id
int64 5
1.93M
| title
stringlengths 0
128
| description
stringlengths 0
25.5k
| collection_id
int64 0
28.1k
| published_timestamp
timestamp[s] | canonical_url
stringlengths 14
581
| tag_list
stringlengths 0
120
| body_markdown
stringlengths 0
716k
| user_username
stringlengths 2
30
|
---|---|---|---|---|---|---|---|---|
1,913,784 | 3. Finale of complete SASS 🤣(longer) | You can read last 2 post from here. ❤part1 - SASS one ❤part2 - SASS two official sass playground❤ ... | 0 | 2024-07-06T12:55:15 | https://dev.to/aryan015/3-finale-of-complete-sass-longer-2gpe | css, tailwindcss, scss, sass | You can read last 2 post from here.
[❤part1 - SASS one](https://dev.to/aryan015/scss-complete-guide-part-one-4d03)
[❤part2 - SASS two](https://dev.to/aryan015/aryans-scss-complete-guide-part-2-1p1i)
[official sass playground❤](https://sass-lang.com/playground)
## `@extend`
The `@extend` directive lets you share a set of CSS properties from one selector to another. The @extend directive is useful if you have almost identically styled elements that only differ in some small details.
[source:w3schools](https://www.w3schools.com/sass/sass_extend.php)
`SCSS`
```scss
.button-basic {
border: none;
padding: 15px 30px;
text-align: center;
font-size: 16px;
cursor: pointer;
}
.button-report {
@extend .button-basic;
background-color: red;
}
.button-submit {
@extend .button-basic;
background-color: green;
color: white;
}
```
`css`
```css
.button-basic, .button-report, .button-submit {
border: none;
padding: 15px 30px;
text-align: center;
font-size: 16px;
cursor: pointer;
}
.button-report {
background-color: red;
}
.button-submit {
background-color: green;
color: white;
}
```
By using the @extend directive, you do not need to specify several classes for an element in your HTML code, like this: `<button class="button-basic button-report">Report this</button>`. You just need to specify .button-report to get both sets of styles.
`note:`This will keep your code DRY.
## SASS String
### `quote` and `unquote`
Just quote the string
```scss
@use "sass:string"
//string.quote(string)
//string.unquote(string)
@debug quote(.widget:hover) // ".widget:hover"
@debug unquote(".widget:hover") // .widget:hover
```
### `str-index`
It return the first occurance with one base indexing.
```scss
@use "sass:string"
//string.quote(string,substring)
@debug str-index()
```
### `str-insert`
Insert at specified index
```scss
@use "sass:string"
//str-insert(str,insert,index)
@debug str-insert("hello ","world",7) //hello world
```
### `str-length`
find the length of the string
```scss
@use "sass:string"
//str-length(string)
@debug str-length("hello world!") //12
```
### `str-slice`
slice works different in sass. It also includes last index.
```scss
@use "sass:string"
//str-slice(string,start,end)
@debug str-slice('hello',1,2) //he
```
### `to-lower-case` and `to-upper-case`
```scss
@use "sass:string"
//to-lower-case(string)
@debug to-lower-case("HELLO") //hello
//to-upper-case(string)
@debug to-upper-case("hello") //HELLO
```
### `unique-id()`
Returns a unique randomly generated unquoted string (guaranteed to be unique within the current sass session).
## SASS Nuber
### `abs`
It invert the values.
```scss
//abs(number)
@debug abs(-15); //15
```
### `ceil` and `floor`
Returns the uppermost and lowermost value respectively.
```scss
//ceil(number)
//floor(number)
@debug ceil(15.5); //16
@debug floor(15.2); //15
```
### `comparable`
Checks whether units are same or not
```scss
//comparable(num1,num2)
@debug comparable(15px, 10%); //false
@debug comparable(20mm,10cm); //true
```
### `max` and `min`
return the highest value among comma seperated
```scss
@debug max(1,2,3,4,5); //5
@debug min(-1,2,3,4); //-1
```
### `percentage`
multiplies value with 100
```scss
@debug percentage(1.2); //120
```
### random
It generates a random number. It has two version. With para and without parameter.
#### `random()`
```scss
//generate a random number btw 0(inclusive) to 1(exclusive)
@debug random(); //0.1234
```
#### `random(number)`
```scss
//generate a random number 0(inclusive) to number(exclusive)
@debug random(6); //0.1234
```
### `round`
Round the number to the nearest integer.
```scss
//round(number)
@debug round(12.5); //13
```
## List (datatype)
### `append` function
append an item to end fo the list
```scss
//append(list,item,seperator)
//seperator auto,space,comma
@debug append((a, b, c),d,space); // a b c d
@debug append((a, b, c),d,comma); // a,b,c,d
```
### `index` function
- Returns the index position for the value in list.
- returns `null` if not found
```scss
//index(list,item-to-find)
@debug index((a b c),b); //2
@debug index((a,b,c),b); //2
```
### `is-bracketed` function
Checks whether the list has square brackets.
```scss
//is-bracketed(list)
@debug is-bracketed((1,2,3)); //false
@debug is-bracketed([1,2,3]); //true
```
### `join` function
```scss
//join(list1,list2,seperator)
@debug join((a b c),(d e f), comma); // a,b,c,d,e,f
```
### `length` function
returns the no of elements in a list
```scss
//length(list)
@debug length((a b c)); //3
```
### `list-seperator` function
returns the list seperator (there are two seperator exists either comma or space)
```scss
//list-seperator(list)
@debug list-separator((a b c)); //space
@debug list-separator((a,b,c)); //comma
```
### `nth` and `set-nth` function
returns the n-th element in the list.
```scss
//nth(list,n)
@debug nth((a b c), 2); //b
@debug nth((a,b,c), 2); //c
//set-nth(list,n,value)
@debug set-nth((a b c), 2 , x); //a x c
```
### `zip` function
create a multidimensional list
```scss
@debug zip(1px 2px 3px,solid dashed dotted,red green orange);
//result:1px solid red,2px dashed green, 3px dotted orange
// it will ommit the list if parameter is missing
@debug zip(1px 2px, solid); //1px solid
```
## `map` datatype
In Sass, the map data type represents one or more key/value pairs.
### `map-get`
```scss
$font-sizes: ("small": 12px, "normal": 18px, "large": 24px)
map-get($font-sizes, "small")
Result: 12px
```
### `map-has-key` function
checks whether a key has same pair
```scss
$font-sizes: ("small": 12px, "normal": 18px, "large": 24px);
map-has-key($font-sizes, "big");
//Result: false
```
### `map-keys` function
returns a list of all keys in map.
```scss
$font-sizes: ("small": 12px, "normal": 18px, "large": 24px);
@debug map-keys($font-sizes);
//Result: "small", "normal, "large"
```
### `map-merge` function
merges map with othe map.(map1+map2)
```scss
//map-merge(map1,map2)
$font-sizes: ("small": 12px, "normal": 18px, "large": 24px);
$font-sizes2: ("x-large": 30px, "xx-large": 36px);
@debug map-merge($font-sizes, $font-sizes2);
// Result: "small": 12px, "normal": 18px, "large": 24px, "x-large": 30px, "xx-large": 36px
```
### `map-remove` function
Removes the specified keys from map.
```scss
$font-size:("small":12px,"large":50px,"normal":20px);
@debug map-remove($font-sizes, "small", "large");
//Result: ("normal": 20px)
```
### `map-values` function
returns all values from `map`.
## colors
### `red`,`green` and `blue` function
Returns the red value of color as a number between 0 and 255.
```scss
//red(color) return red value
@debug red(#fff); //255 🤷♀️🤷♀️
//green(color)
@debug green(#7fffd4); //255
@debug green(blue); //0
//blue(color) (homework)
```
### opacity function
return alpha value of a color
```scss
@debug opacity(rgba(127,255,212,0.5)); //0.5
```
## If you reached here (you are certified css developer)❤
[source - w3school](https://www.w3schools.com/sass/default.php)
[❤ - linkedin](https://www.linkedin.com/in/aryan-khandelwal-779b5723a/) follow if you want to
| aryan015 |
1,913,783 | How does Javascript work under the hood? | Okay, So today we shall learn about How Javascript works. Firstly, JavaScript is a lightweight,... | 0 | 2024-07-06T12:54:45 | https://dev.to/ronak_navadia_0611/how-does-javascript-work-under-the-hood-3ofh | javascript, webdev, coding, programming | Okay, So today we shall learn about How Javascript works.
Firstly, JavaScript is a lightweight, cross-platform, single-threaded, and interpreted compiled programming language.
So, Everything inside Javascript happens inside a place known as execution context.
Now the question arises in your mind what is execution context?
Execution Context:-
The environment that handles the code transformation and code execution by scanning the script file (JS file).
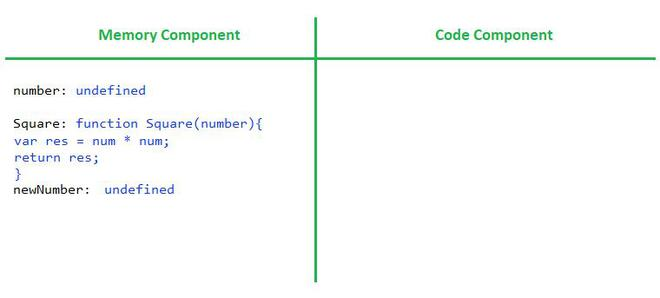
As shown above, the Execution context is divided into 2 parts.
1. Memory component (variable environment)
This is a place where all the variables and functions are stored in key-value pairs.
2. Code component (thread of execution)
This is a place where code is executed one line at a time.
Now lets see how execution context works?
consider the below example
```
var n = 3;
function cube(num) {
var result = num * num * num;
return result;
}
var cube3 = cube(n);
var cube5 = cube(5);
```
Now execution context is created with memory and code components as shown in the above image
The first phase is the memory creation phase and the second phase is the code execution phase.
### Memory creation phase
In this phase, JS will allocate the memory to all the variables and functions
- allocate memory to n variable and store value as undefined.
- allocate memory to the cube function and store the whole
function code.
- allocate memory to cube3 variable and store value as undefined.
- allocate memory to cube5 variable and store value as undefined.
Now after the memory creation let's see how the code is executed in the code execution phase.
## Code execution phase
Javascript goes through the whole JS program line by line and executes the code.
- assign value 2 to n
now it goes to the line where the square function is invoked. so whenever any function is invoked a new execution context is created same as mentioned in the image.
so for the square(n) a new execution context is created with memory and code components
here again, memory is allocated to num and as undefined and then it runs the code execution phase for the function in which it assigns num a value i.e. passed from square(n) where n = 3. so num will assign 3. After that ans will be assigned num * num * num i.e. 27.
Now whenever anything is returned from the function its value is assigned to the variable that invoked the function. here in our case, cube3 will have 27 as ans is assigned 27.
Note here that whenever an execution context is done with its task it is deleted from the call stack.
The same thing happens for the 2nd function cube 5.
Thank you so much for being with me.
| ronak_navadia_0611 |
1,913,781 | BitPower: Exploring the Future of Decentralized Finance | Introduction In the era of rapid development of modern financial technology, decentralized finance... | 0 | 2024-07-06T12:53:13 | https://dev.to/wgac_0f8ada999859bdd2c0e5/bitpower-exploring-the-future-of-decentralized-finance-1al8 | Introduction
In the era of rapid development of modern financial technology, decentralized finance (DeFi) has become an emerging force that subverts traditional financial services. As an innovative project in this field, BitPower is committed to providing users with more transparent, efficient and secure financial services through blockchain technology and smart contracts. This article will introduce in detail the basic concepts, core technologies, security, and applications and advantages of BitPower in the financial field.
Basic Concepts
BitPower is a decentralized lending platform developed based on blockchain technology, aiming to break the barriers of the traditional financial system and provide more flexible and low-cost financial services. BitPower is built on the Ethereum network (EVM) and uses smart contract technology to realize asset deposits and lending. Participants can deposit digital assets on the platform to earn interest or obtain loans by mortgaging assets.
Core Technology
Smart Contract
The core of BitPower lies in smart contract technology. A smart contract is a self-executing code that can automatically perform predefined operations when specific conditions are met. On the BitPower platform, all deposit and lending operations are controlled by smart contracts to ensure the security and transparency of transactions.
Decentralization
BitPower adopts a decentralized architecture without a central control agency. All transaction records are stored on the blockchain and maintained by all nodes in the network. This decentralized approach not only improves the system's anti-attack capabilities, but also ensures the transparency and immutability of the data.
Consensus Mechanism
The BitPower platform uses the consensus mechanism of the blockchain to ensure the validity and consistency of transactions. Through consensus algorithms such as Proof of Work (PoW) or Proof of Stake (PoS), nodes in the network can reach a consensus to ensure that every transaction is legal and accurate.
Security
BitPower attaches great importance to the security of the platform and uses a variety of technical means to ensure the security of users' assets. First, the code of the smart contract is strictly audited and tested to ensure its reliability and security. Secondly, the platform uses cryptography technology to encrypt transaction data to prevent unauthorized access and tampering. In addition, the decentralized architecture makes the platform have no single point of failure, further improving the security of the system.
Applications and Advantages
Efficient and Convenient
Compared with traditional financial services, the lending services provided by BitPower are more efficient and convenient. Users only need to operate through the blockchain wallet without cumbersome approval processes to realize the deposit and lending of assets.
Transparency and fairness
All transaction records on the BitPower platform are open and transparent, and users can check the flow of assets at any time. This transparency not only enhances user trust, but also helps prevent fraud and improper behavior.
Lower cost
Because intermediaries are removed, BitPower's operating costs are greatly reduced, so it can provide users with lower lending rates and higher deposit returns. This is of great significance to those who have difficulty in obtaining services in the traditional financial system, especially users in developing countries.
Conclusion
As an innovative project in the field of decentralized finance, BitPower provides users with a safe, transparent and efficient lending platform through blockchain and smart contract technology. It not only optimizes the process and cost of financial services, but also provides more people with the opportunity to participate in the global financial market. With the continuous advancement of technology and the continuous expansion of applications, BitPower is expected to play a greater role in the future and lead the development trend of decentralized finance.@BitPower | wgac_0f8ada999859bdd2c0e5 |
|
1,913,779 | Let's understand the differences: href="", href="#", and href="javascript:void(0)" | Empty HREF: href="" Using an empty href attribute (href="") reloads the current page. This... | 0 | 2024-07-06T12:51:46 | https://dev.to/readwanmd/lets-understand-the-differences-href-href-and-hrefjavascriptvoid0-a2c | html, javascript |
## Empty HREF: href=""
Using an empty href attribute (href="") reloads the current page. This is like clicking the refresh button of the browser.
## href="#"
The href=”#” attribute makes the page scroll to the top. If you don’t want this, you can stop the behavior with JavaScript:
```html
<a href=”#” onclick=”return false;”>Hey</a>
<!--! and there ane many ways with js to achive this -->
href="javascript:void(0)"
```
Sometimes you will see href="javascript:void(0);" inside an `<a>` tag. This makes a link that does nothing when clicked. Other ways to do this are:
```html
href="javascript:{}"
href="javascript:null"
```
These links do nothing it’s best to avoid them.
## href="#any_id"
The href="#any_id" attribute does nothing unless have an element with an ID of any_idon the page. By clicking the link will scroll to that element.
To avoid any scroll use a different id value that doesn't exist on the page.
**Best Practices:**
Use a Button or Span: If your link doesn’t navigate anywhere, you can use <button> or <span> instead of an `<a>` element as well as you can style these elements as needed using raw CSS or CSS framework.
Lastly, let’s summarize these
- `href="#"` scrolls the current page to the top.
- `href="javascript:void(0)"` does nothing.
- `href="#any_id"` does nothing unless there is an element with the specific ID.
_And finally,
I’m appreciative that you read my piece._ | readwanmd |
1,913,778 | Responsive Là Gì? Thiết Kế Website Chuẩn Responsive Là Như Thế Nào? | Responsive Web Design (RWD) là một kỹ thuật thiết kế và phát triển website nhằm đảm bảo trang web có... | 0 | 2024-07-06T12:51:36 | https://dev.to/terus_technique/responsive-la-gi-thiet-ke-website-chuan-responsive-la-nhu-the-nao-2mo9 | website, digitalmarketing, seo, terus |
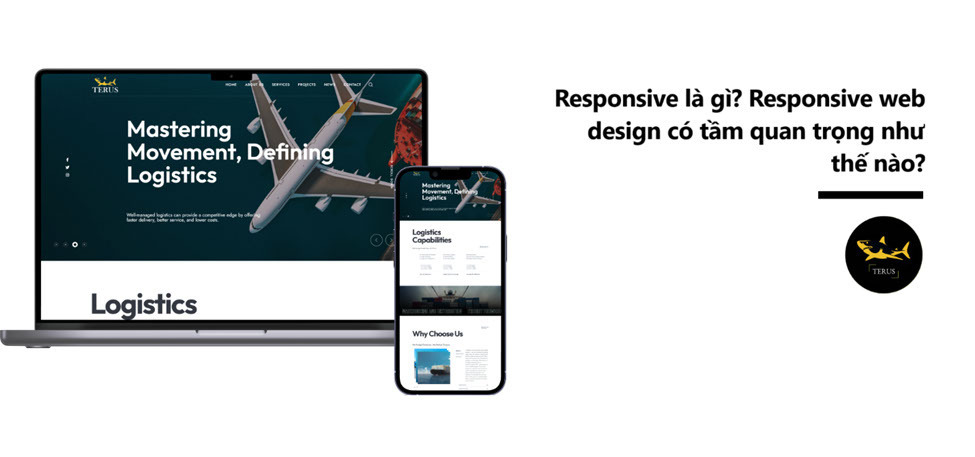
Responsive Web Design (RWD) là một kỹ thuật thiết kế và phát triển website nhằm đảm bảo trang web có thể hiển thị tối ưu trên mọi thiết bị, từ máy tính để bàn đến các thiết bị di động như smartphone, máy tính bảng. Responsive design sử dụng các kỹ thuật như CSS media queries, layout flexible, fluid images/videos để tự động điều chỉnh giao diện phù hợp với kích thước màn hình của từng thiết bị.
Responsive design đáp ứng nhu cầu thực tế của người dùng, vì ngày nay hầu hết truy cập website đều qua các thiết bị di động. Nó mang lại nhiều lợi ích kinh tế, như giảm chi phí bảo trì các phiên bản website riêng biệt, tăng hiệu quả SEO, và giảm tỷ lệ thoát trang. Responsive cũng được Google khuyến khích áp dụng, vì nó tạo trải nghiệm tốt hơn cho người dùng.
Responsive design cải thiện trải nghiệm người dùng, vì website tự động thích ứng với màn hình của mọi thiết bị. Nó còn hiệu quả về chi phí, vì chỉ cần duy trì một phiên bản website. Responsive giúp giảm tỷ lệ thoát trang và thời gian truy cập. Hơn nữa, nó yêu cầu ít bảo trì hơn so với phát triển các trang web riêng biệt cho từng thiết bị.
Một [thiết kế website chuẩn responsive](https://terusvn.com/thiet-ke-website-tai-hcm/) giúp doanh nghiệp thích ứng với xu hướng sử dụng thiết bị di động ngày càng phổ biến. Nó giúp tiết kiệm ngân sách vì chỉ cần duy trì một website. Responsive còn tối ưu hóa SEO, giúp website xếp hạng cao hơn trên kết quả tìm kiếm Google. Điều này cuối cùng sẽ giúp giảm tỷ lệ thoát trang.
Responsive design mang lại trải nghiệm người dùng tốt hơn, vì website tự động điều chỉnh giao diện phù hợp với từng thiết bị. Người dùng có thể dễ dàng truy cập và tương tác với website trên mọi thiết bị.
Một số lưu ý khi thiết kế web responsive bao gồm: phân tích hành vi người dùng, không nên bắt đầu với phiên bản desktop, thử nghiệm trước khi đưa vào sử dụng, chú ý nút CTA, sử dụng hình ảnh có độ phân giải linh hoạt, và tùy chỉnh email.
Responsive web design đóng vai trò quan trọng trong việc tối ưu hóa trải nghiệm người dùng, giảm chi phí, tăng hiệu quả SEO và giảm tỷ lệ thoát trang cho doanh nghiệp. Áp dụng responsive design là một yêu cầu thiết yếu trong [thiết kế website hiện đại](https://terusvn.com/thiet-ke-website-tai-hcm/).
Tìm hiểu thêm về [Responsive Là Gì? Thiết Kế Website Chuẩn Responsive Là Như Thế Nào?](https://terusvn.com/thiet-ke-website/responsive-la-gi/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,765 | BitPower: Exploring the Future of Decentralized Finance | Introduction In the era of rapid development of modern financial technology, decentralized finance... | 0 | 2024-07-06T12:46:51 | https://dev.to/weq_24a494dd3a467ace6aca5/bitpower-exploring-the-future-of-decentralized-finance-33e | Introduction
In the era of rapid development of modern financial technology, decentralized finance (DeFi) has become an emerging force that subverts traditional financial services. As an innovative project in this field, BitPower is committed to providing users with more transparent, efficient and secure financial services through blockchain technology and smart contracts. This article will introduce in detail the basic concepts, core technologies, security, and applications and advantages of BitPower in the financial field.
Basic Concepts
BitPower is a decentralized lending platform developed based on blockchain technology, aiming to break the barriers of the traditional financial system and provide more flexible and low-cost financial services. BitPower is built on the Ethereum network (EVM) and uses smart contract technology to realize asset deposits and lending. Participants can deposit digital assets on the platform to earn interest or obtain loans by mortgaging assets.
Core Technology
Smart Contract
The core of BitPower lies in smart contract technology. A smart contract is a self-executing code that can automatically perform predefined operations when specific conditions are met. On the BitPower platform, all deposit and lending operations are controlled by smart contracts to ensure the security and transparency of transactions.
Decentralization
BitPower adopts a decentralized architecture without a central control agency. All transaction records are stored on the blockchain and maintained by all nodes in the network. This decentralized approach not only improves the system's anti-attack capabilities, but also ensures the transparency and immutability of the data.
Consensus Mechanism
The BitPower platform uses the consensus mechanism of the blockchain to ensure the validity and consistency of transactions. Through consensus algorithms such as Proof of Work (PoW) or Proof of Stake (PoS), nodes in the network can reach a consensus to ensure that every transaction is legal and accurate.
Security
BitPower attaches great importance to the security of the platform and uses a variety of technical means to ensure the security of users' assets. First, the code of the smart contract is strictly audited and tested to ensure its reliability and security. Secondly, the platform uses cryptography technology to encrypt transaction data to prevent unauthorized access and tampering. In addition, the decentralized architecture makes the platform have no single point of failure, further improving the security of the system.
Applications and Advantages
Efficient and Convenient
Compared with traditional financial services, the lending services provided by BitPower are more efficient and convenient. Users only need to operate through the blockchain wallet without cumbersome approval processes to realize the deposit and lending of assets.
Transparency and fairness
All transaction records on the BitPower platform are open and transparent, and users can check the flow of assets at any time. This transparency not only enhances user trust, but also helps prevent fraud and improper behavior.
Lower cost
Because intermediaries are removed, BitPower's operating costs are greatly reduced, so it can provide users with lower lending rates and higher deposit returns. This is of great significance to those who have difficulty in obtaining services in the traditional financial system, especially users in developing countries.
Conclusion
As an innovative project in the field of decentralized finance, BitPower provides users with a safe, transparent and efficient lending platform through blockchain and smart contract technology. It not only optimizes the process and cost of financial services, but also provides more people with the opportunity to participate in the global financial market. With the continuous advancement of technology and the continuous expansion of applications, BitPower is expected to play a greater role in the future and lead the development trend of decentralized finance.@BitPower | weq_24a494dd3a467ace6aca5 |
|
1,913,764 | UI/UX là gì? Tiêu Chuẩn Đánh Giá Website Chuẩn UI/UX | UI/UX là viết tắt của User Interface (Giao diện người dùng) và User Experience (Trải nghiệm người... | 0 | 2024-07-06T12:45:19 | https://dev.to/terus_technique/uiux-la-gi-tieu-chuan-danh-gia-website-chuan-uiux-42ic | website, digitalmarketing, seo, terus |
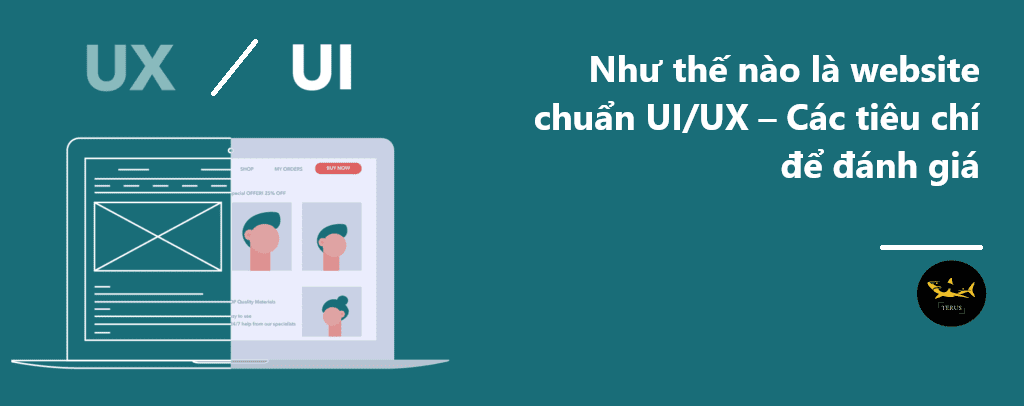
UI/UX là viết tắt của User Interface (Giao diện người dùng) và User Experience (Trải nghiệm người dùng). UI Design liên quan đến việc thiết kế các thành phần trực quan của một sản phẩm như màu sắc, typography, layout,... nhằm tạo ra một giao diện thân thiện và dễ sử dụng. Trong khi đó, UX Design tập trung vào việc thiết kế trải nghiệm người dùng, tối ưu hóa quy trình sử dụng sản phẩm để người dùng có thể đạt được mục tiêu của mình một cách dễ dàng và hiệu quả.
Một website chuẩn UI/UX cần đáp ứng các tiêu chuẩn sau:
Bố cục hợp lý trên giao diện website: Sắp xếp các thành phần trực quan một cách logic, dễ nhìn, dễ sử dụng.
Có các khoảng trống tinh tế: Sử dụng hợp lý các khoảng trắng để tạo sự thoáng đãng, giảm sự xung đột giữa các phần tử.
Các nút kêu gọi (CTA) phải hài hòa với phần nội dung: Các nút CTA cần được thiết kế nổi bật nhưng không lấn át phần nội dung chính.
Lựa chọn màu sắc website có chủ đích: Sử dụng bảng màu phù hợp với thương hiệu, truyền tải thông điệp đúng.
Font chữ sử dụng phù hợp: Lựa chọn font chữ dễ đọc, phù hợp với tổng thể thiết kế.
Các điều hướng phải có tính toán từ trước: Thiết kế menu, liên kết giữa các trang một cách logic, dễ sử dụng.
Website chuẩn Responsive hoạt động trên mọi thiết bị: Giao diện website phải linh hoạt, thích ứng tốt trên các thiết bị khác nhau.
Thông điệp truyền đi rõ ràng: Nội dung và các yếu tố trực quan phải truyền tải thông điệp chính xác, dễ hiểu.
Việc sử dụng [dịch vụ thiết kế website chuẩn UI/UX](https://terusvn.com/thiet-ke-website-tai-hcm/) mang lại nhiều lợi ích:
Đáp ứng tiêu chuẩn đánh giá chất lượng tốt: Người dùng sẽ có trải nghiệm tích cực, dễ sử dụng.
Truyền tải được thông điệp: Nội dung và thương hiệu được truyền tải một cách hiệu quả.
Điều hướng và tính khả dụng tốt: Người dùng dễ dàng tìm thấy thông tin, thực hiện các thao tác mong muốn.
Sự rõ ràng và mạch lạc: Website tạo cảm giác chuyên nghiệp, gây ấn tượng với người dùng.
[Dịch vụ thiết kế website chuẩn UI/UX](https://terusvn.com/thiet-ke-website-tai-hcm/) tại Terus bao gồm các bước như: Nghiên cứu người dùng, Xây dựng sơ đồ trang web, Thiết kế giao diện, Phát triển website, Kiểm thử và hoàn thiện.
Đối với Terus, việc thiết kế một website chuẩn UI/UX là hết sức quan trọng, giúp tối ưu trải nghiệm người dùng, truyền tải thông điệp hiệu quả và tăng cạnh tranh trong thời đại số hóa hiện nay. Các tiêu chuẩn đánh giá như bố cục, màu sắc, điều hướng, tính responsive... góp phần quan trọng vào việc xây dựng một website chất lượng.
Tìm hiểu thêm về [UI/UX là gì? Tiêu Chuẩn Đánh Giá Website Chuẩn UI/UX](https://terusvn.com/thiet-ke-website/nhu-the-nao-la-website-chuan-ui-ux/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,763 | BitPower Security Overview | What is BitPower? BitPower is a decentralized lending platform that uses blockchain and smart... | 0 | 2024-07-06T12:44:31 | https://dev.to/aimm_y/bitpower-security-overview-pln | What is BitPower?
BitPower is a decentralized lending platform that uses blockchain and smart contract technology to provide secure lending services.
Security Features
Smart Contract
Automatically execute transactions and reduce human intervention.
Open source code, anyone can view it.
Decentralization
Users interact directly with the platform without intermediaries.
Funds flow freely between user wallets.
Asset Collateral
Borrowers use crypto assets as collateral to ensure loan security.
When the value of the collateralized assets decreases, the smart contract automatically liquidates to protect the interests of both parties.
Data Transparency
All transaction records are public and can be viewed by anyone.
Users can view transactions and assets at any time.
Multi-Signature
Multi-Signature technology is used to ensure transaction security.
Advantages
High security: Smart contracts and blockchain technology ensure platform security.
Transparency and Trust: Open source code and public transaction records increase transparency.
Risk Control: Collateral and automatic liquidation mechanisms reduce risks.
Conclusion
BitPower provides a secure and transparent decentralized lending platform through smart contracts and blockchain technology. Join BitPower and experience secure lending services!@BitPower | aimm_y |
|
1,913,558 | Buy verified cash app account | https://dmhelpshop.com/product/buy-verified-cash-app-account/ Buy verified cash app account Cash... | 0 | 2024-07-06T09:36:59 | https://dev.to/ranaler355/buy-verified-cash-app-account-20gl | webdev, javascript, beginners, programming | https://dmhelpshop.com/product/buy-verified-cash-app-account/
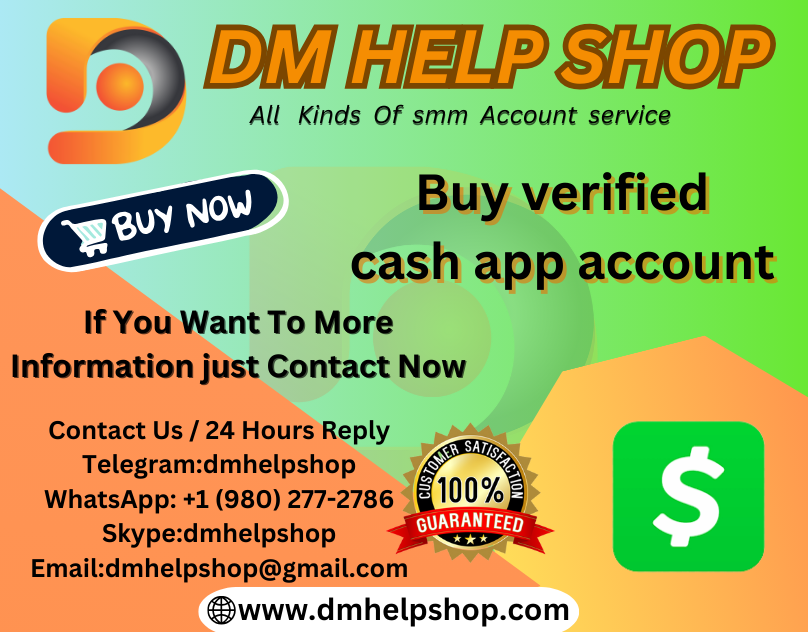
Buy verified cash app account
Cash app has emerged as a dominant force in the realm of mobile banking within the USA, offering unparalleled convenience for digital money transfers, deposits, and trading. As the foremost provider of fully verified cash app accounts, we take pride in our ability to deliver accounts with substantial limits. Bitcoin enablement, and an unmatched level of security.
Our commitment to facilitating seamless transactions and enabling digital currency trades has garnered significant acclaim, as evidenced by the overwhelming response from our satisfied clientele. Those seeking buy verified cash app account with 100% legitimate documentation and unrestricted access need look no further. Get in touch with us promptly to acquire your verified cash app account and take advantage of all the benefits it has to offer.
Why dmhelpshop is the best place to buy USA cash app accounts?
It’s crucial to stay informed about any updates to the platform you’re using. If an update has been released, it’s important to explore alternative options. Contact the platform’s support team to inquire about the status of the cash app service.
Clearly communicate your requirements and inquire whether they can meet your needs and provide the buy verified cash app account promptly. If they assure you that they can fulfill your requirements within the specified timeframe, proceed with the verification process using the required documents.
Our account verification process includes the submission of the following documents: [List of specific documents required for verification].
Genuine and activated email verified
Registered phone number (USA)
Selfie verified
SSN (social security number) verified
Driving license
BTC enable or not enable (BTC enable best)
100% replacement guaranteed
100% customer satisfaction
When it comes to staying on top of the latest platform updates, it’s crucial to act fast and ensure you’re positioned in the best possible place. If you’re considering a switch, reaching out to the right contacts and inquiring about the status of the buy verified cash app account service update is essential.
Clearly communicate your requirements and gauge their commitment to fulfilling them promptly. Once you’ve confirmed their capability, proceed with the verification process using genuine and activated email verification, a registered USA phone number, selfie verification, social security number (SSN) verification, and a valid driving license.
Additionally, assessing whether BTC enablement is available is advisable, buy verified cash app account, with a preference for this feature. It’s important to note that a 100% replacement guarantee and ensuring 100% customer satisfaction are essential benchmarks in this process.
How to use the Cash Card to make purchases?
To activate your Cash Card, open the Cash App on your compatible device, locate the Cash Card icon at the bottom of the screen, and tap on it. Then select “Activate Cash Card” and proceed to scan the QR code on your card. Alternatively, you can manually enter the CVV and expiration date. How To Buy Verified Cash App Accounts.
After submitting your information, including your registered number, expiration date, and CVV code, you can start making payments by conveniently tapping your card on a contactless-enabled payment terminal. Consider obtaining a buy verified Cash App account for seamless transactions, especially for business purposes. Buy verified cash app account.
Why we suggest to unchanged the Cash App account username?
To activate your Cash Card, open the Cash App on your compatible device, locate the Cash Card icon at the bottom of the screen, and tap on it. Then select “Activate Cash Card” and proceed to scan the QR code on your card.
Alternatively, you can manually enter the CVV and expiration date. After submitting your information, including your registered number, expiration date, and CVV code, you can start making payments by conveniently tapping your card on a contactless-enabled payment terminal. Consider obtaining a verified Cash App account for seamless transactions, especially for business purposes. Buy verified cash app account. Purchase Verified Cash App Accounts.
Selecting a username in an app usually comes with the understanding that it cannot be easily changed within the app’s settings or options. This deliberate control is in place to uphold consistency and minimize potential user confusion, especially for those who have added you as a contact using your username. In addition, purchasing a Cash App account with verified genuine documents already linked to the account ensures a reliable and secure transaction experience.
Buy verified cash app accounts quickly and easily for all your financial needs.
As the user base of our platform continues to grow, the significance of verified accounts cannot be overstated for both businesses and individuals seeking to leverage its full range of features. How To Buy Verified Cash App Accounts.
For entrepreneurs, freelancers, and investors alike, a verified cash app account opens the door to sending, receiving, and withdrawing substantial amounts of money, offering unparalleled convenience and flexibility. Whether you’re conducting business or managing personal finances, the benefits of a verified account are clear, providing a secure and efficient means to transact and manage funds at scale.
When it comes to the rising trend of purchasing buy verified cash app account, it’s crucial to tread carefully and opt for reputable providers to steer clear of potential scams and fraudulent activities. How To Buy Verified Cash App Accounts. With numerous providers offering this service at competitive prices, it is paramount to be diligent in selecting a trusted source.
This article serves as a comprehensive guide, equipping you with the essential knowledge to navigate the process of procuring buy verified cash app account, ensuring that you are well-informed before making any purchasing decisions. Understanding the fundamentals is key, and by following this guide, you’ll be empowered to make informed choices with confidence.
Is it safe to buy Cash App Verified Accounts?
Cash App, being a prominent peer-to-peer mobile payment application, is widely utilized by numerous individuals for their transactions. However, concerns regarding its safety have arisen, particularly pertaining to the purchase of “verified” accounts through Cash App. This raises questions about the security of Cash App’s verification process.
Unfortunately, the answer is negative, as buying such verified accounts entails risks and is deemed unsafe. Therefore, it is crucial for everyone to exercise caution and be aware of potential vulnerabilities when using Cash App. How To Buy Verified Cash App Accounts.
Cash App has emerged as a widely embraced platform for purchasing Instagram Followers using PayPal, catering to a diverse range of users. This convenient application permits individuals possessing a PayPal account to procure authenticated Instagram Followers.
Leveraging the Cash App, users can either opt to procure followers for a predetermined quantity or exercise patience until their account accrues a substantial follower count, subsequently making a bulk purchase. Although the Cash App provides this service, it is crucial to discern between genuine and counterfeit items. If you find yourself in search of counterfeit products such as a Rolex, a Louis Vuitton item, or a Louis Vuitton bag, there are two viable approaches to consider.
Why you need to buy verified Cash App accounts personal or business?
The Cash App is a versatile digital wallet enabling seamless money transfers among its users. However, it presents a concern as it facilitates transfer to both verified and unverified individuals.
To address this, the Cash App offers the option to become a verified user, which unlocks a range of advantages. Verified users can enjoy perks such as express payment, immediate issue resolution, and a generous interest-free period of up to two weeks. With its user-friendly interface and enhanced capabilities, the Cash App caters to the needs of a wide audience, ensuring convenient and secure digital transactions for all.
If you’re a business person seeking additional funds to expand your business, we have a solution for you. Payroll management can often be a challenging task, regardless of whether you’re a small family-run business or a large corporation. How To Buy Verified Cash App Accounts.
Improper payment practices can lead to potential issues with your employees, as they could report you to the government. However, worry not, as we offer a reliable and efficient way to ensure proper payroll management, avoiding any potential complications. Our services provide you with the funds you need without compromising your reputation or legal standing. With our assistance, you can focus on growing your business while maintaining a professional and compliant relationship with your employees. Purchase Verified Cash App Accounts.
A Cash App has emerged as a leading peer-to-peer payment method, catering to a wide range of users. With its seamless functionality, individuals can effortlessly send and receive cash in a matter of seconds, bypassing the need for a traditional bank account or social security number. Buy verified cash app account.
This accessibility makes it particularly appealing to millennials, addressing a common challenge they face in accessing physical currency. As a result, ACash App has established itself as a preferred choice among diverse audiences, enabling swift and hassle-free transactions for everyone. Purchase Verified Cash App Accounts.
How to verify Cash App accounts
To ensure the verification of your Cash App account, it is essential to securely store all your required documents in your account. This process includes accurately supplying your date of birth and verifying the US or UK phone number linked to your Cash App account.
As part of the verification process, you will be asked to submit accurate personal details such as your date of birth, the last four digits of your SSN, and your email address. If additional information is requested by the Cash App community to validate your account, be prepared to provide it promptly. Upon successful verification, you will gain full access to managing your account balance, as well as sending and receiving funds seamlessly. Buy verified cash app account.
How cash used for international transaction?
Experience the seamless convenience of this innovative platform that simplifies money transfers to the level of sending a text message. It effortlessly connects users within the familiar confines of their respective currency regions, primarily in the United States and the United Kingdom.
No matter if you’re a freelancer seeking to diversify your clientele or a small business eager to enhance market presence, this solution caters to your financial needs efficiently and securely. Embrace a world of unlimited possibilities while staying connected to your currency domain. Buy verified cash app account.
Understanding the currency capabilities of your selected payment application is essential in today’s digital landscape, where versatile financial tools are increasingly sought after. In this era of rapid technological advancements, being well-informed about platforms such as Cash App is crucial.
As we progress into the digital age, the significance of keeping abreast of such services becomes more pronounced, emphasizing the necessity of staying updated with the evolving financial trends and options available. Buy verified cash app account.
Offers and advantage to buy cash app accounts cheap?
With Cash App, the possibilities are endless, offering numerous advantages in online marketing, cryptocurrency trading, and mobile banking while ensuring high security. As a top creator of Cash App accounts, our team possesses unparalleled expertise in navigating the platform.
We deliver accounts with maximum security and unwavering loyalty at competitive prices unmatched by other agencies. Rest assured, you can trust our services without hesitation, as we prioritize your peace of mind and satisfaction above all else.
Enhance your business operations effortlessly by utilizing the Cash App e-wallet for seamless payment processing, money transfers, and various other essential tasks. Amidst a myriad of transaction platforms in existence today, the Cash App e-wallet stands out as a premier choice, offering users a multitude of functions to streamline their financial activities effectively. Buy verified cash app account.
Trustbizs.com stands by the Cash App’s superiority and recommends acquiring your Cash App accounts from this trusted source to optimize your business potential.
How Customizable are the Payment Options on Cash App for Businesses?
Discover the flexible payment options available to businesses on Cash App, enabling a range of customization features to streamline transactions. Business users have the ability to adjust transaction amounts, incorporate tipping options, and leverage robust reporting tools for enhanced financial management.
Explore trustbizs.com to acquire verified Cash App accounts with LD backup at a competitive price, ensuring a secure and efficient payment solution for your business needs. Buy verified cash app account.
Discover Cash App, an innovative platform ideal for small business owners and entrepreneurs aiming to simplify their financial operations. With its intuitive interface, Cash App empowers businesses to seamlessly receive payments and effectively oversee their finances. Emphasizing customization, this app accommodates a variety of business requirements and preferences, making it a versatile tool for all.
Where To Buy Verified Cash App Accounts
When considering purchasing a verified Cash App account, it is imperative to carefully scrutinize the seller’s pricing and payment methods. Look for pricing that aligns with the market value, ensuring transparency and legitimacy. Buy verified cash app account.
Equally important is the need to opt for sellers who provide secure payment channels to safeguard your financial data. Trust your intuition; skepticism towards deals that appear overly advantageous or sellers who raise red flags is warranted. It is always wise to prioritize caution and explore alternative avenues if uncertainties arise.
The Importance Of Verified Cash App Accounts
In today’s digital age, the significance of verified Cash App accounts cannot be overstated, as they serve as a cornerstone for secure and trustworthy online transactions.
By acquiring verified Cash App accounts, users not only establish credibility but also instill the confidence required to participate in financial endeavors with peace of mind, thus solidifying its status as an indispensable asset for individuals navigating the digital marketplace.
When considering purchasing a verified Cash App account, it is imperative to carefully scrutinize the seller’s pricing and payment methods. Look for pricing that aligns with the market value, ensuring transparency and legitimacy. Buy verified cash app account.
Equally important is the need to opt for sellers who provide secure payment channels to safeguard your financial data. Trust your intuition; skepticism towards deals that appear overly advantageous or sellers who raise red flags is warranted. It is always wise to prioritize caution and explore alternative avenues if uncertainties arise.
Conclusion
Enhance your online financial transactions with verified Cash App accounts, a secure and convenient option for all individuals. By purchasing these accounts, you can access exclusive features, benefit from higher transaction limits, and enjoy enhanced protection against fraudulent activities. Streamline your financial interactions and experience peace of mind knowing your transactions are secure and efficient with verified Cash App accounts.
Choose a trusted provider when acquiring accounts to guarantee legitimacy and reliability. In an era where Cash App is increasingly favored for financial transactions, possessing a verified account offers users peace of mind and ease in managing their finances. Make informed decisions to safeguard your financial assets and streamline your personal transactions effectively.
Contact Us / 24 Hours Reply
Telegram:dmhelpshop
WhatsApp: +1 (980) 277-2786
Skype:dmhelpshop
Email:[email protected] | ranaler355 |
1,913,762 | Latest Newsletter: Surrounded By Paperclip Maximisers (Issue #171) | US EU French and UK Politics, music industry & AI, money problems in developing nations | 0 | 2024-07-06T12:44:13 | https://dev.to/mjgs/latest-newsletter-surrounded-by-paperclip-maximisers-issue-171-2ef2 | javascript, tech, webdev, discuss | ---
title: Latest Newsletter: Surrounded By Paperclip Maximisers (Issue #171)
published: true
description: US EU French and UK Politics, music industry & AI, money problems in developing nations
tags: javascript, tech, webdev, discuss
---
Latest Newsletter: Surrounded By Paperclip Maximisers (Issue #171)
US EU French and UK Politics, music industry & AI, money problems in developing nations
https://markjgsmith.substack.com/p/saturday-6th-july-2024-surrounded
Would love to hear any comments and feedback you have.
[@markjgsmith](https://twitter.com/markjgsmith) | mjgs |
1,913,760 | Networking: A Journey through the Internet Galaxy | Imagine the vast expanse of the internet as an enormous galaxy filled with countless stars, planets,... | 0 | 2024-07-06T12:42:28 | https://dev.to/siashish/networking-a-journey-through-the-internet-galaxy-3eib | networkingbasics, networkdiagram, computernetworking, networktutorial |
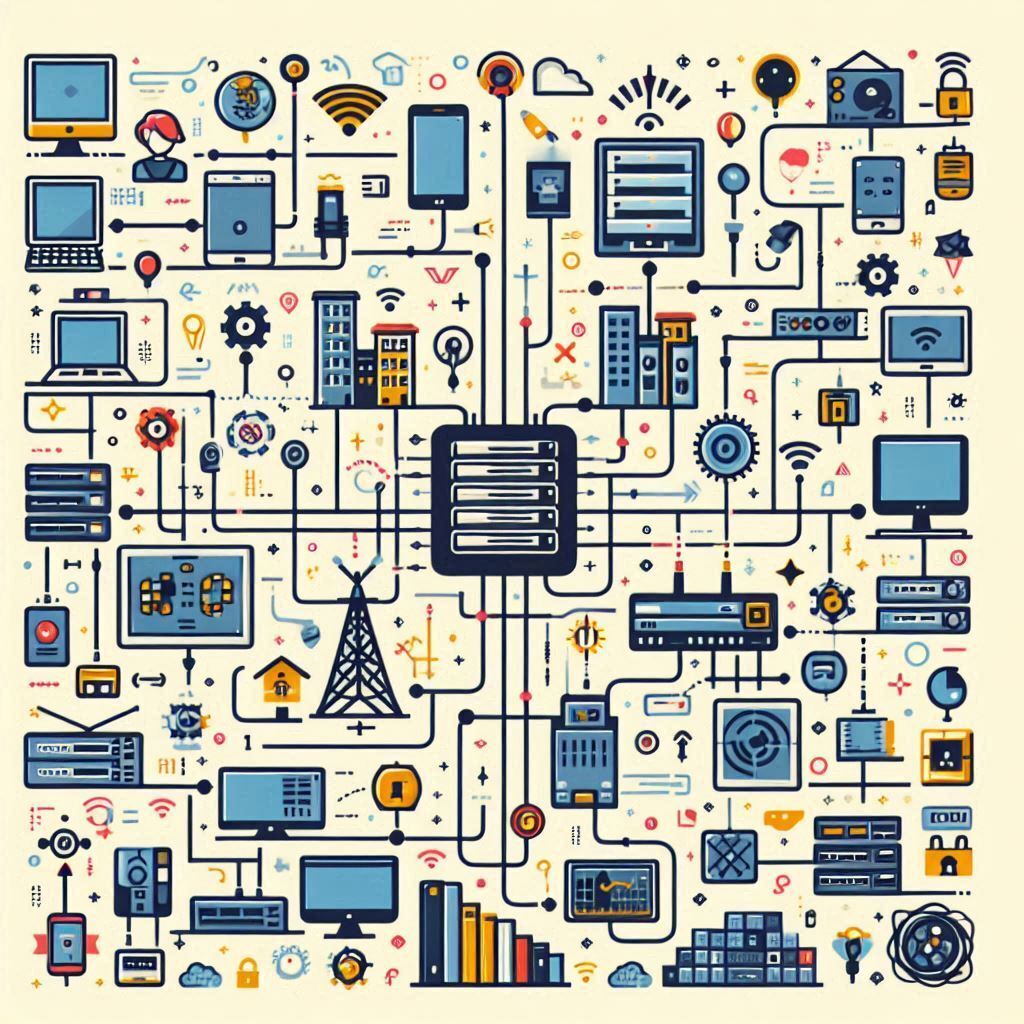
Imagine the vast expanse of the internet as an enormous galaxy filled with countless stars, planets, and mysterious phenomena. Just like space travel, navigating the world of networking can be both exciting and a little overwhelming. Let's embark on a journey through this Internet Galaxy to explore the basics of networking, using some celestial analogies to guide us.
## The Network Nebula: Understanding the Basics
### 1. The Big Bang: The Birth of the Internet
In the beginning, there was ARPANET. This primordial network, created in the late 1960s, marked the inception of what we now know as the internet. Think of it as the Big Bang, the moment that set everything in motion. From this single point, the internet expanded rapidly, connecting more and more computers, like galaxies forming stars.
### 2. Nodes: The Stars of the Network
In our Internet Galaxy, computers and devices are like stars—each one a node in the network. These nodes can be anything from personal computers and smartphones to servers and IoT devices. Nodes are the fundamental building blocks of the network, each one sending and receiving data, much like stars exchanging energy.
### 3. Connections: The Cosmic Web
The connections between nodes are akin to the cosmic web, the intricate network of filaments that connects galaxies across the universe. In networking terms, these connections are made up of cables, wireless signals, and other communication methods that allow data to travel from one node to another. Think of Ethernet cables as the fiber of space, and Wi-Fi signals as waves of light traveling across the void.
## The Protocol Planets: Rules of the Network
### 4. IP Addresses: Your Galactic Coordinates
Every node in the Internet Galaxy needs an address to be found. This is where IP (Internet Protocol) addresses come into play. An IP address is like your cosmic coordinate, a unique identifier that tells other nodes where you are in the galaxy. There are two versions: IPv4, which has been around for decades, and the newer IPv6, designed to accommodate the ever-growing number of devices.
### 5. DNS: The Stargate of the Internet
Imagine trying to find a star without a map—nearly impossible, right? DNS (Domain Name System) is like the stargate that translates human-friendly domain names (like www.example.com) into IP addresses. This system allows you to navigate the galaxy of the internet without needing to remember complex numeric coordinates.
### 6. Protocols: The Laws of the Universe
Protocols are the laws that govern how data is transmitted and received across the network. They ensure that all nodes communicate effectively and understand each other. Some of the key protocols in our Internet Galaxy include:
- **TCP/IP (Transmission Control Protocol/Internet Protocol):** The foundation of internet communication, ensuring reliable data transmission.
- **HTTP/HTTPS (Hypertext Transfer Protocol/Secure):** The protocol used for transferring web pages.
- **FTP (File Transfer Protocol):** Used for transferring files between computers.
## The Constellations of Connectivity
### 7. Local Area Networks (LANs): Your Solar System
A Local Area Network (LAN) is like your immediate solar system, connecting devices within a limited area such as a home, office, or school. These networks are usually fast and secure, allowing seamless communication between nearby nodes.
### 8. Wide Area Networks (WANs): The Galactic Superhighway
Wide Area Networks (WANs) span much larger areas, connecting multiple LANs across cities, countries, or even continents. The internet itself is the largest WAN, a vast intergalactic superhighway that connects millions of smaller networks.
### 9. Routers and Switches: The Space Traffic Controllers
Routers and switches are the traffic controllers of the Internet Galaxy. Routers direct data packets between different networks, ensuring they reach their correct destination. Switches, on the other hand, manage data traffic within a single network, ensuring efficient communication between nodes.
## The Dark Matter: Network Security
### 10. Firewalls: The Galactic Shields
In the vastness of space, there are threats lurking in the dark. Firewalls act as shields, protecting your network from unauthorized access and potential cyber-attacks. They monitor incoming and outgoing traffic, filtering out anything that seems suspicious.
### 11. Encryption: The Secret Codes
Encryption is like encoding your messages in a secret language that only authorized nodes can understand. It ensures that even if data is intercepted, it cannot be read by anyone without the decryption key. SSL/TLS protocols, used in HTTPS, are common encryption methods that protect sensitive information.
### 12. VPNs: The Wormholes of Security
Virtual Private Networks (VPNs) create secure tunnels through the Internet Galaxy, allowing data to travel safely between nodes. They mask your IP address and encrypt your data, making it difficult for anyone to track your online activities.
## Conclusion: Exploring the Infinite Internet Galaxy
Our journey through the Internet Galaxy has just begun. As technology evolves, new stars, planets, and phenomena will continue to emerge, expanding the horizons of networking. Understanding these basics equips you with the knowledge to navigate this vast digital universe and explore its endless possibilities. So, strap in, set your coordinates, and get ready to embark on your own adventures in the ever-expanding cosmos of the internet!
Feel free to reach out if you have any questions or need further guidance. Safe travels through the Internet Galaxy!
Originally published at [https://ashishsingh.in](https://ashishsingh.in/networking-a-journey-through-the-internet-galaxy/) | siashish |
1,913,759 | Chunking an Array in JavaScript: Four Ways Compared | Chunking an array means splitting it into smaller arrays of a specified size. This is useful for data... | 0 | 2024-07-06T12:42:24 | https://dev.to/readwanmd/chunking-an-array-in-javascript-four-ways-compared-48ok | javascript | Chunking an array means splitting it into smaller arrays of a specified size. This is useful for data processing, pagination, and more. We’ll explore four methods to chunk an array and compare their performance.
First, let’s create an array of numbers from 1 to 10:
```javascript
const arr = Array.from({ length: 10 }, (_, i) => i + 1);
```
Array.from() is used to generate an array with elements from 1 to 10.
Now, we’ll look at four ways to chunk this array.
## Method 1: Using a For Loop
```javascript
function chunkArr(arr, size) {
let res = [];
for (let i = 0; i < arr.length; i += size) {
res.push(arr.slice(i, size + i));
}
return res;
}
console.time("for");
console.log(chunkArr(arr, 2));
console.timeEnd("for");
```
**Output:**
```
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ], [ 7, 8 ], [ 9, 10 ] ]
for: 4.363ms
```
**Explanation:** This function iterates through the array in steps of the specified chunk size. It slices the array at each step and adds the resulting sub-array to the result array (res). The performance measurement shows it took about 4.363 milliseconds.
## Method 2: Using `Array.reduce()`
```javascript
function chunkArr2(arr, size) {
if (size <= 0) throw new Error('Chunk size must be a positive integer');
return arr.reduce((acc, _, i) => {
if (i % size === 0) acc.push(arr.slice(i, i + size));
return acc;
}, []);
}
console.time("reduce");
console.log(chunkArr2(arr, 2));
console.timeEnd("reduce");
```
**Output:**
```
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ], [ 7, 8 ], [ 9, 10 ] ]
reduce: 0.069ms
```
**Explanation:** Here Array.reduce() is used to build the chunked array. It checks if the current index is a multiple of the chunk size and slices the array accordingly. This method is significantly faster, taking only about 0.069 milliseconds.
**Method 3: Using `Array.splice()`**
```javascript
let [list, chunkSize] = [arr, 2];
console.time('splice');
list = […Array(Math.ceil(list.length / chunkSize))].map(_ => list.splice(0, chunkSize));
console.timeEnd('splice');
console.log(list);
```
**Output:**
```
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ], [ 7, 8 ], [ 9, 10 ] ]
splice: 0.048ms
```
**Explanation:** This approach uses Array.splice() in combination with Array.map() to chunk the array. It creates a new array with the required number of chunks and uses splice() to remove and collect chunks from the original array. This method is also very fast, taking about 0.048 milliseconds.
**Method 4: Recursive Approach**
```javascript
const chunk = function(array, size) {
if (!array.length) {
return [];
}
const head = array.slice(0, size);
const tail = array.slice(size);
return [head, …chunk(tail, size)];
};
console.time(‘recursive’);
console.log(chunk(arr, 2));
console.timeEnd(‘recursive’);
```
**Output:**
```
[ [ 1, 2 ], [ 3, 4 ], [ 5, 6 ], [ 7, 8 ], [ 9, 10 ] ]
recursive: 4.372ms
```
**Explanation:** This recursive method splits the array into the first chunk (head) and the remaining elements (tail). It then recursively processes the tail, concatenating the results. While more elegant, this method is slower than the reduce and splice methods, taking about 4.372 milliseconds.
**Conclusion**
All four methods successfully chunk the array into sub-arrays of the specified size. However, their performance varies significantly:
1. For Loop: 4.363ms
2. Reduce: 0.069ms
3. Splice: 0.048ms
4. Recursive: 4.372ms
The splice and reduce methods are the fastest, making them preferable for performance-critical applications. While functional, the for loop and recursive methods are slower and might be less suitable for large datasets.
Depending on your needs and readability preferences, you can choose a suitable method for chunking arrays in your JavaScript projects.
You can find more approach [here](https://stackoverflow.com/questions/8495687/split-array-into-chunks?page=1&tab=scoredesc#tab-top)
**And Finally,**
Please don’t hesitate to point out any mistakes in my writing and any errors in my logic.
I’m appreciative that you read my piece. | readwanmd |
1,913,756 | Bảo Mật Website Là Gì? Cách Để Bảo Mật Website Hiệu Quả | Bảo mật website là một khái niệm rất quan trọng trong thời đại số ngày nay. Nó đề cập đến các biện... | 0 | 2024-07-06T12:38:43 | https://dev.to/terus_technique/bao-mat-website-la-gi-cach-de-bao-mat-website-hieu-qua-36n3 | website, digitalmarketing, seo, terus |
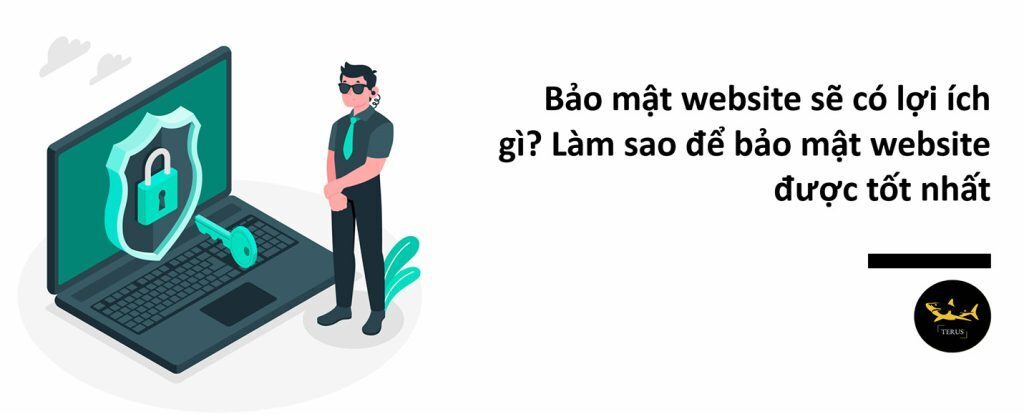
Bảo mật website là một khái niệm rất quan trọng trong thời đại số ngày nay. Nó đề cập đến các biện pháp và quy trình được áp dụng để bảo vệ website và thông tin của người dùng khỏi các mối đe dọa như hack, tấn công từ chối dịch vụ (DDoS), mã độc và các hình thức xâm phạm bảo mật khác.
Một [chính sách bảo mật website](https://terusvn.com/thiet-ke-website-tai-hcm/) là một tài liệu mô tả các biện pháp bảo vệ website và quyền riêng tư của người dùng. Nó thường bao gồm các thông tin như: loại thông tin được thu thập, cách thông tin được sử dụng và chia sẻ, quyền của người dùng đối với thông tin cá nhân, và những hành động sẽ được thực hiện để bảo vệ dữ liệu.
Có nhiều lý do quan trọng để xây dựng chính sách bảo mật website. Trước hết, nó giúp tạo dựng niềm tin của khách hàng, đặc biệt là trong các giao dịch trực tuyến. Thứ hai, nhiều ứng dụng và dịch vụ nhất định yêu cầu người dùng chấp nhận chính sách bảo mật trước khi sử dụng. Cuối cùng, chính sách bảo mật website cung cấp sự bảo vệ pháp lý cho doanh nghiệp.
Một chính sách bảo mật website hiệu quả cần bao gồm các thông tin như: loại thông tin được thu thập, chính sách cookie, các trường hợp dữ liệu có thể được chia sẻ, quyền của người dùng đối với dữ liệu cá nhân, yêu cầu về độ tuổi, và thông tin liên hệ khi có lo ngại về quyền riêng tư.
Ngoài việc xây dựng chính sách bảo mật, có một số biện pháp khác để bảo mật website hiệu quả hơn, bao gồm: luôn cập nhật phần mềm, sử dụng mật khẩu mạnh, triển khai mã hóa SSL/TLS, thường xuyên backup website, và sử dụng tường lửa ứng dụng web (WAF).
Đối với các [website thương mại điện tử](https://terusvn.com/thiet-ke-website-tai-hcm/), yêu cầu về an ninh bảo mật còn nghiêm ngặt hơn, bao gồm: bảo mật giao dịch tài chính, bảo vệ dữ liệu khách hàng, và triển khai các biện pháp ngăn chặn các cuộc tấn công trực tuyến.
Việc bảo mật website mang lại nhiều lợi ích lâu dài cho doanh nghiệp. Nó giúp tăng niềm tin của khách hàng, tuân thủ các quy định pháp lý, bảo vệ danh tiếng và tránh các rủi ro tài chính do vi phạm bảo mật. Trong thời đại số, bảo mật website là một yếu tố then chốt để duy trì sự thành công và phát triển của doanh nghiệp trong dài hạn.
Tìm hiểu thêm về [Bảo Mật Website Là Gì? Cách Để Bảo Mật Website Hiệu Quả](https://terusvn.com/thiet-ke-website/bao-mat-website-se-co-loi-ich-gi/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,731 | JavaScript Tips and Tricks for Developers | JavaScript is a versatile language with numerous features that can make coding more efficient and... | 0 | 2024-07-06T12:23:44 | https://dev.to/mdhassanpatwary/javascript-tips-and-tricks-for-developers-597p | webdev, javascript, beginners, programming | JavaScript is a versatile language with numerous features that can make coding more efficient and enjoyable. Here are some useful tips and tricks every developer should know, complete with code snippets and detailed descriptions to help you understand and implement them easily.
## 1. Swapping Values
Swapping values in an array or between variables can be done in multiple ways. Here are three methods:
**Using a Temporary Variable**
Using a temporary variable is a straightforward method for swapping values.
```
let array = [1, 2, 3, 4, 5];
let temp = array[0];
array[0] = array[4];
array[4] = temp;
console.log(array); // Output: [5, 2, 3, 4, 1]
```
**Using Array Destructuring**
Array destructuring is a concise and modern way to swap values.
```
[array[0], array[4]] = [array[4], array[0]];
console.log(array); // Output: [5, 2, 3, 4, 1]
```
**Using Arithmetic Operations**
This method uses arithmetic operations to swap values without a temporary variable.
```
let a = 1, b = 2;
b = a + (a = b) - b;
console.log(a, b); // Output: 2 1
```
## 2. Copy to Clipboard
Copying text to the clipboard can be done using the following function, which creates a temporary `textarea` element, selects its content, and executes the copy command.
```
function copyToClipBoard(str) {
const element = document.createElement("textarea");
element.value = str;
document.body.appendChild(element);
element.select();
document.execCommand("copy");
document.body.removeChild(element);
}
function handleClick() {
let text = document.querySelector("#text");
copyToClipBoard(text.innerText);
}
```
When `handleClick` is called, it copies the text content of the element with the id `text` to the clipboard.
## 3. Destructuring Aliases
Destructuring objects with aliases allows you to rename properties when extracting them, making your code cleaner and more readable.
```
const language = {
name: "JavaScript",
founded: 1995,
founder: "Brendan Eich",
};
const { name: languageName, founder: creatorName } = language;
console.log(languageName, creatorName); // Output: JavaScript Brendan Eich
```
## 4. Get Value as Data Type
You can retrieve the value of an input element as a specific data type, such as a number, using `valueAsNumber`.
```
const element = document.querySelector("#number").valueAsNumber;
console.log(typeof element); // Output: number
```
## 5. Remove Duplicates from Array
Removing duplicates from an array can be easily achieved using a `Set`, which only stores unique values.
```
const array = [1, 2, 2, 2, 3, 5, 6, 5];
console.log([...new Set(array)]); // Output: [1, 2, 3, 5, 6]
```
## 6. Compare Two Arrays by Value
To check if two arrays have the same elements in the same order, you can use the following function:
```
const hasSameElements = (a, b) => {
return a.length === b.length && a.every((v, i) => v === b[i]);
};
console.log(hasSameElements([1, 2], [1, 2])); // Output: true
console.log(hasSameElements([1, 2], [2, 1])); // Output: false
```
## 7. Shuffling Array
Shuffle an array using `sort` and `Math.random` to randomize the order of elements.
```
const numbers = [1, 2, 3, 4, 5, 6];
console.log(numbers.sort(() => Math.random() - 0.5)); // Output: [3, 5, 1, 6, 4, 2] (example, output may vary)
```
## 8. Comma Operator
The comma operator allows you to include multiple expressions in a context where only one is expected. It evaluates each expression from left to right and returns the value of the last expression.
```
let x = 1;
x = (x++, x);
console.log(x); // Output: 2
let y = (2, 3);
console.log(y); // Output: 3
let a = [[1, 2, 3, 4], [3, 4, 5], [5, 6], [7]];
for (let i = 0, j = 3; i <= 3; i++, j--) {
console.log("a[" + i + "][" + j + "] = " + a[i][j]);
// Output:
// a[0][3] = 4
// a[1][2] = 5
// a[2][1] = 6
// a[3][0] = 7
}
```
## Additional Tips
**Converting NodeList to Array**
You can convert a `NodeList` to an array using the spread operator or `Array.from()`.
```
const nodeList = document.querySelectorAll("div");
const nodeArray = [...nodeList]; // or Array.from(nodeList);
console.log(Array.isArray(nodeArray)); // Output: true
```
**Using Default Parameters**
Default parameters allow you to initialize a function parameter with a default value if no value is provided.
```
function greet(name = "Guest") {
console.log(`Hello, ${name}!`);
}
greet(); // Output: Hello, Guest!
greet("John"); // Output: Hello, John!
```
**Finding Unique Elements in Arrays**
Use the `filter` method along with `indexOf` and `lastIndexOf` to find unique elements in an array.
```
const numbers = [1, 2, 2, 3, 4, 4, 5];
const uniqueNumbers = numbers.filter((value, index, self) => {
return self.indexOf(value) === self.lastIndexOf(value);
});
console.log(uniqueNumbers); // Output: [1, 3, 5]
```
These tips and tricks can help you write more efficient and cleaner code in JavaScript. Keep experimenting and exploring to find more ways to enhance your coding skills! | mdhassanpatwary |
1,913,753 | Comprehensive Guide to Mercedes Workshop Manuals: A Vital Resource for DIY Enthusiasts and Professional Mechanics | Mercedes-Benz, a name synonymous with luxury, innovation, and performance, has a rich history of... | 0 | 2024-07-06T12:36:00 | https://dev.to/workshop_manuals_d1d139b7/comprehensive-guide-to-mercedes-workshop-manuals-a-vital-resource-for-diy-enthusiasts-and-professional-mechanics-4aok | Mercedes-Benz, a name synonymous with luxury, innovation, and performance, has a rich history of producing some of the world's most sought-after vehicles. Whether you're a proud owner of a classic model or the latest release, maintaining and repairing a Mercedes requires specialized knowledge and tools. This is where **[Mercedes workshop manuals](https://workshopmanuals.org/)** come into play. These comprehensive guides are indispensable for both DIY enthusiasts and professional mechanics, providing detailed instructions and insights into every aspect of vehicle maintenance and repair.
### The Importance of Mercedes Workshop Manuals
Mercedes workshop manuals are meticulously crafted documents that offer step-by-step guidance on maintaining, diagnosing, and repairing various models of Mercedes vehicles. These manuals are essential for several reasons:
**Accuracy and Detail:** Unlike generic repair guides, Mercedes workshop manuals are tailored specifically to the make and model of your vehicle. They provide precise instructions and detailed illustrations, ensuring that every repair or maintenance task is performed correctly.
**Time and Cost Efficiency:** Having access to a comprehensive workshop manual can save significant time and money. Instead of relying on trial and error or expensive professional services, you can confidently tackle repairs and maintenance tasks on your own.
**Preserving Vehicle Value:** Proper maintenance is crucial for preserving the value of your Mercedes. Regular upkeep, guided by accurate workshop manuals, ensures that your vehicle remains in top condition, which is especially important for resale value.
**Enhancing Safety:** Accurate repair and maintenance practices are vital for vehicle safety. Mercedes workshop manuals ensure that all procedures are performed correctly, reducing the risk of errors that could compromise the safety of your vehicle.
### Contents of a Mercedes Workshop Manual
A typical Mercedes workshop manual is a comprehensive resource that covers a wide range of topics. Here are some key sections you can expect to find:
**Introduction:** This section provides an overview of the manual's contents, including a table of contents and a guide to using the manual effectively.
**General Information:** Here, you'll find essential information about your vehicle, including its specifications, maintenance schedules, and recommended fluids and lubricants.
**Engine:** This section covers everything related to the engine, including detailed diagrams, component descriptions, and step-by-step instructions for tasks such as oil changes, belt replacements, and engine overhauls.
**Transmission:** Whether your Mercedes has an automatic or manual transmission, this section provides detailed guidance on maintenance, troubleshooting, and repairs.
**Suspension and Steering:** Proper suspension and steering maintenance are crucial for a smooth and safe driving experience. This section includes information on inspecting, servicing, and repairing these critical systems.
**Brakes:** Safety is paramount when it comes to brakes. The manual provides detailed instructions on brake system maintenance, including pad replacement, fluid checks, and system bleeding.
**Electrical Systems:** Modern Mercedes vehicles are equipped with complex electrical systems. This section covers everything from battery maintenance to troubleshooting electronic components and wiring diagrams.
**Body and Interior:** This section provides guidance on maintaining and repairing the vehicle's body and interior, including seats, dashboard components, and exterior trim.
**Diagnostics and Troubleshooting:** One of the most valuable sections, this part of the manual helps you diagnose and troubleshoot common issues, using detailed flowcharts and diagnostic procedures.
### Types of Mercedes Workshop Manuals
There are different types of Mercedes workshop manuals available, each catering to specific needs:
**Factory Service Manuals (FSMs):** These are the official manuals produced by Mercedes-Benz. They offer the most comprehensive and accurate information, covering every aspect of vehicle maintenance and repair. FSMs are typically used by professional mechanics but are also invaluable for dedicated DIY enthusiasts.
**Haynes and Chilton Manuals:** These are aftermarket manuals that provide a good balance of detail and accessibility. They are user-friendly and include a wide range of common maintenance and repair tasks, making them suitable for DIYers.
**Online Workshop Manuals:** With the advent of digital technology, many workshop manuals are now available online. These digital versions are easily accessible and often come with interactive features, such as video tutorials and enhanced search capabilities.
### How to Use a Mercedes Workshop Manual Effectively
To get the most out of your Mercedes workshop manual, follow these tips:
**Read the Manual Thoroughly:** Before starting any repair or maintenance task, read through the relevant sections of the manual. Familiarize yourself with the procedures, tools required, and any safety precautions.
**Use the Right Tools:** Ensure you have the necessary tools and equipment before beginning any task. The manual will often list the required tools for each procedure.
**Follow the Instructions Step-by-Step:** Adhere strictly to the instructions provided in the manual. Skipping steps or taking shortcuts can lead to mistakes and potentially cause damage to your vehicle.
**Keep the Manual Handy:** While working on your vehicle, keep the manual within easy reach. This allows you to refer to it frequently and ensure you're following the correct procedures.
**Stay Safe:** Always prioritize safety. Follow all safety guidelines provided in the manual, and use personal protective equipment (PPE) as needed.
### Conclusion
Mercedes workshop manuals are invaluable resources that empower both DIY enthusiasts and professional mechanics to maintain and repair their vehicles with confidence. By providing detailed, accurate, and model-specific information, these manuals ensure that every task is performed correctly, efficiently, and safely. Whether you're performing routine maintenance or tackling a complex repair, having a Mercedes workshop manual at your disposal is essential for keeping your vehicle in optimal condition. Invest in one today and experience the satisfaction of maintaining your Mercedes-Benz to the highest standards. | workshop_manuals_d1d139b7 |
|
1,913,751 | BitPower Security Overview | What is BitPower? BitPower is a decentralized lending platform that uses blockchain and smart... | 0 | 2024-07-06T12:34:42 | https://dev.to/aimm_x_54a3484700fbe0d3be/bitpower-security-overview-27bg | What is BitPower?
BitPower is a decentralized lending platform that uses blockchain and smart contract technology to provide secure lending services.
Security Features
Smart Contract
Automatically execute transactions and reduce human intervention.
Open source code, anyone can view it.
Decentralization
Users interact directly with the platform without intermediaries.
Funds flow freely between user wallets.
Asset Collateral
Borrowers use crypto assets as collateral to ensure loan security.
When the value of the collateralized assets decreases, the smart contract automatically liquidates to protect the interests of both parties.
Data Transparency
All transaction records are public and can be viewed by anyone.
Users can view transactions and assets at any time.
Multi-Signature
Multi-Signature technology is used to ensure transaction security.
Advantages
High security: Smart contracts and blockchain technology ensure platform security.
Transparency and Trust: Open source code and public transaction records increase transparency.
Risk Control: Collateral and automatic liquidation mechanisms reduce risks.
Conclusion
BitPower provides a secure and transparent decentralized lending platform through smart contracts and blockchain technology. Join BitPower and experience secure lending services!@BitPower | aimm_x_54a3484700fbe0d3be |
|
1,912,771 | Federated Airflow with SQS | Simple Queueing Services (SQS) is one of the most simple and effective services AWS has to offer. ... | 0 | 2024-07-06T12:34:09 | https://dev.to/aws-heroes/federated-airflow-with-sqs-36bg | Simple Queueing Services (SQS) is one of the most simple and effective services AWS has to offer. The thing I like most about it is it's versatility, performing equality well in high volume pub-sub event processing and more general low-volume orchestration. In this post we'll review how we can use SQS to create non-monolithic Airflow architecture, a double-click into my [previous post](https://dev.to/aws-heroes/the-wrath-of-unicron-when-airflow-gets-scary-27kg) on the subject.
## More Airflows = More Happy ##
Airflow is a great tool and at this point fairly ubiquitous in the data engineering community. However the more complex the environment the more difficult it will be to develop/deploy, and ultimately the less stable it will be.
I generally recommend the following principles when architecting with Airflow:
* Small maintainable data product repos
* Multiple purposeful Airflow environments
* Airflow environments communicating through events
So this all sounds good, but what about dependencies :grimacing:??? Organizations are going to have some dependencies that cross domain/product boundaries. We'd stand to lose that Airflow dependency goodness if the products are running in separate Airflow environments. A good example of this would be a "customer master", which might be used in several independently developed data products.
## SQS to the rescue ##
Luckily this problem is VERY easily solved using SQS, and we've put together [this little demo repository](https://github.com/datafuturesco/template-sns-demo) to help you get started.
### Step 1: Setup ###
Assuming you already have two MWAA environments or self-hosted Airflow infrastructure you will need to [create an SNS topic](https://github.com/datafuturesco/template-sns-demo/blob/main/dags/create_sns_topic.py) and [create and subscribe an SQS subscription](https://github.com/datafuturesco/template-sns-demo/blob/main/dags/create_sqs_queue.py). We decided to be cute and package these as DAG's, but you can lift the code or create from the console.
You will then need to attach a policy to the role used by your Airflow environment. Note that this policy is fairly permissive as they will enable the steps above to be run through Airflow.
```json
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "AllowSNSActions",
"Effect": "Allow",
"Action": [
"sns:CreateTopic",
"sns:DeleteTopic",
"sns:ListTopics",
"sns:ListSubscriptionsByTopic",
"sns:GetSubscriptionAttributes",
"sns:Subscribe",
"sns:SetSubscriptionAttributes",
"sns:ConfirmSubscription",
"sns:Publish"
],
"Resource": "arn:aws:sns:*:{AccountID}:*"
},
{
"Sid": "AllowSQSActions",
"Effect": "Allow",
"Action": [
"sqs:CreateQueue",
"sqs:DeleteQueue",
"sqs:GetQueueUrl",
"sqs:GetQueueAttributes",
"sqs:SetQueueAttributes",
"sqs:ListQueues",
"sqs:ReceiveMessage",
"sqs:DeleteMessage"
],
"Resource": "arn:aws:sqs:*:{AccountID}:*"
}
]
}
```
### Step 2: Create SNS Publish DAG ###
In the following DAG we create a simulated upstream dependency(consider this your _customer master_ build step). We use the `SnsPublishOperator` to notify downstream dependencies after our dummy step is complete.
:warning: Note that if you did not build your SNS/SQS resources using the DAGS, you will need to manually set your Airflow variables with the appropriate ARN's.
```python
from airflow import DAG
from airflow.operators.bash import BashOperator
from airflow.providers.amazon.aws.operators.sns import SnsPublishOperator
from airflow.operators.python import PythonOperator
from airflow.models import Variable
from airflow.utils.dates import days_ago
from datetime import datetime
import boto3
default_args = {
'owner': 'airflow',
'start_date': days_ago(1)
}
# Define DAG to display the cross-dag dependency using SNS topic publish
with DAG(
'sns_publish_dummy',
default_args=default_args,
description='A simple DAG to publish a message to an SNS topic',
schedule_interval=None,
catchup=False
) as dag:
# Dummy task to show upward dag dependency success
dummy_sleep_task = BashOperator(
task_id='sleep_task',
bash_command='sleep 10'
)
# SNS Publish operator to publish message to SNS topic after the upward tasks are successful
publish_to_sns = SnsPublishOperator(
task_id='publish_to_sns',
target_arn=Variable.get("sns_test_arn"), # SNS topic arn to which you want to publish the message
message='This is a test message from Airflow',
subject='Test SNS Message'
)
dummy_sleep_task >> publish_to_sns
if __name__ == "__main__":
dag.cli()
```
### Step 3: Create SQS Subscribe DAG ###
This DAG will simulate the downstream dependency, perhaps a _customer profile_ job. Leveraging the `SqsSensor`, it simply waits for the upstream job to complete, and then runs it's own dummy step. Note that the `mode='reschedule'` is required to enable this polling/waiting functionality.
```python
from airflow import DAG
from airflow.providers.amazon.aws.sensors.sqs import SqsSensor
from airflow.operators.python import PythonOperator
from airflow.models import Variable
from airflow.utils.dates import days_ago
from datetime import timedelta
import boto3
default_args = {
'owner': 'airflow',
'start_date': days_ago(1)
}
def print_sqs_message():
print("Hello, SQS read and delete successful!! ")
# Define DAG to show cross-dag dependency using SQS sensor operator
with DAG(
'sqs_sensor_example',
default_args=default_args,
description='A simple DAG to sense and print messages from SQS',
schedule_interval=None
) as dag:
# SQS sensor operator waiting to receive message in the provided SQS queue from SNS topic
sense_sqs_queue = SqsSensor(
task_id='sense_sqs_queue',
sqs_queue=Variable.get("sqs_queue_test_url"), # Airflow variable name for the SQS queue url
aws_conn_id='aws_default',
max_messages=1,
wait_time_seconds=20,
visibility_timeout=30,
mode='reschedule' # the task waits for any message to be received in the specified queue
)
print_message = PythonOperator(
task_id='print_message',
python_callable=print_sqs_message
)
sense_sqs_queue >> print_message
if __name__ == "__main__":
dag.cli()
```
### Testing, Testing###
Once your environment is setup, simply start your SQS subscriber DAG. It will patiently wait polling SQS for a completion state.
When you are ready start your SNS publisher DAG. Once complete your subscriber will start it's dummy step and complete.
## Bringing it all together..##
Big picture, leveraging SQS you can enable a pragmatic, data-mesh inspired infrastructure like has been illustrated below. No Airflow-based single point of failure, observed domain/product boundaries, and team autonomy.
As a bonus you have also enabled evolutionary architecture. If some team wants to transition from Airflow to Step Functions, or Prefect they are empowered to do so, so long as they continue interacting through SNS/SQS.
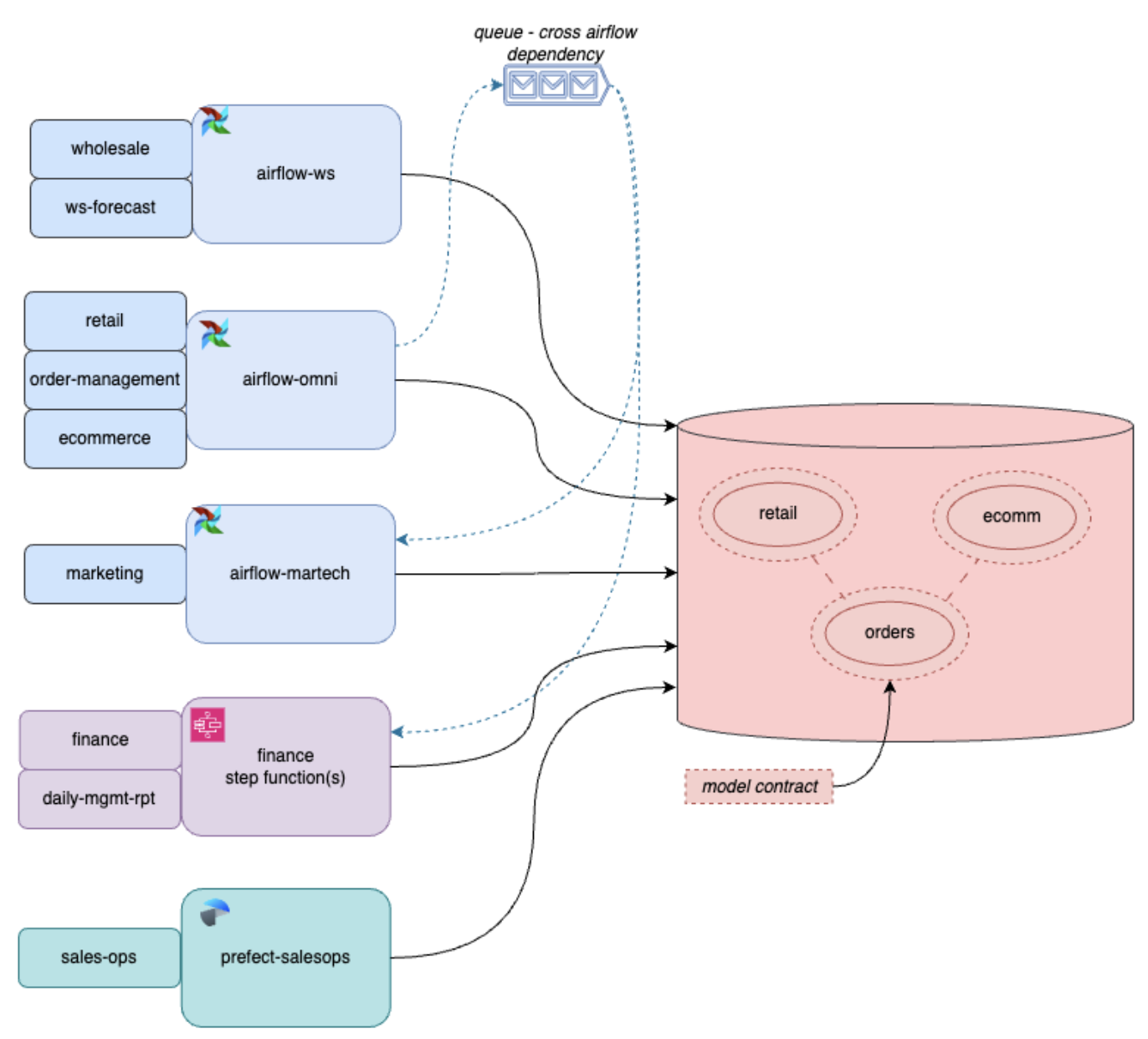
I'd like to give special thanks to @deekshagunde for contributing to this article and preparing the demo repo.
| elliott_cordo |
|
1,913,734 | Building a Sleek C++ Calculator: Harnessing the Power of Modular Functions | In the world of programming, creating practical applications that demonstrate elegance and efficiency... | 0 | 2024-07-06T12:30:51 | https://dev.to/moksh57/building-a-sleek-c-calculator-harnessing-the-power-of-modular-functions-25ib | cpp, programming | In the world of programming, creating practical applications that demonstrate elegance and efficiency is a testament to skillful craftsmanship. One such project that exemplifies this is building a calculator using C++ and modular functions. In this blog post, we'll explore the journey of constructing a functional calculator from scratch, focusing on the principles of modular programming.
**Why Build a Calculator?**
While a calculator may seem like a simple tool, its construction offers valuable insights into fundamental programming concepts such as functions, control flow, and data handling. By building a calculator, we not only practice these concepts but also learn how to structure our code in a modular and reusable manner—a cornerstone of software engineering.
**Getting Started: Preparation and Environment Setup**
Before diving into the implementation, it's essential to set up your C++ development environment. Choose an IDE or text editor with a C++ compiler that suits your preferences. This ensures a smooth development process and efficient coding workflow.
**Step-by-Step Guide to Building the Calculator**
Designing the Functionality: Begin by outlining the basic functionalities your calculator will support. This typically includes arithmetic operations such as addition, subtraction, multiplication, and division. Plan how users will interact with the calculator through a simple user interface.
- **Implementing Modular Functions:** Modular programming involves breaking down complex tasks into smaller, independent modules or functions. Create separate functions for each arithmetic operation to maintain clarity and reusability in your code.
- **Handling User Input and Output:** Develop mechanisms to receive user input for numbers and operation selection. Use conditional statements (if-else or switch-case) to direct the program flow based on user choices. Ensure robust error handling to manage unexpected inputs effectively.
- **Testing and Validation:** Once the core functionalities are implemented, rigorously test the calculator to ensure accuracy and reliability. Verify edge cases such as division by zero or non-numeric inputs. Debug any issues to achieve a stable and user-friendly application.
**Conclusion**
Building a C++ calculator using modular functions not only enhances your programming skills but also reinforces essential software development principles. By organizing code into reusable modules, you promote maintainable and scalable application development practices.
Ready to embark on this coding journey? Explore further by implementing additional features or refining the calculator's UI to expand its functionality and usability.
Read more:
https://mokshelearning.blogspot.com/2024/07/Build-a-Sleek-Calculator-with-Modular-Functions.html]
| moksh57 |
1,913,727 | Tech at Its Best: Leading Operating Systems | In the fast-paced world of tech, picking the right operating system can feel like choosing your... | 0 | 2024-07-06T12:21:27 | https://dev.to/ifavecontents/tech-at-its-best-leading-operating-systems-1hl5 | linux, techtrends, windows, futureoftech | In the fast-paced world of tech, picking the right operating system can feel like choosing your Hogwarts house—crucial and life-altering. Whether you’re binge-watching the latest series, crushing enemies in your favorite game, or juggling spreadsheets at work, the [best operating systems](https://ifave.com/category/The-World/Best-operating-systems) have got your back. From sleek interfaces to rock-solid security, these systems are the unsung heroes of our digital lives. Let’s dive into the coolest operating systems that are making waves and see what all the fuss is about.
**The Dominant Players**
When you think of operating systems, a few heavy hitters come to mind. Windows, macOS, and Linux are the big guns, each with its own loyal fanbase and unique flair.
**Windows** is like the Swiss Army knife of operating systems. It’s versatile, user-friendly, and supports a massive range of software. Whether you’re gaming, working, or just browsing, Windows 10 and the latest Windows 11 have you covered with top-notch security and performance boosts.
**macOS** is the James Bond of operating systems—sleek, sophisticated, and always on point. If you’re into design, video editing, or music, macOS is your best buddy, offering seamless integration with Apple’s ecosystem and a suite of powerful tools to unleash your creativity.
**Linux** is the playground for tech geeks and DIY enthusiasts. It’s all about customization and control. With distributions like Ubuntu, Fedora, and Debian, Linux is perfect for everyone from newbies to coding wizards. Its open-source nature means it’s constantly evolving, thanks to a passionate community of developers.
**The Innovators**
Let’s not forget the underdogs who are shaking things up in their own niches.
**Chrome OS** is Google’s answer to simplicity. It’s perfect for students and anyone who loves living in the cloud. Lightweight and efficient, Chrome OS makes getting things done a breeze, without bogging you down with unnecessary bells and whistles.
**Android and iOS** might be primarily for mobile devices, but their impact on tech is undeniable. Android’s open-source flexibility and iOS’s seamless hardware integration keep pushing mobile computing to new heights.
**The Future of Operating Systems**
As tech marches on, the best operating systems are those that can keep up. We’re heading towards systems that are more secure, more efficient, and smarter, thanks to cloud integration and artificial intelligence. Plus, with virtual and augmented reality on the horizon, we might soon see operating systems designed for mind-blowing immersive experiences.
Picking the right operating system is like finding the perfect pair of shoes—get it right, and everything just fits. Whether you’re a Windows warrior, a macOS maestro, or a Linux legend, the [best operating systems](https://ifave.com/category/The-World/Best-operating-systems) help you get the most out of your tech. As the digital world evolves, these systems will keep innovating, ensuring we’re always ready for whatever comes next. So, whether you’re setting up a new computer, thinking about switching things up, or just curious about the latest trends, knowing about the best operating systems is your ticket to staying ahead in the tech game. | ifavecontents |
1,913,738 | Essential Tools for Mastering Data Science and Machine Learning | A good craftsman needs good tools. This is also true in Data Science and Machine Learning. There are... | 0 | 2024-07-06T12:30:35 | https://dev.to/moubarakmohame4/essential-tools-for-mastering-data-science-and-machine-learning-352b | machinelearning, python, datascience, data | A good craftsman needs good tools. This is also true in Data Science and Machine Learning. There are three main categories of tools:
- **Integrated Tools** that include everything necessary for a project: loading data, analyzing it, creating models, evaluating them, deploying, and generating reports.
- **Auto ML Tools** that simplify the process for non-experts by automating most of the different phases.
- **Development Tools** which allow for more customization, provided you code everything, for example in Python.
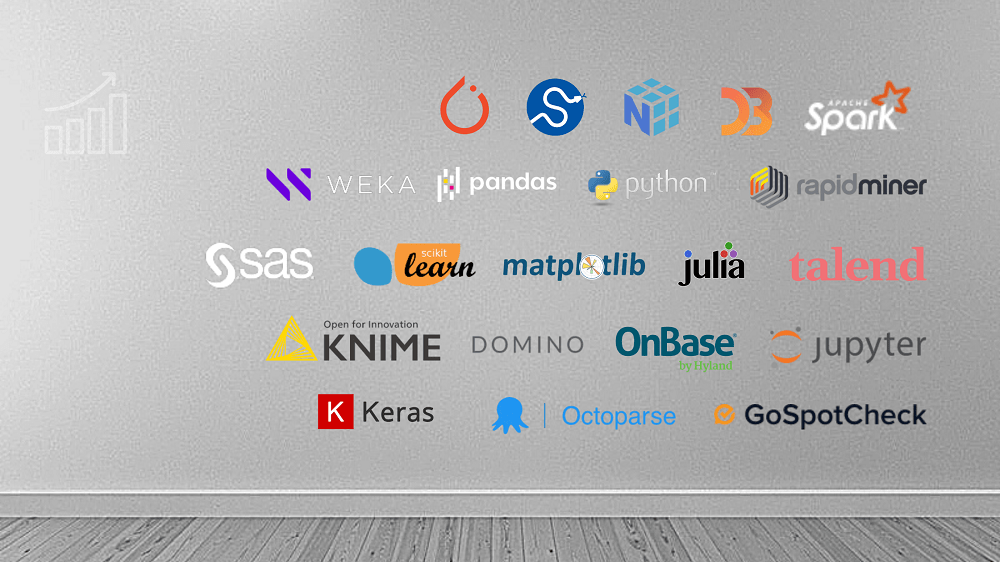
**1. Integrated Tools**
There are many tools in the first category, each requiring specific learning to use them effectively. Additionally, each has its strengths and weaknesses, so it is important to choose the right software based on the intended goal. Examples include: Dataiku DSS, SAS, QlikView, Tableau, Power BI, etc.
Some are very statistics-oriented (SAS), others focus on data visualization (Tableau), but all cover at least part of the process. These are mainly downloadable applications accessible via a web interface.
Most of these software tools are paid. Free versions often exist, but they are limited in functionality and usually restricted to personal use. To be compliant, it is important to contact the main providers.
**2. Auto ML**
Auto ML tools are generally accessible via a web interface. The user inputs their raw data and desired task: classification, regression, etc. The application then takes care of the rest: selecting data preparation methods, models, and parameters. The best-created model is then returned to the user. These tools have the huge advantage of being usable without any knowledge of Machine Learning. However, they lack flexibility and are often disliked by Data Scientists who prefer to have control over their modeling process.
However, they allow for a quick initial model, and a Data Scientist can then spend time on other models and optimizing the Auto ML results. Most cloud providers have their own tools.
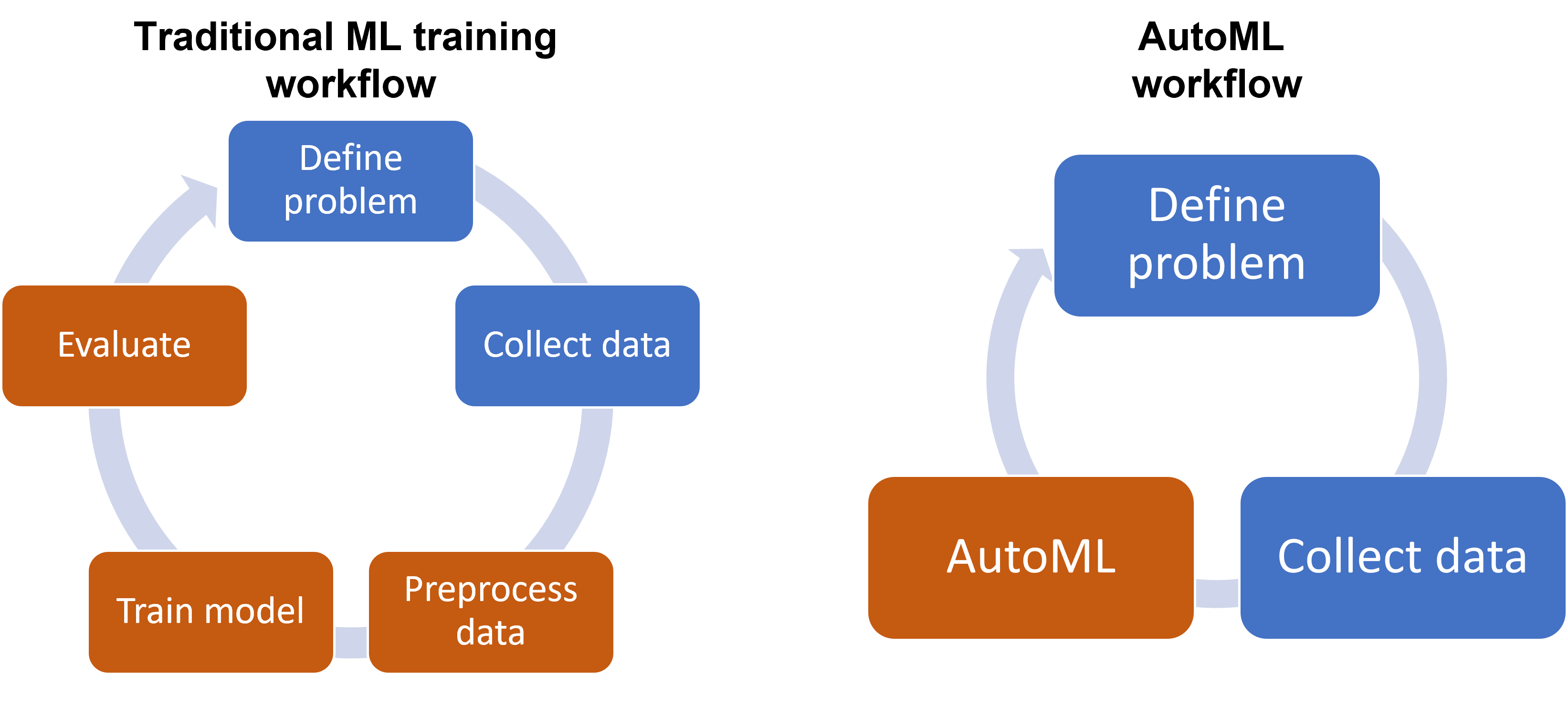
**3. Development Tools**
It is entirely possible to code Machine Learning algorithms in all programming languages. Many frameworks or libraries exist, ready to be incorporated into projects. Classic development tools are therefore perfectly usable. Code editors like Atom, Visual Studio Code, or Sublime Text can all serve a project.
Moreover, code has the advantage of being easily shared and synchronized among multiple people thanks to version control systems like Git. Additionally, CI/CD (Continuous Integration/Continuous Deployment) tools like GitLab CI, Jenkins, or Ansible can facilitate deployment and updates. Again, each cloud provider offers additional tools, such as the series of Amazon Web Services (AWS) services starting with "Code": CodeCommit, CodeBuild... or Azure DevOps.
These tools are increasingly used today. | moubarakmohame4 |
1,913,737 | UI/UX Là Gì? Thiết Kế UI Và UX Khác Nhau Như Thế Nào? | UI đề cập đến mọi thành phần hình ảnh, giao diện mà người dùng nhìn thấy và tương tác khi sử dụng... | 0 | 2024-07-06T12:30:04 | https://dev.to/terus_technique/uiux-la-gi-thiet-ke-ui-va-ux-khac-nhau-nhu-the-nao-1ff9 | website, digitalmarketing, seo, terus |
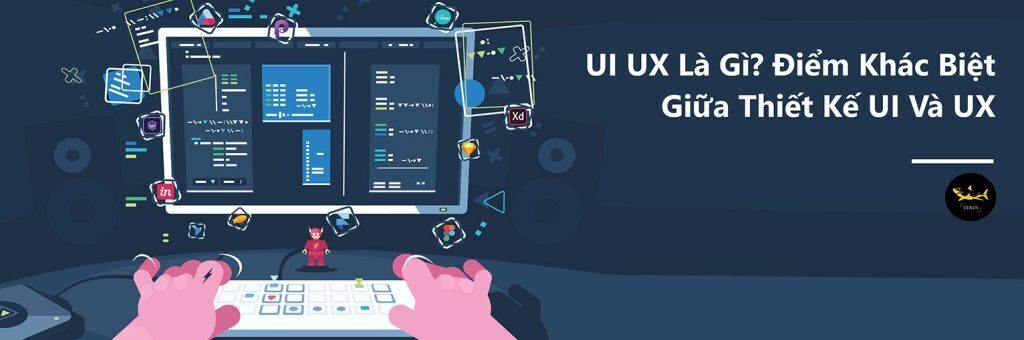
UI đề cập đến mọi thành phần hình ảnh, giao diện mà người dùng nhìn thấy và tương tác khi sử dụng một sản phẩm hoặc dịch vụ, cụ thể là website. Đây là phần trực quan và bề ngoài của sản phẩm, bao gồm các chi tiết như màu sắc, bố cục, nút bấm, menu, biểu tượng... Mục tiêu chính của UI là tạo ra một giao diện người dùng thân thiện và nổi bật.
Trong khi đó, UX tập trung vào việc [thiết kế website với trải nghiệm người dùng tối ưu](https://terusvn.com/thiet-ke-website-tai-hcm/), nhằm đem lại cảm giác thoải mái, dễ sử dụng và hài lòng cho người dùng khi tương tác với wesbite. UX nghiên cứu hành vi, nhu cầu và mong đợi của người dùng để từ đó xây dựng một trải nghiệm tối ưu, tạo ra giá trị cho người dùng. Nó bao gồm các khía cạnh như dễ sử dụng, tính hiệu quả, tính thẩm mỹ, tính hữu dụng...
Mặc dù UI và UX có những điểm khác biệt, nhưng chúng lại đóng vai trò bổ sung cho nhau. Một [website có thiết kế UI đẹp mắt](https://terusvn.com/thiet-ke-website-tai-hcm/) nhưng lại không mang lại trải nghiệm tốt cho người dùng thì sẽ không thể thành công. Ngược lại, một sản phẩm có UX tuyệt vời nhưng giao diện UI lại kém hấp dẫn cũng khó đạt được sự chấp nhận rộng rãi. Vì thế, việc kết hợp cả hai yếu tố UI và UX một cách hài hòa và hiệu quả là hết sức quan trọng.
Trong quá trình thiết kế website, nhóm UI/UX thường sẽ bao gồm các chuyên gia như: nhà thiết kế giao diện, nhà nghiên cứu UX, nhà phát triển front-end... Họ cùng phối hợp với các bên liên quan khác để xây dựng một website hoàn hảo từ cả hai khía cạnh UI và UX. Vai trò của nhóm UI/UX là vô cùng quan trọng, quyết định đến thành công hay thất bại của sản phẩm.
UI và UX là hai khái niệm khác biệt nhưng lại hỗ trợ và bổ sung cho nhau. Sự kết hợp giữa thiết kế giao diện người dùng (UI) và trải nghiệm người dùng (UX) là chìa khóa để tạo ra những sản phẩm và dịch vụ, đặc biệt là website thực sự thành công, đem lại giá trị gia tăng cho người dùng.
Tìm hiểu thêm về [UI/UX Là Gì? Thiết Kế UI Và UX Khác Nhau Như Thế Nào?](https://terusvn.com/thiet-ke-website/ui-ux-la-gi/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,729 | Understanding the Unary Plus Operator (+) in JavaScript | JavaScript is full of interesting and useful operators, one of which is the unary plus operator (+).... | 0 | 2024-07-06T12:28:26 | https://dev.to/mktdev/understanding-the-unary-plus-operator-in-javascript-4amo | webdev, javascript | JavaScript is full of interesting and useful operators, one of which is the unary plus operator (+). You might have come across it in code snippets like +new Date(), but do you know what it really does? In this post, we'll explore the unary plus operator and its various applications.
**What is the Unary Plus Operator?**
The unary plus operator (+) is used to convert its operand into a number. This operator is particularly handy when you need to ensure that a value is treated as a numeric type.
**Example: Converting a Date to a Timestamp**
Consider the following example:
The unary plus operator (+) is used to convert its operand into a number. This operator is particularly handy when you need to ensure that a value is treated as a numeric type.
Example: Converting a Date to a Timestamp
Consider the following example:
```
+new Date(); // Returns the current timestamp in milliseconds
```
Here, new Date() creates a new Date object representing the current date and time. The unary plus operator converts this Date object to its numeric representation, which is the number of milliseconds since January 1, 1970, 00:00:00 UTC (the Unix epoch).
**Other Uses of the Unary Plus Operator**
The unary plus operator isn't just limited to dates. It can be used to convert various types of values to numbers.
- Converting Strings to Numbers
```
let str = "123";
let num = +str; // num is now 123 (number)
```
- Converting Boolean Values to Numbers
```
let boolTrue = true;
let boolFalse = false;
let numTrue = +boolTrue; // numTrue is now 1
let numFalse = +boolFalse; // numFalse is now 0
```
- Converting null to a Number
```
let nullValue = null;
let numNull = +nullValue; // numNull is now 0
```
- Converting undefined to a Number
```
let undefinedValue = undefined;
let numUndefined = +undefinedValue; // numUndefined is NaN
```
- Converting an Object to a Number
```
let obj = {};
let numObj = +obj; // numObj is NaN, because {} cannot be converted to a number
```
- Converting Arrays to Numbers
```
let emptyArray = [];
let singleElementArray = [5];
let multipleElementArray = [1, 2, 3];
let numEmptyArray = +emptyArray; // numEmptyArray is 0
let numSingleElementArray = +singleElementArray; // numSingleElementArray is 5
let numMultipleElementArray = +multipleElementArray; // numMultipleElementArray is NaN
```
- Using in Expressions
```
let a = "5";
let b = 10;
let result = +a + b; // result is 15, because +a converts "5" to 5
```
**Conclusion**
The unary plus operator (+) is a versatile tool in JavaScript that allows for quick type conversion to a number. Whether you're dealing with strings, booleans, null, undefined, or even arrays, this operator can help ensure your values are in the numeric format you need.
Next time you see +new Date() or need to convert a value to a number, you'll know exactly what the unary plus operator is doing and how to use it effectively in your code.
Happy coding!
| mktdev |
1,913,735 | BitPower: Exploring the Future of Decentralized Finance | In the era of rapid development of modern financial technology, decentralized finance (DeFi) has... | 0 | 2024-07-06T12:28:19 | https://dev.to/sang_ce3ded81da27406cb32c/bitpower-exploring-the-future-of-decentralized-finance-56j9 | In the era of rapid development of modern financial technology, decentralized finance (DeFi) has become an emerging force that subverts traditional financial services. As an innovative project in this field, BitPower is committed to providing users with more transparent, efficient and secure financial services through blockchain technology and smart contracts. This article will introduce in detail the basic concepts, core technologies, security, and applications and advantages of BitPower in the financial field.
## Basic Concepts
BitPower is a decentralized lending platform developed based on blockchain technology, aiming to break the barriers of the traditional financial system and provide more flexible and low-cost financial services. BitPower is built on the Ethereum network (EVM) and uses smart contract technology to realize asset deposits and lending. Participants can deposit digital assets on the platform to earn interest or obtain loans by mortgaging assets.
## Core Technology
### Smart Contract
The core of BitPower lies in smart contract technology. A smart contract is a self-executing code that can automatically perform predefined operations when certain conditions are met. On the BitPower platform, all deposit and lending operations are controlled by smart contracts to ensure the security and transparency of transactions.
### Decentralization
BitPower adopts a decentralized architecture with no central control agency. All transaction records are stored on the blockchain and maintained by all nodes in the network. This decentralized approach not only improves the system's anti-attack ability, but also ensures the transparency and immutability of data.
### Consensus Mechanism
The BitPower platform uses the consensus mechanism of the blockchain to ensure the validity and consistency of transactions. Through consensus algorithms such as Proof of Work (PoW) or Proof of Stake (PoS), nodes in the network can reach a consensus to ensure that every transaction is legal and accurate.
## Security
BitPower attaches great importance to the security of the platform and uses a variety of technical means to ensure the security of users' assets. First, the code of the smart contract is strictly audited and tested to ensure its reliability and security. Secondly, the platform uses cryptography technology to encrypt transaction data to prevent unauthorized access and tampering. In addition, the decentralized architecture makes the platform have no single point of failure, further improving the security of the system.
## Applications and Advantages
### Efficient and Convenient
Compared with traditional financial services, the lending services provided by BitPower are more efficient and convenient. Users can deposit and lend assets simply through blockchain wallets without cumbersome approval processes.
### Transparency and fairness
All transaction records on the BitPower platform are open and transparent, and users can view the flow of assets at any time. This transparency not only enhances user trust, but also helps prevent fraud and improper behavior.
### Lower cost
By removing intermediaries, BitPower's operating costs are greatly reduced, which can provide users with lower lending rates and higher deposit returns. This is of great significance to those who have difficulty in obtaining services in the traditional financial system, especially users in developing countries.
## Conclusion
As an innovative project in the field of decentralized finance, BitPower provides users with a safe, transparent and efficient lending platform through blockchain and smart contract technology. It not only optimizes the process and cost of financial services, but also provides more people with the opportunity to participate in the global financial market. With the continuous advancement of technology and the continuous expansion of applications, BitPower is expected to play a greater role in the future and lead the development trend of decentralized finance.@BitPower | sang_ce3ded81da27406cb32c |
|
1,913,732 | BitPower Security Overview | What is BitPower? BitPower is a decentralized lending platform that uses blockchain and smart... | 0 | 2024-07-06T12:26:14 | https://dev.to/aimm/bitpower-security-overview-2b7a | What is BitPower?
BitPower is a decentralized lending platform that uses blockchain and smart contract technology to provide secure lending services.
Security Features
Smart Contract
Automatically execute transactions and reduce human intervention.
Open source code, anyone can view it.
Decentralization
Users interact directly with the platform without intermediaries.
Funds flow freely between user wallets.
Asset Collateral
Borrowers use crypto assets as collateral to ensure loan security.
When the value of the collateralized assets decreases, the smart contract automatically liquidates to protect the interests of both parties.
Data Transparency
All transaction records are public and can be viewed by anyone.
Users can view transactions and assets at any time.
Multi-Signature
Multi-Signature technology is used to ensure transaction security.
Advantages
High security: Smart contracts and blockchain technology ensure platform security.
Transparency and Trust: Open source code and public transaction records increase transparency.
Risk Control: Collateral and automatic liquidation mechanisms reduce risks.
Conclusion
BitPower provides a secure and transparent decentralized lending platform through smart contracts and blockchain technology. Join BitPower and experience secure lending services! | aimm |
|
1,913,726 | Deploying Keycloak to a VPS Using Docker-compose, Nginx, Certbot, and SSL | In this article, I would like to share how to deploy Keycloak to a VPS using Docker-compose, Nginx,... | 0 | 2024-07-06T12:21:23 | https://dev.to/surkoff/deploying-keycloak-on-a-vps-using-docker-compose-nginx-certbot-and-ssl-1pa8 | keycloak, vps, ssl, certbot | In this article, I would like to share how to deploy Keycloak to a VPS using Docker-compose, Nginx, Certbot, and SSL.
## Key points:
- Keycloak v.25.0.1
- SSL protection for Keycloak
- Certbot v.2.11.0 for obtaining and renewing SSL certificates
- Nginx v.1.27.0 as a reverse proxy
- Postgres v.14 to replace the default internal H2 DB of Keycloak
- Automatic realm import during deployment
- Docker-compose for deployment automation
- .env file for managing environment variables
For those who might not be familiar, Keycloak is a powerful access management system with SSO support that can significantly simplify user management and authentication.
The desire to deploy your own Keycloak can arise both for experimenting with your projects and for handling your usual backend tasks. This happened to me as well. I decided to kill two birds with one stone. However, I couldn't find a comprehensive guide. I don't need Keycloak locally, but setting it up on a separate, always-available server with backup and the ability to export/import realms, etc., is excellent. Plus, the deployment process is automated, making it easier to switch to another VPS provider.
Everyone has their own motivation, but let's get to the point.
## Introduction
#### What is Keycloak?
Keycloak is an open-source identity and access management solution. It provides features such as SSO (Single Sign-On), user management, authentication, and authorization.
#### Why Docker-compose?
Docker-compose makes it easy to manage multi-component applications like Keycloak and simplifies the deployment and scaling process. This guide uses containers for Keycloak, Certbot, Nginx, and the Postgres database.
#### Why Nginx and Certbot?
Nginx will act as a reverse proxy, ensuring security and performance, while Certbot will help obtain and automatically renew SSL certificates from Let's Encrypt, saving us a couple of thousand rubles on certificates for our domain, which is nice.
Let's get started!
## Step 1: Preparing the Environment
#### Cloning the Repository
First, clone the repository with ready-made configurations to our VPS, which I have carefully prepared for you. It contains docker-compose.yml for managing deployment, nginx configs, and an environment variables file needed for docker-compose.
```
git clone [email protected]:s-rb/keycloak-dockerized-ssl-nginx.git
cd keycloak-dockerized-ssl-nginx
```
#### Editing the .env File
Open the .env file and edit the following variables:
- `KEYCLOAK_ADMIN_PASSWORD` - Admin password for accessing Keycloak
- `KC_DB_PASSWORD` - Password for Keycloak service access to the Postgres DB (should match `POSTGRES_PASSWORD` if a separate user is not created)
- `POSTGRES_PASSWORD` - Admin password for Postgres
Replace `password` with your values unless you want anyone to connect to your services ;)
Example of a complete environment variables file:
```
KEYCLOAK_ADMIN=admin
KEYCLOAK_ADMIN_PASSWORD=password
PROXY_ADDRESS_FORWARDING=true
KC_PROXY=edge
KC_DB=postgres
KC_DB_URL=jdbc:postgresql://keycloak-postgres:5432/keycloak
KC_DB_USERNAME=keycloak
KC_DB_PASSWORD=password
POSTGRES_DB=keycloak
POSTGRES_USER=keycloak
POSTGRES_PASSWORD=password
```
## Step 2: Domain Registration and DNS Setup
This step can be done before the first step - it does not depend on it. In the following instructions, we assume you have registered your domain (e.g., surkoff.com) and we want Keycloak to be accessible at my-keycloak.surkoff.com.
#### Domain Registration
Register a domain with any registrar, for example, REG.RU.
#### Creating an `A` Record for the Subdomain
Create an `A` record pointing to your server's IP. For example, for the subdomain my-keycloak.surkoff.com, specify your server's IP.
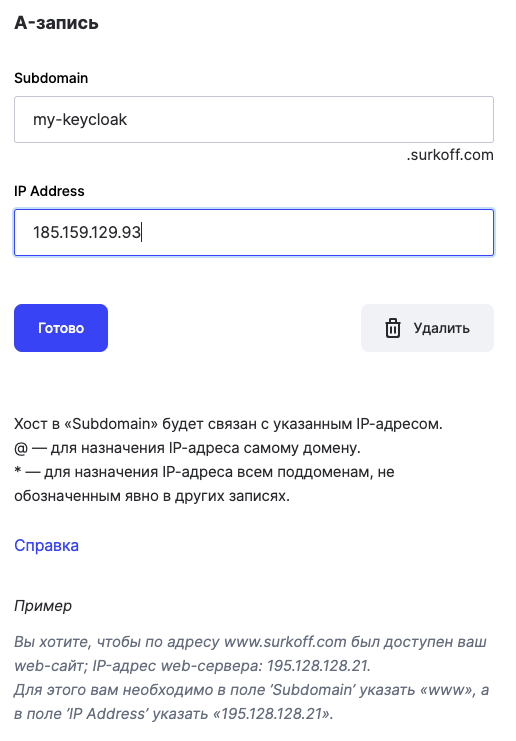
#### Checking the DNS Record
Ensure the DNS record is correctly configured:
```
ping my-keycloak.surkoff.com
```
The response should show your server's IP address.
## Step 3: Configuring Nginx
#### Nginx Configuration
In the nginx configs - `default.conf_with_ssl`, `default.conf_without_ssl` edit and specify your domain:
- `server_name` section
- path to the certificate `ssl_certificate`
- path to the key `ssl_certificate_key`
Example configuration with SSL:
```
server {
listen 443 ssl;
server_name my-keycloak.surkoff.com;
ssl_certificate /etc/letsencrypt/live/my-keycloak.surkoff.com/fullchain.pem;
ssl_certificate_key /etc/letsencrypt/live/my-keycloak.surkoff.com/privkey.pem;
location / {
proxy_pass http://keycloak:8080;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for;
proxy_set_header X-Forwarded-Proto $scheme;
}
}
```
## Step 4: Obtaining an SSL Certificate
#### Obtaining a Test Certificate
Use the configuration without SSL:
```
cp nginx/conf.d/default.conf_without_ssl nginx/conf.d/default.conf
docker-compose up -d
```
Obtain a test certificate (replace the domain and email with your own):
```
docker exec certbot certbot certonly --webroot --webroot-path=/data/letsencrypt -d my-keycloak.surkoff.com --email [email protected] --agree-tos --no-eff-email --staging
```
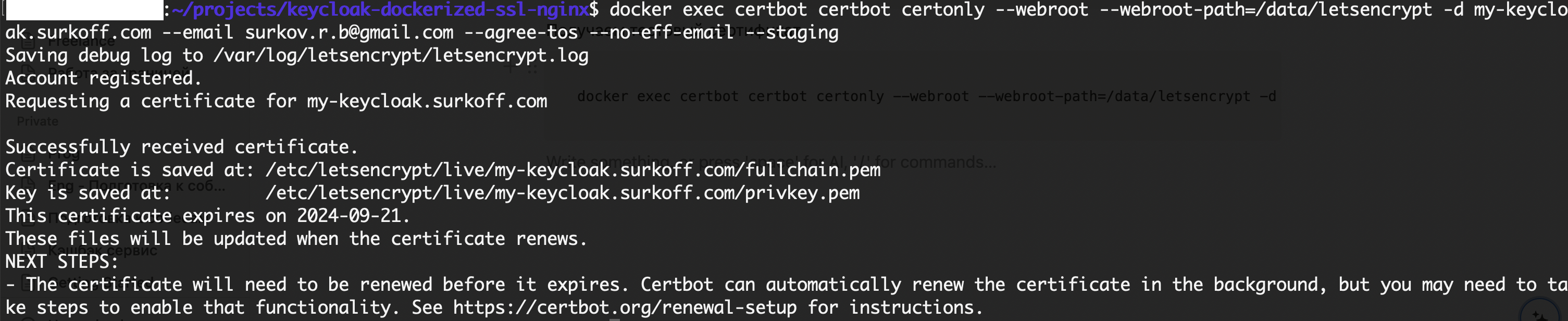
#### Checking the Certificate
```
docker exec certbot certbot certificates
```

#### Deleting the Test Certificate (replace the domain with your own)
```
docker exec certbot certbot delete --cert-name my-keycloak.surkoff.com
```
#### Obtaining a Real Certificate (replace email and domain with your own)
```
docker exec certbot certbot certonly --webroot --webroot-path=/data/letsencrypt -d my-keycloak.surkoff.com --email [email protected] --agree-tos --no-eff-email
```
## Step 5: Final Configuration and Launch
#### Updating Nginx Configuration to Use SSL
```
docker-compose down
cp nginx/conf.d/default.conf_with_ssl nginx/conf.d/default.conf
docker-compose up -d
```
#### Checking Access to Keycloak
Open a browser and go to my-keycloak.surkoff.com (your domain).
You should see the admin login page where you can log in using the username and password you specified in the .env file.
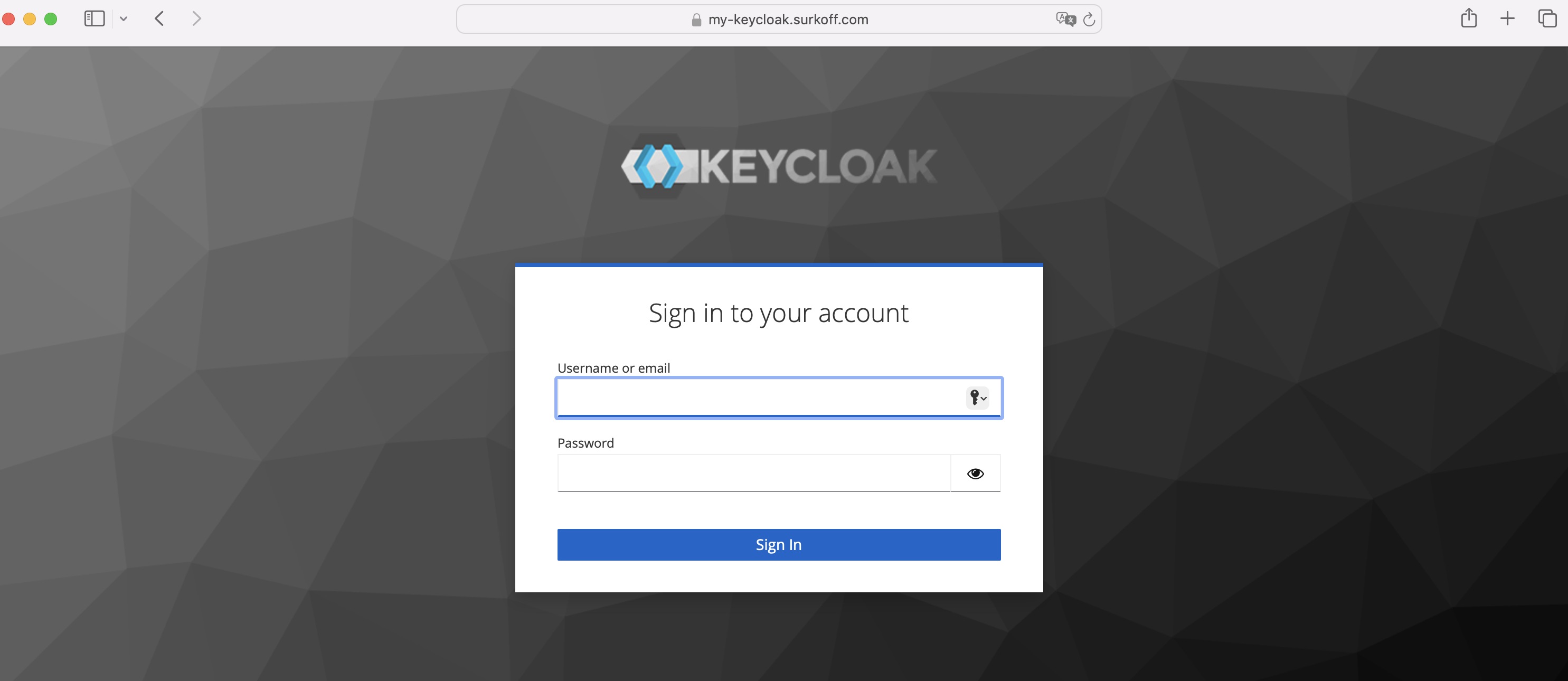
For configuring Keycloak, there are interesting articles on other resources, which we won't cover in this publication.
## Automatic Certificate Renewal
To automatically renew certificates and restart Nginx, create the `renew_and_reload.sh` script (already available in the repository):
```
#!/bin/bash
# Renew certificates
docker exec certbot certbot renew --webroot --webroot-path=/data/letsencrypt
# Restart Nginx
docker restart nginx
```
Make the script executable:
```
chmod +x renew_and_reload.sh
```
Add it to crontab for regular execution:
```
crontab -e
```
Add a line to crontab, remembering to specify the path to the script:
```
0 0 1 * * /path/to/renew_and_reload.sh
```
## Importing Realms
If you want to import a realm at startup, you can place it in the `keycloak/config/` folder, and it will be imported when the application starts.
### Conclusion
That's it! Now you have a deployed latest Keycloak with SSL on your VPS. I hope this article was helpful! If you have any questions or suggestions, feel free to write to me.
The source code is available at [the link here](https://github.com/s-rb/keycloak-dockerized-ssl-nginx) | surkoff |
1,913,725 | BitPower's Security: A Modern Financial Miracle | In a changing world, financial security has become more important than ever. BitPower, as a... | 0 | 2024-07-06T12:21:04 | https://dev.to/pingz_iman_38e5b3b23e011f/bitpowers-security-a-modern-financial-miracle-2efa |
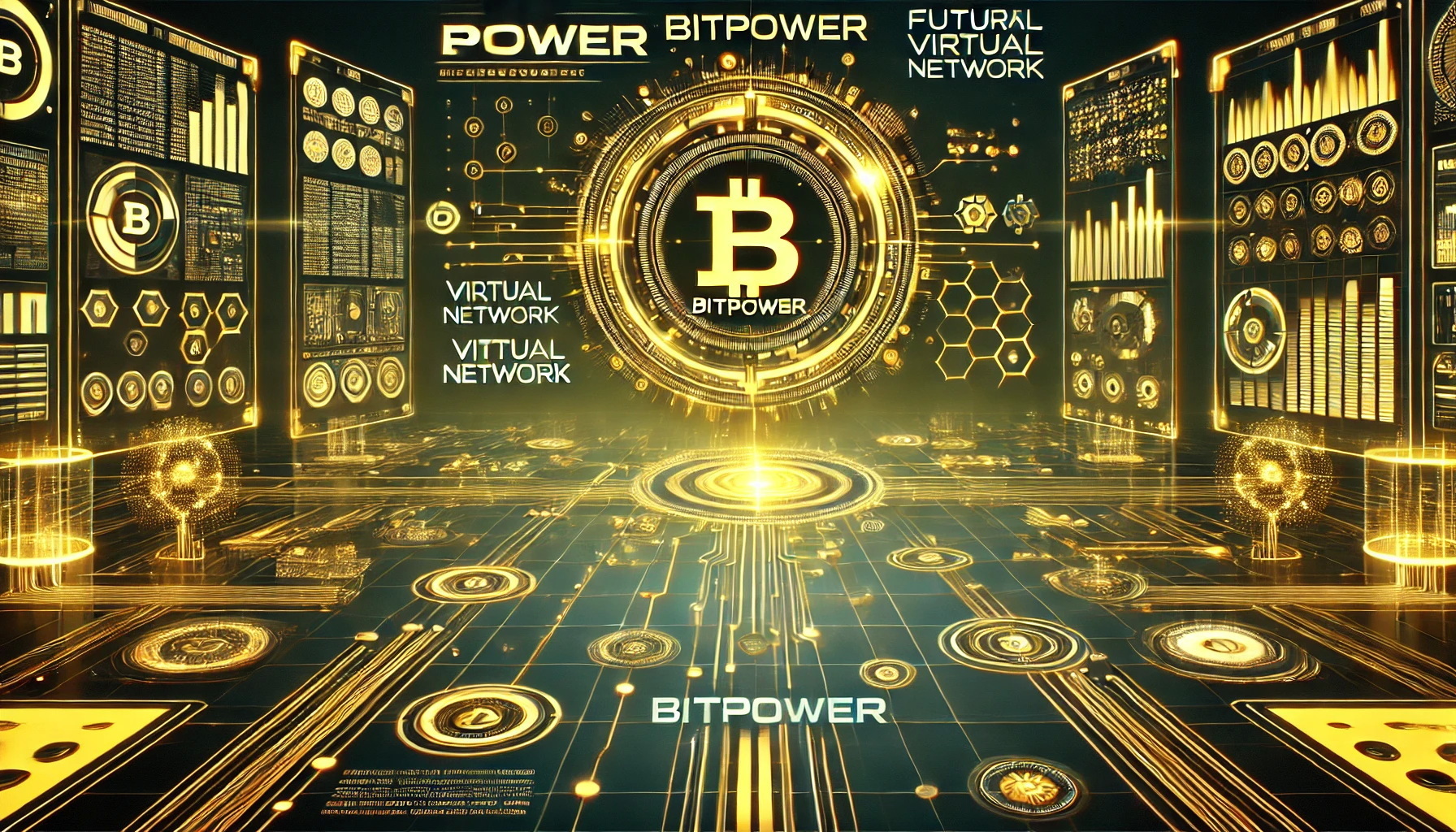
In a changing world, financial security has become more important than ever. BitPower, as a decentralized financial platform based on blockchain technology, provides unparalleled security and stable returns. By using advanced smart contracts and cryptocurrencies, BitPower has not only changed the landscape of traditional finance, but also provided users with unprecedented opportunities for income.
First, BitPower's security is based on the core characteristics of blockchain technology. Blockchain is a distributed ledger technology whose data is stored on thousands of nodes around the world, each of which keeps a complete record of transactions. This decentralized structure means that no one can tamper with the data alone, ensuring the transparency and immutability of transactions.
Second, BitPower uses smart contract technology to automatically execute transactions and agreements. A smart contract is a self-executing code that automatically triggers transactions when preset conditions are met. Through smart contracts, BitPower has achieved fully automated financial operations without intermediaries, reducing the risk of human error and fraud. Once deployed on the blockchain, these contracts cannot be changed, ensuring the stability and security of the system.
The use of cryptocurrency is also a highlight of BitPower's security. Cryptocurrency transactions use complex encryption algorithms to protect users' privacy and asset security. Every transaction of the user is encrypted by cryptography technology, and no personal information will be leaked even on the public network. In addition, the user's funds are directly stored in their own digital wallets, and they have full control over their assets and are not controlled by any third party.
BitPower not only excels in security, but its income mechanism is also very attractive. The platform automatically matches borrowing and lending needs and calculates interest in real time through a decentralized market liquidity pool model. This mechanism not only improves the efficiency of capital utilization, but also provides users with stable income. For example, users can obtain high returns in different cycles of daily, weekly, and monthly by providing liquidity on BitPower. Specifically, the daily yield is 100.4%, 104% for seven days, 109.5% for fourteen days, and 124% for twenty-eight days. These returns are automatically returned to the user's wallet through smart contracts, ensuring the timeliness and security of the income.
In this era of uncertainty, BitPower provides users with a safe, transparent, and high-yield financial platform. Through blockchain technology, smart contracts, and cryptocurrencies, BitPower not only protects users' assets, but also brings them stable and considerable income. This is a miracle of modern finance and a truly game-changing platform. Whether you are a novice or an old hand, BitPower will be your new choice for financial investment. @Bitpower | pingz_iman_38e5b3b23e011f |
|
1,913,724 | HTML, CSS Interview Question&Answer | 1) What Do You Understand By The Universal Selector? Answer: The universal selector (*) in CSS... | 27,972 | 2024-07-06T12:20:49 | https://dev.to/shemanto_sharkar/html-css-interview-questionanswer-31gd | webdev, beginners, programming, career | **1) What Do You Understand By The Universal Selector?**
**Answer:**
The universal selector (`*`) in CSS applies styles to all elements on a web page. It is used to set a common style for all elements without needing to specify each one individually.
**2) Differentiate Between The Use Of ID Selector And Class Selector.**
**Answer:**
- **ID Selector (`#`)**: Used to style a single, unique element. Each ID must be unique within a document.
- **Class Selector (`.`)**: Used to style multiple elements that share the same class. Multiple elements can have the same class.
**3) How Can You Use CSS To Control Image Repetition?**
**Answer:**
CSS property `background-repeat` controls image repetition. For example:
- `background-repeat: repeat;` repeats the background image both horizontally and vertically.
- `background-repeat: no-repeat;` prevents the background image from repeating.
- `background-repeat: repeat-x;` repeats the image horizontally.
- `background-repeat: repeat-y;` repeats the image vertically.
**4) Are The HTML Tags And Elements The Same Thing?**
**Answer:**
No, HTML tags and elements are not the same.
- **Tags**: The code surrounded by angle brackets, such as `<div>`.
- **Elements**: The combination of the opening tag, content, and closing tag, such as `<div>Content</div>`.
**5) Difference Between Inline, Block, And Inline-Block Elements. Is It Possible To Change An Inline Element Into A Block-Level Element?**
**Answer:**
- **Inline Elements**: Do not start on a new line and only take up as much width as necessary (e.g., `<span>`, `<a>`).
- **Block Elements**: Start on a new line and take up the full width available (e.g., `<div>`, `<p>`).
- **Inline-Block Elements**: Behave like inline elements but can have width and height set like block elements.
Yes, it is possible to change an inline element into a block-level element using CSS:
```css
.element {
display: block;
}
``` | shemanto_sharkar |
1,913,723 | Thiết Kế Website Tại Bắc Ninh Tăng Tỷ Lệ Chuyển Đổi | Terus là một công ty chuyên cung cấp dịch vụ thiết kế và phát triển website chuyên nghiệp, uy tín... | 0 | 2024-07-06T12:20:29 | https://dev.to/terus_technique/thiet-ke-website-tai-bac-ninh-tang-ty-le-chuyen-doi-3432 | website, digitalmarketing, seo, terus |
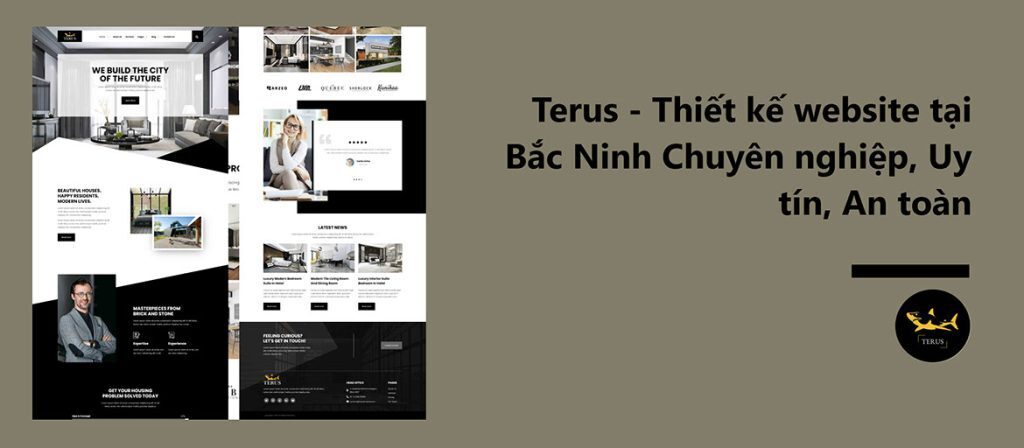
Terus là một công ty chuyên cung cấp dịch vụ thiết kế và phát triển [website chuyên nghiệp, uy tín](https://terusvn.com/thiet-ke-website-tai-hcm/) tại khu vực Bắc Ninh. Chúng tôi tự hào là đơn vị hàng đầu trong lĩnh vực này với đội ngũ chuyên gia có nhiều năm kinh nghiệm.
hững điểm nổi bật trong thiết kế website tại Bắc Ninh của Terus:
Hỗ trợ đa ngôn ngữ: Đáp ứng nhu cầu của khách hàng quốc tế bằng cách cung cấp tính năng đa ngôn ngữ trên website.
Chuyên gia về các lĩnh vực tại Bắc Ninh: Đội ngũ nhân viên của Terus có kiến thức chuyên sâu về các ngành công nghiệp và sản xuất chính tại Bắc Ninh, giúp thiết kế website phù hợp với từng lĩnh vực.
Thiết kế lấy người dùng làm trung tâm: Với phương pháp thiết kế lấy trải nghiệm người dùng (UX) làm ưu tiên, Terus cam kết nâng cao chất lượng trải nghiệm người dùng trên website.
Tích hợp với thương mại điện tử: Các website do Terus thiết kế được tích hợp sẵn với các nền tảng thương mại điện tử phổ biến, giúp doanh nghiệp dễ dàng bán hàng trực tuyến.
Ưu tiên thiết kế cho thiết bị di động: Terus áp dụng phương pháp thiết kế ưu tiên cho trải nghiệm trên các thiết bị di động, đảm bảo website hoạt động tối ưu trên mọi nền tảng.
Terus cung cấp các mẫu website đa dạng, phù hợp với các ngành nghề tại Bắc Ninh. Ngoài ra, Terus cũng cung cấp dịch vụ thiết kế website theo yêu cầu riêng của từng khách hàng.
Với kinh nghiệm phong phú, đội ngũ chuyên gia và quy trình thiết kế chuyên nghiệp, Terus cam kết mang đến những [giải pháp website chất lượng cao, tối ưu](https://terusvn.com/thiet-ke-website-tai-hcm/) trải nghiệm người dùng và tăng năng suất kinh doanh cho khách hàng tại Bắc Ninh.
Tìm hiểu thêm về [Thiết Kế Website Tại Bắc Ninh Tăng Tỷ Lệ Chuyển Đổi](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-bac-ninh/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,908,121 | Build Your Own YouTube Thumbnail Downloader! RoR+SerpApi - Part 1 | Part 1 - Basic Working Example (Done) Part 2 - Refactor and Deployment In this... | 0 | 2024-07-06T12:15:21 | https://dev.to/lipin/build-your-own-youtube-thumbnail-downloader-rorserpapi-part-1-3f15 | tutorial, rails, beginners, api | ## Part 1 - Basic Working Example (Done)
## Part 2 - Refactor and Deployment
In this short tutorial we'll build a simple YouTube thumbnail downloader in Ruby on Rails with SerpApi for finding video thumbnails.
## Prerequisites:-
Ruby version 3.2.2
Ruby on Rails version 7.1.3.4
SerpApi access. You can get the api key by singing up [here](https://serpapi.com/).
The versions don't matter. You should have both ruby and rails installed in your system.
Let's begin by scaffolding our rails app.
`$ rails new YT-Thumbnail-Downloader`
Go to the project directory `$ cd YT-Thumbnail-Downloader`.
Type `$ rails s` in the terminal and open localhost:3000 in the browser. You should see the default home page for the rails app.
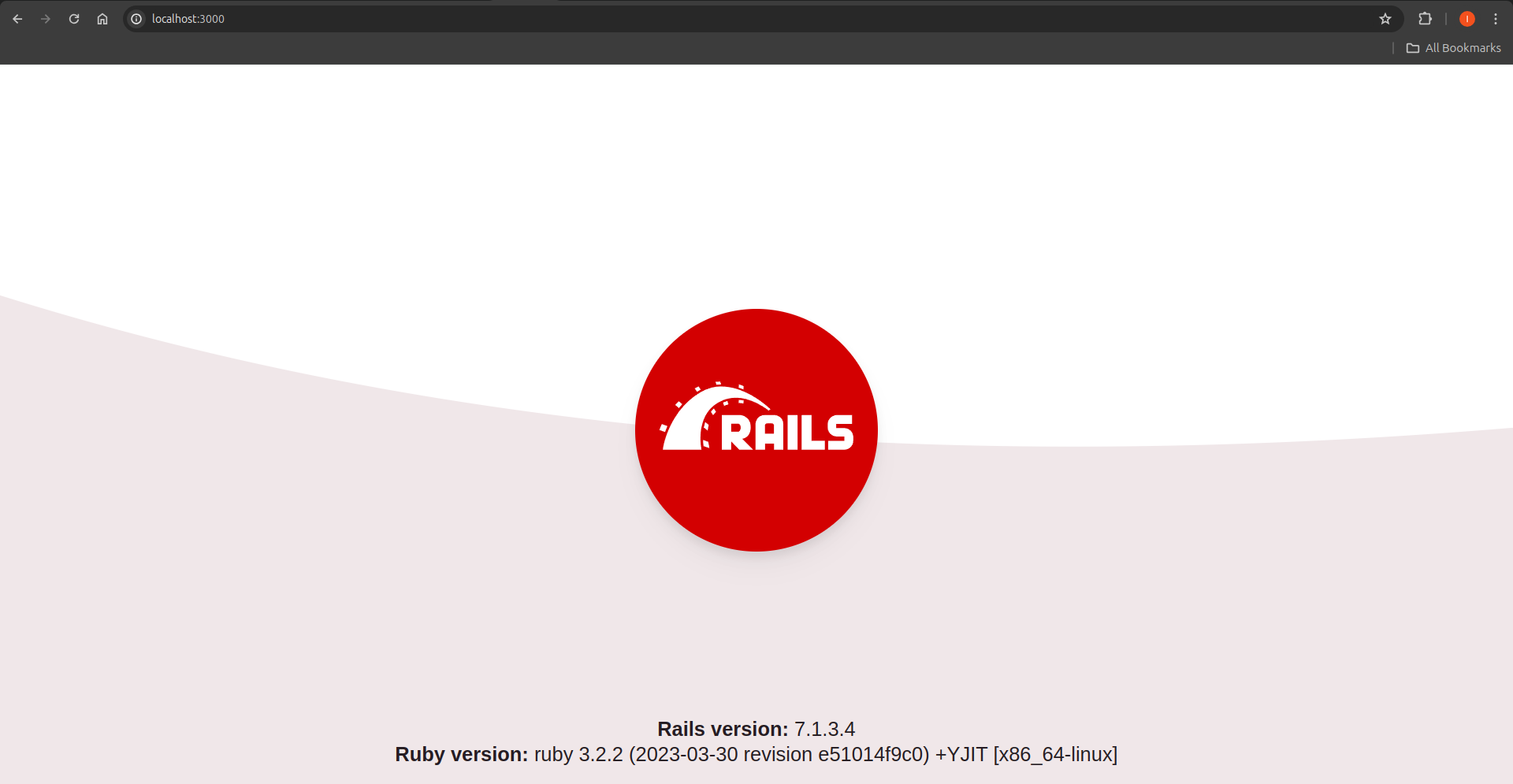
If you can see the rails default page then everything is working fine.
## The Structure of the App.
We'll build a basic working app in part 1 and refactor our code in part 2 of the article.
The app will have one controller and a single view template. There's no model for now. We'll simply make a call to the YouTube Video API from SerpApi and serve the result on the home page.
## Controller
We can use the rails generate command to create controllers. But in this article we'll do it manually.
In your rails app directory inside the app/controller create a home_controller.rb file.
`$ touch app/controller/home_controller.rb`
Now create the index action for home controller.
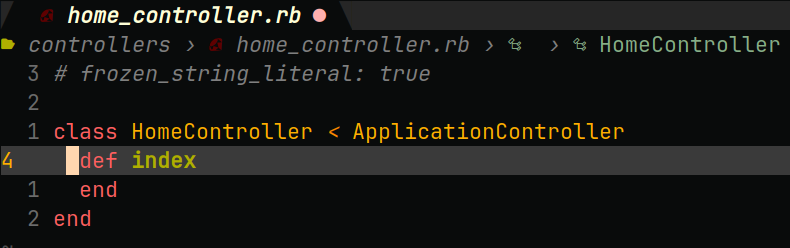
We'll use only one controller action for the app logic to keep things simple.
## SerpApi
Next, we need to make a call to the api. For that grab your api key from SerpApi. You can find your key in your account dashboard.
To work with api, we can use several ruby gems. But for this article we'll stick with the standard HTTP library.
From your SerpApi account select youtube video api from the left panel and scroll down the api examples.
Copy the [example link](https://serpapi.com/youtube-video-api) from from there. It should look like this. https://serpapi.com/search.json?engine=youtube_video&v=vFcS080VYQ0
Clicking on the link will take you the results page. The json response here gives the data of youtube video beign scraped by the SerpApi.
If you look closely, you can find the thumbnail of the video with a link to the high resolution image. Parsing this thumnail is all we need to do.
Let's look at the link from SerpApi. The link returns a json response, it has an engine that defines its purpose (engine=youtube_video). In our case its youtube video api. Don't confuse it with the parent YouTube api. The last query in the link is the id of the video(v=vFcS080VYQ0). Every YouTube video has an ID. We only need to switch this id in the api call to fetch the thumbnail. Interesting video on the topic [here](https://www.youtube.com/watch?v=gocwRvLhDf8).
Let's add all this in our controller.
## Making the API call
To make the api call we'll use the standard ruby http library. First save the link in variable url.

To make authenticated call to the SerpApi you need to add the api key at the end of this url.
`https://serpapi.com/search.json?engine=youtube_video&v=vFcS080VYQ0&api_key={your api key goes here}`
Ideally, you should use something like dotenv for rails to save your api keys. We'll do it in the second part of the article.
Let's start with making the api call. For that, you need to use URI module.

Like so. Next we need to save the response.
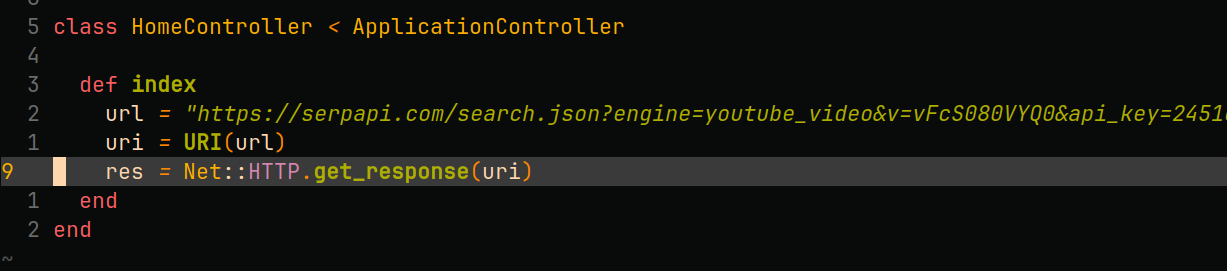
To check wether the api is connected, insert raise right below the response.
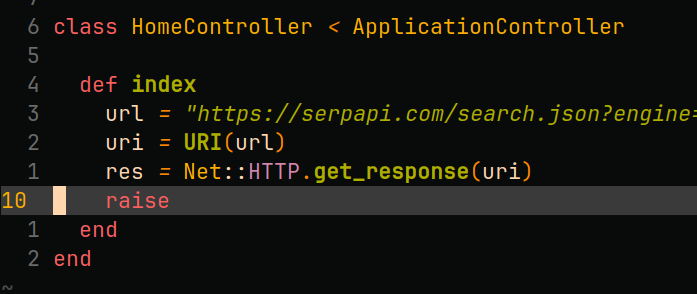
Restart the server and you should see the error page. Below in the console make the call to api by typing `$ res`.
If you see HTTP 200 OK that means your api is working!
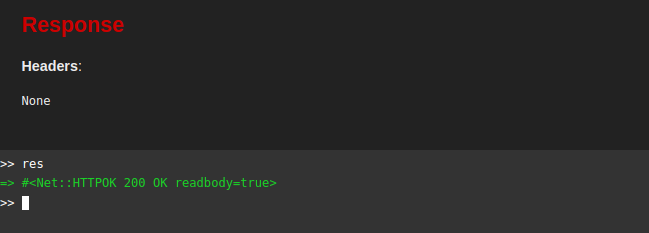
If you type `$ res.body` inside console, you'll see hash response. We need to save this data inside a variable and parse the thumbnail.
## Parsing and Displaying The Thumbnail
We have the data of the YouTube video and all that we need to do now is to extract the thumbnail.
To do this we'll store the response body in a data variable and parse it for thumbnail. Check out the code in below screen shot.
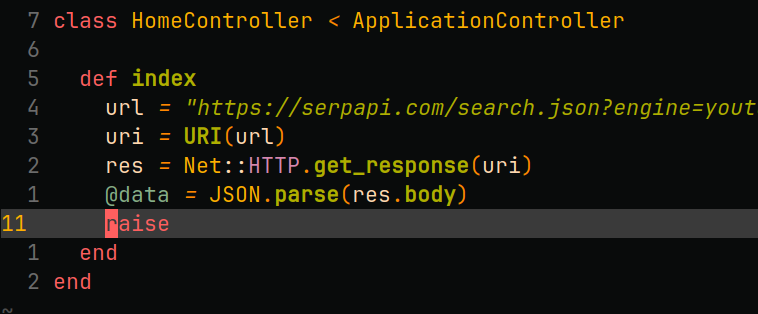
Insert raise below the `$ @data` variable and go to the browser and refresh the page.
In the console type this line `$ @data`. To parse this data, simply parse it as you would parse any other array of data from a json response.
The api gives us all the data for the present video and its channel. To see the number of subscribers you can do `$ @data["channel"]["subscribers"]`.
But want the thumbnail. For that, use `$ @data["thumbnail"]`. This should return an image link.
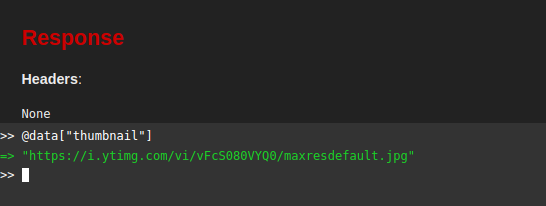
Like so.
Copy and paste this link to see the thumbnail.
Let's store this image link to a variable and render the thumbnail.
## The Views
Create the 'home' folder for the views template. `$ mkdir app/views/home`.
Next create a view index template for the index action of the home controller `$ touch app/views/home/index.html.erb`. Make sure its inside the home folder.
The templates are based on the controller action. Here the controller is 'home' with only one action that is 'index'. The erb is the template extension for rails.
Remove `$ raise` from your controller.
Open up the index view template and create h1 tag with the contents `$ <h1>YT Thumbnial Downloader!</h1>`.
Before refreshing your home page, make sure you update your route.rb file's root route to `$ root 'home#index'.
Go to your app home page localhost:3000 and refresh the page.
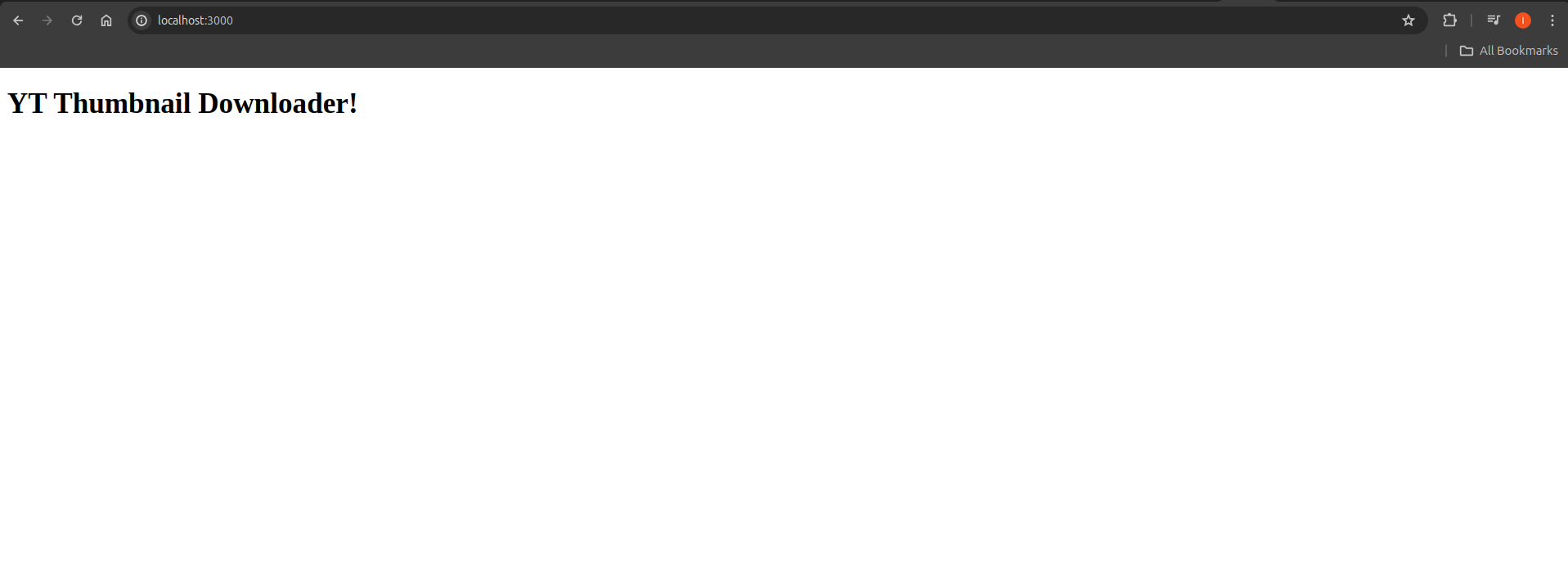
You should see the same page as above.
All that we need to do is to render the thumbnail image on the home page of our app.
We'll need to create another variable `$ @image` in our home controller `$ @image = @data["thumbnail"]`.
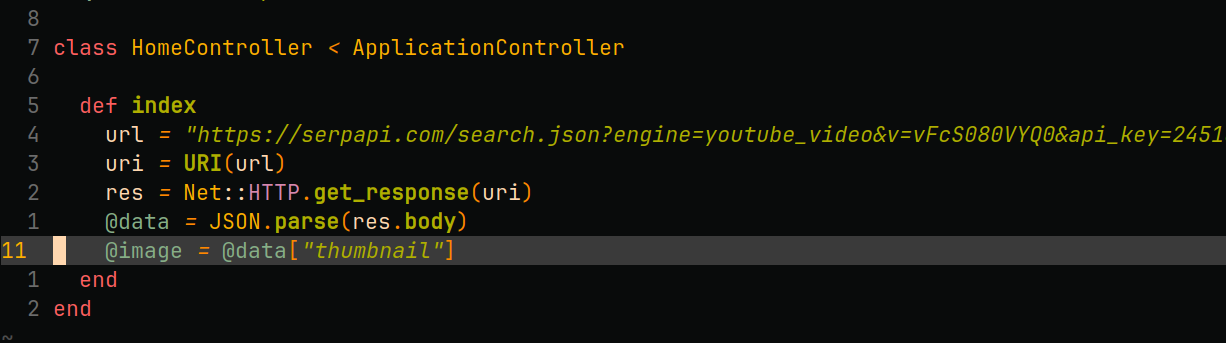
`@` in front of a variable in ruby makes it a global instance that you can access globally in your app.
Now render the image in your views `$ <img src="<%= @image %>" alt="youtube thumbnail image">`
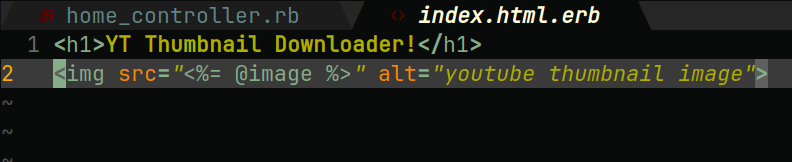
Refresh the home page and you should see the video thumbnail.
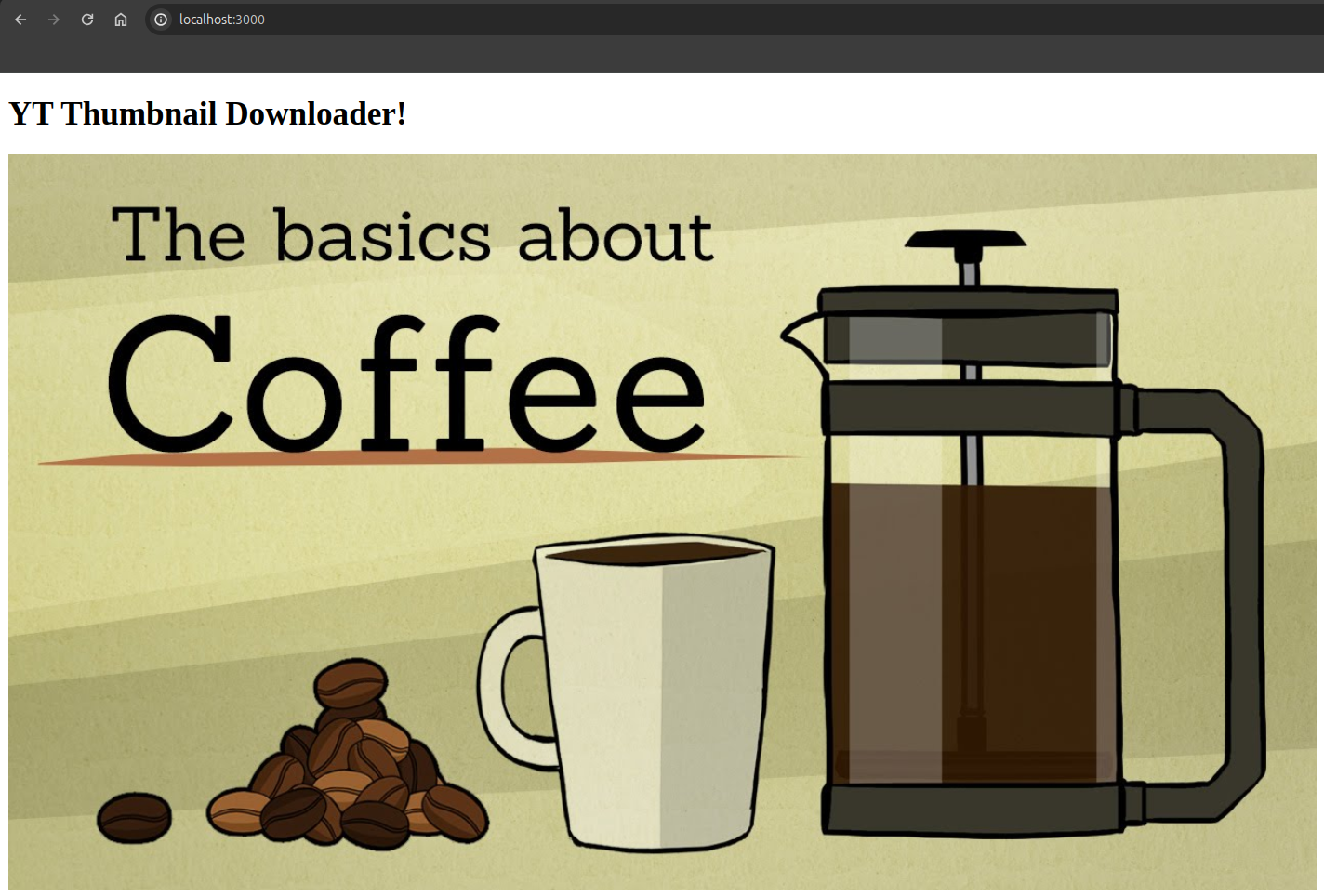
Right now the video id of the youtube video is hard coded. We need to accept the client input and dynamically change the thumbnail.
## The Client Interaction
We need a form in our views to accept user input in our app's frontend.
We'll use form_with helper from rails. Checkout the screenshot below with the completed form for our app.
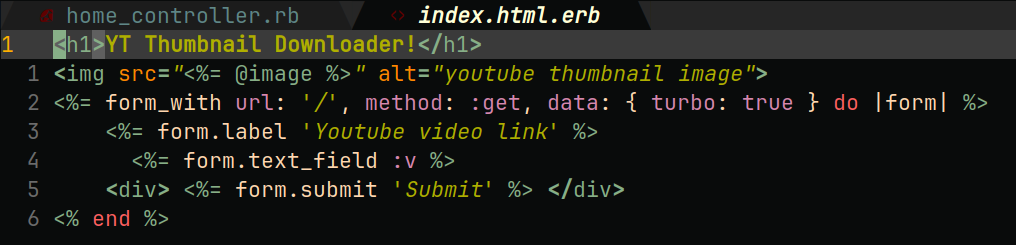
We'll use form_with to send the input from the client/frontend to the home controller.
You can use model or url for form_with. Since, we aren't using any model in our app we'll stick with url.
The line below h1 tag in the above screen shot is the image tag that will display the thumbnail image.
The first line of form_with will direct the form input to the root route. `$ url: '/'`. The method is get, remember that we are making a get request to SerpApi. Next, the data turbo value set to true is optional. In rails the turbo is true by defualt. If you face any problem with the form you can try toggle the value to false.
The form.label will show up on the forntend to let the user know where to input the YouTube video link. Whereas, form.text_field allows for data transfer between the controller and the view.
And form.submit will submit the form.
Now all that we need to do is to update our home controller so that we can complete the app.
Take a look at the screenshot below.
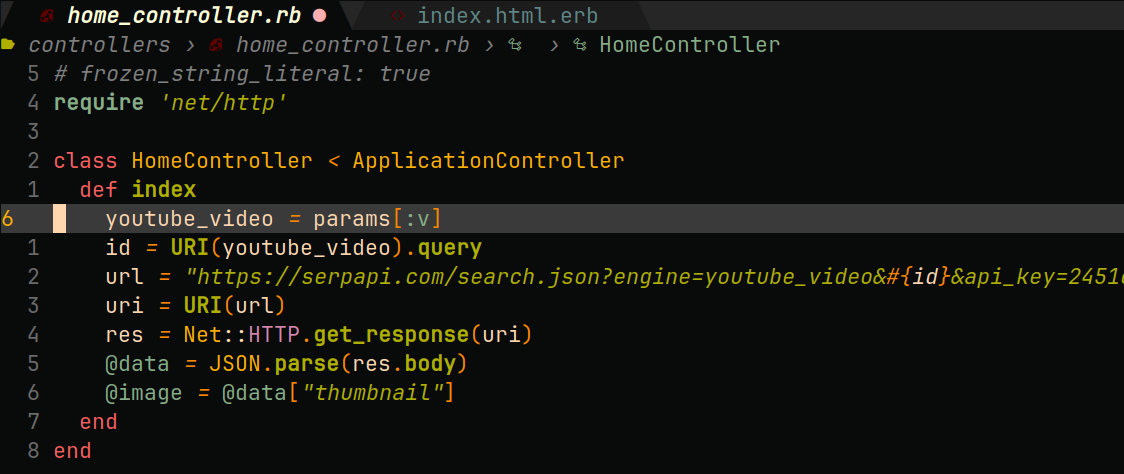
The youtube video link is passed as params. The :v could be named anything. It stores the actual link, which is then again stored inside variable 'id'. Using URI.query method gives us the youtube video id. String after the "?" in a url is a query.
Replace the video id in the SerpApi link with the vriable 'id' as its shown in the screenshot.
And that's it! Now you can copy any YouTube video link and get its thumbnail.
Test this YouTube video link: [Harnessing the True Power of Regular Expressions in Ruby](https://www.youtube.com/watch?v=JfwS4ibJFDw)
You should get this thumbnail:
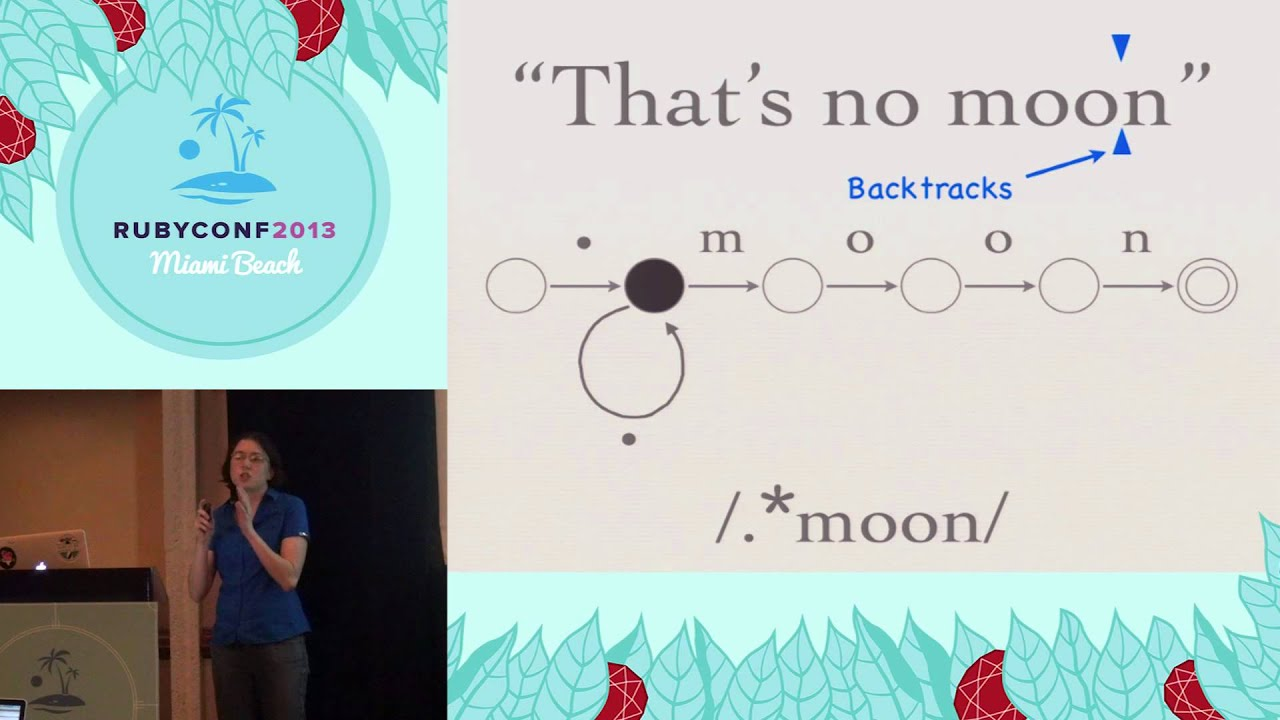
Will cover refactoring and deployment in part 2.
This app works only when the video link is copied from the YouTube. If you get a shared link then this setup won't work. Read more on YouTube video id [here]
(https://gist.github.com/jakebellacera/d81bbf12b99448188f183141e6696817).
Cover Photo by <a href="https://unsplash.com/@vmxhu?utm_content=creditCopyText&utm_medium=referral&utm_source=unsplash">Szabo Viktor</a> on <a href="https://unsplash.com/photos/youtube-website-screengrab-4QmSdCP4bhM?utm_content=creditCopyText&utm_medium=referral&utm_source=unsplash">Unsplash</a> | lipin |
1,913,720 | Exciting New Features in ECMAScript 2024 : In-Place Resizable ArrayBuffers and Enhanced SharedArrayBuffers 🚀 | ECMAScript 2024 brings several exciting enhancements to ArrayBuffer and SharedArrayBuffer, making... | 0 | 2024-07-06T12:13:16 | https://dev.to/rajusaha/exciting-new-features-in-ecmascript-2024-in-place-resizable-arraybuffers-and-enhanced-sharedarraybuffers-94c | javascript, webdev, programming, es15 | ECMAScript 2024 brings several exciting enhancements to ArrayBuffer and SharedArrayBuffer, making memory management in JavaScript more efficient and flexible. In this post, we’ll dive into these new features and provide examples to help you get started.
#### In-Place Resizable ArrayBuffers
Previously, ArrayBuffers had fixed sizes. Resizing required allocating a new buffer and copying data, which was inefficient and could fragment the address space on 32-bit systems. ECMAScript 2024 introduces in-place resizable ArrayBuffers, allowing dynamic resizing without creating new buffers or copying data.
##### New Constructor and Methods
The ArrayBuffer constructor now accepts an additional parameter for `maxByteLength`. Here’s the updated constructor and new methods:
- `new ArrayBuffer(byteLength: number, options?: {maxByteLength?: number})`
- `ArrayBuffer.prototype.resize(newByteLength: number)`
- `ArrayBuffer.prototype.resizable()`
- `ArrayBuffer.prototype.maxByteLength()`
Example: Resizing an ArrayBuffer 🖊️
```javascript
// Create a resizable ArrayBuffer with an initial size of 10 bytes and a max size of 20 bytes
let buffer = new ArrayBuffer(10, { maxByteLength: 20 });
console.log(buffer.byteLength); // 10
// Resize the ArrayBuffer to 15 bytes
buffer.resize(15);
console.log(buffer.byteLength); // 15
// Attempting to resize beyond the max size throws an error
try {
buffer.resize(25);
} catch (e) {
console.error(e); // RangeError: ArrayBuffer length exceeds max length
}
```
#### ArrayBuffer.prototype.transfer
The new `transfer `methods allow efficient data transfer between ArrayBuffers. This is particularly useful for transferring data via `structuredClone()` or within the same agent (e.g., main thread or web worker).
Example
```javascript
let buffer1 = new ArrayBuffer(8);
let buffer2 = buffer1.transfer();
console.log(buffer1.byteLength); // 0 (buffer1 is now detached)
console.log(buffer2.byteLength); // 8
```
#### SharedArrayBuffer Enhancements
SharedArrayBuffer allows multiple JavaScript contexts (threads or processes) to share the same memory. This is crucial for applications that need efficient memory sharing for large data across different agents. With ECMAScript 2024, SharedArrayBuffers can also be resized, but with some limitations:
- _SharedArrayBuffers can only grow; they cannot shrink_.
- _SharedArrayBuffers are not transferrable and therefore do not have the .transfer() method that ArrayBuffers have_.
##### New Constructor and Methods
The SharedArrayBuffer constructor now accepts an additional parameter for maxByteLength. Here’s the updated constructor and new method:
- `new SharedArrayBuffer(byteLength: number, options?: {maxByteLength?: number})`
- `SharedArrayBuffer.prototype.grow(newByteLength: number)`
Example 🖊️
```javascript
const buffer = new SharedArrayBuffer(8, { maxByteLength: 16 });
if (buffer.growable) {
buffer.grow(12);
console.log(buffer.byteLength); // 12
}
```
The new features in ECMAScript 2024 make `ArrayBuffer `and `SharedArrayBuffer `more powerful and flexible. In-place resizing eliminates the need for costly buffer reallocations, and the new transfer methods facilitate efficient data movement. Enhanced SharedArrayBuffer capabilities continue to support efficient memory sharing across JavaScript contexts, with the added ability to resize (though only to grow and not shrink).
Reference Link : [Ecma International approves ECMAScript 2024: What’s new?](https://2ality.com/2024/06/ecmascript-2024.html#new-features-for-arraybuffers-and-sharedarraybuffers) | rajusaha |
1,913,718 | Essential Apps: Must-Haves for Your Smartphone | Hey there, fellow smartphone addict! Let’s face it – our phones are like our right-hand (wo)man. They... | 0 | 2024-07-06T12:12:21 | https://dev.to/ifavecontents/essential-apps-must-haves-for-your-smartphone-4idf | productivityapps, musthaveapps, smartphoneessentials, bestapps | Hey there, fellow smartphone addict! Let’s face it – our phones are like our right-hand (wo)man. They help us keep up with work, stay in touch with friends, and even entertain us when we're bored. But with a bazillion apps out there, how do you know which ones are actually worth your time? Don’t worry, I’ve got you covered. Here are the [best apps](https://ifave.com/category/The-World/Best-apps) that’ll make your life easier, healthier, and a whole lot more fun.
**Productivity Boosters**
Trying to keep your life together? These apps are like having a personal assistant in your pocket:
1. **Todoist:** Your ultimate task manager. It's like having a nagging mom reminding you of your to-do list, but in a good way.
2. **Evernote:** For when you need to jot down genius ideas or grocery lists. Think of it as your second brain.
3. **Trello:** Organize your chaos with boards, lists, and cards. It’s like Pinterest for your projects.
**Health and Wellness**
Getting your life together also means taking care of yourself. These apps are your digital wellness gurus:
1. **MyFitnessPal:** Track what you eat and how much you move. It's like having a fitness coach who doesn’t judge your late-night snacks.
2. **Headspace:** Meditation made easy. Perfect for those “I need a break before I lose it” moments.
3. **Sleep Cycle:** An alarm clock that wakes you up gently. No more feeling like you got hit by a truck when you wake up.
**Entertainment**
Need a break? These apps are here to entertain you:
1. **Spotify:** Your personal DJ. Discover new tunes or stick to your old favorites.
2. **Netflix:** Binge-watch your favorite shows anytime, anywhere. Enough said.
3. **TikTok:** Get lost in a rabbit hole of short, addictive videos. Warning: You might lose track of time.
**Social Connectivity**
Stay in the loop with these must-have social apps:
1. **WhatsApp:** Text, call, or video chat with anyone, anywhere. It’s like a global chatroom.
2. **Instagram:** Share your life in photos and videos, or just scroll through to see what everyone else is up to.
3. **Facebook:** Keep up with news, events, and your social circle. Basically, your digital town square.
**Security and Privacy**
Keep your stuff safe with these top-notch security apps:
1. **LastPass:** Manage all your passwords without pulling your hair out. Think of it as a digital vault.
2. **NordVPN:** Surf the web securely and privately. It’s like having an invisibility cloak for your online data.
3. **Signal:** Chat with peace of mind knowing your messages are encrypted. Your secrets are safe here.
**Financial Management**
Take control of your finances with these handy apps:
1. **Mint:** Budget, track expenses, and see where your money is going. Your wallet will thank you.
2. **Venmo:** Send and receive money with a tap. Perfect for splitting bills without the awkwardness.
3. **Robinhood:** Invest in stocks, ETFs, and cryptocurrencies. It’s like having Wall Street in your pocket.
**Learning and Development**
Feed your brain with these educational gems:
1. **Duolingo:** Learn a new language in a fun way. It's like a game, but you get smarter.
2. **Coursera:** Take online courses from top universities. Your resume will love this.
3. **Khan Academy:** Free lessons on just about anything. The ultimate brain food.
**Travel and Navigation**
Hit the road with these travel essentials:
1. **Google Maps:** Never get lost again. Your ultimate guide to anywhere and everywhere.
2. **TripAdvisor:** Plan the perfect trip with reviews and recommendations. Travel smart, not hard.
3. **Airbnb:** Find unique places to stay, from cozy apartments to luxury villas. Your home away from home.
There you have it – the [best apps](https://ifave.com/category/The-World/Best-apps) that will make your smartphone smarter. From productivity and health to entertainment and travel, these must-haves will keep you organized, entertained, and on top of your game. So go on, download these essential apps and transform the way you use your phone. Happy app-ing! | ifavecontents |
1,913,717 | Thiết Kế Website Tại Biên Hòa Chất Lượng Tối Ưu | Thiết kế website là một nhu cầu quan trọng đối với các doanh nghiệp tại Biên Hòa, bởi vì nó mang lại... | 0 | 2024-07-06T12:12:16 | https://dev.to/terus_technique/thiet-ke-website-tai-bien-hoa-chat-luong-toi-uu-9pe | website, digitalmarketing, seo, terus |
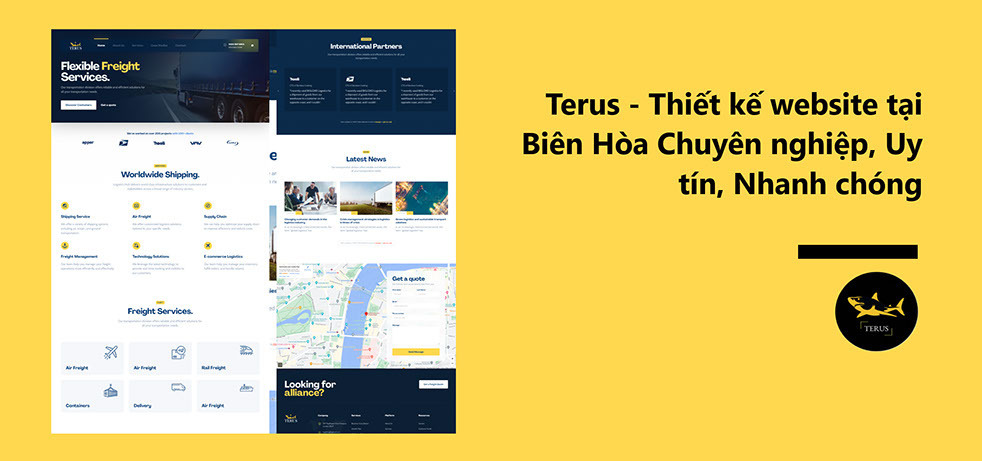
Thiết kế website là một nhu cầu quan trọng đối với các doanh nghiệp tại Biên Hòa, bởi vì nó mang lại nhiều lợi ích thiết thực. Trước hết, website giúp doanh nghiệp trưng bày sản phẩm và dịch vụ của mình một cách trực quan và hiệu quả. Nó cũng là công cụ xây dựng và củng cố thương hiệu, tạo sự khác biệt với các đối thủ cạnh tranh. Một website chuyên nghiệp, dễ sử dụng sẽ giúp tăng khả năng tiếp cận của khách hàng, mang lại sự thuận tiện và tiện lợi.
Hơn nữa, website còn là cơ hội để doanh nghiệp phát triển năng lực thương mại điện tử, tiếp cận được những thị trường mới. Nó cũng tạo kênh tương tác và nhận phản hồi trực tiếp từ khách hàng, giúp doanh nghiệp hiểu rõ hơn nhu cầu và mong muốn của khách hàng. Nhìn chung, một [website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/) sẽ mang lại lợi thế cạnh tranh đáng kể cho doanh nghiệp.
Những điểm nổi bật của website tại Biên Hòa do Terus thiết kế
Terus là công ty chuyên về [thiết kế website tại Biên Hòa](https://terusvn.com/thiet-ke-website-tai-hcm/), với nhiều điểm nổi bật so với các dịch vụ khác. Trước tiên, Terus tùy chỉnh website cho từng ngành công nghiệp cụ thể, đáp ứng đúng nhu cầu và đặc thù của từng doanh nghiệp. Ngoài ra, website do Terus thiết kế còn tích hợp được đặc điểm của địa phương Biên Hòa và phản ánh đúng bản sắc của doanh nghiệp.
Một ưu điểm khác là website đáp ứng tốt cho thiết bị di động, giúp người dùng truy cập dễ dàng từ mọi nơi. Terus cũng chú trọng đến việc bản địa hóa website, tích hợp các tính năng và ngôn ngữ phù hợp với thị trường Biên Hòa. Đặc biệt, họ luôn đảm bảo hiệu suất và tốc độ tải website ở mức tối ưu, cùng với việc lưu trữ an toàn và bảo vệ dữ liệu khách hàng.
Qua từng bước, Terus cam kết mang lại cho khách hàng một website chuyên nghiệp, hiệu quả và phù hợp với nhu cầu kinh doanh.
Việc thiết kế website tại Biên Hòa là một nhu cầu thiết yếu đối với các doanh nghiệp tại địa phương này. Terus là một công ty có nhiều kinh nghiệm và đặc điểm nổi bật trong lĩnh vực này, với quy trình thiết kế chi tiết và cam kết mang lại sản phẩm chất lượng cao. Dịch vụ của Terus sẽ giúp doanh nghiệp xây dựng thương hiệu, tiếp cận khách hàng và phát triển kinh doanh hiệu quả hơn.
Tìm hiểu thêm về [Thiết Kế Website Tại Biên Hòa Chất Lượng Tối Ưu](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-bien-hoa/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,716 | THE RELIABLE WAY TO RECLAIM STOLEN USDC\BNB\DANIEL MEULI WEB RECOVERY | One morning, while casually surfing the web in search of opportunities for financial growth, I... | 0 | 2024-07-06T12:11:32 | https://dev.to/rayleigh_neufeld_a6be5d72/the-reliable-way-to-reclaim-stolen-usdcbnbdaniel-meuli-web-recovery-19bm | cryptocurrency | One morning, while casually surfing the web in search of opportunities for financial growth, I stumbled upon multiple enticing ads promoting an investment platform promising daily profits. Intrigued by the allure of achieving financial freedom, I decided to take the plunge and invest a modest sum. To my delight, the returns began flowing in steadily, convincing me of the legitimacy of the venture. Encouraged by the initial success, I gradually increased my investment, pouring in a substantial portion of my savings totaling approximately $135,000. However, what initially seemed like a pathway to prosperity soon unraveled into a nightmare of deceit and betrayal. Without warning, the investment platform's website vanished into thin air, rendering it inaccessible and leaving me in a state of disbelief. As the realization of being swindled sank in, I found myself plunged into a state of perplexity, grappling with the harsh reality of my financial loss. Determined to seek recourse and reclaim my stolen funds, I embarked on a frantic quest for solutions, scouring the web for avenues of genuine recovery. Fortune smiled upon me when I stumbled upon Daniel Meuli Web Recovery, a beacon of hope amidst the darkness of my despair. Prompted by a referral from a fellow victim who had been rescued by this remarkable specialist, I wasted no time in reaching out to them for assistance. With a sense of urgency, I relayed my predicament to the experts at Daniel Meuli Web Recovery, providing them with pertinent details about the fraudulent scheme that I had faced. Displaying unparalleled expertise and utilizing cutting-edge tracing software, they sprang into action with remarkable efficiency. In a matter of mere hours, Daniel Meuli Web Recovery orchestrated a feat of technological wizardry, successfully tracing and retrieving my stolen funds with precision and finesse. Their swift intervention not only salvaged my financial stability but also spared me from the brink of bankruptcy, averting a potential catastrophe. I cannot overstate the gratitude and admiration I harbor for Daniel Meuli Web Recovery. Their skill, and commitment to their clients represent top-notch quality in the field of financial recovery. In a world full of lies and cheating, they serve as a strong fortress of honesty and dependability, providing vital help to people caught up in financial scams. To sum up, my experience with Daniel Meuli Web Recovery shows how determination and resilience can overcome tough times. For anyone dealing with financial trickery, I highly recommend Daniel Meuli Web Recovery as the best solution to get back what belongs to you. Rely on their know-how, and you can find a way out of the mess caused by deceit. \\ Telegram. At Danielmeuli \\ Website. https://danielmeulirecoverywizard.online \\ WhatsApp. +393512013528
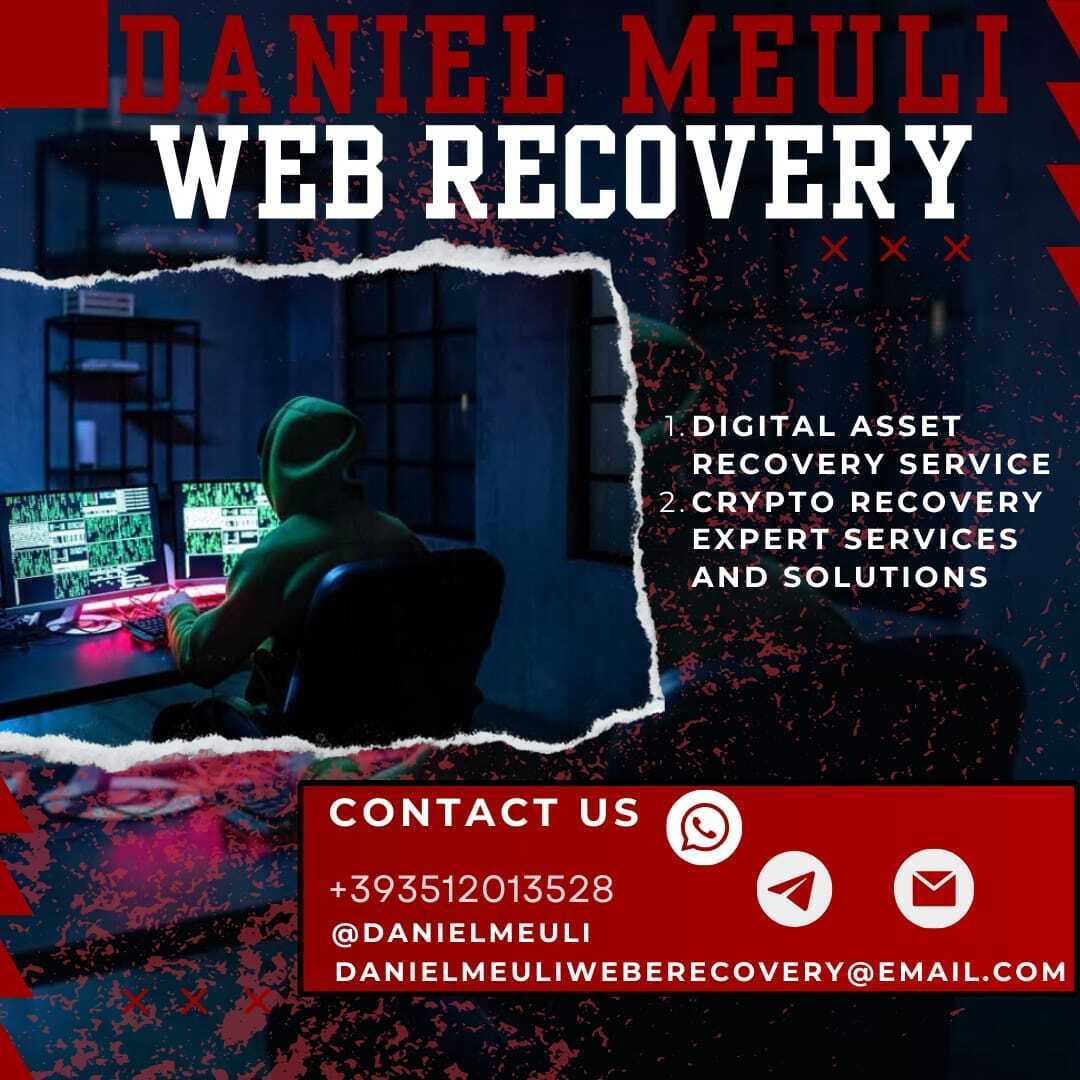 | rayleigh_neufeld_a6be5d72 |
1,913,708 | Diabetic Retinopathy | Diabetic retinopathy is an eye condition that can cause vision loss and blindness in people who have... | 0 | 2024-07-06T12:04:09 | https://dev.to/pouyasonej/diabetic-retinopathy-5dkh | Diabetic retinopathy is an eye condition that can cause vision loss and blindness in people who have diabetes. It affects blood vessels in the retina (the light-sensitive layer of tissue in the back of your eye). | pouyasonej |
|
1,913,714 | BitPower Security Overview | What is BitPower? BitPower is a decentralized lending platform that uses blockchain and smart... | 0 | 2024-07-06T12:08:13 | https://dev.to/aimm_w_1761d19cef7fa886fd/bitpower-security-overview-4mk4 | What is BitPower?
BitPower is a decentralized lending platform that uses blockchain and smart contract technology to provide secure lending services.
Security Features
Smart Contract
Automatically execute transactions and reduce human intervention.
Open source code, anyone can view it.
Decentralization
Users interact directly with the platform without intermediaries.
Funds flow freely between user wallets.
Asset Collateral
Borrowers use crypto assets as collateral to ensure loan security.
When the value of the collateralized assets decreases, the smart contract automatically liquidates to protect the interests of both parties.
Data Transparency
All transaction records are public and can be viewed by anyone.
Users can view transactions and assets at any time.
Multi-Signature
Multi-Signature technology is used to ensure transaction security.
Advantages
High security: Smart contracts and blockchain technology ensure platform security.
Transparency and Trust: Open source code and public transaction records increase transparency.
Risk Control: Collateral and automatic liquidation mechanisms reduce risks.
Conclusion
BitPower provides a secure and transparent decentralized lending platform through smart contracts and blockchain technology. Join BitPower and experience secure lending services! | aimm_w_1761d19cef7fa886fd |
|
1,913,712 | -Object oriented programming -Callback hell -Async/Sync js -Promise | Object oriented programming contains 4 pillars Object oriented programming - We group... | 0 | 2024-07-06T12:07:54 | https://dev.to/husniddin6939/-object-oriented-programming-callback-hell-asyncsync-js-promise-1lik |
## Object oriented programming contains 4 pillars
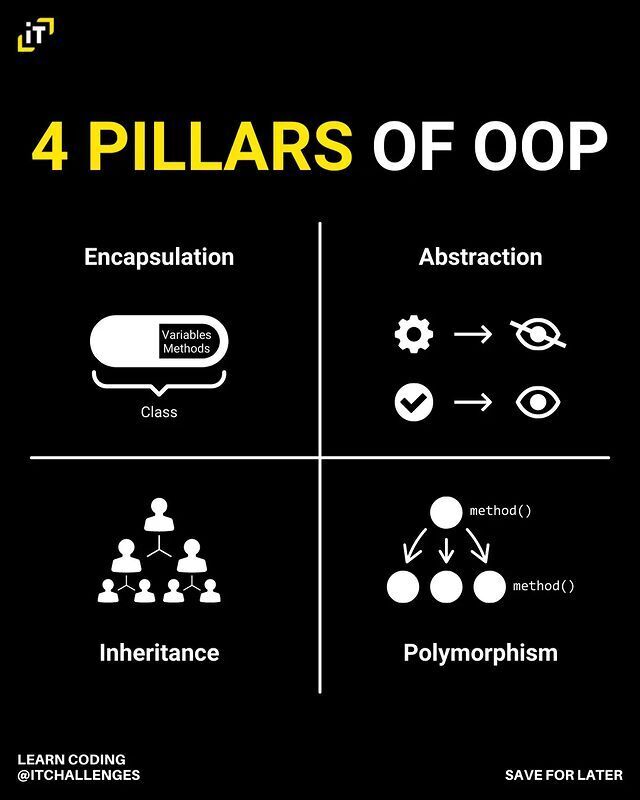
Object oriented programming - We group related variables and function that operate on them into objects and it is what we call encapsulation.
Let's show an example of this in action.
```
function getSalery(baseSalery, overtime, rate){
return baseSalery + (overtime*rate);
}
let employee = {
baseSalery:24000,
overtime:20,
rate:10,
getSalery: function(){
return this.baseSalery + (this.overtime*rate);
}
};
employee.getSalery();
```
When type code in Object oriented way, our functions end up having fewer parametrs so...
> The best functions are those with no parametrs
it is easier to use and mountain it in fewer parametr functions.
##ABSTRUCTION
Imagine abstriction think of a mobile phone on our hand as an object, this devise has a lots of tiny elements on the inside and we only and simply use it by tuchching and we don't care what happened inside, all complexity hidden from us - This is abstraction in practise.
We can use the same technique in objects, we can hide same of the proporties and methods from the outside and throught this way we echiave a couple of benifits.
1. Using an undarstanding an object with a few properties and methods is easier than an object with several properties and methods.
2.Secondly, it helps us reduce the impact of change. Let's imagine if we change inner or private methods, none of these changes will leak to the outside coz we don't have any code that touches these method outside of their content object, we may delete a method or change its parametrs but none of these changes will impact the rest of the aplications code.
So with abstraction we reduce the impact of change.
## Inheritance
| husniddin6939 |
|
1,913,710 | Enhancing Your Driving Experience with Advanced Automotive Technology | In the rapidly evolving world of automotive technology, keeping up with the latest trends and... | 0 | 2024-07-06T12:06:01 | https://dev.to/ronny_odhiambo_dfd50cf0ee/enhancing-your-driving-experience-with-advanced-automotive-technology-53i3 | car, luxury | In the rapidly evolving world of automotive technology, keeping up with the latest trends and upgrades can significantly enhance your driving experience. From high-tech multimedia head units to efficient engine types, there are numerous ways to upgrade your vehicle. In this article, we’ll explore the benefits of integrating a Lexus LX570 2007-2015 to 2020-2021 Tesla-Style Android Multimedia Head Unit, delve into the importance of the Auto Engine Petrol system, and discuss the advantages of a vertical screen double din car stereo.
Transform Your Lexus with a Tesla-
##
Style Android Multimedia Head Unit
The [Lexus LX570 2007-2015 to 2020-2021 Tesla-Style Android Multimedia Head Unit](https://railanewtone4.wixsite.com/digital-products/product-page/lexu-lx570-tesla) is an excellent upgrade for those looking to modernize their vehicle’s infotainment system. This advanced head unit brings a host of features that enhance both convenience and entertainment:
Seamless Integration: Designed specifically for Lexus LX570 models, this head unit fits perfectly into the existing dashboard, maintaining the sleek, luxurious feel of the vehicle.
Android Operating System: With the power of Android, you have access to a wide range of apps from the Google Play Store, allowing you to customize your multimedia experience.
Large Vertical Screen: The [vertical screen](https://railanewtone4.wixsite.com/digital-products/product-page/nissan-patrol-screen) provides a tablet-like interface, making navigation, media playback, and other functions more intuitive and visually appealing.
Connectivity Options: Enjoy various connectivity options, including Bluetooth, Wi-Fi, and USB, making it easier to integrate your smartphone and other devices with the head unit.
The Importance of Auto Engine Petrol Systems
The engine is the heart of any vehicle, and the Auto Engine Petrol system remains a popular choice for many car enthusiasts. Here’s why petrol engines continue to be a reliable option:
Performance: [Petrol engines](https://railanewtone4.wixsite.com/digital-products/product-page/auto-engine-petrol) are known for their superior performance, delivering quick acceleration and high top speeds. This makes them ideal for drivers who enjoy a spirited driving experience.
Efficiency: Modern petrol engines have become increasingly efficient, offering a good balance between power and fuel economy.
Maintenance: Petrol engines are generally easier and less expensive to maintain compared to their diesel counterparts. The availability of parts and experienced mechanics further adds to their appeal.
Advantages of a Vertical Screen Double Din Car Stereo
Upgrading your car stereo can dramatically improve your in-car entertainment and navigation experience. A vertical screen double din car stereo offers several benefits:
Enhanced Usability: The vertical orientation of the screen mimics modern smartphones and tablets, making it easier for users to interact with the stereo.
Better Viewing Angle: The vertical layout provides a better viewing angle for maps and other navigation tools, reducing the need to take your eyes off the road.
Aesthetic Appeal: A vertical screen [double din stereo ](https://railanewtone4.wixsite.com/digital-products/product-page/car-audio-gps)adds a contemporary touch to your car’s interior, giving it a more high-tech and sophisticated look.
Multi-Functionality: These stereos typically come packed with features such as GPS navigation, media playback, hands-free calling, and more, all accessible from the large, easy-to-use screen.
Finding the Right Upgrades for Your Vehicle
When considering upgrades like the Lexus LX570 2007-2015 to 2020-2021 Tesla-Style Android Multimedia Head Unit or a vertical screen double din car stereo, it’s essential to ensure compatibility with your vehicle. Consult with professionals or refer to your car’s manual to make informed decisions. Additionally, consider the reputation of the manufacturer and the availability of customer support to ensure you get a reliable product.
Conclusion
Upgrading your vehicle with the latest automotive technology can significantly enhance your driving experience. Whether it’s installing a Tesla-Style Android Multimedia Head Unit in your Lexus LX570, appreciating the performance of an Auto Engine Petrol system, or enjoying the advanced features of a vertical screen [double din car stereo](https://hiresaudio.co.ke/product-category/double-din/), these improvements can make your time on the road more enjoyable and efficient. By staying informed about the latest trends and choosing the right upgrades, you can ensure your vehicle remains at the cutting edge of automotive innovation.
| ronny_odhiambo_dfd50cf0ee |
1,913,709 | BitPower's Security: A Modern Financial Miracle | In a changing world, financial security has become more important than ever. BitPower, as a... | 0 | 2024-07-06T12:04:31 | https://dev.to/pings_iman_934c7bc4590ba4/bitpowers-security-a-modern-financial-miracle-39d8 |
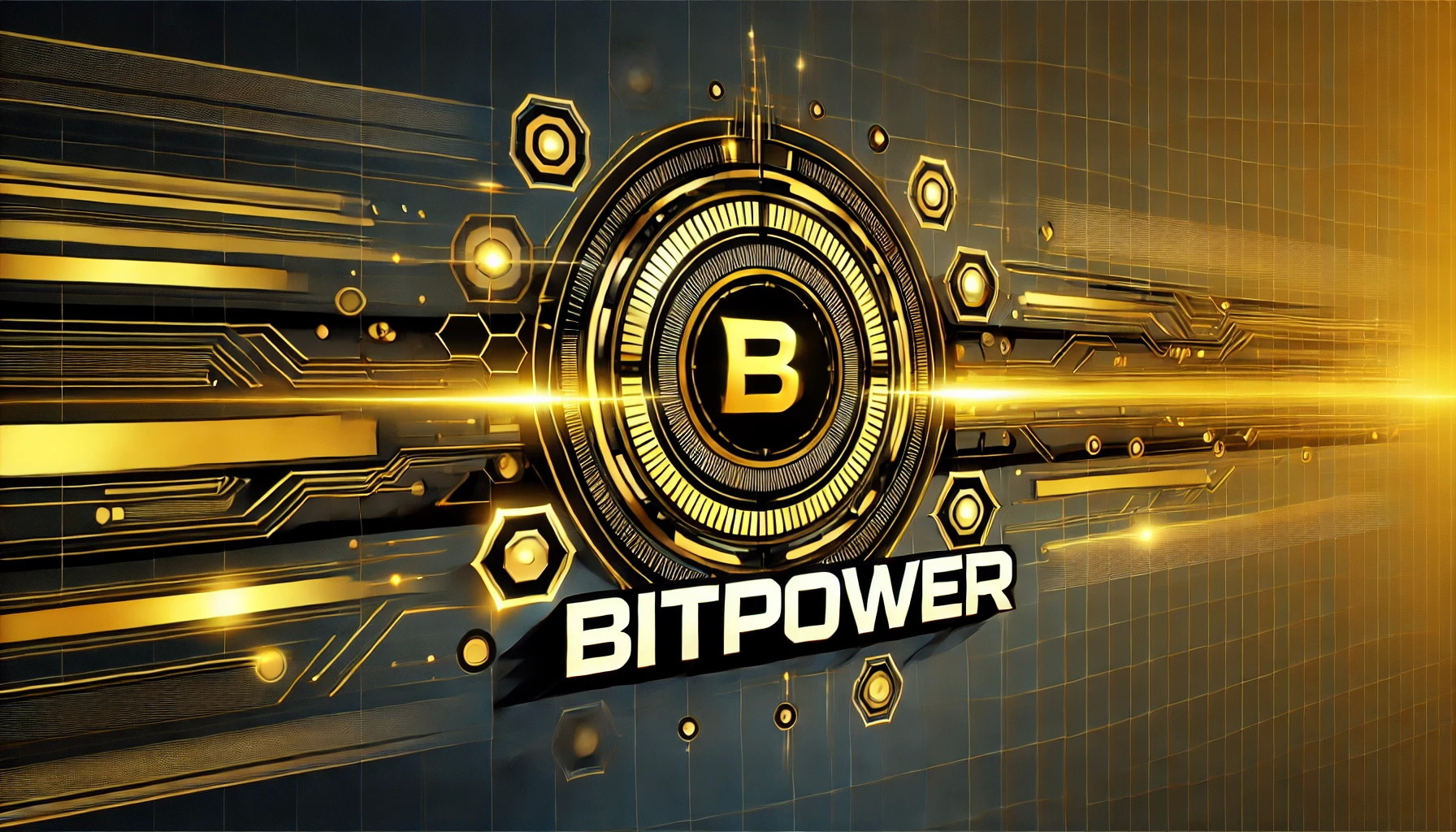
In a changing world, financial security has become more important than ever. BitPower, as a decentralized financial platform based on blockchain technology, provides unparalleled security and stable returns. By using advanced smart contracts and cryptocurrencies, BitPower has not only changed the landscape of traditional finance, but also provided users with unprecedented opportunities for income.
First, BitPower's security is based on the core characteristics of blockchain technology. Blockchain is a distributed ledger technology whose data is stored on thousands of nodes around the world, each of which keeps a complete record of transactions. This decentralized structure means that no one can tamper with the data alone, ensuring the transparency and immutability of transactions.
Second, BitPower uses smart contract technology to automatically execute transactions and agreements. A smart contract is a self-executing code that automatically triggers transactions when preset conditions are met. Through smart contracts, BitPower has achieved fully automated financial operations without intermediaries, reducing the risk of human error and fraud. Once deployed on the blockchain, these contracts cannot be changed, ensuring the stability and security of the system.
The use of cryptocurrency is also a highlight of BitPower's security. Cryptocurrency transactions use complex encryption algorithms to protect users' privacy and asset security. Every transaction of the user is encrypted by cryptography technology, and no personal information will be leaked even on the public network. In addition, the user's funds are directly stored in their own digital wallets, and they have full control over their assets and are not controlled by any third party.
BitPower not only excels in security, but its income mechanism is also very attractive. The platform automatically matches borrowing and lending needs and calculates interest in real time through a decentralized market liquidity pool model. This mechanism not only improves the efficiency of capital utilization, but also provides users with stable income. For example, users can obtain high returns in different cycles of daily, weekly, and monthly by providing liquidity on BitPower. Specifically, the daily yield is 100.4%, 104% for seven days, 109.5% for fourteen days, and 124% for twenty-eight days. These returns are automatically returned to the user's wallet through smart contracts, ensuring the timeliness and security of the income.
In this era of uncertainty, BitPower provides users with a safe, transparent, and high-yield financial platform. Through blockchain technology, smart contracts, and cryptocurrencies, BitPower not only protects users' assets, but also brings them stable and considerable income. This is a miracle of modern finance and a truly game-changing platform. Whether you are a novice or an old hand, BitPower will be your new choice for financial investment. @Bitpower
#BTC #ETH #SC #DeFi | pings_iman_934c7bc4590ba4 |
|
1,913,706 | best acupuncture and acupressure clinic in bangalore | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than... | 0 | 2024-07-06T12:01:04 | https://dev.to/ayushyaa/best-acupuncture-and-acupressure-clinic-in-bangalore-33b7 | ayushyaaclinic, acupuncture | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than Ayushyaa Acupuncture Clinic, the best acupuncture clinic in Bangalore. Our clinic is renowned for offering top-notch acupuncture and acupressure treatments that cater to a wide range of health issues. In this blog, we will explore what makes Ayushyaa clinic the preferred choice for those seeking natural and effective healthcare solutions.
Why Choose Ayushyaa Acupuncture Clinic?
Expertise and Experience
Ayushyaa Acupuncture clinic Bangalore a team of highly skilled and experienced practitioners who are dedicated to providing the best care possible. Our experts are well-versed in traditional acupuncture and modern techniques, ensuring that each patient receives personalized treatment tailored to their specific needs.
Comprehensive Treatments
At Ayushyaa Acupuncture Clinic, we offer a variety of treatments, including:
• Acupuncture: Utilizing fine needles to stimulate specific points on the body, acupuncture helps in balancing the body’s energy flow, alleviating pain, and promoting overall wellness.
• Acupressure: Similar to acupuncture but without needles, acupressure involves applying pressure to specific points to relieve pain and stress, boost energy levels, and improve health.
• Herbal Therapy: Complementing our acupuncture and acupressure treatments, we offer herbal remedies to support the healing process and enhance overall well-being.
Patient-Centric Approach
Our clinic is committed to providing a patient-centric approach, focusing on the individual needs and health goals of each patient. We take the time to understand your concerns, conduct thorough assessments, and design customized treatment plans that ensure optimal results.best acupressure clinic in Bangalore.
Modern Facilities
Ayushyaa Acupuncture Clinic is equipped with state-of-the-art facilities, creating a comfortable and serene environment for our patients. Our modern equipment and clean, calming spaces ensure that you have a relaxing and therapeutic experience during each visit.
Benefits of Acupuncture and Acupressure
Pain Relief
One of the primary reasons people seek acupuncture and acupressure treatments is for pain relief. Whether you suffer from chronic pain, migraines, or joint pain, our treatments can provide significant relief and improve your quality of life.
Stress Reduction
In today’s fast-paced world, stress is a common concern for many individuals. Acupuncture and acupressure treatments at Ayushyaa Acupuncture Clinic help in reducing stress levels, promoting relaxation, and enhancing mental clarity.
Improved Sleep
Many of our patients have reported improved sleep patterns and better sleep quality after undergoing acupuncture and acupressure treatments. By balancing the body’s energy and reducing stress, these treatments promote restful and rejuvenating sleep.
Enhanced Immune Function
Regular acupuncture and acupressure sessions can boost your immune system, helping you stay healthy and ward off illnesses. Our treatments stimulate the body’s natural healing abilities, enhancing overall health and vitality.
Conclusion
If you are looking for the top acupuncture in Bangalore, Ayushyaa Acupuncture Clinic is your ideal destination. Our experienced practitioners, comprehensive treatments, and patient-centric approach ensure that you receive the highest quality care. Visit us today and embark on a journey towards better health and well-being through the natural and effective treatments of acupuncture and acupressure.
For more information or to schedule an appointment, contact Ayushyaa Acupuncture Clinic today. Experience the [best acupuncture and acupressure clinic in Bangalore ](https://www.ayushyaaclinic.com/index)and take the first step towards a healthier, happier life.
| ayushyaa |
1,913,704 | Thiết Kế Website Tại An Giang Tối Ưu Doanh Thu | Lý do các doanh nghiệp ở An Giang cần thiết kế website Khả năng hiển thị và tiếp cận trực tuyến:... | 0 | 2024-07-06T11:59:48 | https://dev.to/terus_technique/thiet-ke-website-tai-an-giang-toi-uu-doanh-thu-4i3a | website, digitalmarketing, seo, terus |
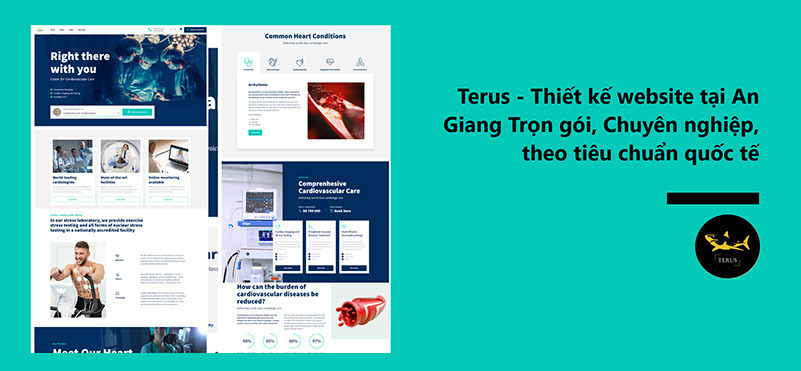
Lý do các doanh nghiệp ở An Giang cần thiết kế website
Khả năng hiển thị và tiếp cận trực tuyến: Một website chuyên nghiệp giúp doanh nghiệp mở rộng phạm vi hoạt động và tiếp cận khách hàng tiềm năng trên môi trường internet.
Uy tín và xây dựng thương hiệu: Sự hiện diện của một website chuyên nghiệp góp phần nâng cao uy tín và xây dựng thương hiệu doanh nghiệp trong mắt khách hàng.
Tiếp cận thông tin: Khách hàng có thể dễ dàng tìm kiếm và tiếp cận thông tin về doanh nghiệp, sản phẩm/dịch vụ, địa chỉ, liên hệ, ...
Sự gắn kết và tương tác với khách hàng: Website giúp doanh nghiệp tăng cường sự gắn kết và tương tác với khách hàng thông qua các tính năng như đặt hàng, đăng ký, ...
Lợi thế cạnh tranh: Sự hiện diện trực tuyến và cung cấp thông tin đầy đủ trên website giúp doanh nghiệp nâng cao năng lực cạnh tranh.
Các tính năng nổi bật của website tại An Giang do Terus thiết kế
Bản địa hóa và hội nhập văn hóa: Các website được thiết kế phù hợp với văn hóa và đặc thù địa phương An Giang.
Chú trọng các ngành trọng tâm: Terus tập trung vào các ngành công nghiệp then chốt của địa phương như nông nghiệp, du lịch, thủy sản, ...
Hỗ trợ đa ngôn ngữ: Người dùng có thể dễ dàng chuyển đổi giữa các ngôn ngữ như tiếng Việt, tiếng Anh, ... ngay trên website.
Tích hợp blog và thông báo sự kiện: Các website được tích hợp blog chia sẻ thông tin về hoạt động doanh nghiệp và sự kiện địa phương.
Tích hợp với nền tảng đặt chỗ địa phương: Website được kết nối với các nền tảng đặt chỗ, đặt dịch vụ của địa phương.
Chiến lược SEO Local: Terus xây dựng chiến lược SEO Local nhằm nâng cao khả năng hiển thị của website trên kết quả tìm kiếm tại khu vực An Giang.
Terus đã thiết kế và xây dựng nhiều [mẫu website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/) cho các doanh nghiệp tại An Giang, bao gồm các ngành như nông nghiệp, thủy sản, du lịch, công nghiệp, ...
Với kinh nghiệm và năng lực chuyên sâu, Terus cung cấp dịch vụ thiết kế website tại An Giang trọn gói, chuyên nghiệp và chuẩn quốc tế. Các website của Terus được xây dựng với nhiều tính năng nổi bật, đáp ứng đầy đủ nhu cầu của doanh nghiệp tại An Giang, từ bản địa hóa đến tích hợp các công cụ hiện đại.
Quy trình thiết kế của Terus đảm bảo sự tham gia và phản hồi của khách hàng trong suốt quá trình, đồng thời tuân thủ các tiêu chuẩn chất lượng cao. Với [dịch vụ thiết kế website tại An Giang](https://terusvn.com/thiet-ke-website-tai-hcm/) của Terus, các doanh nghiệp có thể nâng cao uy tín, mở rộng phạm vi hoạt động và tăng cường tương tác với khách hàng một cách hiệu quả.
Tìm hiểu thêm về [Thiết Kế Website Tại An Giang Tối Ưu Doanh Thu](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-an-giang/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,703 | Top Search Engines for Pentesters | Server Shodan Onyphe Censys IVRE Dorks Google WiFi... | 0 | 2024-07-06T11:59:32 | https://dev.to/falselight/top-search-engines-for-pentesters-2hab | security, web, webdev, testing | ## Server
1. **[Shodan](https://www.shodan.io)**
2. **[Onyphe](https://www.onyphe.io)**
3. **[Censys](https://censys.io)**
4. **[IVRE](https://ivre.rocks)**
## Dorks
1. **[Google](https://www.google.com)**
## WiFi Networks
1. **[Wigle](https://www.wigle.net)**
## Code Search
1. **[grep.app](https://grep.app)**
2. **[Searchcode](https://searchcode.com)**
3. **[PublicWWW](https://publicwww.com)**
## Threat Intelligence
1. **[BinaryEdge](https://app.binaryedge.io)**
2. **[GreyNoise](https://viz.greynoise.io)**
3. **[FOFA](https://fofa.info)**
4. **[ZoomEye](https://www.zoomeye.org)**
5. **[LeakIX](https://leakix.net)**
6. **[Socradar](https://socradar.io)**
7. **[URLScan](https://urlscan.io)**
8. **[Pulsedive](https://pulsedive.com)**
9. **[ThreatMiner](https://www.threatminer.org)**
10. **[Spyse](https://spyse.com)**
11. **[SecurityTrails](https://securitytrails.com)**
12. **[Rapid7](https://www.rapid7.com)**
13. **[RiskIQ](https://www.riskiq.com)**
## Web Tools
1. **[⚡ Interactive Online Web 🛠️ Tools](https://qit.tools/)**
## Email Addresses
1. **[Hunter](https://hunter.io)**
## OSINT
1. **[IntelX](https://intelx.io)**
2. **[SpiderFoot](https://www.spiderfoot.net)**
3. **[Maltego](https://www.maltego.com)**
## Attack Surface
1. **[Netlas](https://app.netlas.io)**
2. **[FullHunt](https://fullhunt.io)**
3. **[BinaryEdge](https://binaryedge.io)**
4. **[NexVision](https://nexvision.io)**
## Certificate Search
1. **[crt.sh](https://crt.sh)**
2. **[CertSpotter](https://sslmate.com/certspotter/)**
## Vulnerabilities & Data Breaches
1. **[Vulners](https://vulners.com)** (Vulnerabilities)
2. **[Have I Been Pwned](https://haveibeenpwned.com)** (Data Breaches)
## Web Security
1. **[Netcraft](https://www.netcraft.com)**
## DNS Reconnaissance
1. **[DNSDumpster](https://dnsdumpster.com)**
| falselight |
1,913,702 | Unlocking Innovation: The Role of an Android Engineer in Shaping Tomorrow's Technology | In the dynamic landscape of technology, the role of an Android engineer stands as a pivotal force... | 0 | 2024-07-06T11:59:03 | https://dev.to/michaeljason_eb570f1a51d6/unlocking-innovation-the-role-of-an-android-engineer-in-shaping-tomorrows-technology-40c2 |
In the dynamic landscape of technology, the role of an Android engineer stands as a pivotal force driving innovation and transforming ideas into reality. From crafting intuitive user interfaces to optimizing performance across a myriad of devices, Android engineers play a crucial role in shaping the digital experiences that define our daily lives. [Hire offshore android engineer](https://www.appsierra.com/blog/offshore-android-development) for your company for better android development.
Embracing Versatility: The Android Engineer’s Toolbox
At the heart of every successful Android engineer lies a versatile toolkit of skills and knowledge. Mastery of programming languages like Java and Kotlin, coupled with deep familiarity with Android SDK and development tools, empowers engineers to build robust applications that meet the demands of a diverse user base.
Designing for Impact: The Art of User-Centric Development
Beyond technical prowess, Android engineers excel in understanding and translating user needs into compelling mobile experiences. They collaborate closely with designers to create interfaces that are not only visually engaging but also intuitive and user-friendly. This collaborative approach ensures that every tap, swipe, and interaction delights users and enhances usability.
Innovating with Agility: Agile Methodologies and Continuous Integration
In an industry marked by rapid change, Android engineers embrace agile methodologies to iterate quickly and adapt to evolving requirements. Continuous integration and deployment practices ensure that innovations reach users swiftly, enhancing user satisfaction and maintaining competitive advantage in the market.
Bridging Worlds: The Nexus of Technology and User Experience
The intersection of technology and user experience defines the Android engineer’s mission. By leveraging Google’s Material Design principles and staying abreast of UX trends, engineers create cohesive, visually appealing interfaces that resonate with modern users. This seamless blend of aesthetics and functionality sets the stage for unforgettable digital experiences.
Collaborative Spirit: Nurturing Innovation in Team Environments
The journey of an Android engineer thrives on collaboration and knowledge-sharing. Whether in startup environments or established tech giants, engineers contribute to vibrant teams where diverse perspectives fuel creativity and drive breakthrough solutions. This collaborative spirit fosters an ecosystem where ideas flourish and innovation thrives.
Looking Ahead: The Future of Android Engineering
As technology continues to evolve, the role of an Android engineer will evolve alongside it. Emerging technologies such as augmented reality (AR), machine learning, and IoT present new frontiers for innovation. Android engineers, equipped with their expertise and passion for exploration, will lead the charge in harnessing these technologies to create transformative experiences.
Conclusion: Empowering Tomorrow’s Digital Frontier
In conclusion, the impact of an Android engineer extends far beyond lines of code and pixels on a screen. It embodies a commitment to excellence, a passion for innovation, and a dedication to shaping the future of technology. As we look ahead to the possibilities that lie ahead, one thing remains clear: the Android engineer will continue to be at the forefront of innovation, driving change and enriching lives through transformative digital experiences.
This guest post celebrates the indispensable role of Android engineers in shaping tomorrow’s technology landscape—a testament to their skills, vision, and unwavering dedication to pushing the boundaries of what’s possible. | michaeljason_eb570f1a51d6 |
|
1,913,700 | Best Acupuncture and Acupressure Clinic in Bangalore: Ayushyaa Acupuncture Clinic | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than... | 0 | 2024-07-06T11:58:23 | https://dev.to/ayushyaa/best-acupuncture-and-acupressure-clinic-in-bangalore-ayushyaa-acupuncture-clinic-51dm | ayushyaaclinic, acupuncture | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than Ayushyaa Acupuncture Clinic, the best acupuncture clinic in Bangalore. Our clinic is renowned for offering top-notch acupuncture and acupressure treatments that cater to a wide range of health issues. In this blog, we will explore what makes Ayushyaa clinic the preferred choice for those seeking natural and effective healthcare solutions.
Why Choose Ayushyaa Acupuncture Clinic?
Expertise and Experience
Ayushyaa Acupuncture clinic Bangalore a team of highly skilled and experienced practitioners who are dedicated to providing the best care possible. Our experts are well-versed in traditional acupuncture and modern techniques, ensuring that each patient receives personalized treatment tailored to their specific needs.
Comprehensive Treatments
At Ayushyaa Acupuncture Clinic, we offer a variety of treatments, including:
• Acupuncture: Utilizing fine needles to stimulate specific points on the body, acupuncture helps in balancing the body’s energy flow, alleviating pain, and promoting overall wellness.
• Acupressure: Similar to acupuncture but without needles, acupressure involves applying pressure to specific points to relieve pain and stress, boost energy levels, and improve health.
• Herbal Therapy: Complementing our acupuncture and acupressure treatments, we offer herbal remedies to support the healing process and enhance overall well-being.
Patient-Centric Approach
Our clinic is committed to providing a patient-centric approach, focusing on the individual needs and health goals of each patient. We take the time to understand your concerns, conduct thorough assessments, and design customized treatment plans that ensure optimal results.[best acupressure clinic in Bangalore.](https://www.ayushyaaclinic.com/index
)
Modern Facilities
Ayushyaa Acupuncture Clinic is equipped with state-of-the-art facilities, creating a comfortable and serene environment for our patients. Our modern equipment and clean, calming spaces ensure that you have a relaxing and therapeutic experience during each visit.
Benefits of Acupuncture and Acupressure
Pain Relief
One of the primary reasons people seek acupuncture and acupressure treatments is for pain relief. Whether you suffer from chronic pain, migraines, or joint pain, our treatments can provide significant relief and improve your quality of life.
Stress Reduction
In today’s fast-paced world, stress is a common concern for many individuals. Acupuncture and acupressure treatments at Ayushyaa Acupuncture Clinic help in reducing stress levels, promoting relaxation, and enhancing mental clarity.
Improved Sleep
Many of our patients have reported improved sleep patterns and better sleep quality after undergoing acupuncture and acupressure treatments. By balancing the body’s energy and reducing stress, these treatments promote restful and rejuvenating sleep.
Enhanced Immune Function
Regular acupuncture and acupressure sessions can boost your immune system, helping you stay healthy and ward off illnesses. Our treatments stimulate the body’s natural healing abilities, enhancing overall health and vitality.
Conclusion
If you are looking for the top acupuncture in Bangalore, Ayushyaa Acupuncture Clinic is your ideal destination. Our experienced practitioners, comprehensive treatments, and patient-centric approach ensure that you receive the highest quality care. Visit us today and embark on a journey towards better health and well-being through the natural and effective treatments of acupuncture and acupressure.
For more information or to schedule an appointment, contact Ayushyaa Acupuncture Clinic today. Experience the best acupuncture and acupressure clinic in Bangalore and take the first step towards a healthier, happier life.
| ayushyaa |
1,913,699 | BitPower's Security: A Modern Financial Miracle | In a changing world, financial security has become more important than ever. BitPower, as a... | 0 | 2024-07-06T11:57:20 | https://dev.to/pingd_iman_9228b54c026437/bitpowers-security-a-modern-financial-miracle-16k5 |
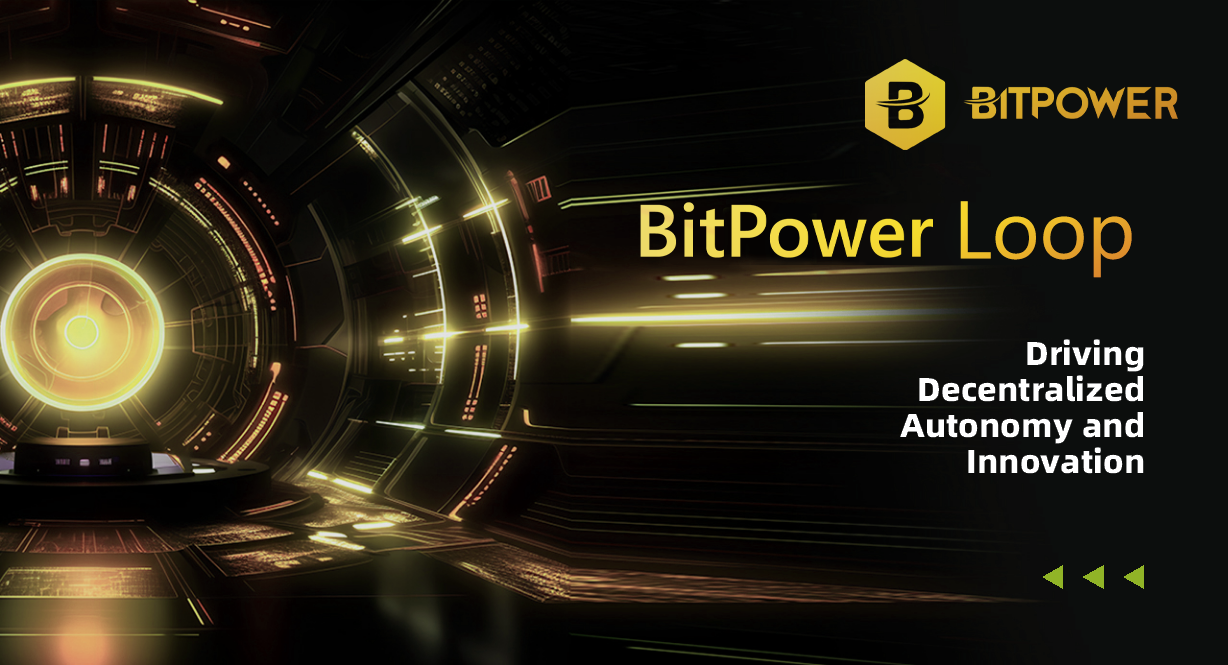
In a changing world, financial security has become more important than ever. BitPower, as a decentralized financial platform based on blockchain technology, provides unparalleled security and stable returns. By using advanced smart contracts and cryptocurrencies, BitPower has not only changed the landscape of traditional finance, but also provided users with unprecedented opportunities for income.
First, BitPower's security is based on the core characteristics of blockchain technology. Blockchain is a distributed ledger technology whose data is stored on thousands of nodes around the world, each of which keeps a complete record of transactions. This decentralized structure means that no one can tamper with the data alone, ensuring the transparency and immutability of transactions.
Second, BitPower uses smart contract technology to automatically execute transactions and agreements. A smart contract is a self-executing code that automatically triggers transactions when preset conditions are met. Through smart contracts, BitPower has achieved fully automated financial operations without intermediaries, reducing the risk of human error and fraud. Once deployed on the blockchain, these contracts cannot be changed, ensuring the stability and security of the system.
The use of cryptocurrency is also a highlight of BitPower's security. Cryptocurrency transactions use complex encryption algorithms to protect users' privacy and asset security. Every transaction of the user is encrypted by cryptography technology, and no personal information will be leaked even on the public network. In addition, the user's funds are directly stored in their own digital wallets, and they have full control over their assets and are not controlled by any third party.
BitPower not only excels in security, but its income mechanism is also very attractive. The platform automatically matches borrowing and lending needs and calculates interest in real time through a decentralized market liquidity pool model. This mechanism not only improves the efficiency of capital utilization, but also provides users with stable income. For example, users can obtain high returns in different cycles of daily, weekly, and monthly by providing liquidity on BitPower. Specifically, the daily yield is 100.4%, 104% for seven days, 109.5% for fourteen days, and 124% for twenty-eight days. These returns are automatically returned to the user's wallet through smart contracts, ensuring the timeliness and security of the income.
In this era of uncertainty, BitPower provides users with a safe, transparent, and high-yield financial platform. Through blockchain technology, smart contracts, and cryptocurrencies, BitPower not only protects users' assets, but also brings them stable and considerable income. This is a miracle of modern finance and a truly game-changing platform. Whether you are a novice or an old hand, BitPower will be your new choice for financial investment. @Bitpower #BTC #ETH #SC #DeFi | pingd_iman_9228b54c026437 |
|
1,913,697 | Mastering iOS Native Bridging in React Native | In recent years, Swift has become the preferred language for iOS development, including for native... | 0 | 2024-07-06T11:56:55 | https://dev.to/ajmal_hasan/android-native-bridging-in-react-native-364m | reactnative, javascript, ios, mobile | In recent years, Swift has become the preferred language for iOS development, including for native bridging in React Native. Swift offers modern syntax, better safety, and more powerful features compared to Objective-C.
Let’s explore how to create a native module in Swift for React Native, covering data passing, promises, callbacks, events, and UI components.
---
## Setting Up a Basic Native Module in Swift
### Step 1:
Open the iOS project in Xcode.
`open ios/SwiftNativeModulesExample.xcworkspace`
---
### Step 2: Create a Swift File
1. In Xcode, right-click on the SwiftNativeModulesExample directory, select New File..., choose Swift File, and name it NativeModule.
2. Add Bridging Header:
When prompted to create a bridging header, click “Create Bridging Header”. This file allows Swift and Objective-C code to interoperate.
Ensure your NativeModule.swift file is created inside the SwiftNativeModulesExample target.
---
### Step 3: Implement the Native Module in Swift:
```
//
// NativeModule.swift
// RN_NativeModules
//
// Created by Ajmal Hasan on 06/07/24.
//
import Foundation
import React
// Declares the class as an Objective-C class and makes it accessible to React Native
@objc(NativeModule)
class NativeModule: NSObject {
// Indicates whether this module should be initialized on the main queue
// Set to true if setup on the main thread is required (e.g., when sending constants to iOS or making UI using UIKit in iOS)
// Set to false to run this module on the main thread before any JS code executes
@objc static func requiresMainQueueSetup() -> Bool {
return true
}
// A method that logs a message to the console
@objc func logMessage(_ message: String) {
NSLog("%@", message) // Logs the message to the Xcode console
}
// A method that adds two numbers and returns the result via a callback
// Callbacks are used for handling asynchronous operations
@objc func add(_ a: Int, b: Int, callback: RCTResponseSenderBlock) {
let result = a + b
callback([NSNull(), result]) // Calls the callback with the result
}
// A method that subtracts two numbers and returns the result via a promise
// Promises provide a more readable and convenient way to handle asynchronous operations
@objc func subtract(_ a: Int, b: Int, resolve: RCTPromiseResolveBlock, reject: RCTPromiseRejectBlock) {
if a >= b {
resolve(a - b) // Resolves the promise with the result if `a` is greater than or equal to `b`
} else {
let error = NSError(domain: "SubtractError", code: 200, userInfo: nil)
reject("subtract_error", "a is less than b", error) // Rejects the promise with an error if `a` is less than `b`
}
}
// A method that sends an event to JavaScript
// Events allow native modules to send data to JavaScript asynchronously, without waiting for a direct method call
@objc public func myMethod(_ param: String) {
print(param) // Prints the parameter to the console
RNEventEmitter.emitter.sendEvent(withName: "onReady", body: [param]) // Sends an event to React Native with the name "onReady" and the parameter as the body
}
}
```
Explanation:
1. `@objc(NativeModule)`: This annotation makes the Swift class available to Objective-C, allowing React Native to call its methods.
2. `requiresMainQueueSetup`: This static method indicates if the module requires initialization on the main thread. Returning true is necessary if the module needs to interact with UI components or send constants to iOS. Returning false means it can be initialized on a background thread.
3. `logMessage`: A simple method to log a message to the Xcode console using NSLog.
4. `add`: This method takes two integers, adds them, and returns the result via a callback. Callbacks are a common pattern in JavaScript to handle asynchronous operations.
5. `subtract`: This method takes two integers, subtracts them, and returns the result via a promise. Promises provide a more readable and convenient way to handle asynchronous operations compared to callbacks.
6. `myMethod`: This method sends an event to JavaScript using an event emitter. Events allow native modules to send data to JavaScript asynchronously, without waiting for a direct method call.
---
Step 4: This file defines the event emitter class in Swift.
```
// RNEventEmitter.swift
import React
@objc(RNEventEmitter)
open class RNEventEmitter:RCTEventEmitter{
public static var emitter: RCTEventEmitter! // global variable
// constructor
override init(){
super.init()
RNEventEmitter.emitter = self
}
open override func supportedEvents() -> [String] {
["onReady", "onPending", "onFailure"] // etc.
}
}
```
**Explanation:**
1. `@objc(RNEventEmitter)`: This annotation makes the Swift class available to Objective-C, allowing React Native to call its methods.
2. `public static var emitter`: A shared instance to access the emitter from other parts of the native module.
3. `init()`: Initializes the emitter and sets the shared instance.
4. `supportedEvents`: Overrides the method to define the supported events. This method should return an array of event names that can be sent to JavaScript.
---
### Step 5: Register the Module:
Create a new Objective-C file to register the Swift module.
```
//
// NativeModuleBridge.m
// RN_NativeModules
//
#import <React/RCTBridgeModule.h>
#import <React/RCTEventEmitter.h>
// Interface declaration for the NativeModule to expose it to React Native
@interface RCT_EXTERN_MODULE(NativeModule, NSObject)
RCT_EXTERN_METHOD(logMessage:(NSString *)message)
RCT_EXTERN_METHOD(add:(NSInteger)a b:(NSInteger)b callback:(RCTResponseSenderBlock)callback)
RCT_EXTERN_METHOD(subtract:(NSInteger)a b:(NSInteger)b resolve:(RCTPromiseResolveBlock)resolve reject:(RCTPromiseRejectBlock)reject)
RCT_EXTERN_METHOD(myMethod:(NSString *)param)
@end
// Interface declaration for the RNEventEmitter to expose it to React Native
@interface RCT_EXTERN_MODULE(RNEventEmitter, RCTEventEmitter)
RCT_EXTERN_METHOD(supportedEvents)
@end
```
**Explanation:**
1. `RCT_EXTERN_MODULE(RNEventEmitter, RCTEventEmitter)`: This macro declares the RNEventEmitter class to React Native.
2. `RCT_EXTERN_METHOD(supportedEvents)`: Exposes the supportedEvents method to React Native, making it accessible from JavaScript.
---
### Step 6:
Using the Native Module in JavaScript
```
import {
Button,
NativeEventEmitter,
NativeModules,
StyleSheet,
View,
} from 'react-native';
import React, {useEffect} from 'react';
const {NativeModule} = NativeModules;
const eventEmittedFromIOS = new NativeEventEmitter(NativeModules.RNEventEmitter);
const IOSNativeModules = () => {
useEffect(() => {
// 1. NativeModule.swift(also added bridging header by default)
// 2. NativeModuleBridge.m
// will print log in xcode after running from xcode
NativeModule.logMessage('Hello from React Native!');
// will print log in RN bundler/xcode
NativeModule.add(3, 4, (error, result) => {
if (error) {
console.error(error);
} else {
console.log(result); // Logs 7
}
});
// will print log in RN bundler/xcode
async function subtractNumbers(a, b) {
try {
const result = await NativeModule.subtract(a, b);
console.log(result); // Logs the result
} catch (error) {
console.error(error);
}
}
subtractNumbers(10, 5);
}, []);
const sendDataToIOS = () => {
// iOS Files Modified for NativeModules(sending data from RN to iOS):
// 1. NativeModule.swift(also added bridging header by default)
// 2. NativeModuleBridge.m
NativeModule.myMethod('THIS IS SENT FROM RN TO IOS');
};
useEffect(() => {
// iOS Files Modified for NativeModules(sending data from iOS to RN):
// 1. RNEventEmitter.swift
// 2. NativeModuleBridge.m
// 3. NativeModule.swift
const eventListener = eventEmittedFromIOS.addListener('onReady', string => {
console.log('sendDataToIOS to IOS then from IOS to RN: ', string);
});
return () => eventListener.remove();
}, []);
return (
<View style={styles.main}>
<Button title="Send Data From RN To IOS" onPress={sendDataToIOS} />
</View>
);
};
export default IOSNativeModules;
const styles = StyleSheet.create({
main: {flex: 1, justifyContent: 'center', alignItems: 'center'},
});
```
[ANDROID PART ->](https://dev.to/ajmal_hasan/android-native-bridging-in-react-native-272o)
| ajmal_hasan |
1,913,696 | Mastering Android Native Bridging in React Native | React Native allows you to write native code in Java and access it from JavaScript. This can be... | 0 | 2024-07-06T11:56:54 | https://dev.to/ajmal_hasan/android-native-bridging-in-react-native-272o | reactnative, android, javascript, mobile | React Native allows you to write native code in Java and access it from JavaScript. This can be particularly useful when you need to access native functionality that isn’t available out of the box in React Native.
## Key Concepts
1. Bridging: Allows JavaScript and native code to communicate.
2. Native Modules: Java classes that are exposed to JavaScript.
3. Native UI Components: Native views that can be used in React Native’s JavaScript code.
4. Events: Mechanism to send data from native code to JavaScript.
## Practical Example
Let’s create a simple example where we:
1. Create a native module in Java.
2. Call this module from JavaScript.
3. Pass data from JavaScript to native code and vice versa.
4. Handle events from native to JavaScript.
5. Create a native UI component.
---
## ANDROID PART:
### Step 1: Create the Native Module Class
First, create a native module in Java(ie Java class).
[](
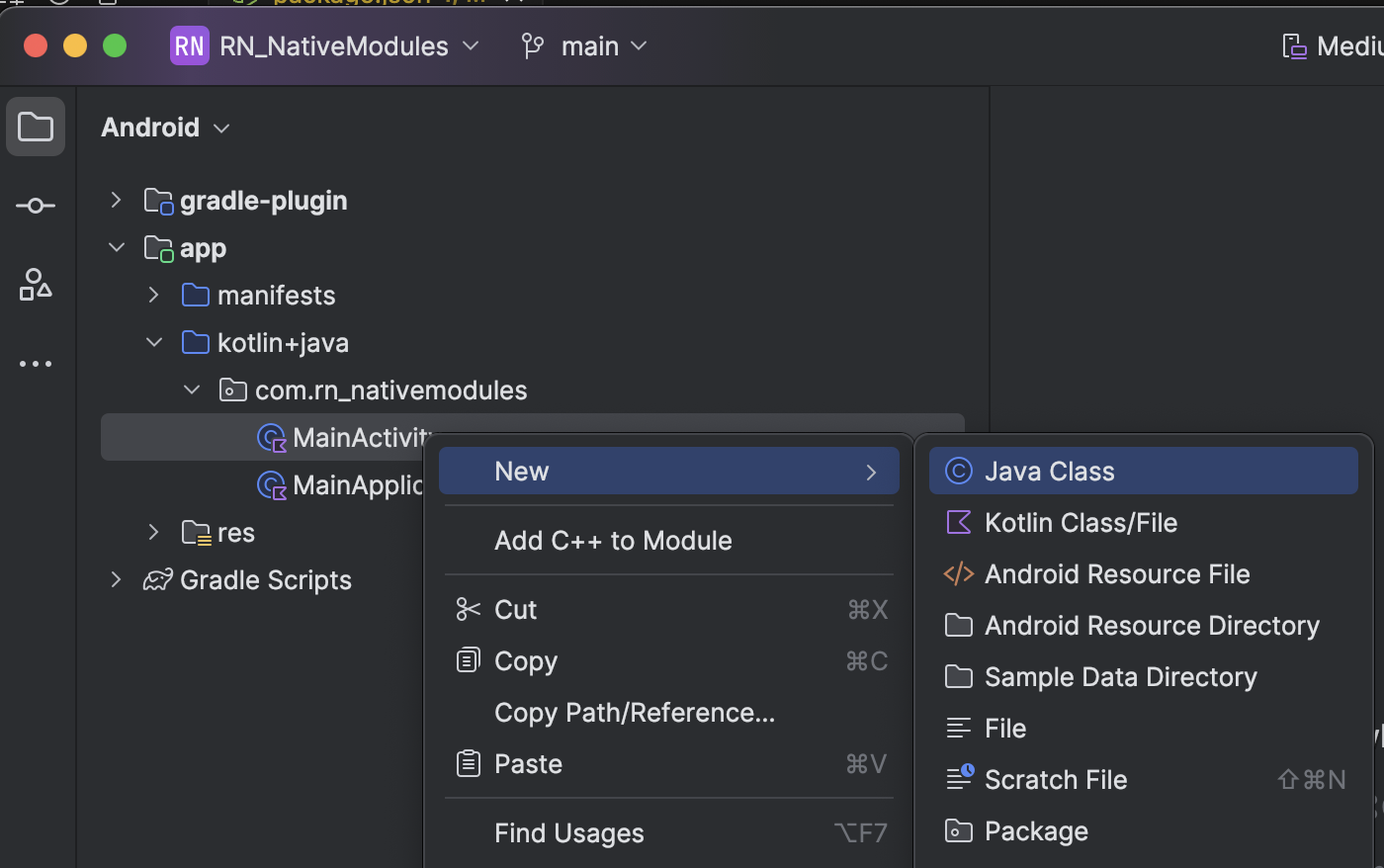)
Create a new Java class in the android/app/src/main/java/com/yourappname directory:
```
package com.rn_nativemodules;
import android.util.Log;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import com.facebook.react.bridge.Arguments;
import com.facebook.react.bridge.Promise;
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.bridge.ReactContext;
import com.facebook.react.bridge.ReactContextBaseJavaModule;
import com.facebook.react.bridge.ReactMethod;
import com.facebook.react.bridge.WritableMap;
import com.facebook.react.bridge.WritableNativeMap;
import com.facebook.react.modules.core.DeviceEventManagerModule;
// This class serves as a bridge between the native Android code and the React Native JavaScript code
// It extends ReactContextBaseJavaModule, which is a base class for native modules in React Native
public class MyNativeModule extends ReactContextBaseJavaModule {
// Define the module name that will be used to reference this module in React Native
private static final String MODULE_NAME ="MyNativeModule";
private ReactApplicationContext reactContext;
// Constructor
public MyNativeModule(@Nullable ReactApplicationContext reactContext) {
super(reactContext);
this.reactContext = reactContext;
}
// Return the name of the module
@NonNull
@Override
public String getName() {
return MODULE_NAME;
}
// Define native methods that can be called from React Native using @ReactMethod annotation
// Method to log a message received from React Native
@ReactMethod
public void myMethod(String param){
System.out.println("GET DATA FROM RN <--- "+param);
}
// Method to send data back to React Native with a promise
@ReactMethod
public void myMethodWithPromise(String param, Promise promise){
// Check if the parameter is not null
if (param != null) {
// Resolve the promise with a success message
promise.resolve("SEND DATA TO RN ---> " + param);
} else {
// Reject the promise with an error code and message
promise.reject("ERROR_CODE", "Error message explaining failure");
}
}
// You can define more native methods here to be called from React Native
// For example, adding and removing event listeners
// Method to send an event to React Native
private void sendEvent(ReactContext reactContext, String eventName, WritableMap params) {
// Access the device event emitter module and emit an event with parameters
reactContext
.getJSModule(DeviceEventManagerModule.RCTDeviceEventEmitter.class)
.emit(eventName, params);
}
// Example method triggered from React Native to send an event
@ReactMethod
public void triggerEvent() {
// Create parameters to send with the event
WritableMap params = new WritableNativeMap();
params.putString("message", "Hello from native code!");
// Call the sendEvent method to emit "MyEvent" with params to React Native
sendEvent(getReactApplicationContext(), "MyEvent", params);
}
}
```
**Summary:**
**1. Creating the Native Module:**
• Class Definition: Extend ReactContextBaseJavaModule to create a native module.
• Module Name: Define a unique module name using the getName() method.
• Constructor: Initialize the module with ReactApplicationContext.
**2. Defining Native Methods:**
• Simple Methods: Use @ReactMethod annotation to define methods that can be called from JavaScript.
• Logging: Create methods to log messages from JavaScript.
• Promise Handling: Implement methods that use promises for asynchronous operations, resolving or rejecting based on logic.
• Event Emission: Create methods to send events from native code to JavaScript using DeviceEventManagerModule.RCTDeviceEventEmitter.
**3. Example Methods:**
• myMethod(String param): Logs a message.
• myMethodWithPromise(String param, Promise promise): Resolves or rejects a promise based on the input.
• triggerEvent(): Emits an event to JavaScript with predefined parameters.
---
### Step 2: Register the Module
Next, you need to register the module in a Package class:
```
package com.rn_nativemodules;
import androidx.annotation.NonNull;
import com.facebook.react.ReactPackage;
import com.facebook.react.bridge.NativeModule;
import com.facebook.react.bridge.ReactApplicationContext;
import com.facebook.react.uimanager.ViewManager;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
// This class implements the ReactPackage interface, which is responsible for providing NativeModules and ViewManagers to React Native
// It acts as a package manager for bridging native code with React Native
public class MyNativeModulePackage implements ReactPackage {
// Method to create and return NativeModules to be used by React Native
@NonNull
@Override
public List<NativeModule> createNativeModules(@NonNull ReactApplicationContext reactApplicationContext) {
List<NativeModule> modules = new ArrayList<>();
// Add your custom NativeModule implementations to the list
modules.add(new MyNativeModule(reactApplicationContext)); // Add MyNativeModule as a NativeModule
return modules;
}
```
---
### Step 3: Add the Package to the Main Application
Add your package to the list of packages in your MainApplication.java:
```
// android/app/src/main/java/com/yourappname/MainApplication.java
import com.yourappname.MyNativeModulePackage; // Add this import
@Override
protected List<ReactPackage> getPackages() {
@SuppressWarnings("UnnecessaryLocalVariable")
List<ReactPackage> packages = new PackageList(this).getPackages();
packages.add(new MyNativeModulePackage()); // Add this line
return packages;
}
```
---
## JAVASCRIPT PART:
### Step 1: Import and Use NativeModules
```
import {
Button,
DeviceEventEmitter,
NativeModules,
requireNativeComponent,
StyleSheet,
View,
} from 'react-native';
import React, {useEffect} from 'react';
const {MyNativeModule} = NativeModules;
// Android Files Modified for NativeModules:
// 1. MyNativeModule.java
// 2. MyNativeModulePackage.java
// 3. MainApplication.java (getPackages())
const AndroidNativeModules = () => {
// Define a function to send data to the native Android module
const sendDataToAndroid = () => {
// Call the native method without using a promise
MyNativeModule.myMethod('THIS IS SENT FROM RN TO ANDROID');
};
// Define a function to get data from the native Android module
const getDataFromAndroid = () => {
// Call the native method with a promise
MyNativeModule.myMethodWithPromise('Sent From RN To A then from A to RN')
// Handle the resolved promise (success case)
.then(result => {
// Log the success result
console.log('Success:', result);
})
// Handle the rejected promise (error case)
.catch(error => {
// Log the error information
console.error('Error:', error.code, error.message);
});
};
// To handle events, we need to use RCTDeviceEventEmitter on the native side and DeviceEventEmitter on the JavaScript side.
useEffect(() => {
const subscription = DeviceEventEmitter.addListener('MyEvent', params => {
console.log('Received event with message:', params.message);
});
// Clean up subscription
return () => {
subscription.remove();
};
}, []);
const triggerNativeEvent = () => {
MyNativeModule.triggerEvent();
};
return (
<View style={styles.main}>
<Button
title="Send Data From RN To Android"
onPress={sendDataToAndroid} // check log in android logcat
/>
<Button
title="Send Data To Android From RN"
onPress={getDataFromAndroid} // check log in react native logcat
/>
<Button
title="Receive event From RN From Android"
onPress={triggerNativeEvent}
/>
</View>
);
};
export default AndroidNativeModules;
const styles = StyleSheet.create({
main: {justifyContent: 'space-evenly', alignItems: 'center', flex: 1},
});
```
---
## Creating a Native UI Component
Creating a native UI component involves creating a ViewManager.
### Step 1: Create the ViewManager
```
package com.rn_nativemodules;
import android.widget.TextView;
import com.facebook.react.uimanager.SimpleViewManager;
import com.facebook.react.uimanager.ThemedReactContext;
import com.facebook.react.uimanager.annotations.ReactProp;
public class MyCustomViewManager extends SimpleViewManager<TextView> {
public static final String REACT_CLASS = "MyCustomView";
@Override
public String getName() {
return REACT_CLASS;
}
@Override
protected TextView createViewInstance(ThemedReactContext reactContext) {
return new TextView(reactContext);
}
@ReactProp(name = "text")
public void setText(TextView view, String text) {
view.setText(text);
}
}
```
### Step 2: Register the ViewManager
```
// android/app/src/main/java/com/yourappname/MyPackage.java
// Existing code...
@Override
public List<ViewManager> createViewManagers(ReactApplicationContext reactContext) {
List<ViewManager> viewManagers = new ArrayList<>();
viewManagers.add(new MyCustomViewManager());
return viewManagers;
}
```
### Step 3: Use the Custom View in JavaScript
```
import { requireNativeComponent } from 'react-native';
const MyCustomView = requireNativeComponent('MyCustomView');
const App = () => {
return (
<MyCustomView style={{ width: 200, height: 50 }} text="Hello from custom view!" />
);
};
export default App;
```
## Final UI:
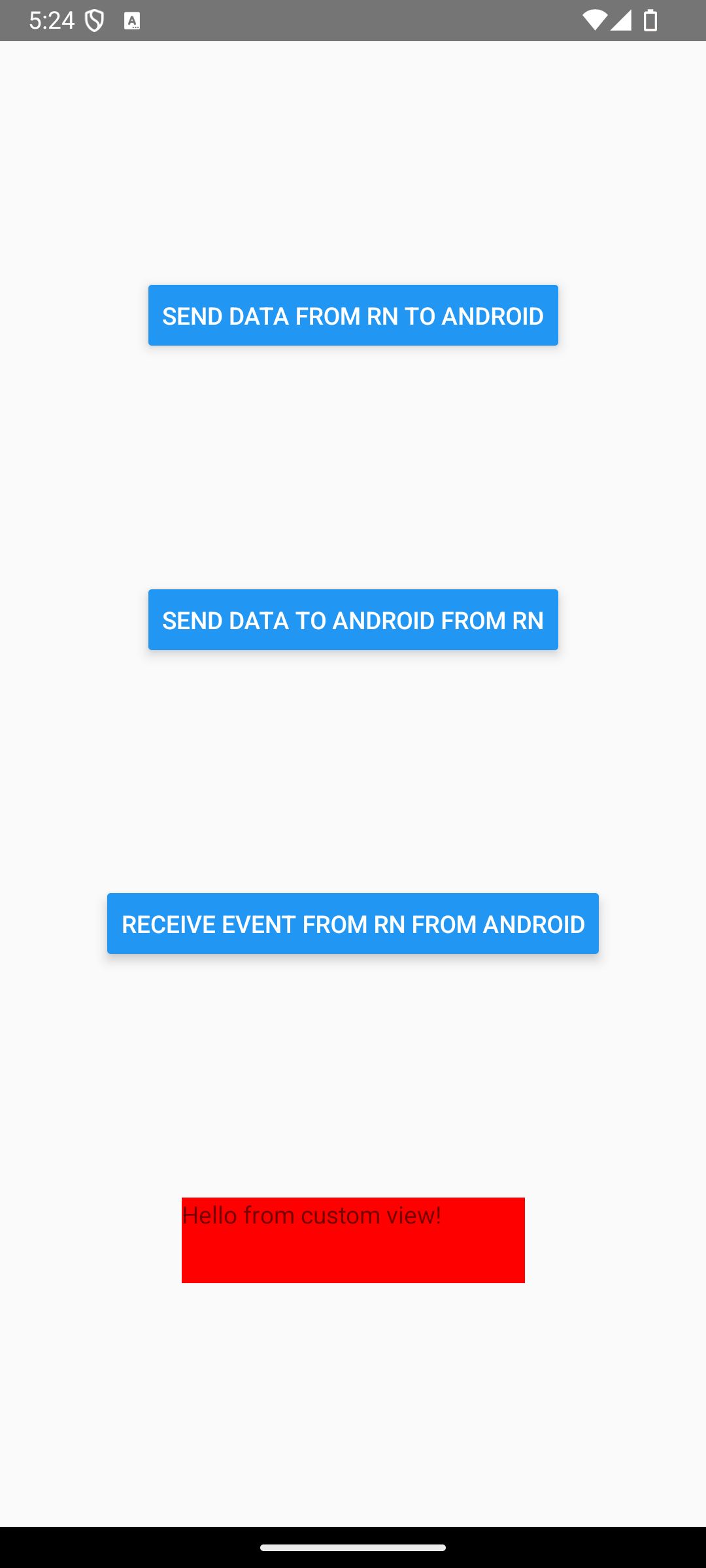
[iOS Part -> ](https://dev.to/ajmal_hasan/android-native-bridging-in-react-native-364m)
| ajmal_hasan |
1,913,695 | BitPower's revenue introduction: | BitPower Loop is a decentralized financial platform that provides an efficient and secure income... | 0 | 2024-07-06T11:56:16 | https://dev.to/xin_l_9aced9191ff93f0bf12/bitpowers-revenue-introduction-2ih6 | BitPower Loop is a decentralized financial platform that provides an efficient and secure income mechanism through smart contracts. The platform allows users to participate directly as depositors or borrowers, and obtain floating interest rate income through decentralized liquidity provision and smart contract operations. The annualized rate of return of BitPower Loop is significantly higher than that of traditional financial products, attracting a large number of investors.
BitPower Loop's income mainly comes from two parts: recurring income and commission sharing. Recurring income is the return obtained by users by providing liquidity, while commission sharing is obtained by recommending new users to participate in the platform. This model not only increases the liquidity of the platform, but also encourages users to actively participate. In particular, when users recommend friends, the referrer can obtain corresponding commission rewards based on the recurring income of their referrals, up to 17 levels of rewards.
Through secure and reliable smart contracts, BitPower Loop ensures the transparency and immutability of all transactions, providing users with a trustworthy income platform. At the same time, the characteristics of global services make it competitive internationally. In short, BitPower Loop has become a leader in the field of decentralized finance with its high returns, transparency and security.
@BitPower | xin_l_9aced9191ff93f0bf12 |
|
1,913,694 | Ayushyaa Acupuncture Clinic in bangalore | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than... | 0 | 2024-07-06T11:55:19 | https://dev.to/ayushyaa/ayushyaa-acupuncture-clinic-in-bangalore-3p6h | ayushyaaclinic, acupuncture | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than Ayushyaa Acupuncture Clinic, the best acupuncture clinic in Bangalore. Our clinic is renowned for offering top-notch acupuncture and acupressure treatments that cater to a wide range of health issues. In this blog, we will explore what makes Ayushyaa clinic the preferred choice for those seeking natural and effective healthcare solutions.
Why Choose Ayushyaa Acupuncture Clinic?
Expertise and Experience
Ayushyaa Acupuncture clinic Bangalore a team of highly skilled and experienced practitioners who are dedicated to providing the best care possible. Our experts are well-versed in traditional acupuncture and modern techniques, ensuring that each patient receives personalized treatment tailored to their specific needs.
Comprehensive Treatments
At Ayushyaa Acupuncture Clinic, we offer a variety of treatments, including:
• Acupuncture: Utilizing fine needles to stimulate specific points on the body, acupuncture helps in balancing the body’s energy flow, alleviating pain, and promoting overall wellness.
• Acupressure: Similar to acupuncture but without needles, acupressure involves applying pressure to specific points to relieve pain and stress, boost energy levels, and improve health.
• Herbal Therapy: Complementing our acupuncture and acupressure treatments, we offer herbal remedies to support the healing process and enhance overall well-being.
Patient-Centric Approach
Our clinic is committed to providing a patient-centric approach, focusing on the individual needs and health goals of each patient. We take the time to understand your concerns, conduct thorough assessments, and design customized treatment plans that ensure optimal results.best acupressure clinic in Bangalore.
Modern Facilities
Ayushyaa Acupuncture Clinic is equipped with state-of-the-art facilities, creating a comfortable and serene environment for our patients. Our modern equipment and clean, calming spaces ensure that you have a relaxing and therapeutic experience during each visit.
Benefits of Acupuncture and Acupressure
Pain Relief
One of the primary reasons people seek acupuncture and acupressure treatments is for pain relief. Whether you suffer from chronic pain, migraines, or joint pain, our treatments can provide significant relief and improve your quality of life.
Stress Reduction
In today’s fast-paced world, stress is a common concern for many individuals. Acupuncture and acupressure treatments at Ayushyaa Acupuncture Clinic help in reducing stress levels, promoting relaxation, and enhancing mental clarity.
Improved Sleep
Many of our patients have reported improved sleep patterns and better sleep quality after undergoing acupuncture and acupressure treatments. By balancing the body’s energy and reducing stress, these treatments promote restful and rejuvenating sleep.
Enhanced Immune Function
Regular acupuncture and acupressure sessions can boost your immune system, helping you stay healthy and ward off illnesses. Our treatments stimulate the body’s natural healing abilities, enhancing overall health and vitality.
Conclusion
If you are looking for the [top acupuncture in Bangalore](https://www.ayushyaaclinic.com/index), Ayushyaa Acupuncture Clinic is your ideal destination. Our experienced practitioners, comprehensive treatments, and patient-centric approach ensure that you receive the highest quality care. Visit us today and embark on a journey towards better health and well-being through the natural and effective treatments of acupuncture and acupressure.
For more information or to schedule an appointment, contact Ayushyaa Acupuncture Clinic today. Experience the best acupuncture and acupressure clinic in Bangalore and take the first step towards a healthier, happier life.
| ayushyaa |
1,913,693 | Best Acupuncture and Acupressure Clinic in Bangalore | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than... | 0 | 2024-07-06T11:52:07 | https://dev.to/ayushyaa/best-acupuncture-and-acupressure-clinic-in-bangalore-5emi | ayushyaaclinic, acupuncture | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than Ayushyaa Acupuncture Clinic, the best acupuncture clinic in Bangalore. Our clinic is renowned for offering top-notch acupuncture and acupressure treatments that cater to a wide range of health issues. In this blog, we will explore what makes Ayushyaa clinic the preferred choice for those seeking natural and effective healthcare solutions.
Why Choose Ayushyaa Acupuncture Clinic?
Expertise and Experience
Ayushyaa [Acupuncture clinic Bangalore](https://www.ayushyaaclinic.com/index) a team of highly skilled and experienced practitioners who are dedicated to providing the best care possible. Our experts are well-versed in traditional acupuncture and modern techniques, ensuring that each patient receives personalized treatment tailored to their specific needs.
Comprehensive Treatments
At Ayushyaa Acupuncture Clinic, we offer a variety of treatments, including:
• Acupuncture: Utilizing fine needles to stimulate specific points on the body, acupuncture helps in balancing the body’s energy flow, alleviating pain, and promoting overall wellness.
• Acupressure: Similar to acupuncture but without needles, acupressure involves applying pressure to specific points to relieve pain and stress, boost energy levels, and improve health.
• Herbal Therapy: Complementing our acupuncture and acupressure treatments, we offer herbal remedies to support the healing process and enhance overall well-being.
Patient-Centric Approach
Our clinic is committed to providing a patient-centric approach, focusing on the individual needs and health goals of each patient. We take the time to understand your concerns, conduct thorough assessments, and design customized treatment plans that ensure optimal results.best acupressure clinic in Bangalore.
Modern Facilities
Ayushyaa Acupuncture Clinic is equipped with state-of-the-art facilities, creating a comfortable and serene environment for our patients. Our modern equipment and clean, calming spaces ensure that you have a relaxing and therapeutic experience during each visit.
Benefits of Acupuncture and Acupressure
Pain Relief
One of the primary reasons people seek acupuncture and acupressure treatments is for pain relief. Whether you suffer from chronic pain, migraines, or joint pain, our treatments can provide significant relief and improve your quality of life.
Stress Reduction
In today’s fast-paced world, stress is a common concern for many individuals. Acupuncture and acupressure treatments at Ayushyaa Acupuncture Clinic help in reducing stress levels, promoting relaxation, and enhancing mental clarity.
Improved Sleep
Many of our patients have reported improved sleep patterns and better sleep quality after undergoing acupuncture and acupressure treatments. By balancing the body’s energy and reducing stress, these treatments promote restful and rejuvenating sleep.
Enhanced Immune Function
Regular acupuncture and acupressure sessions can boost your immune system, helping you stay healthy and ward off illnesses. Our treatments stimulate the body’s natural healing abilities, enhancing overall health and vitality.
Conclusion
If you are looking for the top acupuncture in Bangalore, Ayushyaa Acupuncture Clinic is your ideal destination. Our experienced practitioners, comprehensive treatments, and patient-centric approach ensure that you receive the highest quality care. Visit us today and embark on a journey towards better health and well-being through the natural and effective treatments of acupuncture and acupressure.
For more information or to schedule an appointment, contact Ayushyaa Acupuncture Clinic today. Experience the best acupuncture and acupressure clinic in Bangalore and take the first step towards a healthier, happier life.
| ayushyaa |
1,908,753 | 1. Introduction | In this series I will recreate my current neovim configuration, which I recently build from... | 27,945 | 2024-07-03T09:12:43 | https://dev.to/stroiman/1-introduction-851 | In this series I will recreate my current neovim configuration, which I recently build from scratch.
This introduction is different from other similar tutorials I have seen in two ways
- I my configuration to be **re-sourceable**
- I will focus on improving a specific workflow.
In this process, I have read a lot of documentation, to understand what really happens in all the plugins I use. I will not only show how I set it up, but also try to recreate _my_ process of learning, helping you not just recreate my configuration, but also have a better foundation for making your own decisions about how this should work for you.
I will not cover all basic vim/neovim functionality and options, but I have a companion series, [Concepts of Vim and Neovim](https://dev.to/stroiman/series/27946), where I explain some more fundamental concepts in depth, e.g. the use of leader keys, autocommands and autogroups, etc.
So if you are vim/neovim savvy, you can just read this series, but if you are new to neovim, or perhaps like me until recently, copied someone else's profile and made a few adaptions, the companion series can be helpful.
Note: The complete series is not written yet. I will publish articles as I get them written.
## I Want my Configuration to be Re-Sourceable
In most other neovim tutorials, they often quit and restart neovim after making changes.
That is something that annoys me. For almost all the years I've been using vim, I've been able to just reload my configuration after I made changes, and have the changes take effect. Often I make a change because I detect an commonly repeating editing pattern. I just want to be able to make a change to optimise that specific task immediately.
This change is made while I was in the progress of editing production code, so of course I want to be able to jump right back to where i was and continue.
Having to quit and reopen vim completely breaks the flow, and should be avoided as much as possible.
Plugin managers generally aren't written with this goal in mind - so in order to get this to work properly, I need to deviate a bit from the idiomatic way the plugin manager works.
### A word of warning.
It can never be guaranteed that re-sourcing the configuration will work correctly all the time. Executing the configuration is not a _special_ command, it merely runs the code in the script in the current state of vim/neovim.
Therefore, you can easily have undesired state that will not be removed. In particular, if you remove a plug that has already been loaded, it will stay loaded and cause trouble. A specific plugin may also not work well with reloading.
Also, as I deviate from the idiomatic way of using the plugin manager, my approach could have unintended effects in the future. But I do rely on publicly documented features, so they _should_ continue to work.
## Focus on Improving Workflow
Many tutorials follow the steps:
- Set some default options
- Add a plugin manager
- Add completion and lsp plugins
- Add more awesome plugins and show how fancy they are
I rather start with
- I am working with my neovim configuration, let's make it easy to quickly edit and apply changes.
- Some stuff annoyed me in this process, let me fix those.
- My configuration is getting bigger, I want to modularise it.
- I want to make it easier to navigate the files in my configuration.
This is the order in which I was making changes to the configuration myself, and I think it will be much more helpful and a better learning experience, as it becomes more apparent _why_ I make each change; which process does this setting support.
I also think that it will help you get into the mindset, that when you discover a pattern in _your_ workflow, you will try making the change to fix it.
## Inspiration
I learned from others, and I want to give credit to them. Also, they may use fancy plugins I don't find to be helpful, but maybe you would.
The first two resources were helpful in my early vim journey, and I highly recommend at least checking out VimCasts.
- [VimCasts](http://vimcasts.org/) by Drew Neil still has a lot of _very helpful_ information about basic vim functionality, e.g. did you know that you can [paste in _insert_-mode](http://vimcasts.org/episodes/pasting-from-insert-mode/) using <kbd><Ctrl>+r 0</kbd>?
- [Learn VimScript the Hard Way](https://learnvimscriptthehardway.stevelosh.com/) by Steve Losh. Although learning vimscript is not the most helpful if you use neovim, you should learn lua instead, understanding the principles about the package folder structure is still helpful. E.g. if you want to change the default tab-settings for a specific filetype, the easiest is _still_ to add a vim file in the `ftplugin/` folder.
Newer videos specifically about setting up neovim in lua that helped in my neovim journey
- [Neovim RC From Scratch](https://www.youtube.com/watch?v=w7i4amO_zaE&t=1166s) by [ThePrimeagen](https://www.youtube.com/@ThePrimeagen)
- [Neovim for Newbs](https://www.youtube.com/playlist?list=PLsz00TDipIffreIaUNk64KxTIkQaGguqn)'s by [TypeCraft](https://www.typecraft.dev/)
- [Josean Martinez](https://www.youtube.com/@joseanmartinez) often publishes great videos, not just about neovim, but also configuring the terminal and tmux.
## A little bit of history
I have been using vim for almost 15 years. My original configuration was very small, and adapted, very much inspired by VimCasts. I started using it for Rails development (I think it was actually because of the extremely great [rails plugin](https://github.com/tpope/vim-rails) by [Tim Pope](https://github.com/tpope) that I started with vim in the first place). To get it working, I needed to copy code I didn't understand, but I had a great editor for rails.
I started learning more about vim, started to understand what happened in my config, started to get my own ideas about how to improve the workflow, and particularly reading Learn Vimscript ... made me start writing my own plugins in vimscript, replaced pathogen with vundle, and later vim-plug.
Eventually I started checking out neovim and used it in parallel with vim. The same config was symlinked betweed the two my configuration had `if`/`else` statements as I started to add neovim replacements to vim plugins, and still have it usable in both editors. But eventually I dropped legacy vim altogether.
As more and more plugins started to be developed in lua, my _one large vim config file_ started to have inline lua code in my vimscript initialisation to configure the new plugins. And I started to realise that _soon_ I need to create replace my `init.vim` with `init.lua`.
Eventually, about a years ago, I did it. The original version was very much copy/paste from ThePrimeagen's video mentioned above. This was later adjusted, after watching videos by TypeCraft and Josean Martinez.
But all that time, my neovim lua config was a copy of other people's configurations, and in the process I had made a mess, and re-sourcing everything didn't work well. It also dependend on [lsp-zero](https://github.com/VonHeikemen/lsp-zero.nvim), which is a wrapper around other plugins, that I later learned and understood more.
During that year, I learned to understand more about lua, plugin managers, etc.
It was about time that I wrote a configuration that was mine from scratch, not code copied from someone else.
## But first ...
I started this configuration from scratch, while I already har a working configuration. Before starting, I wanted to make sure I could easily switch between configuration back and forth between the original working, and new work-in-progress configuration. In the next article in the series, I will show how I created a sandbox for the new configuration. | stroiman |
|
1,913,690 | New Window.ai API, 11 System Design blogs, Open Source tools, Web3 domains all in this week | Hello friends, Welcome to the iHateReading newsletter, this newsletter is interesting and important... | 0 | 2024-07-06T11:51:39 | https://dev.to/shreyvijayvargiya/new-windowai-api-11-system-design-blogs-open-source-tools-web3-domains-all-in-this-week-m0f | news, watercooler, webdev, programming | Hello friends,
Welcome to the iHateReading newsletter, this newsletter is interesting and important because we share trendy news, blogs, and tools as well as put some interesting web links to inspire the developer within you.
I would love to hear your opinion and views regarding this newsletter. Yesterday I got a review that this newsletter is sometimes difficult to grasp because of too much going in one letter and to address this issue we will divide the newsletter into categories more often.
Read - [https://ihatereading.in/newsletter](https://ihatereading.in/newsletter)
iHateReading | shreyvijayvargiya |
1,913,689 | BitPower: Exploring the Future of Decentralized Finance | In the era of rapid development of modern financial technology, decentralized finance (DeFi) has... | 0 | 2024-07-06T11:51:29 | https://dev.to/asfg_f674197abb5d7428062d/bitpower-exploring-the-future-of-decentralized-finance-ie4 | In the era of rapid development of modern financial technology, decentralized finance (DeFi) has become an emerging force that subverts traditional financial services. As an innovative project in this field, BitPower is committed to providing users with more transparent, efficient and secure financial services through blockchain technology and smart contracts. This article will introduce in detail the basic concepts, core technologies, security, and applications and advantages of BitPower in the financial field.
## Basic Concepts
BitPower is a decentralized lending platform developed based on blockchain technology, aiming to break the barriers of the traditional financial system and provide more flexible and low-cost financial services. BitPower is built on the Ethereum network (EVM) and uses smart contract technology to realize asset deposits and lending. Participants can deposit digital assets on the platform to earn interest or obtain loans by mortgaging assets.
## Core Technology
### Smart Contract
The core of BitPower lies in smart contract technology. A smart contract is a self-executing code that can automatically perform predefined operations when certain conditions are met. On the BitPower platform, all deposit and lending operations are controlled by smart contracts to ensure the security and transparency of transactions.
### Decentralization
BitPower adopts a decentralized architecture with no central control agency. All transaction records are stored on the blockchain and maintained by all nodes in the network. This decentralized approach not only improves the system's anti-attack ability, but also ensures the transparency and immutability of data.
### Consensus Mechanism
The BitPower platform uses the consensus mechanism of the blockchain to ensure the validity and consistency of transactions. Through consensus algorithms such as Proof of Work (PoW) or Proof of Stake (PoS), nodes in the network can reach a consensus to ensure that every transaction is legal and accurate.
## Security
BitPower attaches great importance to the security of the platform and uses a variety of technical means to ensure the security of users' assets. First, the code of the smart contract is strictly audited and tested to ensure its reliability and security. Secondly, the platform uses cryptography technology to encrypt transaction data to prevent unauthorized access and tampering. In addition, the decentralized architecture makes the platform have no single point of failure, further improving the security of the system.
## Applications and Advantages
### Efficient and Convenient
Compared with traditional financial services, the lending services provided by BitPower are more efficient and convenient. Users can deposit and lend assets simply through blockchain wallets without cumbersome approval processes.
### Transparency and fairness
All transaction records on the BitPower platform are open and transparent, and users can view the flow of assets at any time. This transparency not only enhances user trust, but also helps prevent fraud and improper behavior.
### Lower cost
By removing intermediaries, BitPower's operating costs are greatly reduced, which can provide users with lower lending rates and higher deposit returns. This is of great significance to those who have difficulty in obtaining services in the traditional financial system, especially users in developing countries.
## Conclusion
As an innovative project in the field of decentralized finance, BitPower provides users with a safe, transparent and efficient lending platform through blockchain and smart contract technology. It not only optimizes the process and cost of financial services, but also provides more people with the opportunity to participate in the global financial market. With the continuous advancement of technology and the continuous expansion of applications, BitPower is expected to play a greater role in the future and lead the development trend of decentralized finance. | asfg_f674197abb5d7428062d |
|
1,913,688 | Exploring India’s Rich Heritage Through Iconic Train Journeys | India is a land of diverse cultures, vibrant traditions, and a rich historical legacy. Exploring... | 0 | 2024-07-06T11:49:37 | https://dev.to/heritagetrainsinindia/exploring-indias-rich-heritage-through-iconic-train-journeys-1aob | India is a land of diverse cultures, vibrant traditions, and a rich historical legacy.
Exploring [heritage trains in India](https://www.maharajaexpress.co.uk/heritage-train-tours/
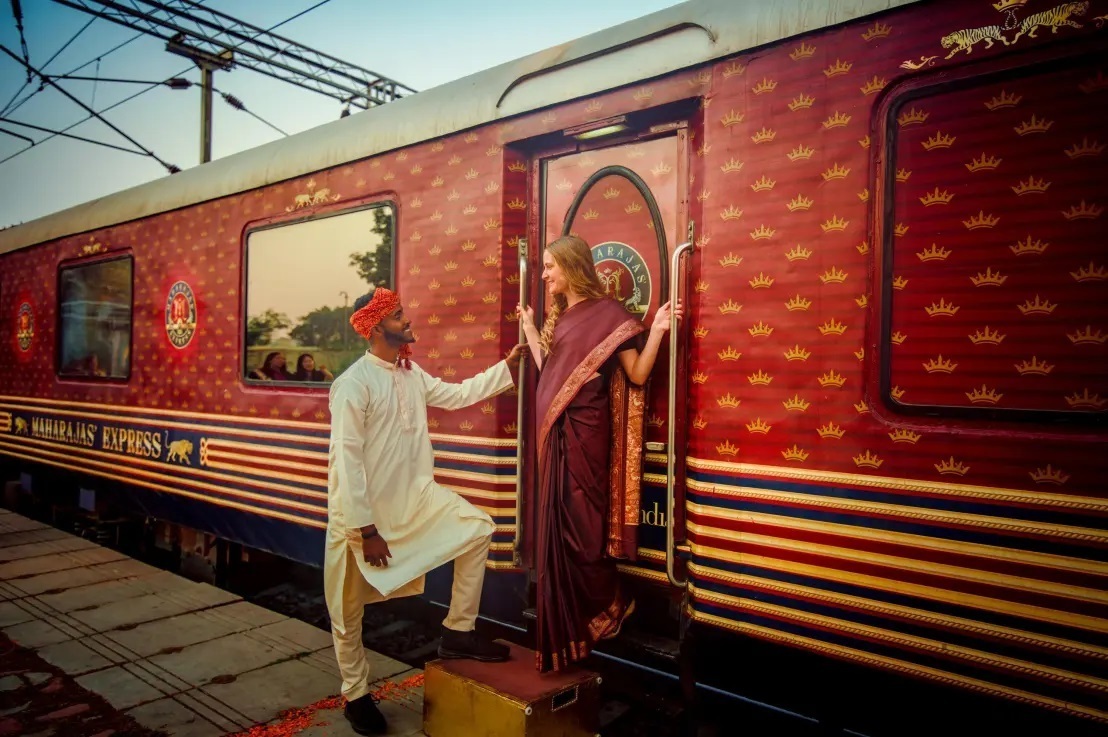) offers a unique way to delve into the heart of this fascinating country. These iconic train journeys provide a window into India’s past while offering modern comforts and luxury.
The Romance of Train Travel in India
Traveling by train in India is not just a mode of transport; it is an experience. The rhythmic chugging of the train, the scenic landscapes passing by, and the blend of diverse cultures make train travel a nostalgic and romantic experience. A heritage train ride takes this a step further, offering travelers a chance to journey back in time while enjoying unparalleled luxury.
Iconic Heritage Train Routes
Palace on Wheels
The Palace on Wheels is a symbol of royal luxury on rails. Launched in 1982, this train takes travelers on a week-long journey through Rajasthan’s royal cities.
Route Overview
The journey begins in Delhi, the capital city, and travels through Jaipur, the Pink City, Jodhpur, the Blue City, Udaipur, the City of Lakes, and other notable destinations. Each stop offers a unique glimpse into Rajasthan’s regal history.
Highlights
Jaipur: Explore the Amer Fort, City Palace, and Hawa Mahal.
Udaipur: Enjoy the serene beauty of Lake Pichola and the grandeur of City Palace.
Jodhpur: Visit the imposing Mehrangarh Fort and the charming old city.
Maharaja Express
The Maharaja Express is one of the most luxurious trains in the world. It offers several itineraries, each designed to showcase the best of India’s cultural and historical treasures.
Routes and Key Destinations
Heritage of India: This itinerary covers Mumbai, Udaipur, Jodhpur, Bikaner, Jaipur, Ranthambore, Agra, and Delhi.
Treasures of India: A shorter journey covering Delhi, Agra, Ranthambore, and Jaipur.
Onboard Experience
Travelers enjoy spacious cabins, fine dining, and personalized service, making it an unforgettable journey.
Deccan Odyssey
The Deccan Odyssey takes travelers through the cultural gems of Maharashtra and beyond.
Route Overview
Starting in Mumbai, the train travels through picturesque landscapes and historical sites, including Goa and the Ajanta-Ellora caves.
Key Stops and Experiences
Mumbai: Explore the Gateway of India and the bustling city life.
Goa: Relax on the beautiful beaches and explore Portuguese heritage.
Ajanta-Ellora: Marvel at the ancient rock-cut caves and their intricate carvings.
Toy Trains of India
India’s toy trains offer a quaint and charming journey through picturesque settings.
Notable Routes
Darjeeling Himalayan Railway: Travel through the scenic tea gardens of Darjeeling.
Kalka-Shimla Railway: Enjoy the breathtaking views of the Himalayas on this UNESCO World Heritage site.
Nilgiri Mountain Railway: Experience the lush landscapes of Tamil Nadu.
Historical Significance
These toy trains, built during the British era, continue to operate, offering a unique travel experience.
Cultural Experiences Along the Way
Heritage trains in India offer not just luxury but also a deep cultural immersion. Travelers can interact with local communities, witness traditional performances, and participate in cultural activities. Onboard, the trains host cultural events, providing a taste of India’s rich traditions.
Heritage and Historical Sites
Each heritage train journey is a passage through India’s historical tapestry.
UNESCO World Heritage Sites
Taj Mahal: The epitome of love and architectural brilliance.
Khajuraho Temples: Renowned for their stunning erotic sculptures.
Ajanta-Ellora Caves: A marvel of ancient rock-cut architecture.
Cultural Significance
Visiting these sites offers travelers a deep understanding of India’s historical and cultural evolution.
Culinary Delights
Indian cuisine is as diverse as its culture. Heritage trains offer an array of regional delicacies.
Regional Cuisines
Rajasthan: Savor traditional Rajasthani dishes like Dal Baati Churma.
Maharashtra: Enjoy the flavors of Vada Pav and Puran Poli.
Goa: Relish Goan seafood and Portuguese-inspired dishes.
Onboard Dining Experience
The onboard dining experience is a culinary journey, with gourmet meals prepared by expert chefs, reflecting the regional flavors of India.
Luxury and Comfort
Heritage trains in India are synonymous with luxury. These trains are equipped with modern amenities, ensuring a comfortable and opulent journey.
Onboard Facilities
Luxurious Cabins: Elegantly designed with modern comforts.
Fine Dining: Multi-cuisine restaurants offering gourmet meals.
Recreational Areas: Lounges, bars, and spas for relaxation.
Unique Aspects
From personalized service to themed decor, every detail is crafted to provide an unparalleled travel experience.
Practical Tips for Travelers
Traveling on heritage trains in India requires some planning.
Booking Tips
Book well in advance, especially during peak travel seasons.
Check for special packages and offers.
Best Times to Travel
Winter months (October to March) are ideal for most routes.
Monsoon season offers lush landscapes but can have travel disruptions.
Packing Essentials
Comfortable clothing and footwear.
Essential travel documents and a camera to capture memories.
Embarking on a heritage train ride through India is more than a journey; it is an exploration of the country’s soul. These iconic train journeys offer a perfect blend of luxury, history, and culture, making them an unforgettable experience for any traveler. | heritagetrainsinindia |
|
1,913,687 | LeetCode Day27 Greedy Algorithms Part 5 | 56. Merge Intervals Given an array of intervals where intervals[i] = [starti, endi], merge... | 0 | 2024-07-06T11:48:14 | https://dev.to/flame_chan_llll/leetcode-day27-greedy-algorithms-part-5-4gof | leetcode, java, algorithms | # 56. Merge Intervals
Given an array of intervals where intervals[i] = [starti, endi], merge all overlapping intervals, and return an array of the non-overlapping intervals that cover all the intervals in the input.
Example 1:
Input: intervals = [[1,3],[2,6],[8,10],[15,18]]
Output: [[1,6],[8,10],[15,18]]
Explanation: Since intervals [1,3] and [2,6] overlap, merge them into [1,6].
Example 2:
Input: intervals = [[1,4],[4,5]]
Output: [[1,5]]
Explanation: Intervals [1,4] and [4,5] are considered overlapping.
Constraints:
1 <= intervals.length <= 10^4
intervals[i].length == 2
0 <= starti <= endi <= 10^4
[Original Page](https://leetcode.com/problems/merge-intervals/description/)
```
public int[][] merge(int[][] intervals) {
if(intervals.length <= 1){
return intervals;
}
Arrays.sort(intervals, (a,b)->{
return Integer.compare(a[0], b[0]);
});
List<int[]> list = new ArrayList();
for(int i=1; i<intervals.length; i++){
if(intervals[i-1][1] >= intervals[i][0]){
intervals[i][0] = intervals[i-1][0];
intervals[i][1] = Math.max(intervals[i-1][1], intervals[i][1]);
} else{
list.add(intervals[i-1]);
}
}
list.add(intervals[intervals.length-1]);
return list.toArray(new int[list.size()][]);
}
```
# 738. Monotone Increasing Digits
An integer has monotone increasing digits if and only if each pair of adjacent digits x and y satisfy x <= y.
Given an integer n, return the largest number that is less than or equal to n with monotone increasing digits.
Example 1:
Input: n = 10
Output: 9
Example 2:
Input: n = 1234
Output: 1234
Example 3:
Input: n = 332
Output: 299
Constraints:
0 <= n <= 10^9
```
public int monotoneIncreasingDigits(int n) {
if(n<10){
return n;
}
String str = Integer.toString(n);
char[] arr = new char[str.length()];
arr[0] = str.charAt(0);
int pos = -1;
for(int i=1; i<str.length(); i++){
char num = str.charAt(i);
if(num < arr[i-1]){
int j;
if(pos == -1){
j = 0;
}else{
j = pos;
}
for(;j<arr.length; j++){
if(j==0||j==pos){
arr[j] = (char) (arr[j]-1);
}else{
arr[j] = '9';
}
}
break;
}
else if(num > arr[i-1]){
pos = i;
}
arr[i] = str.charAt(i);
}
if(arr[0] <=0){
// cost space by using String
str = new String(arr, 1,arr.length);
}else{
str = new String(arr);
}
return Integer.valueOf(str);
}
```
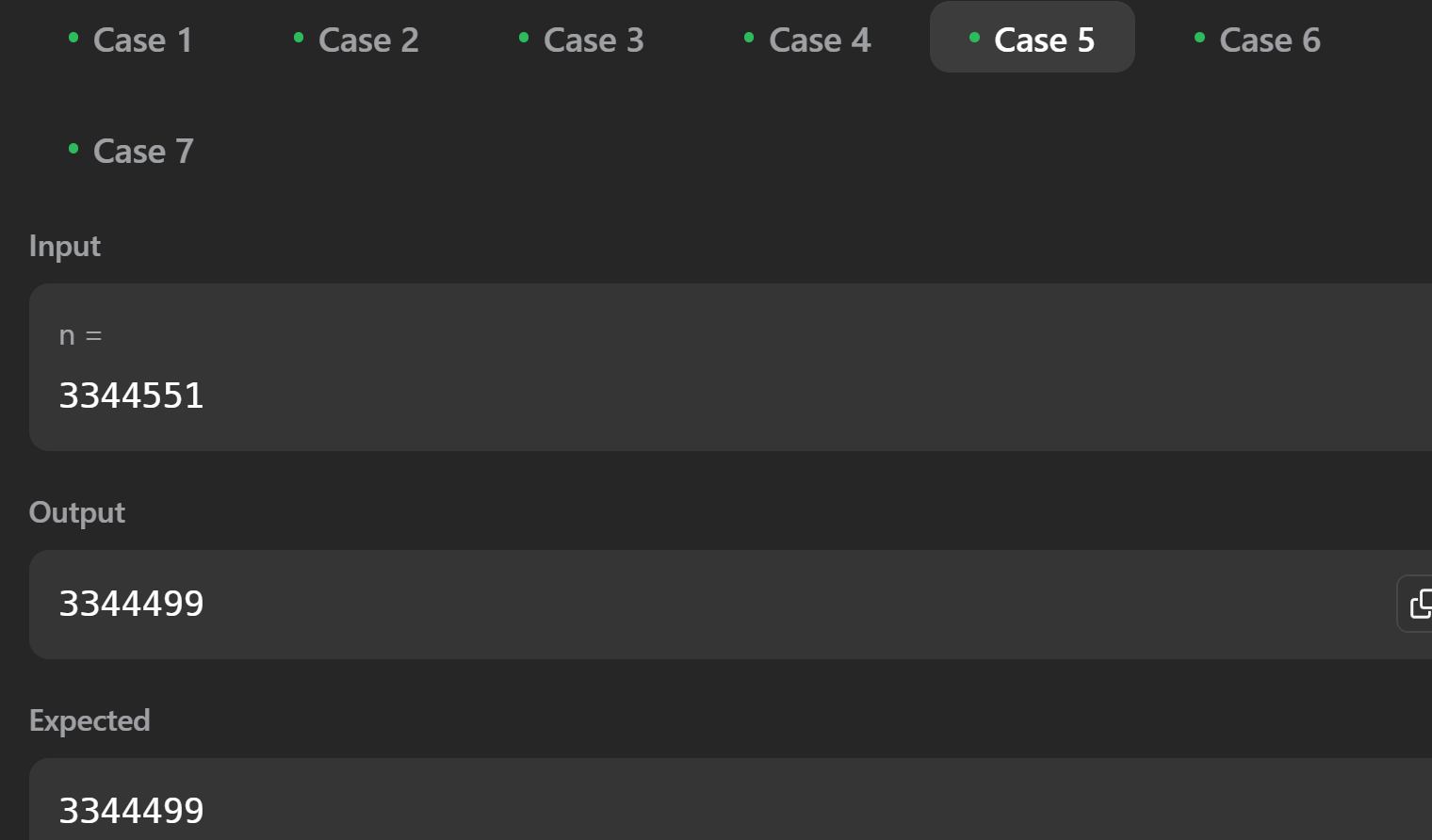
| flame_chan_llll |
1,913,686 | Discover the Best Acupuncture and Acupressure Clinic in Bangalore: Ayushyaa Acupuncture Clinic | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than... | 0 | 2024-07-06T11:47:39 | https://dev.to/ayushyaa/discover-the-best-acupuncture-and-acupressure-clinic-in-bangalore-ayushyaa-acupuncture-clinic-4f5e | ayushyaclinic, acupuncture | Are you seeking a holistic approach to health and wellness in Bangalore? Look no further than Ayushyaa Acupuncture Clinic, the [best acupuncture clinic in Bangalore](https://www.ayushyaaclinic.com/index). Our clinic is renowned for offering top-notch acupuncture and acupressure treatments that cater to a wide range of health issues. In this blog, we will explore what makes Ayushyaa clinic the preferred choice for those seeking natural and effective healthcare solutions.
Why Choose Ayushyaa Acupuncture Clinic?
Expertise and Experience
Ayushyaa Acupuncture clinic Bangalore a team of highly skilled and experienced practitioners who are dedicated to providing the best care possible. Our experts are well-versed in traditional acupuncture and modern techniques, ensuring that each patient receives personalized treatment tailored to their specific needs.
Comprehensive Treatments
At Ayushyaa Acupuncture Clinic, we offer a variety of treatments, including:
• Acupuncture: Utilizing fine needles to stimulate specific points on the body, acupuncture helps in balancing the body’s energy flow, alleviating pain, and promoting overall wellness.
• Acupressure: Similar to acupuncture but without needles, acupressure involves applying pressure to specific points to relieve pain and stress, boost energy levels, and improve health.
• Herbal Therapy: Complementing our acupuncture and acupressure treatments, we offer herbal remedies to support the healing process and enhance overall well-being.
Patient-Centric Approach
Our clinic is committed to providing a patient-centric approach, focusing on the individual needs and health goals of each patient. We take the time to understand your concerns, conduct thorough assessments, and design customized treatment plans that ensure optimal results.best acupressure clinic in Bangalore.
Modern Facilities
Ayushyaa Acupuncture Clinic is equipped with state-of-the-art facilities, creating a comfortable and serene environment for our patients. Our modern equipment and clean, calming spaces ensure that you have a relaxing and therapeutic experience during each visit.
Benefits of Acupuncture and Acupressure
Pain Relief
One of the primary reasons people seek acupuncture and acupressure treatments is for pain relief. Whether you suffer from chronic pain, migraines, or joint pain, our treatments can provide significant relief and improve your quality of life.
Stress Reduction
In today’s fast-paced world, stress is a common concern for many individuals. Acupuncture and acupressure treatments at Ayushyaa Acupuncture Clinic help in reducing stress levels, promoting relaxation, and enhancing mental clarity.
Improved Sleep
Many of our patients have reported improved sleep patterns and better sleep quality after undergoing acupuncture and acupressure treatments. By balancing the body’s energy and reducing stress, these treatments promote restful and rejuvenating sleep.
Enhanced Immune Function
Regular acupuncture and acupressure sessions can boost your immune system, helping you stay healthy and ward off illnesses. Our treatments stimulate the body’s natural healing abilities, enhancing overall health and vitality.
Conclusion
If you are looking for the top acupuncture in Bangalore, Ayushyaa Acupuncture Clinic is your ideal destination. Our experienced practitioners, comprehensive treatments, and patient-centric approach ensure that you receive the highest quality care. Visit us today and embark on a journey towards better health and well-being through the natural and effective treatments of acupuncture and acupressure.
For more information or to schedule an appointment, contact Ayushyaa Acupuncture Clinic today. Experience the best acupuncture and acupressure clinic in Bangalore and take the first step towards a healthier, happier life.
| ayushyaa |
1,913,202 | How I used js to dynamically create and delete pods in kubernetes | First of all: Why? Imagine you have a system where your user needs to execute something... | 0 | 2024-07-06T11:47:17 | https://dev.to/eletroswing/how-i-used-js-to-dynamically-create-and-delete-pods-in-kubernetes-1d5e | kubernetes, node, javascript, azure | # First of all: Why?
Imagine you have a system where your user needs to execute something private on your server quickly and securely, like deploying bots or what Next.js does. This article is perfect for such cases.
# But, how do I do it on localhost?
To develop this code, we will use two approaches: production and development. We will use Minikube for the development environment and Azure for the production environment.
# How can we start?
We need to define a development path to avoid getting lost. We will need a way to communicate with Kubernetes, as well as to create and delete pods.
# Development
We will use the libraries `@kubernetes/client-node` and `@azure`.
## First
We start by creating the Kubernetes API. To do this, we define a function `getKubernetesApi`, and in it, we initiate the Kubernetes configuration, something like:
```javascript
import { ContainerServiceClient } from "@azure/arm-containerservice";
import { DefaultAzureCredential } from "@azure/identity";
import k8s from "kubernetes-client";
async function getKubernetesClient() {
const kc = new k8s.KubeConfig();
}
```
Then we define a try catch block, where we test if the environment is production to configure Kubernetes with Azure, or load the default configuration in the development environment. We create and return the client, something like:
```js
async function getKubernetesClient() {
const kc = new k8s.KubeConfig();
try {
if (process.env.NODE_ENV === "production") {
const credentials = new DefaultAzureCredential();
const containerServiceClient = new ContainerServiceClient(credentials);
const response = await containerServiceClient.managedClusters.listClusterAdminCredentials();
kc.loadFromString(response.kubeconfigs[0].value);
} else {
kc.loadFromDefault();
}
const k8sApi = kc.makeApiClient(k8s.CoreV1Api);
return k8sApi;
} catch (error) {
console.error("Error while getting Kubernetes API", error);
return;
}
}
```
## Second
Next, we define the functions to create and delete pods using the obtained client. For this, we receive data such as the pod name, pod namespace, and metadata for the pod, something like:
```js
async function createPod(metadata, namespace) {
try {
const k8sApi = await getKubernetesClient();
return await k8sApi.createNamespacedPod(namespace, metadata);
} catch (error) {
console.error("Error while creating pod", error);
return;
}
}
async function deletePod(name, namespace) {
try {
const k8sApi = await getKubernetesClient();
return await k8sApi.deleteNamespacedPod(name, namespace);
} catch (error) {
console.error("Error while deleting pod", error);
return;
}
}
```
## Third, but not less important
Finally, we export the useful functions to be used in the code and ensure that they cannot be altered during execution. This can be done as:
```js
export default Object.freeze({
createPod,
deletePod,
getKubernetesClient
});
```
## Final Result
```js
import { ContainerServiceClient } from "@azure/arm-containerservice";
import { DefaultAzureCredential } from "@azure/identity";
import k8s from "kubernetes-client";
async function getKubernetesClient() {
const kc = new k8s.KubeConfig();
try {
if (process.env.NODE_ENV === "production") {
const credentials = new DefaultAzureCredential();
const containerServiceClient = new ContainerServiceClient(credentials);
const response = await containerServiceClient.managedClusters.listClusterAdminCredentials();
kc.loadFromString(response.kubeconfigs[0].value);
} else {
kc.loadFromDefault();
}
const k8sApi = kc.makeApiClient(k8s.CoreV1Api);
return k8sApi;
} catch (error) {
console.error("Error while getting Kubernetes API", error);
return;
}
}
async function createPod(metadata, namespace) {
try {
const k8sApi = await getKubernetesClient();
return await k8sApi.createNamespacedPod(namespace, metadata);
} catch (error) {
console.error("Error while creating pod", error);
return;
}
}
async function deletePod(name, namespace) {
try {
const k8sApi = await getKubernetesClient();
return await k8sApi.deleteNamespacedPod(name, namespace);
} catch (error) {
console.error("Error while deleting pod", error);
return;
}
}
export default Object.freeze({
createPod,
deletePod,
getKubernetesClient
});
``` | eletroswing |
1,913,683 | I Need A Full Tutorial On How To Master Next js | I need documentation on how to master Next.js from zero to hero. Why am I asking this with... | 0 | 2024-07-06T11:44:36 | https://dev.to/kilanibarhoom/i-need-a-full-tutorial-on-how-to-master-next-js-3h2b | nextjs, frontend, backend | I need documentation on how to master **Next.js** from zero to hero.
## Why am I asking this with all the YouTube tutorials?
Well, the tutorials don't explain everything needed to write a full website that is ready to be deployed and used.
I come from **React.js** as I think I've reached a point where I did a good job learning a good amount of it, and I know what to look for when I need help, like for caching I use react-query. But here in Next.js every tutorial I watch or docs I read, they just explain a specific thing, for example, if they are explaining something and they come across something else, they just say that they have a full course on 'this'. Please guys is there a full tutorial to be able to comfortably write a full app and deploy it with all the good practices?
**_Free is always better_**
| kilanibarhoom |
1,913,682 | Estimating Pi using the Monte Carlo Method | Check out this Pen I made! | 0 | 2024-07-06T11:43:06 | https://dev.to/yemon/estimating-pi-using-the-monte-carlo-method-2f79 | codepen | Check out this Pen I made!
{% codepen https://codepen.io/yemon/pen/oNrvReg %} | yemon |
1,913,681 | Thiết Kế Website Tại Đà Nẵng, Chuyên Nghiệp, Uy Tín | Đà Nẵng là một trong những thành phố du lịch hàng đầu của Việt Nam, với nhiều điểm tham quan, khách... | 0 | 2024-07-06T11:40:52 | https://dev.to/terus_technique/thiet-ke-website-tai-da-nang-chuyen-nghiep-uy-tin-4i28 | website, digitalmarketing, seo, terus |
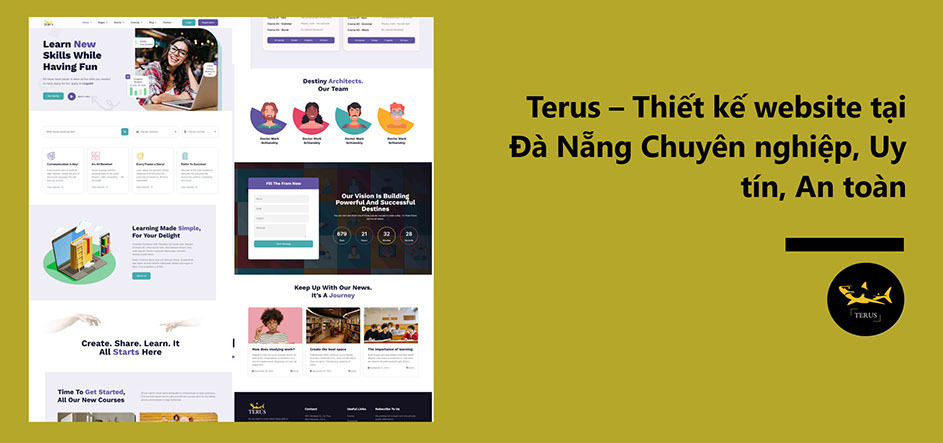
Đà Nẵng là một trong những thành phố du lịch hàng đầu của Việt Nam, với nhiều điểm tham quan, khách sạn và dịch vụ hấp dẫn. Vì vậy, các doanh nghiệp ở Đà Nẵng cần phát triển một website chuyên nghiệp để quảng bá và thu hút khách du lịch. Terus, một công ty [thiết kế website tại Đà Nẵng](https://terusvn.com/thiet-ke-website-tai-hcm/), đã phát triển các giải pháp website độc đáo để đáp ứng nhu cầu này.
Các tính năng đặc biệt của website do Terus thiết kế cho Đà Nẵng bao gồm:
Tích hợp liền mạch các điểm tham quan địa phương, giúp du khách dễ dàng khám phá và lên kế hoạch cho chuyến đi.
Hỗ trợ đa ngôn ngữ và nội dung phong phú, để thu hút khách du lịch trong và ngoài nước.
Bản đồ tương tác và các tính năng dựa trên vị trí, giúp du khách dễ dàng tìm kiếm và đặt dịch vụ.
Thiết kế ưu tiên thiết bị di động, thuận tiện cho khách du lịch khi di chuyển.
Tích hợp với các nền tảng đặt phòng và đặt chỗ địa phương, giúp du khách dễ dàng hoàn tất các giao dịch trên website.
Tích hợp truyền thông xã hội và nội dung do người dùng tạo, để tăng sự tương tác và chia sẻ trải nghiệm của khách du lịch.
Một website tại Đà Nẵng cần đáp ứng các yêu cầu sau:
Thiết kế rõ ràng, hấp dẫn và dễ sử dụng.
Đáp ứng và thân thiện với thiết bị di động.
Điều hướng trực quan, giúp du khách dễ dàng xem và tìm kiếm thông tin.
Nội dung có liên quan và chất lượng cao, để cung cấp thông tin hữu ích cho du khách.
Thông tin liên hệ và lời gọi hành động rõ ràng, để du khách có thể dễ dàng liên hệ và đặt dịch vụ.
Tối ưu hóa công cụ tìm kiếm (SEO), để website dễ dàng được tìm thấy trên các công cụ tìm kiếm.
Tốc độ tải nhanh, để đảm bảo trải nghiệm tốt cho du khách.
Các biện pháp bảo mật, để bảo vệ thông tin của du khách.
Phân tích và theo dõi, để đo lường hiệu quả của website và cải thiện không ngừng.
Terus đã thực hiện nhiều dự án website tại Đà Nẵng, với các ví dụ như: website khách sạn, website du lịch, website nhà hàng, v.v. Khách hàng có thể tham khảo các mẫu website này và liên hệ với Terus để được tư vấn và bắt đầu dự án thiết kế website tại Đà Nẵng.
Việc [thiết kế một website chuyên nghiệp và ưu việt](https://terusvn.com/thiet-ke-website-tai-hcm/) là rất cần thiết cho các doanh nghiệp ở Đà Nẵng để thu hút và phục vụ khách du lịch. Terus, với kinh nghiệm và giải pháp độc đáo, cam kết mang lại cho khách hàng những website ấn tượng và hiệu quả tại Đà Nẵng.
Tìm hiểu thêm về [Thiết Kế Website Tại Đà Nẵng, Chuyên Nghiệp, Uy Tín](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-da-nang/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,680 | BitPower's revenue introduction: | BitPower Loop is a decentralized financial platform that provides an efficient and secure income... | 0 | 2024-07-06T11:40:51 | https://dev.to/bao_xin_145cb69d4d8d82453/bitpowers-revenue-introduction-aep |
BitPower Loop is a decentralized financial platform that provides an efficient and secure income mechanism through smart contracts. The platform allows users to participate directly as depositors or borrowers, and obtain floating interest rate income through decentralized liquidity provision and smart contract operations. The annualized rate of return of BitPower Loop is significantly higher than that of traditional financial products, attracting a large number of investors.
BitPower Loop's income mainly comes from two parts: recurring income and commission sharing. Recurring income is the return obtained by users by providing liquidity, while commission sharing is obtained by recommending new users to participate in the platform. This model not only increases the liquidity of the platform, but also encourages users to actively participate. In particular, when users recommend friends, the referrer can obtain corresponding commission rewards based on the recurring income of their referrals, up to 17 levels of rewards.
Through secure and reliable smart contracts, BitPower Loop ensures the transparency and immutability of all transactions, providing users with a trustworthy income platform. At the same time, the characteristics of global services make it competitive internationally. In short, BitPower Loop has become a leader in the field of decentralized finance with its high returns,
transparency and security. | bao_xin_145cb69d4d8d82453 |
|
1,913,679 | Thiết kế website Rạch Giá Kiên Giang Chất Lượng | Với nhu cầu ngày càng gia tăng của các doanh nghiệp tại Rạch Giá và Kiên Giang muốn có sự hiện diện... | 0 | 2024-07-06T11:37:08 | https://dev.to/terus_technique/thiet-ke-website-rach-gia-kien-giang-chat-luong-25ki | website, digitalmarketing, seo, terus |
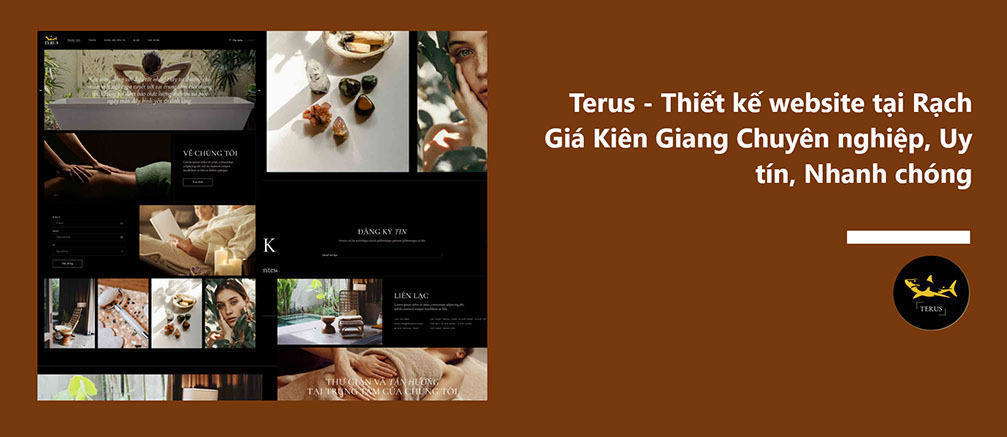
Với nhu cầu ngày càng gia tăng của các doanh nghiệp tại Rạch Giá và Kiên Giang muốn có sự hiện diện kỹ thuật số và gia tăng phạm vi tiếp cận khách hàng, việc thiết kế một website chuyên nghiệp trở nên vô cùng quan trọng. Terus, đơn vị chuyên [thiết kế website tại địa phương](https://terusvn.com/thiet-ke-website-tai-hcm/), đã và đang cung cấp dịch vụ này với nhiều ưu điểm nổi bật.
Lý do doanh nghiệp nên thiết kế website tại Rạch Giá, Kiên Giang:
Hiện diện và hiển thị kỹ thuật số - website là kênh trực tuyến để doanh nghiệp thể hiện hình ảnh, giới thiệu sản phẩm/dịch vụ và tiếp cận khách hàng.
Tăng phạm vi tiếp cận khách hàng - website mang đến cơ hội tiếp cận khách hàng ở phạm vi rộng hơn, không giới hạn bởi không gian địa lý.
Uy tín và chuyên nghiệp - website chuyên nghiệp thể hiện được sự uy tín, chất lượng và chuyên môn của doanh nghiệp.
Công cụ tiếp thị hiệu quả - website là một kênh tiếp thị mạnh mẽ, góp phần gia tăng doanh số và khách hàng.
Sẵn sàng và thuận tiện 24/7 - website hoạt động liên tục, đáp ứng nhu cầu của khách hàng bất kể thời gian.
Lợi thế cạnh tranh - website chuyên nghiệp giúp doanh nghiệp nổi bật hơn so với đối thủ.
Hỗ trợ khách hàng hiệu quả - website cung cấp thông tin, hỗ trợ khách hàng một cách chuyên nghiệp.
Terus, với kinh nghiệm [thiết kế website tại Rạch Giá, Kiên Giang](https://terusvn.com/thiet-ke-website-tai-hcm/), mang đến các giải pháp website chuyên biệt, bao gồm:
Phương pháp thiết kế bản địa hóa - website được thiết kế phù hợp với đặc thù địa phương.
Giới thiệu về du lịch địa phương - website tích hợp thông tin du lịch, giới thiệu điểm đến tại Rạch Giá và Kiên Giang.
Thiết kế đáp ứng cho người dùng di động - website hoạt động tối ưu trên mọi thiết bị.
Tích hợp khả năng đa ngôn ngữ - website có thể được hiển thị bằng nhiều ngôn ngữ.
Tích hợp các công cụ kinh doanh địa phương - website tích hợp các công cụ như bản đồ, đặt lịch, đặt hàng...
Tối ưu hóa công cụ tìm kiếm (SEO) - website được tối ưu để tăng khả năng hiển thị trên kết quả tìm kiếm địa phương.
Hệ thống quản lý nội dung thân thiện - website có giao diện quản lý nội dung dễ sử dụng.
Với các ưu điểm về phương pháp thiết kế, tính năng website, và quy trình chuyên nghiệp, Terus cam kết mang lại những giải pháp website hiệu quả, góp phần gia tăng sự hiện diện kỹ thuật số, tăng phạm vi tiếp cận khách hàng và nâng cao năng lực cạnh tranh cho doanh nghiệp tại Rạch Giá, Kiên Giang.
Tìm hiểu thêm về [Thiết kế website Rạch Giá Kiên Giang Chất Lượng](https://terusvn.com/thiet-ke-website/thiet-ke-website-rach-gia-kien-giang/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,678 | Comparing Frontend development tools HTML and CSS | The word HTML means (HyperText Markup Language) which serves as the backbone of web pages,... | 0 | 2024-07-06T11:36:41 | https://dev.to/milkky98/comparing-frontend-development-tools-html-and-css-1050 | webdev, beginners, programming, python |
The word HTML means (HyperText Markup Language) which serves as the backbone of web pages, providing the user interface, structure and content through elements like headings, paragraphs, and lists. It defines the skeleton of a webpage.
Example elements are <head> <div> <footer> <p><B>
CSS (Cascading Style Sheets) complements HTML by adding style and visual presentation to web pages. Which have it own elements with It controls layout, colors, fonts, and other design elements, enhancing user experience and making content visually appealing. It help to actualize customer color display desires.
**Why ReactJS Stands Out in Modern Web Development**
React.js is a JavaScript library used for building user interfaces, particularly single-page applications where data changes over time
At HNG, ReactJS is a preferred choice due to its component-based architecture, enabling developers to build scalable and interactive user interfaces efficiently. React's virtual DOM (Document Object Model) optimizes rendering performance by selectively updating only the necessary parts of the page, resulting in faster load times and smoother user interactions.
Its declarative approach simplifies code maintenance and collaboration among developers, fostering productivity and code stability.
In conclusion, while HTML and CSS form the fundamental building blocks of web development, ReactJS elevates frontend development with its powerful capabilities in creating dynamic and responsive user interfaces, aligning well with the robust requirements of modern web applications.
The major reason for joining<a href="https://hng.tech/internship">HNG internship</a>program is to acquire skills and mentorship, but it often functions more as a platform for experts to troubleshoot and test their abilities in frontend development. However, there is ample opportunity to learn, especially when working with frameworks like React.js, Vue.js, or Angular, please provide structured and example to approaches each tasks, which will encourage we beginners and other to partakes in this great opportunities <a href="https://hng.tech/hire">website</a>.Thank you for this great privilege.
For more content like this, you can follow me on<a href="https://www.linkedin.com/in/milkky98">linkedin</a>
Share your opinion on HTML/CSS/ReactJs let build a greater future
Best regards. | milkky98 |
1,913,676 | BitPower's Security: A Modern Financial Miracle | In a changing world, financial security has become more important than ever. BitPower, as a... | 0 | 2024-07-06T11:33:45 | https://dev.to/ping_iman_72b37390ccd083e/bitpowers-security-a-modern-financial-miracle-3mbe |
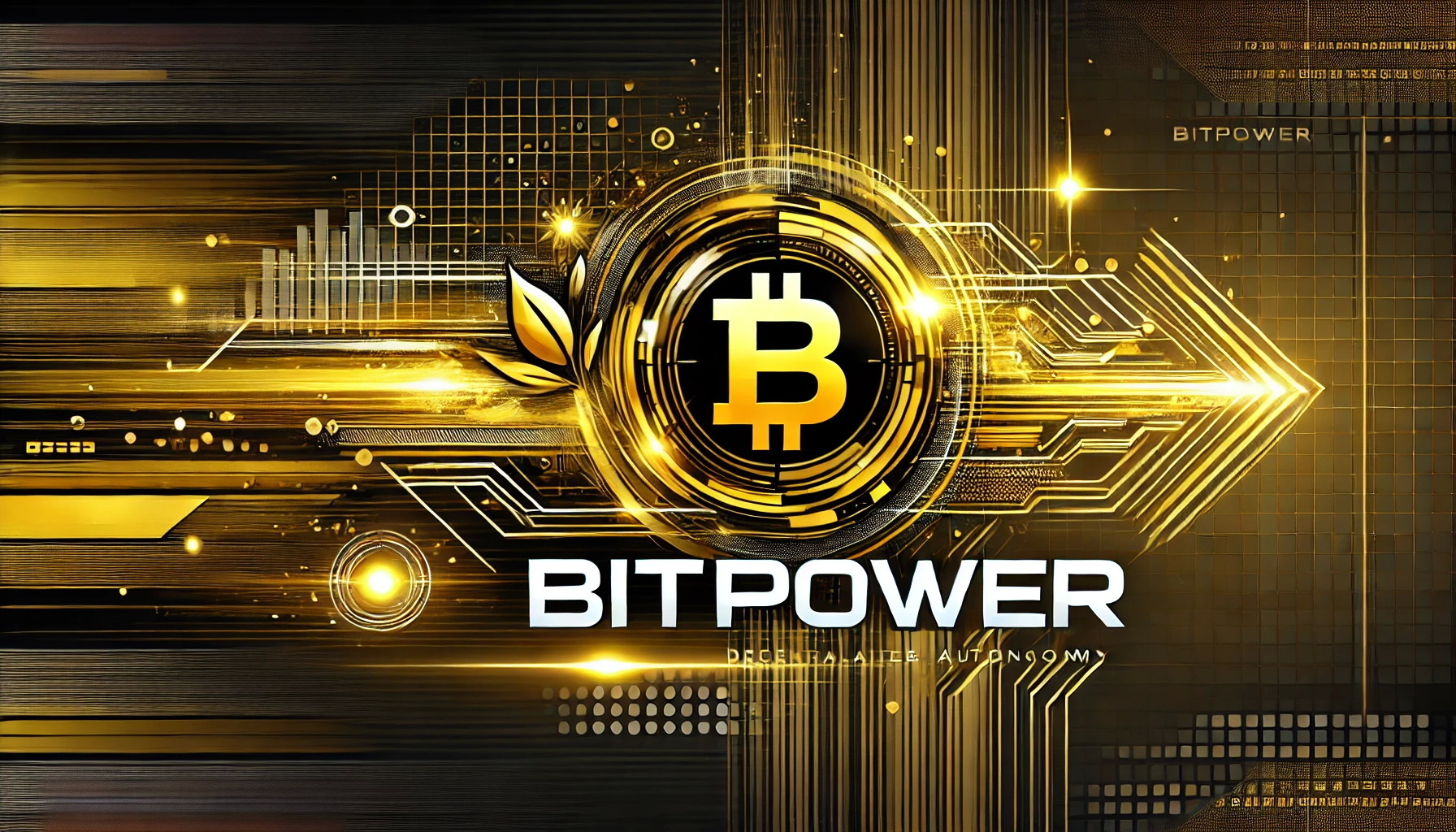
In a changing world, financial security has become more important than ever. BitPower, as a decentralized financial platform based on blockchain technology, provides unparalleled security and stable returns. By using advanced smart contracts and cryptocurrencies, BitPower has not only changed the landscape of traditional finance, but also provided users with unprecedented opportunities for income.
First, BitPower's security is based on the core characteristics of blockchain technology. Blockchain is a distributed ledger technology whose data is stored on thousands of nodes around the world, each of which keeps a complete record of transactions. This decentralized structure means that no one can tamper with the data alone, ensuring the transparency and immutability of transactions.
Second, BitPower uses smart contract technology to automatically execute transactions and agreements. A smart contract is a self-executing code that automatically triggers transactions when preset conditions are met. Through smart contracts, BitPower has achieved fully automated financial operations without intermediaries, reducing the risk of human error and fraud. Once deployed on the blockchain, these contracts cannot be changed, ensuring the stability and security of the system.
The use of cryptocurrency is also a highlight of BitPower's security. Cryptocurrency transactions use complex encryption algorithms to protect users' privacy and asset security. Every transaction of the user is encrypted by cryptography technology, and no personal information will be leaked even on the public network. In addition, the user's funds are directly stored in their own digital wallets, and they have full control over their assets and are not controlled by any third party.
BitPower not only excels in security, but its income mechanism is also very attractive. The platform automatically matches borrowing and lending needs and calculates interest in real time through a decentralized market liquidity pool model. This mechanism not only improves the efficiency of capital utilization, but also provides users with stable income. For example, users can obtain high returns in different cycles of daily, weekly, and monthly by providing liquidity on BitPower. Specifically, the daily yield is 100.4%, 104% for seven days, 109.5% for fourteen days, and 124% for twenty-eight days. These returns are automatically returned to the user's wallet through smart contracts, ensuring the timeliness and security of the income.
In this era of uncertainty, BitPower provides users with a safe, transparent, and high-yield financial platform. Through blockchain technology, smart contracts, and cryptocurrencies, BitPower not only protects users' assets, but also brings them stable and considerable income. This is a miracle of modern finance and a truly game-changing platform. Whether you are a novice or a veteran, BitPower will be your new choice for financial investment.
#BTC #ETH #SC #DeFi | ping_iman_72b37390ccd083e |
|
1,913,675 | Thiết Kế Website Tại Nhơn Trạch Hiệu Quả, Tăng Lợi Nhuận | Trong kỷ nguyên kỹ thuật số, việc sở hữu một website chuyên nghiệp trở nên vô cùng quan trọng đối... | 0 | 2024-07-06T11:32:56 | https://dev.to/terus_technique/thiet-ke-website-tai-nhon-trach-hieu-qua-tang-loi-nhuan-42p3 | website, digitalmarketing, seo, terus |
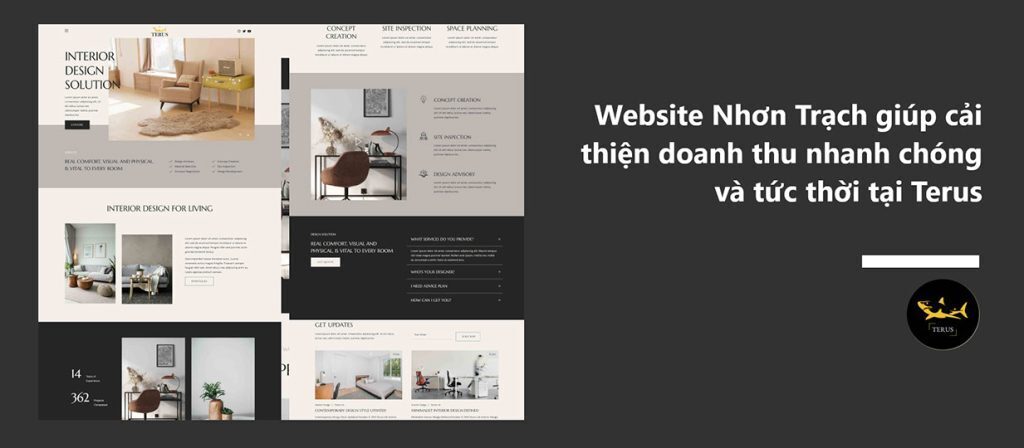
Trong kỷ nguyên kỹ thuật số, việc sở hữu một website chuyên nghiệp trở nên vô cùng quan trọng đối với các doanh nghiệp ở Nhơn Trạch. Terus, một công ty [thiết kế website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/), đã góp phần nâng cao sự hiện diện trực tuyến và tăng cường khả năng tiếp cận khách hàng cho nhiều khách hàng tại khu vực này.
Lý Do Doanh Nghiệp Ở Nhơn Trạch Nên Đầu Tư Vào Thiết Kế Website
Tăng cường hiện diện và phạm vi tiếp cận trực tuyến: Một website chuyên nghiệp giúp mở rộng phạm vi tiếp cận của doanh nghiệp, cho phép tiếp cận với khách hàng ở mọi nơi, không chỉ giới hạn trong một khu vực địa lý cụ thể.
Tiếp thị và xây dựng thương hiệu hiệu quả: Một website ấn tượng góp phần tạo dựng hình ảnh chuyên nghiệp và gia tăng nhận diện thương hiệu, từ đó thu hút và giữ chân khách hàng tiềm năng.
Sự thuận tiện và khả năng tiếp cận của khách hàng: Khách hàng có thể dễ dàng tìm kiếm, truy cập và tương tác với doanh nghiệp thông qua website, tăng cường trải nghiệm của họ.
Xây dựng niềm tin và sự tín nhiệm: Một website chuyên nghiệp thể hiện sự chuyên nghiệp, tin cậy và cam kết phục vụ khách hàng của doanh nghiệp.
Trưng bày Sản phẩm và Dịch vụ: Website cho phép doanh nghiệp giới thiệu và trình bày sản phẩm, dịch vụ một cách sinh động, thu hút khách hàng.
Những Tính Năng Nổi Bật Của Website Terus Tại Nhơn Trạch
Thiết kế theo yêu cầu riêng của khách hàng: Terus tập trung vào việc hiểu và đáp ứng nhu cầu cụ thể của từng doanh nghiệp, tạo ra những giải pháp website độc đáo.
Đáp ứng và thân thiện với thiết bị di động: Website được thiết kế tối ưu hóa cho mọi thiết bị, đảm bảo trải nghiệm người dùng tốt trên mọi nền tảng.
Giao diện thân thiện với người dùng: Giao diện website được thiết kế đơn giản, dễ sử dụng, giúp khách hàng dễ dàng tìm kiếm và tương tác.
Hình ảnh và Đa phương tiện Thu hút: Sử dụng hình ảnh, video, infographic chất lượng cao để truyền tải thông điệp một cách sinh động.
Hệ thống quản lý nội dung (CMS): Cho phép doanh nghiệp dễ dàng cập nhật, quản lý và chỉnh sửa nội dung website.
Tối ưu hóa công cụ tìm kiếm (SEO): Các kỹ thuật SEO tối ưu giúp website được hiển thị tốt trên các công cụ tìm kiếm.
Tích hợp các công cụ và tính năng kinh doanh: Tích hợp các tính năng như giỏ hàng, thanh toán, đăng ký..., hỗ trợ hoạt động kinh doanh.
Phân tích và theo dõi hiệu suất: Cung cấp các công cụ phân tích để theo dõi và đánh giá hiệu quả hoạt động của website.
Terus đã thiết kế nhiều website chuyên nghiệp cho các doanh nghiệp tại Nhơn Trạch, phản ánh phong cách, ngành nghề và nhu cầu riêng của từng khách hàng. Các mẫu website này thể hiện sự sáng tạo, chuyên nghiệp và tính ứng dụng cao.
Terus tự hào là [đơn vị thiết kế website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/), sáng tạo và hiệu quả tại Nhơn Trạch. Với các tính năng nổi bật và cam kết phục vụ khách hàng, Terus sẽ giúp các doanh nghiệp xây dựng sự hiện diện số vững chắc, thu hút và giữ chân khách hàng.
Tìm hiểu thêm về [Thiết Kế Website Tại Nhơn Trạch Hiệu Quả, Tăng Lợi Nhuận](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-nhon-trach/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,617 | HOW TO HOST A STATIC WEBSTIE ON AZURE BLOB STORAGE | TO HOST A STATIC WEBSITE ON AZURE YOU WILL BE NEEDING THE FOLLOWING; Download and Install Visual... | 0 | 2024-07-06T11:29:19 | https://dev.to/monnanka/how-to-host-a-static-webstie-on-azure-blob-storage-57ch | **TO HOST A STATIC WEBSITE ON AZURE YOU WILL BE NEEDING THE FOLLOWING;**
1. Download and Install Visual Studio
2.Download and create a folder that will house your static website
3.Create a storage account on Azure Portal
4. Install two Extensions on the Visual Studio
i. Azure Storage Account
ii. Azure Account
**STEP 1**
_Download and install Visual Studio_
. goto your browser and search **Visual Studio Download**
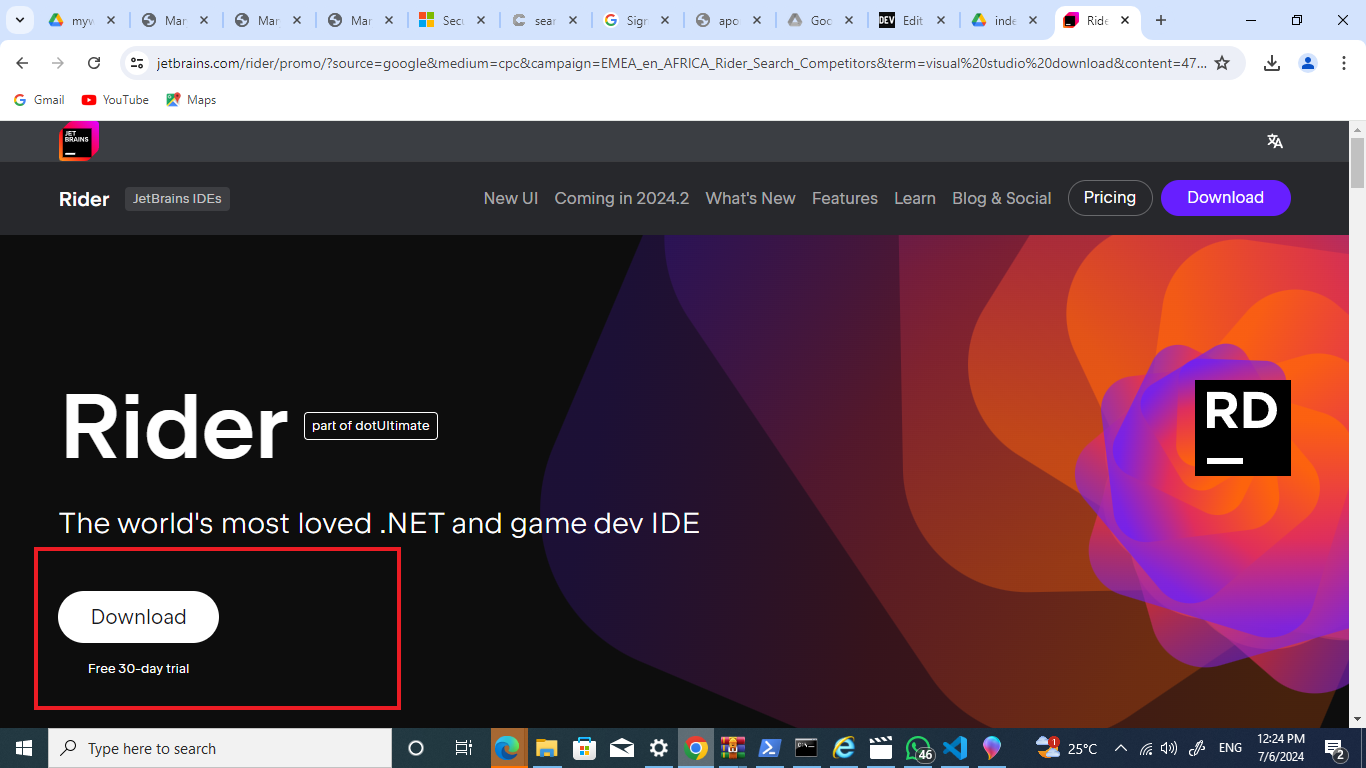
-
**STEP 2**
Download and create the folder that houses your static website from the google drive, if the folder is Zip try and unzip the folder
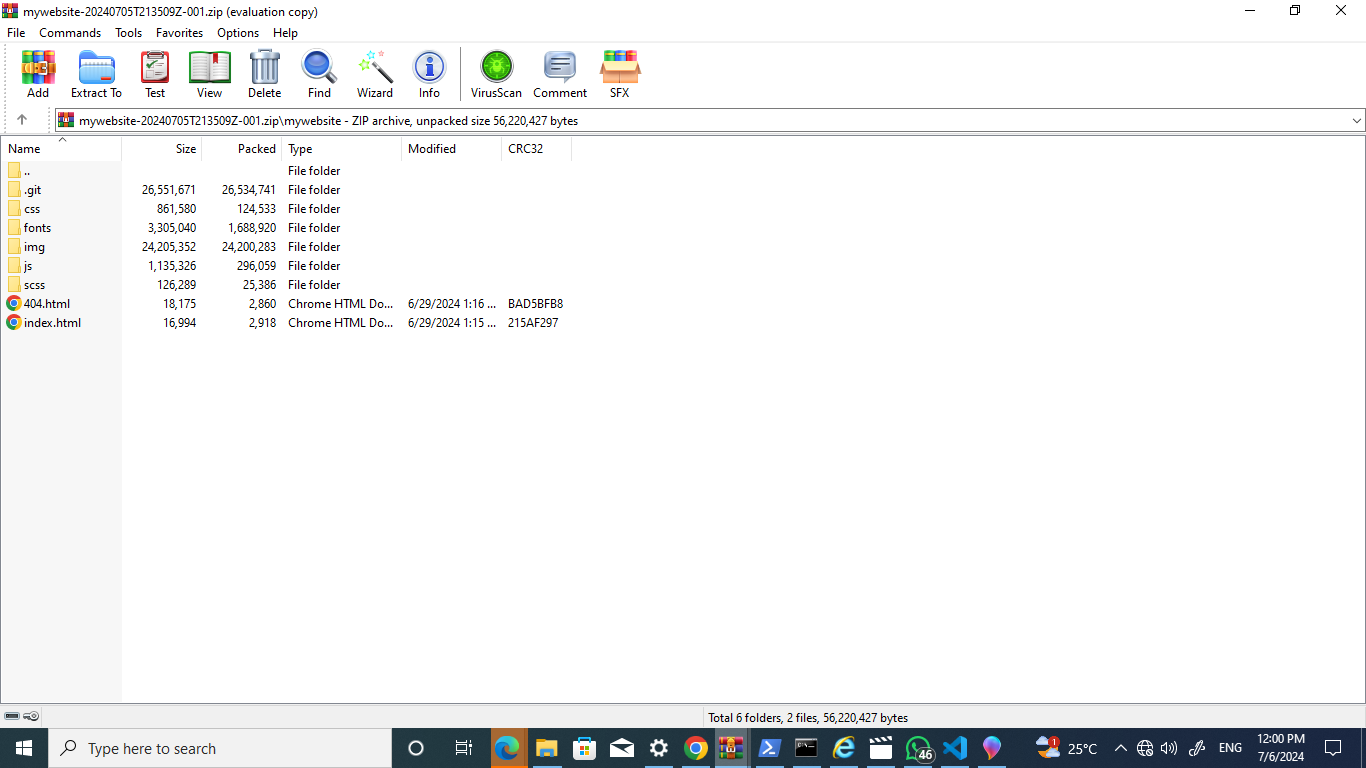
Open the folder
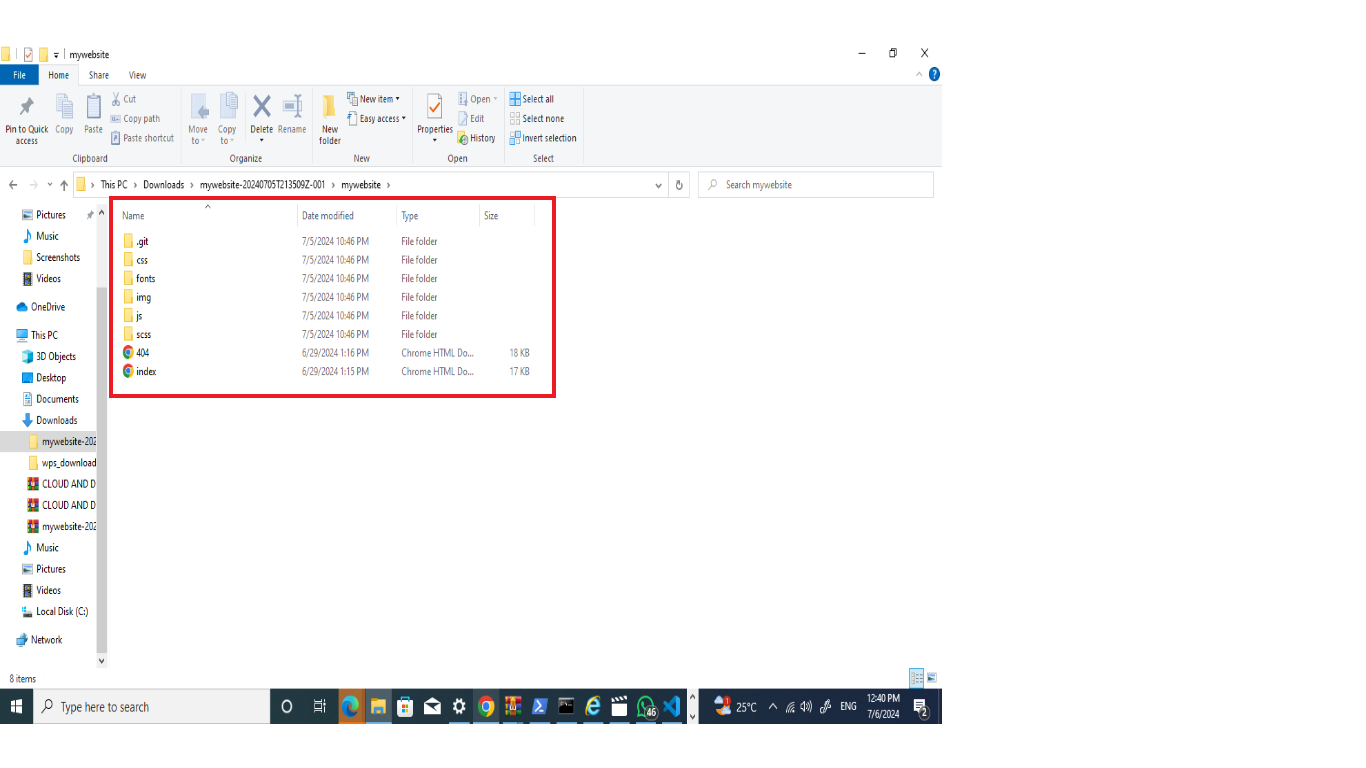
**STEP 3**
.Logon to Azure Portal
. click on create storage Account
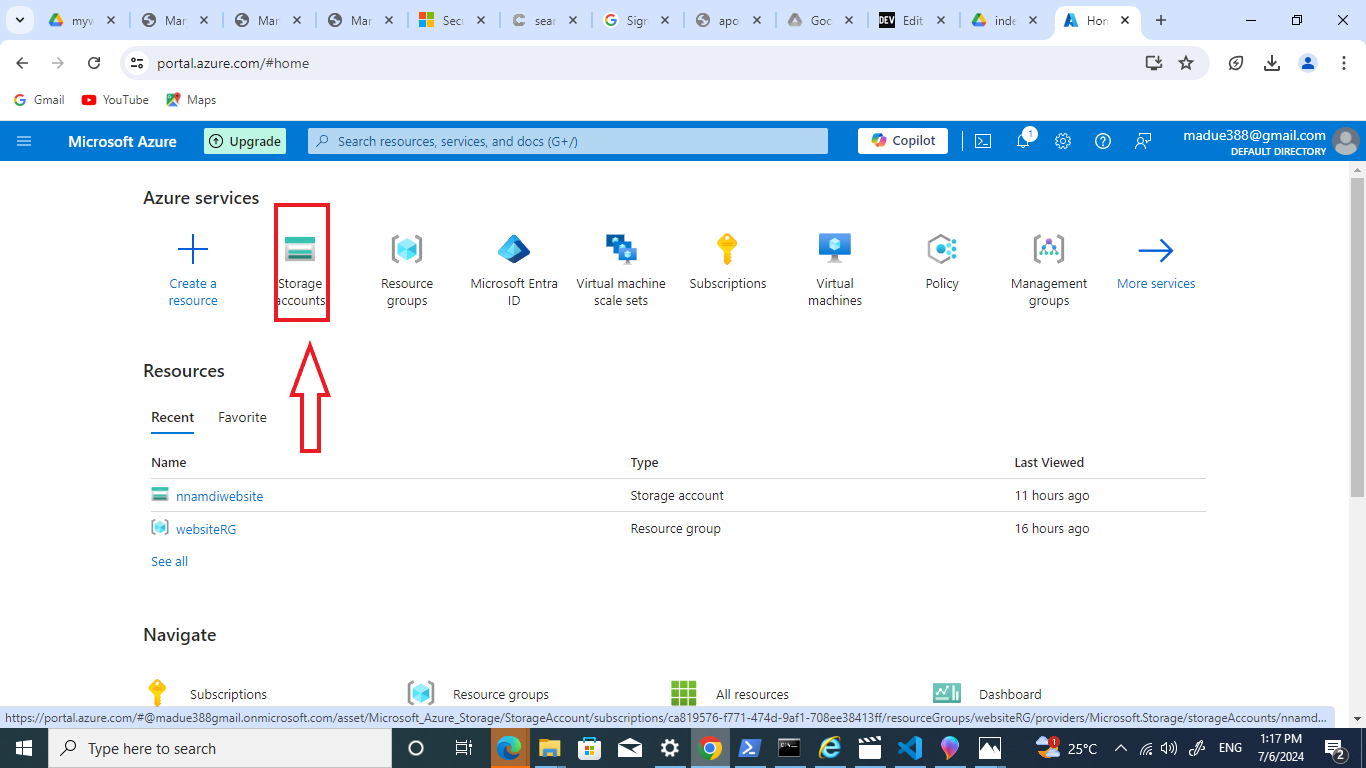
-
| monnanka |
|
1,913,674 | Thiết Kế Website Tại Đà Lạt Thu Hút Khách Hàng | Đà Lạt, với vẻ đẹp tươi mát và khí hậu ôn hòa, đã thu hút ngày càng nhiều doanh nghiệp đến đây mở... | 0 | 2024-07-06T11:28:29 | https://dev.to/terus_technique/thiet-ke-website-tai-da-lat-thu-hut-khach-hang-35i3 | website, digitalmarketing, seo, terus |
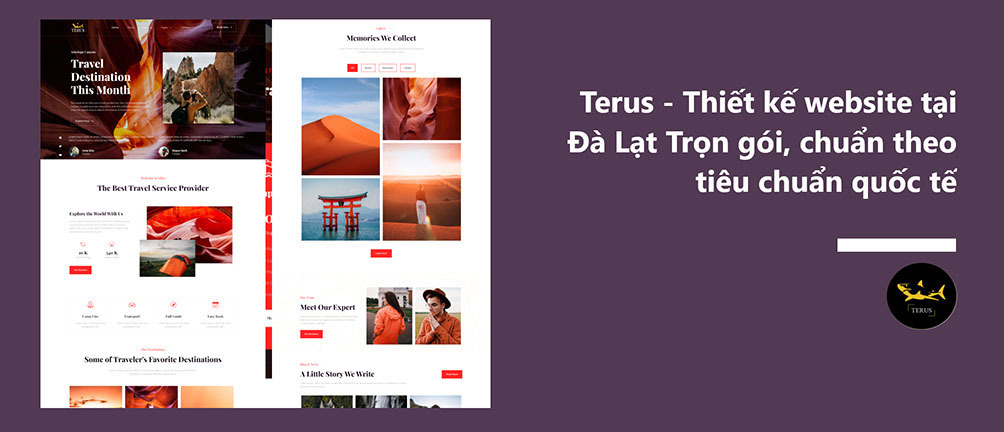
Đà Lạt, với vẻ đẹp tươi mát và khí hậu ôn hòa, đã thu hút ngày càng nhiều doanh nghiệp đến đây mở rộng hoạt động kinh doanh. Tuy nhiên, để thành công trong môi trường cạnh tranh, các doanh nghiệp tại đây cần phải có một sự hiện diện mạnh mẽ trên môi trường trực tuyến. Một website chuyên nghiệp không chỉ là một công cụ thiết yếu để tăng khả năng tiếp cận khách hàng tiềm năng, mà còn là cách để xây dựng uy tín, niềm tin và thu hút khách hàng.
Terus, một công ty [thiết kế website chuyên nghiệp tại Đà Lạt](https://terusvn.com/thiet-ke-website-tai-hcm/), sẵn sàng hỗ trợ các doanh nghiệp trong việc xây dựng một website hiệu quả, đáp ứng đầy đủ các yêu cầu và nhu cầu của doanh nghiệp.
Một trong những lợi ích chính mà một website chuyên nghiệp mang lại cho doanh nghiệp là tăng cường khả năng hiện diện và hiển thị trực tuyến. Điều này không chỉ giúp doanh nghiệp tiếp cận được với nhiều khách hàng tiềm năng hơn, mà còn tạo ra một kênh tiếp thị hiệu quả, giúp khách hàng dễ dàng tìm kiếm và tiếp cận với sản phẩm, dịch vụ của doanh nghiệp.
Ngoài ra, một website chuyên nghiệp còn giúp doanh nghiệp trình bày một cách đầy đủ và thu hút các sản phẩm, dịch vụ của mình, từ đó tạo được niềm tin và sự tín nhiệm từ khách hàng. Điều này rất quan trọng, đặc biệt là với các doanh nghiệp mới thành lập hoặc chưa có được uy tín trên thị trường.
Terus cam kết mang đến các [dịch vụ thiết kế website tại Đà Lạt với chất lượng cao](https://terusvn.com/thiet-ke-website-tai-hcm/) và giá cả cạnh tranh. Đội ngũ nhân viên của Terus không chỉ có chuyên môn cao, mà còn có kiến thức sâu rộng về thị trường và đặc điểm của doanh nghiệp tại Đà Lạt. Từ đó, Terus có thể thiết kế website phù hợp với nhu cầu riêng của từng doanh nghiệp, đồng thời đảm bảo tính thân thiện với người dùng và tính ứng dụng cao.
Ngoài việc thiết kế website, Terus còn cung cấp các dịch vụ digital marketing, giải pháp quản lý, và thiết kế phần mềm. Với đội ngũ chuyên gia và kinh nghiệm phong phú, Terus cam kết mang lại những giải pháp toàn diện, giúp doanh nghiệp tại Đà Lạt tăng cường hiệu quả hoạt động và thúc đẩy sự tăng trưởng bền vững.
Tìm hiểu thêm về [Thiết Kế Website Tại Đà Lạt Thu Hút Khách Hàng](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-da-lat/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,672 | Editing Masterclass: Best Photo Software for Creatives | In today’s photography world, the right tools can make a massive difference. Whether you're a pro... | 0 | 2024-07-06T11:26:06 | https://dev.to/ifavecontents/editing-masterclass-best-photo-software-for-creatives-384l | photoediting, creativetools, photographytips, editingsoftware | In today’s photography world, the right tools can make a massive difference. Whether you're a pro with years under your belt or just starting out with a camera, finding the [best photo editing software](https://ifave.com/category/The-World/Best-photo-editing-software) is a game-changer. With so many options, picking the perfect one can feel like finding a needle in a haystack. This guide will dive into some of the top photo editing tools, helping you make a choice that fits your creative vibe.
**Why Photo Editing Software Matters**
Photo editing software is like the magic wand of photography. It turns your average photos into jaw-dropping masterpieces. The right software not only polishes your images but also lets your creativity run wild. From simple tweaks like adjusting brightness to complex edits like adding layers and masks, the possibilities are endless. And with cool features like AI and machine learning, these tools are smarter and more user-friendly than ever.
**Top Contenders in the Market**
1. **Adobe Photoshop:** Known as the granddaddy of photo editing, Photoshop offers a ton of tools for both editing and design. With layers, masks, and a truckload of filters, it’s the go-to for many pros.
2. **Lightroom:** Another Adobe gem, Lightroom is perfect for managing and editing photos in bulk. Its easy-to-use interface and non-destructive editing make it a favorite for those who want to keep things simple and efficient.
3. **Affinity Photo:** Challenging Adobe’s reign, Affinity Photo packs a punch with powerful features at a fraction of the cost. It’s compatible with Photoshop files and offers professional-grade tools.
4. **Capture One:** This one’s a hit among studio photographers, thanks to its stellar color grading capabilities. Features like tethered shooting and an advanced color editor make it stand out.
5. **GIMP:** If you’re on a budget, GIMP (GNU Image Manipulation Program) is your best bet. It’s free and open-source, with features that rival Photoshop. It might take a bit to learn, but it's a solid option for cost-conscious creatives.
**Choosing the Right Software**
Picking the best photo editing software depends on what you need and how much you’re willing to spend. Think about what features are most important to you and how you plan to use the software. If you need quick and easy photo management, Lightroom might be your best buddy. If you’re diving into heavy-duty design work, Photoshop or Affinity Photo could be your perfect match.
**Hands-On Learning and Community Support**
To get the most out of your software, dive into hands-on learning. Many platforms offer tutorials, webinars, and user guides to help you become a pro. Joining online communities and forums is also a great way to get tips and support from fellow photographers. Sites like YouTube, Reddit, and dedicated photography forums are treasure troves of knowledge and inspiration.
**Experiment and Find Your Style**
Photo editing is as much about creativity as it is about technical know-how. Don’t be afraid to play around with different styles and techniques to find what clicks with you. Experiment with color grading, try out various filters, and dive into advanced features like blending modes and composite editing. The more you experiment, the more you'll develop your unique style.
**Maintaining a Non-Destructive Workflow**
When editing photos, it’s crucial to maintain a non-destructive workflow. This means making adjustments in a way that preserves the original image, allowing you to revert back if needed. Tools like Lightroom and Capture One are designed with non-destructive editing in mind, making them ideal for those who value flexibility and control over their edits.
**Backup and File Management**
As your photo library grows, efficient file management and backup practices become essential. Consider using external hard drives, cloud storage, or dedicated photo management software to organize and protect your work. Regular backups ensure that you won't lose your precious photos due to hardware failure or accidental deletion.
**Keeping Up with Trends**
The world of photo editing is always evolving, with new trends and techniques popping up regularly. Stay updated by following photography blogs, attending workshops, and participating in industry events. Keeping up with trends not only helps you stay relevant but also sparks your creativity.
Investing in the greatest photo editing software can elevate your photography to new heights. By understanding the strengths and capabilities of each option, you can make an informed choice that enhances your creative workflow. Whether you opt for the industry giants or explore lesser-known gems, the right software will empower you to bring your vision to life, ensuring your photos are nothing short of extraordinary.
Remember, mastering photo editing is an ongoing journey. Keep learning, experimenting, and staying updated with the latest advancements to keep your skills sharp and creativity flowing. With the [greatest photo editing software](https://ifave.com/category/The-World/Best-photo-editing-software) at your fingertips, the only limit is your imagination. Happy editing! | ifavecontents |
1,913,671 | The Future of Travel Planning: Visual Search Technology | In a world where wanderlust knows no bounds, travel has become an integral part of our lives. Whether... | 27,673 | 2024-07-06T11:24:48 | https://dev.to/rapidinnovation/the-future-of-travel-planning-visual-search-technology-2hi9 | In a world where wanderlust knows no bounds, travel has become an integral
part of our lives. Whether it's discovering hidden gems, immersing in new
cultures, or escaping routine, travel holds a special place in our hearts.
However, planning a trip can be challenging, from choosing the perfect
destination to crafting a detailed itinerary. But what if technology could
simplify and enhance this process?
## Welcome to the Future of Travel Planning
Innovation takes center stage with visual search technology, a game-changer in
the travel and hospitality industry. This blog explores the evolution of
travel planning, the rise of visual search, its practical applications, and
how rapid innovation is shaping the future for entrepreneurs and innovators.
## The Evolution of Travel Planning
Travel planning has always been a meticulous process, starting with the
question: Where to next? Traditionally, travelers relied on guidebooks, travel
agencies, and online resources. However, the internet and social media have
created information overload, leading to decision fatigue.
## The Emergence of Visual Search
Visual search is a technological marvel that allows users to search using
images instead of text. Initially embraced by e-commerce, its potential
extends far beyond online shopping, revolutionizing how we explore and
discover the world.
## Practical Applications of Visual Search in Travel & Hospitality
Visual search enables travelers to explore destinations through images,
bridging the gap between visual inspiration and practical planning. Imagine
scrolling through stunning travel photos and accessing detailed information
with a simple tap. This technology also provides real-time information during
journeys, streamlining the entire trip planning process.
## The Future Unveiled: Tailored Recommendations and Community-Driven
Exploration
Visual search technology offers tailored recommendations based on individual
preferences, creating unique travel experiences. It also fosters community-
driven exploration, where travelers share discoveries and inspire others.
## Rapid Innovation and the Entrepreneur's Vision
Innovation drives progress in travel planning. Entrepreneurs and innovators
have the opportunity to create tools that empower travelers, making journeys
smoother and more immersive. By harnessing technology, we can simplify the
travel planning process and enhance the overall experience.
## Join the Journey
The future of travel planning is exciting and promising. Together, we can
unlock the full potential of visual search technology, cater to travelers'
unique needs, and redefine how we explore our remarkable planet. Let's embrace
rapid innovation and create a future where travel planning is an enriching
journey of discovery.
📣📣Drive innovation with intelligent AI and secure blockchain technology! Check
out how we can help your business grow!
[Blockchain App Development](https://www.rapidinnovation.io/service-
development/blockchain-app-development-company-in-usa)
[Blockchain App Development](https://www.rapidinnovation.io/service-
development/blockchain-app-development-company-in-usa)
[AI Software Development](https://www.rapidinnovation.io/ai-software-
development-company-in-usa)
[AI Software Development](https://www.rapidinnovation.io/ai-software-
development-company-in-usa)
## URLs
* <https://www.rapidinnovation.io/post/unlocking-the-future-of-travel-planning-app-the-power-of-visual-search>
## Hashtags
#TravelTech
#VisualSearch
#FutureOfTravel
#InnovativeTravel
#ExploreTheWorld
| rapidinnovation |
|
1,913,670 | Thiết Kế Website Tại Vũng Tàu Uy Tín, Chuyên Nghiệp | Vũng Tàu là một thành phố du lịch sầm uất, với nhiều điểm đến hấp dẫn và sự kiện địa phương tấp nập.... | 0 | 2024-07-06T11:23:55 | https://dev.to/terus_technique/thiet-ke-website-tai-vung-tau-uy-tin-chuyen-nghiep-2pcl | website, digitalmarketing, seo, terus |
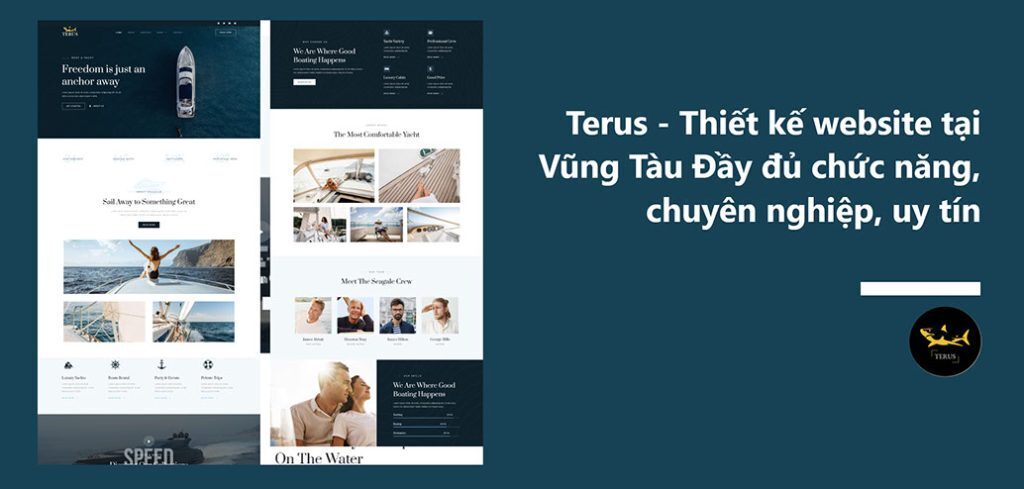
Vũng Tàu là một thành phố du lịch sầm uất, với nhiều điểm đến hấp dẫn và sự kiện địa phương tấp nập. Để tối đa hóa sức hút của thành phố này, các doanh nghiệp tại Vũng Tàu đang có nhu cầu [thiết kế website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/), đáp ứng đầy đủ các chức năng cần thiết.
Thiết kế website tại Vũng Tàu trở nên quan trọng hơn bao giờ hết, vì một website hiệu quả có thể giúp các doanh nghiệp:
Giới thiệu thông tin, sản phẩm/dịch vụ của doanh nghiệp một cách toàn diện
Tăng tương tác và trao đổi với khách hàng tiềm năng
Cung cấp hướng dẫn, thông tin về điểm đến và sự kiện địa phương
Tích hợp các tính năng đặt chỗ, đặt tour du lịch tại Vũng Tàu
Xây dựng cộng đồng địa phương và chia sẻ thông tin hữu ích
Với website tại Vũng Tàu do Terus thiết kế, các doanh nghiệp sẽ nhận được những tính năng độc đáo như:
Hướng dẫn điểm đến toàn diện ngay trên trang chủ
Bản đồ và hành trình tương tác để khách hàng dễ dàng lập kế hoạch
Tính năng đặt chỗ ở và du lịch mới nhất
Blog chia sẻ lịch sự kiện và lễ hội địa phương
Khuyến nghị về ăn uống và ẩm thực tại Vũng Tàu
Thông tin chi tiết về cộng đồng địa phương
Terus cam kết sẽ [thiết kế website tại Vũng Tàu](https://terusvn.com/thiet-ke-website-tai-hcm/) với quy trình chuyên nghiệp, đầy đủ các bước từ tư vấn, thiết kế demo, hoàn thiện giao diện, tối ưu chỉ số, cho đến bàn giao và hướng dẫn sử dụng. Các website Terus thiết kế trước đây cũng đã được đánh giá cao về tính ưu việt, trực quan và tích hợp đầy đủ các tính năng cần thiết.
Đối với doanh nghiệp tại Vũng Tàu, việc hợp tác với Terus trong thiết kế website sẽ mang lại nhiều lợi ích. Ngoài việc có một website chuyên nghiệp, doanh nghiệp còn có thể tận dụng các tính năng độc đáo do Terus tích hợp, giúp tăng trải nghiệm người dùng và thu hút khách hàng hiệu quả hơn. Quy trình thiết kế website chi tiết và chuyên nghiệp cũng đảm bảo doanh nghiệp sẽ có một sản phẩm hoàn thiện, đáp ứng đầy đủ yêu cầu.
Với kinh nghiệm và chuyên môn sâu rộng, Terus cam kết sẽ mang đến cho các doanh nghiệp tại Vũng Tàu những website chất lượng, đầy đủ tính năng và uy tín. Đây chính là giải pháp hiệu quả để tăng sức cạnh tranh, kết nối khách hàng và quảng bá hình ảnh thương hiệu của doanh nghiệp trong bối cảnh du lịch Vũng Tàu ngày càng phát triển.
Tìm hiểu thêm về [Thiết Kế Website Tại Vũng Tàu Uy Tín, Chuyên Nghiệp](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-vung-tau/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,569 | Role of SEO in Website development | SEO (Search Engine Optimization) plays a crucial role in website development by ensuring that a... | 0 | 2024-07-06T10:03:14 | https://dev.to/gulshan_one_29a06d8b2ba37/role-of-seo-in-website-development-18ll | seo, websitedevelopment | SEO (Search Engine Optimization) plays a crucial role in website development by ensuring that a website is not only user-friendly but also search engine-friendly. Integrating SEO into the website development process from the beginning can lead to better search engine rankings, increased organic traffic, and an overall better user experience. Here are the key aspects of SEO in [website development](https://phoenixedgemarketing.com/website-designing-company/):
**1. Site Structure and Navigation**
Clean URL Structure: Use descriptive, keyword-rich URLs that are easy for both users and search engines to understand.
Logical Site Hierarchy: Organize content in a logical, hierarchical manner with clear categories and subcategories, making it easier for search engines to crawl and index the site.
Breadcrumbs: Implement breadcrumbs to help users and search engines understand the site’s structure and navigate more easily.
**2. Mobile-Friendliness**
Responsive Design: Ensure the website is responsive, providing a seamless experience across all devices, which is critical for both user experience and search engine rankings.
Mobile Usability: Optimize for mobile usability by ensuring that buttons are easily clickable, text is readable without zooming, and content fits within the screen without horizontal scrolling.
**3. Page Speed Optimization**
Fast Loading Times: Optimize images, use efficient coding practices, and leverage browser caching to ensure fast loading times, which is a ranking factor for search engines.
Minimalistic Design: Avoid heavy design elements that can slow down the site and impact user experience.
**4. Content Optimization
**
Keyword Research: Conduct thorough keyword research to understand what terms your target audience is searching for and incorporate these keywords naturally into your content.
Quality Content: Develop high-quality, original content that provides value to users and satisfies their search intent.
Content Structure: Use headings (H1, H2, H3) to structure content, making it easier for both users and search engines to read and understand.
**5. Meta Tags and Descriptions
**Title Tags: Create unique, descriptive, and keyword-rich title tags for each page to help search engines understand the page’s content and relevance.
Meta Descriptions: Write compelling meta descriptions that include target keywords and encourage users to click through from the search results.
**6. Image Optimization
**
Alt Text: Use descriptive alt text for images to help search engines understand the content of the images and improve accessibility for users with visual impairments.
Image Compression: Compress images to reduce file size without sacrificing quality, which helps improve page load times.
**7. Internal Linking
**
Logical Internal Links: Use internal links to connect related content, helping search engines crawl the site more effectively and improving user navigation.
Anchor Text: Use descriptive anchor text that includes relevant keywords to provide context to both users and search engines.
**8. XML Sitemaps and Robots.txt
**
XML Sitemaps: Create and submit an XML sitemap to search engines to help them discover and index all the pages on your site.
Robots.txt: Use a robots.txt file to control which pages search engines should crawl and index.
**9. Security
**
HTTPS: Implement HTTPS to secure your website, which is a ranking factor for search engines and provides a secure connection for users.
Regular Security Audits: Perform regular security audits to identify and fix vulnerabilities that could negatively impact your site’s performance and user trust.
**10. Analytics and Monitoring
**
Google Analytics: Install Google Analytics to track user behavior, traffic sources, and conversions, providing valuable insights for ongoing SEO efforts.
Google Search Console: Use Google Search Console to monitor your site’s performance in search results, identify issues, and optimize accordingly.
**11. Schema Markup
**
Structured Data: Implement schema markup to help search engines understand the content and context of your pages, potentially enhancing search visibility with rich snippets.
By integrating SEO best practices into website development, you create a strong foundation for your site that enhances its visibility, usability, and overall effectiveness. This proactive approach helps ensure that your website meets the needs of both users and search engines, leading to better performance and higher search engine rankings. | gulshan_one_29a06d8b2ba37 |
1,913,658 | 10 Captivating Programming Challenges to Elevate Your Coding Skills 🚀 | The article is about a curated collection of 10 captivating programming challenges from the LabEx platform. These challenges cover a wide range of topics, including building responsive layouts, creating interactive games, deploying machine learning models, and more. The article provides a brief overview of each challenge, highlighting the key skills and concepts that users will learn, and includes direct links to the challenges. Whether you're a seasoned developer or a coding enthusiast, this compilation promises to push the boundaries of your skills and ignite your passion for programming. The article aims to inspire readers to dive into these engaging challenges and elevate their coding abilities to new heights. | 27,689 | 2024-07-06T11:20:57 | https://dev.to/labex/10-captivating-programming-challenges-to-elevate-your-coding-skills-eb6 | css, coding, programming, tutorial |
Embark on an exhilarating journey through a curated collection of 10 programming challenges from the LabEx platform. These challenges cover a diverse range of topics, from building responsive layouts and interactive games to deploying machine learning models on the web. Whether you're a seasoned developer or a coding enthusiast, this compilation promises to push the boundaries of your skills and ignite your passion for programming. 🔥

## 1. Implement Atomic Flex Layout with CSS (Challenge)
Dive into the world of Atomic CSS, a popular CSS construction method, and learn how to implement an attribute-based (Attributify) atomic CSS layout. This challenge will have you honing your CSS skills to achieve the desired layout using the flex attribute. [Explore the challenge](https://labex.io/labs/300041)
## 2. Creating a Minesweeper Game With JavaScript (Challenge)
Revisit the classic game of Minesweeper and take on the challenge of developing a web-based version. Unleash your JavaScript prowess to bring this addictive game to life, complete with a visually appealing interface. [Tackle the challenge](https://labex.io/labs/299493)
## 3. Create a Swiper Carousel Web App (Challenge)
Embark on a cosmic-themed journey and learn how to create a Swiper carousel web app. Dive into the world of interactive carousels and craft a visually stunning layout that showcases fascinating facts about the universe. [Dive into the challenge](https://labex.io/labs/299491)
## 4. Build a Tic-Tac-Toe Web App (Challenge)
Revisit the timeless classic of Tic-Tac-Toe and take on the challenge of creating a web-based version using HTML, CSS, and JavaScript. Implement the game logic step by step and bring this engaging two-player game to life. [Explore the challenge](https://labex.io/labs/299482)
## 5. Deploying MobileNet With TensorFlow.js and Flask (Challenge)
Venture into the realm of machine learning and learn how to deploy a pre-trained MobileNetV2 model using TensorFlow.js within a Flask web application. Discover the power of running machine learning models directly in the browser and create an interactive web application that classifies images on the fly. [Dive into the challenge](https://labex.io/labs/299451)
## 6. Building a React GitHub Heatmap Contributions (Challenge)
Explore the world of data visualization and create a heatmap calendar in React, resembling GitHub's contribution graph. Dive into the intricacies of integrating and using a calendar heatmap in a React application. [Tackle the challenge](https://labex.io/labs/299487)
## 7. Responsive Flexible Card Layout (Challenge)
Put your CSS Flexbox skills to the test and create a responsive card layout that adjusts to different screen sizes and orientations. Embrace the challenge of using various Flexbox properties to achieve the desired layout. [Explore the challenge](https://labex.io/labs/300066)
## 8. Create Responsive Modal Boxes (Challenge)
Dive into the world of event handling and learn how to implement a show or hide operation for a modal box component. Tackle the challenge of dealing with event bubbling and ensure a seamless user experience. [Dive into the challenge](https://labex.io/labs/299872)
## 9. Build a Sliding Puzzle Game With JavaScript (Challenge)
Unleash your JavaScript prowess and build a simple Sliding Puzzle game. Craft a functioning 3x3 sliding puzzle game with numbered tiles, a timer, and controls to start, pause, and reset the game. [Explore the challenge](https://labex.io/labs/299481)
## 10. Parsing and Highlighting Text Content (Challenge)
Embark on a challenge that simulates the process of extracting parameter values from a URL string and highlighting text based on those values. Dive into the intricacies of text manipulation and content highlighting. [Tackle the challenge](https://labex.io/labs/295863)
Dive into these captivating programming challenges and elevate your coding skills to new heights! 💻 Each challenge offers a unique opportunity to expand your knowledge, hone your problem-solving abilities, and push the boundaries of your creativity. 🌟 Embrace the journey, and let these challenges be your stepping stones to becoming a more proficient and versatile programmer. 🚀
---
## Want to learn more?
- 🌳 Learn the latest [CSS Skill Trees](https://labex.io/skilltrees/css)
- 📖 Read More [CSS Tutorials](https://labex.io/tutorials/category/css)
- 🚀 Practice thousands of programming labs on [LabEx](https://labex.io)
Join our [Discord](https://discord.gg/J6k3u69nU6) or tweet us [@WeAreLabEx](https://twitter.com/WeAreLabEx) ! 😄 | labby |
1,913,640 | Luxury Car Rentals in Doha: Experience the Best | At A Star Limousine, we believe in offering our clients the ultimate experience in luxury car rentals... | 0 | 2024-07-06T11:20:26 | https://dev.to/astarlimousineqa_e50e8bc9/luxury-car-rentals-in-doha-experience-the-best-15nj | At [A Star Limousine](https://www.astarlimousineqa.com/), we believe in offering our clients the ultimate experience in luxury car rentals in Doha. Whether you are visiting for business or pleasure, our fleet of high-end vehicles, coupled with our professional chauffeur service, ensures that your journey is as comfortable and stylish as possible. In this blog, we will explore the various options available for those looking to rent a car in Qatar, highlighting our unparalleled services and the benefits of choosing A Star Limousine.
Why Choose A Star Limousine for Car Rental in Doha?
When it comes to car rental in Doha, A Star Limousine stands out for several reasons:
Diverse Fleet of Luxury Vehicles: Our collection includes the latest models from top brands, ensuring that you have access to the best vehicles on the market.
Personalized Chauffeur Services: Experience the convenience of having a professional driver who knows the city well, ensuring you reach your destination safely and on time.
Affordable Rates: Despite our focus on luxury, we offer competitive pricing, making it possible for you to enjoy a premium experience without breaking the bank.
Rent a Car in Qatar: Options and Benefits
Luxury Car Rentals
Renting a luxury car in Qatar allows you to travel in style and comfort. Our vehicles are equipped with the latest technology and amenities, ensuring a smooth and enjoyable ride. Whether you need a car for a special occasion or a business trip, A Star Limousine has the perfect vehicle for you.
Car Rental Near Me: Convenience at Your Fingertips
Finding a car rental near you in Doha has never been easier. With multiple locations across the city, A Star Limousine ensures that you can access our services wherever you are. Our online booking system is user-friendly, allowing you to reserve your vehicle quickly and efficiently.
Affordable Options: Cheap Rent a Car in Qatar
At A Star Limousine, we understand that luxury doesn't have to come with a hefty price tag. We offer a range of affordable options for those looking for a cheap rent a car in Qatar. Despite the lower cost, these vehicles still meet our high standards of quality and comfort.
Pre-Owned Vehicles: Used Cars in Qatar
For those interested in purchasing a vehicle, A Star Limousine also offers a selection of used cars in Qatar. Our pre-owned vehicles are thoroughly inspected and maintained, ensuring that you get a reliable car at a fraction of the price of a new one.
The Process: How to Rent a Car in Qatar
Renting a car in Qatar with A Star Limousine is a straightforward process:
Browse Our Fleet: Visit our website to view our selection of luxury vehicles.
Make a Reservation: Use our online booking system to reserve your chosen vehicle.
Pick Up Your Car: Collect your vehicle from one of our convenient locations.
Enjoy Your Ride: Experience the best in luxury car rentals as you explore Doha.
Conclusion
A Star Limousine is dedicated to providing the best luxury car rental experience in Doha. With our diverse fleet, professional chauffeur services, and competitive pricing, we make it easy for you to enjoy the best that Qatar has to offer. Whether you need a car for a day or an extended period, we have the perfect solution for you. Rent a car in Qatar with A Star Limousine and experience the difference.
For more information or to make a reservation,[please visit our website](https://www.astarlimousineqa.com/rent-a-car-qatar/)
| astarlimousineqa_e50e8bc9 |
|
1,913,619 | Thiết Kế Website Tại Vĩnh Phúc Tăng Doanh Thu | Tại sao các doanh nghiệp nên có website tại Vĩnh Phúc? Nâng cao khả năng hiển thị trực tuyến: Một... | 0 | 2024-07-06T11:19:41 | https://dev.to/terus_technique/thiet-ke-website-tai-vinh-phuc-tang-doanh-thu-50dh | website, digitalmarketing, seo, terus |
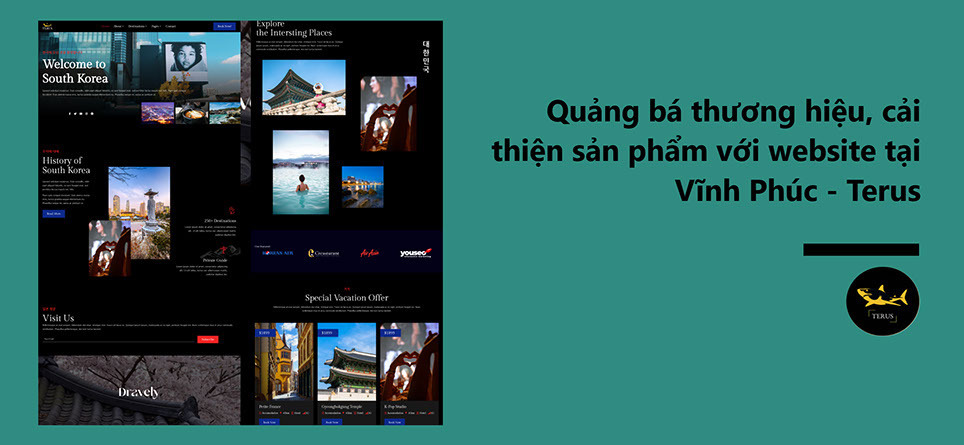
Tại sao các doanh nghiệp nên có website tại Vĩnh Phúc?
Nâng cao khả năng hiển thị trực tuyến: Một [website chuyên nghiệp](https://terusvn.com/thiet-ke-website-tai-hcm/) giúp doanh nghiệp có sự hiện diện mạnh mẽ trên internet, từ đó tiếp cận và thu hút được nhiều khách hàng tiềm năng hơn.
Mở rộng phạm vi tiếp cận thị trường: Website cho phép doanh nghiệp vươn xa hơn, không chỉ giới hạn trong phạm vi địa phương mà có thể tiếp cận với khách hàng trên toàn quốc hoặc thậm chí quốc tế.
Gắn kết khách hàng hiệu quả: Một website được thiết kế tốt sẽ giúp doanh nghiệp tương tác và giao tiếp với khách hàng một cách chuyên nghiệp, tạo niềm tin và trải nghiệm tích cực.
Sẵn sàng và thuận tiện 24/7: Khác với cửa hàng vật lý, website của doanh nghiệp luôn sẵn sàng hoạt động 24 giờ mỗi ngày, 7 ngày trong tuần, mang lại sự tiện lợi tối đa cho khách hàng.
Trưng bày những ưu đãi độc đáo: Website cung cấp một không gian trực tuyến lý tưởng để doanh nghiệp giới thiệu, quảng bá và trưng bày các sản phẩm, dịch vụ, chương trình khuyến mãi độc đáo.
Những điểm nổi bật của dịch vụ thiết kế website tại Vĩnh Phúc của Terus
Thiết kế website phù hợp với địa phương: Terus hiểu rõ thị trường Vĩnh Phúc và thiết kế website phù hợp với nhu cầu và thói quen của người dùng tại đây.
Tích hợp liền mạch thông tin của doanh nghiệp: Website của Terus sẽ tích hợp toàn bộ thông tin, hình ảnh, dữ liệu của doanh nghiệp một cách đồng bộ và chuyên nghiệp.
Các tính năng tương tác sáng tạo: Terus tích hợp các tính năng tương tác độc đáo như chatbot, video, AR/VR, v.v. để tăng trải nghiệm người dùng.
Tích hợp thương mại điện tử: Website của Terus có thể kết nối với hệ thống bán hàng trực tuyến của doanh nghiệp, giúp họ tiếp cận và bán hàng hiệu quả hơn.
Thiết kế lấy người dùng làm trung tâm: Terus luôn đặt nhu cầu và trải nghiệm của người dùng làm ưu tiên hàng đầu khi thiết kế website.
Với kinh nghiệm phong phú, đội ngũ chuyên gia và quy trình thiết kế chặt chẽ, Terus cam kết mang đến những [giải pháp website chuyên nghiệp, hiệu quả](https://terusvn.com/thiet-ke-website-tai-hcm/) và phù hợp với nhu cầu của doanh nghiệp tại Vĩnh Phúc. Liên hệ ngay với Terus để được tư vấn và báo giá dịch vụ thiết kế website phù hợp.
Tìm hiểu thêm về [Thiết Kế Website Tại Vĩnh Phúc Tăng Doanh Thu](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-vinh-phuc/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,910,500 | HNG STAGE 1 TASK: Linux User Creation Bash Script Task | Introduction Managing user accounts and groups efficiently is a critical task for any SysOps... | 0 | 2024-07-06T11:19:24 | https://dev.to/ayocloud247/hng-stage-1-task-linux-user-creation-bash-script-task-61a | **Introduction**
Managing user accounts and groups efficiently is a critical task for any SysOps engineer. It is essential to automate this process to ensure consistency, security, and ease of management. In this article, we will explore a bash script that automates the creation of users and groups based on a provided text file. This script sets up home directories, generates random passwords, and logs all actions performed.
**Script Overview**
Our bash script, create_users.sh, reads a text file where each line is formatted as username;groups, creates users, assigns them to groups, and logs the actions. The script also handles error scenarios for existing users and sets appropriate permissions for home directories and password files.
_Initialization and Variable Declaration_
```
#!/bin/bash
log_message_file="/var/log/user_management.log"
passwords_file="/var/secure/user_passwords.txt"
```
I defined the log and password files, ensuring they exist.
_Fucntion to Verify User and Group_
```
# Check if a user exists
user_exists() {
local username=$1
if getent passwd "$username" > /dev/null 2>&1; then
return 0 # User exists
else
return 1 # User does not exist
fi
}
# Check if a group exists
group_exists() {
local group_name=$1
if getent group "$group_name" > /dev/null 2>&1; then
return 0 # Group exists
else
return 1 # Group does not exist
fi
}
```
I declare functions to check if the users and groups exists. They will be called in later
_Function for Password Generation and Logging_
```
# Generate a random password
generate_password() {
openssl rand -base64 12
}
# Log actions to /var/log/user_management.log
log() {
local MESSAGE="$1"
echo "$(date +'%Y-%m-%d %H:%M:%S') - $MESSAGE" | sudo tee -a $log_message_file > /dev/null
}
```
The first function generates a random password and the second function sends a log of all actions to the specified file
```
# Assign the file name from the command line argument
user_group=$1
```
Here, a variable is assigned to the command line argument as a stand in for the filename
_Log and Password File Creation_
```
# Check if the log file exist
if [ ! -f "$log_messsage_file" ]; then
# Create the log file
sudo touch "$log_message_file"
log "$log_message_file has been created."
else
log "$log_message_file exists already"
fi
# Check and create the passwords_file
if [ ! -f "$passwords_file" ]; then
# Create the file and set permissions
sudo mkdir -p /var/secure/
sudo touch "$passwords_file"
log "$passwords_file has been created."
# Set ownership permissions for passwords_file
sudo chmod 600 "$passwords_file"
log "Updated passwords_file permission to file owner"
else
log "$passwords_file exists already"
fi
```
Here, the log file and password file are checked for any sign of existence and created if none is found
_Looping Through Users and Groups_
```
while IFS=';' read -r username groups; do
# Extract the user name
username=$(echo "$username" | xargs)
```
Using IFS, I separate the value in our text file using the ";" as the delimiter and extract the username and groups
_Checking for Users and Password Creation_
```
# Check if the user exists
if user_exists "$username"; then
log "$username exists already"
continue
else
# Generate a random password for the user
password=$(generate_password)
# Create the user with home directory and set password
sudo useradd -m -s /bin/bash "$username"
echo "$username:$password" | sudo chpasswd
log "Successfully Created User: $username"
fi
```
This code checks if the user already exists; otherwise, it creates the user and gives it a randomized password.
_Checking for User Group_
```
# check that the user has its own group
if ! group_exists "$username"; then
sudo groupadd "$username"
log "Successfully created group: $username"
sudo usermod -aG "$username" "$username"
log "User: $username added to Group: $username"
else
log "User: $username added to Group: $username"
fi
```
It checks if the user created has its own group and adds it to its group if it does not.
_Extracting The Groups_
```
# Extract the groups and remove any spaces
groups=$(echo "$groups" | tr -d ' ')
# Split the groups by comma
IFS=',' read -r -a group_count <<< "$groups"
```
From the text file, we extract the groups, spilt the values by the comma "," delimiter and append the values into the _group_count _array.
_Creation of Groups and Attachment of Users_
```
# Create the groups and add the user to each group
for group in "${group_count[@]}"; do
# Check if the group already exists
if ! group_exists "$group"; then
# Create the group if it does not exist
sudo groupadd "$group"
log "Successfully created Group: $group"
else
log "Group: $group already exists"
fi
# Add the user to the group
sudo usermod -aG "$group" "$username"
done
```
By looping through the array, we check if the group exists, create it if it does not and add the users to their respective groups
_Setting Appropriate Permission_
```
# Set permissions for home directory
sudo chmod 700 "/home/$username"
sudo chown "$username:$username" "/home/$username"
log "Updated permissions for home directory: '/home/$username' of User: $username to '$username:$username'"
```
Here, we set the permission and ownership for the home directory of the created user(s)
_Storing Username and Password in Password file_
```
# Store username and password in secure file
echo "$username,$password" | sudo tee -a "$passwords_file" > /dev/null
log "Stored username and password in $passwords_file"
done < "$user_group"
```
Here, we store the user and password in the specified file
To learn more about the HNG internship and what they do, check out [HNG Internship](https://hng.tech/internship). You can also visit [HNG Hire](https://hng.tech/hire) to scout the best talents.
Thank You. | ayocloud247 |
|
1,913,618 | Tech Trends: Leading Mobile Phone Brands Today | In today's fast-paced digital world, keeping up with the latest in mobile tech is crucial. The mobile... | 0 | 2024-07-06T11:17:45 | https://dev.to/ifavecontents/tech-trends-leading-mobile-phone-brands-today-451 | mobiletech, smartphonebrands, techtrends, mobileinnovation | In today's fast-paced digital world, keeping up with the latest in mobile tech is crucial. The mobile phone market is brimming with innovation, giving us loads of choices from [top mobile phone brands](https://ifave.com/category/The-World/Top-mobile-phone-brands). These brands are constantly raising the bar with cutting-edge features and sleek designs, making it hard to pick a favorite.
When we think of the top mobile phone brands, Apple, Samsung, and Google are the big shots. Apple is famous for its seamless integration of hardware and software, delivering a user experience that's hard to beat. Samsung, with its vast range of devices, has something for everyone, from tech geeks to regular users. And then there's Google, which focuses on giving us the purest Android experience with its Pixel series, known for its stellar cameras.
**Apple**
Apple's iPhone lineup continues to set the bar high. The latest iPhone models boast impressive performance, advanced camera systems, and a secure, user-friendly interface. Features like Face ID and powerful A-series chips make Apple a frontrunner in the smartphone race.
**Samsung**
Samsung's Galaxy series offers something for everyone, from the budget-friendly Galaxy A series to the premium Galaxy S and Note series. Samsung's commitment to innovation shines through its pioneering work with foldable phones like the Galaxy Z Fold and Z Flip, captivating tech enthusiasts worldwide.
**Google**
Google's Pixel phones are celebrated for their exceptional camera performance, thanks to Google's computational photography skills. The Pixel lineup offers a clean Android experience, ensuring users get timely updates and a bloatware-free interface. The latest Pixel devices also showcase impressive AI features, making them a hit among tech-savvy consumers.
**Other Notable Brands**
While Apple, Samsung, and Google dominate, other brands like OnePlus, Xiaomi, and Huawei are making big waves. OnePlus has built a loyal fan base by offering flagship-level features at competitive prices. Xiaomi is expanding its global presence, known for high-quality devices with impressive specs. Huawei, despite facing challenges, remains a key player with its innovative tech and strong focus on camera performance.
**Emerging Contenders**
Beyond these giants, brands like Oppo, Vivo, and Realme are making their mark, especially in Asian markets. Oppo's focus on camera innovation and stylish designs makes it a hit with younger consumers. Vivo, with its emphasis on audio-visual experiences and features like in-display fingerprint sensors, is attracting a growing customer base. Realme, a relatively new player, is quickly gaining a reputation by offering powerful specs at budget-friendly prices.
**Innovations Driving the Market**
The fierce competition among top mobile phone brands has led to rapid tech advancements. 5G connectivity is becoming standard, promising faster speeds, better streaming, and improved online gaming. Foldable phones, once a sci-fi dream, are now a reality with Samsung and Huawei leading the way. These devices offer the convenience of a phone with the screen size of a tablet, appealing to both productivity-focused users and multimedia enthusiasts.
**Sustainability and Ethical Practices**
As we all become more eco-conscious, top mobile phone brands are focusing on sustainability and ethical practices. Apple is reducing its carbon footprint by using recycled materials and aiming for carbon neutrality. Samsung is also committed to sustainable practices, minimizing plastic use, and incorporating eco-friendly materials in its packaging. Fairphone takes it a step further, ensuring fair labor practices and prioritizing repairability and longevity.
**The Role of Software**
In the smartphone world, software is king. Apple's iOS and Google's Android are the dominant operating systems, each with unique features and ecosystems. Apple's iOS is known for its seamless integration with other Apple products, intuitive user interface, and robust security. Android, on the other hand, offers more customization, a wide range of devices, and an open-source nature that appeals to tech enthusiasts. Brands like Samsung and OnePlus add their custom touches to Android, offering unique features and optimizations.
**Consumer Preferences and Trends**
Understanding what consumers want is key for these brands. There's a growing demand for phones with top-notch cameras as smartphone photography becomes more popular. Battery life and fast-charging technologies are also high priorities, ensuring our devices keep up with our busy lives. Plus, there's increasing interest in mid-range smartphones that balance performance and price.
As the mobile tech landscape keeps evolving, these [top mobile phone brands](https://ifave.com/category/The-World/Top-mobile-phone-brands) stay ahead, driving innovation and setting new standards. Their commitment to delivering cutting-edge features, sustainable practices, and great user experiences means we have a wide array of options, each catering to different needs and preferences. | ifavecontents |
1,913,616 | Thiết Kế Website Tại Tây Ninh Chuyên Ngiệp, Thu Hút | Các doanh nghiệp ở Tây Ninh ngày càng nhận thức được tầm quan trọng của việc có một website chuyên... | 0 | 2024-07-06T11:15:44 | https://dev.to/terus_technique/thiet-ke-website-tai-tay-ninh-chuyen-ngiep-thu-hut-4kb1 | website, digitalmarketing, seo, terus |
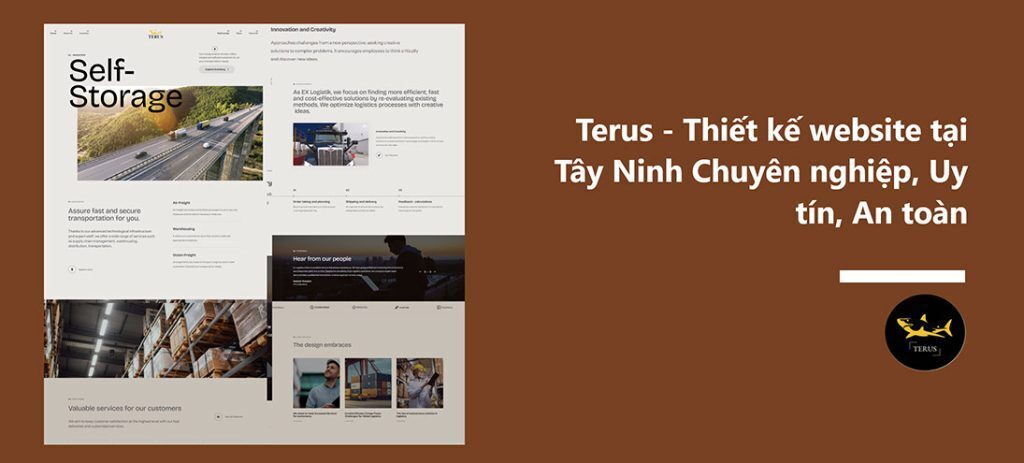
Các doanh nghiệp ở Tây Ninh ngày càng nhận thức được tầm quan trọng của việc có một website chuyên nghiệp. Việc thiết lập một sự hiện diện trực tuyến mạnh mẽ là rất cần thiết cho các công ty tại khu vực này. Một website không chỉ mở rộng phạm vi tiếp cận thị trường của doanh nghiệp, mà còn cung cấp một nền tảng để trưng bày sản phẩm và dịch vụ, xây dựng niềm tin và sự tín nhiệm với khách hàng, tạo điều kiện tương tác, và thúc đẩy doanh số bán hàng cũng như chuyển đổi trực tuyến.
Terus, một công ty chuyên [thiết kế website tại Tây Ninh](https://terusvn.com/thiet-ke-website-tai-hcm/), cung cấp các giải pháp website toàn diện nhằm đáp ứng nhu cầu của các doanh nghiệp địa phương. Các sản phẩm website của Terus tại Tây Ninh được thiết kế dựa trên việc nghiên cứu sâu sắc về khách hàng tiềm năng trong khu vực này. Các tính năng như bản đồ tương tác và các tính năng dựa trên vị trí giúp tăng trải nghiệm người dùng. Ngoài ra, các website còn được thiết kế đa ngôn ngữ để đáp ứng nhu cầu đa dạng của doanh nghiệp. Hệ thống đặt chỗ và đặt hàng trực tuyến cũng được tích hợp để tạo sự tiện lợi cho khách hàng.
Terus cam kết mang đến các website được tối ưu hóa và phản hồi tốt trên thiết bị di động, giúp doanh nghiệp tiếp cận khách hàng hiệu quả hơn. Việc tích hợp các nền tảng truyền thông xã hội vào website cũng là một điểm nhấn, tăcường kết nối giữa doanh nghiệp và khách hàng.
Quy trình [thiết kế website tại Tây Ninh](https://terusvn.com/thiet-ke-website-tai-hcm/) của Terus bao gồm các bước chính như: Nhận yêu cầu và tư vấn, thiết kế bản demo website, hoàn thiện giao diện và triển khai tính năng, tối ưu các chỉ số chuẩn Insight của Terus, chạy thử sản phẩm và hoàn thiện, cuối cùng là bàn giao và hướng dẫn sử dụng cho khách hàng.
Với những ưu điểm nổi bật về thiết kế website chuẩn Insight, tích hợp các tính năng hiện đại, và quy trình triển khai chuyên nghiệp, Terus đã và đang khẳng định vị thế là đơn vị thiết kế website uy tín tại Tây Ninh. Các doanh nghiệp tại khu vực này có thể hoàn toàn tin tưởng vào chất lượng dịch vụ mà Terus mang lại.
Tìm hiểu thêm về [Thiết Kế Website Tại Tây Ninh Chuyên Ngiệp, Thu Hút](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-tay-ninh/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,584 | Comparing React and Next.js Frontend Technology | Frontend development is continuously developing, and two notable technologies in this space are React... | 0 | 2024-07-06T11:09:04 | https://dev.to/bolex_tife_b8a4494/comparing-react-and-nextjs-frontend-technology-49a3 | Frontend development is continuously developing, and two notable technologies in this space are React and Next.js. In this article, I will compare React and Next.js, highlighting their differences, strengths, and weaknesses. Additionally, I’ll share my expectations for the HNG Internship and my thoughts on working with React.
**What is React?**
React is an open-source JavaScript library developed by Facebook for building user interfaces, primarily for single-page applications (SPAs). It allows developers to create large web applications that can change data without reloading the page. React's goal is to be simple, fast, and scalable.
**Merits of React**
1. Component-Based Architecture:
- > Code Reusability: React’s component-based architecture promotes reusability, making code easier to maintain and scale.
- >Modularity: Components can be developed, tested, and deployed independently.
2. Virtual DOM:
-> Performance: The virtual DOM minimizes direct manipulation of the real DOM, leading to better performance in updating the UI.
- >Efficiency: React efficiently updates and renders only the necessary components when the application state changes.
3. Ecosystem and Community:
->Large Community: React has a vast and active community, providing a wealth of resources, libraries, and tools.
- >Rich Ecosystem: With tools like React Router for routing and Redux for state management, React offers a comprehensive ecosystem for building complex applications.
**Demerits of React**
->State Management: Managing state in large applications can become complex, often necessitating the use of additional libraries like Redux or Context API.
- >Setup and Configuration: React projects often require significant setup and configuration, including build tools like Webpack and Babel.
-> JSX Syntax:React’s JSX syntax can be unfamiliar to new developers, requiring a learning period to get accustomed to it.
_What is Next.js?_
Next.js is a React framework developed by Vercel. It extends React’s capabilities by providing a robust solution for building production-ready applications with server-side rendering (SSR), static site generation (SSG), and other powerful features.
**Merit of Next.js**
-> Server-Side Rendering: SSR improves performance by rendering pages on the server, leading to faster initial load times and better SEO.
- >Static Site Generation: SSG generates static HTML at build time, providing optimal performance and scalability.
-> Built-in Routing: Next.js provides a file-based routing system out of the box, simplifying the creation of dynamic routes.
- >API Routes: Next.js allows developers to create API routes within the same project, enabling fullstack development with ease.
**Demerits of Next.js**
- >SSR and SSG: Understanding and effectively utilizing SSR and SSG can require additional learning, especially for developers new to these concepts.
For simple applications, Next.js might introduce unnecessary complexity and overhead compared to a plain React setup.
**My Expectations for the HNG Internship**
As I embark on the HNG Internship, I am eager to immerse myself in a collaborative and fast-paced learning environment. I Believe this program will provides a fantastic opportunity to work on real-world projects, sharpen my skills, and gain practical experience.
**Working with React at HNG**
React is a powerful and widely-used library for building user interfaces. I am thrilled to deepen my understanding of React during the internship.
I look forward to mastering its features, exploring best practices, and contributing to impactful projects.
To learn more about the HNG Internship,
Visit: https://hng.tech/internship
If you are looking to hire talented developers,
Check out HNG Hire https://hng.tech/hire
OR
https://hng.tech/premium
**Conclusion**
React is best suited for developers who need a flexible library for building user interfaces and prefer to set up their own build tools and configurations. It’s ideal for SPAs and dynamic web applications.Next.js is a better choice for developers looking for an opinionated framework that simplifies server-side rendering, static site generation, and provides built-in routing. It’s particularly beneficial for SEO-friendly websites and applications that require both client-side and server-side rendering capabilities.
As I commence on this journey with the HNG Internship, I am excited to utilize these technologies, enhance my skills, and contribute to innovative solutions. The future of frontend development is gleaming, and I am eager to be a part of it.
| bolex_tife_b8a4494 |
|
1,912,900 | Building an AI-Powered Chat Interface Using FastAPI and Gemini | In this blog, we'll walk through creating a WebSocket endpoint using FastAPI to handle real-time chat... | 0 | 2024-07-06T11:06:27 | https://dev.to/muhammadnizamani/building-an-ai-powered-chat-interface-using-fastapi-and-gemini-2j14 | python, fastapi, google, ai | In this blog, we'll walk through creating a WebSocket endpoint using FastAPI to handle real-time chat messages. WebSockets provide a full-duplex communication channel over a single TCP connection, which is perfect for applications requiring real-time updates like chat applications. For frontend we will create a simple html from with one text field, one send button, and you will be able to see the text you text as you and text from gemini as AI.
here are is demo of that product
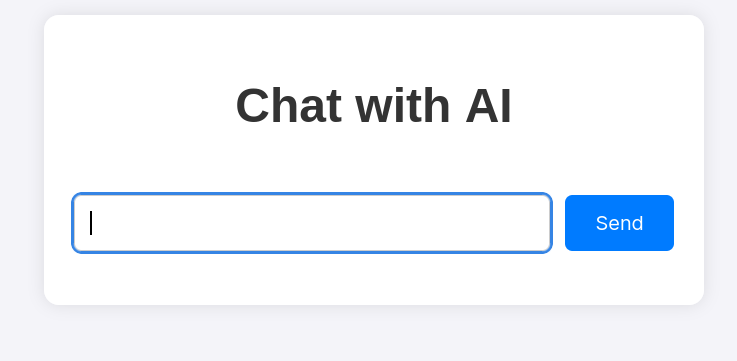
Here I am talking to the AI and tell it about the me and my friends play dota 2.
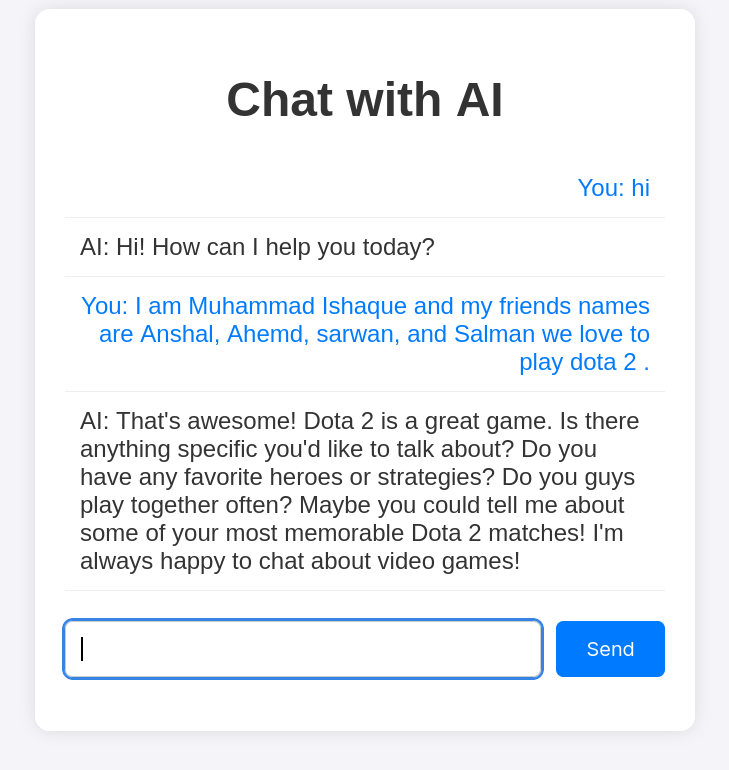
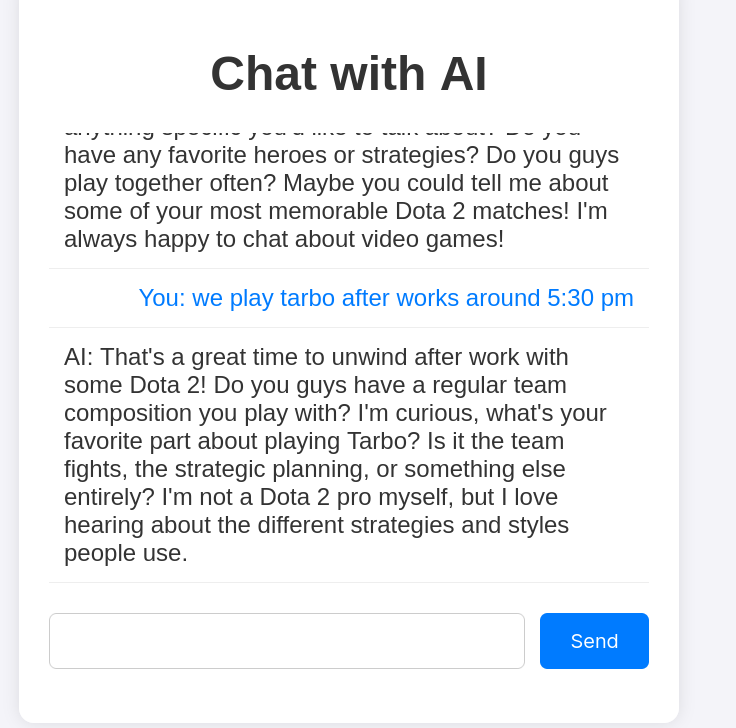
I am creating simple and cool things for beginners.
**let's start**
First create project in new directory then install following Package
```
pip install fastapi
pip install google-generativeai
pip install python-dotenv
```
Go to this following link to get gemini api key
https://aistudio.google.com/app/apikey
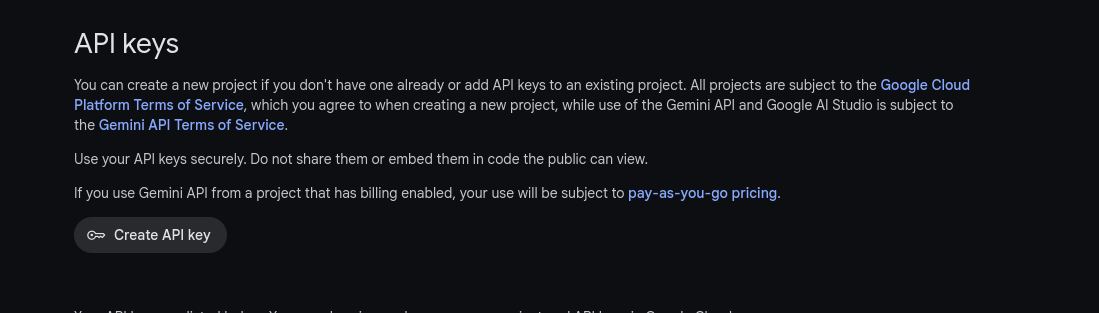
in the above picture you can see that *create API key * click on it and get your api key
Now, create a file named .env in your project directory and add your key like this:
API_KEY = "here you copy paste the API key "
This is blog I will show you how can add LLM chat using fastapi
first import all libraries
```
import os
import google.generativeai as genai
from fastapi import FastAPI, WebSocket, WebSocketDisconnect
from fastapi.responses import HTMLResponse
from fastapi.middleware.cors import CORSMiddleware
from fastapi import APIRouter
from dotenv import load_dotenv
```
then load you API key
```
load_dotenv()
API_KEY = os.getenv("API_KEY")
```
Now we are going to create fastapi app which
```
app = FastAPI(
title="AI Chat API",
docs_url='/',
description="This API allows you to chat with an AI model using WebSocket connections.",
version="1.0.0"
)
```
after that you need to add some permisson and in the cast I am open it for all but in the production you cannot do this.
```
app.add_middleware(
CORSMiddleware,
allow_origins=["*"],
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
```
creating router and loading the model name gemini-1.5-flash
```
router = APIRouter(prefix="/chat", tags=["Chat"])
genai.configure(api_key=API_KEY)
model = genai.GenerativeModel('gemini-1.5-flash')
```
**Setting Up the WebSocket Endpoint**
We'll start by defining our WebSocket endpoint. This endpoint will allow clients to send messages to our AI model and receive streamed responses.
```
@router.websocket("/ws")
async def websocket_endpoint(websocket: WebSocket):
"""
WebSocket endpoint for handling chat messages.
This WebSocket endpoint allows clients to send messages to the AI model
and receive streamed responses.
To use this endpoint, establish a WebSocket connection to `/ws`.
- Send a message to the WebSocket.
- Receive a response from the AI model.
- If the message "exit" is sent, the chat session will end.
"""
await websocket.accept()
chat = model.start_chat(history=[])
try:
while True:
data = await websocket.receive_text()
if data.lower().startswith("you: "):
user_message = data[5:]
if user_message.lower() == "exit":
await websocket.send_text("AI: Ending chat session.")
break
response = chat.send_message(user_message, stream=True)
full_response = ""
for chunk in response:
full_response += chunk.text
await websocket.send_text("AI: " + full_response)
else:
await websocket.send_text("AI: Please start your message with 'You: '")
except WebSocketDisconnect:
print("Client disconnected")
finally:
await websocket.close()
```
**Setting Up the Chat Interface**
We'll start by defining an HTTP endpoint that serves an HTML page. This page contains a form for sending messages and a script for handling WebSocket communication.
Here's the code for the HTTP endpoint:
Note: I am here just showing you how to js is working I have not include the CSS that make chat box like shown above for that check following repo on github and please give it start:
https://github.com/GoAndPyMasters/fastapichatbot/tree/main
```
@router.get("/")
async def get():
return HTMLResponse("""
<html>
<head>
<title>Chat</title>
</head>
<body>
<h1>Chat with AI</h1>
<form action="" onsubmit="sendMessage(event)">
<input type="text" id="messageText" autocomplete="off"/>
<button>Send</button>
</form>
<ul id="messages">
</ul>
<script>
var ws = new WebSocket("ws://0.0.0.0:8080/ws");
ws.onmessage = function(event) {
var messages = document.getElementById('messages')
var message = document.createElement('li')
var content = document.createTextNode(event.data)
message.appendChild(content)
messages.appendChild(message)
};
function sendMessage(event) {
var input = document.getElementById("messageText")
ws.send("You: " + input.value)
input.value = ''
event.preventDefault()
}
</script>
</body>
</html>
""")
```
Now to run the fastapi APP use following command
```
uvicorn main:app --host 0.0.0.0 --port 8000
```
after that use will see
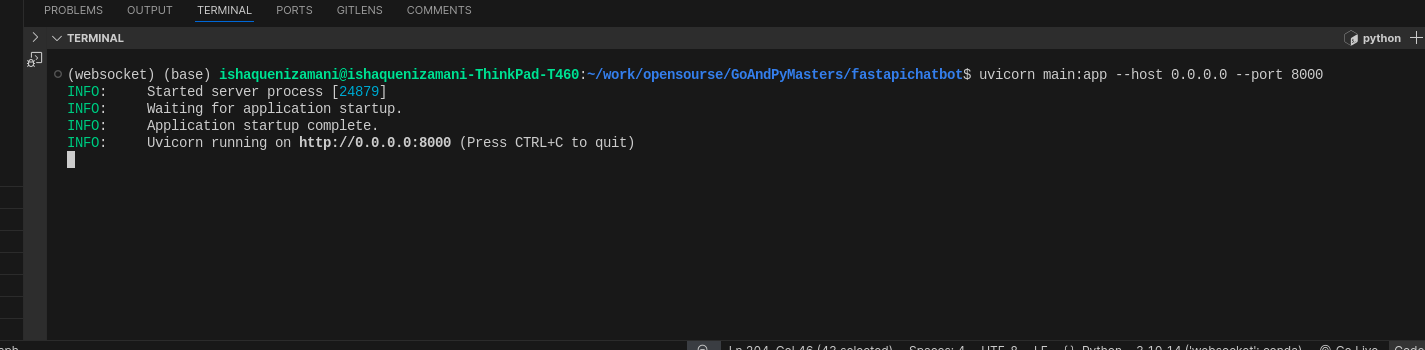
then ctrl+click on this link **`http://0.0.0.0:8000 `**
then show the docs like this
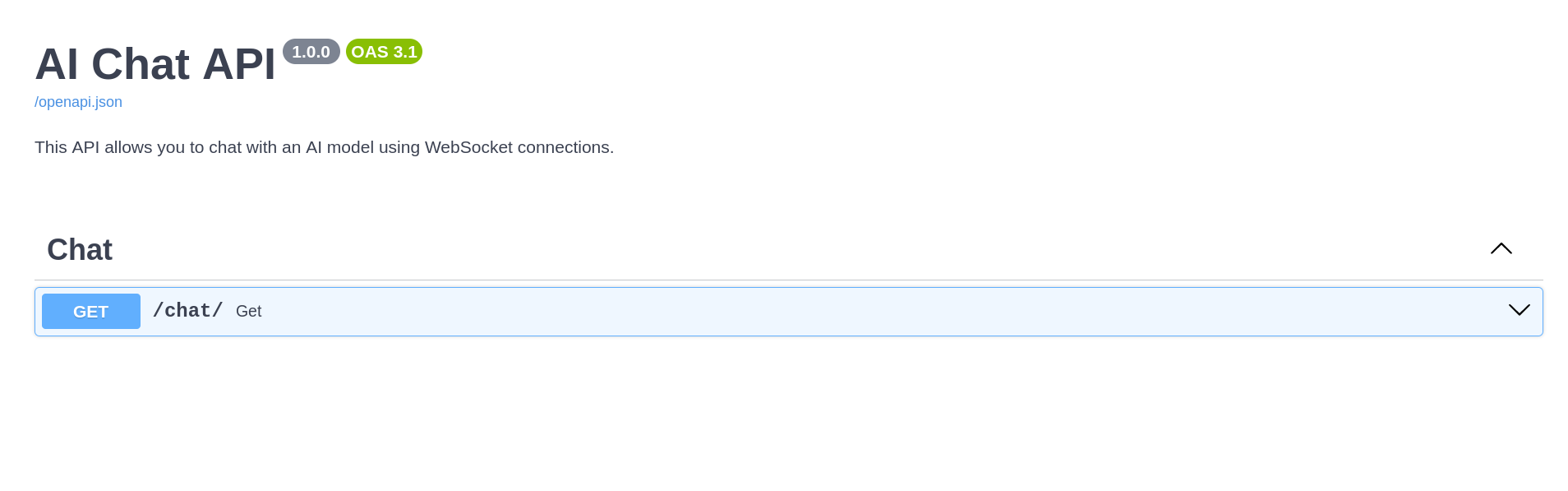
then go to the url and write /chat/ like as shown in the image
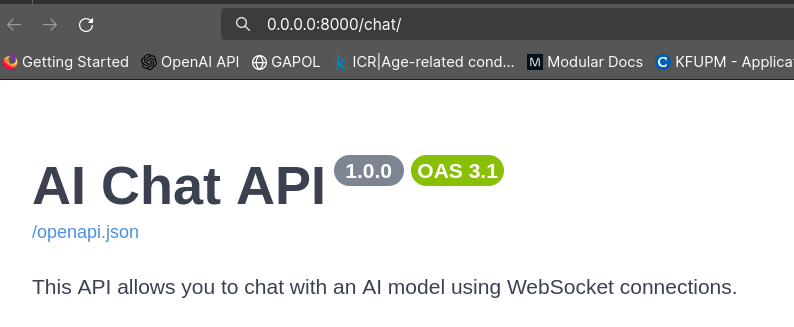
and then you are ready to chat
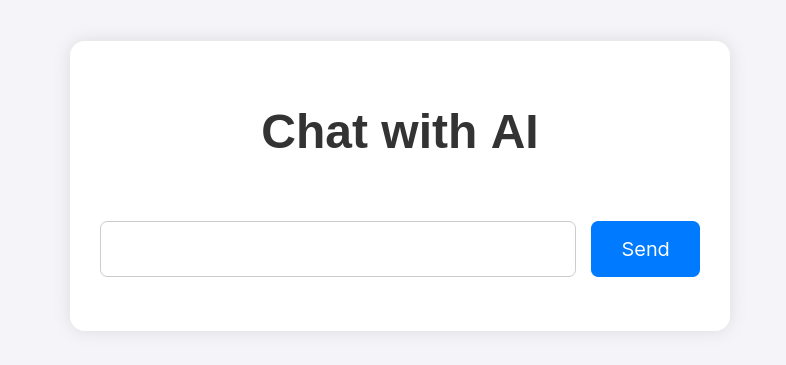
**Conclusion**
In this blog, we demonstrated how to create a real-time chat application using FastAPI and WebSockets, powered by a generative AI model. By following the steps outlined, you can set up a fully functional WebSocket endpoint and a basic HTML interface to interact with the AI. This combination allows for seamless, real-time communication, making it a powerful solution for chat applications and other real-time systems. With the provided code and instructions, you're equipped to build and customize your own AI-powered chat interface.
check the code on following repo on github
https://github.com/GoAndPyMasters/fastapichatbot/tree/main
here is my github profile
https://github.com/MuhammadNizamani
If you need any help contact with on linkedin
https://www.linkedin.com/in/muhammad-ishaque-nizamani-109a13194/
Please like and comment it will give me motivation to write new things
Thanks for Reading | muhammadnizamani |
1,913,613 | Code Craft: Top Programming Languages to Learn | Hey there, future coding whiz! Ready to dive into the world of programming and figure out which... | 0 | 2024-07-06T11:01:53 | https://dev.to/ifavecontents/code-craft-top-programming-languages-to-learn-20pp | programming, coding |
Hey there, future coding whiz! Ready to dive into the world of programming and figure out which languages are hot and worth your time? Whether you’re just starting out or looking to add some new skills to your tech arsenal, knowing the [top programming languages](https://ifave.com/category/The-World/Top-programming-languages) is a game-changer. So, let’s cut to the chase and explore the coolest and most useful languages you should consider learning.
**JavaScript**
First up, we have JavaScript, the rockstar of web development. This bad boy makes websites come alive with all those fancy animations and interactive features. If you’re dreaming of creating the next viral web app, JavaScript is your best buddy. Plus, with frameworks like React, Angular, and Vue.js, you’ll be building slick interfaces that users will love.
**Python**
Next on the list is Python, the Swiss Army knife of programming languages. It’s super easy to read and write, making it perfect for newbies and seasoned pros alike. Whether you’re into web development with Django, dabbling in machine learning with TensorFlow, or diving into data science, Python’s got your back. Plus, it’s got a friendly, helpful community that’s always there when you need a hand.
**Java**
Java is like that dependable friend who’s always there when you need them. It’s been around forever and is still going strong, especially in big business environments. Java’s great for building large, secure applications that can handle tons of users. Its object-oriented nature and vast libraries mean you can tackle pretty much any project with confidence.
**C#**
Now, if you’re a fan of Windows or love gaming, C# is the language for you. Created by Microsoft, C# is used for everything from desktop apps to web services, and it’s the go-to language for developing games with the Unity engine. It’s versatile, powerful, and perfect for bringing your creative ideas to life.
**Swift**
Last but not least, we’ve got Swift, Apple’s brainchild for iOS development. If you’re dreaming of building the next hit iPhone or iPad app, Swift is the way to go. It’s fast, safe, and a blast to work with. Plus, Apple’s ecosystem is massive, so the opportunities are endless.
So, there you have it, a rundown of the top programming languages that can skyrocket your tech career. Whether you’re vibing with the simplicity of Python, the versatility of JavaScript, or the enterprise muscle of Java, learning these [best programming languages](https://ifave.com/category/The-World/Top-programming-languages) will open up a world of possibilities. So, roll up your sleeves, pick a language, and start coding your way to success. Happy coding! | ifavecontents |
1,913,610 | Thiết Kế Website Tại Ninh Bình Tối Ưu Chi Phí | Ninh Bình, một vùng đất đầy tiềm năng, đang ngày càng thu hút sự quan tâm của nhiều doanh nghiệp và... | 0 | 2024-07-06T10:52:56 | https://dev.to/terus_technique/thiet-ke-website-tai-ninh-binh-toi-uu-chi-phi-fjk | website, digitalmarketing, seo, terus |
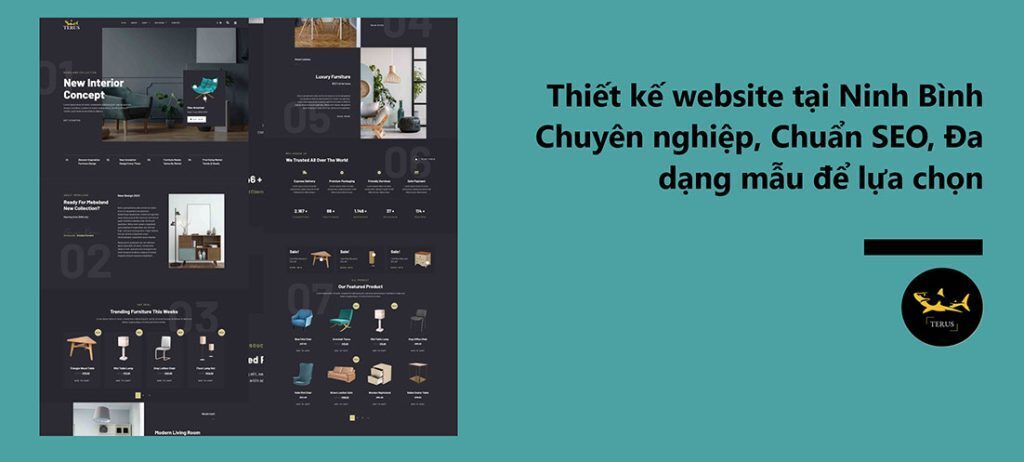
Ninh Bình, một vùng đất đầy tiềm năng, đang ngày càng thu hút sự quan tâm của nhiều doanh nghiệp và du khách. Với những danh lam thắng cảnh tuyệt vời, Ninh Bình không chỉ là điểm đến hấp dẫn về du lịch mà còn là nơi phát triển của nhiều ngành nghề khác như xây dựng, dịch vụ nhà hàng khách sạn. Trong bối cảnh đó, việc thiết kế website chuyên nghiệp, chuẩn SEO trở nên vô cùng quan trọng.
[Thiết kế website tại Ninh Bình](https://terusvn.com/thiet-ke-website-tai-hcm/) không chỉ giúp tạo sự hiện diện trực tuyến đầy ấn tượng mà còn thể hiện được vẻ đẹp thiên nhiên, nâng cao trải nghiệm khách hàng và thúc đẩy doanh nghiệp phát triển. Một website chất lượng không chỉ cung cấp thông tin toàn diện về doanh nghiệp mà còn tích hợp các tính năng tương tác trực tiếp như đặt chỗ, đặt hàng, v.v.
Terus tự tin là một trong những đơn vị hàng đầu trong lĩnh vực thiết kế website tại Ninh Bình, cam kết mang đến những sản phẩm chuyên nghiệp, chuẩn SEO và phù hợp với nhu cầu của từng khách hàng. Quy trình thiết kế website của Terus gồm 6 bước chính: Nhận yêu cầu và tư vấn, Thiết kế bản website demo, Hoàn thiện giao diện và triển khai tính năng, Tối ưu các chỉ mục chuẩn Insight, Chạy thử sản phẩm và hoàn thiện, Bàn giao và hướng dẫn.
Các mẫu website tại Ninh Bình của Terus đều được thiết kế với hình ảnh ấn tượng, tính năng tương tác trực tiếp và nội dung nghiên cứu kỹ lưỡng theo insight của khách hàng. Đặc biệt, các website này còn được tối ưu về giao diện thân thiện với thiết bị di động, trình bày thông tin doanh nghiệp toàn diện và tích hợp hệ thống đặt chỗ, đặt hàng.
Với đội ngũ nhân sự am hiểu sâu về thiết kế website, Terus cam kết mang đến những [giải pháp website chuyên nghiệp, chuẩn SEO](https://terusvn.com/thiet-ke-website-tai-hcm/) và phù hợp với từng doanh nghiệp tại Ninh Bình. Dịch vụ thiết kế website của Terus không chỉ giúp doanh nghiệp tạo sự hiện diện trực tuyến mạnh mẽ mà còn nâng cao trải nghiệm khách hàng, thúc đẩy doanh số và tăng cường lợi thế cạnh tranh trong thị trường.
Tìm hiểu thêm về [Thiết Kế Website Tại Ninh Bình Tối Ưu Chi Phí](https://terusvn.com/thiet-ke-website/thiet-ke-website-tai-ninh-binh/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,880,598 | Ibuprofeno.py💊| #134: Explica este código Python | Explica este código Python Dificultad: Fácil x = {1,2,3} y =... | 25,824 | 2024-07-06T11:00:00 | https://dev.to/duxtech/ibuprofenopy-134-explica-este-codigo-python-1541 | python, beginners, spanish, learning | ## **<center>Explica este código Python</center>**
#### <center>**Dificultad:** <mark>Fácil</mark></center>
```py
x = {1,2,3}
y = {3,4,5}
print(x.difference(y))
```
* **A.** `{1, 2}`
* **B.** `{3}`
* **C.** `{4, 5}`
* **D.** `{1, 2, 4, 5}`
---
{% details **Respuesta:** %}
👉 **A.** `{1, 2}`
En nuestro ejemplo, la diferencia de conjuntos consiste en extraer todos los elementos que estén en `x` pero que no estén en `y`.
La diferencia de conjuntos no es conmutativa, no es lo mismo `x.difference(y)` que `y.difference(x)`:
```py
x = {1,2,3}
y = {3,4,5}
print(y.difference(x)) # {4, 5}
```
{% enddetails %} | duxtech |
1,913,612 | Effective Hair Fall Treatment in Hyderabad - Restore Your Hair with Skinora | Discover advanced hair fall treatments in Hyderabad at Skinora. Our expert dermatologists offer... | 0 | 2024-07-06T10:59:28 | https://dev.to/skinora/effective-hair-fall-treatment-in-hyderabad-restore-your-hair-with-skinora-4a1i | Discover advanced hair fall treatments in Hyderabad at Skinora. Our expert dermatologists offer personalized solutions to help you regain healthy, luscious hair. Book your consultation today and say goodbye to hair loss | skinora |
|
1,913,602 | 10 More VS Code Vim Tricks to Code Faster ⚡ | Around a year ago, I published the article “10 VS Code Vim Tricks to Boost Your Productivity ⚡”,... | 27,327 | 2024-07-06T10:57:13 | https://dev.to/ansonh/10-more-vs-code-vim-tricks-to-become-a-faster-coder-ndi | vscode, vim, tutorial, productivity | Around a year ago, I published the article “[10 VS Code Vim Tricks to Boost Your Productivity ⚡](https://dev.to/ansonh/10-vs-code-vim-tricks-to-boost-your-productivity-1b0n)**”,** which I shared various tips for the [VSCodeVim](https://marketplace.visualstudio.com/items?itemName=vscodevim.vim) extension. Turns out that the article was a hit, so allow me to share a few more VS Code Vim tips that I have up my sleeves 😆.
**Prerequisites**:
- You’ve installed the [VSCodeVim](https://marketplace.visualstudio.com/items?itemName=vscodevim.vim) extension
- Basic understanding of Vim keybindings. This guide is NOT for total beginners!
---
## Table of Contents
([Previous 10 Tips](https://dev.to/ansonh/10-vs-code-vim-tricks-to-boost-your-productivity-1b0n))
- Navigation
- [#1 - Tabs Management](#1-tabs-management)
- [#2 - Splits Management](#2-splits-management)
- [#3 - Multi-Cursor](#3-multicursor)
- [#4 - Quick Search](#4-quick-search)
- Vim Features
- [#5 - Emulated Plugins](#5-emulated-plugins)
- [#6 - Macros](#6-macros)
- Git
- [#7 - Peek Diff](#7-peek-diff)
- [#8 - Revert Hunk or Selected Lines](#8-revert-hunk-or-selected-lines)
- [#9 - Jump to Previous/Next Changes](#9-jump-to-previousnext-changes)
- [#10 - Open File on Remote](#10-open-file-on-remote)
(Full Settings: [`settings.json`](https://gist.github.com/AnsonH/e6b5222c3f0e588253a3e8fb0d55e147) | [`keybindings.json`](https://gist.github.com/AnsonH/dd027ae954fb82124fd90849d36da8b1))
---
## Navigation
### #1 - Tabs Management
I prefix all my custom keybindings for managing [editor tabs](https://code.visualstudio.com/docs/getstarted/userinterface#_tabs) with the `Shift` key so that it’s easy to remember. For tab navigation:
- `Shift + h` - Focus the left tab
- `Shift + l` - Focus the right tab
- `Shift + q` - Close current tab
Notice how intuitive they are, since `h` and `l` keys in Vim means left and right, and `q` means “quit”.

To define the custom keybindings, open `settings.json` by pressing "Ctrl+Shift+P" and search for “Preferences: Open User Settings (JSON)”. Then, add the following item:
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["H"], // Focus previous tab at the left
"commands": ["workbench.action.previousEditor"]
},
{
"before": ["L"], // Focus next tab at the right
"commands": ["workbench.action.nextEditor"]
},
{
"before": ["Q"], // Close current tab
"after": ["<C-w>", "q"]
}
]
}
```
As for reorganizing the order of tabs:
- `Shift + LeftArrow` - Move tab leftwards
- `Shift + RightArrow` - Move tab rightwards

Setting up this keybinding is a bit tricky. VS Code has default keybindings where “Shift + LeftArrow” will select one character at the cursor’s left side (similar for “Shift + RightArrow”).
Actually we don’t need that since Vim’s Visual Mode can achieve the same thing via `vh` and `vl` keybindings. Thus, we need to disable VS Code’s default keybindings for `Shift + Left/Right`.
Open `keybindings.json` via “Ctrl + Shift + P” → “Preferences: Open Keyboard Shortcuts (JSON)” and append the following items:
```jsonc
[
{
"key": "shift+left",
"command": "-cursorColumnSelectLeft",
"when": "editorColumnSelection && textInputFocus"
},
{
"key": "shift+left",
"command": "-cursorLeftSelect",
"when": "textInputFocus && vim.mode != 'Visual'"
},
{
"key": "shift+left",
"command": "workbench.action.moveEditorLeftInGroup",
"when": "vim.mode == 'Normal'"
},
{
"key": "shift+right",
"command": "-cursorColumnSelectRight",
"when": "editorColumnSelection && textInputFocus"
},
{
"key": "shift+right",
"command": "-cursorRightSelect",
"when": "textInputFocus && vim.mode != 'Visual'"
},
{
"key": "shift+right",
"command": "workbench.action.moveEditorRightInGroup",
"when": "vim.mode == 'Normal'"
}
]
```
([🔼 Table of Contents](#table-of-contents))
### #2 - Splits Management
I prefix all splits-related keybindings with `Ctrl` key, similar to [Tip #1](#1-tabs-management) where I prefix all tabs-related keybindings with `Shift` key.
To create splits easily, I’ve defined 2 custom keybindings:
- `|` (`Shift + \`) - Split tab vertically
- `_` - Split tab horizontally
I picked these two symbols because it looks like the split direction (`|` is a vertical stroke, `_` is a horizontal stroke).
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["|"], // Split tab vertically
"after": ["<C-w>", "v"]
},
{
"before": ["_"], // Split tab horizontally
"after": ["<C-w>", "s"]
}
]
}
```
(💡 `<C-w>` is a Vim keybinding syntax that means `Ctrl + w`)
The `Shift + q` keybinding we saw from [Tip #1](#1-tabs-management) can also close a split if it only has one tab 👇

To navigate around splits, I don’t like Vim’s `<C-w> h/j/k/l` keybindings because I have to press `Ctrl + w` every time. To reduce the number of keystrokes, I use `Ctrl + h/j/k/l` instead:
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<C-h>"], // Focus split window at left
"commands": ["workbench.action.focusLeftGroup"]
},
{
"before": ["<C-j>"], // Focus split window at right
"commands": ["workbench.action.focusBelowGroup"]
},
{
"before": ["<C-k>"], // Focus split window at above
"commands": ["workbench.action.focusAboveGroup"]
},
{
"before": ["<C-l>"], // Focus split window at below
"commands": ["workbench.action.focusRightGroup"]
}
]
}
```

⚠️ For Windows/Linux users, the `Ctrl + k` keybinding may not work properly. You may see this message at the bottom bar:
> (Ctrl + K) was pressed. Waiting for second key of chord…
This is because VS Code has a lot of default keyboard shortcuts that starts with `Ctrl + k` (e.g. `Ctrl+K Ctrl+S` to open keyboard shortcuts). As a fallback, you can use Vim’s original keybinding of `Ctrl+w k`.
([🔼 Table of Contents](#table-of-contents))
### #3 - Multi-Cursor
VS Code’s [multi-cursor editing](https://code.visualstudio.com/docs/editor/codebasics#_multiple-selections-multicursor) is also supported in VS Code Vim.
Below are the built-in VS Code keybindings to enter multi-cursor mode:
- MacOS - `Cmd + d`
- Windows/Linux - `Ctrl + d`
Unfortunately for **Windows/Linux** users, pressing `Ctrl + d` will instead scroll down the file, because it clashes with Vim’s keybinding of `<C-d>` for scrolling down a file. As a workaround, I remapped `Ctrl + d` to `Alt + d` to enter multi-cursor mode. To do that, append these to `keybindings.json`:
```jsonc
[
{
"key": "ctrl+d",
"command": "-editor.action.addSelectionToNextFindMatch",
"when": "editorFocus"
},
{
"key": "alt+d",
"command": "editor.action.addSelectionToNextFindMatch",
"when": "editorFocus"
}
]
```
Alternative to `Cmd/Alt + d`, VS Code Vim also has a built-in keybinding of `gb` to enter multi-cursor mode. Here’s a demo in MacOS for both the `Cmd + d` and `gb` keybindings:

VS Code Vim treats multi-cursor mode as a variant of **Visual Mode**, so you need to use Visual Mode keybindings to perform multi-cursor actions, such as:
- `Shift + i` - Move to beginning of selection and enter Insert Mode
- `Shift + a` - Move to end of selection and enter Insert Mode
- `s` or `c` - Delete word and enter Insert Mode
For example, I need to press `Shift + a` in order to start typing at the end of the word (unlike in Normal Mode where I only need to type `a`):

### #4 - Quick Search
To quickly search text from files, I’ve created the `<leader>f` keybinding:
💡 `<leader>` stands for [Leader Key](https://stackoverflow.com/q/1764263/11067496), which is commonly used as a prefix for user-defined shortcuts. By default it's the backslash key (`\`), but we commonly map it to Space key (see `vim.leader` option below)
```jsonc
{
"vim.leader": "<space>",
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "f"], // Search text
"commands": ["workbench.action.findInFiles"]
}
]
}
```

I also created a keybinding of `<leader>s` to search all symbols in a workspace. This will save you a lot of time from locating the definition of a symbol.
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "s"], // Search symbol
"commands": ["workbench.action.showAllSymbols"]
}
]
}
```
For example, I want to directly jump to a React component called `SaveButton`. To do that, I launch the symbol search via `<leader>s` and enter the component name:

💡 Tip: If you place your cursor under a word (e.g. `SaveButton`) and press `<leader>s`, VS Code will automatically fill that word in the search bar 👇

([🔼 Table of Contents](#table-of-contents))
---
## Vim Features
### #5 - Emulated Plugins
VS Code Vim emulates quite a number of Vim plugins. While I’m not going to walkthrough every plugin here ([see docs for the full list](https://github.com/VSCodeVim/Vim#-emulated-plugins)), I want to highlight a plugin called [vim-surround](https://github.com/VSCodeVim/Vim#vim-surround).
This plugin will save you tons of time when working with **surrounding characters** like paranthesis, brackets, quotes, and XML tags. Here’s a quick cheatsheet:
| Surround Command | Description |
| -------------------------- | -------------------------------------------------------- |
| `y s <motion> <desired>` | Add desired surround around text defined by <motion> |
| `d s <existing>` | Delete existing surround |
| `c s <existing> <desired>` | Change existing surround to desired |
| `S <desired>` | Surround when in visual modes (surrounds full selection) |
For a full guide, please refer to [vim-surround’s GitHub README](https://github.com/tpope/vim-surround).
Examples:
- `ysw)` - Add `()` surround around a word
- `ds]` - Delete the `[]` that surrounds the cursor
- `cs)]` - Changes the `()` surrounding to `[]`
- `S}` - Surrounds the selected text with `{}` block

([🔼 Table of Contents](#table-of-contents))
### #6 - Macros
[Macro](https://vim.fandom.com/wiki/Macros) is a powerful feature in Vim that lets you record a series of commands and replay them. It’s useful to perform similar code changes for many times.
For example, let’s say I have a JavaScript object and I want to turn each value from a string to an array of strings. It’d be very tedious to manually edit line-by-line:
```jsx
const data = {
item1: "one", // -> item1: ["one"]
item2: "two", // -> item2: ["two"]
...
}
```
Here’s the anatomy of macros:
- Record a macro: `q<register><commands>q`.
- Begin a macro recording with `q` followed by `<register>` (i.e. a single letter, which is like the name of a save slot). For example, `qa` means I record a macro to a slot named `a`.
- `<commands>` is the series of Vim keybindings you wish to perform.
- End the recording by pressing `q`.
- To play a macro: `@<register>`.
- `<register>` is the single letter that you just recorded your macro to. For example, `@a` will play the commands that I’ve previously recorded via `qa<commands>q`.
- You can play the macro multiple times. For example, `4@a` plays the macro 4 times.
Back to our example. We basically want to repeat the action of adding a `[]` bracket around the string value. Let’s record our macro:
1. Place our cursor at start of the object key name.
2. `qa` - Begin recording the macro to slot `a`.
3. `f"` - Move the cursor to the first double quote.
4. `yst,]` - A [vim-surround](https://github.com/tpope/vim-surround) keybinding that we saw from [Tip #5](#5-emulated-plugins). It means adding a `[]` bracket for the text from the current cursor’s position (first double quote) till the first comma (i.e. `t,`).
5. `j0w` - Places the cursor in an appropriate location before the macro ends. We move one line down with `j`, then move to beginning of first word with `0w`.
- 💡 The cursor’s end position matters because it affects the repeatability of the macro.
6. `q` - Stops the macro recording.
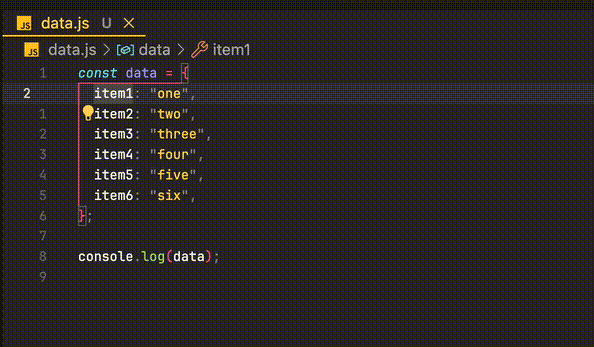
To confirm the macro works, let’s replay it once via `@a`. Note how the cursor perfectly lands at the start of next line (`item3`), so that we can replay the macro consecutively via `4@a` (i.e. play 4 times):
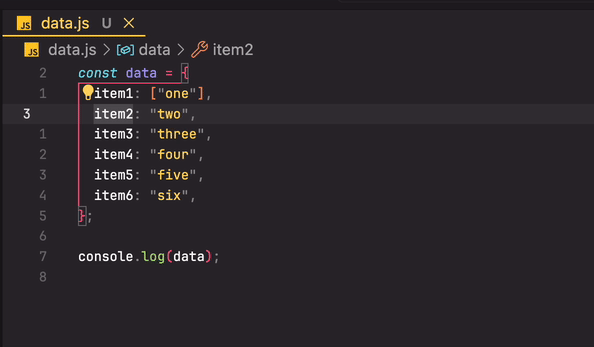
([🔼 Table of Contents](#table-of-contents))
---
## Git
All my custom Git keybindings are prefixed with `<leader>g`, where `g` stands for **g**it. I highly recommend grouping related keybindings with the same prefix so that it’s easier to remember 👍.
### #7 - Peek Diff
If your cursor is located inside a [Git hunk](https://stackoverflow.com/q/37620729), I can press `<leader>gp` to peek the diff changes (`gp` = **g**it **p**eek).
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "g", "p"], // Peek Git diff for the changed line
"commands": ["editor.action.dirtydiff.next"]
}
]
}
```

([🔼 Table of Contents](#table-of-contents))
### #8 - Revert Hunk or Selected Lines
To revert a Git hunk (i.e. discard unstaged changes) in Normal Mode:
1. Place my cursor inside the Git hunk
2. Press `<leader>gr` (`gr` = **g**it **r**evert)
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "g", "r"], // Revert hunk
"commands": ["git.revertSelectedRanges"]
}
]
}
```
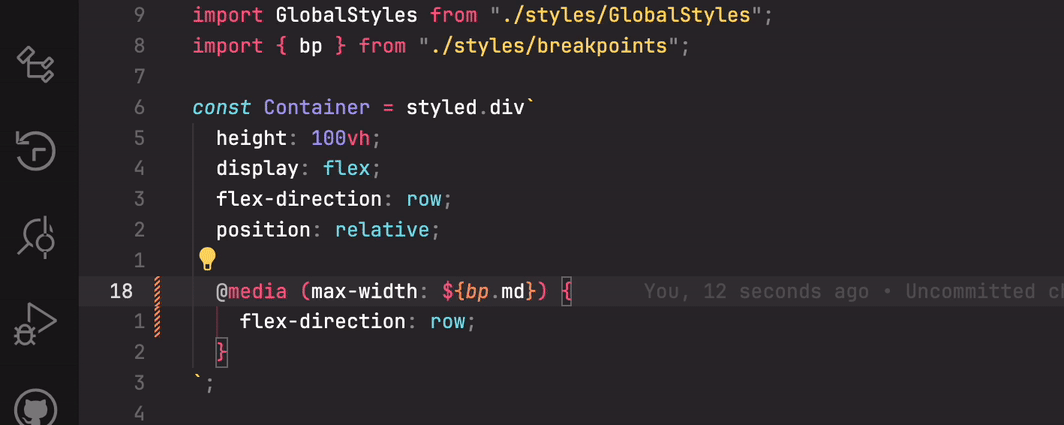
To discard multiple hunks at the same time, I’ve also defined the same keybinding for Visual Mode:
```jsonc
{
"vim.visualModeKeyBindings": [
{
"before": ["<leader>", "g", "r"], // Revert hunk
"commands": ["git.revertSelectedRanges"]
}
]
}
```
Then, I can first select multiple hunks in Visual Mode, then use `<leader>gr` to revert them:

([🔼 Table of Contents](#table-of-contents))
### #9 - Jump to Previous/Next Changes
Sometimes I have many Git changes across a long file. To quickly jump between changes:
- `<leader>gj` - Jump to next Git change (`j` = down)
- `<leader>gk` - Jump to previous Git change (`k` = up)
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "g", "j"], // Jump to next Git change
"commands": ["workbench.action.editor.nextChange"]
},
{
"before": ["<leader>", "g", "k"], // Jump to previous Git change
"commands": ["workbench.action.editor.previousChange"]
}
]
}
```

([🔼 Table of Contents](#table-of-contents))
### #10 - Open File on Remote
If your Git repo has a remote upstream (e.g. GitHub, GitLab), you can easily get a sharable URL to the file you’re currently opening.
1. Prerequisite: Install [GitLens extension](https://marketplace.visualstudio.com/items?itemName=eamodio.gitlens)
2. Add these keybindings to `settings.json`
```jsonc
{
"vim.normalModeKeyBindings": [
{
"before": ["<leader>", "g", "g"], // Open file in GitHub
"commands": ["gitlens.openFileOnRemote"]
}
],
"vim.visualModeKeyBindings": [
{
"before": ["<leader>", "g", "g"], // Open file in GitHub
"commands": ["gitlens.openFileOnRemote"]
}
]
}
```
3. Move your cursor on a line you wish to open on remote, or enter Visual Mode to select multiple lines
4. Press `<leader>gg` (mnemonic: last `g` stands for **G**itHub)
To jump to a specific line on remote, use the keybinding in Normal Mode 👇

To select multiple lines on remote, use Visual Mode 👇
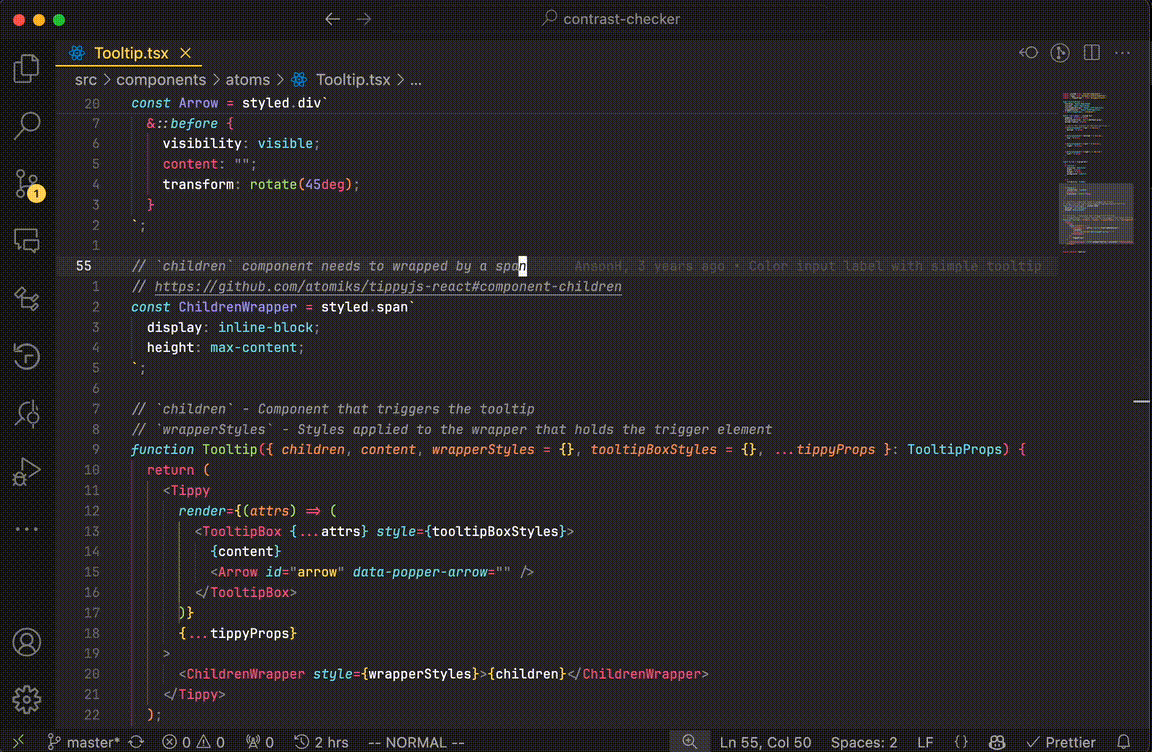
([🔼 Table of Contents](#table-of-contents))
---
For the **full** settings code, they can be found below 👇
- [`settings.json`](https://gist.github.com/AnsonH/e6b5222c3f0e588253a3e8fb0d55e147)
- [`keybindings.json`](https://gist.github.com/AnsonH/dd027ae954fb82124fd90849d36da8b1)
(I used `.jsonc` instead of `.json` in the Gists. Otherwise, the syntax highlighting will add an ugly red background to all comments since theoretically comments are not allowed in JSON 😢)
Thanks for reading. Don’t forget to like and share this post if you found it useful, cheers! 🙌
- [My GitHub](https://github.com/AnsonH)
- [Personal website](https://www.ansonh.com/)
| ansonh |
1,913,611 | Кеширования в React - все ли так однозначно? | Все мы знаем про useCallback(), useMemo(), и memo, которые используются для оптимизации... | 0 | 2024-07-06T10:55:05 | https://dev.to/andreyalexeev98/kieshirovaniia-v-react-vsie-li-tak-odnoznachno-51dc | react, memoization, cash, performance | Все мы знаем про `useCallback()`, `useMemo()`, и `memo`, которые используются для оптимизации производительности в React-приложениях. В этой статье я углублюсь в их работу и создам краткую шпаргалку, чтобы использовать их осмысленно и эффективно.
_Начнем издалека..._
## Зачем вообще использовать кеширование?
Когда мы пишем маленькое приложение в виде учебного TODO листа или нескольких страниц с небольшим числом компонентов, может показаться, что кеширование не нужно. Интерфейс отзывчивый, а React настолько классный и оптимизированный, что не трогает DOM дерево так, как чистый JavaScript, делая точечные перерисовки. Но всё меняется, когда проекты становятся большими и сложными (много разметки, локиги, зависимостей и условных рендеров), а ваше приложение начинают открывать на устройствах с менее мощным железом, чем ваше рабочее.
Кеширование в React используется для оптимизации производительности приложений. Оно позволяет снизить нагрузку на CPU и видеокарту, используя больше ресурсов памяти. В современных устройствах зачастую больше доступной памяти, чем ресурсов CPU или видеокарты, поэтому кеширование становится эффективным методом оптимизации. Перерисовки компонентов требуют значительных вычислительных ресурсов для выполнения различных операций, таких как создание виртуального DOM, сравнение его с предыдущим состоянием и обновление реального DOM. Это может существенно замедлить работу приложения, особенно если речь идет о сложных вычислениях или большом количестве элементов. Кеширование же позволяет избежать этих затрат за счет хранения результатов предыдущих вычислений в памяти и использования их при необходимости. Таким образом, благодаря кешированию можно значительно улучшить производительность приложения, сократив время, затрачиваемое на перерисовки, и улучшив общую отзывчивость пользовательского интерфейса.
Во-первых, важно понимать, что если абсолютно все функции обернуть в useCallback и useMemo, а также все компоненты в memo, это только увеличит нагрузку на память устройства. В этих "обёртках" скрывается реализация React, которая "под капотом" проверяет, нужно ли использовать закешированные данные или пора сбросить и перевыполнить операцию. Это делается через сравнение старых зависимостей (из массива зависимостей в случае хуков и пропсов для memo), при изменении которых и выполняется алгоритм сброса кеша.
## Хук useCallback()
Использую, когда необходимо передать функцию как callback пропсом в другой компонент. Он также полезен, если функция выполняет действительно сложные вычисления. Например, это может быть перебор большого массива, фильтрация данных, выполнение сложных математических расчетов или обработка данных из API. useCallback предотвращает создание новой функции при каждом рендере, что особенно важно для производительности, когда функция передается в дочерние компоненты, используемые в качестве обработчиков событий или callback'ов в компонентах, которые часто обновляются.
## Хук useMemo()
Использую для мемоизации результатов выполнения сложных вычислений, таких как перебор большого массива данных, сортировка, фильтрация, обработка данных из API или сложные математические расчеты. useMemo возвращает мемоизированное значение, которое пересчитывается только при изменении зависимостей, указанных в массиве зависимостей.
Несмотря на то, что useMemo не следует путать с React.memo, иногда можно встретить использование useMemo для мемоизации результатов рендера функционального компонента. Однако это не является распространённой практикой, так как сообщество React обычно использует React.memo для мемоизации компонентов. Следование общепринятым практикам делает ваш код более читабельным и понятным для других разработчиков. Например, функцию, возвращающую JSX, лучше оборачивать в React.memo, поскольку это более ожидаемо и понятно для тех, кто будет читать ваш код.
Подобно тому, как для функций изменения состояния, созданных с помощью useState, принято использовать префикс set (например, setCount), использование React.memo для компонентов является стандартом в сообществе React. Это помогает сохранять консистентность кода и облегчает его поддержку и понимание.
## Метод React.memo
Использую для обёртки компонентов, пропсы которых нечасто меняются. Это особенно полезно для переиспользуемых компонентов из `shared/ui` (вашего UI kit). Если компонент в качестве `props.children` принимает сложную структуру данных (другой компонент), а не примитив (строку или число), такой компонент не следует мемоизировать. Мемоизация занимает память, и сравнение примитивов затрачивает мало ресурсов. Однако в случае сложных объектов это дорого по ресурсам, так как сложные структуры данных (объекты, массивы) сравниваются по ссылке. Даже если такой объект в `props.children` аналогичен предыдущему, передается ссылка на другую ячейку памяти, и memo не сработает — компонент перерисуется, и ресурсы будут потрачены на проверку старого и нового значения.
Используйте мемоизацию с умом. Удачи, работяги! | andreyalexeev98 |
1,907,283 | rygf | eghth | 0 | 2024-07-01T07:09:47 | https://dev.to/jasmeen_sandhu_ceb8f18ac9/rygf-5ai | eghth | jasmeen_sandhu_ceb8f18ac9 |
|
1,913,608 | Some Lesser-Known Scalability Issues in Data-Intensive Applications | Working with many big data teams over the years, something that always shows up across all of them... | 0 | 2024-07-06T10:48:12 | https://dev.to/er_dward/some-lesser-known-scalability-issues-in-data-intensive-applications-d8c | beginners, programming, devops, learning |
Working with many big data teams over the years, something that always shows up across all of them when they reach a certain scale is that they encounter a range of unexpected, lesser-known scalability issues. These problems aren't always obvious from the beginning but become pain points as systems grow. From hotspots in distributed databases and the thundering herd problem to data skew in search engines and write amplification in logging systems, these issues can seriously affect performance and reliability. Each of these challenges requires specific strategies to manage and mitigate, ensuring that your systems can handle increasing loads without degrading. Let's explore these lesser-known issues, understand their impacts with real-world examples I have seen or read about, and look at how to address them effectively.
### TL;DR
Scaling applications isn’t just about throwing more servers at the problem. There are some tricky, lesser-known issues that can sneak up on you and cause big headaches. In this post, we’ll look at hotspots, the thundering herd problem, data skew, write amplification, latency amplification, eventual consistency pitfalls, and cold start latency. I’ll share what these are, real-world examples, and how to fix them.
### 1. Hotspots in Distributed Systems
#### What Are Hotspots?
Hotspots happen when certain parts of your system get way more traffic than others, creating bottlenecks.
#### Example
Let’s use MongoDB, a popular NoSQL database, as an example. Suppose you have a collection of user profiles. If certain user profiles are accessed way more than others (say, those of influencers with millions of followers), the MongoDB shards containing these profiles can get overwhelmed. This uneven load can cause performance issues, as those particular shards experience much higher traffic than others.
#### How to Mitigate
- **Sharding**: Spread data evenly across nodes using a shard key that ensures even distribution. For MongoDB, choose a shard key that avoids creating hotspots.
- **Caching**: Implement a caching layer like Redis in front of MongoDB to handle frequent reads of hot data.
- **Load Balancing**: Use load balancers to distribute traffic evenly across multiple MongoDB nodes, ensuring no single node becomes a bottleneck.
### 2. Thundering Herd Problem
#### What Is this THP?
This issue occurs when many processes wake up at once and hit the same task, overwhelming the system.
#### Example
Picture a scenario with a popular e-commerce site that uses a cache to speed up product searches. When the cache expires, suddenly all incoming requests bypass the cache and hit the backend database simultaneously. This sudden surge can overwhelm the database, leading to slow responses or even outages.
#### How to Mitigate
- **Request Coalescing**: Combine multiple requests for the same resource into a single request. For instance, only allow one request to refresh the cache while the others wait for the result.
- **Rate Limiting**: Implement rate limiting to control the flow of requests to the backend database, preventing it from being overwhelmed.
- **Staggered Expiry**: Configure cache expiration times to be staggered rather than simultaneous, reducing the likelihood of a thundering herd.
### 3. Data Skew in Distributed Processing
#### What Does This Mean?
Data skew happens when data isn’t evenly distributed across nodes, making some nodes work much harder than others. This I believe is quite common, because most of us love to spin up systems and expect data is evenly accessed at scale, lets see an example.
#### Example
Consider Elasticsearch or Solr, which are commonly used for search functionality. These systems distribute data across multiple shards. If one shard ends up with a lot more data than others, maybe because certain keywords or products are much more popular, that shard will have to handle more queries. This can slow down search responses and put a heavier load on specific nodes.
Imagine you're running an e-commerce site with Elasticsearch. If most users search for a few popular products, the shards containing these products get hit harder. The nodes managing these shards can become bottlenecks, affecting your entire search performance.
#### How to Mitigate
- **Partitioning Strategies**: Use strategies like hash partitioning to distribute data evenly across shards. In Elasticsearch, choosing a good shard key is crucial.
- **Replica Shards**: Add replica shards to distribute the read load more evenly. Elasticsearch allows for replicas to share the load of search queries.
- **Adaptive Load Balancing**: Implement dynamic load balancing to adjust the distribution of queries based on current loads. Elasticsearch provides tools to monitor shard load and re-balance as needed.
### 4. Write Amplification
#### What Is It?
Write amplification occurs when a single write operation causes multiple writes throughout the system.
#### Example
In a typical logging setup using Elasticsearch, writing a log entry might involve writing to a log file, updating an Elasticsearch index, and sending notifications to monitoring systems. This single log entry can lead to multiple writes, increasing the load on your system.
#### How to Mitigate
- **Batching**: Combine multiple write operations into a single batch to reduce the number of writes. Elasticsearch supports bulk indexing, which can significantly reduce write amplification.
- **Efficient Data Structures**: Use data structures that minimize the number of writes required. Elasticsearch’s underlying data structure (based on Lucene) is optimized for write-heavy operations, but using it effectively (like tuning the refresh interval) can further reduce amplification.
### 5. Latency Amplification
#### What Does This Mean?
Small delays in one part of your system can snowball, causing significant overall latency.
#### Example
In a microservices architecture, a single slow microservice can delay the entire request chain. Imagine a web application where an API call to fetch user details involves calls to multiple microservices. If one microservice has a 100ms delay, and there are several such calls, the total delay can add up to several seconds, degrading user experience.
#### How to Mitigate
- **Async Processing**: Decouple operations with asynchronous processing using message queues like RabbitMQ or Kafka. This can help avoid blocking calls and reduce the cumulative latency.
- **Optimized Querying**: Speed up database queries with indexing and optimization techniques. In our example, ensure that each microservice query is optimized to return results quickly.
- **Circuit Breakers**: Implement circuit breakers to prevent slow microservices from affecting the entire request chain.
### 6. Eventual Consistency Pitfalls
#### What Does This Mean?
In distributed systems, achieving immediate consistency is often impractical, so eventual consistency is used. However, this can lead to issues if not managed correctly.
#### Example
An e-commerce site might show inconsistent stock levels because updates to the inventory database are only eventually consistent. This could lead to situations where customers are shown inaccurate stock information, potentially resulting in overselling or customer dissatisfaction.
#### How to Mitigate
- **Conflict Resolution**: Implement strategies to handle conflicts that arise from eventual consistency. Using [CRDTs](https://pypi.org/project/crdts/0.0.3/) (Conflict-free Replicated Data Types) can help resolve conflicts automatically.
- **Consistency Guarantees**: Clearly define and communicate the consistency guarantees provided by your system to manage user expectations. For example, explain to users that stock levels might take a few seconds to update.
### 7. Cold Start Latency
#### What Is It?
Cold start latency is the delay that happens when an application or function takes time to initialize.
#### Example
In serverless architectures like AWS Lambda, functions that haven't been used in a while need to be re-initialized. This can cause a noticeable delay in response time, which is particularly problematic for time-sensitive applications.
#### How to Mitigate
- **Warm-Up Strategies**: Keep functions warm by invoking them periodically. AWS Lambda offers provisioned concurrency to keep functions warm and ready to handle requests immediately.
- **Optimized Initialization**: Reduce the initialization time of your functions by optimizing the startup processes and minimizing dependencies loaded during startup. This can involve reducing the size of the deployment package or lazy-loading certain dependencies only when needed.
Something I have noticed about all these issues is that they often stem from the fundamental challenges of distributing and managing data across systems. Whether it’s the uneven load of hotspots and data skew, the cascading delays of latency amplification, or the operational overhead of write amplification and cold starts, these problems highlight the importance of thoughtful architecture and proactive monitoring. Addressing them effectively requires a combination of good design practices, efficient use of technology, and continuous performance tuning. | er_dward |
1,913,607 | Thiết Kế Website Tại Thủ Dầu Một Thu Hút Khách Hàng Tiềm Năng | Ngày nay, việc sở hữu một website chuyên nghiệp và hiệu quả đóng vai trò then chốt trong chiến lược... | 0 | 2024-07-06T10:46:24 | https://dev.to/terus_technique/thiet-ke-website-tai-thu-dau-mot-thu-hut-khach-hang-tiem-nang-4dbi | website, digitalmarketing, seo, terus |
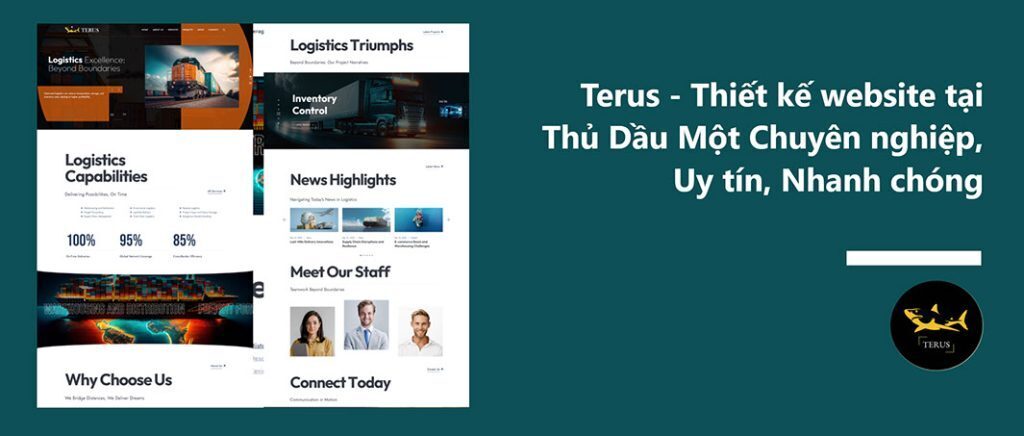
Ngày nay, việc sở hữu một website chuyên nghiệp và hiệu quả đóng vai trò then chốt trong chiến lược phát triển của các doanh nghiệp tại Thủ Dầu Một. Một website được thiết kế và xây dựng phù hợp có thể mang lại nhiều lợi ích quan trọng cho doanh nghiệp.
Tạo ấn tượng đầu tiên mạnh mẽ: Website là cửa ngõ đầu tiên giúp khách hàng tiếp cận và hiểu biết về doanh nghiệp. Một website đẹp mắt, dễ sử dụng và chuyên nghiệp sẽ tạo ấn tượng tốt đẹp về thương hiệu, tăng độ tin cậy và thu hút khách hàng tiềm năng.
Xây dựng niềm tin và sự tín nhiệm: Một website chuyên nghiệp, cung cấp đầy đủ thông tin về doanh nghiệp, sản phẩm/dịch vụ và phản hồi của khách hàng sẽ giúp khách hàng cảm thấy an tâm và tin tưởng khi giao dịch với doanh nghiệp.
Nâng cao trải nghiệm người dùng: Một website được thiết kế tối ưu, dễ điều hướng và đáp ứng nhanh chóng nhu cầu của khách hàng sẽ mang lại trải nghiệm tích cực, từ đó tăng khả năng tương tác và chuyển đổi thành khách hàng.
Tại Terus, chúng tôi cam kết mang lại những [website chuyên nghiệp, chuẩn SEO](https://terusvn.com/thiet-ke-website-tai-hcm/) và đáp ứng tối ưu nhu cầu của doanh nghiệp tại Thủ Dầu Một:
Hỗ trợ đa ngôn ngữ: Cho phép doanh nghiệp tiếp cận khách hàng trên nhiều thị trường bằng các ngôn ngữ khác nhau.
Tích hợp thông tin doanh nghiệp địa phương: Kết hợp thông tin về vị trí, liên hệ, sản phẩm/dịch vụ, khuyến mãi... phù hợp với từng khu vực.
Nổi bật nét độc đáo của doanh nghiệp: Thiết kế website tùy biến, phản ánh đúng bản sắc và văn hoá của doanh nghiệp.
Tích hợp đánh giá của khách hàng: Khách hàng có thể chia sẻ trải nghiệm và đánh giá trực tiếp trên website, tăng độ tin cậy.
Xây dựng chiến lược SEO: Tối ưu website để doanh nghiệp dễ dàng xuất hiện trên kết quả tìm kiếm và thu hút khách hàng tiềm năng.
Tích hợp kênh mạng xã hội: Kết nối website với các nền tảng mạng xã hội phù hợp, tăng tương tác và lan tỏa thương hiệu.
Quảng cáo sự kiện và ưu đãi: Giúp doanh nghiệp quảng bá các hoạt động, chương trình khuyến mãi tại địa phương một cách hiệu quả.
Terus cam kết mang lại những mẫu website chuyên nghiệp, chuẩn SEO và phù hợp với từng doanh nghiệp tại Thủ Dầu Một. Chúng tôi cũng cung cấp [dịch vụ thiết kế website theo yêu cầu](https://terusvn.com/thiet-ke-website-tai-hcm/), đáp ứng nhu cầu riêng biệt của từng khách hàng.
Với đội ngũ chuyên gia thiết kế, lập trình và marketing giàu kinh nghiệm, Terus tự hào mang lại những giải pháp website toàn diện, giúp doanh nghiệp tại Thủ Dầu Một tăng tương tác, thu hút khách hàng và thúc đẩy doanh số. Hãy liên hệ với chúng tôi để được tư vấn và triển khai dịch vụ thiết kế website phù hợp nhất.
Tìm hiểu thêm về [Thiết Kế Website Tại Thủ Dầu Một Thu Hút Khách Hàng Tiềm Năng](https://terusvn.com/thiet-ke-website/thiet-ke-website-tai-thu-dau-mot/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,606 | Exploring Cultural Marvels with the Deccan Odyssey | Embark on a journey through India’s rich tapestry of culture and history aboard the renowned Deccan... | 0 | 2024-07-06T10:45:35 | https://dev.to/deccanodysseycultural/exploring-cultural-marvels-with-the-deccan-odyssey-51m1 | Embark on a journey through India’s rich tapestry of culture and history aboard the renowned Deccan Odyssey.
This luxury train promises not just a mode of travel, but an immersive experience into the [cultural odyssey](https://www.deccanodyssey.co.uk/cultural-odyssey#cultural-odyssey-itinerary) heartlands of Maharashtra and Goa. Join us as we delve into the itinerary that promises to unveil the hidden gems and iconic landmarks of these regions.
1. Overview of the Deccan Odyssey
The Deccan Odyssey stands as a pinnacle of luxury travel in India, blending modern comforts with a nostalgic charm reminiscent of the era of royalty. Its reputation as a luxury travel experience in India precedes it, offering travelers an opportunity to traverse Maharashtra and Goa in unparalleled style. The route covers a carefully curated selection of destinations that epitomize the cultural odyssey richness of Western India.
2. Cultural Highlights of the Deccan Odyssey Itinerary
Day 1: Mumbai Upon boarding in Mumbai, the bustling metropolis welcomes you with its blend of colonial architecture and modern skyscrapers. Introduction to Mumbai’s cultural landmarks such as the Gateway of India and the Elephanta Caves sets the tone for the journey ahead.
Day 2: Nashik Nashik, known for its ancient temples and vineyards, offers a serene retreat into spirituality and viticulture. Explore the intricacies of Nashik’s cultural heritage while indulging in a wine-tasting experience unique to this region.
Day 3: Aurangabad Aurangabad unveils the UNESCO World Heritage sites of Ellora and Ajanta Caves, showcasing ancient rock-cut architecture and exquisite Buddhist art. The cultural significance of Ajanta is profound, offering insights into India’s artistic past.
Day 4: Ajanta Caves A journey to the Ajanta Caves reveals a treasure trove of Buddhist cave paintings and sculptures dating back to the 2nd century BCE. Exploration of the Ajanta Caves’ Buddhist art highlights India’s cultural odyssey diversity and artistic prowess.
Day 5: Kolhapur Kolhapur, steeped in history and tradition, beckons with its royal palaces and temples dedicated to Hindu goddesses. Discover Kolhapur’s historic sites and savor its delectable cuisine, known for its rich flavors and royal heritage.
Day 6: Goa The coastal paradise of Goa welcomes travelers with its pristine beaches, vibrant markets, and a blend of Portuguese and Indian cultures. Goa’s blend of Portuguese and Indian cultures offers a unique perspective on colonial history and local traditions.
Day 7: Sindhudurg Sindhudurg’s coastal fort and pristine beaches provide a tranquil conclusion to the journey. Overview of Sindhudurg’s fort and coastal culture encapsulates the maritime heritage of Maharashtra.
3. Onboard Experience of Cultural Immersion
Beyond the destinations, the Deccan Odyssey ensures a holistic cultural odyssey immersion. The onboard cultural programs feature performances by local artists, showcasing traditional music and dance forms. Culinary enthusiasts can indulge in regional delicacies prepared by skilled chefs, enhancing the journey’s cultural odyssey tapestry.
4. Tips for Cultural Travelers
For travelers seeking to maximize their cultural odyssey experience aboard the Deccan Odyssey, consider these practical tips:
Pack comfortable clothing suitable for both day tours and onboard activities.
Respect local customs and traditions, ensuring a harmonious interaction with local communities.
Embrace the opportunity to engage with fellow travelers and exchange cultural odyssey insights.
This journey aboard the Deccan Odyssey promises an enriching exploration of India’s cultural odyssey diversity, from ancient caves to vibrant coastal towns. Discover the magic of Maharashtra and Goa through this meticulously crafted itinerary, where every moment resonates with history, art, and the essence of India.
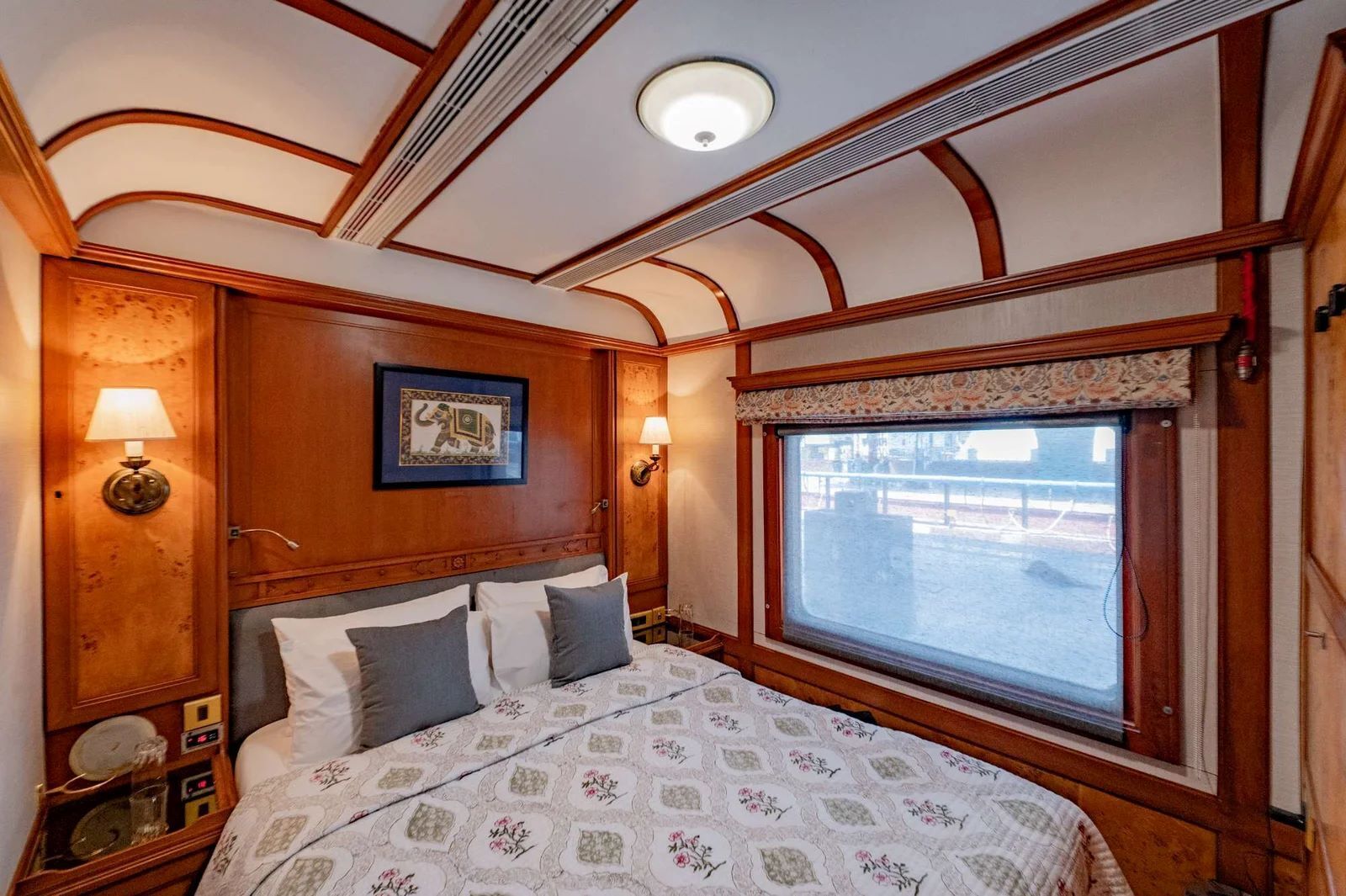 | deccanodysseycultural |
|
1,913,605 | Car Rental Service In Delhi - Rent A Car | We Provided swift Dzire Car Rental hire in delhi,Sedan Car,4 seater car Delhi,local sightseeing and... | 0 | 2024-07-06T10:45:22 | https://dev.to/sgholidaystour/car-rental-service-in-delhi-rent-a-car-25e9 | carrentalindelhi, caronrentindelhi, dzirecarhireindelhi, cabhireindelhi | We Provided swift Dzire Car Rental hire in delhi,Sedan Car,4 seater car Delhi,[](https://www.sgholidaystour.com/swift-dzire-car-hire-in-delhi)local sightseeing and Delhi,Noida,Gurgaon,Local Corporate,Airport Pickup & Drop service. Swift Dzire Car Service,Driver proper Unform,Music System,Medical Kit,Ice Box,Luggage Diggi & Carrier,Neat & Clean Vehicle.
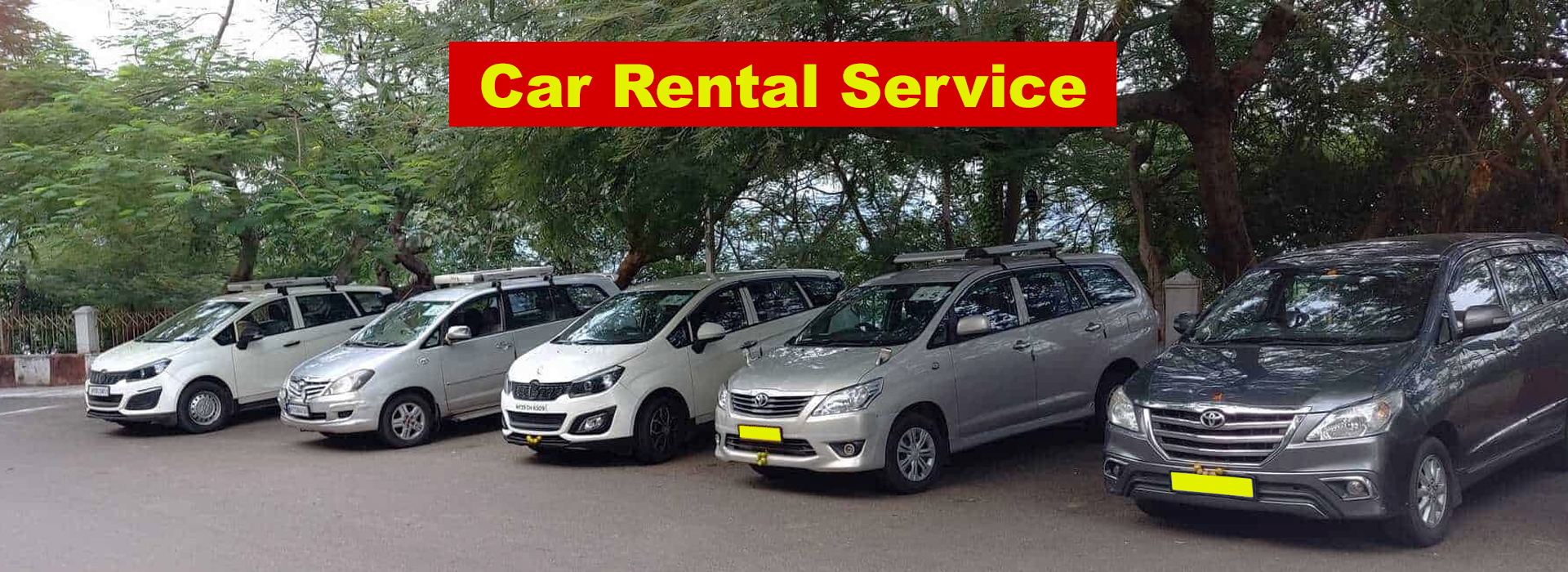 | sgholidaystour |
1,913,604 | Thiết Kế Website Tại Thái Bình Chất Lượng Cao | Doanh nghiệp tại Thái Bình ngày càng nhận thức rõ tầm quan trọng của việc sở hữu một website chuyên... | 0 | 2024-07-06T10:42:30 | https://dev.to/terus_technique/thiet-ke-website-tai-thai-binh-chat-luong-cao-dml | website, digitalmarketing, seo, terus |
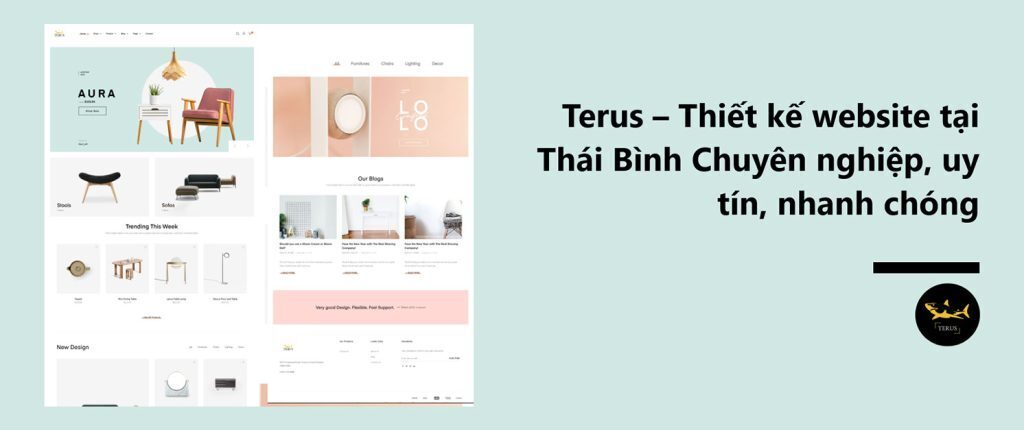
Doanh nghiệp tại Thái Bình ngày càng nhận thức rõ tầm quan trọng của việc sở hữu một website chuyên nghiệp, hiện đại. Một website không chỉ là nơi giới thiệu về sản phẩm, dịch vụ mà còn là công cụ tiếp thị, tương tác với khách hàng hiệu quả. Tuy nhiên, việc thiết kế một website chuyên nghiệp, đáp ứng được nhu cầu của doanh nghiệp và người dùng lại là một thách thức không nhỏ. Đây chính là lý do các doanh nghiệp tại Thái Bình ngày càng lựa chọn các đơn vị thiết kế website uy tín, chuyên nghiệp như Terus.
Terus - Nhà cung cấp [dịch vụ thiết kế website chuyên nghiệp tại Thái Bình](https://terusvn.com/thiet-ke-website-tai-hcm/)
Terus là đơn vị thiết kế website hàng đầu tại Thái Bình, sở hữu đội ngũ chuyên gia có nhiều năm kinh nghiệm trong lĩnh vực này. Với phương châm "Thiết kế website chuyên nghiệp, uy tín và nhanh chóng", Terus mang đến cho khách hàng những giải pháp website toàn diện, đáp ứng được mọi nhu cầu của doanh nghiệp.
Với những ưu điểm nổi bật như: Thiết kế website theo phong cách hiện đại, thân thiện với người dùng, tối ưu hóa trải nghiệm người dùng, tích hợp đầy đủ các tính năng cần thiết, đảm bảo an toàn và bảo mật, hỗ trợ kỹ thuật 24/7,... Terus đã và đang trở thành lựa chọn số 1 của hàng trăm doanh nghiệp tại Thái Bình.
Các mẫu website do Terus thiết kế tại Thái Bình đều mang đậm dấu ấn riêng, phản ánh rõ nét bản sắc và chiến lược của từng doanh nghiệp. Ngoài ra, Terus còn cung cấp dịch vụ thiết kế website chuẩn Insight - một khái niệm độc đáo, tích hợp đầy đủ các giải pháp marketing số, giúp tăng tương tác, thu hút khách hàng một cách hiệu quả.
Để bắt đầu với [dịch vụ thiết kế website chuyên nghiệp tại Thái Bình](https://terusvn.com/thiet-ke-website-tai-hcm/) của Terus, khách hàng chỉ cần liên hệ trực tiếp qua các kênh như website, email, hotline,... Đội ngũ tư vấn sẽ hỗ trợ khách hàng từ khâu lên ý tưởng cho đến khi website chính thức đi vào hoạt động.
Trong bối cảnh cạnh tranh ngày càng gay gắt, việc sở hữu một website chuyên nghiệp, hiện đại là yếu tố then chốt giúp doanh nghiệp tại Thái Bình nâng cao thương hiệu, tiếp cận hiệu quả hơn với khách hàng. Với những giải pháp website toàn diện và uy tín, Terus chắc chắn sẽ là lựa chọn hàng đầu của các doanh nghiệp tại Thái Bình.
Tìm hiểu thêm [Thiết Kế Website Tại Thái Bình Chất Lượng Cao](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-thai-binh/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,603 | Vectors(cpp) Complete Guide ❤by Aryan | warning: use using namespace std: i always use 1st based indexing Introduction #include... | 0 | 2024-07-06T10:42:12 | https://dev.to/aryan015/vectorscpp-complete-guide-by-aryan-1511 | cpp, programming, computerscience, 100daysofcode | `warning:` use `using namespace std`:
` i always use 1st based indexing`
## Introduction
`#include <vector>`
⛓ Vectors are arrays with super power (part of STL). You can sort it, push, pull data with just in-built(not recommended) functions.🧡🧡 It has one more superpower it size is very dynamic which means you can push any number of items even after size is specified.🧡 It just double the vector size.
| initial size | (after adding) after size |
| --- | --- |
| 0 | 1 |
| 2 | 4 |
`note`: Drawback of these vector is that memory wasteage. (`getaway`: always know no of items).
## Syntax
### Declaration
Usually you wont be needing vector without `int`(mainly).🧡
```cpp
// with specified size
//(size,value)
//std::vector<datatype> arr_name(size);
std::vector<int> file(5,12) // 5 elements with each 12
std::vector<int> v(100); // Initializes 100 elements with zeros
std::vector<int> v(existingVector) //create a copy of existingVector
```
```cpp
// without size
// (initialize with zero size)
// std::vector<string> name;
std::vector<string> str; //contains string value
std::vector<bool> str2; //contains bool value
std::vector<float> data = {1,2,3,4,5}; //vector with 5 value
```
## built-in functions
import following header
`#include <algorithm>`
initial vector
`std::vector<int> v = {1,5,4,3,2}`
### `begin` and `end` function
These are the pointer use to point the first and last element address
```cpp
auto first = v.begin();
auto last = v.end();
cout<<"first: "<<*first<< " "<<"last: "<<*last<<ednl;
```
output
```sh
first: 1 last:2
```
### SORT function
```cpp
//ascending order
std::vector<int> v = {1,5,4,3,2};
std::sort(v.begin(),v.end())
```
```cpp
//descending order
std:sort(v.begin(),v.end(),std::greater<int>());
// method 2
//reverse iterator
//use reverse iterator function
//reverse iterator allows you to traverse vector in reverse order
std::sort(v.rbegin(),v.rend())
```
output
```cpp
1 2 3 4 5
5 4 3 2 1
```
### `Size` function
know the no of elements in an array.
```cpp
v.size(); // 5
```
### `push_back` function
append item at the end of the array
```cpp
std::vector<int> v = {1,5,4,3,2};
v.push_back(5)
```
output
```cpp
1 5 4 3 2 5
```
### `pop_back` function
remove the last item from vector
```cpp
v.pop_back(); //remove last item
```
output
```sh
1 5 4 3
```
### `back` `front` function
returns the last element
```cpp
v.back() //3
v.front() //1
```
### `insert` function
insert item at given position
```cpp
std::vector<int> v = {1,2,3,4};
//v.insert(position_pointer,size,value);
// Insert 100 at position 4th (using an iterator)
std::vector<int>::iterator it = myVector.begin() + 3;
//(alternative)auto it = myVector.begin() + 3;
myVector.insert(it,1,100);
```
output
```sh
1 2 3 100 4
```
### `erase` function
This function helps erase element from specific position.
```cpp
v.erase(position) // remove ele from position
v.erase(v.begin()) //remove the first element
v.erase(v.begin() + 1) //remove 2nd element
v.erase(v.end()) //revome last element
```
`note:`erase function always expect normal iterator
```cpp
//remove second last element
auto itlast = v.rbegin();
itlast++; // increase the iterator
v.erase(itlast.base()) // remove second last element
```
`note:` convert the reverse iterator to normal one.
### `resize` function
You can resize vector
### `clear` function
You can clear function to remove all elements
### `empty` function
```cpp
//check if it is empty or not
if(v.empty())
cout<<"yes"<<endl;
```
## Ranged base loop
traverse vector using `for` loop
```cpp
for (int num : v) {
cout << num << " ";
}
```
## Accessing Values
put the index you want to access
```cpp
v[index]
```
## How to pass vector to a function
### by value
```cpp
void func(vector<int> v){
return 0
}
int main(){
vector<int> vect = {1,2,3};
func(vect);
}
```
### by reference
```cpp
void func(vector<int>& vect){
return 0;
}
int main(){
vector<int> vect = {1,2,3};
func(vect);
return 0;
}
```
#### Thank you for wasting your time
[Linkedin](https://www.linkedin.com/in/aryan-khandelwal-779b5723a/)🍑
[Github](https://github.com/ak-khandelwal) ❤
❤Please follow for more such content on dev: @aryan015 | aryan015 |
1,913,601 | WHY DIGITAL MARKETING IS BEST CHOICE FOR YOUR CAREER | The digital landscape is continuously expanding, and businesses of all sizes are increasingly... | 0 | 2024-07-06T10:38:49 | https://dev.to/jessan_roy_28f8b62ba7c328/why-digital-marketing-is-best-choice-for-your-career-efo | The digital landscape is continuously expanding, and businesses of all sizes are increasingly recognizing the importance of having a strong online presence. This has created a high demand for skilled digital marketers who can help organizations reach their target audience and achieve their marketing goals.
Digital Marketing has many types, such as search engine optimization (SEO), social media marketing, content marketing, email marketing, pay-per-click (PPC) advertising, and more. This diversity offers individuals the opportunity to specialize in specific areas or develop a well-rounded skill set that can be applied across different industries.d a subheading.
Entry-level digital marketing positions in India can range from INR 2 to 4 lakhs per year. With a few years of experience, you can expect a mid-level digital marketing role with a salary range of around INR 4 to 8 lakhs per year.
Senior-level digital marketing professionals with a considerable amount of experience and expertise can earn salaries ranging from INR 10 to 20 lakhs per year or even higher, depending on their role and the organization they work for. As you gaining the experience in this feild your work value and salary is increasing in that way.
Digital Marketing this the best career choice for everyone without any degree, Just doing the course for developing your skills and create the strategy of your own. Choosing digital marketing as a career will help you in each way without remotely working. Just sit develop skills, Make strategy and earn.
Read More: https://vkdigitalconsultancy.com/career-in-digital-marketing/
Know More About VK Digital Consultancy
V K Digital Consultancy is a one-stop branding solution for your business. This is a digital era, digital appearance is the necessity of any business. Understanding this wide scope and interest, we started this business and started to help many business owners to grow their digital presence.
Our enthusiastic, dedicated, and expert team manages your social media, manages your online reputation, and keeps your customers updated about your business. Mrs. Vaishnavi Kadam, founder and team leader of VK Digital Consultancy is running this business very successfully, making customers satisfied with reliable branding solutions. | jessan_roy_28f8b62ba7c328 |
|
1,913,600 | 10 DIGITAL MARKETING WAYS TO EARN ONLINE | Here are 10 Digital Marketing Ways to Earn Online 1.) Affiliate Marketing: Promote products or... | 0 | 2024-07-06T10:38:42 | https://dev.to/jessan_roy_28f8b62ba7c328/10-digital-marketing-ways-to-earn-online-17 | Here are 10 Digital Marketing Ways to Earn Online
1.) Affiliate Marketing: Promote products or services from other companies and earn a commission for each sale or lead generated through your affiliate links.
2.) Content Marketing: Create valuable and engaging content, such as blog posts, videos, or infographics, and monetize it through ads, sponsorships, or affiliate marketing.
3.) Email Marketing: Build an email list and send targeted campaigns to promote products, services, or your own digital products like ebooks, courses, or webinars.
4.) Social Media Marketing: Leverage platforms like Facebook, Instagram, Twitter, and TikTok to promote products, offer sponsored posts, or sell your own products/services.
5.) Search Engine Optimization (SEO): Optimize websites or content for search engines to increase organic traffic, which can be monetized through ads, affiliate marketing, or selling products.
6.) Pay-Per-Click (PPC) Advertising: Create and manage ad campaigns on platforms like Google Ads or Bing Ads, and earn money by driving traffic to affiliate offers or selling products.
7.) E-commerce: Set up an online store and sell physical or digital products directly to consumers.
8.) Dropshipping: Start an e-commerce business without holding inventory. Instead, work with suppliers to fulfill orders as they come in.
9.) Freelance Services: Offer your digital marketing skills as a freelancer, providing services like social media management, content writing, SEO, or paid advertising management.
10.) Consulting and Coaching: Share your expertise in digital marketing with businesses or individuals and charge for your consulting or coaching services.
Read More: https://vkdigitalconsultancy.com/10-digital-marketing-ways-to-earn-online/
Know More About VK Digital Consultancy
V K Digital Consultancy is a one-stop branding solution for your business. This is a digital era, digital appearance is the necessity of any business. Understanding this wide scope and interest, we started this business and started to help many business owners to grow their digital presence.
Our enthusiastic, dedicated, and expert team manages your social media, manages your online reputation, and keeps your customers updated about your business. Mrs. Vaishnavi Kadam, founder and team leader of VK Digital Consultancy is running this business very successfully, making customers satisfied with reliable branding solutions. | jessan_roy_28f8b62ba7c328 |
|
1,913,599 | Understanding Adapters in Java for Android Development | Adapters are a crucial part of Android development, especially when dealing with user interfaces.... | 0 | 2024-07-06T10:36:49 | https://dev.to/ankittmeena/understanding-adapters-in-java-for-android-development-2o3a | android, adapter, java, mobile | Adapters are a crucial part of Android development, especially when dealing with user interfaces. They act as a bridge between a data source and a user interface component, enabling the display of dynamic content in views like ListView, GridView, and RecyclerView. This article explores the concept of adapters in Java for Android Studio, illustrating their importance and usage with practical examples.
## Why Are Adapters Important in Android?
**Data Binding:** Adapters facilitate the binding of data from data sources (like arrays, databases, or web services) to UI components.
**Dynamic Content:** They allow for the dynamic display of content, making it easy to update the UI as data changes.
**Reusability:** Adapters enable the reuse of UI components by decoupling data handling from the presentation layer.
## Types of Adapters in Android
**1. ArrayAdapter**
ArrayAdapter is a simple adapter used to bind arrays to ListView or GridView.
```
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, dataArray);
ListView listView = findViewById(R.id.listView);
listView.setAdapter(adapter);
```
**2. BaseAdapter**
BaseAdapter provides a flexible way to create custom adapters by extending it and implementing the required methods.
```
public class CustomAdapter extends BaseAdapter {
private Context context;
private List<Item> items;
public CustomAdapter(Context context, List<Item> items) {
this.context = context;
this.items = items;
}
@Override
public int getCount() {
return items.size();
}
@Override
public Object getItem(int position) {
return items.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.item_layout, parent, false);
}
Item currentItem = items.get(position);
TextView textView = convertView.findViewById(R.id.textView);
textView.setText(currentItem.getName());
return convertView;
}
}
```
**3. RecyclerView.Adapter**
RecyclerView.Adapter is the most powerful and flexible adapter in Android, used for RecyclerViews. It provides better performance and more customization options.
```
public class MyRecyclerViewAdapter extends RecyclerView.Adapter<MyRecyclerViewAdapter.ViewHolder> {
private List<String> mData;
private LayoutInflater mInflater;
public MyRecyclerViewAdapter(Context context, List<String> data) {
this.mInflater = LayoutInflater.from(context);
this.mData = data;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = mInflater.inflate(R.layout.recyclerview_item, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
String item = mData.get(position);
holder.myTextView.setText(item);
}
@Override
public int getItemCount() {
return mData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
TextView myTextView;
ViewHolder(View itemView) {
super(itemView);
myTextView = itemView.findViewById(R.id.tvItem);
}
}
}
```
## Implementing Adapters in Android Studio
To use adapters in your Android project, follow these steps:
****
**Define the Data Source:** Determine where your data is coming from (array, database, etc.).
**Create the Adapter:** Choose the appropriate adapter type (ArrayAdapter, BaseAdapter, RecyclerView.Adapter) and implement it.
**Bind the Adapter to the View:** Attach the adapter to your UI component (ListView, GridView, RecyclerView).
Example: Using RecyclerView with a Custom Adapter
Add RecyclerView to Layout:
```
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
```
RecyclerView recyclerView = findViewById(R.id.recyclerView);
MyRecyclerViewAdapter adapter = new MyRecyclerViewAdapter(this, dataList);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(adapter);
## Conclusion
Adapters are indispensable in Android development, enabling the dynamic display of data in various UI components. Understanding and implementing adapters efficiently can greatly enhance the user experience of your Android applications. Whether you're using ArrayAdapter for simple lists, BaseAdapter for more customization, or RecyclerView.Adapter for advanced performance, and mastering adapters will elevate your Android development skills.
| ankittmeena |
1,913,598 | Thiết Kế Website Tại Thanh Hóa Đầy Đủ Tính Năng | Thanh Hóa, một thành phố năng động và đang phát triển nhanh chóng, đang trở thành một điểm đến hấp... | 0 | 2024-07-06T10:36:13 | https://dev.to/terus_technique/thiet-ke-website-tai-thanh-hoa-day-du-tinh-nang-2a73 | website, digitalmarketing, seo, terus |
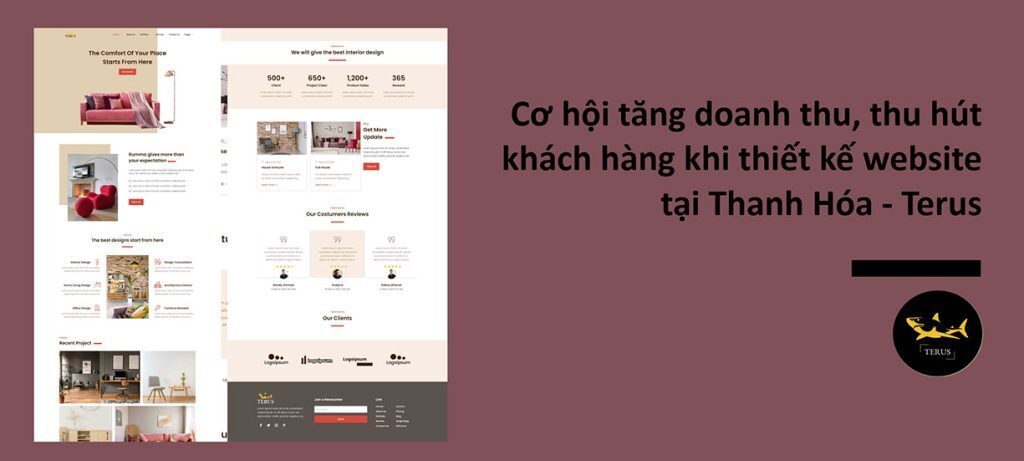
Thanh Hóa, một thành phố năng động và đang phát triển nhanh chóng, đang trở thành một điểm đến hấp dẫn cho các doanh nghiệp muốn mở rộng hoạt động của mình. Với nhiều lợi thế như chi phí thấp, nguồn nhân lực trẻ và năng động, Thanh Hóa đang trở thành một lựa chọn lý tưởng cho các doanh nghiệp muốn thiết kế và phát triển website của mình.
Công ty Terus, với nhiều năm kinh nghiệm trong lĩnh vực thiết kế và phát triển website, đã trở thành một trong những lựa chọn hàng đầu cho các doanh nghiệp tại Thanh Hóa. Terus hiểu rõ đặc thù địa phương và có đội ngũ chuyên gia giàu kinh nghiệm, cam kết mang đến những [giải pháp website tối ưu và thân thiện](https://terusvn.com/thiet-ke-website-tai-hcm/) với người dùng.
Một trong những ưu điểm nổi bật của dịch vụ thiết kế website của Terus tại Thanh Hóa là sự hiểu biết sâu sắc về thị trường và đối tượng khách hàng địa phương. Terus sử dụng phương pháp tiếp cận tùy chỉnh, đảm bảo rằng mỗi website được thiết kế phù hợp với nhu cầu và mục tiêu của từng doanh nghiệp. Ngoài ra, Terus cũng cung cấp dịch vụ bản địa hóa và hỗ trợ đa ngôn ngữ, giúp các doanh nghiệp tiếp cận được với khách hàng địa phương và quốc tế một cách hiệu quả.
Đặc biệt, Terus chú trọng vào việc thiết kế website thân thiện với thiết bị di động, đáp ứng nhu cầu truy cập ngày càng tăng từ các thiết bị di động. Terus cũng xây dựng chiến lược SEO Local để đảm bảo các website của khách hàng được tối ưu hóa cho thị trường địa phương, tăng khả năng hiển thị trên các công cụ tìm kiếm.
Ngoài ra, Terus cam kết cung cấp dịch vụ hỗ trợ và bảo trì liên tục, giúp các doanh nghiệp luôn cập nhật và duy trì website của mình một cách hiệu quả. Quá trình thiết kế website tại Thanh Hóa của Terus bao gồm các bước như: Nhận yêu cầu và tư vấn, thiết kế bản website demo, hoàn thiện giao diện và triển khai tính năng, tối ưu chỉ số Insight, chạy thử sản phẩm và bàn giao hướng dẫn.
Các doanh nghiệp tại Thanh Hóa có thể lựa chọn các mẫu website mẫu của Terus hoặc yêu cầu thiết kế website theo nhu cầu riêng. Terus cam kết mang đến những [giải pháp website chuyên nghiệp, hiệu quả](https://terusvn.com/thiet-ke-website-tai-hcm/) và phù hợp với từng doanh nghiệp.
Terus là một lựa chọn đáng tin cậy cho các doanh nghiệp tại Thanh Hóa muốn thiết kế và phát triển website của mình. Với sự hiểu biết sâu sắc về thị trường địa phương, phương pháp tiếp cận tùy chỉnh và cam kết về chất lượng dịch vụ, Terus mang đến những giải pháp website đáp ứng tối đa nhu cầu của khách hàng.
Tìm hiểu thêm về [Thiết Kế Website Tại Thanh Hóa Đầy Đủ Tính Năng](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-thanh-hoa/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,595 | Thiết Kế Website Tại Nha Trang Đẹp Mắt, Thu Hút | Ngày nay, một website hiện đại, chuyên nghiệp là một yếu tố không thể thiếu đối với mỗi doanh... | 0 | 2024-07-06T10:32:41 | https://dev.to/terus_technique/thiet-ke-website-tai-nha-trang-dep-mat-thu-hut-ce0 | website, digitalmarketing, seo, terus |
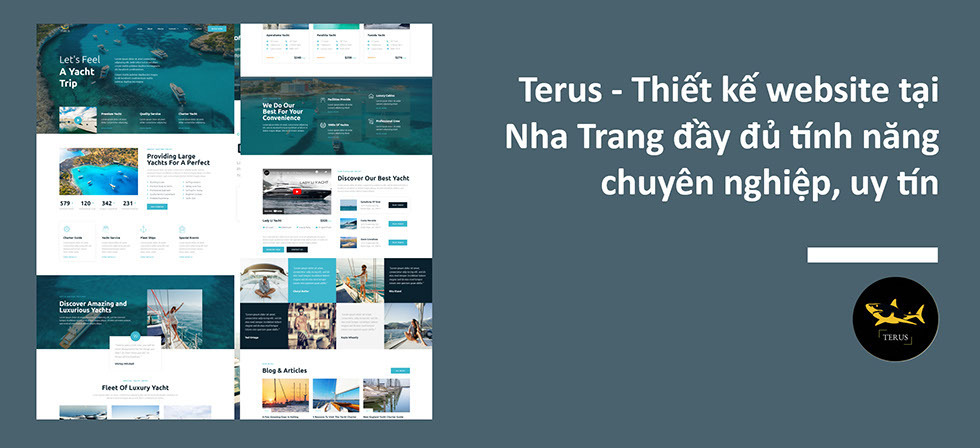
Ngày nay, một website hiện đại, chuyên nghiệp là một yếu tố không thể thiếu đối với mỗi doanh nghiệp. Đặc biệt tại Nha Trang - một thành phố du lịch sầm uất, việc có một website ấn tượng và đầy đủ tính năng là rất quan trọng để thu hút khách hàng tiềm năng, nâng cao hiệu quả kinh doanh.
Terus, một công ty [thiết kế website chuyên nghiệp tại Nha Trang](https://terusvn.com/thiet-ke-website-tai-hcm/), cam kết mang đến những giải pháp website toàn diện, đáp ứng tối đa nhu cầu của các doanh nghiệp tại địa phương. Với đội ngũ nhân sự giàu kinh nghiệm, quy trình thiết kế khoa học và đa dạng dịch vụ, Terus tự hào là [đơn vị thiết kế website hàng đầu tại Nha Trang](https://terusvn.com/thiet-ke-website-tai-hcm/).
Một số nét nổi bật trong dịch vụ thiết kế website của Terus tại Nha Trang:
Giao diện website độc đáo, chuyên nghiệp: Terus cam kết mang đến những giao diện website tại Nha Trang được thiết kế tỉ mỉ, phù hợp với từng ngành nghề, đem lại trải nghiệm tuyệt vời cho người dùng.
Đa dạng tính năng tích hợp: Ngoài giao diện ấn tượng, các website do Terus thiết kế còn được tích hợp nhiều tính năng hiện đại như: kết nối mạng xã hội, thanh toán trực tuyến, đặt lịch hẹn, quản lý sản phẩm/dịch vụ... giúp doanh nghiệp tăng tương tác và tiện ích cho khách hàng.
Sẵn sàng thiết kế theo yêu cầu: Nhận thức rõ mỗi doanh nghiệp có những nhu cầu và mong muốn riêng, Terus luôn sẵn sàng lắng nghe và thiết kế website theo yêu cầu cụ thể của khách hàng.
Kho mẫu website khổng lồ: Terus sở hữu kho mẫu website tại Nha Trang vô cùng phong phú, đa dạng các ngành nghề, giúp khách hàng dễ dàng lựa chọn phù hợp.
Module riêng cho các website tại Nha Trang: Ngoài các tính năng tiêu chuẩn, Terus còn cung cấp nhiều module riêng biệt như: đặt phòng khách sạn, quản lý tour du lịch, đặt vé máy bay... nhằm đáp ứng nhu cầu đa dạng của các doanh nghiệp tại Nha Trang.
Bên cạnh các dịch vụ thiết kế website, Terus còn cung cấp các giải pháp Digital Marketing, phần mềm quản lý doanh nghiệp... nhằm hỗ trợ khách hàng toàn diện trong việc xây dựng và vận hành website.
Với quy trình chuyên nghiệp, đội ngũ năng lực và cam kết dịch vụ chất lượng, Terus tự tin mang đến những website ưu việt, góp phần thúc đẩy sự phát triển của các doanh nghiệp tại Nha Trang.
Tìm hiểu thêm về [Thiết Kế Website Tại Nha Trang Đẹp Mắt, Thu Hút](https://terusvn.com/thiet-ke-website/terus-thiet-ke-website-tai-nha-trang/)
Digital Marketing:
· [Dịch vụ Facebook Ads](https://terusvn.com/digital-marketing/dich-vu-facebook-ads-tai-terus/)
· [Dịch vụ Google Ads](https://terusvn.com/digital-marketing/dich-vu-quang-cao-google-tai-terus/)
· [Dịch vụ SEO Tổng Thể](https://terusvn.com/seo/dich-vu-seo-tong-the-uy-tin-hieu-qua-tai-terus/)
Thiết kế website:
· [Dịch vụ Thiết kế website chuẩn Insight](https://terusvn.com/thiet-ke-website/dich-vu-thiet-ke-website-chuan-insight-chuyen-nghiep-uy-tin-tai-terus/)
· [Dịch vụ Thiết kế website](https://terusvn.com/thiet-ke-website-tai-hcm/) | terus_technique |
1,913,594 | Top 10 Hidden Treks in Uttarakhand That Will Take Your Breath Away | Nestled in the lap of the Himalayas, Uttarakhand is a popular destination for trekking in India.... | 0 | 2024-07-06T10:32:14 | https://dev.to/trekthehimalayas/top-10-hidden-treks-in-uttarakhand-that-will-take-your-breath-away-1pe8 | trekking, adventure, himalayas | Nestled in the lap of the Himalayas, Uttarakhand is a popular destination for trekking in India. While popular routes like the Valley of Flowers and Kedarkantha draw large crowds, the state harbours numerous lesser-known trails that promise solitude, stunning nature, and an authentic connection with the mountains. These hidden treks offer an unparalleled experience, showcasing the untouched beauty of Uttarakhand's diverse landscapes, from dense forests and serene meadows to majestic peaks and glacial rivers. In this article, we have compiled a list of Top 10 hidden treks in Uttarakhand that are perfect for the offbeat traveller.
[**1. Ranthan Kharak Trek**
](https://trekthehimalayas.com/ranthan-kharak-trek)
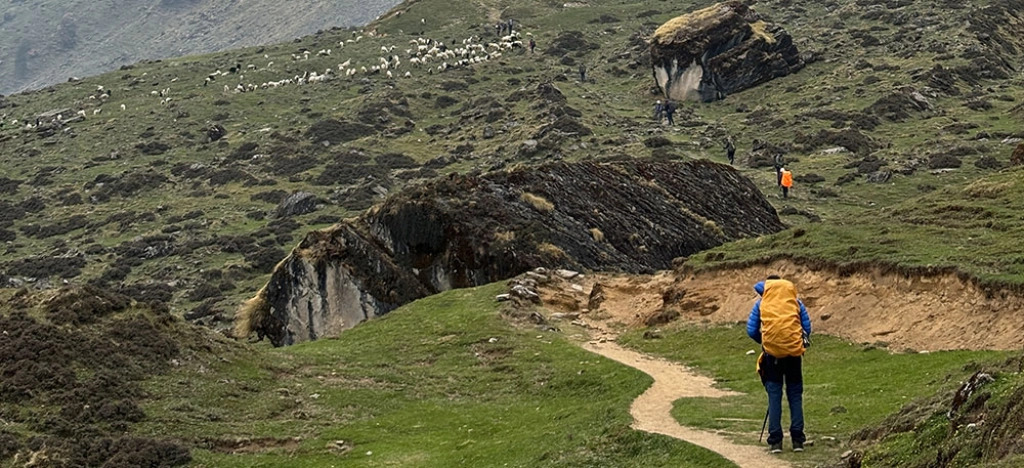
While most of the popular destinations for trekking in Uttarakhand fall in the Garhwal region, the Kumaon region boasts equally stunning mountainscapes and lush greenery that will captivate your heart. The Ranthan Kharak Trek in Kumaon is one such offbeat adventure that encapsulates the region's vivid natural beauty. This trek offers breathtaking views of majestic mountain ranges, including Nanda Devi, Nanda Devi East, Nanda Kot, Mt. Mrigthuni, Mt. Dangthal, and the Panchachuli Range. As you traverse dense forests and serene clearings, or 'Kharaks,' you'll witness the rich biodiversity and vibrant flora unique to Kumaon. The journey through picturesque villages like Namik and Bajimanian Kharak and camping in scenic clearings provide an immersive experience of nature's tranquillity. The trek's challenging terrain and the opportunity to engage with local communities make Ranthan Kharak an essential trek for those who seek solitude and a deep connection with the pristine wilderness of Uttarakhand.
2. Phulara Ridge Trek](https://trekthehimalayas.com/phulara-ridge-trek)
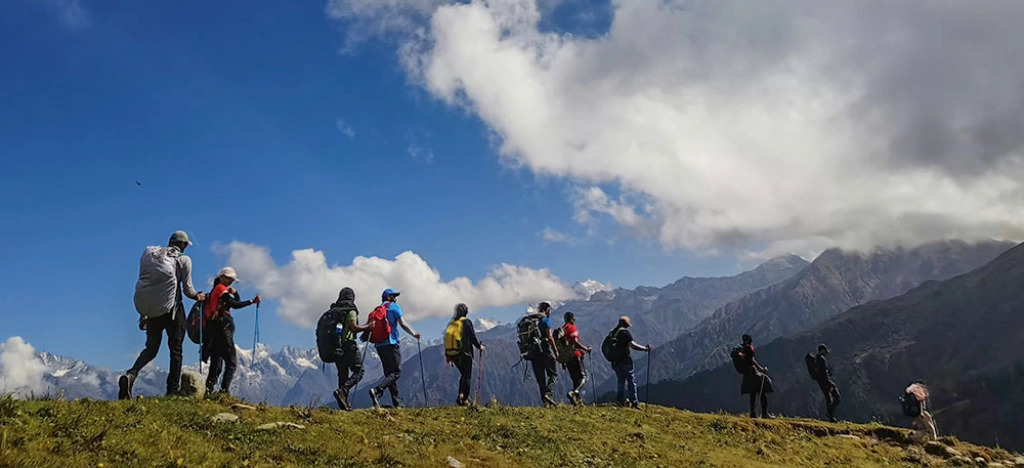
Nestled in the pristine Govind Pashu Vihar National Park, the Phulara Ridge Trek is a hidden gem that offers a unique and unforgettable experience of trekking in Uttarakhand. Starting from the quaint village of Sankri, this 25 km trek is a journey through diverse landscapes, from dense forests and lush meadows to the breathtaking ridge walk that sets it apart. The ridge offers an exhilarating 2-hour walk with panoramic views of snow-capped peaks, including the majestic Dev Kayara and Swargarohini ranges.
As you traverse the ridge, the mesmerizing contrast of verdant meadows on one side and captivating valleys on the other is simply awe-inspiring. The trek is a feast for the senses, with the vibrant colours of blooming wildflowers, the melodies of Himalayan birds like the Monal pheasant, and the serene beauty of the Pushtara meadows, where folds of green hills stretch as far as the eye can see. Ideal for both families and solo adventurers, this trek is best experienced from March to June or September to December, offering a delightful blend of natural beauty, cultural immersion in local Himalayan villages, and the thrill of walking along the edge of the world.
[3. Satopanth Lake Trek](https://trekthehimalayas.com/Satopanth-Lake-Trek)
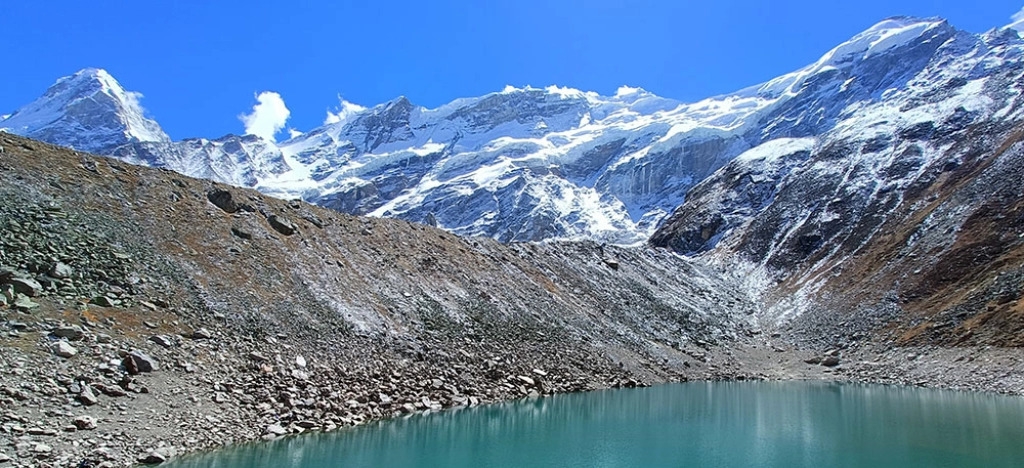
The Satopanth Lake Trek, set at an altitude of 4,600 meters in the Garhwal Himalayas, offers an unparalleled journey through pristine natural beauty and spiritual significance. Beginning from the sacred town of Badrinath, the trek meanders through the picturesque Mana Village, the last inhabited settlement before the Tibetan border. The trail, adorned with the stunning Vasundhara Falls and the mesmerizing Sahastradhara, leads trekkers through lush meadows, serene forests, and rugged moraines to the emerald waters of Satopanth Lake. This triangular lake, believed to be a meditation site for the Trimurti—Brahma, Vishnu, and Mahesh—nestles amidst towering peaks like Chaukhamba, Neelkanth, and Balakun. The tranquil ambiance of the lake, coupled with breathtaking mountain vistas, ancient legends, and the chance to camp under starlit skies, makes the Satopanth Lake Trek an unforgettable adventure for both seasoned trekkers and enthusiastic beginners.
4. Panwali Kantha Trek
](https://trekthehimalayas.com/panwali-kantha)
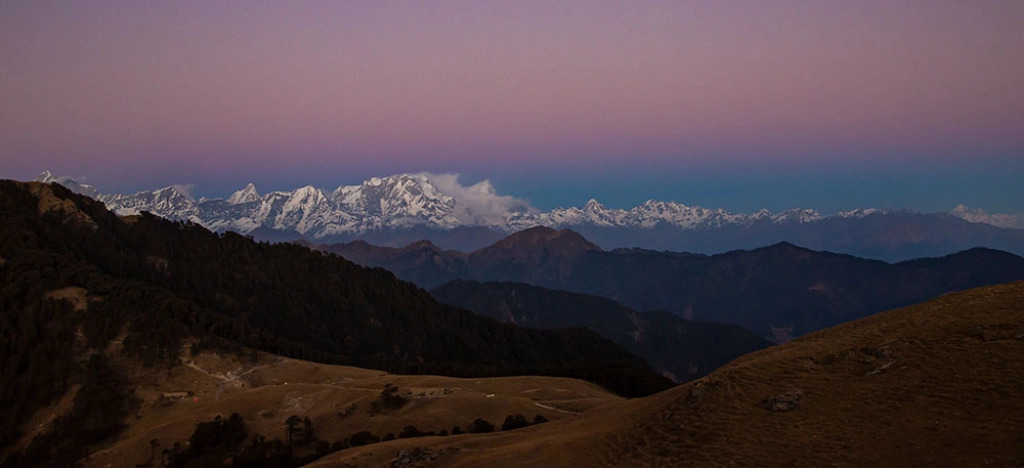
The Panwali Kantha Trek, nestled at an altitude of 3,300 meters in the Garhwal Himalayas, is a hidden gem that offers an unparalleled experience for nature enthusiasts and trekkers alike. This high-altitude meadow trek unveils stunning vistas of the Char Dham mountain ranges, including the majestic Gangotri, Yamunotri, Kedarnath, and Badrinath peaks. The journey through verdant landscapes is enriched with diverse flora, including the vibrant Rhododendron blooms in spring and an array of Himalayan herbs and flowers. Wildlife sightings are a delightful bonus, with the possibility of encountering creatures like the elusive musk deer and Himalayan bears.
The trek culminates at the picturesque Panwali Kantha, where the panoramic views of peaks such as Chaukhamba, Neelkanth, and Thalaysagar leave trekkers spellbound. Each season paints the trek with its unique palette, from the lush greens of monsoon to the snowy splendour of winter. Ending at the sacred Trijuginarayan Temple, believed to be the site of Lord Shiva and Parvati's wedding, the Panwali Kantha Trek is a harmonious blend of natural beauty, wildlife, and spiritual significance, making it a must-do adventure in the Himalayas.
**[5. Kedartal Trek
](https://trekthehimalayas.com/kedar-tal-trek)**
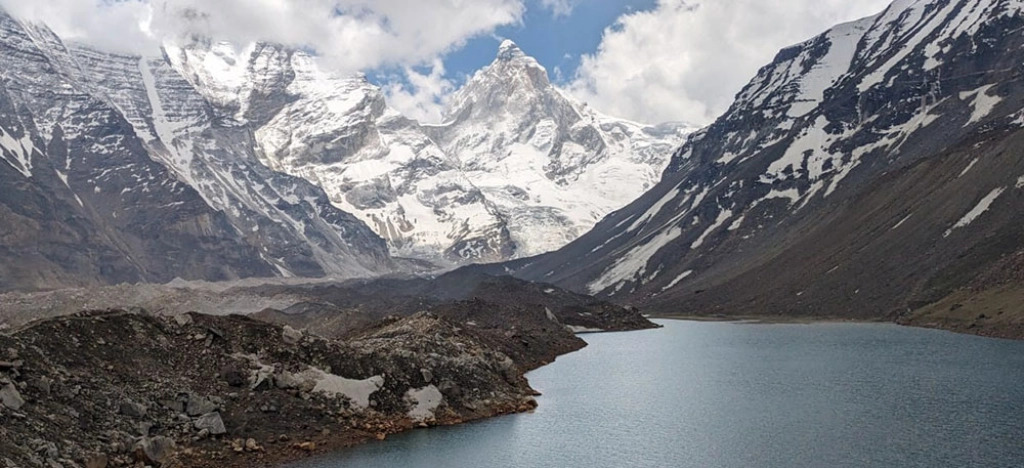
The Kedartal Trek, nestled in the heart of Uttarakhand, is a captivating journey through the majestic Gangotri Range. This seven-day adventure, marked by moderate to difficult trails, takes trekkers to an altitude of 15,500 feet, covering approximately 37 kilometers. The highlight is the emerald Kedartal Lake, reflecting the stunning Thalaysagar peak, renowned for its pristine beauty after Shivling Peak. The trek, starting from the sacred town of Gangotri, weaves through dense forests, rocky paths, and vibrant meadows, offering breathtaking views of towering peaks like Manda, Bhagirathi, and Mt. Meru. The route is challenging, with steep ascents and technical sections like the spider walls, requiring careful preparation and guidance. The journey includes an acclimatization day at Gangotri, ensuring trekkers adapt to the high altitude. Along the way, the landscape transforms from dense Bhojpatra forests to serene meadows, culminating in the surreal beauty of Kedartal. This trek, ideal for experienced adventurers, promises a profound connection with nature, solitude, and a thrilling experience of trekking in Uttarhand.
**[6. Dodital Darwa Pass Trek](https://trekthehimalayas.com/dodital-darwa-pass-trek)**
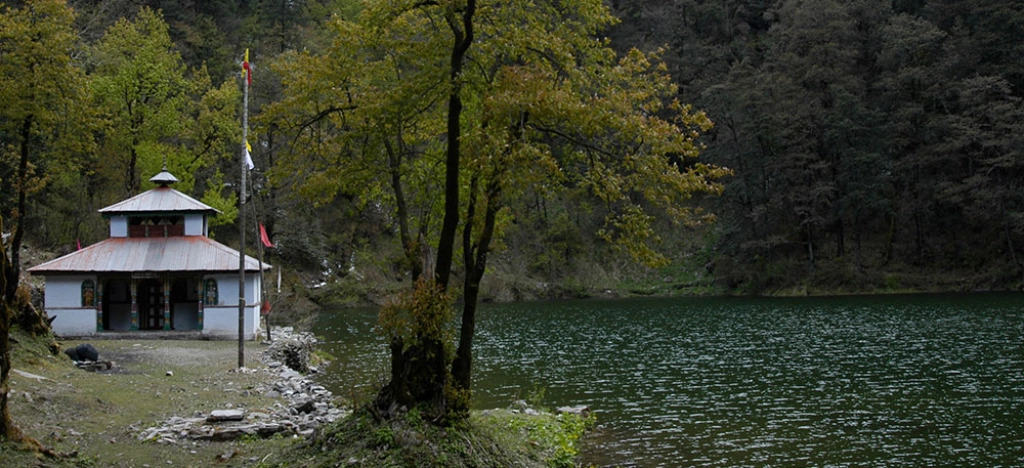
The Dodital-Darwa Pass Trek is a mesmerizing journey through the pristine landscapes of Uttarakhand, India. Nestled at an altitude of 13,780 feet, this 49 km trek unveils a hidden paradise in the Himalayas, perfect for both novice and seasoned trekkers. The trek begins in the quaint village of Sangamchatti, winding through verdant forests, charming villages, and serene campsites like Bebra and Manjhi. The emerald Dodital Lake, surrounded by deodar trees and believed to be the birthplace of Lord Ganesha, offers a tranquil respite. From the summit of Darwa Pass, trekkers are rewarded with breathtaking panoramic views of the snow-capped Himalayan peaks, including the majestic Bandarpoonch Massif. The trek's easy to moderate grade, combined with its rich biodiversity and cultural heritage, makes Dodital-Darwa Pass a must-do for nature lovers and adventure enthusiasts alike, promising an unforgettable experience of serenity and natural splendour.
[
**7. Pangarchulla Peak Trek
**](https://trekthehimalayas.com/pangarchulla-peak-trek)
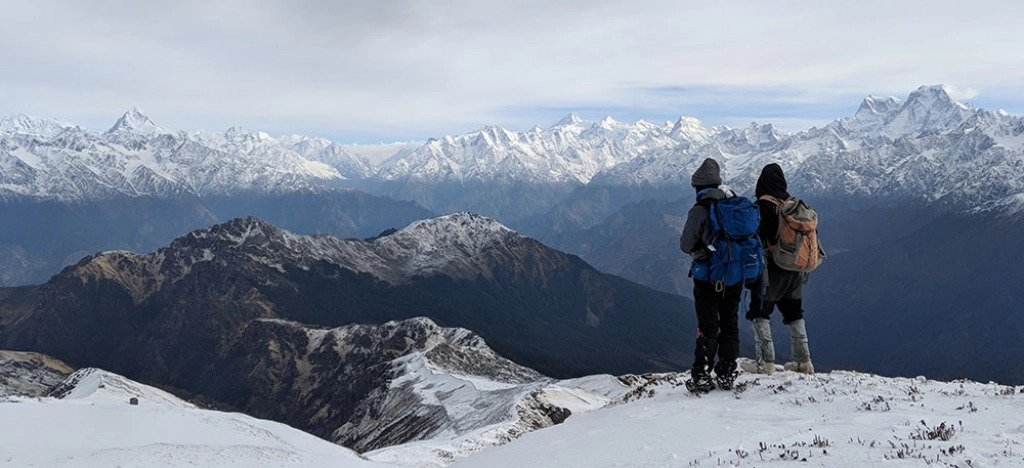
The Pangarchulla Peak Trek is a thrilling adventure nestled in the enchanting Garhwal Himalayas of Uttarakhand. This seven-day journey, ascending to a formidable height of 15,100 feet, offers a perfect blend of challenge and natural splendour, making it a must-do for mountaineering enthusiasts. Starting from the spiritual town of Haridwar, the trek leads you through the serene locales of Joshimath and the quaint Dhak village, unveiling the rustic charm of cliffside villages and terraced farmlands. As you ascend, the trail opens up to verdant meadows and dense forests of Oak, Rhododendron, and Birch, eventually leading to the snow-laden summit of Pangarchulla. Here, the panoramic views of majestic peaks like Nanda Devi, Chaukhamba, and Trishul are nothing short of mesmerizing. Each step on this trek, whether it’s the steep ridges, the expansive meadows, or the glittering snow, promises an unforgettable experience, painting a vivid tapestry of the pristine beauty and the exhilarating thrill of the Himalayas.
[**8. Pindari Glacier Trek**](https://trekthehimalayas.com/pindari-glacier-trek)
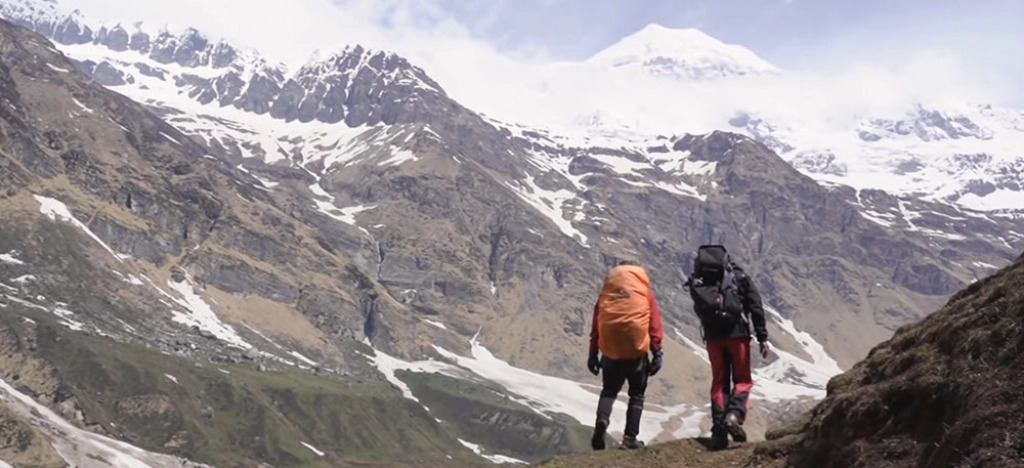
The Pindari Glacier Trek, nestled in the Kumaon Himalayas is one of the best treks in Uttarakhand that seamlessly blends natural splendor and adventurous challenge. Over the course of seven days, trekkers traverse approximately 50 km through an array of stunning landscapes, from lush rhododendron forests to high-altitude meadows and cascading rivers, culminating at the awe-inspiring glacier at 12,300 feet. This moderately graded trek, renowned for its accessibility and breathtaking views of peaks like Maiktoli, Panwali Dwar, and the towering Nanda Devi, offers a perfect introduction to the pristine beauty of the Himalayas.
Starting from the quaint village of Kharkiya, the route weaves through charming hamlets such as Khati and Dwali, leading to the mesmerizing zero point of the Pindari Glacier. Along the way, trekkers encounter diverse flora and fauna, cross serene streams, and are greeted with panoramic vistas that capture the heart and soul of the Himalayas. With experienced guides ensuring safety and comfort, the Pindari Glacier Trek promises an unforgettable adventure, rich with cultural encounters and the sheer joy of exploring one of India's most accessible and picturesque glaciers.
**[9. Bagini Glacier Trek
](https://trekthehimalayas.com/bagini-glacier-and-changbang-base-camp)**
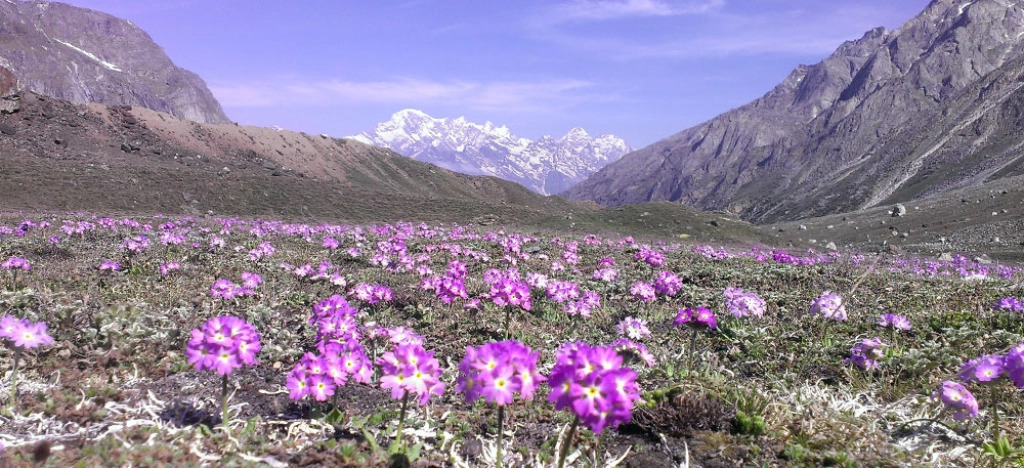
The Bagini Glacier Trek is located in the Nanda Devi National Park and is a captivating adventure for trekking enthusiasts. Spanning nine days and covering approximately 43 km, this moderate trek ascends to an altitude of 14,816 ft, offering breathtaking views of the Garhwal Himalayas. The circular trail begins at Jumma Village and winds through lush forests, rocky paths, and charming mountain hamlets like Ruing and Dronagiri. Trekkers are rewarded with stunning vistas of majestic peaks such as Changabang, Trishuli, and Dunagiri, and the tranquility of the Bagini Glacier Base Camp. Each day brings new experiences, from the historical echoes of Dronagiri to the surreal sunrise over the Changabang base. The journey is not only a test of endurance but also an immersion into the untouched beauty and solitude of the Himalayas, making it a must-do trek for those seeking a blend of adventure and natural splendour.
**[10. Bali Pass Trek
](https://trekthehimalayas.com/bali-pass-trek)**
The Bali Pass Trek is a hidden gem nestled in the heart of Uttarakhand, offering an exhilarating adventure for seasoned trekkers. This 8-day trek, covering approximately 60 km, takes you through the enchanting landscapes of the Ruinsara Tal, Har Ki Dun Valley, and the mystical Yamunotri Dham, linking the Har Ki Dun and Ruinsara Valleys. The journey, starting from Sankri and ending at Jankichatti, presents a blend of thrilling climbs, serene alpine meadows, dense forest covers, and captivating alpine lakes, all set against the backdrop of majestic peaks like Bandarpoonch and Swargrohini.
This trek, with its challenging terrain, steep ascents and descents, and technical paths, is perfect for those looking to push their limits and experience the raw beauty of the Himalayas. Along the way, trekkers encounter ancient villages, lush meadows, and the pristine Ruinsara Tal, culminating in the breathtaking views from the summit of the Bali Pass at 16,200 ft. Despite its difficulty, the trek rewards adventurers with unparalleled panoramic vistas and a profound sense of accomplishment, making it a must-do for any true trek enthusiast.
These ten offbeat treks offer more than just physical challenges; they provide a journey into the heart of nature's untouched beauty. From the mystical passes to the secluded valleys and glacial lakes, each trek is a gateway to unparalleled experiences and breathtaking vistas. As you traverse these lesser-known routes, you'll not only test your limits but also find solace in the profound connection with nature, leaving you with memories that linger long after the journey ends.
| trekthehimalayas |
1,913,593 | Generative Reroll Game Development Using LLMs | Continuously running an LLM until it generates a game you like. Let's call this Generative Reroll... | 0 | 2024-07-06T10:30:13 | https://dev.to/abagames/generative-reroll-game-development-using-llms-22m3 | Continuously running an LLM until it generates a game you like. Let's call this Generative Reroll Game Development.
With the emergence of high-performance LLMs like [Claude 3.5 Sonnet](https://www.anthropic.com/news/claude-3-5-sonnet), it has become possible to have LLMs create simple game ideas and even implement them. Many of the games that come out of LLMs are mediocre, unbalanced, or incorrectly implemented. However, by repeatedly having the LLM generate games, you can occasionally obtain code that exhibits interesting behavior, just one step away from being a game.
For example:
- [claude-one-button-game-creation](https://github.com/abagames/claude-one-button-game-creation)
When given the theme "fragile pillars" to the above prompt, it proposes the following game:
> Pillar Paraglider: Control a paraglider flying through a course of fragile pillars. The paraglider constantly descends. Press the button to ascend, but each press also sends a shockwave that can damage nearby pillars. Core mechanic: Balancing ascent with pillar preservation.
After elaborating on and implementing this idea, the following game was created. This is Claude's output as-is:
- [Claude's original Pillar Paraglider](https://abagames.github.io/claude-one-button-game-creation/?sample_before)
Pressing the button makes the red player character ascend while simultaneously emitting a circular shockwave around it. The shockwave destroys pillars. While it seems overly influenced by Flappy Bird, it has implemented some interesting behavior as a game.
However, this game clearly has several issues:
- It's too easy. You can nullify pillars with shockwaves by continuously pressing the button. Nothing happens when the player character reaches the top or bottom of the screen, so you almost never get a game over.
- The scoring system is simplistic. The score is just based on the distance traveled, so risky actions don't lead to higher scores.
- It doesn't realize the original core mechanic. It says "balancing ascent with pillar preservation," so the original intent was probably to make a game where you avoid destroying pillars with shockwaves. However, it's not implemented that way. Also, if we faithfully implement that mechanic, it would result in a stressful game where you play with a player character that has a large hitbox.
In Generative Reroll Game Development, the important process is how to improve these imperfect games with interesting behaviors into games that are also fun to play.
For this game, I made the following changes:
- The circular shockwave emitting in all directions is too powerful, so we gave the player character a direction and made the shockwave emit only in a limited angle in front of it. Also, the shockwave now destroys only the part of the pillar it hits, not the entire pillar. Additionally, the game ends if the player character touches the top or bottom of the screen.
- Score is given for each destroyed part. Also, continuous destruction increases the score, encouraging risky behavior of advancing towards pillars for higher scores.
- I ignore the core mechanic. Generally, a game about "destroying" is more fun than one about "avoiding destruction."
As a result, the game became as follows:
- [Improved Pillar Paraglider](https://abagames.github.io/claude-one-button-game-creation/?sample_after)
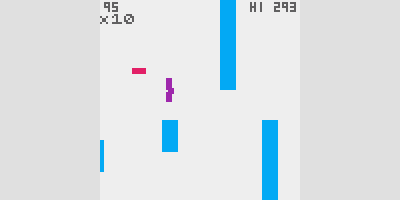
It became a game about making holes in pillars with shockwaves to progress. While it might feel a bit too difficult, it has achieved a more appropriate balance of risk and reward than the initial version, increasing its completeness as a game.
The code differences between the improved version and the original are as follows:
- [Diff between improved version and original](https://github.com/abagames/claude-one-button-game-creation/commit/9e04ca08aa426f33909e99b6eb5d19d6ec55e446)
Looking at this, it seems like nothing remains of the original code, but in reality, it's much easier than creating from scratch because you can modify it while referring to the original framework.
The completed version with sound and title became:
- [Pillar Paraglider, renamed to WAVY BIRD](https://abagames.github.io/claude-one-button-game-creation/?wavybird)
This is the current state of Generative Reroll Game Development using LLMs. If asked whether this is easier than a person coming up with ideas normally and implementing them, it's not particularly easy. However, the development process of facing challenges from computers, such as selecting games proposed by LLMs and figuring out how to make them interesting, has a different kind of appeal compared to conventional development processes. It's also important to enjoy the process of developing and improving prompts for generation alongside game development in Generative Reroll Game Development.
In the future, we might be able to simply tell an LLM "make an interesting game" without thinking, and it will implement and return a nice game. At present, it's normal to need dozens of rerolls to get a game with interesting behavior, and the hit rate is undeniably low. However, with previous LLMs, it was common for only mediocre games that we've seen somewhere before to come out, and getting anything with interesting behavior was virtually impossible. The fact that we can now generate something that feels at least somewhat novel, thanks to the evolution of LLMs over the past year or so, is a good sign.
If LLMs continue to evolve at this rate, the quality of ideas will improve, and they will be able to implement ideas more accurately as code. They might also be able to modify the code in response to pointed out issues like those mentioned above. This would allow us to create playable games with fewer rerolls and simpler improvements. The future development of Generative Reroll Game Development is exciting to anticipate. | abagames |
|
1,913,591 | SEO Strategies for Local Businesses | In today’s digital era, establishing a strong online presence is crucial for the success of any... | 0 | 2024-07-06T10:29:33 | https://dev.to/jessan_roy_28f8b62ba7c328/seo-strategies-for-local-businesses-pim | In today’s digital era, establishing a strong online presence is crucial for the success of any business, especially for local enterprises aiming to connect with their nearby audience. Search Engine Optimization (SEO) plays a pivotal role in enhancing online visibility and driving organic traffic to your website. In this blog, we’ll explore effective SEO strategies tailored for local businesses, helping them thrive in the competitive digital landscape.
Read More: https://vkdigitalconsultancy.com/seo-strategies-for-local-businesses/
1) Optimize Your Google My Business Listing :
Google My Business (GMB) is a game-changer for local businesses. Ensure that your GMB profile is complete and accurate.. Include relevant information such as business hours, address, phone number, and high-quality images. Encourage satisfied customers to leave positive reviews, as these can significantly impact local search rankings.
2) Local Keyword Research :
Conduct thorough research to identify keywords that potential customers in your locality might use to find products or services similar to yours. Use these keywords strategically in your website content, meta tags, and other on-page elements to improve your site’s relevance for local searches.
3) Mobile Optimization :
A significant portion of local searches occurs on mobile devices. Optimize your website for mobile users by ensuring a responsive design, fast loading times, and a user-friendly interface. Google prioritizes mobile-friendly websites in its search rankings, making this optimization crucial for local businesses.
4) Local Content Marketing :
Create content that resonates with your local audience. Develop blog posts, articles, and other content that addresses local concerns, events, or news. This not only helps in building a connection with the community but also improves your chances of appearing in local search results.
5) Local Link Building :
Building a robust network of high-quality backlinks is crucial to achieving success in SEO.. Focus on building quality local backlinks from reputable businesses, local directories, and community organizations. This not only enhances your website’s authority but also signals to search engines that your business is relevant to the local community.
6) Social Media Engagement :
Leverage social media platforms to engage with your local audience. Share relevant content, interact with customers, and encourage user-generated content.
Engagement metrics like social media likes, shares, and comments have the potential to enhance your local search visibility and rankings.
7) Local Schema Markup :
Implementing local schema markup on your website provides search engines with additional information about your business, such as your location, operating hours, and customer reviews. This helps search engines better understand your content and improves the chances of showing up in local search results.
8) Monitor and Analyze Performance :
Consistently overseeing your website’s performance through tools like Google Analytics and Google Search Console. Keep a close eye on essential metrics like organic traffic, user engagement, and conversion rates… Analyzing this data allows you to identify what’s working well and make informed adjustments to your SEO strategy.
Know More About VK Digital Consultancy
V K Digital Consultancy is a one-stop branding solution for your business. This is a digital era, digital appearance is the necessity of any business. Understanding this wide scope and interest, we started this business and started to help many business owners to grow their digital presence.
Our enthusiastic, dedicated, and expert team manages your social media, manages your online reputation, and keeps your customers updated about your business. Mrs. Vaishnavi Kadam, founder and team leader of VK Digital Consultancy is running this business very successfully, making customers satisfied with reliable branding solutions. | jessan_roy_28f8b62ba7c328 |
|
1,913,590 | Why Your Resort Needs to Dive into Digital Marketing (and How it Makes Waves!) | Imagine this: crystal clear waters, swaying palm trees, and happy guests soaking up the sunshine at... | 0 | 2024-07-06T10:29:20 | https://dev.to/jessan_roy_28f8b62ba7c328/why-your-resort-needs-to-dive-into-digital-marketing-and-how-it-makes-waves-4dd9 | Imagine this: crystal clear waters, swaying palm trees, and happy guests soaking up the sunshine at your resort. Sounds idyllic, right? But how do you get those guests to discover your slice of paradise? That’s where digital marketing comes in, your trusty surfboard to ride the waves of online success.
In the past, resorts relied on brochures and travel agents to spread the word. But today, travelers are planning their getaways online. So, if your resort isn’t visible in the digital world, it’s like having a hidden treasure – no one will find it!
Read More: https://vkdigitalconsultancy.com/why-your-resort-needs-to-dive-into-digital-marketing/
Here’s how digital marketing can turn your resort from undiscovered gem to a tourist hotspot :
1) Reach a Global Audience :
Gone are the days of limiting yourself to local advertising. Digital marketing lets you showcase your resort to potential guests worldwide. Think of it as casting a net across the entire ocean instead of a tiny pond!
2) Target the Perfect Guests :
Not everyone is looking for the same vacation experience. With digital marketing, you can target your ads and promotions to people who are most likely to love your resort. Imagine showing dreamy poolside cocktails to sunseekers and thrilling water activities to adventure lovers – that’s the power of targeting!
3) Boost Bookings with a User-Friendly Website :
Your website is your resort’s digital storefront. Digital marketing helps create a beautiful, user-friendly website that showcases your amenities, rooms, and stunning location. Think of it as having a 24/7 open house where guests can explore and book their stay with ease.
4) Get More Bang for Your Buck :
Digital marketing is often more cost-effective than traditional advertising. You can track your campaigns and see exactly where your money is going, allowing you to optimize your spending for maximum impact.
5) Show Off the Good Times :
Digital marketing lets you share captivating photos and videos of your resort. Give potential guests a taste of the amazing experiences that await them – think sparkling pools, delicious food, and breathtaking views.
6) Build Relationships with Guests :
Social media platforms like Instagram and Facebook are fantastic tools for connecting with potential and existing guests. Share interesting content, respond to comments, and run contests to build a community around your resort.
7) Learn What Guests Want :
Digital marketing tools provide valuable data on how people find your resort and what interests them. Use this information to tailor your offerings and improve the guest experience.
Digital marketing isn’t just about fancy ads; it’s about creating a strong online presence that showcases the unique charm of your resort. So, are you ready to make waves and attract a wave of new guests? Dive into the world of digital marketing and watch your resort become the destination everyone’s talking about!
Know More About VK Digital Consultancy
V K Digital Consultancy is a one-stop branding solution for your business. This is a digital era, digital appearance is the necessity of any business. Understanding this wide scope and interest, we started this business and started to help many business owners to grow their digital presence.
Our enthusiastic, dedicated, and expert team manages your social media, manages your online reputation, and keeps your customers updated about your business. Mrs. Vaishnavi Kadam, founder and team leader of VK Digital Consultancy is running this business very successfully, making customers satisfied with reliable branding solutions. | jessan_roy_28f8b62ba7c328 |