update
Browse files- README.md +44 -0
- image-outpainting_result.png +0 -0
- main.py +7 -14
README.md
ADDED
@@ -0,0 +1,44 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
Model from: https://huggingface.co/JunhaoZhuang/PowerPaint_v2
|
2 |
+
|
3 |
+
Tokens (P_ctxt, P_shape, P_obj) added by PowerPaint has been integrated into the text_encoder and tokenizer.
|
4 |
+
|
5 |
+
Unlike PowerPaint_v1, PowerPaint_v2 uses a method similar to BrushNet, so it can be applied to any sd1.5 type basic model.
|
6 |
+
|
7 |
+
Clone demo code and models:
|
8 |
+
|
9 |
+
```bash
|
10 |
+
git lfs install
|
11 |
+
git clone https://huggingface.co/Sanster/PowerPaint_v2
|
12 |
+
```
|
13 |
+
|
14 |
+
Run `main.py`:
|
15 |
+
|
16 |
+
```bash
|
17 |
+
python3 main.py runwayml/stable-diffusion-v1-5
|
18 |
+
```
|
19 |
+
|
20 |
+
The demo code will generate following results:
|
21 |
+
|
22 |
+
| Original Image | Mask |
|
23 |
+
| ---------------------------------------------------------------------------------------------------------------------------------------------- | ----------------------------------------------------------------------------------------------------------------------------------------------- |
|
24 |
+
| 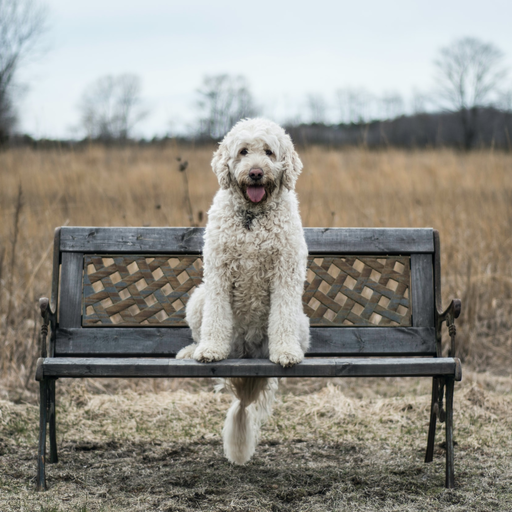 | 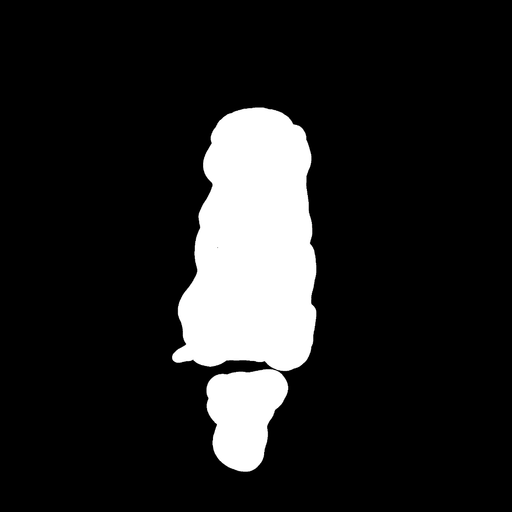 |
|
25 |
+
|
26 |
+
**Object Removal Task**
|
27 |
+
|
28 |
+

|
29 |
+
|
30 |
+
**Shape Guided Task**
|
31 |
+
|
32 |
+

|
33 |
+
|
34 |
+
**Context aware Task**
|
35 |
+
|
36 |
+

|
37 |
+
|
38 |
+
**Inpaint Task**
|
39 |
+
|
40 |
+

|
41 |
+
|
42 |
+
**Outpaint Task**
|
43 |
+
|
44 |
+

|
image-outpainting_result.png
CHANGED
![]() |
![]() |
main.py
CHANGED
@@ -1,11 +1,11 @@
|
|
|
|
1 |
import cv2
|
2 |
import numpy as np
|
3 |
import torch
|
4 |
-
from PIL import Image,
|
5 |
from transformers import CLIPTextModel, CLIPTokenizer
|
6 |
from diffusers.utils import load_image
|
7 |
from diffusers import DPMSolverMultistepScheduler
|
8 |
-
from safetensors.torch import load_model
|
9 |
|
10 |
from powerpaint_v2.BrushNet_CA import BrushNetModel
|
11 |
from powerpaint_v2.pipeline_PowerPaint_Brushnet_CA import (
|
@@ -90,24 +90,17 @@ def predict(
|
|
90 |
height=W,
|
91 |
).images[0]
|
92 |
return result
|
93 |
-
m_img = (
|
94 |
-
input_image["mask"].convert("RGB").filter(ImageFilter.GaussianBlur(radius=3))
|
95 |
-
)
|
96 |
-
m_img = np.asarray(m_img) / 255.0
|
97 |
-
img_np = np.asarray(input_image["image"].convert("RGB")) / 255.0
|
98 |
-
ours_np = np.asarray(result) / 255.0
|
99 |
-
ours_np = ours_np * m_img + (1 - m_img) * img_np
|
100 |
-
result_paste = Image.fromarray(np.uint8(ours_np * 255))
|
101 |
-
return result_paste
|
102 |
|
103 |
|
|
|
|
|
104 |
text_encoder_brushnet = CLIPTextModel.from_pretrained(
|
105 |
"text_encoder_brushnet",
|
106 |
variant="fp16",
|
107 |
torch_dtype=torch.float16,
|
108 |
)
|
109 |
unet = UNet2DConditionModel.from_pretrained(
|
110 |
-
|
111 |
subfolder="unet",
|
112 |
variant="fp16",
|
113 |
torch_dtype=torch.float16,
|
@@ -118,7 +111,7 @@ brushnet = BrushNetModel.from_pretrained(
|
|
118 |
torch_dtype=torch.float16,
|
119 |
)
|
120 |
pipe = StableDiffusionPowerPaintBrushNetPipeline.from_pretrained(
|
121 |
-
|
122 |
torch_dtype=torch.float16,
|
123 |
safety_checker=None,
|
124 |
unet=unet,
|
@@ -170,7 +163,7 @@ tasks = [
|
|
170 |
{
|
171 |
"task": "image-outpainting",
|
172 |
"guidance_scale": 7.5,
|
173 |
-
"prompt": "
|
174 |
"negative_prompt": negative_prompt,
|
175 |
},
|
176 |
]
|
|
|
1 |
+
import sys
|
2 |
import cv2
|
3 |
import numpy as np
|
4 |
import torch
|
5 |
+
from PIL import Image, ImageOps
|
6 |
from transformers import CLIPTextModel, CLIPTokenizer
|
7 |
from diffusers.utils import load_image
|
8 |
from diffusers import DPMSolverMultistepScheduler
|
|
|
9 |
|
10 |
from powerpaint_v2.BrushNet_CA import BrushNetModel
|
11 |
from powerpaint_v2.pipeline_PowerPaint_Brushnet_CA import (
|
|
|
90 |
height=W,
|
91 |
).images[0]
|
92 |
return result
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
93 |
|
94 |
|
95 |
+
# base_model_name = "runwayml/stable-diffusion-v1-5"
|
96 |
+
base_model_name = sys.argv[1]
|
97 |
text_encoder_brushnet = CLIPTextModel.from_pretrained(
|
98 |
"text_encoder_brushnet",
|
99 |
variant="fp16",
|
100 |
torch_dtype=torch.float16,
|
101 |
)
|
102 |
unet = UNet2DConditionModel.from_pretrained(
|
103 |
+
base_model_name,
|
104 |
subfolder="unet",
|
105 |
variant="fp16",
|
106 |
torch_dtype=torch.float16,
|
|
|
111 |
torch_dtype=torch.float16,
|
112 |
)
|
113 |
pipe = StableDiffusionPowerPaintBrushNetPipeline.from_pretrained(
|
114 |
+
base_model_name,
|
115 |
torch_dtype=torch.float16,
|
116 |
safety_checker=None,
|
117 |
unet=unet,
|
|
|
163 |
{
|
164 |
"task": "image-outpainting",
|
165 |
"guidance_scale": 7.5,
|
166 |
+
"prompt": "",
|
167 |
"negative_prompt": negative_prompt,
|
168 |
},
|
169 |
]
|