
{i}
</button>
);
});
};
export class App extends Component {
constructor(props) {
super(props);
this.state = {
input: ['0']
};
};
inputNum = num => {
const lastItem = this.state.input.slice(this.state.input.length - 1)[0];
const beforeLastItem = this.state.input.slice(0, this.state.input.length - 1);
const secondToLastItem = this.state.input.slice(this.state.input.length - 2)[0];
const integer = (num * 1);
let newInput = null;
if (this.state.input[0] === '0' && integer === 0) return;
if (this.state.input[0] === '0' && integer > 0) {
newInput = [num];
} else {
if (operatorCheck(lastItem)) {
newInput = [...this.state.input, num]
}
if (!operatorCheck(lastItem) || decimalCheck([lastItem]) || (operatorCheck(secondToLastItem) && lastItem === '-')) {
newInput = [...beforeLastItem, [lastItem + num].join('')]
}
}
this.setState({
input: newInput
});
};
inputOperator = operator => {
const lastItem = this.state.input.slice(this.state.input.length - 1)[0];
if (operator === "-" || operator !== lastItem) {
this.setState({
input: [...this.state.input, operator]
});
}
};
inputDecimal = decimal => {
const lastItem = this.state.input.slice(this.state.input.length - 1);
const beforeLastItem = this.state.input.slice(0, this.state.input.length - 1);
if (!decimalCheck(lastItem) && (lastItem * 1) % 1 === 0) {
this.setState({
input: [...beforeLastItem, lastItem + decimal]
});
};
};
equate = () => {
const formattedInput = this.state.input.map(str => {
return operatorCheck(str) ? str : (str * 1);
});
const solution = `${calculate(formattedInput)}`;
this.setState({
input: [solution]
});
};
clear = () => {
this.setState({
input: ['0']
});
};
render() {
return (
<div className="App">
<div
id="display"
className="display">
{this.state.input}
</div>
<div className="pad">
<NumPad func={this.inputNum} />
<button className="decimal pad-btn" id="decimal" onClick={() => this.inputDecimal('.')}>.</button>
<button className="equals pad-btn" id="equals" onClick={() => this.equate()}>=</button>
<button className="clear pad-btn" id="clear" onClick={() => this.clear()}>CE</button>
<button className="divide operators pad-btn" id="divide" onClick={() => this.inputOperator("/")}>÷</button>
<button className="multiply operators pad-btn" id="multiply" onClick={() => this.inputOperator("*")}>×</button>
<button className="subtract operators pad-btn" id="subtract" onClick={() => this.inputOperator("-")}>-</button>
<button className="add operators pad-btn" id="add" onClick={() => this.inputOperator("+")}>+</button>
</div>
</div>
)
}
};
export default App; | 72b39679dad0ab56355a148a1c4541c8db094304 | [
"JavaScript"
] | 1 | JavaScript | AndrewZamora/javascript-calculator | 3bf652973c2e0e90c5f81b46e24ef4aed622c6e5 | f0ebb362abe53a0d4e1ca6606a6b015643481cbb | |
refs/heads/master | <repo_name>Melo93/AngularTable<file_sep>/src/app/generic-table/filter.pipe.ts
import {Pipe, PipeTransform} from '@angular/core';
@Pipe({
name: 'filter'
})
export class FilterPipe implements PipeTransform {
transform(value: any[], search: string, column?: string): any[] {
if (!value) {
return [];
}
if (!search) {
return value;
}
search = search.toLocaleLowerCase();
if(column === ''){
return value.filter(
val => Object.keys(val).some(i => {
if (typeof val[i] === 'string') {
return val[i].toLowerCase().includes(search.toLocaleLowerCase());
}
}));
} else {
return value.filter(
val => val[column].toLowerCase().includes(search.toLocaleLowerCase())
);
}
}
}
<file_sep>/src/app/pager.service.ts
import {Injectable} from '@angular/core';
@Injectable()
export class PagerService {
constructor() {}
getPager(totalItems: number, currentPage: number = 1, pageSize: number = 2, maxPages: number = 10) {
const totalPages = Math.ceil(totalItems / pageSize);
if (currentPage < 1) {
currentPage = 1;
} else if (currentPage > totalPages) {
currentPage = totalPages;
}
let startPage: number;
let endPage: number;
if (totalPages <= maxPages) {
// pagine totali inferiori a maxPages, quindi mostra tutte le pagine
startPage = 1;
endPage = totalPages;
// console.log('pagine totali inferiori a maxpages: ' + startPage + ' - ' + endPage);
} else {
// pagine totali più del massimo, quindi calcola le pagine iniziale e finale
const maxPagesBeforeCurrentPage = Math.floor(maxPages / 2);
const maxPagesAfterCurrentPage = Math.ceil(maxPages / 2) - 1;
// console.log('pagine totali più del massimo' + maxPagesBeforeCurrentPage + ' - ' + maxPagesAfterCurrentPage);
if (currentPage <= maxPagesBeforeCurrentPage) {
// pagina corrente vicino alla pagina iniziale
startPage = 1;
endPage = maxPages;
// console.log('Pagina vicino ad iniziale ' + startPage + ' - ' + endPage);
} else if (currentPage + maxPagesAfterCurrentPage >= totalPages) {
// pagina corrente vicino alla pagina finale
startPage = totalPages - maxPages + 1;
endPage = totalPages;
// console.log('Pagina vicino ad finale ' + startPage + ' - ' + endPage);
} else {
// pagina corrente nel mezzo
startPage = currentPage - maxPagesBeforeCurrentPage;
endPage = currentPage + maxPagesAfterCurrentPage;
// console.log('Pagina nel mezzo ' + startPage + ' ' + endPage);
}
}
// calcola index iniziale e finale
// tslint:disable-next-line:no-debugger
debugger;
const startIndex = (currentPage - 1) * pageSize;
const intPageSize: number = +pageSize;
const endIndex = Math.min(startIndex + intPageSize - 1, totalItems - 1);
// Creo l'array delle Pagine
const pages = Array.from(Array((endPage + 1) - startPage).keys()).map(i => startPage + i);
// Setto le proprietà dell'oggetto da restituire alle view
return {
totalItems,
currentPage,
pageSize,
totalPages,
startPage,
endPage,
startIndex,
endIndex,
pages
};
}
}
<file_sep>/src/app/table/table.component.ts
import {Component, EventEmitter, OnInit, Output} from '@angular/core';
import {Header} from '../header';
import {TableContent} from '../table-content';
import car from '../car.json';
import header from '../header.json';
@Component({
selector: 'app-table',
templateUrl: './table.component.html',
styleUrls: ['./table.component.css']
})
export class TableComponent implements OnInit {
data: any = car;
header: Header[] = header;
tableContent: TableContent;
constructor() {
}
ngOnInit() {
this.tableContent = {
header: this.header,
data: this.data
};
}
}
<file_sep>/src/app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import {NgbModule} from '@ng-bootstrap/ng-bootstrap';
import { AppComponent } from './app.component';
import { GenericTableComponent } from './generic-table/generic-table.component';
import { TableComponent } from './table/table.component';
import {FormsModule} from '@angular/forms';
import {PagerService} from './pager.service';
import { FilterPipe } from './generic-table/filter.pipe';
import { AngularFontAwesomeModule } from 'angular-font-awesome';
@NgModule({
declarations: [
AppComponent,
GenericTableComponent,
TableComponent,
FilterPipe
],
imports: [
BrowserModule,
FormsModule,
NgbModule,
AngularFontAwesomeModule
],
providers: [PagerService],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>/src/app/table-content.ts
export class TableContent {
header: any;
data: any;
}
<file_sep>/src/app/header.ts
export class Header {
columnValue: string;
columnLabel: string;
}
<file_sep>/src/app/generic-table/generic-table.component.ts
import {Component, EventEmitter, Input, OnInit, Output} from '@angular/core';
import {TableContent} from '../table-content';
import * as _ from 'lodash';
import {PagerService} from '../pager.service';
import {Action} from 'rxjs/internal/scheduler/Action';
@Component({
selector: 'app-generic-table',
templateUrl: './generic-table.component.html',
styleUrls: ['./generic-table.component.css']
})
export class GenericTableComponent implements OnInit {
@Input() searchBar ? = false;
@Input() tableContent: TableContent;
@Output('onClickAction') event = new EventEmitter();
columnToSearch = '';
search: string;
icon = 'sort';
clicked = false;
//paginazione
maxPageNumberSelector: number;
responsePageObject: any = {};
paginatedData: any[];
isPaginated = false;
lodash = _;
constructor(private pagerService: PagerService) {
}
ngOnInit() {
}
setPage(page: number) {
this.responsePageObject = this.pagerService.getPager(this.tableContent.data.length, page, this.maxPageNumberSelector);
this.paginatedData = this.tableContent.data.slice(this.responsePageObject.startIndex, this.responsePageObject.endIndex + 1);
}
onClickAction(action: Action<any>, model: any, url?: string) {
this.event.emit({action, model});
}
onChange($event: Event) {
console.log(this.maxPageNumberSelector);
this.isPaginated = true;
this.setPage(1);
}
orderBy(key: string) {
if (!this.clicked) {
if (this.isPaginated) {
this.paginatedData = this.tableContent.data.slice(this.responsePageObject.startIndex, this.responsePageObject.endIndex + 1);
}
this.icon = 'sort-up';
this.clicked = true;
this.tableContent.data = _.orderBy(this.tableContent.data, [key], ['asc']);
} else {
if (this.isPaginated) {
this.paginatedData = this.tableContent.data.slice(this.responsePageObject.startIndex, this.responsePageObject.endIndex + 1);
}
this.icon = 'sort-down';
this.clicked = false;
this.tableContent.data = _.orderBy(this.tableContent.data, [key], ['desc']);
}
}
}
<file_sep>/src/app/table-content.spec.ts
import { TableContent } from './table-content';
describe('TableContent', () => {
it('should create an instance', () => {
expect(new TableContent()).toBeTruthy();
});
});
| bd89898e0847b4731ae38267e7f1e04bc1ef4e25 | [
"TypeScript"
] | 8 | TypeScript | Melo93/AngularTable | 215bd71f4a0f173b8b0d4297ee16e04bc0455548 | 8c48690cf4751bb8b1c803f9beac189a9b74e9e4 | |
refs/heads/master | <repo_name>brainlife/app-checkOrientation<file_sep>/main
#!/bin/bash
#PBS -l nodes=1:ppn=8,walltime=0:05:00
#PBS -N checkOrientation
set -e
set -x
input_nii=$(jq -r .input config.json)
orientation=$(singularity exec -e docker://brainlife/fsl:5.0.9 fslorient $input_nii | tail -1)
cat <<EOF > product.json
{"orientation" : "$orientation"}
EOF
<file_sep>/README.md
# app-checkOrientation
This application checks and reports the orientation (neurological or radiological) of a nifti file.
| 08ecd37ce137c90d3d1bd15f9fdfe5c8fa522b1d | [
"Markdown",
"Shell"
] | 2 | Shell | brainlife/app-checkOrientation | acfdfb045e66cb6c18c549be836d2afa8a352c42 | 35adb984b82e189cd3ebac8b9bb312fac959775f | |
refs/heads/master | <repo_name>prem-0009/this-might-be-a-domb-idea<file_sep>/main.js
1
const para = document.querySelector('p')
//changing the font color of tag p
para.style.color = 'blue'
//heading color
document.querySelector('h1').style.fontSize = '5em'
//change the text inside tag p
para.innerText = 'Lorem ipsum dolor amet viral meh selfies drinking vinegar, intelligentsia poke flannel twee paleo enamel pin cray. Banjo celiac crucifix, kickstarter la croix air plant jianbing hashtag vinyl hell of man bun selvage schlitz banh mi. Tacos hella raclette quinoa blog, williamsburg adaptogen tbh. Hexagon af stumptown lumbersexual synth gentrify quinoa enamel pin celiac master cleanse. Truffaut typewriter shoreditch, semiotics iceland mixtape taxidermy umami distillery austin hashtag. Food truck synth wayfarers, street art banh mi actually authentic. Bitters tousled tattooed vegan neutra pug hell of fixie chia unicorn letterpress.'
//making 13th item transparent
document.querySelector('#item-13').style.opacity = '20%'
//change the 3rd item text
document.querySelector('#item-3').innerText = 'I say, "Hi!"'
//
const image = document.querySelector('img')
image.src = 'http://www.tioxic.com/wp-content/uploads/trex_4.jpg'
image.height = 300;
const myImage = document.querySelector('#addImage')
myImage.src = 'https://images.freeimages.com/images/large-previews/5b7/on-the-road-7-1384791.jpg'
myImage.height = 300;
const newList = document.querySelector('#item-16')
newList.className = 'item';
newList.innerText = `Won't get fooled again.`; | cb3c523dba30d9b9afa6a2fee2cfdf2c0d1b2cdb | [
"JavaScript"
] | 1 | JavaScript | prem-0009/this-might-be-a-domb-idea | 50b880ce4e92482debeaad18974f96e5372c35c5 | 0c983972aeea13f9dc5dc13cfa5e1debe18bcbcd | |
refs/heads/master | <file_sep>import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { OsobaComponent } from './osoba/osoba.component';
import { AutoComponent } from './auto/auto.component';
import { TestComponent } from './test/test.component';
import { OsobaEditComponent } from './osoba-edit/osoba-edit.component';
import { OsobaListComponent } from './osoba-list/osoba-list.component';
@NgModule({
declarations: [
AppComponent,
OsobaComponent,
AutoComponent,
TestComponent,
OsobaEditComponent,
OsobaListComponent
],
imports: [
BrowserModule,
FormsModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
<file_sep>export class Osoba {
public Id: number;
public Meno: string;
public Priezvisko: string;
constructor(Id: number, Meno: string, Priezvisko: string) {
this.Id = Id;
this.Meno = Meno;
this.Priezvisko = Priezvisko;
}
}
export class Auto {
public Id: number;
public Ecv: string;
public Poznamka: string;
constructor(Id: number, Ecv: string, Poznamka: string) {
this.Id = Id;
this.Ecv = Ecv;
this.Poznamka = Poznamka;
}
}
<file_sep>import { Component } from '@angular/core';
import { Osoba } from '../app.model';
@Component({
selector: 'app-osoba',
templateUrl: './osoba.component.html',
styleUrls: ['./osoba.component.css']
})
export class OsobaComponent {
osoba: Osoba = new Osoba(-1, 'Miška', 'Bačíková');
}
<file_sep>import { Component } from '@angular/core';
import { Osoba } from '../app.model';
@Component({
selector: 'app-osoba-list',
templateUrl: './osoba-list.component.html',
styleUrls: ['./osoba-list.component.css']
})
export class OsobaListComponent {
osoby: Osoba[] = [
new Osoba(-1, 'Miška', 'Bačíková'),
new Osoba(-1, 'Tomáš', 'Čižmár'),
new Osoba(-1, 'Stano', 'Zavila'),
new Osoba(-1, 'Martinka', 'Révayová')
];
}
<file_sep>import { Component } from '@angular/core';
import { Osoba } from '../app.model';
@Component({
selector: 'app-osoba-edit',
templateUrl: './osoba-edit.component.html',
styleUrls: ['./osoba-edit.component.css']
})
export class OsobaEditComponent {
osoba: Osoba = new Osoba(-1, 'Miška', 'Bačíková');
osobaToString(): string {
// return this.osoba.Id + '. ' + this.osoba.Meno + ' ' + this.osoba.Priezvisko;
return `${this.osoba.Id}. ${this.osoba.Meno} ${this.osoba.Priezvisko}`;
}
}
<file_sep>import { Component } from '@angular/core';
@Component({
selector: 'app-test',
template: `
Obsah premennej: {{premenna}}
<input type="text" [(ngModel)]="premenna">
`,
styleUrls: ['./test.component.css']
})
export class TestComponent {
premenna = 'daco';
}
| a8aa56418b2bc56ec555a3342fe954ede80b8655 | [
"TypeScript"
] | 6 | TypeScript | CDE-services/angularKybernetika2019 | e6114f202094a1606476fd01e1429773913d4376 | 9d8d15cdc8d026372a7bb741aa38a3ccb0484a1e | |
refs/heads/master | <repo_name>snretuerma/cmsc128-ay2015-16-assign001-js<file_sep>/Assignment01.js
/* CMSC 128: Introduction to Software Engineering
** Assign 001: Programming a Number Library
** Author: <NAME>
*/
// function for conveting number in digits to words
function numToWords(number){
var values1 = { // mapping of values in a single variable for 0 to 19
0: "",
1: "one",
2: "two",
3: "three",
4: "four",
5: "five",
6: "six",
7: "seven",
8: "eight",
9: "nine",
10: "ten",
11: "eleven",
12: "twelve",
13: "thirteen",
14: "fourteen",
15: "fifteen",
16: "sixteen",
17: "seventeen",
18: "eighteen",
19: "nineteen"
};
var values2 = { // for values 20 to 90
2: "twenty",
3: "thirty",
4: "forty",
5: "fifty",
6: "sixty",
7: "seventy",
8: "eighty",
9: "ninety"
}
var numberString = number.toString(); // convert the input to string
var digitCount = numberString.length; // get the count of the digits
var index1 = digitCount; // variables that will be used as reference in for accessing the array
var index2 = digitCount - 3;
var substringArray = []; // initialize an array for the substrings
var word = "";
if(digitCount%3!=0){ // grouping the digits to 3, this case is for the digits that are not divisible by 3
for(var i = 0; i< digitCount-3; i+=3){
var substring = numberString.slice(index2, index1);
substringArray.push(substring);
index1 = index2;
index2 = index1 - 3;
}
substringArray.push(numberString.slice(0, digitCount%3));
}
else{ // if the digit count is divisible by 3
for(var i = 0; i< digitCount; i+=3){
var substring = numberString.slice(index2, index1);
substringArray.push(substring);
index1 = index2;
index2 = index1 - 3;
}
}
var output = "";
for(var i = substringArray.length; i > 0; i--){ // use the substrings from the previous step and convert it to words
if(i == 3){
var word = ""; // this gets the millons part of the input
var hundred = Math.floor(substringArray[i-1]/100);
if(hundred>0){
word+=values1[hundred] + " hundred "
}
var temp = substringArray[i-1]%100;
if(temp<20){ // case for numbers that are less than 20
tens = temp;
word+=values1[tens] + " "
}
else{ // case for numbers that are greater than 20
tens = Math.floor((substringArray[i-1]%100)/10);
word+=values2[tens] + " "
var ones = Math.floor((substringArray[i-1]%100)%10);
word+=values1[ones] + " "
}
output += word + " million ";
}
if(i == 2){ // repeat the steps done for the thousandths and hundredths place if it exists
var word = "";
var hundred = Math.floor(substringArray[i-1]/100);
if(hundred>0){
word+=values1[hundred] + " hundred "
}
var temp = substringArray[i-1]%100;
if(temp<20){
tens = temp;
word+=values1[tens] + " "
}
else{
tens = Math.floor((substringArray[i-1]%100)/10);
word+=values2[tens] + " "
var ones = Math.floor((substringArray[i-1]%100)%10);
word+=values1[ones] + " "
}
if(substringArray[i-1] != 0){ // catches the case for 000 in the thousandths place
output += word + " thousand "
}
}
if(i == 1){
var word = "";
var hundred = Math.floor(substringArray[i-1]/100);
if(hundred>0){
word+=values1[hundred] + " hundred "
}
var temp = substringArray[i-1]%100;
if(temp<20){
tens = temp;
word+=values1[tens] + " "
}
else{
tens = Math.floor((substringArray[i-1]%100)/10);
word+=values2[tens] + " "
var ones = Math.floor((substringArray[i-1]%100)%10);
word+=values1[ones] + " "
}
output += word
}
}
return(output); // returns the output to the calling function
}
// function for changing word representation of a number to digits
function wordsToNum(words){
var valueMap = { // map of values
"one": 1,
"two": 2,
"three": 3,
"four": 4,
"five": 5,
"six": 6,
"seven": 7,
"eight": 8,
"nine": 9,
"ten": 10,
"eleven": 11,
"twelve": 12,
"thirteen": 13,
"fourteen": 14,
"fifteen": 15,
"sixteen": 16,
"seventeen": 17,
"eighteen": 18,
"nineteen": 19,
"twenty": 20,
"thirty": 30,
"forty": 40,
"fifty": 50,
"sixty": 60,
"seventy": 70,
"eighty": 80,
"ninety": 90,
"hundred": 100,
"thousand": 1000,
"million": 1000000
}
var splitresult = " ";
output = 0;
splitresult = words.split(" "); // split the input by space
var numberValue = 0; // variable declaration
var oldValue = 0;
var temp = 0;
var answer = 0;
for(var i = 0; i < (splitresult.length); i++){
numberValue = valueMap[splitresult[i]]; // get the numerical value of the word from the map
if(oldValue == 0){
oldValue = numberValue //if the old value is 0
}
else if(oldValue > numberValue){ // check if the old value is bigger than the current value
oldValue = oldValue + numberValue; // add the current value to the old value
}
else{
oldValue = oldValue * numberValue; // if it is bigger, multiply it, this signifies the change of place value (it is multiplied to a place value, 100, 1000 1000000)
}
if(oldValue>=1000){ // if the value is not a hundredths place, add it to the total
answer = answer + oldValue;
oldValue = 0; // reset the value of the old value variable to mark a change of place value
}
}
answer = answer+oldValue; // add the last 3 places in the answer
return(answer); // return the resulting number to the calling function
}
// function for getting the numerical representation of words to its digit with a currency appended to it depending on the passed currency
function wordsToCurrency(words,currency){
var valid = ["JPY", "PHP", "USD"]; // array for the checker of the currency inpur is valid
var value = wordsToNum(words); // use the wordsToNum function to
var check = valid.indexOf(currency) // checks if the input currency is in the list of valid currencies
if(check!=-1){
output = currency+value; // append the currency to the output of wordsToNum function
return (output); // return the currency to the calling function
}
}
// function for separating a number by a number of jumps depending on user input. the delimiter is provided by the user
function numberDelimited(number, delimiter, jumps){
var string = number.toString();
var length = string.length;
var symbol = delimiter;
var ind1 = length; // for the manipulation of input
var ind2 = ind1-jumps;
var word = "";
if(length%jumps!=0){ // if the number can't be divided exactly by the number of jumps
for(var i = 0; i <length-jumps; i+=jumps){ // divide the string from the right to left
var substring = string.slice(ind2, ind1); // slice the substring by the jumps using 2 variables for index reference
word = word.concat(symbol,substring); // concatenate the symbol to the substring formed
ind1 = ind2; // update the indices
ind2 = ind1-jumps;
}
word = string.slice(0, length%jumps).concat("", word) // for the the excess substring
}
else{ // if the string can be divided exactly by the jumps
for(var i = 0; i <length-jumps; i+=jumps){
var substring = string.slice(ind2, ind1);
word = word.concat(symbol,substring);
ind1 = ind2;
ind2 = ind1-jumps;
}
word = string.slice(0, jumps).concat("", word)
}
return(word); // return the output to the calling function
}
/*
** References used:
** http://blog.cordiner.net/2010/01/02/parsing-english-numbers-with-perl/
*/
<file_sep>/README.md
# cmsc128-ay2015-16-assign001-js
CMSC 128: Introduction to Software Engineering
2nd Semester AY 2015 – 2016
Section AB
Assign 001: Programming a Number Library
A number library using JavaScript that contains 4 functions
numToWords, wordsToNum, wordsToCurrency, numberDelimited
| 5698ec0b5afaa775e6f6320867673ef3fbb20959 | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | snretuerma/cmsc128-ay2015-16-assign001-js | 3c83fd8413d2a67b49e2cc2b8ce47c3f49a2cadb | cb62ccf441ed3d1ea813dffdb3f7131b79d7e6bf | |
refs/heads/master | <repo_name>Hs-RuiSun/hello-jenkins<file_sep>/src/test/java/com/rui/sun/HelloJenkinsTest.java
package com.rui.sun;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class HelloJenkinsTest {
@Test
void testPrint() {
assertEquals("hello", "hello");
}
} | cfe7c05a05ca825e9e5d206a6b874952c99f95d2 | [
"Java"
] | 1 | Java | Hs-RuiSun/hello-jenkins | 0aa7a6d036d32f1f437a87dda01f9a694c63e2ff | 70c326f1cb8eb756f9c319a79e1a61fa28f0cf84 | |
refs/heads/main | <file_sep>class Car(object):
def __init__(self,model,color,company,speed_limit):
self.color=color
self.company=company
self.speed_limit=speed_limit
self.model=model
def start(self):
print('started')
def stop(self):
print('stopped')
def accelarate(self):
print('accelarating')
"accelarator functionality here"
def change_gear(self,gear_type):
print('gear changed')
"gear related functionality here"
audi=Car('A6','red','audi',80)
print(audi.color)
print(audi.speed_limit)
print(audi.model)
print(audi.start()) | c4b0d6ab69e03b69620f3b5ff8cbecb0e6fd72ea | [
"Python"
] | 1 | Python | Anthonyne/c100 | ec1c232f0be0ef2355654b165f4220f100908d7e | 231b0e37eb59be7986140e0914f654a0937c09fc | |
refs/heads/master | <repo_name>pcqnt/docker-mailserver<file_sep>/target/scripts/startup/fixes-stack.sh
#! /bin/bash
function fix
{
_notify 'tasklog' 'Post-configuration checks'
for FUNC in "${FUNCS_FIX[@]}"
do
${FUNC} || _defunc
done
_notify 'inf' 'Removing leftover PID files from a stop/start'
find /var/run/ -not -name 'supervisord.pid' -name '*.pid' -delete
touch /dev/shm/supervisor.sock
}
function _fix_var_mail_permissions
{
_notify 'task' 'Checking /var/mail permissions'
# fix permissions, but skip this if 3 levels deep the user id is already set
if find /var/mail -maxdepth 3 -a \( \! -user 5000 -o \! -group 5000 \) | read -r
then
_notify 'inf' 'Fixing /var/mail permissions'
chown -R 5000:5000 /var/mail
else
_notify 'inf' 'Permissions in /var/mail look OK'
fi
return 0
}
function _fix_var_amavis_permissions
{
local AMAVIS_STATE_DIR='/var/mail-state/lib-amavis'
[[ ${ONE_DIR} -eq 0 ]] && AMAVIS_STATE_DIR="/var/lib/amavis"
[[ ! -e ${AMAVIS_STATE_DIR} ]] && return 0
_notify 'inf' 'Checking and fixing Amavis permissions'
chown -hR amavis:amavis "${AMAVIS_STATE_DIR}"
return 0
}
function _fix_cleanup_clamav
{
_notify 'task' 'Cleaning up disabled Clamav'
rm -f /etc/logrotate.d/clamav-*
rm -f /etc/cron.d/clamav-freshclam
}
function _fix_cleanup_spamassassin
{
_notify 'task' 'Cleaning up disabled SpamAssassin'
rm -f /etc/cron.daily/spamassassin
}
<file_sep>/docs/content/config/advanced/override-defaults/user-patches.md
---
title: 'Custom User Changes & Patches | Scripting'
---
If you'd like to change, patch or alter files or behavior of `docker-mailserver`, you can use a script.
In case you cloned this repository, you can copy the file `user-patches.sh.dist` under `config/` with `#!sh cp config/user-patches.sh.dist config/user-patches.sh` in order to create the `user-patches.sh` script. In case you are managing your directory structure yourself, create a `config/` directory and the `user-patches.sh` file yourself.
``` sh
# 1. Either create the config/ directory yourself
# or let docker-mailserver create it on initial
# startup
~/somewhere $ mkdir config && cd config
# 2. Create the user-patches.sh and edit it
~/somewhere/config $ touch user-patches.sh
~/somewhere/config $ vi user-patches.sh
```
The contents could look like this
``` sh
#! /bin/bash
cat >/etc/amavis/conf.d/50-user << "END"
use strict;
$undecipherable_subject_tag = undef;
$admin_maps_by_ccat{+CC_UNCHECKED} = undef;
#------------ Do not modify anything below this line -------------
1; # ensure a defined return
END
...
```
And you're done. The user patches script runs right before starting daemons. That means, all the other configuration is in place, so the script can make final adjustments.
!!! note
Many "patches" can already be done with the Docker Compose-/Stack-file. Adding hostnames to `/etc/hosts` is done with the `#!yaml extra_hosts:` section, `sysctl` commands can be managed with the `#!yaml sysctls:` section, etc.
<file_sep>/CHANGELOG.md
# Changelog
## `v10.1.2`
This is bug fix release. It reverts [a regression](https://github.com/docker-mailserver/docker-mailserver/issues/2154) introduced with [#2104](https://github.com/docker-mailserver/docker-mailserver/pull/2104).
## `v10.1.1`
This release mainly improves on `v10.1.0` with small bugfixes/improvements and dependency updates
- **[feat]** Add logwatch maillog.conf file to support /var/log/mail/ ([#2112](https://github.com/docker-mailserver/docker-mailserver/pull/2112))
- **[docs]** `CONTRIBUTORS.md` now also shows every code contributor from the past ([#2143](https://github.com/docker-mailserver/docker-mailserver/pull/2143))
- **[improve]** Avoid chmod +x when not needed ([#2127](https://github.com/docker-mailserver/docker-mailserver/pull/2127))
- **[improve]** check-for-changes: performance improvements ([#2104](https://github.com/docker-mailserver/docker-mailserver/pull/2104))
- **[dependency]** Update various dependencies through docs and base image
- **[security]** This release contains also [security fixes for OpenSSL](https://www.openssl.org/news/secadv/20210824.txt)
## `v10.1.0`
This release mainly improves on `v10.0.0` with many bugfixes.
- **[docs]** Various documentation updates ([#2105](https://github.com/docker-mailserver/docker-mailserver/pull/2105), [#2045](https://github.com/docker-mailserver/docker-mailserver/pull/2045), [#2043](https://github.com/docker-mailserver/docker-mailserver/pull/2043), [#2035](https://github.com/docker-mailserver/docker-mailserver/pull/2035), [#2001](https://github.com/docker-mailserver/docker-mailserver/pull/2001))
- **[misc]** Fixed a lot of small bugs, updated dependencies and improved functionality ([#2095](https://github.com/docker-mailserver/docker-mailserver/pull/2095), [#2047](https://github.com/docker-mailserver/docker-mailserver/pull/2047), [#2046](https://github.com/docker-mailserver/docker-mailserver/pull/2046), [#2041](https://github.com/docker-mailserver/docker-mailserver/pull/2041), [#1980](https://github.com/docker-mailserver/docker-mailserver/pull/1980), [#2030](https://github.com/docker-mailserver/docker-mailserver/pull/2030), [#2024](https://github.com/docker-mailserver/docker-mailserver/pull/2024), [#2001](https://github.com/docker-mailserver/docker-mailserver/pull/2001), [#2000](https://github.com/docker-mailserver/docker-mailserver/pull/2000), [#2059](https://github.com/docker-mailserver/docker-mailserver/pull/2059))
- **[feat]** Added dovecot-fts-xapian ([#2064](https://github.com/docker-mailserver/docker-mailserver/pull/2064))
- **[security]** Switch GPG keyserver ([#2051](https://github.com/docker-mailserver/docker-mailserver/pull/2051))
## `v10.0.0`
This release improves on `9.1.0` in many aspect, including general fixes, Fail2Ban, LDAP and documentation. This release contains breaking changes.
- **[general]** Fixed many prose errors (spelling, grammar, indentation).
- **[general]** Documentation is better integrated into the development process and it's visibility within the project increased ([#1878](https://github.com/docker-mailserver/docker-mailserver/pull/1878)).
- **[general]** Added `stop_grace_period:` to example Compose file and supervisord ([#1896](https://github.com/docker-mailserver/docker-mailserver/pull/1896) [#1945](https://github.com/docker-mailserver/docker-mailserver/pull/1945))
- **[general]** `./setup.sh email list` was enhanced, now showing information neatly ([#1898](https://github.com/docker-mailserver/docker-mailserver/pull/1898))
- **[general]** Added update check and notification ([#1976](https://github.com/docker-mailserver/docker-mailserver/pull/1976), [#1951](https://github.com/docker-mailserver/docker-mailserver/pull/1951))
- **[general]** Moved environment variables to the documentation and improvements ([#1948](https://github.com/docker-mailserver/docker-mailserver/pull/1948), [#1947](https://github.com/docker-mailserver/docker-mailserver/pull/1947), [#1931](https://github.com/docker-mailserver/docker-mailserver/pull/1931))
- **[security]** Major Fail2Ban improvements (cleanup, update and breaking changes, see below)
- **[fix]** `./setup.sh email del ...` now works properly
- **[code]** Added color variables to `setup.sh` and improved the script as a whole ([#1879](https://github.com/docker-mailserver/docker-mailserver/pull/1879), [#1886](https://github.com/docker-mailserver/docker-mailserver/pull/1886))
- **[ldap]** Added `LDAP_QUERY_FILTER_SENDERS` ([#1902](https://github.com/docker-mailserver/docker-mailserver/pull/1902))
- **[ldap]** Use dovecots LDAP `uris` connect option instead of `hosts` ([#1901](https://github.com/docker-mailserver/docker-mailserver/pull/1901))
- **[ldap]** Complete rework of LDAP documentation ([#1921](https://github.com/docker-mailserver/docker-mailserver/pull/1921))
- **[docs]** PRs that contain changes to docs will now be commented with a preview link ([#1988](https://github.com/docker-mailserver/docker-mailserver/pull/1988))
### Breaking Changes
- **[security]** Fail2Ban adjustments:
- Fail2ban v0.11.2 is now used ([#1965](https://github.com/docker-mailserver/docker-mailserver/pull/1965)).
- The previous F2B config (from an old Debian release) has been replaced with the latest default config for F2B shipped by Debian 10.
- The new default blocktype is now `DROP`, not `REJECT` ([#1914](https://github.com/docker-mailserver/docker-mailserver/pull/1914)).
- A ban now applies to all ports (`iptables-allports`), not just the ones that were "attacked" ([#1914](https://github.com/docker-mailserver/docker-mailserver/pull/1914)).
- Fail2ban 0.11 is totally compatible to 0.10, but the database got some new tables and fields (auto-converted during the first start), so once updated to DMS 10.0.0, you have to remove the database `mailstate:/lib-fail2ban/fail2ban.sqlite3` if you would need to downgrade to DMS 9.1.0 for some reason.
- **[ldap]** Removed `SASLAUTHD_LDAP_SSL`. Instead provide a protocol in `SASLAUTHD_LDAP_SERVER` and adjust `SASLAUTHD_LDAP_` default values ([#1989](https://github.com/docker-mailserver/docker-mailserver/pull/1989)).
- **[general]** Removed `stable` release tag ([#1975](https://github.com/docker-mailserver/docker-mailserver/pull/1975)):
- Scheduled builds are now based off `edge`.
- Instead of `stable`, please use the latest version tag available (_or the `latest` tag_).
- The `stable` image tag will be removed from DockerHub in the near future.
- **[setup]** Removed `./setup config ssl` command (_deprecated since v9_). `SSL_TYPE=self-signed` remains supported however. ([`dc8f49de`](https://github.com/docker-mailserver/docker-mailserver/commit/dc8f49de548e2c2e2aa321841585153a99cd3858), [#2021](https://github.com/docker-mailserver/docker-mailserver/pull/2021))
## `v9.1.0`
This release marks the breakpoint where the wiki was transferred to a [reworked documentation](https://docker-mailserver.github.io/docker-mailserver/edge/)
- **[feat]** Introduce ENABLE_AMAVIS env ([#1866](https://github.com/docker-mailserver/docker-mailserver/pull/1866))
- **[docs]** Move wiki to gh-pages ([#1826](https://github.com/docker-mailserver/docker-mailserver/pull/1826)) - Special thanks to @polarathene 👨🏻💻
- You can [edit the docs](https://github.com/docker-mailserver/docker-mailserver/tree/master/docs/content) now directly with your code changes
- Documentation is now versioned related to docker image versions and viewable here: <https://docker-mailserver.github.io/docker-mailserver/edge/>
## `v9.0.1`
A small update on the notification function which was made more stable as well as minor fixes.
- **[fix]** `_notify` cannot fail anymore - non-zero returns lead to unintended behavior in the past when `DMS_DEBUG` was not set or `0`
- **[refactor]** `check-for-changes.sh` now uses `_notify`
## `v9.0.0`
- **[feat]** Support extra `user_attributes` in accounts configuration ([#1792](https://github.com/docker-mailserver/docker-mailserver/pull/1792))
- **[feat]** Add possibility to use a custom dkim selector ([#1811](https://github.com/docker-mailserver/docker-mailserver/pull/1811))
- **[feat]** TLS: Dual (aka hybrid) certificate support! (eg ECDSA certificate with an RSA fallback for broader compatibility) ([#1801](https://github.com/docker-mailserver/docker-mailserver/pull/1801)).
- This feature is presently only for `SSL_TYPE=manual`, all you need to do is provide your fallback certificate to the `SSL_ALT_CERT_PATH` and `SSL_ALT_KEY_PATH` ENV vars, just like your primary certificate would be setup for manual mode.
- **[security]** TLS: You can now use ECDSA certificates! ([#1802](https://github.com/docker-mailserver/docker-mailserver/pull/1802))
- Warning: ECDSA may not be supported by legacy systems (most pre-2014). You can provide an RSA certificate as a fallback.
- **[fix]** TLS: For some docker-compose setups when restarting the docker-mailserver container, internal config state may have been persisted despite making changes that should reconfigure TLS (eg changing `SSL_TYPE` or replacing the certificate file) ([#1801](https://github.com/docker-mailserver/docker-mailserver/pull/1801)).
- **[refactor]** Split `start-mailserver.sh` ([#1820](https://github.com/docker-mailserver/docker-mailserver/pull/1820))
- **[fix]** Linting now uses local path to remove the sudo dependency ([#1831](https://github.com/docker-mailserver/docker-mailserver/pull/1831)).
### Breaking Changes
- **[security]** TLS: `TLS_LEVEL=modern` has changed the server-side preference order to 128-bit before 256-bit encryption ([#1802](https://github.com/docker-mailserver/docker-mailserver/pull/1802)).
- NOTE: This is still very secure but may result in misleading lower scores/grades from security audit websites.
- **[security]** TLS: `TLS_LEVEL=modern` removed support for AES-CBC cipher suites and follows best practices by supporting only AEAD cipher suites ([#1802](https://github.com/docker-mailserver/docker-mailserver/pull/1802)).
- NOTE: As TLS 1.2 is the minimum required for modern already, AEAD cipher suites should already be supported and preferred.
- **[security]** TLS: `TLS_LEVEL=intermediate` has removed support for cipher suites using RSA for key exchange (only available with an RSA certificate) ([#1802](https://github.com/docker-mailserver/docker-mailserver/pull/1802)).
- NOTE: This only affects Dovecot which supported 5 extra cipher suites using AES-CBC and AES-GCM. Your users MUA clients should be unaffected, preferring ECDHE or DHE for key exchange.
- **[refactor]** Complete refactoring of opendkim script ([#1812](https://github.com/docker-mailserver/docker-mailserver/pull/1812)).
- NOTE: Use `./setup.sh config dkim help` to see the new syntax.
## `v8.0.1`
This release is a hotfix for #1781.
- **[spam]** `bl.spamcop.net` was removed from the list of spam lists since the domain expired and became unusable
## `v8.0.0`
The transfer of the old repository to the new organization has completed. This release marks the new starting point for `docker-mailserver` in the `docker-mailserver` organization. Various improvements were made, small bugs fixed and the complete CI was transferred.
- **[general]** transferred the whole repository to `docker-mailserver/docker-mailserver`
- **[general]** adjusted `README.md` and split off `ENVIRONMENT.md`
- **[ci]** usage of the GitHub Container Registry
- **[ci]** switched from TravisCI to **GitHub Actions for CI/CD**
- now building images for `amd64` and `arm/v7` and `arm/64`
- integrated stale issues action to automatically close stale issues
- adjusted issue templates
- **[build]** completely refactored and improved the `Dockerfile`
- **[build]** improved the `Makefile`
- **[image improvement]** added a proper init process
- **[image improvement]** improved logging significantly
- **[image improvement]** major LDAP improvements
- **[bugfixes]** miscellaneous bug fixes and improvements
### Breaking changes of release `8.0.0`
- **[image improvement]** log-level now defaults to `warn`
- **[image improvement]** DKIM default key size now 4096
- **[general]** the `:latest` tag is now the latest release and `:edge` represents the latest push on `master`
- **[general]** URL changed from `tomav/...` to `docker-mailserver/...`
## `v7.2.0`
- **[scripts]** refactored `target/bin/`
- **[scripts]** redesigned environment variable use
- **[general]** added Code of Conduct
- **[general]** added missing Dovecot descriptions
- **[tests]** enhanced and refactored all tests
## `v7.1.0`
- **[scripts]** use of default variables has changed slightly (consult [environment variables](./ENVIRONMENT.md))
- **[scripts]** Added coherent coding style and linting
- **[scripts]** Added option to use non-default network interface
- **[general]** new contributing guidelines were added
- **[general]** SELinux is now supported
<file_sep>/docs/content/config/best-practices/dmarc.md
---
title: 'Best Practices | DMARC'
hide:
- toc # Hide Table of Contents for this page
---
!!! note
DMARC Guide: https://github.com/internetstandards/toolbox-wiki/blob/master/DMARC-how-to.md
## Enabling DMARC
In `docker-mailserver`, DMARC is pre-configured out-of the box. The only thing you need to do in order to enable it, is to add new TXT entry to your DNS.
In contrast with [DKIM][docs-dkim], DMARC DNS entry does not require any keys, but merely setting the [configuration values](https://github.com/internetstandards/toolbox-wiki/blob/master/DMARC-how-to.md#overview-of-dmarc-configuration-tags). You can either handcraft the entry by yourself or use one of available generators (like https://dmarcguide.globalcyberalliance.org/).
Typically something like this should be good to start with (don't forget to replace `@domain.com` to your actual domain)
```
_dmarc.domain.com. IN TXT "v=DMARC1; p=none; rua=mailto:<EMAIL>; ruf=mailto:<EMAIL>; sp=none; ri=86400"
```
Or a bit more strict policies (mind `p=quarantine` and `sp=quarantine`):
```
_dmarc IN TXT "v=DMARC1; p=quarantine; rua=mailto:<EMAIL>; ruf=mailto:<EMAIL>; fo=0; adkim=r; aspf=r; pct=100; rf=afrf; ri=86400; sp=quarantine"
```
DMARC status is not being displayed instantly in Gmail for instance. If you want to check it directly after DNS entries, you can use some services around the Internet such as https://dmarcguide.globalcyberalliance.org/ or https://ondmarc.redsift.com/. In other case, email clients will show "DMARC: PASS" in ~1 day or so.
Reference: [#1511][github-issue-1511]
[docs-dkim]: ./dkim.md
[github-issue-1511]: https://github.com/docker-mailserver/docker-mailserver/issues/1511
| 3699131ab7eaf1eef489cd0814a0b53d950d3d45 | [
"Markdown",
"Shell"
] | 4 | Shell | pcqnt/docker-mailserver | b8c9581cd6014115295b1d96ce29329dfc893d52 | 88f086b48332f11052baa117e6f2b41d6fcecd19 | |
refs/heads/master | <file_sep>// 接收post数据
// 中间件 body paser 第三方
var express=require("express")
var bodyParser=require("body-parser")
var app=express()
// 使用中间件 use
// 处理json数据
app.use(bodyParser.json())
// 处理字符串
app.use(bodyParser.urlencoded({extended:false}))
app.get("/",(req,res)=>{
res.send("守夜")
})
app.post("/dopost",(req,res)=>{
console.log(req.body)
res.send({"data":"收到"+req.body.username})
})
app.listen(3000) | 80b0fbfbf1f84fc7fa9ec54f73554898d1cd3ac9 | [
"JavaScript"
] | 1 | JavaScript | langjiu990419/langjiu | a5a71012155cf12861b33233df984ed3e2d57c27 | 98a9529737cacdf075e0a3f4d6843d586721ed28 | |
refs/heads/master | <file_sep>---
author: <NAME>, <NAME>
title: flatr -- living community management
date: March 27, 2018
---
## Motivation
- we both live in shared flats
- these get pretty messy from time to time
- old solution don't work very well
Disadvantages of regular cleaning schedules:
- missing overview
- requires a pen on hand
- can't be checked remotely
- _"I'll do it Tomorrow / on Sunday!"_
- static
The solution: `flatr`
- portable mobile & web-application
- can be checked from any smartphone
- sends reminders
- tasks need to be done in a short timeframe
- fair distribution of tasks
The basic priciple:
1. A user gets a push notification on their smartphone _(not implemented)_
- containing a specifc timeframe
2. The task gets done
3. Task gets marked as done in the application
A penalty for overdue tasks could be easily introduced
Our system is highly configurable:
- Title
- Description
- Frequency in times / week
- Completion time in hours
- Users per Task
Task overview can be printed as PDF (through the browser print menu)
To prevent unauthorized access, we have setup an invite system
- not everyone should be able to join a flat
- first user can invite other users
- these users get a Email with an invite link
- then they are able to signup for a new account
## Screenshots:



## Project organization
| | |
|---------------------+----------------------------------------------------------------|
| GitHub organisation | [github.com/flatrapp](https://github.com/flatrapp) |
| API doc in `RAML` | [flatrapp.github.io/api](https://flatrapp.github.io/api) |
| Core | [github.com/flatrapp/core](https://github.com/flatrapp/core) |
| Shell | [github.com/flatrapp/shell](https://github.com/flatrapp/shell) |
## Backend
### Technologies
- Haskell
- SQLite
- Nix
Why nix as a build tool - and not stack or cabal?
- If `nix-build` works once, it always works
- Handles ALL dependencies, even C libraries and system packages
Haskell
- Advanced strong typesystem
- Statically typed
- Compiled
- => safe
- Fast
- pure

No `null` or `undefined`
```haskell
foo :: Maybe a
foo = ??
test (Just bar) = print bar
test Nothing = print "Not there"
```
You can have trust in your software
- compiles => doesn't crash
- works & refactor => still works
Runtime problems:
- inverted if conditionals
- mail library couldn't handle TLS
```haskell
-- sqlAssertIsNotThere
case mEntity of
Nothing -> errorJson errStatus
Just _entity -> return ()
```
```diff
-userIsVerified = isJust . userVerifyCode
+userIsVerified = isNothing . userVerifyCode
```
```diff
- if hashedPw == userPassword user then do
+ if hashedPw /= userPassword user then do
```
----
Parser of json fails if schema is invalid or conditions don't match
```bash
$ http POST localhost:8080/users email="foobarexample.org" firstName="Daniel" lastName="Schäfer" absent:=false password="<PASSWORD>"
HTTP/1.1 400 Bad Request
Access-Control-Allow-Origin: *
Content-Type: application/json; charset=utf-8
Date: Tue, 27 Mar 2018 04:11:29 GMT
Server: Warp/3.2.13
Transfer-Encoding: chunked
```
```json
{
"error": {
"code": "bad_request",
"message": "Failed to parse json: Error in $: email does not match required regex."
}
}
```
---
Json objects
```haskell
data Registration =
Registration { email :: Maybe Text
, firstName :: Text
, lastName :: Text
, password :: <PASSWORD>
, invitationCode :: Maybe Text
, absent :: Bool
} deriving (Show, Generic)
```
## Frontend
- Using Bootstrap v4 for best UI experience
- Implements `flatr` `JSON-API` according to specification
- Reference implementation
- Should work in any modern browser (uses ES5)
We use elm-lang:
- Completely pure functional language
- Strong typesystem
- Compiles to JavaScript
- Extremly fast
- Very similar to Haskell
Benefits of using `Elm`
- If it compiles, it works!
- A function with the same input will produce the same output
- Build the HTML with Elm functions
- Immutable state handling
- Clear and obvious data flow
----
Typesafety in `HTML`:
```haskell
view : Html msg
view =
Grid.container []
[ Grid.row []
[ Grid.col []
[ text "This is valid!"
]
]
]
```
This will not compile, because a Grid has to contain rows of columns:
```haskell
view : Html msg
view =
Grid.container []
[ Grid.col []
[ Grid.row []
[ text "This is invalid!"
]
]
]
```
Disadvantages of using `Elm`
- Fairly young language, still developing
- No widespread usage, less support
- Requires to relearn basic programming concepts
- Takes long to get used to
- Not made for _rapid prototyping_
Should you choose Elm?
- Yes
- If you like stable software that has few bugs
- If you like typesafety and a good compiler
- Want to get desktop-class programming on the web
- No
- If you're in a hurry
- If the software is just a prototype
- If you need to work with a lot of JavaScript code
- _If you need to do a Web assignment_
## Statistics
Backend:
| Part | Lines |
|-------------------+-------|
| Haskell Logic | 947 |
| Imports | 217 |
| Type declarations | 162 |
| SUM Code | 1326 |
| Comments | 79 |
| Blank lines | 208 |
Frontend:
| Part | Lines |
|-----------------+-------|
| Elm Logic | 837 |
| API Calls | 930 |
| HTML Components | 1853 |
| SUM | 3620 |
## TODO & Future Plans
TODO:
- Sending Emails is still buggy (doesn't work if we try to link statically and the c libraries aren't properly linked)
- Further improve the tasks assignment algorithm
Future Plans:
- Create a Android & iOS native App
- Add more modules
- Shopping List
- Expenses
----
This report is free & open source (CC BY-SA 4.0)
Download sources at [https://github.com/flatrapp/documentation](https://github.com/flatrapp/documentation)
<file_sep>#! /usr/bin/env nix-shell
#! nix-shell -i bash -p pandoc texlive.combined.scheme-small
if [ ! -d "reveal.js" ]; then
wget https://github.com/hakimel/reveal.js/archive/master.tar.gz
tar -xzvf master.tar.gz
mv reveal.js-master reveal.js
rm master.tar.gz
fi
pandoc -t revealjs -s -o presentation.html presentation.md -V revealjs-url=./reveal.js/ -V theme=solarized
pandoc report.md --pdf-engine=pdflatex -o report.pdf
| 27e6a12072d755c2516ba76d02be99a4f42270af | [
"Markdown",
"Shell"
] | 2 | Markdown | flatrapp/documentation | 29d54f54c1219395ec859abc3800afce35377a17 | 9d60705beea11e655b700007b908b74f2cc6553c | |
refs/heads/main | <repo_name>Seanxwy/TinyMCE.Blazor<file_sep>/src/TinyMCE.Blazor/Components/Editor.razor.Method.cs
using Microsoft.AspNetCore.Components;
using Microsoft.JSInterop;
using System.Threading.Tasks;
namespace TinyMCE.Blazor
{
public partial class Editor : ComponentBase
{
[Inject] private IJSRuntime Js { get; set; }
public async Task CreateTinyMCE()
{
await Js.InvokeVoidAsync("TinyMCEBlazor.createTinyMCE", _ref, DotNetObjectReference.Create(this), this.Id, this.Value, this.TinyMCEOption, this.Width, this.Height);
}
public ValueTask<string> GetValue()
{
return Js.InvokeAsync<string>("TinyMCEBlazor.getValue", _ref, this.Id);
}
public ValueTask<string> GetHTML()
{
return Js.InvokeAsync<string>("TinyMCEBlazor.getHTML", _ref, this.Id);
}
public async Task SetValue(string value)
{
await Js.InvokeVoidAsync("TinyMCEBlazor.setValue", _ref, this.Id, value);
}
public async Task InsertValue(string value)
{
await Js.InvokeVoidAsync("TinyMCEBlazor.insertValue", _ref, this.Id, value);
}
public async Task Destroy()
{
await Js.InvokeVoidAsync("TinyMCEBlazor.destroy", _ref);
}
public async Task SetHeight(string value, bool stop = false)
{
await Js.InvokeVoidAsync("TinyMCEBlazor.setHeight", _ref, value, stop);
}
}
}<file_sep>/src/TinyMCE.Blazor/Components/Editor.razor.cs
using Microsoft.AspNetCore.Components;
using System.Threading.Tasks;
using System.Collections.Generic;
using System;
using TinyMCE.Blazor.Models;
namespace TinyMCE.Blazor
{
public partial class Editor : ComponentBase, IDisposable
{
/// <summary>
/// Markdown Content
/// </summary>
[Parameter]
public string Value
{
get => _value;
set
{
if (_value != value)
{
_value = value;
_waitingUpdate = true;
}
}
}
[Parameter] public EventCallback<string> ValueChanged { get; set; }
/// <summary>
/// Html Content
/// </summary>
[Parameter]
public string Html { get; set; }
[Parameter]
public string Id { get; set; } = null;
[Parameter] public EventCallback<string> HtmlChanged { get; set; }
[Parameter]
public TinyMCEOption TinyMCEOption { get; set; } = new TinyMCEOption();
[Parameter]
public string Height {
get => _heightValue;
set {
SetHeight(value);
_heightValue = value;
}
}
[Parameter]
public string Width { get; set; }
private ElementReference _ref;
private bool _editorRendered = false;
private bool _waitingUpdate = false;
private string _value, _heightValue = "500";
private bool _afterFirstRender = false;
protected override async Task OnAfterRenderAsync(bool firstRender)
{
await base.OnAfterRenderAsync(firstRender);
if (firstRender)
{
//Console.WriteLine("edit is first render");
await CreateTinyMCE();
}
}
protected override async Task OnInitializedAsync()
{
//Console.WriteLine("edit is initialized");
await base.OnInitializedAsync();
this.Id = Id ?? Guid.NewGuid().ToString();
}
protected override async Task OnParametersSetAsync()
{
//Console.WriteLine("edit is on parameters");
await base.OnParametersSetAsync();
if (_waitingUpdate && _editorRendered)
{
_waitingUpdate = false;
await SetValue(_value);
//Console.WriteLine("edit is on parameters set value");
}
}
public async ValueTask<string> GetValueAsync()
{
if (_editorRendered)
{
return await GetValue();
}
return string.Empty;
}
public void Dispose()
{
//Console.WriteLine("edit is dispose");
Destroy();
}
}
}
<file_sep>/README.md
# TinyMCE.Blazor
<file_sep>/src/TinyMCE.Blazor/Models/TinyMCEOption.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace TinyMCE.Blazor.Models
{
public class TinyMCEOption
{
public bool InlineMode { get; set; }
public string Plugins { get; set; } = "paste autolink link wordcount";
public string Toolbar { get; set; } = "undo redo | styleselect | bold italic | alignleft aligncenter alignright alignjustify | outdent indent | link";
public bool ShowMenuBar { get; set; } = false;
public bool PasteAsText { get; set; }
public bool PasteDataImage { get; set; }
}
}
| 5e9c8541247d5c3e3261896ceb84a0614a7b7832 | [
"Markdown",
"C#"
] | 4 | C# | Seanxwy/TinyMCE.Blazor | 71834c9cb43c2f9338d3c33fbc2138a95f0a8eaa | d1d085fefdb44fc4a8669cb9bde21abc06695d22 | |
refs/heads/master | <repo_name>yorrickslr/guacamole<file_sep>/tutorial/beginner/0_window/main.cpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2018 <NAME> *
* Contact: <EMAIL> *
* <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
#include <functional>
#include <gua/guacamole.hpp>
#include <gua/renderer/TriMeshLoader.hpp>
#include <gua/utils/Trackball.hpp>
/* scenegraph overview for "main_scenegraph"
/
└ screen
└ camera
*/
int main(int argc, char** argv) {
// initialize guacamole
gua::init(argc, argv);
// initialize scenegraph
gua::SceneGraph graph("main_scenegraph");
auto screen = graph.add_node<gua::node::ScreenNode>("/", "screen");
screen->data.set_size(gua::math::vec2(1.28f, 0.72f)); // real world size of screen
screen->translate(0, 0, 1.0);
// resolution to be used for camera resolution and windows size
auto resolution = gua::math::vec2ui(1280, 720);
// set up camera and connect to screen in scenegraph
auto camera = graph.add_node<gua::node::CameraNode>("/screen", "cam");
camera->translate(0, 0, 2.0);
camera->config.set_resolution(resolution);
camera->config.set_screen_path("/screen");
camera->config.set_scene_graph_name("main_scenegraph");
camera->config.set_output_window_name("main_window");
// set up window
auto window = std::make_shared<gua::GlfwWindow>();
gua::WindowDatabase::instance()->add("main_window", window);
window->config.set_enable_vsync(false);
window->config.set_size(resolution);
window->config.set_resolution(resolution);
window->config.set_stereo_mode(gua::StereoMode::MONO);
window->on_resize.connect([&](gua::math::vec2ui const& new_size) {
window->config.set_resolution(new_size);
camera->config.set_resolution(new_size);
screen->data.set_size(
gua::math::vec2(0.001 * new_size.x, 0.001 * new_size.y));
});
window->open();
gua::Renderer renderer;
// application loop
gua::events::MainLoop loop;
// registers the function given in ticker.on_tick to
// be called by the given loop in the given interval
gua::events::Ticker ticker(loop, 1.0 / 500.0);
// log fps and handle close events
size_t ctr{};
ticker.on_tick.connect([&]() {
// log fps every 150th tick
if (ctr++ % 150 == 0) {
gua::Logger::LOG_WARNING
<< "Frame time: " << 1000.f / window->get_rendering_fps()
<< " ms, fps: " << window->get_rendering_fps() << std::endl;
}
window->process_events();
if (window->should_close()) {
// stop rendering and close the window
renderer.stop();
window->close();
loop.stop();
} else {
// draw our scenegrapgh
renderer.queue_draw({&graph});
}
});
loop.start();
return 0;
}
<file_sep>/plugins/guacamole-spoints/include/gua/spoints/SPointsSync.hpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2013 <NAME> *
* Contact: <EMAIL> / <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
#ifndef GUA_SPOINTS_SYNC_HPP
#define GUA_SPOINTS_SYNC_HPP
#include <gua/spoints/platform.hpp>
#include <memory>
#include <queue>
#include <gua/math/math.hpp>
#include <gua/utils.hpp>
#include <fstream>
#include <iostream>
namespace gua
{
class GUA_SPOINTS_DLL SPointsSync
{
public:
SPointsSync();
~SPointsSync(){};
void set_sync_length(int new_sync_length);
int get_sync_length();
scm::math::mat<double, 4u, 4u> get_synchronized(scm::math::mat<double, 4u, 4u> const& matrix, bool is_left);
scm::math::mat<double, 4u, 4u> get_synchronized(scm::math::mat<double, 4u, 4u> const& matrix);
// unsigned long id = (unsigned long)this;
// std::ofstream* file = new std::ofstream();
private:
std::shared_ptr<std::queue<scm::math::mat<double, 4u, 4u>>> sync_queue_l_ = std::make_shared<std::queue<scm::math::mat<double, 4u, 4u>>>(std::queue<scm::math::mat<double, 4u, 4u>>());
std::shared_ptr<std::queue<scm::math::mat<double, 4u, 4u>>> sync_queue_r_ = std::make_shared<std::queue<scm::math::mat<double, 4u, 4u>>>(std::queue<scm::math::mat<double, 4u, 4u>>());
int sync_length_;
};
} // namespace gua
#endif // GUA_SPOINTS_SYNC_HPP
<file_sep>/tutorial/beginner/4_lights/main.cpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2018 <NAME> *
* Contact: <EMAIL> *
* <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
#include <functional>
#include <gua/guacamole.hpp>
#include <gua/renderer/TriMeshLoader.hpp>
/* scenegraph overview for "main_scenegraph"
/
└ screen
└ camera
└ transform
└ sphere_geometry
└ monkey_geometry
└ teapot_geometry
└ light_transform
case:1
└ point_light_transform
└ point_light0
└ point_light1
└ point_light2
case:2
└ spot_light_transform
└ spot_light0
└ spot_light1
└ spot_light2
case:3
└ sun_light_transform
└ sun_light
*/
int main(int argc, char** argv) {
// initialize guacamole
gua::init(argc, argv);
// initialize scenegraph
gua::SceneGraph graph("main_scenegraph");
// initialize trimeshloader for model loading
gua::TriMeshLoader loader;
// create a transform node
// which will be attached to the scenegraph
auto transform = graph.add_node<gua::node::TransformNode>("/", "transform");
// the model will be attached to the transform node
auto sphere_geometry(loader.create_geometry_from_file(
"sphere_geometry", "../data/objects/sphere.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
sphere_geometry->scale(0.4);
sphere_geometry->translate(0.6,0.0,0.0);
auto monkey_geometry(loader.create_geometry_from_file(
"monkey_geometry", "../data/objects/monkey.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
monkey_geometry->scale(0.5);
auto teapot_geometry(loader.create_geometry_from_file(
"teapot_geometry", "../data/objects/teapot.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
teapot_geometry->scale(0.5);
teapot_geometry->translate(-0.6,0.0,0.0);
auto plane_geometry(loader.create_geometry_from_file(
"plane_geometry", "../data/objects/plane.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE)
);
plane_geometry->rotate(10.0f, gua::math::vec3(1.0f,0.0f,0.0f));
plane_geometry->translate(0.0, -0.2, 0.0);
// plane_geometry->scale(gua::math::vec3(2.0f,0.0f,0.0f));
graph.add_node("/transform", sphere_geometry);
graph.add_node("/transform", monkey_geometry);
graph.add_node("/transform", teapot_geometry);
graph.add_node("/transform", plane_geometry);
/* LIGHTS */
// create some transform nodes to append the different lights to
// main light transform node
auto light_transform = graph.add_node<gua::node::TransformNode>("/","light_transform");
// one transform node for every type
auto point_light_transform = std::make_shared<gua::node::TransformNode>();
auto spot_light_transform = std::make_shared<gua::node::TransformNode>();
auto sun_light_transform = std::make_shared<gua::node::TransformNode>();
// create some point lights and configure them
auto point_light0 = point_light_transform->add_child<gua::node::LightNode>("point_light0");
point_light0->data.set_type(gua::node::LightNode::Type::POINT);
point_light0->data.set_brightness(40.0f);
point_light0->data.set_falloff(2.0f);
point_light0->data.set_softness(1.5f);
point_light0->data.set_enable_shadows(true);
// float r = float(std::rand()) / float(RAND_MAX);
// float g = float(std::rand()) / float(RAND_MAX);
// float b = float(std::rand()) / float(RAND_MAX);
// point_light0->data.set_color(gua::utils::Color3f(0.5f+r,g,b));
point_light0->data.set_color(gua::utils::Color3f(1.0f,0.0f,0.0f));
point_light0->scale(10.0f);
point_light0->translate(2.0f,2.0f,2.0f);
auto point_light1 = point_light_transform->add_child<gua::node::LightNode>("point_light1");
point_light1->data.set_type(gua::node::LightNode::Type::POINT);
point_light1->data.set_brightness(25.0f);
point_light1->data.set_falloff(0.5f);
point_light1->data.set_softness(2.0f);
point_light1->data.set_enable_shadows(true);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// point_light1->data.set_color(gua::utils::Color3f(r,0.5f+g,b));
point_light1->data.set_color(gua::utils::Color3f(0.0f,1.0f,0.0f));
point_light1->scale(4.0f);
point_light1->translate(-1.5f,-2.0f,1.0f);
auto point_light2 = point_light_transform->add_child<gua::node::LightNode>("point_light2");
point_light2->data.set_type(gua::node::LightNode::Type::POINT);
point_light2->data.set_brightness(110.0f);
point_light2->data.set_falloff(1.0f);
point_light2->data.set_softness(1.0f);
point_light2->data.set_enable_shadows(true);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// point_light2->data.set_color(gua::utils::Color3f(r,g,0.5f+b));
point_light2->data.set_color(gua::utils::Color3f(0.0f,0.0f,1.0f));
point_light2->scale(10.0f);
point_light2->translate(-0.5f,5.0f,0.0f);
// add the point lights to the scenegraph, you can later switch the light via keyboard input
auto temp = graph.add_node<gua::node::TransformNode>(light_transform, point_light_transform);
// create some spot lights and configure them
auto spot_light0 = spot_light_transform->add_child<gua::node::LightNode>("spot_light0");
spot_light0->data.set_type(gua::node::LightNode::Type::SPOT);
spot_light0->data.set_brightness(50.0f);
spot_light0->data.set_falloff(2.0f);
spot_light0->data.set_softness(1.5f);
spot_light0->data.set_enable_shadows(true);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// spot_light0->data.set_color(gua::utils::Color3f(0.5f+r,g,b));
spot_light0->data.set_color(gua::utils::Color3f(1.0f,0.0f,0.0f));
spot_light0->scale(1.5f);
spot_light0->rotate(45.0f, gua::math::vec3(0.0f,1.0f,0.0f));
spot_light0->translate(1.0f,0.0f,1.0f);
auto spot_light1 = spot_light_transform->add_child<gua::node::LightNode>("spot_light1");
spot_light1->data.set_type(gua::node::LightNode::Type::SPOT);
spot_light1->data.set_brightness(35.0f);
spot_light1->data.set_falloff(0.5f);
spot_light1->data.set_softness(2.0f);
spot_light1->data.set_enable_shadows(true);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// spot_light1->data.set_color(gua::utils::Color3f(r,0.5f+g,b));
spot_light1->data.set_color(gua::utils::Color3f(0.0f,0.6f,0.2f));
spot_light1->scale(2.0f);
spot_light1->rotate(63.43f, gua::math::vec3(1.0f,0.0f,0.0f));
spot_light1->translate(-0.6f,-1.f,0.5f);
auto spot_light2 = spot_light_transform->add_child<gua::node::LightNode>("spot_light2");
spot_light2->data.set_type(gua::node::LightNode::Type::SPOT);
spot_light2->data.set_brightness(120.0f);
spot_light2->data.set_falloff(0.8f);
spot_light2->data.set_softness(1.0f);
spot_light2->data.set_enable_shadows(true);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// spot_light2->data.set_color(gua::utils::Color3f(r,g,0.5f+b));
spot_light2->data.set_color(gua::utils::Color3f(0.0f,0.0f,1.0f));
spot_light2->scale(5.0f);
spot_light2->rotate(-90.0f, gua::math::vec3(1.0f,0.0f,0.0f));
spot_light2->translate(0.0f,5.0f,0.0f);
// add a sun light
auto sun_light = sun_light_transform->add_child<gua::node::LightNode>("sun_light");
sun_light->data.set_type(gua::node::LightNode::Type::SUN);
sun_light->data.set_brightness(3.0f);
// sun_light->data.set_falloff(2.0f);
// sun_light->data.set_softness(1.5f);
sun_light->data.set_enable_shadows(true);
sun_light->data.set_shadow_map_size(1024);
// r = float(std::rand()) / float(RAND_MAX);
// g = float(std::rand()) / float(RAND_MAX);
// b = float(std::rand()) / float(RAND_MAX);
// sun_light->data.set_color(gua::utils::Color3f(0.5f+r,g,b));
sun_light->data.set_color(gua::utils::Color3f(1.0f,0.7f,0.07f));
sun_light->scale(1.0f);
sun_light->translate(70.0f,70.0f,1.0f);
auto screen = graph.add_node<gua::node::ScreenNode>("/", "screen");
screen->data.set_size(gua::math::vec2(1.28f, 0.72f)); // real world size of screen
screen->translate(0, 0, 1.0);
// resolution to be used for camera resolution and windows size
auto resolution = gua::math::vec2ui(1280, 720);
// set up camera and connect to screen in scenegraph
auto camera = graph.add_node<gua::node::CameraNode>("/screen", "cam");
camera->translate(0, 0, 2.0);
camera->config.set_resolution(resolution);
camera->config.set_screen_path("/screen");
camera->config.set_scene_graph_name("main_scenegraph");
camera->config.set_output_window_name("main_window");
// set up window
auto window = std::make_shared<gua::GlfwWindow>();
gua::WindowDatabase::instance()->add("main_window", window);
window->config.set_enable_vsync(false);
window->config.set_size(resolution);
window->config.set_resolution(resolution);
window->config.set_stereo_mode(gua::StereoMode::MONO);
window->on_resize.connect([&](gua::math::vec2ui const& new_size) {
window->config.set_resolution(new_size);
camera->config.set_resolution(new_size);
screen->data.set_size(
gua::math::vec2(0.001 * new_size.x, 0.001 * new_size.y));
});
window->open();
// add keyboard input
window->on_key_press.connect([&](int key, int scancode, int action, int mods) {
if(action == 0) return;
switch(key) {
case '1':
std::cout << "SWITCHED TO POINT LIGHTS" << std::endl;
light_transform->clear_children();
graph.add_node<gua::node::TransformNode>(light_transform, point_light_transform);
break;
case '2':
std::cout << "SWITCHED TO SPOT LIGHTS" << std::endl;
light_transform->clear_children();
graph.add_node<gua::node::TransformNode>(light_transform, spot_light_transform);
break;
case '3':
std::cout << "SWITCHED TO SUN LIGHT" << std::endl;
light_transform->clear_children();
graph.add_node<gua::node::TransformNode>(light_transform, sun_light_transform);
break;
}
});
gua::Renderer renderer;
// application loop
gua::events::MainLoop loop;
gua::events::Ticker ticker(loop, 1.0 / 500.0);
// log fps and handle close events
size_t ctr{};
ticker.on_tick.connect([&]() {
// use a timer for an ongoing transformation
auto time = gua::Timer::get_now()*0.0000000001;
auto trans = (std::cos(time*17000000000.0f)+1.0f)/2.0f;
sun_light_transform->rotate(time, gua::math::vec3(-1.0f,1.0f,0.0f));
sun_light->data.set_color(gua::utils::Color3f(1.0f,trans,0.07f));
// log fps every 150th tick
if (ctr++ % 150 == 0) {
// change light color every 150th tick
// float r = float(std::rand()) / float(RAND_MAX);
// float g = float(std::rand()) / float(RAND_MAX);
// float b = float(std::rand()) / float(RAND_MAX);
// point_light->data.color = gua::utils::Color3f(r,g,b);
gua::Logger::LOG_WARNING
<< "Frame time: " << 1000.f / window->get_rendering_fps()
<< " ms, fps: " << window->get_rendering_fps()
<< "\nCOS(TIME): " << trans << std::endl;
}
window->process_events();
if (window->should_close()) {
// stop rendering and close the window
renderer.stop();
window->close();
loop.stop();
} else {
// draw our scenegrapgh
renderer.queue_draw({&graph});
}
});
loop.start();
return 0;
}
<file_sep>/tutorial/beginner/3_input/main.cpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2018 <NAME> *
* Contact: <EMAIL> *
* <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
#include <functional>
#include <gua/guacamole.hpp>
#include <gua/renderer/TriMeshLoader.hpp>
#include <gua/utils/Trackball.hpp>
/* scenegraph overview for "main_scenegraph"
/
└ screen
└ camera
└ transform
└ teapot_geometry
*/
/* to use the mouse input for trackball navigation,
we need a callback to forward the interaction to the trackball */
void mouse_button(gua::utils::Trackball& trackball, int mousebutton, int action, int mods) {
gua::utils::Trackball::button_type button;
gua::utils::Trackball::state_type state;
switch (mousebutton) {
// left mb for rotation
case 0: button = gua::utils::Trackball::left; break;
// middle mb for translation (shift on x/y plane) on screen pane
case 2: button = gua::utils::Trackball::middle; break;
// right mb for zoom or shift on z axis
case 1: button = gua::utils::Trackball::right; break;
};
switch (action) {
case 0: state = gua::utils::Trackball::released; break;
case 1: state = gua::utils::Trackball::pressed; break;
};
// forward button and state along with the mouse position to the trackball class
trackball.mouse(button, state, trackball.posx(), trackball.posy());
}
int main(int argc, char** argv) {
// initialize guacamole
gua::init(argc, argv);
// initialize scenegraph
gua::SceneGraph graph("main_scenegraph");
// initialize trimeshloader for model loading
gua::TriMeshLoader loader;
// create a transform node
// which will be attached to the scenegraph
auto transform = graph.add_node<gua::node::TransformNode>("/", "transform");
// the model will be attached to the transform node
auto teapot_geometry(loader.create_geometry_from_file(
"teapot_geometry", "../data/objects/teapot.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
// teapot_geometry->scale(0.15);
teapot_geometry->translate(-0.6,0.0,0.0);
graph.add_node("/transform", teapot_geometry);
auto screen = graph.add_node<gua::node::ScreenNode>("/", "screen");
screen->data.set_size(gua::math::vec2(1.28f, 0.72f)); // real world size of screen
screen->translate(0, 0, 1.0);
// initialize trackball for mouse interaction
// trackball(zoom_factor, shift_factor, rotation_factor)
gua::utils::Trackball trackball(0.01, 0.002, 0.2);
// initialize navigator node for key input and keyboard navigation
// resolution to be used for camera resolution and windows size
auto resolution = gua::math::vec2ui(1280, 720);
// set up camera and connect to screen in scenegraph
auto camera = graph.add_node<gua::node::CameraNode>("/screen", "cam");
camera->translate(0, 0, 2.0);
camera->config.set_resolution(resolution);
camera->config.set_screen_path("/screen");
camera->config.set_scene_graph_name("main_scenegraph");
camera->config.set_output_window_name("main_window");
// set up window
auto window = std::make_shared<gua::GlfwWindow>();
gua::WindowDatabase::instance()->add("main_window", window);
window->config.set_enable_vsync(false);
window->config.set_size(resolution);
window->config.set_resolution(resolution);
window->config.set_stereo_mode(gua::StereoMode::MONO);
window->on_resize.connect([&](gua::math::vec2ui const& new_size) {
window->config.set_resolution(new_size);
camera->config.set_resolution(new_size);
screen->data.set_size(
gua::math::vec2(0.001 * new_size.x, 0.001 * new_size.y));
});
// connect mouse movement in window to trackball motion
window->on_move_cursor.connect(
[&](gua::math::vec2 const& pos) { trackball.motion(pos.x, pos.y); });
// connect mouse input to our mouse button callback.
// the placeholders will be replaced by the mousebutton,
// action and mods arguments of the callback funktion
window->on_button_press.connect(
std::bind(mouse_button, std::ref(trackball), std::placeholders::_1,
std::placeholders::_2, std::placeholders::_3));
// add a simple keyboard movement;
// as we need the camera, lambda function is good way to go
window->on_key_press.connect([&](int key, int scancode, int action, int mods) {
if(action == 0) return;
switch(key) {
case 'W':
camera->translate(0.0,0.0,0.5);
break;
case 'S':
camera->translate(0.0,0.0,-0.5);
break;
case 'A':
camera->translate(0.5,0.0,0.0);
break;
case 'D':
camera->translate(-0.5,0.0,0.0);
break;
}
});
window->open();
gua::Renderer renderer;
// application loop
gua::events::MainLoop loop;
gua::events::Ticker ticker(loop, 1.0 / 500.0);
// log fps and handle close events
size_t ctr{};
ticker.on_tick.connect([&]() {
// log fps every 150th tick
if (ctr++ % 150 == 0) {
gua::Logger::LOG_WARNING
<< "Frame time: " << 1000.f / window->get_rendering_fps()
<< " ms, fps: " << window->get_rendering_fps() << std::endl;
}
// apply trackball matrix to the object
gua::math::mat4 modelmatrix =
scm::math::make_translation(trackball.shiftx(), trackball.shifty(),
trackball.distance()) *
gua::math::mat4(trackball.rotation());
transform->set_transform(modelmatrix);
window->process_events();
if (window->should_close()) {
// stop rendering and close the window
renderer.stop();
window->close();
loop.stop();
} else {
// draw our scenegrapgh
renderer.queue_draw({&graph});
}
});
loop.start();
return 0;
}
<file_sep>/tutorial/intermediate/CMakeLists.txt
# guacamole tutorial
<file_sep>/tutorial/beginner/5_pipeline/main.cpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2018 <NAME> *
* Contact: <EMAIL> *
* <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
#include <functional>
#include <gua/guacamole.hpp>
#include <gua/renderer/TriMeshLoader.hpp>
#include <gua/utils/Trackball.hpp>
#include <gua/renderer/DebugViewPass.hpp>
/* scenegraph overview for "main_scenegraph"
/
└ screen
└ camera
└ transform
└ sphere_geometry
└ monkey_geometry
└ teapot_geometry
└ light_transform
└ sun_light
*/
/* to use the mouse input for trackball navigation,
we need a callback to forward the interaction to the trackball */
void mouse_button(gua::utils::Trackball& trackball, int mousebutton, int action, int mods) {
gua::utils::Trackball::button_type button;
gua::utils::Trackball::state_type state;
switch (mousebutton) {
// left mb for rotation
case 0: button = gua::utils::Trackball::left; break;
// middle mb for translation (shift on x/y plane) on screen pane
case 2: button = gua::utils::Trackball::middle; break;
// right mb for zoom or shift on z axis
case 1: button = gua::utils::Trackball::right; break;
};
switch (action) {
case 0: state = gua::utils::Trackball::released; break;
case 1: state = gua::utils::Trackball::pressed; break;
};
// forward button and state along with the mouse position to the trackball class
trackball.mouse(button, state, trackball.posx(), trackball.posy());
}
int main(int argc, char** argv) {
// initialize guacamole
gua::init(argc, argv);
// initialize scenegraph
gua::SceneGraph graph("main_scenegraph");
// initialize trimeshloader for model loading
gua::TriMeshLoader loader;
// create a transform node
// which will be attached to the scenegraph
auto transform = graph.add_node<gua::node::TransformNode>("/", "transform");
// the model will be attached to the transform node
auto sphere_geometry(loader.create_geometry_from_file(
"sphere_geometry", "../data/objects/sphere.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
sphere_geometry->scale(0.4);
sphere_geometry->translate(0.6,0.0,0.0);
auto monkey_geometry(loader.create_geometry_from_file(
"monkey_geometry", "../data/objects/monkey.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
monkey_geometry->scale(0.5);
auto teapot_geometry(loader.create_geometry_from_file(
"teapot_geometry", "../data/objects/teapot.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE )
);
teapot_geometry->scale(0.5);
teapot_geometry->translate(-0.6,0.0,0.0);
auto plane_geometry(loader.create_geometry_from_file(
"plane_geometry", "../data/objects/plane.obj",
gua::TriMeshLoader::OPTIMIZE_GEOMETRY | gua::TriMeshLoader::NORMALIZE_POSITION |
gua::TriMeshLoader::LOAD_MATERIALS | gua::TriMeshLoader::OPTIMIZE_MATERIALS |
gua::TriMeshLoader::NORMALIZE_SCALE)
);
plane_geometry->rotate(10.0f, gua::math::vec3(1.0f,0.0f,0.0f));
plane_geometry->translate(0.0, -0.2, 0.0);
// plane_geometry->scale(gua::math::vec3(2.0f,0.0f,0.0f));
graph.add_node("/transform", sphere_geometry);
graph.add_node("/transform", monkey_geometry);
graph.add_node("/transform", teapot_geometry);
graph.add_node("/transform", plane_geometry);
/* LIGHTS */
// create some transform nodes to append the different lights to
// main light transform node
auto light_transform = graph.add_node<gua::node::TransformNode>("/","light_transform");
// add a sun light
auto sun_light = graph.add_node<gua::node::LightNode>("/light_transform", "sun_light");
sun_light->data.set_type(gua::node::LightNode::Type::SUN);
sun_light->data.set_color(gua::utils::Color3f(1.5f, 1.2f, 1.f));
sun_light->data.set_shadow_cascaded_splits({0.1f, 1.5, 5.f, 10.f});
sun_light->data.set_shadow_near_clipping_in_sun_direction(100.0f);
sun_light->data.set_shadow_far_clipping_in_sun_direction(100.0f);
sun_light->data.set_max_shadow_dist(30.0f);
sun_light->data.set_shadow_offset(0.0004f);
sun_light->data.set_enable_shadows(true);
sun_light->data.set_shadow_map_size(512);
sun_light->rotate(-65, 1, 0, 0);
sun_light->rotate(-100, 0, 1, 0);
// sun_light_transform->rotate(90.0f, gua::math::vec3(-1.0f,1.0f,0.0f));
auto screen = graph.add_node<gua::node::ScreenNode>("/", "screen");
screen->data.set_size(gua::math::vec2(1.28f, 0.72f)); // real world size of screen
screen->translate(0, 0, 1.0);
// resolution to be used for camera resolution and windows size
auto resolution = gua::math::vec2ui(1280, 720);
// initialize trackball for mouse interaction
// trackball(zoom_factor, shift_factor, rotation_factor)
gua::utils::Trackball trackball(0.01, 0.002, 0.2);
// set up camera and connect to screen in scenegraph
auto camera = graph.add_node<gua::node::CameraNode>("/screen", "cam");
camera->translate(0, 0, 2.0);
camera->config.set_resolution(resolution);
camera->config.set_screen_path("/screen");
camera->config.set_scene_graph_name("main_scenegraph");
camera->config.set_output_window_name("main_window");
// Pipeline Description
// The pipeline description is stored in the camera and later used for
// pipeline creation When creating a camera, a default pipeline description is
// set up It includes several passes affecting the pipeline layout and
// functionality defalut pipeline description includes: TrimeMeshPass,
// LineStripPass, TexturedQuadPassDescription, LightVisibilityPassDescription,
// BBoxPassDescription, ResolvePassDescription, TexturedScreenSpaceQuadPassDescription
// You can create an own pipeline description from scratch or configure the existing description.
auto pipe_desc = camera->get_pipeline_description();
// Let's set a skymap as background for the scene
// Those settings have to be configured in the resolve pass
auto res_pass = pipe_desc->get_resolve_pass();
res_pass->background_mode(gua::ResolvePassDescription::BackgroundMode::SKYMAP_TEXTURE);
res_pass->background_texture("../data/textures/sphericalskymap.jpg");
res_pass->background_color(gua::utils::Color3f(0,0,0));
res_pass->environment_lighting_texture("../data/textures/sphericalskymap.jpg");
res_pass->environment_lighting(gua::utils::Color3f(0.4, 0.4, 0.5));
res_pass->environment_lighting_mode(gua::ResolvePassDescription::EnvironmentLightingMode::AMBIENT_COLOR);
res_pass->ssao_enable(true);
res_pass->tone_mapping_method(gua::ResolvePassDescription::ToneMappingMethod::HEJL);
res_pass->tone_mapping_exposure(1.5f);
res_pass->horizon_fade(0.2f);
res_pass->ssao_intensity(1.5f);
res_pass->ssao_radius(2.f);
res_pass->vignette_coverage(0.2f);
res_pass->vignette_softness(1.f);
res_pass->vignette_color(gua::math::vec4f(0.5, 0.1, 0.3, 1.0));
// adding a pipeline pass /!\ don't forget to include the pass /!\
// for example add a debug view pass.
// Debug View will add overviews of normals, depth, color and position of the scene.
pipe_desc->add_pass(std::make_shared<gua::DebugViewPassDescription>());
// set up window
auto window = std::make_shared<gua::GlfwWindow>();
gua::WindowDatabase::instance()->add("main_window", window);
window->config.set_enable_vsync(false);
window->config.set_size(resolution);
window->config.set_resolution(resolution);
window->config.set_stereo_mode(gua::StereoMode::MONO);
window->on_resize.connect([&](gua::math::vec2ui const& new_size) {
window->config.set_resolution(new_size);
camera->config.set_resolution(new_size);
screen->data.set_size(
gua::math::vec2(0.001 * new_size.x, 0.001 * new_size.y));
});
window->open();
// connect mouse movement in window to trackball motion
window->on_move_cursor.connect(
[&](gua::math::vec2 const& pos) { trackball.motion(pos.x, pos.y); });
// connect mouse input to our mouse button callback.
// the placeholders will be replaced by the mousebutton,
// action and mods arguments of the callback funktion
window->on_button_press.connect(
std::bind(mouse_button, std::ref(trackball), std::placeholders::_1,
std::placeholders::_2, std::placeholders::_3));
// add a simple keyboard movement;
// as we need the camera, lambda function is good way to go
// add keyboard input
window->on_key_press.connect([&](int key, int scancode, int action, int mods) {
});
gua::Renderer renderer;
// application loop
gua::events::MainLoop loop;
gua::events::Ticker ticker(loop, 1.0 / 500.0);
// log fps and handle close events
size_t ctr{};
ticker.on_tick.connect([&]() {
// use a timer for an ongoing transformation
auto time = gua::Timer::get_now()*0.0000000001;
auto trans = (std::cos(time*17000000000.0f)+1.0f)/2.0f;
// sun_light_transform->rotate(time, gua::math::vec3(-1.0f,1.0f,0.0f));
/* sun_light->data.set_color(gua::utils::Color3f(1.0f,trans,0.07f)); */
// log fps every 150th tick
if (ctr++ % 150 == 0) {
// change light color every 150th tick
// float r = float(std::rand()) / float(RAND_MAX);
// float g = float(std::rand()) / float(RAND_MAX);
// float b = float(std::rand()) / float(RAND_MAX);
// point_light->data.color = gua::utils::Color3f(r,g,b);
gua::Logger::LOG_WARNING
<< "Frame time: " << 1000.f / window->get_rendering_fps()
<< " ms, fps: " << window->get_rendering_fps() << std::endl;
}
// apply trackball matrix to the object
gua::math::mat4 modelmatrix =
scm::math::make_translation(trackball.shiftx(), trackball.shifty(),
trackball.distance()) *
gua::math::mat4(trackball.rotation());
transform->set_transform(modelmatrix);
window->process_events();
if (window->should_close()) {
// stop rendering and close the window
renderer.stop();
window->close();
loop.stop();
} else {
// draw our scenegrapgh
renderer.queue_draw({&graph});
}
});
loop.start();
return 0;
}
<file_sep>/tutorial/beginner/CMakeLists.txt
# guacamole tutorial
# input requires GLFW3
IF (${GUACAMOLE_GLFW3})
add_subdirectory(0_window)
add_subdirectory(1_load_trimesh)
add_subdirectory(2_transformations)
add_subdirectory(3_input)
add_subdirectory(4_lights)
add_subdirectory(5_pipeline)
ENDIF (${GUACAMOLE_GLFW3})
<file_sep>/examples/0_getting_started/CMakeLists.txt
# guacamole examples
IF (GUACAMOLE_EXAMPLES)
# input requires GLFW3
IF (${GUACAMOLE_GLFW3})
add_subdirectory(0_window)
ENDIF (${GUACAMOLE_GLFW3})
ENDIF (GUACAMOLE_EXAMPLES)
<file_sep>/tutorial/CMakeLists.txt
# guacamole tutorial
IF (GUACAMOLE_TUTORIAL)
add_subdirectory(beginner)
add_subdirectory(intermediate)
ENDIF (GUACAMOLE_TUTORIAL)
<file_sep>/plugins/guacamole-spoints/src/gua/spoints/SPointsSync.cpp
/******************************************************************************
* guacamole - delicious VR *
* *
* Copyright: (c) 2011-2013 <NAME> *
* Contact: <EMAIL> / <EMAIL> *
* *
* This program is free software: you can redistribute it and/or modify it *
* under the terms of the GNU General Public License as published by the Free *
* Software Foundation, either version 3 of the License, or (at your option) *
* any later version. *
* *
* This program is distributed in the hope that it will be useful, but *
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY *
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License *
* for more details. *
* *
* You should have received a copy of the GNU General Public License along *
* with this program. If not, see <http://www.gnu.org/licenses/>. *
* *
******************************************************************************/
// class header
#include <gua/spoints/SPointsSync.hpp>
// guacamole headers
#include <gua/guacamole.hpp>
#include <gua/platform.hpp>
namespace gua
{
////////////////////////////////////////////////////////////////////////////////
SPointsSync::SPointsSync() : sync_length_{1}
{
// file->open("C:/Users/HMDEyes/SPointsSync.log");
}
//////////////////////////////////////////////////////////////////////////////
scm::math::mat<double, 4u, 4u> SPointsSync::get_synchronized(scm::math::mat<double, 4u, 4u> const& matrix, bool is_left)
{
// *file << "sync lenght is " << sync_length << std::endl;
// *file << "pushing matrix to queue:" << std::endl;
// *file << matrix << std::endl;
if(sync_length_ == 0)
{
return matrix;
}
if(is_left)
{
sync_queue_l_->push(matrix);
if(sync_queue_l_->size() > sync_length_)
{
sync_queue_l_->pop();
}
return sync_queue_l_->front();
}
else
{
sync_queue_r_->push(matrix);
if(sync_queue_r_->size() > sync_length_)
{
sync_queue_r_->pop();
}
return sync_queue_r_->front();
}
}
//////////////////////////////////////////////////////////////////////////////
scm::math::mat<double, 4u, 4u> SPointsSync::get_synchronized(scm::math::mat<double, 4u, 4u> const& matrix) { return get_synchronized(matrix, true); }
//////////////////////////////////////////////////////////////////////////////
void SPointsSync::set_sync_length(int new_sync_length)
{
sync_length_ = new_sync_length;
while(sync_length_ < sync_queue_l_->size())
{
sync_queue_l_->pop();
}
while(sync_length_ < sync_queue_r_->size())
{
sync_queue_r_->pop();
}
}
//////////////////////////////////////////////////////////////////////////////
int SPointsSync::get_sync_length() { return sync_length_; }
} // namespace gua
| 538bc2f9fe7281a174caf664b48e89d3cb9d9d6e | [
"CMake",
"C++"
] | 10 | C++ | yorrickslr/guacamole | 2993eca36a0aa30787ccbd13334b6a71dc096678 | 1401297439c3e7d0caa3e92ca1b391098ddab8ac | |
refs/heads/master | <repo_name>jasoneckert/shellscripts<file_sep>/script3.sh
#!/bin/bash
#This script backs up a directory specified by the first argument (positional parameter)
if [ $# -ne 1 ]
then
echo "Usage is $0 <directory to back up>"
exit 255
fi
echo "Performing backup....."
sleep 3
tar -zcvf ~/backupfile.tar.gz $1
echo "Backup completed successfully to ~/backupfile.tar.gz"
<file_sep>/script4.sh
#!/bin/bash
#This script backs up a directory of your choice
echo -e "What directory do you want to back up?-->\c"
read ANS
echo "Performing backup....."
sleep 3
FILE=`echo $ANS | sed s#/#-#g`
DATE=`date +%F`
tar -zcvf ~/backup-$FILE-$DATE.tar.gz $ANS
echo "Backup completed successfully to ~/backup-$FILE-$DATE.tar.gz"
<file_sep>/script2.sh
#!/bin/bash
#This script backs up a directory of your choice
echo -e "What directory do you want to back up?-->\c"
read ANS
echo "Performing backup....."
sleep 3
tar -zcvf ~/backupfile.tar.gz $ANS
echo "Backup completed successfully to ~/backupfile.tar.gz"
<file_sep>/script5.sh
#!/bin/bash
#This script backs up the Oracle DB every night
rm -f /remoteserver/backup-oracle*
if tar -zcvf /remoteserver/backup-oracle-`date +%F`.tar.gz /oracledb/*
then
echo "Oracle backup completed on `date`" >>/var/log/oraclebackuplog
else
echo "Oracle backup failed on `date`" >>/var/log/oraclebackuplog
mail -s "BACKUP FAILED" <EMAIL> </var/log/oraclebackuplog
fi
<file_sep>/script1.sh
#!/bin/bash
#This script creates a report of our disk configuration
FILENAME=`hostname`
echo "Disk report saved to $FILENAME.report"
echo -e "\n LVM Configuration: \n\n" >>$FILENAME.report
lvscan >>$FILENAME.report
vgscan >>$FILENAME.report
pvscan >>$FILENAME.report
echo -e "\n\n Partition Configuration: \n\n" >>$FILENAME.report
lsblk >>$FILENAME.report
echo -e "\n\n Mounted Filesystem Usage: \n\n" >>$FILENAME.report
df -hT | grep -v tmp >>$FILENAME.report
| 27fb554c0f1ce1e84e9e4ff54e34296c20a89d77 | [
"Shell"
] | 5 | Shell | jasoneckert/shellscripts | 321027abc47185bce2a9e37babfc45c498f484e5 | c99b6e6bd90a36bfde4fcaf58afbdf11b6e1d18a | |
refs/heads/master | <file_sep>import React, { useEffect, useState } from 'react';
import { Link, useHistory } from 'react-router-dom';
import * as api from '../../API/api';
import './style.css';
const initialValues = { nameOfDepositor: "", recipient_account_number: "", amount: 0 };
const Deposit = ({ user, setUser, serverMessage, pathname, setPathname }) => {
const history = useHistory();
const [success_message, set_success_message] = useState("");
const [data_inputs, set_data_inputs] = useState(initialValues);
const [err_message, set_err_message] = useState(false);
const [spinner, setSpinner] = useState(false);
const [self, set_Self] = useState(false);
// handle input field
const handleInputChange = e => {
const target = e.target;
const name = target.name;
const value = target.value;
if (target.type === "number") {
if (value < 0) {
set_data_inputs({ ...data_inputs, [name]: 0 })
} else {
set_data_inputs({ ...data_inputs, [name]: value })
}
} else {
set_data_inputs({ ...data_inputs, [name]: value })
}
set_success_message(""); //reset success message when user is typing
set_err_message(false)
serverMessage.set_server_success_message("")
}
// handleSubmit
const handleSubmit = async (e) => {
e.preventDefault();
set_success_message(null) //reset warning message when user tries to submit form data
serverMessage.set_server_success_message("")
// set_err_message(false)
if (!data_inputs.nameOfDepositor || data_inputs.amount <= 0 || !data_inputs.recipient_account_number) {
return set_err_message(true);
} else {
set_err_message(false)
}
setSpinner(true);
const { data } = await api.deposit(data_inputs);
// check if transfer was successful
if (data) {
setSpinner(false);
set_success_message(data.message);
set_data_inputs(initialValues);
// check if the account number input is correct to return the correct details of user
if (data.self) {
setUser({ ...user, balance: data.userBalance });
set_Self(true);
serverMessage.set_server_success_message(data.message);
}
}
}
useEffect(() => {
// i want to check if pathname is equal to the pathname receiving and updating message from server
if ((pathname === "/user/withdraw" || pathname === "/user/transfer") && serverMessage.server_success_message) {
// reset the server success message to null to stop the message from displaying
serverMessage.set_server_success_message("");
// update the pathname to keep track of page location to make decision on the server success message
setPathname(history.location.pathname);
} else {
setPathname(history.location.pathname);
}
}, []);
return (
<div className='container-fluid'>
<h1>Welcome, {user ? `${user.firstname}` : "user"}!</h1>
<form className='deposit-form' onSubmit={handleSubmit}>
<div className="mb-3">
<label htmlFor="nameOfDepositor" className="form-label">Name of Depositor</label>
<input value={data_inputs.nameOfDepositor} type="text" name='nameOfDepositor' className="form-control" placeholder='enter depositors name' value={data_inputs.nameOfDepositor} onChange={handleInputChange} />
</div>
<div className="mb-3">
<label htmlFor="recipient_account_number" className="form-label">Recipient account number</label>
<input value={data_inputs.recipient_account_number} type="text" name='recipient_account_number' className="form-control" placeholder='enter recipients account number' value={data_inputs.recipient_account_number} onChange={handleInputChange} />
</div>
<div className="mb-3">
<label htmlFor="amount" className="form-label">Enter amount</label>
<input value={data_inputs.amount} type="number" name='amount' className="form-control" placeholder='0' value={data_inputs.amount} onChange={handleInputChange} />
</div>
<button type="submit" className="btn btn-primary">Deposit</button>
{
err_message && (<div className="deposit-warning">Please fill the form. Amount should be greater than 0</div>)
}
<br /><br />
{
// server success message from parent component, this is to solve problem of component rerendering
serverMessage.server_success_message && (<div className='text-success'>{serverMessage.server_success_message}</div>)
}
{
spinner && (<div className="spinner-border text-primary" role="status">
<span className="visually-hidden">Loading...</span>
</div>)
}
{
// success message when deposit is complete
(success_message) ? (<div className='deposit-transfer'>{success_message}</div>) : (<div className='deposit-transfer'>{success_message}</div>)
}
</form>
</div>
)
}
export default Deposit;<file_sep>import React, { useState, useEffect } from 'react';
import { useHistory } from 'react-router-dom';
import "./style.css";
import { transfer } from '../../API/api';
const initialValues = { amount: "", recipient_account_number: "" };
const Transfer = ({ user, setUser, serverMessage, pathname, setPathname }) => {
const history = useHistory();
const [data_inputs, set_data_inputs] = useState(initialValues);
const [message, set_message] = useState("");
const [spinner, setSpinner] = useState(false);
const [show_dialogue_box, set_show_dialogue_box] = useState(false);
const [active_button, set_active_button] = useState(false);
const [err_message, set_err_message] = useState(false);
// handle input change
const handleInputChange = e => {
// only allow input value for amount greater than 0
if (e.target.type === "number") {
if (e.target.value < 0 || !Number(e.target.value)) set_data_inputs({ ...data_inputs, [e.target.name]: '' })
}
set_data_inputs({ ...data_inputs, [e.target.name]: e.target.value });
set_message("");
serverMessage.set_server_success_message("")
}
// handle submit button
const handleSubmit = async (e) => {
e.preventDefault();
// show dialogue box for further decision
set_show_dialogue_box(true);
serverMessage.set_server_success_message("")
};
// handle close dialogue box
const closeDialogueBox = () => {
set_show_dialogue_box(false);
}
// handle continue box
const handleContinueButton = async () => {
// make api call to make transfer
setSpinner(true);
set_message("");
serverMessage.set_server_success_message("")
const res = await transfer(data_inputs);
const { data } = res;
if (data) {
setSpinner(false)
set_message(data.message);
set_show_dialogue_box(false);
if (data.success) {
setUser({ ...user, balance: data.userBalance })
serverMessage.set_server_success_message(data.message)
}
}
};
// handle dialogue box
const confirmBox = () => {
return (
<div className='card confirm-box'>
<p>Dear {user && user.firstname},</p>
<p>You are about to transfer ₦{data_inputs.amount} from your account</p>
<p>to {data_inputs.recipient_account_number}</p>
<p>Click on continue to make the transfer</p>
<div className="btn-group">
<button className='btn btn-danger close-btn' disabled={spinner && true} onClick={closeDialogueBox}>Close</button>
<button className='btn btn-primary continue-btn' disabled={spinner && true} onClick={handleContinueButton}>Continue</button>
</div>
</div>
)
}
// update component when user types into the input field
useEffect(() => {
if (data_inputs.recipient_account_number && data_inputs.amount) {
set_err_message(false)
return
}
else {
set_err_message(true)
}
}, [data_inputs]);
// clear server message if there is any when component mounts
useEffect(() => {
// i want to check if pathname is equal to the pathname receiving and updating message from server
if ((pathname === "/user/withdraw" || pathname === "/user/deposit") && serverMessage.server_success_message) {
// reset the server success message to null to stop the message from displaying
serverMessage.set_server_success_message("");
// update the pathname to keep track of page location to make decision on the server success message
setPathname(history.location.pathname);
} else {
setPathname(history.location.pathname);
}
}, []);
return (
<div className='container-fluid'>
<h1>Welcome, {user ? `${user.firstname}` : "user"}!</h1>
<form className='transfer-form' onSubmit={handleSubmit}>
<div className="mb-3">
<label htmlFor="recipient_account_number" className="form-label">Recipient account number</label>
<input value={data_inputs.recipient_account_number} type="text" name='recipient_account_number' className="form-control" placeholder='enter recipients account number' value={data_inputs.recipient_account_number} onChange={handleInputChange} />
</div>
<div className="mb-3">
<label htmlFor="amount" className="form-label">Enter amount</label>
<input value={data_inputs.amount} type="number" name='amount' className="form-control" placeholder='0' value={data_inputs.amount} onChange={handleInputChange} />
</div>
<button type="submit" className="btn btn-primary transfer-btn" disabled={err_message || spinner && true} >Transfer</button>
{
(!message && err_message || serverMessage.server_success_message) && (<div className="transfer-warning warning">Please fill the form</div>)
}
{
message && (<div className="transfer-warning warning">{message}</div>)
}
{
serverMessage.server_success_message && (<div className="text-success">{serverMessage.server_success_message}</div>)
}
<br /><br />
{
spinner && (<div className="spinner-border text-primary" role="status">
<span className="visually-hidden">Loading...</span>
</div>)
}
</form>
<div className='confirm-box-container'>
{show_dialogue_box ? confirmBox() : null}
</div>
</div>
)
}
export default Transfer;<file_sep>import React, { useEffect, useState } from 'react';
import "./style.css";
import History_action from "../general_action/action";
import History from "../general_action/History";
const Transaction_history = ({user}) => {
const [history, setHistory] = useState([]);
const [account_holder_name, set_acc_holder_name] = useState("");
return (
<div className="container-fluid transaction-history history">
<History_action button_name="Check transactions" type="transactions" setHistory={setHistory} user={user} set_acc_holder_name={set_acc_holder_name} />
{/* <div className="view-deposit-history">view transactions here</div> */}
<History history={history} type="transactions" user={user} name={account_holder_name} />
</div>
)
}
export default Transaction_history;<file_sep>import React from 'react';
import { Route, Redirect } from 'react-router-dom';
const PublicRoutes = ({component: Component, user, ...rest}) => {
return (
<Route {...rest} render={props => {
// check if user is not authenticated yet
if(user) {
return <Redirect to={{pathname: "/", state: {from: props.location}}} />
}
// otherwise return the user to the right page for authentication
return <Component {...props} />
}} />
)
}
export default PublicRoutes;<file_sep>import React, { useEffect, useState } from "react";
import { useHistory } from "react-router-dom";
import { withdraw } from '../../API/api';
import './style.css';
const initial_value = { amount: "", account_number: "" };
const Withdraw = ({ user, setUser, serverMessage, pathname, setPathname }) => {
const history = useHistory();
const [data_input, set_data_input] = useState(initial_value);
const [show_dialogue_box, set_show_dialogue_box] = useState(false);
const [activate_button, set_activate_button] = useState(false);
const [message, setMessage] = useState(null);
const [spinner, setSpinner] = useState(false);
const [toggleBtn, setToggleBtn] = useState(false);
// handle input change
const handleInputChange = e => {
if (e.target.type === "number") {
if (e.target.value < 0 || !Number(e.target.value)) set_data_input({ [e.target.name]: '' })
else {
set_data_input({ ...data_input, [e.target.name]: e.target.value });
}
} else {
set_data_input({ ...data_input, [e.target.name]: e.target.value });
}
serverMessage.set_server_success_message("")
}
// handle form submission
const handleFormSubmission = e => {
e.preventDefault();
set_show_dialogue_box(true);
serverMessage.set_server_success_message("")
}
// handle dialogue box continue button
const handleContinueButton = async () => {
setSpinner(true);
const { data } = await withdraw(data_input);
if (data) {
setSpinner(false)
setMessage(data.message);
serverMessage.set_server_success_message("")
set_show_dialogue_box(false);
set_data_input(initial_value);
if (data.success) {
setUser({ ...user, balance: data.userBalance })
// set message to display to user
// set the response message from server
serverMessage.set_server_success_message(data.message);
}
}
}
useEffect(() => {
if (!data_input.amount) set_activate_button(false)
else set_activate_button(true);
}, [data_input])
// dialogue box to confirm transaction
const confirmBox = () => {
return (
<div className='card confirm-box'>
<p>Dear {user && user.firstname},</p>
<p>You want to withdraw ₦{data_input.amount} {data_input.account_number ? " from " + data_input.account_number + "." : "from your account."}</p>
<p>Click on continue to carry on with the transaction</p>
<div className="btn-group">
<button className='btn btn-danger close-btn' disabled={spinner && true} onClick={closeDialogueBox}>Close</button>
<button className='btn btn-primary continue-btn' disabled={spinner && true} onClick={handleContinueButton}>Continue</button>
</div>
</div>
)
}
// handle close dialogue box
const closeDialogueBox = () => {
set_show_dialogue_box(false);
}
// toggle button handler
const toggle = () => {
setToggleBtn(!toggleBtn);
}
// clear server message if there is any when component mounts
useEffect(() => {
// i want to check if pathname is equal to the pathname receiving and updating message from server
if ((pathname === "/user/transfer" || pathname === "/user/deposit") && serverMessage.server_success_message) {
// reset the server success message to null to stop the message from displaying
serverMessage.set_server_success_message("");
// update the pathname to keep track of page location to make decision on the server success message
setPathname(history.location.pathname);
} else {
setPathname(history.location.pathname);
}
}, []);
return (
<div className='container'>
<div className="admin_statement_decision">
{/* this div enables an admin to check for account holder history, this button is disabled for non admin account holders */
user?.admin_user && (<div>
<h6>Admin, you can also withdraw for non-admin account holder.</h6>
<p>Click the button below to toggle right and display input field</p>
<p>This is where you enter users account number for withdrawal</p>
<div className="check_statement_of_account_toggle">
<div className={`toggle_btn ${toggleBtn ? "toggle" : ""}`} onClick={toggle} ></div>
</div>
</div>)
}
</div>
<form className='withdrawal-form' onSubmit={handleFormSubmission}>
{
// display only for admins and togglebtn is on
toggleBtn && (
<div className="mb-3">
<label htmlFor="amount" className="form-label">Account number</label>
<input value={initial_value.account_number} type="text" name='account_number' className="form-control" placeholder='users account number' value={data_input.account_number} onChange={handleInputChange} />
</div>
)
}
<div className="mb-3">
<label htmlFor="amount" className="form-label">Amount</label>
<input value={initial_value.amount} type="number" name='amount' className="form-control" placeholder='0' value={data_input.amount} onChange={handleInputChange} />
</div>
<button type="submit" className="btn btn-primary withdraw-btn" disabled={activate_button ? false : true}>Withdraw</button>
<br />
{
// success message when withdrawal is complete
(<div className='withdraw-amount'>{message ? message : ""}</div>)
}
{
// server success message from parent component, this is to solve problem of component rerendering
serverMessage.server_success_message && (<div className='text-success'>{serverMessage.server_success_message}</div>)
}
</form>
<br />
{
spinner && (<div className="spinner-border text-success" role="status">
<span className="visually-hidden">Loading...</span>
</div>)
}
<div className='confirm-box-container'>
{show_dialogue_box ? confirmBox() : null}
</div>
</div>
)
}
export default Withdraw;<file_sep>import React, { useContext, useEffect, useState } from 'react';
import { BrowserRouter, Switch, Route } from 'react-router-dom'
// import { AuthContext } from "./Context/AuthContext";
import Navbar from './components/navbar/Navbar';
import Home from './components/home/Home';
import Footer from './components/footer/Footer';
import Signup from './components/signup/Signup';
import Signin from './components/signin/Signin';
import Deposit from './components/deposit/Deposit';
import Withdraw from './components/withdraw/Withdraw';
import Deposit_history from './components/history/deposit_history/Deposit_history';
import Transaction_history from './components/history/transaction_history/Transaction_history';
import Withdrawal_history from './components/history/withdrawal_history/Withdrawal_history';
import Transfer_history from "./components/history/transfer_history/Transfer_history";
import Transfer from "./components/transfer/Transfer";
import Manage_users from './components/manage_users/Manage_users';
import { Not_Found } from './components/not_found/Not_Found';
import * as api from "./API/api";
import PrivateRoutes from './HOC/PrivateRoutes';
import PublicRoutes from './HOC/PublicRoutes';
const App = () => {
// set user from props if user token still persist
const [user, setUser] = useState(null);
const [server_success_message, set_server_success_message] = useState(null);
const [pathname, setPathname] = useState(null);
// component did mount to fetch user if user is signed in with token key;
useEffect(async () => {
const response_payload = await api.authenticatedUser();
// check if meet was unable to get response payload
if(!response_payload) {
// meaning that the request payload is undefined, exit the function
return
} else {
// there is a response payload from server
const { data } = response_payload;
if (data) {
// set the user object with data from response payload
setUser(data.user);
}
}
}, []);
return (
<BrowserRouter>
<div className='container-fluid'>
<Navbar userProp={user} setUser={setUser} />
<Switch>
<Route path="/" exact component={() => <Home props={{ user }} />} />
<PublicRoutes path="/signup" user={user} component={Signup} />
{/* <Route path="/signup" component={Signup} /> */}
<PublicRoutes path="/signin" user={user} component={() => <Signin setUser={setUser} />} />
{/* <Route path="/signin" component={() => <Signin setUser={setUser} />} /> */}
<PrivateRoutes path="/user/deposit" user={user} role={[true]} component={() => <Deposit pathname={pathname} setPathname={setPathname} user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} />
{/* <Route path='/user/deposit' component={() => <Deposit user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} /> */}
<PrivateRoutes path='/user/withdraw' user={user} role={[true, false]} component={() => <Withdraw pathname={pathname} setPathname={setPathname} user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} />
{/* <Route path='/user/withdraw' component={() => <Withdraw user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} /> */}
<PrivateRoutes path='/user/transfer' user={user} role={[true, false]} component={() => <Transfer pathname={pathname} setPathname={setPathname} user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} />
{/* <Route path='/user/Transfer' component={() => <Transfer user={user} setUser={setUser} serverMessage={{server_success_message, set_server_success_message}} />} /> */}
<PrivateRoutes path='/history/deposits' user={user} role={[true, false]} component={() => <Deposit_history user={user} />} />
{/* <Route path='/history/deposits' component={() => <Deposit_history user={user} />} /> */}
<PrivateRoutes path='/history/transactions' user={user} role={[true, false]} component={() => <Transaction_history user={user} />} />
{/* <Route path='/history/transactions' component={() => <Transaction_history user={user} />} /> */}
<PrivateRoutes path="/history/withdrawals" user={user} role={[true, false]} component={() => <Withdrawal_history user={user} />} />
{/* <Route path="/history/withdrawals" component={() => <Withdrawal_history user={user} />} /> */}
<PrivateRoutes path="/history/transfers" user={user} role={[true, false]} component={() => <Transfer_history user={user} /> } />
{/* <Route path="/history/transfers" component={() => <Transfer_history user={user} /> } /> */}
{/* <Route path="/admin/users/manage" component={() => <Manage_users admin={user} />} /> */}
<PrivateRoutes path="/admin/users/manage" user={user} role={[true]} component={() => <Manage_users admin={user} />} />
{/* not found routes */}
<Route path='*' component={Not_Found} />
</Switch>
<Footer />
</div>
</BrowserRouter>
);
}
export default App;
<file_sep>import React, { useState } from 'react';
import './style.css';
import History_action from '../general_action/action';
import History from "../general_action/History";
const Deposit_history = ({user}) => {
const [history, setHistory] = useState([]);
const [account_holder_name, set_acc_holder_name] = useState("");
return (
<div className="container-fluid deposit-history history">
<History_action button_name="Check Deposits" type="deposits" setHistory={setHistory} user={user} set_acc_holder_name={set_acc_holder_name} />
{/* <div className="view-deposit-history">view result here</div> */}
<History history={history} type="deposits" user={user} name={account_holder_name} />
</div>
)
}
export default Deposit_history;<file_sep>import React, { useEffect, useState } from 'react';
import { history } from "../../../API/api"
import "./style.css";
const History_action = (props) => {
const initialValues = { starting_date: "", ending_date: "", user_account_number: "", type: props.type }
// set values for transactions
const [data_inputs, set_data_inputs] = useState(initialValues);
const [activate_button, set_activate_button] = useState(false);
const [message, set_message] = useState(null);
const [spinner, setSpinner] = useState(false);
const [toggleBtn, setToggleBtn] = useState(false);
// handle input values
const handleDataInput = e => {
const target = e.target;
const name = target.name;
const value = target.value;
set_data_inputs({ ...data_inputs, [name]: value });
};
// handle form submission
const handleFormSubmission = async (e) => {
e.preventDefault();
setSpinner(true)
set_message(null);
// clear input account number from previous input for a user account statement
if (!toggleBtn) {
data_inputs.user_account_number = "";
}
const { data } = await history(data_inputs);
if (data) {
setSpinner(false);
// check if there was no history delivered in case for a user with no transaction records yet
if (data.message || !data.historySuccess) return set_message(data.message);
// otherwise pass the history data to the component
const { transactions, name } = data;
props.setHistory(transactions);
props.set_acc_holder_name(name);
}
}
// toggle button handler
const toggle = () => {
setToggleBtn(!toggleBtn)
if (!toggleBtn) {
set_data_inputs({ ...data_inputs, user_account_number: "" });
}
}
return (
<div className="card action_card">
<div className="admin_statement_decision">
{/* this div enables an admin to check for account holder history, this button is disabled for non admin account holders */
props.user?.admin_user && (<div>
<h6>Admin can also check for a non-admin account holder.</h6>
<div className="check_statement_of_account_toggle">
<div className={`toggle_btn ${toggleBtn ? "toggle" : ""}`} onClick={toggle} ></div>
</div>
</div>)
}
</div>
<form onSubmit={handleFormSubmission}>
{
// admin to enter account number of the user he wants to check the statements
toggleBtn && (<div>
<label htmlFor="user_account_number">User account number:</label><br />
<input type="text" name="user_account_number" value={data_inputs.user_account_number} onChange={handleDataInput} placeholder='enter account number' />
</div>)
}
<label htmlFor="date">From:</label> <br />
<input type="date" id="from_date" name="starting_date" value={data_inputs.starting_date} onChange={handleDataInput} /> <br />
<label htmlFor="date">To:</label> <br />
<input type="date" id="to_date" name="ending_date" value={data_inputs.ending_date} onChange={handleDataInput} /><br />
<button className='btn btn-primary history-btn' >{props.button_name}</button>
</form>
{
spinner && (<div className="spinner-border text-primary" role="status">
<span className="visually-hidden">Loading...</span>
</div>)
}
{
// display error messages
message && (<div className='text-danger'>{message}</div>)
}
</div>
)
}
export default History_action;<file_sep>import React, { useState, useEffect } from 'react';
import { Link, useHistory } from 'react-router-dom';
import * as api from '../../API/api';
import './style.css';
const Navbar = ({userProp, setUser}) => {
const history = useHistory();
// handle user unauthentication
const signOut = async () => {
const { data } = await api.logoutUser();
if(data.success) {
setUser(null)
history.push("/signin");
const windowLocation = window.location.reload(); //forces reload on browser to clear cache and store values
}
}
return (
<header>
<div className='top-header'>
<a className="navbar-brand brand" href="/">Veegil Inc.</a>
<div className='header-contents'>
<div className='left-nav'>
<Link className="home-link" to="/">Home</Link>
{
// only display balance when a user is authenticated and logged in
(userProp && userProp.balance) && (<span> Balance: ₦{userProp.balance}</span>)
}
</div>
{
// check if a user exist to disable sign in button
(!userProp) ? (<button className='btn btn-primary'><Link to='/signin'>Sign in</Link></button>) : (
<div className='user-settings'>
<div className='action-area'>
<span>{userProp?.firstname[0]}</span>
<button className='btn btn-light' onClick={signOut}>Sign out</button>
</div>
</div>
)
}
</div>
</div>
{
// check if a user exists before displaying the action nav links
(userProp && userProp._id) && (
<nav className="navbar navbar-expand-lg navbar-light bg-light nav-color">
<div className="container-fluid">
<button className="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNavAltMarkup" aria-controls="navbarNavAltMarkup" aria-expanded="false" aria-label="Toggle navigation">
<span className="navbar-toggler-icon"></span>
</button>
<div className="collapse navbar-collapse" id="navbarNavAltMarkup">
<div className="navbar-nav">
{
// only admins can make deposits to users according to users cash at hand
(userProp && userProp.admin_user) && (<Link className="nav-link active" to="/user/deposit">Deposit</Link>)
}
<Link className="nav-link active" to="/user/withdraw" >Withdraw</Link>
<Link className="nav-link active" to="/user/transfer" >Transfer</Link>
<div className="nav-item dropdown">
<Link className="nav-link active dropdown-toggle" to="#" id="navbarDarkDropdownMenuLink" role="button" data-bs-toggle="dropdown" aria-expanded="false">
History
</Link>
<ul className="dropdown-menu dropdown-menu-dark" aria-labelledby="navbarDarkDropdownMenuLink">
<li><Link className="dropdown-item" to="/history/transactions">All transactions History</Link></li>
<li><Link className="dropdown-item" to="/history/deposits">Deposits History</Link></li>
<li><Link className="dropdown-item" to="/history/withdrawals">Withdrawals History</Link></li>
<li><Link className="dropdown-item" to="/history/transfers">Transfers History</Link></li>
</ul>
</div>
{
/* check if the user is an admin to display the manage users link */
(userProp && userProp.admin_user) && (<Link className='nav-link active' to='/admin/users/manage'>Manage users</Link>)
}
</div>
</div>
</div>
</nav>
)
}
</header>
)
}
export default Navbar;<file_sep>import React, {useState, useEffect} from 'react';
import './style.css';
const Footer = () => {
return (
<footer>
© <NAME> 2021
</footer>
)
}
export default Footer;<file_sep>import React, {useState} from "react";
import "./style.css";
import History_action from "../general_action/action";
import History from "../general_action/History";
const Withdrawal_history = ({user}) => {
const [history, setHistory] = useState([]);
const [account_holder_name, set_acc_holder_name] = useState("");
return (
<div className="container-fluid withdrawal-history history">
<History_action button_name="Check withdrawals" type="withdrawals" setHistory={setHistory} user={user} set_acc_holder_name={set_acc_holder_name} />
{/* <div className="view-deposit-history"><></div> */}
<History history={history} type="withdrawals" user={user} name={account_holder_name} />
</div>
)
}
export default Withdrawal_history;<file_sep>import axios from 'axios';
// "proxy": "http://localhost:5000"
// create an API instance to make calls to the base url
// production
const API = axios.create({ baseURL: "https://veegil-b.herokuapp.com/user", withCredentials: true });
// development
// const API = axios.create({ baseURL: "http://localhost:5000/user", withCredentials: true, });
// axios interceptor when a user tampers his token or the token expires
API.interceptors.response.use(
(response) =>
new Promise((resolve, reject)=>{
resolve(response);
}),
(error) => {
// check if error is coming from front end and not server
if(!error.response) {
return new Promise((resolve, reject)=>{
reject(error);
});
}
// otherwise check if the response is forbidden or unauthentication
if (error.response.status === 401 || error.response.status === 403) {
// check if window location is on auth to terminate browser refresh
if(window.location.pathname == "/signin") {
return
} else {
// redirect user to sign in screen
return window.location='/signin';
}
} else {
return new Promise((resolve, reject)=>{
reject(error);
});
}
}
);
// export const logoutUser = () => API.get('/signout'); dev
export const logoutUser = () => API.get('/signout', {credentials: "include"}); //production
export const signInUser = (formData) => API.post('/signin', formData);
export const createUser = (formData) => API.post('/signup', formData);
export const deposit = (formData) => API.post('/deposit', formData);
export const withdraw = (formData) => API.post('/withdraw', formData);
export const transfer = (formData) => API.post('/transfer', formData);
export const history = (date) => API.post("/history", date);
export const authenticatedUser = () => API.get('/authenticated');
export const getUsers = () => API.get('/admin/manage');
export const delete_a_user = (id) => API.delete(`/admin/manage/${id}`);
<file_sep>import React, { useEffect, useState } from 'react';
import { Link } from 'react-router-dom';
import * as api from '../../API/api';
import './style.css'
// initial values before input
const initialValues = { firstname: "", lastname: "", email: "", confirm_email: "", phone_number: "", password: "", confirm_password: "", admin_user: false, pledge_accuracy: "" };
const Signup = () => {
const [inputData, setInputData] = useState(initialValues); //set initial values of input
const [activate_submit_button, set_activate_submit_button] = useState(false); // keeps the submission button active or inactive
const [password_check, set_password_check] = useState(false); // to confirm input passwords
const [email_check, set_email_check] = useState(false); // to compare input emails
const [warning_message, set_warning_messsage] = useState(null); //activates or deactivates warning messages
const [spinner, setSpinner] = useState(false);
const [signup_success, setSignup_success] = useState(false);
const handleInputChange = e => {
const target = e.target;
const value = target.type === "checkbox" ? target.checked : target.value; // get input values or check values
const name = target.name;
setInputData({ ...inputData, [name]: value });
setSignup_success(false);
};
// check box uncheck handler
const checkProps = ["pledge_accuracy", "admin_user"]
const uncheck = (checkedProps) => {
let i = 0;
while (i < checkedProps.length) {
if (document.getElementById(checkedProps[i]).checked) {
document.getElementById(checkedProps[i]).checked = false;
}
++i
}
}
// handle form data submission
const handleSubmit = async (e) => {
e.preventDefault();
// activate warning message if fields are not correct
if (email_check || password_check) return set_warning_messsage("Please make sure your entries are matching!")
else {
setSpinner(true);
set_warning_messsage(null);
setSignup_success(false);
const { data } = await api.createUser(inputData);
if (data) {
setSpinner(false)
set_warning_messsage(data.message);
// check the message received if sign up was successful
if (data.message === "Signed up successfully") {
setInputData(initialValues);
set_activate_submit_button(false);
setSignup_success(true);
uncheck(checkProps);
}
}
}
}
useEffect(() => {
// check if the fileds have been properly supplied with values
const dataKeys = Object.keys(inputData).filter(value => value !== "admin_user");
const length_of_value = dataKeys.length;
let count = 0;
for (let i = 0; i < dataKeys.length; ++i) {
if (inputData[dataKeys[i]]) count++
}
// check if the count is equal to the input fields containing actual values, set the activate button
if (count === length_of_value) set_activate_submit_button(true)
else set_activate_submit_button(false);
// check if the password inputs are thesame
if (inputData.password && inputData.confirm_password)
if (inputData.confirm_password !== inputData.password) set_password_check(true)
else set_password_check(false);
// check if email inputs are the same
if (inputData.email && inputData.confirm_email)
if (inputData.email !== inputData.confirm_email) set_email_check(true)
else set_email_check(false);
}, [inputData])
return (
<div className='sign-up container-fluid'>
<form className='sign-up-form' onSubmit={handleSubmit}>
<div className="mb-3">
<label htmlFor="firstname" className="form-label">First name</label>
<input value={inputData.firstname} type="text" name='firstname' className="form-control" placeholder='enter your first name' onChange={handleInputChange} required />
</div>
<div className="mb-3">
<label htmlFor="lastname" className="form-label">Last name</label>
<input value={inputData.lastname} type="text" name='lastname' className="form-control" placeholder='enter your last name' onChange={handleInputChange} required />
</div>
<div className="mb-3">
<label htmlFor="inputEmail1" className="form-label">Email address</label>
<input value={inputData.email} type="email" name='email' className="form-control" placeholder='enter email' onChange={handleInputChange} required />
<div id="emailHelp" className="form-text">We'll never share your email with anyone else.</div>
</div>
<div className="mb-3">
<label htmlFor="inputEmail1" className="form-label">Confirm Email address</label>
<input value={inputData.confirm_email} type="email" name='confirm_email' className="form-control" placeholder='confirm email' onChange={handleInputChange} required />
{
// check if email are thesame
email_check && (<div id='email_match'>Email do not match</div>)
}
</div>
<div className="mb-3">
<label htmlFor="phone_number" className="form-label">Phone number</label>
<input value={inputData.phone_number} type="text" className="form-control" name="phone_number" placeholder='enter phone number' onChange={handleInputChange} required />
</div>
<div className="mb-3">
<label htmlFor="lastname" className="form-label">Password</label>
<input value={inputData.password} type="<PASSWORD>" name='password' className="form-control" placeholder='enter password' onChange={handleInputChange} required />
</div>
<div className="mb-3">
<label htmlFor="lastname" className="form-label">Confirm Password</label>
<input value={inputData.confirm_password} type="password" name='confirm_password' className="form-control" placeholder='confirm password' onChange={handleInputChange} required />
{
// check if passwords match
password_check && (<div id="password_match" className="form-text">Password do not match</div>)
}
</div>
<div className="mb-3 form-check">
<input value={inputData.admin_user} type="checkbox" className="form-check-input" id='admin_user' name="admin_user" onChange={handleInputChange} />
<label className="form-check-label" htmlFor="admin_user">Admin user? This is not compulsory, only for admin accounts</label>
</div>
<div className="mb-3 form-check">
<input value={inputData.pledge_accuracy} type="checkbox" className="form-check-input" id='pledge_accuracy' name="pledge_accuracy" onChange={handleInputChange} />
<label className="form-check-label" htmlFor="pledge_acuracy">Pledge accuracy</label>
</div>
<button type="submit" disabled={activate_submit_button ? false : true} className="btn btn-success btn-signup">Sign up</button>
{
// display error or success messages
warning_message && (<div className={signup_success ? "text-success" : "warning"}>{signup_success ? (<span>{warning_message}. <Link to='/signin'>Please sign in </Link> to continue</span>) : warning_message}</div>)
}
<br />
{
spinner && (<div className="spinner-border text-success" role="status">
<span className="visually-hidden">Loading...</span>
</div>)
}
<div className='signup_div'>
<span className='decoration_line'></span>
<span className='decoration_text'>or</span>
<span className='decoration_line'></span>
</div>
<div>Have an account? <Link to='/signin'>Sign in</Link></div>
</form>
<div className='brand-description sign-up-container'>
<h1>Veegil.</h1>
<p>Banking with Better Experience!</p>
</div>
</div>
)
}
export default Signup;<file_sep>import React, { useState, useEffect } from 'react';
import "./style.css"
const History = ({ history, type, user, name }) => {
const displayHistory = history.length ? history.map((item, index) => {
const hour = Number(item.time.split("T")[1].split(".")[0].split(":")[0]) + 1;
const min_sec = item.time.split("T")[1].split(".")[0].split(":");
const time_format = hour < 10 ? "0" + hour + ":" + min_sec.slice(1).join(":") : hour+ ":" + min_sec.slice(1).join(":")
return (
<tbody key={item._id}>
<tr className='table-data'>
<th scope="row">{index + 1}</th>
<td>{item.time.split("T")[0]}</td>
<td>{time_format}</td>
<td>{item.transaction}</td>
<td>{item.type}</td>
<td>₦{item.amount}</td>
{(type === "transactions" || type === "deposits") && <td>{item.nameOfDepositor ? item.nameOfDepositor : "--"}</td>}
{(type === "transactions" || type === "transfers") && <td>{item.recipient_account_number ? item.recipient_account_number : "--"}</td>}
{(type === "transactions" || type === "transfers") && <td>{item.account_holder_name ? item.account_holder_name : "--"}</td>}
</tr>
</tbody>
)
}) : null;
return (
<div className="view-deposit-history">
{history.length ? <h5 className='text-success'>Statement of account for {history.length && name}!</h5> : null }
{history.length ? <p className='history-warning-text text-danger'>This statement is best viewed in a large screen</p> : null}
{history.length ? <div className="table-data">
<table className="table table-dark table-striped">
<thead>
<tr>
<th scope="col">S/n</th>
<th scope="col">Date</th>
<th scope="col">Time</th>
<th scope="col">Transaction</th>
<th scope="col">Type</th>
<th scope="col">Amount</th>
{(type === "transactions" || type === "deposits") && (<th scope="col">Depositor Name</th>)}
{(type === "transactions" || type === "transfers") && (<th scope="col">Receivers acc num.</th>)}
{(type === "transactions" || type === "transfers") && (<th scope="col">Receivers acc name</th>)}
</tr>
</thead>
{displayHistory}
</table>
</div> : null }
</div>
)
}
export default History; | 1b8de124ec9c28adb76fba793ee996bd68aebbae | [
"JavaScript"
] | 14 | JavaScript | KellsCodes/veegil-bank-client | 89ea8e33e0db501f0084b4b89fcb257a773c3de7 | af878660f74e56fca9b6092b39ac72f2733ef68e | |
refs/heads/main | <repo_name>vgurunathaprasad/myHappyParking<file_sep>/parkmycar.php
<?php
echo "park my car page";
?><file_sep>/rentmyparking.php
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$name = "parkings";
$file_name = $name . '.json';
$current_data = file_get_contents($file_name);
$array_data = json_decode($current_data, true);
$extra = array(
'fullName' => $_POST['fullName'],
'contactNumber' => $_POST['contactNumber'],
'emailAddress' => $_POST['emailAddress'],
'parkingLocation' => $_POST['parkingLocation'],
);
$array_data[] = $extra;
$final_data = json_encode($array_data);
if(file_put_contents("$file_name", $final_data)) {
echo $file_name .' data added';
}
else {
echo 'There is some error';
}
}
?> | 4012ae6c4d47bc2d5d92d9e1fa361979fef114b8 | [
"PHP"
] | 2 | PHP | vgurunathaprasad/myHappyParking | 23df4731fc20ae9f1196bb2faaf839fb45c3b1fe | 81286af2d8d5d7fee3a770bb25cb5d2b4cd1df82 |