code
stringlengths 3
1.01M
| language
stringclasses 2
values |
---|---|
/*! supercache - v0.0.1
* Release on: 2014-12-13
* Copyright (c) 2014 Stéphane Bachelier
* Licensed MIT */
!function(a,b){"use strict";"function"==typeof define&&define.amd?define(function(){return b()}):"undefined"!=typeof exports?module.exports=b():a.supercache=b()}(this,function(){"use strict";var a=Object.create(null),b=function(b){a[b.req.url]={content:b.text,parse:b.req.parse[b.req.type]}},c=function(b){var c=a[b];return c&&c.content&&c.parse?c.parse(c.text):null},d=function(){a=null},e=function(a){a&&a.on("response",b)};return{writeToCache:e,readFromCache:c,clearCache:d}}); | Java |
from django.db import models
from django.utils import timezone
from django.contrib import admin
from packages.generic import gmodels
from packages.generic.gmodels import content_file_name,content_file_name_same
from datetime import datetime
from django.core.validators import MaxValueValidator, MinValueValidator
from django.conf import settings as stg
import os
import Image as PImage
from embed_video.fields import EmbedVideoField
# Create your models here.
class Conference(models.Model):
title = models.CharField(max_length=160)
def __str__(self):
return self.title
class Category(models.Model):
title = models.CharField(max_length=160)
position = models.PositiveIntegerField(default='0')
class Meta:
verbose_name_plural = 'categories'
def __str__(self):
return self.title
def save(self, *args, **kwargs):
model = self.__class__
if self.position is None:
# Append
try:
last = model.objects.order_by('-position')[0]
self.position = last.position + 1
except IndexError:
# First row
self.position = 0
return super(Category, self).save(*args, **kwargs)
class Code(models.Model):
title = models.CharField(max_length=250)
file = models.FileField(upload_to=content_file_name_same,blank=True)
git_link = models.URLField(blank=True)
programming_language = models.CharField(max_length=40)
details = models.TextField(max_length=600,blank=True)
def __str__(self):
return str(self.title)
class Publication(models.Model):
title = models.CharField(max_length=160)
authors = models.CharField(max_length=220,null=True)
link = models.URLField(null=True, blank=True)
file = models.FileField(upload_to=content_file_name_same, null=True, blank=True)
short = models.CharField(max_length=50,null=True)
bibtex = models.TextField(max_length=1000)
conference_id = models.ForeignKey(Conference)
year = models.PositiveIntegerField(default=datetime.now().year,
validators=[
MaxValueValidator(datetime.now().year + 2),
MinValueValidator(1800)
])
# def __init__(self, *args, **kwargs):
# super(Publication, self).__init__(*args, **kwargs)
# if int(self.conference_id.i) != 0:
# self.conference = Conference.objects.get(id=int(self.conference_id))
def __str__(self):
return self.title
def fullStr(self):
return "%s, \"%s\", %s, %s " % (self.authors, self.title, self.conference_id.title, self.year)
class Project(models.Model):
title = models.CharField(max_length=200)
text = models.TextField(max_length=500)
mtext = models.TextField(max_length=1000,blank=True)
date_created = models.DateTimeField(default=timezone.now)
category_id = models.ForeignKey(Category)
position = models.PositiveIntegerField(default='0')
publications = models.ManyToManyField(Publication, null=True, blank=True)
def save(self, *args, **kwargs):
model = self.__class__
if self.position is None:
# Append
try:
last = model.objects.order_by('-position')[0]
self.position = last.position + 1
except IndexError:
# First row
self.position = 0
return super(Project, self).save(*args, **kwargs)
def get_images(self):
return [y for y in ProjectImage.objects.filter(entity_id_id__exact=self.id)]
def getFirstImage(self):
try:
p = ProjectImage.objects.filter(entity_id_id__exact=self.id)[0]
except IndexError:
p = None
if None != p:
return p
else:
return "default.png"
def get_videos(self):
return [str(y) for y in ProjectVideo.objects.filter(entity_id_id__exact=self.id)]
def get_publications(self):
return [p for p in self.publications.all()]
class Meta:
ordering = ('position',)
def __str__(self):
return self.title
class ProjectImage(gmodels.GImage):
entity_id = models.ForeignKey(Project)
class ProjectVideo(models.Model):
entity_id = models.ForeignKey(Project)
link = EmbedVideoField(null=True) # same like models.URLField()
def __str__(self):
return str(self.link)
class ProjectImageInline(admin.TabularInline):
model = ProjectImage
extra = 1
readonly_fields = ('image_tag',)
class ProjectVideoInline(admin.TabularInline):
model = ProjectVideo
extra = 1
class ProjectAdmin(admin.ModelAdmin):
inlines = [ProjectImageInline, ProjectVideoInline, ]
class Media:
js = ('admin/js/listreorder.js',)
list_display = ('position',)
list_display_links = ('title',)
list_display = ('title', 'position',)
list_editable = ('position',)
class Article(models.Model):
title = models.CharField(max_length=200)
text = models.TextField(max_length=500)
date_created = models.DateTimeField(default=timezone.now)
category_id = models.ForeignKey(Category)
position = models.PositiveIntegerField(default='0')
def save(self, *args, **kwargs):
model = self.__class__
if self.position is None:
# Append
try:
last = model.objects.order_by('-position')[0]
self.position = last.position + 1
except IndexError:
# First row
self.position = 0
return super(Article, self).save(*args, **kwargs)
def get_images(self):
return [y for y in ArticleImage.objects.filter(entity_id_id__exact=self.id)]
def getFirstImage(self):
try:
p = ArticleImage.objects.filter(entity_id_id__exact=self.id)[0]
except IndexError:
p = None
if None != p:
return p
else:
return "default.png"
class Meta:
ordering = ('position',)
def __str__(self):
return self.title
class ArticleImage(gmodels.GImage):
entity_id = models.ForeignKey(Article)
class ArticleImageInline(admin.TabularInline):
model = ArticleImage
extra = 1
readonly_fields = ('image_tag',)
class ArticleAdmin(admin.ModelAdmin):
inlines = [ArticleImageInline, ]
class Media:
js = ('admin/js/listreorder.js',)
list_display = ('position',)
list_display_links = ('title',)
list_display = ('title', 'position',)
list_editable = ('position',)
class CodeSnippet(models.Model):
title = models.CharField(max_length=160)
programming_language = models.CharField(max_length=120)
text = models.TextField(max_length=500)
code = models.TextField(max_length=1000)
date_created = models.DateTimeField(default=timezone.now)
def __str__(self):
return self.title
| Java |
# Be sure to restart your server when you modify this file.
Rails.application.config.session_store :cookie_store, key: '_web-sockets-tutorial_session'
| Java |
title: 基于CAS实现前后端分离的SSO
date: 2020-05-17 21:15:22
categories: web
---
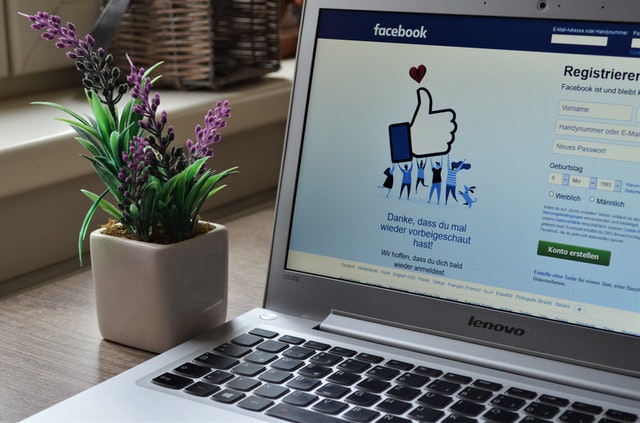
一种基于标准CAS,实现前后端分离的SSO的方案
<!--more-->
# 基础知识
一般同域的SSO,用共享session就可以实现了,常见于各微服务都是自己开发的情况。更普遍的场景是跨域集成的SSO,这时候一般采用标准的CAS方案。一个标准的CAS认证流程如下图,已经画得非常清楚了
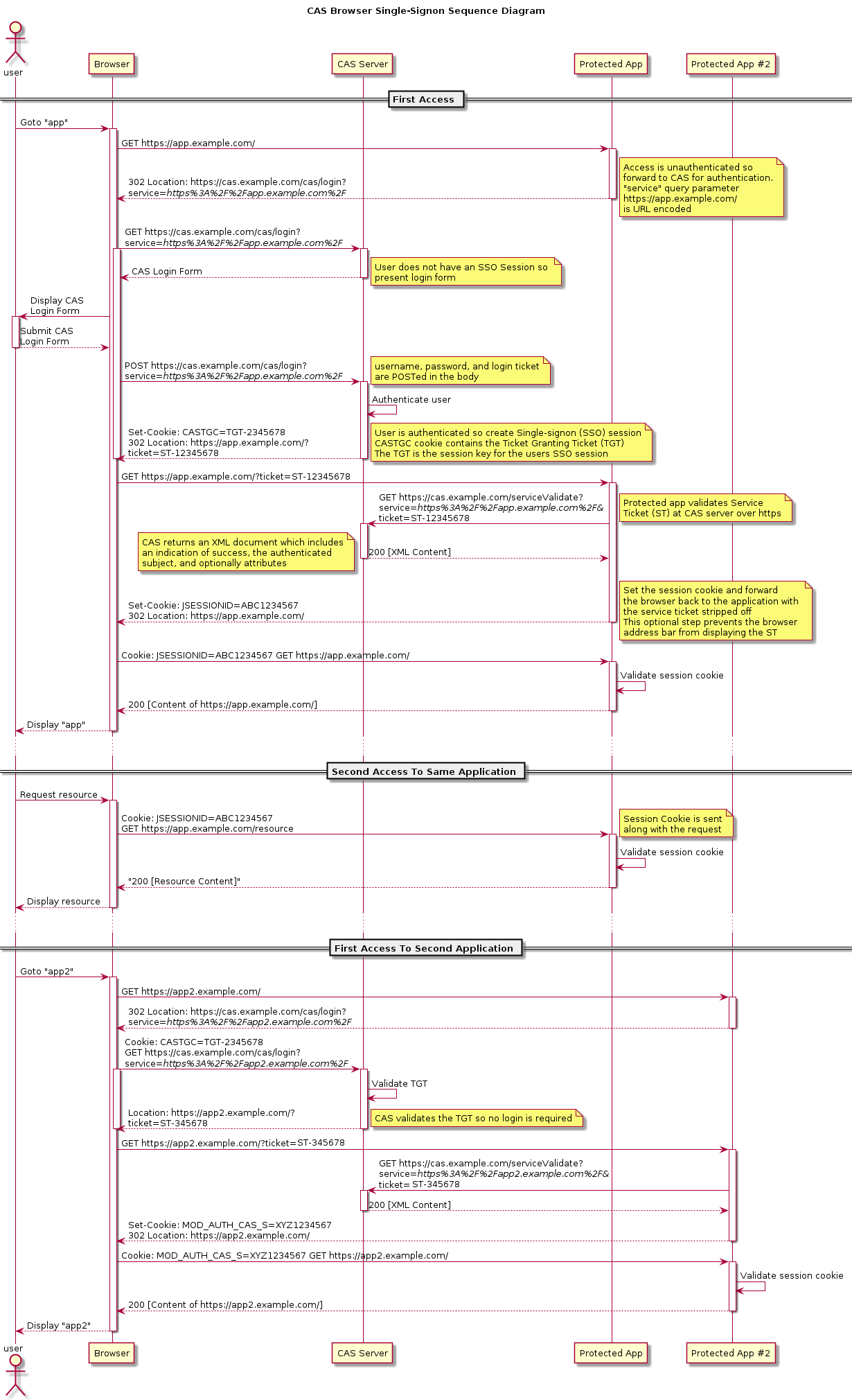
大致上是这样的一个流程,当用户请求应用服务器提供的某个服务`abc`,如果没有登录过,那么会返回一个302,跳转到`cas/login?service=abc`,在CAS登录后,又会302到`abc?ticket=STxxxxx`(这里abc就是前面service填的地址),应用服务器用这个ticket到CAS Server认证,如果OK的话,再一次302到`abc`,完成整个认证过程
## 重要的概念
TGT:Ticket Granting Ticket,这是CAS Server里保存的,用于认证用户已经在CAS登录过
TGC:Ticket Granting Cookie,跟上面的TGT对应,是已经认证过的用户在浏览器里保存的cookie
ST:Service Ticket,这是CAS Server发给应用服务器的凭证,应用服务器再用ST到CAS Server认证
## 常用filter
如果应用服务器是用JAVA写的,那么通常会用到下面几个filter:
一个是AuthenticationFilter,这个filter拦截请求,判断是否认证。如果没有认证,就跳转到CAS Server认证
另一个是TicketFilter,这个filter用于将ST拿到CAS Server认证
# 前后端分离的场景
现在web应用一般采用前后端分离的架构,通过ajax请求后端服务,这样会无法跳转回原来的静态页面,需要在CAS流程的基础上稍加改造
主要思路是让页面在发起业务请求之前,先确保登录完成。在后端提供auth服务用于鉴权,和validate服务用于验证ST,并跳转回初始静态页面,流程如下图
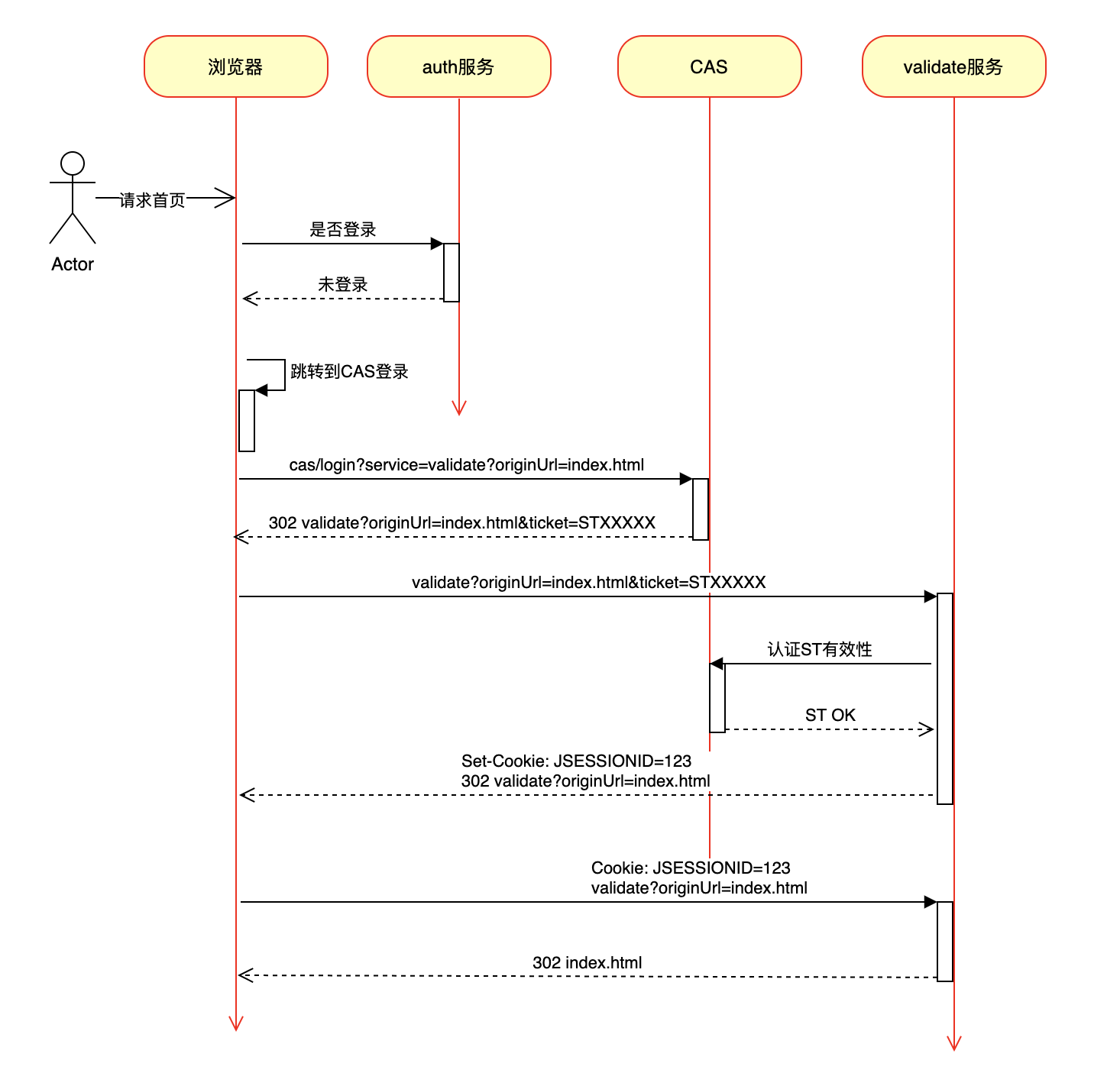
这种方式比起标准的CAS流程,主要差别在于不完全依赖于HTTP response code和浏览器默认行为,在前端javascript中做了控制。比如说普通的情况,后端是返回一个302 `cas/login?service=abc`,在这个方案里,跳转逻辑是直接写在前端javascript里的
这里对service参数做了定制并encoding,传的是`cas/login?service=abc?originUrl=xxx`,于是当CAS Server登录之后,返回的是302 `abc?originUrl=xxx&ticket=STxxxxx`,这里的xxx是验证完成后跳转回的原始静态页面 | Java |
<?php
// +-----------------------------------------------------------------------+
// | Piwigo - a PHP based photo gallery |
// +-----------------------------------------------------------------------+
// | Copyright(C) 2008-2014 Piwigo Team http://piwigo.org |
// | Copyright(C) 2003-2008 PhpWebGallery Team http://phpwebgallery.net |
// | Copyright(C) 2002-2003 Pierrick LE GALL http://le-gall.net/pierrick |
// +-----------------------------------------------------------------------+
// | This program is free software; you can redistribute it and/or modify |
// | it under the terms of the GNU General Public License as published by |
// | the Free Software Foundation |
// | |
// | This program is distributed in the hope that it will be useful, but |
// | WITHOUT ANY WARRANTY; without even the implied warranty of |
// | MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU |
// | General Public License for more details. |
// | |
// | You should have received a copy of the GNU General Public License |
// | along with this program; if not, write to the Free Software |
// | Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, |
// | USA. |
// +-----------------------------------------------------------------------+
/**
* @package functions\___
*/
include_once( PHPWG_ROOT_PATH .'include/functions_plugins.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_user.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_cookie.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_session.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_category.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_html.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_tag.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/functions_url.inc.php' );
include_once( PHPWG_ROOT_PATH .'include/derivative_params.inc.php');
include_once( PHPWG_ROOT_PATH .'include/derivative_std_params.inc.php');
include_once( PHPWG_ROOT_PATH .'include/derivative.inc.php');
include_once( PHPWG_ROOT_PATH .'include/template.class.php');
include_once( PHPWG_ROOT_PATH .'include/cache.class.php');
/**
* returns the current microsecond since Unix epoch
*
* @return int
*/
function micro_seconds()
{
$t1 = explode(' ', microtime());
$t2 = explode('.', $t1[0]);
$t2 = $t1[1].substr($t2[1], 0, 6);
return $t2;
}
/**
* returns a float value coresponding to the number of seconds since
* the unix epoch (1st January 1970) and the microseconds are precised
* e.g. 1052343429.89276600
*
* @return float
*/
function get_moment()
{
return microtime(true);
}
/**
* returns the number of seconds (with 3 decimals precision)
* between the start time and the end time given
*
* @param float $start
* @param float $end
* @return string "$TIME s"
*/
function get_elapsed_time($start, $end)
{
return number_format($end - $start, 3, '.', ' ').' s';
}
/**
* returns the part of the string after the last "."
*
* @param string $filename
* @return string
*/
function get_extension( $filename )
{
return substr( strrchr( $filename, '.' ), 1, strlen ( $filename ) );
}
/**
* returns the part of the string before the last ".".
* get_filename_wo_extension( 'test.tar.gz' ) = 'test.tar'
*
* @param string $filename
* @return string
*/
function get_filename_wo_extension( $filename )
{
$pos = strrpos( $filename, '.' );
return ($pos===false) ? $filename : substr( $filename, 0, $pos);
}
/** no option for mkgetdir() */
define('MKGETDIR_NONE', 0);
/** sets mkgetdir() recursive */
define('MKGETDIR_RECURSIVE', 1);
/** sets mkgetdir() exit script on error */
define('MKGETDIR_DIE_ON_ERROR', 2);
/** sets mkgetdir() add a index.htm file */
define('MKGETDIR_PROTECT_INDEX', 4);
/** sets mkgetdir() add a .htaccess file*/
define('MKGETDIR_PROTECT_HTACCESS', 8);
/** default options for mkgetdir() = MKGETDIR_RECURSIVE | MKGETDIR_DIE_ON_ERROR | MKGETDIR_PROTECT_INDEX */
define('MKGETDIR_DEFAULT', MKGETDIR_RECURSIVE | MKGETDIR_DIE_ON_ERROR | MKGETDIR_PROTECT_INDEX);
/**
* creates directory if not exists and ensures that directory is writable
*
* @param string $dir
* @param int $flags combination of MKGETDIR_xxx
* @return bool
*/
function mkgetdir($dir, $flags=MKGETDIR_DEFAULT)
{
if ( !is_dir($dir) )
{
global $conf;
if (substr(PHP_OS, 0, 3) == 'WIN')
{
$dir = str_replace('/', DIRECTORY_SEPARATOR, $dir);
}
$umask = umask(0);
$mkd = @mkdir($dir, $conf['chmod_value'], ($flags&MKGETDIR_RECURSIVE) ? true:false );
umask($umask);
if ($mkd==false)
{
!($flags&MKGETDIR_DIE_ON_ERROR) or fatal_error( "$dir ".l10n('no write access'));
return false;
}
if( $flags&MKGETDIR_PROTECT_HTACCESS )
{
$file = $dir.'/.htaccess';
file_exists($file) or @file_put_contents( $file, 'deny from all' );
}
if( $flags&MKGETDIR_PROTECT_INDEX )
{
$file = $dir.'/index.htm';
file_exists($file) or @file_put_contents( $file, 'Not allowed!' );
}
}
if ( !is_writable($dir) )
{
!($flags&MKGETDIR_DIE_ON_ERROR) or fatal_error( "$dir ".l10n('no write access'));
return false;
}
return true;
}
/**
* finds out if a string is in ASCII, UTF-8 or other encoding
*
* @param string $str
* @return int *0* if _$str_ is ASCII, *1* if UTF-8, *-1* otherwise
*/
function qualify_utf8($Str)
{
$ret = 0;
for ($i=0; $i<strlen($Str); $i++)
{
if (ord($Str[$i]) < 0x80) continue; # 0bbbbbbb
$ret = 1;
if ((ord($Str[$i]) & 0xE0) == 0xC0) $n=1; # 110bbbbb
elseif ((ord($Str[$i]) & 0xF0) == 0xE0) $n=2; # 1110bbbb
elseif ((ord($Str[$i]) & 0xF8) == 0xF0) $n=3; # 11110bbb
elseif ((ord($Str[$i]) & 0xFC) == 0xF8) $n=4; # 111110bb
elseif ((ord($Str[$i]) & 0xFE) == 0xFC) $n=5; # 1111110b
else return -1; # Does not match any model
for ($j=0; $j<$n; $j++)
{ # n bytes matching 10bbbbbb follow ?
if ((++$i == strlen($Str)) || ((ord($Str[$i]) & 0xC0) != 0x80))
return -1;
}
}
return $ret;
}
/**
* Remove accents from a UTF-8 or ISO-8859-1 string (from wordpress)
*
* @param string $string
* @return string
*/
function remove_accents($string)
{
$utf = qualify_utf8($string);
if ( $utf == 0 )
{
return $string; // ascii
}
if ( $utf > 0 )
{
$chars = array(
// Decompositions for Latin-1 Supplement
"\xc3\x80"=>'A', "\xc3\x81"=>'A',
"\xc3\x82"=>'A', "\xc3\x83"=>'A',
"\xc3\x84"=>'A', "\xc3\x85"=>'A',
"\xc3\x87"=>'C', "\xc3\x88"=>'E',
"\xc3\x89"=>'E', "\xc3\x8a"=>'E',
"\xc3\x8b"=>'E', "\xc3\x8c"=>'I',
"\xc3\x8d"=>'I', "\xc3\x8e"=>'I',
"\xc3\x8f"=>'I', "\xc3\x91"=>'N',
"\xc3\x92"=>'O', "\xc3\x93"=>'O',
"\xc3\x94"=>'O', "\xc3\x95"=>'O',
"\xc3\x96"=>'O', "\xc3\x99"=>'U',
"\xc3\x9a"=>'U', "\xc3\x9b"=>'U',
"\xc3\x9c"=>'U', "\xc3\x9d"=>'Y',
"\xc3\x9f"=>'s', "\xc3\xa0"=>'a',
"\xc3\xa1"=>'a', "\xc3\xa2"=>'a',
"\xc3\xa3"=>'a', "\xc3\xa4"=>'a',
"\xc3\xa5"=>'a', "\xc3\xa7"=>'c',
"\xc3\xa8"=>'e', "\xc3\xa9"=>'e',
"\xc3\xaa"=>'e', "\xc3\xab"=>'e',
"\xc3\xac"=>'i', "\xc3\xad"=>'i',
"\xc3\xae"=>'i', "\xc3\xaf"=>'i',
"\xc3\xb1"=>'n', "\xc3\xb2"=>'o',
"\xc3\xb3"=>'o', "\xc3\xb4"=>'o',
"\xc3\xb5"=>'o', "\xc3\xb6"=>'o',
"\xc3\xb9"=>'u', "\xc3\xba"=>'u',
"\xc3\xbb"=>'u', "\xc3\xbc"=>'u',
"\xc3\xbd"=>'y', "\xc3\xbf"=>'y',
// Decompositions for Latin Extended-A
"\xc4\x80"=>'A', "\xc4\x81"=>'a',
"\xc4\x82"=>'A', "\xc4\x83"=>'a',
"\xc4\x84"=>'A', "\xc4\x85"=>'a',
"\xc4\x86"=>'C', "\xc4\x87"=>'c',
"\xc4\x88"=>'C', "\xc4\x89"=>'c',
"\xc4\x8a"=>'C', "\xc4\x8b"=>'c',
"\xc4\x8c"=>'C', "\xc4\x8d"=>'c',
"\xc4\x8e"=>'D', "\xc4\x8f"=>'d',
"\xc4\x90"=>'D', "\xc4\x91"=>'d',
"\xc4\x92"=>'E', "\xc4\x93"=>'e',
"\xc4\x94"=>'E', "\xc4\x95"=>'e',
"\xc4\x96"=>'E', "\xc4\x97"=>'e',
"\xc4\x98"=>'E', "\xc4\x99"=>'e',
"\xc4\x9a"=>'E', "\xc4\x9b"=>'e',
"\xc4\x9c"=>'G', "\xc4\x9d"=>'g',
"\xc4\x9e"=>'G', "\xc4\x9f"=>'g',
"\xc4\xa0"=>'G', "\xc4\xa1"=>'g',
"\xc4\xa2"=>'G', "\xc4\xa3"=>'g',
"\xc4\xa4"=>'H', "\xc4\xa5"=>'h',
"\xc4\xa6"=>'H', "\xc4\xa7"=>'h',
"\xc4\xa8"=>'I', "\xc4\xa9"=>'i',
"\xc4\xaa"=>'I', "\xc4\xab"=>'i',
"\xc4\xac"=>'I', "\xc4\xad"=>'i',
"\xc4\xae"=>'I', "\xc4\xaf"=>'i',
"\xc4\xb0"=>'I', "\xc4\xb1"=>'i',
"\xc4\xb2"=>'IJ', "\xc4\xb3"=>'ij',
"\xc4\xb4"=>'J', "\xc4\xb5"=>'j',
"\xc4\xb6"=>'K', "\xc4\xb7"=>'k',
"\xc4\xb8"=>'k', "\xc4\xb9"=>'L',
"\xc4\xba"=>'l', "\xc4\xbb"=>'L',
"\xc4\xbc"=>'l', "\xc4\xbd"=>'L',
"\xc4\xbe"=>'l', "\xc4\xbf"=>'L',
"\xc5\x80"=>'l', "\xc5\x81"=>'L',
"\xc5\x82"=>'l', "\xc5\x83"=>'N',
"\xc5\x84"=>'n', "\xc5\x85"=>'N',
"\xc5\x86"=>'n', "\xc5\x87"=>'N',
"\xc5\x88"=>'n', "\xc5\x89"=>'N',
"\xc5\x8a"=>'n', "\xc5\x8b"=>'N',
"\xc5\x8c"=>'O', "\xc5\x8d"=>'o',
"\xc5\x8e"=>'O', "\xc5\x8f"=>'o',
"\xc5\x90"=>'O', "\xc5\x91"=>'o',
"\xc5\x92"=>'OE', "\xc5\x93"=>'oe',
"\xc5\x94"=>'R', "\xc5\x95"=>'r',
"\xc5\x96"=>'R', "\xc5\x97"=>'r',
"\xc5\x98"=>'R', "\xc5\x99"=>'r',
"\xc5\x9a"=>'S', "\xc5\x9b"=>'s',
"\xc5\x9c"=>'S', "\xc5\x9d"=>'s',
"\xc5\x9e"=>'S', "\xc5\x9f"=>'s',
"\xc5\xa0"=>'S', "\xc5\xa1"=>'s',
"\xc5\xa2"=>'T', "\xc5\xa3"=>'t',
"\xc5\xa4"=>'T', "\xc5\xa5"=>'t',
"\xc5\xa6"=>'T', "\xc5\xa7"=>'t',
"\xc5\xa8"=>'U', "\xc5\xa9"=>'u',
"\xc5\xaa"=>'U', "\xc5\xab"=>'u',
"\xc5\xac"=>'U', "\xc5\xad"=>'u',
"\xc5\xae"=>'U', "\xc5\xaf"=>'u',
"\xc5\xb0"=>'U', "\xc5\xb1"=>'u',
"\xc5\xb2"=>'U', "\xc5\xb3"=>'u',
"\xc5\xb4"=>'W', "\xc5\xb5"=>'w',
"\xc5\xb6"=>'Y', "\xc5\xb7"=>'y',
"\xc5\xb8"=>'Y', "\xc5\xb9"=>'Z',
"\xc5\xba"=>'z', "\xc5\xbb"=>'Z',
"\xc5\xbc"=>'z', "\xc5\xbd"=>'Z',
"\xc5\xbe"=>'z', "\xc5\xbf"=>'s',
// Decompositions for Latin Extended-B
"\xc8\x98"=>'S', "\xc8\x99"=>'s',
"\xc8\x9a"=>'T', "\xc8\x9b"=>'t',
// Euro Sign
"\xe2\x82\xac"=>'E',
// GBP (Pound) Sign
"\xc2\xa3"=>'');
$string = strtr($string, $chars);
}
else
{
// Assume ISO-8859-1 if not UTF-8
$chars['in'] = chr(128).chr(131).chr(138).chr(142).chr(154).chr(158)
.chr(159).chr(162).chr(165).chr(181).chr(192).chr(193).chr(194)
.chr(195).chr(196).chr(197).chr(199).chr(200).chr(201).chr(202)
.chr(203).chr(204).chr(205).chr(206).chr(207).chr(209).chr(210)
.chr(211).chr(212).chr(213).chr(214).chr(216).chr(217).chr(218)
.chr(219).chr(220).chr(221).chr(224).chr(225).chr(226).chr(227)
.chr(228).chr(229).chr(231).chr(232).chr(233).chr(234).chr(235)
.chr(236).chr(237).chr(238).chr(239).chr(241).chr(242).chr(243)
.chr(244).chr(245).chr(246).chr(248).chr(249).chr(250).chr(251)
.chr(252).chr(253).chr(255);
$chars['out'] = "EfSZszYcYuAAAAAACEEEEIIIINOOOOOOUUUUYaaaaaaceeeeiiiinoooooouuuuyy";
$string = strtr($string, $chars['in'], $chars['out']);
$double_chars['in'] = array(chr(140), chr(156), chr(198), chr(208), chr(222), chr(223), chr(230), chr(240), chr(254));
$double_chars['out'] = array('OE', 'oe', 'AE', 'DH', 'TH', 'ss', 'ae', 'dh', 'th');
$string = str_replace($double_chars['in'], $double_chars['out'], $string);
}
return $string;
}
if (function_exists('mb_strtolower') && defined('PWG_CHARSET'))
{
/**
* removes accents from a string and converts it to lower case
*
* @param string $term
* @return string
*/
function transliterate($term)
{
return remove_accents( mb_strtolower($term, PWG_CHARSET) );
}
}
else
{
/**
* @ignore
*/
function transliterate($term)
{
return remove_accents( strtolower($term) );
}
}
/**
* simplify a string to insert it into an URL
*
* @param string $str
* @return string
*/
function str2url($str)
{
$str = $safe = transliterate($str);
$str = preg_replace('/[^\x80-\xffa-z0-9_\s\'\:\/\[\],-]/','',$str);
$str = preg_replace('/[\s\'\:\/\[\],-]+/',' ',trim($str));
$res = str_replace(' ','_',$str);
if (empty($res))
{
$res = str_replace(' ','_', $safe);
}
return $res;
}
/**
* returns an array with a list of {language_code => language_name}
*
* @return string[]
*/
function get_languages()
{
$query = '
SELECT id, name
FROM '.LANGUAGES_TABLE.'
ORDER BY name ASC
;';
$result = pwg_query($query);
$languages = array();
while ($row = pwg_db_fetch_assoc($result))
{
if (is_dir(PHPWG_ROOT_PATH.'language/'.$row['id']))
{
$languages[ $row['id'] ] = $row['name'];
}
}
return $languages;
}
/**
* log the visit into history table
*
* @param int $image_id
* @param string $image_type
* @return bool
*/
function pwg_log($image_id = null, $image_type = null)
{
global $conf, $user, $page;
$do_log = $conf['log'];
if (is_admin())
{
$do_log = $conf['history_admin'];
}
if (is_a_guest())
{
$do_log = $conf['history_guest'];
}
$do_log = trigger_change('pwg_log_allowed', $do_log, $image_id, $image_type);
if (!$do_log)
{
return false;
}
$tags_string = null;
if ('tags'==@$page['section'])
{
$tags_string = implode(',', $page['tag_ids']);
}
$query = '
INSERT INTO '.HISTORY_TABLE.'
(
date,
time,
user_id,
IP,
section,
category_id,
image_id,
image_type,
tag_ids
)
VALUES
(
CURRENT_DATE,
CURRENT_TIME,
'.$user['id'].',
\''.$_SERVER['REMOTE_ADDR'].'\',
'.(isset($page['section']) ? "'".$page['section']."'" : 'NULL').',
'.(isset($page['category']['id']) ? $page['category']['id'] : 'NULL').',
'.(isset($image_id) ? $image_id : 'NULL').',
'.(isset($image_type) ? "'".$image_type."'" : 'NULL').',
'.(isset($tags_string) ? "'".$tags_string."'" : 'NULL').'
)
;';
pwg_query($query);
return true;
}
/**
* Computes the difference between two dates.
* returns a DateInterval object or a stdClass with the same attributes
* http://stephenharris.info/date-intervals-in-php-5-2
*
* @param DateTime $date1
* @param DateTime $date2
* @return DateInterval|stdClass
*/
function dateDiff($date1, $date2)
{
if (version_compare(PHP_VERSION, '5.3.0') >= 0)
{
return $date1->diff($date2);
}
$diff = new stdClass();
//Make sure $date1 is ealier
$diff->invert = $date2 < $date1;
if ($diff->invert)
{
list($date1, $date2) = array($date2, $date1);
}
//Calculate R values
$R = ($date1 <= $date2 ? '+' : '-');
$r = ($date1 <= $date2 ? '' : '-');
//Calculate total days
$diff->days = round(abs($date1->format('U') - $date2->format('U'))/86400);
//A leap year work around - consistent with DateInterval
$leap_year = $date1->format('m-d') == '02-29';
if ($leap_year)
{
$date1->modify('-1 day');
}
//Years, months, days, hours
$periods = array('years'=>-1, 'months'=>-1, 'days'=>-1, 'hours'=>-1);
foreach ($periods as $period => &$i)
{
if ($period == 'days' && $leap_year)
{
$date1->modify('+1 day');
}
while ($date1 <= $date2 )
{
$date1->modify('+1 '.$period);
$i++;
}
//Reset date and record increments
$date1->modify('-1 '.$period);
}
list($diff->y, $diff->m, $diff->d, $diff->h) = array_values($periods);
//Minutes, seconds
$diff->s = round(abs($date1->format('U') - $date2->format('U')));
$diff->i = floor($diff->s/60);
$diff->s = $diff->s - $diff->i*60;
return $diff;
}
/**
* converts a string into a DateTime object
*
* @param int|string timestamp or datetime string
* @param string $format input format respecting date() syntax
* @return DateTime|false
*/
function str2DateTime($original, $format=null)
{
if (empty($original))
{
return false;
}
if ($original instanceof DateTime)
{
return $original;
}
if (!empty($format) && version_compare(PHP_VERSION, '5.3.0') >= 0)// from known date format
{
return DateTime::createFromFormat('!'.$format, $original); // ! char to reset fields to UNIX epoch
}
else
{
$t = trim($original, '0123456789');
if (empty($t)) // from timestamp
{
return new DateTime('@'.$original);
}
else // from unknown date format (assuming something like Y-m-d H:i:s)
{
$ymdhms = array();
$tok = strtok($original, '- :/');
while ($tok !== false)
{
$ymdhms[] = $tok;
$tok = strtok('- :/');
}
if (count($ymdhms)<3) return false;
if (!isset($ymdhms[3])) $ymdhms[3] = 0;
if (!isset($ymdhms[4])) $ymdhms[4] = 0;
if (!isset($ymdhms[5])) $ymdhms[5] = 0;
$date = new DateTime();
$date->setDate($ymdhms[0], $ymdhms[1], $ymdhms[2]);
$date->setTime($ymdhms[3], $ymdhms[4], $ymdhms[5]);
return $date;
}
}
}
/**
* returns a formatted and localized date for display
*
* @param int|string timestamp or datetime string
* @param array $show list of components displayed, default is ['day_name', 'day', 'month', 'year']
* THIS PARAMETER IS PLANNED TO CHANGE
* @param string $format input format respecting date() syntax
* @return string
*/
function format_date($original, $show=null, $format=null)
{
global $lang;
$date = str2DateTime($original, $format);
if (!$date)
{
return l10n('N/A');
}
if ($show === null || $show === true)
{
$show = array('day_name', 'day', 'month', 'year');
}
// TODO use IntlDateFormatter for proper i18n
$print = '';
if (in_array('day_name', $show))
$print.= $lang['day'][ $date->format('w') ].' ';
if (in_array('day', $show))
$print.= $date->format('j').' ';
if (in_array('month', $show))
$print.= $lang['month'][ $date->format('n') ].' ';
if (in_array('year', $show))
$print.= $date->format('Y').' ';
if (in_array('time', $show))
{
$temp = $date->format('H:i');
if ($temp != '00:00')
{
$print.= $temp.' ';
}
}
return trim($print);
}
/**
* Format a "From ... to ..." string from two dates
* @param string $from
* @param string $to
* @param boolean $full
* @return string
*/
function format_fromto($from, $to, $full=false)
{
$from = str2DateTime($from);
$to = str2DateTime($to);
if ($from->format('Y-m-d') == $to->format('Y-m-d'))
{
return format_date($from);
}
else
{
if ($full || $from->format('Y') != $to->format('Y'))
{
$from_str = format_date($from);
}
else if ($from->format('m') != $to->format('m'))
{
$from_str = format_date($from, array('day_name', 'day', 'month'));
}
else
{
$from_str = format_date($from, array('day_name', 'day'));
}
$to_str = format_date($to);
return l10n('from %s to %s', $from_str, $to_str);
}
}
/**
* Works out the time since the given date
*
* @param int|string timestamp or datetime string
* @param string $stop year,month,week,day,hour,minute,second
* @param string $format input format respecting date() syntax
* @param bool $with_text append "ago" or "in the future"
* @param bool $with_weeks
* @return string
*/
function time_since($original, $stop='minute', $format=null, $with_text=true, $with_week=true)
{
$date = str2DateTime($original, $format);
if (!$date)
{
return l10n('N/A');
}
$now = new DateTime();
$diff = dateDiff($now, $date);
$chunks = array(
'year' => $diff->y,
'month' => $diff->m,
'week' => 0,
'day' => $diff->d,
'hour' => $diff->h,
'minute' => $diff->i,
'second' => $diff->s,
);
// DateInterval does not contain the number of weeks
if ($with_week)
{
$chunks['week'] = (int)floor($chunks['day']/7);
$chunks['day'] = $chunks['day'] - $chunks['week']*7;
}
$j = array_search($stop, array_keys($chunks));
$print = ''; $i=0;
foreach ($chunks as $name => $value)
{
if ($value != 0)
{
$print.= ' '.l10n_dec('%d '.$name, '%d '.$name.'s', $value);
}
if (!empty($print) && $i >= $j)
{
break;
}
$i++;
}
$print = trim($print);
if ($with_text)
{
if ($diff->invert)
{
$print = l10n('%s ago', $print);
}
else
{
$print = l10n('%s in the future', $print);
}
}
return $print;
}
/**
* transform a date string from a format to another (MySQL to d/M/Y for instance)
*
* @param string $original
* @param string $format_in respecting date() syntax
* @param string $format_out respecting date() syntax
* @param string $default if _$original_ is empty
* @return string
*/
function transform_date($original, $format_in, $format_out, $default=null)
{
if (empty($original)) return $default;
$date = str2DateTime($original, $format_in);
return $date->format($format_out);
}
/**
* append a variable to _$debug_ global
*
* @param string $string
*/
function pwg_debug( $string )
{
global $debug,$t2,$page;
$now = explode( ' ', microtime() );
$now2 = explode( '.', $now[0] );
$now2 = $now[1].'.'.$now2[1];
$time = number_format( $now2 - $t2, 3, '.', ' ').' s';
$debug .= '<p>';
$debug.= '['.$time.', ';
$debug.= $page['count_queries'].' queries] : '.$string;
$debug.= "</p>\n";
}
/**
* Redirects to the given URL (HTTP method).
* once this function called, the execution doesn't go further
* (presence of an exit() instruction.
*
* @param string $url
* @return void
*/
function redirect_http( $url )
{
if (ob_get_length () !== FALSE)
{
ob_clean();
}
// default url is on html format
$url = html_entity_decode($url);
header('Request-URI: '.$url);
header('Content-Location: '.$url);
header('Location: '.$url);
exit();
}
/**
* Redirects to the given URL (HTML method).
* once this function called, the execution doesn't go further
* (presence of an exit() instruction.
*
* @param string $url
* @param string $msg
* @param integer $refresh_time
* @return void
*/
function redirect_html( $url , $msg = '', $refresh_time = 0)
{
global $user, $template, $lang_info, $conf, $lang, $t2, $page, $debug;
if (!isset($lang_info) || !isset($template) )
{
$user = build_user( $conf['guest_id'], true);
load_language('common.lang');
trigger_notify('loading_lang');
load_language('lang', PHPWG_ROOT_PATH.PWG_LOCAL_DIR, array('no_fallback'=>true, 'local'=>true) );
$template = new Template(PHPWG_ROOT_PATH.'themes', get_default_theme());
}
elseif (defined('IN_ADMIN') and IN_ADMIN)
{
$template = new Template(PHPWG_ROOT_PATH.'themes', get_default_theme());
}
if (empty($msg))
{
$msg = nl2br(l10n('Redirection...'));
}
$refresh = $refresh_time;
$url_link = $url;
$title = 'redirection';
$template->set_filenames( array( 'redirect' => 'redirect.tpl' ) );
include( PHPWG_ROOT_PATH.'include/page_header.php' );
$template->set_filenames( array( 'redirect' => 'redirect.tpl' ) );
$template->assign('REDIRECT_MSG', $msg);
$template->parse('redirect');
include( PHPWG_ROOT_PATH.'include/page_tail.php' );
exit();
}
/**
* Redirects to the given URL (automatically choose HTTP or HTML method).
* once this function called, the execution doesn't go further
* (presence of an exit() instruction.
*
* @param string $url
* @param string $msg
* @param integer $refresh_time
* @return void
*/
function redirect( $url , $msg = '', $refresh_time = 0)
{
global $conf;
// with RefeshTime <> 0, only html must be used
if ($conf['default_redirect_method']=='http'
and $refresh_time==0
and !headers_sent()
)
{
redirect_http($url);
}
else
{
redirect_html($url, $msg, $refresh_time);
}
}
/**
* returns available themes
*
* @param bool $show_mobile
* @return array
*/
function get_pwg_themes($show_mobile=false)
{
global $conf;
$themes = array();
$query = '
SELECT
id,
name
FROM '.THEMES_TABLE.'
ORDER BY name ASC
;';
$result = pwg_query($query);
while ($row = pwg_db_fetch_assoc($result))
{
if ($row['id'] == $conf['mobile_theme'])
{
if (!$show_mobile)
{
continue;
}
$row['name'] .= ' ('.l10n('Mobile').')';
}
if (check_theme_installed($row['id']))
{
$themes[ $row['id'] ] = $row['name'];
}
}
// plugins want remove some themes based on user status maybe?
$themes = trigger_change('get_pwg_themes', $themes);
return $themes;
}
/**
* check if a theme is installed (directory exsists)
*
* @param string $theme_id
* @return bool
*/
function check_theme_installed($theme_id)
{
global $conf;
return file_exists($conf['themes_dir'].'/'.$theme_id.'/'.'themeconf.inc.php');
}
/**
* Transforms an original path to its pwg representative
*
* @param string $path
* @param string $representative_ext
* @return string
*/
function original_to_representative($path, $representative_ext)
{
$pos = strrpos($path, '/');
$path = substr_replace($path, 'pwg_representative/', $pos+1, 0);
$pos = strrpos($path, '.');
return substr_replace($path, $representative_ext, $pos+1);
}
/**
* get the full path of an image
*
* @param array $element_info element information from db (at least 'path')
* @return string
*/
function get_element_path($element_info)
{
$path = $element_info['path'];
if ( !url_is_remote($path) )
{
$path = PHPWG_ROOT_PATH.$path;
}
return $path;
}
/**
* fill the current user caddie with given elements, if not already in caddie
*
* @param int[] $elements_id
*/
function fill_caddie($elements_id)
{
global $user;
$query = '
SELECT element_id
FROM '.CADDIE_TABLE.'
WHERE user_id = '.$user['id'].'
;';
$in_caddie = query2array($query, null, 'element_id');
$caddiables = array_diff($elements_id, $in_caddie);
$datas = array();
foreach ($caddiables as $caddiable)
{
$datas[] = array(
'element_id' => $caddiable,
'user_id' => $user['id'],
);
}
if (count($caddiables) > 0)
{
mass_inserts(CADDIE_TABLE, array('element_id','user_id'), $datas);
}
}
/**
* returns the element name from its filename.
* removes file extension and replace underscores by spaces
*
* @param string $filename
* @return string name
*/
function get_name_from_file($filename)
{
return str_replace('_',' ',get_filename_wo_extension($filename));
}
/**
* translation function.
* returns the corresponding value from _$lang_ if existing else the key is returned
* if more than one parameter is provided sprintf is applied
*
* @param string $key
* @param mixed $args,... optional arguments
* @return string
*/
function l10n($key)
{
global $lang, $conf;
if ( ($val=@$lang[$key]) === null)
{
if ($conf['debug_l10n'] and !isset($lang[$key]) and !empty($key))
{
trigger_error('[l10n] language key "'. $key .'" not defined', E_USER_WARNING);
}
$val = $key;
}
if (func_num_args() > 1)
{
$args = func_get_args();
$val = vsprintf($val, array_slice($args, 1));
}
return $val;
}
/**
* returns the printf value for strings including %d
* returned value is concorded with decimal value (singular, plural)
*
* @param string $singular_key
* @param string $plural_key
* @param int $decimal
* @return string
*/
function l10n_dec($singular_key, $plural_key, $decimal)
{
global $lang_info;
return
sprintf(
l10n((
(($decimal > 1) or ($decimal == 0 and $lang_info['zero_plural']))
? $plural_key
: $singular_key
)), $decimal);
}
/**
* returns a single element to use with l10n_args
*
* @param string $key translation key
* @param mixed $args arguments to use on sprintf($key, args)
* if args is a array, each values are used on sprintf
* @return string
*/
function get_l10n_args($key, $args='')
{
if (is_array($args))
{
$key_arg = array_merge(array($key), $args);
}
else
{
$key_arg = array($key, $args);
}
return array('key_args' => $key_arg);
}
/**
* returns a string formated with l10n elements.
* it is usefull to "prepare" a text and translate it later
* @see get_l10n_args()
*
* @param array $key_args one l10n_args element or array of l10n_args elements
* @param string $sep used when translated elements are concatened
* @return string
*/
function l10n_args($key_args, $sep = "\n")
{
if (is_array($key_args))
{
foreach ($key_args as $key => $element)
{
if (isset($result))
{
$result .= $sep;
}
else
{
$result = '';
}
if ($key === 'key_args')
{
array_unshift($element, l10n(array_shift($element))); // translate the key
$result .= call_user_func_array('sprintf', $element);
}
else
{
$result .= l10n_args($element, $sep);
}
}
}
else
{
fatal_error('l10n_args: Invalid arguments');
}
return $result;
}
/**
* returns the corresponding value from $themeconf if existing or an empty string
*
* @param string $key
* @return string
*/
function get_themeconf($key)
{
global $template;
return $template->get_themeconf($key);
}
/**
* Returns webmaster mail address depending on $conf['webmaster_id']
*
* @return string
*/
function get_webmaster_mail_address()
{
global $conf;
$query = '
SELECT '.$conf['user_fields']['email'].'
FROM '.USERS_TABLE.'
WHERE '.$conf['user_fields']['id'].' = '.$conf['webmaster_id'].'
;';
list($email) = pwg_db_fetch_row(pwg_query($query));
$email = trigger_change('get_webmaster_mail_address', $email);
return $email;
}
/**
* Add configuration parameters from database to global $conf array
*
* @param string $condition SQL condition
* @return void
*/
function load_conf_from_db($condition = '')
{
global $conf;
$query = '
SELECT param, value
FROM '.CONFIG_TABLE.'
'.(!empty($condition) ? 'WHERE '.$condition : '').'
;';
$result = pwg_query($query);
if ((pwg_db_num_rows($result) == 0) and !empty($condition))
{
fatal_error('No configuration data');
}
while ($row = pwg_db_fetch_assoc($result))
{
$val = isset($row['value']) ? $row['value'] : '';
// If the field is true or false, the variable is transformed into a boolean value.
if ($val == 'true')
{
$val = true;
}
elseif ($val == 'false')
{
$val = false;
}
$conf[ $row['param'] ] = $val;
}
trigger_notify('load_conf', $condition);
}
/**
* Add or update a config parameter
*
* @param string $param
* @param string $value
* @param boolean $updateGlobal update global *$conf* variable
* @param callable $parser function to apply to the value before save in database
(eg: serialize, json_encode) will not be applied to *$conf* if *$parser* is *true*
*/
function conf_update_param($param, $value, $updateGlobal=false, $parser=null)
{
if ($parser != null)
{
$dbValue = call_user_func($parser, $value);
}
else if (is_array($value) || is_object($value))
{
$dbValue = addslashes(serialize($value));
}
else
{
$dbValue = boolean_to_string($value);
}
$query = '
INSERT INTO
'.CONFIG_TABLE.' (param, value)
VALUES(\''.$param.'\', \''.$dbValue.'\')
ON DUPLICATE KEY UPDATE value = \''.$dbValue.'\'
;';
pwg_query($query);
if ($updateGlobal)
{
global $conf;
$conf[$param] = $value;
}
}
/**
* Delete one or more config parameters
* @since 2.6
*
* @param string|string[] $params
*/
function conf_delete_param($params)
{
global $conf;
if (!is_array($params))
{
$params = array($params);
}
if (empty($params))
{
return;
}
$query = '
DELETE FROM '.CONFIG_TABLE.'
WHERE param IN(\''. implode('\',\'', $params) .'\')
;';
pwg_query($query);
foreach ($params as $param)
{
unset($conf[$param]);
}
}
/**
* Apply *unserialize* on a value only if it is a string
* @since 2.7
*
* @param array|string $value
* @return array
*/
function safe_unserialize($value)
{
if (is_string($value))
{
return unserialize($value);
}
return $value;
}
/**
* Apply *json_decode* on a value only if it is a string
* @since 2.7
*
* @param array|string $value
* @return array
*/
function safe_json_decode($value)
{
if (is_string($value))
{
return json_decode($value, true);
}
return $value;
}
/**
* Prepends and appends strings at each value of the given array.
*
* @param array $array
* @param string $prepend_str
* @param string $append_str
* @return array
*/
function prepend_append_array_items($array, $prepend_str, $append_str)
{
array_walk(
$array,
create_function('&$s', '$s = "'.$prepend_str.'".$s."'.$append_str.'";')
);
return $array;
}
/**
* creates an simple hashmap based on a SQL query.
* choose one to be the key, another one to be the value.
* @deprecated 2.6
*
* @param string $query
* @param string $keyname
* @param string $valuename
* @return array
*/
function simple_hash_from_query($query, $keyname, $valuename)
{
return query2array($query, $keyname, $valuename);
}
/**
* creates an associative array based on a SQL query.
* choose one to be the key
* @deprecated 2.6
*
* @param string $query
* @param string $keyname
* @return array
*/
function hash_from_query($query, $keyname)
{
return query2array($query, $keyname);
}
/**
* creates a numeric array based on a SQL query.
* if _$fieldname_ is empty the returned value will be an array of arrays
* if _$fieldname_ is provided the returned value will be a one dimension array
* @deprecated 2.6
*
* @param string $query
* @param string $fieldname
* @return array
*/
function array_from_query($query, $fieldname=false)
{
if (false === $fieldname)
{
return query2array($query);
}
else
{
return query2array($query, null, $fieldname);
}
}
/**
* Return the basename of the current script.
* The lowercase case filename of the current script without extension
*
* @return string
*/
function script_basename()
{
global $conf;
foreach (array('SCRIPT_NAME', 'SCRIPT_FILENAME', 'PHP_SELF') as $value)
{
if (!empty($_SERVER[$value]))
{
$filename = strtolower($_SERVER[$value]);
if ($conf['php_extension_in_urls'] and get_extension($filename)!=='php')
continue;
$basename = basename($filename, '.php');
if (!empty($basename))
{
return $basename;
}
}
}
return '';
}
/**
* Return $conf['filter_pages'] value for the current page
*
* @param string $value_name
* @return mixed
*/
function get_filter_page_value($value_name)
{
global $conf;
$page_name = script_basename();
if (isset($conf['filter_pages'][$page_name][$value_name]))
{
return $conf['filter_pages'][$page_name][$value_name];
}
elseif (isset($conf['filter_pages']['default'][$value_name]))
{
return $conf['filter_pages']['default'][$value_name];
}
else
{
return null;
}
}
/**
* return the character set used by Piwigo
* @return string
*/
function get_pwg_charset()
{
$pwg_charset = 'utf-8';
if (defined('PWG_CHARSET'))
{
$pwg_charset = PWG_CHARSET;
}
return $pwg_charset;
}
/**
* returns the parent (fallback) language of a language.
* if _$lang_id_ is null it applies to the current language
* @since 2.6
*
* @param string $lang_id
* @return string|null
*/
function get_parent_language($lang_id=null)
{
if (empty($lang_id))
{
global $lang_info;
return !empty($lang_info['parent']) ? $lang_info['parent'] : null;
}
else
{
$f = PHPWG_ROOT_PATH.'language/'.$lang_id.'/common.lang.php';
if (file_exists($f))
{
include($f);
return !empty($lang_info['parent']) ? $lang_info['parent'] : null;
}
}
return null;
}
/**
* includes a language file or returns the content of a language file
*
* tries to load in descending order:
* param language, user language, default language
*
* @param string $filename
* @param string $dirname
* @param mixed options can contain
* @option string language - language to load
* @option bool return - if true the file content is returned
* @option bool no_fallback - if true do not load default language
* @option bool|string force_fallback - force pre-loading of another language
* default language if *true* or specified language
* @option bool local - if true load file from local directory
* @return boolean|string
*/
function load_language($filename, $dirname = '', $options = array())
{
global $user, $language_files;
// keep trace of plugins loaded files for switch_lang_to() function
if (!empty($dirname) && !empty($filename) && !@$options['return']
&& !isset($language_files[$dirname][$filename]))
{
$language_files[$dirname][$filename] = $options;
}
if (!@$options['return'])
{
$filename .= '.php';
}
if (empty($dirname))
{
$dirname = PHPWG_ROOT_PATH;
}
$dirname .= 'language/';
$default_language = defined('PHPWG_INSTALLED') and !defined('UPGRADES_PATH') ?
get_default_language() : PHPWG_DEFAULT_LANGUAGE;
// construct list of potential languages
$languages = array();
if (!empty($options['language']))
{ // explicit language
$languages[] = $options['language'];
}
if (!empty($user['language']))
{ // use language
$languages[] = $user['language'];
}
if (($parent = get_parent_language()) != null)
{ // parent language
// this is only for when the "child" language is missing
$languages[] = $parent;
}
if (isset($options['force_fallback']))
{ // fallback language
// this is only for when the main language is missing
if ($options['force_fallback'] === true)
{
$options['force_fallback'] = $default_language;
}
$languages[] = $options['force_fallback'];
}
if (!@$options['no_fallback'])
{ // default language
$languages[] = $default_language;
}
$languages = array_unique($languages);
// find first existing
$source_file = '';
$selected_language = '';
foreach ($languages as $language)
{
$f = @$options['local'] ?
$dirname.$language.'.'.$filename:
$dirname.$language.'/'.$filename;
if (file_exists($f))
{
$selected_language = $language;
$source_file = $f;
break;
}
}
if (!empty($source_file))
{
if (!@$options['return'])
{
// load forced fallback
if (isset($options['force_fallback']) && $options['force_fallback'] != $selected_language)
{
@include(str_replace($selected_language, $options['force_fallback'], $source_file));
}
// load language content
@include($source_file);
$load_lang = @$lang;
$load_lang_info = @$lang_info;
// access already existing values
global $lang, $lang_info;
if (!isset($lang)) $lang = array();
if (!isset($lang_info)) $lang_info = array();
// load parent language content directly in global
if (!empty($load_lang_info['parent']))
$parent_language = $load_lang_info['parent'];
else if (!empty($lang_info['parent']))
$parent_language = $lang_info['parent'];
else
$parent_language = null;
if (!empty($parent_language) && $parent_language != $selected_language)
{
@include(str_replace($selected_language, $parent_language, $source_file));
}
// merge contents
$lang = array_merge($lang, (array)$load_lang);
$lang_info = array_merge($lang_info, (array)$load_lang_info);
return true;
}
else
{
$content = @file_get_contents($source_file);
//Note: target charset is always utf-8 $content = convert_charset($content, 'utf-8', $target_charset);
return $content;
}
}
return false;
}
/**
* converts a string from a character set to another character set
*
* @param string $str
* @param string $source_charset
* @param string $dest_charset
*/
function convert_charset($str, $source_charset, $dest_charset)
{
if ($source_charset==$dest_charset)
return $str;
if ($source_charset=='iso-8859-1' and $dest_charset=='utf-8')
{
return utf8_encode($str);
}
if ($source_charset=='utf-8' and $dest_charset=='iso-8859-1')
{
return utf8_decode($str);
}
if (function_exists('iconv'))
{
return iconv($source_charset, $dest_charset, $str);
}
if (function_exists('mb_convert_encoding'))
{
return mb_convert_encoding( $str, $dest_charset, $source_charset );
}
return $str; // TODO
}
/**
* makes sure a index.htm protects the directory from browser file listing
*
* @param string $dir
*/
function secure_directory($dir)
{
$file = $dir.'/index.htm';
if (!file_exists($file))
{
@file_put_contents($file, 'Not allowed!');
}
}
/**
* returns a "secret key" that is to be sent back when a user posts a form
*
* @param int $valid_after_seconds - key validity start time from now
* @param string $aditionnal_data_to_hash
* @return string
*/
function get_ephemeral_key($valid_after_seconds, $aditionnal_data_to_hash = '')
{
global $conf;
$time = round(microtime(true), 1);
return $time.':'.$valid_after_seconds.':'
.hash_hmac(
'md5',
$time.substr($_SERVER['REMOTE_ADDR'],0,5).$valid_after_seconds.$aditionnal_data_to_hash,
$conf['secret_key']);
}
/**
* verify a key sent back with a form
*
* @param string $key
* @param string $aditionnal_data_to_hash
* @return bool
*/
function verify_ephemeral_key($key, $aditionnal_data_to_hash = '')
{
global $conf;
$time = microtime(true);
$key = explode( ':', @$key );
if ( count($key)!=3
or $key[0]>$time-(float)$key[1] // page must have been retrieved more than X sec ago
or $key[0]<$time-3600 // 60 minutes expiration
or hash_hmac(
'md5', $key[0].substr($_SERVER['REMOTE_ADDR'],0,5).$key[1].$aditionnal_data_to_hash, $conf['secret_key']
) != $key[2]
)
{
return false;
}
return true;
}
/**
* return an array which will be sent to template to display navigation bar
*
* @param string $url base url of all links
* @param int $nb_elements
* @param int $start
* @param int $nb_element_page
* @param bool $clean_url
* @param string $param_name
* @return array
*/
function create_navigation_bar($url, $nb_element, $start, $nb_element_page, $clean_url = false, $param_name='start')
{
global $conf;
$navbar = array();
$pages_around = $conf['paginate_pages_around'];
$start_str = $clean_url ? '/'.$param_name.'-' : (strpos($url, '?')===false ? '?':'&').$param_name.'=';
if (!isset($start) or !is_numeric($start) or (is_numeric($start) and $start < 0))
{
$start = 0;
}
// navigation bar useful only if more than one page to display !
if ($nb_element > $nb_element_page)
{
$url_start = $url.$start_str;
$cur_page = $navbar['CURRENT_PAGE'] = $start / $nb_element_page + 1;
$maximum = ceil($nb_element / $nb_element_page);
$start = $nb_element_page * round( $start / $nb_element_page );
$previous = $start - $nb_element_page;
$next = $start + $nb_element_page;
$last = ($maximum - 1) * $nb_element_page;
// link to first page and previous page?
if ($cur_page != 1)
{
$navbar['URL_FIRST'] = $url;
$navbar['URL_PREV'] = $previous > 0 ? $url_start.$previous : $url;
}
// link on next page and last page?
if ($cur_page != $maximum)
{
$navbar['URL_NEXT'] = $url_start.($next < $last ? $next : $last);
$navbar['URL_LAST'] = $url_start.$last;
}
// pages to display
$navbar['pages'] = array();
$navbar['pages'][1] = $url;
for ($i = max( floor($cur_page) - $pages_around , 2), $stop = min( ceil($cur_page) + $pages_around + 1, $maximum);
$i < $stop; $i++)
{
$navbar['pages'][$i] = $url.$start_str.(($i - 1) * $nb_element_page);
}
$navbar['pages'][$maximum] = $url_start.$last;
$navbar['NB_PAGE']=$maximum;
}
return $navbar;
}
/**
* return an array which will be sent to template to display recent icon
*
* @param string $date
* @param bool $is_child_date
* @return array
*/
function get_icon($date, $is_child_date = false)
{
global $cache, $user;
if (empty($date))
{
return false;
}
if (!isset($cache['get_icon']['title']))
{
$cache['get_icon']['title'] = l10n(
'photos posted during the last %d days',
$user['recent_period']
);
}
$icon = array(
'TITLE' => $cache['get_icon']['title'],
'IS_CHILD_DATE' => $is_child_date,
);
if (isset($cache['get_icon'][$date]))
{
return $cache['get_icon'][$date] ? $icon : array();
}
if (!isset($cache['get_icon']['sql_recent_date']))
{
// Use MySql date in order to standardize all recent "actions/queries"
$cache['get_icon']['sql_recent_date'] = pwg_db_get_recent_period($user['recent_period']);
}
$cache['get_icon'][$date] = $date > $cache['get_icon']['sql_recent_date'];
return $cache['get_icon'][$date] ? $icon : array();
}
/**
* check token comming from form posted or get params to prevent csrf attacks.
* if pwg_token is empty action doesn't require token
* else pwg_token is compare to server token
*
* @return void access denied if token given is not equal to server token
*/
function check_pwg_token()
{
if (!empty($_REQUEST['pwg_token']))
{
if (get_pwg_token() != $_REQUEST['pwg_token'])
{
access_denied();
}
}
else
{
bad_request('missing token');
}
}
/**
* get pwg_token used to prevent csrf attacks
*
* @return string
*/
function get_pwg_token()
{
global $conf;
return hash_hmac('md5', session_id(), $conf['secret_key']);
}
/*
* breaks the script execution if the given value doesn't match the given
* pattern. This should happen only during hacking attempts.
*
* @param string $param_name
* @param array $param_array
* @param boolean $is_array
* @param string $pattern
* @param boolean $mandatory
*/
function check_input_parameter($param_name, $param_array, $is_array, $pattern, $mandatory=false)
{
$param_value = null;
if (isset($param_array[$param_name]))
{
$param_value = $param_array[$param_name];
}
// it's ok if the input parameter is null
if (empty($param_value))
{
if ($mandatory)
{
fatal_error('[Hacking attempt] the input parameter "'.$param_name.'" is not valid');
}
return true;
}
if ($is_array)
{
if (!is_array($param_value))
{
fatal_error('[Hacking attempt] the input parameter "'.$param_name.'" should be an array');
}
foreach ($param_value as $item_to_check)
{
if (!preg_match($pattern, $item_to_check))
{
fatal_error('[Hacking attempt] an item is not valid in input parameter "'.$param_name.'"');
}
}
}
else
{
if (!preg_match($pattern, $param_value))
{
fatal_error('[Hacking attempt] the input parameter "'.$param_name.'" is not valid');
}
}
}
/**
* get localized privacy level values
*
* @return string[]
*/
function get_privacy_level_options()
{
global $conf;
$options = array();
$label = '';
foreach (array_reverse($conf['available_permission_levels']) as $level)
{
if (0 == $level)
{
$label = l10n('Everybody');
}
else
{
if (strlen($label))
{
$label .= ', ';
}
$label .= l10n( sprintf('Level %d', $level) );
}
$options[$level] = $label;
}
return $options;
}
/**
* return the branch from the version. For example version 2.2.4 is for branch 2.2
*
* @param string $version
* @return string
*/
function get_branch_from_version($version)
{
return implode('.', array_slice(explode('.', $version), 0, 2));
}
/**
* return the device type: mobile, tablet or desktop
*
* @return string
*/
function get_device()
{
$device = pwg_get_session_var('device');
if (is_null($device))
{
include_once(PHPWG_ROOT_PATH.'include/mdetect.php');
$uagent_obj = new uagent_info();
if ($uagent_obj->DetectSmartphone())
{
$device = 'mobile';
}
elseif ($uagent_obj->DetectTierTablet())
{
$device = 'tablet';
}
else
{
$device = 'desktop';
}
pwg_set_session_var('device', $device);
}
return $device;
}
/**
* return true if mobile theme should be loaded
*
* @return bool
*/
function mobile_theme()
{
global $conf;
if (empty($conf['mobile_theme']))
{
return false;
}
if (isset($_GET['mobile']))
{
$is_mobile_theme = get_boolean($_GET['mobile']);
pwg_set_session_var('mobile_theme', $is_mobile_theme);
}
else
{
$is_mobile_theme = pwg_get_session_var('mobile_theme');
}
if (is_null($is_mobile_theme))
{
$is_mobile_theme = (get_device() == 'mobile');
pwg_set_session_var('mobile_theme', $is_mobile_theme);
}
return $is_mobile_theme;
}
/**
* check url format
*
* @param string $url
* @return bool
*/
function url_check_format($url)
{
return filter_var($url, FILTER_VALIDATE_URL, FILTER_FLAG_SCHEME_REQUIRED | FILTER_FLAG_HOST_REQUIRED)!==false;
}
/**
* check email format
*
* @param string $mail_address
* @return bool
*/
function email_check_format($mail_address)
{
return filter_var($mail_address, FILTER_VALIDATE_EMAIL)!==false;
}
/**
* returns the number of available comments for the connected user
*
* @return int
*/
function get_nb_available_comments()
{
global $user;
if (!isset($user['nb_available_comments']))
{
$where = array();
if ( !is_admin() )
$where[] = 'validated=\'true\'';
$where[] = get_sql_condition_FandF
(
array
(
'forbidden_categories' => 'category_id',
'forbidden_images' => 'ic.image_id'
),
'', true
);
$query = '
SELECT COUNT(DISTINCT(com.id))
FROM '.IMAGE_CATEGORY_TABLE.' AS ic
INNER JOIN '.COMMENTS_TABLE.' AS com
ON ic.image_id = com.image_id
WHERE '.implode('
AND ', $where);
list($user['nb_available_comments']) = pwg_db_fetch_row(pwg_query($query));
single_update(USER_CACHE_TABLE,
array('nb_available_comments'=>$user['nb_available_comments']),
array('user_id'=>$user['id'])
);
}
return $user['nb_available_comments'];
}
/**
* Compare two versions with version_compare after having converted
* single chars to their decimal values.
* Needed because version_compare does not understand versions like '2.5.c'.
* @since 2.6
*
* @param string $a
* @param string $b
* @param string $op
*/
function safe_version_compare($a, $b, $op=null)
{
$replace_chars = create_function('$m', 'return ord(strtolower($m[1]));');
// add dot before groups of letters (version_compare does the same thing)
$a = preg_replace('#([0-9]+)([a-z]+)#i', '$1.$2', $a);
$b = preg_replace('#([0-9]+)([a-z]+)#i', '$1.$2', $b);
// apply ord() to any single letter
$a = preg_replace_callback('#\b([a-z]{1})\b#i', $replace_chars, $a);
$b = preg_replace_callback('#\b([a-z]{1})\b#i', $replace_chars, $b);
if (empty($op))
{
return version_compare($a, $b);
}
else
{
return version_compare($a, $b, $op);
}
}
?> | Java |
# Creates graph of restaurant reviews for yelp or trip advisor.
# writes graph to gml file for use in gephi
#
# Rob Churchill
#
# NOTE: I learned to do this in my data science class last semester. If you are looking for plagiarism things, you will almost certainly find similar clustering code.
# I did not copy it, I learned this specific way of doing it, and referred to my previous assignments when doing it for this project. If you would like to see my previous
# assignments, I will provide you them on request. Otherwise, I don't think that it's worth adding a lot of extra files for the sole sake of showing that I haven't plagiarized.
import networkx as nx
import numpy as np
import scipy as sp
import csv
folder = 'data/'
file_names = ['yelp_data.csv', 'trip_advisor_data.csv']
# EDIT this line to change which website you make the graph for. True=yelp, False=TripAdvisor
yelp = False
yelp_dataset = list()
file_name = file_names[1]
if yelp == True:
file_name = file_names[0]
# reads in appropriate file given yelp boolean variable
with open(folder+file_name, 'r') as f:
reader = csv.reader(f)
for line in reader:
yelp_dataset.append(line)
# removes headers
yelp_dataset.remove(yelp_dataset[0])
print len(yelp_dataset)
# create the graph
G = nx.Graph()
for y in yelp_dataset:
# add the nodes if they don't already exist
G.add_node(y[4], type='restaurant')
G.add_node(y[13], type='reviewer')
# add the edge between the reviewer and restaurant, weight is in different position in each file.
if yelp == True:
G.add_edge(y[13], y[4], weight=float(y[2]))
else:
G.add_edge(y[13], y[4], weight=float(y[1]))
print nx.number_of_nodes(G)
print nx.number_of_edges(G)
# write graph to gml file.
nx.write_gml(G, 'ta_graph.gml') | Java |
<?php return unserialize('a:1:{i:0;O:27:"Doctrine\\ORM\\Mapping\\Column":9:{s:4:"name";s:12:"id_formation";s:4:"type";s:7:"integer";s:6:"length";N;s:9:"precision";i:0;s:5:"scale";i:0;s:6:"unique";b:0;s:8:"nullable";b:0;s:7:"options";a:0:{}s:16:"columnDefinition";N;}}'); | Java |
<html>
<head>
<title>User agent detail - SonyEricssonC902c/R3DA Browser/NetFront/3.4 Profile/MIDP-2.1 Configuration/CLDC-1.1 JavaPlatform/JP-8.3.2</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.97.3/css/materialize.min.css">
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="section">
<h1 class="header center orange-text">User agent detail</h1>
<div class="row center">
<h5 class="header light">
SonyEricssonC902c/R3DA Browser/NetFront/3.4 Profile/MIDP-2.1 Configuration/CLDC-1.1 JavaPlatform/JP-8.3.2
</h5>
</div>
</div>
<div class="section">
<table class="striped"><tr><th></th><th colspan="3">General</th><th colspan="5">Device</th><th colspan="3">Bot</th><th colspan="2"></th></tr><tr><th>Provider</th><th>Browser</th><th>Engine</th><th>OS</th><th>Brand</th><th>Model</th><th>Type</th><th>Is mobile</th><th>Is touch</th><th>Is bot</th><th>Name</th><th>Type</th><th>Parse time</th><th>Actions</th></tr><tr><th colspan="14" class="green lighten-3">Source result (test suite)</th></tr><tr><td>ua-parser/uap-core<br /><small>vendor/thadafinser/uap-core/tests/test_device.yaml</small></td><td> </td><td> </td><td> </td><td style="border-left: 1px solid #555">SonyEricsson</td><td>C902c</td><td></td><td></td><td></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td></td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-test">Detail</a>
<!-- Modal Structure -->
<div id="modal-test" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>Testsuite result detail</h4>
<p><pre><code class="php">Array
(
[user_agent_string] => SonyEricssonC902c/R3DA Browser/NetFront/3.4 Profile/MIDP-2.1 Configuration/CLDC-1.1 JavaPlatform/JP-8.3.2
[family] => Ericsson C902c
[brand] => SonyEricsson
[model] => C902c
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><th colspan="14" class="green lighten-3">Providers</th></tr><tr><td>BrowscapPhp<br /><small>6012</small></td><td>NetFront 3.4</td><td>NetFront </td><td> </td><td style="border-left: 1px solid #555"></td><td></td><td>Mobile Device</td><td>yes</td><td></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.05599</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-215ac98d-ccf8-4615-916e-5a819d6a59c9">Detail</a>
<!-- Modal Structure -->
<div id="modal-215ac98d-ccf8-4615-916e-5a819d6a59c9" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>BrowscapPhp result detail</h4>
<p><pre><code class="php">stdClass Object
(
[browser_name_regex] => /^.*netfront\/3\.4.*$/
[browser_name_pattern] => *netfront/3.4*
[parent] => NetFront 3.4
[comment] => Access NetFront 3.4
[browser] => NetFront
[browser_type] => Browser
[browser_bits] => 0
[browser_maker] => Access
[browser_modus] => unknown
[version] => 3.4
[majorver] => 3
[minorver] => 4
[platform] => unknown
[platform_version] => unknown
[platform_description] => unknown
[platform_bits] => 0
[platform_maker] => unknown
[alpha] =>
[beta] =>
[win16] =>
[win32] =>
[win64] =>
[frames] => 1
[iframes] => 1
[tables] => 1
[cookies] => 1
[backgroundsounds] =>
[javascript] => 1
[vbscript] =>
[javaapplets] =>
[activexcontrols] =>
[ismobiledevice] => 1
[istablet] =>
[issyndicationreader] =>
[crawler] =>
[cssversion] => 2
[aolversion] => 0
[device_name] => general Mobile Device
[device_maker] => unknown
[device_type] => Mobile Device
[device_pointing_method] => unknown
[device_code_name] => general Mobile Device
[device_brand_name] => unknown
[renderingengine_name] => NetFront
[renderingengine_version] => unknown
[renderingengine_description] => For Access NetFront.
[renderingengine_maker] => Access
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>DonatjUAParser<br /><small>v0.5.0</small></td><td>SonyEricssonC902c R3DA</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.001</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-f1436016-fdf1-4aea-b4be-1d7c99ab0661">Detail</a>
<!-- Modal Structure -->
<div id="modal-f1436016-fdf1-4aea-b4be-1d7c99ab0661" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>DonatjUAParser result detail</h4>
<p><pre><code class="php">Array
(
[platform] =>
[browser] => SonyEricssonC902c
[version] => R3DA
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>NeutrinoApiCom<br /><small></small></td><td>NetFront 3.4</td><td><i class="material-icons">close</i></td><td>JVM </td><td style="border-left: 1px solid #555">SonyEricsson</td><td>C902</td><td>mobile-browser</td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.18804</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-9b0fa449-ec1b-40c8-8b1c-9486eb3b9cbc">Detail</a>
<!-- Modal Structure -->
<div id="modal-9b0fa449-ec1b-40c8-8b1c-9486eb3b9cbc" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>NeutrinoApiCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[mobile_screen_height] => 320
[is_mobile] => 1
[type] => mobile-browser
[mobile_brand] => SonyEricsson
[mobile_model] => C902
[version] => 3.4
[is_android] =>
[browser_name] => NetFront
[operating_system_family] => JVM
[operating_system_version] =>
[is_ios] =>
[producer] => ACCESS CO.,LTD
[operating_system] => JVM (Platform Micro Edition)
[mobile_screen_width] => 240
[mobile_browser] => Access Netfront
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>PiwikDeviceDetector<br /><small>3.5.2</small></td><td>NetFront 3.4</td><td>NetFront </td><td> </td><td style="border-left: 1px solid #555">Sony Ericsson</td><td>C902c</td><td>feature phone</td><td>yes</td><td></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.006</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-21638055-738d-46ba-a1b1-f5114bc26475">Detail</a>
<!-- Modal Structure -->
<div id="modal-21638055-738d-46ba-a1b1-f5114bc26475" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>PiwikDeviceDetector result detail</h4>
<p><pre><code class="php">Array
(
[client] => Array
(
[type] => browser
[name] => NetFront
[short_name] => NF
[version] => 3.4
[engine] => NetFront
)
[operatingSystem] => Array
(
)
[device] => Array
(
[brand] => SE
[brandName] => Sony Ericsson
[model] => C902c
[device] => 3
[deviceName] => feature phone
)
[bot] =>
[extra] => Array
(
[isBot] =>
[isBrowser] => 1
[isFeedReader] =>
[isMobileApp] =>
[isPIM] =>
[isLibrary] =>
[isMediaPlayer] =>
[isCamera] =>
[isCarBrowser] =>
[isConsole] =>
[isFeaturePhone] => 1
[isPhablet] =>
[isPortableMediaPlayer] =>
[isSmartDisplay] =>
[isSmartphone] =>
[isTablet] =>
[isTV] =>
[isDesktop] =>
[isMobile] => 1
[isTouchEnabled] =>
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>SinergiBrowserDetector<br /><small>6.0.0</small></td>
<td colspan="12" class="center-align red lighten-1">
<strong>No result found</strong>
</td>
</tr><tr><td>UAParser<br /><small>v3.4.5</small></td><td>NetFront 3.4</td><td><i class="material-icons">close</i></td><td> </td><td style="border-left: 1px solid #555">SonyEricsson</td><td>C902c</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.003</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-346c1a98-5fd3-454f-b6c8-350f2f505d8b">Detail</a>
<!-- Modal Structure -->
<div id="modal-346c1a98-5fd3-454f-b6c8-350f2f505d8b" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>UAParser result detail</h4>
<p><pre><code class="php">UAParser\Result\Client Object
(
[ua] => UAParser\Result\UserAgent Object
(
[major] => 3
[minor] => 4
[patch] =>
[family] => NetFront
)
[os] => UAParser\Result\OperatingSystem Object
(
[major] =>
[minor] =>
[patch] =>
[patchMinor] =>
[family] => Other
)
[device] => UAParser\Result\Device Object
(
[brand] => SonyEricsson
[model] => C902c
[family] => Ericsson C902c
)
[originalUserAgent] => SonyEricssonC902c/R3DA Browser/NetFront/3.4 Profile/MIDP-2.1 Configuration/CLDC-1.1 JavaPlatform/JP-8.3.2
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>UserAgentStringCom<br /><small></small></td><td>NetFront 3.4</td><td><i class="material-icons">close</i></td><td> </td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.06401</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-9cdd8b45-a2eb-406b-bd27-7e48af38ffd4">Detail</a>
<!-- Modal Structure -->
<div id="modal-9cdd8b45-a2eb-406b-bd27-7e48af38ffd4" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>UserAgentStringCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[agent_type] => Browser
[agent_name] => NetFront
[agent_version] => 3.4
[os_type] => unknown
[os_name] => unknown
[os_versionName] =>
[os_versionNumber] =>
[os_producer] =>
[os_producerURL] =>
[linux_distibution] => Null
[agent_language] =>
[agent_languageTag] =>
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>WhatIsMyBrowserCom<br /><small></small></td><td>NetFront </td><td> </td><td> </td><td style="border-left: 1px solid #555">Sony Ericsson</td><td>Sony Ericsson C902c</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.57711</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-9795f66f-7271-430e-973a-a5c0e14dc35a">Detail</a>
<!-- Modal Structure -->
<div id="modal-9795f66f-7271-430e-973a-a5c0e14dc35a" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>WhatIsMyBrowserCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[operating_system_name] =>
[simple_sub_description_string] =>
[simple_browser_string] => NetFront 3.4 on Sony Ericsson C902c
[browser_version] => 3.4
[extra_info] => Array
(
)
[operating_platform] => Sony Ericsson C902c
[extra_info_table] => Array
(
)
[layout_engine_name] =>
[detected_addons] => Array
(
)
[operating_system_flavour_code] =>
[hardware_architecture] =>
[operating_system_flavour] =>
[operating_system_frameworks] => Array
(
)
[browser_name_code] => netfront
[operating_system_version] =>
[simple_operating_platform_string] =>
[is_abusive] =>
[layout_engine_version] =>
[browser_capabilities] => Array
(
[0] => MIDP v2.1
[1] => CLDC v1.1
)
[operating_platform_vendor_name] => Sony Ericsson
[operating_system] =>
[operating_system_version_full] =>
[operating_platform_code] => C902c
[browser_name] => NetFront
[operating_system_name_code] =>
[user_agent] => SonyEricssonC902c/R3DA Browser/NetFront/3.4 Profile/MIDP-2.1 Configuration/CLDC-1.1 JavaPlatform/JP-8.3.2
[browser_version_full] =>
[browser] => NetFront 3.4
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>WhichBrowser<br /><small>2.0.10</small></td><td>NetFront 3.4</td><td> </td><td> </td><td style="border-left: 1px solid #555">Sony Ericsson</td><td>C902c</td><td>mobile:feature</td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.014</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-342c8d32-4765-40a8-8a5c-af3a38d19ae4">Detail</a>
<!-- Modal Structure -->
<div id="modal-342c8d32-4765-40a8-8a5c-af3a38d19ae4" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>WhichBrowser result detail</h4>
<p><pre><code class="php">Array
(
[browser] => Array
(
[name] => NetFront
[version] => 3.4
[type] => browser
)
[device] => Array
(
[type] => mobile
[subtype] => feature
[manufacturer] => Sony Ericsson
[model] => C902c
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>Woothee<br /><small>v1.2.0</small></td>
<td colspan="12" class="center-align red lighten-1">
<strong>No result found</strong>
</td>
</tr><tr><td>Wurfl<br /><small>1.6.4</small></td><td>NetFront 3.4</td><td><i class="material-icons">close</i></td><td> </td><td style="border-left: 1px solid #555">SonyEricsson</td><td>C902</td><td>Feature Phone</td><td>yes</td><td></td><td style="border-left: 1px solid #555"></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.038</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-1a1aee36-7ce7-4111-a391-8e2c501f1532">Detail</a>
<!-- Modal Structure -->
<div id="modal-1a1aee36-7ce7-4111-a391-8e2c501f1532" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>Wurfl result detail</h4>
<p><pre><code class="php">Array
(
[virtual] => Array
(
[is_android] => false
[is_ios] => false
[is_windows_phone] => false
[is_app] => false
[is_full_desktop] => false
[is_largescreen] => false
[is_mobile] => true
[is_robot] => false
[is_smartphone] => false
[is_touchscreen] => false
[is_wml_preferred] => false
[is_xhtmlmp_preferred] => false
[is_html_preferred] => true
[advertised_device_os] =>
[advertised_device_os_version] =>
[advertised_browser] => NetFront
[advertised_browser_version] => 3.4
[complete_device_name] => SonyEricsson C902
[form_factor] => Feature Phone
[is_phone] => true
[is_app_webview] => false
)
[all] => Array
(
[brand_name] => SonyEricsson
[model_name] => C902
[unique] => true
[ununiqueness_handler] =>
[is_wireless_device] => true
[device_claims_web_support] => false
[has_qwerty_keyboard] => false
[can_skip_aligned_link_row] => true
[uaprof] => http://wap.sonyericsson.com/UAprof/C902R101-HS.xml
[uaprof2] => http://wap.sonyericsson.com/UAprof/C902R101-3G.xml
[uaprof3] => http://wap.sonyericsson.com/UAprof/C902R101.xml
[nokia_series] => 0
[nokia_edition] => 0
[device_os] =>
[mobile_browser] => Access Netfront
[mobile_browser_version] => 3.4
[device_os_version] =>
[pointing_method] => joystick
[release_date] => 2008_january
[marketing_name] =>
[model_extra_info] =>
[nokia_feature_pack] => 0
[can_assign_phone_number] => true
[is_tablet] => false
[manufacturer_name] =>
[is_bot] => false
[is_google_glass] => false
[proportional_font] => false
[built_in_back_button_support] => false
[card_title_support] => true
[softkey_support] => true
[table_support] => true
[numbered_menus] => false
[menu_with_select_element_recommended] => false
[menu_with_list_of_links_recommended] => true
[icons_on_menu_items_support] => false
[break_list_of_links_with_br_element_recommended] => true
[access_key_support] => false
[wrap_mode_support] => false
[times_square_mode_support] => false
[deck_prefetch_support] => false
[elective_forms_recommended] => true
[wizards_recommended] => false
[image_as_link_support] => false
[insert_br_element_after_widget_recommended] => false
[wml_can_display_images_and_text_on_same_line] => false
[wml_displays_image_in_center] => false
[opwv_wml_extensions_support] => false
[wml_make_phone_call_string] => wtai://wp/mc;
[chtml_display_accesskey] => false
[emoji] => false
[chtml_can_display_images_and_text_on_same_line] => false
[chtml_displays_image_in_center] => false
[imode_region] => none
[chtml_make_phone_call_string] => tel:
[chtml_table_support] => false
[xhtml_honors_bgcolor] => true
[xhtml_supports_forms_in_table] => true
[xhtml_support_wml2_namespace] => false
[xhtml_autoexpand_select] => false
[xhtml_select_as_dropdown] => false
[xhtml_select_as_radiobutton] => false
[xhtml_select_as_popup] => false
[xhtml_display_accesskey] => false
[xhtml_supports_invisible_text] => false
[xhtml_supports_inline_input] => false
[xhtml_supports_monospace_font] => false
[xhtml_supports_table_for_layout] => true
[xhtml_supports_css_cell_table_coloring] => true
[xhtml_format_as_css_property] => true
[xhtml_format_as_attribute] => false
[xhtml_nowrap_mode] => false
[xhtml_marquee_as_css_property] => false
[xhtml_readable_background_color1] => #FFFFFF
[xhtml_readable_background_color2] => #FFFFFF
[xhtml_allows_disabled_form_elements] => false
[xhtml_document_title_support] => true
[xhtml_preferred_charset] => utf8
[opwv_xhtml_extensions_support] => false
[xhtml_make_phone_call_string] => tel:
[xhtmlmp_preferred_mime_type] => application/xhtml+xml
[xhtml_table_support] => true
[xhtml_send_sms_string] => sms:
[xhtml_send_mms_string] => mms:
[xhtml_file_upload] => supported
[cookie_support] => true
[accept_third_party_cookie] => false
[xhtml_supports_iframe] => partial
[xhtml_avoid_accesskeys] => true
[xhtml_can_embed_video] => none
[ajax_support_javascript] => true
[ajax_manipulate_css] => true
[ajax_support_getelementbyid] => true
[ajax_support_inner_html] => true
[ajax_xhr_type] => standard
[ajax_manipulate_dom] => true
[ajax_support_events] => true
[ajax_support_event_listener] => true
[ajax_preferred_geoloc_api] => none
[xhtml_support_level] => 4
[preferred_markup] => html_web_4_0
[wml_1_1] => true
[wml_1_2] => true
[wml_1_3] => true
[html_wi_w3_xhtmlbasic] => true
[html_wi_oma_xhtmlmp_1_0] => true
[html_wi_imode_html_1] => true
[html_wi_imode_html_2] => true
[html_wi_imode_html_3] => true
[html_wi_imode_html_4] => false
[html_wi_imode_html_5] => false
[html_wi_imode_htmlx_1] => false
[html_wi_imode_htmlx_1_1] => false
[html_wi_imode_compact_generic] => false
[html_web_3_2] => false
[html_web_4_0] => true
[voicexml] => false
[multipart_support] => false
[total_cache_disable_support] => false
[time_to_live_support] => false
[resolution_width] => 240
[resolution_height] => 320
[columns] => 16
[max_image_width] => 234
[max_image_height] => 300
[rows] => 16
[physical_screen_width] => 30
[physical_screen_height] => 41
[dual_orientation] => false
[density_class] => 1.0
[wbmp] => true
[bmp] => true
[epoc_bmp] => false
[gif_animated] => true
[jpg] => true
[png] => true
[tiff] => false
[transparent_png_alpha] => false
[transparent_png_index] => false
[svgt_1_1] => false
[svgt_1_1_plus] => false
[greyscale] => false
[gif] => true
[colors] => 262144
[webp_lossy_support] => false
[webp_lossless_support] => false
[post_method_support] => true
[basic_authentication_support] => true
[empty_option_value_support] => true
[emptyok] => false
[nokia_voice_call] => false
[wta_voice_call] => false
[wta_phonebook] => false
[wta_misc] => false
[wta_pdc] => true
[https_support] => true
[phone_id_provided] => false
[max_data_rate] => 3600
[wifi] => false
[sdio] => false
[vpn] => false
[has_cellular_radio] => true
[max_deck_size] => 45000
[max_url_length_in_requests] => 256
[max_url_length_homepage] => 0
[max_url_length_bookmark] => 0
[max_url_length_cached_page] => 0
[max_no_of_connection_settings] => 0
[max_no_of_bookmarks] => 0
[max_length_of_username] => 0
[max_length_of_password] => 0
[max_object_size] => 0
[downloadfun_support] => false
[directdownload_support] => true
[inline_support] => true
[oma_support] => true
[ringtone] => true
[ringtone_3gpp] => false
[ringtone_midi_monophonic] => false
[ringtone_midi_polyphonic] => false
[ringtone_imelody] => true
[ringtone_digiplug] => false
[ringtone_compactmidi] => false
[ringtone_mmf] => false
[ringtone_rmf] => false
[ringtone_xmf] => false
[ringtone_amr] => false
[ringtone_awb] => false
[ringtone_aac] => false
[ringtone_wav] => false
[ringtone_mp3] => false
[ringtone_spmidi] => false
[ringtone_qcelp] => false
[ringtone_voices] => 1
[ringtone_df_size_limit] => 0
[ringtone_directdownload_size_limit] => 0
[ringtone_inline_size_limit] => 0
[ringtone_oma_size_limit] => 0
[wallpaper] => true
[wallpaper_max_width] => 240
[wallpaper_max_height] => 320
[wallpaper_preferred_width] => 240
[wallpaper_preferred_height] => 320
[wallpaper_resize] => none
[wallpaper_wbmp] => false
[wallpaper_bmp] => false
[wallpaper_gif] => true
[wallpaper_jpg] => false
[wallpaper_png] => false
[wallpaper_tiff] => false
[wallpaper_greyscale] => false
[wallpaper_colors] => 2
[wallpaper_df_size_limit] => 0
[wallpaper_directdownload_size_limit] => 0
[wallpaper_inline_size_limit] => 0
[wallpaper_oma_size_limit] => 0
[screensaver] => true
[screensaver_max_width] => 0
[screensaver_max_height] => 0
[screensaver_preferred_width] => 0
[screensaver_preferred_height] => 0
[screensaver_resize] => none
[screensaver_wbmp] => false
[screensaver_bmp] => false
[screensaver_gif] => true
[screensaver_jpg] => false
[screensaver_png] => false
[screensaver_greyscale] => false
[screensaver_colors] => 2
[screensaver_df_size_limit] => 0
[screensaver_directdownload_size_limit] => 0
[screensaver_inline_size_limit] => 0
[screensaver_oma_size_limit] => 0
[picture] => true
[picture_max_width] => 0
[picture_max_height] => 0
[picture_preferred_width] => 0
[picture_preferred_height] => 0
[picture_resize] => none
[picture_wbmp] => false
[picture_bmp] => false
[picture_gif] => true
[picture_jpg] => false
[picture_png] => false
[picture_greyscale] => false
[picture_colors] => 2
[picture_df_size_limit] => 0
[picture_directdownload_size_limit] => 0
[picture_inline_size_limit] => 0
[picture_oma_size_limit] => 0
[video] => true
[oma_v_1_0_forwardlock] => false
[oma_v_1_0_combined_delivery] => false
[oma_v_1_0_separate_delivery] => true
[streaming_video] => true
[streaming_3gpp] => true
[streaming_mp4] => false
[streaming_mov] => false
[streaming_video_size_limit] => 0
[streaming_real_media] => none
[streaming_flv] => false
[streaming_3g2] => false
[streaming_vcodec_h263_0] => 30
[streaming_vcodec_h263_3] => -1
[streaming_vcodec_mpeg4_sp] => 3
[streaming_vcodec_mpeg4_asp] => -1
[streaming_vcodec_h264_bp] => 1.3
[streaming_acodec_amr] => nb
[streaming_acodec_aac] => lc
[streaming_wmv] => none
[streaming_preferred_protocol] => rtsp
[streaming_preferred_http_protocol] => none
[wap_push_support] => true
[connectionless_service_indication] => true
[connectionless_service_load] => false
[connectionless_cache_operation] => false
[connectionoriented_unconfirmed_service_indication] => false
[connectionoriented_unconfirmed_service_load] => false
[connectionoriented_unconfirmed_cache_operation] => false
[connectionoriented_confirmed_service_indication] => false
[connectionoriented_confirmed_service_load] => false
[connectionoriented_confirmed_cache_operation] => false
[utf8_support] => false
[ascii_support] => false
[iso8859_support] => false
[expiration_date] => false
[j2me_cldc_1_0] => false
[j2me_cldc_1_1] => false
[j2me_midp_1_0] => false
[j2me_midp_2_0] => false
[doja_1_0] => false
[doja_1_5] => false
[doja_2_0] => false
[doja_2_1] => false
[doja_2_2] => false
[doja_3_0] => false
[doja_3_5] => false
[doja_4_0] => false
[j2me_jtwi] => false
[j2me_mmapi_1_0] => false
[j2me_mmapi_1_1] => false
[j2me_wmapi_1_0] => false
[j2me_wmapi_1_1] => false
[j2me_wmapi_2_0] => false
[j2me_btapi] => false
[j2me_3dapi] => false
[j2me_locapi] => false
[j2me_nokia_ui] => false
[j2me_motorola_lwt] => false
[j2me_siemens_color_game] => false
[j2me_siemens_extension] => false
[j2me_heap_size] => 0
[j2me_max_jar_size] => 0
[j2me_storage_size] => 0
[j2me_max_record_store_size] => 0
[j2me_screen_width] => 0
[j2me_screen_height] => 0
[j2me_canvas_width] => 0
[j2me_canvas_height] => 0
[j2me_bits_per_pixel] => 0
[j2me_audio_capture_enabled] => false
[j2me_video_capture_enabled] => false
[j2me_photo_capture_enabled] => false
[j2me_capture_image_formats] => none
[j2me_http] => false
[j2me_https] => false
[j2me_socket] => false
[j2me_udp] => false
[j2me_serial] => false
[j2me_gif] => false
[j2me_gif89a] => false
[j2me_jpg] => false
[j2me_png] => false
[j2me_bmp] => false
[j2me_bmp3] => false
[j2me_wbmp] => false
[j2me_midi] => false
[j2me_wav] => false
[j2me_amr] => false
[j2me_mp3] => false
[j2me_mp4] => false
[j2me_imelody] => false
[j2me_rmf] => false
[j2me_au] => false
[j2me_aac] => false
[j2me_realaudio] => false
[j2me_xmf] => false
[j2me_wma] => false
[j2me_3gpp] => false
[j2me_h263] => false
[j2me_svgt] => false
[j2me_mpeg4] => false
[j2me_realvideo] => false
[j2me_real8] => false
[j2me_realmedia] => false
[j2me_left_softkey_code] => -6
[j2me_right_softkey_code] => -7
[j2me_middle_softkey_code] => 0
[j2me_select_key_code] => -5
[j2me_return_key_code] => -11
[j2me_clear_key_code] => -8
[j2me_datefield_no_accepts_null_date] => false
[j2me_datefield_broken] => false
[receiver] => false
[sender] => false
[mms_max_size] => 614400
[mms_max_height] => 1536
[mms_max_width] => 2048
[built_in_recorder] => false
[built_in_camera] => false
[mms_jpeg_baseline] => true
[mms_jpeg_progressive] => false
[mms_gif_static] => true
[mms_gif_animated] => false
[mms_png] => true
[mms_bmp] => true
[mms_wbmp] => true
[mms_amr] => true
[mms_wav] => true
[mms_midi_monophonic] => true
[mms_midi_polyphonic] => false
[mms_midi_polyphonic_voices] => 0
[mms_spmidi] => true
[mms_mmf] => false
[mms_mp3] => false
[mms_evrc] => false
[mms_qcelp] => false
[mms_ota_bitmap] => false
[mms_nokia_wallpaper] => false
[mms_nokia_operatorlogo] => false
[mms_nokia_3dscreensaver] => false
[mms_nokia_ringingtone] => false
[mms_rmf] => false
[mms_xmf] => false
[mms_symbian_install] => false
[mms_jar] => true
[mms_jad] => true
[mms_vcard] => true
[mms_vcalendar] => false
[mms_wml] => false
[mms_wbxml] => false
[mms_wmlc] => false
[mms_video] => false
[mms_mp4] => false
[mms_3gpp] => false
[mms_3gpp2] => false
[mms_max_frame_rate] => 0
[nokiaring] => false
[picturemessage] => false
[operatorlogo] => false
[largeoperatorlogo] => false
[callericon] => false
[nokiavcard] => false
[nokiavcal] => false
[sckl_ringtone] => false
[sckl_operatorlogo] => false
[sckl_groupgraphic] => false
[sckl_vcard] => false
[sckl_vcalendar] => false
[text_imelody] => false
[ems] => false
[ems_variablesizedpictures] => false
[ems_imelody] => false
[ems_odi] => false
[ems_upi] => false
[ems_version] => 0
[siemens_ota] => false
[siemens_logo_width] => 101
[siemens_logo_height] => 29
[siemens_screensaver_width] => 101
[siemens_screensaver_height] => 50
[gprtf] => false
[sagem_v1] => false
[sagem_v2] => false
[panasonic] => false
[sms_enabled] => true
[wav] => true
[mmf] => false
[smf] => false
[mld] => false
[midi_monophonic] => true
[midi_polyphonic] => false
[sp_midi] => true
[rmf] => false
[xmf] => false
[compactmidi] => false
[digiplug] => false
[nokia_ringtone] => false
[imelody] => true
[au] => false
[amr] => true
[awb] => false
[aac] => false
[mp3] => true
[voices] => 1
[qcelp] => false
[evrc] => false
[flash_lite_version] => 2_0
[fl_wallpaper] => true
[fl_screensaver] => true
[fl_standalone] => false
[fl_browser] => true
[fl_sub_lcd] => false
[full_flash_support] => false
[css_supports_width_as_percentage] => true
[css_border_image] => none
[css_rounded_corners] => none
[css_gradient] => none
[css_spriting] => true
[css_gradient_linear] => none
[is_transcoder] => false
[transcoder_ua_header] => user-agent
[rss_support] => false
[pdf_support] => false
[progressive_download] => true
[playback_vcodec_h263_0] => 30
[playback_vcodec_h263_3] => 10
[playback_vcodec_mpeg4_sp] => 3
[playback_vcodec_mpeg4_asp] => -1
[playback_vcodec_h264_bp] => 1.3
[playback_real_media] => none
[playback_3gpp] => true
[playback_3g2] => false
[playback_mp4] => false
[playback_mov] => false
[playback_acodec_amr] => nb
[playback_acodec_aac] => lc
[playback_df_size_limit] => 0
[playback_directdownload_size_limit] => 0
[playback_inline_size_limit] => 0
[playback_oma_size_limit] => 0
[playback_acodec_qcelp] => false
[playback_wmv] => none
[hinted_progressive_download] => false
[html_preferred_dtd] => xhtml_mp1
[viewport_supported] => false
[viewport_width] =>
[viewport_userscalable] =>
[viewport_initial_scale] =>
[viewport_maximum_scale] =>
[viewport_minimum_scale] =>
[mobileoptimized] => false
[handheldfriendly] => false
[canvas_support] => none
[image_inlining] => true
[is_smarttv] => false
[is_console] => false
[nfc_support] => false
[ux_full_desktop] => false
[jqm_grade] => none
[is_sencha_touch_ok] => false
[controlcap_is_smartphone] => default
[controlcap_is_ios] => default
[controlcap_is_android] => default
[controlcap_is_robot] => default
[controlcap_is_app] => default
[controlcap_advertised_device_os] => default
[controlcap_advertised_device_os_version] => default
[controlcap_advertised_browser] => default
[controlcap_advertised_browser_version] => default
[controlcap_is_windows_phone] => default
[controlcap_is_full_desktop] => default
[controlcap_is_largescreen] => default
[controlcap_is_mobile] => default
[controlcap_is_touchscreen] => default
[controlcap_is_wml_preferred] => default
[controlcap_is_xhtmlmp_preferred] => default
[controlcap_is_html_preferred] => default
[controlcap_form_factor] => default
[controlcap_complete_device_name] => default
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr></table>
</div>
<div class="section">
<h1 class="header center orange-text">About this comparison</h1>
<div class="row center">
<h5 class="header light">
The primary goal of this project is simple<br />
I wanted to know which user agent parser is the most accurate in each part - device detection, bot detection and so on...<br />
<br />
The secondary goal is to provide a source for all user agent parsers to improve their detection based on this results.<br />
<br />
You can also improve this further, by suggesting ideas at <a href="https://github.com/ThaDafinser/UserAgentParserComparison">ThaDafinser/UserAgentParserComparison</a><br />
<br />
The comparison is based on the abstraction by <a href="https://github.com/ThaDafinser/UserAgentParser">ThaDafinser/UserAgentParser</a>
</h5>
</div>
</div>
<div class="card">
<div class="card-content">
Comparison created <i>2016-02-13 13:35:44</i> | by
<a href="https://github.com/ThaDafinser">ThaDafinser</a>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-2.1.4.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.97.3/js/materialize.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/list.js/1.1.1/list.min.js"></script>
<script>
$(document).ready(function(){
// the "href" attribute of .modal-trigger must specify the modal ID that wants to be triggered
$('.modal-trigger').leanModal();
});
</script>
</body>
</html> | Java |
<?php
namespace AwsUpload\Tests\Command;
use AwsUpload\Io\Output;
use AwsUpload\AwsUpload;
use AwsUpload\Tests\BaseTestCase;
use AwsUpload\Message\DeleteMessage;
use AwsUpload\Message\ErrorMessage;
use Symfony\Component\Filesystem\Filesystem;
class DeleteTest extends BaseTestCase
{
public function test_noKey_expectedNoProjectMsg()
{
$msg = DeleteMessage::noArgs();
$msg = Output::color($msg);
$this->expectOutputString($msg . "\n");
self::clearArgv();
self::pushToArgv(array('asd.php', 'delete'));
$aws = new AwsUpload();
$aws->setOutput(new \AwsUpload\Io\OutputEcho());
$cmd = new \AwsUpload\Command\DeleteCommand($aws);
$cmd->run();
}
public function test_noValidKey_expectedNoValidKey()
{
$this->expectOutputString("It seems that the key \e[33maaa\e[0m is not valid:\n\n"
. "Please try to use this format:\n"
. " - [project].[environmet]\n\n"
. "Examples of valid key to create a new setting file:\n"
. " - \e[32mmy-site.staging\e[0m\n"
. " - \e[32mmy-site.dev\e[0m\n"
. " - \e[32mmy-site.prod\e[0m\n\n"
. "Tips on choosing the key name:\n"
. " - for [project] and [environmet] try to be: short, sweet, to the point\n"
. " - use only one 'dot' . in the name\n"
. "\n\n");
self::clearArgv();
self::pushToArgv(array('asd.php', 'delete', 'aaa'));
$aws = new AwsUpload();
$aws->setOutput(new \AwsUpload\Io\OutputEcho());
$cmd = new \AwsUpload\Command\DeleteCommand($aws);
$cmd->run();
}
public function test_validKeyNoExists_expectedNoFileFound()
{
$filesystem = new Filesystem();
$filesystem->dumpFile($this->aws_home . '/project-1.dev.json', '{}');
$msg = ErrorMessage::noFileFound('project-2.dev');
$msg = Output::color($msg);
$this->expectOutputString($msg . "\n");
self::clearArgv();
self::pushToArgv(array('asd.php', 'delete', 'project-2.dev'));
$aws = new AwsUpload();
$aws->setOutput(new \AwsUpload\Io\OutputEcho());
$cmd = new \AwsUpload\Command\DeleteCommand($aws);
$cmd->run();
}
}
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>switch: 20 s 🏆</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.10.2 / switch - 1.0.2</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
switch
<small>
1.0.2
<span class="label label-success">20 s 🏆</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-12-02 03:04:00 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-12-02 03:04:00 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
coq 8.10.2 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.08.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.08.1 Official release 4.08.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/vzaliva/coq-switch"
dev-repo: "git://[email protected]:vzaliva/coq-switch.git"
bug-reports: "https://github.com/vzaliva/coq-switch/issues"
authors: ["Vadim Zaliva <[email protected]>"]
license: "MIT"
build: [
[make "-j%{jobs}%"]
]
install: [
[make "install"]
]
depends: [
"coq" {>= "8.10.2"}
"coq-metacoq-template" {>= "1.0~alpha2+8.10" & < "1.0~beta1"}
]
tags: [
"category:Miscellaneous/Coq Extensions"
"date:2020-03-13"
"logpath:Switch"
]
synopsis: "A plugin to implement functionality similar to `switch` statement in C language."
description: """It allows easier dispatch on several constant values
of a type with decidable equality. Given a type, boolean equality
predicate, and list of choices, it will generate (using TemplateCoq)
an inductive type with constructors, one for each of the choices, and
a function mapping values to this type."""
url {
src: "https://github.com/vzaliva/coq-switch/archive/v1.0.2.tar.gz"
checksum: "51ad3d8d53edb2334854d8bc976b75ed"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-switch.1.0.2 coq.8.10.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 4000000; timeout 4h opam install -y --deps-only coq-switch.1.0.2 coq.8.10.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>7 m 29 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 16000000; timeout 4h opam install -y -v coq-switch.1.0.2 coq.8.10.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>20 s</dd>
</dl>
<h2>Installation size</h2>
<p>Total: 120 K</p>
<ul>
<li>108 K <code>../ocaml-base-compiler.4.08.1/lib/coq/user-contrib/Switch/Switch.vo</code></li>
<li>9 K <code>../ocaml-base-compiler.4.08.1/lib/coq/user-contrib/Switch/Switch.glob</code></li>
<li>2 K <code>../ocaml-base-compiler.4.08.1/lib/coq/user-contrib/Switch/Switch.v</code></li>
</ul>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq-switch.1.0.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>relation-extraction: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.13.0 / relation-extraction - 8.5.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
relation-extraction
<small>
8.5.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-12-19 20:43:27 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-12-19 20:43:27 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
conf-gmp 3 Virtual package relying on a GMP lib system installation
coq 8.13.0 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.10.2 The OCaml compiler (virtual package)
ocaml-base-compiler 4.10.2 Official release 4.10.2
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
zarith 1.12 Implements arithmetic and logical operations over arbitrary-precision integers
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/coq-contribs/relation-extraction"
license: "GPL 3"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/RelationExtraction"]
depends: [
"ocaml"
"coq" {>= "8.5" & < "8.6~"}
]
tags: [ "keyword:extraction" "keyword:inductive relations" "keyword:semantics" "category:Computer Science/Semantics and Compilation/Compilation" "date:2011" ]
authors: [ "Catherine Dubois <>" "David Delahaye <>" "Pierre-Nicolas Tollitte <>" ]
bug-reports: "https://github.com/coq-contribs/relation-extraction/issues"
dev-repo: "git+https://github.com/coq-contribs/relation-extraction.git"
synopsis: "Functions extraction from inductive relations"
description: """
This plugin introduces a new set of extraction commands that
generates functional code form inductive specifications."""
flags: light-uninstall
url {
src:
"https://github.com/coq-contribs/relation-extraction/archive/v8.5.0.tar.gz"
checksum: "md5=081bb10e77ea72dfef13df6b9f30248b"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-relation-extraction.8.5.0 coq.8.13.0</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.13.0).
The following dependencies couldn't be met:
- coq-relation-extraction -> coq < 8.6~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-relation-extraction.8.5.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Building the data into separate facilities — orbf_data_quality 0.1 documentation</title>
<link rel="stylesheet" href="_static/classic.css" type="text/css" />
<link rel="stylesheet" href="_static/pygments.css" type="text/css" />
<script type="text/javascript">
var DOCUMENTATION_OPTIONS = {
URL_ROOT: './',
VERSION: '0.1',
COLLAPSE_INDEX: false,
FILE_SUFFIX: '.html',
HAS_SOURCE: true,
SOURCELINK_SUFFIX: '.txt'
};
</script>
<script type="text/javascript" src="_static/jquery.js"></script>
<script type="text/javascript" src="_static/underscore.js"></script>
<script type="text/javascript" src="_static/doctools.js"></script>
<link rel="index" title="Index" href="genindex.html" />
<link rel="search" title="Search" href="search.html" />
<link rel="prev" title="Introduction" href="getting-started.html" />
</head>
<body role="document">
<div class="related" role="navigation" aria-label="related navigation">
<h3>Navigation</h3>
<ul>
<li class="right" style="margin-right: 10px">
<a href="genindex.html" title="General Index"
accesskey="I">index</a></li>
<li class="right" >
<a href="getting-started.html" title="Introduction"
accesskey="P">previous</a> |</li>
<li class="nav-item nav-item-0"><a href="index.html">orbf_data_quality 0.1 documentation</a> »</li>
</ul>
</div>
<div class="document">
<div class="documentwrapper">
<div class="bodywrapper">
<div class="body" role="main">
<div class="section" id="building-the-data-into-separate-facilities">
<h1>Building the data into separate facilities<a class="headerlink" href="#building-the-data-into-separate-facilities" title="Permalink to this headline">¶</a></h1>
<p>The data consists in many facilities, that will be monitored. The data used to update the monitoring algorithms for each facility depends on the supervision trail that has been triggered. In order to efficiently keep track of each facility and of its trail, we transform the collected in a list of facilities objects with specific information stored, related to facilities.</p>
<dl class="class">
<dt id="monitoring_algorithms.facility_monitoring.facility">
<em class="property">class </em><code class="descclassname">monitoring_algorithms.facility_monitoring.</code><code class="descname">facility</code><span class="sig-paren">(</span><em>data</em>, <em>tarifs</em><span class="sig-paren">)</span><a class="headerlink" href="#monitoring_algorithms.facility_monitoring.facility" title="Permalink to this definition">¶</a></dt>
<dd><p>A Facility currently under monitoring is followed in time. Its data is updated as time advances, independtly for each algorithm.</p>
<table class="docutils field-list" frame="void" rules="none">
<col class="field-name" />
<col class="field-body" />
<tbody valign="top">
<tr class="field-odd field"><th class="field-name">Parameters:</th><td class="field-body"><p class="first"><strong>data</strong> : the data for a given facility</p>
<p class="last"><strong>tarifs</strong> : A list of tarifs for the RBF program</p>
</td>
</tr>
</tbody>
</table>
<p class="rubric">Attributes</p>
<table border="1" class="docutils">
<colgroup>
<col width="16%" />
<col width="84%" />
</colgroup>
<tbody valign="top">
<tr class="row-odd"><td>facility_id</td>
<td>(str) The facilities’ unique identifier</td>
</tr>
<tr class="row-even"><td>facility_name</td>
<td>(str) The current name of the facility</td>
</tr>
<tr class="row-odd"><td>facility_type</td>
<td>(str) The administrative type for the facility</td>
</tr>
<tr class="row-even"><td>division</td>
<td>(str) The division in which the facility list_facilities_name</td>
</tr>
<tr class="row-odd"><td>departement</td>
<td>(str) The departement in which the facility lies</td>
</tr>
<tr class="row-even"><td>reports</td>
<td>(list) The data from the reports sent by the facility</td>
</tr>
<tr class="row-odd"><td>last_supervision</td>
<td>(date) The date of the last supervision made in the facility</td>
</tr>
<tr class="row-even"><td>supervisions</td>
<td>(DataFrame) A table of the supervisions made in the facility for each algorithm used</td>
</tr>
</tbody>
</table>
<p class="rubric">Methods</p>
<table border="1" class="longtable docutils">
<colgroup>
<col width="10%" />
<col width="90%" />
</colgroup>
<tbody valign="top">
<tr class="row-odd"><td><code class="xref py py-obj docutils literal"><span class="pre">arima_report_payment</span></code></td>
<td></td>
</tr>
<tr class="row-even"><td><code class="xref py py-obj docutils literal"><span class="pre">make_reports</span></code></td>
<td></td>
</tr>
<tr class="row-odd"><td><code class="xref py py-obj docutils literal"><span class="pre">make_supervision_trail</span></code></td>
<td></td>
</tr>
<tr class="row-even"><td><code class="xref py py-obj docutils literal"><span class="pre">plot_supervision_trail</span></code></td>
<td></td>
</tr>
</tbody>
</table>
</dd></dl>
</div>
</div>
</div>
</div>
<div class="sphinxsidebar" role="navigation" aria-label="main navigation">
<div class="sphinxsidebarwrapper">
<h3><a href="index.html">Table Of Contents</a></h3>
<ul class="current">
<li class="toctree-l1"><a class="reference internal" href="getting-started.html">Introduction</a></li>
<li class="toctree-l1 current"><a class="current reference internal" href="#">Building the data into separate facilities</a><ul class="simple">
</ul>
</li>
<li class="toctree-l1"><a class="reference internal" href="algorithms.html">Defining a Monitoring Algorithm</a></li>
</ul>
<h4>Previous topic</h4>
<p class="topless"><a href="getting-started.html"
title="previous chapter">Introduction</a></p>
<div role="note" aria-label="source link">
<h3>This Page</h3>
<ul class="this-page-menu">
<li><a href="_sources/facilities.rst.txt"
rel="nofollow">Show Source</a></li>
</ul>
</div>
<div id="searchbox" style="display: none" role="search">
<h3>Quick search</h3>
<form class="search" action="search.html" method="get">
<div><input type="text" name="q" /></div>
<div><input type="submit" value="Go" /></div>
<input type="hidden" name="check_keywords" value="yes" />
<input type="hidden" name="area" value="default" />
</form>
</div>
<script type="text/javascript">$('#searchbox').show(0);</script>
</div>
</div>
<div class="clearer"></div>
</div>
<div class="related" role="navigation" aria-label="related navigation">
<h3>Navigation</h3>
<ul>
<li class="right" style="margin-right: 10px">
<a href="genindex.html" title="General Index"
>index</a></li>
<li class="right" >
<a href="getting-started.html" title="Introduction"
>previous</a> |</li>
<li class="nav-item nav-item-0"><a href="index.html">orbf_data_quality 0.1 documentation</a> »</li>
</ul>
</div>
<div class="footer" role="contentinfo">
© Copyright .
Created using <a href="http://sphinx-doc.org/">Sphinx</a> 1.5.6.
</div>
</body>
</html> | Java |
package andexam.ver4_1.c35_setting;
import andexam.ver4_1.*;
import android.app.*;
import android.os.*;
import android.widget.*;
//* 원론적인 방법
public class UserInteraction extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.userinteraction);
}
protected Handler mFinishHandler = new Handler() {
public void handleMessage(android.os.Message msg) {
finish();
}
};
void registerFinish() {
mFinishHandler.sendEmptyMessageDelayed(0, 5 * 1000);
}
void unRegisterFinish() {
mFinishHandler.removeMessages(0);
}
void RefreshFinish() {
unRegisterFinish();
registerFinish();
}
protected void onResume() {
super.onResume();
registerFinish();
}
protected void onPause() {
unRegisterFinish();
super.onPause();
}
public void onUserInteraction() {
super.onUserInteraction();
RefreshFinish();
}
protected void onUserLeaveHint () {
super.onUserLeaveHint();
Toast.makeText(this, "Leave by user", Toast.LENGTH_LONG).show();
}
}
//*/
/* 좀 더 쉬운 방법
public class UserInteraction extends Activity {
long mLastInteraction;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.userinteraction);
}
protected void onResume() {
super.onResume();
mLastInteraction = System.currentTimeMillis();
mFinishHandler.sendEmptyMessageDelayed(0, 1000);
}
protected void onPause() {
super.onPause();
mFinishHandler.removeMessages(0);
}
protected Handler mFinishHandler = new Handler() {
public void handleMessage(android.os.Message msg) {
mFinishHandler.sendEmptyMessageDelayed(0, 1000);
long now = System.currentTimeMillis();
if ((now - mLastInteraction) > 5000) {
finish();
}
}
};
public void onUserInteraction() {
super.onUserInteraction();
mLastInteraction = System.currentTimeMillis();
}
}
//*/
| Java |
#include <iostream>
#include <seqan/sequence.h>
#include <seqan/file.h>
using namespace seqan;
int main()
{
StringSet<String<Dna5> > nucleotideSets;
String<Dna5> seq1 ="ATATANGCGT";
String<Dna5> seq2 ="AAGCATGANT";
String<Dna5> seq3 ="TGAAANTGAC";
appendValue(nucleotideSets,seq1);
appendValue(nucleotideSets,seq2);
appendValue(nucleotideSets,seq3);
String<Dna5> refSeq="GATGCATGAT";
StringSet<String<Dna5> > lesser;
StringSet<String<Dna5> > greater;
for (unsigned i = 0; i < length(nucleotideSets); ++i){
Lexical<> comp(nucleotideSets[i],refSeq);
if (isLess(comp))
appendValue(lesser, nucleotideSets[i]);
if (isGreater(comp))
appendValue(greater, nucleotideSets[i]);
}
//std::cout << "lesser: " << lesser << std::endl;
//std::cout << "greater: " << greater << std::endl;
return 0;
} | Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>User agent detail - Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.97.3/css/materialize.min.css">
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
<link href="../circle.css" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="section">
<h1 class="header center orange-text">User agent detail</h1>
<div class="row center">
<h5 class="header light">
Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
</h5>
</div>
</div>
<div class="section">
<table class="striped"><tr><th></th><th colspan="3">General</th><th colspan="5">Device</th><th colspan="3">Bot</th><th colspan="2"></th></tr><tr><th>Provider</th><th>Browser</th><th>Engine</th><th>OS</th><th>Brand</th><th>Model</th><th>Type</th><th>Is mobile</th><th>Is touch</th><th>Is bot</th><th>Name</th><th>Type</th><th>Parse time</th><th>Actions</th></tr><tr><th colspan="14" class="green lighten-3">Test suite</th></tr><tr><td>WhichBrowser<br /><small>v2.0.18</small><br /><small>vendor/whichbrowser/parser/tests/data/mobile/browser-maxthon.yaml</small></td><td>Maxthon </td><td>Webkit 525.18.1</td><td>iPhone OS 2.2</td><td style="border-left: 1px solid #555"></td><td>iPhone</td><td>mobile:smart</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-027cff01-4a76-491b-ace3-9289fcbc172f">Detail</a>
<!-- Modal Structure -->
<div id="modal-027cff01-4a76-491b-ace3-9289fcbc172f" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>WhichBrowser result detail</h4>
<p><pre><code class="php">Array
(
[headers] => User-Agent: Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
[result] => Array
(
[browser] => Array
(
[name] => Maxthon
[type] => browser
)
[engine] => Array
(
[name] => Webkit
[version] => 525.18.1
)
[os] => Array
(
[name] => iOS
[alias] => iPhone OS
[version] => 2.2
)
[device] => Array
(
[type] => mobile
[subtype] => smart
[manufacturer] => Apple
[model] => iPhone
)
)
[readable] => Maxthon on an Apple iPhone running iPhone OS 2.2
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><th colspan="14" class="green lighten-3">Providers</th></tr><tr><td>BrowscapFull<br /><small>6014</small><br /></td><td>Safari 3.1</td><td>WebKit </td><td>iOS 2.0</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td>Mobile Phone</td><td>yes</td><td>yes</td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.053</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-47a9cd06-e213-4882-bc34-db6aed664223">Detail</a>
<!-- Modal Structure -->
<div id="modal-47a9cd06-e213-4882-bc34-db6aed664223" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>BrowscapFull result detail</h4>
<p><pre><code class="php">stdClass Object
(
[browser_name_regex] => /^mozilla\/5\.0 \(iphone.*cpu iphone os 2..* like mac os x.*\).*applewebkit\/.*\(.*khtml.* like gecko.*\).*version\/3\.1.*mobile\/.*safari\/.*$/
[browser_name_pattern] => mozilla/5.0 (iphone*cpu iphone os 2?* like mac os x*)*applewebkit/*(*khtml* like gecko*)*version/3.1*mobile/*safari/*
[parent] => Mobile Safari 3.1
[comment] => Mobile Safari 3.1
[browser] => Safari
[browser_type] => Browser
[browser_bits] => 32
[browser_maker] => Apple Inc
[browser_modus] => unknown
[version] => 3.1
[majorver] => 3
[minorver] => 1
[platform] => iOS
[platform_version] => 2.0
[platform_description] => iPod, iPhone & iPad
[platform_bits] => 32
[platform_maker] => Apple Inc
[alpha] =>
[beta] =>
[win16] =>
[win32] =>
[win64] =>
[frames] => 1
[iframes] => 1
[tables] => 1
[cookies] => 1
[backgroundsounds] =>
[javascript] => 1
[vbscript] =>
[javaapplets] => 1
[activexcontrols] =>
[ismobiledevice] => 1
[istablet] =>
[issyndicationreader] =>
[crawler] =>
[isfake] =>
[isanonymized] =>
[ismodified] =>
[cssversion] => 3
[aolversion] => 0
[device_name] => iPhone
[device_maker] => Apple Inc
[device_type] => Mobile Phone
[device_pointing_method] => touchscreen
[device_code_name] => iPhone
[device_brand_name] => Apple
[renderingengine_name] => WebKit
[renderingengine_version] => unknown
[renderingengine_description] => For Google Chrome, iOS (including both mobile Safari, WebViews within third-party apps, and web clips), Safari, Arora, Midori, OmniWeb, Shiira, iCab since version 4, Web, SRWare Iron, Rekonq, and in Maxthon 3.
[renderingengine_maker] => Apple Inc
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>BrowscapLite<br /><small>6014</small><br /></td><td>Safari </td><td><i class="material-icons">close</i></td><td>iOS </td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>Mobile Phone</td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.008</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-42bb56ba-b834-47c5-bea0-c0270e9ab371">Detail</a>
<!-- Modal Structure -->
<div id="modal-42bb56ba-b834-47c5-bea0-c0270e9ab371" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>BrowscapLite result detail</h4>
<p><pre><code class="php">stdClass Object
(
[browser_name_regex] => /^mozilla\/5\.0 \(iphone.*cpu.*os.* like mac os x.*\).*applewebkit\/.*\(.*khtml.* like gecko.*\).*version\/.*safari\/.*$/
[browser_name_pattern] => mozilla/5.0 (iphone*cpu*os* like mac os x*)*applewebkit/*(*khtml* like gecko*)*version/*safari/*
[parent] => Mobile Safari Generic
[comment] => Mobile Safari Generic
[browser] => Safari
[browser_type] => unknown
[browser_bits] => 0
[browser_maker] => unknown
[browser_modus] => unknown
[version] => 0.0
[majorver] => 0
[minorver] => 0
[platform] => iOS
[platform_version] => unknown
[platform_description] => unknown
[platform_bits] => 0
[platform_maker] => unknown
[alpha] => false
[beta] => false
[win16] => false
[win32] => false
[win64] => false
[frames] => false
[iframes] => false
[tables] => false
[cookies] => false
[backgroundsounds] => false
[javascript] => false
[vbscript] => false
[javaapplets] => false
[activexcontrols] => false
[ismobiledevice] => 1
[istablet] =>
[issyndicationreader] => false
[crawler] => false
[isfake] => false
[isanonymized] => false
[ismodified] => false
[cssversion] => 0
[aolversion] => 0
[device_name] => unknown
[device_maker] => unknown
[device_type] => Mobile Phone
[device_pointing_method] => unknown
[device_code_name] => unknown
[device_brand_name] => unknown
[renderingengine_name] => unknown
[renderingengine_version] => unknown
[renderingengine_description] => unknown
[renderingengine_maker] => unknown
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>BrowscapPhp<br /><small>6014</small><br /></td><td>Safari 3.1</td><td><i class="material-icons">close</i></td><td>iOS </td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>Mobile Device</td><td>yes</td><td>yes</td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.035</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-ad0041a2-b0f4-43f6-a70d-cad1443caa68">Detail</a>
<!-- Modal Structure -->
<div id="modal-ad0041a2-b0f4-43f6-a70d-cad1443caa68" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>BrowscapPhp result detail</h4>
<p><pre><code class="php">stdClass Object
(
[browser_name_regex] => /^mozilla\/5\.0 \(.*cpu iphone os .* like mac os x.*\).*applewebkit\/.*\(.*khtml.* like gecko.*\).*version\/3\.1.*mobile\/.*safari\/.*$/
[browser_name_pattern] => mozilla/5.0 (*cpu iphone os * like mac os x*)*applewebkit/*(*khtml* like gecko*)*version/3.1*mobile/*safari/*
[parent] => Mobile Safari 3.1
[comment] => Mobile Safari 3.1
[browser] => Safari
[browser_type] => unknown
[browser_bits] => 0
[browser_maker] => Apple Inc
[browser_modus] => unknown
[version] => 3.1
[majorver] => 3
[minorver] => 1
[platform] => iOS
[platform_version] => unknown
[platform_description] => unknown
[platform_bits] => 0
[platform_maker] => unknown
[alpha] => false
[beta] => false
[win16] => false
[win32] => false
[win64] => false
[frames] => false
[iframes] => false
[tables] => false
[cookies] => false
[backgroundsounds] => false
[javascript] => false
[vbscript] => false
[javaapplets] => false
[activexcontrols] => false
[ismobiledevice] => 1
[istablet] =>
[issyndicationreader] => false
[crawler] =>
[isfake] => false
[isanonymized] => false
[ismodified] => false
[cssversion] => 0
[aolversion] => 0
[device_name] => unknown
[device_maker] => unknown
[device_type] => Mobile Device
[device_pointing_method] => touchscreen
[device_code_name] => unknown
[device_brand_name] => unknown
[renderingengine_name] => unknown
[renderingengine_version] => unknown
[renderingengine_description] => unknown
[renderingengine_maker] => unknown
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>DonatjUAParser<br /><small>v0.5.1</small><br /></td><td>Safari 3.1.1</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-15fbc1f0-2615-4d42-b5d9-a30dd647b050">Detail</a>
<!-- Modal Structure -->
<div id="modal-15fbc1f0-2615-4d42-b5d9-a30dd647b050" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>DonatjUAParser result detail</h4>
<p><pre><code class="php">Array
(
[platform] => iPhone
[browser] => Safari
[version] => 3.1.1
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>JenssegersAgent<br /><small>v2.3.3</small><br /></td><td>Safari 3.1.1</td><td><i class="material-icons">close</i></td><td>iOS 2_2</td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.001</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-b85a2b91-6a55-4436-a82c-1ea0d46e2e51">Detail</a>
<!-- Modal Structure -->
<div id="modal-b85a2b91-6a55-4436-a82c-1ea0d46e2e51" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>JenssegersAgent result detail</h4>
<p><pre><code class="php">Array
(
[browserName] => Safari
[browserVersion] => 3.1.1
[osName] => iOS
[osVersion] => 2_2
[deviceModel] => iPhone
[isMobile] => 1
[isRobot] =>
[botName] =>
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>NeutrinoApiCom<br /><small></small><br /></td><td>Mobile Safari 3.1.1</td><td><i class="material-icons">close</i></td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td>mobile-browser</td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.25001</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-8c2a7a4e-3fbf-4df2-8d61-5e730422f67b">Detail</a>
<!-- Modal Structure -->
<div id="modal-8c2a7a4e-3fbf-4df2-8d61-5e730422f67b" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>NeutrinoApiCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[mobile_screen_height] => 480
[is_mobile] => 1
[type] => mobile-browser
[mobile_brand] => Apple
[mobile_model] => iPhone
[version] => 3.1.1
[is_android] =>
[browser_name] => Mobile Safari
[operating_system_family] => iOS
[operating_system_version] => 2.2
[is_ios] => 1
[producer] => Apple Inc.
[operating_system] => iOS
[mobile_screen_width] => 320
[mobile_browser] => Safari
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>PiwikDeviceDetector<br /><small>3.6.1</small><br /></td><td>Maxthon </td><td>WebKit </td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td>smartphone</td><td>yes</td><td></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.002</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-4a941d34-a8d3-4914-9724-346f60ad7046">Detail</a>
<!-- Modal Structure -->
<div id="modal-4a941d34-a8d3-4914-9724-346f60ad7046" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>PiwikDeviceDetector result detail</h4>
<p><pre><code class="php">Array
(
[client] => Array
(
[type] => browser
[name] => Maxthon
[short_name] => MX
[version] =>
[engine] => WebKit
)
[operatingSystem] => Array
(
[name] => iOS
[short_name] => IOS
[version] => 2.2
[platform] =>
)
[device] => Array
(
[brand] => AP
[brandName] => Apple
[model] => iPhone
[device] => 1
[deviceName] => smartphone
)
[bot] =>
[extra] => Array
(
[isBot] =>
[isBrowser] => 1
[isFeedReader] =>
[isMobileApp] =>
[isPIM] =>
[isLibrary] =>
[isMediaPlayer] =>
[isCamera] =>
[isCarBrowser] =>
[isConsole] =>
[isFeaturePhone] =>
[isPhablet] =>
[isPortableMediaPlayer] =>
[isSmartDisplay] =>
[isSmartphone] => 1
[isTablet] =>
[isTV] =>
[isDesktop] =>
[isMobile] => 1
[isTouchEnabled] =>
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>SinergiBrowserDetector<br /><small>6.0.1</small><br /></td><td>Safari 3.1.1</td><td><i class="material-icons">close</i></td><td>iOS 2.2</td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td>iPhone</td><td><i class="material-icons">close</i></td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.001</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-ec1cd248-02b0-457e-8a9d-35bb99af008c">Detail</a>
<!-- Modal Structure -->
<div id="modal-ec1cd248-02b0-457e-8a9d-35bb99af008c" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>SinergiBrowserDetector result detail</h4>
<p><pre><code class="php">Array
(
[browser] => Sinergi\BrowserDetector\Browser Object
(
[userAgent:Sinergi\BrowserDetector\Browser:private] => Sinergi\BrowserDetector\UserAgent Object
(
[userAgentString:Sinergi\BrowserDetector\UserAgent:private] => Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
)
[name:Sinergi\BrowserDetector\Browser:private] => Safari
[version:Sinergi\BrowserDetector\Browser:private] => 3.1.1
[isRobot:Sinergi\BrowserDetector\Browser:private] =>
[isChromeFrame:Sinergi\BrowserDetector\Browser:private] =>
[isFacebookWebView:Sinergi\BrowserDetector\Browser:private] =>
[isCompatibilityMode:Sinergi\BrowserDetector\Browser:private] =>
)
[operatingSystem] => Sinergi\BrowserDetector\Os Object
(
[name:Sinergi\BrowserDetector\Os:private] => iOS
[version:Sinergi\BrowserDetector\Os:private] => 2.2
[isMobile:Sinergi\BrowserDetector\Os:private] => 1
[userAgent:Sinergi\BrowserDetector\Os:private] => Sinergi\BrowserDetector\UserAgent Object
(
[userAgentString:Sinergi\BrowserDetector\UserAgent:private] => Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
)
)
[device] => Sinergi\BrowserDetector\Device Object
(
[name:Sinergi\BrowserDetector\Device:private] => iPhone
[userAgent:Sinergi\BrowserDetector\Device:private] => Sinergi\BrowserDetector\UserAgent Object
(
[userAgentString:Sinergi\BrowserDetector\UserAgent:private] => Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
)
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>UAParser<br /><small>v3.4.5</small><br /></td><td>Maxthon 0</td><td><i class="material-icons">close</i></td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.002</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-3160e405-8a8f-46dd-8f47-5115f06462d2">Detail</a>
<!-- Modal Structure -->
<div id="modal-3160e405-8a8f-46dd-8f47-5115f06462d2" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>UAParser result detail</h4>
<p><pre><code class="php">UAParser\Result\Client Object
(
[ua] => UAParser\Result\UserAgent Object
(
[major] => 0
[minor] =>
[patch] =>
[family] => Maxthon
)
[os] => UAParser\Result\OperatingSystem Object
(
[major] => 2
[minor] => 2
[patch] =>
[patchMinor] =>
[family] => iOS
)
[device] => UAParser\Result\Device Object
(
[brand] => Apple
[model] => iPhone
[family] => iPhone
)
[originalUserAgent] => Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>UserAgentApiCom<br /><small></small><br /></td><td>Safari 525.20</td><td>WebKit 525.18.1</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>Mobile</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0.17801</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-afeb05fb-26b9-4509-b8ac-0c604a9e97d6">Detail</a>
<!-- Modal Structure -->
<div id="modal-afeb05fb-26b9-4509-b8ac-0c604a9e97d6" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>UserAgentApiCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[platform_name] => Apple iPhone
[platform_version] => 2
[platform_type] => Mobile
[browser_name] => Safari
[browser_version] => 525.20
[engine_name] => WebKit
[engine_version] => 525.18.1
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>UserAgentStringCom<br /><small></small><br /></td><td>Maxthon </td><td><i class="material-icons">close</i></td><td>iPhone OS 2.2</td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td></td><td>0.066</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-08a9ddfb-838f-48d7-9ede-1d132306b2ee">Detail</a>
<!-- Modal Structure -->
<div id="modal-08a9ddfb-838f-48d7-9ede-1d132306b2ee" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>UserAgentStringCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[agent_type] => Browser
[agent_name] => Maxthon
[agent_version] => --
[os_type] => Macintosh
[os_name] => iPhone OS
[os_versionName] =>
[os_versionNumber] => 2_2
[os_producer] =>
[os_producerURL] =>
[linux_distibution] => Null
[agent_language] =>
[agent_languageTag] =>
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>WhatIsMyBrowserCom<br /><small></small><br /></td><td>Maxthon </td><td>WebKit 525.18.1</td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.24201</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-5fc1ff22-a74d-481b-9ad1-fcfde73ded9c">Detail</a>
<!-- Modal Structure -->
<div id="modal-5fc1ff22-a74d-481b-9ad1-fcfde73ded9c" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>WhatIsMyBrowserCom result detail</h4>
<p><pre><code class="php">stdClass Object
(
[operating_system_name] => iOS
[simple_sub_description_string] =>
[simple_browser_string] => Maxthon on iOS 2.2
[browser_version] =>
[extra_info] => Array
(
)
[operating_platform] => iPhone
[extra_info_table] => stdClass Object
(
[Mobile Build] => 5G77
)
[layout_engine_name] => WebKit
[detected_addons] => Array
(
)
[operating_system_flavour_code] =>
[hardware_architecture] =>
[operating_system_flavour] =>
[operating_system_frameworks] => Array
(
)
[browser_name_code] => maxthon
[operating_system_version] => 2.2
[simple_operating_platform_string] => Apple iPhone
[is_abusive] =>
[layout_engine_version] => 525.18.1
[browser_capabilities] => Array
(
)
[operating_platform_vendor_name] => Apple
[operating_system] => iOS 2.2
[operating_system_version_full] => 2.2
[operating_platform_code] =>
[browser_name] => Maxthon
[operating_system_name_code] => ios
[user_agent] => Mozilla/5.0 (iPhone; U; CPU iPhone OS 2_2 like Mac OS X; xx) AppleWebKit/525.18.1 (KHTML, like Gecko) Version/3.1.1 Mobile/5G77 Safari/525.20 Mobile Maxthon
[browser_version_full] =>
[browser] => Maxthon
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>WhichBrowser<br /><small>v2.0.18</small><br /></td><td>Maxthon </td><td>Webkit 525.18.1</td><td>iPhone OS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td>mobile:smart</td><td>yes</td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-083a336f-5d73-4505-84f3-c5fc9bb78652">Detail</a>
<!-- Modal Structure -->
<div id="modal-083a336f-5d73-4505-84f3-c5fc9bb78652" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>WhichBrowser result detail</h4>
<p><pre><code class="php">Array
(
[browser] => Array
(
[name] => Maxthon
[type] => browser
)
[engine] => Array
(
[name] => Webkit
[version] => 525.18.1
)
[os] => Array
(
[name] => iOS
[alias] => iPhone OS
[version] => 2.2
)
[device] => Array
(
[type] => mobile
[subtype] => smart
[manufacturer] => Apple
[model] => iPhone
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>Woothee<br /><small>v1.2.0</small><br /></td><td>Safari 3.1.1</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>smartphone</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"></td><td></td><td><i class="material-icons">close</i></td><td>0</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-f00e7198-0e22-49fe-bad0-dbb3a9cde9b9">Detail</a>
<!-- Modal Structure -->
<div id="modal-f00e7198-0e22-49fe-bad0-dbb3a9cde9b9" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>Woothee result detail</h4>
<p><pre><code class="php">Array
(
[name] => Safari
[vendor] => Apple
[version] => 3.1.1
[category] => smartphone
[os] => iPhone
[os_version] => 2.2
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>Wurfl<br /><small>1.7.1.0</small><br /></td><td>Mobile Safari 3.1.1</td><td><i class="material-icons">close</i></td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td>Feature Phone</td><td>yes</td><td>yes</td><td style="border-left: 1px solid #555"></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0.019</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-a2bedf8c-4a95-42a7-96c5-aaf233b2ac50">Detail</a>
<!-- Modal Structure -->
<div id="modal-a2bedf8c-4a95-42a7-96c5-aaf233b2ac50" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>Wurfl result detail</h4>
<p><pre><code class="php">Array
(
[virtual] => Array
(
[is_android] => false
[is_ios] => true
[is_windows_phone] => false
[is_app] => false
[is_full_desktop] => false
[is_largescreen] => false
[is_mobile] => true
[is_robot] => false
[is_smartphone] => false
[is_touchscreen] => true
[is_wml_preferred] => false
[is_xhtmlmp_preferred] => false
[is_html_preferred] => true
[advertised_device_os] => iOS
[advertised_device_os_version] => 2.2
[advertised_browser] => Mobile Safari
[advertised_browser_version] => 3.1.1
[complete_device_name] => Apple iPhone
[device_name] => Apple iPhone
[form_factor] => Feature Phone
[is_phone] => true
[is_app_webview] => false
)
[all] => Array
(
[brand_name] => Apple
[model_name] => iPhone
[unique] => true
[ununiqueness_handler] =>
[is_wireless_device] => true
[device_claims_web_support] => true
[has_qwerty_keyboard] => true
[can_skip_aligned_link_row] => true
[uaprof] =>
[uaprof2] =>
[uaprof3] =>
[nokia_series] => 0
[nokia_edition] => 0
[device_os] => iOS
[mobile_browser] => Safari
[mobile_browser_version] =>
[device_os_version] => 2.2
[pointing_method] => touchscreen
[release_date] => 2008_november
[marketing_name] =>
[model_extra_info] => 2.2
[nokia_feature_pack] => 0
[can_assign_phone_number] => true
[is_tablet] => false
[manufacturer_name] =>
[is_bot] => false
[is_google_glass] => false
[proportional_font] => false
[built_in_back_button_support] => false
[card_title_support] => false
[softkey_support] => false
[table_support] => false
[numbered_menus] => false
[menu_with_select_element_recommended] => false
[menu_with_list_of_links_recommended] => false
[icons_on_menu_items_support] => false
[break_list_of_links_with_br_element_recommended] => false
[access_key_support] => false
[wrap_mode_support] => false
[times_square_mode_support] => false
[deck_prefetch_support] => false
[elective_forms_recommended] => false
[wizards_recommended] => false
[image_as_link_support] => false
[insert_br_element_after_widget_recommended] => false
[wml_can_display_images_and_text_on_same_line] => false
[wml_displays_image_in_center] => false
[opwv_wml_extensions_support] => false
[wml_make_phone_call_string] => none
[chtml_display_accesskey] => false
[emoji] => false
[chtml_can_display_images_and_text_on_same_line] => false
[chtml_displays_image_in_center] => false
[imode_region] => none
[chtml_make_phone_call_string] => tel:
[chtml_table_support] => false
[xhtml_honors_bgcolor] => true
[xhtml_supports_forms_in_table] => false
[xhtml_support_wml2_namespace] => false
[xhtml_autoexpand_select] => false
[xhtml_select_as_dropdown] => false
[xhtml_select_as_radiobutton] => false
[xhtml_select_as_popup] => false
[xhtml_display_accesskey] => false
[xhtml_supports_invisible_text] => false
[xhtml_supports_inline_input] => false
[xhtml_supports_monospace_font] => false
[xhtml_supports_table_for_layout] => true
[xhtml_supports_css_cell_table_coloring] => true
[xhtml_format_as_css_property] => true
[xhtml_format_as_attribute] => false
[xhtml_nowrap_mode] => false
[xhtml_marquee_as_css_property] => false
[xhtml_readable_background_color1] => #D9EFFF
[xhtml_readable_background_color2] => #FFFFFF
[xhtml_allows_disabled_form_elements] => false
[xhtml_document_title_support] => true
[xhtml_preferred_charset] => utf8
[opwv_xhtml_extensions_support] => false
[xhtml_make_phone_call_string] => tel:
[xhtmlmp_preferred_mime_type] => text/html
[xhtml_table_support] => true
[xhtml_send_sms_string] => sms:
[xhtml_send_mms_string] => none
[xhtml_file_upload] => not_supported
[cookie_support] => true
[accept_third_party_cookie] => false
[xhtml_supports_iframe] => full
[xhtml_avoid_accesskeys] => false
[xhtml_can_embed_video] => none
[ajax_support_javascript] => true
[ajax_manipulate_css] => true
[ajax_support_getelementbyid] => true
[ajax_support_inner_html] => true
[ajax_xhr_type] => standard
[ajax_manipulate_dom] => true
[ajax_support_events] => true
[ajax_support_event_listener] => true
[ajax_preferred_geoloc_api] => w3c_api
[xhtml_support_level] => 4
[preferred_markup] => html_web_4_0
[wml_1_1] => false
[wml_1_2] => false
[wml_1_3] => false
[html_wi_w3_xhtmlbasic] => true
[html_wi_oma_xhtmlmp_1_0] => true
[html_wi_imode_html_1] => false
[html_wi_imode_html_2] => false
[html_wi_imode_html_3] => false
[html_wi_imode_html_4] => false
[html_wi_imode_html_5] => false
[html_wi_imode_htmlx_1] => false
[html_wi_imode_htmlx_1_1] => false
[html_wi_imode_compact_generic] => true
[html_web_3_2] => true
[html_web_4_0] => true
[voicexml] => false
[multipart_support] => false
[total_cache_disable_support] => false
[time_to_live_support] => false
[resolution_width] => 320
[resolution_height] => 480
[columns] => 20
[max_image_width] => 320
[max_image_height] => 480
[rows] => 20
[physical_screen_width] => 50
[physical_screen_height] => 74
[dual_orientation] => true
[density_class] => 1.0
[wbmp] => false
[bmp] => true
[epoc_bmp] => false
[gif_animated] => true
[jpg] => true
[png] => true
[tiff] => false
[transparent_png_alpha] => false
[transparent_png_index] => false
[svgt_1_1] => false
[svgt_1_1_plus] => false
[greyscale] => false
[gif] => true
[colors] => 65536
[webp_lossy_support] => false
[webp_lossless_support] => false
[post_method_support] => true
[basic_authentication_support] => true
[empty_option_value_support] => true
[emptyok] => false
[nokia_voice_call] => false
[wta_voice_call] => false
[wta_phonebook] => false
[wta_misc] => false
[wta_pdc] => false
[https_support] => true
[phone_id_provided] => false
[max_data_rate] => 200
[wifi] => true
[sdio] => false
[vpn] => true
[has_cellular_radio] => true
[max_deck_size] => 100000
[max_url_length_in_requests] => 512
[max_url_length_homepage] => 0
[max_url_length_bookmark] => 0
[max_url_length_cached_page] => 0
[max_no_of_connection_settings] => 0
[max_no_of_bookmarks] => 0
[max_length_of_username] => 0
[max_length_of_password] => 0
[max_object_size] => 0
[downloadfun_support] => false
[directdownload_support] => false
[inline_support] => false
[oma_support] => false
[ringtone] => true
[ringtone_3gpp] => false
[ringtone_midi_monophonic] => true
[ringtone_midi_polyphonic] => true
[ringtone_imelody] => false
[ringtone_digiplug] => false
[ringtone_compactmidi] => false
[ringtone_mmf] => false
[ringtone_rmf] => false
[ringtone_xmf] => false
[ringtone_amr] => true
[ringtone_awb] => false
[ringtone_aac] => true
[ringtone_wav] => true
[ringtone_mp3] => true
[ringtone_spmidi] => false
[ringtone_qcelp] => false
[ringtone_voices] => 12
[ringtone_df_size_limit] => 0
[ringtone_directdownload_size_limit] => 0
[ringtone_inline_size_limit] => 0
[ringtone_oma_size_limit] => 0
[wallpaper] => false
[wallpaper_max_width] => 320
[wallpaper_max_height] => 480
[wallpaper_preferred_width] => 320
[wallpaper_preferred_height] => 480
[wallpaper_resize] => none
[wallpaper_wbmp] => false
[wallpaper_bmp] => false
[wallpaper_gif] => false
[wallpaper_jpg] => false
[wallpaper_png] => false
[wallpaper_tiff] => false
[wallpaper_greyscale] => false
[wallpaper_colors] => 2
[wallpaper_df_size_limit] => 0
[wallpaper_directdownload_size_limit] => 0
[wallpaper_inline_size_limit] => 0
[wallpaper_oma_size_limit] => 0
[screensaver] => false
[screensaver_max_width] => 0
[screensaver_max_height] => 0
[screensaver_preferred_width] => 0
[screensaver_preferred_height] => 0
[screensaver_resize] => none
[screensaver_wbmp] => false
[screensaver_bmp] => false
[screensaver_gif] => false
[screensaver_jpg] => false
[screensaver_png] => false
[screensaver_greyscale] => false
[screensaver_colors] => 2
[screensaver_df_size_limit] => 0
[screensaver_directdownload_size_limit] => 0
[screensaver_inline_size_limit] => 0
[screensaver_oma_size_limit] => 0
[picture] => false
[picture_max_width] => 0
[picture_max_height] => 0
[picture_preferred_width] => 0
[picture_preferred_height] => 0
[picture_resize] => none
[picture_wbmp] => false
[picture_bmp] => false
[picture_gif] => false
[picture_jpg] => false
[picture_png] => false
[picture_greyscale] => false
[picture_colors] => 2
[picture_df_size_limit] => 0
[picture_directdownload_size_limit] => 0
[picture_inline_size_limit] => 0
[picture_oma_size_limit] => 0
[video] => true
[oma_v_1_0_forwardlock] => false
[oma_v_1_0_combined_delivery] => false
[oma_v_1_0_separate_delivery] => false
[streaming_video] => true
[streaming_3gpp] => true
[streaming_mp4] => true
[streaming_mov] => false
[streaming_video_size_limit] => 0
[streaming_real_media] => none
[streaming_flv] => false
[streaming_3g2] => false
[streaming_vcodec_h263_0] => 30
[streaming_vcodec_h263_3] => -1
[streaming_vcodec_mpeg4_sp] => 3
[streaming_vcodec_mpeg4_asp] => 3
[streaming_vcodec_h264_bp] => 1.3
[streaming_acodec_amr] => nb
[streaming_acodec_aac] => lc
[streaming_wmv] => none
[streaming_preferred_protocol] => http
[streaming_preferred_http_protocol] => progressive_download
[wap_push_support] => false
[connectionless_service_indication] => false
[connectionless_service_load] => false
[connectionless_cache_operation] => false
[connectionoriented_unconfirmed_service_indication] => false
[connectionoriented_unconfirmed_service_load] => false
[connectionoriented_unconfirmed_cache_operation] => false
[connectionoriented_confirmed_service_indication] => false
[connectionoriented_confirmed_service_load] => false
[connectionoriented_confirmed_cache_operation] => false
[utf8_support] => true
[ascii_support] => false
[iso8859_support] => false
[expiration_date] => false
[j2me_cldc_1_0] => false
[j2me_cldc_1_1] => false
[j2me_midp_1_0] => false
[j2me_midp_2_0] => false
[doja_1_0] => false
[doja_1_5] => false
[doja_2_0] => false
[doja_2_1] => false
[doja_2_2] => false
[doja_3_0] => false
[doja_3_5] => false
[doja_4_0] => false
[j2me_jtwi] => false
[j2me_mmapi_1_0] => false
[j2me_mmapi_1_1] => false
[j2me_wmapi_1_0] => false
[j2me_wmapi_1_1] => false
[j2me_wmapi_2_0] => false
[j2me_btapi] => false
[j2me_3dapi] => false
[j2me_locapi] => false
[j2me_nokia_ui] => false
[j2me_motorola_lwt] => false
[j2me_siemens_color_game] => false
[j2me_siemens_extension] => false
[j2me_heap_size] => 0
[j2me_max_jar_size] => 0
[j2me_storage_size] => 0
[j2me_max_record_store_size] => 0
[j2me_screen_width] => 0
[j2me_screen_height] => 0
[j2me_canvas_width] => 0
[j2me_canvas_height] => 0
[j2me_bits_per_pixel] => 0
[j2me_audio_capture_enabled] => false
[j2me_video_capture_enabled] => false
[j2me_photo_capture_enabled] => false
[j2me_capture_image_formats] => none
[j2me_http] => false
[j2me_https] => false
[j2me_socket] => false
[j2me_udp] => false
[j2me_serial] => false
[j2me_gif] => false
[j2me_gif89a] => false
[j2me_jpg] => false
[j2me_png] => false
[j2me_bmp] => false
[j2me_bmp3] => false
[j2me_wbmp] => false
[j2me_midi] => false
[j2me_wav] => false
[j2me_amr] => false
[j2me_mp3] => false
[j2me_mp4] => false
[j2me_imelody] => false
[j2me_rmf] => false
[j2me_au] => false
[j2me_aac] => false
[j2me_realaudio] => false
[j2me_xmf] => false
[j2me_wma] => false
[j2me_3gpp] => false
[j2me_h263] => false
[j2me_svgt] => false
[j2me_mpeg4] => false
[j2me_realvideo] => false
[j2me_real8] => false
[j2me_realmedia] => false
[j2me_left_softkey_code] => 0
[j2me_right_softkey_code] => 0
[j2me_middle_softkey_code] => 0
[j2me_select_key_code] => 0
[j2me_return_key_code] => 0
[j2me_clear_key_code] => 0
[j2me_datefield_no_accepts_null_date] => false
[j2me_datefield_broken] => false
[receiver] => false
[sender] => false
[mms_max_size] => 0
[mms_max_height] => 0
[mms_max_width] => 0
[built_in_recorder] => false
[built_in_camera] => true
[mms_jpeg_baseline] => false
[mms_jpeg_progressive] => false
[mms_gif_static] => false
[mms_gif_animated] => false
[mms_png] => false
[mms_bmp] => false
[mms_wbmp] => false
[mms_amr] => false
[mms_wav] => false
[mms_midi_monophonic] => false
[mms_midi_polyphonic] => false
[mms_midi_polyphonic_voices] => 0
[mms_spmidi] => false
[mms_mmf] => false
[mms_mp3] => false
[mms_evrc] => false
[mms_qcelp] => false
[mms_ota_bitmap] => false
[mms_nokia_wallpaper] => false
[mms_nokia_operatorlogo] => false
[mms_nokia_3dscreensaver] => false
[mms_nokia_ringingtone] => false
[mms_rmf] => false
[mms_xmf] => false
[mms_symbian_install] => false
[mms_jar] => false
[mms_jad] => false
[mms_vcard] => false
[mms_vcalendar] => false
[mms_wml] => false
[mms_wbxml] => false
[mms_wmlc] => false
[mms_video] => false
[mms_mp4] => false
[mms_3gpp] => false
[mms_3gpp2] => false
[mms_max_frame_rate] => 0
[nokiaring] => false
[picturemessage] => false
[operatorlogo] => false
[largeoperatorlogo] => false
[callericon] => false
[nokiavcard] => false
[nokiavcal] => false
[sckl_ringtone] => false
[sckl_operatorlogo] => false
[sckl_groupgraphic] => false
[sckl_vcard] => false
[sckl_vcalendar] => false
[text_imelody] => false
[ems] => false
[ems_variablesizedpictures] => false
[ems_imelody] => false
[ems_odi] => false
[ems_upi] => false
[ems_version] => 0
[siemens_ota] => false
[siemens_logo_width] => 101
[siemens_logo_height] => 29
[siemens_screensaver_width] => 101
[siemens_screensaver_height] => 50
[gprtf] => false
[sagem_v1] => false
[sagem_v2] => false
[panasonic] => false
[sms_enabled] => true
[wav] => false
[mmf] => false
[smf] => false
[mld] => false
[midi_monophonic] => false
[midi_polyphonic] => false
[sp_midi] => false
[rmf] => false
[xmf] => false
[compactmidi] => false
[digiplug] => false
[nokia_ringtone] => false
[imelody] => false
[au] => false
[amr] => false
[awb] => false
[aac] => true
[mp3] => true
[voices] => 1
[qcelp] => false
[evrc] => false
[flash_lite_version] =>
[fl_wallpaper] => false
[fl_screensaver] => false
[fl_standalone] => false
[fl_browser] => false
[fl_sub_lcd] => false
[full_flash_support] => false
[css_supports_width_as_percentage] => true
[css_border_image] => webkit
[css_rounded_corners] => webkit
[css_gradient] => none
[css_spriting] => true
[css_gradient_linear] => none
[is_transcoder] => false
[transcoder_ua_header] => user-agent
[rss_support] => true
[pdf_support] => true
[progressive_download] => true
[playback_vcodec_h263_0] => 10
[playback_vcodec_h263_3] => -1
[playback_vcodec_mpeg4_sp] => 3
[playback_vcodec_mpeg4_asp] => 2
[playback_vcodec_h264_bp] => 1.2
[playback_real_media] => none
[playback_3gpp] => true
[playback_3g2] => true
[playback_mp4] => true
[playback_mov] => true
[playback_acodec_amr] => nb
[playback_acodec_aac] => lc
[playback_df_size_limit] => 0
[playback_directdownload_size_limit] => 0
[playback_inline_size_limit] => 0
[playback_oma_size_limit] => 0
[playback_acodec_qcelp] => false
[playback_wmv] => none
[hinted_progressive_download] => false
[html_preferred_dtd] => html4
[viewport_supported] => true
[viewport_width] => device_width_token
[viewport_userscalable] => no
[viewport_initial_scale] =>
[viewport_maximum_scale] =>
[viewport_minimum_scale] =>
[mobileoptimized] => false
[handheldfriendly] => false
[canvas_support] => full
[image_inlining] => true
[is_smarttv] => false
[is_console] => false
[nfc_support] => false
[ux_full_desktop] => false
[jqm_grade] => B
[is_sencha_touch_ok] => true
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr><tr><td>Zsxsoft<br /><small>1.3</small><br /></td><td>Maxthon </td><td><i class="material-icons">close</i></td><td>iOS 2.2</td><td style="border-left: 1px solid #555">Apple</td><td>iPhone</td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td style="border-left: 1px solid #555"><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td><i class="material-icons">close</i></td><td>0</td><td>
<!-- Modal Trigger -->
<a class="modal-trigger btn waves-effect waves-light" href="#modal-5d43e024-b46c-44f6-8914-529b05569bc2">Detail</a>
<!-- Modal Structure -->
<div id="modal-5d43e024-b46c-44f6-8914-529b05569bc2" class="modal modal-fixed-footer">
<div class="modal-content">
<h4>Zsxsoft result detail</h4>
<p><pre><code class="php">Array
(
[browser] => Array
(
[link] => http://www.maxthon.com/
[title] => Maxthon
[code] => maxthon
[version] =>
[name] => Maxthon
[image] => img/16/browser/maxthon.png
)
[os] => Array
(
[link] => http://www.apple.com/
[name] => iOS
[version] => 2.2
[code] => mac-3
[x64] =>
[title] => iOS 2.2
[type] => os
[dir] => os
[image] => img/16/os/mac-3.png
)
[device] => Array
(
[link] => http://www.apple.com/iphone
[title] => Apple iPhone
[model] => iPhone
[brand] => Apple
[code] => iphone
[dir] => device
[type] => device
[image] => img/16/device/iphone.png
)
[platform] => Array
(
[link] => http://www.apple.com/iphone
[title] => Apple iPhone
[model] => iPhone
[brand] => Apple
[code] => iphone
[dir] => device
[type] => device
[image] => img/16/device/iphone.png
)
)
</code></pre></p>
</div>
<div class="modal-footer">
<a href="#!" class="modal-action modal-close waves-effect waves-green btn-flat ">close</a>
</div>
</div>
</td></tr></table>
</div>
<div class="section">
<h1 class="header center orange-text">About this comparison</h1>
<div class="row center">
<h5 class="header light">
The primary goal of this project is simple<br />
I wanted to know which user agent parser is the most accurate in each part - device detection, bot detection and so on...<br />
<br />
The secondary goal is to provide a source for all user agent parsers to improve their detection based on this results.<br />
<br />
You can also improve this further, by suggesting ideas at <a href="https://github.com/ThaDafinser/UserAgentParserComparison">ThaDafinser/UserAgentParserComparison</a><br />
<br />
The comparison is based on the abstraction by <a href="https://github.com/ThaDafinser/UserAgentParser">ThaDafinser/UserAgentParser</a>
</h5>
</div>
</div>
<div class="card">
<div class="card-content">
Comparison created <i>2016-05-10 07:55:58</i> | by
<a href="https://github.com/ThaDafinser">ThaDafinser</a>
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-2.1.4.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/materialize/0.97.3/js/materialize.min.js"></script>
<script src="http://cdnjs.cloudflare.com/ajax/libs/list.js/1.2.0/list.min.js"></script>
<script>
$(document).ready(function(){
// the "href" attribute of .modal-trigger must specify the modal ID that wants to be triggered
$('.modal-trigger').leanModal();
});
</script>
</body>
</html> | Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>dpdgraph: 13 s 🏆</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.7.1+2 / dpdgraph - 0.6.2</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
dpdgraph
<small>
0.6.2
<span class="label label-success">13 s 🏆</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-02-24 14:39:15 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-02-24 14:39:15 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.7.1+2 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.05.0 The OCaml compiler (virtual package)
ocaml-base-compiler 4.05.0 Official 4.05.0 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
license: "LGPL 2.1"
homepage: "https://github.com/karmaki/coq-dpdgraph"
build: [
["./configure"]
["echo" "%{jobs}%" "jobs for the linter"]
[make]
]
bug-reports: "https://github.com/karmaki/coq-dpdgraph/issues"
dev-repo: "git+https://github.com/karmaki/coq-dpdgraph.git"
install: [
[make "install" "BINDIR=%{bin}%"]
]
remove: [
["rm" "%{bin}%/dpd2dot" "%{bin}%/dpdusage"]
["rm" "-R" "%{lib}%/coq/user-contrib/dpdgraph"]
]
depends: [
"ocaml" {< "4.08.0"}
"coq" {>= "8.7" & < "8.8~"}
"ocamlgraph"
]
authors: [ "Anne Pacalet" "Yves Bertot"]
synopsis: "Compute dependencies between Coq objects (definitions, theorems) and produce graphs"
flags: light-uninstall
url {
src:
"https://github.com/Karmaki/coq-dpdgraph/releases/download/v0.6.2/coq-dpdgraph-0.6.2.tgz"
checksum: "md5=495ae6444af63129b5ec0001855228d4"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-dpdgraph.0.6.2 coq.8.7.1+2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 4000000; timeout 4h opam install -y --deps-only coq-dpdgraph.0.6.2 coq.8.7.1+2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>19 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 16000000; timeout 4h opam install -y -v coq-dpdgraph.0.6.2 coq.8.7.1+2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>13 s</dd>
</dl>
<h2>Installation size</h2>
<p>Total: 10 M</p>
<ul>
<li>5 M <code>../ocaml-base-compiler.4.05.0/bin/dpdusage</code></li>
<li>5 M <code>../ocaml-base-compiler.4.05.0/bin/dpd2dot</code></li>
<li>95 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/dpdgraph.cmxs</code></li>
<li>11 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/graphdepend.cmi</code></li>
<li>8 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/dpdgraph.cmxa</code></li>
<li>8 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/graphdepend.cmx</code></li>
<li>5 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/searchdepend.cmi</code></li>
<li>5 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/searchdepend.cmx</code></li>
<li>1 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/dpdgraph.vo</code></li>
<li>1 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/dpdgraph.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.05.0/lib/coq/user-contrib/dpdgraph/dpdgraph.v</code></li>
</ul>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq-dpdgraph.0.6.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>kildall: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.11.0 / kildall - 8.9.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
kildall
<small>
8.9.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-12-12 17:32:44 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-12-12 17:32:44 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
coq 8.11.0 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.09.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.09.1 Official release 4.09.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "http://www.lif-sud.univ-mrs.fr/Rapports/24-2005.html"
license: "Unknown"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/Kildall"]
depends: [
"ocaml"
"coq" {>= "8.9" & < "8.10~"}
]
tags: [
"keyword: Kildall"
"keyword: data flow analysis"
"keyword: bytecode verification"
"category: Computer Science/Semantics and Compilation/Semantics"
"date: 2005-07"
]
authors: [
"Solange Coupet-Grimal <[email protected]>"
"William Delobel <[email protected]>"
]
bug-reports: "https://github.com/coq-contribs/kildall/issues"
dev-repo: "git+https://github.com/coq-contribs/kildall.git"
synopsis: "Application of the Generic kildall's Data Flow Analysis Algorithm to a Type and Shape Static Analyses of Bytecode"
description: """
This Library provides a generic data flow analysis
algorithm and a proof of its correctness.
This algorithm is then used to perform type and
shape analysis at bytecode level
on a first order functionnal language."""
flags: light-uninstall
url {
src: "https://github.com/coq-contribs/kildall/archive/v8.9.0.tar.gz"
checksum: "md5=e90951bfbcbdfef76704b8f407b93125"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-kildall.8.9.0 coq.8.11.0</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.11.0).
The following dependencies couldn't be met:
- coq-kildall -> coq < 8.10~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-kildall.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<?xml version="1.0" encoding="ISO-8859-1"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<meta name="dc.language" scheme="ISO639-2" content="eng">
<meta name="dc.creator" content="OACT">
<meta name="lead_content_manager" content="JeffK">
<meta name="coder" content="JeffK">
<meta name="dc.date.created" scheme="ISO8601" content="2005-08-29">
<meta name="dc.date.reviewed" scheme="ISO8601" content="2007-11-19">
<meta name="dc.title" content="Table 6_2060: Life Tables">
<meta name="GENERATOR" content="Quadralay WebWorks Publisher 2003 for FrameMaker 8.0.0.1194" />
<title>Table 6_2060: Life Tables</title>
<link rel="StyleSheet" href="document.css" type="text/css" media="all" />
<link rel="StyleSheet" href="catalog.css" type="text/css" media="all" />
<link rel="Table of Contents" href="TOC.html" />
<link rel="List of Tables" href="LOT.html" />
<link rel="List of Figures" href="LOF.html" />
<link rel="Previous" href="LifeTables_Tbl_6_2050.html" />
<link rel="Next" href="LifeTables_Tbl_6_2070.html" />
</head>
<body>
<table summary="Document title and navigation links" border="0" cellpadding="0" cellspacing="0" width="100%">
<tr>
<td width="60%"><h2 class="pTitle">Life Tables for the<br />United States Social Security<br />
Area 1900-2100<br /></h2></td>
<td><a href="TOC.html"><img id="LongDescNotReq1" src="images/toc.gif" border="0" alt="Contents" /></a></td>
<td><a href="LifeTables_Tbl_6_2050.html"><img id="LongDescNotReq2" src="images/prev.gif" border="0" alt="Previous" /></a></td>
<td><a href="LifeTables_Tbl_6_2070.html"><img id="LongDescNotReq3" src="images/next.gif" border="0" alt="Next" /></a></td>
<td><a href="LOT.html"><img id="LongDescNotReq4" src="images/tables.gif" border="0" alt="List of Tables" /></a></td>
<td><a href="LOF.html"><img id="LongDescNotReq5" src="images/figures.gif" border="0" alt="List of Figures" /></a></td>
</tr>
<tr align="left">
<td colspan="6"><div class="pCellBodyL">ACTUARIAL STUDY NO. 120
<br /><em>by Felicitie C. Bell<br /><em>and Michael L. Miller</em></div></td></em></div></td><br />
</tr>
<tr align="left">
<td colspan="6"><hr /></td>
</tr>
</table>
<a name="wp998290"> </a><p class="pBody">
</p>
<div align="left">
<table border="1" width="100%" id="wp1004907table999968">
<caption><a name="wp1004907"> </a><div class="pTableTitle">
Table 6 — Period Life Tables for the Social Security Area by Calendar Year and Sex (Cont.)
</div>
</caption>
<tr valign="bottom"> <th colspan="7" rowspan="1"><a name="wp1004937"> </a><div class="pCellHeadC">
<b class="cBold">Male</b>
</div>
</th>
<th colspan="1" rowspan="2"> </th>
<th colspan="7" rowspan="1"><a name="wp1004953"> </a><div class="pCellHeadC">
<b class="cBold">Female</b>
</div>
</th>
</tr>
<tr valign="bottom"> <th><a name="wp1004967"> </a><div class="pCellHeadC">
x
</div>
</th>
<th><a name="wp1004969"> </a><div class="pCellHeadC">
q<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004971"> </a><div class="pCellHeadC">
l<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004973"> </a><div class="pCellHeadC">
d<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004975"> </a><div class="pCellHeadC">
L<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004977"> </a><div class="pCellHeadC">
T<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004982"> </a><div class="pCellHeadC">
<sub><sub><sub><img src="images/LifeExp_bold_html.gif" id="wp1171432" alt="Life Expectancy" border="0" hspace="0" vspace="0"/></sub></sub></sub>
</div>
</th>
<th><a name="wp1004986"> </a><div class="pCellHeadC">
x
</div>
</th>
<th><a name="wp1004988"> </a><div class="pCellHeadC">
q<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004990"> </a><div class="pCellHeadC">
l<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004992"> </a><div class="pCellHeadC">
d<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004994"> </a><div class="pCellHeadC">
L<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1004996"> </a><div class="pCellHeadC">
T<sub class="cSubscript">x</sub>
</div>
</th>
<th><a name="wp1015826"> </a><div class="pCellHeadC">
<sub><sub><sub><img src="images/LifeExp_bold_html.gif" id="wp1171432" alt="Life Expectancy" border="0" hspace="0" vspace="0"/></sub></sub></sub>
</div>
</th>
</tr>
<tr valign="bottom"> <th colspan="15" rowspan="1"><a name="wp1005000"> </a><div class="pCellHeadL">
<b class="cBold">Calendar Year 2060 </b>
</div>
</th>
</tr>
<tr> <td><a name="wp1080561"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1080563"> </a><div class="pCellBodyR">
0.00243
</div>
</td>
<td><a name="wp1080565"> </a><div class="pCellBodyR">
100,000
</div>
</td>
<td><a name="wp1080567"> </a><div class="pCellBodyR">
243
</div>
</td>
<td><a name="wp1080569"> </a><div class="pCellBodyR">
99,786
</div>
</td>
<td><a name="wp1080571"> </a><div class="pCellBodyR">
8,018,402
</div>
</td>
<td><a name="wp1080573"> </a><div class="pCellBodyR">
80.18
</div>
</td>
<td> </td>
<td><a name="wp1080577"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1080579"> </a><div class="pCellBodyR">
0.00206
</div>
</td>
<td><a name="wp1080581"> </a><div class="pCellBodyR">
100,000
</div>
</td>
<td><a name="wp1080583"> </a><div class="pCellBodyR">
206
</div>
</td>
<td><a name="wp1080585"> </a><div class="pCellBodyR">
99,821
</div>
</td>
<td><a name="wp1080587"> </a><div class="pCellBodyR">
8,393,390
</div>
</td>
<td><a name="wp1080589"> </a><div class="pCellBodyR">
83.93
</div>
</td>
</tr>
<tr> <td><a name="wp1080531"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1080533"> </a><div class="pCellBodyR">
0.00020
</div>
</td>
<td><a name="wp1080535"> </a><div class="pCellBodyR">
99,757
</div>
</td>
<td><a name="wp1080537"> </a><div class="pCellBodyR">
20
</div>
</td>
<td><a name="wp1080539"> </a><div class="pCellBodyR">
99,747
</div>
</td>
<td><a name="wp1080541"> </a><div class="pCellBodyR">
7,918,616
</div>
</td>
<td><a name="wp1080543"> </a><div class="pCellBodyR">
79.38
</div>
</td>
<td> </td>
<td><a name="wp1080547"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1080549"> </a><div class="pCellBodyR">
0.00017
</div>
</td>
<td><a name="wp1080551"> </a><div class="pCellBodyR">
99,795
</div>
</td>
<td><a name="wp1080553"> </a><div class="pCellBodyR">
17
</div>
</td>
<td><a name="wp1080555"> </a><div class="pCellBodyR">
99,786
</div>
</td>
<td><a name="wp1080557"> </a><div class="pCellBodyR">
8,293,569
</div>
</td>
<td><a name="wp1080559"> </a><div class="pCellBodyR">
83.11
</div>
</td>
</tr>
<tr> <td><a name="wp1080501"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1080503"> </a><div class="pCellBodyR">
0.00014
</div>
</td>
<td><a name="wp1080505"> </a><div class="pCellBodyR">
99,737
</div>
</td>
<td><a name="wp1080507"> </a><div class="pCellBodyR">
14
</div>
</td>
<td><a name="wp1080509"> </a><div class="pCellBodyR">
99,730
</div>
</td>
<td><a name="wp1080511"> </a><div class="pCellBodyR">
7,818,870
</div>
</td>
<td><a name="wp1080513"> </a><div class="pCellBodyR">
78.40
</div>
</td>
<td> </td>
<td><a name="wp1080517"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1080519"> </a><div class="pCellBodyR">
0.00011
</div>
</td>
<td><a name="wp1080521"> </a><div class="pCellBodyR">
99,777
</div>
</td>
<td><a name="wp1080523"> </a><div class="pCellBodyR">
11
</div>
</td>
<td><a name="wp1080525"> </a><div class="pCellBodyR">
99,772
</div>
</td>
<td><a name="wp1080527"> </a><div class="pCellBodyR">
8,193,783
</div>
</td>
<td><a name="wp1080529"> </a><div class="pCellBodyR">
82.12
</div>
</td>
</tr>
<tr> <td><a name="wp1080471"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1080473"> </a><div class="pCellBodyR">
0.00011
</div>
</td>
<td><a name="wp1080475"> </a><div class="pCellBodyR">
99,723
</div>
</td>
<td><a name="wp1080477"> </a><div class="pCellBodyR">
11
</div>
</td>
<td><a name="wp1080479"> </a><div class="pCellBodyR">
99,717
</div>
</td>
<td><a name="wp1080481"> </a><div class="pCellBodyR">
7,719,140
</div>
</td>
<td><a name="wp1080483"> </a><div class="pCellBodyR">
77.41
</div>
</td>
<td> </td>
<td><a name="wp1080487"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1080489"> </a><div class="pCellBodyR">
0.00008
</div>
</td>
<td><a name="wp1080491"> </a><div class="pCellBodyR">
99,766
</div>
</td>
<td><a name="wp1080493"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080495"> </a><div class="pCellBodyR">
99,762
</div>
</td>
<td><a name="wp1080497"> </a><div class="pCellBodyR">
8,094,011
</div>
</td>
<td><a name="wp1080499"> </a><div class="pCellBodyR">
81.13
</div>
</td>
</tr>
<tr> <td><a name="wp1080441"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1080443"> </a><div class="pCellBodyR">
0.00009
</div>
</td>
<td><a name="wp1080445"> </a><div class="pCellBodyR">
99,712
</div>
</td>
<td><a name="wp1080447"> </a><div class="pCellBodyR">
9
</div>
</td>
<td><a name="wp1080449"> </a><div class="pCellBodyR">
99,707
</div>
</td>
<td><a name="wp1080451"> </a><div class="pCellBodyR">
7,619,422
</div>
</td>
<td><a name="wp1080453"> </a><div class="pCellBodyR">
76.41
</div>
</td>
<td> </td>
<td><a name="wp1080457"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1080459"> </a><div class="pCellBodyR">
0.00006
</div>
</td>
<td><a name="wp1080461"> </a><div class="pCellBodyR">
99,758
</div>
</td>
<td><a name="wp1080463"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080465"> </a><div class="pCellBodyR">
99,754
</div>
</td>
<td><a name="wp1080467"> </a><div class="pCellBodyR">
7,994,249
</div>
</td>
<td><a name="wp1080469"> </a><div class="pCellBodyR">
80.14
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1080381"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1080383"> </a><div class="pCellBodyR">
0.00008
</div>
</td>
<td><a name="wp1080385"> </a><div class="pCellBodyR">
99,703
</div>
</td>
<td><a name="wp1080387"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080389"> </a><div class="pCellBodyR">
99,699
</div>
</td>
<td><a name="wp1080391"> </a><div class="pCellBodyR">
7,519,715
</div>
</td>
<td><a name="wp1080393"> </a><div class="pCellBodyR">
75.42
</div>
</td>
<td> </td>
<td><a name="wp1080397"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1080399"> </a><div class="pCellBodyR">
0.00006
</div>
</td>
<td><a name="wp1080401"> </a><div class="pCellBodyR">
99,751
</div>
</td>
<td><a name="wp1080403"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080405"> </a><div class="pCellBodyR">
99,748
</div>
</td>
<td><a name="wp1080407"> </a><div class="pCellBodyR">
7,894,495
</div>
</td>
<td><a name="wp1080409"> </a><div class="pCellBodyR">
79.14
</div>
</td>
</tr>
<tr> <td><a name="wp1080351"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080353"> </a><div class="pCellBodyR">
0.00008
</div>
</td>
<td><a name="wp1080355"> </a><div class="pCellBodyR">
99,695
</div>
</td>
<td><a name="wp1080357"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080359"> </a><div class="pCellBodyR">
99,691
</div>
</td>
<td><a name="wp1080361"> </a><div class="pCellBodyR">
7,420,016
</div>
</td>
<td><a name="wp1080363"> </a><div class="pCellBodyR">
74.43
</div>
</td>
<td> </td>
<td><a name="wp1080367"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080369"> </a><div class="pCellBodyR">
0.00006
</div>
</td>
<td><a name="wp1080371"> </a><div class="pCellBodyR">
99,745
</div>
</td>
<td><a name="wp1080373"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080375"> </a><div class="pCellBodyR">
99,742
</div>
</td>
<td><a name="wp1080377"> </a><div class="pCellBodyR">
7,794,746
</div>
</td>
<td><a name="wp1080379"> </a><div class="pCellBodyR">
78.15
</div>
</td>
</tr>
<tr> <td><a name="wp1080321"> </a><div class="pCellBodyR">
7
</div>
</td>
<td><a name="wp1080323"> </a><div class="pCellBodyR">
0.00007
</div>
</td>
<td><a name="wp1080325"> </a><div class="pCellBodyR">
99,687
</div>
</td>
<td><a name="wp1080327"> </a><div class="pCellBodyR">
7
</div>
</td>
<td><a name="wp1080329"> </a><div class="pCellBodyR">
99,684
</div>
</td>
<td><a name="wp1080331"> </a><div class="pCellBodyR">
7,320,325
</div>
</td>
<td><a name="wp1080333"> </a><div class="pCellBodyR">
73.43
</div>
</td>
<td> </td>
<td><a name="wp1080337"> </a><div class="pCellBodyR">
7
</div>
</td>
<td><a name="wp1080339"> </a><div class="pCellBodyR">
0.00006
</div>
</td>
<td><a name="wp1080341"> </a><div class="pCellBodyR">
99,739
</div>
</td>
<td><a name="wp1080343"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080345"> </a><div class="pCellBodyR">
99,736
</div>
</td>
<td><a name="wp1080347"> </a><div class="pCellBodyR">
7,695,004
</div>
</td>
<td><a name="wp1080349"> </a><div class="pCellBodyR">
77.15
</div>
</td>
</tr>
<tr> <td><a name="wp1080291"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080293"> </a><div class="pCellBodyR">
0.00006
</div>
</td>
<td><a name="wp1080295"> </a><div class="pCellBodyR">
99,680
</div>
</td>
<td><a name="wp1080297"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1080299"> </a><div class="pCellBodyR">
99,677
</div>
</td>
<td><a name="wp1080301"> </a><div class="pCellBodyR">
7,220,641
</div>
</td>
<td><a name="wp1080303"> </a><div class="pCellBodyR">
72.44
</div>
</td>
<td> </td>
<td><a name="wp1080307"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080309"> </a><div class="pCellBodyR">
0.00005
</div>
</td>
<td><a name="wp1080311"> </a><div class="pCellBodyR">
99,733
</div>
</td>
<td><a name="wp1080313"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1080315"> </a><div class="pCellBodyR">
99,731
</div>
</td>
<td><a name="wp1080317"> </a><div class="pCellBodyR">
7,595,267
</div>
</td>
<td><a name="wp1080319"> </a><div class="pCellBodyR">
76.16
</div>
</td>
</tr>
<tr> <td><a name="wp1080261"> </a><div class="pCellBodyR">
9
</div>
</td>
<td><a name="wp1080263"> </a><div class="pCellBodyR">
0.00005
</div>
</td>
<td><a name="wp1080265"> </a><div class="pCellBodyR">
99,674
</div>
</td>
<td><a name="wp1080267"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1080269"> </a><div class="pCellBodyR">
99,672
</div>
</td>
<td><a name="wp1080271"> </a><div class="pCellBodyR">
7,120,964
</div>
</td>
<td><a name="wp1080273"> </a><div class="pCellBodyR">
71.44
</div>
</td>
<td> </td>
<td><a name="wp1080277"> </a><div class="pCellBodyR">
9
</div>
</td>
<td><a name="wp1080279"> </a><div class="pCellBodyR">
0.00005
</div>
</td>
<td><a name="wp1080281"> </a><div class="pCellBodyR">
99,728
</div>
</td>
<td><a name="wp1080283"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1080285"> </a><div class="pCellBodyR">
99,726
</div>
</td>
<td><a name="wp1080287"> </a><div class="pCellBodyR">
7,495,536
</div>
</td>
<td><a name="wp1080289"> </a><div class="pCellBodyR">
75.16
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1080201"> </a><div class="pCellBodyR">
10
</div>
</td>
<td><a name="wp1080203"> </a><div class="pCellBodyR">
0.00003
</div>
</td>
<td><a name="wp1080205"> </a><div class="pCellBodyR">
99,669
</div>
</td>
<td><a name="wp1080207"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1080209"> </a><div class="pCellBodyR">
99,668
</div>
</td>
<td><a name="wp1080211"> </a><div class="pCellBodyR">
7,021,293
</div>
</td>
<td><a name="wp1080213"> </a><div class="pCellBodyR">
70.45
</div>
</td>
<td> </td>
<td><a name="wp1080217"> </a><div class="pCellBodyR">
10
</div>
</td>
<td><a name="wp1080219"> </a><div class="pCellBodyR">
0.00004
</div>
</td>
<td><a name="wp1080221"> </a><div class="pCellBodyR">
99,723
</div>
</td>
<td><a name="wp1080223"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1080225"> </a><div class="pCellBodyR">
99,721
</div>
</td>
<td><a name="wp1080227"> </a><div class="pCellBodyR">
7,395,811
</div>
</td>
<td><a name="wp1080229"> </a><div class="pCellBodyR">
74.16
</div>
</td>
</tr>
<tr> <td><a name="wp1080171"> </a><div class="pCellBodyR">
11
</div>
</td>
<td><a name="wp1080173"> </a><div class="pCellBodyR">
0.00003
</div>
</td>
<td><a name="wp1080175"> </a><div class="pCellBodyR">
99,666
</div>
</td>
<td><a name="wp1080177"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1080179"> </a><div class="pCellBodyR">
99,665
</div>
</td>
<td><a name="wp1080181"> </a><div class="pCellBodyR">
6,921,625
</div>
</td>
<td><a name="wp1080183"> </a><div class="pCellBodyR">
69.45
</div>
</td>
<td> </td>
<td><a name="wp1080187"> </a><div class="pCellBodyR">
11
</div>
</td>
<td><a name="wp1080189"> </a><div class="pCellBodyR">
0.00004
</div>
</td>
<td><a name="wp1080191"> </a><div class="pCellBodyR">
99,719
</div>
</td>
<td><a name="wp1080193"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1080195"> </a><div class="pCellBodyR">
99,717
</div>
</td>
<td><a name="wp1080197"> </a><div class="pCellBodyR">
7,296,089
</div>
</td>
<td><a name="wp1080199"> </a><div class="pCellBodyR">
73.17
</div>
</td>
</tr>
<tr> <td><a name="wp1080141"> </a><div class="pCellBodyR">
12
</div>
</td>
<td><a name="wp1080143"> </a><div class="pCellBodyR">
0.00007
</div>
</td>
<td><a name="wp1080145"> </a><div class="pCellBodyR">
99,663
</div>
</td>
<td><a name="wp1080147"> </a><div class="pCellBodyR">
7
</div>
</td>
<td><a name="wp1080149"> </a><div class="pCellBodyR">
99,660
</div>
</td>
<td><a name="wp1080151"> </a><div class="pCellBodyR">
6,821,960
</div>
</td>
<td><a name="wp1080153"> </a><div class="pCellBodyR">
68.45
</div>
</td>
<td> </td>
<td><a name="wp1080157"> </a><div class="pCellBodyR">
12
</div>
</td>
<td><a name="wp1080159"> </a><div class="pCellBodyR">
0.00005
</div>
</td>
<td><a name="wp1080161"> </a><div class="pCellBodyR">
99,715
</div>
</td>
<td><a name="wp1080163"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1080165"> </a><div class="pCellBodyR">
99,713
</div>
</td>
<td><a name="wp1080167"> </a><div class="pCellBodyR">
7,196,372
</div>
</td>
<td><a name="wp1080169"> </a><div class="pCellBodyR">
72.17
</div>
</td>
</tr>
<tr> <td><a name="wp1080111"> </a><div class="pCellBodyR">
13
</div>
</td>
<td><a name="wp1080113"> </a><div class="pCellBodyR">
0.00014
</div>
</td>
<td><a name="wp1080115"> </a><div class="pCellBodyR">
99,656
</div>
</td>
<td><a name="wp1080117"> </a><div class="pCellBodyR">
14
</div>
</td>
<td><a name="wp1080119"> </a><div class="pCellBodyR">
99,649
</div>
</td>
<td><a name="wp1080121"> </a><div class="pCellBodyR">
6,722,301
</div>
</td>
<td><a name="wp1080123"> </a><div class="pCellBodyR">
67.45
</div>
</td>
<td> </td>
<td><a name="wp1080127"> </a><div class="pCellBodyR">
13
</div>
</td>
<td><a name="wp1080129"> </a><div class="pCellBodyR">
0.00009
</div>
</td>
<td><a name="wp1080131"> </a><div class="pCellBodyR">
99,710
</div>
</td>
<td><a name="wp1080133"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1080135"> </a><div class="pCellBodyR">
99,706
</div>
</td>
<td><a name="wp1080137"> </a><div class="pCellBodyR">
7,096,659
</div>
</td>
<td><a name="wp1080139"> </a><div class="pCellBodyR">
71.17
</div>
</td>
</tr>
<tr> <td><a name="wp1080081"> </a><div class="pCellBodyR">
14
</div>
</td>
<td><a name="wp1080083"> </a><div class="pCellBodyR">
0.00025
</div>
</td>
<td><a name="wp1080085"> </a><div class="pCellBodyR">
99,642
</div>
</td>
<td><a name="wp1080087"> </a><div class="pCellBodyR">
25
</div>
</td>
<td><a name="wp1080089"> </a><div class="pCellBodyR">
99,630
</div>
</td>
<td><a name="wp1080091"> </a><div class="pCellBodyR">
6,622,652
</div>
</td>
<td><a name="wp1080093"> </a><div class="pCellBodyR">
66.46
</div>
</td>
<td> </td>
<td><a name="wp1080097"> </a><div class="pCellBodyR">
14
</div>
</td>
<td><a name="wp1080099"> </a><div class="pCellBodyR">
0.00013
</div>
</td>
<td><a name="wp1080101"> </a><div class="pCellBodyR">
99,702
</div>
</td>
<td><a name="wp1080103"> </a><div class="pCellBodyR">
13
</div>
</td>
<td><a name="wp1080105"> </a><div class="pCellBodyR">
99,695
</div>
</td>
<td><a name="wp1080107"> </a><div class="pCellBodyR">
6,996,953
</div>
</td>
<td><a name="wp1080109"> </a><div class="pCellBodyR">
70.18
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1080021"> </a><div class="pCellBodyR">
15
</div>
</td>
<td><a name="wp1080023"> </a><div class="pCellBodyR">
0.00036
</div>
</td>
<td><a name="wp1080025"> </a><div class="pCellBodyR">
99,618
</div>
</td>
<td><a name="wp1080027"> </a><div class="pCellBodyR">
36
</div>
</td>
<td><a name="wp1080029"> </a><div class="pCellBodyR">
99,600
</div>
</td>
<td><a name="wp1080031"> </a><div class="pCellBodyR">
6,523,022
</div>
</td>
<td><a name="wp1080033"> </a><div class="pCellBodyR">
65.48
</div>
</td>
<td> </td>
<td><a name="wp1080037"> </a><div class="pCellBodyR">
15
</div>
</td>
<td><a name="wp1080039"> </a><div class="pCellBodyR">
0.00018
</div>
</td>
<td><a name="wp1080041"> </a><div class="pCellBodyR">
99,689
</div>
</td>
<td><a name="wp1080043"> </a><div class="pCellBodyR">
18
</div>
</td>
<td><a name="wp1080045"> </a><div class="pCellBodyR">
99,680
</div>
</td>
<td><a name="wp1080047"> </a><div class="pCellBodyR">
6,897,258
</div>
</td>
<td><a name="wp1080049"> </a><div class="pCellBodyR">
69.19
</div>
</td>
</tr>
<tr> <td><a name="wp1079991"> </a><div class="pCellBodyR">
16
</div>
</td>
<td><a name="wp1079993"> </a><div class="pCellBodyR">
0.00047
</div>
</td>
<td><a name="wp1079995"> </a><div class="pCellBodyR">
99,582
</div>
</td>
<td><a name="wp1079997"> </a><div class="pCellBodyR">
47
</div>
</td>
<td><a name="wp1079999"> </a><div class="pCellBodyR">
99,558
</div>
</td>
<td><a name="wp1080001"> </a><div class="pCellBodyR">
6,423,422
</div>
</td>
<td><a name="wp1080003"> </a><div class="pCellBodyR">
64.50
</div>
</td>
<td> </td>
<td><a name="wp1080007"> </a><div class="pCellBodyR">
16
</div>
</td>
<td><a name="wp1080009"> </a><div class="pCellBodyR">
0.00023
</div>
</td>
<td><a name="wp1080011"> </a><div class="pCellBodyR">
99,671
</div>
</td>
<td><a name="wp1080013"> </a><div class="pCellBodyR">
22
</div>
</td>
<td><a name="wp1080015"> </a><div class="pCellBodyR">
99,660
</div>
</td>
<td><a name="wp1080017"> </a><div class="pCellBodyR">
6,797,578
</div>
</td>
<td><a name="wp1080019"> </a><div class="pCellBodyR">
68.20
</div>
</td>
</tr>
<tr> <td><a name="wp1079961"> </a><div class="pCellBodyR">
17
</div>
</td>
<td><a name="wp1079963"> </a><div class="pCellBodyR">
0.00056
</div>
</td>
<td><a name="wp1079965"> </a><div class="pCellBodyR">
99,535
</div>
</td>
<td><a name="wp1079967"> </a><div class="pCellBodyR">
56
</div>
</td>
<td><a name="wp1079969"> </a><div class="pCellBodyR">
99,507
</div>
</td>
<td><a name="wp1079971"> </a><div class="pCellBodyR">
6,323,864
</div>
</td>
<td><a name="wp1079973"> </a><div class="pCellBodyR">
63.53
</div>
</td>
<td> </td>
<td><a name="wp1079977"> </a><div class="pCellBodyR">
17
</div>
</td>
<td><a name="wp1079979"> </a><div class="pCellBodyR">
0.00026
</div>
</td>
<td><a name="wp1079981"> </a><div class="pCellBodyR">
99,648
</div>
</td>
<td><a name="wp1079983"> </a><div class="pCellBodyR">
26
</div>
</td>
<td><a name="wp1079985"> </a><div class="pCellBodyR">
99,636
</div>
</td>
<td><a name="wp1079987"> </a><div class="pCellBodyR">
6,697,919
</div>
</td>
<td><a name="wp1079989"> </a><div class="pCellBodyR">
67.22
</div>
</td>
</tr>
<tr> <td><a name="wp1079931"> </a><div class="pCellBodyR">
18
</div>
</td>
<td><a name="wp1079933"> </a><div class="pCellBodyR">
0.00064
</div>
</td>
<td><a name="wp1079935"> </a><div class="pCellBodyR">
99,479
</div>
</td>
<td><a name="wp1079937"> </a><div class="pCellBodyR">
63
</div>
</td>
<td><a name="wp1079939"> </a><div class="pCellBodyR">
99,448
</div>
</td>
<td><a name="wp1079941"> </a><div class="pCellBodyR">
6,224,357
</div>
</td>
<td><a name="wp1079943"> </a><div class="pCellBodyR">
62.57
</div>
</td>
<td> </td>
<td><a name="wp1079947"> </a><div class="pCellBodyR">
18
</div>
</td>
<td><a name="wp1079949"> </a><div class="pCellBodyR">
0.00027
</div>
</td>
<td><a name="wp1079951"> </a><div class="pCellBodyR">
99,623
</div>
</td>
<td><a name="wp1079953"> </a><div class="pCellBodyR">
27
</div>
</td>
<td><a name="wp1079955"> </a><div class="pCellBodyR">
99,609
</div>
</td>
<td><a name="wp1079957"> </a><div class="pCellBodyR">
6,598,283
</div>
</td>
<td><a name="wp1079959"> </a><div class="pCellBodyR">
66.23
</div>
</td>
</tr>
<tr> <td><a name="wp1079901"> </a><div class="pCellBodyR">
19
</div>
</td>
<td><a name="wp1079903"> </a><div class="pCellBodyR">
0.00069
</div>
</td>
<td><a name="wp1079905"> </a><div class="pCellBodyR">
99,416
</div>
</td>
<td><a name="wp1079907"> </a><div class="pCellBodyR">
69
</div>
</td>
<td><a name="wp1079909"> </a><div class="pCellBodyR">
99,381
</div>
</td>
<td><a name="wp1079911"> </a><div class="pCellBodyR">
6,124,909
</div>
</td>
<td><a name="wp1079913"> </a><div class="pCellBodyR">
61.61
</div>
</td>
<td> </td>
<td><a name="wp1079917"> </a><div class="pCellBodyR">
19
</div>
</td>
<td><a name="wp1079919"> </a><div class="pCellBodyR">
0.00028
</div>
</td>
<td><a name="wp1079921"> </a><div class="pCellBodyR">
99,595
</div>
</td>
<td><a name="wp1079923"> </a><div class="pCellBodyR">
27
</div>
</td>
<td><a name="wp1079925"> </a><div class="pCellBodyR">
99,582
</div>
</td>
<td><a name="wp1079927"> </a><div class="pCellBodyR">
6,498,674
</div>
</td>
<td><a name="wp1079929"> </a><div class="pCellBodyR">
65.25
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1079841"> </a><div class="pCellBodyR">
20
</div>
</td>
<td><a name="wp1079843"> </a><div class="pCellBodyR">
0.00075
</div>
</td>
<td><a name="wp1079845"> </a><div class="pCellBodyR">
99,347
</div>
</td>
<td><a name="wp1079847"> </a><div class="pCellBodyR">
74
</div>
</td>
<td><a name="wp1079849"> </a><div class="pCellBodyR">
99,310
</div>
</td>
<td><a name="wp1079851"> </a><div class="pCellBodyR">
6,025,528
</div>
</td>
<td><a name="wp1079853"> </a><div class="pCellBodyR">
60.65
</div>
</td>
<td> </td>
<td><a name="wp1079857"> </a><div class="pCellBodyR">
20
</div>
</td>
<td><a name="wp1079859"> </a><div class="pCellBodyR">
0.00028
</div>
</td>
<td><a name="wp1079861"> </a><div class="pCellBodyR">
99,568
</div>
</td>
<td><a name="wp1079863"> </a><div class="pCellBodyR">
27
</div>
</td>
<td><a name="wp1079865"> </a><div class="pCellBodyR">
99,554
</div>
</td>
<td><a name="wp1079867"> </a><div class="pCellBodyR">
6,399,092
</div>
</td>
<td><a name="wp1079869"> </a><div class="pCellBodyR">
64.27
</div>
</td>
</tr>
<tr> <td><a name="wp1079811"> </a><div class="pCellBodyR">
21
</div>
</td>
<td><a name="wp1079813"> </a><div class="pCellBodyR">
0.00080
</div>
</td>
<td><a name="wp1079815"> </a><div class="pCellBodyR">
99,273
</div>
</td>
<td><a name="wp1079817"> </a><div class="pCellBodyR">
80
</div>
</td>
<td><a name="wp1079819"> </a><div class="pCellBodyR">
99,233
</div>
</td>
<td><a name="wp1079821"> </a><div class="pCellBodyR">
5,926,218
</div>
</td>
<td><a name="wp1079823"> </a><div class="pCellBodyR">
59.70
</div>
</td>
<td> </td>
<td><a name="wp1079827"> </a><div class="pCellBodyR">
21
</div>
</td>
<td><a name="wp1079829"> </a><div class="pCellBodyR">
0.00028
</div>
</td>
<td><a name="wp1079831"> </a><div class="pCellBodyR">
99,541
</div>
</td>
<td><a name="wp1079833"> </a><div class="pCellBodyR">
28
</div>
</td>
<td><a name="wp1079835"> </a><div class="pCellBodyR">
99,527
</div>
</td>
<td><a name="wp1079837"> </a><div class="pCellBodyR">
6,299,538
</div>
</td>
<td><a name="wp1079839"> </a><div class="pCellBodyR">
63.29
</div>
</td>
</tr>
<tr> <td><a name="wp1079781"> </a><div class="pCellBodyR">
22
</div>
</td>
<td><a name="wp1079783"> </a><div class="pCellBodyR">
0.00083
</div>
</td>
<td><a name="wp1079785"> </a><div class="pCellBodyR">
99,193
</div>
</td>
<td><a name="wp1079787"> </a><div class="pCellBodyR">
82
</div>
</td>
<td><a name="wp1079789"> </a><div class="pCellBodyR">
99,152
</div>
</td>
<td><a name="wp1079791"> </a><div class="pCellBodyR">
5,826,985
</div>
</td>
<td><a name="wp1079793"> </a><div class="pCellBodyR">
58.74
</div>
</td>
<td> </td>
<td><a name="wp1079797"> </a><div class="pCellBodyR">
22
</div>
</td>
<td><a name="wp1079799"> </a><div class="pCellBodyR">
0.00028
</div>
</td>
<td><a name="wp1079801"> </a><div class="pCellBodyR">
99,513
</div>
</td>
<td><a name="wp1079803"> </a><div class="pCellBodyR">
28
</div>
</td>
<td><a name="wp1079805"> </a><div class="pCellBodyR">
99,499
</div>
</td>
<td><a name="wp1079807"> </a><div class="pCellBodyR">
6,200,011
</div>
</td>
<td><a name="wp1079809"> </a><div class="pCellBodyR">
62.30
</div>
</td>
</tr>
<tr> <td><a name="wp1079751"> </a><div class="pCellBodyR">
23
</div>
</td>
<td><a name="wp1079753"> </a><div class="pCellBodyR">
0.00082
</div>
</td>
<td><a name="wp1079755"> </a><div class="pCellBodyR">
99,111
</div>
</td>
<td><a name="wp1079757"> </a><div class="pCellBodyR">
81
</div>
</td>
<td><a name="wp1079759"> </a><div class="pCellBodyR">
99,070
</div>
</td>
<td><a name="wp1079761"> </a><div class="pCellBodyR">
5,727,833
</div>
</td>
<td><a name="wp1079763"> </a><div class="pCellBodyR">
57.79
</div>
</td>
<td> </td>
<td><a name="wp1079767"> </a><div class="pCellBodyR">
23
</div>
</td>
<td><a name="wp1079769"> </a><div class="pCellBodyR">
0.00029
</div>
</td>
<td><a name="wp1079771"> </a><div class="pCellBodyR">
99,485
</div>
</td>
<td><a name="wp1079773"> </a><div class="pCellBodyR">
29
</div>
</td>
<td><a name="wp1079775"> </a><div class="pCellBodyR">
99,471
</div>
</td>
<td><a name="wp1079777"> </a><div class="pCellBodyR">
6,100,512
</div>
</td>
<td><a name="wp1079779"> </a><div class="pCellBodyR">
61.32
</div>
</td>
</tr>
<tr> <td><a name="wp1079721"> </a><div class="pCellBodyR">
24
</div>
</td>
<td><a name="wp1079723"> </a><div class="pCellBodyR">
0.00079
</div>
</td>
<td><a name="wp1079725"> </a><div class="pCellBodyR">
99,029
</div>
</td>
<td><a name="wp1079727"> </a><div class="pCellBodyR">
78
</div>
</td>
<td><a name="wp1079729"> </a><div class="pCellBodyR">
98,991
</div>
</td>
<td><a name="wp1079731"> </a><div class="pCellBodyR">
5,628,763
</div>
</td>
<td><a name="wp1079733"> </a><div class="pCellBodyR">
56.84
</div>
</td>
<td> </td>
<td><a name="wp1079737"> </a><div class="pCellBodyR">
24
</div>
</td>
<td><a name="wp1079739"> </a><div class="pCellBodyR">
0.00030
</div>
</td>
<td><a name="wp1079741"> </a><div class="pCellBodyR">
99,456
</div>
</td>
<td><a name="wp1079743"> </a><div class="pCellBodyR">
29
</div>
</td>
<td><a name="wp1079745"> </a><div class="pCellBodyR">
99,442
</div>
</td>
<td><a name="wp1079747"> </a><div class="pCellBodyR">
6,001,041
</div>
</td>
<td><a name="wp1079749"> </a><div class="pCellBodyR">
60.34
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1079661"> </a><div class="pCellBodyR">
25
</div>
</td>
<td><a name="wp1079663"> </a><div class="pCellBodyR">
0.00074
</div>
</td>
<td><a name="wp1079665"> </a><div class="pCellBodyR">
98,952
</div>
</td>
<td><a name="wp1079667"> </a><div class="pCellBodyR">
74
</div>
</td>
<td><a name="wp1079669"> </a><div class="pCellBodyR">
98,915
</div>
</td>
<td><a name="wp1079671"> </a><div class="pCellBodyR">
5,529,772
</div>
</td>
<td><a name="wp1079673"> </a><div class="pCellBodyR">
55.88
</div>
</td>
<td> </td>
<td><a name="wp1079677"> </a><div class="pCellBodyR">
25
</div>
</td>
<td><a name="wp1079679"> </a><div class="pCellBodyR">
0.00030
</div>
</td>
<td><a name="wp1079681"> </a><div class="pCellBodyR">
99,427
</div>
</td>
<td><a name="wp1079683"> </a><div class="pCellBodyR">
30
</div>
</td>
<td><a name="wp1079685"> </a><div class="pCellBodyR">
99,412
</div>
</td>
<td><a name="wp1079687"> </a><div class="pCellBodyR">
5,901,600
</div>
</td>
<td><a name="wp1079689"> </a><div class="pCellBodyR">
59.36
</div>
</td>
</tr>
<tr> <td><a name="wp1079631"> </a><div class="pCellBodyR">
26
</div>
</td>
<td><a name="wp1079633"> </a><div class="pCellBodyR">
0.00071
</div>
</td>
<td><a name="wp1079635"> </a><div class="pCellBodyR">
98,878
</div>
</td>
<td><a name="wp1079637"> </a><div class="pCellBodyR">
70
</div>
</td>
<td><a name="wp1079639"> </a><div class="pCellBodyR">
98,843
</div>
</td>
<td><a name="wp1079641"> </a><div class="pCellBodyR">
5,430,857
</div>
</td>
<td><a name="wp1079643"> </a><div class="pCellBodyR">
54.92
</div>
</td>
<td> </td>
<td><a name="wp1079647"> </a><div class="pCellBodyR">
26
</div>
</td>
<td><a name="wp1079649"> </a><div class="pCellBodyR">
0.00032
</div>
</td>
<td><a name="wp1079651"> </a><div class="pCellBodyR">
99,397
</div>
</td>
<td><a name="wp1079653"> </a><div class="pCellBodyR">
31
</div>
</td>
<td><a name="wp1079655"> </a><div class="pCellBodyR">
99,381
</div>
</td>
<td><a name="wp1079657"> </a><div class="pCellBodyR">
5,802,188
</div>
</td>
<td><a name="wp1079659"> </a><div class="pCellBodyR">
58.37
</div>
</td>
</tr>
<tr> <td><a name="wp1079601"> </a><div class="pCellBodyR">
27
</div>
</td>
<td><a name="wp1079603"> </a><div class="pCellBodyR">
0.00069
</div>
</td>
<td><a name="wp1079605"> </a><div class="pCellBodyR">
98,808
</div>
</td>
<td><a name="wp1079607"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1079609"> </a><div class="pCellBodyR">
98,774
</div>
</td>
<td><a name="wp1079611"> </a><div class="pCellBodyR">
5,332,014
</div>
</td>
<td><a name="wp1079613"> </a><div class="pCellBodyR">
53.96
</div>
</td>
<td> </td>
<td><a name="wp1079617"> </a><div class="pCellBodyR">
27
</div>
</td>
<td><a name="wp1079619"> </a><div class="pCellBodyR">
0.00033
</div>
</td>
<td><a name="wp1079621"> </a><div class="pCellBodyR">
99,365
</div>
</td>
<td><a name="wp1079623"> </a><div class="pCellBodyR">
33
</div>
</td>
<td><a name="wp1079625"> </a><div class="pCellBodyR">
99,349
</div>
</td>
<td><a name="wp1079627"> </a><div class="pCellBodyR">
5,702,807
</div>
</td>
<td><a name="wp1079629"> </a><div class="pCellBodyR">
57.39
</div>
</td>
</tr>
<tr> <td><a name="wp1079571"> </a><div class="pCellBodyR">
28
</div>
</td>
<td><a name="wp1079573"> </a><div class="pCellBodyR">
0.00069
</div>
</td>
<td><a name="wp1079575"> </a><div class="pCellBodyR">
98,740
</div>
</td>
<td><a name="wp1079577"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1079579"> </a><div class="pCellBodyR">
98,706
</div>
</td>
<td><a name="wp1079581"> </a><div class="pCellBodyR">
5,233,240
</div>
</td>
<td><a name="wp1079583"> </a><div class="pCellBodyR">
53.00
</div>
</td>
<td> </td>
<td><a name="wp1079587"> </a><div class="pCellBodyR">
28
</div>
</td>
<td><a name="wp1079589"> </a><div class="pCellBodyR">
0.00035
</div>
</td>
<td><a name="wp1079591"> </a><div class="pCellBodyR">
99,332
</div>
</td>
<td><a name="wp1079593"> </a><div class="pCellBodyR">
35
</div>
</td>
<td><a name="wp1079595"> </a><div class="pCellBodyR">
99,315
</div>
</td>
<td><a name="wp1079597"> </a><div class="pCellBodyR">
5,603,458
</div>
</td>
<td><a name="wp1079599"> </a><div class="pCellBodyR">
56.41
</div>
</td>
</tr>
<tr> <td><a name="wp1079541"> </a><div class="pCellBodyR">
29
</div>
</td>
<td><a name="wp1079543"> </a><div class="pCellBodyR">
0.00072
</div>
</td>
<td><a name="wp1079545"> </a><div class="pCellBodyR">
98,672
</div>
</td>
<td><a name="wp1079547"> </a><div class="pCellBodyR">
71
</div>
</td>
<td><a name="wp1079549"> </a><div class="pCellBodyR">
98,636
</div>
</td>
<td><a name="wp1079551"> </a><div class="pCellBodyR">
5,134,534
</div>
</td>
<td><a name="wp1079553"> </a><div class="pCellBodyR">
52.04
</div>
</td>
<td> </td>
<td><a name="wp1079557"> </a><div class="pCellBodyR">
29
</div>
</td>
<td><a name="wp1079559"> </a><div class="pCellBodyR">
0.00038
</div>
</td>
<td><a name="wp1079561"> </a><div class="pCellBodyR">
99,298
</div>
</td>
<td><a name="wp1079563"> </a><div class="pCellBodyR">
37
</div>
</td>
<td><a name="wp1079565"> </a><div class="pCellBodyR">
99,279
</div>
</td>
<td><a name="wp1079567"> </a><div class="pCellBodyR">
5,504,143
</div>
</td>
<td><a name="wp1079569"> </a><div class="pCellBodyR">
55.43
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1079481"> </a><div class="pCellBodyR">
30
</div>
</td>
<td><a name="wp1079483"> </a><div class="pCellBodyR">
0.00075
</div>
</td>
<td><a name="wp1079485"> </a><div class="pCellBodyR">
98,601
</div>
</td>
<td><a name="wp1079487"> </a><div class="pCellBodyR">
74
</div>
</td>
<td><a name="wp1079489"> </a><div class="pCellBodyR">
98,564
</div>
</td>
<td><a name="wp1079491"> </a><div class="pCellBodyR">
5,035,898
</div>
</td>
<td><a name="wp1079493"> </a><div class="pCellBodyR">
51.07
</div>
</td>
<td> </td>
<td><a name="wp1079497"> </a><div class="pCellBodyR">
30
</div>
</td>
<td><a name="wp1079499"> </a><div class="pCellBodyR">
0.00041
</div>
</td>
<td><a name="wp1079501"> </a><div class="pCellBodyR">
99,260
</div>
</td>
<td><a name="wp1079503"> </a><div class="pCellBodyR">
40
</div>
</td>
<td><a name="wp1079505"> </a><div class="pCellBodyR">
99,240
</div>
</td>
<td><a name="wp1079507"> </a><div class="pCellBodyR">
5,404,864
</div>
</td>
<td><a name="wp1079509"> </a><div class="pCellBodyR">
54.45
</div>
</td>
</tr>
<tr> <td><a name="wp1079451"> </a><div class="pCellBodyR">
31
</div>
</td>
<td><a name="wp1079453"> </a><div class="pCellBodyR">
0.00078
</div>
</td>
<td><a name="wp1079455"> </a><div class="pCellBodyR">
98,527
</div>
</td>
<td><a name="wp1079457"> </a><div class="pCellBodyR">
77
</div>
</td>
<td><a name="wp1079459"> </a><div class="pCellBodyR">
98,489
</div>
</td>
<td><a name="wp1079461"> </a><div class="pCellBodyR">
4,937,334
</div>
</td>
<td><a name="wp1079463"> </a><div class="pCellBodyR">
50.11
</div>
</td>
<td> </td>
<td><a name="wp1079467"> </a><div class="pCellBodyR">
31
</div>
</td>
<td><a name="wp1079469"> </a><div class="pCellBodyR">
0.00044
</div>
</td>
<td><a name="wp1079471"> </a><div class="pCellBodyR">
99,220
</div>
</td>
<td><a name="wp1079473"> </a><div class="pCellBodyR">
44
</div>
</td>
<td><a name="wp1079475"> </a><div class="pCellBodyR">
99,198
</div>
</td>
<td><a name="wp1079477"> </a><div class="pCellBodyR">
5,305,624
</div>
</td>
<td><a name="wp1079479"> </a><div class="pCellBodyR">
53.47
</div>
</td>
</tr>
<tr> <td><a name="wp1079421"> </a><div class="pCellBodyR">
32
</div>
</td>
<td><a name="wp1079423"> </a><div class="pCellBodyR">
0.00083
</div>
</td>
<td><a name="wp1079425"> </a><div class="pCellBodyR">
98,450
</div>
</td>
<td><a name="wp1079427"> </a><div class="pCellBodyR">
81
</div>
</td>
<td><a name="wp1079429"> </a><div class="pCellBodyR">
98,410
</div>
</td>
<td><a name="wp1079431"> </a><div class="pCellBodyR">
4,838,845
</div>
</td>
<td><a name="wp1079433"> </a><div class="pCellBodyR">
49.15
</div>
</td>
<td> </td>
<td><a name="wp1079437"> </a><div class="pCellBodyR">
32
</div>
</td>
<td><a name="wp1079439"> </a><div class="pCellBodyR">
0.00048
</div>
</td>
<td><a name="wp1079441"> </a><div class="pCellBodyR">
99,176
</div>
</td>
<td><a name="wp1079443"> </a><div class="pCellBodyR">
48
</div>
</td>
<td><a name="wp1079445"> </a><div class="pCellBodyR">
99,152
</div>
</td>
<td><a name="wp1079447"> </a><div class="pCellBodyR">
5,206,426
</div>
</td>
<td><a name="wp1079449"> </a><div class="pCellBodyR">
52.50
</div>
</td>
</tr>
<tr> <td><a name="wp1079391"> </a><div class="pCellBodyR">
33
</div>
</td>
<td><a name="wp1079393"> </a><div class="pCellBodyR">
0.00089
</div>
</td>
<td><a name="wp1079395"> </a><div class="pCellBodyR">
98,369
</div>
</td>
<td><a name="wp1079397"> </a><div class="pCellBodyR">
87
</div>
</td>
<td><a name="wp1079399"> </a><div class="pCellBodyR">
98,325
</div>
</td>
<td><a name="wp1079401"> </a><div class="pCellBodyR">
4,740,436
</div>
</td>
<td><a name="wp1079403"> </a><div class="pCellBodyR">
48.19
</div>
</td>
<td> </td>
<td><a name="wp1079407"> </a><div class="pCellBodyR">
33
</div>
</td>
<td><a name="wp1079409"> </a><div class="pCellBodyR">
0.00053
</div>
</td>
<td><a name="wp1079411"> </a><div class="pCellBodyR">
99,129
</div>
</td>
<td><a name="wp1079413"> </a><div class="pCellBodyR">
53
</div>
</td>
<td><a name="wp1079415"> </a><div class="pCellBodyR">
99,102
</div>
</td>
<td><a name="wp1079417"> </a><div class="pCellBodyR">
5,107,274
</div>
</td>
<td><a name="wp1079419"> </a><div class="pCellBodyR">
51.52
</div>
</td>
</tr>
<tr> <td><a name="wp1079361"> </a><div class="pCellBodyR">
34
</div>
</td>
<td><a name="wp1079363"> </a><div class="pCellBodyR">
0.00095
</div>
</td>
<td><a name="wp1079365"> </a><div class="pCellBodyR">
98,282
</div>
</td>
<td><a name="wp1079367"> </a><div class="pCellBodyR">
94
</div>
</td>
<td><a name="wp1079369"> </a><div class="pCellBodyR">
98,235
</div>
</td>
<td><a name="wp1079371"> </a><div class="pCellBodyR">
4,642,110
</div>
</td>
<td><a name="wp1079373"> </a><div class="pCellBodyR">
47.23
</div>
</td>
<td> </td>
<td><a name="wp1079377"> </a><div class="pCellBodyR">
34
</div>
</td>
<td><a name="wp1079379"> </a><div class="pCellBodyR">
0.00059
</div>
</td>
<td><a name="wp1079381"> </a><div class="pCellBodyR">
99,076
</div>
</td>
<td><a name="wp1079383"> </a><div class="pCellBodyR">
58
</div>
</td>
<td><a name="wp1079385"> </a><div class="pCellBodyR">
99,047
</div>
</td>
<td><a name="wp1079387"> </a><div class="pCellBodyR">
5,008,171
</div>
</td>
<td><a name="wp1079389"> </a><div class="pCellBodyR">
50.55
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1079301"> </a><div class="pCellBodyR">
35
</div>
</td>
<td><a name="wp1079303"> </a><div class="pCellBodyR">
0.00103
</div>
</td>
<td><a name="wp1079305"> </a><div class="pCellBodyR">
98,188
</div>
</td>
<td><a name="wp1079307"> </a><div class="pCellBodyR">
101
</div>
</td>
<td><a name="wp1079309"> </a><div class="pCellBodyR">
98,137
</div>
</td>
<td><a name="wp1079311"> </a><div class="pCellBodyR">
4,543,875
</div>
</td>
<td><a name="wp1079313"> </a><div class="pCellBodyR">
46.28
</div>
</td>
<td> </td>
<td><a name="wp1079317"> </a><div class="pCellBodyR">
35
</div>
</td>
<td><a name="wp1079319"> </a><div class="pCellBodyR">
0.00065
</div>
</td>
<td><a name="wp1079321"> </a><div class="pCellBodyR">
99,018
</div>
</td>
<td><a name="wp1079323"> </a><div class="pCellBodyR">
64
</div>
</td>
<td><a name="wp1079325"> </a><div class="pCellBodyR">
98,986
</div>
</td>
<td><a name="wp1079327"> </a><div class="pCellBodyR">
4,909,124
</div>
</td>
<td><a name="wp1079329"> </a><div class="pCellBodyR">
49.58
</div>
</td>
</tr>
<tr> <td><a name="wp1079271"> </a><div class="pCellBodyR">
36
</div>
</td>
<td><a name="wp1079273"> </a><div class="pCellBodyR">
0.00112
</div>
</td>
<td><a name="wp1079275"> </a><div class="pCellBodyR">
98,087
</div>
</td>
<td><a name="wp1079277"> </a><div class="pCellBodyR">
110
</div>
</td>
<td><a name="wp1079279"> </a><div class="pCellBodyR">
98,032
</div>
</td>
<td><a name="wp1079281"> </a><div class="pCellBodyR">
4,445,738
</div>
</td>
<td><a name="wp1079283"> </a><div class="pCellBodyR">
45.32
</div>
</td>
<td> </td>
<td><a name="wp1079287"> </a><div class="pCellBodyR">
36
</div>
</td>
<td><a name="wp1079289"> </a><div class="pCellBodyR">
0.00071
</div>
</td>
<td><a name="wp1079291"> </a><div class="pCellBodyR">
98,954
</div>
</td>
<td><a name="wp1079293"> </a><div class="pCellBodyR">
70
</div>
</td>
<td><a name="wp1079295"> </a><div class="pCellBodyR">
98,919
</div>
</td>
<td><a name="wp1079297"> </a><div class="pCellBodyR">
4,810,138
</div>
</td>
<td><a name="wp1079299"> </a><div class="pCellBodyR">
48.61
</div>
</td>
</tr>
<tr> <td><a name="wp1079241"> </a><div class="pCellBodyR">
37
</div>
</td>
<td><a name="wp1079243"> </a><div class="pCellBodyR">
0.00122
</div>
</td>
<td><a name="wp1079245"> </a><div class="pCellBodyR">
97,977
</div>
</td>
<td><a name="wp1079247"> </a><div class="pCellBodyR">
119
</div>
</td>
<td><a name="wp1079249"> </a><div class="pCellBodyR">
97,917
</div>
</td>
<td><a name="wp1079251"> </a><div class="pCellBodyR">
4,347,706
</div>
</td>
<td><a name="wp1079253"> </a><div class="pCellBodyR">
44.37
</div>
</td>
<td> </td>
<td><a name="wp1079257"> </a><div class="pCellBodyR">
37
</div>
</td>
<td><a name="wp1079259"> </a><div class="pCellBodyR">
0.00078
</div>
</td>
<td><a name="wp1079261"> </a><div class="pCellBodyR">
98,883
</div>
</td>
<td><a name="wp1079263"> </a><div class="pCellBodyR">
77
</div>
</td>
<td><a name="wp1079265"> </a><div class="pCellBodyR">
98,845
</div>
</td>
<td><a name="wp1079267"> </a><div class="pCellBodyR">
4,711,220
</div>
</td>
<td><a name="wp1079269"> </a><div class="pCellBodyR">
47.64
</div>
</td>
</tr>
<tr> <td><a name="wp1079211"> </a><div class="pCellBodyR">
38
</div>
</td>
<td><a name="wp1079213"> </a><div class="pCellBodyR">
0.00132
</div>
</td>
<td><a name="wp1079215"> </a><div class="pCellBodyR">
97,858
</div>
</td>
<td><a name="wp1079217"> </a><div class="pCellBodyR">
129
</div>
</td>
<td><a name="wp1079219"> </a><div class="pCellBodyR">
97,793
</div>
</td>
<td><a name="wp1079221"> </a><div class="pCellBodyR">
4,249,788
</div>
</td>
<td><a name="wp1079223"> </a><div class="pCellBodyR">
43.43
</div>
</td>
<td> </td>
<td><a name="wp1079227"> </a><div class="pCellBodyR">
38
</div>
</td>
<td><a name="wp1079229"> </a><div class="pCellBodyR">
0.00085
</div>
</td>
<td><a name="wp1079231"> </a><div class="pCellBodyR">
98,806
</div>
</td>
<td><a name="wp1079233"> </a><div class="pCellBodyR">
84
</div>
</td>
<td><a name="wp1079235"> </a><div class="pCellBodyR">
98,764
</div>
</td>
<td><a name="wp1079237"> </a><div class="pCellBodyR">
4,612,375
</div>
</td>
<td><a name="wp1079239"> </a><div class="pCellBodyR">
46.68
</div>
</td>
</tr>
<tr> <td><a name="wp1079181"> </a><div class="pCellBodyR">
39
</div>
</td>
<td><a name="wp1079183"> </a><div class="pCellBodyR">
0.00144
</div>
</td>
<td><a name="wp1079185"> </a><div class="pCellBodyR">
97,729
</div>
</td>
<td><a name="wp1079187"> </a><div class="pCellBodyR">
140
</div>
</td>
<td><a name="wp1079189"> </a><div class="pCellBodyR">
97,658
</div>
</td>
<td><a name="wp1079191"> </a><div class="pCellBodyR">
4,151,995
</div>
</td>
<td><a name="wp1079193"> </a><div class="pCellBodyR">
42.48
</div>
</td>
<td> </td>
<td><a name="wp1079197"> </a><div class="pCellBodyR">
39
</div>
</td>
<td><a name="wp1079199"> </a><div class="pCellBodyR">
0.00092
</div>
</td>
<td><a name="wp1079201"> </a><div class="pCellBodyR">
98,722
</div>
</td>
<td><a name="wp1079203"> </a><div class="pCellBodyR">
91
</div>
</td>
<td><a name="wp1079205"> </a><div class="pCellBodyR">
98,677
</div>
</td>
<td><a name="wp1079207"> </a><div class="pCellBodyR">
4,513,611
</div>
</td>
<td><a name="wp1079209"> </a><div class="pCellBodyR">
45.72
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1079121"> </a><div class="pCellBodyR">
40
</div>
</td>
<td><a name="wp1079123"> </a><div class="pCellBodyR">
0.00156
</div>
</td>
<td><a name="wp1079125"> </a><div class="pCellBodyR">
97,588
</div>
</td>
<td><a name="wp1079127"> </a><div class="pCellBodyR">
152
</div>
</td>
<td><a name="wp1079129"> </a><div class="pCellBodyR">
97,512
</div>
</td>
<td><a name="wp1079131"> </a><div class="pCellBodyR">
4,054,337
</div>
</td>
<td><a name="wp1079133"> </a><div class="pCellBodyR">
41.55
</div>
</td>
<td> </td>
<td><a name="wp1079137"> </a><div class="pCellBodyR">
40
</div>
</td>
<td><a name="wp1079139"> </a><div class="pCellBodyR">
0.00100
</div>
</td>
<td><a name="wp1079141"> </a><div class="pCellBodyR">
98,631
</div>
</td>
<td><a name="wp1079143"> </a><div class="pCellBodyR">
99
</div>
</td>
<td><a name="wp1079145"> </a><div class="pCellBodyR">
98,582
</div>
</td>
<td><a name="wp1079147"> </a><div class="pCellBodyR">
4,414,934
</div>
</td>
<td><a name="wp1079149"> </a><div class="pCellBodyR">
44.76
</div>
</td>
</tr>
<tr> <td><a name="wp1079091"> </a><div class="pCellBodyR">
41
</div>
</td>
<td><a name="wp1079093"> </a><div class="pCellBodyR">
0.00169
</div>
</td>
<td><a name="wp1079095"> </a><div class="pCellBodyR">
97,436
</div>
</td>
<td><a name="wp1079097"> </a><div class="pCellBodyR">
165
</div>
</td>
<td><a name="wp1079099"> </a><div class="pCellBodyR">
97,354
</div>
</td>
<td><a name="wp1079101"> </a><div class="pCellBodyR">
3,956,825
</div>
</td>
<td><a name="wp1079103"> </a><div class="pCellBodyR">
40.61
</div>
</td>
<td> </td>
<td><a name="wp1079107"> </a><div class="pCellBodyR">
41
</div>
</td>
<td><a name="wp1079109"> </a><div class="pCellBodyR">
0.00108
</div>
</td>
<td><a name="wp1079111"> </a><div class="pCellBodyR">
98,533
</div>
</td>
<td><a name="wp1079113"> </a><div class="pCellBodyR">
107
</div>
</td>
<td><a name="wp1079115"> </a><div class="pCellBodyR">
98,479
</div>
</td>
<td><a name="wp1079117"> </a><div class="pCellBodyR">
4,316,352
</div>
</td>
<td><a name="wp1079119"> </a><div class="pCellBodyR">
43.81
</div>
</td>
</tr>
<tr> <td><a name="wp1079061"> </a><div class="pCellBodyR">
42
</div>
</td>
<td><a name="wp1079063"> </a><div class="pCellBodyR">
0.00183
</div>
</td>
<td><a name="wp1079065"> </a><div class="pCellBodyR">
97,271
</div>
</td>
<td><a name="wp1079067"> </a><div class="pCellBodyR">
178
</div>
</td>
<td><a name="wp1079069"> </a><div class="pCellBodyR">
97,183
</div>
</td>
<td><a name="wp1079071"> </a><div class="pCellBodyR">
3,859,471
</div>
</td>
<td><a name="wp1079073"> </a><div class="pCellBodyR">
39.68
</div>
</td>
<td> </td>
<td><a name="wp1079077"> </a><div class="pCellBodyR">
42
</div>
</td>
<td><a name="wp1079079"> </a><div class="pCellBodyR">
0.00116
</div>
</td>
<td><a name="wp1079081"> </a><div class="pCellBodyR">
98,426
</div>
</td>
<td><a name="wp1079083"> </a><div class="pCellBodyR">
114
</div>
</td>
<td><a name="wp1079085"> </a><div class="pCellBodyR">
98,369
</div>
</td>
<td><a name="wp1079087"> </a><div class="pCellBodyR">
4,217,873
</div>
</td>
<td><a name="wp1079089"> </a><div class="pCellBodyR">
42.85
</div>
</td>
</tr>
<tr> <td><a name="wp1079031"> </a><div class="pCellBodyR">
43
</div>
</td>
<td><a name="wp1079033"> </a><div class="pCellBodyR">
0.00197
</div>
</td>
<td><a name="wp1079035"> </a><div class="pCellBodyR">
97,094
</div>
</td>
<td><a name="wp1079037"> </a><div class="pCellBodyR">
191
</div>
</td>
<td><a name="wp1079039"> </a><div class="pCellBodyR">
96,998
</div>
</td>
<td><a name="wp1079041"> </a><div class="pCellBodyR">
3,762,288
</div>
</td>
<td><a name="wp1079043"> </a><div class="pCellBodyR">
38.75
</div>
</td>
<td> </td>
<td><a name="wp1079047"> </a><div class="pCellBodyR">
43
</div>
</td>
<td><a name="wp1079049"> </a><div class="pCellBodyR">
0.00123
</div>
</td>
<td><a name="wp1079051"> </a><div class="pCellBodyR">
98,312
</div>
</td>
<td><a name="wp1079053"> </a><div class="pCellBodyR">
121
</div>
</td>
<td><a name="wp1079055"> </a><div class="pCellBodyR">
98,252
</div>
</td>
<td><a name="wp1079057"> </a><div class="pCellBodyR">
4,119,504
</div>
</td>
<td><a name="wp1079059"> </a><div class="pCellBodyR">
41.90
</div>
</td>
</tr>
<tr> <td><a name="wp1079001"> </a><div class="pCellBodyR">
44
</div>
</td>
<td><a name="wp1079003"> </a><div class="pCellBodyR">
0.00211
</div>
</td>
<td><a name="wp1079005"> </a><div class="pCellBodyR">
96,903
</div>
</td>
<td><a name="wp1079007"> </a><div class="pCellBodyR">
205
</div>
</td>
<td><a name="wp1079009"> </a><div class="pCellBodyR">
96,800
</div>
</td>
<td><a name="wp1079011"> </a><div class="pCellBodyR">
3,665,290
</div>
</td>
<td><a name="wp1079013"> </a><div class="pCellBodyR">
37.82
</div>
</td>
<td> </td>
<td><a name="wp1079017"> </a><div class="pCellBodyR">
44
</div>
</td>
<td><a name="wp1079019"> </a><div class="pCellBodyR">
0.00130
</div>
</td>
<td><a name="wp1079021"> </a><div class="pCellBodyR">
98,191
</div>
</td>
<td><a name="wp1079023"> </a><div class="pCellBodyR">
127
</div>
</td>
<td><a name="wp1079025"> </a><div class="pCellBodyR">
98,128
</div>
</td>
<td><a name="wp1079027"> </a><div class="pCellBodyR">
4,021,252
</div>
</td>
<td><a name="wp1079029"> </a><div class="pCellBodyR">
40.95
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078941"> </a><div class="pCellBodyR">
45
</div>
</td>
<td><a name="wp1078943"> </a><div class="pCellBodyR">
0.00228
</div>
</td>
<td><a name="wp1078945"> </a><div class="pCellBodyR">
96,698
</div>
</td>
<td><a name="wp1078947"> </a><div class="pCellBodyR">
220
</div>
</td>
<td><a name="wp1078949"> </a><div class="pCellBodyR">
96,588
</div>
</td>
<td><a name="wp1078951"> </a><div class="pCellBodyR">
3,568,490
</div>
</td>
<td><a name="wp1078953"> </a><div class="pCellBodyR">
36.90
</div>
</td>
<td> </td>
<td><a name="wp1078957"> </a><div class="pCellBodyR">
45
</div>
</td>
<td><a name="wp1078959"> </a><div class="pCellBodyR">
0.00137
</div>
</td>
<td><a name="wp1078961"> </a><div class="pCellBodyR">
98,064
</div>
</td>
<td><a name="wp1078963"> </a><div class="pCellBodyR">
135
</div>
</td>
<td><a name="wp1078965"> </a><div class="pCellBodyR">
97,997
</div>
</td>
<td><a name="wp1078967"> </a><div class="pCellBodyR">
3,923,124
</div>
</td>
<td><a name="wp1078969"> </a><div class="pCellBodyR">
40.01
</div>
</td>
</tr>
<tr> <td><a name="wp1078911"> </a><div class="pCellBodyR">
46
</div>
</td>
<td><a name="wp1078913"> </a><div class="pCellBodyR">
0.00245
</div>
</td>
<td><a name="wp1078915"> </a><div class="pCellBodyR">
96,478
</div>
</td>
<td><a name="wp1078917"> </a><div class="pCellBodyR">
237
</div>
</td>
<td><a name="wp1078919"> </a><div class="pCellBodyR">
96,359
</div>
</td>
<td><a name="wp1078921"> </a><div class="pCellBodyR">
3,471,902
</div>
</td>
<td><a name="wp1078923"> </a><div class="pCellBodyR">
35.99
</div>
</td>
<td> </td>
<td><a name="wp1078927"> </a><div class="pCellBodyR">
46
</div>
</td>
<td><a name="wp1078929"> </a><div class="pCellBodyR">
0.00146
</div>
</td>
<td><a name="wp1078931"> </a><div class="pCellBodyR">
97,929
</div>
</td>
<td><a name="wp1078933"> </a><div class="pCellBodyR">
143
</div>
</td>
<td><a name="wp1078935"> </a><div class="pCellBodyR">
97,858
</div>
</td>
<td><a name="wp1078937"> </a><div class="pCellBodyR">
3,825,128
</div>
</td>
<td><a name="wp1078939"> </a><div class="pCellBodyR">
39.06
</div>
</td>
</tr>
<tr> <td><a name="wp1078881"> </a><div class="pCellBodyR">
47
</div>
</td>
<td><a name="wp1078883"> </a><div class="pCellBodyR">
0.00261
</div>
</td>
<td><a name="wp1078885"> </a><div class="pCellBodyR">
96,241
</div>
</td>
<td><a name="wp1078887"> </a><div class="pCellBodyR">
251
</div>
</td>
<td><a name="wp1078889"> </a><div class="pCellBodyR">
96,115
</div>
</td>
<td><a name="wp1078891"> </a><div class="pCellBodyR">
3,375,543
</div>
</td>
<td><a name="wp1078893"> </a><div class="pCellBodyR">
35.07
</div>
</td>
<td> </td>
<td><a name="wp1078897"> </a><div class="pCellBodyR">
47
</div>
</td>
<td><a name="wp1078899"> </a><div class="pCellBodyR">
0.00156
</div>
</td>
<td><a name="wp1078901"> </a><div class="pCellBodyR">
97,786
</div>
</td>
<td><a name="wp1078903"> </a><div class="pCellBodyR">
152
</div>
</td>
<td><a name="wp1078905"> </a><div class="pCellBodyR">
97,710
</div>
</td>
<td><a name="wp1078907"> </a><div class="pCellBodyR">
3,727,270
</div>
</td>
<td><a name="wp1078909"> </a><div class="pCellBodyR">
38.12
</div>
</td>
</tr>
<tr> <td><a name="wp1078851"> </a><div class="pCellBodyR">
48
</div>
</td>
<td><a name="wp1078853"> </a><div class="pCellBodyR">
0.00274
</div>
</td>
<td><a name="wp1078855"> </a><div class="pCellBodyR">
95,990
</div>
</td>
<td><a name="wp1078857"> </a><div class="pCellBodyR">
263
</div>
</td>
<td><a name="wp1078859"> </a><div class="pCellBodyR">
95,859
</div>
</td>
<td><a name="wp1078861"> </a><div class="pCellBodyR">
3,279,427
</div>
</td>
<td><a name="wp1078863"> </a><div class="pCellBodyR">
34.16
</div>
</td>
<td> </td>
<td><a name="wp1078867"> </a><div class="pCellBodyR">
48
</div>
</td>
<td><a name="wp1078869"> </a><div class="pCellBodyR">
0.00166
</div>
</td>
<td><a name="wp1078871"> </a><div class="pCellBodyR">
97,634
</div>
</td>
<td><a name="wp1078873"> </a><div class="pCellBodyR">
162
</div>
</td>
<td><a name="wp1078875"> </a><div class="pCellBodyR">
97,553
</div>
</td>
<td><a name="wp1078877"> </a><div class="pCellBodyR">
3,629,560
</div>
</td>
<td><a name="wp1078879"> </a><div class="pCellBodyR">
37.18
</div>
</td>
</tr>
<tr> <td><a name="wp1078821"> </a><div class="pCellBodyR">
49
</div>
</td>
<td><a name="wp1078823"> </a><div class="pCellBodyR">
0.00285
</div>
</td>
<td><a name="wp1078825"> </a><div class="pCellBodyR">
95,727
</div>
</td>
<td><a name="wp1078827"> </a><div class="pCellBodyR">
273
</div>
</td>
<td><a name="wp1078829"> </a><div class="pCellBodyR">
95,591
</div>
</td>
<td><a name="wp1078831"> </a><div class="pCellBodyR">
3,183,568
</div>
</td>
<td><a name="wp1078833"> </a><div class="pCellBodyR">
33.26
</div>
</td>
<td> </td>
<td><a name="wp1078837"> </a><div class="pCellBodyR">
49
</div>
</td>
<td><a name="wp1078839"> </a><div class="pCellBodyR">
0.00177
</div>
</td>
<td><a name="wp1078841"> </a><div class="pCellBodyR">
97,472
</div>
</td>
<td><a name="wp1078843"> </a><div class="pCellBodyR">
173
</div>
</td>
<td><a name="wp1078845"> </a><div class="pCellBodyR">
97,386
</div>
</td>
<td><a name="wp1078847"> </a><div class="pCellBodyR">
3,532,006
</div>
</td>
<td><a name="wp1078849"> </a><div class="pCellBodyR">
36.24
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078761"> </a><div class="pCellBodyR">
50
</div>
</td>
<td><a name="wp1078763"> </a><div class="pCellBodyR">
0.00299
</div>
</td>
<td><a name="wp1078765"> </a><div class="pCellBodyR">
95,454
</div>
</td>
<td><a name="wp1078767"> </a><div class="pCellBodyR">
285
</div>
</td>
<td><a name="wp1078769"> </a><div class="pCellBodyR">
95,312
</div>
</td>
<td><a name="wp1078771"> </a><div class="pCellBodyR">
3,087,978
</div>
</td>
<td><a name="wp1078773"> </a><div class="pCellBodyR">
32.35
</div>
</td>
<td> </td>
<td><a name="wp1078777"> </a><div class="pCellBodyR">
50
</div>
</td>
<td><a name="wp1078779"> </a><div class="pCellBodyR">
0.00190
</div>
</td>
<td><a name="wp1078781"> </a><div class="pCellBodyR">
97,300
</div>
</td>
<td><a name="wp1078783"> </a><div class="pCellBodyR">
185
</div>
</td>
<td><a name="wp1078785"> </a><div class="pCellBodyR">
97,208
</div>
</td>
<td><a name="wp1078787"> </a><div class="pCellBodyR">
3,434,620
</div>
</td>
<td><a name="wp1078789"> </a><div class="pCellBodyR">
35.30
</div>
</td>
</tr>
<tr> <td><a name="wp1078731"> </a><div class="pCellBodyR">
51
</div>
</td>
<td><a name="wp1078733"> </a><div class="pCellBodyR">
0.00315
</div>
</td>
<td><a name="wp1078735"> </a><div class="pCellBodyR">
95,169
</div>
</td>
<td><a name="wp1078737"> </a><div class="pCellBodyR">
300
</div>
</td>
<td><a name="wp1078739"> </a><div class="pCellBodyR">
95,019
</div>
</td>
<td><a name="wp1078741"> </a><div class="pCellBodyR">
2,992,666
</div>
</td>
<td><a name="wp1078743"> </a><div class="pCellBodyR">
31.45
</div>
</td>
<td> </td>
<td><a name="wp1078747"> </a><div class="pCellBodyR">
51
</div>
</td>
<td><a name="wp1078749"> </a><div class="pCellBodyR">
0.00205
</div>
</td>
<td><a name="wp1078751"> </a><div class="pCellBodyR">
97,115
</div>
</td>
<td><a name="wp1078753"> </a><div class="pCellBodyR">
199
</div>
</td>
<td><a name="wp1078755"> </a><div class="pCellBodyR">
97,016
</div>
</td>
<td><a name="wp1078757"> </a><div class="pCellBodyR">
3,337,412
</div>
</td>
<td><a name="wp1078759"> </a><div class="pCellBodyR">
34.37
</div>
</td>
</tr>
<tr> <td><a name="wp1078701"> </a><div class="pCellBodyR">
52
</div>
</td>
<td><a name="wp1078703"> </a><div class="pCellBodyR">
0.00337
</div>
</td>
<td><a name="wp1078705"> </a><div class="pCellBodyR">
94,869
</div>
</td>
<td><a name="wp1078707"> </a><div class="pCellBodyR">
320
</div>
</td>
<td><a name="wp1078709"> </a><div class="pCellBodyR">
94,709
</div>
</td>
<td><a name="wp1078711"> </a><div class="pCellBodyR">
2,897,647
</div>
</td>
<td><a name="wp1078713"> </a><div class="pCellBodyR">
30.54
</div>
</td>
<td> </td>
<td><a name="wp1078717"> </a><div class="pCellBodyR">
52
</div>
</td>
<td><a name="wp1078719"> </a><div class="pCellBodyR">
0.00224
</div>
</td>
<td><a name="wp1078721"> </a><div class="pCellBodyR">
96,916
</div>
</td>
<td><a name="wp1078723"> </a><div class="pCellBodyR">
217
</div>
</td>
<td><a name="wp1078725"> </a><div class="pCellBodyR">
96,807
</div>
</td>
<td><a name="wp1078727"> </a><div class="pCellBodyR">
3,240,397
</div>
</td>
<td><a name="wp1078729"> </a><div class="pCellBodyR">
33.44
</div>
</td>
</tr>
<tr> <td><a name="wp1078671"> </a><div class="pCellBodyR">
53
</div>
</td>
<td><a name="wp1078673"> </a><div class="pCellBodyR">
0.00364
</div>
</td>
<td><a name="wp1078675"> </a><div class="pCellBodyR">
94,549
</div>
</td>
<td><a name="wp1078677"> </a><div class="pCellBodyR">
344
</div>
</td>
<td><a name="wp1078679"> </a><div class="pCellBodyR">
94,377
</div>
</td>
<td><a name="wp1078681"> </a><div class="pCellBodyR">
2,802,938
</div>
</td>
<td><a name="wp1078683"> </a><div class="pCellBodyR">
29.65
</div>
</td>
<td> </td>
<td><a name="wp1078687"> </a><div class="pCellBodyR">
53
</div>
</td>
<td><a name="wp1078689"> </a><div class="pCellBodyR">
0.00246
</div>
</td>
<td><a name="wp1078691"> </a><div class="pCellBodyR">
96,699
</div>
</td>
<td><a name="wp1078693"> </a><div class="pCellBodyR">
238
</div>
</td>
<td><a name="wp1078695"> </a><div class="pCellBodyR">
96,580
</div>
</td>
<td><a name="wp1078697"> </a><div class="pCellBodyR">
3,143,589
</div>
</td>
<td><a name="wp1078699"> </a><div class="pCellBodyR">
32.51
</div>
</td>
</tr>
<tr> <td><a name="wp1078641"> </a><div class="pCellBodyR">
54
</div>
</td>
<td><a name="wp1078643"> </a><div class="pCellBodyR">
0.00397
</div>
</td>
<td><a name="wp1078645"> </a><div class="pCellBodyR">
94,205
</div>
</td>
<td><a name="wp1078647"> </a><div class="pCellBodyR">
374
</div>
</td>
<td><a name="wp1078649"> </a><div class="pCellBodyR">
94,018
</div>
</td>
<td><a name="wp1078651"> </a><div class="pCellBodyR">
2,708,560
</div>
</td>
<td><a name="wp1078653"> </a><div class="pCellBodyR">
28.75
</div>
</td>
<td> </td>
<td><a name="wp1078657"> </a><div class="pCellBodyR">
54
</div>
</td>
<td><a name="wp1078659"> </a><div class="pCellBodyR">
0.00272
</div>
</td>
<td><a name="wp1078661"> </a><div class="pCellBodyR">
96,461
</div>
</td>
<td><a name="wp1078663"> </a><div class="pCellBodyR">
262
</div>
</td>
<td><a name="wp1078665"> </a><div class="pCellBodyR">
96,330
</div>
</td>
<td><a name="wp1078667"> </a><div class="pCellBodyR">
3,047,009
</div>
</td>
<td><a name="wp1078669"> </a><div class="pCellBodyR">
31.59
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078581"> </a><div class="pCellBodyR">
55
</div>
</td>
<td><a name="wp1078583"> </a><div class="pCellBodyR">
0.00436
</div>
</td>
<td><a name="wp1078585"> </a><div class="pCellBodyR">
93,831
</div>
</td>
<td><a name="wp1078587"> </a><div class="pCellBodyR">
409
</div>
</td>
<td><a name="wp1078589"> </a><div class="pCellBodyR">
93,626
</div>
</td>
<td><a name="wp1078591"> </a><div class="pCellBodyR">
2,614,543
</div>
</td>
<td><a name="wp1078593"> </a><div class="pCellBodyR">
27.86
</div>
</td>
<td> </td>
<td><a name="wp1078597"> </a><div class="pCellBodyR">
55
</div>
</td>
<td><a name="wp1078599"> </a><div class="pCellBodyR">
0.00302
</div>
</td>
<td><a name="wp1078601"> </a><div class="pCellBodyR">
96,199
</div>
</td>
<td><a name="wp1078603"> </a><div class="pCellBodyR">
291
</div>
</td>
<td><a name="wp1078605"> </a><div class="pCellBodyR">
96,053
</div>
</td>
<td><a name="wp1078607"> </a><div class="pCellBodyR">
2,950,680
</div>
</td>
<td><a name="wp1078609"> </a><div class="pCellBodyR">
30.67
</div>
</td>
</tr>
<tr> <td><a name="wp1078551"> </a><div class="pCellBodyR">
56
</div>
</td>
<td><a name="wp1078553"> </a><div class="pCellBodyR">
0.00479
</div>
</td>
<td><a name="wp1078555"> </a><div class="pCellBodyR">
93,422
</div>
</td>
<td><a name="wp1078557"> </a><div class="pCellBodyR">
448
</div>
</td>
<td><a name="wp1078559"> </a><div class="pCellBodyR">
93,198
</div>
</td>
<td><a name="wp1078561"> </a><div class="pCellBodyR">
2,520,916
</div>
</td>
<td><a name="wp1078563"> </a><div class="pCellBodyR">
26.98
</div>
</td>
<td> </td>
<td><a name="wp1078567"> </a><div class="pCellBodyR">
56
</div>
</td>
<td><a name="wp1078569"> </a><div class="pCellBodyR">
0.00336
</div>
</td>
<td><a name="wp1078571"> </a><div class="pCellBodyR">
95,908
</div>
</td>
<td><a name="wp1078573"> </a><div class="pCellBodyR">
322
</div>
</td>
<td><a name="wp1078575"> </a><div class="pCellBodyR">
95,747
</div>
</td>
<td><a name="wp1078577"> </a><div class="pCellBodyR">
2,854,627
</div>
</td>
<td><a name="wp1078579"> </a><div class="pCellBodyR">
29.76
</div>
</td>
</tr>
<tr> <td><a name="wp1078521"> </a><div class="pCellBodyR">
57
</div>
</td>
<td><a name="wp1078523"> </a><div class="pCellBodyR">
0.00526
</div>
</td>
<td><a name="wp1078525"> </a><div class="pCellBodyR">
92,974
</div>
</td>
<td><a name="wp1078527"> </a><div class="pCellBodyR">
489
</div>
</td>
<td><a name="wp1078529"> </a><div class="pCellBodyR">
92,730
</div>
</td>
<td><a name="wp1078531"> </a><div class="pCellBodyR">
2,427,719
</div>
</td>
<td><a name="wp1078533"> </a><div class="pCellBodyR">
26.11
</div>
</td>
<td> </td>
<td><a name="wp1078537"> </a><div class="pCellBodyR">
57
</div>
</td>
<td><a name="wp1078539"> </a><div class="pCellBodyR">
0.00371
</div>
</td>
<td><a name="wp1078541"> </a><div class="pCellBodyR">
95,586
</div>
</td>
<td><a name="wp1078543"> </a><div class="pCellBodyR">
355
</div>
</td>
<td><a name="wp1078545"> </a><div class="pCellBodyR">
95,408
</div>
</td>
<td><a name="wp1078547"> </a><div class="pCellBodyR">
2,758,880
</div>
</td>
<td><a name="wp1078549"> </a><div class="pCellBodyR">
28.86
</div>
</td>
</tr>
<tr> <td><a name="wp1078491"> </a><div class="pCellBodyR">
58
</div>
</td>
<td><a name="wp1078493"> </a><div class="pCellBodyR">
0.00574
</div>
</td>
<td><a name="wp1078495"> </a><div class="pCellBodyR">
92,485
</div>
</td>
<td><a name="wp1078497"> </a><div class="pCellBodyR">
531
</div>
</td>
<td><a name="wp1078499"> </a><div class="pCellBodyR">
92,220
</div>
</td>
<td><a name="wp1078501"> </a><div class="pCellBodyR">
2,334,989
</div>
</td>
<td><a name="wp1078503"> </a><div class="pCellBodyR">
25.25
</div>
</td>
<td> </td>
<td><a name="wp1078507"> </a><div class="pCellBodyR">
58
</div>
</td>
<td><a name="wp1078509"> </a><div class="pCellBodyR">
0.00408
</div>
</td>
<td><a name="wp1078511"> </a><div class="pCellBodyR">
95,231
</div>
</td>
<td><a name="wp1078513"> </a><div class="pCellBodyR">
389
</div>
</td>
<td><a name="wp1078515"> </a><div class="pCellBodyR">
95,036
</div>
</td>
<td><a name="wp1078517"> </a><div class="pCellBodyR">
2,663,472
</div>
</td>
<td><a name="wp1078519"> </a><div class="pCellBodyR">
27.97
</div>
</td>
</tr>
<tr> <td><a name="wp1078461"> </a><div class="pCellBodyR">
59
</div>
</td>
<td><a name="wp1078463"> </a><div class="pCellBodyR">
0.00628
</div>
</td>
<td><a name="wp1078465"> </a><div class="pCellBodyR">
91,954
</div>
</td>
<td><a name="wp1078467"> </a><div class="pCellBodyR">
577
</div>
</td>
<td><a name="wp1078469"> </a><div class="pCellBodyR">
91,665
</div>
</td>
<td><a name="wp1078471"> </a><div class="pCellBodyR">
2,242,769
</div>
</td>
<td><a name="wp1078473"> </a><div class="pCellBodyR">
24.39
</div>
</td>
<td> </td>
<td><a name="wp1078477"> </a><div class="pCellBodyR">
59
</div>
</td>
<td><a name="wp1078479"> </a><div class="pCellBodyR">
0.00448
</div>
</td>
<td><a name="wp1078481"> </a><div class="pCellBodyR">
94,842
</div>
</td>
<td><a name="wp1078483"> </a><div class="pCellBodyR">
424
</div>
</td>
<td><a name="wp1078485"> </a><div class="pCellBodyR">
94,630
</div>
</td>
<td><a name="wp1078487"> </a><div class="pCellBodyR">
2,568,436
</div>
</td>
<td><a name="wp1078489"> </a><div class="pCellBodyR">
27.08
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078401"> </a><div class="pCellBodyR">
60
</div>
</td>
<td><a name="wp1078403"> </a><div class="pCellBodyR">
0.00687
</div>
</td>
<td><a name="wp1078405"> </a><div class="pCellBodyR">
91,377
</div>
</td>
<td><a name="wp1078407"> </a><div class="pCellBodyR">
628
</div>
</td>
<td><a name="wp1078409"> </a><div class="pCellBodyR">
91,063
</div>
</td>
<td><a name="wp1078411"> </a><div class="pCellBodyR">
2,151,104
</div>
</td>
<td><a name="wp1078413"> </a><div class="pCellBodyR">
23.54
</div>
</td>
<td> </td>
<td><a name="wp1078417"> </a><div class="pCellBodyR">
60
</div>
</td>
<td><a name="wp1078419"> </a><div class="pCellBodyR">
0.00491
</div>
</td>
<td><a name="wp1078421"> </a><div class="pCellBodyR">
94,418
</div>
</td>
<td><a name="wp1078423"> </a><div class="pCellBodyR">
464
</div>
</td>
<td><a name="wp1078425"> </a><div class="pCellBodyR">
94,186
</div>
</td>
<td><a name="wp1078427"> </a><div class="pCellBodyR">
2,473,806
</div>
</td>
<td><a name="wp1078429"> </a><div class="pCellBodyR">
26.20
</div>
</td>
</tr>
<tr> <td><a name="wp1078371"> </a><div class="pCellBodyR">
61
</div>
</td>
<td><a name="wp1078373"> </a><div class="pCellBodyR">
0.00755
</div>
</td>
<td><a name="wp1078375"> </a><div class="pCellBodyR">
90,749
</div>
</td>
<td><a name="wp1078377"> </a><div class="pCellBodyR">
686
</div>
</td>
<td><a name="wp1078379"> </a><div class="pCellBodyR">
90,406
</div>
</td>
<td><a name="wp1078381"> </a><div class="pCellBodyR">
2,060,041
</div>
</td>
<td><a name="wp1078383"> </a><div class="pCellBodyR">
22.70
</div>
</td>
<td> </td>
<td><a name="wp1078387"> </a><div class="pCellBodyR">
61
</div>
</td>
<td><a name="wp1078389"> </a><div class="pCellBodyR">
0.00541
</div>
</td>
<td><a name="wp1078391"> </a><div class="pCellBodyR">
93,954
</div>
</td>
<td><a name="wp1078393"> </a><div class="pCellBodyR">
508
</div>
</td>
<td><a name="wp1078395"> </a><div class="pCellBodyR">
93,700
</div>
</td>
<td><a name="wp1078397"> </a><div class="pCellBodyR">
2,379,620
</div>
</td>
<td><a name="wp1078399"> </a><div class="pCellBodyR">
25.33
</div>
</td>
</tr>
<tr> <td><a name="wp1078341"> </a><div class="pCellBodyR">
62
</div>
</td>
<td><a name="wp1078343"> </a><div class="pCellBodyR">
0.00837
</div>
</td>
<td><a name="wp1078345"> </a><div class="pCellBodyR">
90,064
</div>
</td>
<td><a name="wp1078347"> </a><div class="pCellBodyR">
754
</div>
</td>
<td><a name="wp1078349"> </a><div class="pCellBodyR">
89,687
</div>
</td>
<td><a name="wp1078351"> </a><div class="pCellBodyR">
1,969,634
</div>
</td>
<td><a name="wp1078353"> </a><div class="pCellBodyR">
21.87
</div>
</td>
<td> </td>
<td><a name="wp1078357"> </a><div class="pCellBodyR">
62
</div>
</td>
<td><a name="wp1078359"> </a><div class="pCellBodyR">
0.00600
</div>
</td>
<td><a name="wp1078361"> </a><div class="pCellBodyR">
93,446
</div>
</td>
<td><a name="wp1078363"> </a><div class="pCellBodyR">
561
</div>
</td>
<td><a name="wp1078365"> </a><div class="pCellBodyR">
93,165
</div>
</td>
<td><a name="wp1078367"> </a><div class="pCellBodyR">
2,285,920
</div>
</td>
<td><a name="wp1078369"> </a><div class="pCellBodyR">
24.46
</div>
</td>
</tr>
<tr> <td><a name="wp1078311"> </a><div class="pCellBodyR">
63
</div>
</td>
<td><a name="wp1078313"> </a><div class="pCellBodyR">
0.00933
</div>
</td>
<td><a name="wp1078315"> </a><div class="pCellBodyR">
89,310
</div>
</td>
<td><a name="wp1078317"> </a><div class="pCellBodyR">
834
</div>
</td>
<td><a name="wp1078319"> </a><div class="pCellBodyR">
88,893
</div>
</td>
<td><a name="wp1078321"> </a><div class="pCellBodyR">
1,879,947
</div>
</td>
<td><a name="wp1078323"> </a><div class="pCellBodyR">
21.05
</div>
</td>
<td> </td>
<td><a name="wp1078327"> </a><div class="pCellBodyR">
63
</div>
</td>
<td><a name="wp1078329"> </a><div class="pCellBodyR">
0.00669
</div>
</td>
<td><a name="wp1078331"> </a><div class="pCellBodyR">
92,885
</div>
</td>
<td><a name="wp1078333"> </a><div class="pCellBodyR">
622
</div>
</td>
<td><a name="wp1078335"> </a><div class="pCellBodyR">
92,574
</div>
</td>
<td><a name="wp1078337"> </a><div class="pCellBodyR">
2,192,754
</div>
</td>
<td><a name="wp1078339"> </a><div class="pCellBodyR">
23.61
</div>
</td>
</tr>
<tr> <td><a name="wp1078281"> </a><div class="pCellBodyR">
64
</div>
</td>
<td><a name="wp1078283"> </a><div class="pCellBodyR">
0.01044
</div>
</td>
<td><a name="wp1078285"> </a><div class="pCellBodyR">
88,476
</div>
</td>
<td><a name="wp1078287"> </a><div class="pCellBodyR">
923
</div>
</td>
<td><a name="wp1078289"> </a><div class="pCellBodyR">
88,015
</div>
</td>
<td><a name="wp1078291"> </a><div class="pCellBodyR">
1,791,054
</div>
</td>
<td><a name="wp1078293"> </a><div class="pCellBodyR">
20.24
</div>
</td>
<td> </td>
<td><a name="wp1078297"> </a><div class="pCellBodyR">
64
</div>
</td>
<td><a name="wp1078299"> </a><div class="pCellBodyR">
0.00748
</div>
</td>
<td><a name="wp1078301"> </a><div class="pCellBodyR">
92,263
</div>
</td>
<td><a name="wp1078303"> </a><div class="pCellBodyR">
690
</div>
</td>
<td><a name="wp1078305"> </a><div class="pCellBodyR">
91,918
</div>
</td>
<td><a name="wp1078307"> </a><div class="pCellBodyR">
2,100,180
</div>
</td>
<td><a name="wp1078309"> </a><div class="pCellBodyR">
22.76
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078221"> </a><div class="pCellBodyR">
65
</div>
</td>
<td><a name="wp1078223"> </a><div class="pCellBodyR">
0.01167
</div>
</td>
<td><a name="wp1078225"> </a><div class="pCellBodyR">
87,553
</div>
</td>
<td><a name="wp1078227"> </a><div class="pCellBodyR">
1,021
</div>
</td>
<td><a name="wp1078229"> </a><div class="pCellBodyR">
87,042
</div>
</td>
<td><a name="wp1078231"> </a><div class="pCellBodyR">
1,703,040
</div>
</td>
<td><a name="wp1078233"> </a><div class="pCellBodyR">
19.45
</div>
</td>
<td> </td>
<td><a name="wp1078237"> </a><div class="pCellBodyR">
65
</div>
</td>
<td><a name="wp1078239"> </a><div class="pCellBodyR">
0.00836
</div>
</td>
<td><a name="wp1078241"> </a><div class="pCellBodyR">
91,573
</div>
</td>
<td><a name="wp1078243"> </a><div class="pCellBodyR">
766
</div>
</td>
<td><a name="wp1078245"> </a><div class="pCellBodyR">
91,190
</div>
</td>
<td><a name="wp1078247"> </a><div class="pCellBodyR">
2,008,262
</div>
</td>
<td><a name="wp1078249"> </a><div class="pCellBodyR">
21.93
</div>
</td>
</tr>
<tr> <td><a name="wp1078191"> </a><div class="pCellBodyR">
66
</div>
</td>
<td><a name="wp1078193"> </a><div class="pCellBodyR">
0.01298
</div>
</td>
<td><a name="wp1078195"> </a><div class="pCellBodyR">
86,531
</div>
</td>
<td><a name="wp1078197"> </a><div class="pCellBodyR">
1,124
</div>
</td>
<td><a name="wp1078199"> </a><div class="pCellBodyR">
85,970
</div>
</td>
<td><a name="wp1078201"> </a><div class="pCellBodyR">
1,615,998
</div>
</td>
<td><a name="wp1078203"> </a><div class="pCellBodyR">
18.68
</div>
</td>
<td> </td>
<td><a name="wp1078207"> </a><div class="pCellBodyR">
66
</div>
</td>
<td><a name="wp1078209"> </a><div class="pCellBodyR">
0.00930
</div>
</td>
<td><a name="wp1078211"> </a><div class="pCellBodyR">
90,807
</div>
</td>
<td><a name="wp1078213"> </a><div class="pCellBodyR">
845
</div>
</td>
<td><a name="wp1078215"> </a><div class="pCellBodyR">
90,385
</div>
</td>
<td><a name="wp1078217"> </a><div class="pCellBodyR">
1,917,072
</div>
</td>
<td><a name="wp1078219"> </a><div class="pCellBodyR">
21.11
</div>
</td>
</tr>
<tr> <td><a name="wp1078161"> </a><div class="pCellBodyR">
67
</div>
</td>
<td><a name="wp1078163"> </a><div class="pCellBodyR">
0.01435
</div>
</td>
<td><a name="wp1078165"> </a><div class="pCellBodyR">
85,408
</div>
</td>
<td><a name="wp1078167"> </a><div class="pCellBodyR">
1,226
</div>
</td>
<td><a name="wp1078169"> </a><div class="pCellBodyR">
84,795
</div>
</td>
<td><a name="wp1078171"> </a><div class="pCellBodyR">
1,530,028
</div>
</td>
<td><a name="wp1078173"> </a><div class="pCellBodyR">
17.91
</div>
</td>
<td> </td>
<td><a name="wp1078177"> </a><div class="pCellBodyR">
67
</div>
</td>
<td><a name="wp1078179"> </a><div class="pCellBodyR">
0.01028
</div>
</td>
<td><a name="wp1078181"> </a><div class="pCellBodyR">
89,962
</div>
</td>
<td><a name="wp1078183"> </a><div class="pCellBodyR">
925
</div>
</td>
<td><a name="wp1078185"> </a><div class="pCellBodyR">
89,500
</div>
</td>
<td><a name="wp1078187"> </a><div class="pCellBodyR">
1,826,687
</div>
</td>
<td><a name="wp1078189"> </a><div class="pCellBodyR">
20.31
</div>
</td>
</tr>
<tr> <td><a name="wp1078131"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1078133"> </a><div class="pCellBodyR">
0.01575
</div>
</td>
<td><a name="wp1078135"> </a><div class="pCellBodyR">
84,182
</div>
</td>
<td><a name="wp1078137"> </a><div class="pCellBodyR">
1,326
</div>
</td>
<td><a name="wp1078139"> </a><div class="pCellBodyR">
83,519
</div>
</td>
<td><a name="wp1078141"> </a><div class="pCellBodyR">
1,445,233
</div>
</td>
<td><a name="wp1078143"> </a><div class="pCellBodyR">
17.17
</div>
</td>
<td> </td>
<td><a name="wp1078147"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1078149"> </a><div class="pCellBodyR">
0.01127
</div>
</td>
<td><a name="wp1078151"> </a><div class="pCellBodyR">
89,037
</div>
</td>
<td><a name="wp1078153"> </a><div class="pCellBodyR">
1,004
</div>
</td>
<td><a name="wp1078155"> </a><div class="pCellBodyR">
88,536
</div>
</td>
<td><a name="wp1078157"> </a><div class="pCellBodyR">
1,737,187
</div>
</td>
<td><a name="wp1078159"> </a><div class="pCellBodyR">
19.51
</div>
</td>
</tr>
<tr> <td><a name="wp1078101"> </a><div class="pCellBodyR">
69
</div>
</td>
<td><a name="wp1078103"> </a><div class="pCellBodyR">
0.01721
</div>
</td>
<td><a name="wp1078105"> </a><div class="pCellBodyR">
82,856
</div>
</td>
<td><a name="wp1078107"> </a><div class="pCellBodyR">
1,426
</div>
</td>
<td><a name="wp1078109"> </a><div class="pCellBodyR">
82,143
</div>
</td>
<td><a name="wp1078111"> </a><div class="pCellBodyR">
1,361,714
</div>
</td>
<td><a name="wp1078113"> </a><div class="pCellBodyR">
16.43
</div>
</td>
<td> </td>
<td><a name="wp1078117"> </a><div class="pCellBodyR">
69
</div>
</td>
<td><a name="wp1078119"> </a><div class="pCellBodyR">
0.01231
</div>
</td>
<td><a name="wp1078121"> </a><div class="pCellBodyR">
88,034
</div>
</td>
<td><a name="wp1078123"> </a><div class="pCellBodyR">
1,084
</div>
</td>
<td><a name="wp1078125"> </a><div class="pCellBodyR">
87,492
</div>
</td>
<td><a name="wp1078127"> </a><div class="pCellBodyR">
1,648,652
</div>
</td>
<td><a name="wp1078129"> </a><div class="pCellBodyR">
18.73
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1078041"> </a><div class="pCellBodyR">
70
</div>
</td>
<td><a name="wp1078043"> </a><div class="pCellBodyR">
0.01888
</div>
</td>
<td><a name="wp1078045"> </a><div class="pCellBodyR">
81,430
</div>
</td>
<td><a name="wp1078047"> </a><div class="pCellBodyR">
1,537
</div>
</td>
<td><a name="wp1078049"> </a><div class="pCellBodyR">
80,661
</div>
</td>
<td><a name="wp1078051"> </a><div class="pCellBodyR">
1,279,571
</div>
</td>
<td><a name="wp1078053"> </a><div class="pCellBodyR">
15.71
</div>
</td>
<td> </td>
<td><a name="wp1078057"> </a><div class="pCellBodyR">
70
</div>
</td>
<td><a name="wp1078059"> </a><div class="pCellBodyR">
0.01349
</div>
</td>
<td><a name="wp1078061"> </a><div class="pCellBodyR">
86,950
</div>
</td>
<td><a name="wp1078063"> </a><div class="pCellBodyR">
1,173
</div>
</td>
<td><a name="wp1078065"> </a><div class="pCellBodyR">
86,364
</div>
</td>
<td><a name="wp1078067"> </a><div class="pCellBodyR">
1,561,160
</div>
</td>
<td><a name="wp1078069"> </a><div class="pCellBodyR">
17.95
</div>
</td>
</tr>
<tr> <td><a name="wp1078011"> </a><div class="pCellBodyR">
71
</div>
</td>
<td><a name="wp1078013"> </a><div class="pCellBodyR">
0.02073
</div>
</td>
<td><a name="wp1078015"> </a><div class="pCellBodyR">
79,892
</div>
</td>
<td><a name="wp1078017"> </a><div class="pCellBodyR">
1,656
</div>
</td>
<td><a name="wp1078019"> </a><div class="pCellBodyR">
79,064
</div>
</td>
<td><a name="wp1078021"> </a><div class="pCellBodyR">
1,198,910
</div>
</td>
<td><a name="wp1078023"> </a><div class="pCellBodyR">
15.01
</div>
</td>
<td> </td>
<td><a name="wp1078027"> </a><div class="pCellBodyR">
71
</div>
</td>
<td><a name="wp1078029"> </a><div class="pCellBodyR">
0.01480
</div>
</td>
<td><a name="wp1078031"> </a><div class="pCellBodyR">
85,778
</div>
</td>
<td><a name="wp1078033"> </a><div class="pCellBodyR">
1,270
</div>
</td>
<td><a name="wp1078035"> </a><div class="pCellBodyR">
85,143
</div>
</td>
<td><a name="wp1078037"> </a><div class="pCellBodyR">
1,474,796
</div>
</td>
<td><a name="wp1078039"> </a><div class="pCellBodyR">
17.19
</div>
</td>
</tr>
<tr> <td><a name="wp1077981"> </a><div class="pCellBodyR">
72
</div>
</td>
<td><a name="wp1077983"> </a><div class="pCellBodyR">
0.02264
</div>
</td>
<td><a name="wp1077985"> </a><div class="pCellBodyR">
78,236
</div>
</td>
<td><a name="wp1077987"> </a><div class="pCellBodyR">
1,771
</div>
</td>
<td><a name="wp1077989"> </a><div class="pCellBodyR">
77,351
</div>
</td>
<td><a name="wp1077991"> </a><div class="pCellBodyR">
1,119,845
</div>
</td>
<td><a name="wp1077993"> </a><div class="pCellBodyR">
14.31
</div>
</td>
<td> </td>
<td><a name="wp1077997"> </a><div class="pCellBodyR">
72
</div>
</td>
<td><a name="wp1077999"> </a><div class="pCellBodyR">
0.01617
</div>
</td>
<td><a name="wp1078001"> </a><div class="pCellBodyR">
84,508
</div>
</td>
<td><a name="wp1078003"> </a><div class="pCellBodyR">
1,366
</div>
</td>
<td><a name="wp1078005"> </a><div class="pCellBodyR">
83,825
</div>
</td>
<td><a name="wp1078007"> </a><div class="pCellBodyR">
1,389,653
</div>
</td>
<td><a name="wp1078009"> </a><div class="pCellBodyR">
16.44
</div>
</td>
</tr>
<tr> <td><a name="wp1077951"> </a><div class="pCellBodyR">
73
</div>
</td>
<td><a name="wp1077953"> </a><div class="pCellBodyR">
0.02456
</div>
</td>
<td><a name="wp1077955"> </a><div class="pCellBodyR">
76,465
</div>
</td>
<td><a name="wp1077957"> </a><div class="pCellBodyR">
1,878
</div>
</td>
<td><a name="wp1077959"> </a><div class="pCellBodyR">
75,526
</div>
</td>
<td><a name="wp1077961"> </a><div class="pCellBodyR">
1,042,495
</div>
</td>
<td><a name="wp1077963"> </a><div class="pCellBodyR">
13.63
</div>
</td>
<td> </td>
<td><a name="wp1077967"> </a><div class="pCellBodyR">
73
</div>
</td>
<td><a name="wp1077969"> </a><div class="pCellBodyR">
0.01757
</div>
</td>
<td><a name="wp1077971"> </a><div class="pCellBodyR">
83,142
</div>
</td>
<td><a name="wp1077973"> </a><div class="pCellBodyR">
1,461
</div>
</td>
<td><a name="wp1077975"> </a><div class="pCellBodyR">
82,411
</div>
</td>
<td><a name="wp1077977"> </a><div class="pCellBodyR">
1,305,828
</div>
</td>
<td><a name="wp1077979"> </a><div class="pCellBodyR">
15.71
</div>
</td>
</tr>
<tr> <td><a name="wp1077921"> </a><div class="pCellBodyR">
74
</div>
</td>
<td><a name="wp1077923"> </a><div class="pCellBodyR">
0.02660
</div>
</td>
<td><a name="wp1077925"> </a><div class="pCellBodyR">
74,587
</div>
</td>
<td><a name="wp1077927"> </a><div class="pCellBodyR">
1,984
</div>
</td>
<td><a name="wp1077929"> </a><div class="pCellBodyR">
73,595
</div>
</td>
<td><a name="wp1077931"> </a><div class="pCellBodyR">
966,969
</div>
</td>
<td><a name="wp1077933"> </a><div class="pCellBodyR">
12.96
</div>
</td>
<td> </td>
<td><a name="wp1077937"> </a><div class="pCellBodyR">
74
</div>
</td>
<td><a name="wp1077939"> </a><div class="pCellBodyR">
0.01908
</div>
</td>
<td><a name="wp1077941"> </a><div class="pCellBodyR">
81,681
</div>
</td>
<td><a name="wp1077943"> </a><div class="pCellBodyR">
1,558
</div>
</td>
<td><a name="wp1077945"> </a><div class="pCellBodyR">
80,902
</div>
</td>
<td><a name="wp1077947"> </a><div class="pCellBodyR">
1,223,417
</div>
</td>
<td><a name="wp1077949"> </a><div class="pCellBodyR">
14.98
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1077861"> </a><div class="pCellBodyR">
75
</div>
</td>
<td><a name="wp1077863"> </a><div class="pCellBodyR">
0.02908
</div>
</td>
<td><a name="wp1077865"> </a><div class="pCellBodyR">
72,603
</div>
</td>
<td><a name="wp1077867"> </a><div class="pCellBodyR">
2,111
</div>
</td>
<td><a name="wp1077869"> </a><div class="pCellBodyR">
71,547
</div>
</td>
<td><a name="wp1077871"> </a><div class="pCellBodyR">
893,374
</div>
</td>
<td><a name="wp1077873"> </a><div class="pCellBodyR">
12.30
</div>
</td>
<td> </td>
<td><a name="wp1077877"> </a><div class="pCellBodyR">
75
</div>
</td>
<td><a name="wp1077879"> </a><div class="pCellBodyR">
0.02092
</div>
</td>
<td><a name="wp1077881"> </a><div class="pCellBodyR">
80,123
</div>
</td>
<td><a name="wp1077883"> </a><div class="pCellBodyR">
1,676
</div>
</td>
<td><a name="wp1077885"> </a><div class="pCellBodyR">
79,284
</div>
</td>
<td><a name="wp1077887"> </a><div class="pCellBodyR">
1,142,516
</div>
</td>
<td><a name="wp1077889"> </a><div class="pCellBodyR">
14.26
</div>
</td>
</tr>
<tr> <td><a name="wp1077831"> </a><div class="pCellBodyR">
76
</div>
</td>
<td><a name="wp1077833"> </a><div class="pCellBodyR">
0.03198
</div>
</td>
<td><a name="wp1077835"> </a><div class="pCellBodyR">
70,492
</div>
</td>
<td><a name="wp1077837"> </a><div class="pCellBodyR">
2,254
</div>
</td>
<td><a name="wp1077839"> </a><div class="pCellBodyR">
69,365
</div>
</td>
<td><a name="wp1077841"> </a><div class="pCellBodyR">
821,827
</div>
</td>
<td><a name="wp1077843"> </a><div class="pCellBodyR">
11.66
</div>
</td>
<td> </td>
<td><a name="wp1077847"> </a><div class="pCellBodyR">
76
</div>
</td>
<td><a name="wp1077849"> </a><div class="pCellBodyR">
0.02308
</div>
</td>
<td><a name="wp1077851"> </a><div class="pCellBodyR">
78,446
</div>
</td>
<td><a name="wp1077853"> </a><div class="pCellBodyR">
1,810
</div>
</td>
<td><a name="wp1077855"> </a><div class="pCellBodyR">
77,541
</div>
</td>
<td><a name="wp1077857"> </a><div class="pCellBodyR">
1,063,231
</div>
</td>
<td><a name="wp1077859"> </a><div class="pCellBodyR">
13.55
</div>
</td>
</tr>
<tr> <td><a name="wp1077801"> </a><div class="pCellBodyR">
77
</div>
</td>
<td><a name="wp1077803"> </a><div class="pCellBodyR">
0.03506
</div>
</td>
<td><a name="wp1077805"> </a><div class="pCellBodyR">
68,238
</div>
</td>
<td><a name="wp1077807"> </a><div class="pCellBodyR">
2,393
</div>
</td>
<td><a name="wp1077809"> </a><div class="pCellBodyR">
67,041
</div>
</td>
<td><a name="wp1077811"> </a><div class="pCellBodyR">
752,462
</div>
</td>
<td><a name="wp1077813"> </a><div class="pCellBodyR">
11.03
</div>
</td>
<td> </td>
<td><a name="wp1077817"> </a><div class="pCellBodyR">
77
</div>
</td>
<td><a name="wp1077819"> </a><div class="pCellBodyR">
0.02530
</div>
</td>
<td><a name="wp1077821"> </a><div class="pCellBodyR">
76,636
</div>
</td>
<td><a name="wp1077823"> </a><div class="pCellBodyR">
1,939
</div>
</td>
<td><a name="wp1077825"> </a><div class="pCellBodyR">
75,667
</div>
</td>
<td><a name="wp1077827"> </a><div class="pCellBodyR">
985,690
</div>
</td>
<td><a name="wp1077829"> </a><div class="pCellBodyR">
12.86
</div>
</td>
</tr>
<tr> <td><a name="wp1077771"> </a><div class="pCellBodyR">
78
</div>
</td>
<td><a name="wp1077773"> </a><div class="pCellBodyR">
0.03831
</div>
</td>
<td><a name="wp1077775"> </a><div class="pCellBodyR">
65,845
</div>
</td>
<td><a name="wp1077777"> </a><div class="pCellBodyR">
2,522
</div>
</td>
<td><a name="wp1077779"> </a><div class="pCellBodyR">
64,584
</div>
</td>
<td><a name="wp1077781"> </a><div class="pCellBodyR">
685,420
</div>
</td>
<td><a name="wp1077783"> </a><div class="pCellBodyR">
10.41
</div>
</td>
<td> </td>
<td><a name="wp1077787"> </a><div class="pCellBodyR">
78
</div>
</td>
<td><a name="wp1077789"> </a><div class="pCellBodyR">
0.02754
</div>
</td>
<td><a name="wp1077791"> </a><div class="pCellBodyR">
74,697
</div>
</td>
<td><a name="wp1077793"> </a><div class="pCellBodyR">
2,057
</div>
</td>
<td><a name="wp1077795"> </a><div class="pCellBodyR">
73,669
</div>
</td>
<td><a name="wp1077797"> </a><div class="pCellBodyR">
910,023
</div>
</td>
<td><a name="wp1077799"> </a><div class="pCellBodyR">
12.18
</div>
</td>
</tr>
<tr> <td><a name="wp1077741"> </a><div class="pCellBodyR">
79
</div>
</td>
<td><a name="wp1077743"> </a><div class="pCellBodyR">
0.04192
</div>
</td>
<td><a name="wp1077745"> </a><div class="pCellBodyR">
63,323
</div>
</td>
<td><a name="wp1077747"> </a><div class="pCellBodyR">
2,654
</div>
</td>
<td><a name="wp1077749"> </a><div class="pCellBodyR">
61,995
</div>
</td>
<td><a name="wp1077751"> </a><div class="pCellBodyR">
620,837
</div>
</td>
<td><a name="wp1077753"> </a><div class="pCellBodyR">
9.80
</div>
</td>
<td> </td>
<td><a name="wp1077757"> </a><div class="pCellBodyR">
79
</div>
</td>
<td><a name="wp1077759"> </a><div class="pCellBodyR">
0.03001
</div>
</td>
<td><a name="wp1077761"> </a><div class="pCellBodyR">
72,640
</div>
</td>
<td><a name="wp1077763"> </a><div class="pCellBodyR">
2,180
</div>
</td>
<td><a name="wp1077765"> </a><div class="pCellBodyR">
71,550
</div>
</td>
<td><a name="wp1077767"> </a><div class="pCellBodyR">
836,355
</div>
</td>
<td><a name="wp1077769"> </a><div class="pCellBodyR">
11.51
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1077681"> </a><div class="pCellBodyR">
80
</div>
</td>
<td><a name="wp1077683"> </a><div class="pCellBodyR">
0.04597
</div>
</td>
<td><a name="wp1077685"> </a><div class="pCellBodyR">
60,668
</div>
</td>
<td><a name="wp1077687"> </a><div class="pCellBodyR">
2,789
</div>
</td>
<td><a name="wp1077689"> </a><div class="pCellBodyR">
59,274
</div>
</td>
<td><a name="wp1077691"> </a><div class="pCellBodyR">
558,841
</div>
</td>
<td><a name="wp1077693"> </a><div class="pCellBodyR">
9.21
</div>
</td>
<td> </td>
<td><a name="wp1077697"> </a><div class="pCellBodyR">
80
</div>
</td>
<td><a name="wp1077699"> </a><div class="pCellBodyR">
0.03286
</div>
</td>
<td><a name="wp1077701"> </a><div class="pCellBodyR">
70,460
</div>
</td>
<td><a name="wp1077703"> </a><div class="pCellBodyR">
2,315
</div>
</td>
<td><a name="wp1077705"> </a><div class="pCellBodyR">
69,303
</div>
</td>
<td><a name="wp1077707"> </a><div class="pCellBodyR">
764,805
</div>
</td>
<td><a name="wp1077709"> </a><div class="pCellBodyR">
10.85
</div>
</td>
</tr>
<tr> <td><a name="wp1077651"> </a><div class="pCellBodyR">
81
</div>
</td>
<td><a name="wp1077653"> </a><div class="pCellBodyR">
0.05089
</div>
</td>
<td><a name="wp1077655"> </a><div class="pCellBodyR">
57,880
</div>
</td>
<td><a name="wp1077657"> </a><div class="pCellBodyR">
2,945
</div>
</td>
<td><a name="wp1077659"> </a><div class="pCellBodyR">
56,407
</div>
</td>
<td><a name="wp1077661"> </a><div class="pCellBodyR">
499,567
</div>
</td>
<td><a name="wp1077663"> </a><div class="pCellBodyR">
8.63
</div>
</td>
<td> </td>
<td><a name="wp1077667"> </a><div class="pCellBodyR">
81
</div>
</td>
<td><a name="wp1077669"> </a><div class="pCellBodyR">
0.03644
</div>
</td>
<td><a name="wp1077671"> </a><div class="pCellBodyR">
68,145
</div>
</td>
<td><a name="wp1077673"> </a><div class="pCellBodyR">
2,483
</div>
</td>
<td><a name="wp1077675"> </a><div class="pCellBodyR">
66,903
</div>
</td>
<td><a name="wp1077677"> </a><div class="pCellBodyR">
695,502
</div>
</td>
<td><a name="wp1077679"> </a><div class="pCellBodyR">
10.21
</div>
</td>
</tr>
<tr> <td><a name="wp1077621"> </a><div class="pCellBodyR">
82
</div>
</td>
<td><a name="wp1077623"> </a><div class="pCellBodyR">
0.05719
</div>
</td>
<td><a name="wp1077625"> </a><div class="pCellBodyR">
54,934
</div>
</td>
<td><a name="wp1077627"> </a><div class="pCellBodyR">
3,142
</div>
</td>
<td><a name="wp1077629"> </a><div class="pCellBodyR">
53,363
</div>
</td>
<td><a name="wp1077631"> </a><div class="pCellBodyR">
443,160
</div>
</td>
<td><a name="wp1077633"> </a><div class="pCellBodyR">
8.07
</div>
</td>
<td> </td>
<td><a name="wp1077637"> </a><div class="pCellBodyR">
82
</div>
</td>
<td><a name="wp1077639"> </a><div class="pCellBodyR">
0.04104
</div>
</td>
<td><a name="wp1077641"> </a><div class="pCellBodyR">
65,662
</div>
</td>
<td><a name="wp1077643"> </a><div class="pCellBodyR">
2,695
</div>
</td>
<td><a name="wp1077645"> </a><div class="pCellBodyR">
64,314
</div>
</td>
<td><a name="wp1077647"> </a><div class="pCellBodyR">
628,599
</div>
</td>
<td><a name="wp1077649"> </a><div class="pCellBodyR">
9.57
</div>
</td>
</tr>
<tr> <td><a name="wp1077591"> </a><div class="pCellBodyR">
83
</div>
</td>
<td><a name="wp1077593"> </a><div class="pCellBodyR">
0.06514
</div>
</td>
<td><a name="wp1077595"> </a><div class="pCellBodyR">
51,792
</div>
</td>
<td><a name="wp1077597"> </a><div class="pCellBodyR">
3,374
</div>
</td>
<td><a name="wp1077599"> </a><div class="pCellBodyR">
50,106
</div>
</td>
<td><a name="wp1077601"> </a><div class="pCellBodyR">
389,797
</div>
</td>
<td><a name="wp1077603"> </a><div class="pCellBodyR">
7.53
</div>
</td>
<td> </td>
<td><a name="wp1077607"> </a><div class="pCellBodyR">
83
</div>
</td>
<td><a name="wp1077609"> </a><div class="pCellBodyR">
0.04686
</div>
</td>
<td><a name="wp1077611"> </a><div class="pCellBodyR">
62,967
</div>
</td>
<td><a name="wp1077613"> </a><div class="pCellBodyR">
2,950
</div>
</td>
<td><a name="wp1077615"> </a><div class="pCellBodyR">
61,491
</div>
</td>
<td><a name="wp1077617"> </a><div class="pCellBodyR">
564,285
</div>
</td>
<td><a name="wp1077619"> </a><div class="pCellBodyR">
8.96
</div>
</td>
</tr>
<tr> <td><a name="wp1077561"> </a><div class="pCellBodyR">
84
</div>
</td>
<td><a name="wp1077563"> </a><div class="pCellBodyR">
0.07449
</div>
</td>
<td><a name="wp1077565"> </a><div class="pCellBodyR">
48,419
</div>
</td>
<td><a name="wp1077567"> </a><div class="pCellBodyR">
3,607
</div>
</td>
<td><a name="wp1077569"> </a><div class="pCellBodyR">
46,615
</div>
</td>
<td><a name="wp1077571"> </a><div class="pCellBodyR">
339,692
</div>
</td>
<td><a name="wp1077573"> </a><div class="pCellBodyR">
7.02
</div>
</td>
<td> </td>
<td><a name="wp1077577"> </a><div class="pCellBodyR">
84
</div>
</td>
<td><a name="wp1077579"> </a><div class="pCellBodyR">
0.05378
</div>
</td>
<td><a name="wp1077581"> </a><div class="pCellBodyR">
60,016
</div>
</td>
<td><a name="wp1077583"> </a><div class="pCellBodyR">
3,227
</div>
</td>
<td><a name="wp1077585"> </a><div class="pCellBodyR">
58,402
</div>
</td>
<td><a name="wp1077587"> </a><div class="pCellBodyR">
502,793
</div>
</td>
<td><a name="wp1077589"> </a><div class="pCellBodyR">
8.38
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1077501"> </a><div class="pCellBodyR">
85
</div>
</td>
<td><a name="wp1077503"> </a><div class="pCellBodyR">
0.08481
</div>
</td>
<td><a name="wp1077505"> </a><div class="pCellBodyR">
44,812
</div>
</td>
<td><a name="wp1077507"> </a><div class="pCellBodyR">
3,801
</div>
</td>
<td><a name="wp1077509"> </a><div class="pCellBodyR">
42,911
</div>
</td>
<td><a name="wp1077511"> </a><div class="pCellBodyR">
293,076
</div>
</td>
<td><a name="wp1077513"> </a><div class="pCellBodyR">
6.54
</div>
</td>
<td> </td>
<td><a name="wp1077517"> </a><div class="pCellBodyR">
85
</div>
</td>
<td><a name="wp1077519"> </a><div class="pCellBodyR">
0.06157
</div>
</td>
<td><a name="wp1077521"> </a><div class="pCellBodyR">
56,789
</div>
</td>
<td><a name="wp1077523"> </a><div class="pCellBodyR">
3,497
</div>
</td>
<td><a name="wp1077525"> </a><div class="pCellBodyR">
55,040
</div>
</td>
<td><a name="wp1077527"> </a><div class="pCellBodyR">
444,391
</div>
</td>
<td><a name="wp1077529"> </a><div class="pCellBodyR">
7.83
</div>
</td>
</tr>
<tr> <td><a name="wp1077471"> </a><div class="pCellBodyR">
86
</div>
</td>
<td><a name="wp1077473"> </a><div class="pCellBodyR">
0.09576
</div>
</td>
<td><a name="wp1077475"> </a><div class="pCellBodyR">
41,011
</div>
</td>
<td><a name="wp1077477"> </a><div class="pCellBodyR">
3,927
</div>
</td>
<td><a name="wp1077479"> </a><div class="pCellBodyR">
39,048
</div>
</td>
<td><a name="wp1077481"> </a><div class="pCellBodyR">
250,165
</div>
</td>
<td><a name="wp1077483"> </a><div class="pCellBodyR">
6.10
</div>
</td>
<td> </td>
<td><a name="wp1077487"> </a><div class="pCellBodyR">
86
</div>
</td>
<td><a name="wp1077489"> </a><div class="pCellBodyR">
0.07007
</div>
</td>
<td><a name="wp1077491"> </a><div class="pCellBodyR">
53,292
</div>
</td>
<td><a name="wp1077493"> </a><div class="pCellBodyR">
3,734
</div>
</td>
<td><a name="wp1077495"> </a><div class="pCellBodyR">
51,425
</div>
</td>
<td><a name="wp1077497"> </a><div class="pCellBodyR">
389,350
</div>
</td>
<td><a name="wp1077499"> </a><div class="pCellBodyR">
7.31
</div>
</td>
</tr>
<tr> <td><a name="wp1077441"> </a><div class="pCellBodyR">
87
</div>
</td>
<td><a name="wp1077443"> </a><div class="pCellBodyR">
0.10712
</div>
</td>
<td><a name="wp1077445"> </a><div class="pCellBodyR">
37,084
</div>
</td>
<td><a name="wp1077447"> </a><div class="pCellBodyR">
3,973
</div>
</td>
<td><a name="wp1077449"> </a><div class="pCellBodyR">
35,098
</div>
</td>
<td><a name="wp1077451"> </a><div class="pCellBodyR">
211,117
</div>
</td>
<td><a name="wp1077453"> </a><div class="pCellBodyR">
5.69
</div>
</td>
<td> </td>
<td><a name="wp1077457"> </a><div class="pCellBodyR">
87
</div>
</td>
<td><a name="wp1077459"> </a><div class="pCellBodyR">
0.07918
</div>
</td>
<td><a name="wp1077461"> </a><div class="pCellBodyR">
49,558
</div>
</td>
<td><a name="wp1077463"> </a><div class="pCellBodyR">
3,924
</div>
</td>
<td><a name="wp1077465"> </a><div class="pCellBodyR">
47,596
</div>
</td>
<td><a name="wp1077467"> </a><div class="pCellBodyR">
337,925
</div>
</td>
<td><a name="wp1077469"> </a><div class="pCellBodyR">
6.82
</div>
</td>
</tr>
<tr> <td><a name="wp1077411"> </a><div class="pCellBodyR">
88
</div>
</td>
<td><a name="wp1077413"> </a><div class="pCellBodyR">
0.11888
</div>
</td>
<td><a name="wp1077415"> </a><div class="pCellBodyR">
33,111
</div>
</td>
<td><a name="wp1077417"> </a><div class="pCellBodyR">
3,936
</div>
</td>
<td><a name="wp1077419"> </a><div class="pCellBodyR">
31,143
</div>
</td>
<td><a name="wp1077421"> </a><div class="pCellBodyR">
176,020
</div>
</td>
<td><a name="wp1077423"> </a><div class="pCellBodyR">
5.32
</div>
</td>
<td> </td>
<td><a name="wp1077427"> </a><div class="pCellBodyR">
88
</div>
</td>
<td><a name="wp1077429"> </a><div class="pCellBodyR">
0.08891
</div>
</td>
<td><a name="wp1077431"> </a><div class="pCellBodyR">
45,634
</div>
</td>
<td><a name="wp1077433"> </a><div class="pCellBodyR">
4,057
</div>
</td>
<td><a name="wp1077435"> </a><div class="pCellBodyR">
43,605
</div>
</td>
<td><a name="wp1077437"> </a><div class="pCellBodyR">
290,330
</div>
</td>
<td><a name="wp1077439"> </a><div class="pCellBodyR">
6.36
</div>
</td>
</tr>
<tr> <td><a name="wp1077381"> </a><div class="pCellBodyR">
89
</div>
</td>
<td><a name="wp1077383"> </a><div class="pCellBodyR">
0.13112
</div>
</td>
<td><a name="wp1077385"> </a><div class="pCellBodyR">
29,175
</div>
</td>
<td><a name="wp1077387"> </a><div class="pCellBodyR">
3,825
</div>
</td>
<td><a name="wp1077389"> </a><div class="pCellBodyR">
27,263
</div>
</td>
<td><a name="wp1077391"> </a><div class="pCellBodyR">
144,876
</div>
</td>
<td><a name="wp1077393"> </a><div class="pCellBodyR">
4.97
</div>
</td>
<td> </td>
<td><a name="wp1077397"> </a><div class="pCellBodyR">
89
</div>
</td>
<td><a name="wp1077399"> </a><div class="pCellBodyR">
0.09934
</div>
</td>
<td><a name="wp1077401"> </a><div class="pCellBodyR">
41,576
</div>
</td>
<td><a name="wp1077403"> </a><div class="pCellBodyR">
4,130
</div>
</td>
<td><a name="wp1077405"> </a><div class="pCellBodyR">
39,511
</div>
</td>
<td><a name="wp1077407"> </a><div class="pCellBodyR">
246,725
</div>
</td>
<td><a name="wp1077409"> </a><div class="pCellBodyR">
5.93
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1077321"> </a><div class="pCellBodyR">
90
</div>
</td>
<td><a name="wp1077323"> </a><div class="pCellBodyR">
0.14401
</div>
</td>
<td><a name="wp1077325"> </a><div class="pCellBodyR">
25,350
</div>
</td>
<td><a name="wp1077327"> </a><div class="pCellBodyR">
3,651
</div>
</td>
<td><a name="wp1077329"> </a><div class="pCellBodyR">
23,525
</div>
</td>
<td><a name="wp1077331"> </a><div class="pCellBodyR">
117,614
</div>
</td>
<td><a name="wp1077333"> </a><div class="pCellBodyR">
4.64
</div>
</td>
<td> </td>
<td><a name="wp1077337"> </a><div class="pCellBodyR">
90
</div>
</td>
<td><a name="wp1077339"> </a><div class="pCellBodyR">
0.11058
</div>
</td>
<td><a name="wp1077341"> </a><div class="pCellBodyR">
37,446
</div>
</td>
<td><a name="wp1077343"> </a><div class="pCellBodyR">
4,141
</div>
</td>
<td><a name="wp1077345"> </a><div class="pCellBodyR">
35,376
</div>
</td>
<td><a name="wp1077347"> </a><div class="pCellBodyR">
207,213
</div>
</td>
<td><a name="wp1077349"> </a><div class="pCellBodyR">
5.53
</div>
</td>
</tr>
<tr> <td><a name="wp1077291"> </a><div class="pCellBodyR">
91
</div>
</td>
<td><a name="wp1077293"> </a><div class="pCellBodyR">
0.15770
</div>
</td>
<td><a name="wp1077295"> </a><div class="pCellBodyR">
21,699
</div>
</td>
<td><a name="wp1077297"> </a><div class="pCellBodyR">
3,422
</div>
</td>
<td><a name="wp1077299"> </a><div class="pCellBodyR">
19,988
</div>
</td>
<td><a name="wp1077301"> </a><div class="pCellBodyR">
94,089
</div>
</td>
<td><a name="wp1077303"> </a><div class="pCellBodyR">
4.34
</div>
</td>
<td> </td>
<td><a name="wp1077307"> </a><div class="pCellBodyR">
91
</div>
</td>
<td><a name="wp1077309"> </a><div class="pCellBodyR">
0.12276
</div>
</td>
<td><a name="wp1077311"> </a><div class="pCellBodyR">
33,306
</div>
</td>
<td><a name="wp1077313"> </a><div class="pCellBodyR">
4,089
</div>
</td>
<td><a name="wp1077315"> </a><div class="pCellBodyR">
31,261
</div>
</td>
<td><a name="wp1077317"> </a><div class="pCellBodyR">
171,837
</div>
</td>
<td><a name="wp1077319"> </a><div class="pCellBodyR">
5.16
</div>
</td>
</tr>
<tr> <td><a name="wp1077261"> </a><div class="pCellBodyR">
92
</div>
</td>
<td><a name="wp1077263"> </a><div class="pCellBodyR">
0.17236
</div>
</td>
<td><a name="wp1077265"> </a><div class="pCellBodyR">
18,277
</div>
</td>
<td><a name="wp1077267"> </a><div class="pCellBodyR">
3,150
</div>
</td>
<td><a name="wp1077269"> </a><div class="pCellBodyR">
16,702
</div>
</td>
<td><a name="wp1077271"> </a><div class="pCellBodyR">
74,101
</div>
</td>
<td><a name="wp1077273"> </a><div class="pCellBodyR">
4.05
</div>
</td>
<td> </td>
<td><a name="wp1077277"> </a><div class="pCellBodyR">
92
</div>
</td>
<td><a name="wp1077279"> </a><div class="pCellBodyR">
0.13599
</div>
</td>
<td><a name="wp1077281"> </a><div class="pCellBodyR">
29,217
</div>
</td>
<td><a name="wp1077283"> </a><div class="pCellBodyR">
3,973
</div>
</td>
<td><a name="wp1077285"> </a><div class="pCellBodyR">
27,231
</div>
</td>
<td><a name="wp1077287"> </a><div class="pCellBodyR">
140,576
</div>
</td>
<td><a name="wp1077289"> </a><div class="pCellBodyR">
4.81
</div>
</td>
</tr>
<tr> <td><a name="wp1077231"> </a><div class="pCellBodyR">
93
</div>
</td>
<td><a name="wp1077233"> </a><div class="pCellBodyR">
0.18812
</div>
</td>
<td><a name="wp1077235"> </a><div class="pCellBodyR">
15,127
</div>
</td>
<td><a name="wp1077237"> </a><div class="pCellBodyR">
2,846
</div>
</td>
<td><a name="wp1077239"> </a><div class="pCellBodyR">
13,704
</div>
</td>
<td><a name="wp1077241"> </a><div class="pCellBodyR">
57,399
</div>
</td>
<td><a name="wp1077243"> </a><div class="pCellBodyR">
3.79
</div>
</td>
<td> </td>
<td><a name="wp1077247"> </a><div class="pCellBodyR">
93
</div>
</td>
<td><a name="wp1077249"> </a><div class="pCellBodyR">
0.15037
</div>
</td>
<td><a name="wp1077251"> </a><div class="pCellBodyR">
25,244
</div>
</td>
<td><a name="wp1077253"> </a><div class="pCellBodyR">
3,796
</div>
</td>
<td><a name="wp1077255"> </a><div class="pCellBodyR">
23,346
</div>
</td>
<td><a name="wp1077257"> </a><div class="pCellBodyR">
113,345
</div>
</td>
<td><a name="wp1077259"> </a><div class="pCellBodyR">
4.49
</div>
</td>
</tr>
<tr> <td><a name="wp1077201"> </a><div class="pCellBodyR">
94
</div>
</td>
<td><a name="wp1077203"> </a><div class="pCellBodyR">
0.20509
</div>
</td>
<td><a name="wp1077205"> </a><div class="pCellBodyR">
12,281
</div>
</td>
<td><a name="wp1077207"> </a><div class="pCellBodyR">
2,519
</div>
</td>
<td><a name="wp1077209"> </a><div class="pCellBodyR">
11,022
</div>
</td>
<td><a name="wp1077211"> </a><div class="pCellBodyR">
43,695
</div>
</td>
<td><a name="wp1077213"> </a><div class="pCellBodyR">
3.56
</div>
</td>
<td> </td>
<td><a name="wp1077217"> </a><div class="pCellBodyR">
94
</div>
</td>
<td><a name="wp1077219"> </a><div class="pCellBodyR">
0.16597
</div>
</td>
<td><a name="wp1077221"> </a><div class="pCellBodyR">
21,448
</div>
</td>
<td><a name="wp1077223"> </a><div class="pCellBodyR">
3,560
</div>
</td>
<td><a name="wp1077225"> </a><div class="pCellBodyR">
19,668
</div>
</td>
<td><a name="wp1077227"> </a><div class="pCellBodyR">
89,999
</div>
</td>
<td><a name="wp1077229"> </a><div class="pCellBodyR">
4.20
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1077141"> </a><div class="pCellBodyR">
95
</div>
</td>
<td><a name="wp1077143"> </a><div class="pCellBodyR">
0.22195
</div>
</td>
<td><a name="wp1077145"> </a><div class="pCellBodyR">
9,762
</div>
</td>
<td><a name="wp1077147"> </a><div class="pCellBodyR">
2,167
</div>
</td>
<td><a name="wp1077149"> </a><div class="pCellBodyR">
8,679
</div>
</td>
<td><a name="wp1077151"> </a><div class="pCellBodyR">
32,673
</div>
</td>
<td><a name="wp1077153"> </a><div class="pCellBodyR">
3.35
</div>
</td>
<td> </td>
<td><a name="wp1077157"> </a><div class="pCellBodyR">
95
</div>
</td>
<td><a name="wp1077159"> </a><div class="pCellBodyR">
0.18175
</div>
</td>
<td><a name="wp1077161"> </a><div class="pCellBodyR">
17,888
</div>
</td>
<td><a name="wp1077163"> </a><div class="pCellBodyR">
3,251
</div>
</td>
<td><a name="wp1077165"> </a><div class="pCellBodyR">
16,263
</div>
</td>
<td><a name="wp1077167"> </a><div class="pCellBodyR">
70,331
</div>
</td>
<td><a name="wp1077169"> </a><div class="pCellBodyR">
3.93
</div>
</td>
</tr>
<tr> <td><a name="wp1077111"> </a><div class="pCellBodyR">
96
</div>
</td>
<td><a name="wp1077113"> </a><div class="pCellBodyR">
0.23840
</div>
</td>
<td><a name="wp1077115"> </a><div class="pCellBodyR">
7,596
</div>
</td>
<td><a name="wp1077117"> </a><div class="pCellBodyR">
1,811
</div>
</td>
<td><a name="wp1077119"> </a><div class="pCellBodyR">
6,690
</div>
</td>
<td><a name="wp1077121"> </a><div class="pCellBodyR">
23,994
</div>
</td>
<td><a name="wp1077123"> </a><div class="pCellBodyR">
3.16
</div>
</td>
<td> </td>
<td><a name="wp1077127"> </a><div class="pCellBodyR">
96
</div>
</td>
<td><a name="wp1077129"> </a><div class="pCellBodyR">
0.19743
</div>
</td>
<td><a name="wp1077131"> </a><div class="pCellBodyR">
14,637
</div>
</td>
<td><a name="wp1077133"> </a><div class="pCellBodyR">
2,890
</div>
</td>
<td><a name="wp1077135"> </a><div class="pCellBodyR">
13,192
</div>
</td>
<td><a name="wp1077137"> </a><div class="pCellBodyR">
54,068
</div>
</td>
<td><a name="wp1077139"> </a><div class="pCellBodyR">
3.69
</div>
</td>
</tr>
<tr> <td><a name="wp1077081"> </a><div class="pCellBodyR">
97
</div>
</td>
<td><a name="wp1077083"> </a><div class="pCellBodyR">
0.25415
</div>
</td>
<td><a name="wp1077085"> </a><div class="pCellBodyR">
5,785
</div>
</td>
<td><a name="wp1077087"> </a><div class="pCellBodyR">
1,470
</div>
</td>
<td><a name="wp1077089"> </a><div class="pCellBodyR">
5,050
</div>
</td>
<td><a name="wp1077091"> </a><div class="pCellBodyR">
17,304
</div>
</td>
<td><a name="wp1077093"> </a><div class="pCellBodyR">
2.99
</div>
</td>
<td> </td>
<td><a name="wp1077097"> </a><div class="pCellBodyR">
97
</div>
</td>
<td><a name="wp1077099"> </a><div class="pCellBodyR">
0.21273
</div>
</td>
<td><a name="wp1077101"> </a><div class="pCellBodyR">
11,747
</div>
</td>
<td><a name="wp1077103"> </a><div class="pCellBodyR">
2,499
</div>
</td>
<td><a name="wp1077105"> </a><div class="pCellBodyR">
10,498
</div>
</td>
<td><a name="wp1077107"> </a><div class="pCellBodyR">
40,876
</div>
</td>
<td><a name="wp1077109"> </a><div class="pCellBodyR">
3.48
</div>
</td>
</tr>
<tr> <td><a name="wp1077051"> </a><div class="pCellBodyR">
98
</div>
</td>
<td><a name="wp1077053"> </a><div class="pCellBodyR">
0.26890
</div>
</td>
<td><a name="wp1077055"> </a><div class="pCellBodyR">
4,315
</div>
</td>
<td><a name="wp1077057"> </a><div class="pCellBodyR">
1,160
</div>
</td>
<td><a name="wp1077059"> </a><div class="pCellBodyR">
3,735
</div>
</td>
<td><a name="wp1077061"> </a><div class="pCellBodyR">
12,254
</div>
</td>
<td><a name="wp1077063"> </a><div class="pCellBodyR">
2.84
</div>
</td>
<td> </td>
<td><a name="wp1077067"> </a><div class="pCellBodyR">
98
</div>
</td>
<td><a name="wp1077069"> </a><div class="pCellBodyR">
0.22735
</div>
</td>
<td><a name="wp1077071"> </a><div class="pCellBodyR">
9,248
</div>
</td>
<td><a name="wp1077073"> </a><div class="pCellBodyR">
2,103
</div>
</td>
<td><a name="wp1077075"> </a><div class="pCellBodyR">
8,197
</div>
</td>
<td><a name="wp1077077"> </a><div class="pCellBodyR">
30,378
</div>
</td>
<td><a name="wp1077079"> </a><div class="pCellBodyR">
3.28
</div>
</td>
</tr>
<tr> <td><a name="wp1077021"> </a><div class="pCellBodyR">
99
</div>
</td>
<td><a name="wp1077023"> </a><div class="pCellBodyR">
0.28235
</div>
</td>
<td><a name="wp1077025"> </a><div class="pCellBodyR">
3,154
</div>
</td>
<td><a name="wp1077027"> </a><div class="pCellBodyR">
891
</div>
</td>
<td><a name="wp1077029"> </a><div class="pCellBodyR">
2,709
</div>
</td>
<td><a name="wp1077031"> </a><div class="pCellBodyR">
8,519
</div>
</td>
<td><a name="wp1077033"> </a><div class="pCellBodyR">
2.70
</div>
</td>
<td> </td>
<td><a name="wp1077037"> </a><div class="pCellBodyR">
99
</div>
</td>
<td><a name="wp1077039"> </a><div class="pCellBodyR">
0.24100
</div>
</td>
<td><a name="wp1077041"> </a><div class="pCellBodyR">
7,146
</div>
</td>
<td><a name="wp1077043"> </a><div class="pCellBodyR">
1,722
</div>
</td>
<td><a name="wp1077045"> </a><div class="pCellBodyR">
6,285
</div>
</td>
<td><a name="wp1077047"> </a><div class="pCellBodyR">
22,181
</div>
</td>
<td><a name="wp1077049"> </a><div class="pCellBodyR">
3.10
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1076961"> </a><div class="pCellBodyR">
100
</div>
</td>
<td><a name="wp1076963"> </a><div class="pCellBodyR">
0.29647
</div>
</td>
<td><a name="wp1076965"> </a><div class="pCellBodyR">
2,264
</div>
</td>
<td><a name="wp1076967"> </a><div class="pCellBodyR">
671
</div>
</td>
<td><a name="wp1076969"> </a><div class="pCellBodyR">
1,928
</div>
</td>
<td><a name="wp1076971"> </a><div class="pCellBodyR">
5,810
</div>
</td>
<td><a name="wp1076973"> </a><div class="pCellBodyR">
2.57
</div>
</td>
<td> </td>
<td><a name="wp1076977"> </a><div class="pCellBodyR">
100
</div>
</td>
<td><a name="wp1076979"> </a><div class="pCellBodyR">
0.25546
</div>
</td>
<td><a name="wp1076981"> </a><div class="pCellBodyR">
5,424
</div>
</td>
<td><a name="wp1076983"> </a><div class="pCellBodyR">
1,385
</div>
</td>
<td><a name="wp1076985"> </a><div class="pCellBodyR">
4,731
</div>
</td>
<td><a name="wp1076987"> </a><div class="pCellBodyR">
15,896
</div>
</td>
<td><a name="wp1076989"> </a><div class="pCellBodyR">
2.93
</div>
</td>
</tr>
<tr> <td><a name="wp1076931"> </a><div class="pCellBodyR">
101
</div>
</td>
<td><a name="wp1076933"> </a><div class="pCellBodyR">
0.31129
</div>
</td>
<td><a name="wp1076935"> </a><div class="pCellBodyR">
1,593
</div>
</td>
<td><a name="wp1076937"> </a><div class="pCellBodyR">
496
</div>
</td>
<td><a name="wp1076939"> </a><div class="pCellBodyR">
1,345
</div>
</td>
<td><a name="wp1076941"> </a><div class="pCellBodyR">
3,882
</div>
</td>
<td><a name="wp1076943"> </a><div class="pCellBodyR">
2.44
</div>
</td>
<td> </td>
<td><a name="wp1076947"> </a><div class="pCellBodyR">
101
</div>
</td>
<td><a name="wp1076949"> </a><div class="pCellBodyR">
0.27078
</div>
</td>
<td><a name="wp1076951"> </a><div class="pCellBodyR">
4,038
</div>
</td>
<td><a name="wp1076953"> </a><div class="pCellBodyR">
1,093
</div>
</td>
<td><a name="wp1076955"> </a><div class="pCellBodyR">
3,491
</div>
</td>
<td><a name="wp1076957"> </a><div class="pCellBodyR">
11,165
</div>
</td>
<td><a name="wp1076959"> </a><div class="pCellBodyR">
2.76
</div>
</td>
</tr>
<tr> <td><a name="wp1076901"> </a><div class="pCellBodyR">
102
</div>
</td>
<td><a name="wp1076903"> </a><div class="pCellBodyR">
0.32685
</div>
</td>
<td><a name="wp1076905"> </a><div class="pCellBodyR">
1,097
</div>
</td>
<td><a name="wp1076907"> </a><div class="pCellBodyR">
359
</div>
</td>
<td><a name="wp1076909"> </a><div class="pCellBodyR">
918
</div>
</td>
<td><a name="wp1076911"> </a><div class="pCellBodyR">
2,537
</div>
</td>
<td><a name="wp1076913"> </a><div class="pCellBodyR">
2.31
</div>
</td>
<td> </td>
<td><a name="wp1076917"> </a><div class="pCellBodyR">
102
</div>
</td>
<td><a name="wp1076919"> </a><div class="pCellBodyR">
0.28703
</div>
</td>
<td><a name="wp1076921"> </a><div class="pCellBodyR">
2,945
</div>
</td>
<td><a name="wp1076923"> </a><div class="pCellBodyR">
845
</div>
</td>
<td><a name="wp1076925"> </a><div class="pCellBodyR">
2,522
</div>
</td>
<td><a name="wp1076927"> </a><div class="pCellBodyR">
7,674
</div>
</td>
<td><a name="wp1076929"> </a><div class="pCellBodyR">
2.61
</div>
</td>
</tr>
<tr> <td><a name="wp1076871"> </a><div class="pCellBodyR">
103
</div>
</td>
<td><a name="wp1076873"> </a><div class="pCellBodyR">
0.34320
</div>
</td>
<td><a name="wp1076875"> </a><div class="pCellBodyR">
738
</div>
</td>
<td><a name="wp1076877"> </a><div class="pCellBodyR">
253
</div>
</td>
<td><a name="wp1076879"> </a><div class="pCellBodyR">
612
</div>
</td>
<td><a name="wp1076881"> </a><div class="pCellBodyR">
1,620
</div>
</td>
<td><a name="wp1076883"> </a><div class="pCellBodyR">
2.19
</div>
</td>
<td> </td>
<td><a name="wp1076887"> </a><div class="pCellBodyR">
103
</div>
</td>
<td><a name="wp1076889"> </a><div class="pCellBodyR">
0.30425
</div>
</td>
<td><a name="wp1076891"> </a><div class="pCellBodyR">
2,099
</div>
</td>
<td><a name="wp1076893"> </a><div class="pCellBodyR">
639
</div>
</td>
<td><a name="wp1076895"> </a><div class="pCellBodyR">
1,780
</div>
</td>
<td><a name="wp1076897"> </a><div class="pCellBodyR">
5,152
</div>
</td>
<td><a name="wp1076899"> </a><div class="pCellBodyR">
2.45
</div>
</td>
</tr>
<tr> <td><a name="wp1076841"> </a><div class="pCellBodyR">
104
</div>
</td>
<td><a name="wp1076843"> </a><div class="pCellBodyR">
0.36036
</div>
</td>
<td><a name="wp1076845"> </a><div class="pCellBodyR">
485
</div>
</td>
<td><a name="wp1076847"> </a><div class="pCellBodyR">
175
</div>
</td>
<td><a name="wp1076849"> </a><div class="pCellBodyR">
398
</div>
</td>
<td><a name="wp1076851"> </a><div class="pCellBodyR">
1,008
</div>
</td>
<td><a name="wp1076853"> </a><div class="pCellBodyR">
2.08
</div>
</td>
<td> </td>
<td><a name="wp1076857"> </a><div class="pCellBodyR">
104
</div>
</td>
<td><a name="wp1076859"> </a><div class="pCellBodyR">
0.32251
</div>
</td>
<td><a name="wp1076861"> </a><div class="pCellBodyR">
1,461
</div>
</td>
<td><a name="wp1076863"> </a><div class="pCellBodyR">
471
</div>
</td>
<td><a name="wp1076865"> </a><div class="pCellBodyR">
1,225
</div>
</td>
<td><a name="wp1076867"> </a><div class="pCellBodyR">
3,372
</div>
</td>
<td><a name="wp1076869"> </a><div class="pCellBodyR">
2.31
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1076781"> </a><div class="pCellBodyR">
105
</div>
</td>
<td><a name="wp1076783"> </a><div class="pCellBodyR">
0.37838
</div>
</td>
<td><a name="wp1076785"> </a><div class="pCellBodyR">
310
</div>
</td>
<td><a name="wp1076787"> </a><div class="pCellBodyR">
117
</div>
</td>
<td><a name="wp1076789"> </a><div class="pCellBodyR">
252
</div>
</td>
<td><a name="wp1076791"> </a><div class="pCellBodyR">
611
</div>
</td>
<td><a name="wp1076793"> </a><div class="pCellBodyR">
1.97
</div>
</td>
<td> </td>
<td><a name="wp1076797"> </a><div class="pCellBodyR">
105
</div>
</td>
<td><a name="wp1076799"> </a><div class="pCellBodyR">
0.34186
</div>
</td>
<td><a name="wp1076801"> </a><div class="pCellBodyR">
990
</div>
</td>
<td><a name="wp1076803"> </a><div class="pCellBodyR">
338
</div>
</td>
<td><a name="wp1076805"> </a><div class="pCellBodyR">
820
</div>
</td>
<td><a name="wp1076807"> </a><div class="pCellBodyR">
2,146
</div>
</td>
<td><a name="wp1076809"> </a><div class="pCellBodyR">
2.17
</div>
</td>
</tr>
<tr> <td><a name="wp1076751"> </a><div class="pCellBodyR">
106
</div>
</td>
<td><a name="wp1076753"> </a><div class="pCellBodyR">
0.39729
</div>
</td>
<td><a name="wp1076755"> </a><div class="pCellBodyR">
193
</div>
</td>
<td><a name="wp1076757"> </a><div class="pCellBodyR">
77
</div>
</td>
<td><a name="wp1076759"> </a><div class="pCellBodyR">
155
</div>
</td>
<td><a name="wp1076761"> </a><div class="pCellBodyR">
359
</div>
</td>
<td><a name="wp1076763"> </a><div class="pCellBodyR">
1.86
</div>
</td>
<td> </td>
<td><a name="wp1076767"> </a><div class="pCellBodyR">
106
</div>
</td>
<td><a name="wp1076769"> </a><div class="pCellBodyR">
0.36237
</div>
</td>
<td><a name="wp1076771"> </a><div class="pCellBodyR">
651
</div>
</td>
<td><a name="wp1076773"> </a><div class="pCellBodyR">
236
</div>
</td>
<td><a name="wp1076775"> </a><div class="pCellBodyR">
533
</div>
</td>
<td><a name="wp1076777"> </a><div class="pCellBodyR">
1,326
</div>
</td>
<td><a name="wp1076779"> </a><div class="pCellBodyR">
2.04
</div>
</td>
</tr>
<tr> <td><a name="wp1076721"> </a><div class="pCellBodyR">
107
</div>
</td>
<td><a name="wp1076723"> </a><div class="pCellBodyR">
0.41716
</div>
</td>
<td><a name="wp1076725"> </a><div class="pCellBodyR">
116
</div>
</td>
<td><a name="wp1076727"> </a><div class="pCellBodyR">
48
</div>
</td>
<td><a name="wp1076729"> </a><div class="pCellBodyR">
92
</div>
</td>
<td><a name="wp1076731"> </a><div class="pCellBodyR">
205
</div>
</td>
<td><a name="wp1076733"> </a><div class="pCellBodyR">
1.76
</div>
</td>
<td> </td>
<td><a name="wp1076737"> </a><div class="pCellBodyR">
107
</div>
</td>
<td><a name="wp1076739"> </a><div class="pCellBodyR">
0.38411
</div>
</td>
<td><a name="wp1076741"> </a><div class="pCellBodyR">
415
</div>
</td>
<td><a name="wp1076743"> </a><div class="pCellBodyR">
160
</div>
</td>
<td><a name="wp1076745"> </a><div class="pCellBodyR">
336
</div>
</td>
<td><a name="wp1076747"> </a><div class="pCellBodyR">
793
</div>
</td>
<td><a name="wp1076749"> </a><div class="pCellBodyR">
1.91
</div>
</td>
</tr>
<tr> <td><a name="wp1076691"> </a><div class="pCellBodyR">
108
</div>
</td>
<td><a name="wp1076693"> </a><div class="pCellBodyR">
0.43802
</div>
</td>
<td><a name="wp1076695"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1076697"> </a><div class="pCellBodyR">
30
</div>
</td>
<td><a name="wp1076699"> </a><div class="pCellBodyR">
53
</div>
</td>
<td><a name="wp1076701"> </a><div class="pCellBodyR">
113
</div>
</td>
<td><a name="wp1076703"> </a><div class="pCellBodyR">
1.66
</div>
</td>
<td> </td>
<td><a name="wp1076707"> </a><div class="pCellBodyR">
108
</div>
</td>
<td><a name="wp1076709"> </a><div class="pCellBodyR">
0.40716
</div>
</td>
<td><a name="wp1076711"> </a><div class="pCellBodyR">
256
</div>
</td>
<td><a name="wp1076713"> </a><div class="pCellBodyR">
104
</div>
</td>
<td><a name="wp1076715"> </a><div class="pCellBodyR">
204
</div>
</td>
<td><a name="wp1076717"> </a><div class="pCellBodyR">
457
</div>
</td>
<td><a name="wp1076719"> </a><div class="pCellBodyR">
1.79
</div>
</td>
</tr>
<tr> <td><a name="wp1076661"> </a><div class="pCellBodyR">
109
</div>
</td>
<td><a name="wp1076663"> </a><div class="pCellBodyR">
0.45992
</div>
</td>
<td><a name="wp1076665"> </a><div class="pCellBodyR">
38
</div>
</td>
<td><a name="wp1076667"> </a><div class="pCellBodyR">
18
</div>
</td>
<td><a name="wp1076669"> </a><div class="pCellBodyR">
29
</div>
</td>
<td><a name="wp1076671"> </a><div class="pCellBodyR">
60
</div>
</td>
<td><a name="wp1076673"> </a><div class="pCellBodyR">
1.57
</div>
</td>
<td> </td>
<td><a name="wp1076677"> </a><div class="pCellBodyR">
109
</div>
</td>
<td><a name="wp1076679"> </a><div class="pCellBodyR">
0.43159
</div>
</td>
<td><a name="wp1076681"> </a><div class="pCellBodyR">
152
</div>
</td>
<td><a name="wp1076683"> </a><div class="pCellBodyR">
65
</div>
</td>
<td><a name="wp1076685"> </a><div class="pCellBodyR">
119
</div>
</td>
<td><a name="wp1076687"> </a><div class="pCellBodyR">
253
</div>
</td>
<td><a name="wp1076689"> </a><div class="pCellBodyR">
1.67
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1076601"> </a><div class="pCellBodyR">
110
</div>
</td>
<td><a name="wp1076603"> </a><div class="pCellBodyR">
0.48291
</div>
</td>
<td><a name="wp1076605"> </a><div class="pCellBodyR">
21
</div>
</td>
<td><a name="wp1076607"> </a><div class="pCellBodyR">
10
</div>
</td>
<td><a name="wp1076609"> </a><div class="pCellBodyR">
16
</div>
</td>
<td><a name="wp1076611"> </a><div class="pCellBodyR">
30
</div>
</td>
<td><a name="wp1076613"> </a><div class="pCellBodyR">
1.48
</div>
</td>
<td> </td>
<td><a name="wp1076617"> </a><div class="pCellBodyR">
110
</div>
</td>
<td><a name="wp1076619"> </a><div class="pCellBodyR">
0.45748
</div>
</td>
<td><a name="wp1076621"> </a><div class="pCellBodyR">
86
</div>
</td>
<td><a name="wp1076623"> </a><div class="pCellBodyR">
39
</div>
</td>
<td><a name="wp1076625"> </a><div class="pCellBodyR">
66
</div>
</td>
<td><a name="wp1076627"> </a><div class="pCellBodyR">
135
</div>
</td>
<td><a name="wp1076629"> </a><div class="pCellBodyR">
1.56
</div>
</td>
</tr>
<tr> <td><a name="wp1076571"> </a><div class="pCellBodyR">
111
</div>
</td>
<td><a name="wp1076573"> </a><div class="pCellBodyR">
0.50706
</div>
</td>
<td><a name="wp1076575"> </a><div class="pCellBodyR">
11
</div>
</td>
<td><a name="wp1076577"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1076579"> </a><div class="pCellBodyR">
8
</div>
</td>
<td><a name="wp1076581"> </a><div class="pCellBodyR">
15
</div>
</td>
<td><a name="wp1076583"> </a><div class="pCellBodyR">
1.39
</div>
</td>
<td> </td>
<td><a name="wp1076587"> </a><div class="pCellBodyR">
111
</div>
</td>
<td><a name="wp1076589"> </a><div class="pCellBodyR">
0.48493
</div>
</td>
<td><a name="wp1076591"> </a><div class="pCellBodyR">
47
</div>
</td>
<td><a name="wp1076593"> </a><div class="pCellBodyR">
23
</div>
</td>
<td><a name="wp1076595"> </a><div class="pCellBodyR">
35
</div>
</td>
<td><a name="wp1076597"> </a><div class="pCellBodyR">
68
</div>
</td>
<td><a name="wp1076599"> </a><div class="pCellBodyR">
1.46
</div>
</td>
</tr>
<tr> <td><a name="wp1076541"> </a><div class="pCellBodyR">
112
</div>
</td>
<td><a name="wp1076543"> </a><div class="pCellBodyR">
0.53241
</div>
</td>
<td><a name="wp1076545"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1076547"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1076549"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1076551"> </a><div class="pCellBodyR">
7
</div>
</td>
<td><a name="wp1076553"> </a><div class="pCellBodyR">
1.31
</div>
</td>
<td> </td>
<td><a name="wp1076557"> </a><div class="pCellBodyR">
112
</div>
</td>
<td><a name="wp1076559"> </a><div class="pCellBodyR">
0.51403
</div>
</td>
<td><a name="wp1076561"> </a><div class="pCellBodyR">
24
</div>
</td>
<td><a name="wp1076563"> </a><div class="pCellBodyR">
12
</div>
</td>
<td><a name="wp1076565"> </a><div class="pCellBodyR">
18
</div>
</td>
<td><a name="wp1076567"> </a><div class="pCellBodyR">
33
</div>
</td>
<td><a name="wp1076569"> </a><div class="pCellBodyR">
1.36
</div>
</td>
</tr>
<tr> <td><a name="wp1076511"> </a><div class="pCellBodyR">
113
</div>
</td>
<td><a name="wp1076513"> </a><div class="pCellBodyR">
0.55903
</div>
</td>
<td><a name="wp1076515"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1076517"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076519"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1076521"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1076523"> </a><div class="pCellBodyR">
1.23
</div>
</td>
<td> </td>
<td><a name="wp1076527"> </a><div class="pCellBodyR">
113
</div>
</td>
<td><a name="wp1076529"> </a><div class="pCellBodyR">
0.54487
</div>
</td>
<td><a name="wp1076531"> </a><div class="pCellBodyR">
12
</div>
</td>
<td><a name="wp1076533"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1076535"> </a><div class="pCellBodyR">
9
</div>
</td>
<td><a name="wp1076537"> </a><div class="pCellBodyR">
15
</div>
</td>
<td><a name="wp1076539"> </a><div class="pCellBodyR">
1.26
</div>
</td>
</tr>
<tr> <td><a name="wp1076481"> </a><div class="pCellBodyR">
114
</div>
</td>
<td><a name="wp1076483"> </a><div class="pCellBodyR">
0.58698
</div>
</td>
<td><a name="wp1076485"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076487"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076489"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076491"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076493"> </a><div class="pCellBodyR">
1.15
</div>
</td>
<td> </td>
<td><a name="wp1076497"> </a><div class="pCellBodyR">
114
</div>
</td>
<td><a name="wp1076499"> </a><div class="pCellBodyR">
0.57756
</div>
</td>
<td><a name="wp1076501"> </a><div class="pCellBodyR">
5
</div>
</td>
<td><a name="wp1076503"> </a><div class="pCellBodyR">
3
</div>
</td>
<td><a name="wp1076505"> </a><div class="pCellBodyR">
4
</div>
</td>
<td><a name="wp1076507"> </a><div class="pCellBodyR">
6
</div>
</td>
<td><a name="wp1076509"> </a><div class="pCellBodyR">
1.17
</div>
</td>
</tr>
<tr> <td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
<td> </td>
</tr>
<tr> <td><a name="wp1076421"> </a><div class="pCellBodyR">
115
</div>
</td>
<td><a name="wp1076423"> </a><div class="pCellBodyR">
0.61633
</div>
</td>
<td><a name="wp1076425"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076427"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076429"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076431"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076433"> </a><div class="pCellBodyR">
1.08
</div>
</td>
<td> </td>
<td><a name="wp1076437"> </a><div class="pCellBodyR">
115
</div>
</td>
<td><a name="wp1076439"> </a><div class="pCellBodyR">
0.61221
</div>
</td>
<td><a name="wp1076441"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1076443"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076445"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1076447"> </a><div class="pCellBodyR">
2
</div>
</td>
<td><a name="wp1076449"> </a><div class="pCellBodyR">
1.08
</div>
</td>
</tr>
<tr> <td><a name="wp1076391"> </a><div class="pCellBodyR">
116
</div>
</td>
<td><a name="wp1076393"> </a><div class="pCellBodyR">
0.64715
</div>
</td>
<td><a name="wp1076395"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076397"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076399"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076401"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076403"> </a><div class="pCellBodyR">
1.01
</div>
</td>
<td> </td>
<td><a name="wp1076407"> </a><div class="pCellBodyR">
116
</div>
</td>
<td><a name="wp1076409"> </a><div class="pCellBodyR">
0.64715
</div>
</td>
<td><a name="wp1076411"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076413"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076415"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076417"> </a><div class="pCellBodyR">
1
</div>
</td>
<td><a name="wp1076419"> </a><div class="pCellBodyR">
1.01
</div>
</td>
</tr>
<tr> <td><a name="wp1076361"> </a><div class="pCellBodyR">
117
</div>
</td>
<td><a name="wp1076363"> </a><div class="pCellBodyR">
0.67951
</div>
</td>
<td><a name="wp1076365"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076367"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076369"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076371"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076373"> </a><div class="pCellBodyR">
0.94
</div>
</td>
<td> </td>
<td><a name="wp1076377"> </a><div class="pCellBodyR">
117
</div>
</td>
<td><a name="wp1076379"> </a><div class="pCellBodyR">
0.67951
</div>
</td>
<td><a name="wp1076381"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076383"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076385"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076387"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076389"> </a><div class="pCellBodyR">
0.94
</div>
</td>
</tr>
<tr> <td><a name="wp1076331"> </a><div class="pCellBodyR">
118
</div>
</td>
<td><a name="wp1076333"> </a><div class="pCellBodyR">
0.71348
</div>
</td>
<td><a name="wp1076335"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076337"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076339"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076341"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076343"> </a><div class="pCellBodyR">
0.88
</div>
</td>
<td> </td>
<td><a name="wp1076347"> </a><div class="pCellBodyR">
118
</div>
</td>
<td><a name="wp1076349"> </a><div class="pCellBodyR">
0.71348
</div>
</td>
<td><a name="wp1076351"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076353"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076355"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076357"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076359"> </a><div class="pCellBodyR">
0.88
</div>
</td>
</tr>
<tr> <td><a name="wp1076301"> </a><div class="pCellBodyR">
119
</div>
</td>
<td><a name="wp1076303"> </a><div class="pCellBodyR">
0.74916
</div>
</td>
<td><a name="wp1076305"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076307"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076309"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076311"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076313"> </a><div class="pCellBodyR">
0.82
</div>
</td>
<td> </td>
<td><a name="wp1076317"> </a><div class="pCellBodyR">
119
</div>
</td>
<td><a name="wp1076319"> </a><div class="pCellBodyR">
0.74916
</div>
</td>
<td><a name="wp1076321"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076323"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076325"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076327"> </a><div class="pCellBodyR">
0
</div>
</td>
<td><a name="wp1076329"> </a><div class="pCellBodyR">
0.82
</div>
</td>
</tr>
</table>
</div>
<p class="pBody">
</p>
<hr />
<div>
<table summary="Document navigation links" border="0" cellpadding="0" cellspacing="0">
<tr>
<td><a href="TOC.html"><img id="LongDescNotReq7" src="images/toc.gif" border="0" alt="Contents" /></a></td>
<td><a href="LifeTables_Tbl_6_2050.html"><img id="LongDescNotReq8" src="images/prev.gif" border="0" alt="Previous" /></a></td>
<td><a href="LifeTables_Tbl_6_2070.html"><img id="LongDescNotReq9" src="images/next.gif" border="0" alt="Next" /></a></td>
<td><a href="LOT.html"><img id="LongDescNotReq10" src="images/tables.gif" border="0" alt="List of Tables" /></a></td>
<td><a href="LOF.html"><img id="LongDescNotReq11" src="images/figures.gif" border="0" alt="List of Figures" /></a></td>
<td width="60%"> <div class="pCellBodyR"><a href="../studylist2000s.html">Return to list of Studies.</a></div></td>
</tr>
</table>
</div>
<br />
</body>
</html>
| Java |
/* Ship.js
KC3改 Ship Object
*/
(function(){
"use strict";
var deferList = {};
window.KC3Ship = function( data, toClone ){
// Default object properties included in stringifications
this.rosterId = 0;
this.masterId = 0;
this.level = 0;
this.exp = [0,0,0];
this.hp = [0,0];
this.afterHp = [0,0];
this.fp = [0,0];
this.tp = [0,0];
this.aa = [0,0];
this.ar = [0,0];
this.ev = [0,0];
this.as = [0,0];
this.ls = [0,0];
this.lk = [0,0];
this.range = 0;
// corresponds to "api_slot" in the API,
// but devs change it to length == 5 someday,
// and item of ex slot still not at 5th.
// extended to 5 slots on 2018-02-16 for Musashi Kai Ni.
this.items = [-1,-1,-1,-1,-1];
// corresponds to "api_slot_ex" in the API,
// which has special meanings on few values:
// 0: ex slot is not available
// -1: ex slot is available but nothing is equipped
this.ex_item = 0;
// "api_onslot" in API, also changed to length 5 now.
this.slots = [0,0,0,0,0];
this.slotnum = 0;
this.speed = 0;
// corresponds to "api_kyouka" in the API,
// represents [fp,tp,aa,ar,lk] in the past.
// expanded to 7-length array since 2017-09-29
// last new twos are [hp,as]
this.mod = [0,0,0,0,0,0,0];
this.fuel = 0;
this.ammo = 0;
this.repair = [0,0,0];
this.stars = 0;
this.morale = 0;
this.lock = 0;
this.sally = 0;
this.akashiMark = false;
this.preExpedCond = [
/* Data Example
["exped300",12,20, 0], // fully supplied
["exped301", 6,10, 0], // not fully supplied
NOTE: this will be used against comparison of pendingConsumption that hardly to detect expedition activities
*/
];
this.pendingConsumption = {
/* Data Example
typeName: type + W {WorldNum} + M {MapNum} + literal underscore + type_id
type_id can be described as sortie/pvp/expedition id
valueStructure: typeName int[3][3] of [fuel, ammo, bauxites] and [fuel, steel, buckets] and [steel]
"sortie3000":[[12,24, 0],[ 0, 0, 0]], // OREL (3 nodes)
"sortie3001":[[ 8,16, 0],[ 0, 0, 0]], // SPARKLE GO 1-1 (2 nodes)
"sortie3002":[[ 4,12, 0],[ 0, 0, 0]], // PVP (1 node+yasen)
"sortie3003":[[ 0, 0, 0],[ 0, 0, 0],[-88]], // 1 node with Jet battle of 36 slot
Practice and Expedition automatically ignore repair consumption.
For every action will be recorded before the sortie.
*/
};
this.lastSortie = ['sortie0'];
// Define properties not included in stringifications
Object.defineProperties(this,{
didFlee: {
value: false,
enumerable: false,
configurable: false,
writable: true
},
mvp: {
value: false,
enumerable: false,
configurable: false,
writable: true
},
// useful when making virtual ship objects.
// requirements:
// * "GearManager.get( itemId )" should get the intended equipment
// * "itemId" is taken from either "items" or "ex_item"
// * "shipId === -1 or 0" should always return a dummy gear
GearManager: {
value: null,
enumerable: false,
configurable: false,
writable: true
}
});
// If specified with data, fill this object
if(typeof data != "undefined"){
// Initialized with raw data
if(typeof data.api_id != "undefined"){
this.rosterId = data.api_id;
this.masterId = data.api_ship_id;
this.level = data.api_lv;
this.exp = data.api_exp;
this.hp = [data.api_nowhp, data.api_maxhp];
this.afterHp = [data.api_nowhp, data.api_maxhp];
this.fp = data.api_karyoku;
this.tp = data.api_raisou;
this.aa = data.api_taiku;
this.ar = data.api_soukou;
this.ev = data.api_kaihi;
this.as = data.api_taisen;
this.ls = data.api_sakuteki;
this.lk = data.api_lucky;
this.range = data.api_leng;
this.items = data.api_slot;
if(typeof data.api_slot_ex != "undefined"){
this.ex_item = data.api_slot_ex;
}
if(typeof data.api_sally_area != "undefined"){
this.sally = data.api_sally_area;
}
this.slotnum = data.api_slotnum;
this.slots = data.api_onslot;
this.speed = data.api_soku;
this.mod = data.api_kyouka;
this.fuel = data.api_fuel;
this.ammo = data.api_bull;
this.repair = [data.api_ndock_time].concat(data.api_ndock_item);
this.stars = data.api_srate;
this.morale = data.api_cond;
this.lock = data.api_locked;
// Initialized with formatted data, deep clone if demanded
} else {
if(!!toClone)
$.extend(true, this, data);
else
$.extend(this, data);
}
if(this.getDefer().length <= 0)
this.checkDefer();
}
};
// Define complex properties on prototype
Object.defineProperties(KC3Ship.prototype,{
bull: {
get: function(){return this.ammo;},
set: function(newAmmo){this.ammo = newAmmo;},
configurable:false,
enumerable :true
}
});
KC3Ship.prototype.getGearManager = function(){ return this.GearManager || KC3GearManager; };
KC3Ship.prototype.exists = function(){ return this.rosterId > 0 && this.masterId > 0; };
KC3Ship.prototype.isDummy = function(){ return ! this.exists(); };
KC3Ship.prototype.master = function(){ return KC3Master.ship( this.masterId ); };
KC3Ship.prototype.name = function(){ return KC3Meta.shipName( this.master().api_name ); };
KC3Ship.prototype.stype = function(){ return KC3Meta.stype( this.master().api_stype ); };
KC3Ship.prototype.equipment = function(slot){
switch(typeof slot) {
case 'number':
case 'string':
/* Number/String => converted as equipment slot key */
return this.getGearManager().get( slot < 0 || slot >= this.items.length ? this.ex_item : this.items[slot] );
case 'boolean':
/* Boolean => return all equipments with ex item if true */
return slot ? this.equipment().concat(this.exItem())
: this.equipment();
case 'undefined':
/* Undefined => returns whole equipment as equip object array */
return this.items
// cloned and fixed to max 4 slots if 5th or more slots not found
.slice(0, Math.max(this.slotnum, 4))
.map(Number.call, Number)
.map(i => this.equipment(i));
case 'function':
/* Function => iterates over given callback for every equipment */
var equipObjs = this.equipment();
equipObjs.forEach((item, index) => {
slot.call(this, item.itemId, index, item);
});
// forEach always return undefined, return equipment for chain use
return equipObjs;
}
};
KC3Ship.prototype.slotSize = function(slotIndex){
// ex-slot is always assumed to be 0 for now
return (slotIndex < 0 || slotIndex >= this.slots.length ? 0 : this.slots[slotIndex]) || 0;
};
KC3Ship.prototype.slotCapacity = function(slotIndex){
// no API data defines the capacity for ex-slot
var maxeq = (this.master() || {}).api_maxeq;
return (Array.isArray(maxeq) ? maxeq[slotIndex] : 0) || 0;
};
KC3Ship.prototype.areAllSlotsFull = function(){
// to leave unfulfilled slots in-game, make bauxite insufficient or use supply button at expedition
var maxeq = (this.master() || {}).api_maxeq;
return Array.isArray(maxeq) ?
maxeq.every((expectedSize, index) => !expectedSize || expectedSize <= this.slotSize(index)) : true;
};
KC3Ship.prototype.isFast = function(){ return (this.speed || this.master().api_soku) >= 10; };
KC3Ship.prototype.getSpeed = function(){ return this.speed || this.master().api_soku; };
KC3Ship.prototype.exItem = function(){ return this.getGearManager().get(this.ex_item); };
KC3Ship.prototype.isStriped = function(){ return (this.hp[1]>0) && (this.hp[0]/this.hp[1] <= 0.5); };
// Current HP < 25% but already in the repair dock not counted
KC3Ship.prototype.isTaiha = function(){ return (this.hp[1]>0) && (this.hp[0]/this.hp[1] <= 0.25) && !this.isRepairing(); };
// To indicate the face grey out ship, retreated or sunk (before her data removed from API)
KC3Ship.prototype.isAbsent = function(){ return (this.didFlee || this.hp[0] <= 0 || this.hp[1] <= 0); };
KC3Ship.prototype.speedName = function(){ return KC3Meta.shipSpeed(this.speed); };
KC3Ship.prototype.rangeName = function(){ return KC3Meta.shipRange(this.range); };
KC3Ship.getMarriedLevel = function(){ return 100; };
KC3Ship.getMaxLevel = function(){ return 175; };
// hard-coded at `Core.swf/vo.UserShipData.VHP` / `main.js#ShipModel.prototype.VHP`
KC3Ship.getMaxHpModernize = function() { return 2; };
// hard-coded at `Core.swf/vo.UserShipData.VAS` / `main.js#ShipModel.prototype.VAS`
KC3Ship.getMaxAswModernize = function() { return 9; };
KC3Ship.prototype.isMarried = function(){ return this.level >= KC3Ship.getMarriedLevel(); };
KC3Ship.prototype.levelClass = function(){
return this.level === KC3Ship.getMaxLevel() ? "married max" :
this.level >= KC3Ship.getMarriedLevel() ? "married" :
this.level >= 80 ? "high" :
this.level >= 50 ? "medium" :
"";
};
/** @return full url of ship face icon according her hp percent. */
KC3Ship.prototype.shipIcon = function(){
return KC3Meta.shipIcon(this.masterId, undefined, true, this.isStriped());
};
KC3Ship.shipIcon = function(masterId, mhp = 0, chp = mhp){
const isStriped = mhp > 0 && (chp / mhp) <= 0.5;
return KC3Meta.shipIcon(masterId, undefined, true, isStriped);
};
/** @return icon file name only without path and extension suffix. */
KC3Ship.prototype.moraleIcon = function(){
return KC3Ship.moraleIcon(this.morale);
};
KC3Ship.moraleIcon = function(morale){
return morale > 49 ? "4" : // sparkle
morale > 29 ? "3" : // normal
morale > 19 ? "2" : // orange face
"1"; // red face
};
/**
* The reason why 53 / 33 is the bound of morale effect being taken:
* on entering battle, morale is subtracted -3 (day) or -2 (night) before its value gets in,
* so +3 value is used as the indeed morale bound for sparkle or fatigue.
*
* @param {Array} valuesArray - values to be returned based on morale section.
* @param {boolean} onBattle - if already on battle, not need to use the bounds mentioned above.
*/
KC3Ship.prototype.moraleEffectLevel = function(valuesArray = [0, 1, 2, 3, 4], onBattle = false){
return onBattle ? (
this.morale > 49 ? valuesArray[4] :
this.morale > 29 ? valuesArray[3] :
this.morale > 19 ? valuesArray[2] :
this.morale >= 0 ? valuesArray[1] :
valuesArray[0]
) : (
this.morale > 52 ? valuesArray[4] :
this.morale > 32 ? valuesArray[3] :
this.morale > 22 ? valuesArray[2] :
this.morale >= 0 ? valuesArray[1] :
valuesArray[0]);
};
KC3Ship.prototype.getDefer = function(){
// returns a new defer if possible
return deferList[this.rosterId] || [];
};
KC3Ship.prototype.checkDefer = function() {
// reset defer if it does not in normal state
var
self= this,
ca = this.getDefer(), // get defer array
cd = ca[0]; // current collection of deferred
if(ca && cd && cd.state() == "pending")
return ca;
//console.debug("replacing",this.rosterId,"cause",!cd ? typeof cd : cd.state());
ca = deferList[this.rosterId] = Array.apply(null,{length:2}).map(function(){return $.Deferred();});
cd = $.when.apply(null,ca);
ca.unshift(cd);
return ca;
};
/* DAMAGE STATUS
Get damage status of the ship, return one of the following string:
* "dummy" if this is a dummy ship
* "taiha" (HP <= 25%)
* "chuuha" (25% < HP <= 50%)
* "shouha" (50% < HP <= 75%)
* "normal" (75% < HP < 100%)
* "full" (100% HP)
--------------------------------------------------------------*/
KC3Ship.prototype.damageStatus = function() {
if (this.hp[1] > 0) {
if (this.hp[0] === this.hp[1]) {
return "full";
}
var hpPercent = this.hp[0] / this.hp[1];
if (hpPercent <= 0.25) {
return "taiha";
} else if (hpPercent <= 0.5) {
return "chuuha";
} else if (hpPercent <= 0.75) {
return "shouha";
} else {
return "normal";
}
} else {
return "dummy";
}
};
KC3Ship.prototype.isSupplied = function(){
if(this.isDummy()){ return true; }
return this.fuel >= this.master().api_fuel_max
&& this.ammo >= this.master().api_bull_max;
};
KC3Ship.prototype.isNeedSupply = function(isEmpty){
if(this.isDummy()){ return false; }
var
fpc = function(x,y){return Math.qckInt("round",(x / y) * 10);},
fuel = fpc(this.fuel,this.master().api_fuel_max),
ammo = fpc(this.ammo,this.master().api_bull_max);
return Math.min(fuel,ammo) <= (ConfigManager.alert_supply) * (!isEmpty);
};
KC3Ship.prototype.onFleet = function(){
var fleetNum = 0;
PlayerManager.fleets.find((fleet, index) => {
if(fleet.ships.some(rid => rid === this.rosterId)){
fleetNum = index + 1;
return true;
}
});
return fleetNum;
};
/**
* @return a tuple for [position in fleet (0-based), fleet total ship amount, fleet number (1-based)].
* return [-1, 0, 0] if this ship is not on any fleet.
*/
KC3Ship.prototype.fleetPosition = function(){
var position = -1,
total = 0,
fleetNum = 0;
if(this.exists()) {
fleetNum = this.onFleet();
if(fleetNum > 0) {
var fleet = PlayerManager.fleets[fleetNum - 1];
position = fleet.ships.indexOf(this.rosterId);
total = fleet.countShips();
}
}
return [position, total, fleetNum];
};
KC3Ship.prototype.isRepairing = function(){
return PlayerManager.repairShips.indexOf(this.rosterId) >= 0;
};
KC3Ship.prototype.isAway = function(){
return this.onFleet() > 1 /* ensures not in main fleet */
&& (KC3TimerManager.exped(this.onFleet()) || {active:false}).active; /* if there's a countdown on expedition, means away */
};
KC3Ship.prototype.isFree = function(){
return !(this.isRepairing() || this.isAway());
};
KC3Ship.prototype.resetAfterHp = function(){
this.afterHp[0] = this.hp[0];
this.afterHp[1] = this.hp[1];
};
KC3Ship.prototype.applyRepair = function(){
this.hp[0] = this.hp[1];
// also keep afterHp consistent
this.resetAfterHp();
this.morale = Math.max(40, this.morale);
this.repair.fill(0);
};
/**
* Return max HP of a ship. Static method for library.
* Especially after marriage, api_taik[1] is hard to reach in game.
* Since 2017-09-29, HP can be modernized, and known max value is within 2.
* @return false if ship ID belongs to abyssal or nonexistence
* @see http://wikiwiki.jp/kancolle/?%A5%B1%A5%C3%A5%B3%A5%F3%A5%AB%A5%C3%A5%B3%A5%AB%A5%EA
* @see https://github.com/andanteyk/ElectronicObserver/blob/develop/ElectronicObserver/Other/Information/kcmemo.md#%E3%82%B1%E3%83%83%E3%82%B3%E3%83%B3%E3%82%AB%E3%83%83%E3%82%B3%E3%82%AB%E3%83%AA%E5%BE%8C%E3%81%AE%E8%80%90%E4%B9%85%E5%80%A4
*/
KC3Ship.getMaxHp = function(masterId, currentLevel, isModernized){
var masterHpArr = KC3Master.isNotRegularShip(masterId) ? [] :
(KC3Master.ship(masterId) || {"api_taik":[]}).api_taik;
var masterHp = masterHpArr[0], maxLimitHp = masterHpArr[1];
var expected = ((currentLevel || KC3Ship.getMaxLevel())
< KC3Ship.getMarriedLevel() ? masterHp :
masterHp > 90 ? masterHp + 9 :
masterHp >= 70 ? masterHp + 8 :
masterHp >= 50 ? masterHp + 7 :
masterHp >= 40 ? masterHp + 6 :
masterHp >= 30 ? masterHp + 5 :
masterHp >= 8 ? masterHp + 4 :
masterHp + 3) || false;
if(isModernized) expected += KC3Ship.getMaxHpModernize();
return maxLimitHp && expected > maxLimitHp ? maxLimitHp : expected;
};
KC3Ship.prototype.maxHp = function(isModernized){
return KC3Ship.getMaxHp(this.masterId, this.level, isModernized);
};
// Since 2017-09-29, asw stat can be modernized, known max value is within 9.
KC3Ship.prototype.maxAswMod = function(){
// the condition `Core.swf/vo.UserShipData.hasTaisenAbility()` also used
var maxAswBeforeMarriage = this.as[1];
var maxModAsw = this.nakedAsw() + KC3Ship.getMaxAswModernize() - (this.mod[6] || 0);
return maxAswBeforeMarriage > 0 ? maxModAsw : 0;
};
/**
* Return total count of aircraft slots of a ship. Static method for library.
* @return -1 if ship ID belongs to abyssal or nonexistence
*/
KC3Ship.getCarrySlots = function(masterId){
var maxeq = KC3Master.isNotRegularShip(masterId) ? undefined :
(KC3Master.ship(masterId) || {}).api_maxeq;
return Array.isArray(maxeq) ? maxeq.sumValues() : -1;
};
KC3Ship.prototype.carrySlots = function(){
return KC3Ship.getCarrySlots(this.masterId);
};
/**
* @param isExslotIncluded - if equipment on ex-slot is counted, here true by default
* @return current equipped pieces of equipment
*/
KC3Ship.prototype.equipmentCount = function(isExslotIncluded = true){
let amount = (this.items.indexOf(-1) + 1 || (this.items.length + 1)) - 1;
// 0 means ex-slot not opened, -1 means opened but none equipped
amount += (isExslotIncluded && this.ex_item > 0) & 1;
return amount;
};
/**
* @param isExslotIncluded - if ex-slot is counted, here true by default
* @return amount of all equippable slots
*/
KC3Ship.prototype.equipmentMaxCount = function(isExslotIncluded = true){
return this.slotnum + ((isExslotIncluded && (this.ex_item === -1 || this.ex_item > 0)) & 1);
};
/**
* @param stypeValue - specific a ship type if not refer to this ship
* @return true if this (or specific) ship is a SS or SSV
*/
KC3Ship.prototype.isSubmarine = function(stypeValue){
if(this.isDummy()) return false;
const stype = stypeValue || this.master().api_stype;
return [13, 14].includes(stype);
};
/**
* @return true if this ship type is CVL, CV or CVB
*/
KC3Ship.prototype.isCarrier = function(){
if(this.isDummy()) return false;
const stype = this.master().api_stype;
return [7, 11, 18].includes(stype);
};
/**
* @return true if this ship is a CVE, which is Light Carrier and her initial ASW stat > 0
*/
KC3Ship.prototype.isEscortLightCarrier = function(){
if(this.isDummy()) return false;
const stype = this.master().api_stype;
// Known implementations: Taiyou series, Gambier Bay series, Zuihou K2B
const minAsw = (this.master().api_tais || [])[0];
return stype === 7 && minAsw > 0;
};
/* REPAIR TIME
Get ship's docking and Akashi times
when optAfterHp is true, return repair time based on afterHp
--------------------------------------------------------------*/
KC3Ship.prototype.repairTime = function(optAfterHp){
var hpArr = optAfterHp ? this.afterHp : this.hp,
HPPercent = hpArr[0] / hpArr[1],
RepairCalc = PS['KanColle.RepairTime'];
var result = { akashi: 0 };
if (HPPercent > 0.5 && HPPercent < 1.00 && this.isFree()) {
var dockTimeMillis = optAfterHp ?
RepairCalc.dockingInSecJSNum(this.master().api_stype, this.level, hpArr[0], hpArr[1]) * 1000 :
this.repair[0];
var repairTime = KC3AkashiRepair.calculateRepairTime(dockTimeMillis);
result.akashi = Math.max(
Math.hrdInt('floor', repairTime, 3, 1), // convert to seconds
20 * 60 // should be at least 20 minutes
);
}
if (optAfterHp) {
result.docking = RepairCalc.dockingInSecJSNum(this.master().api_stype, this.level, hpArr[0], hpArr[1]);
} else {
result.docking = this.isRepairing() ?
Math.ceil(KC3TimerManager.repair(PlayerManager.repairShips.indexOf(this.rosterId)).remainingTime()) / 1000 :
/* RepairCalc. dockingInSecJSNum( this.master().api_stype, this.level, this.hp[0], this.hp[1] ) */
Math.hrdInt('floor', this.repair[0], 3, 1);
}
return result;
};
/* Calculate resupply cost
----------------------------------
0 <= fuelPercent <= 1, < 0 use current fuel
0 <= ammoPercent <= 1, < 0 use current ammo
to calculate bauxite cost: bauxiteNeeded == true
to calculate steel cost per battles: steelNeeded == true
costs of expeditions simulate rounding by adding roundUpFactor(0.4/0.5?) before flooring
returns an object: {fuel: <fuelCost>, ammo: <ammoCost>, steel: <steelCost>, bauxite: <bauxiteCost>}
*/
KC3Ship.prototype.calcResupplyCost = function(fuelPercent, ammoPercent, bauxiteNeeded, steelNeeded, roundUpFactor) {
var self = this;
var result = {
fuel: 0, ammo: 0
};
if(this.isDummy()) {
if(bauxiteNeeded) result.bauxite = 0;
if(steelNeeded) result.steel = 0;
return result;
}
var master = this.master();
var fullFuel = master.api_fuel_max;
var fullAmmo = master.api_bull_max;
var mulRounded = function (a, percent) {
return Math.floor( a * percent + (roundUpFactor || 0) );
};
var marriageConserve = function (v) {
return self.isMarried() && v > 1 ? Math.floor(0.85 * v) : v;
};
result.fuel = fuelPercent < 0 ? fullFuel - this.fuel : mulRounded(fullFuel, fuelPercent);
result.ammo = ammoPercent < 0 ? fullAmmo - this.ammo : mulRounded(fullAmmo, ammoPercent);
// After testing, 85% is applied to resupply value, not max value. and cannot be less than 1
result.fuel = marriageConserve(result.fuel);
result.ammo = marriageConserve(result.ammo);
if(bauxiteNeeded){
var slotsBauxiteCost = (current, max) => (
current < max ? (max - current) * KC3GearManager.carrierSupplyBauxiteCostPerSlot : 0
);
result.bauxite = self.equipment()
.map((g, i) => slotsBauxiteCost(self.slots[i], master.api_maxeq[i]))
.sumValues();
// Bauxite cost to fill slots not affected by marriage.
// via http://kancolle.wikia.com/wiki/Marriage
//result.bauxite = marriageConserve(result.bauxite);
}
if(steelNeeded){
result.steel = this.calcJetsSteelCost();
}
return result;
};
/**
* Calculate steel cost of jet aircraft for 1 battle based on current slot size.
* returns total steel cost for this ship at this time
*/
KC3Ship.prototype.calcJetsSteelCost = function(currentSortieId) {
var totalSteel = 0, consumedSteel = 0;
if(this.isDummy()) { return totalSteel; }
this.equipment().forEach((item, i) => {
// Is Jet aircraft and left slot > 0
if(item.exists() && this.slots[i] > 0 &&
KC3GearManager.jetAircraftType2Ids.includes(item.master().api_type[2])) {
consumedSteel = Math.round(
this.slots[i]
* item.master().api_cost
* KC3GearManager.jetBomberSteelCostRatioPerSlot
) || 0;
totalSteel += consumedSteel;
if(!!currentSortieId) {
let pc = this.pendingConsumption[currentSortieId];
if(!Array.isArray(pc)) {
pc = [[0,0,0],[0,0,0],[0]];
this.pendingConsumption[currentSortieId] = pc;
}
if(!Array.isArray(pc[2])) {
pc[2] = [0];
}
pc[2][0] -= consumedSteel;
}
}
});
if(!!currentSortieId && totalSteel > 0) {
KC3ShipManager.save();
}
return totalSteel;
};
/**
* Get or calculate repair cost of this ship.
* @param currentHp - assumed current HP value if this ship is not damaged effectively.
* @return an object: {fuel: <fuelCost>, steel: <steelCost>}
*/
KC3Ship.prototype.calcRepairCost = function(currentHp){
const result = {
fuel: 0, steel: 0
};
if(this.isDummy()) { return result; }
if(currentHp > 0 && currentHp <= this.hp[1]) {
// formula see http://kancolle.wikia.com/wiki/Docking
const fullFuel = this.master().api_fuel_max;
const hpLost = this.hp[1] - currentHp;
result.fuel = Math.floor(fullFuel * hpLost * 0.032);
result.steel = Math.floor(fullFuel * hpLost * 0.06);
} else {
result.fuel = this.repair[1];
result.steel = this.repair[2];
}
return result;
};
/**
* Naked stats of this ship.
* @return stats without the equipment but with modernization.
*/
KC3Ship.prototype.nakedStats = function(statAttr){
if(this.isDummy()) { return false; }
const stats = {
aa: this.aa[0],
ar: this.ar[0],
as: this.as[0],
ev: this.ev[0],
fp: this.fp[0],
// Naked HP maybe mean HP before marriage
hp: (this.master().api_taik || [])[0] || this.hp[1],
lk: (this.master().api_luck || [])[0] || this.lk[0],
ls: this.ls[0],
tp: this.tp[0],
// Accuracy not shown in-game, so naked value might be plus-minus 0
ht: 0
};
// Limited to currently used stats only,
// all implemented see `KC3Meta.js#statApiNameMap`
const statApiNames = {
"tyku": "aa",
"souk": "ar",
"tais": "as",
"houk": "ev",
"houg": "fp",
"saku": "ls",
"raig": "tp",
"houm": "ht",
"leng": "rn",
"soku": "sp",
};
for(const apiName in statApiNames) {
const equipStats = this.equipmentTotalStats(apiName);
stats[statApiNames[apiName]] -= equipStats;
}
return !statAttr ? stats : stats[statAttr];
};
KC3Ship.prototype.statsBonusOnShip = function(statAttr){
if(this.isDummy()) { return false; }
const stats = {};
const statApiNames = {
"houg": "fp",
"souk": "ar",
"raig": "tp",
"houk": "ev",
"tyku": "aa",
"tais": "as",
"saku": "ls",
"houm": "ht",
"leng": "rn",
"soku": "sp",
};
for(const apiName in statApiNames) {
stats[statApiNames[apiName]] = this.equipmentTotalStats(apiName, true, true, true);
}
return !statAttr ? stats : stats[statAttr];
};
KC3Ship.prototype.equipmentStatsMap = function(apiName, isExslotIncluded = true){
return this.equipment(isExslotIncluded).map(equip => {
if(equip.exists()) {
return equip.master()["api_" + apiName];
}
return undefined;
});
};
KC3Ship.prototype.equipmentTotalStats = function(apiName, isExslotIncluded = true,
isOnShipBonusIncluded = true, isOnShipBonusOnly = false,
includeEquipTypes = null, includeEquipIds = null,
excludeEquipTypes = null, excludeEquipIds = null){
var total = 0;
const bonusDefs = isOnShipBonusIncluded || isOnShipBonusOnly ? KC3Gear.explicitStatsBonusGears() : false;
// Accumulates displayed stats from equipment, and count for special equipment
this.equipment(isExslotIncluded).forEach(equip => {
if(equip.exists()) {
const gearMst = equip.master(),
mstId = gearMst.api_id,
type2 = gearMst.api_type[2];
if(Array.isArray(includeEquipTypes) &&
!includeEquipTypes.includes(type2) ||
Array.isArray(includeEquipIds) &&
!includeEquipIds.includes(mstId) ||
Array.isArray(excludeEquipTypes) &&
excludeEquipTypes.includes(type2) ||
Array.isArray(excludeEquipIds) &&
excludeEquipIds.includes(mstId)
) { return; }
total += gearMst["api_" + apiName] || 0;
if(bonusDefs) KC3Gear.accumulateShipBonusGear(bonusDefs, equip);
}
});
// Add explicit stats bonuses (not masked, displayed on ship) from equipment on specific ship
if(bonusDefs) {
const onShipBonus = KC3Gear.equipmentTotalStatsOnShipBonus(bonusDefs, this, apiName);
total = isOnShipBonusOnly ? onShipBonus : total + onShipBonus;
}
return total;
};
KC3Ship.prototype.equipmentBonusGearAndStats = function(newGearObj){
const newGearMstId = (newGearObj || {}).masterId;
let gearFlag = false;
let synergyFlag = false;
const bonusDefs = KC3Gear.explicitStatsBonusGears();
const synergyGears = bonusDefs.synergyGears;
const allGears = this.equipment(true);
allGears.forEach(g => g.exists() && KC3Gear.accumulateShipBonusGear(bonusDefs, g));
const masterIdList = allGears.map(g => g.masterId)
.filter((value, index, self) => value > 0 && self.indexOf(value) === index);
let bonusGears = masterIdList.map(mstId => bonusDefs[mstId])
.concat(masterIdList.map(mstId => {
const typeDefs = bonusDefs[`t2_${KC3Master.slotitem(mstId).api_type[2]}`];
if (!typeDefs) return; else return $.extend(true, {}, typeDefs);
}));
masterIdList.push(...masterIdList);
// Check if each gear works on the equipped ship
const shipId = this.masterId;
const originId = RemodelDb.originOf(shipId);
const ctype = String(this.master().api_ctype);
const stype = this.master().api_stype;
const checkByShip = (byShip, shipId, originId, stype, ctype) =>
(byShip.ids || []).includes(shipId) ||
(byShip.origins || []).includes(originId) ||
(byShip.stypes || []).includes(stype) ||
(byShip.classes || []).includes(Number(ctype)) ||
(!byShip.ids && !byShip.origins && !byShip.stypes && !byShip.classes);
// Check if ship is eligible for equip bonus and add synergy/id flags
bonusGears = bonusGears.filter((gear, idx) => {
if (!gear) { return false; }
const synergyFlags = [];
const synergyIds = [];
const matchGearByMstId = (g) => g.masterId === masterIdList[idx];
let flag = false;
for (const type in gear) {
if (type === "byClass") {
for (const key in gear[type]) {
if (key == ctype) {
if (Array.isArray(gear[type][key])) {
for (let i = 0; i < gear[type][key].length; i++) {
gear.path = gear.path || [];
gear.path.push(gear[type][key][i]);
}
} else {
gear.path = gear[type][key];
}
}
}
}
else if (type === "byShip") {
if (Array.isArray(gear[type])) {
for (let i = 0; i < gear[type].length; i++) {
if (checkByShip(gear[type][i], shipId, originId, stype, ctype)) {
gear.path = gear.path || [];
gear.path.push(gear[type][i]);
}
}
} else if (checkByShip(gear[type], shipId, originId, stype, ctype)) {
gear.path = gear[type];
}
}
if (gear.path) {
if (typeof gear.path !== "object") { gear.path = gear[type][gear.path]; }
if (!Array.isArray(gear.path)) { gear.path = [gear.path]; }
const count = gear.count;
for (let pathIdx = 0; pathIdx < gear.path.length; pathIdx++) {
const check = gear.path[pathIdx];
if (check.excludes && check.excludes.includes(shipId)) { continue; }
if (check.excludeOrigins && check.excludeOrigins.includes(originId)) { continue; }
if (check.excludeClasses && check.excludeClasses.includes(ctype)) { continue; }
if (check.excludeStypes && check.excludeStypes.includes(stype)) { continue; }
if (check.remodel && RemodelDb.remodelGroup(shipId).indexOf(shipId) < check.remodel) { continue; }
if (check.remodelCap && RemodelDb.remodelGroup(shipId).indexOf(shipId) > check.remodelCap) { continue; }
if (check.origins && !check.origins.includes(originId)) { continue; }
if (check.stypes && !check.stypes.includes(stype)) { continue; }
if (check.classes && !check.classes.includes(ctype)) { continue; }
// Known issue: exact corresponding stars will not be found since identical equipment merged
if (check.minStars && allGears.find(matchGearByMstId).stars < check.minStars) { continue; }
if (check.single) { gear.count = 1; flag = true; }
if (check.multiple) { gear.count = count; flag = true; }
// countCap/minCount take priority
if (check.countCap) { gear.count = Math.min(check.countCap, count); }
if (check.minCount) { gear.count = count; }
// Synergy check
if (check.synergy) {
let synergyCheck = check.synergy;
if (!Array.isArray(synergyCheck)) { synergyCheck = [synergyCheck]; }
for (let checkIdx = 0; checkIdx < synergyCheck.length; checkIdx++) {
const flagList = synergyCheck[checkIdx].flags;
for (let flagIdx = 0; flagIdx < flagList.length; flagIdx++) {
const equipFlag = flagList[flagIdx];
if (equipFlag.endsWith("Nonexist")) {
if (!synergyGears[equipFlag]) { break; }
} else if (synergyGears[equipFlag] > 0) {
if (synergyGears[equipFlag + "Ids"].includes(newGearMstId)) { synergyFlag = true; }
synergyFlags.push(equipFlag);
synergyIds.push(masterIdList.find(id => synergyGears[equipFlag + "Ids"].includes(id)));
}
}
}
flag |= synergyFlags.length > 0;
}
}
}
}
gear.synergyFlags = synergyFlags;
gear.synergyIds = synergyIds;
gear.byType = idx >= Math.floor(masterIdList.length / 2);
gear.id = masterIdList[idx];
return flag;
});
if (!bonusGears.length) { return false; }
// Trim bonus gear object and add icon ids
const byIdGears = bonusGears.filter(g => !g.byType).map(g => g.id);
const result = bonusGears.filter(g => !g.byType || !byIdGears.includes(g.id)).map(gear => {
const obj = {};
obj.count = gear.count;
const g = allGears.find(eq => eq.masterId === gear.id);
obj.name = g.name();
if (g.masterId === newGearMstId) { gearFlag = true; }
obj.icon = g.master().api_type[3];
obj.synergyFlags = gear.synergyFlags.filter((value, index, self) => self.indexOf(value) === index && !!value);
obj.synergyNames = gear.synergyIds.map(id => allGears.find(eq => eq.masterId === id).name());
obj.synergyIcons = obj.synergyFlags.map(flag => {
if (flag.includes("Radar")) { return 11; }
else if (flag.includes("Torpedo")) { return 5; }
else if (flag.includes("LargeGunMount")) { return 3; }
else if (flag.includes("MediumGunMount")) { return 2; }
else if (flag.includes("SmallGunMount")) { return 1; }
else if (flag.includes("skilledLookouts")) { return 32; }
else if (flag.includes("searchlight")) { return 24; }
else if (flag.includes("rotorcraft") || flag.includes("helicopter")) { return 21; }
else if (flag.includes("Boiler")) { return 19; }
return 0; // Unknown synergy type
});
return obj;
});
const stats = this.statsBonusOnShip();
return {
isShow: gearFlag || synergyFlag,
bonusGears: result,
stats: stats,
};
};
// faster naked asw stat method since frequently used
KC3Ship.prototype.nakedAsw = function(){
var asw = this.as[0];
var equipAsw = this.equipmentTotalStats("tais");
return asw - equipAsw;
};
KC3Ship.prototype.nakedLoS = function(){
var los = this.ls[0];
var equipLos = this.equipmentTotalLoS();
return los - equipLos;
};
KC3Ship.prototype.equipmentTotalLoS = function (){
return this.equipmentTotalStats("saku");
};
KC3Ship.prototype.effectiveEquipmentTotalAsw = function(canAirAttack = false, includeImprovedAttack = false, forExped = false){
var equipmentTotalAsw = 0;
if(!forExped) {
// When calculating asw warefare relevant thing,
// asw stat from these known types of equipment not taken into account:
// main gun, recon seaplane, seaplane/carrier fighter, radar, large flying boat, LBAA
// KC Vita counts only carrier bomber, seaplane bomber, sonar (both), depth charges, rotorcraft and as-pby.
// All visible bonuses from equipment not counted towards asw attacks for now.
const noCountEquipType2Ids = [1, 2, 3, 6, 10, 12, 13, 41, 45, 47];
if(!canAirAttack) {
const stype = this.master().api_stype;
const isHayasuiKaiWithTorpedoBomber = this.masterId === 352 && this.hasEquipmentType(2, 8);
// CAV, CVL, BBV, AV, LHA, CVL-like Hayasui Kai
const isAirAntiSubStype = [6, 7, 10, 16, 17].includes(stype) || isHayasuiKaiWithTorpedoBomber;
if(isAirAntiSubStype) {
// exclude carrier bomber, seaplane bomber, rotorcraft, as-pby too if not able to air attack
noCountEquipType2Ids.push(...[7, 8, 11, 25, 26, 57, 58]);
}
}
equipmentTotalAsw = this.equipment(true)
.map(g => g.exists() && g.master().api_tais > 0 &&
noCountEquipType2Ids.includes(g.master().api_type[2]) ? 0 : g.master().api_tais
+ (!!includeImprovedAttack && g.attackPowerImprovementBonus("asw"))
).sumValues();
} else {
// For expeditions, asw from aircraft affected by proficiency and equipped slot size:
// https://wikiwiki.jp/kancolle/%E9%81%A0%E5%BE%81#about_stat
// https://docs.google.com/spreadsheets/d/1o-_-I8GXuJDkSGH0Dhjo7mVYx9Kpay2N2h9H3HMyO_E/htmlview
equipmentTotalAsw = this.equipment(true)
.map((g, i) => g.exists() && g.master().api_tais > 0 &&
// non-aircraft counts its actual asw value, land base plane cannot be used by expedition anyway
!KC3GearManager.carrierBasedAircraftType3Ids.includes(g.master().api_type[3]) ? g.master().api_tais :
// aircraft in 0-size slot take no effect, and
// current formula for 0 proficiency aircraft only,
// max level may give additional asw up to +2
(!this.slotSize(i) ? 0 : Math.floor(g.master().api_tais * (0.65 + 0.1 * Math.sqrt(Math.max(0, this.slotSize(i) - 2)))))
).sumValues()
// unconfirmed: all visible bonuses counted? or just like OASW, only some types counted? or none counted?
+ this.equipmentTotalStats("tais", true, true, true/*, null, null, [1, 6, 7, 8]*/);
}
return equipmentTotalAsw;
};
// estimated basic stats without equipments based on master data and modernization
KC3Ship.prototype.estimateNakedStats = function(statAttr) {
if(this.isDummy()) { return false; }
const shipMst = this.master();
if(!shipMst) { return false; }
const stats = {
fp: shipMst.api_houg[0] + this.mod[0],
tp: shipMst.api_raig[0] + this.mod[1],
aa: shipMst.api_tyku[0] + this.mod[2],
ar: shipMst.api_souk[0] + this.mod[3],
lk: shipMst.api_luck[0] + this.mod[4],
hp: this.maxHp(false) + (this.mod[5] || 0),
};
// shortcuts for following funcs
if(statAttr === "ls") return this.estimateNakedLoS();
if(statAttr === "as") return this.estimateNakedAsw() + (this.mod[6] || 0);
if(statAttr === "ev") return this.estimateNakedEvasion();
return !statAttr ? stats : stats[statAttr];
};
// estimated LoS without equipments based on WhoCallsTheFleetDb
KC3Ship.prototype.estimateNakedLoS = function() {
var losInfo = WhoCallsTheFleetDb.getLoSInfo( this.masterId );
var retVal = WhoCallsTheFleetDb.estimateStat(losInfo, this.level);
return retVal === false ? 0 : retVal;
};
KC3Ship.prototype.estimateNakedAsw = function() {
var aswInfo = WhoCallsTheFleetDb.getStatBound(this.masterId, "asw");
var retVal = WhoCallsTheFleetDb.estimateStat(aswInfo, this.level);
return retVal === false ? 0 : retVal;
};
KC3Ship.prototype.estimateNakedEvasion = function() {
var evaInfo = WhoCallsTheFleetDb.getStatBound(this.masterId, "evasion");
var retVal = WhoCallsTheFleetDb.estimateStat(evaInfo, this.level);
return retVal === false ? 0 : retVal;
};
// estimated the base value (on lv1) of the 3-stats (evasion, asw, los) missing in master data,
// based on current naked stats and max value (on lv99),
// in case whoever wanna submit this in order to collect ship's exact stats quickly.
// NOTE: naked stats needed, so should ensure no equipment equipped or at least no on ship bonus.
// btw, exact evasion and asw values can be found at in-game picture book api data, but los missing still.
KC3Ship.prototype.estimateBaseMasterStats = function() {
if(this.isDummy()) { return false; }
const info = {
// evasion for ship should be `api_kaih`, here uses gear one instead
houk: [0, this.ev[1], this.ev[0]],
tais: [0, this.as[1], this.as[0]],
saku: [0, this.ls[1], this.ls[0]],
};
const level = this.level;
Object.keys(info).forEach(apiName => {
const lv99Stat = info[apiName][1];
const nakedStat = info[apiName][2] - this.equipmentTotalStats(apiName);
info[apiName][3] = nakedStat;
if(level && level > 99) {
info[apiName][0] = false;
} else {
info[apiName][0] = WhoCallsTheFleetDb.estimateStatBase(nakedStat, lv99Stat, level);
// try to get stats on maxed married level too
info[apiName][4] = WhoCallsTheFleetDb.estimateStat({base: info[apiName][0], max: lv99Stat}, KC3Ship.getMaxLevel());
}
});
info.level = level;
info.mstId = this.masterId;
info.equip = this.equipment(true).map(g => g.masterId);
return info;
};
KC3Ship.prototype.equipmentTotalImprovementBonus = function(attackType){
return this.equipment(true)
.map(gear => gear.attackPowerImprovementBonus(attackType))
.sumValues();
};
/**
* Maxed stats of this ship.
* @return stats without the equipment but with modernization at Lv.99,
* stats at Lv.175 can be only estimated by data from known database.
*/
KC3Ship.prototype.maxedStats = function(statAttr, isMarried = this.isMarried()){
if(this.isDummy()) { return false; }
const stats = {
aa: this.aa[1],
ar: this.ar[1],
as: this.as[1],
ev: this.ev[1],
fp: this.fp[1],
hp: this.hp[1],
// Maxed Luck includes full modernized + marriage bonus
lk: this.lk[1],
ls: this.ls[1],
tp: this.tp[1]
};
if(isMarried){
stats.hp = KC3Ship.getMaxHp(this.masterId);
const asBound = WhoCallsTheFleetDb.getStatBound(this.masterId, "asw");
const asw = WhoCallsTheFleetDb.estimateStat(asBound, KC3Ship.getMaxLevel());
if(asw !== false) { stats.as = asw; }
const lsBound = WhoCallsTheFleetDb.getStatBound(this.masterId, "los");
const los = WhoCallsTheFleetDb.estimateStat(lsBound, KC3Ship.getMaxLevel());
if(los !== false) { stats.ls = los; }
const evBound = WhoCallsTheFleetDb.getStatBound(this.masterId, "evasion");
const evs = WhoCallsTheFleetDb.estimateStat(evBound, KC3Ship.getMaxLevel());
if(evs !== false) { stats.ev = evs; }
}
// Unlike stats fp, tp, ar and aa,
// increase final maxed asw since modernized asw is not included in both as[1] and db
if(this.mod[6] > 0) { stats.as += this.mod[6]; }
return !statAttr ? stats : stats[statAttr];
};
/**
* Left modernize-able stats of this ship.
* @return stats to be maxed modernization
*/
KC3Ship.prototype.modernizeLeftStats = function(statAttr){
if(this.isDummy()) { return false; }
const shipMst = this.master();
const stats = {
fp: shipMst.api_houg[1] - shipMst.api_houg[0] - this.mod[0],
tp: shipMst.api_raig[1] - shipMst.api_raig[0] - this.mod[1],
aa: shipMst.api_tyku[1] - shipMst.api_tyku[0] - this.mod[2],
ar: shipMst.api_souk[1] - shipMst.api_souk[0] - this.mod[3],
lk: this.lk[1] - this.lk[0],
// or: shipMst.api_luck[1] - shipMst.api_luck[0] - this.mod[4],
// current stat (hp[1], as[0]) already includes the modded stat
hp: this.maxHp(true) - this.hp[1],
as: this.maxAswMod() - this.nakedAsw()
};
return !statAttr ? stats : stats[statAttr];
};
/**
* Get number of equipments of specific masterId(s).
* @param masterId - slotitem master ID to be matched, can be an Array.
* @return count of specific equipment.
*/
KC3Ship.prototype.countEquipment = function(masterId, isExslotIncluded = true) {
return this.findEquipmentById(masterId, isExslotIncluded)
.reduce((acc, v) => acc + (!!v & 1), 0);
};
/**
* Get number of equipments of specific type(s).
* @param typeIndex - the index of `slotitem.api_type[]`, see `equiptype.json` for more.
* @param typeValue - the expected type value(s) to be matched, can be an Array.
* @return count of specific type(s) of equipment.
*/
KC3Ship.prototype.countEquipmentType = function(typeIndex, typeValue, isExslotIncluded = true) {
return this.findEquipmentByType(typeIndex, typeValue, isExslotIncluded)
.reduce((acc, v) => acc + (!!v & 1), 0);
};
/**
* Get number of some equipment (aircraft usually) equipped on non-0 slot.
*/
KC3Ship.prototype.countNonZeroSlotEquipment = function(masterId, isExslotIncluded = false) {
return this.findEquipmentById(masterId, isExslotIncluded)
.reduce((acc, v, i) => acc + ((!!v && this.slots[i] > 0) & 1), 0);
};
/**
* Get number of some specific types of equipment (aircraft usually) equipped on non-0 slot.
*/
KC3Ship.prototype.countNonZeroSlotEquipmentType = function(typeIndex, typeValue, isExslotIncluded = false) {
return this.findEquipmentByType(typeIndex, typeValue, isExslotIncluded)
.reduce((acc, v, i) => acc + ((!!v && this.slots[i] > 0) & 1), 0);
};
/**
* Get number of drums held by this ship.
*/
KC3Ship.prototype.countDrums = function(){
return this.countEquipment( 75 );
};
/**
* Indicate if some specific equipment equipped.
*/
KC3Ship.prototype.hasEquipment = function(masterId, isExslotIncluded = true) {
return this.findEquipmentById(masterId, isExslotIncluded).some(v => !!v);
};
/**
* Indicate if some specific types of equipment equipped.
*/
KC3Ship.prototype.hasEquipmentType = function(typeIndex, typeValue, isExslotIncluded = true) {
return this.findEquipmentByType(typeIndex, typeValue, isExslotIncluded).some(v => !!v);
};
/**
* Indicate if some specific equipment (aircraft usually) equipped on non-0 slot.
*/
KC3Ship.prototype.hasNonZeroSlotEquipment = function(masterId, isExslotIncluded = false) {
return this.findEquipmentById(masterId, isExslotIncluded)
.some((v, i) => !!v && this.slots[i] > 0);
};
/**
* Indicate if some specific types of equipment (aircraft usually) equipped on non-0 slot.
*/
KC3Ship.prototype.hasNonZeroSlotEquipmentType = function(typeIndex, typeValue, isExslotIncluded = false) {
return this.findEquipmentByType(typeIndex, typeValue, isExslotIncluded)
.some((v, i) => !!v && this.slots[i] > 0);
};
/**
* Indicate if only specific equipment equipped (empty slot not counted).
*/
KC3Ship.prototype.onlyHasEquipment = function(masterId, isExslotIncluded = true) {
const equipmentCount = this.equipmentCount(isExslotIncluded);
return equipmentCount > 0 &&
equipmentCount === this.countEquipment(masterId, isExslotIncluded);
};
/**
* Indicate if only specific types of equipment equipped (empty slot not counted).
*/
KC3Ship.prototype.onlyHasEquipmentType = function(typeIndex, typeValue, isExslotIncluded = true) {
const equipmentCount = this.equipmentCount(isExslotIncluded);
return equipmentCount > 0 &&
equipmentCount === this.countEquipmentType(typeIndex, typeValue, isExslotIncluded);
};
/**
* Simple method to find equipment by Master ID from current ship's equipment.
* @return the mapped Array to indicate equipment found or not at corresponding position,
* max 6-elements including ex-slot.
*/
KC3Ship.prototype.findEquipmentById = function(masterId, isExslotIncluded = true) {
return this.equipment(isExslotIncluded).map(gear =>
Array.isArray(masterId) ? masterId.includes(gear.masterId) :
masterId === gear.masterId
);
};
/**
* Simple method to find equipment by Type ID from current ship's equipment.
* @return the mapped Array to indicate equipment found or not at corresponding position,
* max 6-elements including ex-slot.
*/
KC3Ship.prototype.findEquipmentByType = function(typeIndex, typeValue, isExslotIncluded = true) {
return this.equipment(isExslotIncluded).map(gear =>
gear.exists() && (
Array.isArray(typeValue) ? typeValue.includes(gear.master().api_type[typeIndex]) :
typeValue === gear.master().api_type[typeIndex]
)
);
};
/* FIND DAMECON
Find first available damecon.
search order: extra slot -> 1st slot -> 2ns slot -> 3rd slot -> 4th slot -> 5th slot
return: {pos: <pos>, code: <code>}
pos: 1-5 for normal slots, 0 for extra slot, -1 if not found.
code: 0 if not found, 1 for repair team, 2 for goddess
----------------------------------------- */
KC3Ship.prototype.findDameCon = function() {
const allItems = [ {pos: 0, item: this.exItem() } ];
allItems.push(...this.equipment(false).map((g, i) => ({pos: i + 1, item: g}) ));
const damecon = allItems.filter(x => (
// 42: repair team
// 43: repair goddess
x.item.masterId === 42 || x.item.masterId === 43
)).map(x => (
{pos: x.pos,
code: x.item.masterId === 42 ? 1
: x.item.masterId === 43 ? 2
: 0}
));
return damecon.length > 0 ? damecon[0] : {pos: -1, code: 0};
};
/**
* Static method of the same logic to find available damecon to be used first.
* @param equipArray - the master ID array of ship's all equipment including ex-slot at the last.
* for 5-slot ship, array supposed to be 6 elements; otherwise should be always 5 elements.
* @see KC3Ship.prototype.findDameCon
* @see main.js#ShipModelReplica.prototype.useRepairItem - the repair items using order and the type codes
*/
KC3Ship.findDamecon = function(equipArray = []) {
// push last item from ex-slot to 1st
const sortedMstIds = equipArray.slice(-1);
sortedMstIds.push(...equipArray.slice(0, -1));
const dameconId = sortedMstIds.find(id => id === 42 || id === 43);
// code 1 for repair team, 2 for repair goddess
return dameconId === 42 ? 1 : dameconId === 43 ? 2 : 0;
};
/* CALCULATE TRANSPORT POINT
Retrieve TP object related to the current ship
** TP Object Detail --
value: known value that were calculated for
clear: is the value already clear or not? it's a NaN like.
**
--------------------------------------------------------------*/
KC3Ship.prototype.obtainTP = function() {
var tp = KC3Meta.tpObtained();
if (this.isDummy()) { return tp; }
if (!(this.isAbsent() || this.isTaiha())) {
var tp1,tp2,tp3;
tp1 = String(tp.add(KC3Meta.tpObtained({stype:this.master().api_stype})));
tp2 = String(tp.add(KC3Meta.tpObtained({slots:this.equipment().map(function(slot){return slot.masterId;})})));
tp3 = String(tp.add(KC3Meta.tpObtained({slots:[this.exItem().masterId]})));
// Special case of Kinu Kai 2: Daihatsu embedded :)
if (this.masterId == 487) {
tp.add(KC3Meta.tpObtained({slots:[68]}));
}
}
return tp;
};
/* FIGHTER POWER
Get fighter power of this ship
--------------------------------------------------------------*/
KC3Ship.prototype.fighterPower = function(){
if(this.isDummy()){ return 0; }
return this.equipment().map((g, i) => g.fighterPower(this.slots[i])).sumValues();
};
/* FIGHTER POWER with WHOLE NUMBER BONUS
Get fighter power of this ship as an array
with consideration to whole number proficiency bonus
--------------------------------------------------------------*/
KC3Ship.prototype.fighterVeteran = function(){
if(this.isDummy()){ return 0; }
return this.equipment().map((g, i) => g.fighterVeteran(this.slots[i])).sumValues();
};
/* FIGHTER POWER with LOWER AND UPPER BOUNDS
Get fighter power of this ship as an array
with consideration to min-max bonus
--------------------------------------------------------------*/
KC3Ship.prototype.fighterBounds = function(forLbas = false){
if(this.isDummy()){ return [0, 0]; }
const powerBounds = this.equipment().map((g, i) => g.fighterBounds(this.slots[i], forLbas));
const reconModifier = this.fighterPowerReconModifier(forLbas);
return [
Math.floor(powerBounds.map(b => b[0]).sumValues() * reconModifier),
Math.floor(powerBounds.map(b => b[1]).sumValues() * reconModifier)
];
};
/**
* @return value under verification of LB Recon modifier to LBAS sortie fighter power.
*/
KC3Ship.prototype.fighterPowerReconModifier = function(forLbas = false){
var reconModifier = 1;
this.equipment(function(id, idx, gear){
if(!id || gear.isDummy()){ return; }
const type2 = gear.master().api_type[2];
// LB Recon Aircraft
if(forLbas && type2 === 49){
const los = gear.master().api_saku;
reconModifier = Math.max(reconModifier,
los >= 9 ? 1.18 : 1.15
);
}
});
return reconModifier;
};
/* FIGHTER POWER on Air Defense with INTERCEPTOR FORMULA
Recon plane gives a modifier to total interception power
--------------------------------------------------------------*/
KC3Ship.prototype.interceptionPower = function(){
if(this.isDummy()){ return 0; }
var reconModifier = 1;
this.equipment(function(id, idx, gear){
if(!id || gear.isDummy()){ return; }
const type2 = gear.master().api_type[2];
if(KC3GearManager.landBaseReconnType2Ids.includes(type2)){
const los = gear.master().api_saku;
// Carrier Recon Aircraft
if(type2 === 9){
reconModifier = Math.max(reconModifier,
(los <= 7) ? 1.2 :
(los >= 9) ? 1.3 :
1.2 // unknown
);
// LB Recon Aircraft
} else if(type2 === 49){
reconModifier = Math.max(reconModifier,
(los <= 7) ? 1.18 : // unknown
(los >= 9) ? 1.23 :
1.18
);
// Recon Seaplane, Flying Boat, etc
} else {
reconModifier = Math.max(reconModifier,
(los <= 7) ? 1.1 :
(los >= 9) ? 1.16 :
1.13
);
}
}
});
var interceptionPower = this.equipment()
.map((g, i) => g.interceptionPower(this.slots[i]))
.sumValues();
return Math.floor(interceptionPower * reconModifier);
};
/* SUPPORT POWER
* Get support expedition shelling power of this ship
* http://kancolle.wikia.com/wiki/Expedition/Support_Expedition
* http://wikiwiki.jp/kancolle/?%BB%D9%B1%E7%B4%CF%C2%E2
--------------------------------------------------------------*/
KC3Ship.prototype.supportPower = function(){
if(this.isDummy()){ return 0; }
const fixedFP = this.estimateNakedStats("fp") - 1;
var supportPower = 0;
// for carrier series: CV, CVL, CVB
if(this.isCarrier()){
supportPower = fixedFP;
supportPower += this.equipmentTotalStats("raig");
supportPower += Math.floor(1.3 * this.equipmentTotalStats("baku"));
// will not attack if no dive/torpedo bomber equipped
if(supportPower === fixedFP){
supportPower = 0;
} else {
supportPower = Math.floor(1.5 * supportPower);
supportPower += 55;
}
} else {
// Explicit fire power bonus from equipment on specific ship taken into account:
// http://ja.kancolle.wikia.com/wiki/%E3%82%B9%E3%83%AC%E3%83%83%E3%83%89:2354#13
// so better to use current fp value from `api_karyoku` (including naked fp + all equipment fp),
// to avoid the case that fp bonus not accurately updated in time.
supportPower = 5 + this.fp[0] - 1;
// should be the same value with above if `equipmentTotalStats` works properly
//supportPower = 5 + fixedFP + this.equipmentTotalStats("houg");
}
return supportPower;
};
/**
* Get basic pre-cap shelling fire power of this ship (without pre-cap / post-cap modifiers).
*
* @param {number} combinedFleetFactor - additional power if ship is on a combined fleet.
* @param {boolean} isTargetLand - if the power is applied to a land-installation target.
* @return {number} computed fire power, return 0 if unavailable.
* @see http://kancolle.wikia.com/wiki/Damage_Calculation
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#ua92169d
*/
KC3Ship.prototype.shellingFirePower = function(combinedFleetFactor = 0, isTargetLand = false){
if(this.isDummy()) { return 0; }
let isCarrierShelling = this.isCarrier();
if(!isCarrierShelling) {
// Hayasui Kai gets special when any Torpedo Bomber equipped
isCarrierShelling = this.masterId === 352 && this.hasEquipmentType(2, 8);
}
let shellingPower = this.fp[0];
if(isCarrierShelling) {
if(isTargetLand) {
// https://wikiwiki.jp/kancolle/%E6%88%A6%E9%97%98%E3%81%AB%E3%81%A4%E3%81%84%E3%81%A6#od036af3
// https://wikiwiki.jp/kancolle/%E5%AF%BE%E5%9C%B0%E6%94%BB%E6%92%83#AGCalcCV
// TP from all Torpedo Bombers not taken into account, DV power counted,
// current TB with DV power: TBM-3W+3S
// Regular Dive Bombers make carrier cannot attack against land-installation,
// except following: Ju87C Kai, Prototype Nanzan, F4U-1D, FM-2, Ju87C Kai Ni (variants),
// Suisei Model 12 (634 Air Group w/Type 3 Cluster Bombs)
// DV power from items other than previous ones should not be counted
const tbBaku = this.equipmentTotalStats("baku", true, false, false, [8, 58]);
const dbBaku = this.equipmentTotalStats("baku", true, false, false, [7, 57],
KC3GearManager.antiLandDiveBomberIds);
shellingPower += Math.floor(1.3 * (tbBaku + dbBaku));
} else {
// Should limit to TP from equippable aircraft?
// TP visible bonus from Torpedo Bombers no effect.
// DV visible bonus not implemented yet, unknown.
shellingPower += this.equipmentTotalStats("raig", true, false);
shellingPower += Math.floor(1.3 * this.equipmentTotalStats("baku"), true, false);
}
shellingPower += combinedFleetFactor;
shellingPower += this.equipmentTotalImprovementBonus("airattack");
shellingPower = Math.floor(1.5 * shellingPower);
shellingPower += 50;
} else {
shellingPower += combinedFleetFactor;
shellingPower += this.equipmentTotalImprovementBonus("fire");
}
// 5 is attack power constant also used everywhere
shellingPower += 5;
return shellingPower;
};
/**
* Get pre-cap torpedo power of this ship.
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#n377a90c
*/
KC3Ship.prototype.shellingTorpedoPower = function(combinedFleetFactor = 0){
if(this.isDummy()) { return 0; }
return 5 + this.tp[0] + combinedFleetFactor +
this.equipmentTotalImprovementBonus("torpedo");
};
KC3Ship.prototype.isAswAirAttack = function(){
// check asw attack type, 1530 is Abyssal Submarine Ka-Class
return this.estimateDayAttackType(1530, false)[0] === "AirAttack";
};
/**
* Get pre-cap anti-sub power of this ship.
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#AntiSubmarine
*/
KC3Ship.prototype.antiSubWarfarePower = function(aswDiff = 0){
if(this.isDummy()) { return 0; }
const isAirAttack = this.isAswAirAttack();
const attackMethodConst = isAirAttack ? 8 : 13;
const nakedAsw = this.nakedAsw() + aswDiff;
// only asw stat from partial types of equipment taken into account
const equipmentTotalAsw = this.effectiveEquipmentTotalAsw(isAirAttack);
let aswPower = attackMethodConst;
aswPower += 2 * Math.sqrt(nakedAsw);
aswPower += 1.5 * equipmentTotalAsw;
aswPower += this.equipmentTotalImprovementBonus("asw");
// should move synergy modifier to pre-cap?
let synergyModifier = 1;
// new DC + DCP synergy (x1.1 / x1.25)
const isNewDepthChargeEquipped = this.equipment(true).some(g => g.isDepthCharge());
const isDepthChargeProjectorEquipped = this.equipment(true).some(g => g.isDepthChargeProjector());
if(isNewDepthChargeEquipped && isDepthChargeProjectorEquipped) {
// Large Sonar, like T0 Sonar, not counted here
const isSonarEquipped = this.hasEquipmentType(2, 14);
synergyModifier = isSonarEquipped ? 1.25 : 1.1;
}
// legacy all types of sonar + all DC(P) synergy (x1.15)
synergyModifier *= this.hasEquipmentType(3, 18) && this.hasEquipmentType(3, 17) ? 1.15 : 1;
aswPower *= synergyModifier;
return aswPower;
};
/**
* Get anti-installation power against all possible types of installations.
* Will choose meaningful installation types based on current equipment.
* Special attacks and battle conditions are not taken into consideration.
* @return {array} with element {Object} that has attributes:
* * enemy: Enemy ID to get icon
* * dayPower: Day attack power of ship
* * nightPower: Night attack power of ship
* * modifiers: Known anti-installation modifiers
* * damagedPowers: Day & night attack power tuple on Chuuha ship
* @see estimateInstallationEnemyType for kc3-unique installation types
*/
KC3Ship.prototype.shipPossibleAntiLandPowers = function(){
if(this.isDummy()) { return []; }
let possibleTypes = [];
const hasAntiLandRocket = this.hasEquipment([126, 346, 347, 348, 349]);
const hasT3Shell = this.hasEquipmentType(2, 18);
const hasLandingCraft = this.hasEquipmentType(2, [24, 46]);
// WG42 variants/landing craft-type eligible for all
if (hasAntiLandRocket || hasLandingCraft){
possibleTypes = [1, 2, 3, 4, 5, 6];
}
// T3 Shell eligible for all except Pillbox
else if(hasT3Shell){
possibleTypes = [1, 3, 4, 5, 6];
}
// Return empty if no anti-installation weapon found
else {
return [];
}
// Dummy target enemy IDs, also used for abyssal icons
// 1573: Harbor Princess, 1665: Artillery Imp, 1668: Isolated Island Princess
// 1656: Supply Depot Princess - Damaged, 1699: Summer Harbor Princess
// 1753: Summer Supply Depot Princess
const dummyEnemyList = [1573, 1665, 1668, 1656, 1699, 1753];
const basicPower = this.shellingFirePower();
const resultList = [];
// Fill damage lists for each enemy type
possibleTypes.forEach(installationType => {
const obj = {};
const dummyEnemy = dummyEnemyList[installationType - 1];
// Modifiers from special attacks, battle conditions are not counted for now
const fixedPreConds = ["Shelling", 1, undefined, [], false, false, dummyEnemy],
fixedPostConds = ["Shelling", [], 0, false, false, 0, false, dummyEnemy];
const {power: precap, antiLandModifier, antiLandAdditive} = this.applyPrecapModifiers(basicPower, ...fixedPreConds);
let {power} = this.applyPowerCap(precap, "Day", "Shelling");
const postcapInfo = this.applyPostcapModifiers(power, ...fixedPostConds);
power = postcapInfo.power;
obj.enemy = dummyEnemy;
obj.modifiers = {
antiLandModifier,
antiLandAdditive,
postCapAntiLandModifier: postcapInfo.antiLandModifier,
postCapAntiLandAdditive: postcapInfo.antiLandAdditive
};
obj.dayPower = Math.floor(power);
// Still use day time pre-cap shelling power, without TP value.
// Power constant +5 included, if assumes night contact is triggered.
power = precap;
({power} = this.applyPowerCap(power, "Night", "Shelling"));
({power} = this.applyPostcapModifiers(power, ...fixedPostConds));
obj.nightPower = Math.floor(power);
// Get Chuuha day attack power (in case of nuke setups)
fixedPreConds.push("chuuha");
const {power: damagedPrecap} = this.applyPrecapModifiers(basicPower, ...fixedPreConds);
({power} = this.applyPowerCap(damagedPrecap, "Day", "Shelling"));
({power} = this.applyPostcapModifiers(power, ...fixedPostConds));
obj.damagedPowers = [Math.floor(power)];
// Get Chuuha night power
({power} = this.applyPowerCap(damagedPrecap, "Night", "Shelling"));
({power} = this.applyPostcapModifiers(power, ...fixedPostConds));
obj.damagedPowers.push(Math.floor(power));
resultList.push(obj);
});
return resultList;
};
/**
* Calculate landing craft pre-cap/post-cap bonus depending on installation type.
* @param installationType - kc3-unique installation types
* @return {number} multiplier of landing craft
* @see estimateInstallationEnemyType
* @see http://kancolle.wikia.com/wiki/Partials/Anti-Installation_Weapons
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#antiground
*/
KC3Ship.prototype.calcLandingCraftBonus = function(installationType = 0){
if(this.isDummy() || ![1, 2, 3, 4, 5, 6].includes(installationType)) { return 0; }
// 6 types of Daihatsu Landing Craft with known bonus:
// * 167: Special Type 2 Amphibious Tank
// * 166: Daihatsu Landing Craft (Type 89 Medium Tank & Landing Force)
// * 68 : Daihatsu Landing Craft
// * 230: Toku Daihatsu Landing Craft + 11th Tank Regiment
// * 193: Toku Daihatsu Landing Craft (most bonuses unknown)
// * 355: M4A1 DD
const landingCraftIds = [167, 166, 68, 230, 193, 355];
const landingCraftCounts = landingCraftIds.map(id => this.countEquipment(id));
const landingModifiers = KC3GearManager.landingCraftModifiers[installationType - 1] || {};
const getModifier = (type, forImp = false) => (
(landingModifiers[forImp ? "improvement" : "modifier"] || [])[type] || (forImp ? 0 : 1)
);
let tankBonus = 1;
const landingBonus = [1], landingImprovementBonus = [0];
/**
* Highest landing craft modifier is selected
* (Speculative) Then, highest landing craft improvement modifier is selected
* If two or more of the same equipment present, take average improvement level
*
* Then, multiply total landing modifier with tank modifier
* There are some enemies with recorded modifiers for two or more of same equipment,
* those will take priority
*/
// Arrange equipment in terms of priority
landingCraftCounts.forEach((count, type) => {
let improvement = 0;
this.equipment().forEach((g, i) => {
if(g.exists() && g.masterId === landingCraftIds[type]) {
improvement += g.stars;
}
});
// Two (or more) Type 2 Tank bonus (Currently only for Supply Depot and Pillbox)
if(count > 1 && type === 0 && [2, 4].includes(installationType)) {
tankBonus = installationType === 2 ? 3.2 : 2.5;
tankBonus *= 1 + improvement / count / 30;
}
// Type 2 Tank bonus
else if(count > 0 && type === 0) {
tankBonus = getModifier(type) + getModifier(type, true) * improvement / count;
}
// Bonus for two Type 89 Tank (Pillbox, Supply Depot and Isolated Island)
else if(count > 1 && type === 1 && [2, 3, 4].includes(installationType)) {
landingBonus.push(installationType === 4 ? 2.08 : 3 );
landingImprovementBonus.push((installationType === 4 ? 0.0416 : 0.06) * improvement / count);
}
// Otherwise, match modifier and improvement
else if(count > 0) {
landingBonus.push(getModifier(type));
landingImprovementBonus.push(getModifier(type, true) * improvement / count);
}
});
// Multiply modifiers
return tankBonus * (Math.max(...landingBonus) + Math.max(...landingImprovementBonus));
};
/**
* Get anti land installation power bonus & multiplier of this ship.
* @param targetShipMasterId - target land installation master ID.
* @param precap - type of bonus, false for post-cap, pre-cap by default.
* @param warfareType - to indicate if use different modifiers for phases other than day shelling.
* @see https://kancolle.fandom.com/wiki/Combat/Installation_Type
* @see https://wikiwiki.jp/kancolle/%E5%AF%BE%E5%9C%B0%E6%94%BB%E6%92%83#AGBonus
* @see https://twitter.com/T3_1206/status/994258061081505792
* @see https://twitter.com/KennethWWKK/status/1045315639127109634
* @see https://yy406myon.hatenablog.jp/entry/2018/09/14/213114
* @see https://cdn.discordapp.com/attachments/425302689887289344/614879250419417132/ECrra66VUAAzYMc.jpg_orig.jpg
* @see https://github.com/Nishisonic/UnexpectedDamage/blob/master/UnexpectedDamage.js
* @see estimateInstallationEnemyType
* @see calcLandingCraftBonus
* @return {Array} of [additive damage boost, multiplicative damage boost, precap submarine additive, precap tank additive, precap m4a1dd multiplicative]
*/
KC3Ship.prototype.antiLandWarfarePowerMods = function(targetShipMasterId = 0, precap = true, warfareType = "Shelling"){
if(this.isDummy()) { return [0, 1]; }
const installationType = this.estimateInstallationEnemyType(targetShipMasterId, precap);
if(!installationType) { return [0, 1]; }
const wg42Count = this.countEquipment(126);
const mortarCount = this.countEquipment(346);
const mortarCdCount = this.countEquipment(347);
const type4RocketCount = this.countEquipment(348);
const type4RocketCdCount = this.countEquipment(349);
const hasT3Shell = this.hasEquipmentType(2, 18);
const alDiveBomberCount = this.countEquipment(KC3GearManager.antiLandDiveBomberIds);
let wg42Bonus = 1;
let type4RocketBonus = 1;
let mortarBonus = 1;
let t3Bonus = 1;
let apShellBonus = 1;
let seaplaneBonus = 1;
let alDiveBomberBonus = 1;
let airstrikeBomberBonus = 1;
const submarineBonus = this.isSubmarine() ? 30 : 0;
const landingBonus = this.calcLandingCraftBonus(installationType);
const shikonCount = this.countEquipment(230);
const m4a1ddCount = this.countEquipment(355);
const shikonBonus = 25 * (shikonCount + m4a1ddCount);
const m4a1ddModifier = m4a1ddCount ? 1.4 : 1;
if(precap) {
// [0, 70, 110, 140, 160] additive for each WG42 from PSVita KCKai, unknown for > 4
const wg42Additive = !wg42Count ? 0 : [0, 75, 110, 140, 160][wg42Count] || 160;
const type4RocketAdditive = !type4RocketCount ? 0 : [0, 55, 115, 160, 190][type4RocketCount] || 190;
const type4RocketCdAdditive = !type4RocketCdCount ? 0 : [0, 80, 170][type4RocketCdCount] || 170;
const mortarAdditive = !mortarCount ? 0 : [0, 30, 55, 75, 90][mortarCount] || 90;
const mortarCdAdditive = !mortarCdCount ? 0 : [0, 60, 110, 150][mortarCdCount] || 150;
const rocketsAdditive = wg42Additive + type4RocketAdditive + type4RocketCdAdditive + mortarAdditive + mortarCdAdditive;
switch(installationType) {
case 1: // Soft-skinned, general type of land installation
// 2.5x multiplicative for at least one T3
t3Bonus = hasT3Shell ? 2.5 : 1;
seaplaneBonus = this.hasEquipmentType(2, [11, 45]) ? 1.2 : 1;
wg42Bonus = [1, 1.3, 1.82][wg42Count] || 1.82;
type4RocketBonus = [1, 1.25, 1.25 * 1.5][type4RocketCount + type4RocketCdCount] || 1.875;
mortarBonus = [1, 1.2, 1.2 * 1.3][mortarCount + mortarCdCount] || 1.56;
return [rocketsAdditive,
t3Bonus * seaplaneBonus * wg42Bonus * type4RocketBonus * mortarBonus * landingBonus,
submarineBonus, shikonBonus, m4a1ddModifier];
case 2: // Pillbox, Artillery Imp
// Works even if slot is zeroed
seaplaneBonus = this.hasEquipmentType(2, [11, 45]) ? 1.5 : 1;
alDiveBomberBonus = [1, 1.5, 1.5 * 2.0][alDiveBomberCount] || 3;
// DD/CL bonus
const lightShipBonus = [2, 3].includes(this.master().api_stype) ? 1.4 : 1;
// Multiplicative WG42 bonus
wg42Bonus = [1, 1.6, 2.72][wg42Count] || 2.72;
type4RocketBonus = [1, 1.5, 1.5 * 1.8][type4RocketCount + type4RocketCdCount] || 2.7;
mortarBonus = [1, 1.3, 1.3 * 1.5][mortarCount + mortarCdCount] || 1.95;
apShellBonus = this.hasEquipmentType(2, 19) ? 1.85 : 1;
// Set additive modifier, multiply multiplicative modifiers
return [rocketsAdditive,
seaplaneBonus * alDiveBomberBonus * lightShipBonus
* wg42Bonus * type4RocketBonus * mortarBonus * apShellBonus * landingBonus,
submarineBonus, shikonBonus, m4a1ddModifier];
case 3: // Isolated Island Princess
alDiveBomberBonus = [1, 1.4, 1.4 * 1.75][alDiveBomberCount] || 2.45;
t3Bonus = hasT3Shell ? 1.75 : 1;
wg42Bonus = [1, 1.4, 2.1][wg42Count] || 2.1;
type4RocketBonus = [1, 1.3, 1.3 * 1.65][type4RocketCount + type4RocketCdCount] || 2.145;
mortarBonus = [1, 1.2, 1.2 * 1.4][mortarCount + mortarCdCount] || 1.68;
// Set additive modifier, multiply multiplicative modifiers
return [rocketsAdditive, alDiveBomberBonus * t3Bonus
* wg42Bonus * type4RocketBonus * mortarBonus * landingBonus,
0, shikonBonus, m4a1ddModifier];
case 5: // Summer Harbor Princess
seaplaneBonus = this.hasEquipmentType(2, [11, 45]) ? 1.3 : 1;
alDiveBomberBonus = [1, 1.3, 1.3 * 1.2][alDiveBomberCount] || 1.56;
wg42Bonus = [1, 1.4, 2.1][wg42Count] || 2.1;
t3Bonus = hasT3Shell ? 1.75 : 1;
type4RocketBonus = [1, 1.25, 1.25 * 1.4][type4RocketCount + type4RocketCdCount] || 1.75;
mortarBonus = [1, 1.1, 1.1 * 1.15][mortarCount + mortarCdCount] || 1.265;
apShellBonus = this.hasEquipmentType(2, 19) ? 1.3 : 1;
// Set additive modifier, multiply multiplicative modifiers
return [rocketsAdditive, seaplaneBonus * alDiveBomberBonus * t3Bonus
* wg42Bonus * type4RocketBonus * mortarBonus * apShellBonus * landingBonus,
0, shikonBonus, m4a1ddModifier];
}
} else { // Post-cap types
switch(installationType) {
case 2: // Pillbox, Artillery Imp
// Dive Bomber, Seaplane Bomber, LBAA, Jet Bomber on airstrike phase
airstrikeBomberBonus = warfareType === "Aerial" &&
this.hasEquipmentType(2, [7, 11, 47, 57]) ? 1.55 : 1;
return [0, airstrikeBomberBonus, 0, 0, 1];
case 3: // Isolated Island Princess
airstrikeBomberBonus = warfareType === "Aerial" &&
this.hasEquipmentType(2, [7, 11, 47, 57]) ? 1.7 : 1;
return [0, airstrikeBomberBonus, 0, 0, 1];
case 4: // Supply Depot Princess
wg42Bonus = [1, 1.45, 1.625][wg42Count] || 1.625;
type4RocketBonus = [1, 1.2, 1.2 * 1.4][type4RocketCount + type4RocketCdCount] || 1.68;
mortarBonus = [1, 1.15, 1.15 * 1.2][mortarCount + mortarCdCount] || 1.38;
return [0, wg42Bonus * type4RocketBonus * mortarBonus * landingBonus, 0, 0, 1];
case 6: // Summer Supply Depot Princess (shikon bonus only)
return [0, landingBonus, 0, 0, 1];
}
}
return [0, 1, 0, 0, 1];
};
/**
* Get post-cap airstrike power tuple of this ship.
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#b8c008fa
* @see KC3Gear.prototype.airstrikePower
*/
KC3Ship.prototype.airstrikePower = function(combinedFleetFactor = 0,
isJetAssaultPhase = false, contactPlaneId = 0, isCritical = false){
const totalPower = [0, 0, false];
if(this.isDummy()) { return totalPower; }
// no ex-slot by default since no plane can be equipped on ex-slot for now
this.equipment().forEach((gear, i) => {
if(this.slots[i] > 0 && gear.isAirstrikeAircraft()) {
const power = gear.airstrikePower(this.slots[i], combinedFleetFactor, isJetAssaultPhase);
const isRange = !!power[2];
const capped = [
this.applyPowerCap(power[0], "Day", "Aerial").power,
isRange ? this.applyPowerCap(power[1], "Day", "Aerial").power : 0
];
const postCapped = [
Math.floor(this.applyPostcapModifiers(capped[0], "Aerial", undefined, contactPlaneId, isCritical).power),
isRange ? Math.floor(this.applyPostcapModifiers(capped[1], "Aerial", undefined, contactPlaneId, isCritical).power) : 0
];
totalPower[0] += postCapped[0];
totalPower[1] += isRange ? postCapped[1] : postCapped[0];
totalPower[2] = totalPower[2] || isRange;
}
});
return totalPower;
};
/**
* Get pre-cap night battle power of this ship.
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#b717e35a
*/
KC3Ship.prototype.nightBattlePower = function(isNightContacted = false){
if(this.isDummy()) { return 0; }
// Night contact power bonus based on recon accuracy value: 1: 5, 2: 7, >=3: 9
// but currently only Type 98 Night Recon implemented (acc: 1), so always +5
return (isNightContacted ? 5 : 0) + this.fp[0] + this.tp[0]
+ this.equipmentTotalImprovementBonus("yasen");
};
/**
* Get pre-cap carrier night aerial attack power of this ship.
* This formula is the same with the one above besides slot bonus part and filtered equipment stats.
* @see http://kancolle.wikia.com/wiki/Damage_Calculation
* @see https://wikiwiki.jp/kancolle/%E6%88%A6%E9%97%98%E3%81%AB%E3%81%A4%E3%81%84%E3%81%A6#nightAS
* @see https://wikiwiki.jp/kancolle/%E5%AF%BE%E5%9C%B0%E6%94%BB%E6%92%83#AGCalcCVN
*/
KC3Ship.prototype.nightAirAttackPower = function(isNightContacted = false, isTargetLand = false){
if(this.isDummy()) { return 0; }
const equipTotals = {
fp: 0, tp: 0, dv: 0, slotBonus: 0, improveBonus: 0
};
// Generally, power from only night capable aircraft will be taken into account.
// For Ark Royal (Kai) + Swordfish - Night Aircraft (despite of NOAP), only Swordfish counted.
const isThisArkRoyal = [515, 393].includes(this.masterId);
const isLegacyArkRoyal = isThisArkRoyal && !this.canCarrierNightAirAttack();
this.equipment().forEach((gear, idx) => {
if(gear.exists()) {
const master = gear.master();
const slot = this.slots[idx];
const isNightAircraftType = KC3GearManager.nightAircraftType3Ids.includes(master.api_type[3]);
// Swordfish variants as special torpedo bombers
const isSwordfish = [242, 243, 244].includes(gear.masterId);
// Zero Fighter Model 62 (Fighter-bomber Iwai Squadron)
// Suisei Model 12 (Type 31 Photoelectric Fuze Bombs)
const isSpecialNightPlane = [154, 320].includes(gear.masterId);
const isNightPlane = isLegacyArkRoyal ? isSwordfish :
isNightAircraftType || isSwordfish || isSpecialNightPlane;
if(isNightPlane && slot > 0) {
equipTotals.fp += master.api_houg || 0;
if(!isTargetLand) equipTotals.tp += master.api_raig || 0;
equipTotals.dv += master.api_baku || 0;
if(!isLegacyArkRoyal) {
// Bonus from night aircraft slot which also takes bombing and asw stats into account
equipTotals.slotBonus += slot * (isNightAircraftType ? 3 : 0);
const ftbaPower = master.api_houg + master.api_raig + master.api_baku + master.api_tais;
equipTotals.slotBonus += Math.sqrt(slot) * ftbaPower * (isNightAircraftType ? 0.45 : 0.3);
}
equipTotals.improveBonus += gear.attackPowerImprovementBonus("yasen");
}
}
});
// No effect for both visible fp and tp bonus
let shellingPower = this.estimateNakedStats("fp");
shellingPower += equipTotals.fp + equipTotals.tp + equipTotals.dv;
shellingPower += equipTotals.slotBonus;
shellingPower += equipTotals.improveBonus;
shellingPower += isNightContacted ? 5 : 0;
return shellingPower;
};
/**
* Apply known pre-cap modifiers to attack power.
* @return {Object} capped power and applied modifiers.
* @see http://kancolle.wikia.com/wiki/Damage_Calculation
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#beforecap
* @see https://twitter.com/Nishisonic/status/893030749913227264
*/
KC3Ship.prototype.applyPrecapModifiers = function(basicPower, warfareType = "Shelling",
engagementId = 1, formationId = ConfigManager.aaFormation, nightSpecialAttackType = [],
isNightStart = false, isCombined = false, targetShipMasterId = 0,
damageStatus = this.damageStatus()){
// Engagement modifier
let engagementModifier = (warfareType === "Aerial" ? [] : [0, 1, 0.8, 1.2, 0.6])[engagementId] || 1;
// Formation modifier, about formation IDs:
// ID 1~5: Line Ahead / Double Line / Diamond / Echelon / Line Abreast
// ID 6: new Vanguard formation since 2017-11-17
// ID 11~14: 1st anti-sub / 2nd forward / 3rd diamond / 4th battle
// 0 are placeholders for non-exists ID
let formationModifier = (
warfareType === "Antisub" ?
[0, 0.6, 0.8, 1.2, 1.1 , 1.3, 1, 0, 0, 0, 0, 1.3, 1.1, 1 , 0.7] :
warfareType === "Shelling" ?
[0, 1 , 0.8, 0.7, 0.75, 0.6, 1, 0, 0, 0, 0, 0.8, 1 , 0.7, 1.1] :
warfareType === "Torpedo" ?
[0, 1 , 0.8, 0.7, 0.6 , 0.6, 1, 0, 0, 0, 0, 0.8, 1 , 0.7, 1.1] :
// other warefare types like Aerial Opening Airstrike not affected
[]
)[formationId] || 1;
// TODO Any side Echelon vs Combined Fleet, shelling mod is 0.6?
// Modifier of vanguard formation depends on the position in the fleet
if(formationId === 6) {
const [shipPos, shipCnt] = this.fleetPosition();
// Vanguard formation needs 4 ships at least, fake ID make no sense
if(shipCnt >= 4) {
// Guardian ships counted from 3rd or 4th ship
const isGuardian = shipPos >= Math.floor(shipCnt / 2);
if(warfareType === "Shelling") {
formationModifier = isGuardian ? 1 : 0.5;
} else if(warfareType === "Antisub") {
formationModifier = isGuardian ? 0.6 : 1;
}
}
}
// Non-empty attack type tuple means this supposed to be night battle
const isNightBattle = nightSpecialAttackType.length > 0;
const canNightAntisub = warfareType === "Antisub" && (isNightStart || isCombined);
// No engagement and formation modifier except night starts / combined ASW attack
// Vanguard still applies for night battle
if(isNightBattle && !canNightAntisub) {
engagementModifier = 1;
formationModifier = formationId !== 6 ? 1 : formationModifier;
}
// Damage percent modifier
// http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#m8aa1749
const damageModifier = (!isNightBattle && warfareType === "Torpedo" ? {
// Day time only affect Opening Torpedo in fact, Chuuha cannot Closing at all
// Night time unmentioned, assume to be the same with shelling
"chuuha": 0.8,
"taiha": 0.0
} : (isNightBattle && warfareType === "Torpedo") || warfareType === "Shelling" || warfareType === "Antisub" ? {
"chuuha": 0.7,
"taiha": 0.4
} : // Aerial Opening Airstrike not affected
{})[damageStatus] || 1;
// Night special attack modifier, should not x2 although some types attack 2 times
const nightCutinModifier = nightSpecialAttackType[0] === "Cutin" &&
nightSpecialAttackType[3] > 0 ? nightSpecialAttackType[3] : 1;
// Anti-installation modifiers
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
let antiLandAdditive = 0, antiLandModifier = 1, subAntiLandAdditive = 0, tankAdditive = 0, tankModifier = 1;
if(targetShipType.isLand) {
[antiLandAdditive, antiLandModifier, subAntiLandAdditive, tankAdditive, tankModifier] = this.antiLandWarfarePowerMods(targetShipMasterId, true, warfareType);
}
// Apply modifiers, flooring unknown, multiply and add anti-land modifiers first
let result = (((basicPower + subAntiLandAdditive) * antiLandModifier + tankAdditive) * tankModifier + antiLandAdditive)
* engagementModifier * formationModifier * damageModifier * nightCutinModifier;
// Light Cruiser fit gun bonus, should not applied before modifiers
const stype = this.master().api_stype;
const ctype = this.master().api_ctype;
const isThisLightCruiser = [2, 3, 21].includes(stype);
let lightCruiserBonus = 0;
if(isThisLightCruiser && warfareType !== "Antisub") {
// 14cm, 15.2cm
const singleMountCnt = this.countEquipment([4, 11]);
const twinMountCnt = this.countEquipment([65, 119, 139]);
lightCruiserBonus = Math.sqrt(singleMountCnt) + 2 * Math.sqrt(twinMountCnt);
result += lightCruiserBonus;
}
// Italian Heavy Cruiser (Zara class) fit gun bonus
const isThisZaraClass = ctype === 64;
let italianHeavyCruiserBonus = 0;
if(isThisZaraClass) {
// 203mm/53
const itaTwinMountCnt = this.countEquipment(162);
italianHeavyCruiserBonus = Math.sqrt(itaTwinMountCnt);
result += italianHeavyCruiserBonus;
}
// Night battle anti-sub regular battle condition forced to no damage
const aswLimitation = isNightBattle && warfareType === "Antisub" && !canNightAntisub ? 0 : 1;
result *= aswLimitation;
return {
power: result,
engagementModifier,
formationModifier,
damageModifier,
nightCutinModifier,
antiLandModifier,
antiLandAdditive,
lightCruiserBonus,
italianHeavyCruiserBonus,
aswLimitation,
};
};
/**
* Apply cap to attack power according warfare phase.
* @param {number} precapPower - pre-cap power, see applyPrecapModifiers
* @return {Object} capped power, cap value and is capped flag.
* @see http://kancolle.wikia.com/wiki/Damage_Calculation
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#k5f74647
*/
KC3Ship.prototype.applyPowerCap = function(precapPower,
time = "Day", warfareType = "Shelling"){
const cap = time === "Night" ? 300 :
// increased from 150 to 180 since 2017-03-18
warfareType === "Shelling" ? 180 :
// increased from 100 to 150 since 2017-11-10
warfareType === "Antisub" ? 150 :
150; // default cap for other phases
const isCapped = precapPower > cap;
const power = Math.floor(isCapped ? cap + Math.sqrt(precapPower - cap) : precapPower);
return {
power,
cap,
isCapped
};
};
/**
* Apply known post-cap modifiers to capped attack power.
* @return {Object} capped power and applied modifiers.
* @see http://kancolle.wikia.com/wiki/Damage_Calculation
* @see http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#aftercap
* @see https://github.com/Nishisonic/UnexpectedDamage/blob/master/UnexpectedDamage.js
*/
KC3Ship.prototype.applyPostcapModifiers = function(cappedPower, warfareType = "Shelling",
daySpecialAttackType = [], contactPlaneId = 0, isCritical = false, isAirAttack = false,
targetShipStype = 0, isDefenderArmorCounted = false, targetShipMasterId = 0){
// Artillery spotting modifier, should not x2 although some types attack 2 times
const dayCutinModifier = daySpecialAttackType[0] === "Cutin" && daySpecialAttackType[3] > 0 ?
daySpecialAttackType[3] : 1;
let airstrikeConcatModifier = 1;
// Contact modifier only applied to aerial warfare airstrike power
if(warfareType === "Aerial" && contactPlaneId > 0) {
const contactPlaneAcc = KC3Master.slotitem(contactPlaneId).api_houm;
airstrikeConcatModifier = contactPlaneAcc >= 3 ? 1.2 :
contactPlaneAcc >= 2 ? 1.17 : 1.12;
}
const isNightBattle = daySpecialAttackType.length === 0;
let apshellModifier = 1;
// AP Shell modifier applied to specific target ship types:
// CA, CAV, BB, FBB, BBV, CV, CVB and Land installation
const isTargetShipTypeMatched = [5, 6, 8, 9, 10, 11, 18].includes(targetShipStype);
if(isTargetShipTypeMatched && !isNightBattle) {
const mainGunCnt = this.countEquipmentType(2, [1, 2, 3]);
const apShellCnt = this.countEquipmentType(2, 19);
const secondaryCnt = this.countEquipmentType(2, 4);
const radarCnt = this.countEquipmentType(2, [12, 13]);
if(mainGunCnt >= 1 && secondaryCnt >= 1 && apShellCnt >= 1)
apshellModifier = 1.15;
else if(mainGunCnt >= 1 && apShellCnt >= 1 && radarCnt >= 1)
apshellModifier = 1.1;
else if(mainGunCnt >= 1 && apShellCnt >= 1)
apshellModifier = 1.08;
}
// Standard critical modifier
const criticalModifier = isCritical ? 1.5 : 1;
// Additional aircraft proficiency critical modifier
// Applied to open airstrike and shelling air attack including anti-sub
let proficiencyCriticalModifier = 1;
if(isCritical && (isAirAttack || warfareType === "Aerial")) {
if(daySpecialAttackType[0] === "Cutin" && daySpecialAttackType[1] === 7) {
// special proficiency critical modifier for CVCI
// http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#FAcutin
const firstSlotType = (cutinType => {
switch(cutinType) {
case "CutinFDBTB": return [6, 7, 8];
case "CutinDBDBTB":
case "CutinDBTB": return [7, 8];
default: return [];
}
})(daySpecialAttackType[2]);
const hasNonZeroSlotCaptainPlane = (type2Ids) => {
const firstGear = this.equipment(0);
return this.slots[0] > 0 && firstGear.exists() &&
type2Ids.includes(firstGear.master().api_type[2]);
};
// detail modifier affected by (internal) proficiency under verification
// might be an average value from participants, simply use max modifier (+0.1 / +0.25) here
proficiencyCriticalModifier += 0.1;
proficiencyCriticalModifier += hasNonZeroSlotCaptainPlane(firstSlotType) ? 0.15 : 0;
} else {
// http://wikiwiki.jp/kancolle/?%B4%CF%BA%DC%B5%A1%BD%CF%CE%FD%C5%D9#v3f6d8dd
const expBonus = [0, 1, 2, 3, 4, 5, 7, 10];
this.equipment().forEach((g, i) => {
if(g.isAirstrikeAircraft()) {
const aceLevel = g.ace || 0;
const internalExpLow = KC3Meta.airPowerInternalExpBounds(aceLevel)[0];
let mod = Math.floor(Math.sqrt(internalExpLow) + (expBonus[aceLevel] || 0)) / 100;
if(i > 0) mod /= 2;
proficiencyCriticalModifier += mod;
}
});
}
}
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
// Against PT Imp modifier
let antiPtImpModifier = 1;
if(targetShipType.isPtImp) {
const lightGunBonus = this.countEquipmentType(2, 1) >= 2 ? 1.2 : 1;
const aaGunBonus = this.countEquipmentType(2, 21) >= 2 ? 1.1 : 1;
const secondaryGunBonus = this.countEquipmentType(2, 4) >= 2 ? 1.2 : 1;
const t3Bonus = this.hasEquipmentType(2, 18) ? 1.3 : 1;
antiPtImpModifier = lightGunBonus * aaGunBonus * secondaryGunBonus * t3Bonus;
}
// Anti-installation modifier
let antiLandAdditive = 0, antiLandModifier = 1;
if(targetShipType.isLand) {
[antiLandAdditive, antiLandModifier] = this.antiLandWarfarePowerMods(targetShipMasterId, false, warfareType);
}
// About rounding and position of anti-land modifier:
// http://ja.kancolle.wikia.com/wiki/%E3%82%B9%E3%83%AC%E3%83%83%E3%83%89:925#33
let result = Math.floor(Math.floor(
Math.floor(cappedPower * antiLandModifier + antiLandAdditive) * apshellModifier
) * criticalModifier * proficiencyCriticalModifier
) * dayCutinModifier * airstrikeConcatModifier
* antiPtImpModifier;
// New Depth Charge armor penetration, not attack power bonus
let newDepthChargeBonus = 0;
if(warfareType === "Antisub") {
const type95ndcCnt = this.countEquipment(226);
const type2ndcCnt = this.countEquipment(227);
if(type95ndcCnt > 0 || type2ndcCnt > 0) {
const deShipBonus = this.master().api_stype === 1 ? 1 : 0;
newDepthChargeBonus =
type95ndcCnt * (Math.sqrt(KC3Master.slotitem(226).api_tais - 2) + deShipBonus) +
type2ndcCnt * (Math.sqrt(KC3Master.slotitem(227).api_tais - 2) + deShipBonus);
// Applying this to enemy submarine's armor, result will be capped to at least 1
if(isDefenderArmorCounted) result += newDepthChargeBonus;
}
}
// Remaining ammo percent modifier, applied to final damage, not only attack power
const ammoPercent = Math.floor(this.ammo / this.master().api_bull_max * 100);
const remainingAmmoModifier = ammoPercent >= 50 ? 1 : ammoPercent * 2 / 100;
if(isDefenderArmorCounted) {
result *= remainingAmmoModifier;
}
return {
power: result,
criticalModifier,
proficiencyCriticalModifier,
dayCutinModifier,
airstrikeConcatModifier,
apshellModifier,
antiPtImpModifier,
antiLandAdditive,
antiLandModifier,
newDepthChargeBonus,
remainingAmmoModifier,
};
};
/**
* Collect battle conditions from current battle node if available.
* Do not fall-back to default value here if not available, leave it to appliers.
* @return {Object} an object contains battle properties we concern at.
* @see CalculatorManager.collectBattleConditions
*/
KC3Ship.prototype.collectBattleConditions = function(){
return KC3Calc.collectBattleConditions();
};
/**
* @return extra power bonus for combined fleet battle.
* @see http://wikiwiki.jp/kancolle/?%CF%A2%B9%E7%B4%CF%C2%E2#offense
*/
KC3Ship.prototype.combinedFleetPowerBonus = function(playerCombined, enemyCombined,
warfareType = "Shelling"){
const powerBonus = {
main: 0, escort: 0
};
switch(warfareType) {
case "Shelling":
if(!enemyCombined) {
// CTF
if(playerCombined === 1) { powerBonus.main = 2; powerBonus.escort = 10; }
// STF
if(playerCombined === 2) { powerBonus.main = 10; powerBonus.escort = -5; }
// TCF
if(playerCombined === 3) { powerBonus.main = -5; powerBonus.escort = 10; }
} else {
if(playerCombined === 1) { powerBonus.main = 2; powerBonus.escort = -5; }
if(playerCombined === 2) { powerBonus.main = 2; powerBonus.escort = -5; }
if(playerCombined === 3) { powerBonus.main = -5; powerBonus.escort = -5; }
if(!playerCombined) { powerBonus.main = 5; powerBonus.escort = 5; }
}
break;
case "Torpedo":
if(playerCombined) {
if(!enemyCombined) {
powerBonus.main = -5; powerBonus.escort = -5;
} else {
powerBonus.main = 10; powerBonus.escort = 10;
}
}
break;
case "Aerial":
if(!playerCombined && enemyCombined) {
// differentiated by target enemy fleet, targeting main:
powerBonus.main = -10; powerBonus.escort = -10;
// targeting escort:
//powerBonus.main = -20; powerBonus.escort = -20;
}
break;
}
return powerBonus;
};
// Check if specified equipment (or equip type) can be equipped on this ship.
KC3Ship.prototype.canEquip = function(gearMstId, gearType2) {
return KC3Master.equip_on_ship(this.masterId, gearMstId, gearType2);
};
// check if this ship is capable of equipping Amphibious Tank (Ka-Mi tank only for now, no landing craft variants)
KC3Ship.prototype.canEquipTank = function() {
if(this.isDummy()) { return false; }
return KC3Master.equip_type(this.master().api_stype, this.masterId).includes(46);
};
// check if this ship is capable of equipping Daihatsu (landing craft variants, amphibious tank not counted)
KC3Ship.prototype.canEquipDaihatsu = function() {
if(this.isDummy()) { return false; }
const master = this.master();
// Phase2 method: lookup Daihatsu type2 ID 24 in her master equip types
return KC3Master.equip_type(master.api_stype, this.masterId).includes(24);
// Phase1 method:
/*
// ship types: DD=2, CL=3, BB=9, AV=16, LHA=17, AO=22
// so far only ships with types above can equip daihatsu.
if ([2,3,9,16,17,22].indexOf( master.api_stype ) === -1)
return false;
// excluding Akitsushima(445), Hayasui(460), Commandant Teste(491), Kamoi(162)
// (however their remodels are capable of equipping daihatsu
if ([445, 460, 491, 162].indexOf( this.masterId ) !== -1)
return false;
// only few DDs, CLs and 1 BB are capable of equipping daihatsu
// see comments below.
if ([2, 3, 9].indexOf( master.api_stype ) !== -1 &&
[
// Abukuma K2(200), Tatsuta K2(478), Kinu K2(487), Yura K2(488), Tama K2(547)
200, 478, 487, 488, 547,
// Satsuki K2(418), Mutsuki K2(434), Kisaragi K2(435), Fumizuki(548)
418, 434, 435, 548,
// Kasumi K2(464), Kasumi K2B(470), Arare K2 (198), Ooshio K2(199), Asashio K2D(468), Michishio K2(489), Arashio K2(490)
464, 470, 198, 199, 468, 489, 490,
// Verniy(147), Kawakaze K2(469), Murasame K2(498)
147, 469, 498,
// Nagato K2(541)
541
].indexOf( this.masterId ) === -1)
return false;
return true;
*/
};
/**
* @return true if this ship is capable of equipping (Striking Force) Fleet Command Facility.
*/
KC3Ship.prototype.canEquipFCF = function() {
if(this.isDummy()) { return false; }
const masterId = this.masterId,
stype = this.master().api_stype;
// Phase2 method: lookup FCF type2 ID 34 in her master equip types
return KC3Master.equip_type(stype, masterId).includes(34);
// Phase1 method:
/*
// Excluding DE, DD, XBB, SS, SSV, AO, AR, which can be found at master.stype.api_equip_type[34]
const capableStypes = [3, 4, 5, 6, 7, 8, 9, 10, 11, 16, 17, 18, 20, 21];
// These can be found at `RemodelMain.swf/scene.remodel.view.changeEquip._createType3List()`
// DD Kasumi K2, DD Shiratsuyu K2, DD Murasame K2, AO Kamoi Kai-Bo, DD Yuugumo K2, DD Naganami K2, DD Shiranui K2
const capableShips = [464, 497, 498, 500, 542, 543, 567];
// CVL Kasugamaru
const incapableShips = [521];
return incapableShips.indexOf(masterId) === -1 &&
(capableShips.includes(masterId) || capableStypes.includes(stype));
*/
};
// is this ship able to do OASW unconditionally
KC3Ship.prototype.isOaswShip = function() {
// Isuzu K2, Tatsuta K2, Jervis Kai, Janus Kai, Samuel B.Roberts Kai, Fletcher-Class, Yuubari K2D
return [141, 478, 394, 893, 681, 562, 689, 596, 624, 628, 629, 692].includes(this.masterId);
};
/**
* Test to see if this ship (with equipment) is capable of opening ASW. References:
* @see https://kancolle.fandom.com/wiki/Partials/Opening_ASW
* @see https://wikiwiki.jp/kancolle/%E5%AF%BE%E6%BD%9C%E6%94%BB%E6%92%83#oasw
*/
KC3Ship.prototype.canDoOASW = function (aswDiff = 0) {
if(this.isDummy()) { return false; }
if(this.isOaswShip()) { return true; }
const stype = this.master().api_stype;
const isEscort = stype === 1;
const isLightCarrier = stype === 7;
// is CVE? (Taiyou-class series, Gambier Bay series, Zuihou K2B)
const isEscortLightCarrier = this.isEscortLightCarrier();
// is regular ASW method not supposed to depth charge attack? (CAV, BBV, AV, LHA)
// but unconfirmed for AO and Hayasui Kai
const isAirAntiSubStype = [6, 10, 16, 17].includes(stype);
// is Sonar equipped? also counted large one: Type 0 Sonar
const hasSonar = this.hasEquipmentType(1, 10);
const isHyuugaKaiNi = this.masterId === 554;
const isKagaK2Go = this.masterId === 646;
// lower condition for DE and CVL, even lower if equips Sonar
const aswThreshold = isLightCarrier && hasSonar ? 50
: isEscort ? 60
// May apply to CVL, but only CVE can reach 65 for now (Zuihou K2 modded asw +9 +13x4 = 61)
: isEscortLightCarrier ? 65
// Kaga Kai Ni Go asw starts from 82 on Lv84, let her pass just like Hyuuga K2
: isKagaK2Go ? 80
// Hyuuga Kai Ni can OASW even asw < 100, but lower threshold unknown,
// guessed from her Lv90 naked asw 79 + 12 (1x helicopter, without bonus and mod)
: isHyuugaKaiNi ? 90
: 100;
// ship stats not updated in time when equipment changed, so take the diff if necessary,
// and explicit asw bonus from Sonars taken into account confirmed.
const shipAsw = this.as[0] + aswDiff
// Visible asw bonus from Fighters, Dive Bombers and Torpedo Bombers still not counted,
// confirmed since 2019-06-29: https://twitter.com/trollkin_ball/status/1144714377024532480
// 2019-08-08: https://wikiwiki.jp/kancolle/%E5%AF%BE%E6%BD%9C%E6%94%BB%E6%92%83#trigger_conditions
// but bonus from other aircraft like Rotorcraft not (able to be) confirmed,
// perhaps a similar logic to exclude some types of equipment, see #effectiveEquipmentTotalAsw
// Green (any small?) gun (DE +1 asw from 12cm Single High-angle Gun Mount Model E) not counted,
// confirmed since 2020-06-19: https://twitter.com/99_999999999/status/1273937773225893888
- this.equipmentTotalStats("tais", true, true, true, [1, 6, 7, 8]);
// shortcut on the stricter condition first
if (shipAsw < aswThreshold)
return false;
// For CVE like Taiyou-class, initial asw stat is high enough to reach 50 / 65,
// but for Kasugamaru, since not possible to reach high asw for now, tests are not done.
// For Taiyou-class Kai/Kai Ni, any equippable aircraft with asw should work.
// only Autogyro or PBY equipped will not let CVL anti-sub in day shelling phase,
// but OASW still can be performed. only Sonar equipped can do neither.
// For other CVL possible but hard to reach 50 asw and do OASW with Sonar and high ASW aircraft.
// For CV Kaga K2Go, can perform OASW with any asw aircraft like Taiyou-class Kai+:
// https://twitter.com/noobcyan/status/1299886834919510017
if(isLightCarrier || isKagaK2Go) {
const isTaiyouKaiAfter = RemodelDb.remodelGroup(521).indexOf(this.masterId) > 1
|| RemodelDb.remodelGroup(534).indexOf(this.masterId) > 0;
const hasAswAircraft = this.equipment(true).some(gear => gear.isAswAircraft(false));
return ((isTaiyouKaiAfter || isKagaK2Go) && hasAswAircraft) // ship visible asw irrelevant
|| this.equipment(true).some(gear => gear.isHighAswBomber(false)) // mainly asw 50/65, dive bomber not work
|| (shipAsw >= 100 && hasSonar && hasAswAircraft); // almost impossible for pre-marriage
}
// DE can OASW without Sonar, but total asw >= 75 and equipped total plus asw >= 4
if(isEscort) {
if(hasSonar) return true;
const equipAswSum = this.equipmentTotalStats("tais");
return shipAsw >= 75 && equipAswSum >= 4;
}
// Hyuuga Kai Ni can OASW with 2 Autogyro or 1 Helicopter,
// but her initial naked asw too high to verify the lower threshold.
// Fusou-class Kai Ni can OASW with at least 1 Helicopter + Sonar and asw >= 100.
// https://twitter.com/cat_lost/status/1146075888636710912
// Hyuuga Kai Ni cannot OASW with Sonar only, just like BBV cannot ASW with Depth Charge.
// perhaps all AirAntiSubStype doesn't even they can equip Sonar and asw >= 100?
// at least 1 slot of ASW capable aircraft needed.
if(isAirAntiSubStype) {
return (isHyuugaKaiNi || hasSonar) &&
(this.countEquipmentType(1, 15) >= 2 ||
this.countEquipmentType(1, 44) >= 1);
}
// for other ship types who can do ASW with Depth Charge
return hasSonar;
};
/**
* @return true if this ship can do ASW attack.
*/
KC3Ship.prototype.canDoASW = function(time = "Day") {
if(this.isDummy() || this.isAbsent()) { return false; }
const stype = this.master().api_stype;
const isHayasuiKaiWithTorpedoBomber = this.masterId === 352 && this.hasEquipmentType(2, 8);
const isKagaK2Go = this.masterId === 646;
// CAV, CVL, BBV, AV, LHA, CVL-like Hayasui Kai, Kaga Kai Ni Go
const isAirAntiSubStype = [6, 7, 10, 16, 17].includes(stype) || isHayasuiKaiWithTorpedoBomber || isKagaK2Go;
if(isAirAntiSubStype) {
// CV Kaga Kai Ni Go implemented since 2020-08-27, can do ASW under uncertained conditions (using CVL's currently),
// but any CV form (converted back from K2Go) may ASW either if her asw modded > 0, fixed on the next day
// see https://twitter.com/Synobicort_SC/status/1298998245893394432
const isCvlLike = stype === 7 || isHayasuiKaiWithTorpedoBomber || isKagaK2Go;
// At night, most ship types cannot do ASW,
// only CVL can ASW with depth charge if naked asw is not 0 and not taiha,
// even no plane equipped or survived, such as Taiyou Kai Ni, Hayasui Kai.
// but CVE will attack surface target first if NCVCI met.
// *Some Abyssal AV/BBV can do ASW with air attack at night.
if(time === "Night") return isCvlLike && !this.isTaiha() && this.as[1] > 0;
// For day time, false if CVL or CVL-like chuuha
if(isCvlLike && this.isStriped()) return false;
// and if ASW plane equipped and its slot > 0
return this.equipment().some((g, i) => this.slots[i] > 0 && g.isAswAircraft(isCvlLike));
}
// DE, DD, CL, CLT, CT, AO(*)
// *AO: Hayasui base form and Kamoi Kai-Bo can only depth charge, Kamoi base form cannot asw
const isAntiSubStype = [1, 2, 3, 4, 21, 22].includes(stype);
// if max ASW stat before marriage (Lv99) not 0, can do ASW,
// which also used at `Core.swf/vo.UserShipData.hasTaisenAbility()`
// if as[1] === 0, naked asw stat should be 0, but as[0] may not.
return isAntiSubStype && this.as[1] > 0;
};
/**
* @return true if this ship can do opening torpedo attack.
*/
KC3Ship.prototype.canDoOpeningTorpedo = function() {
if(this.isDummy() || this.isAbsent()) { return false; }
const hasKouhyouteki = this.hasEquipmentType(2, 22);
const isThisSubmarine = this.isSubmarine();
return hasKouhyouteki || (isThisSubmarine && this.level >= 10);
};
/**
* @return {Object} target (enemy) ship category flags defined by us, possible values are:
* `isSurface`, `isSubmarine`, `isLand`, `isPtImp`.
*/
KC3Ship.prototype.estimateTargetShipType = function(targetShipMasterId = 0) {
const targetShip = KC3Master.ship(targetShipMasterId);
// land installation
const isLand = targetShip && targetShip.api_soku === 0;
const isSubmarine = targetShip && this.isSubmarine(targetShip.api_stype);
// regular surface vessel by default
const isSurface = !isLand && !isSubmarine;
// known PT Imp Packs (also belong to surface)
const isPtImp = [1637, 1638, 1639, 1640].includes(targetShipMasterId);
return {
isSubmarine,
isLand,
isSurface,
isPtImp,
};
};
/**
* Divide Abyssal land installation into KC3-unique types:
* Type 0: Not land installation
* Type 1: Soft-skinned, eg: Harbor Princess
* Type 2: Artillery Imp, aka. Pillbox
* Type 3: Isolated Island Princess
* Type 4: Supply Depot Princess
* Type 5: Summer Harbor Princess
* Type 6: Summer Supply Depot Princess
* @param precap - specify true if going to calculate pre-cap modifiers
* @return the numeric type identifier
* @see http://kancolle.wikia.com/wiki/Installation_Type
*/
KC3Ship.prototype.estimateInstallationEnemyType = function(targetShipMasterId = 0, precap = true){
const targetShip = KC3Master.ship(targetShipMasterId);
if(!this.masterId || !targetShip) { return 0; }
if(!this.estimateTargetShipType(targetShipMasterId).isLand) { return 0; }
// Supply Depot Princess
if([1653, 1654, 1655, 1656, 1657, 1658,
1921, 1922, 1923, 1924, 1925, 1926, // B
1933, 1934, 1935, 1936, 1937, 1938 // B Summer Landing Mode
].includes(targetShipMasterId)) {
// Unique case: takes soft-skinned pre-cap but unique post-cap
return precap ? 1 : 4;
}
// Summer Supply Depot Princess
if([1753, 1754].includes(targetShipMasterId)) {
// Same unique case as above
return precap ? 1 : 6;
}
const abyssalIdTypeMap = {
// Summer Harbor Princess
"1699": 5, "1700": 5, "1701": 5, "1702": 5, "1703": 5, "1704": 5,
// Isolated Island Princess
"1668": 3, "1669": 3, "1670": 3, "1671": 3, "1672": 3,
// Artillery Imp
"1665": 2, "1666": 2, "1667": 2,
};
return abyssalIdTypeMap[targetShipMasterId] || 1;
};
/**
* @return false if this ship (and target ship) can attack at day shelling phase.
*/
KC3Ship.prototype.canDoDayShellingAttack = function(targetShipMasterId = 0) {
if(this.isDummy() || this.isAbsent()) { return false; }
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
const isThisSubmarine = this.isSubmarine();
const isHayasuiKaiWithTorpedoBomber = this.masterId === 352 && this.hasEquipmentType(2, 8);
const isThisCarrier = this.isCarrier() || isHayasuiKaiWithTorpedoBomber;
if(isThisCarrier) {
if(this.isTaiha()) return false;
const isNotCvb = this.master().api_stype !== 18;
if(isNotCvb && this.isStriped()) return false;
if(targetShipType.isSubmarine) return this.canDoASW();
// can not attack land installation if dive bomber equipped, except some exceptions
if(targetShipType.isLand && this.equipment().some((g, i) => this.slots[i] > 0 &&
g.master().api_type[2] === 7 &&
!KC3GearManager.antiLandDiveBomberIds.includes(g.masterId)
)) return false;
// can not attack if no bomber with slot > 0 equipped
return this.equipment().some((g, i) => this.slots[i] > 0 && g.isAirstrikeAircraft());
}
// submarines can only landing attack against land installation
if(isThisSubmarine) return this.estimateLandingAttackType(targetShipMasterId) > 0;
// can attack any enemy ship type by default
return true;
};
/**
* @return true if this ship (and target ship) can do closing torpedo attack.
*/
KC3Ship.prototype.canDoClosingTorpedo = function(targetShipMasterId = 0) {
if(this.isDummy() || this.isAbsent()) { return false; }
if(this.isStriped()) return false;
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
if(targetShipType.isSubmarine || targetShipType.isLand) return false;
// DD, CL, CLT, CA, CAV, FBB, BB, BBV, SS, SSV, AV, CT
const isTorpedoStype = [2, 3, 4, 5, 6, 8, 9, 10, 13, 14, 16, 21].includes(this.master().api_stype);
return isTorpedoStype && this.estimateNakedStats("tp") > 0;
};
/**
* Conditions under verification, known for now:
* Flagship is healthy Nelson, Double Line variants formation selected.
* Min 6 ships fleet needed, main fleet only for Combined Fleet.
* 3rd, 5th ship not carrier, no any submarine in fleet.
* No AS/AS+ air battle needed like regular Artillery Spotting.
*
* No PvP sample found for now.
* Can be triggered in 1 battle per sortie, max 3 chances to roll (if yasen).
*
* @return true if this ship (Nelson) can do Nelson Touch cut-in attack.
* @see http://kancolle.wikia.com/wiki/Nelson
* @see https://wikiwiki.jp/kancolle/Nelson
*/
KC3Ship.prototype.canDoNelsonTouch = function() {
if(this.isDummy() || this.isAbsent()) { return false; }
// is this ship Nelson and not even Chuuha
// still okay even 3th and 5th ship are Taiha
if(KC3Meta.nelsonTouchShips.includes(this.masterId) && !this.isStriped()) {
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
// Nelson is flagship of a fleet, which min 6 ships needed
if(fleetNum > 0 && shipPos === 0 && shipCnt >= 6
// not in any escort fleet of Combined Fleet
&& (!PlayerManager.combinedFleet || fleetNum !== 2)) {
// Double Line variants selected
const isDoubleLine = [2, 12].includes(
this.collectBattleConditions().formationId || ConfigManager.aaFormation
);
const fleetObj = PlayerManager.fleets[fleetNum - 1],
// 3th and 5th ship are not carrier or absent?
invalidCombinedShips = [fleetObj.ship(2), fleetObj.ship(4)]
.some(ship => ship.isAbsent() || ship.isCarrier()),
// submarine in any position of the fleet?
hasSubmarine = fleetObj.ship().some(s => s.isSubmarine()),
// no ship(s) sunk or retreated in mid-sortie?
hasSixShips = fleetObj.countShips(true) >= 6;
return isDoubleLine && !invalidCombinedShips && !hasSubmarine && hasSixShips;
}
}
return false;
};
/**
* Most conditions are the same with Nelson Touch, except:
* Flagship is healthy Nagato/Mutsu Kai Ni, Echelon formation selected.
* 2nd ship is a battleship, Chuuha ok, Taiha no good.
*
* Additional ammo consumption for Nagato/Mutsu & 2nd battleship:
* + Math.floor(or ceil?)(total ammo cost of this battle (yasen may included) / 2)
*
* @return true if this ship (Nagato/Mutsu Kai Ni) can do special cut-in attack.
* @see http://kancolle.wikia.com/wiki/Nagato
* @see https://wikiwiki.jp/kancolle/%E9%95%B7%E9%96%80%E6%94%B9%E4%BA%8C
* @see http://kancolle.wikia.com/wiki/Mutsu
* @see https://wikiwiki.jp/kancolle/%E9%99%B8%E5%A5%A5%E6%94%B9%E4%BA%8C
*/
KC3Ship.prototype.canDoNagatoClassCutin = function(flagShipIds = KC3Meta.nagatoClassCutinShips) {
if(this.isDummy() || this.isAbsent()) { return false; }
if(flagShipIds.includes(this.masterId) && !this.isStriped()) {
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
if(fleetNum > 0 && shipPos === 0 && shipCnt >= 6
&& (!PlayerManager.combinedFleet || fleetNum !== 2)) {
const isEchelon = [4, 12].includes(
this.collectBattleConditions().formationId || ConfigManager.aaFormation
);
const fleetObj = PlayerManager.fleets[fleetNum - 1],
// 2nd ship not battle ship?
invalidCombinedShips = [fleetObj.ship(1)].some(ship =>
ship.isAbsent() || ship.isTaiha() ||
![8, 9, 10].includes(ship.master().api_stype)),
hasSubmarine = fleetObj.ship().some(s => s.isSubmarine()),
hasSixShips = fleetObj.countShips(true) >= 6;
return isEchelon && !invalidCombinedShips && !hasSubmarine && hasSixShips;
}
}
return false;
};
/**
* Nagato/Mutsu Kai Ni special cut-in attack modifiers are variant depending on the fleet 2nd ship.
* And there are different modifiers for 2nd ship's 3rd attack.
* @param modifierFor2ndShip - to indicate the returned modifier is used for flagship or 2nd ship.
* @return the modifier, 1 by default for unknown conditions.
* @see https://wikiwiki.jp/kancolle/%E9%95%B7%E9%96%80%E6%94%B9%E4%BA%8C
*/
KC3Ship.prototype.estimateNagatoClassCutinModifier = function(modifierFor2ndShip = false) {
const locatedFleet = PlayerManager.fleets[this.onFleet() - 1];
if(!locatedFleet) return 1;
const flagshipMstId = locatedFleet.ship(0).masterId;
if(!KC3Meta.nagatoClassCutinShips.includes(flagshipMstId)) return 1;
const ship2ndMstId = locatedFleet.ship(1).masterId;
const partnerModifierMap = KC3Meta.nagatoCutinShips.includes(flagshipMstId) ?
(modifierFor2ndShip ? {
"573": 1.4, // Mutsu Kai Ni
"276": 1.35, // Mutsu Kai, base form unverified?
"576": 1.25, // Nelson Kai, base form unverified?
} : {
"573": 1.2, // Mutsu Kai Ni
"276": 1.15, // Mutsu Kai, base form unverified?
"576": 1.1, // Nelson Kai, base form unverified?
}) :
KC3Meta.mutsuCutinShips.includes(flagshipMstId) ?
(modifierFor2ndShip ? {
"541": 1.4, // Nagato Kai Ni
"275": 1.35, // Nagato Kai
"576": 1.25, // Nelson Kai
} : {
"541": 1.2, // Nagato Kai Ni
"275": 1.15, // Nagato Kai
"576": 1.1, // Nelson Kai
}) : {};
const baseModifier = modifierFor2ndShip ? 1.2 : 1.4;
const partnerModifier = partnerModifierMap[ship2ndMstId] || 1;
const apShellModifier = this.hasEquipmentType(2, 19) ? 1.35 : 1;
// Surface Radar modifier not always limited to post-cap and AP Shell synergy now,
// can be applied to night battle (pre-cap) independently?
const surfaceRadarModifier = this.equipment(true).some(gear => gear.isSurfaceRadar()) ? 1.15 : 1;
return baseModifier * partnerModifier * apShellModifier * surfaceRadarModifier;
};
/**
* Most conditions are the same with Nelson Touch, except:
* Flagship is healthy Colorado, Echelon formation selected.
* 2nd and 3rd ships are healthy battleship, neither Taiha nor Chuuha.
*
* The same additional ammo consumption like Nagato/Mutsu cutin for top 3 battleships.
*
* 4 types of smoke animation effects will be used according corresponding position of partener ships,
* see `main.js#CutinColoradoAttack.prototype._getSmoke`.
*
* @return true if this ship (Colorado) can do Colorado special cut-in attack.
* @see http://kancolle.wikia.com/wiki/Colorado
* @see https://wikiwiki.jp/kancolle/Colorado
*/
KC3Ship.prototype.canDoColoradoCutin = function() {
if(this.isDummy() || this.isAbsent()) { return false; }
// is this ship Colorado and not even Chuuha
if(KC3Meta.coloradoCutinShips.includes(this.masterId) && !this.isStriped()) {
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
if(fleetNum > 0 && shipPos === 0 && shipCnt >= 6
&& (!PlayerManager.combinedFleet || fleetNum !== 2)) {
const isEchelon = [4, 12].includes(
this.collectBattleConditions().formationId || ConfigManager.aaFormation
);
const fleetObj = PlayerManager.fleets[fleetNum - 1],
// 2nd and 3rd ship are (F)BB(V) only, not even Chuuha?
validCombinedShips = [fleetObj.ship(1), fleetObj.ship(2)]
.some(ship => !ship.isAbsent() && !ship.isStriped()
&& [8, 9, 10].includes(ship.master().api_stype)),
// submarine in any position of the fleet?
hasSubmarine = fleetObj.ship().some(s => s.isSubmarine()),
// uncertain: ship(s) sunk or retreated in mid-sortie can prevent proc?
hasSixShips = fleetObj.countShips(true) >= 6;
return isEchelon && validCombinedShips && !hasSubmarine && hasSixShips;
}
}
return false;
};
/**
* Colorado special cut-in attack modifiers are variant,
* depending on equipment and 2nd and 3rd ship in the fleet.
* @see https://twitter.com/syoukuretin/status/1132763536222969856
*/
KC3Ship.prototype.estimateColoradoCutinModifier = function(forShipPos = 0) {
const locatedFleet = PlayerManager.fleets[this.onFleet() - 1];
if(!locatedFleet) return 1;
const flagshipMstId = locatedFleet.ship(0).masterId;
if(!KC3Meta.coloradoCutinShips.includes(flagshipMstId)) return 1;
const combinedModifierMaps = [
// No more mods for flagship?
{},
// x1.1 for Big-7 2nd ship
{
"80": 1.1, "275": 1.1, "541": 1.1, // Nagato
"81": 1.1, "276": 1.1, "573": 1.1, // Mutsu
"571": 1.1, "576": 1.1, // Nelson
},
// x1.15 for Big-7 3rd ship
{
"80": 1.15, "275": 1.15, "541": 1.15,
"81": 1.15, "276": 1.15, "573": 1.15,
"571": 1.15, "576": 1.15,
},
];
forShipPos = (forShipPos || 0) % 3;
const baseModifier = [1.3, 1.15, 1.15][forShipPos];
const targetShip = locatedFleet.ship(forShipPos),
targetShipMstId = targetShip.masterId,
targetShipModifier = combinedModifierMaps[forShipPos][targetShipMstId] || 1;
// Bug 'mods of 2nd ship's apshell/radar and on-5th-slot-empty-exslot spread to 3rd ship' not applied here
const apShellModifier = targetShip.hasEquipmentType(2, 19) ? 1.35 : 1;
const surfaceRadarModifier = targetShip.equipment(true).some(gear => gear.isSurfaceRadar()) ? 1.15 : 1;
const ship2ndMstId = locatedFleet.ship(1).masterId,
ship2ndModifier = combinedModifierMaps[1][ship2ndMstId] || 1;
return baseModifier * targetShipModifier
* (forShipPos === 2 && targetShipModifier > 1 ? ship2ndModifier : 1)
* apShellModifier * surfaceRadarModifier;
};
/**
* Most conditions are the same with Nelson Touch, except:
* Flagship is healthy Kongou-class Kai Ni C, Line Ahead formation selected, night battle only.
* 2nd ship is healthy one of the following:
* * Kongou K2C flagship: Hiei K2C / Haruna K2 / Warspite
* * Hiei K2C flagship: Kongou K2C / Kirishima K2
* Surface ships in fleet >= 5 (that means 1 submarine is okay for single fleet)
*
* Since it's a night battle only cutin, have to be escort fleet of any Combined Fleet.
* And it's impossible to be triggered after any other daytime Big-7 special cutin,
* because all ship-combined spcutins only trigger 1-time per sortie?
*
* The additional 30% ammo consumption, see:
* * https://twitter.com/myteaGuard/status/1254040809365618690
* * https://twitter.com/myteaGuard/status/1254048759559778305
*
* @return true if this ship (Kongou-class K2C) can do special cut-in attack.
* @see https://kancolle.fandom.com/wiki/Kongou/Special_Cut-In
* @see https://wikiwiki.jp/kancolle/%E6%AF%94%E5%8F%A1%E6%94%B9%E4%BA%8C%E4%B8%99
*/
KC3Ship.prototype.canDoKongouCutin = function() {
if(this.isDummy() || this.isAbsent()) { return false; }
// is this ship Kongou-class K2C and not even Chuuha
if(KC3Meta.kongouCutinShips.includes(this.masterId) && !this.isStriped()) {
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
if(fleetNum > 0 && shipPos === 0 && shipCnt >= 5
&& (!PlayerManager.combinedFleet || fleetNum === 2)) {
const isLineAhead = [1, 14].includes(
this.collectBattleConditions().formationId || ConfigManager.aaFormation
);
const fleetObj = PlayerManager.fleets[fleetNum - 1],
// 2nd ship is valid partener and not even Chuuha
validCombinedShips = ({
// Kongou K2C: Hiei K2C, Haruna K2, Warspite
"591": [592, 151, 439, 364],
// Hiei K2C: Kongou K2C, Kirishima K2
"592": [591, 152],
}[this.masterId] || []).includes(fleetObj.ship(1).masterId)
&& !fleetObj.ship(1).isStriped(),
// uncertain: ship(s) sunk or retreated in mid-sortie can prevent proc?
hasFiveSurfaceShips = fleetObj.shipsUnescaped().filter(s => !s.isSubmarine()).length >= 5;
return isLineAhead && validCombinedShips && hasFiveSurfaceShips;
}
}
return false;
};
/**
* @return the landing attack kind ID, return 0 if can not attack.
* Since Phase 2, defined by `_getDaihatsuEffectType` at `PhaseHougekiOpening, PhaseHougeki, PhaseHougekiBase`,
* all the ID 1 are replaced by 3, ID 2 except the one at `PhaseHougekiOpening` replaced by 3.
*/
KC3Ship.prototype.estimateLandingAttackType = function(targetShipMasterId = 0) {
const targetShip = KC3Master.ship(targetShipMasterId);
if(!this.masterId || !targetShip) return 0;
const isLand = targetShip.api_soku <= 0;
// new equipment: M4A1 DD
if(this.hasEquipment(355) && isLand) return 6;
// higher priority: Toku Daihatsu + 11th Tank
if(this.hasEquipment(230)) return isLand ? 5 : 0;
// Abyssal hard land installation could be landing attacked
const isTargetLandable =
[1668, 1669, 1670, 1671, 1672, // Isolated Island Princess
1665, 1666, 1667, // Artillery Imp
1653, 1654, 1655, 1656, 1657, 1658, // Supply Depot Princess
// but why Summer Supply Depot Princess not counted?
1809, 1810, 1811, 1812, 1813, 1814, // Supply Depot Princess Vacation Mode
1921, 1922, 1923, 1924, 1925, 1926, // Supply Depot Princess B
1933, 1934, 1935, 1936, 1937, 1938, // Supply Depot Princess B Summer Landing Mode
1815, 1816, 1817, 1818, 1819, 1820, // Anchorage Water Demon Vacation Mode
1556, 1631, 1632, 1633, 1650, 1651, 1652, 1889, 1890, 1891, 1892, 1893, 1894 // Airfield Princess
].includes(targetShipMasterId);
// T2 Tank
if(this.hasEquipment(167)) {
const isThisSubmarine = this.isSubmarine();
if(isThisSubmarine && isLand) return 4;
if(isTargetLandable) return 4;
return 0;
}
if(isTargetLandable) {
// M4A1 DD
if(this.hasEquipment(355)) return 6;
// T89 Tank
if(this.hasEquipment(166)) return 3;
// Toku Daihatsu
if(this.hasEquipment(193)) return 3;
// Daihatsu
if(this.hasEquipment(68)) return 3;
}
return 0;
};
/**
* @param atType - id from `api_hougeki?.api_at_type` which indicates the special attack.
* @param altCutinTerm - different term string for cutin has different variant, like CVCI.
* @param altModifier - different power modifier for cutin has different variant, like CVCI.
* @return known special attack (aka Cut-In) types definition tuple.
* will return an object mapped all IDs and tuples if atType is omitted.
* will return `["SingleAttack", 0]` if no matched ID found.
* @see estimateDayAttackType
*/
KC3Ship.specialAttackTypeDay = function(atType, altCutinTerm, altModifier){
const knownDayAttackTypes = {
1: ["Cutin", 1, "Laser"], // no longer exists now
2: ["Cutin", 2, "DoubleAttack", 1.2],
3: ["Cutin", 3, "CutinMainSecond", 1.1],
4: ["Cutin", 4, "CutinMainRadar", 1.2],
5: ["Cutin", 5, "CutinMainApshell", 1.3],
6: ["Cutin", 6, "CutinMainMain", 1.5],
7: ["Cutin", 7, "CutinCVCI", 1.25],
100: ["Cutin", 100, "CutinNelsonTouch", 2.0],
101: ["Cutin", 101, "CutinNagatoSpecial", 2.27],
102: ["Cutin", 102, "CutinMutsuSpecial", 2.27],
103: ["Cutin", 103, "CutinColoradoSpecial", 2.26],
200: ["Cutin", 200, "CutinZuiunMultiAngle", 1.35],
201: ["Cutin", 201, "CutinAirSeaMultiAngle", 1.3],
};
if(atType === undefined) return knownDayAttackTypes;
const matched = knownDayAttackTypes[atType] || ["SingleAttack", 0];
if(matched[0] === "Cutin") {
if(altCutinTerm) matched[2] = altCutinTerm;
if(altModifier) matched[3] = altModifier;
}
return matched;
};
/**
* Estimate day time attack type of this ship.
* Only according ship type and equipment, ignoring factors such as ship status, target-able.
* @param {number} targetShipMasterId - a Master ID of being attacked ship, used to indicate some
* special attack types. eg: attacking a submarine, landing attack an installation.
* The ID can be just an example to represent this type of target.
* @param {boolean} trySpTypeFirst - specify true if want to estimate special attack type.
* @param {number} airBattleId - air battle result id, to indicate if special attacks can be triggered,
* special attacks require AS / AS +, default is AS+.
* @return {Array} day time attack type constants tuple:
* [name, regular attack id / cutin id / landing id, cutin name, modifier].
* cutin id is from `api_hougeki?.api_at_type` which indicates the special attacks.
* NOTE: Not take 'can not be targeted' into account yet,
* such as: CV/CVB against submarine; submarine against land installation;
* asw aircraft all lost against submarine; torpedo bomber only against land,
* should not pass targetShipMasterId at all for these scenes.
* @see https://github.com/andanteyk/ElectronicObserver/blob/master/ElectronicObserver/Other/Information/kcmemo.md#%E6%94%BB%E6%92%83%E7%A8%AE%E5%88%A5
* @see BattleMain.swf#battle.models.attack.AttackData#setOptionsAtHougeki - client side codes of day attack type.
* @see BattleMain.swf#battle.phase.hougeki.PhaseHougekiBase - client side hints of special cutin attack type.
* @see main.js#PhaseHougeki.prototype._getNormalAttackType - since Phase 2
* @see specialAttackTypeDay
* @see estimateNightAttackType
* @see canDoOpeningTorpedo
* @see canDoDayShellingAttack
* @see canDoASW
* @see canDoClosingTorpedo
*/
KC3Ship.prototype.estimateDayAttackType = function(targetShipMasterId = 0, trySpTypeFirst = false,
airBattleId = 1) {
if(this.isDummy()) { return []; }
// if attack target known, will give different attack according target ship
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
const isThisCarrier = this.isCarrier();
const isThisSubmarine = this.isSubmarine();
// Special cutins do not need isAirSuperiorityBetter
if(trySpTypeFirst) {
// Nelson Touch since 2018-09-15
if(this.canDoNelsonTouch()) {
const isRedT = this.collectBattleConditions().engagementId === 4;
return KC3Ship.specialAttackTypeDay(100, null, isRedT ? 2.5 : 2.0);
}
// Nagato cutin since 2018-11-16
if(this.canDoNagatoClassCutin(KC3Meta.nagatoCutinShips)) {
// To clarify: here only indicates the modifier of flagship's first 2 attacks
return KC3Ship.specialAttackTypeDay(101, null, this.estimateNagatoClassCutinModifier());
}
// Mutsu cutin since 2019-02-27
if(this.canDoNagatoClassCutin(KC3Meta.mutsuCutinShips)) {
return KC3Ship.specialAttackTypeDay(102, null, this.estimateNagatoClassCutinModifier());
}
// Colorado cutin since 2019-05-25
if(this.canDoColoradoCutin()) {
return KC3Ship.specialAttackTypeDay(103, null, this.estimateColoradoCutinModifier());
}
}
const isAirSuperiorityBetter = airBattleId === 1 || airBattleId === 2;
// Special Multi-Angle cutins do not need recon plane and probably higher priority
if(trySpTypeFirst && isAirSuperiorityBetter) {
const isThisIseClassK2 = [553, 554].includes(this.masterId);
const mainGunCnt = this.countEquipmentType(2, [1, 2, 3, 38]);
if(isThisIseClassK2 && mainGunCnt > 0 && !this.isTaiha()) {
// Ise-class Kai Ni Zuiun Multi-Angle Attack since 2019-03-27
const spZuiunCnt = this.countNonZeroSlotEquipment(
// All seaplane bombers named Zuiun capable?
[26, 79, 80, 81, 207, 237, 322, 323]
);
// Zuiun priority to Air/Sea Attack when they are both equipped
if(spZuiunCnt > 1) return KC3Ship.specialAttackTypeDay(200);
// Ise-class Kai Ni Air/Sea Multi-Angle Attack since 2019-03-27
const spSuiseiCnt = this.countNonZeroSlotEquipment(
// Only Suisei named 634th Air Group capable?
[291, 292, 319]
);
if(spSuiseiCnt > 1) return KC3Ship.specialAttackTypeDay(201);
}
}
const hasRecon = this.hasNonZeroSlotEquipmentType(2, [10, 11]);
if(trySpTypeFirst && hasRecon && isAirSuperiorityBetter) {
/*
* To estimate if can do day time special attacks (aka Artillery Spotting).
* In game, special attack types are judged and given by server API result.
* By equip compos, multiply types are possible to be selected to trigger, such as
* CutinMainMain + Double, CutinMainAPShell + CutinMainRadar + CutinMainSecond.
* Here just check by strictness & modifier desc order and return one of them.
*/
const mainGunCnt = this.countEquipmentType(2, [1, 2, 3, 38]);
const apShellCnt = this.countEquipmentType(2, 19);
if(mainGunCnt >= 2 && apShellCnt >= 1) return KC3Ship.specialAttackTypeDay(6);
const secondaryCnt = this.countEquipmentType(2, 4);
if(mainGunCnt >= 1 && secondaryCnt >= 1 && apShellCnt >= 1)
return KC3Ship.specialAttackTypeDay(5);
const radarCnt = this.countEquipmentType(2, [12, 13]);
if(mainGunCnt >= 1 && secondaryCnt >= 1 && radarCnt >= 1)
return KC3Ship.specialAttackTypeDay(4);
if(mainGunCnt >= 1 && secondaryCnt >= 1) return KC3Ship.specialAttackTypeDay(3);
if(mainGunCnt >= 2) return KC3Ship.specialAttackTypeDay(2);
} else if(trySpTypeFirst && isThisCarrier && isAirSuperiorityBetter) {
// day time carrier shelling cut-in
// http://wikiwiki.jp/kancolle/?%C0%EF%C6%AE%A4%CB%A4%C4%A4%A4%A4%C6#FAcutin
// https://twitter.com/_Kotoha07/status/907598964098080768
// https://twitter.com/arielugame/status/908343848459317249
const fighterCnt = this.countNonZeroSlotEquipmentType(2, 6);
const diveBomberCnt = this.countNonZeroSlotEquipmentType(2, 7);
const torpedoBomberCnt = this.countNonZeroSlotEquipmentType(2, 8);
if(diveBomberCnt >= 1 && torpedoBomberCnt >= 1 && fighterCnt >= 1)
return KC3Ship.specialAttackTypeDay(7, "CutinFDBTB", 1.25);
if(diveBomberCnt >= 2 && torpedoBomberCnt >= 1)
return KC3Ship.specialAttackTypeDay(7, "CutinDBDBTB", 1.2);
if(diveBomberCnt >= 1 && torpedoBomberCnt >= 1)
return KC3Ship.specialAttackTypeDay(7, "CutinDBTB", 1.15);
}
// is target a land installation
if(targetShipType.isLand) {
const landingAttackType = this.estimateLandingAttackType(targetShipMasterId);
if(landingAttackType > 0) {
return ["LandingAttack", landingAttackType];
}
// see `main.js#PhaseHougeki.prototype._hasRocketEffect` or same method of `PhaseHougekiBase`,
// and if base attack method is NOT air attack
const hasRocketLauncher = this.hasEquipmentType(2, 37) || this.hasEquipment([346, 347]);
// no such ID -1, just mean higher priority
if(hasRocketLauncher) return ["Rocket", -1];
}
// is this ship Hayasui Kai
if(this.masterId === 352) {
if(targetShipType.isSubmarine) {
// air attack if asw aircraft equipped
const aswEquip = this.equipment().find(g => g.isAswAircraft(false));
return aswEquip ? ["AirAttack", 1] : ["DepthCharge", 2];
}
// air attack if torpedo bomber equipped, otherwise fall back to shelling
if(this.hasEquipmentType(2, 8))
return ["AirAttack", 1];
else
return ["SingleAttack", 0];
}
if(isThisCarrier) {
return ["AirAttack", 1];
}
// only torpedo attack possible if this ship is submarine (but not shelling phase)
if(isThisSubmarine) {
return ["Torpedo", 3];
}
if(targetShipType.isSubmarine) {
const stype = this.master().api_stype;
// CAV, BBV, AV, LHA can only air attack against submarine
return ([6, 10, 16, 17].includes(stype)) ? ["AirAttack", 1] : ["DepthCharge", 2];
}
// default single shelling fire attack
return ["SingleAttack", 0];
};
/**
* @return true if this ship (and target ship) can attack at night.
*/
KC3Ship.prototype.canDoNightAttack = function(targetShipMasterId = 0) {
// no count for escaped ship too
if(this.isDummy() || this.isAbsent()) { return false; }
// no ship can night attack on taiha
if(this.isTaiha()) return false;
const initYasen = this.master().api_houg[0] + this.master().api_raig[0];
const isThisCarrier = this.isCarrier();
// even carrier can do shelling or air attack if her yasen power > 0 (no matter chuuha)
// currently known ships: Graf / Graf Kai, Saratoga, Taiyou Class Kai Ni, Kaga Kai Ni Go
if(isThisCarrier && initYasen > 0) return true;
// carriers without yasen power can do air attack under some conditions:
if(isThisCarrier) {
// only CVB can air attack on chuuha (taiha already excluded)
const isNotCvb = this.master().api_stype !== 18;
if(isNotCvb && this.isStriped()) return false;
// Ark Royal (Kai) can air attack without NOAP if Swordfish variants equipped and slot > 0
if([515, 393].includes(this.masterId)
&& this.hasNonZeroSlotEquipment([242, 243, 244])) return true;
// night aircraft + NOAP equipped
return this.canCarrierNightAirAttack();
}
// can not night attack for any ship type if initial FP + TP is 0
return initYasen > 0;
};
/**
* @return true if a carrier can do air attack at night thank to night aircraft,
* which should be given via `api_n_mother_list`, not judged by client side.
* @see canDoNightAttack - those yasen power carriers not counted in `api_n_mother_list`.
* @see http://wikiwiki.jp/kancolle/?%CC%EB%C0%EF#NightCombatByAircrafts
*/
KC3Ship.prototype.canCarrierNightAirAttack = function() {
if(this.isDummy() || this.isAbsent()) { return false; }
if(this.isCarrier()) {
const hasNightAircraft = this.hasEquipmentType(3, KC3GearManager.nightAircraftType3Ids);
const hasNightAvPersonnel = this.hasEquipment([258, 259]);
// night battle capable carriers: Saratoga Mk.II, Akagi Kai Ni E/Kaga Kai Ni E
const isThisNightCarrier = [545, 599, 610].includes(this.masterId);
// ~~Swordfish variants are counted as night aircraft for Ark Royal + NOAP~~
// Ark Royal + Swordfish variants + NOAP - night aircraft will not get `api_n_mother_list: 1`
//const isThisArkRoyal = [515, 393].includes(this.masterId);
//const isSwordfishArkRoyal = isThisArkRoyal && this.hasEquipment([242, 243, 244]);
// if night aircraft + (NOAP equipped / on Saratoga Mk.2/Akagi K2E/Kaga K2E)
return hasNightAircraft && (hasNightAvPersonnel || isThisNightCarrier);
}
return false;
};
/**
* @param spType - id from `api_hougeki.api_sp_list` which indicates the special attack.
* @param altCutinTerm - different term string for cutin has different variant, like SS TCI, CVNCI, DDCI.
* @param altModifier - different power modifier for cutin has different variant, like SS TCI, CVNCI, DDCI.
* @return known special attack (aka Cut-In) types definition tuple.
* will return an object mapped all IDs and tuples if atType is omitted.
* will return `["SingleAttack", 0]` if no matched ID found.
* @see estimateNightAttackType
*/
KC3Ship.specialAttackTypeNight = function(spType, altCutinTerm, altModifier){
const knownNightAttackTypes = {
1: ["Cutin", 1, "DoubleAttack", 1.2],
2: ["Cutin", 2, "CutinTorpTorpMain", 1.3],
3: ["Cutin", 3, "CutinTorpTorpTorp", 1.5],
4: ["Cutin", 4, "CutinMainMainSecond", 1.75],
5: ["Cutin", 5, "CutinMainMainMain", 2.0],
6: ["Cutin", 6, "CutinCVNCI", 1.25],
7: ["Cutin", 7, "CutinMainTorpRadar", 1.3],
8: ["Cutin", 8, "CutinTorpRadarLookout", 1.2],
100: ["Cutin", 100, "CutinNelsonTouch", 2.0],
101: ["Cutin", 101, "CutinNagatoSpecial", 2.27],
102: ["Cutin", 102, "CutinMutsuSpecial", 2.27],
103: ["Cutin", 103, "CutinColoradoSpecial", 2.26],
104: ["Cutin", 104, "CutinKongouSpecial", 1.9],
};
if(spType === undefined) return knownNightAttackTypes;
const matched = knownNightAttackTypes[spType] || ["SingleAttack", 0];
if(matched[0] === "Cutin") {
if(altCutinTerm) matched[2] = altCutinTerm;
if(altModifier) matched[3] = altModifier;
}
return matched;
};
/**
* Estimate night battle attack type of this ship.
* Also just give possible attack type, no responsibility to check can do attack at night,
* or that ship can be targeted or not, etc.
* @param {number} targetShipMasterId - a Master ID of being attacked ship.
* @param {boolean} trySpTypeFirst - specify true if want to estimate special attack type.
* @return {Array} night battle attack type constants tuple: [name, cutin id, cutin name, modifier].
* cutin id is partially from `api_hougeki.api_sp_list` which indicates the special attacks.
* @see BattleMain.swf#battle.models.attack.AttackData#setOptionsAtNight - client side codes of night attack type.
* @see BattleMain.swf#battle.phase.night.PhaseAttack - client side hints of special cutin attack type.
* @see main.js#PhaseHougekiBase.prototype._getNormalAttackType - since Phase 2
* @see specialAttackTypeNight
* @see estimateDayAttackType
* @see canDoNightAttack
*/
KC3Ship.prototype.estimateNightAttackType = function(targetShipMasterId = 0, trySpTypeFirst = false) {
if(this.isDummy()) { return []; }
const targetShipType = this.estimateTargetShipType(targetShipMasterId);
const isThisCarrier = this.isCarrier();
const isThisSubmarine = this.isSubmarine();
const stype = this.master().api_stype;
const isThisLightCarrier = stype === 7;
const isThisDestroyer = stype === 2;
const isThisKagaK2Go = this.masterId === 646;
const torpedoCnt = this.countEquipmentType(2, [5, 32]);
// simulate server-side night air attack flag: `api_n_mother_list`
const isCarrierNightAirAttack = isThisCarrier && this.canCarrierNightAirAttack();
if(trySpTypeFirst && !targetShipType.isSubmarine) {
// to estimate night special attacks, which should be given by server API result.
// will not trigger if this ship is taiha or targeting submarine.
// carrier night cut-in, NOAP or Saratoga Mk.II/Akagi K2E/Kaga K2E needed
if(isCarrierNightAirAttack) {
// https://kancolle.fandom.com/wiki/Combat#Setups_and_Attack_Types
// http://wikiwiki.jp/kancolle/?%CC%EB%C0%EF#x397cac6
const nightFighterCnt = this.countNonZeroSlotEquipmentType(3, 45);
const nightTBomberCnt = this.countNonZeroSlotEquipmentType(3, 46);
// Zero Fighter Model 62 (Fighter-bomber Iwai Squadron)
const iwaiDBomberCnt = this.countNonZeroSlotEquipment(154);
// Swordfish variants
const swordfishTBomberCnt = this.countNonZeroSlotEquipment([242, 243, 244]);
// new patterns for Suisei Model 12 (Type 31 Photoelectric Fuze Bombs) since 2019-04-30,
// it more likely acts as yet unimplemented Night Dive Bomber type
const photoDBomberCnt = this.countNonZeroSlotEquipment(320);
// might extract this out for estimating unexpected damage actual pattern modifier
const ncvciModifier = (() => {
const otherCnt = photoDBomberCnt + iwaiDBomberCnt + swordfishTBomberCnt;
if(nightFighterCnt >= 2 && nightTBomberCnt >= 1) return 1.25;
if(nightFighterCnt + nightTBomberCnt + otherCnt === 2) return 1.2;
if(nightFighterCnt + nightTBomberCnt + otherCnt >= 3) return 1.18;
return 1; // should not reach here
})();
// first place thank to its highest mod 1.25
if(nightFighterCnt >= 2 && nightTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNFNTB", ncvciModifier);
// 3 planes mod 1.18
if(nightFighterCnt >= 3)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNFNF", ncvciModifier);
if(nightFighterCnt >= 1 && nightTBomberCnt >= 2)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNTBNTB", ncvciModifier);
if(nightFighterCnt >= 2 && iwaiDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNFFBI", ncvciModifier);
if(nightFighterCnt >= 2 && swordfishTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNFSF", ncvciModifier);
if(nightFighterCnt >= 1 && iwaiDBomberCnt >= 2)
return KC3Ship.specialAttackTypeNight(6, "CutinNFFBIFBI", ncvciModifier);
if(nightFighterCnt >= 1 && swordfishTBomberCnt >= 2)
return KC3Ship.specialAttackTypeNight(6, "CutinNFSFSF", ncvciModifier);
if(nightFighterCnt >= 1 && iwaiDBomberCnt >= 1 && swordfishTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFFBISF", ncvciModifier);
if(nightFighterCnt >= 1 && nightTBomberCnt >= 1 && iwaiDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNTBFBI", ncvciModifier);
if(nightFighterCnt >= 1 && nightTBomberCnt >= 1 && swordfishTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNTBSF", ncvciModifier);
if(nightFighterCnt >= 2 && photoDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNFNDB", ncvciModifier);
if(nightFighterCnt >= 1 && photoDBomberCnt >= 2)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNDBNDB", ncvciModifier);
if(nightFighterCnt >= 1 && nightTBomberCnt >= 1 && photoDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNTBNDB", ncvciModifier);
if(nightFighterCnt >= 1 && photoDBomberCnt >= 1 && iwaiDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNDBFBI", ncvciModifier);
if(nightFighterCnt >= 1 && photoDBomberCnt >= 1 && swordfishTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNDBSF", ncvciModifier);
// 2 planes mod 1.2, put here not to mask previous patterns, tho proc rate might be higher
if(nightFighterCnt >= 1 && nightTBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNTB", ncvciModifier);
if(nightFighterCnt >= 1 && photoDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNFNDB", ncvciModifier);
if(nightTBomberCnt >= 1 && photoDBomberCnt >= 1)
return KC3Ship.specialAttackTypeNight(6, "CutinNTBNDB", ncvciModifier);
} else {
// special Nelson Touch since 2018-09-15
if(this.canDoNelsonTouch()) {
const isRedT = this.collectBattleConditions().engagementId === 4;
return KC3Ship.specialAttackTypeNight(100, null, isRedT ? 2.5 : 2.0);
}
// special Nagato Cutin since 2018-11-16
if(this.canDoNagatoClassCutin(KC3Meta.nagatoCutinShips)) {
return KC3Ship.specialAttackTypeNight(101, null, this.estimateNagatoClassCutinModifier());
}
// special Mutsu Cutin since 2019-02-27
if(this.canDoNagatoClassCutin(KC3Meta.mutsuCutinShips)) {
return KC3Ship.specialAttackTypeNight(102, null, this.estimateNagatoClassCutinModifier());
}
// special Colorado Cutin since 2019-05-25
if(this.canDoColoradoCutin()) {
return KC3Ship.specialAttackTypeNight(103, null, this.estimateColoradoCutinModifier());
}
// special Kongou-class K2C Cutin since 2020-04-23
if(this.canDoKongouCutin()) {
// Basic precap modifier is 1.9: https://twitter.com/CC_jabberwock/status/1253677320629399552
const engagementMod = [1, 1, 1, 1.25, 0.75][this.collectBattleConditions().engagementId] || 1.0;
return KC3Ship.specialAttackTypeNight(104, null, 1.9 * engagementMod);
}
// special torpedo radar cut-in for destroyers since 2017-10-25
// http://wikiwiki.jp/kancolle/?%CC%EB%C0%EF#dfcb6e1f
if(isThisDestroyer && torpedoCnt >= 1) {
// according tests, any radar with accuracy stat >= 3 capable,
// even large radars (Kasumi K2 can equip), air radars okay too, see:
// https://twitter.com/nicolai_2501/status/923172168141123584
// https://twitter.com/nicolai_2501/status/923175256092581888
const hasCapableRadar = this.equipment(true).some(gear => gear.isSurfaceRadar());
const hasSkilledLookout = this.hasEquipmentType(2, 39);
const smallMainGunCnt = this.countEquipmentType(2, 1);
// Extra bonus if small main gun is 12.7cm Twin Gun Mount Model D Kai Ni/3
// https://twitter.com/ayanamist_m2/status/944176834551222272
// https://docs.google.com/spreadsheets/d/1_e0M6asJUbu9EEW4PrGCu9hOxZnY7OQEDHH2DUAzjN8/htmlview
const modelDK2SmallGunCnt = this.countEquipment(267),
modelDK3SmallGunCnt = this.countEquipment(366);
// Possible to equip 2 D guns for 4 slots Tashkent
// https://twitter.com/Xe_UCH/status/1011398540654809088
const modelDSmallGunModifier =
([1, 1.25, 1.4][modelDK2SmallGunCnt + modelDK3SmallGunCnt] || 1.4)
* (1 + modelDK3SmallGunCnt * 0.05);
if(hasCapableRadar && smallMainGunCnt >= 1)
return KC3Ship.specialAttackTypeNight(7, null, 1.3 * modelDSmallGunModifier);
if(hasCapableRadar && hasSkilledLookout)
return KC3Ship.specialAttackTypeNight(8, null, 1.2 * modelDSmallGunModifier);
}
// special torpedo cut-in for late model submarine torpedo
const lateTorpedoCnt = this.countEquipment([213, 214, 383]);
const submarineRadarCnt = this.countEquipmentType(2, 51);
if(lateTorpedoCnt >= 1 && submarineRadarCnt >= 1)
return KC3Ship.specialAttackTypeNight(3, "CutinLateTorpRadar", 1.75);
if(lateTorpedoCnt >= 2)
return KC3Ship.specialAttackTypeNight(3, "CutinLateTorpTorp", 1.6);
// although modifier lower than Main CI / Mix CI, but seems be more frequently used
// will not mutex if 5 slots ships can equip torpedo
if(torpedoCnt >= 2) return KC3Ship.specialAttackTypeNight(3);
const mainGunCnt = this.countEquipmentType(2, [1, 2, 3, 38]);
if(mainGunCnt >= 3) return KC3Ship.specialAttackTypeNight(5);
const secondaryCnt = this.countEquipmentType(2, 4);
if(mainGunCnt === 2 && secondaryCnt >= 1)
return KC3Ship.specialAttackTypeNight(4);
if((mainGunCnt === 2 && secondaryCnt === 0 && torpedoCnt === 1) ||
(mainGunCnt === 1 && torpedoCnt === 1))
return KC3Ship.specialAttackTypeNight(2);
// double attack can be torpedo attack animation if topmost slot is torpedo
if((mainGunCnt === 2 && secondaryCnt === 0 && torpedoCnt === 0) ||
(mainGunCnt === 1 && secondaryCnt >= 1) ||
(secondaryCnt >= 2 && torpedoCnt <= 1))
return KC3Ship.specialAttackTypeNight(1);
}
}
if(targetShipType.isLand) {
const landingAttackType = this.estimateLandingAttackType(targetShipMasterId);
if(landingAttackType > 0) {
return ["LandingAttack", landingAttackType];
}
const hasRocketLauncher = this.hasEquipmentType(2, 37);
if(hasRocketLauncher) return ["Rocket", -1];
}
// priority to use server flag
if(isCarrierNightAirAttack) {
return ["AirAttack", 1, true];
}
if(targetShipType.isSubmarine && (isThisLightCarrier || isThisKagaK2Go)) {
return ["DepthCharge", 2];
}
if(isThisCarrier) {
// these abyssal ships can only be shelling attacked,
// see `main.js#PhaseHougekiBase.prototype._getNormalAttackType`
const isSpecialAbyssal = [
1679, 1680, 1681, 1682, 1683, // Lycoris Princess
1711, 1712, 1713, // Jellyfish Princess
].includes[targetShipMasterId];
const isSpecialCarrier = [
432, 353, // Graf & Graf Kai
433 // Saratoga (base form)
].includes(this.masterId);
if(isSpecialCarrier || isSpecialAbyssal) return ["SingleAttack", 0];
// here just indicates 'attack type', not 'can attack or not', see #canDoNightAttack
// Taiyou Kai Ni fell back to shelling attack if no bomber equipped, but ninja changed by devs.
// now she will air attack against surface ships, but no plane appears if no aircraft equipped.
return ["AirAttack", 1];
}
if(isThisSubmarine) {
return ["Torpedo", 3];
}
if(targetShipType.isSubmarine) {
// CAV, BBV, AV, LHA
return ([6, 10, 16, 17].includes(stype)) ? ["AirAttack", 1] : ["DepthCharge", 2];
}
// torpedo attack if any torpedo equipped at top most, otherwise single shelling fire
const topGear = this.equipment().find(gear => gear.exists() &&
[1, 2, 3].includes(gear.master().api_type[1]));
return topGear && topGear.master().api_type[1] === 3 ? ["Torpedo", 3] : ["SingleAttack", 0];
};
/**
* Calculates base value used in day battle artillery spotting process chance.
* Likely to be revamped as formula comes from PSVita and does not include CVCI,
* uncertain about Combined Fleet interaction.
* @see https://kancolle.wikia.com/wiki/User_blog:Shadow27X/Artillery_Spotting_Rate_Formula
* @see KC3Fleet.prototype.artillerySpottingLineOfSight
*/
KC3Ship.prototype.daySpAttackBaseRate = function() {
if (this.isDummy() || !this.onFleet()) { return {}; }
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
const fleet = PlayerManager.fleets[fleetNum - 1];
const fleetLoS = fleet.artillerySpottingLineOfSight();
const adjFleetLoS = Math.floor(Math.sqrt(fleetLoS) + fleetLoS / 10);
const adjLuck = Math.floor(Math.sqrt(this.lk[0]) + 10);
// might exclude equipment on ship LoS bonus for now,
// to include LoS bonus, use `this.equipmentTotalLoS()` instead
const equipLoS = this.equipmentTotalStats("saku", true, false);
// assume to best condition AS+ by default (for non-battle)
const airBattleId = this.collectBattleConditions().airBattleId || 1;
const baseValue = airBattleId === 1 ? adjLuck + 0.7 * (adjFleetLoS + 1.6 * equipLoS) + 10 :
airBattleId === 2 ? adjLuck + 0.6 * (adjFleetLoS + 1.2 * equipLoS) : 0;
return {
baseValue,
isFlagship: shipPos === 0,
equipLoS,
fleetLoS,
dispSeiku: airBattleId
};
};
/**
* Calculates base value used in night battle cut-in process chance.
* @param {number} currentHp - used by simulating from battle prediction or getting different HP value.
* @see https://kancolle.wikia.com/wiki/Combat/Night_Battle#Night_Cut-In_Chance
* @see https://wikiwiki.jp/kancolle/%E5%A4%9C%E6%88%A6#nightcutin1
* @see KC3Fleet.prototype.estimateUsableSearchlight
*/
KC3Ship.prototype.nightSpAttackBaseRate = function(currentHp) {
if (this.isDummy()) { return {}; }
let baseValue = 0;
if (this.lk[0] < 50) {
baseValue += 15 + this.lk[0] + 0.75 * Math.sqrt(this.level);
} else {
baseValue += 65 + Math.sqrt(this.lk[0] - 50) + 0.8 * Math.sqrt(this.level);
}
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
// Flagship bonus
const isFlagship = shipPos === 0;
if (isFlagship) { baseValue += 15; }
// Chuuha bonus
const isChuuhaOrWorse = (currentHp || this.hp[0]) <= (this.hp[1] / 2);
if (isChuuhaOrWorse) { baseValue += 18; }
// Skilled lookout bonus
if (this.hasEquipmentType(2, 39)) { baseValue += 5; }
// Searchlight bonus, large SL unknown for now
const fleetSearchlight = fleetNum > 0 && PlayerManager.fleets[fleetNum - 1].estimateUsableSearchlight();
if (fleetSearchlight) { baseValue += 7; }
// Starshell bonus/penalty
const battleConds = this.collectBattleConditions();
const playerStarshell = battleConds.playerFlarePos > 0;
const enemyStarshell = battleConds.enemyFlarePos > 0;
if (playerStarshell) { baseValue += 4; }
if (enemyStarshell) { baseValue += -10; }
return {
baseValue,
isFlagship,
isChuuhaOrWorse,
fleetSearchlight,
playerStarshell,
enemyStarshell
};
};
/**
* Calculate Nelson Touch process rate, currently only known in day
* @param {boolean} isNight - Nelson Touch has lower modifier at night?
* @return {number} special attack rate
* @see https://twitter.com/Xe_UCH/status/1180283907284979713
*/
KC3Ship.prototype.nelsonTouchRate = function(isNight) {
if (this.isDummy() || isNight) { return false; }
const [shipPos, shipCnt, fleetNum] = this.fleetPosition();
// Nelson Touch prerequisite should be fulfilled before calling this, see also #canDoNelsonTouch
// here only to ensure fleetObj and combinedShips below not undefined if this invoked unexpectedly
if (shipPos !== 0 || shipCnt < 6 || !fleetNum) { return false; }
const fleetObj = PlayerManager.fleets[fleetNum - 1];
const combinedShips = [2, 4].map(pos => fleetObj.ship(pos));
const combinedShipsLevel = combinedShips.reduce((acc, ship) => acc + ship.level, 0);
const combinedShipsPenalty = combinedShips.some(ship => [2, 16].includes(ship.master().api_stype)) ? 10 : 0; // estimate
return (0.08 * this.level + 0.04 * combinedShipsLevel + 0.24 * this.lk[0] + 36 - combinedShipsPenalty) / 100;
};
/**
* Calculate ship day time artillery spotting process rate based on known type factors.
* @param {number} atType - based on api_at_type value of artillery spotting type.
* @param {string} cutinSubType - sub type of cut-in like CVCI.
* @return {number} artillery spotting percentage, false if unable to arty spot or unknown special attack.
* @see daySpAttackBaseRate
* @see estimateDayAttackType
* @see Type factors: https://wikiwiki.jp/kancolle/%E6%88%A6%E9%97%98%E3%81%AB%E3%81%A4%E3%81%84%E3%81%A6#Observation
* @see Type factors for multi-angle cutin: https://wikiwiki.jp/kancolle/%E4%BC%8A%E5%8B%A2%E6%94%B9%E4%BA%8C
*/
KC3Ship.prototype.artillerySpottingRate = function(atType = 0, cutinSubType = "") {
// type 1 laser attack has gone forever, ship not on fleet cannot be evaluated
if (atType < 2 || this.isDummy() || !this.onFleet()) { return false; }
const formatPercent = num => Math.floor(num * 1000) / 10;
// Nelson Touch
if (atType === 100) {
return formatPercent(this.nelsonTouchRate(false));
}
const typeFactor = {
2: 150,
3: 120,
4: 130,
5: 130,
6: 140,
7: ({
"CutinFDBTB" : 125,
"CutinDBDBTB": 140,
"CutinDBTB" : 155,
})[cutinSubType],
// 100~103 might use different formula, see #nelsonTouchRate
200: 120,
201: undefined,
}[atType];
if (!typeFactor) { return false; }
const {baseValue, isFlagship} = this.daySpAttackBaseRate();
return formatPercent(((Math.floor(baseValue) + (isFlagship ? 15 : 0)) / typeFactor) || 0);
};
/**
* Calculate ship night battle special attack (cut-in and double attack) process rate based on known type factors.
* @param {number} spType - based on api_sp_list value of night special attack type.
* @param {string} cutinSubType - sub type of cut-in like CVNCI, submarine cut-in.
* @return {number} special attack percentage, false if unable to perform or unknown special attack.
* @see nightSpAttackBaseRate
* @see estimateNightAttackType
* @see Type factors: https://wikiwiki.jp/kancolle/%E5%A4%9C%E6%88%A6#nightcutin1
*/
KC3Ship.prototype.nightCutinRate = function(spType = 0, cutinSubType = "") {
if (spType < 1 || this.isDummy()) { return false; }
// not sure: DA success rate almost 99%
if (spType === 1) { return 99; }
const typeFactor = {
2: 115,
3: ({ // submarine late torp cutin should be different?
"CutinLateTorpRadar": undefined,
"CutinLateTorpTorp": undefined,
})[cutinSubType] || 122, // regular CutinTorpTorpTorp
4: 130,
5: 140,
6: ({ // CVNCI factors unknown, placeholders
"CutinNFNFNTB": undefined, // should be 3 types, for mod 1.25
"CutinNFNTB" : undefined, // 2 planes for mod 1.2
"CutinNFNDB" : undefined,
"CutinNTBNDB": undefined,
"CutinNFNFNF" : undefined, // 3 planes for mod 1.18
"CutinNFNTBNTB": undefined,
"CutinNFNFFBI" : undefined,
"CutinNFNFSF" : undefined,
"CutinNFFBIFBI": undefined,
"CutinNFSFSF" : undefined,
"CutinNFFBISF" : undefined,
"CutinNFNTBFBI": undefined,
"CutinNFNTBSF" : undefined,
"CutinNFNFNDB" : undefined,
"CutinNFNDBNDB": undefined,
"CutinNFNTBNDB": undefined,
"CutinNFNDBFBI": undefined,
"CutinNFNDBSF" : undefined,
})[cutinSubType],
// This two DD cutins can be rolled after regular cutin, more chance to be processed
7: 130,
8: undefined, // CutinTorpRadarLookout unknown
// 100~104 might be different, even with day one
}[spType];
if (!typeFactor) { return false; }
const {baseValue} = this.nightSpAttackBaseRate();
const formatPercent = num => Math.floor(num * 1000) / 10;
return formatPercent((Math.floor(baseValue) / typeFactor) || 0);
};
/**
* Calculate ship's Taiha rate when taken an overkill damage.
* This is related to the '4n+3 is better than 4n' theory,
* '4n+x' only refer to the rounded Taiha HP threshold, rate is also affected by current HP in fact.
* @param {number} currentHp - expected current hp value, use ship's real current hp by default.
* @param {number} maxHp - expected full hp value, use ship's real max hp by default.
* @return {number} Taiha percentage, 100% for already Taiha or red morale or dummy ship.
* @see https://wikiwiki.jp/kancolle/%E6%88%A6%E9%97%98%E3%81%AB%E3%81%A4%E3%81%84%E3%81%A6#eb18c7e5
*/
KC3Ship.prototype.overkillTaihaRate = function(currentHp = this.hp[0], maxHp = this.hp[1]) {
if (this.isDummy()) { return 100; }
const taihaHp = Math.max(1, Math.floor(0.25 * maxHp));
const battleConds = this.collectBattleConditions();
// already taiha (should get rid of fasly or negative hp value)
// or red morale (hit red morale hp will be left fixed 1)
if (currentHp <= taihaHp || this.moraleEffectLevel([1, 1, 0, 0, 0], battleConds.isOnBattle)) {
return 100;
}
// sum all random cases of taiha
const taihaCases = Array.numbers(0, currentHp - 1).map(rndint => (
(currentHp - Math.floor(currentHp * 0.5 + rndint * 0.3)) <= taihaHp ? 1 : 0
)).sumValues();
// percentage with 2 decimal
return Math.round(taihaCases / currentHp * 10000) / 100;
};
/**
* Get current shelling attack accuracy related info of this ship.
* NOTE: Only attacker accuracy part, not take defender evasion part into account at all, not final hit/critical rate.
* @param {number} formationModifier - see #estimateShellingFormationModifier.
* @param {boolean} applySpAttackModifiers - if special equipment and attack modifiers should be applied.
* @return {Object} accuracy factors of this ship.
* @see http://kancolle.wikia.com/wiki/Combat/Accuracy_and_Evasion
* @see http://wikiwiki.jp/kancolle/?%CC%BF%C3%E6%A4%C8%B2%F3%C8%F2%A4%CB%A4%C4%A4%A4%A4%C6
* @see https://twitter.com/Nishisonic/status/890202416112480256
*/
KC3Ship.prototype.shellingAccuracy = function(formationModifier = 1, applySpAttackModifiers = true) {
if(this.isDummy()) { return {}; }
const byLevel = 2 * Math.sqrt(this.level);
// formula from PSVita is sqrt(1.5 * lk) anyway,
// but verifications have proved this one gets more accurate
// http://ja.kancolle.wikia.com/wiki/%E3%82%B9%E3%83%AC%E3%83%83%E3%83%89:450#68
const byLuck = 1.5 * Math.sqrt(this.lk[0]);
const byEquip = -this.nakedStats("ht");
const byImprove = this.equipment(true)
.map(g => g.accStatImprovementBonus("fire"))
.sumValues();
const byGunfit = this.shellingGunFitAccuracy();
const battleConds = this.collectBattleConditions();
const moraleModifier = this.moraleEffectLevel([1, 0.5, 0.8, 1, 1.2], battleConds.isOnBattle);
const basic = 90 + byLevel + byLuck + byEquip + byImprove;
const beforeSpModifier = basic * formationModifier * moraleModifier + byGunfit;
let artillerySpottingModifier = 1;
// there is trigger chance rate for Artillery Spotting itself, see #artillerySpottingRate
if(applySpAttackModifiers) {
artillerySpottingModifier = (type => {
if(type[0] === "Cutin") {
return ({
// IDs from `api_hougeki.api_at_type`, see #specialAttackTypeDay
"2": 1.1, "3": 1.3, "4": 1.5, "5": 1.3, "6": 1.2,
// modifier for 7 (CVCI) still unknown
// modifiers for [100, 201] (special cutins) still unknown
})[type[1]] || 1;
}
return 1;
})(this.estimateDayAttackType(undefined, true, battleConds.airBattleId));
}
const apShellModifier = (() => {
// AP Shell combined with Large cal. main gun only mainly for battleships
const hasApShellAndMainGun = this.hasEquipmentType(2, 19) && this.hasEquipmentType(2, 3);
if(hasApShellAndMainGun) {
const hasSecondaryGun = this.hasEquipmentType(2, 4);
const hasRadar = this.hasEquipmentType(2, [12, 13]);
if(hasRadar && hasSecondaryGun) return 1.3;
if(hasRadar) return 1.25;
if(hasSecondaryGun) return 1.2;
return 1.1;
}
return 1;
})();
// penalty for combined fleets under verification
const accuracy = Math.floor(beforeSpModifier * artillerySpottingModifier * apShellModifier);
return {
accuracy,
basicAccuracy: basic,
preSpAttackAccuracy: beforeSpModifier,
equipmentStats: byEquip,
equipImprovement: byImprove,
equipGunFit: byGunfit,
moraleModifier,
formationModifier,
artillerySpottingModifier,
apShellModifier
};
};
/**
* @see http://wikiwiki.jp/kancolle/?%CC%BF%C3%E6%A4%C8%B2%F3%C8%F2%A4%CB%A4%C4%A4%A4%A4%C6#hitterm1
* @see http://wikiwiki.jp/kancolle/?%CC%BF%C3%E6%A4%C8%B2%F3%C8%F2%A4%CB%A4%C4%A4%A4%A4%C6#avoidterm1
*/
KC3Ship.prototype.estimateShellingFormationModifier = function(
playerFormationId = ConfigManager.aaFormation,
enemyFormationId = 0,
type = "accuracy") {
let modifier = 1;
switch(type) {
case "accuracy":
// Default is no bonus for regular fleet
// Still unknown for combined fleet formation
// Line Ahead, Diamond:
modifier = 1;
switch(playerFormationId) {
case 2: // Double Line, cancelled by Line Abreast
modifier = enemyFormationId === 5 ? 1.0 : 1.2;
break;
case 4: // Echelon, cancelled by Line Ahead
modifier = enemyFormationId === 1 ? 1.0 : 1.2;
break;
case 5: // Line Abreast, cancelled by Echelon
modifier = enemyFormationId === 4 ? 1.0 : 1.2;
break;
case 6:{// Vanguard, depends on fleet position
const [shipPos, shipCnt] = this.fleetPosition(),
isGuardian = shipCnt >= 4 && shipPos >= Math.floor(shipCnt / 2);
modifier = isGuardian ? 1.2 : 0.8;
break;
}
}
break;
case "evasion":
// Line Ahead, Double Line:
modifier = 1;
switch(playerFormationId) {
case 3: // Diamond
modifier = 1.1;
break;
case 4: // Echelon
// enhanced by Double Line / Echelon?
// mods: https://twitter.com/Xe_UCH/status/1304783506275409920
modifier = enemyFormationId === 2 ? 1.45 : 1.4;
break;
case 5: // Line Abreast, enhanced by Echelon / Line Abreast unknown
modifier = 1.3;
break;
case 6:{// Vanguard, depends on fleet position and ship type
const [shipPos, shipCnt] = this.fleetPosition(),
isGuardian = shipCnt >= 4 && shipPos >= (Math.floor(shipCnt / 2) + 1),
isThisDestroyer = this.master().api_stype === 2;
modifier = isThisDestroyer ?
(isGuardian ? 1.4 : 1.2) :
(isGuardian ? 1.2 : 1.05);
break;
}
}
break;
default:
console.warn("Unknown modifier type:", type);
}
return modifier;
};
/**
* Get current shelling accuracy bonus (or penalty) from equipped guns.
* @see http://kancolle.wikia.com/wiki/Combat/Overweight_Penalty_and_Fit_Gun_Bonus
* @see http://wikiwiki.jp/kancolle/?%CC%BF%C3%E6%A4%C8%B2%F3%C8%F2%A4%CB%A4%C4%A4%A4%A4%C6#fitarms
*/
KC3Ship.prototype.shellingGunFitAccuracy = function(time = "Day") {
if(this.isDummy()) { return 0; }
var result = 0;
// Fit bonus or overweight penalty for ship types:
const stype = this.master().api_stype;
const ctype = this.master().api_ctype;
switch(stype) {
case 2: // for Destroyers
// fit bonus under verification since 2017-06-23
// 12.7cm Single High-angle Gun Mount (Late Model)
const singleHighAngleMountCnt = this.countEquipment(229);
// for Mutsuki class including Satsuki K2
result += (ctype === 28 ? 5 : 0) * Math.sqrt(singleHighAngleMountCnt);
// for Kamikaze class still unknown
break;
case 3:
case 4:
case 21: // for Light Cruisers
// overhaul implemented in-game since 2017-06-23, not fully verified
const singleMountIds = [4, 11];
const twinMountIds = [65, 119, 139];
const tripleMainMountIds = [5, 235];
const singleHighAngleMountId = 229;
const isAganoClass = ctype === 41;
const isOoyodoClass = ctype === 52;
result = -2; // only fit bonus, but -2 fixed (might disappeared?)
// for all CLs
result += 4 * Math.sqrt(this.countEquipment(singleMountIds));
// for twin mount on Agano class / Ooyodo class / general CLs
result += (isAganoClass ? 8 : isOoyodoClass ? 5 : 3) *
Math.sqrt(this.countEquipment(twinMountIds));
// for 15.5cm triple main mount on Ooyodo class
result += (isOoyodoClass ? 7 : 0) *
Math.sqrt(this.countEquipment(tripleMainMountIds));
// for 12.7cm single HA late model on Yura K2
result += (this.masterId === 488 ? 10 : 0) *
Math.sqrt(this.countEquipment(singleHighAngleMountId));
break;
case 5:
case 6: // for Heavy Cruisers
// fit bonus at night battle for 20.3cm variants
if(time === "Night") {
const has203TwinGun = this.hasEquipment(6);
const has203No3TwinGun = this.hasEquipment(50);
const has203No2TwinGun = this.hasEquipment(90);
// 20.3cm priority to No.3, No.2 might also
result += has203TwinGun ? 10 : has203No2TwinGun ? 10 : has203No3TwinGun ? 15 : 0;
}
// for 15.5cm triple main mount on Mogami class
const isMogamiClass = ctype === 9;
if(isMogamiClass) {
const count155TripleMainGun = this.countEquipment(5);
const count155TripleMainGunKai = this.countEquipment(235);
result += 2 * Math.sqrt(count155TripleMainGun) +
5 * Math.sqrt(count155TripleMainGunKai);
}
// for 203mm/53 twin mount on Zara class
const isZaraClass = ctype === 64;
if(isZaraClass) {
result += 1 * Math.sqrt(this.countEquipment(162));
}
break;
case 8:
case 9:
case 10: // for Battleships
// Large cal. main gun gives accuracy bonus if it's fit,
// and accuracy penalty if it's overweight.
const gunCountFitMap = {};
this.equipment(true).forEach(g => {
if(g.itemId && g.masterId && g.master().api_type[2] === 3) {
const fitInfo = KC3Meta.gunfit(this.masterId, g.masterId);
if(fitInfo && !fitInfo.unknown) {
const gunCount = (gunCountFitMap[fitInfo.weight] || [0])[0];
gunCountFitMap[fitInfo.weight] = [gunCount + 1, fitInfo];
}
}
});
$.each(gunCountFitMap, (_, fit) => {
const count = fit[0];
let value = fit[1][time.toCamelCase()] || 0;
if(this.isMarried()) value *= fit[1].married || 1;
result += value * Math.sqrt(count);
});
break;
case 16: // for Seaplane Tender
// Medium cal. guns for partial AVs, no formula summarized
// https://docs.google.com/spreadsheets/d/1wl9v3NqPuRawSuFadokgYh1R1R1W82H51JNC66DH2q8/htmlview
break;
default:
// not found for other ships
}
return result;
};
/**
* Get current shelling attack evasion related info of this ship.
* @param {number} formationModifier - see #estimateShellingFormationModifier
* @return {Object} evasion factors of this ship.
* @see http://kancolle.wikia.com/wiki/Combat/Accuracy_and_Evasion
* @see http://wikiwiki.jp/kancolle/?%CC%BF%C3%E6%A4%C8%B2%F3%C8%F2%A4%CB%A4%C4%A4%A4%A4%C6
*/
KC3Ship.prototype.shellingEvasion = function(formationModifier = 1) {
if(this.isDummy()) { return {}; }
// already naked ev + equipment total ev stats
const byStats = this.ev[0];
const byEquip = this.equipmentTotalStats("houk");
const byLuck = Math.sqrt(2 * this.lk[0]);
const preCap = Math.floor((byStats + byLuck) * formationModifier);
const postCap = preCap >= 65 ? Math.floor(55 + 2 * Math.sqrt(preCap - 65)) :
preCap >= 40 ? Math.floor(40 + 3 * Math.sqrt(preCap - 40)) :
preCap;
const byImprove = this.equipment(true)
.map(g => g.evaStatImprovementBonus("fire"))
.sumValues();
// under verification
const stypeBonus = 0;
const searchlightModifier = this.hasEquipmentType(1, 18) ? 0.2 : 1;
const postCapForYasen = Math.floor(postCap + stypeBonus) * searchlightModifier;
const fuelPercent = Math.floor(this.fuel / this.master().api_fuel_max * 100);
const fuelPenalty = fuelPercent < 75 ? 75 - fuelPercent : 0;
// final hit % = ucap(floor(lcap(attackerAccuracy - defenderEvasion) * defenderMoraleModifier)) + aircraftProficiencyBonus
// capping limits its lower / upper bounds to [10, 96] + 1%, +1 since random number is 0-based, ranged in [0, 99]
// ship morale modifier not applied here since 'evasion' may be looked reduced when sparkle
const battleConds = this.collectBattleConditions();
const moraleModifier = this.moraleEffectLevel([1, 1.4, 1.2, 1, 0.7], battleConds.isOnBattle);
const evasion = Math.floor(postCap + byImprove - fuelPenalty);
const evasionForYasen = Math.floor(postCapForYasen + byImprove - fuelPenalty);
return {
evasion,
evasionForYasen,
preCap,
postCap,
postCapForYasen,
equipmentStats: byEquip,
equipImprovement: byImprove,
fuelPenalty,
moraleModifier,
formationModifier
};
};
KC3Ship.prototype.equipmentAntiAir = function(forFleet) {
return AntiAir.shipEquipmentAntiAir(this, forFleet);
};
KC3Ship.prototype.adjustedAntiAir = function() {
const floor = AntiAir.specialFloor(this);
return floor(AntiAir.shipAdjustedAntiAir(this));
};
KC3Ship.prototype.proportionalShotdownRate = function() {
return AntiAir.shipProportionalShotdownRate(this);
};
KC3Ship.prototype.proportionalShotdown = function(n) {
return AntiAir.shipProportionalShotdown(this, n);
};
// note:
// - fixed shotdown makes no sense if the current ship is not in a fleet.
// - formationId takes one of the following:
// - 1/4/5 (for line ahead / echelon / line abreast)
// - 2 (for double line)
// - 3 (for diamond)
// - 6 (for vanguard)
// - all possible AACIs are considered and the largest AACI modifier
// is used for calculation the maximum number of fixed shotdown
KC3Ship.prototype.fixedShotdownRange = function(formationId) {
if(!this.onFleet()) return false;
const fleetObj = PlayerManager.fleets[ this.onFleet() - 1 ];
return AntiAir.shipFixedShotdownRangeWithAACI(this, fleetObj,
AntiAir.getFormationModifiers(formationId || 1) );
};
KC3Ship.prototype.maxAaciShotdownBonuses = function() {
return AntiAir.shipMaxShotdownAllBonuses( this );
};
/**
* Anti-air Equipment Attack Effect implemented since 2018-02-05 in-game.
* @return a tuple indicates the effect type ID and its term key.
* NOTE: type ID shifted to 1-based since Phase 2, but internal values here unchanged.
* @see `TaskAircraftFlightBase.prototype._getAntiAircraftAbility`
* @see `TaskAirWarAntiAircraft._type` - AA attack animation types
*/
KC3Ship.prototype.estimateAntiAirEffectType = function() {
const aaEquipType = (() => {
// Escaped or sunk ship cannot anti-air
if(this.isDummy() || this.isAbsent()) return -1;
const stype = this.master().api_stype;
// CAV, BBV, CV/CVL/CVB, AV can use Rocket Launcher K2
const isStypeForRockeLaunK2 = [6, 7, 10, 11, 16, 18].includes(stype);
// Type 3 Shell
if(this.hasEquipmentType(2, 18)) {
if(isStypeForRockeLaunK2 && this.hasEquipment(274)) return 5;
return 4;
}
// 12cm 30tube Rocket Launcher Kai Ni
if(isStypeForRockeLaunK2 && this.hasEquipment(274)) return 3;
// 12cm 30tube Rocket Launcher
if(this.hasEquipment(51)) return 2;
// Any HA Mount
if(this.hasEquipmentType(3, 16)) return 1;
// Any AA Machine Gun
if(this.hasEquipmentType(2, 21)) return 0;
// Any Radar plus any Seaplane bomber with AA stat
if(this.hasEquipmentType(3, 11) && this.equipment().some(
g => g.masterId > 0 && g.master().api_type[2] === 11 && g.master().api_tyku > 0
)) return 0;
return -1;
})();
switch(aaEquipType) {
case -1: return [0, "None"];
case 0: return [1, "Normal"];
case 1: return [2, "HighAngleMount"];
case 2: return [3, "RocketLauncher"];
case 3: return [4, "RocketLauncherK2"];
case 4: return [5, "Type3Shell"];
case 5: return [6, "Type3ShellRockeLaunK2"];
default: return [NaN, "Unknown"];
}
};
/**
* To calculate 12cm 30tube Rocket Launcher Kai Ni (Rosa K2) trigger chance (for now),
* we need adjusted AA of ship, number of Rosa K2, ctype and luck stat.
* @see https://twitter.com/noratako5/status/1062027534026428416 - luck modifier
* @see https://twitter.com/kankenRJ/status/979524073934893056 - current formula
* @see http://ja.kancolle.wikia.com/wiki/%E3%82%B9%E3%83%AC%E3%83%83%E3%83%89:2471 - old formula verifying thread
*/
KC3Ship.prototype.calcAntiAirEffectChance = function() {
if(this.isDummy() || this.isAbsent()) return 0;
// Number of 12cm 30tube Rocket Launcher Kai Ni
let rosaCount = this.countEquipment(274);
if(rosaCount === 0) return 0;
// Not tested yet on more than 3 Rosa K2, capped to 3 just in case of exceptions
rosaCount = rosaCount > 3 ? 3 : rosaCount;
const rosaAdjustedAntiAir = 48;
// 70 for Ise-class, 0 otherwise
const classBonus = this.master().api_ctype === 2 ? 70 : 0;
// Rounding to x%
return Math.qckInt("floor",
(this.adjustedAntiAir() + this.lk[0] * 0.9) /
(400 - (rosaAdjustedAntiAir + 30 + 40 * rosaCount + classBonus)) * 100,
0);
};
/**
* Check known possible effects on equipment changed.
* @param {Object} newGearObj - the equipment just equipped, pseudo empty object if unequipped.
* @param {Object} oldGearObj - the equipment before changed, pseudo empty object if there was empty.
* @param {Object} oldShipObj - the cloned old ship instance with stats and item IDs before equipment changed.
*/
KC3Ship.prototype.equipmentChangedEffects = function(newGearObj = {}, oldGearObj = {}, oldShipObj = this) {
if(!this.masterId) return {isShow: false};
const gunFit = newGearObj.masterId ? KC3Meta.gunfit(this.masterId, newGearObj.masterId) : false;
let isShow = gunFit !== false;
const shipAacis = AntiAir.sortedPossibleAaciList(AntiAir.shipPossibleAACIs(this));
isShow = isShow || shipAacis.length > 0;
// NOTE: shipObj here to be returned will be the 'old' ship instance,
// whose stats, like fp, tp, asw, are the values before equipment change.
// but its items array (including ex item) are post-change values.
const shipObj = this;
// To get the 'latest' ship stats, should defer `GunFit` event after `api_get_member/ship3` call,
// and retrieve latest ship instance via KC3ShipManager.get method like this:
// const newShipObj = KC3ShipManager.get(data.shipObj.rosterId);
// It can not be latest ship at the timing of this equipmentChangedEffects invoked,
// because the api call above not executed, KC3ShipManager data not updated yet.
// Or you can compute the simple stat difference manually like this:
const oldEquipAsw = oldGearObj.masterId > 0 ? oldGearObj.master().api_tais : 0;
const newEquipAsw = newGearObj.masterId > 0 ? newGearObj.master().api_tais : 0;
const aswDiff = newEquipAsw - oldEquipAsw
// explicit asw bonus from new equipment
+ shipObj.equipmentTotalStats("tais", true, true, true)
// explicit asw bonus from old equipment
- oldShipObj.equipmentTotalStats("tais", true, true, true);
const oaswPower = shipObj.canDoOASW(aswDiff) ? shipObj.antiSubWarfarePower(aswDiff) : false;
isShow = isShow || (oaswPower !== false);
const antiLandPowers = shipObj.shipPossibleAntiLandPowers();
isShow = isShow || antiLandPowers.length > 0;
const equipBonus = shipObj.equipmentBonusGearAndStats(newGearObj);
isShow = isShow || (equipBonus !== false && equipBonus.isShow);
// Possible TODO:
// can opening torpedo
// can cut-in (fire / air)
// can night attack for CV
// can night cut-in
return {
isShow,
shipObj,
shipOld: oldShipObj,
gearObj: newGearObj.masterId ? newGearObj : false,
gunFit,
shipAacis,
oaswPower,
antiLandPowers: antiLandPowers.length > 0,
equipBonus,
};
};
/* Expedition Supply Change Check */
KC3Ship.prototype.perform = function(command,args) {
try {
args = $.extend({noFuel:0,noAmmo:0},args);
command = command.slice(0,1).toUpperCase() + command.slice(1).toLowerCase();
this["perform"+command].call(this,args);
return true;
} catch (e) {
console.error("Failed when perform" + command, e);
return false;
}
};
KC3Ship.prototype.performSupply = function(args) {
consumePending.call(this,0,{0:0,1:1,2:3,c: 1 - (this.isMarried() && 0.15),i: 0},[0,1,2],args);
};
KC3Ship.prototype.performRepair = function(args) {
consumePending.call(this,1,{0:0,1:2,2:6,c: 1,i: 0},[0,1,2],args);
};
function consumePending(index,mapping,clear,args) {
/*jshint validthis: true */
if(!(this instanceof KC3Ship)) {
throw new Error("Cannot modify non-KC3Ship instance!");
}
var
self = this,
mult = mapping.c,
lastN = Object.keys(this.pendingConsumption).length - mapping.i;
delete mapping.c;
delete mapping.i;
if(args.noFuel) delete mapping['0'];
if(args.noAmmo) delete mapping['1'];
/* clear pending consumption, by iterating each keys */
var
rmd = [0,0,0,0,0,0,0,0],
lsFirst = this.lastSortie[0];
Object.keys(this.pendingConsumption).forEach(function(shipConsumption,iterant){
var
dat = self.pendingConsumption[shipConsumption],
rsc = [0,0,0,0,0,0,0,0],
sid = self.lastSortie.indexOf(shipConsumption);
// Iterate supplied ship part
Object.keys(mapping).forEach(function(key){
var val = dat[index][key] * (mapping[key]===3 ? 5 : mult);
// Calibrate for rounding towards zero
rmd[mapping[key]] += val % 1;
rsc[mapping[key]] += Math.ceil(val) + parseInt(rmd[mapping[key]]);
rmd[mapping[key]] %= 1;
// Checks whether current iteration is last N pending item
if((iterant < lastN) && (clear.indexOf(parseInt(key))>=0))
dat[index][key] = 0;
});
console.log("Ship " + self.rosterId + " consumed", shipConsumption, sid,
[iterant, lastN].join('/'), rsc.map(x => -x), dat[index]);
// Store supplied resource count to database by updating the source
KC3Database.Naverall({
data: rsc
},shipConsumption);
if(dat.every(function(consumptionData){
return consumptionData.every(function(resource){ return !resource; });
})) {
delete self.pendingConsumption[shipConsumption];
}
/* Comment Stopper */
});
var
lsi = 1,
lsk = "";
while(lsi < this.lastSortie.length && this.lastSortie[lsi] != 'sortie0') {
lsk = this.lastSortie[lsi];
if(this.pendingConsumption[ lsk ]){
lsi++;
}else{
this.lastSortie.splice(lsi,1);
}
}
if(this.lastSortie.indexOf(lsFirst) < 0) {
this.lastSortie.unshift(lsFirst);
}
KC3ShipManager.save();
}
/**
* Fill data of this Ship into predefined tooltip HTML elements. Used by Panel/Strategy Room.
* @param tooltipBox - the object of predefined tooltip HTML jq element
* @return return back the jq element of param `tooltipBox`
*/
KC3Ship.prototype.htmlTooltip = function(tooltipBox) {
return KC3Ship.buildShipTooltip(this, tooltipBox);
};
KC3Ship.buildShipTooltip = function(shipObj, tooltipBox) {
//const shipDb = WhoCallsTheFleetDb.getShipStat(shipObj.masterId);
const nakedStats = shipObj.nakedStats(),
maxedStats = shipObj.maxedStats(),
bonusStats = shipObj.statsBonusOnShip(),
maxDiffStats = {},
equipDiffStats = {},
modLeftStats = shipObj.modernizeLeftStats();
Object.keys(maxedStats).map(s => {maxDiffStats[s] = maxedStats[s] - nakedStats[s];});
Object.keys(nakedStats).map(s => {equipDiffStats[s] = nakedStats[s] - (shipObj[s]||[])[0];});
const signedNumber = n => (n > 0 ? '+' : n === 0 ? '\u00b1' : '') + n;
const optionalNumber = (n, pre = '\u21d1', show0 = false) => !n && (!show0 || n !== 0) ? '' : pre + n;
const replaceFilename = (file, newName) => file.slice(0, file.lastIndexOf("/") + 1) + newName;
$(".stat_value span", tooltipBox).css("display", "inline");
$(".ship_full_name .ship_masterId", tooltipBox).text("[{0}]".format(shipObj.masterId));
$(".ship_full_name span.value", tooltipBox).text(shipObj.name());
$(".ship_full_name .ship_yomi", tooltipBox).text(ConfigManager.info_ship_class_name ?
KC3Meta.ctypeName(shipObj.master().api_ctype) :
KC3Meta.shipReadingName(shipObj.master().api_yomi)
);
$(".ship_rosterId span", tooltipBox).text(shipObj.rosterId);
$(".ship_stype", tooltipBox).text(shipObj.stype());
$(".ship_level span.value", tooltipBox).text(shipObj.level);
//$(".ship_level span.value", tooltipBox).addClass(shipObj.levelClass());
$(".ship_hp span.hp", tooltipBox).text(shipObj.hp[0]);
$(".ship_hp span.mhp", tooltipBox).text(shipObj.hp[1]);
$(".ship_morale img", tooltipBox).attr("src",
replaceFilename($(".ship_morale img", tooltipBox).attr("src"), shipObj.moraleIcon() + ".png")
);
$(".ship_morale span.value", tooltipBox).text(shipObj.morale);
$(".ship_exp_next span.value", tooltipBox).text(shipObj.exp[1]);
$(".stat_hp .current", tooltipBox).text(shipObj.hp[1]);
$(".stat_hp .mod", tooltipBox).text(signedNumber(modLeftStats.hp))
.toggle(!!modLeftStats.hp);
$(".stat_fp .current", tooltipBox).text(shipObj.fp[0]);
$(".stat_fp .mod", tooltipBox).text(signedNumber(modLeftStats.fp))
.toggle(!!modLeftStats.fp);
$(".stat_fp .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.fp, optionalNumber(bonusStats.fp)))
.toggle(!!equipDiffStats.fp || !!bonusStats.fp);
$(".stat_ar .current", tooltipBox).text(shipObj.ar[0]);
$(".stat_ar .mod", tooltipBox).text(signedNumber(modLeftStats.ar))
.toggle(!!modLeftStats.ar);
$(".stat_ar .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.ar, optionalNumber(bonusStats.ar)))
.toggle(!!equipDiffStats.ar || !!bonusStats.ar);
$(".stat_tp .current", tooltipBox).text(shipObj.tp[0]);
$(".stat_tp .mod", tooltipBox).text(signedNumber(modLeftStats.tp))
.toggle(!!modLeftStats.tp);
$(".stat_tp .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.tp, optionalNumber(bonusStats.tp)))
.toggle(!!equipDiffStats.tp || !!bonusStats.tp);
$(".stat_ev .current", tooltipBox).text(shipObj.ev[0]);
$(".stat_ev .level", tooltipBox).text(signedNumber(maxDiffStats.ev))
.toggle(!!maxDiffStats.ev);
$(".stat_ev .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.ev, optionalNumber(bonusStats.ev)))
.toggle(!!equipDiffStats.ev || !!bonusStats.ev);
$(".stat_aa .current", tooltipBox).text(shipObj.aa[0]);
$(".stat_aa .mod", tooltipBox).text(signedNumber(modLeftStats.aa))
.toggle(!!modLeftStats.aa);
$(".stat_aa .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.aa, optionalNumber(bonusStats.aa)))
.toggle(!!equipDiffStats.aa || !!bonusStats.aa);
$(".stat_ac .current", tooltipBox).text(shipObj.carrySlots());
const canOasw = shipObj.canDoOASW();
$(".stat_as .current", tooltipBox).text(shipObj.as[0])
.toggleClass("oasw", canOasw);
$(".stat_as .level", tooltipBox).text(signedNumber(maxDiffStats.as))
.toggle(!!maxDiffStats.as);
$(".stat_as .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.as, optionalNumber(bonusStats.as)))
.toggle(!!equipDiffStats.as || !!bonusStats.as);
$(".stat_as .mod", tooltipBox).text(signedNumber(modLeftStats.as))
.toggle(!!modLeftStats.as);
$(".stat_sp", tooltipBox).text(shipObj.speedName())
.addClass(KC3Meta.shipSpeed(shipObj.speed, true));
$(".stat_ls .current", tooltipBox).text(shipObj.ls[0]);
$(".stat_ls .level", tooltipBox).text(signedNumber(maxDiffStats.ls))
.toggle(!!maxDiffStats.ls);
$(".stat_ls .equip", tooltipBox)
.text("({0}{1})".format(nakedStats.ls, optionalNumber(bonusStats.ls)))
.toggle(!!equipDiffStats.ls || !!bonusStats.ls);
$(".stat_rn", tooltipBox).text(shipObj.rangeName())
.toggleClass("RangeChanged", shipObj.range != shipObj.master().api_leng);
$(".stat_lk .current", tooltipBox).text(shipObj.lk[0]);
$(".stat_lk .luck", tooltipBox).text(signedNumber(modLeftStats.lk));
$(".stat_lk .equip", tooltipBox).text("({0})".format(nakedStats.lk))
.toggle(!!equipDiffStats.lk);
if(!(ConfigManager.info_stats_diff & 1)){
$(".equip", tooltipBox).hide();
}
if(!(ConfigManager.info_stats_diff & 2)){
$(".mod,.level,.luck", tooltipBox).hide();
}
// Fill more stats need complex calculations
KC3Ship.fillShipTooltipWideStats(shipObj, tooltipBox, canOasw);
return tooltipBox;
};
KC3Ship.fillShipTooltipWideStats = function(shipObj, tooltipBox, canOasw = false) {
const signedNumber = n => (n > 0 ? '+' : n === 0 ? '\u00b1' : '') + n;
const optionalModifier = (m, showX1) => (showX1 || m !== 1 ? 'x' + m : '');
// show possible critical power and mark capped power with different color
const joinPowerAndCritical = (p, cp, cap) => (cap ? '<span class="power_capped">{0}</span>' : "{0}")
.format(String(Math.qckInt("floor", p, 0))) + (!cp ? "" :
(cap ? '(<span class="power_capped">{0}</span>)' : "({0})")
.format(Math.qckInt("floor", cp, 0))
);
const shipMst = shipObj.master();
const onFleetNum = shipObj.onFleet();
const battleConds = shipObj.collectBattleConditions();
const attackTypeDay = shipObj.estimateDayAttackType();
const warfareTypeDay = {
"Torpedo" : "Torpedo",
"DepthCharge" : "Antisub",
"LandingAttack" : "AntiLand",
"Rocket" : "AntiLand"
}[attackTypeDay[0]] || "Shelling";
const canAsw = shipObj.canDoASW();
if(canAsw){
const aswAttackType = shipObj.estimateDayAttackType(1530, false);
let power = shipObj.antiSubWarfarePower();
let criticalPower = false;
let isCapped = false;
const canShellingAttack = shipObj.canDoDayShellingAttack();
const canOpeningTorp = shipObj.canDoOpeningTorpedo();
const canClosingTorp = shipObj.canDoClosingTorpedo();
if(ConfigManager.powerCapApplyLevel >= 1) {
({power} = shipObj.applyPrecapModifiers(power, "Antisub",
battleConds.engagementId, battleConds.formationId || 5));
}
if(ConfigManager.powerCapApplyLevel >= 2) {
({power, isCapped} = shipObj.applyPowerCap(power, "Day", "Antisub"));
}
if(ConfigManager.powerCapApplyLevel >= 3) {
if(ConfigManager.powerCritical) {
criticalPower = shipObj.applyPostcapModifiers(
power, "Antisub", undefined, undefined,
true, aswAttackType[0] === "AirAttack").power;
}
({power} = shipObj.applyPostcapModifiers(power, "Antisub"));
}
let attackTypeIndicators = !canShellingAttack || !canAsw ?
KC3Meta.term("ShipAttackTypeNone") :
KC3Meta.term("ShipAttackType" + aswAttackType[0]);
if(canOpeningTorp) attackTypeIndicators += ", {0}"
.format(KC3Meta.term("ShipExtraPhaseOpeningTorpedo"));
if(canClosingTorp) attackTypeIndicators += ", {0}"
.format(KC3Meta.term("ShipExtraPhaseClosingTorpedo"));
$(".dayAswPower", tooltipBox).html(
KC3Meta.term("ShipDayAttack").format(
KC3Meta.term("ShipWarfareAntisub"),
joinPowerAndCritical(power, criticalPower, isCapped),
attackTypeIndicators
)
);
} else {
$(".dayAswPower", tooltipBox).html("-");
}
const isAswPowerShown = canAsw && (canOasw && !shipObj.isOaswShip()
|| shipObj.onlyHasEquipmentType(1, [10, 15, 16, 32]));
// Show ASW power if Opening ASW conditions met, or only ASW equipment equipped
if(isAswPowerShown){
$(".dayAttack", tooltipBox).parent().parent().hide();
} else {
$(".dayAswPower", tooltipBox).parent().parent().hide();
}
let combinedFleetBonus = 0;
if(onFleetNum) {
const powerBonus = shipObj.combinedFleetPowerBonus(
battleConds.playerCombinedFleetType, battleConds.isEnemyCombined, warfareTypeDay
);
combinedFleetBonus = onFleetNum === 1 ? powerBonus.main :
onFleetNum === 2 ? powerBonus.escort : 0;
}
let power = warfareTypeDay === "Torpedo" ?
shipObj.shellingTorpedoPower(combinedFleetBonus) :
shipObj.shellingFirePower(combinedFleetBonus);
let criticalPower = false;
let isCapped = false;
const canShellingAttack = warfareTypeDay === "Torpedo" ||
shipObj.canDoDayShellingAttack();
const canOpeningTorp = shipObj.canDoOpeningTorpedo();
const canClosingTorp = shipObj.canDoClosingTorpedo();
const spAttackType = shipObj.estimateDayAttackType(undefined, true, battleConds.airBattleId);
const dayCutinRate = shipObj.artillerySpottingRate(spAttackType[1], spAttackType[2]);
// Apply power cap by configured level
if(ConfigManager.powerCapApplyLevel >= 1) {
({power} = shipObj.applyPrecapModifiers(power, warfareTypeDay,
battleConds.engagementId, battleConds.formationId));
}
if(ConfigManager.powerCapApplyLevel >= 2) {
({power, isCapped} = shipObj.applyPowerCap(power, "Day", warfareTypeDay));
}
if(ConfigManager.powerCapApplyLevel >= 3) {
if(ConfigManager.powerCritical) {
criticalPower = shipObj.applyPostcapModifiers(
power, warfareTypeDay, spAttackType, undefined,
true, attackTypeDay[0] === "AirAttack").power;
}
({power} = shipObj.applyPostcapModifiers(power, warfareTypeDay,
spAttackType));
}
let attackTypeIndicators = !canShellingAttack ? KC3Meta.term("ShipAttackTypeNone") :
spAttackType[0] === "Cutin" ?
KC3Meta.cutinTypeDay(spAttackType[1]) + (dayCutinRate ? " {0}%".format(dayCutinRate) : "") :
KC3Meta.term("ShipAttackType" + attackTypeDay[0]);
if(canOpeningTorp) attackTypeIndicators += ", {0}"
.format(KC3Meta.term("ShipExtraPhaseOpeningTorpedo"));
if(canClosingTorp) attackTypeIndicators += ", {0}"
.format(KC3Meta.term("ShipExtraPhaseClosingTorpedo"));
$(".dayAttack", tooltipBox).html(
KC3Meta.term("ShipDayAttack").format(
KC3Meta.term("ShipWarfare" + warfareTypeDay),
joinPowerAndCritical(power, criticalPower, isCapped),
attackTypeIndicators
)
);
const attackTypeNight = shipObj.estimateNightAttackType();
const canNightAttack = shipObj.canDoNightAttack();
// See functions in previous 2 lines, ships whose night attack is AirAttack,
// but power formula seems be shelling: Taiyou Kai Ni, Shinyou Kai Ni
const hasYasenPower = (shipMst.api_houg || [])[0] + (shipMst.api_raig || [])[0] > 0;
const hasNightFlag = attackTypeNight[0] === "AirAttack" && attackTypeNight[2] === true;
const warfareTypeNight = {
"Torpedo" : "Torpedo",
"DepthCharge" : "Antisub",
"LandingAttack" : "AntiLand",
"Rocket" : "AntiLand"
}[attackTypeNight[0]] || "Shelling";
if(attackTypeNight[0] === "AirAttack" && canNightAttack && (hasNightFlag || !hasYasenPower)){
let power = shipObj.nightAirAttackPower(battleConds.contactPlaneId == 102);
let criticalPower = false;
let isCapped = false;
const spAttackType = shipObj.estimateNightAttackType(undefined, true);
const nightCutinRate = shipObj.nightCutinRate(spAttackType[1], spAttackType[2]);
if(ConfigManager.powerCapApplyLevel >= 1) {
({power} = shipObj.applyPrecapModifiers(power, "Shelling",
battleConds.engagementId, battleConds.formationId, spAttackType,
battleConds.isStartFromNight, battleConds.playerCombinedFleetType > 0));
}
if(ConfigManager.powerCapApplyLevel >= 2) {
({power, isCapped} = shipObj.applyPowerCap(power, "Night", "Shelling"));
}
if(ConfigManager.powerCapApplyLevel >= 3) {
if(ConfigManager.powerCritical) {
criticalPower = shipObj.applyPostcapModifiers(
power, "Shelling", undefined, undefined, true, true).power;
}
({power} = shipObj.applyPostcapModifiers(power, "Shelling"));
}
$(".nightAttack", tooltipBox).html(
KC3Meta.term("ShipNightAttack").format(
KC3Meta.term("ShipWarfareShelling"),
joinPowerAndCritical(power, criticalPower, isCapped),
spAttackType[0] === "Cutin" ?
KC3Meta.cutinTypeNight(spAttackType[1]) + (nightCutinRate ? " {0}%".format(nightCutinRate) : "") :
KC3Meta.term("ShipAttackType" + spAttackType[0])
)
);
} else {
let power = shipObj.nightBattlePower(battleConds.contactPlaneId == 102);
let criticalPower = false;
let isCapped = false;
const spAttackType = shipObj.estimateNightAttackType(undefined, true);
const nightCutinRate = shipObj.nightCutinRate(spAttackType[1], spAttackType[2]);
// Apply power cap by configured level
if(ConfigManager.powerCapApplyLevel >= 1) {
({power} = shipObj.applyPrecapModifiers(power, warfareTypeNight,
battleConds.engagementId, battleConds.formationId, spAttackType,
battleConds.isStartFromNight, battleConds.playerCombinedFleetType > 0));
}
if(ConfigManager.powerCapApplyLevel >= 2) {
({power, isCapped} = shipObj.applyPowerCap(power, "Night", warfareTypeNight));
}
if(ConfigManager.powerCapApplyLevel >= 3) {
if(ConfigManager.powerCritical) {
criticalPower = shipObj.applyPostcapModifiers(
power, warfareTypeNight, undefined, undefined, true).power;
}
({power} = shipObj.applyPostcapModifiers(power, warfareTypeNight));
}
let attackTypeIndicators = !canNightAttack ? KC3Meta.term("ShipAttackTypeNone") :
spAttackType[0] === "Cutin" ?
KC3Meta.cutinTypeNight(spAttackType[1]) + (nightCutinRate ? " {0}%".format(nightCutinRate) : "") :
KC3Meta.term("ShipAttackType" + spAttackType[0]);
$(".nightAttack", tooltipBox).html(
KC3Meta.term("ShipNightAttack").format(
KC3Meta.term("ShipWarfare" + warfareTypeNight),
joinPowerAndCritical(power, criticalPower, isCapped),
attackTypeIndicators
)
);
}
// TODO implement other types of accuracy
const shellingAccuracy = shipObj.shellingAccuracy(
shipObj.estimateShellingFormationModifier(battleConds.formationId, battleConds.enemyFormationId),
true
);
$(".shellingAccuracy", tooltipBox).text(
KC3Meta.term("ShipAccShelling").format(
Math.qckInt("floor", shellingAccuracy.accuracy, 1),
signedNumber(shellingAccuracy.equipmentStats),
signedNumber(Math.qckInt("floor", shellingAccuracy.equipImprovement, 1)),
signedNumber(Math.qckInt("floor", shellingAccuracy.equipGunFit, 1)),
optionalModifier(shellingAccuracy.moraleModifier, true),
optionalModifier(shellingAccuracy.artillerySpottingModifier),
optionalModifier(shellingAccuracy.apShellModifier)
)
);
const shellingEvasion = shipObj.shellingEvasion(
shipObj.estimateShellingFormationModifier(battleConds.formationId, battleConds.enemyFormationId, "evasion")
);
$(".shellingEvasion", tooltipBox).text(
KC3Meta.term("ShipEvaShelling").format(
Math.qckInt("floor", shellingEvasion.evasion, 1),
signedNumber(shellingEvasion.equipmentStats),
signedNumber(Math.qckInt("floor", shellingEvasion.equipImprovement, 1)),
signedNumber(-shellingEvasion.fuelPenalty),
optionalModifier(shellingEvasion.moraleModifier, true)
)
);
const [aaEffectTypeId, aaEffectTypeTerm] = shipObj.estimateAntiAirEffectType();
$(".adjustedAntiAir", tooltipBox).text(
KC3Meta.term("ShipAAAdjusted").format(
"{0}{1}".format(
shipObj.adjustedAntiAir(),
/* Here indicates the type of AA ability, not the real attack animation in-game,
* only special AA effect types will show a banner text in-game,
* like the T3 Shell shoots or Rockets shoots,
* regular AA gun animation triggered by equipping AA gun, Radar+SPB or HA mount.
* btw1, 12cm Rocket Launcher non-Kai belongs to AA guns, no irregular attack effect.
* btw2, flagship will fall-back to the effect user if none has any attack effect.
*/
aaEffectTypeId > 0 ?
" ({0})".format(
aaEffectTypeId === 4 ?
// Show a trigger chance for RosaK2 Defense, still unknown if with Type3 Shell
"{0}:{1}%".format(KC3Meta.term("ShipAAEffect" + aaEffectTypeTerm), shipObj.calcAntiAirEffectChance()) :
KC3Meta.term("ShipAAEffect" + aaEffectTypeTerm)
) : ""
)
)
);
$(".propShotdownRate", tooltipBox).text(
KC3Meta.term("ShipAAShotdownRate").format(
Math.qckInt("floor", shipObj.proportionalShotdownRate() * 100, 1)
)
);
const maxAaciParams = shipObj.maxAaciShotdownBonuses();
if(maxAaciParams[0] > 0){
$(".aaciMaxBonus", tooltipBox).text(
KC3Meta.term("ShipAACIMaxBonus").format(
"+{0} (x{1})".format(maxAaciParams[1], maxAaciParams[2])
)
);
} else {
$(".aaciMaxBonus", tooltipBox).text(
KC3Meta.term("ShipAACIMaxBonus").format(KC3Meta.term("None"))
);
}
// Not able to get following anti-air things if not on a fleet
if(onFleetNum){
const fixedShotdownRange = shipObj.fixedShotdownRange(ConfigManager.aaFormation);
const fleetPossibleAaci = fixedShotdownRange[2];
if(fleetPossibleAaci > 0){
$(".fixedShotdown", tooltipBox).text(
KC3Meta.term("ShipAAFixedShotdown").format(
"{0}~{1} (x{2})".format(fixedShotdownRange[0], fixedShotdownRange[1],
AntiAir.AACITable[fleetPossibleAaci].modifier)
)
);
} else {
$(".fixedShotdown", tooltipBox).text(
KC3Meta.term("ShipAAFixedShotdown").format(fixedShotdownRange[0])
);
}
const propShotdown = shipObj.proportionalShotdown(ConfigManager.imaginaryEnemySlot);
const aaciFixedShotdown = fleetPossibleAaci > 0 ? AntiAir.AACITable[fleetPossibleAaci].fixed : 0;
$.each($(".sd_title .aa_col", tooltipBox), function(idx, col){
$(col).text(KC3Meta.term("ShipAAShotdownTitles").split("/")[idx] || "");
});
$(".bomberSlot span", tooltipBox).text(ConfigManager.imaginaryEnemySlot);
$(".sd_both span", tooltipBox).text(
// Both succeeded
propShotdown + fixedShotdownRange[1] + aaciFixedShotdown + 1
);
$(".sd_prop span", tooltipBox).text(
// Proportional succeeded only
propShotdown + aaciFixedShotdown + 1
);
$(".sd_fixed span", tooltipBox).text(
// Fixed succeeded only
fixedShotdownRange[1] + aaciFixedShotdown + 1
);
$(".sd_fail span", tooltipBox).text(
// Both failed
aaciFixedShotdown + 1
);
} else {
$(".fixedShotdown", tooltipBox).text(
KC3Meta.term("ShipAAFixedShotdown").format("-"));
$.each($(".sd_title .aa_col", tooltipBox), function(idx, col){
$(col).text(KC3Meta.term("ShipAAShotdownTitles").split("/")[idx] || "");
});
}
return tooltipBox;
};
KC3Ship.onShipTooltipOpen = function(event, ui) {
const setStyleVar = (name, value) => {
const shipTooltipStyle = $(ui.tooltip).children().children().get(0).style;
shipTooltipStyle.removeProperty(name);
shipTooltipStyle.setProperty(name, value);
};
// find which width of wide rows overflow, add slide animation to them
// but animation might cost 10% more or less CPU even accelerated with GPU
let maxOverflow = 0;
$(".stat_wide div", ui.tooltip).each(function() {
// scroll width only works if element is visible
const sw = $(this).prop('scrollWidth'),
w = $(this).width(),
over = w - sw;
maxOverflow = Math.min(maxOverflow, over);
// allow overflow some pixels
if(over < -8) { $(this).addClass("use-gpu slide"); }
});
setStyleVar("--maxOverflow", maxOverflow + "px");
// show day shelling power instead of ASW power (if any) on holding Alt key
if(event.altKey && $(".dayAswPower", ui.tooltip).is(":visible")) {
$(".dayAswPower", ui.tooltip).parent().parent().hide();
$(".dayAttack", ui.tooltip).parent().parent().show();
}
// show day ASW power instead of shelling power if can ASW on holding Ctrl/Meta key
if((event.ctrlKey || event.metaKey) && !$(".dayAswPower", ui.tooltip).is(":visible")
&& $(".dayAswPower", ui.tooltip).text() !== "-") {
$(".dayAswPower", ui.tooltip).parent().parent().show();
$(".dayAttack", ui.tooltip).parent().parent().hide();
}
return true;
};
KC3Ship.prototype.deckbuilder = function() {
var itemsInfo = {};
var result = {
id: this.masterId,
lv: this.level,
luck: this.lk[0],
hp: this.hp[0],
asw : this.nakedAsw(),
items: itemsInfo
};
var gearInfo;
for(var i=0; i<5; ++i) {
gearInfo = this.equipment(i).deckbuilder();
if (gearInfo)
itemsInfo["i".concat(i+1)] = gearInfo;
else
break;
}
gearInfo = this.exItem().deckbuilder();
if (gearInfo) {
// #1726 Deckbuilder: if max slot not reach 5, `ix` will not be used,
// which means i? > ship.api_slot_num will be considered as the ex-slot.
var usedSlot = Object.keys(itemsInfo).length;
if(usedSlot < 5) {
itemsInfo["i".concat(usedSlot+1)] = gearInfo;
} else {
itemsInfo.ix = gearInfo;
}
}
return result;
};
})();
| Java |
# This preloads the content types so that they are registered as Tirade::ActiveRecord::Content
APP_CONFIG[:content_types].each do |typ|
typ.to_s.constantize
end if APP_CONFIG[:content_types]
| Java |
---
layout: post
title: Sixth Blog!!
---
This week we have learned some brand new concepts, like iterators, lambda, closure. It’s tricky for me to understand when to add const before passed parameters. Also, I learned that it’s easier to use a for each loop in python to traverse an iterable type of variable instead of using a while loop through iterator. Since I learned Haskell in a class called programming language, it’s relatively easier for me to understand the concept of closure. When writing in Haskell for a basic interpreter, it is important to catch the closure so that the value of a function, for example, is not lost after the return statement. When self-writing a range_iterator, I also learned that python can have nested class, but it can be only called in the syntax of class.nestedclass() instead of this.nestedclass() like in Java.
For me, I think that range is an interesting name for that method because when it is passed in two arguments, it returns a list of integers in that range rather than the range of the two passed parameters. Sometimes, I need to think twice about what range actually returns.
As for exam preparation, what I am trying to do is to review all the materials covered in class, and make a list of questions so that I can ask questions during the study session on Tuesday.
# Tip Of the Week
Same words as always, Never give up, keep learning!!
Computer science is a subject that everybody at all ages is supposed to be encouraged to, and be able to learn. It’s a fascinating subject that can create something powerful.
I also learned that mobile computing is also a studied area in MS and PhD.
| Java |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using YamlDotNet.Serialization;
namespace Knet.Model
{
public class CContext
{
[YamlMember(Alias = "context")]
public CContextDetails ContextDetails { get; set; }
[YamlMember(Alias = "name")]
public string Name { get; set; }
}
}
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>persistent-union-find: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.10.1 / persistent-union-find - 8.8.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
persistent-union-find
<small>
8.8.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-10-02 09:47:12 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-10-02 09:47:12 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
coq 8.10.1 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.05.0 The OCaml compiler (virtual package)
ocaml-base-compiler 4.05.0 Official 4.05.0 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/coq-contribs/persistent-union-find"
license: "Unknown"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/PersistentUnionFind"]
depends: [
"ocaml"
"coq" {>= "8.8" & < "8.9~"}
]
tags: [ "keyword: program verification" "keyword: union-find" "keyword: data structures" "keyword: Tarjan" "category: Computer Science/Decision Procedures and Certified Algorithms/Correctness proofs of algorithms" ]
authors: [ "Jean-Christophe Filliâtre" ]
bug-reports: "https://github.com/coq-contribs/persistent-union-find/issues"
dev-repo: "git+https://github.com/coq-contribs/persistent-union-find.git"
synopsis: "Persistent Union Find"
description: """
http://www.lri.fr/~filliatr/puf/
Correctness proof of the Ocaml implementation of a persistent union-find
data structure. See http://www.lri.fr/~filliatr/puf/ for more details."""
flags: light-uninstall
url {
src:
"https://github.com/coq-contribs/persistent-union-find/archive/v8.8.0.tar.gz"
checksum: "md5=c24293a03ace1caaa86fe3195ad382ef"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-persistent-union-find.8.8.0 coq.8.10.1</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.10.1).
The following dependencies couldn't be met:
- coq-persistent-union-find -> coq < 8.9~ -> ocaml < 4.03.0
base of this switch (use `--unlock-base' to force)
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-persistent-union-find.8.8.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
#!/usr/bin/env python
"""
@file HybridVAControl.py
@author Craig Rafter
@date 19/08/2016
class for fixed time signal control
"""
import signalControl, readJunctionData, traci
from math import atan2, degrees
import numpy as np
from collections import defaultdict
class HybridVAControl(signalControl.signalControl):
def __init__(self, junctionData, minGreenTime=10, maxGreenTime=60, scanRange=250, packetRate=0.2):
super(HybridVAControl, self).__init__()
self.junctionData = junctionData
self.firstCalled = self.getCurrentSUMOtime()
self.lastCalled = self.getCurrentSUMOtime()
self.lastStageIndex = 0
traci.trafficlights.setRedYellowGreenState(self.junctionData.id,
self.junctionData.stages[self.lastStageIndex].controlString)
self.packetRate = int(1000*packetRate)
self.transition = False
self.CAMactive = False
# dict[vehID] = [position, heading, velocity, Tdetect]
self.newVehicleInfo = {}
self.oldVehicleInfo = {}
self.scanRange = scanRange
self.jcnCtrlRegion = self._getJncCtrlRegion()
# print(self.junctionData.id)
# print(self.jcnCtrlRegion)
self.controlledLanes = traci.trafficlights.getControlledLanes(self.junctionData.id)
# dict[laneID] = [heading, shape]
self.laneDetectionInfo = self._getIncomingLaneInfo()
self.stageTime = 0.0
self.minGreenTime = minGreenTime
self.maxGreenTime = maxGreenTime
self.secondsPerMeterTraffic = 0.45
self.nearVehicleCatchDistance = 25
self.extendTime = 1.0 # 5 m in 10 m/s (acceptable journey 1.333)
self.laneInductors = self._getLaneInductors()
def process(self):
# Packets sent on this step
# packet delay + only get packets towards the end of the second
if (not self.getCurrentSUMOtime() % self.packetRate) and (self.getCurrentSUMOtime() % 1000 > 500):
self.CAMactive = True
self._getCAMinfo()
else:
self.CAMactive = False
# Update stage decisions
# If there's no ITS enabled vehicles present use VA ctrl
if len(self.oldVehicleInfo) < 1 and not self.getCurrentSUMOtime() % 1000:
detectTimePerLane = self._getLaneDetectTime()
#print(detectTimePerLane)
# Set adaptive time limit
#print(detectTimePerLane < 3)
if np.any(detectTimePerLane < 2):
extend = self.extendTime
else:
extend = 0.0
self.stageTime = max(self.stageTime + extend, self.minGreenTime)
self.stageTime = min(self.stageTime, self.maxGreenTime)
# If active and on the second, or transition then make stage descision
elif (self.CAMactive and not self.getCurrentSUMOtime() % 1000) or self.transition:
oncomingVeh = self._getOncomingVehicles()
# If new stage get furthest from stop line whose velocity < 5% speed
# limit and determine queue length
if self.transition:
furthestVeh = self._getFurthestStationaryVehicle(oncomingVeh)
if furthestVeh[0] != '':
meteredTime = self.secondsPerMeterTraffic*furthestVeh[1]
self.stageTime = max(self.minGreenTime, meteredTime)
self.stageTime = min(self.stageTime, self.maxGreenTime)
# If we're in this state this should never happen but just in case
else:
self.stageTime = self.minGreenTime
# If currently staging then extend time if there are vehicles close
# to the stop line
else:
nearestVeh = self._getNearestVehicle(oncomingVeh)
# If a vehicle detected
if nearestVeh != '' and nearestVeh[1] <= self.nearVehicleCatchDistance:
if (self.oldVehicleInfo[nearestVeh[0]][2] != 1e6
and self.oldVehicleInfo[nearestVeh[0]][2] > 1.0/self.secondsPerMeterTraffic):
meteredTime = nearestVeh[1]/self.oldVehicleInfo[nearestVeh[0]][2]
else:
meteredTime = self.secondsPerMeterTraffic*nearestVeh[1]
elapsedTime = 0.001*(self.getCurrentSUMOtime() - self.lastCalled)
Tremaining = self.stageTime - elapsedTime
self.stageTime = elapsedTime + max(meteredTime, Tremaining)
self.stageTime = min(self.stageTime, self.maxGreenTime)
# no detectable near vehicle try inductive loop info
elif nearestVeh == '' or nearestVeh[1] <= self.nearVehicleCatchDistance:
detectTimePerLane = self._getLaneDetectTime()
print('Loops2')
# Set adaptive time limit
if np.any(detectTimePerLane < 2):
extend = self.extendTime
else:
extend = 0.0
self.stageTime = max(self.stageTime + extend, self.minGreenTime)
self.stageTime = min(self.stageTime, self.maxGreenTime)
else:
pass
# process stage as normal
else:
pass
# print(self.stageTime)
self.transition = False
if self.transitionObject.active:
# If the transition object is active i.e. processing a transition
pass
elif (self.getCurrentSUMOtime() - self.firstCalled) < (self.junctionData.offset*1000):
# Process offset first
pass
elif (self.getCurrentSUMOtime() - self.lastCalled) < self.stageTime*1000:
# Before the period of the next stage
pass
else:
# Not active, not in offset, stage not finished
if len(self.junctionData.stages) != (self.lastStageIndex)+1:
# Loop from final stage to first stage
self.transitionObject.newTransition(
self.junctionData.id,
self.junctionData.stages[self.lastStageIndex].controlString,
self.junctionData.stages[self.lastStageIndex+1].controlString)
self.lastStageIndex += 1
else:
# Proceed to next stage
#print(0.001*(self.getCurrentSUMOtime() - self.lastCalled))
self.transitionObject.newTransition(
self.junctionData.id,
self.junctionData.stages[self.lastStageIndex].controlString,
self.junctionData.stages[0].controlString)
self.lastStageIndex = 0
#print(0.001*(self.getCurrentSUMOtime() - self.lastCalled))
self.lastCalled = self.getCurrentSUMOtime()
self.transition = True
self.stageTime = 0.0
super(HybridVAControl, self).process()
def _getHeading(self, currentLoc, prevLoc):
dy = currentLoc[1] - prevLoc[1]
dx = currentLoc[0] - prevLoc[0]
if currentLoc[1] == prevLoc[1] and currentLoc[0] == prevLoc[0]:
heading = -1
else:
if dy >= 0:
heading = degrees(atan2(dy, dx))
else:
heading = 360 + degrees(atan2(dy, dx))
# Map angle to make compatible with SUMO heading
if 0 <= heading <= 90:
heading = 90 - heading
elif 90 < heading < 360:
heading = 450 - heading
return heading
def _getJncCtrlRegion(self):
jncPosition = traci.junction.getPosition(self.junctionData.id)
otherJuncPos = [traci.junction.getPosition(x) for x in traci.trafficlights.getIDList() if x != self.junctionData.id]
ctrlRegion = {'N':jncPosition[1]+self.scanRange, 'S':jncPosition[1]-self.scanRange,
'E':jncPosition[0]+self.scanRange, 'W':jncPosition[0]-self.scanRange}
TOL = 10 # Exclusion region around junction boundary
if otherJuncPos != []:
for pos in otherJuncPos:
dx = jncPosition[0] - pos[0]
dy = jncPosition[1] - pos[1]
# North/South Boundary
if abs(dy) < self.scanRange:
if dy < -TOL:
ctrlRegion['N'] = min(pos[1] - TOL, ctrlRegion['N'])
elif dy > TOL:
ctrlRegion['S'] = max(pos[1] + TOL, ctrlRegion['S'])
else:
pass
else:
pass
# East/West Boundary
if abs(dx) < self.scanRange:
if dx < -TOL:
ctrlRegion['E'] = min(pos[0] - TOL, ctrlRegion['E'])
elif dx > TOL:
ctrlRegion['W'] = max(pos[0] + TOL, ctrlRegion['W'])
else:
pass
else:
pass
return ctrlRegion
def _isInRange(self, vehID):
vehPosition = np.array(traci.vehicle.getPosition(vehID))
jcnPosition = np.array(traci.junction.getPosition(self.junctionData.id))
distance = np.linalg.norm(vehPosition - jcnPosition)
if (distance < self.scanRange
and self.jcnCtrlRegion['W'] <= vehPosition[0] <= self.jcnCtrlRegion['E']
and self.jcnCtrlRegion['S'] <= vehPosition[1] <= self.jcnCtrlRegion['N']):
return True
else:
return False
def _getVelocity(self, vehID, vehPosition, Tdetect):
if vehID in self.oldVehicleInfo.keys():
oldX = np.array(self.oldVehicleInfo[vehID][0])
newX = np.array(vehPosition)
dx = np.linalg.norm(newX - oldX)
dt = Tdetect - self.oldVehicleInfo[vehID][3]
velocity = dx/dt
return velocity
else:
return 1e6
def _getCAMinfo(self):
self.oldVehicleInfo = self.newVehicleInfo.copy()
self.newVehicleInfo = {}
Tdetect = 0.001*self.getCurrentSUMOtime()
for vehID in traci.vehicle.getIDList():
if traci.vehicle.getTypeID(vehID) == 'typeITSCV' and self._isInRange(vehID):
vehPosition = traci.vehicle.getPosition(vehID)
vehHeading = traci.vehicle.getAngle(vehID)
vehVelocity = self._getVelocity(vehID, vehPosition, Tdetect)
self.newVehicleInfo[vehID] = [vehPosition, vehHeading, vehVelocity, Tdetect]
def _getIncomingLaneInfo(self):
laneInfo = defaultdict(list)
for lane in list(np.unique(np.array(self.controlledLanes))):
shape = traci.lane.getShape(lane)
width = traci.lane.getWidth(lane)
heading = self._getHeading(shape[1], shape[0])
dx = shape[0][0] - shape[1][0]
dy = shape[0][1] - shape[1][1]
if abs(dx) > abs(dy):
roadBounds = ((shape[0][0], shape[0][1] + width), (shape[1][0], shape[1][1] - width))
else:
roadBounds = ((shape[0][0] + width, shape[0][1]), (shape[1][0] - width, shape[1][1]))
laneInfo[lane] = [heading, roadBounds]
return laneInfo
def _getOncomingVehicles(self):
# Oncoming if (in active lane & heading matches oncoming heading &
# is in lane bounds)
activeLanes = self._getActiveLanes()
vehicles = []
for lane in activeLanes:
for vehID in self.oldVehicleInfo.keys():
# If on correct heading pm 10deg
if (np.isclose(self.oldVehicleInfo[vehID][1], self.laneDetectionInfo[lane][0], atol=10)
# If in lane x bounds
and min(self.laneDetectionInfo[lane][1][0][0], self.laneDetectionInfo[lane][1][1][0]) <
self.oldVehicleInfo[vehID][0][0] <
max(self.laneDetectionInfo[lane][1][0][0], self.laneDetectionInfo[lane][1][1][0])
# If in lane y bounds
and min(self.laneDetectionInfo[lane][1][0][1], self.laneDetectionInfo[lane][1][1][1]) <
self.oldVehicleInfo[vehID][0][1] <
max(self.laneDetectionInfo[lane][1][0][1], self.laneDetectionInfo[lane][1][1][1])):
# Then append vehicle
vehicles.append(vehID)
vehicles = list(np.unique(np.array(vehicles)))
return vehicles
def _getActiveLanes(self):
# Get the current control string to find the green lights
stageCtrlString = self.junctionData.stages[self.lastStageIndex].controlString
activeLanes = []
for i, letter in enumerate(stageCtrlString):
if letter == 'G':
activeLanes.append(self.controlledLanes[i])
# Get a list of the unique active lanes
activeLanes = list(np.unique(np.array(activeLanes)))
return activeLanes
def _getLaneInductors(self):
laneInductors = defaultdict(list)
for loop in traci.inductionloop.getIDList():
loopLane = traci.inductionloop.getLaneID(loop)
if loopLane in self.controlledLanes and 'upstream' not in loop:
laneInductors[loopLane].append(loop)
return laneInductors
def _getFurthestStationaryVehicle(self, vehIDs):
furthestID = ''
maxDistance = -1
jcnPosition = np.array(traci.junction.getPosition(self.junctionData.id))
speedLimit = traci.lane.getMaxSpeed(self._getActiveLanes()[0])
for ID in vehIDs:
vehPosition = np.array(self.oldVehicleInfo[ID][0])
distance = np.linalg.norm(vehPosition - jcnPosition)
if distance > maxDistance and self.oldVehicleInfo[ID][2] < 0.05*speedLimit:
furthestID = ID
maxDistance = distance
return [furthestID, maxDistance]
def _getNearestVehicle(self, vehIDs):
nearestID = ''
minDistance = self.nearVehicleCatchDistance + 1
jcnPosition = np.array(traci.junction.getPosition(self.junctionData.id))
for ID in vehIDs:
vehPosition = np.array(self.oldVehicleInfo[ID][0])
distance = np.linalg.norm(vehPosition - jcnPosition)
if distance < minDistance:
nearestID = ID
minDistance = distance
return [nearestID, minDistance]
def _getLaneDetectTime(self):
activeLanes = self._getActiveLanes()
meanDetectTimePerLane = np.zeros(len(activeLanes))
for i, lane in enumerate(activeLanes):
detectTimes = []
for loop in self.laneInductors[lane]:
detectTimes.append(traci.inductionloop.getTimeSinceDetection(loop))
meanDetectTimePerLane[i] = np.mean(detectTimes)
return meanDetectTimePerLane
| Java |
<!DOCTYPE html>
<!--[if lt IE 9]><html class="no-js lt-ie9" lang="en" dir="ltr"><![endif]-->
<!--[if gt IE 8]><!-->
<html class="no-js" lang="en" dir="ltr">
<!--<![endif]-->
<!-- Usage: /eic/site/ccc-rec.nsf/tpl-eng/template-1col.html?Open&id=3 (optional: ?Open&page=filename.html&id=x) -->
<!-- Created: ; Product Code: 536; Server: stratnotes2.ic.gc.ca -->
<head>
<!-- Title begins / Début du titre -->
<title>
Monsanto Canada Inc. -
Complete profile - Canadian Company Capabilities - Industries and Business - Industry Canada
</title>
<!-- Title ends / Fin du titre -->
<!-- Meta-data begins / Début des métadonnées -->
<meta charset="utf-8" />
<meta name="dcterms.language" title="ISO639-2" content="eng" />
<meta name="dcterms.title" content="" />
<meta name="description" content="" />
<meta name="dcterms.description" content="" />
<meta name="dcterms.type" content="report, data set" />
<meta name="dcterms.subject" content="businesses, industry" />
<meta name="dcterms.subject" content="businesses, industry" />
<meta name="dcterms.issued" title="W3CDTF" content="" />
<meta name="dcterms.modified" title="W3CDTF" content="" />
<meta name="keywords" content="" />
<meta name="dcterms.creator" content="" />
<meta name="author" content="" />
<meta name="dcterms.created" title="W3CDTF" content="" />
<meta name="dcterms.publisher" content="" />
<meta name="dcterms.audience" title="icaudience" content="" />
<meta name="dcterms.spatial" title="ISO3166-1" content="" />
<meta name="dcterms.spatial" title="gcgeonames" content="" />
<meta name="dcterms.format" content="HTML" />
<meta name="dcterms.identifier" title="ICsiteProduct" content="536" />
<!-- EPI-11240 -->
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<!-- MCG-202 -->
<meta content="width=device-width,initial-scale=1" name="viewport">
<!-- EPI-11567 -->
<meta name = "format-detection" content = "telephone=no">
<!-- EPI-12603 -->
<meta name="robots" content="noarchive">
<!-- EPI-11190 - Webtrends -->
<script>
var startTime = new Date();
startTime = startTime.getTime();
</script>
<!--[if gte IE 9 | !IE ]><!-->
<link href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/assets/favicon.ico" rel="icon" type="image/x-icon">
<link rel="stylesheet" href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/css/wet-boew.min.css">
<!--<![endif]-->
<link rel="stylesheet" href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/css/theme.min.css">
<!--[if lt IE 9]>
<link href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/assets/favicon.ico" rel="shortcut icon" />
<link rel="stylesheet" href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/css/ie8-wet-boew.min.css" />
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/js/ie8-wet-boew.min.js"></script>
<![endif]-->
<!--[if lte IE 9]>
<![endif]-->
<noscript><link rel="stylesheet" href="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/css/noscript.min.css" /></noscript>
<!-- Google Tag Manager DO NOT REMOVE OR MODIFY - NE PAS SUPPRIMER OU MODIFIER -->
<script>dataLayer1 = [];</script>
<!-- End Google Tag Manager -->
<!-- EPI-11235 -->
<link rel="stylesheet" href="/eic/home.nsf/css/add_WET_4-0_Canada_Apps.css">
<link href="//netdna.bootstrapcdn.com/font-awesome/4.0.3/css/font-awesome.css" rel="stylesheet">
<link href="/app/ccc/srch/css/print.css" media="print" rel="stylesheet" type="text/css" />
</head>
<body class="home" vocab="http://schema.org/" typeof="WebPage">
<!-- EPIC HEADER BEGIN -->
<!-- Google Tag Manager DO NOT REMOVE OR MODIFY - NE PAS SUPPRIMER OU MODIFIER -->
<noscript><iframe title="Google Tag Manager" src="//www.googletagmanager.com/ns.html?id=GTM-TLGQ9K" height="0" width="0" style="display:none;visibility:hidden"></iframe></noscript>
<script>(function(w,d,s,l,i){w[l]=w[l]||[];w[l].push({'gtm.start': new Date().getTime(),event:'gtm.js'});var f=d.getElementsByTagName(s)[0], j=d.createElement(s),dl=l!='dataLayer1'?'&l='+l:'';j.async=true;j.src='//www.googletagmanager.com/gtm.js?id='+i+dl;f.parentNode.insertBefore(j,f);})(window,document,'script','dataLayer1','GTM-TLGQ9K');</script>
<!-- End Google Tag Manager -->
<!-- EPI-12801 -->
<span typeof="Organization"><meta property="legalName" content="Department_of_Industry"></span>
<ul id="wb-tphp">
<li class="wb-slc">
<a class="wb-sl" href="#wb-cont">Skip to main content</a>
</li>
<li class="wb-slc visible-sm visible-md visible-lg">
<a class="wb-sl" href="#wb-info">Skip to "About this site"</a>
</li>
</ul>
<header role="banner">
<div id="wb-bnr" class="container">
<section id="wb-lng" class="visible-md visible-lg text-right">
<h2 class="wb-inv">Language selection</h2>
<div class="row">
<div class="col-md-12">
<ul class="list-inline mrgn-bttm-0">
<li><a href="nvgt.do?V_TOKEN=1492304605805&V_SEARCH.docsCount=3&V_DOCUMENT.docRank=31097&V_SEARCH.docsStart=31096&V_SEARCH.command=navigate&V_SEARCH.resultsJSP=/prfl.do&lang=fra&redirectUrl=/app/scr/imbs/ccc/rgstrtn//magmi/web/download_file.php?_flId?_flxKy=e1s1&estblmntNo=234567041301&profileId=61&_evId=bck&lang=eng&V_SEARCH.showStricts=false&prtl=1&_flId?_flId?_flxKy=e1s1" title="Français" lang="fr">Français</a></li>
</ul>
</div>
</div>
</section>
<div class="row">
<div class="brand col-xs-8 col-sm-9 col-md-6">
<a href="http://www.canada.ca/en/index.html"><object type="image/svg+xml" tabindex="-1" data="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/assets/sig-blk-en.svg"></object><span class="wb-inv"> Government of Canada</span></a>
</div>
<section class="wb-mb-links col-xs-4 col-sm-3 visible-sm visible-xs" id="wb-glb-mn">
<h2>Search and menus</h2>
<ul class="list-inline text-right chvrn">
<li><a href="#mb-pnl" title="Search and menus" aria-controls="mb-pnl" class="overlay-lnk" role="button"><span class="glyphicon glyphicon-search"><span class="glyphicon glyphicon-th-list"><span class="wb-inv">Search and menus</span></span></span></a></li>
</ul>
<div id="mb-pnl"></div>
</section>
<!-- Site Search Removed -->
</div>
</div>
<nav role="navigation" id="wb-sm" class="wb-menu visible-md visible-lg" data-trgt="mb-pnl" data-ajax-fetch="//cdn.canada.ca/gcweb-cdn-dev/sitemenu/sitemenu-en.html" typeof="SiteNavigationElement">
<h2 class="wb-inv">Topics menu</h2>
<div class="container nvbar">
<div class="row">
<ul class="list-inline menu">
<li><a href="https://www.canada.ca/en/services/jobs.html">Jobs</a></li>
<li><a href="http://www.cic.gc.ca/english/index.asp">Immigration</a></li>
<li><a href="https://travel.gc.ca/">Travel</a></li>
<li><a href="https://www.canada.ca/en/services/business.html">Business</a></li>
<li><a href="https://www.canada.ca/en/services/benefits.html">Benefits</a></li>
<li><a href="http://healthycanadians.gc.ca/index-eng.php">Health</a></li>
<li><a href="https://www.canada.ca/en/services/taxes.html">Taxes</a></li>
<li><a href="https://www.canada.ca/en/services.html">More services</a></li>
</ul>
</div>
</div>
</nav>
<!-- EPIC BODY BEGIN -->
<nav role="navigation" id="wb-bc" class="" property="breadcrumb">
<h2 class="wb-inv">You are here:</h2>
<div class="container">
<div class="row">
<ol class="breadcrumb">
<li><a href="/eic/site/icgc.nsf/eng/home" title="Home">Home</a></li>
<li><a href="/eic/site/icgc.nsf/eng/h_07063.html" title="Industries and Business">Industries and Business</a></li>
<li><a href="/eic/site/ccc-rec.nsf/tpl-eng/../eng/home" >Canadian Company Capabilities</a></li>
</ol>
</div>
</div>
</nav>
</header>
<main id="wb-cont" role="main" property="mainContentOfPage" class="container">
<!-- End Header -->
<!-- Begin Body -->
<!-- Begin Body Title -->
<!-- End Body Title -->
<!-- Begin Body Head -->
<!-- End Body Head -->
<!-- Begin Body Content -->
<br>
<!-- Complete Profile -->
<!-- Company Information above tabbed area-->
<input id="showMore" type="hidden" value='more'/>
<input id="showLess" type="hidden" value='less'/>
<h1 id="wb-cont">
Company profile - Canadian Company Capabilities
</h1>
<div class="profileInfo hidden-print">
<ul class="list-inline">
<li><a href="cccSrch.do?lang=eng&profileId=&prtl=1&key.hitsPerPage=25&searchPage=%252Fapp%252Fccc%252Fsrch%252FcccBscSrch.do%253Flang%253Deng%2526amp%253Bprtl%253D1%2526amp%253Btagid%253D&V_SEARCH.scopeCategory=CCC.Root&V_SEARCH.depth=1&V_SEARCH.showStricts=false&V_SEARCH.sortSpec=title+asc&rstBtn.x=" class="btn btn-link">New Search</a> |</li>
<li><form name="searchForm" method="post" action="/app/ccc/srch/bscSrch.do">
<input type="hidden" name="lang" value="eng" />
<input type="hidden" name="profileId" value="" />
<input type="hidden" name="prtl" value="1" />
<input type="hidden" name="searchPage" value="%2Fapp%2Fccc%2Fsrch%2FcccBscSrch.do%3Flang%3Deng%26amp%3Bprtl%3D1%26amp%3Btagid%3D" />
<input type="hidden" name="V_SEARCH.scopeCategory" value="CCC.Root" />
<input type="hidden" name="V_SEARCH.depth" value="1" />
<input type="hidden" name="V_SEARCH.showStricts" value="false" />
<input id="repeatSearchBtn" class="btn btn-link" type="submit" value="Return to search results" />
</form></li>
<li>| <a href="nvgt.do?V_SEARCH.docsStart=31095&V_DOCUMENT.docRank=31096&V_SEARCH.docsCount=3&lang=eng&prtl=1&sbPrtl=&profile=cmpltPrfl&V_TOKEN=1492304636669&V_SEARCH.command=navigate&V_SEARCH.resultsJSP=%2fprfl.do&estblmntNo=123456002042&profileId=&key.newSearchLabel=">Previous Company</a></li>
<li>| <a href="nvgt.do?V_SEARCH.docsStart=31097&V_DOCUMENT.docRank=31098&V_SEARCH.docsCount=3&lang=eng&prtl=1&sbPrtl=&profile=cmpltPrfl&V_TOKEN=1492304636669&V_SEARCH.command=navigate&V_SEARCH.resultsJSP=%2fprfl.do&estblmntNo=000026400000&profileId=&key.newSearchLabel=">Next Company</a></li>
</ul>
</div>
<details>
<summary>Third-Party Information Liability Disclaimer</summary>
<p>Some of the information on this Web page has been provided by external sources. The Government of Canada is not responsible for the accuracy, reliability or currency of the information supplied by external sources. Users wishing to rely upon this information should consult directly with the source of the information. Content provided by external sources is not subject to official languages, privacy and accessibility requirements.</p>
</details>
<h2>
Monsanto Canada Inc.
</h2>
<div class="row">
<div class="col-md-5">
<h2 class="h5 mrgn-bttm-0">Legal/Operating Name:</h2>
<p>Monsanto Canada Inc.</p>
<div class="mrgn-tp-md"></div>
<p class="mrgn-bttm-0" ><a href="http://www.monsanto.ca/"
target="_blank" title="Website URL">http://www.monsanto.ca/</a></p>
</div>
<div class="col-md-4 mrgn-sm-sm">
<h2 class="h5 mrgn-bttm-0">Mailing Address:</h2>
<address class="mrgn-bttm-md">
900-1 Research Rd<br/>
WINNIPEG,
Manitoba<br/>
R3T 6E3
<br/>
</address>
<h2 class="h5 mrgn-bttm-0">Location Address:</h2>
<address class="mrgn-bttm-md">
900-1 Research Rd<br/>
WINNIPEG,
Manitoba<br/>
R3T 6E3
<br/>
</address>
<p class="mrgn-bttm-0"><abbr title="Telephone">Tel.</abbr>:
(204) 985-1000
</p>
<p class="mrgn-bttm-lg"><abbr title="Facsimile">Fax</abbr>:
(204) 488-9599</p>
</div>
<div class="col-md-3 mrgn-tp-md">
<h2 class="wb-inv">Logo</h2>
<img class="img-responsive text-left" src="https://www.ic.gc.ca/app/ccc/srch/media?estblmntNo=123456183596&graphFileName=Full-Color+Monsanto+&applicationCode=AP&lang=eng" alt="Logo" />
</div>
</div>
<div class="row mrgn-tp-md mrgn-bttm-md">
<div class="col-md-12">
<h2 class="wb-inv">Company Profile</h2>
<br> Headquartered in Winnipeg, Manitoba, Monsanto Canada Inc. is part of the larger global Monsanto family. Monsanto Company is an agricultural company and a leading global provider of technology-based solutions and agricultural products that improve farm productivity and food quality. Monsanto remains focused on enabling both small-holder and large-scale farmers to produce more from their land while conserving more of our worlds natural resources such as water and energy. Learn more about our business and our commitments at www.monsanto.ca.<br>
</div>
</div>
<!-- <div class="wb-tabs ignore-session update-hash wb-eqht-off print-active"> -->
<div class="wb-tabs ignore-session">
<div class="tabpanels">
<details id="details-panel1">
<summary>
Full profile
</summary>
<!-- Tab 1 -->
<h2 class="wb-invisible">
Full profile
</h2>
<!-- Contact Information -->
<h3 class="page-header">
Contact information
</h3>
<section class="container-fluid">
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
Mike
McGuire
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
General Manager
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Management Executive.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(519) 841-5846
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
Trish
Jordan
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
Director
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Management Executive.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(204) 985-1005
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Facsimile:
</strong>
</div>
<div class="col-md-7">
(204) 488-1577
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
John
Dossetor
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
Director
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Government Relations.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(613) 234-5121
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
</section>
<p class="mrgn-tp-lg text-right small hidden-print">
<a href="#wb-cont">top of page</a>
</p>
<!-- Company Description -->
<h3 class="page-header">
Company description
</h3>
<section class="container-fluid">
<div class="row">
<div class="col-md-5">
<strong>
Country of Ownership:
</strong>
</div>
<div class="col-md-7">
Foreign
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Year Established:
</strong>
</div>
<div class="col-md-7">
1951
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Exporting:
</strong>
</div>
<div class="col-md-7">
No
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Primary Industry (NAICS):
</strong>
</div>
<div class="col-md-7">
325320 - Pesticide and Other Agricultural Chemical Manufacturing
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Primary Business Activity:
</strong>
</div>
<div class="col-md-7">
Manufacturer / Processor / Producer
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Number of Employees:
</strong>
</div>
<div class="col-md-7">
35
</div>
</div>
</section>
<!-- Products / Services / Licensing -->
<h3 class="page-header">
Product / Service / Licensing
</h3>
<section class="container-fluid">
<div class="row mrgn-bttm-md">
<div class="col-md-3">
<strong>
Product Name:
</strong>
</div>
<div class="col-md-9">
Seed, Technology, Herbicides <br>
</div>
</div>
</section>
<p class="mrgn-tp-lg text-right small hidden-print">
<a href="#wb-cont">top of page</a>
</p>
<!-- Technology Profile -->
<!-- Market Profile -->
<h3 class="page-header">
Market profile
</h3>
<section class="container-fluid">
<h4>
Geographic markets:
</h4>
<h5>
Actively pursuing:
</h5>
<ul>
<li>Algeria</li>
<li>American Samoa</li>
<li>Antarctica</li>
<li>Argentina</li>
<li>Australia</li>
<li>Bolivia, Plurinational State of</li>
<li>Brazil</li>
<li>Cambodia</li>
<li>Chile</li>
<li>Colombia</li>
<li>Cook Islands</li>
<li>Ecuador</li>
<li>Falkland Islands (Malvinas)</li>
<li>Fiji</li>
<li>French Guiana</li>
<li>French Polynesia</li>
<li>Guam</li>
<li>Guinea</li>
<li>Guyana</li>
<li>Indonesia</li>
<li>Japan</li>
<li>Korea, Democratic People's Republic of</li>
<li>Korea, Republic of</li>
<li>Lao People's Democratic Republic</li>
<li>Macao</li>
<li>Malaysia</li>
<li>Mauritania</li>
<li>New Caledonia</li>
<li>New Zealand</li>
<li>Papua New Guinea</li>
<li>Paraguay</li>
<li>Peru</li>
<li>Philippines</li>
<li>Samoa</li>
<li>Singapore</li>
<li>Solomon Islands</li>
<li>Suriname</li>
<li>Taiwan</li>
<li>Thailand</li>
<li>Tonga</li>
<li>Uruguay</li>
<li>Vanuatu</li>
<li>Venezuela, Bolivarian Republic of</li>
<li>Viet Nam</li>
</ul>
</section>
<p class="mrgn-tp-lg text-right small hidden-print">
<a href="#wb-cont">top of page</a>
</p>
<!-- Sector Information -->
<details class="mrgn-tp-md mrgn-bttm-md">
<summary>
Third-Party Information Liability Disclaimer
</summary>
<p>
Some of the information on this Web page has been provided by external sources. The Government of Canada is not responsible for the accuracy, reliability or currency of the information supplied by external sources. Users wishing to rely upon this information should consult directly with the source of the information. Content provided by external sources is not subject to official languages, privacy and accessibility requirements.
</p>
</details>
</details>
<details id="details-panel2">
<summary>
Contacts
</summary>
<h2 class="wb-invisible">
Contact information
</h2>
<!-- Contact Information -->
<section class="container-fluid">
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
Mike
McGuire
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
General Manager
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Management Executive.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(519) 841-5846
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
Trish
Jordan
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
Director
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Management Executive.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(204) 985-1005
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Facsimile:
</strong>
</div>
<div class="col-md-7">
(204) 488-1577
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
<div class="row mrgn-tp-lg">
<div class="col-md-3">
<strong>
John
Dossetor
</strong></div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Title:
</strong>
</div>
<div class="col-md-7">
<!--if client gender is not null or empty we use gender based job title-->
Director
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Area of Responsibility:
</strong>
</div>
<div class="col-md-7">
Government Relations.
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Telephone:
</strong>
</div>
<div class="col-md-7">
(613) 234-5121
</div>
</div>
<div class="row mrgn-lft-md">
<div class="col-md-5">
<strong>
Email:
</strong>
</div>
<div class="col-md-7">
[email protected]
</div>
</div>
</section>
</details>
<details id="details-panel3">
<summary>
Description
</summary>
<h2 class="wb-invisible">
Company description
</h2>
<section class="container-fluid">
<div class="row">
<div class="col-md-5">
<strong>
Country of Ownership:
</strong>
</div>
<div class="col-md-7">
Foreign
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Year Established:
</strong>
</div>
<div class="col-md-7">
1951
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Exporting:
</strong>
</div>
<div class="col-md-7">
No
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Primary Industry (NAICS):
</strong>
</div>
<div class="col-md-7">
325320 - Pesticide and Other Agricultural Chemical Manufacturing
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Primary Business Activity:
</strong>
</div>
<div class="col-md-7">
Manufacturer / Processor / Producer
</div>
</div>
<div class="row">
<div class="col-md-5">
<strong>
Number of Employees:
</strong>
</div>
<div class="col-md-7">
35
</div>
</div>
</section>
</details>
<details id="details-panel4">
<summary>
Products, services and licensing
</summary>
<h2 class="wb-invisible">
Product / Service / Licensing
</h2>
<section class="container-fluid">
<div class="row mrgn-bttm-md">
<div class="col-md-3">
<strong>
Product Name:
</strong>
</div>
<div class="col-md-9">
Seed, Technology, Herbicides <br>
</div>
</div>
</section>
</details>
<details id="details-panel6">
<summary>
Market
</summary>
<h2 class="wb-invisible">
Market profile
</h2>
<section class="container-fluid">
<h4>
Geographic markets:
</h4>
<h5>
Actively pursuing:
</h5>
<ul>
<li>Algeria</li>
<li>American Samoa</li>
<li>Antarctica</li>
<li>Argentina</li>
<li>Australia</li>
<li>Bolivia, Plurinational State of</li>
<li>Brazil</li>
<li>Cambodia</li>
<li>Chile</li>
<li>Colombia</li>
<li>Cook Islands</li>
<li>Ecuador</li>
<li>Falkland Islands (Malvinas)</li>
<li>Fiji</li>
<li>French Guiana</li>
<li>French Polynesia</li>
<li>Guam</li>
<li>Guinea</li>
<li>Guyana</li>
<li>Indonesia</li>
<li>Japan</li>
<li>Korea, Democratic People's Republic of</li>
<li>Korea, Republic of</li>
<li>Lao People's Democratic Republic</li>
<li>Macao</li>
<li>Malaysia</li>
<li>Mauritania</li>
<li>New Caledonia</li>
<li>New Zealand</li>
<li>Papua New Guinea</li>
<li>Paraguay</li>
<li>Peru</li>
<li>Philippines</li>
<li>Samoa</li>
<li>Singapore</li>
<li>Solomon Islands</li>
<li>Suriname</li>
<li>Taiwan</li>
<li>Thailand</li>
<li>Tonga</li>
<li>Uruguay</li>
<li>Vanuatu</li>
<li>Venezuela, Bolivarian Republic of</li>
<li>Viet Nam</li>
</ul>
</section>
</details>
</div>
</div>
<div class="row">
<div class="col-md-12 text-right">
Last Update Date 2017-03-06
</div>
</div>
<!--
- Artifact ID: CBW - IMBS - CCC Search WAR
- Group ID: ca.gc.ic.strategis.imbs.ccc.search
- Version: 3.26
- Built-By: bamboo
- Build Timestamp: 2017-03-02T21:29:28Z
-->
<!-- End Body Content -->
<!-- Begin Body Foot -->
<!-- End Body Foot -->
<!-- END MAIN TABLE -->
<!-- End body -->
<!-- Begin footer -->
<div class="row pagedetails">
<div class="col-sm-5 col-xs-12 datemod">
<dl id="wb-dtmd">
<dt class=" hidden-print">Date Modified:</dt>
<dd class=" hidden-print">
<span><time>2017-03-02</time></span>
</dd>
</dl>
</div>
<div class="clear visible-xs"></div>
<div class="col-sm-4 col-xs-6">
</div>
<div class="col-sm-3 col-xs-6 text-right">
</div>
<div class="clear visible-xs"></div>
</div>
</main>
<footer role="contentinfo" id="wb-info">
<nav role="navigation" class="container wb-navcurr">
<h2 class="wb-inv">About government</h2>
<!-- EPIC FOOTER BEGIN -->
<!-- EPI-11638 Contact us -->
<ul class="list-unstyled colcount-sm-2 colcount-md-3">
<li><a href="http://www.ic.gc.ca/eic/site/icgc.nsf/eng/h_07026.html#pageid=E048-H00000&from=Industries">Contact us</a></li>
<li><a href="https://www.canada.ca/en/government/dept.html">Departments and agencies</a></li>
<li><a href="https://www.canada.ca/en/government/publicservice.html">Public service and military</a></li>
<li><a href="https://www.canada.ca/en/news.html">News</a></li>
<li><a href="https://www.canada.ca/en/government/system/laws.html">Treaties, laws and regulations</a></li>
<li><a href="https://www.canada.ca/en/transparency/reporting.html">Government-wide reporting</a></li>
<li><a href="http://pm.gc.ca/eng">Prime Minister</a></li>
<li><a href="https://www.canada.ca/en/government/system.html">How government works</a></li>
<li><a href="http://open.canada.ca/en/">Open government</a></li>
</ul>
</nav>
<div class="brand">
<div class="container">
<div class="row">
<nav class="col-md-10 ftr-urlt-lnk">
<h2 class="wb-inv">About this site</h2>
<ul>
<li><a href="https://www.canada.ca/en/social.html">Social media</a></li>
<li><a href="https://www.canada.ca/en/mobile.html">Mobile applications</a></li>
<li><a href="http://www1.canada.ca/en/newsite.html">About Canada.ca</a></li>
<li><a href="http://www.ic.gc.ca/eic/site/icgc.nsf/eng/h_07033.html">Terms and conditions</a></li>
<li><a href="http://www.ic.gc.ca/eic/site/icgc.nsf/eng/h_07033.html#p1">Privacy</a></li>
</ul>
</nav>
<div class="col-xs-6 visible-sm visible-xs tofpg">
<a href="#wb-cont">Top of Page <span class="glyphicon glyphicon-chevron-up"></span></a>
</div>
<div class="col-xs-6 col-md-2 text-right">
<object type="image/svg+xml" tabindex="-1" role="img" data="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/assets/wmms-blk.svg" aria-label="Symbol of the Government of Canada"></object>
</div>
</div>
</div>
</div>
</footer>
<!--[if gte IE 9 | !IE ]><!-->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/js/wet-boew.min.js"></script>
<!--<![endif]-->
<!--[if lt IE 9]>
<script src="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/js/ie8-wet-boew2.min.js"></script>
<![endif]-->
<script src="/utils/scripts/_WET_4-0/apps/themes-dist/gcweb/js/theme.min.js"></script>
<!-- EPI-10519 -->
<span class="wb-sessto"
data-wb-sessto='{"inactivity": 1800000, "reactionTime": 180000, "sessionalive": 1800000, "logouturl": "/app/ccc/srch/cccSrch.do?lang=eng&prtl=1"}'></span>
<script src="/eic/home.nsf/js/jQuery.externalOpensInNewWindow.js"></script>
<!-- EPI-11190 - Webtrends -->
<script src="/eic/home.nsf/js/webtrends.js"></script>
<script>var endTime = new Date();</script>
<noscript>
<div><img alt="" id="DCSIMG" width="1" height="1" src="//wt-sdc.ic.gc.ca/dcs6v67hwe0ei7wsv8g9fv50d_3k6i/njs.gif?dcsuri=/nojavascript&WT.js=No&WT.tv=9.4.0&dcssip=www.ic.gc.ca"/></div>
</noscript>
<!-- /Webtrends -->
<!-- JS deps -->
<script src="/eic/home.nsf/js/jquery.imagesloaded.js"></script>
<!-- EPI-11262 - Util JS -->
<script src="/eic/home.nsf/js/_WET_4-0_utils_canada.min.js"></script>
<!-- EPI-11383 -->
<script src="/eic/home.nsf/js/jQuery.icValidationErrors.js"></script>
<span style="display:none;" id='app-info' data-project-groupid='' data-project-artifactid='' data-project-version='' data-project-build-timestamp='' data-issue-tracking='' data-scm-sha1='' data-scm-sha1-abbrev='' data-scm-branch='' data-scm-commit-date=''></span>
</body></html>
<!-- End Footer -->
<!--
- Artifact ID: CBW - IMBS - CCC Search WAR
- Group ID: ca.gc.ic.strategis.imbs.ccc.search
- Version: 3.26
- Built-By: bamboo
- Build Timestamp: 2017-03-02T21:29:28Z
-->
| Java |
# Phantasy
The idea of Phantasy is to make it as easy as possible to write PHP in a more functional, composable style.
It's essentially broken up into two parts:
* [Core Functions](core.md)
* [Helpful Data Types](DataTypes/README.md)
Note: In these docs, you'll sometimes see functions included from `Phantasy\PHP`, these come from the [phantasy-php](https://github.com/mckayb/phantasy-php) package.
| Java |
<?php
/**
* VirtualMoneyFixture
*
*/
App::uses('VirtualMoney', 'VirtualMoney.Model');
class VirtualMoneyFixture extends CakeTestFixture {
/**
* Import
*
* @var array
*/
public $import = array('model' => 'VirtualMoney.VirtualMoney');
/**
* Records
*
* @var array
*/
public $records = array(
array(
'id' => '5312fecf-5df8-47af-bd52-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '1',
'price' => 1000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-2ffc-4b9c-9bea-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '1',
'price' => 2000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:08'
),
array(
'id' => '5312fecf-b890-46cc-80f4-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '1',
'price' => 3000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-72c0-458b-91d3-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '2',
'price' => 4000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-c9b8-4c89-8175-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '2',
'price' => 5000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-669c-4c15-8610-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '3',
'price' => 6000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-d75c-4cd2-8d19-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '4',
'price' => 7000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
array(
'id' => '5312fecf-5ba4-4825-b39f-3e8831d4c5d0',
'model' => 'User',
'foreign_key' => '5',
'price' => 8000,
'description' => 'null',
'extra' => 'null',
'deleted' => null,
'created' => '2014-03-02 18:50:07'
),
);
}
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>interval: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.7.2 / interval - 4.3.1</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
interval
<small>
4.3.1
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-01-22 06:02:19 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-01-22 06:02:19 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-ocamlbuild base OCamlbuild binary and libraries distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 1 Virtual package relying on perl
coq 8.7.2 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.02.3 The OCaml compiler (virtual package)
ocaml-base-compiler 4.02.3 Official 4.02.3 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://coqinterval.gitlabpages.inria.fr/"
dev-repo: "git+https://gitlab.inria.fr/coqinterval/interval.git"
bug-reports: "https://gitlab.inria.fr/coqinterval/interval/issues"
license: "CeCILL-C"
build: [
["autoconf"] {dev}
["./configure"]
["./remake" "-j%{jobs}%"]
]
install: ["./remake" "install"]
depends: [
"coq" {>= "8.8" & < "8.15~"}
"coq-bignums"
"coq-flocq" {>= "3.1"}
"coq-mathcomp-ssreflect" {>= "1.6"}
"coq-coquelicot" {>= "3.0"}
"conf-autoconf" {build & dev}
("conf-g++" {build} | "conf-clang" {build})
]
tags: [
"keyword:interval arithmetic"
"keyword:decision procedure"
"keyword:floating-point arithmetic"
"keyword:reflexive tactic"
"keyword:Taylor models"
"category:Mathematics/Real Calculus and Topology"
"category:Computer Science/Decision Procedures and Certified Algorithms/Decision procedures"
"logpath:Interval"
"date:2021-11-08"
]
authors: [
"Guillaume Melquiond <[email protected]>"
"Érik Martin-Dorel <[email protected]>"
"Pierre Roux <[email protected]>"
"Thomas Sibut-Pinote <[email protected]>"
]
synopsis: "A Coq tactic for proving bounds on real-valued expressions automatically"
url {
src: "https://coqinterval.gitlabpages.inria.fr/releases/interval-4.3.1.tar.gz"
checksum: "sha512=ca04b178eecc6264116daf52a6b3dc6ca8f8bd9ce704761e574119a2caeceb4367b1c1e7b715b38ec79f4e226d87c3f39efdbde94b2415ef17ce39b0fe9ba9d8"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-interval.4.3.1 coq.8.7.2</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.7.2).
The following dependencies couldn't be met:
- coq-interval -> coq >= 8.8 -> ocaml >= 4.05.0
base of this switch (use `--unlock-base' to force)
Your request can't be satisfied:
- No available version of coq satisfies the constraints
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-interval.4.3.1</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
Ccminer compilation on Debian Stretch is not straight forward, so here are few instruction that worked for me.
Install libssl1.0-dev NOT libssl-dev:
apt-get install libssl1.0-dev
Also you will need gcc-5, which is available in unstable:
nano /etc/apt/sources.list
# Unstable
deb http://debian.linux.edu.lv/debian/ sid main contrib non-free
deb-src http://debian.linux.edu.lv/debian/ sid main contrib non-free
apt-get update
apt-get install gcc-5/unstable g++-5/unstable
To NOT mess up the system it is suggested to setup package pinning:
nano /etc/apt/preferences.d/dist.pref
Package: *
Pin: release l=Debian-Security
Pin-Priority: 1000
Package: *
Pin: release a=stable
Pin-Priority: 900
Package: *
Pin: release a=testing
Pin-Priority: 750
Package: *
Pin: release a=unstable
Pin-Priority: -1
Package: *
Pin: release a=experimental
Pin-Priority: -1
Run ./build.sh.
If it complains something about "relocation R_X86_64_32 against symbol XXXX can not be used when making a shared object":
nano Makefile
find "CFLAGS" and add -fPIC
find "nvcc_FLAGS" and add --compiler-options '-fPIC' --shared
find al occurrences of "$(NVCC) $(JANSSON_INCLUDES)" and add --compiler-options '-fPIC' --shared
make clean && make
That should do it.
| Java |
.foo {
padding-inline-start: 1px;
}
.bar {
padding-inline-end: 1px;
}
| Java |
<div class="commune_descr limited">
<p>
Saint-Germain-la-Montagne est
un village
géographiquement positionné dans le département de Loire en Rhône-Alpes. Elle comptait 201 habitants en 2008.</p>
<p>Si vous pensez emmenager à Saint-Germain-la-Montagne, vous pourrez facilement trouver une maison à vendre. </p>
<p>À proximité de Saint-Germain-la-Montagne sont localisées les villes de
<a href="{{VLROOT}}/immobilier/chauffailles_71120/">Chauffailles</a> localisée à 3 km, 3 998 habitants,
<a href="{{VLROOT}}/immobilier/saint-igny-de-roche_71428/">Saint-Igny-de-Roche</a> localisée à 6 km, 630 habitants,
<a href="{{VLROOT}}/immobilier/saint-clement-de-vers_69186/">Saint-Clément-de-Vers</a> située à 3 km, 221 habitants,
<a href="{{VLROOT}}/immobilier/anglure-sous-dun_71008/">Anglure-sous-Dun</a> localisée à 3 km, 164 habitants,
<a href="{{VLROOT}}/immobilier/saint-igny-de-vers_69209/">Saint-Igny-de-Vers</a> située à 6 km, 592 habitants,
<a href="{{VLROOT}}/immobilier/belmont-de-la-loire_42015/">Belmont-de-la-Loire</a> à 4 km, 1 515 habitants,
entre autres. De plus, Saint-Germain-la-Montagne est située à seulement 29 km de <a href="{{VLROOT}}/immobilier/roanne_42187/">Roanne</a>.</p>
<p>Le parc d'habitations, à Saint-Germain-la-Montagne, était réparti en 2011 en cinq appartements et 168 maisons soit
un marché relativement équilibré.</p>
<p>La commune propose quelques équipements, elle propose entre autres un terrain de tennis et une boucle de randonnée.</p>
</div>
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>metacoq-translations: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.7.2 / metacoq-translations - 1.0~beta1+8.12</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
metacoq-translations
<small>
1.0~beta1+8.12
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-02-10 19:42:23 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-02-10 19:42:23 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.7.2 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.06.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.06.1 Official 4.06.1 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://metacoq.github.io/metacoq"
dev-repo: "git+https://github.com/MetaCoq/metacoq.git#coq-8.12"
bug-reports: "https://github.com/MetaCoq/metacoq/issues"
authors: ["Simon Boulier <[email protected]>"
"Cyril Cohen <[email protected]>"
"Matthieu Sozeau <[email protected]>"
"Nicolas Tabareau <[email protected]>"
"Théo Winterhalter <[email protected]>"
]
license: "MIT"
build: [
["sh" "./configure.sh"]
[make "-j%{jobs}%" "-C" "translations"]
]
install: [
[make "-C" "translations" "install"]
]
depends: [
"ocaml" {>= "4.07.1"}
"coq" {>= "8.12" & < "8.13~"}
"conf-python-3" {build}
"conf-time" {build}
"coq-metacoq-template" {= version}
"coq-metacoq-checker" {= version}
]
synopsis: "Translations built on top of MetaCoq"
description: """
MetaCoq is a meta-programming framework for Coq.
The Translations modules provides implementation of standard translations
from type theory to type theory, e.g. parametricity and the `cross-bool`
translation that invalidates functional extensionality.
"""
url {
src: "https://github.com/MetaCoq/metacoq/archive/v1.0-beta1-8.12.tar.gz"
checksum: "sha256=19fc4475ae81677018e21a1e20503716a47713ec8b2081e7506f5c9390284c7a"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-metacoq-translations.1.0~beta1+8.12 coq.8.7.2</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.7.2).
The following dependencies couldn't be met:
- coq-metacoq-translations -> ocaml >= 4.07.1
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-metacoq-translations.1.0~beta1+8.12</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>mathcomp-bigenough: 36 s 🏆</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / extra-dev</a></li>
<li class="active"><a href="">dev / mathcomp-bigenough - 1.0.1</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
mathcomp-bigenough
<small>
1.0.1
<span class="label label-success">36 s 🏆</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-01-20 06:05:22 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-01-20 06:05:22 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
conf-gmp 3 Virtual package relying on a GMP lib system installation
coq dev Formal proof management system
dune 2.9.1 Fast, portable, and opinionated build system
ocaml 4.10.2 The OCaml compiler (virtual package)
ocaml-base-compiler 4.10.2 Official release 4.10.2
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
zarith 1.12 Implements arithmetic and logical operations over arbitrary-precision integers
# opam file:
opam-version: "2.0"
maintainer: "Cyril Cohen <[email protected]>"
homepage: "https://github.com/math-comp/bigenough"
dev-repo: "git+https://github.com/math-comp/bigenough.git"
bug-reports: "https://github.com/math-comp/bigenough/issues"
license: "CeCILL-B"
synopsis: "A small library to do epsilon - N reasoning"
description: """
The package contains a package to reasoning with big enough objects
(mostly natural numbers). This package is essentially for backward
compatibility purposes as `bigenough` will be subsumed by the near
tactics. The formalization is based on the Mathematical Components
library."""
build: [make "-j%{jobs}%"]
install: [make "install"]
depends: [
"coq" {(>= "8.10" & < "8.16~") | (= "dev")}
"coq-mathcomp-ssreflect" {>= "1.6"}
]
tags: [
"keyword:bigenough"
"keyword:asymptotic reasonning"
"keyword:small scale reflection"
"keyword:mathematical components"
"logpath:mathcomp.bigenough"
]
authors: [
"Cyril Cohen"
]
url {
src: "https://github.com/math-comp/bigenough/archive/1.0.1.tar.gz"
checksum: "sha256=a8ed105271ca7422f87f580ba1c5bb39de9f147ad966e65e02d09010cb3c1e36"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-mathcomp-bigenough.1.0.1 coq.dev</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 4000000; timeout 4h opam install -y --deps-only coq-mathcomp-bigenough.1.0.1 coq.dev</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>4 m 54 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 16000000; timeout 4h opam install -y -v coq-mathcomp-bigenough.1.0.1 coq.dev</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>36 s</dd>
</dl>
<h2>Installation size</h2>
<p>Total: 49 K</p>
<ul>
<li>32 K <code>../ocaml-base-compiler.4.10.2/lib/coq/user-contrib/mathcomp/bigenough/bigenough.vo</code></li>
<li>13 K <code>../ocaml-base-compiler.4.10.2/lib/coq/user-contrib/mathcomp/bigenough/bigenough.glob</code></li>
<li>5 K <code>../ocaml-base-compiler.4.10.2/lib/coq/user-contrib/mathcomp/bigenough/bigenough.v</code></li>
</ul>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq-mathcomp-bigenough.1.0.1</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
/*
***************************************************************************
* *
* Platform Independent *
* Bitmap Image Reader Writer Library *
* *
* Author: Arash Partow - 2002 *
* URL: http://partow.net/programming/bitmap/index.html *
* *
* Note: This library only supports 24-bits per pixel bitmap format files. *
* *
* Copyright notice: *
* Free use of the Platform Independent Bitmap Image Reader Writer Library *
* is permitted under the guidelines and in accordance with the most *
* current version of the Common Public License. *
* http://www.opensource.org/licenses/cpl1.0.php *
* *
***************************************************************************
*/
#ifndef INCLUDE_BITMAP_IMAGE_HPP
#define INCLUDE_BITMAP_IMAGE_HPP
#undef max
#include <algorithm>
#include <cmath>
#include <cstdlib>
#include <fstream>
#include <iostream>
#include <iterator>
#include <limits>
#include <string>
class bitmap_image
{
public:
enum channel_mode {
rgb_mode = 0,
bgr_mode = 1
};
enum color_plane {
blue_plane = 0,
green_plane = 1,
red_plane = 2
};
bitmap_image()
: file_name_(""),
data_ (0),
length_(0),
width_ (0),
height_(0),
row_increment_(0),
bytes_per_pixel_(3),
channel_mode_(bgr_mode)
{}
bitmap_image(const std::string& filename)
: file_name_(filename),
data_ (0),
length_(0),
width_ (0),
height_(0),
row_increment_(0),
bytes_per_pixel_(0),
channel_mode_(bgr_mode)
{
load_bitmap();
}
bitmap_image(const unsigned int width, const unsigned int height)
: file_name_(""),
data_ (0),
length_(0),
width_(width),
height_(height),
row_increment_(0),
bytes_per_pixel_(3),
channel_mode_(bgr_mode)
{
create_bitmap();
}
bitmap_image(const bitmap_image& image)
: file_name_(image.file_name_),
data_(0),
width_(image.width_),
height_(image.height_),
row_increment_(0),
bytes_per_pixel_(3),
channel_mode_(bgr_mode)
{
create_bitmap();
std::copy(image.data_, image.data_ + image.length_, data_);
}
~bitmap_image()
{
delete [] data_;
}
bitmap_image& operator=(const bitmap_image& image)
{
if (this != &image)
{
file_name_ = image.file_name_;
bytes_per_pixel_ = image.bytes_per_pixel_;
width_ = image.width_;
height_ = image.height_;
row_increment_ = 0;
channel_mode_ = image.channel_mode_;
create_bitmap();
std::copy(image.data_, image.data_ + image.length_, data_);
}
return *this;
}
inline bool operator!()
{
return (data_ == 0) ||
(length_ == 0) ||
(width_ == 0) ||
(height_ == 0) ||
(row_increment_== 0);
}
inline void clear(const unsigned char v = 0x00)
{
std::fill(data_,data_ + length_,v);
}
inline unsigned char red_channel(const unsigned int x, const unsigned int y) const
{
return data_[(y * row_increment_) + (x * bytes_per_pixel_ + 2)];
}
inline unsigned char green_channel(const unsigned int x, const unsigned int y) const
{
return data_[(y * row_increment_) + (x * bytes_per_pixel_ + 1)];
}
inline unsigned char blue_channel (const unsigned int x, const unsigned int y) const
{
return data_[(y * row_increment_) + (x * bytes_per_pixel_ + 0)];
}
inline void red_channel(const unsigned int x, const unsigned int y, const unsigned char value)
{
data_[(y * row_increment_) + (x * bytes_per_pixel_ + 2)] = value;
}
inline void green_channel(const unsigned int x, const unsigned int y, const unsigned char value)
{
data_[(y * row_increment_) + (x * bytes_per_pixel_ + 1)] = value;
}
inline void blue_channel (const unsigned int x, const unsigned int y, const unsigned char value)
{
data_[(y * row_increment_) + (x * bytes_per_pixel_ + 0)] = value;
}
inline unsigned char* row(unsigned int row_index) const
{
return data_ + (row_index * row_increment_);
}
inline void get_pixel(const unsigned int x, const unsigned int y,
unsigned char& red,
unsigned char& green,
unsigned char& blue)
{
const unsigned int y_offset = y * row_increment_;
const unsigned int x_offset = x * bytes_per_pixel_;
blue = data_[y_offset + x_offset + 0];
green = data_[y_offset + x_offset + 1];
red = data_[y_offset + x_offset + 2];
}
inline void set_pixel(const unsigned int x, const unsigned int y,
const unsigned char red,
const unsigned char green,
const unsigned char blue)
{
const unsigned int y_offset = y * row_increment_;
const unsigned int x_offset = x * bytes_per_pixel_;
data_[y_offset + x_offset + 0] = blue;
data_[y_offset + x_offset + 1] = green;
data_[y_offset + x_offset + 2] = red;
}
inline bool copy_from(const bitmap_image& image)
{
if (
(image.height_ != height_) ||
(image.width_ != width_ )
)
{
return false;
}
std::copy(image.data_,image.data_ + image.length_,data_);
return true;
}
inline bool copy_from(const bitmap_image& source_image,
const unsigned int& x_offset,
const unsigned int& y_offset)
{
if ((x_offset + source_image.width_ ) > width_ ) { return false; }
if ((y_offset + source_image.height_) > height_) { return false; }
for (unsigned int y = 0; y < source_image.height_; ++y)
{
unsigned char* itr1 = row(y + y_offset) + x_offset * bytes_per_pixel_;
const unsigned char* itr2 = source_image.row(y);
const unsigned char* itr2_end = itr2 + source_image.width_ * bytes_per_pixel_;
std::copy(itr2,itr2_end,itr1);
}
return true;
}
inline bool region(const unsigned int& x,
const unsigned int& y,
const unsigned int& width,
const unsigned int& height,
bitmap_image& dest_image)
{
if ((x + width ) > width_ ) { return false; }
if ((y + height) > height_) { return false; }
if (
(dest_image.width_ < width_ ) ||
(dest_image.height_ < height_)
)
{
dest_image.setwidth_height(width,height);
}
for (unsigned int r = 0; r < height; ++r)
{
unsigned char* itr1 = row(r + y) + x * bytes_per_pixel_;
unsigned char* itr1_end = itr1 + (width * bytes_per_pixel_);
unsigned char* itr2 = dest_image.row(r);
std::copy(itr1,itr1_end,itr2);
}
return true;
}
inline bool set_region(const unsigned int& x,
const unsigned int& y,
const unsigned int& width,
const unsigned int& height,
const unsigned char& value)
{
if ((x + width) > width_) { return false; }
if ((y + height) > height_) { return false; }
for (unsigned int r = 0; r < height; ++r)
{
unsigned char* itr = row(r + y) + x * bytes_per_pixel_;
unsigned char* itr_end = itr + (width * bytes_per_pixel_);
std::fill(itr,itr_end,value);
}
return true;
}
inline bool set_region(const unsigned int& x,
const unsigned int& y,
const unsigned int& width,
const unsigned int& height,
const color_plane color,
const unsigned char& value)
{
if ((x + width) > width_) { return false; }
if ((y + height) > height_) { return false; }
const unsigned int color_plane_offset = offset(color);
for (unsigned int r = 0; r < height; ++r)
{
unsigned char* itr = row(r + y) + x * bytes_per_pixel_ + color_plane_offset;
unsigned char* itr_end = itr + (width * bytes_per_pixel_);
while (itr != itr_end)
{
*itr = value;
itr += bytes_per_pixel_;
}
}
return true;
}
inline bool set_region(const unsigned int& x,
const unsigned int& y,
const unsigned int& width,
const unsigned int& height,
const unsigned char& red,
const unsigned char& green,
const unsigned char& blue)
{
if ((x + width) > width_) { return false; }
if ((y + height) > height_) { return false; }
for (unsigned int r = 0; r < height; ++r)
{
unsigned char* itr = row(r + y) + x * bytes_per_pixel_;
unsigned char* itr_end = itr + (width * bytes_per_pixel_);
while (itr != itr_end)
{
*(itr++) = blue;
*(itr++) = green;
*(itr++) = red;
}
}
return true;
}
void reflective_image(bitmap_image& image)
{
image.setwidth_height(3 * width_, 3 * height_,true);
image.copy_from(*this,width_,height_);
vertical_flip();
image.copy_from(*this,width_,0);
image.copy_from(*this,width_,2 * height_);
vertical_flip();
horizontal_flip();
image.copy_from(*this,0,height_);
image.copy_from(*this,2 * width_,height_);
horizontal_flip();
}
inline unsigned int width() const
{
return width_;
}
inline unsigned int height() const
{
return height_;
}
inline unsigned int bytes_per_pixel() const
{
return bytes_per_pixel_;
}
inline unsigned int pixel_count() const
{
return width_ * height_;
}
inline void setwidth_height(const unsigned int width,
const unsigned int height,
const bool clear = false)
{
delete[] data_;
data_ = 0;
width_ = width;
height_ = height;
create_bitmap();
if (clear)
{
std::fill(data_,data_ + length_,0x00);
}
}
void save_image(const std::string& file_name)
{
std::ofstream stream(file_name.c_str(),std::ios::binary);
if (!stream)
{
std::cout << "bitmap_image::save_image(): Error - Could not open file " << file_name << " for writing!" << std::endl;
return;
}
bitmap_file_header bfh;
bitmap_information_header bih;
bih.width = width_;
bih.height = height_;
bih.bit_count = static_cast<unsigned short>(bytes_per_pixel_ << 3);
bih.clr_important = 0;
bih.clr_used = 0;
bih.compression = 0;
bih.planes = 1;
bih.size = 40;
bih.x_pels_per_meter = 0;
bih.y_pels_per_meter = 0;
bih.size_image = (((bih.width * bytes_per_pixel_) + 3) & 0x0000FFFC) * bih.height;
bfh.type = 19778;
bfh.size = 55 + bih.size_image;
bfh.reserved1 = 0;
bfh.reserved2 = 0;
bfh.off_bits = bih.struct_size() + bfh.struct_size();
write_bfh(stream,bfh);
write_bih(stream,bih);
unsigned int padding = (4 - ((3 * width_) % 4)) % 4;
char padding_data[4] = {0x0,0x0,0x0,0x0};
for (unsigned int i = 0; i < height_; ++i)
{
unsigned char* data_ptr = data_ + (row_increment_ * (height_ - i - 1));
stream.write(reinterpret_cast<char*>(data_ptr),sizeof(unsigned char) * bytes_per_pixel_ * width_);
stream.write(padding_data,padding);
}
stream.close();
}
inline void set_all_ith_bits_low(const unsigned int bitr_index)
{
unsigned char mask = static_cast<unsigned char>(~(1 << bitr_index));
for (unsigned char* itr = data_; itr != data_ + length_; ++itr)
{
*itr &= mask;
}
}
inline void set_all_ith_bits_high(const unsigned int bitr_index)
{
unsigned char mask = static_cast<unsigned char>(1 << bitr_index);
for (unsigned char* itr = data_; itr != data_ + length_; ++itr)
{
*itr |= mask;
}
}
inline void set_all_ith_channels(const unsigned int& channel, const unsigned char& value)
{
for (unsigned char* itr = (data_ + channel); itr < (data_ + length_); itr += bytes_per_pixel_)
{
*itr = value;
}
}
inline void set_channel(const color_plane color,const unsigned char& value)
{
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); itr += bytes_per_pixel_)
{
*itr = value;
}
}
inline void ror_channel(const color_plane color, const unsigned int& ror)
{
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); itr += bytes_per_pixel_)
{
*itr = static_cast<unsigned char>(((*itr) >> ror) | ((*itr) << (8 - ror)));
}
}
inline void set_all_channels(const unsigned char& value)
{
for (unsigned char* itr = data_; itr < (data_ + length_); )
{
*(itr++) = value;
}
}
inline void set_all_channels(const unsigned char& r_value,
const unsigned char& g_value,
const unsigned char& b_value)
{
for (unsigned char* itr = (data_ + 0); itr < (data_ + length_); itr += bytes_per_pixel_)
{
*(itr + 0) = b_value;
*(itr + 1) = g_value;
*(itr + 2) = r_value;
}
}
inline void invert_color_planes()
{
for (unsigned char* itr = data_; itr < (data_ + length_); *itr = ~(*itr), ++itr);
}
inline void add_to_color_plane(const color_plane color,const unsigned char& value)
{
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); (*itr) += value, itr += bytes_per_pixel_);
}
inline void convert_to_grayscale()
{
double r_scaler = 0.299;
double g_scaler = 0.587;
double b_scaler = 0.114;
if (rgb_mode == channel_mode_)
{
double tmp = r_scaler;
r_scaler = b_scaler;
b_scaler = tmp;
}
for (unsigned char* itr = data_; itr < (data_ + length_); )
{
unsigned char gray_value = static_cast<unsigned char>((r_scaler * (*(itr + 2))) +
(g_scaler * (*(itr + 1))) +
(b_scaler * (*(itr + 0))) );
*(itr++) = gray_value;
*(itr++) = gray_value;
*(itr++) = gray_value;
}
}
inline const unsigned char* data()
{
return data_;
}
inline void bgr_to_rgb()
{
if ((bgr_mode == channel_mode_) && (3 == bytes_per_pixel_))
{
reverse_channels();
channel_mode_ = rgb_mode;
}
}
inline void rgb_to_bgr()
{
if ((rgb_mode == channel_mode_) && (3 == bytes_per_pixel_))
{
reverse_channels();
channel_mode_ = bgr_mode;
}
}
inline void reverse()
{
unsigned char* itr1 = data_;
unsigned char* itr2 = (data_ + length_) - bytes_per_pixel_;
while (itr1 < itr2)
{
for (std::size_t i = 0; i < bytes_per_pixel_; ++i)
{
unsigned char* citr1 = itr1 + i;
unsigned char* citr2 = itr2 + i;
unsigned char tmp = *citr1;
*citr1 = *citr2;
*citr2 = tmp;
}
itr1 += bytes_per_pixel_;
itr2 -= bytes_per_pixel_;
}
}
inline void horizontal_flip()
{
for (unsigned int y = 0; y < height_; ++y)
{
unsigned char* itr1 = row(y);
unsigned char* itr2 = itr1 + row_increment_ - bytes_per_pixel_;
while (itr1 < itr2)
{
for (unsigned int i = 0; i < bytes_per_pixel_; ++i)
{
unsigned char* p1 = (itr1 + i);
unsigned char* p2 = (itr2 + i);
unsigned char tmp = *p1;
*p1 = *p2;
*p2 = tmp;
}
itr1 += bytes_per_pixel_;
itr2 -= bytes_per_pixel_;
}
}
}
inline void vertical_flip()
{
for (unsigned int y = 0; y < (height_ / 2); ++y)
{
unsigned char* itr1 = row(y);
unsigned char* itr2 = row(height_ - y - 1);
for (std::size_t x = 0; x < row_increment_; ++x)
{
unsigned char tmp = *(itr1 + x);
*(itr1 + x) = *(itr2 + x);
*(itr2 + x) = tmp;
}
}
}
inline void export_color_plane(const color_plane color, unsigned char* image)
{
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); ++image, itr += bytes_per_pixel_)
{
(*image) = (*itr);
}
}
inline void export_color_plane(const color_plane color, bitmap_image& image)
{
if (
(width_ != image.width_ ) ||
(height_ != image.height_)
)
{
image.setwidth_height(width_,height_);
}
image.clear();
unsigned char* itr1 = (data_ + offset(color));
unsigned char* itr1_end = (data_ + length_);
unsigned char* itr2 = (image.data_ + offset(color));
while (itr1 < itr1_end)
{
(*itr2) = (*itr1);
itr1 += bytes_per_pixel_;
itr2 += bytes_per_pixel_;
}
}
inline void export_response_image(const color_plane color, double* response_image)
{
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); ++response_image, itr += bytes_per_pixel_)
{
(*response_image) = (1.0 * (*itr)) / 256.0;
}
}
inline void export_gray_scale_response_image(double* response_image)
{
for (unsigned char* itr = data_; itr < (data_ + length_); itr += bytes_per_pixel_)
{
unsigned char gray_value = static_cast<unsigned char>((0.299 * (*(itr + 2))) +
(0.587 * (*(itr + 1))) +
(0.114 * (*(itr + 0))));
(*response_image) = (1.0 * gray_value) / 256.0;
}
}
inline void export_rgb(double* red, double* green, double* blue) const
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
(*blue) = (1.0 * (*(itr++))) / 256.0;
(*green) = (1.0 * (*(itr++))) / 256.0;
(*red) = (1.0 * (*(itr++))) / 256.0;
}
}
inline void export_rgb(float* red, float* green, float* blue) const
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
(*blue) = (1.0f * (*(itr++))) / 256.0f;
(*green) = (1.0f * (*(itr++))) / 256.0f;
(*red) = (1.0f * (*(itr++))) / 256.0f;
}
}
inline void export_rgb(unsigned char* red, unsigned char* green, unsigned char* blue) const
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
(*blue) = *(itr++);
(*green) = *(itr++);
(*red) = *(itr++);
}
}
inline void export_ycbcr(double* y, double* cb, double* cr)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++y, ++cb, ++cr)
{
double blue = (1.0 * (*(itr++)));
double green = (1.0 * (*(itr++)));
double red = (1.0 * (*(itr++)));
( *y) = clamp<double>( 16.0 + (1.0/256.0) * ( 65.738 * red + 129.057 * green + 25.064 * blue),1.0,254);
(*cb) = clamp<double>(128.0 + (1.0/256.0) * (- 37.945 * red - 74.494 * green + 112.439 * blue),1.0,254);
(*cr) = clamp<double>(128.0 + (1.0/256.0) * ( 112.439 * red - 94.154 * green - 18.285 * blue),1.0,254);
}
}
inline void export_rgb_normal(double* red, double* green, double* blue) const
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
(*blue) = (1.0 * (*(itr++)));
(*green) = (1.0 * (*(itr++)));
(*red) = (1.0 * (*(itr++)));
}
}
inline void export_rgb_normal(float* red, float* green, float* blue) const
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
(*blue) = (1.0f * (*(itr++)));
(*green) = (1.0f * (*(itr++)));
(*red) = (1.0f * (*(itr++)));
}
}
inline void import_rgb(double* red, double* green, double* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(256.0 * (*blue ));
*(itr++) = static_cast<unsigned char>(256.0 * (*green));
*(itr++) = static_cast<unsigned char>(256.0 * (*red ));
}
}
inline void import_rgb(float* red, float* green, float* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(256.0f * (*blue ));
*(itr++) = static_cast<unsigned char>(256.0f * (*green));
*(itr++) = static_cast<unsigned char>(256.0f * (*red ));
}
}
inline void import_rgb(unsigned char* red, unsigned char* green, unsigned char* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = (*blue );
*(itr++) = (*green);
*(itr++) = (*red );
}
}
inline void import_ycbcr(double* y, double* cb, double* cr)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++y, ++cb, ++cr)
{
double y_ = (*y);
double cb_ = (*cb);
double cr_ = (*cr);
*(itr++) = static_cast<unsigned char>(clamp((298.082 * y_ + 516.412 * cb_ ) / 256.0 - 276.836,0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp((298.082 * y_ - 100.291 * cb_ - 208.120 * cr_ ) / 256.0 + 135.576,0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp((298.082 * y_ + 408.583 * cr_ ) / 256.0 - 222.921,0.0,255.0));
}
}
inline void import_rgb_clamped(double* red, double* green, double* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0 * (*blue ),0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0 * (*green),0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0 * (*red ),0.0,255.0));
}
}
inline void import_rgb_clamped(float* red, float* green, float* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0f * (*blue ),0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0f * (*green),0.0,255.0));
*(itr++) = static_cast<unsigned char>(clamp<double>(256.0f * (*red ),0.0,255.0));
}
}
inline void import_rgb_normal(double* red, double* green, double* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(*blue );
*(itr++) = static_cast<unsigned char>(*green);
*(itr++) = static_cast<unsigned char>(*red );
}
}
inline void import_rgb_normal(float* red, float* green, float* blue)
{
if (bgr_mode != channel_mode_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); ++red, ++green, ++blue)
{
*(itr++) = static_cast<unsigned char>(*blue );
*(itr++) = static_cast<unsigned char>(*green);
*(itr++) = static_cast<unsigned char>(*red );
}
}
inline void subsample(bitmap_image& dest)
{
/*
Half sub-sample of original image.
*/
unsigned int w = 0;
unsigned int h = 0;
bool odd_width = false;
bool odd_height = false;
if (0 == (width_ % 2))
w = width_ / 2;
else
{
w = 1 + (width_ / 2);
odd_width = true;
}
if (0 == (height_ % 2))
h = height_ / 2;
else
{
h = 1 + (height_ / 2);
odd_height = true;
}
unsigned int horizontal_upper = (odd_width) ? (w - 1) : w;
unsigned int vertical_upper = (odd_height) ? (h - 1) : h;
dest.setwidth_height(w,h);
dest.clear();
unsigned char* s_itr[3];
const unsigned char* itr1[3];
const unsigned char* itr2[3];
s_itr[0] = dest.data_ + 0;
s_itr[1] = dest.data_ + 1;
s_itr[2] = dest.data_ + 2;
itr1[0] = data_ + 0;
itr1[1] = data_ + 1;
itr1[2] = data_ + 2;
itr2[0] = data_ + row_increment_ + 0;
itr2[1] = data_ + row_increment_ + 1;
itr2[2] = data_ + row_increment_ + 2;
unsigned int total = 0;
for (unsigned int j = 0; j < vertical_upper; ++j)
{
for (unsigned int i = 0; i < horizontal_upper; ++i)
{
for (unsigned int k = 0; k < bytes_per_pixel_; s_itr[k] += bytes_per_pixel_, ++k)
{
total = 0;
total += *(itr1[k]); itr1[k] += bytes_per_pixel_;
total += *(itr1[k]); itr1[k] += bytes_per_pixel_;
total += *(itr2[k]); itr2[k] += bytes_per_pixel_;
total += *(itr2[k]); itr2[k] += bytes_per_pixel_;
*(s_itr[k]) = static_cast<unsigned char>(total >> 2);
}
}
if (odd_width)
{
for (unsigned int k = 0; k < bytes_per_pixel_; s_itr[k] += bytes_per_pixel_, ++k)
{
total = 0;
total += *(itr1[k]); itr1[k] += bytes_per_pixel_;
total += *(itr2[k]); itr2[k] += bytes_per_pixel_;
*(s_itr[k]) = static_cast<unsigned char>(total >> 1);
}
}
for (unsigned int k = 0; k < bytes_per_pixel_; itr1[k] += row_increment_, ++k);
if (j != (vertical_upper - 1))
{
for (unsigned int k = 0; k < bytes_per_pixel_; itr2[k] += row_increment_, ++k);
}
}
if (odd_height)
{
for (unsigned int i = 0; i < horizontal_upper; ++i)
{
for (unsigned int k = 0; k < bytes_per_pixel_; s_itr[k] += bytes_per_pixel_, ++k)
{
total = 0;
total += *(itr1[k]); itr1[k] += bytes_per_pixel_;
total += *(itr2[k]); itr2[k] += bytes_per_pixel_;
*(s_itr[k]) = static_cast<unsigned char>(total >> 1);
}
}
if (odd_width)
{
for (unsigned int k = 0; k < bytes_per_pixel_; ++k)
{
(*(s_itr[k])) = *(itr1[k]);
}
}
}
}
inline void upsample(bitmap_image& dest)
{
/*
2x up-sample of original image.
*/
dest.setwidth_height(2 * width_ ,2 * height_);
dest.clear();
const unsigned char* s_itr[3];
unsigned char* itr1[3];
unsigned char* itr2[3];
s_itr[0] = data_ + 0;
s_itr[1] = data_ + 1;
s_itr[2] = data_ + 2;
itr1[0] = dest.data_ + 0;
itr1[1] = dest.data_ + 1;
itr1[2] = dest.data_ + 2;
itr2[0] = dest.data_ + dest.row_increment_ + 0;
itr2[1] = dest.data_ + dest.row_increment_ + 1;
itr2[2] = dest.data_ + dest.row_increment_ + 2;
for (unsigned int j = 0; j < height_; ++j)
{
for (unsigned int i = 0; i < width_; ++i)
{
for (unsigned int k = 0; k < bytes_per_pixel_; s_itr[k] += bytes_per_pixel_, ++k)
{
*(itr1[k]) = *(s_itr[k]); itr1[k] += bytes_per_pixel_;
*(itr1[k]) = *(s_itr[k]); itr1[k] += bytes_per_pixel_;
*(itr2[k]) = *(s_itr[k]); itr2[k] += bytes_per_pixel_;
*(itr2[k]) = *(s_itr[k]); itr2[k] += bytes_per_pixel_;
}
}
for (unsigned int k = 0; k < bytes_per_pixel_; ++k)
{
itr1[k] += dest.row_increment_;
itr2[k] += dest.row_increment_;
}
}
}
inline void alpha_blend(const double& alpha, const bitmap_image& image)
{
if (
(image.width_ != width_ ) ||
(image.height_ != height_)
)
{
return;
}
if ((alpha < 0.0) || (alpha > 1.0))
{
return;
}
unsigned char* itr1 = data_;
unsigned char* itr1_end = data_ + length_;
unsigned char* itr2 = image.data_;
double alpha_compliment = 1.0 - alpha;
while (itr1 != itr1_end)
{
*(itr1) = static_cast<unsigned char>((alpha * (*itr2)) + (alpha_compliment * (*itr1)));
++itr1;
++itr2;
}
}
inline double psnr(const bitmap_image& image)
{
if (
(image.width_ != width_ ) ||
(image.height_ != height_)
)
{
return 0.0;
}
unsigned char* itr1 = data_;
unsigned char* itr2 = image.data_;
double mse = 0.0;
while (itr1 != (data_ + length_))
{
double v = (static_cast<double>(*itr1) - static_cast<double>(*itr2));
mse += v * v;
++itr1;
++itr2;
}
if (mse <= 0.0000001)
{
return 1000000.0;
}
else
{
mse /= (3.0 * width_ * height_);
return 20.0 * std::log10(255.0 / std::sqrt(mse));
}
}
inline double psnr(const unsigned int& x,
const unsigned int& y,
const bitmap_image& image)
{
if ((x + image.width()) > width_) { return 0.0; }
if ((y + image.height()) > height_) { return 0.0; }
double mse = 0.0;
const unsigned int height = image.height();
const unsigned int width = image.width();
for (unsigned int r = 0; r < height; ++r)
{
unsigned char* itr1 = row(r + y) + x * bytes_per_pixel_;
unsigned char* itr1_end = itr1 + (width * bytes_per_pixel_);
const unsigned char* itr2 = image.row(r);
while (itr1 != itr1_end)
{
double v = (static_cast<double>(*itr1) - static_cast<double>(*itr2));
mse += v * v;
++itr1;
++itr2;
}
}
if (mse <= 0.0000001)
{
return 1000000.0;
}
else
{
mse /= (3.0 * image.width() * image.height());
return 20.0 * std::log10(255.0 / std::sqrt(mse));
}
}
inline void histogram(const color_plane color, double hist[256])
{
std::fill(hist,hist + 256,0.0);
for (unsigned char* itr = (data_ + offset(color)); itr < (data_ + length_); itr += bytes_per_pixel_)
{
++hist[(*itr)];
}
}
inline void histogram_normalized(const color_plane color, double hist[256])
{
histogram(color,hist);
double* h_itr = hist;
const double* h_end = hist + 256;
const double pixel_count = static_cast<double>(width_ * height_);
while (h_end != h_itr)
{
*(h_itr++) /= pixel_count;
}
}
inline unsigned int offset(const color_plane color)
{
switch (channel_mode_)
{
case rgb_mode : {
switch (color)
{
case red_plane : return 0;
case green_plane : return 1;
case blue_plane : return 2;
default : return std::numeric_limits<int>::max();
}
}
case bgr_mode : {
switch (color)
{
case red_plane : return 2;
case green_plane : return 1;
case blue_plane : return 0;
default : return std::numeric_limits<int>::max();
}
}
default : return std::numeric_limits<unsigned int>::max();
}
}
inline void incremental()
{
unsigned char current_color = 0;
for (unsigned char* itr = data_; itr < (data_ + length_);)
{
(*itr++) = (current_color);
(*itr++) = (current_color);
(*itr++) = (current_color);
++current_color;
}
}
private:
struct bitmap_file_header
{
unsigned short type;
unsigned int size;
unsigned short reserved1;
unsigned short reserved2;
unsigned int off_bits;
unsigned int struct_size()
{
return sizeof(type) +
sizeof(size) +
sizeof(reserved1) +
sizeof(reserved2) +
sizeof(off_bits);
}
};
struct bitmap_information_header
{
unsigned int size;
unsigned int width;
unsigned int height;
unsigned short planes;
unsigned short bit_count;
unsigned int compression;
unsigned int size_image;
unsigned int x_pels_per_meter;
unsigned int y_pels_per_meter;
unsigned int clr_used;
unsigned int clr_important;
unsigned int struct_size()
{
return sizeof(size) +
sizeof(width) +
sizeof(height) +
sizeof(planes) +
sizeof(bit_count) +
sizeof(compression) +
sizeof(size_image) +
sizeof(x_pels_per_meter) +
sizeof(y_pels_per_meter) +
sizeof(clr_used) +
sizeof(clr_important);
}
};
inline bool big_endian()
{
unsigned int v = 0x01;
return (1 != reinterpret_cast<char*>(&v)[0]);
}
inline unsigned short flip(const unsigned short& v)
{
return ((v >> 8) | (v << 8));
}
inline unsigned int flip(const unsigned int& v)
{
return (((v & 0xFF000000) >> 0x18) |
((v & 0x000000FF) << 0x18) |
((v & 0x00FF0000) >> 0x08) |
((v & 0x0000FF00) << 0x08));
}
template<typename T>
inline void read_from_stream(std::ifstream& stream,T& t)
{
stream.read(reinterpret_cast<char*>(&t),sizeof(T));
}
template<typename T>
inline void write_to_stream(std::ofstream& stream,const T& t)
{
stream.write(reinterpret_cast<const char*>(&t),sizeof(T));
}
inline void read_bfh(std::ifstream& stream, bitmap_file_header& bfh)
{
read_from_stream(stream,bfh.type);
read_from_stream(stream,bfh.size);
read_from_stream(stream,bfh.reserved1);
read_from_stream(stream,bfh.reserved2);
read_from_stream(stream,bfh.off_bits);
if (big_endian())
{
bfh.type = flip(bfh.type);
bfh.size = flip(bfh.size);
bfh.reserved1 = flip(bfh.reserved1);
bfh.reserved2 = flip(bfh.reserved2);
bfh.off_bits = flip(bfh.off_bits);
}
}
inline void write_bfh(std::ofstream& stream, const bitmap_file_header& bfh)
{
if (big_endian())
{
write_to_stream(stream,flip(bfh.type ));
write_to_stream(stream,flip(bfh.size ));
write_to_stream(stream,flip(bfh.reserved1));
write_to_stream(stream,flip(bfh.reserved2));
write_to_stream(stream,flip(bfh.off_bits ));
}
else
{
write_to_stream(stream,bfh.type );
write_to_stream(stream,bfh.size );
write_to_stream(stream,bfh.reserved1);
write_to_stream(stream,bfh.reserved2);
write_to_stream(stream,bfh.off_bits );
}
}
inline void read_bih(std::ifstream& stream,bitmap_information_header& bih)
{
read_from_stream(stream,bih.size );
read_from_stream(stream,bih.width );
read_from_stream(stream,bih.height);
read_from_stream(stream,bih.planes);
read_from_stream(stream,bih.bit_count);
read_from_stream(stream,bih.compression);
read_from_stream(stream,bih.size_image);
read_from_stream(stream,bih.x_pels_per_meter);
read_from_stream(stream,bih.y_pels_per_meter);
read_from_stream(stream,bih.clr_used);
read_from_stream(stream,bih.clr_important);
if (big_endian())
{
bih.size = flip(bih.size );
bih.width = flip(bih.width );
bih.height = flip(bih.height );
bih.planes = flip(bih.planes );
bih.bit_count = flip(bih.bit_count);
bih.compression = flip(bih.compression);
bih.size_image = flip(bih.size_image);
bih.x_pels_per_meter = flip(bih.x_pels_per_meter);
bih.y_pels_per_meter = flip(bih.y_pels_per_meter);
bih.clr_used = flip(bih.clr_used);
bih.clr_important = flip(bih.clr_important);
}
}
inline void write_bih(std::ofstream& stream, const bitmap_information_header& bih)
{
if (big_endian())
{
write_to_stream(stream,flip(bih.size));
write_to_stream(stream,flip(bih.width));
write_to_stream(stream,flip(bih.height));
write_to_stream(stream,flip(bih.planes));
write_to_stream(stream,flip(bih.bit_count));
write_to_stream(stream,flip(bih.compression));
write_to_stream(stream,flip(bih.size_image));
write_to_stream(stream,flip(bih.x_pels_per_meter));
write_to_stream(stream,flip(bih.y_pels_per_meter));
write_to_stream(stream,flip(bih.clr_used));
write_to_stream(stream,flip(bih.clr_important));
}
else
{
write_to_stream(stream,bih.size);
write_to_stream(stream,bih.width);
write_to_stream(stream,bih.height);
write_to_stream(stream,bih.planes);
write_to_stream(stream,bih.bit_count);
write_to_stream(stream,bih.compression);
write_to_stream(stream,bih.size_image);
write_to_stream(stream,bih.x_pels_per_meter);
write_to_stream(stream,bih.y_pels_per_meter);
write_to_stream(stream,bih.clr_used);
write_to_stream(stream,bih.clr_important);
}
}
void create_bitmap()
{
length_ = width_ * height_ * bytes_per_pixel_;
row_increment_ = width_ * bytes_per_pixel_;
if (0 != data_)
{
delete[] data_;
}
data_ = new unsigned char[length_];
}
void load_bitmap()
{
std::ifstream stream(file_name_.c_str(),std::ios::binary);
if (!stream)
{
std::cerr << "bitmap_image::load_bitmap() ERROR: bitmap_image - file " << file_name_ << " not found!" << std::endl;
return;
}
bitmap_file_header bfh;
bitmap_information_header bih;
read_bfh(stream,bfh);
read_bih(stream,bih);
if (bfh.type != 19778)
{
stream.close();
std::cerr << "bitmap_image::load_bitmap() ERROR: bitmap_image - Invalid type value " << bfh.type << " expected 19778." << std::endl;
return;
}
if (bih.bit_count != 24)
{
stream.close();
std::cerr << "bitmap_image::load_bitmap() ERROR: bitmap_image - Invalid bit depth " << bih.bit_count << " expected 24." << std::endl;
return;
}
height_ = bih.height;
width_ = bih.width;
bytes_per_pixel_ = bih.bit_count >> 3;
unsigned int padding = (4 - ((3 * width_) % 4)) % 4;
char padding_data[4] = {0,0,0,0};
create_bitmap();
for (unsigned int i = 0; i < height_; ++i)
{
unsigned char* data_ptr = row(height_ - i - 1); // read in inverted row order
stream.read(reinterpret_cast<char*>(data_ptr),sizeof(char) * bytes_per_pixel_ * width_);
stream.read(padding_data,padding);
}
}
inline void reverse_channels()
{
if (3 != bytes_per_pixel_)
return;
for (unsigned char* itr = data_; itr < (data_ + length_); itr += bytes_per_pixel_)
{
unsigned char tmp = *(itr + 0);
*(itr + 0) = *(itr + 2);
*(itr + 2) = tmp;
}
}
template<typename T>
inline T clamp(const T& v, const T& lower_range, const T& upper_range)
{
if (v < lower_range)
return lower_range;
else if (v > upper_range)
return upper_range;
else
return v;
}
std::string file_name_;
unsigned char* data_;
unsigned int length_;
unsigned int width_;
unsigned int height_;
unsigned int row_increment_;
unsigned int bytes_per_pixel_;
channel_mode channel_mode_;
};
struct rgb_store
{
unsigned char red;
unsigned char green;
unsigned char blue;
};
inline void rgb_to_ycbcr(const unsigned int& length, double* red, double* green, double* blue,
double* y, double* cb, double* cr)
{
unsigned int i = 0;
while (i < length)
{
( *y) = 16.0 + ( 65.481 * (*red) + 128.553 * (*green) + 24.966 * (*blue));
(*cb) = 128.0 + ( -37.797 * (*red) + -74.203 * (*green) + 112.000 * (*blue));
(*cr) = 128.0 + ( 112.000 * (*red) + -93.786 * (*green) - 18.214 * (*blue));
++i;
++red; ++green; ++blue;
++y; ++cb; ++cr;
}
}
inline void ycbcr_to_rgb(const unsigned int& length, double* y, double* cb, double* cr,
double* red, double* green, double* blue)
{
unsigned int i = 0;
while (i < length)
{
double y_ = (*y) - 16.0;
double cb_ = (*cb) - 128.0;
double cr_ = (*cr) - 128.0;
(*red) = 0.000456621 * y_ + 0.00625893 * cr_;
(*green) = 0.000456621 * y_ - 0.00153632 * cb_ - 0.00318811 * cr_;
(*blue) = 0.000456621 * y_ + 0.00791071 * cb_;
++i;
++red; ++green; ++blue;
++y; ++cb; ++cr;
}
}
inline void subsample(const unsigned int& width,
const unsigned int& height,
const double* source,
unsigned int& w,
unsigned int& h,
double** dest)
{
/* Single channel. */
w = 0;
h = 0;
bool odd_width = false;
bool odd_height = false;
if (0 == (width % 2))
w = width / 2;
else
{
w = 1 + (width / 2);
odd_width = true;
}
if (0 == (height % 2))
h = height / 2;
else
{
h = 1 + (height / 2);
odd_height = true;
}
unsigned int horizontal_upper = (odd_width) ? w - 1 : w;
unsigned int vertical_upper = (odd_height) ? h - 1 : h;
*dest = new double[w * h];
double* s_itr = *dest;
const double* itr1 = source;
const double* itr2 = source + width;
for (unsigned int j = 0; j < vertical_upper; ++j)
{
for (unsigned int i = 0; i < horizontal_upper; ++i, ++s_itr)
{
(*s_itr) = *(itr1++);
(*s_itr) += *(itr1++);
(*s_itr) += *(itr2++);
(*s_itr) += *(itr2++);
(*s_itr) /= 4.0;
}
if (odd_width)
{
(*(s_itr++)) = ( (*itr1++) + (*itr2++) ) / 2.0;
}
itr1 += width;
if (j != (vertical_upper -1))
{
itr2 += width;
}
}
if (odd_height)
{
for (unsigned int i = 0; i < horizontal_upper; ++i, ++s_itr)
{
(*s_itr) += (*(itr1++));
(*s_itr) += (*(itr1++));
(*s_itr) /= 2.0;
}
if (odd_width)
{
(*(s_itr++)) = (*itr1);
}
}
}
inline void upsample(const unsigned int& width,
const unsigned int& height,
const double* source,
unsigned int& w,
unsigned int& h,
double** dest)
{
/* Single channel. */
w = 2 * width;
h = 2 * height;
*dest = new double[w * h];
const double* s_itr = source;
double* itr1 = *dest;
double* itr2 = *dest + w;
for (unsigned int j = 0; j < height; ++j)
{
for (unsigned int i = 0; i < width; ++i, ++s_itr)
{
*(itr1++) = (*s_itr);
*(itr1++) = (*s_itr);
*(itr2++) = (*s_itr);
*(itr2++) = (*s_itr);
}
itr1 += w;
itr2 += w;
}
}
inline void checkered_pattern(const unsigned int x_width,
const unsigned int y_width,
const unsigned char value,
const bitmap_image::color_plane color,
bitmap_image& image)
{
if (
(x_width >= image.width ()) ||
(y_width >= image.height())
)
{
return;
}
bool setter_x = false;
bool setter_y = true;
const unsigned int color_plane_offset = image.offset(color);
const unsigned int height = image.height();
const unsigned int width = image.width();
for (unsigned int y = 0; y < height; ++y)
{
if (0 == (y % y_width))
{
setter_y = !setter_y;
}
unsigned char* row = image.row(y) + color_plane_offset;
for (unsigned int x = 0; x < width; ++x, row += image.bytes_per_pixel())
{
if (0 == (x % x_width))
{
setter_x = !setter_x;
}
if (setter_x ^ setter_y)
{
*row = value;
}
}
}
}
inline void checkered_pattern(const unsigned int x_width,
const unsigned int y_width,
const unsigned char red,
const unsigned char green,
const unsigned char blue,
bitmap_image& image)
{
if (
(x_width >= image.width ()) ||
(y_width >= image.height())
)
{
return;
}
bool setter_x = false;
bool setter_y = true;
const unsigned int height = image.height();
const unsigned int width = image.width();
for (unsigned int y = 0; y < height; ++y)
{
if (0 == (y % y_width))
{
setter_y = !setter_y;
}
unsigned char* row = image.row(y);
for (unsigned int x = 0; x < width; ++x, row += image.bytes_per_pixel())
{
if (0 == (x % x_width))
{
setter_x = !setter_x;
}
if (setter_x ^ setter_y)
{
*(row + 0) = blue;
*(row + 1) = green;
*(row + 2) = red;
}
}
}
}
inline void plasma(bitmap_image& image,
const double& x, const double& y,
const double& width, const double& height,
const double& c1, const double& c2,
const double& c3, const double& c4,
const double& roughness = 3.0,
const rgb_store colormap[] = 0)
{
// Note: c1,c2,c3,c4 -> [0.0,1.0]
double half_width = ( width / 2.0);
double half_height = (height / 2.0);
if ((width >= 1.0) || (height >= 1.0))
{
double corner1 = (c1 + c2) / 2.0;
double corner2 = (c2 + c3) / 2.0;
double corner3 = (c3 + c4) / 2.0;
double corner4 = (c4 + c1) / 2.0;
double center = (c1 + c2 + c3 + c4) / 4.0 +
((1.0 * ::rand() /(1.0 * RAND_MAX)) - 0.5) * // should use a better rng
((1.0 * half_width + half_height) / (image.width() + image.height()) * roughness);
center = std::min<double>(std::max<double>(0.0,center),1.0);
plasma(image, x, y, half_width, half_height, c1, corner1, center, corner4,roughness,colormap);
plasma(image, x + half_width, y, half_width, half_height, corner1, c2, corner2, center,roughness,colormap);
plasma(image, x + half_width, y + half_height, half_width, half_height, center, corner2, c3, corner3,roughness,colormap);
plasma(image, x, y + half_height, half_width, half_height, corner4, center, corner3, c4,roughness,colormap);
}
else
{
rgb_store color = colormap[static_cast<unsigned int>(1000.0 * ((c1 + c2 + c3 + c4) / 4.0)) % 1000];
image.set_pixel(static_cast<unsigned int>(x),static_cast<unsigned int>(y),color.red,color.green,color.blue);
}
}
inline double psnr_region(const unsigned int& x, const unsigned int& y,
const unsigned int& width, const unsigned int& height,
const bitmap_image& image1, const bitmap_image& image2)
{
if (
(image1.width() != image2.width ()) ||
(image1.height() != image2.height())
)
{
return 0.0;
}
if ((x + width) > image1.width()) { return 0.0; }
if ((y + height) > image1.height()) { return 0.0; }
double mse = 0.0;
for (unsigned int r = 0; r < height; ++r)
{
const unsigned char* itr1 = image1.row(r + y) + x * image1.bytes_per_pixel();
const unsigned char* itr1_end = itr1 + (width * image1.bytes_per_pixel());
const unsigned char* itr2 = image2.row(r + y) + x * image2.bytes_per_pixel();
while (itr1 != itr1_end)
{
double v = (static_cast<double>(*itr1) - static_cast<double>(*itr2));
mse += v * v;
++itr1;
++itr2;
}
}
if (mse <= 0.0000001)
{
return 1000000.0;
}
else
{
mse /= (3.0 * width * height);
return 20.0 * std::log10(255.0 / std::sqrt(mse));
}
}
inline void hierarchical_psnr_r(const double& x, const double& y,
const double& width, const double& height,
const bitmap_image& image1,
bitmap_image& image2,
const double& threshold,
const rgb_store colormap[])
{
if ((width <= 4.0) || (height <= 4.0))
{
double psnr = psnr_region(static_cast<unsigned int>(x),
static_cast<unsigned int>(y),
static_cast<unsigned int>(width),
static_cast<unsigned int>(height),
image1,image2);
if (psnr < threshold)
{
rgb_store c = colormap[static_cast<unsigned int>(1000.0 * (1.0 - (psnr / threshold)))];
image2.set_region(static_cast<unsigned int>(x),
static_cast<unsigned int>(y),
static_cast<unsigned int>(width + 1),
static_cast<unsigned int>(height + 1),
c.red,c.green,c.blue);
}
}
else
{
double half_width = ( width / 2.0);
double half_height = (height / 2.0);
hierarchical_psnr_r(x , y , half_width, half_height,image1,image2,threshold,colormap);
hierarchical_psnr_r(x + half_width, y , half_width, half_height,image1,image2,threshold,colormap);
hierarchical_psnr_r(x + half_width, y + half_height, half_width, half_height,image1,image2,threshold,colormap);
hierarchical_psnr_r(x , y + half_height, half_width, half_height,image1,image2,threshold,colormap);
}
}
inline void hierarchical_psnr(bitmap_image& image1,bitmap_image& image2, const double threshold, const rgb_store colormap[])
{
if (
(image1.width() != image2.width ()) ||
(image1.height() != image2.height())
)
{
return;
}
double psnr = psnr_region(0,0,image1.width(),image1.height(),image1,image2);
if (psnr < threshold)
{
hierarchical_psnr_r(0,0, image1.width(), image1.height(),image1,image2,threshold,colormap);
}
}
class image_drawer
{
public:
image_drawer(bitmap_image& image)
: image_(image),
pen_width_(1),
pen_color_red_ (0),
pen_color_green_(0),
pen_color_blue_ (0)
{}
void rectangle(int x1, int y1, int x2, int y2)
{
line_segment(x1,y1,x2,y1);
line_segment(x2,y1,x2,y2);
line_segment(x2,y2,x1,y2);
line_segment(x1,y2,x1,y1);
}
void triangle(int x1, int y1, int x2, int y2,int x3, int y3)
{
line_segment(x1,y1,x2,y2);
line_segment(x2,y2,x3,y3);
line_segment(x3,y3,x1,y1);
}
void quadix(int x1, int y1, int x2, int y2,int x3, int y3, int x4, int y4)
{
line_segment(x1,y1,x2,y2);
line_segment(x2,y2,x3,y3);
line_segment(x3,y3,x4,y4);
line_segment(x4,y4,x1,y1);
}
void line_segment(int x1, int y1, int x2, int y2)
{
int steep = 0;
int sx = ((x2 - x1) > 0) ? 1 : -1;
int sy = ((y2 - y1) > 0) ? 1 : -1;
int dx = abs(x2 - x1);
int dy = abs(y2 - y1);
if (dy > dx)
{
steep = x1; x1 = y1; y1 = steep; /* swap x1 and y1 */
steep = dx; dx = dy; dy = steep; /* swap dx and dy */
steep = sx; sx = sy; sy = steep; /* swap sx and sy */
steep = 1;
}
int e = 2 * dy - dx;
for (int i = 0; i < dx; ++i)
{
if (steep)
plot_pen_pixel(y1,x1);
else
plot_pen_pixel(x1,y1);
while (e >= 0)
{
y1 += sy;
e -= (dx << 1);
}
x1 += sx;
e += (dy << 1);
}
plot_pen_pixel(x2,y2);
}
void horiztonal_line_segment(int x1, int x2, int y)
{
if (x1 > x2)
{
std::swap(x1,x2);
}
for (int i = 0; i < (x2 - x1); ++i)
{
plot_pen_pixel(x1 + i,y);
}
}
void vertical_line_segment(int y1, int y2, int x)
{
if (y1 > y2)
{
std::swap(y1,y2);
}
for (int i = 0; i < (y2 - y1); ++i)
{
plot_pen_pixel(x, y1 + i);
}
}
void ellipse(int centerx, int centery, int a, int b)
{
int t1 = a * a;
int t2 = t1 << 1;
int t3 = t2 << 1;
int t4 = b * b;
int t5 = t4 << 1;
int t6 = t5 << 1;
int t7 = a * t5;
int t8 = t7 << 1;
int t9 = 0;
int d1 = t2 - t7 + (t4 >> 1);
int d2 = (t1 >> 1) - t8 + t5;
int x = a;
int y = 0;
int negative_tx = centerx - x;
int positive_tx = centerx + x;
int negative_ty = centery - y;
int positive_ty = centery + y;
while (d2 < 0)
{
plot_pen_pixel(positive_tx,positive_ty);
plot_pen_pixel(positive_tx,negative_ty);
plot_pen_pixel(negative_tx,positive_ty);
plot_pen_pixel(negative_tx,negative_ty);
++y;
t9 = t9 + t3;
if (d1 < 0)
{
d1 = d1 + t9 + t2;
d2 = d2 + t9;
}
else
{
x--;
t8 = t8 - t6;
d1 = d1 + (t9 + t2 - t8);
d2 = d2 + (t9 + t5 - t8);
negative_tx = centerx - x;
positive_tx = centerx + x;
}
negative_ty = centery - y;
positive_ty = centery + y;
}
do
{
plot_pen_pixel(positive_tx,positive_ty);
plot_pen_pixel(positive_tx,negative_ty);
plot_pen_pixel(negative_tx,positive_ty);
plot_pen_pixel(negative_tx,negative_ty);
x--;
t8 = t8 - t6;
if (d2 < 0)
{
++y;
t9 = t9 + t3;
d2 = d2 + (t9 + t5 - t8);
negative_ty = centery - y;
positive_ty = centery + y;
}
else
d2 = d2 + (t5 - t8);
negative_tx = centerx - x;
positive_tx = centerx + x;
}
while (x >= 0);
}
void circle(int centerx, int centery, int radius)
{
int x = 0;
int d = (1 - radius) << 1;
while (radius >= 0)
{
plot_pen_pixel(centerx + x,centery + radius);
plot_pen_pixel(centerx + x,centery - radius);
plot_pen_pixel(centerx - x,centery + radius);
plot_pen_pixel(centerx - x,centery - radius);
if ((d + radius) > 0)
d -= ((--radius) << 1) - 1;
if (x > d)
d += ((++x) << 1) + 1;
}
}
void plot_pen_pixel(int x, int y)
{
switch (pen_width_)
{
case 1 : plot_pixel(x,y);
break;
case 2 : {
plot_pixel(x , y );
plot_pixel(x + 1, y );
plot_pixel(x + 1, y + 1);
plot_pixel(x , y + 1);
}
break;
case 3 : {
plot_pixel(x , y - 1);
plot_pixel(x - 1, y - 1);
plot_pixel(x + 1, y - 1);
plot_pixel(x , y );
plot_pixel(x - 1, y );
plot_pixel(x + 1, y );
plot_pixel(x , y + 1);
plot_pixel(x - 1, y + 1);
plot_pixel(x + 1, y + 1);
}
break;
default : plot_pixel(x,y);
break;
}
}
void plot_pixel(int x, int y)
{
image_.set_pixel(x,y,pen_color_red_,pen_color_green_,pen_color_blue_);
}
void pen_width(const unsigned int& width)
{
if ((width > 0) && (width < 4))
{
pen_width_ = width;
}
}
void pen_color(const unsigned char& red,
const unsigned char& green,
const unsigned char& blue)
{
pen_color_red_ = red;
pen_color_green_ = green;
pen_color_blue_ = blue;
}
private:
image_drawer(const image_drawer& id);
image_drawer& operator =(const image_drawer& id);
bitmap_image& image_;
unsigned int pen_width_;
unsigned char pen_color_red_;
unsigned char pen_color_green_;
unsigned char pen_color_blue_;
};
const rgb_store autumn_colormap[1000] = {
{255, 0, 0}, {255, 0, 0}, {255, 1, 0}, {255, 1, 0}, {255, 1, 0},
{255, 1, 0}, {255, 2, 0}, {255, 2, 0}, {255, 2, 0}, {255, 2, 0},
{255, 3, 0}, {255, 3, 0}, {255, 3, 0}, {255, 3, 0}, {255, 4, 0},
{255, 4, 0}, {255, 4, 0}, {255, 4, 0}, {255, 5, 0}, {255, 5, 0},
{255, 5, 0}, {255, 5, 0}, {255, 6, 0}, {255, 6, 0}, {255, 6, 0},
{255, 6, 0}, {255, 7, 0}, {255, 7, 0}, {255, 7, 0}, {255, 7, 0},
{255, 8, 0}, {255, 8, 0}, {255, 8, 0}, {255, 8, 0}, {255, 9, 0},
{255, 9, 0}, {255, 9, 0}, {255, 9, 0}, {255, 10, 0}, {255, 10, 0},
{255, 10, 0}, {255, 10, 0}, {255, 11, 0}, {255, 11, 0}, {255, 11, 0},
{255, 11, 0}, {255, 12, 0}, {255, 12, 0}, {255, 12, 0}, {255, 13, 0},
{255, 13, 0}, {255, 13, 0}, {255, 13, 0}, {255, 14, 0}, {255, 14, 0},
{255, 14, 0}, {255, 14, 0}, {255, 15, 0}, {255, 15, 0}, {255, 15, 0},
{255, 15, 0}, {255, 16, 0}, {255, 16, 0}, {255, 16, 0}, {255, 16, 0},
{255, 17, 0}, {255, 17, 0}, {255, 17, 0}, {255, 17, 0}, {255, 18, 0},
{255, 18, 0}, {255, 18, 0}, {255, 18, 0}, {255, 19, 0}, {255, 19, 0},
{255, 19, 0}, {255, 19, 0}, {255, 20, 0}, {255, 20, 0}, {255, 20, 0},
{255, 20, 0}, {255, 21, 0}, {255, 21, 0}, {255, 21, 0}, {255, 21, 0},
{255, 22, 0}, {255, 22, 0}, {255, 22, 0}, {255, 22, 0}, {255, 23, 0},
{255, 23, 0}, {255, 23, 0}, {255, 23, 0}, {255, 24, 0}, {255, 24, 0},
{255, 24, 0}, {255, 25, 0}, {255, 25, 0}, {255, 25, 0}, {255, 25, 0},
{255, 26, 0}, {255, 26, 0}, {255, 26, 0}, {255, 26, 0}, {255, 27, 0},
{255, 27, 0}, {255, 27, 0}, {255, 27, 0}, {255, 28, 0}, {255, 28, 0},
{255, 28, 0}, {255, 28, 0}, {255, 29, 0}, {255, 29, 0}, {255, 29, 0},
{255, 29, 0}, {255, 30, 0}, {255, 30, 0}, {255, 30, 0}, {255, 30, 0},
{255, 31, 0}, {255, 31, 0}, {255, 31, 0}, {255, 31, 0}, {255, 32, 0},
{255, 32, 0}, {255, 32, 0}, {255, 32, 0}, {255, 33, 0}, {255, 33, 0},
{255, 33, 0}, {255, 33, 0}, {255, 34, 0}, {255, 34, 0}, {255, 34, 0},
{255, 34, 0}, {255, 35, 0}, {255, 35, 0}, {255, 35, 0}, {255, 35, 0},
{255, 36, 0}, {255, 36, 0}, {255, 36, 0}, {255, 37, 0}, {255, 37, 0},
{255, 37, 0}, {255, 37, 0}, {255, 38, 0}, {255, 38, 0}, {255, 38, 0},
{255, 38, 0}, {255, 39, 0}, {255, 39, 0}, {255, 39, 0}, {255, 39, 0},
{255, 40, 0}, {255, 40, 0}, {255, 40, 0}, {255, 40, 0}, {255, 41, 0},
{255, 41, 0}, {255, 41, 0}, {255, 41, 0}, {255, 42, 0}, {255, 42, 0},
{255, 42, 0}, {255, 42, 0}, {255, 43, 0}, {255, 43, 0}, {255, 43, 0},
{255, 43, 0}, {255, 44, 0}, {255, 44, 0}, {255, 44, 0}, {255, 44, 0},
{255, 45, 0}, {255, 45, 0}, {255, 45, 0}, {255, 45, 0}, {255, 46, 0},
{255, 46, 0}, {255, 46, 0}, {255, 46, 0}, {255, 47, 0}, {255, 47, 0},
{255, 47, 0}, {255, 47, 0}, {255, 48, 0}, {255, 48, 0}, {255, 48, 0},
{255, 48, 0}, {255, 49, 0}, {255, 49, 0}, {255, 49, 0}, {255, 50, 0},
{255, 50, 0}, {255, 50, 0}, {255, 50, 0}, {255, 51, 0}, {255, 51, 0},
{255, 51, 0}, {255, 51, 0}, {255, 52, 0}, {255, 52, 0}, {255, 52, 0},
{255, 52, 0}, {255, 53, 0}, {255, 53, 0}, {255, 53, 0}, {255, 53, 0},
{255, 54, 0}, {255, 54, 0}, {255, 54, 0}, {255, 54, 0}, {255, 55, 0},
{255, 55, 0}, {255, 55, 0}, {255, 55, 0}, {255, 56, 0}, {255, 56, 0},
{255, 56, 0}, {255, 56, 0}, {255, 57, 0}, {255, 57, 0}, {255, 57, 0},
{255, 57, 0}, {255, 58, 0}, {255, 58, 0}, {255, 58, 0}, {255, 58, 0},
{255, 59, 0}, {255, 59, 0}, {255, 59, 0}, {255, 59, 0}, {255, 60, 0},
{255, 60, 0}, {255, 60, 0}, {255, 60, 0}, {255, 61, 0}, {255, 61, 0},
{255, 61, 0}, {255, 62, 0}, {255, 62, 0}, {255, 62, 0}, {255, 62, 0},
{255, 63, 0}, {255, 63, 0}, {255, 63, 0}, {255, 63, 0}, {255, 64, 0},
{255, 64, 0}, {255, 64, 0}, {255, 64, 0}, {255, 65, 0}, {255, 65, 0},
{255, 65, 0}, {255, 65, 0}, {255, 66, 0}, {255, 66, 0}, {255, 66, 0},
{255, 66, 0}, {255, 67, 0}, {255, 67, 0}, {255, 67, 0}, {255, 67, 0},
{255, 68, 0}, {255, 68, 0}, {255, 68, 0}, {255, 68, 0}, {255, 69, 0},
{255, 69, 0}, {255, 69, 0}, {255, 69, 0}, {255, 70, 0}, {255, 70, 0},
{255, 70, 0}, {255, 70, 0}, {255, 71, 0}, {255, 71, 0}, {255, 71, 0},
{255, 71, 0}, {255, 72, 0}, {255, 72, 0}, {255, 72, 0}, {255, 72, 0},
{255, 73, 0}, {255, 73, 0}, {255, 73, 0}, {255, 74, 0}, {255, 74, 0},
{255, 74, 0}, {255, 74, 0}, {255, 75, 0}, {255, 75, 0}, {255, 75, 0},
{255, 75, 0}, {255, 76, 0}, {255, 76, 0}, {255, 76, 0}, {255, 76, 0},
{255, 77, 0}, {255, 77, 0}, {255, 77, 0}, {255, 77, 0}, {255, 78, 0},
{255, 78, 0}, {255, 78, 0}, {255, 78, 0}, {255, 79, 0}, {255, 79, 0},
{255, 79, 0}, {255, 79, 0}, {255, 80, 0}, {255, 80, 0}, {255, 80, 0},
{255, 80, 0}, {255, 81, 0}, {255, 81, 0}, {255, 81, 0}, {255, 81, 0},
{255, 82, 0}, {255, 82, 0}, {255, 82, 0}, {255, 82, 0}, {255, 83, 0},
{255, 83, 0}, {255, 83, 0}, {255, 83, 0}, {255, 84, 0}, {255, 84, 0},
{255, 84, 0}, {255, 84, 0}, {255, 85, 0}, {255, 85, 0}, {255, 85, 0},
{255, 86, 0}, {255, 86, 0}, {255, 86, 0}, {255, 86, 0}, {255, 87, 0},
{255, 87, 0}, {255, 87, 0}, {255, 87, 0}, {255, 88, 0}, {255, 88, 0},
{255, 88, 0}, {255, 88, 0}, {255, 89, 0}, {255, 89, 0}, {255, 89, 0},
{255, 89, 0}, {255, 90, 0}, {255, 90, 0}, {255, 90, 0}, {255, 90, 0},
{255, 91, 0}, {255, 91, 0}, {255, 91, 0}, {255, 91, 0}, {255, 92, 0},
{255, 92, 0}, {255, 92, 0}, {255, 92, 0}, {255, 93, 0}, {255, 93, 0},
{255, 93, 0}, {255, 93, 0}, {255, 94, 0}, {255, 94, 0}, {255, 94, 0},
{255, 94, 0}, {255, 95, 0}, {255, 95, 0}, {255, 95, 0}, {255, 95, 0},
{255, 96, 0}, {255, 96, 0}, {255, 96, 0}, {255, 96, 0}, {255, 97, 0},
{255, 97, 0}, {255, 97, 0}, {255, 98, 0}, {255, 98, 0}, {255, 98, 0},
{255, 98, 0}, {255, 99, 0}, {255, 99, 0}, {255, 99, 0}, {255, 99, 0},
{255, 100, 0}, {255, 100, 0}, {255, 100, 0}, {255, 100, 0}, {255, 101, 0},
{255, 101, 0}, {255, 101, 0}, {255, 101, 0}, {255, 102, 0}, {255, 102, 0},
{255, 102, 0}, {255, 102, 0}, {255, 103, 0}, {255, 103, 0}, {255, 103, 0},
{255, 103, 0}, {255, 104, 0}, {255, 104, 0}, {255, 104, 0}, {255, 104, 0},
{255, 105, 0}, {255, 105, 0}, {255, 105, 0}, {255, 105, 0}, {255, 106, 0},
{255, 106, 0}, {255, 106, 0}, {255, 106, 0}, {255, 107, 0}, {255, 107, 0},
{255, 107, 0}, {255, 107, 0}, {255, 108, 0}, {255, 108, 0}, {255, 108, 0},
{255, 108, 0}, {255, 109, 0}, {255, 109, 0}, {255, 109, 0}, {255, 110, 0},
{255, 110, 0}, {255, 110, 0}, {255, 110, 0}, {255, 111, 0}, {255, 111, 0},
{255, 111, 0}, {255, 111, 0}, {255, 112, 0}, {255, 112, 0}, {255, 112, 0},
{255, 112, 0}, {255, 113, 0}, {255, 113, 0}, {255, 113, 0}, {255, 113, 0},
{255, 114, 0}, {255, 114, 0}, {255, 114, 0}, {255, 114, 0}, {255, 115, 0},
{255, 115, 0}, {255, 115, 0}, {255, 115, 0}, {255, 116, 0}, {255, 116, 0},
{255, 116, 0}, {255, 116, 0}, {255, 117, 0}, {255, 117, 0}, {255, 117, 0},
{255, 117, 0}, {255, 118, 0}, {255, 118, 0}, {255, 118, 0}, {255, 118, 0},
{255, 119, 0}, {255, 119, 0}, {255, 119, 0}, {255, 119, 0}, {255, 120, 0},
{255, 120, 0}, {255, 120, 0}, {255, 120, 0}, {255, 121, 0}, {255, 121, 0},
{255, 121, 0}, {255, 122, 0}, {255, 122, 0}, {255, 122, 0}, {255, 122, 0},
{255, 123, 0}, {255, 123, 0}, {255, 123, 0}, {255, 123, 0}, {255, 124, 0},
{255, 124, 0}, {255, 124, 0}, {255, 124, 0}, {255, 125, 0}, {255, 125, 0},
{255, 125, 0}, {255, 125, 0}, {255, 126, 0}, {255, 126, 0}, {255, 126, 0},
{255, 126, 0}, {255, 127, 0}, {255, 127, 0}, {255, 127, 0}, {255, 127, 0},
{255, 128, 0}, {255, 128, 0}, {255, 128, 0}, {255, 128, 0}, {255, 129, 0},
{255, 129, 0}, {255, 129, 0}, {255, 129, 0}, {255, 130, 0}, {255, 130, 0},
{255, 130, 0}, {255, 130, 0}, {255, 131, 0}, {255, 131, 0}, {255, 131, 0},
{255, 131, 0}, {255, 132, 0}, {255, 132, 0}, {255, 132, 0}, {255, 132, 0},
{255, 133, 0}, {255, 133, 0}, {255, 133, 0}, {255, 133, 0}, {255, 134, 0},
{255, 134, 0}, {255, 134, 0}, {255, 135, 0}, {255, 135, 0}, {255, 135, 0},
{255, 135, 0}, {255, 136, 0}, {255, 136, 0}, {255, 136, 0}, {255, 136, 0},
{255, 137, 0}, {255, 137, 0}, {255, 137, 0}, {255, 137, 0}, {255, 138, 0},
{255, 138, 0}, {255, 138, 0}, {255, 138, 0}, {255, 139, 0}, {255, 139, 0},
{255, 139, 0}, {255, 139, 0}, {255, 140, 0}, {255, 140, 0}, {255, 140, 0},
{255, 140, 0}, {255, 141, 0}, {255, 141, 0}, {255, 141, 0}, {255, 141, 0},
{255, 142, 0}, {255, 142, 0}, {255, 142, 0}, {255, 142, 0}, {255, 143, 0},
{255, 143, 0}, {255, 143, 0}, {255, 143, 0}, {255, 144, 0}, {255, 144, 0},
{255, 144, 0}, {255, 144, 0}, {255, 145, 0}, {255, 145, 0}, {255, 145, 0},
{255, 145, 0}, {255, 146, 0}, {255, 146, 0}, {255, 146, 0}, {255, 147, 0},
{255, 147, 0}, {255, 147, 0}, {255, 147, 0}, {255, 148, 0}, {255, 148, 0},
{255, 148, 0}, {255, 148, 0}, {255, 149, 0}, {255, 149, 0}, {255, 149, 0},
{255, 149, 0}, {255, 150, 0}, {255, 150, 0}, {255, 150, 0}, {255, 150, 0},
{255, 151, 0}, {255, 151, 0}, {255, 151, 0}, {255, 151, 0}, {255, 152, 0},
{255, 152, 0}, {255, 152, 0}, {255, 152, 0}, {255, 153, 0}, {255, 153, 0},
{255, 153, 0}, {255, 153, 0}, {255, 154, 0}, {255, 154, 0}, {255, 154, 0},
{255, 154, 0}, {255, 155, 0}, {255, 155, 0}, {255, 155, 0}, {255, 155, 0},
{255, 156, 0}, {255, 156, 0}, {255, 156, 0}, {255, 156, 0}, {255, 157, 0},
{255, 157, 0}, {255, 157, 0}, {255, 157, 0}, {255, 158, 0}, {255, 158, 0},
{255, 158, 0}, {255, 159, 0}, {255, 159, 0}, {255, 159, 0}, {255, 159, 0},
{255, 160, 0}, {255, 160, 0}, {255, 160, 0}, {255, 160, 0}, {255, 161, 0},
{255, 161, 0}, {255, 161, 0}, {255, 161, 0}, {255, 162, 0}, {255, 162, 0},
{255, 162, 0}, {255, 162, 0}, {255, 163, 0}, {255, 163, 0}, {255, 163, 0},
{255, 163, 0}, {255, 164, 0}, {255, 164, 0}, {255, 164, 0}, {255, 164, 0},
{255, 165, 0}, {255, 165, 0}, {255, 165, 0}, {255, 165, 0}, {255, 166, 0},
{255, 166, 0}, {255, 166, 0}, {255, 166, 0}, {255, 167, 0}, {255, 167, 0},
{255, 167, 0}, {255, 167, 0}, {255, 168, 0}, {255, 168, 0}, {255, 168, 0},
{255, 168, 0}, {255, 169, 0}, {255, 169, 0}, {255, 169, 0}, {255, 169, 0},
{255, 170, 0}, {255, 170, 0}, {255, 170, 0}, {255, 171, 0}, {255, 171, 0},
{255, 171, 0}, {255, 171, 0}, {255, 172, 0}, {255, 172, 0}, {255, 172, 0},
{255, 172, 0}, {255, 173, 0}, {255, 173, 0}, {255, 173, 0}, {255, 173, 0},
{255, 174, 0}, {255, 174, 0}, {255, 174, 0}, {255, 174, 0}, {255, 175, 0},
{255, 175, 0}, {255, 175, 0}, {255, 175, 0}, {255, 176, 0}, {255, 176, 0},
{255, 176, 0}, {255, 176, 0}, {255, 177, 0}, {255, 177, 0}, {255, 177, 0},
{255, 177, 0}, {255, 178, 0}, {255, 178, 0}, {255, 178, 0}, {255, 178, 0},
{255, 179, 0}, {255, 179, 0}, {255, 179, 0}, {255, 179, 0}, {255, 180, 0},
{255, 180, 0}, {255, 180, 0}, {255, 180, 0}, {255, 181, 0}, {255, 181, 0},
{255, 181, 0}, {255, 181, 0}, {255, 182, 0}, {255, 182, 0}, {255, 182, 0},
{255, 183, 0}, {255, 183, 0}, {255, 183, 0}, {255, 183, 0}, {255, 184, 0},
{255, 184, 0}, {255, 184, 0}, {255, 184, 0}, {255, 185, 0}, {255, 185, 0},
{255, 185, 0}, {255, 185, 0}, {255, 186, 0}, {255, 186, 0}, {255, 186, 0},
{255, 186, 0}, {255, 187, 0}, {255, 187, 0}, {255, 187, 0}, {255, 187, 0},
{255, 188, 0}, {255, 188, 0}, {255, 188, 0}, {255, 188, 0}, {255, 189, 0},
{255, 189, 0}, {255, 189, 0}, {255, 189, 0}, {255, 190, 0}, {255, 190, 0},
{255, 190, 0}, {255, 190, 0}, {255, 191, 0}, {255, 191, 0}, {255, 191, 0},
{255, 191, 0}, {255, 192, 0}, {255, 192, 0}, {255, 192, 0}, {255, 192, 0},
{255, 193, 0}, {255, 193, 0}, {255, 193, 0}, {255, 193, 0}, {255, 194, 0},
{255, 194, 0}, {255, 194, 0}, {255, 195, 0}, {255, 195, 0}, {255, 195, 0},
{255, 195, 0}, {255, 196, 0}, {255, 196, 0}, {255, 196, 0}, {255, 196, 0},
{255, 197, 0}, {255, 197, 0}, {255, 197, 0}, {255, 197, 0}, {255, 198, 0},
{255, 198, 0}, {255, 198, 0}, {255, 198, 0}, {255, 199, 0}, {255, 199, 0},
{255, 199, 0}, {255, 199, 0}, {255, 200, 0}, {255, 200, 0}, {255, 200, 0},
{255, 200, 0}, {255, 201, 0}, {255, 201, 0}, {255, 201, 0}, {255, 201, 0},
{255, 202, 0}, {255, 202, 0}, {255, 202, 0}, {255, 202, 0}, {255, 203, 0},
{255, 203, 0}, {255, 203, 0}, {255, 203, 0}, {255, 204, 0}, {255, 204, 0},
{255, 204, 0}, {255, 204, 0}, {255, 205, 0}, {255, 205, 0}, {255, 205, 0},
{255, 205, 0}, {255, 206, 0}, {255, 206, 0}, {255, 206, 0}, {255, 207, 0},
{255, 207, 0}, {255, 207, 0}, {255, 207, 0}, {255, 208, 0}, {255, 208, 0},
{255, 208, 0}, {255, 208, 0}, {255, 209, 0}, {255, 209, 0}, {255, 209, 0},
{255, 209, 0}, {255, 210, 0}, {255, 210, 0}, {255, 210, 0}, {255, 210, 0},
{255, 211, 0}, {255, 211, 0}, {255, 211, 0}, {255, 211, 0}, {255, 212, 0},
{255, 212, 0}, {255, 212, 0}, {255, 212, 0}, {255, 213, 0}, {255, 213, 0},
{255, 213, 0}, {255, 213, 0}, {255, 214, 0}, {255, 214, 0}, {255, 214, 0},
{255, 214, 0}, {255, 215, 0}, {255, 215, 0}, {255, 215, 0}, {255, 215, 0},
{255, 216, 0}, {255, 216, 0}, {255, 216, 0}, {255, 216, 0}, {255, 217, 0},
{255, 217, 0}, {255, 217, 0}, {255, 217, 0}, {255, 218, 0}, {255, 218, 0},
{255, 218, 0}, {255, 218, 0}, {255, 219, 0}, {255, 219, 0}, {255, 219, 0},
{255, 220, 0}, {255, 220, 0}, {255, 220, 0}, {255, 220, 0}, {255, 221, 0},
{255, 221, 0}, {255, 221, 0}, {255, 221, 0}, {255, 222, 0}, {255, 222, 0},
{255, 222, 0}, {255, 222, 0}, {255, 223, 0}, {255, 223, 0}, {255, 223, 0},
{255, 223, 0}, {255, 224, 0}, {255, 224, 0}, {255, 224, 0}, {255, 224, 0},
{255, 225, 0}, {255, 225, 0}, {255, 225, 0}, {255, 225, 0}, {255, 226, 0},
{255, 226, 0}, {255, 226, 0}, {255, 226, 0}, {255, 227, 0}, {255, 227, 0},
{255, 227, 0}, {255, 227, 0}, {255, 228, 0}, {255, 228, 0}, {255, 228, 0},
{255, 228, 0}, {255, 229, 0}, {255, 229, 0}, {255, 229, 0}, {255, 229, 0},
{255, 230, 0}, {255, 230, 0}, {255, 230, 0}, {255, 230, 0}, {255, 231, 0},
{255, 231, 0}, {255, 231, 0}, {255, 232, 0}, {255, 232, 0}, {255, 232, 0},
{255, 232, 0}, {255, 233, 0}, {255, 233, 0}, {255, 233, 0}, {255, 233, 0},
{255, 234, 0}, {255, 234, 0}, {255, 234, 0}, {255, 234, 0}, {255, 235, 0},
{255, 235, 0}, {255, 235, 0}, {255, 235, 0}, {255, 236, 0}, {255, 236, 0},
{255, 236, 0}, {255, 236, 0}, {255, 237, 0}, {255, 237, 0}, {255, 237, 0},
{255, 237, 0}, {255, 238, 0}, {255, 238, 0}, {255, 238, 0}, {255, 238, 0},
{255, 239, 0}, {255, 239, 0}, {255, 239, 0}, {255, 239, 0}, {255, 240, 0},
{255, 240, 0}, {255, 240, 0}, {255, 240, 0}, {255, 241, 0}, {255, 241, 0},
{255, 241, 0}, {255, 241, 0}, {255, 242, 0}, {255, 242, 0}, {255, 242, 0},
{255, 242, 0}, {255, 243, 0}, {255, 243, 0}, {255, 243, 0}, {255, 244, 0},
{255, 244, 0}, {255, 244, 0}, {255, 244, 0}, {255, 245, 0}, {255, 245, 0},
{255, 245, 0}, {255, 245, 0}, {255, 246, 0}, {255, 246, 0}, {255, 246, 0},
{255, 246, 0}, {255, 247, 0}, {255, 247, 0}, {255, 247, 0}, {255, 247, 0},
{255, 248, 0}, {255, 248, 0}, {255, 248, 0}, {255, 248, 0}, {255, 249, 0},
{255, 249, 0}, {255, 249, 0}, {255, 249, 0}, {255, 250, 0}, {255, 250, 0},
{255, 250, 0}, {255, 250, 0}, {255, 251, 0}, {255, 251, 0}, {255, 251, 0},
{255, 251, 0}, {255, 252, 0}, {255, 252, 0}, {255, 252, 0}, {255, 252, 0},
{255, 253, 0}, {255, 253, 0}, {255, 253, 0}, {255, 253, 0}, {255, 254, 0},
{255, 254, 0}, {255, 254, 0}, {255, 254, 0}, {255, 255, 0}, {255, 255, 0}
};
const rgb_store copper_colormap[1000] = {
{ 0, 0, 0}, { 0, 0, 0}, { 1, 0, 0}, { 1, 1, 0}, { 1, 1, 1},
{ 2, 1, 1}, { 2, 1, 1}, { 2, 1, 1}, { 3, 2, 1}, { 3, 2, 1},
{ 3, 2, 1}, { 4, 2, 1}, { 4, 2, 2}, { 4, 3, 2}, { 4, 3, 2},
{ 5, 3, 2}, { 5, 3, 2}, { 5, 3, 2}, { 6, 4, 2}, { 6, 4, 2},
{ 6, 4, 3}, { 7, 4, 3}, { 7, 4, 3}, { 7, 5, 3}, { 8, 5, 3},
{ 8, 5, 3}, { 8, 5, 3}, { 9, 5, 3}, { 9, 6, 4}, { 9, 6, 4},
{ 10, 6, 4}, { 10, 6, 4}, { 10, 6, 4}, { 11, 7, 4}, { 11, 7, 4},
{ 11, 7, 4}, { 11, 7, 5}, { 12, 7, 5}, { 12, 8, 5}, { 12, 8, 5},
{ 13, 8, 5}, { 13, 8, 5}, { 13, 8, 5}, { 14, 9, 5}, { 14, 9, 6},
{ 14, 9, 6}, { 15, 9, 6}, { 15, 9, 6}, { 15, 10, 6}, { 16, 10, 6},
{ 16, 10, 6}, { 16, 10, 6}, { 17, 10, 7}, { 17, 11, 7}, { 17, 11, 7},
{ 18, 11, 7}, { 18, 11, 7}, { 18, 11, 7}, { 19, 12, 7}, { 19, 12, 7},
{ 19, 12, 8}, { 19, 12, 8}, { 20, 12, 8}, { 20, 13, 8}, { 20, 13, 8},
{ 21, 13, 8}, { 21, 13, 8}, { 21, 13, 9}, { 22, 14, 9}, { 22, 14, 9},
{ 22, 14, 9}, { 23, 14, 9}, { 23, 14, 9}, { 23, 15, 9}, { 24, 15, 9},
{ 24, 15, 10}, { 24, 15, 10}, { 25, 15, 10}, { 25, 16, 10}, { 25, 16, 10},
{ 26, 16, 10}, { 26, 16, 10}, { 26, 16, 10}, { 26, 17, 11}, { 27, 17, 11},
{ 27, 17, 11}, { 27, 17, 11}, { 28, 17, 11}, { 28, 18, 11}, { 28, 18, 11},
{ 29, 18, 11}, { 29, 18, 12}, { 29, 18, 12}, { 30, 19, 12}, { 30, 19, 12},
{ 30, 19, 12}, { 31, 19, 12}, { 31, 19, 12}, { 31, 20, 12}, { 32, 20, 13},
{ 32, 20, 13}, { 32, 20, 13}, { 33, 20, 13}, { 33, 21, 13}, { 33, 21, 13},
{ 34, 21, 13}, { 34, 21, 13}, { 34, 21, 14}, { 34, 22, 14}, { 35, 22, 14},
{ 35, 22, 14}, { 35, 22, 14}, { 36, 22, 14}, { 36, 23, 14}, { 36, 23, 14},
{ 37, 23, 15}, { 37, 23, 15}, { 37, 23, 15}, { 38, 24, 15}, { 38, 24, 15},
{ 38, 24, 15}, { 39, 24, 15}, { 39, 24, 15}, { 39, 25, 16}, { 40, 25, 16},
{ 40, 25, 16}, { 40, 25, 16}, { 41, 25, 16}, { 41, 26, 16}, { 41, 26, 16},
{ 41, 26, 17}, { 42, 26, 17}, { 42, 26, 17}, { 42, 27, 17}, { 43, 27, 17},
{ 43, 27, 17}, { 43, 27, 17}, { 44, 27, 17}, { 44, 28, 18}, { 44, 28, 18},
{ 45, 28, 18}, { 45, 28, 18}, { 45, 28, 18}, { 46, 29, 18}, { 46, 29, 18},
{ 46, 29, 18}, { 47, 29, 19}, { 47, 29, 19}, { 47, 30, 19}, { 48, 30, 19},
{ 48, 30, 19}, { 48, 30, 19}, { 48, 30, 19}, { 49, 31, 19}, { 49, 31, 20},
{ 49, 31, 20}, { 50, 31, 20}, { 50, 31, 20}, { 50, 32, 20}, { 51, 32, 20},
{ 51, 32, 20}, { 51, 32, 20}, { 52, 32, 21}, { 52, 33, 21}, { 52, 33, 21},
{ 53, 33, 21}, { 53, 33, 21}, { 53, 33, 21}, { 54, 34, 21}, { 54, 34, 21},
{ 54, 34, 22}, { 55, 34, 22}, { 55, 34, 22}, { 55, 34, 22}, { 56, 35, 22},
{ 56, 35, 22}, { 56, 35, 22}, { 56, 35, 22}, { 57, 35, 23}, { 57, 36, 23},
{ 57, 36, 23}, { 58, 36, 23}, { 58, 36, 23}, { 58, 36, 23}, { 59, 37, 23},
{ 59, 37, 23}, { 59, 37, 24}, { 60, 37, 24}, { 60, 37, 24}, { 60, 38, 24},
{ 61, 38, 24}, { 61, 38, 24}, { 61, 38, 24}, { 62, 38, 25}, { 62, 39, 25},
{ 62, 39, 25}, { 63, 39, 25}, { 63, 39, 25}, { 63, 39, 25}, { 63, 40, 25},
{ 64, 40, 25}, { 64, 40, 26}, { 64, 40, 26}, { 65, 40, 26}, { 65, 41, 26},
{ 65, 41, 26}, { 66, 41, 26}, { 66, 41, 26}, { 66, 41, 26}, { 67, 42, 27},
{ 67, 42, 27}, { 67, 42, 27}, { 68, 42, 27}, { 68, 42, 27}, { 68, 43, 27},
{ 69, 43, 27}, { 69, 43, 27}, { 69, 43, 28}, { 70, 43, 28}, { 70, 44, 28},
{ 70, 44, 28}, { 71, 44, 28}, { 71, 44, 28}, { 71, 44, 28}, { 71, 45, 28},
{ 72, 45, 29}, { 72, 45, 29}, { 72, 45, 29}, { 73, 45, 29}, { 73, 46, 29},
{ 73, 46, 29}, { 74, 46, 29}, { 74, 46, 29}, { 74, 46, 30}, { 75, 47, 30},
{ 75, 47, 30}, { 75, 47, 30}, { 76, 47, 30}, { 76, 47, 30}, { 76, 48, 30},
{ 77, 48, 30}, { 77, 48, 31}, { 77, 48, 31}, { 78, 48, 31}, { 78, 49, 31},
{ 78, 49, 31}, { 78, 49, 31}, { 79, 49, 31}, { 79, 49, 31}, { 79, 50, 32},
{ 80, 50, 32}, { 80, 50, 32}, { 80, 50, 32}, { 81, 50, 32}, { 81, 51, 32},
{ 81, 51, 32}, { 82, 51, 33}, { 82, 51, 33}, { 82, 51, 33}, { 83, 52, 33},
{ 83, 52, 33}, { 83, 52, 33}, { 84, 52, 33}, { 84, 52, 33}, { 84, 53, 34},
{ 85, 53, 34}, { 85, 53, 34}, { 85, 53, 34}, { 86, 53, 34}, { 86, 54, 34},
{ 86, 54, 34}, { 86, 54, 34}, { 87, 54, 35}, { 87, 54, 35}, { 87, 55, 35},
{ 88, 55, 35}, { 88, 55, 35}, { 88, 55, 35}, { 89, 55, 35}, { 89, 56, 35},
{ 89, 56, 36}, { 90, 56, 36}, { 90, 56, 36}, { 90, 56, 36}, { 91, 57, 36},
{ 91, 57, 36}, { 91, 57, 36}, { 92, 57, 36}, { 92, 57, 37}, { 92, 58, 37},
{ 93, 58, 37}, { 93, 58, 37}, { 93, 58, 37}, { 93, 58, 37}, { 94, 59, 37},
{ 94, 59, 37}, { 94, 59, 38}, { 95, 59, 38}, { 95, 59, 38}, { 95, 60, 38},
{ 96, 60, 38}, { 96, 60, 38}, { 96, 60, 38}, { 97, 60, 38}, { 97, 61, 39},
{ 97, 61, 39}, { 98, 61, 39}, { 98, 61, 39}, { 98, 61, 39}, { 99, 62, 39},
{ 99, 62, 39}, { 99, 62, 39}, {100, 62, 40}, {100, 62, 40}, {100, 63, 40},
{101, 63, 40}, {101, 63, 40}, {101, 63, 40}, {101, 63, 40}, {102, 64, 41},
{102, 64, 41}, {102, 64, 41}, {103, 64, 41}, {103, 64, 41}, {103, 65, 41},
{104, 65, 41}, {104, 65, 41}, {104, 65, 42}, {105, 65, 42}, {105, 66, 42},
{105, 66, 42}, {106, 66, 42}, {106, 66, 42}, {106, 66, 42}, {107, 67, 42},
{107, 67, 43}, {107, 67, 43}, {108, 67, 43}, {108, 67, 43}, {108, 68, 43},
{108, 68, 43}, {109, 68, 43}, {109, 68, 43}, {109, 68, 44}, {110, 69, 44},
{110, 69, 44}, {110, 69, 44}, {111, 69, 44}, {111, 69, 44}, {111, 70, 44},
{112, 70, 44}, {112, 70, 45}, {112, 70, 45}, {113, 70, 45}, {113, 71, 45},
{113, 71, 45}, {114, 71, 45}, {114, 71, 45}, {114, 71, 45}, {115, 72, 46},
{115, 72, 46}, {115, 72, 46}, {116, 72, 46}, {116, 72, 46}, {116, 73, 46},
{116, 73, 46}, {117, 73, 46}, {117, 73, 47}, {117, 73, 47}, {118, 74, 47},
{118, 74, 47}, {118, 74, 47}, {119, 74, 47}, {119, 74, 47}, {119, 75, 47},
{120, 75, 48}, {120, 75, 48}, {120, 75, 48}, {121, 75, 48}, {121, 76, 48},
{121, 76, 48}, {122, 76, 48}, {122, 76, 49}, {122, 76, 49}, {123, 77, 49},
{123, 77, 49}, {123, 77, 49}, {123, 77, 49}, {124, 77, 49}, {124, 78, 49},
{124, 78, 50}, {125, 78, 50}, {125, 78, 50}, {125, 78, 50}, {126, 79, 50},
{126, 79, 50}, {126, 79, 50}, {127, 79, 50}, {127, 79, 51}, {127, 80, 51},
{128, 80, 51}, {128, 80, 51}, {128, 80, 51}, {129, 80, 51}, {129, 81, 51},
{129, 81, 51}, {130, 81, 52}, {130, 81, 52}, {130, 81, 52}, {130, 82, 52},
{131, 82, 52}, {131, 82, 52}, {131, 82, 52}, {132, 82, 52}, {132, 83, 53},
{132, 83, 53}, {133, 83, 53}, {133, 83, 53}, {133, 83, 53}, {134, 84, 53},
{134, 84, 53}, {134, 84, 53}, {135, 84, 54}, {135, 84, 54}, {135, 85, 54},
{136, 85, 54}, {136, 85, 54}, {136, 85, 54}, {137, 85, 54}, {137, 86, 54},
{137, 86, 55}, {138, 86, 55}, {138, 86, 55}, {138, 86, 55}, {138, 87, 55},
{139, 87, 55}, {139, 87, 55}, {139, 87, 55}, {140, 87, 56}, {140, 88, 56},
{140, 88, 56}, {141, 88, 56}, {141, 88, 56}, {141, 88, 56}, {142, 89, 56},
{142, 89, 57}, {142, 89, 57}, {143, 89, 57}, {143, 89, 57}, {143, 90, 57},
{144, 90, 57}, {144, 90, 57}, {144, 90, 57}, {145, 90, 58}, {145, 91, 58},
{145, 91, 58}, {145, 91, 58}, {146, 91, 58}, {146, 91, 58}, {146, 92, 58},
{147, 92, 58}, {147, 92, 59}, {147, 92, 59}, {148, 92, 59}, {148, 93, 59},
{148, 93, 59}, {149, 93, 59}, {149, 93, 59}, {149, 93, 59}, {150, 94, 60},
{150, 94, 60}, {150, 94, 60}, {151, 94, 60}, {151, 94, 60}, {151, 95, 60},
{152, 95, 60}, {152, 95, 60}, {152, 95, 61}, {153, 95, 61}, {153, 96, 61},
{153, 96, 61}, {153, 96, 61}, {154, 96, 61}, {154, 96, 61}, {154, 97, 61},
{155, 97, 62}, {155, 97, 62}, {155, 97, 62}, {156, 97, 62}, {156, 98, 62},
{156, 98, 62}, {157, 98, 62}, {157, 98, 62}, {157, 98, 63}, {158, 99, 63},
{158, 99, 63}, {158, 99, 63}, {159, 99, 63}, {159, 99, 63}, {159, 100, 63},
{160, 100, 63}, {160, 100, 64}, {160, 100, 64}, {160, 100, 64}, {161, 101, 64},
{161, 101, 64}, {161, 101, 64}, {162, 101, 64}, {162, 101, 65}, {162, 101, 65},
{163, 102, 65}, {163, 102, 65}, {163, 102, 65}, {164, 102, 65}, {164, 102, 65},
{164, 103, 65}, {165, 103, 66}, {165, 103, 66}, {165, 103, 66}, {166, 103, 66},
{166, 104, 66}, {166, 104, 66}, {167, 104, 66}, {167, 104, 66}, {167, 104, 67},
{168, 105, 67}, {168, 105, 67}, {168, 105, 67}, {168, 105, 67}, {169, 105, 67},
{169, 106, 67}, {169, 106, 67}, {170, 106, 68}, {170, 106, 68}, {170, 106, 68},
{171, 107, 68}, {171, 107, 68}, {171, 107, 68}, {172, 107, 68}, {172, 107, 68},
{172, 108, 69}, {173, 108, 69}, {173, 108, 69}, {173, 108, 69}, {174, 108, 69},
{174, 109, 69}, {174, 109, 69}, {175, 109, 69}, {175, 109, 70}, {175, 109, 70},
{175, 110, 70}, {176, 110, 70}, {176, 110, 70}, {176, 110, 70}, {177, 110, 70},
{177, 111, 70}, {177, 111, 71}, {178, 111, 71}, {178, 111, 71}, {178, 111, 71},
{179, 112, 71}, {179, 112, 71}, {179, 112, 71}, {180, 112, 71}, {180, 112, 72},
{180, 113, 72}, {181, 113, 72}, {181, 113, 72}, {181, 113, 72}, {182, 113, 72},
{182, 114, 72}, {182, 114, 73}, {183, 114, 73}, {183, 114, 73}, {183, 114, 73},
{183, 115, 73}, {184, 115, 73}, {184, 115, 73}, {184, 115, 73}, {185, 115, 74},
{185, 116, 74}, {185, 116, 74}, {186, 116, 74}, {186, 116, 74}, {186, 116, 74},
{187, 117, 74}, {187, 117, 74}, {187, 117, 75}, {188, 117, 75}, {188, 117, 75},
{188, 118, 75}, {189, 118, 75}, {189, 118, 75}, {189, 118, 75}, {190, 118, 75},
{190, 119, 76}, {190, 119, 76}, {190, 119, 76}, {191, 119, 76}, {191, 119, 76},
{191, 120, 76}, {192, 120, 76}, {192, 120, 76}, {192, 120, 77}, {193, 120, 77},
{193, 121, 77}, {193, 121, 77}, {194, 121, 77}, {194, 121, 77}, {194, 121, 77},
{195, 122, 77}, {195, 122, 78}, {195, 122, 78}, {196, 122, 78}, {196, 122, 78},
{196, 123, 78}, {197, 123, 78}, {197, 123, 78}, {197, 123, 78}, {198, 123, 79},
{198, 124, 79}, {198, 124, 79}, {198, 124, 79}, {199, 124, 79}, {199, 124, 79},
{199, 125, 79}, {200, 125, 79}, {200, 125, 80}, {200, 125, 80}, {201, 125, 80},
{201, 126, 80}, {201, 126, 80}, {202, 126, 80}, {202, 126, 80}, {202, 126, 81},
{203, 127, 81}, {203, 127, 81}, {203, 127, 81}, {204, 127, 81}, {204, 127, 81},
{204, 128, 81}, {205, 128, 81}, {205, 128, 82}, {205, 128, 82}, {205, 128, 82},
{206, 129, 82}, {206, 129, 82}, {206, 129, 82}, {207, 129, 82}, {207, 129, 82},
{207, 130, 83}, {208, 130, 83}, {208, 130, 83}, {208, 130, 83}, {209, 130, 83},
{209, 131, 83}, {209, 131, 83}, {210, 131, 83}, {210, 131, 84}, {210, 131, 84},
{211, 132, 84}, {211, 132, 84}, {211, 132, 84}, {212, 132, 84}, {212, 132, 84},
{212, 133, 84}, {212, 133, 85}, {213, 133, 85}, {213, 133, 85}, {213, 133, 85},
{214, 134, 85}, {214, 134, 85}, {214, 134, 85}, {215, 134, 85}, {215, 134, 86},
{215, 135, 86}, {216, 135, 86}, {216, 135, 86}, {216, 135, 86}, {217, 135, 86},
{217, 136, 86}, {217, 136, 86}, {218, 136, 87}, {218, 136, 87}, {218, 136, 87},
{219, 137, 87}, {219, 137, 87}, {219, 137, 87}, {220, 137, 87}, {220, 137, 87},
{220, 138, 88}, {220, 138, 88}, {221, 138, 88}, {221, 138, 88}, {221, 138, 88},
{222, 139, 88}, {222, 139, 88}, {222, 139, 89}, {223, 139, 89}, {223, 139, 89},
{223, 140, 89}, {224, 140, 89}, {224, 140, 89}, {224, 140, 89}, {225, 140, 89},
{225, 141, 90}, {225, 141, 90}, {226, 141, 90}, {226, 141, 90}, {226, 141, 90},
{227, 142, 90}, {227, 142, 90}, {227, 142, 90}, {227, 142, 91}, {228, 142, 91},
{228, 143, 91}, {228, 143, 91}, {229, 143, 91}, {229, 143, 91}, {229, 143, 91},
{230, 144, 91}, {230, 144, 92}, {230, 144, 92}, {231, 144, 92}, {231, 144, 92},
{231, 145, 92}, {232, 145, 92}, {232, 145, 92}, {232, 145, 92}, {233, 145, 93},
{233, 146, 93}, {233, 146, 93}, {234, 146, 93}, {234, 146, 93}, {234, 146, 93},
{235, 147, 93}, {235, 147, 93}, {235, 147, 94}, {235, 147, 94}, {236, 147, 94},
{236, 148, 94}, {236, 148, 94}, {237, 148, 94}, {237, 148, 94}, {237, 148, 94},
{238, 149, 95}, {238, 149, 95}, {238, 149, 95}, {239, 149, 95}, {239, 149, 95},
{239, 150, 95}, {240, 150, 95}, {240, 150, 95}, {240, 150, 96}, {241, 150, 96},
{241, 151, 96}, {241, 151, 96}, {242, 151, 96}, {242, 151, 96}, {242, 151, 96},
{242, 152, 97}, {243, 152, 97}, {243, 152, 97}, {243, 152, 97}, {244, 152, 97},
{244, 153, 97}, {244, 153, 97}, {245, 153, 97}, {245, 153, 98}, {245, 153, 98},
{246, 154, 98}, {246, 154, 98}, {246, 154, 98}, {247, 154, 98}, {247, 154, 98},
{247, 155, 98}, {248, 155, 99}, {248, 155, 99}, {248, 155, 99}, {249, 155, 99},
{249, 156, 99}, {249, 156, 99}, {250, 156, 99}, {250, 156, 99}, {250, 156, 100},
{250, 157, 100}, {251, 157, 100}, {251, 157, 100}, {251, 157, 100}, {252, 157, 100},
{252, 158, 100}, {252, 158, 100}, {253, 158, 101}, {253, 158, 101}, {253, 158, 101},
{253, 159, 101}, {253, 159, 101}, {254, 159, 101}, {254, 159, 101}, {254, 159, 101},
{254, 160, 102}, {254, 160, 102}, {254, 160, 102}, {254, 160, 102}, {254, 160, 102},
{255, 161, 102}, {255, 161, 102}, {255, 161, 102}, {255, 161, 103}, {255, 161, 103},
{255, 162, 103}, {255, 162, 103}, {255, 162, 103}, {255, 162, 103}, {255, 162, 103},
{255, 163, 103}, {255, 163, 104}, {255, 163, 104}, {255, 163, 104}, {255, 163, 104},
{255, 164, 104}, {255, 164, 104}, {255, 164, 104}, {255, 164, 105}, {255, 164, 105},
{255, 165, 105}, {255, 165, 105}, {255, 165, 105}, {255, 165, 105}, {255, 165, 105},
{255, 166, 105}, {255, 166, 106}, {255, 166, 106}, {255, 166, 106}, {255, 166, 106},
{255, 167, 106}, {255, 167, 106}, {255, 167, 106}, {255, 167, 106}, {255, 167, 107},
{255, 168, 107}, {255, 168, 107}, {255, 168, 107}, {255, 168, 107}, {255, 168, 107},
{255, 168, 107}, {255, 169, 107}, {255, 169, 108}, {255, 169, 108}, {255, 169, 108},
{255, 169, 108}, {255, 170, 108}, {255, 170, 108}, {255, 170, 108}, {255, 170, 108},
{255, 170, 109}, {255, 171, 109}, {255, 171, 109}, {255, 171, 109}, {255, 171, 109},
{255, 171, 109}, {255, 172, 109}, {255, 172, 109}, {255, 172, 110}, {255, 172, 110},
{255, 172, 110}, {255, 173, 110}, {255, 173, 110}, {255, 173, 110}, {255, 173, 110},
{255, 173, 110}, {255, 174, 111}, {255, 174, 111}, {255, 174, 111}, {255, 174, 111},
{255, 174, 111}, {255, 175, 111}, {255, 175, 111}, {255, 175, 111}, {255, 175, 112},
{255, 175, 112}, {255, 176, 112}, {255, 176, 112}, {255, 176, 112}, {255, 176, 112},
{255, 176, 112}, {255, 177, 113}, {255, 177, 113}, {255, 177, 113}, {255, 177, 113},
{255, 177, 113}, {255, 178, 113}, {255, 178, 113}, {255, 178, 113}, {255, 178, 114},
{255, 178, 114}, {255, 179, 114}, {255, 179, 114}, {255, 179, 114}, {255, 179, 114},
{255, 179, 114}, {255, 180, 114}, {255, 180, 115}, {255, 180, 115}, {255, 180, 115},
{255, 180, 115}, {255, 181, 115}, {255, 181, 115}, {255, 181, 115}, {255, 181, 115},
{255, 181, 116}, {255, 182, 116}, {255, 182, 116}, {255, 182, 116}, {255, 182, 116},
{255, 182, 116}, {255, 183, 116}, {255, 183, 116}, {255, 183, 117}, {255, 183, 117},
{255, 183, 117}, {255, 184, 117}, {255, 184, 117}, {255, 184, 117}, {255, 184, 117},
{255, 184, 117}, {255, 185, 118}, {255, 185, 118}, {255, 185, 118}, {255, 185, 118},
{255, 185, 118}, {255, 186, 118}, {255, 186, 118}, {255, 186, 118}, {255, 186, 119},
{255, 186, 119}, {255, 187, 119}, {255, 187, 119}, {255, 187, 119}, {255, 187, 119},
{255, 187, 119}, {255, 188, 119}, {255, 188, 120}, {255, 188, 120}, {255, 188, 120},
{255, 188, 120}, {255, 189, 120}, {255, 189, 120}, {255, 189, 120}, {255, 189, 121},
{255, 189, 121}, {255, 190, 121}, {255, 190, 121}, {255, 190, 121}, {255, 190, 121},
{255, 190, 121}, {255, 191, 121}, {255, 191, 122}, {255, 191, 122}, {255, 191, 122},
{255, 191, 122}, {255, 192, 122}, {255, 192, 122}, {255, 192, 122}, {255, 192, 122},
{255, 192, 123}, {255, 193, 123}, {255, 193, 123}, {255, 193, 123}, {255, 193, 123},
{255, 193, 123}, {255, 194, 123}, {255, 194, 123}, {255, 194, 124}, {255, 194, 124},
{255, 194, 124}, {255, 195, 124}, {255, 195, 124}, {255, 195, 124}, {255, 195, 124},
{255, 195, 124}, {255, 196, 125}, {255, 196, 125}, {255, 196, 125}, {255, 196, 125},
{255, 196, 125}, {255, 197, 125}, {255, 197, 125}, {255, 197, 125}, {255, 197, 126},
{255, 197, 126}, {255, 198, 126}, {255, 198, 126}, {255, 198, 126}, {255, 198, 126},
{255, 198, 126}, {255, 199, 126}, {255, 199, 127}, {255, 199, 127}, {255, 199, 127}
};
const rgb_store gray_colormap[1000] = {
{255, 255, 255}, {255, 255, 255}, {254, 254, 254}, {254, 254, 254}, {254, 254, 254},
{254, 254, 254}, {253, 253, 253}, {253, 253, 253}, {253, 253, 253}, {253, 253, 253},
{252, 252, 252}, {252, 252, 252}, {252, 252, 252}, {252, 252, 252}, {251, 251, 251},
{251, 251, 251}, {251, 251, 251}, {251, 251, 251}, {250, 250, 250}, {250, 250, 250},
{250, 250, 250}, {250, 250, 250}, {249, 249, 249}, {249, 249, 249}, {249, 249, 249},
{249, 249, 249}, {248, 248, 248}, {248, 248, 248}, {248, 248, 248}, {248, 248, 248},
{247, 247, 247}, {247, 247, 247}, {247, 247, 247}, {247, 247, 247}, {246, 246, 246},
{246, 246, 246}, {246, 246, 246}, {246, 246, 246}, {245, 245, 245}, {245, 245, 245},
{245, 245, 245}, {245, 245, 245}, {244, 244, 244}, {244, 244, 244}, {244, 244, 244},
{244, 244, 244}, {243, 243, 243}, {243, 243, 243}, {243, 243, 243}, {242, 242, 242},
{242, 242, 242}, {242, 242, 242}, {242, 242, 242}, {241, 241, 241}, {241, 241, 241},
{241, 241, 241}, {241, 241, 241}, {240, 240, 240}, {240, 240, 240}, {240, 240, 240},
{240, 240, 240}, {239, 239, 239}, {239, 239, 239}, {239, 239, 239}, {239, 239, 239},
{238, 238, 238}, {238, 238, 238}, {238, 238, 238}, {238, 238, 238}, {237, 237, 237},
{237, 237, 237}, {237, 237, 237}, {237, 237, 237}, {236, 236, 236}, {236, 236, 236},
{236, 236, 236}, {236, 236, 236}, {235, 235, 235}, {235, 235, 235}, {235, 235, 235},
{235, 235, 235}, {234, 234, 234}, {234, 234, 234}, {234, 234, 234}, {234, 234, 234},
{233, 233, 233}, {233, 233, 233}, {233, 233, 233}, {233, 233, 233}, {232, 232, 232},
{232, 232, 232}, {232, 232, 232}, {232, 232, 232}, {231, 231, 231}, {231, 231, 231},
{231, 231, 231}, {230, 230, 230}, {230, 230, 230}, {230, 230, 230}, {230, 230, 230},
{229, 229, 229}, {229, 229, 229}, {229, 229, 229}, {229, 229, 229}, {228, 228, 228},
{228, 228, 228}, {228, 228, 228}, {228, 228, 228}, {227, 227, 227}, {227, 227, 227},
{227, 227, 227}, {227, 227, 227}, {226, 226, 226}, {226, 226, 226}, {226, 226, 226},
{226, 226, 226}, {225, 225, 225}, {225, 225, 225}, {225, 225, 225}, {225, 225, 225},
{224, 224, 224}, {224, 224, 224}, {224, 224, 224}, {224, 224, 224}, {223, 223, 223},
{223, 223, 223}, {223, 223, 223}, {223, 223, 223}, {222, 222, 222}, {222, 222, 222},
{222, 222, 222}, {222, 222, 222}, {221, 221, 221}, {221, 221, 221}, {221, 221, 221},
{221, 221, 221}, {220, 220, 220}, {220, 220, 220}, {220, 220, 220}, {220, 220, 220},
{219, 219, 219}, {219, 219, 219}, {219, 219, 219}, {218, 218, 218}, {218, 218, 218},
{218, 218, 218}, {218, 218, 218}, {217, 217, 217}, {217, 217, 217}, {217, 217, 217},
{217, 217, 217}, {216, 216, 216}, {216, 216, 216}, {216, 216, 216}, {216, 216, 216},
{215, 215, 215}, {215, 215, 215}, {215, 215, 215}, {215, 215, 215}, {214, 214, 214},
{214, 214, 214}, {214, 214, 214}, {214, 214, 214}, {213, 213, 213}, {213, 213, 213},
{213, 213, 213}, {213, 213, 213}, {212, 212, 212}, {212, 212, 212}, {212, 212, 212},
{212, 212, 212}, {211, 211, 211}, {211, 211, 211}, {211, 211, 211}, {211, 211, 211},
{210, 210, 210}, {210, 210, 210}, {210, 210, 210}, {210, 210, 210}, {209, 209, 209},
{209, 209, 209}, {209, 209, 209}, {209, 209, 209}, {208, 208, 208}, {208, 208, 208},
{208, 208, 208}, {208, 208, 208}, {207, 207, 207}, {207, 207, 207}, {207, 207, 207},
{207, 207, 207}, {206, 206, 206}, {206, 206, 206}, {206, 206, 206}, {205, 205, 205},
{205, 205, 205}, {205, 205, 205}, {205, 205, 205}, {204, 204, 204}, {204, 204, 204},
{204, 204, 204}, {204, 204, 204}, {203, 203, 203}, {203, 203, 203}, {203, 203, 203},
{203, 203, 203}, {202, 202, 202}, {202, 202, 202}, {202, 202, 202}, {202, 202, 202},
{201, 201, 201}, {201, 201, 201}, {201, 201, 201}, {201, 201, 201}, {200, 200, 200},
{200, 200, 200}, {200, 200, 200}, {200, 200, 200}, {199, 199, 199}, {199, 199, 199},
{199, 199, 199}, {199, 199, 199}, {198, 198, 198}, {198, 198, 198}, {198, 198, 198},
{198, 198, 198}, {197, 197, 197}, {197, 197, 197}, {197, 197, 197}, {197, 197, 197},
{196, 196, 196}, {196, 196, 196}, {196, 196, 196}, {196, 196, 196}, {195, 195, 195},
{195, 195, 195}, {195, 195, 195}, {195, 195, 195}, {194, 194, 194}, {194, 194, 194},
{194, 194, 194}, {193, 193, 193}, {193, 193, 193}, {193, 193, 193}, {193, 193, 193},
{192, 192, 192}, {192, 192, 192}, {192, 192, 192}, {192, 192, 192}, {191, 191, 191},
{191, 191, 191}, {191, 191, 191}, {191, 191, 191}, {190, 190, 190}, {190, 190, 190},
{190, 190, 190}, {190, 190, 190}, {189, 189, 189}, {189, 189, 189}, {189, 189, 189},
{189, 189, 189}, {188, 188, 188}, {188, 188, 188}, {188, 188, 188}, {188, 188, 188},
{187, 187, 187}, {187, 187, 187}, {187, 187, 187}, {187, 187, 187}, {186, 186, 186},
{186, 186, 186}, {186, 186, 186}, {186, 186, 186}, {185, 185, 185}, {185, 185, 185},
{185, 185, 185}, {185, 185, 185}, {184, 184, 184}, {184, 184, 184}, {184, 184, 184},
{184, 184, 184}, {183, 183, 183}, {183, 183, 183}, {183, 183, 183}, {183, 183, 183},
{182, 182, 182}, {182, 182, 182}, {182, 182, 182}, {181, 181, 181}, {181, 181, 181},
{181, 181, 181}, {181, 181, 181}, {180, 180, 180}, {180, 180, 180}, {180, 180, 180},
{180, 180, 180}, {179, 179, 179}, {179, 179, 179}, {179, 179, 179}, {179, 179, 179},
{178, 178, 178}, {178, 178, 178}, {178, 178, 178}, {178, 178, 178}, {177, 177, 177},
{177, 177, 177}, {177, 177, 177}, {177, 177, 177}, {176, 176, 176}, {176, 176, 176},
{176, 176, 176}, {176, 176, 176}, {175, 175, 175}, {175, 175, 175}, {175, 175, 175},
{175, 175, 175}, {174, 174, 174}, {174, 174, 174}, {174, 174, 174}, {174, 174, 174},
{173, 173, 173}, {173, 173, 173}, {173, 173, 173}, {173, 173, 173}, {172, 172, 172},
{172, 172, 172}, {172, 172, 172}, {172, 172, 172}, {171, 171, 171}, {171, 171, 171},
{171, 171, 171}, {171, 171, 171}, {170, 170, 170}, {170, 170, 170}, {170, 170, 170},
{169, 169, 169}, {169, 169, 169}, {169, 169, 169}, {169, 169, 169}, {168, 168, 168},
{168, 168, 168}, {168, 168, 168}, {168, 168, 168}, {167, 167, 167}, {167, 167, 167},
{167, 167, 167}, {167, 167, 167}, {166, 166, 166}, {166, 166, 166}, {166, 166, 166},
{166, 166, 166}, {165, 165, 165}, {165, 165, 165}, {165, 165, 165}, {165, 165, 165},
{164, 164, 164}, {164, 164, 164}, {164, 164, 164}, {164, 164, 164}, {163, 163, 163},
{163, 163, 163}, {163, 163, 163}, {163, 163, 163}, {162, 162, 162}, {162, 162, 162},
{162, 162, 162}, {162, 162, 162}, {161, 161, 161}, {161, 161, 161}, {161, 161, 161},
{161, 161, 161}, {160, 160, 160}, {160, 160, 160}, {160, 160, 160}, {160, 160, 160},
{159, 159, 159}, {159, 159, 159}, {159, 159, 159}, {159, 159, 159}, {158, 158, 158},
{158, 158, 158}, {158, 158, 158}, {157, 157, 157}, {157, 157, 157}, {157, 157, 157},
{157, 157, 157}, {156, 156, 156}, {156, 156, 156}, {156, 156, 156}, {156, 156, 156},
{155, 155, 155}, {155, 155, 155}, {155, 155, 155}, {155, 155, 155}, {154, 154, 154},
{154, 154, 154}, {154, 154, 154}, {154, 154, 154}, {153, 153, 153}, {153, 153, 153},
{153, 153, 153}, {153, 153, 153}, {152, 152, 152}, {152, 152, 152}, {152, 152, 152},
{152, 152, 152}, {151, 151, 151}, {151, 151, 151}, {151, 151, 151}, {151, 151, 151},
{150, 150, 150}, {150, 150, 150}, {150, 150, 150}, {150, 150, 150}, {149, 149, 149},
{149, 149, 149}, {149, 149, 149}, {149, 149, 149}, {148, 148, 148}, {148, 148, 148},
{148, 148, 148}, {148, 148, 148}, {147, 147, 147}, {147, 147, 147}, {147, 147, 147},
{147, 147, 147}, {146, 146, 146}, {146, 146, 146}, {146, 146, 146}, {145, 145, 145},
{145, 145, 145}, {145, 145, 145}, {145, 145, 145}, {144, 144, 144}, {144, 144, 144},
{144, 144, 144}, {144, 144, 144}, {143, 143, 143}, {143, 143, 143}, {143, 143, 143},
{143, 143, 143}, {142, 142, 142}, {142, 142, 142}, {142, 142, 142}, {142, 142, 142},
{141, 141, 141}, {141, 141, 141}, {141, 141, 141}, {141, 141, 141}, {140, 140, 140},
{140, 140, 140}, {140, 140, 140}, {140, 140, 140}, {139, 139, 139}, {139, 139, 139},
{139, 139, 139}, {139, 139, 139}, {138, 138, 138}, {138, 138, 138}, {138, 138, 138},
{138, 138, 138}, {137, 137, 137}, {137, 137, 137}, {137, 137, 137}, {137, 137, 137},
{136, 136, 136}, {136, 136, 136}, {136, 136, 136}, {136, 136, 136}, {135, 135, 135},
{135, 135, 135}, {135, 135, 135}, {135, 135, 135}, {134, 134, 134}, {134, 134, 134},
{134, 134, 134}, {133, 133, 133}, {133, 133, 133}, {133, 133, 133}, {133, 133, 133},
{132, 132, 132}, {132, 132, 132}, {132, 132, 132}, {132, 132, 132}, {131, 131, 131},
{131, 131, 131}, {131, 131, 131}, {131, 131, 131}, {130, 130, 130}, {130, 130, 130},
{130, 130, 130}, {130, 130, 130}, {129, 129, 129}, {129, 129, 129}, {129, 129, 129},
{129, 129, 129}, {128, 128, 128}, {128, 128, 128}, {128, 128, 128}, {128, 128, 128},
{127, 127, 127}, {127, 127, 127}, {127, 127, 127}, {127, 127, 127}, {126, 126, 126},
{126, 126, 126}, {126, 126, 126}, {126, 126, 126}, {125, 125, 125}, {125, 125, 125},
{125, 125, 125}, {125, 125, 125}, {124, 124, 124}, {124, 124, 124}, {124, 124, 124},
{124, 124, 124}, {123, 123, 123}, {123, 123, 123}, {123, 123, 123}, {123, 123, 123},
{122, 122, 122}, {122, 122, 122}, {122, 122, 122}, {122, 122, 122}, {121, 121, 121},
{121, 121, 121}, {121, 121, 121}, {120, 120, 120}, {120, 120, 120}, {120, 120, 120},
{120, 120, 120}, {119, 119, 119}, {119, 119, 119}, {119, 119, 119}, {119, 119, 119},
{118, 118, 118}, {118, 118, 118}, {118, 118, 118}, {118, 118, 118}, {117, 117, 117},
{117, 117, 117}, {117, 117, 117}, {117, 117, 117}, {116, 116, 116}, {116, 116, 116},
{116, 116, 116}, {116, 116, 116}, {115, 115, 115}, {115, 115, 115}, {115, 115, 115},
{115, 115, 115}, {114, 114, 114}, {114, 114, 114}, {114, 114, 114}, {114, 114, 114},
{113, 113, 113}, {113, 113, 113}, {113, 113, 113}, {113, 113, 113}, {112, 112, 112},
{112, 112, 112}, {112, 112, 112}, {112, 112, 112}, {111, 111, 111}, {111, 111, 111},
{111, 111, 111}, {111, 111, 111}, {110, 110, 110}, {110, 110, 110}, {110, 110, 110},
{110, 110, 110}, {109, 109, 109}, {109, 109, 109}, {109, 109, 109}, {108, 108, 108},
{108, 108, 108}, {108, 108, 108}, {108, 108, 108}, {107, 107, 107}, {107, 107, 107},
{107, 107, 107}, {107, 107, 107}, {106, 106, 106}, {106, 106, 106}, {106, 106, 106},
{106, 106, 106}, {105, 105, 105}, {105, 105, 105}, {105, 105, 105}, {105, 105, 105},
{104, 104, 104}, {104, 104, 104}, {104, 104, 104}, {104, 104, 104}, {103, 103, 103},
{103, 103, 103}, {103, 103, 103}, {103, 103, 103}, {102, 102, 102}, {102, 102, 102},
{102, 102, 102}, {102, 102, 102}, {101, 101, 101}, {101, 101, 101}, {101, 101, 101},
{101, 101, 101}, {100, 100, 100}, {100, 100, 100}, {100, 100, 100}, {100, 100, 100},
{ 99, 99, 99}, { 99, 99, 99}, { 99, 99, 99}, { 99, 99, 99}, { 98, 98, 98},
{ 98, 98, 98}, { 98, 98, 98}, { 98, 98, 98}, { 97, 97, 97}, { 97, 97, 97},
{ 97, 97, 97}, { 96, 96, 96}, { 96, 96, 96}, { 96, 96, 96}, { 96, 96, 96},
{ 95, 95, 95}, { 95, 95, 95}, { 95, 95, 95}, { 95, 95, 95}, { 94, 94, 94},
{ 94, 94, 94}, { 94, 94, 94}, { 94, 94, 94}, { 93, 93, 93}, { 93, 93, 93},
{ 93, 93, 93}, { 93, 93, 93}, { 92, 92, 92}, { 92, 92, 92}, { 92, 92, 92},
{ 92, 92, 92}, { 91, 91, 91}, { 91, 91, 91}, { 91, 91, 91}, { 91, 91, 91},
{ 90, 90, 90}, { 90, 90, 90}, { 90, 90, 90}, { 90, 90, 90}, { 89, 89, 89},
{ 89, 89, 89}, { 89, 89, 89}, { 89, 89, 89}, { 88, 88, 88}, { 88, 88, 88},
{ 88, 88, 88}, { 88, 88, 88}, { 87, 87, 87}, { 87, 87, 87}, { 87, 87, 87},
{ 87, 87, 87}, { 86, 86, 86}, { 86, 86, 86}, { 86, 86, 86}, { 86, 86, 86},
{ 85, 85, 85}, { 85, 85, 85}, { 85, 85, 85}, { 84, 84, 84}, { 84, 84, 84},
{ 84, 84, 84}, { 84, 84, 84}, { 83, 83, 83}, { 83, 83, 83}, { 83, 83, 83},
{ 83, 83, 83}, { 82, 82, 82}, { 82, 82, 82}, { 82, 82, 82}, { 82, 82, 82},
{ 81, 81, 81}, { 81, 81, 81}, { 81, 81, 81}, { 81, 81, 81}, { 80, 80, 80},
{ 80, 80, 80}, { 80, 80, 80}, { 80, 80, 80}, { 79, 79, 79}, { 79, 79, 79},
{ 79, 79, 79}, { 79, 79, 79}, { 78, 78, 78}, { 78, 78, 78}, { 78, 78, 78},
{ 78, 78, 78}, { 77, 77, 77}, { 77, 77, 77}, { 77, 77, 77}, { 77, 77, 77},
{ 76, 76, 76}, { 76, 76, 76}, { 76, 76, 76}, { 76, 76, 76}, { 75, 75, 75},
{ 75, 75, 75}, { 75, 75, 75}, { 75, 75, 75}, { 74, 74, 74}, { 74, 74, 74},
{ 74, 74, 74}, { 74, 74, 74}, { 73, 73, 73}, { 73, 73, 73}, { 73, 73, 73},
{ 72, 72, 72}, { 72, 72, 72}, { 72, 72, 72}, { 72, 72, 72}, { 71, 71, 71},
{ 71, 71, 71}, { 71, 71, 71}, { 71, 71, 71}, { 70, 70, 70}, { 70, 70, 70},
{ 70, 70, 70}, { 70, 70, 70}, { 69, 69, 69}, { 69, 69, 69}, { 69, 69, 69},
{ 69, 69, 69}, { 68, 68, 68}, { 68, 68, 68}, { 68, 68, 68}, { 68, 68, 68},
{ 67, 67, 67}, { 67, 67, 67}, { 67, 67, 67}, { 67, 67, 67}, { 66, 66, 66},
{ 66, 66, 66}, { 66, 66, 66}, { 66, 66, 66}, { 65, 65, 65}, { 65, 65, 65},
{ 65, 65, 65}, { 65, 65, 65}, { 64, 64, 64}, { 64, 64, 64}, { 64, 64, 64},
{ 64, 64, 64}, { 63, 63, 63}, { 63, 63, 63}, { 63, 63, 63}, { 63, 63, 63},
{ 62, 62, 62}, { 62, 62, 62}, { 62, 62, 62}, { 62, 62, 62}, { 61, 61, 61},
{ 61, 61, 61}, { 61, 61, 61}, { 60, 60, 60}, { 60, 60, 60}, { 60, 60, 60},
{ 60, 60, 60}, { 59, 59, 59}, { 59, 59, 59}, { 59, 59, 59}, { 59, 59, 59},
{ 58, 58, 58}, { 58, 58, 58}, { 58, 58, 58}, { 58, 58, 58}, { 57, 57, 57},
{ 57, 57, 57}, { 57, 57, 57}, { 57, 57, 57}, { 56, 56, 56}, { 56, 56, 56},
{ 56, 56, 56}, { 56, 56, 56}, { 55, 55, 55}, { 55, 55, 55}, { 55, 55, 55},
{ 55, 55, 55}, { 54, 54, 54}, { 54, 54, 54}, { 54, 54, 54}, { 54, 54, 54},
{ 53, 53, 53}, { 53, 53, 53}, { 53, 53, 53}, { 53, 53, 53}, { 52, 52, 52},
{ 52, 52, 52}, { 52, 52, 52}, { 52, 52, 52}, { 51, 51, 51}, { 51, 51, 51},
{ 51, 51, 51}, { 51, 51, 51}, { 50, 50, 50}, { 50, 50, 50}, { 50, 50, 50},
{ 50, 50, 50}, { 49, 49, 49}, { 49, 49, 49}, { 49, 49, 49}, { 48, 48, 48},
{ 48, 48, 48}, { 48, 48, 48}, { 48, 48, 48}, { 47, 47, 47}, { 47, 47, 47},
{ 47, 47, 47}, { 47, 47, 47}, { 46, 46, 46}, { 46, 46, 46}, { 46, 46, 46},
{ 46, 46, 46}, { 45, 45, 45}, { 45, 45, 45}, { 45, 45, 45}, { 45, 45, 45},
{ 44, 44, 44}, { 44, 44, 44}, { 44, 44, 44}, { 44, 44, 44}, { 43, 43, 43},
{ 43, 43, 43}, { 43, 43, 43}, { 43, 43, 43}, { 42, 42, 42}, { 42, 42, 42},
{ 42, 42, 42}, { 42, 42, 42}, { 41, 41, 41}, { 41, 41, 41}, { 41, 41, 41},
{ 41, 41, 41}, { 40, 40, 40}, { 40, 40, 40}, { 40, 40, 40}, { 40, 40, 40},
{ 39, 39, 39}, { 39, 39, 39}, { 39, 39, 39}, { 39, 39, 39}, { 38, 38, 38},
{ 38, 38, 38}, { 38, 38, 38}, { 38, 38, 38}, { 37, 37, 37}, { 37, 37, 37},
{ 37, 37, 37}, { 37, 37, 37}, { 36, 36, 36}, { 36, 36, 36}, { 36, 36, 36},
{ 35, 35, 35}, { 35, 35, 35}, { 35, 35, 35}, { 35, 35, 35}, { 34, 34, 34},
{ 34, 34, 34}, { 34, 34, 34}, { 34, 34, 34}, { 33, 33, 33}, { 33, 33, 33},
{ 33, 33, 33}, { 33, 33, 33}, { 32, 32, 32}, { 32, 32, 32}, { 32, 32, 32},
{ 32, 32, 32}, { 31, 31, 31}, { 31, 31, 31}, { 31, 31, 31}, { 31, 31, 31},
{ 30, 30, 30}, { 30, 30, 30}, { 30, 30, 30}, { 30, 30, 30}, { 29, 29, 29},
{ 29, 29, 29}, { 29, 29, 29}, { 29, 29, 29}, { 28, 28, 28}, { 28, 28, 28},
{ 28, 28, 28}, { 28, 28, 28}, { 27, 27, 27}, { 27, 27, 27}, { 27, 27, 27},
{ 27, 27, 27}, { 26, 26, 26}, { 26, 26, 26}, { 26, 26, 26}, { 26, 26, 26},
{ 25, 25, 25}, { 25, 25, 25}, { 25, 25, 25}, { 25, 25, 25}, { 24, 24, 24},
{ 24, 24, 24}, { 24, 24, 24}, { 23, 23, 23}, { 23, 23, 23}, { 23, 23, 23},
{ 23, 23, 23}, { 22, 22, 22}, { 22, 22, 22}, { 22, 22, 22}, { 22, 22, 22},
{ 21, 21, 21}, { 21, 21, 21}, { 21, 21, 21}, { 21, 21, 21}, { 20, 20, 20},
{ 20, 20, 20}, { 20, 20, 20}, { 20, 20, 20}, { 19, 19, 19}, { 19, 19, 19},
{ 19, 19, 19}, { 19, 19, 19}, { 18, 18, 18}, { 18, 18, 18}, { 18, 18, 18},
{ 18, 18, 18}, { 17, 17, 17}, { 17, 17, 17}, { 17, 17, 17}, { 17, 17, 17},
{ 16, 16, 16}, { 16, 16, 16}, { 16, 16, 16}, { 16, 16, 16}, { 15, 15, 15},
{ 15, 15, 15}, { 15, 15, 15}, { 15, 15, 15}, { 14, 14, 14}, { 14, 14, 14},
{ 14, 14, 14}, { 14, 14, 14}, { 13, 13, 13}, { 13, 13, 13}, { 13, 13, 13},
{ 13, 13, 13}, { 12, 12, 12}, { 12, 12, 12}, { 12, 12, 12}, { 11, 11, 11},
{ 11, 11, 11}, { 11, 11, 11}, { 11, 11, 11}, { 10, 10, 10}, { 10, 10, 10},
{ 10, 10, 10}, { 10, 10, 10}, { 9, 9, 9}, { 9, 9, 9}, { 9, 9, 9},
{ 9, 9, 9}, { 8, 8, 8}, { 8, 8, 8}, { 8, 8, 8}, { 8, 8, 8},
{ 7, 7, 7}, { 7, 7, 7}, { 7, 7, 7}, { 7, 7, 7}, { 6, 6, 6},
{ 6, 6, 6}, { 6, 6, 6}, { 6, 6, 6}, { 5, 5, 5}, { 5, 5, 5},
{ 5, 5, 5}, { 5, 5, 5}, { 4, 4, 4}, { 4, 4, 4}, { 4, 4, 4},
{ 4, 4, 4}, { 3, 3, 3}, { 3, 3, 3}, { 3, 3, 3}, { 3, 3, 3},
{ 2, 2, 2}, { 2, 2, 2}, { 2, 2, 2}, { 2, 2, 2}, { 1, 1, 1},
{ 1, 1, 1}, { 1, 1, 1}, { 1, 1, 1}, { 0, 0, 0}, { 0, 0, 0}
};
const rgb_store hot_colormap[1000] = {
{ 11, 0, 0}, { 11, 0, 0}, { 12, 0, 0}, { 13, 0, 0}, { 13, 0, 0},
{ 14, 0, 0}, { 15, 0, 0}, { 15, 0, 0}, { 16, 0, 0}, { 17, 0, 0},
{ 17, 0, 0}, { 18, 0, 0}, { 19, 0, 0}, { 19, 0, 0}, { 20, 0, 0},
{ 21, 0, 0}, { 21, 0, 0}, { 22, 0, 0}, { 23, 0, 0}, { 23, 0, 0},
{ 24, 0, 0}, { 25, 0, 0}, { 25, 0, 0}, { 26, 0, 0}, { 27, 0, 0},
{ 27, 0, 0}, { 28, 0, 0}, { 29, 0, 0}, { 29, 0, 0}, { 30, 0, 0},
{ 31, 0, 0}, { 31, 0, 0}, { 32, 0, 0}, { 33, 0, 0}, { 33, 0, 0},
{ 34, 0, 0}, { 35, 0, 0}, { 35, 0, 0}, { 36, 0, 0}, { 37, 0, 0},
{ 37, 0, 0}, { 38, 0, 0}, { 39, 0, 0}, { 39, 0, 0}, { 40, 0, 0},
{ 41, 0, 0}, { 41, 0, 0}, { 42, 0, 0}, { 43, 0, 0}, { 43, 0, 0},
{ 44, 0, 0}, { 45, 0, 0}, { 45, 0, 0}, { 46, 0, 0}, { 47, 0, 0},
{ 47, 0, 0}, { 48, 0, 0}, { 49, 0, 0}, { 49, 0, 0}, { 50, 0, 0},
{ 51, 0, 0}, { 51, 0, 0}, { 52, 0, 0}, { 53, 0, 0}, { 54, 0, 0},
{ 54, 0, 0}, { 55, 0, 0}, { 56, 0, 0}, { 56, 0, 0}, { 57, 0, 0},
{ 58, 0, 0}, { 58, 0, 0}, { 59, 0, 0}, { 60, 0, 0}, { 60, 0, 0},
{ 61, 0, 0}, { 62, 0, 0}, { 62, 0, 0}, { 63, 0, 0}, { 64, 0, 0},
{ 64, 0, 0}, { 65, 0, 0}, { 66, 0, 0}, { 66, 0, 0}, { 67, 0, 0},
{ 68, 0, 0}, { 68, 0, 0}, { 69, 0, 0}, { 70, 0, 0}, { 70, 0, 0},
{ 71, 0, 0}, { 72, 0, 0}, { 72, 0, 0}, { 73, 0, 0}, { 74, 0, 0},
{ 74, 0, 0}, { 75, 0, 0}, { 76, 0, 0}, { 76, 0, 0}, { 77, 0, 0},
{ 78, 0, 0}, { 78, 0, 0}, { 79, 0, 0}, { 80, 0, 0}, { 80, 0, 0},
{ 81, 0, 0}, { 82, 0, 0}, { 82, 0, 0}, { 83, 0, 0}, { 84, 0, 0},
{ 84, 0, 0}, { 85, 0, 0}, { 86, 0, 0}, { 86, 0, 0}, { 87, 0, 0},
{ 88, 0, 0}, { 88, 0, 0}, { 89, 0, 0}, { 90, 0, 0}, { 90, 0, 0},
{ 91, 0, 0}, { 92, 0, 0}, { 92, 0, 0}, { 93, 0, 0}, { 94, 0, 0},
{ 94, 0, 0}, { 95, 0, 0}, { 96, 0, 0}, { 96, 0, 0}, { 97, 0, 0},
{ 98, 0, 0}, { 98, 0, 0}, { 99, 0, 0}, {100, 0, 0}, {100, 0, 0},
{101, 0, 0}, {102, 0, 0}, {102, 0, 0}, {103, 0, 0}, {104, 0, 0},
{104, 0, 0}, {105, 0, 0}, {106, 0, 0}, {106, 0, 0}, {107, 0, 0},
{108, 0, 0}, {108, 0, 0}, {109, 0, 0}, {110, 0, 0}, {110, 0, 0},
{111, 0, 0}, {112, 0, 0}, {112, 0, 0}, {113, 0, 0}, {114, 0, 0},
{114, 0, 0}, {115, 0, 0}, {116, 0, 0}, {116, 0, 0}, {117, 0, 0},
{118, 0, 0}, {119, 0, 0}, {119, 0, 0}, {120, 0, 0}, {121, 0, 0},
{121, 0, 0}, {122, 0, 0}, {123, 0, 0}, {123, 0, 0}, {124, 0, 0},
{125, 0, 0}, {125, 0, 0}, {126, 0, 0}, {127, 0, 0}, {127, 0, 0},
{128, 0, 0}, {129, 0, 0}, {129, 0, 0}, {130, 0, 0}, {131, 0, 0},
{131, 0, 0}, {132, 0, 0}, {133, 0, 0}, {133, 0, 0}, {134, 0, 0},
{135, 0, 0}, {135, 0, 0}, {136, 0, 0}, {137, 0, 0}, {137, 0, 0},
{138, 0, 0}, {139, 0, 0}, {139, 0, 0}, {140, 0, 0}, {141, 0, 0},
{141, 0, 0}, {142, 0, 0}, {143, 0, 0}, {143, 0, 0}, {144, 0, 0},
{145, 0, 0}, {145, 0, 0}, {146, 0, 0}, {147, 0, 0}, {147, 0, 0},
{148, 0, 0}, {149, 0, 0}, {149, 0, 0}, {150, 0, 0}, {151, 0, 0},
{151, 0, 0}, {152, 0, 0}, {153, 0, 0}, {153, 0, 0}, {154, 0, 0},
{155, 0, 0}, {155, 0, 0}, {156, 0, 0}, {157, 0, 0}, {157, 0, 0},
{158, 0, 0}, {159, 0, 0}, {159, 0, 0}, {160, 0, 0}, {161, 0, 0},
{161, 0, 0}, {162, 0, 0}, {163, 0, 0}, {163, 0, 0}, {164, 0, 0},
{165, 0, 0}, {165, 0, 0}, {166, 0, 0}, {167, 0, 0}, {167, 0, 0},
{168, 0, 0}, {169, 0, 0}, {169, 0, 0}, {170, 0, 0}, {171, 0, 0},
{171, 0, 0}, {172, 0, 0}, {173, 0, 0}, {173, 0, 0}, {174, 0, 0},
{175, 0, 0}, {175, 0, 0}, {176, 0, 0}, {177, 0, 0}, {177, 0, 0},
{178, 0, 0}, {179, 0, 0}, {179, 0, 0}, {180, 0, 0}, {181, 0, 0},
{181, 0, 0}, {182, 0, 0}, {183, 0, 0}, {183, 0, 0}, {184, 0, 0},
{185, 0, 0}, {186, 0, 0}, {186, 0, 0}, {187, 0, 0}, {188, 0, 0},
{188, 0, 0}, {189, 0, 0}, {190, 0, 0}, {190, 0, 0}, {191, 0, 0},
{192, 0, 0}, {192, 0, 0}, {193, 0, 0}, {194, 0, 0}, {194, 0, 0},
{195, 0, 0}, {196, 0, 0}, {196, 0, 0}, {197, 0, 0}, {198, 0, 0},
{198, 0, 0}, {199, 0, 0}, {200, 0, 0}, {200, 0, 0}, {201, 0, 0},
{202, 0, 0}, {202, 0, 0}, {203, 0, 0}, {204, 0, 0}, {204, 0, 0},
{205, 0, 0}, {206, 0, 0}, {206, 0, 0}, {207, 0, 0}, {208, 0, 0},
{208, 0, 0}, {209, 0, 0}, {210, 0, 0}, {210, 0, 0}, {211, 0, 0},
{212, 0, 0}, {212, 0, 0}, {213, 0, 0}, {214, 0, 0}, {214, 0, 0},
{215, 0, 0}, {216, 0, 0}, {216, 0, 0}, {217, 0, 0}, {218, 0, 0},
{218, 0, 0}, {219, 0, 0}, {220, 0, 0}, {220, 0, 0}, {221, 0, 0},
{222, 0, 0}, {222, 0, 0}, {223, 0, 0}, {224, 0, 0}, {224, 0, 0},
{225, 0, 0}, {226, 0, 0}, {226, 0, 0}, {227, 0, 0}, {228, 0, 0},
{228, 0, 0}, {229, 0, 0}, {230, 0, 0}, {230, 0, 0}, {231, 0, 0},
{232, 0, 0}, {232, 0, 0}, {233, 0, 0}, {234, 0, 0}, {234, 0, 0},
{235, 0, 0}, {236, 0, 0}, {236, 0, 0}, {237, 0, 0}, {238, 0, 0},
{238, 0, 0}, {239, 0, 0}, {240, 0, 0}, {240, 0, 0}, {241, 0, 0},
{242, 0, 0}, {242, 0, 0}, {243, 0, 0}, {244, 0, 0}, {244, 0, 0},
{245, 0, 0}, {246, 0, 0}, {246, 0, 0}, {247, 0, 0}, {248, 0, 0},
{248, 0, 0}, {249, 0, 0}, {250, 0, 0}, {251, 0, 0}, {251, 0, 0},
{252, 0, 0}, {253, 0, 0}, {253, 0, 0}, {254, 0, 0}, {255, 0, 0},
{255, 0, 0}, {255, 1, 0}, {255, 2, 0}, {255, 2, 0}, {255, 3, 0},
{255, 4, 0}, {255, 4, 0}, {255, 5, 0}, {255, 6, 0}, {255, 6, 0},
{255, 7, 0}, {255, 8, 0}, {255, 8, 0}, {255, 9, 0}, {255, 10, 0},
{255, 10, 0}, {255, 11, 0}, {255, 12, 0}, {255, 12, 0}, {255, 13, 0},
{255, 14, 0}, {255, 14, 0}, {255, 15, 0}, {255, 16, 0}, {255, 16, 0},
{255, 17, 0}, {255, 18, 0}, {255, 18, 0}, {255, 19, 0}, {255, 20, 0},
{255, 20, 0}, {255, 21, 0}, {255, 22, 0}, {255, 22, 0}, {255, 23, 0},
{255, 24, 0}, {255, 24, 0}, {255, 25, 0}, {255, 26, 0}, {255, 26, 0},
{255, 27, 0}, {255, 28, 0}, {255, 28, 0}, {255, 29, 0}, {255, 30, 0},
{255, 30, 0}, {255, 31, 0}, {255, 32, 0}, {255, 32, 0}, {255, 33, 0},
{255, 34, 0}, {255, 34, 0}, {255, 35, 0}, {255, 36, 0}, {255, 36, 0},
{255, 37, 0}, {255, 38, 0}, {255, 38, 0}, {255, 39, 0}, {255, 40, 0},
{255, 40, 0}, {255, 41, 0}, {255, 42, 0}, {255, 42, 0}, {255, 43, 0},
{255, 44, 0}, {255, 44, 0}, {255, 45, 0}, {255, 46, 0}, {255, 46, 0},
{255, 47, 0}, {255, 48, 0}, {255, 48, 0}, {255, 49, 0}, {255, 50, 0},
{255, 50, 0}, {255, 51, 0}, {255, 52, 0}, {255, 52, 0}, {255, 53, 0},
{255, 54, 0}, {255, 54, 0}, {255, 55, 0}, {255, 56, 0}, {255, 56, 0},
{255, 57, 0}, {255, 58, 0}, {255, 58, 0}, {255, 59, 0}, {255, 60, 0},
{255, 60, 0}, {255, 61, 0}, {255, 62, 0}, {255, 63, 0}, {255, 63, 0},
{255, 64, 0}, {255, 65, 0}, {255, 65, 0}, {255, 66, 0}, {255, 67, 0},
{255, 67, 0}, {255, 68, 0}, {255, 69, 0}, {255, 69, 0}, {255, 70, 0},
{255, 71, 0}, {255, 71, 0}, {255, 72, 0}, {255, 73, 0}, {255, 73, 0},
{255, 74, 0}, {255, 75, 0}, {255, 75, 0}, {255, 76, 0}, {255, 77, 0},
{255, 77, 0}, {255, 78, 0}, {255, 79, 0}, {255, 79, 0}, {255, 80, 0},
{255, 81, 0}, {255, 81, 0}, {255, 82, 0}, {255, 83, 0}, {255, 83, 0},
{255, 84, 0}, {255, 85, 0}, {255, 85, 0}, {255, 86, 0}, {255, 87, 0},
{255, 87, 0}, {255, 88, 0}, {255, 89, 0}, {255, 89, 0}, {255, 90, 0},
{255, 91, 0}, {255, 91, 0}, {255, 92, 0}, {255, 93, 0}, {255, 93, 0},
{255, 94, 0}, {255, 95, 0}, {255, 95, 0}, {255, 96, 0}, {255, 97, 0},
{255, 97, 0}, {255, 98, 0}, {255, 99, 0}, {255, 99, 0}, {255, 100, 0},
{255, 101, 0}, {255, 101, 0}, {255, 102, 0}, {255, 103, 0}, {255, 103, 0},
{255, 104, 0}, {255, 105, 0}, {255, 105, 0}, {255, 106, 0}, {255, 107, 0},
{255, 107, 0}, {255, 108, 0}, {255, 109, 0}, {255, 109, 0}, {255, 110, 0},
{255, 111, 0}, {255, 111, 0}, {255, 112, 0}, {255, 113, 0}, {255, 113, 0},
{255, 114, 0}, {255, 115, 0}, {255, 115, 0}, {255, 116, 0}, {255, 117, 0},
{255, 117, 0}, {255, 118, 0}, {255, 119, 0}, {255, 119, 0}, {255, 120, 0},
{255, 121, 0}, {255, 121, 0}, {255, 122, 0}, {255, 123, 0}, {255, 123, 0},
{255, 124, 0}, {255, 125, 0}, {255, 125, 0}, {255, 126, 0}, {255, 127, 0},
{255, 128, 0}, {255, 128, 0}, {255, 129, 0}, {255, 130, 0}, {255, 130, 0},
{255, 131, 0}, {255, 132, 0}, {255, 132, 0}, {255, 133, 0}, {255, 134, 0},
{255, 134, 0}, {255, 135, 0}, {255, 136, 0}, {255, 136, 0}, {255, 137, 0},
{255, 138, 0}, {255, 138, 0}, {255, 139, 0}, {255, 140, 0}, {255, 140, 0},
{255, 141, 0}, {255, 142, 0}, {255, 142, 0}, {255, 143, 0}, {255, 144, 0},
{255, 144, 0}, {255, 145, 0}, {255, 146, 0}, {255, 146, 0}, {255, 147, 0},
{255, 148, 0}, {255, 148, 0}, {255, 149, 0}, {255, 150, 0}, {255, 150, 0},
{255, 151, 0}, {255, 152, 0}, {255, 152, 0}, {255, 153, 0}, {255, 154, 0},
{255, 154, 0}, {255, 155, 0}, {255, 156, 0}, {255, 156, 0}, {255, 157, 0},
{255, 158, 0}, {255, 158, 0}, {255, 159, 0}, {255, 160, 0}, {255, 160, 0},
{255, 161, 0}, {255, 162, 0}, {255, 162, 0}, {255, 163, 0}, {255, 164, 0},
{255, 164, 0}, {255, 165, 0}, {255, 166, 0}, {255, 166, 0}, {255, 167, 0},
{255, 168, 0}, {255, 168, 0}, {255, 169, 0}, {255, 170, 0}, {255, 170, 0},
{255, 171, 0}, {255, 172, 0}, {255, 172, 0}, {255, 173, 0}, {255, 174, 0},
{255, 174, 0}, {255, 175, 0}, {255, 176, 0}, {255, 176, 0}, {255, 177, 0},
{255, 178, 0}, {255, 178, 0}, {255, 179, 0}, {255, 180, 0}, {255, 180, 0},
{255, 181, 0}, {255, 182, 0}, {255, 182, 0}, {255, 183, 0}, {255, 184, 0},
{255, 184, 0}, {255, 185, 0}, {255, 186, 0}, {255, 186, 0}, {255, 187, 0},
{255, 188, 0}, {255, 188, 0}, {255, 189, 0}, {255, 190, 0}, {255, 190, 0},
{255, 191, 0}, {255, 192, 0}, {255, 192, 0}, {255, 193, 0}, {255, 194, 0},
{255, 195, 0}, {255, 195, 0}, {255, 196, 0}, {255, 197, 0}, {255, 197, 0},
{255, 198, 0}, {255, 199, 0}, {255, 199, 0}, {255, 200, 0}, {255, 201, 0},
{255, 201, 0}, {255, 202, 0}, {255, 203, 0}, {255, 203, 0}, {255, 204, 0},
{255, 205, 0}, {255, 205, 0}, {255, 206, 0}, {255, 207, 0}, {255, 207, 0},
{255, 208, 0}, {255, 209, 0}, {255, 209, 0}, {255, 210, 0}, {255, 211, 0},
{255, 211, 0}, {255, 212, 0}, {255, 213, 0}, {255, 213, 0}, {255, 214, 0},
{255, 215, 0}, {255, 215, 0}, {255, 216, 0}, {255, 217, 0}, {255, 217, 0},
{255, 218, 0}, {255, 219, 0}, {255, 219, 0}, {255, 220, 0}, {255, 221, 0},
{255, 221, 0}, {255, 222, 0}, {255, 223, 0}, {255, 223, 0}, {255, 224, 0},
{255, 225, 0}, {255, 225, 0}, {255, 226, 0}, {255, 227, 0}, {255, 227, 0},
{255, 228, 0}, {255, 229, 0}, {255, 229, 0}, {255, 230, 0}, {255, 231, 0},
{255, 231, 0}, {255, 232, 0}, {255, 233, 0}, {255, 233, 0}, {255, 234, 0},
{255, 235, 0}, {255, 235, 0}, {255, 236, 0}, {255, 237, 0}, {255, 237, 0},
{255, 238, 0}, {255, 239, 0}, {255, 239, 0}, {255, 240, 0}, {255, 241, 0},
{255, 241, 0}, {255, 242, 0}, {255, 243, 0}, {255, 243, 0}, {255, 244, 0},
{255, 245, 0}, {255, 245, 0}, {255, 246, 0}, {255, 247, 0}, {255, 247, 0},
{255, 248, 0}, {255, 249, 0}, {255, 249, 0}, {255, 250, 0}, {255, 251, 0},
{255, 251, 0}, {255, 252, 0}, {255, 253, 0}, {255, 253, 0}, {255, 254, 0},
{255, 255, 0}, {255, 255, 1}, {255, 255, 2}, {255, 255, 3}, {255, 255, 4},
{255, 255, 5}, {255, 255, 6}, {255, 255, 7}, {255, 255, 8}, {255, 255, 9},
{255, 255, 10}, {255, 255, 11}, {255, 255, 12}, {255, 255, 13}, {255, 255, 14},
{255, 255, 15}, {255, 255, 16}, {255, 255, 17}, {255, 255, 18}, {255, 255, 19},
{255, 255, 20}, {255, 255, 21}, {255, 255, 22}, {255, 255, 23}, {255, 255, 24},
{255, 255, 25}, {255, 255, 26}, {255, 255, 27}, {255, 255, 28}, {255, 255, 29},
{255, 255, 30}, {255, 255, 31}, {255, 255, 32}, {255, 255, 33}, {255, 255, 34},
{255, 255, 35}, {255, 255, 36}, {255, 255, 37}, {255, 255, 38}, {255, 255, 39},
{255, 255, 40}, {255, 255, 41}, {255, 255, 42}, {255, 255, 43}, {255, 255, 44},
{255, 255, 45}, {255, 255, 46}, {255, 255, 47}, {255, 255, 48}, {255, 255, 49},
{255, 255, 50}, {255, 255, 51}, {255, 255, 52}, {255, 255, 53}, {255, 255, 54},
{255, 255, 55}, {255, 255, 56}, {255, 255, 57}, {255, 255, 58}, {255, 255, 59},
{255, 255, 60}, {255, 255, 61}, {255, 255, 62}, {255, 255, 63}, {255, 255, 64},
{255, 255, 65}, {255, 255, 66}, {255, 255, 67}, {255, 255, 68}, {255, 255, 69},
{255, 255, 70}, {255, 255, 71}, {255, 255, 72}, {255, 255, 73}, {255, 255, 74},
{255, 255, 75}, {255, 255, 76}, {255, 255, 77}, {255, 255, 78}, {255, 255, 79},
{255, 255, 80}, {255, 255, 81}, {255, 255, 82}, {255, 255, 83}, {255, 255, 84},
{255, 255, 85}, {255, 255, 86}, {255, 255, 87}, {255, 255, 88}, {255, 255, 89},
{255, 255, 90}, {255, 255, 91}, {255, 255, 92}, {255, 255, 93}, {255, 255, 94},
{255, 255, 95}, {255, 255, 96}, {255, 255, 97}, {255, 255, 98}, {255, 255, 99},
{255, 255, 100}, {255, 255, 101}, {255, 255, 102}, {255, 255, 103}, {255, 255, 104},
{255, 255, 105}, {255, 255, 106}, {255, 255, 107}, {255, 255, 108}, {255, 255, 109},
{255, 255, 110}, {255, 255, 111}, {255, 255, 112}, {255, 255, 113}, {255, 255, 114},
{255, 255, 115}, {255, 255, 116}, {255, 255, 117}, {255, 255, 118}, {255, 255, 119},
{255, 255, 120}, {255, 255, 121}, {255, 255, 122}, {255, 255, 123}, {255, 255, 124},
{255, 255, 125}, {255, 255, 126}, {255, 255, 127}, {255, 255, 128}, {255, 255, 129},
{255, 255, 130}, {255, 255, 131}, {255, 255, 132}, {255, 255, 133}, {255, 255, 134},
{255, 255, 135}, {255, 255, 136}, {255, 255, 137}, {255, 255, 138}, {255, 255, 139},
{255, 255, 140}, {255, 255, 141}, {255, 255, 142}, {255, 255, 143}, {255, 255, 144},
{255, 255, 145}, {255, 255, 146}, {255, 255, 147}, {255, 255, 148}, {255, 255, 149},
{255, 255, 150}, {255, 255, 151}, {255, 255, 152}, {255, 255, 153}, {255, 255, 154},
{255, 255, 155}, {255, 255, 157}, {255, 255, 158}, {255, 255, 159}, {255, 255, 160},
{255, 255, 161}, {255, 255, 162}, {255, 255, 163}, {255, 255, 164}, {255, 255, 165},
{255, 255, 166}, {255, 255, 167}, {255, 255, 168}, {255, 255, 169}, {255, 255, 170},
{255, 255, 171}, {255, 255, 172}, {255, 255, 173}, {255, 255, 174}, {255, 255, 175},
{255, 255, 176}, {255, 255, 177}, {255, 255, 178}, {255, 255, 179}, {255, 255, 180},
{255, 255, 181}, {255, 255, 182}, {255, 255, 183}, {255, 255, 184}, {255, 255, 185},
{255, 255, 186}, {255, 255, 187}, {255, 255, 188}, {255, 255, 189}, {255, 255, 190},
{255, 255, 191}, {255, 255, 192}, {255, 255, 193}, {255, 255, 194}, {255, 255, 195},
{255, 255, 196}, {255, 255, 197}, {255, 255, 198}, {255, 255, 199}, {255, 255, 200},
{255, 255, 201}, {255, 255, 202}, {255, 255, 203}, {255, 255, 204}, {255, 255, 205},
{255, 255, 206}, {255, 255, 207}, {255, 255, 208}, {255, 255, 209}, {255, 255, 210},
{255, 255, 211}, {255, 255, 212}, {255, 255, 213}, {255, 255, 214}, {255, 255, 215},
{255, 255, 216}, {255, 255, 217}, {255, 255, 218}, {255, 255, 219}, {255, 255, 220},
{255, 255, 221}, {255, 255, 222}, {255, 255, 223}, {255, 255, 224}, {255, 255, 225},
{255, 255, 226}, {255, 255, 227}, {255, 255, 228}, {255, 255, 229}, {255, 255, 230},
{255, 255, 231}, {255, 255, 232}, {255, 255, 233}, {255, 255, 234}, {255, 255, 235},
{255, 255, 236}, {255, 255, 237}, {255, 255, 238}, {255, 255, 239}, {255, 255, 240},
{255, 255, 241}, {255, 255, 242}, {255, 255, 243}, {255, 255, 244}, {255, 255, 245},
{255, 255, 246}, {255, 255, 247}, {255, 255, 248}, {255, 255, 249}, {255, 255, 250},
{255, 255, 251}, {255, 255, 252}, {255, 255, 253}, {255, 255, 254}, {255, 255, 255}
};
const rgb_store hsv_colormap[1000] = {
{255, 0, 0}, {255, 2, 0}, {255, 3, 0}, {255, 5, 0}, {255, 6, 0},
{255, 8, 0}, {255, 9, 0}, {255, 11, 0}, {255, 12, 0}, {255, 14, 0},
{255, 15, 0}, {255, 17, 0}, {255, 18, 0}, {255, 20, 0}, {255, 21, 0},
{255, 23, 0}, {255, 24, 0}, {255, 26, 0}, {255, 27, 0}, {255, 29, 0},
{255, 30, 0}, {255, 32, 0}, {255, 33, 0}, {255, 35, 0}, {255, 36, 0},
{255, 38, 0}, {255, 39, 0}, {255, 41, 0}, {255, 42, 0}, {255, 44, 0},
{255, 45, 0}, {255, 47, 0}, {255, 48, 0}, {255, 50, 0}, {255, 51, 0},
{255, 53, 0}, {255, 54, 0}, {255, 56, 0}, {255, 57, 0}, {255, 59, 0},
{255, 60, 0}, {255, 62, 0}, {255, 63, 0}, {255, 65, 0}, {255, 66, 0},
{255, 68, 0}, {255, 69, 0}, {255, 71, 0}, {255, 72, 0}, {255, 74, 0},
{255, 75, 0}, {255, 77, 0}, {255, 78, 0}, {255, 80, 0}, {255, 81, 0},
{255, 83, 0}, {255, 84, 0}, {255, 86, 0}, {255, 87, 0}, {255, 89, 0},
{255, 90, 0}, {255, 92, 0}, {255, 93, 0}, {255, 95, 0}, {255, 96, 0},
{255, 98, 0}, {255, 100, 0}, {255, 101, 0}, {255, 103, 0}, {255, 104, 0},
{255, 106, 0}, {255, 107, 0}, {255, 109, 0}, {255, 110, 0}, {255, 112, 0},
{255, 113, 0}, {255, 115, 0}, {255, 116, 0}, {255, 118, 0}, {255, 119, 0},
{255, 121, 0}, {255, 122, 0}, {255, 124, 0}, {255, 125, 0}, {255, 127, 0},
{255, 128, 0}, {255, 130, 0}, {255, 131, 0}, {255, 133, 0}, {255, 134, 0},
{255, 136, 0}, {255, 137, 0}, {255, 139, 0}, {255, 140, 0}, {255, 142, 0},
{255, 143, 0}, {255, 145, 0}, {255, 146, 0}, {255, 148, 0}, {255, 149, 0},
{255, 151, 0}, {255, 152, 0}, {255, 154, 0}, {255, 155, 0}, {255, 157, 0},
{255, 158, 0}, {255, 160, 0}, {255, 161, 0}, {255, 163, 0}, {255, 164, 0},
{255, 166, 0}, {255, 167, 0}, {255, 169, 0}, {255, 170, 0}, {255, 172, 0},
{255, 173, 0}, {255, 175, 0}, {255, 176, 0}, {255, 178, 0}, {255, 179, 0},
{255, 181, 0}, {255, 182, 0}, {255, 184, 0}, {255, 185, 0}, {255, 187, 0},
{255, 188, 0}, {255, 190, 0}, {255, 191, 0}, {255, 193, 0}, {255, 194, 0},
{255, 196, 0}, {255, 197, 0}, {255, 199, 0}, {255, 201, 0}, {255, 202, 0},
{255, 204, 0}, {255, 205, 0}, {255, 207, 0}, {255, 208, 0}, {255, 210, 0},
{255, 211, 0}, {255, 213, 0}, {255, 214, 0}, {255, 216, 0}, {255, 217, 0},
{255, 219, 0}, {255, 220, 0}, {255, 222, 0}, {255, 223, 0}, {255, 225, 0},
{255, 226, 0}, {255, 228, 0}, {255, 229, 0}, {255, 231, 0}, {255, 232, 0},
{255, 234, 0}, {255, 235, 0}, {255, 237, 0}, {255, 238, 0}, {255, 239, 0},
{254, 240, 0}, {254, 242, 0}, {253, 243, 0}, {253, 244, 0}, {252, 245, 0},
{252, 246, 0}, {251, 247, 0}, {251, 248, 0}, {250, 249, 0}, {250, 250, 0},
{249, 251, 0}, {249, 252, 0}, {248, 253, 0}, {248, 254, 0}, {247, 255, 0},
{246, 255, 0}, {245, 255, 0}, {243, 255, 0}, {242, 255, 0}, {240, 255, 0},
{239, 255, 0}, {237, 255, 0}, {236, 255, 0}, {234, 255, 0}, {233, 255, 0},
{231, 255, 0}, {230, 255, 0}, {228, 255, 0}, {227, 255, 0}, {225, 255, 0},
{224, 255, 0}, {222, 255, 0}, {221, 255, 0}, {219, 255, 0}, {218, 255, 0},
{216, 255, 0}, {215, 255, 0}, {213, 255, 0}, {211, 255, 0}, {210, 255, 0},
{208, 255, 0}, {207, 255, 0}, {205, 255, 0}, {204, 255, 0}, {202, 255, 0},
{201, 255, 0}, {199, 255, 0}, {198, 255, 0}, {196, 255, 0}, {195, 255, 0},
{193, 255, 0}, {192, 255, 0}, {190, 255, 0}, {189, 255, 0}, {187, 255, 0},
{186, 255, 0}, {184, 255, 0}, {183, 255, 0}, {181, 255, 0}, {180, 255, 0},
{178, 255, 0}, {177, 255, 0}, {175, 255, 0}, {174, 255, 0}, {172, 255, 0},
{171, 255, 0}, {169, 255, 0}, {168, 255, 0}, {166, 255, 0}, {165, 255, 0},
{163, 255, 0}, {162, 255, 0}, {160, 255, 0}, {159, 255, 0}, {157, 255, 0},
{156, 255, 0}, {154, 255, 0}, {153, 255, 0}, {151, 255, 0}, {150, 255, 0},
{148, 255, 0}, {147, 255, 0}, {145, 255, 0}, {144, 255, 0}, {142, 255, 0},
{141, 255, 0}, {139, 255, 0}, {138, 255, 0}, {136, 255, 0}, {135, 255, 0},
{133, 255, 0}, {132, 255, 0}, {130, 255, 0}, {129, 255, 0}, {127, 255, 0},
{126, 255, 0}, {124, 255, 0}, {123, 255, 0}, {121, 255, 0}, {120, 255, 0},
{118, 255, 0}, {117, 255, 0}, {115, 255, 0}, {114, 255, 0}, {112, 255, 0},
{110, 255, 0}, {109, 255, 0}, {107, 255, 0}, {106, 255, 0}, {104, 255, 0},
{103, 255, 0}, {101, 255, 0}, {100, 255, 0}, { 98, 255, 0}, { 97, 255, 0},
{ 95, 255, 0}, { 94, 255, 0}, { 92, 255, 0}, { 91, 255, 0}, { 89, 255, 0},
{ 88, 255, 0}, { 86, 255, 0}, { 85, 255, 0}, { 83, 255, 0}, { 82, 255, 0},
{ 80, 255, 0}, { 79, 255, 0}, { 77, 255, 0}, { 76, 255, 0}, { 74, 255, 0},
{ 73, 255, 0}, { 71, 255, 0}, { 70, 255, 0}, { 68, 255, 0}, { 67, 255, 0},
{ 65, 255, 0}, { 64, 255, 0}, { 62, 255, 0}, { 61, 255, 0}, { 59, 255, 0},
{ 58, 255, 0}, { 56, 255, 0}, { 55, 255, 0}, { 53, 255, 0}, { 52, 255, 0},
{ 50, 255, 0}, { 49, 255, 0}, { 47, 255, 0}, { 46, 255, 0}, { 44, 255, 0},
{ 43, 255, 0}, { 41, 255, 0}, { 40, 255, 0}, { 38, 255, 0}, { 37, 255, 0},
{ 35, 255, 0}, { 34, 255, 0}, { 32, 255, 0}, { 31, 255, 0}, { 29, 255, 0},
{ 28, 255, 0}, { 26, 255, 0}, { 25, 255, 0}, { 23, 255, 0}, { 22, 255, 0},
{ 20, 255, 0}, { 19, 255, 0}, { 17, 255, 0}, { 16, 255, 0}, { 14, 255, 0},
{ 12, 255, 0}, { 11, 255, 0}, { 9, 255, 0}, { 8, 255, 0}, { 7, 255, 1},
{ 7, 255, 2}, { 6, 255, 3}, { 6, 255, 4}, { 5, 255, 5}, { 5, 255, 6},
{ 4, 255, 7}, { 4, 255, 8}, { 3, 255, 9}, { 3, 255, 10}, { 2, 255, 11},
{ 2, 255, 12}, { 1, 255, 13}, { 1, 255, 14}, { 0, 255, 15}, { 0, 255, 16},
{ 0, 255, 18}, { 0, 255, 19}, { 0, 255, 21}, { 0, 255, 22}, { 0, 255, 24},
{ 0, 255, 25}, { 0, 255, 27}, { 0, 255, 28}, { 0, 255, 30}, { 0, 255, 31},
{ 0, 255, 33}, { 0, 255, 34}, { 0, 255, 36}, { 0, 255, 37}, { 0, 255, 39},
{ 0, 255, 40}, { 0, 255, 42}, { 0, 255, 43}, { 0, 255, 45}, { 0, 255, 46},
{ 0, 255, 48}, { 0, 255, 49}, { 0, 255, 51}, { 0, 255, 52}, { 0, 255, 54},
{ 0, 255, 55}, { 0, 255, 57}, { 0, 255, 58}, { 0, 255, 60}, { 0, 255, 61},
{ 0, 255, 63}, { 0, 255, 64}, { 0, 255, 66}, { 0, 255, 67}, { 0, 255, 69},
{ 0, 255, 70}, { 0, 255, 72}, { 0, 255, 73}, { 0, 255, 75}, { 0, 255, 76},
{ 0, 255, 78}, { 0, 255, 79}, { 0, 255, 81}, { 0, 255, 82}, { 0, 255, 84},
{ 0, 255, 86}, { 0, 255, 87}, { 0, 255, 89}, { 0, 255, 90}, { 0, 255, 92},
{ 0, 255, 93}, { 0, 255, 95}, { 0, 255, 96}, { 0, 255, 98}, { 0, 255, 99},
{ 0, 255, 101}, { 0, 255, 102}, { 0, 255, 104}, { 0, 255, 105}, { 0, 255, 107},
{ 0, 255, 108}, { 0, 255, 110}, { 0, 255, 111}, { 0, 255, 113}, { 0, 255, 114},
{ 0, 255, 116}, { 0, 255, 117}, { 0, 255, 119}, { 0, 255, 120}, { 0, 255, 122},
{ 0, 255, 123}, { 0, 255, 125}, { 0, 255, 126}, { 0, 255, 128}, { 0, 255, 129},
{ 0, 255, 131}, { 0, 255, 132}, { 0, 255, 134}, { 0, 255, 135}, { 0, 255, 137},
{ 0, 255, 138}, { 0, 255, 140}, { 0, 255, 141}, { 0, 255, 143}, { 0, 255, 144},
{ 0, 255, 146}, { 0, 255, 147}, { 0, 255, 149}, { 0, 255, 150}, { 0, 255, 152},
{ 0, 255, 153}, { 0, 255, 155}, { 0, 255, 156}, { 0, 255, 158}, { 0, 255, 159},
{ 0, 255, 161}, { 0, 255, 162}, { 0, 255, 164}, { 0, 255, 165}, { 0, 255, 167},
{ 0, 255, 168}, { 0, 255, 170}, { 0, 255, 171}, { 0, 255, 173}, { 0, 255, 174},
{ 0, 255, 176}, { 0, 255, 177}, { 0, 255, 179}, { 0, 255, 180}, { 0, 255, 182},
{ 0, 255, 183}, { 0, 255, 185}, { 0, 255, 187}, { 0, 255, 188}, { 0, 255, 190},
{ 0, 255, 191}, { 0, 255, 193}, { 0, 255, 194}, { 0, 255, 196}, { 0, 255, 197},
{ 0, 255, 199}, { 0, 255, 200}, { 0, 255, 202}, { 0, 255, 203}, { 0, 255, 205},
{ 0, 255, 206}, { 0, 255, 208}, { 0, 255, 209}, { 0, 255, 211}, { 0, 255, 212},
{ 0, 255, 214}, { 0, 255, 215}, { 0, 255, 217}, { 0, 255, 218}, { 0, 255, 220},
{ 0, 255, 221}, { 0, 255, 223}, { 0, 255, 224}, { 0, 255, 226}, { 0, 255, 227},
{ 0, 255, 229}, { 0, 255, 230}, { 0, 255, 232}, { 0, 255, 233}, { 0, 255, 235},
{ 0, 255, 236}, { 0, 255, 238}, { 0, 255, 239}, { 0, 255, 241}, { 0, 255, 242},
{ 0, 255, 244}, { 0, 255, 245}, { 0, 255, 247}, { 0, 255, 248}, { 0, 255, 250},
{ 0, 255, 251}, { 0, 255, 253}, { 0, 255, 254}, { 0, 254, 255}, { 0, 253, 255},
{ 0, 251, 255}, { 0, 250, 255}, { 0, 248, 255}, { 0, 247, 255}, { 0, 245, 255},
{ 0, 244, 255}, { 0, 242, 255}, { 0, 241, 255}, { 0, 239, 255}, { 0, 238, 255},
{ 0, 236, 255}, { 0, 235, 255}, { 0, 233, 255}, { 0, 232, 255}, { 0, 230, 255},
{ 0, 229, 255}, { 0, 227, 255}, { 0, 225, 255}, { 0, 224, 255}, { 0, 222, 255},
{ 0, 221, 255}, { 0, 219, 255}, { 0, 218, 255}, { 0, 216, 255}, { 0, 215, 255},
{ 0, 213, 255}, { 0, 212, 255}, { 0, 210, 255}, { 0, 209, 255}, { 0, 207, 255},
{ 0, 206, 255}, { 0, 204, 255}, { 0, 203, 255}, { 0, 201, 255}, { 0, 200, 255},
{ 0, 198, 255}, { 0, 197, 255}, { 0, 195, 255}, { 0, 194, 255}, { 0, 192, 255},
{ 0, 191, 255}, { 0, 189, 255}, { 0, 188, 255}, { 0, 186, 255}, { 0, 185, 255},
{ 0, 183, 255}, { 0, 182, 255}, { 0, 180, 255}, { 0, 179, 255}, { 0, 177, 255},
{ 0, 176, 255}, { 0, 174, 255}, { 0, 173, 255}, { 0, 171, 255}, { 0, 170, 255},
{ 0, 168, 255}, { 0, 167, 255}, { 0, 165, 255}, { 0, 164, 255}, { 0, 162, 255},
{ 0, 161, 255}, { 0, 159, 255}, { 0, 158, 255}, { 0, 156, 255}, { 0, 155, 255},
{ 0, 153, 255}, { 0, 152, 255}, { 0, 150, 255}, { 0, 149, 255}, { 0, 147, 255},
{ 0, 146, 255}, { 0, 144, 255}, { 0, 143, 255}, { 0, 141, 255}, { 0, 140, 255},
{ 0, 138, 255}, { 0, 137, 255}, { 0, 135, 255}, { 0, 134, 255}, { 0, 132, 255},
{ 0, 131, 255}, { 0, 129, 255}, { 0, 128, 255}, { 0, 126, 255}, { 0, 124, 255},
{ 0, 123, 255}, { 0, 121, 255}, { 0, 120, 255}, { 0, 118, 255}, { 0, 117, 255},
{ 0, 115, 255}, { 0, 114, 255}, { 0, 112, 255}, { 0, 111, 255}, { 0, 109, 255},
{ 0, 108, 255}, { 0, 106, 255}, { 0, 105, 255}, { 0, 103, 255}, { 0, 102, 255},
{ 0, 100, 255}, { 0, 99, 255}, { 0, 97, 255}, { 0, 96, 255}, { 0, 94, 255},
{ 0, 93, 255}, { 0, 91, 255}, { 0, 90, 255}, { 0, 88, 255}, { 0, 87, 255},
{ 0, 85, 255}, { 0, 84, 255}, { 0, 82, 255}, { 0, 81, 255}, { 0, 79, 255},
{ 0, 78, 255}, { 0, 76, 255}, { 0, 75, 255}, { 0, 73, 255}, { 0, 72, 255},
{ 0, 70, 255}, { 0, 69, 255}, { 0, 67, 255}, { 0, 66, 255}, { 0, 64, 255},
{ 0, 63, 255}, { 0, 61, 255}, { 0, 60, 255}, { 0, 58, 255}, { 0, 57, 255},
{ 0, 55, 255}, { 0, 54, 255}, { 0, 52, 255}, { 0, 51, 255}, { 0, 49, 255},
{ 0, 48, 255}, { 0, 46, 255}, { 0, 45, 255}, { 0, 43, 255}, { 0, 42, 255},
{ 0, 40, 255}, { 0, 39, 255}, { 0, 37, 255}, { 0, 36, 255}, { 0, 34, 255},
{ 0, 33, 255}, { 0, 31, 255}, { 0, 30, 255}, { 0, 28, 255}, { 0, 26, 255},
{ 0, 25, 255}, { 0, 23, 255}, { 0, 22, 255}, { 0, 20, 255}, { 0, 19, 255},
{ 0, 17, 255}, { 0, 16, 255}, { 1, 15, 255}, { 1, 14, 255}, { 2, 13, 255},
{ 2, 12, 255}, { 3, 11, 255}, { 3, 10, 255}, { 4, 9, 255}, { 4, 8, 255},
{ 5, 7, 255}, { 5, 6, 255}, { 6, 5, 255}, { 6, 4, 255}, { 7, 3, 255},
{ 7, 2, 255}, { 8, 1, 255}, { 8, 0, 255}, { 10, 0, 255}, { 11, 0, 255},
{ 13, 0, 255}, { 14, 0, 255}, { 16, 0, 255}, { 17, 0, 255}, { 19, 0, 255},
{ 20, 0, 255}, { 22, 0, 255}, { 23, 0, 255}, { 25, 0, 255}, { 26, 0, 255},
{ 28, 0, 255}, { 29, 0, 255}, { 31, 0, 255}, { 32, 0, 255}, { 34, 0, 255},
{ 35, 0, 255}, { 37, 0, 255}, { 38, 0, 255}, { 40, 0, 255}, { 41, 0, 255},
{ 43, 0, 255}, { 44, 0, 255}, { 46, 0, 255}, { 47, 0, 255}, { 49, 0, 255},
{ 50, 0, 255}, { 52, 0, 255}, { 53, 0, 255}, { 55, 0, 255}, { 56, 0, 255},
{ 58, 0, 255}, { 59, 0, 255}, { 61, 0, 255}, { 62, 0, 255}, { 64, 0, 255},
{ 65, 0, 255}, { 67, 0, 255}, { 68, 0, 255}, { 70, 0, 255}, { 72, 0, 255},
{ 73, 0, 255}, { 75, 0, 255}, { 76, 0, 255}, { 78, 0, 255}, { 79, 0, 255},
{ 81, 0, 255}, { 82, 0, 255}, { 84, 0, 255}, { 85, 0, 255}, { 87, 0, 255},
{ 88, 0, 255}, { 90, 0, 255}, { 91, 0, 255}, { 93, 0, 255}, { 94, 0, 255},
{ 96, 0, 255}, { 97, 0, 255}, { 99, 0, 255}, {100, 0, 255}, {102, 0, 255},
{103, 0, 255}, {105, 0, 255}, {106, 0, 255}, {108, 0, 255}, {109, 0, 255},
{111, 0, 255}, {112, 0, 255}, {114, 0, 255}, {115, 0, 255}, {117, 0, 255},
{118, 0, 255}, {120, 0, 255}, {121, 0, 255}, {123, 0, 255}, {124, 0, 255},
{126, 0, 255}, {127, 0, 255}, {129, 0, 255}, {130, 0, 255}, {132, 0, 255},
{133, 0, 255}, {135, 0, 255}, {136, 0, 255}, {138, 0, 255}, {139, 0, 255},
{141, 0, 255}, {142, 0, 255}, {144, 0, 255}, {145, 0, 255}, {147, 0, 255},
{148, 0, 255}, {150, 0, 255}, {151, 0, 255}, {153, 0, 255}, {154, 0, 255},
{156, 0, 255}, {157, 0, 255}, {159, 0, 255}, {160, 0, 255}, {162, 0, 255},
{163, 0, 255}, {165, 0, 255}, {166, 0, 255}, {168, 0, 255}, {169, 0, 255},
{171, 0, 255}, {173, 0, 255}, {174, 0, 255}, {176, 0, 255}, {177, 0, 255},
{179, 0, 255}, {180, 0, 255}, {182, 0, 255}, {183, 0, 255}, {185, 0, 255},
{186, 0, 255}, {188, 0, 255}, {189, 0, 255}, {191, 0, 255}, {192, 0, 255},
{194, 0, 255}, {195, 0, 255}, {197, 0, 255}, {198, 0, 255}, {200, 0, 255},
{201, 0, 255}, {203, 0, 255}, {204, 0, 255}, {206, 0, 255}, {207, 0, 255},
{209, 0, 255}, {210, 0, 255}, {212, 0, 255}, {213, 0, 255}, {215, 0, 255},
{216, 0, 255}, {218, 0, 255}, {219, 0, 255}, {221, 0, 255}, {222, 0, 255},
{224, 0, 255}, {225, 0, 255}, {227, 0, 255}, {228, 0, 255}, {230, 0, 255},
{231, 0, 255}, {233, 0, 255}, {234, 0, 255}, {236, 0, 255}, {237, 0, 255},
{239, 0, 255}, {240, 0, 255}, {242, 0, 255}, {243, 0, 255}, {245, 0, 255},
{246, 0, 255}, {247, 0, 254}, {248, 0, 253}, {248, 0, 252}, {249, 0, 251},
{249, 0, 250}, {250, 0, 249}, {250, 0, 248}, {251, 0, 247}, {251, 0, 246},
{252, 0, 245}, {252, 0, 244}, {253, 0, 243}, {253, 0, 242}, {254, 0, 241},
{254, 0, 240}, {255, 0, 239}, {255, 0, 238}, {255, 0, 236}, {255, 0, 235},
{255, 0, 233}, {255, 0, 232}, {255, 0, 230}, {255, 0, 229}, {255, 0, 227},
{255, 0, 226}, {255, 0, 224}, {255, 0, 223}, {255, 0, 221}, {255, 0, 220},
{255, 0, 218}, {255, 0, 217}, {255, 0, 215}, {255, 0, 214}, {255, 0, 212},
{255, 0, 211}, {255, 0, 209}, {255, 0, 208}, {255, 0, 206}, {255, 0, 205},
{255, 0, 203}, {255, 0, 202}, {255, 0, 200}, {255, 0, 199}, {255, 0, 197},
{255, 0, 196}, {255, 0, 194}, {255, 0, 193}, {255, 0, 191}, {255, 0, 190},
{255, 0, 188}, {255, 0, 187}, {255, 0, 185}, {255, 0, 184}, {255, 0, 182},
{255, 0, 181}, {255, 0, 179}, {255, 0, 178}, {255, 0, 176}, {255, 0, 175},
{255, 0, 173}, {255, 0, 172}, {255, 0, 170}, {255, 0, 169}, {255, 0, 167},
{255, 0, 166}, {255, 0, 164}, {255, 0, 163}, {255, 0, 161}, {255, 0, 160},
{255, 0, 158}, {255, 0, 157}, {255, 0, 155}, {255, 0, 154}, {255, 0, 152},
{255, 0, 151}, {255, 0, 149}, {255, 0, 148}, {255, 0, 146}, {255, 0, 145},
{255, 0, 143}, {255, 0, 141}, {255, 0, 140}, {255, 0, 138}, {255, 0, 137},
{255, 0, 135}, {255, 0, 134}, {255, 0, 132}, {255, 0, 131}, {255, 0, 129},
{255, 0, 128}, {255, 0, 126}, {255, 0, 125}, {255, 0, 123}, {255, 0, 122},
{255, 0, 120}, {255, 0, 119}, {255, 0, 117}, {255, 0, 116}, {255, 0, 114},
{255, 0, 113}, {255, 0, 111}, {255, 0, 110}, {255, 0, 108}, {255, 0, 107},
{255, 0, 105}, {255, 0, 104}, {255, 0, 102}, {255, 0, 101}, {255, 0, 99},
{255, 0, 98}, {255, 0, 96}, {255, 0, 95}, {255, 0, 93}, {255, 0, 92},
{255, 0, 90}, {255, 0, 89}, {255, 0, 87}, {255, 0, 86}, {255, 0, 84},
{255, 0, 83}, {255, 0, 81}, {255, 0, 80}, {255, 0, 78}, {255, 0, 77},
{255, 0, 75}, {255, 0, 74}, {255, 0, 72}, {255, 0, 71}, {255, 0, 69},
{255, 0, 68}, {255, 0, 66}, {255, 0, 65}, {255, 0, 63}, {255, 0, 62},
{255, 0, 60}, {255, 0, 59}, {255, 0, 57}, {255, 0, 56}, {255, 0, 54},
{255, 0, 53}, {255, 0, 51}, {255, 0, 50}, {255, 0, 48}, {255, 0, 47},
{255, 0, 45}, {255, 0, 44}, {255, 0, 42}, {255, 0, 40}, {255, 0, 39},
{255, 0, 37}, {255, 0, 36}, {255, 0, 34}, {255, 0, 33}, {255, 0, 31},
{255, 0, 30}, {255, 0, 28}, {255, 0, 27}, {255, 0, 25}, {255, 0, 24}
};
const rgb_store jet_colormap[1000] = {
{ 29, 0, 102}, { 23, 0, 107}, { 17, 0, 112}, { 12, 0, 117}, { 6, 0, 122},
{ 0, 0, 127}, { 0, 0, 128}, { 0, 0, 129}, { 0, 0, 129}, { 0, 0, 130},
{ 0, 0, 131}, { 0, 0, 132}, { 0, 0, 133}, { 0, 0, 133}, { 0, 0, 134},
{ 0, 0, 135}, { 0, 0, 136}, { 0, 0, 137}, { 0, 0, 138}, { 0, 0, 140},
{ 0, 0, 141}, { 0, 0, 142}, { 0, 0, 143}, { 0, 0, 145}, { 0, 0, 146},
{ 0, 0, 147}, { 0, 0, 148}, { 0, 0, 150}, { 0, 0, 151}, { 0, 0, 152},
{ 0, 0, 153}, { 0, 0, 154}, { 0, 0, 156}, { 0, 0, 157}, { 0, 0, 158},
{ 0, 0, 159}, { 0, 0, 160}, { 0, 0, 161}, { 0, 0, 163}, { 0, 0, 164},
{ 0, 0, 165}, { 0, 0, 166}, { 0, 0, 168}, { 0, 0, 169}, { 0, 0, 170},
{ 0, 0, 171}, { 0, 0, 173}, { 0, 0, 174}, { 0, 0, 175}, { 0, 0, 176},
{ 0, 0, 178}, { 0, 0, 179}, { 0, 0, 180}, { 0, 0, 181}, { 0, 0, 183},
{ 0, 0, 184}, { 0, 0, 185}, { 0, 0, 186}, { 0, 0, 188}, { 0, 0, 189},
{ 0, 0, 190}, { 0, 0, 191}, { 0, 0, 193}, { 0, 0, 194}, { 0, 0, 195},
{ 0, 0, 196}, { 0, 0, 197}, { 0, 0, 198}, { 0, 0, 200}, { 0, 0, 201},
{ 0, 0, 202}, { 0, 0, 203}, { 0, 0, 204}, { 0, 0, 206}, { 0, 0, 207},
{ 0, 0, 208}, { 0, 0, 209}, { 0, 0, 211}, { 0, 0, 212}, { 0, 0, 213},
{ 0, 0, 214}, { 0, 0, 216}, { 0, 0, 217}, { 0, 0, 218}, { 0, 0, 219},
{ 0, 0, 221}, { 0, 0, 222}, { 0, 0, 223}, { 0, 0, 225}, { 0, 0, 226},
{ 0, 0, 227}, { 0, 0, 228}, { 0, 0, 230}, { 0, 0, 231}, { 0, 0, 232},
{ 0, 0, 233}, { 0, 0, 234}, { 0, 0, 234}, { 0, 0, 235}, { 0, 0, 236},
{ 0, 0, 237}, { 0, 0, 238}, { 0, 0, 239}, { 0, 0, 239}, { 0, 0, 240},
{ 0, 0, 241}, { 0, 0, 242}, { 0, 0, 243}, { 0, 0, 244}, { 0, 0, 246},
{ 0, 0, 247}, { 0, 0, 248}, { 0, 0, 249}, { 0, 0, 250}, { 0, 0, 251},
{ 0, 0, 253}, { 0, 0, 254}, { 0, 0, 254}, { 0, 0, 254}, { 0, 0, 254},
{ 0, 0, 254}, { 0, 0, 254}, { 0, 0, 255}, { 0, 0, 255}, { 0, 0, 255},
{ 0, 0, 255}, { 0, 0, 255}, { 0, 0, 255}, { 0, 1, 255}, { 0, 1, 255},
{ 0, 2, 255}, { 0, 3, 255}, { 0, 3, 255}, { 0, 4, 255}, { 0, 5, 255},
{ 0, 6, 255}, { 0, 6, 255}, { 0, 7, 255}, { 0, 8, 255}, { 0, 9, 255},
{ 0, 10, 255}, { 0, 11, 255}, { 0, 12, 255}, { 0, 13, 255}, { 0, 14, 255},
{ 0, 15, 255}, { 0, 16, 255}, { 0, 17, 255}, { 0, 18, 255}, { 0, 19, 255},
{ 0, 21, 255}, { 0, 22, 255}, { 0, 23, 255}, { 0, 24, 255}, { 0, 25, 255},
{ 0, 26, 255}, { 0, 27, 255}, { 0, 28, 255}, { 0, 29, 255}, { 0, 30, 255},
{ 0, 31, 255}, { 0, 32, 255}, { 0, 34, 255}, { 0, 35, 255}, { 0, 36, 255},
{ 0, 37, 255}, { 0, 38, 255}, { 0, 39, 255}, { 0, 40, 255}, { 0, 41, 255},
{ 0, 42, 255}, { 0, 43, 255}, { 0, 44, 255}, { 0, 45, 255}, { 0, 46, 255},
{ 0, 48, 255}, { 0, 49, 255}, { 0, 50, 255}, { 0, 51, 255}, { 0, 52, 255},
{ 0, 53, 255}, { 0, 54, 255}, { 0, 55, 255}, { 0, 56, 255}, { 0, 57, 255},
{ 0, 58, 255}, { 0, 58, 255}, { 0, 59, 255}, { 0, 60, 255}, { 0, 60, 255},
{ 0, 61, 255}, { 0, 62, 255}, { 0, 63, 255}, { 0, 63, 255}, { 0, 64, 255},
{ 0, 65, 255}, { 0, 66, 255}, { 0, 67, 255}, { 0, 68, 255}, { 0, 69, 255},
{ 0, 71, 255}, { 0, 72, 255}, { 0, 73, 255}, { 0, 74, 255}, { 0, 75, 255},
{ 0, 76, 255}, { 0, 77, 255}, { 0, 78, 255}, { 0, 79, 255}, { 0, 80, 255},
{ 0, 81, 255}, { 0, 82, 255}, { 0, 84, 255}, { 0, 85, 255}, { 0, 86, 255},
{ 0, 87, 255}, { 0, 88, 255}, { 0, 89, 255}, { 0, 90, 255}, { 0, 91, 255},
{ 0, 92, 255}, { 0, 93, 255}, { 0, 94, 255}, { 0, 95, 255}, { 0, 96, 255},
{ 0, 98, 255}, { 0, 99, 255}, { 0, 100, 255}, { 0, 101, 255}, { 0, 102, 255},
{ 0, 103, 255}, { 0, 104, 255}, { 0, 105, 255}, { 0, 106, 255}, { 0, 107, 255},
{ 0, 108, 255}, { 0, 109, 255}, { 0, 111, 255}, { 0, 112, 255}, { 0, 113, 255},
{ 0, 114, 255}, { 0, 115, 255}, { 0, 116, 255}, { 0, 117, 255}, { 0, 118, 255},
{ 0, 119, 255}, { 0, 120, 255}, { 0, 121, 255}, { 0, 122, 255}, { 0, 123, 255},
{ 0, 125, 255}, { 0, 126, 255}, { 0, 127, 255}, { 0, 128, 255}, { 0, 129, 255},
{ 0, 130, 255}, { 0, 131, 255}, { 0, 132, 255}, { 0, 133, 255}, { 0, 134, 255},
{ 0, 135, 255}, { 0, 136, 255}, { 0, 138, 255}, { 0, 139, 255}, { 0, 140, 255},
{ 0, 141, 255}, { 0, 142, 255}, { 0, 143, 255}, { 0, 144, 255}, { 0, 145, 255},
{ 0, 146, 255}, { 0, 147, 255}, { 0, 148, 255}, { 0, 149, 255}, { 0, 150, 255},
{ 0, 150, 255}, { 0, 151, 255}, { 0, 152, 255}, { 0, 153, 255}, { 0, 153, 255},
{ 0, 154, 255}, { 0, 155, 255}, { 0, 155, 255}, { 0, 156, 255}, { 0, 157, 255},
{ 0, 158, 255}, { 0, 159, 255}, { 0, 161, 255}, { 0, 162, 255}, { 0, 163, 255},
{ 0, 164, 255}, { 0, 165, 255}, { 0, 166, 255}, { 0, 167, 255}, { 0, 168, 255},
{ 0, 169, 255}, { 0, 170, 255}, { 0, 171, 255}, { 0, 172, 255}, { 0, 173, 255},
{ 0, 175, 255}, { 0, 176, 255}, { 0, 177, 255}, { 0, 178, 255}, { 0, 179, 255},
{ 0, 180, 255}, { 0, 181, 255}, { 0, 182, 255}, { 0, 183, 255}, { 0, 184, 255},
{ 0, 185, 255}, { 0, 186, 255}, { 0, 188, 255}, { 0, 189, 255}, { 0, 190, 255},
{ 0, 191, 255}, { 0, 192, 255}, { 0, 193, 255}, { 0, 194, 255}, { 0, 195, 255},
{ 0, 196, 255}, { 0, 197, 255}, { 0, 198, 255}, { 0, 199, 255}, { 0, 200, 255},
{ 0, 202, 255}, { 0, 203, 255}, { 0, 204, 255}, { 0, 205, 255}, { 0, 206, 255},
{ 0, 207, 255}, { 0, 208, 255}, { 0, 209, 255}, { 0, 210, 255}, { 0, 211, 255},
{ 0, 212, 255}, { 0, 213, 255}, { 0, 215, 255}, { 0, 216, 255}, { 0, 217, 255},
{ 0, 218, 254}, { 0, 219, 253}, { 0, 220, 252}, { 0, 221, 252}, { 0, 222, 251},
{ 0, 223, 250}, { 0, 224, 250}, { 0, 225, 249}, { 0, 226, 248}, { 0, 227, 247},
{ 0, 229, 247}, { 1, 230, 246}, { 2, 231, 245}, { 3, 232, 244}, { 3, 233, 243},
{ 4, 234, 242}, { 5, 235, 241}, { 5, 236, 240}, { 6, 237, 239}, { 7, 238, 238},
{ 8, 239, 238}, { 8, 240, 237}, { 9, 241, 236}, { 10, 242, 236}, { 10, 242, 235},
{ 11, 243, 235}, { 11, 244, 234}, { 12, 245, 234}, { 13, 245, 233}, { 13, 246, 232},
{ 14, 247, 232}, { 15, 247, 231}, { 15, 248, 231}, { 16, 249, 230}, { 17, 249, 229},
{ 18, 250, 228}, { 18, 251, 227}, { 19, 251, 226}, { 20, 252, 225}, { 21, 253, 224},
{ 22, 253, 224}, { 23, 254, 223}, { 23, 254, 222}, { 24, 255, 221}, { 25, 255, 220},
{ 26, 255, 219}, { 27, 255, 218}, { 28, 255, 218}, { 29, 255, 217}, { 30, 255, 216},
{ 30, 255, 215}, { 31, 255, 214}, { 32, 255, 214}, { 33, 255, 213}, { 34, 255, 212},
{ 35, 255, 211}, { 36, 255, 210}, { 37, 255, 209}, { 38, 255, 208}, { 39, 255, 207},
{ 39, 255, 207}, { 40, 255, 206}, { 41, 255, 205}, { 42, 255, 204}, { 43, 255, 203},
{ 44, 255, 202}, { 45, 255, 201}, { 46, 255, 200}, { 47, 255, 199}, { 48, 255, 198},
{ 48, 255, 198}, { 49, 255, 197}, { 50, 255, 196}, { 51, 255, 195}, { 52, 255, 194},
{ 53, 255, 193}, { 54, 255, 192}, { 55, 255, 191}, { 55, 255, 191}, { 56, 255, 190},
{ 57, 255, 189}, { 58, 255, 188}, { 59, 255, 187}, { 60, 255, 186}, { 60, 255, 186},
{ 61, 255, 185}, { 62, 255, 184}, { 63, 255, 183}, { 64, 255, 182}, { 65, 255, 181},
{ 65, 255, 181}, { 66, 255, 180}, { 67, 255, 179}, { 68, 255, 178}, { 69, 255, 177},
{ 70, 255, 176}, { 71, 255, 175}, { 72, 255, 174}, { 73, 255, 173}, { 74, 255, 172},
{ 74, 255, 172}, { 75, 255, 171}, { 76, 255, 170}, { 77, 255, 169}, { 78, 255, 168},
{ 79, 255, 167}, { 80, 255, 166}, { 81, 255, 165}, { 82, 255, 164}, { 83, 255, 163},
{ 83, 255, 163}, { 84, 255, 162}, { 84, 255, 162}, { 85, 255, 161}, { 85, 255, 161},
{ 86, 255, 160}, { 87, 255, 159}, { 87, 255, 159}, { 88, 255, 158}, { 88, 255, 158},
{ 89, 255, 157}, { 89, 255, 157}, { 90, 255, 156}, { 91, 255, 155}, { 92, 255, 154},
{ 93, 255, 153}, { 94, 255, 152}, { 95, 255, 151}, { 96, 255, 150}, { 97, 255, 149},
{ 97, 255, 149}, { 98, 255, 148}, { 99, 255, 147}, {100, 255, 146}, {101, 255, 145},
{102, 255, 144}, {102, 255, 143}, {103, 255, 142}, {104, 255, 141}, {105, 255, 140},
{106, 255, 140}, {107, 255, 139}, {107, 255, 138}, {108, 255, 137}, {109, 255, 136},
{110, 255, 135}, {111, 255, 134}, {112, 255, 134}, {113, 255, 133}, {114, 255, 132},
{114, 255, 131}, {115, 255, 130}, {116, 255, 130}, {117, 255, 129}, {118, 255, 128},
{119, 255, 127}, {120, 255, 126}, {121, 255, 125}, {122, 255, 124}, {123, 255, 123},
{123, 255, 123}, {124, 255, 122}, {125, 255, 121}, {126, 255, 120}, {127, 255, 119},
{128, 255, 118}, {129, 255, 117}, {130, 255, 116}, {130, 255, 115}, {131, 255, 114},
{132, 255, 114}, {133, 255, 113}, {134, 255, 112}, {134, 255, 111}, {135, 255, 110},
{136, 255, 109}, {137, 255, 108}, {138, 255, 107}, {139, 255, 107}, {140, 255, 106},
{140, 255, 105}, {141, 255, 104}, {142, 255, 103}, {143, 255, 102}, {144, 255, 102},
{145, 255, 101}, {146, 255, 100}, {147, 255, 99}, {148, 255, 98}, {149, 255, 97},
{149, 255, 97}, {150, 255, 96}, {151, 255, 95}, {152, 255, 94}, {153, 255, 93},
{154, 255, 92}, {155, 255, 91}, {156, 255, 90}, {157, 255, 89}, {157, 255, 89},
{158, 255, 88}, {158, 255, 88}, {159, 255, 87}, {159, 255, 87}, {160, 255, 86},
{161, 255, 85}, {161, 255, 85}, {162, 255, 84}, {162, 255, 84}, {163, 255, 83},
{163, 255, 83}, {164, 255, 82}, {165, 255, 81}, {166, 255, 80}, {167, 255, 79},
{168, 255, 78}, {169, 255, 77}, {170, 255, 76}, {171, 255, 75}, {172, 255, 74},
{172, 255, 74}, {173, 255, 73}, {174, 255, 72}, {175, 255, 71}, {176, 255, 70},
{177, 255, 69}, {178, 255, 68}, {179, 255, 67}, {180, 255, 66}, {181, 255, 65},
{181, 255, 65}, {182, 255, 64}, {183, 255, 63}, {184, 255, 62}, {185, 255, 61},
{186, 255, 60}, {186, 255, 60}, {187, 255, 59}, {188, 255, 58}, {189, 255, 57},
{190, 255, 56}, {191, 255, 55}, {191, 255, 55}, {192, 255, 54}, {193, 255, 53},
{194, 255, 52}, {195, 255, 51}, {196, 255, 50}, {197, 255, 49}, {198, 255, 48},
{198, 255, 48}, {199, 255, 47}, {200, 255, 46}, {201, 255, 45}, {202, 255, 44},
{203, 255, 43}, {204, 255, 42}, {205, 255, 41}, {206, 255, 40}, {207, 255, 39},
{207, 255, 39}, {208, 255, 38}, {209, 255, 37}, {210, 255, 36}, {211, 255, 35},
{212, 255, 34}, {213, 255, 33}, {214, 255, 32}, {214, 255, 31}, {215, 255, 30},
{216, 255, 30}, {217, 255, 29}, {218, 255, 28}, {218, 255, 27}, {219, 255, 26},
{220, 255, 25}, {221, 255, 24}, {222, 255, 23}, {223, 255, 23}, {224, 255, 22},
{224, 255, 21}, {225, 255, 20}, {226, 255, 19}, {227, 255, 18}, {228, 255, 18},
{229, 255, 17}, {230, 255, 16}, {231, 255, 15}, {231, 255, 15}, {232, 255, 14},
{232, 255, 13}, {233, 255, 13}, {234, 255, 12}, {234, 255, 11}, {235, 255, 11},
{235, 255, 10}, {236, 255, 10}, {236, 255, 9}, {237, 255, 8}, {238, 254, 8},
{238, 253, 7}, {239, 252, 6}, {240, 251, 5}, {241, 250, 5}, {242, 249, 4},
{243, 248, 3}, {244, 247, 3}, {245, 246, 2}, {246, 246, 1}, {247, 245, 0},
{247, 243, 0}, {248, 242, 0}, {249, 242, 0}, {250, 241, 0}, {250, 240, 0},
{251, 239, 0}, {252, 238, 0}, {252, 237, 0}, {253, 236, 0}, {254, 235, 0},
{255, 234, 0}, {255, 233, 0}, {255, 232, 0}, {255, 231, 0}, {255, 230, 0},
{255, 229, 0}, {255, 228, 0}, {255, 227, 0}, {255, 226, 0}, {255, 225, 0},
{255, 224, 0}, {255, 223, 0}, {255, 222, 0}, {255, 221, 0}, {255, 220, 0},
{255, 219, 0}, {255, 218, 0}, {255, 217, 0}, {255, 216, 0}, {255, 215, 0},
{255, 214, 0}, {255, 213, 0}, {255, 212, 0}, {255, 211, 0}, {255, 210, 0},
{255, 209, 0}, {255, 208, 0}, {255, 207, 0}, {255, 206, 0}, {255, 205, 0},
{255, 204, 0}, {255, 203, 0}, {255, 202, 0}, {255, 201, 0}, {255, 200, 0},
{255, 199, 0}, {255, 198, 0}, {255, 197, 0}, {255, 196, 0}, {255, 195, 0},
{255, 194, 0}, {255, 193, 0}, {255, 192, 0}, {255, 191, 0}, {255, 190, 0},
{255, 189, 0}, {255, 188, 0}, {255, 187, 0}, {255, 186, 0}, {255, 185, 0},
{255, 184, 0}, {255, 183, 0}, {255, 182, 0}, {255, 180, 0}, {255, 179, 0},
{255, 178, 0}, {255, 177, 0}, {255, 176, 0}, {255, 176, 0}, {255, 175, 0},
{255, 175, 0}, {255, 174, 0}, {255, 173, 0}, {255, 173, 0}, {255, 172, 0},
{255, 171, 0}, {255, 171, 0}, {255, 170, 0}, {255, 169, 0}, {255, 168, 0},
{255, 167, 0}, {255, 166, 0}, {255, 165, 0}, {255, 164, 0}, {255, 163, 0},
{255, 162, 0}, {255, 161, 0}, {255, 160, 0}, {255, 159, 0}, {255, 158, 0},
{255, 157, 0}, {255, 156, 0}, {255, 155, 0}, {255, 154, 0}, {255, 153, 0},
{255, 152, 0}, {255, 151, 0}, {255, 150, 0}, {255, 150, 0}, {255, 149, 0},
{255, 147, 0}, {255, 146, 0}, {255, 146, 0}, {255, 145, 0}, {255, 144, 0},
{255, 143, 0}, {255, 142, 0}, {255, 141, 0}, {255, 140, 0}, {255, 139, 0},
{255, 138, 0}, {255, 137, 0}, {255, 136, 0}, {255, 135, 0}, {255, 134, 0},
{255, 133, 0}, {255, 132, 0}, {255, 131, 0}, {255, 130, 0}, {255, 129, 0},
{255, 128, 0}, {255, 127, 0}, {255, 126, 0}, {255, 125, 0}, {255, 124, 0},
{255, 123, 0}, {255, 122, 0}, {255, 121, 0}, {255, 120, 0}, {255, 119, 0},
{255, 118, 0}, {255, 117, 0}, {255, 116, 0}, {255, 115, 0}, {255, 114, 0},
{255, 113, 0}, {255, 112, 0}, {255, 111, 0}, {255, 109, 0}, {255, 108, 0},
{255, 107, 0}, {255, 106, 0}, {255, 105, 0}, {255, 104, 0}, {255, 103, 0},
{255, 102, 0}, {255, 101, 0}, {255, 100, 0}, {255, 99, 0}, {255, 98, 0},
{255, 97, 0}, {255, 96, 0}, {255, 95, 0}, {255, 94, 0}, {255, 93, 0},
{255, 92, 0}, {255, 91, 0}, {255, 91, 0}, {255, 90, 0}, {255, 90, 0},
{255, 89, 0}, {255, 88, 0}, {255, 88, 0}, {255, 87, 0}, {255, 86, 0},
{255, 86, 0}, {255, 85, 0}, {255, 84, 0}, {255, 83, 0}, {255, 82, 0},
{255, 81, 0}, {255, 80, 0}, {255, 79, 0}, {255, 78, 0}, {255, 77, 0},
{255, 76, 0}, {255, 75, 0}, {255, 74, 0}, {255, 73, 0}, {255, 72, 0},
{255, 71, 0}, {255, 70, 0}, {255, 69, 0}, {255, 68, 0}, {255, 67, 0},
{255, 66, 0}, {255, 65, 0}, {255, 64, 0}, {255, 63, 0}, {255, 62, 0},
{255, 61, 0}, {255, 60, 0}, {255, 59, 0}, {255, 58, 0}, {255, 57, 0},
{255, 56, 0}, {255, 55, 0}, {255, 54, 0}, {255, 54, 0}, {255, 53, 0},
{255, 51, 0}, {255, 50, 0}, {255, 49, 0}, {255, 48, 0}, {255, 47, 0},
{255, 46, 0}, {255, 45, 0}, {255, 44, 0}, {255, 43, 0}, {255, 42, 0},
{255, 41, 0}, {255, 40, 0}, {255, 39, 0}, {255, 38, 0}, {255, 37, 0},
{255, 36, 0}, {255, 35, 0}, {255, 34, 0}, {255, 33, 0}, {255, 32, 0},
{255, 31, 0}, {255, 30, 0}, {255, 29, 0}, {255, 28, 0}, {255, 27, 0},
{255, 26, 0}, {255, 25, 0}, {255, 24, 0}, {254, 23, 0}, {254, 22, 0},
{254, 21, 0}, {254, 20, 0}, {254, 19, 0}, {254, 18, 0}, {253, 17, 0},
{251, 16, 0}, {250, 15, 0}, {249, 14, 0}, {248, 13, 0}, {247, 12, 0},
{246, 11, 0}, {244, 10, 0}, {243, 9, 0}, {242, 8, 0}, {241, 7, 0},
{240, 6, 0}, {239, 6, 0}, {239, 5, 0}, {238, 4, 0}, {237, 4, 0},
{236, 3, 0}, {235, 3, 0}, {234, 2, 0}, {234, 1, 0}, {233, 1, 0},
{232, 0, 0}, {231, 0, 0}, {230, 0, 0}, {228, 0, 0}, {227, 0, 0},
{226, 0, 0}, {225, 0, 0}, {223, 0, 0}, {222, 0, 0}, {221, 0, 0},
{219, 0, 0}, {218, 0, 0}, {217, 0, 0}, {216, 0, 0}, {214, 0, 0},
{213, 0, 0}, {212, 0, 0}, {211, 0, 0}, {209, 0, 0}, {208, 0, 0},
{207, 0, 0}, {206, 0, 0}, {204, 0, 0}, {203, 0, 0}, {202, 0, 0},
{201, 0, 0}, {200, 0, 0}, {198, 0, 0}, {197, 0, 0}, {196, 0, 0},
{195, 0, 0}, {194, 0, 0}, {193, 0, 0}, {191, 0, 0}, {190, 0, 0},
{189, 0, 0}, {188, 0, 0}, {186, 0, 0}, {185, 0, 0}, {184, 0, 0},
{183, 0, 0}, {181, 0, 0}, {180, 0, 0}, {179, 0, 0}, {178, 0, 0},
{176, 0, 0}, {175, 0, 0}, {174, 0, 0}, {173, 0, 0}, {171, 0, 0},
{170, 0, 0}, {169, 0, 0}, {168, 0, 0}, {166, 0, 0}, {165, 0, 0},
{164, 0, 0}, {163, 0, 0}, {161, 0, 0}, {160, 0, 0}, {159, 0, 0},
{158, 0, 0}, {157, 0, 0}, {156, 0, 0}, {154, 0, 0}, {153, 0, 0},
{152, 0, 0}, {151, 0, 0}, {150, 0, 0}, {148, 0, 0}, {147, 0, 0},
{146, 0, 0}, {145, 0, 0}, {143, 0, 0}, {142, 0, 0}, {141, 0, 0},
{140, 0, 0}, {138, 0, 0}, {137, 0, 0}, {136, 0, 0}, {135, 0, 0},
{134, 0, 0}, {133, 0, 0}, {133, 0, 0}, {132, 0, 0}, {131, 0, 0},
{130, 0, 0}, {129, 0, 0}, {129, 0, 0}, {128, 0, 0}, {127, 0, 0},
{122, 0, 9}, {117, 0, 18}, {112, 0, 27}, {107, 0, 36}, {102, 0, 45}
};
const rgb_store prism_colormap[1000] = {
{255, 0, 0}, {255, 2, 0}, {255, 4, 0}, {255, 6, 0}, {255, 8, 0},
{255, 10, 0}, {255, 11, 0}, {255, 13, 0}, {255, 15, 0}, {255, 17, 0},
{255, 19, 0}, {255, 21, 0}, {255, 23, 0}, {255, 25, 0}, {255, 27, 0},
{255, 29, 0}, {255, 31, 0}, {255, 33, 0}, {255, 34, 0}, {255, 36, 0},
{255, 38, 0}, {255, 40, 0}, {255, 42, 0}, {255, 44, 0}, {255, 46, 0},
{255, 48, 0}, {255, 50, 0}, {255, 52, 0}, {255, 54, 0}, {255, 56, 0},
{255, 57, 0}, {255, 59, 0}, {255, 61, 0}, {255, 63, 0}, {255, 65, 0},
{255, 67, 0}, {255, 69, 0}, {255, 71, 0}, {255, 73, 0}, {255, 75, 0},
{255, 77, 0}, {255, 78, 0}, {255, 80, 0}, {255, 82, 0}, {255, 84, 0},
{255, 86, 0}, {255, 88, 0}, {255, 90, 0}, {255, 92, 0}, {255, 94, 0},
{255, 96, 0}, {255, 98, 0}, {255, 100, 0}, {255, 101, 0}, {255, 103, 0},
{255, 105, 0}, {255, 107, 0}, {255, 109, 0}, {255, 111, 0}, {255, 113, 0},
{255, 115, 0}, {255, 117, 0}, {255, 119, 0}, {255, 121, 0}, {255, 123, 0},
{255, 124, 0}, {255, 126, 0}, {255, 128, 0}, {255, 130, 0}, {255, 132, 0},
{255, 134, 0}, {255, 136, 0}, {255, 138, 0}, {255, 140, 0}, {255, 142, 0},
{255, 144, 0}, {255, 145, 0}, {255, 147, 0}, {255, 149, 0}, {255, 151, 0},
{255, 153, 0}, {255, 155, 0}, {255, 157, 0}, {255, 159, 0}, {255, 161, 0},
{255, 163, 0}, {255, 165, 0}, {255, 167, 0}, {255, 168, 0}, {255, 170, 0},
{255, 172, 0}, {255, 174, 0}, {255, 176, 0}, {255, 178, 0}, {255, 180, 0},
{255, 182, 0}, {255, 184, 0}, {255, 186, 0}, {255, 188, 0}, {255, 190, 0},
{255, 191, 0}, {255, 193, 0}, {255, 195, 0}, {255, 197, 0}, {255, 199, 0},
{255, 201, 0}, {255, 203, 0}, {255, 205, 0}, {255, 207, 0}, {255, 209, 0},
{255, 211, 0}, {255, 212, 0}, {255, 214, 0}, {255, 216, 0}, {255, 218, 0},
{255, 220, 0}, {255, 222, 0}, {255, 224, 0}, {255, 226, 0}, {255, 228, 0},
{255, 230, 0}, {255, 232, 0}, {255, 234, 0}, {255, 235, 0}, {255, 237, 0},
{255, 239, 0}, {255, 241, 0}, {255, 243, 0}, {255, 245, 0}, {255, 247, 0},
{255, 249, 0}, {255, 251, 0}, {255, 253, 0}, {255, 255, 0}, {252, 255, 0},
{248, 255, 0}, {244, 255, 0}, {240, 255, 0}, {237, 255, 0}, {233, 255, 0},
{229, 255, 0}, {225, 255, 0}, {221, 255, 0}, {217, 255, 0}, {214, 255, 0},
{210, 255, 0}, {206, 255, 0}, {202, 255, 0}, {198, 255, 0}, {195, 255, 0},
{191, 255, 0}, {187, 255, 0}, {183, 255, 0}, {179, 255, 0}, {175, 255, 0},
{172, 255, 0}, {168, 255, 0}, {164, 255, 0}, {160, 255, 0}, {156, 255, 0},
{152, 255, 0}, {149, 255, 0}, {145, 255, 0}, {141, 255, 0}, {137, 255, 0},
{133, 255, 0}, {129, 255, 0}, {126, 255, 0}, {122, 255, 0}, {118, 255, 0},
{114, 255, 0}, {110, 255, 0}, {106, 255, 0}, {103, 255, 0}, { 99, 255, 0},
{ 95, 255, 0}, { 91, 255, 0}, { 87, 255, 0}, { 83, 255, 0}, { 80, 255, 0},
{ 76, 255, 0}, { 72, 255, 0}, { 68, 255, 0}, { 64, 255, 0}, { 60, 255, 0},
{ 57, 255, 0}, { 53, 255, 0}, { 49, 255, 0}, { 45, 255, 0}, { 41, 255, 0},
{ 38, 255, 0}, { 34, 255, 0}, { 30, 255, 0}, { 26, 255, 0}, { 22, 255, 0},
{ 18, 255, 0}, { 15, 255, 0}, { 11, 255, 0}, { 7, 255, 0}, { 3, 255, 0},
{ 0, 254, 1}, { 0, 250, 5}, { 0, 247, 8}, { 0, 243, 12}, { 0, 239, 16},
{ 0, 235, 20}, { 0, 231, 24}, { 0, 227, 28}, { 0, 224, 31}, { 0, 220, 35},
{ 0, 216, 39}, { 0, 212, 43}, { 0, 208, 47}, { 0, 204, 51}, { 0, 201, 54},
{ 0, 197, 58}, { 0, 193, 62}, { 0, 189, 66}, { 0, 185, 70}, { 0, 181, 74},
{ 0, 178, 77}, { 0, 174, 81}, { 0, 170, 85}, { 0, 166, 89}, { 0, 162, 93},
{ 0, 159, 96}, { 0, 155, 100}, { 0, 151, 104}, { 0, 147, 108}, { 0, 143, 112},
{ 0, 139, 116}, { 0, 136, 119}, { 0, 132, 123}, { 0, 128, 127}, { 0, 124, 131},
{ 0, 120, 135}, { 0, 116, 139}, { 0, 113, 142}, { 0, 109, 146}, { 0, 105, 150},
{ 0, 101, 154}, { 0, 97, 158}, { 0, 93, 162}, { 0, 90, 165}, { 0, 86, 169},
{ 0, 82, 173}, { 0, 78, 177}, { 0, 74, 181}, { 0, 70, 185}, { 0, 67, 188},
{ 0, 63, 192}, { 0, 59, 196}, { 0, 55, 200}, { 0, 51, 204}, { 0, 47, 208},
{ 0, 44, 211}, { 0, 40, 215}, { 0, 36, 219}, { 0, 32, 223}, { 0, 28, 227},
{ 0, 25, 230}, { 0, 21, 234}, { 0, 17, 238}, { 0, 13, 242}, { 0, 9, 246},
{ 0, 5, 250}, { 0, 2, 253}, { 2, 0, 255}, { 4, 0, 255}, { 7, 0, 255},
{ 9, 0, 255}, { 12, 0, 255}, { 14, 0, 255}, { 17, 0, 255}, { 19, 0, 255},
{ 22, 0, 255}, { 25, 0, 255}, { 27, 0, 255}, { 30, 0, 255}, { 32, 0, 255},
{ 35, 0, 255}, { 37, 0, 255}, { 40, 0, 255}, { 42, 0, 255}, { 45, 0, 255},
{ 47, 0, 255}, { 50, 0, 255}, { 53, 0, 255}, { 55, 0, 255}, { 58, 0, 255},
{ 60, 0, 255}, { 63, 0, 255}, { 65, 0, 255}, { 68, 0, 255}, { 70, 0, 255},
{ 73, 0, 255}, { 76, 0, 255}, { 78, 0, 255}, { 81, 0, 255}, { 83, 0, 255},
{ 86, 0, 255}, { 88, 0, 255}, { 91, 0, 255}, { 93, 0, 255}, { 96, 0, 255},
{ 99, 0, 255}, {101, 0, 255}, {104, 0, 255}, {106, 0, 255}, {109, 0, 255},
{111, 0, 255}, {114, 0, 255}, {116, 0, 255}, {119, 0, 255}, {122, 0, 255},
{124, 0, 255}, {127, 0, 255}, {129, 0, 255}, {132, 0, 255}, {134, 0, 255},
{137, 0, 255}, {139, 0, 255}, {142, 0, 255}, {144, 0, 255}, {147, 0, 255},
{150, 0, 255}, {152, 0, 255}, {155, 0, 255}, {157, 0, 255}, {160, 0, 255},
{162, 0, 255}, {165, 0, 255}, {167, 0, 255}, {170, 0, 255}, {171, 0, 251},
{173, 0, 247}, {174, 0, 244}, {175, 0, 240}, {176, 0, 236}, {178, 0, 232},
{179, 0, 228}, {180, 0, 224}, {181, 0, 221}, {183, 0, 217}, {184, 0, 213},
{185, 0, 209}, {187, 0, 205}, {188, 0, 201}, {189, 0, 198}, {190, 0, 194},
{192, 0, 190}, {193, 0, 186}, {194, 0, 182}, {196, 0, 178}, {197, 0, 175},
{198, 0, 171}, {199, 0, 167}, {201, 0, 163}, {202, 0, 159}, {203, 0, 155},
{204, 0, 152}, {206, 0, 148}, {207, 0, 144}, {208, 0, 140}, {210, 0, 136},
{211, 0, 132}, {212, 0, 129}, {213, 0, 125}, {215, 0, 121}, {216, 0, 117},
{217, 0, 113}, {218, 0, 110}, {220, 0, 106}, {221, 0, 102}, {222, 0, 98},
{224, 0, 94}, {225, 0, 90}, {226, 0, 87}, {227, 0, 83}, {229, 0, 79},
{230, 0, 75}, {231, 0, 71}, {233, 0, 67}, {234, 0, 64}, {235, 0, 60},
{236, 0, 56}, {238, 0, 52}, {239, 0, 48}, {240, 0, 44}, {241, 0, 41},
{243, 0, 37}, {244, 0, 33}, {245, 0, 29}, {247, 0, 25}, {248, 0, 21},
{249, 0, 18}, {250, 0, 14}, {252, 0, 10}, {253, 0, 6}, {254, 0, 2},
{255, 1, 0}, {255, 3, 0}, {255, 5, 0}, {255, 7, 0}, {255, 8, 0},
{255, 10, 0}, {255, 12, 0}, {255, 14, 0}, {255, 16, 0}, {255, 18, 0},
{255, 20, 0}, {255, 22, 0}, {255, 24, 0}, {255, 26, 0}, {255, 28, 0},
{255, 29, 0}, {255, 31, 0}, {255, 33, 0}, {255, 35, 0}, {255, 37, 0},
{255, 39, 0}, {255, 41, 0}, {255, 43, 0}, {255, 45, 0}, {255, 47, 0},
{255, 49, 0}, {255, 51, 0}, {255, 52, 0}, {255, 54, 0}, {255, 56, 0},
{255, 58, 0}, {255, 60, 0}, {255, 62, 0}, {255, 64, 0}, {255, 66, 0},
{255, 68, 0}, {255, 70, 0}, {255, 72, 0}, {255, 74, 0}, {255, 75, 0},
{255, 77, 0}, {255, 79, 0}, {255, 81, 0}, {255, 83, 0}, {255, 85, 0},
{255, 87, 0}, {255, 89, 0}, {255, 91, 0}, {255, 93, 0}, {255, 95, 0},
{255, 96, 0}, {255, 98, 0}, {255, 100, 0}, {255, 102, 0}, {255, 104, 0},
{255, 106, 0}, {255, 108, 0}, {255, 110, 0}, {255, 112, 0}, {255, 114, 0},
{255, 116, 0}, {255, 118, 0}, {255, 119, 0}, {255, 121, 0}, {255, 123, 0},
{255, 125, 0}, {255, 127, 0}, {255, 129, 0}, {255, 131, 0}, {255, 133, 0},
{255, 135, 0}, {255, 137, 0}, {255, 139, 0}, {255, 141, 0}, {255, 142, 0},
{255, 144, 0}, {255, 146, 0}, {255, 148, 0}, {255, 150, 0}, {255, 152, 0},
{255, 154, 0}, {255, 156, 0}, {255, 158, 0}, {255, 160, 0}, {255, 162, 0},
{255, 163, 0}, {255, 165, 0}, {255, 167, 0}, {255, 169, 0}, {255, 171, 0},
{255, 173, 0}, {255, 175, 0}, {255, 177, 0}, {255, 179, 0}, {255, 181, 0},
{255, 183, 0}, {255, 185, 0}, {255, 186, 0}, {255, 188, 0}, {255, 190, 0},
{255, 192, 0}, {255, 194, 0}, {255, 196, 0}, {255, 198, 0}, {255, 200, 0},
{255, 202, 0}, {255, 204, 0}, {255, 206, 0}, {255, 208, 0}, {255, 209, 0},
{255, 211, 0}, {255, 213, 0}, {255, 215, 0}, {255, 217, 0}, {255, 219, 0},
{255, 221, 0}, {255, 223, 0}, {255, 225, 0}, {255, 227, 0}, {255, 229, 0},
{255, 230, 0}, {255, 232, 0}, {255, 234, 0}, {255, 236, 0}, {255, 238, 0},
{255, 240, 0}, {255, 242, 0}, {255, 244, 0}, {255, 246, 0}, {255, 248, 0},
{255, 250, 0}, {255, 252, 0}, {255, 253, 0}, {254, 255, 0}, {250, 255, 0},
{247, 255, 0}, {243, 255, 0}, {239, 255, 0}, {235, 255, 0}, {231, 255, 0},
{227, 255, 0}, {224, 255, 0}, {220, 255, 0}, {216, 255, 0}, {212, 255, 0},
{208, 255, 0}, {204, 255, 0}, {201, 255, 0}, {197, 255, 0}, {193, 255, 0},
{189, 255, 0}, {185, 255, 0}, {181, 255, 0}, {178, 255, 0}, {174, 255, 0},
{170, 255, 0}, {166, 255, 0}, {162, 255, 0}, {159, 255, 0}, {155, 255, 0},
{151, 255, 0}, {147, 255, 0}, {143, 255, 0}, {139, 255, 0}, {136, 255, 0},
{132, 255, 0}, {128, 255, 0}, {124, 255, 0}, {120, 255, 0}, {116, 255, 0},
{113, 255, 0}, {109, 255, 0}, {105, 255, 0}, {101, 255, 0}, { 97, 255, 0},
{ 93, 255, 0}, { 90, 255, 0}, { 86, 255, 0}, { 82, 255, 0}, { 78, 255, 0},
{ 74, 255, 0}, { 70, 255, 0}, { 67, 255, 0}, { 63, 255, 0}, { 59, 255, 0},
{ 55, 255, 0}, { 51, 255, 0}, { 47, 255, 0}, { 44, 255, 0}, { 40, 255, 0},
{ 36, 255, 0}, { 32, 255, 0}, { 28, 255, 0}, { 25, 255, 0}, { 21, 255, 0},
{ 17, 255, 0}, { 13, 255, 0}, { 9, 255, 0}, { 5, 255, 0}, { 2, 255, 0},
{ 0, 253, 2}, { 0, 249, 6}, { 0, 245, 10}, { 0, 241, 14}, { 0, 237, 18},
{ 0, 234, 21}, { 0, 230, 25}, { 0, 226, 29}, { 0, 222, 33}, { 0, 218, 37},
{ 0, 214, 41}, { 0, 211, 44}, { 0, 207, 48}, { 0, 203, 52}, { 0, 199, 56},
{ 0, 195, 60}, { 0, 191, 64}, { 0, 188, 67}, { 0, 184, 71}, { 0, 180, 75},
{ 0, 176, 79}, { 0, 172, 83}, { 0, 168, 87}, { 0, 165, 90}, { 0, 161, 94},
{ 0, 157, 98}, { 0, 153, 102}, { 0, 149, 106}, { 0, 145, 110}, { 0, 142, 113},
{ 0, 138, 117}, { 0, 134, 121}, { 0, 130, 125}, { 0, 126, 129}, { 0, 123, 132},
{ 0, 119, 136}, { 0, 115, 140}, { 0, 111, 144}, { 0, 107, 148}, { 0, 103, 152},
{ 0, 100, 155}, { 0, 96, 159}, { 0, 92, 163}, { 0, 88, 167}, { 0, 84, 171},
{ 0, 80, 175}, { 0, 77, 178}, { 0, 73, 182}, { 0, 69, 186}, { 0, 65, 190},
{ 0, 61, 194}, { 0, 57, 198}, { 0, 54, 201}, { 0, 50, 205}, { 0, 46, 209},
{ 0, 42, 213}, { 0, 38, 217}, { 0, 34, 221}, { 0, 31, 224}, { 0, 27, 228},
{ 0, 23, 232}, { 0, 19, 236}, { 0, 15, 240}, { 0, 11, 244}, { 0, 8, 247},
{ 0, 4, 251}, { 0, 0, 255}, { 3, 0, 255}, { 5, 0, 255}, { 8, 0, 255},
{ 10, 0, 255}, { 13, 0, 255}, { 15, 0, 255}, { 18, 0, 255}, { 20, 0, 255},
{ 23, 0, 255}, { 26, 0, 255}, { 28, 0, 255}, { 31, 0, 255}, { 33, 0, 255},
{ 36, 0, 255}, { 38, 0, 255}, { 41, 0, 255}, { 43, 0, 255}, { 46, 0, 255},
{ 48, 0, 255}, { 51, 0, 255}, { 54, 0, 255}, { 56, 0, 255}, { 59, 0, 255},
{ 61, 0, 255}, { 64, 0, 255}, { 66, 0, 255}, { 69, 0, 255}, { 71, 0, 255},
{ 74, 0, 255}, { 77, 0, 255}, { 79, 0, 255}, { 82, 0, 255}, { 84, 0, 255},
{ 87, 0, 255}, { 89, 0, 255}, { 92, 0, 255}, { 94, 0, 255}, { 97, 0, 255},
{100, 0, 255}, {102, 0, 255}, {105, 0, 255}, {107, 0, 255}, {110, 0, 255},
{112, 0, 255}, {115, 0, 255}, {117, 0, 255}, {120, 0, 255}, {123, 0, 255},
{125, 0, 255}, {128, 0, 255}, {130, 0, 255}, {133, 0, 255}, {135, 0, 255},
{138, 0, 255}, {140, 0, 255}, {143, 0, 255}, {145, 0, 255}, {148, 0, 255},
{151, 0, 255}, {153, 0, 255}, {156, 0, 255}, {158, 0, 255}, {161, 0, 255},
{163, 0, 255}, {166, 0, 255}, {168, 0, 255}, {171, 0, 253}, {172, 0, 250},
{173, 0, 246}, {174, 0, 242}, {176, 0, 238}, {177, 0, 234}, {178, 0, 230},
{179, 0, 227}, {181, 0, 223}, {182, 0, 219}, {183, 0, 215}, {185, 0, 211},
{186, 0, 208}, {187, 0, 204}, {188, 0, 200}, {190, 0, 196}, {191, 0, 192},
{192, 0, 188}, {193, 0, 185}, {195, 0, 181}, {196, 0, 177}, {197, 0, 173},
{199, 0, 169}, {200, 0, 165}, {201, 0, 162}, {202, 0, 158}, {204, 0, 154},
{205, 0, 150}, {206, 0, 146}, {208, 0, 142}, {209, 0, 139}, {210, 0, 135},
{211, 0, 131}, {213, 0, 127}, {214, 0, 123}, {215, 0, 119}, {216, 0, 116},
{218, 0, 112}, {219, 0, 108}, {220, 0, 104}, {222, 0, 100}, {223, 0, 96},
{224, 0, 93}, {225, 0, 89}, {227, 0, 85}, {228, 0, 81}, {229, 0, 77},
{230, 0, 74}, {232, 0, 70}, {233, 0, 66}, {234, 0, 62}, {236, 0, 58},
{237, 0, 54}, {238, 0, 51}, {239, 0, 47}, {241, 0, 43}, {242, 0, 39},
{243, 0, 35}, {245, 0, 31}, {246, 0, 28}, {247, 0, 24}, {248, 0, 20},
{250, 0, 16}, {251, 0, 12}, {252, 0, 8}, {253, 0, 5}, {255, 0, 1},
{255, 2, 0}, {255, 3, 0}, {255, 5, 0}, {255, 7, 0}, {255, 9, 0},
{255, 11, 0}, {255, 13, 0}, {255, 15, 0}, {255, 17, 0}, {255, 19, 0},
{255, 21, 0}, {255, 23, 0}, {255, 25, 0}, {255, 26, 0}, {255, 28, 0},
{255, 30, 0}, {255, 32, 0}, {255, 34, 0}, {255, 36, 0}, {255, 38, 0},
{255, 40, 0}, {255, 42, 0}, {255, 44, 0}, {255, 46, 0}, {255, 47, 0},
{255, 49, 0}, {255, 51, 0}, {255, 53, 0}, {255, 55, 0}, {255, 57, 0},
{255, 59, 0}, {255, 61, 0}, {255, 63, 0}, {255, 65, 0}, {255, 67, 0},
{255, 69, 0}, {255, 70, 0}, {255, 72, 0}, {255, 74, 0}, {255, 76, 0},
{255, 78, 0}, {255, 80, 0}, {255, 82, 0}, {255, 84, 0}, {255, 86, 0},
{255, 88, 0}, {255, 90, 0}, {255, 92, 0}, {255, 93, 0}, {255, 95, 0},
{255, 97, 0}, {255, 99, 0}, {255, 101, 0}, {255, 103, 0}, {255, 105, 0},
{255, 107, 0}, {255, 109, 0}, {255, 111, 0}, {255, 113, 0}, {255, 114, 0},
{255, 116, 0}, {255, 118, 0}, {255, 120, 0}, {255, 122, 0}, {255, 124, 0},
{255, 126, 0}, {255, 128, 0}, {255, 130, 0}, {255, 132, 0}, {255, 134, 0},
{255, 136, 0}, {255, 137, 0}, {255, 139, 0}, {255, 141, 0}, {255, 143, 0},
{255, 145, 0}, {255, 147, 0}, {255, 149, 0}, {255, 151, 0}, {255, 153, 0},
{255, 155, 0}, {255, 157, 0}, {255, 159, 0}, {255, 160, 0}, {255, 162, 0},
{255, 164, 0}, {255, 166, 0}, {255, 168, 0}, {255, 170, 0}, {255, 172, 0},
{255, 174, 0}, {255, 176, 0}, {255, 178, 0}, {255, 180, 0}, {255, 181, 0},
{255, 183, 0}, {255, 185, 0}, {255, 187, 0}, {255, 189, 0}, {255, 191, 0},
{255, 193, 0}, {255, 195, 0}, {255, 197, 0}, {255, 199, 0}, {255, 201, 0},
{255, 203, 0}, {255, 204, 0}, {255, 206, 0}, {255, 208, 0}, {255, 210, 0},
{255, 212, 0}, {255, 214, 0}, {255, 216, 0}, {255, 218, 0}, {255, 220, 0},
{255, 222, 0}, {255, 224, 0}, {255, 226, 0}, {255, 227, 0}, {255, 229, 0},
{255, 231, 0}, {255, 233, 0}, {255, 235, 0}, {255, 237, 0}, {255, 239, 0},
{255, 241, 0}, {255, 243, 0}, {255, 245, 0}, {255, 247, 0}, {255, 248, 0},
{255, 250, 0}, {255, 252, 0}, {255, 254, 0}, {253, 255, 0}, {249, 255, 0},
{245, 255, 0}, {241, 255, 0}, {237, 255, 0}, {234, 255, 0}, {230, 255, 0},
{226, 255, 0}, {222, 255, 0}, {218, 255, 0}, {214, 255, 0}, {211, 255, 0},
{207, 255, 0}, {203, 255, 0}, {199, 255, 0}, {195, 255, 0}, {191, 255, 0},
{188, 255, 0}, {184, 255, 0}, {180, 255, 0}, {176, 255, 0}, {172, 255, 0},
{168, 255, 0}, {165, 255, 0}, {161, 255, 0}, {157, 255, 0}, {153, 255, 0},
{149, 255, 0}, {145, 255, 0}, {142, 255, 0}, {138, 255, 0}, {134, 255, 0},
{130, 255, 0}, {126, 255, 0}, {123, 255, 0}, {119, 255, 0}, {115, 255, 0},
{111, 255, 0}, {107, 255, 0}, {103, 255, 0}, {100, 255, 0}, { 96, 255, 0},
{ 92, 255, 0}, { 88, 255, 0}, { 84, 255, 0}, { 80, 255, 0}, { 77, 255, 0},
{ 73, 255, 0}, { 69, 255, 0}, { 65, 255, 0}, { 61, 255, 0}, { 57, 255, 0},
{ 54, 255, 0}, { 50, 255, 0}, { 46, 255, 0}, { 42, 255, 0}, { 38, 255, 0},
{ 34, 255, 0}, { 31, 255, 0}, { 27, 255, 0}, { 23, 255, 0}, { 19, 255, 0},
{ 15, 255, 0}, { 11, 255, 0}, { 8, 255, 0}, { 4, 255, 0}, { 0, 255, 0}
};
const rgb_store vga_colormap[1000] = {
{255, 255, 255}, {254, 254, 254}, {253, 253, 253}, {252, 252, 252}, {251, 251, 251},
{250, 250, 250}, {249, 249, 249}, {248, 248, 248}, {247, 247, 247}, {246, 246, 246},
{245, 245, 245}, {244, 244, 244}, {244, 244, 244}, {243, 243, 243}, {242, 242, 242},
{241, 241, 241}, {240, 240, 240}, {239, 239, 239}, {238, 238, 238}, {237, 237, 237},
{236, 236, 236}, {235, 235, 235}, {234, 234, 234}, {233, 233, 233}, {232, 232, 232},
{231, 231, 231}, {230, 230, 230}, {229, 229, 229}, {228, 228, 228}, {227, 227, 227},
{226, 226, 226}, {225, 225, 225}, {224, 224, 224}, {223, 223, 223}, {222, 222, 222},
{221, 221, 221}, {221, 221, 221}, {220, 220, 220}, {219, 219, 219}, {218, 218, 218},
{217, 217, 217}, {216, 216, 216}, {215, 215, 215}, {214, 214, 214}, {213, 213, 213},
{212, 212, 212}, {211, 211, 211}, {210, 210, 210}, {209, 209, 209}, {208, 208, 208},
{207, 207, 207}, {206, 206, 206}, {205, 205, 205}, {204, 204, 204}, {203, 203, 203},
{202, 202, 202}, {201, 201, 201}, {200, 200, 200}, {199, 199, 199}, {199, 199, 199},
{198, 198, 198}, {197, 197, 197}, {196, 196, 196}, {195, 195, 195}, {194, 194, 194},
{193, 193, 193}, {192, 192, 192}, {192, 190, 190}, {193, 187, 187}, {194, 184, 184},
{195, 181, 181}, {195, 179, 179}, {196, 176, 176}, {197, 173, 173}, {198, 170, 170},
{199, 167, 167}, {200, 164, 164}, {201, 161, 161}, {202, 159, 159}, {203, 156, 156},
{204, 153, 153}, {205, 150, 150}, {206, 147, 147}, {207, 144, 144}, {208, 141, 141},
{209, 138, 138}, {210, 136, 136}, {211, 133, 133}, {212, 130, 130}, {213, 127, 127},
{214, 124, 124}, {215, 121, 121}, {216, 118, 118}, {217, 115, 115}, {217, 113, 113},
{218, 110, 110}, {219, 107, 107}, {220, 104, 104}, {221, 101, 101}, {222, 98, 98},
{223, 95, 95}, {224, 92, 92}, {225, 90, 90}, {226, 87, 87}, {227, 84, 84},
{228, 81, 81}, {229, 78, 78}, {230, 75, 75}, {231, 72, 72}, {232, 69, 69},
{233, 67, 67}, {234, 64, 64}, {235, 61, 61}, {236, 58, 58}, {237, 55, 55},
{238, 52, 52}, {239, 49, 49}, {239, 47, 47}, {240, 44, 44}, {241, 41, 41},
{242, 38, 38}, {243, 35, 35}, {244, 32, 32}, {245, 29, 29}, {246, 26, 26},
{247, 24, 24}, {248, 21, 21}, {249, 18, 18}, {250, 15, 15}, {251, 12, 12},
{252, 9, 9}, {253, 6, 6}, {254, 3, 3}, {255, 1, 1}, {255, 3, 0},
{255, 7, 0}, {255, 11, 0}, {255, 15, 0}, {255, 18, 0}, {255, 22, 0},
{255, 26, 0}, {255, 30, 0}, {255, 34, 0}, {255, 38, 0}, {255, 41, 0},
{255, 45, 0}, {255, 49, 0}, {255, 53, 0}, {255, 57, 0}, {255, 60, 0},
{255, 64, 0}, {255, 68, 0}, {255, 72, 0}, {255, 76, 0}, {255, 80, 0},
{255, 83, 0}, {255, 87, 0}, {255, 91, 0}, {255, 95, 0}, {255, 99, 0},
{255, 103, 0}, {255, 106, 0}, {255, 110, 0}, {255, 114, 0}, {255, 118, 0},
{255, 122, 0}, {255, 126, 0}, {255, 129, 0}, {255, 133, 0}, {255, 137, 0},
{255, 141, 0}, {255, 145, 0}, {255, 149, 0}, {255, 152, 0}, {255, 156, 0},
{255, 160, 0}, {255, 164, 0}, {255, 168, 0}, {255, 172, 0}, {255, 175, 0},
{255, 179, 0}, {255, 183, 0}, {255, 187, 0}, {255, 191, 0}, {255, 195, 0},
{255, 198, 0}, {255, 202, 0}, {255, 206, 0}, {255, 210, 0}, {255, 214, 0},
{255, 217, 0}, {255, 221, 0}, {255, 225, 0}, {255, 229, 0}, {255, 233, 0},
{255, 237, 0}, {255, 240, 0}, {255, 244, 0}, {255, 248, 0}, {255, 252, 0},
{254, 255, 0}, {250, 255, 0}, {247, 255, 0}, {243, 255, 0}, {239, 255, 0},
{235, 255, 0}, {231, 255, 0}, {227, 255, 0}, {224, 255, 0}, {220, 255, 0},
{216, 255, 0}, {212, 255, 0}, {208, 255, 0}, {204, 255, 0}, {201, 255, 0},
{197, 255, 0}, {193, 255, 0}, {189, 255, 0}, {185, 255, 0}, {181, 255, 0},
{178, 255, 0}, {174, 255, 0}, {170, 255, 0}, {166, 255, 0}, {162, 255, 0},
{159, 255, 0}, {155, 255, 0}, {151, 255, 0}, {147, 255, 0}, {143, 255, 0},
{139, 255, 0}, {136, 255, 0}, {132, 255, 0}, {128, 255, 0}, {124, 255, 0},
{120, 255, 0}, {116, 255, 0}, {113, 255, 0}, {109, 255, 0}, {105, 255, 0},
{101, 255, 0}, { 97, 255, 0}, { 93, 255, 0}, { 90, 255, 0}, { 86, 255, 0},
{ 82, 255, 0}, { 78, 255, 0}, { 74, 255, 0}, { 70, 255, 0}, { 67, 255, 0},
{ 63, 255, 0}, { 59, 255, 0}, { 55, 255, 0}, { 51, 255, 0}, { 47, 255, 0},
{ 44, 255, 0}, { 40, 255, 0}, { 36, 255, 0}, { 32, 255, 0}, { 28, 255, 0},
{ 25, 255, 0}, { 21, 255, 0}, { 17, 255, 0}, { 13, 255, 0}, { 9, 255, 0},
{ 5, 255, 0}, { 2, 255, 0}, { 0, 255, 2}, { 0, 255, 6}, { 0, 255, 10},
{ 0, 255, 14}, { 0, 255, 18}, { 0, 255, 21}, { 0, 255, 25}, { 0, 255, 29},
{ 0, 255, 33}, { 0, 255, 37}, { 0, 255, 41}, { 0, 255, 44}, { 0, 255, 48},
{ 0, 255, 52}, { 0, 255, 56}, { 0, 255, 60}, { 0, 255, 64}, { 0, 255, 67},
{ 0, 255, 71}, { 0, 255, 75}, { 0, 255, 79}, { 0, 255, 83}, { 0, 255, 87},
{ 0, 255, 90}, { 0, 255, 94}, { 0, 255, 98}, { 0, 255, 102}, { 0, 255, 106},
{ 0, 255, 110}, { 0, 255, 113}, { 0, 255, 117}, { 0, 255, 121}, { 0, 255, 125},
{ 0, 255, 129}, { 0, 255, 132}, { 0, 255, 136}, { 0, 255, 140}, { 0, 255, 144},
{ 0, 255, 148}, { 0, 255, 152}, { 0, 255, 155}, { 0, 255, 159}, { 0, 255, 163},
{ 0, 255, 167}, { 0, 255, 171}, { 0, 255, 175}, { 0, 255, 178}, { 0, 255, 182},
{ 0, 255, 186}, { 0, 255, 190}, { 0, 255, 194}, { 0, 255, 198}, { 0, 255, 201},
{ 0, 255, 205}, { 0, 255, 209}, { 0, 255, 213}, { 0, 255, 217}, { 0, 255, 221},
{ 0, 255, 224}, { 0, 255, 228}, { 0, 255, 232}, { 0, 255, 236}, { 0, 255, 240},
{ 0, 255, 244}, { 0, 255, 247}, { 0, 255, 251}, { 0, 255, 255}, { 0, 251, 255},
{ 0, 247, 255}, { 0, 244, 255}, { 0, 240, 255}, { 0, 236, 255}, { 0, 232, 255},
{ 0, 228, 255}, { 0, 224, 255}, { 0, 221, 255}, { 0, 217, 255}, { 0, 213, 255},
{ 0, 209, 255}, { 0, 205, 255}, { 0, 201, 255}, { 0, 198, 255}, { 0, 194, 255},
{ 0, 190, 255}, { 0, 186, 255}, { 0, 182, 255}, { 0, 178, 255}, { 0, 175, 255},
{ 0, 171, 255}, { 0, 167, 255}, { 0, 163, 255}, { 0, 159, 255}, { 0, 155, 255},
{ 0, 152, 255}, { 0, 148, 255}, { 0, 144, 255}, { 0, 140, 255}, { 0, 136, 255},
{ 0, 132, 255}, { 0, 129, 255}, { 0, 125, 255}, { 0, 121, 255}, { 0, 117, 255},
{ 0, 113, 255}, { 0, 110, 255}, { 0, 106, 255}, { 0, 102, 255}, { 0, 98, 255},
{ 0, 94, 255}, { 0, 90, 255}, { 0, 87, 255}, { 0, 83, 255}, { 0, 79, 255},
{ 0, 75, 255}, { 0, 71, 255}, { 0, 67, 255}, { 0, 64, 255}, { 0, 60, 255},
{ 0, 56, 255}, { 0, 52, 255}, { 0, 48, 255}, { 0, 44, 255}, { 0, 41, 255},
{ 0, 37, 255}, { 0, 33, 255}, { 0, 29, 255}, { 0, 25, 255}, { 0, 21, 255},
{ 0, 18, 255}, { 0, 14, 255}, { 0, 10, 255}, { 0, 6, 255}, { 0, 2, 255},
{ 2, 0, 255}, { 5, 0, 255}, { 9, 0, 255}, { 13, 0, 255}, { 17, 0, 255},
{ 21, 0, 255}, { 25, 0, 255}, { 28, 0, 255}, { 32, 0, 255}, { 36, 0, 255},
{ 40, 0, 255}, { 44, 0, 255}, { 47, 0, 255}, { 51, 0, 255}, { 55, 0, 255},
{ 59, 0, 255}, { 63, 0, 255}, { 67, 0, 255}, { 70, 0, 255}, { 74, 0, 255},
{ 78, 0, 255}, { 82, 0, 255}, { 86, 0, 255}, { 90, 0, 255}, { 93, 0, 255},
{ 97, 0, 255}, {101, 0, 255}, {105, 0, 255}, {109, 0, 255}, {113, 0, 255},
{116, 0, 255}, {120, 0, 255}, {124, 0, 255}, {128, 0, 255}, {132, 0, 255},
{136, 0, 255}, {139, 0, 255}, {143, 0, 255}, {147, 0, 255}, {151, 0, 255},
{155, 0, 255}, {159, 0, 255}, {162, 0, 255}, {166, 0, 255}, {170, 0, 255},
{174, 0, 255}, {178, 0, 255}, {181, 0, 255}, {185, 0, 255}, {189, 0, 255},
{193, 0, 255}, {197, 0, 255}, {201, 0, 255}, {204, 0, 255}, {208, 0, 255},
{212, 0, 255}, {216, 0, 255}, {220, 0, 255}, {224, 0, 255}, {227, 0, 255},
{231, 0, 255}, {235, 0, 255}, {239, 0, 255}, {243, 0, 255}, {247, 0, 255},
{250, 0, 255}, {254, 0, 255}, {252, 0, 252}, {248, 0, 248}, {244, 0, 244},
{240, 0, 240}, {237, 0, 237}, {233, 0, 233}, {229, 0, 229}, {225, 0, 225},
{221, 0, 221}, {217, 0, 217}, {214, 0, 214}, {210, 0, 210}, {206, 0, 206},
{202, 0, 202}, {198, 0, 198}, {195, 0, 195}, {191, 0, 191}, {187, 0, 187},
{183, 0, 183}, {179, 0, 179}, {175, 0, 175}, {172, 0, 172}, {168, 0, 168},
{164, 0, 164}, {160, 0, 160}, {156, 0, 156}, {152, 0, 152}, {149, 0, 149},
{145, 0, 145}, {141, 0, 141}, {137, 0, 137}, {133, 0, 133}, {129, 0, 129},
{126, 0, 126}, {122, 0, 122}, {118, 0, 118}, {114, 0, 114}, {110, 0, 110},
{106, 0, 106}, {103, 0, 103}, { 99, 0, 99}, { 95, 0, 95}, { 91, 0, 91},
{ 87, 0, 87}, { 83, 0, 83}, { 80, 0, 80}, { 76, 0, 76}, { 72, 0, 72},
{ 68, 0, 68}, { 64, 0, 64}, { 60, 0, 60}, { 57, 0, 57}, { 53, 0, 53},
{ 49, 0, 49}, { 45, 0, 45}, { 41, 0, 41}, { 38, 0, 38}, { 34, 0, 34},
{ 30, 0, 30}, { 26, 0, 26}, { 22, 0, 22}, { 18, 0, 18}, { 15, 0, 15},
{ 11, 0, 11}, { 7, 0, 7}, { 3, 0, 3}, { 0, 0, 0}, { 2, 2, 2},
{ 4, 4, 4}, { 6, 6, 6}, { 8, 8, 8}, { 10, 10, 10}, { 12, 12, 12},
{ 14, 14, 14}, { 16, 16, 16}, { 18, 18, 18}, { 20, 20, 20}, { 21, 21, 21},
{ 23, 23, 23}, { 25, 25, 25}, { 27, 27, 27}, { 29, 29, 29}, { 31, 31, 31},
{ 33, 33, 33}, { 35, 35, 35}, { 37, 37, 37}, { 39, 39, 39}, { 41, 41, 41},
{ 43, 43, 43}, { 44, 44, 44}, { 46, 46, 46}, { 48, 48, 48}, { 50, 50, 50},
{ 52, 52, 52}, { 54, 54, 54}, { 56, 56, 56}, { 58, 58, 58}, { 60, 60, 60},
{ 62, 62, 62}, { 64, 64, 64}, { 65, 65, 65}, { 67, 67, 67}, { 69, 69, 69},
{ 71, 71, 71}, { 73, 73, 73}, { 75, 75, 75}, { 77, 77, 77}, { 79, 79, 79},
{ 81, 81, 81}, { 83, 83, 83}, { 85, 85, 85}, { 87, 87, 87}, { 88, 88, 88},
{ 90, 90, 90}, { 92, 92, 92}, { 94, 94, 94}, { 96, 96, 96}, { 98, 98, 98},
{100, 100, 100}, {102, 102, 102}, {104, 104, 104}, {106, 106, 106}, {108, 108, 108},
{110, 110, 110}, {111, 111, 111}, {113, 113, 113}, {115, 115, 115}, {117, 117, 117},
{119, 119, 119}, {121, 121, 121}, {123, 123, 123}, {125, 125, 125}, {127, 127, 127},
{128, 126, 126}, {128, 124, 124}, {128, 123, 123}, {128, 121, 121}, {128, 119, 119},
{128, 117, 117}, {128, 115, 115}, {128, 113, 113}, {128, 111, 111}, {128, 109, 109},
{128, 107, 107}, {128, 105, 105}, {128, 103, 103}, {128, 101, 101}, {128, 100, 100},
{128, 98, 98}, {128, 96, 96}, {128, 94, 94}, {128, 92, 92}, {128, 90, 90},
{128, 88, 88}, {128, 86, 86}, {128, 84, 84}, {128, 82, 82}, {128, 80, 80},
{128, 78, 78}, {128, 77, 77}, {128, 75, 75}, {128, 73, 73}, {128, 71, 71},
{128, 69, 69}, {128, 67, 67}, {128, 65, 65}, {128, 63, 63}, {128, 61, 61},
{128, 59, 59}, {128, 57, 57}, {128, 56, 56}, {128, 54, 54}, {128, 52, 52},
{128, 50, 50}, {128, 48, 48}, {128, 46, 46}, {128, 44, 44}, {128, 42, 42},
{128, 40, 40}, {128, 38, 38}, {128, 36, 36}, {128, 34, 34}, {128, 33, 33},
{128, 31, 31}, {128, 29, 29}, {128, 27, 27}, {128, 25, 25}, {128, 23, 23},
{128, 21, 21}, {128, 19, 19}, {128, 17, 17}, {128, 15, 15}, {128, 13, 13},
{128, 11, 11}, {128, 10, 10}, {128, 8, 8}, {128, 6, 6}, {128, 4, 4},
{128, 2, 2}, {128, 0, 0}, {128, 2, 0}, {128, 4, 0}, {128, 6, 0},
{128, 8, 0}, {128, 10, 0}, {128, 11, 0}, {128, 13, 0}, {128, 15, 0},
{128, 17, 0}, {128, 19, 0}, {128, 21, 0}, {128, 23, 0}, {128, 25, 0},
{128, 27, 0}, {128, 29, 0}, {128, 31, 0}, {128, 33, 0}, {128, 34, 0},
{128, 36, 0}, {128, 38, 0}, {128, 40, 0}, {128, 42, 0}, {128, 44, 0},
{128, 46, 0}, {128, 48, 0}, {128, 50, 0}, {128, 52, 0}, {128, 54, 0},
{128, 56, 0}, {128, 57, 0}, {128, 59, 0}, {128, 61, 0}, {128, 63, 0},
{128, 65, 0}, {128, 67, 0}, {128, 69, 0}, {128, 71, 0}, {128, 73, 0},
{128, 75, 0}, {128, 77, 0}, {128, 78, 0}, {128, 80, 0}, {128, 82, 0},
{128, 84, 0}, {128, 86, 0}, {128, 88, 0}, {128, 90, 0}, {128, 92, 0},
{128, 94, 0}, {128, 96, 0}, {128, 98, 0}, {128, 100, 0}, {128, 101, 0},
{128, 103, 0}, {128, 105, 0}, {128, 107, 0}, {128, 109, 0}, {128, 111, 0},
{128, 113, 0}, {128, 115, 0}, {128, 117, 0}, {128, 119, 0}, {128, 121, 0},
{128, 123, 0}, {128, 124, 0}, {128, 126, 0}, {127, 128, 0}, {125, 128, 0},
{123, 128, 0}, {121, 128, 0}, {119, 128, 0}, {117, 128, 0}, {115, 128, 0},
{113, 128, 0}, {111, 128, 0}, {110, 128, 0}, {108, 128, 0}, {106, 128, 0},
{104, 128, 0}, {102, 128, 0}, {100, 128, 0}, { 98, 128, 0}, { 96, 128, 0},
{ 94, 128, 0}, { 92, 128, 0}, { 90, 128, 0}, { 88, 128, 0}, { 87, 128, 0},
{ 85, 128, 0}, { 83, 128, 0}, { 81, 128, 0}, { 79, 128, 0}, { 77, 128, 0},
{ 75, 128, 0}, { 73, 128, 0}, { 71, 128, 0}, { 69, 128, 0}, { 67, 128, 0},
{ 65, 128, 0}, { 64, 128, 0}, { 62, 128, 0}, { 60, 128, 0}, { 58, 128, 0},
{ 56, 128, 0}, { 54, 128, 0}, { 52, 128, 0}, { 50, 128, 0}, { 48, 128, 0},
{ 46, 128, 0}, { 44, 128, 0}, { 43, 128, 0}, { 41, 128, 0}, { 39, 128, 0},
{ 37, 128, 0}, { 35, 128, 0}, { 33, 128, 0}, { 31, 128, 0}, { 29, 128, 0},
{ 27, 128, 0}, { 25, 128, 0}, { 23, 128, 0}, { 21, 128, 0}, { 20, 128, 0},
{ 18, 128, 0}, { 16, 128, 0}, { 14, 128, 0}, { 12, 128, 0}, { 10, 128, 0},
{ 8, 128, 0}, { 6, 128, 0}, { 4, 128, 0}, { 2, 128, 0}, { 0, 128, 0},
{ 0, 128, 2}, { 0, 128, 3}, { 0, 128, 5}, { 0, 128, 7}, { 0, 128, 9},
{ 0, 128, 11}, { 0, 128, 13}, { 0, 128, 15}, { 0, 128, 17}, { 0, 128, 19},
{ 0, 128, 21}, { 0, 128, 23}, { 0, 128, 25}, { 0, 128, 26}, { 0, 128, 28},
{ 0, 128, 30}, { 0, 128, 32}, { 0, 128, 34}, { 0, 128, 36}, { 0, 128, 38},
{ 0, 128, 40}, { 0, 128, 42}, { 0, 128, 44}, { 0, 128, 46}, { 0, 128, 47},
{ 0, 128, 49}, { 0, 128, 51}, { 0, 128, 53}, { 0, 128, 55}, { 0, 128, 57},
{ 0, 128, 59}, { 0, 128, 61}, { 0, 128, 63}, { 0, 128, 65}, { 0, 128, 67},
{ 0, 128, 69}, { 0, 128, 70}, { 0, 128, 72}, { 0, 128, 74}, { 0, 128, 76},
{ 0, 128, 78}, { 0, 128, 80}, { 0, 128, 82}, { 0, 128, 84}, { 0, 128, 86},
{ 0, 128, 88}, { 0, 128, 90}, { 0, 128, 92}, { 0, 128, 93}, { 0, 128, 95},
{ 0, 128, 97}, { 0, 128, 99}, { 0, 128, 101}, { 0, 128, 103}, { 0, 128, 105},
{ 0, 128, 107}, { 0, 128, 109}, { 0, 128, 111}, { 0, 128, 113}, { 0, 128, 114},
{ 0, 128, 116}, { 0, 128, 118}, { 0, 128, 120}, { 0, 128, 122}, { 0, 128, 124},
{ 0, 128, 126}, { 0, 127, 128}, { 0, 125, 128}, { 0, 123, 128}, { 0, 121, 128},
{ 0, 119, 128}, { 0, 118, 128}, { 0, 116, 128}, { 0, 114, 128}, { 0, 112, 128},
{ 0, 110, 128}, { 0, 108, 128}, { 0, 106, 128}, { 0, 104, 128}, { 0, 102, 128},
{ 0, 100, 128}, { 0, 98, 128}, { 0, 96, 128}, { 0, 95, 128}, { 0, 93, 128},
{ 0, 91, 128}, { 0, 89, 128}, { 0, 87, 128}, { 0, 85, 128}, { 0, 83, 128},
{ 0, 81, 128}, { 0, 79, 128}, { 0, 77, 128}, { 0, 75, 128}, { 0, 74, 128},
{ 0, 72, 128}, { 0, 70, 128}, { 0, 68, 128}, { 0, 66, 128}, { 0, 64, 128},
{ 0, 62, 128}, { 0, 60, 128}, { 0, 58, 128}, { 0, 56, 128}, { 0, 54, 128},
{ 0, 52, 128}, { 0, 51, 128}, { 0, 49, 128}, { 0, 47, 128}, { 0, 45, 128},
{ 0, 43, 128}, { 0, 41, 128}, { 0, 39, 128}, { 0, 37, 128}, { 0, 35, 128},
{ 0, 33, 128}, { 0, 31, 128}, { 0, 29, 128}, { 0, 28, 128}, { 0, 26, 128},
{ 0, 24, 128}, { 0, 22, 128}, { 0, 20, 128}, { 0, 18, 128}, { 0, 16, 128},
{ 0, 14, 128}, { 0, 12, 128}, { 0, 10, 128}, { 0, 8, 128}, { 0, 7, 128},
{ 0, 5, 128}, { 0, 3, 128}, { 0, 1, 128}, { 1, 0, 128}, { 3, 0, 128},
{ 5, 0, 128}, { 7, 0, 128}, { 9, 0, 128}, { 11, 0, 128}, { 13, 0, 128},
{ 15, 0, 128}, { 16, 0, 128}, { 18, 0, 128}, { 20, 0, 128}, { 22, 0, 128},
{ 24, 0, 128}, { 26, 0, 128}, { 28, 0, 128}, { 30, 0, 128}, { 32, 0, 128},
{ 34, 0, 128}, { 36, 0, 128}, { 38, 0, 128}, { 39, 0, 128}, { 41, 0, 128},
{ 43, 0, 128}, { 45, 0, 128}, { 47, 0, 128}, { 49, 0, 128}, { 51, 0, 128},
{ 53, 0, 128}, { 55, 0, 128}, { 57, 0, 128}, { 59, 0, 128}, { 60, 0, 128},
{ 62, 0, 128}, { 64, 0, 128}, { 66, 0, 128}, { 68, 0, 128}, { 70, 0, 128},
{ 72, 0, 128}, { 74, 0, 128}, { 76, 0, 128}, { 78, 0, 128}, { 80, 0, 128},
{ 82, 0, 128}, { 83, 0, 128}, { 85, 0, 128}, { 87, 0, 128}, { 89, 0, 128},
{ 91, 0, 128}, { 93, 0, 128}, { 95, 0, 128}, { 97, 0, 128}, { 99, 0, 128},
{101, 0, 128}, {103, 0, 128}, {105, 0, 128}, {106, 0, 128}, {108, 0, 128},
{110, 0, 128}, {112, 0, 128}, {114, 0, 128}, {116, 0, 128}, {118, 0, 128},
{120, 0, 128}, {122, 0, 128}, {124, 0, 128}, {126, 0, 128}, {128, 0, 128}
};
const rgb_store yarg_colormap[1000] = {
{ 0, 0, 0}, { 0, 0, 0}, { 1, 1, 1}, { 1, 1, 1}, { 1, 1, 1},
{ 1, 1, 1}, { 2, 2, 2}, { 2, 2, 2}, { 2, 2, 2}, { 2, 2, 2},
{ 3, 3, 3}, { 3, 3, 3}, { 3, 3, 3}, { 3, 3, 3}, { 4, 4, 4},
{ 4, 4, 4}, { 4, 4, 4}, { 4, 4, 4}, { 5, 5, 5}, { 5, 5, 5},
{ 5, 5, 5}, { 5, 5, 5}, { 6, 6, 6}, { 6, 6, 6}, { 6, 6, 6},
{ 6, 6, 6}, { 7, 7, 7}, { 7, 7, 7}, { 7, 7, 7}, { 7, 7, 7},
{ 8, 8, 8}, { 8, 8, 8}, { 8, 8, 8}, { 8, 8, 8}, { 9, 9, 9},
{ 9, 9, 9}, { 9, 9, 9}, { 9, 9, 9}, { 10, 10, 10}, { 10, 10, 10},
{ 10, 10, 10}, { 10, 10, 10}, { 11, 11, 11}, { 11, 11, 11}, { 11, 11, 11},
{ 11, 11, 11}, { 12, 12, 12}, { 12, 12, 12}, { 12, 12, 12}, { 13, 13, 13},
{ 13, 13, 13}, { 13, 13, 13}, { 13, 13, 13}, { 14, 14, 14}, { 14, 14, 14},
{ 14, 14, 14}, { 14, 14, 14}, { 15, 15, 15}, { 15, 15, 15}, { 15, 15, 15},
{ 15, 15, 15}, { 16, 16, 16}, { 16, 16, 16}, { 16, 16, 16}, { 16, 16, 16},
{ 17, 17, 17}, { 17, 17, 17}, { 17, 17, 17}, { 17, 17, 17}, { 18, 18, 18},
{ 18, 18, 18}, { 18, 18, 18}, { 18, 18, 18}, { 19, 19, 19}, { 19, 19, 19},
{ 19, 19, 19}, { 19, 19, 19}, { 20, 20, 20}, { 20, 20, 20}, { 20, 20, 20},
{ 20, 20, 20}, { 21, 21, 21}, { 21, 21, 21}, { 21, 21, 21}, { 21, 21, 21},
{ 22, 22, 22}, { 22, 22, 22}, { 22, 22, 22}, { 22, 22, 22}, { 23, 23, 23},
{ 23, 23, 23}, { 23, 23, 23}, { 23, 23, 23}, { 24, 24, 24}, { 24, 24, 24},
{ 24, 24, 24}, { 25, 25, 25}, { 25, 25, 25}, { 25, 25, 25}, { 25, 25, 25},
{ 26, 26, 26}, { 26, 26, 26}, { 26, 26, 26}, { 26, 26, 26}, { 27, 27, 27},
{ 27, 27, 27}, { 27, 27, 27}, { 27, 27, 27}, { 28, 28, 28}, { 28, 28, 28},
{ 28, 28, 28}, { 28, 28, 28}, { 29, 29, 29}, { 29, 29, 29}, { 29, 29, 29},
{ 29, 29, 29}, { 30, 30, 30}, { 30, 30, 30}, { 30, 30, 30}, { 30, 30, 30},
{ 31, 31, 31}, { 31, 31, 31}, { 31, 31, 31}, { 31, 31, 31}, { 32, 32, 32},
{ 32, 32, 32}, { 32, 32, 32}, { 32, 32, 32}, { 33, 33, 33}, { 33, 33, 33},
{ 33, 33, 33}, { 33, 33, 33}, { 34, 34, 34}, { 34, 34, 34}, { 34, 34, 34},
{ 34, 34, 34}, { 35, 35, 35}, { 35, 35, 35}, { 35, 35, 35}, { 35, 35, 35},
{ 36, 36, 36}, { 36, 36, 36}, { 36, 36, 36}, { 37, 37, 37}, { 37, 37, 37},
{ 37, 37, 37}, { 37, 37, 37}, { 38, 38, 38}, { 38, 38, 38}, { 38, 38, 38},
{ 38, 38, 38}, { 39, 39, 39}, { 39, 39, 39}, { 39, 39, 39}, { 39, 39, 39},
{ 40, 40, 40}, { 40, 40, 40}, { 40, 40, 40}, { 40, 40, 40}, { 41, 41, 41},
{ 41, 41, 41}, { 41, 41, 41}, { 41, 41, 41}, { 42, 42, 42}, { 42, 42, 42},
{ 42, 42, 42}, { 42, 42, 42}, { 43, 43, 43}, { 43, 43, 43}, { 43, 43, 43},
{ 43, 43, 43}, { 44, 44, 44}, { 44, 44, 44}, { 44, 44, 44}, { 44, 44, 44},
{ 45, 45, 45}, { 45, 45, 45}, { 45, 45, 45}, { 45, 45, 45}, { 46, 46, 46},
{ 46, 46, 46}, { 46, 46, 46}, { 46, 46, 46}, { 47, 47, 47}, { 47, 47, 47},
{ 47, 47, 47}, { 47, 47, 47}, { 48, 48, 48}, { 48, 48, 48}, { 48, 48, 48},
{ 48, 48, 48}, { 49, 49, 49}, { 49, 49, 49}, { 49, 49, 49}, { 50, 50, 50},
{ 50, 50, 50}, { 50, 50, 50}, { 50, 50, 50}, { 51, 51, 51}, { 51, 51, 51},
{ 51, 51, 51}, { 51, 51, 51}, { 52, 52, 52}, { 52, 52, 52}, { 52, 52, 52},
{ 52, 52, 52}, { 53, 53, 53}, { 53, 53, 53}, { 53, 53, 53}, { 53, 53, 53},
{ 54, 54, 54}, { 54, 54, 54}, { 54, 54, 54}, { 54, 54, 54}, { 55, 55, 55},
{ 55, 55, 55}, { 55, 55, 55}, { 55, 55, 55}, { 56, 56, 56}, { 56, 56, 56},
{ 56, 56, 56}, { 56, 56, 56}, { 57, 57, 57}, { 57, 57, 57}, { 57, 57, 57},
{ 57, 57, 57}, { 58, 58, 58}, { 58, 58, 58}, { 58, 58, 58}, { 58, 58, 58},
{ 59, 59, 59}, { 59, 59, 59}, { 59, 59, 59}, { 59, 59, 59}, { 60, 60, 60},
{ 60, 60, 60}, { 60, 60, 60}, { 60, 60, 60}, { 61, 61, 61}, { 61, 61, 61},
{ 61, 61, 61}, { 62, 62, 62}, { 62, 62, 62}, { 62, 62, 62}, { 62, 62, 62},
{ 63, 63, 63}, { 63, 63, 63}, { 63, 63, 63}, { 63, 63, 63}, { 64, 64, 64},
{ 64, 64, 64}, { 64, 64, 64}, { 64, 64, 64}, { 65, 65, 65}, { 65, 65, 65},
{ 65, 65, 65}, { 65, 65, 65}, { 66, 66, 66}, { 66, 66, 66}, { 66, 66, 66},
{ 66, 66, 66}, { 67, 67, 67}, { 67, 67, 67}, { 67, 67, 67}, { 67, 67, 67},
{ 68, 68, 68}, { 68, 68, 68}, { 68, 68, 68}, { 68, 68, 68}, { 69, 69, 69},
{ 69, 69, 69}, { 69, 69, 69}, { 69, 69, 69}, { 70, 70, 70}, { 70, 70, 70},
{ 70, 70, 70}, { 70, 70, 70}, { 71, 71, 71}, { 71, 71, 71}, { 71, 71, 71},
{ 71, 71, 71}, { 72, 72, 72}, { 72, 72, 72}, { 72, 72, 72}, { 72, 72, 72},
{ 73, 73, 73}, { 73, 73, 73}, { 73, 73, 73}, { 74, 74, 74}, { 74, 74, 74},
{ 74, 74, 74}, { 74, 74, 74}, { 75, 75, 75}, { 75, 75, 75}, { 75, 75, 75},
{ 75, 75, 75}, { 76, 76, 76}, { 76, 76, 76}, { 76, 76, 76}, { 76, 76, 76},
{ 77, 77, 77}, { 77, 77, 77}, { 77, 77, 77}, { 77, 77, 77}, { 78, 78, 78},
{ 78, 78, 78}, { 78, 78, 78}, { 78, 78, 78}, { 79, 79, 79}, { 79, 79, 79},
{ 79, 79, 79}, { 79, 79, 79}, { 80, 80, 80}, { 80, 80, 80}, { 80, 80, 80},
{ 80, 80, 80}, { 81, 81, 81}, { 81, 81, 81}, { 81, 81, 81}, { 81, 81, 81},
{ 82, 82, 82}, { 82, 82, 82}, { 82, 82, 82}, { 82, 82, 82}, { 83, 83, 83},
{ 83, 83, 83}, { 83, 83, 83}, { 83, 83, 83}, { 84, 84, 84}, { 84, 84, 84},
{ 84, 84, 84}, { 84, 84, 84}, { 85, 85, 85}, { 85, 85, 85}, { 85, 85, 85},
{ 86, 86, 86}, { 86, 86, 86}, { 86, 86, 86}, { 86, 86, 86}, { 87, 87, 87},
{ 87, 87, 87}, { 87, 87, 87}, { 87, 87, 87}, { 88, 88, 88}, { 88, 88, 88},
{ 88, 88, 88}, { 88, 88, 88}, { 89, 89, 89}, { 89, 89, 89}, { 89, 89, 89},
{ 89, 89, 89}, { 90, 90, 90}, { 90, 90, 90}, { 90, 90, 90}, { 90, 90, 90},
{ 91, 91, 91}, { 91, 91, 91}, { 91, 91, 91}, { 91, 91, 91}, { 92, 92, 92},
{ 92, 92, 92}, { 92, 92, 92}, { 92, 92, 92}, { 93, 93, 93}, { 93, 93, 93},
{ 93, 93, 93}, { 93, 93, 93}, { 94, 94, 94}, { 94, 94, 94}, { 94, 94, 94},
{ 94, 94, 94}, { 95, 95, 95}, { 95, 95, 95}, { 95, 95, 95}, { 95, 95, 95},
{ 96, 96, 96}, { 96, 96, 96}, { 96, 96, 96}, { 96, 96, 96}, { 97, 97, 97},
{ 97, 97, 97}, { 97, 97, 97}, { 98, 98, 98}, { 98, 98, 98}, { 98, 98, 98},
{ 98, 98, 98}, { 99, 99, 99}, { 99, 99, 99}, { 99, 99, 99}, { 99, 99, 99},
{100, 100, 100}, {100, 100, 100}, {100, 100, 100}, {100, 100, 100}, {101, 101, 101},
{101, 101, 101}, {101, 101, 101}, {101, 101, 101}, {102, 102, 102}, {102, 102, 102},
{102, 102, 102}, {102, 102, 102}, {103, 103, 103}, {103, 103, 103}, {103, 103, 103},
{103, 103, 103}, {104, 104, 104}, {104, 104, 104}, {104, 104, 104}, {104, 104, 104},
{105, 105, 105}, {105, 105, 105}, {105, 105, 105}, {105, 105, 105}, {106, 106, 106},
{106, 106, 106}, {106, 106, 106}, {106, 106, 106}, {107, 107, 107}, {107, 107, 107},
{107, 107, 107}, {107, 107, 107}, {108, 108, 108}, {108, 108, 108}, {108, 108, 108},
{108, 108, 108}, {109, 109, 109}, {109, 109, 109}, {109, 109, 109}, {110, 110, 110},
{110, 110, 110}, {110, 110, 110}, {110, 110, 110}, {111, 111, 111}, {111, 111, 111},
{111, 111, 111}, {111, 111, 111}, {112, 112, 112}, {112, 112, 112}, {112, 112, 112},
{112, 112, 112}, {113, 113, 113}, {113, 113, 113}, {113, 113, 113}, {113, 113, 113},
{114, 114, 114}, {114, 114, 114}, {114, 114, 114}, {114, 114, 114}, {115, 115, 115},
{115, 115, 115}, {115, 115, 115}, {115, 115, 115}, {116, 116, 116}, {116, 116, 116},
{116, 116, 116}, {116, 116, 116}, {117, 117, 117}, {117, 117, 117}, {117, 117, 117},
{117, 117, 117}, {118, 118, 118}, {118, 118, 118}, {118, 118, 118}, {118, 118, 118},
{119, 119, 119}, {119, 119, 119}, {119, 119, 119}, {119, 119, 119}, {120, 120, 120},
{120, 120, 120}, {120, 120, 120}, {120, 120, 120}, {121, 121, 121}, {121, 121, 121},
{121, 121, 121}, {122, 122, 122}, {122, 122, 122}, {122, 122, 122}, {122, 122, 122},
{123, 123, 123}, {123, 123, 123}, {123, 123, 123}, {123, 123, 123}, {124, 124, 124},
{124, 124, 124}, {124, 124, 124}, {124, 124, 124}, {125, 125, 125}, {125, 125, 125},
{125, 125, 125}, {125, 125, 125}, {126, 126, 126}, {126, 126, 126}, {126, 126, 126},
{126, 126, 126}, {127, 127, 127}, {127, 127, 127}, {127, 127, 127}, {127, 127, 127},
{128, 128, 128}, {128, 128, 128}, {128, 128, 128}, {128, 128, 128}, {129, 129, 129},
{129, 129, 129}, {129, 129, 129}, {129, 129, 129}, {130, 130, 130}, {130, 130, 130},
{130, 130, 130}, {130, 130, 130}, {131, 131, 131}, {131, 131, 131}, {131, 131, 131},
{131, 131, 131}, {132, 132, 132}, {132, 132, 132}, {132, 132, 132}, {132, 132, 132},
{133, 133, 133}, {133, 133, 133}, {133, 133, 133}, {133, 133, 133}, {134, 134, 134},
{134, 134, 134}, {134, 134, 134}, {135, 135, 135}, {135, 135, 135}, {135, 135, 135},
{135, 135, 135}, {136, 136, 136}, {136, 136, 136}, {136, 136, 136}, {136, 136, 136},
{137, 137, 137}, {137, 137, 137}, {137, 137, 137}, {137, 137, 137}, {138, 138, 138},
{138, 138, 138}, {138, 138, 138}, {138, 138, 138}, {139, 139, 139}, {139, 139, 139},
{139, 139, 139}, {139, 139, 139}, {140, 140, 140}, {140, 140, 140}, {140, 140, 140},
{140, 140, 140}, {141, 141, 141}, {141, 141, 141}, {141, 141, 141}, {141, 141, 141},
{142, 142, 142}, {142, 142, 142}, {142, 142, 142}, {142, 142, 142}, {143, 143, 143},
{143, 143, 143}, {143, 143, 143}, {143, 143, 143}, {144, 144, 144}, {144, 144, 144},
{144, 144, 144}, {144, 144, 144}, {145, 145, 145}, {145, 145, 145}, {145, 145, 145},
{145, 145, 145}, {146, 146, 146}, {146, 146, 146}, {146, 146, 146}, {147, 147, 147},
{147, 147, 147}, {147, 147, 147}, {147, 147, 147}, {148, 148, 148}, {148, 148, 148},
{148, 148, 148}, {148, 148, 148}, {149, 149, 149}, {149, 149, 149}, {149, 149, 149},
{149, 149, 149}, {150, 150, 150}, {150, 150, 150}, {150, 150, 150}, {150, 150, 150},
{151, 151, 151}, {151, 151, 151}, {151, 151, 151}, {151, 151, 151}, {152, 152, 152},
{152, 152, 152}, {152, 152, 152}, {152, 152, 152}, {153, 153, 153}, {153, 153, 153},
{153, 153, 153}, {153, 153, 153}, {154, 154, 154}, {154, 154, 154}, {154, 154, 154},
{154, 154, 154}, {155, 155, 155}, {155, 155, 155}, {155, 155, 155}, {155, 155, 155},
{156, 156, 156}, {156, 156, 156}, {156, 156, 156}, {156, 156, 156}, {157, 157, 157},
{157, 157, 157}, {157, 157, 157}, {157, 157, 157}, {158, 158, 158}, {158, 158, 158},
{158, 158, 158}, {159, 159, 159}, {159, 159, 159}, {159, 159, 159}, {159, 159, 159},
{160, 160, 160}, {160, 160, 160}, {160, 160, 160}, {160, 160, 160}, {161, 161, 161},
{161, 161, 161}, {161, 161, 161}, {161, 161, 161}, {162, 162, 162}, {162, 162, 162},
{162, 162, 162}, {162, 162, 162}, {163, 163, 163}, {163, 163, 163}, {163, 163, 163},
{163, 163, 163}, {164, 164, 164}, {164, 164, 164}, {164, 164, 164}, {164, 164, 164},
{165, 165, 165}, {165, 165, 165}, {165, 165, 165}, {165, 165, 165}, {166, 166, 166},
{166, 166, 166}, {166, 166, 166}, {166, 166, 166}, {167, 167, 167}, {167, 167, 167},
{167, 167, 167}, {167, 167, 167}, {168, 168, 168}, {168, 168, 168}, {168, 168, 168},
{168, 168, 168}, {169, 169, 169}, {169, 169, 169}, {169, 169, 169}, {169, 169, 169},
{170, 170, 170}, {170, 170, 170}, {170, 170, 170}, {171, 171, 171}, {171, 171, 171},
{171, 171, 171}, {171, 171, 171}, {172, 172, 172}, {172, 172, 172}, {172, 172, 172},
{172, 172, 172}, {173, 173, 173}, {173, 173, 173}, {173, 173, 173}, {173, 173, 173},
{174, 174, 174}, {174, 174, 174}, {174, 174, 174}, {174, 174, 174}, {175, 175, 175},
{175, 175, 175}, {175, 175, 175}, {175, 175, 175}, {176, 176, 176}, {176, 176, 176},
{176, 176, 176}, {176, 176, 176}, {177, 177, 177}, {177, 177, 177}, {177, 177, 177},
{177, 177, 177}, {178, 178, 178}, {178, 178, 178}, {178, 178, 178}, {178, 178, 178},
{179, 179, 179}, {179, 179, 179}, {179, 179, 179}, {179, 179, 179}, {180, 180, 180},
{180, 180, 180}, {180, 180, 180}, {180, 180, 180}, {181, 181, 181}, {181, 181, 181},
{181, 181, 181}, {181, 181, 181}, {182, 182, 182}, {182, 182, 182}, {182, 182, 182},
{183, 183, 183}, {183, 183, 183}, {183, 183, 183}, {183, 183, 183}, {184, 184, 184},
{184, 184, 184}, {184, 184, 184}, {184, 184, 184}, {185, 185, 185}, {185, 185, 185},
{185, 185, 185}, {185, 185, 185}, {186, 186, 186}, {186, 186, 186}, {186, 186, 186},
{186, 186, 186}, {187, 187, 187}, {187, 187, 187}, {187, 187, 187}, {187, 187, 187},
{188, 188, 188}, {188, 188, 188}, {188, 188, 188}, {188, 188, 188}, {189, 189, 189},
{189, 189, 189}, {189, 189, 189}, {189, 189, 189}, {190, 190, 190}, {190, 190, 190},
{190, 190, 190}, {190, 190, 190}, {191, 191, 191}, {191, 191, 191}, {191, 191, 191},
{191, 191, 191}, {192, 192, 192}, {192, 192, 192}, {192, 192, 192}, {192, 192, 192},
{193, 193, 193}, {193, 193, 193}, {193, 193, 193}, {193, 193, 193}, {194, 194, 194},
{194, 194, 194}, {194, 194, 194}, {195, 195, 195}, {195, 195, 195}, {195, 195, 195},
{195, 195, 195}, {196, 196, 196}, {196, 196, 196}, {196, 196, 196}, {196, 196, 196},
{197, 197, 197}, {197, 197, 197}, {197, 197, 197}, {197, 197, 197}, {198, 198, 198},
{198, 198, 198}, {198, 198, 198}, {198, 198, 198}, {199, 199, 199}, {199, 199, 199},
{199, 199, 199}, {199, 199, 199}, {200, 200, 200}, {200, 200, 200}, {200, 200, 200},
{200, 200, 200}, {201, 201, 201}, {201, 201, 201}, {201, 201, 201}, {201, 201, 201},
{202, 202, 202}, {202, 202, 202}, {202, 202, 202}, {202, 202, 202}, {203, 203, 203},
{203, 203, 203}, {203, 203, 203}, {203, 203, 203}, {204, 204, 204}, {204, 204, 204},
{204, 204, 204}, {204, 204, 204}, {205, 205, 205}, {205, 205, 205}, {205, 205, 205},
{205, 205, 205}, {206, 206, 206}, {206, 206, 206}, {206, 206, 206}, {207, 207, 207},
{207, 207, 207}, {207, 207, 207}, {207, 207, 207}, {208, 208, 208}, {208, 208, 208},
{208, 208, 208}, {208, 208, 208}, {209, 209, 209}, {209, 209, 209}, {209, 209, 209},
{209, 209, 209}, {210, 210, 210}, {210, 210, 210}, {210, 210, 210}, {210, 210, 210},
{211, 211, 211}, {211, 211, 211}, {211, 211, 211}, {211, 211, 211}, {212, 212, 212},
{212, 212, 212}, {212, 212, 212}, {212, 212, 212}, {213, 213, 213}, {213, 213, 213},
{213, 213, 213}, {213, 213, 213}, {214, 214, 214}, {214, 214, 214}, {214, 214, 214},
{214, 214, 214}, {215, 215, 215}, {215, 215, 215}, {215, 215, 215}, {215, 215, 215},
{216, 216, 216}, {216, 216, 216}, {216, 216, 216}, {216, 216, 216}, {217, 217, 217},
{217, 217, 217}, {217, 217, 217}, {217, 217, 217}, {218, 218, 218}, {218, 218, 218},
{218, 218, 218}, {218, 218, 218}, {219, 219, 219}, {219, 219, 219}, {219, 219, 219},
{220, 220, 220}, {220, 220, 220}, {220, 220, 220}, {220, 220, 220}, {221, 221, 221},
{221, 221, 221}, {221, 221, 221}, {221, 221, 221}, {222, 222, 222}, {222, 222, 222},
{222, 222, 222}, {222, 222, 222}, {223, 223, 223}, {223, 223, 223}, {223, 223, 223},
{223, 223, 223}, {224, 224, 224}, {224, 224, 224}, {224, 224, 224}, {224, 224, 224},
{225, 225, 225}, {225, 225, 225}, {225, 225, 225}, {225, 225, 225}, {226, 226, 226},
{226, 226, 226}, {226, 226, 226}, {226, 226, 226}, {227, 227, 227}, {227, 227, 227},
{227, 227, 227}, {227, 227, 227}, {228, 228, 228}, {228, 228, 228}, {228, 228, 228},
{228, 228, 228}, {229, 229, 229}, {229, 229, 229}, {229, 229, 229}, {229, 229, 229},
{230, 230, 230}, {230, 230, 230}, {230, 230, 230}, {230, 230, 230}, {231, 231, 231},
{231, 231, 231}, {231, 231, 231}, {232, 232, 232}, {232, 232, 232}, {232, 232, 232},
{232, 232, 232}, {233, 233, 233}, {233, 233, 233}, {233, 233, 233}, {233, 233, 233},
{234, 234, 234}, {234, 234, 234}, {234, 234, 234}, {234, 234, 234}, {235, 235, 235},
{235, 235, 235}, {235, 235, 235}, {235, 235, 235}, {236, 236, 236}, {236, 236, 236},
{236, 236, 236}, {236, 236, 236}, {237, 237, 237}, {237, 237, 237}, {237, 237, 237},
{237, 237, 237}, {238, 238, 238}, {238, 238, 238}, {238, 238, 238}, {238, 238, 238},
{239, 239, 239}, {239, 239, 239}, {239, 239, 239}, {239, 239, 239}, {240, 240, 240},
{240, 240, 240}, {240, 240, 240}, {240, 240, 240}, {241, 241, 241}, {241, 241, 241},
{241, 241, 241}, {241, 241, 241}, {242, 242, 242}, {242, 242, 242}, {242, 242, 242},
{242, 242, 242}, {243, 243, 243}, {243, 243, 243}, {243, 243, 243}, {244, 244, 244},
{244, 244, 244}, {244, 244, 244}, {244, 244, 244}, {245, 245, 245}, {245, 245, 245},
{245, 245, 245}, {245, 245, 245}, {246, 246, 246}, {246, 246, 246}, {246, 246, 246},
{246, 246, 246}, {247, 247, 247}, {247, 247, 247}, {247, 247, 247}, {247, 247, 247},
{248, 248, 248}, {248, 248, 248}, {248, 248, 248}, {248, 248, 248}, {249, 249, 249},
{249, 249, 249}, {249, 249, 249}, {249, 249, 249}, {250, 250, 250}, {250, 250, 250},
{250, 250, 250}, {250, 250, 250}, {251, 251, 251}, {251, 251, 251}, {251, 251, 251},
{251, 251, 251}, {252, 252, 252}, {252, 252, 252}, {252, 252, 252}, {252, 252, 252},
{253, 253, 253}, {253, 253, 253}, {253, 253, 253}, {253, 253, 253}, {254, 254, 254},
{254, 254, 254}, {254, 254, 254}, {254, 254, 254}, {255, 255, 255}, {255, 255, 255}
};
#endif
| Java |
import { Component, OnInit, Input } from '@angular/core';
@Component({
selector: 'app-autocodeparameterrender',
templateUrl: './autocodeparameterrender.component.html',
styleUrls: ['./autocodeparameterrender.component.css', './_autocodeparameterrender.component.scss', '../_app.general.scss']
})
export class AutocodeparameterrenderComponent implements OnInit {
@Input() data: any;
constructor() { }
ngOnInit() {
}
}
| Java |
## intro
This image is intended for git repository one way sync. It makes target
repository a mirror of source repository, including branches and tags.
__Make sure that target service allows force pushing to all branches.__
__Also note that any changes in target repository will be lost__
## supported env vars
### NFQ_SOURCE_REPO (required)
Repository URL to clone changes from
### NFQ_TARGET_REPO (required)
Repository URL to push changes to
### NFQ_SECRET_SOURCE_KEY (optional)
SSH private key prodived as string. Used in all git source repo operations
### NFQ_SECRET_DESTINATION_KEY (optional)
SSH private key prodived as string. Used in all git destination repo operations
| Java |
var watchers = Object.create(null);
var wait = function (linkid, callback) {
watchers[linkid] = cross("options", "/:care-" + linkid).success(function (res) {
if (watchers[linkid]) wait(linkid, callback);
var a = JSAM.parse(res.responseText);
if (isFunction(callback)) a.forEach(b => callback(b));
}).error(function () {
if (watchers[linkid]) wait(linkid, callback);
});
};
var kill = function (linkid) {
var r = watchers[linkid];
delete watchers[linkid];
if (r) r.abort();
};
var block_size = 1024;
var cast = function (linkid, data) {
data = encode(data);
var inc = 0, size = block_size;
return new Promise(function (ok, oh) {
var run = function () {
if (inc > data.length) return ok();
cross("options", "/:cast-" + linkid + "?" + data.slice(inc, inc + size)).success(run).error(oh);
inc += size;
};
run();
}).then(function () {
if (inc === data.length) {
return cast(linkid, '');
}
});
};
var encode = function (data) {
var str = encodeURIComponent(data.replace(/\-/g, '--')).replace(/%/g, '-');
return str;
};
var decode = function (params) {
params = params.replace(/\-\-|\-/g, a => a === '-' ? '%' : '-');
params = decodeURIComponent(params);
return params;
};
function serve(listener) {
return new Promise(function (ok, oh) {
cross("options", "/:link").success(function (res) {
var responseText = res.responseText;
wait(responseText, listener);
ok(responseText);
}).error(oh);
})
}
function servd(getdata) {
return serve(function (linkid) {
cast(linkid, JSAM.stringify(getdata()));
});
}
function servp(linkto) {
return new Promise(function (ok, oh) {
var blocks = [], _linkid;
serve(function (block) {
blocks.push(block);
if (block.length < block_size) {
var data = decode(blocks.join(''));
ok(JSAM.parse(data));
kill(_linkid);
}
}).then(function (linkid) {
cast(linkto, linkid);
_linkid = linkid;
}, oh);
});
}
serve.servd = servd;
serve.servp = servp;
serve.kill = kill;
| Java |
/**
* This cli.js test file tests the `gnode` wrapper executable via
* `child_process.spawn()`. Generator syntax is *NOT* enabled for these
* test cases.
*/
var path = require('path');
var assert = require('assert');
var semver = require('semver');
var spawn = require('child_process').spawn;
// node executable
var node = process.execPath || process.argv[0];
var gnode = path.resolve(__dirname, '..', 'bin', 'gnode');
// chdir() to the "test" dir, so that relative test filenames work as expected
process.chdir(path.resolve(__dirname, 'cli'));
describe('command line interface', function () {
this.slow(1000);
this.timeout(2000);
cli([ '-v' ], 'should output the version number', function (child, done) {
buffer(child.stdout, function (err, data) {
assert(semver.valid(data.trim()));
done();
});
});
cli([ '--help' ], 'should output the "help" display', function (child, done) {
buffer(child.stdout, function (err, data) {
assert(/^Usage\: (node|iojs)/.test(data));
done();
});
});
cli([ 'check.js' ], 'should quit with a SUCCESS exit code', function (child, done) {
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
done();
});
});
cli([ 'nonexistant.js' ], 'should quit with a FAILURE exit code', function (child, done) {
child.on('exit', function (code) {
assert(code != 0, 'gnode quit with exit code: ' + code);
done();
});
});
cli([ 'argv.js', '1', 'foo' ], 'should have a matching `process.argv`', function (child, done) {
buffer(child.stdout, function (err, data) {
if (err) return done(err);
data = JSON.parse(data);
assert('argv.js' == path.basename(data[1]));
assert('1' == data[2]);
assert('foo' == data[3]);
done();
});
});
cli([ '--harmony_generators', 'check.js' ], 'should not output the "unrecognized flag" warning', function (child, done) {
var async = 2;
buffer(child.stderr, function (err, data) {
if (err) return done(err);
assert(!/unrecognized flag/.test(data), 'got stderr data: ' + JSON.stringify(data));
--async || done();
});
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
--async || done();
});
});
cli([], 'should work properly over stdin', function (child, done) {
child.stdin.end(
'var assert = require("assert");' +
'function *test () {};' +
'var t = test();' +
'assert("function" == typeof t.next);' +
'assert("function" == typeof t.throw);'
);
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
done();
});
});
if (!/^v0.8/.test(process.version)) cli(['-p', 'function *test () {yield 3}; test().next().value;'], 'should print result with -p', function (child, done) {
var async = 2
buffer(child.stdout, function (err, data) {
if (err) return done(err);
assert('3' == data.trim(), 'gnode printed ' + data);
--async || done();
});
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
--async || done();
});
});
cli(['-e', 'function *test () {yield 3}; console.log(test().next().value);'], 'should print result with -e', function (child, done) {
var async = 2
buffer(child.stdout, function (err, data) {
if (err) return done(err);
assert('3' == data.trim(), 'expected 3, got: ' + data);
--async || done();
});
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
--async || done();
});
});
cli(['--harmony_generators', '-e', 'function *test () {yield 3}; console.log(test().next().value);'], 'should print result with -e', function (child, done) {
var async = 2
buffer(child.stdout, function (err, data) {
if (err) return done(err);
assert('3' == data.trim(), 'gnode printed ' + data);
--async || done();
});
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
--async || done();
});
});
cli(['-e', 'console.log(JSON.stringify(process.argv))', 'a', 'b', 'c'], 'should pass additional arguments after -e', function (child, done) {
var async = 2
buffer(child.stdout, function (err, data) {
if (err) return done(err);
data = JSON.parse(data)
assert.deepEqual(['a', 'b', 'c'], data.slice(2))
--async || done();
});
child.on('exit', function (code) {
assert(code == 0, 'gnode quit with exit code: ' + code);
--async || done();
});
});
});
function cli (argv, name, fn) {
describe('gnode ' + argv.join(' '), function () {
it(name, function (done) {
var child = spawn(node, [ gnode ].concat(argv));
fn(child, done);
});
});
}
function buffer (stream, fn) {
var buffers = '';
stream.setEncoding('utf8');
stream.on('data', ondata);
stream.on('end', onend);
stream.on('error', onerror);
function ondata (b) {
buffers += b;
}
function onend () {
stream.removeListener('error', onerror);
fn(null, buffers);
}
function onerror (err) {
fn(err);
}
}
| Java |
<?php
namespace Insales\AppBundle\Lib;
use Symfony\Component\DependencyInjection\ContainerAware;
use Insales\AppBundle\Services\Myapp;
/*
* Класс который сохраняется в сессию и хранит данные об авторизации
*/
class Application
{
protected $authorized;
protected $shop;
protected $password;
protected $api_secret;
protected $api_key;
protected $api_host;
protected $api_autologin_path;
protected $auth_token;
protected $salt;
public function __construct($shop, $password, $api_secret, $api_key, $api_host, $api_autologin_path)
{
$this->authorized = false;
$this->shop = $shop;
$this->password = $password;
$this->api_secret = $api_secret;
$this->api_key = $api_key;
$this->api_host = $api_host;
$this->api_autologin_path = $api_autologin_path;
}
public function authorizationUrl()
{
$this->storeAuthToken();
return "http://$this->shop/admin/applications/$this->api_key/login?token=$this->salt&login=http://$this->api_host/$this->api_autologin_path";
}
public function getShop()
{
return $this->shop;
}
public function isAuthorized()
{
return $this->authorized;
}
public function storeAuthToken()
{
if (strlen($this->auth_token) == 0)
{
$this->auth_token = Myapp::generatePassword($this->password, $this->salt());
}
return $this->auth_token;
}
public function authorize($token)
{
$this->authorized = false;
if ($this->auth_token && $this->auth_token == $token)
{
$this->authorized = true;
}
return $this->authorized;
}
public function salt()
{
if (strlen($this->salt) == 0)
{
$this->salt = md5("Twulvyeik".getmypid().time()."thithAwn");
}
return $this->salt;
}
}
| Java |
using System;
namespace CUDAnshita {
/// <summary>
/// C# interface exception.
/// </summary>
public class CudaException : Exception {
public CudaException() {
}
public CudaException(string message) : base(message) {
}
public CudaException(string message, Exception innerException) : base(message, innerException) {
}
public static void Check(cudaError error, string message) {
if (error != cudaError.cudaSuccess) {
message = string.Format("{0}: {1}", error.ToString(), message);
Exception exception = new CudaException(message);
exception.Data.Add("cudaError", error);
throw exception;
}
}
public static void Check(CUresult error, string message) {
if (error != CUresult.CUDA_SUCCESS) {
message = string.Format("{0}: {1}", error.ToString(), message);
Exception exception = new CudaException(message);
exception.Data.Add("CUresult", error);
throw exception;
}
}
}
}
| Java |
"""Module containing character feature extractors."""
import string
from unstyle.features.featregister import register_feat
@register_feat
def characterSpace(text):
"""Return the total number of characters."""
return len(text)
@register_feat
def letterSpace(text):
"""Return the total number of letters (excludes spaces and punctuation)"""
count = 0
alphabet = string.ascii_lowercase + string.ascii_uppercase
for char in text:
if char in alphabet:
count += 1
return count
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>gappa: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.11.2 / gappa - 1.3.2</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
gappa
<small>
1.3.2
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-12-25 14:20:56 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-12-25 14:20:56 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
coq 8.11.2 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.11.2 The OCaml compiler (virtual package)
ocaml-base-compiler 4.11.2 Official release 4.11.2
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://gappa.gitlabpages.inria.fr/"
dev-repo: "git+https://gitlab.inria.fr/gappa/coq.git"
bug-reports: "https://gitlab.inria.fr/gappa/coq/issues"
license: "LGPL 2.1"
build: [
["./configure"]
["./remake" "-j%{jobs}%"]
]
install: ["./remake" "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/Gappa"]
depends: [
"ocaml"
"coq" {>= "8.4pl4" & < "8.7~" & != "8.4.6~camlp4" & != "8.5.0~camlp4" & != "8.5.2~camlp4"}
"coq-flocq" {>= "2.5" & < "3.0~"}
]
tags: [
"keyword:floating-point arithmetic"
"keyword:interval arithmetic"
"keyword:decision procedure"
"category:Computer Science/Decision Procedures and Certified Algorithms/Decision procedures"
]
authors: [ "Guillaume Melquiond <[email protected]>" ]
synopsis: "A Coq tactic for discharging goals about floating-point arithmetic and round-off errors using the Gappa prover"
flags: light-uninstall
url {
src: "https://gappa.gitlabpages.inria.fr/releases/gappalib-coq-1.3.2.tar.gz"
checksum: "md5=d0374859edfc92f490ac291bbcea3341"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-gappa.1.3.2 coq.8.11.2</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.11.2).
The following dependencies couldn't be met:
- coq-gappa -> coq < 8.7~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-gappa.1.3.2</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
from django.db import models
from django.contrib.auth.models import User
from police.models import Stationdata
class general_diary(models.Model):
ref_id = models.CharField(max_length=40,unique=True,default="00000")
firstname = models.CharField(max_length=20)
lastname = models.CharField(max_length=20)
mobile = models.CharField(max_length=10)
email = models.CharField(max_length=80)
address = models.TextField()
DOB = models.DateField('date of birth')
idType_1 = models.CharField(max_length=10)
idType_1_value = models.CharField(max_length=15)
idType_2 = models.CharField(max_length=20)
idType_2_value = models.CharField(max_length=15)
StationCode = models.ForeignKey(Stationdata)
Subject = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
detail = models.TextField()
Time = models.DateTimeField('Occurence')
Place = models.CharField(max_length=200)
Loss = models.CharField(max_length=200)
OTP = models.BooleanField(default=False)
def __str__(self): # __unicode__ on Python 2
return self.Subject
class Fir(models.Model):
ref_id = models.CharField(max_length=40,unique=True,default="00000")
firstname = models.CharField(max_length=20)
lastname = models.CharField(max_length=20)
mobile = models.CharField(max_length=10)
email = models.CharField(max_length=80)
address = models.TextField()
DOB = models.DateField('date of birth')
idType_1 = models.CharField(max_length=10)
idType_1_value = models.CharField(max_length=15)
idType_2 = models.CharField(max_length=20)
idType_2_value = models.CharField(max_length=15)
StationCode = models.ForeignKey(Stationdata)
Subject = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
detail = models.TextField()
Suspect = models.CharField(max_length=500)
Time = models.DateTimeField('Occurence')
Place = models.CharField(max_length=200)
Witness = models.CharField(max_length=500)
Loss = models.CharField(max_length=200)
OTP = models.BooleanField(default=False)
def __str__(self): # __unicode__ on Python 2
return self.Subject
class lookup_table(models.Model):
ref_id = models.CharField(max_length=40,unique=True,default="00000")
hashmap = models.CharField(max_length=70,unique=True,default="00000")
type = models.CharField(max_length=5,default="GD")
def __str__(self): # __unicode__ on Python 2
return self.hashmap | Java |
var group___s_t_m32_f4xx___h_a_l___driver =
[
[ "CRC", "group___c_r_c.html", "group___c_r_c" ],
[ "GPIOEx", "group___g_p_i_o_ex.html", "group___g_p_i_o_ex" ],
[ "AHB3 Peripheral Clock Enable Disable", "group___r_c_c_ex___a_h_b3___clock___enable___disable.html", null ],
[ "HAL", "group___h_a_l.html", "group___h_a_l" ],
[ "ADC", "group___a_d_c.html", "group___a_d_c" ],
[ "ADCEx", "group___a_d_c_ex.html", "group___a_d_c_ex" ],
[ "CORTEX", "group___c_o_r_t_e_x.html", "group___c_o_r_t_e_x" ],
[ "DMA", "group___d_m_a.html", "group___d_m_a" ],
[ "DMAEx", "group___d_m_a_ex.html", "group___d_m_a_ex" ],
[ "FLASH", "group___f_l_a_s_h.html", "group___f_l_a_s_h" ],
[ "FLASHEx", "group___f_l_a_s_h_ex.html", "group___f_l_a_s_h_ex" ],
[ "GPIO", "group___g_p_i_o.html", "group___g_p_i_o" ],
[ "I2C", "group___i2_c.html", "group___i2_c" ],
[ "I2S", "group___i2_s.html", "group___i2_s" ],
[ "I2SEx", "group___i2_s_ex.html", "group___i2_s_ex" ],
[ "IRDA", "group___i_r_d_a.html", "group___i_r_d_a" ],
[ "IWDG", "group___i_w_d_g.html", "group___i_w_d_g" ],
[ "NAND", "group___n_a_n_d.html", null ],
[ "NOR", "group___n_o_r.html", null ],
[ "PWR", "group___p_w_r.html", "group___p_w_r" ],
[ "PWREx", "group___p_w_r_ex.html", "group___p_w_r_ex" ],
[ "RCC", "group___r_c_c.html", "group___r_c_c" ],
[ "RCCEx", "group___r_c_c_ex.html", "group___r_c_c_ex" ],
[ "RTC", "group___r_t_c.html", "group___r_t_c" ],
[ "RTCEx", "group___r_t_c_ex.html", "group___r_t_c_ex" ],
[ "SAI", "group___s_a_i.html", "group___s_a_i" ],
[ "SAIEx", "group___s_a_i_ex.html", "group___s_a_i_ex" ],
[ "SMARTCARD", "group___s_m_a_r_t_c_a_r_d.html", "group___s_m_a_r_t_c_a_r_d" ],
[ "SPI", "group___s_p_i.html", "group___s_p_i" ],
[ "TIM", "group___t_i_m.html", "group___t_i_m" ],
[ "TIMEx", "group___t_i_m_ex.html", "group___t_i_m_ex" ],
[ "UART", "group___u_a_r_t.html", "group___u_a_r_t" ],
[ "USART", "group___u_s_a_r_t.html", "group___u_s_a_r_t" ],
[ "WWDG", "group___w_w_d_g.html", "group___w_w_d_g" ],
[ "FMC_LL", "group___f_m_c___l_l.html", "group___f_m_c___l_l" ],
[ "FSMC_LL", "group___f_s_m_c___l_l.html", null ]
]; | Java |
import React from 'react'
import { compose } from 'redux'
import { connect } from 'react-redux'
import { Field, FieldArray, reduxForm } from 'redux-form'
import { amount, autocomplete, debitCredit } from './Fields'
import { Button, FontIcon } from 'react-toolbox'
import { getAutocomplete } from 'modules/autocomplete'
import { create } from 'modules/entities/journalEntryCreatedEvents'
import classes from './JournalEntryForm.scss'
import { fetch as fetchLedgers } from 'modules/entities/ledgers'
const validate = (values) => {
const errors = {}
return errors
}
const renderRecords = ({ fields, meta: { touched, error, submitFailed }, ledgers, financialFactId }) => (
<div>
<div>
<Button onClick={() => fields.push({})}> <FontIcon value='add' /></Button>
{(touched || submitFailed) && error && <span>{error}</span>}
</div>
<div className={classes.recordsList}>
{fields.map((record, index) =>
<div key={index}>
<Button
onClick={() => fields.remove(index)}> <FontIcon value='remove' /></Button>
<div className={classes.journalEntryField}>
<Field name={`${record}.ledger`}
component={autocomplete}
label='Ledger'
source={ledgers}
multiple={false}
/>
</div>
<div className={classes.journalEntryField}>
<Field
name={`${record}.debitCredit`}
component={debitCredit}
label='Debit/credit' />
</div>
<div className={classes.journalEntryField}>
<Field
name={`${record}.amount`}
component={amount}
label='Amount' />
</div>
</div>
)}
</div>
</div>
)
const JournalEntryForm = (props) => {
const { ledgers, handleSubmit, submitting, reset } = props
return (
<div className={classes.journalEntryForm}>
<form onSubmit={handleSubmit}>
<FieldArray name='records' component={renderRecords} ledgers={ledgers} />
<Button type='submit' disabled={submitting}>Submit</Button>
<Button type='button' disabled={submitting} onClick={reset}>Clear</Button>
</form>
</div>
)
}
const onSubmit = (values) => {
return create({
values: {
...values
}
})
}
const mapDispatchToProps = {
onSubmit,
fetchLedgers
}
const mapStateToProps = (state) => {
return {
ledgers: getAutocomplete(state, 'ledgers')
}
}
export default compose(
connect(mapStateToProps, mapDispatchToProps),
reduxForm({
form: 'JournalEntryForm',
validate
}))(JournalEntryForm)
| Java |
#ifndef __CORPUS_H
#define __CORPUS_H
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
#include <unordered_map>
#include "types.h"
using std::vector;
using std::string;
using std::unordered_map;
class Corpus
{
public:
Corpus(const char *vocabPath, const char *dataPath);
Corpus(const Corpus &from, int start, int end);
// this is wdn, store words in each document
vector<vector<TWord>> dw;
vector<string> vocab;
unordered_map<string, TWord> word2id;
TDoc D;
TWord W;
TSize T;
};
#endif
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>algebra: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.9.1 / algebra - 8.5.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
algebra
<small>
8.5.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2021-11-05 07:20:37 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2021-11-05 07:20:37 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 1 Virtual package relying on perl
coq 8.9.1 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.09.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.09.1 Official release 4.09.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/coq-contribs/algebra"
license: "LGPL 2"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/Algebra"]
depends: [
"ocaml"
"coq" {>= "8.5" & < "8.6~"}
]
tags: [ "keyword:algebra" "category:Mathematics/Algebra" "date:1999-03" ]
authors: [ "Loïc Pottier <>" ]
bug-reports: "https://github.com/coq-contribs/algebra/issues"
dev-repo: "git+https://github.com/coq-contribs/algebra.git"
synopsis: "Basics notions of algebra"
flags: light-uninstall
url {
src: "https://github.com/coq-contribs/algebra/archive/v8.5.0.tar.gz"
checksum: "md5=9ef1b5f1f670d56a55aef37d8c511df9"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-algebra.8.5.0 coq.8.9.1</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.9.1).
The following dependencies couldn't be met:
- coq-algebra -> coq < 8.6~ -> ocaml < 4.06.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-algebra.8.5.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>presburger: 1 m 0 s 🏆</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.9.0 / presburger - 8.9.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
presburger
<small>
8.9.0
<span class="label label-success">1 m 0 s 🏆</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-03-06 09:57:06 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-03-06 09:57:06 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
camlp5 7.14 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-perl 2 Virtual package relying on perl
coq 8.9.0 Formal proof management system
num 1.4 The legacy Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.09.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.09.1 Official release 4.09.1
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.9.3 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/coq-contribs/presburger"
license: "LGPL 2.1"
build: [make "-j%{jobs}%"]
install: [make "install"]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/Presburger"]
depends: [
"ocaml"
"coq" {>= "8.9" & < "8.10~"}
]
tags: [
"keyword: integers"
"keyword: arithmetic"
"keyword: decision procedure"
"keyword: Presburger"
"category: Mathematics/Logic/Foundations"
"category: Mathematics/Arithmetic and Number Theory/Miscellaneous"
"category: Computer Science/Decision Procedures and Certified Algorithms/Decision procedures"
"date: March 2002"
]
authors: [
"Laurent Théry"
]
bug-reports: "https://github.com/coq-contribs/presburger/issues"
dev-repo: "git+https://github.com/coq-contribs/presburger.git"
synopsis: "Presburger's algorithm"
description: """
A formalization of Presburger's algorithm as stated in
the initial paper by Presburger."""
flags: light-uninstall
url {
src: "https://github.com/coq-contribs/presburger/archive/v8.9.0.tar.gz"
checksum: "md5=f3c4e9c316149a8bc03bde86b6c4a2bf"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-presburger.8.9.0 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 4000000; timeout 4h opam install -y --deps-only coq-presburger.8.9.0 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>10 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 16000000; timeout 4h opam install -y -v coq-presburger.8.9.0 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>1 m 0 s</dd>
</dl>
<h2>Installation size</h2>
<p>Total: 3 M</p>
<ul>
<li>180 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Lift.vo</code></li>
<li>161 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Normal.vo</code></li>
<li>153 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Lift.glob</code></li>
<li>149 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Zdivides.vo</code></li>
<li>126 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Sort.vo</code></li>
<li>122 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceEq.glob</code></li>
<li>121 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/GroundN.vo</code></li>
<li>118 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceCong.vo</code></li>
<li>111 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Elim.vo</code></li>
<li>111 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Zdivides.glob</code></li>
<li>109 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceEq.vo</code></li>
<li>108 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Process.vo</code></li>
<li>105 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Factor.vo</code></li>
<li>90 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Form.vo</code></li>
<li>78 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Nat.vo</code></li>
<li>71 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceCong.glob</code></li>
<li>65 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/prestac.cmxs</code></li>
<li>63 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Cong.vo</code></li>
<li>58 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Normal.glob</code></li>
<li>56 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Nat.glob</code></li>
<li>45 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Process.glob</code></li>
<li>39 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Form.glob</code></li>
<li>38 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac_ex.vo</code></li>
<li>38 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac.vo</code></li>
<li>35 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/GroundN.glob</code></li>
<li>34 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Sort.glob</code></li>
<li>31 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Zdivides.v</code></li>
<li>31 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Elim.glob</code></li>
<li>28 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Lift.v</code></li>
<li>26 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Factor.glob</code></li>
<li>22 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Cong.glob</code></li>
<li>22 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Normal.v</code></li>
<li>20 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceEq.v</code></li>
<li>19 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Nat.v</code></li>
<li>16 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/ReduceCong.v</code></li>
<li>14 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Elim.v</code></li>
<li>13 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Form.v</code></li>
<li>12 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/GroundN.v</code></li>
<li>12 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Process.v</code></li>
<li>10 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/prestac.cmi</code></li>
<li>9 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Sort.v</code></li>
<li>8 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Factor.v</code></li>
<li>7 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/prestac.cmx</code></li>
<li>7 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Cong.v</code></li>
<li>3 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/sTactic.vo</code></li>
<li>3 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Option.vo</code></li>
<li>2 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/sTactic.v</code></li>
<li>2 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac_ex.glob</code></li>
<li>2 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac_ex.v</code></li>
<li>2 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac.v</code></li>
<li>2 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Option.v</code></li>
<li>1 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/sTactic.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/Option.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.09.1/lib/coq/user-contrib/Presburger/PresTac.glob</code></li>
</ul>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq-presburger.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
import { ChangeDetectionStrategy, Component, HostBinding, Input, OnDestroy, OnInit } from '@angular/core';
import { animate, style, transition, trigger } from '@angular/animations';
import { select, Store } from '@ngrx/store';
import { ICasesState, selectCaseById, selectSelectedCase } from '../../reducers/cases.reducer';
import { CloseModalAction, RenameCaseAction, SaveCaseAsAction } from '../../actions/cases.actions';
import { take, tap } from 'rxjs/operators';
import { cloneDeep } from '../../../../core/utils/rxjs/operators/cloneDeep';
import { ICase } from '../../models/case.model';
import { AutoSubscription, AutoSubscriptions } from 'auto-subscriptions';
import * as moment from 'moment';
const animationsDuring = '0.2s';
const animations: any[] = [
trigger('modalContent', [
transition(':enter', [style({
'backgroundColor': '#27b2cf',
transform: 'translate(0, -100%)'
}), animate(animationsDuring, style({ 'backgroundColor': 'white', transform: 'translate(0, 0)' }))]),
transition(':leave', [style({
'backgroundColor': 'white',
transform: 'translate(0, 0)'
}), animate(animationsDuring, style({ 'backgroundColor': '#27b2cf', transform: 'translate(0, -100%)' }))])
])
];
@Component({
selector: 'ansyn-save-case',
templateUrl: './save-case.component.html',
styleUrls: ['./save-case.component.less'],
changeDetection: ChangeDetectionStrategy.OnPush,
animations
})
@AutoSubscriptions()
export class SaveCaseComponent implements OnInit, OnDestroy {
@Input() caseId: string;
caseName: string;
@HostBinding('@modalContent')
get modalContent() {
return true;
};
constructor(
protected store: Store<ICasesState>
) {
}
@AutoSubscription
caseName$ = () => this.store.pipe(
select(selectCaseById(this.caseId)),
tap( (_case) => {
this.caseName = _case ? _case.name : moment(new Date()).format('DD/MM/YYYY HH:mm:ss').toLocaleString();
})
);
private cloneDeepOneTime(selector) {
return this.store.pipe(
select(selector),
take(1),
cloneDeep()
)
}
ngOnDestroy(): void {
}
ngOnInit(): void {}
close(): void {
this.store.dispatch(new CloseModalAction());
}
saveNewCase() {
return this.cloneDeepOneTime(selectSelectedCase).pipe(
tap((selectedCase: ICase) => {
this.store.dispatch(new SaveCaseAsAction({ ...selectedCase, name: this.caseName }));
})
)
}
renameCase() {
return this.cloneDeepOneTime(selectCaseById(this.caseId)).pipe(
tap( (_case: ICase) => {
const oldName = _case.name;
this.store.dispatch(new RenameCaseAction({case: _case, oldName: oldName, newName: this.caseName }));
})
)
}
onSubmitCase() {
const obs = this.caseId ? this.renameCase() : this.saveNewCase();
obs.pipe(
tap(this.close.bind(this))
).subscribe()
}
}
| Java |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>msets-extra: Not compatible 👼</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.14.1 / msets-extra - 1.0.0</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
msets-extra
<small>
1.0.0
<span class="label label-info">Not compatible 👼</span>
</small>
</h1>
<p>📅 <em><script>document.write(moment("2022-02-20 11:08:53 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2022-02-20 11:08:53 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-threads base
base-unix base
conf-findutils 1 Virtual package relying on findutils
conf-gmp 4 Virtual package relying on a GMP lib system installation
coq 8.14.1 Formal proof management system
dune 2.9.3 Fast, portable, and opinionated build system
ocaml 4.13.1 The OCaml compiler (virtual package)
ocaml-base-compiler 4.13.1 Official release 4.13.1
ocaml-config 2 OCaml Switch Configuration
ocaml-options-vanilla 1 Ensure that OCaml is compiled with no special options enabled
ocamlfind 1.9.3 A library manager for OCaml
zarith 1.12 Implements arithmetic and logical operations over arbitrary-precision integers
# opam file:
opam-version: "2.0"
maintainer: "[email protected]"
homepage: "https://github.com/thtuerk/MSetsExtra"
dev-repo: "git+https://github.com/thtuerk/MSetsExtra.git"
bug-reports: "https://github.com/thtuerk/MSetsExtra/issues"
authors: ["FireEye Formal Methods Team <[email protected]>" "Thomas Tuerk <[email protected]>"]
license: "LGPL 2.1"
build: [
["./configure.sh"]
[make "-j%{jobs}%"]
]
install: [
[make "install"]
]
remove: ["rm" "-R" "%{lib}%/coq/user-contrib/MSetsExtra"]
depends: [
"ocaml"
"coq" {>= "8.4.5" & < "8.5~"}
"coq-mathcomp-ssreflect" {>= "1.6"}
]
tags: [ "keyword:finite sets"
"keyword:fold with abort"
"keyword:extracting efficient code"
"keyword:data structures"
"category:Computer Science/Data Types and Data Structures"
"category:Miscellaneous/Extracted Programs/Data structures"
"date:2016-10-04" ]
synopsis: "Extensions of MSets for Efficient Execution"
description: """
Coq's MSet library provides various, reasonably efficient finite set
implementations. Nevertheless, FireEye was struggling with performance
issues. This library contains extensions to Coq's MSet library that
helped the FireEye Formal Methods team ([email protected]),
solve these performance issues. There are
- Fold With Abort
efficient folding with possibility to start late and stop early
- Interval Sets
a memory efficient representation of sets of numbers
- Unsorted Lists with Duplicates"""
flags: light-uninstall
url {
src: "https://github.com/thtuerk/MSetsExtra/archive/1.0.0.tar.gz"
checksum: "md5=297a95b8ca4157760862e5812e8addd3"
}
</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install 🏜️</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-msets-extra.1.0.0 coq.8.14.1</code></dd>
<dt>Return code</dt>
<dd>5120</dd>
<dt>Output</dt>
<dd><pre>[NOTE] Package coq is already installed (current version is 8.14.1).
The following dependencies couldn't be met:
- coq-msets-extra -> coq < 8.5~ -> ocaml < 4.03.0
base of this switch (use `--unlock-base' to force)
No solution found, exiting
</pre></dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq; opam install -y --show-action --unlock-base coq-msets-extra.1.0.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Install 🚀</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>0 s</dd>
</dl>
<h2>Installation size</h2>
<p>No files were installed.</p>
<h2>Uninstall 🧹</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
Sources are on <a href="https://github.com/coq-bench">GitHub</a> © Guillaume Claret 🐣
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| Java |
var _ = require('lodash');
var localConfig = {};
try {
localConfig = require('./config.local');
} catch (e) {
console.log(e.code === 'MODULE_NOT_FOUND');
if (e.code !== 'MODULE_NOT_FOUND' || e.message.indexOf('config.local') < 0) {
//config.local module is broken, rethrow the exception
throw e;
}
}
var path = require('path');
var defaultConfig = {
dataSources: [
{
type: 'local',
base: 'tmp/',
endpoints: ['nodes.json', 'graph.json'],
interval: 3000
}
// {
// type: 'remote',
// base: 'http://example.com/ffmap/',
// endpoints: ['nodes.json', 'graph.json'],
// interval: 30000
// },
],
frontend: {
path: path.join(__dirname, 'public')
},
database: {
host: 'rethinkdb',
port: '28015',
db: 'ffmap'
},
port: 8000
};
module.exports = _.defaults({
defaults: defaultConfig
}, localConfig, defaultConfig);
| Java |
* overview tutorial of deep reinforcement learning
* [http://people.eecs.berkeley.edu/~pabbeel/nips-tutorial-policy-optimization-Schulman-Abbeel.pdf](Deep Reinforcement Learning through Policy Optimization)
| Java |
[](https://travis-ci.org/david-grs/static_any)
[](https://ci.appveyor.com/project/david-grs/static-any/branch/master)
[](https://coveralls.io/github/david-grs/static_any?branch=master)
static\_any\<S\>
================
A container for generic (as *general*) data type — like boost.any. However:
- It is **~10x faster** than boost.any, mainly because there is no memory allocation
- As it lies on the stack, it is **cache-friendly**, close to your other class attributes
- There is a very **small space overhead**: a fixed overhead of 8 bytes
static\_any\<S\> is also **safe**:
- operations meet the strong exception guarantee
- compile time check during the assignment, to ensure that its buffer is big enough to store the value
- runtime check before any conversions, to ensure that the stored type is the one's requested by the user
Example
-------
```c++
static_any<32> a;
static_assert(sizeof(a) == 32 + 8, "any has a fixed overhead of 8 bytes");
a = 1234;
ASSERT_EQ(1234, a.get<int>());
a = std::string("foobar");
ASSERT_EQ("foobar", a.get<std::string>());
try {
// This will throw as a std::string has been stored
std::cout << any_cast<int>(a);
}
catch(bad_any_cast& ex) {
}
struct A : std::array<char, 32> {};
a = A();
struct B : A { char c; };
// Does not build: sizeof(B) is too big for static_any<16>
a = B();
```
---
static\_any\_t\<S\>
===================
A container similar to static\_any\<S\>, but for trivially copyable types only. The differences:
- **No space overhead**
- **Faster**
- **Unsafe**: there is no check when you try to access your data
---
Benchmarks
==========
As the main advantage of static\_any(\_t\<S\>) is speed, here is a comparison of assign/get operations on a POD/non-POD types — an integer and a std::string — between
boost.any, QVariant, static\_any\<S\> and static\_any\_t\<S\>.
**assign a double**
```
Test Time (ns)
--------------------------
qvariant 36
boost.any 57
std::any 4
static_any<8> 4
static_any_t<8> 0
```
**get a double**
```
Test Time (ns)
--------------------------
qvariant 9
boost.any 12
std::any 9
static_any<8> 9
static_any_t<8> 6
```
**assign a small struct**
```
Test Time (ns)
--------------------------
qvariant 294
boost.any 47
std::any 54
static_any<8> 12
static_any_t<8> 0
```
**get a small struct**
```
Test Time (ns)
--------------------------
qvariant 12
boost.any 48
std::any 6
static_any<8> 4
static_any_t<8> 0
```
**assign a string**
```
Test Time (ns)
--------------------------
qvariant 400
boost.any 143
std::any 139
static_any<32> 92
```
**get a string**
```
Test Time (ns)
--------------------------
qvariant 31
boost.any 64
std::any 30
static_any<32> 4
```
What I used for this benchmark:
- Boost 1.54
- Qt5
- GCC 5.3.0
- CPU i7-3537U
The code is available in *benchmark.cpp*. There is a dependency on *geiger*, a benchmarking library I developed. In order to build and run the benchmark, first install geiger:
```
git clone https://github.com/david-grs/geiger
cd geiger && mkdir build && cd build
cmake .. && make install
```
Then pass *-DBUILD_BENCHMARK=1* to your cmake command.
| Java |
const frisby = require('frisby')
const config = require('config')
const URL = 'http://localhost:3000'
let blueprint
for (const product of config.get('products')) {
if (product.fileForRetrieveBlueprintChallenge) {
blueprint = product.fileForRetrieveBlueprintChallenge
break
}
}
describe('Server', () => {
it('GET responds with index.html when visiting application URL', done => {
frisby.get(URL)
.expect('status', 200)
.expect('header', 'content-type', /text\/html/)
.expect('bodyContains', 'dist/juice-shop.min.js')
.done(done)
})
it('GET responds with index.html when visiting application URL with any path', done => {
frisby.get(URL + '/whatever')
.expect('status', 200)
.expect('header', 'content-type', /text\/html/)
.expect('bodyContains', 'dist/juice-shop.min.js')
.done(done)
})
it('GET a restricted file directly from file system path on server via Directory Traversal attack loads index.html instead', done => {
frisby.get(URL + '/public/images/../../ftp/eastere.gg')
.expect('status', 200)
.expect('bodyContains', '<meta name="description" content="An intentionally insecure JavaScript Web Application">')
.done(done)
})
it('GET a restricted file directly from file system path on server via URL-encoded Directory Traversal attack loads index.html instead', done => {
frisby.get(URL + '/public/images/%2e%2e%2f%2e%2e%2fftp/eastere.gg')
.expect('status', 200)
.expect('bodyContains', '<meta name="description" content="An intentionally insecure JavaScript Web Application">')
.done(done)
})
})
describe('/public/images/tracking', () => {
it('GET tracking image for "Score Board" page access challenge', done => {
frisby.get(URL + '/public/images/tracking/scoreboard.png')
.expect('status', 200)
.expect('header', 'content-type', 'image/png')
.done(done)
})
it('GET tracking image for "Administration" page access challenge', done => {
frisby.get(URL + '/public/images/tracking/administration.png')
.expect('status', 200)
.expect('header', 'content-type', 'image/png')
.done(done)
})
it('GET tracking background image for "Geocities Theme" challenge', done => {
frisby.get(URL + '/public/images/tracking/microfab.gif')
.expect('status', 200)
.expect('header', 'content-type', 'image/gif')
.done(done)
})
})
describe('/encryptionkeys', () => {
it('GET serves a directory listing', done => {
frisby.get(URL + '/encryptionkeys')
.expect('status', 200)
.expect('header', 'content-type', /text\/html/)
.expect('bodyContains', '<title>listing directory /encryptionkeys</title>')
.done(done)
})
it('GET a non-existing file in will return a 404 error', done => {
frisby.get(URL + '/encryptionkeys/doesnotexist.md')
.expect('status', 404)
.done(done)
})
it('GET the Premium Content AES key', done => {
frisby.get(URL + '/encryptionkeys/premium.key')
.expect('status', 200)
.done(done)
})
it('GET a key file whose name contains a "/" fails with a 403 error', done => {
frisby.fetch(URL + '/encryptionkeys/%2fetc%2fos-release%2500.md', {}, { urlEncode: false })
.expect('status', 403)
.expect('bodyContains', 'Error: File names cannot contain forward slashes!')
.done(done)
})
})
describe('Hidden URL', () => {
it('GET the second easter egg by visiting the Base64>ROT13-decrypted URL', done => {
frisby.get(URL + '/the/devs/are/so/funny/they/hid/an/easter/egg/within/the/easter/egg')
.expect('status', 200)
.expect('header', 'content-type', /text\/html/)
.expect('bodyContains', '<title>Welcome to Planet Orangeuze</title>')
.done(done)
})
it('GET the premium content by visiting the ROT5>Base64>z85>ROT5-decrypted URL', done => {
frisby.get(URL + '/this/page/is/hidden/behind/an/incredibly/high/paywall/that/could/only/be/unlocked/by/sending/1btc/to/us')
.expect('status', 200)
.expect('header', 'content-type', 'image/gif')
.done(done)
})
it('GET Geocities theme CSS is accessible directly from file system path', done => {
frisby.get(URL + '/css/geo-bootstrap/swatch/bootstrap.css')
.expect('status', 200)
.expect('bodyContains', 'Designed and built with all the love in the world @twitter by @mdo and @fat.')
.done(done)
})
it('GET Klingon translation file for "Extra Language" challenge', done => {
frisby.get(URL + '/i18n/tlh_AA.json')
.expect('status', 200)
.expect('header', 'content-type', /application\/json/)
.done(done)
})
it('GET blueprint file for "Retrieve Blueprint" challenge', done => {
frisby.get(URL + '/public/images/products/' + blueprint)
.expect('status', 200)
.done(done)
})
})
| Java |
Subsets and Splits